text
stringlengths 46
74.6k
|
---|
Introducing Wallet Selector: A New Tool for the NEAR Ecosystem
COMMUNITY
April 7, 2022
Web3 users looking for an easy way to interact with the NEAR blockchain using their wallet of choice now have a slew of options. Wallet Selector, a new easy-to-navigate pop-up allows users to select their preferred wallet to easily interact with the NEAR protocol.
Launched in March 2022 by the NEAR Foundation, this simple modal will appear whenever users are given the option to “Connect Wallet” to the NEAR blockchain.
Wallet Selector provides developers and builders with new options to connect their projects to NEAR, and in future, will allow mobile apps to connect to the NEAR blockchain seamlessly in just a few clicks.
NEAR Wallet Selector
Unlocking the wallet ecosystem
At launch, Wallet Selector will feature three supported wallets:
NEAR Wallet – a simple, easy to use web-based wallet.
Sender Wallet – a browser extension available Chrome, Brave, and other browsers.
Ledger Wallet – the secure hardware wallet that allows users to store their wallet in a secure place.
Meteor Wallet – the simple and secure wallet to manage your crypto, access DeFi, and explore NEAR.
Thanks to NEAR’s open and inclusive approach, other wallet developers will be able to contribute to the NEAR ecosystem.
Developers looking to add their wallets, there is documentation and instructions on the NEAR Github repository.
|
---
id: smartcontract
title: Smart Contract
---
Smart contracts are pieces of **executable code** that live in a NEAR account. They can **store data**, **perform transactions** in the account’s name, and **expose methods** so other accounts can interact with them.

Developers can choose between using Javascript or Rust to write smart contracts in NEAR. Indistinctly from the language chosen, the contract will be compiled into WebAssembly, from which point it can be deployed and executed on the NEAR platform.
:::tip Want to build a smart contract?
Check our [**Quickstart Guide**](../../2.build/2.smart-contracts/quickstart.md) to build your first smart contract in NEAR.
:::
---
## What can Smart Contract Do?
Smart contracts have complete control over the account, and thus can perform any action on its behalf. For example, contracts can:
- Transfer $NEAR Tokens
- Call methods on themselves or **other contracts**
- Create new accounts and deploy contracts on them
- Update their own code
Besides, smart contracts can store data in the account's storage. This allows contract's to create almost any type of application, from simple games to complex financial systems.
:::danger What contracts cannot do
- Smart contracts cannot **access the internet**, so they cannot make HTTP requests or access external data
- Smart contracts cannot **execute automatically**, they need to be called by an external account
:::
---
## What are Contract's Used for?
Smart contracts are useful to create **decentralized applications**. Some traditional examples include:
- [Decentralized Autonomous Organizations](https://near.org/nearcatalog.near/widget/Index?cat=dao), where users create and vote proposals
- [Marketplaces](https://near.org/nearcatalog.near/widget/Index?cat=marketplaces), where users create and commercialize digital art pieces
- [Decentralized exchanges](https://near.org/nearcatalog.near/widget/Index?cat=exchanges), where users can trade different currencies
- [And many more...](https://near.org/nearcatalog.near/widget/Index)
For instance, you can easily create a crowdfunding contract that accepts $NEAR. If the goal is met in time, the creator can claim the funds. Otherwise, the backers are refunded.
---
## Contract's Storage
Smart contract stores data in the account's state. The contract's storage is organized as **key-value pairs** encoded using either [JSON](https://www.json.org/json-en.html) or [Borsh](https://borsh.io) serialization. This allows to store any type of data efficiently, from simple numbers to complex objects.
Since the data occupies space in the network, smart contracts need to **pay for the storage they use**. For this, accounts automatically lock a portion of their balance each time new data is stored in the contract. This means that:
- If data is added to the contract's storage the account's **balance decreases ↓**.
- If data is deleted the account's **balance increases ↑**.
Currently, it cost approximately **1 Ⓝ** to store **100kb** of data.
:::tip
Serializing and deserializing the storage happens automatically through our SDK tools, so you can focus on coding the logic of the contract
:::
|
---
id: context-object
title: QueryAPI Context object
sidebar_label: Context object
---
## Overview
The `context` object is a global object made available to developers building indexers with [QueryAPI](intro.md). It provides helper methods to interact with the resources spun up alongside the indexers, such as the GraphQL Endpoint and the database. There are also helper methods to allow specific API calls.
:::caution Under development
The formatting and changes in this document are still in progress. These changes are not fully featured yet but will be by the time they hit production. Specifically, [auto-complete](#auto-complete) and the `context.db.TableName.methodName` format.
:::
## Main Methods
:::tip
All methods are asynchronous, hence why all examples have the `await` keyword in front of the function call.
:::
### GraphQL
When an indexer is published, the SQL DDL written under the `schema.sql` tab is used to spin up a Postgres database. This DB is integrated with Hasura to provide a GraphQL endpoint. This endpoint can be used to interact with your database.
Making calls to the Hasura GraphQL endpoint requires an API call, which is restricted in the environment. So, the GraphQL method allows calls to the endpoint related to the indexer.
:::tip
The GraphQL method was previously the only way to interact with the database. Now, the [DB methods](#db) provide a more accessible functionality. The GraphQL method is better suited for more complex queries and mutations. Also, more information about GraphQL calls can be [found here](https://graphql.org/learn/queries/).
:::
#### Input
```js
await context.graphql(operation, variables)
```
The operation is a string formatted to match a GraphQL query. The variables are any data objects used in the query.
#### Example
```js
const mutationData = {
post: {
account_id: accountId,
block_height: blockHeight,
block_timestamp: blockTimestamp,
content: content,
receipt_id: receiptId,
},
};
// Call GraphQL mutation to insert a new post
await context.graphql(
`mutation createPost($post: dataplatform_near_social_feed_posts_insert_input!){
insert_dataplatform_near_social_feed_posts_one(
object: $post
) {
account_id
block_height
}
}`,
mutationData
);
```
The above is a snippet from the social feed indexer. In this example, you have a mutation (which mutates or changes data in the database, instead of a query that merely reads) called `createPost`. The mutation name can be anything. You specify a variable `post` and execute a graphQL method, which inserts the `post` object and returns `account_id` and `block_height` from the newly inserted object. Finally, you pass in `mutationData` as the variable, which is automatically linked to `post` since it's the only field.
:::tip
You can find other examples of `context.graphql` in the [social_feed indexers](../../../3.tutorials/near-components/indexer-tutorials/feed-indexer.md).
:::
### Set
Each indexer, by default, has a table called `indexer_storage`. It has a field for `key` and `value`, functioning like a key-value store. This table can, of course, be removed from the DDL before publishing. However, it kept the `set` method as an easy way to set some value for a key in that table. This method is used in the default code to set the `height` on each invocation.
#### Input
```js
await context.set(key, value)
```
#### Example
```js
const h = block.header().height;
await context.set("height", h);
```
### Log
Your indexing code is executed on a cloud compute instance. Therefore, things like `console.log` are surfaced to the machine itself. To surface `console.log` statements not only to the machine but also back to you under _Indexer Status_, QueryAPI needs to write these logs to the logging table, which is separate from the developer's schema. This [happens in the runner](how-works.md), and QueryAPI maps `console.log` statements in the developer's code to call this function instead, so you can simply use `console.log`, and QueryAPI takes care of the rest.
## DB
The DB object is a sub-object under `context`. It is accessed through `context.db`. Previously, the GraphQL method was the only way to interact with the database. However, writing GraphQL queries and mutations is pretty complicated and overkill for simple interactions. So, simpler interactions are instead made available through the `db` sub-object. This object is built by reading your schema, parsing its information, and generating methods for each table. See below for what methods are generated for each table. The format to access the below methods is as follows: `context.db.[tableName].[methodName]`. Concrete examples are also given below.
:::info Note
One thing to note is that the process where the code is read is not fully featured.
If an `ALTER TABLE ALTER COLUMN` statement is used in the SQL schema, for example, it will fail to parse.
Should this failure occur, the context object will still be generated but `db` methods will be unavailable. An error will appear on the page saying `types could not be generated`. A more detailed error can be viewed in the browser's console.
:::
### DB Methods
These DB methods are generated when the schema is read. The tables in the schema are parsed, and methods are set under each table name. This makes using the object more intuitive and declarative.
If the schema is invalid for generating types, then an error will pop up both on screen and in the console. Here's an example:

### Methods
:::note
Except for [upsert](#upsert), all of the below methods are used in [social_feed_test indexer](https://near.org/dev-queryapi.dataplatform.near/widget/QueryApi.App?selectedIndexerPath=darunrs.near/social_feed_test). However, keep in mind the current code uses the outdated call structure. An upcoming change will switch to the new method of `context.db.TableName.methodName` instead of `context.db.methodName_tableName`.
:::
### Insert
This method allows inserting one or more objects into the table preceding the function call. The inserted objects are then returned back with all of their information. Later on, we may implement returning specific fields but for now, we are returning them all. This goes for all methods.
#### Input
```js
await context.db.TableName.insert(objects)
```
Objects can be a single object or an array of them.
#### Example
```js
const insertPostData = {
account_id: accountId,
block_height: blockHeight,
block_timestamp: blockTimestamp,
content: content,
receipt_id: receiptId
};
// Insert new post to Posts table
await context.db.Posts.insert(insertPostData);
```
In this example, you insert a single object. But, if you want to insert multiple objects, then you just pass in an array with multiple objects. Such as `[ insertPostDataA, insertPostDataB ]`.
### Select
This method returns rows that match the criteria included in the call. For now, QueryAPI only supports explicit matches. For example, providing `{ colA: valueA, colB: valueB }` means that rows where `colA` and `colB` match those **EXACT** values will be returned.
There is also a `limit` parameter which specifies the maximum amount of objects to get. There are no guarantees on ordering. If there are 10 and the limit is 5, any of them might be returned.
#### Input
```js
await context.db.TableName.select(fieldsToMatch, limit = null)
```
The `fieldsToMatch` is an object that contains `column names: value`, where the value will need to be an exact match for that column. The `limit` parameter defaults to `null`, which means no limit. If a value is provided, it overrides the `null` value and is set to whatever was passed in. All matching rows up to the limit are returned.
#### Example
```js
const posts = await context.db.Posts.select(
{
account_id: postAuthor,
block_height: postBlockHeight
},
1
);
```
In this example, any rows in the `posts` table where the `account_id` column value matches `postAuthor` **AND** `block_height` matches `postBlockheight` will be returned.
### Update
This method updates all rows that match the `whereObj` values by setting the `updateObj` values. It then returns all impacted rows. The `whereObj` is subject to the same restrictions as the select’s `whereObj`.
#### Input
```js
await context.db.TableName.update(whereObj, updateObj)
```
#### Example
```js
await context.db.Posts.update(
{id: post.id},
{last_comment_timestamp: currentTimestamp});
```
In this example, any rows in the `posts` table where the `id` column matches the value `post.id` will have their `last_comment_timestamp` column value overwritten to the value of `currentTimestamp`. All impacted rows are then returned.
### Upsert
Upsert is a combination of insert and update. First, the insert operation is performed. However, if the object already exists, the update portion is called instead. As a result, the input to the function are objects to be inserted, a `conflictColumns` object, which specifies which column values must conflict for the update operation to occur, and an `updateColumns` which specifies which columns have their values overwritten by the incoming object’s values. The `conflictColumns` and `updateColumns` parameters are both arrays. As usual, all impacted rows are returned.
#### Input
```js
await context.db.upsert(objects, conflictColumns, updateColumns)
```
The Objects parameter is either one or an array of objects. The other two parameters are arrays of strings. The strings should correspond to column names for that table.
#### Example
```js
const insertPostDataA = {
id: postId
account_id: accountIdA,
block_height: blockHeightA,
block_timestamp: blockTimeStampA
};
const insertPostDataB = {
id: postId
account_id: accountIdB,
block_height: blockHeightB,
block_timestamp: blockTimeStampB
};
// Insert new post to Posts table
await context.db.Posts.upsert(
[ insertPostDataA, insertPostDataB ],
[‘account_id’, ‘id’],
[‘block_height’, ‘block_timestamp’);
```
In this example, two objects are being inserted. However, if a row already exists in the table where the `account_id` and `id` are the same, then `block_height` and `block_timestamp` would be overwritten in those rows to the value in the object in the call which is conflicting.
### Delete
This method deletes all objects in the row that match the object passed in. Caution should be taken when using this method. It currently only supports **AND** and exact match, just like in the [select method](#select). That doubles as a safety measure against accidentally deleting a bunch of data. All deleted rows are returned so you can always insert them back if you get back more rows than expected. (Or reindex your indexer if needed)
#### Input
```js
await context.db.TableName.delete(whereObj)
```
As stated, only a single object is allowed.
#### Example
```js
await context.db.delete_post_likes(
{
account_id: likeAuthorAccountId,
post_id: postId
}
);
```
In this example, any rows where `account_id` and `post_id` match the supplied value are deleted. All deleted rows are returned.
### Auto Complete
:::tip
Autocomplete works while writing the schema and before publishing to the chain. In other words, you don't need to publish the indexer to get autocomplete.
:::
As mentioned, the [DB methods](#db-methods) are generated when the schema is read.
In addition to that, TypeScript types are generated which represent the table itself. These types are set as the parameter types. This provides autocomplete and strong typing in the IDE. These restrictions are not enforced on the runner side and are instead mainly as a suggestion to help guide the developer to use the methods in a way that is deemed correct by QueryAPI.
:::info Types
You can also generate types manually. Clicking the `<>` button generates the types. It can be useful for debugging and iterative development while writing the schema.

:::
#### Compatibility
By default, current indexers have a `context` object included as a parameter in the top-level `async function getBlock`. This prevents autocomplete, as the local `context` object shadows the global one, preventing access to it. Users need to manually remove the `context` parameter from their indexers to get the autocomplete feature. For example:
```js
async function getBlock(block: Block, context) {
```
should become
```js
async function getBlock(block: Block) {
```
#### Examples
Here are some screenshots that demonstrate autocomplete on methods, strong typing, and field names:





