path
stringlengths 5
300
| repo_name
stringlengths 6
76
| content
stringlengths 26
1.05M
|
---|---|---|
popcorn.js | kaltura/popcorn-js | (function(global, document) {
// Popcorn.js does not support archaic browsers
if ( !document.addEventListener ) {
global.Popcorn = {
isSupported: false
};
var methods = ( "byId forEach extend effects error guid sizeOf isArray nop position disable enable destroy" +
"addTrackEvent removeTrackEvent getTrackEvents getTrackEvent getLastTrackEventId " +
"timeUpdate plugin removePlugin compose effect xhr getJSONP getScript" ).split(/\s+/);
while ( methods.length ) {
global.Popcorn[ methods.shift() ] = function() {};
}
return;
}
var
AP = Array.prototype,
OP = Object.prototype,
forEach = AP.forEach,
slice = AP.slice,
hasOwn = OP.hasOwnProperty,
toString = OP.toString,
// Copy global Popcorn (may not exist)
_Popcorn = global.Popcorn,
// Ready fn cache
readyStack = [],
readyBound = false,
readyFired = false,
// Non-public internal data object
internal = {
events: {
hash: {},
apis: {}
}
},
// Non-public `requestAnimFrame`
// http://paulirish.com/2011/requestanimationframe-for-smart-animating/
requestAnimFrame = (function(){
return global.requestAnimationFrame ||
global.webkitRequestAnimationFrame ||
global.mozRequestAnimationFrame ||
global.oRequestAnimationFrame ||
global.msRequestAnimationFrame ||
function( callback, element ) {
global.setTimeout( callback, 16 );
};
}()),
// Non-public `getKeys`, return an object's keys as an array
getKeys = function( obj ) {
return Object.keys ? Object.keys( obj ) : (function( obj ) {
var item,
list = [];
for ( item in obj ) {
if ( hasOwn.call( obj, item ) ) {
list.push( item );
}
}
return list;
})( obj );
},
Abstract = {
// [[Put]] props from dictionary onto |this|
// MUST BE CALLED FROM WITHIN A CONSTRUCTOR:
// Abstract.put.call( this, dictionary );
put: function( dictionary ) {
// For each own property of src, let key be the property key
// and desc be the property descriptor of the property.
Object.getOwnPropertyNames( dictionary ).forEach(function( key ) {
this[ key ] = dictionary[ key ];
}, this);
}
},
// Declare constructor
// Returns an instance object.
Popcorn = function( entity, options ) {
// Return new Popcorn object
return new Popcorn.p.init( entity, options || null );
};
// Popcorn API version, automatically inserted via build system.
Popcorn.version = "@VERSION";
// Boolean flag allowing a client to determine if Popcorn can be supported
Popcorn.isSupported = true;
// Instance caching
Popcorn.instances = [];
// Declare a shortcut (Popcorn.p) to and a definition of
// the new prototype for our Popcorn constructor
Popcorn.p = Popcorn.prototype = {
init: function( entity, options ) {
var matches, nodeName,
self = this;
// Supports Popcorn(function () { /../ })
// Originally proposed by Daniel Brooks
if ( typeof entity === "function" ) {
// If document ready has already fired
if ( document.readyState === "complete" ) {
entity( document, Popcorn );
return;
}
// Add `entity` fn to ready stack
readyStack.push( entity );
// This process should happen once per page load
if ( !readyBound ) {
// set readyBound flag
readyBound = true;
var DOMContentLoaded = function() {
readyFired = true;
// Remove global DOM ready listener
document.removeEventListener( "DOMContentLoaded", DOMContentLoaded, false );
// Execute all ready function in the stack
for ( var i = 0, readyStackLength = readyStack.length; i < readyStackLength; i++ ) {
readyStack[ i ].call( document, Popcorn );
}
// GC readyStack
readyStack = null;
};
// Register global DOM ready listener
document.addEventListener( "DOMContentLoaded", DOMContentLoaded, false );
}
return;
}
if ( typeof entity === "string" ) {
try {
matches = document.querySelector( entity );
} catch( e ) {
throw new Error( "Popcorn.js Error: Invalid media element selector: " + entity );
}
}
// Get media element by id or object reference
this.media = matches || entity;
// inner reference to this media element's nodeName string value
nodeName = ( this.media.nodeName && this.media.nodeName.toLowerCase() ) || "video";
// Create an audio or video element property reference
this[ nodeName ] = this.media;
this.options = Popcorn.extend( {}, options ) || {};
// Resolve custom ID or default prefixed ID
this.id = this.options.id || Popcorn.guid( nodeName );
// Throw if an attempt is made to use an ID that already exists
if ( Popcorn.byId( this.id ) ) {
throw new Error( "Popcorn.js Error: Cannot use duplicate ID (" + this.id + ")" );
}
this.isDestroyed = false;
this.data = {
// data structure of all
running: {
cue: []
},
// Executed by either timeupdate event or in rAF loop
timeUpdate: Popcorn.nop,
// Allows disabling a plugin per instance
disabled: {},
// Stores DOM event queues by type
events: {},
// Stores Special event hooks data
hooks: {},
// Store track event history data
history: [],
// Stores ad-hoc state related data]
state: {
volume: this.media.volume
},
// Store track event object references by trackId
trackRefs: {},
// Playback track event queues
trackEvents: new TrackEvents( this )
};
// Register new instance
Popcorn.instances.push( this );
// function to fire when video is ready
var isReady = function() {
// chrome bug: http://code.google.com/p/chromium/issues/detail?id=119598
// it is possible the video's time is less than 0
// this has the potential to call track events more than once, when they should not
// start: 0, end: 1 will start, end, start again, when it should just start
// just setting it to 0 if it is below 0 fixes this issue
if ( self.media.currentTime < 0 ) {
self.media.currentTime = 0;
}
self.media.removeEventListener( "loadedmetadata", isReady, false );
var duration, videoDurationPlus,
runningPlugins, runningPlugin, rpLength, rpNatives;
// Adding padding to the front and end of the arrays
// this is so we do not fall off either end
duration = self.media.duration;
// Check for no duration info (NaN)
videoDurationPlus = duration != duration ? Number.MAX_VALUE : duration + 1;
Popcorn.addTrackEvent( self, {
start: videoDurationPlus,
end: videoDurationPlus
});
if ( !self.isDestroyed ) {
self.data.durationChange = function() {
var newDuration = self.media.duration,
newDurationPlus = newDuration + 1,
byStart = self.data.trackEvents.byStart,
byEnd = self.data.trackEvents.byEnd;
// Remove old padding events
byStart.pop();
byEnd.pop();
// Remove any internal tracking of events that have end times greater than duration
// otherwise their end events will never be hit.
for ( var k = byEnd.length - 1; k > 0; k-- ) {
if ( byEnd[ k ].end > newDuration ) {
self.removeTrackEvent( byEnd[ k ]._id );
}
}
// Remove any internal tracking of events that have end times greater than duration
// otherwise their end events will never be hit.
for ( var i = 0; i < byStart.length; i++ ) {
if ( byStart[ i ].end > newDuration ) {
self.removeTrackEvent( byStart[ i ]._id );
}
}
// References to byEnd/byStart are reset, so accessing it this way is
// forced upon us.
self.data.trackEvents.byEnd.push({
start: newDurationPlus,
end: newDurationPlus
});
self.data.trackEvents.byStart.push({
start: newDurationPlus,
end: newDurationPlus
});
};
// Listen for duration changes and adjust internal tracking of event timings
self.media.addEventListener( "durationchange", self.data.durationChange, false );
}
if ( self.options.frameAnimation ) {
// if Popcorn is created with frameAnimation option set to true,
// requestAnimFrame is used instead of "timeupdate" media event.
// This is for greater frame time accuracy, theoretically up to
// 60 frames per second as opposed to ~4 ( ~every 15-250ms)
self.data.timeUpdate = function () {
Popcorn.timeUpdate( self, {} );
// fire frame for each enabled active plugin of every type
Popcorn.forEach( Popcorn.manifest, function( key, val ) {
runningPlugins = self.data.running[ val ];
// ensure there are running plugins on this type on this instance
if ( runningPlugins ) {
rpLength = runningPlugins.length;
for ( var i = 0; i < rpLength; i++ ) {
runningPlugin = runningPlugins[ i ];
rpNatives = runningPlugin._natives;
rpNatives && rpNatives.frame &&
rpNatives.frame.call( self, {}, runningPlugin, self.currentTime() );
}
}
});
self.emit( "timeupdate" );
!self.isDestroyed && requestAnimFrame( self.data.timeUpdate );
};
!self.isDestroyed && requestAnimFrame( self.data.timeUpdate );
} else {
self.data.timeUpdate = function( event ) {
Popcorn.timeUpdate( self, event );
};
if ( !self.isDestroyed ) {
self.media.addEventListener( "timeupdate", self.data.timeUpdate, false );
}
}
};
Object.defineProperty( this, "error", {
get: function() {
return self.media.error;
}
});
// http://www.whatwg.org/specs/web-apps/current-work/#dom-media-readystate
//
// If media is in readyState (rS) >= 1, we know the media's duration,
// which is required before running the isReady function.
// If rS is 0, attach a listener for "loadedmetadata",
// ( Which indicates that the media has moved from rS 0 to 1 )
//
// This has been changed from a check for rS 2 because
// in certain conditions, Firefox can enter this code after dropping
// to rS 1 from a higher state such as 2 or 3. This caused a "loadeddata"
// listener to be attached to the media object, an event that had
// already triggered and would not trigger again. This left Popcorn with an
// instance that could never start a timeUpdate loop.
if ( self.media.readyState >= 1 ) {
isReady();
} else {
self.media.addEventListener( "loadedmetadata", isReady, false );
}
return this;
}
};
// Extend constructor prototype to instance prototype
// Allows chaining methods to instances
Popcorn.p.init.prototype = Popcorn.p;
Popcorn.byId = function( str ) {
var instances = Popcorn.instances,
length = instances.length,
i = 0;
for ( ; i < length; i++ ) {
if ( instances[ i ].id === str ) {
return instances[ i ];
}
}
return null;
};
Popcorn.forEach = function( obj, fn, context ) {
if ( !obj || !fn ) {
return {};
}
context = context || this;
var key, len;
// Use native whenever possible
if ( forEach && obj.forEach === forEach ) {
return obj.forEach( fn, context );
}
if ( toString.call( obj ) === "[object NodeList]" ) {
for ( key = 0, len = obj.length; key < len; key++ ) {
fn.call( context, obj[ key ], key, obj );
}
return obj;
}
for ( key in obj ) {
if ( hasOwn.call( obj, key ) ) {
fn.call( context, obj[ key ], key, obj );
}
}
return obj;
};
Popcorn.extend = function( obj ) {
var dest = obj, src = slice.call( arguments, 1 );
Popcorn.forEach( src, function( copy ) {
for ( var prop in copy ) {
dest[ prop ] = copy[ prop ];
}
});
return dest;
};
// A Few reusable utils, memoized onto Popcorn
Popcorn.extend( Popcorn, {
noConflict: function( deep ) {
if ( deep ) {
global.Popcorn = _Popcorn;
}
return Popcorn;
},
error: function( msg ) {
throw new Error( msg );
},
guid: function( prefix ) {
Popcorn.guid.counter++;
return ( prefix ? prefix : "" ) + ( +new Date() + Popcorn.guid.counter );
},
sizeOf: function( obj ) {
var size = 0;
for ( var prop in obj ) {
size++;
}
return size;
},
isArray: Array.isArray || function( array ) {
return toString.call( array ) === "[object Array]";
},
nop: function() {},
position: function( elem ) {
if ( !elem.parentNode ) {
return null;
}
var clientRect = elem.getBoundingClientRect(),
bounds = {},
doc = elem.ownerDocument,
docElem = document.documentElement,
body = document.body,
clientTop, clientLeft, scrollTop, scrollLeft, top, left;
// Determine correct clientTop/Left
clientTop = docElem.clientTop || body.clientTop || 0;
clientLeft = docElem.clientLeft || body.clientLeft || 0;
// Determine correct scrollTop/Left
scrollTop = ( global.pageYOffset && docElem.scrollTop || body.scrollTop );
scrollLeft = ( global.pageXOffset && docElem.scrollLeft || body.scrollLeft );
// Temp top/left
top = Math.ceil( clientRect.top + scrollTop - clientTop );
left = Math.ceil( clientRect.left + scrollLeft - clientLeft );
for ( var p in clientRect ) {
bounds[ p ] = Math.round( clientRect[ p ] );
}
return Popcorn.extend({}, bounds, { top: top, left: left });
},
disable: function( instance, plugin ) {
if ( instance.data.disabled[ plugin ] ) {
return;
}
instance.data.disabled[ plugin ] = true;
if ( plugin in Popcorn.registryByName &&
instance.data.running[ plugin ] ) {
for ( var i = instance.data.running[ plugin ].length - 1, event; i >= 0; i-- ) {
event = instance.data.running[ plugin ][ i ];
event._natives.end.call( instance, null, event );
instance.emit( "trackend",
Popcorn.extend({}, event, {
plugin: event.type,
type: "trackend"
})
);
}
}
return instance;
},
enable: function( instance, plugin ) {
if ( !instance.data.disabled[ plugin ] ) {
return;
}
instance.data.disabled[ plugin ] = false;
if ( plugin in Popcorn.registryByName &&
instance.data.running[ plugin ] ) {
for ( var i = instance.data.running[ plugin ].length - 1, event; i >= 0; i-- ) {
event = instance.data.running[ plugin ][ i ];
event._natives.start.call( instance, null, event );
instance.emit( "trackstart",
Popcorn.extend({}, event, {
plugin: event.type,
type: "trackstart",
track: event
})
);
}
}
return instance;
},
destroy: function( instance ) {
var events = instance.data.events,
trackEvents = instance.data.trackEvents,
singleEvent, item, fn, plugin;
// Iterate through all events and remove them
for ( item in events ) {
singleEvent = events[ item ];
for ( fn in singleEvent ) {
delete singleEvent[ fn ];
}
events[ item ] = null;
}
// remove all plugins off the given instance
for ( plugin in Popcorn.registryByName ) {
Popcorn.removePlugin( instance, plugin );
}
// Remove all data.trackEvents #1178
trackEvents.byStart.length = 0;
trackEvents.byEnd.length = 0;
if ( !instance.isDestroyed ) {
instance.data.timeUpdate && instance.media.removeEventListener( "timeupdate", instance.data.timeUpdate, false );
instance.isDestroyed = true;
}
Popcorn.instances.splice( Popcorn.instances.indexOf( instance ), 1 );
}
});
// Memoized GUID Counter
Popcorn.guid.counter = 1;
// Factory to implement getters, setters and controllers
// as Popcorn instance methods. The IIFE will create and return
// an object with defined methods
Popcorn.extend(Popcorn.p, (function() {
var methods = "load play pause currentTime playbackRate volume duration preload playbackRate " +
"autoplay loop controls muted buffered readyState seeking paused played seekable ended",
ret = {};
// Build methods, store in object that is returned and passed to extend
Popcorn.forEach( methods.split( /\s+/g ), function( name ) {
ret[ name ] = function( arg ) {
var previous;
if ( typeof this.media[ name ] === "function" ) {
// Support for shorthanded play(n)/pause(n) jump to currentTime
// If arg is not null or undefined and called by one of the
// allowed shorthandable methods, then set the currentTime
// Supports time as seconds or SMPTE
if ( arg != null && /play|pause/.test( name ) ) {
this.media.currentTime = Popcorn.util.toSeconds( arg );
}
this.media[ name ]();
return this;
}
if ( arg != null ) {
// Capture the current value of the attribute property
previous = this.media[ name ];
// Set the attribute property with the new value
this.media[ name ] = arg;
// If the new value is not the same as the old value
// emit an "attrchanged event"
if ( previous !== arg ) {
this.emit( "attrchange", {
attribute: name,
previousValue: previous,
currentValue: arg
});
}
return this;
}
return this.media[ name ];
};
});
return ret;
})()
);
Popcorn.forEach( "enable disable".split(" "), function( method ) {
Popcorn.p[ method ] = function( plugin ) {
return Popcorn[ method ]( this, plugin );
};
});
Popcorn.extend(Popcorn.p, {
// Rounded currentTime
roundTime: function() {
return Math.round( this.media.currentTime );
},
// Attach an event to a single point in time
exec: function( id, time, fn ) {
var length = arguments.length,
eventType = "trackadded",
trackEvent, sec, options;
// Check if first could possibly be a SMPTE string
// p.cue( "smpte string", fn );
// try/catch avoid awful throw in Popcorn.util.toSeconds
// TODO: Get rid of that, replace with NaN return?
try {
sec = Popcorn.util.toSeconds( id );
} catch ( e ) {}
// If it can be converted into a number then
// it's safe to assume that the string was SMPTE
if ( typeof sec === "number" ) {
id = sec;
}
// Shift arguments based on use case
//
// Back compat for:
// p.cue( time, fn );
if ( typeof id === "number" && length === 2 ) {
fn = time;
time = id;
id = Popcorn.guid( "cue" );
} else {
// Support for new forms
// p.cue( "empty-cue" );
if ( length === 1 ) {
// Set a time for an empty cue. It's not important what
// the time actually is, because the cue is a no-op
time = -1;
} else {
// Get the TrackEvent that matches the given id.
trackEvent = this.getTrackEvent( id );
if ( trackEvent ) {
// remove existing cue so a new one can be added via trackEvents.add
this.data.trackEvents.remove( id );
TrackEvent.end( this, trackEvent );
// Update track event references
Popcorn.removeTrackEvent.ref( this, id );
eventType = "cuechange";
// p.cue( "my-id", 12 );
// p.cue( "my-id", function() { ... });
if ( typeof id === "string" && length === 2 ) {
// p.cue( "my-id", 12 );
// The path will update the cue time.
if ( typeof time === "number" ) {
// Re-use existing TrackEvent start callback
fn = trackEvent._natives.start;
}
// p.cue( "my-id", function() { ... });
// The path will update the cue function
if ( typeof time === "function" ) {
fn = time;
// Re-use existing TrackEvent start time
time = trackEvent.start;
}
}
} else {
if ( length >= 2 ) {
// p.cue( "a", "00:00:00");
if ( typeof time === "string" ) {
try {
sec = Popcorn.util.toSeconds( time );
} catch ( e ) {}
time = sec;
}
// p.cue( "b", 11 );
// p.cue( "b", 11, function() {} );
if ( typeof time === "number" ) {
fn = fn || Popcorn.nop();
}
// p.cue( "c", function() {});
if ( typeof time === "function" ) {
fn = time;
time = -1;
}
}
}
}
}
options = {
id: id,
start: time,
end: time + 1,
_running: false,
_natives: {
start: fn || Popcorn.nop,
end: Popcorn.nop,
type: "cue"
}
};
if ( trackEvent ) {
options = Popcorn.extend( trackEvent, options );
}
if ( eventType === "cuechange" ) {
// Supports user defined track event id
options._id = options.id || options._id || Popcorn.guid( options._natives.type );
this.data.trackEvents.add( options );
TrackEvent.start( this, options );
this.timeUpdate( this, null, true );
// Store references to user added trackevents in ref table
Popcorn.addTrackEvent.ref( this, options );
this.emit( eventType, Popcorn.extend({}, options, {
id: id,
type: eventType,
previousValue: {
time: trackEvent.start,
fn: trackEvent._natives.start
},
currentValue: {
time: time,
fn: fn || Popcorn.nop
},
track: trackEvent
}));
} else {
// Creating a one second track event with an empty end
Popcorn.addTrackEvent( this, options );
}
return this;
},
// Mute the calling media, optionally toggle
mute: function( toggle ) {
var event = toggle == null || toggle === true ? "muted" : "unmuted";
// If `toggle` is explicitly `false`,
// unmute the media and restore the volume level
if ( event === "unmuted" ) {
this.media.muted = false;
this.media.volume = this.data.state.volume;
}
// If `toggle` is either null or undefined,
// save the current volume and mute the media element
if ( event === "muted" ) {
this.data.state.volume = this.media.volume;
this.media.muted = true;
}
// Trigger either muted|unmuted event
this.emit( event );
return this;
},
// Convenience method, unmute the calling media
unmute: function( toggle ) {
return this.mute( toggle == null ? false : !toggle );
},
// Get the client bounding box of an instance element
position: function() {
return Popcorn.position( this.media );
},
// Toggle a plugin's playback behaviour (on or off) per instance
toggle: function( plugin ) {
return Popcorn[ this.data.disabled[ plugin ] ? "enable" : "disable" ]( this, plugin );
},
// Set default values for plugin options objects per instance
defaults: function( plugin, defaults ) {
// If an array of default configurations is provided,
// iterate and apply each to this instance
if ( Popcorn.isArray( plugin ) ) {
Popcorn.forEach( plugin, function( obj ) {
for ( var name in obj ) {
this.defaults( name, obj[ name ] );
}
}, this );
return this;
}
if ( !this.options.defaults ) {
this.options.defaults = {};
}
if ( !this.options.defaults[ plugin ] ) {
this.options.defaults[ plugin ] = {};
}
Popcorn.extend( this.options.defaults[ plugin ], defaults );
return this;
}
});
Popcorn.Events = {
UIEvents: "blur focus focusin focusout load resize scroll unload",
MouseEvents: "mousedown mouseup mousemove mouseover mouseout mouseenter mouseleave click dblclick",
Events: "loadstart progress suspend emptied stalled play pause error " +
"loadedmetadata loadeddata waiting playing canplay canplaythrough " +
"seeking seeked timeupdate ended ratechange durationchange volumechange"
};
Popcorn.Events.Natives = Popcorn.Events.UIEvents + " " +
Popcorn.Events.MouseEvents + " " +
Popcorn.Events.Events;
internal.events.apiTypes = [ "UIEvents", "MouseEvents", "Events" ];
// Privately compile events table at load time
(function( events, data ) {
var apis = internal.events.apiTypes,
eventsList = events.Natives.split( /\s+/g ),
idx = 0, len = eventsList.length, prop;
for( ; idx < len; idx++ ) {
data.hash[ eventsList[idx] ] = true;
}
apis.forEach(function( val, idx ) {
data.apis[ val ] = {};
var apiEvents = events[ val ].split( /\s+/g ),
len = apiEvents.length,
k = 0;
for ( ; k < len; k++ ) {
data.apis[ val ][ apiEvents[ k ] ] = true;
}
});
})( Popcorn.Events, internal.events );
Popcorn.events = {
isNative: function( type ) {
return !!internal.events.hash[ type ];
},
getInterface: function( type ) {
if ( !Popcorn.events.isNative( type ) ) {
return false;
}
var eventApi = internal.events,
apis = eventApi.apiTypes,
apihash = eventApi.apis,
idx = 0, len = apis.length, api, tmp;
for ( ; idx < len; idx++ ) {
tmp = apis[ idx ];
if ( apihash[ tmp ][ type ] ) {
api = tmp;
break;
}
}
return api;
},
// Compile all native events to single array
all: Popcorn.Events.Natives.split( /\s+/g ),
// Defines all Event handling static functions
fn: {
trigger: function( type, data ) {
var eventInterface, evt, clonedEvents,
events = this.data.events[ type ];
// setup checks for custom event system
if ( events ) {
eventInterface = Popcorn.events.getInterface( type );
if ( eventInterface ) {
evt = document.createEvent( eventInterface );
evt.initEvent( type, true, true, global, 1 );
this.media.dispatchEvent( evt );
return this;
}
// clone events in case callbacks remove callbacks themselves
clonedEvents = events.slice();
// iterate through all callbacks
while ( clonedEvents.length ) {
clonedEvents.shift().call( this, data );
}
}
return this;
},
listen: function( type, fn ) {
var self = this,
hasEvents = true,
eventHook = Popcorn.events.hooks[ type ],
origType = type,
clonedEvents,
tmp;
if ( typeof fn !== "function" ) {
throw new Error( "Popcorn.js Error: Listener is not a function" );
}
// Setup event registry entry
if ( !this.data.events[ type ] ) {
this.data.events[ type ] = [];
// Toggle if the previous assumption was untrue
hasEvents = false;
}
// Check and setup event hooks
if ( eventHook ) {
// Execute hook add method if defined
if ( eventHook.add ) {
eventHook.add.call( this, {}, fn );
}
// Reassign event type to our piggyback event type if defined
if ( eventHook.bind ) {
type = eventHook.bind;
}
// Reassign handler if defined
if ( eventHook.handler ) {
tmp = fn;
fn = function wrapper( event ) {
eventHook.handler.call( self, event, tmp );
};
}
// assume the piggy back event is registered
hasEvents = true;
// Setup event registry entry
if ( !this.data.events[ type ] ) {
this.data.events[ type ] = [];
// Toggle if the previous assumption was untrue
hasEvents = false;
}
}
// Register event and handler
this.data.events[ type ].push( fn );
// only attach one event of any type
if ( !hasEvents && Popcorn.events.all.indexOf( type ) > -1 ) {
this.media.addEventListener( type, function( event ) {
if ( self.data.events[ type ] ) {
// clone events in case callbacks remove callbacks themselves
clonedEvents = self.data.events[ type ].slice();
// iterate through all callbacks
while ( clonedEvents.length ) {
clonedEvents.shift().call( self, event );
}
}
}, false );
}
return this;
},
unlisten: function( type, fn ) {
var ind,
events = this.data.events[ type ];
if ( !events ) {
return; // no listeners = nothing to do
}
if ( typeof fn === "string" ) {
// legacy support for string-based removal -- not recommended
for ( var i = 0; i < events.length; i++ ) {
if ( events[ i ].name === fn ) {
// decrement i because array length just got smaller
events.splice( i--, 1 );
}
}
return this;
} else if ( typeof fn === "function" ) {
while( ind !== -1 ) {
ind = events.indexOf( fn );
if ( ind !== -1 ) {
events.splice( ind, 1 );
}
}
return this;
}
// if we got to this point, we are deleting all functions of this type
this.data.events[ type ] = null;
return this;
}
},
hooks: {
canplayall: {
bind: "canplaythrough",
add: function( event, callback ) {
var state = false;
if ( this.media.readyState ) {
// always call canplayall asynchronously
setTimeout(function() {
callback.call( this, event );
}.bind(this), 0 );
state = true;
}
this.data.hooks.canplayall = {
fired: state
};
},
// declare special handling instructions
handler: function canplayall( event, callback ) {
if ( !this.data.hooks.canplayall.fired ) {
// trigger original user callback once
callback.call( this, event );
this.data.hooks.canplayall.fired = true;
}
}
}
}
};
// Extend Popcorn.events.fns (listen, unlisten, trigger) to all Popcorn instances
// Extend aliases (on, off, emit)
Popcorn.forEach( [ [ "trigger", "emit" ], [ "listen", "on" ], [ "unlisten", "off" ] ], function( key ) {
Popcorn.p[ key[ 0 ] ] = Popcorn.p[ key[ 1 ] ] = Popcorn.events.fn[ key[ 0 ] ];
});
// Internal Only - construct simple "TrackEvent"
// data type objects
function TrackEvent( track ) {
Abstract.put.call( this, track );
}
// Determine if a TrackEvent's "start" and "trackstart" must be called.
TrackEvent.start = function( instance, track ) {
if ( track.end > instance.media.currentTime &&
track.start <= instance.media.currentTime && !track._running ) {
track._running = true;
instance.data.running[ track._natives.type ].push( track );
if ( !instance.data.disabled[ track._natives.type ] ) {
track._natives.start.call( instance, null, track );
instance.emit( "trackstart",
Popcorn.extend( {}, track, {
plugin: track._natives.type,
type: "trackstart",
track: track
})
);
}
}
};
// Determine if a TrackEvent's "end" and "trackend" must be called.
TrackEvent.end = function( instance, track ) {
var runningPlugins;
if ( ( track.end <= instance.media.currentTime ||
track.start > instance.media.currentTime ) && track._running ) {
runningPlugins = instance.data.running[ track._natives.type ];
track._running = false;
runningPlugins.splice( runningPlugins.indexOf( track ), 1 );
if ( !instance.data.disabled[ track._natives.type ] ) {
track._natives.end.call( instance, null, track );
instance.emit( "trackend",
Popcorn.extend( {}, track, {
plugin: track._natives.type,
type: "trackend",
track: track
})
);
}
}
};
// Internal Only - construct "TrackEvents"
// data type objects that are used by the Popcorn
// instance, stored at p.data.trackEvents
function TrackEvents( parent ) {
this.parent = parent;
this.byStart = [{
start: -1,
end: -1
}];
this.byEnd = [{
start: -1,
end: -1
}];
this.animating = [];
this.startIndex = 0;
this.endIndex = 0;
this.previousUpdateTime = -1;
Object.defineProperty( this, "count", {
get: function() {
return this.byStart.length;
}
});
}
function isMatch( obj, key, value ) {
return obj[ key ] && obj[ key ] === value;
}
TrackEvents.prototype.where = function( params ) {
return ( this.parent.getTrackEvents() || [] ).filter(function( event ) {
var key, value;
// If no explicit params, match all TrackEvents
if ( !params ) {
return true;
}
// Filter keys in params against both the top level properties
// and the _natives properties
for ( key in params ) {
value = params[ key ];
if ( isMatch( event, key, value ) || isMatch( event._natives, key, value ) ) {
return true;
}
}
return false;
});
};
TrackEvents.prototype.add = function( track ) {
// Store this definition in an array sorted by times
var byStart = this.byStart,
byEnd = this.byEnd,
startIndex, endIndex;
// Push track event ids into the history
if ( track && track._id ) {
this.parent.data.history.push( track._id );
}
track.start = Popcorn.util.toSeconds( track.start, this.parent.options.framerate );
track.end = Popcorn.util.toSeconds( track.end, this.parent.options.framerate );
for ( startIndex = byStart.length - 1; startIndex >= 0; startIndex-- ) {
if ( track.start >= byStart[ startIndex ].start ) {
byStart.splice( startIndex + 1, 0, track );
break;
}
}
for ( endIndex = byEnd.length - 1; endIndex >= 0; endIndex-- ) {
if ( track.end > byEnd[ endIndex ].end ) {
byEnd.splice( endIndex + 1, 0, track );
break;
}
}
// update startIndex and endIndex
if ( startIndex <= this.parent.data.trackEvents.startIndex &&
track.start <= this.parent.data.trackEvents.previousUpdateTime ) {
this.parent.data.trackEvents.startIndex++;
}
if ( endIndex <= this.parent.data.trackEvents.endIndex &&
track.end < this.parent.data.trackEvents.previousUpdateTime ) {
this.parent.data.trackEvents.endIndex++;
}
};
TrackEvents.prototype.remove = function( removeId, state ) {
if ( removeId instanceof TrackEvent ) {
removeId = removeId.id;
}
if ( typeof removeId === "object" ) {
// Filter by key=val and remove all matching TrackEvents
this.where( removeId ).forEach(function( event ) {
// |this| refers to the calling Popcorn "parent" instance
this.removeTrackEvent( event._id );
}, this.parent );
return this;
}
var start, end, animate, historyLen, track,
length = this.byStart.length,
index = 0,
indexWasAt = 0,
byStart = [],
byEnd = [],
animating = [],
history = [],
comparable = {};
state = state || {};
while ( --length > -1 ) {
start = this.byStart[ index ];
end = this.byEnd[ index ];
// Padding events will not have _id properties.
// These should be safely pushed onto the front and back of the
// track event array
if ( !start._id ) {
byStart.push( start );
byEnd.push( end );
}
// Filter for user track events (vs system track events)
if ( start._id ) {
// If not a matching start event for removal
if ( start._id !== removeId ) {
byStart.push( start );
}
// If not a matching end event for removal
if ( end._id !== removeId ) {
byEnd.push( end );
}
// If the _id is matched, capture the current index
if ( start._id === removeId ) {
indexWasAt = index;
// cache the track event being removed
track = start;
}
}
// Increment the track index
index++;
}
// Reset length to be used by the condition below to determine
// if animating track events should also be filtered for removal.
// Reset index below to be used by the reverse while as an
// incrementing counter
length = this.animating.length;
index = 0;
if ( length ) {
while ( --length > -1 ) {
animate = this.animating[ index ];
// Padding events will not have _id properties.
// These should be safely pushed onto the front and back of the
// track event array
if ( !animate._id ) {
animating.push( animate );
}
// If not a matching animate event for removal
if ( animate._id && animate._id !== removeId ) {
animating.push( animate );
}
// Increment the track index
index++;
}
}
// Update
if ( indexWasAt <= this.startIndex ) {
this.startIndex--;
}
if ( indexWasAt <= this.endIndex ) {
this.endIndex--;
}
this.byStart = byStart;
this.byEnd = byEnd;
this.animating = animating;
historyLen = this.parent.data.history.length;
for ( var i = 0; i < historyLen; i++ ) {
if ( this.parent.data.history[ i ] !== removeId ) {
history.push( this.parent.data.history[ i ] );
}
}
// Update ordered history array
this.parent.data.history = history;
};
// Helper function used to retrieve old values of properties that
// are provided for update.
function getPreviousProperties( oldOptions, newOptions ) {
var matchProps = {};
for ( var prop in oldOptions ) {
if ( hasOwn.call( newOptions, prop ) && hasOwn.call( oldOptions, prop ) ) {
matchProps[ prop ] = oldOptions[ prop ];
}
}
return matchProps;
}
// Internal Only - Adds track events to the instance object
Popcorn.addTrackEvent = function( obj, track ) {
var temp;
if ( track instanceof TrackEvent ) {
return;
}
track = new TrackEvent( track );
// Determine if this track has default options set for it
// If so, apply them to the track object
if ( track && track._natives && track._natives.type &&
( obj.options.defaults && obj.options.defaults[ track._natives.type ] ) ) {
// To ensure that the TrackEvent Invariant Policy is enforced,
// First, copy the properties of the newly created track event event
// to a temporary holder
temp = Popcorn.extend( {}, track );
// Next, copy the default onto the newly created trackevent, followed by the
// temporary holder.
Popcorn.extend( track, obj.options.defaults[ track._natives.type ], temp );
}
if ( track._natives ) {
// Supports user defined track event id
track._id = track.id || track._id || Popcorn.guid( track._natives.type );
// Trigger _setup method if exists
if ( track._natives._setup ) {
track._natives._setup.call( obj, track );
obj.emit( "tracksetup", Popcorn.extend( {}, track, {
plugin: track._natives.type,
type: "tracksetup",
track: track
}));
}
}
obj.data.trackEvents.add( track );
TrackEvent.start( obj, track );
this.timeUpdate( obj, null, true );
// Store references to user added trackevents in ref table
if ( track._id ) {
Popcorn.addTrackEvent.ref( obj, track );
}
obj.emit( "trackadded", Popcorn.extend({}, track,
track._natives ? { plugin: track._natives.type } : {}, {
type: "trackadded",
track: track
}));
};
// Internal Only - Adds track event references to the instance object's trackRefs hash table
Popcorn.addTrackEvent.ref = function( obj, track ) {
obj.data.trackRefs[ track._id ] = track;
return obj;
};
Popcorn.removeTrackEvent = function( obj, removeId ) {
var track = obj.getTrackEvent( removeId );
if ( !track ) {
return;
}
// If a _teardown function was defined,
// enforce for track event removals
if ( track._natives._teardown ) {
track._natives._teardown.call( obj, track );
}
obj.data.trackEvents.remove( removeId );
// Update track event references
Popcorn.removeTrackEvent.ref( obj, removeId );
if ( track._natives ) {
// Fire a trackremoved event
obj.emit( "trackremoved", Popcorn.extend({}, track, {
plugin: track._natives.type,
type: "trackremoved",
track: track
}));
}
};
// Internal Only - Removes track event references from instance object's trackRefs hash table
Popcorn.removeTrackEvent.ref = function( obj, removeId ) {
delete obj.data.trackRefs[ removeId ];
return obj;
};
// Return an array of track events bound to this instance object
Popcorn.getTrackEvents = function( obj ) {
var trackevents = [],
refs = obj.data.trackEvents.byStart,
length = refs.length,
idx = 0,
ref;
for ( ; idx < length; idx++ ) {
ref = refs[ idx ];
// Return only user attributed track event references
if ( ref._id ) {
trackevents.push( ref );
}
}
return trackevents;
};
// Internal Only - Returns an instance object's trackRefs hash table
Popcorn.getTrackEvents.ref = function( obj ) {
return obj.data.trackRefs;
};
// Return a single track event bound to this instance object
Popcorn.getTrackEvent = function( obj, trackId ) {
return obj.data.trackRefs[ trackId ];
};
// Internal Only - Returns an instance object's track reference by track id
Popcorn.getTrackEvent.ref = function( obj, trackId ) {
return obj.data.trackRefs[ trackId ];
};
Popcorn.getLastTrackEventId = function( obj ) {
return obj.data.history[ obj.data.history.length - 1 ];
};
Popcorn.timeUpdate = function( obj, event ) {
var currentTime = obj.media.currentTime,
previousTime = obj.data.trackEvents.previousUpdateTime,
tracks = obj.data.trackEvents,
end = tracks.endIndex,
start = tracks.startIndex,
byStartLen = tracks.byStart.length,
byEndLen = tracks.byEnd.length,
registryByName = Popcorn.registryByName,
trackstart = "trackstart",
trackend = "trackend",
byEnd, byStart, byAnimate, natives, type, runningPlugins;
// Playbar advancing
if ( previousTime <= currentTime ) {
while ( tracks.byEnd[ end ] && tracks.byEnd[ end ].end <= currentTime ) {
byEnd = tracks.byEnd[ end ];
natives = byEnd._natives;
type = natives && natives.type;
// If plugin does not exist on this instance, remove it
if ( !natives ||
( !!registryByName[ type ] ||
!!obj[ type ] ) ) {
if ( byEnd._running === true ) {
byEnd._running = false;
runningPlugins = obj.data.running[ type ];
runningPlugins.splice( runningPlugins.indexOf( byEnd ), 1 );
if ( !obj.data.disabled[ type ] ) {
natives.end.call( obj, event, byEnd );
obj.emit( trackend,
Popcorn.extend({}, byEnd, {
plugin: type,
type: trackend,
track: byEnd
})
);
}
}
end++;
} else {
// remove track event
Popcorn.removeTrackEvent( obj, byEnd._id );
return;
}
}
while ( tracks.byStart[ start ] && tracks.byStart[ start ].start <= currentTime ) {
byStart = tracks.byStart[ start ];
natives = byStart._natives;
type = natives && natives.type;
// If plugin does not exist on this instance, remove it
if ( !natives ||
( !!registryByName[ type ] ||
!!obj[ type ] ) ) {
if ( byStart.end > currentTime &&
byStart._running === false ) {
byStart._running = true;
obj.data.running[ type ].push( byStart );
if ( !obj.data.disabled[ type ] ) {
natives.start.call( obj, event, byStart );
obj.emit( trackstart,
Popcorn.extend({}, byStart, {
plugin: type,
type: trackstart,
track: byStart
})
);
}
}
start++;
} else {
// remove track event
Popcorn.removeTrackEvent( obj, byStart._id );
return;
}
}
// Playbar receding
} else if ( previousTime > currentTime ) {
while ( tracks.byStart[ start ] && tracks.byStart[ start ].start > currentTime ) {
byStart = tracks.byStart[ start ];
natives = byStart._natives;
type = natives && natives.type;
// if plugin does not exist on this instance, remove it
if ( !natives ||
( !!registryByName[ type ] ||
!!obj[ type ] ) ) {
if ( byStart._running === true ) {
byStart._running = false;
runningPlugins = obj.data.running[ type ];
runningPlugins.splice( runningPlugins.indexOf( byStart ), 1 );
if ( !obj.data.disabled[ type ] ) {
natives.end.call( obj, event, byStart );
obj.emit( trackend,
Popcorn.extend({}, byStart, {
plugin: type,
type: trackend,
track: byStart
})
);
}
}
start--;
} else {
// remove track event
Popcorn.removeTrackEvent( obj, byStart._id );
return;
}
}
while ( tracks.byEnd[ end ] && tracks.byEnd[ end ].end > currentTime ) {
byEnd = tracks.byEnd[ end ];
natives = byEnd._natives;
type = natives && natives.type;
// if plugin does not exist on this instance, remove it
if ( !natives ||
( !!registryByName[ type ] ||
!!obj[ type ] ) ) {
if ( byEnd.start <= currentTime &&
byEnd._running === false ) {
byEnd._running = true;
obj.data.running[ type ].push( byEnd );
if ( !obj.data.disabled[ type ] ) {
natives.start.call( obj, event, byEnd );
obj.emit( trackstart,
Popcorn.extend({}, byEnd, {
plugin: type,
type: trackstart,
track: byEnd
})
);
}
}
end--;
} else {
// remove track event
Popcorn.removeTrackEvent( obj, byEnd._id );
return;
}
}
}
tracks.endIndex = end;
tracks.startIndex = start;
tracks.previousUpdateTime = currentTime;
//enforce index integrity if trackRemoved
tracks.byStart.length < byStartLen && tracks.startIndex--;
tracks.byEnd.length < byEndLen && tracks.endIndex--;
};
// Map and Extend TrackEvent functions to all Popcorn instances
Popcorn.extend( Popcorn.p, {
getTrackEvents: function() {
return Popcorn.getTrackEvents.call( null, this );
},
getTrackEvent: function( id ) {
return Popcorn.getTrackEvent.call( null, this, id );
},
getLastTrackEventId: function() {
return Popcorn.getLastTrackEventId.call( null, this );
},
removeTrackEvent: function( id ) {
Popcorn.removeTrackEvent.call( null, this, id );
return this;
},
removePlugin: function( name ) {
Popcorn.removePlugin.call( null, this, name );
return this;
},
timeUpdate: function( event ) {
Popcorn.timeUpdate.call( null, this, event );
return this;
},
destroy: function() {
Popcorn.destroy.call( null, this );
return this;
}
});
// Plugin manifests
Popcorn.manifest = {};
// Plugins are registered
Popcorn.registry = [];
Popcorn.registryByName = {};
// An interface for extending Popcorn
// with plugin functionality
Popcorn.plugin = function( name, definition, manifest ) {
if ( Popcorn.protect.natives.indexOf( name.toLowerCase() ) >= 0 ) {
Popcorn.error( "'" + name + "' is a protected function name" );
return;
}
// Provides some sugar, but ultimately extends
// the definition into Popcorn.p
var isfn = typeof definition === "function",
blacklist = [ "start", "end", "type", "manifest" ],
methods = [ "_setup", "_teardown", "start", "end", "frame" ],
plugin = {},
setup;
// combines calls of two function calls into one
var combineFn = function( first, second ) {
first = first || Popcorn.nop;
second = second || Popcorn.nop;
return function() {
first.apply( this, arguments );
second.apply( this, arguments );
};
};
// If `manifest` arg is undefined, check for manifest within the `definition` object
// If no `definition.manifest`, an empty object is a sufficient fallback
Popcorn.manifest[ name ] = manifest = manifest || definition.manifest || {};
// apply safe, and empty default functions
methods.forEach(function( method ) {
definition[ method ] = safeTry( definition[ method ] || Popcorn.nop, name );
});
var pluginFn = function( setup, options ) {
if ( !options ) {
return this;
}
// When the "ranges" property is set and its value is an array, short-circuit
// the pluginFn definition to recall itself with an options object generated from
// each range object in the ranges array. (eg. { start: 15, end: 16 } )
if ( options.ranges && Popcorn.isArray(options.ranges) ) {
Popcorn.forEach( options.ranges, function( range ) {
// Create a fresh object, extend with current options
// and start/end range object's properties
// Works with in/out as well.
var opts = Popcorn.extend( {}, options, range );
// Remove the ranges property to prevent infinitely
// entering this condition
delete opts.ranges;
// Call the plugin with the newly created opts object
this[ name ]( opts );
}, this);
// Return the Popcorn instance to avoid creating an empty track event
return this;
}
// Storing the plugin natives
var natives = options._natives = {},
compose = "",
originalOpts, manifestOpts;
Popcorn.extend( natives, setup );
options._natives.type = options._natives.plugin = name;
options._running = false;
natives.start = natives.start || natives[ "in" ];
natives.end = natives.end || natives[ "out" ];
if ( options.once ) {
natives.end = combineFn( natives.end, function() {
this.removeTrackEvent( options._id );
});
}
// extend teardown to always call end if running
natives._teardown = combineFn(function() {
var args = slice.call( arguments ),
runningPlugins = this.data.running[ natives.type ];
// end function signature is not the same as teardown,
// put null on the front of arguments for the event parameter
args.unshift( null );
// only call end if event is running
args[ 1 ]._running &&
runningPlugins.splice( runningPlugins.indexOf( options ), 1 ) &&
natives.end.apply( this, args );
args[ 1 ]._running = false;
this.emit( "trackend",
Popcorn.extend( {}, options, {
plugin: natives.type,
type: "trackend",
track: Popcorn.getTrackEvent( this, options.id || options._id )
})
);
}, natives._teardown );
// extend teardown to always trigger trackteardown after teardown
natives._teardown = combineFn( natives._teardown, function() {
this.emit( "trackteardown", Popcorn.extend( {}, options, {
plugin: name,
type: "trackteardown",
track: Popcorn.getTrackEvent( this, options.id || options._id )
}));
});
// default to an empty string if no effect exists
// split string into an array of effects
options.compose = options.compose || [];
if ( typeof options.compose === "string" ) {
options.compose = options.compose.split( " " );
}
options.effect = options.effect || [];
if ( typeof options.effect === "string" ) {
options.effect = options.effect.split( " " );
}
// join the two arrays together
options.compose = options.compose.concat( options.effect );
options.compose.forEach(function( composeOption ) {
// if the requested compose is garbage, throw it away
compose = Popcorn.compositions[ composeOption ] || {};
// extends previous functions with compose function
methods.forEach(function( method ) {
natives[ method ] = combineFn( natives[ method ], compose[ method ] );
});
});
// Ensure a manifest object, an empty object is a sufficient fallback
options._natives.manifest = manifest;
// Checks for expected properties
if ( !( "start" in options ) ) {
options.start = options[ "in" ] || 0;
}
if ( !options.end && options.end !== 0 ) {
options.end = options[ "out" ] || Number.MAX_VALUE;
}
// Use hasOwn to detect non-inherited toString, since all
// objects will receive a toString - its otherwise undetectable
if ( !hasOwn.call( options, "toString" ) ) {
options.toString = function() {
var props = [
"start: " + options.start,
"end: " + options.end,
"id: " + (options.id || options._id)
];
// Matches null and undefined, allows: false, 0, "" and truthy
if ( options.target != null ) {
props.push( "target: " + options.target );
}
return name + " ( " + props.join(", ") + " )";
};
}
// Resolves 239, 241, 242
if ( !options.target ) {
// Sometimes the manifest may be missing entirely
// or it has an options object that doesn't have a `target` property
manifestOpts = "options" in manifest && manifest.options;
options.target = manifestOpts && "target" in manifestOpts && manifestOpts.target;
}
if ( !options._id && options._natives ) {
// ensure an initial id is there before setup is called
options._id = Popcorn.guid( options._natives.type );
}
if ( options instanceof TrackEvent ) {
if ( options._natives ) {
// Supports user defined track event id
options._id = options.id || options._id || Popcorn.guid( options._natives.type );
// Trigger _setup method if exists
if ( options._natives._setup ) {
options._natives._setup.call( this, options );
this.emit( "tracksetup", Popcorn.extend( {}, options, {
plugin: options._natives.type,
type: "tracksetup",
track: options
}));
}
}
this.data.trackEvents.add( options );
TrackEvent.start( this, options );
this.timeUpdate( this, null, true );
// Store references to user added trackevents in ref table
if ( options._id ) {
Popcorn.addTrackEvent.ref( this, options );
}
} else {
// Create new track event for this instance
Popcorn.addTrackEvent( this, options );
}
// Future support for plugin event definitions
// for all of the native events
Popcorn.forEach( setup, function( callback, type ) {
// Don't attempt to create events for certain properties:
// "start", "end", "type", "manifest". Fixes #1365
if ( blacklist.indexOf( type ) === -1 ) {
this.on( type, callback );
}
}, this );
return this;
};
// Extend Popcorn.p with new named definition
// Assign new named definition
Popcorn.p[ name ] = plugin[ name ] = function( id, options ) {
var length = arguments.length,
trackEvent, defaults, mergedSetupOpts, previousOpts, newOpts;
// Shift arguments based on use case
//
// Back compat for:
// p.plugin( options );
if ( id && !options ) {
options = id;
id = null;
} else {
// Get the trackEvent that matches the given id.
trackEvent = this.getTrackEvent( id );
// If the track event does not exist, ensure that the options
// object has a proper id
if ( !trackEvent ) {
options.id = id;
// If the track event does exist, merge the updated properties
} else {
newOpts = options;
previousOpts = getPreviousProperties( trackEvent, newOpts );
// Call the plugins defined update method if provided. Allows for
// custom defined updating for a track event to be defined by the plugin author
if ( trackEvent._natives._update ) {
this.data.trackEvents.remove( trackEvent );
// It's safe to say that the intent of Start/End will never change
// Update them first before calling update
if ( hasOwn.call( options, "start" ) ) {
trackEvent.start = options.start;
}
if ( hasOwn.call( options, "end" ) ) {
trackEvent.end = options.end;
}
TrackEvent.end( this, trackEvent );
if ( isfn ) {
definition.call( this, trackEvent );
}
trackEvent._natives._update.call( this, trackEvent, options );
this.data.trackEvents.add( trackEvent );
TrackEvent.start( this, trackEvent );
} else {
// This branch is taken when there is no explicitly defined
// _update method for a plugin. Which will occur either explicitly or
// as a result of the plugin definition being a function that _returns_
// a definition object.
//
// In either case, this path can ONLY be reached for TrackEvents that
// already exist.
// Directly update the TrackEvent instance.
// This supports TrackEvent invariant enforcement.
Popcorn.extend( trackEvent, options );
this.data.trackEvents.remove( id );
// If a _teardown function was defined,
// enforce for track event removals
if ( trackEvent._natives._teardown ) {
trackEvent._natives._teardown.call( this, trackEvent );
}
// Update track event references
Popcorn.removeTrackEvent.ref( this, id );
if ( isfn ) {
pluginFn.call( this, definition.call( this, trackEvent ), trackEvent );
} else {
// Supports user defined track event id
trackEvent._id = trackEvent.id || trackEvent._id || Popcorn.guid( trackEvent._natives.type );
if ( trackEvent._natives && trackEvent._natives._setup ) {
trackEvent._natives._setup.call( this, trackEvent );
this.emit( "tracksetup", Popcorn.extend( {}, trackEvent, {
plugin: trackEvent._natives.type,
type: "tracksetup",
track: trackEvent
}));
}
this.data.trackEvents.add( trackEvent );
TrackEvent.start( this, trackEvent );
this.timeUpdate( this, null, true );
// Store references to user added trackevents in ref table
Popcorn.addTrackEvent.ref( this, trackEvent );
}
// Fire an event with change information
this.emit( "trackchange", {
id: trackEvent.id,
type: "trackchange",
previousValue: previousOpts,
currentValue: trackEvent,
track: trackEvent
});
return this;
}
if ( trackEvent._natives.type !== "cue" ) {
// Fire an event with change information
this.emit( "trackchange", {
id: trackEvent.id,
type: "trackchange",
previousValue: previousOpts,
currentValue: newOpts,
track: trackEvent
});
}
return this;
}
}
this.data.running[ name ] = this.data.running[ name ] || [];
// Merge with defaults if they exist, make sure per call is prioritized
defaults = ( this.options.defaults && this.options.defaults[ name ] ) || {};
mergedSetupOpts = Popcorn.extend( {}, defaults, options );
pluginFn.call( this, isfn ? definition.call( this, mergedSetupOpts ) : definition,
mergedSetupOpts );
return this;
};
// if the manifest parameter exists we should extend it onto the definition object
// so that it shows up when calling Popcorn.registry and Popcorn.registryByName
if ( manifest ) {
Popcorn.extend( definition, {
manifest: manifest
});
}
// Push into the registry
var entry = {
fn: plugin[ name ],
definition: definition,
base: definition,
parents: [],
name: name
};
Popcorn.registry.push(
Popcorn.extend( plugin, entry, {
type: name
})
);
Popcorn.registryByName[ name ] = entry;
return plugin;
};
// Storage for plugin function errors
Popcorn.plugin.errors = [];
// Returns wrapped plugin function
function safeTry( fn, pluginName ) {
return function() {
// When Popcorn.plugin.debug is true, do not suppress errors
if ( Popcorn.plugin.debug ) {
return fn.apply( this, arguments );
}
try {
return fn.apply( this, arguments );
} catch ( ex ) {
// Push plugin function errors into logging queue
Popcorn.plugin.errors.push({
plugin: pluginName,
thrown: ex,
source: fn.toString()
});
// Trigger an error that the instance can listen for
// and react to
this.emit( "pluginerror", Popcorn.plugin.errors );
}
};
}
// Debug-mode flag for plugin development
// True for Popcorn development versions, false for stable/tagged versions
Popcorn.plugin.debug = ( Popcorn.version === "@" + "VERSION" );
// removePlugin( type ) removes all tracks of that from all instances of popcorn
// removePlugin( obj, type ) removes all tracks of type from obj, where obj is a single instance of popcorn
Popcorn.removePlugin = function( obj, name ) {
// Check if we are removing plugin from an instance or from all of Popcorn
if ( !name ) {
// Fix the order
name = obj;
obj = Popcorn.p;
if ( Popcorn.protect.natives.indexOf( name.toLowerCase() ) >= 0 ) {
Popcorn.error( "'" + name + "' is a protected function name" );
return;
}
var registryLen = Popcorn.registry.length,
registryIdx;
// remove plugin reference from registry
for ( registryIdx = 0; registryIdx < registryLen; registryIdx++ ) {
if ( Popcorn.registry[ registryIdx ].name === name ) {
Popcorn.registry.splice( registryIdx, 1 );
delete Popcorn.registryByName[ name ];
delete Popcorn.manifest[ name ];
// delete the plugin
delete obj[ name ];
// plugin found and removed, stop checking, we are done
return;
}
}
}
var byStart = obj.data.trackEvents.byStart,
byEnd = obj.data.trackEvents.byEnd,
animating = obj.data.trackEvents.animating,
idx, sl;
// remove all trackEvents
for ( idx = 0, sl = byStart.length; idx < sl; idx++ ) {
if ( byStart[ idx ] && byStart[ idx ]._natives && byStart[ idx ]._natives.type === name ) {
byStart[ idx ]._natives._teardown && byStart[ idx ]._natives._teardown.call( obj, byStart[ idx ] );
byStart.splice( idx, 1 );
// update for loop if something removed, but keep checking
idx--; sl--;
if ( obj.data.trackEvents.startIndex <= idx ) {
obj.data.trackEvents.startIndex--;
obj.data.trackEvents.endIndex--;
}
}
// clean any remaining references in the end index
// we do this seperate from the above check because they might not be in the same order
if ( byEnd[ idx ] && byEnd[ idx ]._natives && byEnd[ idx ]._natives.type === name ) {
byEnd.splice( idx, 1 );
}
}
//remove all animating events
for ( idx = 0, sl = animating.length; idx < sl; idx++ ) {
if ( animating[ idx ] && animating[ idx ]._natives && animating[ idx ]._natives.type === name ) {
animating.splice( idx, 1 );
// update for loop if something removed, but keep checking
idx--; sl--;
}
}
};
Popcorn.compositions = {};
// Plugin inheritance
Popcorn.compose = function( name, definition, manifest ) {
// If `manifest` arg is undefined, check for manifest within the `definition` object
// If no `definition.manifest`, an empty object is a sufficient fallback
Popcorn.manifest[ name ] = manifest = manifest || definition.manifest || {};
// register the effect by name
Popcorn.compositions[ name ] = definition;
};
Popcorn.plugin.effect = Popcorn.effect = Popcorn.compose;
var rnaiveExpr = /^(?:\.|#|\[)/;
// Basic DOM utilities and helpers API. See #1037
Popcorn.dom = {
debug: false,
// Popcorn.dom.find( selector, context )
//
// Returns the first element that matches the specified selector
// Optionally provide a context element, defaults to `document`
//
// eg.
// Popcorn.dom.find("video") returns the first video element
// Popcorn.dom.find("#foo") returns the first element with `id="foo"`
// Popcorn.dom.find("foo") returns the first element with `id="foo"`
// Note: Popcorn.dom.find("foo") is the only allowed deviation
// from valid querySelector selector syntax
//
// Popcorn.dom.find(".baz") returns the first element with `class="baz"`
// Popcorn.dom.find("[preload]") returns the first element with `preload="..."`
// ...
// See https://developer.mozilla.org/En/DOM/Document.querySelector
//
//
find: function( selector, context ) {
var node = null;
// Default context is the `document`
context = context || document;
if ( selector ) {
// If the selector does not begin with "#", "." or "[",
// it could be either a nodeName or ID w/o "#"
if ( !rnaiveExpr.test( selector ) ) {
// Try finding an element that matches by ID first
node = document.getElementById( selector );
// If a match was found by ID, return the element
if ( node !== null ) {
return node;
}
}
// Assume no elements have been found yet
// Catch any invalid selector syntax errors and bury them.
try {
node = context.querySelector( selector );
} catch ( e ) {
if ( Popcorn.dom.debug ) {
throw new Error(e);
}
}
}
return node;
}
};
// Cache references to reused RegExps
var rparams = /\?/,
// XHR Setup object
setup = {
ajax: null,
url: "",
data: "",
dataType: "",
success: Popcorn.nop,
type: "GET",
async: true,
contentType: "application/x-www-form-urlencoded; charset=UTF-8"
};
Popcorn.xhr = function( options ) {
var settings;
options.dataType = options.dataType && options.dataType.toLowerCase() || null;
if ( options.dataType &&
( options.dataType === "jsonp" || options.dataType === "script" ) ) {
Popcorn.xhr.getJSONP(
options.url,
options.success,
options.dataType === "script"
);
return;
}
// Merge the "setup" defaults and custom "options"
// into a new plain object.
settings = Popcorn.extend( {}, setup, options );
// Create new XMLHttpRequest object
settings.ajax = new XMLHttpRequest();
if ( settings.ajax ) {
if ( settings.type === "GET" && settings.data ) {
// append query string
settings.url += ( rparams.test( settings.url ) ? "&" : "?" ) + settings.data;
// Garbage collect and reset settings.data
settings.data = null;
}
// Open the request
settings.ajax.open( settings.type, settings.url, settings.async );
// For POST, set the content-type request header
if ( settings.type === "POST" ) {
settings.ajax.setRequestHeader(
"Content-Type", settings.contentType
);
}
settings.ajax.send( settings.data || null );
return Popcorn.xhr.httpData( settings );
}
};
Popcorn.xhr.httpData = function( settings ) {
var data, json = null,
parser, xml = null;
settings.ajax.onreadystatechange = function() {
if ( settings.ajax.readyState === 4 ) {
try {
json = JSON.parse( settings.ajax.responseText );
} catch( e ) {
//suppress
}
data = {
xml: settings.ajax.responseXML,
text: settings.ajax.responseText,
json: json
};
// Normalize: data.xml is non-null in IE9 regardless of if response is valid xml
if ( !data.xml || !data.xml.documentElement ) {
data.xml = null;
try {
parser = new DOMParser();
xml = parser.parseFromString( settings.ajax.responseText, "text/xml" );
if ( !xml.getElementsByTagName( "parsererror" ).length ) {
data.xml = xml;
}
} catch ( e ) {
// data.xml remains null
}
}
// If a dataType was specified, return that type of data
if ( settings.dataType ) {
data = data[ settings.dataType ];
}
settings.success.call( settings.ajax, data );
}
};
return data;
};
Popcorn.xhr.getJSONP = function( url, success, isScript ) {
var head = document.head || document.getElementsByTagName( "head" )[ 0 ] || document.documentElement,
script = document.createElement( "script" ),
isFired = false,
params = [],
rjsonp = /(=)\?(?=&|$)|\?\?/,
replaceInUrl, prefix, paramStr, callback, callparam;
if ( !isScript ) {
// is there a calback already in the url
callparam = url.match( /(callback=[^&]*)/ );
if ( callparam !== null && callparam.length ) {
prefix = callparam[ 1 ].split( "=" )[ 1 ];
// Since we need to support developer specified callbacks
// and placeholders in harmony, make sure matches to "callback="
// aren't just placeholders.
// We coded ourselves into a corner here.
// JSONP callbacks should never have been
// allowed to have developer specified callbacks
if ( prefix === "?" ) {
prefix = "jsonp";
}
// get the callback name
callback = Popcorn.guid( prefix );
// replace existing callback name with unique callback name
url = url.replace( /(callback=[^&]*)/, "callback=" + callback );
} else {
callback = Popcorn.guid( "jsonp" );
if ( rjsonp.test( url ) ) {
url = url.replace( rjsonp, "$1" + callback );
}
// split on first question mark,
// this is to capture the query string
params = url.split( /\?(.+)?/ );
// rebuild url with callback
url = params[ 0 ] + "?";
if ( params[ 1 ] ) {
url += params[ 1 ] + "&";
}
url += "callback=" + callback;
}
// Define the JSONP success callback globally
window[ callback ] = function( data ) {
// Fire success callbacks
success && success( data );
isFired = true;
};
}
script.addEventListener( "load", function() {
// Handling remote script loading callbacks
if ( isScript ) {
// getScript
success && success();
}
// Executing for JSONP requests
if ( isFired ) {
// Garbage collect the callback
delete window[ callback ];
}
// Garbage collect the script resource
head.removeChild( script );
}, false );
script.src = url;
head.insertBefore( script, head.firstChild );
return;
};
Popcorn.getJSONP = Popcorn.xhr.getJSONP;
Popcorn.getScript = Popcorn.xhr.getScript = function( url, success ) {
return Popcorn.xhr.getJSONP( url, success, true );
};
Popcorn.util = {
// Simple function to parse a timestamp into seconds
// Acceptable formats are:
// HH:MM:SS.MMM
// HH:MM:SS;FF
// Hours and minutes are optional. They default to 0
toSeconds: function( timeStr, framerate ) {
// Hours and minutes are optional
// Seconds must be specified
// Seconds can be followed by milliseconds OR by the frame information
var validTimeFormat = /^([0-9]+:){0,2}[0-9]+([.;][0-9]+)?$/,
errorMessage = "Invalid time format",
digitPairs, lastIndex, lastPair, firstPair,
frameInfo, frameTime;
if ( typeof timeStr === "number" ) {
return timeStr;
}
if ( typeof timeStr === "string" &&
!validTimeFormat.test( timeStr ) ) {
Popcorn.error( errorMessage );
}
digitPairs = timeStr.split( ":" );
lastIndex = digitPairs.length - 1;
lastPair = digitPairs[ lastIndex ];
// Fix last element:
if ( lastPair.indexOf( ";" ) > -1 ) {
frameInfo = lastPair.split( ";" );
frameTime = 0;
if ( framerate && ( typeof framerate === "number" ) ) {
frameTime = parseFloat( frameInfo[ 1 ], 10 ) / framerate;
}
digitPairs[ lastIndex ] = parseInt( frameInfo[ 0 ], 10 ) + frameTime;
}
firstPair = digitPairs[ 0 ];
return {
1: parseFloat( firstPair, 10 ),
2: ( parseInt( firstPair, 10 ) * 60 ) +
parseFloat( digitPairs[ 1 ], 10 ),
3: ( parseInt( firstPair, 10 ) * 3600 ) +
( parseInt( digitPairs[ 1 ], 10 ) * 60 ) +
parseFloat( digitPairs[ 2 ], 10 )
}[ digitPairs.length || 1 ];
}
};
// alias for exec function
Popcorn.p.cue = Popcorn.p.exec;
// Protected API methods
Popcorn.protect = {
natives: getKeys( Popcorn.p ).map(function( val ) {
return val.toLowerCase();
})
};
// Setup logging for deprecated methods
Popcorn.forEach({
// Deprecated: Recommended
"listen": "on",
"unlisten": "off",
"trigger": "emit",
"exec": "cue"
}, function( recommend, api ) {
var original = Popcorn.p[ api ];
// Override the deprecated api method with a method of the same name
// that logs a warning and defers to the new recommended method
Popcorn.p[ api ] = function() {
if ( typeof console !== "undefined" && console.warn ) {
console.warn(
"Deprecated method '" + api + "', " +
(recommend == null ? "do not use." : "use '" + recommend + "' instead." )
);
// Restore api after first warning
Popcorn.p[ api ] = original;
}
return Popcorn.p[ recommend ].apply( this, [].slice.call( arguments ) );
};
});
// Exposes Popcorn to global context
global.Popcorn = Popcorn;
})(window, window.document);
|
docs/src/sections/JumbotronSection.js | jesenko/react-bootstrap | import React from 'react';
import Anchor from '../Anchor';
import PropTable from '../PropTable';
import ReactPlayground from '../ReactPlayground';
import Samples from '../Samples';
export default function JumbotronSection() {
return (
<div className="bs-docs-section">
<h2 className="page-header">
<Anchor id="jumbotron">Jumbotron</Anchor> <small>Jumbotron</small>
</h2>
<p>A lightweight, flexible component that can optionally extend the entire viewport to showcase key content on your site.</p>
<ReactPlayground codeText={Samples.Jumbotron} />
<h3><Anchor id="jumbotron-props">Props</Anchor></h3>
<PropTable component="Jumbotron"/>
</div>
);
}
|
src/components/detailsComponents/CompanyInfo.js | markgreenburg/acquisitiontracker | // @flow
import React from 'react';
import PropTypes from 'prop-types';
import Nav from './Nav';
import CompanyName from './CompanyName';
import CompanyBody from './CompanyBody';
const CompanyInfo = props => (
<div className="row company-info-row">
<div className="col-xs-12 company-info-content">
<div className="row company-header-row">
<Nav changeTab={props.changeTab} />
<CompanyName
deal={props.deal}
handleChange={props.handleChange}
handleSubmit={props.handleSubmit}
/>
</div>
<CompanyBody
deal={props.deal}
contacts={props.contacts}
handleChange={props.handleChange}
handleHqChange={props.handleHqChange}
handleSubmit={props.handleSubmit}
addContact={props.addContact}
editContact={props.editContact}
deleteContact={props.deleteContact}
/>
</div>
</div>
);
CompanyInfo.propTypes = {
deal: PropTypes.objectOf(PropTypes.any).isRequired,
changeTab: PropTypes.func.isRequired,
handleHqChange: PropTypes.func.isRequired,
handleChange: PropTypes.func.isRequired,
handleSubmit: PropTypes.func.isRequired,
addContact: PropTypes.func.isRequired,
editContact: PropTypes.func.isRequired,
deleteContact: PropTypes.func.isRequired,
contacts: PropTypes.arrayOf(PropTypes.object).isRequired,
};
export default CompanyInfo;
|
public/js/components/chat/tabs1.react.js | IsuruDilhan/Coupley | import React from 'react';
import List from 'material-ui/lib/lists/list';
import ListItem from 'material-ui/lib/lists/list-item';
import TextField from 'material-ui/lib/text-field';
import Divider from 'material-ui/lib/divider';
import IconButton from 'material-ui/lib/icon-button';
import Tabs from 'material-ui/lib/tabs/tabs';
import Tab from 'material-ui/lib/tabs/tab';
import Slider from 'material-ui/lib/slider';
import Dialog from 'material-ui/lib/dialog';
import FlatButton from 'material-ui/lib/flat-button';
import RaisedButton from 'material-ui/lib/raised-button';
import PreviousChat from './ChatPrevious.react';
import ThreadActions from '../../actions/Thread/ThreadActions';
import ThreadStore from '../../stores/ThreadStore';
import LoginStore from '../../stores/LoginStore';
var SearchCeck = true;
const ListStyle = {
width: 300,
height:635,
};
const searchconvo = {
marginTop:'-18',
paddingLeft:20,
};
const initMessagges = {
user1:LoginStore.getUsername(),
};
function validateStatusText(textStatus) {
if (textStatus.length > 250) {
return {
error: '*search is too long',
};
} else if (textStatus.length == 0) {
console.log('empty');
return {
error: '*search cannot be empty',
};
} else {
return true;
}
};
const PreviousChatContainer = React.createClass({
handleOpen: function () {
this.setState({ open: true });
},
handleClose: function () {
this.setState({ open: false });
},
getInitialState: function () {
return {
results:ThreadStore.getpreviousmessage(initMessagges),
statusText: '',
value: 'a',
open: false,
};
},
componentDidMount: function () {
ThreadStore.addChangeListener(this._onChange);
ThreadActions.getpreviousmessage(initMessagges);
},
handleChange:function (event, index, value) {
this.setState({
value: event,
});
},
_onChange: function () {
if (SearchCeck) {
this.setState({ results:ThreadStore.getpreviousmessage(initMessagges) });
} else if (!SearchCeck) {
this.setState({ results:ThreadStore.getsearchconv() });
}
},
previousMList: function () {
return this.state.results.map((result) => {
return (<PreviousChat key={result.id} id={result.thread_id} firstname={result.firstname} message={result.message} created_at={result.created_at}/>);
});
},
SearchConv:function () {
let ThisUser = this.refs.SearchM.getValue();
let ToSearch = {
user1:LoginStore.getUsername(),
user2:ThisUser,
};
if (validateStatusText(ThisUser).error) {
console.log('menna error');
this.setState({
statusText: validateStatusText(ThisUser).error,
});
val = false;
} else {
console.log('error na');
ThreadActions.getseachconv(ToSearch);
SearchCeck = false;
this.setState({
statusText: '',
});
}
{this.SearchResult();}
{this.clearText();}
},
clearText:function () {
document.getElementById('SearchField').value = '';
},
EnterKey(e) {
if (e.key === 'Enter') {
console.log('enter una');
{this.SearchConv();}
}
},
SearchResult:function () {
this.setState({ results:ThreadStore.getsearchconv() });
return this.state.results.map((result) => {
return (<PreviousChat key={result.id} id={result.id} firstname={result.firstname} message={result.message} created_at={result.created_at}/>);
});
},
render: function () {
const actions = [
<FlatButton
label="Cancel"
secondary={true}
onTouchTap={this.handleClose}
/>,
<FlatButton
label="Submit"
primary={true}
keyboardFocused={true}
onTouchTap={this.handleClose}
/>,
];
return (
<List style={ListStyle} zDepth={1}>
<Tabs>
<Tab label="Conversations" >
<div>
<Divider inset={false} />
<TextField hintText="Username" floatingLabelText="Search Conversation" style={searchconvo}
ref="SearchM" errorText={this.state.statusText} onKeyPress={this.EnterKey} id="SearchField"/>
<Divider inset={false} />
<div>
{this.previousMList()}
</div>
</div>
</Tab>
<Tab label="Message Request" >
<div>
<Divider inset={false} />
<TextField hintText="Username" floatingLabelText="Search Conversation" style={searchconvo} ref="SearchM" errorText={this.state.statusText} onKeyPress={this.EnterKey} id="SearchField"/>
<Divider inset={false} />
<div>
<div>
<RaisedButton label="Dialog" onTouchTap={this.handleOpen} />
<Dialog
title="Dialog With Actions"
actions={actions}
modal={false}
open={this.state.open}
onRequestClose={this.handleClose}
>
The actions in this window were passed in as an array of React objects.
</Dialog>
</div>
</div>
</div>
</Tab>
</Tabs>
</List>
);
},
});
export default PreviousChatContainer;
|
src/routes/user/UserList.js | hhj679/mybition-web | import React from 'react'
import PropTypes from 'prop-types'
import { Table, Modal } from 'antd'
import styles from './UserList.less'
import classnames from 'classnames'
import AnimTableBody from '../../components/DataTable/AnimTableBody'
import { DropOption } from '../../components'
import { Link } from 'dva/router'
const confirm = Modal.confirm
function list ({ loading, dataSource, pagination, onPageChange, onDeleteItem, onEditItem, isMotion, location }) {
const handleMenuClick = (record, e) => {
if (e.key === '1') {
onEditItem(record)
} else if (e.key === '2') {
confirm({
title: 'Are you sure delete this record?',
onOk () {
onDeleteItem(record.id)
},
})
}
}
const columns = [
{
title: 'Avatar',
dataIndex: 'avatar',
key: 'avatar',
width: 64,
className: styles.avatar,
render: (text) => <img alt={'avatar'} width={24} src={text} />,
}, {
title: 'Name',
dataIndex: 'name',
key: 'name',
render: (text, record) => <Link to={`user/${record.id}`}>{text}</Link>,
}, {
title: 'NickName',
dataIndex: 'nickName',
key: 'nickName',
}, {
title: 'Age',
dataIndex: 'age',
key: 'age',
}, {
title: 'Gender',
dataIndex: 'isMale',
key: 'isMale',
render: (text) => <span>{text
? 'Male'
: 'Female'}</span>,
}, {
title: 'Phone',
dataIndex: 'phone',
key: 'phone',
}, {
title: 'Email',
dataIndex: 'email',
key: 'email',
}, {
title: 'Address',
dataIndex: 'address',
key: 'address',
}, {
title: 'CreateTime',
dataIndex: 'createTime',
key: 'createTime',
}, {
title: 'Operation',
key: 'operation',
width: 100,
render: (text, record) => {
return <DropOption onMenuClick={e => handleMenuClick(record, e)} menuOptions={[{ key: '1', name: '编辑' }, { key: '2', name: '删除' }]} />
},
},
]
const getBodyWrapperProps = {
page: location.query.page,
current: pagination.current,
}
const getBodyWrapper = body => { return isMotion ? <AnimTableBody {...getBodyWrapperProps} body={body} /> : body }
return (
<div>
<Table
className={classnames({ [styles.table]: true, [styles.motion]: isMotion })}
bordered
scroll={{ x: 1200 }}
columns={columns}
dataSource={dataSource}
loading={loading}
onChange={onPageChange}
pagination={pagination}
simple
rowKey={record => record.id}
getBodyWrapper={getBodyWrapper}
/>
</div>
)
}
list.propTypes = {
loading: PropTypes.bool,
dataSource: PropTypes.array,
pagination: PropTypes.object,
onPageChange: PropTypes.func,
onDeleteItem: PropTypes.func,
onEditItem: PropTypes.func,
isMotion: PropTypes.bool,
location: PropTypes.object,
}
export default list
|
src/svg-icons/image/camera-alt.js | mtsandeep/material-ui | import React from 'react';
import pure from 'recompose/pure';
import SvgIcon from '../../SvgIcon';
let ImageCameraAlt = (props) => (
<SvgIcon {...props}>
<circle cx="12" cy="12" r="3.2"/><path d="M9 2L7.17 4H4c-1.1 0-2 .9-2 2v12c0 1.1.9 2 2 2h16c1.1 0 2-.9 2-2V6c0-1.1-.9-2-2-2h-3.17L15 2H9zm3 15c-2.76 0-5-2.24-5-5s2.24-5 5-5 5 2.24 5 5-2.24 5-5 5z"/>
</SvgIcon>
);
ImageCameraAlt = pure(ImageCameraAlt);
ImageCameraAlt.displayName = 'ImageCameraAlt';
ImageCameraAlt.muiName = 'SvgIcon';
export default ImageCameraAlt;
|
ajax/libs/yui/3.17.1-rc-1/datatable-body/datatable-body.js | iamJoeTaylor/cdnjs | YUI.add('datatable-body', function (Y, NAME) {
/**
View class responsible for rendering the `<tbody>` section of a table. Used as
the default `bodyView` for `Y.DataTable.Base` and `Y.DataTable` classes.
@module datatable
@submodule datatable-body
@since 3.5.0
**/
var Lang = Y.Lang,
isArray = Lang.isArray,
isNumber = Lang.isNumber,
isString = Lang.isString,
fromTemplate = Lang.sub,
htmlEscape = Y.Escape.html,
toArray = Y.Array,
bind = Y.bind,
YObject = Y.Object,
valueRegExp = /\{value\}/g,
EV_CONTENT_UPDATE = 'contentUpdate',
shiftMap = {
above: [-1, 0],
below: [1, 0],
next: [0, 1],
prev: [0, -1],
previous: [0, -1]
};
/**
View class responsible for rendering the `<tbody>` section of a table. Used as
the default `bodyView` for `Y.DataTable.Base` and `Y.DataTable` classes.
Translates the provided `modelList` into a rendered `<tbody>` based on the data
in the constituent Models, altered or amended by any special column
configurations.
The `columns` configuration, passed to the constructor, determines which
columns will be rendered.
The rendering process involves constructing an HTML template for a complete row
of data, built by concatenating a customized copy of the instance's
`CELL_TEMPLATE` into the `ROW_TEMPLATE` once for each column. This template is
then populated with values from each Model in the `modelList`, aggregating a
complete HTML string of all row and column data. A `<tbody>` Node is then created from the markup and any column `nodeFormatter`s are applied.
Supported properties of the column objects include:
* `key` - Used to link a column to an attribute in a Model.
* `name` - Used for columns that don't relate to an attribute in the Model
(`formatter` or `nodeFormatter` only) if the implementer wants a
predictable name to refer to in their CSS.
* `cellTemplate` - Overrides the instance's `CELL_TEMPLATE` for cells in this
column only.
* `formatter` - Used to customize or override the content value from the
Model. These do not have access to the cell or row Nodes and should
return string (HTML) content.
* `nodeFormatter` - Used to provide content for a cell as well as perform any
custom modifications on the cell or row Node that could not be performed by
`formatter`s. Should be used sparingly for better performance.
* `emptyCellValue` - String (HTML) value to use if the Model data for a
column, or the content generated by a `formatter`, is the empty string,
`null`, or `undefined`.
* `allowHTML` - Set to `true` if a column value, `formatter`, or
`emptyCellValue` can contain HTML. This defaults to `false` to protect
against XSS.
* `className` - Space delimited CSS classes to add to all `<td>`s in a column.
A column `formatter` can be:
* a function, as described below.
* a string which can be:
* the name of a pre-defined formatter function
which can be located in the `Y.DataTable.BodyView.Formatters` hash using the
value of the `formatter` property as the index.
* A template that can use the `{value}` placeholder to include the value
for the current cell or the name of any field in the underlaying model
also enclosed in curly braces. Any number and type of these placeholders
can be used.
Column `formatter`s are passed an object (`o`) with the following properties:
* `value` - The current value of the column's associated attribute, if any.
* `data` - An object map of Model keys to their current values.
* `record` - The Model instance.
* `column` - The column configuration object for the current column.
* `className` - Initially empty string to allow `formatter`s to add CSS
classes to the cell's `<td>`.
* `rowIndex` - The zero-based row number.
* `rowClass` - Initially empty string to allow `formatter`s to add CSS
classes to the cell's containing row `<tr>`.
They may return a value or update `o.value` to assign specific HTML content. A
returned value has higher precedence.
Column `nodeFormatter`s are passed an object (`o`) with the following
properties:
* `value` - The current value of the column's associated attribute, if any.
* `td` - The `<td>` Node instance.
* `cell` - The `<div>` liner Node instance if present, otherwise, the `<td>`.
When adding content to the cell, prefer appending into this property.
* `data` - An object map of Model keys to their current values.
* `record` - The Model instance.
* `column` - The column configuration object for the current column.
* `rowIndex` - The zero-based row number.
They are expected to inject content into the cell's Node directly, including
any "empty" cell content. Each `nodeFormatter` will have access through the
Node API to all cells and rows in the `<tbody>`, but not to the `<table>`, as
it will not be attached yet.
If a `nodeFormatter` returns `false`, the `o.td` and `o.cell` Nodes will be
`destroy()`ed to remove them from the Node cache and free up memory. The DOM
elements will remain as will any content added to them. _It is highly
advisable to always return `false` from your `nodeFormatter`s_.
@class BodyView
@namespace DataTable
@extends View
@since 3.5.0
**/
Y.namespace('DataTable').BodyView = Y.Base.create('tableBody', Y.View, [], {
// -- Instance properties -------------------------------------------------
/**
HTML template used to create table cells.
@property CELL_TEMPLATE
@type {String}
@default '<td {headers} class="{className}">{content}</td>'
@since 3.5.0
**/
CELL_TEMPLATE: '<td {headers} class="{className}">{content}</td>',
/**
CSS class applied to even rows. This is assigned at instantiation.
For DataTable, this will be `yui3-datatable-even`.
@property CLASS_EVEN
@type {String}
@default 'yui3-table-even'
@since 3.5.0
**/
//CLASS_EVEN: null
/**
CSS class applied to odd rows. This is assigned at instantiation.
When used by DataTable instances, this will be `yui3-datatable-odd`.
@property CLASS_ODD
@type {String}
@default 'yui3-table-odd'
@since 3.5.0
**/
//CLASS_ODD: null
/**
HTML template used to create table rows.
@property ROW_TEMPLATE
@type {String}
@default '<tr id="{rowId}" data-yui3-record="{clientId}" class="{rowClass}">{content}</tr>'
@since 3.5.0
**/
ROW_TEMPLATE : '<tr id="{rowId}" data-yui3-record="{clientId}" class="{rowClass}">{content}</tr>',
/**
The object that serves as the source of truth for column and row data.
This property is assigned at instantiation from the `host` property of
the configuration object passed to the constructor.
@property host
@type {Object}
@default (initially unset)
@since 3.5.0
**/
//TODO: should this be protected?
//host: null,
/**
HTML templates used to create the `<tbody>` containing the table rows.
@property TBODY_TEMPLATE
@type {String}
@default '<tbody class="{className}">{content}</tbody>'
@since 3.6.0
**/
TBODY_TEMPLATE: '<tbody class="{className}"></tbody>',
// -- Public methods ------------------------------------------------------
/**
Returns the `<td>` Node from the given row and column index. Alternately,
the `seed` can be a Node. If so, the nearest ancestor cell is returned.
If the `seed` is a cell, it is returned. If there is no cell at the given
coordinates, `null` is returned.
Optionally, include an offset array or string to return a cell near the
cell identified by the `seed`. The offset can be an array containing the
number of rows to shift followed by the number of columns to shift, or one
of "above", "below", "next", or "previous".
<pre><code>// Previous cell in the previous row
var cell = table.getCell(e.target, [-1, -1]);
// Next cell
var cell = table.getCell(e.target, 'next');
var cell = table.getCell(e.target, [0, 1];</pre></code>
@method getCell
@param {Number[]|Node} seed Array of row and column indexes, or a Node that
is either the cell itself or a descendant of one.
@param {Number[]|String} [shift] Offset by which to identify the returned
cell Node
@return {Node}
@since 3.5.0
**/
getCell: function (seed, shift) {
var tbody = this.tbodyNode,
row, cell, index, rowIndexOffset;
if (seed && tbody) {
if (isArray(seed)) {
row = tbody.get('children').item(seed[0]);
cell = row && row.get('children').item(seed[1]);
} else if (seed._node) {
cell = seed.ancestor('.' + this.getClassName('cell'), true);
}
if (cell && shift) {
rowIndexOffset = tbody.get('firstChild.rowIndex');
if (isString(shift)) {
if (!shiftMap[shift]) {
Y.error('Unrecognized shift: ' + shift, null, 'datatable-body');
}
shift = shiftMap[shift];
}
if (isArray(shift)) {
index = cell.get('parentNode.rowIndex') +
shift[0] - rowIndexOffset;
row = tbody.get('children').item(index);
index = cell.get('cellIndex') + shift[1];
cell = row && row.get('children').item(index);
}
}
}
return cell || null;
},
/**
Returns the generated CSS classname based on the input. If the `host`
attribute is configured, it will attempt to relay to its `getClassName`
or use its static `NAME` property as a string base.
If `host` is absent or has neither method nor `NAME`, a CSS classname
will be generated using this class's `NAME`.
@method getClassName
@param {String} token* Any number of token strings to assemble the
classname from.
@return {String}
@protected
@since 3.5.0
**/
getClassName: function () {
var host = this.host,
args;
if (host && host.getClassName) {
return host.getClassName.apply(host, arguments);
} else {
args = toArray(arguments);
args.unshift(this.constructor.NAME);
return Y.ClassNameManager.getClassName
.apply(Y.ClassNameManager, args);
}
},
/**
Returns the Model associated to the row Node or id provided. Passing the
Node or id for a descendant of the row also works.
If no Model can be found, `null` is returned.
@method getRecord
@param {String|Node} seed Row Node or `id`, or one for a descendant of a row
@return {Model}
@since 3.5.0
**/
getRecord: function (seed) {
var modelList = this.get('modelList'),
tbody = this.tbodyNode,
row = null,
record;
if (tbody) {
if (isString(seed)) {
seed = tbody.one('#' + seed);
}
if (seed && seed._node) {
row = seed.ancestor(function (node) {
return node.get('parentNode').compareTo(tbody);
}, true);
record = row &&
modelList.getByClientId(row.getData('yui3-record'));
}
}
return record || null;
},
/**
Returns the `<tr>` Node from the given row index, Model, or Model's
`clientId`. If the rows haven't been rendered yet, or if the row can't be
found by the input, `null` is returned.
@method getRow
@param {Number|String|Model} id Row index, Model instance, or clientId
@return {Node}
@since 3.5.0
**/
getRow: function (id) {
var tbody = this.tbodyNode,
row = null;
if (tbody) {
if (id) {
id = this._idMap[id.get ? id.get('clientId') : id] || id;
}
row = isNumber(id) ?
tbody.get('children').item(id) :
tbody.one('#' + id);
}
return row;
},
/**
Creates the table's `<tbody>` content by assembling markup generated by
populating the `ROW\_TEMPLATE`, and `CELL\_TEMPLATE` templates with content
from the `columns` and `modelList` attributes.
The rendering process happens in three stages:
1. A row template is assembled from the `columns` attribute (see
`_createRowTemplate`)
2. An HTML string is built up by concatenating the application of the data in
each Model in the `modelList` to the row template. For cells with
`formatter`s, the function is called to generate cell content. Cells
with `nodeFormatter`s are ignored. For all other cells, the data value
from the Model attribute for the given column key is used. The
accumulated row markup is then inserted into the container.
3. If any column is configured with a `nodeFormatter`, the `modelList` is
iterated again to apply the `nodeFormatter`s.
Supported properties of the column objects include:
* `key` - Used to link a column to an attribute in a Model.
* `name` - Used for columns that don't relate to an attribute in the Model
(`formatter` or `nodeFormatter` only) if the implementer wants a
predictable name to refer to in their CSS.
* `cellTemplate` - Overrides the instance's `CELL_TEMPLATE` for cells in
this column only.
* `formatter` - Used to customize or override the content value from the
Model. These do not have access to the cell or row Nodes and should
return string (HTML) content.
* `nodeFormatter` - Used to provide content for a cell as well as perform
any custom modifications on the cell or row Node that could not be
performed by `formatter`s. Should be used sparingly for better
performance.
* `emptyCellValue` - String (HTML) value to use if the Model data for a
column, or the content generated by a `formatter`, is the empty string,
`null`, or `undefined`.
* `allowHTML` - Set to `true` if a column value, `formatter`, or
`emptyCellValue` can contain HTML. This defaults to `false` to protect
against XSS.
* `className` - Space delimited CSS classes to add to all `<td>`s in a
column.
Column `formatter`s are passed an object (`o`) with the following
properties:
* `value` - The current value of the column's associated attribute, if
any.
* `data` - An object map of Model keys to their current values.
* `record` - The Model instance.
* `column` - The column configuration object for the current column.
* `className` - Initially empty string to allow `formatter`s to add CSS
classes to the cell's `<td>`.
* `rowIndex` - The zero-based row number.
* `rowClass` - Initially empty string to allow `formatter`s to add CSS
classes to the cell's containing row `<tr>`.
They may return a value or update `o.value` to assign specific HTML
content. A returned value has higher precedence.
Column `nodeFormatter`s are passed an object (`o`) with the following
properties:
* `value` - The current value of the column's associated attribute, if
any.
* `td` - The `<td>` Node instance.
* `cell` - The `<div>` liner Node instance if present, otherwise, the
`<td>`. When adding content to the cell, prefer appending into this
property.
* `data` - An object map of Model keys to their current values.
* `record` - The Model instance.
* `column` - The column configuration object for the current column.
* `rowIndex` - The zero-based row number.
They are expected to inject content into the cell's Node directly, including
any "empty" cell content. Each `nodeFormatter` will have access through the
Node API to all cells and rows in the `<tbody>`, but not to the `<table>`,
as it will not be attached yet.
If a `nodeFormatter` returns `false`, the `o.td` and `o.cell` Nodes will be
`destroy()`ed to remove them from the Node cache and free up memory. The
DOM elements will remain as will any content added to them. _It is highly
advisable to always return `false` from your `nodeFormatter`s_.
@method render
@chainable
@since 3.5.0
**/
render: function () {
var table = this.get('container'),
data = this.get('modelList'),
displayCols = this.get('columns'),
tbody = this.tbodyNode ||
(this.tbodyNode = this._createTBodyNode());
// Needed for mutation
this._createRowTemplate(displayCols);
if (data) {
tbody.setHTML(this._createDataHTML(displayCols));
this._applyNodeFormatters(tbody, displayCols);
}
if (tbody.get('parentNode') !== table) {
table.appendChild(tbody);
}
this.bindUI();
return this;
},
/**
Refreshes the provided row against the provided model and the Array of
columns to be updated.
@method refreshRow
@param {Node} row
@param {Model} model Y.Model representation of the row
@param {String[]} colKeys Array of column keys
@chainable
*/
refreshRow: function (row, model, colKeys) {
var col,
cell,
len = colKeys.length,
i;
for (i = 0; i < len; i++) {
col = this.getColumn(colKeys[i]);
if (col !== null) {
cell = row.one('.' + this.getClassName('col', col._id || col.key));
this.refreshCell(cell, model);
}
}
return this;
},
/**
Refreshes the given cell with the provided model data and the provided
column configuration.
Uses the provided column formatter if aviable.
@method refreshCell
@param {Node} cell Y.Node pointer to the cell element to be updated
@param {Model} [model] Y.Model representation of the row
@param {Object} [col] Column configuration object for the cell
@chainable
*/
refreshCell: function (cell, model, col) {
var content,
formatterFn,
formatterData,
data = model.toJSON();
cell = this.getCell(cell);
/* jshint -W030 */
model || (model = this.getRecord(cell));
col || (col = this.getColumn(cell));
/* jshint +W030 */
if (col.nodeFormatter) {
formatterData = {
cell: cell.one('.' + this.getClassName('liner')) || cell,
column: col,
data: data,
record: model,
rowIndex: this._getRowIndex(cell.ancestor('tr')),
td: cell,
value: data[col.key]
};
keep = col.nodeFormatter.call(host,formatterData);
if (keep === false) {
// Remove from the Node cache to reduce
// memory footprint. This also purges events,
// which you shouldn't be scoping to a cell
// anyway. You've been warned. Incidentally,
// you should always return false. Just sayin.
cell.destroy(true);
}
} else if (col.formatter) {
if (!col._formatterFn) {
col = this._setColumnsFormatterFn([col])[0];
}
formatterFn = col._formatterFn || null;
if (formatterFn) {
formatterData = {
value : data[col.key],
data : data,
column : col,
record : model,
className: '',
rowClass : '',
rowIndex : this._getRowIndex(cell.ancestor('tr'))
};
// Formatters can either return a value ...
content = formatterFn.call(this.get('host'), formatterData);
// ... or update the value property of the data obj passed
if (content === undefined) {
content = formatterData.value;
}
}
if (content === undefined || content === null || content === '') {
content = col.emptyCellValue || '';
}
} else {
content = data[col.key] || col.emptyCellValue || '';
}
cell.setHTML(col.allowHTML ? content : Y.Escape.html(content));
return this;
},
/**
Returns column data from this.get('columns'). If a Y.Node is provided as
the key, will try to determine the key from the classname
@method getColumn
@param {String|Node} name
@return {Object} Returns column configuration
*/
getColumn: function (name) {
if (name && name._node) {
// get column name from node
name = name.get('className').match(
new RegExp( this.getClassName('col') +'-([^ ]*)' )
)[1];
}
if (this.host) {
return this.host._columnMap[name] || null;
}
var displayCols = this.get('columns'),
col = null;
Y.Array.some(displayCols, function (_col) {
if ((_col._id || _col.key) === name) {
col = _col;
return true;
}
});
return col;
},
// -- Protected and private methods ---------------------------------------
/**
Handles changes in the source's columns attribute. Redraws the table data.
@method _afterColumnsChange
@param {EventFacade} e The `columnsChange` event object
@protected
@since 3.5.0
**/
// TODO: Preserve existing DOM
// This will involve parsing and comparing the old and new column configs
// and reacting to four types of changes:
// 1. formatter, nodeFormatter, emptyCellValue changes
// 2. column deletions
// 3. column additions
// 4. column moves (preserve cells)
_afterColumnsChange: function () {
this.render();
},
/**
Handles modelList changes, including additions, deletions, and updates.
Modifies the existing table DOM accordingly.
@method _afterDataChange
@param {EventFacade} e The `change` event from the ModelList
@protected
@since 3.5.0
**/
_afterDataChange: function (e) {
var type = (e.type.match(/:(add|change|remove)$/) || [])[1],
index = e.index,
displayCols = this.get('columns'),
col,
changed = e.changed && Y.Object.keys(e.changed),
key,
row,
i,
len;
for (i = 0, len = displayCols.length; i < len; i++ ) {
col = displayCols[i];
// since nodeFormatters typcially make changes outside of it's
// cell, we need to see if there are any columns that have a
// nodeFormatter and if so, we need to do a full render() of the
// tbody
if (col.hasOwnProperty('nodeFormatter')) {
this.render();
this.fire(EV_CONTENT_UPDATE);
return;
}
}
// TODO: if multiple rows are being added/remove/swapped, can we avoid the restriping?
switch (type) {
case 'change':
for (i = 0, len = displayCols.length; i < len; i++) {
col = displayCols[i];
key = col.key;
if (col.formatter && !e.changed[key]) {
changed.push(key);
}
}
this.refreshRow(this.getRow(e.target), e.target, changed);
break;
case 'add':
// we need to make sure we don't have an index larger than the data we have
index = Math.min(index, this.get('modelList').size() - 1);
// updates the columns with formatter functions
this._setColumnsFormatterFn(displayCols);
row = Y.Node.create(this._createRowHTML(e.model, index, displayCols));
this.tbodyNode.insert(row, index);
this._restripe(index);
break;
case 'remove':
this.getRow(index).remove(true);
// we removed a row, so we need to back up our index to stripe
this._restripe(index - 1);
break;
default:
this.render();
}
// Event fired to tell users when we are done updating after the data
// was changed
this.fire(EV_CONTENT_UPDATE);
},
/**
Toggles the odd/even classname of the row after the given index. This method
is used to update rows after a row is inserted into or removed from the table.
Note this event is delayed so the table is only restriped once when multiple
rows are updated at one time.
@protected
@method _restripe
@param {Number} [index] Index of row to start restriping after
@since 3.11.0
*/
_restripe: function (index) {
var task = this._restripeTask,
self;
// index|0 to force int, avoid NaN. Math.max() to avoid neg indexes.
index = Math.max((index|0), 0);
if (!task) {
self = this;
this._restripeTask = {
timer: setTimeout(function () {
// Check for self existence before continuing
if (!self || self.get('destroy') || !self.tbodyNode || !self.tbodyNode.inDoc()) {
self._restripeTask = null;
return;
}
var odd = [self.CLASS_ODD, self.CLASS_EVEN],
even = [self.CLASS_EVEN, self.CLASS_ODD],
index = self._restripeTask.index;
self.tbodyNode.get('childNodes')
.slice(index)
.each(function (row, i) { // TODO: each vs batch
row.replaceClass.apply(row, (index + i) % 2 ? even : odd);
});
self._restripeTask = null;
}, 0),
index: index
};
} else {
task.index = Math.min(task.index, index);
}
},
/**
Handles replacement of the modelList.
Rerenders the `<tbody>` contents.
@method _afterModelListChange
@param {EventFacade} e The `modelListChange` event
@protected
@since 3.6.0
**/
_afterModelListChange: function () {
var handles = this._eventHandles;
if (handles.dataChange) {
handles.dataChange.detach();
delete handles.dataChange;
this.bindUI();
}
if (this.tbodyNode) {
this.render();
}
},
/**
Iterates the `modelList`, and calls any `nodeFormatter`s found in the
`columns` param on the appropriate cell Nodes in the `tbody`.
@method _applyNodeFormatters
@param {Node} tbody The `<tbody>` Node whose columns to update
@param {Object[]} displayCols The column configurations
@protected
@since 3.5.0
**/
_applyNodeFormatters: function (tbody, displayCols) {
var host = this.host || this,
data = this.get('modelList'),
formatters = [],
linerQuery = '.' + this.getClassName('liner'),
rows, i, len;
// Only iterate the ModelList again if there are nodeFormatters
for (i = 0, len = displayCols.length; i < len; ++i) {
if (displayCols[i].nodeFormatter) {
formatters.push(i);
}
}
if (data && formatters.length) {
rows = tbody.get('childNodes');
data.each(function (record, index) {
var formatterData = {
data : record.toJSON(),
record : record,
rowIndex : index
},
row = rows.item(index),
i, len, col, key, cells, cell, keep;
if (row) {
cells = row.get('childNodes');
for (i = 0, len = formatters.length; i < len; ++i) {
cell = cells.item(formatters[i]);
if (cell) {
col = formatterData.column = displayCols[formatters[i]];
key = col.key || col.id;
formatterData.value = record.get(key);
formatterData.td = cell;
formatterData.cell = cell.one(linerQuery) || cell;
keep = col.nodeFormatter.call(host,formatterData);
if (keep === false) {
// Remove from the Node cache to reduce
// memory footprint. This also purges events,
// which you shouldn't be scoping to a cell
// anyway. You've been warned. Incidentally,
// you should always return false. Just sayin.
cell.destroy(true);
}
}
}
}
});
}
},
/**
Binds event subscriptions from the UI and the host (if assigned).
@method bindUI
@protected
@since 3.5.0
**/
bindUI: function () {
var handles = this._eventHandles,
modelList = this.get('modelList'),
changeEvent = modelList.model.NAME + ':change';
if (!handles.columnsChange) {
handles.columnsChange = this.after('columnsChange',
bind('_afterColumnsChange', this));
}
if (modelList && !handles.dataChange) {
handles.dataChange = modelList.after(
['add', 'remove', 'reset', changeEvent],
bind('_afterDataChange', this));
}
},
/**
Iterates the `modelList` and applies each Model to the `_rowTemplate`,
allowing any column `formatter` or `emptyCellValue` to override cell
content for the appropriate column. The aggregated HTML string is
returned.
@method _createDataHTML
@param {Object[]} displayCols The column configurations to customize the
generated cell content or class names
@return {String} The markup for all Models in the `modelList`, each applied
to the `_rowTemplate`
@protected
@since 3.5.0
**/
_createDataHTML: function (displayCols) {
var data = this.get('modelList'),
html = '';
if (data) {
data.each(function (model, index) {
html += this._createRowHTML(model, index, displayCols);
}, this);
}
return html;
},
/**
Applies the data of a given Model, modified by any column formatters and
supplemented by other template values to the instance's `_rowTemplate` (see
`_createRowTemplate`). The generated string is then returned.
The data from Model's attributes is fetched by `toJSON` and this data
object is appended with other properties to supply values to {placeholders}
in the template. For a template generated from a Model with 'foo' and 'bar'
attributes, the data object would end up with the following properties
before being used to populate the `_rowTemplate`:
* `clientID` - From Model, used the assign the `<tr>`'s 'id' attribute.
* `foo` - The value to populate the 'foo' column cell content. This
value will be the value stored in the Model's `foo` attribute, or the
result of the column's `formatter` if assigned. If the value is '',
`null`, or `undefined`, and the column's `emptyCellValue` is assigned,
that value will be used.
* `bar` - Same for the 'bar' column cell content.
* `foo-className` - String of CSS classes to apply to the `<td>`.
* `bar-className` - Same.
* `rowClass` - String of CSS classes to apply to the `<tr>`. This
will be the odd/even class per the specified index plus any additional
classes assigned by column formatters (via `o.rowClass`).
Because this object is available to formatters, any additional properties
can be added to fill in custom {placeholders} in the `_rowTemplate`.
@method _createRowHTML
@param {Model} model The Model instance to apply to the row template
@param {Number} index The index the row will be appearing
@param {Object[]} displayCols The column configurations
@return {String} The markup for the provided Model, less any `nodeFormatter`s
@protected
@since 3.5.0
**/
_createRowHTML: function (model, index, displayCols) {
var data = model.toJSON(),
clientId = model.get('clientId'),
values = {
rowId : this._getRowId(clientId),
clientId: clientId,
rowClass: (index % 2) ? this.CLASS_ODD : this.CLASS_EVEN
},
host = this.host || this,
i, len, col, token, value, formatterData;
for (i = 0, len = displayCols.length; i < len; ++i) {
col = displayCols[i];
value = data[col.key];
token = col._id || col.key;
values[token + '-className'] = '';
if (col._formatterFn) {
formatterData = {
value : value,
data : data,
column : col,
record : model,
className: '',
rowClass : '',
rowIndex : index
};
// Formatters can either return a value
value = col._formatterFn.call(host, formatterData);
// or update the value property of the data obj passed
if (value === undefined) {
value = formatterData.value;
}
values[token + '-className'] = formatterData.className;
values.rowClass += ' ' + formatterData.rowClass;
}
// if the token missing OR is the value a legit value
if (!values.hasOwnProperty(token) || data.hasOwnProperty(col.key)) {
if (value === undefined || value === null || value === '') {
value = col.emptyCellValue || '';
}
values[token] = col.allowHTML ? value : htmlEscape(value);
}
}
// replace consecutive whitespace with a single space
values.rowClass = values.rowClass.replace(/\s+/g, ' ');
return fromTemplate(this._rowTemplate, values);
},
/**
Locates the row within the tbodyNode and returns the found index, or Null
if it is not found in the tbodyNode
@param {Node} row
@return {Number} Index of row in tbodyNode
*/
_getRowIndex: function (row) {
var tbody = this.tbodyNode,
index = 1;
if (tbody && row) {
//if row is not in the tbody, return
if (row.ancestor('tbody') !== tbody) {
return null;
}
// increment until we no longer have a previous node
/*jshint boss: true*/
while (row = row.previous()) { // NOTE: assignment
/*jshint boss: false*/
index++;
}
}
return index;
},
/**
Creates a custom HTML template string for use in generating the markup for
individual table rows with {placeholder}s to capture data from the Models
in the `modelList` attribute or from column `formatter`s.
Assigns the `_rowTemplate` property.
@method _createRowTemplate
@param {Object[]} displayCols Array of column configuration objects
@protected
@since 3.5.0
**/
_createRowTemplate: function (displayCols) {
var html = '',
cellTemplate = this.CELL_TEMPLATE,
i, len, col, key, token, headers, tokenValues, formatter;
this._setColumnsFormatterFn(displayCols);
for (i = 0, len = displayCols.length; i < len; ++i) {
col = displayCols[i];
key = col.key;
token = col._id || key;
formatter = col._formatterFn;
// Only include headers if there are more than one
headers = (col._headers || []).length > 1 ?
'headers="' + col._headers.join(' ') + '"' : '';
tokenValues = {
content : '{' + token + '}',
headers : headers,
className: this.getClassName('col', token) + ' ' +
(col.className || '') + ' ' +
this.getClassName('cell') +
' {' + token + '-className}'
};
if (!formatter && col.formatter) {
tokenValues.content = col.formatter.replace(valueRegExp, tokenValues.content);
}
if (col.nodeFormatter) {
// Defer all node decoration to the formatter
tokenValues.content = '';
}
html += fromTemplate(col.cellTemplate || cellTemplate, tokenValues);
}
this._rowTemplate = fromTemplate(this.ROW_TEMPLATE, {
content: html
});
},
/**
Parses the columns array and defines the column's _formatterFn if there
is a formatter available on the column
@protected
@method _setColumnsFormatterFn
@param {Object[]} displayCols Array of column configuration objects
@return {Object[]} Returns modified displayCols configuration Array
*/
_setColumnsFormatterFn: function (displayCols) {
var Formatters = Y.DataTable.BodyView.Formatters,
formatter,
col,
i,
len;
for (i = 0, len = displayCols.length; i < len; i++) {
col = displayCols[i];
formatter = col.formatter;
if (!col._formatterFn && formatter) {
if (Lang.isFunction(formatter)) {
col._formatterFn = formatter;
} else if (formatter in Formatters) {
col._formatterFn = Formatters[formatter].call(this.host || this, col);
}
}
}
return displayCols;
},
/**
Creates the `<tbody>` node that will store the data rows.
@method _createTBodyNode
@return {Node}
@protected
@since 3.6.0
**/
_createTBodyNode: function () {
return Y.Node.create(fromTemplate(this.TBODY_TEMPLATE, {
className: this.getClassName('data')
}));
},
/**
Destroys the instance.
@method destructor
@protected
@since 3.5.0
**/
destructor: function () {
(new Y.EventHandle(YObject.values(this._eventHandles))).detach();
},
/**
Holds the event subscriptions needing to be detached when the instance is
`destroy()`ed.
@property _eventHandles
@type {Object}
@default undefined (initially unset)
@protected
@since 3.5.0
**/
//_eventHandles: null,
/**
Returns the row ID associated with a Model's clientId.
@method _getRowId
@param {String} clientId The Model clientId
@return {String}
@protected
**/
_getRowId: function (clientId) {
return this._idMap[clientId] || (this._idMap[clientId] = Y.guid());
},
/**
Map of Model clientIds to row ids.
@property _idMap
@type {Object}
@protected
**/
//_idMap,
/**
Initializes the instance. Reads the following configuration properties in
addition to the instance attributes:
* `columns` - (REQUIRED) The initial column information
* `host` - The object to serve as source of truth for column info and
for generating class names
@method initializer
@param {Object} config Configuration data
@protected
@since 3.5.0
**/
initializer: function (config) {
this.host = config.host;
this._eventHandles = {
modelListChange: this.after('modelListChange',
bind('_afterModelListChange', this))
};
this._idMap = {};
this.CLASS_ODD = this.getClassName('odd');
this.CLASS_EVEN = this.getClassName('even');
}
/**
The HTML template used to create a full row of markup for a single Model in
the `modelList` plus any customizations defined in the column
configurations.
@property _rowTemplate
@type {String}
@default (initially unset)
@protected
@since 3.5.0
**/
//_rowTemplate: null
},{
/**
Hash of formatting functions for cell contents.
This property can be populated with a hash of formatting functions by the developer
or a set of pre-defined functions can be loaded via the `datatable-formatters` module.
See: [DataTable.BodyView.Formatters](./DataTable.BodyView.Formatters.html)
@property Formatters
@type Object
@since 3.8.0
@static
**/
Formatters: {}
});
}, '@VERSION@', {"requires": ["datatable-core", "view", "classnamemanager"]});
|
frontend/app_v2/src/common/icons/Checkmark.js | First-Peoples-Cultural-Council/fv-web-ui | import React from 'react'
import PropTypes from 'prop-types'
/**
* @summary Checkmark
* @component
*
* @param {object} props
*
* @returns {node} jsx markup
*/
function Checkmark({ styling }) {
return (
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 24 24" className={styling}>
<path d="M16.707 5.293a1 1 0 010 1.414l-8 8a1 1 0 01-1.414 0l-4-4a1 1 0 011.414-1.414L8 12.586l7.293-7.293a1 1 0 011.414 0z" />
</svg>
)
}
// PROPTYPES
const { string } = PropTypes
Checkmark.propTypes = {
styling: string,
}
export default Checkmark
|
ajax/libs/react-cookie/0.3.4/react-cookie.min.js | yinghunglai/cdnjs | (function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s})({1:[function(require,module,exports){var cookie=require("cookie");var _rawCookie={};var _res=undefined;function load(name,doNotParse){var cookies={};if(typeof document!=="undefined"){cookies=cookie.parse(document.cookie)}var cookieVal=cookies&&cookies[name]||_rawCookie[name];if(!doNotParse){try{cookieVal=JSON.parse(cookieVal)}catch(e){}}return cookieVal}function save(name,val,opt){_rawCookie[name]=val;if(typeof val==="object"){_rawCookie[name]=JSON.stringify(val)}if(typeof document!=="undefined"){document.cookie=cookie.serialize(name,_rawCookie[name],opt)}if(_res&&_res.cookie){_res.cookie(name,val,opt)}}function remove(name,path){delete _rawCookie[name];if(typeof document!=="undefined"){var removeCookie=name+"=; expires=Thu, 01 Jan 1970 00:00:01 GMT;";if(path){removeCookie+=" path="+path}document.cookie=removeCookie}if(_res&&_res.clearCookie){var opt=path?{path:path}:undefined;_res.clearCookie(name,opt)}}function setRawCookie(rawCookie){_rawCookie=cookie.parse(rawCookie)}function plugToRequest(req,res){if(req){if(req.cookie){_rawCookie=req.cookie}else if(req.headers&&req.headers.cookie){setRawCookie(req.headers.cookie)}}_res=res}var reactCookie={load:load,save:save,remove:remove,setRawCookie:setRawCookie,plugToRequest:plugToRequest};if(typeof window!=="undefined"){window["reactCookie"]=reactCookie}module.exports=reactCookie},{cookie:2}],2:[function(require,module,exports){exports.parse=parse;exports.serialize=serialize;var decode=decodeURIComponent;var encode=encodeURIComponent;function parse(str,options){var obj={};var opt=options||{};var pairs=str.split(/; */);var dec=opt.decode||decode;pairs.forEach(function(pair){var eq_idx=pair.indexOf("=");if(eq_idx<0){return}var key=pair.substr(0,eq_idx).trim();var val=pair.substr(++eq_idx,pair.length).trim();if('"'==val[0]){val=val.slice(1,-1)}if(undefined==obj[key]){obj[key]=tryDecode(val,dec)}});return obj}function serialize(name,val,options){var opt=options||{};var enc=opt.encode||encode;var pairs=[name+"="+enc(val)];if(null!=opt.maxAge){var maxAge=opt.maxAge-0;if(isNaN(maxAge))throw new Error("maxAge should be a Number");pairs.push("Max-Age="+maxAge)}if(opt.domain)pairs.push("Domain="+opt.domain);if(opt.path)pairs.push("Path="+opt.path);if(opt.expires)pairs.push("Expires="+opt.expires.toUTCString());if(opt.httpOnly)pairs.push("HttpOnly");if(opt.secure)pairs.push("Secure");return pairs.join("; ")}function tryDecode(str,decode){try{return decode(str)}catch(e){return str}}},{}]},{},[1]); |
ajax/libs/cellx/1.6.73/cellx.js | honestree/cdnjs | (function (global, factory) {
typeof exports === 'object' && typeof module !== 'undefined' ? module.exports = factory() :
typeof define === 'function' && define.amd ? define(factory) :
(global.cellx = factory());
}(this, (function () { 'use strict';
var ErrorLogger = {
_handler: null,
/**
* @typesign (handler: (...msg));
*/
setHandler: function setHandler(handler) {
this._handler = handler;
},
/**
* @typesign (...msg);
*/
log: function log() {
this._handler.apply(this, arguments);
}
};
var uidCounter = 0;
/**
* @typesign () -> string;
*/
function nextUID() {
return String(++uidCounter);
}
var Symbol = Function('return this;')().Symbol;
if (!Symbol) {
Symbol = function Symbol(key) {
return '__' + key + '_' + Math.floor(Math.random() * 1e9) + '_' + nextUID() + '__';
};
Symbol.iterator = Symbol('Symbol.iterator');
}
var Symbol$1 = Symbol;
var UID = Symbol$1('cellx.uid');
var CELLS = Symbol$1('cellx.cells');
var global$1 = Function('return this;')();
/**
* @typesign (a, b) -> boolean;
*/
var is = Object.is || function is(a, b) {
if (a === 0 && b === 0) {
return 1 / a == 1 / b;
}
return a === b || (a != a && b != b);
};
var hasOwn = Object.prototype.hasOwnProperty;
/**
* @typesign (target: Object, source: Object) -> Object;
*/
function mixin(target, source) {
var names = Object.getOwnPropertyNames(source);
for (var i = 0, l = names.length; i < l; i++) {
var name = names[i];
Object.defineProperty(target, name, Object.getOwnPropertyDescriptor(source, name));
}
return target;
}
var extend;
/**
* @typesign (description: {
* Extends?: Function,
* Implements?: Array<Object|Function>,
* Static?: Object,
* constructor?: Function,
* [key: string]
* }) -> Function;
*/
function createClass(description) {
var parent;
if (description.Extends) {
parent = description.Extends;
delete description.Extends;
} else {
parent = Object;
}
var constr;
if (hasOwn.call(description, 'constructor')) {
constr = description.constructor;
delete description.constructor;
} else {
constr = parent == Object ?
function() {} :
function() {
return parent.apply(this, arguments);
};
}
var proto = constr.prototype = Object.create(parent.prototype);
if (description.Implements) {
description.Implements.forEach((function(implementation) {
if (typeof implementation == 'function') {
Object.keys(implementation).forEach((function(name) {
Object.defineProperty(constr, name, Object.getOwnPropertyDescriptor(implementation, name));
}));
mixin(proto, implementation.prototype);
} else {
mixin(proto, implementation);
}
}));
delete description.Implements;
}
Object.keys(parent).forEach((function(name) {
Object.defineProperty(constr, name, Object.getOwnPropertyDescriptor(parent, name));
}));
if (description.Static) {
mixin(constr, description.Static);
delete description.Static;
}
if (constr.extend === void 0) {
constr.extend = extend;
}
mixin(proto, description);
Object.defineProperty(proto, 'constructor', {
configurable: true,
writable: true,
value: constr
});
return constr;
}
/**
* @this {Function}
*
* @typesign (description: {
* Implements?: Array<Object|Function>,
* Static?: Object,
* constructor?: Function,
* [key: string]
* }) -> Function;
*/
extend = function extend(description) {
description.Extends = this;
return createClass(description);
};
var Map = global$1.Map;
if (!Map) {
var entryStub = {
value: void 0
};
Map = createClass({
constructor: function Map(entries) {
this._entries = Object.create(null);
this._objectStamps = {};
this._first = null;
this._last = null;
this.size = 0;
if (entries) {
for (var i = 0, l = entries.length; i < l; i++) {
this.set(entries[i][0], entries[i][1]);
}
}
},
has: function has(key) {
return !!this._entries[this._getValueStamp(key)];
},
get: function get(key) {
return (this._entries[this._getValueStamp(key)] || entryStub).value;
},
set: function set(key, value) {
var entries = this._entries;
var keyStamp = this._getValueStamp(key);
if (entries[keyStamp]) {
entries[keyStamp].value = value;
} else {
var entry = entries[keyStamp] = {
key: key,
keyStamp: keyStamp,
value: value,
prev: this._last,
next: null
};
if (this.size++) {
this._last.next = entry;
} else {
this._first = entry;
}
this._last = entry;
}
return this;
},
delete: function delete_(key) {
var keyStamp = this._getValueStamp(key);
var entry = this._entries[keyStamp];
if (!entry) {
return false;
}
if (--this.size) {
var prev = entry.prev;
var next = entry.next;
if (prev) {
prev.next = next;
} else {
this._first = next;
}
if (next) {
next.prev = prev;
} else {
this._last = prev;
}
} else {
this._first = null;
this._last = null;
}
delete this._entries[keyStamp];
delete this._objectStamps[keyStamp];
return true;
},
clear: function clear() {
var entries = this._entries;
for (var stamp in entries) {
delete entries[stamp];
}
this._objectStamps = {};
this._first = null;
this._last = null;
this.size = 0;
},
_getValueStamp: function _getValueStamp(value) {
switch (typeof value) {
case 'undefined': {
return 'undefined';
}
case 'object': {
if (value === null) {
return 'null';
}
break;
}
case 'boolean': {
return '?' + value;
}
case 'number': {
return '+' + value;
}
case 'string': {
return ',' + value;
}
}
return this._getObjectStamp(value);
},
_getObjectStamp: function _getObjectStamp(obj) {
if (!hasOwn.call(obj, UID)) {
if (!Object.isExtensible(obj)) {
var stamps = this._objectStamps;
var stamp;
for (stamp in stamps) {
if (hasOwn.call(stamps, stamp) && stamps[stamp] == obj) {
return stamp;
}
}
stamp = nextUID();
stamps[stamp] = obj;
return stamp;
}
Object.defineProperty(obj, UID, {
value: nextUID()
});
}
return obj[UID];
},
forEach: function forEach(cb, context) {
var entry = this._first;
while (entry) {
cb.call(context, entry.value, entry.key, this);
do {
entry = entry.next;
} while (entry && !this._entries[entry.keyStamp]);
}
},
toString: function toString() {
return '[object Map]';
}
});
[
['keys', function keys(entry) {
return entry.key;
}],
['values', function values(entry) {
return entry.value;
}],
['entries', function entries(entry) {
return [entry.key, entry.value];
}]
].forEach((function(settings) {
var getStepValue = settings[1];
Map.prototype[settings[0]] = function() {
var entries = this._entries;
var entry;
var done = false;
var map = this;
return {
next: function() {
if (!done) {
if (entry) {
do {
entry = entry.next;
} while (entry && !entries[entry.keyStamp]);
} else {
entry = map._first;
}
if (entry) {
return {
value: getStepValue(entry),
done: false
};
}
done = true;
}
return {
value: void 0,
done: true
};
}
};
};
}));
}
if (!Map.prototype[Symbol$1.iterator]) {
Map.prototype[Symbol$1.iterator] = Map.prototype.entries;
}
var Map$1 = Map;
var KEY_IS_EMITTER_EVENT = Symbol$1('cellx.EventEmitter~isEmitterEvent');
var KEY_INNER = Symbol$1('cellx.EventEmitter.inner');
/**
* @typedef {{
* target?: Object,
* type: string,
* bubbles?: boolean,
* isPropagationStopped?: boolean
* }} cellx~Event
*/
/**
* @class cellx.EventEmitter
* @extends {Object}
* @typesign new EventEmitter() -> cellx.EventEmitter;
*/
var EventEmitter = createClass({
Static: {
KEY_INNER: KEY_INNER
},
constructor: function EventEmitter() {
/**
* @type {Object<Array<{
* listener: (evt: cellx~Event) -> ?boolean,
* context
* }>>}
*/
this._events = new Map$1();
},
/**
* @typesign (type?: string) -> Array<{ listener: (evt: cellx~Event) -> ?boolean, context }> |
* Object<Array<{ listener: (evt: cellx~Event) -> ?boolean, context }>>;
*/
getEvents: function getEvents(type) {
if (type) {
var events = this._events && this._events.get(type);
if (!events) {
return [];
}
return events._isEmitterEvent === KEY_IS_EMITTER_EVENT ? [events] : events;
}
var resultEvents = Object.create(null);
this._events.forEach((function(events, type) {
resultEvents[type] = events._isEmitterEvent === KEY_IS_EMITTER_EVENT ? [events] : events;
}));
return resultEvents;
},
/**
* @typesign (
* type: string,
* listener: (evt: cellx~Event) -> ?boolean,
* context?
* ) -> cellx.EventEmitter;
*
* @typesign (
* listeners: Object<(evt: cellx~Event) -> ?boolean>,
* context?
* ) -> cellx.EventEmitter;
*/
on: function on(type, listener, context) {
if (typeof type == 'object') {
context = arguments.length >= 2 ? listener : this;
var listeners = type;
for (type in listeners) {
this._on(type, listeners[type], context);
}
} else {
this._on(type, listener, arguments.length >= 3 ? context : this);
}
return this;
},
/**
* @typesign (
* type: string,
* listener: (evt: cellx~Event) -> ?boolean,
* context?
* ) -> cellx.EventEmitter;
*
* @typesign (
* listeners: Object<(evt: cellx~Event) -> ?boolean>,
* context?
* ) -> cellx.EventEmitter;
*
* @typesign () -> cellx.EventEmitter;
*/
off: function off(type, listener, context) {
var argCount = arguments.length;
if (argCount) {
if (typeof type == 'object') {
context = argCount >= 2 ? listener : this;
var listeners = type;
for (type in listeners) {
this._off(type, listeners[type], context);
}
} else {
this._off(type, listener, argCount >= 3 ? context : this);
}
} else if (this._events) {
this._events.clear();
}
return this;
},
/**
* @typesign (
* type: string,
* listener: (evt: cellx~Event) -> ?boolean,
* context
* );
*/
_on: function _on(type, listener, context) {
var index = type.indexOf(':');
if (index != -1) {
this['_' + type.slice(index + 1)].on(type.slice(0, index), listener, context);
} else {
var events = (this._events || (this._events = new Map$1())).get(type);
var evt = {
_isEmitterEvent: KEY_IS_EMITTER_EVENT,
listener: listener,
context: context
};
if (!events) {
this._events.set(type, evt);
} else if (events._isEmitterEvent === KEY_IS_EMITTER_EVENT) {
this._events.set(type, [events, evt]);
} else {
events.push(evt);
}
}
},
/**
* @typesign (
* type: string,
* listener: (evt: cellx~Event) -> ?boolean,
* context
* );
*/
_off: function _off(type, listener, context) {
var index = type.indexOf(':');
if (index != -1) {
this['_' + type.slice(index + 1)].off(type.slice(0, index), listener, context);
} else {
var events = this._events && this._events.get(type);
if (!events) {
return;
}
if (events._isEmitterEvent === KEY_IS_EMITTER_EVENT) {
if (
(events.listener == listener || events.listener[KEY_INNER] === listener) &&
events.context === context
) {
this._events.delete(type);
}
} else {
var eventCount = events.length;
if (eventCount == 1) {
var evt = events[0];
if ((evt.listener == listener || evt.listener[KEY_INNER] === listener) && evt.context === context) {
this._events.delete(type);
}
} else {
for (var i = eventCount; i;) {
var evt2 = events[--i];
if (
(evt2.listener == listener || evt2.listener[KEY_INNER] === listener) &&
evt2.context === context
) {
events.splice(i, 1);
break;
}
}
}
}
}
},
/**
* @typesign (
* type: string,
* listener: (evt: cellx~Event) -> ?boolean,
* context?
* ) -> cellx.EventEmitter;
*/
once: function once(type, listener, context) {
if (arguments.length < 3) {
context = this;
}
function wrapper() {
this._off(type, wrapper, context);
return listener.apply(this, arguments);
}
wrapper[KEY_INNER] = listener;
this._on(type, wrapper, context);
return this;
},
/**
* @typesign (evt: cellx~Event) -> cellx~Event;
* @typesign (type: string) -> cellx~Event;
*/
emit: function emit(evt) {
if (typeof evt == 'string') {
evt = {
target: this,
type: evt
};
} else if (!evt.target) {
evt.target = this;
} else if (evt.target != this) {
throw new TypeError('Event cannot be emitted on this object');
}
this._handleEvent(evt);
return evt;
},
/**
* @typesign (evt: cellx~Event);
*
* For override:
* @example
* function View(el) {
* this.element = el;
* el._view = this;
* }
*
* View.prototype = Object.create(EventEmitter.prototype);
* View.prototype.constructor = View;
*
* View.prototype.getParent = function() {
* var node = this.element;
*
* while (node = node.parentNode) {
* if (node._view) {
* return node._view;
* }
* }
*
* return null;
* };
*
* View.prototype._handleEvent = function(evt) {
* EventEmitter.prototype._handleEvent.call(this, evt);
*
* if (evt.bubbles !== false && !evt.isPropagationStopped) {
* var parent = this.getParent();
*
* if (parent) {
* parent._handleEvent(evt);
* }
* }
* };
*/
_handleEvent: function _handleEvent(evt) {
var events = this._events && this._events.get(evt.type);
if (!events) {
return;
}
if (events._isEmitterEvent === KEY_IS_EMITTER_EVENT) {
if (this._tryEventListener(events, evt) === false) {
evt.isPropagationStopped = true;
}
} else {
var eventCount = events.length;
if (eventCount == 1) {
if (this._tryEventListener(events[0], evt) === false) {
evt.isPropagationStopped = true;
}
} else {
events = events.slice();
for (var i = 0; i < eventCount; i++) {
if (this._tryEventListener(events[i], evt) === false) {
evt.isPropagationStopped = true;
}
}
}
}
},
_tryEventListener: function _tryEventListener(emEvt, evt) {
try {
return emEvt.listener.call(emEvt.context, evt);
} catch (err) {
this._logError(err);
}
},
/**
* @typesign (...msg);
*/
_logError: function _logError() {
ErrorLogger.log.apply(ErrorLogger, arguments);
}
});
var ObservableCollectionMixin = EventEmitter.extend({
constructor: function ObservableCollectionMixin() {
/**
* @type {Map<*, uint>}
*/
this._valueCounts = new Map$1();
},
/**
* @typesign (evt: cellx~Event);
*/
_onItemChange: function _onItemChange(evt) {
this._handleEvent(evt);
},
/**
* @typesign (value);
*/
_registerValue: function _registerValue(value) {
var valueCounts = this._valueCounts;
var valueCount = valueCounts.get(value);
if (valueCount) {
valueCounts.set(value, valueCount + 1);
} else {
valueCounts.set(value, 1);
if (this.adoptsValueChanges && value instanceof EventEmitter) {
value.on('change', this._onItemChange, this);
}
}
},
/**
* @typesign (value);
*/
_unregisterValue: function _unregisterValue(value) {
var valueCounts = this._valueCounts;
var valueCount = valueCounts.get(value);
if (valueCount > 1) {
valueCounts.set(value, valueCount - 1);
} else {
valueCounts.delete(value);
if (this.adoptsValueChanges && value instanceof EventEmitter) {
value.off('change', this._onItemChange, this);
}
}
}
});
/**
* @class cellx.ObservableMap
* @extends {cellx.EventEmitter}
* @implements {cellx.ObservableCollectionMixin}
*
* @typesign new ObservableMap(entries?: Object|cellx.ObservableMap|Map|Array<{ 0, 1 }>, opts?: {
* adoptsValueChanges?: boolean
* }) -> cellx.ObservableMap;
*/
var ObservableMap = EventEmitter.extend({
Implements: [ObservableCollectionMixin],
constructor: function ObservableMap(entries, opts) {
EventEmitter.call(this);
ObservableCollectionMixin.call(this);
this._entries = new Map$1();
this.size = 0;
/**
* @type {boolean}
*/
this.adoptsValueChanges = !!(opts && opts.adoptsValueChanges);
if (entries) {
var mapEntries = this._entries;
if (entries instanceof ObservableMap || entries instanceof Map$1) {
entries._entries.forEach((function(value, key) {
this._registerValue(value);
mapEntries.set(key, value);
}), this);
} else if (Array.isArray(entries)) {
for (var i = 0, l = entries.length; i < l; i++) {
var entry = entries[i];
this._registerValue(entry[1]);
mapEntries.set(entry[0], entry[1]);
}
} else {
for (var key in entries) {
this._registerValue(entries[key]);
mapEntries.set(key, entries[key]);
}
}
this.size = mapEntries.size;
}
},
/**
* @typesign (key) -> boolean;
*/
has: function has(key) {
return this._entries.has(key);
},
/**
* @typesign (value) -> boolean;
*/
contains: function contains(value) {
return this._valueCounts.has(value);
},
/**
* @typesign (key) -> *;
*/
get: function get(key) {
return this._entries.get(key);
},
/**
* @typesign (key, value) -> cellx.ObservableMap;
*/
set: function set(key, value) {
var entries = this._entries;
var hasKey = entries.has(key);
var oldValue;
if (hasKey) {
oldValue = entries.get(key);
if (is(value, oldValue)) {
return this;
}
this._unregisterValue(oldValue);
}
this._registerValue(value);
entries.set(key, value);
if (!hasKey) {
this.size++;
}
this.emit({
type: 'change',
subtype: hasKey ? 'update' : 'add',
key: key,
oldValue: oldValue,
value: value
});
return this;
},
/**
* @typesign (key) -> boolean;
*/
delete: function delete_(key) {
var entries = this._entries;
if (!entries.has(key)) {
return false;
}
var value = entries.get(key);
this._unregisterValue(value);
entries.delete(key);
this.size--;
this.emit({
type: 'change',
subtype: 'delete',
key: key,
oldValue: value,
value: void 0
});
return true;
},
/**
* @typesign () -> cellx.ObservableMap;
*/
clear: function clear() {
if (!this.size) {
return this;
}
if (this.adoptsValueChanges) {
this._valueCounts.forEach((function(value) {
if (value instanceof EventEmitter) {
value.off('change', this._onItemChange, this);
}
}), this);
}
this._entries.clear();
this._valueCounts.clear();
this.size = 0;
this.emit({
type: 'change',
subtype: 'clear'
});
return this;
},
/**
* @typesign (
* cb: (value, key, map: cellx.ObservableMap),
* context?
* );
*/
forEach: function forEach(cb, context) {
this._entries.forEach((function(value, key) {
cb.call(context, value, key, this);
}), this);
},
/**
* @typesign () -> { next: () -> { value, done: boolean } };
*/
keys: function keys() {
return this._entries.keys();
},
/**
* @typesign () -> { next: () -> { value, done: boolean } };
*/
values: function values() {
return this._entries.values();
},
/**
* @typesign () -> { next: () -> { value: { 0, 1 }, done: boolean } };
*/
entries: function entries() {
return this._entries.entries();
},
/**
* @typesign () -> cellx.ObservableMap;
*/
clone: function clone() {
return new this.constructor(this, {
adoptsValueChanges: this.adoptsValueChanges
});
}
});
ObservableMap.prototype[Symbol$1.iterator] = ObservableMap.prototype.entries;
var slice = Array.prototype.slice;
var push$1 = Array.prototype.push;
var splice = Array.prototype.splice;
var map$1 = Array.prototype.map;
/**
* @typesign (a, b) -> -1|1|0;
*/
function defaultComparator(a, b) {
if (a < b) { return -1; }
if (a > b) { return 1; }
return 0;
}
/**
* @class cellx.ObservableList
* @extends {cellx.EventEmitter}
* @implements {cellx.ObservableCollectionMixin}
*
* @typesign new ObservableList(items?: Array|cellx.ObservableList, opts?: {
* adoptsValueChanges?: boolean,
* comparator?: (a, b) -> int,
* sorted?: boolean
* }) -> cellx.ObservableList;
*/
var ObservableList = EventEmitter.extend({
Implements: [ObservableCollectionMixin],
constructor: function ObservableList(items, opts) {
EventEmitter.call(this);
ObservableCollectionMixin.call(this);
if (!opts) {
opts = {};
}
this._items = [];
this.length = 0;
/**
* @type {boolean}
*/
this.adoptsValueChanges = !!opts.adoptsValueChanges;
/**
* @type {?(a, b) -> int}
*/
this.comparator = null;
this.sorted = false;
if (opts.sorted || (opts.comparator && opts.sorted !== false)) {
this.comparator = opts.comparator || defaultComparator;
this.sorted = true;
}
if (items) {
this._addRange(items);
}
},
/**
* @typesign (index: ?int, allowedEndIndex?: boolean) -> ?uint;
*/
_validateIndex: function _validateIndex(index, allowedEndIndex) {
if (index === void 0) {
return index;
}
if (index < 0) {
index += this.length;
if (index < 0) {
throw new RangeError('Index out of valid range');
}
} else if (index >= (this.length + (allowedEndIndex ? 1 : 0))) {
throw new RangeError('Index out of valid range');
}
return index;
},
/**
* @typesign (value) -> boolean;
*/
contains: function contains(value) {
return this._valueCounts.has(value);
},
/**
* @typesign (value, fromIndex?: int) -> int;
*/
indexOf: function indexOf(value, fromIndex) {
return this._items.indexOf(value, this._validateIndex(fromIndex));
},
/**
* @typesign (value, fromIndex?: int) -> int;
*/
lastIndexOf: function lastIndexOf(value, fromIndex) {
return this._items.lastIndexOf(value, fromIndex === void 0 ? -1 : this._validateIndex(fromIndex));
},
/**
* @typesign (index: int) -> *;
*/
get: function get(index) {
return this._items[this._validateIndex(index, true)];
},
/**
* @typesign (index: int, count?: uint) -> Array;
*/
getRange: function getRange(index, count) {
index = this._validateIndex(index, true);
var items = this._items;
if (count === void 0) {
return items.slice(index);
}
if (index + count > items.length) {
throw new RangeError('Sum of "index" and "count" out of valid range');
}
return items.slice(index, index + count);
},
/**
* @typesign (index: int, value) -> cellx.ObservableList;
*/
set: function set(index, value) {
if (this.sorted) {
throw new TypeError('Cannot set to sorted list');
}
index = this._validateIndex(index);
var items = this._items;
if (is(value, items[index])) {
return this;
}
this._unregisterValue(items[index]);
this._registerValue(value);
items[index] = value;
this.emit('change');
return this;
},
/**
* @typesign (index: int, values: Array|cellx.ObservableList) -> cellx.ObservableList;
*/
setRange: function setRange(index, values) {
if (this.sorted) {
throw new TypeError('Cannot set to sorted list');
}
index = this._validateIndex(index);
if (values instanceof ObservableList) {
values = values._items;
}
var valueCount = values.length;
if (!valueCount) {
return this;
}
if (index + valueCount > this.length) {
throw new RangeError('Sum of "index" and "values.length" out of valid range');
}
var items = this._items;
var changed = false;
for (var i = index + valueCount; i > index;) {
var value = values[--i - index];
if (!is(value, items[i])) {
this._unregisterValue(items[i]);
this._registerValue(value);
items[i] = value;
changed = true;
}
}
if (changed) {
this.emit('change');
}
return this;
},
/**
* @typesign (value) -> cellx.ObservableList;
*/
add: function add(value) {
if (this.sorted) {
this._insertValue(value);
} else {
this._registerValue(value);
this._items.push(value);
}
this.length++;
this.emit('change');
return this;
},
/**
* @typesign (values: Array|cellx.ObservableList) -> cellx.ObservableList;
*/
addRange: function addRange(values) {
if (values.length) {
this._addRange(values);
this.emit('change');
}
return this;
},
/**
* @typesign (values: Array|cellx.ObservableList);
*/
_addRange: function _addRange(values) {
if (values instanceof ObservableList) {
values = values._items;
}
if (this.sorted) {
for (var i = 0, l = values.length; i < l; i++) {
this._insertValue(values[i]);
}
this.length += values.length;
} else {
for (var j = values.length; j;) {
this._registerValue(values[--j]);
}
this.length = push$1.apply(this._items, values);
}
},
/**
* @typesign (value);
*/
_insertValue: function _insertValue(value) {
this._registerValue(value);
var items = this._items;
var comparator = this.comparator;
var low = 0;
var high = items.length;
while (low != high) {
var mid = (low + high) >> 1;
if (comparator(value, items[mid]) < 0) {
high = mid;
} else {
low = mid + 1;
}
}
items.splice(low, 0, value);
},
/**
* @typesign (index: int, value) -> cellx.ObservableList;
*/
insert: function insert(index, value) {
if (this.sorted) {
throw new TypeError('Cannot insert to sorted list');
}
index = this._validateIndex(index, true);
this._registerValue(value);
this._items.splice(index, 0, value);
this.length++;
this.emit('change');
return this;
},
/**
* @typesign (index: int, values: Array|cellx.ObservableList) -> cellx.ObservableList;
*/
insertRange: function insertRange(index, values) {
if (this.sorted) {
throw new TypeError('Cannot insert to sorted list');
}
index = this._validateIndex(index, true);
if (values instanceof ObservableList) {
values = values._items;
}
var valueCount = values.length;
if (!valueCount) {
return this;
}
for (var i = valueCount; i;) {
this._registerValue(values[--i]);
}
splice.apply(this._items, [index, 0].concat(values));
this.length += valueCount;
this.emit('change');
return this;
},
/**
* @typesign (value, fromIndex?: int) -> boolean;
*/
remove: function remove(value, fromIndex) {
var index = this._items.indexOf(value, this._validateIndex(fromIndex));
if (index == -1) {
return false;
}
this._unregisterValue(value);
this._items.splice(index, 1);
this.length--;
this.emit('change');
return true;
},
/**
* @typesign (value, fromIndex?: int) -> boolean;
*/
removeAll: function removeAll(value, fromIndex) {
var index = this._validateIndex(fromIndex);
var items = this._items;
var changed = false;
while ((index = items.indexOf(value, index)) != -1) {
this._unregisterValue(value);
items.splice(index, 1);
changed = true;
}
if (changed) {
this.length = items.length;
this.emit('change');
}
return changed;
},
/**
* @typesign (values: Array|cellx.ObservableList, fromIndex?: int) -> boolean;
*/
removeEach: function removeEach(values, fromIndex) {
fromIndex = this._validateIndex(fromIndex);
if (values instanceof ObservableList) {
values = values._items;
}
var items = this._items;
var changed = false;
for (var i = 0, l = values.length; i < l; i++) {
var value = values[i];
var index = items.indexOf(value, fromIndex);
if (index != -1) {
this._unregisterValue(value);
items.splice(index, 1);
changed = true;
}
}
if (changed) {
this.length = items.length;
this.emit('change');
}
return changed;
},
/**
* @typesign (values: Array|cellx.ObservableList, fromIndex?: int) -> boolean;
*/
removeAllEach: function removeAllEach(values, fromIndex) {
fromIndex = this._validateIndex(fromIndex);
if (values instanceof ObservableList) {
values = values._items;
}
var items = this._items;
var changed = false;
for (var i = 0, l = values.length; i < l; i++) {
var value = values[i];
for (var index = fromIndex; (index = items.indexOf(value, index)) != -1;) {
this._unregisterValue(value);
items.splice(index, 1);
changed = true;
}
}
if (changed) {
this.length = items.length;
this.emit('change');
}
return changed;
},
/**
* @typesign (index: int) -> *;
*/
removeAt: function removeAt(index) {
var value = this._items.splice(this._validateIndex(index), 1)[0];
this._unregisterValue(value);
this.length--;
this.emit('change');
return value;
},
/**
* @typesign (index: int, count?: uint) -> Array;
*/
removeRange: function removeRange(index, count) {
index = this._validateIndex(index, true);
var items = this._items;
if (count === void 0) {
count = items.length - index;
} else if (index + count > items.length) {
throw new RangeError('Sum of "index" and "count" out of valid range');
}
if (!count) {
return [];
}
for (var i = index + count; i > index;) {
this._unregisterValue(items[--i]);
}
var values = items.splice(index, count);
this.length -= count;
this.emit('change');
return values;
},
/**
* @typesign () -> cellx.ObservableList;
*/
clear: function clear() {
if (!this.length) {
return this;
}
if (this.adoptsValueChanges) {
this._valueCounts.forEach((function(value) {
if (value instanceof EventEmitter) {
value.off('change', this._onItemChange, this);
}
}), this);
}
this._items.length = 0;
this._valueCounts.clear();
this.length = 0;
this.emit({
type: 'change',
subtype: 'clear'
});
return this;
},
/**
* @typesign (separator?: string) -> string;
*/
join: function join(separator) {
return this._items.join(separator);
},
/**
* @typesign (
* cb: (item, index: uint, list: cellx.ObservableList),
* context?
* );
*/
forEach: null,
/**
* @typesign (
* cb: (item, index: uint, list: cellx.ObservableList) -> *,
* context?
* ) -> Array;
*/
map: null,
/**
* @typesign (
* cb: (item, index: uint, list: cellx.ObservableList) -> ?boolean,
* context?
* ) -> Array;
*/
filter: null,
/**
* @typesign (
* cb: (item, index: uint, list: cellx.ObservableList) -> ?boolean,
* context?
* ) -> *;
*/
find: function(cb, context) {
var items = this._items;
for (var i = 0, l = items.length; i < l; i++) {
var item = items[i];
if (cb.call(context, item, i, this)) {
return item;
}
}
},
/**
* @typesign (
* cb: (item, index: uint, list: cellx.ObservableList) -> ?boolean,
* context?
* ) -> int;
*/
findIndex: function(cb, context) {
var items = this._items;
for (var i = 0, l = items.length; i < l; i++) {
if (cb.call(context, items[i], i, this)) {
return i;
}
}
return -1;
},
/**
* @typesign (
* cb: (item, index: uint, list: cellx.ObservableList) -> ?boolean,
* context?
* ) -> boolean;
*/
every: null,
/**
* @typesign (
* cb: (item, index: uint, list: cellx.ObservableList) -> ?boolean,
* context?
* ) -> boolean;
*/
some: null,
/**
* @typesign (
* cb: (accumulator, item, index: uint, list: cellx.ObservableList) -> *,
* initialValue?
* ) -> *;
*/
reduce: null,
/**
* @typesign (
* cb: (accumulator, item, index: uint, list: cellx.ObservableList) -> *,
* initialValue?
* ) -> *;
*/
reduceRight: null,
/**
* @typesign () -> cellx.ObservableList;
*/
clone: function clone() {
return new this.constructor(this, {
adoptsValueChanges: this.adoptsValueChanges,
comparator: this.comparator,
sorted: this.sorted
});
},
/**
* @typesign () -> Array;
*/
toArray: function toArray() {
return this._items.slice();
},
/**
* @typesign () -> string;
*/
toString: function toString() {
return this._items.join();
}
});
['forEach', 'map', 'filter', 'every', 'some'].forEach((function(name) {
ObservableList.prototype[name] = function(cb, context) {
return this._items[name]((function(item, index) {
return cb.call(context, item, index, this);
}), this);
};
}));
['reduce', 'reduceRight'].forEach((function(name) {
ObservableList.prototype[name] = function(cb, initialValue) {
var items = this._items;
var list = this;
function wrapper(accumulator, item, index) {
return cb(accumulator, item, index, list);
}
return arguments.length >= 2 ? items[name](wrapper, initialValue) : items[name](wrapper);
};
}));
[
['keys', function keys(index) {
return index;
}],
['values', function values(index, item) {
return item;
}],
['entries', function entries(index, item) {
return [index, item];
}]
].forEach((function(settings) {
var getStepValue = settings[1];
ObservableList.prototype[settings[0]] = function() {
var items = this._items;
var index = 0;
var done = false;
return {
next: function() {
if (!done) {
if (index < items.length) {
return {
value: getStepValue(index, items[index++]),
done: false
};
}
done = true;
}
return {
value: void 0,
done: true
};
}
};
};
}));
ObservableList.prototype[Symbol$1.iterator] = ObservableList.prototype.values;
/**
* @typesign (cb: ());
*/
var nextTick;
/* istanbul ignore next */
if (global$1.process && process.toString() == '[object process]' && process.nextTick) {
nextTick = process.nextTick;
} else if (global$1.setImmediate) {
nextTick = function nextTick(cb) {
setImmediate(cb);
};
} else if (global$1.Promise && Promise.toString().indexOf('[native code]') != -1) {
var prm = Promise.resolve();
nextTick = function nextTick(cb) {
prm.then((function() {
cb();
}));
};
} else {
var queue;
global$1.addEventListener('message', (function() {
if (queue) {
var track = queue;
queue = null;
for (var i = 0, l = track.length; i < l; i++) {
try {
track[i]();
} catch (err) {
ErrorLogger.log(err);
}
}
}
}));
nextTick = function nextTick(cb) {
if (queue) {
queue.push(cb);
} else {
queue = [cb];
postMessage('__tic__', '*');
}
};
}
var nextTick$1 = nextTick;
function noop() {}
var EventEmitterProto = EventEmitter.prototype;
var MAX_SAFE_INTEGER = Number.MAX_SAFE_INTEGER || 0x1fffffffffffff;
var KEY_INNER$1 = EventEmitter.KEY_INNER;
var errorIndexCounter = 0;
var pushingIndexCounter = 0;
var releasePlan = new Map$1();
var releasePlanIndex = MAX_SAFE_INTEGER;
var releasePlanToIndex = -1;
var releasePlanned = false;
var currentlyRelease = false;
var currentCell = null;
var error = { original: null };
var releaseVersion = 1;
var transactionLevel = 0;
var pendingReactions = [];
var afterReleaseCallbacks;
function release() {
if (!releasePlanned) {
return;
}
releasePlanned = false;
currentlyRelease = true;
var queue = releasePlan.get(releasePlanIndex);
for (;;) {
var cell = queue && queue.shift();
if (!cell) {
if (releasePlanIndex == releasePlanToIndex) {
break;
}
queue = releasePlan.get(++releasePlanIndex);
continue;
}
var level = cell._level;
var changeEvent = cell._changeEvent;
if (!changeEvent) {
if (level > releasePlanIndex || cell._levelInRelease == -1) {
if (!queue.length) {
if (releasePlanIndex == releasePlanToIndex) {
break;
}
queue = releasePlan.get(++releasePlanIndex);
}
continue;
}
cell.pull();
level = cell._level;
changeEvent = cell._changeEvent;
if (level > releasePlanIndex) {
if (!queue.length) {
queue = releasePlan.get(++releasePlanIndex);
}
continue;
}
}
cell._levelInRelease = -1;
if (changeEvent) {
var oldReleasePlanIndex = releasePlanIndex;
cell._fixedValue = cell._value;
cell._changeEvent = null;
cell._handleEvent(changeEvent);
var pushingIndex = cell._pushingIndex;
var slaves = cell._slaves;
for (var i = 0, l = slaves.length; i < l; i++) {
var slave = slaves[i];
if (slave._level <= level) {
slave._level = level + 1;
}
if (pushingIndex >= slave._pushingIndex) {
slave._pushingIndex = pushingIndex;
slave._changeEvent = null;
slave._addToRelease();
}
}
if (releasePlanIndex != oldReleasePlanIndex) {
queue = releasePlan.get(releasePlanIndex);
continue;
}
}
if (!queue.length) {
if (releasePlanIndex == releasePlanToIndex) {
break;
}
queue = releasePlan.get(++releasePlanIndex);
}
}
releasePlanIndex = MAX_SAFE_INTEGER;
releasePlanToIndex = -1;
currentlyRelease = false;
releaseVersion++;
if (afterReleaseCallbacks) {
var callbacks = afterReleaseCallbacks;
afterReleaseCallbacks = null;
for (var j = 0, m = callbacks.length; j < m; j++) {
callbacks[j]();
}
}
}
/**
* @typesign (value);
*/
function defaultPut(value, push$$1) {
push$$1(value);
}
var config = {
asynchronous: true
};
/**
* @class cellx.Cell
* @extends {cellx.EventEmitter}
*
* @example
* var a = new Cell(1);
* var b = new Cell(2);
* var c = new Cell(function() {
* return a.get() + b.get();
* });
*
* c.on('change', function() {
* console.log('c = ' + c.get());
* });
*
* console.log(c.get());
* // => 3
*
* a.set(5);
* b.set(10);
* // => 'c = 15'
*
* @typesign new Cell(value?, opts?: {
* debugKey?: string,
* owner?: Object,
* get?: (value) -> *,
* validate?: (value, oldValue),
* merge: (value, oldValue) -> *,
* put?: (value, push: (value), fail: (err), oldValue),
* reap?: (),
* onChange?: (evt: cellx~Event) -> ?boolean,
* onError?: (evt: cellx~Event) -> ?boolean
* }) -> cellx.Cell;
*
* @typesign new Cell(pull: (push: (value), fail: (err), next) -> *, opts?: {
* debugKey?: string,
* owner?: Object,
* get?: (value) -> *,
* validate?: (value, oldValue),
* merge: (value, oldValue) -> *,
* put?: (value, push: (value), fail: (err), oldValue),
* reap?: (),
* onChange?: (evt: cellx~Event) -> ?boolean,
* onError?: (evt: cellx~Event) -> ?boolean
* }) -> cellx.Cell;
*/
var Cell = EventEmitter.extend({
Static: {
_nextTick: nextTick$1,
/**
* @typesign (cnfg: { asynchronous?: boolean });
*/
configure: function configure(cnfg) {
if (cnfg.asynchronous !== void 0) {
if (releasePlanned) {
release();
}
config.asynchronous = cnfg.asynchronous;
}
},
/**
* @typesign (cb: (), context?) -> ();
*/
autorun: function autorun(cb, context) {
var cell = new Cell(function() {
if (transactionLevel) {
var index = pendingReactions.indexOf(this);
if (index > -1) {
pendingReactions.splice(index, 1);
}
pendingReactions.push(this);
} else {
cb.call(context);
}
}, { onChange: noop });
return function disposer() {
cell.dispose();
};
},
/**
* @typesign ();
*/
forceRelease: function forceRelease() {
if (releasePlanned) {
release();
}
},
/**
* @typesign (cb: ());
*/
transaction: function transaction(cb) {
if (!transactionLevel++ && releasePlanned) {
release();
}
var success;
try {
cb();
success = true;
} catch (err) {
ErrorLogger.log(err);
for (var iterator = releasePlan.values(), step; !(step = iterator.next()).done;) {
var queue = step.value;
for (var i = queue.length; i;) {
var cell = queue[--i];
cell._value = cell._fixedValue;
cell._levelInRelease = -1;
cell._changeEvent = null;
}
}
releasePlan.clear();
releasePlanIndex = MAX_SAFE_INTEGER;
releasePlanToIndex = -1;
releasePlanned = false;
pendingReactions.length = 0;
success = false;
}
if (!--transactionLevel && success) {
for (var i = 0, l = pendingReactions.length; i < l; i++) {
var reaction = pendingReactions[i];
if (reaction instanceof Cell) {
reaction.pull();
} else {
EventEmitterProto._handleEvent.call(reaction[1], reaction[0]);
}
}
pendingReactions.length = 0;
if (releasePlanned) {
release();
}
}
},
/**
* @typesign (cb: ());
*/
afterRelease: function afterRelease(cb) {
(afterReleaseCallbacks || (afterReleaseCallbacks = [])).push(cb);
}
},
constructor: function Cell(value, opts) {
EventEmitter.call(this);
if (!opts) {
opts = {};
}
var cell = this;
this.debugKey = opts.debugKey;
this.owner = opts.owner || this;
this._pull = typeof value == 'function' ? value : null;
this._get = opts.get || null;
this._validate = opts.validate || null;
this._merge = opts.merge || null;
this._put = opts.put || defaultPut;
var push$$1 = this.push;
var fail = this.fail;
this.push = function(value) { push$$1.call(cell, value); };
this.fail = function(err) { fail.call(cell, err); };
this._onFulfilled = this._onRejected = null;
this._reap = opts.reap || null;
if (this._pull) {
this._fixedValue = this._value = void 0;
} else {
if (this._validate) {
this._validate(value, void 0);
}
if (this._merge) {
value = this._merge(value, void 0);
}
this._fixedValue = this._value = value;
if (value instanceof EventEmitter) {
value.on('change', this._onValueChange, this);
}
}
this._inited = false;
this._currentlyPulling = false;
this._active = false;
this._hasFollowers = false;
this._errorIndex = 0;
this._pushingIndex = 0;
this._version = 0;
/**
* Ведущие ячейки.
* @type {?Array<cellx.Cell>}
*/
this._masters = void 0;
/**
* Ведомые ячейки.
* @type {Array<cellx.Cell>}
*/
this._slaves = [];
this._level = 0;
this._levelInRelease = -1;
this._pending = false;
this._selfPendingStatusCell = null;
this._pendingStatusCell = null;
this._error = null;
this._selfErrorCell = null;
this._errorCell = null;
this._fulfilled = false;
this._rejected = false;
this._changeEvent = null;
this._canCancelChange = true;
this._lastErrorEvent = null;
if (opts.onChange) {
this.on('change', opts.onChange);
}
if (opts.onError) {
this.on('error', opts.onError);
}
},
_handleEvent: function _handleEvent(evt) {
if (transactionLevel) {
pendingReactions.push([evt, this]);
} else {
EventEmitterProto._handleEvent.call(this, evt);
}
},
/**
* @override
*/
on: function on(type, listener, context) {
if (releasePlanned) {
release();
}
this._activate();
if (typeof type == 'object') {
EventEmitterProto.on.call(this, type, arguments.length >= 2 ? listener : this.owner);
} else {
EventEmitterProto.on.call(this, type, listener, arguments.length >= 3 ? context : this.owner);
}
this._hasFollowers = true;
return this;
},
/**
* @override
*/
off: function off(type, listener, context) {
if (releasePlanned) {
release();
}
var argCount = arguments.length;
if (argCount) {
if (typeof type == 'object') {
EventEmitterProto.off.call(this, type, argCount >= 2 ? listener : this.owner);
} else {
EventEmitterProto.off.call(this, type, listener, argCount >= 3 ? context : this.owner);
}
} else {
EventEmitterProto.off.call(this);
}
if (!this._slaves.length && !this._events.has('change') && !this._events.has('error') && this._hasFollowers) {
this._hasFollowers = false;
this._deactivate();
if (this._reap) {
this._reap.call(this.owner);
}
}
return this;
},
/**
* @typesign (
* listener: (evt: cellx~Event) -> ?boolean,
* context?
* ) -> cellx.Cell;
*/
addChangeListener: function addChangeListener(listener, context) {
return this.on('change', listener, arguments.length >= 2 ? context : this.owner);
},
/**
* @typesign (
* listener: (evt: cellx~Event) -> ?boolean,
* context?
* ) -> cellx.Cell;
*/
removeChangeListener: function removeChangeListener(listener, context) {
return this.off('change', listener, arguments.length >= 2 ? context : this.owner);
},
/**
* @typesign (
* listener: (evt: cellx~Event) -> ?boolean,
* context?
* ) -> cellx.Cell;
*/
addErrorListener: function addErrorListener(listener, context) {
return this.on('error', listener, arguments.length >= 2 ? context : this.owner);
},
/**
* @typesign (
* listener: (evt: cellx~Event) -> ?boolean,
* context?
* ) -> cellx.Cell;
*/
removeErrorListener: function removeErrorListener(listener, context) {
return this.off('error', listener, arguments.length >= 2 ? context : this.owner);
},
/**
* @typesign (
* listener: (err: ?Error, evt: cellx~Event) -> ?boolean,
* context?
* ) -> cellx.Cell;
*/
subscribe: function subscribe(listener, context) {
function wrapper(evt) {
return listener.call(this, evt.error || null, evt);
}
wrapper[KEY_INNER$1] = listener;
if (arguments.length < 2) {
context = this.owner;
}
return this
.on('change', wrapper, context)
.on('error', wrapper, context);
},
/**
* @typesign (
* listener: (err: ?Error, evt: cellx~Event) -> ?boolean,
* context?