|
---
id: random
title: Random Numbers
---
When writing smart contracts in NEAR you have access to a `random seed` that enables you to create random numbers/strings
within your contract.
This `random seed` is **deterministic and verifiable**: it comes from the validator that produced the block signing the previous
block-hash with their private key.
The way the random seed is created implies two things:
- Only the validator mining the transaction **can predict** which random number will come out. **No one else** could predict it because nobody knows the validator's private key (except the validator itself).
- The validator **cannot interfere** with the random number being created. This is because they need to sign the previous block, over which (with a high probability) they had no control.
However, notice that this still leaves room for three types of attacks from the validator:
1. [Frontrunning](./frontrunning.md), which we cover in another page
2. Gaming the input
3. Refusing to mine the block.
----
## Gaming the Input
Imagine you have a method that takes an input and gives a reward based on it. For example, you ask the user to choose a number,
and if it the same as your `random seed` you give them money.
Since the validator knows which `random seed` will come out, it can create a transaction with that specific input and win the prize.
----
## Refusing to Mine the Block
One way to fix the "gaming the input" problem is to force the user to send the input first, and then decide the result on a different block.
Let's call these two stages: "bet" and "resolve".
In this way, a validator cannot game the input, since the `random` number against which it will be compared is computed in a different block.
However, something that the validator can still do to increase their chance of winning is:
1. Create a "bet" transaction with an account.
2. When it's their turn to validate, decide if they want to "resolve" or not.
If the validator, on their turn, sees that generating a random number makes them win, they can add the transaction to the block. And if they
see that they will not, they can skip the transaction.
While this does not ensure that the validator will win (other good validators could mine the transaction), it can improve their chance of winning.
Imagine a flip-coin game, where you choose `heads` or `tails` in the "bet" stage, and later resolve if you won or not. If you are a validator
you can send a first transaction choosing either input.
Then, on your turn to validate, you can check if your chosen input came out. If not, you can simply skip the transaction. This brings your
probability of winning from `1/2` to `3/4`, that's a 25% increase!
These odds, of course, dilute in games with more possible outcomes.
<details>
<summary>How does the math work here?</summary>
Imagine you always bet for `heads`.
In a fair coin-flip game you have 50-50 percent chance of winning, this is because after the coin is flipped there are two possible outcomes:
`H` and `T`, and you only win in one (`H`).
However, if you can choose to flip again if `tails` comes out, now there are 4 scenarios: `H H` `T H` `H T` `T T`, and in 3 of those
you win (all the ones including an `H`)!!!.
</details>
|
NEAR at Davos for World Economic Forum
COMMUNITY
May 20, 2022
Each year, decision makers and stakeholders from across the globe and many different fields descend on Davos, Switzerland. They come for the World Economic Forum, to discuss the most pressing issues facing the global economy. For WEF 2022, topics will include the pandemic recovery, inflation, climate change, new technologies, and more.
This year, NEAR community members and partners will be attending WEF. Why will NEAR be there? Great question!
NEAR is heading to WEF to lend its voice and expertise on matters related to blockchain, crypto, and Web3. NEAR and its partners also have some other cool plans for WEF. And given that WEF is such an influential gathering, with much media coverage, the NEAR ecosystem will gain global exposure both during and after the forum.
So, let’s take a look at what you can expect from NEAR at Davos.
NEAR, CNBC and Filecoin partner for The Sanctuary
NEAR is a featured Partner of The Sanctuary, a space hosted by CNBC and Filecoin Foundation. Community members will be joining a list of featured Partners that includes the New York Times, Financial Times, CoinDesk, Global Blockchain Business Council, and others.
The Sanctuary sessions will focus on: Sustainability, Diversity Equity & Inclusion (DEI), and Cryptocurrency & Web3. NEAR and other Sanctuary partners will discuss a number of topics facing Web3, including regulatory frameworks, decentralized app ecosystems, and ensuring the Open Web is a welcome space for anyone and everyone.
NEAR Wallet demo and brunch
The NEAR community will also host a brunch at The Sanctuary on May 25th from 8:30-10:30am. During coffee and brunch, NEAR Foundation CEO Marieke Flament will introduce Davos attendees to the NEAR ecosystem.
NEAR Co-founder Illia Polosukhin will then lead a session on how to open a wallet on NEAR. He will also explain how to make a transaction and more NEAR wallet functionality.
The Davos Challenge: Walk for Ukraine
Sweatcoin, pioneers of the “movement economy”—in which users can earn by moving—is joining NEAR at Davos. They will be featured in the official WEF Davos app, and will be hosting “The Davos Challenge: Walk for Ukraine”.
Sweatcoin is encouraging Davos delegates, plus millions of other Sweatcoin users, to track their steps and donate the sweatcoins generated to the Free Ukraine Foundation. Users simply download the free Sweatcoin app (iOs and Android) and start walking. Delegate and user steps will be counted and start to generate sweatcoins, which they can then donate to the campaign at the end of the week.
“There’s typically a lot of walking involved at an event like Davos,” says Sweatcoin’s Chief Marketing Officer, Jessica Butcher. “So, why not put the sweatcoins to good use and donate them to a great cause!”
“As the Russian invasion in Ukraine continues, millions of Ukrainians are facing a humanitarian crisis and are in need of urgent assistance,” she adds. “Together, we can support communities impacted by this violence against the Ukrainian people.”
The Davos Challenge: Walk for Ukraine will take place on WEF’s opening night, Sunday, May 22nd. For more details on the challenge, head over to Sweatcoin.
Tune into CNBC for NEAR @ Davos
Few people get to attend the Davos forum in person. WEF attendance is limited to 2,000 of the world’s most influential figures across business, politics, technology, social impact, and more.
However, the NEAR community and other Web3 developers and entrepreneurs can check out the schedule and stay tuned for the recap.
|
Music and Blockchain: Building a Record Label on the Blockchain
NEAR FOUNDATION
April 14, 2022
Blockchain technology and Web3 are on their way to transform everything—particularly the world of art and music. Right now, the music industry is very much centralized, with Web2 streaming platforms (despite being extremely popular) often not getting the best deal for artists.
Blockchain solutions are working to solve this. Decentralized streaming platforms, like the NEAR-based Amplify.Art and Tamago, will give musicians more artistic control (and revenue). Music NFTs, already popular on NEAR, are also helping artists create a closer connection with fans.
Even the way live events sell tickets is finding its place in web3. Mintbase, an NFT minting platform built on NEAR, is experimenting with smart ticketing—selling tickets as NFTs to prove authenticity and prevent fraud.
One of the next logical steps is reenvisioning the record label. By decentralizing music, the hope is that a more fair and less byzantine system can take root, allowing musicians and other industry professionals flourish on more equal terms.
This future is already unfolding on the NEAR ecosystem, where musicians can learn to build on a super fast, low cost, and secure blockchain.
Reimagining record labels on NEAR
DAOrecords, a label built on NEAR, is a good example of the creative reimagining of a blockchain record label. Brainchild of hip hop musician, producer, and Web3 proponent, Vandal, DAOrecords is using blockchain to give artists their fair share through NFTs, automated royalty-splitting, and live events in metaverse venues.
DAOrecords will launch their alpha product in May through SoundSplash, a Metaverse event series. Until then, the label is helping artists navigate Music NFTs and Web3. And it’s not just DAO Records that is helping to create a hub for music NFTs on the NEAR ecosystem.
Last year, Ed Young (co-founder of The Source) and the Universal Hip Hop Museum (UHHM) partnered for NFT.hiphop, a collection of art/music NFTs featuring hip hop artists. The collection featured illustrations of the most famous hip hop artists, created by André LeRoy Davis. Mintbase also got into the tokenized music game with its release of Deadmau5 and Portugal. The Man’s collaborative NFT single “this is fine” in December 2021.
While NFT.hiphop and the Mintbase-Deadmau5 projects are creative experiments, DAOrecords is using NFTs to revolutionize the record label. Music NFTs are simply smart contracts that give fans ownership of songs, artwork, merchandise, and other unique assets. With original NFT collections, the idea is to create more personal connections between artists and fans.
Making royalty splits transparent
Vandal formed DAOrecords, in large part, to improve the distribution of music sales and royalties to artists. Like many music industry professionals, Vandal recognized the longstanding issue of artist royalties, which streaming platforms only amplified. After record labels, managers, and others take their cut of music sales and streams, musicians are left with meager income.. This model means smaller independent artists often struggle to hit billions of streams to earn a liveable wage.
“As an independent artist it can be really hard to compete in an industry that relies so heavily on streams,” Vandal says. He adds that we need to re-examine our relationship to streaming: artists are unable to know their audiences and have little connection with them. He says NFTs can be the new collectibles, replacing CDs or vinyl records, and bringing musicians and fans closer together.
Vandal adds that “blockchain can provide a layer of transparency and trust,” using music NFTs as an example. Everything is baked into smart contracts, and artists know exactly where funds will be allocated. Joining a music DAO can help artists participate in decentralized governance, request funds, and ultimately see every activity recorded on the blockchain.
DAOrecords is also heavily involved in the metaverse—the next, more interactive generation of the Internet. The label’s affiliated artists have been performing at metaverse events since April 2020, most recently at the Metaverse Music Festival in Decentraland last October.
Why build on NEAR’s blockchain?
NEAR’s super fast transactions and ease-of-use are obvious attractions to Web3 labels. But Vandal also says that creating a label on NEAR was perfect because of the variety of people using it.
“There is a sense of community and family with everyone doing different things,” he says. “It [has] always felt very much like a family.”
Beyond the ecosystems’ diversity and sense of community, Vandal chose NEAR after experimenting with Ethereum to mint music NFTs. The network worked well but he was put off by the high gas costs. NEAR’s fast and cheap platform was a better alternative. Vandal was also drawn to the tools available on NEAR, especially automated splits for contracts.
For song and album royalty splits, NEAR’s speed, efficiency, and cost is a natural draw for music industry folks. Jordan Gray, an electronic musician and co-founder of Astro DAO, says quick automated royalty payments are being transformed on NEAR.
In the music industry’s current royalty model, artists can wait months to get their cut from a Web2 streaming platform. NEAR’s platform can immediately split royalties for up to 40 people, as small as a fraction of a cent. This is something bigger blockchains, like Ethereum, can’t do because of cost.
“I don’t think anybody has taken advantage of it yet, or found the perfect formula,” says Gray. “But I think that is a key component to taking a part of the music industry and automating it—making them more efficient so artists can get more money for the music they create.”
Eventually, even listeners will receive rewards, it is hoped. By curating playlists of their favorite artists, Vandal says they will receive quick payments from artists depending on how well their selections do online.
Rethinking record contracts
For well over 100 years, music artists have signed contracts with record labels. This is set to change on Web3 platforms like NEAR.
Vandal says DAOrecords and others are moving away from the typical model of signing artists with paper contracts. Musicians will be in full control of their work with non-exclusive, non-IP (intellectual property) systems where they can release their own music, or even set up their own platforms, with the label.
These platforms will resemble a WordPress template on which artists will have their own “landing pages,” where fans can fully interact with them. Eventually, artists will create their own labels using web3 technology, he adds.
DAOrecords and others are also reimagining the way funds are handled. Vandal says money raised from NFT sales will be reinvested into the artists so they can do what they do best—create.
DAORecords’ approach to contracts is one path forward but there will be others. And if things get complex, artists can tap into the NEAR’s Legal Guild, which provides legal expertise and support to projects, DAOs, and entrepreneurs using the NEAR blockchain.
“Our main mission is to see how we can reimagine what a record label is,” Vandal says.
If all goes according to plan, and the future of music does find a secure place on the blockchain, then artists will be able to have a greater say in creative control, through music NFTs, and a better chance of receiving the money they truly deserve. Traditional streaming platforms will evolve into something more inclusive and fair, and live metaverse shows will be more viable. |
---
sidebar_position: 1
---
# Public Method Types
Methods can be called externally by using the `pub` identifier within the [`#[near_bindgen]` macro](../contract-structure/near-bindgen.md) which will expose the method in the compiled WASM bytecode.
It is important to only mark methods that should be called externally as public. If you need a contract to call itself, you can mark the function as public but add the [`#[private]` annotation](private-methods.md) so that it will panic if called from anything but the contract itself.
A basic usage of this would look like the following:
```rust
#[near_bindgen]
impl MyContractStructure {
pub fn some_method(&mut self) {
// .. method logic here
}
}
```
Where this would expose `some_method` from the WASM binary and allow it to be called externally.
<details>
<summary>Expand to see generated code</summary>
```rust
#[cfg(target_arch = "wasm32")]
#[no_mangle]
pub extern "C" fn some_method() {
near_sdk::env::setup_panic_hook();
if near_sdk::env::attached_deposit() != 0 {
near_sdk::env::panic("Method some_method doesn\'t accept deposit".as_bytes());
}
let mut contract: MyContractStructure = near_sdk::env::state_read().unwrap_or_default();
contract.some_method();
near_sdk::env::state_write(&contract);
}
```
</details>
## Exposing trait implementations
Functions can also be exposed through trait implementations. This can be useful if implementing a shared interface or standard for a contract. This code generation is handled very similarly to basic `pub` functions, but the `#[near_bindgen]` macro only needs to be attached to the trait implementation, not the trait itself:
```rust
pub trait MyTrait {
fn trait_method(&mut self);
}
#[near_bindgen]
impl MyTrait for MyContractStructure {
fn trait_method(&mut self) {
// .. method logic here
}
}
```
In this example, the generated code will be the same as the previous example, except with a different method name.
<details>
<summary>Expand to see generated code</summary>
```rust
#[cfg(target_arch = "wasm32")]
#[no_mangle]
pub extern "C" fn trait_method() {
near_sdk::env::setup_panic_hook();
if near_sdk::env::attached_deposit() != 0 {
near_sdk::env::panic("Method trait_method doesn\'t accept deposit".as_bytes());
}
let mut contract: MyContractStructure = near_sdk::env::state_read().unwrap_or_default();
contract.trait_method();
near_sdk::env::state_write(&contract);
}
```
</details>
|
---
sidebar_position: 3
sidebar_label: Chunk
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
# `Chunk` Structure
## Definition
`Chunk` of a [`Block`](block.mdx) is a part of a [`Block`](block.mdx) from a [Shard](shard.mdx). The collection of Chunks of the Block forms the NEAR Protocol [`Block`](block.mdx)
Chunk contains all the structures that make the Block:
- [Transactions](transaction.mdx)
- [Receipts](receipt.mdx)
- [ChunkHeader](#chunkheaderview)
## `IndexerChunkView`
<Tabs>
<TabItem value="rust" label="Rust">
```rust links=1
pub struct ChunkView {
pub author: AccountId,
pub header: ChunkHeaderView,
pub transactions: Vec<IndexerTransactionWithOutcome>,
pub receipts: Vec<ReceiptView>,
}
```
</TabItem>
<TabItem value="typescript" label="TypeScript">
```ts links=1
export interface Chunk {
author: string;
header: ChunkHeader;
transactions: IndexerTransactionWithOutcome[];
receipts: Receipt[],
}
```
</TabItem>
</Tabs>
## `ChunkHeaderView`
<Tabs>
<TabItem value="rust" label="value">
```rust links=1
pub struct ChunkHeaderView {
pub chunk_hash: CryptoHash,
pub prev_block_hash: CryptoHash,
pub outcome_root: CryptoHash,
pub prev_state_root: StateRoot,
pub encoded_merkle_root: CryptoHash,
pub encoded_length: u64,
pub height_created: BlockHeight,
pub height_included: BlockHeight,
pub shard_id: ShardId,
pub gas_used: Gas,
pub gas_limit: Gas,
/// TODO(2271): deprecated.
#[serde(with = "u128_dec_format")]
pub rent_paid: Balance,
/// TODO(2271): deprecated.
#[serde(with = "u128_dec_format")]
pub validator_reward: Balance,
#[serde(with = "u128_dec_format")]
pub balance_burnt: Balance,
pub outgoing_receipts_root: CryptoHash,
pub tx_root: CryptoHash,
pub validator_proposals: Vec<ValidatorStakeView>,
pub signature: Signature,
}
```
</TabItem>
<TabItem value="typescript" value="TypeScript">
```ts links=1
export interface ChunkHeader {
balanceBurnt: number;
chunkHash: string;
encodedLength: number;
encodedMerkleRoot: string;
gasLimit: number;
gasUsed: number;
heightCreated: number;
heightIncluded: number;
outcomeRoot: string;
outgoingReceiptsRoot: string;
prevBlockHash: string;
prevStateRoot: string;
rentPaid: string;
shardId: number;
signature: string;
txRoot: string;
validatorProposals: ValidatorProposal[];
validatorReward: string;
};
```
</TabItem>
</Tabs>
|
```rust
#[near_bindgen]
impl Contract {
#[payable]
pub fn send_tokens(&mut self, receiver_id: AccountId, amount: U128) -> Promise {
assert_eq!(env::attached_deposit(), 1, "Requires attached deposit of exactly 1 yoctoNEAR");
let promise = ext(self.ft_contract.clone())
.with_attached_deposit(YOCTO_NEAR)
.ft_transfer(receiver_id, amount, None);
return promise.then( // Create a promise to callback query_greeting_callback
Self::ext(env::current_account_id())
.with_static_gas(Gas(30*TGAS))
.external_call_callback()
)
}
#[private] // Public - but only callable by env::current_account_id()
pub fn external_call_callback(&self, #[callback_result] call_result: Result<(), PromiseError>) {
// Check if the promise succeeded
if call_result.is_err() {
log!("There was an error contacting external contract");
}
}
}
```
_This snippet assumes that the contract is already holding some FTs and that you want to send them to another account._ |
The Beginner’s Guide to the NEAR Blockchain
COMMUNITY
January 17, 2019
Welcome! This guide is intended to help anyone interested in understanding what NEAR actually is. That includes new users, curious community members, prospective partners and new core contributors to the NEAR Collective. I’ll provide a plain-English understanding of what we do, why we do it, and how you can learn what you need in order to get directly involved.
We’ll keep this guide updated over time as well so you can be sure it’s as current as possible.
My assumption is that you’re familiar with the high level of what a blockchain is so I’m not going to be using any of those block-and-line diagrams here. I’ll make no assumption about your level of technicality — you could be a highly technical engineer but you could be a less technical designer or marketer or community manager as well.
The Basics
So what is NEAR (aka “the NEAR Platform”)? NEAR is a decentralized development platform built on top of the NEAR Protocol, which is a public, sharded, developer-friendly, proof-of-stake blockchain. Put another way, NEAR is like a public community-run cloud platform. That means it is a highly scalable, low cost platform for developers to create decentralized apps on top of. While it’s built on top of the NEAR Protocol blockchain, the NEAR Platform also contains a wide range of tooling from explorers to CLI tools to wallet apps to interoperability components which help developers build much more easily and the ecosystem to scale more widely.
Whereas most other “scalable” blockchains use approaches that centralize processing on high-end hardware to provide a temporary boost in throughput, NEAR Protocol’s approach allows the platform’s capacity to scale nearly linearly up to billions of transactions in a fully decentralized way.
NEAR is being built by the NEAR Collective, a global collection of people and organizations who are collaboratively building this massive open source project. Everyone in this Collective is fanatically focused on enabling usability improvements for both developers and their end-users so the next wave of apps can cross the chasm to a more general audience that has thus far been unable to consistently work with blockchain-based apps built on today’s platforms.
This Collective contains a number of extraordinary teams and includes championship-level competitive programmers who have built some of the only at-scale sharded database systems in the world. In a space dominated by academic research projects and failures to launch, NEAR has a team well accustomed to shipping. It is also backed by financial and community contributions by the best names in the crypto industry.
Why are we doing this? Because this is an opportunity to build the ground floor for a far better Internet which puts the user in control of their money, their data and their identity. With the potential for creating composable open-state services, it’s a chance to kick start the biggest wave of innovation — and business progress — since the Internet. This vision is the biggest sort — there are few opportunities in the world larger than the one we are tackling.
Let’s be clear: NEAR is not a side chain, an ERC20 token or a highly specialized task-specific blockchain… it is nothing less than a brand new and fundamentally reimagined layer 1 protocol designed to independently power the base of the emerging Open Web stack.
Ok… Let’s back up and assume you’re still getting up to speed on what the heck a public, sharded, developer-friendly, proof-of-stake blockchain really is.
From 10,000 feet…
The NEAR Collective is basically building the infrastructure for a new Internet which makes it harder for giant companies to steal your data and for bad guy countries to shut it down. People have been trying to figure this stuff out with similar technologies since 2008 but it’s been slow going.
You’ve heard of Bitcoin, that digital currency everyone thinks is only used by criminals and third-world dictators. Well, despite a really bad reputation and a lot of hiccups along the way, ten years later they still haven’t been able to kill Bitcoin so you know it’s built on very resilient technology. We’re basically trying to use that same kind of technology to power a brand new Internet which is just as hard to kill or screw up.
A few other projects, especially one called Ethereum, tried doing this a few years ago and got a really good start but ultimately got totally bogged down in the growing pains of early technology and they were too slow and expensive to get mainstream adoption. Now, a lot of really smart people are working on a way to speed this up and keep costs low while making sure this new Internet is just as hard to mess up as Bitcoin.
You can read more about this journey in the Evolution of the Open Web.
From 1,000 feet…
We aren’t building the only blockchain which is taking on the scaling and cost problems but NEAR has a team that’s all aces and we’re coming at this in a slightly different way.
To set the stage, we’re building a “base-layer blockchain”, meaning that it’s on the same level of the infrastructure as projects like Ethereum, EOS or Polkadot. That means everything else will be built on top of NEAR.
It’s a general-purpose platform that allows developers to create and deploy decentralized applications on top of it. A decent analogy is that it’s sort of like Amazon’s AWS platform, which is where most of the applications you know and love host their servers, except the NEAR platform isn’t actually run and controlled by one company, it’s run and controlled by thousands or even millions of people. You can call it a “community-operated cloud” but we usually prefer to simply call it a “decentralized application platform.”
The Arc of Technology
It’s worth checking in quickly with how we got here because it will help you understand the context of the current ecosystem. A recent post goes into greater detail but here’s the quick version:
Bitcoin is the original “programmable money” or “digital gold”. It has been doing a pretty good job of fulfilling those functions but its use so far as a more general-purpose computing platform (like we’re building) is mostly an accident. Essentially, developers saw they could hack together some basic programs on top of the limited functionality that Bitcoin provided and they began using Bitcoin as the base for some of these new applications because it’s now highly trusted and secure.
Unfortunately, transactions are very costly and, because this was definitely NOT what the Bitcoin platform was meant for, the functionality is very limited. The platform there is slow (roughly 4 transactions per second), costly, and a massive waste of global energy.
Ethereum, back in 2014, tried to directly address this use case by creating a platform that was, from day 1, intended to use the same blockchain technology to build a global virtual computer which any application could be built on top of.
So, if Bitcoin was really just a basic calculator, Ethereum was a fancy TI-83 graphing calculator on which you could write some interesting, if basic, games. While it put lots of good ideas into place, it is also rather slow (14 transactions per second) and still quite costly for developers to use. They’ve tried to upgrade this but are now having difficulty pivoting because of how much technical work, value storage and community growth has already occurred in their legacy model.
“Layer 2” scaling solutions, including “state channels” and “side chains”, have popped up to try and improve the performance and cost of these slower (but rather secure) platforms by taking some of the work off the main chain and doing it elsewhere. They exist for both Bitcoin and Ethereum but haven’t achieved the adoption we hoped.
The first serious challenger blockchains launched in 2017–2018 with a wide variety of approaches to helping the scaling problem. They generally tried centralizing more of the hardware (eg EOS) but most of the approaches are still ultimately bounded by a fixed limit because every single one of the “nodes” that make up the network are repeating the exact same work, whether there are 21 of them or 1,000. So these approaches have been able to achieve throughputs of thousands (or more) transactions per second but often sacrifice decentralization to do so.
Next generation scalable blockchains like NEAR represent the new wave. In this case, NEAR breaks free from the idea that every single node which participates in the network has to run all of the code because that essentially creates one big wasteful bottleneck and slows down all of the other approaches.
To fix this, NEAR uses a technique called “sharding” from the database world (technical explanation) which splits the network so that much of the computation is actually being done in parallel. This allows the network’s capacity to scale up as the number of nodes in the network increases so there isn’t a theoretical limit on the network’s capacity.
Unlike a lot of other sharding approaches, which still require nodes to be run on increasingly complex hardware (reducing the ability of more people to participate in the network), NEAR’s technique allows nodes to stay small enough to run on simple cloud-hosted instances.
But it’s not all about scaling. In fact, for scaling to even be a benefit, developers need to be able to create apps that people actually use and current blockchains make this difficult on both the developer and the end-user. Many of these issues have to be addressed by setting up the protocol properly from the beginning and few projects who focus on scalability have taken this properly into account.
For example, many scalability solutions require developers to build and provision their own blockchain (or “app chain”), which is a massive amount of work and maintenance, and it seems equally as unnecessary for most teams as building and on-premise server farm would be for most traditional web developers. By comparison, NEAR allows developers to just deploy their app without thinking too much about how the infrastructure around it operates or scales, which is more like the modern clouds like Amazon AWS or GCP or Azure which drive almost all of today’s web applications.
A few quick notes…
There are a few kinds of projects that sort of fit into the landscape but won’t be covered much further here:
Currencies: Fundamentally, any token can operate as a currency because you can use it as a unit of account, a medium of exchange and/or a store of value. But a number of blockchains have been created to specifically act either as currencies (Bitcoin, Zcash, Monero…) or operate directly in the world of currencies (Ripple, Stellar, Libra). These are not general computation platforms, which we’re targeting here, so I’ll leave it at that.
Private Chains: Some blockchains, like Linux Foundation’s Hyperledger project or R3 Corda, are pitched to big companies as a more secure sort of blockchain because they allow those companies to control all of the nodes of the network. While NEAR also has the ability to provide privacy in its network shards, I’ll ignore this category of chains as well because the biggest security advantages come from broad public decentralization not building an oligopoly of a few big companies controlling the chain.
“DAGs”: This one is the trickiest because these chains play in the blockchain world and are, essentially, doing the same sort of thing — creating an immutable (append-only) ledger. They are called “DAGs” because of the computer science term “Directed Acyclic Graph”, which is the actual data structure that makes them up. Generally speaking, these projects, like IOTA, tend to build a complicated mess of transactions among lots of small devices, which functions a lot like a blockchain. It’s scalable but has a lot of security and implementation challenges. I won’t dig deeply into it here but don’t forget about DAGs because many chains, including NEAR, use a sort of smaller DAG somewhere in the process of building their blocks.
More about NEAR
So, as we said before, the collection of teams that make up the NEAR Collective is building the NEAR Platform, which is built on top of the NEAR Protocol, which is a sharded, developer-friendly, proof-of-stake blockchain that developers can build decentralized apps on top of.
Let’s dig into what the NEAR Protocol actually does…
A Decentralized Network
As mentioned above, NEAR is similar in principle to the “cloud-based” infrastructure that developers currently build applications on top of except the cloud is no longer controlled by a single company running a giant data center — that data center is actually made up of all the people around the world who are operating nodes of that decentralized network. Instead of a “company-operated cloud” it is a “community-operated cloud”.
Here are a couple of perspectives on why this decentralization is useful:
Developers/Entrepreneurs: It’s good to have an application decentralized this way because, as a developer, you aren’t at the mercy of a single company like Amazon or even a government who might want to shut the app down… both of which have bedeviled companies since the very beginning. During the development process, you also get access to a few extra things “for free” like payments and cryptography, which can be a challenge to set up in a traditional application.
End Users: It’s better to have a decentralized application in some cases because the code is all open source (so you know exactly what it’s doing) and it can’t be changed once it has been launched (so there’s no chance they’re going to do sketchy things with your money or data). Even better, the app is actively disincentivized from hoarding your data.
Let’s dig a bit deeper into how this network is run.
The NEAR Token
So who actually runs all those individual “nodes” that make up this decentralized network? Anyone who feels properly incentivized to do so! The incentives for this permissionless network are powered by the NEAR token.
The NEAR token is how people who use applications on the network pay to submit transactions to the nodes who actually run the network. The token is thus a utility — if you hold it, you can use applications hosted on the network.
This is a little different from today’s web, where applications are owned by single developers or corporations who pay their cloud hosting bills on behalf of their users. Some aspects of the NEAR protocol allow developers to do this as well but, for simplicity, we will assume users generally pay directly for their use of the network.
Because NEAR is a permissionless protocol, anyone can run one of the nodes which operate the network by validating transactions which have been submitted to the network. But running infrastructure, even simple code that you can run from a laptop, costs some money and time, so few people would do it for free. Thus, in exchange for performing that service, you earn a portion of the transaction fees paid by users during each block where you are validating transactions.
How does the network make sure you’re actually running the code you’re supposed to and not just freeloading and earning income? You are required to “stake” your tokens (which basically means putting them in escrow) as a gesture of good faith. If you perform any malicious behavior (like trying to hack the system or mess with other people’s transactions), you will lose your stake. The system figures this out by coming to “consensus” among the nodes in each period and determining how the code should have been run so it’s easy to identify who did so improperly.
Luckily, you really don’t have to think about this stuff because, as long as you download and start up the standard node program from a reputable source, it all happens behind the scenes by the application code you downloaded and so you aren’t likely to lose your stake.
How do you make money as a “company”?
Good question. For starters, NEAR is not a company!
One of the biggest stumbling blocks people seem to have with blockchains is figuring out how they work as if they were traditional businesses. And that’s totally valid since the 2017 bubble saw all manner of convoluted monetization schemes which actually didn’t make any sense and usually didn’t require a token anyway.
The key to understanding this is realizing that the entire economics supporting the NEAR network are embedded at the protocol level and allow anyone to participate in the protocol by running a validating node themselves. Users of the network pay costs to use this network and the providers of the network capacity receive rewards from this activity. There is no shadowy company behind it all which is secretly trying to sell subscriptions or anything like that. The protocol has self-sustaining economics.
The people who build the early technology are rewarded with participation in the initial allocation of tokens and funded by fiat contributions from early financial backers.
What is the NEAR Collective?
NEAR Collective is the globally distributed group of teams, made up of many individual organizations and contributors, who self-organize in order to bring this technology to life. It is not a business or anything nearly so formal. Think of it instead like the groups of people who run large open-source software projects.
One of the Collective’s projects is writing the initial code and the reference implementation for the open source NEAR network, sort of like building the rocket boosters on the space shuttle. Their job is to do the necessary R&D work to help the blockchain get into orbit. The code for that chain is open source so literally anyone can contribute to or run it.
It’s important to stress that networks like NEAR are designed to be totally decentralized. This means they ultimately operate completely on their own and can’t actually be censored, shut down or otherwise messed with by third parties… not even the teams who initially built them! So, while members of this collective are here to get the ball rolling on building the reference implementation, they quickly become nonessential to the operation of the network once it has started running. In fact, once it’s launch-ready, anyone could modify and run the NEAR Protocol code to start up their own blockchain because it’s all open source and any changes would have to be democratically accepted by the independent validators who run it.
That said, the core teams can (and hopefully will) stick around to keep updating the system and performing bug fixes. After the network has been launched, any ongoing development work will hopefully be supported by the governance of the network through grant funding or other means.
One Collective member worth noting is the NEAR Foundation, a nonprofit entity whose entire goal is to build a vibrant and active long-term ecosystem around the blockchain and which has commissioned the development of the reference implementation of that chain. The Foundation helps coordinate some of the early development work and governance activities.
The NEAR blockchain is only one of the NEAR Collective’s projects, so there are plenty of other areas where we can help the ecosystem going forward.
What is the Path Ahead?
In the early phases of this market, projects focused on strutting a bunch of vanity metrics in order to get as many retail investors on board for a big ICO (initial coin offering). That meant trying to have the biggest Telegram community, the most big-name advisors, the best-looking proof-of-concept projects from big businesses and so on.
The ICO boom finished in 2018 so, luckily, we’re able to focus more on what actually matters. In this case, it’s all about community and adoption.
We have extraordinary technical teams who are working to make sure the technology is implemented properly but everything they build will be open source. That means anyone in the world could, theoretically, just copy the code and run their own NEAR blockchain. Now, it’s not quite as easy as that and our teams’ expertise puts this version ahead should anyone try that, but ultimately technology is merely a time-based advantage. The real traction comes from building a great community.
The ecosystem is most useful when there are lots of applications and lots of users who want to use those applications. Because we see a world where the major development paradigm is to build in a decentralized fashion, there is *lots* of ground to cover between here and there. So the key metric we’re shooting for is adoption and usage of the platform.
A lot of projects are focusing on targeting developers to build apps on top of their platforms. That’s obviously important since this is a highly technical field but it’s not the only thing that matters. Specifically, if you look at the history of major development platforms, the ones that are truly successful need to support real, at-scale businesses not just a bunch of side projects.
So building an ecosystem that has the kind of functional breadth and technical depth to create at-scale businesses for the long term is everything to us. That requires significant efforts on the dimensions of community building, education and overall user experience. And plenty of cool technical tools as well, of course!
Luckily, if we succeed, we have the opportunity to drive the greatest wealth creation since the original Internet by helping developers and entrepreneurs everywhere access new markets and build new kinds of businesses on top of the NEAR Protocol. The upside of what we’re doing is super exciting!
Why is NEAR going to succeed?
You can take this from two perspectives — what makes the NEAR Protocol likely to actually get to market and what makes it better to developers/end-users once we get it there? Let’s explore both.
Execution Matters
What says the NEAR team is going to out-execute the competition and get into the market with the right technology on the right timeline?
For starters… NEAR has already launched its MainNet genesis! And it handed off operation to the community as planned :). But also…
If you hop over to the NEAR project website and you’ll see the best team in the industry and, importantly, a team who has shipped sharded systems before in production settings. Having that kind of experienced team behind it already sets the NEAR Protocol apart from almost every other network out there.
This technology is complicated! Technically speaking, sharding is a scaling approach that’s being seriously attempted by roughly a dozen chains, including the market-leading Ethereum… but they’ve taken years to put together their proposal and expect to take more years to implement it. So it’s non-trivial to get this started.
To round things out, the NEAR team has the support of the best financial and non-financial backers in the space and a cadre of informal advisors who span everything from economics and mechanism design to cryptography and blockchain design.
Killer Features for Everyone
This market — and the technology — is still in the “roll up your sleeves, it’s going to be messy” phase. Every major stakeholder group has challenges:
For developers, that means the tools are really hard to use and hundreds of teams are building hundreds of different things that point in all different directions, including some projects inventing their own programming languages. In short, it’s chaos and it’s painful to develop on existing systems.
For end users, the path to getting started with crypto projects and the new web is incredibly long. In the normal web world, you lose a huge portion of users for each step you make them go through before achieving their goal. Right now, to get a brand new user from zero to playing a crypto-based game or sending a friend money, it takes literally *dozens* of steps… and it’s incredibly slow and somewhat expensive to perform these steps. Not ideal.
For Validators, the people who run the nodes which actually operate the network, their options for receiving tokens from their communities are very limited by existing protocol designs so they are forced to compete with each other based entirely on price, which commoditizes their offerings and makes them less attractive to prospective delegators who might lend them tokens.
All of these problems require a fanatical focus on user experience and user needs as the driving force for creating technology instead of starting by driving technology forward and then seeing what happens. We’ve been developing the NEAR Protocol and many supporting tools with a focus on these experiences while stepping forward to engage the broader community on solving these issues. It’s not going to be fast or easy, but it *is* the priority for us.
We describe the NEAR platform as “developer-friendly” or “usable” because it implements approaches at the protocol level which address each of those problems:
For developers, NEAR’s contract-based account model allows them to build advanced permissions into their apps and sign transactions on behalf of users. They also have access to a set of tools that make app composition straightforward and the opportunity for a protocol-level fee rebate which compensates them for creating well-used apps and critical system infrastructure.
For end users, the flexible account model allows them to benefit from “progressive UX”, which means they don’t have to touch wallets or tokens until they are ready because the application is hiding them behind the scenes. For their end-users, the way our accounts are set up allows for smoother onboarding experiences which don’t require as many steps or as many annoying wallet pop-ups.
For validators, having access to “delegation” at the contract level means that they can create infinitely diversified offerings of their services, thus making NEAR a very attractive place to run validating nodes.
What are the use cases for the NEAR blockchain?
One of the hottest questions is what the use cases are for blockchains precisely because it hasn’t been definitively answered. Sure, it’s being used in everything from supply chain tracking to cross border payments, but most of these cases are still early enough that they haven’t achieved mainstream adoption. We’re still in a phase of trying new things to see what new primitives they unlock and how they extend.
In our case, we’re talking to hundreds of existing businesses and opportunistic entrepreneurs about how a truly scalable, usable blockchain can unlock new business opportunities. I won’t spoil the surprises by getting into that here but suffice it to say, there are a few burning areas where people are begging us to solve their problems and existing blockchains have been too slow or expensive or painful for users. A couple of areas to start include gaming and decentralized finance but that’s just the beginning. In many cases, it starts by taking the things people already do on less performant chains — like Open Finance on Ethereum — and scaling them out to more potential users and uses by providing a more versatile and performant chain.
In the long run, just like with the original Internet revolution, the earliest use cases are likely just going to bridge the gap until people invent entirely new business models. So, while we’re excited about addressing things in the short term, we’re especially excited about building a toolkit that future entrepreneurs can combine with their creativity to change the world in ways we can’t even imagine now.
This is where new ideas around composability of microservices and open/transferrable state (a paradigm we call the Open Web) are really exciting. How different will it be to create businesses which sit atop a portfolio of open services rather than gated APIs and centralized platforms? These are new development paradigms that we’ve only scratched the surface of so far.
What should I learn next?
If you’ve gotten this far, you’re probably curious to learn more. There are a lot of resources out there but I’ll recommend a reasonable path through them. Blockchain is a highly technical field that covers just about every discipline under the sun so it can feel like drinking from a firehose without proper guidance.
If you’re pretty new to blockchain, start here to get a strong overview of the ecosystem and its development:
Deconstructing the blockchain ecosystem (2018, slides)
The state of blockchain product, design and development (2018)
After that, it’s a good idea to start diving a little deeper into the functional disciplines. The next layer deeper is to explore the NEAR Protocol White Paper, which is a human-readable deconstruction of every aspect of the project. You can find it along with the other technical papers at:
https://pages.near.org/technology
Technical Stuff
No matter what role you have, you’ll need to get up to speed technically because the lingo is going to come up repeatedly. That doesn’t necessarily mean you have to understand all the computer science underneath it but you should know the basics.
Generally:
What are decentralized applications and how do they work (slides)
Using blockchain in “regular” web and mobile apps (slides)
An overview of “all you need to know” in blockchain development by Haseeb Qureshi
NEAR specific:
Overview:
Check out the NEAR Whitepaper for the first layer of depth into the technical aspects of this project.
From there, this blog (at https://pages.near.org/blog) is the best record of technical specs as they grow and change over time. Subscribe for updates at https://pages.near.org/newsletter.
A good primer is on the Bison Trails blog.
Economics:
Learn about how NEAR Economics work in the Introduction to NEAR Economics post
Dive deeper into the NEAR Economics Paper
If you are curious about acquiring tokens in the future, any information about that will be available on the tokens page.
Technical:
The NEAR Whiteboard Series on YouTube has founders Alex and Illia do technical deep-dives with the founders of over 30 other projects including Ethereum, Cosmos, Nervos, Celo, IOTA, and so on.
Differences between the NEAR approach and Ethereum 2.0 sharding (written with Vitalik’s feedback)
Our sharding talk at Blockchain@Berkeley
Our work with Vlad Zamfir on a sharding POC during the Ethereum SF hackathon
Design
We’re focusing on building a great developer and end-user experience. And here are a few resources that will be helpful from a design perspective.
Improving blockchain developer experience (slides)
The design of blockchain-based applications (DApps) (slides)
Why the future will be driven by “UX Equilibrium”
Check out the San Francisco Blockchain Product, Design and Development meetup group to get a sense of the kind of broader community we’re hoping to build across functional disciplines
Bonus: Look for YouTube talks from the folks at Consensys, Coinbase and IDEO Colab.
General Resources
Podcasts
Podcasts are one of the best ways to educate yourself because the back catalogs can be very informative. I recommend taking up a time-consuming endurance sport where you can listen to them for hours on end and then consuming the following:
Laura Shin’s Unchained (http://unchainedpodcast.co/) and Unconfirmed (https://unconfirmed.libsyn.com/) for plain-English interviews
Epicenter (https://epicenter.tv/) for a bit more technical dives into various systems.
Zero Knowledge (https://www.zeroknowledge.fm/) is another on the more technical side.
Newsletters
There aren’t too many great newsletters and you should avoid anything that is primarily price or trading focused. That said, check out:
Token Economy (http://weekly.tokeneconomy.co/) is a good weekly roundup.
It’s highly Bitcoin-biased but I do enjoy reading Anthony Pompliano’s Off the Chain daily (https://offthechain.substack.com/) because it doesn’t have a lot of BS.
The CB Insights newsletter (https://www.cbinsights.com/newsletter) is more broad-ranging but generally has one general-interest blockchain story per issue.
Writing
The team from a16z Crypto has excellent writing on everything from legal issues around tokens to the future of web 3 with open state. See their main blog at https://a16zcrypto.com/content but also check out the original a16z blog before 2018 when they migrated to the new site for the legacy content, which is great too.
Vitalik from the Ethereum Foundation has been a prolific writer over the years and just about everything he puts out is interesting. Check out his blog at https://vitalik.ca/ and Ethereum-related research at https://ethresear.ch/.
People and Community at NEAR
To stay in the loop on an ongoing basis, follow us on Twitter at https://twitter.com/nearprotocol
To join the more in-depth community discussion and talk to the team, jump into our chat Channels: http://near.chat
To learn more and stay in the loop as we publish more content about the protocol and the industry in general, subscribe to our updates and newsletter (https://pages.near.org/newsletter) and subscribe to our YouTube channel (https://www.youtube.com/nearprotocol)
Thanks for your interest in the project! If you have more questions, suggestions for high-quality resources or just want to engage in the conversation more, Discord is your best bet. We’ll see you there 🙂 |
NEAR Releases JavaScript SDK, Bringing Web3 to 20 Million Developers
DEVELOPERS
August 8, 2022
Blockchain development has just become a whole lot easier for Web2 developers. NEAR is thrilled to unveil its much anticipated JavaScript Software Development Kit (JS SDK) at ETHToronto, the official hackathon of the Blockchain Futurist Conference, in a significant move to democratize Web3 development.
With NEAR’s JS SDK, more than 20 million developers that make JavaScript the world’s most popular coding language can finally join the internet revolution and quickly build fast, scalable, and user-friendly decentralized applications. Having that many more contributors in the space could bring about a fascinating renaissance to the open web.
Although Rust and Solidity are the most prominent programming languages for Layer 1 blockchains, there are fewer than 2.5 million developers worldwide who use these languages. So, opening up the doors nearly 10x is a major step towards mass adoption. JS runs in every browser, the basics can be taught in an afternoon, it requires no compiler setup, and it comes pre-loaded with useful libraries like RegExp, Math, and Array. The NEAR JS SDK brings this same capability and ease of use to developing smart contracts, unlocking the power of blockchain to a much, much wider audience.
Commenting on the launch, Illia Polosukhin, Founder of NEAR, says: “Developers can spend less time learning a new language and more time building their application in a language they already know. Millions of developers already know how to program in JavaScript; enabling this group to build novel applications on NEAR is a critical step in achieving our vision of a billion users interacting with NEAR.”
Learn, build, and deploy in minutes
NEAR’s commitment to putting simplicity and usability first is reinforced by this release, making the learning curve for blockchain far less steep than it has ever been. Javascript developers can now set up their first dApp in the same amount of time it takes to spin up a React app.
The JS SDK release has everything you need to dive right into blockchain development including the contract framework itself, JavaScript and TypeScript contract examples, and example tests. It is implemented in TypeScript, which offers developers implicit, strict, and structural typing, along with type annotations built into your favorite IDE.
Developers can get started building their first JavaScript decentralized application by following this quick start guide or heading to Pagoda’s landing page to learn more.
A Closer Look at the JS SDK
The JS SDK provides each contract its own instance of a JS engine running inside it. This design allows developers to write contracts in pure JavaScript or TypeScript, and access all the same features, like oracles, available to Rust contracts.
To get started, run npx create-near-app in your terminal and select JavaScript for your smart contract. Once everything is installed you can test, compile, and deploy your newly created project with ease.
For a more detailed look at the contract structure and other configurations check out this dApp QuickStart Guide on NEAR’s Official Documentation site.
About Pagoda
The JS SDK is developed by Pagoda, the engineering team that builds and maintains NEAR Protocol and some of the most important tools needed for decentralized application (dApp) development. Developers can use the Pagoda suite of tools to build, deploy, test, and interact with smart contracts on NEAR. For more information on the JS SDK, visit Pagoda or join the NEAR Discord and checkout the #engineering channels where resources like live support are available every day.
|
Rove World v2 Simplifies Web3 Ticketing and Loyalty for Brands
NEAR FOUNDATION
April 12, 2023
Rove, one of the most user-friendly entertainment ecosystems in Web3, is now in collaboration with NEAR. Their next release, Rove World v2, will act as a one-stop mobile-based ticketing solution and loyalty rewards program for brands. The update will also feature an in-app store that will include major brands, sports leagues, and musical artists.
Rove and Rove World v2 removes many of the key friction points that occur when brands and creators try to engage their audience with Web3 technology. Notable brands are already on board, as Rove has collaborated with the likes of Tommy Hilfiger on the Web2 side, alongside some of Web3’s largest brands such as Claynosaurz and MonkeDAO, with more exciting partnerships and activations to be announced soon.
“A multichain approach was always at the forefront of our long-term growth strategy for Rove,” said Jason Desimone, Founder of Rove. “Having built and launched the initial version of the Rove World mobile app, our goal is to now expand the reach and traction of Rove to other ‘Layer 1’ blockchain communities and ecosystems that align with our vision and will help us achieve our goals with the launch of Rove v2.”
Let’s take a look at how Rove World V2 is a game-changer for branded NFTs on NEAR.
How Rove is helping brands amp up their NFTs
The Rove ecosystem is a solution to many of the core issues facing brand and entertainment NFT campaigns, launches, and projects. That’s because Web3 itself is often difficult or daunting for the end user, who amongst other things might have questions about the value or utility of NFT ownership.
What Rove brings to the table is a user-friendly mobile app where NFTs are minted in mere seconds and with minimal steps. Brands can also easily add utility to the NFTs which are easily accessible by the user. Rove’s vision aligns seamlessly with NEAR’s Web2.5 strategy of making the onboarding of the next billion users into Web3 as easy as possible.
Brand and entertainment NFT experiences built atop Rove and NEAR lets users log in seamlessly through familiar means like social media log-in, Apple ID, or facial ID. NFTs are easily sent and received on the Rove app via usernames, again with a similar Web2 interface to that of Venmo.
Rove World v2 release lets brands create buzz
The Rove World v2 release will be a major part of Rove’s suite of tools that make it easy for brands to get started with blockchain technology. Their approach is to allow brands to engage with NFTs in a collaborative manner that benefits creators, brands, and end users alike. Rove World v2 on Near is a huge leap in that direction.
The main feature is the in-app store that brands can now leverage in combination with Rove’s flagship app for curated NFT launches and blockchain-based ticketing. Not only will attendees be able to claim NFTs instantly at the tap of their phone, but brands can also now leverage Rove World v2 to offer seamless in-app purchases via the mobile store.
NEAR and entertainment continue to shine with Rove
Rove has many brands in the pipeline across the fashion, film, music, and sports verticals that want a seamless NFT experience for their customers. This not only brings more notable and forward-thinking brands into NEAR’s orbit but also showcases how the NEAR ecosystem continues to make major strides in the sports and entertainment verticals.
Eventually, Rove will serve as a central ticketing and NFT project on NEAR. Users can easily create assets, mint the NFT, and distribute it however they’d like. The entire experience will be on a single mobile app, bridging the technical gap between users and brands while giving a fairer share to creators than mainstream NFT marketplaces.
All assets on Rove and Rove World v2 also have some form of utility. Anyone who owns an NFT on Rove can stake that asset for Rove credits that unlock various perks. It’s a great example of how Rove is making all of Web3 simple, including things like staking.
Ticketing and NFT distribution for brands and creators are about to get a whole lot easier for everyone thanks to Rove. And with Rove World v2’s in-app store, major brands are lining up to be at the forefront of making web3 truly accessible, user-friendly, and financially fair.
“NEAR’s emphasis on onboarding the next billion users into Web3 with a specialized focus on entertainment, sports, and culture fit identically with our approach,” said Rove’s Desimone. “Not to mention NEAR’s industry-leading technology which enables a seamless migration of the easy-to-use features of the Rove World mobile app onto the NEAR blockchain.” |
NEAR Ecosystem @ Korea BUIDL Week 2024
COMMUNITY
March 18, 2024
MARCH 26 – APRIL 2, 2024
As the sun rises in the east, a new dawn breaks for NEAR. Fresh from ETHDenver, where we planted a firm flag in the ground to own the Chain Abstraction narrative, the NEAR Ecosystem arrives at Korea BUIDL Week 2024 riding a wave of momentum and armed with exciting updates and alpha destined to reshape the blockchain landscape.
Chain Abstraction – a groundbreaking approach that abstracts away the complexities of different blockchain architectures – was a central focus for us at ETHDenver. We captivated attendees with live demonstrations, showcasing Chain Abstraction’s potential to enable developers to build seamlessly across multiple networks without the need for custom integrations or bridges.
By boldly staking our claim on this game-changing technology, we made a powerful statement at ETHDenver, signaling our intent to lead the charge in unlocking unprecedented levels of cross-chain communication and composability.
Now, as we descend upon Korea BUIDL Week, we are poised to take Chain Abstraction to the global stage, introducing this transformative narrative and its robust, developer-friendly tooling to an international audience of innovators, entrepreneurs, and industry leaders.
Developers, builders, researchers, and enthusiasts – join us for a week of events as we continue to push the boundaries of what’s possible.
NEAR is Everything Everywhere All at Once at Korea BUIDL Week
We’re hitting the ground running with NEAR Foundation’s second Chain Abstraction Day (apply-to-attend) in our year long series, taking place March 26, 2024 during Korea BUIDL Week. We’re doubling down and taking everyone to the moon!
Our co-founder and CEO, Illia Polosukhin, will be dropping some major alpha at BUIDL Asia 2024, sharing insights into NEAR’s vision for Chain Abstraction and how it’s poised to unlock unprecedented levels of interoperability and composability across decentralized networks.
But that’s not all – we’re bringing the heat with dedicated workshops, content, and a massive hackathon bounty prize up for grabs at ETH Seoul, challenging the brilliant minds of the blockchain world to push the boundaries of Chain Abstraction. Developers, builders, and innovators: get ready to bring your A-game and compete for this lucrative reward.
Don’t miss your shot at NEAR’s massive hackathon bounty prizes at ETHSeoul – check out the link to see what lucrative rewards await the winners. Register for the hackathon today and let your skills shine on the global stage.
And if that’s not enough, NEAR is also proud to support SheFi Summit Seoul, empowering women builders in Web3. This groundbreaking event showcases the incredible female talent in our ecosystem, with NEAR women taking the stage to share their visionary work.
Abstractooors, you won’t want to miss the fun! |
# Math API
```rust
random_seed(register_id: u64)
```
Returns random seed that can be used for pseudo-random number generation in deterministic way.
###### Panics
- If the size of the registers exceed the set limit `MemoryAccessViolation`;
---
```rust
sha256(value_len: u64, value_ptr: u64, register_id: u64)
```
Hashes the random sequence of bytes using sha256 and returns it into `register_id`.
###### Panics
- If `value_len + value_ptr` points outside the memory or the registers use more memory than the limit with `MemoryAccessViolation`.
---
```rust
keccak256(value_len: u64, value_ptr: u64, register_id: u64)
```
Hashes the random sequence of bytes using keccak256 and returns it into `register_id`.
###### Panics
- If `value_len + value_ptr` points outside the memory or the registers use more memory than the limit with `MemoryAccessViolation`.
---
```rust
keccak512(value_len: u64, value_ptr: u64, register_id: u64)
```
Hashes the random sequence of bytes using keccak512 and returns it into `register_id`.
###### Panics
- If `value_len + value_ptr` points outside the memory or the registers use more memory than the limit with `MemoryAccessViolation`.
|
Community Update: MainNet Phase I 🚀 , Hack the Rainbow 🌈 , OWC Demo Day
COMMUNITY
September 15, 2020
Hello citizens of NEARverse! This is NiMA coming to you from a NEARby planet in the Milky Chain …
These coming two weeks will be packed with a ton of exciting news and events for all stakeholders in the NEAR community and our wider ecosystem of projects, validators, and token holders.
Starting on September 24th, NEAR will be transitioning into Phase I, aka “MainNet Restricted”, which will prepare NEAR for becoming a fully permissionless community-governed network. Read the section below on Phase I to find out what this means for you!
This week we will also be kicking off Hack the Rainbow hackathon and webinar series. Even if you are not planning to participate in the hackathon, we invite you to join us on Crowdcast to watch and participate in some of the exciting panels and workshops that we have planned for you!
3, 2, 1 … ready for Phase I
Phase I, as previously described in this post, is the final stage on our roadmap before NEAR fully transitions to a permissionless community-governed network. The decision to upgrade the network to Phase II is up to validators and token holders (via delegation). NEAR Foundation (NF) will stop running its PoA nodes and will only facilitate this transition by temporarily delegating 40 million NEAR tokens to a set of validators composed of NVAB and the first 30 validators from the community who will be invited to participate!
You can read more about the details of validation/delegation during Phase I in this post by Stefano, our chief staking sorcerer.
As a token holder you have the option to already delegate your tokens to the validator of your choice. At launch this will only be possible for more technically savvy community members who are familiar with near-cli (command line tool). As we derisk the process, delegation will also be offered via NEAR Wallet graphical user interface (GUI) and 3rd party validator services.
Important to note: there will be no staking rewards during Phase I, but your participation will support further decentralisation of the governance process during this transitory phase. It will also allow you to familiarise yourself with NEAR validators early on before the launch of Phase II.
What’s next?
As soon as 2/3rd of all staked funds (during Phase I) vote to unlock transfers, lock-up smart contracts begin unlocking tokens as scheduled. A separate update of nearcore software will enable inflationary staking rewards. It’s worth mentioning that staking rewards are immediately transferable and have no lock-up. All tokens in circulation, regardless of lock-up schedule, can be staked to earn staking reward! 🚀
Hack the Rainbow: workshops, panels, bounties and more 🎉
Hack the Rainbow 🌈 is officially starting on Sep 15th with a series of talks and workshops hosted on Crowdcast!
If you’re a builder/hacker, you can sign-up on Gitcoin to start working on your project or meet other hackers to form teams. In case you’re not sure what to work on during the hackathon … worry no more! We have put together a list of cool ideas on Notion which you can use as a starting point for your own hackathon project.
You can see the full list of webinars on Crowdcast, but here are some of the highlights:
Grilling the Rainbow with Justin Drake (Ethereum 2.0 research lead) and Illia Polosukhin (NEAR co-founder)
DAOs, DAOs, DAOs with Burrrata (Aragon/RaidGuild), James (Moloch/Abridged), …
DeFi: Food, Farming, and Philanthropy moderated by Simona Pop with star 💫 guest $ALEX
Several demos and workshops with our partner projects: Balancer, ChainLink, Sia/SkyNet, …
Introduction to Rainbow 🌈 Bridge, cross-chain demo, NEAR 101 and 102, …
We would like to give a special shout-out to Aaron from Vital Point AI Guild who has put together this amazing overview of his project using Textile, NEAR, and Moloch! Feel free to reach out to Aaron to start BUIDLing the future of decentralised community platforms ✌️
Engineering Updates
Our engineering team is focused on improving bridge devX, working on upgradeability of the bridge and adding native support for EVM (Ethereum Virtual Machine) to NEAR. This should make onboarding Ethereum applications much easier, and together with the ETH<>NEAR bridge, will position NEAR as one of the best choices for near-term scaling of DApps in the blockchain space.
Generalized token locker for the Rainbow bridge is implemented;
Implicit account creation to match DevX and UX of Ethereum ecosystem is implemented;
Runtime documentation is improved;
We are working combining Wasmer and Wasmtime runtimes;
Native EVM support is ready and is currently undergoing review and testing;
Edu updates
NEAR 101 “NEAR for Web Developers” shipped. Slides and recordings available (bit.ly/near-101)
NEAR 102 “NEAR for Ethereum Developers” available next week (will bebit.ly/near-102)
“Show and Tell: Rainbow Bridge” makes explaining the bridge more fun
As always looking for YOUR input to our educational priorities. If you have any suggestions, ideas or preferences and would like them to be heard, email me directly at[email protected] or find me on Telegram (amgando) or Discord (Sherif#5201)
Open Web Collective (OWC) Demo Day 🎉
Are you joining the most anticipated blockchain Demo Day?
Attend theOpen Web Collective Demo Day, organised byNEAR, and get an exclusive look into the future of blockchain projects and DeFi. Nine pioneering startups will showcase their DApps and pitch their projects to top crypto investors!
Join us on September 23, 2020 at 8am PST. Save your free spot now 👉https://www.crowdcast.io/e/ace93fjx/register
The Open Web Collective is an innovative blockchain accelerator designed by NEAR to help passionate entrepreneurs grow their startups into scalable & successful companies. Our founders have worked with leading partners and investors such as IDEO, Deloitte, Pantera Capital and more and have already closed funding rounds of more than $3.5M. Join the Demo Day and be at the forefront of blockchain developments.
If you’re an entrepreneur or working at a blockchain startup and would like to participate in our program, please reach out. Connect with us onTwitter,Medium,YouTube, and Email.
This week we are watching, reading, and listening to …
Jake Brukhman, founder of CoinFund and Crypto OG, talks to Sasha about various models in the fast-paced crypto funding and governance space.
You can listen to this episode of Building the Open Web podcast on Spotify or Apple Podcasts.
That’s it for this update. See you in the next one!
Your friends,
NiMA and the NEAR team |
How to Convert Web 2 Games to Web 3
DEVELOPERS
July 1, 2022
Gaming has become one of the fastest growing sectors of the Web3 world. On NEAR alone, there are nearly 100 projects building gaming titles in the ecosystem. But harnessing the unique properties of blockchain can be a challenge. Which is why an ecosystem project, in collaboration with the NEAR Foundation has created a handy tutorial for anyone considering converting their games to Web3.
If you’ve ever tried to find a simple answer to the question of “How do I start converting my mobile game to NEAR blockchain?”, then this Web2 to Web3 tutorial is for you.
The tutorial was created by Sigma Software Group, a consultancy group with Swedish and Ukrainian roots, as a result of its own work converting a free to play large-scale mobile AR game called Black Snow, which makes players the heroes of an epic sci-fi movie at their physical or virtual locations.
Through this conversion, Black Snow is utilising NFTs to radically reimagine the gaming experience for players. So the team have decided to share its knowledge with other gaming companies looking to explore similar conversions.
Essentially, there is no way of just “converting” your game to use a blockchain, although NEAR strives to make the transition and onboarding to web3 ecosystem as natural and hassle free as possible. Thus, even the very first step to add NFTs into a mobile game presents a multi-faceted challenge that includes game mechanics, architecture, engineering, and live ops.
A step-by-step guide
The tutorial begins with explanations of the very core concepts, which you’ll need to understand before starting the move to web3. It highlights both the benefits and constraints of moving to blockchain.
Then, you’ll find a sequence of practical steps along with code examples to guide you through converting a regular mobile game to a game with NFTs in the NEAR ecosystem.
These steps cover the technical aspects of how to choose libraries, frameworks and which third-party solutions can be used to implement the blockchain architecture.
The tutorial describes how NFTs are used to represent ownership of an asset in web3 world, how to implement them technically, and on which moment you need to stay focused during this process. Further, it covers aspects on NFT usage and minting as well as NFT marketplace integration.
The tutorial also provides a number of tips and tricks, which are not that obvious for any newcomer to blockchain, concerning security, scalability, availability, and costs of a web3 application. |
---
sidebar_position: 3
---
# Creating Accounts
You might want to create an account from a contract for many reasons. One example:
You want to [progressively onboard](https://www.youtube.com/watch?v=7mO4yN1zjbs&t=2s) users, hiding the whole concept of NEAR from them at the beginning, and automatically create accounts for them (these could be sub-accounts of your main contract, such as `user123.some-cool-game.near`).
Since an account with no balance is almost unusable, you probably want to combine this with the token transfer from [the last page](./token-tx.md). You will also need to give the account an access key. Here's a way do it:
```rust
Promise::new("subaccount.example.near".parse().unwrap())
.create_account()
.add_full_access_key(env::signer_account_pk())
.transfer(250_000_000_000_000_000_000_000); // 2.5e23yN, 0.25N
```
In the context of a full contract:
```rust
use near_sdk::{env, near_bindgen, AccountId, Balance, Promise};
const INITIAL_BALANCE: Balance = 250_000_000_000_000_000_000_000; // 2.5e23yN, 0.25N
#[near_bindgen]
pub struct Contract {}
#[near_bindgen]
impl Contract {
#[private]
pub fn create_subaccount(prefix: AccountId) -> Promise {
let subaccount_id = AccountId::new_unchecked(
format!("{}.{}", prefix, env::current_account_id())
);
Promise::new(subaccount_id)
.create_account()
.add_full_access_key(env::signer_account_pk())
.transfer(INITIAL_BALANCE)
}
}
```
Things to note:
* `add_full_access_key` – This example passes in the public key of the human or app that signed the original transaction that resulted in this function call ([`signer_account_pk`](https://docs.rs/near-sdk/3.1.0/near_sdk/env/fn.signer_account_id.html)). You could also use [`add_access_key`](https://docs.rs/near-sdk/latest/near_sdk/struct.Promise.html#method.add_access_key) to add a Function Call access key that only permits the account to make calls to a predefined set of contract functions.
* `#[private]` – if you have a function that spends your contract's funds, you probably want to protect it in some way. This example does so with a perhaps-too-simple [`#[private]`](../contract-interface/private-methods.md) macro.
* `INITIAL_BALANCE` uses the [`Balance`](https://docs.rs/near-sdk/3.1.0/near_sdk/type.Balance.html) type from near-sdk-rs. Today this is a simple alias for `u128`, but in the future may be expanded to have additional functionality, similar to recent [changes to the `Gas` type](https://github.com/near/near-sdk-rs/pull/471).
|
---
id: token-loss
title: Avoiding Token Loss
sidebar_label: Avoiding Token Loss
---
:::warning
Careful! Losing tokens means losing money!
:::
Token loss is possible under multiple scenarios. These scenarios can be grouped into a few related classes:
1. Improper key management
2. Refunding deleted accounts
3. Failed function calls in batches
---
## Improper key management
Improper key management may lead to token loss. Mitigating such scenarios may be done by issuing backup keys
allowing for recovery of accounts whose keys have been lost or deleted.
### Loss of `FullAccess` key
A user may lose their private key of a `FullAccess` key pair for an account with no other keys.
No one will be able to recover the funds. Funds will remain locked in the account forever.
### Loss of `FunctionCall` access key
An account may have its one and only `FunctionCall` access key deleted.
No one will be able to recover the funds. Funds will remain locked in the account forever.
---
## Refunding deleted accounts
When a refund receipt is issued for an account, if that account no longer exists, the funds will be dispersed among
validators proportional to their stake in the current epoch.
### Deleting account with non-existent beneficiary
When you delete an account, you must assign a beneficiary.
Once deleted, a transfer receipt is generated and sent to the beneficiary account.
If the beneficiary account does not exist, a refund receipt will be generated and sent back to the original account.
Since the original account has already been deleted, the funds will be dispersed among validators.
### Account with zero balance is garbage-collected, just before it receives refund
If an account `A` transfers all of its funds to another account `B` and account `B` does not exist,
a refund receipt will be generated for account `A`. During the period of this round trip,
account `A` is vulnerable to deletion by garbage collection activities on the network.
If account `A` is deleted before the refund receipt arrives, the funds will be dispersed among validators.
---
## Failed function calls in batches
:::warning
When designing a smart contract, you should always consider the asynchronous nature of NEAR Protocol.
:::
If a contract function `f1` calls two (or more) other functions `f2` and `f3`, and at least one of these functions, `f2` and `f3` fails, then tokens will be refunded from the function that failed, but tokens will be appropriately credited to the function(s) which succeed.
The successful call's tokens may be considered lost depending on your use case if a single failure in the batch means the whole batch failed.
|
Case Study: Bernoulli-Locke-Locke’s David Palmer on the DAO-Owned SailGP Team
NEAR FOUNDATION
June 14, 2023
NEAR is a home to a number of amazing apps and projects. These developers, founders, and entrepreneurs are using NEAR to effortlessly create and distribute innovative decentralized apps, while helping build a more open web — free from centralized platforms. In recent installments, NEAR Foundation explored the BOS with Pagoda’s Chief Product Officer Alex Chiocchi, heard from OnMachina co-founder Jonathan Bryce on building decentralized storage, and dove into Move-to-Earn with Sweat Economy’s Oleg Fomenko.
In the latest case study video, Bernouilli-Locke founder David Palmer details how his group is implementing a DAO for team ownership and governance for SailGP boat racing, by leveraging the power of NEAR. |
---
title: Core Experience
description: Overview of the core experience on NEAR BOS
sidebar_position: 5
---
# Core Experience
Coming Soon! |
---
id: utils
title: Utilities
sidebar_label: Utilities
---
### NEAR => yoctoNEAR {#near--yoctonear}
```js
// converts NEAR amount into yoctoNEAR (10^-24)
const { utils } = nearAPI;
const amountInYocto = utils.format.parseNearAmount("1");
```
[<span className="typedoc-icon typedoc-icon-function"></span> Function `parseNearAmount`](https://near.github.io/near-api-js/functions/_near_js_utils.format.parseNearAmount.html)
### YoctoNEAR => NEAR {#yoctonear--near}
```js
// converts yoctoNEAR (10^-24) amount into NEAR
const { utils } = nearAPI;
const amountInNEAR = utils.format.formatNearAmount("1000000000000000000000000");
```
[<span className="typedoc-icon typedoc-icon-function"></span> Function `formatNearAmount`](https://near.github.io/near-api-js/functions/_near_js_utils.format.formatNearAmount.html)
|
# Data Types
## type CryptoHash = [u8; 32]
A sha256 or keccak256 hash.
## AccountId = String
Account identifier. Provides access to user's state.
## type MerkleHash = CryptoHash
Hash used by a struct implementing the Merkle tree.
## type ValidatorId = usize
Validator identifier in current group.
## type ValidatorMask = [bool]
Mask which validators participated in multi sign.
## type StorageUsage = u64
StorageUsage is used to count the amount of storage used by a contract.
## type StorageUsageChange = i64
StorageUsageChange is used to count the storage usage within a single contract call.
## type Nonce = u64
Nonce for transactions.
## type BlockIndex = u64
Index of the block.
## type ShardId = u64
Shard index, from 0 to NUM_SHARDS - 1.
## type Balance = u128
Balance is type for storing amounts of tokens.
## Gas = u64
Gas is a type for storing amount of gas.
|
NEAR Community Update: January 17th, 2020
COMMUNITY
January 17, 2020
Welcome to our first Community Update of 2020. This is a weekly summary of our progress and community. As you might realise, we decided to change the structure of our community update. If you have any thoughts, please let us know what you think. Want something mentioned? Tweet us at @NEARProtocol or send us a message.
Content
Tune in to Ep. 32 of NEAR Protocol’s Whiteboard Series with @celoHQ to hear from Time Moreton
Evgeny’s tweet thread explaining transactions in NEAR
Have a read of Flux’ use case series. The best part? — They publish new ones every Friday
Our must watch
Alex’ sharding jam with Dean Eigenmann and Ryan Zurrer
Call for Participation
We want to hear from you! Will you take 15 seconds to fill out this survey so we can make more of the kind of content you love?
Stardust: One of our beta program projects just released their integration guide. If you have experience with nodejs and would like to help out by providing some feedback, please reach out to Canaan: [email protected].
Community
Illia, Amos and several NEAR core developers just came back from an event series and hackathon in China. Have a look at the pictures on our Discord.
We did a complete overhaul of our community channels. Please join us in our new Telegram channels for development and validators.
Engineering
108 PRs across 31 repos by 28 authors. Featured repos: nearcore, near-bindgen-as, near-explorer, near-bridge, nearlib, near-runtime-ts, near-wallet, create-near-app, near-fiddle-api and near-shell
Responding to view requests from a different actor than the actor that produces blocks to ensure requests do not interfere with chain maintenance in nearcore
Limiting the number of blocks / chunks per height to prevent spamming in nearcore
New infrastructure to execute smart contracts from python tests in nearcore
Release 0.4.12 of nearcore
Added near dev-deploy which allows developer to deploy contract easily on throwaway account (similar to studio) during development in near-shell
We made our tooling compatible with GitPod (for now onlyhttps://github.com/nearprotocol/near-chess converted)
Added account list in near-explorer
Added EthRelay in near-bridge
Use near-bindgen-as in near-runtime-ts
Expose batch action promise API in near-runtime-ts
Stake Wars is on hold until the implementation of Doomslug is fully finalized and our internal testing becomes all green.
Upcoming Events
We are going to be at ETHDenver between the 14th and 16th of February
And at the same time, you can meet us at SF Developer Week Expo and Hackathon
Are you a developer, student, or entrepreneur interested in joining us at DevWeek2020? Fill out this brief survey for a chance to win two free tickets!
@One of our events in Shanghai earlier this month; kudos to our co-hosts HashQuark and IOSG
https://upscri.be/633436/ |
NEAR Community in Focus: Web3 vs Web2 Communities
COMMUNITY
June 13, 2022
Each new wave of cultural and technological change brings with it a redefinition of community. As the world shifts from the centralized Web2 paradigm to the burgeoning world of Web3, communities are undergoing rapid redefinitions. In this shift, communities are reimagining how they organize, what they do, and how individuals can ultimately benefit.
“Web2 communities happen around a product rather than an organic network of like-minded people gearing up to contribute towards a long-term goal,” says Max Goodman, founder of Gyde, a low-cost resource directory designed to help all manner of communities in Web3.
“User interaction is primarily with marketing officials or customer service or other users, not key decision-makers in the organization,” Max continues. “Web2, as a rule, does not share value with builders and users. Instead, it extracts value.”
Goodman touches on one of the most important differentiators between Web2 and Web3 communities. But combating an extractive, centralized model is only the tip of the iceberg. Here’s a deeper look at how Web3 communities are evolving, and the role that builders on NEAR are playing.
Correcting for Web2 biases against the underclass
Goodman is building Gyde as a response to one of Web2 biggest issues: how entities approach community. In Web2, biases can exclude certain marginalized groups or members of the underclass. Google Maps and other location-based search applications, for instance, don’t show relevant or helpful services those living in poverty. This top-down approach is something Web3 projects, particularly Decentralized Autonomous Organizations (DAOs), are turning on its head.
“I’m bullish on cooperative, non-hierarchical, ‘impact DAOs,’ that focus on social good,” Goodman continues. “We can now break free of traditional hierarchical structures and move towards communities or networks, rather than top-down entities. This can be done without replicating existing problematic behaviors such as excluding traditionally marginalized folks from participation.”
With Gyde, Goodman is striving to build a viable alternative to traditional Web2 map-based search applications. One that is based on the needs and values of individual communities, not what any centralized entity dictates. Goodman also plans to implement an impact DAO, so that community members can earn tokens for adding new locations like food banks or non-profit services, in addition to those who post helpful reviews.
“We’re building community in-person first,” explains Goodman. “We’re onboarding traditionally excluded people into crypto, onto NEAR specifically, with one-on-one educational meetings. And the best part is that this helps solve the non-profit problem of connecting with their clients fast. We like to call it map-to-earn.”
Impact DAOs and Web3 projects like Gyde are addressing the needs of marginalized communities and the “Unexotic Underclass”. Communities that Web2 has largely ignored.
“It’s not uncommon to believe that poor people do not understand technology or advanced topics,” Goodman points out. “But homeless people were mining Bitcoin to pay for pizza with their laptops in San Francisco in 2013. Society oftentimes implicitly excludes the unexotic underclass by requiring IDs for work. A NEAR address does not care.”
From consumers to participants in musical communities
Goodman and Gyde hint at another defining difference between Web2 and Web3 communities: the economic model and resource distribution. Web2 companies tend to view individuals as consumers or users. In Web3, people are more akin to creators, contributors, and stakeholders on a more level economic playing field.
“What communities want, need, and what brings them together hasn’t really changed in millennia,” says Tim Exile, founder of collaborative music platform Endless.fm. “What’s changed is the technology and the products people built on top of that technology. Web2 hasis been effective at leveraging a broad, one-to-many cost dynamic. That’s why we see a skewed distribution of producers and consumers in the ‘going viral’ attention economy.”
With Endless.fm, Exile is attempting to re-define economic models for Web3 creative communities, building on the NEAR blockchain. With Endless.fm, individuals can enter collaborative “jam sessions” with musicians. The can sample music,and create music NFTs in what Exile and others have referred to as the “creator-owned economy”. The beauty of Web3 community is that it erases hierarchical boundaries between music creators and consumers, allowing for a more open economy.
“Industrial media is very binary—either you’re a producer or a consumer,” Exile explains. “But in Web3, everyone is a participant. And those who are the most intrinsically motivated to lean in and participate in communities can be rewarded and empowered more economically.”
Exile explains that the most fundamental shift occurring within music-driven communities is that association and context are becoming more valuable than pre-packaged experiences. Rather than paying $30 to attend a concert, people can create music socially with other community members in real-time, mint their own music NFTs, and decide on the price or value of their music on the open market.
“With Endless, we’re unbundling music in a way that’s much more about creating context than it is about creating content,” he says. “In Web3 communities, ultimately the value is really driven by relationships, interactions, histories, and stories. And that’s what we’re building towards.”
Empowering sports clubs and communities
Sports teams, clubs, and communities are other areas where Web3 is poised to disrupt where Web2 could not. In Web2, the dynamic is very similar to what Exile describes in music: a one-to-many distribution model that’s not all that participatory.
“The NFT wave has been very popular amongst the top one percent of professional athletes,” says Kyle Ludon, VP of product management at Stack Sports. “But the truth is that 99 percent of athletes aren’t professional, and these people aren’t being addressed with this new wave of technology.”
Ludon points to the projects that Stack Sports is building on NEAR using NFT technology as a way to build sports communities from the ground up. A parent who attends their child’s soccer game and captures a picture of their first goal, for instance, can upload that image and mint it as an NFT “trading card.” They can even add unique design elements to the digital collectible. Aside from being cool digital keepsakes, Ludon projects that NFTs can open doors for communities like little league teams, cycling clubs, and social or passion-based athletic groups.
“Athletes and parents can share NFTs of their special moments and even trade them amongst each other. You might even create a DAO around your club or team, use the NFT as a form of membership, and even implement voting or governance,” posits Ludon.
This is already coming to fruition with SailGP’s partnership with NEAR, in which DAO will eventually own a sailing team. In Web2, sports are more about corporations, franchises, and elite athletes selling products or experiences via hierarchically designed communities on platforms like Facebook. In Web3, athletes and enthusiasts can mint and exchange NFTs, create their own DAOs, and directly organize with like-minded enthusiasts.
A distributed notion of society
These are just a few examples of how the nature and definitions of community are changing in real-time. Endless.fm, Stack Sports, and Gyde are all using the NEAR blockchain to make communities more inclusive, collaborative, and economically prosperous.
“In Web3, no one really cares that you’re labeled as marginalized in general society,” summarizes Goodman from Gyde. “They care about your skills and ability to communicate. We’re embracing a distributed notion of community, which will create larger opportunities for participation in the Web3 economy.” |
NEAR Transparency Report: December 2
NEAR FOUNDATION
December 2, 2022
As part of the Foundation’s commitment to transparency, each week it will publish data to help the NEAR community understand the health of the ecosystem. This will be on top of the quarterly reports, and the now monthly funding reports.
You can find the quarterly reports here.
You can find monthly reports on funding here.
Last week’s transparency report can be found here.
The importance of transparency
The NEAR Foundation has always held transparency as one of its core beliefs. Being open to the community, investors, builders and creators is one of the core tenets of being a Web3 project. But it’s become apparent the Foundation needs to do more.
The Foundation hears the frustration from the community, and it wants to be more pro-active in when and how it communicates.
New Accounts and Active Accounts
New Accounts are new wallets being created on the NEAR blockchain. In November the number of new accounts has been declining. This week, new account creation has averaged 16,000 new accounts per day, down from a monthly average of 24,000 wallets per 24 hours.
Looking at the quarter, the highest day for new account creation in Q3 was September 13 where 130,000 new wallets were created in one day. Collectively, these numbers equate to 22,454,000 total wallets on the NEAR blockchain.
The Daily Number of Active Accounts is a measure of how many wallets on NEAR are making transactions on chain. Over the last week, the number of daily active accounts has been on average, above 50,000, remaining consistent across the week.
Historically, as the chart below shows, this is a decline from highs of more than 100,000 active accounts on the network. The highest number of active accounts on any one day in Q3 this year was logged on September 14, where 183,000 accounts were active.
New Contracts and Active Contracts
Contracts on NEAR are simply programs stored on a blockchain that run when predetermined conditions are met. The Daily Number of New Contracts is a valuable metric for understanding the health and growth of an ecosystem.
The more active contracts there are, the more projects are actively engaging with the NEAR protocol. The chart below shows a cyclical rhythm to new contracts, with rises and falls a common occurrence. Over the last seven days, the number of new contracts has risen from a low of 12 new contracts on November 27 to a high off 44 new contracts on November 30.
Active contracts is a measure of contracts that execute in a 24 hour period. This number has remained consistent throughout the last week with an average of 500 active contracts on the NEAR network. Taking a historical perspective on these numbers, the average has declined in Q3, with previous highs in active contract activity coming in the third week of September 2022.
Used Gas and Gas Fee
Gas Fees are a catch all term for the cost of making transactions on the NEAR network. These fees are paid to validators for their services to the blockchain. Without these fees, there would be no incentive for anyone to keep the network secure.
Over the last few weeks, the daily amount of gas, expressed here as PetaGas, which is the equivalent of 0.1 $NEAR, has risen. To learn more about Gas on NEAR, there is an excellent explainer in the NEAR White Paper. The Daily Amount of Used Gas has increased, briefly topping 8,000 PetaGas, before returning to the weekly average of just over 7,000 PetaGas.
The Daily Amount of Gas correlates with the Daily Gas Fee used on the network. Over the last week, there has been an uptick in the amount of Gas used, which can be brought on by a number of different factors. One of the most common is increased activity among users of the network.
Daily Transactions
The daily number of transactions is a record of how many times the blockchain logged a transaction. The earliest data available is from the third and fourth weeks of November. The data shows that daily transactions had been trending upwards and at the end of November, were averaging more than 500,000 transactions per day. Since then, the number has declined sharply and now sits below 400,000.
These reports will be generated each week and published on Friday.
|
Introduction to NEAR Protocol’s Economics
COMMUNITY
April 23, 2020
Intro to NEAR Protocol’s Economics
NEAR is a developer platform for the Open Web. This means that NEAR has a set of protocols and tools that enable people to build a new wave of community-owned applications.
The main protocol, NEAR Protocol, is a blockchain to power the security, economy, and incentives of NEAR’s developer platform.
Open Web is a paradigm shift of how applications and businesses are built. In the current paradigm, a company with investors and shareholders started by founders provides some service to its users. Over time, though, the divide between what users want and what generates more revenue usually grows. At the same time, companies try to reduce external dependencies to defend against the chances that one of its suppliers fails or gets acquired by competition.
The principles of Open Web are as follows:
Governed by community
Hackable and composable
Open and permissionless
User-first and sovereign
Open markets
To ensure these principles are followed, a combination of efforts is required:
A trustless and permissionless base layer that can be leveraged by all parties for various contracts, marketplaces, rooting sovereign data storage and more.
An independent currency that can be operated in a permissionless way and that can have a monetary policy to incentivize the behaviors of the parties.
The ability of new teams to create their own community-driven economies around their applications.
Backend for high-risk and low-trust serverless decentralized applications.
NEAR Protocol sits at the bottom of the stack, providing a permission-less base layer, independent currency and predetermined monetary policy and marketplace of computing resources.
This article explores the economic principles governing NEAR Protocol, and how they keep aligned the interests of its community.
Native cryptocurrency Ⓝ
$NEAR (Ⓝ) is the native cryptocurrency used in NEAR Protocol and, as the lifeblood of the network, has several different use cases. As the native currency, it secures the network, provides a unit of account and medium of exchange for native resources and third-party applications, and, over the long term, aims to become a unit used by individuals as well as by contracts and decentralized finance (DeFi) applications.
Security of the Network
NEAR Protocol is a proof-of-stake network which means that Sybil resistance from various attacks is done by staking Ⓝ. Staked Ⓝ represent a “medallion” for service providers that supply a decentralized infrastructure of servers that are maintaining state and processing transactions to NEAR users and applications. In exchange for this service, node providers receive rewards in Ⓝ.
Network Usage Fees
The utility of the network is provided by storing application data and providing a way to change it by issuing transactions. The network charges transaction fees for processing the changes to this stored data. The NEAR network collects and automatically burns these fees, such that higher usage of the network will increase the incentives to run validating nodes (as they receive higher real yield).
On the other hand, Ⓝ is also used as collateral for storing data on the blockchain. Having 1 Ⓝ on an account allows the user to store some amount of data (the specific amount depends on the available storage but will increase over time).
Medium of Exchange
Because Ⓝ is readily available on the protocol level, it can be easily used to transfer value across NEAR applications and accounts. This monetary function enables the creation of applications that use Ⓝ to charge for various functions, access to data, or performing other complex transactions. Ⓝ is exchanged within the network in a peer-to-peer fashion, across various applications and accounts, without the need for trusted third parties to clear and settle transactions.
Unit of Account
As mentioned in the “Network Usage Fees” section, Ⓝ is used to price the computation and storage of the NEAR infrastructure, which is offered by node operators. At the same time, applications and external parties can use Ⓝ as a unit of account for their application services or to measure the amount of the exchange in cases of Ⓝ as medium of exchange.
Genesis and inflation
At the launch, the NEAR network will have 1 billion Ⓝ. Each Ⓝ is divisible into 1024yocto Ⓝ.
Ⓝ Issuance and inflation
NEAR Protocol’s issuance of tokens, or inflation, is necessary to pay network operators, also called Validators. There is fixed issuance around 5% of the total supply each year, 90% of which goes to Validators in exchange for computing, storage, and securing the transactions happening on the network.
As mentioned above, all transaction fees collected by the network get burned. Therefore, the issuance of Ⓝ is actually ~5% minus transaction fees. This means that, as the network grows in usage, issuance can become negative, introducing negative inflation in the protocol. Since the smallest unit of account for Ⓝ is yocto Ⓝ, the system can keep its exchange price resolution as small as infinitesimal fractions of the U.S. dollar, even with a reduction of the overall supply by two or three orders of magnitude.
avg # of tx/day Min Ⓝ in fees/day Ⓝ mint/day Annual inflation
1,000 0.1 136,986 5.000%
10,000 1 136,985 5.000%
100,000 10 136,976 5.000%
1,000,000 100 136,886 4.996%
10,000,000 1,000 135,986 4.964%
100,000,000 10,000 126,986 4.635%
1,000,000,000 100,000 36,986 1.350%
1,500,000,000 150,000 -13,014 -0.475%
2,000,000,000 200,000 -63,014 -2.300%
Expected inflation per day given different number of tx
This table includes a few assumptions. But the main point is to show how inflation gets affected by usage.
Note that even though 1 billion transactions per day is a pretty large number for existing blockchains, that’s about ~11k tx per second of sustained load (also these tx are benchmarked as payment tx; more complex smart contract calls will require more gas and cost more).
Bitcoin pioneered cryptocurrencies and the idea of fixed supply. At the same time, constant reduction in security rewards available to miners creates interesting issues long term with the longest chain consensus (for more details read this paper). In NEAR Protocol, we addressed this issue by guaranteeing a constant security reward rate to Validators. And instead of issuance declining independent of the usage, NEAR’s issuance declines with more usage of the network.
Also note that in comparison to Bitcoin and Ethereum, token holders can avoid inflation by delegating/validating with their stake, preserving their percentage or even increasing it (see details in the “Validators” section).
Network Usage
Transaction fees
Each transaction takes some amount of bandwidth to be included in the block and computation to be executed. We combine both of these resources into a unified measure called “gas” that Validators must spend to execute a transaction. NEAR uses WebAssembly Virtual Machine (VM) to execute transactions and provides a mapping from each VM operation to the amount of gas it spends. The goal for gas is to represent a unified measure of resources spent to receive, execute, and propagate a transaction on default hardware.
Users who want to send such a transaction must pay a transaction fee, which is computed based on the amount of gas that the transaction will require multiplied by the current gas price.
Gas price is defined systemwide and changes from block to block in a predictable manner. Specifically, if the previous block used more than ½ of the gas limit for that block, the gas price is increased by a small margin. Otherwise, the gas price will decrease. There is also a minimum gas price which provides a floor for the price.
Minimum gas price is selected to provide a cheap transaction price for users and developers, with the expectation of collecting ~0.1 Ⓝ per full chunk (part of the block from a shard, see Nightshade for more detail). This is designed to make blockchain usage more accessible. Instead of the economy of the scarcity of transaction processing (how it works in Bitcoin and Ethereum), the goal of this system is to hit the economy of scale by increasing the number of shards.
Validators when including transactions do not use fees for ordering, instead, there is a deterministic ordering based on transaction hash. As explained in the “Issuance” section, fees that are collected from transactions are burned instead of being redistributed within the network of Validators.
This is done for a few reasons:
In the Proof-of-Stake network, every validator does the same amount of work by executing and validating transactions; the block producer is not all that special to be rewarded. In the case of a sharded network, it’s even more complicated to distribute these fees, as there are also receipts between shards where gas is bought when a transaction arrives but executed by different Validators.
Validators are still incentivized from burning transaction fees as that directly increases their yield. For simple example, let’s say total supply is 100, 50 of which is staked. Let’s 5 tokens are paid in epoch reward, then if there are no fees – yield is (55/105)/(50/100)-1~=4.76%. While if there were 5 tokens of fees burned, yield would be (55/100)/(50/100)-1~=10%.
This also incentivizes locking of tokens in other applications by offsetting the issuance.
Storage
Storing data on the blockchain has a long-playing role. Networks like Bitcoin and Ethereum misprice storage by only allocating reward to miners who mined specific transactions instead of future miners who will need to continue storing this data while they are mining.
In NEAR, Ⓝ also represents the right to store some amount of data. Token holders have the right to occupy some amount of the blockchain’s overall space.
For example, if Alice has a balance of 1 Ⓝ, she can store roughly 10 kilobytes on her account. This means that users need to maintain a fraction of Ⓝ as a minimum balance if they want to have their account, similar to how checking accounts in banks require a minimum balance.
This allows contracts which are maintaining important state to pay to Validators proportionally to the amount of data they are securing. For example, an important contract of the stable coin that would maintain the balances of millions of users will accordingly need to have a reserve of Ⓝ to cover the amount of storage it will require on the blockchain.
Storage Ⓝ locked for Storage % of Total Supply
10 MB 1,049 0.0001%
100 MB 10,486 0.0010%
1 GB 107,374 0.0107%
10 GB 1,073,742 0.107%
100 GB 10,737,418 1.07%
1 TB 109,951,163 11.00%
Amount of Ⓝ locked at different amounts of total storage.
For reference, Ethereum is at ~100 GB of storage right now, the USDT contract takes ~100 MB. Contrast that with the total amount locked in Ethereum’s DeFi is ~2.5% of ETH.
There are many ways a developer who doesn’t have a large amount of Ⓝ can still occupy space. For example, a developer can borrow Ⓝ from either off-chain entities or via on-chain lending protocol. Paying back with revenue that his application generates.
Additionally, this can also provide a good opportunity for developers to issue their own token, where they allocate a portion to the Ⓝ token holders who are interested in supporting this application. They attract more support and get Ⓝ required to launch their application and maintain its state, while their token captures revenue from the application usage.
Validators
Rewards
Validators as a group are paid fixed 90% of around 5% of total supply annualized (other 10% go to Protocol Treasury). For example, in the first year Validators will receive around 45,000,000 Ⓝ. Rewards are distributed per epoch — every half a day. Which is on average ~61,640 Ⓝ of reward per epoch to be allocated between Validators.
Each validator receives a reward proportional to their participation. As a validator stakes, how many seats they take is determined via simple auction. After each epoch finishes, the validator will be evaluated based on how many blocks and chunks they produced versus what they were expected to produce.
In the event it’s below 90% of what’s expected, the validator is considered to be offline/unstable, won’t get any rewards, and will be removed from the coming epoch’s validation (i.e., force unstaked). Validators with at least 90% online presence will receive rewards that grow linearly, with 100% of the reward given for those with a 99% or above online presence. Read details of uptime and reward computation here.
For example, if there are 100 seats, a validator that has one seat – will get ~615 Ⓝ per epoch if they are above 99% of uptime. If the validator was 95% uptime, they will receive 55% of their reward – ~342 Ⓝ.
% staked / amount staked
10% 25% 50% 70% 90% 100%
number of tx / day 100,000,000 250,000,000 500,000,000 700,000,000 900,000,000 1,000,000,000
1,000 38.10% 12.38% 3.81% 1.36% 0.00% -0.48%
10,000 38.10% 12.38% 3.81% 1.36% 0.00% -0.48%
100,000 38.10% 12.38% 3.81% 1.36% 0.00% -0.48%
1,000,000 38.10% 12.38% 3.81% 1.36% 0.00% -0.47%
10,000,000 38.14% 12.42% 3.85% 1.40% 0.03% -0.44%
100,000,000 38.58% 12.77% 4.17% 1.71% 0.35% -0.13%
1,000,000,000 43.07% 16.43% 7.55% 5.01% 3.60% 3.11%
1,500,000,000 45.69% 18.56% 9.52% 6.94% 5.50% 5.00%
2,000,000,000 48.41% 20.78% 11.57% 8.93% 7.47% 6.96%
Annualized yield at different amounts staked and number of transactions per day.
Selection
NEAR is a sharded blockchain designed to scale throughput as usage grows. In the beginning, the system starts with one shard and 100 validator seats. Based on the stake offered, the protocol proportionally assigns these seats, and redistributes the rewards. Therefore, if the protocol will have 40% of the total supply at stake, each seat will require 4 million Ⓝ stake.
However, the number of seats will increase linearly with the number of shards: when 8 shards are running, 800 seats will be available. And every seat will require 500,000 Ⓝ stake, lowering the barriers of entry for more Validators.
A simple auction takes place to actually determine the seat price. Let’s say you observe the following set of proposals (and rollovers from Validators of previous epoch):
Validator Stake # Seats
V1 1,000,000 48
V2 500,000 24
V3 300,000 14
V4 300,000 14
V5 20,000 0
Example proposals by Validators and number of seats they would get at the selection process
The seat price given this proposal is determined by finding an integer number that if you divide each validator’s stake with rounding down (e.g., for V5, 20,000 / 20,500 rounding down will be 0), you will get a required number of seats. This determines who will get their seat(s) and who’s funds will be returned back. In the case of the table above, the seat price will be 20,500 if there are 100 seats, and that gives the last column’s number of seats to each validator.
The protocol automatically measures the availability of Validators: if a node fails to be online above a certain percentage when it should be creating new blocks, it loses the status of validator and will be required to submit a staking transaction again. The penalty for low-quality nodes is missing epoch rewards.
In the beginning, a small number of shards will require a larger stake, preferring professional Validators and organizations that will allocate significant resources to NEAR Protocol.
As the network and its usage grow, the number of seats will increase with the number of shards. Therefore, the minimum stake necessary to become a validator will be lower and the seat price selection mechanics will allow for a long tail of Validators to come on board.
This long tail of non-professional Validators might individually have higher risk of failure. But together, they will provide greater decentralization and fault tolerance. At the same time, Validators with a larger stake will need to commit more computational resources when they have to validate multiple seats across different shards.
Delegation
Validators can receive Ⓝ to stake from third parties via Delegation. NEAR Protocol enables delegation via smart contracts. Validators that want to accept delegation can create a special contract and allow users who don’t want to run their own nodes to deposit their Ⓝ into it. By doing so, funds deposited to that contract are available to the creator of the contract to use as part of their stake.
In the beginning, there will be a reference delegation smart contract. In the future, we can expect Validators to come up with their own staking contracts that provide various taxation benefits, liquidity, and yet-to-see features. The goal is to allow professional Validators who might not have funds to participate, increase the total stake and thus the security of the protocol, and improve the redistribution of rewards across the ecosystem.
Protocol Treasury
We believe in sustainable development. We allocate 10% of epoch rewards to the treasury. This treasury account is designed to continue sponsoring protocol and ecosystem development. Over the long term, it should be managed by a decentralized governance process.
Before that is fully established, we allocate the custody of this to the NEAR Foundation. As decentralized governance progresses and clearly shows that it can effectively manage execution, we strongly suggest for the network to hard fork and change the custody of the treasury to a new entity.
Contract rewards
As one of the steps to create a sustainable path for contract independent operation and possibly developer income, NEAR Protocol allocates 30% of transaction fees to the contracts these transactions touched.
For example, if Alice sent a transaction to smart contract A with 0.0001 Ⓝ fee, and contract A doesn’t call any other contracts, it will receive a reward of 0.00003 Ⓝ (which is 30% of 0.0001 Ⓝ). On the other hand, if contract A is using contract B for 50% of its functionality (measured by gas usage), A and B will split the contract reward, each one receiving 0.000015 Ⓝ. This way popular libraries can receive a flow of funds from all the client applications that are using them.
Developers of the contracts can program various ways how these funds can be used. For example, they can be kept on the contract to allow it to allocate more storage space. DAO managing this application can decide what to do with these funds. Or there might be some third-party token buy-back & burn mechanics for contracts that have their own token.
Account Name Auction
NEAR Protocol uses human-readable account names. This allows for much better user experience for regular blockchain use cases. We also expect that these accounts will be used across various novel use cases (e.g., DNS, certification, and applications) as they represent the user’s digital identity that is also connected to finances, data ownership, and cryptography—and, in the future, supporting more of Decentralized Identifier (DID) standard.
We split account names into two groups by length: those longer than 32 characters and those shorter. We expect that shorter accounts names will have way more value for users compared to longer ones. Longer account names can register on a first-come-first-serve basis.
To give everyone a fair chance to acquire short account names, a naming auction will start shortly after MainNet launch.
Each week’s account names—such that sha256(account_id) % 52 is equal to the week since the launch of the auction—will open for bidding. Auctions will run for seven days after the first bid, and anyone can bid for a given name. A bid consists of a bid and mask, allowing the bidder to hide the amount that they are bidding. After the seven days run out, participants must reveal their bid and mask within the next seven days. The winner of the auction pays the second-largest price.
Proceeds of the auctions then get burned by the naming contract, benefiting all the token holders.
Conclusion
NEAR Protocol provides a powerful cryptocurrency Ⓝ, which links together Validators, developers, users and token holders into one ecosystem.
Each role has different goals:
Users want security for their assets and data.
Developers want more adoption and capture a sustainable source of revenue.
Validators want to receive higher income for providing validation services.
Token holders want their tokens to hold value long term.
The goal of the economic design is to align these interests and incentivize the growth of the ecosystem. For example, as the price of Bitcoin grows, so does the security of the network. In other words, BTC becomes a better store of value as it becomes more expensive, and more people want to store their money there. On the other hand, Bitcoin is missing developers and hasn’t yet attracted active development.
With NEAR Protocol, as demand for tokens grows from new users and developers and increases usage of applications, we see the alignment of incentives between everyone.
Validators will receive a higher income in Ⓝ because there is less staked and more tokens burned (increasing their yield). As more people use the network, their individual usage fees can be kept low while validators are still compensated for storing more data overall, which leads to the network becoming more secure and users (who are also token holders) receiving more security for their assets and data stored on the chain. The network also becomes more attractive as a place for developers to store data and assets because it has higher security and more users.
Add it all up, and growth of the demand from users and developers and the reduction of supply via staking, transaction fee burning, and state staking leads to the NEAR token balancing good utility for users and developers with what validators need to help make the network increasingly more secure. |
```js
import { Wallet } from './near-wallet';
const DAO_CONTRACT_ADDRESS = "nearweek-news-contribution.sputnik-dao.near";
const wallet = new Wallet({ createAccessKeyFor: DAO_CONTRACT_ADDRESS });
await wallet.viewMethod({
method: 'get_proposals',
args: { from_index: 9262, limit: 2 },
contractId: DAO_CONTRACT_ADDRESS
});
```
_The `Wallet` object comes from our [quickstart template](https://github.com/near-examples/hello-near-examples/blob/main/frontend/near-wallet.js)_ |
Get in my Beta Program! | July 12, 2019
COMMUNITY
July 12, 2019
We’ve just announced our funding! We had a big press release this week announcing we’ve raised $12.1 million dollars in a round led by Metastable and Accomplice. We’re using this to power our growth to become the greatest blockchain the world has ever seen. We’re doing this in the form of a new Beta Program and we’ve opened new positions across the board. If you think you could see yourself working at Near, shoot us an email or application. Check out open positions here.
With the funding we’ve also released the Nightshade Paper (link), which is the most comprehensive overview to date of our approach to sharding, validation and consensus in the NEAR Protocol.
COMMUNITY AND EVENTS
Our main update on the community front is that we’ve opened applications for our developer beta program. We’re looking for high-quality dApp projects to launch alongside the NEAR Protocol on Day 1 of mainnet, as well as receiving tokens, technical support and a dedicated adviser from NEAR. If you have a blockchain project idea, learn more and apply for the beta program here.
With our funding announcement, we have received a lot of press – including from CoinDesk, TheBlock, Coin Telegraph, and of course, the Daily HODL.There are mentions of us in the media as far afield as Russia and China.
There were also some wonderful posts by Near investors, including IDEO Lab, Greenfield and Xspring.
Illia is back in China (I know, wasn’t he just there?) to present at StakingCon and give a workshop on developing blockchain smart contracts in Beijing.
(Caption: Illia presenting with Chinese slides to developers in Beijing. One day his Chinese will be good enough to do the whole presentation in Chinese! Until then we have interpreters.)
Coming up, we’ll be presenting a multi-part series on Crypto-Prototyping at Dweb Camp July 18-21 south of San Francisco. There are some tickets still available so register here.
WRITING AND CONTENT
Videos
Saito Whiteboard Session: https://youtu.be/KGOZ7udYz0A
Fireside Chat with Chase Freo from Alto.io: https://youtu.be/sZCJarxVZpg
Alex Skidanov on Usability and Scalability at IBM Blockchain Dev Summit: https://youtu.be/U9WBW3R06oI
Blog
WASM for Blockchain in 2019 by Maksym Zavershynskyi — https://medium.com/nearprotocol/wasm-for-blockchain-2019-d093bfeb6133
NEAR fundraise and beta program announcement — https://pages.near.org/blog/fundraise/
We’ve published the sharding paper with more details on the approach NEAR Protocol is built upon: https://pages.near.org/downloads/Nightshade.pdf
ENGINEERING HIGHLIGHTS
A lot of our recent efforts have been dedicated to preparing for our announcement of funding. We’re still dealing with updating our libraries with new user flows.
Over 30 PRs across 12 repos and 14 authors. Featured repos: nearcore, nearlib, near-shell, and near-wallet.
Beta Program has launched: https://pages.near.org/beta
Published the sharding paper with more details on the approach NEAR Protocol is built upon: https://pages.near.org/downloads/Nightshade.pdf
We’ve opened new positions: https://pages.near.org/careers
Application Layer
Integration testing near-shell and wallet
Added blockchain interface to the runtime in rust
Blockchain
Stabilizing cross-shard transactions and receipts.
Implemented validator kick out
HOW YOU CAN GET INVOLVED
Join us: there are new jobs we’re hiring across the board!
If you want to work with one of the most talented teams in the world right now to solve incredibly hard problems, check out our careers page for openings. And tell your friends!
Learn more about NEAR in The Beginner’s Guide to NEAR Protocol. Stay up to date with what we’re building by following us on Twitter for updates, joining the conversation on Discord and subscribing to our newsletter to receive updates right to your inbox.
Reminder: you can help out significantly by adding your thoughts in our 2-minute Developer Survey.
https://upscri.be/633436/ |
# VMConfig
Config of wasm operations.
## ext_costs:
_type: [ExtCostsConfig](ExtCostsConfig.md)_
Costs for runtime externals
## grow_mem_cost
_type: u32_
Gas cost of a growing memory by single page.
## regular_op_cost
_type: u32_
Gas cost of a regular operation.
## max_gas_burnt
_type: Gas_
Max amount of gas that can be used, excluding gas attached to promises.
## max_stack_height
_type: u32_
How tall the stack is allowed to grow?
## initial_memory_pages
_type: u32_
## max_memory_pages
_type: u32_
The initial number of memory pages.
What is the maximal memory pages amount is allowed to have for
a contract.
## registers_memory_limit
_type: u64_
Limit of memory used by registers.
## max_register_size
_type: u64_
Maximum number of bytes that can be stored in a single register.
## max_number_registers
_type: u64_
Maximum number of registers that can be used simultaneously.
## max_number_logs
_type: u64_
Maximum number of log entries.
## max_log_len
_type: u64_
Maximum length of a single log, in bytes.
|
---
id: tech-resources
title: Tech Resources
sidebar_label: Tech Resources
sidebar_position: 5
---
# Technical Resources
:::info on this page
* Theoretical documentation: includes resources for smart contract development, technical documentation directory, NEAR core development resources, and The Graph documentation for building subgraphs on NEAR.
* Synchronous support: offers developer office hours every weekday where DevRel and Community team members are available to answer questions.
* Asynchronous support: includes video tutorials on YouTube, active dev support on the NEAR Discord, and dev support on Telegram.
* Specific documentation related to gaming: includes resources for developing gaming projects on NEAR, such as how to and game economies.
:::
We understand that the tech/dev side is fundamental to developing web3 projects and taking them to the next level. Below are a collection of some of the most commonly referenced and most sought after technichal resources to help you get started.
## Theoretical Documentation
* [Wallet Selector repo for integrating NEAR Wallets into your dApp](https://github.com/near/wallet-selector)
* [Guide for Wallet Selector](https://github.com/near/wallet-selector/blob/dev/packages/core/docs/guides/custom-wallets.md)
* [NEAR SDK resources for smart contract development](https://docs.near.org/sdk/welcome)
* [Technical Documentation Directory](https://docs.near.org/)
* [Near spec website](http://nomicon.io/)
* [Understanding NEAR Keys](https://www.vitalpoint.ai/understanding-near-keys/) - deep dive into NEAR accounts, contracts, and keys.
* [Create a NEAR App](https://github.com/near/create-near-app)
* [NEAR Core Development](https://near.github.io/nearcore/) resources - useful if you want to develop at protocol level, or run a node.
* [The Graph documentation for building subgraphs on NEAR](https://thegraph.com/docs/en/supported-networks/near/)
* [Kurtosis - local development and testing environments for developers in the NEAR ecosystem](https://www.kurtosistech.com/)
## How to Run Nodes
* Local Node using near: If you want to run a node locally for testing (and don't have the space to download all the data) [https://github.com/near/nearup](https://github.com/near/nearup)
* Run RPC node using near: https://near-nodes.io/rpc/run-rpc-node-without-nearup (replace with validator node https://near-nodes.io/validator/validator-bootcamp for validator, custody partners or archival node for exchanges https://near-nodes.io/archival/run-archival-node-without-nearup)
## Indexer
* near lake: https://github.com/near/near-lake-indexer
* docs: https://near-indexers.io/
## NFTs
examples: [Recur](https://www.recur.com/), [Sweat](https://sweateconomy.com/)
* Specification: [NEP-171](https://nomicon.io/Standards/Tokens/NonFungibleToken/Core#nep-171) (similar to ERC-721)
* NFT resources: [RUST](https://docs.near.org/tutorials/nfts/introduction), [JavaScript](https://docs.near.org/tutorials/nfts/js/introduction)
* Tutorial: [NFT Contracts](https://docs.near.org/develop/relevant-contracts/nft)
* Code example: https://github.com/near-examples/NFT
## FTs
examples: [Sweat](https://sweateconomy.com/), [Circle](https://www.circle.com/en/)
* Specification: [NEP-141](https://nomicon.io/Standards/Tokens/FungibleToken/Core#nep-141) (similar to ERC-20)
* FT resource: [Introduction to FTs](https://docs.near.org/tutorials/fts/introduction), [FT Contracts Specs](https://docs.near.org/integrator/fungible-tokens)
* Tutorial: [FT Contracts](https://docs.near.org/develop/relevant-contracts/ft)
* Code example: https://github.com/near-examples/FT
<!-- ## Synchronous support: Video, Audio, Office-hours
* [Developer Office Hours](https://near.org/office-hours/) - Every weekday NEAR hosts “Office Hours” where DevRel and Community team members are available to field any questions you may have about building on NEAR -->
## Asynchronous support
* [Video Tutorials on YouTube](https://www.youtube.com/c/NEARProtocol)
* [Active dev support on the NEAR Discord](https://discord.com/channels/490367152054992913/542945453533036544)
* [Dev support Telegram](https://t.me/neardev)
## Specific Documentation related to Gaming
* [How-To Article](https://github.com/vgrichina/near-lands/blob/main/HOWTO.md)
* [Game Economies](https://github.com/vgrichina/near-lands/blob/main/GAME-ECONOMIES.md)
|
---
id: storage
title: State
sidebar_label: State (Storage)
hide_table_of_contents: true
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
import {CodeTabs, Language, Github} from "@site/src/components/codetabs"
import {ExplainCode, Block, File} from '@site/src/components/CodeExplainer/code-explainer';
NEAR accounts store data for their contracts. The storage starts **empty** until a contract is deployed and the state is initialized. The contract's code and state are independent: updating the code does not erase the state.
<ExplainCode languages={["js", "rust"]} >
<Block highlights={{"js": "3-6,10-11"}} fname="auction">
### Defining the State
The attributes of the `class` marked as the contract define the data that will be stored
The contract can store all native types (e.g. `number`, `string`, `Array`, `Map`) as well as complex objects
For example, our Auction contract stores when the auction ends, and an object representing the highest bid
**Note:** The SDK also provides [collections](./collections.md) to efficiently store collections of data
</Block>
<Block highlights={{"rust": "6-9,14,15"}} fname="auction">
### Defining the State
The attributes of the `struct` marked as the contract define the data that will be stored
The contract can store all native types (e.g. `u8`, `string`, `HashMap`, `Vector`) as well as complex objects
For example, our Auction contract stores when the auction ends, and an object representing the highest bid
**Note:** The structures that will be saved need a special macro, that tells the SDK to store them [serialized in Borsh](./serialization.md)
**Note:** The SDK also provides [collections](./collections.md) to efficiently store collections of data
</Block>
<Block highlights={{"rust": "4"}} fname="auction">
#### [*] Note
The `structs` that will be persisted need to be marked with a macro, so the SDK knows to [serialize them in Borsh](./serialization.md) before writing them to the state
</Block>
<Block highlights={{"js":"", "rust": ""}} fname="auction">
#### [!] Important
Contracts pay for their storage by locking part of their balance
It currently costs ~**1Ⓝ** to store **100KB** of data
</Block>
<Block highlights={{"js": "", "rust": ""}} fname="auction" >
### Initializing the State
After the contract is deployed, its state is empty and needs to be initialized with
some initial values
There are two ways to initialize a state:
1. By creating an initilization function
2. By setting default values
</Block>
<Block highlights={{"js": "8,13-17"}} fname="auction">
### I. Initialization Functions
An option to initialize the state is to create an `initialization` function, which needs to be called before executing any other function
In our Auction example, the contract has an initialization function that sets when the auction ends. Note the `@initialization` decorator, and the forced initialization on `NearBindgen`
**Note:** It is a good practice to mark initialization functions as private. We will cover function types in the [functions section](./functions.md)
</Block>
<Block highlights={{"js": "10-11"}} fname="auction">
#### [!] Important
In TS/JS you still **must** set default values for the attributes, so the SDK can infer their types
</Block>
<Block highlights={{"rust": "12,22-30"}} fname="auction">
### I. Initialization Functions
An option to initialize the state is to create an `initialization` function, which needs to be called before executing any other function
In our Auction example, the contract has an initialization function that sets when the auction ends. The contract derives the `PanicOnDefault`, which forces the user to call the init method denoted by the `#[init]` macro
**Note:** It is a good practice to mark initialization functions as private. We will cover function types in the [functions section](./functions.md)
</Block>
<Block highlights={{"js": "5"}} fname="hello">
### II. Default State
Another option to initialize the state is to set default values for the attributes of the class
Such is the case for our "Hello World" contract, which stores a `greeting` with the default value `"Hello"`
The first time the contract is called (somebody executes `get_greeting` or `set_greeting`), the default values will be stored in the state, and the state will be considered initialized
**Note:** The state can only be initialized once
</Block>
<Block highlights={{"rust": "10-16"}} fname="hello">
### II. Default State
Another option to initialize the state is to create a `Default` version of our contract's `struct`.
For example, our "Hello World" contract has a default state with a `greeting` set to `"Hello"`
The first time the contract executes, the `Default` will be stored in the state, and the state will be considered initialized
**Note:** The state can only be initialized once
</Block>
<Block highlights={{"js": "", "rust":""}} fname="hello">
### Lifecycle of the State
When a function is called, the contract's state is loaded from the storage and put into memory
The state is actually [stored serialized](./serialization.md), and the SDK takes a bit of time to deserialize it before the method can access it
When the method finishes executing successfully, all the changes to the state are serialized, and saved back to the storage
</Block>
<Block highlights={{"js": "", "rust":""}} fname="hello">
### State and Code
In NEAR, the contract's code and contract's storage are **independent**
Updating the code of a contract does **not erase** the state, and can indeed lead to unexpected behavior or errors
Make sure to read the [updating a contract](../release/upgrade.md) if you ran into issues
</Block>
<File
language="js"
fname="auction"
url="https://github.com/near-examples/auction-examples/blob/main/contract-ts/src/contract.ts"
start="2"
end="51"
/>
<File
language="js"
fname="hello"
url="https://github.com/near-examples/hello-near-examples/blob/main/contract-ts/src/contract.ts"
start="2"
end="18"
/>
<File
language="rust"
fname="auction"
url="https://github.com/near-examples/auction-examples/blob/main/contract-rs/src/lib.rs"
start="2"
end="68"
/>
<File
language="rust"
fname="hello"
url="https://github.com/near-examples/hello-near-examples/blob/main/contract-rs/src/lib.rs"
start="2"
end="32"
/>
</ExplainCode> |
---
id: growth
title: Growth & Marketing
sidebar_label: Growth & Marketing
sidebar_position: 1
---
import {EcosystemResources, CommunitySpaces, NearWeek, EventFunding, MarketingDAO, LegalResources, IDOPlatforms } from '@site/src/components/GrowthCards';
# Growth & marketing
:::info On this page
* Overview of the NEAR Protocol and its support for growth.
* Different funding programs available to developers building on NEAR, such as MarketingDAO.
* Events and opportunities to connect with the NEAR community.
:::
## NEAR Foundation's Role Around Marketing Support
Welcome to the NEAR Protocol Growth & Marketing Resources Wiki Page! This one-stop hub is designed to provide projects building on NEAR with the available growth & marketing resources, opportunities, and initiatives in the ecosystem to help you maximize your project's visibility and success. As you navigate the vibrant NEAR ecosystem, you'll find various community marketing outlets, AMA/Twitter Spaces initiatives, podcasts, and much more to propel your project's growth.
Marketing and community engagement play a pivotal role in the success of any project, so be sure to explore, learn, and leverage these resources to build a strong presence, foster meaningful connections, and drive your project forward!
For those integrating with or building within the NEAR ecosystem and needing NEAR branding, please refer to the official [branding guidelines](https://near.org/brand/).
## Ecosystem Resources
<EcosystemResources />
## Community Spaces/AMA Resources
<CommunitySpaces />
## [NEARWEEK](https://nearweek.com/)
NEARWEEK has established itself as a prominent news & amplification platform in the NEAR ecosystem, having built out a suite of services and tools for teams to effectively promote their projects. These include:
<NearWeek />
## MarketingDAO
The MarketingDAO is a Near Community-run organization of globally distributed marketing professionals, creatives, and NEAR enthusiasts who engage with the Near Community to facilitate the allocation of Community funds for marketing activities under $10 000. The Marketing DAO was established as a key pillar on the road to decentralization of the Near Ecosystem and empowers the NEAR Community to access funding for marketing activities furthering the overall growth and expansion of the NEAR ecosystem.
Interested in Applying? Please read:
<MarketingDAO />
## GTM/Event Funding
If you are planning a GTM push or looking to host an event in partnership with NEAR - a side event alongside a major crypto event, a launch event, etc. - and want to explore funding opportunities from NEAR Foundation to support this, please follow the workflow outlined below:
*Ideally, please try to complete and submit with 4 weeks overhead to allow for review*
<EventFunding />
## Community Events T&Cs
Please note that the [NEAR Community Events Terms and Conditions](https://shortlink.near.foundation/wiki-community-events-tandc-pdf) apply to participants of community events (such as quizzes, AMAs, contests, competitions, Twitter Spaces etc.) hosted by the NEAR Foundation.
## Legal
There are several entities in the NEAR ecosystem that can provide legal support in the web3 space:
<LegalResources />
## IDO Platforms
<IDOPlatforms />
|
Korea BUIDL Week Recap: One Account to Rule Them All
COMMUNITY
April 3, 2024
Last week, Korea BUIDL Week was the epicenter of cutting-edge thought leadership in Web3. From cross-chain innovations to the convergence of AI and blockchain, the week brought together industry pioneers to explore the technologies shaping the decentralized future.
Chain Abstraction Day Tackles Interoperability
Chain Abstraction Day kicked Korea BUIDL Week off by tackling the need for seamless blockchain interoperability. NEAR’s Co-founder and CEO, Illia Polosukhin, made a compelling case for “Why Web3 Needs Chain Abstraction” by highlighting how abstracting away individual chains can unlock Web3’s full potential. Mike Purvis of Proximity Labs then painted a vision of “Ushering in the Era of Bridgeless Cross-Chain DeFi,” while Riqi Ras presented “Bitcoin Beyond Gold”, showcasing snippets of a testnet transaction where a NEAR account signed transactions on Bitcoin. This proof-of-concept highlighted the potential of chain signatures to not just interact with Bitcoin, but even enable trading of Bitcoin Ordinals natively using the cross-chain signing capabilities.
Blockchain Brain Trust: Vitalik Envisions Account Abstraction, Illia Unleashes Chain Signatures
At BUIDL Asia, anticipation was high for Vitalik Buterin’s talk on “The Future of Account Abstraction.” By abstracting user accounts, he explored enabling frictionless cross-chain experiences. “The next 10 years are going to be about really upgrading the ecosystem at the user level,” Buterin said at the event. Illia Polosukhin then took center stage, announcing NEAR’s groundbreaking “Chain Signatures” for managing assets across chains via a single account. Illia demonstrated how Chain Signatures allow signing transactions on multiple chains from a single NEAR account, paving the way for seamless cross-chain dApp development.
Illia and Vitalik later joined forces for a can’t-miss fireside chat dissecting the “AI/Crypto Fact vs Fiction.” As two towering minds in their respective fields, they offered unparalleled insights into AI’s transformative potential for Web3. The lively discussion separated hype from reality, exploring how AI could enhance privacy, scalability, and usability of decentralized systems. Vitalik and Illia also examined the risks of centralized AI models and the importance of developing decentralized AI aligned with Web3’s core values.
ETH Seoul Dives Deep into Chain Signatures and Decentralized Intelligence
The event baton was then passed to ETH Seoul, where Mike Purvis expounded on “Chain Signatures: Cross-Chain Without Bridges.” Purvis explained how this technology enables secure cross-chain communication, eliminating the need for centralized bridges. He demonstrated how it could pave the way for seamless blockchain interoperability and conducted a workshop showcasing potential use cases. Boris Polania made a case for “Decentralized Intelligence” discussing how combining blockchain transparency with AI data analysis could revolutionize collective decision-making processes. The conference featured comprehensive developer tracks put together by DevHub, covering everything from building on NEAR to integrating Chain Signatures. ETH Seoul also hosted a hackathon where teams built innovative decentralized apps, with members of the NEAR ecosystem serving as judges to evaluate and provide feedback on the projects.
DeFiBrain, an AI-powered DeFi investment assistant that harnesses NEAR to discover and analyze promising DeFi products, won NEAR’s Chain Signatures bounty and ETH Seoul’s first prize. DeFiBrain impressed with its advanced AI models and seamless NEAR integration. The first prize of $5,000 for the NEARDevHub’s “AI Governance is NEAR” bounty was awarded to Connor AI, which integrates AI into DAOs to automate execution processes and empower decentralized decision-making. Connor AI was commended for its novel AI-DAO approach.
Vitalik Outlines Ethereum’s Scaling Roadmap, Illia Tackles AI Misconceptions
Not to be outdone, Vitalik outlined his vision for Ethereum’s future in “Dencun: Where We Go From Here.” During his talk, he primarily focused on EIP 4844, also known as “Blobs”, which represents a significant upgrade for Ethereum scaling. This upgrade allows Layer 2 networks to post batched transactions at a much lower cost. Consequently, Vitalik issued a call-to-action, encouraging developers to build solutions for today’s needs. Closing the event, Illia Polosukhin delivered a talk cheekily titled “Secrets About AI that Web3 Doesn’t Want You To Know,” likely pulling back the curtain on AI’s game-changing implications. Illia’s provocative talk challenged misconceptions about the limitations of current AI while hinting at potential breakthroughs that could supercharge decentralized applications.
SheFi Emphasizes Cultivating Inclusive Web3 Communities
Finally, SheFi spotlighted building inclusive Web3 communities. NEAR’s Yadira Blocker led the discussion on cultivating Web3 culture and community – a critical topic as the ecosystem grows. The panel emphasized genuine communities are earned through authentic connections, not purchased. It also discussed how global Web3 communities localize efforts while valuing in-person meetups to drive progress collaboratively. Unlike Web2, community heavily influences Web3 marketing and adoption.
From multi-chain architectures to AI convergence, Korea BUIDL Week demonstrated that Web3 is rapidly evolving towards a future of seamless cross-chain functionality, enhanced intelligence, and open participation. The insights shared will undoubtedly help shape the coming decades of decentralized innovation. |
---
id: notifications
title: Social Notifications
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
import {WidgetEditor} from "@site/src/components/widget-editor"
Applications such as [NEAR Social](https://near.social) and the [NEAR Dev Portal](https://dev.near.org/) allow components to send notifications to their users.
Notifications are great to inform users in real time that something has happened, and can be [easily incorporated into any web app](../../../3.tutorials/near-components/push-notifications.md).
---
## Sending Notifications
Notifications are implemented as a particular case of [indexed actions](./social.md#socialindex).
This means that to send a notification we just need to `index` the `notify` action for the indexer, with a `key` and a `value`.
- The `key` tells **which account** to notify.
- The `value` includes the [notification type](#notification-types) and the item being notified of.
<WidgetEditor>
```js
const notifyMe = () => {
Social.set({
index: {
notify: JSON.stringify({
key: context.accountId,
value: "docs notification"
})
}
});
}
return <>
{context.accountId?
<button onClick={notifyMe}> Get Notification </button>
:
<p> Please login to be notified</p>
}
</>
```
</WidgetEditor>
In this example, the account executing the code is notifying `mob.near` that they liked their social post created at the block height `102169725`.
---
## Notification Types
Since notifications are indexed actions, anyone can create their own kind of notification.
While there is no standard for notifications, we recommend using the following types:
<Tabs>
<TabItem value="Custom" default>
```js
Social.set({
index: JSON.stringify({
notify: {
key: "mob.near",
value: {
type: "custom",
message: "A message in the notification",
widget: "The widget to open when clicking on the notification",
params: { parameters: "to pass to the widget", ... },
},
}
})
});
```
**Note**: currently, only near.org implements custom notifications
</TabItem>
<TabItem value="Like">
```js
Social.set({
index: JSON.stringify({
notify: {
key: "mob.near",
value: {
type: "like",
item: {
type: "social",
path: "mob.near/post/main",
blockHeight: 102169725
}
}
}
})
})
```
**Reference**: [LikeButton](https://near.org/near/widget/ComponentDetailsPage?src=near/widget/LikeButton&tab=source)
</TabItem>
<TabItem value="Comment">
```js
Social.set({
index: JSON.stringify({
notify: {
key: "nearhacks.near",
value: {
type: "comment",
item: {
type: "social",
path: "nearhacks.near/post/main",
blockHeight: 102224773
}
}
}
})
})
```
**Reference**: [CommentButton](https://near.org/near/widget/ComponentDetailsPage?src=near/widget/Comments.Compose&tab=source)
</TabItem>
<TabItem value="Follow">
```js
Social.set({
index: JSON.stringify({
notify: {
key: "mob.near",
value: {
type: "follow",
}
}
})
})
```
**Reference**: [FollowButton](https://near.org/near/widget/ComponentDetailsPage?src=near/widget/FollowButton&tab=source)
</TabItem>
</Tabs>
:::caution
There is no standard for notifications, but you can contribute to create one [in this public discussion](https://github.com/NearSocial/standards/pull/19/files).
:::
---
## Parsing Notifications
In order to get all notifications for an user, we make a call to the Social indexer.
<WidgetEditor id='1' height="190px">
```js
// login to see your notifications
const accountId = context.accountId || 'influencer.testnet'
const index = Social.index("notify", accountId, {limit: 2, order: "desc", subscribe: true});
return <>
<h4> Notifications for {accountId} </h4>
{index.map(e => <> {JSON.stringify(e, null, 2)} <br/></>) }
</>
```
</WidgetEditor>
:::caution
Please notice that anyone can create a notification for the user, and thus some form of filtering might be needed.
:::
:::tip
You can also check how the [Notifications Page](https://near.org/near/widget/ComponentDetailsPage?src=near/widget/NotificationsPage&tab=source) is implemented.
:::
|
---
NEP: 364
Title: Efficient signature verification and hashing precompile functions
Author: Blas Rodriguez Irizar <rodrigblas@gmail.com>
DiscussionsTo: https://github.com/nearprotocol/neps/pull/364
Status: Draft
Type: Runtime Spec
Category: Contract
Created: 15-Jun-2022
---
## Summary
This NEP introduces the request of adding into the NEAR runtime a pre-compiled
function used to verify signatures that can help IBC compatible light clients run on-chain.
## Motivation
Signature verification and hashing are ubiquitous operations in light clients,
especially in PoS consensus mechanisms. Based on Polkadot's consensus mechanism
there will be a need for verification of ~200 signatures every minute
(Polkadot’s authority set is ~300 signers and it may be increased in the future: https://polkadot.polkassembly.io/referendum/16).
Therefore, a mechanism to perform these operations cost-effectively in terms
of gas and speed would be highly beneficial to have. Currently, NEAR does not have any native signature verification toolbox.
This implies that a light client operating inside NEAR will have to import a library
compiled to WASM as mentioned in [Zulip](https://near.zulipchat.com/#narrow/stream/295302-general/topic/light_client).
Polkadot uses [three different cryptographic schemes](https://wiki.polkadot.network/docs/learn-keys)
for its keys/accounts, which also translates into different signature types. However, for this NEP the focus is on:
- The vanilla ed25519 implementation uses Schnorr signatures.
## Rationale and alternatives
Add a signature verification signatures function into the runtime as host functions.
- ED25519 signature verification function using `ed25519_dalek` crates into NEAR runtime as pre-compiled functions.
Benchmarks were run using a signature verifier smart contract on-chain importing the aforementioned functions from
widespread used crypto Rust crates. The biggest pitfall of these functions running wasm code instead of native
is performance and gas cost. Our [benchmarks](https://github.com/blasrodri/near-test) show the following results:
```log
near call sitoula-test.testnet verify_ed25519 '{"signature_p1": [145,193,203,18,114,227,14,117,33,213,121,66,130,14,25,4,36,120,46,142,226,215,7,66,122,112,97,30,249,135,61,165], "signature_p2": [221,249,252,23,105,40,56,70,31,152,236,141,154,122,207,20,75,118,79,90,168,6,221,122,213,29,126,196,216,104,191,6], "msg": [107,97,106,100,108,102,107,106,97,108,107,102,106,97,107,108,102,106,100,107,108,97,100,106,102,107,108,106,97,100,115,107], "iterations": 10}' --accountId sitoula-test.testnet --gas 300000000000000
# transaction id DZMuFHisupKW42w3giWxTRw5nhBviPu4YZLgKZ6cK4Uq
```
With `iterations = 130` **all these calls return ExecutionError**: `'Exceeded the maximum amount of gas allowed to burn per contract.'`
With iterations = 50 these are the results:
```text
ed25519: tx id 6DcJYfkp9fGxDGtQLZ2m6PEDBwKHXpk7Lf5VgDYLi9vB (299 Tgas)
```
- Performance in wall clock time when you compile the signature validation library directly from rust to native.
Here are the results on an AMD Ryzen 9 5900X 12-Core Processor machine:
```text
# 10k signature verifications
ed25519: took 387ms
```
- Performance in wall clock time when you compile the library into wasm first and then use the single-pass compiler in Wasmer 1 to then compile to native.
```text
ed25519: took 9926ms
```
As an extra data point, when passing `--enable-simd` instead of `--singlepass`
```text
ed25519: took 3085ms
```
Steps to reproduce:
commit: `31cf97fb2e155d238308f062c4b92bae716ac19f` in `https://github.com/blasrodri/near-test`
```sh
# wasi singlepass
cargo wasi build --bin benches --release
wasmer compile --singlepass ./target/wasm32-wasi/release/benches.wasi.wasm -o benches_singlepass
wasmer run ./benches_singlepass
```
```sh
# rust native
cargo run --bin benches --release
```
Overall: the difference between the two versions (native vs wasi + singlepass is)
```text
ed25519: 25.64x slower
```
### What is the impact of not doing this?
Costs of running IBC-compatible trustless bridges would be very high. Plus, the fact that signature verification
is such an expensive operation will force the contract to split the process of batch verification of signatures
into multiple transactions.
### Why is this design the best in the space of possible designs?
Adding existing proved and vetted crypto crates into the runtime is a safe workaround. It will boost performance
between 20-25x according to our benchmarks. This will both reduce operating costs significantly and will also
enable the contract to verify all the signatures in one transaction, which will simplify the contract design.
### What other designs have been considered and what is the rationale for not choosing them?
One possible alternative would be to improve the runtime implementation so that it can compile WASM code to a sufficiently
fast machine code. Even when it may not be as fast as LLVM native produced code it could still be acceptable for
these types of use cases (CPU intensive functions) and will certainly avoid the need of adding host functions.
The effort of adding such a feature will be significantly higher than adding these host functions one by one.
But on the other side, it will decrease the need of including more host functions in the future.
Another alternative is to deal with the high cost of computing/verifying these signatures in some other manner.
Decreasing the overall cost of gas and increasing the limits of gas available to attach to the contract could be a possibility.
Introducing such modification for some contracts, and not for some others can be rather arbitrary
and not straightforward in the implementation, but an alternative nevertheless.
## Specification
This NEP aims to introduce the following host function:
```rust
extern "C"{
/// Verify an ED25519 signature given a message and a public key.
/// Ed25519 is a public-key signature system with several attractive features
///
/// Proof of Stake Validator sets can contain different signature schemes.
/// Ed25519 is one of the most used ones across blockchains, and hence it's importance to be added.
/// For further reference, visit: https://ed25519.cr.yp.to
/// # Returns
/// - 1 if the signature was properly verified
/// - 0 if the signature failed to be verified
///
/// # Cost
///
/// Each input can either be in memory or in a register. Set the length of the input to `u64::MAX`
/// to declare that the input is a register number and not a pointer.
/// Each input has a gas cost input_cost(num_bytes) that depends on whether it is from memory
/// or from a register. It is either read_memory_base + num_bytes * read_memory_byte in the
/// former case or read_register_base + num_bytes * read_register_byte in the latter. This function
/// is labeled as `input_cost` below.
///
/// `input_cost(num_bytes_signature) + input_cost(num_bytes_message) + input_cost(num_bytes_public_key)
/// ed25519_verify_base + ed25519_verify_byte * num_bytes_message`
///
/// # Errors
///
/// If the signature size is not equal to 64 bytes, or public key length is not equal to 32 bytes, contract execution is terminated with an error.
fn ed25519_verify(
sig_len: u64,
sig_ptr: u64,
msg_len: u64,
msg_ptr: u64,
pub_key_len: u64,
pub_key_ptr: u64,
) -> u64;
```
And a `rust-sdk` possible implementation could look like this:
```rs
pub fn ed25519_verify(sig: &ed25519::Signature, msg: &[u8], pub_key: &ed25519::Public) -> bool;
```
Once this NEP is approved and integrated, these functions will be available in the `near_sdk` crate in the
`env` module.
[This blog post](https://hdevalence.ca/blog/2020-10-04-its-25519am) describes the various ways in which the existing Ed25519 implementations differ in practice. The behavior that this proposal uses, which is shared by Go `crypto/ed25519`, Rust `ed25519-dalek` (using `verify` function with `legacy_compatibility` feature turned off) and several others, makes the following decisions:
- The encoding of the values `R` and `s` must be canonical, while the encoding of `A` doesn't need to.
- The verification equation is `R = [s]B − [k]A`.
- No additional checks are performed. In particular, the points outside of the order-l subgroup are accepted, as are the points in the torsion subgroup.
Note that this implementation only refers to the `verify` function in the
crate `ed25519-dalek` and **not** `verify_strict` or `verify_batch`.
## Security Implications (Optional)
We have chosen this crate because it is already integrated into `nearcore`.
## Unresolved Issues (Optional)
- What parts of the design do you expect to resolve through the NEP process before this gets merged?
Both the function signatures and crates are up for discussion.
## Future possibilities
I currently do not envision any extension in this regard.
## Copyright
Copyright and related rights waived via [CC0](https://creativecommons.org/publicdomain/zero/1.0/).
|
---
title: 2.4 The Product Stack
description: Investigating the infrastructure that creates a sustainable crypto ecosystem
---
# 2.4 The Product Stack Around This Landscape
The crypto ‘Product Stack’ is perhaps the most improved facet for crypto in recent years. In the 2017 - 2018 market, minimal infrastructure existed, such that one could speak of ‘blockchain’ but not much about the infrastructure needed to make a blockchain into a sustainable ecosystem.
In the past four years, infrastructure for L1 ecosystems has come a long way. Such infrastructure includes:
* **Oracles:** Ways of bringing off-chain data into smart contracts.
* **Indexers:** Ways of tracking actions on chain and between smart contracts.
* **Storage Solutions (IPFS, Arweave, Machina):** Ways of storing data on-chain or in a decentralized manner.
* **Bridges**: Ways of moving assets between chains, either in a permissionless and decentralized manner, or in a centralized / multi-sig design structure.
* **Custody Providers and On-Ramps:** Ways of having a centralized institution hold assets, market make, and participate in products, on behalf of retail and other institutions.
To continue the geopolitical analogy the crypto product stack is much like the services and infrastructure layer of a city or country: It is the roads, raw materials, picks and shovels, and resource distribution machinery that helps a city build different buildings and venues around the area. And, following this analogy, there is a certain amount of composability between these products, such that together they provide for more advanced dApps and products that can be built on top of an L1.
## Oracles
**“Blockchain oracles are entities that connect [blockchains](https://blog.chain.link/what-is-a-blockchain-and-how-can-it-impact-the-world/) to external systems, thereby enabling [smart contracts](https://chain.link/education/smart-contracts) to execute based upon inputs and outputs from the real world.”**