* ) -> cellx.Cell;
*/
unsubscribe: function unsubscribe(listener, context) {
if (arguments.length < 2) {
context = this.owner;
}
return this
.off('change', listener, context)
.off('error', listener, context);
},
/**
* @typesign (slave: cellx.Cell);
*/
_registerSlave: function _registerSlave(slave) {
this._activate();
this._slaves.push(slave);
this._hasFollowers = true;
},
/**
* @typesign (slave: cellx.Cell);
*/
_unregisterSlave: function _unregisterSlave(slave) {
this._slaves.splice(this._slaves.indexOf(slave), 1);
if (!this._slaves.length && !this._events.has('change') && !this._events.has('error')) {
this._hasFollowers = false;
this._deactivate();
if (this._reap) {
this._reap.call(this.owner);
}
}
},
/**
* @typesign ();
*/
_activate: function _activate() {
if (!this._pull || this._active || this._masters === null) {
return;
}
var masters = this._masters;
if (this._version < releaseVersion) {
var value = this._tryPull();
if (masters || this._masters || !this._inited) {
if (value === error) {
this._fail(error.original, false);
} else {
this._push(value, false, false);
}
}
masters = this._masters;
}
if (masters) {
var i = masters.length;
do {
masters[--i]._registerSlave(this);
} while (i);
this._active = true;
}
},
/**
* @typesign ();
*/
_deactivate: function _deactivate() {
if (!this._active) {
return;
}
var masters = this._masters;
var i = masters.length;
do {
masters[--i]._unregisterSlave(this);
} while (i);
if (this._levelInRelease != -1 && !this._changeEvent) {
this._levelInRelease = -1;
}
this._active = false;
},
/**
* @typesign ();
*/
_addToRelease: function _addToRelease() {
var level = this._level;
if (level <= this._levelInRelease) {
return;
}
var queue;
(releasePlan.get(level) || (releasePlan.set(level, (queue = [])), queue)).push(this);
if (releasePlanIndex > level) {
releasePlanIndex = level;
}
if (releasePlanToIndex < level) {
releasePlanToIndex = level;
}
this._levelInRelease = level;
if (!releasePlanned && !currentlyRelease) {
releasePlanned = true;
if (!transactionLevel && !config.asynchronous) {
release();
} else {
Cell._nextTick(release);
}
}
},
/**
* @typesign (evt: cellx~Event);
*/
_onValueChange: function _onValueChange(evt) {
this._pushingIndex = ++pushingIndexCounter;
if (this._changeEvent) {
evt.prev = this._changeEvent;
this._changeEvent = evt;
if (this._value === this._fixedValue) {
this._canCancelChange = false;
}
} else {
evt.prev = null;
this._changeEvent = evt;
this._canCancelChange = false;
this._addToRelease();
}
},
/**
* @typesign () -> *;
*/
get: function get() {
if (releasePlanned && this._pull) {
release();
}
if (this._pull && !this._active && this._version < releaseVersion && this._masters !== null) {
var oldMasters = this._masters;
var value = this._tryPull();
var masters = this._masters;
if (oldMasters || masters || !this._inited) {
if (masters && this._hasFollowers) {
var i = masters.length;
do {
masters[--i]._registerSlave(this);
} while (i);
this._active = true;
}
if (value === error) {
this._fail(error.original, false);
} else {
this._push(value, false, false);
}
}
}
if (currentCell) {
var currentCellMasters = currentCell._masters;
var level = this._level;
if (currentCellMasters) {
if (currentCellMasters.indexOf(this) == -1) {
currentCellMasters.push(this);
if (currentCell._level <= level) {
currentCell._level = level + 1;
}
}
} else {
currentCell._masters = [this];
currentCell._level = level + 1;
}
}
return this._get ? this._get(this._value) : this._value;
},
/**
* @typesign () -> boolean;
*/
pull: function pull() {
if (!this._pull) {
return false;
}
if (releasePlanned) {
release();
}
var hasFollowers = this._hasFollowers;
var oldMasters;
var oldLevel;
if (hasFollowers) {
oldMasters = this._masters;
oldLevel = this._level;
}
var value = this._tryPull();
if (hasFollowers) {
var masters = this._masters;
var newMasterCount = 0;
if (masters) {
var i = masters.length;
do {
var master = masters[--i];
if (!oldMasters || oldMasters.indexOf(master) == -1) {
master._registerSlave(this);
newMasterCount++;
}
} while (i);
}
if (oldMasters && (masters ? masters.length - newMasterCount : 0) < oldMasters.length) {
for (var j = oldMasters.length; j;) {
var oldMaster = oldMasters[--j];
if (!masters || masters.indexOf(oldMaster) == -1) {
oldMaster._unregisterSlave(this);
}
}
}
this._active = !!(masters && masters.length);
if (currentlyRelease && this._level > oldLevel) {
this._addToRelease();
return false;
}
}
if (value === error) {
this._fail(error.original, false);
return true;
}
return this._push(value, false, true);
},
/**
* @typesign () -> *;
*/
_tryPull: function _tryPull() {
if (this._currentlyPulling) {
throw new TypeError('Circular pulling detected');
}
this._pending = true;
this._fulfilled = this._rejected = false;
if (this._selfPendingStatusCell) {
this._selfPendingStatusCell.set(true);
}
var prevCell = currentCell;
currentCell = this;
this._currentlyPulling = true;
this._masters = null;
this._level = 0;
try {
return this._pull.call(this.owner, this.push, this.fail, this._value);
} catch (err) {
error.original = err;
return error;
} finally {
currentCell = prevCell;
this._version = releaseVersion + currentlyRelease;
var pendingStatusCell = this._pendingStatusCell;
if (pendingStatusCell && pendingStatusCell._active) {
pendingStatusCell.pull();
}
var errorCell = this._errorCell;
if (errorCell && errorCell._active) {
errorCell.pull();
}
this._currentlyPulling = false;
}
},
/**
* @typesign () -> ?Error;
*/
getError: function getError() {
if (!this._errorCell) {
var debugKey = this.debugKey;
this._selfErrorCell = new Cell(this._error, debugKey ? { debugKey: debugKey + '._selfErrorCell' } : null);
this._errorCell = new Cell(function() {
this.get();
var err = this._selfErrorCell.get();
var index;
if (err) {
index = this._errorIndex;
if (index == errorIndexCounter) {
return err;
}
}
var masters = this._masters;
if (masters) {
var i = masters.length;
do {
var master = masters[--i];
var masterError = master.getError();
if (masterError) {
var masterErrorIndex = master._errorIndex;
if (masterErrorIndex == errorIndexCounter) {
return masterError;
}
if (!err || index < masterErrorIndex) {
err = masterError;
index = masterErrorIndex;
}
}
} while (i);
}
return err;
}, debugKey ? { debugKey: debugKey + '._errorCell', owner: this } : { owner: this });
}
return this._errorCell.get();
},
/**
* @typesign (value) -> cellx.Cell;
*/
set: function set(value) {
var oldValue = this._value;
if (this._validate) {
this._validate(value, oldValue);
}
if (this._merge) {
value = this._merge(value, oldValue);
}
this._put.call(this.owner, value, this.push, this.fail, oldValue);
return this;
},
/**
* @typesign (value) -> cellx.Cell;
*/
push: function push(value) {
this._push(value, true, false);
return this;
},
/**
* @typesign (value, external: boolean, pulling: boolean) -> boolean;
*/
_push: function _push(value, external, pulling) {
this._inited = true;
var oldValue = this._value;
if (external && currentlyRelease) {
if (is(value, oldValue)) {
this._setError(null);
this._fulfill(value);
return false;
}
var cell = this;
(afterReleaseCallbacks || (afterReleaseCallbacks = [])).push((function() {
cell._push(value, true, false);
}));
return true;
}
if (external || !currentlyRelease && pulling) {
this._pushingIndex = ++pushingIndexCounter;
}
this._setError(null);
if (is(value, oldValue)) {
if (external) {
this._fulfill(value);
}
return false;
}
this._value = value;
if (oldValue instanceof EventEmitter) {
oldValue.off('change', this._onValueChange, this);
}
if (value instanceof EventEmitter) {
value.on('change', this._onValueChange, this);
}
if (this._hasFollowers || transactionLevel) {
if (this._changeEvent) {
if (is(value, this._fixedValue) && this._canCancelChange) {
this._levelInRelease = -1;
this._changeEvent = null;
} else {
this._changeEvent = {
target: this,
type: 'change',
oldValue: oldValue,
value: value,
prev: this._changeEvent
};
}
} else {
this._changeEvent = {
target: this,
type: 'change',
oldValue: oldValue,
value: value,
prev: null
};
this._canCancelChange = true;
this._addToRelease();
}
} else {
if (external || !currentlyRelease && pulling) {
releaseVersion++;
}
this._fixedValue = value;
this._version = releaseVersion + currentlyRelease;
}
if (external) {
this._fulfill(value);
}
return true;
},
_fulfill: function _fulfill(value) {
if (!this._pending) {
return;
}
this._pending = false;
this._fulfilled = true;
if (this._selfPendingStatusCell) {
this._selfPendingStatusCell.set(false);
}
if (this._onFulfilled) {
this._onFulfilled(value);
}
},
/**
* @typesign (err) -> cellx.Cell;
*/
fail: function fail(err) {
this._fail(err, true);
return this;
},
/**
* @typesign (err, external: boolean);
*/
_fail: function _fail(err, external) {
this._logError(err);
if (!(err instanceof Error)) {
err = new Error(String(err));
}
this._setError(err);
if (external) {
this._reject(err);
}
},
/**
* @typesign (err: ?Error);
*/
_setError: function _setError(err) {
if (!err && !this._error) {
return;
}
this._error = err;
if (this._selfErrorCell) {
this._selfErrorCell.set(err);
}
if (err) {
this._errorIndex = ++errorIndexCounter;
this._handleErrorEvent({
type: 'error',
error: err
});
}
},
/**
* @typesign (evt: cellx~Event{ error: Error });
*/
_handleErrorEvent: function _handleErrorEvent(evt) {
if (this._lastErrorEvent === evt) {
return;
}
this._lastErrorEvent = evt;
this._handleEvent(evt);
var slaves = this._slaves;
for (var i = 0, l = slaves.length; i < l; i++) {
slaves[i]._handleErrorEvent(evt);
}
},
_reject: function _reject(err) {
if (!this._pending) {
return;
}
this._pending = false;
this._rejected = true;
if (this._selfPendingStatusCell) {
this._selfPendingStatusCell.set(false);
}
if (this._onRejected) {
this._onRejected(err);
}
},
/**
* @typesign () -> boolean;
*/
isPending: function isPending() {
if (!this._pendingStatusCell) {
var debugKey = this.debugKey;
if (this._pull && this._pull.length) {
this._selfPendingStatusCell = new Cell(
this._pending,
debugKey ? { debugKey: debugKey + '._selfPendingStatusCell' } : null
);
}
this._pendingStatusCell = new Cell(function() {
if (this._selfPendingStatusCell && this._selfPendingStatusCell.get()) {
return true;
}
this.get();
var masters = this._masters;
if (masters) {
var i = masters.length;
do {
if (masters[--i].isPending()) {
return true;
}
} while (i);
}
return false;
}, debugKey ? { debugKey: debugKey + '._pendingStatusCell', owner: this } : { owner: this });
}
return this._pendingStatusCell.get();
},
/**
* @typesign (onFulfilled: ?(value) -> *, onRejected?: (err) -> *) -> Promise;
*/
then: function then(onFulfilled, onRejected) {
if (releasePlanned) {
release();
}
if (!this._pull || this._fulfilled) {
return Promise.resolve(this._get ? this._get(this._value) : this._value).then(onFulfilled);
}
if (this._rejected) {
return Promise.reject(this._error).catch(onRejected);
}
var cell = this;
var promise = new Promise(function(resolve, reject) {
cell._onFulfilled = function onFulfilled(value) {
cell._onFulfilled = cell._onRejected = null;
resolve(cell._get ? cell._get(value) : value);
};
cell._onRejected = function onRejected(err) {
cell._onFulfilled = cell._onRejected = null;
reject(err);
};
}).then(onFulfilled, onRejected);
if (!this._pending) {
this.pull();
}
return promise;
},
/**
* @typesign (onRejected: (err) -> *) -> Promise;
*/
catch: function catch_(onRejected) {
return this.then(null, onRejected);
},
/**
* @override
*/
_logError: function _logError() {
var msg = slice.call(arguments);
if (this.debugKey) {
msg.unshift('[' + this.debugKey + ']');
}
EventEmitterProto._logError.apply(this, msg);
},
/**
* @typesign () -> cellx.Cell;
*/
dispose: function dispose() {
var slaves = this._slaves;
for (var i = 0, l = slaves.length; i < l; i++) {
slaves[i].dispose();
}
return this.off();
}
});
Cell.prototype[Symbol$1.iterator] = function() {
return this._value[Symbol$1.iterator]();
};
/**
* @typesign (...msg);
*/
function logError() {
var console = global$1.console;
(console && console.error || noop).call(console || global$1, map$1.call(arguments, (function(arg) {
return arg === Object(arg) && arg.stack || arg;
})).join(' '));
}
ErrorLogger.setHandler(logError);
/**
* @typesign (value?, opts?: {
* debugKey?: string,
* owner?: Object,
* validate?: (value, oldValue),
* put?: (value, push: (value), fail: (err), oldValue),
* reap?: (),
* onChange?: (evt: cellx~Event) -> ?boolean,
* onError?: (evt: cellx~Event) -> ?boolean
* }) -> cellx;
*
* @typesign (pull: (push: (value), fail: (err), next) -> *, opts?: {
* debugKey?: string
* owner?: Object,
* validate?: (value, oldValue),
* put?: (value, push: (value), fail: (err), oldValue),
* reap?: (),
* onChange?: (evt: cellx~Event) -> ?boolean,
* onError?: (evt: cellx~Event) -> ?boolean
* }) -> cellx;
*/
function cellx(value, opts) {
if (!opts) {
opts = {};
}
var initialValue = value;
function cx(value) {
var owner = this;
if (!owner || owner == global$1) {
owner = cx;
}
if (!hasOwn.call(owner, CELLS)) {
Object.defineProperty(owner, CELLS, {
value: new Map$1()
});
}
var cell = owner[CELLS].get(cx);
if (!cell) {
if (value === 'dispose' && arguments.length >= 2) {
return;
}
opts = Object.create(opts);
opts.owner = owner;
cell = new Cell(initialValue, opts);
owner[CELLS].set(cx, cell);
}
switch (arguments.length) {
case 0: {
return cell.get();
}
case 1: {
cell.set(value);
return value;
}
default: {
var method = value;
switch (method) {
case 'bind': {
cx = cx.bind(owner);
cx.constructor = cellx;
return cx;
}
case 'unwrap': {
return cell;
}
default: {
var result = Cell.prototype[method].apply(cell, slice.call(arguments, 1));
return result === cell ? cx : result;
}
}
}
}
}
cx.constructor = cellx;
if (opts.onChange || opts.onError) {
cx.call(opts.owner || global$1);
}
return cx;
}
cellx.configure = function(config) {
Cell.configure(config);
};
cellx.ErrorLogger = ErrorLogger;
cellx.EventEmitter = EventEmitter;
cellx.ObservableCollectionMixin = ObservableCollectionMixin;
cellx.ObservableMap = ObservableMap;
cellx.ObservableList = ObservableList;
cellx.Cell = Cell;
cellx.autorun = Cell.autorun;
cellx.transact = cellx.transaction = Cell.transaction;
cellx.KEY_UID = UID;
cellx.KEY_CELLS = CELLS;
/**
* @typesign (
* entries?: Object|Array<{ 0, 1 }>|cellx.ObservableMap,
* opts?: { adoptsValueChanges?: boolean }
* ) -> cellx.ObservableMap;
*
* @typesign (
* entries?: Object|Array<{ 0, 1 }>|cellx.ObservableMap,
* adoptsValueChanges?: boolean
* ) -> cellx.ObservableMap;
*/
function map$$1(entries, opts) {
return new ObservableMap(entries, typeof opts == 'boolean' ? { adoptsValueChanges: opts } : opts);
}
cellx.map = map$$1;
/**
* @typesign (items?: Array|cellx.ObservableList, opts?: {
* adoptsValueChanges?: boolean,
* comparator?: (a, b) -> int,
* sorted?: boolean
* }) -> cellx.ObservableList;
*
* @typesign (items?: Array|cellx.ObservableList, adoptsValueChanges?: boolean) -> cellx.ObservableList;
*/
function list(items, opts) {
return new ObservableList(items, typeof opts == 'boolean' ? { adoptsValueChanges: opts } : opts);
}
cellx.list = list;
/**
* @typesign (obj: cellx.EventEmitter, name: string, value) -> cellx.EventEmitter;
*/
function defineObservableProperty(obj, name, value) {
var privateName = '_' + name;
obj[privateName] = value instanceof Cell ? value : new Cell(value, { owner: obj });
Object.defineProperty(obj, name, {
configurable: true,
enumerable: true,
get: function() {
return this[privateName].get();
},
set: function(value) {
this[privateName].set(value);
}
});
return obj;
}
cellx.defineObservableProperty = defineObservableProperty;
/**
* @typesign (obj: cellx.EventEmitter, props: Object) -> cellx.EventEmitter;
*/
function defineObservableProperties(obj, props) {
Object.keys(props).forEach((function(name) {
defineObservableProperty(obj, name, props[name]);
}));
return obj;
}
cellx.defineObservableProperties = defineObservableProperties;
/**
* @typesign (obj: cellx.EventEmitter, name: string, value) -> cellx.EventEmitter;
* @typesign (obj: cellx.EventEmitter, props: Object) -> cellx.EventEmitter;
*/
function define(obj, name, value) {
if (arguments.length == 3) {
defineObservableProperty(obj, name, value);
} else {
defineObservableProperties(obj, name);
}
return obj;
}
cellx.define = define;
cellx.JS = cellx.js = {
is: is,
Symbol: Symbol$1,
Map: Map$1
};
cellx.Utils = cellx.utils = {
logError: logError,
nextUID: nextUID,
mixin: mixin,
createClass: createClass,
nextTick: nextTick$1,
noop: noop
};
cellx.cellx = cellx; // for destructuring
return cellx;
})));
|
packages/material-ui-icons/src/LocalHospitalOutlined.js | kybarg/material-ui | import React from 'react';
import createSvgIcon from './utils/createSvgIcon';
export default createSvgIcon(
<path d="M19 3H5c-1.1 0-1.99.9-1.99 2L3 19c0 1.1.9 2 2 2h14c1.1 0 2-.9 2-2V5c0-1.1-.9-2-2-2zm0 16H5V5h14v14zm-8.5-2h3v-3.5H17v-3h-3.5V7h-3v3.5H7v3h3.5z" />
, 'LocalHospitalOutlined');
|
src/svg-icons/content/sort.js | kasra-co/material-ui | import React from 'react';
import pure from 'recompose/pure';
import SvgIcon from '../../SvgIcon';
let ContentSort = (props) => (
<SvgIcon {...props}>
<path d="M3 18h6v-2H3v2zM3 6v2h18V6H3zm0 7h12v-2H3v2z"/>
</SvgIcon>
);
ContentSort = pure(ContentSort);
ContentSort.displayName = 'ContentSort';
ContentSort.muiName = 'SvgIcon';
export default ContentSort;
|
packages/material-ui-icons/src/PhonelinkErase.js | AndriusBil/material-ui | import React from 'react';
import pure from 'recompose/pure';
import SvgIcon from 'material-ui/SvgIcon';
let PhonelinkErase = props =>
<SvgIcon {...props}>
<path d="M13 8.2l-1-1-4 4-4-4-1 1 4 4-4 4 1 1 4-4 4 4 1-1-4-4 4-4zM19 1H9c-1.1 0-2 .9-2 2v3h2V4h10v16H9v-2H7v3c0 1.1.9 2 2 2h10c1.1 0 2-.9 2-2V3c0-1.1-.9-2-2-2z" />
</SvgIcon>;
PhonelinkErase = pure(PhonelinkErase);
PhonelinkErase.muiName = 'SvgIcon';
export default PhonelinkErase;
|
src/TextField/TextFieldLabel.spec.js | ruifortes/material-ui | /* eslint-env mocha */
import React from 'react';
import {shallow} from 'enzyme';
import {expect} from 'chai';
import TextFieldLabel from './TextFieldLabel';
import getMuiTheme from '../styles/getMuiTheme';
describe('<TextFieldLabel>', () => {
it('uses focus styles', () => {
const wrapper = shallow(
<TextFieldLabel
muiTheme={getMuiTheme()}
shrink={false}
style={{color: 'regularcolor'}}
shrinkStyle={{color: 'focuscolor'}}
/>
);
expect(wrapper.type()).to.equal('label');
expect(wrapper.prop('style').color).to.equal('regularcolor');
wrapper.setProps({shrink: true});
expect(wrapper.prop('style').color).to.equal('focuscolor');
});
});
|
ajax/libs/zxcvbn/1.0.3/zxcvbn.min.js | jackdoyle/cdnjs | (function(){var aC,aM,aK,aB,ah,ag,af,ae,ad,ac,ab,t,ap,aL,ao,r,o,m,l,k,i;ab=function(n){var p,q;q=[];for(p in n){q.push(p)}return 0===q.length};ap=function(n,p){return n.push.apply(n,p)};k=function(n,p){var w,u,s,v,q;v=n.split("");q=[];u=0;for(s=v.length;u<s;u++){w=v[u],q.push(p[w]||w)}return q.join("")};r=function(n,p){var v,s,q,u;u=[];v=0;for(s=p.length;v<s;v++){q=p[v],ap(u,q(n))}return u.sort(function(x,w){return x.i-w.i||x.j-w.j})};ad=function(B,C){var A,y,x,z,w,v,s,u,q,p,n;p=[];z=B.length;v=B.toLowerCase();for(A=x=0;0<=z?x<z:x>z;A=0<=z?++x:--x){y=w=u=A;for(q=z;u<=q?w<q:w>q;y=u<=q?++w:--w){if(v.slice(A,+y+1||9000000000) in C){n=v.slice(A,+y+1||9000000000),s=C[n],p.push({pattern:"dictionary",i:A,j:y,token:B.slice(A,+y+1||9000000000),matched_word:n,rank:s})}}}return p};aK=function(n){var p,v,s,q,u;q={};p=1;v=0;for(s=n.length;v<s;v++){u=n[v],q[u]=p,p+=1}return q};aM=function(n,p){return function(v){var s,q,u;u=ad(v,p);v=0;for(s=u.length;v<s;v++){q=u[v],q.dictionary_name=n}return u}};ao={a:["4","@"],b:["8"],c:["(","{","[","<"],e:["3"],g:["6","9"],i:["1","!","|"],l:["1","|","7"],o:["0"],s:["$","5"],t:["+","7"],x:["%"],z:["2"]};o=function(n){var p,x,v,u,w;u={};w=n.split("");p=0;for(x=w.length;p<x;p++){n=w[p],u[n]=!0}n={};for(v in ao){x=ao[v];var s=w=void 0,q=void 0,q=[],s=0;for(w=x.length;s<w;s++){p=x[s],p in u&&q.push(p)}p=q;0<p.length&&(n[v]=p)}return n};t=function(C){var D,B,z,y,A,x,w,u,v,s,q,p,n;A=function(){var E;E=[];for(y in C){E.push(y)}return E}();n=[[]];B=function(M){var L,K,J,I,H,E,G,F;K=[];E={};J=0;for(H=M.length;J<H;J++){G=M[J],L=function(){var O,N,P;P=[];F=N=0;for(O=G.length;N<O;F=++N){y=G[F],P.push([y,F])}return P}(),L.sort(),I=function(){var N,P,O;O=[];F=P=0;for(N=L.length;P<N;F=++P){y=L[F],O.push(y+","+F)}return O}().join("-"),I in E||(E[I]=!0,K.push(G))}return K};z=function(Q){var P,O,N,L,M,K,J,I,H,G,F,R,E;if(Q.length){O=Q[0];R=Q.slice(1);I=[];G=C[O];Q=0;for(M=G.length;Q<M;Q++){L=G[Q];J=0;for(K=n.length;J<K;J++){E=n[J];P=-1;N=H=0;for(F=E.length;0<=F?H<F:H>F;N=0<=F?++H:--H){if(E[N][0]===L){P=N;break}}-1===P?(P=E.concat([[L,O]]),I.push(P)):(N=E.slice(0),N.splice(P,1),N.push([L,O]),I.push(E),I.push(N))}}n=B(I);return z(R)}};z(A);p=[];A=0;for(w=n.length;A<w;A++){s=n[A];q={};v=0;for(u=s.length;v<u;v++){D=s[v],x=D[0],D=D[1],q[x]=D}p.push(q)}return p};l=function(F,G,E){var C,B,D,A,z,x,y,w,v,u,p,q,s;p=[];for(x=0;x<F.length-1;){y=x+1;v=null;for(q=s=0;;){C=F.charAt(y-1);z=!1;A=-1;B=G[C]||[];if(y<F.length){D=F.charAt(y);w=0;for(u=B.length;w<u;w++){if(C=B[w],A+=1,C&&-1!==C.indexOf(D)){z=!0;1===C.indexOf(D)&&(q+=1);v!==A&&(s+=1,v=A);break}}}if(z){y+=1}else{2<y-x&&p.push({pattern:"spatial",i:x,j:y-1,token:F.slice(x,y),graph:E,turns:s,shifted_count:q});x=y;break}}}return p};aC={lower:"abcdefghijklmnopqrstuvwxyz",upper:"ABCDEFGHIJKLMNOPQRSTUVWXYZ",digits:"01234567890"};m=function(n,p){var s,q;q=[];for(s=1;1<=p?s<=p:s>=p;1<=p?++s:--s){q.push(n)}return q.join("")};aL=function(n,p){var s,q;for(q=[];;){s=n.match(p);if(!s){break}s.i=s.index;s.j=s.index+s[0].length-1;q.push(s);n=n.replace(s[0],m(" ",s[0].length))}return q};ac=/\d{3,}/;i=/19\d\d|200\d|201\d/;ae=function(D){var E,C,A,z,B,y,x,v,w,u,s,q,n,p;z=[];n=aL(D,/\d{4,8}/);v=0;for(w=n.length;v<w;v++){y=n[v];x=[y.i,y.j];y=x[0];x=x[1];A=D.slice(y,+x+1||9000000000);E=A.length;C=[];6>=A.length&&(C.push({daymonth:A.slice(2),year:A.slice(0,2),i:y,j:x}),C.push({daymonth:A.slice(0,E-2),year:A.slice(E-2),i:y,j:x}));6<=A.length&&(C.push({daymonth:A.slice(4),year:A.slice(0,4),i:y,j:x}),C.push({daymonth:A.slice(0,E-4),year:A.slice(E-4),i:y,j:x}));A=[];s=0;for(u=C.length;s<u;s++){switch(E=C[s],E.daymonth.length){case 2:A.push({day:E.daymonth[0],month:E.daymonth[1],year:E.year,i:E.i,j:E.j});break;case 3:A.push({day:E.daymonth.slice(0,2),month:E.daymonth[2],year:E.year,i:E.i,j:E.j});A.push({day:E.daymonth[0],month:E.daymonth.slice(1,3),year:E.year,i:E.i,j:E.j});break;case 4:A.push({day:E.daymonth.slice(0,2),month:E.daymonth.slice(2,4),year:E.year,i:E.i,j:E.j})}}u=0;for(C=A.length;u<C;u++){E=A[u],B=parseInt(E.day),q=parseInt(E.month),p=parseInt(E.year),B=aB(B,q,p),s=B[0],p=B[1],B=p[0],q=p[1],p=p[2],s&&z.push({pattern:"date",i:E.i,j:E.j,token:D.slice(y,+x+1||9000000000),separator:"",day:B,month:q,year:p})}}return z};ag=/(\d{1,2})(\s|-|\/|\\|_|\.)(\d{1,2})\2(19\d{2}|200\d|201\d|\d{2})/;ah=/(19\d{2}|200\d|201\d|\d{2})(\s|-|\/|\\|_|\.)(\d{1,2})\2(\d{1,2})/;af=function(z){var A,y,w,v,x,u,s,p,q,n;u=[];p=aL(z,ag);w=0;for(v=p.length;w<v;w++){x=p[w],q=function(){var C,B,E,D;E=[1,3,4];D=[];B=0;for(C=E.length;B<C;B++){y=E[B],D.push(parseInt(x[y]))}return D}(),x.day=q[0],x.month=q[1],x.year=q[2],x.sep=x[2],u.push(x)}p=aL(z,ah);v=0;for(w=p.length;v<w;v++){x=p[v],q=function(){var C,B,E,D;E=[4,3,1];D=[];B=0;for(C=E.length;B<C;B++){y=E[B],D.push(parseInt(x[y]))}return D}(),x.day=q[0],x.month=q[1],x.year=q[2],x.sep=x[2],u.push(x)}p=[];v=0;for(w=u.length;v<w;v++){x=u[v],A=aB(x.day,x.month,x.year),q=A[0],n=A[1],A=n[0],s=n[1],n=n[2],q&&p.push({pattern:"date",i:x.i,j:x.j,token:z.slice(x.i,+x.j+1||9000000000),separator:x.sep,day:A,month:s,year:n})}return p};aB=function(n,p,q){12<=p&&31>=p&&12>=n&&(p=[p,n],n=p[0],p=p[1]);return 31<n||12<p||!(1900<=q&&2019>=q)?[!1,[]]:[!0,[n,p,q]]};var h,f,e,c,an,ax,aq,aO,ay,ar,a,az,at,b,aN,aA,aH,au,am,d,aE,av;aH=function(n,p){var u,s,q;if(p>n){return 0}if(0===p){return 1}for(u=s=q=1;1<=p?s<=p:s>=p;u=1<=p?++s:--s){q*=n,q/=u,n-=1}return q};aN=function(n){return Math.log(n)/Math.log(2)};aA=function(B,C){var A,y,x,z,w,v,s,u,q,p,n;y=an(B);n=[];A=[];z=v=0;for(p=B.length;0<=p?v<p:v>p;z=0<=p?++v:--v){n[z]=(n[z-1]||0)+aN(y);A[z]=null;u=0;for(s=C.length;u<s;u++){q=C[u],q.j===z&&(w=[q.i,q.j],x=w[0],w=w[1],x=(n[x-1]||0)+ax(q),x<n[w]&&(n[w]=x,A[w]=q))}}v=[];for(z=B.length-1;0<=z;){(q=A[z])?(v.push(q),z=q.i-1):z-=1}v.reverse();s=function(D,E){return{pattern:"bruteforce",i:D,j:E,token:B.slice(D,+E+1||9000000000),entropy:aN(Math.pow(y,E-D+1)),cardinality:y}};z=0;u=[];p=0;for(A=v.length;p<A;p++){q=v[p],w=[q.i,q.j],x=w[0],w=w[1],0<x-z&&u.push(s(z,x-1)),z=w+1,u.push(q)}z<B.length&&u.push(s(z,B.length-1));v=u;q=n[B.length-1]||0;z=az(q);return{password:B,entropy:am(q,3),match_sequence:v,crack_time:am(z,3),crack_time_display:a(z),score:aq(z)}};am=function(n,p){return Math.round(n*Math.pow(10,p))/Math.pow(10,p)};az=function(n){return 0.00005*Math.pow(2,n)};aq=function(n){return n<Math.pow(10,2)?0:n<Math.pow(10,4)?1:n<Math.pow(10,6)?2:n<Math.pow(10,8)?3:4};ax=function(n){var p;if(null!=n.entropy){return n.entropy}p=function(){switch(n.pattern){case"repeat":return au;case"sequence":return d;case"digits":return ar;case"year":return av;case"date":return aO;case"spatial":return aE;case"dictionary":return ay}}();return n.entropy=p(n)};au=function(n){var p;p=an(n.token);return aN(p*n.token.length)};d=function(n){var p;p=n.token.charAt(0);p="a"===p||"1"===p?1:p.match(/\d/)?aN(10):p.match(/[a-z]/)?aN(26):aN(26)+1;n.ascending||(p+=1);return p+aN(n.token.length)};ar=function(n){return aN(Math.pow(10,n.token.length))};av=function(){return aN(119)};aO=function(n){var p;p=100>n.year?aN(37200):aN(44268);n.separator&&(p+=2);return p};aE=function(z){var A,y,w,v,x,u,s,p,q,n;"qwerty"===(w=z.graph)||"dvorak"===w?(q=g,y=aG):(q=aw,y=j);s=0;A=z.token.length;n=z.turns;for(w=x=2;2<=A?x<=A:x>=A;w=2<=A?++x:--x){p=Math.min(n,w-1);for(v=u=1;1<=p?u<=p:u>=p;v=1<=p?++u:--u){s+=aH(w-1,v-1)*q*Math.pow(y,v)}}y=aN(s);if(z.shifted_count){A=z.shifted_count;z=z.token.length-z.shifted_count;w=v=s=0;for(x=Math.min(A,z);0<=x?v<=x:v>=x;w=0<=x?++v:--v){s+=aH(A+z,w)}y+=aN(s)}return y};ay=function(n){n.base_entropy=aN(n.rank);n.uppercase_entropy=b(n);n.l33t_entropy=at(n);return n.base_entropy+n.uppercase_entropy+n.l33t_entropy};c=/^[A-Z][^A-Z]+$/;e=/^[^A-Z]+[A-Z]$/;f=/^[^a-z]+$/;h=/^[^A-Z]+$/;b=function(n){var p,x,v,u,w,s,q;q=n.token;if(q.match(h)){return 0}v=[c,e,f];n=0;for(p=v.length;n<p;n++){if(u=v[n],q.match(u)){return 1}}p=function(){var z,y,B,A;B=q.split("");A=[];y=0;for(z=B.length;y<z;y++){x=B[y],x.match(/[A-Z]/)&&A.push(x)}return A}().length;n=function(){var z,y,B,A;B=q.split("");A=[];y=0;for(z=B.length;y<z;y++){x=B[y],x.match(/[a-z]/)&&A.push(x)}return A}().length;v=u=w=0;for(s=Math.min(p,n);0<=s?u<=s:u>=s;v=0<=s?++u:--u){w+=aH(p+n,v)}return aN(w)};at=function(z){var A,y,w,v,x,u,s,p,q,n;if(!z.l33t){return 0}u=0;s=z.sub;for(q in s){n=s[q];A=function(){var B,D,E,C;E=z.token.split("");C=[];B=0;for(D=E.length;B<D;B++){w=E[B],w===q&&C.push(w)}return C}().length;y=function(){var B,D,E,C;E=z.token.split("");C=[];B=0;for(D=E.length;B<D;B++){w=E[B],w===n&&C.push(w)}return C}().length;v=x=0;for(p=Math.min(y,A);0<=p?x<=p:x>=p;v=0<=p?++x:--x){u+=aH(y+A,v)}}return aN(u)||1};an=function(x){var y,w,u,s,v,q,p,n;v=[!1,!1,!1,!1,!1];s=v[0];n=v[1];w=v[2];p=v[3];v=v[4];q=x.split("");x=0;for(u=q.length;x<u;x++){y=q[x],y=y.charCodeAt(0),48<=y&&57>=y?w=!0:65<=y&&90>=y?n=!0:97<=y&&122>=y?s=!0:127>=y?p=!0:v=!0}x=0;w&&(x+=10);n&&(x+=26);s&&(x+=26);p&&(x+=33);v&&(x+=100);return x};a=function(n){return 60>n?"instant":3600>n?1+Math.ceil(n/60)+" minutes":86400>n?1+Math.ceil(n/3600)+" hours":2678400>n?1+Math.ceil(n/86400)+" days":32140800>n?1+Math.ceil(n/2678400)+" months":3214080000>n?1+Math.ceil(n/32140800)+" years":"centuries"};var al={"!":["`~",null,null,"2@","qQ",null],'"':[";:","[{","]}",null,null,"/?"],"#":["2@",null,null,"4$","eE","wW"],$:["3#",null,null,"5%","rR","eE"],"%":["4$",null,null,"6^","tT","rR"],"&":["6^",null,null,"8*","uU","yY"],"'":[";:","[{","]}",null,null,"/?"],"(":["8*",null,null,"0)","oO","iI"],")":["9(",null,null,"-_","pP","oO"],"*":["7&",null,null,"9(","iI","uU"],"+":["-_",null,null,null,"]}","[{"],",":["mM","kK","lL",".>",null,null],"-":["0)",null,null,"=+","[{","pP"],".":[",<","lL",";:","/?",null,null],"/":[".>",";:","'\"",null,null,null],"0":["9(",null,null,"-_","pP","oO"],1:["`~",null,null,"2@","qQ",null],2:["1!",null,null,"3#","wW","qQ"],3:["2@",null,null,"4$","eE","wW"],4:["3#",null,null,"5%","rR","eE"],5:["4$",null,null,"6^","tT","rR"],6:["5%",null,null,"7&","yY","tT"],7:["6^",null,null,"8*","uU","yY"],8:["7&",null,null,"9(","iI","uU"],9:["8*",null,null,"0)","oO","iI"],":":"lL,pP,[{,'\",/?,.>".split(","),";":"lL,pP,[{,'\",/?,.>".split(","),"<":["mM","kK","lL",".>",null,null],"=":["-_",null,null,null,"]}","[{"],">":[",<","lL",";:","/?",null,null],"?":[".>",";:","'\"",null,null,null],"@":["1!",null,null,"3#","wW","qQ"],A:[null,"qQ","wW","sS","zZ",null],B:["vV","gG","hH","nN",null,null],C:["xX","dD","fF","vV",null,null],D:"sS,eE,rR,fF,cC,xX".split(","),E:"wW,3#,4$,rR,dD,sS".split(","),F:"dD,rR,tT,gG,vV,cC".split(","),G:"fF,tT,yY,hH,bB,vV".split(","),H:"gG,yY,uU,jJ,nN,bB".split(","),I:"uU,8*,9(,oO,kK,jJ".split(","),J:"hH,uU,iI,kK,mM,nN".split(","),K:"jJ iI oO lL ,< mM".split(" "),L:"kK oO pP ;: .> ,<".split(" "),M:["nN","jJ","kK",",<",null,null],N:["bB","hH","jJ","mM",null,null],O:"iI,9(,0),pP,lL,kK".split(","),P:"oO,0),-_,[{,;:,lL".split(","),Q:[null,"1!","2@","wW","aA",null],R:"eE,4$,5%,tT,fF,dD".split(","),S:"aA,wW,eE,dD,xX,zZ".split(","),T:"rR,5%,6^,yY,gG,fF".split(","),U:"yY,7&,8*,iI,jJ,hH".split(","),V:["cC","fF","gG","bB",null,null],W:"qQ,2@,3#,eE,sS,aA".split(","),X:["zZ","sS","dD","cC",null,null],Y:"tT,6^,7&,uU,hH,gG".split(","),Z:[null,"aA","sS","xX",null,null],"[":"pP,-_,=+,]},'\",;:".split(","),"\\":["]}",null,null,null,null,null],"]":["[{","=+",null,"\\|",null,"'\""],"^":["5%",null,null,"7&","yY","tT"],_:["0)",null,null,"=+","[{","pP"],"`":[null,null,null,"1!",null,null],a:[null,"qQ","wW","sS","zZ",null],b:["vV","gG","hH","nN",null,null],c:["xX","dD","fF","vV",null,null],d:"sS,eE,rR,fF,cC,xX".split(","),e:"wW,3#,4$,rR,dD,sS".split(","),f:"dD,rR,tT,gG,vV,cC".split(","),g:"fF,tT,yY,hH,bB,vV".split(","),h:"gG,yY,uU,jJ,nN,bB".split(","),i:"uU,8*,9(,oO,kK,jJ".split(","),j:"hH,uU,iI,kK,mM,nN".split(","),k:"jJ iI oO lL ,< mM".split(" "),l:"kK oO pP ;: .> ,<".split(" "),m:["nN","jJ","kK",",<",null,null],n:["bB","hH","jJ","mM",null,null],o:"iI,9(,0),pP,lL,kK".split(","),p:"oO,0),-_,[{,;:,lL".split(","),q:[null,"1!","2@","wW","aA",null],r:"eE,4$,5%,tT,fF,dD".split(","),s:"aA,wW,eE,dD,xX,zZ".split(","),t:"rR,5%,6^,yY,gG,fF".split(","),u:"yY,7&,8*,iI,jJ,hH".split(","),v:["cC","fF","gG","bB",null,null],w:"qQ,2@,3#,eE,sS,aA".split(","),x:["zZ","sS","dD","cC",null,null],y:"tT,6^,7&,uU,hH,gG".split(","),z:[null,"aA","sS","xX",null,null],"{":"pP,-_,=+,]},'\",;:".split(","),"|":["]}",null,null,null,null,null],"}":["[{","=+",null,"\\|",null,"'\""],"~":[null,null,null,"1!",null,null]},ak={"*":["/",null,null,null,"-","+","9","8"],"+":["9","*","-",null,null,null,null,"6"],"-":["*",null,null,null,null,null,"+","9"],".":["0","2","3",null,null,null,null,null],"/":[null,null,null,null,"*","9","8","7"],"0":[null,"1","2","3",".",null,null,null],1:[null,null,"4","5","2","0",null,null],2:["1","4","5","6","3",".","0",null],3:["2","5","6",null,null,null,".","0"],4:[null,null,"7","8","5","2","1",null],5:"4,7,8,9,6,3,2,1".split(","),6:["5","8","9","+",null,null,"3","2"],7:[null,null,null,"/","8","5","4",null],8:["7",null,"/","*","9","6","5","4"],9:["8","/","*","-","+",null,"6","5"]},aF,aj,aG,g,j,aw,aJ,aI,aD,ai;aF=[aM("passwords",aK("password,123456,12345678,1234,qwerty,12345,dragon,pussy,baseball,football,letmein,monkey,696969,abc123,mustang,shadow,master,111111,2000,jordan,superman,harley,1234567,fuckme,hunter,fuckyou,trustno1,ranger,buster,tigger,soccer,fuck,batman,test,pass,killer,hockey,charlie,love,sunshine,asshole,6969,pepper,access,123456789,654321,maggie,starwars,silver,dallas,yankees,123123,666666,hello,orange,biteme,freedom,computer,sexy,thunder,ginger,hammer,summer,corvette,fucker,austin,1111,merlin,121212,golfer,cheese,princess,chelsea,diamond,yellow,bigdog,secret,asdfgh,sparky,cowboy,camaro,matrix,falcon,iloveyou,guitar,purple,scooter,phoenix,aaaaaa,tigers,porsche,mickey,maverick,cookie,nascar,peanut,131313,money,horny,samantha,panties,steelers,snoopy,boomer,whatever,iceman,smokey,gateway,dakota,cowboys,eagles,chicken,dick,black,zxcvbn,ferrari,knight,hardcore,compaq,coffee,booboo,bitch,bulldog,xxxxxx,welcome,player,ncc1701,wizard,scooby,junior,internet,bigdick,brandy,tennis,blowjob,banana,monster,spider,lakers,rabbit,enter,mercedes,fender,yamaha,diablo,boston,tiger,marine,chicago,rangers,gandalf,winter,bigtits,barney,raiders,porn,badboy,blowme,spanky,bigdaddy,chester,london,midnight,blue,fishing,000000,hannah,slayer,11111111,sexsex,redsox,thx1138,asdf,marlboro,panther,zxcvbnm,arsenal,qazwsx,mother,7777777,jasper,winner,golden,butthead,viking,iwantu,angels,prince,cameron,girls,madison,hooters,startrek,captain,maddog,jasmine,butter,booger,golf,rocket,theman,liverpoo,flower,forever,muffin,turtle,sophie,redskins,toyota,sierra,winston,giants,packers,newyork,casper,bubba,112233,lovers,mountain,united,driver,helpme,fucking,pookie,lucky,maxwell,8675309,bear,suckit,gators,5150,222222,shithead,fuckoff,jaguar,hotdog,tits,gemini,lover,xxxxxxxx,777777,canada,florida,88888888,rosebud,metallic,doctor,trouble,success,stupid,tomcat,warrior,peaches,apples,fish,qwertyui,magic,buddy,dolphins,rainbow,gunner,987654,freddy,alexis,braves,cock,2112,1212,cocacola,xavier,dolphin,testing,bond007,member,voodoo,7777,samson,apollo,fire,tester,beavis,voyager,porno,rush2112,beer,apple,scorpio,skippy,sydney,red123,power,beaver,star,jackass,flyers,boobs,232323,zzzzzz,scorpion,doggie,legend,ou812,yankee,blazer,runner,birdie,bitches,555555,topgun,asdfasdf,heaven,viper,animal,2222,bigboy,4444,private,godzilla,lifehack,phantom,rock,august,sammy,cool,platinum,jake,bronco,heka6w2,copper,cumshot,garfield,willow,cunt,slut,69696969,kitten,super,jordan23,eagle1,shelby,america,11111,free,123321,chevy,bullshit,broncos,horney,surfer,nissan,999999,saturn,airborne,elephant,shit,action,adidas,qwert,1313,explorer,police,christin,december,wolf,sweet,therock,online,dickhead,brooklyn,cricket,racing,penis,0000,teens,redwings,dreams,michigan,hentai,magnum,87654321,donkey,trinity,digital,333333,cartman,guinness,123abc,speedy,buffalo,kitty,pimpin,eagle,einstein,nirvana,vampire,xxxx,playboy,pumpkin,snowball,test123,sucker,mexico,beatles,fantasy,celtic,cherry,cassie,888888,sniper,genesis,hotrod,reddog,alexande,college,jester,passw0rd,bigcock,lasvegas,slipknot,3333,death,1q2w3e,eclipse,1q2w3e4r,drummer,montana,music,aaaa,carolina,colorado,creative,hello1,goober,friday,bollocks,scotty,abcdef,bubbles,hawaii,fluffy,horses,thumper,5555,pussies,darkness,asdfghjk,boobies,buddha,sandman,naughty,honda,azerty,6666,shorty,money1,beach,loveme,4321,simple,poohbear,444444,badass,destiny,vikings,lizard,assman,nintendo,123qwe,november,xxxxx,october,leather,bastard,101010,extreme,password1,pussy1,lacrosse,hotmail,spooky,amateur,alaska,badger,paradise,maryjane,poop,mozart,video,vagina,spitfire,cherokee,cougar,420420,horse,enigma,raider,brazil,blonde,55555,dude,drowssap,lovely,1qaz2wsx,booty,snickers,nipples,diesel,rocks,eminem,westside,suzuki,passion,hummer,ladies,alpha,suckme,147147,pirate,semperfi,jupiter,redrum,freeuser,wanker,stinky,ducati,paris,babygirl,windows,spirit,pantera,monday,patches,brutus,smooth,penguin,marley,forest,cream,212121,flash,maximus,nipple,vision,pokemon,champion,fireman,indian,softball,picard,system,cobra,enjoy,lucky1,boogie,marines,security,dirty,admin,wildcats,pimp,dancer,hardon,fucked,abcd1234,abcdefg,ironman,wolverin,freepass,bigred,squirt,justice,hobbes,pearljam,mercury,domino,9999,rascal,hitman,mistress,bbbbbb,peekaboo,naked,budlight,electric,sluts,stargate,saints,bondage,bigman,zombie,swimming,duke,qwerty1,babes,scotland,disney,rooster,mookie,swordfis,hunting,blink182,8888,samsung,bubba1,whore,general,passport,aaaaaaaa,erotic,liberty,arizona,abcd,newport,skipper,rolltide,balls,happy1,galore,christ,weasel,242424,wombat,digger,classic,bulldogs,poopoo,accord,popcorn,turkey,bunny,mouse,007007,titanic,liverpool,dreamer,everton,chevelle,psycho,nemesis,pontiac,connor,eatme,lickme,cumming,ireland,spiderma,patriots,goblue,devils,empire,asdfg,cardinal,shaggy,froggy,qwer,kawasaki,kodiak,phpbb,54321,chopper,hooker,whynot,lesbian,snake,teen,ncc1701d,qqqqqq,airplane,britney,avalon,sugar,sublime,wildcat,raven,scarface,elizabet,123654,trucks,wolfpack,pervert,redhead,american,bambam,woody,shaved,snowman,tiger1,chicks,raptor,1969,stingray,shooter,france,stars,madmax,sports,789456,simpsons,lights,chronic,hahaha,packard,hendrix,service,spring,srinivas,spike,252525,bigmac,suck,single,popeye,tattoo,texas,bullet,taurus,sailor,wolves,panthers,japan,strike,pussycat,chris1,loverboy,berlin,sticky,tarheels,russia,wolfgang,testtest,mature,catch22,juice,michael1,nigger,159753,alpha1,trooper,hawkeye,freaky,dodgers,pakistan,machine,pyramid,vegeta,katana,moose,tinker,coyote,infinity,pepsi,letmein1,bang,hercules,james1,tickle,outlaw,browns,billybob,pickle,test1,sucks,pavilion,changeme,caesar,prelude,darkside,bowling,wutang,sunset,alabama,danger,zeppelin,pppppp,2001,ping,darkstar,madonna,qwe123,bigone,casino,charlie1,mmmmmm,integra,wrangler,apache,tweety,qwerty12,bobafett,transam,2323,seattle,ssssss,openup,pandora,pussys,trucker,indigo,storm,malibu,weed,review,babydoll,doggy,dilbert,pegasus,joker,catfish,flipper,fuckit,detroit,cheyenne,bruins,smoke,marino,fetish,xfiles,stinger,pizza,babe,stealth,manutd,gundam,cessna,longhorn,presario,mnbvcxz,wicked,mustang1,victory,21122112,awesome,athena,q1w2e3r4,holiday,knicks,redneck,12341234,gizmo,scully,dragon1,devildog,triumph,bluebird,shotgun,peewee,angel1,metallica,madman,impala,lennon,omega,access14,enterpri,search,smitty,blizzard,unicorn,tight,asdf1234,trigger,truck,beauty,thailand,1234567890,cadillac,castle,bobcat,buddy1,sunny,stones,asian,butt,loveyou,hellfire,hotsex,indiana,panzer,lonewolf,trumpet,colors,blaster,12121212,fireball,precious,jungle,atlanta,gold,corona,polaris,timber,theone,baller,chipper,skyline,dragons,dogs,licker,engineer,kong,pencil,basketba,hornet,barbie,wetpussy,indians,redman,foobar,travel,morpheus,target,141414,hotstuff,photos,rocky1,fuck_inside,dollar,turbo,design,hottie,202020,blondes,4128,lestat,avatar,goforit,random,abgrtyu,jjjjjj,cancer,q1w2e3,smiley,express,virgin,zipper,wrinkle1,babylon,consumer,monkey1,serenity,samurai,99999999,bigboobs,skeeter,joejoe,master1,aaaaa,chocolat,christia,stephani,tang,1234qwer,98765432,sexual,maxima,77777777,buckeye,highland,seminole,reaper,bassman,nugget,lucifer,airforce,nasty,warlock,2121,dodge,chrissy,burger,snatch,pink,gang,maddie,huskers,piglet,photo,dodger,paladin,chubby,buckeyes,hamlet,abcdefgh,bigfoot,sunday,manson,goldfish,garden,deftones,icecream,blondie,spartan,charger,stormy,juventus,galaxy,escort,zxcvb,planet,blues,david1,ncc1701e,1966,51505150,cavalier,gambit,ripper,oicu812,nylons,aardvark,whiskey,bing,plastic,anal,babylon5,loser,racecar,insane,yankees1,mememe,hansolo,chiefs,fredfred,freak,frog,salmon,concrete,zxcv,shamrock,atlantis,wordpass,rommel,1010,predator,massive,cats,sammy1,mister,stud,marathon,rubber,ding,trunks,desire,montreal,justme,faster,irish,1999,jessica1,alpine,diamonds,00000,swinger,shan,stallion,pitbull,letmein2,ming,shadow1,clitoris,fuckers,jackoff,bluesky,sundance,renegade,hollywoo,151515,wolfman,soldier,ling,goddess,manager,sweety,titans,fang,ficken,niners,bubble,hello123,ibanez,sweetpea,stocking,323232,tornado,content,aragorn,trojan,christop,rockstar,geronimo,pascal,crimson,google,fatcat,lovelove,cunts,stimpy,finger,wheels,viper1,latin,greenday,987654321,creampie,hiphop,snapper,funtime,duck,trombone,adult,cookies,mulder,westham,latino,jeep,ravens,drizzt,madness,energy,kinky,314159,slick,rocker,55555555,mongoose,speed,dddddd,catdog,cheng,ghost,gogogo,tottenha,curious,butterfl,mission,january,shark,techno,lancer,lalala,chichi,orion,trixie,delta,bobbob,bomber,kang,1968,spunky,liquid,beagle,granny,network,kkkkkk,1973,biggie,beetle,teacher,toronto,anakin,genius,cocks,dang,karate,snakes,bangkok,fuckyou2,pacific,daytona,infantry,skywalke,sailing,raistlin,vanhalen,huang,blackie,tarzan,strider,sherlock,gong,dietcoke,ultimate,shai,sprite,ting,artist,chai,chao,devil,python,ninja,ytrewq,superfly,456789,tian,jing,jesus1,freedom1,drpepper,chou,hobbit,shen,nolimit,mylove,biscuit,yahoo,shasta,sex4me,smoker,pebbles,pics,philly,tong,tintin,lesbians,cactus,frank1,tttttt,chun,danni,emerald,showme,pirates,lian,dogg,xiao,xian,tazman,tanker,toshiba,gotcha,rang,keng,jazz,bigguy,yuan,tomtom,chaos,fossil,racerx,creamy,bobo,musicman,warcraft,blade,shuang,shun,lick,jian,microsoft,rong,feng,getsome,quality,1977,beng,wwwwww,yoyoyo,zhang,seng,harder,qazxsw,qian,cong,chuan,deng,nang,boeing,keeper,western,1963,subaru,sheng,thuglife,teng,jiong,miao,mang,maniac,pussie,a1b2c3,zhou,zhuang,xing,stonecol,spyder,liang,jiang,memphis,ceng,magic1,logitech,chuang,sesame,shao,poison,titty,kuan,kuai,mian,guan,hamster,guai,ferret,geng,duan,pang,maiden,quan,velvet,nong,neng,nookie,buttons,bian,bingo,biao,zhong,zeng,zhun,ying,zong,xuan,zang,0.0.000,suan,shei,shui,sharks,shang,shua,peng,pian,piao,liao,meng,miami,reng,guang,cang,ruan,diao,luan,qing,chui,chuo,cuan,nuan,ning,heng,huan,kansas,muscle,weng,1passwor,bluemoon,zhui,zhua,xiang,zheng,zhen,zhei,zhao,zhan,yomama,zhai,zhuo,zuan,tarheel,shou,shuo,tiao,leng,kuang,jiao,13579,basket,qiao,qiong,qiang,chuai,nian,niao,niang,huai,22222222,zhuan,zhuai,shuan,shuai,stardust,jumper,66666666,charlott,qwertz,bones,waterloo,2002,11223344,oldman,trains,vertigo,246810,black1,swallow,smiles,standard,alexandr,parrot,user,1976,surfing,pioneer,apple1,asdasd,auburn,hannibal,frontier,panama,welcome1,vette,blue22,shemale,111222,baggins,groovy,global,181818,1979,blades,spanking,byteme,lobster,dawg,japanese,1970,1964,2424,polo,coco,deedee,mikey,1972,171717,1701,strip,jersey,green1,capital,putter,vader,seven7,banshee,grendel,dicks,hidden,iloveu,1980,ledzep,147258,female,bugger,buffett,molson,2020,wookie,sprint,jericho,102030,ranger1,trebor,deepthroat,bonehead,molly1,mirage,models,1984,2468,showtime,squirrel,pentium,anime,gator,powder,twister,connect,neptune,engine,eatshit,mustangs,woody1,shogun,septembe,pooh,jimbo,russian,sabine,voyeur,2525,363636,camel,germany,giant,qqqq,nudist,bone,sleepy,tequila,fighter,obiwan,makaveli,vacation,walnut,1974,ladybug,cantona,ccbill,satan,rusty1,passwor1,columbia,kissme,motorola,william1,1967,zzzz,skater,smut,matthew1,valley,coolio,dagger,boner,bull,horndog,jason1,penguins,rescue,griffey,8j4ye3uz,californ,champs,qwertyuiop,portland,colt45,xxxxxxx,xanadu,tacoma,carpet,gggggg,safety,palace,italia,picturs,picasso,thongs,tempest,asd123,hairy,foxtrot,nimrod,hotboy,343434,1111111,asdfghjkl,goose,overlord,stranger,454545,shaolin,sooners,socrates,spiderman,peanuts,13131313,andrew1,filthy,ohyeah,africa,intrepid,pickles,assass,fright,potato,hhhhhh,kingdom,weezer,424242,pepsi1,throat,looker,puppy,butch,sweets,megadeth,analsex,nymets,ddddddd,bigballs,oakland,oooooo,qweasd,chucky,carrot,chargers,discover,dookie,condor,horny1,sunrise,sinner,jojo,megapass,martini,assfuck,ffffff,mushroom,jamaica,7654321,77777,cccccc,gizmodo,tractor,mypass,hongkong,1975,blue123,pissing,thomas1,redred,basketball,satan666,dublin,bollox,kingkong,1971,22222,272727,sexx,bbbb,grizzly,passat,defiant,bowler,knickers,monitor,wisdom,slappy,thor,letsgo,robert1,brownie,098765,playtime,lightnin,atomic,goku,llllll,qwaszx,cosmos,bosco,knights,beast,slapshot,assword,frosty,dumbass,mallard,dddd,159357,titleist,aussie,golfing,doobie,loveit,werewolf,vipers,1965,blabla,surf,sucking,tardis,thegame,legion,rebels,sarah1,onelove,loulou,toto,blackcat,0007,tacobell,soccer1,jedi,method,poopie,boob,breast,kittycat,belly,pikachu,thunder1,thankyou,celtics,frogger,scoobydo,sabbath,coltrane,budman,jackal,zzzzz,licking,gopher,geheim,lonestar,primus,pooper,newpass,brasil,heather1,husker,element,moomoo,beefcake,zzzzzzzz,shitty,smokin,jjjj,anthony1,anubis,backup,gorilla,fuckface,lowrider,punkrock,traffic,delta1,amazon,fatass,dodgeram,dingdong,qqqqqqqq,breasts,boots,honda1,spidey,poker,temp,johnjohn,147852,asshole1,dogdog,tricky,crusader,syracuse,spankme,speaker,meridian,amadeus,harley1,falcons,turkey50,kenwood,keyboard,ilovesex,1978,shazam,shalom,lickit,jimbob,roller,fatman,sandiego,magnus,cooldude,clover,mobile,plumber,texas1,tool,topper,mariners,rebel,caliente,celica,oxford,osiris,orgasm,punkin,porsche9,tuesday,breeze,bossman,kangaroo,latinas,astros,scruffy,qwertyu,hearts,jammer,java,1122,goodtime,chelsea1,freckles,flyboy,doodle,nebraska,bootie,kicker,webmaster,vulcan,191919,blueeyes,321321,farside,rugby,director,pussy69,power1,hershey,hermes,monopoly,birdman,blessed,blackjac,southern,peterpan,thumbs,fuckyou1,rrrrrr,a1b2c3d4,coke,bohica,elvis1,blacky,sentinel,snake1,richard1,1234abcd,guardian,candyman,fisting,scarlet,dildo,pancho,mandingo,lucky7,condom,munchkin,billyboy,summer1,sword,skiing,site,sony,thong,rootbeer,assassin,fffff,fitness,durango,postal,achilles,kisses,warriors,plymouth,topdog,asterix,hallo,cameltoe,fuckfuck,eeeeee,sithlord,theking,avenger,backdoor,chevrole,trance,cosworth,houses,homers,eternity,kingpin,verbatim,incubus,1961,blond,zaphod,shiloh,spurs,mighty,aliens,charly,dogman,omega1,printer,aggies,deadhead,bitch1,stone55,pineappl,thekid,rockets,camels,formula,oracle,pussey,porkchop,abcde,clancy,mystic,inferno,blackdog,steve1,alfa,grumpy,flames,puffy,proxy,valhalla,unreal,herbie,engage,yyyyyy,010101,pistol,celeb,gggg,portugal,a12345,newbie,mmmm,1qazxsw2,zorro,writer,stripper,sebastia,spread,links,metal,1221,565656,funfun,trojans,cyber,hurrican,moneys,1x2zkg8w,zeus,tomato,lion,atlantic,usa123,trans,aaaaaaa,homerun,hyperion,kevin1,blacks,44444444,skittles,fart,gangbang,fubar,sailboat,oilers,buster1,hithere,immortal,sticks,pilot,lexmark,jerkoff,maryland,cheers,possum,cutter,muppet,swordfish,sport,sonic,peter1,jethro,rockon,asdfghj,pass123,pornos,ncc1701a,bootys,buttman,bonjour,1960,bears,362436,spartans,tinman,threesom,maxmax,1414,bbbbb,camelot,chewie,gogo,fusion,saint,dilligaf,nopass,hustler,hunter1,whitey,beast1,yesyes,spank,smudge,pinkfloy,patriot,lespaul,hammers,formula1,sausage,scooter1,orioles,oscar1,colombia,cramps,exotic,iguana,suckers,slave,topcat,lancelot,magelan,racer,crunch,british,steph,456123,skinny,seeking,rockhard,filter,freaks,sakura,pacman,poontang,newlife,homer1,klingon,watcher,walleye,tasty,sinatra,starship,steel,starbuck,poncho,amber1,gonzo,catherin,candle,firefly,goblin,scotch,diver,usmc,huskies,kentucky,kitkat,beckham,bicycle,yourmom,studio,33333333,splash,jimmy1,12344321,sapphire,mailman,raiders1,ddddd,excalibu,illini,imperial,lansing,maxx,gothic,golfball,facial,front242,macdaddy,qwer1234,vectra,cowboys1,crazy1,dannyboy,aquarius,franky,ffff,sassy,pppp,pppppppp,prodigy,noodle,eatpussy,vortex,wanking,billy1,siemens,phillies,groups,chevy1,cccc,gggggggg,doughboy,dracula,nurses,loco,lollipop,utopia,chrono,cooler,nevada,wibble,summit,1225,capone,fugazi,panda,qazwsxed,puppies,triton,9876,nnnnnn,momoney,iforgot,wolfie,studly,hamburg,81fukkc,741852,catman,china,gagging,scott1,oregon,qweqwe,crazybab,daniel1,cutlass,holes,mothers,music1,walrus,1957,bigtime,xtreme,simba,ssss,rookie,bathing,rotten,maestro,turbo1,99999,butthole,hhhh,yoda,shania,phish,thecat,rightnow,baddog,greatone,gateway1,abstr,napster,brian1,bogart,hitler,wildfire,jackson1,1981,beaner,yoyo,0.0.0.000,super1,select,snuggles,slutty,phoenix1,technics,toon,raven1,rayray,123789,1066,albion,greens,gesperrt,brucelee,hehehe,kelly1,mojo,1998,bikini,woofwoof,yyyy,strap,sites,central,f**k,nyjets,punisher,username,vanilla,twisted,bunghole,viagra,veritas,pony,titts,labtec,jenny1,masterbate,mayhem,redbull,govols,gremlin,505050,gmoney,rovers,diamond1,trident,abnormal,deskjet,cuddles,bristol,milano,vh5150,jarhead,1982,bigbird,bizkit,sixers,slider,star69,starfish,penetration,tommy1,john316,caligula,flicks,films,railroad,cosmo,cthulhu,br0d3r,bearbear,swedish,spawn,patrick1,reds,anarchy,groove,fuckher,oooo,airbus,cobra1,clips,delete,duster,kitty1,mouse1,monkeys,jazzman,1919,262626,swinging,stroke,stocks,sting,pippen,labrador,jordan1,justdoit,meatball,females,vector,cooter,defender,nike,bubbas,bonkers,kahuna,wildman,4121,sirius,static,piercing,terror,teenage,leelee,microsof,mechanic,robotech,rated,chaser,salsero,macross,quantum,tsunami,daddy1,cruise,newpass6,nudes,hellyeah,1959,zaq12wsx,striker,spice,spectrum,smegma,thumb,jjjjjjjj,mellow,cancun,cartoon,sabres,samiam,oranges,oklahoma,lust,denali,nude,noodles,brest,hooter,mmmmmmmm,warthog,blueblue,zappa,wolverine,sniffing,jjjjj,calico,freee,rover,pooter,closeup,bonsai,emily1,keystone,iiii,1955,yzerman,theboss,tolkien,megaman,rasta,bbbbbbbb,hal9000,goofy,gringo,gofish,gizmo1,samsam,scuba,onlyme,tttttttt,corrado,clown,clapton,bulls,jayhawk,wwww,sharky,seeker,ssssssss,pillow,thesims,lighter,lkjhgf,melissa1,marcius2,guiness,gymnast,casey1,goalie,godsmack,lolo,rangers1,poppy,clemson,clipper,deeznuts,holly1,eeee,kingston,yosemite,sucked,sex123,sexy69,pic\\'s,tommyboy,masterbating,gretzky,happyday,frisco,orchid,orange1,manchest,aberdeen,ne1469,boxing,korn,intercourse,161616,1985,ziggy,supersta,stoney,amature,babyboy,bcfields,goliath,hack,hardrock,frodo,scout,scrappy,qazqaz,tracker,active,craving,commando,cohiba,cyclone,bubba69,katie1,mpegs,vsegda,irish1,sexy1,smelly,squerting,lions,jokers,jojojo,meathead,ashley1,groucho,cheetah,champ,firefox,gandalf1,packer,love69,tyler1,typhoon,tundra,bobby1,kenworth,village,volley,wolf359,0420,000007,swimmer,skydive,smokes,peugeot,pompey,legolas,redhot,rodman,redalert,grapes,4runner,carrera,floppy,ou8122,quattro,cloud9,davids,nofear,busty,homemade,mmmmm,whisper,vermont,webmaste,wives,insertion,jayjay,philips,topher,temptress,midget,ripken,havefun,canon,celebrity,ghetto,ragnarok,usnavy,conover,cruiser,dalshe,nicole1,buzzard,hottest,kingfish,misfit,milfnew,warlord,wassup,bigsexy,blackhaw,zippy,tights,kungfu,labia,meatloaf,area51,batman1,bananas,636363,ggggg,paradox,queens,adults,aikido,cigars,hoosier,eeyore,moose1,warez,interacial,streaming,313131,pertinant,pool6123,mayday,animated,banker,baddest,gordon24,ccccc,fantasies,aisan,deadman,homepage,ejaculation,whocares,iscool,jamesbon,1956,1pussy,womam,sweden,skidoo,spock,sssss,pepper1,pinhead,micron,allsop,amsterda,gunnar,666999,february,fletch,george1,sapper,sasha1,luckydog,lover1,magick,popopo,ultima,cypress,businessbabe,brandon1,vulva,vvvv,jabroni,bigbear,yummy,010203,searay,secret1,sinbad,sexxxx,soleil,software,piccolo,thirteen,leopard,legacy,memorex,redwing,rasputin,134679,anfield,greenbay,catcat,feather,scanner,pa55word,contortionist,danzig,daisy1,hores,exodus,iiiiii,1001,subway,snapple,sneakers,sonyfuck,picks,poodle,test1234,llll,junebug,marker,mellon,ronaldo,roadkill,amanda1,asdfjkl,beaches,great1,cheerleaers,doitnow,ozzy,boxster,brighton,housewifes,kkkk,mnbvcx,moocow,vides,1717,bigmoney,blonds,1000,storys,stereo,4545,420247,seductive,sexygirl,lesbean,justin1,124578,cabbage,canadian,gangbanged,dodge1,dimas,malaka,puss,probes,coolman,nacked,hotpussy,erotica,kool,implants,intruder,bigass,zenith,woohoo,womans,tango,pisces,laguna,maxell,andyod22,barcelon,chainsaw,chickens,flash1,orgasms,magicman,profit,pusyy,pothead,coconut,chuckie,clevelan,builder,budweise,hotshot,horizon,experienced,mondeo,wifes,1962,stumpy,smiths,slacker,pitchers,passwords,laptop,allmine,alliance,bbbbbbb,asscock,halflife,88888,chacha,saratoga,sandy1,doogie,qwert40,transexual,close-up,ib6ub9,volvo,jacob1,iiiii,beastie,sunnyday,stoned,sonics,starfire,snapon,pictuers,pepe,testing1,tiberius,lisalisa,lesbain,litle,retard,ripple,austin1,badgirl,golfgolf,flounder,royals,dragoon,dickie,passwor,majestic,poppop,trailers,nokia,bobobo,br549,minime,mikemike,whitesox,1954,3232,353535,seamus,solo,sluttey,pictere,titten,lback,1024,goodluck,fingerig,gallaries,goat,passme,oasis,lockerroom,logan1,rainman,treasure,custom,cyclops,nipper,bucket,homepage-,hhhhh,momsuck,indain,2345,beerbeer,bimmer,stunner,456456,tootsie,testerer,reefer,1012,harcore,gollum,545454,chico,caveman,fordf150,fishes,gaymen,saleen,doodoo,pa55w0rd,presto,qqqqq,cigar,bogey,helloo,dutch,kamikaze,wasser,vietnam,visa,japanees,0123,swords,slapper,peach,masterbaiting,redwood,1005,ametuer,chiks,fucing,sadie1,panasoni,mamas,rambo,unknown,absolut,dallas1,housewife,keywest,kipper,18436572,1515,zxczxc,303030,shaman,terrapin,masturbation,mick,redfish,1492,angus,goirish,hardcock,forfun,galary,freeporn,duchess,olivier,lotus,pornographic,ramses,purdue,traveler,crave,brando,enter1,killme,moneyman,welder,windsor,wifey,indon,yyyyy,taylor1,4417,picher,pickup,thumbnils,johnboy,jets,ameteur,amateurs,apollo13,hambone,goldwing,5050,sally1,doghouse,padres,pounding,quest,truelove,underdog,trader,climber,bolitas,hohoho,beanie,beretta,wrestlin,stroker,sexyman,jewels,johannes,mets,rhino,bdsm,balloons,grils,happy123,flamingo,route66,devo,outkast,paintbal,magpie,llllllll,twilight,critter,cupcake,nickel,bullseye,knickerless,videoes,binladen,xerxes,slim,slinky,pinky,thanatos,meister,menace,retired,albatros,balloon,goten,5551212,getsdown,donuts,nwo4life,tttt,comet,deer,dddddddd,deeznutz,nasty1,nonono,enterprise,eeeee,misfit99,milkman,vvvvvv,1818,blueboy,bigbutt,tech,toolman,juggalo,jetski,barefoot,50spanks,gobears,scandinavian,cubbies,nitram,kings,bilbo,yumyum,zzzzzzz,stylus,321654,shannon1,server,squash,starman,steeler,phrases,techniques,laser,135790,athens,cbr600,chemical,fester,gangsta,fucku2,droopy,objects,passwd,lllll,manchester,vedder,clit,chunky,darkman,buckshot,buddah,boobed,henti,winter1,bigmike,beta,zidane,talon,slave1,pissoff,thegreat,lexus,matador,readers,armani,goldstar,5656,fmale,fuking,fucku,ggggggg,sauron,diggler,pacers,looser,pounded,premier,triangle,cosmic,depeche,norway,helmet,mustard,misty1,jagger,3x7pxr,silver1,snowboar,penetrating,photoes,lesbens,lindros,roadking,rockford,1357,143143,asasas,goodboy,898989,chicago1,ferrari1,galeries,godfathe,gawker,gargoyle,gangster,rubble,rrrr,onetime,pussyman,pooppoop,trapper,cinder,newcastl,boricua,bunny1,boxer,hotred,hockey1,edward1,moscow,mortgage,bigtit,snoopdog,joshua1,july,1230,assholes,frisky,sanity,divine,dharma,lucky13,akira,butterfly,hotbox,hootie,howdy,earthlink,kiteboy,westwood,1988,blackbir,biggles,wrench,wrestle,slippery,pheonix,penny1,pianoman,thedude,jenn,jonjon,jones1,roadrunn,arrow,azzer,seahawks,diehard,dotcom,tunafish,chivas,cinnamon,clouds,deluxe,northern,boobie,momomo,modles,volume,23232323,bluedog,wwwwwww,zerocool,yousuck,pluto,limewire,joung,awnyce,gonavy,haha,films+pic+galeries,girsl,fuckthis,girfriend,uncencored,a123456,chrisbln,combat,cygnus,cupoi,netscape,hhhhhhhh,eagles1,elite,knockers,1958,tazmania,shonuf,pharmacy,thedog,midway,arsenal1,anaconda,australi,gromit,gotohell,787878,66666,carmex2,camber,gator1,ginger1,fuzzy,seadoo,lovesex,rancid,uuuuuu,911911,bulldog1,heater,monalisa,mmmmmmm,whiteout,virtual,jamie1,japanes,james007,2727,2469,blam,bitchass,zephyr,stiffy,sweet1,southpar,spectre,tigger1,tekken,lakota,lionking,jjjjjjj,megatron,1369,hawaiian,gymnastic,golfer1,gunners,7779311,515151,sanfran,optimus,panther1,love1,maggie1,pudding,aaron1,delphi,niceass,bounce,house1,killer1,momo,musashi,jammin,2003,234567,wp2003wp,submit,sssssss,spikes,sleeper,passwort,kume,meme,medusa,mantis,reebok,1017,artemis,harry1,cafc91,fettish,oceans,oooooooo,mango,ppppp,trainer,uuuu,909090,death1,bullfrog,hokies,holyshit,eeeeeee,jasmine1,&,&,spinner,jockey,babyblue,gooner,474747,cheeks,pass1234,parola,okokok,poseidon,989898,crusher,cubswin,nnnn,kotaku,mittens,whatsup,vvvvv,iomega,insertions,bengals,biit,yellow1,012345,spike1,sowhat,pitures,pecker,theend,hayabusa,hawkeyes,florian,qaz123,usarmy,twinkle,chuckles,hounddog,hover,hothot,europa,kenshin,kojak,mikey1,water1,196969,wraith,zebra,wwwww,33333,simon1,spider1,snuffy,philippe,thunderb,teddy1,marino13,maria1,redline,renault,aloha,handyman,cerberus,gamecock,gobucks,freesex,duffman,ooooo,nuggets,magician,longbow,preacher,porno1,chrysler,contains,dalejr,navy,buffy1,hedgehog,hoosiers,honey1,hott,heyhey,dutchess,everest,wareagle,ihateyou,sunflowe,3434,senators,shag,spoon,sonoma,stalker,poochie,terminal,terefon,maradona,1007,142536,alibaba,america1,bartman,astro,goth,chicken1,cheater,ghost1,passpass,oral,r2d2c3po,civic,cicero,myxworld,kkkkk,missouri,wishbone,infiniti,1a2b3c,1qwerty,wonderboy,shojou,sparky1,smeghead,poiuy,titanium,lantern,jelly,1213,bayern,basset,gsxr750,cattle,fishing1,fullmoon,gilles,dima,obelix,popo,prissy,ramrod,bummer,hotone,dynasty,entry,konyor,missy1,282828,xyz123,426hemi,404040,seinfeld,pingpong,lazarus,marine1,12345a,beamer,babyface,greece,gustav,7007,ccccccc,faggot,foxy,gladiato,duckie,dogfood,packers1,longjohn,radical,tuna,clarinet,danny1,novell,bonbon,kashmir,kiki,mortimer,modelsne,moondog,vladimir,insert,1953,zxc123,supreme,3131,sexxx,softail,poipoi,pong,mars,martin1,rogue,avalanch,audia4,55bgates,cccccccc,came11,figaro,dogboy,dnsadm,dipshit,paradigm,othello,operator,tripod,chopin,coucou,cocksuck,borussia,heritage,hiziad,homerj,mullet,whisky,4242,speedo,starcraf,skylar,spaceman,piggy,tiger2,legos,jezebel,joker1,mazda,727272,chester1,rrrrrrrr,dundee,lumber,ppppppp,tranny,aaliyah,admiral,comics,delight,buttfuck,homeboy,eternal,kilroy,violin,wingman,walmart,bigblue,blaze,beemer,beowulf,bigfish,yyyyyyy,woodie,yeahbaby,0123456,tbone,syzygy,starter,linda1,merlot,mexican,11235813,banner,bangbang,badman,barfly,grease,charles1,ffffffff,doberman,dogshit,overkill,coolguy,claymore,demo,nomore,hhhhhhh,hondas,iamgod,enterme,electron,eastside,minimoni,mybaby,wildbill,wildcard,ipswich,200000,bearcat,zigzag,yyyyyyyy,sweetnes,369369,skyler,skywalker,pigeon,tipper,asdf123,alphabet,asdzxc,babybaby,banane,guyver,graphics,chinook,florida1,flexible,fuckinside,ursitesux,tototo,adam12,christma,chrome,buddie,bombers,hippie,misfits,292929,woofer,wwwwwwww,stubby,sheep,sparta,stang,spud,sporty,pinball,just4fun,maxxxx,rebecca1,fffffff,freeway,garion,rrrrr,sancho,outback,maggot,puddin,987456,hoops,mydick,19691969,bigcat,shiner,silverad,templar,lamer,juicy,mike1,maximum,1223,10101010,arrows,alucard,haggis,cheech,safari,dog123,orion1,paloma,qwerasdf,presiden,vegitto,969696,adonis,cookie1,newyork1,buddyboy,hellos,heineken,eraser,moritz,millwall,visual,jaybird,1983,beautifu,zodiac,steven1,sinister,slammer,smashing,slick1,sponge,teddybea,ticklish,jonny,1211,aptiva,applepie,bailey1,guitar1,canyon,gagged,fuckme1,digital1,dinosaur,98765,90210,clowns,cubs,deejay,nigga,naruto,boxcar,icehouse,hotties,electra,widget,1986,2004,bluefish,bingo1,*****,stratus,sultan,storm1,44444,4200,sentnece,sexyboy,sigma,smokie,spam,pippo,temppass,manman,1022,bacchus,aztnm,axio,bamboo,hakr,gregor,hahahaha,5678,camero1,dolphin1,paddle,magnet,qwert1,pyon,porsche1,tripper,noway,burrito,bozo,highheel,hookem,eddie1,entropy,kkkkkkkk,kkkkkkk,illinois,1945,1951,24680,21212121,100000,stonecold,taco,subzero,sexxxy,skolko,skyhawk,spurs1,sputnik,testpass,jiggaman,1224,hannah1,525252,4ever,carbon,scorpio1,rt6ytere,madison1,loki,coolness,coldbeer,citadel,monarch,morgan1,washingt,1997,bella1,yaya,superb,taxman,studman,3636,pizzas,tiffany1,lassie,larry1,joseph1,mephisto,reptile,razor,1013,hammer1,gypsy,grande,camper,chippy,cat123,chimera,fiesta,glock,domain,dieter,dragonba,onetwo,nygiants,password2,quartz,prowler,prophet,towers,ultra,cocker,corleone,dakota1,cumm,nnnnnnn,boxers,heynow,iceberg,kittykat,wasabi,vikings1,beerman,splinter,snoopy1,pipeline,mickey1,mermaid,micro,meowmeow,redbird,baura,chevys,caravan,frogman,diving,dogger,draven,drifter,oatmeal,paris1,longdong,quant4307s,rachel1,vegitta,cobras,corsair,dadada,mylife,bowwow,hotrats,eastwood,moonligh,modena,illusion,iiiiiii,jayhawks,swingers,shocker,shrimp,sexgod,squall,poiu,tigers1,toejam,tickler,julie1,jimbo1,jefferso,michael2,rodeo,robot,1023,annie1,bball,happy2,charter,flasher,falcon1,fiction,fastball,gadget,scrabble,diaper,dirtbike,oliver1,paco,macman,poopy,popper,postman,ttttttt,acura,cowboy1,conan,daewoo,nemrac58,nnnnn,nextel,bobdylan,eureka,kimmie,kcj9wx5n,killbill,musica,volkswag,wage,windmill,wert,vintage,iloveyou1,itsme,zippo,311311,starligh,smokey1,snappy,soulmate,plasma,krusty,just4me,marius,rebel1,1123,audi,fick,goaway,rusty2,dogbone,doofus,ooooooo,oblivion,mankind,mahler,lllllll,pumper,puck,pulsar,valkyrie,tupac,compass,concorde,cougars,delaware,niceguy,nocturne,bob123,boating,bronze,herewego,hewlett,houhou,earnhard,eeeeeeee,mingus,mobydick,venture,verizon,imation,1950,1948,1949,223344,bigbig,wowwow,sissy,spiker,snooker,sluggo,player1,jsbach,jumbo,medic,reddevil,reckless,123456a,1125,1031,astra,gumby,757575,585858,chillin,fuck1,radiohea,upyours,trek,coolcool,classics,choochoo,nikki1,nitro,boytoy,excite,kirsty,wingnut,wireless,icu812,1master,beatle,bigblock,wolfen,summer99,sugar1,tartar,sexysexy,senna,sexman,soprano,platypus,pixies,telephon,laura1,laurent,rimmer,1020,12qwaszx,hamish,halifax,fishhead,forum,dododo,doit,paramedi,lonesome,mandy1,uuuuu,uranus,ttttt,bruce1,helper,hopeful,eduard,dusty1,kathy1,moonbeam,muscles,monster1,monkeybo,windsurf,vvvvvvv,vivid,install,1947,187187,1941,1952,susan1,31415926,sinned,sexxy,smoothie,snowflak,playstat,playa,playboy1,toaster,jerry1,marie1,mason1,merlin1,roger1,roadster,112358,1121,andrea1,bacardi,hardware,789789,5555555,captain1,fergus,sascha,rrrrrrr,dome,onion,lololo,qqqqqqq,undertak,uuuuuuuu,uuuuuuu,cobain,cindy1,coors,descent,nimbus,nomad,nanook,norwich,bombay,broker,hookup,kiwi,winners,jackpot,1a2b3c4d,1776,beardog,bighead,bird33,0987,spooge,pelican,peepee,titan,thedoors,jeremy1,altima,baba,hardone,5454,catwoman,finance,farmboy,farscape,genesis1,salomon,loser1,r2d2,pumpkins,chriss,cumcum,ninjas,ninja1,killers,miller1,islander,jamesbond,intel,19841984,2626,bizzare,blue12,biker,yoyoma,sushi,shitface,spanker,steffi,sphinx,please1,paulie,pistons,tiburon,maxwell1,mdogg,rockies,armstron,alejandr,arctic,banger,audio,asimov,753951,4you,chilly,care1839,flyfish,fantasia,freefall,sandrine,oreo,ohshit,macbeth,madcat,loveya,qwerqwer,colnago,chocha,cobalt,crystal1,dabears,nevets,nineinch,broncos1,epsilon,kestrel,winston1,warrior1,iiiiiiii,iloveyou2,1616,woowoo,sloppy,specialk,tinkerbe,jellybea,reader,redsox1,1215,1112,arcadia,baggio,555666,cayman,cbr900rr,gabriell,glennwei,sausages,disco,pass1,lovebug,macmac,puffin,vanguard,trinitro,airwolf,aaa111,cocaine,cisco,datsun,bricks,bumper,eldorado,kidrock,wizard1,whiskers,wildwood,istheman,25802580,bigones,woodland,wolfpac,strawber,3030,sheba1,sixpack,peace1,physics,tigger2,toad,megan1,meow,ringo,amsterdam,717171,686868,5424,canuck,football1,footjob,fulham,seagull,orgy,lobo,mancity,vancouve,vauxhall,acidburn,derf,myspace1,boozer,buttercu,hola,minemine,munch,1dragon,biology,bestbuy,bigpoppa,blackout,blowfish,bmw325,bigbob,stream,talisman,tazz,sundevil,3333333,skate,shutup,shanghai,spencer1,slowhand,pinky1,tootie,thecrow,jubilee,jingle,matrix1,manowar,messiah,resident,redbaron,romans,andromed,athlon,beach1,badgers,guitars,harald,harddick,gotribe,6996,7grout,5wr2i7h8,635241,chase1,fallout,fiddle,fenris,francesc,fortuna,fairlane,felix1,gasman,fucks,sahara,sassy1,dogpound,dogbert,divx1,manila,pornporn,quasar,venom,987987,access1,clippers,daman,crusty,nathan1,nnnnnnnn,bruno1,budapest,kittens,kerouac,mother1,waldo1,whistler,whatwhat,wanderer,idontkno,1942,1946,bigdawg,bigpimp,zaqwsx,414141,3000gt,434343,serpent,smurf,pasword,thisisit,john1,robotics,redeye,rebelz,1011,alatam,asians,bama,banzai,harvest,575757,5329,fatty,fender1,flower2,funky,sambo,drummer1,dogcat,oedipus,osama,prozac,private1,rampage,concord,cinema,cornwall,cleaner,ciccio,clutch,corvet07,daemon,bruiser,boiler,hjkl,egghead,mordor,jamess,iverson3,bluesman,zouzou,090909,1002,stone1,4040,sexo,smith1,sperma,sneaky,polska,thewho,terminat,krypton,lekker,johnson1,johann,rockie,aspire,goodie,cheese1,fenway,fishon,fishin,fuckoff1,girls1,doomsday,pornking,ramones,rabbits,transit,aaaaa1,boyz,bookworm,bongo,bunnies,buceta,highbury,henry1,eastern,mischief,mopar,ministry,vienna,wildone,bigbooty,beavis1,xxxxxx1,yogibear,000001,0815,zulu,420000,sigmar,sprout,stalin,lkjhgfds,lagnaf,rolex,redfox,referee,123123123,1231,angus1,ballin,attila,greedy,grunt,747474,carpedie,caramel,foxylady,gatorade,futbol,frosch,saiyan,drums,donner,doggy1,drum,doudou,nutmeg,quebec,valdepen,tosser,tuscl,comein,cola,deadpool,bremen,hotass,hotmail1,eskimo,eggman,koko,kieran,katrin,kordell1,komodo,mone,munich,vvvvvvvv,jackson5,2222222,bergkamp,bigben,zanzibar,xxx123,sunny1,373737,slayer1,snoop,peachy,thecure,little1,jennaj,rasta69,1114,aries,havana,gratis,calgary,checkers,flanker,salope,dirty1,draco,dogface,luv2epus,rainbow6,qwerty123,umpire,turnip,vbnm,tucson,troll,codered,commande,neon,nico,nightwin,boomer1,bushido,hotmail0,enternow,keepout,karen1,mnbv,viewsoni,volcom,wizards,1995,berkeley,woodstoc,tarpon,shinobi,starstar,phat,toolbox,julien,johnny1,joebob,riders,reflex,120676,1235,angelus,anthrax,atlas,grandam,harlem,hawaii50,655321,cabron,challeng,callisto,firewall,firefire,flyer,flower1,gambler,frodo1,sam123,scania,dingo,papito,passmast,ou8123,randy1,twiggy,travis1,treetop,addict,admin1,963852,aceace,cirrus,bobdole,bonjovi,bootsy,boater,elway7,kenny1,moonshin,montag,wayne1,white1,jazzy,jakejake,1994,1991,2828,bluejays,belmont,sensei,southpark,peeper,pharao,pigpen,tomahawk,teensex,leedsutd,jeepster,jimjim,josephin,melons,matthias,robocop,1003,1027,antelope,azsxdc,gordo,hazard,granada,8989,7894,ceasar,cabernet,cheshire,chelle,candy1,fergie,fidelio,giorgio,fuckhead,dominion,qawsed,trucking,chloe1,daddyo,nostromo,boyboy,booster,bucky,honolulu,esquire,dynamite,mollydog,windows1,waffle,wealth,vincent1,jabber,jaguars,javelin,irishman,idefix,bigdog1,blue42,blanked,blue32,biteme1,bearcats,yessir,sylveste,sunfire,tbird,stryker,3ip76k2,sevens,pilgrim,tenchi,titman,leeds,lithium,linkin,marijuan,mariner,markie,midnite,reddwarf,1129,123asd,12312312,allstar,albany,asdf12,aspen,hardball,goldfing,7734,49ers,carnage,callum,carlos1,fitter,fandango,gofast,gamma,fucmy69,scrapper,dogwood,django,magneto,premium,9999999,abc1234,newyear,bookie,bounty,brown1,bologna,elway,killjoy,klondike,mouser,wayer,impreza,insomnia,24682468,2580,24242424,billbill,bellaco,blues1,blunts,teaser,sf49ers,shovel,solitude,spikey,pimpdadd,timeout,toffee,lefty,johndoe,johndeer,mega,manolo,ratman,robin1,1124,1210,1028,1226,babylove,barbados,gramma,646464,carpente,chaos1,fishbone,fireblad,frogs,screamer,scuba1,ducks,doggies,dicky,obsidian,rams,tottenham,aikman,comanche,corolla,cumslut,cyborg,boston1,houdini,helmut,elvisp,keksa12,monty1,wetter,watford,wiseguy,1989,1987,20202020,biatch,beezer,bigguns,blueball,bitchy,wyoming,yankees2,wrestler,stupid1,sealteam,sidekick,simple1,smackdow,sporting,spiral,smeller,plato,tophat,test2,toomuch,jello,junkie,maxim,maxime,meadow,remingto,roofer,124038,1018,1269,1227,123457,arkansas,aramis,beaker,barcelona,baltimor,googoo,goochi,852456,4711,catcher,champ1,fortress,fishfish,firefigh,geezer,rsalinas,samuel1,saigon,scooby1,dick1,doom,dontknow,magpies,manfred,vader1,universa,tulips,mygirl,bowtie,holycow,honeys,enforcer,waterboy,1992,23skidoo,bimbo,blue11,birddog,zildjian,030303,stinker,stoppedby,sexybabe,speakers,slugger,spotty,smoke1,polopolo,perfect1,torpedo,lakeside,jimmys,junior1,masamune,1214,april1,grinch,767676,5252,cherries,chipmunk,cezer121,carnival,capecod,finder,fearless,goats,funstuff,gideon,savior,seabee,sandro,schalke,salasana,disney1,duckman,pancake,pantera1,malice,love123,qwert123,tracer,creation,cwoui,nascar24,hookers,erection,ericsson,edthom,kokoko,kokomo,mooses,inter,1michael,1993,19781978,25252525,shibby,shamus,skibum,sheepdog,sex69,spliff,slipper,spoons,spanner,snowbird,toriamos,temp123,tennesse,lakers1,jomama,mazdarx7,recon,revolver,1025,1101,barney1,babycake,gotham,gravity,hallowee,616161,515000,caca,cannabis,chilli,fdsa,getout,fuck69,gators1,sable,rumble,dolemite,dork,duffer,dodgers1,onions,logger,lookout,magic32,poon,twat,coventry,citroen,civicsi,cocksucker,coochie,compaq1,nancy1,buzzer,boulder,butkus,bungle,hogtied,hotgirls,heidi1,eggplant,mustang6,monkey12,wapapapa,wendy1,volleyba,vibrate,blink,birthday4,xxxxx1,stephen1,suburban,sheeba,start1,soccer10,starcraft,soccer12,peanut1,plastics,penthous,peterbil,tetsuo,torino,tennis1,termite,lemmein,lakewood,jughead,melrose,megane,redone,angela1,goodgirl,gonzo1,golden1,gotyoass,656565,626262,capricor,chains,calvin1,getmoney,gabber,runaway,salami,dungeon,dudedude,opus,paragon,panhead,pasadena,opendoor,odyssey,magellan,printing,prince1,trustme,nono,buffet,hound,kajak,killkill,moto,winner1,vixen,whiteboy,versace,voyager1,indy,jackjack,bigal,beech,biggun,blake1,blue99,big1,synergy,success1,336699,sixty9,shark1,simba1,sebring,spongebo,spunk,springs,sliver,phialpha,password9,pizza1,pookey,tickling,lexingky,lawman,joe123,mike123,romeo1,redheads,apple123,backbone,aviation,green123,carlitos,byebye,cartman1,camden,chewy,camaross,favorite6,forumwp,ginscoot,fruity,sabrina1,devil666,doughnut,pantie,oldone,paintball,lumina,rainbow1,prosper,umbrella,ajax,951753,achtung,abc12345,compact,corndog,deerhunt,darklord,dank,nimitz,brandy1,hetfield,holein1,hillbill,hugetits,evolutio,kenobi,whiplash,wg8e3wjf,istanbul,invis,1996,bigjohn,bluebell,beater,benji,bluejay,xyzzy,suckdick,taichi,stellar,shaker,semper,splurge,squeak,pearls,playball,pooky,titfuck,joemama,johnny5,marcello,maxi,rhubarb,ratboy,reload,1029,1030,1220,bbking,baritone,gryphon,57chevy,494949,celeron,fishy,gladiator,fucker1,roswell,dougie,dicker,diva,donjuan,nympho,racers,truck1,trample,acer,cricket1,climax,denmark,cuervo,notnow,nittany,neutron,bosco1,buffa,breaker,hello2,hydro,kisskiss,kittys,montecar,modem,mississi,20012001,bigdick1,benfica,yahoo1,striper,tabasco,supra,383838,456654,seneca,shuttle,penguin1,pathfind,testibil,thethe,jeter2,marma,mark1,metoo,republic,rollin,redleg,redbone,redskin,1245,anthony7,altoids,barley,asswipe,bauhaus,bbbbbb1,gohome,harrier,golfpro,goldeney,818181,6666666,5000,5rxypn,cameron1,checker,calibra,freefree,faith1,fdm7ed,giraffe,giggles,fringe,scamper,rrpass1,screwyou,dimples,pacino,ontario,passthie,oberon,quest1,postov1000,puppydog,puffer,qwerty7,tribal,adam25,a1234567,collie,cleopatr,davide,namaste,buffalo1,bonovox,bukkake,burner,bordeaux,burly,hun999,enters,mohawk,vgirl,jayden,1812,1943,222333,bigjim,bigd,zoom,wordup,ziggy1,yahooo,workout,young1,xmas,zzzzzz1,surfer1,strife,sunlight,tasha1,skunk,sprinter,peaches1,pinetree,plum,pimping,theforce,thedon,toocool,laddie,lkjh,jupiter1,matty,redrose,1200,102938,antares,austin31,goose1,737373,78945612,789987,6464,calimero,caster,casper1,cement,chevrolet,chessie,caddy,canucks,fellatio,f00tball,gateway2,gamecube,rugby1,scheisse,dshade,dixie1,offshore,lucas1,macaroni,manga,pringles,puff,trouble1,ussy,coolhand,colonial,colt,darthvad,cygnusx1,natalie1,newark,hiking,errors,elcamino,koolaid,knight1,murphy1,volcano,idunno,2005,2233,blueberr,biguns,yamahar1,zapper,zorro1,0911,3006,sixsix,shopper,sextoy,snowboard,speedway,pokey,playboy2,titi,toonarmy,lambda,joecool,juniper,max123,mariposa,met2002,reggae,ricky1,1236,1228,1016,all4one,baberuth,asgard,484848,5683,6669,catnip,charisma,capslock,cashmone,galant,frenchy,gizmodo1,girlies,screwy,doubled,divers,dte4uw,dragonfl,treble,twinkie,tropical,crescent,cococo,dabomb,daffy,dandfa,cyrano,nathanie,boners,helium,hellas,espresso,killa,kikimora,w4g8at,ilikeit,iforget,1944,20002000,birthday1,beatles1,blue1,bigdicks,beethove,blacklab,blazers,benny1,woodwork,0069,0101,taffy,4567,shodan,pavlov,pinnacle,petunia,tito,teenie,lemonade,lalakers,lebowski,lalalala,ladyboy,jeeper,joyjoy,mercury1,mantle,mannn,rocknrol,riversid,123aaa,11112222,121314,1021,1004,1120,allen1,ambers,amstel,alice1,alleycat,allegro,ambrosia,gspot,goodsex,hattrick,harpoon,878787,8inches,4wwvte,cassandr,charlie123,gatsby,generic,gareth,fuckme2,samm,seadog,satchmo,scxakv,santafe,dipper,outoutout,madmad,london1,qbg26i,pussy123,tzpvaw,vamp,comp,cowgirl,coldplay,dawgs,nt5d27,novifarm,notredam,newness,mykids,bryan1,bouncer,hihihi,honeybee,iceman1,hotlips,dynamo,kappa,kahlua,muffy,mizzou,wannabe,wednesda,whatup,waterfal,willy1,bear1,billabon,youknow,yyyyyy1,zachary1,01234567,070462,zurich,superstar,stiletto,strat,427900,sigmachi,shells,sexy123,smile1,sophie1,stayout,somerset,playmate,pinkfloyd,phish1,payday,thebear,telefon,laetitia,kswbdu,jerky,metro,revoluti,1216,1201,1204,1222,1115,archange,barry1,handball,676767,chewbacc,furball,gocubs,fullback,gman,dewalt,dominiqu,diver1,dhip6a,olemiss,mandrake,mangos,pretzel,pusssy,tripleh,vagabond,clovis,dandan,csfbr5yy,deadspin,ninguna,ncc74656,bootsie,bp2002,bourbon,bumble,heyyou,houston1,hemlock,hippo,hornets,horseman,excess,extensa,muffin1,virginie,werdna,idontknow,jack1,1bitch,151nxjmt,bendover,bmwbmw,zaq123,wxcvbn,supernov,tahoe,shakur,sexyone,seviyi,smart1,speed1,pepito,phantom1,playoffs,terry1,terrier,laser1,lite,lancia,johngalt,jenjen,midori,maserati,matteo,miami1,riffraff,ronald1,1218,1026,123987,1015,1103,armada,architec,austria,gotmilk,cambridg,camero,flex,foreplay,getoff,glacier,glotest,froggie,gerbil,rugger,sanity72,donna1,orchard,oyster,palmtree,pajero,m5wkqf,magenta,luckyone,treefrog,vantage,usmarine,tyvugq,uptown,abacab,aaaaaa1,chuck1,darkange,cyclones,navajo,bubba123,iawgk2,hrfzlz,dylan1,enrico,encore,eclipse1,mutant,mizuno,mustang2,video1,viewer,weed420,whales,jaguar1,1990,159159,1love,bears1,bigtruck,bigboss,blitz,xqgann,yeahyeah,zeke,zardoz,stickman,3825,sentra,shiva,skipper1,singapor,southpaw,sonora,squid,slamdunk,slimjim,placid,photon,placebo,pearl1,test12,therock1,tiger123,leinad,legman,jeepers,joeblow,mike23,redcar,rhinos,rjw7x4,1102,13576479,112211,gwju3g,greywolf,7bgiqk,7878,535353,4snz9g,candyass,cccccc1,catfight,cali,fister,fosters,finland,frankie1,gizzmo,royalty,rugrat,dodo,oemdlg,out3xf,paddy,opennow,puppy1,qazwsxedc,ramjet,abraxas,cn42qj,dancer1,death666,nudity,nimda2k,buick,bobb,braves1,henrik,hooligan,everlast,karachi,mortis,monies,motocros,wally1,willie1,inspiron,1test,2929,bigblack,xytfu7,yackwin,zaq1xsw2,yy5rbfsc,100100,0660,tahiti,takehana,332211,3535,sedona,seawolf,skydiver,spleen,slash,spjfet,special1,slimshad,sopranos,spock1,penis1,patches1,thierry,thething,toohot,limpone,mash4077,matchbox,masterp,maxdog,ribbit,rockin,redhat,1113,14789632,1331,allday,aladin,andrey,amethyst,baseball1,athome,goofy1,greenman,goofball,ha8fyp,goodday,778899,charon,chappy,caracas,cardiff,capitals,canada1,cajun,catter,freddy1,favorite2,forme,forsaken,feelgood,gfxqx686,saskia,sanjose,salsa,dilbert1,dukeduke,downhill,longhair,locutus,lockdown,malachi,mamacita,lolipop,rainyday,pumpkin1,punker,prospect,rambo1,rainbows,quake,trinity1,trooper1,citation,coolcat,default,deniro,d9ungl,daddys,nautica,nermal,bukowski,bubbles1,bogota,buds,hulk,hitachi,ender,export,kikiki,kcchiefs,kram,morticia,montrose,mongo,waqw3p,wizzard,whdbtp,whkzyc,154ugeiu,1fuck,binky,bigred1,blubber,becky1,year2005,wonderfu,xrated,0001,tampabay,survey,tammy1,stuffer,3mpz4r,3000,3some,sierra1,shampoo,shyshy,slapnuts,standby,spartan1,sprocket,stanley1,poker1,theshit,lavalamp,light1,laserjet,jediknig,jjjjj1,mazda626,menthol,margaux,medic1,rhino1,1209,1234321,amigos,apricot,asdfgh1,hairball,hatter,grimace,7xm5rq,6789,cartoons,capcom,cashflow,carrots,fanatic,format,girlie,safeway,dogfart,dondon,outsider,odin,opiate,lollol,love12,mallrats,prague,primetime21,pugsley,r29hqq,valleywa,airman,abcdefg1,darkone,cummer,natedogg,nineball,ndeyl5,natchez,newone,normandy,nicetits,buddy123,buddys,homely,husky,iceland,hr3ytm,highlife,holla,earthlin,exeter,eatmenow,kimkim,k2trix,kernel,money123,moonman,miles1,mufasa,mousey,whites,warhamme,jackass1,2277,20spanks,blobby,blinky,bikers,blackjack,becca,blue23,xman,wyvern,085tzzqi,zxzxzx,zsmj2v,suede,t26gn4,sugars,tantra,swoosh,4226,4271,321123,383pdjvl,shane1,shelby1,spades,smother,sparhawk,pisser,photo1,pebble,peavey,pavement,thistle,kronos,lilbit,linux,melanie1,marbles,redlight,1208,1138,1008,alchemy,aolsucks,alexalex,atticus,auditt,b929ezzh,goodyear,gubber,863abgsg,7474,797979,464646,543210,4zqauf,4949,ch5nmk,carlito,chewey,carebear,checkmat,cheddar,chachi,forgetit,forlife,giants1,getit,gerhard,galileo,g3ujwg,ganja,rufus1,rushmore,discus,dudeman,olympus,oscars,osprey,madcow,locust,loyola,mammoth,proton,rabbit1,ptfe3xxp,pwxd5x,purple1,punkass,prophecy,uyxnyd,tyson1,aircraft,access99,abcabc,colts,civilwar,claudia1,contour,dddddd1,cypher,dapzu455,daisydog,noles,hoochie,hoser,eldiablo,kingrich,mudvayne,motown,mp8o6d,vipergts,italiano,2055,2211,bloke,blade1,yamato,zooropa,yqlgr667,050505,zxcvbnm1,zw6syj,suckcock,tango1,swampy,445566,333666,380zliki,sexpot,sexylady,sixtynin,sickboy,spiffy,skylark,sparkles,pintail,phreak,teller,timtim,thighs,latex,letsdoit,lkjhg,landmark,lizzard,marlins,marauder,metal1,manu,righton,1127,alain,alcat,amigo,basebal1,azertyui,azrael,hamper,gotenks,golfgti,hawkwind,h2slca,grace1,6chid8,789654,canine,casio,cazzo,cbr900,cabrio,calypso,capetown,feline,flathead,fisherma,flipmode,fungus,g9zns4,giggle,gabriel1,fuck123,saffron,dogmeat,dreamcas,dirtydog,douche,dresden,dickdick,destiny1,pappy,oaktree,luft4,puta,ramada,trumpet1,vcradq,tulip,tracy71,tycoon,aaaaaaa1,conquest,chitown,creepers,cornhole,danman,dada,density,d9ebk7,darth,nirvana1,nestle,brenda1,bonanza,hotspur,hufmqw,electro,erasure,elisabet,etvww4,ewyuza,eric1,kenken,kismet,klaatu,milamber,willi,isacs155,igor,1million,1letmein,x35v8l,yogi,ywvxpz,xngwoj,zippy1,020202,****,stonewal,sentry,sexsexsex,sonysony,smirnoff,star12,solace,star1,pkxe62,pilot1,pommes,paulpaul,tical,tictac,lighthou,lemans,kubrick,letmein22,letmesee,jys6wz,jonesy,jjjjjj1,jigga,redstorm,riley1,14141414,1126,allison1,badboy1,asthma,auggie,hardwood,gumbo,616913,57np39,56qhxs,4mnveh,fatluvr69,fqkw5m,fidelity,feathers,fresno,godiva,gecko,gibson1,gogators,general1,saxman,rowing,sammys,scotts,scout1,sasasa,samoht,dragon69,ducky,dragonball,driller,p3wqaw,papillon,oneone,openit,optimist,longshot,rapier,pussy2,ralphie,tuxedo,undertow,copenhag,delldell,culinary,deltas,mytime,noname,noles1,bucker,bopper,burnout,ibilltes,hihje863,hitter,ekim,espana,eatme69,elpaso,express1,eeeeee1,eatme1,karaoke,mustang5,wellingt,willem,waterski,webcam,jasons,infinite,iloveyou!,jakarta,belair,bigdad,beerme,yoshi,yinyang,x24ik3,063dyjuy,0000007,ztmfcq,stopit,stooges,symow8,strato,2hot4u,skins,shakes,sex1,snacks,softtail,slimed123,pizzaman,tigercat,tonton,lager,lizzy,juju,john123,jesse1,jingles,martian,mario1,rootedit,rochard,redwine,requiem,riverrat,1117,1014,1205,amor,amiga,alpina,atreides,banana1,bahamut,golfman,happines,7uftyx,5432,5353,5151,4747,foxfire,ffvdj474,foreskin,gayboy,gggggg1,gameover,glitter,funny1,scoobydoo,saxophon,dingbat,digimon,omicron,panda1,loloxx,macintos,lululu,lollypop,racer1,queen1,qwertzui,upnfmc,tyrant,trout1,9skw5g,aceman,acls2h,aaabbb,acapulco,aggie,comcast,cloudy,cq2kph,d6o8pm,cybersex,davecole,darian,crumbs,davedave,dasani,mzepab,myporn,narnia,booger1,bravo1,budgie,btnjey,highlander,hotel6,humbug,ewtosi,kristin1,kobe,knuckles,keith1,katarina,muff,muschi,montana1,wingchun,wiggle,whatthe,vette1,vols,virago,intj3a,ishmael,jachin,illmatic,199999,2010,blender,bigpenis,bengal,blue1234,zaqxsw,xray,xxxxxxx1,zebras,yanks,tadpole,stripes,3737,4343,3728,4444444,368ejhih,solar,sonne,sniffer,sonata,squirts,playstation,pktmxr,pescator,texaco,lesbos,l8v53x,jo9k2jw2,jimbeam,jimi,jupiter2,jurassic,marines1,rocket1,14725836,12345679,1219,123098,1233,alessand,althor,arch,alpha123,basher,barefeet,balboa,bbbbb1,badabing,gopack,golfnut,gsxr1000,gregory1,766rglqy,8520,753159,8dihc6,69camaro,666777,cheeba,chino,cheeky,camel1,fishcake,flubber,gianni,gnasher23,frisbee,fuzzy1,fuzzball,save13tx,russell1,sandra1,scrotum,scumbag,sabre,samdog,dripping,dragon12,dragster,orwell,mainland,maine,qn632o,poophead,rapper,porn4life,rapunzel,velocity,vanessa1,trueblue,vampire1,abacus,902100,crispy,chooch,d6wnro,dabulls,dehpye,navyseal,njqcw4,nownow,nigger1,nightowl,nonenone,nightmar,bustle,buddy2,boingo,bugman,bosshog,hybrid,hillside,hilltop,hotlegs,hzze929b,hhhhh1,hellohel,evilone,edgewise,e5pftu,eded,embalmer,excalibur,elefant,kenzie,killah,kleenex,mouses,mounta1n,motors,mutley,muffdive,vivitron,w00t88,iloveit,jarjar,incest,indycar,17171717,1664,17011701,222777,2663,beelch,benben,yitbos,yyyyy1,zzzzz1,stooge,tangerin,taztaz,stewart1,summer69,system1,surveyor,stirling,3qvqod,3way,456321,sizzle,simhrq,sparty,ssptx452,sphere,persian,ploppy,pn5jvw,poobear,pianos,plaster,testme,tiff,thriller,master12,rockey,1229,1217,1478,1009,anastasi,amonra,argentin,albino,azazel,grinder,6uldv8,83y6pv,8888888,4tlved,515051,carsten,flyers88,ffffff1,firehawk,firedog,flashman,ggggg1,godspeed,galway,giveitup,funtimes,gohan,giveme,geryfe,frenchie,sayang,rudeboy,sandals,dougal,drag0n,dga9la,desktop,onlyone,otter,pandas,mafia,luckys,lovelife,manders,qqh92r,qcmfd454,radar1,punani,ptbdhw,turtles,undertaker,trs8f7,ugejvp,abba,911turbo,acdc,abcd123,crash1,colony,delboy,davinci,notebook,nitrox,borabora,bonzai,brisbane,heeled,hooyah,hotgirl,i62gbq,horse1,hpk2qc,epvjb6,mnbvc,mommy1,munster,wiccan,2369,bettyboo,blondy,bismark,beanbag,bjhgfi,blackice,yvtte545,ynot,yess,zlzfrh,wolvie,007bond,******,tailgate,tanya1,sxhq65,stinky1,3234412,3ki42x,seville,shimmer,sienna,shitshit,skillet,sooners1,solaris,smartass,pedros,pennywis,pfloyd,tobydog,thetruth,letme1n,mario66,micky,rocky2,rewq,reindeer,1128,1207,1104,1432,aprilia,allstate,bagels,baggies,barrage,guru,72d5tn,606060,4wcqjn,chance1,flange,fartman,geil,gbhcf2,fussball,fuaqz4,gameboy,geneviev,rotary,seahawk,saab,samadams,devlt4,ditto,drevil,drinker,deuce,dipstick,octopus,ottawa,losangel,loverman,porky,q9umoz,rapture,pussy4me,triplex,ue8fpw,turbos,aaa340,churchil,crazyman,cutiepie,ddddd1,dejavu,cuxldv,nbvibt,nikon,niko,nascar1,bubba2,boobear,boogers,bullwink,bulldawg,horsemen,escalade,eagle2,dynamic,efyreg,minnesot,mogwai,msnxbi,mwq6qlzo,werder,verygood,voodoo1,iiiiii1,159951,1624,1911a1,2244,bellagio,bedlam,belkin,bill1,xirt2k,??????,susieq,sundown,sukebe,swifty,2fast4u,sexe,shroom,seaweed,skeeter1,snicker,spanky1,spook,phaedrus,pilots,peddler,thumper1,tiger7,tmjxn151,thematri,l2g7k3,letmeinn,jeffjeff,johnmish,mantra,mike69,mazda6,riptide,robots,1107,1130,142857,11001001,1134,armored,allnight,amatuers,bartok,astral,baboon,balls1,bassoon,hcleeb,happyman,granite,graywolf,golf1,gomets,8vjzus,7890,789123,8uiazp,5757,474jdvff,551scasi,50cent,camaro1,cherry1,chemist,firenze,fishtank,freewill,glendale,frogfrog,ganesh,scirocco,devilman,doodles,okinawa,olympic,orpheus,ohmygod,paisley,pallmall,lunchbox,manhatta,mahalo,mandarin,qwqwqw,qguvyt,pxx3eftp,rambler,poppy1,turk182,vdlxuc,tugboat,valiant,uwrl7c,chris123,cmfnpu,decimal,debbie1,dandy,daedalus,natasha1,nissan1,nancy123,nevermin,napalm,newcastle,bonghit,ibxnsm,hhhhhh1,holger,edmonton,equinox,dvader,kimmy,knulla,mustafa,monsoon,mistral,morgana,monica1,mojave,monterey,mrbill,vkaxcs,victor1,violator,vfdhif,wilson1,wavpzt,wildstar,winter99,iqzzt580,imback,1914,19741974,1monkey,1q2w3e4r5t,2500,2255,bigshow,bigbucks,blackcoc,zoomer,wtcacq,wobble,xmen,xjznq5,yesterda,yhwnqc,zzzxxx,393939,2fchbg,skinhead,skilled,shadow12,seaside,sinful,silicon,smk7366,snapshot,sniper1,soccer11,smutty,peepers,plokij,pdiddy,pimpdaddy,thrust,terran,topaz,today1,lionhear,littlema,lauren1,lincoln1,lgnu9d,juneau,methos,rogue1,romulus,redshift,1202,1469,12locked,arizona1,alfarome,al9agd,aol123,altec,apollo1,arse,baker1,bbb747,axeman,astro1,hawthorn,goodfell,hawks1,gstring,hannes,8543852,868686,4ng62t,554uzpad,5401,567890,5232,catfood,fire1,flipflop,fffff1,fozzie,fluff,fzappa,rustydog,scarab,satin,ruger,samsung1,destin,diablo2,dreamer1,detectiv,doqvq3,drywall,paladin1,papabear,offroad,panasonic,nyyankee,luetdi,qcfmtz,pyf8ah,puddles,pussyeat,ralph1,princeto,trivia,trewq,tri5a3,advent,9898,agyvorc,clarkie,coach1,courier,christo,chowder,cyzkhw,davidb,dad2ownu,daredevi,de7mdf,nazgul,booboo1,bonzo,butch1,huskers1,hgfdsa,hornyman,elektra,england1,elodie,kermit1,kaboom,morten,mocha,monday1,morgoth,weewee,weenie,vorlon,wahoo,ilovegod,insider,jayman,1911,1dallas,1900,1ranger,201jedlz,2501,1qaz,bignuts,bigbad,beebee,billows,belize,wvj5np,wu4etd,yamaha1,wrinkle5,zebra1,yankee1,zoomzoom,09876543,0311,?????,stjabn,tainted,3tmnej,skooter,skelter,starlite,spice1,stacey1,smithy,pollux,peternorth,pixie,piston,poets,toons,topspin,kugm7b,legends,jeepjeep,joystick,junkmail,jojojojo,jonboy,midland,mayfair,riches,reznor,rockrock,reboot,renee1,roadway,rasta220,1411,1478963,1019,archery,andyandy,barks,bagpuss,auckland,gooseman,hazmat,gucci,grammy,happydog,7kbe9d,7676,6bjvpe,5lyedn,5858,5291,charlie2,c7lrwu,candys,chateau,ccccc1,cardinals,fihdfv,fortune12,gocats,gaelic,fwsadn,godboy,gldmeo,fx3tuo,fubar1,generals,gforce,rxmtkp,rulz,sairam,dunhill,dogggg,ozlq6qwm,ov3ajy,lockout,makayla,macgyver,mallorca,prima,pvjegu,qhxbij,prelude1,totoro,tusymo,trousers,tulane,turtle1,tracy1,aerosmit,abbey1,clticic,cooper1,comets,delpiero,cyprus,dante1,dave1,nounours,nexus6,nogard,norfolk,brent1,booyah,bootleg,bulls23,bulls1,booper,heretic,icecube,hellno,hounds,honeydew,hooters1,hoes,hevnm4,hugohugo,epson,evangeli,eeeee1,eyphed".split(","))),aM("english",aK("you,i,to,the,a,and,that,it,of,me,what,is,in,this,know,i'm,for,no,have,my,don't,just,not,do,be,on,your,was,we,it's,with,so,but,all,well,are,he,oh,about,right,you're,get,here,out,going,like,yeah,if,her,she,can,up,want,think,that's,now,go,him,at,how,got,there,one,did,why,see,come,good,they,really,as,would,look,when,time,will,okay,back,can't,mean,tell,i'll,from,hey,were,he's,could,didn't,yes,his,been,or,something,who,because,some,had,then,say,ok,take,an,way,us,little,make,need,gonna,never,we're,too,she's,i've,sure,them,more,over,our,sorry,where,what's,let,thing,am,maybe,down,man,has,uh,very,by,there's,should,anything,said,much,any,life,even,off,doing,thank,give,only,thought,help,two,talk,people,god,still,wait,into,find,nothing,again,things,let's,doesn't,call,told,great,before,better,ever,night,than,away,first,believe,other,feel,everything,work,you've,fine,home,after,last,these,day,keep,does,put,around,stop,they're,i'd,guy,isn't,always,listen,wanted,mr,guys,huh,those,big,lot,happened,thanks,won't,trying,kind,wrong,through,talking,made,new,being,guess,hi,care,bad,mom,remember,getting,we'll,together,dad,leave,place,understand,wouldn't,actually,hear,baby,nice,father,else,stay,done,wasn't,their,course,might,mind,every,enough,try,hell,came,someone,you'll,own,family,whole,another,house,yourself,idea,ask,best,must,coming,old,looking,woman,which,years,room,left,knew,tonight,real,son,hope,name,same,went,um,hmm,happy,pretty,saw,girl,sir,show,friend,already,saying,next,three,job,problem,minute,found,world,thinking,haven't,heard,honey,matter,myself,couldn't,exactly,having,ah,probably,happen,we've,hurt,boy,both,while,dead,gotta,alone,since,excuse,start,kill,hard,you'd,today,car,ready,until,without,wants,hold,wanna,yet,seen,deal,took,once,gone,called,morning,supposed,friends,head,stuff,most,used,worry,second,part,live,truth,school,face,forget,true,business,each,cause,soon,knows,few,telling,wife,who's,use,chance,run,move,anyone,person,bye,somebody,dr,heart,such,miss,married,point,later,making,meet,anyway,many,phone,reason,damn,lost,looks,bring,case,turn,wish,tomorrow,kids,trust,check,change,end,late,anymore,five,least,town,aren't,ha,working,year,makes,taking,means,brother,play,hate,ago,says,beautiful,gave,fact,crazy,party,sit,open,afraid,between,important,rest,fun,kid,word,watch,glad,everyone,days,sister,minutes,everybody,bit,couple,whoa,either,mrs,feeling,daughter,wow,gets,asked,under,break,promise,door,set,close,hand,easy,question,tried,far,walk,needs,mine,though,times,different,killed,hospital,anybody,alright,wedding,shut,able,die,perfect,stand,comes,hit,story,ya,mm,waiting,dinner,against,funny,husband,almost,pay,answer,four,office,eyes,news,child,shouldn't,half,side,yours,moment,sleep,read,where's,started,men,sounds,sonny,pick,sometimes,em,bed,also,date,line,plan,hours,lose,hands,serious,behind,inside,high,ahead,week,wonderful,fight,past,cut,quite,number,he'll,sick,it'll,game,eat,nobody,goes,along,save,seems,finally,lives,worried,upset,carly,met,book,brought,seem,sort,safe,living,children,weren't,leaving,front,shot,loved,asking,running,clear,figure,hot,felt,six,parents,drink,absolutely,how's,daddy,alive,sense,meant,happens,special,bet,blood,ain't,kidding,lie,full,meeting,dear,seeing,sound,fault,water,ten,women,buy,months,hour,speak,lady,jen,thinks,christmas,body,order,outside,hang,possible,worse,company,mistake,ooh,handle,spend,totally,giving,control,here's,marriage,realize,president,unless,sex,send,needed,taken,died,scared,picture,talked,ass,hundred,changed,completely,explain,playing,certainly,sign,boys,relationship,loves,hair,lying,choice,anywhere,future,weird,luck,she'll,turned,known,touch,kiss,crane,questions,obviously,wonder,pain,calling,somewhere,throw,straight,cold,fast,words,food,none,drive,feelings,they'll,worked,marry,light,drop,cannot,sent,city,dream,protect,twenty,class,surprise,its,sweetheart,poor,looked,mad,except,gun,y'know,dance,takes,appreciate,especially,situation,besides,pull,himself,hasn't,act,worth,sheridan,amazing,top,given,expect,rather,involved,swear,piece,busy,law,decided,happening,movie,we'd,catch,country,less,perhaps,step,fall,watching,kept,darling,dog,win,air,honor,personal,moving,till,admit,problems,murder,he'd,evil,definitely,feels,information,honest,eye,broke,missed,longer,dollars,tired,evening,human,starting,red,entire,trip,club,niles,suppose,calm,imagine,fair,caught,blame,street,sitting,favor,apartment,court,terrible,clean,learn,works,frasier,relax,million,accident,wake,prove,smart,message,missing,forgot,interested,table,nbsp,become,mouth,pregnant,middle,ring,careful,shall,team,ride,figured,wear,shoot,stick,follow,angry,instead,write,stopped,early,ran,war,standing,forgive,jail,wearing,kinda,lunch,cristian,eight,greenlee,gotten,hoping,phoebe,thousand,ridge,paper,tough,tape,state,count,boyfriend,proud,agree,birthday,seven,they've,history,share,offer,hurry,feet,wondering,decision,building,ones,finish,voice,herself,would've,list,mess,deserve,evidence,cute,dress,interesting,hotel,quiet,concerned,road,staying,beat,sweetie,mention,clothes,finished,fell,neither,mmm,fix,respect,spent,prison,attention,holding,calls,near,surprised,bar,keeping,gift,hadn't,putting,dark,self,owe,using,ice,helping,normal,aunt,lawyer,apart,certain,plans,jax,girlfriend,floor,whether,everything's,present,earth,box,cover,judge,upstairs,sake,mommy,possibly,worst,station,acting,accept,blow,strange,saved,conversation,plane,mama,yesterday,lied,quick,lately,stuck,report,difference,rid,store,she'd,bag,bought,doubt,listening,walking,cops,deep,dangerous,buffy,sleeping,chloe,rafe,shh,record,lord,moved,join,card,crime,gentlemen,willing,window,return,walked,guilty,likes,fighting,difficult,soul,joke,favorite,uncle,promised,public,bother,island,seriously,cell,lead,knowing,broken,advice,somehow,paid,losing,push,helped,killing,usually,earlier,boss,beginning,liked,innocent,doc,rules,cop,learned,thirty,risk,letting,speaking,officer,ridiculous,support,afternoon,born,apologize,seat,nervous,across,song,charge,patient,boat,how'd,hide,detective,planning,nine,huge,breakfast,horrible,age,awful,pleasure,driving,hanging,picked,sell,quit,apparently,dying,notice,congratulations,chief,one's,month,visit,could've,c'mon,letter,decide,double,sad,press,forward,fool,showed,smell,seemed,spell,memory,pictures,slow,seconds,hungry,board,position,hearing,roz,kitchen,ma'am,force,fly,during,space,should've,realized,experience,kick,others,grab,mother's,discuss,third,cat,fifty,responsible,fat,reading,idiot,yep,suddenly,agent,destroy,bucks,track,shoes,scene,peace,arms,demon,low,livvie,consider,papers,medical,incredible,witch,drunk,attorney,tells,knock,ways,gives,department,nose,skye,turns,keeps,jealous,drug,sooner,cares,plenty,extra,tea,won,attack,ground,whose,outta,weekend,matters,wrote,type,father's,gosh,opportunity,impossible,books,waste,pretend,named,jump,eating,proof,complete,slept,career,arrest,breathe,perfectly,warm,pulled,twice,easier,goin,dating,suit,romantic,drugs,comfortable,finds,checked,fit,divorce,begin,ourselves,closer,ruin,although,smile,laugh,treat,god's,fear,what'd,guy's,otherwise,excited,mail,hiding,cost,stole,pacey,noticed,fired,excellent,lived,bringing,pop,bottom,note,sudden,bathroom,flight,honestly,sing,foot,games,remind,bank,charges,witness,finding,places,tree,dare,hardly,that'll,interest,steal,silly,contact,teach,shop,plus,colonel,fresh,trial,invited,roll,radio,reach,heh,choose,emergency,dropped,credit,obvious,cry,locked,loving,positive,nuts,agreed,prue,goodbye,condition,guard,fuckin,grow,cake,mood,dad's,total,crap,crying,belong,lay,partner,trick,pressure,ohh,arm,dressed,cup,lies,bus,taste,neck,south,something's,nurse,raise,lots,carry,group,whoever,drinking,they'd,breaking,file,lock,wine,closed,writing,spot,paying,study,assume,asleep,man's,turning,legal,viki,bedroom,shower,nikolas,camera,fill,reasons,forty,bigger,nope,breath,doctors,pants,level,movies,gee,area,folks,ugh,continue,focus,wild,truly,desk,convince,client,threw,band,hurts,spending,allow,grand,answers,shirt,chair,allowed,rough,doin,sees,government,ought,empty,round,hat,wind,shows,aware,dealing,pack,meaning,hurting,ship,subject,guest,mom's,pal,match,arrested,salem,confused,surgery,expecting,deacon,unfortunately,goddamn,lab,passed,bottle,beyond,whenever,pool,opinion,held,common,starts,jerk,secrets,falling,played,necessary,barely,dancing,health,tests,copy,cousin,planned,dry,ahem,twelve,simply,tess,skin,often,fifteen,speech,names,issue,orders,nah,final,results,code,believed,complicated,umm,research,nowhere,escape,biggest,restaurant,grateful,usual,burn,address,within,someplace,screw,everywhere,train,film,regret,goodness,mistakes,details,responsibility,suspect,corner,hero,dumb,terrific,further,gas,whoo,hole,memories,o'clock,following,ended,nobody's,teeth,ruined,split,airport,bite,stenbeck,older,liar,showing,project,cards,desperate,themselves,pathetic,damage,spoke,quickly,scare,marah,afford,vote,settle,mentioned,due,stayed,rule,checking,tie,hired,upon,heads,concern,blew,natural,alcazar,champagne,connection,tickets,happiness,form,saving,kissing,hated,personally,suggest,prepared,build,leg,onto,leaves,downstairs,ticket,it'd,taught,loose,holy,staff,sea,duty,convinced,throwing,defense,kissed,legs,according,loud,practice,saturday,babies,army,where'd,warning,miracle,carrying,flying,blind,ugly,shopping,hates,someone's,sight,bride,coat,account,states,clearly,celebrate,brilliant,wanting,add,forrester,lips,custody,center,screwed,buying,size,toast,thoughts,student,stories,however,professional,reality,birth,lexie,attitude,advantage,grandfather,sami,sold,opened,grandma,beg,changes,someday,grade,roof,brothers,signed,ahh,marrying,powerful,grown,grandmother,fake,opening,expected,eventually,must've,ideas,exciting,covered,familiar,bomb,bout,television,harmony,color,heavy,schedule,records,capable,practically,including,correct,clue,forgotten,immediately,appointment,social,nature,deserves,threat,bloody,lonely,ordered,shame,local,jacket,hook,destroyed,scary,investigation,above,invite,shooting,port,lesson,criminal,growing,caused,victim,professor,followed,funeral,nothing's,considering,burning,strength,loss,view,gia,sisters,everybody's,several,pushed,written,somebody's,shock,pushing,heat,chocolate,greatest,miserable,corinthos,nightmare,brings,zander,character,became,famous,enemy,crash,chances,sending,recognize,healthy,boring,feed,engaged,percent,headed,lines,treated,purpose,knife,rights,drag,san,fan,badly,hire,paint,pardon,built,behavior,closet,warn,gorgeous,milk,survive,forced,operation,offered,ends,dump,rent,remembered,lieutenant,trade,thanksgiving,rain,revenge,physical,available,program,prefer,baby's,spare,pray,disappeared,aside,statement,sometime,meat,fantastic,breathing,laughing,itself,tip,stood,market,affair,ours,depends,main,protecting,jury,national,brave,large,jack's,interview,fingers,murdered,explanation,process,picking,based,style,pieces,blah,assistant,stronger,aah,pie,handsome,unbelievable,anytime,nearly,shake,everyone's,oakdale,cars,wherever,serve,pulling,points,medicine,facts,waited,lousy,circumstances,stage,disappointed,weak,trusted,license,nothin,community,trash,understanding,slip,cab,sounded,awake,friendship,stomach,weapon,threatened,mystery,official,regular,river,vegas,understood,contract,race,basically,switch,frankly,issues,cheap,lifetime,deny,painting,ear,clock,weight,garbage,why'd,tear,ears,dig,selling,setting,indeed,changing,singing,tiny,particular,draw,decent,avoid,messed,filled,touched,score,people's,disappear,exact,pills,kicked,harm,recently,fortune,pretending,raised,insurance,fancy,drove,cared,belongs,nights,shape,lorelai,base,lift,stock,sonny's,fashion,timing,guarantee,chest,bridge,woke,source,patients,theory,original,burned,watched,heading,selfish,oil,drinks,failed,period,doll,committed,elevator,freeze,noise,exist,science,pair,edge,wasting,sat,ceremony,pig,uncomfortable,peg,guns,staring,files,bike,weather,name's,mostly,stress,permission,arrived,thrown,possibility,example,borrow,release,ate,notes,hoo,library,property,negative,fabulous,event,doors,screaming,xander,term,what're,meal,fellow,apology,anger,honeymoon,wet,bail,parking,non,protection,fixed,families,chinese,campaign,map,wash,stolen,sensitive,stealing,chose,lets,comfort,worrying,whom,pocket,mateo,bleeding,students,shoulder,ignore,fourth,neighborhood,fbi,talent,tied,garage,dies,demons,dumped,witches,training,rude,crack,model,bothering,radar,grew,remain,soft,meantime,gimme,connected,kinds,cast,sky,likely,fate,buried,hug,brother's,concentrate,prom,messages,east,unit,intend,crew,ashamed,somethin,manage,guilt,weapons,terms,interrupt,guts,tongue,distance,conference,treatment,shoe,basement,sentence,purse,glasses,cabin,universe,towards,repeat,mirror,wound,travers,tall,reaction,odd,engagement,therapy,letters,emotional,runs,magazine,jeez,decisions,soup,daughter's,thrilled,society,managed,stake,chef,moves,extremely,entirely,moments,expensive,counting,shots,kidnapped,square,son's,cleaning,shift,plate,impressed,smells,trapped,male,tour,aidan,knocked,charming,attractive,argue,puts,whip,language,embarrassed,settled,package,laid,animals,hitting,disease,bust,stairs,alarm,pure,nail,nerve,incredibly,walks,dirt,stamp,sister's,becoming,terribly,friendly,easily,damned,jobs,suffering,disgusting,stopping,deliver,riding,helps,federal,disaster,bars,dna,crossed,rate,create,trap,claim,california,talks,eggs,effect,chick,threatening,spoken,introduce,confession,embarrassing,bags,impression,gate,year's,reputation,attacked,among,knowledge,presents,inn,europe,chat,suffer,argument,talkin,crowd,homework,fought,coincidence,cancel,accepted,rip,pride,solve,hopefully,pounds,pine,mate,illegal,generous,streets,con,separate,outfit,maid,bath,punch,mayor,freaked,begging,recall,enjoying,bug,woman's,prepare,parts,wheel,signal,direction,defend,signs,painful,yourselves,rat,maris,amount,that'd,suspicious,flat,cooking,button,warned,sixty,pity,parties,crisis,coach,row,yelling,leads,awhile,pen,confidence,offering,falls,image,farm,pleased,panic,hers,gettin,role,refuse,determined,hell's,grandpa,progress,testify,passing,military,choices,uhh,gym,cruel,wings,bodies,mental,gentleman,coma,cutting,proteus,guests,girl's,expert,benefit,faces,cases,led,jumped,toilet,secretary,sneak,mix,firm,halloween,agreement,privacy,dates,anniversary,smoking,reminds,pot,created,twins,swing,successful,season,scream,considered,solid,options,commitment,senior,ill,else's,crush,ambulance,wallet,discovered,officially,til,rise,reached,eleven,option,laundry,former,assure,stays,skip,fail,accused,wide,challenge,popular,learning,discussion,clinic,plant,exchange,betrayed,bro,sticking,university,members,lower,bored,mansion,soda,sheriff,suite,handled,busted,senator,load,happier,younger,studying,romance,procedure,ocean,section,sec,commit,assignment,suicide,minds,swim,ending,bat,yell,llanview,league,chasing,seats,proper,command,believes,humor,hopes,fifth,winning,solution,leader,theresa's,sale,lawyers,nor,material,latest,highly,escaped,audience,parent,tricks,insist,dropping,cheer,medication,higher,flesh,district,routine,century,shared,sandwich,handed,false,beating,appear,warrant,family's,awfully,odds,article,treating,thin,suggesting,fever,sweat,silent,specific,clever,sweater,request,prize,mall,tries,mile,fully,estate,union,sharing,assuming,judgment,goodnight,divorced,despite,surely,steps,jet,confess,math,listened,comin,answered,vulnerable,bless,dreaming,rooms,chip,zero,potential,pissed,nate,kills,tears,knees,chill,carly's,brains,agency,harvard,degree,unusual,wife's,joint,packed,dreamed,cure,covering,newspaper,lookin,coast,grave,egg,direct,cheating,breaks,quarter,mixed,locker,husband's,gifts,awkward,toy,thursday,rare,policy,kid's,joking,competition,classes,assumed,reasonable,dozen,curse,quartermaine,millions,dessert,rolling,detail,alien,served,delicious,closing,vampires,released,ancient,wore,value,tail,secure,salad,murderer,hits,toward,spit,screen,offense,dust,conscience,bread,answering,admitted,lame,invitation,grief,smiling,path,stands,bowl,pregnancy,hollywood,prisoner,delivery,guards,virus,shrink,influence,freezing,concert,wreck,partners,massimo,chain,birds,life's,wire,technically,presence,blown,anxious,cave,version,holidays,cleared,wishes,survived,caring,candles,bound,related,charm,yup,pulse,jumping,jokes,frame,boom,vice,performance,occasion,silence,opera,nonsense,frightened,downtown,americans,slipped,dimera,blowing,world's,session,relationships,kidnapping,actual,spin,civil,roxy,packing,education,blaming,wrap,obsessed,fruit,torture,personality,location,effort,daddy's,commander,trees,there'll,owner,fairy,per,other's,necessarily,county,contest,seventy,print,motel,fallen,directly,underwear,grams,exhausted,believing,particularly,freaking,carefully,trace,touching,messing,committee,recovery,intention,consequences,belt,sacrifice,courage,officers,enjoyed,lack,attracted,appears,bay,yard,returned,remove,nut,carried,today's,testimony,intense,granted,violence,heal,defending,attempt,unfair,relieved,political,loyal,approach,slowly,plays,normally,buzz,alcohol,actor,surprises,psychiatrist,pre,plain,attic,who'd,uniform,terrified,sons,pet,cleaned,zach,threaten,teaching,mum,motion,fella,enemies,desert,collection,incident,failure,satisfied,imagination,hooked,headache,forgetting,counselor,andie,acted,opposite,highest,equipment,badge,italian,visiting,naturally,frozen,commissioner,sakes,labor,appropriate,trunk,armed,thousands,received,dunno,costume,temporary,sixteen,impressive,zone,kicking,junk,hon,grabbed,unlike,understands,describe,clients,owns,affect,witnesses,starving,instincts,happily,discussing,deserved,strangers,leading,intelligence,host,authority,surveillance,cow,commercial,admire,questioning,fund,dragged,barn,object,deeply,amp,wrapped,wasted,tense,route,reports,hoped,fellas,election,roommate,mortal,fascinating,chosen,stops,shown,arranged,abandoned,sides,delivered,becomes,arrangements,agenda,began,theater,series,literally,propose,honesty,underneath,forces,services,sauce,promises,lecture,eighty,torn,shocked,relief,explained,counter,circle,victims,transfer,response,channel,identity,differently,campus,spy,ninety,interests,guide,deck,biological,pheebs,ease,creep,will's,waitress,skills,telephone,ripped,raising,scratch,rings,prints,wave,thee,arguing,figures,ephram,asks,reception,pin,oops,diner,annoying,agents,taggert,goal,mass,ability,sergeant,julian's,international,gig,blast,basic,tradition,towel,earned,rub,president's,habit,customers,creature,bermuda,actions,snap,react,prime,paranoid,wha,handling,eaten,therapist,comment,charged,tax,sink,reporter,beats,priority,interrupting,gain,fed,warehouse,shy,pattern,loyalty,inspector,events,pleasant,media,excuses,threats,permanent,guessing,financial,demand,assault,tend,praying,motive,los,unconscious,trained,museum,tracks,range,nap,mysterious,unhappy,tone,switched,rappaport,award,sookie,neighbor,loaded,gut,childhood,causing,swore,piss,hundreds,balance,background,toss,mob,misery,valentine's,thief,squeeze,lobby,hah,goa'uld,geez,exercise,ego,drama,al's,forth,facing,booked,boo,songs,sandburg,eighteen,d'you,bury,perform,everyday,digging,creepy,compared,wondered,trail,liver,hmmm,drawn,device,magical,journey,fits,discussed,supply,moral,helpful,attached,timmy's,searching,flew,depressed,aisle,underground,pro,daughters,cris,amen,vows,proposal,pit,neighbors,darn,cents,arrange,annulment,uses,useless,squad,represent,product,joined,afterwards,adventure,resist,protected,net,fourteen,celebrating,piano,inch,flag,debt,violent,tag,sand,gum,dammit,teal'c,hip,celebration,below,reminded,claims,tonight's,replace,phones,paperwork,emotions,typical,stubborn,stable,sheridan's,pound,papa,lap,designed,current,bum,tension,tank,suffered,steady,provide,overnight,meanwhile,chips,beef,wins,suits,boxes,salt,cassadine,collect,boy's,tragedy,therefore,spoil,realm,profile,degrees,wipe,surgeon,stretch,stepped,nephew,neat,limo,confident,anti,perspective,designer,climb,title,suggested,punishment,finest,ethan's,springfield,occurred,hint,furniture,blanket,twist,surrounded,surface,proceed,lip,fries,worries,refused,niece,gloves,soap,signature,disappoint,crawl,convicted,zoo,result,pages,lit,flip,counsel,doubts,crimes,accusing,when's,shaking,remembering,phase,hallway,halfway,bothered,useful,makeup,madam,gather,concerns,cia,cameras,blackmail,symptoms,rope,ordinary,imagined,concept,cigarette,supportive,memorial,explosion,yay,woo,trauma,ouch,leo's,furious,cheat,avoiding,whew,thick,oooh,boarding,approve,urgent,shhh,misunderstanding,minister,drawer,sin,phony,joining,jam,interfere,governor,chapter,catching,bargain,tragic,schools,respond,punish,penthouse,hop,thou,remains,rach,ohhh,insult,doctor's,bugs,beside,begged,absolute,strictly,stefano,socks,senses,ups,sneaking,yah,serving,reward,polite,checks,tale,physically,instructions,fooled,blows,tabby,internal,bitter,adorable,y'all,tested,suggestion,string,jewelry,debate,com,alike,pitch,fax,distracted,shelter,lessons,foreign,average,twin,friend's,damnit,constable,circus,audition,tune,shoulders,mud,mask,helpless,feeding,explains,dated,robbery,objection,behave,valuable,shadows,courtroom,confusing,tub,talented,struck,smarter,mistaken,italy,customer,bizarre,scaring,punk,motherfucker,holds,focused,alert,activity,vecchio,reverend,highway,foolish,compliment,bastards,attend,scheme,aid,worker,wheelchair,protective,poetry,gentle,script,reverse,picnic,knee,intended,construction,cage,wednesday,voices,toes,stink,scares,pour,effects,cheated,tower,time's,slide,ruining,recent,jewish,filling,exit,cottage,corporate,upside,supplies,proves,parked,instance,grounds,diary,complaining,basis,wounded,thing's,politics,confessed,pipe,merely,massage,data,chop,budget,brief,spill,prayer,costs,betray,begins,arrangement,waiter,scam,rats,fraud,flu,brush,anyone's,adopted,tables,sympathy,pill,pee,web,seventeen,landed,expression,entrance,employee,drawing,cap,bracelet,principal,pays,jen's,fairly,facility,dru,deeper,arrive,unique,tracking,spite,shed,recommend,oughta,nanny,naive,menu,grades,diet,corn,authorities,separated,roses,patch,dime,devastated,description,tap,subtle,include,citizen,bullets,beans,ric,pile,las,executive,confirm,toe,strings,parade,harbor,charity's,bow,borrowed,toys,straighten,steak,status,remote,premonition,poem,planted,honored,youth,specifically,meetings,exam,convenient,traveling,matches,laying,insisted,apply,units,technology,dish,aitoro,sis,kindly,grandson,donor,temper,teenager,strategy,richard's,proven,iron,denial,couples,backwards,tent,swell,noon,happiest,episode,drives,thinkin,spirits,potion,fence,affairs,acts,whatsoever,rehearsal,proved,overheard,nuclear,lemme,hostage,faced,constant,bench,tryin,taxi,shove,sets,moron,limits,impress,entitled,needle,limit,lad,intelligent,instant,forms,disagree,stinks,rianna,recover,paul's,losers,groom,gesture,developed,constantly,blocks,bartender,tunnel,suspects,sealed,removed,legally,illness,hears,dresses,aye,vehicle,thy,teachers,sheet,receive,psychic,night's,denied,knocking,judging,bible,behalf,accidentally,waking,ton,superior,seek,rumor,natalie's,manners,homeless,hollow,desperately,critical,theme,tapes,referring,personnel,item,genoa,gear,majesty,fans,exposed,cried,tons,spells,producer,launch,instinct,belief,quote,motorcycle,convincing,appeal,advance,greater,fashioned,aids,accomplished,mommy's,grip,bump,upsetting,soldiers,scheduled,production,needing,invisible,forgiveness,feds,complex,compare,bothers,tooth,territory,sacred,mon,jessica's,inviting,inner,earn,compromise,cocktail,tramp,temperature,signing,landing,jabot,intimate,dignity,dealt,souls,informed,gods,entertainment,dressing,cigarettes,blessing,billion,alistair,upper,manner,lightning,leak,heaven's,fond,corky,alternative,seduce,players,operate,modern,liquor,fingerprints,enchantment,butters,stuffed,stavros,rome,filed,emotionally,division,conditions,uhm,transplant,tips,passes,oxygen,nicely,lunatic,hid,drill,designs,complain,announcement,visitors,unfortunate,slap,prayers,plug,organization,opens,oath,o'neill,mutual,graduate,confirmed,broad,yacht,spa,remembers,fried,extraordinary,bait,appearance,abuse,warton,sworn,stare,safely,reunion,plot,burst,aha,might've,experiment,dive,commission,cells,aboard,returning,independent,expose,environment,buddies,trusting,smaller,mountains,booze,sweep,sore,scudder,properly,parole,manhattan,effective,ditch,decides,canceled,bra,antonio's,speaks,spanish,reaching,glow,foundation,women's,wears,thirsty,skull,ringing,dorm,dining,bend,unexpected,systems,sob,pancakes,michael's,harsh,flattered,existence,ahhh,troubles,proposed,fights,favourite,eats,driven,computers,rage,luke's,causes,border,undercover,spoiled,sloane,shine,rug,identify,destroying,deputy,deliberately,conspiracy,clothing,thoughtful,similar,sandwiches,plates,nails,miracles,investment,fridge,drank,contrary,beloved,allergic,washed,stalking,solved,sack,misses,hope's,forgiven,erica's,cuz,bent,approval,practical,organized,maciver,involve,industry,fuel,dragging,cooked,possession,pointing,foul,editor,dull,beneath,ages,horror,heels,grass,faking,deaf,stunt,portrait,painted,jealousy,hopeless,fears,cuts,conclusion,volunteer,scenario,satellite,necklace,men's,crashed,chapel,accuse,restraining,jason's,humans,homicide,helicopter,formal,firing,shortly,safer,devoted,auction,videotape,tore,stores,reservations,pops,appetite,anybody's,wounds,vanquish,symbol,prevent,patrol,ironic,flow,fathers,excitement,anyhow,tearing,sends,sam's,rape,laughed,function,core,charmed,whatever's,sub,lucy's,dealer,cooperate,bachelor,accomplish,wakes,struggle,spotted,sorts,reservation,ashes,yards,votes,tastes,supposedly,loft,intentions,integrity,wished,towels,suspected,slightly,qualified,log,investigating,inappropriate,immediate,companies,backed,pan,owned,lipstick,lawn,compassion,cafeteria,belonged,affected,scarf,precisely,obsession,management,loses,lighten,jake's,infection,granddaughter,explode,chemistry,balcony,this'll,storage,spying,publicity,exists,employees,depend,cue,cracked,conscious,aww,ally,ace,accounts,absurd,vicious,tools,strongly,rap,invented,forbid,directions,defendant,bare,announce,alcazar's,screwing,salesman,robbed,leap,lakeview,insanity,injury,genetic,document,why's,reveal,religious,possibilities,kidnap,gown,entering,chairs,wishing,statue,setup,serial,punished,dramatic,dismissed,criminals,seventh,regrets,raped,quarters,produce,lamp,dentist,anyways,anonymous,added,semester,risks,regarding,owes,magazines,machines,lungs,explaining,delicate,child's,tricked,oldest,liv,eager,doomed,cafe,bureau,adoption,traditional,surrender,stab,sickness,scum,loop,independence,generation,floating,envelope,entered,combination,chamber,worn,vault,sorel,pretended,potatoes,plea,photograph,payback,misunderstood,kiddo,healing,cascade,capeside,application,stabbed,remarkable,cabinet,brat,wrestling,sixth,scale,privilege,passionate,nerves,lawsuit,kidney,disturbed,crossing,cozy,associate,tire,shirts,required,posted,oven,ordering,mill,journal,gallery,delay,clubs,risky,nest,monsters,honorable,grounded,favour,culture,closest,brenda's,breakdown,attempted,tony's,placed,conflict,bald,actress,abandon,steam,scar,pole,duh,collar,worthless,standards,resources,photographs,introduced,injured,graduation,enormous,disturbing,disturb,distract,deals,conclusions,vodka,situations,require,mid,measure,dishes,crawling,congress,children's,briefcase,wiped,whistle,sits,roast,rented,pigs,greek,flirting,existed,deposit,damaged,bottles,vanessa's,types,topic,riot,overreacting,minimum,logical,impact,hostile,embarrass,casual,beacon,amusing,altar,values,recognized,maintain,goods,covers,claus,battery,survival,skirt,shave,prisoners,porch,med,ghosts,favors,drops,dizzy,chili,begun,beaten,advise,transferred,strikes,rehab,raw,photographer,peaceful,leery,heavens,fortunately,fooling,expectations,draft,citizens,weakness,ski,ships,ranch,practicing,musical,movement,individual,homes,executed,examine,documents,cranes,column,bribe,task,species,sail,rum,resort,prescription,operating,hush,fragile,forensics,expense,drugged,differences,cows,conduct,comic,bells,avenue,attacking,assigned,visitor,suitcase,sources,sorta,scan,payment,motor,mini,manticore,inspired,insecure,imagining,hardest,clerk,yea,wrist,what'll,tube,starters,silk,pump,pale,nicer,haul,flies,demands,boot,arts,african,there'd,limited,how're,elders,connections,quietly,pulls,idiots,factor,erase,denying,attacks,ankle,amnesia,accepting,ooo,heartbeat,gal,devane,confront,backing,phrase,operations,minus,meets,legitimate,hurricane,fixing,communication,boats,auto,arrogant,supper,studies,slightest,sins,sayin,recipe,pier,paternity,humiliating,genuine,catholic,snack,rational,pointed,minded,guessed,grace's,display,dip,brooke's,advanced,weddings,unh,tumor,teams,reported,humiliated,destruction,copies,closely,bid,aspirin,academy,wig,throughout,spray,occur,logic,eyed,equal,drowning,contacts,shakespeare,ritual,perfume,kelly's,hiring,hating,generally,error,elected,docks,creatures,visions,thanking,thankful,sock,replaced,nineteen,nick's,fork,comedy,analysis,yale,throws,teenagers,studied,stressed,slice,rolls,requires,plead,ladder,kicks,detectives,assured,alison's,widow,tomorrow's,tissue,tellin,shallow,responsibilities,repay,rejected,permanently,girlfriends,deadly,comforting,ceiling,bonus,verdict,maintenance,jar,insensitive,factory,aim,triple,spilled,respected,recovered,messy,interrupted,halliwell,car's,bleed,benefits,wardrobe,takin,significant,objective,murders,doo,chart,backs,workers,waves,underestimate,ties,registered,multiple,justify,harmless,frustrated,fold,enzo,convention,communicate,bugging,attraction,arson,whack,salary,rumors,residence,party's,obligation,medium,liking,laura's,development,develop,dearest,david's,danny's,congratulate,vengeance,switzerland,severe,rack,puzzle,puerto,guidance,fires,courtesy,caller,blamed,tops,repair,quiz,prep,now's,involves,headquarters,curiosity,codes,circles,barbecue,troops,sunnydale,spinning,scores,pursue,psychotic,cough,claimed,accusations,shares,resent,money's,laughs,gathered,freshman,envy,drown,cristian's,bartlet,asses,sofa,scientist,poster,islands,highness,dock,apologies,welfare,victor's,theirs,stat,stall,spots,somewhat,ryan's,realizes,psych,fools,finishing,album,wee,understandable,unable,treats,theatre,succeed,stir,relaxed,makin,inches,gratitude,faithful,bin,accent,zip,witter,wandering,regardless,que,locate,inevitable,gretel,deed,crushed,controlling,taxes,smelled,settlement,robe,poet,opposed,marked,greenlee's,gossip,gambling,determine,cuba,cosmetics,cent,accidents,surprising,stiff,sincere,shield,rushed,resume,reporting,refrigerator,reference,preparing,nightmares,mijo,ignoring,hunch,fog,fireworks,drowned,crown,cooperation,brass,accurate,whispering,sophisticated,religion,luggage,investigate,hike,explore,emotion,creek,crashing,contacted,complications,ceo,acid,shining,rolled,righteous,reconsider,inspiration,goody,geek,frightening,festival,ethics,creeps,courthouse,camping,assistance,affection,vow,smythe,protest,lodge,haircut,forcing,essay,chairman,baked,apologized,vibe,respects,receipt,mami,includes,hats,exclusive,destructive,define,defeat,adore,adopt,voted,tracked,signals,shorts,rory's,reminding,relative,ninth,floors,dough,creations,continues,cancelled,cabot,barrel,adam's,snuck,slight,reporters,rear,pressing,novel,newspapers,magnificent,madame,lazy,glorious,fiancee,candidate,brick,bits,australia,activities,visitation,scholarship,sane,previous,kindness,ivy's,shoulda,rescued,mattress,maria's,lounge,lifted,label,importantly,glove,enterprises,driver's,disappointment,condo,cemetery,beings,admitting,yelled,waving,screech,satisfaction,requested,reads,plants,nun,nailed,described,dedicated,certificate,centuries,annual,worm,tick,resting,primary,polish,marvelous,fuss,funds,defensive,cortlandt,compete,chased,provided,pockets,luckily,lilith,filing,depression,conversations,consideration,consciousness,worlds,innocence,indicate,grandmother's,forehead,bam,appeared,aggressive,trailer,slam,retirement,quitting,pry,person's,narrow,levels,kay's,inform,encourage,dug,delighted,daylight,danced,currently,confidential,billy's,ben's,aunts,washing,vic,tossed,spectra,rick's,permit,marrow,lined,implying,hatred,grill,efforts,corpse,clues,sober,relatives,promotion,offended,morgue,larger,infected,humanity,eww,emily's,electricity,electrical,distraction,cart,broadcast,wired,violation,suspended,promising,harassment,glue,gathering,d'angelo,cursed,controlled,calendar,brutal,assets,warlocks,wagon,unpleasant,proving,priorities,observation,mustn't,lease,grows,flame,domestic,disappearance,depressing,thrill,sitter,ribs,offers,naw,flush,exception,earrings,deadline,corporal,collapsed,update,snapped,smack,orleans,offices,melt,figuring,delusional,coulda,burnt,actors,trips,tender,sperm,specialist,scientific,realise,pork,popped,planes,kev,interrogation,institution,included,esteem,communications,choosing,choir,undo,pres,prayed,plague,manipulate,lifestyle,insulting,honour,detention,delightful,coffeehouse,chess,betrayal,apologizing,adjust,wrecked,wont,whipped,rides,reminder,psychological,principle,monsieur,injuries,fame,faint,confusion,christ's,bon,bake,nearest,korea,industries,execution,distress,definition,creating,correctly,complaint,blocked,trophy,tortured,structure,rot,risking,pointless,household,heir,handing,eighth,dumping,cups,chloe's,alibi,absence,vital,tokyo,thus,struggling,shiny,risked,refer,mummy,mint,joey's,involvement,hose,hobby,fortunate,fleischman,fitting,curtain,counseling,addition,wit,transport,technical,rode,puppet,opportunities,modeling,memo,irresponsible,humiliation,hiya,freakin,fez,felony,choke,blackmailing,appreciated,tabloid,suspicion,recovering,rally,psychology,pledge,panicked,nursery,louder,jeans,investigator,identified,homecoming,helena's,height,graduated,frustrating,fabric,distant,buys,busting,buff,wax,sleeve,products,philosophy,irony,hospitals,dope,declare,autopsy,workin,torch,substitute,scandal,prick,limb,leaf,lady's,hysterical,growth,goddamnit,fetch,dimension,day's,crowded,clip,climbing,bonding,approved,yeh,woah,ultimately,trusts,returns,negotiate,millennium,majority,lethal,length,iced,deeds,bore,babysitter,questioned,outrageous,medal,kiriakis,insulted,grudge,established,driveway,deserted,definite,capture,beep,wires,suggestions,searched,owed,originally,nickname,lighting,lend,drunken,demanding,costanza,conviction,characters,bumped,weigh,touches,tempted,shout,resolve,relate,poisoned,pip,phoebe's,pete's,occasionally,molly's,meals,maker,invitations,haunted,fur,footage,depending,bogus,autograph,affects,tolerate,stepping,spontaneous,sleeps,probation,presentation,performed,manny,identical,fist,cycle,associates,aaron's,streak,spectacular,sector,lasted,isaac's,increase,hostages,heroin,havin,habits,encouraging,cult,consult,burgers,boyfriends,bailed,baggage,association,wealthy,watches,versus,troubled,torturing,teasing,sweetest,stations,sip,shawn's,rag,qualities,postpone,pad,overwhelmed,malkovich,impulse,hut,follows,classy,charging,barbara's,angel's,amazed,scenes,rising,revealed,representing,policeman,offensive,mug,hypocrite,humiliate,hideous,finals,experiences,d'ya,courts,costumes,captured,bluffing,betting,bein,bedtime,alcoholic,vegetable,tray,suspicions,spreading,splendid,shouting,roots,pressed,nooo,liza's,jew,intent,grieving,gladly,fling,eliminate,disorder,courtney's,cereal,arrives,aaah,yum,technique,statements,sonofabitch,servant,roads,republican,paralyzed,orb,lotta,locks,guaranteed,european,dummy,discipline,despise,dental,corporation,carries,briefing,bluff,batteries,atmosphere,whatta,tux,sounding,servants,rifle,presume,kevin's,handwriting,goals,gin,fainted,elements,dried,cape,allright,allowing,acknowledge,whacked,toxic,skating,reliable,quicker,penalty,panel,overwhelming,nearby,lining,importance,harassing,fatal,endless,elsewhere,dolls,convict,bold,ballet,whatcha,unlikely,spiritual,shutting,separation,recording,positively,overcome,goddam,failing,essence,dose,diagnosis,cured,claiming,bully,airline,ahold,yearbook,various,tempting,shelf,rig,pursuit,prosecution,pouring,possessed,partnership,miguel's,lindsay's,countries,wonders,tsk,thorough,spine,rath,psychiatric,meaningless,latte,jammed,ignored,fiance,exposure,exhibit,evidently,duties,contempt,compromised,capacity,cans,weekends,urge,theft,suing,shipment,scissors,responding,refuses,proposition,noises,matching,located,ink,hormones,hiv,hail,grandchildren,godfather,gently,establish,crane's,contracts,compound,buffy's,worldwide,smashed,sexually,sentimental,senor,scored,patient's,nicest,marketing,manipulated,jaw,intern,handcuffs,framed,errands,entertaining,discovery,crib,carriage,barge,awards,attending,ambassador,videos,tab,spends,slipping,seated,rubbing,rely,reject,recommendation,reckon,ratings,headaches,float,embrace,corners,whining,sweating,sole,skipped,restore,receiving,population,pep,mountie,motives,mama's,listens,korean,heroes,heart's,cristobel,controls,cheerleader,balsom,unnecessary,stunning,shipping,scent,santa's,quartermaines,praise,pose,montega,luxury,loosen,kyle's,keri's,info,hum,haunt,gracious,git,forgiving,fleet,errand,emperor,cakes,blames,abortion,worship,theories,strict,sketch,shifts,plotting,physician,perimeter,passage,pals,mere,mattered,lonigan,longest,jews,interference,eyewitness,enthusiasm,encounter,diapers,craig's,artists,strongest,shaken,serves,punched,projects,portal,outer,nazi,hal's,colleagues,catches,bearing,backyard,academic,winds,terrorists,sabotage,pea,organs,needy,mentor,measures,listed,lex,cuff,civilization,caribbean,articles,writes,woof,who'll,viki's,valid,rarely,rabbi,prank,performing,obnoxious,mates,improve,hereby,gabby,faked,cellar,whitelighter,void,substance,strangle,sour,skill,senate,purchase,native,muffins,interfering,hoh,gina's,demonic,colored,clearing,civilian,buildings,boutique,barrington,trading,terrace,smoked,seed,righty,relations,quack,published,preliminary,petey,pact,outstanding,opinions,knot,ketchup,items,examined,disappearing,cordy,coin,circuit,assist,administration,walt,uptight,ticking,terrifying,tease,tabitha's,syd,swamp,secretly,rejection,reflection,realizing,rays,pennsylvania,partly,mentally,marone,jurisdiction,frasier's,doubted,deception,crucial,congressman,cheesy,arrival,visited,supporting,stalling,scouts,scoop,ribbon,reserve,raid,notion,income,immune,grandma's,expects,edition,destined,constitution,classroom,bets,appreciation,appointed,accomplice,whitney's,wander,shoved,sewer,scroll,retire,paintings,lasts,fugitive,freezer,discount,cranky,crank,clearance,bodyguard,anxiety,accountant,abby's,whoops,volunteered,terrorist,tales,talents,stinking,resolved,remotely,protocol,livvie's,garlic,decency,cord,beds,asa's,areas,altogether,uniforms,tremendous,restaurants,rank,profession,popping,philadelphia,outa,observe,lung,largest,hangs,feelin,experts,enforcement,encouraged,economy,dudes,donation,disguise,diane's,curb,continued,competitive,businessman,bites,antique,advertising,ads,toothbrush,retreat,represents,realistic,profits,predict,nora's,lid,landlord,hourglass,hesitate,frank's,focusing,equally,consolation,boyfriend's,babbling,aged,troy's,tipped,stranded,smartest,sabrina's,rhythm,replacement,repeating,puke,psst,paycheck,overreacted,macho,leadership,kendall's,juvenile,john's,images,grocery,freshen,disposal,cuffs,consent,caffeine,arguments,agrees,abigail's,vanished,unfinished,tobacco,tin,syndrome,ripping,pinch,missiles,isolated,flattering,expenses,dinners,cos,colleague,ciao,buh,belthazor,belle's,attorneys,amber's,woulda,whereabouts,wars,waitin,visits,truce,tripped,tee,tasted,stu,steer,ruling,poisoning,nursing,manipulative,immature,husbands,heel,granddad,delivering,deaths,condoms,automatically,anchor,trashed,tournament,throne,raining,prices,pasta,needles,leaning,leaders,judges,ideal,detector,coolest,casting,batch,approximately,appointments,almighty,achieve,vegetables,sum,spark,ruled,revolution,principles,perfection,pains,momma,mole,interviews,initiative,hairs,getaway,employment,den,cracking,counted,compliments,behold,verge,tougher,timer,tapped,taped,stakes,specialty,snooping,shoots,semi,rendezvous,pentagon,passenger,leverage,jeopardize,janitor,grandparents,forbidden,examination,communist,clueless,cities,bidding,arriving,adding,ungrateful,unacceptable,tutor,soviet,shaped,serum,scuse,savings,pub,pajamas,mouths,modest,methods,lure,irrational,depth,cries,classified,bombs,beautifully,arresting,approaching,vessel,variety,traitor,sympathetic,smug,smash,rental,prostitute,premonitions,mild,jumps,inventory,ing,improved,grandfather's,developing,darlin,committing,caleb's,banging,asap,amendment,worms,violated,vent,traumatic,traced,tow,swiss,sweaty,shaft,recommended,overboard,literature,insight,healed,grasp,fluid,experiencing,crappy,crab,connecticut,chunk,chandler's,awww,applied,witnessed,traveled,stain,shack,reacted,pronounce,presented,poured,occupied,moms,marriages,jabez,invested,handful,gob,gag,flipped,fireplace,expertise,embarrassment,disappears,concussion,bruises,brakes,anything's,week's,twisting,tide,swept,summon,splitting,settling,scientists,reschedule,regard,purposes,ohio,notch,mike's,improvement,hooray,grabbing,extend,exquisite,disrespect,complaints,colin's,armor,voting,thornhart,sustained,straw,slapped,simon's,shipped,shattered,ruthless,reva's,refill,recorded,payroll,numb,mourning,marijuana,manly,jerry's,involving,hunk,entertain,earthquake,drift,dreadful,doorstep,confirmation,chops,bridget's,appreciates,announced,vague,tires,stressful,stem,stashed,stash,sensed,preoccupied,predictable,noticing,madly,halls,gunshot,embassy,dozens,dinner's,confuse,cleaners,charade,chalk,cappuccino,breed,bouquet,amulet,addiction,who've,warming,unlock,transition,satisfy,sacrificed,relaxing,lone,input,hampshire,girlfriend's,elaborate,concerning,completed,channels,category,cal,blocking,blend,blankets,america's,addicted,yuck,voters,professionals,positions,monica's,mode,initial,hunger,hamburger,greeting,greet,gravy,gram,dreamt,dice,declared,collecting,caution,brady's,backpack,agreeing,writers,whale,tribe,taller,supervisor,sacrifices,radiation,poo,phew,outcome,ounce,missile,meter,likewise,irrelevant,gran,felon,feature,favorites,farther,fade,experiments,erased,easiest,disk,convenience,conceived,compassionate,challenged,cane,blair's,backstage,agony,adores,veins,tweek,thieves,surgical,strangely,stetson,recital,proposing,productive,meaningful,marching,immunity,hassle,goddamned,frighten,directors,dearly,comments,closure,cease,ambition,wisconsin,unstable,sweetness,salvage,richer,refusing,raging,pumping,pressuring,petition,mortals,lowlife,jus,intimidated,intentionally,inspire,forgave,eric's,devotion,despicable,deciding,dash,comfy,breach,bo's,bark,alternate,aaaah,switching,swallowed,stove,slot,screamed,scars,russians,relevant,poof,pipes,persons,pawn,losses,legit,invest,generations,farewell,experimental,difficulty,curtains,civilized,championship,caviar,boost,token,tends,temporarily,superstition,supernatural,sunk,sadness,reduced,recorder,psyched,presidential,owners,motivated,microwave,lands,karen's,hallelujah,gap,fraternity,engines,dryer,cocoa,chewing,additional,acceptable,unbelievably,survivor,smiled,smelling,sized,simpler,sentenced,respectable,remarks,registration,premises,passengers,organ,occasional,khasinau,indication,gutter,grabs,goo,fulfill,flashlight,ellenor,courses,blooded,blessings,beware,beth's,bands,advised,water's,uhhh,turf,swings,slips,shocking,resistance,privately,olivia's,mirrors,lyrics,locking,instrument,historical,heartless,fras,decades,comparison,childish,cassie's,cardiac,admission,utterly,tuscany,ticked,suspension,stunned,statesville,sadly,resolution,reserved,purely,opponent,noted,lowest,kiddin,jerks,hitch,flirt,fare,extension,establishment,equals,dismiss,delayed,decade,christening,casket,c'mere,breakup,brad's,biting,antibiotics,accusation,abducted,witchcraft,whoever's,traded,thread,spelling,so's,school's,runnin,remaining,punching,protein,printed,paramedics,newest,murdering,mine's,masks,lawndale,intact,ins,initials,heights,grampa,democracy,deceased,colleen's,choking,charms,careless,bushes,buns,bummed,accounting,travels,taylor's,shred,saves,saddle,rethink,regards,references,precinct,persuade,patterns,meds,manipulating,llanfair,leash,kenny's,housing,hearted,guarantees,flown,feast,extent,educated,disgrace,determination,deposition,coverage,corridor,burial,bookstore,boil,abilities,vitals,veil,trespassing,teaches,sidewalk,sensible,punishing,overtime,optimistic,occasions,obsessing,oak,notify,mornin,jeopardy,jaffa,injection,hilarious,distinct,directed,desires,curve,confide,challenging,cautious,alter,yada,wilderness,where're,vindictive,vial,tomb,teeny,subjects,stroll,sittin,scrub,rebuild,rachel's,posters,parallel,ordeal,orbit,o'brien,nuns,max's,jennifer's,intimacy,inheritance,fails,exploded,donate,distracting,despair,democratic,defended,crackers,commercials,bryant's,ammunition,wildwind,virtue,thoroughly,tails,spicy,sketches,sights,sheer,shaving,seize,scarecrow,refreshing,prosecute,possess,platter,phillip's,napkin,misplaced,merchandise,membership,loony,jinx,heroic,frankenstein,fag,efficient,devil's,corps,clan,boundaries,attract,ambitious,virtually,syrup,solitary,resignation,resemblance,reacting,pursuing,premature,pod,liz's,lavery,journalist,honors,harvey's,genes,flashes,erm,contribution,company's,client's,cheque,charts,cargo,awright,acquainted,wrapping,untie,salute,ruins,resign,realised,priceless,partying,myth,moonlight,lightly,lifting,kasnoff,insisting,glowing,generator,flowing,explosives,employer,cutie,confronted,clause,buts,breakthrough,blouse,ballistic,antidote,analyze,allowance,adjourned,vet,unto,understatement,tucked,touchy,toll,subconscious,sequence,screws,sarge,roommates,reaches,rambaldi,programs,offend,nerd,knives,kin,irresistible,inherited,incapable,hostility,goddammit,fuse,frat,equation,curfew,centered,blackmailed,allows,alleged,walkin,transmission,text,starve,sleigh,sarcastic,recess,rebound,procedures,pinned,parlor,outfits,livin,issued,institute,industrial,heartache,head's,haired,fundraiser,doorman,documentary,discreet,dilucca,detect,cracks,cracker,considerate,climbed,catering,author,apophis,zoey,vacuum,urine,tunnels,todd's,tanks,strung,stitches,sordid,sark,referred,protector,portion,phoned,pets,paths,mat,lengths,kindergarten,hostess,flaw,flavor,discharge,deveraux,consumed,confidentiality,automatic,amongst,viktor,victim's,tactics,straightened,specials,spaghetti,soil,prettier,powerless,por,poems,playin,playground,parker's,paranoia,nsa,mainly,mac's,joe's,instantly,havoc,exaggerating,evaluation,eavesdropping,doughnuts,diversion,deepest,cutest,companion,comb,bela,behaving,avoided,anyplace,agh,accessory,zap,whereas,translate,stuffing,speeding,slime,polls,personalities,payments,musician,marital,lurking,lottery,journalism,interior,imaginary,hog,guinea,greetings,game's,fairwinds,ethical,equipped,environmental,elegant,elbow,customs,cuban,credibility,credentials,consistent,collapse,cloth,claws,chopped,challenges,bridal,boards,bedside,babysitting,authorized,assumption,ant,youngest,witty,vast,unforgivable,underworld,tempt,tabs,succeeded,sophomore,selfless,secrecy,runway,restless,programming,professionally,okey,movin,metaphor,messes,meltdown,lecter,incoming,hence,gasoline,gained,funding,episodes,diefenbaker,contain,comedian,collected,cam,buckle,assembly,ancestors,admired,adjustment,acceptance,weekly,warmth,throats,seduced,ridge's,reform,rebecca's,queer,poll,parenting,noses,luckiest,graveyard,gifted,footsteps,dimeras,cynical,assassination,wedded,voyage,volunteers,verbal,unpredictable,tuned,stoop,slides,sinking,show's,rio,rigged,regulations,region,promoted,plumbing,lingerie,layer,katie's,hankey,greed,everwood,essential,elope,dresser,departure,dat,dances,coup,chauffeur,bulletin,bugged,bouncing,website,tubes,temptation,supported,strangest,sorel's,slammed,selection,sarcasm,rib,primitive,platform,pending,partial,packages,orderly,obsessive,nevertheless,nbc,murderers,motto,meteor,inconvenience,glimpse,froze,fiber,execute,etc,ensure,drivers,dispute,damages,crop,courageous,consulate,closes,bosses,bees,amends,wuss,wolfram,wacky,unemployed,traces,town's,testifying,tendency,syringe,symphony,stew,startled,sorrow,sleazy,shaky,screams,rsquo,remark,poke,phone's,philip's,nutty,nobel,mentioning,mend,mayor's,iowa,inspiring,impulsive,housekeeper,germans,formed,foam,fingernails,economic,divide,conditioning,baking,whine,thug,starved,sedative,rose's,reversed,publishing,programmed,picket,paged,nowadays,newman's,mines,margo's,invasion,homosexual,homo,hips,forgets,flipping,flea,flatter,dwell,dumpster,consultant,choo,banking,assignments,apartments,ants,affecting,advisor,vile,unreasonable,tossing,thanked,steals,souvenir,screening,scratched,rep,psychopath,proportion,outs,operative,obstruction,obey,neutral,lump,lily's,insists,ian's,harass,gloat,flights,filth,extended,electronic,edgy,diseases,didn,coroner,confessing,cologne,cedar,bruise,betraying,bailing,attempting,appealing,adebisi,wrath,wandered,waist,vain,traps,transportation,stepfather,publicly,presidents,poking,obligated,marshal,lexie's,instructed,heavenly,halt,employed,diplomatic,dilemma,crazed,contagious,coaster,cheering,carved,bundle,approached,appearances,vomit,thingy,stadium,speeches,robbing,reflect,raft,qualify,pumped,pillows,peep,pageant,packs,neo,neglected,m'kay,loneliness,liberal,intrude,indicates,helluva,gardener,freely,forresters,err,drooling,continuing,betcha,alan's,addressed,acquired,vase,supermarket,squat,spitting,spaces,slaves,rhyme,relieve,receipts,racket,purchased,preserve,pictured,pause,overdue,officials,nod,motivation,morgendorffer,lucky's,lacking,kidnapper,introduction,insect,hunters,horns,feminine,eyeballs,dumps,disc,disappointing,difficulties,crock,convertible,context,claw,clamp,canned,cambias,bathtub,avanya,artery,weep,warmer,vendetta,tenth,suspense,summoned,stuff's,spiders,sings,reiber,raving,pushy,produced,poverty,postponed,ohhhh,noooo,mold,mice,laughter,incompetent,hugging,groceries,frequency,fastest,drip,differ,daphne's,communicating,body's,beliefs,bats,bases,auntie,adios,wraps,willingly,weirdest,voila,timmih,thinner,swelling,swat,steroids,sensitivity,scrape,rehearse,quarterback,organic,matched,ledge,justified,insults,increased,heavily,hateful,handles,feared,doorway,decorations,colour,chatting,buyer,buckaroo,bedrooms,batting,askin,ammo,tutoring,subpoena,span,scratching,requests,privileges,pager,mart,kel,intriguing,idiotic,hotels,grape,enlighten,dum,door's,dixie's,demonstrate,dairy,corrupt,combined,brunch,bridesmaid,barking,architect,applause,alongside,ale,acquaintance,yuh,wretched,superficial,sufficient,sued,soak,smoothly,sensing,restraint,quo,pow,posing,pleading,pittsburgh,peru,payoff,participate,organize,oprah,nemo,morals,loans,loaf,lists,laboratory,jumpy,intervention,ignorant,herbal,hangin,germs,generosity,flashing,country's,convent,clumsy,chocolates,captive,bianca's,behaved,apologise,vanity,trials,stumbled,republicans,represented,recognition,preview,poisonous,perjury,parental,onboard,mugged,minding,linen,learns,knots,interviewing,inmates,ingredients,humour,grind,greasy,goons,estimate,elementary,edmund's,drastic,database,coop,comparing,cocky,clearer,bruised,brag,bind,axe,asset,apparent,ann's,worthwhile,whoop,wedding's,vanquishing,tabloids,survivors,stenbeck's,sprung,spotlight,shops,sentencing,sentences,revealing,reduce,ram,racist,provoke,piper's,pining,overly,oui,ops,mop,louisiana,locket,king's,jab,imply,impatient,hovering,hotter,fest,endure,dots,doren,dim,diagnosed,debts,cultures,crawled,contained,condemned,chained,brit,breaths,adds,weirdo,warmed,wand,utah,troubling,tok'ra,stripped,strapped,soaked,skipping,sharon's,scrambled,rattle,profound,musta,mocking,mnh,misunderstand,merit,loading,linked,limousine,kacl,investors,interviewed,hustle,forensic,foods,enthusiastic,duct,drawers,devastating,democrats,conquer,concentration,comeback,clarify,chores,cheerleaders,cheaper,charlie's,callin,blushing,barging,abused,yoga,wrecking,wits,waffles,virginity,vibes,uninvited,unfaithful,underwater,tribute,strangled,state's,scheming,ropes,responded,residents,rescuing,rave,priests,postcard,overseas,orientation,ongoing,o'reily,newly,neil's,morphine,lotion,limitations,lesser,lectures,lads,kidneys,judgement,jog,itch,intellectual,installed,infant,indefinitely,grenade,glamorous,genetically,freud,faculty,engineering,doh,discretion,delusions,declaration,crate,competent,commonwealth,catalog,bakery,attempts,asylum,argh,applying,ahhhh,yesterday's,wedge,wager,unfit,tripping,treatments,torment,superhero,stirring,spinal,sorority,seminar,scenery,repairs,rabble,pneumonia,perks,owl,override,ooooh,moo,mija,manslaughter,mailed,love's,lime,lettuce,intimidate,instructor,guarded,grieve,grad,globe,frustration,extensive,exploring,exercises,eve's,doorbell,devices,deal's,dam,cultural,ctu,credits,commerce,chinatown,chemicals,baltimore,authentic,arraignment,annulled,altered,allergies,wanta,verify,vegetarian,tunes,tourist,tighter,telegram,suitable,stalk,specimen,spared,solving,shoo,satisfying,saddam,requesting,publisher,pens,overprotective,obstacles,notified,negro,nasedo,judged,jill's,identification,grandchild,genuinely,founded,flushed,fluids,floss,escaping,ditched,demon's,decorated,criticism,cramp,corny,contribute,connecting,bunk,bombing,bitten,billions,bankrupt,yikes,wrists,ultrasound,ultimatum,thirst,spelled,sniff,scope,ross's,room's,retrieve,releasing,reassuring,pumps,properties,predicted,neurotic,negotiating,needn't,multi,monitors,millionaire,microphone,mechanical,lydecker,limp,incriminating,hatchet,gracias,gordie,fills,feeds,egypt,doubting,dedication,decaf,dawson's,competing,cellular,biopsy,whiz,voluntarily,visible,ventilator,unpack,unload,universal,tomatoes,targets,suggests,strawberry,spooked,snitch,schillinger,sap,reassure,providing,prey,pressure's,persuasive,mystical,mysteries,mri,moment's,mixing,matrimony,mary's,mails,lighthouse,liability,kgb,jock,headline,frankie's,factors,explosive,explanations,dispatch,detailed,curly,cupid,condolences,comrade,cassadines,bulb,brittany's,bragging,awaits,assaulted,ambush,adolescent,adjusted,abort,yank,whit,verse,vaguely,undermine,tying,trim,swamped,stitch,stan's,stabbing,slippers,skye's,sincerely,sigh,setback,secondly,rotting,rev,retail,proceedings,preparation,precaution,pox,pcpd,nonetheless,melting,materials,mar,liaison,hots,hooking,headlines,hag,ganz,fury,felicity,fangs,expelled,encouragement,earring,dreidel,draws,dory,donut,dog's,dis,dictate,dependent,decorating,coordinates,cocktails,bumps,blueberry,believable,backfired,backfire,apron,anticipated,adjusting,activated,vous,vouch,vitamins,vista,urn,uncertain,ummm,tourists,tattoos,surrounding,sponsor,slimy,singles,sibling,shhhh,restored,representative,renting,reign,publish,planets,peculiar,parasite,paddington,noo,marries,mailbox,magically,lovebirds,listeners,knocks,kane's,informant,grain,exits,elf,drazen,distractions,disconnected,dinosaurs,designing,dashwood,crooked,conveniently,contents,argued,wink,warped,underestimated,testified,tacky,substantial,steve's,steering,staged,stability,shoving,seizure,reset,repeatedly,radius,pushes,pitching,pairs,opener,mornings,mississippi,matthew's,mash,investigations,invent,indulge,horribly,hallucinating,festive,eyebrows,expand,enjoys,dictionary,dialogue,desperation,dealers,darkest,daph,critic,consulting,cartman's,canal,boragora,belts,bagel,authorization,auditions,associated,ape,amy's,agitated,adventures,withdraw,wishful,wimp,vehicles,vanish,unbearable,tonic,tom's,tackle,suffice,suction,slaying,singapore,safest,rosanna's,rocking,relive,rates,puttin,prettiest,oval,noisy,newlyweds,nauseous,moi,misguided,mildly,midst,maps,liable,kristina's,judgmental,introducing,individuals,hunted,hen,givin,frequent,fisherman,fascinated,elephants,dislike,diploma,deluded,decorate,crummy,contractions,carve,careers,bottled,bonded,bahamas,unavailable,twenties,trustworthy,translation,traditions,surviving,surgeons,stupidity,skies,secured,salvation,remorse,rafe's,princeton,preferably,pies,photography,operational,nuh,northwest,nausea,napkins,mule,mourn,melted,mechanism,mashed,julia's,inherit,holdings,hel,greatness,golly,excused,edges,dumbo,drifting,delirious,damaging,cubicle,compelled,comm,colleges,cole's,chooses,checkup,chad's,certified,candidates,boredom,bob's,bandages,baldwin's,bah,automobile,athletic,alarms,absorbed,absent,windshield,who're,whaddya,vitamin,transparent,surprisingly,sunglasses,starring,slit,sided,schemes,roar,relatively,reade,quarry,prosecutor,prognosis,probe,potentially,pitiful,persistent,perception,percentage,peas,oww,nosy,neighbourhood,nagging,morons,molecular,meters,masterpiece,martinis,limbo,liars,jax's,irritating,inclined,hump,hoynes,haw,gauge,functions,fiasco,educational,eatin,donated,destination,dense,cubans,continent,concentrating,commanding,colorful,clam,cider,brochure,behaviour,barto,bargaining,awe,artistic,welcoming,weighing,villain,vein,vanquished,striking,stains,sooo,smear,sire,simone's,secondary,roughly,rituals,resentment,psychologist,preferred,pint,pension,passive,overhear,origin,orchestra,negotiations,mounted,morality,landingham,labs,kisser,jackson's,icy,hoot,holling,handshake,grilled,functioning,formality,elevators,edward's,depths,confirms,civilians,bypass,briefly,boathouse,binding,acres,accidental,westbridge,wacko,ulterior,transferring,tis,thugs,tangled,stirred,stefano's,sought,snag,smallest,sling,sleaze,seeds,rumour,ripe,remarried,reluctant,regularly,puddle,promote,precise,popularity,pins,perceptive,miraculous,memorable,maternal,lucinda's,longing,lockup,locals,librarian,job's,inspection,impressions,immoral,hypothetically,guarding,gourmet,gabe,fighters,fees,features,faxed,extortion,expressed,essentially,downright,digest,der,crosses,cranberry,city's,chorus,casualties,bygones,buzzing,burying,bikes,attended,allah,all's,weary,viewing,viewers,transmitter,taping,takeout,sweeping,stepmother,stating,stale,seating,seaborn,resigned,rating,prue's,pros,pepperoni,ownership,occurs,nicole's,newborn,merger,mandatory,malcolm's,ludicrous,jan's,injected,holden's,henry's,heating,geeks,forged,faults,expressing,eddie's,drue,dire,dief,desi,deceiving,centre,celebrities,caterer,calmed,businesses,budge,ashley's,applications,ankles,vending,typing,tribbiani,there're,squared,speculation,snowing,shades,sexist,scudder's,scattered,sanctuary,rewrite,regretted,regain,raises,processing,picky,orphan,mural,misjudged,miscarriage,memorize,marshall's,mark's,licensed,lens,leaking,launched,larry's,languages,judge's,jitters,invade,interruption,implied,illegally,handicapped,glitch,gittes,finer,fewer,engineered,distraught,dispose,dishonest,digs,dahlia's,dads,cruelty,conducting,clinical,circling,champions,canceling,butterflies,belongings,barbrady,amusement,allegations,alias,aging,zombies,where've,unborn,tri,swearing,stables,squeezed,spaulding's,slavery,sew,sensational,revolutionary,resisting,removing,radioactive,races,questionable,privileged,portofino,par,owning,overlook,overhead,orson,oddly,nazis,musicians,interrogate,instruments,imperative,impeccable,icu,hurtful,hors,heap,harley's,graduating,graders,glance,endangered,disgust,devious,destruct,demonstration,creates,crazier,countdown,coffee's,chump,cheeseburger,cat's,burglar,brotherhood,berries,ballroom,assumptions,ark,annoyed,allies,allergy,advantages,admirer,admirable,addresses,activate,accompany,wed,victoria's,valve,underpants,twit,triggered,teacher's,tack,strokes,stool,starr's,sham,seasons,sculpture,scrap,sailed,retarded,resourceful,remarkably,refresh,ranks,pressured,precautions,pointy,obligations,nightclub,mustache,month's,minority,mind's,maui,lace,isabella's,improving,iii,hunh,hubby,flare,fierce,farmers,dont,dokey,divided,demise,demanded,dangerously,crushing,considerable,complained,clinging,choked,chem,cheerleading,checkbook,cashmere,calmly,blush,believer,aspect,amazingly,alas,acute,a's,yak,whores,what've,tuition,trey's,tolerance,toilets,tactical,tacos,stairwell,spur,spirited,slower,sewing,separately,rubbed,restricted,punches,protects,partially,ole,nuisance,niagara,motherfuckers,mingle,mia's,kynaston,knack,kinkle,impose,hosting,harry's,gullible,grid,godmother,funniest,friggin,folding,financially,filming,fashions,eater,dysfunctional,drool,distinguished,defence,defeated,cruising,crude,criticize,corruption,contractor,conceive,clone,circulation,cedars,caliber,brighter,blinded,birthdays,bio,bill's,banquet,artificial,anticipate,annoy,achievement,whim,whichever,volatile,veto,vested,uncle's,supports,successfully,shroud,severely,rests,representation,quarantine,premiere,pleases,parent's,painless,pads,orphans,orphanage,offence,obliged,nip,niggers,negotiation,narcotics,nag,mistletoe,meddling,manifest,lookit,loo,lilah,investigated,intrigued,injustice,homicidal,hayward's,gigantic,exposing,elves,disturbance,disastrous,depended,demented,correction,cooped,colby's,cheerful,buyers,brownies,beverage,basics,attorney's,atm,arvin,arcade,weighs,upsets,unethical,tidy,swollen,sweaters,swap,stupidest,sensation,scalpel,rail,prototype,props,prescribed,pompous,poetic,ploy,paws,operates,objections,mushrooms,mulwray,monitoring,manipulation,lured,lays,lasting,kung,keg,jell,internship,insignificant,inmate,incentive,gandhi,fulfilled,flooded,expedition,evolution,discharged,disagreement,dine,dean's,crypt,coroner's,cornered,copied,confrontation,cds,catalogue,brightest,beethoven,banned,attendant,athlete,amaze,airlines,yogurt,wyndemere,wool,vocabulary,vcr,tulsa,tags,tactic,stuffy,slug,sexuality,seniors,segment,revelation,respirator,pulp,prop,producing,processed,pretends,polygraph,perp,pennies,ordinarily,opposition,olives,necks,morally,martyr,martial,lisa's,leftovers,joints,jimmy's,irs,invaded,imported,hopping,homey,hints,helicopters,heed,heated,heartbroken,gulf,greatly,forge,florist,firsthand,fiend,expanding,emma's,defenses,crippled,cousin's,corrected,conniving,conditioner,clears,chemo,bubbly,bladder,beeper,baptism,apb,answer's,anna's,angles,ache,womb,wiring,wench,weaknesses,volunteering,violating,unlocked,unemployment,tummy,tibet,threshold,surrogate,submarine,subid,stray,stated,startle,specifics,snob,slowing,sled,scoot,robbers,rightful,richest,quid,qfxmjrie,puffs,probable,pitched,pierced,pencils,paralysis,nuke,managing,makeover,luncheon,lords,linksynergy,jury's,jacuzzi,ish,interstate,hitched,historic,hangover,gasp,fracture,flock,firemen,drawings,disgusted,darned,coal,clams,chez,cables,broadcasting,brew,borrowing,banged,achieved,wildest,weirder,unauthorized,stunts,sleeves,sixties,shush,shalt,senora,rises,retro,quits,pupils,politicians,pegged,painfully,paging,outlet,omelet,observed,ned's,memorized,lawfully,jackets,interpretation,intercept,ingredient,grownup,glued,gaining,fulfilling,flee,enchanted,dvd,delusion,daring,conservative,conducted,compelling,charitable,carton,bronx,bridesmaids,bribed,boiling,bathrooms,bandage,awareness,awaiting,assign,arrogance,antiques,ainsley,turkeys,travelling,trashing,tic,takeover,sync,supervision,stockings,stalked,stabilized,spacecraft,slob,skates,sirs,sedated,robes,reviews,respecting,rat's,psyche,prominent,prizes,presumptuous,prejudice,platoon,permitted,paragraph,mush,mum's,movements,mist,missions,mints,mating,mantan,lorne,lord's,loads,listener,legendary,itinerary,hugs,hepatitis,heave,guesses,gender,flags,fading,exams,examining,elizabeth's,egyptian,dumbest,dishwasher,dimera's,describing,deceive,cunning,cripple,cove,convictions,congressional,confided,compulsive,compromising,burglary,bun,bumpy,brainwashed,benes,arnie,alvy,affirmative,adrenaline,adamant,watchin,waitresses,uncommon,treaty,transgenic,toughest,toby's,surround,stormed,spree,spilling,spectacle,soaking,significance,shreds,sewers,severed,scarce,scamming,scalp,sami's,salem's,rewind,rehearsing,pretentious,potions,possessions,planner,placing,periods,overrated,obstacle,notices,nerds,meems,medieval,mcmurphy,maturity,maternity,masses,maneuver,lyin,loathe,lawyer's,irv,investigators,hep,grin,gospel,gals,formation,fertility,facilities,exterior,epidemic,eloping,ecstatic,ecstasy,duly,divorcing,distribution,dignan,debut,costing,coaching,clubhouse,clot,clocks,classical,candid,bursting,breather,braces,bennett's,bending,australian,attendance,arsonist,applies,adored,accepts,absorb,vacant,uuh,uphold,unarmed,turd,topolsky,thrilling,thigh,terminate,tempo,sustain,spaceship,snore,sneeze,smuggling,shrine,sera,scott's,salty,salon,ramp,quaint,prostitution,prof,policies,patronize,patio,nasa,morbid,marlo's,mamma,locations,licence,kettle,joyous,invincible,interpret,insecurities,insects,inquiry,infamous,impulses,illusions,holed,glen's,fragments,forrester's,exploit,economics,drivin,des,defy,defenseless,dedicate,cradle,cpr,coupon,countless,conjure,confined,celebrated,cardboard,booking,blur,bleach,ban,backseat,austin's,alternatives,afterward,accomplishment,wordsworth,wisely,wildlife,valet,vaccine,urges,unnatural,unlucky,truths,traumatized,tit,tennessee,tasting,swears,strawberries,steaks,stats,skank,seducing,secretive,screwdriver,schedules,rooting,rightfully,rattled,qualifies,puppets,provides,prospects,pronto,prevented,powered,posse,poorly,polling,pedestal,palms,muddy,morty,miniature,microscope,merci,margin,lecturing,inject,incriminate,hygiene,hospital's,grapefruit,gazebo,funnier,freight,flooding,equivalent,eliminated,elaine's,dios,deacon's,cuter,continental,container,cons,compensation,clap,cbs,cavity,caves,capricorn,canvas,calculations,bossy,booby,bacteria,aides,zende,winthrop,wider,warrants,valentines,undressed,underage,truthfully,tampered,suffers,stored,statute,speechless,sparkling,sod,socially,sidelines,shrek,sank,roy's,raul's,railing,puberty,practices,pesky,parachute,outrage,outdoors,operated,openly,nominated,motions,moods,lunches,litter,kidnappers,itching,intuition,index,imitation,icky,humility,hassling,gallons,firmly,excessive,evolved,employ,eligible,elections,elderly,drugstore,dosage,disrupt,directing,dipping,deranged,debating,cuckoo,cremated,craziness,cooperating,compatible,circumstantial,chimney,bonnie's,blinking,biscuits,belgium,arise,analyzed,admiring,acquire,accounted,willow's,weeping,volumes,views,triad,trashy,transaction,tilt,soothing,slumber,slayers,skirts,siren,ship's,shindig,sentiment,sally's,rosco,riddance,rewarded,quaid,purity,proceeding,pretzels,practiced,politician,polar,panicking,overall,occupation,naming,minimal,mckechnie,massacre,marah's,lovin,leaked,layers,isolation,intruding,impersonating,ignorance,hoop,hamburgers,gwen's,fruits,footprints,fluke,fleas,festivities,fences,feisty,evacuate,emergencies,diabetes,detained,democrat,deceived,creeping,craziest,corpses,conned,coincidences,charleston,bums,brussels,bounced,bodyguards,blasted,bitterness,baloney,ashtray,apocalypse,advances,zillion,watergate,wallpaper,viable,tory's,tenants,telesave,sympathize,sweeter,swam,sup,startin,stages,spencer's,sodas,snowed,sleepover,signor,seein,reviewing,reunited,retainer,restroom,rested,replacing,repercussions,reliving,reef,reconciliation,reconcile,recognise,prevail,preaching,planting,overreact,oof,omen,o'neil,numerous,noose,moustache,morning's,manicure,maids,mah,lorelei's,landlady,hypothetical,hopped,homesick,hives,hesitation,herbs,hectic,heartbreak,haunting,gangs,frown,fingerprint,extract,expired,exhausting,exchanged,exceptional,everytime,encountered,disregard,daytime,cooperative,constitutional,cling,chevron,chaperone,buenos,blinding,bitty,beads,battling,badgering,anticipation,advocate,zander's,waterfront,upstanding,unprofessional,unity,unhealthy,undead,turmoil,truthful,toothpaste,tippin,thoughtless,tagataya,stretching,strategic,spun,shortage,shooters,sheriff's,shady,senseless,sailors,rewarding,refuge,rapid,rah,pun,propane,pronounced,preposterous,pottery,portable,pigeons,pastry,overhearing,ogre,obscene,novels,negotiable,mtv,morgan's,monthly,loner,leisure,leagues,jogging,jaws,itchy,insinuating,insides,induced,immigration,hospitality,hormone,hilda's,hearst,grandpa's,frequently,forthcoming,fists,fifties,etiquette,endings,elevated,editing,dunk,distinction,disabled,dibs,destroys,despises,desired,designers,deprived,dancers,dah,cuddy,crust,conductor,communists,cloak,circumstance,chewed,casserole,bora,bidder,bearer,assessment,artoo,applaud,appalling,amounts,admissions,withdrawal,weights,vowed,virgins,vigilante,vatican,undone,trench,touchdown,throttle,thaw,tha,testosterone,tailor,symptom,swoop,suited,suitcases,stomp,sticker,stakeout,spoiling,snatched,smoochy,smitten,shameless,restraints,researching,renew,relay,regional,refund,reclaim,rapids,raoul,rags,puzzles,purposely,punks,prosecuted,plaid,pineapple,picturing,pickin,pbs,parasites,offspring,nyah,mysteriously,multiply,mineral,masculine,mascara,laps,kramer's,jukebox,interruptions,hoax,gunfire,gays,furnace,exceptions,engraved,elbows,duplicate,drapes,designated,deliberate,deli,decoy,cub,cryptic,crowds,critics,coupla,convert,conventional,condemn,complicate,combine,colossal,clerks,clarity,cassadine's,byes,brushed,bride's,banished,arrests,argon,andy's,alarmed,worships,versa,uncanny,troop,treasury,transformation,terminated,telescope,technicality,sydney's,sundae,stumble,stripping,shuts,separating,schmuck,saliva,robber,retain,remained,relentless,reconnect,recipes,rearrange,ray's,rainy,psychiatrists,producers,policemen,plunge,plugged,patched,overload,ofc,obtained,obsolete,o'malley,numbered,number's,nay,moth,module,mkay,mindless,menus,lullaby,lotte,leavin,layout,knob,killin,karinsky,irregular,invalid,hides,grownups,griff,flaws,flashy,flaming,fettes,evicted,epic,encoded,dread,dil,degrassi,dealings,dangers,cushion,console,concluded,casey's,bowel,beginnings,barged,apes,announcing,amanda's,admits,abroad,abide,abandoning,workshop,wonderfully,woak,warfare,wait'll,wad,violate,turkish,tim's,ter,targeted,susan's,suicidal,stayin,sorted,slamming,sketchy,shoplifting,shapes,selected,sarah's,retiring,raiser,quizmaster,pursued,pupkin,profitable,prefers,politically,phenomenon,palmer's,olympics,needless,nature's,mutt,motherhood,momentarily,migraine,lizzie's,lilo,lifts,leukemia,leftover,law's,keepin,idol,hinks,hellhole,h'mm,gowns,goodies,gallon,futures,friction,finale,farms,extraction,entertained,electronics,eighties,earth's,dmv,darker,daniel's,cum,conspiring,consequence,cheery,caps,calf,cadet,builds,benign,barney's,aspects,artillery,apiece,allison's,aggression,adjustments,abusive,abduction,wiping,whipping,welles,unspeakable,unlimited,unidentified,trivial,transcripts,threatens,textbook,tenant,supervise,superstitious,stricken,stretched,story's,stimulating,steep,statistics,spielberg,sodium,slices,shelves,scratches,saudi,sabotaged,roxy's,retrieval,repressed,relation,rejecting,quickie,promoting,ponies,peeking,paw,paolo,outraged,observer,o'connell,moping,moaning,mausoleum,males,licked,kovich,klutz,iraq,interrogating,interfered,intensive,insulin,infested,incompetence,hyper,horrified,handedly,hacked,guiding,glamour,geoff,gekko,fraid,fractured,formerly,flour,firearms,fend,executives,examiner,evaluate,eloped,duke's,disoriented,delivers,dashing,crystals,crossroads,crashdown,court's,conclude,coffees,cockroach,climate,chipped,camps,brushing,boulevard,bombed,bolts,begs,baths,baptized,astronaut,assurance,anemia,allegiance,aiming,abuela,abiding,workplace,withholding,weave,wearin,weaker,warnings,usa,tours,thesis,terrorism,suffocating,straws,straightforward,stench,steamed,starboard,sideways,shrinks,shortcut,sean's,scram,roasted,roaming,riviera,respectfully,repulsive,recognizes,receiver,psychiatry,provoked,penitentiary,peed,pas,painkillers,oink,norm,ninotchka,muslim,montgomery's,mitzvah,milligrams,mil,midge,marshmallows,markets,macy's,looky,lapse,kubelik,knit,jeb,investments,intellect,improvise,implant,hometown,hanged,handicap,halo,governor's,goa'ulds,giddy,gia's,geniuses,fruitcake,footing,flop,findings,fightin,fib,editorial,drinkin,doork,discovering,detour,danish,cuddle,crashes,coordinate,combo,colonnade,collector,cheats,cetera,canadians,bip,bailiff,auditioning,assed,amused,alienate,algebra,alexi,aiding,aching,woe,wah,unwanted,typically,tug,topless,tongues,tiniest,them's,symbols,superiors,soy,soften,sheldrake,sensors,seller,seas,ruler,rival,rips,renowned,recruiting,reasoning,rawley,raisins,racial,presses,preservation,portfolio,oversight,organizing,obtain,observing,nessa,narrowed,minions,midwest,meth,merciful,manages,magistrate,lawsuits,labour,invention,intimidating,infirmary,indicated,inconvenient,imposter,hugged,honoring,holdin,hades,godforsaken,fumes,forgery,foremost,foolproof,folder,folded,flattery,fingertips,financing,fifteenth,exterminator,explodes,eccentric,drained,dodging,documented,disguised,developments,currency,crafts,constructive,concealed,compartment,chute,chinpokomon,captains,capitol,calculated,buses,bodily,astronauts,alimony,accustomed,accessories,abdominal,zen,zach's,wrinkle,wallow,viv,vicinity,venue,valued,valium,valerie's,upgrade,upcoming,untrue,uncover,twig,twelfth,trembling,treasures,torched,toenails,timed,termites,telly,taunting,taransky,tar,talker,succubus,statues,smarts,sliding,sizes,sighting,semen,seizures,scarred,savvy,sauna,saddest,sacrificing,rubbish,riled,ricky's,rican,revive,recruit,ratted,rationally,provenance,professors,prestigious,pms,phonse,perky,pedal,overdose,organism,nasal,nanites,mushy,movers,moot,missus,midterm,merits,melodramatic,manure,magnetic,knockout,knitting,jig,invading,interpol,incapacitated,idle,hotline,horse's,highlight,hauling,hair's,gunpoint,greenwich,grail,ganza,framing,formally,fleeing,flap,flannel,fin,fibers,faded,existing,email,eavesdrop,dwelling,dwarf,donations,detected,desserts,dar,corporations,constellation,collision,chic,calories,businessmen,buchanan's,breathtaking,bleak,blacked,batter,balanced,ante,aggravated,agencies,abu,yanked,wuh,withdrawn,wigand,whoah,wham,vocal,unwind,undoubtedly,unattractive,twitch,trimester,torrance,timetable,taxpayers,strained,stationed,stared,slapping,sincerity,signatures,siding,siblings,shit's,shenanigans,shacking,seer,satellites,sappy,samaritan,rune,regained,rebellion,proceeds,privy,power's,poorer,politely,paste,oysters,overruled,olaf,nightcap,networks,necessity,mosquito,millimeter,michelle's,merrier,massachusetts,manuscript,manufacture,manhood,lunar,lug,lucked,loaned,kilos,ignition,hurl,hauled,harmed,goodwill,freshmen,forming,fenmore,fasten,farce,failures,exploding,erratic,elm,drunks,ditching,d'artagnan,crops,cramped,contacting,coalition,closets,clientele,chimp,cavalry,casa,cabs,bled,bargained,arranging,archives,anesthesia,amuse,altering,afternoons,accountable,abetting,wrinkles,wolek,waved,unite,uneasy,unaware,ufo,toot,toddy,tens,tattooed,tad's,sway,stained,spauldings,solely,sliced,sirens,schibetta,scatter,rumours,roger's,robbie's,rinse,remo,remedy,redemption,queen's,progressive,pleasures,picture's,philosopher,pacey's,optimism,oblige,natives,muy,measuring,measured,masked,mascot,malicious,mailing,luca,lifelong,kosher,koji,kiddies,judas,isolate,intercepted,insecurity,initially,inferior,incidentally,ifs,hun,heals,headlights,guided,growl,grilling,glazed,gem,gel,gaps,fundamental,flunk,floats,fiery,fairness,exercising,excellency,evenings,ere,enrolled,disclosure,det,department's,damp,curling,cupboard,counterfeit,cooling,condescending,conclusive,clicked,cleans,cholesterol,chap,cashed,brow,broccoli,brats,blueprints,blindfold,biz,billing,barracks,attach,aquarium,appalled,altitude,alrighty,aimed,yawn,xander's,wynant,winslow's,welcomed,violations,upright,unsolved,unreliable,toots,tighten,symbolic,sweatshirt,steinbrenner,steamy,spouse,sox,sonogram,slowed,slots,sleepless,skeleton,shines,roles,retaliate,representatives,rephrase,repeated,renaissance,redeem,rapidly,rambling,quilt,quarrel,prying,proverbial,priced,presiding,presidency,prescribe,prepped,pranks,possessive,plaintiff,philosophical,pest,persuaded,perk,pediatrics,paige's,overlooked,outcast,oop,odor,notorious,nightgown,mythology,mumbo,monitored,mediocre,master's,mademoiselle,lunchtime,lifesaver,legislation,leaned,lambs,lag,killings,interns,intensity,increasing,identities,hounding,hem,hellmouth,goon,goner,ghoul,germ,gardening,frenzy,foyer,food's,extras,extinct,exhibition,exaggerate,everlasting,enlightened,drilling,doubles,digits,dialed,devote,defined,deceitful,d'oeuvres,csi,cosmetic,contaminated,conspired,conning,colonies,cerebral,cavern,cathedral,carving,butting,boiled,blurry,beams,barf,babysit,assistants,ascension,architecture,approaches,albums,albanian,aaaaah,wildly,whoopee,whiny,weiskopf,walkie,vultures,veteran,vacations,upfront,unresolved,tile,tampering,struggled,stockholders,specially,snaps,sleepwalking,shrunk,sermon,seeks,seduction,scenarios,scams,ridden,revolve,repaired,regulation,reasonably,reactor,quotes,preserved,phenomenal,patrolling,paranormal,ounces,omigod,offs,nonstop,nightfall,nat,militia,meeting's,logs,lineup,libby's,lava,lashing,labels,kilometers,kate's,invites,investigative,innocents,infierno,incision,import,implications,humming,highlights,haunts,greeks,gloss,gloating,general's,frannie,flute,fled,fitted,finishes,fiji,fetal,feeny,entrapment,edit,dyin,download,discomfort,dimensions,detonator,dependable,deke,decree,dax,cot,confiscated,concludes,concede,complication,commotion,commence,chulak,caucasian,casually,canary,brainer,bolie,ballpark,arm's,anwar,anatomy,analyzing,accommodations,yukon,youse,wring,wharf,wallowing,uranium,unclear,treason,transgenics,thrive,think's,thermal,territories,tedious,survives,stylish,strippers,sterile,squeezing,squeaky,sprained,solemn,snoring,sic,shifting,shattering,shabby,seams,scrawny,rotation,risen,revoked,residue,reeks,recite,reap,ranting,quoting,primal,pressures,predicament,precision,plugs,pits,pinpoint,petrified,petite,persona,pathological,passports,oughtta,nods,nighter,navigate,nashville,namely,museums,morale,milwaukee,meditation,mathematics,martin's,malta,logan's,latter,kippie,jackie's,intrigue,intentional,insufferable,incomplete,inability,imprisoned,hup,hunky,how've,horrifying,hearty,headmaster,hath,har,hank's,handbook,hamptons,grazie,goof,george's,funerals,fuck's,fraction,forks,finances,fetched,excruciating,enjoyable,enhanced,enhance,endanger,efficiency,dumber,drying,diabolical,destroyer,desirable,defendants,debris,darts,cuisine,cucumber,cube,crossword,contestant,considers,comprehend,club's,clipped,classmates,choppers,certificates,carmen's,canoe,candlelight,building's,brutally,brutality,boarded,bathrobe,backward,authorize,audrey's,atom,assemble,appeals,airports,aerobics,ado,abbott's,wholesome,whiff,vessels,vermin,varsity,trophies,trait,tragically,toying,titles,tissues,testy,team's,tasteful,surge,sun's,studios,strips,stocked,stephen's,staircase,squares,spinach,sow,southwest,southeast,sookie's,slayer's,sipping,singers,sidetracked,seldom,scrubbing,scraping,sanctity,russell's,ruse,robberies,rink,ridin,retribution,reinstated,refrain,rec,realities,readings,radiant,protesting,projector,posed,plutonium,plaque,pilar's,payin,parting,pans,o'reilly,nooooo,motorcycles,motherfucking,mein,measly,marv,manic,line's,lice,liam,lenses,lama,lalita,juggling,jerking,jamie's,intro,inevitably,imprisonment,hypnosis,huddle,horrendous,hobbies,heavier,heartfelt,harlin,hairdresser,grub,gramps,gonorrhea,gardens,fussing,fragment,fleeting,flawless,flashed,fetus,exclusively,eulogy,equality,enforce,distinctly,disrespectful,denies,crossbow,crest,cregg,crabs,cowardly,countess,contrast,contraction,contingency,consulted,connects,confirming,condone,coffins,cleansing,cheesecake,certainty,captain's,cages,c'est,briefed,brewing,bravest,bosom,boils,binoculars,bachelorette,aunt's,atta,assess,appetizer,ambushed,alerted,woozy,withhold,weighed,vulgar,viral,utmost,unusually,unleashed,unholy,unhappiness,underway,uncovered,unconditional,typewriter,typed,twists,sweeps,supervised,supermodel,suburbs,subpoenaed,stringing,snyder's,snot,skeptical,skateboard,shifted,secret's,scottish,schoolgirl,romantically,rocked,revoir,reviewed,respiratory,reopen,regiment,reflects,refined,puncture,pta,prone,produces,preach,pools,polished,pods,planetarium,penicillin,peacefully,partner's,nurturing,nation's,more'n,monastery,mmhmm,midgets,marklar,machinery,lodged,lifeline,joanna's,jer,jellyfish,infiltrate,implies,illegitimate,hutch,horseback,henri,heist,gents,frickin,freezes,forfeit,followers,flakes,flair,fathered,fascist,eternally,eta,epiphany,enlisted,eleventh,elect,effectively,dos,disgruntled,discrimination,discouraged,delinquent,decipher,danvers,dab,cubes,credible,coping,concession,cnn,clash,chills,cherished,catastrophe,caretaker,bulk,bras,branches,bombshell,birthright,billionaire,awol,ample,alumni,affections,admiration,abbotts,zelda's,whatnot,watering,vinegar,vietnamese,unthinkable,unseen,unprepared,unorthodox,underhanded,uncool,transmitted,traits,timeless,thump,thermometer,theoretically,theoretical,testament,tapping,tagged,tac,synthetic,syndicate,swung,surplus,supplier,stares,spiked,soviets,solves,smuggle,scheduling,scarier,saucer,reinforcements,recruited,rant,quitter,prudent,projection,previously,powdered,poked,pointers,placement,peril,penetrate,penance,patriotic,passions,opium,nudge,nostrils,nevermind,neurological,muslims,mow,momentum,mockery,mobster,mining,medically,magnitude,maggie's,loudly,listing,killer's,kar,jim's,insights,indicted,implicate,hypocritical,humanly,holiness,healthier,hammered,haldeman,gunman,graphic,gloom,geography,gary's,freshly,francs,formidable,flunked,flawed,feminist,faux,ewww,escorted,escapes,emptiness,emerge,drugging,dozer,doc's,directorate,diana's,derevko,deprive,deodorant,cryin,crusade,crocodile,creativity,controversial,commands,coloring,colder,cognac,clocked,clippings,christine's,chit,charades,chanting,certifiable,caterers,brute,brochures,briefs,bran,botched,blinders,bitchin,bauer's,banter,babu,appearing,adequate,accompanied,abrupt,abdomen,zones,wooo,woken,winding,vip,venezuela,unanimous,ulcer,tread,thirteenth,thankfully,tame,tabby's,swine,swimsuit,swans,suv,stressing,steaming,stamped,stabilize,squirm,spokesman,snooze,shuffle,shredded,seoul,seized,seafood,scratchy,savor,sadistic,roster,rica,rhetorical,revlon,realist,reactions,prosecuting,prophecies,prisons,precedent,polyester,petals,persuasion,paddles,o'leary,nuthin,neighbour,negroes,naval,mute,muster,muck,minnesota,meningitis,matron,mastered,markers,maris's,manufactured,lot's,lockers,letterman,legged,launching,lanes,journals,indictment,indicating,hypnotized,housekeeping,hopelessly,hmph,hallucinations,grader,goldilocks,girly,furthermore,frames,flask,expansion,envelopes,engaging,downside,doves,doorknob,distinctive,dissolve,discourage,disapprove,diabetic,departed,deliveries,decorator,deaq,crossfire,criminally,containment,comrades,complimentary,commitments,chum,chatter,chapters,catchy,cashier,cartel,caribou,cardiologist,bull's,buffer,brawl,bowls,booted,boat's,billboard,biblical,barbershop,awakening,aryan,angst,administer,acquitted,acquisition,aces,accommodate,zellie,yield,wreak,witch's,william's,whistles,wart,vandalism,vamps,uterus,upstate,unstoppable,unrelated,understudy,tristin,transporting,transcript,tranquilizer,trails,trafficking,toxins,tonsils,timing's,therapeutic,tex,subscription,submitted,stephanie's,stempel,spotting,spectator,spatula,soho,softer,snotty,slinging,showered,sexiest,sensual,scoring,sadder,roam,rimbaud,rim,rewards,restrain,resilient,remission,reinstate,rehash,recollection,rabies,quinn's,presenting,preference,prairie,popsicle,plausible,plantation,pharmaceutical,pediatric,patronizing,patent,participation,outdoor,ostrich,ortolani,oooooh,omelette,neighbor's,neglect,nachos,movie's,mixture,mistrial,mio,mcginty's,marseilles,mare,mandate,malt,luv,loophole,literary,liberation,laughin,lacey's,kevvy,jah,irritated,intends,initiation,initiated,initiate,influenced,infidelity,indigenous,inc,idaho,hypothermia,horrific,hive,heroine,groupie,grinding,graceful,government's,goodspeed,gestures,gah,frantic,extradition,evil's,engineers,echelon,earning,disks,discussions,demolition,definitive,dawnie,dave's,date's,dared,dan's,damsel,curled,courtyard,constitutes,combustion,collective,collateral,collage,col,chant,cassette,carol's,carl's,calculating,bumping,britain,bribes,boardwalk,blinds,blindly,bleeds,blake's,bickering,beasts,battlefield,bankruptcy,backside,avenge,apprehended,annie's,anguish,afghanistan,acknowledged,abusing,youthful,yells,yanking,whomever,when'd,waterfall,vomiting,vine,vengeful,utility,unpacking,unfamiliar,undying,tumble,trolls,treacherous,todo,tipping,tantrum,tanked,summons,strategies,straps,stomped,stinkin,stings,stance,staked,squirrels,sprinkles,speculate,specialists,sorting,skinned,sicko,sicker,shootin,shep,shatter,seeya,schnapps,s'posed,rows,rounded,ronee,rite,revolves,respectful,resource,reply,rendered,regroup,regretting,reeling,reckoned,rebuilding,randy's,ramifications,qualifications,pulitzer,puddy,projections,preschool,pots,potassium,plissken,platonic,peter's,permalash,performer,peasant,outdone,outburst,ogh,obscure,mutants,mugging,molecules,misfortune,miserably,miraculously,medications,medals,margaritas,manpower,lovemaking,long's,logo,logically,leeches,latrine,lamps,lacks,kneel,johnny's,jenny's,inflict,impostor,icon,hypocrisy,hype,hosts,hippies,heterosexual,heightened,hecuba's,hecuba,healer,habitat,gunned,grooming,groo,groin,gras,gory,gooey,gloomy,frying,friendships,fredo,foil,fishermen,firepower,fess,fathom,exhaustion,evils,epi,endeavor,ehh,eggnog,dreaded,drafted,dimensional,detached,deficit,d'arcy,crotch,coughing,coronary,cookin,contributed,consummate,congrats,concerts,companionship,caved,caspar,bulletproof,bris,brilliance,breakin,brash,blasting,beak,arabia,analyst,aluminum,aloud,alligator,airtight,advising,advertise,adultery,administered,aches,abstract,aahh,wronged,wal,voluntary,ventilation,upbeat,uncertainty,trot,trillion,tricia's,trades,tots,tol,tightly,thingies,tending,technician,tarts,surreal,summer's,strengths,specs,specialize,spat,spade,slogan,sloane's,shrew,shaping,seth's,selves,seemingly,schoolwork,roomie,requirements,redundant,redo,recuperating,recommendations,ratio,rabid,quart,pseudo,provocative,proudly,principal's,pretenses,prenatal,pillar,photographers,photographed,pharmaceuticals,patron,pacing,overworked,originals,nicotine,newsletter,neighbours,murderous,miller's,mileage,mechanics,mayonnaise,massages,maroon,lucrative,losin,lil,lending,legislative,kat,juno,iran,interrogated,instruction,injunction,impartial,homing,heartbreaker,harm's,hacks,glands,giver,fraizh,flows,flips,flaunt,excellence,estimated,espionage,englishman,electrocuted,eisenhower,dusting,ducking,drifted,donna's,donating,dom,distribute,diem,daydream,cylon,curves,crutches,crates,cowards,covenant,converted,contributions,composed,comfortably,cod,cockpit,chummy,chitchat,childbirth,charities,businesswoman,brood,brewery,bp's,blatant,bethy,barring,bagged,awakened,assumes,assembled,asbestos,arty,artwork,arc,anthony's,aka,airplanes,accelerated,worshipped,winnings,why're,whilst,wesley's,volleyball,visualize,unprotected,unleash,unexpectedly,twentieth,turnpike,trays,translated,tones,three's,thicker,therapists,takeoff,sums,stub,streisand,storm's,storeroom,stethoscope,stacked,sponsors,spiteful,solutions,sneaks,snapping,slaughtered,slashed,simplest,silverware,shits,secluded,scruples,scrubs,scraps,scholar,ruptured,rubs,roaring,relying,reflected,refers,receptionist,recap,reborn,raisin,rainforest,rae's,raditch,radiator,pushover,pout,plastered,pharmacist,petroleum,perverse,perpetrator,passages,ornament,ointment,occupy,nineties,napping,nannies,mousse,mort,morocco,moors,momentary,modified,mitch's,misunderstandings,marina's,marcy's,marched,manipulator,malfunction,loot,limbs,latitude,lapd,laced,kivar,kickin,interface,infuriating,impressionable,imposing,holdup,hires,hick,hesitated,hebrew,hearings,headphones,hammering,groundwork,grotesque,greenhouse,gradually,graces,genetics,gauze,garter,gangsters,g's,frivolous,freelance,freeing,fours,forwarding,feud,ferrars,faulty,fantasizing,extracurricular,exhaust,empathy,educate,divorces,detonate,depraved,demeaning,declaring,deadlines,dea,daria's,dalai,cursing,cufflink,crows,coupons,countryside,coo,consultation,composer,comply,comforted,clive,claustrophobic,chef's,casinos,caroline's,capsule,camped,cairo,busboy,bred,bravery,bluth,biography,berserk,bennetts,baskets,attacker,aplastic,angrier,affectionate,zit,zapped,yorker,yarn,wormhole,weaken,vat,unrealistic,unravel,unimportant,unforgettable,twain,tv's,tush,turnout,trio,towed,tofu,textbooks,territorial,suspend,supplied,superbowl,sundays,stutter,stewardess,stepson,standin,sshh,specializes,spandex,souvenirs,sociopath,snails,slope,skeletons,shivering,sexier,sequel,sensory,selfishness,scrapbook,romania,riverside,rites,ritalin,rift,ribbons,reunite,remarry,relaxation,reduction,realization,rattling,rapist,quad,pup,psychosis,promotions,presumed,prepping,posture,poses,pleasing,pisses,piling,photographic,pfft,persecuted,pear,part's,pantyhose,padded,outline,organizations,operatives,oohh,obituary,northeast,nina's,neural,negotiator,nba,natty,nathan's,minimize,merl,menopause,mennihan,marty's,martimmys,makers,loyalties,literal,lest,laynie,lando,justifies,josh's,intimately,interact,integrated,inning,inexperienced,impotent,immortality,imminent,ich,horrors,hooky,holders,hinges,heartbreaking,handcuffed,gypsies,guacamole,grovel,graziella,goggles,gestapo,fussy,functional,filmmaker,ferragamo,feeble,eyesight,explosions,experimenting,enzo's,endorsement,enchanting,eee,ed's,duration,doubtful,dizziness,dismantle,disciplinary,disability,detectors,deserving,depot,defective,decor,decline,dangling,dancin,crumble,criteria,creamed,cramping,cooled,conceal,component,competitors,clockwork,clark's,circuits,chrissakes,chrissake,chopping,cabinets,buttercup,brooding,bonfire,blurt,bluestar,bloated,blackmailer,beforehand,bathed,bathe,barcode,banjo,banish,badges,babble,await,attentive,artifacts,aroused,antibodies,animosity,administrator,accomplishments,ya'll,wrinkled,wonderland,willed,whisk,waltzing,waitressing,vis,vin,vila,vigilant,upbringing,unselfish,unpopular,unmarried,uncles,trendy,trajectory,targeting,surroundings,stun,striped,starbucks,stamina,stalled,staking,stag,spoils,snuff,snooty,snide,shrinking,senorita,securities,secretaries,scrutiny,scoundrel,saline,salads,sails,rundown,roz's,roommate's,riddles,responses,resistant,requirement,relapse,refugees,recommending,raspberry,raced,prosperity,programme,presumably,preparations,posts,pom,plight,pleaded,pilot's,peers,pecan,particles,pantry,overturned,overslept,ornaments,opposing,niner,nfl,negligent,negligence,nailing,mutually,mucho,mouthed,monstrous,monarchy,minsk,matt's,mateo's,marking,manufacturing,manager's,malpractice,maintaining,lowly,loitering,logged,lingering,light's,lettin,lattes,kim's,kamal,justification,juror,junction,julie's,joys,johnson's,jillefsky,jacked,irritate,intrusion,inscription,insatiable,infect,inadequate,impromptu,icing,hmmmm,hefty,grammar,generate,gdc,gasket,frightens,flapping,firstborn,fire's,fig,faucet,exaggerated,estranged,envious,eighteenth,edible,downward,dopey,doesn,disposition,disposable,disasters,disappointments,dipped,diminished,dignified,diaries,deported,deficiency,deceit,dealership,deadbeat,curses,coven,counselors,convey,consume,concierge,clutches,christians,cdc,casbah,carefree,callous,cahoots,caf,brotherly,britches,brides,bop,bona,bethie,beige,barrels,ballot,ave,autographed,attendants,attachment,attaboy,astonishing,ashore,appreciative,antibiotic,aneurysm,afterlife,affidavit,zuko,zoning,work's,whats,whaddaya,weakened,watermelon,vasectomy,unsuspecting,trial's,trailing,toula,topanga,tonio,toasted,tiring,thereby,terrorized,tenderness,tch,tailing,syllable,sweats,suffocated,sucky,subconsciously,starvin,staging,sprouts,spineless,sorrows,snowstorm,smirk,slicery,sledding,slander,simmer,signora,sigmund,siege,siberia,seventies,sedate,scented,sampling,sal's,rowdy,rollers,rodent,revenue,retraction,resurrection,resigning,relocate,releases,refusal,referendum,recuperate,receptive,ranking,racketeering,queasy,proximity,provoking,promptly,probability,priors,princes,prerogative,premed,pornography,porcelain,poles,podium,pinched,pig's,pendant,packet,owner's,outsiders,outpost,orbing,opportunist,olanov,observations,nurse's,nobility,neurologist,nate's,nanobot,muscular,mommies,molested,misread,melon,mediterranean,mea,mastermind,mannered,maintained,mackenzie's,liberated,lesions,lee's,laundromat,landscape,lagoon,labeled,jolt,intercom,inspect,insanely,infrared,infatuation,indulgent,indiscretion,inconsiderate,incidents,impaired,hurrah,hungarian,howling,honorary,herpes,hasta,harassed,hanukkah,guides,groveling,groosalug,geographic,gaze,gander,galactica,futile,fridays,flier,fixes,fide,fer,feedback,exploiting,exorcism,exile,evasive,ensemble,endorse,emptied,dreary,dreamy,downloaded,dodged,doctored,displayed,disobeyed,disneyland,disable,diego's,dehydrated,defect,customary,csc,criticizing,contracted,contemplating,consists,concepts,compensate,commonly,colours,coins,coconuts,cockroaches,clogged,cincinnati,churches,chronicle,chilling,chaperon,ceremonies,catalina's,cant,cameraman,bulbs,bucklands,bribing,brava,bracelets,bowels,bobby's,bmw,bluepoint,baton,barred,balm,audit,astronomy,aruba,appetizers,appendix,antics,anointed,analogy,almonds,albuquerque,abruptly,yore,yammering,winch,white's,weston's,weirdness,wangler,vibrations,vendor,unmarked,unannounced,twerp,trespass,tres,travesty,transported,transfusion,trainee,towelie,topics,tock,tiresome,thru,theatrical,terrain,suspect's,straightening,staggering,spaced,sonar,socializing,sitcom,sinus,sinners,shambles,serene,scraped,scones,scepter,sarris,saberhagen,rouge,rigid,ridiculously,ridicule,reveals,rents,reflecting,reconciled,rate's,radios,quota,quixote,publicist,pubes,prune,prude,provider,propaganda,prolonged,projecting,prestige,precrime,postponing,pluck,perpetual,permits,perish,peppermint,peeled,particle,parliament,overdo,oriented,optional,nutshell,notre,notions,nostalgic,nomination,mulan,mouthing,monkey's,mistook,mis,milhouse,mel's,meddle,maybourne,martimmy,loon,lobotomy,livelihood,litigation,lippman,likeness,laurie's,kindest,kare,kaffee,jocks,jerked,jeopardizing,jazzed,investing,insured,inquisition,inhale,ingenious,inflation,incorrect,igby,ideals,holier,highways,hereditary,helmets,heirloom,heinous,haste,harmsway,hardship,hanky,gutters,gruesome,groping,governments,goofing,godson,glare,garment,founding,fortunes,foe,finesse,figuratively,ferrie,fda,external,examples,evacuation,ethnic,est,endangerment,enclosed,emphasis,dyed,dud,dreading,dozed,dorky,dmitri,divert,dissertation,discredit,director's,dialing,describes,decks,cufflinks,crutch,creator,craps,corrupted,coronation,contemporary,consumption,considerably,comprehensive,cocoon,cleavage,chile,carriers,carcass,cannery,bystander,brushes,bruising,bribery,brainstorm,bolted,binge,bart's,barracuda,baroness,ballistics,b's,astute,arroway,arabian,ambitions,alexandra's,afar,adventurous,adoptive,addicts,addictive,accessible,yadda,wilson's,wigs,whitelighters,wematanye,weeds,wedlock,wallets,walker's,vulnerability,vroom,vibrant,vertical,vents,uuuh,urgh,upped,unsettling,unofficial,unharmed,underlying,trippin,trifle,tracing,tox,tormenting,timothy's,threads,theaters,thats,tavern,taiwan,syphilis,susceptible,summary,suites,subtext,stickin,spices,sores,smacked,slumming,sixteenth,sinks,signore,shitting,shameful,shacked,sergei,septic,seedy,security's,searches,righteousness,removal,relish,relevance,rectify,recruits,recipient,ravishing,quickest,pupil,productions,precedence,potent,pooch,pledged,phoebs,perverted,peeing,pedicure,pastrami,passionately,ozone,overlooking,outnumbered,outlook,oregano,offender,nukes,novelty,nosed,nighty,nifty,mugs,mounties,motivate,moons,misinterpreted,miners,mercenary,mentality,mas,marsellus,mapped,malls,lupus,lumbar,lovesick,longitude,lobsters,likelihood,leaky,laundering,latch,japs,jafar,instinctively,inspires,inflicted,inflammation,indoors,incarcerated,imagery,hundredth,hula,hemisphere,handkerchief,hand's,gynecologist,guittierez,groundhog,grinning,graduates,goodbyes,georgetown,geese,fullest,ftl,floral,flashback,eyelashes,eyelash,excluded,evening's,evacuated,enquirer,endlessly,encounters,elusive,disarm,detest,deluding,dangle,crabby,cotillion,corsage,copenhagen,conjugal,confessional,cones,commandment,coded,coals,chuckle,christmastime,christina's,cheeseburgers,chardonnay,ceremonial,cept,cello,celery,carter's,campfire,calming,burritos,burp,buggy,brundle,broflovski,brighten,bows,borderline,blinked,bling,beauties,bauers,battered,athletes,assisting,articulate,alot,alienated,aleksandr,ahhhhh,agreements,agamemnon,accountants,zat,y'see,wrongful,writer's,wrapper,workaholic,wok,winnebago,whispered,warts,vikki's,verified,vacate,updated,unworthy,unprecedented,unanswered,trend,transformed,transform,trademark,tote,tonane,tolerated,throwin,throbbing,thriving,thrills,thorns,thereof,there've,terminator,tendencies,tarot,tailed,swab,sunscreen,stretcher,stereotype,spike's,soggy,sobbing,slopes,skis,skim,sizable,sightings,shucks,shrapnel,sever,senile,sections,seaboard,scripts,scorned,saver,roxanne's,resemble,red's,rebellious,rained,putty,proposals,prenup,positioned,portuguese,pores,pinching,pilgrims,pertinent,peeping,pamphlet,paints,ovulating,outbreak,oppression,opposites,occult,nutcracker,nutcase,nominee,newt,newsstand,newfound,nepal,mocked,midterms,marshmallow,manufacturer,managers,majesty's,maclaren,luscious,lowered,loops,leans,laurence's,krudski,knowingly,keycard,katherine's,junkies,juilliard,judicial,jolinar,jase,irritable,invaluable,inuit,intoxicating,instruct,insolent,inexcusable,induce,incubator,illustrious,hydrogen,hunsecker,hub,houseguest,honk,homosexuals,homeroom,holly's,hindu,hernia,harming,handgun,hallways,hallucination,gunshots,gums,guineas,groupies,groggy,goiter,gingerbread,giggling,geometry,genre,funded,frontal,frigging,fledged,fedex,feat,fairies,eyeball,extending,exchanging,exaggeration,esteemed,ergo,enlist,enlightenment,encyclopedia,drags,disrupted,dispense,disloyal,disconnect,dimitri,desks,dentists,delhi,delacroix,degenerate,deemed,decay,daydreaming,cushions,cuddly,corroborate,contender,congregation,conflicts,confessions,complexion,completion,compensated,cobbler,closeness,chilled,checkmate,channing,carousel,calms,bylaws,bud's,benefactor,belonging,ballgame,baiting,backstabbing,assassins,artifact,armies,appoint,anthropology,anthropologist,alzheimer's,allegedly,alex's,airspace,adversary,adolf,actin,acre,aced,accuses,accelerant,abundantly,abstinence,abc,zsa,zissou,zandt,yom,yapping,wop,witchy,winter's,willows,whee,whadaya,want's,walter's,waah,viruses,vilandra,veiled,unwilling,undress,undivided,underestimating,ultimatums,twirl,truckload,tremble,traditionally,touring,touche,toasting,tingling,tiles,tents,tempered,sussex,sulking,stunk,stretches,sponges,spills,softly,snipers,slid,sedan,screens,scourge,rooftop,rog,rivalry,rifles,riana,revolting,revisit,resisted,rejects,refreshments,redecorating,recurring,recapture,raysy,randomly,purchases,prostitutes,proportions,proceeded,prevents,pretense,prejudiced,precogs,pouting,poppie,poofs,pimple,piles,pediatrician,patrick's,pathology,padre,packets,paces,orvelle,oblivious,objectivity,nikki's,nighttime,nervosa,navigation,moist,moan,minors,mic,mexicans,meurice,melts,mau,mats,matchmaker,markings,maeby,lugosi,lipnik,leprechaun,kissy,kafka,italians,introductions,intestines,intervene,inspirational,insightful,inseparable,injections,informal,influential,inadvertently,illustrated,hussy,huckabees,hmo,hittin,hiss,hemorrhaging,headin,hazy,haystack,hallowed,haiti,haa,grudges,grenades,granilith,grandkids,grading,gracefully,godsend,gobbles,fyi,future's,fun's,fret,frau,fragrance,fliers,firms,finchley,fbi's,farts,eyewitnesses,expendable,existential,endured,embraced,elk,ekg,dude's,dragonfly,dorms,domination,directory,depart,demonstrated,delaying,degrading,deduction,darlings,dante's,danes,cylons,counsellor,cortex,cop's,coordinator,contraire,consensus,consciously,conjuring,congratulating,compares,commentary,commandant,cokes,centimeters,cc's,caucus,casablanca,buffay,buddy's,brooch,bony,boggle,blood's,bitching,bistro,bijou,bewitched,benevolent,bends,bearings,barren,arr,aptitude,antenna,amish,amazes,alcatraz,acquisitions,abomination,worldly,woodstock,withstand,whispers,whadda,wayward,wayne's,wailing,vinyl,variables,vanishing,upscale,untouchable,unspoken,uncontrollable,unavoidable,unattended,tuning,trite,transvestite,toupee,timid,timers,themes,terrorizing,teamed,taipei,t's,swana,surrendered,suppressed,suppress,stumped,strolling,stripe,storybook,storming,stomachs,stoked,stationery,springtime,spontaneity,sponsored,spits,spins,soiree,sociology,soaps,smarty,shootout,shar,settings,sentiments,senator's,scramble,scouting,scone,runners,rooftops,retract,restrictions,residency,replay,remainder,regime,reflexes,recycling,rcmp,rawdon,ragged,quirky,quantico,psychologically,prodigal,primo,pounce,potty,portraits,pleasantries,plane's,pints,phd,petting,perceive,patrons,parameters,outright,outgoing,onstage,officer's,o'connor,notwithstanding,noah's,nibble,newmans,neutralize,mutilated,mortality,monumental,ministers,millionaires,mentions,mcdonald's,mayflower,masquerade,mangy,macreedy,lunatics,luau,lover's,lovable,louie's,locating,lizards,limping,lasagna,largely,kwang,keepers,juvie,jaded,ironing,intuitive,intensely,insure,installation,increases,incantation,identifying,hysteria,hypnotize,humping,heavyweight,happenin,gung,griet,grasping,glorified,glib,ganging,g'night,fueled,focker,flunking,flimsy,flaunting,fixated,fitzwallace,fictional,fearing,fainting,eyebrow,exonerated,ether,ers,electrician,egotistical,earthly,dusted,dues,donors,divisions,distinguish,displays,dismissal,dignify,detonation,deploy,departments,debrief,dazzling,dawn's,dan'l,damnedest,daisies,crushes,crucify,cordelia's,controversy,contraband,contestants,confronting,communion,collapsing,cocked,clock's,clicks,cliche,circular,circled,chord,characteristics,chandelier,casualty,carburetor,callers,bup,broads,breathes,boca,bobbie's,bloodshed,blindsided,blabbing,binary,bialystock,bashing,ballerina,ball's,aviva,avalanche,arteries,appliances,anthem,anomaly,anglo,airstrip,agonizing,adjourn,abandonment,zack's,you's,yearning,yams,wrecker,word's,witnessing,winged,whence,wept,warsaw,warp,warhead,wagons,visibility,usc,unsure,unions,unheard,unfreeze,unfold,unbalanced,ugliest,troublemaker,tolerant,toddler,tiptoe,threesome,thirties,thermostat,tampa,sycamore,switches,swipe,surgically,supervising,subtlety,stung,stumbling,stubs,struggles,stride,strangling,stamp's,spruce,sprayed,socket,snuggle,smuggled,skulls,simplicity,showering,shhhhh,sensor,sci,sac,sabotaging,rumson,rounding,risotto,riots,revival,responds,reserves,reps,reproduction,repairman,rematch,rehearsed,reelection,redi,recognizing,ratty,ragging,radiology,racquetball,racking,quieter,quicksand,pyramids,pulmonary,puh,publication,prowl,provisions,prompt,premeditated,prematurely,prancing,porcupine,plated,pinocchio,perceived,peeked,peddle,pasture,panting,overweight,oversee,overrun,outing,outgrown,obsess,o'donnell,nyu,nursed,northwestern,norma's,nodding,negativity,negatives,musketeers,mugger,mounting,motorcade,monument,merrily,matured,massimo's,masquerading,marvellous,marlena's,margins,maniacs,mag,lumpy,lovey,louse,linger,lilies,libido,lawful,kudos,knuckle,kitchen's,kennedy's,juices,judgments,joshua's,jars,jams,jamal's,jag,itches,intolerable,intermission,interaction,institutions,infectious,inept,incentives,incarceration,improper,implication,imaginative,ight,hussein,humanitarian,huckleberry,horatio,holster,heiress,heartburn,hayley's,hap,gunna,guitarist,groomed,greta's,granting,graciously,glee,gentleman's,fulfillment,fugitives,fronts,founder,forsaking,forgives,foreseeable,flavors,flares,fixation,figment,fickle,featuring,featured,fantasize,famished,faith's,fades,expiration,exclamation,evolve,euro,erasing,emphasize,elevator's,eiffel,eerie,earful,duped,dulles,distributor,distorted,dissing,dissect,dispenser,dilated,digit,differential,diagnostic,detergent,desdemona,debriefing,dazzle,damper,cylinder,curing,crowbar,crispina,crafty,crackpot,courting,corrections,cordial,copying,consuming,conjunction,conflicted,comprehension,commie,collects,cleanup,chiropractor,charmer,chariot,charcoal,chaplain,challenger,census,cd's,cauldron,catatonic,capabilities,calculate,bullied,buckets,brilliantly,breathed,boss's,booths,bombings,boardroom,blowout,blower,blip,blindness,blazing,birthday's,biologically,bibles,biased,beseech,barbaric,band's,balraj,auditorium,audacity,assisted,appropriations,applicants,anticipating,alcoholics,airhead,agendas,aft,admittedly,adapt,absolution,abbot,zing,youre,yippee,wittlesey,withheld,willingness,willful,whammy,webber's,weakest,washes,virtuous,violently,videotapes,vials,vee,unplugged,unpacked,unfairly,und,turbulence,tumbling,troopers,tricking,trenches,tremendously,travelled,travelers,traitors,torches,tommy's,tinga,thyroid,texture,temperatures,teased,tawdry,tat,taker,sympathies,swiped,swallows,sundaes,suave,strut,structural,stone's,stewie,stepdad,spewing,spasm,socialize,slither,sky's,simulator,sighted,shutters,shrewd,shocks,sherry's,sgc,semantics,scout's,schizophrenic,scans,savages,satisfactory,rya'c,runny,ruckus,royally,roadblocks,riff,rewriting,revoke,reversal,repent,renovation,relating,rehearsals,regal,redecorate,recovers,recourse,reconnaissance,receives,ratched,ramali,racquet,quince,quiche,puppeteer,puking,puffed,prospective,projected,problemo,preventing,praises,pouch,posting,postcards,pooped,poised,piled,phoney,phobia,performances,patty's,patching,participating,parenthood,pardner,oppose,oozing,oils,ohm,ohhhhh,nypd,numbing,novelist,nostril,nosey,nominate,noir,neatly,nato,naps,nappa,nameless,muzzle,muh,mortuary,moronic,modesty,mitz,missionary,mimi's,midwife,mercenaries,mcclane,maxie's,matuka,mano,mam,maitre,lush,lumps,lucid,loosened,loosely,loins,lawnmower,lane's,lamotta,kroehner,kristen's,juggle,jude's,joins,jinxy,jessep,jaya,jamming,jailhouse,jacking,ironically,intruders,inhuman,infections,infatuated,indoor,indigestion,improvements,implore,implanted,id's,hormonal,hoboken,hillbilly,heartwarming,headway,headless,haute,hatched,hartmans,harping,hari,grapevine,graffiti,gps,gon,gogh,gnome,ged,forties,foreigners,fool's,flyin,flirted,fingernail,fdr,exploration,expectation,exhilarating,entrusted,enjoyment,embark,earliest,dumper,duel,dubious,drell,dormant,docking,disqualified,disillusioned,dishonor,disbarred,directive,dicey,denny's,deleted,del's,declined,custodial,crunchy,crises,counterproductive,correspondent,corned,cords,cor,coot,contributing,contemplate,containers,concur,conceivable,commissioned,cobblepot,cliffs,clad,chief's,chickened,chewbacca,checkout,carpe,cap'n,campers,calcium,buyin,buttocks,bullies,brown's,brigade,brain's,braid,boxed,bouncy,blueberries,blubbering,bloodstream,bigamy,bel,beeped,bearable,bank's,awarded,autographs,attracts,attracting,asteroid,arbor,arab,apprentice,announces,andie's,ammonia,alarming,aidan's,ahoy,ahm,zan,wretch,wimps,widows,widower,whirlwind,whirl,weather's,warms,war's,wack,villagers,vie,vandelay,unveiling,uno,undoing,unbecoming,ucla,turnaround,tribunal,togetherness,tickles,ticker,tended,teensy,taunt,system's,sweethearts,superintendent,subcommittee,strengthen,stomach's,stitched,standpoint,staffers,spotless,splits,soothe,sonnet,smothered,sickening,showdown,shouted,shepherds,shelters,shawl,seriousness,separates,sen,schooled,schoolboy,scat,sats,sacramento,s'mores,roped,ritchie's,resembles,reminders,regulars,refinery,raggedy,profiles,preemptive,plucked,pheromones,particulars,pardoned,overpriced,overbearing,outrun,outlets,onward,oho,ohmigod,nosing,norwegian,nightly,nicked,neanderthal,mosquitoes,mortified,moisture,moat,mime,milky,messin,mecha,markinson,marivellas,mannequin,manderley,maid's,madder,macready,maciver's,lookie,locusts,lisbon,lifetimes,leg's,lanna,lakhi,kholi,joke's,invasive,impersonate,impending,immigrants,ick,i's,hyperdrive,horrid,hopin,hombre,hogging,hens,hearsay,haze,harpy,harboring,hairdo,hafta,hacking,gun's,guardians,grasshopper,graded,gobble,gatehouse,fourteenth,foosball,floozy,fitzgerald's,fished,firewood,finalize,fever's,fencing,felons,falsely,fad,exploited,euphemism,entourage,enlarged,ell,elitist,elegance,eldest,duo,drought,drokken,drier,dredge,dramas,dossier,doses,diseased,dictator,diarrhea,diagnose,despised,defuse,defendant's,d'amour,crowned,cooper's,continually,contesting,consistently,conserve,conscientious,conjured,completing,commune,commissioner's,collars,coaches,clogs,chenille,chatty,chartered,chamomile,casing,calculus,calculator,brittle,breached,boycott,blurted,birthing,bikinis,bankers,balancing,astounding,assaulting,aroma,arbitration,appliance,antsy,amnio,alienating,aliases,aires,adolescence,administrative,addressing,achieving,xerox,wrongs,workload,willona,whistling,werewolves,wallaby,veterans,usin,updates,unwelcome,unsuccessful,unseemly,unplug,undermining,ugliness,tyranny,tuesdays,trumpets,transference,traction,ticks,tete,tangible,tagging,swallowing,superheroes,sufficiently,studs,strep,stowed,stow,stomping,steffy,stature,stairway,sssh,sprain,spouting,sponsoring,snug,sneezing,smeared,slop,slink,slew,skid,simultaneously,simulation,sheltered,shakin,sewed,sewage,seatbelt,scariest,scammed,scab,sanctimonious,samir,rushes,rugged,routes,romanov,roasting,rightly,retinal,rethinking,resulted,resented,reruns,replica,renewed,remover,raiding,raided,racks,quantity,purest,progressing,primarily,presidente,prehistoric,preeclampsia,postponement,portals,poppa,pop's,pollution,polka,pliers,playful,pinning,pharaoh,perv,pennant,pelvic,paved,patented,paso,parted,paramedic,panels,pampered,painters,padding,overjoyed,orthodox,organizer,one'll,octavius,occupational,oakdale's,nous,nite,nicknames,neurosurgeon,narrows,mitt,misled,mislead,mishap,milltown,milking,microscopic,meticulous,mediocrity,meatballs,measurements,mandy's,malaria,machete,lydecker's,lurch,lorelai's,linda's,layin,lavish,lard,knockin,khruschev,kelso's,jurors,jumpin,jugular,journalists,jour,jeweler,jabba,intersection,intellectually,integral,installment,inquiries,indulging,indestructible,indebted,implicated,imitate,ignores,hyperventilating,hyenas,hurrying,huron,horizontal,hermano,hellish,heheh,header,hazardous,hart's,harshly,harper's,handout,handbag,grunemann,gots,glum,gland,glances,giveaway,getup,gerome,furthest,funhouse,frosting,franchise,frail,fowl,forwarded,forceful,flavored,flank,flammable,flaky,fingered,finalists,fatherly,famine,fags,facilitate,exempt,exceptionally,ethic,essays,equity,entrepreneur,enduring,empowered,employers,embezzlement,eels,dusk,duffel,downfall,dotted,doth,doke,distressed,disobey,disappearances,disadvantage,dinky,diminish,diaphragm,deuces,deployed,delia's,davidson's,curriculum,curator,creme,courteous,correspondence,conquered,comforts,coerced,coached,clots,clarification,cite,chunks,chickie,chick's,chases,chaperoning,ceramic,ceased,cartons,capri,caper,cannons,cameron's,calves,caged,bustin,bungee,bulging,bringin,brie,boomhauer,blowin,blindfolded,blab,biscotti,bird's,beneficial,bastard's,ballplayer,bagging,automated,auster,assurances,aschen,arraigned,anonymity,annex,animation,andi,anchorage,alters,alistair's,albatross,agreeable,advancement,adoring,accurately,abduct,wolfi,width,weirded,watchers,washroom,warheads,voltage,vincennes,villains,victorian,urgency,upward,understandably,uncomplicated,uhuh,uhhhh,twitching,trig,treadmill,transactions,topped,tiffany's,they's,thermos,termination,tenorman,tater,tangle,talkative,swarm,surrendering,summoning,substances,strive,stilts,stickers,stationary,squish,squashed,spraying,spew,sparring,sorrel's,soaring,snout,snort,sneezed,slaps,skanky,singin,sidle,shreck,shortness,shorthand,shepherd's,sharper,shamed,sculptures,scanning,saga,sadist,rydell,rusik,roulette,rodi's,rockefeller,revised,resumes,restoring,respiration,reiber's,reek,recycle,recount,reacts,rabbit's,purge,purgatory,purchasing,providence,prostate,princesses,presentable,poultry,ponytail,plotted,playwright,pinot,pigtails,pianist,phillippe,philippines,peddling,paroled,owww,orchestrated,orbed,opted,offends,o'hara,noticeable,nominations,nancy's,myrtle's,music's,mope,moonlit,moines,minefield,metaphors,memoirs,mecca,maureen's,manning's,malignant,mainframe,magicks,maggots,maclaine,lobe,loathing,linking,leper,leaps,leaping,lashed,larch,larceny,lapses,ladyship,juncture,jiffy,jane's,jakov,invoke,interpreted,internally,intake,infantile,increasingly,inadmissible,implement,immense,howl,horoscope,hoof,homage,histories,hinting,hideaway,hesitating,hellbent,heddy,heckles,hat's,harmony's,hairline,gunpowder,guidelines,guatemala,gripe,gratifying,grants,governess,gorge,goebbels,gigolo,generated,gears,fuzz,frigid,freddo,freddie's,foresee,filters,filmed,fertile,fellowship,feeling's,fascination,extinction,exemplary,executioner,evident,etcetera,estimates,escorts,entity,endearing,encourages,electoral,eaters,earplugs,draped,distributors,disrupting,disagrees,dimes,devastate,detain,deposits,depositions,delicacy,delays,darklighter,dana's,cynicism,cyanide,cutters,cronus,convoy,continuous,continuance,conquering,confiding,concentrated,compartments,companions,commodity,combing,cofell,clingy,cleanse,christmases,cheered,cheekbones,charismatic,cabaret,buttle,burdened,buddhist,bruenell,broomstick,brin,brained,bozos,bontecou,bluntman,blazes,blameless,bizarro,benny's,bellboy,beaucoup,barry's,barkeep,bali,bala,bacterial,axis,awaken,astray,assailant,aslan,arlington,aria,appease,aphrodisiac,announcements,alleys,albania,aitoro's,activation,acme,yesss,wrecks,woodpecker,wondrous,window's,wimpy,willpower,widowed,wheeling,weepy,waxing,waive,vulture,videotaped,veritable,vascular,variations,untouched,unlisted,unfounded,unforeseen,two's,twinge,truffles,triggers,traipsing,toxin,tombstone,titties,tidal,thumping,thor's,thirds,therein,testicles,tenure,tenor,telephones,technicians,tarmac,talby,tackled,systematically,swirling,suicides,suckered,subtitles,sturdy,strangler,stockbroker,stitching,steered,staple,standup,squeal,sprinkler,spontaneously,splendor,spiking,spender,sovereign,snipe,snip,snagged,slum,skimming,significantly,siddown,showroom,showcase,shovels,shotguns,shoelaces,shitload,shifty,shellfish,sharpest,shadowy,sewn,seizing,seekers,scrounge,scapegoat,sayonara,satan's,saddled,rung,rummaging,roomful,romp,retained,residual,requiring,reproductive,renounce,reggie's,reformed,reconsidered,recharge,realistically,radioed,quirks,quadrant,punctual,public's,presently,practising,pours,possesses,poolhouse,poltergeist,pocketbook,plural,plots,pleasure's,plainly,plagued,pity's,pillars,picnics,pesto,pawing,passageway,partied,para,owing,openings,oneself,oats,numero,nostalgia,nocturnal,nitwit,nile,nexus,neuro,negotiated,muss,moths,mono,molecule,mixer,medicines,meanest,mcbeal,matinee,margate,marce,manipulations,manhunt,manger,magicians,maddie's,loafers,litvack,lightheaded,lifeguard,lawns,laughingstock,kodak,kink,jewellery,jessie's,jacko,itty,inhibitor,ingested,informing,indignation,incorporate,inconceivable,imposition,impersonal,imbecile,ichabod,huddled,housewarming,horizons,homicides,hobo,historically,hiccups,helsinki,hehe,hearse,harmful,hardened,gushing,gushie,greased,goddamit,gigs,freelancer,forging,fonzie,fondue,flustered,flung,flinch,flicker,flak,fixin,finalized,fibre,festivus,fertilizer,fenmore's,farted,faggots,expanded,exonerate,exceeded,evict,establishing,enormously,enforced,encrypted,emdash,embracing,embedded,elliot's,elimination,dynamics,duress,dupres,dowser,doormat,dominant,districts,dissatisfied,disfigured,disciplined,discarded,dibbs,diagram,detailing,descend,depository,defining,decorative,decoration,deathbed,death's,dazzled,da's,cuttin,cures,crowding,crepe,crater,crammed,costly,cosmopolitan,cortlandt's,copycat,coordinated,conversion,contradict,containing,constructed,confidant,condemning,conceited,computer's,commute,comatose,coleman's,coherent,clinics,clapping,circumference,chuppah,chore,choksondik,chestnuts,catastrophic,capitalist,campaigning,cabins,briault,bottomless,boop,bonnet,board's,bloomingdale's,blokes,blob,bids,berluti,beret,behavioral,beggars,bar's,bankroll,bania,athos,assassinate,arsenic,apperantly,ancestor,akron,ahhhhhh,afloat,adjacent,actresses,accordingly,accents,abe's,zipped,zeros,zeroes,zamir,yuppie,youngsters,yorkers,writ,wisest,wipes,wield,whyn't,weirdos,wednesdays,villages,vicksburg,variable,upchuck,untraceable,unsupervised,unpleasantness,unpaid,unhook,unconscionable,uncalled,turks,tumors,trappings,translating,tragedies,townie,timely,tiki,thurgood,things'll,thine,tetanus,terrorize,temptations,teamwork,tanning,tampons,tact,swarming,surfaced,supporter,stuart's,stranger's,straitjacket,stint,stimulation,steroid,statistically,startling,starry,squander,speculating,source's,sollozzo,sobriety,soar,sneaked,smithsonian,slugs,slaw,skit,skedaddle,sinker,similarities,silky,shortcomings,shipments,sheila's,severity,sellin,selective,seattle's,seasoned,scrubbed,scrooge,screwup,scrapes,schooling,scarves,saturdays,satchel,sandburg's,sandbox,salesmen,rooming,romances,revolving,revere,resulting,reptiles,reproach,reprieve,recreational,rearranging,realtor,ravine,rationalize,raffle,quoted,punchy,psychobabble,provocation,profoundly,problematic,prescriptions,preferable,praised,polishing,poached,plow,pledges,planetary,plan's,pirelli,perverts,peaked,pastures,pant,oversized,overdressed,outdid,outdated,oriental,ordinance,orbs,opponents,occurrence,nuptials,nominees,nineteenth,nefarious,mutiny,mouthpiece,motels,mopping,moon's,mongrel,monetary,mommie,missin,metaphorically,merv,mertin,memos,memento,melodrama,melancholy,measles,meaner,marches,mantel,maneuvers,maneuvering,mailroom,machine's,luring,listenin,lion's,lifeless,liege,licks,libraries,liberties,levon,legwork,lanka,lacked,kneecaps,kippur,kiddie,kaput,justifiable,jigsaw,issuing,islamic,insistent,insidious,innuendo,innit,inhabitants,individually,indicator,indecent,imaginable,illicit,hymn,hurling,humane,hospitalized,horseshit,hops,hondo,hemorrhoid,hella,healthiest,haywire,hamsters,halibut,hairbrush,hackers,guam,grouchy,grisly,griffin's,gratuitous,glutton,glimmer,gibberish,ghastly,geologist,gentler,generously,generators,geeky,gaga,furs,fuhrer,fronting,forklift,foolin,fluorescent,flats,flan,financed,filmmaking,fight's,faxes,faceless,extinguisher,expressions,expel,etched,entertainer,engagements,endangering,empress,egos,educator,ducked,dual,dramatically,dodgeball,dives,diverted,dissolved,dislocated,discrepancy,discovers,dink,devour,destroyers,derail,deputies,dementia,decisive,daycare,daft,cynic,crumbling,cowardice,cow's,covet,cornwallis,corkscrew,cookbook,conditioned,commendation,commandments,columns,coincidental,cobwebs,clouded,clogging,clicking,clasp,citizenship,chopsticks,chefs,chaps,catherine's,castles,cashing,carat,calmer,burgundy,bulldog's,brightly,brazen,brainwashing,bradys,bowing,booties,bookcase,boned,bloodsucking,blending,bleachers,bleached,belgian,bedpan,bearded,barrenger,bachelors,awwww,atop,assures,assigning,asparagus,arabs,apprehend,anecdote,amoral,alterations,alli,aladdin,aggravation,afoot,acquaintances,accommodating,accelerate,yakking,wreckage,worshipping,wladek,willya,willies,wigged,whoosh,whisked,wavelength,watered,warpath,warehouses,volts,vitro,violates,viewed,vicar,valuables,users,urging,uphill,unwise,untimely,unsavory,unresponsive,unpunished,unexplained,unconventional,tubby,trolling,treasurer,transfers,toxicology,totaled,tortoise,tormented,toothache,tingly,tina's,timmiihh,tibetan,thursdays,thoreau,terrifies,temperature's,temperamental,telegrams,ted's,technologies,teaming,teal'c's,talkie,takers,table's,symbiote,swirl,suffocate,subsequently,stupider,strapping,store's,steckler,standardized,stampede,stainless,springing,spreads,spokesperson,speeds,someway,snowflake,sleepyhead,sledgehammer,slant,slams,situation's,showgirl,shoveling,shmoopy,sharkbait,shan't,seminars,scrambling,schizophrenia,schematics,schedule's,scenic,sanitary,sandeman,saloon,sabbatical,rural,runt,rummy,rotate,reykjavik,revert,retrieved,responsive,rescheduled,requisition,renovations,remake,relinquish,rejoice,rehabilitation,recreation,reckoning,recant,rebuilt,rebadow,reassurance,reassigned,rattlesnake,ramble,racism,quor,prowess,prob,primed,pricey,predictions,prance,pothole,pocus,plains,pitches,pistols,persist,perpetrated,penal,pekar,peeling,patter,pastime,parmesan,paper's,papa's,panty,pail,pacemaker,overdrive,optic,operas,ominous,offa,observant,nothings,noooooo,nonexistent,nodded,nieces,neia,neglecting,nauseating,mutton,mutated,musket,munson's,mumbling,mowing,mouthful,mooseport,monologue,momma's,moly,mistrust,meetin,maximize,masseuse,martha's,marigold,mantini,mailer,madre,lowlifes,locksmith,livid,liven,limos,licenses,liberating,lhasa,lenin,leniency,leering,learnt,laughable,lashes,lasagne,laceration,korben,katan,kalen,jordan's,jittery,jesse's,jammies,irreplaceable,intubate,intolerant,inhaler,inhaled,indifferent,indifference,impound,imposed,impolite,humbly,holocaust,heroics,heigh,gunk,guillotine,guesthouse,grounding,groundbreaking,groom's,grips,grant's,gossiping,goatee,gnomes,gellar,fusion's,fumble,frutt,frobisher,freudian,frenchman,foolishness,flagged,fixture,femme,feeder,favored,favorable,fatso,fatigue,fatherhood,farmer's,fantasized,fairest,faintest,factories,eyelids,extravagant,extraterrestrial,extraordinarily,explicit,escalator,eros,endurance,encryption,enchantment's,eliminating,elevate,editors,dysfunction,drivel,dribble,dominican,dissed,dispatched,dismal,disarray,dinnertime,devastation,dermatologist,delicately,defrost,debutante,debacle,damone,dainty,cuvee,culpa,crucified,creeped,crayons,courtship,counsel's,convene,continents,conspicuous,congresswoman,confinement,conferences,confederate,concocted,compromises,comprende,composition,communism,comma,collectors,coleslaw,clothed,clinically,chug,chickenshit,checkin,chaotic,cesspool,caskets,cancellation,calzone,brothel,boomerang,bodega,bloods,blasphemy,black's,bitsy,bink,biff,bicentennial,berlini,beatin,beards,barbas,barbarians,backpacking,audiences,artist's,arrhythmia,array,arousing,arbitrator,aqui,appropriately,antagonize,angling,anesthetic,altercation,alice's,aggressor,adversity,adopting,acne,accordance,acathla,aaahhh,wreaking,workup,workings,wonderin,wolf's,wither,wielding,whopper,what'm,what'cha,waxed,vibrating,veterinarian,versions,venting,vasey,valor,validate,urged,upholstery,upgraded,untied,unscathed,unsafe,unlawful,uninterrupted,unforgiving,undies,uncut,twinkies,tucking,tuba,truffle,truck's,triplets,treatable,treasured,transmit,tranquility,townspeople,torso,tomei,tipsy,tinsel,timeline,tidings,thirtieth,tensions,teapot,tasks,tantrums,tamper,talky,swayed,swapping,sven,sulk,suitor,subjected,stylist,stroller,storing,stirs,statistical,standoff,staffed,squadron,sprinklers,springsteen,specimens,sparkly,song's,snowy,snobby,snatcher,smoother,smith's,sleepin,shrug,shortest,shoebox,shel,sheesh,shee,shackles,setbacks,sedatives,screeching,scorched,scanned,satyr,sammy's,sahib,rosemary's,rooted,rods,roadblock,riverbank,rivals,ridiculed,resentful,repellent,relates,registry,regarded,refugee,recreate,reconvene,recalled,rebuttal,realmedia,quizzes,questionnaire,quartet,pusher,punctured,pucker,propulsion,promo,prolong,professionalism,prized,premise,predators,portions,pleasantly,planet's,pigsty,physicist,phil's,penniless,pedestrian,paychecks,patiently,paternal,parading,pa's,overactive,ovaries,orderlies,oracles,omaha,oiled,offending,nudie,neonatal,neighborly,nectar,nautical,naught,moops,moonlighting,mobilize,mite,misleading,milkshake,mickey's,metropolitan,menial,meats,mayan,maxed,marketplace,mangled,magua,lunacy,luckier,llanview's,livestock,liters,liter,licorice,libyan,legislature,lasers,lansbury,kremlin,koreans,kooky,knowin,kilt,junkyard,jiggle,jest,jeopardized,jags,intending,inkling,inhalation,influences,inflated,inflammatory,infecting,incense,inbound,impractical,impenetrable,iffy,idealistic,i'mma,hypocrites,hurtin,humbled,hosted,homosexuality,hologram,hokey,hocus,hitchhiking,hemorrhoids,headhunter,hassled,harts,hardworking,haircuts,hacksaw,guerrilla,genitals,gazillion,gatherings,ganza's,gammy,gamesphere,fugue,fuels,forests,footwear,folly,folds,flexibility,flattened,flashlights,fives,filet,field's,famously,extenuating,explored,exceed,estrogen,envisioned,entails,emerged,embezzled,eloquent,egomaniac,dummies,duds,ducts,drowsy,drones,dragon's,drafts,doree,donovon,donny's,docked,dixon's,distributed,disorders,disguises,disclose,diggin,dickie's,detachment,deserting,depriving,demographic,delegation,defying,deductible,decorum,decked,daylights,daybreak,dashboard,darien,damnation,d'angelo's,cuddling,crunching,crickets,crazies,crayon,councilman,coughed,coordination,conundrum,contractors,contend,considerations,compose,complimented,compliance,cohaagen,clutching,cluster,clued,climbs,clader,chuck's,chromosome,cheques,checkpoint,chats,channeling,ceases,catholics,cassius,carver's,carasco,capped,capisce,cantaloupe,cancelling,campsite,camouflage,cambodia,burglars,bureaucracy,breakfasts,branding,bra'tac,book's,blueprint,bleedin,blaze's,blabbed,bisexual,bile,big's,beverages,beneficiary,battery's,basing,avert,avail,autobiography,atone,army's,arlyn,ares,architectural,approves,apothecary,anus,antiseptic,analytical,amnesty,alphabetical,alignment,aligned,aleikuum,advisory,advisors,advisement,adulthood,acquiring,accessed,zombie's,zadir,wrestled,wobbly,withnail,wheeled,whattaya,whacking,wedged,wanders,walkman,visionary,virtues,vincent's,vega's,vaginal,usage,unnamed,uniquely,unimaginable,undeniable,unconditionally,uncharted,unbridled,tweezers,tvmegasite,trumped,triumphant,trimming,tribes,treading,translates,tranquilizers,towing,tout,toontown,thunk,taps,taboo,suture,suppressing,succeeding,submission,strays,stonewall,stogie,stepdaughter,stalls,stace,squint,spouses,splashed,speakin,sounder,sorrier,sorrel,sorcerer,sombrero,solemnly,softened,socialist,snobs,snippy,snare,smoothing,slump,slimeball,slaving,sips,singular,silently,sicily,shiller,shayne's,shareholders,shakedown,sensations,seagulls,scrying,scrumptious,screamin,saucy,santoses,santos's,sanctions,roundup,roughed,rosary,robechaux,roadside,riley's,retrospect,resurrected,restoration,reside,researched,rescind,reproduce,reprehensible,repel,rendering,remodeling,religions,reconsidering,reciprocate,ratchet,rambaldi's,railroaded,raccoon,quasi,psychics,psat,promos,proclamation,problem's,prob'ly,pristine,printout,priestess,prenuptial,prediction,precedes,pouty,potter's,phoning,petersburg,peppy,pariah,parched,parcel,panes,overloaded,overdoing,operators,oldies,obesity,nymphs,nother,notebooks,nook,nikolai,nearing,nearer,mutation,municipal,monstrosity,minister's,milady,mieke,mephesto,memory's,melissa's,medicated,marshals,manilow,mammogram,mainstream,madhouse,m'lady,luxurious,luck's,lucas's,lotsa,loopy,logging,liquids,lifeboat,lesion,lenient,learner,lateral,laszlo,larva,kross,kinks,jinxed,involuntary,inventor,interim,insubordination,inherent,ingrate,inflatable,independently,incarnate,inane,imaging,hypoglycemia,huntin,humorous,humongous,hoodlum,honoured,honking,hitler's,hemorrhage,helpin,hearing's,hathor,hatching,hangar,halftime,guise,guggenheim,grrr,grotto,grandson's,grandmama,gorillas,godless,girlish,ghouls,gershwin,frosted,friday's,forwards,flutter,flourish,flagpole,finely,finder's,fetching,fatter,fated,faithfully,faction,fabrics,exposition,expo,exploits,exert,exclude,eviction,everwood's,evasion,espn,escorting,escalate,enticing,enroll,enhancement,endowed,enchantress,emerging,elopement,drills,drat,downtime,downloading,dorks,doorways,doctorate,divulge,dissociative,diss,disgraceful,disconcerting,dirtbag,deteriorating,deteriorate,destinies,depressive,dented,denim,defeating,decruz,decidedly,deactivate,daydreams,czar,curls,culprit,cues,crybaby,cruelest,critique,crippling,cretin,cranberries,cous,coupled,corvis,copped,convicts,converts,contingent,contests,complement,commend,commemorate,combinations,coastguard,cloning,cirque,churning,chock,chivalry,chemotherapy,charlotte's,chancellor's,catalogues,cartwheels,carpets,carols,canister,camera's,buttered,bureaucratic,bundt,buljanoff,bubbling,brokers,broaden,brimstone,brainless,borneo,bores,boing,bodied,billie's,biceps,beijing,bead,badmouthing,bad's,avec,autopilot,attractions,attire,atoms,atheist,ascertain,artificially,archbishop,aorta,amps,ampata,amok,alloy,allied,allenby,align,albeit,aired,aint,adjoining,accosted,abyss,absolve,aborted,aaagh,aaaaaah,your's,yonder,yellin,yearly,wyndham,wrongdoing,woodsboro,wigging,whup,wasteland,warranty,waltzed,walnuts,wallace's,vividly,vibration,verses,veggie,variation,validation,unnecessarily,unloaded,unicorns,understated,undefeated,unclean,umbrellas,tyke,twirling,turpentine,turnover,tupperware,tugger,triangles,triage,treehouse,tract,toil,tidbit,tickled,thud,threes,thousandth,thingie,terminally,temporal,teething,tassel,talkies,syndication,syllables,swoon,switchboard,swerved,suspiciously,superiority,successor,subsequentlyne,subsequent,subscribe,strudel,stroking,strictest,steven's,stensland,stefan's,starsky,starin,stannart,squirming,squealing,sorely,solidarity,softie,snookums,sniveling,snail,smidge,smallpox,sloth,slab,skulking,singled,simian,silo,sightseeing,siamese,shudder,shoppers,shax,sharpen,shannen,semtex,sellout,secondhand,season's,seance,screenplay,scowl,scorn,scandals,santiago's,safekeeping,sacked,russe,rummage,rosie's,roshman,roomies,roaches,rinds,retrace,retires,resuscitate,restrained,residential,reservoir,rerun,reputations,rekall,rejoin,refreshment,reenactment,recluse,ravioli,raves,ranked,rampant,rama,rallies,raking,purses,punishable,punchline,puked,provincial,prosky,prompted,processor,previews,prepares,poughkeepsie,poppins,polluted,placenta,pissy,petulant,peterson's,perseverance,persecution,pent,peasants,pears,pawns,patrols,pastries,partake,paramount,panky,palate,overzealous,overthrow,overs,oswald's,oskar,originated,orchids,optical,onset,offenses,obstructing,objectively,obituaries,obedient,obedience,novice,nothingness,nitrate,newer,nets,mwah,musty,mung,motherly,mooning,monique's,momentous,moby,mistaking,mistakenly,minutemen,milos,microchip,meself,merciless,menelaus,mazel,mauser,masturbate,marsh's,manufacturers,mahogany,lysistrata,lillienfield,likable,lightweight,liberate,leveled,letdown,leer,leeloo,larynx,lardass,lainey,lagged,lab's,klorel,klan,kidnappings,keyed,karmic,jive,jiggy,jeebies,isabel's,irate,iraqi,iota,iodine,invulnerable,investor,intrusive,intricate,intimidation,interestingly,inserted,insemination,inquire,innate,injecting,inhabited,informative,informants,incorporation,inclination,impure,impasse,imbalance,illiterate,i'ma,i'ii,hurled,hunts,hispanic,hematoma,help's,helen's,headstrong,harmonica,hark,handmade,handiwork,gymnasium,growling,governors,govern,gorky,gook,girdle,getcha,gesundheit,gazing,gazette,garde,galley,funnel,fred's,fossils,foolishly,fondness,flushing,floris,firearm,ferocious,feathered,fateful,fancies,fakes,faker,expressway,expire,exec,ever'body,estates,essentials,eskimos,equations,eons,enlightening,energetic,enchilada,emmi,emissary,embolism,elsinore,ecklie,drenched,drazi,doped,dogging,documentation,doable,diverse,disposed,dislikes,dishonesty,disengage,discouraging,diplomat,diplomacy,deviant,descended,derailed,depleted,demi,deformed,deflect,defines,defer,defcon,deactivated,crips,creditors,counters,corridors,cordy's,conversation's,constellations,congressmen,congo,complimenting,colombian,clubbing,clog,clint's,clawing,chromium,chimes,chicken's,chews,cheatin,chaste,ceremony's,cellblock,ceilings,cece,caving,catered,catacombs,calamari,cabbie,bursts,bullying,bucking,brulee,brits,brisk,breezes,brandon's,bounces,boudoir,blockbuster,binks,better'n,beluga,bellied,behrani,behaves,bedding,battalion,barriers,banderas,balmy,bakersfield,badmouth,backers,avenging,atat,aspiring,aromatherapy,armpit,armoire,anythin,another's,anonymously,anniversaries,alonzo's,aftershave,affordable,affliction,adrift,admissible,adieu,activist,acquittal,yucky,yearn,wrongly,wino,whitter,whirlpool,wendigo,watchdog,wannabes,walkers,wakey,vomited,voicemail,verb,vans,valedictorian,vacancy,uttered,up's,unwed,unrequited,unnoticed,unnerving,unkind,unjust,uniformed,unconfirmed,unadulterated,unaccounted,uglier,tyler's,twix,turnoff,trough,trolley,trampled,tramell,traci's,tort,toads,titled,timbuktu,thwarted,throwback,thon,thinker,thimble,tasteless,tarantula,tammy's,tamale,takeovers,symposium,symmetry,swish,supposing,supporters,suns,sully,streaking,strands,statutory,starlight,stargher,starch,stanzi,stabs,squeamish,spokane,splattered,spiritually,spilt,sped,speciality,spacious,soundtrack,smacking,slain,slag,slacking,skywire,skips,skeet,skaara,simpatico,shredding,showin,shortcuts,shite,shielding,sheep's,shamelessly,serafine,sentimentality,sect,secretary's,seasick,scientifically,scholars,schemer,scandalous,saturday's,salts,saks,sainted,rustic,rugs,riedenschneider,ric's,rhyming,rhetoric,revolt,reversing,revel,retractor,retards,retaliation,resurrect,remiss,reminiscing,remanded,reluctance,relocating,relied,reiben,regions,regains,refuel,refresher,redoing,redheaded,redeemed,recycled,reassured,rearranged,rapport,qumar,prowling,promotional,promoter,preserving,prejudices,precarious,powwow,pondering,plunger,plunged,pleasantville,playpen,playback,pioneers,physicians,phlegm,perfected,pancreas,pakistani,oxide,ovary,output,outbursts,oppressed,opal's,ooohhh,omoroca,offed,o'toole,nurture,nursemaid,nosebleed,nixon's,necktie,muttering,munchies,mucking,mogul,mitosis,misdemeanor,miscarried,minx,millionth,migraines,midler,methane,metabolism,merchants,medicinal,margaret's,manifestation,manicurist,mandelbaum,manageable,mambo,malfunctioned,mais,magnesium,magnanimous,loudmouth,longed,lifestyles,liddy,lickety,leprechauns,lengthy,komako,koji's,klute,kennel,kathy's,justifying,jerusalem,israelis,isle,irreversible,inventing,invariably,intervals,intergalactic,instrumental,instability,insinuate,inquiring,ingenuity,inconclusive,incessant,improv,impersonation,impeachment,immigrant,id'd,hyena,humperdinck,humm,hubba,housework,homeland,holistic,hoffa,hither,hissy,hippy,hijacked,hero's,heparin,hellooo,heat's,hearth,hassles,handcuff,hairstyle,hadda,gymnastics,guys'll,gutted,gulp,gulls,guard's,gritty,grievous,gravitational,graft,gossamer,gooder,glory's,gere,gash,gaming,gambled,galaxies,gadgets,fundamentals,frustrations,frolicking,frock,frilly,fraser's,francais,foreseen,footloose,fondly,fluent,flirtation,flinched,flight's,flatten,fiscal,fiercely,felicia's,fashionable,farting,farthest,farming,facade,extends,exposer,exercised,evading,escrow,errr,enzymes,energies,empathize,embryos,embodiment,ellsberg,electromagnetic,ebola,earnings,dulcinea,dreamin,drawbacks,drains,doyle's,doubling,doting,doose's,doose,doofy,dominated,dividing,diversity,disturbs,disorderly,disliked,disgusts,devoid,detox,descriptions,denominator,demonstrating,demeanor,deliriously,decode,debauchery,dartmouth,d'oh,croissant,cravings,cranked,coworkers,councilor,council's,convergence,conventions,consistency,consist,conquests,conglomerate,confuses,confiscate,confines,confesses,conduit,compress,committee's,commanded,combed,colonel's,coated,clouding,clamps,circulating,circa,cinch,chinnery,celebratory,catalogs,carpenters,carnal,carla's,captures,capitan,capability,canin,canes,caitlin's,cadets,cadaver,cable's,bundys,bulldozer,buggers,bueller,bruno's,breakers,brazilian,branded,brainy,booming,bookstores,bloodbath,blister,bittersweet,biologist,billed,betty's,bellhop,beeping,beaut,beanstalk,beady,baudelaire,bartenders,bargains,ballad,backgrounds,averted,avatar's,atmospheric,assert,assassinated,armadillo,archive,appreciating,appraised,antlers,anterior,alps,aloof,allowances,alleyway,agriculture,agent's,affleck,acknowledging,achievements,accordion,accelerator,abracadabra,abject,zinc,zilch,yule,yemen,xanax,wrenching,wreath,wouldn,witted,widely,wicca,whorehouse,whooo,whips,westchester,websites,weaponry,wasn,walsh's,vouchers,vigorous,viet,victimized,vicodin,untested,unsolicited,unofficially,unfocused,unfettered,unfeeling,unexplainable,uneven,understaffed,underbelly,tutorial,tuberculosis,tryst,trois,trix,transmitting,trampoline,towering,topeka,tirade,thieving,thang,tentacles,teflon,teachings,tablets,swimmin,swiftly,swayzak,suspecting,supplying,suppliers,superstitions,superhuman,subs,stubbornness,structures,streamers,strattman,stonewalling,stimulate,stiffs,station's,stacking,squishy,spout,splice,spec,sonrisa,smarmy,slows,slicing,sisterly,sierra's,sicilian,shrill,shined,shift's,seniority,seine,seeming,sedley,seatbelts,scour,scold,schoolyard,scarring,sash,sark's,salieri,rustling,roxbury,richly,rexy,rex's,rewire,revved,retriever,respective,reputable,repulsed,repeats,rendition,remodel,relocated,reins,reincarnation,regression,reconstruction,readiness,rationale,rance,rafters,radiohead,radio's,rackets,quarterly,quadruple,pumbaa,prosperous,propeller,proclaim,probing,privates,pried,prewedding,premeditation,posturing,posterity,posh,pleasurable,pizzeria,pish,piranha,pimps,penmanship,penchant,penalties,pelvis,patriotism,pasa,papaya,packaging,overturn,overture,overstepped,overcoat,ovens,outsmart,outed,orient,ordained,ooohh,oncologist,omission,olly,offhand,odour,occurring,nyazian,notarized,nobody'll,nightie,nightclubs,newsweek,nesting,navel,nationwide,nabbed,naah,mystique,musk,mover,mortician,morose,moratorium,monster's,moderate,mockingbird,mobsters,misconduct,mingling,mikey's,methinks,metaphysical,messengered,merge,merde,medallion,mathematical,mater,mason's,masochist,martouf,martians,marinara,manray,manned,mammal,majorly,magnifying,mackerel,mabel's,lyme,lurid,lugging,lonnegan,loathsome,llantano,liszt,listings,limiting,liberace,leprosy,latinos,lanterns,lamest,laferette,ladybird,kraut,kook,kits,kipling,joyride,inward,intestine,innocencia,inhibitions,ineffectual,indisposed,incurable,incumbent,incorporated,inconvenienced,inanimate,improbable,implode,idea's,hypothesis,hydrant,hustling,hustled,huevos,how'm,horseshoe,hooey,hoods,honcho,hinge,hijack,heroism,hermit,heimlich,harvesting,hamunaptra,haladki,haiku,haggle,haaa,gutsy,grunting,grueling,grit,grifter,grievances,gribbs,greevy,greeted,green's,grandstanding,godparents,glows,glistening,glider,gimmick,genocide,gaping,fraiser,formalities,foreigner,forecast,footprint,folders,foggy,flaps,fitty,fiends,femmes,fearful,fe'nos,favours,fabio,eyeing,extort,experimentation,expedite,escalating,erect,epinephrine,entitles,entice,enriched,enable,emissions,eminence,eights,ehhh,educating,eden's,earthquakes,earthlings,eagerly,dunville,dugout,draining,doublemeat,doling,disperse,dispensing,dispatches,dispatcher,discoloration,disapproval,diners,dieu,diddly,dictates,diazepam,descendants,derogatory,deposited,delights,defies,decoder,debates,dealio,danson,cutthroat,crumbles,crud,croissants,crematorium,craftsmanship,crafted,could'a,correctional,cordless,cools,contradiction,constitute,conked,confine,concealing,composite,complicates,communique,columbian,cockamamie,coasters,clusters,clobbered,clipping,clipboard,clergy,clemenza,cleanser,circumcision,cindy's,chisel,character's,chanukah,certainaly,centerpiece,cellmate,cartoonist,cancels,cadmium,buzzed,busiest,bumstead,bucko,browsing,broth,broader,break's,braver,boundary,boggling,bobbing,blurred,birkhead,bethesda,benet,belvedere,bellies,begrudge,beckworth,bebe's,banky,baldness,bagpipes,baggy,babysitters,aversion,auxiliary,attributes,attain,astonished,asta,assorted,aspirations,arnold's,area's,appetites,apparel,apocalyptic,apartment's,announcer,angina,amiss,ambulances,allo,alleviate,alibis,algeria,alaskan,airway,affiliated,aerial,advocating,adrenalin,admires,adhesive,actively,accompanying,zeta,yoyou,yoke,yachts,wreaked,wracking,woooo,wooing,wised,winnie's,wind's,wilshire,wedgie,watson's,warden's,waging,violets,vincey,victorious,victories,velcro,vastly,valves,valley's,uplifting,untrustworthy,unmitigated,universities,uneventful,undressing,underprivileged,unburden,umbilical,twigs,tweet,tweaking,turquoise,trustees,truckers,trimmed,triggering,treachery,trapping,tourism,tosses,torching,toothpick,toga,toasty,toasts,tiamat,thickens,ther,tereza,tenacious,temperament,televised,teldar,taxis,taint,swill,sweatin,sustaining,surgery's,surgeries,succeeds,subtly,subterranean,subject's,subdural,streep,stopwatch,stockholder,stillwater,steamer,stang's,stalkers,squished,squeegee,splinters,spliced,splat,spied,specialized,spaz,spackle,sophistication,snapshots,smoky,smite,sluggish,slithered,skin's,skeeters,sidewalks,sickly,shrugs,shrubbery,shrieking,shitless,shithole,settin,servers,serge,sentinels,selfishly,segments,scarcely,sawdust,sanitation,sangria,sanctum,samantha's,sahjhan,sacrament,saber,rustle,rupture,rump,roving,rousing,rosomorf,rosario's,rodents,robust,rigs,riddled,rhythms,revelations,restart,responsibly,repression,reporter's,replied,repairing,renoir,remoray,remedial,relocation,relies,reinforcement,refundable,redirect,recheck,ravenwood,rationalizing,ramus,ramsey's,ramelle,rails,radish,quivering,pyjamas,puny,psychos,prussian,provocations,prouder,protestors,protesters,prohibited,prohibit,progression,prodded,proctologist,proclaimed,primordial,pricks,prickly,predatory,precedents,praising,pragmatic,powerhouse,posterior,postage,porthos,populated,poly,pointe,pivotal,pinata,persistence,performers,pentangeli,pele,pecs,pathetically,parka,parakeet,panicky,pandora's,pamphlets,paired,overthruster,outsmarted,ottoman,orthopedic,oncoming,oily,offing,nutritious,nuthouse,nourishment,nietzsche,nibbling,newlywed,newcomers,need's,nautilus,narcissist,myths,mythical,mutilation,mundane,mummy's,mummies,mumble,mowed,morvern,mortem,mortal's,mopes,mongolian,molasses,modification,misplace,miscommunication,miney,militant,midlife,mens,menacing,memorizing,memorabilia,membrane,massaging,masking,maritime,mapping,manually,magnets,ma's,luxuries,lows,lowering,lowdown,lounging,lothario,longtime,liposuction,lieutenant's,lidocaine,libbets,lewd,levitate,leslie's,leeway,lectured,lauren's,launcher,launcelot,latent,larek,lagos,lackeys,kumbaya,kryptonite,knapsack,keyhole,kensington,katarangura,kann,junior's,juiced,jugs,joyful,jihad,janitor's,jakey,ironclad,invoice,intertwined,interlude,interferes,insurrection,injure,initiating,infernal,india's,indeedy,incur,incorrigible,incantations,imprint,impediment,immersion,immensely,illustrate,ike's,igloo,idly,ideally,hysterectomy,hyah,house's,hour's,hounded,hooch,honeymoon's,hollering,hogs,hindsight,highs,high's,hiatus,helix,heirs,heebie,havesham,hassan's,hasenfuss,hankering,hangers,hakuna,gutless,gusto,grubbing,grrrr,greg's,grazed,gratification,grandeur,gorak,godammit,gnawing,glanced,gladiators,generating,galahad,gaius,furnished,funeral's,fundamentally,frostbite,frees,frazzled,fraulein,fraternizing,fortuneteller,formaldehyde,followup,foggiest,flunky,flickering,flashbacks,fixtures,firecrackers,fines,filly,figger,fetuses,fella's,feasible,fates,eyeliner,extremities,extradited,expires,experimented,exiting,exhibits,exhibited,exes,excursion,exceedingly,evaporate,erupt,equilibrium,epileptic,ephram's,entrails,entities,emporium,egregious,eggshells,easing,duwayne,drone,droll,dreyfuss,drastically,dovey,doubly,doozy,donkeys,donde,dominate,distrust,distributing,distressing,disintegrate,discreetly,disagreements,diff,dick's,devised,determines,descending,deprivation,delegate,dela,degradation,decision's,decapitated,dealin,deader,dashed,darkroom,dares,daddies,dabble,cycles,cushy,currents,cupcakes,cuffed,croupier,croak,criticized,crapped,coursing,cornerstone,copyright,coolers,continuum,contaminate,cont,consummated,construed,construct,condos,concoction,compulsion,committees,commish,columnist,collapses,coercion,coed,coastal,clemency,clairvoyant,circulate,chords,chesterton,checkered,charlatan,chaperones,categorically,cataracts,carano,capsules,capitalize,cache,butcher's,burdon,bullshitting,bulge,buck's,brewed,brethren,bren,breathless,breasted,brainstorming,bossing,borealis,bonsoir,bobka,boast,blimp,bleu,bleep,bleeder,blackouts,bisque,binford's,billboards,bernie's,beecher's,beatings,bayberry,bashed,bartlet's,bapu,bamboozled,ballon,balding,baklava,baffled,backfires,babak,awkwardness,attributed,attest,attachments,assembling,assaults,asphalt,arthur's,arthritis,armenian,arbitrary,apologizes,anyhoo,antiquated,alcante,agency's,advisable,advertisement,adventurer,abundance,aahhh,aaahh,zatarc,yous,york's,yeti,yellowstone,yearbooks,yakuza,wuddya,wringing,woogie,womanhood,witless,winging,whatsa,wetting,wessex,wendy's,way's,waterproof,wastin,washington's,wary,voom,volition,volcanic,vogelman,vocation,visually,violinist,vindicated,vigilance,viewpoint,vicariously,venza,vasily,validity,vacuuming,utensils,uplink,unveil,unloved,unloading,uninhibited,unattached,ukraine,typo,tweaked,twas,turnips,tunisia,tsch,trinkets,tribune,transmitters,translator,train's,toured,toughen,toting,topside,topical,toothed,tippy,tides,theology,terrors,terrify,tentative,technologically,tarnish,target's,tallest,tailored,tagliati,szpilman,swimmers,swanky,susie's,surly,supple,sunken,summation,suds,suckin,substantially,structured,stockholm,stepmom,squeaking,springfield's,spooks,splashmore,spanked,souffle,solitaire,solicitation,solarium,smooch,smokers,smog,slugged,slobbering,skylight,skimpy,situated,sinuses,simplify,silenced,sideburns,sid's,shutdown,shrinkage,shoddy,shhhhhh,shelling,shelled,shareef,shangri,shakey's,seuss,servicing,serenade,securing,scuffle,scrolls,scoff,scholarships,scanners,sauerkraut,satisfies,satanic,sars,sardines,sarcophagus,santino,sandi's,salvy,rusted,russells,ruby's,rowboat,routines,routed,rotating,rolfsky,ringside,rigging,revered,retreated,respectability,resonance,resembling,reparations,reopened,renewal,renegotiate,reminisce,reluctantly,reimburse,regimen,regaining,rectum,recommends,recognizable,realism,reactive,rawhide,rappaport's,raincoat,quibble,puzzled,pursuits,purposefully,puns,pubic,psychotherapy,prosecution's,proofs,proofing,professor's,prevention,prescribing,prelim,positioning,pore,poisons,poaching,pizza's,pertaining,personalized,personable,peroxide,performs,pentonville,penetrated,peggy's,payphone,payoffs,participated,park's,parisian,palp,paleontology,overhaul,overflowing,organised,oompa,ojai,offenders,oddest,objecting,o'hare,o'daniel,notches,noggin,nobody'd,nitrogen,nightstand,niece's,nicky's,neutralized,nervousness,nerdy,needlessly,navigational,narrative,narc,naquadah,nappy,nantucket,nambla,myriad,mussolini,mulberry,mountaineer,mound,motherfuckin,morrie,monopolizing,mohel,mistreated,misreading,misbehave,miramax,minstrel,minivan,milligram,milkshakes,milestone,middleweight,michelangelo,metamorphosis,mesh,medics,mckinnon's,mattresses,mathesar,matchbook,matata,marys,marco's,malucci,majored,magilla,magic's,lymphoma,lowers,lordy,logistics,linens,lineage,lindenmeyer,limelight,libel,leery's,leased,leapt,laxative,lather,lapel,lamppost,laguardia,labyrinth,kindling,key's,kegs,kegger,kawalsky,juries,judo,jokin,jesminder,janine's,izzy,israeli,interning,insulation,institutionalized,inspected,innings,innermost,injun,infallible,industrious,indulgence,indonesia,incinerator,impossibility,imports,impart,illuminate,iguanas,hypnotic,hyped,huns,housed,hostilities,hospitable,hoses,horton's,homemaker,history's,historian,hirschmuller,highlighted,hideout,helpers,headset,guardianship,guapo,guantanamo,grubby,greyhound,grazing,granola,granddaddy,gotham's,goren,goblet,gluttony,glucose,globes,giorno,gillian's,getter,geritol,gassed,gang's,gaggle,freighter,freebie,frederick's,fractures,foxhole,foundations,fouled,foretold,forcibly,folklore,floorboards,floods,floated,flippers,flavour,flaked,firstly,fireflies,feedings,fashionably,fascism,farragut,fallback,factions,facials,exterminate,exited,existent,exiled,exhibiting,excites,everything'll,evenin,evaluated,ethically,entree,entirety,ensue,enema,empath,embryo,eluded,eloquently,elle,eliminates,eject,edited,edema,echoes,earns,dumpling,drumming,droppings,drazen's,drab,dolled,doll's,doctrine,distasteful,disputing,disputes,displeasure,disdain,disciples,diamond's,develops,deterrent,detection,dehydration,defied,defiance,decomposing,debated,dawned,darken,daredevil,dailies,cyst,custodian,crusts,crucifix,crowning,crier,crept,credited,craze,crawls,coveted,couple's,couldn,corresponding,correcting,corkmaster,copperfield,cooties,coopers,cooperated,controller,contraption,consumes,constituents,conspire,consenting,consented,conquers,congeniality,computerized,compute,completes,complains,communicator,communal,commits,commendable,colonels,collide,coladas,colada,clout,clooney,classmate,classifieds,clammy,claire's,civility,cirrhosis,chink,chemically,characterize,censor,catskills,cath,caterpillar,catalyst,carvers,carts,carpool,carelessness,career's,cardio,carbs,captivity,capeside's,capades,butabi,busmalis,bushel,burping,buren,burdens,bunks,buncha,bulldozers,browse,brockovich,bria,breezy,breeds,breakthroughs,bravado,brandy's,bracket,boogety,bolshevik,blossoms,bloomington,blooming,bloodsucker,blockade,blight,blacksmith,betterton,betrayer,bestseller,bennigan's,belittle,beeps,bawling,barts,bartending,barbed,bankbooks,back's,babs,babish,authors,authenticity,atropine,astronomical,assertive,arterial,armbrust,armageddon,aristotle,arches,anyanka,annoyance,anemic,anck,anago,ali's,algiers,airways,airwaves,air's,aimlessly,ails,ahab,afflicted,adverse,adhere,accuracy,aaargh,aaand,zest,yoghurt,yeast,wyndham's,writings,writhing,woven,workable,winking,winded,widen,whooping,whiter,whip's,whatya,whacko,we's,wazoo,wasp,waived,vlad,virile,vino,vic's,veterinary,vests,vestibule,versed,venetian,vaughn's,vanishes,vacancies,urkel,upwards,uproot,unwarranted,unscheduled,unparalleled,undertaking,undergrad,tweedle,turtleneck,turban,trickery,travolta,transylvania,transponder,toyed,townhouse,tonto,toed,tion,tier,thyself,thunderstorm,thnk,thinning,thinkers,theatres,thawed,tether,tempus,telegraph,technicalities,tau'ri,tarp,tarnished,tara's,taggert's,taffeta,tada,tacked,systolic,symbolize,swerve,sweepstakes,swami,swabs,suspenders,surfers,superwoman,sunsets,sumo,summertime,succulent,successes,subpoenas,stumper,stosh,stomachache,stewed,steppin,stepatech,stateside,starvation,staff's,squads,spicoli,spic,sparing,soulless,soul's,sonnets,sockets,snit,sneaker,snatching,smothering,slush,sloman,slashing,sitters,simpson's,simpleton,signify,signal's,sighs,sidra,sideshow,sickens,shunned,shrunken,showbiz,shopped,shootings,shimmering,shakespeare's,shagging,seventeenth,semblance,segue,sedation,scuzzlebutt,scumbags,scribble,screwin,scoundrels,scarsdale,scamp,scabs,saucers,sanctioned,saintly,saddened,runaways,runaround,rumored,rudimentary,rubies,rsvp,rots,roman's,ripley's,rheya,revived,residing,resenting,researcher,repertoire,rehashing,rehabilitated,regrettable,regimental,refreshed,reese's,redial,reconnecting,rebirth,ravenous,raping,ralph's,railroads,rafting,rache,quandary,pylea,putrid,punitive,puffing,psychopathic,prunes,protests,protestant,prosecutors,proportional,progressed,prod,probate,prince's,primate,predicting,prayin,practitioner,possessing,pomegranate,polgara,plummeting,planners,planing,plaintiffs,plagues,pitt's,pithy,photographer's,philharmonic,petrol,perversion,personals,perpetrators,perm,peripheral,periodic,perfecto,perched,pees,peeps,pedigree,peckish,pavarotti,partnered,palette,pajama,packin,pacifier,oyez,overstepping,outpatient,optimum,okama,obstetrician,nutso,nuance,noun,noting,normalcy,normal's,nonnegotiable,nomak,nobleman,ninny,nines,nicey,newsflash,nevermore,neutered,nether,nephew's,negligee,necrosis,nebula,navigating,narcissistic,namesake,mylie,muses,munitions,motivational,momento,moisturizer,moderation,mmph,misinformed,misconception,minnifield,mikkos,methodical,mechanisms,mebbe,meager,maybes,matchmaking,masry,markovic,manifesto,malakai,madagascar,m'am,luzhin,lusting,lumberjack,louvre,loopholes,loaning,lightening,liberals,lesbo,leotard,leafs,leader's,layman's,launder,lamaze,kubla,kneeling,kilo,kibosh,kelp,keith's,jumpsuit,joy's,jovi,joliet,jogger,janover,jakovasaurs,irreparable,intervened,inspectors,innovation,innocently,inigo,infomercial,inexplicable,indispensable,indicative,incognito,impregnated,impossibly,imperfect,immaculate,imitating,illnesses,icarus,hunches,hummus,humidity,housewives,houmfort,hothead,hostiles,hooves,hoopla,hooligans,homos,homie,hisself,himalayas,hidy,hickory,heyyy,hesitant,hangout,handsomest,handouts,haitian,hairless,gwennie,guzzling,guinevere,grungy,grunge,grenada,gout,gordon's,goading,gliders,glaring,geology,gems,gavel,garments,gardino,gannon's,gangrene,gaff,gabrielle's,fundraising,fruitful,friendlier,frequencies,freckle,freakish,forthright,forearm,footnote,footer,foot's,flops,flamenco,fixer,firm's,firecracker,finito,figgered,fezzik,favourites,fastened,farfetched,fanciful,familiarize,faire,failsafe,fahrenheit,fabrication,extravaganza,extracted,expulsion,exploratory,exploitation,explanatory,exclusion,evolutionary,everglades,evenly,eunuch,estas,escapade,erasers,entries,enforcing,endorsements,enabling,emptying,emperor's,emblem,embarassing,ecosystem,ebby,ebay,dweeb,dutiful,dumplings,drilled,drafty,doug's,dolt,dollhouse,displaced,dismissing,disgraced,discrepancies,disbelief,disagreeing,disagreed,digestion,didnt,deviled,deviated,deterioration,departmental,departing,demoted,demerol,delectable,deco,decaying,decadent,dears,daze,dateless,d'algout,cultured,cultivating,cryto,crusades,crumpled,crumbled,cronies,critters,crew's,crease,craves,cozying,cortland,corduroy,cook's,consumers,congratulated,conflicting,confidante,condensed,concessions,compressor,compressions,compression,complicating,complexity,compadre,communicated,coerce,coding,coating,coarse,clown's,clockwise,clerk's,classier,clandestine,chums,chumash,christopher's,choreography,choirs,chivalrous,chinpoko,chilean,chihuahua,cheerio,charred,chafing,celibacy,casts,caste,cashier's,carted,carryin,carpeting,carp,carotid,cannibals,candor,caen,cab's,butterscotch,busts,busier,bullcrap,buggin,budding,brookside,brodski,bristow's,brig,bridesmaid's,brassiere,brainwash,brainiac,botrelle,boatload,blimey,blaring,blackness,bipolar,bipartisan,bins,bimbos,bigamist,biebe,biding,betrayals,bestow,bellerophon,beefy,bedpans,battleship,bathroom's,bassinet,basking,basin,barzini,barnyard,barfed,barbarian,bandit,balances,baker's,backups,avid,augh,audited,attribute,attitudes,at's,astor,asteroids,assortment,associations,asinine,asalaam,arouse,architects,aqua,applejack,apparatus,antiquities,annoys,angela's,anew,anchovies,anchors,analysts,ampule,alphabetically,aloe,allure,alameida,aisles,airfield,ahah,aggressively,aggravate,aftermath,affiliation,aesthetic,advertised,advancing,adept,adage,accomplices,accessing,academics,aagh,zoned,zoey's,zeal,yokel,y'ever,wynant's,wringer,witwer,withdrew,withdrawing,withdrawals,windward,wimbledon,wily,willfully,whorfin,whimsical,whimpering,welding,weddin,weathered,wealthiest,weakening,warmest,wanton,waif,volant,vivo,vive,visceral,vindication,vikram,vigorously,verification,veggies,urinate,uproar,upload,unwritten,unwrap,unsung,unsubstantiated,unspeakably,unscrupulous,unraveling,unquote,unqualified,unfulfilled,undetectable,underlined,unconstitutional,unattainable,unappreciated,ummmm,ulcers,tylenol,tweak,tutu,turnin,turk's,tucker's,tuatha,tropez,trends,trellis,traffic's,torque,toppings,tootin,toodles,toodle,tivo,tinkering,thursday's,thrives,thorne's,thespis,thereafter,theatrics,thatherton,texts,testicle,terr,tempers,teammates,taxpayer,tavington,tampon,tackling,systematic,syndicated,synagogue,swelled,sweeney's,sutures,sustenance,surfaces,superstars,sunflowers,sumatra,sublet,subjective,stubbins,strutting,strewn,streams,stowaway,stoic,sternin,stereotypes,steadily,star's,stalker's,stabilizing,sprang,spotter,spiraling,spinster,spell's,speedometer,specified,speakeasy,sparked,soooo,songwriter,soiled,sneakin,smithereens,smelt,smacks,sloan's,slaughterhouse,slang,slacks,skids,sketching,skateboards,sizzling,sixes,sirree,simplistic,sift,side's,shouts,shorted,shoelace,sheeit,shaw's,shards,shackled,sequestered,selmak,seduces,seclusion,seasonal,seamstress,seabeas,scry,scripted,scotia,scoops,scooped,schillinger's,scavenger,saturation,satch,salaries,safety's,s'more,s'il,rudeness,rostov,romanian,romancing,robo,robert's,rioja,rifkin,rieper,revise,reunions,repugnant,replicating,replacements,repaid,renewing,remembrance,relic,relaxes,rekindle,regulate,regrettably,registering,regenerate,referenced,reels,reducing,reconstruct,reciting,reared,reappear,readin,ratting,rapes,rancho,rancher,rammed,rainstorm,railroading,queers,punxsutawney,punishes,pssst,prudy,proudest,protectors,prohibits,profiling,productivity,procrastinating,procession,proactive,priss,primaries,potomac,postmortem,pompoms,polio,poise,piping,pickups,pickings,physiology,philanthropist,phenomena,pheasant,perfectionist,peretti,people'll,peninsula,pecking,peaks,pave,patrolman,participant,paralegal,paragraphs,paparazzi,pankot,pampering,pain's,overstep,overpower,ovation,outweigh,outlawed,orion's,openness,omnipotent,oleg,okra,okie,odious,nuwanda,nurtured,niles's,newsroom,netherlands,nephews,neeson,needlepoint,necklaces,neato,nationals,muggers,muffler,mousy,mourned,mosey,morn,mormon,mopey,mongolians,moldy,moderately,modelling,misinterpret,minneapolis,minion,minibar,millenium,microfilm,metals,mendola,mended,melissande,me's,mathematician,masturbating,massacred,masbath,marler's,manipulates,manifold,malp,maimed,mailboxes,magnetism,magna,m'lord,m'honey,lymph,lunge,lull,luka,lt's,lovelier,loser's,lonigan's,lode,locally,literacy,liners,linear,lefferts,leezak,ledgers,larraby,lamborghini,laloosh,kundun,kozinski,knockoff,kissin,kiosk,khasinau's,kennedys,kellman,karlo,kaleidoscope,jumble,juggernaut,joseph's,jiminy,jesuits,jeffy,jaywalking,jailbird,itsy,irregularities,inventive,introduces,interpreter,instructing,installing,inquest,inhabit,infraction,informer,infarction,incidence,impulsively,impressing,importing,impersonated,impeach,idiocy,hyperbole,hydra,hurray,hungary,humped,huhuh,hsing,hotspot,horsepower,hordes,hoodlums,honky,hitchhiker,hind,hideously,henchmen,heaving,heathrow,heather's,heathcliff,healthcare,headgear,headboard,hazing,hawking,harem,handprint,halves,hairspray,gutiurrez,greener,grandstand,goosebumps,good's,gondola,gnaw,gnat,glitches,glide,gees,gasping,gases,garrison's,frolic,fresca,freeways,frayed,fortnight,fortitude,forgetful,forefathers,foley's,foiled,focuses,foaming,flossing,flailing,fitzgeralds,firehouse,finders,filmmakers,fiftieth,fiddler,fellah,feats,fawning,farquaad,faraway,fancied,extremists,extremes,expresses,exorcist,exhale,excel,evaluations,ethros,escalated,epilepsy,entrust,enraged,ennui,energized,endowment,encephalitis,empties,embezzling,elster,ellie's,ellen's,elixir,electrolytes,elective,elastic,edged,econ,eclectic,eagle's,duplex,dryers,drexl,dredging,drawback,drafting,don'ts,docs,dobisch,divorcee,ditches,distinguishing,distances,disrespected,disprove,disobeying,disobedience,disinfectant,discs,discoveries,dips,diplomas,dingy,digress,dignitaries,digestive,dieting,dictatorship,dictating,devoured,devise,devane's,detonators,detecting,desist,deserter,derriere,deron,derive,derivative,delegates,defects,defeats,deceptive,debilitating,deathwok,dat's,darryl's,dago,daffodils,curtsy,cursory,cuppa,cumin,cultivate,cujo,cubic,cronkite,cremation,credence,cranking,coverup,courted,countin,counselling,cornball,converting,contentment,contention,contamination,consortium,consequently,consensual,consecutive,compressed,compounds,compost,components,comparative,comparable,commenting,color's,collections,coleridge,coincidentally,cluett,cleverly,cleansed,cleanliness,clea,clare's,citizen's,chopec,chomp,cholera,chins,chime,cheswick,chessler,cheapest,chatted,cauliflower,catharsis,categories,catchin,caress,cardigan,capitalism,canopy,cana,camcorder,calorie,cackling,cabot's,bystanders,buttoned,buttering,butted,buries,burgel,bullpen,buffoon,brogna,brah,bragged,boutros,boosted,bohemian,bogeyman,boar,blurting,blurb,blowup,bloodhound,blissful,birthmark,biotech,bigot,bestest,benefited,belted,belligerent,bell's,beggin,befall,beeswax,beer's,becky's,beatnik,beaming,bazaar,bashful,barricade,banners,bangers,baja,baggoli,badness,awry,awoke,autonomy,automobiles,attica,astoria,assessing,ashram,artsy,artful,aroun,armpits,arming,arithmetic,annihilate,anise,angiogram,andre's,anaesthetic,amorous,ambiguous,ambiance,alligators,afforded,adoration,admittance,administering,adama,aclu,abydos,absorption,zonked,zhivago,zealand,zazu,youngster,yorkin,wrongfully,writin,wrappers,worrywart,woops,wonderfalls,womanly,wickedness,wichita,whoopie,wholesale,wholeheartedly,whimper,which'll,wherein,wheelchairs,what'ya,west's,wellness,welcomes,wavy,warren's,warranted,wankers,waltham,wallop,wading,wade's,wacked,vogue,virginal,vill,vets,vermouth,vermeil,verger,verbs,verbally,ventriss,veneer,vecchio's,vampira,utero,ushers,urgently,untoward,unshakable,unsettled,unruly,unrest,unmanned,unlocks,unified,ungodly,undue,undermined,undergoing,undergo,uncooperative,uncontrollably,unbeatable,twitchy,tunh,tumbler,tubs,truest,troublesome,triumphs,triplicate,tribbey,trent's,transmissions,tortures,torpedoes,torah,tongaree,tommi,tightening,thunderbolt,thunderbird,thorazine,thinly,theta,theres,testifies,terre,teenaged,technological,tearful,taxing,taldor,takashi,tach,symbolizes,symbolism,syllabus,swoops,swingin,swede,sutra,suspending,supplement,sunday's,sunburn,succumbed,subtitled,substituting,subsidiary,subdued,stuttering,stupor,stumps,strummer,strides,strategize,strangulation,stooped,stipulation,stingy,stigma,stewart's,statistic,startup,starlet,stapled,squeaks,squawking,spoilsport,splicing,spiel,spencers,specifications,spawned,spasms,spaniard,sous,softener,sodding,soapbox,snow's,smoldering,smithbauer,slogans,slicker,slasher,skittish,skepticism,simulated,similarity,silvio,signifies,signaling,sifting,sickest,sicilians,shuffling,shrivel,shortstop,sensibility,sender,seminary,selecting,segretti,seeping,securely,scurrying,scrunch,scrote,screwups,schoolteacher,schibetta's,schenkman,sawing,savin,satine,saps,sapiens,salvaging,salmonella,safeguard,sacrilege,rumpus,ruffle,rube,routing,roughing,rotted,roshman's,rondall,road's,ridding,rickshaw,rialto,rhinestone,reversible,revenues,retina,restrooms,resides,reroute,requisite,repress,replicate,repetition,removes,relationship's,regent,regatta,reflective,rednecks,redeeming,rectory,recordings,reasoned,rayed,ravell,raked,rainstorm's,raincheck,raids,raffi,racked,query,quantities,pushin,prototypes,proprietor,promotes,prometheus,promenade,projectile,progeny,profess,prodding,procure,primetime,presuming,preppy,prednisone,predecessor,potted,posttraumatic,poppies,poorhouse,pool's,polaroid,podiatrist,plucky,plowed,pledging,playroom,playhouse,play's,plait,placate,pitchfork,pissant,pinback,picketing,photographing,pharoah,petrak,petal,persecuting,perchance,penny's,pellets,peeved,peerless,payable,pauses,pathways,pathologist,pat's,parchment,papi,pagliacci,owls,overwrought,overwhelmingly,overreaction,overqualified,overheated,outward,outlines,outcasts,otherworldly,originality,organisms,opinionated,oodles,oftentimes,octane,occured,obstinate,observatory,o'er,nutritionist,nutrition,numbness,nubile,notification,notary,nooooooo,nodes,nobodies,nepotism,neighborhoods,neanderthals,musicals,mushu,murphy's,multimedia,mucus,mothering,mothballs,monogrammed,monk's,molesting,misspoke,misspelled,misconstrued,miscellaneous,miscalculated,minimums,mince,mildew,mighta,middleman,metabolic,messengers,mementos,mellowed,meditate,medicare,mayol,maximilian,mauled,massaged,marmalade,mardi,mannie,mandates,mammals,malaysia,makings,major's,maim,lundegaard,lovingly,lout,louisville,loudest,lotto,loosing,loompa,looming,longs,lodging,loathes,littlest,littering,linebacker,lifelike,li'l,legalities,lavery's,laundered,lapdog,lacerations,kopalski,knobs,knitted,kittridge,kidnaps,kerosene,katya,karras,jungles,juke,joes,jockeys,jeremy's,jefe,janeiro,jacqueline's,ithaca,irrigation,iranoff,invoices,invigorating,intestinal,interactive,integration,insolence,insincere,insectopia,inhumane,inhaling,ingrates,infrastructure,infestation,infants,individuality,indianapolis,indeterminate,indefinite,inconsistent,incomprehensible,inaugural,inadequacy,impropriety,importer,imaginations,illuminating,ignited,ignite,iggy,i'da,hysterics,hypodermic,hyperventilate,hypertension,hyperactive,humoring,hotdogs,honeymooning,honed,hoist,hoarding,hitching,hinted,hill's,hiker,hijo,hightail,highlands,hemoglobin,helo,hell'd,heinie,hanoi,hags,gush,guerrillas,growin,grog,grissom's,gregory's,grasped,grandparent,granddaughters,gouged,goblins,gleam,glades,gigantor,get'em,geriatric,geared,gawk,gawd,gatekeeper,gargoyles,gardenias,garcon,garbo,gallows,gabe's,gabby's,gabbing,futon,fulla,frightful,freshener,freedoms,fountains,fortuitous,formulas,forceps,fogged,fodder,foamy,flogging,flaun,flared,fireplaces,firefighters,fins,filtered,feverish,favell,fattest,fattening,fate's,fallow,faculties,fabricated,extraordinaire,expressly,expressive,explorers,evade,evacuating,euclid,ethanol,errant,envied,enchant,enamored,enact,embarking,election's,egocentric,eeny,dussander,dunwitty,dullest,dru's,dropout,dredged,dorsia,dormitory,doot,doornail,dongs,dogged,dodgy,do's,ditty,dishonorable,discriminating,discontinue,dings,dilly,diffuse,diets,dictation,dialysis,deteriorated,delly,delightfully,definitions,decreased,declining,deadliest,daryll,dandruff,cynthia's,cush,cruddy,croquet,crocodiles,cringe,crimp,credo,cranial,crackling,coyotes,courtside,coupling,counteroffer,counterfeiting,corrupting,corrective,copter,copping,conway's,conveyor,contusions,contusion,conspirator,consoling,connoisseur,conjecture,confetti,composure,competitor,compel,commanders,coloured,collector's,colic,coldest,coincide,coddle,cocksuckers,coax,coattails,cloned,cliff's,clerical,claustrophobia,classrooms,clamoring,civics,churn,chugga,chromosomes,christened,chopper's,chirping,chasin,characterized,chapped,chalkboard,centimeter,caymans,catheter,caspian,casings,cartilage,carlton's,card's,caprica,capelli,cannolis,cannoli,canals,campaigns,camogli,camembert,butchers,butchered,busboys,bureaucrats,bungalow,buildup,budweiser,buckled,bubbe,brownstone,bravely,brackley,bouquets,botox,boozing,boosters,bodhi,blunders,blunder,blockage,blended,blackberry,bitch's,birthplace,biocyte,biking,bike's,betrays,bestowed,bested,beryllium,beheading,beginner's,beggar,begbie,beamed,bayou,bastille,bask,barstool,barricades,baron's,barbecues,barbecued,barb's,bandwagon,bandits,ballots,ballads,backfiring,bacarra,avoidance,avenged,autopsies,austrian,aunties,attache,atrium,associating,artichoke,arrowhead,arrivals,arose,armory,appendage,apostrophe,apostles,apathy,antacid,ansel,anon,annul,annihilation,andrew's,anderson's,anastasia's,amuses,amped,amicable,amendments,amberg,alluring,allotted,alfalfa,alcoholism,airs,ailing,affinity,adversaries,admirers,adlai,adjective,acupuncture,acorn,abnormality,aaaahhhh,zooming,zippity,zipping,zeroed,yuletide,yoyodyne,yengeese,yeahhh,xena,wrinkly,wracked,wording,withered,winks,windmills,widow's,whopping,wholly,wendle,weigart,weekend's,waterworks,waterford,waterbed,watchful,wantin,wally's,wail,wagging,waal,waaah,vying,voter,ville,vertebrae,versatile,ventures,ventricle,varnish,vacuumed,uugh,utilities,uptake,updating,unreachable,unprovoked,unmistakable,unky,unfriendly,unfolding,undesirable,undertake,underpaid,uncuff,unchanged,unappealing,unabomber,ufos,tyres,typhoid,tweek's,tuxedos,tushie,turret,turds,tumnus,tude,truman's,troubadour,tropic,trinium,treaters,treads,transpired,transient,transgression,tournaments,tought,touchdowns,totem,tolstoy,thready,thins,thinners,thas,terrible's,television's,techs,teary,tattaglia,tassels,tarzana,tape's,tanking,tallahassee,tablecloths,synonymous,synchronize,symptomatic,symmetrical,sycophant,swimmingly,sweatshop,surrounds,surfboard,superpowers,sunroom,sunflower,sunblock,sugarplum,sudan,subsidies,stupidly,strumpet,streetcar,strategically,strapless,straits,stooping,stools,stifler,stems,stealthy,stalks,stairmaster,staffer,sshhh,squatting,squatters,spores,spelt,spectacularly,spaniel,soulful,sorbet,socked,society's,sociable,snubbed,snub,snorting,sniffles,snazzy,snakebite,smuggler,smorgasbord,smooching,slurping,sludge,slouch,slingshot,slicer,slaved,skimmed,skier,sisterhood,silliest,sideline,sidarthur,shrink's,shipwreck,shimmy,sheraton,shebang,sharpening,shanghaied,shakers,sendoff,scurvy,scoliosis,scaredy,scaled,scagnetti,saxophone,sawchuk,saviour,saugus,saturated,sasquatch,sandbag,saltines,s'pose,royalties,routinely,roundabout,roston,rostle,riveting,ristle,righ,rifling,revulsion,reverently,retrograde,restriction,restful,resolving,resents,rescinded,reptilian,repository,reorganize,rentals,rent's,renovating,renal,remedies,reiterate,reinvent,reinmar,reibers,reechard,recuse,recorders,record's,reconciling,recognizance,recognised,reclaiming,recitation,recieved,rebate,reacquainted,rations,rascals,raptors,railly,quintuplets,quahog,pygmies,puzzling,punctuality,psychoanalysis,psalm,prosthetic,proposes,proms,proliferation,prohibition,probie,printers,preys,pretext,preserver,preppie,prag,practise,postmaster,portrayed,pollen,polled,poachers,plummet,plumbers,pled,plannin,pitying,pitfalls,piqued,pinecrest,pinches,pillage,pigheaded,pied,physique,pessimistic,persecute,perjure,perch,percentile,pentothal,pensky,penises,peking,peini,peacetime,pazzi,pastels,partisan,parlour,parkway,parallels,paperweight,pamper,palsy,palaces,pained,overwhelm,overview,overalls,ovarian,outrank,outpouring,outhouse,outage,ouija,orbital,old's,offset,offer's,occupying,obstructed,obsessions,objectives,obeying,obese,o'riley,o'neal,o'higgins,nylon,notoriously,nosebleeds,norman's,norad,noooooooo,nononono,nonchalant,nominal,nome,nitrous,nippy,neurosis,nekhorvich,necronomicon,nativity,naquada,nano,nani,n'est,mystik,mystified,mums,mumps,multinational,muddle,mothership,moped,monumentally,monogamous,mondesi,molded,mixes,misogynistic,misinterpreting,miranda's,mindlock,mimic,midtown,microphones,mending,megaphone,meeny,medicating,meanings,meanie,masseur,maru,marshal's,markstrom,marklars,mariachi,margueritas,manifesting,maintains,mail's,maharajah,lurk,lulu's,lukewarm,loveliest,loveable,lordship,looting,lizardo,liquored,lipped,lingers,limey,limestone,lieutenants,lemkin,leisurely,laureate,lathe,latched,lars,lapping,ladle,kuala,krevlorneswath,kosygin,khakis,kenaru,keats,kath,kaitlan,justin's,julliard,juliet's,journeys,jollies,jiff,jaundice,jargon,jackals,jabot's,invoked,invisibility,interacting,instituted,insipid,innovative,inflamed,infinitely,inferiority,inexperience,indirectly,indications,incompatible,incinerated,incinerate,incidental,incendiary,incan,inbred,implicitly,implicating,impersonator,impacted,ida's,ichiro,iago,hypo,hurricanes,hunks,host's,hospice,horsing,hooded,honey's,homestead,hippopotamus,hindus,hiked,hetson,hetero,hessian,henslowe,hendler,hellstrom,hecate,headstone,hayloft,hater,hast,harold's,harbucks,handguns,hallucinate,halliwell's,haldol,hailing,haggling,hadj,gynaecologist,gumball,gulag,guilder,guaranteeing,groundskeeper,ground's,grindstone,grimoir,grievance,griddle,gribbit,greystone,graceland,gooders,goeth,glossy,glam,giddyup,gentlemanly,gels,gelatin,gazelle,gawking,gaulle,gate's,ganged,fused,fukes,fromby,frenchmen,franny,foursome,forsley,foreman's,forbids,footwork,foothold,fonz,fois,foie,floater,flinging,flicking,fittest,fistfight,fireballs,filtration,fillings,fiddling,festivals,fertilization,fennyman,felonious,felonies,feces,favoritism,fatten,fanfare,fanatics,faceman,extensions,executions,executing,excusing,excepted,examiner's,ex's,evaluating,eugh,erroneous,enzyme,envoy,entwined,entrances,ensconced,enrollment,england's,enemy's,emit,emerges,embankment,em's,ellison's,electrons,eladio,ehrlichman,easterland,dylan's,dwellers,dueling,dubbed,dribbling,drape,doze,downtrodden,doused,dosed,dorleen,dopamine,domesticated,dokie,doggone,disturbances,distort,displeased,disown,dismount,disinherited,disarmed,disapproves,disabilities,diperna,dioxide,dined,diligent,dicaprio,diameter,dialect,detonated,destitute,designate,depress,demolish,demographics,degraded,deficient,decoded,debatable,dealey,darsh,dapper,damsels,damning,daisy's,dad'll,d'oeuvre,cutter's,curlers,curie,cubed,cryo,critically,crikey,crepes,crackhead,countrymen,count's,correlation,cornfield,coppers,copilot,copier,coordinating,cooing,converge,contributor,conspiracies,consolidated,consigliere,consecrated,configuration,conducts,condoning,condemnation,communities,commoner,commies,commented,comical,combust,comas,colds,clod,clique,clay's,clawed,clamped,cici,christianity,choosy,chomping,chimps,chigorin,chianti,cheval,chet's,cheep,checkups,check's,cheaters,chase's,charted,celibate,cautiously,cautionary,castell,carpentry,caroling,carjacking,caritas,caregiver,cardiology,carb,capturing,canteen,candlesticks,candies,candidacy,canasta,calendars,cain't,caboose,buster's,burro,burnin,buon,bunking,bumming,bullwinkle,budgets,brummel,brooms,broadcasts,britt's,brews,breech,breathin,braslow,bracing,bouts,botulism,bosnia,boorish,bluenote,bloodless,blayne,blatantly,blankie,birdy,bene,beetles,bedbugs,becuase,becks,bearers,bazooka,baywatch,bavarian,baseman,bartender's,barrister,barmaid,barges,bared,baracus,banal,bambino,baltic,baku,bakes,badminton,bacon's,backpacks,authorizing,aurelius,attentions,atrocious,ativan,athame,asunder,astound,assuring,aspirins,asphyxiation,ashtrays,aryans,artistry,arnon,aren,approximate,apprehension,appraisal,applauding,anya's,anvil,antiquing,antidepressants,annoyingly,amputate,altruistic,alotta,allegation,alienation,algerian,algae,alerting,airport's,aided,agricultural,afterthought,affront,affirm,adapted,actuality,acoustics,acoustic,accumulate,accountability,abysmal,absentee,zimm,yves,yoohoo,ymca,yeller,yakushova,wuzzy,wriggle,worrier,workmen,woogyman,womanizer,windpipe,windex,windbag,willy's,willin,widening,whisking,whimsy,wendall,weeny,weensy,weasels,watery,watcha,wasteful,waski,washcloth,wartime,waaay,vowel,vouched,volkswagen,viznick,visuals,visitor's,veteran's,ventriloquist,venomous,vendors,vendettas,veils,vehicular,vayhue,vary,varies,van's,vamanos,vadimus,uuhh,upstage,uppity,upheaval,unsaid,unlocking,universally,unintentionally,undisputed,undetected,undergraduate,undergone,undecided,uncaring,unbearably,twos,tween,tuscan,turkey's,tumor's,tryout,trotting,tropics,trini,trimmings,trickier,tree's,treatin,treadstone,trashcan,transports,transistor,transcendent,tramps,toxicity,townsfolk,torturous,torrid,toothpicks,tombs,tolerable,toenail,tireless,tiptoeing,tins,tinkerbell,tink,timmay,tillinghouse,tidying,tibia,thumbing,thrusters,thrashing,thompson's,these'll,testicular,terminology,teriyaki,tenors,tenacity,tellers,telemetry,teas,tea's,tarragon,taliban,switchblade,swicker,swells,sweatshirts,swatches,swatch,swapped,suzanne's,surging,supremely,suntan,sump'n,suga,succumb,subsidize,subordinate,stumbles,stuffs,stronghold,stoppin,stipulate,stewie's,stenographer,steamroll,stds,stately,stasis,stagger,squandered,splint,splendidly,splatter,splashy,splashing,spectra's,specter,sorry's,sorcerers,soot,somewheres,somber,solvent,soldier's,soir,snuggled,snowmobile,snowball's,sniffed,snake's,snags,smugglers,smudged,smirking,smearing,slings,sleet,sleepovers,sleek,slackers,skirmish,siree,siphoning,singed,sincerest,signifying,sidney's,sickened,shuffled,shriveled,shorthanded,shittin,shish,shipwrecked,shins,shingle,sheetrock,shawshank,shamu,sha're,servitude,sequins,seinfeld's,seat's,seascape,seam,sculptor,scripture,scrapings,scoured,scoreboard,scorching,sciences,sara's,sandpaper,salvaged,saluting,salud,salamander,rugrats,ruffles,ruffled,rudolph's,router,roughnecks,rougher,rosslyn,rosses,rosco's,roost,roomy,romping,romeo's,robs,roadie,ride's,riddler,rianna's,revolutionize,revisions,reuniting,retake,retaining,restitution,restaurant's,resorts,reputed,reprimanded,replies,renovate,remnants,refute,refrigerated,reforms,reeled,reefs,reed's,redundancies,rectangle,rectal,recklessly,receding,reassignment,rearing,reapers,realms,readout,ration,raring,ramblings,racetrack,raccoons,quoi,quell,quarantined,quaker,pursuant,purr,purging,punters,pulpit,publishers,publications,psychologists,psychically,provinces,proust,protocols,prose,prophets,project's,priesthood,prevailed,premarital,pregnancies,predisposed,precautionary,poppin,pollute,pollo,podunk,plums,plaything,plateau,pixilated,pivot,pitting,piranhas,pieced,piddles,pickled,picker,photogenic,phosphorous,phases,pffft,petey's,pests,pestilence,pessimist,pesos,peruvian,perspiration,perps,penticoff,pedals,payload,passageways,pardons,paprika,paperboy,panics,pancamo,pam's,paleontologist,painting's,pacifist,ozzie,overwhelms,overstating,overseeing,overpaid,overlap,overflow,overdid,outspoken,outlive,outlaws,orthodontist,orin,orgies,oreos,ordover,ordinates,ooooooh,oooohhh,omelettes,officiate,obtuse,obits,oakwood,nymph,nutritional,nuremberg,nozzle,novocaine,notable,noooooooooo,node,nipping,nilly,nikko,nightstick,nicaragua,neurology,nelson's,negate,neatness,natured,narrowly,narcotic,narcissism,napoleon's,nana's,namun,nakatomi,murky,muchacho,mouthwash,motzah,motherfucker's,mortar,morsel,morrison's,morph,morlocks,moreover,mooch,monoxide,moloch,molest,molding,mohra,modus,modicum,mockolate,mobility,missionaries,misdemeanors,miscalculation,minorities,middies,metric,mermaids,meringue,mercilessly,merchandising,ment,meditating,me'n,mayakovsky,maximillian,martinique,marlee,markovski,marissa's,marginal,mansions,manitoba,maniacal,maneuvered,mags,magnificence,maddening,lyrical,lutze,lunged,lovelies,lou's,lorry,loosening,lookee,liver's,liva,littered,lilac,lightened,lighted,licensing,lexington,lettering,legality,launches,larvae,laredo,landings,lancelot's,laker,ladyship's,laces,kurzon,kurtzweil,kobo,knowledgeable,kinship,kind've,kimono,kenji,kembu,keanu,kazuo,kayaking,juniors,jonesing,joad,jilted,jiggling,jewelers,jewbilee,jeffrey's,jamey's,jacqnoud,jacksons,jabs,ivories,isnt,irritation,iraqis,intellectuals,insurmountable,instances,installments,innocuous,innkeeper,inna,influencing,infantery,indulged,indescribable,incorrectly,incoherent,inactive,inaccurate,improperly,impervious,impertinent,imperfections,imhotep,ideology,identifies,i'il,hymns,huts,hurdles,hunnert,humpty,huffy,hourly,horsies,horseradish,hooo,honours,honduras,hollowed,hogwash,hockley,hissing,hiromitsu,hierarchy,hidin,hereafter,helpmann,haughty,happenings,hankie,handsomely,halliwells,haklar,haise,gunsights,gunn's,grossly,grossed,grope,grocer,grits,gripping,greenpeace,granddad's,grabby,glorificus,gizzard,gilardi,gibarian,geminon,gasses,garnish,galloping,galactic,gairwyn,gail's,futterman,futility,fumigated,fruitless,friendless,freon,fraternities,franc,fractions,foxes,foregone,forego,foliage,flux,floored,flighty,fleshy,flapjacks,fizzled,fittings,fisherman's,finalist,ficus,festering,ferragamo's,federation,fatalities,farbman,familial,famed,factual,fabricate,eyghon,extricate,exchanges,exalted,evolving,eventful,esophagus,eruption,envision,entre,enterprising,entail,ensuring,enrolling,endor,emphatically,eminent,embarrasses,electroshock,electronically,electrodes,efficiently,edinburgh,ecstacy,ecological,easel,dwarves,duffle,drumsticks,drake's,downstream,downed,dollface,divas,distortion,dissent,dissection,dissected,disruptive,disposing,disparaging,disorientation,disintegrated,discounts,disarming,dictated,devoting,deviation,detective's,dessaline,deprecating,deplorable,delve,deity,degenerative,deficiencies,deduct,decomposed,deceased's,debbie's,deathly,dearie,daunting,dankova,czechoslovakia,cyclotron,cyberspace,cutbacks,cusp,culpable,cuddled,crypto,crumpets,cruises,cruisers,cruelly,crowns,crouching,cristo,crip,criminology,cranium,cramming,cowering,couric,counties,cosy,corky's,cordesh,conversational,conservatory,conklin's,conducive,conclusively,competitions,compatibility,coeur,clung,cloud's,clotting,cleanest,classify,clambake,civilizations,cited,cipher,cinematic,chlorine,chipping,china's,chimpanzee,chests,checkpoints,cheapen,chainsaws,censure,censorship,cemeteries,celebrates,ceej,cavities,catapult,cassettes,cartridge,caravaggio,carats,captivating,cancers,campuses,campbell's,calrissian,calibre,calcutta,calamity,butt's,butlers,busybody,bussing,bureau's,bunion,bundy's,bulimic,bulgaria,budging,brung,browbeat,brokerage,brokenhearted,brecher,breakdowns,braun's,bracebridge,boyhood,botanical,bonuses,boning,blowhard,bloc,blisters,blackboard,blackbird,births,birdies,bigotry,biggy,bibliography,bialy,bhamra,bethlehem,bet's,bended,belgrade,begat,bayonet,bawl,battering,baste,basquiat,barrymore,barrington's,barricaded,barometer,balsom's,balled,ballast,baited,badenweiler,backhand,aztec,axle,auschwitz,astrophysics,ascenscion,argumentative,arguably,arby's,arboretum,aramaic,appendicitis,apparition,aphrodite,anxiously,antagonistic,anomalies,anne's,angora,anecdotes,anand,anacott,amniotic,amenities,ambience,alonna,aleck,albert's,akashic,airing,ageless,afro,affiliates,advertisers,adobe,adjustable,acrobat,accommodation,accelerating,absorbing,abouts,abortions,abnormalities,aawwww,aaaaarrrrrrggghhh,zuko's,zoloft,zendi,zamboni,yuppies,yodel,y'hear,wyck,wrangle,wounding,worshippers,worker's,worf,wombosi,wittle,withstanding,wisecracks,williamsburg,wilder's,wiggly,wiggling,wierd,whittlesley,whipper,whattya,whatsamatter,whatchamacallit,whassup,whad'ya,weighted,weakling,waxy,waverly,wasps,warhol,warfarin,waponis,wampum,walled,wadn't,waco,vorash,vogler's,vizzini,visas,virtucon,viridiana,veve,vetoed,vertically,veracity,ventricular,ventilated,varicose,varcon,vandalized,vampire's,vamos,vamoose,val's,vaccinated,vacationing,usted,urinal,uppers,upkeep,unwittingly,unsigned,unsealed,unplanned,unhinged,unhand,unfathomable,unequivocally,unearthed,unbreakable,unanimously,unadvisedly,udall,tynacorp,twisty,tuxes,tussle,turati,tunic,tubing,tsavo,trussed,troublemakers,trollop,trip's,trinket,trilogy,tremors,trekkie,transsexual,transitional,transfusions,tractors,toothbrushes,toned,toke,toddlers,titan's,tita,tinted,timon,timeslot,tightened,thundering,thorpey,thoracic,this'd,thespian,therapist's,theorem,thaddius,texan,tenuous,tenths,tenement,telethon,teleprompter,technicolor,teaspoon,teammate,teacup,taunted,tattle,tardiness,taraka,tappy,tapioca,tapeworm,tanith,tandem,talons,talcum,tais,tacks,synchronized,swivel,swig,swaying,swann's,suppression,supplements,superpower,summed,summarize,sumbitch,sultry,sulfur,sues,subversive,suburbia,substantive,styrofoam,stylings,struts,strolls,strobe,streaks,strategist,stockpile,stewardesses,sterilized,sterilize,stealin,starred,stakeouts,stad,squawk,squalor,squabble,sprinkled,sportsmanship,spokes,spiritus,spectators,specialties,sparklers,spareribs,sowing,sororities,sorbonne,sonovabitch,solicit,softy,softness,softening,socialite,snuggling,snatchers,snarling,snarky,snacking,smythe's,smears,slumped,slowest,slithering,sleepers,sleazebag,slayed,slaughtering,skynet,skidded,skated,sivapathasundaram,sitter's,sitcoms,sissies,sinai,silliness,silences,sidecar,sicced,siam,shylock,shtick,shrugged,shriek,shredder,shoves,should'a,shorten,shortcake,shockingly,shirking,shelly's,shedding,shaves,shatner,sharpener,shapely,shafted,sexless,sequencing,septum,semitic,selflessness,sega,sectors,seabea,scuff,screwball,screened,scoping,scooch,scolding,scholarly,schnitzel,schemed,scalper,sayings,saws,sashimi,santy,sankara,sanest,sanatorium,sampled,samoan,salzburg,saltwater,salma,salesperson,sakulos,safehouse,sabers,rwanda,ruth's,runes,rumblings,rumbling,ruijven,roxie's,round's,ringers,rigorous,righto,rhinestones,reviving,retrieving,resorted,reneging,remodelling,reliance,relentlessly,relegated,relativity,reinforced,reigning,regurgitate,regulated,refills,referencing,reeking,reduces,recreated,reclusive,recklessness,recanted,ranges,ranchers,rallied,rafer,racy,quintet,quaking,quacks,pulses,provision,prophesied,propensity,pronunciation,programmer,profusely,procedural,problema,principals,prided,prerequisite,preferences,preceded,preached,prays,postmark,popsicles,poodles,pollyanna,policing,policeman's,polecat,polaroids,polarity,pokes,poignant,poconos,pocketful,plunging,plugging,pleeease,pleaser,platters,pitied,pinetti,piercings,phyllis's,phooey,phonies,pestering,periscope,perennial,perceptions,pentagram,pelts,patronized,parliamentary,paramour,paralyze,paraguay,parachutes,pancreatic,pales,paella,paducci,oxymoron,owatta,overpass,overgrown,overdone,overcrowded,overcompensating,overcoming,ostracized,orphaned,organise,organisation,ordinate,orbiting,optometrist,oprah's,operandi,oncology,on's,omoc,omens,okayed,oedipal,occupants,obscured,oboe,nuys,nuttier,nuptial,nunheim,noxious,nourish,notepad,notation,nordic,nitroglycerin,niki's,nightmare's,nightlife,nibblet,neuroses,neighbour's,navy's,nationally,nassau,nanosecond,nabbit,mythic,murdock's,munchkins,multiplied,multimillion,mulroney,mulch,mucous,muchas,moxie,mouth's,mountaintop,mounds,morlin,mongorians,moneymaker,moneybags,monde,mom'll,molto,mixup,mitchell's,misgivings,misery's,minerals,mindset,milo's,michalchuk,mesquite,mesmerized,merman,mensa,megan's,media's,meaty,mbwun,materialize,materialistic,mastery,masterminded,mastercard,mario's,marginally,mapuhe,manuscripts,manny's,malvern,malfunctioning,mahatma,mahal,magnify,macnamara,macinerney,machinations,macarena,macadamia,lysol,luxembourg,lurks,lumpur,luminous,lube,lovelorn,lopsided,locator,lobbying,litback,litany,linea,limousines,limo's,limes,lighters,liechtenstein,liebkind,lids,libya,levity,levelheaded,letterhead,lester's,lesabre,leron,lepers,legions,lefts,leftenant,learner's,laziness,layaway,laughlan,lascivious,laryngitis,laptops,lapsed,laos,landok,landfill,laminated,laden,ladders,labelled,kyoto,kurten,kobol,koala,knucklehead,knowed,knotted,kit's,kinsa,kiln,kickboxing,karnovsky,karat,kacl's,judiciary,judaism,journalistic,jolla,joked,jimson,jettison,jet's,jeric,jeeves,jay's,jawed,jankis,janitors,janice's,jango,jamaican,jalopy,jailbreak,jackers,jackasses,j'ai,ivig,invalidate,intoxicated,interstellar,internationally,intercepting,intercede,integrate,instructors,insinuations,insignia,inn's,inflicting,infiltrated,infertile,ineffective,indies,indie,impetuous,imperialist,impaled,immerse,immaterial,imbeciles,imam,imagines,idyllic,idolized,icebox,i'd've,hypochondriac,hyphen,hydraulic,hurtling,hurried,hunchback,hums,humid,hullo,hugger,hubby's,howard's,hostel,horsting,horned,hoooo,homies,homeboys,hollywood's,hollandaise,hoity,hijinks,heya,hesitates,herrero,herndorff,hemp,helplessly,heeyy,heathen,hearin,headband,harv,harrassment,harpies,harmonious,harcourt,harbors,hannah's,hamstring,halstrom,hahahahaha,hackett's,hacer,gunmen,guff,grumbling,grimlocks,grift,greets,grandmothers,grander,granddaughter's,gran's,grafts,governing,gordievsky,gondorff,godorsky,goddesses,glscripts,gillman's,geyser,gettysburg,geological,gentlemen's,genome,gauntlet,gaudy,gastric,gardeners,gardener's,gandolf,gale's,gainful,fuses,fukienese,fucker's,frizzy,freshness,freshening,freb,fraught,frantically,fran's,foxbooks,fortieth,forked,forfeited,forbidding,footed,foibles,flunkies,fleur,fleece,flatbed,flagship,fisted,firefight,fingerpaint,fined,filibuster,fiancee's,fhloston,ferrets,fenceline,femur,fellow's,fatigues,farmhouse,fanucci,fantastically,familiars,falafel,fabulously,eyesore,extracting,extermination,expedient,expectancy,exiles,executor,excluding,ewwww,eviscerated,eventual,evac,eucalyptus,ethnicity,erogenous,equestrian,equator,epidural,enrich,endeavors,enchante,embroidered,embarassed,embarass,embalming,emails,elude,elspeth,electrocute,electrified,eigth,eheh,eggshell,eeyy,echinacea,eases,earpiece,earlobe,dwarfs,dumpsters,dumbshit,dumbasses,duloc,duisberg,drummed,drinkers,dressy,drainage,dracula's,dorma,dolittle,doily,divvy,diverting,ditz,dissuade,disrespecting,displacement,displace,disorganized,dismantled,disgustingly,discriminate,discord,disapproving,dinero,dimwit,diligence,digitally,didja,diddy,dickless,diced,devouring,devlin's,detach,destructing,desperado,desolate,designation,derek's,deposed,dependency,dentist's,demonstrates,demerits,delirium,degrade,deevak,deemesa,deductions,deduce,debriefed,deadbeats,dazs,dateline,darndest,damnable,dalliance,daiquiri,d'agosta,cuvee's,cussing,curate,cryss,cripes,cretins,creature's,crapper,crackerjack,cower,coveting,couriers,countermission,cotswolds,cornholio,copa,convinces,convertibles,conversationalist,contributes,conspirators,consorting,consoled,conservation,consarn,confronts,conformity,confides,confidentially,confederacy,concise,competence,commited,commissioners,commiserate,commencing,comme,commandos,comforter,comeuppance,combative,comanches,colosseum,colling,collaboration,coli,coexist,coaxing,cliffside,clayton's,clauses,cia's,chuy,chutes,chucked,christian's,chokes,chinaman,childlike,childhoods,chickening,chicano,chenowith,chassis,charmingly,changin,championships,chameleon,ceos,catsup,carvings,carlotta's,captioning,capsize,cappucino,capiche,cannonball,cannibal,candlewell,cams,call's,calculation,cakewalk,cagey,caesar's,caddie,buxley,bumbling,bulky,bulgarian,bugle,buggered,brussel,brunettes,brumby,brotha,bros,bronck,brisket,bridegroom,breathing's,breakout,braveheart,braided,bowled,bowed,bovary,bordering,bookkeeper,bluster,bluh,blue's,blot,bloodline,blissfully,blarney,binds,billionaires,billiard,bide,bicycles,bicker,berrisford,bereft,berating,berate,bendy,benches,bellevue,belive,believers,belated,beikoku,beens,bedspread,bed's,bear's,bawdy,barrett's,barreling,baptize,banya,balthazar,balmoral,bakshi,bails,badgered,backstreet,backdrop,awkwardly,avoids,avocado,auras,attuned,attends,atheists,astaire,assuredly,art's,arrivederci,armaments,arises,argyle,argument's,argentine,appetit,appendectomy,appealed,apologetic,antihistamine,antigua,anesthesiologist,amulets,algonquin,alexander's,ales,albie,alarmist,aiight,agility,aforementioned,adstream,adolescents,admirably,adjectives,addison's,activists,acquaint,acids,abound,abominable,abolish,abode,abfc,aaaaaaah,zorg,zoltan,zoe's,zekes,zatunica,yama,wussy,wrcw,worded,wooed,woodrell,wiretap,windowsill,windjammer,windfall,whitey's,whitaker's,whisker,whims,whatiya,whadya,westerns,welded,weirdly,weenies,webster's,waunt,washout,wanto,waning,vitality,vineyards,victimless,vicki's,verdad,veranda,vegan,veer,vandaley,vancouver,vancomycin,valise,validated,vaguest,usefulness,upshot,uprising,upgrading,unzip,unwashed,untrained,unsuitable,unstuck,unprincipled,unmentionables,unjustly,unit's,unfolds,unemployable,uneducated,unduly,undercut,uncovering,unconsciousness,unconsciously,unbeknownst,unaffected,ubiquitous,tyndareus,tutors,turncoat,turlock,tulle,tuesday's,tryouts,truth's,trouper,triplette,trepkos,tremor,treeger,treatment's,traveller,traveler's,trapeze,traipse,tradeoff,trach,torin,tommorow,tollan,toity,timpani,tilted,thumbprint,throat's,this's,theater's,thankless,terrestrial,tenney's,tell'em,telepathy,telemarketing,telekinesis,teevee,teeming,tc's,tarred,tankers,tambourine,talentless,taki,takagi,swooped,switcheroo,swirly,sweatpants,surpassed,surgeon's,supermarkets,sunstroke,suitors,suggestive,sugarcoat,succession,subways,subterfuge,subservient,submitting,subletting,stunningly,student's,strongbox,striptease,stravanavitch,stradling,stoolie,stodgy,stocky,stimuli,stigmata,stifle,stealer,statewide,stark's,stardom,stalemate,staggered,squeezes,squatter,squarely,sprouted,spool,spirit's,spindly,spellman's,speedos,specify,specializing,spacey,soups,soundly,soulmates,somethin's,somebody'll,soliciting,solenoid,sobering,snowflakes,snowballs,snores,slung,slimming,slender,skyscrapers,skulk,skivvies,skillful,skewered,skewer,skaters,sizing,sistine,sidebar,sickos,shushing,shunt,shugga,shone,shol'va,shiv,shifter,sharply,sharpened,shareholder,shapeshifter,shadowing,shadoe,serviced,selwyn,selectman,sefelt,seared,seamen,scrounging,scribbling,scotty's,scooping,scintillating,schmoozing,schenectady,scene's,scattering,scampi,scallops,sat's,sapphires,sans,sanitarium,sanded,sanction,safes,sacrificial,rudely,roust,rosebush,rosasharn,rondell,roadhouse,riveted,rile,ricochet,rhinoceros,rewrote,reverence,revamp,retaliatory,rescues,reprimand,reportedly,replicators,replaceable,repeal,reopening,renown,remo's,remedied,rembrandt,relinquishing,relieving,rejoicing,reincarnated,reimbursed,refinement,referral,reevaluate,redundancy,redid,redefine,recreating,reconnected,recession,rebelling,reassign,rearview,reappeared,readily,rayne,ravings,ravage,ratso,rambunctious,rallying,radiologist,quiver,quiero,queef,quark,qualms,pyrotechnics,pyro,puritan,punky,pulsating,publisher's,psychosomatic,provisional,proverb,protested,proprietary,promiscuous,profanity,prisoner's,prioritize,preying,predisposition,precocious,precludes,preceding,prattling,prankster,povich,potting,postpartum,portray,porter's,porridge,polluting,pogo,plowing,plating,plankton,pistachio,pissin,pinecone,pickpocket,physicists,physicals,pesticides,peruse,pertains,personified,personalize,permitting,perjured,perished,pericles,perfecting,percentages,pepys,pepperdine,pembry,peering,peels,pedophile,patties,pathogen,passkey,parrots,paratroopers,paratrooper,paraphernalia,paralyzing,panned,pandering,paltry,palpable,painkiller,pagers,pachyderm,paced,overtaken,overstay,overestimated,overbite,outwit,outskirts,outgrow,outbid,origins,ordnance,ooze,ooops,oomph,oohhh,omni,oldie,olas,oddball,observers,obscurity,obliterate,oblique,objectionable,objected,oars,o'keefe,nygma,nyet,nouveau,notting,nothin's,noches,nnno,nitty,nighters,nigger's,niche,newsstands,newfoundland,newborns,neurosurgery,networking,nellie's,nein,neighboring,negligible,necron,nauseated,nastiest,nasedo's,narrowing,narrator,narcolepsy,napa,nala,nairobi,mutilate,muscled,murmur,mulva,multitude,multiplex,mulling,mules,mukada,muffled,mueller's,motorized,motif,mortgages,morgues,moonbeams,monogamy,mondays,mollusk,molester,molestation,molars,modifications,modeled,moans,misuse,misprint,mismatched,mirth,minnow,mindful,mimosas,millander,mikhail,mescaline,mercutio,menstrual,menage,mellowing,medicaid,mediator,medevac,meddlesome,mcgarry's,matey,massively,massacres,marky,many's,manifests,manifested,manicures,malevolent,malaysian,majoring,madmen,mache,macarthur's,macaroons,lydell,lycra,lunchroom,lunching,lozenges,lorenzo's,looped,look's,lolly,lofty,lobbyist,litigious,liquidate,linoleum,lingk,lincoln's,limitless,limitation,limber,lilacs,ligature,liftoff,lifeboats,lemmiwinks,leggo,learnin,lazarre,lawyered,landmarks,lament,lambchop,lactose,kringle,knocker,knelt,kirk's,kins,kiev,keynote,kenyon's,kenosha,kemosabe,kazi,kayak,kaon,kama,jussy,junky,joyce's,journey's,jordy,jo's,jimmies,jetson,jeriko,jean's,janet's,jakovasaur,jailed,jace,issacs,isotopes,isabela,irresponsibility,ironed,intravenous,intoxication,intermittent,insufficient,insinuated,inhibitors,inherits,inherently,ingest,ingenue,informs,influenza,inflexible,inflame,inevitability,inefficient,inedible,inducement,indignant,indictments,indentured,indefensible,inconsistencies,incomparable,incommunicado,in's,improvising,impounded,illogical,ignoramus,igneous,idlewild,hydrochloric,hydrate,hungover,humorless,humiliations,humanoid,huhh,hugest,hudson's,hoverdrone,hovel,honor's,hoagie,hmmph,hitters,hitchhike,hit's,hindenburg,hibernating,hermione,herds,henchman,helloooo,heirlooms,heaviest,heartsick,headshot,headdress,hatches,hastily,hartsfield's,harrison's,harrisburg,harebrained,hardships,hapless,hanen,handsomer,hallows,habitual,habeas,guten,gus's,gummy,guiltier,guidebook,gstaad,grunts,gruff,griss,grieved,grids,grey's,greenville,grata,granny's,gorignak,goosed,goofed,goat's,gnarly,glowed,glitz,glimpses,glancing,gilmores,gilligan's,gianelli,geraniums,georgie's,genitalia,gaydar,gart,garroway,gardenia,gangbusters,gamblers,gamble's,galls,fuddy,frumpy,frowning,frothy,fro'tak,friars,frere,freddy's,fragrances,founders,forgettin,footsie,follicles,foes,flowery,flophouse,floor's,floatin,flirts,flings,flatfoot,firefighter,fingerprinting,fingerprinted,fingering,finald,film's,fillet,file's,fianc,femoral,fellini,federated,federales,faze,fawkes,fatally,fascists,fascinates,farfel,familiarity,fambly,falsified,fait,fabricating,fables,extremist,exterminators,extensively,expectant,excusez,excrement,excercises,excavation,examinations,evian,evah,etins,esther's,esque,esophageal,equivalency,equate,equalizer,environmentally,entrees,enquire,enough's,engine's,endorsed,endearment,emulate,empathetic,embodies,emailed,eggroll,edna's,economist,ecology,eased,earmuffs,eared,dyslexic,duper,dupe,dungeons,duncan's,duesouth,drunker,drummers,druggie,dreadfully,dramatics,dragnet,dragline,dowry,downplay,downers,doritos,dominatrix,doers,docket,docile,diversify,distracts,disruption,disloyalty,disinterested,disciple,discharging,disagreeable,dirtier,diplomats,dinghy,diner's,dimwitted,dimoxinil,dimmy,dietary,didi,diatribe,dialects,diagrams,diagnostics,devonshire,devising,deviate,detriment,desertion,derp,derm,dept,depressants,depravity,dependence,denounced,deniability,demolished,delinquents,defiled,defends,defamation,deepcore,deductive,decrease,declares,declarations,decimated,decimate,deb's,deadbolt,dauthuille,dastardly,darla's,dans,daiquiris,daggers,dachau,d'ah,cymbals,customized,curved,curiouser,curdled,cupid's,cults,cucamonga,cruller,cruces,crow's,crosswalk,crossover,crinkle,crescendo,cremate,creeper,craftsman,cox's,counteract,counseled,couches,coronet,cornea,cornbread,corday,copernicus,conveyed,contrition,contracting,contested,contemptible,consultants,constructing,constipated,conqueror,connor's,conjoined,congenital,confounded,condescend,concubine,concoct,conch,concerto,conceded,compounded,compensating,comparisons,commoners,committment,commencement,commandeered,comely,coined,cognitive,codex,coddled,cockfight,cluttered,clunky,clownfish,cloaked,cliches,clenched,cleft,cleanin,cleaner's,civilised,circumcised,cimmeria,cilantro,chutzpah,chutney,chucking,chucker,chronicles,chiseled,chicka,chicago's,chattering,charting,characteristic,chaise,chair's,cervix,cereals,cayenne,carrey,carpal,carnations,caricature,cappuccinos,candy's,candied,cancer's,cameo,calluses,calisthenics,cadre,buzzsaw,bushy,burners,bundled,bum's,budington,buchanans,brock's,britons,brimming,breeders,breakaway,braids,bradley's,boycotting,bouncers,botticelli,botherin,boosting,bookkeeping,booga,bogyman,bogged,bluepoint's,bloodthirsty,blintzes,blanky,blak,biosphere,binturong,billable,bigboote,bewildered,betas,bernard's,bequeath,beirut,behoove,beheaded,beginners,beginner,befriend,beet,bedpost,bedded,bay's,baudelaires,barty,barreled,barboni,barbeque,bangin,baltus,bailout,bag's,backstabber,baccarat,awning,awaited,avenues,austen,augie,auditioned,auctions,astrology,assistant's,assassinations,aspiration,armenians,aristocrat,arguillo,archway,archaeologist,arcane,arabic,apricots,applicant,apologising,antennas,annyong,angered,andretti,anchorman,anchored,amritsar,amour,amidst,amid,americana,amenable,ambassadors,ambassador's,amazement,allspice,alannis,airliner,airfare,airbags,ahhhhhhhhh,ahhhhhhhh,ahhhhhhh,agitator,afternoon's,afghan,affirmation,affiliate,aegean,adrenal,actor's,acidosis,achy,achoo,accessorizing,accentuate,academically,abuses,abrasions,abilene,abductor,aaaahhh,zuzu,zoot,zeroing,zelner,zeldy,yo's,yevgeny,yeup,yeska,yellows,yeesh,yeahh,yamuri,yaks,wyatt's,wspr,writing's,wrestlers,wouldn't've,workmanship,woodsman,winnin,winked,wildness,widespread,whoring,whitewash,whiney,when're,wheezer,wheelman,wheelbarrow,whaling,westerburg,wegener's,weekdays,weeding,weaving,watermelons,watcher's,washboard,warmly,wards,waltzes,walt's,walkway,waged,wafting,voulez,voluptuous,vitone,vision's,villa's,vigilantes,videotaping,viciously,vices,veruca,vermeer,verifying,ventured,vaya,vaults,vases,vasculitis,varieties,vapor,valets,upriver,upholstered,upholding,unwavering,unused,untold,unsympathetic,unromantic,unrecognizable,unpredictability,unmask,unleashing,unintentional,unilaterally,unglued,unequivocal,underside,underrated,underfoot,unchecked,unbutton,unbind,unbiased,unagi,uhhhhh,turnovers,tugging,trouble's,triads,trespasses,treehorn,traviata,trappers,transplants,transforming,trannie,tramping,trainers,traders,tracheotomy,tourniquet,tooty,toothless,tomarrow,toasters,tine,tilting,thruster,thoughtfulness,thornwood,therapies,thanksgiving's,tha's,terri's,tengo,tenfold,telltale,telephoto,telephoned,telemarketer,teddy's,tearin,tastic,tastefully,tasking,taser,tamed,tallow,taketh,taillight,tadpoles,tachibana,syringes,sweated,swarthy,swagger,surrey,surges,surf's,supermodels,superhighway,sunup,sun'll,summaries,sumerian,sulu,sulphur,sullivan's,sulfa,suis,sugarless,sufficed,substituted,subside,submerged,subdue,styling,strolled,stringy,strengthens,street's,straightest,straightens,storyteller,storefront,stopper,stockpiling,stimulant,stiffed,steyne,sternum,stereotypical,stepladder,stepbrother,steers,steeple,steelheads,steakhouse,statue's,stathis,stankylecartmankennymr,standoffish,stalwart,stallions,stacy's,squirted,squeaker,squad's,spuds,spritz,sprig,sprawl,spousal,sportsman,sphincter,spenders,spearmint,spatter,sparrows,spangled,southey,soured,sonuvabitch,somethng,societies,snuffed,snowfall,snowboarding,sniffs,snafu,smokescreen,smilin,slurred,slurpee,slums,slobs,sleepwalker,sleds,slays,slayage,skydiving,sketched,skateboarding,skanks,sixed,siri,sired,siphoned,siphon,singer's,simpering,silencer,sigfried,siena,sidearm,siddons,sickie,siberian,shuteye,shuk,shuffleboard,shrubberies,shrouded,showmanship,shower's,shouldn't've,shortwave,shoplift,shooter's,shiatsu,sheriffs,shak,shafts,serendipity,serena's,sentries,sentance,sensuality,semesters,seething,sedition,secular,secretions,searing,scuttlebutt,sculpt,scowling,scouring,scorecard,schwarzenegger,schoolers,schmucks,scepters,scaly,scalps,scaling,scaffolding,sauces,sartorius,santen,sampler,salivating,salinger,sainthood,said's,saget,saddens,rygalski,rusting,rumson's,ruination,rueland,rudabaga,rubles,rowr,rottweiler,rotations,roofies,romantics,rollerblading,roldy,rob's,roadshow,rike,rickets,rible,rheza,revisiting,revisited,reverted,retrospective,retentive,resurface,restores,respite,resounding,resorting,resolutions,resists,repulse,repressing,repaying,reneged,relays,relayed,reinforce,regulator,registers,refunds,reflections,rediscover,redecorated,recruitment,reconstructive,reconstructed,recommitted,recollect,recoil,recited,receptor,receptacle,receivers,reassess,reanimation,realtors,razinin,ravaged,ratios,rationalization,ratified,ratatouille,rashum,rasczak,rarer,rapping,rancheros,rampler,rain's,railway,racehorse,quotient,quizzing,quips,question's,quartered,qualification,purring,pummeling,puede,publicized,psychedelic,proximo,proteins,protege,prospectus,pronouncing,pronoun,prolonging,program's,proficient,procreation,proclamations,prio,principled,prides,pricing,presbyterian,preoccupation,prego,preferential,predicts,precog,prattle,pounced,potshots,potpourri,portsmouth,porque,poppie's,poms,pomeranian,pomegranates,polynesian,polymer,polenta,plying,plume,plumber's,pluie,plough,plesac,playoff,playmates,planter,plantains,plaintiff's,pituitary,pisano's,pillowcase,piddle,pickers,phys,photocopied,philistine,pfeiffer's,peyton's,petitioned,persuading,perpetuate,perpetually,periodically,perilous,pensacola,pawned,pausing,pauper,patterned,pats,patronage,passover,partition,parter,parlez,parlay,parkinson's,parades,paperwork's,pally,pairing,ovulation,overtake,overstate,overpowering,overpowered,overconfident,overbooked,ovaltine,ouzo,outweighs,outings,outfit's,out's,ottos,orrin,originate,orifice,orangutan,optimal,optics,opportunistic,ooww,oopsy,ooooooooh,ooohhhh,onyx,onslaught,oldsmobile,ocular,ocean's,obstruct,obscenely,o'dwyer,o'brien's,nutjob,nunur,notifying,nostrand,nonny,nonfat,noblest,nimble,nikes,nicht,newsworthy,network's,nestled,nessie,necessities,nearsighted,ne'er,nazareth,navidad,nastier,nasa's,narco,nakedness,muted,mummified,multiplying,mudda,mtv's,mozzarella,moxica,motorists,motivator,motility,mothafucka,mortmain,mortgaged,mortally,moroccan,mores,moonshine,mongers,moe's,modify,mobster's,mobilization,mobbed,mitigating,mistah,misrepresented,mishke,misfortunes,misdirection,mischievous,mirrored,mineshaft,mimosa,millers,millaney,miho,midday,microwaves,mick's,metzenbaum,metres,merc,mentoring,medicine's,mccovey,maya's,mau's,masterful,masochistic,martie,marliston,market's,marijawana,marie's,marian's,manya,manuals,mantumbi,mannheim,mania,mane,mami's,malarkey,magnifique,magics,magician's,madrona,madox,madison's,machida,m'mm,m'hm,m'hidi,lyric,luxe,luther's,lusty,lullabies,loveliness,lotions,looka,lompoc,loader,litterbug,litigator,lithe,liquorice,lins,linguistics,linds,limericks,lightbulb,lewises,letch,lemec,lecter's,leavenworth,leasing,leases,layover,layered,lavatory,laurels,launchers,laude,latvian,lateness,lasky's,laparotomy,landlord's,laboring,la's,kumquat,kuato,kroff,krispy,kree,krauts,kona,knuckleheads,knighthood,kiva,kitschy,kippers,kip's,kimbrow,kike,keypad,keepsake,kebab,keane's,kazakhstan,karloff,justices,junket,juicer,judy's,judgemental,jsut,jointed,jogs,jezzie,jetting,jekyll,jehovah's,jeff's,jeeze,jeeter,jeesus,jeebs,janeane,jalapeno,jails,jailbait,jagged,jackin,jackhammer,jacket's,ixnay,ivanovich,issue's,isotope,island's,irritates,irritability,irrevocable,irrefutable,irma's,irked,invoking,intricacies,interferon,intents,inte,insubordinate,instructive,instinctive,inspector's,inserting,inscribed,inquisitive,inlay,injuns,inhibited,infringement,information's,infer,inebriated,indignity,indecisive,incisors,incacha,inauguration,inalienable,impresses,impregnate,impregnable,implosion,immersed,ikea,idolizes,ideological,idealism,icepick,hypothyroidism,hypoglycemic,hyde's,hutz,huseni,humvee,hummingbird,hugely,huddling,housekeeper's,honing,hobnobbing,hobnob,histrionics,histamine,hirohito,hippocratic,hindquarters,hinder,himalayan,hikita,hikes,hightailed,hieroglyphics,heyy,heuh,heretofore,herbalist,her's,henryk,henceforth,hehey,hedriks,heartstrings,headmistress,headlight,harvested,hardheaded,happend,handlers,handlebars,hagitha,habla,gyroscope,guys'd,guy'd,guttersnipe,grump,growed,grovelling,grooves,groan,greenbacks,greats,gravedigger,grating,grasshoppers,grappling,graph,granger's,grandiose,grandest,gram's,grains,grafted,gradual,grabthar's,goop,gooood,goood,gooks,godsakes,goaded,gloria's,glamorama,giveth,gingham,ghostbusters,germane,georgy,geisha,gazzo,gazelles,gargle,garbled,galgenstein,galapagos,gaffe,g'day,fyarl,furnish,furies,fulfills,frowns,frowned,frommer's,frighteningly,fresco,freebies,freakshow,freakishly,fraudulent,fragrant,forewarned,foreclose,forearms,fordson,ford's,fonics,follies,foghorn,fly's,flushes,fluffy's,flitting,flintstone,flemmer,flatline,flamboyant,flabby,fishbowl,firsts,finger's,financier,figs,fidgeting,fictitious,fevers,feur,ferns,feminism,fema,feigning,faxing,fatigued,fathoms,fatherless,fares,fancier,fanatical,fairs,factored,eyelid,eyeglasses,eye's,expresso,exponentially,expletive,expectin,excruciatingly,evidentiary,ever'thing,evelyn's,eurotrash,euphoria,eugene's,eubie,ethiopian,ethiopia,estrangement,espanol,erupted,ernie's,erlich,eres,epitome,epitaph,environments,environmentalists,entrap,enthusiastically,entertainers,entangled,enclose,encased,empowering,empires,emphysema,embers,embargo,emasculating,elizabethan,elephant's,eighths,egyptians,effigy,editions,echoing,eardrum,dyslexia,duplicitous,duplicated,dumpty,dumbledore,dufus,dudley's,duddy,duck's,duchamp,drunkenness,drumlin,drowns,droid,drinky,drifts,drawbridge,dramamine,downey's,douggie,douchebag,dostoyevsky,dorian's,doodling,don'tcha,domo,domineering,doings,dogcatcher,documenting,doctoring,doctoral,dockers,divides,ditzy,dissimilar,dissecting,disparage,disliking,disintegrating,dishwalla,dishonored,dishing,disengaged,discretionary,discard,disavowed,directives,dippy,diorama,dimmed,diminishing,dilate,dijon,digitalis,diggory,dicing,diagnosing,devout,devola,developmental,deter,destiny's,desolation,descendant,derived,derevko's,deployment,dennings,denials,deliverance,deliciously,delicacies,degenerates,degas,deflector,defile,deference,defenders,deduced,decrepit,decreed,decoding,deciphered,dazed,dawdle,dauphine,daresay,dangles,dampen,damndest,customer's,curricular,cucumbers,cucaracha,cryogenically,cruella,crowd's,croaks,croaked,criticise,crit,crisper,creepiest,creep's,credit's,creams,crawford's,crackle,crackin,covertly,cover's,county's,counterintelligence,corrosive,corpsman,cordially,cops'll,convulsions,convoluted,convincingly,conversing,contradictions,conga,confucius,confrontational,confab,condolence,conditional,condition's,condiments,composing,complicit,compiled,compile,compiegne,commuter,commodus,commissions,comings,cometh,combining,colossus,collusion,collared,cockeyed,coastline,clobber,clemonds,clashes,clarithromycin,clarified,cinq,cienega,chronological,christmasy,christmassy,chloroform,chippie,childless,chested,chemistry's,cheerios,cheeco,checklist,chaz,chauvinist,char,chang's,chandlers,chamois,chambermaid,chakras,chak,censored,cemented,cellophane,celestial,celebrations,caveat,catholicism,cataloguing,cartmanland,carples,carny,carded,caramels,captors,caption,cappy,caped,canvassing,cannibalism,canada's,camille's,callback,calibrated,calamine,cal's,cabo,bypassed,buzzy,buttermilk,butterfingers,bushed,burlesque,bunsen,bung,bulimia,bukatari,buildin,budged,bronck's,brom,brobich,bringer,brine,brendell,brawling,bratty,brasi,braking,braised,brackett's,braced,boyish,boundless,botch,borough,boosh,bookies,bonbons,bois,bodes,bobunk,bluntly,blossoming,bloopers,bloomers,bloodstains,bloodhounds,blitzen,blinker,blech,blasts,blanca's,bitterly,biter,biometric,bioethics,bilk,bijan,bigoted,bicep,betrothed,bergdorf's,bereaved,bequeathed,belo,bellowing,belching,beholden,befriended,beached,bawk,battled,batmobile,batman's,baseline,baseball's,barcodes,barch,barbie's,barbecuing,bandanna,baldy,bailey's,baghdad,backwater,backtrack,backdraft,ayuh,awgh,augustino,auctioned,attaching,attaches,atrophy,atrocity,atley,athletics,atchoo,asymmetrical,asthmatic,assoc,assists,ascending,ascend,articulated,arrr,armstrong's,armchair,arisen,archeology,archeological,arachnids,aptly,applesauce,appetizing,antisocial,antagonizing,anorexia,anini,angie's,andersons,anarchist,anagram,amputation,amherst,alleluia,algorithms,albemarle,ajar,airlock,airbag,aims,aimless,ailments,agua,agonized,agitate,aggravating,affirming,aerosol,aerosmith,aeroplane,acing,accumulated,accomplishing,accolades,accidently,academia,abuser,abstain,abso,abnormally,aberration,abandons,aaww,aaaaahh,zlotys,zesty,zerzura,zapruder,zany,zantopia,yugoslavia,youo,yoru,yipe,yeow,yello,yelburton,yeess,yaah,y'knowwhati'msayin,wwhat,wussies,wrenched,would'a,worryin,wormser,wooooo,wookiee,wolfe's,wolchek,woes,wishin,wiseguys,winston's,winky,wine's,windbreaker,wiggy,wieners,wiedersehen,whoopin,whittled,whey,whet,wherefore,wharvey,welts,welt,wellstone,weee,wednesday's,wedges,wavered,watchit,wastebasket,ward's,wank,wango,wallet's,wall's,waken,waiver,waitressed,wacquiem,wabbit,vrykolaka,voula,vote's,volt,volga,volcanoes,vocals,vitally,visualizing,viscous,virgo,virg,violet's,viciousness,vewy,vespers,vertes,verily,vegetarians,vater,vaseline,varied,vaporize,vannacutt,vallens,valenti's,vacated,uterine,usta,ussher,urns,urinating,urchin,upping,upheld,unwitting,untreated,untangle,untamed,unsanitary,unraveled,unopened,unisex,uninvolved,uninteresting,unintelligible,unimaginative,undisclosed,undeserving,undermines,undergarments,unconcerned,unbroken,ukrainian,tyrants,typist,tykes,tybalt,twosome,twits,tutti,turndown,tularemia,tuberculoma,tsimshian,truffaut,truer,truant,trove,triumphed,tripe,trigonometry,trifled,trifecta,tricycle,trickle,tribulations,trevor's,tremont,tremoille,treaties,trawler,translators,transcends,trafficker,touchin,tonnage,tomfoolery,tolls,tokens,tinkered,tinfoil,tightrope,ticket's,thth,thousan,thoracotomy,theses,thesaurus,theologian,themed,thawing,thatta,thar,textiles,testimonies,tessio,terminating,temps,taxidermist,tator,tarkin,tangent,tactile,tachycardia,t'akaya,synthesize,symbolically,swelco,sweetbreads,swedes,swatting,swastika,swamps,suze,supernova,supercollider,sunbathing,summarily,suffocation,sueleen,succinct,subtitle,subsided,submissive,subjecting,subbing,subatomic,stupendous,stunted,stubble,stubbed,striving,streetwalker,strategizing,straining,straightaway,storyline,stoli,stock's,stipulated,stimulus,stiffer,stickup,stens,steamroller,steadwell,steadfast,stave,statutes,stateroom,stans,stacey's,sshhhh,squishing,squinting,squealed,sprouting,sprimp,spreadsheets,sprawled,spotlights,spooning,spoiler,spirals,spinner's,speedboat,spectacles,speakerphone,spar,spaniards,spacing,sovereignty,southglen,souse,soundproof,soothsayer,soon's,sommes,somethings,solidify,soars,snorted,snorkeling,snitches,sniping,sniper's,snifter,sniffin,snickering,sneer,snarl,smila,slinking,sleuth,slater's,slated,slanted,slanderous,slammin,skyscraper,skimp,skilosh,skeletal,skag,siteid,sirloin,singe,simulate,signaled,sighing,sidekicks,sicken,shrubs,shrub,showstopper,shot's,shostakovich,shoreline,shoppin,shoplifter,shop's,shoe's,shoal,shitter,shirt's,shimokawa,sherborne,sheds,shawna's,shavadai,sharpshooters,sharking,shane's,shakespearean,shagged,shaddup,sexism,sexes,sesterces,serotonin,sequences,sentient,sensuous,seminal,selections,seismic,seashell,seaplane,sealing,seahaven,seagrave,scuttled,scullery,scow,scots,scorcher,scorch,schotzie,schnoz,schmooze,schlep,schizo,schindler's,scents,scalping,scalped,scallop,scalding,sayeth,saybrooke,sawed,savoring,sardine,sandy's,sandstorm,sandalwood,samoa,samo,salutations,salad's,saki,sailor's,sagman,s'okay,rudy's,rsvp'd,royale,rousted,rootin,roofs,romper,romanovs,rollercoaster,rolfie,rockers,rock's,robinsons,ritzy,ritualistic,ringwald,rhymed,rheingold,rewrites,revolved,revolutionaries,revoking,reviewer,reverts,retrofit,retort,retinas,resurfaced,respirations,respectively,resolute,resin,reprobate,replaying,repayment,repaint,renquist,renege,renders,rename,remarked,relapsing,rekindled,rejuvenating,rejuvenated,reinstating,reinstatement,reigns,referendums,recriminations,recitals,rechecked,reception's,recaptured,rebounds,reassemble,rears,reamed,realty,reader's,reacquaint,rayanne,ravish,rava,rathole,raspail,rarest,rapists,rants,ramone,ragnar,radiating,radial,racketeer,quotation,quittin,quitters,quintessential,quincy's,queremos,quellek,quelle,quasimodo,quarterbacks,quarter's,pyromaniac,puttanesca,puritanical,purged,purer,puree,punishments,pungent,pummel,puedo,pudge,puce,psychotherapist,psycho's,prosecutorial,prosciutto,propositioning,propellers,pronouns,progresses,procured,procrastination,processes,probationary,primping,primates,priest's,preventative,prevails,presided,preserves,preservatives,prefix,predecessors,preachy,prancer,praetorians,practicality,powders,potus,pot's,postop,positives,poser,portolano,portokalos,poolside,poltergeists,pocketed,poach,plunder,plummeted,plucking,plop,plimpton,plethora,playthings,player's,playboys,plastique,plainclothes,pious,pinpointed,pinkus,pinks,pilgrimage,pigskin,piffle,pictionary,piccata,photocopy,phobias,persia,permissible,perils,perignon,perfumes,peon,penned,penalized,peg's,pecks,pecked,paving,patriarch,patents,patently,passable,participants,parasitic,parasailing,paramus,paramilitary,parabolic,parable,papier,paperback,paintbrush,pacer,paaiint,oxen,owen's,overtures,overthink,overstayed,overrule,overlapping,overestimate,overcooked,outlandish,outgrew,outdoorsy,outdo,outbound,ostensibly,originating,orchestrate,orally,oppress,opposable,opponent's,operation's,oooohh,oomupwah,omitted,okeydokey,okaaay,ohashi,offerings,of'em,od'd,occurrences,occupant,observable,obscenities,obligatory,oakie,o'malley's,o'gar,nyah's,nurection,nun's,nougat,nostradamus,norther,norcom,nooch,nonviolent,nonsensical,nominating,nomadic,noel's,nkay,nipped,nimbala,nigeria,nigel's,nicklaus,newscast,nervously,nell's,nehru,neckline,nebbleman,navigator,nasdaq,narwhal,nametag,n'n't,mycenae,myanmar,muzak,muumuu,murderer's,mumbled,mulvehill,multiplication,multiples,muggings,muffet,mozart's,mouthy,motorbike,motivations,motivates,motaba,mortars,mordred,mops,moocher,moniker,mongi,mondo,monday's,moley,molds,moisturize,mohair,mocky,mmkay,mistuh,missis,mission's,misdeeds,minuscule,minty,mined,mincemeat,milton's,milt,millennia,mikes,miggs,miffed,mieke's,midwestern,methadone,metaphysics,messieur,merging,mergers,menopausal,menagerie,meee,mckenna's,mcgillicuddy,mayflowers,maxim's,matrimonial,matisse,matick,masculinity,mascots,masai,marzipan,marika,maplewood,manzelle,manufactures,manticore's,mannequins,manhole,manhandle,manatee,mallory's,malfunctions,mainline,magua's,madwoman,madeline's,machiavelli,lynley,lynching,lynched,lurconis,lujack,lubricant,looove,loons,loom,loofah,longevity,lonelyhearts,lollipops,loca,llama,liquidation,lineswoman,lindsey's,lindbergh,lilith's,lila's,lifers,lichen,liberty's,lias,lexter,levee,letter's,lessen,lepner,leonard's,lemony,leggy,leafy,leaflets,leadeth,lazerus,lazare,lawford,languishing,langford's,landslide,landlords,lagoda,ladman,lad's,kuwait,kundera,krist's,krinkle,krendler,kreigel,kowolski,kosovo,knockdown,knifed,kneed,kneecap,kids'll,kevlar,kennie,keeled,kazootie,kaufman's,katzenmoyer,kasdan,karl's,karak,kapowski,kakistos,jumpers,julyan,juanito,jockstrap,jobless,jiggly,jesuit,jaunt,jarring,jabbering,israelites,irrigate,irrevocably,irrationally,ironies,ions,invitro,inventions,intrigues,intimated,interview's,intervening,interchangeable,intently,intentioned,intelligently,insulated,institutional,instill,instigator,instigated,instep,inopportune,innuendoes,inheriting,inflate,infiltration,infects,infamy,inducing,indiscretions,indiscreet,indio,indignities,indict,indecision,incurred,incubation,inconspicuous,inappropriately,impunity,impudent,improves,impotence,implicates,implausible,imperfection,impatience,immutable,immobilize,illustration,illumination,idiot's,idealized,idealist,icelandic,iambic,hysterically,hyperspace,hygienist,hydraulics,hydrated,huzzah,husks,hurricane's,hunt's,hunched,huffed,hubris,hubbub,hovercraft,houngan,hotel's,hosed,horoscopes,hoppy,hopelessness,hoodwinked,honourable,honorably,honeysuckle,homeowners,homegirl,holiest,hoisted,hoho,ho's,hippity,hildie,hikers,hieroglyphs,hexton,herein,helicopter's,heckle,heats,heartbeat's,heaping,healthilizer,headmaster's,headfirst,hawk's,haviland's,hatsue,harlot,hardwired,hanno's,hams,hamilton's,halothane,hairstyles,hails,hailed,haagen,haaaaa,gyno,gutting,gurl,gumshoe,gummi,gull,guerilla,gttk,grover's,grouping,groundless,groaning,gristle,grills,graynamore,grassy,graham's,grabbin,governmental,goodes,goggle,godlike,glittering,glint,gliding,gleaming,glassy,girth,gimbal,gilmore's,gibson's,giblets,gert,geometric,geographical,genealogy,gellers,geller's,geezers,geeze,garshaw,gargantuan,garfunkel,gardner's,garcia's,garb,gangway,gandarium,gamut,galoshes,gallivanting,galleries,gainfully,gack,gachnar,fusionlips,fusilli,furiously,fulfil,fugu,frugal,fron,friendship's,fricking,frederika,freckling,frauds,fraternal,fountainhead,forthwith,forgo,forgettable,foresight,foresaw,footnotes,fondling,fondled,fondle,folksy,fluttering,flutie,fluffing,floundering,florin,florentine,flirtatious,flexing,flatterer,flaring,fizz,fixating,five's,fishnet,firs,firestorm,finchy,figurehead,fifths,fiendish,fertilize,ferment,fending,fellahs,feeny's,feelers,feeders,fatality,fascinate,fantabulous,falsify,fallopian,faithless,fairy's,fairer,fair's,fainter,failings,facto,facets,facetious,eyepatch,exxon,extraterrestrials,extradite,extracurriculars,extinguish,expunged,exports,expenditure,expelling,exorbitant,exigent,exhilarated,exertion,exerting,exemption,excursions,excludes,excessively,excercise,exceeds,exceeding,everbody,evaporated,euthanasia,euros,europeans,escargot,escapee,erases,epizootics,epithelials,ephrum,enthusiast,entanglements,enslaved,enslave,engrossed,endeavour,enables,enabled,empowerment,employer's,emphatic,emeralds,embroiled,embraces,ember,embellished,emancipated,ello,elisa's,elevates,ejaculate,ego's,effeminate,economically,eccentricities,easygoing,earshot,durp,dunks,dunes,dullness,dulli,dulled,drumstick,dropper,driftwood,dregs,dreck,dreamboat,draggin,downsizing,dost,doofer,donowitz,dominoes,dominance,doe's,diversions,distinctions,distillery,distended,dissolving,dissipate,disraeli,disqualify,disowned,dishwashing,discusses,discontent,disclosed,disciplining,discerning,disappoints,dinged,diluted,digested,dicking,diablos,deux,detonating,destinations,despising,designer's,deserts,derelict,depressor,depose,deport,dents,demonstrations,deliberations,defused,deflection,deflecting,decryption,decoys,decoupage,decompress,decibel,decadence,dealer's,deafening,deadlock,dawning,dater,darkened,darcy's,dappy,dancing's,damon's,dallying,dagon,d'etat,czechoslovakians,cuticles,cuteness,curacao,cupboards,cumulative,culottes,culmination,culminating,csi's,cruisin,crosshairs,cronyn,croc,criminalistics,crimean,creatively,creaming,crapping,cranny,cowed,countermeasures,corsica,corinne's,corey's,cooker,convened,contradicting,continuity,constitutionally,constipation,consort,consolidate,consisted,connection's,confining,confidences,confessor,confederates,condensation,concluding,conceiving,conceivably,concealment,compulsively,complainin,complacent,compiling,compels,communing,commonplace,commode,commission's,commissary,comming,commensurate,columnists,colonoscopy,colonists,collagen,collaborate,colchicine,coddling,clump,clubbed,clowning,closet's,clones,clinton's,clinic's,cliffhanger,classification,clang,citrus,cissy,circuitry,chronology,christophe,choosers,choker,chloride,chippewa,chip's,chiffon,chesty,chesapeake,chernobyl,chants,channeled,champagne's,chalet,chaka,cervical,cellphone,cellmates,caverns,catwalk,cathartic,catcher's,cassandra's,caseload,carpenter's,carolyn's,carnivorous,carjack,carbohydrates,capt,capitalists,canvass,cantonese,canisters,candlestick,candlelit,canaries,camry,camel's,calzones,calitri,caldy,cabin's,byline,butterball,bustier,burmese,burlap,burgeoning,bureaucrat,buffoons,buenas,bryan's,brookline,bronzed,broiled,broda,briss,brioche,briar,breathable,brea,brays,brassieres,braille,brahms,braddock's,boysenberry,bowman's,bowline,boutiques,botticelli's,boooo,boonies,booklets,bookish,boogeyman,boogey,bomb's,boldly,bogs,bogas,boardinghouse,bluuch,blundering,bluffs,bluer,blowed,blotto,blotchy,blossomed,blooms,bloodwork,bloodied,blithering,blinks,blathering,blasphemous,blacking,bison,birdson,bings,bilateral,bfmid,bfast,berserker,berkshires,bequest,benjamins,benevolence,benched,benatar,belthazor's,bellybutton,belabor,bela's,behooves,beddy,beaujolais,beattle,baxworth,batted,baseless,baring,barfing,barbi,bannish,bankrolled,banek,ballsy,ballpoint,balkans,balconies,bakers,bahama,baffling,badder,badda,bada,bactine,backgammon,baako,aztreonam,aztecs,awed,avon,autobiographical,autistic,authoritah,auspicious,august's,auditing,audible,auctioning,attitude's,atrocities,athlete's,astronomer,assessed,ascot,aristocratic,arid,argues,arachtoids,arachnid,aquaman,apropos,aprons,apprised,apprehensive,apex,anythng,antivenin,antichrist,antennae,anorexic,anoint,annum,annihilated,animal's,anguished,angioplasty,angio,amply,ampicillin,amphetamines,amino,american's,ambiguity,ambient,amarillo,alyssa's,alternator,alcove,albacore,alarm's,alabaster,airlifted,ahta,agrabah,affidavits,advocacy,advises,adversely,admonished,admonish,adler's,addled,addendum,acknowledgement,accuser,accompli,acclaim,acceleration,abut,abundant,absurdity,absolved,abrusso,abreast,abrasive,aboot,abductions,abducting,abbots,aback,ababwa,aand,aaahhhh,zorin,zinthar,zinfandel,zimbabwe,zillions,zephyrs,zatarcs,zacks,youuu,youths,yokels,yech,yardstick,yammer,y'understand,wynette,wrung,wrought,wreaths,wowed,wouldn'ta,worshiped,worming,wormed,workday,wops,woolly,wooh,woodsy,woodshed,woodchuck,wojadubakowski,withering,witching,wiseass,wiretaps,winner's,wining,willoby,wiccaning,whupped,whoopi,whoomp,wholesaler,whiteness,whiner,whatchya,wharves,whah,wetlands,westward,wenus,weirdoes,weds,webs,weaver's,wearer,weaning,watusi,wastes,warlock's,warfield's,waponi,waiting's,waistband,waht,wackos,vouching,votre,voight's,voiced,vivica,viveca,vivant,vivacious,visor,visitin,visage,virgil's,violins,vinny,vinci's,villas,vigor,video's,vicrum,vibrator,vetted,versailles,vernon's,venues,ventriloquism,venison,venerable,varnsen,variant,variance,vaporized,vapid,vanstock,vandals,vader's,vaccination,uuuuh,utilize,ushering,usda,usable,urur,urologist,urination,urinary,upstart,uprooted,unsubtitled,unspoiled,unseat,unseasonably,unseal,unsatisfying,unnerve,unlikable,unleaded,university's,universe's,uninsured,uninspired,uniformity,unicycle,unhooked,ungh,unfunny,unfreezing,unflattering,unfairness,unexpressed,unending,unencumbered,unearth,undiscovered,undisciplined,undertaken,understan,undershirt,underlings,underline,undercurrent,uncontrolled,uncivilized,uncharacteristic,umpteenth,uglies,u're,tut's,turner's,turbine,tunnel's,tuney,trustee,trumps,truckasaurus,trubshaw,trouser,trippy,tringle,trifling,trickster,triangular,trespassers,trespasser,traverse,traumas,trattoria,trashes,transgressions,tranquil,trampling,trainees,tracy's,tp'ed,toxoplasmosis,tounge,tortillas,torrent,torpedoed,topsy,topple,topnotch,top's,tonsil,tippin's,tions,timmuh,timithious,tilney,tighty,tightness,tightens,tidbits,ticketed,thyme,thrones,threepio,thoughtfully,thornhart's,thorkel,thommo,thing'll,theological,thel,theh,thefts,that've,thanksgivings,tetherball,testikov,terraforming,terminus,tepid,tendonitis,tenboom,telex,teleport,telepathic,teenybopper,taxicab,taxed,taut,tattered,tattaglias,tapered,tantric,tanneke,takedown,tailspin,tacs,tacit,tablet,tablecloth,systemic,syria,syphon,synthesis,symbiotic,swooping,swizzle,swiping,swindled,swilling,swerving,sweatshops,swayzak's,swaddling,swackhammer,svetkoff,suzie's,surpass,supossed,superdad,super's,sumptuous,sula,suit's,sugary,sugar's,sugai,suey,subvert,suburb,substantiate,subsidy,submersible,sublimating,subjugation,styx,stymied,stuntman,studded,strychnine,strikingly,strenuous,streetlights,strassmans,stranglehold,strangeness,straddling,straddle,stowaways,stotch,stockbrokers,stifling,stepford,stepdad's,steerage,steena,staunch,statuary,starlets,stanza,stanley's,stagnant,staggeringly,ssshhh,squaw,spurt,spungeon,sprightly,sprays,sportswear,spoonful,splittin,splitsville,spirituality,spiny,spider's,speedily,speculative,specialise,spatial,spastic,spas,sparrin,soybean,souvlaki,southie,southampton,sourpuss,soupy,soup's,soundstage,sophie's,soothes,somebody'd,solicited,softest,sociopathic,socialized,socialism,snyders,snowmobiles,snowballed,snatches,smugness,smoothest,smashes,slurp,slur,sloshed,sleight,skyrocket,skied,skewed,sizeable,sixpence,sipowicz,singling,simulations,simulates,similarly,silvery,silverstone,siesta,siempre,sidewinder,shyness,shuvanis,showoff,shortsighted,shopkeeper,shoehorn,shithouse,shirtless,shipshape,shingles,shifu,shes,sherman's,shelve,shelbyville,sheepskin,shat,sharpens,shaquille,shaq,shanshu,shania's,set's,servings,serpico,sequined,sensibilities,seizes,seesaw,seep,seconded,sebastian's,seashells,scrapped,scrambler,scorpions,scopes,schnauzer,schmo,schizoid,scampered,scag,savagely,saudis,satire,santas,sanskrit,sandovals,sanding,sandal,salient,saleswoman,sagging,s'cuse,rutting,ruthlessly,runoff,runneth,rulers,ruffians,rubes,roughriders,rotates,rotated,roswell's,rosalita,rookies,ron's,rollerblades,rohypnol,rogues,robinson's,roasts,roadies,river's,ritten,rippling,ripples,ring's,rigor,rigoletto,richardo,ribbed,revolutions,revlon's,reverend's,retreating,retractable,rethought,retaliated,retailers,reshoot,reserving,reseda,researchers,rescuer,reread,requisitions,repute,reprogram,representations,report's,replenish,repetitive,repetitious,repentance,reorganizing,renton,renee's,remodeled,religiously,relics,reinventing,reinvented,reheat,rehabilitate,registrar,regeneration,refueling,refrigerators,refining,reenter,redress,recruiter,recliner,reciprocal,reappears,razors,rawdy,rashes,rarity,ranging,rajeski,raison,raisers,rainier,ragtime,rages,radar's,quinine,questscape,queller,quartermaine's,pyre,pygmalion,pushers,pusan,purview,purification,pumpin,puller,pubescent,psychiatrist's,prudes,provolone,protestants,prospero,propriety,propped,prom's,procrastinate,processors,processional,princely,preyed,preventive,pretrial,preside,premiums,preface,preachers,pounder,ports,portrays,portrayal,portent,populations,poorest,pooling,poofy,pontoon,pompeii,polymerization,polloi,policia,poacher,pluses,pleasuring,pleads,playgrounds,platitudes,platforms,plateaued,plate's,plantations,plaguing,pittance,pitcher's,pinky's,pinheads,pincushion,pimply,pimped,piggyback,pierce's,piecing,physiological,physician's,phosphate,phillipe,philipse,philby,phased,pharaohs,petyr,petitioner,peshtigo,pesaram,perspectives,persnickety,perpetrate,percolating,pepto,pensions,penne,penell,pemmican,peeks,pedaling,peacemaker,pawnshop,patting,pathologically,patchouli,pasts,pasties,passin,parlors,panda's,panache,paltrow,palamon,padlock,paddy's,paddling,oversleep,overheating,overdosed,overcharge,overcame,overblown,outset,outrageously,outfitted,orsini's,ornery,origami,orgasmic,orga,order's,opportune,ooow,oooooooooh,oohhhh,olympian,olfactory,okum,ohhhhhh,ogres,odysseus,odorless,occupations,occupancy,obscenity,obliterated,nyong,nymphomaniac,nutsack,numa,ntozake,novocain,nough,noth,nosh,norwegians,northstar,nonnie,nonissue,nodules,nightmarish,nightline,nighthawk,niggas,nicu,nicolae,nicknamed,niceties,newsman,neverland,negatively,needra,nedry,necking,navour,nauseam,nauls,narim,nanda,namath,nagged,nads,naboo,n'sync,mythological,mysticism,myslexia,mutator,mustafi,mussels,muskie,musketeer,murtaugh,murderess,murder's,murals,munching,mumsy,muley,mouseville,mosque,mosh,mortifying,morgendorffers,moola,montel,mongoloid,molten,molestered,moldings,mocarbies,mo'ss,mixers,misrell,misnomer,misheard,mishandled,miscreant,misconceptions,miniscule,minimalist,millie's,millgate,migrate,michelangelo's,mettle,metricconverter,methodology,meter's,meteors,mesozoic,menorah,mengele,mendy's,membranes,melding,meanness,mcneil's,mcgruff,mcarnold,matzoh,matted,mathematically,materialized,mated,masterpieces,mastectomy,massager,masons,marveling,marta's,marquee,marooned,marone's,marmaduke,marick,marcie's,manhandled,mangoes,manatees,managerial,man'll,maltin,maliciously,malfeasance,malahide,maketh,makeshift,makeovers,maiming,magazine's,machismo,maarten,lutheran,lumpectomy,lumbering,luigi's,luge,lubrication,lording,lorca,lookouts,loogie,loners,london's,loin,lodgings,locomotive,lobes,loathed,lissen,linus,lighthearted,ligament,lifetime's,lifer,lier,lido,lickin,lewen,levitation,lestercorp,lessee,lentils,lena's,lemur,lein,legislate,legalizing,lederhosen,lawmen,laundry's,lasskopf,lardner,landscapes,landfall,lambeau,lamagra,lagging,ladonn,lactic,lacquer,laborers,labatier,kwan's,krit,krabappel,kpxy,kooks,knobby,knickknacks,klutzy,kleynach,klendathu,kinross,kinko's,kinkaid,kind'a,kimberly's,kilometer,khruschev's,khaki,keyboards,kewl,ketch,kesher,ken's,karikos,karenina,kanamits,junshi,juno's,jumbled,jujitsu,judith's,jt's,joust,journeyed,jotted,jonathan's,jizz,jingling,jigalong,jerseys,jerries,jellybean,jellies,jeeps,jeannie's,javna,jamestown,james's,jamboree,jail's,islanders,irresistable,irene's,ious,investigation's,investigates,invaders,inundated,introductory,interviewer,interrupts,interpreting,interplanetary,internist,intercranial,inspections,inspecting,inseminated,inquisitor,inland,infused,infuriate,influx,inflating,infidelities,inference,inexpensive,industrialist,incessantly,inception,incensed,incase,incapacitate,inca,inasmuch,inaccuracies,imus,improvised,imploding,impeding,impediments,immaturity,ills,illegible,idols,iditarod,identifiable,id'n,icicles,ibuprofen,i'i'm,hymie,hydrolase,hybrids,hunsecker's,hunker,humps,humons,humidor,humdinger,humbling,humankind,huggin,huffing,households,housecleaning,hothouse,hotcakes,hosty,hootenanny,hootchie,hoosegow,honouring,honks,honeymooners,homophobic,homily,homeopathic,hoffman's,hnnn,hitchhikers,hissed,hispanics,hillnigger,hexavalent,hewwo,heston's,hershe,herodotus,hermey,hergott,heresy,henny,hennigans,henhouse,hemolytic,hells,helipad,heifer,hebrews,hebbing,heaved,heartland,heah,headlock,hatchback,harvard's,harrowing,harnessed,harding's,happy's,hannibal's,hangovers,handi,handbasket,handbags,halloween's,hall's,halfrek,halfback,hagrid,hacene,gyges,guys're,gut's,gundersons,gumption,guardia,gruntmaster,grubs,group's,grouch,grossie,grosser,groped,grins,grime,grigio,griff's,greaseball,gravesite,gratuity,graphite,granma,grandfathers,grandbaby,gradski,gracing,got's,gossips,goonie,gooble,goobers,goners,golitsyn,gofer,godsake,goddaughter,gnats,gluing,glub,global's,glares,gizmos,givers,ginza,gimmie,gimmee,georgia's,gennero,gazpacho,gazed,gato,gated,gassy,gargling,gandhiji,galvanized,gallery's,gallbladder,gabriel's,gaaah,furtive,furthering,fungal,fumigation,fudd,fucka,fronkonsteen,fromby's,frills,fresher,freezin,freewald,freeloader,franklin's,framework,frailty,fortified,forger,forestry,foreclosure,forbade,foray,football's,foolhardy,fondest,fomin,followin,follower,follicle,flue,flowering,flotation,flopping,floodgates,flogged,flog,flicked,flenders,fleabag,flanks,fixings,fixable,fistful,firewater,firestarter,firelight,fingerbang,finalizing,fillin,filipov,fido,fiderer,feminists,felling,feldberg,feign,favorably,fave,faunia,faun,fatale,fasting,farkus,fared,fallible,faithfulness,factoring,facilitated,fable,eyeful,extramarital,extracts,extinguished,exterminated,exposes,exporter,exponential,exhumed,exhume,exasperated,eviscerate,evidenced,evanston,estoy,estimating,esmerelda,esme,escapades,erosion,erie,equitable,epsom,epoxy,enticed,enthused,entendre,ensued,enhances,engulfed,engrossing,engraving,endorphins,enamel,emptive,empirical,emmys,emission,eminently,embody,embezzler,embarressed,embarrassingly,embalmed,emancipation,eludes,eling,elevation,electorate,elated,eirie,egotitis,effecting,eerily,eeew,eecom,editorials,edict,eczema,ecumenical,ecklie's,earthy,earlobes,eally,dyeing,dwells,dvds,duvet,duncans,dulcet,duckling,droves,droppin,drools,drey'auc,dreamers,dowser's,downriver,downgraded,doping,doodie,dominicans,dominating,domesticity,dollop,doesnt,doer,dobler,divulged,divisional,diversionary,distancing,dissolves,dissipated,displaying,dispensers,dispensation,disorienting,disneyworld,dismissive,dismantling,disingenuous,disheveled,disfiguring,discourse,discontinued,disallowed,dinning,dimming,diminutive,diligently,dilettante,dilation,diggity,diggers,dickensian,diaphragms,diagnoses,dewy,developer,devastatingly,determining,destabilize,desecrate,derives,deposing,denzel,denouncing,denominations,denominational,deniece,demony,delving,delt,delicates,deigned,degrassi's,degeneration,defraud,deflower,defibrillator,defiantly,deferred,defenceless,defacing,dedicating,deconstruction,decompose,deciphering,decibels,deceptively,deceptions,decapitation,debutantes,debonair,deadlier,dawdling,davic,databases,darwinism,darnit,darks,danke,danieljackson,dangled,daimler,cytoxan,cylinders,cutout,cutlery,cuss,cushing's,curveball,curiously,curfews,cummerbund,cuckoo's,crunches,crucifixion,crouched,croix,criterion,crisps,cripples,crilly,cribs,crewman,cretaceous,creepin,creeds,credenza,creak,crawly,crawlin,crawlers,crated,crasher,crackheads,coworker,counterpart,councillor,coun,couldn't've,cots,costanza's,cosgrove's,corwins,corset,correspondents,coriander,copiously,convenes,contraceptives,continuously,contingencies,contaminating,consul,constantinople,conniption,connie's,conk,conjugate,condiment,concurrently,concocting,conclave,concert's,con's,comprehending,compliant,complacency,compilation,competitiveness,commendatore,comedies,comedians,comebacks,combines,com'on,colonized,colonization,collided,collectively,collarbone,collaborating,collaborated,colitis,coldly,coiffure,coffers,coeds,codependent,cocksucking,cockney,cockles,clutched,cluett's,cloverleaf,closeted,cloistered,clinched,clicker,cleve,clergyman,cleats,clarifying,clapped,citations,cinnabar,cinco,chunnel,chumps,chucks,christof,cholinesterase,choirboy,chocolatey,chlamydia,chili's,chigliak,cheesie,cheeses,chechnya,chauvinistic,chasm,chartreuse,charnier,chapil,chapel's,chalked,chadway,cerveza,cerulean,certifiably,celsius,cellulite,celled,ceiling's,cavalry's,cavalcade,catty,caters,cataloging,casy,castrated,cassio,cashman's,cashews,carwash,cartouche,carnivore,carcinogens,carasco's,carano's,capulet,captives,captivated,capt'n,capsized,canoes,cannes,candidate's,cancellations,camshaft,campin,callate,callar,calendar's,calculators,cair,caffeinated,cadavers,cacophony,cackle,byproduct,bwana,buzzes,buyout,buttoning,busload,burglaries,burbs,bura,buona,bunions,bungalows,bundles,bunches,bullheaded,buffs,bucyk,buckling,bruschetta,browbeating,broomsticks,broody,bromly,brolin,brigadier,briefings,bridgeport,brewskies,breathalyzer,breakups,breadth,bratwurst,brania,branching,braiding,brags,braggin,bradywood,bozo's,bottomed,bottom's,bottling,botany,boston's,bossa,bordello,booo,bookshelf,boogida,bondsman,bolsheviks,bolder,boggles,boarder,boar's,bludgeoned,blowtorch,blotter,blips,blends,blemish,bleaching,blainetologists,blading,blabbermouth,bismarck,bishops,biscayne,birdseed,birdcage,bionic,biographies,biographical,bimmel,biloxi,biggly,bianchinni,bette's,betadine,berg's,berenson,belus,belt's,belly's,belloq,bella's,belfast,behavior's,begets,befitting,beethoven's,beepers,beelzebub,beefed,bedroom's,bedrock,bedridden,bedevere,beckons,beckett's,beauty's,beaded,baubles,bauble,battlestar,battleground,battle's,bathrobes,basketballs,basements,barroom,barnacle,barkin,barked,barium,baretta,bangles,bangler,banality,bambang,baltar,ballplayers,baio,bahrain,bagman,baffles,backstroke,backroom,bachelor's,babysat,babylonian,baboons,aviv,avez,averse,availability,augmentation,auditory,auditor,audiotape,auctioneer,atten,attained,attackers,atcha,astonishment,asshole's,assembler,arugula,arsonist's,arroz,arigato,arif,ardent,archaic,approximation,approving,appointing,apartheid,antihistamines,antarctica,annoyances,annals,annabelle's,angrily,angelou,angelo's,anesthesiology,android,anatomically,anarchists,analyse,anachronism,amiable,amex,ambivalent,amassed,amaretto,alumnus,alternating,alternates,alteration,aloft,alluding,allen's,allahu,alight,alfred's,alfie,airlift,aimin,ailment,aground,agile,ageing,afterglow,africans,affronte,affectionately,aerobic,adviser,advil,adventist,advancements,adrenals,admiral's,administrators,adjutant,adherence,adequately,additives,additions,adapting,adaptable,actualization,activating,acrost,ached,accursed,accoutrements,absconded,aboveboard,abou,abetted,abbot's,abbey's,aargh,aaaahh,zuzu's,zuwicky,zolda,zits,ziploc,zakamatak,yutz,yumm,youve,yolk,yippie,yields,yiddish,yesterdays,yella,yearns,yearnings,yearned,yawning,yalta,yahtzee,yacht's,y'mean,y'are,xand,wuthering,wreaks,woul,worsened,worrisome,workstation,workiiing,worcestershire,woop,wooooooo,wooded,wonky,womanizing,wolodarsky,wnkw,wnat,wiwith,withdraws,wishy,wisht,wipers,wiper,winos,winery,windthorne,windsurfing,windermere,wiggles,wiggled,wiggen,whys,whwhat,whuh,whos,whore's,whodunit,whoaaa,whittling,whitesnake,whirling,whereof,wheezing,wheeze,whatley's,whatd'ya,whataya,whammo,whackin,wets,westbound,wellll,wellesley,welch's,weirdo's,weightless,weevil,wedgies,webbing,weasly,weapon's,wean,wayside,waxes,wavelengths,waturi,washy,washrooms,warton's,wandell,wakeup,waitaminute,waddya,wabash,waaaah,vornac,voir,voicing,vocational,vocalist,vixens,vishnoor,viscount,virulent,virtuoso,vindictiveness,vinceres,vince's,villier,viii,vigeous,viennese,viceroy,vestigial,vernacular,venza's,ventilate,vented,venereal,vell,vegetative,veering,veered,veddy,vaslova,valosky,vailsburg,vaginas,vagas,vacation's,uuml,urethra,upstaged,uploading,upgrades,unwrapping,unwieldy,untenable,untapped,unsatisfied,unsatisfactory,unquenchable,unnerved,unmentionable,unlovable,unknowns,universes,uninformed,unimpressed,unhappily,unguarded,unexplored,underpass,undergarment,underdeveloped,undeniably,uncompromising,unclench,unclaimed,uncharacteristically,unbuttoned,unblemished,unas,umpa,ululd,uhhhm,tweeze,tutsami,tusk,tushy,tuscarora,turkle,turghan,turbulent,turbinium,tuffy,tubers,tsun,trucoat,troxa,trou,tropicana,triquetra,tripled,trimmers,triceps,tribeca,trespassed,traya,travellers,traumatizing,transvestites,transatlantic,tran's,trainors,tradin,trackers,townies,tourelles,toughness,toucha,totals,totalled,tossin,tortious,topshop,topes,tonics,tongs,tomsk,tomorrows,toiling,toddle,tobs,tizzy,tiramisu,tippers,timmi,timbre,thwap,thusly,ththe,thruway,thrusts,throwers,throwed,throughway,thrice,thomas's,thickening,thia,thermonuclear,therapy's,thelwall,thataway,th's,textile,texans,terry's,terrifically,tenets,tendons,tendon,telescopic,teleportation,telepathically,telekinetic,teetering,teaspoons,teamsters,taunts,tatoo,tarantulas,tapas,tanzania,tanned,tank's,tangling,tangerine,tamales,tallied,tailors,tai's,tahitian,tag's,tactful,tackles,tachy,tablespoon,tableau,syrah,syne,synchronicity,synch,synaptic,synapses,swooning,switchman,swimsuits,swimmer's,sweltering,swelling's,sweetly,sweeper,suvolte,suss,suslov,surname,surfed,supremacy,supposition,suppertime,supervillains,superman's,superfluous,superego,sunspots,sunnydale's,sunny's,sunning,sunless,sundress,sump,suki,suffolk,sue's,suckah,succotash,substation,subscriptions,submarines,sublevel,subbasement,styled,studious,studio's,striping,stresses,strenuously,streamlined,strains,straights,stony,stonewalled,stonehenge,stomper,stipulates,stinging,stimulated,stillness,stilettos,stewards,stevesy,steno,sten,stemmed,steenwyck,statesmen,statehood,stargates,standstill,stammering,staedert,squiggly,squiggle,squashing,squaring,spurred,sprints,spreadsheet,spramp,spotters,sporto,spooking,sponsorship,splendido,spittin,spirulina,spiky,speculations,spectral,spate,spartacus,spans,spacerun,sown,southbound,sorr,sorcery,soonest,sono,sondheim,something'll,someth,somepin,someone'll,solicitor,sofas,sodomy,sobs,soberly,sobered,soared,soapy,snowmen,snowbank,snowballing,snorkel,snivelling,sniffling,snakeskin,snagging,smush,smooter,smidgen,smackers,smackdown,slumlord,slugging,slossum,slimmer,slighted,sleepwalk,sleazeball,skokie,skirmishes,skipper's,skeptic,sitka,sitarides,sistah,sipped,sindell,simpletons,simp,simony,simba's,silkwood,silks,silken,silicone,sightless,sideboard,shuttles,shrugging,shrouds,showy,shoveled,shouldn'ta,shoplifters,shitstorm,shipyard,shielded,sheldon's,sheeny,shaven,shapetype,shankar,shaming,shallows,shale,shading,shackle,shabbily,shabbas,severus,settlements,seppuku,senility,semite,semiautomatic,semester's,selznick,secretarial,sebacio,sear,seamless,scuzzy,scummy,scud,scrutinized,scrunchie,scriptures,scribbled,scouted,scotches,scolded,scissor,schooner,schmidt's,schlub,scavenging,scarin,scarfing,scarecrow's,scant,scallions,scald,scabby,say's,savour,savored,sarcoidosis,sandbar,saluted,salted,salish,saith,sailboats,sagittarius,sagan,safeguards,sacre,saccharine,sacamano,sabe,rushdie,rumpled,rumba,rulebook,rubbers,roughage,rotterdam,roto,rotisserie,rosebuds,rootie,roosters,roosevelt's,rooney's,roofy,roofie,romanticize,roma's,rolodex,rolf's,roland's,rodney's,robotic,robin's,rittle,ristorante,rippin,rioting,rinsing,ringin,rincess,rickety,rewritten,revising,reveling,rety,retreats,retest,retaliating,resumed,restructuring,restrict,restorative,reston,restaurateur,residences,reshoots,resetting,resentments,rescuers,rerouted,reprogramming,reprisals,reprisal,repossess,repartee,renzo,renfield,remore,remitting,remeber,reliability,relaxants,rejuvenate,rejections,rehu,regularity,registrar's,regionals,regimes,regenerated,regency,refocus,referrals,reeno,reelected,redevelopment,recycles,recrimination,recombinant,reclining,recanting,recalling,reattach,reassigning,realises,reactors,reactionary,rbis,razor's,razgul,raved,rattlesnakes,rattles,rashly,raquetball,rappers,rapido,ransack,rankings,rajah,raisinettes,raheem,radisson,radishes,radically,radiance,rabbi's,raban,quoth,qumari,quints,quilts,quilting,quien,queue,quarreled,qualifying,pygmy,purty,puritans,purblind,puppy's,punctuation,punchbowl,puget,publically,psychotics,psychopaths,psychoanalyze,pruning,provasik,protruding,protracted,protons,protections,protectin,prospector,prosecutor's,propping,proportioned,prophylactic,propelled,proofed,prompting,prompter,professed,procreate,proclivities,prioritizing,prinze,princess's,pricked,press'll,presets,prescribes,preocupe,prejudicial,prefex,preconceived,precipice,preamble,pram,pralines,pragmatist,powering,powerbar,pottie,pottersville,potsie,potholes,potency,posses,posner's,posies,portkey,porterhouse,pornographers,poring,poppycock,poppet,poppers,poopsie,pomponi,pokin,poitier,poes,podiatry,plush,pleeze,pleadings,playbook,platelets,plane'arium,placebos,place'll,pj's,pixels,pitted,pistachios,pisa,pirated,pirate's,pinochle,pineapples,pinafore,pimples,piggly,piggies,pie's,piddling,picon,pickpockets,picchu,physiologically,physic,photo's,phobic,philosophies,philosophers,philly's,philandering,phenomenally,pheasants,phasing,phantoms,pewter,petticoat,petronis,petitioning,perturbed,perth,persists,persians,perpetuating,permutat,perishable,periphery,perimeters,perfumed,percocet,per'sus,pepperjack,pensioners,penalize,pelting,pellet,peignoir,pedicures,pedestrians,peckers,pecans,payback's,pay's,pawning,paulsson,pattycake,patrolmen,patrolled,patois,pathos,pasted,passer,partnerships,parp,parishioners,parishioner,parcheesi,parachuting,pappa,paperclip,papayas,paolo's,pantheon,pantaloons,panhandle,pampers,palpitations,paler,palantine,paintballing,pago,owow,overtired,overstress,oversensitive,overnights,overexcited,overanxious,overachiever,outwitted,outvoted,outnumber,outlived,outlined,outlast,outlander,outfield,out've,ortolani's,orphey,ornate,ornamental,orienteering,orchestrating,orator,oppressive,operator's,openers,opec,ooky,oliver's,olde,okies,okee,ohhhhhhhhh,ohhhhhhhh,ogling,offline,offbeat,oceanographic,obsessively,obeyed,oaths,o'leary's,o'hana,o'bannon,o'bannion,numpce,nummy,nuked,nuff,nuances,nourishing,noticeably,notably,nosedive,northeastern,norbu,nomlies,nomine,nomads,noge,nixed,niro,nihilist,nightshift,newmeat,nevis,nemo's,neighborhood's,neglectful,neediness,needin,necromancer,neck's,ncic,nathaniel's,nashua,naphthalene,nanotechnology,nanocytes,nanite,naivete,nacho,n'yeah,mystifying,myhnegon,mutating,muskrat,musing,museum's,muppets,mumbles,mulled,muggy,muerto,muckraker,muchachos,mris,move's,mourners,mountainside,moulin,mould,motherless,motherfuck,mosquitos,morphed,mopped,moodoo,montage,monsignor,moncho,monarchs,mollem,moisturiser,moil,mohicans,moderator,mocks,mobs,mizz,mites,mistresses,misspent,misinterpretation,mishka,miscarry,minuses,minotaur,minoan,mindee,mimicking,millisecond,milked,militants,migration,mightn't,mightier,mierzwiak,midwives,micronesia,microchips,microbes,michele's,mhmm,mezzanine,meyerling,meticulously,meteorite,metaphorical,mesmerizing,mershaw,meir,meg's,meecrob,medicate,medea,meddled,mckinnons,mcgewan,mcdunnough,mcats,mbien,maytag,mayors,matzah,matriarch,matic,mathematicians,masturbated,masselin,marxist,martyrs,martini's,martialed,marten's,marlboros,marksmanship,marishka,marion's,marinate,marge's,marchin,manifestations,manicured,mandela,mamma's,mame,malnourished,malk,malign,majorek,maidens,mahoney's,magnon,magnificently,maestro's,macking,machiavellian,macdougal,macchiato,macaws,macanaw,m'self,lynx,lynn's,lyman's,lydells,lusts,lures,luna's,ludwig's,lucite,lubricants,louise's,lopper,lopped,loneliest,lonelier,lomez,lojack,localized,locale,loath,lloyd's,literate,liquidated,liquefy,lippy,linguistic,limps,lillian's,likin,lightness,liesl,liebchen,licious,libris,libation,lhamo,lewis's,leveraged,leticia's,leotards,leopards,leonid,leonardo's,lemmings,leland's,legitimacy,leanin,laxatives,lavished,latka,later's,larval,lanyard,lans,lanky,landscaping,landmines,lameness,lakeshore,laddies,lackluster,lacerated,labored,laboratories,l'amour,kyrgyzstan,kreskin,krazy,kovitch,kournikova,kootchy,konoss,know's,knknow,knickety,knackety,kmart,klicks,kiwanis,kitty's,kitties,kites,kissable,kirby's,kingdoms,kindergartners,kimota,kimble's,kilter,kidnet,kidman,kid'll,kicky,kickbacks,kickback,kickass,khrushchev,kholokov,kewpie,kent's,keno,kendo,keller's,kcdm,katrina's,katra,kareoke,kaia,kafelnikov,kabob,ka's,junjun,jumba,julep,jordie,jondy,jolson,jinnah,jeweler's,jerkin,jenoff,jefferson's,jaye's,jawbone,janitorial,janiro,janie's,iron's,ipecac,invigorated,inverted,intruded,intros,intravenously,interruptus,interrogations,interracial,interpretive,internment,intermediate,intermediary,interject,interfacing,interestin,insuring,instilled,instantaneous,insistence,insensitivity,inscrutable,inroads,innards,inlaid,injector,initiatives,inhe,ingratitude,infuriates,infra,informational,infliction,infighting,induction,indonesian,indochina,indistinguishable,indicators,indian's,indelicate,incubators,incrimination,increments,inconveniencing,inconsolable,incite,incestuous,incas,incarnation,incarcerate,inbreeding,inaccessible,impudence,impressionists,implemented,impeached,impassioned,impacts,imipenem,idling,idiosyncrasies,icicle,icebreaker,icebergs,i'se,hyundai,hypotensive,hydrochloride,huuh,hushed,humus,humph,hummm,hulking,hubcaps,hubald,http,howya,howbout,how'll,houseguests,housebroken,hotwire,hotspots,hotheaded,horticulture,horrace,horde,horace's,hopsfield,honto,honkin,honeymoons,homophobia,homewrecker,hombres,hollow's,hollers,hollerin,hokkaido,hohh,hogwarts,hoedown,hoboes,hobbling,hobble,hoarse,hinky,himmler,hillcrest,hijacking,highlighters,hiccup,hibernation,hexes,heru'ur,hernias,herding,heppleman,henderson's,hell're,heine's,heighten,heheheheheh,heheheh,hedging,heckling,heckled,heavyset,heatshield,heathens,heartthrob,headpiece,headliner,he'p,hazelnut,hazards,hayseed,haveo,hauls,hattie's,hathor's,hasten,harriers,harridan,harpoons,harlin's,hardens,harcesis,harbouring,hangouts,hangman,handheld,halkein,haleh,halberstam,hairpin,hairnet,hairdressers,hacky,haah,haaaa,h'yah,gyms,gusta,gushy,gusher,gurgling,gunnery,guilted,guilt's,gruel,grudging,grrrrrr,grouse,grossing,grosses,groomsmen,griping,gretchen's,gregorian,gray's,gravest,gratified,grated,graphs,grandad,goulash,goopy,goonies,goona,goodman's,goodly,goldwater,godliness,godawful,godamn,gobs,gob's,glycerin,glutes,glowy,glop,globetrotters,glimpsed,glenville,glaucoma,girlscout,giraffes,gimp,gilbey,gil's,gigglepuss,ghora,gestating,geologists,geographically,gelato,gekko's,geishas,geek's,gearshift,gear's,gayness,gasped,gaslighting,garretts,garba,gams,gags,gablyczyck,g'head,fungi,fumigating,fumbling,fulton's,fudged,fuckwad,fuck're,fuchsia,fruition,freud's,fretting,freshest,frenchies,freezers,fredrica,fraziers,francesca's,fraidy,foxholes,fourty,fossilized,forsake,formulate,forfeits,foreword,foreclosed,foreal,foraging,footsies,focussed,focal,florists,flopped,floorshow,floorboard,flinching,flecks,flavours,flaubert,flatware,flatulence,flatlined,flashdance,flail,flagging,fizzle,fiver,fitzy,fishsticks,finster,finetti,finelli,finagle,filko,filipino,figurines,figurative,fifi,fieldstone,fibber,fiance's,feuds,feta,ferrini,female's,feedin,fedora,fect,feasting,favore,fathering,farrouhk,farmin,far's,fanny's,fajita,fairytale,fairservice,fairgrounds,fads,factoid,facet,facedown,fabled,eyeballin,extortionist,exquisitely,exporting,explicitly,expenditures,expedited,expands,exorcise,existentialist,exhaustive,execs,exculpatory,excommunicated,exacerbate,everthing,eventuality,evander,eustace,euphoric,euphemisms,eton,esto,estimation,estamos,establishes,erred,environmentalist,entrepreneurial,entitle,enquiries,enormity,engages,enfants,enen,endive,end's,encyclopedias,emulating,emts,employee's,emphasized,embossed,embittered,embassies,eliot,elicit,electrolyte,ejection,effortless,effectiveness,edvard,educators,edmonton's,ecuador,ectopic,ecirc,easely,earphones,earmarks,earmarked,earl's,dysentery,dwindling,dwight's,dweller,dusky,durslar,durned,dunois,dunking,dunked,dumdum,dullard,dudleys,duce,druthers,druggist,drug's,drossos,drosophila,drooled,driveways,drippy,dreamless,drawstring,drang,drainpipe,dragoons,dozing,down's,dour,dougie's,dotes,dorsal,dorkface,doorknobs,doohickey,donnell's,donnatella,doncha,don's,dominates,domicile,dokos,dobermans,djez,dizzying,divola,dividends,ditsy,distaste,disservice,disregarded,dispensed,dismay,dislodged,dislodge,disinherit,disinformation,discrete,discounting,disciplines,disapproved,dirtball,dinka,dimly,dilute,dilucca's,digesting,diello,diddling,dictatorships,dictators,diagonal,diagnostician,devours,devilishly,detract,detoxing,detours,detente,destructs,desecrated,descends,derris,deplore,deplete,depicts,depiction,depicted,denver's,denounce,demure,demolitions,demean,deluge,dell's,delish,deliberation,delbruck,delaford,deities,degaulle,deftly,deft,deformity,deflate,definatly,defense's,defector,deducted,decrypted,decontamination,decker's,decapitate,decanter,deadline's,dardis,danger's,dampener,damme,daddy'll,dabbling,dabbled,d'etre,d'argent,d'alene,d'agnasti,czechs,czechoslovakian,cyrillic,cymbal,cyberdyne,cutoffs,cuticle,cut's,curvaceous,curiousity,curfew's,culturally,cued,cubby,cruised,crucible,crowing,crowed,croutons,cropped,croaker,cristobel's,criminy,crested,crescentis,cred,cream's,crashers,crapola,cranwell,coverin,cousteau,courtrooms,counterattack,countenance,counselor's,cottages,cosmically,cosign,cosa,corroboration,corresponds,correspond,coroners,coro,cornflakes,corbett's,copy's,copperpot,copperhead,copacetic,coordsize,convulsing,contradicted,contract's,continuation,consults,consultations,constraints,conjures,congenial,confluence,conferring,confederation,condominium,concourse,concealer,compulsory,complexities,comparatively,compactor,commodities,commercialism,colleague's,collaborator,cokey,coiled,cognizant,cofell's,cobweb,co's,cnbc,clyde's,clunkers,clumsily,clucking,cloves,cloven,cloths,clothe,clop,clods,clocking,clings,climbers,clef,clearances,clavicle,claudia's,classless,clashing,clanking,clanging,clamping,civvies,citywide,citing,circulatory,circuited,circ,chung's,chronisters,chromic,choppy,choos,chongo,chloroformed,chilton's,chillun,chil,chicky,cheetos,cheesed,chatterbox,charlies,chaperoned,channukah,chamberlain's,chairman's,chaim,cessation,cerebellum,centred,centerpieces,centerfold,cellars,ceecee,ccedil,cavorting,cavemen,cavaliers,cauterized,caustic,cauldwell,catting,cathy's,caterine,castor's,cassiopeia,cascade's,carves,cartwheel,cartridges,carpeted,carob,carlsbad,caressing,carelessly,careening,carcinoma,capricious,capitalistic,capillaries,capes,candle's,candidly,canaan,camaraderie,calumet,callously,calligraphy,calfskin,cake's,caddies,cabinet's,buzzers,buttholes,butler's,busywork,busses,burps,burgomeister,buoy,bunny's,bunkhouse,bungchow,bulkhead,builders,bugler,buffets,buffed,buckaroo's,brutish,brusque,browser,bronchitis,bromden,brolly,brody's,broached,brewskis,brewski,brewin,brewers,brean,breadwinner,brana,brackets,bozz,bountiful,bounder,bouncin,bosoms,borgnine,bopping,bootlegs,booing,bons,boneyard,bombosity,bolting,bolivia,boilerplate,boba,bluey,blowback,blouses,bloodsuckers,bloodstained,blonde's,bloat,bleeth,blazed,blaine's,blackhawk,blackface,blackest,blackened,blacken,blackballed,blabs,blabbering,birdbrain,bipartisanship,biodegradable,binghamton,biltmore,billiards,bilked,big'uns,bidwell's,bidet,bessie's,besotted,beset,berth,bernheim,benson's,beni,benegas,bendiga,belushi,beltway,bellboys,belittling,belinda's,behinds,behemoth,begone,beeline,beehive,bedsheets,beckoning,beaute,beaudine,beastly,beachfront,be's,bauk,bathes,batak,bastion,baser,baseballs,barker's,barber's,barbella,bans,bankrolling,bangladesh,bandaged,bamba,bally's,bagpipe,bagger,baerly,backlog,backin,babying,azkaban,ayatollah,axes,awwwww,awakens,aviary,avery's,autonomic,authorizes,austero,aunty,augustine's,attics,atreus,astronomers,astounded,astonish,assertion,asserting,assailants,asha's,artemus,arses,arousal,armin,arintero,argon's,arduous,archers,archdiocese,archaeology,arbitrarily,ararat,appropriated,appraiser,applicable,apathetic,anybody'd,anxieties,anwar's,anticlimactic,antar,ankle's,anima,anglos,angleman,anesthetist,androscoggin,andromeda,andover,andolini,andale,anan,amway,amuck,amphibian,amniocentesis,amnesiac,ammonium,americano,amara,alway,alvah,alum,altruism,alternapalooza,alphabetize,alpaca,almanac,ally's,allus,alluded,allocation,alliances,allergist,alleges,alexandros,alec's,alaikum,alabam,akimbo,airy,ahab's,agoraphobia,agides,aggrhh,agatha's,aftertaste,affiliations,aegis,adoptions,adjuster,addictions,adamantium,acumen,activator,activates,acrylic,accomplishes,acclaimed,absorbs,aberrant,abbu,aarp,aaaaargh,aaaaaaaaaaaaa,a'ight,zucchini,zoos,zookeeper,zirconia,zippers,zequiel,zephyr's,zellary,zeitgeist,zanuck,zambia,zagat,ylang,yielded,yes'm,yenta,yegg,yecchh,yecch,yayo,yawp,yawns,yankin,yahdah,yaaah,y'got,xeroxed,wwooww,wristwatch,wrangled,wouldst,worthiness,wort,worshiping,worsen,wormy,wormtail,wormholes,woosh,woodworking,wonka,womens,wolverines,wollsten,wolfing,woefully,wobbling,witter's,wisp,wiry,wire's,wintry,wingding,windstorm,windowtext,wiluna,wilting,wilted,willick,willenholly,wildflowers,wildebeest,wilco,wiggum,wields,widened,whyyy,whoppers,whoaa,whizzing,whizz,whitest,whitefish,whistled,whist,whinny,whereupon,whereby,wheelies,wheaties,whazzup,whatwhatwhaaat,whato,whatdya,what'dya,whar,whacks,wexler's,wewell,wewe,wetsuit,wetland,westport,welluh,weight's,weeps,webpage,waylander,wavin,watercolors,wassail,wasnt,warships,warns,warneford,warbucks,waltons,wallbanger,waiving,waitwait,vowing,voucher,vornoff,vork,vorhees,voldemort,vivre,vittles,vishnu,vips,vindaloo,videogames,victors,vicky's,vichyssoise,vicarious,vet's,vesuvius,verve,verguenza,venturing,ventura's,venezuelan,ven't,velveteen,velour,velociraptor,vegetation,vaudeville,vastness,vasectomies,vapors,vanderhof,valmont,validates,valiantly,valerian,vacuums,vaccines,uzbekistan,usurp,usernum,us'll,urinals,unyielding,unwillingness,unvarnished,unturned,untouchables,untangled,unsecured,unscramble,unreturned,unremarkable,unregistered,unpublished,unpretentious,unopposed,unnerstand,unmade,unlicensed,unites,union's,uninhabited,unimpeachable,unilateral,unicef,unfolded,unfashionable,undisturbed,underwriting,underwrite,underlining,underling,underestimates,underappreciated,undamaged,uncouth,uncork,uncontested,uncommonly,unclog,uncircumcised,unchallenged,uncas,unbuttoning,unapproved,unamerican,unafraid,umpteen,umhmm,uhwhy,uhmm,ughuh,ughh,ufo's,typewriters,twitches,twitched,twirly,twinkling,twink,twinges,twiddling,twiddle,tutored,tutelage,turners,turnabout,ture,tunisian,tumultuous,tumour,tumblin,tryed,truckin,trubshaw's,trowel,trousseau,trivialize,trifles,tribianni,trib,triangulation,trenchcoat,trembled,traumatize,transplanted,translations,transitory,transients,transfuse,transforms,transcribing,transcend,tranq,trampy,traipsed,trainin,trail's,trafalgar,trachea,traceable,touristy,toughie,totality,totaling,toscanini,tortola,tortilla,tories,toreador,tooo,tonka,tommorrow,tollbooth,tollans,toidy,togs,togas,tofurkey,toddling,toddies,tobruk,toasties,toadstool,to've,tive,tingles,timin,timey,timetables,tightest,tide's,tibetans,thunderstorms,thuggee,thrusting,thrombus,throes,throated,thrifty,thoroughbred,thornharts,thinnest,thicket,thetas,thesulac,tethered,testimonial,testaburger,tersenadine,terrif,teresa's,terdlington,tepui,tenured,tentacle,temping,temperance,temp's,teller's,televisions,telefono,tele,teddies,tector,taxidermy,taxi's,taxation,tastebuds,tasker's,tartlets,tartabull,tard,tar'd,tantamount,tans,tangy,tangles,tamer,talmud,taiwan's,tabula,tabletops,tabithia,tabernacle,szechwan,syrian,synthedyne,synopsis,synonyms,swaps,swahili,svenjolly,svengali,suvs,sush,survivalists,surmise,surfboards,surefire,suprise,supremacists,suppositories,supervisors,superstore,supermen,supercop,supercilious,suntac,sunburned,summercliff,sullied,suite's,sugared,sufficiency,suerte,suckle,sucker's,sucka,succumbing,subtleties,substantiated,subsidiaries,subsides,subliminal,subhuman,stst,strowman,stroked,stroganoff,strikers,strengthening,streetlight,straying,strainer,straighter,straightener,storytelling,stoplight,stockade,stirrups,stink's,sting's,stimulates,stifler's,stewing,stetson's,stereotyping,ster,stepmommy,stephano,steeped,statesman,stashing,starshine,stand's,stamping,stamford,stairwells,stabilization,squatsie,squandering,squalid,squabbling,squab,sprinkling,spring's,spreader,spongy,spongebob,spokeswoman,spokesmen,splintered,spittle,spitter,spiced,spews,spendin,spect,speckled,spearchucker,spatulas,sparse,sparking,spares,spaceboy,soybeans,southtown,southside,southport,southland,soused,sotheby's,soshi,sorter,sorrowful,sorceress,sooth,songwriters,some'in,solstice,soliloquy,sods,sodomized,sode,sociologist,sobriki,soaping,snows,snowcone,snowcat,snitching,snitched,sneering,snausages,snaking,smoothed,smoochies,smolensk,smarten,smallish,slushy,slurring,sluman,slobber,slithers,slippin,sleuthing,sleeveless,slade's,skinner's,skinless,skillfully,sketchbook,skagnetti,sista,sioux,sinning,sinjin,singularly,sinewy,sinclair's,simultaneous,silverlake,silva's,siguto,signorina,signature's,signalling,sieve,sids,sidearms,shyster,shying,shunning,shtud,shrooms,shrieks,shorting,shortbread,shopkeepers,shmuck,shmancy,shizzit,shitheads,shitfaced,shitbag,shipmates,shiftless,sherpa,shelving,shelley's,sheik,shedlow,shecky,sheath,shavings,shatters,sharifa,shampoos,shallots,shafter,sha'nauc,sextant,settlers,setter,seti,serviceable,serrated,serbian,sequentially,sepsis,senores,sendin,semis,semanski,seller's,selflessly,selects,selectively,seinfelds,seers,seer's,seeps,see's,seductress,sedimentary,sediment,second's,secaucus,seater,seashore,sealant,seaborn's,scuttling,scusa,sculpting,scrunched,scrimmage,screenwriter,scotsman,scorer,sclerosis,scissorhands,schreber,scholastic,schmancy,schlong,scathing,scandinavia,scamps,scalloped,savoir,savagery,sasha's,sarong,sarnia,santangel,samool,samba,salons,sallow,salino,safecracker,sadism,saddles,sacrilegious,sabrini,sabath,s'aright,ruttheimer,russia's,rudest,rubbery,rousting,rotarian,roslin,rosey,rosa's,roomed,romari,romanticism,romanica,rolltop,rolfski,rod's,rockland,rockettes,roared,riverfront,rinpoche,ringleader,rims,riker's,riffing,ricans,ribcage,riana's,rhythmic,rhah,rewired,retroactive,retrial,reting,reticulum,resuscitated,resuming,restricting,restorations,restock,resilience,reservoirs,resembled,resale,requisitioned,reprogrammed,reproducing,repressive,replicant,repentant,repellant,repays,repainting,reorganization,renounced,renegotiating,rendez,renamed,reminiscent,remem,remade,relived,relinquishes,reliant,relearn,relaxant,rekindling,rehydrate,regulatory,regiments,regan's,refueled,refrigeration,refreshingly,reflector,refine,refilling,reexamine,reeseman,redness,redirected,redeemable,redder,redcoats,rectangles,recoup,reconstituted,reciprocated,recipients,recessed,recalls,rebounded,reassessing,realy,reality's,realisation,realer,reachin,re'kali,rawlston,ravages,rattlers,rasa,raps,rappaports,ramoray,ramming,ramadan,raindrops,rahesh,radioactivity,radials,racists,racin,rabartu,quotas,quintus,quiches,ques,queries,quench,quel,quarrels,quarreling,quaintly,quagmire,quadrants,pylon,putumayo,put'em,purifier,purified,pureed,punitis,pullout,pukin,pudgy,puddings,puckering,puccini,pterodactyl,psychodrama,pseudonym,psats,proximal,providers,protestations,protectee,prospered,prosaic,propositioned,prolific,progressively,proficiency,professions,prodigious,proclivity,probed,probabilities,pro's,prison's,printouts,principally,prig,prevision,prevailing,presumptive,pressers,preset,presentations,preposition,preparatory,preliminaries,preempt,preemie,predetermined,preconceptions,precipitate,prancan,powerpuff,powerfully,potties,potters,potpie,poseur,portraying,portico,porthole,portfolios,poops,pooping,pone,pomp,pomade,polyps,polymerized,politic,politeness,polisher,polack,pokers,pocketknife,poatia,plebeian,playgroup,platonically,plato's,platitude,platelet,plastering,plasmapheresis,plaques,plaids,placemats,place's,pizzazz,piracy,pipelines,pip's,pintauro,pinstripes,pinpoints,pinkner,pincer,pimento,pillaged,pileup,pilates,pigment,pigmen,pieter,pieeee,picturesque,piano's,phrasing,phrased,photojournalist,photocopies,phosphorus,phonograph,phoebes,phoe,philistines,philippine,philanderer,pheromone,phasers,pharaoh's,pfff,pfeffernuesse,petrov,petitions,peterman's,peso,pervs,perspire,personify,perservere,perplexed,perpetrating,perp's,perkiness,perjurer,periodontist,perfunctory,performa,perdido,percodan,penzance,pentameter,pentagon's,pentacle,pensive,pensione,pennybaker,pennbrooke,penhall,pengin,penetti,penetrates,pegs,pegnoir,peeve,peephole,pectorals,peckin,peaky,peaksville,payout,paxcow,paused,pauline's,patted,pasteur,passe,parochial,parkland,parkishoff,parkers,pardoning,paraplegic,paraphrasing,parapet,paperers,papered,panoramic,pangs,paneling,pander,pandemonium,pamela's,palooza,palmed,palmdale,palisades,palestinian,paleolithic,palatable,pakistanis,pageants,packaged,pacify,pacified,oyes,owwwww,overthrown,overt,oversexed,overriding,overrides,overpaying,overdrawn,overcompensate,overcomes,overcharged,outtakes,outmaneuver,outlying,outlining,outfoxed,ousted,oust,ouse,ould,oughtn't,ough,othe,ostentatious,oshun,oscillation,orthopedist,organizational,organization's,orca,orbits,or'derves,opting,ophthalmologist,operatic,operagirl,oozes,oooooooh,only's,onesie,omnis,omelets,oktoberfest,okeydoke,ofthe,ofher,obstetrics,obstetrical,obeys,obeah,o'rourke,o'reily's,o'henry,nyquil,nyanyanyanyah,nuttin,nutsy,nutrients,nutball,nurhachi,numbskull,nullifies,nullification,nucking,nubbin,ntnt,nourished,notoriety,northland,nonspecific,nonfiction,noing,noinch,nohoho,nobler,nitwits,nitric,nips,nibs,nibbles,newton's,newsprint,newspaperman,newspaper's,newscaster,never's,neuter,neuropathy,netherworld,nests,nerf,neee,neediest,neath,navasky,naturalization,nat's,narcissists,napped,nando,nags,nafta,myocardial,mylie's,mykonos,mutilating,mutherfucker,mutha,mutations,mutates,mutate,musn't,muskets,murray's,murchy,mulwray's,multitasking,muldoon's,mujeeb,muerte,mudslinging,muckraking,mrsa,mown,mousie,mousetrap,mourns,mournful,motivating,motherland,motherf,mostro,mosaic,morphing,morphate,mormons,moralistic,moored,moochy,mooching,monotonous,monorail,monopolize,monogram,monocle,molehill,molar,moland,mofet,modestly,mockup,moca,mobilizing,mitzvahs,mitre,mistreating,misstep,misrepresentation,misjudge,misinformation,miserables,misdirected,miscarriages,minute's,miniskirt,minimizing,mindwarped,minced,milquetoast,millimeters,miguelito,migrating,mightily,midsummer,midstream,midriff,mideast,midas,microbe,metropolis,methuselah,mesdames,mescal,mercury's,menudo,menu's,mentors,men'll,memorial's,memma,melvins,melanie's,megaton,megara,megalomaniac,meeee,medulla,medivac,mediate,meaninglessness,mcnuggets,mccarthyism,maypole,may've,mauve,maturing,matter's,mateys,mate's,mastering,masher,marxism,martimmy's,marshack,marseille,markles,marketed,marketable,mansiere,manservant,manse,manhandling,manco's,manana,maman,malnutrition,mallomars,malkovich's,malcontent,malaise,makeup's,majesties,mainsail,mailmen,mahandra,magnolias,magnified,magev,maelstrom,madcap,mack's,machu,macfarlane's,macado,ma'm,m'boy,m'appelle,lying's,lustrous,lureen,lunges,lumped,lumberyard,lulled,luego,lucks,lubricated,loveseat,loused,lounger,loski,lorre,loora,looong,loonies,lonnegan's,lola's,loire,loincloth,logistical,lofts,lodges,lodgers,lobbing,loaner,livered,lithuania,liqueur,linkage,ling's,lillienfield's,ligourin,lighter's,lifesaving,lifeguards,lifeblood,library's,liberte,liaisons,liabilities,let'em,lesbianism,lenny's,lennart,lence,lemonlyman,legz,legitimize,legalized,legalization,leadin,lazars,lazarro,layoffs,lawyering,lawson's,lawndale's,laugher,laudanum,latte's,latrines,lations,laters,lastly,lapels,lansing's,lan's,lakefront,lait,lahit,lafortunata,lachrymose,laborer,l'italien,l'il,kwaini,kuzmich,kuato's,kruczynski,kramerica,krakatoa,kowtow,kovinsky,koufax,korsekov,kopek,knoxville,knowakowski,knievel,knacks,klux,klein's,kiran,kiowas,kinshasa,kinkle's,kincaid's,killington,kidnapper's,kickoff,kickball,khan's,keyworth,keymaster,kevie,keveral,kenyons,keggers,keepsakes,kechner,keaty,kavorka,katmandu,katan's,karajan,kamerev,kamal's,kaggs,juvi,jurisdictional,jujyfruit,judeo,jostled,joni's,jonestown,jokey,joists,joint's,johnnie's,jocko,jimmied,jiggled,jig's,jests,jessy,jenzen,jensen's,jenko,jellyman,jeet,jedediah,jealitosis,jaya's,jaunty,jarmel,jankle,jagoff,jagielski,jacky,jackrabbits,jabbing,jabberjaw,izzat,iuml,isolating,irreverent,irresponsibly,irrepressible,irregularity,irredeemable,investigator's,inuvik,intuitions,intubated,introspective,intrinsically,intra,intimates,interval,intersections,interred,interned,interminable,interloper,intercostal,interchange,integer,intangible,instyle,instrumentation,instigate,instantaneously,innumerable,inns,injustices,ining,inhabits,ings,ingrown,inglewood,ingestion,ingesting,infusion,infusing,infringing,infringe,inflection,infinitum,infact,inexplicably,inequities,ineligible,industry's,induces,indubitably,indisputable,indirect,indescribably,independents,indentation,indefinable,incursion,incontrovertible,inconsequential,incompletes,incoherently,inclement,inciting,incidentals,inarticulate,inadequacies,imprudent,improvisation,improprieties,imprison,imprinted,impressively,impostors,importante,implicit,imperious,impale,immortalized,immodest,immobile,imbued,imbedded,imbecilic,illustrates,illegals,iliad,idn't,idiom,icons,hysteric,hypotenuse,hygienic,hyeah,hushpuppies,hunhh,hungarians,humpback,humored,hummed,humiliates,humidifier,huggy,huggers,huckster,html,hows,howlin,hoth,hotbed,hosing,hosers,horsehair,homegrown,homebody,homebake,holographic,holing,holies,hoisting,hogwallop,hogan's,hocks,hobbits,hoaxes,hmmmmm,hisses,hippos,hippest,hindrance,hindi,him's,hillbillies,hilarity,highball,hibiscus,heyday,heurh,hershey's,herniated,hermaphrodite,hera,hennifer,hemlines,hemline,hemery,helplessness,helmsley,hellhound,heheheheh,heey,heeey,hedda,heck's,heartbeats,heaped,healers,headstart,headsets,headlong,headlining,hawkland,havta,havana's,haulin,hastened,hasn,harvey'll,harpo,hardass,haps,hanta,hansom,hangnail,handstand,handrail,handoff,hander,han's,hamlet's,hallucinogen,hallor,halitosis,halen,hahah,hado,haberdashery,gypped,guy'll,guni,gumbel,gulch,gues,guerillas,guava,guatemalan,guardrail,guadalajara,grunther,grunick,grunemann's,growers,groppi,groomer,grodin,gris,gripes,grinds,grimaldi's,grifters,griffins,gridlock,gretch,greevey,greasing,graveyards,grandkid,grainy,graced,governed,gouging,gordie's,gooney,googly,golfers,goldmuff,goldenrod,goingo,godly,gobbledygook,gobbledegook,goa'uld's,glues,gloriously,glengarry,glassware,glamor,glaciers,ginseng,gimmicks,gimlet,gilded,giggly,gig's,giambetti,ghoulish,ghettos,ghandi,ghali,gether,get's,gestation,geriatrics,gerbils,gerace's,geosynchronous,georgio,geopolitical,genus,gente,genital,geneticist,generation's,generates,gendarme,gelbman,gazillionth,gayest,gauging,gastro,gaslight,gasbag,garters,garish,garas,garages,gantu,gangy,gangly,gangland,gamer,galling,galilee,galactica's,gaiety,gadda,gacy,futuristic,futs,furrowed,funny's,funnies,funkytown,fundraisers,fundamentalist,fulcrum,fugimotto,fuente,fueling,fudging,fuckup,fuckeen,frutt's,frustrates,froufrou,froot,frontiers,fromberge,frog's,frizzies,fritters,fringes,frightfully,frigate,friendliest,freeloading,freelancing,fredonia,freakazoid,fraternization,frankfurter,francine's,franchises,framers,fostered,fortune's,fornication,fornicating,formulating,formations,forman's,forgeries,forethought,forage,footstool,foisting,focussing,focking,foal,flutes,flurries,fluffed,flourished,florida's,floe,flintstones,fleischman's,fledgling,fledermaus,flayed,flay,flawlessly,flatters,flashbang,flapped,flanking,flamer,fission,fishies,firmer,fireproof,fireman's,firebug,firebird,fingerpainting,finessed,findin,financials,finality,fillets,fighter's,fiercest,fiefdom,fibrosis,fiberglass,fibbing,feudal,festus,fervor,fervent,fentanyl,fenelon,fenders,fedorchuk,feckless,feathering,fearsome,fauna,faucets,farmland,farewells,fantasyland,fanaticism,faltered,fallacy,fairway,faggy,faberge,extremism,extorting,extorted,exterminating,exhumation,exhilaration,exhausts,exfoliate,exemptions,excesses,excels,exasperating,exacting,evoked,evocative,everyman,everybody'd,evasions,evangelical,establishments,espressos,esoteric,esmail,errrr,erratically,eroding,erode,ernswiler,episcopalian,ephemeral,epcot,entrenched,entomology,entomologist,enthralled,ensuing,ensenada,enriching,enrage,enlisting,enhancer,enhancements,endorsing,endear,encrusted,encino,enacted,employing,emperors,empathic,embodied,embezzle,embarked,emanates,elton's,eloquence,eloi,elmwood,elliptical,ellenor's,elemental,electricians,electing,elapsed,eking,egomaniacal,eggo,egging,effected,effacing,eeww,edits,editor's,edging,ectoplasm,economical,ecch,eavesdropped,eastbound,earwig,e'er,durable,dunbar's,dummkopf,dugray,duchaisne,duality,drusilla's,drunkard,drudge,drucilla's,droop,droids,drips,dripped,dribbles,drew's,dressings,drazens,downy,downsize,downpour,dowager,dote,dosages,dorothy's,doppler,doppelganger,dopes,doorman's,doohicky,doof,dontcha,donovon's,doneghy,domi,domes,dojo,documentaries,divinity,divining,divest,diuretics,diuretic,distrustful,distortions,dissident,disrupts,disruptions,disproportionate,dispensary,disparity,dismemberment,dismember,disinfect,disillusionment,disheartening,discriminated,discourteous,discotheque,discolored,disassembled,disabling,dirtiest,diphtheria,dinks,dimpled,digg,diffusion,differs,didya,dickweed,dickwad,dickson's,diatribes,diathesis,diabetics,dewars,deviants,detrimental,detonates,detests,detestable,detaining,despondent,desecration,descriptive,derision,derailing,deputized,depressors,depo,depicting,depict,dependant,dentures,denominators,demur,demonstrators,demonology,delts,dellarte,delinquency,delacour,deflated,definitively,defib,defected,defaced,deeded,decorators,debit,deaqon,davola,datin,dasilva's,darwinian,darling's,darklighters,dandelions,dandelion,dancer's,dampened,dame's,damaskinos,dama,dalrimple,dagobah,dack,d'peshu,d'hoffryn,d'astier,cystic,cynics,cybernetic,cutoff,cutesy,cutaway,customarily,curtain's,cursive,curmudgeon,curdle,cuneiform,cultivated,culpability,culo,cuisinart,cuffing,crypts,cryptid,cryogenic,crux,crunched,crumblers,crudely,crosscheck,croon,crissake,crime's,cribbage,crevasse,creswood,creepo,creases,creased,creaky,cranks,cran,craftsmen,crafting,crabgrass,cowboy's,coveralls,couple'a,councilors,coughs,cotton's,cosmology,coslaw,corresponded,corporeal,corollary,cornucopia,cornering,corks,cordoned,coolly,coolin,cooley's,coolant,cookbooks,converging,contrived,contrite,contributors,contradictory,contra,contours,contented,contenders,contemplated,contact's,constrictor,congressman's,congestion,confrontations,confound,conform,confit,confiscating,conferred,condoned,conditioners,concussions,concentric,conceding,coms,comprised,comprise,comprendo,composers,commuted,commercially,commentator,commentaries,commemorating,commander's,comers,comedic,combustible,combusted,columbo,columbia's,colourful,colonials,collingswood,coliseum,coldness,cojones,coitus,cohesive,cohesion,cohen's,coffey's,codicil,cochran's,coasting,clydesdale,cluttering,clunker,clunk,clumsiness,clumps,clotted,clothesline,clinches,clincher,cleverness,clench,clein,cleave,cleanses,claymores,clarisse,clarissa's,clammed,civilisation,ciudad,circumvent,circulated,circuit's,cinnamon's,cind,church's,chugging,chronically,christsakes,chris's,choque,chompers,choco,chiseling,chirpy,chirp,chinks,chingachgook,chigger,chicklet,chickenpox,chickadee,chewin,chessboard,cherub,chemo's,chauffeur's,chaucer,chariots,chargin,characterizing,chanteuse,chandeliers,chamdo,chalupa,chagrined,chaff,certs,certify,certification,certainties,cerreno,cerebrum,cerebro,century's,centennial,censured,cemetary,cellist,celine's,cedar's,cayo,caterwauling,caterpillars,categorized,catchers,cataclysmic,cassidy's,casitas,casino's,cased,carvel,cartographers,carting,cartels,carriages,carrear,carr's,carolling,carolinas,carolers,carnie,carne,cardiovascular,cardiogram,carbuncle,caramba,capulets,capping,canyons,canines,candaules,canape,canadiens,campaigned,cambodian,camberwell,caldecott,calamitous,caff,cadillacs,cachet,cabeza,cabdriver,byzantium,buzzkill,buzzards,buzz's,buyer's,butai,bustling,businesswomen,bunyan,bungled,bumpkins,bummers,bulletins,bullet's,bulldoze,bulbous,bug's,buffybot,budgeted,budda,bubut,bubbies,brunei,brrrrr,brownout,brouhaha,bronzing,bronchial,broiler,broadening,briskly,briefcases,bricked,breezing,breeher,breckinridge,breakwater,breakable,breadstick,bravenet,braved,brass's,brandies,brandeis,branched,brainwaves,brainiest,braggart,bradlee,boys're,boys'll,boys'd,boyd's,boutonniere,bottle's,bossed,bosomy,bosnian,borans,boosts,boombox,bookshelves,bookmark,booklet,bookends,bontecou's,bongos,boneless,bone's,bond's,bombarding,bombarded,bollo,boinked,boink,boilers,bogart's,bobbo,bobbin,bluest,bluebells,blowjobs,bloodshot,blondie's,blockhead,blockbusters,blithely,blim,bleh,blather,blasters,blankly,bladders,blackhawks,blackbeard,bjorn,bitte,bippy,bios,biohazard,biogenetics,biochemistry,biochemist,bilingual,bilge,bigmouth,bighorn,bigglesworth,bicuspids,beususe,betaseron,besmirch,besieged,bernece,bergman's,bereavement,bentonville,benthic,benjie,benji's,benefactors,benchley,benching,bembe,bellyaching,bellhops,belie,beleaguered,being's,behrle,beginnin,begining,beenie,beefs,beechwood,bee's,bedbug,becau,beaverhausen,beakers,beacon's,bazillion,baudouin,bat's,bartlett's,barrytown,barringtons,baroque,baronet,barneys,barbs,barbers,barbatus,baptists,bankrupted,banker's,bamn,bambi's,ballon's,balinese,bakeries,bailiffs,backslide,baby'd,baaad,b'fore,awwwk,aways,awakes,averages,avengers,avatars,autonomous,automotive,automaton,automatics,autism,authoritative,authenticated,authenticate,aught,audition's,aubyn,attired,attagirl,atrophied,atonement,atherton's,asystole,astroturf,assimilated,assimilate,assertiveness,assemblies,assassin's,artiste,article's,artichokes,arsehole,arrears,arquillians,arnie's,aright,archenemy,arched,arcade's,aquatic,apps,appraise,applauded,appendages,appeased,apostle,apollo's,antwerp,antler,antiquity,antin,antidepressant,antibody,anthropologists,anthology,anthea,antagonism,ant's,anspaugh,annually,anka,angola,anesthetics,anda,ancients,anchoring,anaphylactic,anaheim,ana's,amtrak,amscray,amputated,amounted,americas,amended,ambivalence,amalio,amah,altoid,alriiight,alphabetized,alpena,alouette,allowable,allora,alliteration,allenwood,alleging,allegiances,aligning,algerians,alerts,alchemist,alcerro,alastor,airway's,airmen,ahaha,ah'm,agitators,agitation,aforethought,afis,aesthetics,aerospace,aerodynamics,advertises,advert,advantageous,admonition,administration's,adirondacks,adenoids,adebisi's,acupuncturist,acula,actuarial,activators,actionable,acme's,acknowledges,achmed,achingly,acetate,accusers,accumulation,accorded,acclimated,acclimate,absurdly,absorbent,absolvo,absolutes,absences,abraham's,aboriginal,ablaze,abdomenizer,aaaaaaaaah,aaaaaaaaaa,a'right".split(","))),aM("male_names",aK("james,john,robert,michael,william,david,richard,charles,joseph,thomas,christopher,daniel,paul,mark,donald,george,kenneth,steven,edward,brian,ronald,anthony,kevin,jason,matthew,gary,timothy,jose,larry,jeffrey,frank,scott,eric,stephen,andrew,raymond,gregory,joshua,jerry,dennis,walter,patrick,peter,harold,douglas,henry,carl,arthur,ryan,roger,joe,juan,jack,albert,jonathan,justin,terry,gerald,keith,samuel,willie,ralph,lawrence,nicholas,roy,benjamin,bruce,brandon,adam,harry,fred,wayne,billy,steve,louis,jeremy,aaron,randy,eugene,carlos,russell,bobby,victor,ernest,phillip,todd,jesse,craig,alan,shawn,clarence,sean,philip,chris,johnny,earl,jimmy,antonio,danny,bryan,tony,luis,mike,stanley,leonard,nathan,dale,manuel,rodney,curtis,norman,marvin,vincent,glenn,jeffery,travis,jeff,chad,jacob,melvin,alfred,kyle,francis,bradley,jesus,herbert,frederick,ray,joel,edwin,don,eddie,ricky,troy,randall,barry,bernard,mario,leroy,francisco,marcus,micheal,theodore,clifford,miguel,oscar,jay,jim,tom,calvin,alex,jon,ronnie,bill,lloyd,tommy,leon,derek,darrell,jerome,floyd,leo,alvin,tim,wesley,dean,greg,jorge,dustin,pedro,derrick,dan,zachary,corey,herman,maurice,vernon,roberto,clyde,glen,hector,shane,ricardo,sam,rick,lester,brent,ramon,tyler,gilbert,gene,marc,reginald,ruben,brett,angel,nathaniel,rafael,edgar,milton,raul,ben,cecil,duane,andre,elmer,brad,gabriel,ron,roland,jared,adrian,karl,cory,claude,erik,darryl,neil,christian,javier,fernando,clinton,ted,mathew,tyrone,darren,lonnie,lance,cody,julio,kurt,allan,clayton,hugh,max,dwayne,dwight,armando,felix,jimmie,everett,ian,ken,bob,jaime,casey,alfredo,alberto,dave,ivan,johnnie,sidney,byron,julian,isaac,clifton,willard,daryl,virgil,andy,salvador,kirk,sergio,seth,kent,terrance,rene,eduardo,terrence,enrique,freddie,stuart,fredrick,arturo,alejandro,joey,nick,luther,wendell,jeremiah,evan,julius,donnie,otis,trevor,luke,homer,gerard,doug,kenny,hubert,angelo,shaun,lyle,matt,alfonso,orlando,rex,carlton,ernesto,pablo,lorenzo,omar,wilbur,blake,horace,roderick,kerry,abraham,rickey,ira,andres,cesar,johnathan,malcolm,rudolph,damon,kelvin,rudy,preston,alton,archie,marco,wm,pete,randolph,garry,geoffrey,jonathon,felipe,bennie,gerardo,ed,dominic,loren,delbert,colin,guillermo,earnest,benny,noel,rodolfo,myron,edmund,salvatore,cedric,lowell,gregg,sherman,devin,sylvester,roosevelt,israel,jermaine,forrest,wilbert,leland,simon,irving,owen,rufus,woodrow,kristopher,levi,marcos,gustavo,lionel,marty,gilberto,clint,nicolas,laurence,ismael,orville,drew,ervin,dewey,al,wilfred,josh,hugo,ignacio,caleb,tomas,sheldon,erick,frankie,darrel,rogelio,terence,alonzo,elias,bert,elbert,ramiro,conrad,noah,grady,phil,cornelius,lamar,rolando,clay,percy,dexter,bradford,merle,darin,amos,terrell,moses,irvin,saul,roman,darnell,randal,tommie,timmy,darrin,brendan,toby,van,abel,dominick,emilio,elijah,cary,domingo,aubrey,emmett,marlon,emanuel,jerald,edmond,emil,dewayne,otto,teddy,reynaldo,bret,jess,trent,humberto,emmanuel,stephan,louie,vicente,lamont,garland,micah,efrain,heath,rodger,demetrius,ethan,eldon,rocky,pierre,eli,bryce,antoine,robbie,kendall,royce,sterling,grover,elton,cleveland,dylan,chuck,damian,reuben,stan,leonardo,russel,erwin,benito,hans,monte,blaine,ernie,curt,quentin,agustin,jamal,devon,adolfo,tyson,wilfredo,bart,jarrod,vance,denis,damien,joaquin,harlan,desmond,elliot,darwin,gregorio,kermit,roscoe,esteban,anton,solomon,norbert,elvin,nolan,carey,rod,quinton,hal,brain,rob,elwood,kendrick,darius,moises,marlin,fidel,thaddeus,cliff,marcel,ali,raphael,bryon,armand,alvaro,jeffry,dane,joesph,thurman,ned,sammie,rusty,michel,monty,rory,fabian,reggie,kris,isaiah,gus,avery,loyd,diego,adolph,millard,rocco,gonzalo,derick,rodrigo,gerry,rigoberto,alphonso,ty,rickie,noe,vern,elvis,bernardo,mauricio,hiram,donovan,basil,nickolas,scot,vince,quincy,eddy,sebastian,federico,ulysses,heriberto,donnell,denny,gavin,emery,romeo,jayson,dion,dante,clement,coy,odell,jarvis,bruno,issac,dudley,sanford,colby,carmelo,nestor,hollis,stefan,donny,art,linwood,beau,weldon,galen,isidro,truman,delmar,johnathon,silas,frederic,irwin,merrill,charley,marcelino,carlo,trenton,kurtis,aurelio,winfred,vito,collin,denver,leonel,emory,pasquale,mohammad,mariano,danial,landon,dirk,branden,adan,numbers,clair,buford,german,bernie,wilmer,emerson,zachery,jacques,errol,josue,edwardo,wilford,theron,raymundo,daren,tristan,robby,lincoln,jame,genaro,octavio,cornell,hung,arron,antony,herschel,alva,giovanni,garth,cyrus,cyril,ronny,stevie,lon,kennith,carmine,augustine,erich,chadwick,wilburn,russ,myles,jonas,mitchel,mervin,zane,jamel,lazaro,alphonse,randell,major,johnie,jarrett,ariel,abdul,dusty,luciano,seymour,scottie,eugenio,mohammed,valentin,arnulfo,lucien,ferdinand,thad,ezra,aldo,rubin,royal,mitch,earle,abe,marquis,lanny,kareem,jamar,boris,isiah,emile,elmo,aron,leopoldo,everette,josef,eloy,dorian,rodrick,reinaldo,lucio,jerrod,weston,hershel,lemuel,lavern,burt,jules,gil,eliseo,ahmad,nigel,efren,antwan,alden,margarito,refugio,dino,osvaldo,les,deandre,normand,kieth,ivory,trey,norberto,napoleon,jerold,fritz,rosendo,milford,sang,deon,christoper,alfonzo,lyman,josiah,brant,wilton,rico,jamaal,dewitt,brenton,yong,olin,faustino,claudio,judson,gino,edgardo,alec,jarred,donn,trinidad,tad,porfirio,odis,lenard,chauncey,tod,mel,marcelo,kory,augustus,keven,hilario,bud,sal,orval,mauro,dannie,zachariah,olen,anibal,milo,jed,thanh,amado,lenny,tory,richie,horacio,brice,mohamed,delmer,dario,mac,jonah,jerrold,robt,hank,sung,rupert,rolland,kenton,damion,chi,antone,waldo,fredric,bradly,kip,burl,tyree,jefferey,ahmed,willy,stanford,oren,moshe,mikel,enoch,brendon,quintin,jamison,florencio,darrick,tobias,minh,hassan,giuseppe,demarcus,cletus,tyrell,lyndon,keenan,werner,theo,geraldo,columbus,chet,bertram,markus,huey,hilton,dwain,donte,tyron,omer,isaias,hipolito,fermin,chung,adalberto,jamey,teodoro,mckinley,maximo,sol,raleigh,lawerence,abram,rashad,emmitt,daron,chong,samual,otha,miquel,eusebio,dong,domenic,darron,wilber,renato,hoyt,haywood,ezekiel,chas,florentino,elroy,clemente,arden,neville,edison,deshawn,carrol,shayne,nathanial,jordon,danilo,claud,val,sherwood,raymon,rayford,cristobal,ambrose,titus,hyman,felton,ezequiel,erasmo,lonny,len,ike,milan,lino,jarod,herb,andreas,rhett,jude,douglass,cordell,oswaldo,ellsworth,virgilio,toney,nathanael,del,benedict,mose,hong,isreal,garret,fausto,asa,arlen,zack,modesto,francesco,manual,jae,gaylord,gaston,filiberto,deangelo,michale,granville,wes,malik,zackary,tuan,nicky,cristopher,antione,malcom,korey,jospeh,colton,waylon,von,hosea,shad,santo,rudolf,rolf,rey,renaldo,marcellus,lucius,kristofer,harland,arnoldo,rueben,leandro,kraig,jerrell,jeromy,hobert,cedrick,arlie,winford,wally,luigi,keneth,jacinto,graig,franklyn,edmundo,sid,leif,jeramy,willian,vincenzo,shon,michal,lynwood,jere,hai,elden,darell,broderick,alonso".split(","))),aM("female_names",aK("mary,patricia,linda,barbara,elizabeth,jennifer,maria,susan,margaret,dorothy,lisa,nancy,karen,betty,helen,sandra,donna,carol,ruth,sharon,michelle,laura,sarah,kimberly,deborah,jessica,shirley,cynthia,angela,melissa,brenda,amy,anna,rebecca,virginia,kathleen,pamela,martha,debra,amanda,stephanie,carolyn,christine,marie,janet,catherine,frances,ann,joyce,diane,alice,julie,heather,teresa,doris,gloria,evelyn,jean,cheryl,mildred,katherine,joan,ashley,judith,rose,janice,kelly,nicole,judy,christina,kathy,theresa,beverly,denise,tammy,irene,jane,lori,rachel,marilyn,andrea,kathryn,louise,sara,anne,jacqueline,wanda,bonnie,julia,ruby,lois,tina,phyllis,norma,paula,diana,annie,lillian,emily,robin,peggy,crystal,gladys,rita,dawn,connie,florence,tracy,edna,tiffany,carmen,rosa,cindy,grace,wendy,victoria,edith,kim,sherry,sylvia,josephine,thelma,shannon,sheila,ethel,ellen,elaine,marjorie,carrie,charlotte,monica,esther,pauline,emma,juanita,anita,rhonda,hazel,amber,eva,debbie,april,leslie,clara,lucille,jamie,joanne,eleanor,valerie,danielle,megan,alicia,suzanne,michele,gail,bertha,darlene,veronica,jill,erin,geraldine,lauren,cathy,joann,lorraine,lynn,sally,regina,erica,beatrice,dolores,bernice,audrey,yvonne,annette,june,marion,dana,stacy,ana,renee,ida,vivian,roberta,holly,brittany,melanie,loretta,yolanda,jeanette,laurie,katie,kristen,vanessa,alma,sue,elsie,beth,jeanne,vicki,carla,tara,rosemary,eileen,terri,gertrude,lucy,tonya,ella,stacey,wilma,gina,kristin,jessie,natalie,agnes,vera,charlene,bessie,delores,melinda,pearl,arlene,maureen,colleen,allison,tamara,joy,georgia,constance,lillie,claudia,jackie,marcia,tanya,nellie,minnie,marlene,heidi,glenda,lydia,viola,courtney,marian,stella,caroline,dora,jo,vickie,mattie,maxine,irma,mabel,marsha,myrtle,lena,christy,deanna,patsy,hilda,gwendolyn,jennie,nora,margie,nina,cassandra,leah,penny,kay,priscilla,naomi,carole,olga,billie,dianne,tracey,leona,jenny,felicia,sonia,miriam,velma,becky,bobbie,violet,kristina,toni,misty,mae,shelly,daisy,ramona,sherri,erika,katrina,claire,lindsey,lindsay,geneva,guadalupe,belinda,margarita,sheryl,cora,faye,ada,natasha,sabrina,isabel,marguerite,hattie,harriet,molly,cecilia,kristi,brandi,blanche,sandy,rosie,joanna,iris,eunice,angie,inez,lynda,madeline,amelia,alberta,genevieve,monique,jodi,janie,kayla,sonya,jan,kristine,candace,fannie,maryann,opal,alison,yvette,melody,luz,susie,olivia,flora,shelley,kristy,mamie,lula,lola,verna,beulah,antoinette,candice,juana,jeannette,pam,kelli,whitney,bridget,karla,celia,latoya,patty,shelia,gayle,della,vicky,lynne,sheri,marianne,kara,jacquelyn,erma,blanca,myra,leticia,pat,krista,roxanne,angelica,robyn,adrienne,rosalie,alexandra,brooke,bethany,sadie,bernadette,traci,jody,kendra,nichole,rachael,mable,ernestine,muriel,marcella,elena,krystal,angelina,nadine,kari,estelle,dianna,paulette,lora,mona,doreen,rosemarie,desiree,antonia,janis,betsy,christie,freda,meredith,lynette,teri,cristina,eula,leigh,meghan,sophia,eloise,rochelle,gretchen,cecelia,raquel,henrietta,alyssa,jana,gwen,jenna,tricia,laverne,olive,tasha,silvia,elvira,delia,kate,patti,lorena,kellie,sonja,lila,lana,darla,mindy,essie,mandy,lorene,elsa,josefina,jeannie,miranda,dixie,lucia,marta,faith,lela,johanna,shari,camille,tami,shawna,elisa,ebony,melba,ora,nettie,tabitha,ollie,winifred,kristie,marina,alisha,aimee,rena,myrna,marla,tammie,latasha,bonita,patrice,ronda,sherrie,addie,francine,deloris,stacie,adriana,cheri,abigail,celeste,jewel,cara,adele,rebekah,lucinda,dorthy,effie,trina,reba,sallie,aurora,lenora,etta,lottie,kerri,trisha,nikki,estella,francisca,josie,tracie,marissa,karin,brittney,janelle,lourdes,laurel,helene,fern,elva,corinne,kelsey,ina,bettie,elisabeth,aida,caitlin,ingrid,iva,eugenia,christa,goldie,maude,jenifer,therese,dena,lorna,janette,latonya,candy,consuelo,tamika,rosetta,debora,cherie,polly,dina,jewell,fay,jillian,dorothea,nell,trudy,esperanza,patrica,kimberley,shanna,helena,cleo,stefanie,rosario,ola,janine,mollie,lupe,alisa,lou,maribel,susanne,bette,susana,elise,cecile,isabelle,lesley,jocelyn,paige,joni,rachelle,leola,daphne,alta,ester,petra,graciela,imogene,jolene,keisha,lacey,glenna,gabriela,keri,ursula,lizzie,kirsten,shana,adeline,mayra,jayne,jaclyn,gracie,sondra,carmela,marisa,rosalind,charity,tonia,beatriz,marisol,clarice,jeanine,sheena,angeline,frieda,lily,shauna,millie,claudette,cathleen,angelia,gabrielle,autumn,katharine,jodie,staci,lea,christi,justine,elma,luella,margret,dominique,socorro,martina,margo,mavis,callie,bobbi,maritza,lucile,leanne,jeannine,deana,aileen,lorie,ladonna,willa,manuela,gale,selma,dolly,sybil,abby,ivy,dee,winnie,marcy,luisa,jeri,magdalena,ofelia,meagan,audra,matilda,leila,cornelia,bianca,simone,bettye,randi,virgie,latisha,barbra,georgina,eliza,leann,bridgette,rhoda,haley,adela,nola,bernadine,flossie,ila,greta,ruthie,nelda,minerva,lilly,terrie,letha,hilary,estela,valarie,brianna,rosalyn,earline,catalina,ava,mia,clarissa,lidia,corrine,alexandria,concepcion,tia,sharron,rae,dona,ericka,jami,elnora,chandra,lenore,neva,marylou,melisa,tabatha,serena,avis,allie,sofia,jeanie,odessa,nannie,harriett,loraine,penelope,milagros,emilia,benita,allyson,ashlee,tania,esmeralda,karina,eve,pearlie,zelma,malinda,noreen,tameka,saundra,hillary,amie,althea,rosalinda,lilia,alana,clare,alejandra,elinor,lorrie,jerri,darcy,earnestine,carmella,noemi,marcie,liza,annabelle,louisa,earlene,mallory,carlene,nita,selena,tanisha,katy,julianne,lakisha,edwina,maricela,margery,kenya,dollie,roxie,roslyn,kathrine,nanette,charmaine,lavonne,ilene,tammi,suzette,corine,kaye,chrystal,lina,deanne,lilian,juliana,aline,luann,kasey,maryanne,evangeline,colette,melva,lawanda,yesenia,nadia,madge,kathie,ophelia,valeria,nona,mitzi,mari,georgette,claudine,fran,alissa,roseann,lakeisha,susanna,reva,deidre,chasity,sheree,elvia,alyce,deirdre,gena,briana,araceli,katelyn,rosanne,wendi,tessa,berta,marva,imelda,marietta,marci,leonor,arline,sasha,madelyn,janna,juliette,deena,aurelia,josefa,augusta,liliana,lessie,amalia,savannah,anastasia,vilma,natalia,rosella,lynnette,corina,alfreda,leanna,amparo,coleen,tamra,aisha,wilda,karyn,queen,maura,mai,evangelina,rosanna,hallie,erna,enid,mariana,lacy,juliet,jacklyn,freida,madeleine,mara,cathryn,lelia,casandra,bridgett,angelita,jannie,dionne,annmarie,katina,beryl,millicent,katheryn,diann,carissa,maryellen,liz,lauri,helga,gilda,rhea,marquita,hollie,tisha,tamera,angelique,francesca,kaitlin,lolita,florine,rowena,reyna,twila,fanny,janell,ines,concetta,bertie,alba,brigitte,alyson,vonda,pansy,elba,noelle,letitia,deann,brandie,louella,leta,felecia,sharlene,lesa,beverley,isabella,herminia,terra,celina,tori,octavia,jade,denice,germaine,michell,cortney,nelly,doretha,deidra,monika,lashonda,judi,chelsey,antionette,margot,adelaide,nan,leeann,elisha,dessie,libby,kathi,gayla,latanya,mina,mellisa,kimberlee,jasmin,renae,zelda,elda,justina,gussie,emilie,camilla,abbie,rocio,kaitlyn,edythe,ashleigh,selina,lakesha,geri,allene,pamala,michaela,dayna,caryn,rosalia,sun,jacquline,rebeca,marybeth,krystle,iola,dottie,belle,griselda,ernestina,elida,adrianne,demetria,delma,jaqueline,arleen,virgina,retha,fatima,tillie,eleanore,cari,treva,wilhelmina,rosalee,maurine,latrice,jena,taryn,elia,debby,maudie,jeanna,delilah,catrina,shonda,hortencia,theodora,teresita,robbin,danette,delphine,brianne,nilda,danna,cindi,bess,iona,winona,vida,rosita,marianna,racheal,guillermina,eloisa,celestine,caren,malissa,lona,chantel,shellie,marisela,leora,agatha,soledad,migdalia,ivette,christen,janel,veda,pattie,tessie,tera,marilynn,lucretia,karrie,dinah,daniela,alecia,adelina,vernice,shiela,portia,merry,lashawn,dara,tawana,oma,verda,alene,zella,sandi,rafaela,maya,kira,candida,alvina,suzan,shayla,lyn,lettie,samatha,oralia,matilde,larissa,vesta,renita,india,delois,shanda,phillis,lorri,erlinda,cathrine,barb,zoe,isabell,ione,gisela,roxanna,mayme,kisha,ellie,mellissa,dorris,dalia,bella,annetta,zoila,reta,reina,lauretta,kylie,christal,pilar,charla,elissa,tiffani,tana,paulina,leota,breanna,jayme,carmel,vernell,tomasa,mandi,dominga,santa,melodie,lura,alexa,tamela,mirna,kerrie,venus,felicita,cristy,carmelita,berniece,annemarie,tiara,roseanne,missy,cori,roxana,pricilla,kristal,jung,elyse,haydee,aletha,bettina,marge,gillian,filomena,zenaida,harriette,caridad,vada,una,aretha,pearline,marjory,marcela,flor,evette,elouise,alina,damaris,catharine,belva,nakia,marlena,luanne,lorine,karon,dorene,danita,brenna,tatiana,louann,julianna,andria,philomena,lucila,leonora,dovie,romona,mimi,jacquelin,gaye,tonja,misti,chastity,stacia,roxann,micaela,nikita,mei,velda,marlys,johnna,aura,ivonne,hayley,nicki,majorie,herlinda,yadira,perla,gregoria,antonette,shelli,mozelle,mariah,joelle,cordelia,josette,chiquita,trista,laquita,georgiana,candi,shanon,hildegard,valentina,stephany,magda,karol,gabriella,tiana,roma,richelle,oleta,jacque,idella,alaina,suzanna,jovita,tosha,nereida,marlyn,kyla,delfina,tena,stephenie,sabina,nathalie,marcelle,gertie,darleen,thea,sharonda,shantel,belen,venessa,rosalina,ona,genoveva,clementine,rosalba,renate,renata,georgianna,floy,dorcas,ariana,tyra,theda,mariam,juli,jesica,vikki,verla,roselyn,melvina,jannette,ginny,debrah,corrie,asia,violeta,myrtis,latricia,collette,charleen,anissa,viviana,twyla,nedra,latonia,lan,hellen,fabiola,annamarie,adell,sharyn,chantal,niki,maud,lizette,lindy,kia,kesha,jeana,danelle,charline,chanel,valorie,lia,dortha,cristal,leone,leilani,gerri,debi,andra,keshia,ima,eulalia,easter,dulce,natividad,linnie,kami,georgie,catina,brook,alda,winnifred,sharla,ruthann,meaghan,magdalene,lissette,adelaida,venita,trena,shirlene,shameka,elizebeth,dian,shanta,latosha,carlotta,windy,rosina,mariann,leisa,jonnie,dawna,cathie,astrid,laureen,janeen,holli,fawn,vickey,teressa,shante,rubye,marcelina,chanda,terese,scarlett,marnie,lulu,lisette,jeniffer,elenor,dorinda,donita,carman,bernita,altagracia,aleta,adrianna,zoraida,nicola,lyndsey,janina,ami,starla,phylis,phuong,kyra,charisse,blanch,sanjuanita,rona,nanci,marilee,maranda,brigette,sanjuana,marita,kassandra,joycelyn,felipa,chelsie,bonny,mireya,lorenza,kyong,ileana,candelaria,sherie,lucie,leatrice,lakeshia,gerda,edie,bambi,marylin,lavon,hortense,garnet,evie,tressa,shayna,lavina,kyung,jeanetta,sherrill,shara,phyliss,mittie,anabel,alesia,thuy,tawanda,joanie,tiffanie,lashanda,karissa,enriqueta,daria,daniella,corinna,alanna,abbey,roxane,roseanna,magnolia,lida,joellen,era,coral,carleen,tresa,peggie,novella,nila,maybelle,jenelle,carina,nova,melina,marquerite,margarette,josephina,evonne,cinthia,albina,toya,tawnya,sherita,myriam,lizabeth,lise,keely,jenni,giselle,cheryle,ardith,ardis,alesha,adriane,shaina,linnea,karolyn,felisha,dori,darci,artie,armida,zola,xiomara,vergie,shamika,nena,nannette,maxie,lovie,jeane,jaimie,inge,farrah,elaina,caitlyn,felicitas,cherly,caryl,yolonda,yasmin,teena,prudence,pennie,nydia,mackenzie,orpha,marvel,lizbeth,laurette,jerrie,hermelinda,carolee,tierra,mirian,meta,melony,kori,jennette,jamila,ena,anh,yoshiko,susannah,salina,rhiannon,joleen,cristine,ashton,aracely,tomeka,shalonda,marti,lacie,kala,jada,ilse,hailey,brittani,zona,syble,sherryl,nidia,marlo,kandice,kandi,deb,alycia,ronna,norene,mercy,ingeborg,giovanna,gemma,christel,audry,zora,vita,trish,stephaine,shirlee,shanika,melonie,mazie,jazmin,inga,hoa,hettie,geralyn,fonda,estrella,adella,sarita,rina,milissa,maribeth,golda,evon,ethelyn,enedina,cherise,chana,velva,tawanna,sade,mirta,karie,jacinta,elna,davina,cierra,ashlie,albertha,tanesha,nelle,mindi,lorinda,larue,florene,demetra,dedra,ciara,chantelle,ashly,suzy,rosalva,noelia,lyda,leatha,krystyna,kristan,karri,darline,darcie,cinda,cherrie,awilda,almeda,rolanda,lanette,jerilyn,gisele,evalyn,cyndi,cleta,carin,zina,zena,velia,tanika,charissa,talia,margarete,lavonda,kaylee,kathlene,jonna,irena,ilona,idalia,candis,candance,brandee,anitra,alida,sigrid,nicolette,maryjo,linette,hedwig,christiana,alexia,tressie,modesta,lupita,lita,gladis,evelia,davida,cherri,cecily,ashely,annabel,agustina,wanita,shirly,rosaura,hulda,eun,yetta,verona,thomasina,sibyl,shannan,mechelle,lue,leandra,lani,kylee,kandy,jolynn,ferne,eboni,corene,alysia,zula,nada,moira,lyndsay,lorretta,jammie,hortensia,gaynell,adria,vina,vicenta,tangela,stephine,norine,nella,liana,leslee,kimberely,iliana,glory,felica,emogene,elfriede,eden,eartha,carma,bea,ocie,lennie,kiara,jacalyn,carlota,arielle,otilia,kirstin,kacey,johnetta,joetta,jeraldine,jaunita,elana,dorthea,cami,amada,adelia,vernita,tamar,siobhan,renea,rashida,ouida,nilsa,meryl,kristyn,julieta,danica,breanne,aurea,anglea,sherron,odette,malia,lorelei,leesa,kenna,kathlyn,fiona,charlette,suzie,shantell,sabra,racquel,myong,mira,martine,lucienne,lavada,juliann,elvera,delphia,christiane,charolette,carri,asha,angella,paola,ninfa,leda,lai,eda,stefani,shanell,palma,machelle,lissa,kecia,kathryne,karlene,julissa,jettie,jenniffer,hui,corrina,carolann,alena,rosaria,myrtice,marylee,liane,kenyatta,judie,janey,elmira,eldora,denna,cristi,cathi,zaida,vonnie,viva,vernie,rosaline,mariela,luciana,lesli,karan,felice,deneen,adina,wynona,tarsha,sheron,shanita,shani,shandra,randa,pinkie,nelida,marilou,lyla,laurene,laci,joi,janene,dorotha,daniele,dani,carolynn,carlyn,berenice,ayesha,anneliese,alethea,thersa,tamiko,rufina,oliva,mozell,marylyn,kristian,kathyrn,kasandra,kandace,janae,domenica,debbra,dannielle,arcelia,aja,zenobia,sharen,sharee,lavinia,kum,kacie,jackeline,huong,felisa,emelia,eleanora,cythia,cristin,claribel,anastacia,zulma,zandra,yoko,tenisha,susann,sherilyn,shay,shawanda,romana,mathilda,linsey,keiko,joana,isela,gretta,georgetta,eugenie,desirae,delora,corazon,antonina,anika,willene,tracee,tamatha,nichelle,mickie,maegan,luana,lanita,kelsie,edelmira,bree,afton,teodora,tamie,shena,meg,linh,keli,kaci,danyelle,arlette,albertine,adelle,tiffiny,simona,nicolasa,nichol,nia,nakisha,mee,maira,loreen,kizzy,fallon,christene,bobbye,vincenza,tanja,rubie,roni,queenie,margarett,kimberli,irmgard,idell,hilma,evelina,esta,emilee,dennise,dania,carie,wai,risa,rikki,particia,mui,masako,luvenia,loree,loni,lien,gigi,florencia,denita,billye,tomika,sharita,rana,nikole,neoma,margarite,madalyn,lucina,laila,kali,jenette,gabriele,evelyne,elenora,clementina,alejandrina,zulema,violette,vannessa,thresa,retta,pia,patience,noella,nickie,jonell,chaya,camelia,bethel,anya,suzann,shu,mila,lilla,laverna,keesha,kattie,georgene,eveline,estell,elizbeth,vivienne,vallie,trudie,stephane,magaly,madie,kenyetta,karren,janetta,hermine,drucilla,debbi,celestina,candie,britni,beckie,amina,zita,yun,yolande,vivien,vernetta,trudi,sommer,pearle,patrina,ossie,nicolle,loyce,letty,larisa,katharina,joselyn,jonelle,jenell,iesha,heide,florinda,florentina,flo,elodia,dorine,brunilda,brigid,ashli,ardella,twana,thu,tarah,shavon,serina,rayna,ramonita,nga,margurite,lucrecia,kourtney,kati,jesenia,crista,ayana,alica,alia,vinnie,suellen,romelia,rachell,olympia,michiko,kathaleen,jolie,jessi,janessa,hana,elease,carletta,britany,shona,salome,rosamond,regena,raina,ngoc,nelia,louvenia,lesia,latrina,laticia,larhonda,jina,jacki,emmy,deeann,coretta,arnetta,thalia,shanice,neta,mikki,micki,lonna,leana,lashunda,kiley,joye,jacqulyn,ignacia,hyun,hiroko,henriette,elayne,delinda,dahlia,coreen,consuela,conchita,celine,babette,ayanna,anette,albertina,shawnee,shaneka,quiana,pamelia,min,merri,merlene,margit,kiesha,kiera,kaylene,jodee,jenise,erlene,emmie,dalila,daisey,casie,belia,babara,versie,vanesa,shelba,shawnda,nikia,naoma,marna,margeret,madaline,lawana,kindra,jutta,jazmine,janett,hannelore,glendora,gertrud,garnett,freeda,frederica,florance,flavia,carline,beverlee,anjanette,valda,tamala,shonna,sha,sarina,oneida,merilyn,marleen,lurline,lenna,katherin,jin,jeni,hae,gracia,glady,farah,enola,ema,dominque,devona,delana,cecila,caprice,alysha,alethia,vena,theresia,tawny,shakira,samara,sachiko,rachele,pamella,marni,mariel,maren,malisa,ligia,lera,latoria,larae,kimber,kathern,karey,jennefer,janeth,halina,fredia,delisa,debroah,ciera,angelika,andree,altha,yen,vivan,terresa,tanna,suk,sudie,soo,signe,salena,ronni,rebbecca,myrtie,malika,maida,loan,leonarda,kayleigh,ethyl,ellyn,dayle,cammie,brittni,birgit,avelina,asuncion,arianna,akiko,venice,tyesha,tonie,tiesha,takisha,steffanie,sindy,meghann,manda,macie,kellye,kellee,joslyn,inger,indira,glinda,glennis,fernanda,faustina,eneida,elicia,dot,digna,dell,arletta,willia,tammara,tabetha,sherrell,sari,rebbeca,pauletta,natosha,nakita,mammie,kenisha,kazuko,kassie,earlean,daphine,corliss,clotilde,carolyne,bernetta,augustina,audrea,annis,annabell,yan,tennille,tamica,selene,rosana,regenia,qiana,markita,macy,leeanne,laurine,kym,jessenia,janita,georgine,genie,emiko,elvie,deandra,dagmar,corie,collen,cherish,romaine,porsha,pearlene,micheline,merna,margorie,margaretta,lore,jenine,hermina,fredericka,elke,drusilla,dorathy,dione,celena,brigida,angeles,allegra,tamekia,synthia,sook,slyvia,rosann,reatha,raye,marquetta,margart,layla,kymberly,kiana,kayleen,katlyn,karmen,joella,irina,emelda,eleni,detra,clemmie,cheryll,chantell,cathey,arnita,arla,angle,angelic,alyse,zofia,thomasine,tennie,sherly,sherley,sharyl,remedios,petrina,nickole,myung,myrle,mozella,louanne,lisha,latia,krysta,julienne,jeanene,jacqualine,isaura,gwenda,earleen,cleopatra,carlie,audie,antonietta,alise,verdell,tomoko,thao,talisha,shemika,savanna,santina,rosia,raeann,odilia,nana,minna,magan,lynelle,karma,joeann,ivana,inell,ilana,hye,hee,gudrun,dreama,crissy,chante,carmelina,arvilla,annamae,alvera,aleida,yanira,vanda,tianna,tam,stefania,shira,nicol,nancie,monserrate,melynda,melany,lovella,laure,kacy,jacquelynn,hyon,gertha,eliana,christena,christeen,charise,caterina,carley,candyce,arlena,ammie,willette,vanita,tuyet,syreeta,penney,nyla,maryam,marya,magen,ludie,loma,livia,lanell,kimberlie,julee,donetta,diedra,denisha,deane,dawne,clarine,cherryl,bronwyn,alla,valery,tonda,sueann,soraya,shoshana,shela,sharleen,shanelle,nerissa,meridith,mellie,maye,maple,magaret,lili,leonila,leonie,leeanna,lavonia,lavera,kristel,kathey,kathe,jann,ilda,hildred,hildegarde,genia,fumiko,evelin,ermelinda,elly,dung,doloris,dionna,danae,berneice,annice,alix,verena,verdie,shawnna,shawana,shaunna,rozella,randee,ranae,milagro,lynell,luise,loida,lisbeth,karleen,junita,jona,isis,hyacinth,hedy,gwenn,ethelene,erline,donya,domonique,delicia,dannette,cicely,branda,blythe,bethann,ashlyn,annalee,alline,yuko,vella,trang,towanda,tesha,sherlyn,narcisa,miguelina,meri,maybell,marlana,marguerita,madlyn,lory,loriann,leonore,leighann,laurice,latesha,laronda,katrice,kasie,kaley,jadwiga,glennie,gearldine,francina,epifania,dyan,dorie,diedre,denese,demetrice,delena,cristie,cleora,catarina,carisa,barbera,almeta,trula,tereasa,solange,sheilah,shavonne,sanora,rochell,mathilde,margareta,maia,lynsey,lawanna,launa,kena,keena,katia,glynda,gaylene,elvina,elanor,danuta,danika,cristen,cordie,coletta,clarita,carmon,brynn,azucena,aundrea,angele,verlie,verlene,tamesha,silvana,sebrina,samira,reda,raylene,penni,norah,noma,mireille,melissia,maryalice,laraine,kimbery,karyl,karine,kam,jolanda,johana,jesusa,jaleesa,jacquelyne,iluminada,hilaria,hanh,gennie,francie,floretta,exie,edda,drema,delpha,bev,barbar,assunta,ardell,annalisa,alisia,yukiko,yolando,wonda,wei,waltraud,veta,temeka,tameika,shirleen,shenita,piedad,ozella,mirtha,marilu,kimiko,juliane,jenice,janay,jacquiline,hilde,fae,elois,echo,devorah,chau,brinda,betsey,arminda,aracelis,apryl,annett,alishia,veola,usha,toshiko,theola,tashia,talitha,shery,renetta,reiko,rasheeda,obdulia,mika,melaine,meggan,marlen,marget,marceline,mana,magdalen,librada,lezlie,latashia,lasandra,kelle,isidra,isa,inocencia,gwyn,francoise,erminia,erinn,dimple,devora,criselda,armanda,arie,ariane,angelena,aliza,adriene,adaline,xochitl,twanna,tomiko,tamisha,taisha,susy,siu,rutha,rhona,noriko,natashia,merrie,marinda,mariko,margert,loris,lizzette,leisha,kaila,joannie,jerrica,jene,jannet,janee,jacinda,herta,elenore,doretta,delaine,daniell,claudie,britta,apolonia,amberly,alease,yuri,yuk,wen,waneta,ute,tomi,sharri,sandie,roselle,reynalda,raguel,phylicia,patria,olimpia,odelia,mitzie,minda,mignon,mica,mendy,marivel,maile,lynetta,lavette,lauryn,latrisha,lakiesha,kiersten,kary,josphine,jolyn,jetta,janise,jacquie,ivelisse,glynis,gianna,gaynelle,danyell,danille,dacia,coralee,cher,ceola,arianne,aleshia,yung,williemae,trinh,thora,tai,svetlana,sherika,shemeka,shaunda,roseline,ricki,melda,mallie,lavonna,latina,laquanda,lala,lachelle,klara,kandis,johna,jeanmarie,jaye,grayce,gertude,emerita,ebonie,clorinda,ching,chery,carola,breann,blossom,bernardine,becki,arletha,argelia,ara,alita,yulanda,yon,yessenia,tobi,tasia,sylvie,shirl,shirely,shella,shantelle,sacha,rebecka,providencia,paulene,misha,miki,marline,marica,lorita,latoyia,lasonya,kerstin,kenda,keitha,kathrin,jaymie,gricelda,ginette,eryn,elina,elfrieda,danyel,cheree,chanelle,barrie,aurore,annamaria,alleen,ailene,aide,yasmine,vashti,treasa,tiffaney,sheryll,sharie,shanae,sau,raisa,neda,mitsuko,mirella,milda,maryanna,maragret,mabelle,luetta,lorina,letisha,latarsha,lanelle,lajuana,krissy,karly,karena,jessika,jerica,jeanelle,jalisa,jacelyn,izola,euna,etha,domitila,dominica,daina,creola,carli,camie,brittny,ashanti,anisha,aleen,adah,yasuko,valrie,tona,tinisha,thi,terisa,taneka,simonne,shalanda,serita,ressie,refugia,olene,margherita,mandie,maire,lyndia,luci,lorriane,loreta,leonia,lavona,lashawnda,lakia,kyoko,krystina,krysten,kenia,kelsi,jeanice,isobel,georgiann,genny,felicidad,eilene,deloise,conception,clora,cherilyn,calandra,armandina,anisa,ula,tiera,theressa,stephania,sima,shyla,shonta,shera,shaquita,shala,rossana,nohemi,nery,moriah,melita,melida,melani,marylynn,marisha,mariette,malorie,madelene,ludivina,loria,lorette,loralee,lianne,lavenia,laurinda,lashon,kit,kimi,keila,katelynn,kai,jone,joane,jayna,janella,hue,hertha,francene,elinore,despina,delsie,deedra,clemencia,carolin,bulah,brittanie,bok,blondell,bibi,beaulah,beata,annita,agripina,virgen,valene,twanda,tommye,toi,tarra,tari,tammera,shakia,sadye,ruthanne,rochel,rivka,pura,nenita,natisha,merrilee,melodee,marvis,lucilla,leena,laveta,larita,lanie,keren,ileen,georgeann,genna,frida,ewa,eufemia,emely,ela,edyth,deonna,deadra,darlena,chanell,cathern,cassondra,cassaundra,bernarda,berna,arlinda,anamaria,vertie,valeri,torri,tatyana,stasia,sherise,sherill,sanda,ruthe,rosy,robbi,ranee,quyen,pearly,palmira,onita,nisha,niesha,nida,nam,merlyn,mayola,marylouise,marth,margene,madelaine,londa,leontine,leoma,leia,lauralee,lanora,lakita,kiyoko,keturah,katelin,kareen,jonie,johnette,jenee,jeanett,izetta,hiedi,heike,hassie,giuseppina,georgann,fidela,fernande,elwanda,ellamae,eliz,dusti,dotty,cyndy,coralie,celesta,argentina,alverta,xenia,wava,vanetta,torrie,tashina,tandy,tambra,tama,stepanie,shila,shaunta,sharan,shaniqua,shae,setsuko,serafina,sandee,rosamaria,priscila,olinda,nadene,muoi,michelina,mercedez,maryrose,marcene,mao,magali,mafalda,lannie,kayce,karoline,kamilah,kamala,justa,joline,jennine,jacquetta,iraida,georgeanna,franchesca,emeline,elane,ehtel,earlie,dulcie,dalene,classie,chere,charis,caroyln,carmina,carita,bethanie,ayako,arica,alysa,alessandra,akilah,adrien,zetta,youlanda,yelena,yahaira,wendolyn,tijuana,terina,teresia,suzi,sherell,shavonda,shaunte,sharda,shakita,sena,ryann,rubi,riva,reginia,rachal,parthenia,pamula,monnie,monet,michaele,melia,malka,maisha,lisandra,lekisha,lean,lakendra,krystin,kortney,kizzie,kittie,kera,kendal,kemberly,kanisha,julene,jule,johanne,jamee,halley,gidget,galina,fredricka,fleta,fatimah,eusebia,elza,eleonore,dorthey,doria,donella,dinorah,delorse,claretha,christinia,charlyn,bong,belkis,azzie,andera,aiko,adena,yer,yajaira,wan,vania,ulrike,toshia,tifany,stefany,shizue,shenika,shawanna,sharolyn,sharilyn,shaquana,shantay,rozanne,roselee,remona,reanna,raelene,phung,petronila,natacha,nancey,myrl,miyoko,miesha,merideth,marvella,marquitta,marhta,marchelle,lizeth,libbie,lahoma,ladawn,kina,katheleen,katharyn,karisa,kaleigh,junie,julieann,johnsie,janean,jaimee,jackqueline,hisako,herma,helaine,gwyneth,gita,eustolia,emelina,elin,edris,donnette,donnetta,dierdre,denae,darcel,clarisa,cinderella,chia,charlesetta,charita,celsa,cassy,cassi,carlee,bruna,brittaney,brande,billi,bao,antonetta,angla,angelyn,analisa,alane,wenona,wendie,veronique,vannesa,tobie,tempie,sumiko,sulema,sparkle,somer,sheba,sharice,shanel,shalon,rosio,roselia,renay,rema,reena,ozie,oretha,oralee,oda,ngan,nakesha,milly,marybelle,margrett,maragaret,manie,lurlene,lillia,lieselotte,lavelle,lashaunda,lakeesha,kaycee,kalyn,joya,joette,jenae,janiece,illa,grisel,glayds,genevie,gala,fredda,eleonor,debera,deandrea,corrinne,cordia,contessa,colene,cleotilde,chantay,cecille,beatris,azalee,arlean,ardath,anjelica,anja,alfredia,aleisha,zada,yuonne,willodean,vennie,vanna,tyisha,tova,torie,tonisha,tilda,tien,sirena,sherril,shanti,senaida,samella,robbyn,renda,reita,phebe,paulita,nobuko,nguyet,neomi,mikaela,melania,maximina,marg,maisie,lynna,lilli,lashaun,lakenya,lael,kirstie,kathline,kasha,karlyn,karima,jovan,josefine,jennell,jacqui,jackelyn,hyo,hien,grazyna,florrie,floria,eleonora,dwana,dorla,delmy,deja,dede,dann,crysta,clelia,claris,chieko,cherlyn,cherelle,charmain,chara,cammy,bee,arnette,ardelle,annika,amiee,amee,allena,yvone,yuki,yoshie,yevette,yael,willetta,voncile,venetta,tula,tonette,timika,temika,telma,teisha,taren,stacee,shawnta,saturnina,ricarda,pok,pasty,onie,nubia,marielle,mariella,marianela,mardell,luanna,loise,lisabeth,lindsy,lilliana,lilliam,lelah,leigha,leanora,kristeen,khalilah,keeley,kandra,junko,joaquina,jerlene,jani,jamika,hsiu,hermila,genevive,evia,eugena,emmaline,elfreda,elene,donette,delcie,deeanna,darcey,cuc,clarinda,cira,chae,celinda,catheryn,casimira,carmelia,camellia,breana,bobette,bernardina,bebe,basilia,arlyne,amal,alayna,zonia,zenia,yuriko,yaeko,wynell,willena,vernia,tora,terrilyn,terica,tenesha,tawna,tajuana,taina,stephnie,sona,sina,shondra,shizuko,sherlene,sherice,sharika,rossie,rosena,rima,ria,rheba,renna,natalya,nancee,melodi,meda,matha,marketta,maricruz,marcelene,malvina,luba,louetta,leida,lecia,lauran,lashawna,laine,khadijah,katerine,kasi,kallie,julietta,jesusita,jestine,jessia,jeffie,janyce,isadora,georgianne,fidelia,evita,eura,eulah,estefana,elsy,eladia,dodie,dia,denisse,deloras,delila,daysi,crystle,concha,claretta,charlsie,charlena,carylon,bettyann,asley,ashlea,amira,agueda,agnus,yuette,vinita,victorina,tynisha,treena,toccara,tish,thomasena,tegan,soila,shenna,sharmaine,shantae,shandi,september,saran,sarai,sana,rosette,rolande,regine,otelia,olevia,nicholle,necole,naida,myrta,myesha,mitsue,minta,mertie,margy,mahalia,madalene,loura,lorean,lesha,leonida,lenita,lavone,lashell,lashandra,lamonica,kimbra,katherina,karry,kanesha,jong,jeneva,jaquelyn,hwa,gilma,ghislaine,gertrudis,fransisca,fermina,ettie,etsuko,ellan,elidia,edra,dorethea,doreatha,denyse,deetta,daine,cyrstal,corrin,cayla,carlita,camila,burma,bula,buena,barabara,avril,alaine,zana,wilhemina,wanetta,veronika,verline,vasiliki,tonita,tisa,teofila,tayna,taunya,tandra,takako,sunni,suanne,sixta,sharell,seema,rosenda,robena,raymonde,pei,pamila,ozell,neida,mistie,micha,merissa,maurita,maryln,maryetta,marcell,malena,makeda,lovetta,lourie,lorrine,lorilee,laurena,lashay,larraine,laree,lacresha,kristle,krishna,keva,keira,karole,joie,jinny,jeannetta,jama,heidy,gilberte,gema,faviola,evelynn,enda,elli,ellena,divina,dagny,collene,codi,cindie,chassidy,chasidy,catrice,catherina,cassey,caroll,carlena,candra,calista,bryanna,britteny,beula,bari,audrie,audria,ardelia,annelle,angila,alona,allyn".split(","))),aM("surnames",aK("smith,johnson,williams,jones,brown,davis,miller,wilson,moore,taylor,anderson,jackson,white,harris,martin,thompson,garcia,martinez,robinson,clark,rodriguez,lewis,lee,walker,hall,allen,young,hernandez,king,wright,lopez,hill,green,adams,baker,gonzalez,nelson,carter,mitchell,perez,roberts,turner,phillips,campbell,parker,evans,edwards,collins,stewart,sanchez,morris,rogers,reed,cook,morgan,bell,murphy,bailey,rivera,cooper,richardson,cox,howard,ward,torres,peterson,gray,ramirez,watson,brooks,sanders,price,bennett,wood,barnes,ross,henderson,coleman,jenkins,perry,powell,long,patterson,hughes,flores,washington,butler,simmons,foster,gonzales,bryant,alexander,griffin,diaz,hayes,myers,ford,hamilton,graham,sullivan,wallace,woods,cole,west,owens,reynolds,fisher,ellis,harrison,gibson,mcdonald,cruz,marshall,ortiz,gomez,murray,freeman,wells,webb,simpson,stevens,tucker,porter,hicks,crawford,boyd,mason,morales,kennedy,warren,dixon,ramos,reyes,burns,gordon,shaw,holmes,rice,robertson,hunt,daniels,palmer,mills,nichols,grant,ferguson,stone,hawkins,dunn,perkins,hudson,spencer,gardner,stephens,payne,pierce,berry,matthews,arnold,wagner,willis,watkins,olson,carroll,duncan,snyder,hart,cunningham,lane,andrews,ruiz,harper,fox,riley,armstrong,carpenter,weaver,greene,elliott,chavez,sims,peters,kelley,franklin,lawson,fields,gutierrez,schmidt,carr,vasquez,castillo,wheeler,chapman,oliver,montgomery,richards,williamson,johnston,banks,meyer,bishop,mccoy,howell,alvarez,morrison,hansen,fernandez,garza,burton,nguyen,jacobs,reid,fuller,lynch,garrett,romero,welch,larson,frazier,burke,hanson,mendoza,moreno,bowman,medina,fowler,brewer,hoffman,carlson,silva,pearson,holland,fleming,jensen,vargas,byrd,davidson,hopkins,may,herrera,wade,soto,walters,neal,caldwell,lowe,jennings,barnett,graves,jimenez,horton,shelton,barrett,obrien,castro,sutton,mckinney,lucas,miles,rodriquez,chambers,holt,lambert,fletcher,watts,bates,hale,rhodes,pena,beck,newman,haynes,mcdaniel,mendez,bush,vaughn,parks,dawson,santiago,norris,hardy,steele,curry,powers,schultz,barker,guzman,page,munoz,ball,keller,chandler,weber,walsh,lyons,ramsey,wolfe,schneider,mullins,benson,sharp,bowen,barber,cummings,hines,baldwin,griffith,valdez,hubbard,salazar,reeves,warner,stevenson,burgess,santos,tate,cross,garner,mann,mack,moss,thornton,mcgee,farmer,delgado,aguilar,vega,glover,manning,cohen,harmon,rodgers,robbins,newton,blair,higgins,ingram,reese,cannon,strickland,townsend,potter,goodwin,walton,rowe,hampton,ortega,patton,swanson,goodman,maldonado,yates,becker,erickson,hodges,rios,conner,adkins,webster,malone,hammond,flowers,cobb,moody,quinn,pope,osborne,mccarthy,guerrero,estrada,sandoval,gibbs,gross,fitzgerald,stokes,doyle,saunders,wise,colon,gill,alvarado,greer,padilla,waters,nunez,ballard,schwartz,mcbride,houston,christensen,klein,pratt,briggs,parsons,mclaughlin,zimmerman,french,buchanan,moran,copeland,pittman,brady,mccormick,holloway,brock,poole,logan,bass,marsh,drake,wong,jefferson,park,morton,abbott,sparks,norton,huff,massey,figueroa,carson,bowers,roberson,barton,tran,lamb,harrington,boone,cortez,clarke,mathis,singleton,wilkins,cain,underwood,hogan,mckenzie,collier,luna,phelps,mcguire,bridges,wilkerson,nash,summers,atkins,wilcox,pitts,conley,marquez,burnett,cochran,chase,davenport,hood,gates,ayala,sawyer,vazquez,dickerson,hodge,acosta,flynn,espinoza,nicholson,monroe,morrow,whitaker,oconnor,skinner,ware,molina,kirby,huffman,gilmore,dominguez,oneal,lang,combs,kramer,hancock,gallagher,gaines,shaffer,short,wiggins,mathews,mcclain,fischer,wall,small,melton,hensley,bond,dyer,grimes,contreras,wyatt,baxter,snow,mosley,shepherd,larsen,hoover,beasley,petersen,whitehead,meyers,garrison,shields,horn,savage,olsen,schroeder,hartman,woodard,mueller,kemp,deleon,booth,patel,calhoun,wiley,eaton,cline,navarro,harrell,humphrey,parrish,duran,hutchinson,hess,dorsey,bullock,robles,beard,dalton,avila,rich,blackwell,york,johns,blankenship,trevino,salinas,campos,pruitt,callahan,montoya,hardin,guerra,mcdowell,stafford,gallegos,henson,wilkinson,booker,merritt,atkinson,orr,decker,hobbs,tanner,knox,pacheco,stephenson,glass,rojas,serrano,marks,hickman,english,sweeney,strong,mcclure,conway,roth,maynard,farrell,lowery,hurst,nixon,weiss,trujillo,ellison,sloan,juarez,winters,mclean,boyer,villarreal,mccall,gentry,carrillo,ayers,lara,sexton,pace,hull,leblanc,browning,velasquez,leach,chang,sellers,herring,noble,foley,bartlett,mercado,landry,durham,walls,barr,mckee,bauer,rivers,bradshaw,pugh,velez,rush,estes,dodson,morse,sheppard,weeks,camacho,bean,barron,livingston,middleton,spears,branch,blevins,chen,kerr,mcconnell,hatfield,harding,solis,frost,giles,blackburn,pennington,woodward,finley,mcintosh,koch,mccullough,blanchard,rivas,brennan,mejia,kane,benton,buckley,valentine,maddox,russo,mcknight,buck,moon,mcmillan,crosby,berg,dotson,mays,roach,church,chan,richmond,meadows,faulkner,oneill,knapp,kline,ochoa,jacobson,gay,hendricks,horne,shepard,hebert,cardenas,mcintyre,waller,holman,donaldson,cantu,morin,gillespie,fuentes,tillman,bentley,peck,key,salas,rollins,gamble,dickson,battle,santana,cabrera,cervantes,howe,hinton,hurley,spence,zamora,yang,mcneil,suarez,petty,gould,mcfarland,sampson,carver,bray,macdonald,stout,hester,melendez,dillon,farley,hopper,galloway,potts,joyner,stein,aguirre,osborn,mercer,bender,franco,rowland,sykes,pickett,sears,mayo,dunlap,hayden,wilder,mckay,coffey,mccarty,ewing,cooley,vaughan,bonner,cotton,holder,stark,ferrell,cantrell,fulton,lott,calderon,pollard,hooper,burch,mullen,fry,riddle,levy,odonnell,britt,daugherty,berger,dillard,alston,frye,riggs,chaney,odom,duffy,fitzpatrick,valenzuela,mayer,alford,mcpherson,acevedo,barrera,cote,reilly,compton,mooney,mcgowan,craft,clemons,wynn,nielsen,baird,stanton,snider,rosales,bright,witt,hays,holden,rutledge,kinney,clements,castaneda,slater,hahn,burks,delaney,pate,lancaster,sharpe,whitfield,talley,macias,burris,ratliff,mccray,madden,kaufman,goff,cash,bolton,mcfadden,levine,byers,kirkland,kidd,workman,carney,mcleod,holcomb,england,finch,sosa,haney,franks,sargent,nieves,downs,rasmussen,bird,hewitt,foreman,valencia,oneil,delacruz,vinson,dejesus,hyde,forbes,gilliam,guthrie,wooten,huber,barlow,boyle,mcmahon,buckner,rocha,puckett,langley,knowles,cooke,velazquez,whitley,vang,shea,rouse,hartley,mayfield,elder,rankin,hanna,cowan,lucero,arroyo,slaughter,haas,oconnell,minor,boucher,archer,boggs,dougherty,andersen,newell,crowe,wang,friedman,bland,swain,holley,pearce,childs,yarbrough,galvan,proctor,meeks,lozano,mora,rangel,bacon,villanueva,schaefer,rosado,helms,boyce,goss,stinson,lake,ibarra,hutchins,covington,crowley,hatcher,mackey,bunch,womack,polk,dodd,childress,childers,camp,villa,dye,springer,mahoney,dailey,belcher,lockhart,griggs,costa,brandt,walden,moser,tatum,mccann,akers,lutz,pryor,orozco,mcallister,lugo,davies,shoemaker,rutherford,newsome,magee,chamberlain,blanton,simms,godfrey,flanagan,crum,cordova,escobar,downing,sinclair,donahue,krueger,mcginnis,gore,farris,webber,corbett,andrade,starr,lyon,yoder,hastings,mcgrath,spivey,krause,harden,crabtree,kirkpatrick,arrington,ritter,mcghee,bolden,maloney,gagnon,dunbar,ponce,pike,mayes,beatty,mobley,kimball,butts,montes,eldridge,braun,hamm,gibbons,moyer,manley,herron,plummer,elmore,cramer,rucker,pierson,fontenot,field,rubio,goldstein,elkins,wills,novak,hickey,worley,gorman,katz,dickinson,broussard,woodruff,crow,britton,nance,lehman,bingham,zuniga,whaley,shafer,coffman,steward,delarosa,nix,neely,mata,davila,mccabe,kessler,hinkle,welsh,pagan,goldberg,goins,crouch,cuevas,quinones,mcdermott,hendrickson,samuels,denton,bergeron,lam,ivey,locke,haines,snell,hoskins,byrne,arias,roe,corbin,beltran,chappell,downey,dooley,tuttle,couch,payton,mcelroy,crockett,groves,cartwright,dickey,mcgill,dubois,muniz,tolbert,dempsey,cisneros,sewell,latham,vigil,tapia,rainey,norwood,stroud,meade,tipton,kuhn,hilliard,bonilla,teague,gunn,greenwood,correa,reece,poe,pineda,phipps,frey,kaiser,ames,gunter,schmitt,milligan,espinosa,bowden,vickers,lowry,pritchard,costello,piper,mcclellan,lovell,sheehan,hatch,dobson,singh,jeffries,hollingsworth,sorensen,meza,fink,donnelly,burrell,tomlinson,colbert,billings,ritchie,helton,sutherland,peoples,mcqueen,thomason,givens,crocker,vogel,robison,dunham,coker,swartz,keys,ladner,richter,hargrove,edmonds,brantley,albright,murdock,boswell,muller,quintero,padgett,kenney,daly,connolly,inman,quintana,lund,barnard,villegas,simons,land,huggins,tidwell,sanderson,bullard,mcclendon,duarte,draper,marrero,dwyer,abrams,stover,goode,fraser,crews,bernal,godwin,conklin,mcneal,baca,esparza,crowder,bower,brewster,mcneill,rodrigues,leal,coates,raines,mccain,mccord,miner,holbrook,swift,dukes,carlisle,aldridge,ackerman,starks,ricks,holliday,ferris,hairston,sheffield,lange,fountain,doss,betts,kaplan,carmichael,bloom,ruffin,penn,kern,bowles,sizemore,larkin,dupree,seals,metcalf,hutchison,henley,farr,mccauley,hankins,gustafson,curran,ash,waddell,ramey,cates,pollock,cummins,messer,heller,lin,funk,cornett,palacios,galindo,cano,hathaway,singer,pham,enriquez,salgado,pelletier,painter,wiseman,blount,feliciano,temple,houser,doherty,mead,mcgraw,swan,capps,blanco,blackmon,thomson,mcmanus,burkett,post,gleason,ott,dickens,cormier,voss,rushing,rosenberg,hurd,dumas,benitez,arellano,marin,caudill,bragg,jaramillo,huerta,gipson,colvin,biggs,vela,platt,cassidy,tompkins,mccollum,dolan,daley,crump,sneed,kilgore,grove,grimm,davison,brunson,prater,marcum,devine,stratton,rosas,choi,tripp,ledbetter,hightower,feldman,epps,yeager,posey,scruggs,cope,stubbs,richey,overton,trotter,sprague,cordero,butcher,stiles,burgos,woodson,horner,bassett,purcell,haskins,akins,ziegler,spaulding,hadley,grubbs,sumner,murillo,zavala,shook,lockwood,driscoll,dahl,thorpe,redmond,putnam,mcwilliams,mcrae,romano,joiner,sadler,hedrick,hager,hagen,fitch,coulter,thacker,mansfield,langston,guidry,ferreira,corley,conn,rossi,lackey,baez,saenz,mcnamara,mcmullen,mckenna,mcdonough,link,engel,browne,roper,peacock,eubanks,drummond,stringer,pritchett,parham,mims,landers,ham,grayson,schafer,egan,timmons,ohara,keen,hamlin,finn,cortes,mcnair,nadeau,moseley,michaud,rosen,oakes,kurtz,jeffers,calloway,beal,bautista,winn,suggs,stern,stapleton,lyles,laird,montano,dawkins,hagan,goldman,bryson,barajas,lovett,segura,metz,lockett,langford,hinson,eastman,hooks,smallwood,shapiro,crowell,whalen,triplett,chatman,aldrich,cahill,youngblood,ybarra,stallings,sheets,reeder,connelly,bateman,abernathy,winkler,wilkes,masters,hackett,granger,gillis,schmitz,sapp,napier,souza,lanier,gomes,weir,otero,ledford,burroughs,babcock,ventura,siegel,dugan,bledsoe,atwood,wray,varner,spangler,anaya,staley,kraft,fournier,belanger,wolff,thorne,bynum,burnette,boykin,swenson,purvis,pina,khan,duvall,darby,xiong,kauffman,healy,engle,benoit,valle,steiner,spicer,shaver,randle,lundy,dow,chin,calvert,staton,neff,kearney,darden,oakley,medeiros,mccracken,crenshaw,block,perdue,dill,whittaker,tobin,washburn,hogue,goodrich,easley,bravo,dennison,shipley,kerns,jorgensen,crain,villalobos,maurer,longoria,keene,coon,witherspoon,staples,pettit,kincaid,eason,madrid,echols,lusk,stahl,currie,thayer,shultz,mcnally,seay,north,maher,gagne,barrow,nava,moreland,honeycutt,hearn,diggs,caron,whitten,westbrook,stovall,ragland,munson,meier,looney,kimble,jolly,hobson,goddard,culver,burr,presley,negron,connell,tovar,huddleston,ashby,salter,root,pendleton,oleary,nickerson,myrick,judd,jacobsen,bain,adair,starnes,matos,busby,herndon,hanley,bellamy,doty,bartley,yazzie,rowell,parson,gifford,cullen,christiansen,benavides,barnhart,talbot,mock,crandall,connors,bonds,whitt,gage,bergman,arredondo,addison,lujan,dowdy,jernigan,huynh,bouchard,dutton,rhoades,ouellette,kiser,herrington,hare,blackman,babb,allred,rudd,paulson,ogden,koenig,geiger,begay,parra,lassiter,hawk,esposito,cho,waldron,ransom,prather,chacon,vick,sands,roark,parr,mayberry,greenberg,coley,bruner,whitman,skaggs,shipman,leary,hutton,romo,medrano,ladd,kruse,askew,schulz,alfaro,tabor,mohr,gallo,bermudez,pereira,bliss,reaves,flint,comer,woodall,naquin,guevara,delong,carrier,pickens,brand,tilley,schaffer,lim,knutson,fenton,doran,chu,vogt,vann,prescott,mclain,landis,corcoran,zapata,hyatt,hemphill,faulk,dove,boudreaux,aragon,whitlock,trejo,tackett,shearer,saldana,hanks,mckinnon,koehler,bourgeois,keyes,goodson,foote,lunsford,goldsmith,flood,winslow,sams,reagan,mccloud,hough,esquivel,naylor,loomis,coronado,ludwig,braswell,bearden,fagan,ezell,edmondson,cyr,cronin,nunn,lemon,guillory,grier,dubose,traylor,ryder,dobbins,coyle,aponte,whitmore,smalls,rowan,malloy,cardona,braxton,borden,humphries,carrasco,ruff,metzger,huntley,hinojosa,finney,madsen,hills,ernst,dozier,burkhart,bowser,peralta,daigle,whittington,sorenson,saucedo,roche,redding,fugate,avalos,waite,lind,huston,hay,hawthorne,hamby,boyles,boles,regan,faust,crook,beam,barger,hinds,gallardo,willoughby,willingham,eckert,busch,zepeda,worthington,tinsley,hoff,hawley,carmona,varela,rector,newcomb,kinsey,dube,whatley,ragsdale,bernstein,becerra,yost,mattson,felder,cheek,handy,grossman,gauthier,escobedo,braden,beckman,mott,hillman,flaherty,dykes,doe,stockton,stearns,lofton,coats,cavazos,beavers,barrios,parish,mosher,cardwell,coles,burnham,weller,lemons,beebe,aguilera,parnell,harman,couture,alley,schumacher,redd,dobbs,blum,blalock,merchant,ennis,denson,cottrell,brannon,bagley,aviles,watt,sousa,rosenthal,rooney,dietz,blank,paquette,mcclelland,duff,velasco,lentz,grubb,burrows,barbour,ulrich,shockley,rader,beyer,mixon,layton,altman,weathers,stoner,squires,shipp,priest,lipscomb,cutler,caballero,zimmer,willett,thurston,storey,medley,epperson,shah,mcmillian,baggett,torrez,laws,hirsch,dent,poirier,peachey,farrar,creech,barth,trimble,dupre,albrecht,sample,lawler,crisp,conroy,wetzel,nesbitt,murry,jameson,wilhelm,patten,minton,matson,kimbrough,iverson,guinn,croft,toth,pulliam,nugent,newby,littlejohn,dias,canales,bernier,baron,singletary,renteria,pruett,mchugh,mabry,landrum,brower,stoddard,cagle,stjohn,scales,kohler,kellogg,hopson,gant,tharp,gann,zeigler,pringle,hammons,fairchild,deaton,chavis,carnes,rowley,matlock,kearns,irizarry,carrington,starkey,lopes,jarrell,craven,baum,spain,littlefield,linn,humphreys,etheridge,cuellar,chastain,bundy,speer,skelton,quiroz,pyle,portillo,ponder,moulton,machado,liu,killian,hutson,hitchcock,dowling,cloud,burdick,spann,pedersen,levin,leggett,hayward,hacker,dietrich,beaulieu,barksdale,wakefield,snowden,briscoe,bowie,berman,ogle,mcgregor,laughlin,helm,burden,wheatley,schreiber,pressley,parris,alaniz,agee,urban,swann,snodgrass,schuster,radford,monk,mattingly,harp,girard,cheney,yancey,wagoner,ridley,lombardo,lau,hudgins,gaskins,duckworth,coe,coburn,willey,prado,newberry,magana,hammonds,elam,whipple,slade,serna,ojeda,liles,dorman,diehl,upton,reardon,michaels,goetz,eller,bauman,baer,layne,hummel,brenner,amaya,adamson,ornelas,dowell,cloutier,castellanos,wing,wellman,saylor,orourke,moya,montalvo,kilpatrick,durbin,shell,oldham,garvin,foss,branham,bartholomew,templeton,maguire,holton,rider,monahan,mccormack,beaty,anders,streeter,nieto,nielson,moffett,lankford,keating,heck,gatlin,delatorre,callaway,adcock,worrell,unger,robinette,nowak,jeter,brunner,steen,parrott,overstreet,nobles,montanez,clevenger,brinkley,trahan,quarles,pickering,pederson,jansen,grantham,gilchrist,crespo,aiken,schell,schaeffer,lorenz,leyva,harms,dyson,wallis,pease,leavitt,cavanaugh,batts,warden,seaman,rockwell,quezada,paxton,linder,houck,fontaine,durant,caruso,adler,pimentel,mize,lytle,cleary,cason,acker,switzer,isaacs,higginbotham,han,waterman,vandyke,stamper,sisk,shuler,riddick,mcmahan,levesque,hatton,bronson,bollinger,arnett,okeefe,gerber,gannon,farnsworth,baughman,silverman,satterfield,mccrary,kowalski,grigsby,greco,cabral,trout,rinehart,mahon,linton,gooden,curley,baugh,wyman,weiner,schwab,schuler,morrissey,mahan,bunn,thrasher,spear,waggoner,qualls,purdy,mcwhorter,mauldin,gilman,perryman,newsom,menard,martino,graf,billingsley,artis,simpkins,salisbury,quintanilla,gilliland,fraley,foust,crouse,scarborough,ngo,grissom,fultz,marlow,markham,madrigal,lawton,barfield,whiting,varney,schwarz,gooch,arce,wheat,truong,poulin,hurtado,selby,gaither,fortner,culpepper,coughlin,brinson,boudreau,barkley,bales,stepp,holm,tan,schilling,morrell,kahn,heaton,gamez,causey,turpin,shanks,schrader,meek,isom,hardison,carranza,yanez,scroggins,schofield,runyon,ratcliff,murrell,moeller,irby,currier,butterfield,yee,ralston,pullen,pinson,estep,carbone,hawks,ellington,casillas,spurlock,sikes,motley,mccartney,kruger,isbell,houle,burk,tomlin,quigley,neumann,lovelace,fennell,cheatham,bustamante,skidmore,hidalgo,forman,culp,bowens,betancourt,aquino,robb,rea,milner,martel,gresham,wiles,ricketts,dowd,collazo,bostic,blakely,sherrod,kenyon,gandy,ebert,deloach,allard,sauer,robins,olivares,gillette,chestnut,bourque,paine,hite,hauser,devore,crawley,chapa,talbert,poindexter,meador,mcduffie,mattox,kraus,harkins,choate,wren,sledge,sanborn,kinder,geary,cornwell,barclay,abney,seward,rhoads,howland,fortier,benner,vines,tubbs,troutman,rapp,mccurdy,deluca,westmoreland,havens,guajardo,ely,clary,seal,meehan,herzog,guillen,ashcraft,waugh,renner,milam,elrod,churchill,breaux,bolin,asher,windham,tirado,pemberton,nolen,noland,knott,emmons,cornish,christenson,brownlee,barbee,waldrop,pitt,olvera,lombardi,gruber,gaffney,eggleston,banda,archuleta,slone,prewitt,pfeiffer,nettles,mena,mcadams,henning,gardiner,cromwell,chisholm,burleson,vest,oglesby,mccarter,lumpkin,grey,wofford,vanhorn,thorn,teel,swafford,stclair,stanfield,ocampo,herrmann,hannon,arsenault,roush,mcalister,hiatt,gunderson,forsythe,duggan,delvalle,cintron,wilks,weinstein,uribe,rizzo,noyes,mclendon,gurley,bethea,winstead,maples,guyton,giordano,alderman,valdes,polanco,pappas,lively,grogan,griffiths,arevalo,whitson,sowell,rendon,fernandes,farrow,benavidez,ayres,alicea,stump,smalley,seitz,schulte,gilley,gallant,canfield,wolford,omalley,mcnutt,mcnulty,mcgovern,hardman,harbin,cowart,chavarria,brink,beckett,bagwell,armstead,anglin,abreu,reynoso,krebs,jett,hoffmann,greenfield,forte,burney,broome,sisson,trammell,partridge,mace,lomax,lemieux,gossett,frantz,fogle,cooney,broughton,pence,paulsen,muncy,mcarthur,hollins,beauchamp,withers,osorio,mulligan,hoyle,foy,dockery,cockrell,begley,amador,roby,rains,lindquist,gentile,everhart,bohannon,wylie,sommers,purnell,fortin,dunning,breeden,vail,phelan,phan,marx,cosby,colburn,boling,biddle,ledesma,gaddis,denney,chow,bueno,berrios,wicker,tolliver,thibodeaux,nagle,lavoie,fisk,crist,barbosa,reedy,march,locklear,kolb,himes,behrens,beckwith,weems,wahl,shorter,shackelford,rees,muse,cerda,valadez,thibodeau,saavedra,ridgeway,reiter,mchenry,majors,lachance,keaton,ferrara,clemens,blocker,applegate,paz,needham,mojica,kuykendall,hamel,escamilla,doughty,burchett,ainsworth,vidal,upchurch,thigpen,strauss,spruill,sowers,riggins,ricker,mccombs,harlow,buffington,sotelo,olivas,negrete,morey,macon,logsdon,lapointe,bigelow,bello,westfall,stubblefield,peak,lindley,hein,hawes,farrington,breen,birch,wilde,steed,sepulveda,reinhardt,proffitt,minter,messina,mcnabb,maier,keeler,gamboa,donohue,basham,shinn,crooks,cota,borders,bills,bachman,tisdale,tavares,schmid,pickard,gulley,fonseca,delossantos,condon,batista,wicks,wadsworth,martell,littleton,ison,haag,folsom,brumfield,broyles,brito,mireles,mcdonnell,leclair,hamblin,gough,fanning,binder,winfield,whitworth,soriano,palumbo,newkirk,mangum,hutcherson,comstock,carlin,beall,bair,wendt,watters,walling,putman,otoole,morley,mares,lemus,keener,hundley,dial,damico,billups,strother,mcfarlane,lamm,eaves,crutcher,caraballo,canty,atwell,taft,siler,rust,rawls,rawlings,prieto,mcneely,mcafee,hulsey,hackney,galvez,escalante,delagarza,crider,charlton,bandy,wilbanks,stowe,steinberg,renfro,masterson,massie,lanham,haskell,hamrick,fort,dehart,burdette,branson,bourne,babin,aleman,worthy,tibbs,smoot,slack,paradis,mull,luce,houghton,gantt,furman,danner,christianson,burge,ashford,arndt,almeida,stallworth,shade,searcy,sager,noonan,mclemore,mcintire,maxey,lavigne,jobe,ferrer,falk,coffin,byrnes,aranda,apodaca,stamps,rounds,peek,olmstead,lewandowski,kaminski,dunaway,bruns,brackett,amato,reich,mcclung,lacroix,koontz,herrick,hardesty,flanders,cousins,cato,cade,vickery,shank,nagel,dupuis,croteau,cotter,cable,stuckey,stine,porterfield,pauley,nye,moffitt,knudsen,hardwick,goforth,dupont,blunt,barrows,barnhill,shull,rash,loftis,lemay,kitchens,horvath,grenier,fuchs,fairbanks,culbertson,calkins,burnside,beattie,ashworth,albertson,wertz,vaught,vallejo,turk,tuck,tijerina,sage,peterman,marroquin,marr,lantz,hoang,demarco,daily,cone,berube,barnette,wharton,stinnett,slocum,scanlon,sander,pinto,mancuso,lima,headley,epstein,counts,clarkson,carnahan,boren,arteaga,adame,zook,whittle,whitehurst,wenzel,saxton,reddick,puente,handley,haggerty,earley,devlin,chaffin,cady,acuna,solano,sigler,pollack,pendergrass,ostrander,janes,francois,crutchfield,chamberlin,brubaker,baptiste,willson,reis,neeley,mullin,mercier,lira,layman,keeling,higdon,espinal,chapin,warfield,toledo,pulido,peebles,nagy,montague,mello,lear,jaeger,hogg,graff,furr,soliz,poore,mendenhall,mclaurin,maestas,gable,barraza,tillery,snead,pond,neill,mcculloch,mccorkle,lightfoot,hutchings,holloman,harness,dorn,council,bock,zielinski,turley,treadwell,stpierre,starling,somers,oswald,merrick,easterling,bivens,truitt,poston,parry,ontiveros,olivarez,moreau,medlin,lenz,knowlton,fairley,cobbs,chisolm,bannister,woodworth,toler,ocasio,noriega,neuman,moye,milburn,mcclanahan,lilley,hanes,flannery,dellinger,danielson,conti,blodgett,beers,weatherford,strain,karr,hitt,denham,custer,coble,clough,casteel,bolduc,batchelor,ammons,whitlow,tierney,staten,sibley,seifert,schubert,salcedo,mattison,laney,haggard,grooms,dix,dees,cromer,cooks,colson,caswell,zarate,swisher,shin,ragan,pridgen,mcvey,matheny,lafleur,franz,ferraro,dugger,whiteside,rigsby,mcmurray,lehmann,jacoby,hildebrand,hendrick,headrick,goad,fincher,drury,borges,archibald,albers,woodcock,trapp,soares,seaton,monson,luckett,lindberg,kopp,keeton,hsu,healey,garvey,gaddy,fain,burchfield,wentworth,strand,stack,spooner,saucier,sales,ricci,plunkett,pannell,ness,leger,hoy,freitas,fong,elizondo,duval,beaudoin,urbina,rickard,partin,moe,mcgrew,mcclintock,ledoux,forsyth,faison,devries,bertrand,wasson,tilton,scarbrough,leung,irvine,garber,denning,corral,colley,castleberry,bowlin,bogan,beale,baines,trice,rayburn,parkinson,pak,nunes,mcmillen,leahy,kimmel,higgs,fulmer,carden,bedford,taggart,spearman,register,prichard,morrill,koonce,heinz,hedges,guenther,grice,findley,dover,creighton,boothe,bayer,arreola,vitale,valles,raney,osgood,hanlon,burley,bounds,worden,weatherly,vetter,tanaka,stiltner,nevarez,mosby,montero,melancon,harter,hamer,goble,gladden,gist,ginn,akin,zaragoza,towns,tarver,sammons,royster,oreilly,muir,morehead,luster,kingsley,kelso,grisham,glynn,baumann,alves,yount,tamayo,paterson,oates,menendez,longo,hargis,gillen,desantis,breedlove,sumpter,scherer,rupp,reichert,heredia,creel,cohn,clemmons,casas,bickford,belton,bach,williford,whitcomb,tennant,sutter,stull,sessions,mccallum,langlois,keel,keegan,dangelo,dancy,damron,clapp,clanton,bankston,oliveira,mintz,mcinnis,martens,mabe,laster,jolley,hildreth,hefner,glaser,duckett,demers,brockman,blais,alcorn,agnew,toliver,tice,seeley,najera,musser,mcfall,laplante,galvin,fajardo,doan,coyne,copley,clawson,cheung,barone,wynne,woodley,tremblay,stoll,sparrow,sparkman,schweitzer,sasser,samples,roney,legg,heim,farias,colwell,christman,bratcher,winchester,upshaw,southerland,sorrell,sells,mount,mccloskey,martindale,luttrell,loveless,lovejoy,linares,latimer,embry,coombs,bratton,bostick,venable,tuggle,toro,staggs,sandlin,jefferies,heckman,griffis,crayton,clem,browder,thorton,sturgill,sprouse,royer,rousseau,ridenour,pogue,perales,peeples,metzler,mesa,mccutcheon,mcbee,hornsby,heffner,corrigan,armijo,vue,plante,peyton,paredes,macklin,hussey,hodgson,granados,frias,becnel,batten,almanza,turney,teal,sturgeon,meeker,mcdaniels,limon,keeney,kee,hutto,holguin,gorham,fishman,fierro,blanchette,rodrigue,reddy,osburn,oden,lerma,kirkwood,keefer,haugen,hammett,chalmers,brinkman,baumgartner,valerio,tellez,steffen,shumate,sauls,ripley,kemper,jacks,guffey,evers,craddock,carvalho,blaylock,banuelos,balderas,wooden,wheaton,turnbull,shuman,pointer,mosier,mccue,ligon,kozlowski,johansen,ingle,herr,briones,snipes,rickman,pipkin,pantoja,orosco,moniz,lawless,kunkel,hibbard,galarza,enos,bussey,schott,salcido,perreault,mcdougal,mccool,haight,garris,ferry,easton,conyers,atherton,wimberly,utley,spellman,smithson,slagle,ritchey,rand,petit,osullivan,oaks,nutt,mcvay,mccreary,mayhew,knoll,jewett,harwood,cardoza,ashe,arriaga,zeller,wirth,whitmire,stauffer,rountree,redden,mccaffrey,martz,larose,langdon,humes,gaskin,faber,devito,cass,almond,wingfield,wingate,villareal,tyner,smothers,severson,reno,pennell,maupin,leighton,janssen,hassell,hallman,halcomb,folse,fitzsimmons,fahey,cranford,bolen,battles,battaglia,wooldridge,trask,rosser,regalado,mcewen,keefe,fuqua,echevarria,caro,boynton,andrus,viera,vanmeter,taber,spradlin,seibert,provost,prentice,oliphant,laporte,hwang,hatchett,hass,greiner,freedman,covert,chilton,byars,wiese,venegas,swank,shrader,roberge,mullis,mortensen,mccune,marlowe,kirchner,keck,isaacson,hostetler,halverson,gunther,griswold,fenner,durden,blackwood,ahrens,sawyers,savoy,nabors,mcswain,mackay,loy,lavender,lash,labbe,jessup,fullerton,cruse,crittenden,correia,centeno,caudle,canady,callender,alarcon,ahern,winfrey,tribble,styles,salley,roden,musgrove,minnick,fortenberry,carrion,bunting,batiste,whited,underhill,stillwell,rauch,pippin,perrin,messenger,mancini,lister,kinard,hartmann,fleck,broadway,wilt,treadway,thornhill,spalding,rafferty,pitre,patino,ordonez,linkous,kelleher,homan,galbraith,feeney,curtin,coward,camarillo,buss,bunnell,bolt,beeler,autry,alcala,witte,wentz,stidham,shively,nunley,meacham,martins,lemke,lefebvre,hynes,horowitz,hoppe,holcombe,dunne,derr,cochrane,brittain,bedard,beauregard,torrence,strunk,soria,simonson,shumaker,scoggins,oconner,moriarty,kuntz,ives,hutcheson,horan,hales,garmon,fitts,bohn,atchison,wisniewski,vanwinkle,sturm,sallee,prosser,moen,lundberg,kunz,kohl,keane,jorgenson,jaynes,funderburk,freed,durr,creamer,cosgrove,batson,vanhoose,thomsen,teeter,smyth,redmon,orellana,maness,heflin,goulet,frick,forney,bunker,asbury,aguiar,talbott,southard,mowery,mears,lemmon,krieger,hickson,elston,duong,delgadillo,dayton,dasilva,conaway,catron,bruton,bradbury,bordelon,bivins,bittner,bergstrom,beals,abell,whelan,tejada,pulley,pino,norfleet,nealy,maes,loper,gatewood,frierson,freund,finnegan,cupp,covey,catalano,boehm,bader,yoon,walston,tenney,sipes,rawlins,medlock,mccaskill,mccallister,marcotte,maclean,hughey,henke,harwell,gladney,gilson,dew,chism,caskey,brandenburg,baylor,villasenor,veal,thatcher,stegall,shore,petrie,nowlin,navarrete,muhammad,lombard,loftin,lemaster,kroll,kovach,kimbrell,kidwell,hershberger,fulcher,eng,cantwell,bustos,boland,bobbitt,binkley,wester,weis,verdin,tiller,sisco,sharkey,seymore,rosenbaum,rohr,quinonez,pinkston,nation,malley,logue,lessard,lerner,lebron,krauss,klinger,halstead,haller,getz,burrow,alger,shores,pfeifer,perron,nelms,munn,mcmaster,mckenney,manns,knudson,hutchens,huskey,goebel,flagg,cushman,click,castellano,carder,bumgarner,wampler,spinks,robson,neel,mcreynolds,mathias,maas,loera,kasper,jenson,florez,coons,buckingham,brogan,berryman,wilmoth,wilhite,thrash,shephard,seidel,schulze,roldan,pettis,obryan,maki,mackie,hatley,frazer,fiore,chesser,bui,bottoms,bisson,benefield,allman,wilke,trudeau,timm,shifflett,rau,mundy,milliken,mayers,leake,kohn,huntington,horsley,hermann,guerin,fryer,frizzell,foret,flemming,fife,criswell,carbajal,bozeman,boisvert,angulo,wallen,tapp,silvers,ramsay,oshea,orta,moll,mckeever,mcgehee,linville,kiefer,ketchum,howerton,groce,gass,fusco,corbitt,betz,bartels,amaral,aiello,yoo,weddle,sperry,seiler,runyan,raley,overby,osteen,olds,mckeown,matney,lauer,lattimore,hindman,hartwell,fredrickson,fredericks,espino,clegg,carswell,cambell,burkholder,woodbury,welker,totten,thornburg,theriault,stitt,stamm,stackhouse,scholl,saxon,rife,razo,quinlan,pinkerton,olivo,nesmith,nall,mattos,lafferty,justus,giron,geer,fielder,drayton,dortch,conners,conger,boatwright,billiot,barden,armenta,tibbetts,steadman,slattery,rinaldi,raynor,pinckney,pettigrew,milne,matteson,halsey,gonsalves,fellows,durand,desimone,cowley,cowles,brill,barham,barela,barba,ashmore,withrow,valenti,tejeda,spriggs,sayre,salerno,peltier,peel,merriman,matheson,lowman,lindstrom,hyland,giroux,earls,dugas,dabney,collado,briseno,baxley,whyte,wenger,vanover,vanburen,thiel,schindler,schiller,rigby,pomeroy,passmore,marble,manzo,mahaffey,lindgren,laflamme,greathouse,fite,calabrese,bayne,yamamoto,wick,townes,thames,reinhart,peeler,naranjo,montez,mcdade,mast,markley,marchand,leeper,kellum,hudgens,hennessey,hadden,gainey,coppola,borrego,bolling,beane,ault,slaton,poland,pape,null,mulkey,lightner,langer,hillard,glasgow,ethridge,enright,derosa,baskin,weinberg,turman,somerville,pardo,noll,lashley,ingraham,hiller,hendon,glaze,cothran,cooksey,conte,carrico,abner,wooley,swope,summerlin,sturgis,sturdivant,stott,spurgeon,spillman,speight,roussel,popp,nutter,mckeon,mazza,magnuson,lanning,kozak,jankowski,heyward,forster,corwin,callaghan,bays,wortham,usher,theriot,sayers,sabo,poling,loya,lieberman,laroche,labelle,howes,harr,garay,fogarty,everson,durkin,dominquez,chaves,chambliss,witcher,vieira,vandiver,terrill,stoker,schreiner,moorman,liddell,lew,lawhorn,krug,irons,hylton,hollenbeck,herrin,hembree,goolsby,goodin,gilmer,foltz,dinkins,daughtry,caban,brim,briley,bilodeau,wyant,vergara,tallent,swearingen,stroup,scribner,quillen,pitman,monaco,mccants,maxfield,martinson,holtz,flournoy,brookins,brody,baumgardner,straub,sills,roybal,roundtree,oswalt,mcgriff,mcdougall,mccleary,maggard,gragg,gooding,godinez,doolittle,donato,cowell,cassell,bracken,appel,zambrano,reuter,perea,nakamura,monaghan,mickens,mcclinton,mcclary,marler,kish,judkins,gilbreath,freese,flanigan,felts,erdmann,dodds,chew,brownell,boatright,barreto,slayton,sandberg,saldivar,pettway,odum,narvaez,moultrie,montemayor,merrell,lees,keyser,hoke,hardaway,hannan,gilbertson,fogg,dumont,deberry,coggins,buxton,bucher,broadnax,beeson,araujo,appleton,amundson,aguayo,ackley,yocum,worsham,shivers,sanches,sacco,robey,rhoden,pender,ochs,mccurry,madera,luong,knotts,jackman,heinrich,hargrave,gault,comeaux,chitwood,caraway,boettcher,bernhardt,barrientos,zink,wickham,whiteman,thorp,stillman,settles,schoonover,roque,riddell,pilcher,phifer,novotny,macleod,hardee,haase,grider,doucette,clausen,bevins,beamon,badillo,tolley,tindall,soule,snook,seale,pitcher,pinkney,pellegrino,nowell,nemeth,mondragon,mclane,lundgren,ingalls,hudspeth,hixson,gearhart,furlong,downes,dibble,deyoung,cornejo,camara,brookshire,boyette,wolcott,surratt,sellars,segal,salyer,reeve,rausch,labonte,haro,gower,freeland,fawcett,eads,driggers,donley,collett,bromley,boatman,ballinger,baldridge,volz,trombley,stonge,shanahan,rivard,rhyne,pedroza,matias,jamieson,hedgepeth,hartnett,estevez,eskridge,denman,chiu,chinn,catlett,carmack,buie,bechtel,beardsley,bard,ballou,ulmer,skeen,robledo,rincon,reitz,piazza,munger,moten,mcmichael,loftus,ledet,kersey,groff,fowlkes,folk,crumpton,clouse,bettis,villagomez,timmerman,strom,santoro,roddy,penrod,musselman,macpherson,leboeuf,harless,haddad,guido,golding,fulkerson,fannin,dulaney,dowdell,cottle,ceja,cate,bosley,benge,albritton,voigt,trowbridge,soileau,seely,rohde,pearsall,paulk,orth,nason,mota,mcmullin,marquardt,madigan,hoag,gillum,gabbard,fenwick,eck,danforth,cushing,cress,creed,cazares,casanova,bey,bettencourt,barringer,baber,stansberry,schramm,rutter,rivero,oquendo,necaise,mouton,montenegro,miley,mcgough,marra,macmillan,lamontagne,jasso,horst,hetrick,heilman,gaytan,gall,fortney,dingle,desjardins,dabbs,burbank,brigham,breland,beaman,arriola,yarborough,wallin,toscano,stowers,reiss,pichardo,orton,michels,mcnamee,mccrory,leatherman,kell,keister,horning,hargett,guay,ferro,deboer,dagostino,carper,blanks,beaudry,towle,tafoya,stricklin,strader,soper,sonnier,sigmon,schenk,saddler,pedigo,mendes,lunn,lohr,lahr,kingsbury,jarman,hume,holliman,hofmann,haworth,harrelson,hambrick,flick,edmunds,dacosta,crossman,colston,chaplin,carrell,budd,weiler,waits,valentino,trantham,tarr,solorio,roebuck,powe,plank,pettus,palm,pagano,mink,luker,leathers,joslin,hartzell,gambrell,deutsch,cepeda,carty,caputo,brewington,bedell,ballew,applewhite,warnock,walz,urena,tudor,reel,pigg,parton,mickelson,meagher,mclellan,mcculley,mandel,leech,lavallee,kraemer,kling,kipp,kehoe,hochstetler,harriman,gregoire,grabowski,gosselin,gammon,fancher,edens,desai,brannan,armendariz,woolsey,whitehouse,whetstone,ussery,towne,testa,tallman,studer,strait,steinmetz,sorrells,sauceda,rolfe,paddock,mitchem,mcginn,mccrea,lovato,hazen,gilpin,gaynor,fike,devoe,delrio,curiel,burkhardt,bode,backus,zinn,watanabe,wachter,vanpelt,turnage,shaner,schroder,sato,riordan,quimby,portis,natale,mckoy,mccown,kilmer,hotchkiss,hesse,halbert,gwinn,godsey,delisle,chrisman,canter,arbogast,angell,acree,yancy,woolley,wesson,weatherspoon,trainor,stockman,spiller,sipe,rooks,reavis,propst,porras,neilson,mullens,loucks,llewellyn,kumar,koester,klingensmith,kirsch,kester,honaker,hodson,hennessy,helmick,garrity,garibay,fee,drain,casarez,callis,botello,aycock,avant,wingard,wayman,tully,theisen,szymanski,stansbury,segovia,rainwater,preece,pirtle,padron,mincey,mckelvey,mathes,larrabee,kornegay,klug,ingersoll,hecht,germain,eggers,dykstra,deering,decoteau,deason,dearing,cofield,carrigan,bonham,bahr,aucoin,appleby,almonte,yager,womble,wimmer,weimer,vanderpool,stancil,sprinkle,romine,remington,pfaff,peckham,olivera,meraz,maze,lathrop,koehn,hazelton,halvorson,hallock,haddock,ducharme,dehaven,caruthers,brehm,bosworth,bost,bias,beeman,basile,bane,aikens,wold,walther,tabb,suber,strawn,stocker,shirey,schlosser,riedel,rembert,reimer,pyles,peele,merriweather,letourneau,latta,kidder,hixon,hillis,hight,herbst,henriquez,haygood,hamill,gabel,fritts,eubank,dawes,correll,cha,bushey,buchholz,brotherton,botts,barnwell,auger,atchley,westphal,veilleux,ulloa,stutzman,shriver,ryals,prior,pilkington,moyers,marrs,mangrum,maddux,lockard,laing,kuhl,harney,hammock,hamlett,felker,doerr,depriest,carrasquillo,carothers,bogle,bischoff,bergen,albanese,wyckoff,vermillion,vansickle,thibault,tetreault,stickney,shoemake,ruggiero,rawson,racine,philpot,paschal,mcelhaney,mathison,legrand,lapierre,kwan,kremer,jiles,hilbert,geyer,faircloth,ehlers,egbert,desrosiers,dalrymple,cotten,cashman,cadena,breeding,boardman,alcaraz,ahn,wyrick,therrien,tankersley,strickler,puryear,plourde,pattison,pardue,mcginty,mcevoy,landreth,kuhns,koon,hewett,giddens,emerick,eades,deangelis,cosme,ceballos,birdsong,benham,bemis,armour,anguiano,welborn,tsosie,storms,shoup,sessoms,samaniego,rood,rojo,rhinehart,raby,northcutt,myer,munguia,morehouse,mcdevitt,mallett,lozada,lemoine,kuehn,hallett,grim,gillard,gaylor,garman,gallaher,feaster,faris,darrow,dardar,coney,carreon,braithwaite,boylan,boyett,bixler,bigham,benford,barragan,barnum,zuber,wyche,westcott,vining,stoltzfus,simonds,shupe,sabin,ruble,rittenhouse,richman,perrone,mulholland,millan,lomeli,kite,jemison,hulett,holler,hickerson,herold,hazelwood,griffen,gause,forde,eisenberg,dilworth,charron,chaisson,brodie,bristow,breunig,brace,boutwell,bentz,belk,bayless,batchelder,baran,baeza,zimmermann,weathersby,volk,toole,theis,tedesco,searle,schenck,satterwhite,ruelas,rankins,partida,nesbit,morel,menchaca,levasseur,kaylor,johnstone,hulse,hollar,hersey,harrigan,harbison,guyer,gish,giese,gerlach,geller,geisler,falcone,elwell,doucet,deese,darr,corder,chafin,byler,bussell,burdett,brasher,bowe,bellinger,bastian,barner,alleyne,wilborn,weil,wegner,wales,tatro,spitzer,smithers,schoen,resendez,parisi,overman,obrian,mudd,moy,mclaren,maggio,lindner,lalonde,lacasse,laboy,killion,kahl,jessen,jamerson,houk,henshaw,gustin,graber,durst,duenas,davey,cundiff,conlon,colunga,coakley,chiles,capers,buell,bricker,bissonnette,birmingham,bartz,bagby,zayas,volpe,treece,toombs,thom,terrazas,swinney,skiles,silveira,shouse,senn,ramage,nez,moua,langham,kyles,holston,hoagland,herd,feller,denison,carraway,burford,bickel,ambriz,abercrombie,yamada,weidner,waddle,verduzco,thurmond,swindle,schrock,sanabria,rosenberger,probst,peabody,olinger,nazario,mccafferty,mcbroom,mcabee,mazur,matherne,mapes,leverett,killingsworth,heisler,griego,gosnell,frankel,franke,ferrante,fenn,ehrlich,christopherso,chasse,chancellor,caton,brunelle,bly,bloomfield,babbitt,azevedo,abramson,ables,abeyta,youmans,wozniak,wainwright,stowell,smitherman,samuelson,runge,rothman,rosenfeld,peake,owings,olmos,munro,moreira,leatherwood,larkins,krantz,kovacs,kizer,kindred,karnes,jaffe,hubbell,hosey,hauck,goodell,erdman,dvorak,doane,cureton,cofer,buehler,bierman,berndt,banta,abdullah,warwick,waltz,turcotte,torrey,stith,seger,sachs,quesada,pinder,peppers,pascual,paschall,parkhurst,ozuna,oster,nicholls,lheureux,lavalley,kimura,jablonski,haun,gourley,gilligan,derby,croy,cotto,cargill,burwell,burgett,buckman,booher,adorno,wrenn,whittemore,urias,szabo,sayles,saiz,rutland,rael,pharr,pelkey,ogrady,nickell,musick,moats,mather,massa,kirschner,kieffer,kellar,hendershot,gott,godoy,gadson,furtado,fiedler,erskine,dutcher,dever,daggett,chevalier,brake,ballesteros,amerson,wingo,waldon,trott,silvey,showers,schlegel,rue,ritz,pepin,pelayo,parsley,palermo,moorehead,mchale,lett,kocher,kilburn,iglesias,humble,hulbert,huckaby,hix,haven,hartford,hardiman,gurney,grigg,grasso,goings,fillmore,farber,depew,dandrea,dame,cowen,covarrubias,burrus,bracy,ardoin,thompkins,standley,radcliffe,pohl,persaud,parenteau,pabon,newson,newhouse,napolitano,mulcahy,malave,keim,hooten,hernandes,heffernan,hearne,greenleaf,glick,fuhrman,fetter,faria,dishman,dickenson,crites,criss,clapper,chenault,castor,casto,bugg,bove,bonney,ard,anderton,allgood,alderson,woodman,warrick,toomey,tooley,tarrant,summerville,stebbins,sokol,searles,schutz,schumann,scheer,remillard,raper,proulx,palmore,monroy,messier,melo,melanson,mashburn,manzano,lussier,jenks,huneycutt,hartwig,grimsley,fulk,fielding,fidler,engstrom,eldred,dantzler,crandell,calder,brumley,breton,brann,bramlett,boykins,bianco,bancroft,almaraz,alcantar,whitmer,whitener,welton,vineyard,rahn,paquin,mizell,mcmillin,mckean,marston,maciel,lundquist,liggins,lampkin,kranz,koski,kirkham,jiminez,hazzard,harrod,graziano,grammer,gendron,garrido,fordham,englert,dryden,demoss,deluna,crabb,comeau,brummett,blume,benally,wessel,vanbuskirk,thorson,stumpf,stockwell,reams,radtke,rackley,pelton,niemi,newland,nelsen,morrissette,miramontes,mcginley,mccluskey,marchant,luevano,lampe,lail,jeffcoat,infante,hinman,gaona,erb,eady,desmarais,decosta,dansby,choe,breckenridge,bostwick,borg,bianchi,alberts,wilkie,whorton,vargo,tait,soucy,schuman,ousley,mumford,lum,lippert,leath,lavergne,laliberte,kirksey,kenner,johnsen,izzo,hiles,gullett,greenwell,gaspar,galbreath,gaitan,ericson,delapaz,croom,cottingham,clift,bushnell,bice,beason,arrowood,waring,voorhees,truax,shreve,shockey,schatz,sandifer,rubino,rozier,roseberry,pieper,peden,nester,nave,murphey,malinowski,macgregor,lafrance,kunkle,kirkman,hipp,hasty,haddix,gervais,gerdes,gamache,fouts,fitzwater,dillingham,deming,deanda,cedeno,cannady,burson,bouldin,arceneaux,woodhouse,whitford,wescott,welty,weigel,torgerson,toms,surber,sunderland,sterner,setzer,riojas,pumphrey,puga,metts,mcgarry,mccandless,magill,lupo,loveland,llamas,leclerc,koons,kahler,huss,holbert,heintz,haupt,grimmett,gaskill,ellingson,dorr,dingess,deweese,desilva,crossley,cordeiro,converse,conde,caldera,cairns,burmeister,burkhalter,brawner,bott,youngs,vierra,valladares,shrum,shropshire,sevilla,rusk,rodarte,pedraza,nino,merino,mcminn,markle,mapp,lajoie,koerner,kittrell,kato,hyder,hollifield,heiser,hazlett,greenwald,fant,eldredge,dreher,delafuente,cravens,claypool,beecher,aronson,alanis,worthen,wojcik,winger,whitacre,wellington,valverde,valdivia,troupe,thrower,swindell,suttles,suh,stroman,spires,slate,shealy,sarver,sartin,sadowski,rondeau,rolon,rascon,priddy,paulino,nolte,munroe,molloy,mciver,lykins,loggins,lenoir,klotz,kempf,hupp,hollowell,hollander,haynie,harkness,harker,gottlieb,frith,eddins,driskell,doggett,densmore,charette,cassady,byrum,burcham,buggs,benn,whitted,warrington,vandusen,vaillancourt,steger,siebert,scofield,quirk,purser,plumb,orcutt,nordstrom,mosely,michalski,mcphail,mcdavid,mccraw,marchese,mannino,lefevre,largent,lanza,kress,isham,hunsaker,hoch,hildebrandt,guarino,grijalva,graybill,ewell,ewald,cusick,crumley,coston,cathcart,carruthers,bullington,bowes,blain,blackford,barboza,yingling,weiland,varga,silverstein,sievers,shuster,shumway,runnels,rumsey,renfroe,provencher,polley,mohler,middlebrooks,kutz,koster,groth,glidden,fazio,deen,chipman,chenoweth,champlin,cedillo,carrero,carmody,buckles,brien,boutin,bosch,berkowitz,altamirano,wilfong,wiegand,waites,truesdale,toussaint,tobey,tedder,steelman,sirois,schnell,robichaud,richburg,plumley,pizarro,piercy,ortego,oberg,neace,mertz,mcnew,matta,lapp,lair,kibler,howlett,hollister,hofer,hatten,hagler,falgoust,engelhardt,eberle,dombrowski,dinsmore,daye,casares,braud,balch,autrey,wendel,tyndall,strobel,stoltz,spinelli,serrato,rochester,reber,rathbone,palomino,nickels,mayle,mathers,mach,loeffler,littrell,levinson,leong,lemire,lejeune,lazo,lasley,koller,kennard,hoelscher,hintz,hagerman,greaves,fore,eudy,engler,corrales,cordes,brunet,bidwell,bennet,tyrrell,tharpe,swinton,stribling,southworth,sisneros,savoie,samons,ruvalcaba,ries,ramer,omara,mosqueda,millar,mcpeak,macomber,luckey,litton,lehr,lavin,hubbs,hoard,hibbs,hagans,futrell,exum,evenson,culler,carbaugh,callen,brashear,bloomer,blakeney,bigler,addington,woodford,unruh,tolentino,sumrall,stgermain,smock,sherer,rayner,pooler,oquinn,nero,mcglothlin,linden,kowal,kerrigan,ibrahim,harvell,hanrahan,goodall,geist,fussell,fung,ferebee,eley,eggert,dorsett,dingman,destefano,colucci,clemmer,burnell,brumbaugh,boddie,berryhill,avelar,alcantara,winder,winchell,vandenberg,trotman,thurber,thibeault,stlouis,stilwell,sperling,shattuck,sarmiento,ruppert,rumph,renaud,randazzo,rademacher,quiles,pearman,palomo,mercurio,lowrey,lindeman,lawlor,larosa,lander,labrecque,hovis,holifield,henninger,hawkes,hartfield,hann,hague,genovese,garrick,fudge,frink,eddings,dinh,cribbs,calvillo,bunton,brodeur,bolding,blanding,agosto,zahn,wiener,trussell,tew,tello,teixeira,speck,sharma,shanklin,sealy,scanlan,santamaria,roundy,robichaux,ringer,rigney,prevost,polson,nord,moxley,medford,mccaslin,mcardle,macarthur,lewin,lasher,ketcham,keiser,heine,hackworth,grose,grizzle,gillman,gartner,frazee,fleury,edson,edmonson,derry,cronk,conant,burress,burgin,broom,brockington,bolick,boger,birchfield,billington,baily,bahena,armbruster,anson,yoho,wilcher,tinney,timberlake,thoma,thielen,sutphin,stultz,sikora,serra,schulman,scheffler,santillan,rego,preciado,pinkham,mickle,luu,lomas,lizotte,lent,kellerman,keil,johanson,hernadez,hartsfield,haber,gorski,farkas,eberhardt,duquette,delano,cropper,cozart,cockerham,chamblee,cartagena,cahoon,buzzell,brister,brewton,blackshear,benfield,aston,ashburn,arruda,wetmore,weise,vaccaro,tucci,sudduth,stromberg,stoops,showalter,shears,runion,rowden,rosenblum,riffle,renfrow,peres,obryant,leftwich,lark,landeros,kistler,killough,kerley,kastner,hoggard,hartung,guertin,govan,gatling,gailey,fullmer,fulford,flatt,esquibel,endicott,edmiston,edelstein,dufresne,dressler,dickman,chee,busse,bonnett,berard,arena,yoshida,velarde,veach,vanhouten,vachon,tolson,tolman,tennyson,stites,soler,shutt,ruggles,rhone,pegues,ong,neese,muro,moncrief,mefford,mcphee,mcmorris,mceachern,mcclurg,mansour,mader,leija,lecompte,lafountain,labrie,jaquez,heald,hash,hartle,gainer,frisby,farina,eidson,edgerton,dyke,durrett,duhon,cuomo,cobos,cervantez,bybee,brockway,borowski,binion,beery,arguello,amaro,acton,yuen,winton,wigfall,weekley,vidrine,vannoy,tardiff,shoop,shilling,schick,safford,prendergast,pellerin,osuna,nissen,nalley,moller,messner,messick,merrifield,mcguinness,matherly,marcano,mahone,lemos,lebrun,jara,hoffer,herren,hecker,haws,haug,gwin,gober,gilliard,fredette,favela,echeverria,downer,donofrio,desrochers,crozier,corson,bechtold,argueta,aparicio,zamudio,westover,westerman,utter,troyer,thies,tapley,slavin,shirk,sandler,roop,raymer,radcliff,otten,moorer,millet,mckibben,mccutchen,mcavoy,mcadoo,mayorga,mastin,martineau,marek,madore,leflore,kroeger,kennon,jimerson,hostetter,hornback,hendley,hance,guardado,granado,gowen,goodale,flinn,fleetwood,fitz,durkee,duprey,dipietro,dilley,clyburn,brawley,beckley,arana,weatherby,vollmer,vestal,tunnell,trigg,tingle,takahashi,sweatt,storer,snapp,shiver,rooker,rathbun,poisson,perrine,perri,pastor,parmer,parke,pare,palmieri,nottingham,midkiff,mecham,mccomas,mcalpine,lovelady,lillard,lally,knopp,kile,kiger,haile,gupta,goldsberry,gilreath,fulks,friesen,franzen,flack,findlay,ferland,dreyer,dore,dennard,deckard,debose,crim,coulombe,cork,chancey,cantor,branton,bissell,barns,woolard,witham,wasserman,spiegel,shoffner,scholz,ruch,rossman,petry,palacio,paez,neary,mortenson,millsap,miele,menke,mckim,mcanally,martines,manor,lemley,larochelle,klaus,klatt,kaufmann,kapp,helmer,hedge,halloran,glisson,frechette,fontana,eagan,distefano,danley,creekmore,chartier,chaffee,carillo,burg,bolinger,berkley,benz,basso,bash,barrier,zelaya,woodring,witkowski,wilmot,wilkens,wieland,verdugo,urquhart,tsai,timms,swiger,swaim,sussman,pires,molnar,mcatee,lowder,loos,linker,landes,kingery,hufford,higa,hendren,hammack,hamann,gillam,gerhardt,edelman,eby,delk,deans,curl,constantine,cleaver,claar,casiano,carruth,carlyle,brophy,bolanos,bibbs,bessette,beggs,baugher,bartel,averill,andresen,amin,adames,via,valente,turnbow,tse,swink,sublett,stroh,stringfellow,ridgway,pugliese,poteat,ohare,neubauer,murchison,mingo,lemmons,kwon,kellam,kean,jarmon,hyden,hudak,hollinger,henkel,hemingway,hasson,hansel,halter,haire,ginsberg,gillispie,fogel,flory,etter,elledge,eckman,deas,currin,crafton,coomer,colter,claxton,bulter,braddock,bowyer,binns,bellows,baskerville,barros,ansley,woolf,wight,waldman,wadley,tull,trull,tesch,stouffer,stadler,slay,shubert,sedillo,santacruz,reinke,poynter,neri,neale,mowry,moralez,monger,mitchum,merryman,manion,macdougall,lux,litchfield,ley,levitt,lepage,lasalle,khoury,kavanagh,karns,ivie,huebner,hodgkins,halpin,garica,eversole,dutra,dunagan,duffey,dillman,dillion,deville,dearborn,damato,courson,coulson,burdine,bousquet,bonin,bish,atencio,westbrooks,wages,vaca,tye,toner,tillis,swett,struble,stanfill,solorzano,slusher,sipple,sim,silvas,shults,schexnayder,saez,rodas,rager,pulver,plaza,penton,paniagua,meneses,mcfarlin,mcauley,matz,maloy,magruder,lohman,landa,lacombe,jaimes,hom,holzer,holst,heil,hackler,grundy,gilkey,farnham,durfee,dunton,dunston,duda,dews,craver,corriveau,conwell,colella,chambless,bremer,boutte,bourassa,blaisdell,backman,babineaux,audette,alleman,towner,taveras,tarango,sullins,suiter,stallard,solberg,schlueter,poulos,pimental,owsley,okelley,nations,moffatt,metcalfe,meekins,medellin,mcglynn,mccowan,marriott,marable,lennox,lamoureux,koss,kerby,karp,isenberg,howze,hockenberry,highsmith,harbour,hallmark,gusman,greeley,giddings,gaudet,gallup,fleenor,eicher,edington,dimaggio,dement,demello,decastro,bushman,brundage,brooker,bourg,blackstock,bergmann,beaton,banister,argo,appling,wortman,watterson,villalpando,tillotson,tighe,sundberg,sternberg,stamey,shipe,seeger,scarberry,sattler,sain,rothstein,poteet,plowman,pettiford,penland,partain,pankey,oyler,ogletree,ogburn,moton,merkel,lucier,lakey,kratz,kinser,kershaw,josephson,imhoff,hendry,hammon,frisbie,friedrich,frawley,fraga,forester,eskew,emmert,drennan,doyon,dandridge,cawley,carvajal,bracey,belisle,batey,ahner,wysocki,weiser,veliz,tincher,sansone,sankey,sandstrom,rohrer,risner,pridemore,pfeffer,persinger,peery,oubre,nowicki,musgrave,murdoch,mullinax,mccary,mathieu,livengood,kyser,klink,kimes,kellner,kavanaugh,kasten,imes,hoey,hinshaw,hake,gurule,grube,grillo,geter,gatto,garver,garretson,farwell,eiland,dunford,decarlo,corso,colman,collard,cleghorn,chasteen,cavender,carlile,calvo,byerly,brogdon,broadwater,breault,bono,bergin,behr,ballenger,amick,tamez,stiffler,steinke,simmon,shankle,schaller,salmons,sackett,saad,rideout,ratcliffe,rao,ranson,plascencia,petterson,olszewski,olney,olguin,nilsson,nevels,morelli,montiel,monge,michaelson,mertens,mcchesney,mcalpin,mathewson,loudermilk,lineberry,liggett,kinlaw,kight,jost,hereford,hardeman,halpern,halliday,hafer,gaul,friel,freitag,forsberg,evangelista,doering,dicarlo,dendy,delp,deguzman,dameron,curtiss,cosper,cauthen,cao,bradberry,bouton,bonnell,bixby,bieber,beveridge,bedwell,barhorst,bannon,baltazar,baier,ayotte,attaway,arenas,abrego,turgeon,tunstall,thaxton,thai,tenorio,stotts,sthilaire,shedd,seabolt,scalf,salyers,ruhl,rowlett,robinett,pfister,perlman,parkman,nunnally,norvell,napper,modlin,mckellar,mcclean,mascarenas,leibowitz,ledezma,kuhlman,kobayashi,hunley,holmquist,hinkley,hartsell,gribble,gravely,fifield,eliason,doak,crossland,carleton,bridgeman,bojorquez,boggess,auten,woosley,whiteley,wexler,twomey,tullis,townley,standridge,santoyo,rueda,riendeau,revell,pless,ottinger,nigro,nickles,mulvey,menefee