### Why Do Oracles Matter? The On-Chain, Off-Chain, Cross-Chain Trichotomy
_“Oracles expand the types of digital agreements that blockchains can support by offering a universal gateway to off-chain resources while still upholding the valuable security properties of blockchains. Major industries benefit from combining oracles and smart contracts including asset prices for finance, weather information for insurance, randomness for gaming, IoT sensors for supply chain, ID verification for government, and much more.” [(Chainlink)](https://chain.link/education/blockchain-oracles)_
**On-Chain Data:** Data held on-chain in storage or refers to a limited amount of data stored in smart contracts or decentralized storage, that is most often utilized for calling specific commands (so called, ‘function calls’).
**Off-Chain Data:** Refers to the massive world of data that has emerged since the inception of the internet, and is usually stored in a centralized repository or ‘data center’. For crypto in its current state, the bulk of off-chain data coming on-chain is used for price feeds, insurance claims, game algorithms, and limited amounts of physical world data (weather, temperature, etc.) used for prediction markets, insurance claims, and so forth.
**Cross-Chain Oracles:** Read and Write Information Between Different Blockchains and off-chain data sources.
Oracles are the necessary communication technology capable of transmitting information, into a blockchain ecosystem, out of a blockchain ecosystem, and between blockchain ecosystems. They are fundamental for a number of use-cases and products that can be built on top of an L1.
**The Tech Tree for Oracles:**
The reason why Oracles matter for crypto, is because of the enhanced products that can thrive from off-chain data feeds. Below is a list of the most popular industry clusters that drastically grew thanks to Oracles:
* **Oracles for Price Feeds and DeFi. Synthetic Products. Derivatives:** Off-chain data for Decentralized Finance applications range from simple price feeds (price oracles) pulling data from centralized exchanges, to data on synthetic products including stocks, bonds, and treasuries. The uses range from concentrated liquidity for AMM’s to lending rates to users. For many builders, having reliable Oracle infrastructure is the most important component of building a successful DeFi ecosystem.
* **Data for Insurance Claims. **Decentralized insurance for events taking place off-chain is heavily reliant on Oracles for providing timely claim data from the off-chain world. This refers to agriculture, weather, thermostat, shipping logs, and so forth.
* **Dynamic NFTs:** Dynamic NFTs refer to NFTs that can change appearance, value or distribution, based on external / off-chain events. Oracles ensure that such NFT’s can operate dynamically by correlated the off-chain / external event with the NFT such that when the event happens, the NFT is able to update.
* **Gaming**: Any mathematical algorithm or ‘Verifiable Randomness’ as it pertains to the generation of rewards in loot boxes, random match customizations (PVP), or synchronizations with real world events, is all dependent on Oracles communicating such off-chain data, on-chain to the game in question.
* **Enterprise Solutions:** Enterprise blockchain solutions tend to center around the potential of leveraging existing business software and data (ERP system data, supply chain data, insurance claim data and so forth), and uploading that data on-chain to create automated and value laden business networks. Applicable off-chain data fields include: Supply chain, ERP data, IoT Sensor Data, AR/VR data, AI data / activities, biological data, etc.
* **ReFi:** Regenerative Finance lives at the intersection of environmental data, and financial markets. Oracles bridge the majority of environmental data on-chain either for informing such markets, or creating entirely blockchain native assets (like Carbon Credits). Such areas include: Remote sensing data, carbon methodologies, carbon credits, biodiversity credits, real-time deforestation data, and so forth.
* **DeSci:** Decentralized Science refers to the infrastructure supporting the financing of scientific research and coordinated research among decentralized communities. Oracle’s in the future will most likely be used to connect off-chain activities including: Academic research, clinical trials, photos, experimental data, and so forth, with releases in value or triggers to funding.

### Centralized and Decentralized Oracles:
The idea of smart-contract based execution is that permissionless code can execute decisions in the place of centralized stakeholders. Oracles can challenge this capability in the following manner: If the data input for the self-executing code, is itself dependent on a centralized third party, then we have only kicked the can down the road. This raises a serious question about the nature of the Oracle itself - and to what extent an Oracle is decentralized, or centralized.
**Top Solutions To Date:**
* Chainlink.
* BAND.
* Seda (formerly Flux).
* API3 - Decentralized API’s.
## Indexers
A second essential product is known as an indexer. An indexer is a way of collecting, organizing, storing, and displaying on-chain data. Indexers fetch raw data from nodes and process them in such a manner whereby they can be queried quickly and easily by a user or smart contract.

Indexing is useful for handling on-chain data, specifically as it refers to trading, account activity, and data spread across multiple blocks. Its high level value proposition as part of the product stack is to allow users to benefit from the history of data processed on chain and across blocks, as well as to better organize and operationalize such data.
**Top Solutions to Date:**
* The Graph - Decentralized Indexing Service.
* Certain dApps (Mintbase) or Protocols (NEAR) may build their own indexing service to emphasize certain queries.
## Storage Solutions
Storage protocols fulfill the need of holding data that cannot be stored on the blockchain, in a decentralized repository. Most often the CID (the cryptographic hash of the storage entry) is stored in the transaction meta-data on-chain, such that it can be used as a reference point to larger data stored off-chain (images, videos, text documents, etc.). With high costs for storage on-chain (either in deposits or capacity), data intensive solutions can benefit from offloading data to a decentralized storage protocol.
Once more, similar to the question of centralization with Oracles, having centralized storage is a limited value proposition when the goal is to decentralize digital system management. A number of decentralized storage solutions exist - some requiring token payment (File coin) for data storage, and others simply using inscription and multiple servers in different locations.
**Top Solutions To Date:**
* IPFS / Filecoin
* Arweave
* Sia Coin
## Bridges
Bridges are extremely important infrastructure for moving value and data between blockchains. While this has most commonly been used in reference to the metric of _Total Value Locked _moving between chains, the premise also applies to users.
Bridging infrastructure allows value to flow from one ecosystem into another, or from one rollup into another. Certain ecosystems even have bridging protocols built into their design such as Cosmos’ IBC network (inter-blockchain communication network).
What matters beyond _what_ a bridge in crypto does, is the design of the bridge: _To what degree is it centralized or controlled by a few people vs. decentralized and operational without the sign off or control of a few people?_
**NEAR’s Rainbow Bridge:**
Fully decentralized, with value being approved by the different validator nodes on each respective chain.
**Cosmos Bridge Design: IBC**
Specific chain nodes connected to the bridge provide consensus.
**Wormhole - Solana:**
Consensus comes from multi-sig of trusted parties.
**Polygon Bridge Design:**
Multi-sig based bridge, whereby less than 10 entities have full control over the ability for assets to move between chains.
Bridge security is a crucial component in bringing certain assets into an ecosystem - specifically stable coins such as USDC and Tether (USDT). If these assets are centralized, the party can block transfers in or out of an ecosystem in a crisis scenario (as USDC did with USDC on Solana during the FTX meltdown). What is important to takeaway from this is that an ecosystem is only as strong as the value flowing in and out of the ecosystem - much like a country is only as strong as its imports and exports.
## Custody Providers and On-Ramps
Amidst the failure of Celsius, BlockFi, FTX, and many others, it may be easy to overlook the clear value proposition of custody providers and on-ramps.
Custody providers like Fireblox and Finoa provide institutions and investors with asset storage - both hot and cold - such that these larger capital players can hold tokens from a specific ecosystem. What is often overlooked is that while an ecosystem may be extremely well developed with solid products, not having infrastructure for incumbent financial players and institutions will seriously limit the capacity for the ecosystem to grow.
On-Ramps, such as centralized exchanges and payment processors, as well as neo-banks create liquid markets for ecosystem tokens. This is perhaps one of the largest factors in the success of Ethereum: Not the fact that the solutions built were incredible and durable, but rather that they were the first ones that retail and institutions had access to.
Any ecosystem would do well to prioritize custody and on-ramp infrastructure in a timely manner with the development of the projects inside of their ecosystem. With custodians and on-ramps ecosystems can benefit from more users, larger investors into products, and liquid markets for launched tokens. Without such players projects inside of ecosystems usually struggle to survive.
## Conclusion
The product stack and infrastructure support for any L1 ecosystem is important for the further development of that ecosystem and the many different types of products inside of it. Ecosystem building as a whole, continues to be analogous to country building, insofar as crucial infrastructure must be in place so as to optimize development and streamline the number of users, investors, and liquid markets, for increasingly complex products. Storage, Indexing, Oracles, Bridges, and Custodians form the core backbone of the emergent product stack in crypto from which many other solutions can come to life.
|
NEAR’s BOS Impact at We Are Developers 2023 World Congress
NEAR FOUNDATION
July 27, 2023
The excitement is palpable as NEAR hits the ground in the vibrant city of Berlin for the We Are Developers World Congress 2023. With a packed agenda and a bustling venue, the stage is set for a memorable exploration into the Blockchain Operating System (BOS).
Amidst a crowd of enthusiastic developers, NEAR is showcasing the immense potential of BOS. The team looks forward to sparking curiosity, fostering innovation, and expanding horizons within the global developer community, setting the tone for a transformative two days.
Let’s take a quick look at what happened on Day 1 with NEAR at We Are Developers, before taking a look at what’s on the schedule for Day 2, July 28th.
On the agenda: NEAR’s engaging BOS offerings
The conference kicked off with “Exploring BOS: The Blockchain Operating System by NEAR”, scheduled for Thursday, July 27, at 3:30 PM and led by Andrej Šarić, senior NEAR developer. The presentation dissectd how BOS is redefining the application-building landscape while highlighting user-friendly onboarding and seamless multi-chain interactions.
Friday, July 28, will see the enlightening “Mastering BOS: A Developer Workshop”, helmed by Andrej along with fellow senior developer, Alen Meštrov. A must-attend for developers keen to enhance their BOS acumen, this comprehensive workshop promises an in-depth, immersive learning experience, centered around the innovative capabilities of BOS.
Additionally, the “Tekuno Activation: Devs Without Limits” is adding an exciting gamified element to the conference, running throughout both days (more on this below). Attendees are encouraged to collect PODs at the NEAR booth, as well as during both BOS sessions, culminating in the exclusive “We Are Devs” POD and a shot at winning a ticket to NEARCON 2023.
Making waves: the buzz at the NEAR booth
NEAR’s BOS booth is serving as a dynamic hotspot of interactivity and engagement throughout the event. Attendees enthusiastically participated in the “How Big of a Dev BOS(s) are you really?” quiz, testing their knowledge about blockchain development while adding a dose of fun to their conference experience.
In addition to being a learning hub, the booth continues to be a place for connection. Visitors had the opportunity to meet some of the brilliant minds behind NEAR, fostering meaningful interactions, exchanging ideas, and discussing BOS’s transformative potential. The booth was also brimming with awesome NEAR merchandise for everyone who stopped by.
Tekuno activation: “Devs Without Limits” POD
The feature stealing the spotlight at the NEAR booth is the Tekuno activation. Offering the exclusive “Devs Without Limits” NFT collection, attendees can collect PODs at the booth, the “Mastering BOS” workshop, and the “Exploring BOS” talk. Successfully gathering these PODs unlocks the ultimate “We Are Devs” POD and offers the chance to win a free ticket to NEARCON 2023.
With the We Are Developers World Congress 2023 in full swing, the NEAR team continues to engage passionately with attendees and fellow developers. Every conversation, presentation, and interaction amplifies the buzz around the transformative power of the Blockchain Operating System (BOS).
There’s plenty more to explore and experience as the event unfolds. If you haven’t yet visited the NEAR booth or attended our sessions, seize the opportunity to dive into BOS and discover its revolutionary impact on the application-building process. And don’t forget to partake in all the fun and rewards, like a chance to win NEARCON tickets with Tekuno PODs.
|
---
id: block-chunk
title: Block / Chunk
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
The RPC API enables you to query the network and get details about specific blocks or chunks.
---
## Block details {#block-details}
> Queries network and returns block for given height or hash. You can also use `finality` param to return latest block details.
**Note**: You may choose to search by a specific block _or_ finality, you can not choose both.
- method: `block`
- params:
- [`finality`](/api/rpc/setup#using-finality-param) _OR_ [`block_id`](/api/rpc/setup#using-block_id-param)
`finality` example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "block",
"params": {
"finality": "final"
}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.block({
finality: "final",
});
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=block \
params:='{
"finality": "final"
}'
```
</TabItem>
</Tabs>
`[block_id]`
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "block",
"params": {
"block_id": 17821130
}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.block({blockId: 17821130});
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=block \
params:='{
"block_id": 17821130
}'
```
</TabItem>
</Tabs>
`[block_hash]`
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "block",
"params": {
"block_id": "7nsuuitwS7xcdGnD9JgrE22cRB2vf2VS4yh1N9S71F4d"
}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.block(
{blockId: "7nsuuitwS7xcdGnD9JgrE22cRB2vf2VS4yh1N9S71F4d"}
);
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=block \
params:='{
"block_id": "7nsuuitwS7xcdGnD9JgrE22cRB2vf2VS4yh1N9S71F4d"
}'
```
</TabItem>
</Tabs>
<details>
<summary>Example response:</summary>
<p>
```json
{
"jsonrpc": "2.0",
"result": {
"author": "bitcat.pool.f863973.m0",
"header": {
"height": 17821130,
"epoch_id": "7Wr3GFJkYeCxjVGz3gDaxvAMUzXuzG8MjFXTFoAXB6ZZ",
"next_epoch_id": "A5AdnxEn7mfHieQ5fRxx9AagCkHNJz6wr61ppEXiWvvh",
"hash": "CLo31YCUhzz8ZPtS5vXLFskyZgHV5qWgXinBQHgu9Pyd",
"prev_hash": "2yUTTubrv1gJhTUVnHXh66JG3qxStBqySoN6wzRzgdVD",
"prev_state_root": "5rSz37fySS8XkVgEy3FAZwUncX4X1thcSpuvCgA6xmec",
"chunk_receipts_root": "9ETNjrt6MkwTgSVMMbpukfxRshSD1avBUUa4R4NuqwHv",
"chunk_headers_root": "HMpEoBhPvThWZvppLwrXQSSfumVdaDW7WfZoCAPtjPfo",
"chunk_tx_root": "7tkzFg8RHBmMw1ncRJZCCZAizgq4rwCftTKYLce8RU8t",
"outcome_root": "7tkzFg8RHBmMw1ncRJZCCZAizgq4rwCftTKYLce8RU8t",
"chunks_included": 1,
"challenges_root": "11111111111111111111111111111111",
"timestamp": 1601280114229875635,
"timestamp_nanosec": "1601280114229875635",
"random_value": "ACdUSF3nehbMTwT7qjUB6Mm4Ynck5TVAWbNH3DR1cjQ7",
"validator_proposals": [],
"chunk_mask": [true],
"gas_price": "100000000",
"rent_paid": "0",
"validator_reward": "0",
"total_supply": "1042339182040791154864822502764857",
"challenges_result": [],
"last_final_block": "AaxTqjYND5WAKbV2UZaFed6DH1DShN9fEemtnpTsv3eR",
"last_ds_final_block": "2yUTTubrv1gJhTUVnHXh66JG3qxStBqySoN6wzRzgdVD",
"next_bp_hash": "3ZNEoFYh2CQeJ9dc1pLBeUd1HWG8657j2c1v72ENE45Q",
"block_merkle_root": "H3912Nkw6rtamfjsjmafe2uV2p1XmUKDou5ywgxb1gJr",
"approvals": [
"ed25519:4hNtc9vLhn2PQhktWtLKJV9g8SBfpm6NBT1w4syNFqoKE7ZMts2WwKA9x1ZUSBGVKYCuDGEqogLvwCF25G7e1UR3",
"ed25519:2UNmbTqysMMevVPqJEKSq57hkcxVFcAMdGq7CFhpW65yBKFxYwpoziiWsAtARusLn9Sy1eXM7DkGTXwAqFiSooS6",
"ed25519:4sumGoW9dnQCsJRpzkd4FQ5NSJypGQRCppWp7eQ9tpsEcJXjHZN8GVTCyeEk19WmbbMEJ5KBNypryyHzaH2gBxd4",
"ed25519:3fP2dri6GjYkmHgEqQWWP9GcoQEgakbaUtfr3391tXtYBgxmiJUEymRe54m7D8bQrSJ3LhKD8gTFT7qqdemRnizR",
"ed25519:3mwdqSWNm6RiuZAoZhD6pqsirC2cL48nEZAGoKixpqbrsBpAzqV3W2paH4KtQQ4JPLvk5pnzojaint2kNBCcUyq1",
"ed25519:D4hMnxqLyQW4Wo29MRNMej887GH46yJXDKNN4es8UDSi9shJ9Y4FcGqkxdV4AZhn1yUjwN5LwfgAgY6fyczk5L3",
null,
"ed25519:4WCVm4dn88VJxTkUgcvdS7vs34diBqtQY4XWMRctSN1NpbgdkwwVyxg7d2SbGC22SuED7w4nrToMhcpJXrkhkDmF",
"ed25519:JqtC7TFP7U14s7YhRKQEqwbc2RUxoctq75mrBdX91f7DuCWsPpe6ZTTnfHPmuJPjTzFHVZTsaQJWzwfSrrgNpnc",
"ed25519:ngGUpWc2SyHmMCkWGTNNNfvZAJQ5z7P92JCmDqB7JW3j8fNH6LobvFFXb2zVdssibJKgnjwBj8CRe6qiZtuYQZM",
"ed25519:5kzW6RbjukyJZiw9NTzTPPsQdoqN6EecafjVFEoWmTxQ4uSv1uSXhQYcHK2eq4m84oMmPABQDz2mm73Qx8mDdCQX",
"ed25519:5wHnuuxwJJiZ4bXNq5cESnr4YovFU2yaUcuHRDUw3DnLoxkqc15CsegoyUSQKEwtCZ4yETv8Z9QcD6Wr9zHV4AUk",
"ed25519:3F9XzWBxto31e8RAcBShAJBzJPgSJQsWbPXR38AfQnJn6AiveGz3JjebQm9Ye63BrnNA57QrPshwknxpzSrcNEZW",
"ed25519:2g5s4SKsHt9PMdekkDqVtwwtz14v4edhqdBX1MYA8tB6nDpj3vDCDCTy9pEU8dX31PoQe5ygnf88aTZukMBMK1Yt",
"ed25519:3Xz4jqhdyS3qs6xTmWdgjwt5gJraU5czMA89hPhmvbAN4aA7SUKL1HkevpmutRQqqxe7c7uCFeGiDHvDcxhhmD8W",
null,
"ed25519:55xs3vwPEys39egf9Z8SNyn1JsHPRMgj9HCX1GE7GJsVTcAuutQUCo91E12ZdXkuToYRXb9KzoT8n9XQRCNuLpwY",
null,
"ed25519:28JrFw7KnhnQPN89qZnnw17KDBjS6CDN7zB1hTg7KGg8qQPoCzakz9DNnaSnx39ji7e2fQSpZt4cNJaD7K7Yu7yo",
"ed25519:41hAr5qhtvUYpdD2NK9qqTVnpG325ZoAiwrcmk1MJH7fdpxm7oSKXvXZqh7bTmPhv61hH2RpHnhcGuN4QqLzK2zt",
"ed25519:4QacMsQ5FJgvecAYDFq8QBh19BBjh4qU8oeD5bV7p6Zhhu3e6r2iSHTvDBU2Q62RZAaWQQkkEwDUC9rsXdkGVhAt",
"ed25519:27smtCZ3WobEvBuD5DggY6kkGxjB9qRVY6kPixgwqvBT1eKbRVoV8cLj1z51S8RTcp7YzAr1vhHJUHgksatR9Udz",
"ed25519:4wspCWoAbhYxb3th2eX6ZXvKep1Fsco7mFP5zBodXBR8Wr344ANXSUCri3gUgNCCSoQ2CKSdqDEsvE6Y2jQ9hmbB",
"ed25519:46XpYf9ZB9gjDfdnJLHqqhYJpQCuvCgB9tzKWS88GANMCb2j9BM3KXyjaEzynSsaPK8VrKFXQuTsTzgQSeo9cWGW",
null,
"ed25519:Y5ehsrhEpTRGjG6fHJHsEXj2NYPGMmKguiJHXP7TqsCWHBvNzaJbieR7UDp78hJ1ib7C18J5MB2kCzTXBCF9c3b",
"ed25519:3P9363Dc8Kqvgjt3TsNRncUrncCHid7aSRnuySjF4JYmQbApkAxomyMu8xm9Rgo3mj9rqXb16PM7Xjn7hKP6TyVr",
null,
null,
"ed25519:65ATjGsigZ3vMp7sGcp1c4ptxoqhHPkBeAaZ5GWJguVDLyrRLPJrtXhLGjH9DpXd7CZswjyMYq5aRtorLnmmJ7GW",
null,
"ed25519:5SvqSViXbtsLoFMdtCufyyDgZnrEK7LheFi38X5M2ic17gfV5cz37r85RyixjUv98MbAmgVdmkxVFDGfSbeoHW7X",
null,
null,
"ed25519:2n3fQiBEiDKkB84biXWyQmvnupKX7B8faugY37jVi8hVXuWLggJmaEjqub511RCYwFnwW1RBxYpuJQ455KaniCd4",
"ed25519:2K9xKFLJ2fW74tddXtghFGFurKWomAqaJmkKYVZKHQT6zHe5wNSYT3vzMotLQcez5JD1Ta57N9zQ4H1RysB2s5DZ",
null,
null,
"ed25519:3qeCRtcLAqLtQ2YSQLcHDa26ykKX1BvAhP9jshLLYapxSEGGgZJY8sU72p9E78AkXwHP3X2Eq74jvts7gTRzNgMg",
null,
"ed25519:2czSQCF8wBDomEeSdDRH4gFoyJrp2ppZqR6JDaDGoYpaFkpWxZf2oGDkKfQLZMbfvU6LXkQjJssVHcLCJRMzG8co"
],
"signature": "ed25519:58sdWd6kxzhQdCGvHzxqvdtDLJzqspe74f3gytnqdxDLHf4eesXi7B3nYq2YaosCHZJYmcR4HPHKSoFm3WE4MbxT",
"latest_protocol_version": 35
},
"chunks": [
{
"chunk_hash": "EBM2qg5cGr47EjMPtH88uvmXHDHqmWPzKaQadbWhdw22",
"prev_block_hash": "2yUTTubrv1gJhTUVnHXh66JG3qxStBqySoN6wzRzgdVD",
"outcome_root": "11111111111111111111111111111111",
"prev_state_root": "HqWDq3f5HJuWnsTfwZS6jdAUqDjGFSTvjhb846vV27dx",
"encoded_merkle_root": "9zYue7drR1rhfzEEoc4WUXzaYRnRNihvRoGt1BgK7Lkk",
"encoded_length": 8,
"height_created": 17821130,
"height_included": 17821130,
"shard_id": 0,
"gas_used": 0,
"gas_limit": 1000000000000000,
"rent_paid": "0",
"validator_reward": "0",
"balance_burnt": "0",
"outgoing_receipts_root": "H4Rd6SGeEBTbxkitsCdzfu9xL9HtZ2eHoPCQXUeZ6bW4",
"tx_root": "11111111111111111111111111111111",
"validator_proposals": [],
"signature": "ed25519:4iPgpYAcPztAvnRHjfpegN37Rd8dTJKCjSd1gKAPLDaLcHUySJHjexMSSfC5iJVy28vqF9VB4psz13x2nt92cbR7"
}
]
},
"id": "dontcare"
}
```
</p>
</details>
#### What Could Go Wrong?? {#what-could-go-wrong}
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `block` method:
<table className="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan="2">HANDLER_ERROR</td>
<td>UNKNOWN_BLOCK</td>
<td>The requested block has not been produced yet or it has been garbage-collected (cleaned up to save space on the RPC node)</td>
<td>
<ul>
<li>Check that the requested block is legit</li>
<li>If the block had been produced more than 5 epochs ago, try to send your request to <a href="https://near-nodes.io/intro/node-types#archival-node">an archival node</a></li>
</ul>
</td>
</tr>
<tr>
<td>NOT_SYNCED_YET</td>
<td>The node is still syncing and the requested block is not in the database yet</td>
<td>
<ul>
<li>Wait until the node finish syncing</li>
<li>Send a request to a different node which is synced</li>
</ul>
</td>
</tr>
<tr className="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
## Changes in Block {#changes-in-block}
> Returns changes in block for given block height or hash. You can also use `finality` param to return latest block details.
**Note**: You may choose to search by a specific block _or_ finality, you can not choose both.
- method: `EXPERIMENTAL_changes_in_block`
- params:
- [`finality`](/api/rpc/setup#using-finality-param) _OR_ [`block_id`](/api/rpc/setup#using-block_id-param)
`finality`
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "EXPERIMENTAL_changes_in_block",
"params": {
"finality": "final"
}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.experimental_changes_in_block({
finality: "final",
});
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=EXPERIMENTAL_changes_in_block \
params:='{
"finality": "final"
}'
```
</TabItem>
</Tabs>
`[block_id]`
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "EXPERIMENTAL_changes_in_block",
"params": {
"block_id": 17821135
}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.experimental_changes_in_block(
17821135
);
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=EXPERIMENTAL_changes_in_block \
params:='{
"block_id": 17821135
}'
```
</TabItem>
</Tabs>
`block_hash`
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "EXPERIMENTAL_changes_in_block",
"params": {
"block_id": "81k9ked5s34zh13EjJt26mxw5npa485SY4UNoPi6yYLo"
}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.experimental_changes_in_block(
"81k9ked5s34zh13EjJt26mxw5npa485SY4UNoPi6yYLo"
);
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=EXPERIMENTAL_changes_in_block \
params:='{
"block_id": "81k9ked5s34zh13EjJt26mxw5npa485SY4UNoPi6yYLo"
}'
```
</TabItem>
</Tabs>
<details>
<summary>Example response: </summary>
<p>
```json
{
"jsonrpc": "2.0",
"result": {
"block_hash": "81k9ked5s34zh13EjJt26mxw5npa485SY4UNoPi6yYLo",
"changes": [
{
"type": "account_touched",
"account_id": "lee.testnet"
},
{
"type": "contract_code_touched",
"account_id": "lee.testnet"
},
{
"type": "access_key_touched",
"account_id": "lee.testnet"
}
]
},
"id": "dontcare"
}
```
</p>
</details>
#### What Could Go Wrong?? {#what-could-go-wrong-1}
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `EXPERIMENTAL_changes_in_block` method:
<table className="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan="2">HANDLER_ERROR</td>
<td>UNKNOWN_BLOCK</td>
<td>The requested block has not been produced yet or it has been garbage-collected (cleaned up to save space on the RPC node)</td>
<td>
<ul>
<li>Check that the requested block is legit</li>
<li>If the block had been produced more than 5 epochs ago, try to send your request to <a href="https://near-nodes.io/intro/node-types#archival-node">an archival node</a></li>
</ul>
</td>
</tr>
<tr>
<td>NOT_SYNCED_YET</td>
<td>The node is still syncing and the requested block is not in the database yet</td>
<td>
<ul>
<li>Wait until the node finish syncing</li>
<li>Send a request to a different node which is synced</li>
</ul>
</td>
</tr>
<tr className="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
## Chunk Details {#chunk-details}
> Returns details of a specific chunk. You can run a [block details](/api/rpc/setup#block-details) query to get a valid chunk hash.
- method: `chunk`
- params:
- `chunk_id` _OR_ [`block_id`, `shard_id`](/api/rpc/setup#using-block_id-param)
`chunk_id` example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "chunk",
"params": {"chunk_id": "EBM2qg5cGr47EjMPtH88uvmXHDHqmWPzKaQadbWhdw22"}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.chunk({
chunk_id: "EBM2qg5cGr47EjMPtH88uvmXHDHqmWPzKaQadbWhdw22"
});
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 method=chunk params:='{"chunk_id": "EBM2qg5cGr47EjMPtH88uvmXHDHqmWPzKaQadbWhdw22"}' id=dontcare
```
</TabItem>
</Tabs>
`block_id`, `shard_id` example:
<Tabs>
<TabItem value="json" label="JSON" default>
```json
{
"jsonrpc": "2.0",
"id": "dontcare",
"method": "chunk",
"params": {"block_id": 58934027, "shard_id": 0}
}
```
</TabItem>
<TabItem value="js" label="🌐 JavaScript" label="JavaScript">
```js
const response = await near.connection.provider.chunk({
block_id: 58934027, shard_id: 0
});
```
</TabItem>
<TabItem value="http" label="HTTPie">
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 method=chunk params:='{"block_id": 58934027, "shard_id": 0}' id=dontcare
```
</TabItem>
</Tabs>
<details>
<summary>Example response: </summary>
<p>
```json
{
"jsonrpc": "2.0",
"result": {
"author": "bitcat.pool.f863973.m0",
"header": {
"chunk_hash": "EBM2qg5cGr47EjMPtH88uvmXHDHqmWPzKaQadbWhdw22",
"prev_block_hash": "2yUTTubrv1gJhTUVnHXh66JG3qxStBqySoN6wzRzgdVD",
"outcome_root": "11111111111111111111111111111111",
"prev_state_root": "HqWDq3f5HJuWnsTfwZS6jdAUqDjGFSTvjhb846vV27dx",
"encoded_merkle_root": "9zYue7drR1rhfzEEoc4WUXzaYRnRNihvRoGt1BgK7Lkk",
"encoded_length": 8,
"height_created": 17821130,
"height_included": 17821130,
"shard_id": 0,
"gas_used": 0,
"gas_limit": 1000000000000000,
"rent_paid": "0",
"validator_reward": "0",
"balance_burnt": "0",
"outgoing_receipts_root": "H4Rd6SGeEBTbxkitsCdzfu9xL9HtZ2eHoPCQXUeZ6bW4",
"tx_root": "11111111111111111111111111111111",
"validator_proposals": [],
"signature": "ed25519:4iPgpYAcPztAvnRHjfpegN37Rd8dTJKCjSd1gKAPLDaLcHUySJHjexMSSfC5iJVy28vqF9VB4psz13x2nt92cbR7"
},
"transactions": [],
"receipts": []
},
"id": "dontcare"
}
```
</p>
</details>
#### What Could Go Wrong?? {#what-could-go-wrong-2}
When API request fails, RPC server returns a structured error response with a limited number of well-defined error variants, so client code can exhaustively handle all the possible error cases. Our JSON-RPC errors follow [verror](https://github.com/joyent/node-verror) convention for structuring the error response:
```json
{
"error": {
"name": <ERROR_TYPE>,
"cause": {
"info": {..},
"name": <ERROR_CAUSE>
},
"code": -32000,
"data": String,
"message": "Server error",
},
"id": "dontcare",
"jsonrpc": "2.0"
}
```
> **Heads up**
>
> The fields `code`, `data`, and `message` in the structure above are considered legacy ones and might be deprecated in the future. Please, don't rely on them.
Here is the exhaustive list of the error variants that can be returned by `chunk` method:
<table className="custom-stripe">
<thead>
<tr>
<th>
ERROR_TYPE<br />
<code>error.name</code>
</th>
<th>ERROR_CAUSE<br /><code>error.cause.name</code></th>
<th>Reason</th>
<th>Solution</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan="4">HANDLER_ERROR</td>
<td>UNKNOWN_BLOCK</td>
<td>The requested block has not been produced yet or it has been garbage-collected (cleaned up to save space on the RPC node)</td>
<td>
<ul>
<li>Check that the requested block is legit</li>
<li>If the block had been produced more than 5 epochs ago, try to send your request to <a href="https://near-nodes.io/intro/node-types#archival-node">an archival node</a></li>
</ul>
</td>
</tr>
<tr>
<td>UNKNOWN_CHUNK</td>
<td>The requested chunk can't be found in a database</td>
<td>
<ul>
<li>Check that the requested chunk is legit</li>
<li>If the chunk had been produced more than 5 epochs ago, try to send your request to an archival node</li>
</ul>
</td>
</tr>
<tr>
<td>INVALID_SHARD_ID</td>
<td>Provided <code>shard_id</code> does not exist</td>
<td>
<ul>
<li>Provide <code>shard_id</code> for an existing shard</li>
</ul>
</td>
</tr>
<tr>
<td>NOT_SYNCED_YET</td>
<td>The node is still syncing and the requested chunk is not in the database yet</td>
<td>
<ul>
<li>Wait until the node finish syncing</li>
<li>Send a request to a different node which is synced</li>
</ul>
</td>
</tr>
<tr className="stripe">
<td>REQUEST_VALIDATION_ERROR</td>
<td>PARSE_ERROR</td>
<td>Passed arguments can't be parsed by JSON RPC server (missing arguments, wrong format, etc.)</td>
<td>
<ul>
<li>Check the arguments passed and pass the correct ones</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
<tr>
<td>INTERNAL_ERROR</td>
<td>INTERNAL_ERROR</td>
<td>Something went wrong with the node itself or overloaded</td>
<td>
<ul>
<li>Try again later</li>
<li>Send a request to a different node</li>
<li>Check <code>error.cause.info</code> for more details</li>
</ul>
</td>
</tr>
</tbody>
</table>
---
|
```bash
near call token.v2.ref-finance.near ft_transfer '{"receiver_id": "alice.near", "amount": "100000000000000000"}' --depositYocto 1 --accountId bob.near
``` |
NEAR at ETHDenver: Building a Multi-Chain Future
COMMUNITY
February 9, 2022
It’s that time of the year when Open Web builders head to the Web3 castle in Denver, Colorado. ETHDenver is upon us, and the NEAR community will be there with a full slate of events (February 15-20) focused on building a multi-chain future.
Why is the NEAR community attending ETHDenver? Good question. The NEAR community believes in a collaborative, decentralized, multi-chain ecosystem of Open Web and Metaverse platforms. Projects like Aurora and Rainbow Bridge, for example, have helped build simple and secure bridges between NEAR and Ethererum, allowing users and developers to freely move assets between the two networks.
The NEAR community is a big believer in a multi-chain future, whereby interoperable blockchains work collaboratively to build simple, scalable, and secure ways to onboard the world to Web3.
NEAR @ETHDenver
The NEAR community is part of ETHDenver’s Kickoff with the NEAR #BUIDLWEEK Lounge. Stop by the NEAR #BUIDLWEEK Lounge (at the Jonas Brothers Building at 1037 N. Broadway), which is open to all from Tuesday, February 15 through February 17 starting at 10am MST. Here you’ll find a whole host of blockchain events, including panels, speakers, workshops, demos, and happy hours all designed to empower and educate attendees. There will be a slew of partnered programming from Brave, CoinFund, Aurora, Octopus Network, and more.
For the full schedule and information about NEAR at #BUIDLWEEK and Main Event, please visit our sign-up page. We also encourage all NEARians attending virtually and in-person to tune into NEAR’s Twitter Spaces.
The NEAR community is also a Cypher Sponsor of ETH Denver. We’ll have additional panels, talks, and workshops for the Open Web community, and will be taking part in the hackathon, which wraps on February 20. The NEAR community also has a number of bounties for ETHDenver hackathon participants, which will be announced through ETHDenver next week.
Check out ETHDenver’s full schedule, where you can learn more about featured NEAR sessions and more.
NEAR #BUIDLWEEK Lounge Program – Let’s Build a Multi-Chain Future
A number of NEAR community partners will be joining us at ETHDenver within the NEAR #BUIDLWEEK Lounge.
On Monday, the 14th at 3:00pm MST, tune in for a YouTube livestream hosted by NEAR Co-Founder Illia Polosukhin on the fundamentals of building on NEAR. Watch as he codes and goes deep into the art of developing on NEAR in real-time.
On Tuesday, February 15, Octopus Network will host talks on appchains, GameFi, game Guilds, NFTs, the Metaverse, as well as community building and network effects. After lunch, join NEAR community teams for sessions on Sharding and Developer Tools ending the day with a Developer Office Hours Happy Hour.
Wednesday the 16th starts off with a “In Rust We Trust Brunch” followed by some programming with Aurora, Meta Pool, and Brave. Meet Aurora’s CEO Alex Shevchenko and learn How to Build on Aurora, the Ethereum scaling solution on NEAR. Daniel Bocanegra, who leads Business Development at Aurora will host a session on “Getting Onboarded to Aurora’s Ecosystem.”Stop by for other panels and presentations by the MoonNoobs DAO platform, Renaissance DAO, API3, and more.
We’ll end the 16th with Brave’s DevRel Leader, Sampson, who will cover, “Opportunities for Gaming and NFTs as the Entry to the Masses”, and then we will move into a Fireside Chat between Luke Mulks, Brave’s VP of Business Operations, and NEAR’s Co-Founder Illia Polosukhin. They’ll be exploring the need to build bridges with ETH-based projects, and why blockchain needs the whole ecosystem working towards building simple, scalable, and secure ways to onboard billions to Web3.
Thursday the 17th is all about DAOversity. Among a range of events showcasing the NEAR community’s commitment to inclusivity, will include the NEAR DAOversity Brunch and Building (Rainbow) Bridges panel, co-hosted by H.E.R. DAO. Join the NEAR community for the brunch, hosted by NEAR Foundation CEO Marieke Flament and Illia Polosukhin, featuring a panel on multi-chain collaborations with founders and project leads building allies across (rainbow) cross-chain bridges. All are welcome!
DAOversity day is also set to feature Research and DAOvelopment programming. This afternoon schedule features a Multi-DAO Workshop as well as presentations on DAOs from Chroncats, Governauts Research Initiative, AstroDAO, and more.
Notable NEAR Talks at ETH Denver
If you’re looking for some cool talks, then don’t miss the speaking engagement by NEAR Co-Founder Illia Polosukhin. Also, check out Josh Quintal’s “Dev Console Session” on Monday the 14th.
Looking for some other NEAR talks to check out? Then be sure to drop in on a talk being held by MoonNoobs’ Justin Friedman, and the Aurora NFTs Panel Discussion, which features the people behind Endemic, Chronicle, and TENKbay.
How to Claim Your POAP
POAP stands for Proof of Attendance Protocol. A POAP basically proves your attendance at ETH Denver and NEAR events.
NEAR will be participating in ETHDenver’s POAP scavenger hunt. Be sure to find our table in the shill zone and we will instruct you on what you have to do to claim your POAP.
Visit the Maker Space NFT Art Gallery
The NEAR #BUIDLWEEK Lounge will also be hosting an NFT Art Gallery. This event is part of our Satellite Maker Space, organized in collaboration with ETH Denver.
One of the highlights of the Satellite Maker Space will be a new augmented reality mobile application called MozARt, which will integrate NFT features on NEAR. They will have a drawing station, where drawings will come to life in the Satellite Maker space.
Register and Learn More
Register for NEAR’s #BUIDLWEEK Lounge at ETH Denver and learn more. There you will find plenty of info on the NEAR community’s agenda, including a schedule of events, what to expect, speaker bios, and hackathon bounties.
You can also find out more about Developer Console, developer platform for creating and maintaining dapps on NEAR, featuring interactive tutorials, scalable RPC service, and operational metrics. Be one of the first to experience RPC as a service! |
Register for NEAR’s May Town Hall: NFTs and NEAR
COMMUNITY
May 16, 2022
Hey there, NEAR World! This month we’re exploring the world of NFTs. Join the community for NEAR’s May Town Hall on May 26, 2022, at 5:00pm GMT (1:00PM EDT/10:00am PDT) for a deep dive into NEAR’s ecosystem of NFT projects and platforms.
NEAR Foundation CEO Marieke Flament will open the Town Hall with updates on the NEAR community and ecosystem, as well as the Foundation’s ongoing effort to onboard millions into Web3.
The Town Hall will also include other exciting news, including details of a major NEAR Protocol upgrade. There will also be updates on strategic partnerships, ecosystem funding and education progress, upcoming crypto events, and a panel dedicated to the ever-evolving world of NFTs.
NEAR Protocol Validator Upgrade
One of the NEAR Foundation’s major missions is to help decentralize NEAR Protocol’s block validation. This is accomplished through regular protocol upgrades.
In the May Town Hall, Pagoda will detail NEAR’s new staking pool contract, which will give validators additional features and functionality. Over the next few months, ecosystem players will work with validators and delegators to help migrate currently staked $NEAR from the old staking pool (poolv1.near) to the new staking pool (pool.near).
Pagoda will share more details, including the pool migration timeline for validators and delegators.
A Panel on NFTs in the NEAR ecosystem
NEAR Foundation CEO Marieke Flament will return to talk briefly about NEAR’s vibrant NFT marketplaces, projects, and creatives. There will also be a panel on NFTs, with surprise guests from across the NFT and NEAR ecosystems.
Register for the May Town Hall
To join the conversation with the global NEAR community, register for the May Town Hall. |
Case Study: Pagoda Chief Product Officer Alex Chiocchi on the BOS
NEAR FOUNDATION
May 31, 2023
NEAR is a home to a number of amazing apps and projects. These developers, founders, and entrepreneurs are using NEAR to effortlessly create and distribute innovative decentralized apps, while helping build a more open web — free from centralized platforms.
To showcase some of the innovative products launching on NEAR, the Case Studies content vertical is making a return. With its new multimedia makeover, Case Studies will demonstrate how these projects are leveraging the power of NEAR’s layer 1 protocol and the Blockchain Operating System (BOS) to distribute their apps and onboard the masses into the open web.
The new NEAR Case Studies series kicks off with a video featuring Pagoda’s Chief Product Officer Alex Chiocchi. For much of the last year Alex has been deeply embedded in the development and launch of the BOS — an OS for an Open Web.
In the BOS Case Study video, Alex explains how the BOS takes advantage of the NEAR Protocol’s scaling architecture, while allowing builders to code in languages they already know, like Javascript. As you’ll learn from Alex, the BOS makes it easy to use the tools you already know to build apps that engage users, while fostering an open web free from centralized platforms.
Stay tuned for more Case Study videos on NEAR, the Blockchain Operating System for an open web. |
Why doesn’t NEAR just replicate Ethereum Serenity design?
DEVELOPERS
November 7, 2018
NEAR Protocol builds a sharded public blockchain that executes smart contracts on a WASM virtual machine. If this sounds like Ethereum 2.0 (aka Serenity), it’s because they actually are very similar. However, based on Serenity’s multi-year roadmap, we believe that with our team and focus, we can deliver significantly faster.
Despite the release not being around the corner, Serenity’s specification is mostly available. As of time of this writing (November 6th, 2018), the spec for the beacon chain is complete, and the spec for the shard chains, while not published yet, is mostly finalized (in the absence of the official spec, I have a rather detailed blog post that describes the design for Ethereum 2.0 shard chains). This blog post also contains some details of the Ethereum’s beacon chain design that are not immediately obvious from the specification, that I learned from an in-depth conversation with Vitalik.
NEAR differs from Serenity in several aspects. Most notably, NEAR uses different consensus algorithms and fork choice rules in both the beacon chain and the shard chains. Given the extensive experience that Ethereum researchers have, strong motivations are required to validate such decisions.
In this post, I will describe the differences between the two protocols and motivations on why we use our own consensus algorithms and fork choice rules rather than those designed by the Ethereum team.
The Beacon Chain
It is highly desirable for the beacon chain not to have forks. In both Ethereum and NEAR, the beacon chains are responsible for selecting validators for shard chains and for snapshotting the state of the shard chains (the so-called cross-linking), with both processes relying on the beacon chain not having forks.
BFT consensus tradeoffs
Achieving zero forkfulness is highly challenging. The majority of modern BFT consensus algorithms do not scale beyond 1000 participants, while the permissionless blockchain networks are expected to scale to millions or possibly billions of people. Therefore, consensus on each block has to be reached by a number of participants that is significantly smaller than the total number of participants in the system. It can be done in two somewhat similar but fundamentally different ways (assuming a proof-of-stake sybil resistance mechanism exists):
Make the stake for becoming a consensus participant (“validator”) so high that only on the order of 1000 participants can participate. Generally, that would be six digit numbers in the US dollars equivalent per validator. In this approach, a fork in the blockchain would result in millions or dozens of millions of dollars slashed. Even if a fork does occur, it will be a significant event, with consequences that are likely to result in a hard fork with some mitigation of the damage. For all practical reasons, such a system can be assumed to have zero forkfulness. It is arguable, however, whether such a system is decentralized. People capable of staking such sums of money within a particular blockchain ecosystem tend to know each other, meaning that the security of the system will be in the hands of a tight-knit group of people. It can result in all sorts of non-slashable misbehavior such as censoring, stalling, etc.
Make the stake for becoming a validator low, and randomly select 1000 validators to create blocks. A new set of validators can be selected for each block, or rotated every few blocks. Assuming that the total number of malicious actors in the system is substantially less than ⅓, the probability of more than ⅓ corrupted validators appearing in a sample is very low. The problem with this approach is that the validators can be corrupted after they are selected (see this blog post with some further analysis). Corrupting a sufficient percentage of a shard is extremely hard, but not impossible. Therefore, a system that uses such an approach cannot rely on the absence of forks.
Footnote: For example, Algorand, that claims to never have forks, uses the latter approach. When answering a direct question about bribing validators, Silvio Micali responded that Algorand assumes that less than 50% of all the validators are corruptible. It is not only an unreasonable assumption but also in my opinion invalidates some of the other Algorand declared properties.
In essence, the design decision comes down to some compromise between centralization and forkfulness. An early design of Casper heavily favored centralization (see this link with a deprecated design, in particular MIN_DEPOSIT_SIZE being set to 1500 ETH). In the present designs NEAR favors forkfulness, while Ethereum’s Casper builds a consensus algorithm that scales to hundreds of thousands of validators, thus avoiding the compromise altogether. The pros and cons of both and why we do not use Casper are as follows.
NEAR’s approach
NEAR’s approach with Thresholded Proof of Stake and a flavor of TxFlow (our custom consensus) favor forkfulness.
With our current constants, each block is backed by approximately 0.1% of all the stake in the system. Thus, assuming the same valuation as Ethereum’s today ($20B) and 5% of all the tokens staked for validation, the cost of corrupting 50% of one block’s validators is around ~$0.5M, which is significantly less than the cost of corrupting the entire system.
Importantly, however, while for each block (produced once a minute) the probability of a fork is not negligible, the probability of reverting a large sequence of blocks is very low. Within one day, the validators (in terms of tokens staked) for each block do not intersect, so the number of tokens slashed to revert a tail of X blocks is linear in X. In particular, reverting all the blocks produced in one day would result in at least ⅓ of the total stake of all validators slashed.
Ethereum’s approach
Despite the fact that the beacon chain spec is published, the exact details of how the validation on the beacon chain is done and which subset of validators finalizes the blocks is not easy to derive from the spec. I had an in-depth conversation with Vitalik to better understand the current design.
To become a validator in Ethereum, it is sufficient to stake 32ETH. The number of validators is capped at approximately 4 million, but the expected value in practice should be around 400K. Shards sample committees from those validators, but on the beacon chain, all validators attest to each block, and all validators participate in Casper (see my blog post for the overview of the shard chains in Ethereum, and an overview of proposing and attesting; from now, I assume the reader is familiar with those concepts).
The attestations on the beacon chain serve multiple purposes, two that are relevant for us are:
The attestations are used for the LMD (latest-message driven) fork choice rule that is used for blocks produced since the last block finalized by Casper;
The attestations are reused for Casper finalization itself (see the Casper FFG paper).
Unlike the previous proposals, all the ~400K validators rather than a sample participate in each Casper finalization. LMD still relies on samples of 1/64 of all the validators.
Update: make sure to read Vitalik’s response here, where he provides more details and clarifications.
The blocks on the beacon chain are produced every 16 seconds (increased from 8 seconds in a recent spec update), and Casper finalization happens every 100 blocks. This effectively means that every 16 seconds, 400K/64 participants create a multisignature on a block, and every ~26 minutes all 400K participants reach a byzantine consensus on a block.
Both sending 400K signatures over network and aggregating them is expensive. To make it feasible, the validators are split into committees. Assuming 400K participants, each committee consists of 4096 participants (with 1024 total committees). Each committee aggregates the BLS signature internally, and propagates it up to the whole validators set, where only the resulting combined signatures from the committees are aggregated into the final BLS signature. The validation of a BLS signature is rather cheap, along with computing an aggregated public key for the 400K validators. I personally estimate the most expensive part will be validating 4K signatures within each committee, but according to Vitalik that should be doable in a couple seconds.
Comparison
While Casper FFG, in practice, indeed provides almost zero forkfulness, there are a few reasons why we chose our consensus instead of adopting Casper FFG:
In Ethereum, the underlying block production mechanism relies on synchronized clocks; I will discuss problems with this reliance below when talk about shard chains;
Casper only finalizes blocks every 26 minutes. Blocks between such finalizations can theoretically have forks — the attestations do not provide theoretical guarantees, and even with ⅔ attestations on a block and less than ⅓ of malicious actors a block could be reverted;
Besides those reasons, NEAR aims to enable network operators to run nodes on mobile phones. To fully leverage the benefits of linear scalability that sharding provides, a blockchain network needs to have significantly more participating nodes than there are in any blockchain network existing today, and the ability to run nodes on (high end) mobile phones taps into a pool of hundreds of millions of devices. With Thresholded Proof of Stake, a participant on the beacon chain only needs to participate in a cheap consensus once per stake per day, while with Ethereum’s approach one would need to be constantly online, participating in heavy computations (validating thousands of BLS signatures every few seconds). Ethereum doesn’t target mobile devices as operating nodes, so for them, such a decision makes sense.
It is also important to note that the majority of participants on Ethereum will stake significantly more than 32ETH, and will thus participate in multiple committees, which might create some bottleneck on networking (a participant that staked 32000 ETH and thus participates in ~1000 committees will have to receive around 1000 x 4096 signatures every 16 seconds).
Overall, the main consideration for NEAR is the ability to run on low end devices, so we chose simpler and cheaper BFT consensus with small committees instead of running a consensus among all the validators. As a result, the beacon chain in NEAR Protocol can in theory have forks, and the rest of the system is designed to work without assuming that the beacon chain has zero forkfulness.
The Shard Chains
NEAR uses its own consensus called TxFlow for shard chains, while Ethereum 2.0 uses the proposers / attesters framework. While TxFlow provides byzantine fault-tolerant consensus under the assumption that less than ⅓ of nodes are malicious in each shard, such an assumption is completely unreasonable for a shard chain, for reasons discussed above.
With that assumption removed, TxFlow and Attestations have very similar properties: blocks are produced relatively quickly, and the probability of forks is reasonably small under normal operation. The major drawback of TxFlow is that it stalls if more than ⅓ of the participants are offline. Ethereum maintains liveness with any number of validators dropping out (though the speed of block production linearly degrades with fewer participants online).
On the other hand, Ethereum shard chains depend crucially on participants having synchronized clocks. The blocks are produced at a regular schedule (one every 8 seconds), and for the system to make progress, the clocks need to be synchronized with an accuracy of a few seconds. I personally do not believe that such synchronization is possible without depending on centralized time servers that become a single point of failure for the system. Also, the security analysis of possible timing attacks when there’s a dependency on a clock appears to be extremely complex.
At NEAR, we have a principled position to not have any dependency on synchronized clocks, and thus cannot use the proposers/attesters framework for the shard chains.
It is also worth mentioning that we are actively researching ways to adjust TxFlow in such a way that it maintains liveness when fewer than ⅔ of validators are online (naturally at an expense of higher forkfulness under such circumstances).
Outro
When designing complex sharded blockchains, many design decisions come down to choosing from multiple suboptimal solutions, such as choosing between centralization and forkfulness in the beacon chain.
We are working closely with Ethereum Foundation on sharding research, and both teams are aware of the pros and cons of different approaches. In this blog post I presented our thinking behind the decisions that differ in our design from Ethereum Serenity.
If you want to stay up to date with what we build at NEAR, use the following channels:
Twitter — https://twitter.com/nearprotocol,
Discord — https://discord.gg/kRY6AFp, we have open conversations on tech, governance and economics of blockchain on our discord.
Our recently launched research forum — http://research.nearprotocol.com/
https://upscri.be/633436/
Huge thanks to Vitalik Buterin for providing detailed explanation on how the beacon chain in Ethereum Serenity works. |
---
id: delegation
title: Token Delegation
sidebar_label: Token Delegation
sidebar_position: 3
---
---
## Overview
This page will describe the mechanics of participating in delegation.
At a high level, anyone can delegate their tokens to a staking pool which is run by a validator. This is usually just called "Staking". This is possible even if your tokens are in a lockup contract (as most tokens are during the network's early release). Delegating to a staking pool allows you to receive some staking rewards (which will activate as part of the Phase II rollout and depend on the exact fees charged by the validator) and to participate in protocol governance by adding weight to the vote of the validator whose pool you have delegated to.
You can participate in delegation by using a website (GUI), using the `view` and `call` methods of the command-line interface (CLI) or via sending RPC calls directly. These are described below.
Reminder: If you haven't already, evaluate your token custody options from [this documentation page](token-custody.md).
### Viewing and Choosing Validators
Currently, the staking pool contracts ([code](https://github.com/near/core-contracts/tree/master/staking-pool)) allow validators to select what fee they will charge on rewards earned by pools they run. In the future, updated versions of the staking pool contracts may include more parameters (which is one of the nice features enabled by NEAR's contract-based delegation).
Several community-run lists of validators list who they are, how much stake they have, what their vote was [for the Phase II transition](https://near.org/blog/mainnet-phase-i/) and more. These can be helpful for selecting where to delegate your tokens. As always, do your own diligence.
| Operator | URL (to copy and paste) |
| ------------------ | ---------------------------------------------------------------------------------------------------------- |
| NEAR Explorer | [https://explorer.near.org/nodes/validators](https://explorer.near.org/nodes/validators) |
| DokiaCapital | [https://staking.dokia.cloud/staking/near/validators](https://staking.dokia.cloud/staking/near/validators) |
| Stardust NEAR Pool | [https://near-staking.com/](https://near-staking.com) |
| YOUR OPERATOR | ADD YOUR LINK HERE |
## GUI-based delegation (via a website or app)
Disclaimer: the list below is community-maintained, and is not an endorsement by NEAR to use any of them. Do your own research before staking your funds with them!
| Provider | URL (to copy and paste) | Added on |
| -------------- | ------------------------------------------------------------------ | ----------------------- |
| NEAR Wallet | [https://help.mynearwallet.com/en/articles/44-how-to-recover-your-mynearwallet-account](https://help.mynearwallet.com/en/articles/44-how-to-recover-your-mynearwallet-account) | Oct 11 2020 |
| DokiaCapital | [https://staking.dokia.cloud](https://staking.dokia.cloud) | Sept 11 2020 |
| Moonlet Wallet | [https://moonlet.io/near-staking](https://moonlet.io/near-staking) | 15 Oct 2020 |
| add here | your link | first come, first serve |
## CLI-based delegation
Disclaimer: the documentation below refers to the Github repository [Core Contracts](https://github.com/near/core-contracts/). Always check the source of the smart contract before delegating your funds to it!
You can use `near-cli` with NEAR Core Contracts to delegation via:
1. Lockup Contract
2. Staking Pool
Before starting, make sure you are running the latest version of [near-cli](https://github.com/near/near-cli), you are familiar with [gas fees](https://docs.near.org/concepts/basics/transactions/gas) and you are sending transactions to the correct NEAR network. By default `near-cli` is configured to work with TestNet. You can change the network to MainNet by issuing the command
```bash
export NODE_ENV=mainnet
```
It will also be helpful to set some variables, which we will use in commands below. On Linux/macOS/Unix:
```
export ACCOUNT_ID=#your account id
export LOCKUP_ID=#your lockup contract address
export POOL_ID=#your staking pool
```
If you're using Windows, you can replace all the `export` commands with `set`:
```
set ACCOUNT_ID=#your account id
```
You can check that you set these correctly with `echo`:
```
echo $ACCOUNT_ID
```
Note that when you set variables with `export` you do not prefix them with the dollar sign, but when you use them elsewhere such as with `echo`, you need the dollar sign.
If you're using a secondary account on a Ledger Nano device, you will also want to create an HD\_PATH variable. For example:
```
export HD_PATH="44'/397'/0'/0'/2'"
```
The default HD path for the NEAR app on Ledger devices is `44'/397'/0'/0'/1'`. See more info on using `near-cli` with Ledger [here](token-custody.md#option-1-ledger-via-cli).
> **Heads up**
>
> Signing a transaction with the wrong Ledger key can help associating multiple accounts of a user, since even failed transactions are recorded to the blockchain and can be subsequently analyzed.
## 1. Lockup Contracts Delegation
The [Lockup Contract](https://github.com/near/core-contracts/tree/master/lockup) is common among NEAR contributors and, essentially, anyone who didn't acquire tokens through an exchange. This contract acts as an escrow that locks and holds an owner's tokens for a lockup period (such as vesting). You can learn more about lockups and their implementation in the [this documentation page](lockups.md).
The owner may want to stake these tokens (including locked ones) to help secure the network and also earn staking rewards that are distributed to the validator. The lockup contract doesn't allow to directly stake from its account, so the owner delegates the tokens using the contract built-in functions.
> **heads up**
>
> The commands below are tested with [build 877e2db](https://github.com/near/core-contracts/tree/877e2db699a02b81fc46f5034642a2ebd06d9f19) of the Core Contracts.
>
> They also use formatting only available on Linux/macOS/Unix. If you are using Windows, you will need to replace dollar variables, `$LOCKUP_ID`, with percent variables, `%LOCKUP_ID%`. And you will need to use all double quotes, so things like `'{"account_id": "'$ACCOUNT_ID'"}'` become `"{\"account_id\": \"%ACCOUNT_ID%\"}\"`.
Before proceeding with the tutorial below, check that you have control of your lockup contract, by issuing the command
```bash
near view $LOCKUP_ID get_owner_account_id ''
```
You should expect a result like:
```
$ near view meerkat.stakewars.testnet get_owner_account_id ''
View call: meerkat.stakewars.testnet.get_owner_account_id()
'meerkat.testnet'
```
Note that this example us using `NEAR_ENV=testnet` with `$LOCKUP_ID` set to `meerkat.stakewars.testnet` and `ACCOUNT_ID` set to `meerkat.testnet`, which matches the returned result above.
You can stake with Lockup contracts in three steps:
1. Set up the staking pool
2. Deposit and stake the tokens
3. Measure the rewards
### a. Set the staking pool
Lockup contracts can stake **to one staking pool at a time**, so this parameter can be changed only while no funds are staked.
You can select the staking pool by calling the method `select_staking_pool` from the lockup contract:
```bash
near call $LOCKUP_ID select_staking_pool '{
"staking_pool_account_id": "'$POOL_ID'"
}' --accountId $ACCOUNT_ID
```
If using a Ledger:
```bash
near call $LOCKUP_ID select_staking_pool '{
"staking_pool_account_id": "'$POOL_ID'"
}' --accountId $ACCOUNT_ID --useLedgerKey $HD_PATH
```
You should expect a result like:
```
Scheduling a call: meerkat.stakewars.testnet.select_staking_pool({"staking_pool_account_id": "zpool.pool.f863973.m0"})
Receipts: HEATt32tHqWdjkZoSxLhd43CYVBCUp1cFHexgWBEeKJg, EUP7tncJfKDPpKYdunx2qySZ6JmV3injBigyFBiuD96J, 99hdXWmaTQE7gR5vucxMWFNMw7ZLtzJ6WNMSVJHPGJxh
Log [meerkat.stakewars.testnet]: Selecting staking pool @zpool.pool.f863973.m0. Going to check whitelist first.
Transaction Id 4Z2t1SeN2rdbJcPvfVGScpwyuGGKobZjT3eqvDu28sBk
To see the transaction in the transaction explorer, please open this url in your browser
https://explorer.testnet.near.org/transactions/4Z2t1SeN2rdbJcPvfVGScpwyuGGKobZjT3eqvDu28sBk
true
```
The `true` statement means that your call was successful, and the lockup contract accepted the `$POOL_ID` parameter.
### b. Deposit and stake the tokens
Lockup contracts can stake their balance, regardless of their vesting schedule. You can proceed in two steps:
1. check the lockup balance
2. stake the balance
3. To know how many tokens you can stake, use the view method `get_balance`:
```
near view $LOCKUP_ID get_balance ''
```
You should expect a result like:
```
$ near view meerkat.stakewars.testnet get_balance ''
View call: meerkat.stakewars.testnet.get_balance()
'100000499656128234500000000'
```
Where the resulting balance (in [yocto](https://www.nist.gov/pml/weights-and-measures/metric-si-prefixes)NEAR) is `100000499656128234500000000` or 100 $NEAR.
> **heads up**
>
> You have to leave 35 $NEAR in the lockup contract for the storage fees. If you have 100 tokens, you can stake up to 65 tokens. If you have 1000 tokens, you can stake up to 965 tokens.
>
> You may want to `npm i -g near-units` to easily convert yoctoNEAR amounts to human-readable representations, and to go from human-typeable numbers to yoctoNEAR. Examples:
>
> ```bash
> near-units -h 100000499656128234500000000 yN
> near-units 965 N
> ```
>
> On Linux/macOS/Unix, you can also use this directly in the following commands:
>
> ```bash
> near call $LOCKUP_ID deposit_and_stake '{
> "amount": "'$(near-units 965N)'"
> }' --accountId $ACCOUNT_ID --gas $(near-units 200Tgas)
> ```
You may want to set your desired amount-to-stake to a variable:
```
export AMOUNT=# your desired amount to stake
```
To stake the balance, use the call method `deposit_and_stake`:
```bash
near call $LOCKUP_ID deposit_and_stake '{
"amount": "'$AMOUNT'"
}' --accountId $ACCOUNT_ID --gas 200000000000000
```
If using a Ledger:
```bash
near call $LOCKUP_ID deposit_and_stake '{
"amount": "'$AMOUNT'"
}' --accountId $ACCOUNT_ID --gas 200000000000000 --useLedgerKey $HD_PATH
```
> **Heads up**
>
> This sets the gas parameter to 200000000000000 [gas units](https://docs.near.org/concepts/basics/transactions/gas), or 200 Tgas (Teragas), as the near-cli default allocation of 30Tgas is too low.
You should expect a result like:
```
Scheduling a call: meerkat.stakewars.testnet.deposit_and_stake({"amount": "65000000000000000000000000"})
Receipts: BFJxgYMbPLpKy4jae3b5ZJfLuAmpcYCBG2oJniDgh1PA, 9sZSqdLvPXPzpRg9VLwaWjJaBUnXm42ntVCEK1UwmYTp, CMLdB4So6ZYPif8qq2s7zFvKw599HMBVcBfQg85AKNQm
Log [meerkat.stakewars.testnet]: Depositing and staking 65000000000000000000000000 to the staking pool @zpool.pool.f863973.m0
Receipts: 3VpzWTG9Y2nbZmuJhmsub9bRLnwHCFtnW5NQqcMBWawF, 4BrEKDPmz3mAhnq5tTFYRQp8y9uRxGFwYffJFkPMdQ5P, HJPqqJDTajFfeEA8vFXTLDpxh4PYZCMJ2YLKpWVtwSMF
Log [meerkat.stakewars.testnet]: Epoch 105: Contract received total rewards of 20383576173536987339742291104 tokens. New total staked balance is 104251712242552700026473040909. Total number of shares 77295581098329153455984430997
Log [meerkat.stakewars.testnet]: Total rewards fee is 1511304065231935217653387665 stake shares.
Log [meerkat.stakewars.testnet]: @meerkat.stakewars.testnet deposited 65000000000000000000000000. New unstaked balance is 65000000000000000000000000
Log [meerkat.stakewars.testnet]: @meerkat.stakewars.testnet staking 64999999999999999999999999. Received 48193095953206307331949682 new staking shares. Total 1 unstaked balance and 48193095953206307331949682 staking shares
Log [meerkat.stakewars.testnet]: Contract total staked balance is 104316712242552700026473040909. Total number of shares 77343774194282359763316380679
Receipt: FsJLXXEa8Jp8UNyiyG3XQhyHHSWzZ2bCjgWr8kPVjGmG
Log [meerkat.stakewars.testnet]: The deposit and stake of 65000000000000000000000000 to @zpool.pool.f863973.m0 succeeded
Transaction Id AW9pFb5RjkCjsyu8ng56XVvckvd3drPBPtnqVo6bJhqh
To see the transaction in the transaction explorer, please open this url in your browser
https://explorer.testnet.near.org/transactions/AW9pFb5RjkCjsyu8ng56XVvckvd3drPBPtnqVo6bJhqh
true
```
The `true` statement at the end means the transaction was successful.
### c. Measure the rewards
Since NEAR automatically re-stakes all staking pool rewards, you have to update your staked balance to know the amount of tokens you earned with your validator.
Use the call method `refresh_staking_pool_balance` to check your new balance:
```bash
near call $LOCKUP_ID refresh_staking_pool_balance '' \
--accountId $ACCOUNT_ID
```
If using a Ledger:
```bash
near call $LOCKUP_ID refresh_staking_pool_balance '' \
--accountId $ACCOUNT_ID --useLedgerKey $HD_PATH
```
You should expect a result like:
```
$ near call $LOCKUP_ID refresh_staking_pool_balance '' \
--accountId $ACCOUNT_ID | grep "current total balance"
Log [meerkat.stakewars.testnet]: The current total balance on the staking pool is 65000000000000000000000000
```
Note that in this example, the result is piped to [grep](https://www.tutorialspoint.com/unix\_commands/grep.htm) (`| grep "current total balance"`) to display only the relevant output.
Please refer to the [Lockup Contract readme](https://github.com/near/core-contracts/tree/master/lockup) if you need to know how to withdraw the staking rewards to your main wallet.
## Unstake and withdraw your lockup tokens
NEAR Protocol automatically re-stakes all the rewards back to the staking pools, so your staked balance increases over time, accruing rewards.
Before starting, it's highly recommended to read the [Lockup contracts documentation](lockups.md) to understand which portion of the tokens is liquid, and which one is still _locked_ even after the three epochs.
If you want to withdraw funds, you have to issue two separate commands:
1. Manually unstake tokens from the staking pool, and wait three epochs
2. Manually withdraw tokens back to your main account
Both these command require the amount in yoctoNEAR, which is the smallest unit of account for NEAR tokens. You can use the table below for the conversion, or install [near-units](https://www.npmjs.com/package/near-units) (`npm i -g near-units`) and use commands like `near-units 1,000N`:
| NEAR | YoctoNEAR | YoctoNEAR |
| -------- | --------- | ------------------------------- |
| `1` | `1*10^24` | `1000000000000000000000000` |
| `10` | `1*10^25` | `10000000000000000000000000` |
| `100` | `1*10^26` | `100000000000000000000000000` |
| `1,000` | `1*10^27` | `1000000000000000000000000000` |
| `10,000` | `1*10^28` | `10000000000000000000000000000` |
As an example, if you want to unstake `10` NEAR tokens from the staking pool, you have to call the method `unstake` with `10000000000000000000000000` (`1*10^24`, 10 power 24, or 10 with 24 zeros) as an argument.
### a. Unstake the tokens
Before unstaking any tokens, use the the view method `get_account` introduced above to know what is the available balance:
```
near view $POOL_ID get_account '{"account_id": "'$LOCKUP_ID'"}'
```
You should expect a result like:
```
$ near view zpool.pool.f863973.m0 get_account '{"account_id": "meerkat.stakewars.testnet"}'
View call: zpool.pool.f863973.m0.get_account({"account_id": "meerkat.stakewars.testnet"})
{
account_id: 'meerkat.stakewars.testnet',
unstaked_balance: '5',
staked_balance: '86236099167204810592552675',
can_withdraw: false
}
```
The result of this method shows:
* An unstaked balance of `5` _yocto_
* A staked balance of `86236099167204810592552675` _yocto_, or `86.23` $NEAR
* `can_withdraw` is `false` (see below)
> **Check your rewards**
>
> From the examples above, the initial stake of 65 NEAR tokens increased to 86.23 tokens, with 21.23 tokens as a reward!
You can unstake an amount of funds that is equal or lower than your `staked_balance`, by calling the metod `unstake`. You may first want to set the amount you want to unstake to a variable `AMOUNT`:
```bash
export AMOUNT=# amount to unstake
```
Check that it looks correct:
```bash
echo $AMOUNT
```
And maybe also using `near-units`:
```bash
near-units -h $AMOUNT yN
```
And now unstake:
```bash
near call $LOCKUP_ID unstake '{
"amount": "'$AMOUNT'"
}' --accountId $ACCOUNT_ID --gas 200000000000000
```
Or with a Ledger:
```bash
near call $LOCKUP_ID unstake '{
"amount": "'$AMOUNT'"
}' --accountId $ACCOUNT_ID --gas 200000000000000 --useLedgerKey $HD_PATH
```
You should expect a result like:
```
Scheduling a call: meerkat.stakewars.testnet.unstake({"amount": "42000000000000000000000000"})
Waiting for confirmation on Ledger...
Using public key: ed25519:5JgsX9jWUTS5ttHeTjuDFgCmB5YX77f7GHxuQfaGVsyj
Waiting for confirmation on Ledger...
Ledger app version: 1.1.3
Receipts: D5fCCWa5b5N8X9VtxsbRvGhSSCQc56Jb312DEbG4YY8Z, 72rgHrMb1zkUU2RiEz2EMCAuMWFgRJTf8eovwAUkuo31, A2dvSgxpDXfNZUrDVdpewk1orVTVxCMiicjk7qyNq3dW
Log [meerkat.stakewars.testnet]: Unstaking 42000000000000000000000000 from the staking pool @zpool.pool.f863973.m0
Receipts: 9MbLXn28nQyL6BopMEUggBD9NA1QfVio9VB3LTNJ6P5b, 5g7F6HehoGZ2DHS7w54xHAjAeHCsHaAsKpVed76MFYru, BKbtF77i1WEqpuFLtEjWerM2LVZW1md9ecQ5UcBpMNXj
Log [meerkat.stakewars.testnet]: Epoch 162: Contract received total rewards of 9488161121527521670023204339 tokens. New total staked balance is 142388816229749953331375665038. Total number of shares 79574075700231185032355056920
Log [meerkat.stakewars.testnet]: Total rewards fee is 530246455678218748147822292 stake shares.
Log [meerkat.stakewars.testnet]: @meerkat.stakewars.testnet unstaking 42000000000000000000000001. Spent 23471725293488513736732361 staking shares. Total 42000000000000000000000002 unstaked balance and 24721370659717793595217321 staking shares
Log [meerkat.stakewars.testnet]: Contract total staked balance is 142346816229749953331375665038. Total number of shares 79550603974937696518618324559
Receipt: 3DXSx8aMvhwtJUB6mhTueNbDiqz5wYu3xzzYkZrgjw1u
Log [meerkat.stakewars.testnet]: Unstaking of 42000000000000000000000000 at @zpool.pool.f863973.m0 succeeded
Transaction Id 2rRpdN8AAoySfWsgE7BRtRfz7VFnyFzRhssfaL87rXGf
To see the transaction in the transaction explorer, please open this url in your browser
https://explorer.testnet.near.org/transactions/2rRpdN8AAoySfWsgE7BRtRfz7VFnyFzRhssfaL87rXGf
true
```
The `true` statement at the end means the transaction was successful.
**Wait three epochs, or \~36 hours before using the withdraw command**
For security reasons, NEAR Protocol keeps your unstaked funds locked for three more epochs (\~36 hours). Use again the view method `get_account` to verify if `can_withdraw` is `true`:
```
near view $POOL_ID get_account '{"account_id": "'$LOCKUP_ID'"}'
```
The result should be as follows:
```
View call: zpool.pool.f863973.m0.get_account({"account_id": "meerkat.stakewars.testnet"})
{
account_id: 'meerkat.stakewars.testnet',
unstaked_balance: '42000000000000000000000006',
staked_balance: '44244760537191121230392104',
can_withdraw: true
}
```
Note that the variable `can_withdraw` is `true`. This means that the `unstaked_balance` of `42000000000000000000000000` yoctoNEAR (42 NEAR tokens) is now available for withdraw.
### b. Withdraw the tokens
Funds can be withdrawn after three epochs (\~36 hours) from the `unstake` command. It is highly recommended to read the [Lockup contracts documentation](lockups.md) to understand which portion of the unstaked tokens is available for transfers, and which is still vesting and unavailable (even after three epochs).
Use the call method `withdraw_all_from_staking_pool`:
```bash
near call $LOCKUP_ID withdraw_all_from_staking_pool '' --accountId $ACCOUNT_ID --gas 200000000000000
```
Or if using a Ledger:
```bash
near call $LOCKUP_ID withdraw_all_from_staking_pool '' --accountId $ACCOUNT_ID --gas 200000000000000 --useLedgerKey $HD_PATH
```
You should expect a result like this one:
```
Scheduling a call: meerkat.stakewars.testnet.withdraw_all_from_staking_pool()
Waiting for confirmation on Ledger...
Using public key: ed25519:5JgsX9jWUTS5ttHeTjuDFgCmB5YX77f7GHxuQfaGVsyj
Waiting for confirmation on Ledger...
Ledger app version: 1.1.3
Receipts: 6dt3HNDrb7jM4F7cRtwgRSfRMWwFmE8BHeZSDghrpQtw, 7pNKTevct7Z4birAPec81LmnSpJSeJXbFq3cBdjFvsnM, A7yJXdaGcFyiBNQimFtHF479Jm6xiXHvfodTbtFf7Rww
Log [meerkat.stakewars.testnet]: Going to query the unstaked balance at the staking pool @zpool.pool.f863973.m0
Receipts: 2ytgB57D6CJe3vkxdVTiVAytygpTteR4sDmpUNxo5Ltk, CZChcT1uDDxqojnZQq6r7zzx6FE3Z6E5fZ3F23Y1dnyM, GjrWhJgbCAAHUNyvw4QX5YKFpeUVNJ9GpuEdmUXFozeG
Log [meerkat.stakewars.testnet]: Withdrawing 42000000000000000000000006 from the staking pool @zpool.pool.f863973.m0
Receipts: 9nHoqnQygqKYyyR5KDofkYy5KiJxbu2eymoGUUc2tSR3, 5RvBSb4BuHxRhc5U6nLgsCjAvJ88hJ2jwkr1ramk37mE, 5PDN1EYXnCJrTSU7zzJtmAuj9vvGSVurh94nvfHDHXpa, GTTGm4sXcSWD2gnohMJzD7TQcQxuSe7AFABZKqxPLgau
Log [meerkat.stakewars.testnet]: Epoch 168: Contract received total rewards of 514182787298700000000 tokens. New total staked balance is 142377784876419564057032272059. Total number of shares 79552334320822393318832070510
Log [meerkat.stakewars.testnet]: Total rewards fee is 28729510739826825657 stake shares.
Log [meerkat.stakewars.testnet]: @meerkat.stakewars.testnet withdrawing 42000000000000000000000006. New unstaked balance is 0
Receipt: rS5KjvzkbaxEH7aG8VXyd9EEEfY7PygdLzSZ3W9qf3w
Log [meerkat.stakewars.testnet]: The withdrawal of 42000000000000000000000006 from @zpool.pool.f863973.m0 succeeded
Transaction Id EjNpoU7BNfEQujckL8JiAP8kYDH1SMLw4wC32tL3uyaH
To see the transaction in the transaction explorer, please open this url in your browser
https://explorer.testnet.near.org/transactions/EjNpoU7BNfEQujckL8JiAP8kYDH1SMLw4wC32tL3uyaH
true
```
The `true` statement confirms the successful withdrawal.
By using again the view method:
```bash
near view $POOL_ID get_account '{"account_id": "'$LOCKUP_ID'"}'
```
The result should be as follows:
```
View call: zpool.pool.f863973.m0.get_account({"account_id": "meerkat.stakewars.testnet"})
{
account_id: 'meerkat.stakewars.testnet',
unstaked_balance: '0',
staked_balance: '44244760947075774431066852',
can_withdraw: true
}
```
At this point the `unstaked_balance` is `0`, and the funds are back in the lockup contract balance.
> **Heads up**
>
> Lockup contracts allow you to transfer only the unlocked portion of your funds. In the example above, out of the 42 NEAR unstaked, only 21.23 can be transferred to another wallet or exchange.
### c. Change staking pools
To change from one staking pool to another, you must first withdraw all deposits in the currently selected staking pool. Then call `unselect_staking_pool` as follows ([docs](https://github.com/near/core-contracts/blob/215d4ed2edb563c47edd961555106b74275c4274/lockup/README.md)):
```bash
near call $LOCKUP_ID unselect_staking_pool --accountId $ACCOUNT_ID --gas 300000000000000
```
Or if using Ledger:
```bash
near call $LOCKUP_ID unselect_staking_pool --accountId $ACCOUNT_ID --useLedgerKey $HD_PATH --gas 300000000000000
```
## Staking Pool Delegation
Any funds that are not stored inside lockup contracts can be directly delegated to a [staking pool](https://github.com/near/core-contracts/tree/master/staking-pool) by using the call method `deposit_and_stake`:
```bash
near call $POOL_ID deposit_and_stake '' --accountId $ACCOUNT_ID --amount 100
```
Or if using Ledger:
```bash
near call $POOL_ID deposit_and_stake '' --accountId $ACCOUNT_ID --useLedgerKey $HD_PATH --amount 100
```
You should expect a result like:
```
Scheduling a call: valeraverim.pool.f863973.m0.deposit_and_stake() with attached 100 NEAR
Receipts: FEfNuuCSttu7m4dKQPUSgpSFJSB86i6T2sYJWSv7PHPJ, GPYhZsyUuJgvr7gefxac4566DVQcyGs4wSFeydkmRX7D, 8hfcJuwsstnFQ1cgU5iUigvfrq1JcbaKURvKf5shaB4g
Log [valeraverim.pool.f863973.m0]: Epoch 106: Contract received total rewards of 545371217890000000000 tokens. New total staked balance is 75030000959768421358700000000. Total number of shares 75029067713856889417103116457
Log [valeraverim.pool.f863973.m0]: Total rewards fee is 54536443439735902364 stake shares.
Log [valeraverim.pool.f863973.m0]: @meerkat.testnet deposited 100000000000000000000000000. New unstaked balance is 100000000000000000000000000
Log [valeraverim.pool.f863973.m0]: @meerkat.testnet staking 99999999999999999999999999. Received 99998756169666008195607157 new staking shares. Total 1 unstaked balance and 99998756169666008195607157 staking shares
Log [valeraverim.pool.f863973.m0]: Contract total staked balance is 75130000959768421358700000000. Total number of shares 75129066470026555425298723614
Transaction Id FDtzMmusJgFbryeVrdQyNvp6XU2xr11tecgqnnSsvyxv
To see the transaction in the transaction explorer, please open this url in your browser
https://explorer.testnet.near.org/transactions/FDtzMmusJgFbryeVrdQyNvp6XU2xr11tecgqnnSsvyxv
''
```
The empty string at the end, `''`, means the call was successful.
If you want to check your staking rewards, use the view method `get_account`:
```bash
near view $POOL_ID get_account '{"account_id": "'$ACCOUNT_ID'"}'
```
You should expect a result like:
```
View call: valeraverim.pool.f863973.m0.get_account({"account_id": "meerkat.testnet"})
{
account_id: 'meerkat.testnet',
unstaked_balance: '1',
staked_balance: '100663740438210643632989745',
can_withdraw: true
}
```
The staked balance in this example is `100663740438210643632989745`, or `100.66` $NEAR (reminder: you can easily convert these using `near-units -h 100663740438210643632989745yN`).
A pool's rewards only compound if it has been "pinged", which means either having a direct action performed on it (like someone delegating or undelegating) or if the `ping` method is called on it once within a particular epoch. This is important to do if you run a pool and want your delegators to see updated reward balances.
Use the call method `ping` to re-calculate the rewards up to the previous epoch:
```bash
near call $POOL_ID ping '{}' --accountId $ACCOUNT_ID
```
Or if using a Ledger:
```bash
near call $POOL_ID ping '{}' --accountId $ACCOUNT_ID --useLedgerKey $HD_PATH
```
You should expect a result like:
```
Scheduling a call: valeraverim.pool.f863973.m0.ping({})
Transaction Id 4mTrz1hDBMTWZx251tX4M5CAo5j7LaxLiPtQrDvQgcZ9
To see the transaction in the transaction explorer, please open this url in your browser
https://explorer.testnet.near.org/transactions/4mTrz1hDBMTWZx251tX4M5CAo5j7LaxLiPtQrDvQgcZ9
''
```
The `''` result means that your call was successful, and the `get_account` view method will provide updated results.
Note that you can ping any pool, not just one you own.
## Additional links
* [Lockup contracts explained](lockups.md)
* [NEAR Core Contracts on Github](https://github.com/near/core-contracts)
* [NEAR block explorer](https://explorer.near.org)
* [near-cli on Github](https://github.com/near/near-cli)
## Fun Facts
1. `ping`ing a pool technically removes 2 epochs of future compounding but it's an extremely small amount -- without considering compounding effect of epochs with 9 hour epochs, the reward per epoch is 5% / (365 \* 24 / 9) + 1 or 1.00005136986
It means this reward on top of reward is what you’re losing, so that's about 1.00000000264 or 0.000000264%... meaning for 10M stake it’s 0.02638862826 NEAR per epoch.
> Got a question?
>
> Ask it on [StackOverflow!](https://stackoverflow.com/questions/tagged/nearprotocol)
|
---
NEP: 199
Title: Non Fungible Token Royalties and Payouts
Author: Thor <@thor314>, Matt Lockyer <@mattlockyer>
DiscussionsTo: https://github.com/near/NEPs/discussions/199
Status: Final
Type: Standards Track
Category: Contract
Created: 03-Mar-2022
Requires: 171, 178
---
## Summary
An interface allowing non-fungible token contracts to request that financial contracts pay-out multiple receivers, enabling flexible royalty implementations.
## Motivation
Currently, NFTs on NEAR support the field `owner_id`, but lack flexibility for ownership and payout mechanics with more complexity, including but not limited to royalties. Financial contracts, such as marketplaces, auction houses, and NFT loan contracts would benefit from a standard interface on NFT producer contracts for querying whom to pay out, and how much to pay.
Therefore, the core goal of this standard is to define a set of methods for financial contracts to call, without specifying how NFT contracts define the divide of payout mechanics, and a standard `Payout` response structure.
## Specification
This Payout extension standard adds two methods to NFT contracts:
- a view method: `nft_payout`, accepting a `token_id` and some `balance`, returning the `Payout` mapping for the given token.
- a call method: `nft_transfer_payout`, accepting all the arguments of`nft_transfer`, plus a field for some `Balance` that calculates the `Payout`, calls `nft_transfer`, and returns the `Payout` mapping.
Financial contracts MUST validate several invariants on the returned
`Payout`:
1. The returned `Payout` MUST be no longer than the given maximum length (`max_len_payout` parameter) if provided. Payouts of excessive length can become prohibitively gas-expensive. Financial contracts can specify the maximum length of payout the contract is willing to respect with the `max_len_payout` field on `nft_transfer_payout`.
2. The balances MUST add up to less than or equal to the `balance` argument in `nft_transfer_payout`. If the balance adds up to less than the `balance` argument, the financial contract MAY claim the remainder for itself.
3. The sum of the balances MUST NOT overflow. This is technically identical to 2, but financial contracts should be expected to handle this possibility.
Financial contracts MAY specify their own maximum length payout to respect.
At minimum, financial contracts MUST NOT set their maximum length below 10.
If the Payout contains any addresses that do not exist, the financial contract MAY keep those wasted payout funds.
Financial contracts MAY take a cut of the NFT sale price as commission, subtracting their cut from the total token sale price, and calling `nft_transfer_payout` with the remainder.
## Example Flow
```text
┌─────────────────────────────────────────────────┐
│Token Owner approves marketplace for token_id "0"│
├─────────────────────────────────────────────────┘
│ nft_approve("0",market.near,<SaleArgs>)
▼
┌───────────────────────────────────────────────┐
│Marketplace sells token to user.near for 10N │
├───────────────────────────────────────────────┘
│ nft_transfer_payout(user.near,"0",0,"10000000",5)
▼
┌───────────────────────────────────────────────┐
│NFT contract returns Payout data │
├───────────────────────────────────────────────┘
│ Payout(<who_gets_paid_and_how_much)
▼
┌───────────────────────────────────────────────┐
│Market validates and pays out addresses │
└───────────────────────────────────────────────┘
```
## Reference Implementation
```rust
/// A mapping of NEAR accounts to the amount each should be paid out, in
/// the event of a token-sale. The payout mapping MUST be shorter than the
/// maximum length specified by the financial contract obtaining this
/// payout data. Any mapping of length 10 or less MUST be accepted by
/// financial contracts, so 10 is a safe upper limit.
#[derive(Serialize, Deserialize)]
#[serde(crate = "near_sdk::serde")]
pub struct Payout {
pub payout: HashMap<AccountId, U128>,
}
pub trait Payouts {
/// Given a `token_id` and NEAR-denominated balance, return the `Payout`.
/// struct for the given token. Panic if the length of the payout exceeds
/// `max_len_payout.`
fn nft_payout(&self, token_id: String, balance: U128, max_len_payout: Option<u32>) -> Payout;
/// Given a `token_id` and NEAR-denominated balance, transfer the token
/// and return the `Payout` struct for the given token. Panic if the
/// length of the payout exceeds `max_len_payout.`
#[payable]
fn nft_transfer_payout(
&mut self,
receiver_id: AccountId,
token_id: String,
approval_id: Option<u64>,
memo: Option<String>,
balance: U128,
max_len_payout: Option<u32>,
) -> Payout {
assert_one_yocto();
let payout = self.nft_payout(token_id, balance);
self.nft_transfer(receiver_id, token_id, approval_id, memo);
payout
}
}
```
## Fallback on error
In the case where either the `max_len_payout` causes a panic, or a malformed `Payout` is returned, the caller contract should transfer all funds to the original token owner selling the token.
## Potential pitfalls
The payout must include all accounts that should receive funds. Thus it is a mistake to assume that the original token owner will receive funds if they are not included in the payout.
NFT and financial contracts vary in implementation. This means that some extra CPU cycles may occur in one NFT contract and not another. Furthermore, a financial contract may accept fungible tokens, native NEAR, or another entity as payment. Transferring native NEAR tokens is less expensive in gas than sending fungible tokens. For these reasons, the maximum length of payouts may vary according to the customization of the smart contracts.
## Drawbacks
There is an introduction of trust that the contract calling `nft_transfer_payout` will indeed pay out to all intended parties. However, since the calling contract will typically be something like a marketplace used by end users, malicious actors might be found out more easily and might have less incentive.
There is an assumption that NFT contracts will understand the limits of gas and not allow for a number of payouts that cannot be achieved.
## Future possibilities
In the future, the NFT contract itself may be able to place an NFT transfer is a state that is "pending transfer" until all payouts have been awarded. This would keep all the information inside the NFT and remove trust.
## Errata
- Version `2.1.0` adds a memo parameter to `nft_transfer_payout`, which previously forced implementers of `2.0.0` to pass `None` to the inner `nft_transfer`. Also refactors `max_len_payout` to be an option type.
- Version `2.0.0` contains the intended `approval_id` of `u64` instead of the stringified `U64` version. This was an oversight, but since the standard was live for a few months before noticing, the team thought it best to bump the major version.
## Copyright
Copyright and related rights waived via [CC0](https://creativecommons.org/publicdomain/zero/1.0/).
|
Keypom Brings Frictionless Onboarding to Near and Web3
NEAR FOUNDATION
February 23, 2023
A key roadblock to mass Web3 adoption is onboarding. People want to get into Web3 and crypto, but they don’t know how. Seed phrases, private keys, smart contract transactions, technical knowledge and crypto jargon are massive barriers to Web3 entry.
With Keypom, that’s about to change. Keypom is a new zero friction onboarding protocol with a companion no-code app, built on Near.
Now users, regardless of their background, can experience the value of Near’s blockchain technology whether or not they have a wallet. Envisioned as a community-driven, public good — or a public utility — Keypom’s APIs (the Keypom Protocol) will be completely fee-free. The Keypom App will also allow non-developers to take full advantage of Keypom’s powerful wallet-less onboarding experiences.
How Keypom’s frictionless onboarding works
Debuting at NEARCON 2022, Keypom’s onboarding solution is entirely on-chain and non-custodial. It’s run completely on the Near blockchain via Near smart contracts — not on any centralized databases.
Keypom allows Near and Web3 to function more like Web2, where developers and end-users alike can use applications without Web3’s usually confusing, tedious, time-consuming onboarding. For those looking to explore this technology, Keypom will offer an App, which is currently set to release at ETH Denver 2023. This app will give users and organizations the ability to easily create links to invite users to experience aspects of the Near blockchain without having to set up a wallet first.
On other blockchains, users are commonly onboarded with pre-created, pre-loaded wallets. Keypom operates on the basis of Near’s unique access key model, which allows for much more flexibility in the end user-experience.
With Keypom, users are given a special type of access key that can be used to experience a crypto application and later be turned into a wallet. This key can be embedded within a simple Web2 style link that, once clicked, allows users to enter into a crypto experience, entirely on-chain and non-custodially.
Currently, Keypom experiences include receiving $NEAR, NFTs, Tickets to an event, or even dropping the user into a trial account with Near tokens to spend on a preset selection of apps. Once a user has enjoyed an experience with a Keypom link, they can upgrade to a full account and enjoy the entire Near ecosystem.
For organizations that have experienced Keypom and choose to develop on top of their protocol, the Keypom SDK and Wallet Selector plugin allows for a seamless integration of Keypom’s technology into their own apps. Companies will be able to customize every aspect of the user experience to tailor their needs, all while using carefully designed plug-and-play libraries meant to abstract complicated processes for the developer.
Low-cost, customizable linkdrops
Typically, linkdrops have been used to onboard developers and end-users looking to build on Near. The cost of creating 1,000 accounts via traditional means has been approximately 1,003 $NEAR. With Keypom, the costs for onboarding 1,000 users can fall to about 3.5 $NEAR.
“We imagine a world where decentralized applications use the Keypom Protocol to create truly immersive user experiences and specialize in specific use-cases spanning across the entire ecosystem,” says Keypom’s Ben Kurrek. “Companies can build anything from event ticketing to subscription-based payments and multi-sig tooling. While the Keypom App would still allow for users to experience these use-cases, it would be at a much smaller scope.”
Keypom can be used, for instance, to automatically register users into a Decentralized Autonomous Organization (DAO) as part of the onboarding process. Another use case is for events ticketing. With Keypom, users wouldn’t need a wallet to enter an event, and would also be able to receive a POAP (Proof of Attendance Protocol) NFT.
Perhaps one of the most powerful use cases is the ability for applications to use the Keypom protocol to give their users a free trial. After choosing an account name, the user is instantly signed in and can begin exploring and experiencing the app. Just like with gift cards and other vouchers, the funds can only be used on that dApp thus preventing the possibility for a rug to occur. Once the trial is over, the user can onboard fully with the added option of a payback condition for the funds that the dApp fronted.
In this new model, Keypom introduces a paradigm shift in Web3 where users experience crypto first and only focus on onboarding once they’ve seen the benefit and gained value.
“We leave it to those building atop the Keypom Protocol to specialize and create applications however they see fit, such as charging custom fees,” says Keypom’s Matt Lockyer. “We’ve created a world where there are no limits and we simply provide the technology that unlocks these experiences.”
For a full explanation on what is possible with Keypom’s customizable linkdrops, check out the Keypom Protocol README. Keypom developer documentation can be found here and Keypom Executive Summary here.
|
NEAR & Gaming: Putting Gamers in the Driver’s Seat
COMMUNITY
August 17, 2022
Video games are portals to alternate realities. They are the means of escape for the more than three billion players worldwide who value the journey and experience of gameplay. Unfortunately, the journey through imaginative landscapes has been threatened by the prevalence of microtransactions and in-game currencies offering less and less to players.
As industry-wide revenues are forecasted to surpass $200B in 2022, many gamers are looking for a more prominent voice in where and how they invest their time and money in virtual worlds. Blockchain and Web3 have thrown gas on the fire of gaming innovation with their underlying technology and the ease with which people can interact with each other on a global scale.
Never before has there been as wide an array of platforms, developers, publishers, and gamers as exists now. Blockchains like NEAR have generated mass appeal in crypto gaming, boosted by incentives in play-to-earn mechanics. Players can now monetize their time and attention to find, craft, and seamlessly generate NFT assets of in-game digital items while establishing connections along the way. Self-governing inclusive communities in the form of decentralized autonomous organizations, or DAOs, serve as a foundation for gamers empowered to take on leadership roles.
From arcade to massively multiplayer to casual mobile gaming, NEAR is powering the next generation of games through blockchain technologies like NFTs, DeFi, and DAOs, unlocking substantial rewards for players’ time invested. A radical transformation in gameplay, the Web3 approach gives power and control to the players. Beyond helming the controls, significant players in the gaming industry are truly putting gamers in the driver’s seat.
Building the future of gaming, one block at a time
OPGames, a Web3 arcade gaming platform, fuses the worlds of gaming and NFTs with a vision of revolutionizing game development and publishing. Their HTML5 arcade is a profound transformation in gameplay and development that shifts power to the players. OPGames’ developers utilize fractionalized NFTs to grant the community the ability to own a piece of the games they play.
Arcadia, OPGames’ community-owned gaming universe, invites players to step into virtual worlds by discovering games, joining tournaments, and experiencing limitless gaming in entirely new ways. Their end-to-end solution promotes game discovery and seamless distribution in a novel way.
Through collective ownership, gamers’ time and attention are effectively monetized through NFT price appreciation as any game grows in success. The OPGames DAO establishes a relationship between players and developers, with real dialogue about how the game evolves over time. Together, these facets combine to make the perfect toolbox for game developers to jump from Web2 to Web3.
The OPGames DAO gives the community a direct line of communication with game developers. This opens up dialogue on upcoming enhancements, new levels, or the latest in-game assets that players can buy, own, and trade through gameplay. Most gaming platforms lockdown digital collectibles in their ecosystem, but OPGames users are given the freedom to bring accomplishments and skins from other games. This model affords players more meaningful rewards for the time they invest in virtual worlds.
Arcadia is an inclusive gaming universe that lowers the barriers of game ownership and truly serves as a platform for everyone. Gamers, game creators, and communities alike can share fun experiences through an ever-expanding roster of games with an ecosystem feature set that continues to evolve.
A GameFi ecosystem for the players, by the players
Vorto Gaming is a prominent blockchain gaming company with a mission of creating a new gaming economy owned by the players. Vorto Network’s marketplace allows users to browse, buy, sell, and trade NFTs and other in-game assets. Their “the more you play, the more you earn” model empowers players to monetize their time and attention to find, craft, and generate NFT assets of in-game digital items.
Hash Rush, the first title launched on the Vorto Network, is a massively multiplayer real-time strategy game featuring play-and-earn mechanics. By establishing Crypto Crystal mining colonies, players play-to-earn as they mine, complete quests, and defeat enemies. Through tokenization and P2P trading, the gaming industry’s status quo model is flipped. “Instead of players working for the game, the game should work for them,” says CEO Kris Vavoids.
In early 2022, Vorto revealed an exclusive partnership with Gold Town Games in developing a blockchain cricket game, Cricket Star Manager. Inspired by sports simulation titles and play-to-earn games, Cricket Star Manager lets players create their own cricket team and compete for the highest title in their country. For the first time, the cricket community has an opportunity to earn real money from their passion through gaming.
Vorto has created a platform that allows gamers to easily manage their digital game items, whether collecting, selling, or trading. Their APIs allow game studios access to item creation, inventory management, and transaction reporting, while gamers benefit from an online storefront with a peer-to-peer marketplace. Not only does this bring an unprecedented level of autonomy to gamers, but it also puts them in control of their achievements and virtual legacy on and off the platform.
Bringing Web3 fun to Web2 games
playEmber is bringing Web3 to the hyper-casual and casual gaming space, with ambitions to onboard at least one million new crypto gamers. The first wave of play-to-earn games were lousy offerings, or as CEO Hugo Furneaux bluntly says, “a bit crap.”
“Rather than fun games, they seemed to be reverse engineered gamified staking platforms, giving the player a sub-optimal gaming experience,” he adds.
playEmber’s player-centric focus is rooted in three tenants: fun, interoperability, and ownership. They recognize that if the main target is to earn, the core of gaming’s appeal–escapism through joy and pure fun–is lost.
“We think that is key to putting gamers back in the driving seat,” says Furneaux. “It shouldn’t be a job, it should be a passion.”
By bringing blockchain gaming to existing mobile gamers, their everyday experience is enhanced by enabling the interoperability of game assets. Players have power by playing multiple games with the same avatar and storing and trading their game achievements and progress. For gaming studios, a new audience of Web3 gamers is unlocked without the complexities of worrying about core elements like smart contracts, tokens, and NFTs.
Players make substantial investments in virtual worlds with their time and resources. As such, gamers should retain ownership of the characters, weapons, avatars, and NFTs they’ve invested and improved upon. For playEmber, this is non-negotiable. Their OG NFT collection partners with many of the top NEAR collections that allow followers to play, earn, reward, and compete in thousands of mobile games.
CMO Jon Hook says that a key focus is an ongoing commitment to greater inclusivity and governance within the greater community. “We are looking forward to welcoming key strategic studio partners into our DAO to inform and decide future product developments,” he says.
Having a seat at the table is a powerful and exciting way to empower players to control their future.
Leveling up Web3 gaming on NEAR
Thanks to NEAR’s robust framework and infrastructure, gaming in the Web3 era is limited only by the collective imaginations of developers and players. NEAR’s robust, stable, and feature-rich blockchain also achieves a level of energy efficiency and impact unheard of in other blockchains. Its scaling technology divides computation across parallel “shards,” which optimize the already super cheap, high-speed, and outstandingly secure platform. So with NEAR, developers now have the toolkit to level up worldbuilding and gameplay experiences.
Extremely versatile, DAOs are one component of NEAR’s commitment to facilitating the network’s decentralization to a worldwide creative community and beyond. Through their democratic, flexible, and collaborative foundations, DAOs empower like-minded individuals to come together and decide how the gameplay journey will unfold.
Building the next hit Web3 game on NEAR is possible through supporting documentation and open source repositories available to all. Any developer can build on existing concepts and choose different elements to get their next idea from concept to fruition. And a low barrier to entry for developers, creators, and players translates to massive exposure and accessibility to the global gaming community.
As gamers are put in the driver’s seat in the era of Web3, the industry’s development and evolution know no limits on the road ahead.
|
---
sidebar_position: 5
sidebar_label: "Add simple frontend"
title: "Add a simple frontend to the crossword puzzle that checks the solution's hash"
---
import {Github} from "@site/src/components/codetabs"
import nearReactFriends from '/docs/assets/crosswords/near-and-react--dakila.near--rodolf_dtbbx.png';
# Add a simple frontend
This will be the final section in this chapter, where we'll add a simple frontend using React and [`near-api-js`](https://docs.near.org/tools/near-api-js/quick-reference) to communicate with the smart contract.
<figure>
<img src={nearReactFriends} alt="Two characters hanging out, NEAR and React. Art created by dakila.near" width="600"/>
<figcaption>Dynamic duo of NEAR as the backend and React as a frontend.<br/>Art by <a href="https://twitter.com/rodolf_dtbbx" target="_blank">dakila.near</a></figcaption>
</figure>
<br/>
There will be three main files we'll be working with:
1. `src/index.js` will be the entry point, where NEAR network configuration will be set up, and the view-only call to `get_solution` will happen.
2. `src/App.js` is then called and sets up the crossword table and checks to see if a solution has been found.
3. `src/utils.js` is used to make a view-only call to the blockchain to get the solution, and other helper functions.
## Entry point
We'll go over a pattern that may look familiar to folks who have surveyed the [NEAR examples site](https://github.com/near-examples). We'll start with an asynchronous JavaScript function that sets up desired logic, then pass that to the React app.
<Github language="js" start="3" end="22" url="https://github.com/near-examples/crossword-tutorial-chapter-1/blob/3e497b4815600b8382614f76c7812520710f704d/src/index.js" />
Let's talk through the code above, starting with the imports.
We import from:
- `config.js` which, at the moment, is a common pattern. This file contains details on the different networks. (Which RPC endpoint to hit, which NEAR Wallet site to redirect to, which NEAR Explorer as well…)
- `utils.js` for that view-only function call that will call `get_solution` to retrieve the correct solution hash when a person has completed the crossword puzzle correctly.
- `hardcoded-data.js` is a file containing info on the crossword puzzle clues. This chapter has covered the crossword puzzle where the solution is **near nomicon ref finance**, and according to the chapter overview we've committed to serving *one* puzzle. We'll improve our smart contract later, allowing for multiple crossword puzzles, but for now it's hardcoded here.
Next, we define an asynchronous function called `initCrossword` that will be called before passing data to the React app. It's often useful to set up a connection with the blockchain here, but in our case all we need to do is retrieve the crossword puzzle solution as a hash. Note that we're attempting to pass this environment variable `NEAR_ENV` into our configuration file. `NEAR_ENV` is used to designate the blockchain network (testnet, betanet, mainnet) and is also [used in NEAR CLI](https://docs.near.org/develop/deploy).
Lastly, we'll call `initCrossword` and, when everything is complete, pass data to the React app contained in `App.js`.
## React app
Here's a large portion of the `App.js` file, which will make use of a fork of a React crossword library by Jared Reisinger.
<Github language="js" start="3" end="54" url="https://github.com/near-examples/crossword-tutorial-chapter-1/blob/3e497b4815600b8382614f76c7812520710f704d/src/App.js" />
We'll discuss a few key points in the code above, but seeing as we're here to focus on a frontend connection to the blockchain, will brush over other parts that are library-specific.
The two imports worth highlighting are:
- `parseSolutionSeedPhrase` from the utility file we'll cover shortly. This will take the solution entered by the user and put it in the correct order according to the rules discussed in [the chapter overview](00-overview.md#how-it-works).
- `sha256` will take the ordered solution from above and hash it. Then we'll compare that hash with the one retrieved from the smart contract.
```js
const [solutionFound, setSolutionFound] = useState(false);
```
We're using [React Hooks](https://reactjs.org/docs/hooks-state.html) here, setting up the variable `solutionFound` that will be changed when the player of the crossword puzzle enters the final letter of the crossword puzzle, having entries for all the letters on the board.
The `onCrosswordComplete` and `checkSolution` blocks of code fire events to check the final solution entered by the user, hash it, and compare it to the `solutionHash` that was passed in from the view-only call in `index.js` earlier.
Finally, we return the [JSX](https://reactjs.org/docs/introducing-jsx.html) for our app and render the crossword puzzle! In this basic case we'll change this heading to indicate when the user has completed the puzzle successfully:
```html
<h3>Status: { solutionFound }</h3>
```
## Utility functions
We'll be using two utility functions here:
- `parseSolutionSeedPhrase` which will take a completed crossword puzzle and place the answers in the proper order. (Ascending by number, across answers come before down ones.)
- `viewMethodOnContract` makes the view-only call to the smart contract to retrieve the solution hash.
We'll only focus on the second method:
<Github language="js" start="8" end="12" url="https://github.com/near-examples/crossword-tutorial-chapter-1/blob/3e497b4815600b8382614f76c7812520710f704d/src/utils.js" />
This API doesn't look warm and friendly yet. You caught us! We'd love some help to improve our API as [detailed in this issue](https://github.com/near/near-api-js/issues/612), but for now, this is a concise way to get data from a view-only method.
We haven't had the frontend call a mutable method for our project yet. We'll get into that in the coming chapters when we'll want to have a prize sent to the first person to solve the puzzle.
## Run the React app
Let's run our frontend on testnet! We won't add any new concepts at this point in the chapter, but note that the [near examples](https://github.com/near-examples) typically create an account for you automatically with a NodeJS command. We covered the important pattern of creating a subaccount and deploying the smart contract to it, so let's stick with that pattern as we start up our frontend.
```bash
# Go into the directory containing the Rust smart contract we've been working on
cd contract
# Build (for Windows it's build.bat)
./build.sh
# Create fresh account if you wish, which is good practice
near delete crossword.friend.testnet friend.testnet
near create-account crossword.friend.testnet --masterAccount friend.testnet
# Deploy
near deploy crossword.friend.testnet --wasmFile res/my_crossword.wasm \
--initFunction 'new' \
--initArgs '{"solution": "69c2feb084439956193f4c21936025f14a5a5a78979d67ae34762e18a7206a0f"}'
# Return to the project root and start the React app
cd ..
env CONTRACT_NAME=crossword.friend.testnet npm run start
```
The last line sends the environment variable `CONTRACT_NAME` into the NodeJS script. This is picked up in the `config.js` file that's used to set up the contract account and network configuration:
<Github language="js" start="1" end="2" url="https://github.com/near-examples/crossword-tutorial-chapter-1/blob/3e497b4815600b8382614f76c7812520710f704d/src/config.js" />
After running the last command to start the React app, you'll be given a link to a local website, like `https://localhost:1234`. When you visit the site you'll see the simple frontend that interacts with our smart contract:

Again, the full code for this chapter is [available here](https://github.com/near-examples/crossword-tutorial-chapter-1).
|
---
sidebar_position: 2
---
# Callbacks
NEAR Protocol is a sharded, proof-of-stake blockchain that behaves differently than proof-of-work blockchains. When interacting with a native Rust (compiled to Wasm) smart contract, cross-contract calls are asynchronous. Callbacks are used to either get the result of a cross-contract call or tell if a cross-contract call has succeeded or failed.
There are two techniques to write cross-contract calls: [high-level](https://github.com/near/near-sdk-rs/blob/master/examples/cross-contract-calls/high-level/src/lib.rs) and [low-level](https://github.com/near/near-sdk-rs/blob/master/examples/cross-contract-calls/low-level/src/lib.rs). This document will mostly focus on the high-level approach. There are two examples in the Rust SDK repository that demonstrate these, as linked above. Note that these examples use cross-contract calls "to itself." We'll show two examples demonstrating the high-level approach.
## Calculator example
There is a helper macro that allows you to make cross-contract calls with the syntax `#[ext_contract(...)]`. It takes a Rust Trait and converts it to a module with static methods. Each of these static methods takes positional arguments defined by the Trait, then the `receiver_id`, the attached deposit and the amount of gas and returns a new `Promise`.
:::info
If the function returns the promise, then it will delegate the return value and status of transaction execution, but if you return a unit type (`()`, `void`, `nothing`), then the `Promise` result will not influence the transaction status.
:::
For example, let's define a calculator contract Trait:
```rust
#[ext_contract(ext_calculator)]
trait Calculator {
fn mult(&self, a: U64, b: U64) -> U128;
fn sum(&self, a: U128, b: U128) -> U128;
}
```
It's equivalent to the following code:
```rust
mod ext_calculator {
pub fn mult(a: U64, b: U64, receiver_id: &AccountId, deposit: Balance, gas: Gas) -> Promise {
Promise::new(receiver_id.clone())
.function_call(
b"mult",
json!({ "a": a, "b": b }).to_string().as_bytes(),
deposit,
gas,
)
}
pub fn sum(a: U128, b: U128, receiver_id: &AccountId, deposit: Balance, gas: Gas) -> Promise {
// ...
}
}
```
Let's assume the calculator is deployed on `calc.near`, we can use the following:
```rust
#[near]
impl Contract {
pub fn sum_a_b(&mut self, a: U128, b: U128) -> Promise {
let calculator_account_id: AccountId = "calc.near".parse().unwrap();
// Call the method `sum` on the calculator contract.
// Any unused GAS will be attached since the default GAS weight is 1.
// Attached deposit is defaulted to 0.
ext_calculator::ext(calculator_account_id)
.sum(a, b)
}
}
```
## Allowlist example
Next we'll look at a simple cross-contract call that is made to an allowlist smart contract, returning whether an account is in the list or not.
The common pattern with cross-contract calls is to call a method on an external smart contract, use `.then` syntax to specify a callback, and then retrieve the result or status of the promise. The callback will typically live inside the same, calling smart contract. There's a special macro used for the callback function, which is [#[private]](https://docs.rs/near-sdk-core/latest/near_sdk_core/struct.AttrSigInfo.html#structfield.is_private). We'll see this pattern in the example below.
The following example demonstrates two common approaches to callbacks using the high-level cross-contract approach. When writing high-level cross-contract calls, special [traits](https://doc.rust-lang.org/rust-by-example/trait.html) are set up as interfaces for the smart contract being called.
```rust
#[ext_contract(ext_allowlist)]
pub trait ExtAllowlist {
fn is_allowlisted(staking_pool_account_id: AccountId) -> bool;
}
```
After creating the trait, we'll show two simple functions that will make a cross-contract call to an allowlist smart contract, asking if the account `mike.testnet` is allowlisted. These methods will both return `true` using different approaches. First we'll look at the methods, then we'll look at the differences in callbacks. Note that for simplicity in this example, the values are hardcoded.
```rust
pub const XCC_GAS: Gas = Gas(20_000_000_000_000);
fn get_allowlist_contract() -> AccountId {
"allowlist.demo.testnet".parse().unwrap()
}
fn get_account_to_check() -> AccountId {
"mike.testnet".parse().unwrap()
}
```
```rust
#[near]
impl Contract {
pub fn xcc_use_promise_result() -> Promise {
// Call the method `is_allowlisted` on the allowlisted contract. Static GAS is only attached to the callback.
// Any unused GAS will be split between the function call and the callback since both have a default unused GAS weight of 1
// Attached deposit is defaulted to 0 for both the function call and the callback.
ext_allowlist::ext(get_allowlist_contract())
.is_allowlisted(get_account_to_check())
.then(
Self::ext(env::current_account_id())
.with_static_gas(XCC_GAS)
.callback_promise_result()
)
}
pub fn xcc_use_arg_macro(&mut self) -> Promise {
// Call the method `is_allowlisted` on the allowlisted contract. Attach static GAS equal to XCC_GAS only for the callback.
// Any unused GAS will be split between the function call and the callback since both have a default unused GAS weight of 1
// Attached deposit is defaulted to 0 for both the function call and the callback.
ext_allowlist::ext(get_allowlist_contract())
.is_allowlisted(get_account_to_check())
.then(
Self::ext(env::current_account_id())
.with_static_gas(XCC_GAS)
.callback_arg_macro()
)
}
```
The syntax begins with `ext_allowlist::ext()` showing that we're using the trait to call the method on the account passed into `ext()`. We then use `with_static_gas()` to specify a base amount of GAS to attach to the call. We then call the method `is_allow_listed()` and pass in the parameters we'd like to attach.
There are a couple things to note when doing these function calls:
1. You can attach a deposit of Ⓝ, in yoctoⓃ to the call by specifying the `.with_attached_deposit()` method but it is defaulted to 0 (1 Ⓝ = 1000000000000000000000000 yoctoⓃ, or 1^24 yoctoⓃ).
2. You can attach a static amount of GAS by specifying the `.with_static_gas()` method but it is defaulted to 0.
3. You can attach an unused GAS weight by specifying the `.with_unused_gas_weight()` method but it is defaulted to 1. The unused GAS will be split amongst all the functions in the current execution depending on their weights. If there is only 1 function, any weight above 1 will result in all the unused GAS being attached to that function. If you specify a weight of 0, however, the unused GAS will **not** be attached to that function. If you have two functions, one with a weight of 3, and one with a weight of 1, the first function will get `3/4` of the unused GAS and the other function will get `1/4` of the unused GAS.
The two methods in the snippet above are very similar, except they will call separate callbacks in the smart contract, `callback_promise_result` and `callback_arg_macro`. These two callbacks show how a value can be obtained.
```rust
#[private]
pub fn callback_arg_macro(#[callback_unwrap] val: bool) -> bool {
val
}
#[private]
pub fn callback_promise_result() -> bool {
assert_eq!(env::promise_results_count(), 1, "ERR_TOO_MANY_RESULTS");
match env::promise_result(0) {
PromiseResult::NotReady => unreachable!(),
PromiseResult::Successful(val) => {
if let Ok(is_allowlisted) = near_sdk::serde_json::from_slice::<bool>(&val) {
is_allowlisted
} else {
env::panic_str("ERR_WRONG_VAL_RECEIVED")
}
},
PromiseResult::Failed => env::panic_str("ERR_CALL_FAILED"),
}
}
```
The first method uses a macro on the argument to cast the value into what's desired. In this approach, if the value is unable to be casted, it will panic. If you'd like to gracefully handle the error, you can either use the first approach, or use the `#[callback_result]` macro instead. An example of this can be seen below.
```rust
#[private]
pub fn handle_callbacks(
// New pattern, will gracefully handle failed callback results
#[callback_result] b: Result<u8, near_sdk::PromiseError>,
) {
if b.is_err() {
// ...
}
}
```
The second method gets the value from the promise result and is essentially the expanded version of the `#[callback_result]` macro.
And that's it! Understanding how to make a cross-contract call and receive a result is an important part of developing smart contracts on NEAR. Two interesting references for using cross-contract calls can be found in the [fungible token](https://github.com/near-examples/FT) and [non-fungible token](https://github.com/near-examples/NFT) examples.
|
NEAR FastAuth Competition Terms and Conditions
NEAR FOUNDATION
May 31, 2023
Introduction:
The following terms and conditions (the “Terms”) govern the use of the NEAR Foundation Fast Auth Competition (the “Competition”) provided by NEAR Foundation (“we,” “us,” “our” or the “Company”). By participating in the Competition, you (“you” or “Participant”) agree to be bound by these Terms. If you do not agree to these Terms, you may not be eligible to participate in the Competition or receive any rewards. For more information on FastAuth, please visit the link here.
Eligibility:
The Competition is available only to individuals who are at least 18 years old. By participating in the Competition, you represent and warrant that you are at least 18 years old.
Participation in the Competition:
We are excited to provide NEAR users with the ability to win, you acknowledge and agree that any information you provide may be used by us in connection with the Competition. To participate, users must follow these steps:
Participants must submit a video of their screen while opening a NEAR wallet using FastAuth. The video should be in the form of a screen recording. All videos must be legible and any sensitive information can be blacked out.
The Participant must submit the video to their personal Twitter account using the hashtags #FastAuthTimeTrial and #NEARistheBOS by June 9th, 13:00 UTC.
Videos submitted after the deadline will not be accepted.
The time taken to open the wallet, will be taken into consideration.
Winners will be notified via Twitter and announced on the Near social media channels on June 12th.
Competition Prize:
Five Selected winners will be announced and contacted via email. The Competition prize consists of: 100 NEAR tokens for each winner.
5. Disclaimer:
The Prize is awarded ‘as is’ and without warranty of any kind, express or implied, including without limitation any warranty of merchantability or fitness for a particular purpose. The Competition organizers and affiliated companies make no representations or warranties regarding the prize or its use and are not responsible for any claims arising out of or in connection with the prize or its use. The Competition event and prizes are solely sponsored and organized by us, and any reference to third-party companies or organizations is for informational purposes only.
Limitation of Liability:
The Competition organizers, sponsors, and affiliated companies are not responsible for any injury, loss, or damage to property or person, including death, arising out of participation in the Competition or use or misuse of the prize.
Intellectual Property:
The Service, including all content provided by the expert(s) or other participants, is protected by copyright and other intellectual property laws. You may not reproduce, distribute, or create derivative works from any content provided by the Service without our prior written consent.
Modification of Terms:
We reserve the right to modify these Terms at any time without notice. Your continued use of the Service following any changes to these Terms constitutes your acceptance of the revised Terms.
Governing Law:
These Terms and your use of the Service are governed by the laws of Switzerland without regard to its conflict of laws provisions.
Dispute Resolution:
Any disputes arising out of or in connection with these Terms or the Service will be resolved through binding arbitration in accordance with the rules of the Switzerland Arbitration Association.
Entire Agreement:
These Terms constitute the entire agreement between you and us with respect to the Competition and supersede all prior or contemporaneous agreements and understandings, whether written or oral.
Contact Information:
If you have any questions or comments about these Terms, please contact us at [email protected].
|
---
id: blog
title: Onchain Blog
---
# Blogchain
[](https://blogchain.mintbase.xyz/)
[](https://vercel.com/new/clone?repository-url=https%3A%2F%2Fgithub.com%2FMintbase%2Ftemplates%2Ftree%2Fmain%2Fblogchain)
Blogchain makes your content unstoppable. Transform your blogs into smart contracts and posts into NFTs.