,mcshane,mcloughlin,mckinzie,markey,lockridge,lipsey,knisley,knepper,kitts,kiel,jinks,hathcock,godin,gallego,fikes,fecteau,estabrook,ellinger,dunlop,dudek,countryman,chauvin,chatham,bullins,brownfield,boughton,bloodworth,bibb,baucom,barbieri,aubin,armitage,alessi,absher,abbate,zito,woolery,wiggs,wacker,tynes,tolle,telles,tarter,swarey,strode,stockdale,stalnaker,spina,schiff,saari,risley,rameriz,rakes,pettaway,penner,paulus,palladino,omeara,montelongo,melnick,mehta,mcgary,mccourt,mccollough,marchetti,manzanares,lowther,leiva,lauderdale,lafontaine,kowalczyk,knighton,joubert,jaworski,ide,huth,hurdle,housley,hackman,gulick,gordy,gilstrap,gehrke,gebhart,gaudette,foxworth,essex,endres,dunkle,cimino,caddell,brauer,braley,bodine,blackmore,belden,backer,ayer,andress,wisner,vuong,valliere,twigg,tso,tavarez,strahan,steib,staub,sowder,seiber,schutt,scharf,schade,rodriques,risinger,renshaw,rahman,presnell,piatt,nieman,nevins,mcilwain,mcgaha,mccully,mccomb,massengale,macedo,lesher,kearse,jauregui,husted,hudnall,holmberg,hertel,hardie,glidewell,frausto,fassett,dalessandro,dahlgren,corum,constantino,conlin,colquitt,colombo,claycomb,cardin,buller,boney,bocanegra,biggers,benedetto,araiza,andino,albin,zorn,werth,weisman,walley,vanegas,ulibarri,towe,tedford,teasley,suttle,steffens,stcyr,squire,singley,sifuentes,shuck,schram,sass,rieger,ridenhour,rickert,richerson,rayborn,rabe,raab,pendley,pastore,ordway,moynihan,mellott,mckissick,mcgann,mccready,mauney,marrufo,lenhart,lazar,lafave,keele,kautz,jardine,jahnke,jacobo,hord,hardcastle,hageman,giglio,gehring,fortson,duque,duplessis,dicken,derosier,deitz,dalessio,cram,castleman,candelario,callison,caceres,bozarth,biles,bejarano,bashaw,avina,armentrout,alverez,acord,waterhouse,vereen,vanlandingham,uhl,strawser,shotwell,severance,seltzer,schoonmaker,schock,schaub,schaffner,roeder,rodrigez,riffe,rhine,rasberry,rancourt,railey,quade,pursley,prouty,perdomo,oxley,osterman,nickens,murphree,mounts,merida,maus,mattern,masse,martinelli,mangan,lutes,ludwick,loney,laureano,lasater,knighten,kissinger,kimsey,kessinger,honea,hollingshead,hockett,heyer,heron,gurrola,gove,glasscock,gillett,galan,featherstone,eckhardt,duron,dunson,dasher,culbreth,cowden,cowans,claypoole,churchwell,chabot,caviness,cater,caston,callan,byington,burkey,boden,beckford,atwater,archambault,alvey,alsup,whisenant,weese,voyles,verret,tsang,tessier,sweitzer,sherwin,shaughnessy,revis,remy,prine,philpott,peavy,paynter,parmenter,ovalle,offutt,nightingale,newlin,nakano,myatt,muth,mohan,mcmillon,mccarley,mccaleb,maxson,marinelli,maley,liston,letendre,kain,huntsman,hirst,hagerty,gulledge,greenway,grajeda,gorton,goines,gittens,frederickson,fanelli,embree,eichelberger,dunkin,dixson,dillow,defelice,chumley,burleigh,borkowski,binette,biggerstaff,berglund,beller,audet,arbuckle,allain,alfano,youngman,wittman,weintraub,vanzant,vaden,twitty,stollings,standifer,sines,shope,scalise,saville,posada,pisano,otte,nolasco,napoli,mier,merkle,mendiola,melcher,mejias,mcmurry,mccalla,markowitz,manis,mallette,macfarlane,lough,looper,landin,kittle,kinsella,kinnard,hobart,herald,helman,hellman,hartsock,halford,hage,gordan,glasser,gayton,gattis,gastelum,gaspard,frisch,fitzhugh,eckstein,eberly,dowden,despain,crumpler,crotty,cornelison,chouinard,chamness,catlin,cann,bumgardner,budde,branum,bradfield,braddy,borst,birdwell,bazan,banas,bade,arango,ahearn,addis,zumwalt,wurth,wilk,widener,wagstaff,urrutia,terwilliger,tart,steinman,staats,sloat,rives,riggle,revels,reichard,prickett,poff,pitzer,petro,pell,northrup,nicks,moline,mielke,maynor,mallon,magness,lingle,lindell,lieb,lesko,lebeau,lammers,lafond,kiernan,ketron,jurado,holmgren,hilburn,hayashi,hashimoto,harbaugh,guillot,gard,froehlich,feinberg,falco,dufour,drees,doney,diep,delao,daves,dail,crowson,coss,congdon,carner,camarena,butterworth,burlingame,bouffard,bloch,bilyeu,barta,bakke,baillargeon,avent,aquilar,ake,aho,zeringue,yarber,wolfson,vogler,voelker,truss,troxell,thrift,strouse,spielman,sistrunk,sevigny,schuller,schaaf,ruffner,routh,roseman,ricciardi,peraza,pegram,overturf,olander,odaniel,neu,millner,melchor,maroney,machuca,macaluso,livesay,layfield,laskowski,kwiatkowski,kilby,hovey,heywood,hayman,havard,harville,haigh,hagood,grieco,glassman,gebhardt,fleischer,fann,elson,eccles,cunha,crumb,blakley,bardwell,abshire,woodham,wines,welter,wargo,varnado,tutt,traynor,swaney,svoboda,stricker,stoffel,stambaugh,sickler,shackleford,selman,seaver,sansom,sanmiguel,royston,rourke,rockett,rioux,puleo,pitchford,nardi,mulvaney,middaugh,malek,leos,lathan,kujawa,kimbro,killebrew,houlihan,hinckley,herod,hepler,hamner,hammel,hallowell,gonsalez,gingerich,gambill,funkhouser,fricke,fewell,falkner,endsley,dulin,drennen,deaver,dambrosio,chadwell,castanon,burkes,brune,brisco,brinker,bowker,boldt,berner,beaumont,beaird,bazemore,barrick,albano,younts,wunderlich,weidman,vanness,toland,theobald,stickler,steiger,stanger,spies,spector,sollars,smedley,seibel,scoville,saito,rye,rummel,rowles,rouleau,roos,rogan,roemer,ream,raya,purkey,priester,perreira,penick,paulin,parkins,overcash,oleson,neves,muldrow,minard,midgett,michalak,melgar,mcentire,mcauliffe,marte,lydon,lindholm,leyba,langevin,lagasse,lafayette,kesler,kelton,kao,kaminsky,jaggers,humbert,huck,howarth,hinrichs,higley,gupton,guimond,gravois,giguere,fretwell,fontes,feeley,faucher,eichhorn,ecker,earp,dole,dinger,derryberry,demars,deel,copenhaver,collinsworth,colangelo,cloyd,claiborne,caulfield,carlsen,calzada,caffey,broadus,brenneman,bouie,bodnar,blaney,blanc,beltz,behling,barahona,yockey,winkle,windom,wimer,villatoro,trexler,teran,taliaferro,sydnor,swinson,snelling,smtih,simonton,simoneaux,simoneau,sherrer,seavey,scheel,rushton,rupe,ruano,rippy,reiner,reiff,rabinowitz,quach,penley,odle,nock,minnich,mckown,mccarver,mcandrew,longley,laux,lamothe,lafreniere,kropp,krick,kates,jepson,huie,howse,howie,henriques,haydon,haught,hartzog,harkey,grimaldo,goshorn,gormley,gluck,gilroy,gillenwater,giffin,fluker,feder,eyre,eshelman,eakins,detwiler,delrosario,davisson,catalan,canning,calton,brammer,botelho,blakney,bartell,averett,askins,aker,zak,worcester,witmer,wiser,winkelman,widmer,whittier,weitzel,wardell,wagers,ullman,tupper,tingley,tilghman,talton,simard,seda,scheller,sala,rundell,rost,roa,ribeiro,rabideau,primm,pinon,peart,ostrom,ober,nystrom,nussbaum,naughton,murr,moorhead,monti,monteiro,melson,meissner,mclin,mcgruder,marotta,makowski,majewski,madewell,lunt,lukens,leininger,lebel,lakin,kepler,jaques,hunnicutt,hungerford,hoopes,hertz,heins,halliburton,grosso,gravitt,glasper,gallman,gallaway,funke,fulbright,falgout,eakin,dostie,dorado,dewberry,derose,cutshall,crampton,costanzo,colletti,cloninger,claytor,chiang,canterbury,campagna,burd,brokaw,broaddus,bretz,brainard,binford,bilbrey,alpert,aitken,ahlers,zajac,woolfolk,witten,windle,wayland,tramel,tittle,talavera,suter,straley,specht,sommerville,soloman,skeens,sigman,sibert,shavers,schuck,schmit,sartain,sabol,rosenblatt,rollo,rashid,rabb,province,polston,nyberg,northrop,navarra,muldoon,mikesell,mcdougald,mcburney,mariscal,lui,lozier,lingerfelt,legere,latour,lagunas,lacour,kurth,killen,kiely,kayser,kahle,isley,huertas,hower,hinz,haugh,gumm,galicia,fortunato,flake,dunleavy,duggins,doby,digiovanni,devaney,deltoro,cribb,corpuz,coronel,coen,charbonneau,caine,burchette,blakey,blakemore,bergquist,beene,beaudette,bayles,ballance,bakker,bailes,asberry,arwood,zucker,willman,whitesell,wald,walcott,vancleave,trump,strasser,simas,shick,schleicher,schaal,saleh,rotz,resnick,rainer,partee,ollis,oller,oday,munday,mong,millican,merwin,mazzola,mansell,magallanes,llanes,lewellen,lepore,kisner,keesee,jeanlouis,ingham,hornbeck,hawn,hartz,harber,haffner,gutshall,guth,grays,gowan,finlay,finkelstein,eyler,enloe,dungan,diez,dearman,cull,crosson,chronister,cassity,campion,callihan,butz,breazeale,blumenthal,berkey,batty,batton,arvizu,alderete,aldana,albaugh,abernethy,wolter,wille,tweed,tollefson,thomasson,teter,testerman,sproul,spates,southwick,soukup,skelly,senter,sealey,sawicki,sargeant,rossiter,rosemond,repp,pifer,ormsby,nickelson,naumann,morabito,monzon,millsaps,millen,mcelrath,marcoux,mantooth,madson,macneil,mackinnon,louque,leister,lampley,kushner,krouse,kirwan,jessee,janson,jahn,jacquez,islas,hutt,holladay,hillyer,hepburn,hensel,harrold,gingrich,geis,gales,fults,finnell,ferri,featherston,epley,ebersole,eames,dunigan,drye,dismuke,devaughn,delorenzo,damiano,confer,collum,clower,clow,claussen,clack,caylor,cawthon,casias,carreno,bluhm,bingaman,bewley,belew,beckner,auld,amey,wolfenbarger,wilkey,wicklund,waltman,villalba,valero,valdovinos,ung,ullrich,tyus,twyman,trost,tardif,tanguay,stripling,steinbach,shumpert,sasaki,sappington,sandusky,reinhold,reinert,quijano,pye,placencia,pinkard,phinney,perrotta,pernell,parrett,oxendine,owensby,orman,nuno,mori,mcroberts,mcneese,mckamey,mccullum,markel,mardis,maines,lueck,lubin,lefler,leffler,larios,labarbera,kershner,josey,jeanbaptiste,izaguirre,hermosillo,haviland,hartshorn,hafner,ginter,getty,franck,fiske,dufrene,doody,davie,dangerfield,dahlberg,cuthbertson,crone,coffelt,chidester,chesson,cauley,caudell,cantara,campo,caines,bullis,bucci,brochu,bogard,bickerstaff,benning,arzola,antonelli,adkinson,zellers,wulf,worsley,woolridge,whitton,westerfield,walczak,vassar,truett,trueblood,trawick,townsley,topping,tobar,telford,steverson,stagg,sitton,sill,sergent,schoenfeld,sarabia,rutkowski,rubenstein,rigdon,prentiss,pomerleau,plumlee,philbrick,peer,patnode,oloughlin,obregon,nuss,morell,mikell,mele,mcinerney,mcguigan,mcbrayer,lor,lollar,lakes,kuehl,kinzer,kamp,joplin,jacobi,howells,holstein,hedden,hassler,harty,halle,greig,gouge,goodrum,gerhart,geier,geddes,gast,forehand,ferree,fendley,feltner,esqueda,encarnacion,eichler,egger,edmundson,eatmon,doud,donohoe,donelson,dilorenzo,digiacomo,diggins,delozier,dejong,danford,crippen,coppage,cogswell,clardy,cioffi,cabe,brunette,bresnahan,bramble,blomquist,blackstone,biller,bevis,bevan,bethune,benbow,baty,basinger,balcom,andes,aman,aguero,adkisson,yandell,wilds,whisenhunt,weigand,weeden,voight,villar,trottier,tillett,suazo,setser,scurry,schuh,schreck,schauer,samora,roane,rinker,reimers,ratchford,popovich,parkin,natal,melville,mcbryde,magdaleno,loehr,lockman,lingo,leduc,larocca,lao,lamere,laclair,krall,korte,koger,jalbert,hughs,higbee,henton,heaney,haith,gump,greeson,goodloe,gholston,gasper,gagliardi,fregoso,farthing,fabrizio,ensor,elswick,elgin,eklund,eaddy,drouin,dorton,dizon,derouen,deherrera,davy,dampier,cullum,culley,cowgill,cardoso,cardinale,brodsky,broadbent,brimmer,briceno,branscum,bolyard,boley,bennington,beadle,baur,ballentine,azure,aultman,arciniega,aguila,aceves,yepez,yap,woodrum,wethington,weissman,veloz,trusty,troup,trammel,tarpley,stivers,steck,sprayberry,spraggins,spitler,spiers,sohn,seagraves,schiffman,rudnick,rizo,riccio,rennie,quackenbush,puma,plott,pearcy,parada,paiz,munford,moskowitz,mease,mcnary,mccusker,lozoya,longmire,loesch,lasky,kuhlmann,krieg,koziol,kowalewski,konrad,kindle,jowers,jolin,jaco,hua,horgan,hine,hileman,hepner,heise,heady,hawkinson,hannigan,haberman,guilford,grimaldi,garton,gagliano,fruge,follett,fiscus,ferretti,ebner,easterday,eanes,dirks,dimarco,depalma,deforest,cruce,craighead,christner,candler,cadwell,burchell,buettner,brinton,brazier,brannen,brame,bova,bomar,blakeslee,belknap,bangs,balzer,athey,armes,alvis,alverson,alvardo,yeung,wheelock,westlund,wessels,volkman,threadgill,thelen,tague,symons,swinford,sturtevant,straka,stier,stagner,segarra,seawright,rutan,roux,ringler,riker,ramsdell,quattlebaum,purifoy,poulson,permenter,peloquin,pasley,pagel,osman,obannon,nygaard,newcomer,munos,motta,meadors,mcquiston,mcniel,mcmann,mccrae,mayne,matte,legault,lechner,kucera,krohn,kratzer,koopman,jeske,horrocks,hock,hibbler,hesson,hersh,harvin,halvorsen,griner,grindle,gladstone,garofalo,frampton,forbis,eddington,diorio,dingus,dewar,desalvo,curcio,creasy,cortese,cordoba,connally,cluff,cascio,capuano,canaday,calabro,bussard,brayton,borja,bigley,arnone,arguelles,acuff,zamarripa,wooton,widner,wideman,threatt,thiele,templin,teeters,synder,swint,swick,sturges,stogner,stedman,spratt,siegfried,shetler,scull,savino,sather,rothwell,rook,rone,rhee,quevedo,privett,pouliot,poche,pickel,petrillo,pellegrini,peaslee,partlow,otey,nunnery,morelock,morello,meunier,messinger,mckie,mccubbin,mccarron,lerch,lavine,laverty,lariviere,lamkin,kugler,krol,kissel,keeter,hubble,hickox,hetzel,hayner,hagy,hadlock,groh,gottschalk,goodsell,gassaway,garrard,galligan,fye,firth,fenderson,feinstein,etienne,engleman,emrick,ellender,drews,doiron,degraw,deegan,dart,crissman,corr,cookson,coil,cleaves,charest,chapple,chaparro,castano,carpio,byer,bufford,bridgewater,bridgers,brandes,borrero,bonanno,aube,ancheta,abarca,abad,yim,wooster,wimbush,willhite,willams,wigley,weisberg,wardlaw,vigue,vanhook,unknow,torre,tasker,tarbox,strachan,slover,shamblin,semple,schuyler,schrimsher,sayer,salzman,rubalcava,riles,reneau,reichel,rayfield,rabon,pyatt,prindle,poss,polito,plemmons,pesce,perrault,pereyra,ostrowski,nilsen,niemeyer,munsey,mundell,moncada,miceli,meader,mcmasters,mckeehan,matsumoto,marron,marden,lizarraga,lingenfelter,lewallen,langan,lamanna,kovac,kinsler,kephart,keown,kass,kammerer,jeffreys,hysell,householder,hosmer,hardnett,hanner,guyette,greening,glazer,ginder,fromm,fluellen,finkle,fey,fessler,essary,eisele,duren,dittmer,crochet,cosentino,cogan,coelho,cavin,carrizales,campuzano,brough,bopp,bookman,blouin,beesley,battista,bascom,bakken,badgett,arneson,anselmo,ahumada,woodyard,wolters,wireman,willison,warman,waldrup,vowell,vantassel,vale,twombly,toomer,tennison,teets,tedeschi,swanner,stutz,stelly,sheehy,schermerhorn,scala,sandidge,salters,salo,saechao,roseboro,rolle,ressler,renz,renn,redford,raposa,rainbolt,pelfrey,orndorff,oney,nolin,nimmons,ney,nardone,myhre,morman,menjivar,mcglone,mccammon,maxon,marciano,manus,lowrance,lorenzen,lonergan,lollis,littles,lindahl,lamas,lach,kuster,krawczyk,knuth,knecht,kirkendall,keitt,keever,kantor,jarboe,hoye,houchens,holter,holsinger,hickok,helwig,helgeson,hassett,harner,hamman,hames,hadfield,goree,goldfarb,gaughan,gaudreau,gantz,gallion,frady,foti,flesher,ferrin,faught,engram,donegan,desouza,degroot,cutright,crowl,criner,coan,clinkscales,chewning,chavira,catchings,carlock,bulger,buenrostro,bramblett,brack,boulware,bookout,bitner,birt,baranowski,baisden,augustin,allmon,acklin,yoakum,wilbourn,whisler,weinberger,washer,vasques,vanzandt,vanatta,troxler,tomes,tindle,tims,throckmorton,thach,stpeter,stlaurent,stenson,spry,spitz,songer,snavely,sly,shroyer,shortridge,shenk,sevier,seabrook,scrivner,saltzman,rosenberry,rockwood,robeson,roan,reiser,ramires,raber,posner,popham,piotrowski,pinard,peterkin,pelham,peiffer,peay,nadler,musso,millett,mestas,mcgowen,marques,marasco,manriquez,manos,mair,lipps,leiker,krumm,knorr,kinslow,kessel,kendricks,kelm,ito,irick,ickes,hurlburt,horta,hoekstra,heuer,helmuth,heatherly,hampson,hagar,haga,greenlaw,grau,godbey,gingras,gillies,gibb,gayden,gauvin,garrow,fontanez,florio,finke,fasano,ezzell,ewers,eveland,eckenrode,duclos,drumm,dimmick,delancey,defazio,dashiell,cusack,crowther,crigger,cray,coolidge,coldiron,cleland,chalfant,cassel,camire,cabrales,broomfield,brittingham,brisson,brickey,braziel,brazell,bragdon,boulanger,bos,boman,bohannan,beem,barre,baptist,azar,ashbaugh,armistead,almazan,adamski,zendejas,winburn,willaims,wilhoit,westberry,wentzel,wendling,visser,vanscoy,vankirk,vallee,tweedy,thornberry,sweeny,spradling,spano,smelser,shim,sechrist,schall,scaife,rugg,rothrock,roesler,riehl,ridings,render,ransdell,radke,pinero,petree,pendergast,peluso,pecoraro,pascoe,panek,oshiro,navarrette,murguia,moores,moberg,michaelis,mcwhirter,mcsweeney,mcquade,mccay,mauk,mariani,marceau,mandeville,maeda,lunde,ludlow,loeb,lindo,linderman,leveille,leith,larock,lambrecht,kulp,kinsley,kimberlin,kesterson,hoyos,helfrich,hanke,grisby,goyette,gouveia,glazier,gile,gerena,gelinas,gasaway,funches,fujimoto,flynt,fenske,fellers,fehr,eslinger,escalera,enciso,duley,dittman,dineen,diller,devault,dao,collings,clymer,clowers,chavers,charland,castorena,castello,camargo,bunce,bullen,boyes,borchers,borchardt,birnbaum,birdsall,billman,benites,bankhead,ange,ammerman,adkison,winegar,wickman,warr,warnke,villeneuve,veasey,vassallo,vannatta,vadnais,twilley,towery,tomblin,tippett,theiss,talkington,talamantes,swart,swanger,streit,stines,stabler,spurling,sobel,sine,simmers,shippy,shiflett,shearin,sauter,sanderlin,rusch,runkle,ruckman,rorie,roesch,richert,rehm,randel,ragin,quesenberry,puentes,plyler,plotkin,paugh,oshaughnessy,ohalloran,norsworthy,niemann,nader,moorefield,mooneyham,modica,miyamoto,mickel,mebane,mckinnie,mazurek,mancilla,lukas,lovins,loughlin,lotz,lindsley,liddle,levan,lederman,leclaire,lasseter,lapoint,lamoreaux,lafollette,kubiak,kirtley,keffer,kaczmarek,housman,hiers,hibbert,herrod,hegarty,hathorn,greenhaw,grafton,govea,futch,furst,franko,forcier,foran,flickinger,fairfield,eure,emrich,embrey,edgington,ecklund,eckard,durante,deyo,delvecchio,dade,currey,creswell,cottrill,casavant,cartier,cargile,capel,cammack,calfee,burse,burruss,brust,brousseau,bridwell,braaten,borkholder,bloomquist,bjork,bartelt,arp,amburgey,yeary,yao,whitefield,vinyard,vanvalkenburg,twitchell,timmins,tapper,stringham,starcher,spotts,slaugh,simonsen,sheffer,sequeira,rosati,rhymes,reza,quint,pollak,peirce,patillo,parkerson,paiva,nilson,nevin,narcisse,nair,mitton,merriam,merced,meiners,mckain,mcelveen,mcbeth,marsden,marez,manke,mahurin,mabrey,luper,krull,kees,iles,hunsicker,hornbuckle,holtzclaw,hirt,hinnant,heston,hering,hemenway,hegwood,hearns,halterman,guiterrez,grote,granillo,grainger,glasco,gilder,garren,garlock,garey,fryar,fredricks,fraizer,foxx,foshee,ferrel,felty,everitt,evens,esser,elkin,eberhart,durso,duguay,driskill,doster,dewall,deveau,demps,demaio,delreal,deleo,deem,darrah,cumberbatch,culberson,cranmer,cordle,colgan,chesley,cavallo,castellon,castelli,carreras,carnell,carlucci,bontrager,blumberg,blasingame,becton,ayon,artrip,andujar,alkire,alder,agan,zukowski,zuckerman,zehr,wroblewski,wrigley,woodside,wigginton,westman,westgate,werts,washam,wardlow,walser,waiters,tadlock,stringfield,stimpson,stickley,standish,spurlin,spindler,speller,spaeth,sotomayor,sok,sluder,shryock,shepardson,shatley,scannell,santistevan,rosner,rhode,resto,reinhard,rathburn,prisco,poulsen,pinney,phares,pennock,pastrana,oviedo,ostler,noto,nauman,mulford,moise,moberly,mirabal,metoyer,metheny,mentzer,meldrum,mcinturff,mcelyea,mcdougle,massaro,lumpkins,loveday,lofgren,loe,lirette,lesperance,lefkowitz,ledger,lauzon,lain,lachapelle,kurz,klassen,keough,kempton,kaelin,jeffords,huot,hsieh,hoyer,horwitz,hopp,hoeft,hennig,haskin,gourdine,golightly,girouard,fulgham,fritsch,freer,frasher,foulk,firestone,fiorentino,fedor,ensley,englehart,eells,ebel,dunphy,donahoe,dileo,dibenedetto,dabrowski,crick,coonrod,conder,coddington,chunn,choy,chaput,cerna,carreiro,calahan,braggs,bourdon,bollman,bittle,behm,bauder,batt,barreras,aubuchon,anzalone,adamo,zerbe,wirt,willcox,westberg,weikel,waymire,vroman,vinci,vallejos,truesdell,troutt,trotta,tollison,toles,tichenor,symonds,surles,strayer,stgeorge,sroka,sorrentino,solares,snelson,silvestri,sikorski,shawver,schumaker,schorr,schooley,scates,satterlee,satchell,sacks,rymer,roselli,robitaille,riegel,regis,reames,provenzano,priestley,plaisance,pettey,palomares,oman,nowakowski,nace,monette,minyard,mclamb,mchone,mccarroll,masson,magoon,maddy,lundin,loza,licata,leonhardt,lema,landwehr,kircher,kinch,karpinski,johannsen,hussain,houghtaling,hoskinson,hollaway,holeman,hobgood,hilt,hiebert,gros,goggin,geissler,gadbois,gabaldon,fleshman,flannigan,fairman,epp,eilers,dycus,dunmire,duffield,dowler,deloatch,dehaan,deemer,clayborn,christofferso,chilson,chesney,chatfield,carron,canale,brigman,branstetter,bosse,borton,bonar,blau,biron,barroso,arispe,zacharias,zabel,yaeger,woolford,whetzel,weakley,veatch,vandeusen,tufts,troxel,troche,traver,townsel,tosh,talarico,swilley,sterrett,stenger,speakman,sowards,sours,souders,souder,soles,sobers,snoddy,smither,sias,shute,shoaf,shahan,schuetz,scaggs,santini,rosson,rolen,robidoux,rentas,recio,pixley,pawlowski,pawlak,paull,overbey,orear,oliveri,oldenburg,nutting,naugle,mote,mossman,moor,misner,milazzo,michelson,mcentee,mccullar,mccree,mcaleer,mazzone,mandell,manahan,malott,maisonet,mailloux,lumley,lowrie,louviere,lipinski,lindemann,leppert,leopold,leasure,labarge,kubik,knisely,knepp,kenworthy,kennelly,kelch,karg,kanter,hyer,houchin,hosley,hosler,hollon,holleman,heitman,hebb,haggins,gwaltney,guin,goulding,gorden,geraci,georges,gathers,frison,feagin,falconer,espada,erving,erikson,eisenhauer,eder,ebeling,durgin,dowdle,dinwiddie,delcastillo,dedrick,crimmins,covell,cournoyer,coria,cohan,cataldo,carpentier,canas,campa,brode,brashears,blaser,bicknell,berk,bednar,barwick,ascencio,althoff,almodovar,alamo,zirkle,zabala,wolverton,winebrenner,wetherell,westlake,wegener,weddington,vong,tuten,trosclair,tressler,theroux,teske,swinehart,swensen,sundquist,southall,socha,sizer,silverberg,shortt,shimizu,sherrard,shaeffer,scheid,scheetz,saravia,sanner,rubinstein,rozell,romer,rheaume,reisinger,randles,pullum,petrella,payan,papp,nordin,norcross,nicoletti,nicholes,newbold,nakagawa,mraz,monteith,milstead,milliner,mellen,mccardle,luft,liptak,lipp,leitch,latimore,larrison,landau,laborde,koval,izquierdo,hymel,hoskin,holte,hoefer,hayworth,hausman,harrill,harrel,hardt,gully,groover,grinnell,greenspan,graver,grandberry,gorrell,goldenberg,goguen,gilleland,garr,fuson,foye,feldmann,everly,dyess,dyal,dunnigan,downie,dolby,deatherage,cosey,cheever,celaya,caver,cashion,caplinger,cansler,byrge,bruder,breuer,breslin,brazelton,botkin,bonneau,bondurant,bohanan,bogue,boes,bodner,boatner,blatt,bickley,belliveau,beiler,beier,beckstead,bachmann,atkin,altizer,alloway,allaire,albro,abron,zellmer,yetter,yelverton,wiltshire,wiens,whidden,viramontes,vanwormer,tarantino,tanksley,sumlin,strauch,strang,stice,spahn,sosebee,sigala,shrout,seamon,schrum,schneck,schantz,ruddy,romig,roehl,renninger,reding,pyne,polak,pohlman,pasillas,oldfield,oldaker,ohanlon,ogilvie,norberg,nolette,nies,neufeld,nellis,mummert,mulvihill,mullaney,monteleone,mendonca,meisner,mcmullan,mccluney,mattis,massengill,manfredi,luedtke,lounsbury,liberatore,leek,lamphere,laforge,kuo,koo,jourdan,ismail,iorio,iniguez,ikeda,hubler,hodgdon,hocking,heacock,haslam,haralson,hanshaw,hannum,hallam,haden,garnes,garces,gammage,gambino,finkel,faucett,fahy,ehrhardt,eggen,dusek,durrant,dubay,dones,dey,depasquale,delucia,degraff,decamp,davalos,cullins,conard,clouser,clontz,cifuentes,chappel,chaffins,celis,carwile,byram,bruggeman,bressler,brathwaite,brasfield,bradburn,boose,boon,bodie,blosser,blas,bise,bertsch,bernardi,bernabe,bengtson,barrette,astorga,alday,albee,abrahamson,yarnell,wiltse,wile,wiebe,waguespack,vasser,upham,tyre,turek,traxler,torain,tomaszewski,tinnin,tiner,tindell,teed,styron,stahlman,staab,skiba,shih,sheperd,seidl,secor,schutte,sanfilippo,ruder,rondon,rearick,procter,prochaska,pettengill,pauly,neilsen,nally,mutter,mullenax,morano,meads,mcnaughton,mcmurtry,mcmath,mckinsey,matthes,massenburg,marlar,margolis,malin,magallon,mackin,lovette,loughran,loring,longstreet,loiselle,lenihan,laub,kunze,kull,koepke,kerwin,kalinowski,kagan,innis,innes,holtzman,heinemann,harshman,haider,haack,guss,grondin,grissett,greenawalt,gravel,goudy,goodlett,goldston,gokey,gardea,galaviz,gafford,gabrielson,furlow,fritch,fordyce,folger,elizalde,ehlert,eckhoff,eccleston,ealey,dubin,diemer,deschamps,delapena,decicco,debolt,daum,cullinan,crittendon,crase,cossey,coppock,coots,colyer,cluck,chamberland,burkhead,bumpus,buchan,borman,bork,boe,birkholz,berardi,benda,behnke,barter,auer,amezquita,wotring,wirtz,wingert,wiesner,whitesides,weyant,wainscott,venezia,varnell,tussey,thurlow,tabares,stiver,stell,starke,stanhope,stanek,sisler,sinnott,siciliano,shehan,selph,seager,scurlock,scranton,santucci,santangelo,saltsman,ruel,ropp,rogge,rettig,renwick,reidy,reider,redfield,quam,premo,peet,parente,paolucci,palmquist,orme,ohler,ogg,netherton,mutchler,morita,mistretta,minnis,middendorf,menzel,mendosa,mendelson,meaux,mcspadden,mcquaid,mcnatt,manigault,maney,mager,lukes,lopresti,liriano,lipton,letson,lechuga,lazenby,lauria,larimore,kwok,kwak,krupp,krupa,krum,kopec,kinchen,kifer,kerney,kerner,kennison,kegley,kays,karcher,justis,johson,jellison,janke,huskins,holzman,hinojos,hefley,hatmaker,harte,halloway,hallenbeck,goodwyn,glaspie,geise,fullwood,fryman,frew,frakes,fraire,farrer,enlow,engen,ellzey,eckles,earles,ealy,dunkley,drinkard,dreiling,draeger,dinardo,dills,desroches,desantiago,curlee,crumbley,critchlow,coury,courtright,coffield,cleek,charpentier,cardone,caples,cantin,buntin,bugbee,brinkerhoff,brackin,bourland,bohl,bogdan,blassingame,beacham,banning,auguste,andreasen,amann,almon,alejo,adelman,abston,zeno,yerger,wymer,woodberry,windley,whiteaker,westfield,weibel,wanner,waldrep,villani,vanarsdale,utterback,updike,triggs,topete,tolar,tigner,thoms,tauber,tarvin,tally,swiney,sweatman,studebaker,stennett,starrett,stannard,stalvey,sonnenberg,smithey,sieber,sickles,shinault,segars,sanger,salmeron,rothe,rizzi,rine,ricard,restrepo,ralls,ragusa,quiroga,pero,pegg,pavlik,papenfuss,oropeza,okane,neer,nee,mudge,mozingo,molinaro,mcvicker,mcgarvey,mcfalls,mccraney,matus,magers,llanos,livermore,liss,linehan,leto,leitner,laymon,lawing,lacourse,kwong,kollar,kneeland,keo,kennett,kellett,kangas,janzen,hutter,huse,huling,hoss,hohn,hofmeister,hewes,hern,harjo,habib,gust,guice,grullon,greggs,grayer,granier,grable,gowdy,giannini,getchell,gartman,garnica,ganey,gallimore,fray,fetters,fergerson,farlow,fagundes,exley,esteves,enders,edenfield,easterwood,drakeford,dipasquale,desousa,deshields,deeter,dedmon,debord,daughtery,cutts,courtemanche,coursey,copple,coomes,collis,coll,cogburn,clopton,choquette,chaidez,castrejon,calhoon,burbach,bulloch,buchman,bruhn,bohon,blough,bien,baynes,barstow,zeman,zackery,yardley,yamashita,wulff,wilken,wiliams,wickersham,wible,whipkey,wedgeworth,walmsley,walkup,vreeland,verrill,valera,umana,traub,swingle,summey,stroupe,stockstill,steffey,stefanski,statler,stapp,speights,solari,soderberg,shunk,shorey,shewmaker,sheilds,schiffer,schank,schaff,sagers,rochon,riser,rickett,reale,raglin,polen,plata,pitcock,percival,palen,pahl,orona,oberle,nocera,navas,nault,mullings,moos,montejano,monreal,minick,middlebrook,meece,mcmillion,mccullen,mauck,marshburn,maillet,mahaney,magner,maclin,lucey,litteral,lippincott,leite,leis,leaks,lamarre,kost,jurgens,jerkins,jager,hurwitz,hughley,hotaling,horstman,hohman,hocker,hively,hipps,hile,hessler,hermanson,hepworth,henn,helland,hedlund,harkless,haigler,gutierez,grindstaff,glantz,giardina,gerken,gadsden,finnerty,feld,farnum,encinas,drakes,dennie,cutlip,curtsinger,couto,cortinas,corby,chiasson,carle,carballo,brindle,borum,bober,blagg,birk,berthiaume,beahm,batres,basnight,backes,axtell,aust,atterberry,alvares,alt,alegria,yow,yip,woodell,wojciechowski,winfree,winbush,wiest,wesner,wamsley,wakeman,verner,truex,trafton,toman,thorsen,theus,tellier,tallant,szeto,strope,stills,sorg,simkins,shuey,shaul,servin,serio,serafin,salguero,saba,ryerson,rudder,ruark,rother,rohrbaugh,rohrbach,rohan,rogerson,risher,rigg,reeser,pryce,prokop,prins,priebe,prejean,pinheiro,petrone,petri,penson,pearlman,parikh,natoli,murakami,mullikin,mullane,motes,morningstar,monks,mcveigh,mcgrady,mcgaughey,mccurley,masi,marchan,manske,maez,lusby,linde,lile,likens,licon,leroux,lemaire,legette,lax,laskey,laprade,laplant,kolar,kittredge,kinley,kerber,kanagy,jetton,janik,ippolito,inouye,hunsinger,howley,howery,horrell,holthaus,hiner,hilson,hilderbrand,hasan,hartzler,harnish,harada,hansford,halligan,hagedorn,gwynn,gudino,greenstein,greear,gracey,goudeau,gose,goodner,ginsburg,gerth,gerner,fyfe,fujii,frier,frenette,folmar,fleisher,fleischmann,fetzer,eisenman,earhart,dupuy,dunkelberger,drexler,dillinger,dilbeck,dewald,demby,deford,dake,craine,como,chesnut,casady,carstens,carrick,carino,carignan,canchola,cale,bushong,burman,buono,brownlow,broach,britten,brickhouse,boyden,boulton,borne,borland,bohrer,blubaugh,bever,berggren,benevides,arocho,arends,amezcua,almendarez,zalewski,witzel,winkfield,wilhoite,vara,vangundy,vanfleet,vanetten,vandergriff,urbanski,troiano,thibodaux,straus,stoneking,stjean,stillings,stange,speicher,speegle,sowa,smeltzer,slawson,simmonds,shuttleworth,serpa,senger,seidman,schweiger,schloss,schimmel,schechter,sayler,sabb,sabatini,ronan,rodiguez,riggleman,richins,reep,reamer,prunty,porath,plunk,piland,philbrook,pettitt,perna,peralez,pascale,padula,oboyle,nivens,nickols,murph,mundt,munden,montijo,mcmanis,mcgrane,mccrimmon,manzi,mangold,malick,mahar,maddock,losey,litten,liner,leff,leedy,leavell,ladue,krahn,kluge,junker,iversen,imler,hurtt,huizar,hubbert,howington,hollomon,holdren,hoisington,hise,heiden,hauge,hartigan,gutirrez,griffie,greenhill,gratton,granata,gottfried,gertz,gautreaux,furry,furey,funderburg,flippen,fitzgibbon,dyar,drucker,donoghue,dildy,devers,detweiler,despres,denby,degeorge,cueto,cranston,courville,clukey,cirillo,chon,chivers,caudillo,catt,butera,bulluck,buckmaster,braunstein,bracamonte,bourdeau,bonnette,bobadilla,boaz,blackledge,beshears,bernhard,bergeson,baver,barthel,balsamo,bak,aziz,awad,authement,altom,altieri,abels,zigler,zhu,younker,yeomans,yearwood,wurster,winget,whitsett,wechsler,weatherwax,wathen,warriner,wanamaker,walraven,viens,vandemark,vancamp,uchida,triana,tinoco,terpstra,tellis,tarin,taranto,takacs,studdard,struthers,strout,stiller,spataro,soderquist,sliger,silberman,shurtleff,sheetz,ritch,reif,raybon,ratzlaff,radley,putt,putney,pinette,piner,petrin,parise,osbourne,nyman,northington,noblitt,nishimura,neher,nalls,naccarato,mucha,mounce,miron,millis,meaney,mcnichols,mckinnis,mcjunkin,mcduffy,manrique,mannion,mangual,malveaux,mains,lumsden,lohmann,lipe,lightsey,lemasters,leist,laxton,laverriere,latorre,lamons,kral,kopf,knauer,kitt,kaul,karas,kamps,jusino,islam,hullinger,huges,hornung,hiser,hempel,helsel,hassinger,hargraves,hammes,hallberg,gutman,gumbs,gruver,graddy,gonsales,goncalves,glennon,gilford,geno,freshour,flippo,fifer,fason,farrish,fallin,ewert,estepp,escudero,ensminger,emberton,elms,ellerbe,eide,dysart,dougan,dierking,dicus,detrick,deroche,depue,demartino,delosreyes,dalke,culbreath,crownover,crisler,crass,corsi,chagnon,centers,cavanagh,casson,carollo,cadwallader,burnley,burciaga,burchard,broadhead,bolte,berens,bellman,bellard,baril,antonucci,wolfgram,winsor,wimbish,wier,wallach,viveros,vento,varley,vanslyke,vangorder,touchstone,tomko,tiemann,throop,tamura,talmadge,swayze,sturdevant,strauser,stolz,stenberg,stayton,spohn,spillers,spillane,sluss,slavens,simonetti,shofner,shead,senecal,seales,schueler,schley,schacht,sauve,sarno,salsbury,rothschild,rosier,rines,reveles,rein,redus,redfern,reck,ranney,raggs,prout,prill,preble,prager,plemons,pilon,piccirillo,pewitt,pesina,pecora,otani,orsini,oestreich,odea,ocallaghan,northup,niehaus,newberg,nasser,narron,monarrez,mishler,mcsherry,mcelfresh,mayon,mauer,mattice,marrone,marmolejo,marini,malm,machen,lunceford,loewen,liverman,litwin,linscott,levins,lenox,legaspi,leeman,leavy,lannon,lamson,lambdin,labarre,knouse,klemm,kleinschmidt,kirklin,keels,juliano,howser,hosier,hopwood,holyfield,hodnett,hirsh,heimann,heckel,harger,hamil,hajek,gurganus,gunning,grange,gonzalas,goggins,gerow,gaydos,garduno,ganley,galey,farner,engles,emond,emert,ellenburg,edick,duell,dorazio,dimond,diederich,depuy,dempster,demaria,dehoyos,dearth,dealba,czech,crose,crespin,cogdill,clinard,cipriano,chretien,cerny,ceniceros,celestin,caple,cacho,burrill,buhr,buckland,branam,boysen,bovee,boos,boler,blom,blasko,beyers,belz,belmonte,bednarz,beckmann,beaudin,bazile,barbeau,balentine,abrahams,zielke,yunker,yeates,wrobel,wike,whisnant,wherry,wagnon,vogan,vansant,vannest,vallo,ullery,towles,towell,thill,taormina,tannehill,taing,storrs,stickles,stetler,sparling,solt,silcox,sheard,shadle,seman,selleck,schlemmer,scher,sapien,sainz,roye,romain,rizzuto,resch,rentz,rasch,ranieri,purtell,primmer,portwood,pontius,pons,pletcher,pledger,pirkle,pillsbury,pentecost,paxson,ortez,oles,mullett,muirhead,mouzon,mork,mollett,mohn,mitcham,melillo,medders,mcmiller,mccleery,mccaughey,mak,maciejewski,macaulay,lute,lipman,lewter,larocque,langton,kriner,knipp,killeen,karn,kalish,kaczor,jonson,jerez,jarrard,janda,hymes,hollman,hollandsworth,holl,hobdy,hennen,hemmer,hagins,haddox,guitierrez,guernsey,gorsuch,gholson,genova,gazaway,gauna,gammons,freels,fonville,fetterman,fava,farquhar,farish,fabela,escoto,eisen,dossett,dority,dorfman,demmer,dehn,dawley,darbonne,damore,damm,crosley,cron,crompton,crichton,cotner,cordon,conerly,colvard,clauson,cheeseman,cavallaro,castille,cabello,burgan,buffum,bruss,brassfield,bowerman,bothwell,borgen,bonaparte,bombard,boivin,boissonneault,bogner,bodden,boan,bittinger,bickham,bedolla,bale,bainbridge,aybar,avendano,ashlock,amidon,almanzar,akridge,ackermann,zager,worrall,winans,wilsey,wightman,westrick,wenner,warne,warford,verville,utecht,upson,tuma,tseng,troncoso,trollinger,torbert,taulbee,sutterfield,stough,storch,stonebraker,stolle,stilson,stiefel,steptoe,stepney,stender,stemple,staggers,spurrier,spinney,spengler,smartt,skoog,silvis,sieg,shuford,selfridge,seguin,sedgwick,sease,scotti,schroer,schlenker,schill,savarese,sapienza,sanson,sandefur,salamone,rusnak,rudisill,rothermel,roca,resendiz,reliford,rasco,raiford,quisenberry,quijada,pullins,puccio,postell,poppe,pinter,piche,petrucci,pellegrin,pelaez,paton,pasco,parkes,paden,pabst,olmsted,newlon,mynatt,mower,morrone,moree,moffat,mixson,minner,millette,mederos,mcgahan,mcconville,maughan,massingill,marano,macri,lovern,lichtenstein,leonetti,lehner,lawley,laramie,lappin,lahti,lago,lacayo,kuester,kincade,juhl,jiron,jessop,jarosz,jain,hults,hoge,hodgins,hoban,hinkson,hillyard,herzig,hervey,henriksen,hawker,hause,hankerson,gregson,golliday,gilcrease,gessner,gerace,garwood,garst,gaillard,flinchum,fishel,fishback,filkins,fentress,fabre,ethier,eisner,ehrhart,efird,drennon,dominy,domingue,dipaolo,dinan,dimartino,deskins,dengler,defreitas,defranco,dahlin,cutshaw,cuthbert,croyle,crothers,critchfield,cowie,costner,coppedge,copes,ciccone,caufield,capo,cambron,cambridge,buser,burnes,buhl,buendia,brindley,brecht,bourgoin,blackshire,birge,benninger,bembry,beil,begaye,barrentine,banton,balmer,baity,auerbach,ambler,alexandre,ackerson,zurcher,zell,wynkoop,wallick,waid,vos,vizcaino,vester,veale,vandermark,vanderford,tuthill,trivette,thiessen,tewksbury,tao,tabron,swasey,swanigan,stoughton,stoudt,stimson,stecker,stead,spady,souther,smoak,sklar,simcox,sidwell,seybert,sesco,seeman,schwenk,schmeling,rossignol,robillard,robicheaux,riveria,rippeon,ridgley,remaley,rehkop,reddish,rauscher,quirion,pusey,pruden,pressler,potvin,pospisil,paradiso,pangburn,palmateer,ownby,otwell,osterberg,osmond,olsson,oberlander,nusbaum,novack,nokes,nicastro,nehls,naber,mulhern,motter,moretz,milian,mckeel,mcclay,mccart,matsuda,martucci,marple,marko,marciniak,manes,mancia,macrae,lybarger,lint,lineberger,levingston,lecroy,lattimer,laseter,kulick,krier,knutsen,klem,kinne,kinkade,ketterman,kerstetter,kersten,karam,joshi,jent,jefcoat,hillier,hillhouse,hettinger,henthorn,henline,helzer,heitzman,heineman,heenan,haughton,haris,harbert,haman,grinstead,gremillion,gorby,giraldo,gioia,gerardi,geraghty,gaunt,gatson,gardin,gans,gammill,friedlander,frahm,fossett,fosdick,forbush,fondren,fleckenstein,fitchett,filer,feliz,feist,ewart,esters,elsner,edgin,easterly,dussault,durazo,devereaux,deshotel,deckert,dargan,cornman,conkle,condit,claunch,clabaugh,cheesman,chea,charney,casella,carone,carbonell,canipe,campana,calles,cabezas,cabell,buttram,bustillos,buskirk,boyland,bourke,blakeley,berumen,berrier,belli,behrendt,baumbach,bartsch,baney,arambula,alldredge,allbritton,ziemba,zanders,youngquist,yoshioka,yohe,wunder,woodfin,wojtowicz,winkel,wilmore,willbanks,wesolowski,wendland,walko,votaw,vanek,uriarte,urbano,turnipseed,triche,trautman,towler,tokarz,temples,tefft,teegarden,syed,swigart,stoller,stapler,stansfield,smit,smelley,sicard,shulman,shew,shear,sheahan,sharpton,selvidge,schlesinger,savell,sandford,sabatino,rosenbloom,roepke,rish,rhames,renken,reger,quarterman,puig,prasad,poplar,pizano,pigott,phair,petrick,patt,pascua,paramore,papineau,olivieri,ogren,norden,noga,nisbet,munk,morvant,moro,moloney,merz,meltzer,mellinger,mehl,mcnealy,mckernan,mchaney,mccleskey,mcandrews,mayton,markert,maresca,maner,mandujano,malpass,macintyre,lytton,lyall,lummus,longshore,longfellow,lokey,locher,leverette,lepe,lefever,leeson,lederer,lampert,lagrone,kreider,korth,knopf,kleist,keltner,kelling,kaspar,kappler,josephs,huckins,holub,hofstetter,hoehn,higginson,hennings,heid,havel,hauer,harnden,hargreaves,hanger,guild,guidi,grate,grandy,grandstaff,goza,goodridge,goodfellow,goggans,godley,giusti,gilyard,geoghegan,galyon,gaeta,funes,font,flanary,fales,erlandson,ellett,edinger,dziedzic,duerr,draughn,donoho,dimatteo,devos,dematteo,degnan,darlington,danis,dahlstrom,dahlke,czajkowski,cumbie,culbert,crosier,croley,corry,clinger,chalker,cephas,caywood,capehart,cales,cadiz,bussiere,burriss,burkart,brundidge,bronstein,bradt,boydston,bostrom,borel,bolles,blay,blackwelder,bissett,bevers,bester,bernardino,benefiel,belote,beedle,beckles,baysinger,bassler,bartee,barlett,bargas,barefield,baptista,arterburn,armas,apperson,amoroso,amedee,zullo,zellner,yelton,willems,wilkin,wiggin,widman,welk,weingarten,walla,viers,vess,verdi,veazey,vannote,tullos,trudell,trower,trosper,trimm,trew,tousignant,topp,tocco,thoreson,terhune,tatom,suniga,sumter,steeves,stansell,soltis,sloss,slaven,shisler,shanley,servantes,selders,segrest,seese,seeber,schaible,savala,sartor,rutt,rumbaugh,ruis,roten,roessler,ritenour,riney,restivo,renard,rakestraw,rake,quiros,pullin,prudhomme,primeaux,prestridge,presswood,ponte,polzin,poarch,pittenger,piggott,pickell,phaneuf,parvin,parmley,palmeri,ozment,ormond,ordaz,ono,olea,obanion,oakman,novick,nicklas,nemec,nappi,mund,morfin,mera,melgoza,melby,mcgoldrick,mcelwain,mcchristian,mccaw,marquart,marlatt,markovich,mahr,lupton,lucus,lorusso,lerman,leddy,leaman,leachman,lavalle,laduke,kummer,koury,konopka,koh,koepp,kloss,klock,khalil,kernan,kappel,jakes,inoue,hutsell,howle,honore,hockman,hockaday,hiltz,hetherington,hesser,hershman,heffron,headen,haskett,hartline,harned,guillemette,guglielmo,guercio,greenbaum,goris,glines,gilmour,gardella,gadd,gabler,gabbert,fuselier,freudenburg,fragoso,follis,flemings,feltman,febus,farren,fallis,evert,ekstrom,eastridge,dyck,dufault,dubreuil,drapeau,domingues,dolezal,dinkel,didonato,devitt,demott,daughtrey,daubert,das,creason,crary,costilla,chipps,cheatwood,carmean,canton,caffrey,burgher,buker,brunk,brodbeck,brantner,bolivar,boerner,bodkin,biel,bencomo,bellino,beliveau,beauvais,beaupre,baylis,baskett,barcus,baltz,asay,arney,arcuri,ankney,agostini,addy,zwilling,zubia,zollinger,zeitz,yanes,winship,winningham,wickline,webre,waddington,vosburgh,verrett,varnum,vandeventer,vacca,usry,towry,touchet,tookes,tonkin,timko,tibbitts,thedford,tarleton,talty,talamantez,tafolla,sugg,strecker,steffan,spiva,slape,shatzer,seyler,seamans,schmaltz,schipper,sasso,ruppe,roudebush,riemer,richarson,revilla,reichenbach,ratley,railsback,quayle,poplin,poorman,ponton,pollitt,poitras,piscitelli,piedra,pew,perera,penwell,pelt,parkhill,paladino,ore,oram,olmo,oliveras,olivarria,ogorman,naron,muncie,mowbray,morones,moretti,monn,mitts,minks,minarik,mimms,milliron,millington,millhouse,messersmith,mcnett,mckinstry,mcgeorge,mcdill,mcateer,mazzeo,matchett,mahood,mabery,lundell,louden,losoya,lisk,lezama,leib,lebo,lanoue,lanford,lafortune,kump,krone,kreps,kott,kopecky,kolodziej,kinman,kimmons,kelty,kaster,karlson,kania,joyal,jenner,jasinski,jandreau,isenhour,hunziker,huhn,houde,houchins,holtman,hodo,heyman,hentges,hedberg,hayne,haycraft,harshbarger,harshaw,harriss,haring,hansell,hanford,handler,hamblen,gunnell,groat,gorecki,gochenour,gleeson,genest,geiser,fulghum,friese,fridley,freeborn,frailey,flaugher,fiala,ettinger,etheredge,espitia,eriksen,engelbrecht,engebretson,elie,eickhoff,edney,edelen,eberhard,eastin,eakes,driggs,doner,donaghy,disalvo,deshong,dahms,dahlquist,curren,cripe,cree,creager,corle,conatser,commons,coggin,coder,coaxum,closson,clodfelter,classen,chittenden,castilleja,casale,cartee,carriere,canup,canizales,burgoon,bunger,bugarin,buchanon,bruning,bruck,brookes,broadwell,brier,brekke,breese,bracero,bowley,bowersox,bose,bogar,blauser,blacker,bjorklund,baumer,basler,baize,baden,auman,amundsen,amore,alvarenga,adamczyk,yerkes,yerby,yamaguchi,worthey,wolk,wixom,wiersma,wieczorek,whiddon,weyer,wetherington,wein,watchman,warf,wansley,vesely,velazco,vannorman,valasquez,utz,urso,turco,turbeville,trivett,toothaker,toohey,tondreau,thaler,sylvain,swindler,swigert,swider,stiner,stever,steffes,stampley,stair,smidt,skeete,silvestre,shutts,shealey,seigler,schweizer,schuldt,schlichting,scherr,saulsberry,saner,rosin,rosato,roling,rohn,rix,rister,remley,remick,recinos,ramm,raabe,pursell,poythress,poli,pokorny,pettry,petrey,petitt,penman,payson,paquet,pappalardo,outland,orenstein,nuttall,nuckols,nott,nimmo,murtagh,mousseau,moulder,mooneyhan,moak,minch,miera,mercuri,meighan,mcnelly,mcguffin,mccreery,mcclaskey,mainor,luongo,lundstrom,loughman,lobb,linhart,lever,leu,leiter,lehoux,lehn,lares,lapan,langhorne,lamon,ladwig,ladson,kuzma,kreitzer,knop,keech,kea,kadlec,jhonson,jantz,inglis,husk,hulme,housel,hofman,hillery,heidenreich,heaps,haslett,harting,hartig,hamler,halton,hallum,gutierres,guida,guerrier,grossi,gress,greenhalgh,gravelle,gow,goslin,gonyea,gipe,gerstner,gasser,garceau,gannaway,gama,gallop,gaiser,fullilove,foutz,fossum,flannagan,farrior,faller,ericksen,entrekin,enochs,englund,ellenberger,eastland,earwood,dudash,drozd,desoto,delph,dekker,dejohn,degarmo,defeo,defalco,deblois,dacus,cudd,crossen,crooms,cronan,costin,cordray,comerford,colegrove,coldwell,claassen,chartrand,castiglione,carte,cardella,carberry,capp,capobianco,cangelosi,buch,brunell,brucker,brockett,brizendine,brinegar,brimer,brase,bosque,bonk,bolger,bohanon,bohan,blazek,berning,bergan,bennette,beauchemin,battiste,barra,balogh,avallone,aubry,ashcroft,asencio,arledge,anchondo,alvord,acheson,zaleski,yonker,wyss,wycoff,woodburn,wininger,winders,willmon,wiechmann,westley,weatherholt,warnick,wardle,warburton,volkert,villanveva,veit,vass,vanallen,tung,toribio,toothman,tiggs,thornsberry,thome,tepper,teeple,tebo,tassone,tann,stucker,stotler,stoneman,stehle,stanback,stallcup,spurr,speers,spada,solum,smolen,sinn,silvernail,sholes,shives,shain,secrest,seagle,schuette,schoch,schnieders,schild,schiavone,schiavo,scharff,santee,sandell,salvo,rollings,rivenburg,ritzman,rist,reynosa,retana,regnier,rarick,ransome,rall,propes,prall,poyner,ponds,poitra,pippins,pinion,phu,perillo,penrose,pendergraft,pelchat,patenaude,palko,odoms,oddo,novoa,noone,newburn,negri,nantz,mosser,moshier,molter,molinari,moler,millman,meurer,mendel,mcray,mcnicholas,mcnerney,mckillip,mcilvain,mcadory,marmol,marinez,manzer,mankin,makris,majeski,maffei,luoma,luman,luebke,luby,lomonaco,loar,litchford,lintz,licht,levenson,legge,lanigan,krom,kreger,koop,kober,klima,kitterman,kinkead,kimbell,kilian,kibbe,kendig,kemmer,kash,jenkin,inniss,hurlbut,hunsucker,huckabee,hoxie,hoglund,hockensmith,hoadley,hinkel,higuera,herrman,heiner,hausmann,haubrich,hassen,hanlin,hallinan,haglund,hagberg,gullo,gullion,groner,greenwalt,gobert,glowacki,glessner,gines,gildersleeve,gildea,gerke,gebhard,gatton,gately,galasso,fralick,fouse,fluharty,faucette,fairfax,evanoff,elser,ellard,egerton,ector,ebling,dunkel,duhart,drysdale,dostal,dorey,dolph,doles,dismukes,digregorio,digby,dewees,deramus,denniston,dennett,deloney,delaughter,cuneo,cumberland,crotts,crosswhite,cremeans,creasey,cottman,cothern,costales,cosner,corpus,colligan,cobble,clutter,chupp,chevez,chatmon,chaires,caplan,caffee,cabana,burrough,burditt,buckler,brunswick,brouillard,broady,bowlby,bouley,borgman,boltz,boddy,blackston,birdsell,bedgood,bate,bartos,barriga,barna,barcenas,banach,baccus,auclair,ashman,arter,arendt,ansell,allums,allender,alber,albarran,adelson,zoll,wysong,wimbley,wildes,whitis,whitehill,whicker,weymouth,weldy,wark,wareham,waddy,viveiros,vath,vandoren,vanderhoof,unrein,uecker,tsan,trepanier,tregre,torkelson,tobler,tineo,timmer,swopes,swofford,sweeten,swarts,summerfield,sumler,stucky,strozier,stigall,stickel,stennis,stelzer,steely,slayden,skillern,shurtz,shelor,shellenbarger,shand,shabazz,seo,scroggs,schwandt,schrecengost,schoenrock,schirmer,sandridge,ruzicka,rozek,rowlands,roser,rosendahl,romanowski,rolston,riggio,reichman,redondo,reay,rawlinson,raskin,raine,quandt,purpura,pruneda,prevatte,prettyman,pinedo,pierro,pidgeon,phillippi,pfeil,penix,peasley,paro,ospina,ortegon,ogata,ogara,normandin,nordman,nims,nassar,motz,morlan,mooring,moles,moir,mizrahi,mire,minaya,millwood,mikula,messmer,meikle,mctaggart,mcgonagle,mcewan,mccasland,mccane,mccaffery,mcalexander,mattocks,matranga,martone,markland,maravilla,manno,mancha,mallery,magno,lorentz,locklin,livingstone,lipford,lininger,lepley,leming,lemelin,leadbetter,lawhon,lattin,langworthy,lampman,lambeth,lamarr,lahey,krajewski,klopp,kinnison,kestner,kennell,karim,jozwiak,jakubowski,ivery,iliff,iddings,hudkins,houseman,holz,holderman,hoehne,highfill,hiett,heskett,heldt,hedman,hayslett,hatchell,hasse,hamon,hamada,hakala,haislip,haffey,hackbarth,guo,gullickson,guerrette,greenblatt,goudreau,gongora,godbout,glaude,gills,gillison,gigliotti,gargano,gallucci,galli,galante,frasure,fodor,fizer,fishburn,finkbeiner,finck,fager,estey,espiritu,eppinger,epperly,emig,eckley,dray,dorsch,dille,devita,deslauriers,demery,delorme,delbosque,dauphin,dantonio,curd,crume,cozad,cossette,comacho,climer,chadbourne,cespedes,cayton,castaldo,carpino,carls,capozzi,canela,buzard,busick,burlison,brinkmann,bridgeforth,bourbeau,bornstein,bonfiglio,boice,boese,biondi,bilski,betton,berwick,berlanga,behan,becraft,barrientez,banh,balke,balderrama,bahe,bachand,armer,arceo,aliff,alatorre,zermeno,younce,yeoman,yamasaki,wroten,woodby,winer,willits,wilcoxon,wehmeyer,waterbury,wass,wann,wachtel,vizcarra,veitch,vanderbilt,vallone,vallery,ureno,tyer,tipps,tiedeman,theberge,texeira,taub,tapscott,stutts,stults,stukes,spink,sottile,smithwick,slane,simeone,silvester,siegrist,shiffer,sheedy,sheaffer,severin,sellman,scotto,schupp,schueller,schreier,schoolcraft,schoenberger,schnabel,sangster,samford,saliba,ryles,ryans,rossetti,rodriguz,risch,riel,rezendes,rester,rencher,recker,rathjen,profitt,poteete,polizzi,perrigo,patridge,osby,orvis,opperman,oppenheim,onorato,olaughlin,ohagan,ogles,oehler,obyrne,nuzzo,nickle,nease,neagle,navarette,nagata,musto,morison,montz,mogensen,mizer,miraglia,migliore,menges,mellor,mcnear,mcnab,mcloud,mcelligott,mccollom,maynes,marquette,markowski,marcantonio,maldanado,macey,lundeen,longino,lisle,linthicum,limones,lesure,lesage,lauver,laubach,latshaw,lary,lapham,lacoste,lacher,kutcher,knickerbocker,klos,klingler,kleiman,kittleson,kimbrel,kemmerer,kelson,keese,kallas,jurgensen,junkins,juergens,jolliff,jelks,janicki,jang,ingles,huguley,huggard,howton,hone,holford,hogle,hipple,heimbach,heider,heidel,havener,hattaway,harrah,hanscom,hankinson,hamdan,gridley,goulette,goulart,goodrow,girardi,gent,gautreau,gandara,gamblin,galipeau,fyffe,furrow,fulp,fricks,frase,frandsen,fout,foulks,fouche,foskey,forgey,foor,fobbs,finklea,fincham,figueiredo,festa,ferrier,fellman,eslick,eilerman,eckart,eaglin,dunfee,dumond,drewry,douse,dimick,diener,dickert,deines,declue,daw,dattilo,danko,custodio,cuccia,crunk,crispin,corp,corea,coppin,considine,coniglio,conboy,cockrum,clute,clewis,christiano,channell,cerrato,cecere,catoe,castillon,castile,carstarphen,carmouche,caperton,buteau,bumpers,brey,brazeal,brassard,braga,bradham,bourget,borrelli,borba,boothby,bohr,bohm,boehme,bodin,bloss,blocher,bizzell,bieker,berthelot,bernardini,berends,benard,belser,baze,bartling,barrientes,barras,barcia,banfield,aurand,artman,arnott,arend,amon,almaguer,allee,albarado,alameda,abdo,zuehlke,zoeller,yokoyama,yocom,wyllie,woolum,wint,winland,wilner,wilmes,whitlatch,westervelt,walthall,walkowiak,walburn,viviano,vanderhoff,valez,ugalde,trumbull,todaro,tilford,tidd,tibbits,terranova,templeman,tannenbaum,talmage,tabarez,swearengin,swartwood,svendsen,strum,strack,storie,stockard,steinbeck,starns,stanko,stankiewicz,stacks,stach,sproles,spenser,smotherman,slusser,sinha,silber,siefert,siddiqui,shuff,sherburne,seldon,seddon,schweigert,schroeter,schmucker,saffold,rutz,rundle,rosinski,rosenow,rogalski,ridout,rhymer,replogle,raygoza,ratner,rascoe,rahm,quast,pressnell,predmore,pou,porto,pleasants,pigford,pavone,patnaude,parramore,papadopoulos,palmatier,ouzts,oshields,ortis,olmeda,olden,okamoto,norby,nitz,niebuhr,nevius,neiman,neidig,neece,murawski,mroz,moylan,moultry,mosteller,moring,morganti,mook,moffet,mettler,merlo,mengel,mendelsohn,meli,melchior,mcmeans,mcfaddin,mccullers,mccollister,mccloy,mcclaine,maury,maser,martelli,manthey,malkin,maio,magwood,maginnis,mabon,luton,lusher,lucht,lobato,levis,letellier,legendre,latson,larmon,largo,landreneau,landgraf,lamberson,kurland,kresge,korman,korando,klapper,kitson,kinyon,kincheloe,kawamoto,kawakami,jenney,jeanpierre,ivers,issa,ince,hollier,hollars,hoerner,hodgkinson,hiott,hibbitts,herlihy,henricks,heavner,hayhurst,harvill,harewood,hanselman,hanning,gustavson,grizzard,graybeal,gravley,gorney,goll,goehring,godines,gobeil,glickman,giuliano,gimbel,geib,gayhart,gatti,gains,gadberry,frei,fraise,fouch,forst,forsman,folden,fogleman,fetty,feely,fabry,eury,estill,epling,elamin,echavarria,dutil,duryea,dumais,drago,downard,douthit,doolin,dobos,dison,dinges,diebold,desilets,deshazo,depaz,degennaro,dall,cyphers,cryer,croce,crisman,credle,coriell,copp,compos,colmenero,cogar,carnevale,campanella,caley,calderone,burtch,brouwer,brehmer,brassell,brafford,bourquin,bourn,bohnert,blewett,blass,blakes,bhakta,besser,berge,bellis,balfour,avera,applin,ammon,alsop,aleshire,akbar,zoller,zapien,wymore,wyble,wolken,wix,wickstrom,whobrey,whigham,westerlund,welsch,weisser,weisner,weinstock,wehner,watlington,wakeland,wafer,victorino,veltri,veith,urich,uresti,umberger,twedt,tuohy,tschida,trumble,troia,trimmer,topps,tonn,tiernan,threet,thrall,thetford,teneyck,tartaglia,strohl,streater,strausbaugh,stradley,stonecipher,steadham,stansel,stalcup,stabile,sprenger,spradley,speier,southwood,sorrels,slezak,skow,sirmans,simental,sifford,sievert,shover,sheley,selzer,scriven,schwindt,schwan,schroth,saylors,saragosa,sant,salaam,saephan,routt,rousey,ros,rolfes,rieke,rieder,richeson,redinger,rasnick,rapoza,rambert,quist,pyron,pullman,przybylski,pridmore,pooley,pines,perkinson,perine,perham,pecor,peavler,partington,panton,oliverio,olague,ohman,ohearn,noyola,nicolai,nebel,murtha,mowrey,moroney,morgenstern,morant,monsour,moffit,mijares,meriwether,mendieta,melendrez,mejorado,mckittrick,mckey,mckenny,mckelvy,mcelvain,mccoin,mazzarella,mazon,maurin,matthies,maston,maske,marzano,marmon,marburger,mangus,mangino,mallet,luo,losada,londono,lobdell,lipson,lesniak,leighty,lei,lavallie,lareau,laperle,lape,laforce,laffey,kuehner,kravitz,kowalsky,kohr,kinsman,keppler,kennemer,keiper,kaler,jun,jelinek,jarnagin,isakson,hypes,hutzler,huls,horak,hitz,hice,herrell,henslee,heitz,heiss,heiman,hasting,hartwick,harmer,hammontree,hakes,guse,guillotte,groleau,greve,greenough,golub,golson,goldschmidt,golder,godbolt,gilmartin,gies,gibby,geren,genthner,gendreau,gemmill,gaymon,galyean,galeano,friar,folkerts,fleeman,fitzgibbons,ferranti,felan,farrand,eoff,enger,engels,ducksworth,duby,drumheller,douthitt,donis,dixion,dittrich,dials,descoteaux,depaul,denker,demuth,demelo,delacerda,deforge,danos,dalley,daigneault,cybulski,cothren,corns,corkery,copas,clubb,clore,chitty,chichester,chace,catanzaro,castonguay,cassella,carlberg,cammarata,calle,cajigas,byas,buzbee,busey,burling,bufkin,brzezinski,brun,brickner,brabham,boller,bockman,bleich,blakeman,bisbee,bier,bezanson,bevilacqua,besaw,berrian,bequette,beauford,baumgarten,baudoin,batie,basaldua,bardin,bangert,banes,backlund,avitia,artz,archey,apel,amico,alam,aden,zebrowski,yokota,wormley,wootton,womac,wiltz,wigington,whitehorn,whisman,weisgerber,weigle,weedman,watkin,wasilewski,wadlington,wadkins,viverette,vidaurri,vidales,vezina,vanleer,vanhoy,vanguilder,vanbrunt,updegraff,tylor,trinkle,touchette,tilson,tilman,tengan,tarkington,surrett,summy,streetman,straughter,steere,spruell,spadaro,solley,smathers,silvera,siems,shreffler,sholar,selden,schaper,samayoa,ruggeri,rowen,rosso,rosenbalm,roose,ronquillo,rogowski,rexford,repass,renzi,renick,rehberg,ranck,raffa,rackers,raap,puglisi,prinz,pounders,pon,pompa,plasencia,pipkins,petrosky,pelley,pauls,pauli,parkison,parisien,pangle,pancoast,palazzolo,owenby,overbay,orris,orlowski,nipp,newbern,nedd,nealon,najar,mysliwiec,myres,musson,murrieta,munsell,mumma,muldowney,moyle,mowen,morejon,moodie,monier,mikkelsen,miers,metzinger,melin,mcquay,mcpeek,mcneeley,mcglothin,mcghie,mcdonell,mccumber,mccranie,mcbean,mayhugh,marts,marenco,manges,lynam,lupien,luff,luebbert,loh,loflin,lococo,loch,lis,linke,lightle,lewellyn,leishman,lebow,lebouef,leanos,lanz,landy,landaverde,lacefield,kyler,kuebler,kropf,kroeker,kluesner,klass,kimberling,kilkenny,kiker,ketter,kelemen,keasler,kawamura,karst,kardos,igo,huseman,huseby,hurlbert,huard,hottinger,hornberger,hopps,holdsworth,hensen,heilig,heeter,harpole,haak,gutowski,gunnels,grimmer,gravatt,granderson,gotcher,gleaves,genao,garfinkel,frerichs,foushee,flanery,finnie,feldt,fagin,ewalt,ellefson,eiler,eckhart,eastep,digirolamo,didomenico,devera,delavega,defilippo,debusk,daub,damiani,cupples,crofoot,courter,coto,costigan,corning,corman,corlett,cooperman,collison,coghlan,cobbins,coady,coachman,clothier,cipolla,chmielewski,chiodo,chatterton,chappelle,chairez,ceron,casperson,casler,casados,carrow,carlino,carico,cardillo,caouette,canto,canavan,cambra,byard,buterbaugh,buse,bucy,buckwalter,bubb,bryd,brissette,brault,bradwell,boshears,borchert,blansett,biondo,biehl,bessey,belles,beeks,beekman,beaufort,bayliss,bardsley,avilla,astudillo,ardito,antunez,aderholt,abate,yowell,yin,yearby,wurst,woolverton,woolbright,wildermuth,whittenburg,whitely,wetherbee,wenz,welliver,welling,wason,warlick,voorhies,vivier,villines,verde,veiga,varghese,vanwyk,vanwingerden,vanhorne,umstead,twiggs,tusing,trego,tompson,tinkle,thoman,thole,tatman,tartt,suda,studley,strock,strawbridge,stokely,stec,stalter,speidel,spafford,sontag,sokolowski,skillman,skelley,skalski,sison,sippel,sinquefield,siegle,sher,sharrow,setliff,sellner,selig,seibold,seery,scriber,schull,schrupp,schippers,saulsbury,sao,santillo,sanor,rubalcaba,roosa,ronk,robbs,roache,riebe,reinoso,quin,preuss,pottorff,pontiff,plouffe,picou,picklesimer,pettyjohn,petti,penaloza,parmelee,pardee,palazzo,overholt,ogawa,ofarrell,nolting,noda,nickson,nevitt,neveu,navarre,murrow,munz,mulloy,monzo,milliman,metivier,merlino,mcpeters,mckissack,mckeen,mcgurk,mcfee,mcfarren,mcelwee,mceachin,mcdonagh,mccarville,mayhall,mattoon,martello,marconi,marbury,manzella,maly,malec,maitland,maheu,maclennan,lyke,luera,lowenstein,losh,lopiccolo,longacre,loman,loden,loaiza,lieber,libbey,lenhardt,lefebre,lauterbach,lauritsen,lass,larocco,larimer,lansford,lanclos,lamay,lal,kulikowski,kriebel,kosinski,kleinman,kleiner,kleckner,kistner,kissner,kissell,keisler,keeble,keaney,kale,joly,jimison,ikner,hursey,hruska,hove,hou,hosking,hoose,holle,hoeppner,hittle,hitchens,hirth,hinerman,higby,hertzog,hentz,hensler,heier,hegg,hassel,harpe,hara,hain,hagopian,grimshaw,grado,gowin,gowans,googe,goodlow,goering,gleaton,gidley,giannone,gascon,garneau,gambrel,galaz,fuentez,frisina,fresquez,fraher,feuerstein,felten,everman,ertel,erazo,ensign,endo,ellerman,eichorn,edgell,ebron,eaker,dundas,duncanson,duchene,ducan,dombroski,doman,dickison,dewoody,deloera,delahoussaye,dejean,degroat,decaro,dearmond,dashner,dales,crossett,cressey,cowger,cornette,corbo,coplin,coover,condie,cokley,ceaser,cannaday,callanan,cadle,buscher,bullion,bucklin,bruening,bruckner,brose,branan,bradway,botsford,bortz,borelli,bonetti,bolan,boerger,bloomberg,bingman,bilger,berns,beringer,beres,beets,beede,beaudet,beachum,baughn,bator,bastien,basquez,barreiro,barga,baratta,balser,baillie,axford,attebery,arakaki,annunziata,andrzejewski,ament,amendola,adcox,abril,zenon,zeitler,zambrana,ybanez,yagi,wolak,wilcoxson,whitesel,whitehair,weyand,westendorf,welke,weinmann,weesner,weekes,wedel,weatherall,warthen,vose,villalta,viator,vaz,valtierra,urbanek,tulley,trojanowski,trapani,toups,torpey,tomita,tindal,tieman,tevis,tedrow,taul,tash,tammaro,sylva,swiderski,sweeting,sund,stutler,stich,sterns,stegner,stalder,splawn,speirs,southwell,soltys,smead,slye,skipworth,sipos,simmerman,sidhu,shuffler,shingleton,shadwick,sermons,seefeldt,scipio,schwanke,schreffler,schiro,scheiber,sandoz,samsel,ruddell,royse,rouillard,rotella,rosalez,romriell,rizer,riner,rickards,rhoton,rhem,reppert,rayl,raulston,raposo,rainville,radel,quinney,purdie,pizzo,pincus,petrus,pendelton,pendarvis,peltz,peguero,peete,patricio,patchett,parrino,papke,palafox,ottley,ostby,oritz,ogan,odegaard,oatman,noell,nicoll,newhall,newbill,netzer,nettleton,neblett,murley,mungo,mulhall,mosca,morissette,morford,monsen,mitzel,miskell,minder,mehaffey,mcquillen,mclennan,mcgrail,mccreight,mayville,maysonet,maust,mathieson,mastrangelo,maskell,manz,malmberg,makela,madruga,lotts,longnecker,logston,littell,liska,lindauer,lillibridge,levron,letchworth,lesh,leffel,leday,leamon,kulas,kula,kucharski,kromer,kraatz,konieczny,konen,komar,kivett,kirts,kinnear,kersh,keithley,keifer,judah,jimenes,jeppesen,jansson,huntsberry,hund,huitt,huffine,hosford,holmstrom,hollen,hodgin,hirschman,hiltner,hilliker,hibner,hennis,helt,heidelberg,heger,heer,hartness,hardrick,halladay,gula,guillaume,guerriero,grunewald,grosse,griffeth,grenz,grassi,grandison,ginther,gimenez,gillingham,gillham,gess,gelman,gearheart,gaskell,gariepy,gamino,gallien,galentine,fuquay,froman,froelich,friedel,foos,fomby,focht,flythe,fiqueroa,filson,filip,fierros,fett,fedele,fasching,farney,fargo,everts,etzel,elzey,eichner,eger,eatman,ducker,duchesne,donati,domenech,dollard,dodrill,dinapoli,denn,delfino,delcid,delaune,delatte,deems,daluz,cusson,cullison,cuadrado,crumrine,cruickshank,crosland,croll,criddle,crepeau,coutu,couey,cort,coppinger,collman,cockburn,coca,clayborne,claflin,cissell,chowdhury,chicoine,chenier,causby,caulder,cassano,casner,cardiel,brunton,bruch,broxton,brosius,brooking,branco,bracco,bourgault,bosserman,bonet,bolds,bolander,bohman,boelter,blohm,blea,blaise,bischof,beus,bellew,bastarache,bast,bartolome,barcomb,barco,balk,balas,bakos,avey,atnip,ashbrook,arno,arbour,aquirre,appell,aldaco,alban,ahlstrom,abadie,zylstra,zick,yother,wyse,wunsch,whitty,weist,vrooman,villalon,vidrio,vavra,vasbinder,vanmatre,vandorn,ugarte,turberville,tuel,trogdon,toupin,toone,tolleson,tinkham,tinch,tiano,teston,teer,tawney,taplin,tant,tansey,swayne,sutcliffe,sunderman,strothers,stromain,stork,stoneburner,stolte,stolp,stoehr,stingley,stegman,stangl,spinella,spier,soules,sommerfield,sipp,simek,siders,shufelt,shue,shor,shires,shellenberger,sheely,sepe,seaberg,schwing,scherrer,scalzo,sasse,sarvis,santora,sansbury,salls,saleem,ryland,rybicki,ruggieri,rothenberg,rosenstein,roquemore,rollison,rodden,rivet,ridlon,riche,riccardi,reiley,regner,rech,rayo,raff,radabaugh,quon,quill,privette,prange,pickrell,perino,penning,pankratz,orlandi,nyquist,norrell,noren,naples,nale,nakashima,musselwhite,murrin,murch,mullinix,mullican,mullan,morneau,mondor,molinar,minjares,minix,minchew,milewski,mikkelson,mifflin,merkley,meis,meas,mcroy,mcphearson,mcneel,mcmunn,mcmorrow,mcdorman,mccroskey,mccoll,mcclusky,mcclaran,mccampbell,mazzariello,mauzy,mauch,mastro,martinek,marsala,marcantel,mahle,luciani,lubbers,lobel,linch,liller,legros,layden,lapine,lansberry,lage,laforest,labriola,koga,knupp,klimek,kittinger,kirchoff,kinzel,killinger,kilbourne,ketner,kepley,kemble,kells,kear,kaya,karsten,kaneshiro,kamm,joines,joachim,jacobus,iler,holgate,hoar,hisey,hird,hilyard,heslin,herzberg,hennigan,hegland,hartl,haner,handel,gualtieri,greenly,grasser,goetsch,godbold,gilland,gidney,gibney,giancola,gettinger,garzon,galle,galgano,gaier,gaertner,fuston,freel,fortes,fiorillo,figgs,fenstermacher,fedler,facer,fabiano,evins,euler,esquer,enyeart,elem,eich,edgerly,durocher,durgan,duffin,drolet,drewes,dotts,dossantos,dockins,dirksen,difiore,dierks,dickerman,dery,denault,demaree,delmonte,delcambre,daulton,darst,dahle,curnutt,cully,culligan,cueva,crosslin,croskey,cromartie,crofts,covin,coutee,coppa,coogan,condrey,concannon,coger,cloer,clatterbuck,cieslak,chumbley,choudhury,chiaramonte,charboneau,carneal,cappello,campisi,callicoat,burgoyne,bucholz,brumback,brosnan,brogden,broder,brendle,breece,bown,bou,boser,bondy,bolster,boll,bluford,blandon,biscoe,bevill,bence,battin,basel,bartram,barnaby,barmore,balbuena,badgley,backstrom,auyeung,ater,arrellano,arant,ansari,alling,alejandre,alcock,alaimo,aguinaldo,aarons,zurita,zeiger,zawacki,yutzy,yarger,wygant,wurm,wuest,witherell,wisneski,whitby,whelchel,weisz,weisinger,weishaar,wehr,waxman,waldschmidt,walck,waggener,vosburg,villela,vercher,venters,vanscyoc,vandyne,valenza,utt,urick,ungar,ulm,tumlin,tsao,tryon,trudel,treiber,tober,tipler,tillson,tiedemann,thornley,tetrault,temme,tarrance,tackitt,sykora,sweetman,swatzell,sutliff,suhr,sturtz,strub,strayhorn,stormer,steveson,stengel,steinfeldt,spiro,spieker,speth,spero,soza,souliere,soucie,snedeker,slifer,skillings,situ,siniard,simeon,signorelli,siggers,shultis,shrewsbury,shippee,shimp,shepler,sharpless,shadrick,severt,severs,semon,semmes,seiter,segers,sclafani,sciortino,schroyer,schrack,schoenberg,schober,scheidt,scheele,satter,sartori,sarratt,salvaggio,saladino,sakamoto,saine,ryman,rumley,ruggerio,rucks,roughton,robards,ricca,rexroad,resler,reny,rentschler,redrick,redick,reagle,raymo,raker,racette,pyburn,pritt,presson,pressman,pough,pisani,perz,perras,pelzer,pedrosa,palos,palmisano,paille,orem,orbison,oliveros,nourse,nordquist,newbury,nelligan,nawrocki,myler,mumaw,morphis,moldenhauer,miyashiro,mignone,mickelsen,michalec,mesta,mcree,mcqueary,mcninch,mcneilly,mclelland,mclawhorn,mcgreevy,mcconkey,mattes,maselli,marten,marcucci,manseau,manjarrez,malbrough,machin,mabie,lynde,lykes,lueras,lokken,loken,linzy,lillis,lilienthal,levey,legler,leedom,lebowitz,lazzaro,larabee,lapinski,langner,langenfeld,lampkins,lamotte,lambright,lagarde,ladouceur,labounty,lablanc,laberge,kyte,kroon,kron,kraker,kouba,kirwin,kincer,kimbler,kegler,keach,katzman,katzer,kalman,jimmerson,jenning,janus,iacovelli,hust,huson,husby,humphery,hufnagel,honig,holsey,holoman,hohl,hogge,hinderliter,hildebrant,hemby,helle,heintzelman,heidrick,hearon,hazelip,hauk,hasbrouck,harton,hartin,harpster,hansley,hanchett,haar,guthridge,gulbranson,guill,guerrera,grund,grosvenor,grist,grell,grear,granberry,gonser,giunta,giuliani,gillon,gillmore,gillan,gibbon,gettys,gelb,gano,galliher,fullen,frese,frates,foxwell,fleishman,fleener,fielden,ferrera,fells,feemster,fauntleroy,evatt,espy,eno,emmerich,edler,eastham,dunavant,duca,drinnon,dowe,dorgan,dollinger,dipalma,difranco,dietrick,denzer,demarest,delee,delariva,delany,decesare,debellis,deavers,deardorff,dawe,darosa,darley,dalzell,dahlen,curto,cupps,cunniff,cude,crivello,cripps,cresswell,cousar,cotta,compo,clyne,clayson,cearley,catania,carini,cantero,buttrey,buttler,burpee,bulkley,buitron,buda,bublitz,bryer,bryden,brouillette,brott,brookman,bronk,breshears,brennen,brannum,brandl,braman,bracewell,boyter,bomberger,bogen,boeding,blauvelt,blandford,biermann,bielecki,bibby,berthold,berkman,belvin,bellomy,beland,behne,beecham,becher,bax,bassham,barret,baley,auxier,atkison,ary,arocha,arechiga,anspach,algarin,alcott,alberty,ager,ackman,abdallah,zwick,ziemer,zastrow,zajicek,yokum,yokley,wittrock,winebarger,wilker,wilham,whitham,wetzler,westling,westbury,wendler,wellborn,weitzman,weitz,wallner,waldroup,vrabel,vowels,volker,vitiello,visconti,villicana,vibbert,vesey,vannatter,vangilder,vandervort,vandegrift,vanalstyne,vallecillo,usrey,tynan,turpen,tuller,trisler,townson,tillmon,threlkeld,thornell,terrio,taunton,tarry,tardy,swoboda,swihart,sustaita,suitt,stuber,strine,stookey,stmartin,stiger,stainbrook,solem,smail,sligh,siple,sieben,shumake,shriner,showman,sheen,sheckler,seim,secrist,scoggin,schultheis,schmalz,schendel,schacher,savard,saulter,santillanes,sandiford,sande,salzer,salvato,saltz,sakai,ryckman,ryant,ruck,rittenberry,ristau,richart,rhynes,reyer,reulet,reser,redington,reddington,rebello,reasor,raftery,rabago,raasch,quintanar,pylant,purington,provencal,prioleau,prestwood,pothier,popa,polster,politte,poffenberger,pinner,pietrzak,pettie,penaflor,pellot,pellham,paylor,payeur,papas,paik,oyola,osbourn,orzechowski,oppenheimer,olesen,oja,ohl,nuckolls,nordberg,noonkester,nold,nitta,niblett,neuhaus,nesler,nanney,myrie,mutch,mosquera,morena,montalto,montagna,mizelle,mincy,millikan,millay,miler,milbourn,mikels,migues,miesner,mershon,merrow,meigs,mealey,mcraney,mcmartin,mclachlan,mcgeehan,mcferren,mcdole,mccaulley,mcanulty,maziarz,maul,mateer,martinsen,marson,mariotti,manna,mance,malbon,magnusson,maclachlan,macek,lurie,luc,lown,loranger,lonon,lisenby,linsley,lenk,leavens,lauritzen,lathem,lashbrook,landman,lamarche,lamantia,laguerre,lagrange,kogan,klingbeil,kist,kimpel,kime,kier,kerfoot,kennamer,kellems,kammer,kamen,jepsen,jarnigan,isler,ishee,hux,hungate,hummell,hultgren,huffaker,hruby,hornick,hooser,hooley,hoggan,hirano,hilley,higham,heuser,henrickson,henegar,hellwig,hedley,hasegawa,hartt,hambright,halfacre,hafley,guion,guinan,grunwald,grothe,gries,greaney,granda,grabill,gothard,gossman,gosser,gossard,gosha,goldner,gobin,ginyard,gilkes,gilden,gerson,gephart,gengler,gautier,gassett,garon,galusha,gallager,galdamez,fulmore,fritsche,fowles,foutch,footman,fludd,ferriera,ferrero,ferreri,fenimore,fegley,fegan,fearn,farrier,fansler,fane,falzone,fairweather,etherton,elsberry,dykema,duppstadt,dunnam,dunklin,duet,dudgeon,dubuc,doxey,donmoyer,dodgen,disanto,dingler,dimattia,dilday,digennaro,diedrich,derossett,depp,demasi,degraffenreid,deakins,deady,davin,daigre,daddario,czerwinski,cullens,cubbage,cracraft,combest,coletti,coghill,claybrooks,christofferse,chiesa,chason,chamorro,celentano,cayer,carolan,carnegie,capetillo,callier,cadogan,caba,byrom,byrns,burrowes,burket,burdge,burbage,buchholtz,brunt,brungardt,brunetti,brumbelow,brugger,broadhurst,brigance,brandow,bouknight,bottorff,bottomley,bosarge,borger,bombardier,boggan,blumer,blecha,birney,birkland,betances,beran,belin,belgrave,bealer,bauch,bashir,bartow,baro,barnhouse,barile,ballweg,baisley,bains,baehr,badilla,bachus,bacher,bachelder,auzenne,aten,astle,allis,agarwal,adger,adamek,ziolkowski,zinke,zazueta,zamorano,younkin,wittig,witman,winsett,winkles,wiedman,whitner,whitcher,wetherby,westra,westhoff,wehrle,wagaman,voris,vicknair,veasley,vaugh,vanderburg,valletta,tunney,trumbo,truluck,trueman,truby,trombly,tourville,tostado,titcomb,timpson,tignor,thrush,thresher,thiede,tews,tamplin,taff,tacker,syverson,sylvestre,summerall,stumbaugh,strouth,straker,stradford,stokley,steinhoff,steinberger,spigner,soltero,snively,sletten,sinkler,sinegal,simoes,siller,sigel,shire,shinkle,shellman,sheller,sheats,sharer,selvage,sedlak,schriver,schimke,scheuerman,schanz,savory,saulters,sauers,sais,rusin,rumfelt,ruhland,rozar,rosborough,ronning,rolph,roloff,robie,rimer,riehle,ricco,rhein,retzlaff,reisman,reimann,rayes,raub,raminez,quesinberry,pua,procopio,priolo,printz,prewett,preas,prahl,poovey,ploof,platz,plaisted,pinzon,pineiro,pickney,petrovich,perl,pehrson,peets,pavon,pautz,pascarella,paras,paolini,pafford,oyer,ovellette,outten,outen,orduna,odriscoll,oberlin,nosal,niven,nisbett,nevers,nathanson,mukai,mozee,mowers,motyka,morency,montford,mollica,molden,mitten,miser,millender,midgette,messerly,melendy,meisel,meidinger,meany,mcnitt,mcnemar,mcmakin,mcgaugh,mccaa,mauriello,maudlin,matzke,mattia,matsumura,masuda,mangels,maloof,malizia,mahmoud,maglione,maddix,lucchesi,lochner,linquist,lietz,leventhal,lemanski,leiser,laury,lauber,lamberth,kuss,kulik,kuiper,krout,kotter,kort,kohlmeier,koffler,koeller,knipe,knauss,kleiber,kissee,kirst,kirch,kilgo,kerlin,kellison,kehl,kalb,jorden,jantzen,inabinet,ikard,husman,hunsberger,hundt,hucks,houtz,houseknecht,hoots,hogsett,hogans,hintze,hession,henault,hemming,helsley,heinen,heffington,heberling,heasley,hazley,hazeltine,hayton,hayse,hawke,haston,harward,harrow,hanneman,hafford,hadnot,guerro,grahm,gowins,gordillo,goosby,glatt,gibbens,ghent,gerrard,germann,gebo,gean,garling,gardenhire,garbutt,gagner,furguson,funchess,fujiwara,fujita,friley,frigo,forshee,folkes,filler,fernald,ferber,feingold,faul,farrelly,fairbank,failla,espey,eshleman,ertl,erhart,erhardt,erbe,elsea,ells,ellman,eisenhart,ehmann,earnhardt,duplantis,dulac,ducote,draves,dosch,dolce,divito,dimauro,derringer,demeo,demartini,delima,dehner,degen,defrancisco,defoor,dedeaux,debnam,cypert,cutrer,cusumano,custis,croker,courtois,costantino,cormack,corbeil,copher,conlan,conkling,cogdell,cilley,chapdelaine,cendejas,castiglia,cashin,carstensen,caprio,calcote,calaway,byfield,butner,bushway,burritt,browner,brobst,briner,bridger,brickley,brendel,bratten,bratt,brainerd,brackman,bowne,bouck,borunda,bordner,bonenfant,boer,boehmer,bodiford,bleau,blankinship,blane,blaha,bitting,bissonette,bigby,bibeau,bermudes,berke,bergevin,bergerson,bendel,belville,bechard,bearce,beadles,batz,bartlow,ayoub,avans,aumiller,arviso,arpin,arnwine,armwood,arent,arehart,arcand,antle,ambrosino,alongi,alm,allshouse,ahart,aguon,ziebarth,zeledon,zakrzewski,yuhas,yingst,yedinak,wommack,winnett,wingler,wilcoxen,whitmarsh,wayt,watley,warkentin,voll,vogelsang,voegele,vivanco,vinton,villafane,viles,ver,venne,vanwagoner,vanwagenen,vanleuven,vanauken,uselton,uren,trumbauer,tritt,treadaway,tozier,tope,tomczak,tomberlin,tomasini,tollett,toller,titsworth,tirrell,tilly,tavera,tarnowski,tanouye,swarthout,sutera,surette,styers,styer,stipe,stickland,stembridge,stearn,starkes,stanberry,stahr,spino,spicher,sperber,speece,sonntag,sneller,smalling,slowik,slocumb,sliva,slemp,slama,sitz,sisto,sisemore,sindelar,shipton,shillings,sheeley,sharber,shaddix,severns,severino,sensabaugh,seder,seawell,seamons,schrantz,schooler,scheffer,scheerer,scalia,saum,santibanez,sano,sanjuan,sampley,sailer,sabella,sabbagh,royall,rottman,rivenbark,rikard,ricketson,rickel,rethman,reily,reddin,reasoner,rast,ranallo,quintal,pung,pucci,proto,prosperie,prim,preusser,preslar,powley,postma,pinnix,pilla,pietsch,pickerel,pica,pharris,petway,petillo,perin,pereda,pennypacker,pennebaker,pedrick,patin,patchell,parodi,parman,pantano,padua,padro,osterhout,orner,olivar,ohlson,odonoghue,oceguera,oberry,novello,noguera,newquist,newcombe,neihoff,nehring,nees,nebeker,mundo,mullenix,morrisey,moronta,morillo,morefield,mongillo,molino,minto,midgley,michie,menzies,medved,mechling,mealy,mcshan,mcquaig,mcnees,mcglade,mcgarity,mcgahey,mcduff,mayweather,mastropietro,masten,maranto,maniscalco,maize,mahmood,maddocks,maday,macha,maag,luken,lopp,lolley,llanas,litz,litherland,lindenberg,lieu,letcher,lentini,lemelle,leet,lecuyer,leber,laursen,larrick,lantigua,langlinais,lalli,lafever,labat,labadie,krogman,kohut,knarr,klimas,klar,kittelson,kirschbaum,kintzel,kincannon,kimmell,killgore,kettner,kelsch,karle,kapoor,johansson,jenkinson,janney,iraheta,insley,hyslop,huckstep,holleran,hoerr,hinze,hinnenkamp,hilger,higgin,hicklin,heroux,henkle,helfer,heikkinen,heckstall,heckler,heavener,haydel,haveman,haubert,harrop,harnois,hansard,hanover,hammitt,haliburton,haefner,hadsell,haakenson,guynn,guizar,grout,grosz,gomer,golla,godby,glanz,glancy,givan,giesen,gerst,gayman,garraway,gabor,furness,frisk,fremont,frary,forand,fessenden,ferrigno,fearon,favreau,faulks,falbo,ewen,eurich,etchison,esterly,entwistle,ellingsworth,eisenbarth,edelson,eckel,earnshaw,dunneback,doyal,donnellan,dolin,dibiase,deschenes,dermody,degregorio,darnall,dant,dansereau,danaher,dammann,dames,czarnecki,cuyler,custard,cummingham,cuffie,cuffee,cudney,cuadra,crigler,creger,coughlan,corvin,cortright,corchado,connery,conforti,condron,colosimo,colclough,cohee,ciotti,chien,chacko,cevallos,cavitt,cavins,castagna,cashwell,carrozza,carrara,capra,campas,callas,caison,caggiano,bynoe,buswell,burpo,burnam,burges,buerger,buelow,bueche,bruni,brummitt,brodersen,briese,breit,brakebill,braatz,boyers,boughner,borror,borquez,bonelli,bohner,blaker,blackmer,bissette,bibbins,bhatt,bhatia,bessler,bergh,beresford,bensen,benningfield,bellantoni,behler,beehler,beazley,beauchesne,bargo,bannerman,baltes,balog,ballantyne,axelson,apgar,aoki,anstett,alejos,alcocer,albury,aichele,ackles,zerangue,zehner,zank,zacarias,youngberg,yorke,yarbro,wydra,worthley,wolbert,wittmer,witherington,wishart,winkleman,willilams,willer,wiedeman,whittingham,whitbeck,whetsel,wheless,westerberg,welcher,wegman,waterfield,wasinger,warfel,wannamaker,walborn,wada,vogl,vizcarrondo,vitela,villeda,veras,venuti,veney,ulrey,uhlig,turcios,tremper,torian,torbett,thrailkill,terrones,teitelbaum,teems,swoope,sunseri,stutes,stthomas,strohm,stroble,striegel,streicher,stodola,stinchcomb,steves,steppe,steller,staudt,starner,stamant,stam,stackpole,sprankle,speciale,spahr,sowders,sova,soluri,soderlund,slinkard,sjogren,sirianni,siewert,sickels,sica,shugart,shoults,shive,shimer,shier,shepley,sheeran,sevin,seto,segundo,sedlacek,scuderi,schurman,schuelke,scholten,schlater,schisler,schiefelbein,schalk,sanon,sabala,ruyle,ruybal,rueb,rowsey,rosol,rocheleau,rishel,rippey,ringgold,rieves,ridinger,retherford,rempe,reith,rafter,raffaele,quinto,putz,purdom,puls,pulaski,propp,principato,preiss,prada,polansky,poch,plath,pittard,pinnock,pfarr,pfannenstiel,penniman,pauling,patchen,paschke,parkey,pando,ouimet,ottman,ostlund,ormiston,occhipinti,nowacki,norred,noack,nishida,nilles,nicodemus,neth,nealey,myricks,murff,mungia,motsinger,moscato,morado,monnier,molyneux,modzelewski,miura,minich,militello,milbrandt,michalik,meserve,mendivil,melara,mcnish,mcelhannon,mccroy,mccrady,mazzella,maule,mattera,mathena,matas,mascorro,marinello,marguez,manwaring,manhart,mangano,maggi,lymon,luter,luse,lukasik,luiz,ludlum,luczak,lowenthal,lossett,lorentzen,loredo,longworth,lomanto,lisi,lish,lipsky,linck,liedtke,levering,lessman,lemond,lembo,ledonne,leatham,laufer,lanphear,langlais,lamphear,lamberton,lafon,lade,lacross,kyzer,krok,kring,krell,krehbiel,kratochvil,krach,kovar,kostka,knudtson,knaack,kliebert,klahn,kirkley,kimzey,kerrick,kennerson,keesler,karlin,janousek,imel,icenhour,hyler,hudock,houpt,holquin,holiman,holahan,hodapp,hillen,hickmon,hersom,henrich,helvey,heidt,heideman,hedstrom,hedin,hebron,hayter,harn,hardage,halsted,hahne,hagemann,guzik,guel,groesbeck,gritton,grego,graziani,grasty,graney,gouin,gossage,golston,goheen,godina,glade,giorgi,giambrone,gerrity,gerrish,gero,gerling,gaulke,garlick,galiano,gaiter,gahagan,gagnier,friddle,fredericksen,franqui,follansbee,foerster,flury,fitzmaurice,fiorini,finlayson,fiecke,fickes,fichter,ferron,farrel,fackler,eyman,escarcega,errico,erler,erby,engman,engelmann,elsass,elliston,eddleman,eadie,dummer,drost,dorrough,dorrance,doolan,donalson,domenico,ditullio,dittmar,dishon,dionisio,dike,devinney,desir,deschamp,derrickson,delamora,deitch,dechant,danek,dahmen,curci,cudjoe,croxton,creasman,craney,crader,cowling,coulston,cortina,corlew,corl,copland,convery,cohrs,clune,clausing,cipriani,cianciolo,chubb,chittum,chenard,charlesworth,charlebois,champine,chamlee,chagoya,casselman,cardello,capasso,cannella,calderwood,byford,buttars,bushee,burrage,buentello,brzozowski,bryner,brumit,brookover,bronner,bromberg,brixey,brinn,briganti,bremner,brawn,branscome,brannigan,bradsher,bozek,boulay,bormann,bongiorno,bollin,bohler,bogert,bodenhamer,blose,bivona,billips,bibler,benfer,benedetti,belue,bellanger,belford,behn,barnhardt,baltzell,balling,balducci,bainter,babineau,babich,baade,attwood,asmus,asaro,artiaga,applebaum,anding,amar,amaker,allsup,alligood,alers,agin,agar,achenbach,abramowitz,abbas,aasen,zehnder,yopp,yelle,yeldell,wynter,woodmansee,wooding,woll,winborne,willsey,willeford,widger,whiten,whitchurch,whang,weissinger,weinman,weingartner,weidler,waltrip,wagar,wafford,vitagliano,villalvazo,villacorta,vigna,vickrey,vicini,ventimiglia,vandenbosch,valvo,valazquez,utsey,urbaniak,unzueta,trombetta,trevizo,trembley,tremaine,traverso,tores,tolan,tillison,tietjen,teachout,taube,tatham,tarwater,tarbell,sydow,swims,swader,striplin,stoltenberg,steinhauer,steil,steigerwald,starkweather,stallman,squier,sparacino,spadafora,shiflet,shibata,shevlin,sherrick,sessums,servais,senters,seevers,seelye,searfoss,seabrooks,scoles,schwager,schrom,schmeltzer,scheffel,sawin,saterfiel,sardina,sanroman,sandin,salamanca,saladin,sabia,rustin,rushin,ruley,rueter,rotter,rosenzweig,rohe,roder,riter,rieth,ried,ridder,rennick,remmers,remer,relyea,reilley,reder,rasheed,rakowski,rabin,queener,pursel,prowell,pritts,presler,pouncy,porche,porcaro,pollman,pleas,planas,pinkley,pinegar,pilger,philson,petties,perrodin,pendergrast,patao,pasternak,passarelli,pasko,parshall,panos,panella,palombo,padillo,oyama,overlock,overbeck,otterson,orrell,ornellas,opitz,okelly,obando,noggle,nicosia,netto,negrin,natali,nakayama,nagao,nadel,musial,murrill,murrah,munsch,mucci,mrozek,moyes,mowrer,moris,morais,moorhouse,monico,mondy,moncayo,miltenberger,milsap,milone,millikin,milardo,micheals,micco,meyerson,mericle,mendell,meinhardt,meachum,mcleroy,mcgray,mcgonigal,maultsby,matis,matheney,matamoros,marro,marcil,marcial,mantz,mannings,maltby,malchow,maiorano,mahn,mahlum,maglio,maberry,lustig,luellen,longwell,longenecker,lofland,locascio,linney,linneman,lighty,levell,levay,lenahan,lemen,lehto,lebaron,lanctot,lamy,lainez,laffoon,labombard,kujawski,kroger,kreutzer,korhonen,kondo,kollman,kohan,kogut,knaus,kivi,kittel,kinner,kindig,kindel,kiesel,kibby,khang,kettler,ketterer,kepner,kelliher,keenum,kanode,kail,juhasz,jowett,jolicoeur,jeon,iser,ingrassia,imai,hutchcraft,humiston,hulings,hukill,huizenga,hugley,hornyak,hodder,hisle,hillenbrand,hille,higuchi,hertzler,herdon,heppner,hepp,heitmann,heckart,hazlewood,hayles,hayek,hawkin,haugland,hasler,harbuck,happel,hambly,hambleton,hagaman,guzzi,gullette,guinyard,grogg,grise,griffing,goto,gosney,goley,goldblatt,gledhill,girton,giltner,gillock,gilham,gilfillan,giblin,gentner,gehlert,gehl,garten,garney,garlow,garett,galles,galeana,futral,fuhr,friedland,franson,fransen,foulds,follmer,foland,flax,flavin,firkins,fillion,figueredo,ferrill,fenster,fenley,fauver,farfan,eustice,eppler,engelman,engelke,emmer,elzy,ellwood,ellerbee,elks,ehret,ebbert,durrah,dupras,dubuque,dragoo,donlon,dolloff,dibella,derrico,demko,demar,darrington,czapla,crooker,creagh,cranor,craner,crabill,coyer,cowman,cowherd,cottone,costillo,coster,costas,cosenza,corker,collinson,coello,clingman,clingerman,claborn,chmura,chausse,chaudhry,chapell,chancy,cerrone,caverly,caulkins,carn,campfield,campanelli,callaham,cadorette,butkovich,buske,burrier,burkley,bunyard,buckelew,buchheit,broman,brescia,brasel,boyster,booe,bonomo,bondi,bohnsack,blomberg,blanford,bilderback,biggins,bently,behrends,beegle,bedoya,bechtol,beaubien,bayerl,baumgart,baumeister,barratt,barlowe,barkman,barbagallo,baldree,baine,baggs,bacote,aylward,ashurst,arvidson,arthurs,arrieta,arrey,arreguin,arrant,arner,arizmendi,anker,amis,amend,alphin,allbright,aikin,zupan,zuchowski,zeolla,zanchez,zahradnik,zahler,younan,yeater,yearta,yarrington,yantis,woomer,wollard,wolfinger,woerner,witek,wishon,wisener,wingerter,willet,wilding,wiedemann,weisel,wedeking,waybright,wardwell,walkins,waldorf,voth,voit,virden,viloria,villagran,vasta,vashon,vaquera,vantassell,vanderlinden,vandergrift,vancuren,valenta,underdahl,tygart,twining,twiford,turlington,tullius,tubman,trowell,trieu,transue,tousant,torgersen,tooker,tome,toma,tocci,tippins,tinner,timlin,tillinghast,tidmore,teti,tedrick,tacey,swanberg,sunde,summitt,summerford,summa,stratman,strandberg,storck,stober,steitz,stayer,stauber,staiger,sponaugle,spofford,sparano,spagnola,sokoloski,snay,slough,skowronski,sieck,shimkus,sheth,sherk,shankles,shahid,sevy,senegal,seiden,seidell,searls,searight,schwalm,schug,schilke,schier,scheck,sawtelle,santore,sanks,sandquist,sanden,saling,saathoff,ryberg,rustad,ruffing,rudnicki,ruane,rozzi,rowse,rosenau,rodes,risser,riggin,riess,riese,rhoten,reinecke,reigle,reichling,redner,rebelo,raynes,raimondi,rahe,rada,querry,quellette,pulsifer,prochnow,prato,poulton,poudrier,policastro,polhemus,polasek,poissant,pohlmann,plotner,pitkin,pita,pinkett,piekarski,pichon,pfau,petroff,petermann,peplinski,peller,pecinovsky,pearse,pattillo,patague,parlier,parenti,parchman,pane,paff,ortner,oros,nolley,noakes,nigh,nicolosi,nicolay,newnam,netter,nass,napoles,nakata,nakamoto,morlock,moraga,montilla,mongeau,molitor,mohney,mitchener,meyerhoff,medel,mcniff,mcmonagle,mcglown,mcglinchey,mcgarrity,mccright,mccorvey,mcconnel,mccargo,mazzei,matula,mastroianni,massingale,maring,maricle,mans,mannon,mannix,manney,manalo,malo,malan,mahony,madril,mackowiak,macko,macintosh,lurry,luczynski,lucke,lucarelli,losee,lorence,loiacono,lohse,loder,lipari,linebarger,lindamood,limbaugh,letts,leleux,leep,leeder,leard,laxson,lawry,laverdiere,laughton,lastra,kurek,kriss,krishnan,kretschmer,krebsbach,kontos,knobel,knauf,klick,kleven,klawitter,kitchin,kirkendoll,kinkel,kingrey,kilbourn,kensinger,kennerly,kamin,justiniano,jurek,junkin,judon,jordahl,jeanes,jarrells,iwamoto,ishida,immel,iman,ihle,hyre,hurn,hunn,hultman,huffstetler,huffer,hubner,howey,hooton,holts,holscher,holen,hoggatt,hilaire,herz,henne,helstrom,hellickson,heinlein,heckathorn,heckard,headlee,hauptman,haughey,hatt,harring,harford,hammill,hamed,halperin,haig,hagwood,hagstrom,gunnells,gundlach,guardiola,greeno,greenland,gonce,goldsby,gobel,gisi,gillins,gillie,germano,geibel,gauger,garriott,garbarino,gajewski,funari,fullbright,fuell,fritzler,freshwater,freas,fortino,forbus,flohr,flemister,fisch,finks,fenstermaker,feldstein,farhat,fankhauser,fagg,fader,exline,emigh,eguia,edman,eckler,eastburn,dunmore,dubuisson,dubinsky,drayer,doverspike,doubleday,doten,dorner,dolson,dohrmann,disla,direnzo,dipaola,dines,diblasi,dewolf,desanti,dennehy,demming,delker,decola,davilla,daughtridge,darville,darland,danzy,dagenais,culotta,cruzado,crudup,croswell,coverdale,covelli,couts,corbell,coplan,coolbaugh,conyer,conlee,conigliaro,comiskey,coberly,clendening,clairmont,cienfuegos,chojnacki,chilcote,champney,cassara,casazza,casado,carew,carbin,carabajal,calcagni,cail,busbee,burts,burbridge,bunge,bundick,buhler,bucholtz,bruen,broce,brite,brignac,brierly,bridgman,braham,bradish,boyington,borjas,bonn,bonhomme,bohlen,bogardus,bockelman,blick,blackerby,bizier,biro,binney,bertolini,bertin,berti,bento,beno,belgarde,belding,beckel,becerril,bazaldua,bayes,bayard,barrus,barris,baros,bara,ballow,bakewell,baginski,badalamenti,backhaus,avilez,auvil,atteberry,ardon,anzaldua,anello,amsler,ambrosio,althouse,alles,alberti,alberson,aitchison,aguinaga,ziemann,zickefoose,zerr,zeck,zartman,zahm,zabriskie,yohn,yellowhair,yeaton,yarnall,yaple,wolski,wixon,willner,willms,whitsitt,wheelwright,weyandt,wess,wengerd,weatherholtz,wattenbarger,walrath,walpole,waldrip,voges,vinzant,viars,veres,veneziano,veillon,vawter,vaughns,vanwart,vanostrand,valiente,valderas,uhrig,tunison,tulloch,trostle,treaster,traywick,toye,tomson,tomasello,tomasek,tippit,tinajero,tift,tienda,thorington,thieme,thibeau,thakkar,tewell,telfer,sweetser,stratford,stracener,stoke,stiverson,stelling,spatz,spagnoli,sorge,slevin,slabaugh,simson,shupp,shoultz,shotts,shiroma,shetley,sherrow,sheffey,shawgo,shamburger,sester,segraves,seelig,scioneaux,schwartzkopf,schwabe,scholes,schluter,schlecht,schillaci,schildgen,schieber,schewe,schecter,scarpelli,scaglione,sautter,santelli,salmi,sabado,ryer,rydberg,ryba,rushford,runk,ruddick,rotondo,rote,rosenfield,roesner,rocchio,ritzer,rippel,rimes,riffel,richison,ribble,reynold,resh,rehn,ratti,rasor,rasnake,rappold,rando,radosevich,pulice,prichett,pribble,poynor,plowden,pitzen,pittsley,pitter,philyaw,philipps,pestana,perro,perone,pera,peil,pedone,pawlowicz,pattee,parten,parlin,pariseau,paredez,paek,pacifico,otts,ostrow,osornio,oslund,orso,ooten,onken,oniel,onan,ollison,ohlsen,ohlinger,odowd,niemiec,neubert,nembhard,neaves,neathery,nakasone,myerson,muto,muntz,munez,mumme,mumm,mujica,muise,muench,morriss,molock,mishoe,minier,metzgar,mero,meiser,meese,mcsween,mcquire,mcquinn,mcpheeters,mckeller,mcilrath,mcgown,mcdavis,mccuen,mcclenton,maxham,matsui,marriner,marlette,mansur,mancino,maland,majka,maisch,maheux,madry,madriz,mackley,macke,lydick,lutterman,luppino,lundahl,lovingood,loudon,longmore,liefer,leveque,lescarbeau,lemmer,ledgerwood,lawver,lawrie,lattea,lasko,lahman,kulpa,kukowski,kukla,kubota,kubala,krizan,kriz,krikorian,kravetz,kramp,kowaleski,knobloch,klosterman,kloster,klepper,kirven,kinnaman,kinnaird,killam,kiesling,kesner,keebler,keagle,karls,kapinos,kantner,kaba,junious,jefferys,jacquet,izzi,ishii,irion,ifill,hotard,horman,hoppes,hopkin,hokanson,hoda,hocutt,hoaglin,hites,hirai,hindle,hinch,hilty,hild,hier,hickle,hibler,henrichs,hempstead,helmers,hellard,heims,heidler,hawbaker,harkleroad,harari,hanney,hannaford,hamid,haltom,hallford,guilliams,guerette,gryder,groseclose,groen,grimley,greenidge,graffam,goucher,goodenough,goldsborough,gloster,glanton,gladson,gladding,ghee,gethers,gerstein,geesey,geddie,gayer,gaver,gauntt,gartland,garriga,garoutte,fronk,fritze,frenzel,forgione,fluitt,flinchbaugh,flach,fiorito,finan,finamore,fimbres,fillman,figeroa,ficklin,feher,feddersen,fambro,fairbairn,eves,escalona,elsey,eisenstein,ehrenberg,eargle,drane,dogan,dively,dewolfe,dettman,desiderio,desch,dennen,denk,demaris,delsignore,dejarnette,deere,dedman,daws,dauphinais,danz,dantin,dannenberg,dalby,currence,culwell,cuesta,croston,crossno,cromley,crisci,craw,coryell,condra,colpitts,colas,clink,clevinger,clermont,cistrunk,cirilo,chirico,chiarello,cephus,cecena,cavaliere,caughey,casimir,carwell,carlon,carbonaro,caraveo,cantley,callejas,cagney,cadieux,cabaniss,bushard,burlew,buras,budzinski,bucklew,bruneau,brummer,brueggemann,brotzman,bross,brittian,brimage,briles,brickman,breneman,breitenstein,brandel,brackins,boydstun,botta,bosket,boros,borgmann,bordeau,bonifacio,bolten,boehman,blundell,bloodsaw,bjerke,biffle,bickett,bickers,beville,bergren,bergey,benzing,belfiore,beirne,beckert,bebout,baumert,battey,barrs,barriere,barcelo,barbe,balliet,baham,babst,auton,asper,asbell,arzate,argento,arel,araki,arai,antley,amodeo,ammann,allensworth,aldape,akey,abeita,zweifel,zeiler,zamor,zalenski,yzaguirre,yousef,yetman,wyer,woolwine,wohlgemuth,wohlers,wittenberg,wingrove,wimsatt,willimas,wilkenson,wildey,wilderman,wilczynski,wigton,whorley,wellons,welle,weirich,weideman,weide,weast,wasmund,warshaw,walson,waldner,walch,walberg,wagener,wageman,vrieze,vossen,vorce,voorhis,vonderheide,viruet,vicari,verne,velasques,vautour,vartanian,varona,vankeuren,vandine,vandermeer,ursery,underdown,uhrich,uhlman,tworek,twine,twellman,tweedie,tutino,turmelle,tubb,trivedi,triano,trevathan,treese,treanor,treacy,traina,topham,toenjes,tippetts,tieu,thomure,thatch,tetzlaff,tetterton,teamer,tappan,talcott,tagg,szczepanski,syring,surace,sulzer,sugrue,sugarman,suess,styons,stwart,stupka,strey,straube,strate,stoddart,stockbridge,stjames,steimle,steenberg,stamand,staller,stahly,stager,spurgin,sprow,sponsler,speas,spainhour,sones,smits,smelcer,slovak,slaten,singleterry,simien,sidebottom,sibrian,shellhammer,shelburne,shambo,sepeda,seigel,scogin,scianna,schmoll,schmelzer,scheu,schachter,savant,sauseda,satcher,sandor,sampsell,rugh,rufener,rotenberry,rossow,rossbach,rollman,rodrique,rodreguez,rodkey,roda,rini,riggan,rients,riedl,rhines,ress,reinbold,raschke,rardin,racicot,quillin,pushard,primrose,pries,pressey,precourt,pratts,postel,poppell,plumer,pingree,pieroni,pflug,petre,petrarca,peterka,perkin,pergande,peranio,penna,paulhus,pasquariello,parras,parmentier,pamplin,oviatt,osterhoudt,ostendorf,osmun,ortman,orloff,orban,onofrio,olveda,oltman,okeeffe,ocana,nunemaker,novy,noffsinger,nish,niday,nethery,nemitz,neidert,nadal,nack,muszynski,munsterman,mulherin,mortimore,morter,montesino,montalvan,montalbano,momon,moman,mogan,minns,millward,milling,michelsen,mewborn,metayer,mensch,meloy,meggs,meaders,mcsorley,mcmenamin,mclead,mclauchlin,mcguffey,mcguckin,mcglaughlin,mcferron,mcentyre,mccrum,mccawley,mcbain,mayhue,matzen,matton,marsee,marrin,marland,markum,mantilla,manfre,makuch,madlock,macauley,luzier,luthy,lufkin,lucena,loudin,lothrop,lorch,loll,loadholt,lippold,lichtman,liberto,liakos,lewicki,levett,lentine,leja,legree,lawhead,lauro,lauder,lanman,lank,laning,lalor,krob,kriger,kriegel,krejci,kreisel,kozel,konkel,kolstad,koenen,kocsis,knoblock,knebel,klopfer,klee,kilday,kesten,kerbs,kempker,keathley,kazee,kaur,kamer,kamaka,kallenbach,jehle,jaycox,jardin,jahns,ivester,hyppolite,hyche,huppert,hulin,hubley,horsey,hornak,holzwarth,holmon,hollabaugh,holaway,hodes,hoak,hinesley,hillwig,hillebrand,highfield,heslop,herrada,hendryx,hellums,heit,heishman,heindel,hayslip,hayford,hastie,hartgrove,hanus,hakim,hains,hadnott,gundersen,gulino,guidroz,guebert,gressett,graydon,gramling,grahn,goupil,gorelick,goodreau,goodnough,golay,goers,glatz,gillikin,gieseke,giammarino,getman,gensler,gazda,garibaldi,gahan,funderburke,fukuda,fugitt,fuerst,fortman,forsgren,formica,flink,fitton,feltz,fekete,feit,fehrenbach,farone,farinas,faries,fagen,ewin,esquilin,esch,enderle,ellery,ellers,ekberg,egli,effinger,dymond,dulle,dula,duhe,dudney,dowless,dower,dorminey,dopp,dooling,domer,disher,dillenbeck,difilippo,dibernardo,deyoe,devillier,denley,deland,defibaugh,deeb,debow,dauer,datta,darcangelo,daoust,damelio,dahm,dahlman,curlin,cupit,culton,cuenca,cropp,croke,cremer,crace,cosio,corzine,coombe,coman,colone,coloma,collingwood,coderre,cocke,cobler,claybrook,cincotta,cimmino,christoff,chisum,chillemi,chevere,chachere,cervone,cermak,cefalu,cauble,cather,caso,carns,carcamo,carbo,capoccia,capello,capell,canino,cambareri,calvi,cabiness,bushell,burtt,burstein,burkle,bunner,bundren,buechler,bryand,bruso,brownstein,brouse,brodt,brisbin,brightman,brenes,breitenbach,brazzell,brazee,bramwell,bramhall,bradstreet,boyton,bowland,boulter,bossert,bonura,bonebrake,bonacci,boeck,blystone,birchard,bilal,biddy,bibee,bevans,bethke,bertelsen,berney,bergfeld,benware,bellon,bellah,batterton,barberio,bamber,bagdon,badeaux,averitt,augsburger,ates,arvie,aronowitz,arens,araya,angelos,andrada,amell,amante,almy,almquist,alls,aispuro,aguillon,agudelo,aceto,abalos,zdenek,zaremba,zaccaria,youssef,wrona,wrede,wotton,woolston,wolpert,wollman,wince,wimberley,willmore,willetts,wikoff,wieder,wickert,whitenack,wernick,welte,welden,weisenberger,weich,wallington,walder,vossler,vore,vigo,vierling,victorine,verdun,vencill,vazguez,vassel,vanzile,vanvliet,vantrease,vannostrand,vanderveer,vanderveen,vancil,uyeda,umphrey,uhler,uber,tutson,turrentine,tullier,tugwell,trundy,tripodi,tomer,tomasi,tomaselli,tokarski,tisher,tibbets,thweatt,tharrington,tesar,telesco,teasdale,tatem,taniguchi,suriel,sudler,stutsman,sturman,strite,strelow,streight,strawder,stransky,strahl,stours,stong,stinebaugh,stillson,steyer,stelle,steffensmeier,statham,squillante,spiess,spargo,southward,soller,soden,snuggs,snellgrove,smyers,smiddy,slonaker,skyles,skowron,sivils,siqueiros,siers,siddall,shontz,shingler,shiley,shibley,sherard,shelnutt,shedrick,shasteen,sereno,selke,scovil,scola,schuett,schuessler,schreckengost,schranz,schoepp,schneiderman,schlanger,schiele,scheuermann,schertz,scheidler,scheff,schaner,schamber,scardina,savedra,saulnier,sater,sarro,sambrano,salomone,sabourin,ruud,rutten,ruffino,ruddock,rowser,roussell,rosengarten,rominger,rollinson,rohman,roeser,rodenberg,roberds,ridgell,rhodus,reynaga,rexrode,revelle,rempel,remigio,reising,reiling,reetz,rayos,ravenscroft,ravenell,raulerson,rasmusson,rask,rase,ragon,quesnel,quashie,puzo,puterbaugh,ptak,prost,prisbrey,principe,pricer,pratte,pouncey,portman,pontious,pomerantz,planck,pilkenton,pilarski,phegley,pertuit,penta,pelc,peffer,pech,peagler,pavelka,pavao,patman,paskett,parrilla,pardini,papazian,panter,palin,paley,paetzold,packett,pacheo,ostrem,orsborn,olmedo,okamura,oiler,oglesbee,oatis,nuckles,notter,nordyke,nogueira,niswander,nibert,nesby,neloms,nading,naab,munns,mullarkey,moudy,moret,monnin,molder,modisette,moczygemba,moctezuma,mischke,miro,mings,milot,milledge,milhorn,milera,mieles,mickley,micek,metellus,mersch,merola,mercure,mencer,mellin,mell,meinke,mcquillan,mcmurtrie,mckillop,mckiernan,mckendrick,mckamie,mcilvaine,mcguffie,mcgonigle,mcgarrah,mcfetridge,mcenaney,mcdow,mccutchan,mccallie,mcadam,maycock,maybee,mattei,massi,masser,masiello,marshell,marmo,marksberry,markell,marchal,manross,manganaro,mally,mallow,mailhot,magyar,madero,madding,maddalena,macfarland,lynes,lugar,luckie,lucca,lovitt,loveridge,loux,loth,loso,lorenzana,lorance,lockley,lockamy,littler,litman,litke,liebel,lichtenberger,licea,leverich,letarte,lesesne,leno,legleiter,leffew,laurin,launius,laswell,lassen,lasala,laraway,laramore,landrith,lancon,lanahan,laiche,laford,lachermeier,kunst,kugel,kuck,kuchta,kube,korus,koppes,kolbe,koerber,kochan,knittel,kluck,kleve,kleine,kitch,kirton,kirker,kintz,kinghorn,kindell,kimrey,kilduff,kilcrease,kicklighter,kibble,kervin,keplinger,keogh,kellog,keeth,kealey,kazmierczak,karner,kamel,kalina,kaczynski,juel,jerman,jeppson,jawad,jasik,jaqua,janusz,janco,inskeep,inks,ingold,hyndman,hymer,hunte,hunkins,humber,huffstutler,huffines,hudon,hudec,hovland,houze,hout,hougland,hopf,holsapple,holness,hollenbach,hoffmeister,hitchings,hirata,hieber,hickel,hewey,herriman,hermansen,herandez,henze,heffelfinger,hedgecock,hazlitt,hazelrigg,haycock,harren,harnage,harling,harcrow,hannold,hanline,hanel,hanberry,hammersley,hamernik,hajduk,haithcock,haff,hadaway,haan,gullatt,guilbault,guidotti,gruner,grisson,grieves,granato,grabert,gover,gorka,glueck,girardin,giesler,gersten,gering,geers,gaut,gaulin,gaskamp,garbett,gallivan,galland,gaeth,fullenkamp,fullam,friedrichs,freire,freeney,fredenburg,frappier,fowkes,foree,fleurant,fleig,fleagle,fitzsimons,fischetti,fiorenza,finneran,filippi,figueras,fesler,fertig,fennel,feltmann,felps,felmlee,fannon,familia,fairall,fadden,esslinger,enfinger,elsasser,elmendorf,ellisor,einhorn,ehrman,egner,edmisten,edlund,ebinger,dyment,dykeman,durling,dunstan,dunsmore,dugal,duer,drescher,doyel,dossey,donelan,dockstader,dobyns,divis,dilks,didier,desrosier,desanto,deppe,delosh,delange,defrank,debo,dauber,dartez,daquila,dankert,dahn,cygan,cusic,curfman,croghan,croff,criger,creviston,crays,cravey,crandle,crail,crago,craghead,cousineau,couchman,cothron,corella,conine,coller,colberg,cogley,coatney,coale,clendenin,claywell,clagon,cifaldi,choiniere,chickering,chica,chennault,chavarin,chattin,chaloux,challis,cesario,cazarez,caughman,catledge,casebolt,carrel,carra,carlow,capote,canez,camillo,caliendo,calbert,bylsma,buskey,buschman,burkhard,burghardt,burgard,buonocore,bunkley,bungard,bundrick,bumbrey,buice,buffkin,brundige,brockwell,brion,briant,bredeson,bransford,brannock,brakefield,brackens,brabant,bowdoin,bouyer,bothe,boor,bonavita,bollig,blurton,blunk,blanke,blanck,birden,bierbaum,bevington,beutler,betters,bettcher,bera,benway,bengston,benesh,behar,bedsole,becenti,beachy,battersby,basta,bartmess,bartle,bartkowiak,barsky,barrio,barletta,barfoot,banegas,baldonado,azcona,avants,austell,aungst,aune,aumann,audia,atterbury,asselin,asmussen,ashline,asbill,arvizo,arnot,ariola,ardrey,angstadt,anastasio,amsden,amerman,alred,allington,alewine,alcina,alberico,ahlgren,aguas,agrawal,agosta,adolphsen,acey,aburto,abler,zwiebel,zepp,zentz,ybarbo,yarberry,yamauchi,yamashiro,wurtz,wronski,worster,wootten,wongus,woltz,wolanski,witzke,withey,wisecarver,wingham,wineinger,winegarden,windholz,wilgus,wiesen,wieck,widrick,wickliffe,whittenberg,westby,werley,wengert,wendorf,weimar,weick,weckerly,watrous,wasden,walford,wainright,wahlstrom,wadlow,vrba,voisin,vives,vivas,vitello,villescas,villavicencio,villanova,vialpando,vetrano,vensel,vassell,varano,vanriper,vankleeck,vanduyne,vanderpol,vanantwerp,valenzula,udell,turnquist,tuff,trickett,tramble,tingey,timbers,tietz,thiem,tercero,tenner,tenaglia,teaster,tarlton,taitt,tabon,sward,swaby,suydam,surita,suman,suddeth,stumbo,studivant,strobl,streich,stoodley,stoecker,stillwagon,stickle,stellmacher,stefanik,steedley,starbird,stainback,stacker,speir,spath,sommerfeld,soltani,solie,sojka,sobota,sobieski,sobczak,smullen,sleeth,slaymaker,skolnick,skoglund,sires,singler,silliman,shrock,shott,shirah,shimek,shepperd,sheffler,sheeler,sharrock,sharman,shalash,seyfried,seybold,selander,seip,seifried,sedor,sedlock,sebesta,seago,scutt,scrivens,sciacca,schultze,schoemaker,schleifer,schlagel,schlachter,schempp,scheider,scarboro,santi,sandhu,salim,saia,rylander,ryburn,rutigliano,ruocco,ruland,rudloff,rott,rosenburg,rosenbeck,romberger,romanelli,rohloff,rohlfing,rodda,rodd,ritacco,rielly,rieck,rickles,rickenbacker,respass,reisner,reineck,reighard,rehbein,rega,reddix,rawles,raver,rattler,ratledge,rathman,ramsburg,raisor,radovich,radigan,quail,puskar,purtee,priestly,prestidge,presti,pressly,pozo,pottinger,portier,porta,porcelli,poplawski,polin,poeppelman,pocock,plump,plantz,placek,piro,pinnell,pinkowski,pietz,picone,philbeck,pflum,peveto,perret,pentz,payer,patlan,paterno,papageorge,overmyer,overland,osier,orwig,orum,orosz,oquin,opie,ochsner,oathout,nygard,norville,northway,niver,nicolson,newhart,neitzel,nath,nanez,murnane,mortellaro,morreale,morino,moriarity,morgado,moorehouse,mongiello,molton,mirza,minnix,millspaugh,milby,miland,miguez,mickles,michaux,mento,melugin,melito,meinecke,mehr,meares,mcneece,mckane,mcglasson,mcgirt,mcgilvery,mcculler,mccowen,mccook,mcclintic,mccallon,mazzotta,maza,mayse,mayeda,matousek,matley,martyn,marney,marnell,marling,manuelito,maltos,malson,mahi,maffucci,macken,maass,lyttle,lynd,lyden,lukasiewicz,luebbers,lovering,loveall,longtin,lobue,loberg,lipka,lightbody,lichty,levert,lettieri,letsinger,lepak,lemmond,lembke,leitz,lasso,lasiter,lango,landsman,lamirande,lamey,laber,kuta,kulesza,krenz,kreiner,krein,kreiger,kraushaar,kottke,koser,kornreich,kopczynski,konecny,koff,koehl,kocian,knaub,kmetz,kluender,klenke,kleeman,kitzmiller,kirsh,kilman,kildow,kielbasa,ketelsen,kesinger,kehr,keef,kauzlarich,karter,kahre,jobin,jinkins,jines,jeffress,jaquith,jaillet,jablonowski,ishikawa,irey,ingerson,indelicato,huntzinger,huisman,huett,howson,houge,hosack,hora,hoobler,holtzen,holtsclaw,hollingworth,hollin,hoberg,hobaugh,hilker,hilgefort,higgenbotham,heyen,hetzler,hessel,hennessee,hendrie,hellmann,heft,heesch,haymond,haymon,haye,havlik,havis,haverland,haus,harstad,harriston,harju,hardegree,hammell,hamaker,halbrook,halberg,guptill,guntrum,gunderman,gunder,gularte,guarnieri,groll,grippo,greely,gramlich,goewey,goetzinger,goding,giraud,giefer,giberson,gennaro,gemmell,gearing,gayles,gaudin,gatz,gatts,gasca,garn,gandee,gammel,galindez,galati,gagliardo,fulop,fukushima,friedt,fretz,frenz,freeberg,fravel,fountaine,forry,forck,fonner,flippin,flewelling,flansburg,filippone,fettig,fenlon,felter,felkins,fein,favero,faulcon,farver,farless,fahnestock,facemire,faas,eyer,evett,esses,escareno,ensey,ennals,engelking,empey,ellithorpe,effler,edling,edgley,durrell,dunkerson,draheim,domina,dombrosky,doescher,dobbin,divens,dinatale,dieguez,diede,devivo,devilbiss,devaul,determan,desjardin,deshaies,delpozo,delorey,delman,delapp,delamater,deibert,degroff,debelak,dapolito,dano,dacruz,dacanay,cushenberry,cruze,crosbie,cregan,cousino,corrao,corney,cookingham,conry,collingsworth,coldren,cobian,coate,clauss,christenberry,chmiel,chauez,charters,chait,cesare,cella,caya,castenada,cashen,cantrelle,canova,campione,calixte,caicedo,byerley,buttery,burda,burchill,bulmer,bulman,buesing,buczek,buckholz,buchner,buchler,buban,bryne,brunkhorst,brumsey,brumer,brownson,brodnax,brezinski,brazile,braverman,branning,boye,boulden,bough,bossard,bosak,borth,borgmeyer,borge,blowers,blaschke,blann,blankenbaker,bisceglia,billingslea,bialek,beverlin,besecker,berquist,benigno,benavente,belizaire,beisner,behrman,beausoleil,baylon,bayley,bassi,basnett,basilio,basden,basco,banerjee,balli,bagnell,bady,averette,arzu,archambeault,arboleda,arbaugh,arata,antrim,amrhein,amerine,alpers,alfrey,alcon,albus,albertini,aguiniga,aday,acquaviva,accardi,zygmont,zych,zollner,zobel,zinck,zertuche,zaragosa,zale,zaldivar,yeadon,wykoff,woullard,wolfrum,wohlford,wison,wiseley,wisecup,winchenbach,wiltsie,whittlesey,whitelow,whiteford,wever,westrich,wertman,wensel,wenrich,weisbrod,weglarz,wedderburn,weatherhead,wease,warring,wadleigh,voltz,vise,villano,vicario,vermeulen,vazques,vasko,varughese,vangieson,vanfossen,vanepps,vanderploeg,vancleve,valerius,uyehara,unsworth,twersky,turrell,tuner,tsui,trunzo,trousdale,trentham,traughber,torgrimson,toppin,tokar,tobia,tippens,tigue,thiry,thackston,terhaar,tenny,tassin,tadeo,sweigart,sutherlin,sumrell,suen,stuhr,strzelecki,strosnider,streiff,stottlemyer,storment,storlie,stonesifer,stogsdill,stenzel,stemen,stellhorn,steidl,stecklein,statton,stangle,spratling,spoor,spight,spelman,spece,spanos,spadoni,southers,sola,sobol,smyre,slaybaugh,sizelove,sirmons,simington,silversmith,siguenza,sieren,shelman,sharples,sharif,sessler,serrata,serino,serafini,semien,selvey,seedorf,seckman,seawood,scoby,scicchitano,schorn,schommer,schnitzer,schleusner,schlabach,schiel,schepers,schaber,scally,sautner,sartwell,santerre,sandage,salvia,salvetti,salsman,sallis,salais,saeger,sabat,saar,ruther,russom,ruoff,rumery,rubottom,rozelle,rowton,routon,rotolo,rostad,roseborough,rorick,ronco,roher,roberie,robare,ritts,rison,rippe,rinke,ringwood,righter,rieser,rideaux,rickerson,renfrew,releford,reinsch,reiman,reifsteck,reidhead,redfearn,reddout,reaux,rado,radebaugh,quinby,quigg,provo,provenza,provence,pridgeon,praylow,powel,poulter,portner,pontbriand,poirrier,poirer,platero,pixler,pintor,pigman,piersall,piel,pichette,phou,pharis,phalen,petsche,perrier,penfield,pelosi,pebley,peat,pawloski,pawlik,pavlick,pavel,patz,patout,pascucci,pasch,parrinello,parekh,pantaleo,pannone,pankow,pangborn,pagani,pacelli,orsi,oriley,orduno,oommen,olivero,okada,ocon,ocheltree,oberman,nyland,noss,norling,nolton,nobile,nitti,nishimoto,nghiem,neuner,neuberger,neifert,negus,nagler,mullally,moulden,morra,morquecho,moots,mizzell,mirsky,mirabito,minardi,milholland,mikus,mijangos,michener,michalek,methvin,merrit,menter,meneely,meiers,mehring,mees,mcwhirt,mcwain,mcphatter,mcnichol,mcnaught,mclarty,mcivor,mcginness,mcgaughy,mcferrin,mcfate,mcclenny,mcclard,mccaskey,mccallion,mcamis,mathisen,marton,marsico,marchi,mani,mangione,macaraeg,lupi,lunday,lukowski,lucious,locicero,loach,littlewood,litt,lipham,linley,lindon,lightford,lieser,leyendecker,lewey,lesane,lenzi,lenart,leisinger,lehrman,lefebure,lazard,laycock,laver,launer,lastrapes,lastinger,lasker,larkey,lanser,lanphere,landey,lampton,lamark,kumm,kullman,krzeminski,krasner,koran,koning,kohls,kohen,kobel,kniffen,knick,kneip,knappenberger,klumpp,klausner,kitamura,kisling,kirshner,kinloch,kingman,kimery,kestler,kellen,keleher,keehn,kearley,kasprzak,kampf,kamerer,kalis,kahan,kaestner,kadel,kabel,junge,juckett,joynt,jorstad,jetter,jelley,jefferis,jeansonne,janecek,jaffee,izzard,istre,isherwood,ipock,iannuzzi,hypolite,humfeld,hotz,hosein,honahni,holzworth,holdridge,holdaway,holaday,hodak,hitchman,hippler,hinchey,hillin,hiler,hibdon,hevey,heth,hepfer,henneman,hemsley,hemmings,hemminger,helbert,helberg,heinze,heeren,heber,haver,hauff,haswell,harvison,hartson,harshberger,harryman,harries,hane,hamsher,haggett,hagemeier,haecker,haddon,haberkorn,guttman,guttierrez,guthmiller,guillet,guilbert,gugino,grumbles,griffy,gregerson,grana,goya,goranson,gonsoulin,goettl,goertz,godlewski,glandon,gilsdorf,gillogly,gilkison,giard,giampaolo,gheen,gettings,gesell,gershon,gaumer,gartrell,garside,garrigan,garmany,garlitz,garlington,gamet,furlough,funston,funaro,frix,frasca,francoeur,forshey,foose,flatley,flagler,fils,fillers,fickett,feth,fennelly,fencl,felch,fedrick,febres,fazekas,farnan,fairless,ewan,etsitty,enterline,elsworth,elliff,eleby,eldreth,eidem,edgecomb,edds,ebarb,dworkin,dusenberry,durrance,duropan,durfey,dungy,dundon,dumbleton,dubon,dubberly,droz,drinkwater,dressel,doughtie,doshier,dorrell,dople,doonan,donadio,dollison,doig,ditzler,dishner,discher,dimaio,digman,difalco,devino,devens,derosia,deppen,depaola,deniz,denardo,demos,demay,delgiudice,davi,danielsen,dally,dais,dahmer,cutsforth,cusimano,curington,cumbee,cryan,crusoe,crowden,crete,cressman,crapo,cowens,coupe,councill,coty,cotnoir,correira,copen,consiglio,combes,coffer,cockrill,coad,clogston,clasen,chesnutt,charrier,chadburn,cerniglia,cebula,castruita,castilla,castaldi,casebeer,casagrande,carta,carrales,carnley,cardon,capshaw,capron,cappiello,capito,canney,candela,caminiti,califano,calabria,caiazzo,cahall,buscemi,burtner,burgdorf,burdo,buffaloe,buchwald,brwon,brunke,brummond,brumm,broe,brocious,brocato,briski,brisker,brightwell,bresett,breiner,brazeau,braz,brayman,brandis,bramer,bradeen,boyko,bossi,boshart,bortle,boniello,bomgardner,bolz,bolenbaugh,bohling,bohland,bochenek,blust,bloxham,blowe,blish,blackwater,bjelland,biros,biederman,bickle,bialaszewski,bevil,beumer,bettinger,besse,bernett,bermejo,bement,belfield,beckler,baxendale,batdorf,bastin,bashore,bascombe,bartlebaugh,barsh,ballantine,bahl,badon,autin,astin,askey,ascher,arrigo,arbeiter,antes,angers,amburn,amarante,alvidrez,althaus,allmond,alfieri,aldinger,akerley,akana,aikins,ader,acebedo,accardo,abila,aberle,abele,abboud,zollars,zimmerer,zieman,zerby,zelman,zellars,yoshimura,yonts,yeats,yant,yamanaka,wyland,wuensche,worman,wordlaw,wohl,winslett,winberg,wilmeth,willcutt,wiers,wiemer,wickwire,wichman,whitting,whidbee,westergard,wemmer,wellner,weishaupt,weinert,weedon,waynick,wasielewski,waren,walworth,wallingford,walke,waechter,viviani,vitti,villagrana,vien,vicks,venema,varnes,varnadoe,varden,vanpatten,vanorden,vanderzee,vandenburg,vandehey,valls,vallarta,valderrama,valade,urman,ulery,tusa,tuft,tripoli,trimpe,trickey,tortora,torrens,torchia,toft,tjaden,tison,tindel,thurmon,thode,tardugno,tancredi,taketa,taillon,tagle,sytsma,symes,swindall,swicegood,swartout,sundstrom,sumners,sulton,studstill,stroop,stonerock,stmarie,stlawrence,stemm,steinhauser,steinert,steffensen,stefaniak,starck,stalzer,spidle,spake,sowinski,sosnowski,sorber,somma,soliday,soldner,soja,soderstrom,soder,sockwell,sobus,sloop,sinkfield,simerly,silguero,sigg,siemers,siegmund,shum,sholtis,shkreli,sheikh,shattles,sharlow,shambaugh,shaikh,serrao,serafino,selley,selle,seel,sedberry,secord,schunk,schuch,schor,scholze,schnee,schmieder,schleich,schimpf,scherf,satterthwaite,sasson,sarkisian,sarinana,sanzone,salvas,salone,salido,saiki,sahr,rusher,rusek,ruppel,rubel,rothfuss,rothenberger,rossell,rosenquist,rosebrook,romito,romines,rolan,roker,roehrig,rockhold,rocca,robuck,riss,rinaldo,riggenbach,rezentes,reuther,renolds,rench,remus,remsen,reller,relf,reitzel,reiher,rehder,redeker,ramero,rahaim,radice,quijas,qualey,purgason,prum,proudfoot,prock,probert,printup,primer,primavera,prenatt,pratico,polich,podkowka,podesta,plattner,plasse,plamondon,pittmon,pippenger,pineo,pierpont,petzold,petz,pettiway,petters,petroski,petrik,pesola,pershall,perlmutter,penepent,peevy,pechacek,peaden,pazos,pavia,pascarelli,parm,parillo,parfait,paoletti,palomba,palencia,pagaduan,oxner,overfield,overcast,oullette,ostroff,osei,omarah,olenick,olah,odem,nygren,notaro,northcott,nodine,nilges,neyman,neve,neuendorf,neisler,neault,narciso,naff,muscarella,morrisette,morphew,morein,montville,montufar,montesinos,monterroso,mongold,mojarro,moitoso,mirarchi,mirando,minogue,milici,miga,midyett,michna,meuser,messana,menzie,menz,mendicino,melone,mellish,meller,melle,meints,mechem,mealer,mcwilliam,mcwhite,mcquiggan,mcphillips,mcpartland,mcnellis,mcmackin,mclaughin,mckinny,mckeithan,mcguirk,mcgillivray,mcgarr,mcgahee,mcfaul,mcfadin,mceuen,mccullah,mcconico,mcclaren,mccaul,mccalley,mccalister,mazer,mayson,mayhan,maugeri,mauger,mattix,mattews,maslowski,masek,martir,marsch,marquess,maron,markwell,markow,marinaro,marcinek,mannella,mallen,majeed,mahnke,mahabir,magby,magallan,madere,machnik,lybrand,luque,lundholm,lueders,lucian,lubinski,lowy,loew,lippard,linson,lindblad,lightcap,levitsky,levens,leonardi,lenton,lengyel,leitzel,leicht,leaver,laubscher,lashua,larusso,larrimore,lanterman,lanni,lanasa,lamoureaux,lambros,lamborn,lamberti,lall,lafuente,laferriere,laconte,kyger,kupiec,kunzman,kuehne,kuder,kubat,krogh,kreidler,krawiec,krauth,kratky,kottwitz,korb,kono,kolman,kolesar,koeppel,knapper,klingenberg,kjos,keppel,kennan,keltz,kealoha,kasel,karney,kanne,kamrowski,kagawa,johnosn,jilek,jarvie,jarret,jansky,jacquemin,jacox,jacome,iriarte,ingwersen,imboden,iglesia,huyser,hurston,hursh,huntoon,hudman,hoying,horsman,horrigan,hornbaker,horiuchi,hopewell,hommel,homeyer,holzinger,holmer,hipsher,hinchman,hilts,higginbottom,hieb,heyne,hessling,hesler,hertlein,herford,heras,henricksen,hennemann,henery,hendershott,hemstreet,heiney,heckert,heatley,hazell,hazan,hayashida,hausler,hartsoe,harth,harriott,harriger,harpin,hardisty,hardge,hannaman,hannahs,hamp,hammersmith,hamiton,halsell,halderman,hagge,habel,gusler,gushiken,gurr,gummer,gullick,grunden,grosch,greenburg,greb,greaver,gratz,grajales,gourlay,gotto,gorley,goodpasture,godard,glorioso,gloor,glascock,gizzi,giroir,gibeault,gauldin,gauer,gartin,garrels,gamber,gallogly,gade,fusaro,fripp,freyer,freiberg,franzoni,fragale,foston,forti,forness,folts,followell,foard,flom,flett,fleitas,flamm,fino,finnen,finchum,filippelli,fickel,feucht,feiler,feenstra,feagins,faver,faulkenberry,farabaugh,fandel,faler,faivre,fairey,facey,exner,evensen,erion,erben,epting,epping,ephraim,engberg,elsen,ellingwood,eisenmann,eichman,ehle,edsall,durall,dupler,dunker,dumlao,duford,duffie,dudding,dries,doung,dorantes,donahoo,domenick,dollins,dobles,dipiazza,dimeo,diehm,dicicco,devenport,desormeaux,derrow,depaolo,demas,delpriore,delosantos,degreenia,degenhardt,defrancesco,defenbaugh,deets,debonis,deary,dazey,dargie,dambrosia,dalal,dagen,cuen,crupi,crossan,crichlow,creque,coutts,counce,coram,constante,connon,collelo,coit,cocklin,coblentz,cobey,coard,clutts,clingan,clampitt,claeys,ciulla,cimini,ciampa,christon,choat,chiou,chenail,chavous,catto,catalfamo,casterline,cassinelli,caspers,carroway,carlen,carithers,cappel,calo,callow,cagley,cafferty,byun,byam,buttner,buth,burtenshaw,burget,burfield,buresh,bunt,bultman,bulow,buchta,buchmann,brunett,bruemmer,brueggeman,britto,briney,brimhall,bribiesca,bresler,brazan,brashier,brar,brandstetter,boze,boonstra,bluitt,blomgren,blattner,blasi,bladen,bitterman,bilby,bierce,biello,bettes,bertone,berrey,bernat,berberich,benshoof,bendickson,bellefeuille,bednarski,beddingfield,beckerman,beaston,bavaro,batalla,basye,baskins,bartolotta,bartkowski,barranco,barkett,banaszak,bame,bamberger,balsley,ballas,balicki,badura,aymond,aylor,aylesworth,axley,axelrod,aubert,armond,ariza,apicella,anstine,ankrom,angevine,andreotti,alto,alspaugh,alpaugh,almada,allinder,alequin,aguillard,agron,agena,afanador,ackerley,abrev,abdalla,aaronson,zynda,zucco,zipp,zetina,zenz,zelinski,youngren,yochum,yearsley,yankey,woodfork,wohlwend,woelfel,wiste,wismer,winzer,winker,wilkison,wigger,wierenga,whipps,westray,wesch,weld,weible,wedell,weddell,wawrzyniak,wasko,washinton,wantz,walts,wallander,wain,wahlen,wachowiak,voshell,viteri,vire,villafuerte,vieyra,viau,vescio,verrier,verhey,vause,vandermolen,vanderhorst,valois,valla,valcourt,vacek,uzzle,umland,ulman,ulland,turvey,tuley,trembath,trabert,towsend,totman,toews,tisch,tisby,tierce,thivierge,tenenbaum,teagle,tacy,tabler,szewczyk,swearngin,suire,sturrock,stubbe,stronach,stoute,stoudemire,stoneberg,sterba,stejskal,steier,stehr,steckel,stearman,steakley,stanforth,stancill,srour,sprowl,spevak,sokoloff,soderman,snover,sleeman,slaubaugh,sitzman,simes,siegal,sidoti,sidler,sider,sidener,siddiqi,shireman,shima,sheroan,shadduck,seyal,sentell,sennett,senko,seligman,seipel,seekins,seabaugh,scouten,schweinsberg,schwartzberg,schurr,schult,schrick,schoening,schmitmeyer,schlicher,schlager,schack,schaar,scavuzzo,scarpa,sassano,santigo,sandavol,sampsel,samms,samet,salzano,salyards,salva,saidi,sabir,saam,runions,rundquist,rousselle,rotunno,rosch,romney,rohner,roff,rockhill,rocamora,ringle,riggie,ricklefs,rexroat,reves,reuss,repka,rentfro,reineke,recore,recalde,rease,rawling,ravencraft,ravelo,rappa,randol,ramsier,ramerez,rahimi,rahim,radney,racey,raborn,rabalais,quebedeaux,pujol,puchalski,prothro,proffit,prigge,prideaux,prevo,portales,porco,popovic,popek,popejoy,pompei,plude,platner,pizzuto,pizer,pistone,piller,pierri,piehl,pickert,piasecki,phong,philipp,peugh,pesqueira,perrett,perfetti,percell,penhollow,pelto,pellett,pavlak,paulo,pastorius,parsell,parrales,pareja,parcell,pappan,pajak,owusu,ovitt,orrick,oniell,olliff,olberding,oesterling,odwyer,ocegueda,obermiller,nylander,nulph,nottage,northam,norgard,nodal,niel,nicols,newhard,nellum,neira,nazzaro,nassif,narducci,nalbandian,musil,murga,muraoka,mumper,mulroy,mountjoy,mossey,moreton,morea,montoro,montesdeoca,montealegre,montanye,montandon,moisan,mohl,modeste,mitra,minson,minjarez,milbourne,michaelsen,metheney,mestre,mescher,mervis,mennenga,melgarejo,meisinger,meininger,mcwaters,mckern,mckendree,mchargue,mcglothlen,mcgibbon,mcgavock,mcduffee,mcclurkin,mccausland,mccardell,mccambridge,mazzoni,mayen,maxton,mawson,mauffray,mattinson,mattila,matsunaga,mascia,marse,marotz,marois,markin,markee,marcinko,marcin,manville,mantyla,manser,manry,manderscheid,mallari,malecha,malcomb,majerus,macinnis,mabey,lyford,luth,lupercio,luhman,luedke,lovick,lossing,lookabaugh,longway,loisel,logiudice,loffredo,lobaugh,lizaola,livers,littlepage,linnen,limmer,liebsch,liebman,leyden,levitan,levison,levier,leven,levalley,lettinga,lessley,lessig,lepine,leight,leick,leggio,leffingwell,leffert,lefevers,ledlow,leaton,leander,leaming,lazos,laviolette,lauffer,latz,lasorsa,lasch,larin,laporta,lanter,langstaff,landi,lamica,lambson,lambe,lamarca,laman,lamagna,lajeunesse,lafontant,lafler,labrum,laakso,kush,kuether,kuchar,kruk,kroner,kroh,kridler,kreuzer,kovats,koprowski,kohout,knicely,knell,klutts,kindrick,kiddy,khanna,ketcher,kerschner,kerfien,kensey,kenley,kenan,kemplin,kellerhouse,keesling,keas,kaplin,kanady,kampen,jutras,jungers,jeschke,janowski,janas,iskra,imperato,ikerd,igoe,hyneman,hynek,husain,hurrell,hultquist,hullett,hulen,huberty,hoyte,hossain,hornstein,hori,hopton,holms,hollmann,holdman,holdeman,holben,hoffert,himel,hillsman,herdt,hellyer,heister,heimer,heidecker,hedgpeth,hedgepath,hebel,heatwole,hayer,hausner,haskew,haselden,hartranft,harsch,harres,harps,hardimon,halm,hallee,hallahan,hackley,hackenberg,hachey,haapala,guynes,gunnerson,gunby,gulotta,gudger,groman,grignon,griebel,gregori,greenan,grauer,gourd,gorin,gorgone,gooslin,goold,goltz,goldberger,glotfelty,glassford,gladwin,giuffre,gilpatrick,gerdts,geisel,gayler,gaunce,gaulding,gateley,gassman,garson,garron,garand,gangestad,gallow,galbo,gabrielli,fullington,fucci,frum,frieden,friberg,frasco,francese,fowle,foucher,fothergill,foraker,fonder,foisy,fogal,flurry,flenniken,fitzhenry,fishbein,finton,filmore,filice,feola,felberbaum,fausnaught,fasciano,farquharson,faires,estridge,essman,enriques,emmick,ekker,ekdahl,eisman,eggleton,eddinger,eakle,eagar,durio,dunwoody,duhaime,duenes,duden,dudas,dresher,dresel,doutt,donlan,donathan,domke,dobrowolski,dingee,dimmitt,dimery,dilullo,deveaux,devalle,desper,desnoyers,desautels,derouin,derbyshire,denmon,demski,delucca,delpino,delmont,deller,dejulio,deibler,dehne,deharo,degner,defore,deerman,decuir,deckman,deasy,dease,deaner,dawdy,daughdrill,darrigo,darity,dalbey,dagenhart,daffron,curro,curnutte,curatolo,cruikshank,crosswell,croslin,croney,crofton,criado,crecelius,coscia,conniff,commodore,coltharp,colonna,collyer,collington,cobbley,coache,clonts,cloe,cliett,clemans,chrisp,chiarini,cheatam,cheadle,chand,chadd,cervera,cerulli,cerezo,cedano,cayetano,cawthorne,cavalieri,cattaneo,cartlidge,carrithers,carreira,carranco,cargle,candanoza,camburn,calender,calderin,calcagno,cahn,cadden,byham,buttry,burry,burruel,burkitt,burgio,burgener,buescher,buckalew,brymer,brumett,brugnoli,brugman,brosnahan,bronder,broeckel,broderson,brisbon,brinsfield,brinks,bresee,bregman,branner,brambila,brailsford,bouska,boster,borucki,bortner,boroughs,borgeson,bonier,bomba,bolender,boesch,boeke,bloyd,bley,binger,bilbro,biery,bichrest,bezio,bevel,berrett,bermeo,bergdoll,bercier,benzel,bentler,belnap,bellini,beitz,behrend,bednarczyk,bearse,bartolini,bartol,barretta,barbero,barbaro,banvelos,bankes,ballengee,baldon,ausmus,atilano,atienza,aschenbrenner,arora,armstong,aquilino,appleberry,applebee,apolinar,antos,andrepont,ancona,amesquita,alvino,altschuler,allin,alire,ainslie,agular,aeschliman,accetta,abdulla,abbe,zwart,zufelt,zirbel,zingaro,zilnicki,zenteno,zent,zemke,zayac,zarrella,yoshimoto,yearout,womer,woltman,wolin,wolery,woldt,witts,wittner,witherow,winward,winrow,wiemann,wichmann,whitwell,whitelaw,wheeless,whalley,wessner,wenzl,wene,weatherbee,waye,wattles,wanke,walkes,waldeck,vonruden,voisine,vogus,vittetoe,villalva,villacis,venturini,venturi,venson,vanloan,vanhooser,vanduzer,vandever,vanderwal,vanderheyden,vanbeek,vanbebber,vallance,vales,vahle,urbain,upshur,umfleet,tsuji,trybus,triolo,trimarchi,trezza,trenholm,tovey,tourigny,torry,torrain,torgeson,tomey,tischler,tinkler,tinder,ticknor,tibbles,tibbals,throneberry,thormahlen,thibert,thibeaux,theurer,templet,tegeler,tavernier,taubman,tamashiro,tallon,tallarico,taboada,sypher,sybert,swyers,switalski,swedberg,suther,surprenant,sullen,sulik,sugden,suder,suchan,strube,stroope,strittmatter,streett,straughn,strasburg,stjacques,stimage,stimac,stifter,stgelais,steinhart,stehlik,steffenson,steenbergen,stanbery,stallone,spraggs,spoto,spilman,speno,spanbauer,spalla,spagnolo,soliman,solan,sobolik,snelgrove,snedden,smale,sliter,slankard,sircy,shutter,shurtliff,shur,shirkey,shewmake,shams,shadley,shaddox,sgro,serfass,seppala,segawa,segalla,seaberry,scruton,scism,schwein,schwartzman,schwantes,schomer,schoenborn,schlottmann,schissler,scheurer,schepis,scheidegger,saunier,sauders,sassman,sannicolas,sanderfur,salser,sagar,saffer,saeed,sadberry,saban,ryce,rybak,rumore,rummell,rudasill,rozman,rota,rossin,rosell,rosel,romberg,rojero,rochin,robideau,robarge,roath,risko,ringel,ringdahl,riera,riemann,ribas,revard,renegar,reinwald,rehman,redel,raysor,rathke,rapozo,rampton,ramaker,rakow,raia,radin,raco,rackham,racca,racanelli,rabun,quaranta,purves,pundt,protsman,prezioso,presutti,presgraves,poydras,portnoy,portalatin,pontes,poehler,poblete,poat,plumadore,pleiman,pizana,piscopo,piraino,pinelli,pillai,picken,picha,piccoli,philen,petteway,petros,peskin,perugini,perrella,pernice,peper,pensinger,pembleton,passman,parrent,panetta,pallas,palka,pais,paglia,padmore,ottesen,oser,ortmann,ormand,oriol,orick,oler,okafor,ohair,obert,oberholtzer,nowland,nosek,nordeen,nolf,nogle,nobriga,nicley,niccum,newingham,neumeister,neugebauer,netherland,nerney,neiss,neis,neider,neeld,nailor,mustain,mussman,musante,murton,murden,munyon,muldrew,motton,moscoso,moschella,moroz,morelos,morace,moone,montesano,montemurro,montas,montalbo,molander,mleczko,miyake,mitschke,minger,minelli,minear,millener,mihelich,miedema,miah,metzer,mery,merrigan,merck,mennella,membreno,melecio,melder,mehling,mehler,medcalf,meche,mealing,mcqueeney,mcphaul,mcmickle,mcmeen,mcmains,mclees,mcgowin,mcfarlain,mcdivitt,mccotter,mcconn,mccaster,mcbay,mcbath,mayoral,mayeux,matsuo,masur,massman,marzette,martensen,marlett,markgraf,marcinkowski,marchbanks,mansir,mandez,mancil,malagon,magnani,madonia,madill,madia,mackiewicz,macgillivray,macdowell,mabee,lundblad,lovvorn,lovings,loreto,linz,linnell,linebaugh,lindstedt,lindbloom,limberg,liebig,lickteig,lichtenberg,licari,lewison,levario,levar,lepper,lenzen,lenderman,lemarr,leinen,leider,legrande,lefort,lebleu,leask,leacock,lazano,lawalin,laven,laplaca,lant,langsam,langone,landress,landen,lande,lamorte,lairsey,laidlaw,laffin,lackner,lacaze,labuda,labree,labella,labar,kyer,kuyper,kulinski,kulig,kuhnert,kuchera,kubicek,kruckeberg,kruchten,krider,kotch,kornfeld,koren,koogler,koll,kole,kohnke,kohli,kofoed,koelling,kluth,klump,klopfenstein,klippel,klinge,klett,klemp,kleis,klann,kitzman,kinnan,kingsberry,kilmon,killpack,kilbane,kijowski,kies,kierstead,kettering,kesselman,kennington,keniston,kehrer,kearl,keala,kassa,kasahara,kantz,kalin,kaina,jupin,juntunen,juares,joynes,jovel,joos,jiggetts,jervis,jerabek,jennison,jaso,janz,izatt,ishibashi,iannotti,hymas,huneke,hulet,hougen,horvat,horstmann,hopple,holtkamp,holsten,hohenstein,hoefle,hoback,hiney,hiemstra,herwig,herter,herriott,hermsen,herdman,herder,herbig,helling,helbig,heitkamp,heinrichs,heinecke,heileman,heffley,heavrin,heaston,haymaker,hauenstein,hartlage,harig,hardenbrook,hankin,hamiter,hagens,hagel,grizzell,griest,griese,grennan,graden,gosse,gorder,goldin,goatley,gillespi,gilbride,giel,ghoston,gershman,geisinger,gehringer,gedeon,gebert,gaxiola,gawronski,gathright,gatchell,gargiulo,garg,galang,gadison,fyock,furniss,furby,funnell,frizell,frenkel,freeburg,frankhouser,franchi,foulger,formby,forkey,fonte,folson,follette,flavell,finegan,filippini,ferencz,ference,fennessey,feggins,feehan,fazzino,fazenbaker,faunce,farraj,farnell,farler,farabee,falkowski,facio,etzler,ethington,esterline,esper,esker,erxleben,engh,emling,elridge,ellenwood,elfrink,ekhoff,eisert,eifert,eichenlaub,egnor,eggebrecht,edlin,edberg,eble,eber,easler,duwe,dutta,dutremble,dusseault,durney,dunworth,dumire,dukeman,dufner,duey,duble,dreese,dozal,douville,ditmore,distin,dimuzio,dildine,dieterich,dieckman,didonna,dhillon,dezern,devereux,devall,detty,detamore,derksen,deremer,deras,denslow,deno,denicola,denbow,demma,demille,delira,delawder,delara,delahanty,dejonge,deininger,dedios,dederick,decelles,debus,debruyn,deborde,deak,dauenhauer,darsey,dansie,dalman,dakin,dagley,czaja,cybart,cutchin,currington,curbelo,croucher,crinklaw,cremin,cratty,cranfield,crafford,cowher,couvillion,couturier,corter,coombes,contos,consolini,connaughton,conely,collom,cockett,clepper,cleavenger,claro,clarkin,ciriaco,ciesla,cichon,ciancio,cianci,chynoweth,chrzanowski,christion,cholewa,chipley,chilcott,cheyne,cheslock,chenevert,charlot,chagolla,chabolla,cesena,cerutti,cava,caul,cassone,cassin,cassese,casaus,casali,cartledge,cardamone,carcia,carbonneau,carboni,carabello,capozzoli,capella,cannata,campoverde,campeau,cambre,camberos,calvery,calnan,calmes,calley,callery,calise,cacciotti,cacciatore,butterbaugh,burgo,burgamy,burell,bunde,bumbalough,buel,buechner,buchannon,brunn,brost,broadfoot,brittan,brevard,breda,brazel,brayboy,brasier,boyea,boxx,boso,bosio,boruff,borda,bongiovanni,bolerjack,boedeker,blye,blumstein,blumenfeld,blinn,bleakley,blatter,blan,bjornson,bisignano,billick,bieniek,bhatti,bevacqua,berra,berenbaum,bensinger,bennefield,belvins,belson,bellin,beighley,beecroft,beaudreau,baynard,bautch,bausch,basch,bartleson,barthelemy,barak,balzano,balistreri,bailer,bagnall,bagg,auston,augustyn,aslinger,ashalintubbi,arjona,arebalo,appelbaum,angert,angelucci,andry,andersson,amorim,amavisca,alward,alvelo,alvear,alumbaugh,alsobrook,allgeier,allende,aldrete,akiyama,ahlquist,adolphson,addario,acoff,abelson,abasta,zulauf,zirkind,zeoli,zemlicka,zawislak,zappia,zanella,yelvington,yeatman,yanni,wragg,wissing,wischmeier,wirta,wiren,wilmouth,williard,willert,willaert,wildt,whelpley,weingart,weidenbach,weidemann,weatherman,weakland,watwood,wattley,waterson,wambach,walzer,waldow,waag,vorpahl,volkmann,vitolo,visitacion,vincelette,viggiano,vieth,vidana,vert,verges,verdejo,venzon,velardi,varian,vargus,vandermeulen,vandam,vanasse,vanaman,utzinger,uriostegui,uplinger,twiss,tumlinson,tschanz,trunnell,troung,troublefield,trojacek,treloar,tranmer,touchton,torsiello,torina,tootle,toki,toepfer,tippie,thronson,thomes,tezeno,texada,testani,tessmer,terrel,terlizzi,tempel,temblador,tayler,tawil,tasch,tames,talor,talerico,swinderman,sweetland,swager,sulser,sullens,subia,sturgell,stumpff,stufflebeam,stucki,strohmeyer,strebel,straughan,strackbein,stobaugh,stetz,stelter,steinmann,steinfeld,stecher,stanwood,stanislawski,stander,speziale,soppe,soni,sobotka,smuin,slee,skerrett,sjoberg,sittig,simonelli,simo,silverio,silveria,silsby,sillman,sienkiewicz,shomo,shoff,shoener,shiba,sherfey,shehane,sexson,setton,sergi,selvy,seiders,seegmiller,sebree,seabury,scroggin,sconyers,schwalb,schurg,schulenberg,schuld,schrage,schow,schon,schnur,schneller,schmidtke,schlatter,schieffer,schenkel,scheeler,schauwecker,schartz,schacherer,scafe,sayegh,savidge,saur,sarles,sarkissian,sarkis,sarcone,sagucio,saffell,saenger,sacher,rylee,ruvolo,ruston,ruple,rulison,ruge,ruffo,ruehl,rueckert,rudman,rudie,rubert,rozeboom,roysden,roylance,rothchild,rosse,rosecrans,rodi,rockmore,robnett,roberti,rivett,ritzel,rierson,ricotta,ricken,rezac,rendell,reitman,reindl,reeb,reddic,reddell,rebuck,reali,raso,ramthun,ramsden,rameau,ralphs,rago,racz,quinteros,quinter,quinley,quiggle,purvines,purinton,purdum,pummill,puglia,puett,ptacek,przybyla,prowse,prestwich,pracht,poutre,poucher,portera,polinsky,poage,platts,pineau,pinckard,pilson,pilling,pilkins,pili,pikes,pigram,pietila,pickron,philippi,philhower,pflueger,pfalzgraf,pettibone,pett,petrosino,persing,perrino,perotti,periera,peri,peredo,peralto,pennywell,pennel,pellegren,pella,pedroso,paulos,paulding,pates,pasek,paramo,paolino,panganiban,paneto,paluch,ozaki,ownbey,overfelt,outman,opper,onstad,oland,okuda,oertel,oelke,normandeau,nordby,nordahl,noecker,noblin,niswonger,nishioka,nett,negley,nedeau,natera,nachman,naas,musich,mungin,mourer,mounsey,mottola,mothershed,moskal,mosbey,morini,moreles,montaluo,moneypenny,monda,moench,moates,moad,missildine,misiewicz,mirabella,minott,mincks,milum,milani,mikelson,mestayer,mertes,merrihew,merlos,meritt,melnyk,medlen,meder,mcvea,mcquarrie,mcquain,mclucas,mclester,mckitrick,mckennon,mcinnes,mcgrory,mcgranahan,mcglamery,mcgivney,mcgilvray,mccuiston,mccuin,mccrystal,mccolley,mcclerkin,mcclenon,mccamey,mcaninch,mazariegos,maynez,mattioli,mastronardi,masone,marzett,marsland,margulies,margolin,malatesta,mainer,maietta,magrath,maese,madkins,madeiros,madamba,mackson,maben,lytch,lundgreen,lumb,lukach,luick,luetkemeyer,luechtefeld,ludy,ludden,luckow,lubinsky,lowes,lorenson,loran,lopinto,looby,lones,livsey,liskey,lisby,lintner,lindow,lindblom,liming,liechty,leth,lesniewski,lenig,lemonds,leisy,lehrer,lehnen,lehmkuhl,leeth,leeks,lechler,lebsock,lavere,lautenschlage,laughridge,lauderback,laudenslager,lassonde,laroque,laramee,laracuente,lapeyrouse,lampron,lamers,laino,lague,lafromboise,lafata,lacount,lachowicz,kysar,kwiecien,kuffel,kueter,kronenberg,kristensen,kristek,krings,kriesel,krey,krebbs,kreamer,krabbe,kossman,kosakowski,kosak,kopacz,konkol,koepsell,koening,koen,knerr,knapik,kluttz,klocke,klenk,klemme,klapp,kitchell,kita,kissane,kirkbride,kirchhoff,kinter,kinsel,kingsland,kimmer,kimler,killoran,kieser,khalsa,khalaf,kettel,kerekes,keplin,kentner,kennebrew,kenison,kellough,keatts,keasey,kauppi,katon,kanner,kampa,kall,kaczorowski,kaczmarski,juarbe,jordison,jobst,jezierski,jeanbart,jarquin,jagodzinski,ishak,isett,infantino,imburgia,illingworth,hysmith,hynson,hydrick,hurla,hunton,hunnell,humbertson,housand,hottle,hosch,hoos,honn,hohlt,hodel,hochmuth,hixenbaugh,hislop,hisaw,hintzen,hilgendorf,hilchey,higgens,hersman,herrara,hendrixson,hendriks,hemond,hemmingway,heminger,helgren,heisey,heilmann,hehn,hegna,heffern,hawrylak,haverty,hauger,haslem,harnett,harb,happ,hanzlik,hanway,hanby,hanan,hamric,hammaker,halas,hagenbuch,habeck,gwozdz,gunia,guadarrama,grubaugh,grivas,griffieth,grieb,grewell,gregorich,grazier,graeber,graciano,gowens,goodpaster,gondek,gohr,goffney,godbee,gitlin,gisler,gillyard,gillooly,gilchrest,gilbo,gierlach,giebler,giang,geske,gervasio,gertner,gehling,geeter,gaus,gattison,gatica,gathings,gath,gassner,gassert,garabedian,gamon,gameros,galban,gabourel,gaal,fuoco,fullenwider,fudala,friscia,franceschini,foronda,fontanilla,florey,flore,flegle,flecha,fisler,fischbach,fiorita,figura,figgins,fichera,ferra,fawley,fawbush,fausett,farnes,farago,fairclough,fahie,fabiani,evanson,eutsey,eshbaugh,ertle,eppley,englehardt,engelhard,emswiler,elling,elderkin,eland,efaw,edstrom,edgemon,ecton,echeverri,ebright,earheart,dynes,dygert,dyches,dulmage,duhn,duhamel,dubrey,dubray,dubbs,drey,drewery,dreier,dorval,dorough,dorais,donlin,donatelli,dohm,doetsch,dobek,disbrow,dinardi,dillahunty,dillahunt,diers,dier,diekmann,diangelo,deskin,deschaine,depaoli,denner,demyan,demont,demaray,delillo,deleeuw,deibel,decato,deblasio,debartolo,daubenspeck,darner,dardon,danziger,danials,damewood,dalpiaz,dallman,dallaire,cunniffe,cumpston,cumbo,cubero,cruzan,cronkhite,critelli,crimi,creegan,crean,craycraft,cranfill,coyt,courchesne,coufal,corradino,corprew,colville,cocco,coby,clinch,clickner,clavette,claggett,cirigliano,ciesielski,christain,chesbro,chavera,chard,casteneda,castanedo,casseus,caruana,carnero,cappelli,capellan,canedy,cancro,camilleri,calero,cada,burghart,burbidge,bulfer,buis,budniewski,bruney,brugh,brossard,brodmerkel,brockmann,brigmond,briere,bremmer,breck,breau,brautigam,brasch,brandenberger,bragan,bozell,bowsher,bosh,borgia,borey,boomhower,bonneville,bonam,bolland,boise,boeve,boettger,boersma,boateng,bliven,blazier,blahnik,bjornstad,bitton,biss,birkett,billingsly,biagioni,bettle,bertucci,bertolino,bermea,bergner,berber,bensley,bendixen,beltrami,bellone,belland,behringer,begum,bayona,batiz,bassin,baskette,bartolomeo,bartolo,bartholow,barkan,barish,barett,bardo,bamburg,ballerini,balla,balis,bakley,bailon,bachicha,babiarz,ayars,axton,axel,awong,awalt,auslander,ausherman,aumick,atha,atchinson,aslett,askren,arrowsmith,arras,arnhold,armagost,arey,arcos,archibeque,antunes,antilla,andras,amyx,amison,amero,alzate,alper,aller,alioto,aigner,agtarap,agbayani,adami,achorn,aceuedo,acedo,abundis,aber,abee,zuccaro,ziglar,zier,ziebell,zieba,zamzow,zahl,yurko,yurick,yonkers,yerian,yeaman,yarman,yann,yahn,yadon,yadao,woodbridge,wolske,wollenberg,wojtczak,wnuk,witherite,winther,winick,widell,wickens,whichard,wheelis,wesely,wentzell,wenthold,wemple,weisenburger,wehling,weger,weaks,wassink,walquist,wadman,wacaster,waage,voliva,vlcek,villafana,vigliotti,viger,viernes,viands,veselka,versteeg,vero,verhoeven,vendetti,velardo,vatter,vasconcellos,varn,vanwagner,vanvoorhis,vanhecke,vanduyn,vandervoort,vanderslice,valone,vallier,vails,uvalle,ursua,urenda,uphoff,tustin,turton,turnbough,turck,tullio,tuch,truehart,tropea,troester,trippe,tricarico,trevarthen,trembly,trabue,traber,tosi,toal,tinley,tingler,timoteo,tiffin,ticer,thorman,therriault,theel,tessman,tekulve,tejera,tebbs,tavernia,tarpey,tallmadge,takemoto,szot,sylvest,swindoll,swearinger,swantek,swaner,swainston,susi,surrette,sullenger,sudderth,suddarth,suckow,strege,strassburg,stoval,stotz,stoneham,stilley,stille,stierwalt,stfleur,steuck,stermer,stclaire,stano,staker,stahler,stablein,srinivasan,squillace,sprvill,sproull,sprau,sporer,spore,spittler,speelman,sparr,sparkes,spang,spagnuolo,sosinski,sorto,sorkin,sondag,sollers,socia,snarr,smrekar,smolka,slyter,slovinsky,sliwa,slavik,slatter,skiver,skeem,skala,sitzes,sitsler,sitler,sinko,simser,siegler,sideris,shrewsberry,shoopman,shoaff,shindler,shimmin,shill,shenkel,shemwell,shehorn,severa,semones,selsor,sekulski,segui,sechrest,schwer,schwebach,schur,schmiesing,schlick,schlender,schebler,schear,schapiro,sauro,saunder,sauage,satterly,saraiva,saracino,saperstein,sanmartin,sanluis,sandt,sandrock,sammet,sama,salk,sakata,saini,sackrider,russum,russi,russaw,rozzell,roza,rowlette,rothberg,rossano,rosebrock,romanski,romanik,romani,roiger,roig,roehr,rodenberger,rodela,rochford,ristow,rispoli,rigo,riesgo,riebel,ribera,ribaudo,reys,resendes,repine,reisdorf,reisch,rebman,rasmus,raske,ranum,rames,rambin,raman,rajewski,raffield,rady,radich,raatz,quinnie,pyper,puthoff,prow,proehl,pribyl,pretti,prete,presby,poyer,powelson,porteous,poquette,pooser,pollan,ploss,plewa,placide,pion,pinnick,pinales,pillot,pille,pilato,piggee,pietrowski,piermarini,pickford,piccard,phenix,pevey,petrowski,petrillose,pesek,perrotti,peppler,peppard,penfold,pellitier,pelland,pehowic,pedretti,paules,passero,pasha,panza,pallante,palau,pakele,pacetti,paavola,overy,overson,outler,osegueda,oplinger,oldenkamp,ohern,oetting,odums,nowlen,nowack,nordlund,noblett,nobbe,nierman,nichelson,niblock,newbrough,nemetz,needleman,navin,nastasi,naslund,naramore,nakken,nakanishi,najarro,mushrush,muma,mulero,morganfield,moreman,morain,moquin,monterrosa,monsivais,monroig,monje,monfort,moffa,moeckel,mobbs,misiak,mires,mirelez,mineo,mineau,milnes,mikeska,michelin,michalowski,meszaros,messineo,meshell,merten,meola,menton,mends,mende,memmott,melius,mehan,mcnickle,mcmorran,mclennon,mcleish,mclaine,mckendry,mckell,mckeighan,mcisaac,mcie,mcguinn,mcgillis,mcfatridge,mcfarling,mcelravy,mcdonalds,mcculla,mcconnaughy,mcconnaughey,mcchriston,mcbeath,mayr,matyas,matthiesen,matsuura,matinez,mathys,matarazzo,masker,masden,mascio,martis,marrinan,marinucci,margerum,marengo,manthe,mansker,manoogian,mankey,manigo,manier,mangini,maltese,malsam,mallo,maliszewski,mainolfi,maharaj,maggart,magar,maffett,macmaster,macky,macdonnell,lyvers,luzzi,lutman,lovan,lonzo,longerbeam,lofthouse,loethen,lodi,llorens,lizama,litscher,lisowski,lipski,lipsett,lipkin,linzey,lineman,limerick,limas,lige,lierman,liebold,liberti,leverton,levene,lesueur,lenser,lenker,legnon,lefrancois,ledwell,lavecchia,laurich,lauricella,lannigan,landor,lamprecht,lamountain,lamore,lammert,lamboy,lamarque,lamacchia,lalley,lagace,lacorte,lacomb,kyllonen,kyker,kuschel,kupfer,kunde,kucinski,kubacki,kroenke,krech,koziel,kovacich,kothari,koth,kotek,kostelnik,kosloski,knoles,knabe,kmiecik,klingman,kliethermes,kleffman,klees,klaiber,kittell,kissling,kisinger,kintner,kinoshita,kiener,khouri,kerman,kelii,keirn,keezer,kaup,kathan,kaser,karlsen,kapur,kandoll,kammel,kahele,justesen,jonason,johnsrud,joerling,jochim,jespersen,jeong,jenness,jedlicka,jakob,isaman,inghram,ingenito,iadarola,hynd,huxtable,huwe,hurless,humpal,hughston,hughart,huggett,hugar,huether,howdyshell,houtchens,houseworth,hoskie,holshouser,holmen,holloran,hohler,hoefler,hodsdon,hochman,hjort,hippert,hippe,hinzman,hillock,hilden,heyn,heyden,heyd,hergert,henrikson,henningsen,hendel,helget,helf,helbing,heintzman,heggie,hege,hecox,heatherington,heare,haxton,haverstock,haverly,hatler,haselton,hase,hartzfeld,harten,harken,hargrow,haran,hanton,hammar,hamamoto,halper,halko,hackathorn,haberle,haake,gunnoe,gunkel,gulyas,guiney,guilbeau,guider,guerrant,gudgel,guarisco,grossen,grossberg,gropp,groome,grobe,gremminger,greenley,grauberger,grabenstein,gowers,gostomski,gosier,goodenow,gonzoles,goliday,goettle,goens,goates,glymph,glavin,glassco,gladfelter,glackin,githens,girgis,gimpel,gilbreth,gilbeau,giffen,giannotti,gholar,gervasi,gertsch,gernatt,gephardt,genco,gehr,geddis,gase,garrott,garrette,gapinski,ganter,ganser,gangi,gangemi,gallina,galdi,gailes,gaetano,gadomski,gaccione,fuschetto,furtick,furfaro,fullman,frutos,fruchter,frogge,freytag,freudenthal,fregoe,franzone,frankum,francia,franceschi,forys,forero,folkers,flug,flitter,flemons,fitzer,firpo,finizio,filiault,figg,fichtner,fetterolf,ferringer,feil,fayne,farro,faddis,ezzo,ezelle,eynon,evitt,eutsler,euell,escovedo,erne,eriksson,enriguez,empson,elkington,eisenmenger,eidt,eichenberger,ehrmann,ediger,earlywine,eacret,duzan,dunnington,ducasse,dubiel,drovin,drager,drage,donham,donat,dolinger,dokken,doepke,dodwell,docherty,distasio,disandro,diniz,digangi,didion,dezzutti,detmer,deshon,derrigo,dentler,demoura,demeter,demeritt,demayo,demark,demario,delzell,delnero,delgrosso,dejarnett,debernardi,dearmas,dashnaw,daris,danks,danker,dangler,daignault,dafoe,dace,curet,cumberledge,culkin,crowner,crocket,crawshaw,craun,cranshaw,cragle,courser,costella,cornforth,corkill,coopersmith,conzemius,connett,connely,condict,condello,comley,cohoon,coday,clugston,clowney,clippard,clinkenbeard,clines,clelland,clapham,clancey,clabough,cichy,cicalese,chua,chittick,chisom,chisley,chinchilla,cheramie,cerritos,cercone,cena,cawood,cavness,catanzarite,casada,carvell,carmicheal,carll,cardozo,caplin,candia,canby,cammon,callister,calligan,calkin,caillouet,buzzelli,bute,bustillo,bursey,burgeson,bupp,bulson,buist,buffey,buczkowski,buckbee,bucio,brueckner,broz,brookhart,brong,brockmeyer,broberg,brittenham,brisbois,bridgmon,breyer,brede,breakfield,breakey,brauner,branigan,brandewie,branche,brager,brader,bovell,bouthot,bostock,bosma,boseman,boschee,borthwick,borneman,borer,borek,boomershine,boni,bommarito,bolman,boleware,boisse,boehlke,bodle,blash,blasco,blakesley,blacklock,blackley,bittick,birks,birdin,bircher,bilbao,bick,biby,bertoni,bertino,bertini,berson,bern,berkebile,bergstresser,benne,benevento,belzer,beltre,bellomo,bellerose,beilke,begeman,bebee,beazer,beaven,beamish,baymon,baston,bastidas,basom,basey,bartles,baroni,barocio,barnet,barclift,banville,balthazor,balleza,balkcom,baires,bailie,baik,baggott,bagen,bachner,babington,babel,asmar,arvelo,artega,arrendondo,arreaga,arrambide,arquette,aronoff,arico,argentieri,arevalos,archbold,apuzzo,antczak,ankeny,angelle,angelini,anfinson,amer,amarillas,altier,altenburg,alspach,alosa,allsbrook,alexopoulos,aleem,aldred,albertsen,akerson,agler,adley,addams,acoba,achille,abplanalp,abella,abare,zwolinski,zollicoffer,zins,ziff,zenner,zender,zelnick,zelenka,zeches,zaucha,zauala,zangari,zagorski,youtsey,yasso,yarde,yarbough,woolever,woodsmall,woodfolk,wobig,wixson,wittwer,wirtanen,winson,wingerd,wilkening,wilhelms,wierzbicki,wiechman,weyrick,wessell,wenrick,wenning,weltz,weinrich,weiand,wehunt,wareing,walth,waibel,wahlquist,vona,voelkel,vitek,vinsant,vincente,vilar,viel,vicars,vermette,verma,venner,veazie,vayda,vashaw,varon,vardeman,vandevelde,vanbrocklin,vaccarezza,urquidez,urie,urbach,uram,ungaro,umali,ulsh,tutwiler,turnbaugh,tumminello,tuite,tueller,trulove,troha,trivino,trisdale,trippett,tribbett,treptow,tremain,travelstead,trautwein,trautmann,tram,traeger,tonelli,tomsic,tomich,tomasulo,tomasino,tole,todhunter,toborg,tischer,tirpak,tircuit,tinnon,tinnel,tines,timbs,tilden,tiede,thumm,throgmorton,thorndike,thornburgh,thoren,thomann,therrell,thau,thammavong,tetrick,tessitore,tesreau,teicher,teaford,tauscher,tauer,tanabe,talamo,takeuchi,taite,tadych,sweeton,swecker,swartzentrube,swarner,surrell,surbaugh,suppa,sumbry,suchy,stuteville,studt,stromer,strome,streng,stonestreet,stockley,stmichel,stfort,sternisha,stensrud,steinhardt,steinback,steichen,stauble,stasiak,starzyk,stango,standerfer,stachowiak,springston,spratlin,spracklen,sponseller,spilker,spiegelman,spellacy,speiser,spaziani,spader,spackman,sorum,sopha,sollis,sollenberger,solivan,solheim,sokolsky,sogge,smyser,smitley,sloas,slinker,skora,skiff,skare,siverd,sivels,siska,siordia,simmering,simko,sime,silmon,silano,sieger,siebold,shukla,shreves,shoun,shortle,shonkwiler,shoals,shimmel,shiel,shieh,sherbondy,shenkman,shein,shearon,shean,shatz,shanholtz,shafran,shaff,shackett,sgroi,sewall,severy,sethi,sessa,sequra,sepulvado,seper,senteno,sendejo,semmens,seipp,segler,seegers,sedwick,sedore,sechler,sebastiano,scovel,scotton,scopel,schwend,schwarting,schutter,schrier,schons,scholtes,schnetzer,schnelle,schmutz,schlichter,schelling,schams,schamp,scarber,scallan,scalisi,scaffidi,saxby,sawrey,sauvageau,sauder,sarrett,sanzo,santizo,santella,santander,sandez,sandel,sammon,salsedo,salge,sagun,safi,sader,sacchetti,sablan,saade,runnion,runkel,rumbo,ruesch,ruegg,ruckle,ruchti,rubens,rubano,rozycki,roupe,roufs,rossel,rosmarin,rosero,rosenwald,ronca,romos,rolla,rohling,rohleder,roell,roehm,rochefort,roch,robotham,rivenburgh,riopel,riederer,ridlen,rias,rhudy,reynard,retter,respess,reppond,repko,rengifo,reinking,reichelt,reeh,redenius,rebolledo,rauh,ratajczak,rapley,ranalli,ramie,raitt,radloff,radle,rabbitt,quay,quant,pusateri,puffinberger,puerta,provencio,proano,privitera,prenger,prellwitz,pousson,potier,portz,portlock,porth,portela,portee,porchia,pollick,polinski,polfer,polanski,polachek,pluta,plourd,plauche,pitner,piontkowski,pileggi,pierotti,pico,piacente,phinisee,phaup,pfost,pettinger,pettet,petrich,peto,persley,persad,perlstein,perko,pere,penders,peifer,peco,pawley,pash,parrack,parady,papen,pangilinan,pandolfo,palone,palmertree,padin,ottey,ottem,ostroski,ornstein,ormonde,onstott,oncale,oltremari,olcott,olan,oishi,oien,odonell,odonald,obeso,obeirne,oatley,nusser,novo,novicki,nitschke,nistler,nikkel,niese,nierenberg,nield,niedzwiecki,niebla,niebel,nicklin,neyhart,newsum,nevares,nageotte,nagai,mutz,murata,muralles,munnerlyn,mumpower,muegge,muckle,muchmore,moulthrop,motl,moskos,mortland,morring,mormile,morimoto,morikawa,morgon,mordecai,montour,mont,mongan,monell,miyasato,mish,minshew,mimbs,millin,milliard,mihm,middlemiss,miano,mesick,merlan,mendonsa,mench,melonson,melling,meachem,mctighe,mcnelis,mcmurtrey,mckesson,mckenrick,mckelvie,mcjunkins,mcgory,mcgirr,mcgeever,mcfield,mcelhinney,mccrossen,mccommon,mccannon,mazyck,mawyer,maull,matute,mathies,maschino,marzan,martinie,marrotte,marmion,markarian,marinacci,margolies,margeson,marak,maraia,maracle,manygoats,manker,mank,mandich,manderson,maltz,malmquist,malacara,majette,magnan,magliocca,madina,madara,macwilliams,macqueen,maccallum,lyde,lyday,lutrick,lurz,lurvey,lumbreras,luhrs,luhr,lowrimore,lowndes,lourenco,lougee,lorona,longstreth,loht,lofquist,loewenstein,lobos,lizardi,lionberger,limoli,liljenquist,liguori,liebl,liburd,leukhardt,letizia,lesinski,lepisto,lenzini,leisenring,leipold,leier,leggitt,legare,leaphart,lazor,lazaga,lavey,laue,laudermilk,lauck,lassalle,larsson,larison,lanzo,lantzy,lanners,langtry,landford,lancour,lamour,lambertson,lalone,lairson,lainhart,lagreca,lacina,labranche,labate,kurtenbach,kuipers,kuechle,kubo,krinsky,krauser,kraeger,kracht,kozeliski,kozar,kowalik,kotler,kotecki,koslosky,kosel,koob,kolasinski,koizumi,kohlman,koffman,knutt,knore,knaff,kmiec,klamm,kittler,kitner,kirkeby,kiper,kindler,kilmartin,kilbride,kerchner,kendell,keddy,keaveney,kearsley,karlsson,karalis,kappes,kapadia,kallman,kallio,kalil,kader,jurkiewicz,jitchaku,jillson,jeune,jarratt,jarchow,janak,ivins,ivans,isenhart,inocencio,inoa,imhof,iacono,hynds,hutching,hutchin,hulsman,hulsizer,hueston,huddleson,hrbek,howry,housey,hounshell,hosick,hortman,horky,horine,hootman,honeywell,honeyestewa,holste,holien,holbrooks,hoffmeyer,hoese,hoenig,hirschfeld,hildenbrand,higson,higney,hibert,hibbetts,hewlin,hesley,herrold,hermon,hepker,henwood,helbling,heinzman,heidtbrink,hedger,havey,hatheway,hartshorne,harpel,haning,handelman,hamalainen,hamad,halasz,haigwood,haggans,hackshaw,guzzo,gundrum,guilbeault,gugliuzza,guglielmi,guderian,gruwell,grunow,grundman,gruen,grotzke,grossnickle,groomes,grode,grochowski,grob,grein,greif,greenwall,greenup,grassl,grannis,grandfield,grames,grabski,grabe,gouldsberry,gosch,goodling,goodermote,gonzale,golebiowski,goldson,godlove,glanville,gillin,gilkerson,giessler,giambalvo,giacomini,giacobbe,ghio,gergen,gentz,genrich,gelormino,gelber,geitner,geimer,gauthreaux,gaultney,garvie,gareau,garbacz,ganoe,gangwer,gandarilla,galyen,galt,galluzzo,galardo,gager,gaddie,gaber,gabehart,gaarder,fusilier,furnari,furbee,fugua,fruth,frohman,friske,frilot,fridman,frescas,freier,frayer,franzese,frankenberry,frain,fosse,foresman,forbess,flook,fletes,fleer,fleek,fleegle,fishburne,fiscalini,finnigan,fini,filipiak,figueira,fiero,ficek,fiaschetti,ferren,ferrando,ferman,fergusson,fenech,feiner,feig,faulds,fariss,falor,falke,ewings,eversley,everding,etling,essen,erskin,enstrom,engebretsen,eitel,eichberger,ehler,eekhoff,edrington,edmonston,edgmon,edes,eberlein,dwinell,dupee,dunklee,dungey,dunagin,dumoulin,duggar,duenez,dudzic,dudenhoeffer,ducey,drouillard,dreibelbis,dreger,dreesman,draughon,downen,dorminy,dombeck,dolman,doebler,dittberner,dishaw,disanti,dinicola,dinham,dimino,dilling,difrancesco,dicello,dibert,deshazer,deserio,descoteau,deruyter,dering,depinto,dente,demus,demattos,demarsico,delude,dekok,debrito,debois,deakin,dayley,dawsey,dauria,datson,darty,darsow,darragh,darensbourg,dalleva,dalbec,dadd,cutcher,cung,cuello,cuadros,crute,crutchley,crispino,crislip,crisco,crevier,creekmur,crance,cragg,crager,cozby,coyan,coxon,covalt,couillard,costley,costilow,cossairt,corvino,corigliano,cordaro,corbridge,corban,coor,conkel,conary,coltrain,collopy,colgin,colen,colbath,coiro,coffie,cochrum,cobbett,clopper,cliburn,clendenon,clemon,clementi,clausi,cirino,cina,churchman,chilcutt,cherney,cheetham,cheatom,chatelain,chalifour,cesa,cervenka,cerullo,cerreta,cerbone,cecchini,ceccarelli,cawthorn,cavalero,castner,castlen,castine,casimiro,casdorph,cartmill,cartmell,carro,carriger,carias,caravella,cappas,capen,cantey,canedo,camuso,campanaro,cambria,calzado,callejo,caligiuri,cafaro,cadotte,cacace,byrant,busbey,burtle,burres,burnworth,burggraf,burback,bunte,bunke,bulle,bugos,budlong,buckhalter,buccellato,brummet,bruff,brubeck,brouk,broten,brosky,broner,brislin,brimm,brillhart,bridgham,brideau,brennecke,breer,breeland,bredesen,brackney,brackeen,boza,boyum,bowdry,bowdish,bouwens,bouvier,bougie,bouche,bottenfield,bostian,bossie,bosler,boschert,boroff,borello,bonser,bonfield,bole,boldue,bogacz,boemer,bloxom,blickenstaff,blessinger,bleazard,blatz,blanchet,blacksher,birchler,binning,binkowski,biltz,bilotta,bilagody,bigbee,bieri,biehle,bidlack,betker,bethers,bethell,bero,bernacchi,bermingham,berkshire,benvenuto,bensman,benoff,bencivenga,beman,bellow,bellany,belflower,belch,bekker,bejar,beisel,beichner,beedy,beas,beanblossom,bawek,baus,baugus,battie,battershell,bateson,basque,basford,bartone,barritt,barko,bann,bamford,baltrip,balon,balliew,ballam,baldus,ayling,avelino,ashwell,ashland,arseneau,arroyos,armendarez,arita,argust,archuletta,arcement,antonacci,anthis,antal,annan,anderman,amster,amiri,amadon,alveraz,altomari,altmann,altenhofen,allers,allbee,allaway,aleo,alcoser,alcorta,akhtar,ahuna,agramonte,agard,adkerson,achord,abdi,abair,zurn,zoellner,zirk,zion,zarro,zarco,zambo,zaiser,zaino,zachry,youd,yonan,yniguez,yepes,yellock,yellen,yeatts,yearling,yatsko,yannone,wyler,woodridge,wolfrom,wolaver,wolanin,wojnar,wojciak,wittmann,wittich,wiswell,wisser,wintersteen,wineland,willford,wiginton,wigfield,wierman,wice,wiater,whitsel,whitbread,wheller,wettstein,werling,wente,wenig,wempe,welz,weinhold,weigelt,weichman,wedemeyer,weddel,wayment,waycaster,wauneka,watzka,watton,warnell,warnecke,warmack,warder,wands,waldvogel,waldridge,wahs,wagganer,waddill,vyas,vought,votta,voiles,virga,viner,villella,villaverde,villaneda,viele,vickroy,vicencio,vetere,vermilyea,verley,verburg,ventresca,veno,venard,venancio,velaquez,veenstra,vasil,vanzee,vanwie,vantine,vant,vanschoyck,vannice,vankampen,vanicek,vandersloot,vanderpoel,vanderlinde,vallieres,uzzell,uzelac,uranga,uptain,updyke,uong,untiedt,umbrell,umbaugh,umbarger,ulysse,ullmann,ullah,tutko,turturro,turnmire,turnley,turcott,turbyfill,turano,tuminello,tumbleson,tsou,truscott,trulson,troutner,trone,trinklein,tremmel,tredway,trease,traynham,traw,totty,torti,torregrossa,torok,tomkins,tomaino,tkach,tirey,tinsman,timpe,tiefenauer,tiedt,tidball,thwaites,thulin,throneburg,thorell,thorburn,thiemann,thieman,thesing,tham,terrien,telfair,taybron,tasson,tasso,tarro,tanenbaum,taddeo,taborn,tabios,szekely,szatkowski,sylve,swineford,swartzfager,swanton,swagerty,surrency,sunderlin,sumerlin,suero,suddith,sublette,stumpe,stueve,stuckert,strycker,struve,struss,strubbe,strough,strothmann,strahle,stoutner,stooksbury,stonebarger,stokey,stoffer,stimmel,stief,stephans,stemper,steltenpohl,stellato,steinle,stegeman,steffler,steege,steckman,stapel,stansbery,stanaland,stahley,stagnaro,stachowski,squibb,sprunger,sproule,sprehe,spreen,sprecher,sposato,spivery,souter,sopher,sommerfeldt,soffer,snowberger,snape,smylie,smyer,slaydon,slatton,slaght,skovira,skeans,sjolund,sjodin,siragusa,singelton,silis,siebenaler,shuffield,shobe,shiring,shimabukuro,shilts,sherbert,shelden,sheil,shedlock,shearn,shaub,sharbono,shapley,shands,shaheen,shaffner,servantez,sentz,seney,selin,seitzinger,seider,sehr,sego,segall,sebastien,scimeca,schwenck,schweiss,schwark,schwalbe,schucker,schronce,schrag,schouten,schoppe,schomaker,schnarr,schmied,schmader,schlicht,schlag,schield,schiano,scheve,scherbarth,schaumburg,schauman,scarpino,savinon,sassaman,saporito,sanville,santilli,santaana,salzmann,salman,sagraves,safran,saccone,rutty,russett,rupard,rumbley,ruffins,ruacho,rozema,roxas,routson,rourk,rought,rotunda,rotermund,rosman,rork,rooke,rolin,rohm,rohlman,rohl,roeske,roecker,rober,robenson,riso,rinne,riina,rigsbee,riggles,riester,rials,rhinehardt,reynaud,reyburn,rewis,revermann,reutzel,retz,rende,rendall,reistad,reinders,reichardt,rehrig,rehrer,recendez,reamy,rauls,ratz,rattray,rasband,rapone,ragle,ragins,radican,raczka,rachels,raburn,rabren,raboin,quesnell,quaintance,puccinelli,pruner,prouse,prosise,proffer,prochazka,probasco,previte,portell,porcher,popoca,pomroy,poma,polsky,polsgrove,polidore,podraza,plymale,plescia,pleau,platte,pizzi,pinchon,picot,piccione,picazo,philibert,phebus,pfohl,petell,pesso,pesante,pervis,perrins,perley,perkey,pereida,penate,peloso,pellerito,peffley,peddicord,pecina,peale,payette,paxman,pawlikowski,pavy,patry,patmon,patil,pater,patak,pasqua,pasche,partyka,parody,parmeter,pares,pardi,paonessa,panozzo,panameno,paletta,pait,oyervides,ossman,oshima,ortlieb,orsak,onley,oldroyd,okano,ohora,offley,oestreicher,odonovan,odham,odegard,obst,obriant,obrecht,nuccio,nowling,nowden,novelli,nost,norstrom,nordgren,nopper,noller,nisonger,niskanen,nienhuis,nienaber,neuwirth,neumeyer,neice,naugher,naiman,nagamine,mustin,murrietta,murdaugh,munar,muhlbauer,mroczkowski,mowdy,mouw,mousel,mountcastle,moscowitz,mosco,morro,moresi,morago,moomaw,montroy,montpas,montieth,montanaro,mongelli,mollison,mollette,moldovan,mohar,mitchelle,mishra,misenheimer,minshall,minozzi,minniefield,milhous,migliaccio,migdal,mickell,meyering,methot,mester,mesler,meriweather,mensing,mensah,menge,mendibles,meloche,melnik,mellas,meinert,mehrhoff,medas,meckler,mctague,mcspirit,mcshea,mcquown,mcquiller,mclarney,mckiney,mckearney,mcguyer,mcfarlan,mcfadyen,mcdanial,mcdanel,mccurtis,mccrohan,mccorry,mcclune,mccant,mccanna,mccandlish,mcaloon,mayall,maver,maune,matza,matsuzaki,matott,mathey,mateos,masoner,masino,marzullo,marz,marsolek,marquard,marchetta,marberry,manzione,manthei,manka,mangram,mangle,mangel,mandato,mancillas,mammen,malina,maletta,malecki,majkut,mages,maestre,macphail,maco,macneill,macadam,lysiak,lyne,luxton,luptak,lundmark,luginbill,lovallo,louthan,lousteau,loupe,lotti,lopresto,lonsdale,longsworth,lohnes,loghry,logemann,lofaro,loeber,locastro,livings,litzinger,litts,liotta,lingard,lineback,lindhorst,lill,lide,lickliter,liberman,lewinski,levandowski,leimbach,leifer,leidholt,leiby,leibel,leibee,lehrke,lehnherr,lego,leese,leen,ledo,lech,leblond,leahey,lazzari,lawrance,lawlis,lawhorne,lawes,lavigna,lavell,lauzier,lauter,laumann,latsha,latourette,latona,latney,laska,larner,larmore,larke,larence,lapier,lanzarin,lammey,lamke,laminack,lamastus,lamaster,lacewell,labarr,laabs,kutch,kuper,kuna,kubis,krzemien,krupinski,krepps,kreeger,kraner,krammer,kountz,kothe,korpela,komara,kolenda,kolek,kohnen,koelzer,koelsch,kocurek,knoke,knauff,knaggs,knab,kluver,klose,klien,klahr,kitagawa,kissler,kirstein,kinnon,kinnebrew,kinnamon,kimmins,kilgour,kilcoyne,kiester,kiehm,kesselring,kerestes,kenniston,kennamore,kenebrew,kelderman,keitel,kefauver,katzenberger,katt,kast,kassel,kamara,kalmbach,kaizer,kaiwi,kainz,jurczyk,jumonville,juliar,jourdain,johndrow,johanning,johannesen,joffrion,jobes,jerde,jentzsch,jenkens,jendro,jellerson,jefferds,jaure,jaquish,janeway,jago,iwasaki,ishman,isaza,inmon,inlow,inclan,ildefonso,iezzi,ianni,iacovetto,hyldahl,huxhold,huser,humpherys,humburg,hult,hullender,hulburt,huckabay,howeth,hovermale,hoven,houtman,hourigan,hosek,hopgood,homrich,holstine,holsclaw,hokama,hoffpauir,hoffner,hochstein,hochstatter,hochberg,hjelm,hiscox,hinsley,hineman,hineline,hinck,hilbun,hewins,herzing,hertzberg,hertenstein,herrea,herington,henrie,henman,hengst,hemmen,helmke,helgerson,heinsohn,heigl,hegstad,heggen,hegge,hefti,heathcock,haylett,haupert,haufler,hatala,haslip,hartless,hartje,hartis,harpold,harmsen,harbach,hanten,hanington,hammen,hameister,hallstrom,habersham,habegger,gussman,gundy,guitterez,guisinger,guilfoyle,groulx,grismer,griesbach,grawe,grall,graben,goulden,gornick,gori,gookin,gonzalaz,gonyer,gonder,golphin,goller,goergen,glosson,glor,gladin,girdler,gillim,gillians,gillaspie,gilhooly,gildon,gignac,gibler,gibbins,giardino,giampietro,gettman,gerringer,gerrald,gerlich,georgiou,georgi,geiselman,gehman,gangl,gamage,gallian,gallen,gallatin,galea,gainor,gahr,furbush,fulfer,fuhrmann,fritter,friis,friedly,freudenberger,freemon,fratus,frans,foulke,fosler,forquer,fontan,folwell,foeller,fodge,fobes,florek,fliss,flesner,flegel,fitzloff,fiser,firmin,firestine,finfrock,fineberg,fiegel,fickling,fesperman,fernadez,felber,feimster,feazel,favre,faughn,fatula,fasone,farron,faron,farino,falvey,falkenberg,faley,faletti,faeth,fackrell,espe,eskola,escott,esaw,erps,erker,erath,enfield,emfinger,embury,embleton,emanuele,elvers,ellwanger,ellegood,eichinger,egge,egeland,edgett,echard,eblen,eastmond,duteau,durland,dure,dunlavy,dungee,dukette,dugay,duboise,dubey,dsouza,druck,dralle,doubek,dorta,dorch,dorce,dopson,dolney,dockter,distler,dippel,dichiara,dicerbo,dewindt,dewan,deveney,devargas,deutscher,deuel,detter,dess,derrington,deroberts,dern,deponte,denogean,denardi,denard,demary,demarais,delucas,deloe,delmonico,delisi,delio,delduca,deihl,dehmer,decoste,dechick,decatur,debruce,debold,debell,deats,daunt,daquilante,dambrosi,damas,dalin,dahman,dahlem,daffin,dacquel,cutrell,cusano,curtner,currens,curnow,cuppett,cummiskey,cullers,culhane,crull,crossin,cropsey,cromie,crofford,criscuolo,crisafulli,crego,creeden,covello,covel,corse,correra,cordner,cordier,coplen,copeman,contini,conteras,consalvo,conduff,compher,colliver,colan,cohill,cohenour,cogliano,codd,cockayne,clum,clowdus,clarida,clance,clairday,clagg,citron,citino,ciriello,cicciarelli,chrostowski,christley,chrisco,chrest,chisler,chieffo,cherne,cherico,cherian,cheirs,chauhan,chamblin,cerra,cepero,cellini,celedon,cejka,cavagnaro,cauffman,catanese,castrillo,castrellon,casserly,caseres,carthen,carse,carragher,carpentieri,carmony,carmer,carlozzi,caradine,cappola,capece,capaldi,cantres,cantos,canevari,canete,calcaterra,cadigan,cabbell,byrn,bykowski,butchko,busler,bushaw,buschmann,burow,buri,burgman,bunselmeyer,bunning,buhrman,budnick,buckson,buckhannon,brunjes,brumleve,bruckman,brouhard,brougham,brostrom,broerman,brocks,brison,brining,brindisi,brereton,breon,breitling,breedon,brasseaux,branaman,bramon,brackenridge,boyan,boxley,bouman,bouillion,botting,botti,bosshart,borup,borner,bordonaro,bonsignore,bonsall,bolter,bojko,bohne,bohlmann,bogdon,boen,bodenschatz,bockoven,bobrow,blondin,blissett,bligen,blasini,blankenburg,bjorkman,bistline,bisset,birdow,biondolillo,bielski,biele,biddix,biddinger,bianchini,bevens,bevard,betancur,bernskoetter,bernet,bernardez,berliner,berland,berkheimer,berent,bensch,benesch,belleau,bedingfield,beckstrom,beckim,bechler,beachler,bazzell,basa,bartoszek,barsch,barrell,barnas,barnaba,barillas,barbier,baltodano,baltierra,balle,balint,baldi,balderson,balderama,baldauf,balcazar,balay,baiz,bairos,azim,aversa,avellaneda,ausburn,auila,augusto,atwill,artiles,arterberry,arnow,arnaud,arnall,arenz,arduini,archila,arakawa,appleman,aplin,antonini,anstey,anglen,andros,amweg,amstutz,amari,amadeo,alteri,aloi,allebach,aley,alamillo,airhart,ahrendt,aegerter,adragna,admas,adderly,adderley,addair,abelar,abbamonte,abadi,zurek,zundel,zuidema,zuelke,zuck,zogg,zody,zets,zech,zecca,zavaleta,zarr,yousif,yoes,yoast,yeagley,yaney,yanda,yackel,wyles,wyke,woolman,woollard,woodis,woodin,wonderly,wombles,woloszyn,wollam,wnek,wittie,withee,wissman,wisham,wintle,winokur,wilmarth,willhoite,wildner,wikel,wieser,wien,wicke,wiatrek,whitehall,whetstine,wheelus,weyrauch,weyers,westerling,wendelken,welner,weinreb,weinheimer,weilbacher,weihe,weider,wecker,wead,watler,watkinson,wasmer,waskiewicz,wasik,warneke,wares,wangerin,wamble,walken,waker,wakeley,wahlgren,wahlberg,wagler,wachob,vorhies,vonseggern,vittitow,vink,villarruel,villamil,villamar,villalovos,vidmar,victorero,vespa,vertrees,verissimo,veltman,vecchione,veals,varrone,varma,vanveen,vanterpool,vaneck,vandyck,vancise,vanausdal,vanalphen,valdiviezo,urton,urey,updegrove,unrue,ulbrich,tysinger,twiddy,tunson,trueheart,troyan,trier,traweek,trafford,tozzi,toulouse,tosto,toste,torez,tooke,tonini,tonge,tomerlin,tolmie,tobe,tippen,tierno,tichy,thuss,thran,thornbury,thone,theunissen,thelmon,theall,textor,teters,tesh,tench,tekautz,tehrani,teat,teare,tavenner,tartaglione,tanski,tanis,tanguma,tangeman,taney,tammen,tamburri,tamburello,talsma,tallie,takeda,taira,taheri,tademy,taddei,taaffe,szymczak,szczepaniak,szafranski,swygert,swem,swartzlander,sutley,supernaw,sundell,sullivant,suderman,sudbury,suares,stueber,stromme,streeper,streck,strebe,stonehouse,stoia,stohr,stodghill,stirewalt,sterry,stenstrom,stene,steinbrecher,stear,stdenis,stanphill,staniszewski,stanard,stahlhut,stachowicz,srivastava,spong,spomer,spinosa,spindel,spera,soward,sopp,sooter,sonnek,soland,sojourner,soeder,sobolewski,snellings,smola,smetana,smeal,smarr,sloma,sligar,skenandore,skalsky,sissom,sirko,simkin,silverthorn,silman,sikkink,signorile,siddens,shumsky,shrider,shoulta,shonk,shomaker,shippey,shimada,shillingburg,shifflet,shiels,shepheard,sheerin,shedden,sheckles,sharrieff,sharpley,shappell,shaneyfelt,shampine,shaefer,shaddock,shadd,sforza,severtson,setzler,sepich,senne,senatore,sementilli,selway,selover,sellick,seigworth,sefton,seegars,sebourn,seaquist,sealock,seabreeze,scriver,scinto,schumer,schulke,schryver,schriner,schramek,schoon,schoolfield,schonberger,schnieder,schnider,schlitz,schlather,schirtzinger,scherman,schenker,scheiner,scheible,schaus,schakel,schaad,saxe,savely,savary,sardinas,santarelli,sanschagrin,sanpedro,sandine,sandigo,sandgren,sanderford,sandahl,salzwedel,salzar,salvino,salvatierra,salminen,salierno,salberg,sahagun,saelee,sabel,rynearson,ryker,rupprecht,runquist,rumrill,ruhnke,rovira,rottenberg,rosoff,rosete,rosebrough,roppolo,roope,romas,roley,rohrback,rohlfs,rogriguez,roel,rodriguiz,rodewald,roback,rizor,ritt,rippee,riolo,rinkenberger,riggsby,rigel,rieman,riedesel,rideau,ricke,rhinebolt,rheault,revak,relford,reinsmith,reichmann,regula,redlinger,rayno,raycroft,raus,raupp,rathmann,rastorfer,rasey,raponi,rantz,ranno,ranes,ramnauth,rahal,raddatz,quattrocchi,quang,pullis,pulanco,pryde,prohaska,primiano,prez,prevatt,prechtl,pottle,potenza,portes,porowski,poppleton,pontillo,politz,politi,poggi,plonka,plaskett,placzek,pizzuti,pizzaro,pisciotta,pippens,pinkins,pinilla,pini,pingitore,piercey,piccola,piccioni,picciano,philps,philp,philo,philmon,philbin,pflieger,pezzullo,petruso,petrea,petitti,peth,peshlakai,peschel,persico,persichetti,persechino,perris,perlow,perico,pergola,penniston,pembroke,pellman,pekarek,peirson,pearcey,pealer,pavlicek,passino,pasquarello,pasion,parzych,parziale,parga,papalia,papadakis,paino,pacini,oyen,ownes,owczarzak,outley,ouelette,ottosen,otting,ostwinkle,osment,oshita,osario,orlow,oriordan,orefice,orantes,oran,orahood,opel,olpin,oliveria,okon,okerlund,okazaki,ohta,offerman,nyce,nutall,northey,norcia,noor,niehoff,niederhauser,nickolson,nguy,neylon,newstrom,nevill,netz,nesselrodt,nemes,neally,nauyen,nascimento,nardella,nanni,myren,murchinson,munter,mundschenk,mujalli,muckleroy,moussa,mouret,moulds,mottram,motte,morre,montreuil,monton,montellano,monninger,monhollen,mongeon,monestime,monegro,mondesir,monceaux,mola,moga,moening,moccia,misko,miske,mishaw,minturn,mingione,milstein,milla,milks,michl,micheletti,michals,mesia,merson,meras,menifee,meluso,mella,melick,mehlman,meffert,medoza,mecum,meaker,meahl,mczeal,mcwatters,mcomber,mcmonigle,mckiddy,mcgranor,mcgeary,mcgaw,mcenery,mcelderry,mcduffey,mccuistion,mccrudden,mccrossin,mccosh,mccolgan,mcclish,mcclenahan,mcclam,mccartt,mccarrell,mcbane,maybury,mayben,maulden,mauceri,matko,mathie,matheis,mathai,masucci,massiah,martorano,martnez,martindelcamp,marschke,marovich,markiewicz,marinaccio,marhefka,marcrum,manton,mannarino,manlove,mangham,manasco,malpica,mallernee,malinsky,malhotra,maish,maisel,mainville,maharrey,magid,maertz,mada,maclaughlin,macina,macdermott,macallister,macadangdang,maack,lynk,lydic,luyando,lutke,lupinacci,lunz,lundsten,lujano,luhn,luecke,luebbe,ludolph,luckman,lucker,luckenbill,luckenbach,lucido,lowney,lowitz,lovaglio,louro,louk,loudy,louderback,lorick,lorenzini,lorensen,lorenc,lomuscio,loguidice,lockner,lockart,lochridge,litaker,lisowe,liptrap,linnane,linhares,lindfors,lindenmuth,lincourt,liew,liebowitz,levengood,leskovec,lesch,leoni,lennard,legner,leaser,leas,leadingham,lazarski,layland,laurito,laulu,laughner,laughman,laughery,laube,latiolais,lasserre,lasser,larrow,larrea,lapsley,lantrip,lanthier,langwell,langelier,landaker,lampi,lamond,lamblin,lambie,lakins,laipple,lagrimas,lafrancois,laffitte,laday,lacko,lacava,labianca,kutsch,kuske,kunert,kubly,kuamoo,krummel,krise,krenek,kreiser,krausz,kraska,krakowski,kradel,kozik,koza,kotowski,koslow,korber,kojima,kochel,knabjian,klunder,klugh,klinkhammer,kliewer,klever,kleber,klages,klaas,kizziar,kitchel,kishimoto,kirschenman,kirschenbaum,kinnick,kinn,kiner,kindla,kindall,kincaide,kilson,killins,kightlinger,kienzle,kiah,khim,ketcherside,kerl,kelsoe,kelker,keizer,keir,kawano,kawa,kaveney,kasparek,kaplowitz,kantrowitz,kant,kanoff,kano,kamalii,kalt,kaleta,kalbach,kalauli,kalata,kalas,kaigler,kachel,juran,jubb,jonker,jonke,jolivette,joles,joas,jividen,jeffus,jeanty,jarvi,jardon,janvier,janosko,janoski,janiszewski,janish,janek,iwanski,iuliano,irle,ingmire,imber,ijames,iiams,ihrig,ichikawa,hynum,hutzel,hutts,huskin,husak,hurndon,huntsinger,hulette,huitron,huguenin,hugg,hugee,huelskamp,huch,howen,hovanec,hoston,hostettler,horsfall,horodyski,holzhauer,hollimon,hollender,hogarth,hoffelmeyer,histand,hissem,hisel,hirayama,hinegardner,hinde,hinchcliffe,hiltbrand,hilsinger,hillstrom,hiley,hickenbottom,hickam,hibley,heying,hewson,hetland,hersch,herlong,herda,henzel,henshall,helson,helfen,heinbach,heikkila,heggs,hefferon,hebard,heathcote,hearl,heaberlin,hauth,hauschild,haughney,hauch,hattori,hasley,hartpence,harroun,harelson,hardgrove,hardel,hansbrough,handshoe,handly,haluska,hally,halling,halfhill,halferty,hakanson,haist,hairgrove,hahner,hagg,hafele,haaland,guttierez,gutknecht,gunnarson,gunlock,gummersheimer,gullatte,guity,guilmette,guhl,guenette,guardino,groshong,grober,gripp,grillot,grilli,greulich,gretzinger,greenwaldt,graven,grassman,granberg,graeser,graeff,graef,grabow,grabau,gotchy,goswick,gosa,gordineer,gorczyca,goodchild,golz,gollihue,goldwire,goldbach,goffredo,glassburn,glaeser,gillilan,gigante,giere,gieger,gidcumb,giarrusso,giannelli,gettle,gesualdi,geschke,gerwig,gervase,geoffrion,gentilcore,genther,gemes,gemberling,gelles,geitz,geeslin,gedney,gebauer,gawron,gavia,gautney,gaustad,gasmen,gargus,ganske,ganger,galvis,gallinger,gallichio,galletta,gaede,gadlin,gaby,gabrielsen,gaboriault,furlan,furgerson,fujioka,fugett,fuehrer,frint,frigon,frevert,frautschi,fraker,fradette,foulkes,forslund,forni,fontenette,fones,folz,folmer,follman,folkman,flourney,flickner,flemmings,fleischacker,flander,flament,fithian,fiorello,fiorelli,fioravanti,fieck,ficke,fiallos,fiacco,feuer,ferrington,fernholz,feria,fergurson,feick,febles,favila,faulkingham,fath,farnam,falter,fakhouri,fairhurst,fahs,estrello,essick,espree,esmond,eskelson,escue,escatel,erebia,epperley,epler,enyart,engelbert,enderson,emch,elisondo,elford,ekman,eick,eichmann,ehrich,ehlen,edwardson,edley,edghill,edel,eastes,easterbrooks,eagleson,eagen,eade,dyle,dutkiewicz,dunnagan,duncil,duling,drumgoole,droney,dreyfus,dragan,dowty,doscher,dornan,doremus,doogan,donaho,donahey,dombkowski,dolton,dolen,dobratz,diveley,dittemore,ditsch,disque,dishmon,disch,dirickson,dippolito,dimuccio,dilger,diefenderfer,dicola,diblasio,dibello,devan,dettmer,deschner,desbiens,derusha,denkins,demonbreun,demchak,delucchi,delprete,deloy,deliz,deline,delap,deiter,deignan,degiacomo,degaetano,defusco,deboard,debiase,deaville,deadwyler,davanzo,daughton,darter,danser,dandrade,dando,dampeer,dalziel,dalen,dain,dague,czekanski,cutwright,cutliff,curle,cuozzo,cunnington,cunnigham,cumings,crowston,crittle,crispell,crisostomo,crear,creach,craigue,crabbs,cozzi,cozza,coxe,cowsert,coviello,couse,coull,cottier,costagliola,corra,corpening,cormany,corless,corkern,conteh,conkey,conditt,conaty,colomb,collura,colledge,colins,colgate,coleson,colemon,coffland,coccia,clougherty,clewell,cleckley,cleaveland,clarno,civils,cillo,cifelli,ciesluk,christison,chowning,chouteau,choung,childres,cherrington,chenette,cheeves,cheairs,chaddock,cernoch,cerino,cazier,castel,casselberry,caserta,carvey,carris,carmant,cariello,cardarelli,caras,caracciolo,capitano,cantoni,cantave,cancio,campillo,callens,caldero,calamia,cahee,cahan,cahalan,cabanilla,cabal,bywater,bynes,byassee,busker,bushby,busack,burtis,burrola,buroker,burnias,burlock,burham,burak,bulla,buffin,buening,budney,buchannan,buchalter,brule,brugler,broxson,broun,brosh,brissey,brisby,brinlee,brinkmeyer,brimley,brickell,breth,breger,brees,brank,braker,bozak,bowlds,bowersock,bousman,boushie,botz,bordwell,bonkowski,bonine,bonifay,bonesteel,boldin,bohringer,bohlander,boecker,bocook,bocock,boblett,bobbett,boas,boarman,bleser,blazejewski,blaustein,blausey,blancarte,blaize,blackson,blacketer,blackard,bisch,birchett,billa,bilder,bierner,bienvenu,bielinski,bialas,biagini,beynon,beyl,bettini,betcher,bessent,beshara,besch,bernd,bergemann,bergeaux,berdan,bens,benedicto,bendall,beltron,beltram,bellville,beisch,behney,beechler,beckum,batzer,batte,bastida,bassette,basley,bartosh,bartolone,barraclough,barnick,barket,barkdoll,baringer,barella,barbian,barbati,bannan,balles,baldo,balasubramani,baig,bahn,bachmeier,babyak,baas,baars,ayuso,avinger,avella,ausbrooks,aull,augello,atkeson,atkerson,atherley,athan,assad,asebedo,arrison,armon,armfield,arkin,archambeau,antonellis,angotti,amorose,amini,amborn,amano,aluarez,allgaier,allegood,alen,aldama,aird,ahsing,ahmann,aguado,agostino,agostinelli,adwell,adsit,adelstein,actis,acierno,achee,abbs,abbitt,zwagerman,zuercher,zinno,zettler,zeff,zavalza,zaugg,zarzycki,zappulla,zanotti,zachman,zacher,yundt,yslas,younes,yontz,yglesias,yeske,yeargin,yauger,yamane,xang,wylam,wrobleski,wratchford,woodlee,wolsey,wolfinbarger,wohlenhaus,wittler,wittenmyer,witkop,wishman,wintz,winkelmann,windus,winborn,wims,wiltrout,willmott,williston,wilemon,wilbourne,wiedyk,widmann,wickland,wickes,wichert,whitsell,whisenand,whidby,wetz,westmeyer,wertheim,wernert,werle,werkheiser,weldin,weissenborn,weingard,weinfeld,weihl,weightman,weichel,wehrheim,wegrzyn,wegmann,waszak,wankum,walthour,waltermire,walstad,waldren,walbert,walawender,wahlund,wahlert,wahlers,wach,vuncannon,vredenburgh,vonk,vollmar,voisinet,vlahos,viscardi,vires,vipperman,violante,vidro,vessey,vesper,veron,vergari,verbeck,venturino,velastegui,vegter,varas,vanwey,vanvranken,vanvalkenbur,vanorsdale,vanoli,vanochten,vanier,vanevery,vane,vanduser,vandersteen,vandell,vandall,vallot,vallon,vallez,vallely,vadenais,uthe,usery,unga,ultsch,ullom,tyminski,twogood,tursi,turay,tungate,truxillo,trulock,trovato,troise,tripi,trinks,trimboli,trickel,trezise,trefry,treen,trebilcock,travieso,trachtenberg,touhey,tougas,tortorella,tormey,torelli,torborg,toran,tomek,tomassi,tollerson,tolden,toda,tobon,tjelmeland,titmus,tilbury,tietje,thurner,thum,thrope,thornbrough,thibaudeau,thackeray,tesoro,territo,ternes,teich,tecson,teater,teagarden,tatsch,tarallo,tapanes,tanberg,tamm,sylvis,swenor,swedlund,sutfin,sura,sundt,sundin,summerson,sumatzkuku,sultemeier,sulivan,suggitt,suermann,sturkie,sturgess,stumph,stuemke,struckhoff,strose,stroder,stricklen,strick,streib,strei,strawther,stratis,strahm,stortz,storrer,storino,stohler,stohl,stockel,stinnette,stile,stieber,steffenhagen,stefanowicz,steever,steagall,statum,stapley,stanish,standiford,standen,stamos,stahlecker,stadtler,spratley,spraker,sposito,spickard,spehar,spees,spearing,spangle,spallone,soulard,sora,sopko,sood,sonnen,solly,solesbee,soldano,sobey,sobczyk,snedegar,sneddon,smolinski,smolik,slota,slavick,skorupski,skolnik,skirvin,skeels,skains,skahan,skaar,siwiec,siverly,siver,sivak,sirk,sinton,sinor,sincell,silberstein,sieminski,sidelinger,shurman,shunnarah,shirer,shidler,sherlin,shepperson,shemanski,sharum,shartrand,shapard,shanafelt,shamp,shader,shackelton,seyer,seroka,sernas,seright,serano,sengupta,selinger,seith,seidler,seehusen,seefried,scovell,scorzelli,sconiers,schwind,schwichtenber,schwerin,schwenke,schwaderer,schussler,schuneman,schumpert,schultheiss,schroll,schroepfer,schroeden,schrimpf,schook,schoof,schomburg,schoenfeldt,schoener,schnoor,schmick,schlereth,schindele,schildt,schildknecht,schemmel,scharfenberg,schanno,schane,schaer,schad,scearce,scardino,sawka,sawinski,savoca,savery,saults,sarpy,saris,sardinha,sarafin,sankar,sanjurjo,sanderfer,sanagustin,samudio,sammartino,samas,salz,salmen,salkeld,salamon,sakurai,sakoda,safley,sada,sachse,ryden,ryback,russow,russey,ruprecht,rumple,ruffini,rudzinski,rudel,rudden,rovero,routledge,roussin,rousse,rouser,rougeau,rosica,romey,romaniello,rolfs,rogoff,rogne,rodriquz,rodrequez,rodin,rocray,rocke,riviere,rivette,riske,risenhoover,rindfleisch,rinaudo,rimbey,riha,righi,ridner,ridling,riden,rhue,reyome,reynoldson,reusch,rensing,rensch,rennels,renderos,reininger,reiners,reigel,rehmer,regier,reff,redlin,recchia,reaume,reagor,rawe,rattigan,raska,rashed,ranta,ranft,randlett,ramiez,ramella,rallis,rajan,raisbeck,raimondo,raible,ragone,rackliffe,quirino,quiring,quero,quaife,pyke,purugganan,pursifull,purkett,purdon,pulos,puccia,provance,propper,preis,prehn,prata,prasek,pranger,pradier,portor,portley,porte,popiel,popescu,pomales,polowy,pollett,politis,polit,poley,pohler,poggio,podolak,poag,plymel,ploeger,planty,piskura,pirrone,pirro,piroso,pinsky,pilant,pickerill,piccolomini,picart,piascik,phann,petruzzelli,petosa,persson,perretta,perkowski,perilli,percifield,perault,peppel,pember,pelotte,pelcher,peixoto,pehl,peatross,pearlstein,peacher,payden,paya,pawelek,pavey,pauda,pathak,parrillo,parness,parlee,paoli,pannebaker,palomar,palo,palmberg,paganelli,paffrath,padovano,padden,pachucki,ovando,othman,osowski,osler,osika,orsburn,orlowsky,oregel,oppelt,opfer,opdyke,onell,olivos,okumura,okoro,ogas,oelschlaeger,oder,ocanas,obrion,obarr,oare,nyhus,nyenhuis,nunnelley,nunamaker,nuckels,noyd,nowlan,novakovich,noteboom,norviel,nortz,norment,norland,nolt,nolie,nixson,nitka,nissley,nishiyama,niland,niewiadomski,niemeier,nieland,nickey,nicholsen,neugent,neto,nerren,neikirk,neigh,nedrow,neave,nazaire,navaro,navalta,nasworthy,nasif,nalepa,nakao,nakai,nadolny,myklebust,mussel,murthy,muratore,murat,mundie,mulverhill,muilenburg,muetzel,mudra,mudgett,mrozinski,moura,mottinger,morson,moretto,morentin,mordan,mooreland,mooers,monts,montone,montondo,montiero,monie,monat,monares,mollo,mollet,molacek,mokry,mohrmann,mohabir,mogavero,moes,moceri,miyoshi,mitzner,misra,mirr,minish,minge,minckler,milroy,mille,mileski,milanesi,miko,mihok,mihalik,mieczkowski,messerli,meskill,mesenbrink,merton,merryweather,merkl,menser,menner,menk,menden,menapace,melbourne,mekus,meinzer,meers,mctigue,mcquitty,mcpheron,mcmurdie,mcleary,mclafferty,mckinzy,mckibbin,mckethan,mcintee,mcgurl,mceachran,mcdowall,mcdermitt,mccuaig,mccreedy,mccoskey,mcclosky,mcclintick,mccleese,mccanless,mazzucco,mazzocco,mazurkiewicz,mazariego,mayhorn,maxcy,mavity,mauzey,maulding,matuszewski,mattsson,mattke,matsushita,matsuno,matsko,matkin,mathur,masterman,massett,massart,massari,mashni,martella,marren,margotta,marder,marczak,maran,maradiaga,manwarren,manter,mantelli,manso,mangone,manfredonia,malden,malboeuf,malanga,makara,maison,maisano,mairs,mailhiot,magri,madron,madole,mackall,macduff,macartney,lynds,lusane,luffman,louth,loughmiller,lougheed,lotspeich,lorenzi,loosli,longe,longanecker,lonero,lohmeyer,loeza,lobstein,lobner,lober,littman,litalien,lippe,lints,lijewski,ligas,liebert,liebermann,liberati,lezcano,levinthal,lessor,lesieur,lenning,lengel,lempke,lemp,lemar,leitzke,leinweber,legrone,lege,leder,lawnicki,lauth,laun,laughary,lassley,lashway,larrivee,largen,lare,lanouette,lanno,langille,langen,lamonte,lalin,laible,lafratta,laforte,lacuesta,lacer,labore,laboe,labeau,kwasniewski,kunselman,kuhr,kuchler,krugman,kruckenberg,krotzer,kroemer,krist,krigbaum,kreke,kreisman,kreisler,kreft,krasnow,kras,krag,kouyate,kough,kotz,kostura,korner,kornblum,korczynski,koppa,kopczyk,konz,komorowski,kollen,kolander,koepnick,koehne,kochis,knoch,knippers,knaebel,klipp,klinedinst,klimczyk,klier,klement,klaphake,kisler,kinzie,kines,kindley,kimple,kimm,kimbel,kilker,kilborn,kibbey,khong,ketchie,kerbow,kennemore,kennebeck,kenneally,kenndy,kenmore,kemnitz,kemler,kemery,kelnhofer,kellstrom,kellis,kellams,keiter,keirstead,keeny,keelin,keefauver,keams,kautzman,kaus,katayama,kasson,kassim,kasparian,kase,karwoski,kapuscinski,kaneko,kamerling,kamada,kalka,kalar,kakacek,kaczmarczyk,jurica,junes,journell,jolliffe,johnsey,jindra,jimenz,jette,jesperson,jerido,jenrette,jencks,jech,jayroe,jayo,javens,jaskot,jaros,jaquet,janowiak,jaegers,jackel,izumi,irelan,inzunza,imoto,imme,iglehart,iannone,iannacone,huyler,hussaini,hurlock,hurlbutt,huprich,humphry,hulslander,huelsman,hudelson,hudecek,hsia,hreha,hoyland,howk,housholder,housden,houff,horkey,honan,homme,holtzberg,hollyfield,hollings,hollenbaugh,hokenson,hogrefe,hogland,hoel,hodgkin,hochhalter,hjelle,hittson,hinderman,hinchliffe,hime,hilyer,hilby,hibshman,heydt,hewell,heward,hetu,hestand,heslep,herridge,herner,hernande,hermandez,hermance,herbold,heon,henthorne,henion,henao,heming,helmkamp,hellberg,heidgerken,heichel,hehl,hegedus,heckathorne,hearron,haymer,haycook,havlicek,hausladen,haseman,hartsook,hartog,harns,harne,harmann,haren,hanserd,hanners,hanekamp,hamra,hamley,hamelin,hamblet,hakimi,hagle,hagin,haehn,haeck,hackleman,haacke,gulan,guirand,guiles,guggemos,guerrieri,guerreiro,guereca,gudiel,guccione,gubler,gruenwald,gritz,grieser,grewe,grenon,gregersen,grefe,grech,grecco,gravette,grassia,granholm,graner,grandi,grahan,gradowski,gradney,graczyk,gouthier,gottschall,goracke,gootee,goodknight,goodine,gonzalea,gonterman,gonalez,gomm,goleman,goldtooth,goldstone,goldey,golan,goen,goeller,goel,goecke,godek,goan,glunz,gloyd,glodowski,glinski,glawe,girod,girdley,gindi,gillings,gildner,giger,giesbrecht,gierke,gier,giboney,giaquinto,giannakopoulo,giaimo,giaccio,giacalone,gessel,gerould,gerlt,gerhold,geralds,genson,genereux,gellatly,geigel,gehrig,gehle,geerdes,geagan,gawel,gavina,gauss,gatwood,gathman,gaster,garske,garratt,garms,garis,gansburg,gammell,gambale,gamba,galimore,gadway,gadoury,furrer,furino,fullard,fukui,fryou,friesner,friedli,friedl,friedberg,freyermuth,fremin,fredell,fraze,franken,foth,fote,fortini,fornea,formanek,forker,forgette,folan,foister,foglesong,flinck,flewellen,flaten,flaig,fitgerald,fischels,firman,finstad,finkelman,finister,fina,fetterhoff,ferriter,ferch,fennessy,feltus,feltes,feinman,farve,farry,farrall,farag,falzarano,falck,falanga,fakhoury,fairbrother,fagley,faggins,facteau,ewer,ewbank,evola,evener,eustis,estwick,estel,essa,espinola,escutia,eschmann,erpelding,ernsberger,erling,entz,engelhart,enbody,emick,elsinger,ellinwood,ellingsen,ellicott,elkind,eisinger,eisenbeisz,eischen,eimer,eigner,eichhorst,ehmke,egleston,eggett,efurd,edgeworth,eckels,ebey,eberling,eagleton,dwiggins,dweck,dunnings,dunnavant,dumler,duman,dugue,duerksen,dudeck,dreisbach,drawdy,drawbaugh,draine,draggoo,dowse,dovel,doughton,douds,doubrava,dort,dorshorst,dornier,doolen,donavan,dominik,domingez,dolder,dold,dobies,diskin,disano,dirden,diponio,dipirro,dimock,diltz,dillabough,diley,dikes,digges,digerolamo,diel,dicharry,dicecco,dibartolomeo,diamant,dewire,devone,dessecker,dertinger,derousselle,derk,depauw,depalo,denherder,demeyer,demetro,demastus,delvillar,deloye,delosrios,delgreco,delarge,delangel,dejongh,deitsch,degiorgio,degidio,defreese,defoe,decambra,debenedetto,deaderick,daza,dauzat,daughenbaugh,dato,dass,darwish,dantuono,danton,dammeyer,daloia,daleo,dagg,dacey,curts,cuny,cunneen,culverhouse,cucinella,cubit,crumm,crudo,crowford,crout,crotteau,crossfield,crooke,crom,critz,cristaldi,crickmore,cribbin,cremeens,crayne,cradduck,couvertier,cottam,cossio,correy,cordrey,coplon,copass,coone,coody,contois,consla,connelley,connard,congleton,condry,coltey,colindres,colgrove,colfer,colasurdo,cochell,cobbin,clouthier,closs,cloonan,clizbe,clennon,clayburn,claybourn,clausell,clasby,clagett,ciskowski,cirrincione,cinque,cinelli,cimaglia,ciaburri,christiani,christeson,chladek,chizmar,chinnici,chiarella,chevrier,cheves,chernow,cheong,chelton,chanin,cham,chaligoj,celestino,cayce,cavey,cavaretta,caughron,catmull,catapano,cashaw,carullo,carualho,carthon,cartelli,carruba,carrere,carolus,carlstrom,carfora,carello,carbary,caplette,cannell,cancilla,campell,cammarota,camilo,camejo,camarata,caisse,cacioppo,cabbagestalk,cabatu,cabanas,byles,buxbaum,butland,burrington,burnsed,burningham,burlingham,burgy,buitrago,bueti,buehring,buday,bucknell,buchbinder,bucey,bruster,brunston,brouillet,brosious,broomes,brodin,broddy,brochard,britsch,britcher,brierley,brezina,bressi,bressette,breslow,brenden,breier,brei,braymer,brasuell,branscomb,branin,brandley,brahler,bracht,bracamontes,brabson,boyne,boxell,bowery,bovard,boutelle,boulette,bottini,botkins,bosen,boscia,boscarino,borich,boreman,bordoy,bordley,bordenet,boquet,boocks,bolner,boissy,boilard,bohnen,bohall,boening,boccia,boccella,bobe,blyth,biviano,bitto,bisel,binstock,bines,billiter,bigsby,bighorse,bielawski,bickmore,bettin,bettenhausen,besson,beseau,berton,berroa,berntson,bernas,berisford,berhow,bergsma,benyo,benyard,bente,bennion,benko,belsky,bellavance,belasco,belardo,beidler,behring,begnaud,bega,befort,beek,bedore,beddard,becknell,beardslee,beardall,beagan,bayly,bauza,bautz,bausman,baumler,batterson,battenfield,bassford,basse,basemore,baruch,bartholf,barman,baray,barabas,banghart,banez,balsam,ballester,ballagh,baldock,bagnoli,bagheri,bacus,bacho,baccam,axson,averhart,aver,austill,auberry,athans,atcitty,atay,astarita,ascolese,artzer,arrasmith,argenbright,aresco,aranjo,appleyard,appenzeller,apilado,antonetti,antis,annas,angwin,andris,andries,andreozzi,ando,andis,anderegg,amyot,aminov,amelung,amelio,amason,alviar,allendorf,aldredge,alcivar,alaya,alapai,airington,aina,ailor,ahrns,ahmadi,agresta,affolter,aeschlimann,adney,aderhold,adachi,ackiss,aben,abdelhamid,abar,aase,zorilla,zordan,zollman,zoch,zipfel,zimmerle,zike,ziel,zens,zelada,zaman,zahner,zadora,zachar,zaborowski,zabinski,yzquierdo,yoshizawa,yori,yielding,yerton,yehl,yeargain,yeakley,yamaoka,yagle,yablonski,wynia,wyne,wyers,wrzesinski,wrye,wriston,woolums,woolen,woodlock,woodle,wonser,wombacher,wollschlager,wollen,wolfley,wolfer,wisse,wisell,wirsing,winstanley,winsley,winiecki,winiarski,winge,winesett,windell,winberry,willyard,willemsen,wilkosz,wilensky,wikle,wiford,wienke,wieneke,wiederhold,wiebold,widick,wickenhauser,whitrock,whisner,whinery,wherley,whedbee,wheadon,whary,wessling,wessells,wenninger,wendroth,wende,wellard,weirick,weinkauf,wehrman,weech,weathersbee,warncke,wardrip,walstrom,walkowski,walcutt,waight,wagman,waggett,wadford,vowles,vormwald,vondran,vohs,vitt,vitalo,viser,vinas,villena,villaneuva,villafranca,villaflor,vilain,vicory,viana,vian,verucchi,verra,venzke,venske,veley,veile,veeder,vaske,vasconez,vargason,varble,vanwert,vantol,vanscooter,vanmetre,vanmaanen,vanhise,vaneaton,vandyk,vandriel,vandorp,vandewater,vandervelden,vanderstelt,vanderhoef,vanderbeck,vanbibber,vanalstine,vanacore,valdespino,vaill,vailes,vagliardo,ursini,urrea,urive,uriegas,umphress,ucci,uballe,tynon,twiner,tutton,tudela,tuazon,troisi,tripplett,trias,trescott,treichel,tredo,tranter,tozer,toxey,tortorici,tornow,topolski,topia,topel,topalian,tonne,tondre,tola,toepke,tisdell,tiscareno,thornborrow,thomison,thilges,theuret,therien,thagard,thacher,texter,terzo,tenpenny,tempesta,teetz,teaff,tavella,taussig,tatton,tasler,tarrence,tardie,tarazon,tantillo,tanney,tankson,tangen,tamburo,tabone,szilagyi,syphers,swistak,swiatkowski,sweigert,swayzer,swapp,svehla,sutphen,sutch,susa,surma,surls,sundermeyer,sundeen,sulek,sughrue,sudol,sturms,stupar,stum,stuckman,strole,strohman,streed,strebeck,strausser,strassel,stpaul,storts,storr,stommes,stmary,stjulien,stika,stiggers,sthill,stevick,sterman,stepanek,stemler,stelman,stelmack,steinkamp,steinbock,stcroix,stcharles,staudinger,stanly,stallsworth,stalley,srock,spritzer,spracklin,spinuzzi,spidell,speyrer,sperbeck,spendlove,speckman,spargur,spangenberg,spaid,sowle,soulier,sotolongo,sostre,sorey,sonier,somogyi,somera,soldo,soderholm,snoots,snooks,snoke,snodderly,snee,smithhart,smillie,smay,smallman,sliwinski,slentz,sledd,slager,skogen,skog,skarda,skalicky,siwek,sitterson,sisti,sissel,sinopoli,similton,simila,simenson,silvertooth,silos,siggins,sieler,siburt,sianez,shurley,shular,shuecraft,shreeves,shollenberger,shoen,shishido,shipps,shipes,shinall,sherfield,shawe,sharrett,sharrard,shankman,sessum,serviss,servello,serice,serda,semler,semenza,selmon,sellen,seley,seidner,seib,sehgal,seelbach,sedivy,sebren,sebo,seanez,seagroves,seagren,seabron,schwertner,schwegel,schwarzer,schrunk,schriefer,schreder,schrank,schopp,schonfeld,schoenwetter,schnall,schnackenberg,schnack,schmutzler,schmierer,schmidgall,schlup,schloemer,schlitt,schermann,scherff,schellenberg,schain,schaedler,schabel,scaccia,saye,saurez,sasseen,sasnett,sarti,sarra,sarber,santoy,santeramo,sansoucy,sando,sandles,sandau,samra,samaha,salizar,salam,saindon,sagaser,saeteun,sadusky,sackman,sabater,saas,ruthven,ruszkowski,rusche,rumpf,ruhter,ruhenkamp,rufo,rudge,ruddle,rowlee,rowand,routhier,rougeot,rotramel,rotan,rosten,rosillo,rookard,roode,rongstad,rollie,roider,roffe,roettger,rodick,rochez,rochat,rivkin,rivadeneira,riston,risso,rinderknecht,riis,riggsbee,rieker,riegle,riedy,richwine,richmon,ricciuti,riccardo,ricardson,rhew,revier,remsberg,remiszewski,rembold,rella,reinken,reiland,reidel,reichart,rehak,redway,rednour,redifer,redgate,redenbaugh,redburn,readus,raybuck,rauhuff,rauda,ratte,rathje,rappley,rands,ramseyer,ramseur,ramsdale,ramo,ramariz,raitz,raisch,rainone,rahr,ragasa,rafalski,radunz,quenzer,queja,queenan,pyun,putzier,puskas,purrington,puri,punt,pullar,pruse,pring,primeau,prevette,preuett,prestage,pownell,pownall,potthoff,potratz,poth,poter,posthuma,posen,porritt,popkin,poormon,polidoro,polcyn,pokora,poer,pluviose,plock,pleva,placke,pioli,pingleton,pinchback,pieretti,piccone,piatkowski,philley,phibbs,phay,phagan,pfund,peyer,pettersen,petter,petrucelli,petropoulos,petras,petix,pester,pepperman,pennick,penado,pelot,pelis,peeden,pechon,peal,pazmino,patchin,pasierb,parran,parilla,pardy,parcells,paragas,paradee,papin,panko,pangrazio,pangelinan,pandya,pancheri,panas,palmiter,pallares,palinkas,palek,pagliaro,packham,pacitti,ozier,overbaugh,oursler,ouimette,otteson,otsuka,othon,osmundson,oroz,orgill,ordeneaux,orama,oppy,opheim,onkst,oltmanns,olstad,olofson,ollivier,olejniczak,okura,okuna,ohrt,oharra,oguendo,ogier,offermann,oetzel,oechsle,odoherty,oddi,ockerman,occhiogrosso,obryon,obremski,nyreen,nylund,nylen,nyholm,nuon,nuanes,norrick,noris,nordell,norbury,nooner,nomura,nole,nolden,nofsinger,nocito,niedbala,niebergall,nicolini,nevils,neuburger,nemerofsky,nemecek,nazareno,nastri,nast,nagorski,myre,muzzey,mutschler,muther,musumeci,muranaka,muramoto,murad,murach,muns,munno,muncrief,mugrage,muecke,mozer,moyet,mowles,mottern,mosman,mosconi,morine,morge,moravec,morad,mones,moncur,monarez,molzahn,moglia,moesch,mody,modisett,mitnick,mithcell,mitchiner,mistry,misercola,mirabile,minvielle,mino,minkler,minifield,minichiello,mindell,minasian,milteer,millwee,millstein,millien,mikrut,mihaly,miggins,michard,mezo,metzner,mesquita,merriwether,merk,merfeld,mercik,mercadante,menna,mendizabal,mender,melusky,melquist,mellado,meler,melendes,mekeel,meiggs,megginson,meck,mcwherter,mcwayne,mcsparren,mcrea,mcneff,mcnease,mcmurrin,mckeag,mchughes,mcguiness,mcgilton,mcelreath,mcelhone,mcelhenney,mceldowney,mccurtain,mccure,mccosker,mccory,mccormic,mccline,mccleave,mcclatchey,mccarney,mccanse,mcallen,mazzie,mazin,mazanec,mayette,mautz,maun,mattas,mathurin,mathiesen,massmann,masri,masias,mascolo,mascetti,mascagni,marzolf,maruska,martain,marszalek,marolf,marmas,marlor,markwood,marinero,marier,marich,marcom,marciante,marchman,marchio,marbach,manzone,mantey,mannina,manhardt,manaois,malmgren,mallonee,mallin,mallary,malette,makinson,makins,makarewicz,mainwaring,maiava,magro,magouyrk,magett,maeder,madyun,maduena,maden,madeira,mackins,mackel,macinnes,macia,macgowan,lyssy,lyerly,lyalls,lutter,lunney,luksa,ludeman,lucidi,lucci,lowden,lovier,loughridge,losch,lorson,lorenzano,lorden,lorber,lopardo,loosier,loomer,longsdorf,longchamps,loncar,loker,logwood,loeffelholz,lockmiller,livoti,linford,linenberger,lindloff,lindenbaum,limoges,liley,lighthill,lightbourne,lieske,leza,levandoski,leuck,lepere,leonhart,lenon,lemma,lemler,leising,leinonen,lehtinen,lehan,leetch,leeming,ledyard,ledwith,ledingham,leclere,leck,lebert,leandry,lazzell,layo,laye,laxen,lawther,lawerance,lavoy,lavertu,laverde,latouche,latner,lathen,laskin,lashbaugh,lascala,larroque,larick,laraia,laplume,lanzilotta,lannom,landrigan,landolt,landess,lamkins,lalla,lalk,lakeman,lakatos,laib,lahay,lagrave,lagerquist,lafoy,lafleche,lader,labrada,kwiecinski,kutner,kunshier,kulakowski,kujak,kuehnle,kubisiak,krzyminski,krugh,krois,kritikos,krill,kriener,krewson,kretzschmar,kretz,kresse,kreiter,kreischer,krebel,krans,kraling,krahenbuhl,kouns,kotson,kossow,kopriva,konkle,kolter,kolk,kolich,kohner,koeppen,koenigs,kock,kochanski,kobus,knowling,knouff,knoerzer,knippel,kloberdanz,kleinert,klarich,klaassen,kisamore,kirn,kiraly,kipps,kinson,kinneman,kington,kine,kimbriel,kille,kibodeaux,khamvongsa,keylon,kever,keser,kertz,kercheval,kendrix,kendle,kempt,kemple,keesey,keatley,kazmierski,kazda,kazarian,kawashima,katsch,kasun,kassner,kassem,kasperski,kasinger,kaschak,karels,kantola,kana,kamai,kalthoff,kalla,kalani,kahrs,kahanek,kacher,jurasek,jungels,jukes,juelfs,judice,juda,josselyn,jonsson,jonak,joens,jobson,jegede,jeanjacques,jaworowski,jaspers,jannsen,janner,jankowiak,jank,janiak,jackowski,jacklin,jabbour,iyer,iveson,isner,iniquez,ingwerson,ingber,imbrogno,ille,ikehara,iannelli,hyson,huxford,huseth,hurns,hurney,hurles,hunnings,humbarger,hulan,huisinga,hughett,hughen,hudler,hubiak,hricko,hoversten,hottel,hosaka,horsch,hormann,hordge,honzell,homburg,holten,holme,hollopeter,hollinsworth,hollibaugh,holberg,hohmann,hoenstine,hodell,hodde,hiter,hirko,hinzmann,hinrichsen,hinger,hincks,hilz,hilborn,highley,higashi,hieatt,hicken,heverly,hesch,hervert,hershkowitz,herreras,hermanns,herget,henriguez,hennon,hengel,helmlinger,helmig,heldman,heizer,heinitz,heifner,heidorn,heglin,heffler,hebner,heathman,heaslip,hazlip,haymes,hayase,hawver,havermale,havas,hauber,hashim,hasenauer,harvel,hartney,hartel,harsha,harpine,harkrider,harkin,harer,harclerode,hanzely,hanni,hannagan,hampel,hammerschmidt,hamar,hallums,hallin,hainline,haid,haggart,hafen,haer,hadiaris,hadad,hackford,habeeb,guymon,guttery,gunnett,guillette,guiliano,guilbeaux,guiher,guignard,guerry,gude,gucman,guadian,grzybowski,grzelak,grussendorf,grumet,gruenhagen,grudzinski,grossmann,grof,grisso,grisanti,griffitts,griesbaum,grella,gregston,graveline,grandusky,grandinetti,gramm,goynes,gowing,goudie,gosman,gort,gorsline,goralski,goodstein,goodroe,goodlin,goodheart,goodhart,gonzelez,gonthier,goldsworthy,goldade,goettel,goerlitz,goepfert,goehner,goben,gobeille,gliem,gleich,glasson,glascoe,gladwell,giusto,girdner,gipple,giller,giesing,giammona,ghormley,germon,geringer,gergely,gerberich,gepner,gens,genier,gemme,gelsinger,geigle,gebbia,gayner,gavitt,gatrell,gastineau,gasiewski,gascoigne,garro,garin,ganong,ganga,galpin,gallus,galizia,gajda,gahm,gagen,gaffigan,furno,furnia,furgason,fronczak,frishman,friess,frierdich,freestone,franta,frankovich,fors,forres,forrer,florido,flis,flicek,flens,flegal,finkler,finkenbinder,finefrock,filpo,filion,fierman,fieldman,ferreyra,fernendez,fergeson,fera,fencil,feith,feight,federici,federer,fechtner,feagan,fausnaugh,faubert,fata,farman,farinella,fantauzzi,fanara,falso,falardeau,fagnani,fabro,excell,ewton,evey,everetts,evarts,etherington,estremera,estis,estabrooks,essig,esplin,espenschied,ernzen,eppes,eppard,entwisle,emison,elison,elguezabal,eledge,elbaz,eisler,eiden,eichorst,eichert,egle,eggler,eggimann,edey,eckerman,echelberger,ebbs,ebanks,dziak,dyche,dyce,dusch,duross,durley,durate,dunsworth,dumke,dulek,duhl,duggin,dufford,dudziak,ducrepin,dubree,dubre,dubie,dubas,droste,drisko,drewniak,doxtator,dowtin,downum,doubet,dottle,dosier,doshi,dorst,dorset,dornbusch,donze,donica,domanski,domagala,dohse,doerner,doerfler,doble,dobkins,dilts,digiulio,digaetano,dietzel,diddle,dickel,dezarn,devoy,devoss,devilla,devere,deters,desvergnes,deshay,desena,deross,depedro,densley,demorest,demore,demora,demirjian,demerchant,dematteis,demateo,delgardo,delfavero,delaurentis,delamar,delacy,deitrich,deisher,degracia,degraaf,defries,defilippis,decoursey,debruin,debiasi,debar,dearden,dealy,dayhoff,davino,darvin,darrisaw,darbyshire,daquino,daprile,danh,danahy,dalsanto,dallavalle,dagel,dadamo,dacy,dacunha,dabadie,czyz,cutsinger,curney,cuppernell,cunliffe,cumby,cullop,cullinane,cugini,cudmore,cuda,cucuzza,cuch,crumby,crouser,critton,critchley,cremona,cremar,crehan,creary,crasco,crall,crabbe,cozzolino,cozier,coyner,couvillier,counterman,coulthard,coudriet,cottom,corzo,cornutt,corkran,corda,copelin,coonan,consolo,conrow,conran,connerton,conkwright,condren,comly,comisky,colli,collet,colello,colbeck,colarusso,coiner,cohron,codere,cobia,clure,clowser,clingenpeel,clenney,clendaniel,clemenson,cleere,cleckler,claybaugh,clason,cirullo,ciraulo,ciolek,ciampi,christopherse,chovanec,chopra,chol,chiem,chestnutt,chesterman,chernoff,chermak,chelette,checketts,charpia,charo,chargois,champman,challender,chafins,cerruto,celi,cazenave,cavaluzzi,cauthon,caudy,catino,catano,cassaro,cassarino,carrano,carozza,carow,carmickle,carlyon,carlew,cardena,caputi,capley,capalbo,canseco,candella,campton,camposano,calleros,calleja,callegari,calica,calarco,calais,caillier,cahue,cadenhead,cadenas,cabera,buzzo,busto,bussmann,busenbark,burzynski,bursley,bursell,burle,burkleo,burkette,burczyk,bullett,buikema,buenaventura,buege,buechel,budreau,budhram,bucknam,brye,brushwood,brumbalow,brulotte,bruington,bruderer,brougher,bromfield,broege,brodhead,brocklesby,broadie,brizuela,britz,brisendine,brilla,briggeman,brierton,bridgeford,breyfogle,brevig,breuninger,bresse,bresette,brelsford,breitbach,brayley,braund,branscom,brandner,brahm,braboy,brabble,bozman,boyte,boynes,boyken,bowell,bowan,boutet,bouse,boulet,boule,bottcher,bosquez,borrell,boria,bordes,borchard,bonson,bonino,bonas,bonamico,bolstad,bolser,bollis,bolich,bolf,boker,boileau,bohac,bogucki,bogren,boeger,bodziony,bodo,bodley,boback,blyther,blenker,blazina,blase,blamer,blacknall,blackmond,bitz,biser,biscardi,binz,bilton,billotte,billafuerte,bigford,biegler,bibber,bhandari,beyersdorf,bevelle,bettendorf,bessard,bertsche,berne,berlinger,berish,beranek,bentson,bentsen,benskin,benoy,benoist,benitz,belongia,belmore,belka,beitzel,beiter,beitel,behrns,becka,beaudion,beary,beare,beames,beabout,beaber,bazzano,bazinet,baucum,batrez,baswell,bastos,bascomb,bartha,barstad,barrilleaux,barretto,barresi,barona,barkhurst,barke,bardales,barczak,barca,barash,banfill,balonek,balmes,balko,balestrieri,baldino,baldelli,baken,baiza,bahner,baek,badour,badley,badia,backmon,bacich,bacca,ayscue,aynes,ausiello,auringer,auiles,aspinwall,askwith,artiga,arroliga,arns,arman,arellanes,aracena,antwine,antuna,anselmi,annen,angelino,angeli,angarola,andrae,amodio,ameen,alwine,alverio,altro,altobello,altemus,alquicira,allphin,allemand,allam,alessio,akpan,akerman,aiona,agyeman,agredano,adamik,adamczak,acrey,acevado,abreo,abrahamsen,abild,zwicker,zweig,zuvich,zumpano,zuluaga,zubek,zornes,zoglmann,ziminski,zimbelman,zhanel,zenor,zechman,zauner,zamarron,zaffino,yusuf,ytuarte,yett,yerkovich,yelder,yasuda,yapp,yaden,yackley,yaccarino,wytch,wyre,wussow,worthing,wormwood,wormack,wordell,woodroof,woodington,woodhams,wooddell,wollner,wojtkowski,wojcicki,wogan,wlodarczyk,wixted,withington,withem,wisler,wirick,winterhalter,winski,winne,winemiller,wimett,wiltfong,willibrand,willes,wilkos,wilbon,wiktor,wiggers,wigg,wiegmann,wickliff,wiberg,whittler,whittenton,whitling,whitledge,whitherspoon,whiters,whitecotton,whitebird,wheary,wetherill,westmark,westaby,wertenberger,wentland,wenstrom,wenker,wellen,weier,wegleitner,wedekind,wawers,wassel,warehime,wandersee,waltmon,waltersheid,walbridge,wakely,wakeham,wajda,waithe,waidelich,wahler,wahington,wagster,wadel,vuyovich,vuolo,vulich,vukovich,volmer,vollrath,vollbrecht,vogelgesang,voeller,vlach,vivar,vitullo,vitanza,visker,visalli,viray,vinning,viniard,villapando,villaman,vier,viar,viall,verstraete,vermilya,verdon,venn,velten,velis,vanoven,vanorder,vanlue,vanheel,vanderwoude,vanderheide,vandenheuvel,vandenbos,vandeberg,vandal,vanblarcom,vanaken,vanacker,vallian,valine,valent,vaine,vaile,vadner,uttech,urioste,urbanik,unrath,unnasch,underkofler,uehara,tyrer,tyburski,twaddle,turntine,tunis,tullock,tropp,troilo,tritsch,triola,trigo,tribou,tribley,trethewey,tress,trela,treharne,trefethen,trayler,trax,traut,tranel,trager,traczyk,towsley,torrecillas,tornatore,tork,torivio,toriello,tooles,tomme,tolosa,tolen,toca,titterington,tipsword,tinklenberg,tigney,tigert,thygerson,thurn,thur,thorstad,thornberg,thoresen,thomaston,tholen,thicke,theiler,thebeau,theaux,thaker,tewani,teufel,tetley,terrebonne,terrano,terpening,tela,teig,teichert,tegethoff,teele,tatar,tashjian,tarte,tanton,tanimoto,tamimi,tamas,talman,taal,szydlowski,szostak,swoyer,swerdlow,sweeden,sweda,swanke,swander,suyama,suriano,suri,surdam,suprenant,sundet,summerton,sult,suleiman,suffridge,suby,stych,studeny,strupp,struckman,strief,strictland,stremcha,strehl,stramel,stoy,stoutamire,storozuk,stordahl,stopher,stolley,stolfi,stoeger,stockhausen,stjulian,stivanson,stinton,stinchfield,stigler,stieglitz,stgermaine,steuer,steuber,steuart,stepter,stepnowski,stepanian,steimer,stefanelli,stebner,stears,steans,stayner,staubin,statz,stasik,starn,starmer,stargel,stanzione,stankovich,stamour,staib,stadelman,stadel,stachura,squadrito,springstead,spragg,spigelmyer,spieler,spaur,sovocool,soundara,soulia,souffrant,sorce,sonkin,sodhi,soble,sniffen,smouse,smittle,smithee,smedick,slowinski,slovacek,slominski,skowronek,skokan,skanes,sivertson,sinyard,sinka,sinard,simonin,simonian,simmions,silcott,silberg,siefken,siddon,shuttlesworth,shubin,shubeck,shiro,shiraki,shipper,shina,shilt,shikles,shideler,shenton,shelvey,shellito,shelhorse,shawcroft,shatto,shanholtzer,shamonsky,shadden,seymer,seyfarth,setlock,serratos,serr,sepulueda,senay,semmel,semans,selvig,selkirk,selk,seligson,seldin,seiple,seiersen,seidling,seidensticker,secker,searson,scordo,scollard,scoggan,scobee,sciandra,scialdone,schwimmer,schwieger,schweer,schwanz,schutzenhofer,schuetze,schrodt,schriever,schriber,schremp,schrecongost,schraeder,schonberg,scholtz,scholle,schoettle,schoenemann,schoene,schnitker,schmuhl,schmith,schlotterbeck,schleppenbach,schlee,schickel,schibi,schein,scheide,scheibe,scheib,schaumberg,schardein,schaalma,scantlin,scantlebury,sayle,sausedo,saurer,sassone,sarracino,saric,sanz,santarpia,santano,santaniello,sangha,sandvik,sandoral,sandobal,sandercock,sanantonio,salviejo,salsberry,salois,salazer,sagon,saglibene,sagel,sagal,saetern,saefong,sadiq,sabori,saballos,rygiel,rushlow,runco,rulli,ruller,ruffcorn,ruess,ruebush,rudlong,rudin,rudgers,rudesill,ruderman,rucki,rucinski,rubner,rubinson,rubiano,roznowski,rozanski,rowson,rower,rounsaville,roudabush,rotundo,rothell,rotchford,rosiles,roshak,rosetti,rosenkranz,rorer,rollyson,rokosz,rojek,roitman,rohrs,rogel,roewe,rodriges,rodocker,rodgerson,rodan,rodak,rocque,rochholz,robicheau,robbinson,roady,ritchotte,ripplinger,rippetoe,ringstaff,ringenberg,rinard,rigler,rightmire,riesen,riek,ridges,richner,richberg,riback,rial,rhyner,rhees,resse,renno,rendleman,reisz,reisenauer,reinschmidt,reinholt,reinard,reifsnyder,rehfeld,reha,regester,reffitt,redler,rediske,reckner,reckart,rebolloso,rebollar,reasonover,reasner,reaser,reano,reagh,raval,ratterman,ratigan,rater,rasp,raneses,randolf,ramil,ramdas,ramberg,rajaniemi,raggio,ragel,ragain,rade,radaker,racioppi,rabinovich,quickle,quertermous,queal,quartucci,quander,quain,pynes,putzel,purl,pulizzi,pugliares,prusak,prueter,protano,propps,primack,prieur,presta,preister,prawl,pratley,pozzo,powless,povey,pottorf,pote,postley,porzio,portney,ponzi,pontoriero,ponto,pont,poncedeleon,polimeni,polhamus,polan,poetker,poellnitz,podgurski,plotts,pliego,plaugher,plantenberg,plair,plagmann,pizzitola,pittinger,pitcavage,pischke,piontek,pintar,pinnow,pinneo,pinley,pingel,pinello,pimenta,pillard,piker,pietras,piere,phillps,pfleger,pfahl,pezzuti,petruccelli,petrello,peteet,pescatore,peruzzi,perusse,perotta,perona,perini,perelman,perciful,peppin,pennix,pennino,penalosa,pemble,pelz,peltzer,pelphrey,pelote,pellum,pellecchia,pelikan,peitz,pebworth,peary,pawlicki,pavelich,paster,pasquarella,paskey,paseur,paschel,parslow,parrow,parlow,parlett,parler,pargo,parco,paprocki,panepinto,panebianco,pandy,pandey,pamphile,pamintuan,pamer,paluso,paleo,paker,pagett,paczkowski,ozburn,ovington,overmeyer,ouellet,osterlund,oslin,oseguera,osaki,orrock,ormsbee,orlikowski,organista,oregan,orebaugh,orabuena,openshaw,ontiveroz,ondo,omohundro,ollom,ollivierre,olivencia,oley,olazabal,okino,offenberger,oestmann,ocker,obar,oakeson,nuzum,nurre,nowinski,novosel,norquist,nordlie,noorani,nonnemacher,nolder,njoku,niznik,niwa,niss,ninneman,nimtz,niemczyk,nieder,nicolo,nichlos,niblack,newtown,newill,newcom,neverson,neuhart,neuenschwande,nestler,nenno,nejman,neiffer,neidlinger,neglia,nazarian,navor,nary,narayan,nangle,nakama,naish,naik,nadolski,muscato,murphrey,murdick,murchie,muratalla,munnis,mundwiller,muncey,munce,mullenbach,mulhearn,mulcahey,muhammed,muchow,mountford,moudry,mosko,morvay,morrical,morr,moros,mormann,morgen,moredock,morden,mordarski,moravek,morandi,mooradian,montejo,montegut,montan,monsanto,monford,moncus,molinas,molek,mohd,moehrle,moehring,modzeleski,modafferi,moala,moake,miyahira,mitani,mischel,minges,minella,mimes,milles,milbrett,milanes,mikolajczyk,mikami,meucci,metler,methven,metge,messmore,messerschmidt,mesrobian,meservey,merseal,menor,menon,menear,melott,melley,melfi,meinhart,megivern,megeath,meester,meeler,meegan,medoff,medler,meckley,meath,mearns,mcquigg,mcpadden,mclure,mckellips,mckeithen,mcglathery,mcginnes,mcghan,mcdonel,mccullom,mccraken,mccrackin,mcconathy,mccloe,mcclaughry,mcclaflin,mccarren,mccaig,mcaulay,mcaffee,mazzuca,maytubby,mayner,maymi,mattiello,matthis,matthees,matthai,mathiason,mastrogiovann,masteller,mashack,marucci,martorana,martiniz,marter,martellaro,marsteller,marris,marrara,maroni,marolda,marocco,maritn,maresh,maready,marchione,marbut,maranan,maragno,mapps,manrriquez,mannis,manni,mangina,manganelli,mancera,mamon,maloch,mallozzi,maller,majchrzak,majano,mainella,mahanna,maertens,madon,macumber,macioce,machuga,machlin,machala,mabra,lybbert,luvert,lutts,luttrull,lupez,lukehart,ludewig,luchsinger,lovecchio,louissaint,loughney,lostroh,lorton,lopeman,loparo,londo,lombera,lokietek,loiko,lohrenz,lohan,lofties,locklar,lockaby,lobianco,llano,livesey,litster,liske,linsky,linne,lindbeck,licudine,leyua,levie,leonelli,lenzo,lenze,lents,leitao,leidecker,leibold,lehne,legan,lefave,leehy,ledue,lecount,lecea,leadley,lazzara,lazcano,lazalde,lavi,lavancha,lavan,latu,latty,lato,larranaga,lapidus,lapenta,langridge,langeveld,langel,landowski,landgren,landfried,lamattina,lallier,lairmore,lahaie,lagazo,lagan,lafoe,lafluer,laflame,lafevers,lada,lacoss,lachney,labreck,labreche,labay,kwasnik,kuzyk,kutzner,kushnir,kusek,kurtzman,kurian,kulhanek,kuklinski,kueny,kuczynski,kubitz,kruschke,krous,krompel,kritz,krimple,kriese,krenzer,kreis,kratzke,krane,krage,kraebel,kozub,kozma,kouri,koudelka,kotcher,kotas,kostic,kosh,kosar,kopko,kopka,kooy,konigsberg,konarski,kolmer,kohlmeyer,kobbe,knoop,knoedler,knocke,knipple,knippenberg,knickrehm,kneisel,kluss,klossner,klipfel,klawiter,klasen,kittles,kissack,kirtland,kirschenmann,kirckof,kiphart,kinstler,kinion,kilton,killman,kiehl,kief,kett,kesling,keske,kerstein,kepple,keneipp,kempson,kempel,kehm,kehler,keeran,keedy,kebert,keast,kearbey,kawaguchi,kaupu,kauble,katzenbach,katcher,kartes,karpowicz,karpf,karban,kanzler,kanarek,kamper,kaman,kalsow,kalafut,kaeser,kaercher,kaeo,kaeding,jurewicz,julson,jozwick,jollie,johnigan,johll,jochum,jewkes,jestes,jeska,jereb,jaurez,jarecki,jansma,janosik,jandris,jamin,jahr,jacot,ivens,itson,isenhower,iovino,ionescu,ingrum,ingels,imrie,imlay,ihlenfeld,ihde,igou,ibach,huyett,huppe,hultberg,hullihen,hugi,hueso,huesman,hsiao,hronek,hovde,housewright,houlahan,hougham,houchen,hostler,hoster,hosang,hornik,hornes,horio,honyumptewa,honeyman,honer,hommerding,holsworth,hollobaugh,hollinshead,hollands,hollan,holecek,holdorf,hokes,hogston,hoesly,hodkinson,hodgman,hodgens,hochstedler,hochhauser,hobbie,hoare,hnat,hiskey,hirschy,hinostroza,hink,hing,hillmer,hillian,hillerman,hietala,hierro,hickling,hickingbottom,heye,heubusch,hesselschward,herriot,hernon,hermida,hermans,hentschel,henningson,henneke,henk,heninger,heltsley,helmle,helminiak,helmes,hellner,hellmuth,helke,heitmeyer,heird,heinle,heinicke,heinandez,heimsoth,heibel,hegyi,heggan,hefel,heeralall,hedrington,heacox,hazlegrove,hazelett,haymore,havenhill,hautala,hascall,harvie,hartrick,hartling,harrer,harles,hargenrader,hanshew,hanly,hankla,hanisch,hancox,hammann,hambelton,halseth,hallisey,halleck,hallas,haisley,hairr,hainey,hainer,hailstock,haertel,guzek,guyett,guster,gussler,gurwitz,gurka,gunsolus,guinane,guiden,gugliotti,guevin,guevarra,guerard,gudaitis,guadeloupe,gschwind,grupe,grumbach,gruenes,gruenberg,grom,grodski,groden,grizzel,gritten,griswald,grishaber,grinage,grimwood,grims,griffon,griffies,gribben,gressley,gren,greenstreet,grealish,gravett,grantz,granfield,granade,gowell,gossom,gorsky,goring,goodnow,goodfriend,goodemote,golob,gollnick,golladay,goldwyn,goldsboro,golds,goldrick,gohring,gohn,goettsch,goertzen,goelz,godinho,goans,glumac,gleisner,gleen,glassner,glanzer,gladue,gjelaj,givhan,girty,girone,girgenti,giorgianni,gilpatric,gillihan,gillet,gilbar,gierut,gierhart,gibert,gianotti,giannetto,giambanco,gharing,geurts,gettis,gettel,gest,germani,gerdis,gerbitz,geppert,gennings,gemmer,gelvin,gellert,gehler,geddings,gearon,geach,gazaille,gayheart,gauld,gaukel,gaudio,gathing,gasque,garstka,garsee,garringer,garofano,garo,garnsey,garigen,garcias,garbe,ganoung,ganfield,ganaway,gamero,galuska,galster,gallacher,galinski,galimi,galik,galeazzi,galdo,galdames,galas,galanis,gaglio,gaeddert,gadapee,fussner,furukawa,fuhs,fuerte,fuerstenberg,fryrear,froese,fringer,frieson,friesenhahn,frieler,friede,freymuth,freyman,freudenberg,freman,fredricksen,frech,frasch,frantum,frankin,franca,frago,fragnoli,fouquet,fossen,foskett,forner,formosa,formisano,fooks,fons,folino,flott,flesch,flener,flemmons,flanagin,flamino,flamand,fitzerald,findling,filsinger,fillyaw,fillinger,fiechter,ferre,ferdon,feldkamp,fazzio,favia,faulconer,faughnan,faubel,fassler,faso,farrey,farrare,farnworth,farland,fairrow,faille,faherty,fagnant,fabula,fabbri,eylicio,esteve,estala,espericueta,escajeda,equia,enrriquez,enomoto,enmon,engemann,emmerson,emmel,emler,elstad,ellwein,ellerson,eliott,eliassen,elchert,eisenbeis,eisel,eikenberry,eichholz,ehmer,edgerson,echenique,eberley,eans,dziuk,dykhouse,dworak,dutt,dupas,duntz,dunshee,dunovant,dunnaway,dummermuth,duerson,ducotey,duchon,duchesneau,ducci,dubord,duberry,dubach,drummonds,droege,drish,drexel,dresch,dresbach,drenner,drechsler,dowen,dotter,dosreis,doser,dorward,dorin,dorf,domeier,doler,doleman,dolbow,dolbin,dobrunz,dobransky,dobberstein,dlouhy,diosdado,dingmann,dimmer,dimarino,dimaria,dillenburg,dilaura,dieken,dickhaus,dibbles,dibben,diamante,dewilde,dewaard,devich,devenney,devaux,dettinger,desroberts,dershem,dersch,derita,derickson,depina,deorio,deoliveira,denzler,dentremont,denoble,demshar,demond,demint,demichele,demel,delzer,delval,delorbe,delli,delbridge,delanoy,delancy,delahoya,dekle,deitrick,deis,dehnert,degrate,defrance,deetz,deeg,decoster,decena,dearment,daughety,datt,darrough,danzer,danielovich,dandurand,dancause,dalo,dalgleish,daisley,dadlani,daddona,daddio,dacpano,cyprian,cutillo,curz,curvin,cuna,cumber,cullom,cudworth,cubas,crysler,cryderman,crummey,crumbly,crookshanks,croes,criscione,crespi,cresci,creaser,craton,cowin,cowdrey,coutcher,cotterman,cosselman,cosgriff,cortner,corsini,corporan,corniel,cornick,cordts,copening,connick,conlisk,conelli,comito,colten,colletta,coldivar,colclasure,colantuono,colaizzi,coggeshall,cockman,cockfield,cobourn,cobo,cobarrubias,clyatt,cloney,clonch,climes,cleckner,clearo,claybourne,clavin,claridge,claffey,ciufo,cisnero,cipollone,cieslik,ciejka,cichocki,cicchetti,cianflone,chrusciel,christesen,chmielowiec,chirino,chillis,chhoun,chevas,chehab,chaviano,chavaria,chasten,charbonnet,chanley,champoux,champa,chalifoux,cerio,cedotal,cech,cavett,cavendish,catoire,castronovo,castellucci,castellow,castaner,casso,cassels,cassatt,cassar,cashon,cartright,carros,carrisalez,carrig,carrejo,carnicelli,carnett,carlise,carhart,cardova,cardell,carchi,caram,caquias,capper,capizzi,capano,cannedy,campese,calvello,callon,callins,callies,callicutt,calix,calin,califf,calderaro,caldeira,cadriel,cadmus,cadman,caccamise,buttermore,butay,bustamente,busa,burmester,burkard,burhans,burgert,bure,burdin,bullman,bulin,buelna,buehner,budin,buco,buckhanon,bryars,brutger,brus,brumitt,brum,bruer,brucato,broyhill,broy,brownrigg,brossart,brookings,broden,brocklehurst,brockert,bristo,briskey,bringle,bries,bressman,branyan,brands,bramson,brammell,brallier,bozich,boysel,bowthorpe,bowron,bowin,boutilier,boulos,boullion,boughter,bottiglieri,borruso,borreggine,borns,borkoski,borghese,borenstein,boran,booton,bonvillain,bonini,bonello,bolls,boitnott,boike,bohnet,bohnenkamp,bohmer,boeson,boeneke,bodey,bocchino,bobrowski,bobic,bluestein,bloomingdale,blogg,blewitt,blenman,bleck,blaszak,blankenbeckle,blando,blanchfield,blancato,blalack,blakenship,blackett,bisping,birkner,birckhead,bingle,bineau,billiel,bigness,bies,bierer,bhalla,beyerlein,betesh,besler,berzins,bertalan,berntsen,bergo,berganza,bennis,benney,benkert,benjamen,benincasa,bengochia,bendle,bendana,benchoff,benbrook,belsito,belshaw,belinsky,belak,beigert,beidleman,behen,befus,beel,bedonie,beckstrand,beckerle,beato,bauguess,baughan,bauerle,battis,batis,bastone,bassetti,bashor,bary,bartunek,bartoletti,barro,barno,barnicle,barlage,barkus,barkdull,barcellos,barbarino,baranski,baranick,bankert,banchero,bambrick,bamberg,bambenek,balthrop,balmaceda,ballman,balistrieri,balcomb,balboni,balbi,bagner,bagent,badasci,bacot,bache,babione,babic,babers,babbs,avitabile,avers,avena,avance,ausley,auker,audas,aubut,athearn,atcheson,astorino,asplund,aslanian,askari,ashmead,asby,asai,arterbury,artalejo,arqueta,arquero,arostegui,arnell,armeli,arista,arender,arca,arballo,aprea,applen,applegarth,apfel,antonello,antolin,antkowiak,angis,angione,angerman,angelilli,andujo,andrick,anderberg,amigon,amalfitano,alviso,alvez,altice,altes,almarez,allton,allston,allgeyer,allegretti,aliaga,algood,alberg,albarez,albaladejo,akre,aitkin,ahles,ahlberg,agnello,adinolfi,adamis,abramek,abolt,abitong,zurawski,zufall,zubke,zizzo,zipperer,zinner,zinda,ziller,zill,zevallos,zesati,zenzen,zentner,zellmann,zelinsky,zboral,zarcone,zapalac,zaldana,zakes,zaker,zahniser,zacherl,zabawa,zabaneh,youree,younis,yorty,yonce,yero,yerkey,yeck,yeargan,yauch,yashinski,yambo,wrinn,wrightsman,worton,wortley,worland,woolworth,woolfrey,woodhead,woltjer,wolfenden,wolden,wolchesky,wojick,woessner,witters,witchard,wissler,wisnieski,wisinski,winnike,winkowski,winkels,wingenter,wineman,winegardner,wilridge,wilmont,willians,williamsen,wilhide,wilhelmsen,wilhelmi,wildrick,wilden,wiland,wiker,wigglesworth,wiebusch,widdowson,wiant,wiacek,whittet,whitelock,whiteis,whiley,westrope,westpfahl,westin,wessman,wessinger,wesemann,wesby,wertheimer,weppler,wenke,wengler,wender,welp,weitzner,weissberg,weisenborn,weipert,weiman,weidmann,wehrsig,wehrenberg,weemes,weeman,wayner,waston,wasicek,wascom,wasco,warmath,warbritton,waltner,wallenstein,waldoch,waldal,wala,waide,wadlinger,wadhams,vullo,voorheis,vonbargen,volner,vollstedt,vollman,vold,voge,vittorio,violett,viney,vinciguerra,vinal,villata,villarrvel,vilanova,vigneault,vielma,veyna,vessella,versteegh,verderber,venier,venditti,velotta,vejarano,vecchia,vecchi,vastine,vasguez,varella,vanry,vannah,vanhyning,vanhuss,vanhoff,vanhoesen,vandivort,vandevender,vanderlip,vanderkooi,vandebrink,vancott,vallien,vallas,vallandingham,valiquette,valasek,vahey,vagott,uyematsu,urbani,uran,umbach,tyon,tyma,twyford,twombley,twohig,tutterrow,turnes,turkington,turchi,tunks,tumey,tumbaga,tuinstra,tsukamoto,tschetter,trussel,trubey,trovillion,troth,trostel,tron,trinka,trine,triarsi,treto,trautz,tragesser,tooman,toolson,tonozzi,tomkiewicz,tomasso,tolin,tolfree,toelle,tisor,tiry,tinstman,timmermann,tickner,tiburcio,thunberg,thronton,thompsom,theil,thayne,thaggard,teschner,tensley,tenery,tellman,tellado,telep,teigen,teator,teall,tayag,tavis,tattersall,tassoni,tarshis,tappin,tappe,tansley,talone,talford,tainter,taha,taguchi,tacheny,tabak,szymczyk,szwaja,szopinski,syvertsen,swogger,switcher,swist,swierczek,swiech,swickard,swiatek,swezey,swepson,sweezy,swaringen,swanagan,swailes,swade,sveum,svenningsen,svec,suttie,supry,sunga,summerhill,summars,sulit,stys,stutesman,stupak,stumpo,stuller,stuekerjuerge,stuckett,stuckel,stuchlik,stuard,strutton,strop,stromski,stroebel,strehlow,strause,strano,straney,stoyle,stormo,stopyra,stoots,stonis,stoltenburg,stoiber,stoessel,stitzer,stien,stichter,stezzi,stewert,stepler,steinkraus,stegemann,steeples,steenburg,steeley,staszak,stasko,starkson,stanwick,stanke,stanifer,stangel,stai,squiers,spraglin,spragins,spraberry,spoelstra,spisak,spirko,spille,spidel,speyer,speroni,spenst,spartz,sparlin,sparacio,spaman,spainhower,souers,souchet,sosbee,sorn,sorice,sorbo,soqui,solon,soehl,sodergren,sobie,smucker,smsith,smoley,smolensky,smolenski,smolder,smethers,slusar,slowey,slonski,slemmons,slatkin,slates,slaney,slagter,slacum,skutnik,skrzypek,skibbe,sjostrom,sjoquist,sivret,sitko,sisca,sinnett,sineath,simoni,simar,simao,silvestro,silleman,silha,silfies,silberhorn,silacci,sigrist,sieczkowski,sieczka,shure,shulz,shugrue,shrode,shovlin,shortell,shonka,shiyou,shiraishi,shiplett,sheu,shermer,sherick,sheeks,shantz,shakir,shaheed,shadoan,shadid,shackford,shabot,seung,seufert,setty,setters,servis,serres,serrell,serpas,sensenig,senft,semenec,semas,semaan,selvera,sellmeyer,segar,seever,seeney,seeliger,seehafer,seebach,sebben,seaward,seary,searl,searby,scordino,scolieri,scolaro,schwiebert,schwartze,schwaner,schuur,schupbach,schumacker,schum,schudel,schubbe,schroader,schramel,schollmeyer,schoenherr,schoeffler,schoeder,schnurr,schnorr,schneeman,schnake,schnaible,schmaus,schlotter,schinke,schimming,schimek,schikora,scheulen,scherping,schermer,scherb,schember,schellhase,schedler,schanck,schaffhauser,schaffert,schadler,scarola,scarfo,scarff,scantling,scaff,sayward,sayas,saxbury,savel,savastano,sault,satre,sarkar,santellan,sandmeier,sampica,salvesen,saltis,salloum,salling,salce,salatino,salata,salamy,sadowsky,sadlier,sabbatini,sabatelli,sabal,sabados,rydzewski,rybka,rybczyk,rusconi,rupright,rufino,ruffalo,rudiger,rudig,ruda,rubyor,royea,roxberry,rouzer,roumeliotis,rossmann,rosko,rosene,rosenbluth,roseland,rosasco,rosano,rosal,rorabaugh,romie,romaro,rolstad,rollow,rohrich,roghair,rogala,roets,roen,roemmich,roelfs,roeker,roedl,roedel,rodeheaver,roddenberry,rockstad,rocchi,robirds,robben,robasciotti,robaina,rizzotto,rizzio,ritcher,rissman,riseden,ripa,rion,rintharamy,rinehimer,rinck,riling,rietschlin,riesenberg,riemenschneid,rieland,rickenbaugh,rickenbach,rhody,revells,reutter,respress,resnik,remmel,reitmeyer,reitan,reister,reinstein,reino,reinkemeyer,reifschneider,reierson,reichle,rehmeier,rehl,reeds,rede,recar,rebeiro,raybourn,rawl,rautio,raugust,raudenbush,raudales,rattan,rapuano,rapoport,rantanen,ransbottom,raner,ramkissoon,rambousek,raio,rainford,radakovich,rabenhorst,quivers,quispe,quinoes,quilici,quattrone,quates,quance,quale,purswell,purpora,pulera,pulcher,puckhaber,pryer,pruyne,pruit,prudencio,prows,protzman,prothero,prosperi,prospal,privott,pritchet,priem,prest,prell,preer,pree,preddy,preda,pravata,pradhan,potocki,postier,postema,posadas,poremba,popichak,ponti,pomrenke,pomarico,pollok,polkinghorn,polino,pock,plater,plagman,pipher,pinzone,pinkleton,pillette,pillers,pilapil,pignone,pignatelli,piersol,piepho,picton,pickrel,pichard,picchi,piatek,pharo,phanthanouvon,pettingill,pettinato,petrovits,pethtel,petersheim,pershing,perrez,perra,pergram,peretz,perego,perches,pennello,pennella,pendry,penaz,pellish,pecanty,peare,paysour,pavlovich,pavick,pavelko,paustian,patzer,patete,patadia,paszkiewicz,pase,pasculli,pascascio,parrotte,parajon,paparo,papandrea,paone,pantaleon,panning,paniccia,panarello,palmeter,pallan,palardy,pahmeier,padget,padel,oxborrow,oveson,outwater,ottaway,otake,ostermeyer,osmer,osinski,osiecki,oroak,orndoff,orms,orkin,ordiway,opatz,onsurez,onishi,oliger,okubo,okoye,ohlmann,offord,offner,offerdahl,oesterle,oesch,odonnel,odeh,odebralski,obie,obermeier,oberhausen,obenshain,obenchain,nute,nulty,norrington,norlin,nore,nordling,nordhoff,norder,nordan,norals,nogales,noboa,nitsche,niermann,nienhaus,niedringhaus,niedbalski,nicolella,nicolais,nickleberry,nicewander,newfield,neurohr,neumeier,netterville,nersesian,nern,nerio,nerby,nerbonne,neitz,neidecker,neason,nead,navratil,naves,nastase,nasir,nasca,narine,narimatsu,nard,narayanan,nappo,namm,nalbone,nakonechny,nabarro,myott,muthler,muscatello,murriel,murin,muoio,mundel,munafo,mukherjee,muffoletto,muessig,muckey,mucher,mruk,moyd,mowell,mowatt,moutray,motzer,moster,morgenroth,morga,morataya,montross,montezuma,monterroza,montemarano,montello,montbriand,montavon,montaque,monigold,monforte,molgard,moleski,mohsin,mohead,mofield,moerbe,moeder,mochizuki,miyazaki,miyasaki,mital,miskin,mischler,minniear,minero,milosevic,mildenhall,mielsch,midden,michonski,michniak,michitsch,michelotti,micheli,michelfelder,michand,metelus,merkt,merando,meranda,mentz,meneley,menaker,melino,mehaffy,meehl,meech,meczywor,mcweeney,mcumber,mcredmond,mcneer,mcnay,mcmikle,mcmaken,mclaurine,mclauglin,mclaney,mckune,mckinnies,mckague,mchattie,mcgrapth,mcglothen,mcgath,mcfolley,mcdannell,mccurty,mccort,mcclymonds,mcclimon,mcclamy,mccaughan,mccartan,mccan,mccadden,mcburnie,mcburnett,mcbryar,mcannally,mcalevy,mcaleese,maytorena,mayrant,mayland,mayeaux,mauter,matthewson,mathiew,matern,matera,maslow,mashore,masaki,maruco,martorell,martenez,marrujo,marrison,maroun,markway,markos,markoff,markman,marello,marbry,marban,maphis,manuele,mansel,manganello,mandrell,mandoza,manard,manago,maltba,mallick,mallak,maline,malikowski,majure,majcher,maise,mahl,maffit,maffeo,madueno,madlem,madariaga,macvane,mackler,macconnell,macchi,maccarone,lyng,lynchard,lunning,luneau,lunden,lumbra,lumbert,lueth,ludington,luckado,lucchini,lucatero,luallen,lozeau,lowen,lovera,lovelock,louck,lothian,lorio,lorimer,lorge,loretto,longhenry,lonas,loiseau,lohrman,logel,lockie,llerena,livington,liuzzi,liscomb,lippeatt,liou,linhardt,lindelof,lindbo,limehouse,limage,lillo,lilburn,liggons,lidster,liddick,lich,liberato,leysath,lewelling,lesney,leser,lescano,leonette,lentsch,lenius,lemmo,lemming,lemcke,leggette,legerski,legard,leever,leete,ledin,lecomte,lecocq,leakes,leab,lazarz,layous,lawrey,lawery,lauze,lautz,laughinghouse,latulippe,lattus,lattanzio,lascano,larmer,laris,larcher,laprise,lapin,lapage,lano,langseth,langman,langland,landstrom,landsberg,landsaw,landram,lamphier,lamendola,lamberty,lakhani,lajara,lagrow,lagman,ladewig,laderman,ladden,lacrue,laclaire,lachut,lachner,kwit,kvamme,kvam,kutscher,kushi,kurgan,kunsch,kundert,kulju,kukene,kudo,kubin,kubes,kuberski,krystofiak,kruppa,krul,krukowski,kruegel,kronemeyer,krock,kriston,kretzer,krenn,kralik,krafft,krabill,kozisek,koverman,kovatch,kovarik,kotlowski,kosmala,kosky,kosir,kosa,korpi,kornbluth,koppen,kooistra,kohlhepp,kofahl,koeneman,koebel,koczur,kobrin,kobashigawa,koba,knuteson,knoff,knoble,knipper,knierim,kneisley,klusman,kloc,klitzing,klinko,klinefelter,klemetson,kleinpeter,klauser,klatte,klaren,klare,kissam,kirkhart,kirchmeier,kinzinger,kindt,kincy,kincey,kimoto,killingworth,kilcullen,kilbury,kietzman,kienle,kiedrowski,kidane,khamo,khalili,ketterling,ketchem,kessenich,kessell,kepp,kenon,kenning,kennady,kendzior,kemppainen,kellermann,keirns,keilen,keiffer,kehew,keelan,keawe,keator,kealy,keady,kathman,kastler,kastanes,kassab,karpin,karau,karathanasis,kaps,kaplun,kapaun,kannenberg,kanipe,kander,kandel,kanas,kanan,kamke,kaltenbach,kallenberger,kallam,kafton,kafer,kabler,kaaihue,jundt,jovanovich,jojola,johnstad,jodon,joachin,jinright,jessick,jeronimo,jenne,jelsma,jeannotte,jeangilles,jaworsky,jaubert,jarry,jarrette,jarreau,jarett,janos,janecka,janczak,jalomo,jagoda,jagla,jacquier,jaber,iwata,ivanoff,isola,iserman,isais,isaacks,inverso,infinger,ibsen,hyser,hylan,hybarger,hwee,hutchenson,hutchcroft,husar,hurlebaus,hunsley,humberson,hulst,hulon,huhtala,hugill,hugghins,huffmaster,huckeba,hrabovsky,howden,hoverson,houts,houskeeper,housh,hosten,horras,horchler,hopke,hooke,honie,holtsoi,holsomback,holoway,holmstead,hoistion,hohnstein,hoheisel,hoguet,hoggle,hogenson,hoffstetter,hoffler,hofe,hoefling,hoague,hizer,hirschfield,hironaka,hiraldo,hinote,hingston,hinaman,hillie,hillesheim,hilderman,hiestand,heyser,heys,hews,hertler,herrandez,heppe,henle,henkensiefken,henigan,henandez,henagan,hemberger,heman,helser,helmich,hellinger,helfrick,heldenbrand,heinonen,heineck,heikes,heidkamp,heglar,heffren,heelan,hedgebeth,heckmann,heckaman,hechmer,hazelhurst,hawken,haverkamp,havatone,hausauer,hasch,harwick,hartse,harrower,harle,hargroder,hardway,hardinger,hardemon,harbeck,hant,hamre,hamberg,hallback,haisten,hailstone,hahl,hagner,hagman,hagemeyer,haeussler,hackwell,haby,haataja,gverrero,gustovich,gustave,guske,gushee,gurski,gurnett,gura,gunto,gunselman,gugler,gudmundson,gudinas,guarneri,grumbine,gruis,grotz,grosskopf,grosman,grosbier,grinter,grilley,grieger,grewal,gressler,greaser,graus,grasman,graser,grannan,granath,gramer,graboski,goyne,gowler,gottwald,gottesman,goshay,gorr,gorovitz,gores,goossens,goodier,goodhue,gonzeles,gonzalos,gonnella,golomb,golick,golembiewski,goeke,godzik,goar,glosser,glendenning,glendening,glatter,glas,gittings,gitter,gisin,giscombe,gimlin,gillitzer,gillick,gilliand,gilb,gigler,gidden,gibeau,gibble,gianunzio,giannattasio,gertelman,gerosa,gerold,gerland,gerig,gerecke,gerbino,genz,genovesi,genet,gelrud,geitgey,geiszler,gehrlein,gawrys,gavilanes,gaulden,garthwaite,garmoe,gargis,gara,gannett,galligher,galler,galleher,gallahan,galford,gahn,gacek,gabert,fuster,furuya,furse,fujihara,fuhriman,frueh,fromme,froemming,friskney,frietas,freiler,freelove,freber,frear,frankl,frankenfield,franey,francke,foxworthy,formella,foringer,forgue,fonnesbeck,fonceca,folland,fodera,fode,floresca,fleurent,fleshner,flentge,fleischhacker,fleeger,flecher,flam,flaim,fivecoat,firebaugh,fioretti,finucane,filley,figuroa,figuerda,fiddelke,feurtado,fetterly,fessel,femia,feild,fehling,fegett,fedde,fechter,fawver,faulhaber,fatchett,fassnacht,fashaw,fasel,farrugia,farran,farness,farhart,fama,falwell,falvo,falkenstein,falin,failor,faigin,fagundo,fague,fagnan,fagerstrom,faden,eytchison,eyles,everage,evangelist,estrin,estorga,esponda,espindola,escher,esche,escarsega,escandon,erven,erding,eplin,enix,englade,engdahl,enck,emmette,embery,emberson,eltzroth,elsayed,ellerby,ellens,elhard,elfers,elazegui,eisermann,eilertson,eiben,ehrhard,ehresman,egolf,egnew,eggins,efron,effland,edminster,edgeston,eckstrom,eckhard,eckford,echoles,ebsen,eatherly,eastlick,earnheart,dykhuizen,dyas,duttweiler,dutka,dusenbury,dusenbery,durre,durnil,durnell,durie,durhan,durando,dupriest,dunsmoor,dunseith,dunnum,dunman,dunlevy,duma,dulude,dulong,duignan,dugar,dufek,ducos,duchaine,duch,dubow,drowne,dross,drollinger,droke,driggars,drawhorn,drach,drabek,doyne,doukas,dorvil,dorow,doroski,dornak,dormer,donnelson,donivan,dondero,dompe,dolle,doakes,diza,divirgilio,ditore,distel,disimone,disbro,dipiero,dingson,diluzio,dillehay,digiorgio,diflorio,dietzler,dietsch,dieterle,dierolf,dierker,dicostanzo,dicesare,dexheimer,dewitte,dewing,devoti,devincentis,devary,deutschman,dettloff,detienne,destasio,dest,despard,desmet,deslatte,desfosses,derise,derenzo,deppner,depolo,denoyer,denoon,denno,denne,deniston,denike,denes,demoya,demick,demicco,demetriou,demange,delva,delorge,delley,delisio,delhoyo,delgrande,delgatto,delcour,delair,deinert,degruy,degrave,degeyter,defino,deffenbaugh,deener,decook,decant,deboe,deblanc,deatley,dearmitt,deale,deaguiar,dayan,daus,dauberman,datz,dase,dary,dartt,darocha,dari,danowski,dancel,dami,dallmann,dalere,dalba,dakan,daise,dailing,dahan,dagnan,daggs,dagan,czarkowski,czaplinski,cutten,curtice,curenton,curboy,cura,culliton,culberth,cucchiara,cubbison,csaszar,crytser,crotzer,crossgrove,crosser,croshaw,crocco,critzer,creveling,cressy,creps,creese,cratic,craigo,craigen,craib,cracchiolo,crable,coykendall,cowick,coville,couzens,coutch,cousens,cousain,counselman,coult,cotterell,cott,cotham,corsaut,corriere,corredor,cornet,corkum,coreas,cordoza,corbet,corathers,conwill,contreas,consuegra,constanza,conolly,conedy,comins,combee,colosi,colom,colmenares,collymore,colleran,colina,colaw,colatruglio,colantro,colantonio,cohea,cogill,codner,codding,cockram,cocanougher,cobine,cluckey,clucas,cloward,cloke,clisham,clinebell,cliffe,clendenen,cisowski,cirelli,ciraolo,ciocca,cintora,ciesco,cibrian,chupka,chugg,christmann,choma,chiverton,chirinos,chinen,chimenti,chima,cheuvront,chesla,chesher,chesebro,chern,chehebar,cheatum,chastine,chapnick,chapelle,chambley,cercy,celius,celano,cayea,cavicchi,cattell,catanach,catacutan,castelluccio,castellani,cassmeyer,cassetta,cassada,caspi,cashmore,casebier,casanas,carrothers,carrizal,carriveau,carretero,carradine,carosella,carnine,carloni,carkhuff,cardosi,cardo,carchidi,caravello,caranza,carandang,cantrall,canpos,canoy,cannizzaro,canion,canida,canham,cangemi,cange,cancelliere,canard,camarda,calverley,calogero,callendar,calame,cadrette,cachero,caccavale,cabreros,cabrero,cabrara,cabler,butzer,butte,butrick,butala,bustios,busser,busic,bushorn,busher,burmaster,burkland,burkins,burkert,burgueno,burgraff,burel,burck,burby,bumford,bulock,bujnowski,buggie,budine,bucciero,bubier,brzoska,brydges,brumlow,brosseau,brooksher,brokke,broeker,brittin,bristle,briano,briand,brettschneide,bresnan,brentson,brenneis,brender,brazle,brassil,brasington,branstrom,branon,branker,brandwein,brandau,bralley,brailey,brague,brade,bozzi,bownds,bowmer,bournes,bour,bouchey,botto,boteler,borroel,borra,boroski,boothroyd,boord,bonga,bonato,bonadonna,bolejack,boldman,boiser,boggio,bogacki,boerboom,boehnlein,boehle,bodah,bobst,boak,bluemel,blockmon,blitch,blincoe,bleier,blaydes,blasius,bittel,binsfeld,bindel,bilotti,billiott,bilbrew,bihm,biersner,bielat,bidrowski,bickler,biasi,bhola,bhat,bewick,betzen,bettridge,betti,betsch,besley,beshero,besa,bertoli,berstein,berrien,berrie,berrell,bermel,berenguer,benzer,bensing,benedix,bemo,belile,beilman,behunin,behrmann,bedient,becht,beaule,beaudreault,bealle,beagley,bayuk,bayot,bayliff,baugess,battistoni,batrum,basinski,basgall,bartolomei,bartnik,bartl,bartko,bartholomay,barthlow,bartgis,barsness,barski,barlette,barickman,bargen,bardon,barcliff,barbu,barakat,baracani,baraban,banos,banko,bambach,balok,balogun,bally,baldini,balck,balcer,balash,baim,bailor,bahm,bahar,bagshaw,baggerly,badie,badal,backues,babino,aydelott,awbrey,aversano,avansino,auyon,aukamp,aujla,augenstein,astacio,asplin,asato,asano,aruizu,artale,arrick,arneecher,armelin,armbrester,armacost,arkell,argrave,areizaga,apolo,anzures,anzualda,antwi,antillon,antenor,annand,anhalt,angove,anglemyer,anglada,angiano,angeloni,andaya,ancrum,anagnos,ammirati,amescua,ambrosius,amacker,amacher,amabile,alvizo,alvernaz,alvara,altobelli,altobell,althauser,alterman,altavilla,alsip,almeyda,almeter,alman,allscheid,allaman,aliotta,aliberti,alghamdi,albiston,alberding,alarie,alano,ailes,ahsan,ahrenstorff,ahler,aerni,ackland,achor,acero,acebo,abshier,abruzzo,abrom,abood,abnet,abend,abegg,abbruzzese,aaberg,zysk,zutell,zumstein,zummo,zuhlke,zuehlsdorff,zuch,zucconi,zortman,zohn,zingone,zingg,zingale,zima,zientek,zieg,zervas,zerger,zenk,zeldin,zeiss,zeiders,zediker,zavodny,zarazua,zappone,zappala,zapanta,zaniboni,zanchi,zampedri,zaller,zakrajsek,zagar,zadrozny,zablocki,zable,yust,yunk,youngkin,yosten,yockers,yochim,yerke,yerena,yanos,wysinger,wyner,wrisley,woznicki,wortz,worsell,wooters,woon,woolcock,woodke,wonnacott,wolnik,wittstock,witting,witry,witfield,witcraft,wissmann,wissink,wisehart,wiscount,wironen,wipf,winterrowd,wingett,windon,windish,windisch,windes,wiltbank,willmarth,wiler,wieseler,wiedmaier,wiederstein,wiedenheft,wieberg,wickware,wickkiser,wickell,whittmore,whitker,whitegoat,whitcraft,whisonant,whisby,whetsell,whedon,westry,westcoat,wernimont,wentling,wendlandt,wencl,weisgarber,weininger,weikle,weigold,weigl,weichbrodt,wehrli,wehe,weege,weare,watland,wassmann,warzecha,warrix,warrell,warnack,waples,wantland,wanger,wandrei,wanat,wampole,waltjen,walterscheid,waligora,walding,waldie,walczyk,wakins,waitman,wair,wainio,wahpekeche,wahlman,wagley,wagenknecht,wadle,waddoups,wadding,vuono,vuillemot,vugteveen,vosmus,vorkink,vories,vondra,voelz,vlashi,vitelli,vitali,viscarra,vinet,vimont,villega,villard,vignola,viereck,videtto,vicoy,vessell,vescovi,verros,vernier,vernaglia,vergin,verdone,verdier,verastequi,vejar,vasile,vasi,varnadore,vardaro,vanzanten,vansumeren,vanschuyver,vanleeuwen,vanhowe,vanhoozer,vaness,vandewalker,vandevoorde,vandeveer,vanderzwaag,vanderweide,vanderhyde,vandellen,vanamburg,vanalst,vallin,valk,valentini,valcarcel,valasco,valadao,vacher,urquijo,unterreiner,unsicker,unser,unrau,undercoffler,uffelman,uemura,ueda,tyszko,tyska,tymon,tyce,tyacke,twinam,tutas,tussing,turmel,turkowski,turkel,turchetta,tupick,tukes,tufte,tufo,tuey,tuell,tuckerman,tsutsumi,tsuchiya,trossbach,trivitt,trippi,trippensee,trimbach,trillo,triller,trible,tribby,trevisan,tresch,tramonte,traff,trad,tousey,totaro,torregrosa,torralba,tolly,tofil,tofani,tobiassen,tiogangco,tino,tinnes,tingstrom,tingen,tindol,tifft,tiffee,tiet,thuesen,thruston,throndson,thornsbury,thornes,thiery,thielman,thie,theilen,thede,thate,thane,thalacker,thaden,teuscher,terracina,terell,terada,tepfer,tenneson,temores,temkin,telleria,teaque,tealer,teachey,tavakoli,tauras,taucher,tartaglino,tarpy,tannery,tani,tams,tamlin,tambe,tallis,talamante,takayama,takaki,taibl,taffe,tadesse,tade,tabeling,tabag,szoke,szoc,szala,szady,sysak,sylver,syler,swonger,swiggett,swensson,sweis,sweers,sweene,sweany,sweaney,swartwout,swamy,swales,susman,surman,sundblad,summerset,summerhays,sumerall,sule,sugimoto,subramanian,sturch,stupp,stunkard,stumpp,struiksma,stropes,stromyer,stromquist,strede,strazza,strauf,storniolo,storjohann,stonum,stonier,stonecypher,stoneberger,stollar,stokke,stokan,stoetzel,stoeckel,stockner,stockinger,stockert,stockdill,stobbe,stitzel,stitely,stirgus,stigers,stettner,stettler,sterlin,sterbenz,stemp,stelluti,steinmeyer,steininger,steinauer,steigerwalt,steider,stavrou,staufenberger,stassi,stankus,stanaway,stammer,stakem,staino,stahlnecker,stagnitta,staelens,staal,srsen,sprott,sprigg,sprenkle,sprenkel,spreitzer,spraque,sprandel,sporn,spivak,spira,spiewak,spieth,spiering,sperow,speh,specking,spease,spead,sparger,spanier,spall,sower,southcott,sosna,soran,sookram,sonders,solak,sohr,sohl,sofranko,soderling,sochor,sobon,smutz,smudrick,smithj,smid,slosser,sliker,slenker,sleger,slaby,skousen,skilling,skibinski,skees,skane,skafidas,sivic,sivertsen,sivers,sitra,sito,siracusa,sinicki,simpers,simley,simbeck,silberberg,siever,siegwarth,sidman,siddle,sibbett,shumard,shubrooks,shough,shorb,shoptaw,sholty,shoffstall,shiverdecker,shininger,shimasaki,shifrin,shiffler,sheston,sherr,shere,shepeard,shelquist,sheler,shauf,sharrar,sharpnack,shamsiddeen,shambley,shallenberger,shadler,shaban,sferra,seys,sexauer,sevey,severo,setlak,seta,sesko,sersen,serratore,serdula,senechal,seldomridge,seilhamer,seifer,seidlitz,sehnert,sedam,sebron,seber,sebek,seavers,scullark,scroger,scovill,sciascia,sciarra,schweers,schwarze,schummer,schultes,schuchardt,schuchard,schrieber,schrenk,schreifels,schowalter,schoultz,scholer,schofill,schoff,schnuerer,schnettler,schmitke,schmiege,schloop,schlinger,schlessman,schlesser,schlageter,schiess,schiefer,schiavoni,scherzer,scherich,schechtman,schebel,scharpman,schaich,schaap,scappaticci,scadlock,savocchia,savini,savers,savageau,sauvage,sause,sauerwein,sary,sarwary,sarnicola,santone,santoli,santalucia,santacruce,sansoucie,sankoff,sanes,sandri,sanderman,sammartano,salmonson,salmela,salmans,sallaz,salis,sakuma,sakowski,sajdak,sahm,sagredo,safrit,sackey,sabio,sabino,rybolt,ruzzo,ruthstrom,ruta,russin,russak,rusko,ruskin,rusiecki,ruscher,rupar,rumberger,rullan,ruliffson,ruhlman,rufenacht,ruelle,rudisell,rudi,rucci,rublee,ruberto,rubeck,rowett,rottinghaus,roton,rothgeb,rothgaber,rothermich,rostek,rossini,roskelley,rosing,rosi,rosewell,rosberg,roon,ronin,romesburg,romelus,rolley,rollerson,rollefson,rolins,rolens,rois,rohrig,rohrbacher,rohland,rohen,rogness,roes,roering,roehrick,roebke,rodregez,rodabaugh,rockingham,roblee,robel,roadcap,rizzolo,riviezzo,rivest,riveron,risto,rissler,rippentrop,ripka,rinn,ringuette,ringering,rindone,rindels,rieffer,riedman,riede,riecke,riebow,riddlebarger,rhome,rhodd,rhatigan,rhame,reyers,rewitzer,revalee,retzer,rettinger,reschke,requa,reper,reopell,renzelman,renne,renker,renk,renicker,rendina,rendel,remund,remmele,remiasz,remaklus,remak,reitsma,reitmeier,reiswig,reishus,reining,reim,reidinger,reick,reiche,regans,reffett,reesor,reekie,redpath,redditt,rechtzigel,recht,rearden,raynoso,raxter,ratkowski,rasulo,rassmussen,rassel,raser,rappleye,rappe,randrup,randleman,ramson,rampey,radziewicz,quirarte,quintyne,quickel,quattrini,quakenbush,quaile,pytel,pushaw,pusch,purslow,punzo,pullam,pugmire,puello,przekop,pruss,pruiett,provow,prophete,procaccini,pritz,prillaman,priess,pretlow,prestia,presha,prescod,preast,praytor,prashad,praino,pozzi,pottenger,potash,porada,popplewell,ponzo,ponter,pommier,polland,polidori,polasky,pola,poisso,poire,pofahl,podolsky,podell,plueger,plowe,plotz,plotnik,ploch,pliska,plessner,plaut,platzer,plake,pizzino,pirog,piquette,pipho,pioche,pintos,pinkert,pinet,pilkerton,pilch,pilarz,pignataro,piermatteo,picozzi,pickler,pickette,pichler,philogene,phare,phang,pfrogner,pfisterer,pettinelli,petruzzi,petrovic,petretti,petermeier,pestone,pesterfield,pessin,pesch,persky,perruzza,perrott,perritt,perretti,perrera,peroutka,peroni,peron,peret,perdew,perazzo,peppe,peno,penberthy,penagos,peles,pelech,peiper,peight,pefferman,peddie,peckenpaugh,pean,payen,pavloski,pavlica,paullin,patteson,passon,passey,passalacqua,pasquini,paskel,partch,parriott,parrella,parraz,parmely,parizo,papelian,papasergi,pantojz,panto,panich,panchal,palys,pallone,palinski,pali,palevic,pagels,paciorek,pacho,pacella,paar,ozbun,overweg,overholser,ovalles,outcalt,otterbein,otta,ostergren,osher,osbon,orzech,orwick,orrico,oropesa,ormes,orillion,onorati,onnen,omary,olding,okonski,okimoto,ohlrich,ohayon,oguin,ogley,oftedahl,offen,ofallon,oeltjen,odam,ockmond,ockimey,obermeyer,oberdorf,obanner,oballe,oard,oakden,nyhan,nydam,numan,noyer,notte,nothstein,notestine,noser,nork,nolde,nishihara,nishi,nikolic,nihart,nietupski,niesen,niehus,nidiffer,nicoulin,nicolaysen,nicklow,nickl,nickeson,nichter,nicholl,ngyun,newsham,newmann,neveux,neuzil,neumayer,netland,nessen,nesheim,nelli,nelke,necochea,nazari,navorro,navarez,navan,natter,natt,nater,nasta,narvaiz,nardelli,napp,nakahara,nairn,nagg,nager,nagano,nafziger,naffziger,nadelson,muzzillo,murri,murrey,murgia,murcia,muno,munier,mulqueen,mulliniks,mulkins,mulik,muhs,muffley,moynahan,mounger,mottley,motil,moseman,moseby,mosakowski,mortell,morrisroe,morrero,mormino,morland,morger,morgenthaler,moren,morelle,morawski,morasca,morang,morand,moog,montney,montera,montee,montane,montagne,mons,monohan,monnett,monkhouse,moncure,momphard,molyneaux,molles,mollenkopf,molette,mohs,mohmand,mohlke,moessner,moers,mockus,moccio,mlinar,mizzelle,mittler,mitri,mitchusson,mitchen,mistrot,mistler,misch,miriello,minkin,mininger,minerich,minehart,minderman,minden,minahan,milonas,millon,millholland,milleson,millerbernd,millage,militante,milionis,milhoan,mildenberger,milbury,mikolajczak,miklos,mikkola,migneault,mifsud,mietus,mieszala,mielnicki,midy,michon,michioka,micheau,michaeli,micali,methe,metallo,messler,mesch,merow,meroney,mergenthaler,meres,menuey,menousek,menning,menn,menghini,mendia,memmer,melot,mellenthin,melland,meland,meixner,meisenheimer,meineke,meinders,mehrens,mehlig,meglio,medsker,medero,mederios,meabon,mcwright,mcright,mcreath,mcrary,mcquirter,mcquerry,mcquary,mcphie,mcnurlen,mcnelley,mcnee,mcnairy,mcmanamy,mcmahen,mckowen,mckiver,mckinlay,mckearin,mcirvin,mcintrye,mchorse,mchaffie,mcgroarty,mcgoff,mcgivern,mceniry,mcelhiney,mcdiarmid,mccullars,mccubbins,mccrimon,mccovery,mccommons,mcclour,mccarrick,mccarey,mccallen,mcbrien,mcarthy,mayone,maybin,maxam,maurais,maughn,matzek,matts,matin,mathre,mathia,mateen,matava,masso,massar,massanet,masingale,mascaro,marthaler,martes,marso,marshman,marsalis,marrano,marolt,marold,markins,margulis,mardirosian,marchiano,marchak,marandola,marana,manues,mante,mansukhani,mansi,mannan,maniccia,mangine,manery,mandigo,mancell,mamo,malstrom,malouf,malenfant,maldenado,malandruccolo,malak,malabanan,makino,maisonave,mainord,maino,mainard,maillard,mahmud,mahdi,mahapatra,mahaley,mahaffy,magouirk,maglaras,magat,maga,maffia,madrazo,madrano,maditz,mackert,mackellar,mackell,macht,macchia,maccarthy,maahs,lytal,luzar,luzader,lutjen,lunger,lunan,luma,lukins,luhmann,luers,ludvigsen,ludlam,ludemann,luchini,lucente,lubrano,lubow,luber,lubeck,lowing,loven,loup,louge,losco,lorts,lormand,lorenzetti,longford,longden,longbrake,lokhmatov,loge,loeven,loeser,locey,locatelli,litka,lista,lisonbee,lisenbee,liscano,liranzo,liquori,liptrot,lionetti,linscomb,linkovich,linington,lingefelt,lindler,lindig,lindall,lincks,linander,linan,limburg,limbrick,limbach,likos,lighthall,liford,lietzke,liebe,liddicoat,lickley,lichter,liapis,lezo,lewan,levitz,levesgue,leverson,levander,leuthauser,letbetter,lesuer,lesmeister,lesly,lerer,leppanen,lepinski,lenherr,lembrick,lelonek,leisten,leiss,leins,leingang,leinberger,leinbach,leikam,leidig,lehtonen,lehnert,lehew,legier,lefchik,lecy,leconte,lecher,lebrecht,leaper,lawter,lawrenz,lavy,laur,lauderbaugh,lauden,laudato,latting,latsko,latini,lassere,lasseigne,laspina,laso,laslie,laskowitz,laske,lasenby,lascola,lariosa,larcade,lapete,laperouse,lanuza,lanting,lantagne,lansdale,lanphier,langmaid,langella,lanese,landrus,lampros,lamens,laizure,laitinen,laigle,lahm,lagueux,lagorio,lagomarsino,lagasca,lagana,lafont,laflen,lafavor,lafarge,laducer,ladnier,ladesma,lacognata,lackland,lacerte,labuff,laborin,labine,labauve,kuzio,kusterer,kussman,kusel,kusch,kurutz,kurdyla,kupka,kunzler,kunsman,kuni,kuney,kunc,kulish,kuliga,kulaga,kuilan,kuhre,kuhnke,kuemmerle,kueker,kudla,kudelka,kubinski,kubicki,kubal,krzyzanowski,krupicka,krumwiede,krumme,kropidlowski,krokos,kroell,kritzer,kribs,kreitlow,kreisher,kraynak,krass,kranzler,kramb,kozyra,kozicki,kovalik,kovalchik,kovacevic,kotula,kotrba,koteles,kosowski,koskela,kosiba,koscinski,kosch,korab,kopple,kopper,koppelman,koppel,konwinski,kolosky,koloski,kolinsky,kolinski,kolbeck,kolasa,koepf,koda,kochevar,kochert,kobs,knust,knueppel,knoy,knieriem,knier,kneller,knappert,klitz,klintworth,klinkenberg,klinck,kleindienst,kleeb,klecker,kjellberg,kitsmiller,kisor,kisiel,kise,kirbo,kinzle,kingsford,kingry,kimpton,kimel,killmon,killick,kilgallon,kilcher,kihn,kiggins,kiecker,kher,khaleel,keziah,kettell,ketchen,keshishian,kersting,kersch,kerins,kercher,kenefick,kemph,kempa,kelsheimer,kelln,kellenberger,kekahuna,keisling,keirnan,keimig,kehn,keal,kaupp,kaufhold,kauffmann,katzenberg,katona,kaszynski,kaszuba,kassebaum,kasa,kartye,kartchner,karstens,karpinsky,karmely,karel,karasek,kapral,kaper,kanelos,kanahele,kampmann,kampe,kalp,kallus,kallevig,kallen,kaliszewski,kaleohano,kalchthaler,kalama,kalahiki,kaili,kahawai,kagey,justiss,jurkowski,jurgensmeyer,juilfs,jopling,jondahl,jomes,joice,johannessen,joeckel,jezewski,jezek,jeswald,jervey,jeppsen,jenniges,jennett,jemmott,jeffs,jaurequi,janisch,janick,jacek,jacaruso,iwanicki,ishihara,isenberger,isbister,iruegas,inzer,inyart,inscore,innocenti,inglish,infantolino,indovina,inaba,imondi,imdieke,imbert,illes,iarocci,iannucci,huver,hutley,husser,husmann,hupf,huntsberger,hunnewell,hullum,huit,huish,hughson,huft,hufstetler,hueser,hudnell,hovden,housen,houghtling,hossack,hoshaw,horsford,horry,hornbacher,hoppenstedt,hopkinson,honza,homann,holzmeister,holycross,holverson,holtzlander,holroyd,holmlund,holderness,holderfield,holck,hojnacki,hohlfeld,hohenberger,hoganson,hogancamp,hoffses,hoerauf,hoell,hoefert,hodum,hoder,hockenbury,hoage,hisserich,hislip,hirons,hippensteel,hippen,hinkston,hindes,hinchcliff,himmel,hillberry,hildring,hiester,hiefnar,hibberd,hibben,heyliger,heyl,heyes,hevia,hettrick,hert,hersha,hernandz,herkel,herber,henscheid,hennesy,henly,henegan,henebry,hench,hemsath,hemm,hemken,hemann,heltzel,hellriegel,hejny,heinl,heinke,heidinger,hegeman,hefferan,hedglin,hebdon,hearnen,heape,heagy,headings,headd,hazelbaker,havlick,hauschildt,haury,hassenfritz,hasenbeck,haseltine,hartstein,hartry,hartnell,harston,harpool,harmen,hardister,hardey,harders,harbolt,harbinson,haraway,haque,hansmann,hanser,hansch,hansberry,hankel,hanigan,haneline,hampe,hamons,hammerstone,hammerle,hamme,hammargren,hamelton,hamberger,hamasaki,halprin,halman,hallihan,haldane,haifley,hages,hagadorn,hadwin,habicht,habermehl,gyles,gutzman,gutekunst,gustason,gusewelle,gurnsey,gurnee,gunterman,gumina,gulliver,gulbrandson,guiterez,guerino,guedry,gucwa,guardarrama,guagliano,guadagno,grulke,groote,groody,groft,groeneweg,grochow,grippe,grimstead,griepentrog,greenfeld,greenaway,grebe,graziosi,graw,gravina,grassie,granzow,grandjean,granby,gramacy,gozalez,goyer,gotch,gosden,gorny,gormont,goodgion,gonya,gonnerman,gompert,golish,goligoski,goldmann,goike,goetze,godeaux,glaza,glassel,glaspy,glander,giumarro,gitelman,gisondi,gismondi,girvan,girten,gironda,giovinco,ginkel,gilster,giesy,gierman,giddins,giardini,gianino,ghea,geurin,gett,getson,gerrero,germond,gentsy,genta,gennette,genito,genis,gendler,geltz,geiss,gehret,gegenheimer,geffert,geeting,gebel,gavette,gavenda,gaumond,gaudioso,gatzke,gatza,gattshall,gaton,gatchel,gasperi,gaska,gasiorowski,garritson,garrigus,garnier,garnick,gardinier,gardenas,garcy,garate,gandolfi,gamm,gamel,gambel,gallmon,gallemore,gallati,gainous,gainforth,gahring,gaffey,gaebler,gadzinski,gadbury,gabri,gaba,fyke,furtaw,furnas,furcron,funn,funck,fulwood,fulvio,fullmore,fukumoto,fuest,fuery,frymire,frush,frohlich,froedge,frodge,fritzinger,fricker,frericks,frein,freid,freggiaro,fratto,franzi,franciscus,fralix,fowble,fotheringham,foslien,foshie,fortmann,forsey,forkner,foppiano,fontanetta,fonohema,fogler,fockler,fluty,flusche,flud,flori,flenory,fleharty,fleeks,flaxman,fiumara,fitzmorris,finnicum,finkley,fineran,fillhart,filipi,fijal,fieldson,ficarra,festerman,ferryman,ferner,fergason,ferell,fennern,femmer,feldmeier,feeser,feenan,federick,fedak,febbo,feazell,fazzone,fauth,fauset,faurote,faulker,faubion,fatzinger,fasick,fanguy,fambrough,falks,fahl,faaita,exler,ewens,estrado,esten,esteen,esquivez,espejo,esmiol,esguerra,esco,ertz,erspamer,ernstes,erisman,erhard,ereaux,ercanbrack,erbes,epple,entsminger,entriken,enslow,ennett,engquist,englebert,englander,engesser,engert,engeman,enge,enerson,emhoff,emge,elting,ellner,ellenberg,ellenbecker,elio,elfert,elawar,ekstrand,eison,eismont,eisenbrandt,eiseman,eischens,ehrgott,egley,egert,eddlemon,eckerson,eckersley,eckberg,echeverry,eberts,earthman,earnhart,eapen,eachus,dykas,dusi,durning,durdan,dunomes,duncombe,dume,dullen,dullea,dulay,duffett,dubs,dubard,drook,drenth,drahos,dragone,downin,downham,dowis,dowhower,doward,dovalina,dopazo,donson,donnan,dominski,dollarhide,dolinar,dolecki,dolbee,doege,dockus,dobkin,dobias,divoll,diviney,ditter,ditman,dissinger,dismang,dirlam,dinneen,dini,dingwall,diloreto,dilmore,dillaman,dikeman,diiorio,dighton,diffley,dieudonne,dietel,dieringer,diercks,dienhart,diekrager,diefendorf,dicke,dicamillo,dibrito,dibona,dezeeuw,dewhurst,devins,deviney,deupree,detherage,despino,desmith,desjarlais,deshner,desha,desanctis,derring,derousse,derobertis,deridder,derego,derden,deprospero,deprofio,depping,deperro,denty,denoncourt,dencklau,demler,demirchyan,demichiel,demesa,demere,demaggio,delung,deluise,delmoral,delmastro,delmas,delligatti,delle,delasbour,delarme,delargy,delagrange,delafontaine,deist,deiss,deighan,dehoff,degrazia,degman,defosses,deforrest,deeks,decoux,decarolis,debuhr,deberg,debarr,debari,dearmon,deare,deardurff,daywalt,dayer,davoren,davignon,daviau,dauteuil,dauterive,daul,darnley,darakjy,dapice,dannunzio,danison,daniello,damario,dalonzo,dallis,daleske,dalenberg,daiz,dains,daines,dagnese,dady,dadey,czyzewski,czapor,czaplewski,czajka,cyganiewicz,cuttino,cutrona,cussins,cusanelli,cuperus,cundy,cumiskey,cumins,cuizon,cuffia,cuffe,cuffari,cuccaro,cubie,cryder,cruson,crounse,cromedy,cring,creer,credeur,crea,cozort,cozine,cowee,cowdery,couser,courtway,courington,cotman,costlow,costell,corton,corsaro,corrieri,corrick,corradini,coron,coren,corbi,corado,copus,coppenger,cooperwood,coontz,coonce,contrera,connealy,conell,comtois,compere,commins,commings,comegys,colyar,colo,collister,collick,collella,coler,colborn,cohran,cogbill,coffen,cocuzzo,clynes,closter,clipp,clingingsmith,clemence,clayman,classon,clas,clarey,clague,ciubal,citrino,citarella,cirone,cipponeri,cindrich,cimo,ciliberto,cichowski,ciccarello,cicala,chura,chubbuck,chronis,christlieb,chizek,chittester,chiquito,chimento,childree,chianese,chevrette,checo,chastang,chargualaf,chapmon,chantry,chahal,chafetz,cezar,ceruantes,cerrillo,cerrano,cerecedes,cerami,cegielski,cavallero,catinella,cassata,caslin,casano,casacchia,caruth,cartrette,carten,carodine,carnrike,carnall,carmicle,carlan,carlacci,caris,cariaga,cardine,cardimino,cardani,carbonara,capua,capponi,cappellano,caporale,canupp,cantrel,cantone,canterberry,cannizzo,cannan,canelo,caneer,candill,candee,campbel,caminero,camble,caluya,callicott,calk,caito,caffie,caden,cadavid,cacy,cachu,cachola,cabreja,cabiles,cabada,caamano,byran,byon,buyck,bussman,bussie,bushner,burston,burnison,burkman,burkhammer,bures,burdeshaw,bumpass,bullinger,bullers,bulgrin,bugay,budak,buczynski,buckendorf,buccieri,bubrig,brynteson,brunz,brunmeier,brunkow,brunetto,brunelli,brumwell,bruggman,brucki,brucculeri,brozovich,browing,brotman,brocker,broadstreet,brix,britson,brinck,brimmage,brierre,bridenstine,brezenski,brezee,brevik,brentlinger,brentley,breidenbach,breckel,brech,brazzle,braughton,brauch,brattin,brattain,branhan,branford,braner,brander,braly,braegelmann,brabec,boyt,boyack,bowren,bovian,boughan,botton,botner,bosques,borzea,borre,boron,bornhorst,borgstrom,borella,bontempo,bonniwell,bonnes,bonillo,bonano,bolek,bohol,bohaty,boffa,boetcher,boesen,boepple,boehler,boedecker,boeckx,bodi,boal,bloodsworth,bloodgood,blome,blockett,blixt,blanchett,blackhurst,blackaby,bjornberg,bitzer,bittenbender,bitler,birchall,binnicker,binggeli,billett,bilberry,biglow,bierly,bielby,biegel,berzas,berte,bertagnolli,berreth,bernhart,bergum,berentson,berdy,bercegeay,bentle,bentivegna,bentham,benscoter,benns,bennick,benjamine,beneze,benett,beneke,bendure,bendix,bendick,benauides,belman,bellus,bellott,bellefleur,bellas,beljan,belgard,beith,beinlich,beierle,behme,beevers,beermann,beeching,bedward,bedrosian,bedner,bedeker,bechel,becera,beaubrun,beardmore,bealmear,bazin,bazer,baumhoer,baumgarner,bauknecht,battson,battiest,basulto,baster,basques,basista,basiliere,bashi,barzey,barz,bartus,bartucca,bartek,barrero,barreca,barnoski,barndt,barklow,baribeau,barette,bares,barentine,bareilles,barbre,barberi,barbagelata,baraw,baratto,baranoski,baptise,bankson,bankey,bankard,banik,baltzley,ballen,balkey,balius,balderston,bakula,bakalar,baffuto,baerga,badoni,backous,bachtel,bachrach,baccari,babine,babilonia,baar,azbill,azad,aycox,ayalla,avolio,austerberry,aughtry,aufderheide,auch,attanasio,athayde,atcher,asselta,aslin,aslam,ashwood,ashraf,ashbacher,asbridge,asakura,arzaga,arriaza,arrez,arrequin,arrants,armiger,armenteros,armbrister,arko,argumedo,arguijo,ardolino,arcia,arbizo,aravjo,aper,anzaldo,antu,antrikin,antonetty,antinoro,anthon,antenucci,anstead,annese,ankrum,andreason,andrado,andaverde,anastos,anable,amspoker,amrine,amrein,amorin,amel,ambrosini,alsbrook,alnutt,almasi,allessio,allateef,aldous,alderink,aldaz,akmal,akard,aiton,aites,ainscough,aikey,ahrends,ahlm,aguada,agans,adelmann,addesso,adaway,adamaitis,ackison,abud,abendroth,abdur,abdool,aamodt,zywiec,zwiefelhofer,zwahlen,zunino,zuehl,zmuda,zmolek,zizza,ziska,zinser,zinkievich,zinger,zingarelli,ziesmer,ziegenfuss,ziebol,zettlemoyer,zettel,zervos,zenke,zembower,zelechowski,zelasko,zeise,zeek,zeeb,zarlenga,zarek,zaidi,zahnow,zahnke,zaharis,zacate,zabrocki,zaborac,yurchak,yuengling,younie,youngers,youell,yott,yoshino,yorks,yordy,yochem,yerico,yerdon,yeiser,yearous,yearick,yeaney,ybarro,yasutake,yasin,yanke,yanish,yanik,yamazaki,yamat,yaggi,ximenez,wyzard,wynder,wyly,wykle,wutzke,wuori,wuertz,wuebker,wrightsel,worobel,worlie,worford,worek,woolson,woodrome,woodly,woodling,wontor,wondra,woltemath,wollmer,wolinski,wolfert,wojtanik,wojtak,wohlfarth,woeste,wobbleton,witz,wittmeyer,witchey,wisotzkey,wisnewski,wisman,wirch,wippert,wineberg,wimpee,wilusz,wiltsey,willig,williar,willers,willadsen,wildhaber,wilday,wigham,wiewel,wieting,wietbrock,wiesel,wiesehan,wiersema,wiegert,widney,widmark,wickson,wickings,wichern,whtie,whittie,whitlinger,whitfill,whitebread,whispell,whetten,wheeley,wheeles,wheelen,whatcott,weyland,weter,westrup,westphalen,westly,westland,wessler,wesolick,wesler,wesche,werry,wero,wernecke,werkhoven,wellspeak,wellings,welford,welander,weissgerber,weisheit,weins,weill,weigner,wehrmann,wehrley,wehmeier,wege,weers,weavers,watring,wassum,wassman,wassil,washabaugh,wascher,warth,warbington,wanca,wammack,wamboldt,walterman,walkington,walkenhorst,walinski,wakley,wagg,wadell,vuckovich,voogd,voller,vokes,vogle,vogelsberg,vodicka,vissering,vipond,vincik,villalona,vickerman,vettel,veteto,vesperman,vesco,vertucci,versaw,verba,ventris,venecia,vendela,venanzi,veldhuizen,vehrs,vaughen,vasilopoulos,vascocu,varvel,varno,varlas,varland,vario,vareschi,vanwyhe,vanweelden,vansciver,vannaman,vanluven,vanloo,vanlaningham,vankomen,vanhout,vanhampler,vangorp,vangorden,vanella,vandresar,vandis,vandeyacht,vandewerker,vandevsen,vanderwall,vandercook,vanderberg,vanbergen,valko,valesquez,valeriano,valen,vachula,vacha,uzee,uselman,urizar,urion,urben,upthegrove,unzicker,unsell,unick,umscheid,umin,umanzor,ullo,ulicki,uhlir,uddin,tytler,tymeson,tyger,twisdale,twedell,tweddle,turrey,tures,turell,tupa,tuitt,tuberville,tryner,trumpower,trumbore,troglen,troff,troesch,trivisonno,tritto,tritten,tritle,trippany,tringali,tretheway,treon,trejos,tregoning,treffert,traycheff,travali,trauth,trauernicht,transou,trane,trana,toves,tosta,torp,tornquist,tornes,torchio,toor,tooks,tonks,tomblinson,tomala,tollinchi,tolles,tokich,tofte,todman,titze,timpone,tillema,tienken,tiblier,thyberg,thursby,thurrell,thurm,thruman,thorsted,thorley,thomer,thoen,thissen,theimer,thayn,thanpaeng,thammavongsa,thalman,texiera,texidor,teverbaugh,teska,ternullo,teplica,tepe,teno,tenholder,tenbusch,tenbrink,temby,tejedor,teitsworth,teichmann,tehan,tegtmeyer,tees,teem,tays,taubert,tauares,taschler,tartamella,tarquinio,tarbutton,tappendorf,tapija,tansil,tannahill,tamondong,talahytewa,takashima,taecker,tabora,tabin,tabbert,szymkowski,szymanowski,syversen,syrett,synnott,sydnes,swimm,sweney,swearegene,swartzel,swanstrom,svedin,suryan,supplice,supnet,suoboda,sundby,sumaya,sumabat,sulzen,sukovaty,sukhu,sugerman,sugalski,sudweeks,sudbeck,sucharski,stutheit,stumfoll,stuffle,struyk,strutz,strumpf,strowbridge,strothman,strojny,strohschein,stroffolino,stribble,strevel,strenke,stremming,strehle,stranak,stram,stracke,stoudamire,storks,stopp,stonebreaker,stolt,stoica,stofer,stockham,stockfisch,stjuste,stiteler,stiman,stillions,stillabower,stierle,sterlace,sterk,stepps,stenquist,stenner,stellman,steines,steinbaugh,steinbacher,steiling,steidel,steffee,stavinoha,staver,stastny,stasiuk,starrick,starliper,starlin,staniford,staner,standre,standefer,standafer,stanczyk,stallsmith,stagliano,staehle,staebler,stady,stadtmiller,squyres,spurbeck,sprunk,spranger,spoonamore,spoden,spilde,spezio,speros,sperandio,specchio,spearin,spayer,spallina,spadafino,sovie,sotello,sortor,sortino,soros,sorola,sorbello,sonner,sonday,somes,soloway,soens,soellner,soderblom,sobin,sniezek,sneary,smyly,smutnick,smoots,smoldt,smitz,smitreski,smallen,smades,slunaker,sluka,slown,slovick,slocomb,slinger,slife,sleeter,slanker,skufca,skubis,skrocki,skov,skjei,skilton,skarke,skalka,skalak,skaff,sixkiller,sitze,siter,sisko,sirman,sirls,sinotte,sinon,sincock,sincebaugh,simmoms,similien,silvius,silton,silloway,sikkema,sieracki,sienko,siemon,siemer,siefker,sieberg,siebens,siebe,sicurella,sicola,sickle,shumock,shumiloff,shuffstall,shuemaker,shuart,shroff,shreeve,shostak,shortes,shorr,shivley,shintaku,shindo,shimomura,shiigi,sherow,sherburn,shepps,shenefield,shelvin,shelstad,shelp,sheild,sheaman,shaulis,sharrer,sharps,sharpes,shappy,shapero,shanor,shandy,seyller,severn,sessom,sesley,servidio,serrin,sero,septon,septer,sennott,sengstock,senff,senese,semprini,semone,sembrat,selva,sella,selbig,seiner,seif,seidt,sehrt,seemann,seelbinder,sedlay,sebert,seaholm,seacord,seaburg,scungio,scroggie,scritchfield,scrimpsher,scrabeck,scorca,scobey,scivally,schwulst,schwinn,schwieson,schwery,schweppe,schwartzenbur,schurz,schumm,schulenburg,schuff,schuerholz,schryer,schrager,schorsch,schonhardt,schoenfelder,schoeck,schoeb,schnitzler,schnick,schnautz,schmig,schmelter,schmeichel,schluneger,schlosberg,schlobohm,schlenz,schlembach,schleisman,schleining,schleiff,schleider,schink,schilz,schiffler,schiavi,scheuer,schemonia,scheman,schelb,schaul,schaufelberge,scharer,schardt,scharbach,schabacker,scee,scavone,scarth,scarfone,scalese,sayne,sayed,savitz,satterlund,sattazahn,satow,sastre,sarr,sarjeant,sarff,sardella,santoya,santoni,santai,sankowski,sanft,sandow,sandoe,sandhaus,sandefer,sampey,samperi,sammarco,samia,samek,samay,samaan,salvadore,saltness,salsgiver,saller,salaz,salano,sakal,saka,saintlouis,saile,sahota,saggese,sagastume,sadri,sadak,sachez,saalfrank,saal,saadeh,rynn,ryley,ryle,rygg,rybarczyk,ruzich,ruyter,ruvo,rupel,ruopp,rundlett,runde,rundall,runck,rukavina,ruggiano,rufi,ruef,rubright,rubbo,rowbottom,rotner,rotman,rothweiler,rothlisberger,rosseau,rossean,rossa,roso,rosiek,roshia,rosenkrans,rosener,rosencrantz,rosencrans,rosello,roques,rookstool,rondo,romasanta,romack,rokus,rohweder,roethler,roediger,rodwell,rodrigus,rodenbeck,rodefer,rodarmel,rockman,rockholt,rochow,roches,roblin,roblez,roble,robers,roat,rizza,rizvi,rizk,rixie,riveiro,rius,ritschard,ritrovato,risi,rishe,rippon,rinks,ringley,ringgenberg,ringeisen,rimando,rilley,rijos,rieks,rieken,riechman,riddley,ricord,rickabaugh,richmeier,richesin,reyolds,rexach,requena,reppucci,reposa,renzulli,renter,remondini,reither,reisig,reifsnider,reifer,reibsome,reibert,rehor,rehmann,reedus,redshaw,reczek,recupero,recor,reckard,recher,realbuto,razer,rayman,raycraft,rayas,rawle,raviscioni,ravetto,ravenelle,rauth,raup,rattliff,rattley,rathfon,rataj,rasnic,rappleyea,rapaport,ransford,rann,rampersad,ramis,ramcharan,rainha,rainforth,ragans,ragains,rafidi,raffety,raducha,radsky,radler,radatz,raczkowski,rabenold,quraishi,quinerly,quercia,quarnstrom,pusser,puppo,pullan,pulis,pugel,puca,pruna,prowant,provines,pronk,prinkleton,prindall,primas,priesmeyer,pridgett,prevento,preti,presser,presnall,preseren,presas,presa,prchal,prattis,pratillo,praska,prak,powis,powderly,postlewait,postle,posch,porteus,porraz,popwell,popoff,poplaski,poniatoski,pollina,polle,polhill,poletti,polaski,pokorney,pointdexter,poinsette,ploszaj,plitt,pletz,pletsch,plemel,pleitez,playford,plaxco,platek,plambeck,plagens,placido,pisarski,pinuelas,pinnette,pinick,pinell,pinciaro,pinal,pilz,piltz,pillion,pilkinton,pikul,piepenburg,piening,piehler,piedrahita,piechocki,picknell,pickelsimer,pich,picariello,phoeuk,phillipson,philbert,pherigo,phelka,peverini,petrash,petramale,petraglia,pery,personius,perrington,perrill,perpall,perot,perman,peragine,pentland,pennycuff,penninger,pennachio,pendexter,penalver,pelzel,pelter,pelow,pelo,peli,peinado,pedley,pecue,pecore,pechar,peairs,paynes,payano,pawelk,pavlock,pavlich,pavich,pavek,pautler,paulik,patmore,patella,patee,patalano,passini,passeri,paskell,parrigan,parmar,parayno,paparelli,pantuso,pante,panico,panduro,panagos,pama,palmo,pallotta,paling,palamino,pake,pajtas,pailthorpe,pahler,pagon,paglinawan,pagley,paget,paetz,paet,padley,pacleb,pachelo,paccione,pabey,ozley,ozimek,ozawa,owney,outram,ouillette,oudekerk,ostrosky,ostermiller,ostermann,osterloh,osterfeld,ossenfort,osoria,oshell,orsino,orscheln,orrison,ororke,orellano,orejuela,ordoyne,opsahl,opland,onofre,onaga,omahony,olszowka,olshan,ollig,oliff,olien,olexy,oldridge,oldfather,olalde,okun,okumoto,oktavec,okin,ohme,ohlemacher,ohanesian,odneal,odgers,oderkirk,odden,ocain,obradovich,oakey,nussey,nunziato,nunoz,nunnenkamp,nuncio,noviello,novacek,nothstine,northum,norsen,norlander,norkus,norgaard,norena,nored,nobrega,niziolek,ninnemann,nievas,nieratko,nieng,niedermeyer,niedermaier,nicolls,newham,newcome,newberger,nevills,nevens,nevel,neumiller,netti,nessler,neria,nemet,nelon,nellon,neller,neisen,neilly,neifer,neid,neering,neehouse,neef,needler,nebergall,nealis,naumoff,naufzinger,narum,narro,narramore,naraine,napps,nansteel,namisnak,namanny,nallie,nakhle,naito,naccari,nabb,myracle,myhand,mwakitwile,muzzy,muscolino,musco,muscente,muscat,muscara,musacchia,musa,murrish,murfin,muray,munnelly,munley,munivez,mundine,mundahl,munari,mullennex,mullendore,mulkhey,mulinix,mulders,muhl,muenchow,muellner,mudget,mudger,muckenfuss,muchler,mozena,movius,mouldin,motola,mosseri,mossa,moselle,mory,morsell,morrish,morles,morie,morguson,moresco,morck,moppin,moosman,montuori,montono,montogomery,montis,monterio,monter,monsalve,mongomery,mongar,mondello,moncivais,monard,monagan,molt,mollenhauer,moldrem,moldonado,molano,mokler,moisant,moilanen,mohrman,mohamad,moger,mogel,modine,modin,modic,modha,mlynek,miya,mittiga,mittan,mitcheltree,misfeldt,misener,mirchandani,miralles,miotke,miosky,mintey,mins,minassian,minar,mimis,milon,milloy,millison,milito,milfort,milbradt,mikulich,mikos,miklas,mihelcic,migliorisi,migliori,miesch,midura,miclette,michela,micale,mezey,mews,mewes,mettert,mesker,mesich,mesecher,merthie,mersman,mersereau,merrithew,merriott,merring,merenda,merchen,mercardo,merati,mentzel,mentis,mentel,menotti,meno,mengle,mendolia,mellick,mellett,melichar,melhorn,melendres,melchiorre,meitzler,mehtani,mehrtens,meditz,medeiras,meckes,mcteer,mctee,mcparland,mcniell,mcnealey,mcmanaway,mcleon,mclay,mclavrin,mcklveen,mckinzey,mcken,mckeand,mckale,mcilwraith,mcilroy,mcgreal,mcgougan,mcgettigan,mcgarey,mcfeeters,mcelhany,mcdaris,mccomis,mccomber,mccolm,mccollins,mccollin,mccollam,mccoach,mcclory,mcclennon,mccathern,mccarthey,mccarson,mccarrel,mccargar,mccandles,mccamish,mccally,mccage,mcbrearty,mcaneny,mcanallen,mcalarney,mcaferty,mazzo,mazy,mazurowski,mazique,mayoras,mayden,maxberry,mauller,matusiak,mattsen,matthey,matkins,mathiasen,mathe,mateus,matalka,masullo,massay,mashak,mascroft,martinex,martenson,marsiglia,marsella,maroudas,marotte,marner,markes,maret,mareno,marean,marcinkiewicz,marchel,marasigan,manzueta,manzanilla,manternach,manring,manquero,manoni,manne,mankowski,manjarres,mangen,mangat,mandonado,mandia,mancias,manbeck,mamros,maltez,mallia,mallar,malla,malen,malaspina,malahan,malagisi,malachowski,makowsky,makinen,makepeace,majkowski,majid,majercin,maisey,mainguy,mailliard,maignan,mahlman,maha,magsamen,magpusao,magnano,magley,magedanz,magarelli,magaddino,maenner,madnick,maddrey,madaffari,macnaughton,macmullen,macksey,macknight,macki,macisaac,maciejczyk,maciag,machenry,machamer,macguire,macdaniel,maccormack,maccabe,mabbott,mabb,lynott,lycan,lutwin,luscombe,lusco,lusardi,luria,lunetta,lundsford,lumas,luisi,luevanos,lueckenhoff,ludgate,ludd,lucherini,lubbs,lozado,lourens,lounsberry,loughrey,loughary,lotton,losser,loshbaugh,loseke,loscalzo,lortz,loperena,loots,loosle,looman,longstaff,longobardi,longbottom,lomay,lomasney,lohrmann,lohmiller,logalbo,loetz,loeffel,lodwick,lodrigue,lockrem,llera,llarena,littrel,littmann,lisser,lippa,lipner,linnemann,lingg,lindemuth,lindeen,lillig,likins,lieurance,liesmann,liesman,liendo,lickert,lichliter,leyvas,leyrer,lewy,leubner,lesslie,lesnick,lesmerises,lerno,lequire,lepera,lepard,lenske,leneau,lempka,lemmen,lemm,lemere,leinhart,leichner,leicher,leibman,lehmberg,leggins,lebeda,leavengood,leanard,lazaroff,laventure,lavant,lauster,laumea,latigo,lasota,lashure,lasecki,lascurain,lartigue,larouche,lappe,laplaunt,laplace,lanum,lansdell,lanpher,lanoie,lankard,laniado,langowski,langhorn,langfield,langfeldt,landt,landerman,landavazo,lampo,lampke,lamper,lamery,lambey,lamadrid,lallemand,laisure,laigo,laguer,lagerman,lageman,lagares,lacosse,lachappelle,laborn,labonne,kuzia,kutt,kutil,kurylo,kurowski,kuriger,kupcho,kulzer,kulesa,kules,kuhs,kuhne,krutz,krus,krupka,kronberg,kromka,kroese,krizek,krivanek,kringel,kreiss,kratofil,krapp,krakowsky,kracke,kozlow,kowald,kover,kovaleski,kothakota,kosten,koskinen,kositzke,korff,korbar,kopplin,koplin,koos,konyn,konczak,komp,komo,kolber,kolash,kolakowski,kohm,kogen,koestner,koegler,kodama,kocik,kochheiser,kobler,kobara,knezevich,kneifl,knapchuck,knabb,klugman,klosner,klingel,klimesh,klice,kley,kleppe,klemke,kleinmann,kleinhans,kleinberg,kleffner,kleckley,klase,kisto,kissick,kisselburg,kirschman,kirks,kirkner,kirkey,kirchman,kinville,kinnunen,kimmey,kimmerle,kimbley,kilty,kilts,killmeyer,killilea,killay,kiest,kierce,kiepert,kielman,khalid,kewal,keszler,kesson,kesich,kerwood,kerksiek,kerkhoff,kerbo,keranen,keomuangtai,kenter,kennelley,keniry,kendzierski,kempner,kemmis,kemerling,kelsay,kelchner,kela,keithly,keipe,kegg,keer,keahey,kaywood,kayes,kawahara,kasuboski,kastendieck,kassin,kasprzyk,karraker,karnofski,karman,karger,karge,karella,karbowski,kapphahn,kannel,kamrath,kaminer,kamansky,kalua,kaltz,kalpakoff,kalkbrenner,kaku,kaib,kaehler,kackley,kaber,justo,juris,jurich,jurgenson,jurez,junor,juniel,juncker,jugo,jubert,jowell,jovanovic,joosten,joncas,joma,johnso,johanns,jodoin,jockers,joans,jinwright,jinenez,jimeson,jerrett,jergens,jerden,jerdee,jepperson,jendras,jeanfrancois,jazwa,jaussi,jaster,jarzombek,jarencio,janocha,jakab,jadlowiec,jacobsma,jach,izaquirre,iwaoka,ivaska,iturbe,israelson,isles,isachsen,isaak,irland,inzerillo,insogna,ingegneri,ingalsbe,inciong,inagaki,icenogle,hyett,hyers,huyck,hutti,hutten,hutnak,hussar,hurrle,hurford,hurde,hupper,hunkin,hunkele,hunke,humann,huhtasaari,hugel,hufft,huegel,hrobsky,hren,hoyles,hovsepian,hovenga,hovatter,houdek,hotze,hossler,hossfeld,hosseini,horten,hort,horr,horgen,horen,hoopii,hoon,hoogland,hontz,honnold,homewood,holway,holtgrewe,holtan,holstrom,holstege,hollway,hollingshed,hollenback,hollard,holberton,hoines,hogeland,hofstad,hoetger,hoen,hoaglund,hirota,hintermeister,hinnen,hinders,hinderer,hinchee,himelfarb,himber,hilzer,hilling,hillers,hillegas,hildinger,hignight,highman,hierholzer,heyde,hettich,hesketh,herzfeld,herzer,hershenson,hershberg,hernando,hermenegildo,hereth,hererra,hereda,herbin,heraty,herard,hepa,henschel,henrichsen,hennes,henneberger,heningburg,henig,hendron,hendericks,hemple,hempe,hemmingsen,hemler,helvie,helmly,helmbrecht,heling,helin,helfrey,helble,helaire,heizman,heisser,heiny,heinbaugh,heidemann,heidema,heiberger,hegel,heerdt,heeg,heefner,heckerman,heckendorf,heavin,headman,haynesworth,haylock,hayakawa,hawksley,haverstick,haut,hausen,hauke,haubold,hattan,hattabaugh,hasstedt,hashem,haselhorst,harrist,harpst,haroldsen,harmison,harkema,harison,hariri,harcus,harcum,harcharik,hanzel,hanvey,hantz,hansche,hansberger,hannig,hanken,hanhardt,hanf,hanauer,hamberlin,halward,halsall,hals,hallquist,hallmon,halk,halbach,halat,hajdas,hainsworth,haik,hahm,hagger,haggar,hader,hadel,haddick,hackmann,haasch,haaf,guzzetta,guzy,gutterman,gutmann,gutkowski,gustine,gursky,gurner,gunsolley,gumpert,gulla,guilmain,guiliani,guier,guers,guerero,guerena,guebara,guadiana,grunder,grothoff,grosland,grosh,groos,grohs,grohmann,groepper,grodi,grizzaffi,grissinger,grippi,grinde,griffee,grether,greninger,greigo,gregorski,greger,grega,greenberger,graza,grattan,grasse,grano,gramby,gradilla,govin,goutremout,goulas,gotay,gosling,gorey,gordner,goossen,goodwater,gonzaga,gonyo,gonska,gongalves,gomillion,gombos,golonka,gollman,goldtrap,goldammer,golas,golab,gola,gogan,goffman,goeppinger,godkin,godette,glore,glomb,glauner,glassey,glasner,gividen,giuffrida,gishal,giovanelli,ginoza,ginns,gindlesperger,gindhart,gillem,gilger,giggey,giebner,gibbson,giacomo,giacolone,giaccone,giacchino,ghere,gherardini,gherardi,gfeller,getts,gerwitz,gervin,gerstle,gerfin,geremia,gercak,gener,gencarelli,gehron,gehrmann,geffers,geery,geater,gawlik,gaudino,garsia,garrahan,garrabrant,garofolo,garigliano,garfinkle,garelick,gardocki,garafola,gappa,gantner,ganther,gangelhoff,gamarra,galstad,gally,gallik,gallier,galimba,gali,galassi,gaige,gadsby,gabbin,gabak,fyall,furney,funez,fulwider,fulson,fukunaga,fujikawa,fugere,fuertes,fuda,fryson,frump,frothingham,froning,froncillo,frohling,froberg,froats,fritchman,frische,friedrichsen,friedmann,friddell,frid,fresch,frentzel,freno,frelow,freimuth,freidel,freehan,freeby,freeburn,fredieu,frederiksen,fredeen,frazell,frayser,fratzke,frattini,franze,franich,francescon,framer,fragman,frack,foxe,fowlston,fosberg,fortna,fornataro,forden,foots,foody,fogt,foglia,fogerty,fogelson,flygare,flowe,flinner,flem,flath,flater,flahaven,flad,fjeld,fitanides,fistler,fishbaugh,firsching,finzel,finical,fingar,filosa,filicetti,filby,fierst,fierra,ficklen,ficher,fersner,ferrufino,ferrucci,fero,ferlenda,ferko,fergerstrom,ferge,fenty,fent,fennimore,fendt,femat,felux,felman,feldhaus,feisthamel,feijoo,feiertag,fehrman,fehl,feezell,feeback,fedigan,fedder,fechner,feary,fayson,faylor,fauteux,faustini,faure,fauci,fauber,fattig,farruggio,farrens,faraci,fantini,fantin,fanno,fannings,faniel,fallaw,falker,falkenhagen,fajen,fahrner,fabel,fabacher,eytcheson,eyster,exford,exel,evetts,evenstad,evanko,euresti,euber,etcitty,estler,essner,essinger,esplain,espenshade,espaillat,escribano,escorcia,errington,errett,errera,erlanger,erenrich,erekson,erber,entinger,ensworth,ensell,enno,ennen,englin,engblom,engberson,encinias,enama,emel,elzie,elsbree,elman,ellebracht,elkan,elfstrom,elerson,eleazer,eleam,eldrige,elcock,einspahr,eike,eidschun,eickman,eichele,eiche,ehlke,eguchi,eggink,edouard,edgehill,eckes,eblin,ebberts,eavenson,earvin,eardley,eagon,eader,dzubak,dylla,dyckman,dwire,dutrow,dutile,dusza,dustman,dusing,duryee,durupan,durtschi,durtsche,durell,dunny,dunnegan,dunken,dumm,dulak,duker,dukelow,dufort,dufilho,duffee,duett,dueck,dudzinski,dudasik,duckwall,duchemin,dubrow,dubis,dubicki,duba,drust,druckman,drinnen,drewett,drewel,dreitzler,dreckman,drappo,draffen,drabant,doyen,dowding,doub,dorson,dorschner,dorrington,dorney,dormaier,dorff,dorcy,donges,donelly,donel,domangue,dols,dollahite,dolese,doldo,doiley,dohrman,dohn,doheny,doceti,dobry,dobrinski,dobey,divincenzo,dischinger,dirusso,dirocco,dipiano,diop,dinitto,dinehart,dimsdale,diminich,dimalanta,dillavou,dilello,difusco,diffey,diffenderfer,diffee,difelice,difabio,dietzman,dieteman,diepenbrock,dieckmann,dicampli,dibari,diazdeleon,diallo,dewitz,dewiel,devoll,devol,devincent,devier,devendorf,devalk,detten,detraglia,dethomas,detemple,desler,desharnais,desanty,derocco,dermer,derks,derito,derhammer,deraney,dequattro,depass,depadua,denyes,denyer,dentino,denlinger,deneal,demory,demopoulos,demontigny,demonte,demeza,delsol,delrosso,delpit,delpapa,delouise,delone,delo,delmundo,delmore,dellapaolera,delfin,delfierro,deleonardis,delenick,delcarlo,delcampo,delcamp,delawyer,delaroca,delaluz,delahunt,delaguardia,dekeyser,dekay,dejaeger,dejackome,dehay,dehass,degraffenried,degenhart,degan,deever,deedrick,deckelbaum,dechico,dececco,decasas,debrock,debona,debeaumont,debarros,debaca,dearmore,deangelus,dealmeida,dawood,davney,daudt,datri,dasgupta,darring,darracott,darcus,daoud,dansbury,dannels,danielski,danehy,dancey,damour,dambra,dalcour,dahlheimer,dadisman,dacunto,dacamara,dabe,cyrulik,cyphert,cwik,cussen,curles,curit,curby,curbo,cunas,cunard,cunanan,cumpton,culcasi,cucinotta,cucco,csubak,cruthird,crumwell,crummitt,crumedy,crouthamel,cronce,cromack,crisafi,crimin,cresto,crescenzo,cremonese,creedon,crankshaw,cozzens,coval,courtwright,courcelle,coupland,counihan,coullard,cotrell,cosgrave,cornelio,corish,cordoua,corbit,coppersmith,coonfield,conville,contrell,contento,conser,conrod,connole,congrove,conery,condray,colver,coltman,colflesh,colcord,colavito,colar,coile,coggan,coenen,codling,coda,cockroft,cockrel,cockerill,cocca,coberley,clouden,clos,clish,clinkscale,clester,clammer,cittadino,citrano,ciresi,cillis,ciccarelli,ciborowski,ciarlo,ciardullo,chritton,chopp,chirco,chilcoat,chevarie,cheslak,chernak,chay,chatterjee,chatten,chatagnier,chastin,chappuis,channey,champlain,chalupsky,chalfin,chaffer,chadek,chadderton,cestone,cestero,cestari,cerros,cermeno,centola,cedrone,cayouette,cavan,cavaliero,casuse,castricone,castoreno,casten,castanada,castagnola,casstevens,cassanova,caspari,casher,cashatt,casco,casassa,casad,carville,cartland,cartegena,carsey,carsen,carrino,carrilo,carpinteyro,carmley,carlston,carlsson,cariddi,caricofe,carel,cardy,carducci,carby,carangelo,capriotti,capria,caprario,capelo,canul,cantua,cantlow,canny,cangialosi,canepa,candland,campolo,campi,camors,camino,camfield,camelo,camarero,camaeho,calvano,calliste,caldarella,calcutt,calcano,caissie,cager,caccamo,cabotage,cabble,byman,buzby,butkowski,bussler,busico,bushovisky,busbin,busard,busalacchi,burtman,burrous,burridge,burrer,burno,burin,burgette,burdock,burdier,burckhard,bunten,bungay,bundage,bumby,bultema,bulinski,bulan,bukhari,buganski,buerkle,buen,buehl,budzynski,buckham,bryk,brydon,bruyere,brunsvold,brunnett,brunker,brunfield,brumble,brue,brozina,brossman,brosey,brookens,broersma,brodrick,brockmeier,brockhouse,brisky,brinkly,brincefield,brighenti,brigante,brieno,briede,bridenbaugh,brickett,breske,brener,brenchley,breitkreutz,breitbart,breister,breining,breighner,breidel,brehon,breheny,breard,breakell,brazill,braymiller,braum,brau,brashaw,bransom,brandolino,brancato,branagan,braff,brading,bracker,brackenbury,bracher,braasch,boylen,boyda,boyanton,bowlus,bowditch,boutot,bouthillette,boursiquot,bourjolly,bouret,boulerice,bouer,bouchillon,bouchie,bottin,boteilho,bosko,bosack,borys,bors,borla,borjon,borghi,borah,booten,boore,bonuz,bonne,bongers,boneta,bonawitz,bonanni,bomer,bollen,bollard,bolla,bolio,boisseau,boies,boiani,bohorquez,boghossian,boespflug,boeser,boehl,boegel,bodrick,bodkins,bodenstein,bodell,bockover,bocci,bobbs,boals,boahn,boadway,bluma,bluett,bloor,blomker,blevens,blethen,bleecker,blayney,blaske,blasetti,blancas,blackner,bjorkquist,bjerk,bizub,bisono,bisges,bisaillon,birr,birnie,bires,birdtail,birdine,bina,billock,billinger,billig,billet,bigwood,bigalk,bielicki,biddick,biccum,biafore,bhagat,beza,beyah,bevier,bevell,beute,betzer,betthauser,bethay,bethard,beshaw,bertholf,bertels,berridge,bernot,bernath,bernabei,berkson,berkovitz,berkich,bergsten,berget,berezny,berdin,beougher,benthin,benhaim,benenati,benejan,bemiss,beloate,bellucci,bellotti,belling,bellido,bellaire,bellafiore,bekins,bekele,beish,behnken,beerly,beddo,becket,becke,bebeau,beauchaine,beaucage,beadling,beacher,bazar,baysmore".split(",")))];aJ=aF.concat([function(J){var K,I,G,F,H,E,D,B,C,A,z,y,x,w,v,s;A=[];y=t(o(J));G=0;for(F=y.length;G<F;G++){w=y[G];if(ab(w)){break}D=0;for(H=aF.length;D<H;D++){E=aF[D];B=k(J,w);x=E(B);z=0;for(E=x.length;z<E;z++){if(B=x[z],s=J.slice(B.i,+B.j+1||9000000000),s.toLowerCase()!==B.matched_word){C={};for(v in w){K=w[v],-1!==s.indexOf(v)&&(C[v]=K)}B.l33t=!0;B.token=s;B.sub=C;s=B;var u=void 0,u=[];for(I in C){K=C[I],u.push(I+" -> "+K)}s.sub_display=u.join(", ");A.push(B)}}}}return A},function(n){var p,w,u,s,v,q;v=aL(n,ac);q=[];u=0;for(s=v.length;u<s;u++){p=v[u],w=[p.i,p.j],p=w[0],w=w[1],q.push({pattern:"digits",i:p,j:w,token:n.slice(p,+w+1||9000000000)})}return q},function(n){var p,w,u,s,v,q;v=aL(n,i);q=[];u=0;for(s=v.length;u<s;u++){p=v[u],w=[p.i,p.j],p=w[0],w=w[1],q.push({pattern:"year",i:p,j:w,token:n.slice(p,+w+1||9000000000)})}return q},function(n){return ae(n).concat(af(n))},function(n){var p,s,q;q=[];for(p=0;p<n.length;){for(s=p+1;;){if(n.slice(s-1,+s+1||9000000000),n.charAt(s-1)===n.charAt(s)){s+=1}else{2<s-p&&q.push({pattern:"repeat",i:p,j:s-1,token:n.slice(p,s),repeated_char:n.charAt(p)});break}}p=s}return q},function(D){var E,C,A,z,B,y,x,v,w,u,s,q,p;v=[];for(B=0;B<D.length;){y=B+1;q=p=w=null;for(s in aC){if(u=aC[s],A=function(){var H,G,F,n;F=[D.charAt(B),D.charAt(y)];n=[];H=0;for(G=F.length;H<G;H++){E=F[H],n.push(u.indexOf(E))}return n}(),z=A[0],A=A[1],-1<z&&-1<A&&(z=A-z,1===z||-1===z)){w=u;p=s;q=z;break}}if(w){for(;;){if(z=D.slice(y-1,+y+1||9000000000),x=z[0],C=z[1],A=function(){var n,H,G,F;G=[x,C];F=[];n=0;for(H=G.length;n<H;n++){E=G[n],F.push(u.indexOf(E))}return F}(),z=A[0],A=A[1],A-z===q){y+=1}else{2<y-B&&v.push({pattern:"sequence",i:B,j:y-1,token:D.slice(B,y),sequence_name:p,sequence_space:w.length,ascending:1===q});break}}}B=y}return v},function(n){var p,s,q;q=[];for(s in aj){p=aj[s],ap(q,l(n,p,s))}return q}]);aj={qwerty:al,dvorak:{"!":["`~",null,null,"2@","'\"",null],'"':[null,"1!","2@",",<","aA",null],"#":["2@",null,null,"4$",".>",",<"],$:["3#",null,null,"5%","pP",".>"],"%":["4$",null,null,"6^","yY","pP"],"&":["6^",null,null,"8*","gG","fF"],"'":[null,"1!","2@",",<","aA",null],"(":["8*",null,null,"0)","rR","cC"],")":["9(",null,null,"[{","lL","rR"],"*":["7&",null,null,"9(","cC","gG"],"+":["/?","]}",null,"\\|",null,"-_"],",":"'\",2@,3#,.>,oO,aA".split(","),"-":["sS","/?","=+",null,null,"zZ"],".":",< 3# 4$ pP eE oO".split(" "),"/":"lL,[{,]},=+,-_,sS".split(","),"0":["9(",null,null,"[{","lL","rR"],1:["`~",null,null,"2@","'\"",null],2:["1!",null,null,"3#",",<","'\""],3:["2@",null,null,"4$",".>",",<"],4:["3#",null,null,"5%","pP",".>"],5:["4$",null,null,"6^","yY","pP"],6:["5%",null,null,"7&","fF","yY"],7:["6^",null,null,"8*","gG","fF"],8:["7&",null,null,"9(","cC","gG"],9:["8*",null,null,"0)","rR","cC"],":":[null,"aA","oO","qQ",null,null],";":[null,"aA","oO","qQ",null,null],"<":"'\",2@,3#,.>,oO,aA".split(","),"=":["/?","]}",null,"\\|",null,"-_"],">":",< 3# 4$ pP eE oO".split(" "),"?":"lL,[{,]},=+,-_,sS".split(","),"@":["1!",null,null,"3#",",<","'\""],A:[null,"'\"",",<","oO",";:",null],B:["xX","dD","hH","mM",null,null],C:"gG,8*,9(,rR,tT,hH".split(","),D:"iI,fF,gG,hH,bB,xX".split(","),E:"oO,.>,pP,uU,jJ,qQ".split(","),F:"yY,6^,7&,gG,dD,iI".split(","),G:"fF,7&,8*,cC,hH,dD".split(","),H:"dD,gG,cC,tT,mM,bB".split(","),I:"uU,yY,fF,dD,xX,kK".split(","),J:["qQ","eE","uU","kK",null,null],K:["jJ","uU","iI","xX",null,null],L:"rR,0),[{,/?,sS,nN".split(","),M:["bB","hH","tT","wW",null,null],N:"tT,rR,lL,sS,vV,wW".split(","),O:"aA ,< .> eE qQ ;:".split(" "),P:".>,4$,5%,yY,uU,eE".split(","),Q:[";:","oO","eE","jJ",null,null],R:"cC,9(,0),lL,nN,tT".split(","),S:"nN,lL,/?,-_,zZ,vV".split(","),T:"hH,cC,rR,nN,wW,mM".split(","),U:"eE,pP,yY,iI,kK,jJ".split(","),V:["wW","nN","sS","zZ",null,null],W:["mM","tT","nN","vV",null,null],X:["kK","iI","dD","bB",null,null],Y:"pP,5%,6^,fF,iI,uU".split(","),Z:["vV","sS","-_",null,null,null],"[":["0)",null,null,"]}","/?","lL"],"\\":["=+",null,null,null,null,null],"]":["[{",null,null,null,"=+","/?"],"^":["5%",null,null,"7&","fF","yY"],_:["sS","/?","=+",null,null,"zZ"],"`":[null,null,null,"1!",null,null],a:[null,"'\"",",<","oO",";:",null],b:["xX","dD","hH","mM",null,null],c:"gG,8*,9(,rR,tT,hH".split(","),d:"iI,fF,gG,hH,bB,xX".split(","),e:"oO,.>,pP,uU,jJ,qQ".split(","),f:"yY,6^,7&,gG,dD,iI".split(","),g:"fF,7&,8*,cC,hH,dD".split(","),h:"dD,gG,cC,tT,mM,bB".split(","),i:"uU,yY,fF,dD,xX,kK".split(","),j:["qQ","eE","uU","kK",null,null],k:["jJ","uU","iI","xX",null,null],l:"rR,0),[{,/?,sS,nN".split(","),m:["bB","hH","tT","wW",null,null],n:"tT,rR,lL,sS,vV,wW".split(","),o:"aA ,< .> eE qQ ;:".split(" "),p:".>,4$,5%,yY,uU,eE".split(","),q:[";:","oO","eE","jJ",null,null],r:"cC,9(,0),lL,nN,tT".split(","),s:"nN,lL,/?,-_,zZ,vV".split(","),t:"hH,cC,rR,nN,wW,mM".split(","),u:"eE,pP,yY,iI,kK,jJ".split(","),v:["wW","nN","sS","zZ",null,null],w:["mM","tT","nN","vV",null,null],x:["kK","iI","dD","bB",null,null],y:"pP,5%,6^,fF,iI,uU".split(","),z:["vV","sS","-_",null,null,null],"{":["0)",null,null,"]}","/?","lL"],"|":["=+",null,null,null,null,null],"}":["[{",null,null,null,"=+","/?"],"~":[null,null,null,"1!",null,null]},keypad:ak,mac_keypad:{"*":["/",null,null,null,null,null,"-","9"],"+":["6","9","-",null,null,null,null,"3"],"-":["9","/","*",null,null,null,"+","6"],".":["0","2","3",null,null,null,null,null],"/":["=",null,null,null,"*","-","9","8"],"0":[null,"1","2","3",".",null,null,null],1:[null,null,"4","5","2","0",null,null],2:["1","4","5","6","3",".","0",null],3:["2","5","6","+",null,null,".","0"],4:[null,null,"7","8","5","2","1",null],5:"4,7,8,9,6,3,2,1".split(","),6:["5","8","9","-","+",null,"3","2"],7:[null,null,null,"=","8","5","4",null],8:["7",null,"=","/","9","6","5","4"],9:"8,=,/,*,-,+,6,5".split(","),"=":[null,null,null,null,"/","9","8","7"]}};aI=function(n){var p,v,s,q,u;p=0;for(s in n){u=n[s],p+=function(){var x,w,y;y=[];x=0;for(w=u.length;x<w;x++){(q=u[x])&&y.push(q)}return y}().length}return p/=function(){var w;w=[];for(v in n){w.push(v)}return w}().length};aG=aI(al);j=aI(ak);g=function(){var n;n=[];for(aD in al){n.push(aD)}return n}().length;aw=function(){var n;n=[];for(aD in ak){n.push(aD)}return n}().length;ai=function(){return(new Date).getTime()};aI=function(n,p){var s,q;null==p&&(p=[]);q=ai();s=r(n,aJ.concat([aM("user_inputs",aK(p.map(function(u){return u.toLowerCase()})))]));s=aA(n,s);s.calc_time=ai()-q;return s};"undefined"!==typeof window&&null!==window?(window.zxcvbn=aI,"function"===typeof window.zxcvbn_load_hook&&window.zxcvbn_load_hook()):"undefined"!==typeof exports&&null!==exports&&(exports.zxcvbn=aI)})(); |
example/examples/MapStyle.js | phantomlds/react-native-maps | import React from 'react';
import {
StyleSheet,
View,
Dimensions,
} from 'react-native';
import MapView from 'react-native-maps';
const { width, height } = Dimensions.get('window');
const ASPECT_RATIO = width / height;
const LATITUDE = 37.78825;
const LONGITUDE = -122.4324;
const LATITUDE_DELTA = 0.0922;
const LONGITUDE_DELTA = LATITUDE_DELTA * ASPECT_RATIO;
const customStyle = [
{
elementType: 'geometry',
stylers: [
{
color: '#242f3e',
},
],
},
{
elementType: 'labels.text.fill',
stylers: [
{
color: '#746855',
},
],
},
{
elementType: 'labels.text.stroke',
stylers: [
{
color: '#242f3e',
},
],
},
{
featureType: 'administrative.locality',
elementType: 'labels.text.fill',
stylers: [
{
color: '#d59563',
},
],
},
{
featureType: 'poi',
elementType: 'labels.text.fill',
stylers: [
{
color: '#d59563',
},
],
},
{
featureType: 'poi.park',
elementType: 'geometry',
stylers: [
{
color: '#263c3f',
},
],
},
{
featureType: 'poi.park',
elementType: 'labels.text.fill',
stylers: [
{
color: '#6b9a76',
},
],
},
{
featureType: 'road',
elementType: 'geometry',
stylers: [
{
color: '#38414e',
},
],
},
{
featureType: 'road',
elementType: 'geometry.stroke',
stylers: [
{
color: '#212a37',
},
],
},
{
featureType: 'road',
elementType: 'labels.text.fill',
stylers: [
{
color: '#9ca5b3',
},
],
},
{
featureType: 'road.highway',
elementType: 'geometry',
stylers: [
{
color: '#746855',
},
],
},
{
featureType: 'road.highway',
elementType: 'geometry.stroke',
stylers: [
{
color: '#1f2835',
},
],
},
{
featureType: 'road.highway',
elementType: 'labels.text.fill',
stylers: [
{
color: '#f3d19c',
},
],
},
{
featureType: 'transit',
elementType: 'geometry',
stylers: [
{
color: '#2f3948',
},
],
},
{
featureType: 'transit.station',
elementType: 'labels.text.fill',
stylers: [
{
color: '#d59563',
},
],
},
{
featureType: 'water',
elementType: 'geometry',
stylers: [
{
color: '#17263c',
},
],
},
{
featureType: 'water',
elementType: 'labels.text.fill',
stylers: [
{
color: '#515c6d',
},
],
},
{
featureType: 'water',
elementType: 'labels.text.stroke',
stylers: [
{
color: '#17263c',
},
],
},
];
class MapStyle extends React.Component {
constructor(props) {
super(props);
this.state = {
};
}
render() {
return (
<View style={styles.container}>
<MapView
provider={this.props.provider}
style={styles.map}
initialRegion={{
latitude: LATITUDE,
longitude: LONGITUDE,
latitudeDelta: LATITUDE_DELTA,
longitudeDelta: LONGITUDE_DELTA,
}}
customMapStyle={customStyle}
/>
</View>
);
}
}
MapStyle.propTypes = {
provider: MapView.ProviderPropType,
};
const styles = StyleSheet.create({
container: {
...StyleSheet.absoluteFillObject,
justifyContent: 'flex-end',
alignItems: 'center',
},
map: {
...StyleSheet.absoluteFillObject,
},
});
module.exports = MapStyle;
|
src/components/Header/Header.js | ihnat/work-hard | /*! React Starter Kit | MIT License | http://www.reactstarterkit.com/ */
import React, { Component } from 'react';
import styles from './Header.css';
import withStyles from '../../decorators/withStyles';
import Link from '../Link';
import Navigation from '../Navigation';
@withStyles(styles)
class Header extends Component {
render() {
return (
<div className="Header">
<div className="Header-container">
<a className="Header-brand" href="/" onClick={Link.handleClick}>
<img className="Header-brandImg" src={require('./logo-small.png')} width="38" height="38" alt="React" />
<span className="Header-brandTxt">Your Company</span>
</a>
<Navigation className="Header-nav" />
<div className="Header-banner">
<h1 className="Header-bannerTitle">React</h1>
<p className="Header-bannerDesc">Complex web apps made easy</p>
</div>
</div>
</div>
);
}
}
export default Header;
|
examples/pinterest/app.js | bs1180/react-router | import React from 'react'
import { render } from 'react-dom'
import { createHistory, useBasename } from 'history'
import { Router, Route, IndexRoute, Link } from 'react-router'
const history = useBasename(createHistory)({
basename: '/pinterest'
})
const PICTURES = [
{ id: 0, src: 'http://placekitten.com/601/601' },
{ id: 1, src: 'http://placekitten.com/610/610' },
{ id: 2, src: 'http://placekitten.com/620/620' }
]
const Modal = React.createClass({
styles: {
position: 'fixed',
top: '20%',
right: '20%',
bottom: '20%',
left: '20%',
padding: 20,
boxShadow: '0px 0px 150px 130px rgba(0, 0, 0, 0.5)',
overflow: 'auto',
background: '#fff'
},
render() {
return (
<div style={this.styles}>
<p><Link to={this.props.returnTo}>Back</Link></p>
{this.props.children}
</div>
)
}
})
const App = React.createClass({
componentWillReceiveProps(nextProps) {
// if we changed routes...
if ((
nextProps.location.key !== this.props.location.key &&
nextProps.location.state &&
nextProps.location.state.modal
)) {
// save the old children (just like animation)
this.previousChildren = this.props.children
}
},
render() {
let { location } = this.props
let isModal = (
location.state &&
location.state.modal &&
this.previousChildren
)
return (
<div>
<h1>Pinterest Style Routes</h1>
<div>
{isModal ?
this.previousChildren :
this.props.children
}
{isModal && (
<Modal isOpen={true} returnTo={location.state.returnTo}>
{this.props.children}
</Modal>
)}
</div>
</div>
)
}
})
const Index = React.createClass({
render() {
return (
<div>
<p>
The url `/pictures/:id` can be rendered anywhere in the app as a modal.
Simply put `modal: true` in the `state` prop of links.
</p>
<p>
Click on an item and see its rendered as a modal, then copy/paste the
url into a different browser window (with a different session, like
Chrome -> Firefox), and see that the image does not render inside the
overlay. One URL, two session dependent screens :D
</p>
<div>
{PICTURES.map(picture => (
<Link key={picture.id} to={`/pictures/${picture.id}`} state={{ modal: true, returnTo: this.props.location.pathname }}>
<img style={{ margin: 10 }} src={picture.src} height="100" />
</Link>
))}
</div>
<p><Link to="/some/123/deep/456/route">Go to some deep route</Link></p>
</div>
)
}
})
const Deep = React.createClass({
render() {
return (
<div>
<p>You can link from anywhere really deep too</p>
<p>Params stick around: {this.props.params.one} {this.props.params.two}</p>
<p>
<Link to={`/pictures/0`} state={{ modal: true, returnTo: this.props.location.pathname }}>
Link to picture with Modal
</Link><br/>
<Link to={`/pictures/0`}>
Without modal
</Link>
</p>
</div>
)
}
})
const Picture = React.createClass({
render() {
return (
<div>
<img src={PICTURES[this.props.params.id].src} style={{ height: '80%' }} />
</div>
)
}
})
render((
<Router history={history}>
<Route path="/" component={App}>
<IndexRoute component={Index}/>
<Route path="/pictures/:id" component={Picture}/>
<Route path="/some/:one/deep/:two/route" component={Deep}/>
</Route>
</Router>
), document.getElementById('example'))
|
addons/info/src/components/types/Shape.js | rhalff/storybook | import PropTypes from 'prop-types';
import React from 'react';
import { HighlightButton } from '@storybook/components';
import PrettyPropType from './PrettyPropType';
import PropertyLabel from './PropertyLabel';
import { TypeInfo, getPropTypes } from './proptypes';
const MARGIN_SIZE = 15;
class Shape extends React.Component {
constructor(props) {
super(props);
this.state = {
minimized: false,
};
}
handleToggle = () => {
this.setState({
minimized: !this.state.minimized,
});
};
handleMouseEnter = () => {
this.setState({ hover: true });
};
handleMouseLeave = () => {
this.setState({ hover: false });
};
render() {
const { propType, depth } = this.props;
const propTypes = getPropTypes(propType);
return (
<span>
<HighlightButton
onMouseEnter={this.handleMouseEnter}
onMouseLeave={this.handleMouseLeave}
highlight={this.state.hover}
onClick={this.handleToggle}
>
{'{'}
</HighlightButton>
<HighlightButton onClick={this.handleToggle}>...</HighlightButton>
{!this.state.minimized &&
Object.keys(propTypes).map(childProperty => (
<div key={childProperty} style={{ marginLeft: depth * MARGIN_SIZE }}>
<PropertyLabel
property={childProperty}
required={propTypes[childProperty].required}
/>
<PrettyPropType depth={depth + 1} propType={propTypes[childProperty]} />
,
</div>
))}
<HighlightButton
onMouseEnter={this.handleMouseEnter}
onMouseLeave={this.handleMouseLeave}
highlight={this.state.hover}
onClick={this.handleToggle}
>
{'}'}
</HighlightButton>
</span>
);
}
}
Shape.propTypes = {
propType: TypeInfo,
depth: PropTypes.number.isRequired,
};
Shape.defaultProps = {
propType: null,
};
export default Shape;
|
components/fields/forked-react-dates/src/components/DayPickerSingleDateController.js | Mudano/m-dash-ui | import React from 'react';
import PropTypes from 'prop-types';
import momentPropTypes from 'react-moment-proptypes';
import { forbidExtraProps, nonNegativeInteger } from 'airbnb-prop-types';
import moment from 'moment';
import values from 'object.values';
import isTouchDevice from 'is-touch-device';
import { DayPickerPhrases } from '../defaultPhrases';
import getPhrasePropTypes from '../utils/getPhrasePropTypes';
import isSameDay from '../utils/isSameDay';
import isAfterDay from '../utils/isAfterDay';
import getVisibleDays from '../utils/getVisibleDays';
import isDayVisible from '../utils/isDayVisible';
import toISODateString from '../utils/toISODateString';
import toISOMonthString from '../utils/toISOMonthString';
import ScrollableOrientationShape from '../shapes/ScrollableOrientationShape';
import DayOfWeekShape from '../shapes/DayOfWeekShape';
import {
HORIZONTAL_ORIENTATION,
VERTICAL_SCROLLABLE,
DAY_SIZE,
} from '../../constants';
import DayPicker from './DayPicker';
const propTypes = forbidExtraProps({
date: momentPropTypes.momentObj,
onDateChange: PropTypes.func,
focused: PropTypes.bool,
onFocusChange: PropTypes.func,
onClose: PropTypes.func,
keepOpenOnDateSelect: PropTypes.bool,
minimumNights: PropTypes.number,
isOutsideRange: PropTypes.func,
isDayBlocked: PropTypes.func,
isDayHighlighted: PropTypes.func,
// DayPicker props
renderMonth: PropTypes.func,
enableOutsideDays: PropTypes.bool,
numberOfMonths: PropTypes.number,
orientation: ScrollableOrientationShape,
withPortal: PropTypes.bool,
initialVisibleMonth: PropTypes.func,
firstDayOfWeek: DayOfWeekShape,
hideKeyboardShortcutsPanel: PropTypes.bool,
daySize: nonNegativeInteger,
navPrev: PropTypes.node,
navNext: PropTypes.node,
onPrevMonthClick: PropTypes.func,
onNextMonthClick: PropTypes.func,
onOutsideClick: PropTypes.func,
renderDay: PropTypes.func,
renderCalendarInfo: PropTypes.func,
// accessibility
onBlur: PropTypes.func,
isFocused: PropTypes.bool,
showKeyboardShortcuts: PropTypes.bool,
// i18n
monthFormat: PropTypes.string,
phrases: PropTypes.shape(getPhrasePropTypes(DayPickerPhrases)),
isRTL: PropTypes.bool,
});
const defaultProps = {
date: undefined, // TODO: use null
onDateChange() {},
focused: false,
onFocusChange() {},
onClose() {},
keepOpenOnDateSelect: false,
minimumNights: 1,
isOutsideRange() {},
isDayBlocked() {},
isDayHighlighted() {},
// DayPicker props
renderMonth: null,
enableOutsideDays: false,
numberOfMonths: 1,
orientation: HORIZONTAL_ORIENTATION,
withPortal: false,
hideKeyboardShortcutsPanel: false,
initialVisibleMonth: null,
firstDayOfWeek: null,
daySize: DAY_SIZE,
navPrev: null,
navNext: null,
onPrevMonthClick() {},
onNextMonthClick() {},
onOutsideClick() {},
renderDay: null,
renderCalendarInfo: null,
// accessibility
onBlur() {},
isFocused: false,
showKeyboardShortcuts: false,
// i18n
monthFormat: 'MMMM YYYY',
phrases: DayPickerPhrases,
isRTL: false,
};
export default class DayPickerSingleDateController extends React.Component {
constructor(props) {
super(props);
this.isTouchDevice = false;
this.today = moment();
this.modifiers = {
today: day => this.isToday(day),
blocked: day => this.isBlocked(day),
'blocked-calendar': day => props.isDayBlocked(day),
'blocked-out-of-range': day => props.isOutsideRange(day),
'highlighted-calendar': day => props.isDayHighlighted(day),
valid: day => !this.isBlocked(day),
hovered: day => this.isHovered(day),
selected: day => this.isSelected(day),
};
const { currentMonth, visibleDays } = this.getStateForNewMonth(props);
this.state = {
hoverDate: null,
currentMonth,
visibleDays,
};
this.onDayMouseEnter = this.onDayMouseEnter.bind(this);
this.onDayMouseLeave = this.onDayMouseLeave.bind(this);
this.onDayClick = this.onDayClick.bind(this);
this.onPrevMonthClick = this.onPrevMonthClick.bind(this);
this.onNextMonthClick = this.onNextMonthClick.bind(this);
this.getFirstFocusableDay = this.getFirstFocusableDay.bind(this);
}
componentDidMount() {
this.isTouchDevice = isTouchDevice();
}
componentWillReceiveProps(nextProps) {
const {
date,
focused,
isOutsideRange,
isDayBlocked,
isDayHighlighted,
initialVisibleMonth,
numberOfMonths,
enableOutsideDays,
} = nextProps;
let { visibleDays } = this.state;
if (isOutsideRange !== this.props.isOutsideRange) {
this.modifiers['blocked-out-of-range'] = day => isOutsideRange(day);
}
if (isDayBlocked !== this.props.isDayBlocked) {
this.modifiers['blocked-calendar'] = day => isDayBlocked(day);
}
if (isDayHighlighted !== this.props.isDayHighlighted) {
this.modifiers['highlighted-calendar'] = day => isDayHighlighted(day);
}
if (
initialVisibleMonth !== this.props.initialVisibleMonth ||
numberOfMonths !== this.props.numberOfMonths ||
enableOutsideDays !== this.props.enableOutsideDays
) {
const newMonthState = this.getStateForNewMonth(nextProps);
const currentMonth = newMonthState.currentMonth;
visibleDays = newMonthState.visibleDays;
this.setState({
currentMonth,
visibleDays,
});
}
const didDateChange = date !== this.props.date;
const didFocusChange = focused !== this.props.focused;
let modifiers = {};
if (didDateChange) {
modifiers = this.deleteModifier(modifiers, this.props.date, 'selected');
modifiers = this.addModifier(modifiers, date, 'selected');
}
if (didFocusChange) {
values(visibleDays).forEach((days) => {
Object.keys(days).forEach((day) => {
const momentObj = moment(day);
if (this.isBlocked(momentObj)) {
modifiers = this.addModifier(modifiers, momentObj, 'blocked');
} else {
modifiers = this.deleteModifier(modifiers, momentObj, 'blocked');
}
if (isOutsideRange(momentObj)) {
modifiers = this.addModifier(modifiers, momentObj, 'blocked-out-of-range');
} else {
modifiers = this.deleteModifier(modifiers, momentObj, 'blocked-out-of-range');
}
if (isDayBlocked(momentObj)) {
modifiers = this.addModifier(modifiers, momentObj, 'blocked-calendar');
} else {
modifiers = this.deleteModifier(modifiers, momentObj, 'blocked-calendar');
}
if (isDayHighlighted(momentObj)) {
modifiers = this.addModifier(modifiers, momentObj, 'highlighted-calendar');
} else {
modifiers = this.deleteModifier(modifiers, momentObj, 'highlighted-calendar');
}
});
});
}
const today = moment();
if (!isSameDay(this.today, today)) {
modifiers = this.deleteModifier(modifiers, this.today, 'today');
modifiers = this.addModifier(modifiers, today, 'today');
this.today = today;
}
if (Object.keys(modifiers).length > 0) {
this.setState({
visibleDays: {
...visibleDays,
...modifiers,
},
});
}
}
componentWillUpdate() {
this.today = moment();
}
onDayClick(day, e) {
if (e) e.preventDefault();
if (this.isBlocked(day)) return;
const {
onDateChange,
keepOpenOnDateSelect,
onFocusChange,
onClose,
} = this.props;
onDateChange(day);
if (!keepOpenOnDateSelect) {
onFocusChange({ focused: null });
onClose({ date: day });
}
}
onDayMouseEnter(day) {
if (this.isTouchDevice) return;
const { hoverDate, visibleDays } = this.state;
let modifiers = this.deleteModifier({}, hoverDate, 'hovered');
modifiers = this.addModifier(modifiers, day, 'hovered');
this.setState({
hoverDate: day,
visibleDays: {
...visibleDays,
...modifiers,
},
});
}
onDayMouseLeave() {
const { hoverDate, visibleDays } = this.state;
if (this.isTouchDevice || !hoverDate) return;
const modifiers = this.deleteModifier({}, hoverDate, 'hovered');
this.setState({
hoverDate: null,
visibleDays: {
...visibleDays,
...modifiers,
},
});
}
onPrevMonthClick() {
const { onPrevMonthClick, numberOfMonths, enableOutsideDays } = this.props;
const { currentMonth, visibleDays } = this.state;
const newVisibleDays = {};
Object.keys(visibleDays).sort().slice(0, numberOfMonths + 1).forEach((month) => {
newVisibleDays[month] = visibleDays[month];
});
const prevMonth = currentMonth.clone().subtract(1, 'month');
const prevMonthVisibleDays = getVisibleDays(prevMonth, 1, enableOutsideDays);
this.setState({
currentMonth: prevMonth,
visibleDays: {
...newVisibleDays,
...this.getModifiers(prevMonthVisibleDays),
},
});
onPrevMonthClick();
}
onNextMonthClick() {
const { onNextMonthClick, numberOfMonths, enableOutsideDays } = this.props;
const { currentMonth, visibleDays } = this.state;
const newVisibleDays = {};
Object.keys(visibleDays).sort().slice(1).forEach((month) => {
newVisibleDays[month] = visibleDays[month];
});
const nextMonth = currentMonth.clone().add(numberOfMonths, 'month');
const nextMonthVisibleDays = getVisibleDays(nextMonth, 1, enableOutsideDays);
this.setState({
currentMonth: currentMonth.clone().add(1, 'month'),
visibleDays: {
...newVisibleDays,
...this.getModifiers(nextMonthVisibleDays),
},
});
onNextMonthClick();
}
getFirstFocusableDay(newMonth) {
const { date, numberOfMonths } = this.props;
let focusedDate = newMonth.clone().startOf('month');
if (date) {
focusedDate = date.clone();
}
if (this.isBlocked(focusedDate)) {
const days = [];
const lastVisibleDay = newMonth.clone().add(numberOfMonths - 1, 'months').endOf('month');
let currentDay = focusedDate.clone();
while (!isAfterDay(currentDay, lastVisibleDay)) {
currentDay = currentDay.clone().add(1, 'day');
days.push(currentDay);
}
const viableDays = days.filter(day => !this.isBlocked(day) && isAfterDay(day, focusedDate));
if (viableDays.length > 0) focusedDate = viableDays[0];
}
return focusedDate;
}
getModifiers(visibleDays) {
const modifiers = {};
Object.keys(visibleDays).forEach((month) => {
modifiers[month] = {};
visibleDays[month].forEach((day) => {
modifiers[month][toISODateString(day)] = this.getModifiersForDay(day);
});
});
return modifiers;
}
getModifiersForDay(day) {
return new Set(Object.keys(this.modifiers).filter(modifier => this.modifiers[modifier](day)));
}
getStateForNewMonth(nextProps) {
const { initialVisibleMonth, date, numberOfMonths, enableOutsideDays } = nextProps;
const initialVisibleMonthThunk = initialVisibleMonth || (date ? () => date : () => this.today);
const currentMonth = initialVisibleMonthThunk();
const visibleDays =
this.getModifiers(getVisibleDays(currentMonth, numberOfMonths, enableOutsideDays));
return { currentMonth, visibleDays };
}
addModifier(updatedDays, day, modifier) {
const { numberOfMonths: numberOfVisibleMonths, enableOutsideDays, orientation } = this.props;
const { currentMonth: firstVisibleMonth, visibleDays } = this.state;
let currentMonth = firstVisibleMonth;
let numberOfMonths = numberOfVisibleMonths;
if (orientation !== VERTICAL_SCROLLABLE) {
currentMonth = currentMonth.clone().subtract(1, 'month');
numberOfMonths += 2;
}
if (!day || !isDayVisible(day, currentMonth, numberOfMonths, enableOutsideDays)) {
return updatedDays;
}
const iso = toISODateString(day);
let updatedDaysAfterAddition = { ...updatedDays };
if (enableOutsideDays) {
const monthsToUpdate = Object.keys(visibleDays).filter(monthKey => (
Object.keys(visibleDays[monthKey]).indexOf(iso) > -1
));
updatedDaysAfterAddition = monthsToUpdate.reduce((days, monthIso) => {
const month = updatedDays[monthIso] || visibleDays[monthIso];
const modifiers = new Set(month[iso]);
modifiers.add(modifier);
return {
...days,
[monthIso]: {
...month,
[iso]: modifiers,
},
};
}, updatedDaysAfterAddition);
} else {
const monthIso = toISOMonthString(day);
const month = updatedDays[monthIso] || visibleDays[monthIso];
const modifiers = new Set(month[iso]);
modifiers.add(modifier);
updatedDaysAfterAddition = {
...updatedDaysAfterAddition,
[monthIso]: {
...month,
[iso]: modifiers,
},
};
}
return updatedDaysAfterAddition;
}
deleteModifier(updatedDays, day, modifier) {
const { numberOfMonths: numberOfVisibleMonths, enableOutsideDays, orientation } = this.props;
const { currentMonth: firstVisibleMonth, visibleDays } = this.state;
let currentMonth = firstVisibleMonth;
let numberOfMonths = numberOfVisibleMonths;
if (orientation !== VERTICAL_SCROLLABLE) {
currentMonth = currentMonth.clone().subtract(1, 'month');
numberOfMonths += 2;
}
if (!day || !isDayVisible(day, currentMonth, numberOfMonths, enableOutsideDays)) {
return updatedDays;
}
const iso = toISODateString(day);
let updatedDaysAfterDeletion = { ...updatedDays };
if (enableOutsideDays) {
const monthsToUpdate = Object.keys(visibleDays).filter(monthKey => (
Object.keys(visibleDays[monthKey]).indexOf(iso) > -1
));
updatedDaysAfterDeletion = monthsToUpdate.reduce((days, monthIso) => {
const month = updatedDays[monthIso] || visibleDays[monthIso];
const modifiers = new Set(month[iso]);
modifiers.delete(modifier);
return {
...days,
[monthIso]: {
...month,
[iso]: modifiers,
},
};
}, updatedDaysAfterDeletion);
} else {
const monthIso = toISOMonthString(day);
const month = updatedDays[monthIso] || visibleDays[monthIso];
const modifiers = new Set(month[iso]);
modifiers.delete(modifier);
updatedDaysAfterDeletion = {
...updatedDaysAfterDeletion,
[monthIso]: {
...month,
[iso]: modifiers,
},
};
}
return updatedDaysAfterDeletion;
}
isBlocked(day) {
const { isDayBlocked, isOutsideRange } = this.props;
return isDayBlocked(day) || isOutsideRange(day);
}
isHovered(day) {
const { hoverDate } = this.state || {};
return isSameDay(day, hoverDate);
}
isSelected(day) {
return isSameDay(day, this.props.date);
}
isToday(day) {
return isSameDay(day, this.today);
}
render() {
const {
numberOfMonths,
orientation,
monthFormat,
renderMonth,
navPrev,
navNext,
withPortal,
focused,
enableOutsideDays,
hideKeyboardShortcutsPanel,
daySize,
firstDayOfWeek,
renderDay,
renderCalendarInfo,
isFocused,
isRTL,
phrases,
} = this.props;
const { currentMonth, visibleDays } = this.state;
return (
<DayPicker
orientation={orientation}
enableOutsideDays={enableOutsideDays}
modifiers={visibleDays}
numberOfMonths={numberOfMonths}
onDayClick={this.onDayClick}
onDayMouseEnter={this.onDayMouseEnter}
onDayMouseLeave={this.onDayMouseLeave}
onPrevMonthClick={this.onPrevMonthClick}
onNextMonthClick={this.onNextMonthClick}
monthFormat={monthFormat}
withPortal={withPortal}
hidden={!focused}
hideKeyboardShortcutsPanel={hideKeyboardShortcutsPanel}
initialVisibleMonth={() => currentMonth}
firstDayOfWeek={firstDayOfWeek}
navPrev={navPrev}
navNext={navNext}
renderMonth={renderMonth}
renderDay={renderDay}
renderCalendarInfo={renderCalendarInfo}
isFocused={isFocused}
getFirstFocusableDay={this.getFirstFocusableDay}
onBlur={this.onDayPickerBlur}
phrases={phrases}
daySize={daySize}
isRTL={isRTL}
/>
);
}
}
DayPickerSingleDateController.propTypes = propTypes;
DayPickerSingleDateController.defaultProps = defaultProps;
|
solr/example/solr-webapp/webapp/js/require.js | frne/symfony-solr-playground | /*
MIT License
-----------
Copyright (c) 2010-2011, The Dojo Foundation
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
*/
/** vim: et:ts=4:sw=4:sts=4
* @license RequireJS 1.0.6 Copyright (c) 2010-2012, The Dojo Foundation All Rights Reserved.