:::tip Mintbase Templates
This is part of the [Mintbase Templates](https://templates.mintbase.xyz/), a collection of templates that you can use to scaffold your own project
:::
---
## Project Walkthrough
Within the framework of blogchain, every blog manifests as an nft contract deployed from the Mintbase contract factory, while each individual blog post is uniquely represented as a non-fungible token (NFT).
*NOTE: As a standard on Mintbase as we use the latest versions of Next.js we recommend using pnpm, but the package manager is up to your personal choice.*
---
## Run the project
```bash
# install
pnpm i
#run project
pnpm run dev
```
---
## Create a Blog (deploy contract)
### Step 1: check if the contract (blog) name already exists
Using [@mintbase-js/data](https://docs.mintbase.xyz/dev/mintbase-sdk-ref/data/api/checkstorename) checkStoreName method we can check if the store already exists.
```typescript
const { data: checkStore } = await checkStoreName(
data.name,
NEAR_NETWORKS.TESTNET
);
if (checkStore?.nft_contracts.length === 0) {
(...)
}
```
<hr class="subsection" />
### Step 2: if contract name doesn't exist execute the deploy contract action with the instantiated wallet
Create deploy contract args using [mintbase-js/sdk](https://docs.mintbase.xyz/dev/mintbase-sdk-ref/sdk/deploycontract) deployContract method. This will deploy an NFT contract from the [mintbase contract factory](https://github.com/Mintbase/mb-contracts/tree/main/mb-factory-v1)
```typescript
const wallet = await selector.wallet();
const deployArgs = deployContract({
name: data.name,
ownerId: activeAccountId,
factoryContractId: MINTBASE_CONTRACTS.testnet,
metadata: {
symbol: "",
},
});
```
We can then execute the deploy contract by passing in the wallet. If you wan't to learn about wallet connection check out the [wallet starter template](https://templates.mintbase.xyz/templates/starter-next)
```typescript
await execute({ wallet }, deployArgs);
```
---
## Create a Blog Post (mint an NFT)
### Step 1: call storage method to upload file inserted by the user to arweave
Using [@mintbase-js/storage](https://docs.mintbase.xyz/dev/mintbase-sdk-ref/storage#uploadreference-referenceobject-referenceobject-arweaveresponse) uploadReference method we upload the nft image to arweave.
```typescript
const metadata = {
title: data.title,
media: data.media,
};
const referenceJson = await uploadReference(metadata);
const reference = referenceJson.id;
```
<hr class="subsection" />
### Step 2: mint the nft in the contract (blog)
Create mint args using [mintbase-js/sdk](https://docs.mintbase.xyz/dev/mintbase-sdk-ref/sdk/mint) mint method.
```typescript
const wallet = await selector.wallet();
const mintCall = mint({
noMedia: true,
metadata: {
reference: reference,
title: data.title,
description: postContent,
extra: "blogpost",
},
contractAddress: data.contract,
ownerId: activeAccountId,
});
```
We can then execute the mint nft method
```typescript
await execute({ wallet }, mintCall);
```
:::note
We populate the 'extra' field with the value 'blogpost' to subsequently filter the displayed NFTs and blogs in blogchain, ensuring that only blogs are included.
:::
---
## Get Data
### Get blog posts (nfts) from a blog (smart contract)
Using [Mintbase GraphQL Indexer](https://docs.mintbase.xyz/dev/mintbase-graph) we can fetch the nfts from a specific smart contract - to filter by blog post we use 'blogpost' as an extra field as explained in the previous step.
```typescript
export const GET_BLOG_POSTS =
`
query GET_BLOG_POSTS($contractId: String!) {
mb_views_nft_tokens(
where: {extra: {_eq: "blogpost"}, _and: {nft_contract_id: {_eq: $contractId}}}
) {
metadata_id
title
description
media
minted_timestamp
}
}
`;
```
<hr class="subsection" />
### Get user blog posts (nfts)
```typescript
export const GET_USER_POSTS =
`
query GET_USER_POSTS($accountId: String!) {
mb_views_nft_tokens(
where: {extra: {_eq: "blogpost"}, _and: {nft_contract_owner_id: {_eq: $accountId}}}
) {
metadata_id
title
description
media
minted_timestamp
}
}
`;
```
<hr class="subsection" />
### Get user blogs (smart contracts)
```typescript
export const GET_USER_BLOGS =
`
query GET_USER_BLOGS($accountId: String!) {
nft_contracts(where: {owner_id: {_eq: $accountId}}) {
id
}
}
`;
```
<hr class="subsection" />
### Get latest blogs (smart contracts)
```typescript
export const GET_LATEST_UPDATED_BLOGS =
`
query GET_LATEST_UPDATED_BLOGS {
mb_views_nft_metadata(
where: {extra: {_eq: "blogpost"}}
distinct_on: [nft_contract_id, nft_contract_created_at]
limit: 6
order_by: [{nft_contract_created_at: desc}, {nft_contract_id: desc}]
) {
nft_contract_id
nft_contract_owner_id
}
}
`;
```
<hr class="subsection" />
### Get latest blog posts (nfts)
```typescript
export const GET_LATEST_POSTS =
`
query GET_LATEST_POSTS {
mb_views_nft_tokens(
where: {extra: {_eq: "blogpost"}}
limit: 10
order_by: {minted_timestamp: desc}
) {
metadata_id
title
description
media
minted_timestamp
minter
nft_contract_id
}
}`;
```
<hr class="subsection" />
### Get blog post (nft) data
```typescript
export const GET_POST_METADATA =
`
query GET_POST_METADATA($metadataId: String!) {
mb_views_nft_tokens(where: {metadata_id: {_eq: $metadataId}}) {
metadata_id
title
description
media
minted_timestamp
minter
nft_contract_id
}
}`;
```
Presently, this template exclusively functions within the testnet environment. To transition to a different network the configuration must be changed in ```<MintbaseWalletContextProvider>``` and every 'testnet' instance.
---
## Extending
This project is setup using Next.js + @mintbase/js
You can use this project as a reference to build your own, and use or remove any library you think it would suit your needs.
:::info Get in touch
You can get in touch with the mintbase team using the following channels:
- Support: [Join the Telegram](https://tg.me/mintdev)
- Twitter: [@mintbase](https://twitter.com/mintbase)
::: |
```js
const keypomContract = "v2.keypom.near";
const dropAmount = "10000000000000000000000";
Near.call([
{
contractName: keypomContract,
methodName: "create_drop",
args: {
public_keys: state.publicKeys,
deposit_per_use: dropAmount,
},
deposit: "23000000000000000000000", // state.publicKeys.length * dropAmount + 3000000000000000000000,
gas: "100000000000000",
},
]);
```
|
---
sidebar_position: 2
sidebar_label: "Solution as seed phrase"
title: "Replacing the solution hash with an access key"
---
import puzzleFrontrun from '/docs/assets/crosswords/puzzle-frontrun.png';
import padlockSafe from '/docs/assets/crosswords/safe-with-access-key--soulless.near--ZeroSerotonin__.png';
# Replacing our solution hash
So far in this tutorial, the user sends the plaintext solution to the crossword puzzle smart contract, where it's hashed and compared with the known answer.
This works, but we might want to be more careful and avoid sending the plaintext solution.
## Why?
Blockchains rely on many computers processing transactions. When you send a transaction to the blockchain, it doesn't immediately get processed. In some Layer 1 blockchains it can take minutes or longer. On NEAR transactions settle within a couple seconds, but nonetheless there's a small period of waiting time.
When we previously sent the crossword puzzle solution in plain text (via the parameter `solution` to `submit_solution`) it means it's visible to everyone before it gets processed.
At the time of this writing, there haven't been outstanding incidents of validators "front-running" transactions, but it's something to be aware of. Front-running is when a validator sees a transaction that might be profitable and does it themselves.
There have been several incidents of this and it continues to be an issue.
<figure>
<img src={puzzleFrontrun} alt="Tweet talking about a puzzle where tens of thousands of dollars were taken because of a frontrun attack" width="600"/>
<figcaption>Real-life example of a puzzle being front-run.<br/>Read <a href="https://twitter.com/_anishagnihotri/status/1444113372715356162" target="_blank">Anish Agnihotri's thread</a></figcaption>
</figure>
<br/>
## How?
We're doing to do something unique — and frankly unusual — with our crossword puzzle. We're going to use function-call access keys in a new way.
Our crossword puzzle smart contract will add a function-call access key to itself. The private key is derived from the solution, used as a seed phrase.
:::info What's a seed phrase, again?
A private key is essentially a very large number. So large that the number of possible private keys is approaching the estimated number of atoms in the known universe.
It would be pretty long if we wrote it down, so it's often made human-readable with numbers and letters. However, even the human-readable version is hard to memorize and prone to mistakes.
A seed phrase is a series of words (usually 12 or 24 words) that create a private key. (There's actually a [bit more to it](https://learnmeabitcoin.com/technical/mnemonic).)
Seed phrases typically use a [BIP-30 wordlist](https://github.com/bitcoin/bips/blob/master/bip-0039/bip-0039-wordlists.md), but *they do not need to* use a wordlist or have a certain number of words. As long as the words create entropy, a crossword puzzle solution can act as a deterministic seed phrase.
:::
So when we add a new puzzle, we'll use the `AddKey` Action to add a limited, function-call access key can that *only* call the `submit_solution` method.
The first user to solve the puzzle will essentially "discover" the private key and call that method. Think of it like a safe that contains a function-call access key.
<figure>
<img src={padlockSafe} alt="A small safe with a padlock containing words to a seed phrase, and you can see through the safe, showing it holds a function-call access key. Art created by soulless.near."/>
<figcaption className="full-width">Open the safe using answers to the puzzle, revealing the function-call access key.<br/>Art by <a href="https://twitter.com/ZeroSerotonin__" target="_blank">soulless.near</a></figcaption>
</figure><br/>
Our method `submit_solution` no longer needs to hash the plaintext answer, but instead looks at the key that signed this transaction. Cool, huh!
## Onboarding
In the previous chapter we implemented login to the crossword, but this requires a person to have a NEAR account.
If the end user is discovering a key that exists on the crossword contract, they don't even need a NEAR account, right? Well, that's partly accurate, but we'll still need to send the prize in NEAR somewhere.
What if we could make the winner an account on the fly? Is that possible? Yes, and that's what we're going to do in this chapter.
|
Open sourcing NEAR client
DEVELOPERS
November 28, 2018
Today we are open sourcing the main code base of the NEAR Protocol client. You can start exploring code on GitHub right now.
It’s the first milestone in our plan to build a decentralized future. From my previous experience at Google with open sourcing projects (ScikitFlow, TensorFlow), the earlier a project opens the source code, the easier it is to build a truly open source community around it.
Our grand vision of making crypto and blockchain accessible to billions of people starts with a more intuitive UX for both end users and developers. While end-user experience is an important topic, today we’ll focus on developer experience. The tools we’re building are the first step in addressing this.
Our aim for open sourcing the code today is two-fold:
Start iterating on developer experience with real developers;
Enable collaborations with external developers who would be interested in contributing to the client development or ecosystem;
In this post, I’ll briefly describe what is included, how it fits into the bigger picture and how you can get involved.
Typescript
We are using TypeScript as the main programming language, which makes it easy for anyone with a background in JavaScript to build smart contracts. Also, this allows developers to test smart contracts with familiar JavaScript tools.
Near Studio
Building and testing ERC-20 contract in NEAR Studio
We are building a lightweight web-based IDE — NEAR Studio (github), where one can try building smart contracts now. We’ve provided an example fiddle of an ERC-20 contract that you can play with right now. Share your fiddles with us on Discord.
DevNet
Running DevNet and interacting via RPC interface
As the backend to NEAR Studio, we provide a “DevNet”, which is a stripped-down version of the NEAR node that only runs WebAssembly and State Transition without running consensus/networking. This is an analog of the Ganache project in Ethereum. See how to run and use DevNet here.
We are going to add more documentation in the next few days about how to use NEAR Studio and DevNet together to test deploying and transacting with your smart contracts.
If you are interested in the consensus layer or how the NEAR Protocol works overall, feel free to dive deeper into the code. Currently, we have just a minimal amount of architecture in place to facilitate contributions. You can also learn more about our consensus on research portal: Informal spec to TxFlow and Beacon Chain consensus spec.
Upcoming Milestones
Now that codebase is open we are going to be diligent about reporting current progress and upcoming milestones through GitHub issues and Community Updates.
We split our future development into three major milestones:
DevNet: complete developer experience for our blockchain. We are progressing on this milestone pretty well, but there is still more work to be done.
“Minimum Viable Blockchain” (MVB): a fully functioning blockchain that can provide a platform for running experiments on governance, economics and user experience. This version will only have a Beacon chain consensus.
Shard chains: adding sharding of state and processing to MVB.
Even though the release of these milestones is ordered, we are working on them in parallel.
Get Started
Developers, we encourage you to identify problems in the blockchain space you are most interested in (scalability, usability for developers or consumers, etc) and start working on that. The easiest way to start is to join our Discord or create a discussion issue on Github. Or simply find a bug in our code and fix it 🙂
PS. Note on the license. It is currently GPL v3 because of usage of Substrate components. Parity promised to switch the license to Apache v2 when they release POC v3 in a few months. Thus we will update as well to Apache v2 at that moment.
If you want to stay up to date with what we build at NEAR, use the following channels:
Twitter — https://twitter.com/nearprotocol,
Discord — https://discord.gg/kRY6AFp, we have open conversations on tech, governance and economics of blockchain on our discord.
Lastly, please subscribe to our newsletter.
Thanks to Ash Egan, Erik Trautman and Bowen Wang for comments on the draft of this post.
|
So what exactly is Vlad’s Sharding PoC doing?
DEVELOPERS
October 8, 2018
I had a chance to work with Vlad Zamfir and Aditya Asgaonkar on the continuation of Vlad’s Berlin hack this weekend.
Now I can shed some light on what his prototype was actually showcasing in Berlin, what it was not, what was built in SF, and how it all works under the hood.
Image by @ETHSanFrancisco used with their permission
The Proof of Concept (PoC) comprises an implementation of a hierarchical sharding protocol, messaging and message routing between shards, and cross-shard transactions on top of the messaging protocol that guarantees the impossibility of finalization of atomicity failures. More specifically, if a transaction touches multiple shards, it will ultimately either be finalized on all of them, or be orphaned on all of them. The PoC is not yet concerned with Proof of Stake (in particular, it doesn’t implement Casper CBC), wallet or light client.
We published and open sourced the code. Here’s the link to the last commit as of the time we presented the demo (it lacks some visualization improvements that Vlad has locally).
The core component of the PoC is a fork choice rule. It needs to be designed in such a way that for any message sent between two blocks in two shards, either both blocks are in the chosen forks in their corresponding shards, or neither is.
Vlad built the initial fork choice rule between two shards while at Berlin hackathon. The goal for the Eth SF hackathon was twofold: a) extend it to an arbitrary number of shards with arbitrary configuration and b) build messages routing, so that if one shard wants to send a message to another shard that is not its neighbor, it can send the message along the path in the shards hierarchy, the message will be properly routed, and the atomicity guarantees are still satisfied.
The stretch goal was to also design dynamic topology rebalancing to reduce traffic in the network. For example, if it is found that some shards that are a few hops away from each other send a lot of messages to each other, the topology will be adjusted so that those shards are closer to each other.
Here’s our whiteboard’s photo after Vlad’s initial explanation:
It contains some core ideas: parent-child relationship and messaging between shards (the two blockchains at the top left, and two at the middle left), the structure of a block, and how the shards hierarchy can be changed (demonstration on the right).
Let’s dive in into how the fork choice rule, message routing, and dynamic rebalancing are implemented, and what challenges they present.
Fork choice rule
Let’s for now assume a static hierarchy of the shards, and only consider two shards. Each block in each shard is defined by
The shard ID;
A link to the previous block in the shard;
The ID of the parent shard;
The IDs of the child shards;
sent log: A map that maps a neighbor shard ID to a list of messages that have been sent to the neighbor shard ID from any block in the current shard prior to or including this block;
received log: A similar map from shard ID to messages received from other shards;
source map: A map from shard ID to the last block known to the block producer in the corresponding shards, according to the fork choice rule.
The maps in (5) and (6) contain each message in the following format:
Target shard ID; Note that this shard ID is not necessarily the same as the key in the map (either sent log or received log) the entry is associated with. The key is the neighbor shard from which or to which the message is directly sent while the Target shard ID is the final destination of the message.
Target block, or base (each message has a specific block it is sent to in its target shard);
TTL — the maximum distance from the block that will ultimately include the message to the base block;
Payload
On the figure above time goes from left to right, so A1 and B1 are the genesis blocks. On all the figures in this write-up, the timeline in each shard is independent.
There’s a message in the sent log of block A2 that has B2 as its base. That, in particular, means that B2 already existed by the time A2 was published. While not shown on the figure, the message is also stored in the sent log of blocks A3 and A4, and all the future blocks that have A2 in their chain. The message was received at B3 and thus is also in the received log of all blocks that have B3 in their chain.
The shards have a hierarchy, so each shard has a parent except for one, which is the root shard. We assume on the figure above that Shard #1 is the parent of Shard #2.
Observe that there is a fork in shard #2, and so the fork choice rule would kick in to decide on what chain is the accepted chain. The fork choice rule works in the following way: if a shard doesn’t have a parent (like shard #1), then a regular GHOST rule is applied.
Otherwise, first messages that are inconsistent with the selected chain on the parent shard are filtered out, and then the GHOST is run on the messages that are left. You can find all the rules for filtering out messages in the method is_block_filtered in fork_choice.py, but some important rules are:
If in the parent shard there’s a block on the selected chain that sends a message to the child chain, any block that is further than TTL blocks in the future from the base block of the message and still doesn’t have the message in the received log is filtered out. On the figure above blocks B4’, B5’ and B6’ are all filtered out, because the message that targets B2 is not in their received logs, and they are all 2 or more blocks away from B2. After the messages are filtered out, GHOST is executed, and the chain with B5 at its tip is chosen as it is now the longer chain.
If a block on the child shard sent a message to some block that is on the selected chain in the parent shard, and such message is not received within TTL blocks on the parent chain, the block on the child chain gets filtered out.
Any block on the child shard that sends or receives messages to/from a block in the parent shard that is not on the selected chain is filtered out.
Note that blocks are always filtered out in the child shard, no matter in what direction the message that causes the filtering goes.
There are also some rules on what blocks are considered to be valid, those can be found in method is_valid in blocks.py. Most notably, a message on the parent shard cannot attempt to send a message to the child shard that would result in filtering out some blocks from which other blocks in the chain on the parent shard have received messages. Neither can two messages in a sent log target two blocks in the same child shard such that neither block is in the chain of the other.
On the figure above blocks A3’ and A4’ are not published yet. The block A2’ is not on the selected chain in Shard #1, since the chain with a tip at A3 is longer. Thus B2 that sends a message with the base block A2’ is filtered out. However, once A3’ and A4’ get published, for as long as at least one of them contains the message from B2 in their received log, B2 will reappear on the selected chain in the child shard.
From the rule (3) in the definition of the fork choice above it follows that a single message from a parent shard that has as its base a block that is not presently on the selected chain in the child chain can trigger a cascading effect of many blocks being filtered out. In the extreme case, if a message uses the genesis block as its base, all the descendant shards will get all the blocks filtered out. This is prevented by the fact that some messages are sent between the child and the parent shard, and as mentioned above it is illegal to publish a message that would result in filtering out a block from which a message was received or to which a message was sent in any block in the chain.
The fork choice rule above is at the core of atomic transactions. A transaction originates at some shard and sends a message to some other shard, and because of the rules above either a block that sends the message and a block that receives the message are finalized or no block that sends the message and no block that receives the message are finalized. Finalization of an atomicity failure is impossible.
Messages routing
Everything up to here is what Vlad built in Berlin. The EthSF project started from generalizing the setup to arbitrary shards configuration. Then we built messages routing. In the original idea, Vlad assumed that each block is extended with a routing table that contains the next hop for each destination shard, but we got convinced that it is not needed since it can be recomputed realtime from parent and children shard IDs and the source map.
For as long as the topology of shards is static, the idea is rather straightforward: every message contains a target shard ID. A block is only considered valid if, for every new message in the received log that doesn’t have the current shard as its target shard, there’s a corresponding new message in the sent log that is sent to the next hop on the way to the target shard.
Importantly, from the fact that for every message it is guaranteed that either both the source and destination blocks are finalized or neither is finalized it follows that for a multi-hop sequence of messages either all the blocks the messages go through are eventually finalized or none is finalized.
On the figure above the dotted blocks do not exist yet. At some point in the past a message from C2 was sent to shard 2 through shard 1, was received at A3’ (at which point A3’ was on the selected chain, meaning that A4 didn’t exist yet), and immediately forwarded to shard 2, where it was ultimately received at B3. Then A4 was published on shard 1 making A3’ not be on the selected chain any longer. Once this happened, the selected chain on shard 2, which had B3 as its selected tip before, now has B2 as its selected tip, since B3 receives a message from a block not on the selected chain of its shard. Similarly, C2 and C3 are not on the selected chain in shard 3 any longer, and the entire selected chain on shard 3 now consists of just the genesis block C1.
As B3’ gets published and sends a message to shard 3, it gets rerouted via shard 1. In the example above A5 routes it, and C2’ receives. At this point B3’, A5 and C2’ are all on their selected chains. If a couple more blocks get published on top of A3’, then A5 will get orphaned, and with it will B3’ and C2’, while B3, C2, and C3 will now become the selected chains. Importantly, as this happens, at any given instance of time from the perspective of any observer the following is true:
Either all C2, A3’ and B3 are in the selected chains of their shards, or none is (and thus either both hops of the message are acknowledged, or both are not);
Either all B3’, A5 and C2’ are in the selected chains of their shards, or none is.
Dynamic rebalancing
Message routing was finished rather early, and we started working on the dynamic rebalancing. There are three operations that, if all supported, enable reconfiguring from any configuration to any other configuration.
To prove it one can show that using the latter two operations it is always possible to convert any configuration into a configuration in which each shard except for one has exactly one child, then an arbitrary permutation can be applied using the first operation, and that all operations are reversible.
In this PoC we only implemented the second operation, that moves one immediate child of a shard to become a child of another immediate child of the same shard.
I do not have a formal proof in my head that dynamic rebalancing doesn’t cause any fundamental problems with the fork choice rule, and started building it having only a simple intuition that the messages that the parent shard sends to its children have the same atomicity guarantees as any other message, and thus either ultimately the reconfiguration is committed, or rolled back.
Consider a parent shard A that moves its child B to become a child of its another child C. In practice the first obstacle was that once A publishes a block that sends the rebalancing messages to B and C, it doesn’t recognize B as its immediate child any longer, but until C has received the message neither does C. Thus if a message that targets B arrives to any block on A without having a routing table, the source map with parent and child IDs is not sufficient to route the message. At the same time, rerouting such messages to shard C is safe, since it will not arrive to C (won’t appear in the received log) until the block that has the message with the instruction to accept B as a child in its received log.
We addressed the issue of not having sufficient information to route to shard C by introducing the routing table into each block, and Vlad was very happy that he called out the necessity to have the routing table early on.
The second challenge is that in the absence of rebalances the fork choice rule would start from the root shard, and traverse the shards down, using the tip and the selected chain found in the parent shard to filter out messages in the child shard. With dynamic rebalancing, the parent-child relationship is not defined globally anymore. In the current prototype, we compute the tip and the selected chain for the parent shard separately for each block. It doesn’t affect the runtime too much due to caching of the results, but would cause problems if after few reorganizations we end up in a situation in which during some time frame some shard A was the parent of B, and during some other time frame B was the parent of A. With the current implementation it would result in an infinite loop. Vlad presumably found some modification to the fork choice rule that doesn’t suffer from this problem, but he explained it to me at 3:00 am, while I was debugging some consistency violations, so I would lie if I say I understood his idea well. He almost built it though in the morning and it looked promising but didn’t finish fixing all the bugs before the demo, so we went with my infinite loop-prone fork choice rule.
From committing the first implementation of the rebalancing in the evening until almost 6 am there was a lot of debugging and pain figuring out the interaction between the fork choice rule and the rebalancing code and improving the visualization (did I mention that we had an absolutely gorgeous visualizer?), but ultimately rerouting, rebalancing and the visualizer were the core of the PoC.
How to play with it
To run the demo just invoke simulator.py with python3. The only dependencies are numpy, networkx and matplotlib, so no need to run the Dockerfile from the README.
When you run the simulation, you will see something like this:
On the left is the shard topology, and on the right are blockchains in each shard, aligned the same way as the topology on the left (so on the screenshot above the topmost blockchain is shard 0, the two below it are 1 and 2, and the bottommost one is 7).
When you launch it, shards 3 and 4 will be both children of 1, but quickly a rebalancing will happen that will move 3 to become the child of 1. For a split second, you will also see 3 being in some quantum state, that is visualizer being confused by no shard reporting 3 as their child during the time between 1 sending the message and 4 receiving it.
The thick green lines within each shard represent the selected chain.
The thick colored arrows between shards are messages being received. We configured the demo to always send messages between shards 3 and 7 so that as many hops as possible are made, you can disable it be setting RESTRICT_ROUTING to False in config.py.
The thick black arrows are messages with the rebalancing request. In this demo, they will always go from shard 1 to shards 3 and 4.
You can also significantly speed up the demo by setting VALIDITY_CHECKS_OFF to True.
Sometimes (though rather rarely) you will see the think messages lines becoming thin. That means that the blocks with the message were orphaned. For a particular message, you will always either see all its lines think or all thin. It follows from the guarantee that atomicity failure is never finalized, and is never observed from a perspective of any observer at any time. An example of thin lines is the rightmost messages on the screenshot above from shard 7 to shard 6. They are received by a block on shard 6 that is not on the selected chain.
Footnote: If you play with it, it is also worth mentioning that the visualizer has a bug that sometimes shows the message destination incorrectly. It is because the same message can be received by multiple blocks on multiple competing chains, and the visualizer will render the arrows all point at the same block, sometimes resulting a thick line pointing at an orphaned block.
What’s next for the project
If Vlad is right and the fork choice rule does work with dynamic rebalancing, it would provide a foundation to build a sharded blockchain protocol with atomic cross-shard transactions and adaptive topology that adjusts to reduce the number of hops messages traverse on average, while maintaining a reasonable number of neighbors per shard.
We will continue hacking it to support all three rotations and arbitrary rebalances, and then I personally plan to thoroughly test it to increase my own confidence that the fork choice rule works with rebalances. Once that is done, Vlad will hopefully release a formal spec, and anyone in the community will be able to implement it.
So that’s what NEAR will be building now?
While I personally plan to continue working on the PoC, NEAR sharding design won’t use the fork choice rule, messages routing or dynamic rebalancing discussed above. NEAR’s primary goal is to create almost real-time cross-shard transactions while keeping clients sufficiently light that any low-end device can run a node that operates (a part of) the network and processes (a subset of) the transactions. Despite the beauty of Vlad’s design, it doesn’t fit naturally into the architecture we built on top of.
We just started publishing posts on NEAR’s research portal. For the most recent development, read this write-up on our sharded chain consensus algorithm “TxFlow”.
To follow our progress you can use:
NEAR Twitter — https://twitter.com/nearprotocol
My Twitter — https://twitter.com/AlexSkidanov
Discord — https://discord.gg/kRY6AFp,
https://upscri.be/633436/ |
---
id: gas-station
title: Multichain Gas Station Contract
sidebar_label: Multichain Gas Station
---
The [multichain gas station smart contract](https://github.com/near/multichain-gas-station-contract) accepts payments in NEAR tokens in exchange for gas funding on non-NEAR foreign chains. Part of the NEAR Multichain effort, it works in conjunction with the [MPC recovery service](https://github.com/near/mpc-recovery) to generate on-chain signatures.
## What is it?
This smart contract is a piece of the NEAR Multichain project, which makes NEAR Protocol an effortlessly cross-chain network. This contract accepts EVM transaction request payloads and facilitates the signing, gas funding, and relaying of the signed transactions to their destination chains. It works in conjunction with a few different services, including:
- The [MPC recovery service](https://github.com/near/mpc-recovery), also called the _"MPC signer service"_, includes a network of trusted MPC signers, which hold keyshares and cooperatively sign transactions on behalf of the MPC network. It also includes an on-chain component, called the _"MPC signer contract,"_ which accepts on-chain signature requests and returns signatures computed by the MPC network.
- The [multichain relayer server](multichain-server.md) scans this smart contract for signed transaction payloads and emits them to foreign chain RPCs.
## How it works
Currently, relaying one transaction to a foreign chain requires three transactions.
Three transactions are required because of the gas restrictions imposed by the protocol. Currently (pre-NEP-516), the MPC signing function requires a _lot_ of gas, so dividing up the signing process into three parts is required to maximize the amount of gas available to each signing call.
:::info
[NEP-516 (delayed receipts / runtime triggers)](https://github.com/near/NEPs/issues/516) will reduce the required transactions to one.
:::
Transaction breakdown:
1. The first transaction is a call to the `create_transaction` function. This function accepts an EVM transaction request payload and a deposit amount (to pay for gas on the foreign chain) and returns an `id` and a `pending_transactions_count`.
2. The second transaction is a call to the `sign_next` function. This function accepts the `id` returned in step 1 and returns a signed payload. This payload is the gas funding transaction, transferring funds from a paymaster account on the foreign chain to the user's account on the foreign chain. It must be submitted to the foreign chain before the second signed payload.
3. The third transaction is another call to the `sign_next` function, identical to the one before. This function accepts an `id` and returns a signed payload. This payload is the signed user transaction.
Once this service and its supporting services are live, the multichain relayer server will be monitoring this gas station contract and relaying the signed transactions in the proper order as they become available, so it will not be strictly necessary for the users of this contract to ensure that the transactions are properly relayed, unless the user wishes to relay the transactions using their own RPC (e.g. to minimize latency).
## Variable Gas fees
There's a premium on the Gas Station in `NEAR` for what the gas will cost on the foreign chain to account for variation in both the exchange rate between transactions, settlement between chains, and to account for variation in gas costs until the transaction is confirmed.
This is the formula for calculating the gas fee:
`(gas_limit_of_user_transaction + 21000) * gas_price_of_user_transaction * near_tokens_per_foreign_token * 1.2`
:::note
- `21000` is the exact amount of gas necessary to transfer funds on `BSC`.
- `1.2` is an arbitrage fee: charge 20% more than market rate to discourage people from using the Gas Station as an arbitrage/DEX.
:::
## Settlement
Settlement is needed because the Gas Station contract is accumulating NEAR, while the [Paymaster accounts](multichain-server.md#paymaster) on foreign chains are spending native foreign chain gas tokens (`ETH`, `BNB`, `SOL`, etc).
Manual Settlement involves several steps:
1. Withdrawing the NEAR held in the gas station contract and swapping for a token that can be bridged.
This may be the native gas token of the foreign chain, another token like USDC that has wide bridge support, or NEAR.
2. Bridging the token from NEAR to the foreign chain.
- Here's an [overview of bridging related to NEAR](https://knotty-marsupial-f6d.notion.site/NEAR-Bridging-Guides-f4359bd35c794dc184b098f7ed00c4ce).
3. Sending the native gas tokens to the paymaster accounts on the foreign chains.
- A swap from the bridged token to the native gas token before sending to the paymaster accounts is necessary if the token that was bridged was not the foreign chain native gas token
## Contract Interactions
:::tip
You can review the complete smart contract source code in [this GitHub repository](https://github.com/near/multichain-gas-station-contract).
:::
### Setup and Administration
1. Initialize the contract with a call to `new`. The [owner](https://github.com/near/near-sdk-contract-tools/blob/develop/src/owner.rs) is initialized as the predecessor of this transaction. All of the following transactions must be called by the owner.
2. Refresh the MPC contract public key by calling `refresh_signer_public_key`.
3. Set up foreign chain configurations with `add_foreign_chain`.
4. Add paymasters to each foreign chain with `add_paymaster`.
### Usage
Users who wish to get transactions signed and relayed by this contract and its accompanying infrastructure should perform the following steps:
1. Construct an unsigned transaction payload for the foreign chain they wish to interact with, e.g. Ethereum.
2. Call `create_transaction` on this contract, passing in that payload and activating the `use_paymaster` toggle in the case that the user wishes to use a paymaster.
- If the user uses a paymaster, he must attach a sufficient quantity of NEAR tokens to this transaction to pay for the gas + service fee.
- This function call returns an `id` and a `pending_transactions_count`.
3. Call `sign_next`, passing in the id value obtained in the previous step. This transaction should be executed with the maximum allowable quantity of gas (i.e. 300 TGas).
- This transaction will return a signed payload, part of the sequence of transactions necessary to send the user's transaction to the foreign chain.
- Repeat `pending_transactions_count` times.
4. Relay each signed payload to the foreign chain RPC in the order they were requested.
:::tip testnet contract
If you want to try things out, this smart contract is available on `canhazgas.testnet`.
:::
## Limitations
When using the Multichain Gas relayer solution, some limitations should be consider. Here's a list of potential issues you might encounter, and suggested ways to mitigate them:
- Not enough gas for a cross-chain transaction to get included in time.
- **Solution:** overcharge for gas at the gas station and when constructing the transaction include more than the average gas price.
- Slippage violations causing the gas token or foreign chain Fungible Token to get refunded to the user's foreign chain address.
- **Solution:** encourage your users to use high slippage settings in volatile or low liquidity market conditions.
- **Solution:** if such error occurs, make the user aware of what happened and that funds were not lost.
- **Note:** in future versions the solution will support retrying transactions.
- Nonce issues if Paymaster rotation isn't done properly. This issue is a function of concurrent usage, blockchain finality time, and number of paymaster treasury accounts that the [Gas Station](gas-station.md) is rotating through.
- **Solution:** use a blockchain that has faster finality.
- **Solution:** increase the number of paymaster treasury accounts that the gas station rotates through.
|
---
id: using-iframes
title: Using IFrames
---
# Iframes
In this tutorial you'll learn [how to use](#using-iframes-on-bos-vm) the `iframe` VM tag and the [Iframe resizer](#iframe-resizer) library, so you can embed external HTML or use custom DOM elements when building NEAR components.
You can use it along the rest of approved VM tags to simplify your component development.
## Using IFrames on NEAR VM
Iframes can be used to embed external HTML or to use custom DOM
elements, for example `canvas`.
### Properties
The `iframe` tag takes the following properties: `className`, `style`, `src`,
`srcDoc`, `title`, `message`, and `onMessage`. The iframe has a sandbox property
set to `sandbox="allow-scripts"`, which only allows scripts.
:::info
`message` and `onMessage` are used to communicate with this iframe
instance.
:::
| param | description |
|-------|-------------|
| `message` | it's passed to the iframe every time the deep equal is different, or the iframe is recreated. The message is passed using `contentWindow.postMessage(message, "*")` on the iframe. |
| `onMessage(data)` | it's called when the iframe passes a message to `window.top`. Only `event.data` is passed to the `onMessage` |
### Events
The VM exposes the following `<iframe>` events:
- `onLoad()`: support for `onLoad` event without any event info
```js
<iframe onLoad={() => { console.log('iframe loaded') }}>
```
- `onResized()`: support for `onResized` [Iframe Resizer](#iframe-resizer) event with an object only containing the new `width` and `height`
```js
<iframe iframeResizer={{
onResized: ({width, height}) => { console.log('iframe resized', width, height) },
}}>
```
### Example
The following example demonstrates how you can use an iframe to call
`eval`:
```js
State.init({
text: `"b" + "a" + +"a" + "a"`,
});
const code = `
<div>Expression: <pre id="exp" /></div>
<div>Results: <pre id="res" /></div>
<script>
window.top.postMessage("loaded", "*");
window.addEventListener("message", (event) => {
const data = event.data
document.getElementById("exp").innerHTML = JSON.stringify(data);
try {
const result = eval(data.exp);
document.getElementById("res").innerHTML = result;
event.source.postMessage(result, "*");
} catch (e) {
// ignore
}
}, false);
</script>
`;
return (
<div>
<input
value={state.text || ""}
onChange={(e) => State.update({ text: e.target.value })}
/>
Iframes below
<div className="d-flex">
<iframe
className="w-50 border"
srcDoc={code}
message={{ exp: state.text || "" }}
onMessage={(res1) => State.update({ res1 })}
/>
<iframe
className="w-50 border"
srcDoc={code}
message={{ exp: (state.text || "") + " + ' banana'" }}
onMessage={(res2) => State.update({ res2 })}
/>
</div>
Result:{" "}
<pre>
res1 = {JSON.stringify(state.res1)}
res2 = {JSON.stringify(state.res2)}
</pre>
</div>
);
```
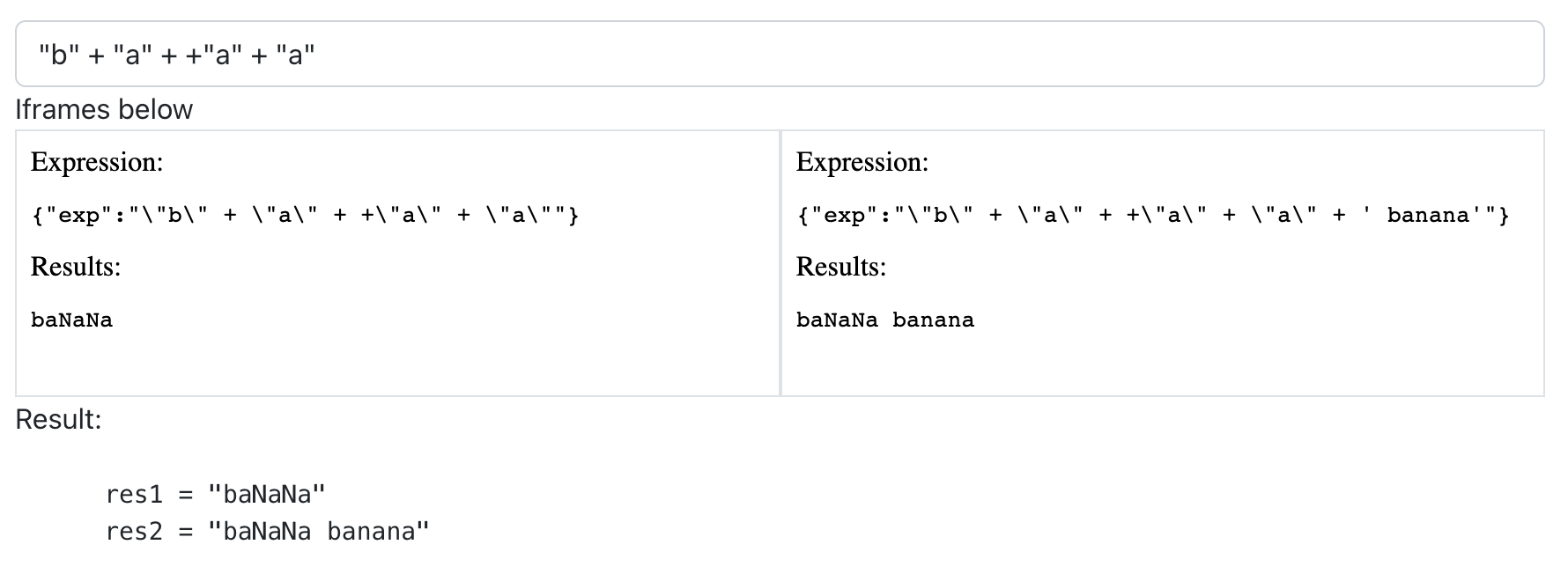
## Iframe Resizer
[Iframe Resizer](https://github.com/davidjbradshaw/iframe-resizer) is a critical library for rendering responsive iframes. This library automatically resizes the `iframe` to match the child content size to avoid scrollbars on the `iframe` itself.
:::caution don't forget
The child page rendered by the `iframe` **must include** this script in order for the resizing to work:
```html
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/iframe-resizer/4.3.6/iframeResizer.contentWindow.js"></script>
```
:::
:::note
NEAR VM uses the React flavor of [this plugin](https://github.com/davidjbradshaw/iframe-resizer-react).
:::
### Basic Example
You can use the Iframe resizer library like this:
```js
return (
<div>
<iframe
iframeResizer
src="https://davidjbradshaw.com/iframe-resizer/example/frame.content.html"
/>
</div>
);
```
If you need to pass in options to override the default behavior:
```js
return (
<div>
<iframe
iframeResizer={{ log: true }}
src="https://davidjbradshaw.com/iframe-resizer/example/frame.content.html"
/>
</div>
);
```
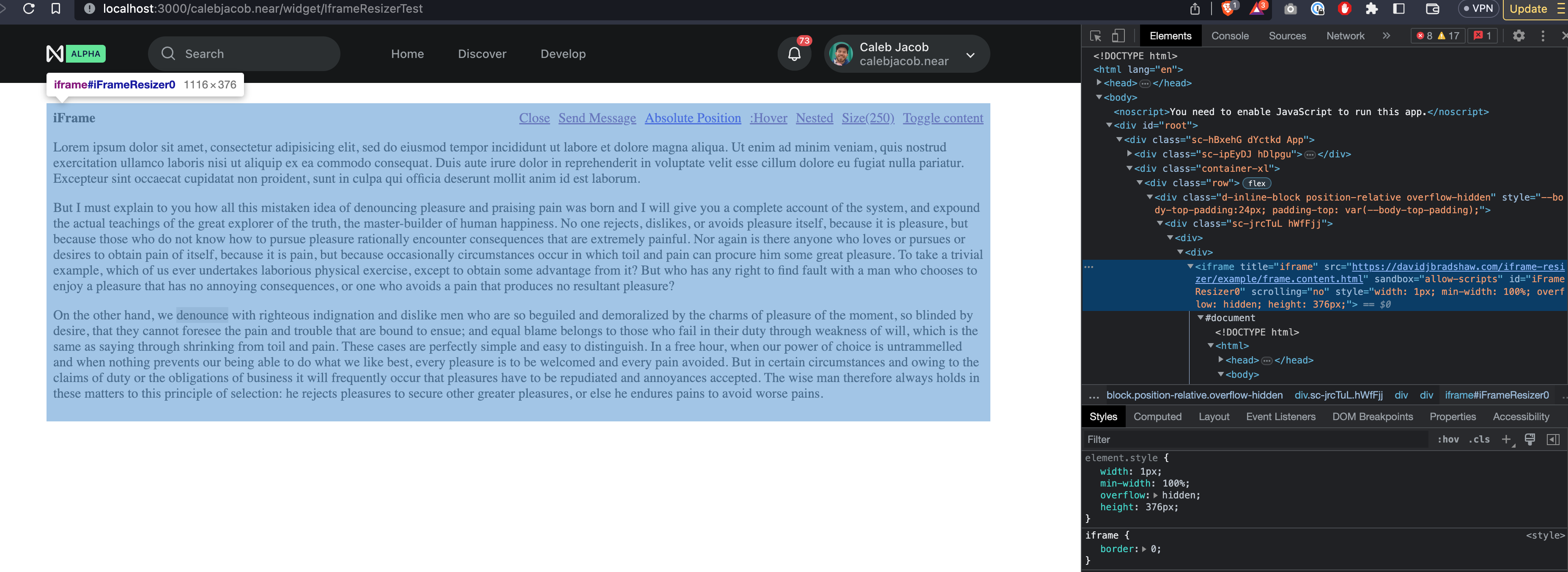
:::tip
You can check [this example](https://near.org/near/widget/ComponentDetailsPage?src=calebjacob.near/widget/IframeResizerTest) to see a complete component using Iframe Resizer.
:::
### `srcDoc` Example
An example of a valid `srcDoc` for a secure Iframe using `iframeResizer`:
```js
const code = `
<script>
// ...your code...
// define message handler
const handleMessage = (m) => {
console.log('received message', m)
document.getElementById("messageText").innerHTML = m;
};
// finally, configure iframe resizer options before importing the script
window.iFrameResizer = {
onMessage: handleMessage
}
</script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/iframe-resizer/4.3.6/iframeResizer.contentWindow.js"></script>
<p id="messageText">loading...</p>
`;
return (
<div>
<iframe
iframeResizer
className="w-100"
srcDoc={code}
message="my message"
/>
</div>
);
```
|
---
id: transaction-execution
title: Lifecycle of a Transaction
---
`Transactions` are constructed by users to express the intent of performing actions in the network. Once in the network, transactions are converted into `Receipts`, which are messages exchanged between network nodes.
On this page, we will explore the lifecycle of a transaction, from its creation to its final status.
:::tip Recommended Reading
To dig deeper into transaction routing, we recommend reading the [nearcore documentation](https://near.github.io/nearcore/architecture/how/tx_routing.html)
:::
---
## Receipts & Finality
Let's walk through the lifecycle of a complex transaction and see how it is processed by the network using blocks as **time units**.
#### Block #1: The Transaction Arrives
After a transaction arrives, the network takes one block to validate it and transform it into a single `Receipt` that contains all the [actions](./transaction-anatomy.md) to be executed.
While creating the `Receipt`, the `signer` gets $NEAR deducted from its balance to **pay for the gas** and **any attached NEAR**.
If the `signer` and `receiver` coincide - e.g. the `signer` is adding a Key - the `Receipt` is immediately processed in this first block and the transaction is considered final.
#### Block #2: The Receipt is Processed
If the `signer` and `receiver` differs - e.g. the `signer` transfers NEAR to the `receiver` - the `Receipt` is processed in a second block.
During this process a `FunctionCall` could span a **cross-contract call**, creating one or multiple new `Receipts`.
#### Block #3...: Function Calls
Each `Receipt` created from the function call take an additional block to be processed. Notice that, if those `Receipts` are `FunctionCall` they could spawn new `Receipts` and so on.
#### Final Block: Gas is Refunded
A final `Receipt` is processed in a new block, refunding any extra gas paid by the user.
:::info
A transaction is considered **final** when all its receipts are processed.
:::
:::tip
Most transactions will just spawn a receipt to process the actions, and a receipt to refund the gas, being final in 1-3 blocks (~1-3 seconds):
- [One block](https://testnet.nearblocks.io/txns/8MAvH96aMfDxPb3kVDrgj8nvJS7CAXP1GgtiivKAMGkF#execution) if the `signer` and `receiver` coincide - e.g. when adding a key
- [Three blocks](https://testnet.nearblocks.io/txns/B7gxJNxav1A9WhWvaNWYLrSTub1Mkfj3tAudoASVM5tG#) if the `signer` and `receiver` differ, since the first block creates the `Receipt`, and the last reimburse gas
Function calls might take longer, as they can spawn multiple receipts. Network congestion can also increase the time to process a receipt and, thus, a transaction.
:::
---
## Transaction Status
As the `Receipts` of a `Transaction` are processed, they get a status:
- `Success`: the actions on the receipt were executed successfully
- `Failed`: an action on the receipt failed
- `Unknown`: the receipt is not known by the network
If an action in a `Receipt` fails, all the actions in that `Receipt` are rolled back. Notice that we are talking about the `Receipt` status, and not the `Transaction` status.
The status of a transaction is determined by its first receipt, which contains all its actions. If any of the actions in the first receipt fail, the transaction is marked as failed.
Notice that, it could happen that a transaction is marked as successful, but some of its receipt fails. This happens when a `FunctionCall` successfully spawns a new receipt, but the consequent function call fails. In this case, the transaction is marked as successful because the original function call was successful.
See the examples below for more details.
<details>
<summary> Status Examples </summary>
#### Example: Transaction with Transfer
1. `bob.near` creates a transaction to transfer 10 NEAR to `alice.near`
2. The transaction is converted into a receipt
3. The conversion fails because `bob.near` does not have enough balance
4. The transaction is marked as failed ⛔
#### Example: Deploying a Contract
1. `bob.near` creates a transaction to:
- create the account `contract.bob.near`
- transfer 5 NEAR to `contract.bob.near`
- deploy a contract in `contract.bob.near`
2. The transaction is transformed into one receipt
3. The account is created, the money transfer and the contract deployed
4. The transaction is marked as successful ✅
#### Example: Deploying a Contract Fails
1. `bob.near` creates a transaction to:
- create the account `contract.bob.near`
- transfer 5 NEAR to `contract.bob.near`
- deploy a contract in `contract.bob.near`
2. The transaction is transformed into one receipt
3. The account is created, but the transfer fails because `bob.near` does not have enough balance
4. The whole process is reverted (i.e. no account is created)
5. The transaction is marked as successful ⛔
#### Example: Calling a Function
1. `bob.near` creates a transaction to call the function `cross-call` in `contract.near`
2. The transaction is transformed into one receipt
3. The function `cross-call` creates a promise to call the function `external-call` in `external.near`
4. The function finishes correctly and the transaction is marked as successful ✅
5. A new receipt is created to call the function `external-call` in `external.near`
5. The function `external-call` fails
6. The original transaction is still marked as successful ✅ because the first receipt was successful
</details>
:::tip
You can check the status of a transactions using the [NearBlocks explorer](https://nearblocks.io/)
:::
|
---
id: bos-components
title: Historical data
sidebar_label: Handling Historical data
---
Building components that handle historical blockchain data require dedicated solutions that manage the data and reduce the latency of requests, as it's not possible to scan the whole blockchain when a user makes a request.
A simple solution for developers building on NEAR is using [QueryAPI](../environment.md), a fully managed solution to build indexer functions, extract on-chain data, store it in a database, and be able to query it using GraphQL endpoints.
:::tip
Learn more about QueryAPI in this [QueryAPI Overview](../environment.md) article.
:::
---
## Tutorials
For a technical implementation deep-dive, check these QueryAPI tutorials:
- [Posts Indexer tutorial](../../../3.tutorials/near-components/indexer-tutorials/posts-indexer.md): this indexer creates a new row in a pre-defined database for every new Social post found on the blockchain.
- [Hype Indexer tutorial](../../../3.tutorials/near-components/indexer-tutorials/hype-indexer.md): this indexer creates a new row in a pre-defined database for every new Social post or comment found on the blockchain that contains either `PEPE` or `DOGE` in the contents.
- [Social Feed Indexer tutorial](../../../3.tutorials/near-components/indexer-tutorials/feed-indexer.md): this indexer keeps track of new posts, comments, and likes on Social, so a social feed can be rendered quickly.
---
## GraphQL queries
Using [QueryAPI's GraphiQL](../../6.data-infrastructure/query-api/index-function.md#mutations-in-graphql) tab, you can access the GraphiQL Explorer that provides a user friendly GraphQL playground, where you can view and create queries and mutations based on the DB schema that you defined for the indexer.

You can easily set some fields and select the returning data
that you want, and the tool will build a query on the mutation panel on the right.
Then you can copy the resulting query, either in your JavaScript code so that you pass actual
data manually, or you pass in the mutation data object as a second parameter.
For example, if you go and add a new mutation, click <kbd>+</kbd>, then you can do a bunch of actions here, such as creating, deleting, or inserting posts into your table.

If you want to test your mutation, using [Debug Mode](../../6.data-infrastructure/query-api/index-function.md#local-debug-mode) you can add a specific
block to the list, and then play it to see how it works.
Based on the indexer logic you defined, you'll get a call to the GraphQL mutation with the object
and data passed into it.
:::tip Video Walkthrough
**Tip:** watch the video on how to [create mutations in GraphQL](https://www.youtube.com/watch?v=VwO6spk8D58&t=781s).
:::
---
## Generate a NEAR component using Playground
Creating a NEAR component from a GraphQL query is simple when using QueryAPI's GraphQL Playground. Just follow these steps:
- go to the GraphiQL tab
- select the query that you want to use
- click on the <kbd>Show GraphiQL Code Exporter</kbd> button
- get some default code here, copy it,
- go to the NEAR sandbox, paste it.
This will set up some boilerplate code to execute the GraphQL query, add the query that you had
in your playground and then call that query, extract the data and render it using the
render data function.
Once you have the NEAR component code, you can test it out by going to [the sandbox](https://near.org/sandbox),
pasting the generated code, and then selecting <kbd>Component Preview</kbd>.
Next, you can create a nice UI over this boilerplate code, and publish your new NEAR component.
#### Component Examples
- [Activity Feed widget](https://near.org/near/widget/ComponentDetailsPage?src=roshaan.near/widget/user-activity-feed&tab=source) running on [near.org](https://near.org)
|
```bash
near view sputnik-dao.near get_dao_list '{}'
```
<details>
<summary>Example response</summary>
<p>
```bash
[
'ref-finance.sputnik-dao.near'
'gaming-dao.sputnik-dao.near',
...
]
```
</p>
</details> |
NEAR Ecosystem Kicks Up a Sandstorm at TOKEN2049 Dubai
COMMUNITY
April 4, 2024
After making waves at last year’s Singapore edition, we’re turning up the heat for TOKEN2049 in Dubai, the city that’s rapidly becoming a hotbed for Fintech and AI innovation. This year, the NEAR ecosystem is buzzing with excitement to introduce discussions on chain abstraction and spotlight some of our most dynamic projects, including Calimero, Sweat Economy, and Aurora, to the forefront of the global tech scene.
Tech, Coffee, & Bubbles!
By day, make your way to our booth to engage with the NEAR collective and the visionaries from Calimero, Sweat Economy, and Aurora. Unpack the ways we’re transforming the Web3 space with breakthroughs in chain abstraction, data availability, frameworks for building decentralized application protocols, and Blockchain as a Service (BaaS). Indulge in the flavors of traditional Arabic coffee and pick up the hottest swag.
Yet, the experience doesn’t stop at coffee.
As the enthusiastic sponsor of TOKEN2049 Dubai’s closing party, NEAR is preparing an unforgettable evening. Anticipate a gala filled with select champagne and spirits, celebrating the milestones and fellowship of the Web3 community.
Can’t-Miss Speaking Engagements
At TOKEN2049 Dubai, NEAR Protocol is diving into the conversations that are shaping the future of Web3. We’re kicking things off with a panel on “AI x Web3: Pioneering Decentralized Intelligence,” featuring NEAR’s own Illia Polosukhin alongside experts like Ahmad Shadid from io.net, Niraj Pant of Ritual, Sean Ren of Sahara, and moderated by Tarun Chitra of Gauntlet. They’ll unpack the exciting crossroads of AI and Web3 in fostering decentralized intelligence. Then, don’t miss our fireside chat, “Sweat Economy’s Billion-User Blueprint for Scaling Consumer Crypto Apps,” where Chris Donovan, COO of NEAR Foundation and Oleg Fomenko, Co-founder & CEO of Sweat Economy open up about their ground-breaking collaboration.
Swing by our booth for a firsthand look at how NEAR is redefining norms with chain abstraction, data availability, and more—all powered by a healthy dose of caffeine.
TOKEN2049 is gearing up to be a dynamic assembly featuring the forefront of Web3 innovation, an event you won’t want to miss. For updates on our activities, side events, and speakers, please visit our Lu.ma page and the TOKEN2049 landing page! |
```bash
near call nft.primitives.near nft_transfer_call '{"receiver_id": "v2.keypom.near", "token_id": <YOUR TOKEN ID>, "msg": <YOUR DROP ID>}' --depositYocto 1 --gas 100000000000000 --accountId bob.near
``` |
```js
const args = {
args: {
owner_id: "bob.near",
total_supply: "1000000000",
metadata: {
spec: "ft-1.0.0",
name: "Test Token",
symbol: "test",
icon: "data:image/gif;base64,R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7",
decimals: 18,
},
},
account_id: "bob.near",
};
Near.call("tkn.near", "create_token", args, 300000000000000, "2234830000000000000000000");
``` |
---
id: storage
title: Million Small Deposits
---
On NEAR, your contract pays for the storage it uses. This means that the more data you store, the more balance you need to cover for storage. If you don't handle these costs correctly (e.g. asking the user to cover their storage usage), then a million little deposits can drain your contract of its funds.
Let's walk through an example:
1. You launch [a guest book app](https://examples.near.org/guest-book-js), deploying your app's smart contract to the account `example.near`
2. Visitors to your app can add messages to the guest book. This means your users will pay a small gas fee to **store** their message to your contract.
3. When a new message comes in, NEAR will check if `example.near` has enough balance to cover the new storage needs. If it does not, the transaction will fail.
Note that this can create an attack surface. If sending data to your guest book is inexpensive to the user while costing the contract owner significantly more, a malicious user can exploit the imbalance to make maintaining the contract prohibitively expensive.
One possible way to tackle this problem is asking the user to attach money to the call to cover the storage used by their message.
:::tip
Remember that you can release the *balance locked for storage* by simply deleting data from the contract.
:::
|
```bash
near call v2.ref-finance.near swap "{\"actions\": [{\"pool_id\": 79, \"token_in\": \"token.v2.ref-finance.near\", \"amount_in\": \"100000000000000000\", \"token_out\": \"wrap.near\", \"min_amount_out\": \"1\"}]}" --gas 300000000000000 --depositYocto 1
--accountId bob.near
```
<details>
<summary>Example response</summary>
<p>
```bash
'5019606679394603179450'
```
</p>
</details> |
---
id: common-errors
title: Common Node Errors and Solutions
sidebar_label: Common Node Errors and Solutions
description: Common Node Errors and Solutions
---
***My node is looking for peers on guildnet, testnet, and mainnet. Why?***
This is a known logging issue. The node should find peers from the same chain. This prints as a warning message as the node checks for peers from the same chain.
***I don’t have the normal number of peers, and see missing blocks?***
Normally, a node should find 12 to 15 peers. If your node’s peer count is low and you see missing blocks, you may try restarting the node to reset your peers.
***My node does not have enough peers and some peers are down.***
Cause: My node has the average number of peers (12-15) and x number of peers are down (such during protocol upgrade when these nodes upgrade late and are kicked), the blocks can be missed.
Solution:
Restart your node and try to find new peers. A bad performing peer that is close to your node may cause your node to miss blocks. If a peer is malicious, you can consider using `"blacklist": ["IP"]` in the `config.json` to ban the peer.
***My node cannot find any peers or has one peer.***
Cause 1: Depending on who the first peer is and if there are other peers close to them, the node is not able to find more peers.
Solution: This can be fixed by a restart of the node.
Cause 2: The bootnodes, defined in the `config.json` can be full and are not accept more connections.
Solution: You can potentially add any node into the `config.json` and use that node as a bootnode to find more peers.
Cause 3: Connected to a node that has just connected to the network and doesn’t have up-to-date peers.
Solution: Restart your node to find a different peer. You can potentially add any node into the config and use that node to find more peers.
***My node can't sync state or stuck in a sync state loop.***
Cause: Potentially use a wrong nearcore release.
Solution: Fix the nearcore release: https://github.com/near/nearcore/releases, and please also check the details on the node setup.
***My node is stuck on a block.***
Cause: This usually occurs in the beginning of an epoch, where the block processing time may increase.
Solution: Restarting the node usually improves the situation.
***My node shows "Mailbox closed" when the node crashes.***
Cause 1: Corrupt State
Solution: There is an issue with the DB. Download the latest snapshot and resync the node, or sync from your backup node if you have a backup node.
Cause 2: Block Height Mismatch
Solution: There is an issue with peers. The node has banned all the peers for a period of time, and is related to the peers not being properly terminated: https://github.com/near/nearcore/issues/5340. For the node operator, search in the log to see there is a thread panic. If there’s panic, then store your DB from a snapshot. If there is no panic, restart your node.
Please report all mailbox related issues using Zendesk and add your log: https://near-node.zendesk.com/hc/en-us/requests/new.
***My node shows "Mailbox closed" when the node is running.***
Cause: Unclear
Solution: Restart the node.
***My node is banning peers for block height fraud.***
Cause: It’s possible that your peer network connection is slow or unstable or that the data center has an issue. The peers don’t send you block headers faster enough.
Solution: If your node is running fine, do nothing. Unless your peers are all banned, and go to the next issue.
***All your peers are banned because of block height fraud.***
Cause: It’s possible that your peer network connection is slow or unstable or that your datacenter has an issue. If you have 0 bps frequently, this usually indicates a connectivity issue.
The peers are not sending your node block headers faster enough. Your node can start banning your peers due to your node falling behind (not being synced).
Solution: Fix your network connection. In the `config.json`, you can change the `ban_window` to 1 second to avoid banning peers.
***If your node is yet not synced, and you’ve proposed and the node shows many different errors (DB not found, Mailbox closed etc).***
Cause: Your node is not synced. Please wait for the node to fully synced before proposing.
Solution: Be fully synced, Wait 2 epochs and then ping again.
***When the main node failsover to the backup node, the back node is having issues and is falling behind and missing all blocks.***
Cause: This issue happens frequently due to the config.json in the backup node isn’t set up properly.
Solution: The backup node should track all shards. The default config.json during initialization has `"tracked_shards": []`. Please update the `config.json` to include `"tracked_shards": [0]` in both the primary and backup node. You must make this update to both the primary node and backup node.
***My node sends many request and has memory usage spike.***
Cause: This is infrequently observed but sometimes happens. A node starts to send too many requests and causes memory usage spikes, then it is stuck after 10 minutes and runs out of memory.
Solution: Restart the node.
***What are the causes of a node’s corrupt state?***
- Late upgrade due to a different protocol version transition
- Incorrectly stopping the node. The node needs to write the latest updates to disk to keep DB in a consistent state.
- Bad snapshots: Please raise the issue on [Zendesk](https://near-node.zendesk.com/hc/en-us/requests/new) to alert us.
- DB migration known issue: If you stop the DB migration, the node will end up in a corrupt state.
***My node shows inconsistent DB State or DB Not Found.***
Cause: Inconsistent DB state, DB not found are usually related to corrupt state (see above).
Solution: Depending on the severity of issue, the node can be restarted to solve for an inconsistent state. If more severe, the node may have to download the latest snapshot and restart.
***My node is missed blocks in the beginning of epochs.***
Cause: Usually blocks can be missed in the beginning of epochs due to garbage collection. However, the node usually catches up during the epoch and is not kicked.
Solution: Waiting for the node to try to catch up. If you are kicked, then try to rejoin.
***What’s a typical incident recovery plan on mainnet with a primary validator node and a secondary node***
1. Copy over node_key.json to secondary node
2. Copy over validator_key.json to secondary node
3. Stop the primary node
4. Stop the secondary node
5. Restart the secondary node
***What happens in a typical validator / RPC node upgrade process?***
1. DB Migration (optional if the release contains a DB migration)
2. Finding Peers
3. Download Headers to 100%
4. State Sync
5. Download Blocks
***What happens in a typical archival node upgrade process?***
Since archival node needs all blocks, it will download blocks directly after downloading headers.
1. DB Migration (optional if the release contains a DB migration)
2. Finding Peers
3. Download Headers to 100%
4. Download Blocks
## Still seeing an issue with your node?
Please find a Bug Report on Zendesk, https://near-node.zendesk.com/hc/en-us/requests/new, to the NEAR team, and attach your logs.
|
NEAR and Circle Announce USDC Support for Multi-Chain Ecosystem
COMMUNITY
September 28, 2022
Big news coming out of the Converge22 conference in San Francisco this week. Circle Internet Financial, a global digital fintech firm and the issuer of USD Coin (USDC), announced a partnership with NEAR that will make USDC widely available on the NEAR ecosystem by the end of 2022.
Having USDC on NEAR is definitely a reason to celebrate. Circle will also make USDC available on other platforms to support a multi-chain ecosystem.
Powering NEAR developers
Bringing USDC to NEAR will help empower developers to integrate stablecoin payments flows into their Javascript and Rust-based decentralized applications. This will unlock a new wave of accessibility for Web2 builders moving into Web3. It will also serve a vital role in NEAR’s mission of Web3 mass adoption, while helping developers build without limits.
“The multi-chain expansion increases USDC’s native availability from eight ecosystems to thirteen, and enables blockchain developers building on USDC and their users to experience greater liquidity and interoperability within the crypto economy,” said Joao Reginatto, VP of Product at Circle. “Extending multi-chain support for USDC opens the door for institutions, exchanges, developers and more to build their innovations and have easier access to a trusted and stable asset.”
Bringing USDC to NEAR is a big win for developers, who will now be able to integrate stablecoin payment flows into Javascript or Rust-based dApps. This will help usher in a new wave of accessibility for Web 2 builders making the shift to Web3.
What to know about stablecoins
A stablecoin is simply a cryptocurrency that is “pegged” to a more traditional currency like USD or EURO. Through various treasury and/or collateral models, they are designed to keep a 1:1 value, or parity, with their pegged counterparts. Stablecoins can also be pegged to credit and/or debt or have no collateral at all, as seen in algorithmic stablecoins.
Their presence in the Web3 ecosystem brings a sense of familiarity for natives and newcomers alike. A stablecoin is also a sort of on-ramp to understanding token economics—the gas that powers Web3 and beyond.
While designed to reduce cryptocurrency volatility, stablecoins present their own set of risks. When centralized, stablecoins can be victims of hacks, theft, or even over-printing which can lead to hyperinflation. If an algorithmic stablecoin experiences algorithmic failure, as happened with Terra and its sister currency, Luna, the stablecoin can fail. If a stablecoin is fractionally reserved instead of fully backed, there can be a bank run that triggers a dramatic stablecoin price drop. |
Music and Blockchain: The Rise of the New Musician
NEAR FOUNDATION
April 18, 2022
New music tools and platforms are popping up on blockchains, like NFTs, music software, Metaverse gatherings, and decentralized streaming platforms. With them, a new type of musician is coming into focus. One exploring novel creative technologies and more equitable revenue streams—on their own terms.
How exactly are musicians navigating Web3 in 2022? Let’s take a closer look at what’s happening on NEAR, where musicians are finding a fast, low cost, and secure blockchain, and an ecosystem of decentralized apps to power their Web3 journey.
New musicians on the blockchain
NEAR x Music (NxM), a guild for musicians and community-building, operates right at the center of blockchain music. Members are already witnessing musicians working in Web3 in several different ways.
Beyond minting NFTs, musicians are using Cryptovoxels, NEARhub, and other virtual or Metaverse spaces for live gatherings. In some cases, musicians use these spaces for music videos and other content.
“There is also more opportunity for musicians to sell their merchandise and music directly to their fan bases rather than depending on platforms as an intermediary,” the NxM Council says. “The blockchain allows you to see who is interacting with and collecting your work.”
Musicians in muti, a creative collective and DAO based in Portugal, are very active on NEAR. Apart from minting audiovisual NFTs, they promote each other’s music and stream original music and DJ sets through their Mutiverse on NEARHub.
“We are working in the digital and physical spaces,” says muti’s Tabea, noting that the collective often organizes events in nature and other non-urban areas. “As NEAR and its platforms develop tools, we’re experimenting with how to integrate the digital with the physical—ticketing, streams from nature into the Metaverse, utility NFTs, etc.”
But, not all musicians are trying to shift the industry paradigm. Some established artists simply want to explore new content distribution and revenue channels.
“Artists are taking advantage of opportunities in Web3 to ride the wave in parallel with whatever they’re already doing in Web2,” says Vandal, founder of DAORecords, a label founded on NEAR.
Origin is a musician and active community member of Amplify.Art, decentralized music platform currently building on NEAR. He points to Cryptopunk rapper Spottie WiFi, real name Miguel Mora, as an artist completely committed to blockchain music. Like David Bowie’s Ziggy Stardust or Damon Albarn’s cartoon band Gorillaz, Mora’s Spottie WiFi persona is an artistic avatar. A cryptovoxel purpose-built for Web3, Spottie WiFi partakes inNFT releases and Metaverse performances.
Other artists, like Emily Lazard, FiFi Rong, and even Deadmau5—who released the NFT single “this is fine” on NEAR—already have sizable followings. For them, Web3 helps grow their audiences and revenue.
“What’s most attractive about Web3 is the independence and control to do whatever you want,” says Origin. “What’s so interesting and exciting is the way you could combine any artform, be it poetry, sculpture, photography, video, graphic design, music, and spoken word into an NFT.”
Like other musicians exploring music NFTs, Origin is excited about the opportunity to earn more per release. With Web3, one neeedn’t make homogenous music just to get millions of streams.
Building and using music software
Musicians will also find new blockchain music software on Web3. And if they have the developer chops, they can design it.
Chiptune musician and developer Peter Salomensen developed WebAssembly Music, a live coding music and synthesizer in Javascript/AssemblyScript (WebAssembly) on NEAR. With WebAssembly Music, Salomensen has minted music NFTs and made them remixable. He also released a piano roll interface for short, editable music NFTs, on which musicians can create and publish their own music.
Endlesss, a Digital Audio Workstation (DAW), gives musicians the instruments and tools to produce music collectively. Founder and developer Tim Exile, a former Warp Records music producer, is on a mission to unbundle music from a product produced by professionals and consumed by the masses.
With the Endlesss app (iOS, MacOS, Windows), music becomes a collective journey. Musicians collaborate live or asynchronously in “Jams”. Think of them as “musical chat groups” in which participants create, share, and remix short bits of songs called “Rifffs”, short bits of songs. Artists share Rifffs that musicians or even fans can then remix and sample, which evolve over time like a musical exquisite corpse.
“That’s why we built Endlesss—to be collaborative and real-time so that it’s conversational,” says Exile. “Every step along anyone’s musical journey from creation, to curation, to discovery, to listening is one that brings you into contact with people.”
Call it the gamification of music collaboration and remix culture. As Exile notes, games aren’t prescriptive. Each level or an entire game can be completed in any number of ways, which is the goal of Endlesss..
“As you jam together, you build an entire history of everything that happened in that jam,” Exile explains. “So, it’s music creation as storytelling because as the jam evolves you listen to how the music evolves.”
“The first thing we’re building on NEAR is to share those Jams with an audience or other creators, and allow people to collect individual Rifffs as NFTs,” Exile adds.
In the future, Vandal expects tighter integration between music software and blockchain platforms. DAWs that could, for instance, export music files directly as minted NFTs.
“You could even mint the stems when you click ‘master’,” says Vandal. “I’ve seen a few products out there shifting over to Web3, and it’s going to be great as a creator when it’s all integrated into the hardware..”
One Endlesss community member even created a hardware controller for the music software. While it’s a proof-of-concept, it demonstrates how hardware could be integrated with blockchain software.
Reinventing remix culture and collaboration
The “Amen break,” a drum break found in countless 20th and 21st century recordings, is perhaps the most famous sample in music history. Best estimates suggest it has appeared on at least 5,500 songs. And yet the drummer who laid down the track, “G. C.” Coleman, a member of the 60s funk and soul band The Winstons, never got a penny from it. Coleman is a cautionary tale of sampled works: that while sampling can lead to great artistic works, the original creators deserve fair compensation.
The music industry clamped down on sampling after The Beastie Boys seminal album Paul’s Boutique (1989). Now, sample clearance can and often does stifle creativity by making it cost-prohibitive.
With blockchain technology, sample clearance and royalty payments could be made easier and cheaper for musicians. Artists and labels could simply make music available to musicians for sampling and remixing through smart contracts, cutting out middlemen, and allowing a new era of sample-based creativity to bloom. While a boon for new musicians, Vandal isn’t sure how this will impact older music that artists want to sample.
“It’s hard to say whether older music will be re-released to include on-chain splits to facilitate royalties,” Vandal says. “If it does, it could positively impact the future of sampling.”
To nurture remix culture, Endlesss will launch a marketplace with a smart contract that features a “remix royalty” for creators. The NEAR blockchain is unique in that a royalty function is baked in at the protocol level, unlike other chains where it can only be added after the fact.
“So, if someone buys a Rifff and starts a new Jam, then the participants in the Jam from which that Riff was purchased will collectively get a royalty,” he says. “Whether that royalty will be 60%, 20%, 80% is hard to say. I have no idea what people will feel is right.”
“The secret part of remix culture has always been recontextualizing,” says Exile. “It’s context to the power of 2: that’s actually where the value gets created. Splice is all-you-can-eat samples, and Spotify all-you-can-eat music, which is great for commodities but bad for culture because it commodifies it. Culture is all about meaning, purpose, and uniqueness, and I would say that all-you-can-eat and culture are incompatible.”
Exile also sees something else fascinating in blockchain-based sampling and remix culture. With smart contracts, musicians and listeners can trace sample attribution through layers upon layers of creation, like descending Wikipedia-style rabbit holes.
This just doesn’t happen with Web2 music software and streaming platforms. In Web3, musicians can be part of a new renaissance in music culture.
“There’s a golden opportunity to monetize culture through meaning rather than commodification,” says Exile. “This is how it should be, I think.”
Redefining the record label and royalties
Labels are facilitators—this won’t change in Web3. But, those labels that wish to operate in Web3 will have to rethink how they function.
“Record labels will most likely be DAOs, providing funding, support, resources, and even minting NFTs and performing other services to artists, as needed,” says Vandal. DAO labels can offer shared ownership, transparency, and a blockchain-inspired business model that appeals to artists.
Amber Stoneman, founder of Minting Music, a music-first NFT production and promotion company, says musicians under contract already have labels managing their NFT releases. Minting Music helps these labels, or artists that decide to forego labels, build NFT marketplaces.
In song publishing, there are multiple forms of ownership. Someone can own the overall master recording, while over 20 other individuals or entities can own rights to lyrics, composition, recording, and more. Song catalogs are bought out from under musicians and sold over and over again.
“In the Web2 space, musicians don’t know who owns their music or where it’s at,” says Stoneman. “We’ve never had technology like the blockchain to track things so clearly and transparently.”
“An artist can sell an NFT and it could make X amount of dollars, and they have just a few creator wallets connected to that smart contract, and the royalty splits are happening in real time,” she adds.
Artists will also be able to easily claim their copyrights in cases of dispute by using NFTs and on-chain data. Muti’s smokedfalmon says listeners and fans could also tip artists for their work.
Both muti’s Tabea and DAORecords’ Vandal believe simplifying royalty payouts with smart contracts will make artists more open and willing to collaborate and create work together. “Fractionalization of the publishing pie with fans or early investors is also an exciting possibility,” says Vandal.
“This is a plus from the community side of things, as Web3 can help in shifting the decision-making,” Tabea says. “It allows a collective, agency, or artist to use a DAO to not only hold and receive funds but integrate the wider community. So, fans and supporters can actually vote on decisions towards the future of the DAO/project, if the collective wants that.”
Creators and community are the future
With blockchain, the future of music is the creator. But no musical creator will exist in a silo or vacuum. Musicians can and will be more integrated with communities. New ones will pop up, morph, evolve, and die, becoming the seeds for new artistic communities.
“The creator proposition is in building, owning, and curating your identity in collaborating with others, on your own terms,” adds Endlesss’ Exile. “And the dynamics will propel people along those collective journeys.”
“Doing this work on NEAR over the last year with guilds and DAOs has given me confidence that I’ve chosen right,” says Vandal. “Community is everything,” Vandal says.
What needs to happen, in his opinion, is a true understanding of decentralization’s power. Sure, the new musician can leverage blockchain for their own benefit, but its capacity for community-building and engagement is potent. This, Vandal believes, can put the music industry on the path to revolution.
“It is definitely an exciting time for musicians,” he says. “We are history in the making.”
Check out the complete series on Blockchain Music:
Part 1: Music and Blockchain: Introducing the Future of Sound
Part 2: Building a Record Label on the Blockchain |
# Marketplace Frontend
[](https://marketplace-template.mintbase.xyz/)
[](https://vercel.com/new/clone?repository-url=https%3A%2F%2Fgithub.com%2FMintbase%2Ftemplates%2Ftree%2Fmain%2Fmarketplace)
Unlock Your NFT Storefront: Clone & Customize Your Path to Blockchain Success with this whitelabel marketplace template!

:::tip Mintbase Templates
This is part of the [Mintbase Templates](https://templates.mintbase.xyz/), a collection of templates that you can use to scaffold your own project
:::
---
## Project Walkthrough
This guide will take you step by step through the process of creating a basic marketplace frontend where you can purchase tokens and filter your selection by store. It uses [mintbase-js/data](https://docs.mintbase.xyz/dev/mintbase-sdk-ref/data) for retrieving data and [mintbase-js/sdk](https://docs.mintbase.xyz/dev/mintbase-sdk-ref/sdk) for executing marketplace methods on the [mintbase marketplace contract](https://github.com/Mintbase/mb-contracts/tree/main/mb-interop-market).
The mintbase-js/data package provides convenient functions for retrieving nft data from the mintbase indexer. In this example, you will be able to view and purchase NFTs from a specific nft contract.
You can find more information on Github: [GitHub link](https://github.com/Mintbase/mintbase-js/tree/beta/packages/data)
A live demo of the marketplace can be found here: [Live demo link](https://marketplace-template.mintbase.xyz/)
### Setup
```bash
# install dependencies
pnpm install
#run the project
pnpm dev
```
<hr class="subsection" />
### Step 1: Connect Wallet
Before proceeding, it is important to have a wallet connection feature implemented in your application in order to interact with the contract. To do this, you can check our guide [Wallet Connection Guide](https://docs.mintbase.xyz/dev/getting-started/add-wallet-connection-to-your-react-app).
<hr class="subsection" />
### Step 2: Get NFTs from Store
In this example, we utilized react-query to manage the loading state when retrieving NFTs from the contract via the storeNfts method. This method returns all listed NFTs from the specified contract, allowing you to display them in the user interface.
```ts
// src/hooks/useStoreNfts.ts
import { useQuery } from 'react-query';
import { storeNfts } from '@mintbase-js/data';
const useStoreNfts = (store?: string) => {
const defaultStores = process.env.NEXT_PUBLIC_STORES || MAINNET_CONFIG.stores;
const formattedStores = defaultStores.split(/[ ,]+/);
const { isLoading, error, data } = useQuery(['storeNfts', store], () => storeNfts(store || formattedStores, true), {
retry: false,
refetchOnWindowFocus: false,
select: mapStoreNfts,
});
return { ...data, error, loading: isLoading };
};
export { useStoreNfts };
```
<hr class="subsection" />
### Step 3: Get Store Data
To control the tabs, we need to retrieve store data using the storeData method. This method returns the data from the specified contract, enabling you to display it in the user interface.
```ts
// src/hooks/useStoreData.ts
import { useQuery } from 'react-query';
import { storeData } from '@mintbase-js/data';
const useStoreData = () => {
const defaultStores = process.env.NEXT_PUBLIC_STORES || MAINNET_CONFIG.stores;
const formattedStores = defaultStores.split(/[ ,]+/);
const { isLoading, error, data } = useQuery('storeData', () => storeData(formattedStores), {
retry: false,
refetchOnWindowFocus: false,
select: mapStoreData,
});
return { ...data, error, loading: isLoading };
};
export { useStoreData };
```
<hr class="subsection" />
### Step 4: Get Metadata from an NFT
To display NFT pricing information, available quantities, and other details in the user interface, it is necessary to access the NFT metadata using the metadataByMetadataId method.
```ts
// src/hooks/useMetadataByMetadataId.ts
import { useQuery } from 'react-query';
import { metadataByMetadataId } from '@mintbase-js/data';
const useMetadataByMetadataId = ({ metadataId }) => {
const { isLoading, data: metadata } = useQuery('metadataByMetadataId', () => metadataByMetadataId(metadataId), {
retry: false,
refetchOnWindowFocus: false,
select: mapMetadata,
});
return { ...metadata, isTokenListLoading: isLoading };
};
export { useMetadataByMetadataId };
```
<hr class="subsection" />
### Step 5: Get Current NEAR Price
To obtain the current price of the NFT in USD, it is necessary to retrieve the current Near price. We accomplish this by using the nearPrice method.
```ts
// src/hooks/useNearPrice.ts
import { useEffect, useState } from 'react';
import { nearPrice } from '@mintbase-js/data';
const useNearPrice = () => {
const [nearPriceData, setNearPriceData] = useState('0');
useEffect(() => {
const getNearPrice = async () => {
const { data: priceData, error } = await nearPrice();
setNearPriceData(error ? '0' : priceData);
};
getNearPrice();
}, []);
return nearPriceData;
};
export { useNearPrice };
```
<hr class="subsection" />
### Step 6: Execute the Contract Call - Buy
The execute method accepts one or more contract call objects and executes them using a specified wallet instance. In this example, we need to use the execute method to execute the "buy" call, allowing the user to purchase the desired NFT.
```ts
const singleBuy = async () => {
const wallet = await selector.wallet();
if (tokenId) {
(await execute(
{ wallet, callbackArgs: callback },
{
...buy({
contractAddress: nftContractId,
tokenId,
affiliateAccount:
process.env.NEXT_PUBLIC_AFFILIATE_ACCOUNT ||
MAINNET_CONFIG.affiliate,
marketId,
price: nearToYocto(currentPrice?.toString()) || "0",
}),
}
)) as FinalExecutionOutcome;
}
};
```
---
## Set ENV variables
Once that's done, copy the `.env.example` file in this directory to `.env.local` (which will be ignored by Git):
```bash
cp .env.example .env.local
```
if you use windows without powershell or cygwin:
```bash
copy .env.example .env.local
```
To get your `api key` visit :
[Mintbase Developers Page for Mainnet](https://www.mintbase.xyz/developer):
[Mintbase Developers Page for testnet](https://testnet.mintbase.xyz/developer):
```
NEXT_PUBLIC_DEVELOPER_KEY=your_mintbase_api_key
```
`NEXT_PUBLIC_NETWORK` could be `testnet` or `mainnet`
```
NEXT_PUBLIC_NETWORK=testnet
```
`NEXT_PUBLIC_STORES` is your store's ids
```
NEXT_PUBLIC_STORES=latium.mintspace2.testnet,mufasa.mintspace2.testnet
```
`NEXT_PUBLIC_AFFILIATE_ACCOUNT` is your near account where your should get your market fee
```
NEXT_PUBLIC_AFFILIATE_ACCOUNT=your_near_account.near
```
## Extending
This project is setup using Next.js + @mintbase/js
You can use this project as a reference to build your own, and use or remove any library you think it would suit your needs.
:::info Get in touch
You can get in touch with the mintbase team using the following channels:
- Support: [Join the Telegram](https://tg.me/mintdev)
- Twitter: [@mintbase](https://twitter.com/mintbase)
::: |
A Deep Dive into DAOs: Exploring Astro DAO
COMMUNITY
March 21, 2022
DAOs are transforming the way things are done—be it in the business world, creative spaces, social arenas, and the charity sector. From artists and political activists to legal services and tech investors, Web3’s answer to a company, organization, or collective has piqued everyone’s interest.
First things first: a DAO is an internet-based formalized community that can self-govern and take on aspects of a company. It is decentralized, meaning there is no top-down hierarchical structure, as there is with most businesses, so decisions can be made by all involved.
A DAO’s rules are enforced automatically because they’re embedded in code and stored in a blockchain. Members are able to vote on what the DAO will do—often via governance tokens. With some DAOs, those who hold the most tokens will call the bigger shots.
What’s NEAR’s role in the DAO world? With its super-fast, easy, and low-cost blockchain, which is moving toward infinite scalability through the unique Nightshade sharding approach, the NEAR protocol is the ideal ecosystem for DAOs.
One such project making use of NEAR to help accelerate the DAO ecosystem is Astro—a DAO designed and maintained for DAO creation. Take a closer look.
What is Astro DAO?
Unveiled in late 2021, Astro is a NEAR-powered platform that lets anyone launch a DAO. Celebrated by users for being simple, it allows anyone to set up a DAO in just a few clicks. “Everyday, easy DAOs” is Astro’s motto, and once you get under its hood, it’s easy to see why. But first, an origin story.
Jordan Gray, who is part of Astro as well as TenK DAO and the NEAR Misfits NFT collection, was working on a decentralized incubator at NEAR last year when he was drawn into helping create Sputnik—a new hub for developing and interacting with DAOs through powerful smart contracts built on the NEAR blockchain. (Smart contracts, by the way, are lines of untamperable cryptographic code that automatically execute contracts between multiple parties.)
With Sputnik, the NEAR community now had a launchpad to help people set up their own DAOs. But Jordan wanted to simplify DAO creation. Sputnik’s smart contracts were powerful but complex for newcomers, or anyone wanting to set up more basic DAOs, for that matter. Jordan worked through smart contracts to essentially strip them down and group them together in the easy-to-use interface that became Astro.
Astro is now a web front end to all the smart contacts that run DAOs using the NEAR network. Jordan stripped it down so Astro’s easy-to-use UI is almost binary in how it lets users set up a DAO: users are given two options on how to launch. For example, whether voting power will be democratic or token-weighted.
“Somebody who is even new to crypto could come and start a DAO, and be able to manage a treasury with their friends and vote on things,” says Gray. “With a few clicks, you can get your own DAO set up.”
Who is using Astro?
In the year since its launch, Astro is gaining traction. Scores of DAOs have been set up using Astro, and they really run the gamut.
A DAO designed for Africa’s blockchain developers? Check. A DAO to publicize NEAR artists and the DAOs’ metaverse activities? Yes. An exhibition in the NFT world to give “visibility to cis women, trans women, non-binaries and transvestite Brazilian artists”? This was also created on the Astro platform.
The list of DAOs launching on Astro is varied and constantly growing. Each new DAO built on Astro takes advantage of how quick and easy it is to use the platform to build on the NEAR blockchain.
One project, Marma J, which leverages web3 tech for social good, chose Astro because of its ease-of-use feature set. The DAO, comprised of council members who vote on proposals with “the goal of spreading love and positivity”—such as raising funds for sick children—is one of the most active projects launched on Astro.
Chloe Lewis, the founder of Marma J, said that NEAR’s low-cost blockchain is what helped the project expand.
“Astro has been much easier to use, not just for us, but more importantly for the community,” said Lewis. “NEAR helps mostly by having a good user interface as well as low transaction fees so our community can onboard simply.”
Michael Kelly, also known as Ozymandius, is a founding member of the Croncats DAO, a platform for scheduling blockchain transactions, and a member of MOVE Capital DAO, which helps early ecosystem projects launch on NEAR Protocol. He said that Astro was easy for those who didn’t necessarily have the technical know-how to set up a DAO on NEAR but were attracted to its benefits.
“This is what we got with Astro,” Ozymandius says. “A clean, user-friendly, and well-powered DAO spin-up factory for projects to have pretty good customization on how they want to handle funds and govern decision making.”
What’s next for Astro?
These are still early days for Astro, and Jordan Gray says things aren’t 100 percent where they need to be just yet. The team behind Astro is still working to make the platform simpler to use. “We’re getting there but we’re not there yet,” Gray says.
Though Sputnik will be updating its smart contracts: v3 is on its way. And when it arrives, it will have new tools and functions built into them. Astro will implement these new smart contracts which, it is hoped, will speed up DAO adoption and innovation on NEAR.
Gray did make it clear that using Astro won’t immediately create a community that many big DAOs have. It just provides the template to quickly automate what DAOs do.
“It’s really just software that allows you to automate some things and have security if you are doing a treasury, or governance,” Jordan adds. “Your community is something other than your DAO.”
|
# Transaction
See [Transactions](../RuntimeSpec/Transactions.md) documentation in the [Runtime Specification](../RuntimeSpec) section.
|
---
id: builtin-components
title: List of Native Components
---
import {WidgetEditor} from "@site/src/components/widget-editor"
A list of all the built-in components to be used on Near Components.
---
## Widget
The predefined component `Widget` allows you to include an existing component into your code, thus enabling to create complex applications by composing components.
<WidgetEditor id='1' height="100px">
```ts
const props = { name: "Anna", amount: 3 };
return <Widget src="influencer.testnet/widget/Greeter" props={props} />;
```
</WidgetEditor>
---
## IpfsImageUpload
A built-in component that enables users to directly upload an image to the InterPlanetary File System (IPFS).
<WidgetEditor id='2' height="200px">
```js
State.init({image: {}})
return <>
<p> Raw State: {JSON.stringify(state.image)} </p>
<IpfsImageUpload image={state.image} />
</>
```
</WidgetEditor>
---
## Files
A built-in component that enables to input files with drag and drop support. Read more about the `Files` component [here](https://www.npmjs.com/package/react-files).
<WidgetEditor id='3' height="220px">
```js
const [img, setImg] = useState(null);
const [msg, setMsg] = useState('Upload an Image')
const uploadFile = (files) => {
setMsg('Uploading...')
const file = fetch(
"https://ipfs.near.social/add",
{
method: "POST",
headers: { Accept: "application/json" },
body: files[0]
}
)
setImg(file.body.cid)
setMsg('Upload an Image')
}
return <>
<Files
multiple={false}
accepts={["image/*"]}
clickable
className="btn btn-outline-primary"
onChange={uploadFile}
>
{msg}
</Files>
{img ? <div><img src={`https://ipfs.near.social/ipfs/${img}`} /></div> : ''}
</>;
```
</WidgetEditor>
---
## Markdown
A component that enables to render Markdown.
<WidgetEditor id='4' height="60px">
```jsx
const markdown = (`
## A title
This is some example **markdown** content, with _styled_ text
`)
return <Markdown text={markdown} />;
```
</WidgetEditor>
:::tip Markdown Editor
Checkout this [Markdown Editor](https://near.social/#/mob.near/widget/MarkdownEditorIframeExample), and [its source code](https://near.social/mob.near/widget/WidgetSource?src=mob.near/widget/MarkdownEditorIframeExample).
:::
---
## OverlayTrigger
Used to display a message or icon when the mouse is over a DOM element.
<WidgetEditor id='5' height="200px">
```javascript
const [show, setShow] = useState(false);
const overlay = (
<div className='border m-3 p-3'>
This is the overlay Message
</div>
);
return (
<OverlayTrigger
show={show}
delay={{ show: 250, hide: 300 }}
placement='auto'
overlay={overlay}
>
<button
className="btn btn-outline-primary"
onMouseEnter={() => setShow(true)}
onMouseLeave={() => setShow(false)}
>
Place Mouse Over Me
</button>
</OverlayTrigger>
);
```
</WidgetEditor>
<details markdown="1">
<summary> Component props </summary>
The OverlayTrigger component has several props that allow you to customize its behavior:
| Prop | Description |
|-------------|------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| `show` | A boolean value that determines whether the overlay is currently visible or not. |
| `trigger` | An array of events that trigger the display of the overlay. In this example, the `trigger` prop is set to `["hover", "focus"]`, which means that the overlay will be displayed when the user hovers over or focuses on the element. |
| `delay` | An object that specifies the delay before the overlay is displayed or hidden. In this example, the `delay` prop is set to `{ show: 250, hide: 300 }`, which means that the overlay will be displayed after a 250-millisecond delay and hidden after a 300-millisecond delay. |
| `placement` | A string that specifies the position of the overlay relative to the trigger element. In this example, the `placement` prop is set to `"auto"`, which means that the position will be automatically determined based on available space. |
| `overlay` | The content that will be displayed in the overlay. In this example, the `overlay` prop is set to a `<div>` element containing the message "This is the overlay message. |
</details>
---
## InfiniteScroll
Infinitely load a grid or list of items. This component allows you to create a simple, lightweight infinite scrolling page or element by supporting both window and scrollable elements.
Read more about the [react-infinite-scroller](https://www.npmjs.com/package/react-infinite-scroller) package.
<WidgetEditor id='6' height="200px">
```js
const allNumbers = Array.from(Array(100).keys())
const [lastNumber, setLastNumber] = useState(0);
const [display, setDisplay] = useState([]);
const loadNumbers = (page) => {
const toDisplay = allNumbers
.slice(0, lastNumber + page*10)
.map(n => <p>{n}</p>)
console.log(lastNumber + page*10)
setDisplay(toDisplay);
setLastNumber(lastNumber + page*10);
};
return (
<InfiniteScroll
loadMore={loadNumbers}
hasMore={lastNumber < allNumbers.length}
useWindow={false}
>
{display}
</InfiniteScroll>
);
```
</WidgetEditor>
---
## TypeAhead
Provides a type-ahead input field for selecting an option from a list of choices. More information about the component can be found [here](https://github.com/ericgio/react-bootstrap-typeahead).
<WidgetEditor id='7' height="300px">
```jsx
const [selected, setSelected] = useState([]);
const options = ["Apple", "Banana", "Cherry", "Durian", "Elderberry"];
return <>
<Typeahead
options={options}
multiple
onChange={v => setSelected(v)}
placeholder='Choose a fruit...'
/>
<hr />
<p> Selected: {selected.join(', ')} </p>
</>;
```
</WidgetEditor>
---
## Styled Components
[Styled Components](https://styled-components.com/) is a popular library for styling React components using CSS-in-JS.
<WidgetEditor id='8' height="80px">
```jsx
const Button = styled.button`
/* Adapt the colors based on primary prop */
background: ${(props) => (props.primary ? "palevioletred" : "white")};
color: ${(props) => (props.primary ? "white" : "palevioletred")};
font-size: 1em;
margin: 1em;
padding: 0.25em 1em;
border: 2px solid palevioletred;
border-radius: 10px;
`;
return (
<div>
<Button>Normal</Button>
<Button primary>Primary</Button>
</div>
);
```
</WidgetEditor>
---
## Tooltip
Displays a message once the mouse hovers over a particular item. This component was imported from [`React-Bootstrap`](https://react-bootstrap-v3.netlify.app/components/tooltips/).
<WidgetEditor id='9' height="120px">
```js
const tooltip = (
<Tooltip id="tooltip">
<strong>Holy guacamole!</strong> Check this info.
</Tooltip>
);
return <>
<OverlayTrigger placement="left" overlay={tooltip}>
<button>Holy guacamole!</button>
</OverlayTrigger>
<OverlayTrigger placement="top" overlay={tooltip}>
<button>Holy guacamole!</button>
</OverlayTrigger>
<OverlayTrigger placement="bottom" overlay={tooltip}>
<button>Holy guacamole!</button>
</OverlayTrigger>
<OverlayTrigger placement="right" overlay={tooltip}>
<button>Holy guacamole!</button>
</OverlayTrigger>
</>
```
</WidgetEditor> |
---
sidebar_position: 4
---
# Architecture
Near node consists roughly of a blockchain layer and a runtime layer.
These layers are designed to be independent from each other: the blockchain layer can in theory support runtime that processes
transactions differently, has a different virtual machine (e.g. RISC-V), has different fees; on the other hand the runtime
is oblivious to where the transactions are coming from. It is not aware whether the
blockchain it runs on is sharded, what consensus it uses, and whether it runs as part of a blockchain at all.
The blockchain layer and the runtime layer share the following components and invariants:
## Transactions and Receipts
Transactions and receipts are a fundamental concept in Near Protocol. Transactions represent actions requested by the
blockchain user, e.g. send assets, create account, execute a method, etc. Receipts, on the other hand is an internal
structure; think of a receipt as a message which is used inside a message-passing system.
Transactions are created outside the Near Protocol node, by the user who sends them via RPC or network communication.
Receipts are created by the runtime from transactions or as the result of processing other receipts.
Blockchain layer cannot create or process transactions and receipts, it can only manipulate them by passing them
around and feeding them to a runtime.
## Account-Based System
Similar to Ethereum, Near Protocol is an account-based system. Which means that each blockchain user is roughly
associated with one or several accounts (there are exceptions though, when users share an account and are separated
through the access keys).
The runtime is essentially a complex set of rules on what to do with accounts based on the information from the
transactions and the receipts. It is therefore deeply aware of the concept of account.
Blockchain layer however is mostly aware of the accounts through the trie (see below) and the validators (see below).
Outside these two it does not operate on the accounts directly.
### Assume every account belongs to its own shard
Every account at NEAR belongs to some shard.
All the information related to this account also belongs to the same shard. The information includes:
- Balance
- Locked balance (for staking)
- Code of the contract
- Key-value storage of the contract
- All Access Keys
Runtime assumes, it's the only information that is available for the contract execution.
While other accounts may belong to the same shards, the Runtime never uses or provides them during contract execution.
We can just assume that every account belongs to its own shard. So there is no reason to intentionally try to collocate accounts.
## Trie
Near Protocol is a stateful blockchain -- there is a state associated with each account and the user actions performed
through transactions mutate that state. The state then is stored as a trie, and both the blockchain layer and the
runtime layer are aware of this technical detail.
The blockchain layer manipulates the trie directly. It partitions the trie between the shards to distribute the load.
It synchronizes the trie between the nodes, and eventually it is responsible for maintaining the consistency of the trie
between the nodes through its consensus mechanism and other game-theoretic methods.
The runtime layer is also aware that the storage that it uses to perform the operations on is a trie. In general it does
not have to know this technical detail and in theory we could have abstracted out the trie as a generic key-value storage.
However, we allow some trie-specific operations that we expose to the smart contract developers so that they utilize
Near Protocol to its maximum efficiency.
## Tokens and gas
Even though tokens is a fundamental concept of the blockchain, it is neatly encapsulated
inside the runtime layer together with the gas, fees, and rewards.
The only way the blockchain layer is aware of the tokens and the gas is through the computation of the exchange rate
and the inflation which is based strictly on the block production mechanics.
## Validators
Both the blockchain layer and the runtime layer are aware of a special group of participants who are
responsible for maintaining the integrity of the Near Protocol. These participants are associated with the
accounts and are rewarded accordingly. The reward part is what the runtime layer is aware of, while everything
around the orchestration of the validators is inside the blockchain layer.
## Blockchain Layer Concepts
Interestingly, the following concepts are for the blockchain layer only and the runtime layer is not aware of them:
- Sharding -- the runtime layer does not know that it is being used in a sharded blockchain, e.g. it does not know
that the trie it works on is only a part of the overall blockchain state;
- Blocks or chunks -- the runtime does not know that the receipts that it processes constitute a chunk and that the output
receipts will be used in other chunks. From the runtime perspective it consumes and outputs batches of transactions and receipts;
- Consensus -- the runtime does not know how consistency of the state is maintained;
- Communication -- the runtime does not know anything about the current network topology. Receipt has only a receiver_id (a recipient account), but knows nothing about the destination shard, so it's a responsibility of the blockchain layer to route a particular receipt.
## Runtime Layer Concepts
- Fees and rewards -- fees and rewards are neatly encapsulated in the runtime layer. The blockchain layer, however,
has an indirect knowledge of them through the computation of the tokens-to-gas exchange rate and the inflation.
|
# action_creation_config
Describes the cost of creating a specific action, `Action`. Includes all variants.
## create_account_cost
_type: Fee_
Base cost of creating an account.
## deploy_contract_cost
_type: Fee_
Base cost of deploying a contract.
## deploy_contract_cost_per_byte
_type: Fee_
Cost per byte of deploying a contract.
## function_call_cost
_type: Fee_
Base cost of calling a function.
## function_call_cost_per_byte
_type: Fee_
Cost per byte of method name and arguments of calling a function.
## transfer_cost
_type: Fee_
Base cost of making a transfer.
NOTE: If the account ID is an implicit account ID (64-length hex account ID), then the cost of the transfer fee
will be `transfer_cost + create_account_cost + add_key_cost.full_access_cost`.
This is needed to account for the implicit account creation costs.
## stake_cost
_type: Fee_
Base cost of staking.
## add_key_cost:
_type: [AccessKeyCreationConfig](AccessKeyCreationConfig.md)_
Base cost of adding a key.
## delete_key_cost
_type: Fee_
Base cost of deleting a key.
## delete_account_cost
_type: Fee_
Base cost of deleting an account.
|
```bash
near call ft.primitives.near ft_transfer '{"receiver_id": "v2.keypom.near", "amount": "1"}' --depositYocto 1 --gas 100000000000000 --accountId bob.near
``` |
---
title: JS SDK CLI
---
The SDK [Command Line Interface](https://github.com/near/near-sdk-js/blob/develop/packages/near-sdk-js/src/cli/cli.ts) (CLI) is a tool that enables to act on different parts of the build process as well as generate validations and an [ABI](https://github.com/near/abi). Among other things, the SDK CLI enables you to:
- Control the different parts of the build process
- Validate your contract and TypeScript code
- Create an ABI JSON file
---
## Overview {#overview}
_Click on a command for more information and examples._
**Commands**
| Command | Description |
| ----------------------------------------- | ------------------------------------------------------------------------------------------------------------------------------ |
| [`near-sdk-js build`](#build) | Build a NEAR JS Smart-contract |
| [`near-sdk-js validateContract`](#validate-contract) | Validate a NEAR JS Smart-contract |
| [`near-sdk-js checkTypescript`](#check-ts) | Run TSC with some CLI flags |
| [`near-sdk-js createJsFileWithRollup`](#create-js-file) | Create an intermediate JavaScript file for later processing with QJSC |
| [`near-sdk-js transpileJsAndBuildWasm`](#transpile-js-to-wasm) | Transpiles the target javascript file into .c and .h using QJSC then compiles that into wasm using clang |
---
## Setup {#setup}
### Installation {#installation}
> Make sure you have a current version of `npm` and `NodeJS` installed.
#### Mac and Linux {#mac-and-linux}
1. Install `npm` and `node` using a [package manager](https://nodejs.org/en/download/package-manager/) like `nvm` as sometimes there are issues using Ledger due to how macOS handles node packages related to USB devices.
2. Ensure you have installed Node version 12 or above.
3. Install `near-cli` globally by running:
```bash
npm install -g near-cli
```
#### Windows {#windows}
> For Windows users, we recommend using Windows Subsystem for Linux (`WSL`).
1. Install `WSL` [[click here]](https://docs.microsoft.com/en-us/windows/wsl/install-manual#downloading-distros)
2. Install `npm` [[click here]](https://www.npmjs.com/get-npm)
3. Install ` Node.js` [ [ click here ]](https://nodejs.org/en/download/package-manager/)
4. Change `npm` default directory [ [ click here ] ](https://docs.npmjs.com/resolving-eacces-permissions-errors-when-installing-packages-globally#manually-change-npms-default-directory)
- This is to avoid any permission issues with `WSL`
5. Open `WSL` and install `near-cli` globally by running:
```bash
npm install -g near-cli
```
:::info heads up
Copy/pasting can be a bit odd using `WSL`.
- "Quick Edit Mode" will allow right-click pasting.
- Depending on your version there may be another checkbox allowing `Ctrl` + `V` pasting as well.

:::
---
## Commands {#commands}
### `near-sdk-js build` {#build}
Build a NEAR JS Smart-contract, specifying the source, target, `package.json`, and `tsconfig.json` files. If none are specified, the default values are used. The argument default values are:
- source: `src/index.js`
- target: `build/contract.wasm`
- packageJson: `package.json`
- tsConfig: `tsconfig.json`
Options default values are set to `false`.
- arguments (optional): `[source] [target] [packageJson] [tsConfig]`
- options: `--verbose --generateABI`
**Example:**
```bash
near-sdk-js build src/main.ts out/main.wasm package.json tsconfig.json --verbose true --generateABI true
```
### `near-sdk-js validateContract` {#validate-contract}
Validate a NEAR JS Smart-contract. Validates the contract by checking that all parameters are initialized in the constructor. Works only for TypeScript.
- arguments: `[source]`
- options: `--verbose`
**Example:**
```bash
near-sdk-js validateContract src/main.ts --verbose true
```
**Example Response:**
```bash
npx near-sdk-js validateContract src/index.ts
[validate] › … awaiting Validating src/index.ts contract...
```
---
### `near-sdk-js checkTypescript` {#check-ts}
Run TSC with some CLI flags.
:::note warning
This command ignores `tsconfig.json`.
:::
- arguments: `[source]`
- options: `--verbose`
**Example:**
```bash
near-sdk-js checkTypescript src/main.ts --verbose true
```
**Example Response:**
```bash
npx near-sdk-js checkTypescript src/index.ts
[checkTypescript] › … awaiting Typechecking src/index.ts with tsc...
```
---
### `near-sdk-js createJsFileWithRollup` {#create-js-file}
Create an intermediate JavaScript file for later processing with QJSC.
- arguments: `[source]` `[target]`
- options: `--verbose`
**Example:**
```bash
near-sdk-js createJsFileWithRollup src/main.ts out/main.js --verbose true
```
**Example Response:**
```bash
npx near-sdk-js createJsFileWithRollup src/index.ts
[createJsFileWithRollup] › … awaiting Creating src/index.ts file with Rollup...
```
### `near-sdk-js transpileJsAndBuildWasm` {#transpile-js-to-wasm}
Create an intermediate JavaScript file for later processing with QJSC.
- arguments: `[source]` `[target]`
- options: `--verbose`
**Example:**
```bash
near-sdk-js transpileJsAndBuildWasm src/main.js out/main.wasm --verbose true
```
**Example Response:**
```bash
npx near-sdk-js transpileJsAndBuildWasm
[transpileJsAndBuildWasm] › ✔ success Generated build/contract.wasm contract successfully!
```
|
# Upgradability
This part of specification describes specifics of upgrading the protocol, and touches on few different parts of the system.
Three different levels of upgradability are:
1. Updating without any changes to underlying data structures or protocol;
2. Updating when underlying data structures changed (config, database or something else internal to the node and probably client specific);
3. Updating with protocol changes that all validating nodes must adjust to.
## Versioning
There are 2 different important versions:
- Version of binary defines it's internal data structures / database and configs. This version is client specific and doesn't need to be matching between nodes.
- Version of the protocol, defining the "language" nodes are speaking.
```rust
/// Latest version of protocol that this binary can work with.
type ProtocolVersion = u32;
```
## Client versioning
Clients should follow [semantic versioning](https://semver.org/).
Specifically:
- MAJOR version defines protocol releases.
- MINOR version defines changes that are client specific but require database migration, change of config or something similar. This includes client-specific features. Client should execute migrations on start, by detecting that information on disk is produced by previous version and auto-migrate it to new one.
- PATCH version defines when bug fixes, which should not require migrations or protocol changes.
Clients can define how current version of data is stored and migrations applied.
General recommendation is to store version in the database and on binary start, check version of database and perform required migrations.
## Protocol Upgrade
Generally, we handle data structure upgradability via enum wrapper around it. See `BlockHeader` structure for example.
### Versioned data structures
Given we expect many data structures to change or get updated as protocol evolves, a few changes are required to support that.
The major one is adding backward compatible `Versioned` data structures like this one:
```rust
enum VersionedBlockHeader {
BlockHeaderV1(BlockHeaderV1),
/// Current version, where `BlockHeader` is used internally for all operations.
BlockHeaderV2(BlockHeader),
}
```
Where `VersionedBlockHeader` will be stored on disk and sent over the wire.
This allows to encode and decode old versions (up to 256 given https://borsh.io specification). If some data structures has more than 256 versions, old versions are probably can be retired and reused.
Internally current version is used. Previous versions either much interfaces / traits that are defined by different components or are up-casted into the next version (saving for hash validation).
### Consensus
| Name | Value |
| - | - |
| `PROTOCOL_UPGRADE_BLOCK_THRESHOLD` | `80%` |
| `PROTOCOL_UPGRADE_NUM_EPOCHS` | `2` |
The way the version will be indicated by validators, will be via
```rust
/// Add `version` into block header.
struct BlockHeaderInnerRest {
...
/// Latest version that current producing node binary is running on.
version: ProtocolVersion,
}
```
The condition to switch to next protocol version is based on % of stake `PROTOCOL_UPGRADE_NUM_EPOCHS` epochs prior indicated about switching to the next version:
```python
def next_epoch_protocol_version(last_block):
"""Determines next epoch's protocol version given last block."""
epoch_info = epoch_manager.get_epoch_info(last_block)
# Find epoch that decides if version should change by walking back.
for _ in PROTOCOL_UPGRADE_NUM_EPOCHS:
epoch_info = epoch_manager.prev_epoch(epoch_info)
# Stop if this is the first epoch.
if epoch_info.prev_epoch_id == GENESIS_EPOCH_ID:
break
versions = collections.defaultdict(0)
# Iterate over all blocks in previous epoch and collect latest version for each validator.
authors = {}
for block in epoch_info:
author_id = epoch_manager.get_block_producer(block.header.height)
if author_id not in authors:
authors[author_id] = block.header.rest.version
# Weight versions with stake of each validator.
for author in authors:
versions[authors[author] += epoch_manager.validators[author].stake
(version, stake) = max(versions.items(), key=lambda x: x[1])
if stake > PROTOCOL_UPGRADE_BLOCK_THRESHOLD * epoch_info.total_block_producer_stake:
return version
# Otherwise return version that was used in that deciding epoch.
return epoch_info.version
```
|
---
id: remix-ide-plugin
sidebar_label: Remix IDE Plugin
---
# Remix IDE Plugin Integration
This tutorial details how to deploy and run NEAR smart contract on Remix IDE. It is a no-setup tool with a GUI for developing NEAR smart contract.