* Available via the MIT or new BSD license.
* see: http://github.com/jrburke/requirejs for details
*/
/*jslint strict: false, plusplus: false, sub: true */
/*global window, navigator, document, importScripts, jQuery, setTimeout, opera */
var requirejs, require, define;
(function () {
//Change this version number for each release.
var version = "1.0.6",
commentRegExp = /(\/\*([\s\S]*?)\*\/|([^:]|^)\/\/(.*)$)/mg,
cjsRequireRegExp = /require\(\s*["']([^'"\s]+)["']\s*\)/g,
currDirRegExp = /^\.\//,
jsSuffixRegExp = /\.js$/,
ostring = Object.prototype.toString,
ap = Array.prototype,
aps = ap.slice,
apsp = ap.splice,
isBrowser = !!(typeof window !== "undefined" && navigator && document),
isWebWorker = !isBrowser && typeof importScripts !== "undefined",
//PS3 indicates loaded and complete, but need to wait for complete
//specifically. Sequence is "loading", "loaded", execution,
// then "complete". The UA check is unfortunate, but not sure how
//to feature test w/o causing perf issues.
readyRegExp = isBrowser && navigator.platform === 'PLAYSTATION 3' ?
/^complete$/ : /^(complete|loaded)$/,
defContextName = "_",
//Oh the tragedy, detecting opera. See the usage of isOpera for reason.
isOpera = typeof opera !== "undefined" && opera.toString() === "[object Opera]",
empty = {},
contexts = {},
globalDefQueue = [],
interactiveScript = null,
checkLoadedDepth = 0,
useInteractive = false,
reservedDependencies = {
require: true,
module: true,
exports: true
},
req, cfg = {}, currentlyAddingScript, s, head, baseElement, scripts, script,
src, subPath, mainScript, dataMain, globalI, ctx, jQueryCheck, checkLoadedTimeoutId;
function isFunction(it) {
return ostring.call(it) === "[object Function]";
}
function isArray(it) {
return ostring.call(it) === "[object Array]";
}
/**
* Simple function to mix in properties from source into target,
* but only if target does not already have a property of the same name.
* This is not robust in IE for transferring methods that match
* Object.prototype names, but the uses of mixin here seem unlikely to
* trigger a problem related to that.
*/
function mixin(target, source, force) {
for (var prop in source) {
if (!(prop in empty) && (!(prop in target) || force)) {
target[prop] = source[prop];
}
}
return req;
}
/**
* Constructs an error with a pointer to an URL with more information.
* @param {String} id the error ID that maps to an ID on a web page.
* @param {String} message human readable error.
* @param {Error} [err] the original error, if there is one.
*
* @returns {Error}
*/
function makeError(id, msg, err) {
var e = new Error(msg + '\nhttp://requirejs.org/docs/errors.html#' + id);
if (err) {
e.originalError = err;
}
return e;
}
/**
* Used to set up package paths from a packagePaths or packages config object.
* @param {Object} pkgs the object to store the new package config
* @param {Array} currentPackages an array of packages to configure
* @param {String} [dir] a prefix dir to use.
*/
function configurePackageDir(pkgs, currentPackages, dir) {
var i, location, pkgObj;
for (i = 0; (pkgObj = currentPackages[i]); i++) {
pkgObj = typeof pkgObj === "string" ? { name: pkgObj } : pkgObj;
location = pkgObj.location;
//Add dir to the path, but avoid paths that start with a slash
//or have a colon (indicates a protocol)
if (dir && (!location || (location.indexOf("/") !== 0 && location.indexOf(":") === -1))) {
location = dir + "/" + (location || pkgObj.name);
}
//Create a brand new object on pkgs, since currentPackages can
//be passed in again, and config.pkgs is the internal transformed
//state for all package configs.
pkgs[pkgObj.name] = {
name: pkgObj.name,
location: location || pkgObj.name,
//Remove leading dot in main, so main paths are normalized,
//and remove any trailing .js, since different package
//envs have different conventions: some use a module name,
//some use a file name.
main: (pkgObj.main || "main")
.replace(currDirRegExp, '')
.replace(jsSuffixRegExp, '')
};
}
}
/**
* jQuery 1.4.3-1.5.x use a readyWait/ready() pairing to hold DOM
* ready callbacks, but jQuery 1.6 supports a holdReady() API instead.
* At some point remove the readyWait/ready() support and just stick
* with using holdReady.
*/
function jQueryHoldReady($, shouldHold) {
if ($.holdReady) {
$.holdReady(shouldHold);
} else if (shouldHold) {
$.readyWait += 1;
} else {
$.ready(true);
}
}
if (typeof define !== "undefined") {
//If a define is already in play via another AMD loader,
//do not overwrite.
return;
}
if (typeof requirejs !== "undefined") {
if (isFunction(requirejs)) {
//Do not overwrite and existing requirejs instance.
return;
} else {
cfg = requirejs;
requirejs = undefined;
}
}
//Allow for a require config object
if (typeof require !== "undefined" && !isFunction(require)) {
//assume it is a config object.
cfg = require;
require = undefined;
}
/**
* Creates a new context for use in require and define calls.
* Handle most of the heavy lifting. Do not want to use an object
* with prototype here to avoid using "this" in require, in case it
* needs to be used in more super secure envs that do not want this.
* Also there should not be that many contexts in the page. Usually just
* one for the default context, but could be extra for multiversion cases
* or if a package needs a special context for a dependency that conflicts
* with the standard context.
*/
function newContext(contextName) {
var context, resume,
config = {
waitSeconds: 7,
baseUrl: "./",
paths: {},
pkgs: {},
catchError: {}
},
defQueue = [],
specified = {
"require": true,
"exports": true,
"module": true
},
urlMap = {},
defined = {},
loaded = {},
waiting = {},
waitAry = [],
urlFetched = {},
managerCounter = 0,
managerCallbacks = {},
plugins = {},
//Used to indicate which modules in a build scenario
//need to be full executed.
needFullExec = {},
fullExec = {},
resumeDepth = 0;
/**
* Trims the . and .. from an array of path segments.
* It will keep a leading path segment if a .. will become
* the first path segment, to help with module name lookups,
* which act like paths, but can be remapped. But the end result,
* all paths that use this function should look normalized.
* NOTE: this method MODIFIES the input array.
* @param {Array} ary the array of path segments.
*/
function trimDots(ary) {
var i, part;
for (i = 0; (part = ary[i]); i++) {
if (part === ".") {
ary.splice(i, 1);
i -= 1;
} else if (part === "..") {
if (i === 1 && (ary[2] === '..' || ary[0] === '..')) {
//End of the line. Keep at least one non-dot
//path segment at the front so it can be mapped
//correctly to disk. Otherwise, there is likely
//no path mapping for a path starting with '..'.
//This can still fail, but catches the most reasonable
//uses of ..
break;
} else if (i > 0) {
ary.splice(i - 1, 2);
i -= 2;
}
}
}
}
/**
* Given a relative module name, like ./something, normalize it to
* a real name that can be mapped to a path.
* @param {String} name the relative name
* @param {String} baseName a real name that the name arg is relative
* to.
* @returns {String} normalized name
*/
function normalize(name, baseName) {
var pkgName, pkgConfig;
//Adjust any relative paths.
if (name && name.charAt(0) === ".") {
//If have a base name, try to normalize against it,
//otherwise, assume it is a top-level require that will
//be relative to baseUrl in the end.
if (baseName) {
if (config.pkgs[baseName]) {
//If the baseName is a package name, then just treat it as one
//name to concat the name with.
baseName = [baseName];
} else {
//Convert baseName to array, and lop off the last part,
//so that . matches that "directory" and not name of the baseName's
//module. For instance, baseName of "one/two/three", maps to
//"one/two/three.js", but we want the directory, "one/two" for
//this normalization.
baseName = baseName.split("/");
baseName = baseName.slice(0, baseName.length - 1);
}
name = baseName.concat(name.split("/"));
trimDots(name);
//Some use of packages may use a . path to reference the
//"main" module name, so normalize for that.
pkgConfig = config.pkgs[(pkgName = name[0])];
name = name.join("/");
if (pkgConfig && name === pkgName + '/' + pkgConfig.main) {
name = pkgName;
}
} else if (name.indexOf("./") === 0) {
// No baseName, so this is ID is resolved relative
// to baseUrl, pull off the leading dot.
name = name.substring(2);
}
}
return name;
}
/**
* Creates a module mapping that includes plugin prefix, module
* name, and path. If parentModuleMap is provided it will
* also normalize the name via require.normalize()
*
* @param {String} name the module name
* @param {String} [parentModuleMap] parent module map
* for the module name, used to resolve relative names.
*
* @returns {Object}
*/
function makeModuleMap(name, parentModuleMap) {
var index = name ? name.indexOf("!") : -1,
prefix = null,
parentName = parentModuleMap ? parentModuleMap.name : null,
originalName = name,
normalizedName, url, pluginModule;
if (index !== -1) {
prefix = name.substring(0, index);
name = name.substring(index + 1, name.length);
}
if (prefix) {
prefix = normalize(prefix, parentName);
}
//Account for relative paths if there is a base name.
if (name) {
if (prefix) {
pluginModule = defined[prefix];
if (pluginModule && pluginModule.normalize) {
//Plugin is loaded, use its normalize method.
normalizedName = pluginModule.normalize(name, function (name) {
return normalize(name, parentName);
});
} else {
normalizedName = normalize(name, parentName);
}
} else {
//A regular module.
normalizedName = normalize(name, parentName);
url = urlMap[normalizedName];
if (!url) {
//Calculate url for the module, if it has a name.
//Use name here since nameToUrl also calls normalize,
//and for relative names that are outside the baseUrl
//this causes havoc. Was thinking of just removing
//parentModuleMap to avoid extra normalization, but
//normalize() still does a dot removal because of
//issue #142, so just pass in name here and redo
//the normalization. Paths outside baseUrl are just
//messy to support.
url = context.nameToUrl(name, null, parentModuleMap);
//Store the URL mapping for later.
urlMap[normalizedName] = url;
}
}
}
return {
prefix: prefix,
name: normalizedName,
parentMap: parentModuleMap,
url: url,
originalName: originalName,
fullName: prefix ? prefix + "!" + (normalizedName || '') : normalizedName
};
}
/**
* Determine if priority loading is done. If so clear the priorityWait
*/
function isPriorityDone() {
var priorityDone = true,
priorityWait = config.priorityWait,
priorityName, i;
if (priorityWait) {
for (i = 0; (priorityName = priorityWait[i]); i++) {
if (!loaded[priorityName]) {
priorityDone = false;
break;
}
}
if (priorityDone) {
delete config.priorityWait;
}
}
return priorityDone;
}
function makeContextModuleFunc(func, relModuleMap, enableBuildCallback) {
return function () {
//A version of a require function that passes a moduleName
//value for items that may need to
//look up paths relative to the moduleName
var args = aps.call(arguments, 0), lastArg;
if (enableBuildCallback &&
isFunction((lastArg = args[args.length - 1]))) {
lastArg.__requireJsBuild = true;
}
args.push(relModuleMap);
return func.apply(null, args);
};
}
/**
* Helper function that creates a require function object to give to
* modules that ask for it as a dependency. It needs to be specific
* per module because of the implication of path mappings that may
* need to be relative to the module name.
*/
function makeRequire(relModuleMap, enableBuildCallback, altRequire) {
var modRequire = makeContextModuleFunc(altRequire || context.require, relModuleMap, enableBuildCallback);
mixin(modRequire, {
nameToUrl: makeContextModuleFunc(context.nameToUrl, relModuleMap),
toUrl: makeContextModuleFunc(context.toUrl, relModuleMap),
defined: makeContextModuleFunc(context.requireDefined, relModuleMap),
specified: makeContextModuleFunc(context.requireSpecified, relModuleMap),
isBrowser: req.isBrowser
});
return modRequire;
}
/*
* Queues a dependency for checking after the loader is out of a
* "paused" state, for example while a script file is being loaded
* in the browser, where it may have many modules defined in it.
*/
function queueDependency(manager) {
context.paused.push(manager);
}
function execManager(manager) {
var i, ret, err, errFile, errModuleTree,
cb = manager.callback,
map = manager.map,
fullName = map.fullName,
args = manager.deps,
listeners = manager.listeners,
cjsModule;
//Call the callback to define the module, if necessary.
if (cb && isFunction(cb)) {
if (config.catchError.define) {
try {
ret = req.execCb(fullName, manager.callback, args, defined[fullName]);
} catch (e) {
err = e;
}
} else {
ret = req.execCb(fullName, manager.callback, args, defined[fullName]);
}
if (fullName) {
//If setting exports via "module" is in play,
//favor that over return value and exports. After that,
//favor a non-undefined return value over exports use.
cjsModule = manager.cjsModule;
if (cjsModule &&
cjsModule.exports !== undefined &&
//Make sure it is not already the exports value
cjsModule.exports !== defined[fullName]) {
ret = defined[fullName] = manager.cjsModule.exports;
} else if (ret === undefined && manager.usingExports) {
//exports already set the defined value.
ret = defined[fullName];
} else {
//Use the return value from the function.
defined[fullName] = ret;
//If this module needed full execution in a build
//environment, mark that now.
if (needFullExec[fullName]) {
fullExec[fullName] = true;
}
}
}
} else if (fullName) {
//May just be an object definition for the module. Only
//worry about defining if have a module name.
ret = defined[fullName] = cb;
//If this module needed full execution in a build
//environment, mark that now.
if (needFullExec[fullName]) {
fullExec[fullName] = true;
}
}
//Clean up waiting. Do this before error calls, and before
//calling back listeners, so that bookkeeping is correct
//in the event of an error and error is reported in correct order,
//since the listeners will likely have errors if the
//onError function does not throw.
if (waiting[manager.id]) {
delete waiting[manager.id];
manager.isDone = true;
context.waitCount -= 1;
if (context.waitCount === 0) {
//Clear the wait array used for cycles.
waitAry = [];
}
}
//Do not need to track manager callback now that it is defined.
delete managerCallbacks[fullName];
//Allow instrumentation like the optimizer to know the order
//of modules executed and their dependencies.
if (req.onResourceLoad && !manager.placeholder) {
req.onResourceLoad(context, map, manager.depArray);
}
if (err) {
errFile = (fullName ? makeModuleMap(fullName).url : '') ||
err.fileName || err.sourceURL;
errModuleTree = err.moduleTree;
err = makeError('defineerror', 'Error evaluating ' +
'module "' + fullName + '" at location "' +
errFile + '":\n' +
err + '\nfileName:' + errFile +
'\nlineNumber: ' + (err.lineNumber || err.line), err);
err.moduleName = fullName;
err.moduleTree = errModuleTree;
return req.onError(err);
}
//Let listeners know of this manager's value.
for (i = 0; (cb = listeners[i]); i++) {
cb(ret);
}
return undefined;
}
/**
* Helper that creates a callack function that is called when a dependency
* is ready, and sets the i-th dependency for the manager as the
* value passed to the callback generated by this function.
*/
function makeArgCallback(manager, i) {
return function (value) {
//Only do the work if it has not been done
//already for a dependency. Cycle breaking
//logic in forceExec could mean this function
//is called more than once for a given dependency.
if (!manager.depDone[i]) {
manager.depDone[i] = true;
manager.deps[i] = value;
manager.depCount -= 1;
if (!manager.depCount) {
//All done, execute!
execManager(manager);
}
}
};
}
function callPlugin(pluginName, depManager) {
var map = depManager.map,
fullName = map.fullName,
name = map.name,
plugin = plugins[pluginName] ||
(plugins[pluginName] = defined[pluginName]),
load;
//No need to continue if the manager is already
//in the process of loading.
if (depManager.loading) {
return;
}
depManager.loading = true;
load = function (ret) {
depManager.callback = function () {
return ret;
};
execManager(depManager);
loaded[depManager.id] = true;
//The loading of this plugin
//might have placed other things
//in the paused queue. In particular,
//a loader plugin that depends on
//a different plugin loaded resource.
resume();
};
//Allow plugins to load other code without having to know the
//context or how to "complete" the load.
load.fromText = function (moduleName, text) {
/*jslint evil: true */
var hasInteractive = useInteractive;
//Indicate a the module is in process of loading.
loaded[moduleName] = false;
context.scriptCount += 1;
//Indicate this is not a "real" module, so do not track it
//for builds, it does not map to a real file.
context.fake[moduleName] = true;
//Turn off interactive script matching for IE for any define
//calls in the text, then turn it back on at the end.
if (hasInteractive) {
useInteractive = false;
}
req.exec(text);
if (hasInteractive) {
useInteractive = true;
}
//Support anonymous modules.
context.completeLoad(moduleName);
};
//No need to continue if the plugin value has already been
//defined by a build.
if (fullName in defined) {
load(defined[fullName]);
} else {
//Use parentName here since the plugin's name is not reliable,
//could be some weird string with no path that actually wants to
//reference the parentName's path.
plugin.load(name, makeRequire(map.parentMap, true, function (deps, cb) {
var moduleDeps = [],
i, dep, depMap;
//Convert deps to full names and hold on to them
//for reference later, when figuring out if they
//are blocked by a circular dependency.
for (i = 0; (dep = deps[i]); i++) {
depMap = makeModuleMap(dep, map.parentMap);
deps[i] = depMap.fullName;
if (!depMap.prefix) {
moduleDeps.push(deps[i]);
}
}
depManager.moduleDeps = (depManager.moduleDeps || []).concat(moduleDeps);
return context.require(deps, cb);
}), load, config);
}
}
/**
* Adds the manager to the waiting queue. Only fully
* resolved items should be in the waiting queue.
*/
function addWait(manager) {
if (!waiting[manager.id]) {
waiting[manager.id] = manager;
waitAry.push(manager);
context.waitCount += 1;
}
}
/**
* Function added to every manager object. Created out here
* to avoid new function creation for each manager instance.
*/
function managerAdd(cb) {
this.listeners.push(cb);
}
function getManager(map, shouldQueue) {
var fullName = map.fullName,
prefix = map.prefix,
plugin = prefix ? plugins[prefix] ||
(plugins[prefix] = defined[prefix]) : null,
manager, created, pluginManager, prefixMap;
if (fullName) {
manager = managerCallbacks[fullName];
}
if (!manager) {
created = true;
manager = {
//ID is just the full name, but if it is a plugin resource
//for a plugin that has not been loaded,
//then add an ID counter to it.
id: (prefix && !plugin ?
(managerCounter++) + '__p@:' : '') +
(fullName || '__r@' + (managerCounter++)),
map: map,
depCount: 0,
depDone: [],
depCallbacks: [],
deps: [],
listeners: [],
add: managerAdd
};
specified[manager.id] = true;
//Only track the manager/reuse it if this is a non-plugin
//resource. Also only track plugin resources once
//the plugin has been loaded, and so the fullName is the
//true normalized value.
if (fullName && (!prefix || plugins[prefix])) {
managerCallbacks[fullName] = manager;
}
}
//If there is a plugin needed, but it is not loaded,
//first load the plugin, then continue on.
if (prefix && !plugin) {
prefixMap = makeModuleMap(prefix);
//Clear out defined and urlFetched if the plugin was previously
//loaded/defined, but not as full module (as in a build
//situation). However, only do this work if the plugin is in
//defined but does not have a module export value.
if (prefix in defined && !defined[prefix]) {
delete defined[prefix];
delete urlFetched[prefixMap.url];
}
pluginManager = getManager(prefixMap, true);
pluginManager.add(function (plugin) {
//Create a new manager for the normalized
//resource ID and have it call this manager when
//done.
var newMap = makeModuleMap(map.originalName, map.parentMap),
normalizedManager = getManager(newMap, true);
//Indicate this manager is a placeholder for the real,
//normalized thing. Important for when trying to map
//modules and dependencies, for instance, in a build.
manager.placeholder = true;
normalizedManager.add(function (resource) {
manager.callback = function () {
return resource;
};
execManager(manager);
});
});
} else if (created && shouldQueue) {
//Indicate the resource is not loaded yet if it is to be
//queued.
loaded[manager.id] = false;
queueDependency(manager);
addWait(manager);
}
return manager;
}
function main(inName, depArray, callback, relModuleMap) {
var moduleMap = makeModuleMap(inName, relModuleMap),
name = moduleMap.name,
fullName = moduleMap.fullName,
manager = getManager(moduleMap),
id = manager.id,
deps = manager.deps,
i, depArg, depName, depPrefix, cjsMod;
if (fullName) {
//If module already defined for context, or already loaded,
//then leave. Also leave if jQuery is registering but it does
//not match the desired version number in the config.
if (fullName in defined || loaded[id] === true ||
(fullName === "jquery" && config.jQuery &&
config.jQuery !== callback().fn.jquery)) {
return;
}
//Set specified/loaded here for modules that are also loaded
//as part of a layer, where onScriptLoad is not fired
//for those cases. Do this after the inline define and
//dependency tracing is done.
specified[id] = true;
loaded[id] = true;
//If module is jQuery set up delaying its dom ready listeners.
if (fullName === "jquery" && callback) {
jQueryCheck(callback());
}
}
//Attach real depArray and callback to the manager. Do this
//only if the module has not been defined already, so do this after
//the fullName checks above. IE can call main() more than once
//for a module.
manager.depArray = depArray;
manager.callback = callback;
//Add the dependencies to the deps field, and register for callbacks
//on the dependencies.
for (i = 0; i < depArray.length; i++) {
depArg = depArray[i];
//There could be cases like in IE, where a trailing comma will
//introduce a null dependency, so only treat a real dependency
//value as a dependency.
if (depArg) {
//Split the dependency name into plugin and name parts
depArg = makeModuleMap(depArg, (name ? moduleMap : relModuleMap));
depName = depArg.fullName;
depPrefix = depArg.prefix;
//Fix the name in depArray to be just the name, since
//that is how it will be called back later.
depArray[i] = depName;
//Fast path CommonJS standard dependencies.
if (depName === "require") {
deps[i] = makeRequire(moduleMap);
} else if (depName === "exports") {
//CommonJS module spec 1.1
deps[i] = defined[fullName] = {};
manager.usingExports = true;
} else if (depName === "module") {
//CommonJS module spec 1.1
manager.cjsModule = cjsMod = deps[i] = {
id: name,
uri: name ? context.nameToUrl(name, null, relModuleMap) : undefined,
exports: defined[fullName]
};
} else if (depName in defined && !(depName in waiting) &&
(!(fullName in needFullExec) ||
(fullName in needFullExec && fullExec[depName]))) {
//Module already defined, and not in a build situation
//where the module is a something that needs full
//execution and this dependency has not been fully
//executed. See r.js's requirePatch.js for more info
//on fullExec.
deps[i] = defined[depName];
} else {
//Mark this dependency as needing full exec if
//the current module needs full exec.
if (fullName in needFullExec) {
needFullExec[depName] = true;
//Reset state so fully executed code will get
//picked up correctly.
delete defined[depName];
urlFetched[depArg.url] = false;
}
//Either a resource that is not loaded yet, or a plugin
//resource for either a plugin that has not
//loaded yet.
manager.depCount += 1;
manager.depCallbacks[i] = makeArgCallback(manager, i);
getManager(depArg, true).add(manager.depCallbacks[i]);
}
}
}
//Do not bother tracking the manager if it is all done.
if (!manager.depCount) {
//All done, execute!
execManager(manager);
} else {
addWait(manager);
}
}
/**
* Convenience method to call main for a define call that was put on
* hold in the defQueue.
*/
function callDefMain(args) {
main.apply(null, args);
}
/**
* jQuery 1.4.3+ supports ways to hold off calling
* calling jQuery ready callbacks until all scripts are loaded. Be sure
* to track it if the capability exists.. Also, since jQuery 1.4.3 does
* not register as a module, need to do some global inference checking.
* Even if it does register as a module, not guaranteed to be the precise
* name of the global. If a jQuery is tracked for this context, then go
* ahead and register it as a module too, if not already in process.
*/
jQueryCheck = function (jqCandidate) {
if (!context.jQuery) {
var $ = jqCandidate || (typeof jQuery !== "undefined" ? jQuery : null);
if ($) {
//If a specific version of jQuery is wanted, make sure to only
//use this jQuery if it matches.
if (config.jQuery && $.fn.jquery !== config.jQuery) {
return;
}
if ("holdReady" in $ || "readyWait" in $) {
context.jQuery = $;
//Manually create a "jquery" module entry if not one already
//or in process. Note this could trigger an attempt at
//a second jQuery registration, but does no harm since
//the first one wins, and it is the same value anyway.
callDefMain(["jquery", [], function () {
return jQuery;
}]);
//Ask jQuery to hold DOM ready callbacks.
if (context.scriptCount) {
jQueryHoldReady($, true);
context.jQueryIncremented = true;
}
}
}
}
};
function findCycle(manager, traced) {
var fullName = manager.map.fullName,
depArray = manager.depArray,
fullyLoaded = true,
i, depName, depManager, result;
if (manager.isDone || !fullName || !loaded[fullName]) {
return result;
}
//Found the cycle.
if (traced[fullName]) {
return manager;
}
traced[fullName] = true;
//Trace through the dependencies.
if (depArray) {
for (i = 0; i < depArray.length; i++) {
//Some array members may be null, like if a trailing comma
//IE, so do the explicit [i] access and check if it has a value.
depName = depArray[i];
if (!loaded[depName] && !reservedDependencies[depName]) {
fullyLoaded = false;
break;
}
depManager = waiting[depName];
if (depManager && !depManager.isDone && loaded[depName]) {
result = findCycle(depManager, traced);
if (result) {
break;
}
}
}
if (!fullyLoaded) {
//Discard the cycle that was found, since it cannot
//be forced yet. Also clear this module from traced.
result = undefined;
delete traced[fullName];
}
}
return result;
}
function forceExec(manager, traced) {
var fullName = manager.map.fullName,
depArray = manager.depArray,
i, depName, depManager, prefix, prefixManager, value;
if (manager.isDone || !fullName || !loaded[fullName]) {
return undefined;
}
if (fullName) {
if (traced[fullName]) {
return defined[fullName];
}
traced[fullName] = true;
}
//Trace through the dependencies.
if (depArray) {
for (i = 0; i < depArray.length; i++) {
//Some array members may be null, like if a trailing comma
//IE, so do the explicit [i] access and check if it has a value.
depName = depArray[i];
if (depName) {
//First, make sure if it is a plugin resource that the
//plugin is not blocked.
prefix = makeModuleMap(depName).prefix;
if (prefix && (prefixManager = waiting[prefix])) {
forceExec(prefixManager, traced);
}
depManager = waiting[depName];
if (depManager && !depManager.isDone && loaded[depName]) {
value = forceExec(depManager, traced);
manager.depCallbacks[i](value);
}
}
}
}
return defined[fullName];
}
/**
* Checks if all modules for a context are loaded, and if so, evaluates the
* new ones in right dependency order.
*
* @private
*/
function checkLoaded() {
var waitInterval = config.waitSeconds * 1000,
//It is possible to disable the wait interval by using waitSeconds of 0.
expired = waitInterval && (context.startTime + waitInterval) < new Date().getTime(),
noLoads = "", hasLoadedProp = false, stillLoading = false,
cycleDeps = [],
i, prop, err, manager, cycleManager, moduleDeps;
//If there are items still in the paused queue processing wait.
//This is particularly important in the sync case where each paused
//item is processed right away but there may be more waiting.
if (context.pausedCount > 0) {
return undefined;
}
//Determine if priority loading is done. If so clear the priority. If
//not, then do not check
if (config.priorityWait) {
if (isPriorityDone()) {
//Call resume, since it could have
//some waiting dependencies to trace.
resume();
} else {
return undefined;
}
}
//See if anything is still in flight.
for (prop in loaded) {
if (!(prop in empty)) {
hasLoadedProp = true;
if (!loaded[prop]) {
if (expired) {
noLoads += prop + " ";
} else {
stillLoading = true;
if (prop.indexOf('!') === -1) {
//No reason to keep looking for unfinished
//loading. If the only stillLoading is a
//plugin resource though, keep going,
//because it may be that a plugin resource
//is waiting on a non-plugin cycle.
cycleDeps = [];
break;
} else {
moduleDeps = managerCallbacks[prop] && managerCallbacks[prop].moduleDeps;
if (moduleDeps) {
cycleDeps.push.apply(cycleDeps, moduleDeps);
}
}
}
}
}
}
//Check for exit conditions.
if (!hasLoadedProp && !context.waitCount) {
//If the loaded object had no items, then the rest of
//the work below does not need to be done.
return undefined;
}
if (expired && noLoads) {
//If wait time expired, throw error of unloaded modules.
err = makeError("timeout", "Load timeout for modules: " + noLoads);
err.requireType = "timeout";
err.requireModules = noLoads;
err.contextName = context.contextName;
return req.onError(err);
}
//If still loading but a plugin is waiting on a regular module cycle
//break the cycle.
if (stillLoading && cycleDeps.length) {
for (i = 0; (manager = waiting[cycleDeps[i]]); i++) {
if ((cycleManager = findCycle(manager, {}))) {
forceExec(cycleManager, {});
break;
}
}
}
//If still waiting on loads, and the waiting load is something
//other than a plugin resource, or there are still outstanding
//scripts, then just try back later.
if (!expired && (stillLoading || context.scriptCount)) {
//Something is still waiting to load. Wait for it, but only
//if a timeout is not already in effect.
if ((isBrowser || isWebWorker) && !checkLoadedTimeoutId) {
checkLoadedTimeoutId = setTimeout(function () {
checkLoadedTimeoutId = 0;
checkLoaded();
}, 50);
}
return undefined;
}
//If still have items in the waiting cue, but all modules have
//been loaded, then it means there are some circular dependencies
//that need to be broken.
//However, as a waiting thing is fired, then it can add items to
//the waiting cue, and those items should not be fired yet, so
//make sure to redo the checkLoaded call after breaking a single
//cycle, if nothing else loaded then this logic will pick it up
//again.
if (context.waitCount) {
//Cycle through the waitAry, and call items in sequence.
for (i = 0; (manager = waitAry[i]); i++) {
forceExec(manager, {});
}
//If anything got placed in the paused queue, run it down.
if (context.paused.length) {
resume();
}
//Only allow this recursion to a certain depth. Only
//triggered by errors in calling a module in which its
//modules waiting on it cannot finish loading, or some circular
//dependencies that then may add more dependencies.
//The value of 5 is a bit arbitrary. Hopefully just one extra
//pass, or two for the case of circular dependencies generating
//more work that gets resolved in the sync node case.
if (checkLoadedDepth < 5) {
checkLoadedDepth += 1;
checkLoaded();
}
}
checkLoadedDepth = 0;
//Check for DOM ready, and nothing is waiting across contexts.
req.checkReadyState();
return undefined;
}
/**
* Resumes tracing of dependencies and then checks if everything is loaded.
*/
resume = function () {
var manager, map, url, i, p, args, fullName;
//Any defined modules in the global queue, intake them now.
context.takeGlobalQueue();
resumeDepth += 1;
if (context.scriptCount <= 0) {
//Synchronous envs will push the number below zero with the
//decrement above, be sure to set it back to zero for good measure.
//require() calls that also do not end up loading scripts could
//push the number negative too.
context.scriptCount = 0;
}
//Make sure any remaining defQueue items get properly processed.
while (defQueue.length) {
args = defQueue.shift();
if (args[0] === null) {
return req.onError(makeError('mismatch', 'Mismatched anonymous define() module: ' + args[args.length - 1]));
} else {
callDefMain(args);
}
}
//Skip the resume of paused dependencies
//if current context is in priority wait.
if (!config.priorityWait || isPriorityDone()) {
while (context.paused.length) {
p = context.paused;
context.pausedCount += p.length;
//Reset paused list
context.paused = [];
for (i = 0; (manager = p[i]); i++) {
map = manager.map;
url = map.url;
fullName = map.fullName;
//If the manager is for a plugin managed resource,
//ask the plugin to load it now.
if (map.prefix) {
callPlugin(map.prefix, manager);
} else {
//Regular dependency.
if (!urlFetched[url] && !loaded[fullName]) {
req.load(context, fullName, url);
//Mark the URL as fetched, but only if it is
//not an empty: URL, used by the optimizer.
//In that case we need to be sure to call
//load() for each module that is mapped to
//empty: so that dependencies are satisfied
//correctly.
if (url.indexOf('empty:') !== 0) {
urlFetched[url] = true;
}
}
}
}
//Move the start time for timeout forward.
context.startTime = (new Date()).getTime();
context.pausedCount -= p.length;
}
}
//Only check if loaded when resume depth is 1. It is likely that
//it is only greater than 1 in sync environments where a factory
//function also then calls the callback-style require. In those
//cases, the checkLoaded should not occur until the resume
//depth is back at the top level.
if (resumeDepth === 1) {
checkLoaded();
}
resumeDepth -= 1;
return undefined;
};
//Define the context object. Many of these fields are on here
//just to make debugging easier.
context = {
contextName: contextName,
config: config,
defQueue: defQueue,
waiting: waiting,
waitCount: 0,
specified: specified,
loaded: loaded,
urlMap: urlMap,
urlFetched: urlFetched,
scriptCount: 0,
defined: defined,
paused: [],
pausedCount: 0,
plugins: plugins,
needFullExec: needFullExec,
fake: {},
fullExec: fullExec,
managerCallbacks: managerCallbacks,
makeModuleMap: makeModuleMap,
normalize: normalize,
/**
* Set a configuration for the context.
* @param {Object} cfg config object to integrate.
*/
configure: function (cfg) {
var paths, prop, packages, pkgs, packagePaths, requireWait;
//Make sure the baseUrl ends in a slash.
if (cfg.baseUrl) {
if (cfg.baseUrl.charAt(cfg.baseUrl.length - 1) !== "/") {
cfg.baseUrl += "/";
}
}
//Save off the paths and packages since they require special processing,
//they are additive.
paths = config.paths;
packages = config.packages;
pkgs = config.pkgs;
//Mix in the config values, favoring the new values over
//existing ones in context.config.
mixin(config, cfg, true);
//Adjust paths if necessary.
if (cfg.paths) {
for (prop in cfg.paths) {
if (!(prop in empty)) {
paths[prop] = cfg.paths[prop];
}
}
config.paths = paths;
}
packagePaths = cfg.packagePaths;
if (packagePaths || cfg.packages) {
//Convert packagePaths into a packages config.
if (packagePaths) {
for (prop in packagePaths) {
if (!(prop in empty)) {
configurePackageDir(pkgs, packagePaths[prop], prop);
}
}
}
//Adjust packages if necessary.
if (cfg.packages) {
configurePackageDir(pkgs, cfg.packages);
}
//Done with modifications, assing packages back to context config
config.pkgs = pkgs;
}
//If priority loading is in effect, trigger the loads now
if (cfg.priority) {
//Hold on to requireWait value, and reset it after done
requireWait = context.requireWait;
//Allow tracing some require calls to allow the fetching
//of the priority config.
context.requireWait = false;
//But first, call resume to register any defined modules that may
//be in a data-main built file before the priority config
//call.
resume();
context.require(cfg.priority);
//Trigger a resume right away, for the case when
//the script with the priority load is done as part
//of a data-main call. In that case the normal resume
//call will not happen because the scriptCount will be
//at 1, since the script for data-main is being processed.
resume();
//Restore previous state.
context.requireWait = requireWait;
config.priorityWait = cfg.priority;
}
//If a deps array or a config callback is specified, then call
//require with those args. This is useful when require is defined as a
//config object before require.js is loaded.
if (cfg.deps || cfg.callback) {
context.require(cfg.deps || [], cfg.callback);
}
},
requireDefined: function (moduleName, relModuleMap) {
return makeModuleMap(moduleName, relModuleMap).fullName in defined;
},
requireSpecified: function (moduleName, relModuleMap) {
return makeModuleMap(moduleName, relModuleMap).fullName in specified;
},
require: function (deps, callback, relModuleMap) {
var moduleName, fullName, moduleMap;
if (typeof deps === "string") {
if (isFunction(callback)) {
//Invalid call
return req.onError(makeError("requireargs", "Invalid require call"));
}
//Synchronous access to one module. If require.get is
//available (as in the Node adapter), prefer that.
//In this case deps is the moduleName and callback is
//the relModuleMap
if (req.get) {
return req.get(context, deps, callback);
}
//Just return the module wanted. In this scenario, the
//second arg (if passed) is just the relModuleMap.
moduleName = deps;
relModuleMap = callback;
//Normalize module name, if it contains . or ..
moduleMap = makeModuleMap(moduleName, relModuleMap);
fullName = moduleMap.fullName;
if (!(fullName in defined)) {
return req.onError(makeError("notloaded", "Module name '" +
moduleMap.fullName +
"' has not been loaded yet for context: " +
contextName));
}
return defined[fullName];
}
//Call main but only if there are dependencies or
//a callback to call.
if (deps && deps.length || callback) {
main(null, deps, callback, relModuleMap);
}
//If the require call does not trigger anything new to load,
//then resume the dependency processing.
if (!context.requireWait) {
while (!context.scriptCount && context.paused.length) {
resume();
}
}
return context.require;
},
/**
* Internal method to transfer globalQueue items to this context's
* defQueue.
*/
takeGlobalQueue: function () {
//Push all the globalDefQueue items into the context's defQueue
if (globalDefQueue.length) {
//Array splice in the values since the context code has a
//local var ref to defQueue, so cannot just reassign the one
//on context.
apsp.apply(context.defQueue,
[context.defQueue.length - 1, 0].concat(globalDefQueue));
globalDefQueue = [];
}
},
/**
* Internal method used by environment adapters to complete a load event.
* A load event could be a script load or just a load pass from a synchronous
* load call.
* @param {String} moduleName the name of the module to potentially complete.
*/
completeLoad: function (moduleName) {
var args;
context.takeGlobalQueue();
while (defQueue.length) {
args = defQueue.shift();
if (args[0] === null) {
args[0] = moduleName;
break;
} else if (args[0] === moduleName) {
//Found matching define call for this script!
break;
} else {
//Some other named define call, most likely the result
//of a build layer that included many define calls.
callDefMain(args);
args = null;
}
}
if (args) {
callDefMain(args);
} else {
//A script that does not call define(), so just simulate
//the call for it. Special exception for jQuery dynamic load.
callDefMain([moduleName, [],
moduleName === "jquery" && typeof jQuery !== "undefined" ?
function () {
return jQuery;
} : null]);
}
//Doing this scriptCount decrement branching because sync envs
//need to decrement after resume, otherwise it looks like
//loading is complete after the first dependency is fetched.
//For browsers, it works fine to decrement after, but it means
//the checkLoaded setTimeout 50 ms cost is taken. To avoid
//that cost, decrement beforehand.
if (req.isAsync) {
context.scriptCount -= 1;
}
resume();
if (!req.isAsync) {
context.scriptCount -= 1;
}
},
/**
* Converts a module name + .extension into an URL path.
* *Requires* the use of a module name. It does not support using
* plain URLs like nameToUrl.
*/
toUrl: function (moduleNamePlusExt, relModuleMap) {
var index = moduleNamePlusExt.lastIndexOf("."),
ext = null;
if (index !== -1) {
ext = moduleNamePlusExt.substring(index, moduleNamePlusExt.length);
moduleNamePlusExt = moduleNamePlusExt.substring(0, index);
}
return context.nameToUrl(moduleNamePlusExt, ext, relModuleMap);
},
/**
* Converts a module name to a file path. Supports cases where
* moduleName may actually be just an URL.
*/
nameToUrl: function (moduleName, ext, relModuleMap) {
var paths, pkgs, pkg, pkgPath, syms, i, parentModule, url,
config = context.config;
//Normalize module name if have a base relative module name to work from.
moduleName = normalize(moduleName, relModuleMap && relModuleMap.fullName);
//If a colon is in the URL, it indicates a protocol is used and it is just
//an URL to a file, or if it starts with a slash or ends with .js, it is just a plain file.
//The slash is important for protocol-less URLs as well as full paths.
if (req.jsExtRegExp.test(moduleName)) {
//Just a plain path, not module name lookup, so just return it.
//Add extension if it is included. This is a bit wonky, only non-.js things pass
//an extension, this method probably needs to be reworked.
url = moduleName + (ext ? ext : "");
} else {
//A module that needs to be converted to a path.
paths = config.paths;
pkgs = config.pkgs;
syms = moduleName.split("/");
//For each module name segment, see if there is a path
//registered for it. Start with most specific name
//and work up from it.
for (i = syms.length; i > 0; i--) {
parentModule = syms.slice(0, i).join("/");
if (paths[parentModule]) {
syms.splice(0, i, paths[parentModule]);
break;
} else if ((pkg = pkgs[parentModule])) {
//If module name is just the package name, then looking
//for the main module.
if (moduleName === pkg.name) {
pkgPath = pkg.location + '/' + pkg.main;
} else {
pkgPath = pkg.location;
}
syms.splice(0, i, pkgPath);
break;
}
}
//Join the path parts together, then figure out if baseUrl is needed.
url = syms.join("/") + (ext || ".js");
url = (url.charAt(0) === '/' || url.match(/^\w+:/) ? "" : config.baseUrl) + url;
}
return config.urlArgs ? url +
((url.indexOf('?') === -1 ? '?' : '&') +
config.urlArgs) : url;
}
};
//Make these visible on the context so can be called at the very
//end of the file to bootstrap
context.jQueryCheck = jQueryCheck;
context.resume = resume;
return context;
}
/**
* Main entry point.
*
* If the only argument to require is a string, then the module that
* is represented by that string is fetched for the appropriate context.
*
* If the first argument is an array, then it will be treated as an array
* of dependency string names to fetch. An optional function callback can
* be specified to execute when all of those dependencies are available.
*
* Make a local req variable to help Caja compliance (it assumes things
* on a require that are not standardized), and to give a short
* name for minification/local scope use.
*/
req = requirejs = function (deps, callback) {
//Find the right context, use default
var contextName = defContextName,
context, config;
// Determine if have config object in the call.
if (!isArray(deps) && typeof deps !== "string") {
// deps is a config object
config = deps;
if (isArray(callback)) {
// Adjust args if there are dependencies
deps = callback;
callback = arguments[2];
} else {
deps = [];
}
}
if (config && config.context) {
contextName = config.context;
}
context = contexts[contextName] ||
(contexts[contextName] = newContext(contextName));
if (config) {
context.configure(config);
}
return context.require(deps, callback);
};
/**
* Support require.config() to make it easier to cooperate with other
* AMD loaders on globally agreed names.
*/
req.config = function (config) {
return req(config);
};
/**
* Export require as a global, but only if it does not already exist.
*/
if (!require) {
require = req;
}
/**
* Global require.toUrl(), to match global require, mostly useful
* for debugging/work in the global space.
*/
req.toUrl = function (moduleNamePlusExt) {
return contexts[defContextName].toUrl(moduleNamePlusExt);
};
req.version = version;
//Used to filter out dependencies that are already paths.
req.jsExtRegExp = /^\/|:|\?|\.js$/;
s = req.s = {
contexts: contexts,
//Stores a list of URLs that should not get async script tag treatment.
skipAsync: {}
};
req.isAsync = req.isBrowser = isBrowser;
if (isBrowser) {
head = s.head = document.getElementsByTagName("head")[0];
//If BASE tag is in play, using appendChild is a problem for IE6.
//When that browser dies, this can be removed. Details in this jQuery bug:
//http://dev.jquery.com/ticket/2709
baseElement = document.getElementsByTagName("base")[0];
if (baseElement) {
head = s.head = baseElement.parentNode;
}
}
/**
* Any errors that require explicitly generates will be passed to this
* function. Intercept/override it if you want custom error handling.
* @param {Error} err the error object.
*/
req.onError = function (err) {
throw err;
};
/**
* Does the request to load a module for the browser case.
* Make this a separate function to allow other environments
* to override it.
*
* @param {Object} context the require context to find state.
* @param {String} moduleName the name of the module.
* @param {Object} url the URL to the module.
*/
req.load = function (context, moduleName, url) {
req.resourcesReady(false);
context.scriptCount += 1;
req.attach(url, context, moduleName);
//If tracking a jQuery, then make sure its ready callbacks
//are put on hold to prevent its ready callbacks from
//triggering too soon.
if (context.jQuery && !context.jQueryIncremented) {
jQueryHoldReady(context.jQuery, true);
context.jQueryIncremented = true;
}
};
function getInteractiveScript() {
var scripts, i, script;
if (interactiveScript && interactiveScript.readyState === 'interactive') {
return interactiveScript;
}
scripts = document.getElementsByTagName('script');
for (i = scripts.length - 1; i > -1 && (script = scripts[i]); i--) {
if (script.readyState === 'interactive') {
return (interactiveScript = script);
}
}
return null;
}
/**
* The function that handles definitions of modules. Differs from
* require() in that a string for the module should be the first argument,
* and the function to execute after dependencies are loaded should
* return a value to define the module corresponding to the first argument's
* name.
*/
define = function (name, deps, callback) {
var node, context;
//Allow for anonymous functions
if (typeof name !== 'string') {
//Adjust args appropriately
callback = deps;
deps = name;
name = null;
}
//This module may not have dependencies
if (!isArray(deps)) {
callback = deps;
deps = [];
}
//If no name, and callback is a function, then figure out if it a
//CommonJS thing with dependencies.
if (!deps.length && isFunction(callback)) {
//Remove comments from the callback string,
//look for require calls, and pull them into the dependencies,
//but only if there are function args.
if (callback.length) {
callback
.toString()
.replace(commentRegExp, "")
.replace(cjsRequireRegExp, function (match, dep) {
deps.push(dep);
});
//May be a CommonJS thing even without require calls, but still
//could use exports, and module. Avoid doing exports and module
//work though if it just needs require.
//REQUIRES the function to expect the CommonJS variables in the
//order listed below.
deps = (callback.length === 1 ? ["require"] : ["require", "exports", "module"]).concat(deps);
}
}
//If in IE 6-8 and hit an anonymous define() call, do the interactive
//work.
if (useInteractive) {
node = currentlyAddingScript || getInteractiveScript();
if (node) {
if (!name) {
name = node.getAttribute("data-requiremodule");
}
context = contexts[node.getAttribute("data-requirecontext")];
}
}
//Always save off evaluating the def call until the script onload handler.
//This allows multiple modules to be in a file without prematurely
//tracing dependencies, and allows for anonymous module support,
//where the module name is not known until the script onload event
//occurs. If no context, use the global queue, and get it processed
//in the onscript load callback.
(context ? context.defQueue : globalDefQueue).push([name, deps, callback]);
return undefined;
};
define.amd = {
multiversion: true,
plugins: true,
jQuery: true
};
/**
* Executes the text. Normally just uses eval, but can be modified
* to use a more environment specific call.
* @param {String} text the text to execute/evaluate.
*/
req.exec = function (text) {
return eval(text);
};
/**
* Executes a module callack function. Broken out as a separate function
* solely to allow the build system to sequence the files in the built
* layer in the right sequence.
*
* @private
*/
req.execCb = function (name, callback, args, exports) {
return callback.apply(exports, args);
};
/**
* Adds a node to the DOM. Public function since used by the order plugin.
* This method should not normally be called by outside code.
*/
req.addScriptToDom = function (node) {
//For some cache cases in IE 6-8, the script executes before the end
//of the appendChild execution, so to tie an anonymous define
//call to the module name (which is stored on the node), hold on
//to a reference to this node, but clear after the DOM insertion.
currentlyAddingScript = node;
if (baseElement) {
head.insertBefore(node, baseElement);
} else {
head.appendChild(node);
}
currentlyAddingScript = null;
};
/**
* callback for script loads, used to check status of loading.
*
* @param {Event} evt the event from the browser for the script
* that was loaded.
*
* @private
*/
req.onScriptLoad = function (evt) {
//Using currentTarget instead of target for Firefox 2.0's sake. Not
//all old browsers will be supported, but this one was easy enough
//to support and still makes sense.
var node = evt.currentTarget || evt.srcElement, contextName, moduleName,
context;
if (evt.type === "load" || (node && readyRegExp.test(node.readyState))) {
//Reset interactive script so a script node is not held onto for
//to long.
interactiveScript = null;
//Pull out the name of the module and the context.
contextName = node.getAttribute("data-requirecontext");
moduleName = node.getAttribute("data-requiremodule");
context = contexts[contextName];
contexts[contextName].completeLoad(moduleName);
//Clean up script binding. Favor detachEvent because of IE9
//issue, see attachEvent/addEventListener comment elsewhere
//in this file.
if (node.detachEvent && !isOpera) {
//Probably IE. If not it will throw an error, which will be
//useful to know.
node.detachEvent("onreadystatechange", req.onScriptLoad);
} else {
node.removeEventListener("load", req.onScriptLoad, false);
}
}
};
/**
* Attaches the script represented by the URL to the current
* environment. Right now only supports browser loading,
* but can be redefined in other environments to do the right thing.
* @param {String} url the url of the script to attach.
* @param {Object} context the context that wants the script.
* @param {moduleName} the name of the module that is associated with the script.
* @param {Function} [callback] optional callback, defaults to require.onScriptLoad
* @param {String} [type] optional type, defaults to text/javascript
* @param {Function} [fetchOnlyFunction] optional function to indicate the script node
* should be set up to fetch the script but do not attach it to the DOM
* so that it can later be attached to execute it. This is a way for the
* order plugin to support ordered loading in IE. Once the script is fetched,
* but not executed, the fetchOnlyFunction will be called.
*/
req.attach = function (url, context, moduleName, callback, type, fetchOnlyFunction) {
var node;
if (isBrowser) {
//In the browser so use a script tag
callback = callback || req.onScriptLoad;
node = context && context.config && context.config.xhtml ?
document.createElementNS("http://www.w3.org/1999/xhtml", "html:script") :
document.createElement("script");
node.type = type || (context && context.config.scriptType) ||
"text/javascript";
node.charset = "utf-8";
//Use async so Gecko does not block on executing the script if something
//like a long-polling comet tag is being run first. Gecko likes
//to evaluate scripts in DOM order, even for dynamic scripts.
//It will fetch them async, but only evaluate the contents in DOM
//order, so a long-polling script tag can delay execution of scripts
//after it. But telling Gecko we expect async gets us the behavior
//we want -- execute it whenever it is finished downloading. Only
//Helps Firefox 3.6+
//Allow some URLs to not be fetched async. Mostly helps the order!
//plugin
node.async = !s.skipAsync[url];
if (context) {
node.setAttribute("data-requirecontext", context.contextName);
}
node.setAttribute("data-requiremodule", moduleName);
//Set up load listener. Test attachEvent first because IE9 has
//a subtle issue in its addEventListener and script onload firings
//that do not match the behavior of all other browsers with
//addEventListener support, which fire the onload event for a
//script right after the script execution. See:
//https://connect.microsoft.com/IE/feedback/details/648057/script-onload-event-is-not-fired-immediately-after-script-execution
//UNFORTUNATELY Opera implements attachEvent but does not follow the script
//script execution mode.
if (node.attachEvent && !isOpera) {
//Probably IE. IE (at least 6-8) do not fire
//script onload right after executing the script, so
//we cannot tie the anonymous define call to a name.
//However, IE reports the script as being in "interactive"
//readyState at the time of the define call.
useInteractive = true;
if (fetchOnlyFunction) {
//Need to use old school onreadystate here since
//when the event fires and the node is not attached
//to the DOM, the evt.srcElement is null, so use
//a closure to remember the node.
node.onreadystatechange = function (evt) {
//Script loaded but not executed.
//Clear loaded handler, set the real one that
//waits for script execution.
if (node.readyState === 'loaded') {
node.onreadystatechange = null;
node.attachEvent("onreadystatechange", callback);
fetchOnlyFunction(node);
}
};
} else {
node.attachEvent("onreadystatechange", callback);
}
} else {
node.addEventListener("load", callback, false);
}
node.src = url;
//Fetch only means waiting to attach to DOM after loaded.
if (!fetchOnlyFunction) {
req.addScriptToDom(node);
}
return node;
} else if (isWebWorker) {
//In a web worker, use importScripts. This is not a very
//efficient use of importScripts, importScripts will block until
//its script is downloaded and evaluated. However, if web workers
//are in play, the expectation that a build has been done so that
//only one script needs to be loaded anyway. This may need to be
//reevaluated if other use cases become common.
importScripts(url);
//Account for anonymous modules
context.completeLoad(moduleName);
}
return null;
};
//Look for a data-main script attribute, which could also adjust the baseUrl.
if (isBrowser) {
//Figure out baseUrl. Get it from the script tag with require.js in it.
scripts = document.getElementsByTagName("script");
for (globalI = scripts.length - 1; globalI > -1 && (script = scripts[globalI]); globalI--) {
//Set the "head" where we can append children by
//using the script's parent.
if (!head) {
head = script.parentNode;
}
//Look for a data-main attribute to set main script for the page
//to load. If it is there, the path to data main becomes the
//baseUrl, if it is not already set.
if ((dataMain = script.getAttribute('data-main'))) {
if (!cfg.baseUrl) {
//Pull off the directory of data-main for use as the
//baseUrl.
src = dataMain.split('/');
mainScript = src.pop();
subPath = src.length ? src.join('/') + '/' : './';
//Set final config.
cfg.baseUrl = subPath;
//Strip off any trailing .js since dataMain is now
//like a module name.
dataMain = mainScript.replace(jsSuffixRegExp, '');
}
//Put the data-main script in the files to load.
cfg.deps = cfg.deps ? cfg.deps.concat(dataMain) : [dataMain];
break;
}
}
}
//See if there is nothing waiting across contexts, and if not, trigger
//resourcesReady.
req.checkReadyState = function () {
var contexts = s.contexts, prop;
for (prop in contexts) {
if (!(prop in empty)) {
if (contexts[prop].waitCount) {
return;
}
}
}
req.resourcesReady(true);
};
/**
* Internal function that is triggered whenever all scripts/resources
* have been loaded by the loader. Can be overridden by other, for
* instance the domReady plugin, which wants to know when all resources
* are loaded.
*/
req.resourcesReady = function (isReady) {
var contexts, context, prop;
//First, set the public variable indicating that resources are loading.
req.resourcesDone = isReady;
if (req.resourcesDone) {
//If jQuery with DOM ready delayed, release it now.
contexts = s.contexts;
for (prop in contexts) {
if (!(prop in empty)) {
context = contexts[prop];
if (context.jQueryIncremented) {
jQueryHoldReady(context.jQuery, false);
context.jQueryIncremented = false;
}
}
}
}
};
//FF < 3.6 readyState fix. Needed so that domReady plugin
//works well in that environment, since require.js is normally
//loaded via an HTML script tag so it will be there before window load,
//where the domReady plugin is more likely to be loaded after window load.
req.pageLoaded = function () {
if (document.readyState !== "complete") {
document.readyState = "complete";
}
};
if (isBrowser) {
if (document.addEventListener) {
if (!document.readyState) {
document.readyState = "loading";
window.addEventListener("load", req.pageLoaded, false);
}
}
}
//Set up default context. If require was a configuration object, use that as base config.
req(cfg);
//If modules are built into require.js, then need to make sure dependencies are
//traced. Use a setTimeout in the browser world, to allow all the modules to register
//themselves. In a non-browser env, assume that modules are not built into require.js,
//which seems odd to do on the server.
if (req.isAsync && typeof setTimeout !== "undefined") {
ctx = s.contexts[(cfg.context || defContextName)];
//Indicate that the script that includes require() is still loading,
//so that require()'d dependencies are not traced until the end of the
//file is parsed (approximated via the setTimeout call).
ctx.requireWait = true;
setTimeout(function () {
ctx.requireWait = false;
if (!ctx.scriptCount) {
ctx.resume();
}
req.checkReadyState();
}, 0);
}
}());
/*!
* jQuery JavaScript Library v1.7.1
* http://jquery.com/
*
* Copyright 2011, John Resig
* Dual licensed under the MIT or GPL Version 2 licenses.
* http://jquery.org/license
*
* Includes Sizzle.js
* http://sizzlejs.com/
* Copyright 2011, The Dojo Foundation
* Released under the MIT, BSD, and GPL Licenses.
*
* Date: Mon Nov 21 21:11:03 2011 -0500
*/
(function( window, undefined ) {
// Use the correct document accordingly with window argument (sandbox)
var document = window.document,
navigator = window.navigator,
location = window.location;
var jQuery = (function() {
// Define a local copy of jQuery
var jQuery = function( selector, context ) {
// The jQuery object is actually just the init constructor 'enhanced'
return new jQuery.fn.init( selector, context, rootjQuery );
},
// Map over jQuery in case of overwrite
_jQuery = window.jQuery,
// Map over the $ in case of overwrite
_$ = window.$,
// A central reference to the root jQuery(document)
rootjQuery,
// A simple way to check for HTML strings or ID strings
// Prioritize #id over <tag> to avoid XSS via location.hash (#9521)
quickExpr = /^(?:[^#<]*(<[\w\W]+>)[^>]*$|#([\w\-]*)$)/,
// Check if a string has a non-whitespace character in it
rnotwhite = /\S/,
// Used for trimming whitespace
trimLeft = /^\s+/,
trimRight = /\s+$/,
// Match a standalone tag
rsingleTag = /^<(\w+)\s*\/?>(?:<\/\1>)?$/,
// JSON RegExp
rvalidchars = /^[\],:{}\s]*$/,
rvalidescape = /\\(?:["\\\/bfnrt]|u[0-9a-fA-F]{4})/g,
rvalidtokens = /"[^"\\\n\r]*"|true|false|null|-?\d+(?:\.\d*)?(?:[eE][+\-]?\d+)?/g,
rvalidbraces = /(?:^|:|,)(?:\s*\[)+/g,
// Useragent RegExp
rwebkit = /(webkit)[ \/]([\w.]+)/,
ropera = /(opera)(?:.*version)?[ \/]([\w.]+)/,
rmsie = /(msie) ([\w.]+)/,
rmozilla = /(mozilla)(?:.*? rv:([\w.]+))?/,
// Matches dashed string for camelizing
rdashAlpha = /-([a-z]|[0-9])/ig,
rmsPrefix = /^-ms-/,
// Used by jQuery.camelCase as callback to replace()
fcamelCase = function( all, letter ) {
return ( letter + "" ).toUpperCase();
},
// Keep a UserAgent string for use with jQuery.browser
userAgent = navigator.userAgent,
// For matching the engine and version of the browser
browserMatch,
// The deferred used on DOM ready
readyList,
// The ready event handler
DOMContentLoaded,
// Save a reference to some core methods
toString = Object.prototype.toString,
hasOwn = Object.prototype.hasOwnProperty,
push = Array.prototype.push,
slice = Array.prototype.slice,
trim = String.prototype.trim,
indexOf = Array.prototype.indexOf,
// [[Class]] -> type pairs
class2type = {};
jQuery.fn = jQuery.prototype = {
constructor: jQuery,
init: function( selector, context, rootjQuery ) {
var match, elem, ret, doc;
// Handle $(""), $(null), or $(undefined)
if ( !selector ) {
return this;
}
// Handle $(DOMElement)
if ( selector.nodeType ) {
this.context = this[0] = selector;
this.length = 1;
return this;
}
// The body element only exists once, optimize finding it
if ( selector === "body" && !context && document.body ) {
this.context = document;
this[0] = document.body;
this.selector = selector;
this.length = 1;
return this;
}
// Handle HTML strings
if ( typeof selector === "string" ) {
// Are we dealing with HTML string or an ID?
if ( selector.charAt(0) === "<" && selector.charAt( selector.length - 1 ) === ">" && selector.length >= 3 ) {
// Assume that strings that start and end with <> are HTML and skip the regex check
match = [ null, selector, null ];
} else {
match = quickExpr.exec( selector );
}
// Verify a match, and that no context was specified for #id
if ( match && (match[1] || !context) ) {
// HANDLE: $(html) -> $(array)
if ( match[1] ) {
context = context instanceof jQuery ? context[0] : context;
doc = ( context ? context.ownerDocument || context : document );
// If a single string is passed in and it's a single tag
// just do a createElement and skip the rest
ret = rsingleTag.exec( selector );
if ( ret ) {
if ( jQuery.isPlainObject( context ) ) {
selector = [ document.createElement( ret[1] ) ];
jQuery.fn.attr.call( selector, context, true );
} else {
selector = [ doc.createElement( ret[1] ) ];
}
} else {
ret = jQuery.buildFragment( [ match[1] ], [ doc ] );
selector = ( ret.cacheable ? jQuery.clone(ret.fragment) : ret.fragment ).childNodes;
}
return jQuery.merge( this, selector );
// HANDLE: $("#id")
} else {
elem = document.getElementById( match[2] );
// Check parentNode to catch when Blackberry 4.6 returns
// nodes that are no longer in the document #6963
if ( elem && elem.parentNode ) {
// Handle the case where IE and Opera return items
// by name instead of ID
if ( elem.id !== match[2] ) {
return rootjQuery.find( selector );
}
// Otherwise, we inject the element directly into the jQuery object
this.length = 1;
this[0] = elem;
}
this.context = document;
this.selector = selector;
return this;
}
// HANDLE: $(expr, $(...))
} else if ( !context || context.jquery ) {
return ( context || rootjQuery ).find( selector );
// HANDLE: $(expr, context)
// (which is just equivalent to: $(context).find(expr)
} else {
return this.constructor( context ).find( selector );
}
// HANDLE: $(function)
// Shortcut for document ready
} else if ( jQuery.isFunction( selector ) ) {
return rootjQuery.ready( selector );
}
if ( selector.selector !== undefined ) {
this.selector = selector.selector;
this.context = selector.context;
}
return jQuery.makeArray( selector, this );
},
// Start with an empty selector
selector: "",
// The current version of jQuery being used
jquery: "1.7.1",
// The default length of a jQuery object is 0
length: 0,
// The number of elements contained in the matched element set
size: function() {
return this.length;
},
toArray: function() {
return slice.call( this, 0 );
},
// Get the Nth element in the matched element set OR
// Get the whole matched element set as a clean array
get: function( num ) {
return num == null ?
// Return a 'clean' array
this.toArray() :
// Return just the object
( num < 0 ? this[ this.length + num ] : this[ num ] );
},
// Take an array of elements and push it onto the stack
// (returning the new matched element set)
pushStack: function( elems, name, selector ) {
// Build a new jQuery matched element set
var ret = this.constructor();
if ( jQuery.isArray( elems ) ) {
push.apply( ret, elems );
} else {
jQuery.merge( ret, elems );
}
// Add the old object onto the stack (as a reference)
ret.prevObject = this;
ret.context = this.context;
if ( name === "find" ) {
ret.selector = this.selector + ( this.selector ? " " : "" ) + selector;
} else if ( name ) {
ret.selector = this.selector + "." + name + "(" + selector + ")";
}
// Return the newly-formed element set
return ret;
},
// Execute a callback for every element in the matched set.
// (You can seed the arguments with an array of args, but this is
// only used internally.)
each: function( callback, args ) {
return jQuery.each( this, callback, args );
},
ready: function( fn ) {
// Attach the listeners
jQuery.bindReady();
// Add the callback
readyList.add( fn );
return this;
},
eq: function( i ) {
i = +i;
return i === -1 ?
this.slice( i ) :
this.slice( i, i + 1 );
},
first: function() {
return this.eq( 0 );
},
last: function() {
return this.eq( -1 );
},
slice: function() {
return this.pushStack( slice.apply( this, arguments ),
"slice", slice.call(arguments).join(",") );
},
map: function( callback ) {
return this.pushStack( jQuery.map(this, function( elem, i ) {
return callback.call( elem, i, elem );
}));
},
end: function() {
return this.prevObject || this.constructor(null);
},
// For internal use only.
// Behaves like an Array's method, not like a jQuery method.
push: push,
sort: [].sort,
splice: [].splice
};
// Give the init function the jQuery prototype for later instantiation
jQuery.fn.init.prototype = jQuery.fn;
jQuery.extend = jQuery.fn.extend = function() {
var options, name, src, copy, copyIsArray, clone,
target = arguments[0] || {},
i = 1,
length = arguments.length,
deep = false;
// Handle a deep copy situation
if ( typeof target === "boolean" ) {
deep = target;
target = arguments[1] || {};
// skip the boolean and the target
i = 2;
}
// Handle case when target is a string or something (possible in deep copy)
if ( typeof target !== "object" && !jQuery.isFunction(target) ) {
target = {};
}
// extend jQuery itself if only one argument is passed
if ( length === i ) {
target = this;
--i;
}
for ( ; i < length; i++ ) {
// Only deal with non-null/undefined values
if ( (options = arguments[ i ]) != null ) {
// Extend the base object
for ( name in options ) {
src = target[ name ];
copy = options[ name ];
// Prevent never-ending loop
if ( target === copy ) {
continue;
}
// Recurse if we're merging plain objects or arrays
if ( deep && copy && ( jQuery.isPlainObject(copy) || (copyIsArray = jQuery.isArray(copy)) ) ) {
if ( copyIsArray ) {
copyIsArray = false;
clone = src && jQuery.isArray(src) ? src : [];
} else {
clone = src && jQuery.isPlainObject(src) ? src : {};
}
// Never move original objects, clone them
target[ name ] = jQuery.extend( deep, clone, copy );
// Don't bring in undefined values
} else if ( copy !== undefined ) {
target[ name ] = copy;
}
}
}
}
// Return the modified object
return target;
};
jQuery.extend({
noConflict: function( deep ) {
if ( window.$ === jQuery ) {
window.$ = _$;
}
if ( deep && window.jQuery === jQuery ) {
window.jQuery = _jQuery;
}
return jQuery;
},
// Is the DOM ready to be used? Set to true once it occurs.
isReady: false,
// A counter to track how many items to wait for before
// the ready event fires. See #6781
readyWait: 1,
// Hold (or release) the ready event
holdReady: function( hold ) {
if ( hold ) {
jQuery.readyWait++;
} else {
jQuery.ready( true );
}
},
// Handle when the DOM is ready
ready: function( wait ) {
// Either a released hold or an DOMready/load event and not yet ready
if ( (wait === true && !--jQuery.readyWait) || (wait !== true && !jQuery.isReady) ) {
// Make sure body exists, at least, in case IE gets a little overzealous (ticket #5443).
if ( !document.body ) {
return setTimeout( jQuery.ready, 1 );
}
// Remember that the DOM is ready
jQuery.isReady = true;
// If a normal DOM Ready event fired, decrement, and wait if need be
if ( wait !== true && --jQuery.readyWait > 0 ) {
return;
}
// If there are functions bound, to execute
readyList.fireWith( document, [ jQuery ] );
// Trigger any bound ready events
if ( jQuery.fn.trigger ) {
jQuery( document ).trigger( "ready" ).off( "ready" );
}
}
},
bindReady: function() {
if ( readyList ) {
return;
}
readyList = jQuery.Callbacks( "once memory" );
// Catch cases where $(document).ready() is called after the
// browser event has already occurred.
if ( document.readyState === "complete" ) {
// Handle it asynchronously to allow scripts the opportunity to delay ready
return setTimeout( jQuery.ready, 1 );
}
// Mozilla, Opera and webkit nightlies currently support this event
if ( document.addEventListener ) {
// Use the handy event callback
document.addEventListener( "DOMContentLoaded", DOMContentLoaded, false );
// A fallback to window.onload, that will always work
window.addEventListener( "load", jQuery.ready, false );
// If IE event model is used
} else if ( document.attachEvent ) {
// ensure firing before onload,
// maybe late but safe also for iframes
document.attachEvent( "onreadystatechange", DOMContentLoaded );
// A fallback to window.onload, that will always work
window.attachEvent( "onload", jQuery.ready );
// If IE and not a frame
// continually check to see if the document is ready
var toplevel = false;
try {
toplevel = window.frameElement == null;
} catch(e) {}
if ( document.documentElement.doScroll && toplevel ) {
doScrollCheck();
}
}
},
// See test/unit/core.js for details concerning isFunction.
// Since version 1.3, DOM methods and functions like alert
// aren't supported. They return false on IE (#2968).
isFunction: function( obj ) {
return jQuery.type(obj) === "function";
},
isArray: Array.isArray || function( obj ) {
return jQuery.type(obj) === "array";
},
// A crude way of determining if an object is a window
isWindow: function( obj ) {
return obj && typeof obj === "object" && "setInterval" in obj;
},
isNumeric: function( obj ) {
return !isNaN( parseFloat(obj) ) && isFinite( obj );
},
type: function( obj ) {
return obj == null ?
String( obj ) :
class2type[ toString.call(obj) ] || "object";
},
isPlainObject: function( obj ) {
// Must be an Object.
// Because of IE, we also have to check the presence of the constructor property.
// Make sure that DOM nodes and window objects don't pass through, as well
if ( !obj || jQuery.type(obj) !== "object" || obj.nodeType || jQuery.isWindow( obj ) ) {
return false;
}
try {
// Not own constructor property must be Object
if ( obj.constructor &&
!hasOwn.call(obj, "constructor") &&
!hasOwn.call(obj.constructor.prototype, "isPrototypeOf") ) {
return false;
}
} catch ( e ) {
// IE8,9 Will throw exceptions on certain host objects #9897
return false;
}
// Own properties are enumerated firstly, so to speed up,
// if last one is own, then all properties are own.
var key;
for ( key in obj ) {}
return key === undefined || hasOwn.call( obj, key );
},
isEmptyObject: function( obj ) {
for ( var name in obj ) {
return false;
}
return true;
},
error: function( msg ) {
throw new Error( msg );
},
parseJSON: function( data ) {
if ( typeof data !== "string" || !data ) {
return null;
}
// Make sure leading/trailing whitespace is removed (IE can't handle it)
data = jQuery.trim( data );
// Attempt to parse using the native JSON parser first
if ( window.JSON && window.JSON.parse ) {
return window.JSON.parse( data );
}
// Make sure the incoming data is actual JSON
// Logic borrowed from http://json.org/json2.js
if ( rvalidchars.test( data.replace( rvalidescape, "@" )
.replace( rvalidtokens, "]" )
.replace( rvalidbraces, "")) ) {
return ( new Function( "return " + data ) )();
}
jQuery.error( "Invalid JSON: " + data );
},
// Cross-browser xml parsing
parseXML: function( data ) {
var xml, tmp;
try {
if ( window.DOMParser ) { // Standard
tmp = new DOMParser();
xml = tmp.parseFromString( data , "text/xml" );
} else { // IE
xml = new ActiveXObject( "Microsoft.XMLDOM" );
xml.async = "false";
xml.loadXML( data );
}
} catch( e ) {
xml = undefined;
}
if ( !xml || !xml.documentElement || xml.getElementsByTagName( "parsererror" ).length ) {
jQuery.error( "Invalid XML: " + data );
}
return xml;
},
noop: function() {},
// Evaluates a script in a global context
// Workarounds based on findings by Jim Driscoll
// http://weblogs.java.net/blog/driscoll/archive/2009/09/08/eval-javascript-global-context
globalEval: function( data ) {
if ( data && rnotwhite.test( data ) ) {
// We use execScript on Internet Explorer
// We use an anonymous function so that context is window
// rather than jQuery in Firefox
( window.execScript || function( data ) {
window[ "eval" ].call( window, data );
} )( data );
}
},
// Convert dashed to camelCase; used by the css and data modules
// Microsoft forgot to hump their vendor prefix (#9572)
camelCase: function( string ) {
return string.replace( rmsPrefix, "ms-" ).replace( rdashAlpha, fcamelCase );
},
nodeName: function( elem, name ) {
return elem.nodeName && elem.nodeName.toUpperCase() === name.toUpperCase();
},
// args is for internal usage only
each: function( object, callback, args ) {
var name, i = 0,
length = object.length,
isObj = length === undefined || jQuery.isFunction( object );
if ( args ) {
if ( isObj ) {
for ( name in object ) {
if ( callback.apply( object[ name ], args ) === false ) {
break;
}
}
} else {
for ( ; i < length; ) {
if ( callback.apply( object[ i++ ], args ) === false ) {
break;
}
}
}
// A special, fast, case for the most common use of each
} else {
if ( isObj ) {
for ( name in object ) {
if ( callback.call( object[ name ], name, object[ name ] ) === false ) {
break;
}
}
} else {
for ( ; i < length; ) {
if ( callback.call( object[ i ], i, object[ i++ ] ) === false ) {
break;
}
}
}
}
return object;
},
// Use native String.trim function wherever possible
trim: trim ?
function( text ) {
return text == null ?
"" :
trim.call( text );
} :
// Otherwise use our own trimming functionality
function( text ) {
return text == null ?
"" :
text.toString().replace( trimLeft, "" ).replace( trimRight, "" );
},
// results is for internal usage only
makeArray: function( array, results ) {
var ret = results || [];
if ( array != null ) {
// The window, strings (and functions) also have 'length'
// Tweaked logic slightly to handle Blackberry 4.7 RegExp issues #6930
var type = jQuery.type( array );
if ( array.length == null || type === "string" || type === "function" || type === "regexp" || jQuery.isWindow( array ) ) {
push.call( ret, array );
} else {
jQuery.merge( ret, array );
}
}
return ret;
},
inArray: function( elem, array, i ) {
var len;
if ( array ) {
if ( indexOf ) {
return indexOf.call( array, elem, i );
}
len = array.length;
i = i ? i < 0 ? Math.max( 0, len + i ) : i : 0;
for ( ; i < len; i++ ) {
// Skip accessing in sparse arrays
if ( i in array && array[ i ] === elem ) {
return i;
}
}
}
return -1;
},
merge: function( first, second ) {
var i = first.length,
j = 0;
if ( typeof second.length === "number" ) {
for ( var l = second.length; j < l; j++ ) {
first[ i++ ] = second[ j ];
}
} else {
while ( second[j] !== undefined ) {
first[ i++ ] = second[ j++ ];
}
}
first.length = i;
return first;
},
grep: function( elems, callback, inv ) {
var ret = [], retVal;
inv = !!inv;
// Go through the array, only saving the items
// that pass the validator function
for ( var i = 0, length = elems.length; i < length; i++ ) {
retVal = !!callback( elems[ i ], i );
if ( inv !== retVal ) {
ret.push( elems[ i ] );
}
}
return ret;
},
// arg is for internal usage only
map: function( elems, callback, arg ) {
var value, key, ret = [],
i = 0,
length = elems.length,
// jquery objects are treated as arrays
isArray = elems instanceof jQuery || length !== undefined && typeof length === "number" && ( ( length > 0 && elems[ 0 ] && elems[ length -1 ] ) || length === 0 || jQuery.isArray( elems ) ) ;
// Go through the array, translating each of the items to their
if ( isArray ) {
for ( ; i < length; i++ ) {
value = callback( elems[ i ], i, arg );
if ( value != null ) {
ret[ ret.length ] = value;
}
}
// Go through every key on the object,
} else {
for ( key in elems ) {
value = callback( elems[ key ], key, arg );
if ( value != null ) {
ret[ ret.length ] = value;
}
}
}
// Flatten any nested arrays
return ret.concat.apply( [], ret );
},
// A global GUID counter for objects
guid: 1,
// Bind a function to a context, optionally partially applying any
// arguments.
proxy: function( fn, context ) {
if ( typeof context === "string" ) {
var tmp = fn[ context ];
context = fn;
fn = tmp;
}
// Quick check to determine if target is callable, in the spec
// this throws a TypeError, but we will just return undefined.
if ( !jQuery.isFunction( fn ) ) {
return undefined;
}
// Simulated bind
var args = slice.call( arguments, 2 ),
proxy = function() {
return fn.apply( context, args.concat( slice.call( arguments ) ) );
};
// Set the guid of unique handler to the same of original handler, so it can be removed
proxy.guid = fn.guid = fn.guid || proxy.guid || jQuery.guid++;
return proxy;
},
// Mutifunctional method to get and set values to a collection
// The value/s can optionally be executed if it's a function
access: function( elems, key, value, exec, fn, pass ) {
var length = elems.length;
// Setting many attributes
if ( typeof key === "object" ) {
for ( var k in key ) {
jQuery.access( elems, k, key[k], exec, fn, value );
}
return elems;
}
// Setting one attribute
if ( value !== undefined ) {
// Optionally, function values get executed if exec is true
exec = !pass && exec && jQuery.isFunction(value);
for ( var i = 0; i < length; i++ ) {
fn( elems[i], key, exec ? value.call( elems[i], i, fn( elems[i], key ) ) : value, pass );
}
return elems;
}
// Getting an attribute
return length ? fn( elems[0], key ) : undefined;
},
now: function() {
return ( new Date() ).getTime();
},
// Use of jQuery.browser is frowned upon.
// More details: http://docs.jquery.com/Utilities/jQuery.browser
uaMatch: function( ua ) {
ua = ua.toLowerCase();
var match = rwebkit.exec( ua ) ||
ropera.exec( ua ) ||
rmsie.exec( ua ) ||
ua.indexOf("compatible") < 0 && rmozilla.exec( ua ) ||
[];
return { browser: match[1] || "", version: match[2] || "0" };
},
sub: function() {
function jQuerySub( selector, context ) {
return new jQuerySub.fn.init( selector, context );
}
jQuery.extend( true, jQuerySub, this );
jQuerySub.superclass = this;
jQuerySub.fn = jQuerySub.prototype = this();
jQuerySub.fn.constructor = jQuerySub;
jQuerySub.sub = this.sub;
jQuerySub.fn.init = function init( selector, context ) {
if ( context && context instanceof jQuery && !(context instanceof jQuerySub) ) {
context = jQuerySub( context );
}
return jQuery.fn.init.call( this, selector, context, rootjQuerySub );
};
jQuerySub.fn.init.prototype = jQuerySub.fn;
var rootjQuerySub = jQuerySub(document);
return jQuerySub;
},
browser: {}
});
// Populate the class2type map
jQuery.each("Boolean Number String Function Array Date RegExp Object".split(" "), function(i, name) {
class2type[ "[object " + name + "]" ] = name.toLowerCase();
});
browserMatch = jQuery.uaMatch( userAgent );
if ( browserMatch.browser ) {
jQuery.browser[ browserMatch.browser ] = true;
jQuery.browser.version = browserMatch.version;
}
// Deprecated, use jQuery.browser.webkit instead
if ( jQuery.browser.webkit ) {
jQuery.browser.safari = true;
}
// IE doesn't match non-breaking spaces with \s
if ( rnotwhite.test( "\xA0" ) ) {
trimLeft = /^[\s\xA0]+/;
trimRight = /[\s\xA0]+$/;
}
// All jQuery objects should point back to these
rootjQuery = jQuery(document);
// Cleanup functions for the document ready method
if ( document.addEventListener ) {
DOMContentLoaded = function() {
document.removeEventListener( "DOMContentLoaded", DOMContentLoaded, false );
jQuery.ready();
};
} else if ( document.attachEvent ) {
DOMContentLoaded = function() {
// Make sure body exists, at least, in case IE gets a little overzealous (ticket #5443).
if ( document.readyState === "complete" ) {
document.detachEvent( "onreadystatechange", DOMContentLoaded );
jQuery.ready();
}
};
}
// The DOM ready check for Internet Explorer
function doScrollCheck() {
if ( jQuery.isReady ) {
return;
}
try {
// If IE is used, use the trick by Diego Perini
// http://javascript.nwbox.com/IEContentLoaded/
document.documentElement.doScroll("left");
} catch(e) {
setTimeout( doScrollCheck, 1 );
return;
}
// and execute any waiting functions
jQuery.ready();
}
return jQuery;
})();
// String to Object flags format cache
var flagsCache = {};
// Convert String-formatted flags into Object-formatted ones and store in cache
function createFlags( flags ) {
var object = flagsCache[ flags ] = {},
i, length;
flags = flags.split( /\s+/ );
for ( i = 0, length = flags.length; i < length; i++ ) {
object[ flags[i] ] = true;
}
return object;
}
/*
* Create a callback list using the following parameters:
*
* flags: an optional list of space-separated flags that will change how
* the callback list behaves
*
* By default a callback list will act like an event callback list and can be
* "fired" multiple times.
*
* Possible flags:
*
* once: will ensure the callback list can only be fired once (like a Deferred)
*
* memory: will keep track of previous values and will call any callback added
* after the list has been fired right away with the latest "memorized"
* values (like a Deferred)
*
* unique: will ensure a callback can only be added once (no duplicate in the list)
*
* stopOnFalse: interrupt callings when a callback returns false
*
*/
jQuery.Callbacks = function( flags ) {
// Convert flags from String-formatted to Object-formatted
// (we check in cache first)
flags = flags ? ( flagsCache[ flags ] || createFlags( flags ) ) : {};
var // Actual callback list
list = [],
// Stack of fire calls for repeatable lists
stack = [],
// Last fire value (for non-forgettable lists)
memory,
// Flag to know if list is currently firing
firing,
// First callback to fire (used internally by add and fireWith)
firingStart,
// End of the loop when firing
firingLength,
// Index of currently firing callback (modified by remove if needed)
firingIndex,
// Add one or several callbacks to the list
add = function( args ) {
var i,
length,
elem,
type,
actual;
for ( i = 0, length = args.length; i < length; i++ ) {
elem = args[ i ];
type = jQuery.type( elem );
if ( type === "array" ) {
// Inspect recursively
add( elem );
} else if ( type === "function" ) {
// Add if not in unique mode and callback is not in
if ( !flags.unique || !self.has( elem ) ) {
list.push( elem );
}
}
}
},
// Fire callbacks
fire = function( context, args ) {
args = args || [];
memory = !flags.memory || [ context, args ];
firing = true;
firingIndex = firingStart || 0;
firingStart = 0;
firingLength = list.length;
for ( ; list && firingIndex < firingLength; firingIndex++ ) {
if ( list[ firingIndex ].apply( context, args ) === false && flags.stopOnFalse ) {
memory = true; // Mark as halted
break;
}
}
firing = false;
if ( list ) {
if ( !flags.once ) {
if ( stack && stack.length ) {
memory = stack.shift();
self.fireWith( memory[ 0 ], memory[ 1 ] );
}
} else if ( memory === true ) {
self.disable();
} else {
list = [];
}
}
},
// Actual Callbacks object
self = {
// Add a callback or a collection of callbacks to the list
add: function() {
if ( list ) {
var length = list.length;
add( arguments );
// Do we need to add the callbacks to the
// current firing batch?
if ( firing ) {
firingLength = list.length;
// With memory, if we're not firing then
// we should call right away, unless previous
// firing was halted (stopOnFalse)
} else if ( memory && memory !== true ) {
firingStart = length;
fire( memory[ 0 ], memory[ 1 ] );
}
}
return this;
},
// Remove a callback from the list
remove: function() {
if ( list ) {
var args = arguments,
argIndex = 0,
argLength = args.length;
for ( ; argIndex < argLength ; argIndex++ ) {
for ( var i = 0; i < list.length; i++ ) {
if ( args[ argIndex ] === list[ i ] ) {
// Handle firingIndex and firingLength
if ( firing ) {
if ( i <= firingLength ) {
firingLength--;
if ( i <= firingIndex ) {
firingIndex--;
}
}
}
// Remove the element
list.splice( i--, 1 );
// If we have some unicity property then
// we only need to do this once
if ( flags.unique ) {
break;
}
}
}
}
}
return this;
},
// Control if a given callback is in the list
has: function( fn ) {
if ( list ) {
var i = 0,
length = list.length;
for ( ; i < length; i++ ) {
if ( fn === list[ i ] ) {
return true;
}
}
}
return false;
},
// Remove all callbacks from the list
empty: function() {
list = [];
return this;
},
// Have the list do nothing anymore
disable: function() {
list = stack = memory = undefined;
return this;
},
// Is it disabled?
disabled: function() {
return !list;
},
// Lock the list in its current state
lock: function() {
stack = undefined;
if ( !memory || memory === true ) {
self.disable();
}
return this;
},
// Is it locked?
locked: function() {
return !stack;
},
// Call all callbacks with the given context and arguments
fireWith: function( context, args ) {
if ( stack ) {
if ( firing ) {
if ( !flags.once ) {
stack.push( [ context, args ] );
}
} else if ( !( flags.once && memory ) ) {
fire( context, args );
}
}
return this;
},
// Call all the callbacks with the given arguments
fire: function() {
self.fireWith( this, arguments );
return this;
},
// To know if the callbacks have already been called at least once
fired: function() {
return !!memory;
}
};
return self;
};
var // Static reference to slice
sliceDeferred = [].slice;
jQuery.extend({
Deferred: function( func ) {
var doneList = jQuery.Callbacks( "once memory" ),
failList = jQuery.Callbacks( "once memory" ),
progressList = jQuery.Callbacks( "memory" ),
state = "pending",
lists = {
resolve: doneList,
reject: failList,
notify: progressList
},
promise = {
done: doneList.add,
fail: failList.add,
progress: progressList.add,
state: function() {
return state;
},
// Deprecated
isResolved: doneList.fired,
isRejected: failList.fired,
then: function( doneCallbacks, failCallbacks, progressCallbacks ) {
deferred.done( doneCallbacks ).fail( failCallbacks ).progress( progressCallbacks );
return this;
},
always: function() {
deferred.done.apply( deferred, arguments ).fail.apply( deferred, arguments );
return this;
},
pipe: function( fnDone, fnFail, fnProgress ) {
return jQuery.Deferred(function( newDefer ) {
jQuery.each( {
done: [ fnDone, "resolve" ],
fail: [ fnFail, "reject" ],
progress: [ fnProgress, "notify" ]
}, function( handler, data ) {
var fn = data[ 0 ],
action = data[ 1 ],
returned;
if ( jQuery.isFunction( fn ) ) {
deferred[ handler ](function() {
returned = fn.apply( this, arguments );
if ( returned && jQuery.isFunction( returned.promise ) ) {
returned.promise().then( newDefer.resolve, newDefer.reject, newDefer.notify );
} else {
newDefer[ action + "With" ]( this === deferred ? newDefer : this, [ returned ] );
}
});
} else {
deferred[ handler ]( newDefer[ action ] );
}
});
}).promise();
},
// Get a promise for this deferred
// If obj is provided, the promise aspect is added to the object
promise: function( obj ) {
if ( obj == null ) {
obj = promise;
} else {
for ( var key in promise ) {
obj[ key ] = promise[ key ];
}
}
return obj;
}
},
deferred = promise.promise({}),
key;
for ( key in lists ) {
deferred[ key ] = lists[ key ].fire;
deferred[ key + "With" ] = lists[ key ].fireWith;
}
// Handle state
deferred.done( function() {
state = "resolved";
}, failList.disable, progressList.lock ).fail( function() {
state = "rejected";
}, doneList.disable, progressList.lock );
// Call given func if any
if ( func ) {
func.call( deferred, deferred );
}
// All done!
return deferred;
},
// Deferred helper
when: function( firstParam ) {
var args = sliceDeferred.call( arguments, 0 ),
i = 0,
length = args.length,
pValues = new Array( length ),
count = length,
pCount = length,
deferred = length <= 1 && firstParam && jQuery.isFunction( firstParam.promise ) ?
firstParam :
jQuery.Deferred(),
promise = deferred.promise();
function resolveFunc( i ) {
return function( value ) {
args[ i ] = arguments.length > 1 ? sliceDeferred.call( arguments, 0 ) : value;
if ( !( --count ) ) {
deferred.resolveWith( deferred, args );
}
};
}
function progressFunc( i ) {
return function( value ) {
pValues[ i ] = arguments.length > 1 ? sliceDeferred.call( arguments, 0 ) : value;
deferred.notifyWith( promise, pValues );
};
}
if ( length > 1 ) {
for ( ; i < length; i++ ) {
if ( args[ i ] && args[ i ].promise && jQuery.isFunction( args[ i ].promise ) ) {
args[ i ].promise().then( resolveFunc(i), deferred.reject, progressFunc(i) );
} else {
--count;
}
}
if ( !count ) {
deferred.resolveWith( deferred, args );
}
} else if ( deferred !== firstParam ) {
deferred.resolveWith( deferred, length ? [ firstParam ] : [] );
}
return promise;
}
});
jQuery.support = (function() {
var support,
all,
a,
select,
opt,
input,
marginDiv,
fragment,
tds,
events,
eventName,
i,
isSupported,
div = document.createElement( "div" ),
documentElement = document.documentElement;
// Preliminary tests
div.setAttribute("className", "t");
div.innerHTML = " <link/><table></table><a href='/a' style='top:1px;float:left;opacity:.55;'>a</a><input type='checkbox'/>";
all = div.getElementsByTagName( "*" );
a = div.getElementsByTagName( "a" )[ 0 ];
// Can't get basic test support
if ( !all || !all.length || !a ) {
return {};
}
// First batch of supports tests
select = document.createElement( "select" );
opt = select.appendChild( document.createElement("option") );
input = div.getElementsByTagName( "input" )[ 0 ];
support = {
// IE strips leading whitespace when .innerHTML is used
leadingWhitespace: ( div.firstChild.nodeType === 3 ),
// Make sure that tbody elements aren't automatically inserted
// IE will insert them into empty tables
tbody: !div.getElementsByTagName("tbody").length,
// Make sure that link elements get serialized correctly by innerHTML
// This requires a wrapper element in IE
htmlSerialize: !!div.getElementsByTagName("link").length,
// Get the style information from getAttribute
// (IE uses .cssText instead)
style: /top/.test( a.getAttribute("style") ),
// Make sure that URLs aren't manipulated
// (IE normalizes it by default)
hrefNormalized: ( a.getAttribute("href") === "/a" ),
// Make sure that element opacity exists
// (IE uses filter instead)
// Use a regex to work around a WebKit issue. See #5145
opacity: /^0.55/.test( a.style.opacity ),
// Verify style float existence
// (IE uses styleFloat instead of cssFloat)
cssFloat: !!a.style.cssFloat,
// Make sure that if no value is specified for a checkbox
// that it defaults to "on".
// (WebKit defaults to "" instead)
checkOn: ( input.value === "on" ),
// Make sure that a selected-by-default option has a working selected property.
// (WebKit defaults to false instead of true, IE too, if it's in an optgroup)
optSelected: opt.selected,
// Test setAttribute on camelCase class. If it works, we need attrFixes when doing get/setAttribute (ie6/7)
getSetAttribute: div.className !== "t",
// Tests for enctype support on a form(#6743)
enctype: !!document.createElement("form").enctype,
// Makes sure cloning an html5 element does not cause problems
// Where outerHTML is undefined, this still works
html5Clone: document.createElement("nav").cloneNode( true ).outerHTML !== "<:nav></:nav>",
// Will be defined later
submitBubbles: true,
changeBubbles: true,
focusinBubbles: false,
deleteExpando: true,
noCloneEvent: true,
inlineBlockNeedsLayout: false,
shrinkWrapBlocks: false,
reliableMarginRight: true
};
// Make sure checked status is properly cloned
input.checked = true;
support.noCloneChecked = input.cloneNode( true ).checked;
// Make sure that the options inside disabled selects aren't marked as disabled
// (WebKit marks them as disabled)
select.disabled = true;
support.optDisabled = !opt.disabled;
// Test to see if it's possible to delete an expando from an element
// Fails in Internet Explorer
try {
delete div.test;
} catch( e ) {
support.deleteExpando = false;
}
if ( !div.addEventListener && div.attachEvent && div.fireEvent ) {
div.attachEvent( "onclick", function() {
// Cloning a node shouldn't copy over any
// bound event handlers (IE does this)
support.noCloneEvent = false;
});
div.cloneNode( true ).fireEvent( "onclick" );
}
// Check if a radio maintains its value
// after being appended to the DOM
input = document.createElement("input");
input.value = "t";
input.setAttribute("type", "radio");
support.radioValue = input.value === "t";
input.setAttribute("checked", "checked");
div.appendChild( input );
fragment = document.createDocumentFragment();
fragment.appendChild( div.lastChild );
// WebKit doesn't clone checked state correctly in fragments
support.checkClone = fragment.cloneNode( true ).cloneNode( true ).lastChild.checked;
// Check if a disconnected checkbox will retain its checked
// value of true after appended to the DOM (IE6/7)
support.appendChecked = input.checked;
fragment.removeChild( input );
fragment.appendChild( div );
div.innerHTML = "";
// Check if div with explicit width and no margin-right incorrectly
// gets computed margin-right based on width of container. For more
// info see bug #3333
// Fails in WebKit before Feb 2011 nightlies
// WebKit Bug 13343 - getComputedStyle returns wrong value for margin-right
if ( window.getComputedStyle ) {
marginDiv = document.createElement( "div" );
marginDiv.style.width = "0";
marginDiv.style.marginRight = "0";
div.style.width = "2px";
div.appendChild( marginDiv );
support.reliableMarginRight =
( parseInt( ( window.getComputedStyle( marginDiv, null ) || { marginRight: 0 } ).marginRight, 10 ) || 0 ) === 0;
}
// Technique from Juriy Zaytsev
// http://perfectionkills.com/detecting-event-support-without-browser-sniffing/
// We only care about the case where non-standard event systems
// are used, namely in IE. Short-circuiting here helps us to
// avoid an eval call (in setAttribute) which can cause CSP
// to go haywire. See: https://developer.mozilla.org/en/Security/CSP
if ( div.attachEvent ) {
for( i in {
submit: 1,
change: 1,
focusin: 1
}) {
eventName = "on" + i;
isSupported = ( eventName in div );
if ( !isSupported ) {
div.setAttribute( eventName, "return;" );
isSupported = ( typeof div[ eventName ] === "function" );
}
support[ i + "Bubbles" ] = isSupported;
}
}
fragment.removeChild( div );
// Null elements to avoid leaks in IE
fragment = select = opt = marginDiv = div = input = null;
// Run tests that need a body at doc ready
jQuery(function() {
var container, outer, inner, table, td, offsetSupport,
conMarginTop, ptlm, vb, style, html,
body = document.getElementsByTagName("body")[0];
if ( !body ) {
// Return for frameset docs that don't have a body
return;
}
conMarginTop = 1;
ptlm = "position:absolute;top:0;left:0;width:1px;height:1px;margin:0;";
vb = "visibility:hidden;border:0;";
style = "style='" + ptlm + "border:5px solid #000;padding:0;'";
html = "<div " + style + "><div></div></div>" +
"<table " + style + " cellpadding='0' cellspacing='0'>" +
"<tr><td></td></tr></table>";
container = document.createElement("div");
container.style.cssText = vb + "width:0;height:0;position:static;top:0;margin-top:" + conMarginTop + "px";
body.insertBefore( container, body.firstChild );
// Construct the test element
div = document.createElement("div");
container.appendChild( div );
// Check if table cells still have offsetWidth/Height when they are set
// to display:none and there are still other visible table cells in a
// table row; if so, offsetWidth/Height are not reliable for use when
// determining if an element has been hidden directly using
// display:none (it is still safe to use offsets if a parent element is
// hidden; don safety goggles and see bug #4512 for more information).
// (only IE 8 fails this test)
div.innerHTML = "<table><tr><td style='padding:0;border:0;display:none'></td><td>t</td></tr></table>";
tds = div.getElementsByTagName( "td" );
isSupported = ( tds[ 0 ].offsetHeight === 0 );
tds[ 0 ].style.display = "";
tds[ 1 ].style.display = "none";
// Check if empty table cells still have offsetWidth/Height
// (IE <= 8 fail this test)
support.reliableHiddenOffsets = isSupported && ( tds[ 0 ].offsetHeight === 0 );
// Figure out if the W3C box model works as expected
div.innerHTML = "";
div.style.width = div.style.paddingLeft = "1px";
jQuery.boxModel = support.boxModel = div.offsetWidth === 2;
if ( typeof div.style.zoom !== "undefined" ) {
// Check if natively block-level elements act like inline-block
// elements when setting their display to 'inline' and giving
// them layout
// (IE < 8 does this)
div.style.display = "inline";
div.style.zoom = 1;
support.inlineBlockNeedsLayout = ( div.offsetWidth === 2 );
// Check if elements with layout shrink-wrap their children
// (IE 6 does this)
div.style.display = "";
div.innerHTML = "<div style='width:4px;'></div>";
support.shrinkWrapBlocks = ( div.offsetWidth !== 2 );
}
div.style.cssText = ptlm + vb;
div.innerHTML = html;
outer = div.firstChild;
inner = outer.firstChild;
td = outer.nextSibling.firstChild.firstChild;
offsetSupport = {
doesNotAddBorder: ( inner.offsetTop !== 5 ),
doesAddBorderForTableAndCells: ( td.offsetTop === 5 )
};
inner.style.position = "fixed";
inner.style.top = "20px";
// safari subtracts parent border width here which is 5px
offsetSupport.fixedPosition = ( inner.offsetTop === 20 || inner.offsetTop === 15 );
inner.style.position = inner.style.top = "";
outer.style.overflow = "hidden";
outer.style.position = "relative";
offsetSupport.subtractsBorderForOverflowNotVisible = ( inner.offsetTop === -5 );
offsetSupport.doesNotIncludeMarginInBodyOffset = ( body.offsetTop !== conMarginTop );
body.removeChild( container );
div = container = null;
jQuery.extend( support, offsetSupport );
});
return support;
})();
var rbrace = /^(?:\{.*\}|\[.*\])$/,
rmultiDash = /([A-Z])/g;
jQuery.extend({
cache: {},
// Please use with caution
uuid: 0,
// Unique for each copy of jQuery on the page
// Non-digits removed to match rinlinejQuery
expando: "jQuery" + ( jQuery.fn.jquery + Math.random() ).replace( /\D/g, "" ),
// The following elements throw uncatchable exceptions if you
// attempt to add expando properties to them.
noData: {
"embed": true,
// Ban all objects except for Flash (which handle expandos)
"object": "clsid:D27CDB6E-AE6D-11cf-96B8-444553540000",
"applet": true
},
hasData: function( elem ) {
elem = elem.nodeType ? jQuery.cache[ elem[jQuery.expando] ] : elem[ jQuery.expando ];
return !!elem && !isEmptyDataObject( elem );
},
data: function( elem, name, data, pvt /* Internal Use Only */ ) {
if ( !jQuery.acceptData( elem ) ) {
return;
}
var privateCache, thisCache, ret,
internalKey = jQuery.expando,
getByName = typeof name === "string",
// We have to handle DOM nodes and JS objects differently because IE6-7
// can't GC object references properly across the DOM-JS boundary
isNode = elem.nodeType,
// Only DOM nodes need the global jQuery cache; JS object data is
// attached directly to the object so GC can occur automatically
cache = isNode ? jQuery.cache : elem,
// Only defining an ID for JS objects if its cache already exists allows
// the code to shortcut on the same path as a DOM node with no cache
id = isNode ? elem[ internalKey ] : elem[ internalKey ] && internalKey,
isEvents = name === "events";
// Avoid doing any more work than we need to when trying to get data on an
// object that has no data at all
if ( (!id || !cache[id] || (!isEvents && !pvt && !cache[id].data)) && getByName && data === undefined ) {
return;
}
if ( !id ) {
// Only DOM nodes need a new unique ID for each element since their data
// ends up in the global cache
if ( isNode ) {
elem[ internalKey ] = id = ++jQuery.uuid;
} else {
id = internalKey;
}
}
if ( !cache[ id ] ) {
cache[ id ] = {};
// Avoids exposing jQuery metadata on plain JS objects when the object
// is serialized using JSON.stringify
if ( !isNode ) {
cache[ id ].toJSON = jQuery.noop;
}
}
// An object can be passed to jQuery.data instead of a key/value pair; this gets
// shallow copied over onto the existing cache
if ( typeof name === "object" || typeof name === "function" ) {
if ( pvt ) {
cache[ id ] = jQuery.extend( cache[ id ], name );
} else {
cache[ id ].data = jQuery.extend( cache[ id ].data, name );
}
}
privateCache = thisCache = cache[ id ];
// jQuery data() is stored in a separate object inside the object's internal data
// cache in order to avoid key collisions between internal data and user-defined
// data.
if ( !pvt ) {
if ( !thisCache.data ) {
thisCache.data = {};
}
thisCache = thisCache.data;
}
if ( data !== undefined ) {
thisCache[ jQuery.camelCase( name ) ] = data;
}
// Users should not attempt to inspect the internal events object using jQuery.data,
// it is undocumented and subject to change. But does anyone listen? No.
if ( isEvents && !thisCache[ name ] ) {
return privateCache.events;
}
// Check for both converted-to-camel and non-converted data property names
// If a data property was specified
if ( getByName ) {
// First Try to find as-is property data
ret = thisCache[ name ];
// Test for null|undefined property data
if ( ret == null ) {
// Try to find the camelCased property
ret = thisCache[ jQuery.camelCase( name ) ];
}
} else {
ret = thisCache;
}
return ret;
},
removeData: function( elem, name, pvt /* Internal Use Only */ ) {
if ( !jQuery.acceptData( elem ) ) {
return;
}
var thisCache, i, l,
// Reference to internal data cache key
internalKey = jQuery.expando,
isNode = elem.nodeType,
// See jQuery.data for more information
cache = isNode ? jQuery.cache : elem,
// See jQuery.data for more information
id = isNode ? elem[ internalKey ] : internalKey;
// If there is already no cache entry for this object, there is no
// purpose in continuing
if ( !cache[ id ] ) {
return;
}
if ( name ) {
thisCache = pvt ? cache[ id ] : cache[ id ].data;
if ( thisCache ) {
// Support array or space separated string names for data keys
if ( !jQuery.isArray( name ) ) {
// try the string as a key before any manipulation
if ( name in thisCache ) {
name = [ name ];
} else {
// split the camel cased version by spaces unless a key with the spaces exists
name = jQuery.camelCase( name );
if ( name in thisCache ) {
name = [ name ];
} else {
name = name.split( " " );
}
}
}
for ( i = 0, l = name.length; i < l; i++ ) {
delete thisCache[ name[i] ];
}
// If there is no data left in the cache, we want to continue
// and let the cache object itself get destroyed
if ( !( pvt ? isEmptyDataObject : jQuery.isEmptyObject )( thisCache ) ) {
return;
}
}
}
// See jQuery.data for more information
if ( !pvt ) {
delete cache[ id ].data;
// Don't destroy the parent cache unless the internal data object
// had been the only thing left in it
if ( !isEmptyDataObject(cache[ id ]) ) {
return;
}
}
// Browsers that fail expando deletion also refuse to delete expandos on
// the window, but it will allow it on all other JS objects; other browsers
// don't care
// Ensure that `cache` is not a window object #10080
if ( jQuery.support.deleteExpando || !cache.setInterval ) {
delete cache[ id ];
} else {
cache[ id ] = null;
}
// We destroyed the cache and need to eliminate the expando on the node to avoid
// false lookups in the cache for entries that no longer exist
if ( isNode ) {
// IE does not allow us to delete expando properties from nodes,
// nor does it have a removeAttribute function on Document nodes;
// we must handle all of these cases
if ( jQuery.support.deleteExpando ) {
delete elem[ internalKey ];
} else if ( elem.removeAttribute ) {
elem.removeAttribute( internalKey );
} else {
elem[ internalKey ] = null;
}
}
},
// For internal use only.
_data: function( elem, name, data ) {
return jQuery.data( elem, name, data, true );
},
// A method for determining if a DOM node can handle the data expando
acceptData: function( elem ) {
if ( elem.nodeName ) {
var match = jQuery.noData[ elem.nodeName.toLowerCase() ];
if ( match ) {
return !(match === true || elem.getAttribute("classid") !== match);
}
}
return true;
}
});
jQuery.fn.extend({
data: function( key, value ) {
var parts, attr, name,
data = null;
if ( typeof key === "undefined" ) {
if ( this.length ) {
data = jQuery.data( this[0] );
if ( this[0].nodeType === 1 && !jQuery._data( this[0], "parsedAttrs" ) ) {
attr = this[0].attributes;
for ( var i = 0, l = attr.length; i < l; i++ ) {
name = attr[i].name;
if ( name.indexOf( "data-" ) === 0 ) {
name = jQuery.camelCase( name.substring(5) );
dataAttr( this[0], name, data[ name ] );
}
}
jQuery._data( this[0], "parsedAttrs", true );
}
}
return data;
} else if ( typeof key === "object" ) {
return this.each(function() {
jQuery.data( this, key );
});
}
parts = key.split(".");
parts[1] = parts[1] ? "." + parts[1] : "";
if ( value === undefined ) {
data = this.triggerHandler("getData" + parts[1] + "!", [parts[0]]);
// Try to fetch any internally stored data first
if ( data === undefined && this.length ) {
data = jQuery.data( this[0], key );
data = dataAttr( this[0], key, data );
}
return data === undefined && parts[1] ?
this.data( parts[0] ) :
data;
} else {
return this.each(function() {
var self = jQuery( this ),
args = [ parts[0], value ];
self.triggerHandler( "setData" + parts[1] + "!", args );
jQuery.data( this, key, value );
self.triggerHandler( "changeData" + parts[1] + "!", args );
});
}
},
removeData: function( key ) {
return this.each(function() {
jQuery.removeData( this, key );
});
}
});
function dataAttr( elem, key, data ) {
// If nothing was found internally, try to fetch any
// data from the HTML5 data-* attribute
if ( data === undefined && elem.nodeType === 1 ) {
var name = "data-" + key.replace( rmultiDash, "-$1" ).toLowerCase();
data = elem.getAttribute( name );
if ( typeof data === "string" ) {
try {
data = data === "true" ? true :
data === "false" ? false :
data === "null" ? null :
jQuery.isNumeric( data ) ? parseFloat( data ) :
rbrace.test( data ) ? jQuery.parseJSON( data ) :
data;
} catch( e ) {}
// Make sure we set the data so it isn't changed later
jQuery.data( elem, key, data );
} else {
data = undefined;
}
}
return data;
}
// checks a cache object for emptiness
function isEmptyDataObject( obj ) {
for ( var name in obj ) {
// if the public data object is empty, the private is still empty
if ( name === "data" && jQuery.isEmptyObject( obj[name] ) ) {
continue;
}
if ( name !== "toJSON" ) {
return false;
}
}
return true;
}
function handleQueueMarkDefer( elem, type, src ) {
var deferDataKey = type + "defer",
queueDataKey = type + "queue",
markDataKey = type + "mark",
defer = jQuery._data( elem, deferDataKey );
if ( defer &&
( src === "queue" || !jQuery._data(elem, queueDataKey) ) &&
( src === "mark" || !jQuery._data(elem, markDataKey) ) ) {
// Give room for hard-coded callbacks to fire first
// and eventually mark/queue something else on the element
setTimeout( function() {
if ( !jQuery._data( elem, queueDataKey ) &&
!jQuery._data( elem, markDataKey ) ) {
jQuery.removeData( elem, deferDataKey, true );
defer.fire();
}
}, 0 );
}
}
jQuery.extend({
_mark: function( elem, type ) {
if ( elem ) {
type = ( type || "fx" ) + "mark";
jQuery._data( elem, type, (jQuery._data( elem, type ) || 0) + 1 );
}
},
_unmark: function( force, elem, type ) {
if ( force !== true ) {
type = elem;
elem = force;
force = false;
}
if ( elem ) {
type = type || "fx";
var key = type + "mark",
count = force ? 0 : ( (jQuery._data( elem, key ) || 1) - 1 );
if ( count ) {
jQuery._data( elem, key, count );
} else {
jQuery.removeData( elem, key, true );
handleQueueMarkDefer( elem, type, "mark" );
}
}
},
queue: function( elem, type, data ) {
var q;
if ( elem ) {
type = ( type || "fx" ) + "queue";
q = jQuery._data( elem, type );
// Speed up dequeue by getting out quickly if this is just a lookup
if ( data ) {
if ( !q || jQuery.isArray(data) ) {
q = jQuery._data( elem, type, jQuery.makeArray(data) );
} else {
q.push( data );
}
}
return q || [];
}
},
dequeue: function( elem, type ) {
type = type || "fx";
var queue = jQuery.queue( elem, type ),
fn = queue.shift(),
hooks = {};
// If the fx queue is dequeued, always remove the progress sentinel
if ( fn === "inprogress" ) {
fn = queue.shift();
}
if ( fn ) {
// Add a progress sentinel to prevent the fx queue from being
// automatically dequeued
if ( type === "fx" ) {
queue.unshift( "inprogress" );
}
jQuery._data( elem, type + ".run", hooks );
fn.call( elem, function() {
jQuery.dequeue( elem, type );
}, hooks );
}
if ( !queue.length ) {
jQuery.removeData( elem, type + "queue " + type + ".run", true );
handleQueueMarkDefer( elem, type, "queue" );
}
}
});
jQuery.fn.extend({
queue: function( type, data ) {
if ( typeof type !== "string" ) {
data = type;
type = "fx";
}
if ( data === undefined ) {
return jQuery.queue( this[0], type );
}
return this.each(function() {
var queue = jQuery.queue( this, type, data );
if ( type === "fx" && queue[0] !== "inprogress" ) {
jQuery.dequeue( this, type );
}
});
},
dequeue: function( type ) {
return this.each(function() {
jQuery.dequeue( this, type );
});
},
// Based off of the plugin by Clint Helfers, with permission.
// http://blindsignals.com/index.php/2009/07/jquery-delay/
delay: function( time, type ) {
time = jQuery.fx ? jQuery.fx.speeds[ time ] || time : time;
type = type || "fx";
return this.queue( type, function( next, hooks ) {
var timeout = setTimeout( next, time );
hooks.stop = function() {
clearTimeout( timeout );
};
});
},
clearQueue: function( type ) {
return this.queue( type || "fx", [] );
},
// Get a promise resolved when queues of a certain type
// are emptied (fx is the type by default)
promise: function( type, object ) {
if ( typeof type !== "string" ) {
object = type;
type = undefined;
}
type = type || "fx";
var defer = jQuery.Deferred(),
elements = this,
i = elements.length,
count = 1,
deferDataKey = type + "defer",
queueDataKey = type + "queue",
markDataKey = type + "mark",
tmp;
function resolve() {
if ( !( --count ) ) {
defer.resolveWith( elements, [ elements ] );
}
}
while( i-- ) {
if (( tmp = jQuery.data( elements[ i ], deferDataKey, undefined, true ) ||
( jQuery.data( elements[ i ], queueDataKey, undefined, true ) ||
jQuery.data( elements[ i ], markDataKey, undefined, true ) ) &&
jQuery.data( elements[ i ], deferDataKey, jQuery.Callbacks( "once memory" ), true ) )) {
count++;
tmp.add( resolve );
}
}
resolve();
return defer.promise();
}
});
var rclass = /[\n\t\r]/g,
rspace = /\s+/,
rreturn = /\r/g,
rtype = /^(?:button|input)$/i,
rfocusable = /^(?:button|input|object|select|textarea)$/i,
rclickable = /^a(?:rea)?$/i,
rboolean = /^(?:autofocus|autoplay|async|checked|controls|defer|disabled|hidden|loop|multiple|open|readonly|required|scoped|selected)$/i,
getSetAttribute = jQuery.support.getSetAttribute,
nodeHook, boolHook, fixSpecified;
jQuery.fn.extend({
attr: function( name, value ) {
return jQuery.access( this, name, value, true, jQuery.attr );
},
removeAttr: function( name ) {
return this.each(function() {
jQuery.removeAttr( this, name );
});
},
prop: function( name, value ) {
return jQuery.access( this, name, value, true, jQuery.prop );
},
removeProp: function( name ) {
name = jQuery.propFix[ name ] || name;
return this.each(function() {
// try/catch handles cases where IE balks (such as removing a property on window)
try {
this[ name ] = undefined;
delete this[ name ];
} catch( e ) {}
});
},
addClass: function( value ) {
var classNames, i, l, elem,
setClass, c, cl;
if ( jQuery.isFunction( value ) ) {
return this.each(function( j ) {
jQuery( this ).addClass( value.call(this, j, this.className) );
});
}
if ( value && typeof value === "string" ) {
classNames = value.split( rspace );
for ( i = 0, l = this.length; i < l; i++ ) {
elem = this[ i ];
if ( elem.nodeType === 1 ) {
if ( !elem.className && classNames.length === 1 ) {
elem.className = value;
} else {
setClass = " " + elem.className + " ";
for ( c = 0, cl = classNames.length; c < cl; c++ ) {
if ( !~setClass.indexOf( " " + classNames[ c ] + " " ) ) {
setClass += classNames[ c ] + " ";
}
}
elem.className = jQuery.trim( setClass );
}
}
}
}
return this;
},
removeClass: function( value ) {
var classNames, i, l, elem, className, c, cl;
if ( jQuery.isFunction( value ) ) {
return this.each(function( j ) {
jQuery( this ).removeClass( value.call(this, j, this.className) );
});
}
if ( (value && typeof value === "string") || value === undefined ) {
classNames = ( value || "" ).split( rspace );
for ( i = 0, l = this.length; i < l; i++ ) {
elem = this[ i ];
if ( elem.nodeType === 1 && elem.className ) {
if ( value ) {
className = (" " + elem.className + " ").replace( rclass, " " );
for ( c = 0, cl = classNames.length; c < cl; c++ ) {
className = className.replace(" " + classNames[ c ] + " ", " ");
}
elem.className = jQuery.trim( className );
} else {
elem.className = "";
}
}
}
}
return this;
},
toggleClass: function( value, stateVal ) {
var type = typeof value,
isBool = typeof stateVal === "boolean";
if ( jQuery.isFunction( value ) ) {
return this.each(function( i ) {
jQuery( this ).toggleClass( value.call(this, i, this.className, stateVal), stateVal );
});
}
return this.each(function() {
if ( type === "string" ) {
// toggle individual class names
var className,
i = 0,
self = jQuery( this ),
state = stateVal,
classNames = value.split( rspace );
while ( (className = classNames[ i++ ]) ) {
// check each className given, space seperated list
state = isBool ? state : !self.hasClass( className );
self[ state ? "addClass" : "removeClass" ]( className );
}
} else if ( type === "undefined" || type === "boolean" ) {
if ( this.className ) {
// store className if set
jQuery._data( this, "__className__", this.className );
}
// toggle whole className
this.className = this.className || value === false ? "" : jQuery._data( this, "__className__" ) || "";
}
});
},
hasClass: function( selector ) {
var className = " " + selector + " ",
i = 0,
l = this.length;
for ( ; i < l; i++ ) {
if ( this[i].nodeType === 1 && (" " + this[i].className + " ").replace(rclass, " ").indexOf( className ) > -1 ) {
return true;
}
}
return false;
},
val: function( value ) {
var hooks, ret, isFunction,
elem = this[0];
if ( !arguments.length ) {
if ( elem ) {
hooks = jQuery.valHooks[ elem.nodeName.toLowerCase() ] || jQuery.valHooks[ elem.type ];
if ( hooks && "get" in hooks && (ret = hooks.get( elem, "value" )) !== undefined ) {
return ret;
}
ret = elem.value;
return typeof ret === "string" ?
// handle most common string cases
ret.replace(rreturn, "") :
// handle cases where value is null/undef or number
ret == null ? "" : ret;
}
return;
}
isFunction = jQuery.isFunction( value );
return this.each(function( i ) {
var self = jQuery(this), val;
if ( this.nodeType !== 1 ) {
return;
}
if ( isFunction ) {
val = value.call( this, i, self.val() );
} else {
val = value;
}
// Treat null/undefined as ""; convert numbers to string
if ( val == null ) {
val = "";
} else if ( typeof val === "number" ) {
val += "";
} else if ( jQuery.isArray( val ) ) {
val = jQuery.map(val, function ( value ) {
return value == null ? "" : value + "";
});
}
hooks = jQuery.valHooks[ this.nodeName.toLowerCase() ] || jQuery.valHooks[ this.type ];
// If set returns undefined, fall back to normal setting
if ( !hooks || !("set" in hooks) || hooks.set( this, val, "value" ) === undefined ) {
this.value = val;
}
});
}
});
jQuery.extend({
valHooks: {
option: {
get: function( elem ) {
// attributes.value is undefined in Blackberry 4.7 but
// uses .value. See #6932
var val = elem.attributes.value;
return !val || val.specified ? elem.value : elem.text;
}
},
select: {
get: function( elem ) {
var value, i, max, option,
index = elem.selectedIndex,
values = [],
options = elem.options,
one = elem.type === "select-one";
// Nothing was selected
if ( index < 0 ) {
return null;
}
// Loop through all the selected options
i = one ? index : 0;
max = one ? index + 1 : options.length;
for ( ; i < max; i++ ) {
option = options[ i ];
// Don't return options that are disabled or in a disabled optgroup
if ( option.selected && (jQuery.support.optDisabled ? !option.disabled : option.getAttribute("disabled") === null) &&
(!option.parentNode.disabled || !jQuery.nodeName( option.parentNode, "optgroup" )) ) {
// Get the specific value for the option
value = jQuery( option ).val();
// We don't need an array for one selects
if ( one ) {
return value;
}
// Multi-Selects return an array
values.push( value );
}
}
// Fixes Bug #2551 -- select.val() broken in IE after form.reset()
if ( one && !values.length && options.length ) {
return jQuery( options[ index ] ).val();
}
return values;
},
set: function( elem, value ) {
var values = jQuery.makeArray( value );
jQuery(elem).find("option").each(function() {
this.selected = jQuery.inArray( jQuery(this).val(), values ) >= 0;
});
if ( !values.length ) {
elem.selectedIndex = -1;
}
return values;
}
}
},
attrFn: {
val: true,
css: true,
html: true,
text: true,
data: true,
width: true,
height: true,
offset: true
},
attr: function( elem, name, value, pass ) {
var ret, hooks, notxml,
nType = elem.nodeType;
// don't get/set attributes on text, comment and attribute nodes
if ( !elem || nType === 3 || nType === 8 || nType === 2 ) {
return;
}
if ( pass && name in jQuery.attrFn ) {
return jQuery( elem )[ name ]( value );
}
// Fallback to prop when attributes are not supported
if ( typeof elem.getAttribute === "undefined" ) {
return jQuery.prop( elem, name, value );
}
notxml = nType !== 1 || !jQuery.isXMLDoc( elem );
// All attributes are lowercase
// Grab necessary hook if one is defined
if ( notxml ) {
name = name.toLowerCase();
hooks = jQuery.attrHooks[ name ] || ( rboolean.test( name ) ? boolHook : nodeHook );
}
if ( value !== undefined ) {
if ( value === null ) {
jQuery.removeAttr( elem, name );
return;
} else if ( hooks && "set" in hooks && notxml && (ret = hooks.set( elem, value, name )) !== undefined ) {
return ret;
} else {
elem.setAttribute( name, "" + value );
return value;
}
} else if ( hooks && "get" in hooks && notxml && (ret = hooks.get( elem, name )) !== null ) {
return ret;
} else {
ret = elem.getAttribute( name );
// Non-existent attributes return null, we normalize to undefined
return ret === null ?
undefined :
ret;
}
},
removeAttr: function( elem, value ) {
var propName, attrNames, name, l,
i = 0;
if ( value && elem.nodeType === 1 ) {
attrNames = value.toLowerCase().split( rspace );
l = attrNames.length;
for ( ; i < l; i++ ) {
name = attrNames[ i ];
if ( name ) {
propName = jQuery.propFix[ name ] || name;
// See #9699 for explanation of this approach (setting first, then removal)
jQuery.attr( elem, name, "" );
elem.removeAttribute( getSetAttribute ? name : propName );
// Set corresponding property to false for boolean attributes
if ( rboolean.test( name ) && propName in elem ) {
elem[ propName ] = false;
}
}
}
}
},
attrHooks: {
type: {
set: function( elem, value ) {
// We can't allow the type property to be changed (since it causes problems in IE)
if ( rtype.test( elem.nodeName ) && elem.parentNode ) {
jQuery.error( "type property can't be changed" );
} else if ( !jQuery.support.radioValue && value === "radio" && jQuery.nodeName(elem, "input") ) {
// Setting the type on a radio button after the value resets the value in IE6-9
// Reset value to it's default in case type is set after value
// This is for element creation
var val = elem.value;
elem.setAttribute( "type", value );
if ( val ) {
elem.value = val;
}
return value;
}
}
},
// Use the value property for back compat
// Use the nodeHook for button elements in IE6/7 (#1954)
value: {
get: function( elem, name ) {
if ( nodeHook && jQuery.nodeName( elem, "button" ) ) {
return nodeHook.get( elem, name );
}
return name in elem ?
elem.value :
null;
},
set: function( elem, value, name ) {
if ( nodeHook && jQuery.nodeName( elem, "button" ) ) {
return nodeHook.set( elem, value, name );
}
// Does not return so that setAttribute is also used
elem.value = value;
}
}
},
propFix: {
tabindex: "tabIndex",
readonly: "readOnly",
"for": "htmlFor",
"class": "className",
maxlength: "maxLength",
cellspacing: "cellSpacing",
cellpadding: "cellPadding",
rowspan: "rowSpan",
colspan: "colSpan",
usemap: "useMap",
frameborder: "frameBorder",
contenteditable: "contentEditable"
},
prop: function( elem, name, value ) {
var ret, hooks, notxml,
nType = elem.nodeType;
// don't get/set properties on text, comment and attribute nodes
if ( !elem || nType === 3 || nType === 8 || nType === 2 ) {
return;
}
notxml = nType !== 1 || !jQuery.isXMLDoc( elem );
if ( notxml ) {
// Fix name and attach hooks
name = jQuery.propFix[ name ] || name;
hooks = jQuery.propHooks[ name ];
}
if ( value !== undefined ) {
if ( hooks && "set" in hooks && (ret = hooks.set( elem, value, name )) !== undefined ) {
return ret;
} else {
return ( elem[ name ] = value );
}
} else {
if ( hooks && "get" in hooks && (ret = hooks.get( elem, name )) !== null ) {
return ret;
} else {
return elem[ name ];
}
}
},
propHooks: {
tabIndex: {
get: function( elem ) {
// elem.tabIndex doesn't always return the correct value when it hasn't been explicitly set
// http://fluidproject.org/blog/2008/01/09/getting-setting-and-removing-tabindex-values-with-javascript/
var attributeNode = elem.getAttributeNode("tabindex");
return attributeNode && attributeNode.specified ?
parseInt( attributeNode.value, 10 ) :
rfocusable.test( elem.nodeName ) || rclickable.test( elem.nodeName ) && elem.href ?
0 :
undefined;
}
}
}
});
// Add the tabIndex propHook to attrHooks for back-compat (different case is intentional)
jQuery.attrHooks.tabindex = jQuery.propHooks.tabIndex;
// Hook for boolean attributes
boolHook = {
get: function( elem, name ) {
// Align boolean attributes with corresponding properties
// Fall back to attribute presence where some booleans are not supported
var attrNode,
property = jQuery.prop( elem, name );
return property === true || typeof property !== "boolean" && ( attrNode = elem.getAttributeNode(name) ) && attrNode.nodeValue !== false ?
name.toLowerCase() :
undefined;
},
set: function( elem, value, name ) {
var propName;
if ( value === false ) {
// Remove boolean attributes when set to false
jQuery.removeAttr( elem, name );
} else {
// value is true since we know at this point it's type boolean and not false
// Set boolean attributes to the same name and set the DOM property
propName = jQuery.propFix[ name ] || name;
if ( propName in elem ) {
// Only set the IDL specifically if it already exists on the element
elem[ propName ] = true;
}
elem.setAttribute( name, name.toLowerCase() );
}
return name;
}
};
// IE6/7 do not support getting/setting some attributes with get/setAttribute
if ( !getSetAttribute ) {
fixSpecified = {
name: true,
id: true
};
// Use this for any attribute in IE6/7
// This fixes almost every IE6/7 issue
nodeHook = jQuery.valHooks.button = {
get: function( elem, name ) {
var ret;
ret = elem.getAttributeNode( name );
return ret && ( fixSpecified[ name ] ? ret.nodeValue !== "" : ret.specified ) ?
ret.nodeValue :
undefined;
},
set: function( elem, value, name ) {
// Set the existing or create a new attribute node
var ret = elem.getAttributeNode( name );
if ( !ret ) {
ret = document.createAttribute( name );
elem.setAttributeNode( ret );
}
return ( ret.nodeValue = value + "" );
}
};
// Apply the nodeHook to tabindex
jQuery.attrHooks.tabindex.set = nodeHook.set;
// Set width and height to auto instead of 0 on empty string( Bug #8150 )
// This is for removals
jQuery.each([ "width", "height" ], function( i, name ) {
jQuery.attrHooks[ name ] = jQuery.extend( jQuery.attrHooks[ name ], {
set: function( elem, value ) {
if ( value === "" ) {
elem.setAttribute( name, "auto" );
return value;
}
}
});
});
// Set contenteditable to false on removals(#10429)
// Setting to empty string throws an error as an invalid value
jQuery.attrHooks.contenteditable = {
get: nodeHook.get,
set: function( elem, value, name ) {
if ( value === "" ) {
value = "false";
}
nodeHook.set( elem, value, name );
}
};
}
// Some attributes require a special call on IE
if ( !jQuery.support.hrefNormalized ) {
jQuery.each([ "href", "src", "width", "height" ], function( i, name ) {
jQuery.attrHooks[ name ] = jQuery.extend( jQuery.attrHooks[ name ], {
get: function( elem ) {
var ret = elem.getAttribute( name, 2 );
return ret === null ? undefined : ret;
}
});
});
}
if ( !jQuery.support.style ) {
jQuery.attrHooks.style = {
get: function( elem ) {
// Return undefined in the case of empty string
// Normalize to lowercase since IE uppercases css property names
return elem.style.cssText.toLowerCase() || undefined;
},
set: function( elem, value ) {
return ( elem.style.cssText = "" + value );
}
};
}
// Safari mis-reports the default selected property of an option
// Accessing the parent's selectedIndex property fixes it
if ( !jQuery.support.optSelected ) {
jQuery.propHooks.selected = jQuery.extend( jQuery.propHooks.selected, {
get: function( elem ) {
var parent = elem.parentNode;
if ( parent ) {
parent.selectedIndex;
// Make sure that it also works with optgroups, see #5701
if ( parent.parentNode ) {
parent.parentNode.selectedIndex;
}
}
return null;
}
});
}
// IE6/7 call enctype encoding
if ( !jQuery.support.enctype ) {
jQuery.propFix.enctype = "encoding";
}
// Radios and checkboxes getter/setter
if ( !jQuery.support.checkOn ) {
jQuery.each([ "radio", "checkbox" ], function() {
jQuery.valHooks[ this ] = {
get: function( elem ) {
// Handle the case where in Webkit "" is returned instead of "on" if a value isn't specified
return elem.getAttribute("value") === null ? "on" : elem.value;
}
};
});
}
jQuery.each([ "radio", "checkbox" ], function() {
jQuery.valHooks[ this ] = jQuery.extend( jQuery.valHooks[ this ], {
set: function( elem, value ) {
if ( jQuery.isArray( value ) ) {
return ( elem.checked = jQuery.inArray( jQuery(elem).val(), value ) >= 0 );
}
}
});
});
var rformElems = /^(?:textarea|input|select)$/i,
rtypenamespace = /^([^\.]*)?(?:\.(.+))?$/,
rhoverHack = /\bhover(\.\S+)?\b/,
rkeyEvent = /^key/,
rmouseEvent = /^(?:mouse|contextmenu)|click/,
rfocusMorph = /^(?:focusinfocus|focusoutblur)$/,
rquickIs = /^(\w*)(?:#([\w\-]+))?(?:\.([\w\-]+))?$/,
quickParse = function( selector ) {
var quick = rquickIs.exec( selector );
if ( quick ) {
// 0 1 2 3
// [ _, tag, id, class ]
quick[1] = ( quick[1] || "" ).toLowerCase();
quick[3] = quick[3] && new RegExp( "(?:^|\\s)" + quick[3] + "(?:\\s|$)" );
}
return quick;
},
quickIs = function( elem, m ) {
var attrs = elem.attributes || {};
return (
(!m[1] || elem.nodeName.toLowerCase() === m[1]) &&
(!m[2] || (attrs.id || {}).value === m[2]) &&
(!m[3] || m[3].test( (attrs[ "class" ] || {}).value ))
);
},
hoverHack = function( events ) {
return jQuery.event.special.hover ? events : events.replace( rhoverHack, "mouseenter$1 mouseleave$1" );
};
/*
* Helper functions for managing events -- not part of the public interface.
* Props to Dean Edwards' addEvent library for many of the ideas.
*/
jQuery.event = {
add: function( elem, types, handler, data, selector ) {
var elemData, eventHandle, events,
t, tns, type, namespaces, handleObj,
handleObjIn, quick, handlers, special;
// Don't attach events to noData or text/comment nodes (allow plain objects tho)
if ( elem.nodeType === 3 || elem.nodeType === 8 || !types || !handler || !(elemData = jQuery._data( elem )) ) {
return;
}
// Caller can pass in an object of custom data in lieu of the handler
if ( handler.handler ) {
handleObjIn = handler;
handler = handleObjIn.handler;
}
// Make sure that the handler has a unique ID, used to find/remove it later
if ( !handler.guid ) {
handler.guid = jQuery.guid++;
}
// Init the element's event structure and main handler, if this is the first
events = elemData.events;
if ( !events ) {
elemData.events = events = {};
}
eventHandle = elemData.handle;
if ( !eventHandle ) {
elemData.handle = eventHandle = function( e ) {
// Discard the second event of a jQuery.event.trigger() and
// when an event is called after a page has unloaded
return typeof jQuery !== "undefined" && (!e || jQuery.event.triggered !== e.type) ?
jQuery.event.dispatch.apply( eventHandle.elem, arguments ) :
undefined;
};
// Add elem as a property of the handle fn to prevent a memory leak with IE non-native events
eventHandle.elem = elem;
}
// Handle multiple events separated by a space
// jQuery(...).bind("mouseover mouseout", fn);
types = jQuery.trim( hoverHack(types) ).split( " " );
for ( t = 0; t < types.length; t++ ) {
tns = rtypenamespace.exec( types[t] ) || [];
type = tns[1];
namespaces = ( tns[2] || "" ).split( "." ).sort();
// If event changes its type, use the special event handlers for the changed type
special = jQuery.event.special[ type ] || {};
// If selector defined, determine special event api type, otherwise given type
type = ( selector ? special.delegateType : special.bindType ) || type;
// Update special based on newly reset type
special = jQuery.event.special[ type ] || {};
// handleObj is passed to all event handlers
handleObj = jQuery.extend({
type: type,
origType: tns[1],
data: data,
handler: handler,
guid: handler.guid,
selector: selector,
quick: quickParse( selector ),
namespace: namespaces.join(".")
}, handleObjIn );
// Init the event handler queue if we're the first
handlers = events[ type ];
if ( !handlers ) {
handlers = events[ type ] = [];
handlers.delegateCount = 0;
// Only use addEventListener/attachEvent if the special events handler returns false
if ( !special.setup || special.setup.call( elem, data, namespaces, eventHandle ) === false ) {
// Bind the global event handler to the element
if ( elem.addEventListener ) {
elem.addEventListener( type, eventHandle, false );
} else if ( elem.attachEvent ) {
elem.attachEvent( "on" + type, eventHandle );
}
}
}
if ( special.add ) {
special.add.call( elem, handleObj );
if ( !handleObj.handler.guid ) {
handleObj.handler.guid = handler.guid;
}
}
// Add to the element's handler list, delegates in front
if ( selector ) {
handlers.splice( handlers.delegateCount++, 0, handleObj );
} else {
handlers.push( handleObj );
}
// Keep track of which events have ever been used, for event optimization
jQuery.event.global[ type ] = true;
}
// Nullify elem to prevent memory leaks in IE
elem = null;
},
global: {},
// Detach an event or set of events from an element
remove: function( elem, types, handler, selector, mappedTypes ) {
var elemData = jQuery.hasData( elem ) && jQuery._data( elem ),
t, tns, type, origType, namespaces, origCount,
j, events, special, handle, eventType, handleObj;
if ( !elemData || !(events = elemData.events) ) {
return;
}
// Once for each type.namespace in types; type may be omitted
types = jQuery.trim( hoverHack( types || "" ) ).split(" ");
for ( t = 0; t < types.length; t++ ) {
tns = rtypenamespace.exec( types[t] ) || [];
type = origType = tns[1];
namespaces = tns[2];
// Unbind all events (on this namespace, if provided) for the element
if ( !type ) {
for ( type in events ) {
jQuery.event.remove( elem, type + types[ t ], handler, selector, true );
}
continue;
}
special = jQuery.event.special[ type ] || {};
type = ( selector? special.delegateType : special.bindType ) || type;
eventType = events[ type ] || [];
origCount = eventType.length;
namespaces = namespaces ? new RegExp("(^|\\.)" + namespaces.split(".").sort().join("\\.(?:.*\\.)?") + "(\\.|$)") : null;
// Remove matching events
for ( j = 0; j < eventType.length; j++ ) {
handleObj = eventType[ j ];
if ( ( mappedTypes || origType === handleObj.origType ) &&
( !handler || handler.guid === handleObj.guid ) &&
( !namespaces || namespaces.test( handleObj.namespace ) ) &&
( !selector || selector === handleObj.selector || selector === "**" && handleObj.selector ) ) {
eventType.splice( j--, 1 );
if ( handleObj.selector ) {
eventType.delegateCount--;
}
if ( special.remove ) {
special.remove.call( elem, handleObj );
}
}
}
// Remove generic event handler if we removed something and no more handlers exist
// (avoids potential for endless recursion during removal of special event handlers)
if ( eventType.length === 0 && origCount !== eventType.length ) {
if ( !special.teardown || special.teardown.call( elem, namespaces ) === false ) {
jQuery.removeEvent( elem, type, elemData.handle );
}
delete events[ type ];
}
}
// Remove the expando if it's no longer used
if ( jQuery.isEmptyObject( events ) ) {
handle = elemData.handle;
if ( handle ) {
handle.elem = null;
}
// removeData also checks for emptiness and clears the expando if empty
// so use it instead of delete
jQuery.removeData( elem, [ "events", "handle" ], true );
}
},
// Events that are safe to short-circuit if no handlers are attached.
// Native DOM events should not be added, they may have inline handlers.
customEvent: {
"getData": true,
"setData": true,
"changeData": true
},
trigger: function( event, data, elem, onlyHandlers ) {
// Don't do events on text and comment nodes
if ( elem && (elem.nodeType === 3 || elem.nodeType === 8) ) {
return;
}
// Event object or event type
var type = event.type || event,
namespaces = [],
cache, exclusive, i, cur, old, ontype, special, handle, eventPath, bubbleType;
// focus/blur morphs to focusin/out; ensure we're not firing them right now
if ( rfocusMorph.test( type + jQuery.event.triggered ) ) {
return;
}
if ( type.indexOf( "!" ) >= 0 ) {
// Exclusive events trigger only for the exact event (no namespaces)
type = type.slice(0, -1);
exclusive = true;
}
if ( type.indexOf( "." ) >= 0 ) {
// Namespaced trigger; create a regexp to match event type in handle()
namespaces = type.split(".");
type = namespaces.shift();
namespaces.sort();
}
if ( (!elem || jQuery.event.customEvent[ type ]) && !jQuery.event.global[ type ] ) {
// No jQuery handlers for this event type, and it can't have inline handlers
return;
}
// Caller can pass in an Event, Object, or just an event type string
event = typeof event === "object" ?
// jQuery.Event object
event[ jQuery.expando ] ? event :
// Object literal
new jQuery.Event( type, event ) :
// Just the event type (string)
new jQuery.Event( type );
event.type = type;
event.isTrigger = true;
event.exclusive = exclusive;
event.namespace = namespaces.join( "." );
event.namespace_re = event.namespace? new RegExp("(^|\\.)" + namespaces.join("\\.(?:.*\\.)?") + "(\\.|$)") : null;
ontype = type.indexOf( ":" ) < 0 ? "on" + type : "";
// Handle a global trigger
if ( !elem ) {
// TODO: Stop taunting the data cache; remove global events and always attach to document
cache = jQuery.cache;
for ( i in cache ) {
if ( cache[ i ].events && cache[ i ].events[ type ] ) {
jQuery.event.trigger( event, data, cache[ i ].handle.elem, true );
}
}
return;
}
// Clean up the event in case it is being reused
event.result = undefined;
if ( !event.target ) {
event.target = elem;
}
// Clone any incoming data and prepend the event, creating the handler arg list
data = data != null ? jQuery.makeArray( data ) : [];
data.unshift( event );
// Allow special events to draw outside the lines
special = jQuery.event.special[ type ] || {};
if ( special.trigger && special.trigger.apply( elem, data ) === false ) {
return;
}
// Determine event propagation path in advance, per W3C events spec (#9951)
// Bubble up to document, then to window; watch for a global ownerDocument var (#9724)
eventPath = [[ elem, special.bindType || type ]];
if ( !onlyHandlers && !special.noBubble && !jQuery.isWindow( elem ) ) {
bubbleType = special.delegateType || type;
cur = rfocusMorph.test( bubbleType + type ) ? elem : elem.parentNode;
old = null;
for ( ; cur; cur = cur.parentNode ) {
eventPath.push([ cur, bubbleType ]);
old = cur;
}
// Only add window if we got to document (e.g., not plain obj or detached DOM)
if ( old && old === elem.ownerDocument ) {
eventPath.push([ old.defaultView || old.parentWindow || window, bubbleType ]);
}
}
// Fire handlers on the event path
for ( i = 0; i < eventPath.length && !event.isPropagationStopped(); i++ ) {
cur = eventPath[i][0];
event.type = eventPath[i][1];
handle = ( jQuery._data( cur, "events" ) || {} )[ event.type ] && jQuery._data( cur, "handle" );
if ( handle ) {
handle.apply( cur, data );
}
// Note that this is a bare JS function and not a jQuery handler
handle = ontype && cur[ ontype ];
if ( handle && jQuery.acceptData( cur ) && handle.apply( cur, data ) === false ) {
event.preventDefault();
}
}
event.type = type;
// If nobody prevented the default action, do it now
if ( !onlyHandlers && !event.isDefaultPrevented() ) {
if ( (!special._default || special._default.apply( elem.ownerDocument, data ) === false) &&
!(type === "click" && jQuery.nodeName( elem, "a" )) && jQuery.acceptData( elem ) ) {
// Call a native DOM method on the target with the same name name as the event.
// Can't use an .isFunction() check here because IE6/7 fails that test.
// Don't do default actions on window, that's where global variables be (#6170)
// IE<9 dies on focus/blur to hidden element (#1486)
if ( ontype && elem[ type ] && ((type !== "focus" && type !== "blur") || event.target.offsetWidth !== 0) && !jQuery.isWindow( elem ) ) {
// Don't re-trigger an onFOO event when we call its FOO() method
old = elem[ ontype ];
if ( old ) {
elem[ ontype ] = null;
}
// Prevent re-triggering of the same event, since we already bubbled it above
jQuery.event.triggered = type;
elem[ type ]();
jQuery.event.triggered = undefined;
if ( old ) {
elem[ ontype ] = old;
}
}
}
}
return event.result;
},
dispatch: function( event ) {
// Make a writable jQuery.Event from the native event object
event = jQuery.event.fix( event || window.event );
var handlers = ( (jQuery._data( this, "events" ) || {} )[ event.type ] || []),
delegateCount = handlers.delegateCount,
args = [].slice.call( arguments, 0 ),
run_all = !event.exclusive && !event.namespace,
handlerQueue = [],
i, j, cur, jqcur, ret, selMatch, matched, matches, handleObj, sel, related;
// Use the fix-ed jQuery.Event rather than the (read-only) native event
args[0] = event;
event.delegateTarget = this;
// Determine handlers that should run if there are delegated events
// Avoid disabled elements in IE (#6911) and non-left-click bubbling in Firefox (#3861)
if ( delegateCount && !event.target.disabled && !(event.button && event.type === "click") ) {
// Pregenerate a single jQuery object for reuse with .is()
jqcur = jQuery(this);
jqcur.context = this.ownerDocument || this;
for ( cur = event.target; cur != this; cur = cur.parentNode || this ) {
selMatch = {};
matches = [];
jqcur[0] = cur;
for ( i = 0; i < delegateCount; i++ ) {
handleObj = handlers[ i ];
sel = handleObj.selector;
if ( selMatch[ sel ] === undefined ) {
selMatch[ sel ] = (
handleObj.quick ? quickIs( cur, handleObj.quick ) : jqcur.is( sel )
);
}
if ( selMatch[ sel ] ) {
matches.push( handleObj );
}
}
if ( matches.length ) {
handlerQueue.push({ elem: cur, matches: matches });
}
}
}
// Add the remaining (directly-bound) handlers
if ( handlers.length > delegateCount ) {
handlerQueue.push({ elem: this, matches: handlers.slice( delegateCount ) });
}
// Run delegates first; they may want to stop propagation beneath us
for ( i = 0; i < handlerQueue.length && !event.isPropagationStopped(); i++ ) {
matched = handlerQueue[ i ];
event.currentTarget = matched.elem;
for ( j = 0; j < matched.matches.length && !event.isImmediatePropagationStopped(); j++ ) {
handleObj = matched.matches[ j ];
// Triggered event must either 1) be non-exclusive and have no namespace, or
// 2) have namespace(s) a subset or equal to those in the bound event (both can have no namespace).
if ( run_all || (!event.namespace && !handleObj.namespace) || event.namespace_re && event.namespace_re.test( handleObj.namespace ) ) {
event.data = handleObj.data;
event.handleObj = handleObj;
ret = ( (jQuery.event.special[ handleObj.origType ] || {}).handle || handleObj.handler )
.apply( matched.elem, args );
if ( ret !== undefined ) {
event.result = ret;
if ( ret === false ) {
event.preventDefault();
event.stopPropagation();
}
}
}
}
}
return event.result;
},
// Includes some event props shared by KeyEvent and MouseEvent
// *** attrChange attrName relatedNode srcElement are not normalized, non-W3C, deprecated, will be removed in 1.8 ***
props: "attrChange attrName relatedNode srcElement altKey bubbles cancelable ctrlKey currentTarget eventPhase metaKey relatedTarget shiftKey target timeStamp view which".split(" "),
fixHooks: {},
keyHooks: {
props: "char charCode key keyCode".split(" "),
filter: function( event, original ) {
// Add which for key events
if ( event.which == null ) {
event.which = original.charCode != null ? original.charCode : original.keyCode;
}
return event;
}
},
mouseHooks: {
props: "button buttons clientX clientY fromElement offsetX offsetY pageX pageY screenX screenY toElement".split(" "),
filter: function( event, original ) {
var eventDoc, doc, body,
button = original.button,
fromElement = original.fromElement;
// Calculate pageX/Y if missing and clientX/Y available
if ( event.pageX == null && original.clientX != null ) {
eventDoc = event.target.ownerDocument || document;
doc = eventDoc.documentElement;
body = eventDoc.body;
event.pageX = original.clientX + ( doc && doc.scrollLeft || body && body.scrollLeft || 0 ) - ( doc && doc.clientLeft || body && body.clientLeft || 0 );
event.pageY = original.clientY + ( doc && doc.scrollTop || body && body.scrollTop || 0 ) - ( doc && doc.clientTop || body && body.clientTop || 0 );
}
// Add relatedTarget, if necessary
if ( !event.relatedTarget && fromElement ) {
event.relatedTarget = fromElement === event.target ? original.toElement : fromElement;
}
// Add which for click: 1 === left; 2 === middle; 3 === right
// Note: button is not normalized, so don't use it
if ( !event.which && button !== undefined ) {
event.which = ( button & 1 ? 1 : ( button & 2 ? 3 : ( button & 4 ? 2 : 0 ) ) );
}
return event;
}
},
fix: function( event ) {
if ( event[ jQuery.expando ] ) {
return event;
}
// Create a writable copy of the event object and normalize some properties
var i, prop,
originalEvent = event,
fixHook = jQuery.event.fixHooks[ event.type ] || {},
copy = fixHook.props ? this.props.concat( fixHook.props ) : this.props;
event = jQuery.Event( originalEvent );
for ( i = copy.length; i; ) {
prop = copy[ --i ];
event[ prop ] = originalEvent[ prop ];
}
// Fix target property, if necessary (#1925, IE 6/7/8 & Safari2)
if ( !event.target ) {
event.target = originalEvent.srcElement || document;
}
// Target should not be a text node (#504, Safari)
if ( event.target.nodeType === 3 ) {
event.target = event.target.parentNode;
}
// For mouse/key events; add metaKey if it's not there (#3368, IE6/7/8)
if ( event.metaKey === undefined ) {
event.metaKey = event.ctrlKey;
}
return fixHook.filter? fixHook.filter( event, originalEvent ) : event;
},
special: {
ready: {
// Make sure the ready event is setup
setup: jQuery.bindReady
},
load: {
// Prevent triggered image.load events from bubbling to window.load
noBubble: true
},
focus: {
delegateType: "focusin"
},
blur: {
delegateType: "focusout"
},
beforeunload: {
setup: function( data, namespaces, eventHandle ) {
// We only want to do this special case on windows
if ( jQuery.isWindow( this ) ) {
this.onbeforeunload = eventHandle;
}
},
teardown: function( namespaces, eventHandle ) {
if ( this.onbeforeunload === eventHandle ) {
this.onbeforeunload = null;
}
}
}
},
simulate: function( type, elem, event, bubble ) {
// Piggyback on a donor event to simulate a different one.
// Fake originalEvent to avoid donor's stopPropagation, but if the
// simulated event prevents default then we do the same on the donor.
var e = jQuery.extend(
new jQuery.Event(),
event,
{ type: type,
isSimulated: true,
originalEvent: {}
}
);
if ( bubble ) {
jQuery.event.trigger( e, null, elem );
} else {
jQuery.event.dispatch.call( elem, e );
}
if ( e.isDefaultPrevented() ) {
event.preventDefault();
}
}
};
// Some plugins are using, but it's undocumented/deprecated and will be removed.
// The 1.7 special event interface should provide all the hooks needed now.
jQuery.event.handle = jQuery.event.dispatch;
jQuery.removeEvent = document.removeEventListener ?
function( elem, type, handle ) {
if ( elem.removeEventListener ) {
elem.removeEventListener( type, handle, false );
}
} :
function( elem, type, handle ) {
if ( elem.detachEvent ) {
elem.detachEvent( "on" + type, handle );
}
};
jQuery.Event = function( src, props ) {
// Allow instantiation without the 'new' keyword
if ( !(this instanceof jQuery.Event) ) {
return new jQuery.Event( src, props );
}
// Event object
if ( src && src.type ) {
this.originalEvent = src;
this.type = src.type;
// Events bubbling up the document may have been marked as prevented
// by a handler lower down the tree; reflect the correct value.
this.isDefaultPrevented = ( src.defaultPrevented || src.returnValue === false ||
src.getPreventDefault && src.getPreventDefault() ) ? returnTrue : returnFalse;
// Event type
} else {
this.type = src;
}
// Put explicitly provided properties onto the event object
if ( props ) {
jQuery.extend( this, props );
}
// Create a timestamp if incoming event doesn't have one
this.timeStamp = src && src.timeStamp || jQuery.now();
// Mark it as fixed
this[ jQuery.expando ] = true;
};
function returnFalse() {
return false;
}
function returnTrue() {
return true;
}
// jQuery.Event is based on DOM3 Events as specified by the ECMAScript Language Binding
// http://www.w3.org/TR/2003/WD-DOM-Level-3-Events-20030331/ecma-script-binding.html
jQuery.Event.prototype = {
preventDefault: function() {
this.isDefaultPrevented = returnTrue;
var e = this.originalEvent;
if ( !e ) {
return;
}
// if preventDefault exists run it on the original event
if ( e.preventDefault ) {
e.preventDefault();
// otherwise set the returnValue property of the original event to false (IE)
} else {
e.returnValue = false;
}
},
stopPropagation: function() {
this.isPropagationStopped = returnTrue;
var e = this.originalEvent;
if ( !e ) {
return;
}
// if stopPropagation exists run it on the original event
if ( e.stopPropagation ) {
e.stopPropagation();
}
// otherwise set the cancelBubble property of the original event to true (IE)
e.cancelBubble = true;
},
stopImmediatePropagation: function() {
this.isImmediatePropagationStopped = returnTrue;
this.stopPropagation();
},
isDefaultPrevented: returnFalse,
isPropagationStopped: returnFalse,
isImmediatePropagationStopped: returnFalse
};
// Create mouseenter/leave events using mouseover/out and event-time checks
jQuery.each({
mouseenter: "mouseover",
mouseleave: "mouseout"
}, function( orig, fix ) {
jQuery.event.special[ orig ] = {
delegateType: fix,
bindType: fix,
handle: function( event ) {
var target = this,
related = event.relatedTarget,
handleObj = event.handleObj,
selector = handleObj.selector,
ret;
// For mousenter/leave call the handler if related is outside the target.
// NB: No relatedTarget if the mouse left/entered the browser window
if ( !related || (related !== target && !jQuery.contains( target, related )) ) {
event.type = handleObj.origType;
ret = handleObj.handler.apply( this, arguments );
event.type = fix;
}
return ret;
}
};
});
// IE submit delegation
if ( !jQuery.support.submitBubbles ) {
jQuery.event.special.submit = {
setup: function() {
// Only need this for delegated form submit events
if ( jQuery.nodeName( this, "form" ) ) {
return false;
}
// Lazy-add a submit handler when a descendant form may potentially be submitted
jQuery.event.add( this, "click._submit keypress._submit", function( e ) {
// Node name check avoids a VML-related crash in IE (#9807)
var elem = e.target,
form = jQuery.nodeName( elem, "input" ) || jQuery.nodeName( elem, "button" ) ? elem.form : undefined;
if ( form && !form._submit_attached ) {
jQuery.event.add( form, "submit._submit", function( event ) {
// If form was submitted by the user, bubble the event up the tree
if ( this.parentNode && !event.isTrigger ) {
jQuery.event.simulate( "submit", this.parentNode, event, true );
}
});
form._submit_attached = true;
}
});
// return undefined since we don't need an event listener
},
teardown: function() {
// Only need this for delegated form submit events
if ( jQuery.nodeName( this, "form" ) ) {
return false;
}
// Remove delegated handlers; cleanData eventually reaps submit handlers attached above
jQuery.event.remove( this, "._submit" );
}
};
}
// IE change delegation and checkbox/radio fix
if ( !jQuery.support.changeBubbles ) {
jQuery.event.special.change = {
setup: function() {
if ( rformElems.test( this.nodeName ) ) {
// IE doesn't fire change on a check/radio until blur; trigger it on click
// after a propertychange. Eat the blur-change in special.change.handle.
// This still fires onchange a second time for check/radio after blur.
if ( this.type === "checkbox" || this.type === "radio" ) {
jQuery.event.add( this, "propertychange._change", function( event ) {
if ( event.originalEvent.propertyName === "checked" ) {
this._just_changed = true;
}
});
jQuery.event.add( this, "click._change", function( event ) {
if ( this._just_changed && !event.isTrigger ) {
this._just_changed = false;
jQuery.event.simulate( "change", this, event, true );
}
});
}
return false;
}
// Delegated event; lazy-add a change handler on descendant inputs
jQuery.event.add( this, "beforeactivate._change", function( e ) {
var elem = e.target;
if ( rformElems.test( elem.nodeName ) && !elem._change_attached ) {
jQuery.event.add( elem, "change._change", function( event ) {
if ( this.parentNode && !event.isSimulated && !event.isTrigger ) {
jQuery.event.simulate( "change", this.parentNode, event, true );
}
});
elem._change_attached = true;
}
});
},
handle: function( event ) {
var elem = event.target;
// Swallow native change events from checkbox/radio, we already triggered them above
if ( this !== elem || event.isSimulated || event.isTrigger || (elem.type !== "radio" && elem.type !== "checkbox") ) {
return event.handleObj.handler.apply( this, arguments );
}
},
teardown: function() {
jQuery.event.remove( this, "._change" );
return rformElems.test( this.nodeName );
}
};
}
// Create "bubbling" focus and blur events
if ( !jQuery.support.focusinBubbles ) {
jQuery.each({ focus: "focusin", blur: "focusout" }, function( orig, fix ) {
// Attach a single capturing handler while someone wants focusin/focusout
var attaches = 0,
handler = function( event ) {
jQuery.event.simulate( fix, event.target, jQuery.event.fix( event ), true );
};
jQuery.event.special[ fix ] = {
setup: function() {
if ( attaches++ === 0 ) {
document.addEventListener( orig, handler, true );
}
},
teardown: function() {
if ( --attaches === 0 ) {
document.removeEventListener( orig, handler, true );
}
}
};
});
}
jQuery.fn.extend({
on: function( types, selector, data, fn, /*INTERNAL*/ one ) {
var origFn, type;
// Types can be a map of types/handlers
if ( typeof types === "object" ) {
// ( types-Object, selector, data )
if ( typeof selector !== "string" ) {
// ( types-Object, data )
data = selector;
selector = undefined;
}
for ( type in types ) {
this.on( type, selector, data, types[ type ], one );
}
return this;
}
if ( data == null && fn == null ) {
// ( types, fn )
fn = selector;
data = selector = undefined;
} else if ( fn == null ) {
if ( typeof selector === "string" ) {
// ( types, selector, fn )
fn = data;
data = undefined;
} else {
// ( types, data, fn )
fn = data;
data = selector;
selector = undefined;
}
}
if ( fn === false ) {
fn = returnFalse;
} else if ( !fn ) {
return this;
}
if ( one === 1 ) {
origFn = fn;
fn = function( event ) {
// Can use an empty set, since event contains the info
jQuery().off( event );
return origFn.apply( this, arguments );
};
// Use same guid so caller can remove using origFn
fn.guid = origFn.guid || ( origFn.guid = jQuery.guid++ );
}
return this.each( function() {
jQuery.event.add( this, types, fn, data, selector );
});
},
one: function( types, selector, data, fn ) {
return this.on.call( this, types, selector, data, fn, 1 );
},
off: function( types, selector, fn ) {
if ( types && types.preventDefault && types.handleObj ) {
// ( event ) dispatched jQuery.Event
var handleObj = types.handleObj;
jQuery( types.delegateTarget ).off(
handleObj.namespace? handleObj.type + "." + handleObj.namespace : handleObj.type,
handleObj.selector,
handleObj.handler
);
return this;
}
if ( typeof types === "object" ) {
// ( types-object [, selector] )
for ( var type in types ) {
this.off( type, selector, types[ type ] );
}
return this;
}
if ( selector === false || typeof selector === "function" ) {
// ( types [, fn] )
fn = selector;
selector = undefined;
}
if ( fn === false ) {
fn = returnFalse;
}
return this.each(function() {
jQuery.event.remove( this, types, fn, selector );
});
},
bind: function( types, data, fn ) {
return this.on( types, null, data, fn );
},
unbind: function( types, fn ) {
return this.off( types, null, fn );
},
live: function( types, data, fn ) {
jQuery( this.context ).on( types, this.selector, data, fn );
return this;
},
die: function( types, fn ) {
jQuery( this.context ).off( types, this.selector || "**", fn );
return this;
},
delegate: function( selector, types, data, fn ) {
return this.on( types, selector, data, fn );
},
undelegate: function( selector, types, fn ) {
// ( namespace ) or ( selector, types [, fn] )
return arguments.length == 1? this.off( selector, "**" ) : this.off( types, selector, fn );
},
trigger: function( type, data ) {
return this.each(function() {
jQuery.event.trigger( type, data, this );
});
},
triggerHandler: function( type, data ) {
if ( this[0] ) {
return jQuery.event.trigger( type, data, this[0], true );
}
},
toggle: function( fn ) {
// Save reference to arguments for access in closure
var args = arguments,
guid = fn.guid || jQuery.guid++,
i = 0,
toggler = function( event ) {
// Figure out which function to execute
var lastToggle = ( jQuery._data( this, "lastToggle" + fn.guid ) || 0 ) % i;
jQuery._data( this, "lastToggle" + fn.guid, lastToggle + 1 );
// Make sure that clicks stop
event.preventDefault();
// and execute the function
return args[ lastToggle ].apply( this, arguments ) || false;
};
// link all the functions, so any of them can unbind this click handler
toggler.guid = guid;
while ( i < args.length ) {
args[ i++ ].guid = guid;
}
return this.click( toggler );
},
hover: function( fnOver, fnOut ) {
return this.mouseenter( fnOver ).mouseleave( fnOut || fnOver );
}
});
jQuery.each( ("blur focus focusin focusout load resize scroll unload click dblclick " +
"mousedown mouseup mousemove mouseover mouseout mouseenter mouseleave " +
"change select submit keydown keypress keyup error contextmenu").split(" "), function( i, name ) {
// Handle event binding
jQuery.fn[ name ] = function( data, fn ) {
if ( fn == null ) {
fn = data;
data = null;
}
return arguments.length > 0 ?
this.on( name, null, data, fn ) :
this.trigger( name );
};
if ( jQuery.attrFn ) {
jQuery.attrFn[ name ] = true;
}
if ( rkeyEvent.test( name ) ) {
jQuery.event.fixHooks[ name ] = jQuery.event.keyHooks;
}
if ( rmouseEvent.test( name ) ) {
jQuery.event.fixHooks[ name ] = jQuery.event.mouseHooks;
}
});
/*!
* Sizzle CSS Selector Engine
* Copyright 2011, The Dojo Foundation
* Released under the MIT, BSD, and GPL Licenses.
* More information: http://sizzlejs.com/
*/
(function(){
var chunker = /((?:\((?:\([^()]+\)|[^()]+)+\)|\[(?:\[[^\[\]]*\]|['"][^'"]*['"]|[^\[\]'"]+)+\]|\\.|[^ >+~,(\[\\]+)+|[>+~])(\s*,\s*)?((?:.|\r|\n)*)/g,
expando = "sizcache" + (Math.random() + '').replace('.', ''),
done = 0,
toString = Object.prototype.toString,
hasDuplicate = false,
baseHasDuplicate = true,
rBackslash = /\\/g,
rReturn = /\r\n/g,
rNonWord = /\W/;
// Here we check if the JavaScript engine is using some sort of
// optimization where it does not always call our comparision
// function. If that is the case, discard the hasDuplicate value.
// Thus far that includes Google Chrome.
[0, 0].sort(function() {
baseHasDuplicate = false;
return 0;
});
var Sizzle = function( selector, context, results, seed ) {
results = results || [];
context = context || document;
var origContext = context;
if ( context.nodeType !== 1 && context.nodeType !== 9 ) {
return [];
}
if ( !selector || typeof selector !== "string" ) {
return results;
}
var m, set, checkSet, extra, ret, cur, pop, i,
prune = true,
contextXML = Sizzle.isXML( context ),
parts = [],
soFar = selector;
// Reset the position of the chunker regexp (start from head)
do {
chunker.exec( "" );
m = chunker.exec( soFar );
if ( m ) {
soFar = m[3];
parts.push( m[1] );
if ( m[2] ) {
extra = m[3];
break;
}
}
} while ( m );
if ( parts.length > 1 && origPOS.exec( selector ) ) {
if ( parts.length === 2 && Expr.relative[ parts[0] ] ) {
set = posProcess( parts[0] + parts[1], context, seed );
} else {
set = Expr.relative[ parts[0] ] ?
[ context ] :
Sizzle( parts.shift(), context );
while ( parts.length ) {
selector = parts.shift();
if ( Expr.relative[ selector ] ) {
selector += parts.shift();
}
set = posProcess( selector, set, seed );
}
}
} else {
// Take a shortcut and set the context if the root selector is an ID
// (but not if it'll be faster if the inner selector is an ID)
if ( !seed && parts.length > 1 && context.nodeType === 9 && !contextXML &&
Expr.match.ID.test(parts[0]) && !Expr.match.ID.test(parts[parts.length - 1]) ) {
ret = Sizzle.find( parts.shift(), context, contextXML );
context = ret.expr ?
Sizzle.filter( ret.expr, ret.set )[0] :
ret.set[0];
}
if ( context ) {
ret = seed ?
{ expr: parts.pop(), set: makeArray(seed) } :
Sizzle.find( parts.pop(), parts.length === 1 && (parts[0] === "~" || parts[0] === "+") && context.parentNode ? context.parentNode : context, contextXML );
set = ret.expr ?
Sizzle.filter( ret.expr, ret.set ) :
ret.set;
if ( parts.length > 0 ) {
checkSet = makeArray( set );
} else {
prune = false;
}
while ( parts.length ) {
cur = parts.pop();
pop = cur;
if ( !Expr.relative[ cur ] ) {
cur = "";
} else {
pop = parts.pop();
}
if ( pop == null ) {
pop = context;
}
Expr.relative[ cur ]( checkSet, pop, contextXML );
}
} else {
checkSet = parts = [];
}
}
if ( !checkSet ) {
checkSet = set;
}
if ( !checkSet ) {
Sizzle.error( cur || selector );
}
if ( toString.call(checkSet) === "[object Array]" ) {
if ( !prune ) {
results.push.apply( results, checkSet );
} else if ( context && context.nodeType === 1 ) {
for ( i = 0; checkSet[i] != null; i++ ) {
if ( checkSet[i] && (checkSet[i] === true || checkSet[i].nodeType === 1 && Sizzle.contains(context, checkSet[i])) ) {
results.push( set[i] );
}
}
} else {
for ( i = 0; checkSet[i] != null; i++ ) {
if ( checkSet[i] && checkSet[i].nodeType === 1 ) {
results.push( set[i] );
}
}
}
} else {
makeArray( checkSet, results );
}
if ( extra ) {
Sizzle( extra, origContext, results, seed );
Sizzle.uniqueSort( results );
}
return results;
};
Sizzle.uniqueSort = function( results ) {
if ( sortOrder ) {
hasDuplicate = baseHasDuplicate;
results.sort( sortOrder );
if ( hasDuplicate ) {
for ( var i = 1; i < results.length; i++ ) {
if ( results[i] === results[ i - 1 ] ) {
results.splice( i--, 1 );
}
}
}
}
return results;
};
Sizzle.matches = function( expr, set ) {
return Sizzle( expr, null, null, set );
};
Sizzle.matchesSelector = function( node, expr ) {
return Sizzle( expr, null, null, [node] ).length > 0;
};
Sizzle.find = function( expr, context, isXML ) {
var set, i, len, match, type, left;
if ( !expr ) {
return [];
}
for ( i = 0, len = Expr.order.length; i < len; i++ ) {
type = Expr.order[i];
if ( (match = Expr.leftMatch[ type ].exec( expr )) ) {
left = match[1];
match.splice( 1, 1 );
if ( left.substr( left.length - 1 ) !== "\\" ) {
match[1] = (match[1] || "").replace( rBackslash, "" );
set = Expr.find[ type ]( match, context, isXML );
if ( set != null ) {
expr = expr.replace( Expr.match[ type ], "" );
break;
}
}
}
}
if ( !set ) {
set = typeof context.getElementsByTagName !== "undefined" ?
context.getElementsByTagName( "*" ) :
[];
}
return { set: set, expr: expr };
};
Sizzle.filter = function( expr, set, inplace, not ) {
var match, anyFound,
type, found, item, filter, left,
i, pass,
old = expr,
result = [],
curLoop = set,
isXMLFilter = set && set[0] && Sizzle.isXML( set[0] );
while ( expr && set.length ) {
for ( type in Expr.filter ) {
if ( (match = Expr.leftMatch[ type ].exec( expr )) != null && match[2] ) {
filter = Expr.filter[ type ];
left = match[1];
anyFound = false;
match.splice(1,1);
if ( left.substr( left.length - 1 ) === "\\" ) {
continue;
}
if ( curLoop === result ) {
result = [];
}
if ( Expr.preFilter[ type ] ) {
match = Expr.preFilter[ type ]( match, curLoop, inplace, result, not, isXMLFilter );
if ( !match ) {
anyFound = found = true;
} else if ( match === true ) {
continue;
}
}
if ( match ) {
for ( i = 0; (item = curLoop[i]) != null; i++ ) {
if ( item ) {
found = filter( item, match, i, curLoop );
pass = not ^ found;
if ( inplace && found != null ) {
if ( pass ) {
anyFound = true;
} else {
curLoop[i] = false;
}
} else if ( pass ) {
result.push( item );
anyFound = true;
}
}
}
}
if ( found !== undefined ) {
if ( !inplace ) {
curLoop = result;
}
expr = expr.replace( Expr.match[ type ], "" );
if ( !anyFound ) {
return [];
}
break;
}
}
}
// Improper expression
if ( expr === old ) {
if ( anyFound == null ) {
Sizzle.error( expr );
} else {
break;
}
}
old = expr;
}
return curLoop;
};
Sizzle.error = function( msg ) {
throw new Error( "Syntax error, unrecognized expression: " + msg );
};
/**
* Utility function for retreiving the text value of an array of DOM nodes
* @param {Array|Element} elem
*/
var getText = Sizzle.getText = function( elem ) {
var i, node,
nodeType = elem.nodeType,
ret = "";
if ( nodeType ) {
if ( nodeType === 1 || nodeType === 9 ) {
// Use textContent || innerText for elements
if ( typeof elem.textContent === 'string' ) {
return elem.textContent;
} else if ( typeof elem.innerText === 'string' ) {
// Replace IE's carriage returns
return elem.innerText.replace( rReturn, '' );
} else {
// Traverse it's children
for ( elem = elem.firstChild; elem; elem = elem.nextSibling) {
ret += getText( elem );
}
}
} else if ( nodeType === 3 || nodeType === 4 ) {
return elem.nodeValue;
}
} else {
// If no nodeType, this is expected to be an array
for ( i = 0; (node = elem[i]); i++ ) {
// Do not traverse comment nodes
if ( node.nodeType !== 8 ) {
ret += getText( node );
}
}
}
return ret;
};
var Expr = Sizzle.selectors = {
order: [ "ID", "NAME", "TAG" ],
match: {
ID: /#((?:[\w\u00c0-\uFFFF\-]|\\.)+)/,
CLASS: /\.((?:[\w\u00c0-\uFFFF\-]|\\.)+)/,
NAME: /\[name=['"]*((?:[\w\u00c0-\uFFFF\-]|\\.)+)['"]*\]/,
ATTR: /\[\s*((?:[\w\u00c0-\uFFFF\-]|\\.)+)\s*(?:(\S?=)\s*(?:(['"])(.*?)\3|(#?(?:[\w\u00c0-\uFFFF\-]|\\.)*)|)|)\s*\]/,
TAG: /^((?:[\w\u00c0-\uFFFF\*\-]|\\.)+)/,
CHILD: /:(only|nth|last|first)-child(?:\(\s*(even|odd|(?:[+\-]?\d+|(?:[+\-]?\d*)?n\s*(?:[+\-]\s*\d+)?))\s*\))?/,
POS: /:(nth|eq|gt|lt|first|last|even|odd)(?:\((\d*)\))?(?=[^\-]|$)/,
PSEUDO: /:((?:[\w\u00c0-\uFFFF\-]|\\.)+)(?:\((['"]?)((?:\([^\)]+\)|[^\(\)]*)+)\2\))?/
},
leftMatch: {},
attrMap: {
"class": "className",
"for": "htmlFor"
},
attrHandle: {
href: function( elem ) {
return elem.getAttribute( "href" );
},
type: function( elem ) {
return elem.getAttribute( "type" );
}
},
relative: {
"+": function(checkSet, part){
var isPartStr = typeof part === "string",
isTag = isPartStr && !rNonWord.test( part ),
isPartStrNotTag = isPartStr && !isTag;
if ( isTag ) {
part = part.toLowerCase();
}
for ( var i = 0, l = checkSet.length, elem; i < l; i++ ) {
if ( (elem = checkSet[i]) ) {
while ( (elem = elem.previousSibling) && elem.nodeType !== 1 ) {}
checkSet[i] = isPartStrNotTag || elem && elem.nodeName.toLowerCase() === part ?
elem || false :
elem === part;
}
}
if ( isPartStrNotTag ) {
Sizzle.filter( part, checkSet, true );
}
},
">": function( checkSet, part ) {
var elem,
isPartStr = typeof part === "string",
i = 0,
l = checkSet.length;
if ( isPartStr && !rNonWord.test( part ) ) {
part = part.toLowerCase();
for ( ; i < l; i++ ) {
elem = checkSet[i];
if ( elem ) {
var parent = elem.parentNode;
checkSet[i] = parent.nodeName.toLowerCase() === part ? parent : false;
}
}
} else {
for ( ; i < l; i++ ) {
elem = checkSet[i];
if ( elem ) {
checkSet[i] = isPartStr ?
elem.parentNode :
elem.parentNode === part;
}
}
if ( isPartStr ) {
Sizzle.filter( part, checkSet, true );
}
}
},
"": function(checkSet, part, isXML){
var nodeCheck,
doneName = done++,
checkFn = dirCheck;
if ( typeof part === "string" && !rNonWord.test( part ) ) {
part = part.toLowerCase();
nodeCheck = part;
checkFn = dirNodeCheck;
}
checkFn( "parentNode", part, doneName, checkSet, nodeCheck, isXML );
},
"~": function( checkSet, part, isXML ) {
var nodeCheck,
doneName = done++,
checkFn = dirCheck;
if ( typeof part === "string" && !rNonWord.test( part ) ) {
part = part.toLowerCase();
nodeCheck = part;
checkFn = dirNodeCheck;
}
checkFn( "previousSibling", part, doneName, checkSet, nodeCheck, isXML );
}
},
find: {
ID: function( match, context, isXML ) {
if ( typeof context.getElementById !== "undefined" && !isXML ) {
var m = context.getElementById(match[1]);
// Check parentNode to catch when Blackberry 4.6 returns
// nodes that are no longer in the document #6963
return m && m.parentNode ? [m] : [];
}
},
NAME: function( match, context ) {
if ( typeof context.getElementsByName !== "undefined" ) {
var ret = [],
results = context.getElementsByName( match[1] );
for ( var i = 0, l = results.length; i < l; i++ ) {
if ( results[i].getAttribute("name") === match[1] ) {
ret.push( results[i] );
}
}
return ret.length === 0 ? null : ret;
}
},
TAG: function( match, context ) {
if ( typeof context.getElementsByTagName !== "undefined" ) {
return context.getElementsByTagName( match[1] );
}
}
},
preFilter: {
CLASS: function( match, curLoop, inplace, result, not, isXML ) {
match = " " + match[1].replace( rBackslash, "" ) + " ";
if ( isXML ) {
return match;
}
for ( var i = 0, elem; (elem = curLoop[i]) != null; i++ ) {
if ( elem ) {
if ( not ^ (elem.className && (" " + elem.className + " ").replace(/[\t\n\r]/g, " ").indexOf(match) >= 0) ) {
if ( !inplace ) {
result.push( elem );
}
} else if ( inplace ) {
curLoop[i] = false;
}
}
}
return false;
},
ID: function( match ) {
return match[1].replace( rBackslash, "" );
},
TAG: function( match, curLoop ) {
return match[1].replace( rBackslash, "" ).toLowerCase();
},
CHILD: function( match ) {
if ( match[1] === "nth" ) {
if ( !match[2] ) {
Sizzle.error( match[0] );
}
match[2] = match[2].replace(/^\+|\s*/g, '');
// parse equations like 'even', 'odd', '5', '2n', '3n+2', '4n-1', '-n+6'
var test = /(-?)(\d*)(?:n([+\-]?\d*))?/.exec(
match[2] === "even" && "2n" || match[2] === "odd" && "2n+1" ||
!/\D/.test( match[2] ) && "0n+" + match[2] || match[2]);
// calculate the numbers (first)n+(last) including if they are negative
match[2] = (test[1] + (test[2] || 1)) - 0;
match[3] = test[3] - 0;
}
else if ( match[2] ) {
Sizzle.error( match[0] );
}
// TODO: Move to normal caching system
match[0] = done++;
return match;
},
ATTR: function( match, curLoop, inplace, result, not, isXML ) {
var name = match[1] = match[1].replace( rBackslash, "" );
if ( !isXML && Expr.attrMap[name] ) {
match[1] = Expr.attrMap[name];
}
// Handle if an un-quoted value was used
match[4] = ( match[4] || match[5] || "" ).replace( rBackslash, "" );
if ( match[2] === "~=" ) {
match[4] = " " + match[4] + " ";
}
return match;
},
PSEUDO: function( match, curLoop, inplace, result, not ) {
if ( match[1] === "not" ) {
// If we're dealing with a complex expression, or a simple one
if ( ( chunker.exec(match[3]) || "" ).length > 1 || /^\w/.test(match[3]) ) {
match[3] = Sizzle(match[3], null, null, curLoop);
} else {
var ret = Sizzle.filter(match[3], curLoop, inplace, true ^ not);
if ( !inplace ) {
result.push.apply( result, ret );
}
return false;
}
} else if ( Expr.match.POS.test( match[0] ) || Expr.match.CHILD.test( match[0] ) ) {
return true;
}
return match;
},
POS: function( match ) {
match.unshift( true );
return match;
}
},
filters: {
enabled: function( elem ) {
return elem.disabled === false && elem.type !== "hidden";
},
disabled: function( elem ) {
return elem.disabled === true;
},
checked: function( elem ) {
return elem.checked === true;
},
selected: function( elem ) {
// Accessing this property makes selected-by-default
// options in Safari work properly
if ( elem.parentNode ) {
elem.parentNode.selectedIndex;
}
return elem.selected === true;
},
parent: function( elem ) {
return !!elem.firstChild;
},
empty: function( elem ) {
return !elem.firstChild;
},
has: function( elem, i, match ) {
return !!Sizzle( match[3], elem ).length;
},
header: function( elem ) {
return (/h\d/i).test( elem.nodeName );
},
text: function( elem ) {
var attr = elem.getAttribute( "type" ), type = elem.type;
// IE6 and 7 will map elem.type to 'text' for new HTML5 types (search, etc)
// use getAttribute instead to test this case
return elem.nodeName.toLowerCase() === "input" && "text" === type && ( attr === type || attr === null );
},
radio: function( elem ) {
return elem.nodeName.toLowerCase() === "input" && "radio" === elem.type;
},
checkbox: function( elem ) {
return elem.nodeName.toLowerCase() === "input" && "checkbox" === elem.type;
},
file: function( elem ) {
return elem.nodeName.toLowerCase() === "input" && "file" === elem.type;
},
password: function( elem ) {
return elem.nodeName.toLowerCase() === "input" && "password" === elem.type;
},
submit: function( elem ) {
var name = elem.nodeName.toLowerCase();
return (name === "input" || name === "button") && "submit" === elem.type;
},
image: function( elem ) {
return elem.nodeName.toLowerCase() === "input" && "image" === elem.type;
},
reset: function( elem ) {
var name = elem.nodeName.toLowerCase();
return (name === "input" || name === "button") && "reset" === elem.type;
},
button: function( elem ) {
var name = elem.nodeName.toLowerCase();
return name === "input" && "button" === elem.type || name === "button";
},
input: function( elem ) {
return (/input|select|textarea|button/i).test( elem.nodeName );
},
focus: function( elem ) {
return elem === elem.ownerDocument.activeElement;
}
},
setFilters: {
first: function( elem, i ) {
return i === 0;
},
last: function( elem, i, match, array ) {
return i === array.length - 1;
},
even: function( elem, i ) {
return i % 2 === 0;
},
odd: function( elem, i ) {
return i % 2 === 1;
},
lt: function( elem, i, match ) {
return i < match[3] - 0;
},
gt: function( elem, i, match ) {
return i > match[3] - 0;
},
nth: function( elem, i, match ) {
return match[3] - 0 === i;
},
eq: function( elem, i, match ) {
return match[3] - 0 === i;
}
},
filter: {
PSEUDO: function( elem, match, i, array ) {
var name = match[1],
filter = Expr.filters[ name ];
if ( filter ) {
return filter( elem, i, match, array );
} else if ( name === "contains" ) {
return (elem.textContent || elem.innerText || getText([ elem ]) || "").indexOf(match[3]) >= 0;
} else if ( name === "not" ) {
var not = match[3];
for ( var j = 0, l = not.length; j < l; j++ ) {
if ( not[j] === elem ) {
return false;
}
}
return true;
} else {
Sizzle.error( name );
}
},
CHILD: function( elem, match ) {
var first, last,
doneName, parent, cache,
count, diff,
type = match[1],
node = elem;
switch ( type ) {
case "only":
case "first":
while ( (node = node.previousSibling) ) {
if ( node.nodeType === 1 ) {
return false;
}
}
if ( type === "first" ) {
return true;
}
node = elem;
case "last":
while ( (node = node.nextSibling) ) {
if ( node.nodeType === 1 ) {
return false;
}
}
return true;
case "nth":
first = match[2];
last = match[3];
if ( first === 1 && last === 0 ) {
return true;
}
doneName = match[0];
parent = elem.parentNode;
if ( parent && (parent[ expando ] !== doneName || !elem.nodeIndex) ) {
count = 0;
for ( node = parent.firstChild; node; node = node.nextSibling ) {
if ( node.nodeType === 1 ) {
node.nodeIndex = ++count;
}
}
parent[ expando ] = doneName;
}
diff = elem.nodeIndex - last;
if ( first === 0 ) {
return diff === 0;
} else {
return ( diff % first === 0 && diff / first >= 0 );
}
}
},
ID: function( elem, match ) {
return elem.nodeType === 1 && elem.getAttribute("id") === match;
},
TAG: function( elem, match ) {
return (match === "*" && elem.nodeType === 1) || !!elem.nodeName && elem.nodeName.toLowerCase() === match;
},
CLASS: function( elem, match ) {
return (" " + (elem.className || elem.getAttribute("class")) + " ")
.indexOf( match ) > -1;
},
ATTR: function( elem, match ) {
var name = match[1],
result = Sizzle.attr ?