## Connect to Remix IDE
[WELLDONE Code](https://docs.welldonestudio.io/code) is the official Remix IDE Plug-in. Please visit the [Remix IDE](https://remix.ethereum.org/) and follow the guide below.
Click **Plugin Manager** button in the left bar and search for **CODE BY WELLDONE STUDIO** and click the Activate button.
<img src={require('/docs/assets/remix-ide-plugin/plugin-manager.png').default} alt='plugin-manager' style={{width: '500px', marginRight: '10px', display: 'inline'}}/>
<img src={require('/docs/assets/remix-ide-plugin/activate-plugin.png').default} alt='active-plugin' style={{width: '300px', display: 'inline'}}/>
## Select a Chain
Click on NEAR(NVM) in the list of chains.
If you click the `Documentation` button, go to WELL DONE Docs, and if you find a problem or have any questions while using it, click the `Make an issue` button to go to the [Github Repository](https://github.com/welldonestudio/welldonestudio.github.io) and feel free to create an issue.
<img src={require('/docs/assets/remix-ide-plugin/select-chain.png').default} alt='select-chain' style={{width: '318px'}}/>
## Install a browser extension wallet
:::info
Other wallets will be supported soon, and WELLDONE Wallet can be used now.
:::
After choosing a chain, click the `Connect to WELLDONE` button to connect to the **WELLDONE Wallet.**
If you haven't installed the WELLDONE Wallet yet, please follow the following [manual](https://docs.welldonestudio.io/wallet/manual/) to install and create a wallet and create an account for the selected chain. Finally, go into the Setting tab of your wallet and activate Developer Mode.
<img src={require('/docs/assets/remix-ide-plugin/wallet-developer-mode.png').default} alt='wallet-developer-mode' style={{width: '318px', marginBottom: '10px'}}/>
And you must click the Refresh button in the upper right corner of the plug-in to apply changes to your wallet.
## Create the Project
In NEAR, you can write smart contracts with Rust, JavaScript, and TypeScript. Because the structure of the contract is different in each language, **WELLDONE Code** provides two features to help developers new to NEAR.
### Select a Template
Create a simple example contract code written in Rust, JavaScript, and TypeScript. You can create a sample contract by selecting the template option and clicking the `Create` button. More templates may be found at [NEAR Samples](https://github.com/near-examples/).
<img src={require('/docs/assets/remix-ide-plugin/template-code-near.png').default} alt='template-code-near' style={{width: '318px'}}/>
### New Project
Automatically generate a contract structure based on the smart contract language you want to use. Select the language option, write a name for the project, and click the `Create` button to create a contract structure that is appropriate for the language.
<img src={require('/docs/assets/remix-ide-plugin/new-project-near.png').default} alt='new-project-near' style={{width: '318px'}}/>
:::info
You can create your own contract projects without using the features above. However, for the remix plugin to build and deploy the contract, it must be built within the directory `near/`. If you start a new project, the structure should look like the following.
:::
#### 1. Writing Contracts in Rust
```
near
└── <YOUR_PROJECT_NAME>
├── Cargo.toml
└── src
└── lib.rs
```
#### 2. Writing Contracts in TypeScript
```
near
└── <YOUR_PROJECT_NAME>
├── package.json
├── babel.config.json
├── tsconfig.json
└── src
└── contract.ts
```
#### 3. Writing Contracts in JavaScript
```
near
└── <YOUR_PROJECT_NAME>
├── package.json
├── babel.config.json
└── src
└── contract.js
```
## Compile the Contract
:::info
Six compilation options are now available in WELLDONE Code: `Rust`, `CARGO-NEAR`, `EMBED-ABI`, `JavaScript`, and `TypeScript`.
We now only support the AMD compilation server, however, we will shortly add support for the ARM compilation server.
:::
**Step 1**: Select the project you want to compile in the **TARGET PROJECT** section.
**Step 2**: Select a compilation option and click the `Compile` button.
**Step 3**: When the compilation is complete, a wasm file is returned.
<img src={require('/docs/assets/remix-ide-plugin/project-compile.png').default} alt='project-compile' style={{width: '318px'}}/>
:::note
You can check the returned wasm file in `near/<YOUR_PROJECT_NAME>/out` directory.
If you need to revise the contract and compile again, delete the `out` directory and click the compile button.
:::
### 1. Rust Compile
Using the `cargo build` command to compile a smart contract written in Rust. Although it offers a stable compile, it is inconvenient to enter the method's parameters directly when executing the contract.
### 2. CARGO-NEAR Compile (for Rust) - `Experimental`
Compile using `cargo near` which is officially being developed by NEAR. If Compile is successful, an executable wasm binary file and a json file containing the ABI of the contract are created together. If you have deployed and imported a compiled contract using `cargo-near`, you can find out the parameter type of the method, making it easier to run the contract.
However, because this feature is still under development, the `near-sdk-rs` version must be specified at **4.1.0** or higher in the `Cargo.toml` file, and unexpected issues may occur during compilation. Please check out the NEAR's [repository](https://github.com/near/abi) for more detail.
### 3. EMBED-ABI Compile (for Rust) - `Experimental`
When using `-embed-abi` option in `cargo-near`, generates a wasm file containing ABI inside. For contracts that have deployed the wasm file compiling with this option, you can get ABI information even when importing the contract through `At Address` button. See the [cargo-near](https://github.com/near/cargo-near) repository for a detailed description of the options.
### 4. JavaScript & TypeScript Compile
Using [`near-sdk-js`](https://github.com/near/near-sdk-js) to compile a smart contract written in JavaScript or TypeScript.
:::note
If you are using JavaScript or TypeScript compile options, you must write the name of the contract file you want to compile as follows to ensure that the compilation runs without error.
- JavaScript: `contract.js`
- TypeScript: `contract.ts`
:::
## Deploy the Contract
:::tip
The WELLDONE Wallet automatically finds and imports networks associated with your wallet address. As a result, before deploying, you should choose whether you want to send a transaction to mainnet or testnet.
:::
**Step 1**: If you have a compiled contract code, then `Deploy` button will be activated.
**Step 2**: Enter the Account ID for which you want to deployed the contract and click the `Deploy` button. If you want to add `init function`, click `Deploy Option` to add the method name and arguments.
<img src={require('/docs/assets/remix-ide-plugin/deploy-option-near.png').default} alt='deploy-option-near' style={{width: '318px'}}/>
**Step 3**: If the AccountId already has a deployed contract, confirm once more.
**Step 4**: Click the `Send Tx` button in the **WELLDONE Wallet** to sign the transaction.
<img src={require('/docs/assets/remix-ide-plugin/deploy-near.png').default} alt='deploy-near' style={{width: '500px'}}/>
**Step 5**: A transaction success log will be printed to the terminal and the contract can be executed if contract deployment is successful.
<img src={require('/docs/assets/remix-ide-plugin/deployed-contract-near.png').default} alt='deployed-contract-near' style={{width: '318px'}}/>
## Execute the Contract
:::info
There are two ways to import contracts.
1. Automatically import contracts deployed through the above process.
2. Import existing deployed contracts through `At Address` button.
:::
**Step 1**: Select the method to run.
**Step 2**: Add parameters as you needed.
**Step 3**: In the case of the `Call` function, You can specify the number of NEAR tokens to attach to a function call and the GAS LIMIT.
**Step 4**: Run the method via clicking `View` or `Call` button. If you are sending a transaction, you must sign the transaction by clicking the `Send Tx` button in the **WELLDONE Wallet**.
<img src={require('/docs/assets/remix-ide-plugin/function-call.png').default} alt='function-call' style={{width: '318px'}}/>
:::info
If you deployed the compiled contract using `CARGO-NEAR` or `EMBED-ABI` options, you can execute the contract more easily using the ABI without directly entering the parameters of the method.
:::
<img src={require('/docs/assets/remix-ide-plugin/cargo-near.png').default} alt='cargo-near' style={{width: '250px', display: 'inline-block'}}/>
<img src={require('/docs/assets/remix-ide-plugin/cargo-near1.png').default} alt='cargo-near1' style={{width: '250px', display: 'inline-block'}}/>
<img src={require('/docs/assets/remix-ide-plugin/cargo-near2.png').default} alt='cargo-near2' style={{width: '250px', display: 'inline-block'}}/> |
---
sidebar_position: 2
---
import {Github} from "@site/src/components/codetabs"
# Integration Tests
## Unit Tests vs. Integration Tests
Unit tests are great for ensuring that functionality works as expected at an insolated, functional-level. This might include checking that function `get_nth_fibonacci(n: u8)` works as expected, handles invalid input gracefully, etc. Unit tests in smart contracts might similarly test public functions, but can get unruly if there are several calls between accounts. As mentioned in the [unit tests](unit-tests.md) section, there is a `VMContext` object used by unit tests to mock some aspects of a transaction. One might, for instance, modify the testing context to have the `predecessor_account_id` of `"bob.near"`. The limits of unit tests become obvious with certain interactions, like transferring tokens. Since `"bob.near"` is simply a string and not an account object, there is no way to write a unit test that confirms that Alice sent Bob 6 NEAR (Ⓝ). Furthermore, there is no way to write a unit test that executes cross-contract calls. Additionally, there is no way of profiling gas usage and the execution of the call (or set of calls) on the blockchain.
Integration tests provide the ability to have end-to-end testing that includes cross-contract calls, proper user accounts, access to state, structured execution outcomes, and more. In NEAR, we can make use of the `workspaces` libraries in both [Rust](https://github.com/near/workspaces-rs) and [JavaScript](https://github.com/near/workspaces-js) for this type of testing on a locally-run blockchain or testnet.
## When to Use Integration Tests
You'll probably want to use integration tests when:
- There are cross-contract calls.
- There are multiple users with balance changes.
- You'd like to gather information about gas usage and execution outcomes on-chain.
- You want to assert the use-case execution flow of your smart contract logic works as expected.
- You want to assert given execution patterns do not work (as expected).
## Setup
Unlike unit tests (which would often live in the `src/lib.rs` file of the contract), integration tests in Rust are located in a separate directory at the same level as `/src`, called `/tests` ([read more](https://doc.rust-lang.org/cargo/reference/cargo-targets.html#integration-tests)). Refer to this folder structure below:
```sh
├── Cargo.toml ⟵ contains `dependencies` for contract and `dev-dependencies` for workspaces-rs tests
├── src
│ └── lib.rs ⟵ contract code
├── target
└── tests ⟵ integration test directory
└── integration-tests.rs ⟵ integration test file
```
:::info
These tests don't have to be placed in their own `/tests` directory. Instead, you can place them in the `/src` directory which can be beneficial since then you can use the non-exported types for serialization within the test case.
:::
A sample configuration for this project's `Cargo.toml` is shown below:
```toml
[package]
name = "fungible-token-wrapper"
version = "0.0.2"
authors = ["Near Inc <hello@nearprotocol.com>"]
edition = "2021"
[dev-dependencies]
anyhow = "1.0"
near-primitives = "0.5.0"
near-sdk = "4.0.0"
near-units = "0.2.0"
serde_json = "1.0"
tokio = { version = "1.14", features = ["full"] }
workspaces = "0.4.1"
# remember to include a line for each contract
fungible-token = { path = "./ft" }
defi = { path = "./test-contract-defi" }
[profile.release]
codegen-units = 1
# Tell `rustc` to optimize for small code size.
opt-level = "z"
lto = true
debug = false
panic = "abort"
overflow-checks = true
[workspace]
# remember to include a member for each contract
members = [
"ft",
"test-contract-defi",
]
```
The `integration-tests.rs` file above will contain the integration tests. These can be run with the following command from the same level as the test `Cargo.toml` file:
cargo test --test integration-tests
## Comparing an Example
### Unit Test
Let's take a look at a very simple unit test and integration test that accomplish the same thing. Normally you wouldn't duplicate efforts like this (as integration tests are intended to be broader in scope), but it will be informative.
We'll be using snippets from the [fungible-token example](https://github.com/near/near-sdk-rs/blob/master/examples/fungible-token) from the `near-sdk-rs` repository to demonstrate simulation tests.
First, note this unit test that tests the functionality of the `test_transfer` method:
<Github language="rust" start="100" end="165" url="https://github.com/near/near-sdk-rs/blob/6d4045251c63ec875dc55f43b065b33a36d94792/examples/fungible-token/ft/src/lib.rs" />
The test above sets up the testing context, instantiates the test environment through `get_context()`, calls the `test_transfer` method, and performs the `storage_deposit()` initialization call (to register with the fungible token contract) and the `ft_transfer()` fungible token transfer call.
Let's look at how this might be written with workspaces tests. The snippet below is a bit longer as it demonstrates a couple of things worth noting.
### Workspaces Test
<Github language="rust" start="25" end="115" url="https://github.com/near/near-sdk-rs/blob/master/examples/fungible-token/tests/workspaces.rs" />
In the test above, the compiled smart contract `.wasm` file (which we compiled into the `/out` directory) for the Fungible Token example is dev-deployed (newly created account) to the environment. The `ft_contract` account is created as a result from the environment which is used to create accounts. This specific file's format has only one test entry point (`main`), and every test is declared with `#[tokio::test]`. Tests do not share state between runs.
Notice the layout within `test_total_supply`. `.call()` obtains its required gas from the account performing it. Unlike the unit test, there is no mocking being performed before the call as the context is provided by the environment initialized during `init()`. Every call interacts with this environment to either fetch or change state.
:::info
**Pitfall**: you must compile your contract before running integration tests. Because workspaces tests use the `.wasm` files to deploy the contracts to the network. If changes are made to the smart contract code, the smart contract wasm should be rebuilt before running these tests again.
:::
:::note
In case you wish to preserve state between runs, you can call multiple tests within one function, passing the worker around from a `workspaces::sandbox()` call.
:::
## Helpful Snippets
### Create an Account
<Github language="rust" start="16" end="21" url="https://github.com/near-examples/rust-counter/blob/6a7af5a32c630e0298c09c24eab87267746552b2/integration-tests/rs/src/tests.rs" />
:::note
You can also create a `dev_account` without having to deploy a contract as follows:
<Github language="rust" start="7" end="8" url="https://github.com/near/workspaces-rs/blob/8f12f3dc3b0251ac3f44ddf6ab6fc63003579139/workspaces/tests/create_account.rs" />
:::
### Create Helper Functions
<Github language="rust" start="148" end="161" url="https://github.com/near-examples/nft-tutorial/blob/7fb267b83899d1f65f1bceb71804430fab62c7a7/integration-tests/rs/src/helpers.rs" />
### Spooning - Pulling Existing State and Contracts from Mainnet/Testnet
This example showcases spooning state from a testnet contract into our local sandbox environment:
<Github language="rust" start="64" end="122" url="https://github.com/near/workspaces-rs/blob/c14fe2aa6cdf586028b2993c6a28240f78484d3e/examples/src/spooning.rs" />
For a full example, see the [examples/src/spooning.rs](https://github.com/near/workspaces-rs/blob/main/examples/src/spooning.rs) example.
### Fast Forwarding - Fast Forward to a Future Block
`workspaces` testing offers support for forwarding the state of the blockchain to the future. This means contracts which require time sensitive data do not need to sit and wait the same amount of time for blocks on the sandbox to be produced. We can simply just call `worker.fast_forward` to get us further in time:
<Github language="rust" start="12" end="44" url="https://github.com/near/workspaces-rs/blob/c14fe2aa6cdf586028b2993c6a28240f78484d3e/examples/src/fast_forward.rs" />
For a full example, take a look at [examples/src/fast_forward.rs](https://github.com/near/workspaces-rs/blob/main/examples/src/fast_forward.rs).
### Handle Errors
<Github language="rust" start="199" end="225" url="https://github.com/near-examples/FT/blob/98b85297a270cbcb8ef3901c29c17701e1cab698/integration-tests/rs/src/tests.rs" />
:::note
Returning `Err(msg)` is also a viable (and arguably simpler) implementation.
:::
### Batch Transactions
```rust title="Batch Transaction - workspace-rs"
let res = contract
.batch(&worker)
.call(
Function::new("ft_transfer_call")
.args_json((defi_contract.id(), transfer_amount, Option::<String>::None, "10"))?
.gas(300_000_000_000_000 / 2)
.deposit(1),
)
.call(
Function::new("storage_unregister")
.args_json((Some(true),))?
.gas(300_000_000_000_000 / 2)
.deposit(1),
)
.transact()
.await?;
```
### Inspecting Logs
```rust title="Logs - workspaces-rs"
assert_eq!(
res.logs()[1],
format!("Closed @{} with {}", contract.id(), initial_balance.0 - transfer_amount.0)
);
```
Examining receipt outcomes:
```rust title="Logs - workspaces-rs"
let outcome = &res.receipt_outcomes()[5];
assert_eq!(outcome.logs[0], "The account of the sender was deleted");
assert_eq!(outcome.logs[2], format!("Account @{} burned {}", contract.id(), 10));
```
### Profiling Gas
`CallExecutionDetails::total_gas_burnt` includes all gas burnt by call execution, including by receipts. This is exposed as a surface level API since it is a much more commonly used concept:
```rust title="Gas (all) - workspaces-rs"
println!("Burnt gas (all): {}", res.total_gas_burnt);
```
If you do actually want gas burnt by transaction itself you can do it like this:
```rust title="Gas (transaction) - workspaces-rs"
println!("Burnt gas (transaction): {}", res.outcome().gas_burnt);
```
If you want to see the gas burnt by each receipt, you can do it like this:
```rust title="Gas (receipt) - workspaces-rs"
for receipt in res.receipt_outcomes() {
println!("Burnt gas (receipt): {}", receipt.gas_burnt);
}
```
|
---
id: welcome
title: "NEAR SDKs"
hide_table_of_contents: false
---
import {FeatureList, Column, Feature} from "@site/src/components/featurelist";
Explore our language-specific SDK documentation
<FeatureList>
<Column title="SDKs">
<Feature url="/sdk/rust/introduction" title="Rust SDK" subtitle="Write Contracts in Rust" image="smartcontract-rust.png" />
</Column>
<Column title=" ">
<Feature url="/sdk/js/introduction" title="JavaScript SDK" subtitle="Write Contracts in JavaScript" image="smartcontract-js.png" />
</Column>
</FeatureList>
|
---
id: validators
title: Validators
---
The NEAR network is decentralized, meaning that multiple people collaborate in order to keep it safe. We call such people **validators**.
In order to make sure that all the transactions in the network are valid, i.e. that nobody is trying to steal money, the validators follow a specific consensus
mechanism.
Currently, there are a few well-known consensus mechanisms to keep a blockchain working correctly and resistant to attacks.
NEAR Protocol uses a version of **Proof-of-Stake**, particularly [Thresholded Proof of Stake](https://near.org/blog/thresholded-proof-of-stake/).
In Proof-of-Stake, users show support to specific network validators by delegating NEAR tokens to them. This process is known as **staking**. The main idea is that, if a validator has a large amount of tokens delegated is because the community trusts them.
### Securing the Network
Validators have two main jobs. The first is to validate and execute transactions, aggregating them in the blocks that form the blockchain. Their second job is to oversee other validators, making sure no one produces an invalid block or creates an alternative chain (eg. with the goal of creating a double spend).
If a validator is caught misbehaving, then they get "slashed", meaning that their stake (or part of it) is burned.
In the NEAR networks, an attempt to manipulate the chain would mean taking control over the majority of the validators at once, so that the malicious activity won't be flagged. However, this would require putting a huge sum of capital at risk, since an unsuccessful attack would mean slashing your staked tokens.
### Validator's Economy
In exchange for servicing the network, validators are rewarded with a target number of NEAR every epoch. The target value is computed in such a way that, on an annualized basis, it will be 4.5% of the total supply.
All transaction fees (minus the part which is allocated as the rebate for contracts) which are collected within each epoch are burned by the system. The inflationary reward is paid out to validators at the same rate regardless of the number of fees collected or burned.
## Intro to Validators
[Validators](https://pages.near.org/papers/the-official-near-white-paper/#economics) are responsible for producing blocks and the security of the network.
Since Validators validate all shards, high requirements are set for running them (an 8-Core CPU with 16GB of RAM and 1 TB SSD of storage). The cost of running a block-producing validator node is estimated to be $330 per month for hosting. Please see our [hardware and cost estimates page](https://near-nodes.io/validator/hardware) for more info.
The current active Validators are available on the Explorer. The minimum seat price to become a block-producing validator is based on the 300th proposal. (If more than 300 proposals are submitted, the threshold will simply be the stake of the 300th proposal, provided that it’s larger than the minimum threshold of 25,500 $NEAR.) The current seat price to become a block-producing validator is updated live on the Explorer. Any validator nodes with stakes higher than the seat price can join the active set of Validators.
<blockquote className="lesson">
<strong>Is there a plan to support GPU compute if certain validator nodes can offer that or is it just CPU?</strong><br /><br />
We don't need GPU support as we are a POS chain and we require very little compute power.
You can read more about our consensus strategy on our <a href="https://github.com/near/wiki/blob/master/Archive/validators/about.md">Validator Quickstart</a> and <a href="https://github.com/near/wiki/blob/master/Archive/validators/faq.md">Staking FA</a>.
</blockquote>
## Chunk-Only Validators
The Chunk-Only Producer is a more accessible role with lower hardware and token requirements. This new role will allow the network's validator number to grow, creating more opportunities to earn rewards and secure the NEAR Ecosystem.
[Chunk-Only Producers](https://pages.near.org/papers/the-official-near-white-paper/#economics) are solely responsible for [producing chunks](https://pages.near.org/papers/nightshade/#nightshade) (parts of the block from a shard, see [Nightshade](https://pages.near.org/papers/nightshade/) for more detail) in one shard (a partition on the network). Because Chunk-Only Producers only need to validate one shard, they can run the validator node on a 8-Core CPU, with 16GB of RAM, and 500 GB SSD of storage.
Like Validators, Chunk-Only Producers will receive, at minimum, 4.5% annual rewards. If less than 100% of the tokens on the network is staked, Chunk-Only Producers stand to earn even more annual rewards. For more details about the Validator’s economics, please check out [NEAR’s Economics Explained](https://near.org/blog/near-protocol-economics/).
## Dedicated Validator Documentation Site
If you'd like to further explore Validators and Nodes in general, you can visit the [Dedicated Validator Documentation Site](https://near-nodes.io/).
<blockquote className="lesson">
<strong>If a developer writes a vulnerable or malicious dApp, is a validator implicitly taking on risk?</strong><br /><br />
No. We have handled the potential damages to the network on the protocol level. For example, we have a lot of limiters that constrain how much data you can pass into a function call or how much compute you can do in one function call, etc.
That said, smart contract developers will need to be responsible for their own dApps, as there is no stage gate or approval process. All vulnerability can only damage the smart contract itself. Luckily, updating smart contracts is very smooth on NEAR, so vulnerabilities can be updated/patched to an account in ways that cannot be done on other blockchains.
</blockquote>
|
This is an example on how you can make your smart contract buy a NFT on some marketplace (Paras this case).
:::info
Please note that in this example the contract will be the owner of the NFT, however, some marketplaces allow you to buy NFT for somebody else.
:::
```rust
const NFT_MARKETPLACE_CONTRACT: &str = "paras-marketplace-v2.testnet";
// Define the contract structure
#[near_bindgen]
#[derive(BorshDeserialize, BorshSerialize)]
pub struct Contract {
nft_marketplace_contract: AccountId
}
impl Default for Contract {
// The default trait with which to initialize the contract
fn default() -> Self {
Self {
nft_marketplace_contract: NFT_MARKETPLACE_CONTRACT.parse().unwrap()
}
}
}
// Validator interface, for cross-contract calls
#[ext_contract(ext_nft_contract)]
trait ExternalNftContract {
fn buy(&self, nft_contract_id: AccountId, token_id: TokenId, ft_token_id: Option<AccountId>, price: Option<U128>) -> Promise;
}
// Implement the contract structure
#[near_bindgen]
impl Contract {
#[payable]
pub fn buy(&mut self, nft_contract_id: AccountId, token_id: TokenId, ft_token_id: Option<AccountId>, price: Option<U128>) -> Promise {
let promise = ext_nft_contract::ext(self.nft_marketplace_contract.clone())
.with_static_gas(Gas(30*TGAS))
.with_attached_deposit(env::attached_deposit())
.buy(nft_contract_id, token_id, ft_token_id, price);
return promise.then( // Create a promise to callback query_greeting_callback
Self::ext(env::current_account_id())
.with_static_gas(Gas(30*TGAS))
.buy_callback()
)
}
#[private] // Public - but only callable by env::current_account_id()
pub fn buy_callback(&self, #[callback_result] call_result: Result<(), PromiseError>) {
// Check if the promise succeeded
if call_result.is_err() {
log!("There was an error contacting NFT contract");
}
}
}
```
|
An Open Invitation to the Harmony Community
COMMUNITY
July 29, 2022
The NEAR Foundation works tirelessly to create a stable ecosystem. A place for developers, founders, and community members to collectively build an Open Web world.
During this tumultuous period for Web3 and the broader global economy, many projects that launched or are actively building on the Harmony network are now facing uncertainty. Which is why NEAR Foundation is extending an open invitation to anyone currently building on Harmony to join the NEAR community, and make this vibrant ecosystem their new home.
As part of this invitation, NEAR Foundation and Proximity Labs have put together sizable relief funds for projects from the Harmony ecosystem. If you’re looking to bring your project over to NEAR, here’s what you need to know.
Why choose NEAR?
The NEAR blockchain is built with simplicity in mind. NEAR uses common coding languages like WebAssembly (WASM) and Rust to build its smart contracts. NEAR is also launching a Javascript SDK, making the switch simple for those who built with Harmony’s own Javascript implementation, or were considering it. Aurora, which is built on NEAR, is 100% EVM-compatible, and NEAR’s super fast transactions run on an infinitely scalable yet carbon neutral protocol. By switching to NEAR, your project would join over 700 apps building on NEAR.
NEAR was built by developers for developers. NEAR Foundation and other ecosystem platforms, like Aurora and Pagoda, have vast offerings of class-leading resources for developers to begin building on NEAR. These include NEAR.University’s training and certification programs—to date, over 5,000 developers trained—and $45M+ in project grants within the NEAR ecosystem.
The NEAR community also offers extensive documentation on building and deploying on the network, and much more.
NEAR’s developer resources
Interested in developing on NEAR? Great! A range of resources are at your disposal:
Developer Tools – NEAR CLIs, SDKs, APIs and more.
Code Examples – Explore how to deploy Rust and AssemblyScript.
Developer Bounties – Bug fixes, developer grants and other incentives.
Grants Program – More than 800 projects funded and over $45 million awarded.
Office Hours – Talk to expert developers about your project.
Education – Learn, watch and build.
Ecosystem – Explore the more than 700 projects already being building in the NEAR ecosystem.
Interested in learning more about how the NEAR Foundation team can help you make the switch? Please email [email protected] with the subject line, “Harmony Migration”, and one of the teams will respond within 24 hours. |
# Function Call
In this section we provide an explanation how the `FunctionCall` action execution works, what are
the inputs and what are the outputs. Suppose runtime received the following ActionReceipt:
```rust
ActionReceipt {
id: "A1",
signer_id: "alice",
signer_public_key: "6934...e248",
receiver_id: "dex",
predecessor_id: "alice",
input_data_ids: [],
output_data_receivers: [],
actions: [FunctionCall { gas: 100000, deposit: 100000u128, method_name: "exchange", args: "{arg1, arg2, ...}", ... }],
}
```
### `input_data_ids` to `PromiseResult`s
`ActionReceipt.input_data_ids` must be satisfied before execution (see
[Receipt Matching](#receipt-matching)). Each of `ActionReceipt.input_data_ids` will be converted to
the `PromiseResult::Successful(Vec<u8>)` if `data_id.data` is `Some(Vec<u8>)` otherwise if
`data_id.data` is `None` promise will be `PromiseResult::Failed`.
## Input
The `FunctionCall` executes in the `receiver_id` account environment.
- a vector of [Promise Results](#promise-results) which can be accessed by a `promise_result`
import [PromisesAPI](Components/BindingsSpec/PromisesAPI.md) `promise_result`)
- the original Transaction `signer_id`, `signer_public_key` data from the ActionReceipt (e.g.
`method_name`, `args`, `predecessor_id`, `deposit`, `prepaid_gas` (which is `gas` in
FunctionCall))
- a general blockchain data (e.g. `block_index`, `block_timestamp`)
- read data from the account storage
A full list of the data available for the contract can be found in [Context
API](Components/BindingsSpec/ContextAPI.md) and [Trie](Components/BindingsSpec/TrieAPI.md)
## Execution
In order to implement this action, the runtime will:
- load the contract code from the `receiver_id` [account](../DataStructures/Account.md#account)’s
storage;
- parse, validate and instrument the contract code (see [Preparation](./Preparation.md));
- optionally, convert the contract code to a different executable format;
- instantiate the WASM module, linking runtime-provided functions defined in the
[Bindings Spec](Components/BindingsSpec/BindingsSpec.md) & running the start function; and
- invoke the function that has been exported from the wasm module with the name matching
that specified in the `FunctionCall.method_name` field.
Note that some of these steps may be executed during the
[`DeployContractAction`](./Actions.md#DeployContractAction) instead. This is largely an
optimization of the `FunctionCall` gas fee, and must not result in an observable behavioral
difference of the `FunctionCall` action.
During the execution of the contract, the runtime will:
- count burnt gas on execution;
- count used gas (which is `burnt gas` + gas attached to the new created receipts);
- measure the increase in account’s storage usage as a result of this call;
- collect logs produced by the contract;
- set the return data; and
- create new receipts through [PromisesAPI](Components/BindingsSpec/PromisesAPI.md).
## Output
The output of the `FunctionCall`:
- storage updates - changes to the account trie storage which will be applied on a successful call
- `burnt_gas`, `used_gas` - see [Runtime Fees](Fees/Fees.md)
- `balance` - unspent account balance (account balance could be spent on deposits of newly created
`FunctionCall`s or [`TransferAction`s](Actions.md#transferaction) to other contracts)
- `storage_usage` - storage_usage after ActionReceipt application
- `logs` - during contract execution, utf8/16 string log records could be created. Logs are not
persistent currently.
- `new_receipts` - new `ActionReceipts` created during the execution. These receipts are going to
be sent to the respective `receiver_id`s (see [Receipt Matching explanation](#receipt-matching))
- result could be either [`ReturnData::Value(Vec<u8>)`](#value-result) or
[`ReturnData::ReceiptIndex(u64)`](#receiptindex-result)`
### Value Result
If applied `ActionReceipt` contains [`output_data_receivers`](Receipts.md#output_data_receivers),
runtime will create `DataReceipt` for each of `data_id` and `receiver_id` and `data` equals
returned value. Eventually, these `DataReceipt` will be delivered to the corresponding receivers.
### ReceiptIndex Result
Successful result could not return any Value, but generates a bunch of new ActionReceipts instead.
One example could be a callback. In this case, we assume the the new Receipt will send its Value
Result to the [`output_data_receivers`](Receipts.md#output_data_receivers) of the current
`ActionReceipt`.
### Errors
As with other actions, errors can be divided into two categories: validation error and execution
error.
#### Validation Error
- If there is zero gas attached to the function call, a
```rust
/// The attached amount of gas in a FunctionCall action has to be a positive number.
FunctionCallZeroAttachedGas,
```
error will be returned
- If the length of the method name to be called exceeds `max_length_method_name`, a genesis
parameter whose current value is `256`, a
```rust
/// The length of the method name exceeded the limit in a Function Call action.
FunctionCallMethodNameLengthExceeded { length: u64, limit: u64 }
```
error is returned.
- If the length of the argument to the function call exceeds `max_arguments_length`, a genesis
parameter whose current value is `4194304` (4MB), a
```rust
/// The length of the arguments exceeded the limit in a Function Call action.
FunctionCallArgumentsLengthExceeded { length: u64, limit: u64 }
```
error is returned.
#### Execution Error
There are three types of errors which may occur when applying a function call action:
`FunctionCallError`, `ExternalError`, and `StorageError`.
* `FunctionCallError` includes everything from around the execution of the wasm binary,
from compiling wasm to native to traps occurred while executing the compiled native binary. More specifically,
it includes the following errors:
```rust
pub enum FunctionCallError {
/// Wasm compilation error
CompilationError(CompilationError),
/// Wasm binary env link error
LinkError {
msg: String,
},
/// Import/export resolve error
MethodResolveError(MethodResolveError),
/// A trap happened during execution of a binary
WasmTrap(WasmTrap),
WasmUnknownError,
HostError(HostError),
}
```
- `CompilationError` includes errors that can occur during the compilation of wasm binary.
- `LinkError` is returned when wasmer runtime is unable to link the wasm module with provided imports.
- `MethodResolveError` occurs when the method in the action cannot be found in the contract code.
- `WasmTrap` error happens when a trap occurs during the execution of the binary. Traps here include
```rust
pub enum WasmTrap {
/// An `unreachable` opcode was executed.
Unreachable,
/// Call indirect incorrect signature trap.
IncorrectCallIndirectSignature,
/// Memory out of bounds trap.
MemoryOutOfBounds,
/// Call indirect out of bounds trap.
CallIndirectOOB,
/// An arithmetic exception, e.g. divided by zero.
IllegalArithmetic,
/// Misaligned atomic access trap.
MisalignedAtomicAccess,
/// Breakpoint trap.
BreakpointTrap,
/// Stack overflow.
StackOverflow,
/// Generic trap.
GenericTrap,
}
```
- `WasmUnknownError` occurs when something inside wasmer goes wrong
- `HostError` includes errors that might be returned during the execution of a host function. Those errors are
```rust
pub enum HostError {
/// String encoding is bad UTF-16 sequence
BadUTF16,
/// String encoding is bad UTF-8 sequence
BadUTF8,
/// Exceeded the prepaid gas
GasExceeded,
/// Exceeded the maximum amount of gas allowed to burn per contract
GasLimitExceeded,
/// Exceeded the account balance
BalanceExceeded,
/// Tried to call an empty method name
EmptyMethodName,
/// Smart contract panicked
GuestPanic { panic_msg: String },
/// IntegerOverflow happened during a contract execution
IntegerOverflow,
/// `promise_idx` does not correspond to existing promises
InvalidPromiseIndex { promise_idx: u64 },
/// Actions can only be appended to non-joint promise.
CannotAppendActionToJointPromise,
/// Returning joint promise is currently prohibited
CannotReturnJointPromise,
/// Accessed invalid promise result index
InvalidPromiseResultIndex { result_idx: u64 },
/// Accessed invalid register id
InvalidRegisterId { register_id: u64 },
/// Iterator `iterator_index` was invalidated after its creation by performing a mutable operation on trie
IteratorWasInvalidated { iterator_index: u64 },
/// Accessed memory outside the bounds
MemoryAccessViolation,
/// VM Logic returned an invalid receipt index
InvalidReceiptIndex { receipt_index: u64 },
/// Iterator index `iterator_index` does not exist
InvalidIteratorIndex { iterator_index: u64 },
/// VM Logic returned an invalid account id
InvalidAccountId,
/// VM Logic returned an invalid method name
InvalidMethodName,
/// VM Logic provided an invalid public key
InvalidPublicKey,
/// `method_name` is not allowed in view calls
ProhibitedInView { method_name: String },
/// The total number of logs will exceed the limit.
NumberOfLogsExceeded { limit: u64 },
/// The storage key length exceeded the limit.
KeyLengthExceeded { length: u64, limit: u64 },
/// The storage value length exceeded the limit.
ValueLengthExceeded { length: u64, limit: u64 },
/// The total log length exceeded the limit.
TotalLogLengthExceeded { length: u64, limit: u64 },
/// The maximum number of promises within a FunctionCall exceeded the limit.
NumberPromisesExceeded { number_of_promises: u64, limit: u64 },
/// The maximum number of input data dependencies exceeded the limit.
NumberInputDataDependenciesExceeded { number_of_input_data_dependencies: u64, limit: u64 },
/// The returned value length exceeded the limit.
ReturnedValueLengthExceeded { length: u64, limit: u64 },
/// The contract size for DeployContract action exceeded the limit.
ContractSizeExceeded { size: u64, limit: u64 },
/// The host function was deprecated.
Deprecated { method_name: String },
}
```
* `ExternalError` includes errors that occur during the execution inside `External`, which is an interface between runtime
and the rest of the system. The possible errors are:
```rust
pub enum ExternalError {
/// Unexpected error which is typically related to the node storage corruption.
/// It's possible the input state is invalid or malicious.
StorageError(StorageError),
/// Error when accessing validator information. Happens inside epoch manager.
ValidatorError(EpochError),
}
```
* `StorageError` occurs when state or storage is corrupted.
|
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
<Tabs groupId="nft-contract-tabs" className="file-tabs">
<TabItem value="NFT Primitive" label="Reference" default>
```js
const tokenData = Near.call(
"nft.primitives.near",
"nft_mint",
{
token_id: "1",
receiver_id: "bob.near",
token_metadata: {
title: "NFT Primitive Token",
description: "Awesome NFT Primitive Token",
media: "string", // URL to associated media, preferably to decentralized, content-addressed storage
}
},
undefined,
10000000000000000000000, // Depends on your NFT metadata
);
```
</TabItem>
<TabItem value="Paras" label="Paras">
```js
const tokenData = Near.call(
"x.paras.near",
"nft_mint",
{
token_series_id: "490641",
receiver_id: "bob.near",
},
undefined,
10000000000000000000000 // Depends on your NFT metadata
);
```
:::note
In order to use `nft_mint` method of the `x.paras.near` contract you have to be a creator of a particular token series.
:::
</TabItem>
<TabItem value="Mintbase" label="Mintbase">
```js
const tokenData = Near.call(
"thomasettorreiv.mintbase1.near",
"nft_batch_mint",
{
num_to_mint: 1,
owner_id: "bob.near",
metadata: {},
},
undefined,
10000000000000000000000
);
```
:::note
In order to use `nft_batch_mint` method of Mintbase store contract your account have to be a in the contract minters list.
:::
</TabItem>
</Tabs> |
NEARCON 2022 is Almost Here: Explore the NEAR Ecosystem in Lisbon!
COMMUNITY
September 1, 2022
Olá amigos! There’s a lot of excitement brewing in the NEAR community for what is gearing up to be one of the year’s premier Web3 events. If you’re not already up to speed, NEARCON is more than just a typical Web3 conference with talks and panels. NEARCON will be a showcase of 700+ ecosystem projects spanning all Web3 verticals and layers in the stunning coastal city of Lisbon, in a converted warehouse space located directly on the water.
NEARCON will be an interactive demonstration of the talent, creativity, intelligence, and practical use cases of the mighty (and growing) ecosystem. It will also be a pretty rad time, with parties, IRL hackathon, food trucks from around the world, fun swag, and more!
Can’t Stop, Won’t Stop
Start the week off strong on September 11th by picking up your badge and swag bag. The NEARCON Registration Party, which is carnival-themed, will feature a bounce house from SWEAT where you can Bounce to earn SWEAT. Oh, and did we mention there will be a ferris wheel to take in the epic views that Lisbon has to offer?
Opening Night at NEARCON kicks off with a soirée of epic proportions hosted by Proximity. You won’t want to miss the future of finance taking over Lisbon in one incredible night. VIP Tickets are FREE, but they must be obtained from one of our 10 fabulous sponsors: Orderly Network, Few and Far, Cornerstone/MetaWeb, Ref.Finance, Meta Pool, Aurigami, Burrow Cash, Trisolaris, Pembrock Finance, and Bastion. Check out their Twitter, Discord, Telegram today. Tickets will be released this week!
Part of the NEARNaut tribe yet? If not, definitely get your NEARNaut NFT from one of NEAR’s marketplaces (Paras or Few and Far) before NEARCON so you can attend the NEARnaut after party. It will be a great vibe and a chance to get acquainted with this community-driven, randomly-generated NFT PFP project built on NEAR. We hear they are also demoing their mutant serums—you might want to upgrade that NEARNaut!
NEAR UA Takes Lisbon
Since March, the NEAR Ukraine Hub (NEAR UA) has made its home in Lisbon and at NEARCON you’ll get to learn all about what they’ve been up to. NEAR UA is a combination of community, project accelerator, fund pool, product lab, event and marketing service, and a hiring service for the NEAR ecosystem. At their booth you will be able to explore hub activity and discuss current projects and opportunities for collaboration. You’ll also be able to grab some swag with Ukrainian symbols to show off when you get home.
On September 13th, NEAR UA is hosting a Ukrainian night called “The Code of Freedom” with headliner ONUKA and opener Miura. You definitely don’t want to miss this or the afterparty thrown by NEARNauts.
At “The Code of Freedom” everyone will have the opportunity to learn about Ukraine, including its unique culture, hip styles, yummy food, and funky music that will make you want to get on your feet and shake it. You’ll get to witness the very “code of freedom” that helps Ukrainians now.
100% of proceeds from ticket sales will directly help Ukrainian hospitals procure modular external fixators and prepare for winter in areas in the Kharkiv region with heat, food, and hygiene. Purchase a ticket to the event and help NEAR UA surpass their goal of €20,000!
A Global Happening
Though the talks and panels will be in English, expect to hear a lot of different languages spoken at NEARCON. There will be over 170 countries represented in attendance! In addition to the NEAR UA, other regional hubs featured at NEARCON will include the Balkans, Kenya, and Vietnam.
Visit the various Regional Hubs to learn how NEAR is operating around the world. Also, expect to find delicious food trucks representing all these regions in the World Lounge. Who doesn’t love food trucks?
Mainstage Revelry
The Mainstage at NEARCON is the crown jewel of the Conference. The Mainstage is where you’ll hear keynote speeches from some of the most ambitious minds in Web3, including the top leaders at NEAR, Pagoda, Aurora, and Sweat Economy. You’ll also be present for a ton of great panels on a wide variety of Web3 topics, including the “Meaning of Money”, “Why We Need Web2.5”, “Navigating Crypto’s Cycles”, “How NEAR and SailGP Are Revolutionizing Professional Sport”, and much more.
House of Hackstravaganza
Hackers can still grab a free conference pass by applying for the hackathon here. If selected, hackers get free admission to NEARCON, and after submitting a successful hack they get a travel stipend paid in NEAR tokens. There will be a registration party/orientation on September 11th when hackers will be onboarded and teams will be assembled.
In addition to receiving hands-on mentoring, hackers will be able to compete for $145k in crypto prizes to be awarded to 10 individuals and teams, including first, second, and third place, as well as winners in categories such as Future of Finance, Web2 to Web3, Governance, and more. Check out the judges and get more information here.
Get Activated
NEARCON will be a hotspot for developer activations, with Pagoda and Aurora leading the way. You will definitely want to stop by their booths and meet their teams, especially if you are participating in the hackathon. Learn about the developer tools the team at Pagoda builds to simplify the dev experience for dapp developers as they build, deploy, test, and launch on NEAR.
Hackers will be invited to an Orientation on the 11th during the Registration Party. Meet with the Pagoda team, learn about the latest dev tools, and maybe find future hackathon team members.
You’ll also get to meet the core Aurora team and learn from them firsthand how to build dapps on Aurora EVM, enhance the UX of blockchain projects with Aurora+, and network with an array of ecosystem projects that are already up and running on Aurora.
Activations aren’t only for devs, though. Creatives will find themselves more than satisfied with the art, music, and creativity present everywhere at NEARCON, both inside and outside of the conference. Come check out artists working on NEAR including Orrin Campbell, Franknines, Strakts, and Zeitwarp. Get your imagination fed!
Women of Web3
Ten winners of the Women in Web3 Changemakers will be announced at NEARCON and it will be meaningful to honor them and make room for more women to join the Web3 ecosystem. Check out Forkast’s interview coverage to learn more about the shortlist of nominees.
There will also be a yummy brunch worth waking up early for on September 12th at Dear Breakfast, which runs from 8:30am to 10:30am. Don’t forget to set your alarm!
Discounts
TAP Air Portugal has partnered with NEAR to get you discounted airfare to NEARCON! Traveling from Paris, London, or some other European destination? Hop on a quick flight to Lisbon on the cheap. Book through flytap.com and insert the event code “IT22TPCG61” in the promotion/congress code box for 10% off your economy or business class tickets. The discount is valid only for trips made ten days before or after NEARCON.
And psst! If you want to save a little cash on your conference ticket, Pagoda and Aurora have discount codes for 35% off. Check their Twitter feeds to find them!
Don’t be afraid to say hi!
The NEARCON gathering is a great chance to see what the future holds for Web3. And if you’re developing your own project on NEAR, or thinking about building one, it’s also a fantastic time to get feedback from NEAR’s creative and supportive community. Don’t hesitate to say hello and introduce yourself. Everyone at NEAR is excited to be hosting NEARCON and will be happy to meet you.
See you in Lisbon! |
---
id: setup
title: Setup
---
In order to use the RPC API you will need to setup the correct RPC endpoints.
<hr className="subsection" />
## RPC Endpoint Setup
- `POST` for all methods
- `JSON RPC 2.0`
- `id: "dontcare"`
- endpoint URL varies by network:
- mainnet `https://rpc.mainnet.near.org`
- testnet `https://rpc.testnet.near.org`
- betanet `https://rpc.betanet.near.org` _(may be unstable)_
- localnet `http://localhost:3030`
### Limits
- Maximum number of requests per IP: 600 req/min
<hr className="subsection" />
## Querying Historical Data
Querying historical data (older than 5 [epochs](../../1.concepts/basics/epoch.md) or ~2.5 days), you may get responses that the data is not available anymore. In that case, archival RPC nodes will come to your rescue:
- mainnet `https://archival-rpc.mainnet.near.org`
- testnet `https://archival-rpc.testnet.near.org`
You can see this interface defined in `nearcore` [here](https://github.com/near/nearcore/blob/bf9ae4ce8c680d3408db1935ebd0ca24c4960884/chain/jsonrpc/client/src/lib.rs#L181).
### Limits
- Maximum number of requests per IP: 600 req/min
---
## Postman Setup {#postman-setup}
An easy way to test the queries in this documentation page is to use an API request tool such as [Postman](https://www.postman.com/).
You only need to configure two things:
1. Make sure you add a header with a key of `Content-Type` and value of `application/json`.

2. Then select the `Body` tab and choose the `raw` radio button and ensure `JSON` is the selected format.

After that is set up, just copy/paste the `JSON object` example snippets below into the `body` of your request, on Postman, and click `send`.
---
## JavaScript Setup {#javascript-setup}
All of the queries listed in this documentation page can be called using [`near-api-js`](https://github.com/near/near-api-js).
- For `near-api-js` installation and setup please refer to `near-api-js` [quick reference documentation](/tools/near-api-js/quick-reference).
- All JavaScript code snippets require a `near` object. For examples of how to instantiate, [**click here**](/tools/near-api-js/quick-reference#connect).
---
## HTTPie Setup {#httpie-setup}
If you prefer to use a command line interface, we have provided RPC examples you can use with [HTTPie](https://httpie.org/). Please note that params take
either an object or array passed as a string.
```bash
http post https://rpc.testnet.near.org jsonrpc=2.0 id=dontcare method=network_info params:='[]'
```
---
## Using `block_id` param {#using-block_id-param}
The `block_id` param can take either the block number (e.g. `27912554`) or the block hash (e.g. `'3Xz2wM9rigMXzA2c5vgCP8wTgFBaePucgUmVYPkMqhRL'` ) as an argument.
:::caution
The block IDs of transactions shown in [NearBlocks Explorer](https://testnet.nearblocks.io) are not necessarily the block ID of the executed transaction. Transactions may execute a block or two after its recorded, and in some cases, can take place over several blocks. Due to this, it is important to to check subsequent blocks to be sure all results related to the queried transaction are discovered.
:::
---
## Using `finality` param {#using-finality-param}
The `finality` param has two options: `optimistic` and `final`.
1. `optimistic` uses the latest block recorded on the node that responded to your query _(< 1 second delay after the transaction is submitted)_
2. `final` is for a block that has been validated on at least 66% of the nodes in the network _(usually takes 2 blocks / approx. 2 second delay)_
|
NEAR Community Update: March 22, 2019
COMMUNITY
March 22, 2019
If you’ve ever observed a duckling gliding across the surface of a pond, you know that what looks like effortless motion above the water is generated because their little feet spin furiously below the surface to keep them moving. For us, it’s definitely been a duck week — lots going on beneath the surface while we continue the march towards MainNet.
A big welcome to Peter DePaulo, who is joining us in Developer Relations. Peter comes with a rich background that spans everything from open source communities to engineering products that serve some of the biggest companies in the world. On his first day, he upgraded our documentation to a much more user-friendly organizational style and his presence will undoubtedly be felt in the community.
The Whiteboard Series continues to pick up steam and is best consumed on our YouTube channel, despite how educational it is in GIF form:
Community and Events
After an exhilarating — and exhausting — couple of months packed full of events, it’s good to have the team back in one place for a hot second to focus on creative ideation and building. It’s also helpful to have the Game Developer Conference in our home town this week as well because we’ve had the opportunity to meet some very interesting projects and show off our usability.
We will be pushing community development efforts in some exciting new directions over the upcoming weeks so stay tuned for that.
If you want to host a local NEAR meetup in your city or see if we can support yours, reach out to [email protected].
Recent Events
[Paris] March 8–10: Events around Eth Paris
[Tokyo] March 8: NEAR at Waseda University
[Tokyo] March 9: NEAR at HashHub
[Beijing] March 11: NEAR at Beijing AI
[Beijing] March 13: NEAR at Tsinghua University
[SF] March 20: Building on a Blockchain DevNet (Workshop)
[San Jose] March 21: Blockchain Developer Meetup
Upcoming Events
[Berlin] Mar 25: NEAR, Fluence and Cosmos DevNet and MainNet Demo Night
[SF] Mar 27: Unblocked SF with Erik Trautman of NEAR Protocol
[SF] Mar 28: Decentralized Computing with WASM @ Github
[Uruguay] Mar 29: Rust Latam conference
[San Mateo] Mar 30–31: Nueva Hacks Hackathon
[Sydney] April 8: EdCon
[Fremont] April 20: Launch Hacks hackathon
If you want to collaborate on events, reach out to [email protected]
Writing and Content
Dr Maksym Zavershynskyi wrote another in-depth post on Rust called Remote Development and Debugging of Rust with CLion. The Whiteboard Series continues strong with several new videos:
Ep9: Stan Kladko of SKALE Labs
Ep10: David Utrobin from MakerDAO
EP11: Guang Yang from Conflux
Engineering Highlights
On the application/development layer, we’re re-skinning the UIs for the wallet and block explorers. On the blockchain layer, we’ve deployed our multi-node AlphaNet (a Proof-of-Authority-style network that runs a full consensus) and are stabilizing for public release.
There were 42 PRs in nearcore from 10 different authors over the last couple of weeks. As always, the code is completely open source on Github.
Blockchain Layer
Stabilizing TestNet “Alpha”: reactive catchup for blocks and bug fixes. Follow the documentation to launch your own network and attach our studio IDE to it.
Updated runtime WASM api to support faster reads and writes
Caching authority computation to speed up startup time
Benchmarks for the client excluding network and consensus.
Improved APIs and integration tests for DevNet while improving stability.
Application/Development Layer
Streamlined command line tools for local development, testing, and deployment
Continued work on improving receipts for cross-contract calls.
Deployed new docs.
How You Can Get Involved
Join us! If you want to work with one of the most talented teams in the world right now to solve incredibly hard problems, check out our careers page for openings.
Learn more about NEAR in The Beginner’s Guide to NEAR Protocol. Stay up to date with what we’re building by following us on Twitter for updates, joining the conversation on Discord and subscribing to our newsletter to receive updates right to your inbox.
Reminder: you can help out significantly by adding your thoughts in our 2-minute Developer Survey.
https://upscri.be/633436/ |
---
sidebar_position: 4
sidebar_label: Shard
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
# `Shard` structure
## Definition
`IndexerShard` struct is ephemeral structure, there is no such entity in `nearcore`. We've introduces it as a container in [`near-indexer-primitives`](https://crates.io/crates/near-indexer-primitives). This container includes:
- shard ID
- [Chunk](chunk.mdx) that might be absent
- [ExecutionOutcomes](execution_outcome.mdx) for [Receipts](receipt.mdx) (these belong to a Shard not to a [Chunk](chunk.mdx) or a [Block](block.mdx))
- [StateChanges](state_change.mdx) for a Shard
## `IndexerShard`
<Tabs>
<TabItem value="rust" label="Rust">
```rust links=1
pub struct IndexerShard {
pub shard_id: types::ShardId,
pub chunk: Option<IndexerChunkView>,
pub receipt_execution_outcomes: Vec<IndexerExecutionOutcomeWithReceipt>,
pub state_changes: views::StateChangesView,
}
```
</TabItem>
<TabItem value="typescript" value="TypeScript">
```ts links=1
export interface Shard {
shard_id: number;
chunk?: Chunk;
receiptExecutionOutcomes: ExecutionOutcomeWithReceipt[];
stateChanges: StateChange[];
};
```
</TabItem>
</Tabs>
|
---
NEP: 0399
Title: Flat Storage
Author: Aleksandr Logunov <alex.logunov@near.org> Min Zhang <min@near.org>
DiscussionsTo: https://github.com/nearprotocol/neps/pull/0399
Status: Draft
Type: Protocol Track
Category: Storage
Created: 30-Sep-2022
---
## Summary
This NEP proposes the idea of Flat Storage, which stores a flattened map of key/value pairs of the current
blockchain state on disk. Note that original Trie (persistent merkelized trie) is not removed, but Flat Storage
allows to make storage reads faster, make storage fees more predictable and potentially decrease them.
## Motivation
Currently, the blockchain state is stored in our storage only in the format of persistent merkelized tries.
Although it is needed to compute state roots and prove the validity of states, reading from it requires a
traversal from the trie root to the leaf node that contains the key value pair, which could mean up to
2 \* key_length disk accesses in the worst case.
In addition, we charge receipts by the number of trie nodes they touched (TTN cost). Note that the number
of touched trie node does not always equal to the key length, it depends on the internal trie structure.
Based on some feedback from contract developers collected in the past, they are interested in predictable fees,
but TTN costs are annoying to predict and can lead to unexpected excess of the gas limit. They are also a burden
for NEAR Protocol client implementations, i.e. nearcore, as exact TTN number must be computed deterministically
by all clients. This prevents storage optimizations that use other strategies than nearcore uses today.
With Flat Storage, number of disk reads is reduced from worst-case 2 \* key_length to exactly 2, storage read gas
fees are simplified by getting rid of TTN cost, and potentially can be further reduced because fewer disk reads
are needed.
## Rationale and alternatives
Q: Why is this design the best in the space of possible designs?
A: Space of possible designs is quite big here, let's show some meaningful examples.
The most straightforward one is just to increase TTN, or align it with biggest possible value, or alternatively increase
the base fees for storage reads and writes. However, technically the biggest TTN could be 4096 as of today. And we tend
to strongly avoid increasing fees, because it may break existing contract calls, not even mentioning that it would greatly
reduce capacity of NEAR blocks, because for current mainnet usecases depth is usually below 20.
We also consider changing tree type from Trie to AVL, B-tree, etc. to make number of traversed nodes more stable and
predictable. But we approached AVL idea, and implementation turned out to be tricky, so we didn't go
much further than POC here: https://github.com/near/nearcore/discussions/4815. Also for most key-value pairs
tree depth will actually increase - for example, if you have 1M keys, depth is always 20, and it would cause increasing
fees as well. Size of intermediate node also increases, because we have to need to store a key there to decide whether
we should go to the left or right child.
Separate idea is to get rid of global state root completely: https://github.com/near/NEPs/discussions/425. Instead,
we could track the latest account state in the block where it was changed. But after closer look, it brings us to
similar questions - if some key was untouched for a long time, it becomes harder to find exact block to find latest
value for it, and we need some tree-shaped structure again. Because ideas like that could be also extremely invasive,
we stopped considering them at this point.
Other ideas around storage include exploring databases other than RocksDB, or moving State to a separate database.
We can tweak State representation, i.e. start trie key from account id to achieve data locality within
the same account. However, Flat Storage main goal is speed up reads and make their costs predictable, and these ideas
are orthogonal to that, although they still can improve storage in other ways.
Q: What other designs have been considered and what is the rationale for not choosing them?
A: There were several ideas on Flat Storage implementation. One of questions is whether we need global flat storage
for all shards or separate flat storages. Due to how sharding works, we have make flat storages separate, because in
the future node may have to catchup new shard while already tracking old shards, and flat storage heads (see
Specification) must be different for these shards.
Flat storage deltas are another tricky part of design, but we cannot avoid them, because at certain points of time
different nodes can disagree what is the current chain head, and they have to support reads for some subset of latest
blocks with decent speed. We can't really fallback to Trie in such cases because storage reads for it are much slower.
Another implementation detail is where to put flat storage head. The planned implementation doesn't rely on that
significantly and can be changed, but for MVP we assume flat storage head = chain final head as a simplest solution.
Q: What is the impact of not doing this?
A: Storage reads will remain inefficiently implemented and cost more than they should, and the gas fees will remain
difficult for the contract developers to predict.
## Specification
The key idea of Flat Storage is to store a direct mapping from trie keys to values in the DB.
Here the values of this mapping can be either the value corresponding to the trie key itself,
or the value ref, a hash that points to the address of the value. If the value itself is stored,
only one disk read is needed to look up a value from flat storage, otherwise two disk reads are needed if the value
ref is stored. We will discuss more in the following section for whether we use values or value refs.
For the purpose of high level discussion, it suffices to say that with Flat Storage,
at most two disk reads are needed to perform a storage read.
The simple design above won't work because there could be forks in the chain. In the following case, FlatStorage
must support key value lookups for states of the blocks on both forks.
```text
/ Block B1 - Block B2 - ...
block A
\ Block C1 - Block C2 - ...
```
The handling of forks will be the main consideration of the following design. More specifically,
the design should satisfy the following requirements,
1. It should support concurrent block processing. Blocks on different forks are processed
concurrently in the nearcore Client code, the struct which responsibility includes receiving blocks from network,
scheduling applying chunks and writing results of that to disk. Flat storage API must be aligned with that.
2. In case of long forks, block processing time should not be too much longer than the average case.
We don’t want this case to be exploitable. It is acceptable that block processing time is 200ms longer,
which may slow down block production, but probably won’t cause missing blocks and chunks.
10s delays are not acceptable and may lead to more forks and instability in the network.
3. The design must be able to decrease storage access cost in all cases,
since we are going to change the storage read fees based on flat storage.
We can't conditionally enable Flat Storage for some blocks and disable it for other, because
the fees we charge must be consistent.
The mapping of key value pairs FlatStorage stored on disk matches the state at some block.
We call this block the head of flat storage, or the flat head. During block processing,
the flat head is set to the last final block. The Doomslug consensus algorithm
guarantees that if a block is final, all future final blocks must be descendants of this block.
In other words, any block that is not built on top of the last final block can be discarded because they
will never be finalized. As a result, if we use the last final block as the flat head, any block
FlatStorage needs to process is a descendant of the flat head.
To support key value lookups for other blocks that are not the flat head, FlatStorage will
store key value changes(deltas) per block for these blocks.
We call these deltas FlatStorageDelta (FSD). Let’s say the flat storage head is at block h,
and we are applying transactions based on block h’. Since h is the last final block,
h is an ancestor of h'. To access the state at block h', we need FSDs of all blocks between h and h'.
Note that all these FSDs must be stored in memory, otherwise, the access of FSDs will trigger
more disk reads and we will have to set storage key read fee higher.
### FSD size estimation
We prefer to store deltas in memory, because memory read is much faster than disk read, and even a single extra RocksDB
access requires increasing storage fees, which is not desirable. To reduce delta size, we will store hashes of trie keys
instead of keys, because deltas are read-only. Now let's carefully estimate FSD size.
We can do so using protocol fees as of today. Assume that flat state stores a mapping from keys to value refs.
Maximal key length is ~2 KiB which is the limit of contract data key size. During wasm execution, we pay
`wasm_storage_write_base` = 64 Ggas per call. Entry size is 68 B for key hash and value ref.
Then the total size of keys changed in a block is at most
`chunk_gas_limit / gas_per_entry * entry_size * num_shards = (1300 Tgas / 64 Ggas) * 68 B * 4 ~= 5.5 MiB`.
Assuming that we can increase RAM requirements by 1 GiB, we can afford to store deltas for 100-200 blocks
simultaneously.
Note that if we store a value instead of value ref, size of FSDs can potentially be much larger.
Because value limit is 4 MiB, we can’t apply previous argument about base cost.
Since `wasm_storage_write_value_byte` = 31 Mgas, values contribution to FSD size can be estimated as
`(1300 Tgas / storage_write_value_byte * num_shards)`, or ~167 MiB. Same estimation for trie keys gives 54 MiB.
The advantage of storing values instead of value refs is that it saves one disk read if the key has been
modified in the recent blocks. It may be beneficial if we get many transactions or receipts touching the same
trie keys in consecutive blocks, but it is hard to estimate the value of such benefits without more data.
We may store only short values ("inlining"), but this idea is orthogonal and can be applied separately.
### Protocol changes
Flat Storage itself doesn't change protocol. We only change impacted storage costs to reflect changes in performance. Below we describe reads and writes separately.
#### Storage Reads
Latest proposal for shipping storage reads is [here](https://github.com/near/nearcore/issues/8006#issuecomment-1473718509).
It solves several issues with costs, but the major impact of flat storage is that essentially for reads
`wasm_touching_trie_node` and `wasm_read_cached_trie_node` are reduced to 0. Reason is that before we had to cover costs
of reading nodes from memory or disk, and with flat storage we make only 2 DB reads.
Latest up-to-date gas and compute costs can be found in nearcore repo.
#### Storage Writes
Storage writes are charged similarly to reads and include TTN as well, because updating the leaf trie
node which stores the value to the trie key requires updating all trie nodes on the path leading to the leaf node.
All writes are committed at once in one db transaction at the end of block processing, outside of runtime after
all receipts in a block are executed. However, at the time of execution, runtime needs to calculate the cost,
which means it needs to know how many trie nodes the write affects, so runtime will issue a read for every write
to calculate the TTN cost for the write. Such reads cannot be replaced by a read in FlatStorage because FlatStorage does
not provide the path to the trie node.
There are multiple proposals on how storage writes can work with FlatStorage.
- Keep it the same. The cost of writes remain the same. Note that this can increase the cost for writes in
some cases, for example, if a contract first read from a key and then writes to the same key in the same chunk.
Without FlatStorage, the key will be cached in the chunk cache after the read, so the write will cost less.
With FlatStorage, the read will go through FlatStorage, the write will not find the key in the chunk cache and
it will cost more.
- Remove the TTN cost from storage write fees. Currently, there are two ideas in this direction.
- Charge based on maximum depth of a contract’s state, instead of per-touch-trie node.
- Charge based on key length only.
Both of the above ideas would allow us to get rid of trie traversal ("reads-for-writes") from the critical path of
block execution. However,
it is unclear at this point what the new cost would look like and whether further optimizations are needed
to bring down the cost for writes in the new cost model.
See https://gov.near.org/t/storage-write-optimizations/30083 for more details.
While storage writes are not fully implemented yet, we may increase parameter compute cost for storage writes implemented
in https://github.com/near/NEPs/pull/455 as an intermediate solution.
### Migration Plan
There are two main questions regarding to how to enable FlatStorage.
1. Whether there should be database migration. The main challenge of enabling FlatStorage will be to build the flat state
column, which requires iterating the entire state. Estimations showed that it takes 10 hours to build
flat state for archival nodes and 5 hours for rpc and validator nodes in 8 threads. The main concern is that if
it takes too long for archival node to migrate,
they may have a hard time catching up later since the block processing speed of archival nodes is not very fast.
Alternatively, we can build the flat state in a background process while the node is running. This provides a better
experience for both archival and validator nodes since the migration process is transient to them. It would require
more implementation effort from our side.
We currently proceed with background migration using 8 threads.
2. Whether there should be a protocol upgrade. The enabling of FlatStorage itself does not require a protocol upgrade, since
it is an internal storage implementation that doesn't change protocol level. However, a protocol upgrade is needed
if we want to adjust fees based on the storage performance with FlatStorage. These two changes can happen in one release,
or we can be release them separately. We propose that the enabling of FlatStorage and the protocol upgrade
to adjust fees should happen in separate release to reduce the risk. The period between the two releases can be
used to test the stability and performance of FlatStorage. Because it is not a protocol change, it is easy to roll back
the change in case any issue arises.
## Reference Implementation
FlatStorage will implement the following structs.
`FlatStorageChunkView`: interface for getting value or value reference from flat storage for
specific shard, block hash and trie key. In current logic we plan to make it part of `Trie`,
and all trie reads will be directed to this object. Though we could work with chunk hashes, we don't,
because block hashes are easier to navigate.
`FlatStorage`: API for interacting with flat storage for fixed shard, including updating head,
adding new delta and creating `FlatStorageChunkView`s.
for example, all block deltas that are stored in flat storage and the flat
storage head. `FlatStorageChunkView` can access `FlatStorage` to get the list of
deltas it needs to apply on top of state of current flat head in order to
compute state of a target block.
`FlatStorageManager`: owns flat storages for all shards, being stored in `NightshadeRuntime`, accepts
updates from `Chain` side, caused by successful processing of chunk or block.
`FlatStorageCreator`: handles flat storage structs creation or initiates background creation (aka migration
process) if flat storage data is not presend on DB yet.
`FlatStateDelta`: a HashMap that contains state changes introduced in a chunk. They can be applied
on top the state at flat storage head to compute state at another block.
The reason for having separate flat storages that there are two modes of block processing,
normal block processing and block catchups.
Since they are performed on different ranges of blocks, flat storage need to be able to support
different range of blocks on different shards. Therefore, we separate the flat storage objects
used for different shards.
### DB columns
`DBCol::FlatState` stores a mapping from trie keys to the value corresponding to the trie keys,
based on the state of the block at flat storage head.
- _Rows_: trie key (`Vec<u8>`)
- _Column type_: `ValueRef`
`DBCol::FlatStateDeltas` stores all existing FSDs as mapping from `(shard_id, block_hash, trie_key)` to the `ValueRef`.
To read the whole delta, we read all values for given key prefix. This delta stores all state changes introduced in the
given shard of the given block.
- _Rows_: `{ shard_id, block_hash, trie_key }`
- _Column type_: `ValueRef`
Note that `FlatStateDelta`s needed are stored in memory, so during block processing this column won't be used
at all. This column is only used to load deltas into memory at `FlatStorage` initialization time when node starts.
`DBCol::FlatStateMetadata` stores miscellaneous data about flat storage layout, including current flat storage
head, current creation status and info about deltas existence. We don't specify exact format here because it is under
discussion and can be tweaked until release.
Similarly, flat head is also stored in `FlatStorage` in memory, so this column is only used to initialize
`FlatStorage` when node starts.
### `FlatStateDelta`
`FlatStateDelta` stores a mapping from trie keys to value refs. If the value is `None`, it means the key is deleted
in the block.
```rust
pub struct FlatStateDelta(HashMap<Vec<u8>, Option<ValueRef>>);
```
```rust
pub fn from_state_changes(changes: &[RawStateChangesWithTrieKey]) -> FlatStateDelta
```
Converts raw state changes to flat state delta. The raw state changes will be returned as part of the result of
`Runtime::apply_transactions`. They will be converted to `FlatStateDelta` to be added
to `FlatStorage` during `Chain::postprocess_block` or `Chain::catch_up_postprocess`.
### `FlatStorageChunkView`
`FlatStorageChunkView` will be created for a shard `shard_id` and a block `block_hash`, and it can perform
key value lookup for the state of shard `shard_id` after block `block_hash` is applied.
```rust
pub struct FlatStorageChunkView {
/// Used to access flat state stored at the head of flat storage.
store: Store,
/// The block for which key-value pairs of its state will be retrieved. The flat state
/// will reflect the state AFTER the block is applied.
block_hash: CryptoHash,
/// Stores the state of the flat storage
flat_storage: FlatStorage,
}
```
`FlatStorageChunkView` will provide the following interface.
```rust
pub fn get_ref(
&self,
key: &[u8],
) -> Result<Option<ValueRef>, StorageError>
```
Returns the value or value reference corresponding to the given `key`
for the state that this `FlatStorageChunkView` object represents, i.e., the state that after
block `self.block_hash` is applied.
### `FlatStorageManager`
`FlatStorageManager` will be stored as part of `ShardTries` and `NightshadeRuntime`. Similar to how `ShardTries` is used to
construct new `Trie` objects given a state root and a shard id, `FlatStorageManager` is used to construct
a new `FlatStorageChunkView` object given a block hash and a shard id.
```rust
pub fn new_flat_storage_chunk_view(
&self,
shard_id: ShardId,
block_hash: Option<CryptoHash>,
) -> FlatStorageChunkView
```
Creates a new `FlatStorageChunkView` to be used for performing key value lookups on the state of shard `shard_id`
after block `block_hash` is applied.
```rust
pub fn get_flat_storage(
&self,
shard_id: ShardId,
) -> Result<FlatStorage, FlatStorageError>
```
Returns the `FlatStorage` for the shard `shard_id`. This function is needed because even though
`FlatStorage` is part of `NightshadeRuntime`, `Chain` also needs access to `FlatStorage` to update flat head.
We will also create a function with the same in `NightshadeRuntime` that calls this function to provide `Chain` to access
to `FlatStorage`.
```rust
pub fn remove_flat_storage(
&self,
shard_id: ShardId,
) -> Result<FlatStorage, FlatStorageError>
```
Removes flat storage for shard if we stopped tracking it.
### `FlatStorage`
`FlatStorage` is created per shard. It provides information to which blocks the flat storage
on the given shard currently supports and what block deltas need to be applied on top the stored
flat state on disk to get the state of the target block.
```rust
fn get_blocks_to_head(
&self,
target_block_hash: &CryptoHash,
) -> Result<Vec<CryptoHash>, FlatStorageError>
```
Returns the list of deltas between blocks `target_block_hash` (inclusive) and flat head (exclusive),
Returns an error if `target_block_hash` is not a direct descendent of the current flat head.
This function will be used in `FlatStorageChunkView::get_ref`. Note that we can't call it once and store during applying
chunk, because in parallel to that some block can be processed and flat head can be updated.
```rust
fn update_flat_head(&self, new_head: &CryptoHash) -> Result<(), FlatStorageError>
```
Updates the head of the flat storage, including updating the flat head in memory and on disk,
update the flat state on disk to reflect the state at the new head, and gc the `FlatStateDelta`s that
are no longer needed from memory and from disk.
```rust
fn add_block(
&self,
block_hash: &CryptoHash,
delta: FlatStateDelta,
) -> Result<StoreUpdate, FlatStorageError>
```
Adds `delta` to `FlatStorage`, returns a `StoreUpdate` object that includes DB transaction to be committed to persist
that change.
```rust
fn get_ref(
&self,
block_hash: &CryptoHash,
key: &[u8],
) -> Result<Option<ValueRef>, FlatStorageError>
```
Returns `ValueRef` from flat storage state on top of `block_hash`. Returns `None` if key is not present, or an error if
block is not supported.
### Thread Safety
We should note that the implementation of `FlatStorage` must be thread safe because it can
be concurrently accessed by multiple threads. A node can process multiple blocks at the same time
if they are on different forks, and chunks from these blocks can trigger storage reads in parallel.
Therefore, `FlatStorage` will be guarded by a `RwLock` so its access can be shared safely:
```rust
pub struct FlatStorage(Arc<RwLock<FlatStorageInner>>);
```
## Drawbacks
The main drawback is that we need to control total size of state updates in blocks after current final head.
current testnet/mainnet load amount of blocks under final head doesn't exceed 5 in 99.99% cases, we still have to
consider extreme cases, because Doomslug consensus doesn't give guarantees / upper limit on that. If we don't consider
this at all and there is no finality for a long time, validator nodes can crash because of too many FSDs of memory, and
chain slows down and stalls, which can have a negative impact on user/validator experience and reputation. For now, we
claim that we support enough deltas in memory for chain to be finalized, and the proper discussions are likely to happen
in NEPs like https://github.com/near/NEPs/pull/460.
Risk of DB corruption slightly increases, and it becomes harder to replay blocks on chain. While `Trie` entries are
essentially immutable (in fact, value for each key is
unique, because key is a value hash), `FlatStorage` is read-modify-write, because values for the same `TrieKey` can be
completely different. We believe that such flat mapping is reasonable to maintain anyway, as for newly discovered state
sync idea. But if some change was applied incorrectly, we may have to recompute the whole flat storage, and for block
hashes before flat head we can't access flat storage at all.
Though Flat Storage significantly reduces amount of storage reads, we have to keep it up-to-date, which results in 1
extra disk write for changed key, and 1 auxiliary disk write + removal for each FSD. Disk requirements also slightly
increase. We think it is acceptable, because actual disk writes are executed in background and are not a bottleneck
for block processing. For storage write in general Flat Storage is even a net improvement, because it removes
necessity to traverse changed nodes during write execution ("reads-for-writes"), and we can apply optimizations
there (see "Storage Writes" section).
Implementing FlatStorage will require a lot of engineering effort and introduce code that will make the codebase more
complicated. In particular, we had to extend `RuntimeAdapter` API with flat storage-related method after thorough
considerations. We are confident that FlatStorage will bring a lot of performance benefit, but we can only measure the exact
improvement after the implementation. We may find that the benefit FlatStorage brings is not
worth the effort, but it is very unlikely.
It will make the state rollback harder in the future when we enable challenges in phase 2 of sharding.
When a challenge is accepted and the state needs to be rolled back to a previous block, the entire flat state needs to
be rebuilt, which could take a long time. Alternatively, we could postpone garbage collection of deltas and add support
of applying them backwards.
Speaking of new sharding phases, once nodes are no longer tracking all shards, Flat Storage must have support for adding
or removing state for some specific shard. Adding new shard is a tricky but natural extension of catchup process. Our
current approach for removal is to iterate over all entries in `DBCol::FlatState` and find out for each trie key to
which shard it belongs to. We would be happy to assume that each shard is represented by set of
contiguous ranges in `DBCol::FlatState` and make removals simpler, but this is still under discussion.
Last but not least, resharding is not supported by current implementation yet.
## Future possibilities
Flat Storage maintains all state keys in sorted order, which seems beneficial. We currently investigate opportunity to
speed up state sync: instead of traversing state part in Trie, we can extract range of keys and values from Flat Storage
and build range of Trie nodes based on it. It is well known that reading Trie nodes is a bottleneck for state sync as
well.
## Changelog
### 1.0.0 - Initial Version
The NEP was approved by Protocol Working Group members on March 16, 2023 ([meeting recording](https://www.youtube.com/watch?v=4VxRoKwLXIs)):
- [Bowen's vote](https://github.com/near/NEPs/pull/399#issuecomment-1467010125)
- [Marcelo's vote](https://github.com/near/NEPs/pull/399#pullrequestreview-1341069564)
- [Marcin's vote](https://github.com/near/NEPs/pull/399#issuecomment-1465977749)
#### Benefits
- The proposal makes serving reads more efficient; making the NEAR protocol cheaper to use and increasing the capacity of the network;
- The proposal makes estimating gas costs for a transaction easier as the fees for reading are no longer a function of the trie structure whose shape the smart contract developer does not know ahead of time and can continuously change.
- The proposal should open doors to enabling future efficiency gains in the protocol and further simplifying gas fee estimations.
- 'Secondary' index over the state data - which would allow further optimisations in the future.
#### Concerns
| # | Concern | Resolution | Status |
| --- | ---------------------------------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- | ------------------------------------------------------------------------------------- |
| 1 | The cache requires additional database storage | There is an upper bound on how much additional storage is needed. The costs for the additional disk storage should be negligible | Not an issue |
| 2 | Additional implementation complexity | Given the benefits of the proposal, I believe the complexity is justified | not an issue |
| 3 | Additional memory requirement | Most node operators are already operating over-provisioned machines which can handle the additional memory requirement. The minimum requirements should be raised but it appears that minimum requirements are already not enough to operate a node | This is a concern but it is not specific to this project |
| 4 | Slowing down the read-update-write workload | This is common pattern in smart contracts so indeed a concern. However, there are future plans on how to address this by serving writes from the flat storage as well which will also reduce the fees of serving writes and make further improvements to the NEAR protocol | This is a concern but hopefully will be addressed in future iterations of the project |
## Copyright
Copyright and related rights waived via [CC0](https://creativecommons.org/publicdomain/zero/1.0/).
|
---
id: state-change
sidebar_position: 9
sidebar_label: StateChange
---
import Tabs from '@theme/Tabs';
import TabItem from '@theme/TabItem';
# `StateChange` Structure
## Definition
This entitiy from `nearcore` describes how account's state has changed and the reason
## `StateChangeWithCauseView`
<Tabs>
<TabItem value="rust" label="Rust">
```rust links=1
pub struct StateChangeWithCauseView {
pub cause: StateChangeCauseView,
#[serde(flatten)]
pub value: StateChangeValueView,
}
pub enum StateChangeCauseView {
NotWritableToDisk,
InitialState,
TransactionProcessing { tx_hash: CryptoHash },
ActionReceiptProcessingStarted { receipt_hash: CryptoHash },
ActionReceiptGasReward { receipt_hash: CryptoHash },
ReceiptProcessing { receipt_hash: CryptoHash },
PostponedReceipt { receipt_hash: CryptoHash },
UpdatedDelayedReceipts,
ValidatorAccountsUpdate,
Migration,
Resharding,
}
pub enum StateChangeValueView {
AccountUpdate {
account_id: AccountId,
#[serde(flatten)]
account: AccountView,
},
AccountDeletion {
account_id: AccountId,
},
AccessKeyUpdate {
account_id: AccountId,
public_key: PublicKey,
access_key: AccessKeyView,
},
AccessKeyDeletion {
account_id: AccountId,
public_key: PublicKey,
},
DataUpdate {
account_id: AccountId,
#[serde(rename = "key_base64", with = "base64_format")]
key: StoreKey,
#[serde(rename = "value_base64", with = "base64_format")]
value: StoreValue,
},
DataDeletion {
account_id: AccountId,
#[serde(rename = "key_base64", with = "base64_format")]
key: StoreKey,
},
ContractCodeUpdate {
account_id: AccountId,
#[serde(rename = "code_base64", with = "base64_format")]
code: Vec<u8>,
},
ContractCodeDeletion {
account_id: AccountId,
},
}
```
</TabItem>
<TabItem value="typescript" label="TypeScript">
```ts links=1
export type StateChange = {
cause: {
receiptHash: string;
type: string;
};
change: {
accountId: string;
keyBase64: string;
valueBase64: string;
},
type: string;
};
```
</TabItem>
</Tabs>
|
---
id: series
title: Customizing the NFT Contract
sidebar_label: Lazy Minting, Collections, and More!
---
import {Github} from "@site/src/components/codetabs"
In this tutorial, you'll learn how to take the [existing NFT contract](https://github.com/near-examples/nft-tutorial) you've been working with and modify it to meet some of the most common needs in the ecosystem. This includes:
- Lazy Minting NFTs
- Creating Collections
- Restricting Minting Access
- Highly Optimizing Storage
- Hacking Enumeration Methods
---
## Introduction
Now that you have a deeper understanding of basic NFT smart contracts, we can start to get creative and implement more unique features. The basic contract works really well for simple use-cases but as you begin to explore the potential of NFTs, you can use it as a foundation to build upon.
A fun analogy would be that you now have a standard muffin recipe and it's now up to you to decide how to alter it to create your own delicious varieties, may I suggest blueberry perhaps.
Below we've created a few of these new varieties by showing potential solutions to the problems outlined above. As we demonstrate how to customize the basic NFT contract, we hope it activates your ingenuity thus introducing you to what's possible and helping you discover the true potential of NFTs. 💪
<hr class="subsection" />
### NFT Collections and Series
NFT Collections help solve two common problems when dealing with the basic NFT contract:
- Storing repeated data.
- Organizing data and code.
The concept of a collection in the NFT space has a very loose meaning and can be interpreted in many different ways. In our case, we'll define a collection as a set of tokens that share **similar metadata**. For example, you could create a painting and want 100 identical copies to be put for sale. In this case, all one hundred pieces would be part of the same *collection*. Each piece would have the same artist, title, description, media etc.
One of the biggest problems with the basic NFT contract is that you store similar data many times. If you mint NFTs, the contract will store the metadata individually for **every single token ID**. We can fix this by introducing the idea of NFT series, or NFT collection.
A series can be thought of as a bucket of token IDs that *all* share similar information. This information is specified when the series is **created** and can be the metadata, royalties, price etc. Rather than storing this information for **every token ID**, you can simply store it once in the series and then associate token IDs with their respective buckets.
<hr class="subsection" />
### Restricted Access
Currently, the NFT contract allows anyone to mint NFTs. While this works well for some projects, the vast majority of dApps and creators want to restrict who can create NFTs on the contract. This is why you'll introduce an allowlist functionality for both series and for NFTs. You'll have two data structures customizable by the contract owner:
- Approved Minters
- Approved Creators
If you're an approved minter, you can freely mint NFTs for any given series. You cannot, however, create new series.
On the other hand, you can also be an approved creator. This allows you to define new series that NFTs can be minted from. It's important to note that if you're an approved creator, you're not automatically an approved minter as well. Each of these permissions need to be given by the owner of the contract and they can be revoked at any time.
<hr class="subsection" />
### Lazy Minting
Lazy minting allows users to mint *on demand*. Rather than minting all the NFTs and spending $NEAR on storage, you can instead mint the tokens **when they are purchased**. This helps to avoid burning unnecessary Gas and saves on storage for when not all the NFTs are purchased. Let's look at a common scenario to help solidify your understanding:
Benji has created an amazing digital painting of the famous Go Team gif. He wants to sell 1000 copies of it for 1 $NEAR each. Using the traditional approach, he would have to mint each copy individually and pay for the storage himself. He would then need to either find or deploy a marketplace contract and pay for the storage to put 1000 copies up for sale. He would need to burn Gas putting each token ID up for sale 1 by 1.
After that, people would purchase the NFTs, and there would be no guarantee that all or even any would be sold. There's a real possibility that nobody buys a single piece of his artwork, and Benji spent all that time, effort and money on nothing.
Lazy minting would allow the NFTs to be *automatically minted on-demand*. Rather than having to purchase NFTs from a marketplace, Benji could specify a price on the NFT contract and a user could directly call the `nft_mint` function whereby the funds would be distributed to Benji's account directly.
Using this model, NFTs would **only** be minted when they're actually purchased and there wouldn't be any upfront fee that Benji would need to pay in order to mint all 1000 NFTs. In addition, it removes the need to have a separate marketplace contract.
With this example laid out, a high level overview of lazy minting is that it gives the ability for someone to mint "on-demand" - they're lazily minting the NFTs instead of having to mint everything up-front even if they're unsure if there's any demand for the NFTs. With this model, you don't have to waste Gas or storage fees because you're only ever minting when someone actually purchases the artwork.
---
## New Contract File Structure
Let's now take a look at how we've implemented solutions to the issues we've discussed so far.
In your locally cloned example of the [`nft-tutorial`](https://github.com/near-examples/nft-tutorial) check out the `main` branch and be sure to pull the most recent version.
```bash
git checkout main && git pull
```
You'll notice that there's a folder at the root of the project called `nft-series`. This is where the smart contract code lives. If you open the `src` folder, it should look similar to the following:
```
src
├── approval.rs
├── enumeration.rs
├── events.rs
├── internal.rs
├── lib.rs
├── metadata.rs
├── nft_core.rs
├── owner.rs
├── royalty.rs
├── series.rs
```
---
## Differences
You'll notice that most of this code is the same, however, there are a few differences between this contract and the basic NFT contract.
### Main Library File
Starting with `lib.rs`, you'll notice that the contract struct has been modified to now store the following information.
```diff
pub owner_id: AccountId,
+ pub approved_minters: LookupSet<AccountId>,
+ pub approved_creators: LookupSet<AccountId>,
pub tokens_per_owner: LookupMap<AccountId, UnorderedSet<TokenId>>,
pub tokens_by_id: UnorderedMap<TokenId, Token>,
- pub token_metadata_by_id: UnorderedMap<TokenId, TokenMetadata>,
+ pub series_by_id: UnorderedMap<SeriesId, Series>,
pub metadata: LazyOption<NFTContractMetadata>,
```
As you can see, we've replaced `token_metadata_by_id` with `series_by_id` and added two lookup sets:
- **series_by_id**: Map a series ID (u64) to its Series object.
- **approved_minters**: Keeps track of accounts that can call the `nft_mint` function.
- **approved_creators**: Keeps track of accounts that can create new series.
<hr class="subsection" />
### Series Object {#series-object}
In addition, we're now keeping track of a new object called a `Series`.
```rust
pub struct Series {
// Metadata including title, num copies etc.. that all tokens will derive from
metadata: TokenMetadata,
// Royalty used for all tokens in the collection
royalty: Option<HashMap<AccountId, u32>>,
// Set of tokens in the collection
tokens: UnorderedSet<TokenId>,
// What is the price of each token in this series? If this is specified, when minting,
// Users will need to attach enough $NEAR to cover the price.
price: Option<Balance>,
// Owner of the collection
owner_id: AccountId,
}
```
This object stores information that each token will inherit from. This includes:
- The [metadata](2-minting.md#metadata-and-token-info).
- The [royalties](6-royalty.md).
- The price.
:::caution
If a price is specified, there will be no restriction on who can mint tokens in the series. In addition, if the `copies` field is specified in the metadata, **only** that number of NFTs can be minted. If the field is omitted, an unlimited amount of tokens can be minted.
:::
We've also added a field `tokens` which keeps track of all the token IDs that have been minted for this series. This allows us to deal with the potential `copies` cap by checking the length of the set. It also allows us to paginate through all the tokens in the series.
<hr class="subsection" />
### Creating Series
`series.rs` is a new file that replaces the old [minting](2-minting.md) logic. This file has been created to combine both the series creation and minting logic into one.
<Github language="rust" start="10" end="56" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/series.rs" />
The function takes in a series ID in the form of a [u64](https://doc.rust-lang.org/std/primitive.u64.html), the metadata, royalties, and the price for tokens in the series. It will then create the [Series object](#series-object) and insert it into the contract's series_by_id data structure. It's important to note that the caller must be an approved creator and they must attach enough $NEAR to cover storage costs.
<hr class="subsection" />
### Minting NFTs
Next, we'll look at the minting function. If you remember from before, this used to take the following parameters:
- Token ID
- Metadata
- Receiver ID
- Perpetual Royalties
With the new and improved minting function, these parameters have been changed to just two:
- The series ID
- The receiver ID.
The mint function might look complicated at first but let's break it down to understand what's happening. The first thing it does is get the [series object](#series-object) from the specified series ID. From there, it will check that the number of copies won't be exceeded if one is specified in the metadata.
It will then store the token information on the contract as explained in the [minting section](2-minting.md#storage-implications) of the tutorial and map the token ID to the series. Once this is finished, a mint log will be emitted and it will ensure that enough deposit has been attached to the call. This amount differs based on whether or not the series has a price.
#### Required Deposit
As we went over in the [minting section](2-minting.md#storage-implications) of this tutorial, all information stored on the contract costs $NEAR. When minting, there is a required deposit to pay for this storage. For *this contract*, a series price can also be specified by the owner when the series is created. This price will be used for **all** NFTs in the series when they are minted. If the price is specified, the deposit must cover both the storage as well as the price.
If a price **is specified** and the user attaches more deposit than what is necessary, the excess is sent to the **series owner**. There is also *no restriction* on who can mint tokens for series that have a price. The caller does **not** need to be an approved minter.
If **no price** was specified in the series and the user attaches more deposit than what is necessary, the excess is *refunded to them*. In addition, the contract makes sure that the caller is an approved minter in this case.
:::info
Notice how the token ID isn't required? This is because the token ID is automatically generated when minting. The ID stored on the contract is `${series_id}:${token_id}` where the token ID is a nonce that increases each time a new token is minted in a series. This not only reduces the amount of information stored on the contract but it also acts as a way to check the specific edition number.
:::
<Github language="rust" start="60" end="147" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/series.rs" />
<hr class="subsection" />
### View Functions
Now that we've introduced the idea of series, more view functions have also been added.
:::info
Notice how we've also created a new struct `JsonSeries` instead of returning the regular `Series` struct. This is because the `Series` struct contains an `UnorderedSet` which cannot be serialized.
The common practice is to return everything **except** the `UnorderedSet` in a separate struct and then have entirely different methods for accessing the data from the `UnorderedSet` itself.
<!-- TODO: add a learn more here call to action -->
:::
<Github language="rust" start="6" end="17" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/enumeration.rs" />
The view functions are listed below.
- **[get_series_total_supply](https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/enumeration.rs#L92)**: Get the total number of series currently on the contract.
- Arguments: None.
- **[get_series](https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/enumeration.rs#L97)**: Paginate through all the series in the contract and return a vector of `JsonSeries` objects.
- Arguments: `from_index: String | null`, `limit: number | null`.
- **[get_series_details](https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/enumeration.rs#L115)**: Get the `JsonSeries` details for a specific series.
- Arguments: `id: number`.
- **[nft_supply_for_series](https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/enumeration.rs#L133)**: View the total number of NFTs minted for a specific series.
- Arguments: `id: number`.
- **[nft_tokens_for_series](https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/enumeration.rs#L146)**: Paginate through all NFTs for a specific series and return a vector of `JsonToken` objects.
- Arguments: `id: number`, `from_index: String | null`, `limit: number | null`.
:::info
Notice how with every pagination function, we've also included a getter to view the total supply. This is so that you can use the `from_index` and `limit` parameters of the pagination functions in conjunction with the total supply so you know where to end your pagination.
:::
<hr class="subsection" />
### Modifying View Calls for Optimizations
Storing information on-chain can be very expensive. As you level up in your smart contract development skills, one area to look into is reducing the amount of information stored. View calls are a perfect example of this optimization.
For example, if you wanted to relay the edition number for a given NFT in its title, you don't necessarily need to store this on-chain for every token. Instead, you could modify the view functions to manually append this information to the title before returning it.
To do this, here's a way of modifying the `nft_token` function as it's central to all enumeration methods.
<Github language="rust" start="156" end="192" url="https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/nft_core.rs" />
For example if a token had a title `"My Amazing Go Team Gif"` and the NFT was edition 42, the new title returned would be `"My Amazing Go Team Gif - 42"`. If the NFT didn't have a title in the metadata, the series and edition number would be returned in the form of `Series {} : Edition {}`.
While this is a small optimization, this idea is extremely powerful as you can potentially save on a ton of storage. As an example: most of the time NFTs don't utilize the following fields in their metadata.
- issued_at
- expires_at
- starts_at
- updated_at
As an optimization, you could change the token metadata that's **stored** on the contract to not include these fields but then when returning the information in `nft_token`, you could simply add them in as `null` values.
<hr class="subsection" />
### Owner File
The last file we'll look at is the owner file found at `owner.rs`. This file simply contains all the functions for getting and setting approved creators and approved minters which can only be called by the contract owner.
:::info
There are some other smaller changes made to the contract that you can check out if you'd like. The most notable are:
- The `Token` and `JsonToken` objects have been [changed](https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/metadata.rs#L40) to reflect the new series IDs.
- All references to `token_metadata_by_id` have been [changed](https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/enumeration.rs#L23) to `tokens_by_id`
- Royalty functions [now](https://github.com/near-examples/nft-tutorial/blob/main/nft-series/src/royalty.rs#L43) calculate the payout objects by using the series' royalties rather than the token's royalties.
:::
---
## Building the Contract
Now that you hopefully have a good understanding of the contract, let's get started building it. Run the following build command to compile the contract to wasm.
```bash
cargo near build
```
---
## Deployment and Initialization
Next, you'll deploy this contract to the network.
```bash
export NFT_CONTRACT_ID=<accountId>
near create-account $NFT_CONTRACT_ID --useFaucet
```
Check if this worked correctly by echoing the environment variable.
```bash
echo $NFT_CONTRACT_ID
```
This should return your `<accountId>`. The next step is to initialize the contract with some default metadata.
```bash
cargo near deploy $NFT_CONTRACT_ID with-init-call new_default_meta json-args '{"owner_id": "'$NFT_CONTRACT_ID'"}' prepaid-gas '100.0 Tgas' attached-deposit '0 NEAR' network-config testnet sign-with-keychain send
```
If you now query for the metadata of the contract, it should return our default metadata.
```bash
near view $NFT_CONTRACT_ID nft_metadata
```
---
## Creating The Series
The next step is to create two different series. One will have a price for lazy minting and the other will simply be a basic series with no price. The first step is to create an owner [sub-account](../../4.tools/cli.md#create-a-sub-account) that you can use to create both series
```bash
near create-account owner.$NFT_CONTRACT_ID --masterAccount $NFT_CONTRACT_ID --initialBalance 3 && export SERIES_OWNER=owner.$NFT_CONTRACT_ID
```
### Basic Series
You'll now need to create the simple series with no price and no royalties. If you try to run the following command before adding the owner account as an approved creator, the contract should throw an error.
```bash
near call $NFT_CONTRACT_ID create_series '{"id": 1, "metadata": {"title": "SERIES!", "description": "testing out the new series contract", "media": "https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif"}}' --accountId $SERIES_OWNER --amount 1
```
The expected output is an error thrown: `ExecutionError: 'Smart contract panicked: only approved creators can add a type`. If you now add the series owner as a creator, it should work.
```bash
near call $NFT_CONTRACT_ID add_approved_creator '{"account_id": "'$SERIES_OWNER'"}' --accountId $NFT_CONTRACT_ID
```
```bash
near call $NFT_CONTRACT_ID create_series '{"id": 1, "metadata": {"title": "SERIES!", "description": "testing out the new series contract", "media": "https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif"}}' --accountId $SERIES_OWNER --amount 1
```
If you now query for the series information, it should work!
```bash
near view $NFT_CONTRACT_ID get_series
```
Which should return something similar to:
```bash
[
{
series_id: 1,
metadata: {
title: 'SERIES!',
description: 'testing out the new series contract',
media: 'https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif',
media_hash: null,
copies: null,
issued_at: null,
expires_at: null,
starts_at: null,
updated_at: null,
extra: null,
reference: null,
reference_hash: null
},
royalty: null,
owner_id: 'owner.nft_contract.testnet'
}
]
```
<hr class="subsection" />
### Series With a Price
Now that you've created the first, simple series, let's create the second one that has a price of 1 $NEAR associated with it.
```bash
near call $NFT_CONTRACT_ID create_series '{"id": 2, "metadata": {"title": "COMPLEX SERIES!", "description": "testing out the new contract with a complex series", "media": "https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif"}, "price": "500000000000000000000000"}' --accountId $SERIES_OWNER --amount 1
```
If you now paginate through the series again, you should see both appear.
```bash
near view $NFT_CONTRACT_ID get_series
```
Which has
```bash
[
{
series_id: 1,
metadata: {
title: 'SERIES!',
description: 'testing out the new series contract',
media: 'https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif',
media_hash: null,
copies: null,
issued_at: null,
expires_at: null,
starts_at: null,
updated_at: null,
extra: null,
reference: null,
reference_hash: null
},
royalty: null,
owner_id: 'owner.nft_contract.testnet'
},
{
series_id: 2,
metadata: {
title: 'COMPLEX SERIES!',
description: 'testing out the new contract with a complex series',
media: 'https://bafybeiftczwrtyr3k7a2k4vutd3amkwsmaqyhrdzlhvpt33dyjivufqusq.ipfs.dweb.link/goteam-gif.gif',
media_hash: null,
copies: null,
issued_at: null,
expires_at: null,
starts_at: null,
updated_at: null,
extra: null,
reference: null,
reference_hash: null
},
royalty: null,
owner_id: 'owner.nft_contract.testnet'
}
]
```
---
## Minting NFTs
Now that you have both series created, it's time to now mint some NFTs. You can either login with an existing NEAR wallet using [`near login`](../../4.tools/cli.md#near-login) or you can create a sub-account of the NFT contract. In our case, we'll use a sub-account.
```bash
near create-account buyer.$NFT_CONTRACT_ID --masterAccount $NFT_CONTRACT_ID --initialBalance 1 && export BUYER_ID=buyer.$NFT_CONTRACT_ID
```
### Lazy Minting
The first workflow you'll test out is [lazy minting](#lazy-minting) NFTs. If you remember, the second series has a price associated with it of 1 $NEAR. This means that there are no minting restrictions and anyone can try and purchase the NFT. Let's try it out.
In order to view the NFT in the NEAR wallet, you'll want the `receiver_id` to be an account you have currently available in the wallet site. Let's export it to an environment variable. Run the following command but replace `YOUR_ACCOUNT_ID_HERE` with your actual NEAR account ID.
```bash
export NFT_RECEIVER_ID=YOUR_ACCOUNT_ID_HERE
```
Now if you try and run the mint command but don't attach enough $NEAR, it should throw an error.
```bash
near call $NFT_CONTRACT_ID nft_mint '{"id": "2", "receiver_id": "'$NFT_RECEIVER_ID'"}' --accountId $BUYER_ID
```
Run the command again but this time, attach 1.5 $NEAR.
```bash
near call $NFT_CONTRACT_ID nft_mint '{"id": "2", "receiver_id": "'$NFT_RECEIVER_ID'"}' --accountId $BUYER_ID --amount 0.6
```
This should output the following logs.
```bash
Receipts: BrJLxCVmxLk3yNFVnwzpjZPDRhiCinNinLQwj9A7184P, 3UwUgdq7i1VpKyw3L5bmJvbUiqvFRvpi2w7TfqmnPGH6
Log [nft_contract.testnet]: EVENT_JSON:{"standard":"nep171","version":"nft-1.0.0","event":"nft_mint","data":[{"owner_id":"benjiman.testnet","token_ids":["2:1"]}]}
Transaction Id FxWLFGuap7SFrUPLskVr7Uxxq8hpDtAG76AvshWppBVC
To see the transaction in the transaction explorer, please open this url in your browser
https://testnet.nearblocks.io/txns/FxWLFGuap7SFrUPLskVr7Uxxq8hpDtAG76AvshWppBVC
''
```
If you check the explorer link, it should show that the owner received on the order of `0.59305 $NEAR`.
<img width="80%" src="/docs/assets/nfts/explorer-payout-series-owner.png" />
<hr class="subsection" />
### Becoming an Approved Minter
If you try to mint the NFT for the simple series with no price, it should throw an error saying you're not an approved minter.
```bash
near call $NFT_CONTRACT_ID nft_mint '{"id": "1", "receiver_id": "'$NFT_RECEIVER_ID'"}' --accountId $BUYER_ID --amount 0.1
```
Go ahead and run the following command to add the buyer account as an approved minter.
```bash
near call $NFT_CONTRACT_ID add_approved_minter '{"account_id": "'$BUYER_ID'"}' --accountId $NFT_CONTRACT_ID
```
If you now run the mint command again, it should work.
```bash
near call $NFT_CONTRACT_ID nft_mint '{"id": "1", "receiver_id": "'$NFT_RECEIVER_ID'"}' --accountId $BUYER_ID --amount 0.1
```
<hr class="subsection" />
### Viewing the NFTs in the Wallet
Now that you've received both NFTs, they should show up in the NEAR wallet. Open the collectibles tab and search for the contract with the title `NFT Series Contract` and you should own two NFTs. One should be the complex series and the other should just be the simple version. Both should have ` - 1` appended to the end of the title because the NFTs are the first editions for each series.
<img width="80%" src="/docs/assets/nfts/series-wallet-collectibles.png" />
Hurray! You've successfully deployed and tested the series contract! **GO TEAM!**.
---
## Conclusion
In this tutorial, you learned how to take the basic NFT contract and iterate on it to create a complex and custom version to meet the needs of the community. You optimized the storage, introduced the idea of collections, created a lazy minting functionality, hacked the enumeration functions to save on storage, and created an allowlist functionality.
You then built the contract and deployed it on chain. Once it was on-chain, you initialized it and created two sets of series. One was complex with a price and the other was a regular series. You lazy minted an NFT and purchased it for `1.5 $NEAR` and then added yourself as an approved minter. You then minted an NFT from the regular series and viewed them both in the NEAR wallet.
Thank you so much for going through this journey with us! I wish you all the best and am eager to see what sorts of neat and unique use-cases you can come up with. If you have any questions, feel free to ask on our [Discord](https://near.chat) or any other social media channels we have. If you run into any issues or have feedback, feel free to use the `Feedback` button on the right.
:::note Versioning for this article
At the time of this writing, this example works with the following versions:
- near-cli: `4.0.13`
- cargo-near `0.6.1`
- NFT standard: [NEP171](https://nomicon.io/Standards/Tokens/NonFungibleToken/Core), version `1.1.0`
:::
|
NEAR Foundation Partners with Vortex Gaming, a Web3 Subsidiary of Korea’s Largest Gaming Community INVEN
NEAR FOUNDATION
June 22, 2023
NEAR Foundation is excited to announce a new partnership with Vortex Gaming, a Web3 subsidiary of the leading game industry INVEN. INVEN is the largest game media·community in Korea, with a monthly active user (MAU) count of 7.2 million.
With the guidance of the NEAR Korea Hub, NEAR Foundation and Vortex Gaming plan to achieve mutual growth by collaborating to support the growth of Vortex Gaming; strengthen the NEAR game ecosystem; conduct offline hackathons and events to foster developer talent; and enhance brand awareness and business networks.
To support Web3 gaming, Vortex Gaming will leverage NEAR’s global layer 1 protocol, known for its user experience and scalability, and the Blockchain Operating System (BOS). The BOS is an OS for an open web, allowing developers to effortlessly build apps for an internet free from centralized platforms.
“Following the leading companies in the gaming industry such as Kakao Games, Wemade, Netmarble’s Marblex, we expect our sustainable game ecosystem to be further enhanced through this partnership with INVEN,” said Marieke Flament, CEO of NEAR Foundation, expressed her delight at INVEN joining the NEAR ecosystem.
Vortex Gaming: an open web community game changer
Vortex Gaming is a content-based game community that builds services optimized for Web3 games. It also offers a global social media platform and features such as Gaming Guild. Vortex’s Gaming Guild will allow members to earn rewards by playing games, participating in tournaments, and contributing to the guild’s community. The guild system also provides a stronger sense of bonding between guild members, which can help strengthen user retention.
Vortex Gaming also aims to break a major stereotype of current Web3 games as being dominated by a few profit-seeking individuals. It does this by offering specialized content that thoroughly explores the in-game economy, the completeness of the game, and gameplay guide. By leveraging its successful experience in building Web2 communities, Vortex Gaming plans to establish a robust user base by incorporating both high quality content and INVEN’s existing wide pool of users.
INVEN is the largest game media·community in Korea. For over 20 years it has been the leading company in the game industry, driving the development as the top online game media platform. Based on content generated in-house, INVEN continues to innovate and expand into industries such as entertainment and Web3.
“The ultimate goal is to build an integrated gamer community that encompasses both Web3 and traditional Web2 gamers by providing high quality Web3 and Web2 content alike,” said Vortex Gaming CEO, HOON JAI LEE. “Gamers and their communities are the most crucial components of the game ecosystem. In addition to offering game content for gamers and their communities, Vortex Gaming envisions establishing a sustainable cycle by incentivizing gamers’ production of content such as character builds and game guides”.
The NEAR Korea Hub, responsible for business expansion across Korea and other Asian regions, has been instrumental in fostering this partnership. The Hub views Vortex’s onboarding as a significant moment that further accelerates the development of the NEAR gaming ecosystem.
“While the strong content competitiveness of leading companies plays a crucial role in attracting many users to the NEAR ecosystem, community is the key driving force in maintaining this competitiveness,”said Scott Lee, Co-CEO of NEAR Korea. “The synergy effects resulting from the onboarding of Vortex Gaming will further strengthen NEAR’s position as the optimal mainnet in the Web3 game ecosystem, accelerating ecosystem development.” |
The Future of DAOs: DAOs at Work and IRL
COMMUNITY
March 31, 2022
In “DAOs of the Near Future” Part I, we explored how DAO tooling, coordination, and standards will be crucial going forward. Now that we can imagine the ways in which DAOs can be improved, let’s explore how they will change things IRL.
As Web3 matures and goes mainstream, existing alongside and working in concert with Web2 platforms, DAOs will impact the world in more physical ways, from work to ReFi and sustainability to automation of business documentation and beyond.
Let’s take a look at how DAOs are already evolving, and how this process will be accelerated for mass Web3 adoption.
The DAO of work
In the last few years, remote employment changed how people work—up to a point. But it’s possible that DAOs will be even more transformative with work in terms of how, when, how long, and under what conditions.
With more transparency in contracts and fund transfers, Marma J Foundation’s Chloe Lewis thinks DAOs will allow community members to work on what they want, when they want to, without worrying about the next paycheck.
“When you can see publicly that there are hundreds of funded DAOs that have open gigs, it’s less stressful to think of working for DAOs as being a reasonable way of life,” Lewis muses. “I also see DAOs using their governance token as a way to fund more projects without really selling or giving away the token through more advanced DeFi opportunities.”
Belonging to a DAO is, in effect, a new type of work. A person isn’t hired so much as they join a DAO in a more participatory way.
With DAOs, work’s traditional top-down command structure can be flipped. This will create a fairer entry for ambitious individuals looking to get rewarded based on participation, output, and unity.
“Coming from both a startup and corporate world, I can say that a DAO is not comparable,” says Trevor Clarke, founder of Croncat, which does decentralized scheduling NEAR blockchain transactions. “A DAO utilizes elements of both, but when a startup or corporation would constantly require higher authority chains to finalize direction, DAOs can simply allow the community to be the decisive force.”
Open Forest Protocol’s Rim Berjack is full-time crypto and notices that when she introduces herself, or interacts with different projects and stakeholders, she identifies herself as her role in the DAO. This isn’t simply an add-on to who she is. It’s necessary since she works as part of a DAO, and the DAO actually makes decisions and implements changes in projects.
“If I were to try and explain that I work for a DAO to my parents, they wouldn’t really understand what that means and not really consider it a ‘job,’,” she says. “But when you think about it simply in terms of effects, if you contribute to something, you produce results, and get compensated for it, that’s work.”
In the current Web3 space, working for a DAO means freedom and creativity, with work being self-motivated and “earned” rather than assigned. A very startup-like ethos. Right now, DAOs offer a lot of equality and very little hierarchy in the decision-making processes.
“Working in a DAO is a testimony to the nature of work in crypto at large, which is much more entrepreneurial, undefined, and therefore creative,” says Berjack. “I was at ETH Denver recently and a Coordinape and Yearn contributor talked about how in DAOs work is defined by the intersection of what you like, what you can do, and what the project needs.”
There is a lot of fluidity and flexibility in DAO governance, and this likely has something to do with the lack of contractual obligations for work. This might change for certain DAOs, like those operating in the DeFi space, who might be working with sensitive financial information. In the future, those DAOs that want a more traditional mode of work will balance it with the free-wheeling, creative Web3 startup ethos.
Beyond flattening work hierarchies, H.E.R. DAO’s Tracey Bowen sees future DAOs shifting the autonomy of work—centering the human rather than the company or machine.
“The DAOs of the future will look markedly different from today,” Bowen says. “Less dogmatic and rigid concerning technical requirements and more geared towards serving the needs of groups and populations across the globe.”
“Equity is the new currency of our future cooperative world,” Bowen continues. “It’s the foundation on which many new Web3 projects are being built, and will be the standard bearer and rating system related to the efficacy of our ideas and the authenticity of our practices.”
Jordan Gray of AstroDAO, a DAO launchpad platform, has a lot of different ideas on how DAOs will impact the world. For one, he thinks DAOs will have a huge impact on how business will be conducted.
“The last time things were really revolutionized in the business world was with spreadsheets—people use them to do any kind of business, computation, or keeping track of things,” says Gray. “I think that DAOs are going to revolutionize business in a very similar way, where many things that happen with spreadsheets are going to be automated, and processes are going to get put in place and cemented into code that allows organizations to work a lot more efficiently.”
“That’s where I see DAOs maturing on the legal front, so that things like that are possible,” he adds. “That’s also where I see a huge opportunity in the future.”
Jessica Zartler, Communications Advisor and Researcher at BlockScience, hopes that people won’t work for DAOs as anthropomorphized entities.
“[Ideally, people will] share collective ownership of the value created in the network and have unconditional basic income,” she says. “This will enable them to choose to work on things they are more passionate about—exploring via natural curiosity and intrinsic motivation.”
Others aren’t so sure how DAOs will change the nature of work. But many Web3 natives, including Luis Freitas, Co-founder of 3XR—a platform for creating customer experiences like VR galleries—believe that DAOs are already changing how people work and cooperate in the NEAR ecosystem and on other blockchains.
“When I started thinking more actively about DAOs in 2020 it was mostly because I was working remotely with a global team on a project owned by us—the team,” says Freitas.
“Now, I’ve started DAOs [DAOLEX and VR DAO] for the exact same reason and I think it has not only changed the way I work but also for everyone involved.”
DAOs IRL
Some might wonder how DAOs will interact with things outside of crypto. This was a question also put to developers of Web1 and Web2 platforms. The truth is that Web3, and DAOs themselves, are already IRL.
“If we understand DAOs as a tool providing two primary functions—a digital stamp (for immutably publicizing decision making) and an on-chain treasury—then there is always the ‘swan’s legs underneath’ of weekly Zoom syncs, discussions on forums, notifying people on Telegram to vote on proposals, that happen off-chain, accompanying what is visible on-chain,” Berjack explains. “So, in that sense, you could say DAOs are never a purely on-chain affair.”
In the more literal sense, the physical and virtual worlds of Web3 are already being connected. OFP is a leader in the ReFi (regenerative finance) space, which is currently experiencing a lot of momentum. OFP acts as a “forest oracle,” bringing data from real forests and trees planted, then verifying them on-chain through the distributed network of validators.
Berjack says that OFP is also planning to progressively decentralize and govern itself as a DAO. And they aren’t the only projects in the ReFi space doing so. The ReFi platform Ecorise’s DAO buying up land, staking it (as an NFT) in their vault, and creating a coin pegged to these natural assets to create an alternative income stream for ecosystem services and regeneration. Klima DAO, which brought an explosive interest to the ReFi space, tokenizes carbon credits and drives up demand in the market, urging carbon credit buyers to pay a higher price for their offsets, which incentivizes companies to reduce emissions.
Block Science’s Zartler echoes this sentiment. Beyond land-based DAOs for carbon capture, soil regeneration, and other sustainability projects, she also envisions DAOs paving the way for cyber-physical commons and local currencies, where there would be real world impact.
“We will also see more NFT/AR tagging of artifacts which could be gameable for incentivizing coordination, like local trash clean up,” says Zartler. “DAOs are also an effective coordination tool to raise capital which could be used for pretty much anything; perhaps creating more land trusts, fighting injustices of the current system like supporting and developing affordable housing networks, sharing more unused resources (vehicles/houses), and enable layers of connection based on shared interest signaling/GPS proximity tech.”
There is also the recent launch of Unchain Fund, a new DAO fund built on NEAR, which is currently collecting donations for Ukraine in multiple tokens, including BSC, ETH, Harmony, NEAR, and Polygon. Created on AstroDAO, a DAO launchpad built on NEAR, Unchain Fund is having real world impact by raising funds for humanitarian purposes in Ukraine, including evacuation, shelter, food, and more. Over 700 volunteers are currently helping out with Unchain Fund in one form or another, and the effort is growing quickly.
Using a funding model similar to Unchain, DAOs could be how community projects organize in the future, helping communities efficiently raise and manage funds for goals.
“A lot of the benefits of crypto are present with the DAOs of today,” says Chloe Lewis, Co-founder of Marma J Foundation. “What’s missing are a lot of the human coordination elements.”
Lewis expects DAOs to evolve into community support platforms. All of the tooling will be built-in—the only other elements will be “the people and the purpose.”
“As Web3 gains traction, I see DAOs expanding and collaborating to manage assets in much more complex ways,” Lewis says. “This will hopefully allow for more individuals to be able to add and extract value to communities in ways that are rewarding for themselves while also supporting the wider communities that they interact with.”
Luis Freitas believes that DAOs are flexible enough to pop up in most things humans and software do, playing important roles in how organizations operate, from households and larger corporations to use cases in warehouses, global logistics, and a myriad of things in between.
“The Digital Assets industry will keep on growing in the years to come,” says Freitas. “My belief is that DAOs need to be at the heart of creating and managing these assets, and to participate in all sorts of digital decision-making processes.”
Faster and cheaper networks will probably yield more DAO experiments. In 3XR, one of Freitas’ DAO experiments, users create 3D spaces with references to other NFTs.
“This is really interesting because the minting can be proposed to DAOs, so in a way DAOs are curating curator’s work,” he says. “This incentivizes DAOs to participate by placing them in the lifetime royalties of the asset they helped create.”
DAOs could also have an impact on team sports. Recently, NEAR announced a new partnership with SailGP, one of the world’s fastest-growing sailing events. As part of this multi-year partnership, SailGP is set to explore the sale of a new team to a DAO that will be launched on the NEAR Protocol. The SailGP team DAO’s community members will have a say in athlete selection, team management, commercialization options, operations, and overall team strategy.
Sail GP and MotorDAO, which is democratizing how racing drivers and teams get funding for high-level competition, could inspire the entry of other competitive sports into Web3. In MotorDAO’s membership-based platform, motorsports fans directly participate in the benefits of ownership, driver sponsorship, events; they can also get retail perks, ticket packages, race day NFT air drops, and more.
Ambitious, yes, but MotorDAO’s model could be used to create similarly functioning DAOs for other industries, like music, film and television, community investment, and more.
To DAO or not to DAO?
It’s interesting to consider a future in which people, instead of telling others what job they have or social media platforms they use, identify themselves by the DAOs in which they participate. Individuals will remain individual, on their terms, but they will also be part of collectives that will have input into how Web3 evolves and integrates into the wider world.
Croncat’s Trevor Clark believes mass DAO adoption will happen when the UX meets people where they already coordinate like DAOs, but just don’t realize it yet.
“Think of the mom groups that organize school activities, exercise groups at public parks, social good initiatives with a deadline—all of these groups are already DAOs utilizing disparate technologies (social media pages, SMS, email, etc) that could benefit from a DAO,” says Clarke. “The key will be unlocking the next generation DAO tools for everyday uses already in existence, similar to how Venmo took over the group dinner bill.”
Will everyone be part of a DAO in the future? Perhaps not. As Astro DAO’s Jordan Gray says, everyone is at different stages of technological adoption—and that’s okay.
“There are still people who make candles and sell candles, or make soap and sell it,” says Gray. “So there’s always going to be people that are at different stages of technology adoption and technology relevant to their lives. I think that there will definitely be folks that are like in that Web2 mindset and they continue cranking away in the mindset.”
Gray says Web3 tools like DAOs present new opportunities, even for people and businesses who have grown used to Web2 and found success in it. “It’s about recognizing that there’s another wave of opportunity hitting and stepping into it,” he says. |
NEAR Community Update: March 13th, 2020
COMMUNITY
March 13, 2020
In this community update, we introduce Flux, a prediction market built on NEAR, to show how we’ll make our internal operations public and share the latest and greatest on content.
Stay Healthy!
In light of the recent global volatility, Near closed its office in San Francisco and canceled all in-person events. Until further notice, we are moving all of our interactions online.
To create community experiences we are curious to know what type of things you want to learn and talk most about. Let us know in this week’s vote, and we’ll share the results on Tuesday.
Vote Here!
Flux
Flux announced their fully redesigned app built on top of their protocol (see the announcement here: https://twitter.com/Flux_Market/status/1235958316708302849?s=20). This app allows users to onboard into Flux via the NEAR wallet in seconds and trade on a range of markets. Use cases include politics, stock-markets, private companies, sports, esports, and even insurance. All trading is done in DAI, a stable coin pegged to the US Dollar.
In addition, ARterra an eSports engagement platform announced a partnership launching tailored eSports markets on top of Flux Protocol (go check it out here: https://twitter.com/ARterra_company/status/1237078763516694529?s=20).
Peter and Jasper — the founders of Flux
Content Highlight
“Not only should it feel like a Web2 backend, it should feel truly serverless.” — Sasha
Abel’s Abstracts recorded a podcast with Sasha on NEAR and the Open Web Collective!
Tweet preview
Web: https://anchor.fm/abelsabstracts
Apple Podcasts: https://apple.co/38B6uNf –
Spotify: https://spoti.fi/3cPDJiS
Join Our Public Meetings
As part of being an open community, we are beginning to make our meetings public. You can see them on this calendar. Join to listen, make suggestions and start discussions (within the scope of the meeting).
We are starting with the following meetings: Middleware Work Group (Wasm, Runtime, RPC, etc.) and Eth Bridge Work Group.
Additionally, we invite you to join our forum discussions: https://commonwealth.im/near/.
Welcome our new team member Huxley!
How You Can Get Involved
Join the NEAR Contributor Program. If you would like to host events, create content or provide developer feedback, we would love to hear from you! To learn more about the program and ways to participate, please head over to the website.
If you’re just getting started, learn more in The Beginner’s Guide to NEAR Protocol or read the official White Paper. Stay up to date with what we’re building by following us on Twitter for updates, joining the conversation on Discord and subscribing to our newsletter to receive updates right to your inbox. |
---
NEP: 366
Title: Meta Transactions
Author: Illia Polosukhin <ilblackdragon@gmail.com>, Egor Uleyskiy (egor.ulieiskii@gmail.com), Alexander Fadeev (fadeevab.com@gmail.com)
DiscussionsTo: https://github.com/nearprotocol/neps/pull/366
Status: Approved
Type: Protocol Track
Category: Runtime
Version: 1.1.0
Created: 19-Oct-2022
LastUpdated: 03-Aug-2023
---
## Summary
In-protocol meta transactions allow third-party accounts to initiate and pay transaction fees on behalf of the account.
## Motivation
NEAR has been designed with simplicity of onboarding in mind. One of the large hurdles right now is that after creating an implicit or even named account the user does not have NEAR to pay gas fees to interact with apps.
For example, apps that pay user for doing work (like NEARCrowd or Sweatcoin) or free-to-play games.
[Aurora Plus](https://aurora.plus) has shown viability of the relayers that can offer some number of free transactions and a subscription model. Shifting the complexity of dealing with fees to the infrastructure from the user space.
## Rationale and alternatives
The proposed design here provides the easiest way for users and developers to onboard and to pay for user transactions.
An alternative is to have a proxy contract deployed on the user account.
This design has severe limitations as it requires the user to deploy such contract and incur additional costs for storage.
## Specification
- **User** (Sender) is the one who is going to send the `DelegateAction` to Receiver via Relayer.
- **Relayer** is the one who publishes the `DelegateAction` to the protocol.
- **User** and Relayer doesn't trust each other.
The main flow of the meta transaction will be as follows:
- User specifies `sender_id` (the user's account id), `receiver_id` (the receiver's account id) and other information (see `DelegateAction` format).
- User signs `DelegateAction` specifying the set of actions that they need to be executed.
- User forms `SignedDelegateAction` with the `DelegateAction` and the signature.
- User forms `DelegateActionMessage` with the `SignedDelegateAction`.
- User sends `DelegateActionMessage` data to the relayer.
- Relayer verifies actions specified in `DelegateAction`: the total cost and whether the user included the reward for the relayer.
- Relayer forms a `Transaction` with `receiver_id` equals to `delegate_action.sender_id` and `actions: [SignedDelegateAction { ... }]`. Signs it with its key. Note that such transactions can contain other actions toward user's account (for example calling a function).
- This transaction is processed normally. A `Receipt` is created with a copy of the actions in the transaction.
- When processing a `SignedDelegateAction`, a number of checks are done (see below), mainly a check to ensure that the `signature` matches the user account's key.
- When a `Receipt` with a valid `SignedDelegateAction` in actions arrives at the user's account, it gets executed. Execution means creation of a new Receipt with `receiver_id: AccountId` and `actions: Action` matching `receiver_id` and `actions` in the `DelegateAction`.
- The new `Receipt` looks like a normal receipt that could have originated from the user's account, with `predeccessor_id` equal to tbe user's account, `signer_id` equal to the relayer's account, `signer_public_key` equal to the relayer's public key.
## Diagram

## Limitations
- If User account exist, then deposit and gas are refunded as usual: gas is refuned to Relayer, deposit is refunded to User.
- If User account doesn't exist then gas is refunded to Relayer, deposit is burnt.
- `DelegateAction` actions mustn't contain another `DelegateAction` (`DelegateAction` can't contain the nested ones).
### DelegateAction
Delegate actions allow an account to initiate a batch of actions on behalf of a receiving account, allowing proxy actions. This can be used to implement meta transactions.
```rust
pub struct DelegateAction {
/// Signer of the delegated actions
sender_id: AccountId,
/// Receiver of the delegated actions.
receiver_id: AccountId,
/// List of actions to be executed.
actions: Vec<Action>,
/// Nonce to ensure that the same delegate action is not sent twice by a relayer and should match for given account's `public_key`.
/// After this action is processed it will increment.
nonce: Nonce,
/// The maximal height of the block in the blockchain below which the given DelegateAction is valid.
max_block_height: BlockHeight,
/// Public key that is used to sign this delegated action.
public_key: PublicKey,
}
```
```rust
pub struct SignedDelegateAction {
delegate_action: DelegateAction,
/// Signature of the `DelegateAction`.
signature: Signature,
}
```
Supporting batches of `actions` means `DelegateAction` can be used to initiate complex steps like creating new accounts, transferring funds, deploying contracts, and executing an initialization function all within the same transaction.
##### Validation
1. Validate `DelegateAction` doesn't contain a nested `DelegateAction` in actions.
2. To ensure that a `DelegateAction` is correct, on receipt the following signature verification is performed: `verify_signature(hash(delegate_action), delegate_action.public_key, signature)`.
3. Verify `transaction.receiver_id` matches `delegate_action.sender_id`.
4. Verify `delegate_action.max_block_height`. The `max_block_height` must be greater than the current block height (at the `DelegateAction` processing time).
5. Verify `delegate_action.sender_id` owns `delegate_action.public_key`.
6. Verify `delegate_action.nonce > sender.access_key.nonce`.
A `message` is formed in the following format:
```rust
struct DelegateActionMessage {
signed_delegate_action: SignedDelegateAction
}
```
The next set of security concerns are addressed by this format:
- `sender_id` is included to ensure that the relayer sets the correct `transaction.receiver_id`.
- `max_block_height` is included to ensure that the `DelegateAction` isn't expired.
- `nonce` is included to ensure that the `DelegateAction` can't be replayed again.
- `public_key` and `sender_id` are needed to ensure that on the right account, work across rotating keys and fetch the correct `nonce`.
The permissions are verified based on the variant of `public_key`:
- `AccessKeyPermission::FullAccess`, all actions are allowed.
- `AccessKeyPermission::FunctionCall`, only a single `FunctionCall` action is allowed in `actions`.
- `DelegateAction.receiver_id` must match to the `account[public_key].receiver_id`
- `DelegateAction.actions[0].method_name` must be in the `account[public_key].method_names`
##### Outcomes
- If the `signature` matches the receiver's account's `public_key`, a new receipt is created from this account with a set of `ActionReceipt { receiver_id, action }` for each action in `actions`.
##### Recommendations
- Because the User doesn't trust the Relayer, the User should verify whether the Relayer has submitted the `DelegateAction` and the execution result.
### Errors
- If the Sender's account doesn't exist
```rust
/// Happens when TX receiver_id doesn't exist
AccountDoesNotExist
```
- If the `signature` does not match the data and the `public_key` of the given key, then the following error will be returned
```rust
/// Signature does not match the provided actions and given signer public key.
DelegateActionInvalidSignature
```
- If the `sender_id` doesn't match the `tx.receiver_id`
```rust
/// Receiver of the transaction doesn't match Sender of the delegate action
DelegateActionSenderDoesNotMatchTxReceiver
```
- If the current block is equal or greater than `max_block_height`
```rust
/// Delegate action has expired
DelegateActionExpired
```
- If the `public_key` does not exist for Sender account
```rust
/// The given public key doesn't exist for Sender account
DelegateActionAccessKeyError
```
- If the `nonce` does match the `public_key` for the `sender_id`
```rust
/// Nonce must be greater sender[public_key].nonce
DelegateActionInvalidNonce
```
- If `nonce` is too large
```rust
/// DelegateAction nonce is larger than the upper bound given by the block height (block_height * 1e6)
DelegateActionNonceTooLarge
```
- If the list of Transaction actions contains several `DelegateAction`
```rust
/// There should be the only one DelegateAction
DelegateActionMustBeOnlyOne
```
See the [DelegateAction specification](/specs/RuntimeSpec/Actions.md#DelegateAction) for details.
## Security Implications
Delegate actions do not override `signer_public_key`, leaving that to the original signer that initiated the transaction (e.g. the relayer in the meta transaction case). Although it is possible to override the `signer_public_key` in the context with one from the `DelegateAction`, there is no clear value in that.
See the **_Validation_** section in [DelegateAction specification](/specs/RuntimeSpec/Actions.md#DelegateAction) for security considerations around what the user signs and the validation of actions with different permissions.
## Drawbacks
- Increases complexity of NEAR's transactional model.
- Meta transactions take an extra block to execute, as they first need to be included by the originating account, then routed to the delegate account, and only after that to the real destination.
- User can't call functions from different contracts in same `DelegateAction`. This is because `DelegateAction` has only one receiver for all inner actions.
- The Relayer must verify most of the parameters before submitting `DelegateAction`, making sure that one of the function calls is the reward action. Either way, this is a risk for Relayer in general.
- User must not trust Relayer’s response and should check execution errors in Blockchain.
## Future possibilities
Supporting ZK proofs instead of just signatures can allow for anonymous transactions, which pay fees to relayers anonymously.
## Changelog
### 1.1.0 - Adjust errors to reflect deployed reality (03-Aug`2023)
- Remove the error variant `DelegateActionCantContainNestedOne` because this would already fail in the parsing stage.
- Rename the error variant `DelegateActionSenderDoesNotMatchReceiver` to `DelegateActionSenderDoesNotMatchTxReceiver` to reflect published types in [near_primitives](https://docs.rs/near-primitives/0.17.0/near_primitives/errors/enum.ActionErrorKind.html#variant.DelegateActionSenderDoesNotMatchTxReceiver).
## Copyright
Copyright and related rights waived via [CC0](https://creativecommons.org/publicdomain/zero/1.0/).
|
Welcome to NEAR, the B.O.S
NEAR FOUNDATION
July 4, 2023
Create an Account
The blockchain space has come a long way since its inception in 2009, when Bitcoin was released. But building mainstream blockchain applications — commonly referred to as Web3 apps, or dApps — still remains a challenge. Building Web3 apps on most chains require learning chain-specific programming languages, or use paradigms that are completely alien to web developers. And even after building a successful Web3 app, projects quickly find there is no simple way to onboard users, especially those that are later in the crypto adoption curve. Interacting with a Web3 app requires the download of a wallet like Metamask and converting fiat to a desired token. As a final barrier to entry, the transaction fees may be so high that the benefit of using the app becomes redundant to the user.
It’s precisely these problems that NEAR Protocol was created to address. In NEAR, web developers can build Web3 apps using languages they know and love such as Javascript and Rust. What’s even better is that these applications can then be used by simply logging in with an email — no blockchain knowledge, add-on wallets, or crypto needed! This amazing user experience is achieved thanks to a robust tech-stack built with simplicity in mind. The combination of developer-friendly smart contracts, Web3 applications, and user-friendly onboarding tools is what makes NEAR the B.O.S (Blockchain Operating System).
NEAR’s B.O.S make it easier than ever for developers to build multi-chain web applications, while providing a common layer for browsing and discovering open web experiences. You no longer have to choose between decentralization and discoverability. Whether you’re a developer new to Web3 and want to play around, or somebody looking to build in the open web, you’ll find a treasure trove of components, code, and a thriving community to help get you started.
Read through this blog to learn how you can also be a B.O.S.
Use languages that you already know
Simplicity of use for developers is at the core of NEAR’s tech stack. With Near you can use Javascript or Rust throughout the whole development process. This includes the creation of smart contracts and their testing, using our friendly API to connect your existing frontend to the NEAR Blockchain, as well as building fully composable applications that talk with any blockchain.
Thanks to our vast library of Javascript and Rust tools, any web developer can easily adopt NEAR technology and be onboarded into the B.O.S.
Develop, build and deploy multi-chain Apps —‚ all in 1 day
Building a traditional web app entails a long list of needs, such as: installing an IDE (such as VSCode), setting up a development environment (e.g. node.js), then of course building the WebApp (lots of Javascript goes here). Even after finishing the application, another hurdle lies ahead — you still need to publish it! This generally means obtaining and setting up a hosting service such as Firebase or Vercel.
A powerful Web3 alternative is to join the B.O.S at near.org. NEAR offers a comprehensive set of tools and capabilities to get developers started quickly. TLDR: Code what you want, fork what you don’t! You can navigate through the component library (currently more than 10,000 components available!), choose the ones you need, and compose them into a full web application.
Do you want to build an exchange that allows people to also have discussions? Simply fork a token-exchange component and the discussion component, and compose them into a single app. It’s that simple. What’s even better is that B.O.S is readily interoperable with multiple blockchains. If you want to build a frontend that talks with Ethereum, NEAR’s environment is set up for you, and it’s not only Ethereum — support for many other networks area always being added!
Thanks to the B.O.S, you can focus on building what you want — simply fork, adapt, and incorporate the work of our community to speed up the process.
Seamless onboarding for Web3
On a typical website, you arrive and login with your email. That’s it — the onboarding journey is over. Now let’s compare that with the usual Web3 onboarding.
On Web3, you come to a blockchain-based app and are asked to install Metamask or an equivalent, which will create an account for you. But here is the catch: to use the account you’ll need to figure out where to buy crypto (and which crypto) to put in the account, at which time you will realize that your account is just a bunch of random numbers and letters. This painful onboarding has hampered Web3 adoption since cryptocurrency’s very beginnings.
To tackle this problem, NEAR offers FastAuth, a technology that directly links your email account to a NEAR account. In this way, users can simply login to any NEAR app using their email, just as every web application allows. Even better, the user will be able to use exactly the same NEAR account if they login into any other application using their email.
What’s even better is that users can enjoy B.O.S apps without the need to obtain crypto! NEAR supports ‘0 balance accounts’, meaning that people do not need to hold crypto in their wallets. Instead, developers can pay for the gas of their users through what is known as a ‘Relayer’. Relayers are super simple to set up, and anyone can create their own. Thanks to the FastAuth and Relayers tech, users in B.O.S experience a simple and seamless web style onboarding. In practice, this means that developers can build Web3 apps ready for mass-adoption from the get-go.
Instantly access thousands of users and devs
The current Web3 landscape is multi-chain and therefore highly fragmented, making it hard to discover the amazing experiences Web3 has to offer. NEAR’s B.O.S fixes this problem by combining a thorough search experience with social collaborative tools. With B.O.S, you can quickly search for Web3 apps and components other developers built, as well as connect with other devs and communities. Moreover, you can leverage its social aspects to shill your application, gather feedback, and expose your work to a large audience.
Shill your project as soon as you finished just as @zhocuy did
Easily bridge Web2 and Web3
B.O.S enables developers to easily supercharge traditional web apps with Web3 experiences through what we call ‘Gateways’. Gateways are simple web apps that work as access points to the Web3 — they have the capability to read components from the B.O.S and render them for their users. The best example of a Gateway is near.org itself! Gateways makes composing React and Web3 components easier than ever, helping to bridge Web2 and web3 development. This way, developers can focus on writing an application using technologies they know (React/Next.js), and effortlessly supercharge them with Web3 components.
All supported by a fast, cheap and robust network
Everything we’ve talked about so far runs atop the NEAR Protocol: a blazing fast, cheap, and robust blockchain. Indeed, transactions can be as low as 0.0008Ⓝ — less than a thousand of dollar’s cent!
On top of that, transactions are objectively fast, and are processed in less than 1.2 seconds (the current processing time is 1.11s). All of this runs atop of the most stable chains ever created. NEAR has enjoyed 100% uptime since its launch, recently crossing the 100 Millon block produced with the network never going down!
Build and discover apps on NEAR: the B.O.S for an Open Web
NEAR’s B.O.S tech-stack is deep yet accessible to everyone. It allows you to develop smart contracts and build composable applications using Javascript and Rust. B.O.S also allows you to easily go multi-chain, extending your reach beyond NEAR’s network and across other major blockchains such as Ethereum. Finally, its social component allows you to publish your application easily on near.org and leverage a growing community of developers and crypto enthusiasts.
Build amazing apps, deploy them, gather feedback, and interact with your community — all in the same platform.
Visit Near.org. Let’s build an open web together! |
# RuntimeConfig
The structure that holds the parameters of the runtime, mostly economics.
## storage_cost_byte_per_block
_type: Balance_
The cost to store one byte of storage per block.
## storage_cost_byte_per_block
_type: Balance_
Costs of different actions that need to be performed when sending and processing transaction
and receipts.
## poke_threshold
_type: BlockIndex_
The minimum number of blocks of storage rent an account has to maintain to prevent forced deletion.
## transaction_costs
_type: [RuntimeFeesConfig](RuntimeFeeConfig.md)_
Costs of different actions that need to be performed when sending and processing transaction and receipts.
## wasm_config
_type: [VMConfig](VMConfig.md),_
Config of wasm operations.
## account_length_baseline_cost_per_block
_type: Balance_
The baseline cost to store account_id of short length per block.
The original formula in NEP#0006 is `1,000 / (3 ^ (account_id.length - 2))` for cost per year.
This value represents `1,000` above adjusted to use per block
|
Knaq and NEAR: A New Era of Fan Engagement with Web3
NEAR FOUNDATION
May 10, 2023
Knaq, the pioneering fan loyalty platform, is poised to transform the way fans interact with their favorite creators on both Web2 and Web3 social media platforms. In collaboration with the NEAR Foundation, Knaq aims to foster more meaningful interactions within creator communities while providing true utility to NFTs and tokens via a summertime soft launch.
By leveraging NEAR’s fast and scalable blockchain, Knaq will offer a seamless user experience through a user-friendly browser extension and redemption marketplace. This exclusive partnership empowers fans and creators, enabling them to benefit from the innovative features and rewards offered by Knaq and its diverse range of brand partners.
With Knaq’s unique vision and NEAR’s robust platform, the stage is set for a new era of fan engagement and loyalty in the creator space.
A perfect match for seamless creator engagement
Loyalty and rewards programs have become an integral part of our daily lives, shaping our experiences with various services and products. Knaq recognizes the potential of this concept and seeks to bring it to social media users who invest time and energy in engaging with their favorite content creators. By partnering with NEAR, Knaq brings the benefits of Web3 and crypto to the creator space.
Knaq’s browser extension and redemption marketplace, powered by NEAR, offers a seamless onboarding process for users as they engage with their favorite creators. This allows easy compatibility with existing crypto wallets and provides an effortless sign-up process using Google OAuth. Fans and creators can link their social media accounts to start earning rewards, while NEAR’s platform facilitates secure and efficient transactions.
The partnership between Knaq and NEAR Foundation brings additional benefits to users in the form of enhanced scalability and accessibility. NEAR’s highly scalable blockchain allows Knaq to handle high volumes of transactions, ensuring that the platform remains fast and reliable even as its user base grows. NEAR’s focus on user-friendly solutions also aligns with Knaq’s goal of making its platform accessible to a wide range of social media users, including those who may be new to Web3 and crypto.
Knaq’s exciting fan and creator features built on NEAR
NEAR’s technology enables Knaq to offer a multitude of exciting features and benefits that elevate fan engagement to new heights. By bridging the gap between Web3 and Web2 content platforms, Knaq delivers an experience that not only rewards fans for their support but also empowers creators to foster stronger connections with their audience.
One of the exciting features enabled by NEAR is the use of NFTs to grant exclusive access to live and virtual events. These NFTs offer fans unique opportunities to interact with their favorite creators and enjoy immersive experiences, while creators benefit from enhanced engagement and revenue generation.
Knaq’s engage-to-earn model rewards users with tokens for their engagement on all social media platforms, starting with YouTube. Built on NEAR, these tokens can be redeemed for various perks within the Knaq app and in real life via Knaq’s brand partners, providing fans with tangible incentives for their continued support. This, in turn, boosts creator engagement and revenues, making the platform a win-win solution for all parties involved.
Through Knaq, fans also get a behind-the-scenes look at their most beloved creators. This feature allows for a deeper connection between creators and their audience, building a stronger sense of community and loyalty.
Lastly, Knaq users have access to all related drops, tokens, and ecosystem incentives, with the first one planned for shortly after launch for early waitlist users. Thanks to NEAR’s robust platform, these initiatives can be seamlessly executed, further enhancing the value and utility of the Knaq experience for both fans and creators.
Knaq and NEAR: Powerhouse team and exciting prospects
The team-building Knaq brings together experienced creators, builders, and investors from both traditional markets and the crypto space. Their diverse backgrounds include working with renowned brands like Starbucks, ULTA Beauty, Buzzfeed, and J.P. Morgan, as well as prominent players in the crypto industry such as Circle, OKX, and Genesis Block. With a strong foundation and support from NEAR, Knaq is well-positioned to make a significant impact in the creator industry.
As part of their commitment to transparency and community engagement, the Knaq team will be fully doxxed in Discord, building trust and openness with users. NEAR’s collaboration has been instrumental in bringing Knaq to life, providing the technological backbone necessary for seamless integration and exceptional user experience.
The exciting features and benefits of Knaq set the stage for a new era of fan engagement and loyalty in the creator space. With innovative rewards and incentives, Knaq is poised to revolutionize the way we interact with creators, paving the way for a more engaging and rewarding experience for fans and creators alike.
Looking ahead, Knaq has planned a soft launch for the summer, with the first month reserved exclusively for those who sign up for the waitlist. This presents an exciting opportunity for early adopters to get a head start on the platform and even participate in a free giveaway post-launch. Creators interested in boosting community engagement are encouraged to reach out and explore the potential of Knaq and NEAR Foundation’s partnership.
To learn more about Knaq’s rewards offerings and follow major upcoming events and announcements, sign up for the waitlist today at knaqapp.com. |
---
sidebar_position: 1
sidebar_label: Table of contents
---
# Structures. Table of Contents
The structures described in this section of the docs are indexer-related more than `nearcore` related. Some of the are artificial and are not a part of `nearcore`
:::note How data flows in NEAR Protocol
Don't skip the article about the [NEAR Data Flow](https://docs.near.org/concepts/data-flow/near-data-flow)
:::
**The main goal of this section is to describe `StreamerMessage` structure from [`near-indexer-primitives`](https://crates.io/crates/near-indexer-primitives)**
- `StreamerMessage`
- [Block](./block.mdx)
- [BlockHeader](./block.mdx#blockheaderview)
- [Shards](./shard.mdx)
- [Chunk](./chunk.mdx)
- [ChunkHeader](./chunk.mdx#chunkheaderview)
- [Transactions](./transaction.mdx)
- [Receipts](./receipt.mdx)
- [ExecutionOutcomes](./execution_outcome.mdx) for [Receipts](./receipt.mdx)
- [StateChanges](./state_change.mdx)
|