Sizzle.attr( elem, name ) :
Expr.attrHandle[ name ] ?
Expr.attrHandle[ name ]( elem ) :
elem[ name ] != null ?
elem[ name ] :
elem.getAttribute( name ),
value = result + "",
type = match[2],
check = match[4];
return result == null ?
type === "!=" :
!type && Sizzle.attr ?
result != null :
type === "=" ?
value === check :
type === "*=" ?
value.indexOf(check) >= 0 :
type === "~=" ?
(" " + value + " ").indexOf(check) >= 0 :
!check ?
value && result !== false :
type === "!=" ?
value !== check :
type === "^=" ?
value.indexOf(check) === 0 :
type === "$=" ?
value.substr(value.length - check.length) === check :
type === "|=" ?
value === check || value.substr(0, check.length + 1) === check + "-" :
false;
},
POS: function( elem, match, i, array ) {
var name = match[2],
filter = Expr.setFilters[ name ];
if ( filter ) {
return filter( elem, i, match, array );
}
}
}
};
var origPOS = Expr.match.POS,
fescape = function(all, num){
return "\\" + (num - 0 + 1);
};
for ( var type in Expr.match ) {
Expr.match[ type ] = new RegExp( Expr.match[ type ].source + (/(?![^\[]*\])(?![^\(]*\))/.source) );
Expr.leftMatch[ type ] = new RegExp( /(^(?:.|\r|\n)*?)/.source + Expr.match[ type ].source.replace(/\\(\d+)/g, fescape) );
}
var makeArray = function( array, results ) {
array = Array.prototype.slice.call( array, 0 );
if ( results ) {
results.push.apply( results, array );
return results;
}
return array;
};
// Perform a simple check to determine if the browser is capable of
// converting a NodeList to an array using builtin methods.
// Also verifies that the returned array holds DOM nodes
// (which is not the case in the Blackberry browser)
try {
Array.prototype.slice.call( document.documentElement.childNodes, 0 )[0].nodeType;
// Provide a fallback method if it does not work
} catch( e ) {
makeArray = function( array, results ) {
var i = 0,
ret = results || [];
if ( toString.call(array) === "[object Array]" ) {
Array.prototype.push.apply( ret, array );
} else {
if ( typeof array.length === "number" ) {
for ( var l = array.length; i < l; i++ ) {
ret.push( array[i] );
}
} else {
for ( ; array[i]; i++ ) {
ret.push( array[i] );
}
}
}
return ret;
};
}
var sortOrder, siblingCheck;
if ( document.documentElement.compareDocumentPosition ) {
sortOrder = function( a, b ) {
if ( a === b ) {
hasDuplicate = true;
return 0;
}
if ( !a.compareDocumentPosition || !b.compareDocumentPosition ) {
return a.compareDocumentPosition ? -1 : 1;
}
return a.compareDocumentPosition(b) & 4 ? -1 : 1;
};
} else {
sortOrder = function( a, b ) {
// The nodes are identical, we can exit early
if ( a === b ) {
hasDuplicate = true;
return 0;
// Fallback to using sourceIndex (in IE) if it's available on both nodes
} else if ( a.sourceIndex && b.sourceIndex ) {
return a.sourceIndex - b.sourceIndex;
}
var al, bl,
ap = [],
bp = [],
aup = a.parentNode,
bup = b.parentNode,
cur = aup;
// If the nodes are siblings (or identical) we can do a quick check
if ( aup === bup ) {
return siblingCheck( a, b );
// If no parents were found then the nodes are disconnected
} else if ( !aup ) {
return -1;
} else if ( !bup ) {
return 1;
}
// Otherwise they're somewhere else in the tree so we need
// to build up a full list of the parentNodes for comparison
while ( cur ) {
ap.unshift( cur );
cur = cur.parentNode;
}
cur = bup;
while ( cur ) {
bp.unshift( cur );
cur = cur.parentNode;
}
al = ap.length;
bl = bp.length;
// Start walking down the tree looking for a discrepancy
for ( var i = 0; i < al && i < bl; i++ ) {
if ( ap[i] !== bp[i] ) {
return siblingCheck( ap[i], bp[i] );
}
}
// We ended someplace up the tree so do a sibling check
return i === al ?
siblingCheck( a, bp[i], -1 ) :
siblingCheck( ap[i], b, 1 );
};
siblingCheck = function( a, b, ret ) {
if ( a === b ) {
return ret;
}
var cur = a.nextSibling;
while ( cur ) {
if ( cur === b ) {
return -1;
}
cur = cur.nextSibling;
}
return 1;
};
}
// Check to see if the browser returns elements by name when
// querying by getElementById (and provide a workaround)
(function(){
// We're going to inject a fake input element with a specified name
var form = document.createElement("div"),
id = "script" + (new Date()).getTime(),
root = document.documentElement;
form.innerHTML = "<a name='" + id + "'/>";
// Inject it into the root element, check its status, and remove it quickly
root.insertBefore( form, root.firstChild );
// The workaround has to do additional checks after a getElementById
// Which slows things down for other browsers (hence the branching)
if ( document.getElementById( id ) ) {
Expr.find.ID = function( match, context, isXML ) {
if ( typeof context.getElementById !== "undefined" && !isXML ) {
var m = context.getElementById(match[1]);
return m ?
m.id === match[1] || typeof m.getAttributeNode !== "undefined" && m.getAttributeNode("id").nodeValue === match[1] ?
[m] :
undefined :
[];
}
};
Expr.filter.ID = function( elem, match ) {
var node = typeof elem.getAttributeNode !== "undefined" && elem.getAttributeNode("id");
return elem.nodeType === 1 && node && node.nodeValue === match;
};
}
root.removeChild( form );
// release memory in IE
root = form = null;
})();
(function(){
// Check to see if the browser returns only elements
// when doing getElementsByTagName("*")
// Create a fake element
var div = document.createElement("div");
div.appendChild( document.createComment("") );
// Make sure no comments are found
if ( div.getElementsByTagName("*").length > 0 ) {
Expr.find.TAG = function( match, context ) {
var results = context.getElementsByTagName( match[1] );
// Filter out possible comments
if ( match[1] === "*" ) {
var tmp = [];
for ( var i = 0; results[i]; i++ ) {
if ( results[i].nodeType === 1 ) {
tmp.push( results[i] );
}
}
results = tmp;
}
return results;
};
}
// Check to see if an attribute returns normalized href attributes
div.innerHTML = "<a href='#'></a>";
if ( div.firstChild && typeof div.firstChild.getAttribute !== "undefined" &&
div.firstChild.getAttribute("href") !== "#" ) {
Expr.attrHandle.href = function( elem ) {
return elem.getAttribute( "href", 2 );
};
}
// release memory in IE
div = null;
})();
if ( document.querySelectorAll ) {
(function(){
var oldSizzle = Sizzle,
div = document.createElement("div"),
id = "__sizzle__";
div.innerHTML = "<p class='TEST'></p>";
// Safari can't handle uppercase or unicode characters when
// in quirks mode.
if ( div.querySelectorAll && div.querySelectorAll(".TEST").length === 0 ) {
return;
}
Sizzle = function( query, context, extra, seed ) {
context = context || document;
// Only use querySelectorAll on non-XML documents
// (ID selectors don't work in non-HTML documents)
if ( !seed && !Sizzle.isXML(context) ) {
// See if we find a selector to speed up
var match = /^(\w+$)|^\.([\w\-]+$)|^#([\w\-]+$)/.exec( query );
if ( match && (context.nodeType === 1 || context.nodeType === 9) ) {
// Speed-up: Sizzle("TAG")
if ( match[1] ) {
return makeArray( context.getElementsByTagName( query ), extra );
// Speed-up: Sizzle(".CLASS")
} else if ( match[2] && Expr.find.CLASS && context.getElementsByClassName ) {
return makeArray( context.getElementsByClassName( match[2] ), extra );
}
}
if ( context.nodeType === 9 ) {
// Speed-up: Sizzle("body")
// The body element only exists once, optimize finding it
if ( query === "body" && context.body ) {
return makeArray( [ context.body ], extra );
// Speed-up: Sizzle("#ID")
} else if ( match && match[3] ) {
var elem = context.getElementById( match[3] );
// Check parentNode to catch when Blackberry 4.6 returns
// nodes that are no longer in the document #6963
if ( elem && elem.parentNode ) {
// Handle the case where IE and Opera return items
// by name instead of ID
if ( elem.id === match[3] ) {
return makeArray( [ elem ], extra );
}
} else {
return makeArray( [], extra );
}
}
try {
return makeArray( context.querySelectorAll(query), extra );
} catch(qsaError) {}
// qSA works strangely on Element-rooted queries
// We can work around this by specifying an extra ID on the root
// and working up from there (Thanks to Andrew Dupont for the technique)
// IE 8 doesn't work on object elements
} else if ( context.nodeType === 1 && context.nodeName.toLowerCase() !== "object" ) {
var oldContext = context,
old = context.getAttribute( "id" ),
nid = old || id,
hasParent = context.parentNode,
relativeHierarchySelector = /^\s*[+~]/.test( query );
if ( !old ) {
context.setAttribute( "id", nid );
} else {
nid = nid.replace( /'/g, "\\$&" );
}
if ( relativeHierarchySelector && hasParent ) {
context = context.parentNode;
}
try {
if ( !relativeHierarchySelector || hasParent ) {
return makeArray( context.querySelectorAll( "[id='" + nid + "'] " + query ), extra );
}
} catch(pseudoError) {
} finally {
if ( !old ) {
oldContext.removeAttribute( "id" );
}
}
}
}
return oldSizzle(query, context, extra, seed);
};
for ( var prop in oldSizzle ) {
Sizzle[ prop ] = oldSizzle[ prop ];
}
// release memory in IE
div = null;
})();
}
(function(){
var html = document.documentElement,
matches = html.matchesSelector || html.mozMatchesSelector || html.webkitMatchesSelector || html.msMatchesSelector;
if ( matches ) {
// Check to see if it's possible to do matchesSelector
// on a disconnected node (IE 9 fails this)
var disconnectedMatch = !matches.call( document.createElement( "div" ), "div" ),
pseudoWorks = false;
try {
// This should fail with an exception
// Gecko does not error, returns false instead
matches.call( document.documentElement, "[test!='']:sizzle" );
} catch( pseudoError ) {
pseudoWorks = true;
}
Sizzle.matchesSelector = function( node, expr ) {
// Make sure that attribute selectors are quoted
expr = expr.replace(/\=\s*([^'"\]]*)\s*\]/g, "='$1']");
if ( !Sizzle.isXML( node ) ) {
try {
if ( pseudoWorks || !Expr.match.PSEUDO.test( expr ) && !/!=/.test( expr ) ) {
var ret = matches.call( node, expr );
// IE 9's matchesSelector returns false on disconnected nodes
if ( ret || !disconnectedMatch ||
// As well, disconnected nodes are said to be in a document
// fragment in IE 9, so check for that
node.document && node.document.nodeType !== 11 ) {
return ret;
}
}
} catch(e) {}
}
return Sizzle(expr, null, null, [node]).length > 0;
};
}
})();
(function(){
var div = document.createElement("div");
div.innerHTML = "<div class='test e'></div><div class='test'></div>";
// Opera can't find a second classname (in 9.6)
// Also, make sure that getElementsByClassName actually exists
if ( !div.getElementsByClassName || div.getElementsByClassName("e").length === 0 ) {
return;
}
// Safari caches class attributes, doesn't catch changes (in 3.2)
div.lastChild.className = "e";
if ( div.getElementsByClassName("e").length === 1 ) {
return;
}
Expr.order.splice(1, 0, "CLASS");
Expr.find.CLASS = function( match, context, isXML ) {
if ( typeof context.getElementsByClassName !== "undefined" && !isXML ) {
return context.getElementsByClassName(match[1]);
}
};
// release memory in IE
div = null;
})();
function dirNodeCheck( dir, cur, doneName, checkSet, nodeCheck, isXML ) {
for ( var i = 0, l = checkSet.length; i < l; i++ ) {
var elem = checkSet[i];
if ( elem ) {
var match = false;
elem = elem[dir];
while ( elem ) {
if ( elem[ expando ] === doneName ) {
match = checkSet[elem.sizset];
break;
}
if ( elem.nodeType === 1 && !isXML ){
elem[ expando ] = doneName;
elem.sizset = i;
}
if ( elem.nodeName.toLowerCase() === cur ) {
match = elem;
break;
}
elem = elem[dir];
}
checkSet[i] = match;
}
}
}
function dirCheck( dir, cur, doneName, checkSet, nodeCheck, isXML ) {
for ( var i = 0, l = checkSet.length; i < l; i++ ) {
var elem = checkSet[i];
if ( elem ) {
var match = false;
elem = elem[dir];
while ( elem ) {
if ( elem[ expando ] === doneName ) {
match = checkSet[elem.sizset];
break;
}
if ( elem.nodeType === 1 ) {
if ( !isXML ) {
elem[ expando ] = doneName;
elem.sizset = i;
}
if ( typeof cur !== "string" ) {
if ( elem === cur ) {
match = true;
break;
}
} else if ( Sizzle.filter( cur, [elem] ).length > 0 ) {
match = elem;
break;
}
}
elem = elem[dir];
}
checkSet[i] = match;
}
}
}
if ( document.documentElement.contains ) {
Sizzle.contains = function( a, b ) {
return a !== b && (a.contains ? a.contains(b) : true);
};
} else if ( document.documentElement.compareDocumentPosition ) {
Sizzle.contains = function( a, b ) {
return !!(a.compareDocumentPosition(b) & 16);
};
} else {
Sizzle.contains = function() {
return false;
};
}
Sizzle.isXML = function( elem ) {
// documentElement is verified for cases where it doesn't yet exist
// (such as loading iframes in IE - #4833)
var documentElement = (elem ? elem.ownerDocument || elem : 0).documentElement;
return documentElement ? documentElement.nodeName !== "HTML" : false;
};
var posProcess = function( selector, context, seed ) {
var match,
tmpSet = [],
later = "",
root = context.nodeType ? [context] : context;
// Position selectors must be done after the filter
// And so must :not(positional) so we move all PSEUDOs to the end
while ( (match = Expr.match.PSEUDO.exec( selector )) ) {
later += match[0];
selector = selector.replace( Expr.match.PSEUDO, "" );
}
selector = Expr.relative[selector] ? selector + "*" : selector;
for ( var i = 0, l = root.length; i < l; i++ ) {
Sizzle( selector, root[i], tmpSet, seed );
}
return Sizzle.filter( later, tmpSet );
};
// EXPOSE
// Override sizzle attribute retrieval
Sizzle.attr = jQuery.attr;
Sizzle.selectors.attrMap = {};
jQuery.find = Sizzle;
jQuery.expr = Sizzle.selectors;
jQuery.expr[":"] = jQuery.expr.filters;
jQuery.unique = Sizzle.uniqueSort;
jQuery.text = Sizzle.getText;
jQuery.isXMLDoc = Sizzle.isXML;
jQuery.contains = Sizzle.contains;
})();
var runtil = /Until$/,
rparentsprev = /^(?:parents|prevUntil|prevAll)/,
// Note: This RegExp should be improved, or likely pulled from Sizzle
rmultiselector = /,/,
isSimple = /^.[^:#\[\.,]*$/,
slice = Array.prototype.slice,
POS = jQuery.expr.match.POS,
// methods guaranteed to produce a unique set when starting from a unique set
guaranteedUnique = {
children: true,
contents: true,
next: true,
prev: true
};
jQuery.fn.extend({
find: function( selector ) {
var self = this,
i, l;
if ( typeof selector !== "string" ) {
return jQuery( selector ).filter(function() {
for ( i = 0, l = self.length; i < l; i++ ) {
if ( jQuery.contains( self[ i ], this ) ) {
return true;
}
}
});
}
var ret = this.pushStack( "", "find", selector ),
length, n, r;
for ( i = 0, l = this.length; i < l; i++ ) {
length = ret.length;
jQuery.find( selector, this[i], ret );
if ( i > 0 ) {
// Make sure that the results are unique
for ( n = length; n < ret.length; n++ ) {
for ( r = 0; r < length; r++ ) {
if ( ret[r] === ret[n] ) {
ret.splice(n--, 1);
break;
}
}
}
}
}
return ret;
},
has: function( target ) {
var targets = jQuery( target );
return this.filter(function() {
for ( var i = 0, l = targets.length; i < l; i++ ) {
if ( jQuery.contains( this, targets[i] ) ) {
return true;
}
}
});
},
not: function( selector ) {
return this.pushStack( winnow(this, selector, false), "not", selector);
},
filter: function( selector ) {
return this.pushStack( winnow(this, selector, true), "filter", selector );
},
is: function( selector ) {
return !!selector && (
typeof selector === "string" ?
// If this is a positional selector, check membership in the returned set
// so $("p:first").is("p:last") won't return true for a doc with two "p".
POS.test( selector ) ?
jQuery( selector, this.context ).index( this[0] ) >= 0 :
jQuery.filter( selector, this ).length > 0 :
this.filter( selector ).length > 0 );
},
closest: function( selectors, context ) {
var ret = [], i, l, cur = this[0];
// Array (deprecated as of jQuery 1.7)
if ( jQuery.isArray( selectors ) ) {
var level = 1;
while ( cur && cur.ownerDocument && cur !== context ) {
for ( i = 0; i < selectors.length; i++ ) {
if ( jQuery( cur ).is( selectors[ i ] ) ) {
ret.push({ selector: selectors[ i ], elem: cur, level: level });
}
}
cur = cur.parentNode;
level++;
}
return ret;
}
// String
var pos = POS.test( selectors ) || typeof selectors !== "string" ?
jQuery( selectors, context || this.context ) :
0;
for ( i = 0, l = this.length; i < l; i++ ) {
cur = this[i];
while ( cur ) {
if ( pos ? pos.index(cur) > -1 : jQuery.find.matchesSelector(cur, selectors) ) {
ret.push( cur );
break;
} else {
cur = cur.parentNode;
if ( !cur || !cur.ownerDocument || cur === context || cur.nodeType === 11 ) {
break;
}
}
}
}
ret = ret.length > 1 ? jQuery.unique( ret ) : ret;
return this.pushStack( ret, "closest", selectors );
},
// Determine the position of an element within
// the matched set of elements
index: function( elem ) {
// No argument, return index in parent
if ( !elem ) {
return ( this[0] && this[0].parentNode ) ? this.prevAll().length : -1;
}
// index in selector
if ( typeof elem === "string" ) {
return jQuery.inArray( this[0], jQuery( elem ) );
}
// Locate the position of the desired element
return jQuery.inArray(
// If it receives a jQuery object, the first element is used
elem.jquery ? elem[0] : elem, this );
},
add: function( selector, context ) {
var set = typeof selector === "string" ?
jQuery( selector, context ) :
jQuery.makeArray( selector && selector.nodeType ? [ selector ] : selector ),
all = jQuery.merge( this.get(), set );
return this.pushStack( isDisconnected( set[0] ) || isDisconnected( all[0] ) ?
all :
jQuery.unique( all ) );
},
andSelf: function() {
return this.add( this.prevObject );
}
});
// A painfully simple check to see if an element is disconnected
// from a document (should be improved, where feasible).
function isDisconnected( node ) {
return !node || !node.parentNode || node.parentNode.nodeType === 11;
}
jQuery.each({
parent: function( elem ) {
var parent = elem.parentNode;
return parent && parent.nodeType !== 11 ? parent : null;
},
parents: function( elem ) {
return jQuery.dir( elem, "parentNode" );
},
parentsUntil: function( elem, i, until ) {
return jQuery.dir( elem, "parentNode", until );
},
next: function( elem ) {
return jQuery.nth( elem, 2, "nextSibling" );
},
prev: function( elem ) {
return jQuery.nth( elem, 2, "previousSibling" );
},
nextAll: function( elem ) {
return jQuery.dir( elem, "nextSibling" );
},
prevAll: function( elem ) {
return jQuery.dir( elem, "previousSibling" );
},
nextUntil: function( elem, i, until ) {
return jQuery.dir( elem, "nextSibling", until );
},
prevUntil: function( elem, i, until ) {
return jQuery.dir( elem, "previousSibling", until );
},
siblings: function( elem ) {
return jQuery.sibling( elem.parentNode.firstChild, elem );
},
children: function( elem ) {
return jQuery.sibling( elem.firstChild );
},
contents: function( elem ) {
return jQuery.nodeName( elem, "iframe" ) ?
elem.contentDocument || elem.contentWindow.document :
jQuery.makeArray( elem.childNodes );
}
}, function( name, fn ) {
jQuery.fn[ name ] = function( until, selector ) {
var ret = jQuery.map( this, fn, until );
if ( !runtil.test( name ) ) {
selector = until;
}
if ( selector && typeof selector === "string" ) {
ret = jQuery.filter( selector, ret );
}
ret = this.length > 1 && !guaranteedUnique[ name ] ? jQuery.unique( ret ) : ret;
if ( (this.length > 1 || rmultiselector.test( selector )) && rparentsprev.test( name ) ) {
ret = ret.reverse();
}
return this.pushStack( ret, name, slice.call( arguments ).join(",") );
};
});
jQuery.extend({
filter: function( expr, elems, not ) {
if ( not ) {
expr = ":not(" + expr + ")";
}
return elems.length === 1 ?
jQuery.find.matchesSelector(elems[0], expr) ? [ elems[0] ] : [] :
jQuery.find.matches(expr, elems);
},
dir: function( elem, dir, until ) {
var matched = [],
cur = elem[ dir ];
while ( cur && cur.nodeType !== 9 && (until === undefined || cur.nodeType !== 1 || !jQuery( cur ).is( until )) ) {
if ( cur.nodeType === 1 ) {
matched.push( cur );
}
cur = cur[dir];
}
return matched;
},
nth: function( cur, result, dir, elem ) {
result = result || 1;
var num = 0;
for ( ; cur; cur = cur[dir] ) {
if ( cur.nodeType === 1 && ++num === result ) {
break;
}
}
return cur;
},
sibling: function( n, elem ) {
var r = [];
for ( ; n; n = n.nextSibling ) {
if ( n.nodeType === 1 && n !== elem ) {
r.push( n );
}
}
return r;
}
});
// Implement the identical functionality for filter and not
function winnow( elements, qualifier, keep ) {
// Can't pass null or undefined to indexOf in Firefox 4
// Set to 0 to skip string check
qualifier = qualifier || 0;
if ( jQuery.isFunction( qualifier ) ) {
return jQuery.grep(elements, function( elem, i ) {
var retVal = !!qualifier.call( elem, i, elem );
return retVal === keep;
});
} else if ( qualifier.nodeType ) {
return jQuery.grep(elements, function( elem, i ) {
return ( elem === qualifier ) === keep;
});
} else if ( typeof qualifier === "string" ) {
var filtered = jQuery.grep(elements, function( elem ) {
return elem.nodeType === 1;
});
if ( isSimple.test( qualifier ) ) {
return jQuery.filter(qualifier, filtered, !keep);
} else {
qualifier = jQuery.filter( qualifier, filtered );
}
}
return jQuery.grep(elements, function( elem, i ) {
return ( jQuery.inArray( elem, qualifier ) >= 0 ) === keep;
});
}
function createSafeFragment( document ) {
var list = nodeNames.split( "|" ),
safeFrag = document.createDocumentFragment();
if ( safeFrag.createElement ) {
while ( list.length ) {
safeFrag.createElement(
list.pop()
);
}
}
return safeFrag;
}
var nodeNames = "abbr|article|aside|audio|canvas|datalist|details|figcaption|figure|footer|" +
"header|hgroup|mark|meter|nav|output|progress|section|summary|time|video",
rinlinejQuery = / jQuery\d+="(?:\d+|null)"/g,
rleadingWhitespace = /^\s+/,
rxhtmlTag = /<(?!area|br|col|embed|hr|img|input|link|meta|param)(([\w:]+)[^>]*)\/>/ig,
rtagName = /<([\w:]+)/,
rtbody = /<tbody/i,
rhtml = /<|&#?\w+;/,
rnoInnerhtml = /<(?:script|style)/i,
rnocache = /<(?:script|object|embed|option|style)/i,
rnoshimcache = new RegExp("<(?:" + nodeNames + ")", "i"),
// checked="checked" or checked
rchecked = /checked\s*(?:[^=]|=\s*.checked.)/i,
rscriptType = /\/(java|ecma)script/i,
rcleanScript = /^\s*<!(?:\[CDATA\[|\-\-)/,
wrapMap = {
option: [ 1, "<select multiple='multiple'>", "</select>" ],
legend: [ 1, "<fieldset>", "</fieldset>" ],
thead: [ 1, "<table>", "</table>" ],
tr: [ 2, "<table><tbody>", "</tbody></table>" ],
td: [ 3, "<table><tbody><tr>", "</tr></tbody></table>" ],
col: [ 2, "<table><tbody></tbody><colgroup>", "</colgroup></table>" ],
area: [ 1, "<map>", "</map>" ],
_default: [ 0, "", "" ]
},
safeFragment = createSafeFragment( document );
wrapMap.optgroup = wrapMap.option;
wrapMap.tbody = wrapMap.tfoot = wrapMap.colgroup = wrapMap.caption = wrapMap.thead;
wrapMap.th = wrapMap.td;
// IE can't serialize <link> and <script> tags normally
if ( !jQuery.support.htmlSerialize ) {
wrapMap._default = [ 1, "div<div>", "</div>" ];
}
jQuery.fn.extend({
text: function( text ) {
if ( jQuery.isFunction(text) ) {
return this.each(function(i) {
var self = jQuery( this );
self.text( text.call(this, i, self.text()) );
});
}
if ( typeof text !== "object" && text !== undefined ) {
return this.empty().append( (this[0] && this[0].ownerDocument || document).createTextNode( text ) );
}
return jQuery.text( this );
},
wrapAll: function( html ) {
if ( jQuery.isFunction( html ) ) {
return this.each(function(i) {
jQuery(this).wrapAll( html.call(this, i) );
});
}
if ( this[0] ) {
// The elements to wrap the target around
var wrap = jQuery( html, this[0].ownerDocument ).eq(0).clone(true);
if ( this[0].parentNode ) {
wrap.insertBefore( this[0] );
}
wrap.map(function() {
var elem = this;
while ( elem.firstChild && elem.firstChild.nodeType === 1 ) {
elem = elem.firstChild;
}
return elem;
}).append( this );
}
return this;
},
wrapInner: function( html ) {
if ( jQuery.isFunction( html ) ) {
return this.each(function(i) {
jQuery(this).wrapInner( html.call(this, i) );
});
}
return this.each(function() {
var self = jQuery( this ),
contents = self.contents();
if ( contents.length ) {
contents.wrapAll( html );
} else {
self.append( html );
}
});
},
wrap: function( html ) {
var isFunction = jQuery.isFunction( html );
return this.each(function(i) {
jQuery( this ).wrapAll( isFunction ? html.call(this, i) : html );
});
},
unwrap: function() {
return this.parent().each(function() {
if ( !jQuery.nodeName( this, "body" ) ) {
jQuery( this ).replaceWith( this.childNodes );
}
}).end();
},
append: function() {
return this.domManip(arguments, true, function( elem ) {
if ( this.nodeType === 1 ) {
this.appendChild( elem );
}
});
},
prepend: function() {
return this.domManip(arguments, true, function( elem ) {
if ( this.nodeType === 1 ) {
this.insertBefore( elem, this.firstChild );
}
});
},
before: function() {
if ( this[0] && this[0].parentNode ) {
return this.domManip(arguments, false, function( elem ) {
this.parentNode.insertBefore( elem, this );
});
} else if ( arguments.length ) {
var set = jQuery.clean( arguments );
set.push.apply( set, this.toArray() );
return this.pushStack( set, "before", arguments );
}
},
after: function() {
if ( this[0] && this[0].parentNode ) {
return this.domManip(arguments, false, function( elem ) {
this.parentNode.insertBefore( elem, this.nextSibling );
});
} else if ( arguments.length ) {
var set = this.pushStack( this, "after", arguments );
set.push.apply( set, jQuery.clean(arguments) );
return set;
}
},
// keepData is for internal use only--do not document
remove: function( selector, keepData ) {
for ( var i = 0, elem; (elem = this[i]) != null; i++ ) {
if ( !selector || jQuery.filter( selector, [ elem ] ).length ) {
if ( !keepData && elem.nodeType === 1 ) {
jQuery.cleanData( elem.getElementsByTagName("*") );
jQuery.cleanData( [ elem ] );
}
if ( elem.parentNode ) {
elem.parentNode.removeChild( elem );
}
}
}
return this;
},
empty: function() {
for ( var i = 0, elem; (elem = this[i]) != null; i++ ) {
// Remove element nodes and prevent memory leaks
if ( elem.nodeType === 1 ) {
jQuery.cleanData( elem.getElementsByTagName("*") );
}
// Remove any remaining nodes
while ( elem.firstChild ) {
elem.removeChild( elem.firstChild );
}
}
return this;
},
clone: function( dataAndEvents, deepDataAndEvents ) {
dataAndEvents = dataAndEvents == null ? false : dataAndEvents;
deepDataAndEvents = deepDataAndEvents == null ? dataAndEvents : deepDataAndEvents;
return this.map( function () {
return jQuery.clone( this, dataAndEvents, deepDataAndEvents );
});
},
html: function( value ) {
if ( value === undefined ) {
return this[0] && this[0].nodeType === 1 ?
this[0].innerHTML.replace(rinlinejQuery, "") :
null;
// See if we can take a shortcut and just use innerHTML
} else if ( typeof value === "string" && !rnoInnerhtml.test( value ) &&
(jQuery.support.leadingWhitespace || !rleadingWhitespace.test( value )) &&
!wrapMap[ (rtagName.exec( value ) || ["", ""])[1].toLowerCase() ] ) {
value = value.replace(rxhtmlTag, "<$1></$2>");
try {
for ( var i = 0, l = this.length; i < l; i++ ) {
// Remove element nodes and prevent memory leaks
if ( this[i].nodeType === 1 ) {
jQuery.cleanData( this[i].getElementsByTagName("*") );
this[i].innerHTML = value;
}
}
// If using innerHTML throws an exception, use the fallback method
} catch(e) {
this.empty().append( value );
}
} else if ( jQuery.isFunction( value ) ) {
this.each(function(i){
var self = jQuery( this );
self.html( value.call(this, i, self.html()) );
});
} else {
this.empty().append( value );
}
return this;
},
replaceWith: function( value ) {
if ( this[0] && this[0].parentNode ) {
// Make sure that the elements are removed from the DOM before they are inserted
// this can help fix replacing a parent with child elements
if ( jQuery.isFunction( value ) ) {
return this.each(function(i) {
var self = jQuery(this), old = self.html();
self.replaceWith( value.call( this, i, old ) );
});
}
if ( typeof value !== "string" ) {
value = jQuery( value ).detach();
}
return this.each(function() {
var next = this.nextSibling,
parent = this.parentNode;
jQuery( this ).remove();
if ( next ) {
jQuery(next).before( value );
} else {
jQuery(parent).append( value );
}
});
} else {
return this.length ?
this.pushStack( jQuery(jQuery.isFunction(value) ? value() : value), "replaceWith", value ) :
this;
}
},
detach: function( selector ) {
return this.remove( selector, true );
},
domManip: function( args, table, callback ) {
var results, first, fragment, parent,
value = args[0],
scripts = [];
// We can't cloneNode fragments that contain checked, in WebKit
if ( !jQuery.support.checkClone && arguments.length === 3 && typeof value === "string" && rchecked.test( value ) ) {
return this.each(function() {
jQuery(this).domManip( args, table, callback, true );
});
}
if ( jQuery.isFunction(value) ) {
return this.each(function(i) {
var self = jQuery(this);
args[0] = value.call(this, i, table ? self.html() : undefined);
self.domManip( args, table, callback );
});
}
if ( this[0] ) {
parent = value && value.parentNode;
// If we're in a fragment, just use that instead of building a new one
if ( jQuery.support.parentNode && parent && parent.nodeType === 11 && parent.childNodes.length === this.length ) {
results = { fragment: parent };
} else {
results = jQuery.buildFragment( args, this, scripts );
}
fragment = results.fragment;
if ( fragment.childNodes.length === 1 ) {
first = fragment = fragment.firstChild;
} else {
first = fragment.firstChild;
}
if ( first ) {
table = table && jQuery.nodeName( first, "tr" );
for ( var i = 0, l = this.length, lastIndex = l - 1; i < l; i++ ) {
callback.call(
table ?
root(this[i], first) :
this[i],
// Make sure that we do not leak memory by inadvertently discarding
// the original fragment (which might have attached data) instead of
// using it; in addition, use the original fragment object for the last
// item instead of first because it can end up being emptied incorrectly
// in certain situations (Bug #8070).
// Fragments from the fragment cache must always be cloned and never used
// in place.
results.cacheable || ( l > 1 && i < lastIndex ) ?
jQuery.clone( fragment, true, true ) :
fragment
);
}
}
if ( scripts.length ) {
jQuery.each( scripts, evalScript );
}
}
return this;
}
});
function root( elem, cur ) {
return jQuery.nodeName(elem, "table") ?
(elem.getElementsByTagName("tbody")[0] ||
elem.appendChild(elem.ownerDocument.createElement("tbody"))) :
elem;
}
function cloneCopyEvent( src, dest ) {
if ( dest.nodeType !== 1 || !jQuery.hasData( src ) ) {
return;
}
var type, i, l,
oldData = jQuery._data( src ),
curData = jQuery._data( dest, oldData ),
events = oldData.events;
if ( events ) {
delete curData.handle;
curData.events = {};
for ( type in events ) {
for ( i = 0, l = events[ type ].length; i < l; i++ ) {
jQuery.event.add( dest, type + ( events[ type ][ i ].namespace ? "." : "" ) + events[ type ][ i ].namespace, events[ type ][ i ], events[ type ][ i ].data );
}
}
}
// make the cloned public data object a copy from the original
if ( curData.data ) {
curData.data = jQuery.extend( {}, curData.data );
}
}
function cloneFixAttributes( src, dest ) {
var nodeName;
// We do not need to do anything for non-Elements
if ( dest.nodeType !== 1 ) {
return;
}
// clearAttributes removes the attributes, which we don't want,
// but also removes the attachEvent events, which we *do* want
if ( dest.clearAttributes ) {
dest.clearAttributes();
}
// mergeAttributes, in contrast, only merges back on the
// original attributes, not the events
if ( dest.mergeAttributes ) {
dest.mergeAttributes( src );
}
nodeName = dest.nodeName.toLowerCase();
// IE6-8 fail to clone children inside object elements that use
// the proprietary classid attribute value (rather than the type
// attribute) to identify the type of content to display
if ( nodeName === "object" ) {
dest.outerHTML = src.outerHTML;
} else if ( nodeName === "input" && (src.type === "checkbox" || src.type === "radio") ) {
// IE6-8 fails to persist the checked state of a cloned checkbox
// or radio button. Worse, IE6-7 fail to give the cloned element
// a checked appearance if the defaultChecked value isn't also set
if ( src.checked ) {
dest.defaultChecked = dest.checked = src.checked;
}
// IE6-7 get confused and end up setting the value of a cloned
// checkbox/radio button to an empty string instead of "on"
if ( dest.value !== src.value ) {
dest.value = src.value;
}
// IE6-8 fails to return the selected option to the default selected
// state when cloning options
} else if ( nodeName === "option" ) {
dest.selected = src.defaultSelected;
// IE6-8 fails to set the defaultValue to the correct value when
// cloning other types of input fields
} else if ( nodeName === "input" || nodeName === "textarea" ) {
dest.defaultValue = src.defaultValue;
}
// Event data gets referenced instead of copied if the expando
// gets copied too
dest.removeAttribute( jQuery.expando );
}
jQuery.buildFragment = function( args, nodes, scripts ) {
var fragment, cacheable, cacheresults, doc,
first = args[ 0 ];
// nodes may contain either an explicit document object,
// a jQuery collection or context object.
// If nodes[0] contains a valid object to assign to doc
if ( nodes && nodes[0] ) {
doc = nodes[0].ownerDocument || nodes[0];
}
// Ensure that an attr object doesn't incorrectly stand in as a document object
// Chrome and Firefox seem to allow this to occur and will throw exception
// Fixes #8950
if ( !doc.createDocumentFragment ) {
doc = document;
}
// Only cache "small" (1/2 KB) HTML strings that are associated with the main document
// Cloning options loses the selected state, so don't cache them
// IE 6 doesn't like it when you put <object> or <embed> elements in a fragment
// Also, WebKit does not clone 'checked' attributes on cloneNode, so don't cache
// Lastly, IE6,7,8 will not correctly reuse cached fragments that were created from unknown elems #10501
if ( args.length === 1 && typeof first === "string" && first.length < 512 && doc === document &&
first.charAt(0) === "<" && !rnocache.test( first ) &&
(jQuery.support.checkClone || !rchecked.test( first )) &&
(jQuery.support.html5Clone || !rnoshimcache.test( first )) ) {
cacheable = true;
cacheresults = jQuery.fragments[ first ];
if ( cacheresults && cacheresults !== 1 ) {
fragment = cacheresults;
}
}
if ( !fragment ) {
fragment = doc.createDocumentFragment();
jQuery.clean( args, doc, fragment, scripts );
}
if ( cacheable ) {
jQuery.fragments[ first ] = cacheresults ? fragment : 1;
}
return { fragment: fragment, cacheable: cacheable };
};
jQuery.fragments = {};
jQuery.each({
appendTo: "append",
prependTo: "prepend",
insertBefore: "before",
insertAfter: "after",
replaceAll: "replaceWith"
}, function( name, original ) {
jQuery.fn[ name ] = function( selector ) {
var ret = [],
insert = jQuery( selector ),
parent = this.length === 1 && this[0].parentNode;
if ( parent && parent.nodeType === 11 && parent.childNodes.length === 1 && insert.length === 1 ) {
insert[ original ]( this[0] );
return this;
} else {
for ( var i = 0, l = insert.length; i < l; i++ ) {
var elems = ( i > 0 ? this.clone(true) : this ).get();
jQuery( insert[i] )[ original ]( elems );
ret = ret.concat( elems );
}
return this.pushStack( ret, name, insert.selector );
}
};
});
function getAll( elem ) {
if ( typeof elem.getElementsByTagName !== "undefined" ) {
return elem.getElementsByTagName( "*" );
} else if ( typeof elem.querySelectorAll !== "undefined" ) {
return elem.querySelectorAll( "*" );
} else {
return [];
}
}
// Used in clean, fixes the defaultChecked property
function fixDefaultChecked( elem ) {
if ( elem.type === "checkbox" || elem.type === "radio" ) {
elem.defaultChecked = elem.checked;
}
}
// Finds all inputs and passes them to fixDefaultChecked
function findInputs( elem ) {
var nodeName = ( elem.nodeName || "" ).toLowerCase();
if ( nodeName === "input" ) {
fixDefaultChecked( elem );
// Skip scripts, get other children
} else if ( nodeName !== "script" && typeof elem.getElementsByTagName !== "undefined" ) {
jQuery.grep( elem.getElementsByTagName("input"), fixDefaultChecked );
}
}
// Derived From: http://www.iecss.com/shimprove/javascript/shimprove.1-0-1.js
function shimCloneNode( elem ) {
var div = document.createElement( "div" );
safeFragment.appendChild( div );
div.innerHTML = elem.outerHTML;
return div.firstChild;
}
jQuery.extend({
clone: function( elem, dataAndEvents, deepDataAndEvents ) {
var srcElements,
destElements,
i,
// IE<=8 does not properly clone detached, unknown element nodes
clone = jQuery.support.html5Clone || !rnoshimcache.test( "<" + elem.nodeName ) ?
elem.cloneNode( true ) :
shimCloneNode( elem );
if ( (!jQuery.support.noCloneEvent || !jQuery.support.noCloneChecked) &&
(elem.nodeType === 1 || elem.nodeType === 11) && !jQuery.isXMLDoc(elem) ) {
// IE copies events bound via attachEvent when using cloneNode.
// Calling detachEvent on the clone will also remove the events
// from the original. In order to get around this, we use some
// proprietary methods to clear the events. Thanks to MooTools
// guys for this hotness.
cloneFixAttributes( elem, clone );
// Using Sizzle here is crazy slow, so we use getElementsByTagName instead
srcElements = getAll( elem );
destElements = getAll( clone );
// Weird iteration because IE will replace the length property
// with an element if you are cloning the body and one of the
// elements on the page has a name or id of "length"
for ( i = 0; srcElements[i]; ++i ) {
// Ensure that the destination node is not null; Fixes #9587
if ( destElements[i] ) {
cloneFixAttributes( srcElements[i], destElements[i] );
}
}
}
// Copy the events from the original to the clone
if ( dataAndEvents ) {
cloneCopyEvent( elem, clone );
if ( deepDataAndEvents ) {
srcElements = getAll( elem );
destElements = getAll( clone );
for ( i = 0; srcElements[i]; ++i ) {
cloneCopyEvent( srcElements[i], destElements[i] );
}
}
}
srcElements = destElements = null;
// Return the cloned set
return clone;
},
clean: function( elems, context, fragment, scripts ) {
var checkScriptType;
context = context || document;
// !context.createElement fails in IE with an error but returns typeof 'object'
if ( typeof context.createElement === "undefined" ) {
context = context.ownerDocument || context[0] && context[0].ownerDocument || document;
}
var ret = [], j;
for ( var i = 0, elem; (elem = elems[i]) != null; i++ ) {
if ( typeof elem === "number" ) {
elem += "";
}
if ( !elem ) {
continue;
}
// Convert html string into DOM nodes
if ( typeof elem === "string" ) {
if ( !rhtml.test( elem ) ) {
elem = context.createTextNode( elem );
} else {
// Fix "XHTML"-style tags in all browsers
elem = elem.replace(rxhtmlTag, "<$1></$2>");
// Trim whitespace, otherwise indexOf won't work as expected
var tag = ( rtagName.exec( elem ) || ["", ""] )[1].toLowerCase(),
wrap = wrapMap[ tag ] || wrapMap._default,
depth = wrap[0],
div = context.createElement("div");
// Append wrapper element to unknown element safe doc fragment
if ( context === document ) {
// Use the fragment we've already created for this document
safeFragment.appendChild( div );
} else {
// Use a fragment created with the owner document
createSafeFragment( context ).appendChild( div );
}
// Go to html and back, then peel off extra wrappers
div.innerHTML = wrap[1] + elem + wrap[2];
// Move to the right depth
while ( depth-- ) {
div = div.lastChild;
}
// Remove IE's autoinserted <tbody> from table fragments
if ( !jQuery.support.tbody ) {
// String was a <table>, *may* have spurious <tbody>
var hasBody = rtbody.test(elem),
tbody = tag === "table" && !hasBody ?
div.firstChild && div.firstChild.childNodes :
// String was a bare <thead> or <tfoot>
wrap[1] === "<table>" && !hasBody ?
div.childNodes :
[];
for ( j = tbody.length - 1; j >= 0 ; --j ) {
if ( jQuery.nodeName( tbody[ j ], "tbody" ) && !tbody[ j ].childNodes.length ) {
tbody[ j ].parentNode.removeChild( tbody[ j ] );
}
}
}
// IE completely kills leading whitespace when innerHTML is used
if ( !jQuery.support.leadingWhitespace && rleadingWhitespace.test( elem ) ) {
div.insertBefore( context.createTextNode( rleadingWhitespace.exec(elem)[0] ), div.firstChild );
}
elem = div.childNodes;
}
}
// Resets defaultChecked for any radios and checkboxes
// about to be appended to the DOM in IE 6/7 (#8060)
var len;
if ( !jQuery.support.appendChecked ) {
if ( elem[0] && typeof (len = elem.length) === "number" ) {
for ( j = 0; j < len; j++ ) {
findInputs( elem[j] );
}
} else {
findInputs( elem );
}
}
if ( elem.nodeType ) {
ret.push( elem );
} else {
ret = jQuery.merge( ret, elem );
}
}
if ( fragment ) {
checkScriptType = function( elem ) {
return !elem.type || rscriptType.test( elem.type );
};
for ( i = 0; ret[i]; i++ ) {
if ( scripts && jQuery.nodeName( ret[i], "script" ) && (!ret[i].type || ret[i].type.toLowerCase() === "text/javascript") ) {
scripts.push( ret[i].parentNode ? ret[i].parentNode.removeChild( ret[i] ) : ret[i] );
} else {
if ( ret[i].nodeType === 1 ) {
var jsTags = jQuery.grep( ret[i].getElementsByTagName( "script" ), checkScriptType );
ret.splice.apply( ret, [i + 1, 0].concat( jsTags ) );
}
fragment.appendChild( ret[i] );
}
}
}
return ret;
},
cleanData: function( elems ) {
var data, id,
cache = jQuery.cache,
special = jQuery.event.special,
deleteExpando = jQuery.support.deleteExpando;
for ( var i = 0, elem; (elem = elems[i]) != null; i++ ) {
if ( elem.nodeName && jQuery.noData[elem.nodeName.toLowerCase()] ) {
continue;
}
id = elem[ jQuery.expando ];
if ( id ) {
data = cache[ id ];
if ( data && data.events ) {
for ( var type in data.events ) {
if ( special[ type ] ) {
jQuery.event.remove( elem, type );
// This is a shortcut to avoid jQuery.event.remove's overhead
} else {
jQuery.removeEvent( elem, type, data.handle );
}
}
// Null the DOM reference to avoid IE6/7/8 leak (#7054)
if ( data.handle ) {
data.handle.elem = null;
}
}
if ( deleteExpando ) {
delete elem[ jQuery.expando ];
} else if ( elem.removeAttribute ) {
elem.removeAttribute( jQuery.expando );
}
delete cache[ id ];
}
}
}
});
function evalScript( i, elem ) {
if ( elem.src ) {
jQuery.ajax({
url: elem.src,
async: false,
dataType: "script"
});
} else {
jQuery.globalEval( ( elem.text || elem.textContent || elem.innerHTML || "" ).replace( rcleanScript, "/*$0*/" ) );
}
if ( elem.parentNode ) {
elem.parentNode.removeChild( elem );
}
}
var ralpha = /alpha\([^)]*\)/i,
ropacity = /opacity=([^)]*)/,
// fixed for IE9, see #8346
rupper = /([A-Z]|^ms)/g,
rnumpx = /^-?\d+(?:px)?$/i,
rnum = /^-?\d/,
rrelNum = /^([\-+])=([\-+.\de]+)/,
cssShow = { position: "absolute", visibility: "hidden", display: "block" },
cssWidth = [ "Left", "Right" ],
cssHeight = [ "Top", "Bottom" ],
curCSS,
getComputedStyle,
currentStyle;
jQuery.fn.css = function( name, value ) {
// Setting 'undefined' is a no-op
if ( arguments.length === 2 && value === undefined ) {
return this;
}
return jQuery.access( this, name, value, true, function( elem, name, value ) {
return value !== undefined ?
jQuery.style( elem, name, value ) :
jQuery.css( elem, name );
});
};
jQuery.extend({
// Add in style property hooks for overriding the default
// behavior of getting and setting a style property
cssHooks: {
opacity: {
get: function( elem, computed ) {
if ( computed ) {
// We should always get a number back from opacity
var ret = curCSS( elem, "opacity", "opacity" );
return ret === "" ? "1" : ret;
} else {
return elem.style.opacity;
}
}
}
},
// Exclude the following css properties to add px
cssNumber: {
"fillOpacity": true,
"fontWeight": true,
"lineHeight": true,
"opacity": true,
"orphans": true,
"widows": true,
"zIndex": true,
"zoom": true
},
// Add in properties whose names you wish to fix before
// setting or getting the value
cssProps: {
// normalize float css property
"float": jQuery.support.cssFloat ? "cssFloat" : "styleFloat"
},
// Get and set the style property on a DOM Node
style: function( elem, name, value, extra ) {
// Don't set styles on text and comment nodes
if ( !elem || elem.nodeType === 3 || elem.nodeType === 8 || !elem.style ) {
return;
}
// Make sure that we're working with the right name
var ret, type, origName = jQuery.camelCase( name ),
style = elem.style, hooks = jQuery.cssHooks[ origName ];
name = jQuery.cssProps[ origName ] || origName;
// Check if we're setting a value
if ( value !== undefined ) {
type = typeof value;
// convert relative number strings (+= or -=) to relative numbers. #7345
if ( type === "string" && (ret = rrelNum.exec( value )) ) {
value = ( +( ret[1] + 1) * +ret[2] ) + parseFloat( jQuery.css( elem, name ) );
// Fixes bug #9237
type = "number";
}
// Make sure that NaN and null values aren't set. See: #7116
if ( value == null || type === "number" && isNaN( value ) ) {
return;
}
// If a number was passed in, add 'px' to the (except for certain CSS properties)
if ( type === "number" && !jQuery.cssNumber[ origName ] ) {
value += "px";
}
// If a hook was provided, use that value, otherwise just set the specified value
if ( !hooks || !("set" in hooks) || (value = hooks.set( elem, value )) !== undefined ) {
// Wrapped to prevent IE from throwing errors when 'invalid' values are provided
// Fixes bug #5509
try {
style[ name ] = value;
} catch(e) {}
}
} else {
// If a hook was provided get the non-computed value from there
if ( hooks && "get" in hooks && (ret = hooks.get( elem, false, extra )) !== undefined ) {
return ret;
}
// Otherwise just get the value from the style object
return style[ name ];
}
},
css: function( elem, name, extra ) {
var ret, hooks;
// Make sure that we're working with the right name
name = jQuery.camelCase( name );
hooks = jQuery.cssHooks[ name ];
name = jQuery.cssProps[ name ] || name;
// cssFloat needs a special treatment
if ( name === "cssFloat" ) {
name = "float";
}
// If a hook was provided get the computed value from there
if ( hooks && "get" in hooks && (ret = hooks.get( elem, true, extra )) !== undefined ) {
return ret;
// Otherwise, if a way to get the computed value exists, use that
} else if ( curCSS ) {
return curCSS( elem, name );
}
},
// A method for quickly swapping in/out CSS properties to get correct calculations
swap: function( elem, options, callback ) {
var old = {};
// Remember the old values, and insert the new ones
for ( var name in options ) {
old[ name ] = elem.style[ name ];
elem.style[ name ] = options[ name ];
}
callback.call( elem );
// Revert the old values
for ( name in options ) {
elem.style[ name ] = old[ name ];
}
}
});
// DEPRECATED, Use jQuery.css() instead
jQuery.curCSS = jQuery.css;
jQuery.each(["height", "width"], function( i, name ) {
jQuery.cssHooks[ name ] = {
get: function( elem, computed, extra ) {
var val;
if ( computed ) {
if ( elem.offsetWidth !== 0 ) {
return getWH( elem, name, extra );
} else {
jQuery.swap( elem, cssShow, function() {
val = getWH( elem, name, extra );
});
}
return val;
}
},
set: function( elem, value ) {
if ( rnumpx.test( value ) ) {
// ignore negative width and height values #1599
value = parseFloat( value );
if ( value >= 0 ) {
return value + "px";
}
} else {
return value;
}
}
};
});
if ( !jQuery.support.opacity ) {
jQuery.cssHooks.opacity = {
get: function( elem, computed ) {
// IE uses filters for opacity
return ropacity.test( (computed && elem.currentStyle ? elem.currentStyle.filter : elem.style.filter) || "" ) ?
( parseFloat( RegExp.$1 ) / 100 ) + "" :
computed ? "1" : "";
},
set: function( elem, value ) {
var style = elem.style,
currentStyle = elem.currentStyle,
opacity = jQuery.isNumeric( value ) ? "alpha(opacity=" + value * 100 + ")" : "",
filter = currentStyle && currentStyle.filter || style.filter || "";
// IE has trouble with opacity if it does not have layout
// Force it by setting the zoom level
style.zoom = 1;
// if setting opacity to 1, and no other filters exist - attempt to remove filter attribute #6652
if ( value >= 1 && jQuery.trim( filter.replace( ralpha, "" ) ) === "" ) {
// Setting style.filter to null, "" & " " still leave "filter:" in the cssText
// if "filter:" is present at all, clearType is disabled, we want to avoid this
// style.removeAttribute is IE Only, but so apparently is this code path...
style.removeAttribute( "filter" );
// if there there is no filter style applied in a css rule, we are done
if ( currentStyle && !currentStyle.filter ) {
return;
}
}
// otherwise, set new filter values
style.filter = ralpha.test( filter ) ?
filter.replace( ralpha, opacity ) :
filter + " " + opacity;
}
};
}
jQuery(function() {
// This hook cannot be added until DOM ready because the support test
// for it is not run until after DOM ready
if ( !jQuery.support.reliableMarginRight ) {
jQuery.cssHooks.marginRight = {
get: function( elem, computed ) {
// WebKit Bug 13343 - getComputedStyle returns wrong value for margin-right
// Work around by temporarily setting element display to inline-block
var ret;
jQuery.swap( elem, { "display": "inline-block" }, function() {
if ( computed ) {
ret = curCSS( elem, "margin-right", "marginRight" );
} else {
ret = elem.style.marginRight;
}
});
return ret;
}
};
}
});
if ( document.defaultView && document.defaultView.getComputedStyle ) {
getComputedStyle = function( elem, name ) {
var ret, defaultView, computedStyle;
name = name.replace( rupper, "-$1" ).toLowerCase();
if ( (defaultView = elem.ownerDocument.defaultView) &&
(computedStyle = defaultView.getComputedStyle( elem, null )) ) {
ret = computedStyle.getPropertyValue( name );
if ( ret === "" && !jQuery.contains( elem.ownerDocument.documentElement, elem ) ) {
ret = jQuery.style( elem, name );
}
}
return ret;
};
}
if ( document.documentElement.currentStyle ) {
currentStyle = function( elem, name ) {
var left, rsLeft, uncomputed,
ret = elem.currentStyle && elem.currentStyle[ name ],
style = elem.style;
// Avoid setting ret to empty string here
// so we don't default to auto
if ( ret === null && style && (uncomputed = style[ name ]) ) {
ret = uncomputed;
}
// From the awesome hack by Dean Edwards
// http://erik.eae.net/archives/2007/07/27/18.54.15/#comment-102291
// If we're not dealing with a regular pixel number
// but a number that has a weird ending, we need to convert it to pixels
if ( !rnumpx.test( ret ) && rnum.test( ret ) ) {
// Remember the original values
left = style.left;
rsLeft = elem.runtimeStyle && elem.runtimeStyle.left;
// Put in the new values to get a computed value out
if ( rsLeft ) {
elem.runtimeStyle.left = elem.currentStyle.left;
}
style.left = name === "fontSize" ? "1em" : ( ret || 0 );
ret = style.pixelLeft + "px";
// Revert the changed values
style.left = left;
if ( rsLeft ) {
elem.runtimeStyle.left = rsLeft;
}
}
return ret === "" ? "auto" : ret;
};
}
curCSS = getComputedStyle || currentStyle;
function getWH( elem, name, extra ) {
// Start with offset property
var val = name === "width" ? elem.offsetWidth : elem.offsetHeight,
which = name === "width" ? cssWidth : cssHeight,
i = 0,
len = which.length;
if ( val > 0 ) {
if ( extra !== "border" ) {
for ( ; i < len; i++ ) {
if ( !extra ) {
val -= parseFloat( jQuery.css( elem, "padding" + which[ i ] ) ) || 0;
}
if ( extra === "margin" ) {
val += parseFloat( jQuery.css( elem, extra + which[ i ] ) ) || 0;
} else {
val -= parseFloat( jQuery.css( elem, "border" + which[ i ] + "Width" ) ) || 0;
}
}
}
return val + "px";
}
// Fall back to computed then uncomputed css if necessary
val = curCSS( elem, name, name );
if ( val < 0 || val == null ) {
val = elem.style[ name ] || 0;
}
// Normalize "", auto, and prepare for extra
val = parseFloat( val ) || 0;
// Add padding, border, margin
if ( extra ) {
for ( ; i < len; i++ ) {
val += parseFloat( jQuery.css( elem, "padding" + which[ i ] ) ) || 0;
if ( extra !== "padding" ) {
val += parseFloat( jQuery.css( elem, "border" + which[ i ] + "Width" ) ) || 0;
}
if ( extra === "margin" ) {
val += parseFloat( jQuery.css( elem, extra + which[ i ] ) ) || 0;
}
}
}
return val + "px";
}
if ( jQuery.expr && jQuery.expr.filters ) {
jQuery.expr.filters.hidden = function( elem ) {
var width = elem.offsetWidth,
height = elem.offsetHeight;
return ( width === 0 && height === 0 ) || (!jQuery.support.reliableHiddenOffsets && ((elem.style && elem.style.display) || jQuery.css( elem, "display" )) === "none");
};
jQuery.expr.filters.visible = function( elem ) {
return !jQuery.expr.filters.hidden( elem );
};
}
var r20 = /%20/g,
rbracket = /\[\]$/,
rCRLF = /\r?\n/g,
rhash = /#.*$/,
rheaders = /^(.*?):[ \t]*([^\r\n]*)\r?$/mg, // IE leaves an \r character at EOL
rinput = /^(?:color|date|datetime|datetime-local|email|hidden|month|number|password|range|search|tel|text|time|url|week)$/i,
// #7653, #8125, #8152: local protocol detection
rlocalProtocol = /^(?:about|app|app\-storage|.+\-extension|file|res|widget):$/,
rnoContent = /^(?:GET|HEAD)$/,
rprotocol = /^\/\//,
rquery = /\?/,
rscript = /<script\b[^<]*(?:(?!<\/script>)<[^<]*)*<\/script>/gi,
rselectTextarea = /^(?:select|textarea)/i,
rspacesAjax = /\s+/,
rts = /([?&])_=[^&]*/,
rurl = /^([\w\+\.\-]+:)(?:\/\/([^\/?#:]*)(?::(\d+))?)?/,
// Keep a copy of the old load method
_load = jQuery.fn.load,
/* Prefilters
* 1) They are useful to introduce custom dataTypes (see ajax/jsonp.js for an example)
* 2) These are called:
* - BEFORE asking for a transport
* - AFTER param serialization (s.data is a string if s.processData is true)
* 3) key is the dataType
* 4) the catchall symbol "*" can be used
* 5) execution will start with transport dataType and THEN continue down to "*" if needed
*/
prefilters = {},
/* Transports bindings
* 1) key is the dataType
* 2) the catchall symbol "*" can be used
* 3) selection will start with transport dataType and THEN go to "*" if needed
*/
transports = {},
// Document location
ajaxLocation,
// Document location segments
ajaxLocParts,
// Avoid comment-prolog char sequence (#10098); must appease lint and evade compression
allTypes = ["*/"] + ["*"];
// #8138, IE may throw an exception when accessing
// a field from window.location if document.domain has been set
try {
ajaxLocation = location.href;
} catch( e ) {
// Use the href attribute of an A element
// since IE will modify it given document.location
ajaxLocation = document.createElement( "a" );
ajaxLocation.href = "";
ajaxLocation = ajaxLocation.href;
}
// Segment location into parts
ajaxLocParts = rurl.exec( ajaxLocation.toLowerCase() ) || [];
// Base "constructor" for jQuery.ajaxPrefilter and jQuery.ajaxTransport
function addToPrefiltersOrTransports( structure ) {
// dataTypeExpression is optional and defaults to "*"
return function( dataTypeExpression, func ) {
if ( typeof dataTypeExpression !== "string" ) {
func = dataTypeExpression;
dataTypeExpression = "*";
}
if ( jQuery.isFunction( func ) ) {
var dataTypes = dataTypeExpression.toLowerCase().split( rspacesAjax ),
i = 0,
length = dataTypes.length,
dataType,
list,
placeBefore;
// For each dataType in the dataTypeExpression
for ( ; i < length; i++ ) {
dataType = dataTypes[ i ];
// We control if we're asked to add before
// any existing element
placeBefore = /^\+/.test( dataType );
if ( placeBefore ) {
dataType = dataType.substr( 1 ) || "*";
}
list = structure[ dataType ] = structure[ dataType ] || [];
// then we add to the structure accordingly
list[ placeBefore ? "unshift" : "push" ]( func );
}
}
};
}
// Base inspection function for prefilters and transports
function inspectPrefiltersOrTransports( structure, options, originalOptions, jqXHR,
dataType /* internal */, inspected /* internal */ ) {
dataType = dataType || options.dataTypes[ 0 ];
inspected = inspected || {};
inspected[ dataType ] = true;
var list = structure[ dataType ],
i = 0,
length = list ? list.length : 0,
executeOnly = ( structure === prefilters ),
selection;
for ( ; i < length && ( executeOnly || !selection ); i++ ) {
selection = list[ i ]( options, originalOptions, jqXHR );
// If we got redirected to another dataType
// we try there if executing only and not done already
if ( typeof selection === "string" ) {
if ( !executeOnly || inspected[ selection ] ) {
selection = undefined;
} else {
options.dataTypes.unshift( selection );
selection = inspectPrefiltersOrTransports(
structure, options, originalOptions, jqXHR, selection, inspected );
}
}
}
// If we're only executing or nothing was selected
// we try the catchall dataType if not done already
if ( ( executeOnly || !selection ) && !inspected[ "*" ] ) {
selection = inspectPrefiltersOrTransports(
structure, options, originalOptions, jqXHR, "*", inspected );
}
// unnecessary when only executing (prefilters)
// but it'll be ignored by the caller in that case
return selection;
}
// A special extend for ajax options
// that takes "flat" options (not to be deep extended)
// Fixes #9887
function ajaxExtend( target, src ) {
var key, deep,
flatOptions = jQuery.ajaxSettings.flatOptions || {};
for ( key in src ) {
if ( src[ key ] !== undefined ) {
( flatOptions[ key ] ? target : ( deep || ( deep = {} ) ) )[ key ] = src[ key ];
}
}
if ( deep ) {
jQuery.extend( true, target, deep );
}
}
jQuery.fn.extend({
load: function( url, params, callback ) {
if ( typeof url !== "string" && _load ) {
return _load.apply( this, arguments );
// Don't do a request if no elements are being requested
} else if ( !this.length ) {
return this;
}
var off = url.indexOf( " " );
if ( off >= 0 ) {
var selector = url.slice( off, url.length );
url = url.slice( 0, off );
}
// Default to a GET request
var type = "GET";
// If the second parameter was provided
if ( params ) {
// If it's a function
if ( jQuery.isFunction( params ) ) {
// We assume that it's the callback
callback = params;
params = undefined;
// Otherwise, build a param string
} else if ( typeof params === "object" ) {
params = jQuery.param( params, jQuery.ajaxSettings.traditional );
type = "POST";
}
}
var self = this;
// Request the remote document
jQuery.ajax({
url: url,
type: type,
dataType: "html",
data: params,
// Complete callback (responseText is used internally)
complete: function( jqXHR, status, responseText ) {
// Store the response as specified by the jqXHR object
responseText = jqXHR.responseText;
// If successful, inject the HTML into all the matched elements
if ( jqXHR.isResolved() ) {
// #4825: Get the actual response in case
// a dataFilter is present in ajaxSettings
jqXHR.done(function( r ) {
responseText = r;
});
// See if a selector was specified
self.html( selector ?
// Create a dummy div to hold the results
jQuery("<div>")
// inject the contents of the document in, removing the scripts
// to avoid any 'Permission Denied' errors in IE
.append(responseText.replace(rscript, ""))
// Locate the specified elements
.find(selector) :
// If not, just inject the full result
responseText );
}
if ( callback ) {
self.each( callback, [ responseText, status, jqXHR ] );
}
}
});
return this;
},
serialize: function() {
return jQuery.param( this.serializeArray() );
},
serializeArray: function() {
return this.map(function(){
return this.elements ? jQuery.makeArray( this.elements ) : this;
})
.filter(function(){
return this.name && !this.disabled &&
( this.checked || rselectTextarea.test( this.nodeName ) ||
rinput.test( this.type ) );
})
.map(function( i, elem ){
var val = jQuery( this ).val();
return val == null ?
null :
jQuery.isArray( val ) ?
jQuery.map( val, function( val, i ){
return { name: elem.name, value: val.replace( rCRLF, "\r\n" ) };
}) :
{ name: elem.name, value: val.replace( rCRLF, "\r\n" ) };
}).get();
}
});
// Attach a bunch of functions for handling common AJAX events
jQuery.each( "ajaxStart ajaxStop ajaxComplete ajaxError ajaxSuccess ajaxSend".split( " " ), function( i, o ){
jQuery.fn[ o ] = function( f ){
return this.on( o, f );
};
});
jQuery.each( [ "get", "post" ], function( i, method ) {
jQuery[ method ] = function( url, data, callback, type ) {
// shift arguments if data argument was omitted
if ( jQuery.isFunction( data ) ) {
type = type || callback;
callback = data;
data = undefined;
}
return jQuery.ajax({
type: method,
url: url,
data: data,
success: callback,
dataType: type
});
};
});
jQuery.extend({
getScript: function( url, callback ) {
return jQuery.get( url, undefined, callback, "script" );
},
getJSON: function( url, data, callback ) {
return jQuery.get( url, data, callback, "json" );
},
// Creates a full fledged settings object into target
// with both ajaxSettings and settings fields.
// If target is omitted, writes into ajaxSettings.
ajaxSetup: function( target, settings ) {
if ( settings ) {
// Building a settings object
ajaxExtend( target, jQuery.ajaxSettings );
} else {
// Extending ajaxSettings
settings = target;
target = jQuery.ajaxSettings;
}
ajaxExtend( target, settings );
return target;
},
ajaxSettings: {
url: ajaxLocation,
isLocal: rlocalProtocol.test( ajaxLocParts[ 1 ] ),
global: true,
type: "GET",
contentType: "application/x-www-form-urlencoded",
processData: true,
async: true,
/*
timeout: 0,
data: null,
dataType: null,
username: null,
password: null,
cache: null,
traditional: false,
headers: {},
*/
accepts: {
xml: "application/xml, text/xml",
html: "text/html",
text: "text/plain",
json: "application/json, text/javascript",
"*": allTypes
},
contents: {
xml: /xml/,
html: /html/,
json: /json/
},
responseFields: {
xml: "responseXML",
text: "responseText"
},
// List of data converters
// 1) key format is "source_type destination_type" (a single space in-between)
// 2) the catchall symbol "*" can be used for source_type
converters: {
// Convert anything to text
"* text": window.String,
// Text to html (true = no transformation)
"text html": true,
// Evaluate text as a json expression
"text json": jQuery.parseJSON,
// Parse text as xml
"text xml": jQuery.parseXML
},
// For options that shouldn't be deep extended:
// you can add your own custom options here if
// and when you create one that shouldn't be
// deep extended (see ajaxExtend)
flatOptions: {
context: true,
url: true
}
},
ajaxPrefilter: addToPrefiltersOrTransports( prefilters ),
ajaxTransport: addToPrefiltersOrTransports( transports ),
// Main method
ajax: function( url, options ) {
// If url is an object, simulate pre-1.5 signature
if ( typeof url === "object" ) {
options = url;
url = undefined;
}
// Force options to be an object
options = options || {};
var // Create the final options object
s = jQuery.ajaxSetup( {}, options ),
// Callbacks context
callbackContext = s.context || s,
// Context for global events
// It's the callbackContext if one was provided in the options
// and if it's a DOM node or a jQuery collection
globalEventContext = callbackContext !== s &&
( callbackContext.nodeType || callbackContext instanceof jQuery ) ?
jQuery( callbackContext ) : jQuery.event,
// Deferreds
deferred = jQuery.Deferred(),
completeDeferred = jQuery.Callbacks( "once memory" ),
// Status-dependent callbacks
statusCode = s.statusCode || {},
// ifModified key
ifModifiedKey,
// Headers (they are sent all at once)
requestHeaders = {},
requestHeadersNames = {},
// Response headers
responseHeadersString,
responseHeaders,
// transport
transport,
// timeout handle
timeoutTimer,
// Cross-domain detection vars
parts,
// The jqXHR state
state = 0,
// To know if global events are to be dispatched
fireGlobals,
// Loop variable
i,
// Fake xhr
jqXHR = {
readyState: 0,
// Caches the header
setRequestHeader: function( name, value ) {
if ( !state ) {
var lname = name.toLowerCase();
name = requestHeadersNames[ lname ] = requestHeadersNames[ lname ] || name;
requestHeaders[ name ] = value;
}
return this;
},
// Raw string
getAllResponseHeaders: function() {
return state === 2 ? responseHeadersString : null;
},
// Builds headers hashtable if needed
getResponseHeader: function( key ) {
var match;
if ( state === 2 ) {
if ( !responseHeaders ) {
responseHeaders = {};
while( ( match = rheaders.exec( responseHeadersString ) ) ) {
responseHeaders[ match[1].toLowerCase() ] = match[ 2 ];
}
}
match = responseHeaders[ key.toLowerCase() ];
}
return match === undefined ? null : match;
},
// Overrides response content-type header
overrideMimeType: function( type ) {
if ( !state ) {
s.mimeType = type;
}
return this;
},
// Cancel the request
abort: function( statusText ) {
statusText = statusText || "abort";
if ( transport ) {
transport.abort( statusText );
}
done( 0, statusText );
return this;
},
url : s.url,
data : s.data
};
// Callback for when everything is done
// It is defined here because jslint complains if it is declared
// at the end of the function (which would be more logical and readable)
function done( status, nativeStatusText, responses, headers ) {
// Called once
if ( state === 2 ) {
return;
}
// State is "done" now
state = 2;
// Clear timeout if it exists
if ( timeoutTimer ) {
clearTimeout( timeoutTimer );
}
// Dereference transport for early garbage collection
// (no matter how long the jqXHR object will be used)
transport = undefined;
// Cache response headers
responseHeadersString = headers || "";
// Set readyState
jqXHR.readyState = status > 0 ? 4 : 0;
var isSuccess,
success,
error,
statusText = nativeStatusText,
response = responses ? ajaxHandleResponses( s, jqXHR, responses ) : undefined,
lastModified,
etag;
// If successful, handle type chaining
if ( status >= 200 && status < 300 || status === 304 ) {
// Set the If-Modified-Since and/or If-None-Match header, if in ifModified mode.
if ( s.ifModified ) {
if ( ( lastModified = jqXHR.getResponseHeader( "Last-Modified" ) ) ) {
jQuery.lastModified[ ifModifiedKey ] = lastModified;
}
if ( ( etag = jqXHR.getResponseHeader( "Etag" ) ) ) {
jQuery.etag[ ifModifiedKey ] = etag;
}
}
// If not modified
if ( status === 304 ) {
statusText = "notmodified";
isSuccess = true;
// If we have data
} else {
try {
success = ajaxConvert( s, response );
statusText = "success";
isSuccess = true;
} catch(e) {
// We have a parsererror
statusText = "parsererror";
error = e;
}
}
} else {
// We extract error from statusText
// then normalize statusText and status for non-aborts
error = statusText;
if ( !statusText || status ) {
statusText = "error";
if ( status < 0 ) {
status = 0;
}
}
}
// Set data for the fake xhr object
jqXHR.status = status;
jqXHR.statusText = "" + ( nativeStatusText || statusText );
// Success/Error
if ( isSuccess ) {
deferred.resolveWith( callbackContext, [ success, statusText, jqXHR ] );
} else {
deferred.rejectWith( callbackContext, [ jqXHR, statusText, error ] );
}
// Status-dependent callbacks
jqXHR.statusCode( statusCode );
statusCode = undefined;
if ( fireGlobals ) {
globalEventContext.trigger( "ajax" + ( isSuccess ? "Success" : "Error" ),
[ jqXHR, s, isSuccess ? success : error ] );
}
// Complete
completeDeferred.fireWith( callbackContext, [ jqXHR, statusText ] );
if ( fireGlobals ) {
globalEventContext.trigger( "ajaxComplete", [ jqXHR, s ] );
// Handle the global AJAX counter
if ( !( --jQuery.active ) ) {
jQuery.event.trigger( "ajaxStop" );
}
}
}
// Attach deferreds
deferred.promise( jqXHR );
jqXHR.success = jqXHR.done;
jqXHR.error = jqXHR.fail;
jqXHR.complete = completeDeferred.add;
// Status-dependent callbacks
jqXHR.statusCode = function( map ) {
if ( map ) {
var tmp;
if ( state < 2 ) {
for ( tmp in map ) {
statusCode[ tmp ] = [ statusCode[tmp], map[tmp] ];
}
} else {
tmp = map[ jqXHR.status ];
jqXHR.then( tmp, tmp );
}
}
return this;
};
// Remove hash character (#7531: and string promotion)
// Add protocol if not provided (#5866: IE7 issue with protocol-less urls)
// We also use the url parameter if available
s.url = ( ( url || s.url ) + "" ).replace( rhash, "" ).replace( rprotocol, ajaxLocParts[ 1 ] + "//" );
// Extract dataTypes list
s.dataTypes = jQuery.trim( s.dataType || "*" ).toLowerCase().split( rspacesAjax );
// Determine if a cross-domain request is in order
if ( s.crossDomain == null ) {
parts = rurl.exec( s.url.toLowerCase() );
s.crossDomain = !!( parts &&
( parts[ 1 ] != ajaxLocParts[ 1 ] || parts[ 2 ] != ajaxLocParts[ 2 ] ||
( parts[ 3 ] || ( parts[ 1 ] === "http:" ? 80 : 443 ) ) !=
( ajaxLocParts[ 3 ] || ( ajaxLocParts[ 1 ] === "http:" ? 80 : 443 ) ) )
);
}
// Convert data if not already a string
if ( s.data && s.processData && typeof s.data !== "string" ) {
s.data = jQuery.param( s.data, s.traditional );
}
// Apply prefilters
inspectPrefiltersOrTransports( prefilters, s, options, jqXHR );
// If request was aborted inside a prefiler, stop there
if ( state === 2 ) {
return false;
}
// We can fire global events as of now if asked to
fireGlobals = s.global;
// Uppercase the type
s.type = s.type.toUpperCase();
// Determine if request has content
s.hasContent = !rnoContent.test( s.type );
// Watch for a new set of requests
if ( fireGlobals && jQuery.active++ === 0 ) {
jQuery.event.trigger( "ajaxStart" );
}
// More options handling for requests with no content
if ( !s.hasContent ) {
// If data is available, append data to url
if ( s.data ) {
s.url += ( rquery.test( s.url ) ? "&" : "?" ) + s.data;
// #9682: remove data so that it's not used in an eventual retry
delete s.data;
}
// Get ifModifiedKey before adding the anti-cache parameter
ifModifiedKey = s.url;
// Add anti-cache in url if needed
if ( s.cache === false ) {
var ts = jQuery.now(),
// try replacing _= if it is there
ret = s.url.replace( rts, "$1_=" + ts );
// if nothing was replaced, add timestamp to the end
s.url = ret + ( ( ret === s.url ) ? ( rquery.test( s.url ) ? "&" : "?" ) + "_=" + ts : "" );
}
}
// Set the correct header, if data is being sent
if ( s.data && s.hasContent && s.contentType !== false || options.contentType ) {
jqXHR.setRequestHeader( "Content-Type", s.contentType );
}
// Set the If-Modified-Since and/or If-None-Match header, if in ifModified mode.
if ( s.ifModified ) {
ifModifiedKey = ifModifiedKey || s.url;
if ( jQuery.lastModified[ ifModifiedKey ] ) {
jqXHR.setRequestHeader( "If-Modified-Since", jQuery.lastModified[ ifModifiedKey ] );
}
if ( jQuery.etag[ ifModifiedKey ] ) {
jqXHR.setRequestHeader( "If-None-Match", jQuery.etag[ ifModifiedKey ] );
}
}
// Set the Accepts header for the server, depending on the dataType
jqXHR.setRequestHeader(
"Accept",
s.dataTypes[ 0 ] && s.accepts[ s.dataTypes[0] ] ?
s.accepts[ s.dataTypes[0] ] + ( s.dataTypes[ 0 ] !== "*" ? ", " + allTypes + "; q=0.01" : "" ) :
s.accepts[ "*" ]
);
// Check for headers option
for ( i in s.headers ) {
jqXHR.setRequestHeader( i, s.headers[ i ] );
}
// Allow custom headers/mimetypes and early abort
if ( s.beforeSend && ( s.beforeSend.call( callbackContext, jqXHR, s ) === false || state === 2 ) ) {
// Abort if not done already
jqXHR.abort();
return false;
}
// Install callbacks on deferreds
for ( i in { success: 1, error: 1, complete: 1 } ) {
jqXHR[ i ]( s[ i ] );
}
// Get transport
transport = inspectPrefiltersOrTransports( transports, s, options, jqXHR );
// If no transport, we auto-abort
if ( !transport ) {
done( -1, "No Transport" );
} else {
jqXHR.readyState = 1;
// Send global event
if ( fireGlobals ) {
globalEventContext.trigger( "ajaxSend", [ jqXHR, s ] );
}
// Timeout
if ( s.async && s.timeout > 0 ) {
timeoutTimer = setTimeout( function(){
jqXHR.abort( "timeout" );
}, s.timeout );
}
try {
state = 1;
transport.send( requestHeaders, done );
} catch (e) {
// Propagate exception as error if not done
if ( state < 2 ) {
done( -1, e );
// Simply rethrow otherwise
} else {
throw e;
}
}
}
return jqXHR;
},
// Serialize an array of form elements or a set of
// key/values into a query string
param: function( a, traditional ) {
var s = [],
add = function( key, value ) {
// If value is a function, invoke it and return its value
value = jQuery.isFunction( value ) ? value() : value;
s[ s.length ] = encodeURIComponent( key ) + "=" + encodeURIComponent( value );
};
// Set traditional to true for jQuery <= 1.3.2 behavior.
if ( traditional === undefined ) {
traditional = jQuery.ajaxSettings.traditional;
}
// If an array was passed in, assume that it is an array of form elements.
if ( jQuery.isArray( a ) || ( a.jquery && !jQuery.isPlainObject( a ) ) ) {
// Serialize the form elements
jQuery.each( a, function() {
add( this.name, this.value );
});
} else {
// If traditional, encode the "old" way (the way 1.3.2 or older
// did it), otherwise encode params recursively.
for ( var prefix in a ) {
buildParams( prefix, a[ prefix ], traditional, add );
}
}
// Return the resulting serialization
return s.join( "&" ).replace( r20, "+" );
}
});
function buildParams( prefix, obj, traditional, add ) {
if ( jQuery.isArray( obj ) ) {
// Serialize array item.
jQuery.each( obj, function( i, v ) {
if ( traditional || rbracket.test( prefix ) ) {
// Treat each array item as a scalar.
add( prefix, v );
} else {
// If array item is non-scalar (array or object), encode its
// numeric index to resolve deserialization ambiguity issues.
// Note that rack (as of 1.0.0) can't currently deserialize
// nested arrays properly, and attempting to do so may cause
// a server error. Possible fixes are to modify rack's
// deserialization algorithm or to provide an option or flag
// to force array serialization to be shallow.
buildParams( prefix + "[" + ( typeof v === "object" || jQuery.isArray(v) ? i : "" ) + "]", v, traditional, add );
}
});
} else if ( !traditional && obj != null && typeof obj === "object" ) {
// Serialize object item.
for ( var name in obj ) {
buildParams( prefix + "[" + name + "]", obj[ name ], traditional, add );
}
} else {
// Serialize scalar item.
add( prefix, obj );
}
}
// This is still on the jQuery object... for now
// Want to move this to jQuery.ajax some day
jQuery.extend({
// Counter for holding the number of active queries
active: 0,
// Last-Modified header cache for next request
lastModified: {},
etag: {}
});
/* Handles responses to an ajax request:
* - sets all responseXXX fields accordingly
* - finds the right dataType (mediates between content-type and expected dataType)
* - returns the corresponding response
*/
function ajaxHandleResponses( s, jqXHR, responses ) {
var contents = s.contents,
dataTypes = s.dataTypes,
responseFields = s.responseFields,
ct,
type,
finalDataType,
firstDataType;
// Fill responseXXX fields
for ( type in responseFields ) {
if ( type in responses ) {
jqXHR[ responseFields[type] ] = responses[ type ];
}
}
// Remove auto dataType and get content-type in the process
while( dataTypes[ 0 ] === "*" ) {
dataTypes.shift();
if ( ct === undefined ) {
ct = s.mimeType || jqXHR.getResponseHeader( "content-type" );
}
}
// Check if we're dealing with a known content-type
if ( ct ) {
for ( type in contents ) {
if ( contents[ type ] && contents[ type ].test( ct ) ) {
dataTypes.unshift( type );
break;
}
}
}
// Check to see if we have a response for the expected dataType
if ( dataTypes[ 0 ] in responses ) {
finalDataType = dataTypes[ 0 ];
} else {
// Try convertible dataTypes
for ( type in responses ) {
if ( !dataTypes[ 0 ] || s.converters[ type + " " + dataTypes[0] ] ) {
finalDataType = type;
break;
}
if ( !firstDataType ) {
firstDataType = type;
}
}
// Or just use first one
finalDataType = finalDataType || firstDataType;
}
// If we found a dataType
// We add the dataType to the list if needed
// and return the corresponding response
if ( finalDataType ) {
if ( finalDataType !== dataTypes[ 0 ] ) {
dataTypes.unshift( finalDataType );
}
return responses[ finalDataType ];
}
}
// Chain conversions given the request and the original response
function ajaxConvert( s, response ) {
// Apply the dataFilter if provided
if ( s.dataFilter ) {
response = s.dataFilter( response, s.dataType );
}
var dataTypes = s.dataTypes,
converters = {},
i,
key,
length = dataTypes.length,
tmp,
// Current and previous dataTypes
current = dataTypes[ 0 ],
prev,
// Conversion expression
conversion,
// Conversion function
conv,
// Conversion functions (transitive conversion)
conv1,
conv2;
// For each dataType in the chain
for ( i = 1; i < length; i++ ) {
// Create converters map
// with lowercased keys
if ( i === 1 ) {
for ( key in s.converters ) {
if ( typeof key === "string" ) {
converters[ key.toLowerCase() ] = s.converters[ key ];
}
}
}
// Get the dataTypes
prev = current;
current = dataTypes[ i ];
// If current is auto dataType, update it to prev
if ( current === "*" ) {
current = prev;
// If no auto and dataTypes are actually different
} else if ( prev !== "*" && prev !== current ) {
// Get the converter
conversion = prev + " " + current;
conv = converters[ conversion ] || converters[ "* " + current ];
// If there is no direct converter, search transitively
if ( !conv ) {
conv2 = undefined;
for ( conv1 in converters ) {
tmp = conv1.split( " " );
if ( tmp[ 0 ] === prev || tmp[ 0 ] === "*" ) {
conv2 = converters[ tmp[1] + " " + current ];
if ( conv2 ) {
conv1 = converters[ conv1 ];
if ( conv1 === true ) {
conv = conv2;
} else if ( conv2 === true ) {
conv = conv1;
}
break;
}
}
}
}
// If we found no converter, dispatch an error
if ( !( conv || conv2 ) ) {
jQuery.error( "No conversion from " + conversion.replace(" "," to ") );
}
// If found converter is not an equivalence
if ( conv !== true ) {
// Convert with 1 or 2 converters accordingly
response = conv ? conv( response ) : conv2( conv1(response) );
}
}
}
return response;
}
var jsc = jQuery.now(),
jsre = /(\=)\?(&|$)|\?\?/i;
// Default jsonp settings
jQuery.ajaxSetup({
jsonp: "callback",
jsonpCallback: function() {
return jQuery.expando + "_" + ( jsc++ );
}
});
// Detect, normalize options and install callbacks for jsonp requests
jQuery.ajaxPrefilter( "json jsonp", function( s, originalSettings, jqXHR ) {
var inspectData = s.contentType === "application/x-www-form-urlencoded" &&
( typeof s.data === "string" );
if ( s.dataTypes[ 0 ] === "jsonp" ||
s.jsonp !== false && ( jsre.test( s.url ) ||
inspectData && jsre.test( s.data ) ) ) {
var responseContainer,
jsonpCallback = s.jsonpCallback =
jQuery.isFunction( s.jsonpCallback ) ? s.jsonpCallback() : s.jsonpCallback,
previous = window[ jsonpCallback ],
url = s.url,
data = s.data,
replace = "$1" + jsonpCallback + "$2";
if ( s.jsonp !== false ) {
url = url.replace( jsre, replace );
if ( s.url === url ) {
if ( inspectData ) {
data = data.replace( jsre, replace );
}
if ( s.data === data ) {
// Add callback manually
url += (/\?/.test( url ) ? "&" : "?") + s.jsonp + "=" + jsonpCallback;
}
}
}
s.url = url;
s.data = data;
// Install callback
window[ jsonpCallback ] = function( response ) {
responseContainer = [ response ];
};
// Clean-up function
jqXHR.always(function() {
// Set callback back to previous value
window[ jsonpCallback ] = previous;
// Call if it was a function and we have a response
if ( responseContainer && jQuery.isFunction( previous ) ) {
window[ jsonpCallback ]( responseContainer[ 0 ] );
}
});
// Use data converter to retrieve json after script execution
s.converters["script json"] = function() {
if ( !responseContainer ) {
jQuery.error( jsonpCallback + " was not called" );
}
return responseContainer[ 0 ];
};
// force json dataType
s.dataTypes[ 0 ] = "json";
// Delegate to script
return "script";
}
});
// Install script dataType
jQuery.ajaxSetup({
accepts: {
script: "text/javascript, application/javascript, application/ecmascript, application/x-ecmascript"
},
contents: {
script: /javascript|ecmascript/
},
converters: {
"text script": function( text ) {
jQuery.globalEval( text );
return text;
}
}
});
// Handle cache's special case and global
jQuery.ajaxPrefilter( "script", function( s ) {
if ( s.cache === undefined ) {
s.cache = false;
}
if ( s.crossDomain ) {
s.type = "GET";
s.global = false;
}
});
// Bind script tag hack transport
jQuery.ajaxTransport( "script", function(s) {
// This transport only deals with cross domain requests
if ( s.crossDomain ) {
var script,
head = document.head || document.getElementsByTagName( "head" )[0] || document.documentElement;
return {
send: function( _, callback ) {
script = document.createElement( "script" );
script.async = "async";
if ( s.scriptCharset ) {
script.charset = s.scriptCharset;
}
script.src = s.url;
// Attach handlers for all browsers
script.onload = script.onreadystatechange = function( _, isAbort ) {
if ( isAbort || !script.readyState || /loaded|complete/.test( script.readyState ) ) {
// Handle memory leak in IE
script.onload = script.onreadystatechange = null;
// Remove the script
if ( head && script.parentNode ) {
head.removeChild( script );
}
// Dereference the script
script = undefined;
// Callback if not abort
if ( !isAbort ) {
callback( 200, "success" );
}
}
};
// Use insertBefore instead of appendChild to circumvent an IE6 bug.
// This arises when a base node is used (#2709 and #4378).
head.insertBefore( script, head.firstChild );
},
abort: function() {
if ( script ) {
script.onload( 0, 1 );
}
}
};
}
});
var // #5280: Internet Explorer will keep connections alive if we don't abort on unload
xhrOnUnloadAbort = window.ActiveXObject ? function() {
// Abort all pending requests
for ( var key in xhrCallbacks ) {
xhrCallbacks[ key ]( 0, 1 );
}
} : false,
xhrId = 0,
xhrCallbacks;
// Functions to create xhrs
function createStandardXHR() {
try {
return new window.XMLHttpRequest();
} catch( e ) {}
}
function createActiveXHR() {
try {
return new window.ActiveXObject( "Microsoft.XMLHTTP" );
} catch( e ) {}
}
// Create the request object
// (This is still attached to ajaxSettings for backward compatibility)
jQuery.ajaxSettings.xhr = window.ActiveXObject ?
/* Microsoft failed to properly
* implement the XMLHttpRequest in IE7 (can't request local files),
* so we use the ActiveXObject when it is available
* Additionally XMLHttpRequest can be disabled in IE7/IE8 so
* we need a fallback.
*/
function() {
return !this.isLocal && createStandardXHR() || createActiveXHR();
} :
// For all other browsers, use the standard XMLHttpRequest object
createStandardXHR;
// Determine support properties
(function( xhr ) {
jQuery.extend( jQuery.support, {
ajax: !!xhr,
cors: !!xhr && ( "withCredentials" in xhr )
});
})( jQuery.ajaxSettings.xhr() );
// Create transport if the browser can provide an xhr
if ( jQuery.support.ajax ) {
jQuery.ajaxTransport(function( s ) {
// Cross domain only allowed if supported through XMLHttpRequest
if ( !s.crossDomain || jQuery.support.cors ) {
var callback;
return {
send: function( headers, complete ) {
// Get a new xhr
var xhr = s.xhr(),
handle,
i;
// Open the socket
// Passing null username, generates a login popup on Opera (#2865)
if ( s.username ) {
xhr.open( s.type, s.url, s.async, s.username, s.password );
} else {
xhr.open( s.type, s.url, s.async );
}
// Apply custom fields if provided
if ( s.xhrFields ) {
for ( i in s.xhrFields ) {
xhr[ i ] = s.xhrFields[ i ];
}
}
// Override mime type if needed
if ( s.mimeType && xhr.overrideMimeType ) {
xhr.overrideMimeType( s.mimeType );
}
// X-Requested-With header
// For cross-domain requests, seeing as conditions for a preflight are
// akin to a jigsaw puzzle, we simply never set it to be sure.
// (it can always be set on a per-request basis or even using ajaxSetup)
// For same-domain requests, won't change header if already provided.
if ( !s.crossDomain && !headers["X-Requested-With"] ) {
headers[ "X-Requested-With" ] = "XMLHttpRequest";
}
// Need an extra try/catch for cross domain requests in Firefox 3
try {
for ( i in headers ) {
xhr.setRequestHeader( i, headers[ i ] );
}
} catch( _ ) {}
// Do send the request
// This may raise an exception which is actually
// handled in jQuery.ajax (so no try/catch here)
xhr.send( ( s.hasContent && s.data ) || null );
// Listener
callback = function( _, isAbort ) {
var status,
statusText,
responseHeaders,
responses,
xml;
// Firefox throws exceptions when accessing properties
// of an xhr when a network error occured
// http://helpful.knobs-dials.com/index.php/Component_returned_failure_code:_0x80040111_(NS_ERROR_NOT_AVAILABLE)
try {
// Was never called and is aborted or complete
if ( callback && ( isAbort || xhr.readyState === 4 ) ) {
// Only called once
callback = undefined;
// Do not keep as active anymore
if ( handle ) {
xhr.onreadystatechange = jQuery.noop;
if ( xhrOnUnloadAbort ) {
delete xhrCallbacks[ handle ];
}
}
// If it's an abort
if ( isAbort ) {
// Abort it manually if needed
if ( xhr.readyState !== 4 ) {
xhr.abort();
}
} else {
status = xhr.status;
responseHeaders = xhr.getAllResponseHeaders();
responses = {};
xml = xhr.responseXML;
// Construct response list
if ( xml && xml.documentElement /* #4958 */ ) {
responses.xml = xml;
}
responses.text = xhr.responseText;
// Firefox throws an exception when accessing
// statusText for faulty cross-domain requests
try {
statusText = xhr.statusText;
} catch( e ) {
// We normalize with Webkit giving an empty statusText
statusText = "";
}
// Filter status for non standard behaviors
// If the request is local and we have data: assume a success
// (success with no data won't get notified, that's the best we
// can do given current implementations)
if ( !status && s.isLocal && !s.crossDomain ) {
status = responses.text ? 200 : 404;
// IE - #1450: sometimes returns 1223 when it should be 204
} else if ( status === 1223 ) {
status = 204;
}
}
}
} catch( firefoxAccessException ) {
if ( !isAbort ) {
complete( -1, firefoxAccessException );
}
}
// Call complete if needed
if ( responses ) {
complete( status, statusText, responses, responseHeaders );
}
};
// if we're in sync mode or it's in cache
// and has been retrieved directly (IE6 & IE7)
// we need to manually fire the callback
if ( !s.async || xhr.readyState === 4 ) {
callback();
} else {
handle = ++xhrId;
if ( xhrOnUnloadAbort ) {
// Create the active xhrs callbacks list if needed
// and attach the unload handler
if ( !xhrCallbacks ) {
xhrCallbacks = {};
jQuery( window ).unload( xhrOnUnloadAbort );
}
// Add to list of active xhrs callbacks
xhrCallbacks[ handle ] = callback;
}
xhr.onreadystatechange = callback;
}
},
abort: function() {
if ( callback ) {
callback(0,1);
}
}
};
}
});
}
var elemdisplay = {},
iframe, iframeDoc,
rfxtypes = /^(?:toggle|show|hide)$/,
rfxnum = /^([+\-]=)?([\d+.\-]+)([a-z%]*)$/i,
timerId,
fxAttrs = [
// height animations
[ "height", "marginTop", "marginBottom", "paddingTop", "paddingBottom" ],
// width animations
[ "width", "marginLeft", "marginRight", "paddingLeft", "paddingRight" ],
// opacity animations
[ "opacity" ]
],
fxNow;
jQuery.fn.extend({
show: function( speed, easing, callback ) {
var elem, display;
if ( speed || speed === 0 ) {
return this.animate( genFx("show", 3), speed, easing, callback );
} else {
for ( var i = 0, j = this.length; i < j; i++ ) {
elem = this[ i ];
if ( elem.style ) {
display = elem.style.display;
// Reset the inline display of this element to learn if it is
// being hidden by cascaded rules or not
if ( !jQuery._data(elem, "olddisplay") && display === "none" ) {
display = elem.style.display = "";
}
// Set elements which have been overridden with display: none
// in a stylesheet to whatever the default browser style is
// for such an element
if ( display === "" && jQuery.css(elem, "display") === "none" ) {
jQuery._data( elem, "olddisplay", defaultDisplay(elem.nodeName) );
}
}
}
// Set the display of most of the elements in a second loop
// to avoid the constant reflow
for ( i = 0; i < j; i++ ) {
elem = this[ i ];
if ( elem.style ) {
display = elem.style.display;
if ( display === "" || display === "none" ) {
elem.style.display = jQuery._data( elem, "olddisplay" ) || "";
}
}
}
return this;
}
},
hide: function( speed, easing, callback ) {
if ( speed || speed === 0 ) {
return this.animate( genFx("hide", 3), speed, easing, callback);
} else {
var elem, display,
i = 0,
j = this.length;
for ( ; i < j; i++ ) {
elem = this[i];
if ( elem.style ) {
display = jQuery.css( elem, "display" );
if ( display !== "none" && !jQuery._data( elem, "olddisplay" ) ) {
jQuery._data( elem, "olddisplay", display );
}
}
}
// Set the display of the elements in a second loop
// to avoid the constant reflow
for ( i = 0; i < j; i++ ) {
if ( this[i].style ) {
this[i].style.display = "none";
}
}
return this;
}
},
// Save the old toggle function
_toggle: jQuery.fn.toggle,
toggle: function( fn, fn2, callback ) {
var bool = typeof fn === "boolean";
if ( jQuery.isFunction(fn) && jQuery.isFunction(fn2) ) {
this._toggle.apply( this, arguments );
} else if ( fn == null || bool ) {
this.each(function() {
var state = bool ? fn : jQuery(this).is(":hidden");
jQuery(this)[ state ? "show" : "hide" ]();
});
} else {
this.animate(genFx("toggle", 3), fn, fn2, callback);
}
return this;
},
fadeTo: function( speed, to, easing, callback ) {
return this.filter(":hidden").css("opacity", 0).show().end()
.animate({opacity: to}, speed, easing, callback);
},
animate: function( prop, speed, easing, callback ) {
var optall = jQuery.speed( speed, easing, callback );
if ( jQuery.isEmptyObject( prop ) ) {
return this.each( optall.complete, [ false ] );
}
// Do not change referenced properties as per-property easing will be lost
prop = jQuery.extend( {}, prop );
function doAnimation() {
// XXX 'this' does not always have a nodeName when running the
// test suite
if ( optall.queue === false ) {
jQuery._mark( this );
}
var opt = jQuery.extend( {}, optall ),
isElement = this.nodeType === 1,
hidden = isElement && jQuery(this).is(":hidden"),
name, val, p, e,
parts, start, end, unit,
method;
// will store per property easing and be used to determine when an animation is complete
opt.animatedProperties = {};
for ( p in prop ) {
// property name normalization
name = jQuery.camelCase( p );
if ( p !== name ) {
prop[ name ] = prop[ p ];
delete prop[ p ];
}
val = prop[ name ];
// easing resolution: per property > opt.specialEasing > opt.easing > 'swing' (default)
if ( jQuery.isArray( val ) ) {
opt.animatedProperties[ name ] = val[ 1 ];
val = prop[ name ] = val[ 0 ];
} else {
opt.animatedProperties[ name ] = opt.specialEasing && opt.specialEasing[ name ] || opt.easing || 'swing';
}
if ( val === "hide" && hidden || val === "show" && !hidden ) {
return opt.complete.call( this );
}
if ( isElement && ( name === "height" || name === "width" ) ) {
// Make sure that nothing sneaks out
// Record all 3 overflow attributes because IE does not
// change the overflow attribute when overflowX and
// overflowY are set to the same value
opt.overflow = [ this.style.overflow, this.style.overflowX, this.style.overflowY ];
// Set display property to inline-block for height/width
// animations on inline elements that are having width/height animated
if ( jQuery.css( this, "display" ) === "inline" &&
jQuery.css( this, "float" ) === "none" ) {
// inline-level elements accept inline-block;
// block-level elements need to be inline with layout
if ( !jQuery.support.inlineBlockNeedsLayout || defaultDisplay( this.nodeName ) === "inline" ) {
this.style.display = "inline-block";
} else {
this.style.zoom = 1;
}
}
}
}
if ( opt.overflow != null ) {
this.style.overflow = "hidden";
}
for ( p in prop ) {
e = new jQuery.fx( this, opt, p );
val = prop[ p ];
if ( rfxtypes.test( val ) ) {
// Tracks whether to show or hide based on private
// data attached to the element
method = jQuery._data( this, "toggle" + p ) || ( val === "toggle" ? hidden ? "show" : "hide" : 0 );
if ( method ) {
jQuery._data( this, "toggle" + p, method === "show" ? "hide" : "show" );
e[ method ]();
} else {
e[ val ]();
}
} else {
parts = rfxnum.exec( val );
start = e.cur();
if ( parts ) {
end = parseFloat( parts[2] );
unit = parts[3] || ( jQuery.cssNumber[ p ] ? "" : "px" );
// We need to compute starting value
if ( unit !== "px" ) {
jQuery.style( this, p, (end || 1) + unit);
start = ( (end || 1) / e.cur() ) * start;
jQuery.style( this, p, start + unit);
}
// If a +=/-= token was provided, we're doing a relative animation
if ( parts[1] ) {
end = ( (parts[ 1 ] === "-=" ? -1 : 1) * end ) + start;
}
e.custom( start, end, unit );
} else {
e.custom( start, val, "" );
}
}
}
// For JS strict compliance
return true;
}
return optall.queue === false ?
this.each( doAnimation ) :
this.queue( optall.queue, doAnimation );
},
stop: function( type, clearQueue, gotoEnd ) {
if ( typeof type !== "string" ) {
gotoEnd = clearQueue;
clearQueue = type;
type = undefined;
}
if ( clearQueue && type !== false ) {
this.queue( type || "fx", [] );
}
return this.each(function() {
var index,
hadTimers = false,
timers = jQuery.timers,
data = jQuery._data( this );
// clear marker counters if we know they won't be
if ( !gotoEnd ) {
jQuery._unmark( true, this );
}
function stopQueue( elem, data, index ) {
var hooks = data[ index ];
jQuery.removeData( elem, index, true );
hooks.stop( gotoEnd );
}
if ( type == null ) {
for ( index in data ) {
if ( data[ index ] && data[ index ].stop && index.indexOf(".run") === index.length - 4 ) {
stopQueue( this, data, index );
}
}
} else if ( data[ index = type + ".run" ] && data[ index ].stop ){
stopQueue( this, data, index );
}
for ( index = timers.length; index--; ) {
if ( timers[ index ].elem === this && (type == null || timers[ index ].queue === type) ) {
if ( gotoEnd ) {
// force the next step to be the last
timers[ index ]( true );
} else {
timers[ index ].saveState();
}
hadTimers = true;
timers.splice( index, 1 );
}
}
// start the next in the queue if the last step wasn't forced
// timers currently will call their complete callbacks, which will dequeue
// but only if they were gotoEnd
if ( !( gotoEnd && hadTimers ) ) {
jQuery.dequeue( this, type );
}
});
}
});
// Animations created synchronously will run synchronously
function createFxNow() {
setTimeout( clearFxNow, 0 );
return ( fxNow = jQuery.now() );
}
function clearFxNow() {
fxNow = undefined;
}
// Generate parameters to create a standard animation
function genFx( type, num ) {
var obj = {};
jQuery.each( fxAttrs.concat.apply([], fxAttrs.slice( 0, num )), function() {
obj[ this ] = type;
});
return obj;
}
// Generate shortcuts for custom animations
jQuery.each({
slideDown: genFx( "show", 1 ),
slideUp: genFx( "hide", 1 ),
slideToggle: genFx( "toggle", 1 ),
fadeIn: { opacity: "show" },
fadeOut: { opacity: "hide" },
fadeToggle: { opacity: "toggle" }
}, function( name, props ) {
jQuery.fn[ name ] = function( speed, easing, callback ) {
return this.animate( props, speed, easing, callback );
};
});
jQuery.extend({
speed: function( speed, easing, fn ) {
var opt = speed && typeof speed === "object" ? jQuery.extend( {}, speed ) : {
complete: fn || !fn && easing ||
jQuery.isFunction( speed ) && speed,
duration: speed,
easing: fn && easing || easing && !jQuery.isFunction( easing ) && easing
};
opt.duration = jQuery.fx.off ? 0 : typeof opt.duration === "number" ? opt.duration :
opt.duration in jQuery.fx.speeds ? jQuery.fx.speeds[ opt.duration ] : jQuery.fx.speeds._default;
// normalize opt.queue - true/undefined/null -> "fx"
if ( opt.queue == null || opt.queue === true ) {
opt.queue = "fx";
}
// Queueing
opt.old = opt.complete;
opt.complete = function( noUnmark ) {
if ( jQuery.isFunction( opt.old ) ) {
opt.old.call( this );
}
if ( opt.queue ) {
jQuery.dequeue( this, opt.queue );
} else if ( noUnmark !== false ) {
jQuery._unmark( this );
}
};
return opt;
},
easing: {
linear: function( p, n, firstNum, diff ) {
return firstNum + diff * p;
},
swing: function( p, n, firstNum, diff ) {
return ( ( -Math.cos( p*Math.PI ) / 2 ) + 0.5 ) * diff + firstNum;
}
},
timers: [],
fx: function( elem, options, prop ) {
this.options = options;
this.elem = elem;
this.prop = prop;
options.orig = options.orig || {};
}
});
jQuery.fx.prototype = {
// Simple function for setting a style value
update: function() {
if ( this.options.step ) {
this.options.step.call( this.elem, this.now, this );
}
( jQuery.fx.step[ this.prop ] || jQuery.fx.step._default )( this );
},
// Get the current size
cur: function() {
if ( this.elem[ this.prop ] != null && (!this.elem.style || this.elem.style[ this.prop ] == null) ) {
return this.elem[ this.prop ];
}
var parsed,
r = jQuery.css( this.elem, this.prop );
// Empty strings, null, undefined and "auto" are converted to 0,
// complex values such as "rotate(1rad)" are returned as is,
// simple values such as "10px" are parsed to Float.
return isNaN( parsed = parseFloat( r ) ) ? !r || r === "auto" ? 0 : r : parsed;
},
// Start an animation from one number to another
custom: function( from, to, unit ) {
var self = this,
fx = jQuery.fx;
this.startTime = fxNow || createFxNow();
this.end = to;
this.now = this.start = from;
this.pos = this.state = 0;
this.unit = unit || this.unit || ( jQuery.cssNumber[ this.prop ] ? "" : "px" );
function t( gotoEnd ) {
return self.step( gotoEnd );
}
t.queue = this.options.queue;
t.elem = this.elem;
t.saveState = function() {
if ( self.options.hide && jQuery._data( self.elem, "fxshow" + self.prop ) === undefined ) {
jQuery._data( self.elem, "fxshow" + self.prop, self.start );
}
};
if ( t() && jQuery.timers.push(t) && !timerId ) {
timerId = setInterval( fx.tick, fx.interval );
}
},
// Simple 'show' function
show: function() {
var dataShow = jQuery._data( this.elem, "fxshow" + this.prop );
// Remember where we started, so that we can go back to it later
this.options.orig[ this.prop ] = dataShow || jQuery.style( this.elem, this.prop );
this.options.show = true;
// Begin the animation
// Make sure that we start at a small width/height to avoid any flash of content
if ( dataShow !== undefined ) {
// This show is picking up where a previous hide or show left off
this.custom( this.cur(), dataShow );
} else {
this.custom( this.prop === "width" || this.prop === "height" ? 1 : 0, this.cur() );
}
// Start by showing the element
jQuery( this.elem ).show();
},
// Simple 'hide' function
hide: function() {
// Remember where we started, so that we can go back to it later
this.options.orig[ this.prop ] = jQuery._data( this.elem, "fxshow" + this.prop ) || jQuery.style( this.elem, this.prop );
this.options.hide = true;
// Begin the animation
this.custom( this.cur(), 0 );
},
// Each step of an animation
step: function( gotoEnd ) {
var p, n, complete,
t = fxNow || createFxNow(),
done = true,
elem = this.elem,
options = this.options;
if ( gotoEnd || t >= options.duration + this.startTime ) {
this.now = this.end;
this.pos = this.state = 1;
this.update();
options.animatedProperties[ this.prop ] = true;
for ( p in options.animatedProperties ) {
if ( options.animatedProperties[ p ] !== true ) {
done = false;
}
}
if ( done ) {
// Reset the overflow
if ( options.overflow != null && !jQuery.support.shrinkWrapBlocks ) {
jQuery.each( [ "", "X", "Y" ], function( index, value ) {
elem.style[ "overflow" + value ] = options.overflow[ index ];
});
}
// Hide the element if the "hide" operation was done
if ( options.hide ) {
jQuery( elem ).hide();
}
// Reset the properties, if the item has been hidden or shown
if ( options.hide || options.show ) {
for ( p in options.animatedProperties ) {
jQuery.style( elem, p, options.orig[ p ] );
jQuery.removeData( elem, "fxshow" + p, true );
// Toggle data is no longer needed
jQuery.removeData( elem, "toggle" + p, true );
}
}
// Execute the complete function
// in the event that the complete function throws an exception
// we must ensure it won't be called twice. #5684
complete = options.complete;
if ( complete ) {
options.complete = false;
complete.call( elem );
}
}
return false;
} else {
// classical easing cannot be used with an Infinity duration
if ( options.duration == Infinity ) {
this.now = t;
} else {
n = t - this.startTime;
this.state = n / options.duration;
// Perform the easing function, defaults to swing
this.pos = jQuery.easing[ options.animatedProperties[this.prop] ]( this.state, n, 0, 1, options.duration );
this.now = this.start + ( (this.end - this.start) * this.pos );
}
// Perform the next step of the animation
this.update();
}
return true;
}
};
jQuery.extend( jQuery.fx, {
tick: function() {
var timer,
timers = jQuery.timers,
i = 0;
for ( ; i < timers.length; i++ ) {
timer = timers[ i ];
// Checks the timer has not already been removed
if ( !timer() && timers[ i ] === timer ) {
timers.splice( i--, 1 );
}
}
if ( !timers.length ) {
jQuery.fx.stop();
}
},
interval: 13,
stop: function() {
clearInterval( timerId );
timerId = null;
},
speeds: {
slow: 600,
fast: 200,
// Default speed
_default: 400
},
step: {
opacity: function( fx ) {
jQuery.style( fx.elem, "opacity", fx.now );
},
_default: function( fx ) {
if ( fx.elem.style && fx.elem.style[ fx.prop ] != null ) {
fx.elem.style[ fx.prop ] = fx.now + fx.unit;
} else {
fx.elem[ fx.prop ] = fx.now;
}
}
}
});
// Adds width/height step functions
// Do not set anything below 0
jQuery.each([ "width", "height" ], function( i, prop ) {
jQuery.fx.step[ prop ] = function( fx ) {
jQuery.style( fx.elem, prop, Math.max(0, fx.now) + fx.unit );
};
});
if ( jQuery.expr && jQuery.expr.filters ) {
jQuery.expr.filters.animated = function( elem ) {
return jQuery.grep(jQuery.timers, function( fn ) {
return elem === fn.elem;
}).length;
};
}
// Try to restore the default display value of an element
function defaultDisplay( nodeName ) {
if ( !elemdisplay[ nodeName ] ) {
var body = document.body,
elem = jQuery( "<" + nodeName + ">" ).appendTo( body ),
display = elem.css( "display" );
elem.remove();
// If the simple way fails,
// get element's real default display by attaching it to a temp iframe
if ( display === "none" || display === "" ) {
// No iframe to use yet, so create it
if ( !iframe ) {
iframe = document.createElement( "iframe" );
iframe.frameBorder = iframe.width = iframe.height = 0;
}
body.appendChild( iframe );
// Create a cacheable copy of the iframe document on first call.
// IE and Opera will allow us to reuse the iframeDoc without re-writing the fake HTML
// document to it; WebKit & Firefox won't allow reusing the iframe document.
if ( !iframeDoc || !iframe.createElement ) {
iframeDoc = ( iframe.contentWindow || iframe.contentDocument ).document;
iframeDoc.write( ( document.compatMode === "CSS1Compat" ? "<!doctype html>" : "" ) + "<html><body>" );
iframeDoc.close();
}
elem = iframeDoc.createElement( nodeName );
iframeDoc.body.appendChild( elem );
display = jQuery.css( elem, "display" );
body.removeChild( iframe );
}
// Store the correct default display
elemdisplay[ nodeName ] = display;
}
return elemdisplay[ nodeName ];
}
var rtable = /^t(?:able|d|h)$/i,
rroot = /^(?:body|html)$/i;
if ( "getBoundingClientRect" in document.documentElement ) {
jQuery.fn.offset = function( options ) {
var elem = this[0], box;
if ( options ) {
return this.each(function( i ) {
jQuery.offset.setOffset( this, options, i );
});
}
if ( !elem || !elem.ownerDocument ) {
return null;
}
if ( elem === elem.ownerDocument.body ) {
return jQuery.offset.bodyOffset( elem );
}
try {
box = elem.getBoundingClientRect();
} catch(e) {}
var doc = elem.ownerDocument,
docElem = doc.documentElement;
// Make sure we're not dealing with a disconnected DOM node
if ( !box || !jQuery.contains( docElem, elem ) ) {
return box ? { top: box.top, left: box.left } : { top: 0, left: 0 };
}
var body = doc.body,
win = getWindow(doc),
clientTop = docElem.clientTop || body.clientTop || 0,
clientLeft = docElem.clientLeft || body.clientLeft || 0,
scrollTop = win.pageYOffset || jQuery.support.boxModel && docElem.scrollTop || body.scrollTop,
scrollLeft = win.pageXOffset || jQuery.support.boxModel && docElem.scrollLeft || body.scrollLeft,
top = box.top + scrollTop - clientTop,
left = box.left + scrollLeft - clientLeft;
return { top: top, left: left };
};
} else {
jQuery.fn.offset = function( options ) {
var elem = this[0];
if ( options ) {
return this.each(function( i ) {
jQuery.offset.setOffset( this, options, i );
});
}
if ( !elem || !elem.ownerDocument ) {
return null;
}
if ( elem === elem.ownerDocument.body ) {
return jQuery.offset.bodyOffset( elem );
}
var computedStyle,
offsetParent = elem.offsetParent,
prevOffsetParent = elem,
doc = elem.ownerDocument,
docElem = doc.documentElement,
body = doc.body,
defaultView = doc.defaultView,
prevComputedStyle = defaultView ? defaultView.getComputedStyle( elem, null ) : elem.currentStyle,
top = elem.offsetTop,
left = elem.offsetLeft;
while ( (elem = elem.parentNode) && elem !== body && elem !== docElem ) {
if ( jQuery.support.fixedPosition && prevComputedStyle.position === "fixed" ) {
break;
}
computedStyle = defaultView ? defaultView.getComputedStyle(elem, null) : elem.currentStyle;
top -= elem.scrollTop;
left -= elem.scrollLeft;
if ( elem === offsetParent ) {
top += elem.offsetTop;
left += elem.offsetLeft;
if ( jQuery.support.doesNotAddBorder && !(jQuery.support.doesAddBorderForTableAndCells && rtable.test(elem.nodeName)) ) {
top += parseFloat( computedStyle.borderTopWidth ) || 0;
left += parseFloat( computedStyle.borderLeftWidth ) || 0;
}
prevOffsetParent = offsetParent;
offsetParent = elem.offsetParent;
}
if ( jQuery.support.subtractsBorderForOverflowNotVisible && computedStyle.overflow !== "visible" ) {
top += parseFloat( computedStyle.borderTopWidth ) || 0;
left += parseFloat( computedStyle.borderLeftWidth ) || 0;
}
prevComputedStyle = computedStyle;
}
if ( prevComputedStyle.position === "relative" || prevComputedStyle.position === "static" ) {
top += body.offsetTop;
left += body.offsetLeft;
}
if ( jQuery.support.fixedPosition && prevComputedStyle.position === "fixed" ) {
top += Math.max( docElem.scrollTop, body.scrollTop );
left += Math.max( docElem.scrollLeft, body.scrollLeft );
}
return { top: top, left: left };
};
}
jQuery.offset = {
bodyOffset: function( body ) {
var top = body.offsetTop,
left = body.offsetLeft;
if ( jQuery.support.doesNotIncludeMarginInBodyOffset ) {
top += parseFloat( jQuery.css(body, "marginTop") ) || 0;
left += parseFloat( jQuery.css(body, "marginLeft") ) || 0;
}
return { top: top, left: left };
},
setOffset: function( elem, options, i ) {
var position = jQuery.css( elem, "position" );
// set position first, in-case top/left are set even on static elem
if ( position === "static" ) {
elem.style.position = "relative";
}
var curElem = jQuery( elem ),
curOffset = curElem.offset(),
curCSSTop = jQuery.css( elem, "top" ),
curCSSLeft = jQuery.css( elem, "left" ),
calculatePosition = ( position === "absolute" || position === "fixed" ) && jQuery.inArray("auto", [curCSSTop, curCSSLeft]) > -1,
props = {}, curPosition = {}, curTop, curLeft;
// need to be able to calculate position if either top or left is auto and position is either absolute or fixed
if ( calculatePosition ) {
curPosition = curElem.position();
curTop = curPosition.top;
curLeft = curPosition.left;
} else {
curTop = parseFloat( curCSSTop ) || 0;
curLeft = parseFloat( curCSSLeft ) || 0;
}
if ( jQuery.isFunction( options ) ) {
options = options.call( elem, i, curOffset );
}
if ( options.top != null ) {
props.top = ( options.top - curOffset.top ) + curTop;
}
if ( options.left != null ) {
props.left = ( options.left - curOffset.left ) + curLeft;
}
if ( "using" in options ) {
options.using.call( elem, props );
} else {
curElem.css( props );
}
}
};
jQuery.fn.extend({
position: function() {
if ( !this[0] ) {
return null;
}
var elem = this[0],
// Get *real* offsetParent
offsetParent = this.offsetParent(),
// Get correct offsets
offset = this.offset(),
parentOffset = rroot.test(offsetParent[0].nodeName) ? { top: 0, left: 0 } : offsetParent.offset();
// Subtract element margins
// note: when an element has margin: auto the offsetLeft and marginLeft
// are the same in Safari causing offset.left to incorrectly be 0
offset.top -= parseFloat( jQuery.css(elem, "marginTop") ) || 0;
offset.left -= parseFloat( jQuery.css(elem, "marginLeft") ) || 0;
// Add offsetParent borders
parentOffset.top += parseFloat( jQuery.css(offsetParent[0], "borderTopWidth") ) || 0;
parentOffset.left += parseFloat( jQuery.css(offsetParent[0], "borderLeftWidth") ) || 0;
// Subtract the two offsets
return {
top: offset.top - parentOffset.top,
left: offset.left - parentOffset.left
};
},
offsetParent: function() {
return this.map(function() {
var offsetParent = this.offsetParent || document.body;
while ( offsetParent && (!rroot.test(offsetParent.nodeName) && jQuery.css(offsetParent, "position") === "static") ) {
offsetParent = offsetParent.offsetParent;
}
return offsetParent;
});
}
});
// Create scrollLeft and scrollTop methods
jQuery.each( ["Left", "Top"], function( i, name ) {
var method = "scroll" + name;
jQuery.fn[ method ] = function( val ) {
var elem, win;
if ( val === undefined ) {
elem = this[ 0 ];
if ( !elem ) {
return null;
}
win = getWindow( elem );
// Return the scroll offset
return win ? ("pageXOffset" in win) ? win[ i ? "pageYOffset" : "pageXOffset" ] :
jQuery.support.boxModel && win.document.documentElement[ method ] ||
win.document.body[ method ] :
elem[ method ];
}
// Set the scroll offset
return this.each(function() {
win = getWindow( this );
if ( win ) {
win.scrollTo(
!i ? val : jQuery( win ).scrollLeft(),
i ? val : jQuery( win ).scrollTop()
);
} else {
this[ method ] = val;
}
});
};
});
function getWindow( elem ) {
return jQuery.isWindow( elem ) ?
elem :
elem.nodeType === 9 ?
elem.defaultView || elem.parentWindow :
false;
}
// Create width, height, innerHeight, innerWidth, outerHeight and outerWidth methods
jQuery.each([ "Height", "Width" ], function( i, name ) {
var type = name.toLowerCase();
// innerHeight and innerWidth
jQuery.fn[ "inner" + name ] = function() {
var elem = this[0];
return elem ?
elem.style ?
parseFloat( jQuery.css( elem, type, "padding" ) ) :
this[ type ]() :
null;
};
// outerHeight and outerWidth
jQuery.fn[ "outer" + name ] = function( margin ) {
var elem = this[0];
return elem ?
elem.style ?
parseFloat( jQuery.css( elem, type, margin ? "margin" : "border" ) ) :
this[ type ]() :
null;
};
jQuery.fn[ type ] = function( size ) {
// Get window width or height
var elem = this[0];
if ( !elem ) {
return size == null ? null : this;
}
if ( jQuery.isFunction( size ) ) {
return this.each(function( i ) {
var self = jQuery( this );
self[ type ]( size.call( this, i, self[ type ]() ) );
});
}
if ( jQuery.isWindow( elem ) ) {
// Everyone else use document.documentElement or document.body depending on Quirks vs Standards mode
// 3rd condition allows Nokia support, as it supports the docElem prop but not CSS1Compat
var docElemProp = elem.document.documentElement[ "client" + name ],
body = elem.document.body;
return elem.document.compatMode === "CSS1Compat" && docElemProp ||
body && body[ "client" + name ] || docElemProp;
// Get document width or height
} else if ( elem.nodeType === 9 ) {
// Either scroll[Width/Height] or offset[Width/Height], whichever is greater
return Math.max(
elem.documentElement["client" + name],
elem.body["scroll" + name], elem.documentElement["scroll" + name],
elem.body["offset" + name], elem.documentElement["offset" + name]
);
// Get or set width or height on the element
} else if ( size === undefined ) {
var orig = jQuery.css( elem, type ),
ret = parseFloat( orig );
return jQuery.isNumeric( ret ) ? ret : orig;
// Set the width or height on the element (default to pixels if value is unitless)
} else {
return this.css( type, typeof size === "string" ? size : size + "px" );
}
};
});
// Expose jQuery to the global object
window.jQuery = window.$ = jQuery;
// Expose jQuery as an AMD module, but only for AMD loaders that
// understand the issues with loading multiple versions of jQuery
// in a page that all might call define(). The loader will indicate
// they have special allowances for multiple jQuery versions by
// specifying define.amd.jQuery = true. Register as a named module,
// since jQuery can be concatenated with other files that may use define,
// but not use a proper concatenation script that understands anonymous
// AMD modules. A named AMD is safest and most robust way to register.
// Lowercase jquery is used because AMD module names are derived from
// file names, and jQuery is normally delivered in a lowercase file name.
// Do this after creating the global so that if an AMD module wants to call
// noConflict to hide this version of jQuery, it will work.
if ( typeof define === "function" && define.amd && define.amd.jQuery ) {
define( "jquery", [], function () { return jQuery; } );
}
})( window );
|
admin/client/App/screens/List/components/UpdateForm.js | webteckie/keystone | import React from 'react';
import ReactDOM from 'react-dom';
import Select from 'react-select';
import Fields from 'FieldTypes';
import InvalidFieldType from '../../../shared/InvalidFieldType';
import { plural } from '../../../../utils/string';
import { BlankState, Button, Form, Modal } from 'elemental';
var UpdateForm = React.createClass({
displayName: 'UpdateForm',
propTypes: {
isOpen: React.PropTypes.bool,
itemIds: React.PropTypes.array,
list: React.PropTypes.object,
onCancel: React.PropTypes.func,
},
getDefaultProps () {
return {
isOpen: false,
};
},
getInitialState () {
return {
fields: [],
};
},
componentDidMount () {
if (this.refs.focusTarget) {
this.refs.focusTarget.focus();
}
},
componentDidUpdate () {
if (this.refs.focusTarget) {
this.refs.focusTarget.focus();
}
},
getOptions () {
const { fields } = this.props.list;
return Object.keys(fields).map(key => ({ value: fields[key].path, label: fields[key].label }));
},
getFieldProps (field) {
var props = Object.assign({}, field);
props.value = this.state.fields[field.path];
props.values = this.state.fields;
props.onChange = this.handleChange;
props.mode = 'create';
props.key = field.path;
return props;
},
updateOptions (fields) {
this.setState({
fields: fields,
}, () => {
ReactDOM.findDOMNode(this.refs.focusTarget).focus();
});
},
handleChange (value) {
console.log('handleChange:', value);
},
handleClose () {
this.setState({
fields: [],
});
this.props.onCancel();
},
renderFields () {
const { list } = this.props;
const { fields } = this.state;
const formFields = [];
let focusRef;
fields.forEach((fieldOption) => {
const field = list.fields[fieldOption.value];
if (typeof Fields[field.type] !== 'function') {
formFields.push(React.createElement(InvalidFieldType, { type: field.type, path: field.path, key: field.path }));
return;
}
var fieldProps = this.getFieldProps(field);
if (!focusRef) {
fieldProps.ref = focusRef = 'focusTarget';
}
formFields.push(React.createElement(Fields[field.type], fieldProps));
});
const fieldsUI = formFields.length ? formFields : (
<BlankState style={{ padding: '3em 2em' }}>
<BlankState.Heading style={{ fontSize: '1.5em' }}>Choose a field above to begin</BlankState.Heading>
</BlankState>
);
return (
<div style={{ borderTop: '1px dashed rgba(0,0,0,0.1)', marginTop: 20, paddingTop: 20 }}>
{fieldsUI}
</div>
);
},
renderForm () {
const { itemIds, list } = this.props;
const itemCount = plural(itemIds, ('* ' + list.singular), ('* ' + list.plural));
const formAction = `${Keystone.adminPath}/${list.path}`;
return (
<Form type="horizontal" encType="multipart/form-data" method="post" action={formAction} noValidate="true">
<input type="hidden" name="action" value="update" />
<input type="hidden" name={Keystone.csrf.key} value={Keystone.csrf.value} />
<Modal.Header text={'Update ' + itemCount} onClose={this.handleClose} showCloseButton />
<Modal.Body>
<Select ref="initialFocusTarget" onChange={this.updateOptions} options={this.getOptions()} value={this.state.fields} key="field-select" multi />
{this.renderFields()}
</Modal.Body>
<Modal.Footer>
<Button type="primary" submit>Update</Button>
<Button type="link-cancel" onClick={this.handleClose}>Cancel</Button>
</Modal.Footer>
</Form>
);
},
render () {
return (
<Modal isOpen={this.props.isOpen} onCancel={this.handleClose} backdropClosesModal>
{this.renderForm()}
</Modal>
);
},
});
module.exports = UpdateForm;
|
src/seasonCalendar/seasonCalendar.js | mistyjae/rc-calendar-extend | /**
* Created by xlm on 2017/7/6.
*/
import React from 'react';
import 'rc-calendar/assets/index.css'
import 'styles/index.css'
import createReactClass from 'create-react-class';
import PropTypes from 'prop-types';
import KeyCode from 'rc-util/lib/KeyCode';
import SeasonPanel from '../season/seasonPanel';
import CalendarMixin from 'rc-calendar/lib/mixin/CalendarMixin';
import CommonMixin from 'rc-calendar/lib/mixin/CommonMixin';
const SeasonCalendar = createReactClass({
propTypes: {
monthCellRender: PropTypes.func,
dateCellRender: PropTypes.func,
},
mixins: [CommonMixin, CalendarMixin],
onKeyDown(event) {
const keyCode = event.keyCode;
const ctrlKey = event.ctrlKey || event.metaKey;
const stateValue = this.state.value;
const { disabledDate } = this.props;
let value = stateValue;
switch (keyCode) {
case KeyCode.DOWN:
value = stateValue.clone();
value.add(3, 'months');
break;
case KeyCode.UP:
value = stateValue.clone();
value.add(-3, 'months');
break;
case KeyCode.LEFT:
value = stateValue.clone();
if (ctrlKey) {
value.add(-1, 'years');
} else {
value.add(-1, 'months');
}
break;
case KeyCode.RIGHT:
value = stateValue.clone();
if (ctrlKey) {
value.add(1, 'years');
} else {
value.add(1, 'months');
}
break;
case KeyCode.ENTER:
if (!disabledDate || !disabledDate(stateValue)) {
this.onSelect(stateValue);
}
event.preventDefault();
return 1;
default:
return undefined;
}
if (value !== stateValue) {
this.setValue(value);
event.preventDefault();
return 1;
}
},
render() {
const props = this.props;
const children = (
<SeasonPanel
locale={props.locale}
disabledDate={props.disabledDate}
style={{ position: 'relative' }}
value={this.state.value}
cellRender={props.monthCellRender}
contentRender={props.monthCellContentRender}
rootPrefixCls={props.prefixCls}
onChange={this.setValue}
onSelect={this.onSelect}
/>
);
return this.renderRoot({
children,
});
},
});
export default SeasonCalendar;
|
ajax/libs/radium/0.18.2/radium.js | pvnr0082t/cdnjs | (function webpackUniversalModuleDefinition(root, factory) {
if(typeof exports === 'object' && typeof module === 'object')
module.exports = factory(require("react"));
else if(typeof define === 'function' && define.amd)
define(["react"], factory);
else if(typeof exports === 'object')
exports["Radium"] = factory(require("react"));
else
root["Radium"] = factory(root["React"]);
})(this, function(__WEBPACK_EXTERNAL_MODULE_2__) {
return /******/ (function(modules) { // webpackBootstrap
/******/ // The module cache
/******/ var installedModules = {};
/******/
/******/ // The require function
/******/ function __webpack_require__(moduleId) {
/******/
/******/ // Check if module is in cache
/******/ if(installedModules[moduleId])
/******/ return installedModules[moduleId].exports;
/******/
/******/ // Create a new module (and put it into the cache)
/******/ var module = installedModules[moduleId] = {
/******/ i: moduleId,
/******/ l: false,
/******/ exports: {}
/******/ };
/******/
/******/ // Execute the module function
/******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__);
/******/
/******/ // Flag the module as loaded
/******/ module.l = true;
/******/
/******/ // Return the exports of the module
/******/ return module.exports;
/******/ }
/******/
/******/
/******/ // expose the modules object (__webpack_modules__)
/******/ __webpack_require__.m = modules;
/******/
/******/ // expose the module cache
/******/ __webpack_require__.c = installedModules;
/******/
/******/ // identity function for calling harmony imports with the correct context
/******/ __webpack_require__.i = function(value) { return value; };
/******/
/******/ // define getter function for harmony exports
/******/ __webpack_require__.d = function(exports, name, getter) {
/******/ if(!__webpack_require__.o(exports, name)) {
/******/ Object.defineProperty(exports, name, {
/******/ configurable: false,
/******/ enumerable: true,
/******/ get: getter
/******/ });
/******/ }
/******/ };
/******/
/******/ // getDefaultExport function for compatibility with non-harmony modules
/******/ __webpack_require__.n = function(module) {
/******/ var getter = module && module.__esModule ?
/******/ function getDefault() { return module['default']; } :
/******/ function getModuleExports() { return module; };
/******/ __webpack_require__.d(getter, 'a', getter);
/******/ return getter;
/******/ };
/******/
/******/ // Object.prototype.hasOwnProperty.call
/******/ __webpack_require__.o = function(object, property) { return Object.prototype.hasOwnProperty.call(object, property); };
/******/
/******/ // __webpack_public_path__
/******/ __webpack_require__.p = "";
/******/
/******/ // Load entry module and return exports
/******/ return __webpack_require__(__webpack_require__.s = 67);
/******/ })
/************************************************************************/
/******/ ([
/* 0 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = function (prefixedValue, value, keepUnprefixed) {
return keepUnprefixed ? [prefixedValue, value] : prefixedValue;
};
module.exports = exports["default"];
/***/ }),
/* 1 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
// shim for using process in browser
var process = module.exports = {};
// cached from whatever global is present so that test runners that stub it
// don't break things. But we need to wrap it in a try catch in case it is
// wrapped in strict mode code which doesn't define any globals. It's inside a
// function because try/catches deoptimize in certain engines.
var cachedSetTimeout;
var cachedClearTimeout;
function defaultSetTimout() {
throw new Error('setTimeout has not been defined');
}
function defaultClearTimeout() {
throw new Error('clearTimeout has not been defined');
}
(function () {
try {
if (typeof setTimeout === 'function') {
cachedSetTimeout = setTimeout;
} else {
cachedSetTimeout = defaultSetTimout;
}
} catch (e) {
cachedSetTimeout = defaultSetTimout;
}
try {
if (typeof clearTimeout === 'function') {
cachedClearTimeout = clearTimeout;
} else {
cachedClearTimeout = defaultClearTimeout;
}
} catch (e) {
cachedClearTimeout = defaultClearTimeout;
}
})();
function runTimeout(fun) {
if (cachedSetTimeout === setTimeout) {
//normal enviroments in sane situations
return setTimeout(fun, 0);
}
// if setTimeout wasn't available but was latter defined
if ((cachedSetTimeout === defaultSetTimout || !cachedSetTimeout) && setTimeout) {
cachedSetTimeout = setTimeout;
return setTimeout(fun, 0);
}
try {
// when when somebody has screwed with setTimeout but no I.E. maddness
return cachedSetTimeout(fun, 0);
} catch (e) {
try {
// When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally
return cachedSetTimeout.call(null, fun, 0);
} catch (e) {
// same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error
return cachedSetTimeout.call(this, fun, 0);
}
}
}
function runClearTimeout(marker) {
if (cachedClearTimeout === clearTimeout) {
//normal enviroments in sane situations
return clearTimeout(marker);
}
// if clearTimeout wasn't available but was latter defined
if ((cachedClearTimeout === defaultClearTimeout || !cachedClearTimeout) && clearTimeout) {
cachedClearTimeout = clearTimeout;
return clearTimeout(marker);
}
try {
// when when somebody has screwed with setTimeout but no I.E. maddness
return cachedClearTimeout(marker);
} catch (e) {
try {
// When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally
return cachedClearTimeout.call(null, marker);
} catch (e) {
// same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error.
// Some versions of I.E. have different rules for clearTimeout vs setTimeout
return cachedClearTimeout.call(this, marker);
}
}
}
var queue = [];
var draining = false;
var currentQueue;
var queueIndex = -1;
function cleanUpNextTick() {
if (!draining || !currentQueue) {
return;
}
draining = false;
if (currentQueue.length) {
queue = currentQueue.concat(queue);
} else {
queueIndex = -1;
}
if (queue.length) {
drainQueue();
}
}
function drainQueue() {
if (draining) {
return;
}
var timeout = runTimeout(cleanUpNextTick);
draining = true;
var len = queue.length;
while (len) {
currentQueue = queue;
queue = [];
while (++queueIndex < len) {
if (currentQueue) {
currentQueue[queueIndex].run();
}
}
queueIndex = -1;
len = queue.length;
}
currentQueue = null;
draining = false;
runClearTimeout(timeout);
}
process.nextTick = function (fun) {
var args = new Array(arguments.length - 1);
if (arguments.length > 1) {
for (var i = 1; i < arguments.length; i++) {
args[i - 1] = arguments[i];
}
}
queue.push(new Item(fun, args));
if (queue.length === 1 && !draining) {
runTimeout(drainQueue);
}
};
// v8 likes predictible objects
function Item(fun, array) {
this.fun = fun;
this.array = array;
}
Item.prototype.run = function () {
this.fun.apply(null, this.array);
};
process.title = 'browser';
process.browser = true;
process.env = {};
process.argv = [];
process.version = ''; // empty string to avoid regexp issues
process.versions = {};
function noop() {}
process.on = noop;
process.addListener = noop;
process.once = noop;
process.off = noop;
process.removeListener = noop;
process.removeAllListeners = noop;
process.emit = noop;
process.binding = function (name) {
throw new Error('process.binding is not supported');
};
process.cwd = function () {
return '/';
};
process.chdir = function (dir) {
throw new Error('process.chdir is not supported');
};
process.umask = function () {
return 0;
};
/***/ }),
/* 2 */
/***/ (function(module, exports) {
module.exports = __WEBPACK_EXTERNAL_MODULE_2__;
/***/ }),
/* 3 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true });
} else {
obj[key] = value;
}return obj;
}
// returns a style object with a single concated prefixed value string
exports.default = function (property, value) {
var replacer = arguments.length <= 2 || arguments[2] === undefined ? function (prefix, value) {
return prefix + value;
} : arguments[2];
return _defineProperty({}, property, ['-webkit-', '-moz-', ''].map(function (prefix) {
return replacer(prefix, value);
}));
};
module.exports = exports['default'];
/***/ }),
/* 4 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
// helper to capitalize strings
exports.default = function (str) {
return str.charAt(0).toUpperCase() + str.slice(1);
};
module.exports = exports["default"];
/***/ }),
/* 5 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = function (value) {
if (Array.isArray(value)) value = value.join(',');
return value.match(/-webkit-|-moz-|-ms-/) !== null;
};
module.exports = exports['default'];
/***/ }),
/* 6 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = cssRuleSetToString;
var _appendPxIfNeeded = __webpack_require__(16);
var _appendPxIfNeeded2 = _interopRequireDefault(_appendPxIfNeeded);
var _camelCasePropsToDashCase = __webpack_require__(54);
var _camelCasePropsToDashCase2 = _interopRequireDefault(_camelCasePropsToDashCase);
var _mapObject = __webpack_require__(19);
var _mapObject2 = _interopRequireDefault(_mapObject);
var _prefixer = __webpack_require__(7);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
function createMarkupForStyles(style) {
return Object.keys(style).map(function (property) {
return property + ': ' + style[property] + ';';
}).join('\n');
}
function cssRuleSetToString(selector, rules, userAgent) {
if (!rules) {
return '';
}
var rulesWithPx = (0, _mapObject2.default)(rules, function (value, key) {
return (0, _appendPxIfNeeded2.default)(key, value);
});
var prefixedRules = (0, _prefixer.getPrefixedStyle)(rulesWithPx, userAgent);
var cssPrefixedRules = (0, _camelCasePropsToDashCase2.default)(prefixedRules);
var serializedRules = createMarkupForStyles(cssPrefixedRules);
return selector + '{' + serializedRules + '}';
}
module.exports = exports['default'];
/***/ }),
/* 7 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
/* WEBPACK VAR INJECTION */(function(global, process) {
Object.defineProperty(exports, "__esModule", {
value: true
});
var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; }; /**
* Based on https://github.com/jsstyles/css-vendor, but without having to
* convert between different cases all the time.
*
*
*/
exports.getPrefixedKeyframes = getPrefixedKeyframes;
exports.getPrefixedStyle = getPrefixedStyle;
var _inlineStylePrefixer = __webpack_require__(26);
var _inlineStylePrefixer2 = _interopRequireDefault(_inlineStylePrefixer);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
function transformValues(style) {
return Object.keys(style).reduce(function (newStyle, key) {
var value = style[key];
if (Array.isArray(value)) {
value = value.join(';' + key + ':');
} else if (value && (typeof value === 'undefined' ? 'undefined' : _typeof(value)) === 'object' && typeof value.toString === 'function') {
value = value.toString();
}
newStyle[key] = value;
return newStyle;
}, {});
}
var _hasWarnedAboutUserAgent = false;
var _lastUserAgent = void 0;
var _cachedPrefixer = void 0;
function getPrefixer(userAgent) {
var actualUserAgent = userAgent || global && global.navigator && global.navigator.userAgent;
if (process.env.NODE_ENV !== 'production') {
if (!actualUserAgent && !_hasWarnedAboutUserAgent) {
/* eslint-disable no-console */
console.warn('Radium: userAgent should be supplied for server-side rendering. See ' + 'https://github.com/FormidableLabs/radium/tree/master/docs/api#radium ' + 'for more information.');
/* eslint-enable no-console */
_hasWarnedAboutUserAgent = true;
}
}
if (!_cachedPrefixer || actualUserAgent !== _lastUserAgent) {
if (actualUserAgent === 'all') {
_cachedPrefixer = {
prefix: _inlineStylePrefixer2.default.prefixAll,
prefixedKeyframes: 'keyframes'
};
} else {
_cachedPrefixer = new _inlineStylePrefixer2.default({ userAgent: actualUserAgent });
}
_lastUserAgent = actualUserAgent;
}
return _cachedPrefixer;
}
function getPrefixedKeyframes(userAgent) {
return getPrefixer(userAgent).prefixedKeyframes;
}
// Returns a new style object with vendor prefixes added to property names
// and values.
function getPrefixedStyle(style, userAgent) {
var styleWithFallbacks = transformValues(style);
var prefixer = getPrefixer(userAgent);
var prefixedStyle = prefixer.prefix(styleWithFallbacks);
return prefixedStyle;
}
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(52), __webpack_require__(1)))
/***/ }),
/* 8 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
var StyleKeeper = function () {
function StyleKeeper(userAgent) {
_classCallCheck(this, StyleKeeper);
this._userAgent = userAgent;
this._listeners = [];
this._cssSet = {};
}
StyleKeeper.prototype.subscribe = function subscribe(listener) {
var _this = this;
if (this._listeners.indexOf(listener) === -1) {
this._listeners.push(listener);
}
return {
// Must be fat arrow to capture `this`
remove: function remove() {
var listenerIndex = _this._listeners.indexOf(listener);
if (listenerIndex > -1) {
_this._listeners.splice(listenerIndex, 1);
}
}
};
};
StyleKeeper.prototype.addCSS = function addCSS(css) {
var _this2 = this;
if (!this._cssSet[css]) {
this._cssSet[css] = true;
this._emitChange();
}
return {
// Must be fat arrow to capture `this`
remove: function remove() {
delete _this2._cssSet[css];
_this2._emitChange();
}
};
};
StyleKeeper.prototype.getCSS = function getCSS() {
return Object.keys(this._cssSet).join('\n');
};
StyleKeeper.prototype._emitChange = function _emitChange() {
this._listeners.forEach(function (listener) {
return listener();
});
};
return StyleKeeper;
}();
exports.default = StyleKeeper;
module.exports = exports['default'];
/***/ }),
/* 9 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
/* WEBPACK VAR INJECTION */(function(process) {
Object.defineProperty(exports, "__esModule", {
value: true
});
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
exports.default = enhanceWithRadium;
var _react = __webpack_require__(2);
var _styleKeeper = __webpack_require__(8);
var _styleKeeper2 = _interopRequireDefault(_styleKeeper);
var _resolveStyles = __webpack_require__(12);
var _resolveStyles2 = _interopRequireDefault(_resolveStyles);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
var KEYS_TO_IGNORE_WHEN_COPYING_PROPERTIES = ['arguments', 'callee', 'caller', 'length', 'name', 'prototype', 'type'];
function copyProperties(source, target) {
Object.getOwnPropertyNames(source).forEach(function (key) {
if (KEYS_TO_IGNORE_WHEN_COPYING_PROPERTIES.indexOf(key) < 0 && !target.hasOwnProperty(key)) {
var descriptor = Object.getOwnPropertyDescriptor(source, key);
Object.defineProperty(target, key, descriptor);
}
});
}
function isStateless(component) {
return !component.render && !(component.prototype && component.prototype.render);
}
function enhanceWithRadium(configOrComposedComponent) {
var _class, _temp;
var config = arguments.length > 1 && arguments[1] !== undefined ? arguments[1] : {};
if (typeof configOrComposedComponent !== 'function') {
var newConfig = _extends({}, config, configOrComposedComponent);
return function (configOrComponent) {
return enhanceWithRadium(configOrComponent, newConfig);
};
}
var component = configOrComposedComponent;
var ComposedComponent = component;
// Handle stateless components
if (isStateless(ComposedComponent)) {
ComposedComponent = function (_Component) {
_inherits(ComposedComponent, _Component);
function ComposedComponent() {
_classCallCheck(this, ComposedComponent);
return _possibleConstructorReturn(this, _Component.apply(this, arguments));
}
ComposedComponent.prototype.render = function render() {
return component(this.props, this.context);
};
return ComposedComponent;
}(_react.Component);
ComposedComponent.displayName = component.displayName || component.name;
}
var RadiumEnhancer = (_temp = _class = function (_ComposedComponent) {
_inherits(RadiumEnhancer, _ComposedComponent);
function RadiumEnhancer() {
_classCallCheck(this, RadiumEnhancer);
var _this2 = _possibleConstructorReturn(this, _ComposedComponent.apply(this, arguments));
_this2.state = _this2.state || {};
_this2.state._radiumStyleState = {};
_this2._radiumIsMounted = true;
return _this2;
}
RadiumEnhancer.prototype.componentWillUnmount = function componentWillUnmount() {
if (_ComposedComponent.prototype.componentWillUnmount) {
_ComposedComponent.prototype.componentWillUnmount.call(this);
}
this._radiumIsMounted = false;
if (this._radiumMouseUpListener) {
this._radiumMouseUpListener.remove();
}
if (this._radiumMediaQueryListenersByQuery) {
Object.keys(this._radiumMediaQueryListenersByQuery).forEach(function (query) {
this._radiumMediaQueryListenersByQuery[query].remove();
}, this);
}
};
RadiumEnhancer.prototype.getChildContext = function getChildContext() {
var superChildContext = _ComposedComponent.prototype.getChildContext ? _ComposedComponent.prototype.getChildContext.call(this) : {};
if (!this.props.radiumConfig) {
return superChildContext;
}
var newContext = _extends({}, superChildContext);
if (this.props.radiumConfig) {
newContext._radiumConfig = this.props.radiumConfig;
}
return newContext;
};
RadiumEnhancer.prototype.render = function render() {
var renderedElement = _ComposedComponent.prototype.render.call(this);
var currentConfig = this.props.radiumConfig || this.context._radiumConfig || config;
if (config && currentConfig !== config) {
currentConfig = _extends({}, config, currentConfig);
}
return (0, _resolveStyles2.default)(this, renderedElement, currentConfig);
};
return RadiumEnhancer;
}(ComposedComponent), _class._isRadiumEnhanced = true, _temp);
// Class inheritance uses Object.create and because of __proto__ issues
// with IE <10 any static properties of the superclass aren't inherited and
// so need to be manually populated.
// See http://babeljs.io/docs/advanced/caveats/#classes-10-and-below-
copyProperties(component, RadiumEnhancer);
if (process.env.NODE_ENV !== 'production') {
// This also fixes React Hot Loader by exposing the original components top
// level prototype methods on the Radium enhanced prototype as discussed in
// https://github.com/FormidableLabs/radium/issues/219.
copyProperties(ComposedComponent.prototype, RadiumEnhancer.prototype);
}
if (RadiumEnhancer.propTypes && RadiumEnhancer.propTypes.style) {
RadiumEnhancer.propTypes = _extends({}, RadiumEnhancer.propTypes, {
style: _react.PropTypes.oneOfType([_react.PropTypes.array, _react.PropTypes.object])
});
}
RadiumEnhancer.displayName = component.displayName || component.name || 'Component';
RadiumEnhancer.contextTypes = _extends({}, RadiumEnhancer.contextTypes, {
_radiumConfig: _react.PropTypes.object,
_radiumStyleKeeper: _react.PropTypes.instanceOf(_styleKeeper2.default)
});
RadiumEnhancer.childContextTypes = _extends({}, RadiumEnhancer.childContextTypes, {
_radiumConfig: _react.PropTypes.object,
_radiumStyleKeeper: _react.PropTypes.instanceOf(_styleKeeper2.default)
});
return RadiumEnhancer;
}
module.exports = exports['default'];
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(1)))
/***/ }),
/* 10 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _getStateKey = __webpack_require__(17);
var _getStateKey2 = _interopRequireDefault(_getStateKey);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
var getState = function getState(state, elementKey, value) {
var key = (0, _getStateKey2.default)(elementKey);
return !!state && !!state._radiumStyleState && !!state._radiumStyleState[key] && state._radiumStyleState[key][value];
};
exports.default = getState;
module.exports = exports['default'];
/***/ }),
/* 11 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _checkPropsPlugin = __webpack_require__(57);
var _checkPropsPlugin2 = _interopRequireDefault(_checkPropsPlugin);
var _keyframesPlugin = __webpack_require__(58);
var _keyframesPlugin2 = _interopRequireDefault(_keyframesPlugin);
var _mergeStyleArrayPlugin = __webpack_require__(59);
var _mergeStyleArrayPlugin2 = _interopRequireDefault(_mergeStyleArrayPlugin);
var _prefixPlugin = __webpack_require__(61);
var _prefixPlugin2 = _interopRequireDefault(_prefixPlugin);
var _removeNestedStylesPlugin = __webpack_require__(62);
var _removeNestedStylesPlugin2 = _interopRequireDefault(_removeNestedStylesPlugin);
var _resolveInteractionStylesPlugin = __webpack_require__(63);
var _resolveInteractionStylesPlugin2 = _interopRequireDefault(_resolveInteractionStylesPlugin);
var _resolveMediaQueriesPlugin = __webpack_require__(64);
var _resolveMediaQueriesPlugin2 = _interopRequireDefault(_resolveMediaQueriesPlugin);
var _visitedPlugin = __webpack_require__(65);
var _visitedPlugin2 = _interopRequireDefault(_visitedPlugin);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
exports.default = {
checkProps: _checkPropsPlugin2.default,
keyframes: _keyframesPlugin2.default,
mergeStyleArray: _mergeStyleArrayPlugin2.default,
prefix: _prefixPlugin2.default,
removeNestedStyles: _removeNestedStylesPlugin2.default,
resolveInteractionStyles: _resolveInteractionStylesPlugin2.default,
resolveMediaQueries: _resolveMediaQueriesPlugin2.default,
visited: _visitedPlugin2.default
};
/* eslint-disable block-scoped-const */
module.exports = exports['default'];
/***/ }),
/* 12 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
/* WEBPACK VAR INJECTION */(function(process) {
Object.defineProperty(exports, "__esModule", {
value: true
});
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; };
var _appendImportantToEachValue = __webpack_require__(53);
var _appendImportantToEachValue2 = _interopRequireDefault(_appendImportantToEachValue);
var _cssRuleSetToString = __webpack_require__(6);
var _cssRuleSetToString2 = _interopRequireDefault(_cssRuleSetToString);
var _getState = __webpack_require__(10);
var _getState2 = _interopRequireDefault(_getState);
var _getStateKey = __webpack_require__(17);
var _getStateKey2 = _interopRequireDefault(_getStateKey);
var _hash = __webpack_require__(18);
var _hash2 = _interopRequireDefault(_hash);
var _mergeStyles = __webpack_require__(56);
var _plugins = __webpack_require__(11);
var _plugins2 = _interopRequireDefault(_plugins);
var _exenv = __webpack_require__(25);
var _exenv2 = _interopRequireDefault(_exenv);
var _react = __webpack_require__(2);
var _react2 = _interopRequireDefault(_react);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
var DEFAULT_CONFIG = {
plugins: [_plugins2.default.mergeStyleArray, _plugins2.default.checkProps, _plugins2.default.resolveMediaQueries, _plugins2.default.resolveInteractionStyles, _plugins2.default.keyframes, _plugins2.default.visited, _plugins2.default.removeNestedStyles, _plugins2.default.prefix, _plugins2.default.checkProps]
};
// Gross
var globalState = {};
// Declare early for recursive helpers.
var resolveStyles = null;
var _shouldResolveStyles = function _shouldResolveStyles(component) {
return component.type && !component.type._isRadiumEnhanced;
};
var _resolveChildren = function _resolveChildren(_ref) {
var children = _ref.children,
component = _ref.component,
config = _ref.config,
existingKeyMap = _ref.existingKeyMap;
if (!children) {
return children;
}
var childrenType = typeof children === 'undefined' ? 'undefined' : _typeof(children);
if (childrenType === 'string' || childrenType === 'number') {
// Don't do anything with a single primitive child
return children;
}
if (childrenType === 'function') {
// Wrap the function, resolving styles on the result
return function () {
var result = children.apply(this, arguments);
if (_react2.default.isValidElement(result)) {
return resolveStyles(component, result, config, existingKeyMap, true);
}
return result;
};
}
if (_react2.default.Children.count(children) === 1 && children.type) {
// If a React Element is an only child, don't wrap it in an array for
// React.Children.map() for React.Children.only() compatibility.
var onlyChild = _react2.default.Children.only(children);
return resolveStyles(component, onlyChild, config, existingKeyMap, true);
}
return _react2.default.Children.map(children, function (child) {
if (_react2.default.isValidElement(child)) {
return resolveStyles(component, child, config, existingKeyMap, true);
}
return child;
});
};
// Recurse over props, just like children
var _resolveProps = function _resolveProps(_ref2) {
var component = _ref2.component,
config = _ref2.config,
existingKeyMap = _ref2.existingKeyMap,
props = _ref2.props;
var newProps = props;
Object.keys(props).forEach(function (prop) {
// We already recurse over children above
if (prop === 'children') {
return;
}
var propValue = props[prop];
if (_react2.default.isValidElement(propValue)) {
newProps = _extends({}, newProps);
newProps[prop] = resolveStyles(component, propValue, config, existingKeyMap, true);
}
});
return newProps;
};
var _buildGetKey = function _buildGetKey(_ref3) {
var componentName = _ref3.componentName,
existingKeyMap = _ref3.existingKeyMap,
renderedElement = _ref3.renderedElement;
// We need a unique key to correlate state changes due to user interaction
// with the rendered element, so we know to apply the proper interactive
// styles.
var originalKey = typeof renderedElement.ref === 'string' ? renderedElement.ref : renderedElement.key;
var key = (0, _getStateKey2.default)(originalKey);
var alreadyGotKey = false;
var getKey = function getKey() {
if (alreadyGotKey) {
return key;
}
alreadyGotKey = true;
if (existingKeyMap[key]) {
var elementName = void 0;
if (typeof renderedElement.type === 'string') {
elementName = renderedElement.type;
} else if (renderedElement.type.constructor) {
elementName = renderedElement.type.constructor.displayName || renderedElement.type.constructor.name;
}
throw new Error('Radium requires each element with interactive styles to have a unique ' + 'key, set using either the ref or key prop. ' + (originalKey ? 'Key "' + originalKey + '" is a duplicate.' : 'Multiple elements have no key specified.') + ' ' + 'Component: "' + componentName + '". ' + (elementName ? 'Element: "' + elementName + '".' : ''));
}
existingKeyMap[key] = true;
return key;
};
return getKey;
};
var _setStyleState = function _setStyleState(component, key, stateKey, value) {
if (!component._radiumIsMounted) {
return;
}
var existing = component._lastRadiumState || component.state && component.state._radiumStyleState || {};
var state = { _radiumStyleState: _extends({}, existing) };
state._radiumStyleState[key] = _extends({}, state._radiumStyleState[key]);
state._radiumStyleState[key][stateKey] = value;
component._lastRadiumState = state._radiumStyleState;
component.setState(state);
};
var _runPlugins = function _runPlugins(_ref4) {
var component = _ref4.component,
config = _ref4.config,
existingKeyMap = _ref4.existingKeyMap,
props = _ref4.props,
renderedElement = _ref4.renderedElement;
// Don't run plugins if renderedElement is not a simple ReactDOMElement or has
// no style.
if (!_react2.default.isValidElement(renderedElement) || typeof renderedElement.type !== 'string' || !props.style) {
return props;
}
var newProps = props;
var plugins = config.plugins || DEFAULT_CONFIG.plugins;
var componentName = component.constructor.displayName || component.constructor.name;
var getKey = _buildGetKey({
renderedElement: renderedElement,
existingKeyMap: existingKeyMap,
componentName: componentName
});
var getComponentField = function getComponentField(key) {
return component[key];
};
var getGlobalState = function getGlobalState(key) {
return globalState[key];
};
var componentGetState = function componentGetState(stateKey, elementKey) {
return (0, _getState2.default)(component.state, elementKey || getKey(), stateKey);
};
var setState = function setState(stateKey, value, elementKey) {
return _setStyleState(component, elementKey || getKey(), stateKey, value);
};
var addCSS = function addCSS(css) {
var styleKeeper = component._radiumStyleKeeper || component.context._radiumStyleKeeper;
if (!styleKeeper) {
if (__isTestModeEnabled) {
return {
remove: function remove() {}
};
}
throw new Error('To use plugins requiring `addCSS` (e.g. keyframes, media queries), ' + 'please wrap your application in the StyleRoot component. Component ' + 'name: `' + componentName + '`.');
}
return styleKeeper.addCSS(css);
};
var newStyle = props.style;
plugins.forEach(function (plugin) {
var result = plugin({
ExecutionEnvironment: _exenv2.default,
addCSS: addCSS,
appendImportantToEachValue: _appendImportantToEachValue2.default,
componentName: componentName,
config: config,
cssRuleSetToString: _cssRuleSetToString2.default,
getComponentField: getComponentField,
getGlobalState: getGlobalState,
getState: componentGetState,
hash: _hash2.default,
mergeStyles: _mergeStyles.mergeStyles,
props: newProps,
setState: setState,
isNestedStyle: _mergeStyles.isNestedStyle,
style: newStyle
}) || {};
newStyle = result.style || newStyle;
newProps = result.props && Object.keys(result.props).length ? _extends({}, newProps, result.props) : newProps;
var newComponentFields = result.componentFields || {};
Object.keys(newComponentFields).forEach(function (fieldName) {
component[fieldName] = newComponentFields[fieldName];
});
var newGlobalState = result.globalState || {};
Object.keys(newGlobalState).forEach(function (key) {
globalState[key] = newGlobalState[key];
});
});
if (newStyle !== props.style) {
newProps = _extends({}, newProps, { style: newStyle });
}
return newProps;
};
// Wrapper around React.cloneElement. To avoid processing the same element
// twice, whenever we clone an element add a special prop to make sure we don't
// process this element again.
var _cloneElement = function _cloneElement(renderedElement, newProps, newChildren) {
// Only add flag if this is a normal DOM element
if (typeof renderedElement.type === 'string') {
newProps = _extends({}, newProps, { 'data-radium': true });
}
return _react2.default.cloneElement(renderedElement, newProps, newChildren);
};
//
// The nucleus of Radium. resolveStyles is called on the rendered elements
// before they are returned in render. It iterates over the elements and
// children, rewriting props to add event handlers required to capture user
// interactions (e.g. mouse over). It also replaces the style prop because it
// adds in the various interaction styles (e.g. :hover).
//
resolveStyles = function resolveStyles(component, // ReactComponent, flow+eslint complaining
renderedElement) {
var config = arguments.length > 2 && arguments[2] !== undefined ? arguments[2] : DEFAULT_CONFIG;
var existingKeyMap = arguments[3];
var shouldCheckBeforeResolve = arguments.length > 4 && arguments[4] !== undefined ? arguments[4] : false;
// ReactElement
existingKeyMap = existingKeyMap || {};
if (!renderedElement ||
// Bail if we've already processed this element. This ensures that only the
// owner of an element processes that element, since the owner's render
// function will be called first (which will always be the case, since you
// can't know what else to render until you render the parent component).
renderedElement.props && renderedElement.props['data-radium'] ||
// Bail if this element is a radium enhanced element, because if it is,
// then it will take care of resolving its own styles.
shouldCheckBeforeResolve && !_shouldResolveStyles(renderedElement)) {
return renderedElement;
}
var newChildren = _resolveChildren({
children: renderedElement.props.children,
component: component,
config: config,
existingKeyMap: existingKeyMap
});
var newProps = _resolveProps({
component: component,
config: config,
existingKeyMap: existingKeyMap,
props: renderedElement.props
});
newProps = _runPlugins({
component: component,
config: config,
existingKeyMap: existingKeyMap,
props: newProps,
renderedElement: renderedElement
});
// If nothing changed, don't bother cloning the element. Might be a bit
// wasteful, as we add the sentinal to stop double-processing when we clone.
// Assume benign double-processing is better than unneeded cloning.
if (newChildren === renderedElement.props.children && newProps === renderedElement.props) {
return renderedElement;
}
return _cloneElement(renderedElement, newProps !== renderedElement.props ? newProps : {}, newChildren);
};
// Only for use by tests
var __isTestModeEnabled = false;
if (process.env.NODE_ENV !== 'production') {
resolveStyles.__clearStateForTests = function () {
globalState = {};
};
resolveStyles.__setTestMode = function (isEnabled) {
__isTestModeEnabled = isEnabled;
};
}
exports.default = resolveStyles;
module.exports = exports['default'];
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(1)))
/***/ }),
/* 13 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
var uppercasePattern = /[A-Z]/g;
var msPattern = /^ms-/;
var cache = {};
function hyphenateStyleName(string) {
return string in cache ? cache[string] : cache[string] = string.replace(uppercasePattern, '-$&').toLowerCase().replace(msPattern, '-ms-');
}
module.exports = hyphenateStyleName;
/***/ }),
/* 14 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = { "Webkit": { "transform": true, "transformOrigin": true, "transformOriginX": true, "transformOriginY": true, "backfaceVisibility": true, "perspective": true, "perspectiveOrigin": true, "transformStyle": true, "transformOriginZ": true, "animation": true, "animationDelay": true, "animationDirection": true, "animationFillMode": true, "animationDuration": true, "animationIterationCount": true, "animationName": true, "animationPlayState": true, "animationTimingFunction": true, "appearance": true, "userSelect": true, "fontKerning": true, "textEmphasisPosition": true, "textEmphasis": true, "textEmphasisStyle": true, "textEmphasisColor": true, "boxDecorationBreak": true, "clipPath": true, "maskImage": true, "maskMode": true, "maskRepeat": true, "maskPosition": true, "maskClip": true, "maskOrigin": true, "maskSize": true, "maskComposite": true, "mask": true, "maskBorderSource": true, "maskBorderMode": true, "maskBorderSlice": true, "maskBorderWidth": true, "maskBorderOutset": true, "maskBorderRepeat": true, "maskBorder": true, "maskType": true, "textDecorationStyle": true, "textDecorationSkip": true, "textDecorationLine": true, "textDecorationColor": true, "filter": true, "fontFeatureSettings": true, "breakAfter": true, "breakBefore": true, "breakInside": true, "columnCount": true, "columnFill": true, "columnGap": true, "columnRule": true, "columnRuleColor": true, "columnRuleStyle": true, "columnRuleWidth": true, "columns": true, "columnSpan": true, "columnWidth": true, "flex": true, "flexBasis": true, "flexDirection": true, "flexGrow": true, "flexFlow": true, "flexShrink": true, "flexWrap": true, "alignContent": true, "alignItems": true, "alignSelf": true, "justifyContent": true, "order": true, "transition": true, "transitionDelay": true, "transitionDuration": true, "transitionProperty": true, "transitionTimingFunction": true, "backdropFilter": true, "scrollSnapType": true, "scrollSnapPointsX": true, "scrollSnapPointsY": true, "scrollSnapDestination": true, "scrollSnapCoordinate": true, "shapeImageThreshold": true, "shapeImageMargin": true, "shapeImageOutside": true, "hyphens": true, "flowInto": true, "flowFrom": true, "regionFragment": true, "textSizeAdjust": true }, "Moz": { "appearance": true, "userSelect": true, "boxSizing": true, "textAlignLast": true, "textDecorationStyle": true, "textDecorationSkip": true, "textDecorationLine": true, "textDecorationColor": true, "tabSize": true, "hyphens": true, "fontFeatureSettings": true, "breakAfter": true, "breakBefore": true, "breakInside": true, "columnCount": true, "columnFill": true, "columnGap": true, "columnRule": true, "columnRuleColor": true, "columnRuleStyle": true, "columnRuleWidth": true, "columns": true, "columnSpan": true, "columnWidth": true }, "ms": { "flex": true, "flexBasis": false, "flexDirection": true, "flexGrow": false, "flexFlow": true, "flexShrink": false, "flexWrap": true, "alignContent": false, "alignItems": false, "alignSelf": false, "justifyContent": false, "order": false, "transform": true, "transformOrigin": true, "transformOriginX": true, "transformOriginY": true, "userSelect": true, "wrapFlow": true, "wrapThrough": true, "wrapMargin": true, "scrollSnapType": true, "scrollSnapPointsX": true, "scrollSnapPointsY": true, "scrollSnapDestination": true, "scrollSnapCoordinate": true, "touchAction": true, "hyphens": true, "flowInto": true, "flowFrom": true, "breakBefore": true, "breakAfter": true, "breakInside": true, "regionFragment": true, "gridTemplateColumns": true, "gridTemplateRows": true, "gridTemplateAreas": true, "gridTemplate": true, "gridAutoColumns": true, "gridAutoRows": true, "gridAutoFlow": true, "grid": true, "gridRowStart": true, "gridColumnStart": true, "gridRowEnd": true, "gridRow": true, "gridColumn": true, "gridColumnEnd": true, "gridColumnGap": true, "gridRowGap": true, "gridArea": true, "gridGap": true, "textSizeAdjust": true } };
module.exports = exports["default"];
/***/ }),
/* 15 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = sortPrefixedStyle;
var _isPrefixedProperty = __webpack_require__(50);
var _isPrefixedProperty2 = _interopRequireDefault(_isPrefixedProperty);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
function sortPrefixedStyle(style) {
return Object.keys(style).sort(function (left, right) {
if ((0, _isPrefixedProperty2.default)(left) && !(0, _isPrefixedProperty2.default)(right)) {
return -1;
} else if (!(0, _isPrefixedProperty2.default)(left) && (0, _isPrefixedProperty2.default)(right)) {
return 1;
}
return 0;
}).reduce(function (sortedStyle, prop) {
sortedStyle[prop] = style[prop];
return sortedStyle;
}, {});
}
module.exports = exports['default'];
/***/ }),
/* 16 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = appendPxIfNeeded;
// Copied from https://github.com/facebook/react/blob/
// 102cd291899f9942a76c40a0e78920a6fe544dc1/
// src/renderers/dom/shared/CSSProperty.js
var isUnitlessNumber = {
animationIterationCount: true,
boxFlex: true,
boxFlexGroup: true,
boxOrdinalGroup: true,
columnCount: true,
flex: true,
flexGrow: true,
flexPositive: true,
flexShrink: true,
flexNegative: true,
flexOrder: true,
gridRow: true,
gridColumn: true,
fontWeight: true,
lineClamp: true,
lineHeight: true,
opacity: true,
order: true,
orphans: true,
tabSize: true,
widows: true,
zIndex: true,
zoom: true,
// SVG-related properties
fillOpacity: true,
stopOpacity: true,
strokeDashoffset: true,
strokeOpacity: true,
strokeWidth: true
};
function appendPxIfNeeded(propertyName, value) {
var needsPxSuffix = !isUnitlessNumber[propertyName] && typeof value === 'number' && value !== 0;
return needsPxSuffix ? value + 'px' : value;
}
module.exports = exports['default'];
/***/ }),
/* 17 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var getStateKey = function getStateKey(elementKey) {
return elementKey === null || elementKey === undefined ? 'main' : elementKey.toString();
};
exports.default = getStateKey;
module.exports = exports['default'];
/***/ }),
/* 18 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = hash;
// a simple djb2 hash based on hash-string:
// https://github.com/MatthewBarker/hash-string/blob/master/source/hash-string.js
// returns a hex-encoded hash
function hash(text) {
if (!text) {
return '';
}
var hashValue = 5381;
var index = text.length - 1;
while (index) {
hashValue = hashValue * 33 ^ text.charCodeAt(index);
index -= 1;
}
return (hashValue >>> 0).toString(16);
}
module.exports = exports['default'];
/***/ }),
/* 19 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = mapObject;
function mapObject(object, mapper) {
return Object.keys(object).reduce(function (result, key) {
result[key] = mapper(object[key], key);
return result;
}, {});
}
module.exports = exports["default"];
/***/ }),
/* 20 */
/***/ (function(module, exports) {
/* WEBPACK VAR INJECTION */(function(__webpack_amd_options__) {/* globals __webpack_amd_options__ */
module.exports = __webpack_amd_options__;
/* WEBPACK VAR INJECTION */}.call(exports, {}))
/***/ }),
/* 21 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _react = __webpack_require__(2);
var _react2 = _interopRequireDefault(_react);
var _enhancer = __webpack_require__(9);
var _enhancer2 = _interopRequireDefault(_enhancer);
var _styleKeeper = __webpack_require__(8);
var _styleKeeper2 = _interopRequireDefault(_styleKeeper);
var _styleSheet = __webpack_require__(55);
var _styleSheet2 = _interopRequireDefault(_styleSheet);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
function _objectWithoutProperties(obj, keys) { var target = {}; for (var i in obj) { if (keys.indexOf(i) >= 0) continue; if (!Object.prototype.hasOwnProperty.call(obj, i)) continue; target[i] = obj[i]; } return target; }
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
function _getStyleKeeper(instance) {
if (!instance._radiumStyleKeeper) {
var userAgent = instance.props.radiumConfig && instance.props.radiumConfig.userAgent || instance.context._radiumConfig && instance.context._radiumConfig.userAgent;
instance._radiumStyleKeeper = new _styleKeeper2.default(userAgent);
}
return instance._radiumStyleKeeper;
}
var StyleRoot = function (_PureComponent) {
_inherits(StyleRoot, _PureComponent);
function StyleRoot() {
_classCallCheck(this, StyleRoot);
var _this = _possibleConstructorReturn(this, _PureComponent.apply(this, arguments));
_getStyleKeeper(_this);
return _this;
}
StyleRoot.prototype.getChildContext = function getChildContext() {
return { _radiumStyleKeeper: _getStyleKeeper(this) };
};
StyleRoot.prototype.render = function render() {
/* eslint-disable no-unused-vars */
// Pass down all props except config to the rendered div.
var _props = this.props,
radiumConfig = _props.radiumConfig,
otherProps = _objectWithoutProperties(_props, ['radiumConfig']);
/* eslint-enable no-unused-vars */
return _react2.default.createElement(
'div',
otherProps,
this.props.children,
_react2.default.createElement(_styleSheet2.default, null)
);
};
return StyleRoot;
}(_react.PureComponent);
StyleRoot.contextTypes = {
_radiumConfig: _react.PropTypes.object,
_radiumStyleKeeper: _react.PropTypes.instanceOf(_styleKeeper2.default)
};
StyleRoot.childContextTypes = {
_radiumStyleKeeper: _react.PropTypes.instanceOf(_styleKeeper2.default)
};
StyleRoot = (0, _enhancer2.default)(StyleRoot);
exports.default = StyleRoot;
module.exports = exports['default'];
/***/ }),
/* 22 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; };
var _class, _temp;
var _cssRuleSetToString = __webpack_require__(6);
var _cssRuleSetToString2 = _interopRequireDefault(_cssRuleSetToString);
var _react = __webpack_require__(2);
var _react2 = _interopRequireDefault(_react);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
var Style = (_temp = _class = function (_PureComponent) {
_inherits(Style, _PureComponent);
function Style() {
_classCallCheck(this, Style);
return _possibleConstructorReturn(this, _PureComponent.apply(this, arguments));
}
Style.prototype._buildStyles = function _buildStyles(styles) {
var _this2 = this;
var userAgent = this.props.radiumConfig && this.props.radiumConfig.userAgent || this.context && this.context._radiumConfig && this.context._radiumConfig.userAgent;
var scopeSelector = this.props.scopeSelector;
var rootRules = Object.keys(styles).reduce(function (accumulator, selector) {
if (_typeof(styles[selector]) !== 'object') {
accumulator[selector] = styles[selector];
}
return accumulator;
}, {});
var rootStyles = Object.keys(rootRules).length ? (0, _cssRuleSetToString2.default)(scopeSelector || '', rootRules, userAgent) : '';
return rootStyles + Object.keys(styles).reduce(function (accumulator, selector) {
var rules = styles[selector];
if (selector === 'mediaQueries') {
accumulator += _this2._buildMediaQueryString(rules);
} else if (_typeof(styles[selector]) === 'object') {
var completeSelector = scopeSelector ? selector.split(',').map(function (part) {
return scopeSelector + ' ' + part.trim();
}).join(',') : selector;
accumulator += (0, _cssRuleSetToString2.default)(completeSelector, rules, userAgent);
}
return accumulator;
}, '');
};
Style.prototype._buildMediaQueryString = function _buildMediaQueryString(stylesByMediaQuery) {
var _this3 = this;
var mediaQueryString = '';
Object.keys(stylesByMediaQuery).forEach(function (query) {
mediaQueryString += '@media ' + query + '{' + _this3._buildStyles(stylesByMediaQuery[query]) + '}';
});
return mediaQueryString;
};
Style.prototype.render = function render() {
if (!this.props.rules) {
return null;
}
var styles = this._buildStyles(this.props.rules);
return _react2.default.createElement('style', { dangerouslySetInnerHTML: { __html: styles } });
};
return Style;
}(_react.PureComponent), _class.propTypes = {
radiumConfig: _react.PropTypes.object,
rules: _react.PropTypes.object,
scopeSelector: _react.PropTypes.string
}, _class.contextTypes = {
_radiumConfig: _react.PropTypes.object
}, _class.defaultProps = {
scopeSelector: ''
}, _temp);
exports.default = Style;
module.exports = exports['default'];
/***/ }),
/* 23 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = keyframes;
var _cssRuleSetToString = __webpack_require__(6);
var _cssRuleSetToString2 = _interopRequireDefault(_cssRuleSetToString);
var _hash = __webpack_require__(18);
var _hash2 = _interopRequireDefault(_hash);
var _prefixer = __webpack_require__(7);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
function keyframes(keyframeRules, name) {
return {
__radiumKeyframes: true,
__process: function __process(userAgent) {
var keyframesPrefixed = (0, _prefixer.getPrefixedKeyframes)(userAgent);
var rules = Object.keys(keyframeRules).map(function (percentage) {
return (0, _cssRuleSetToString2.default)(percentage, keyframeRules[percentage], userAgent);
}).join('\n');
var animationName = (name ? name + '-' : '') + 'radium-animation-' + (0, _hash2.default)(rules);
var css = '@' + keyframesPrefixed + ' ' + animationName + ' {\n' + rules + '\n}\n';
return { css: css, animationName: animationName };
}
};
}
module.exports = exports['default'];
/***/ }),
/* 24 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
/*!
* Bowser - a browser detector
* https://github.com/ded/bowser
* MIT License | (c) Dustin Diaz 2015
*/
!function (root, name, definition) {
if (typeof module != 'undefined' && module.exports) module.exports = definition();else if (true) __webpack_require__(66)(name, definition);else root[name] = definition();
}(undefined, 'bowser', function () {
/**
* See useragents.js for examples of navigator.userAgent
*/
var t = true;
function detect(ua) {
function getFirstMatch(regex) {
var match = ua.match(regex);
return match && match.length > 1 && match[1] || '';
}
function getSecondMatch(regex) {
var match = ua.match(regex);
return match && match.length > 1 && match[2] || '';
}
var iosdevice = getFirstMatch(/(ipod|iphone|ipad)/i).toLowerCase(),
likeAndroid = /like android/i.test(ua),
android = !likeAndroid && /android/i.test(ua),
nexusMobile = /nexus\s*[0-6]\s*/i.test(ua),
nexusTablet = !nexusMobile && /nexus\s*[0-9]+/i.test(ua),
chromeos = /CrOS/.test(ua),
silk = /silk/i.test(ua),
sailfish = /sailfish/i.test(ua),
tizen = /tizen/i.test(ua),
webos = /(web|hpw)os/i.test(ua),
windowsphone = /windows phone/i.test(ua),
samsungBrowser = /SamsungBrowser/i.test(ua),
windows = !windowsphone && /windows/i.test(ua),
mac = !iosdevice && !silk && /macintosh/i.test(ua),
linux = !android && !sailfish && !tizen && !webos && /linux/i.test(ua),
edgeVersion = getFirstMatch(/edge\/(\d+(\.\d+)?)/i),
versionIdentifier = getFirstMatch(/version\/(\d+(\.\d+)?)/i),
tablet = /tablet/i.test(ua),
mobile = !tablet && /[^-]mobi/i.test(ua),
xbox = /xbox/i.test(ua),
result;
if (/opera/i.test(ua)) {
// an old Opera
result = {
name: 'Opera',
opera: t,
version: versionIdentifier || getFirstMatch(/(?:opera|opr|opios)[\s\/](\d+(\.\d+)?)/i)
};
} else if (/opr|opios/i.test(ua)) {
// a new Opera
result = {
name: 'Opera',
opera: t,
version: getFirstMatch(/(?:opr|opios)[\s\/](\d+(\.\d+)?)/i) || versionIdentifier
};
} else if (/SamsungBrowser/i.test(ua)) {
result = {
name: 'Samsung Internet for Android',
samsungBrowser: t,
version: versionIdentifier || getFirstMatch(/(?:SamsungBrowser)[\s\/](\d+(\.\d+)?)/i)
};
} else if (/coast/i.test(ua)) {
result = {
name: 'Opera Coast',
coast: t,
version: versionIdentifier || getFirstMatch(/(?:coast)[\s\/](\d+(\.\d+)?)/i)
};
} else if (/yabrowser/i.test(ua)) {
result = {
name: 'Yandex Browser',
yandexbrowser: t,
version: versionIdentifier || getFirstMatch(/(?:yabrowser)[\s\/](\d+(\.\d+)?)/i)
};
} else if (/ucbrowser/i.test(ua)) {
result = {
name: 'UC Browser',
ucbrowser: t,
version: getFirstMatch(/(?:ucbrowser)[\s\/](\d+(?:\.\d+)+)/i)
};
} else if (/mxios/i.test(ua)) {
result = {
name: 'Maxthon',
maxthon: t,
version: getFirstMatch(/(?:mxios)[\s\/](\d+(?:\.\d+)+)/i)
};
} else if (/epiphany/i.test(ua)) {
result = {
name: 'Epiphany',
epiphany: t,
version: getFirstMatch(/(?:epiphany)[\s\/](\d+(?:\.\d+)+)/i)
};
} else if (/puffin/i.test(ua)) {
result = {
name: 'Puffin',
puffin: t,
version: getFirstMatch(/(?:puffin)[\s\/](\d+(?:\.\d+)?)/i)
};
} else if (/sleipnir/i.test(ua)) {
result = {
name: 'Sleipnir',
sleipnir: t,
version: getFirstMatch(/(?:sleipnir)[\s\/](\d+(?:\.\d+)+)/i)
};
} else if (/k-meleon/i.test(ua)) {
result = {
name: 'K-Meleon',
kMeleon: t,
version: getFirstMatch(/(?:k-meleon)[\s\/](\d+(?:\.\d+)+)/i)
};
} else if (windowsphone) {
result = {
name: 'Windows Phone',
windowsphone: t
};
if (edgeVersion) {
result.msedge = t;
result.version = edgeVersion;
} else {
result.msie = t;
result.version = getFirstMatch(/iemobile\/(\d+(\.\d+)?)/i);
}
} else if (/msie|trident/i.test(ua)) {
result = {
name: 'Internet Explorer',
msie: t,
version: getFirstMatch(/(?:msie |rv:)(\d+(\.\d+)?)/i)
};
} else if (chromeos) {
result = {
name: 'Chrome',
chromeos: t,
chromeBook: t,
chrome: t,
version: getFirstMatch(/(?:chrome|crios|crmo)\/(\d+(\.\d+)?)/i)
};
} else if (/chrome.+? edge/i.test(ua)) {
result = {
name: 'Microsoft Edge',
msedge: t,
version: edgeVersion
};
} else if (/vivaldi/i.test(ua)) {
result = {
name: 'Vivaldi',
vivaldi: t,
version: getFirstMatch(/vivaldi\/(\d+(\.\d+)?)/i) || versionIdentifier
};
} else if (sailfish) {
result = {
name: 'Sailfish',
sailfish: t,
version: getFirstMatch(/sailfish\s?browser\/(\d+(\.\d+)?)/i)
};
} else if (/seamonkey\//i.test(ua)) {
result = {
name: 'SeaMonkey',
seamonkey: t,
version: getFirstMatch(/seamonkey\/(\d+(\.\d+)?)/i)
};
} else if (/firefox|iceweasel|fxios/i.test(ua)) {
result = {
name: 'Firefox',
firefox: t,
version: getFirstMatch(/(?:firefox|iceweasel|fxios)[ \/](\d+(\.\d+)?)/i)
};
if (/\((mobile|tablet);[^\)]*rv:[\d\.]+\)/i.test(ua)) {
result.firefoxos = t;
}
} else if (silk) {
result = {
name: 'Amazon Silk',
silk: t,
version: getFirstMatch(/silk\/(\d+(\.\d+)?)/i)
};
} else if (/phantom/i.test(ua)) {
result = {
name: 'PhantomJS',
phantom: t,
version: getFirstMatch(/phantomjs\/(\d+(\.\d+)?)/i)
};
} else if (/slimerjs/i.test(ua)) {
result = {
name: 'SlimerJS',
slimer: t,
version: getFirstMatch(/slimerjs\/(\d+(\.\d+)?)/i)
};
} else if (/blackberry|\bbb\d+/i.test(ua) || /rim\stablet/i.test(ua)) {
result = {
name: 'BlackBerry',
blackberry: t,
version: versionIdentifier || getFirstMatch(/blackberry[\d]+\/(\d+(\.\d+)?)/i)
};
} else if (webos) {
result = {
name: 'WebOS',
webos: t,
version: versionIdentifier || getFirstMatch(/w(?:eb)?osbrowser\/(\d+(\.\d+)?)/i)
};
/touchpad\//i.test(ua) && (result.touchpad = t);
} else if (/bada/i.test(ua)) {
result = {
name: 'Bada',
bada: t,
version: getFirstMatch(/dolfin\/(\d+(\.\d+)?)/i)
};
} else if (tizen) {
result = {
name: 'Tizen',
tizen: t,
version: getFirstMatch(/(?:tizen\s?)?browser\/(\d+(\.\d+)?)/i) || versionIdentifier
};
} else if (/qupzilla/i.test(ua)) {
result = {
name: 'QupZilla',
qupzilla: t,
version: getFirstMatch(/(?:qupzilla)[\s\/](\d+(?:\.\d+)+)/i) || versionIdentifier
};
} else if (/chromium/i.test(ua)) {
result = {
name: 'Chromium',
chromium: t,
version: getFirstMatch(/(?:chromium)[\s\/](\d+(?:\.\d+)?)/i) || versionIdentifier
};
} else if (/chrome|crios|crmo/i.test(ua)) {
result = {
name: 'Chrome',
chrome: t,
version: getFirstMatch(/(?:chrome|crios|crmo)\/(\d+(\.\d+)?)/i)
};
} else if (android) {
result = {
name: 'Android',
version: versionIdentifier
};
} else if (/safari|applewebkit/i.test(ua)) {
result = {
name: 'Safari',
safari: t
};
if (versionIdentifier) {
result.version = versionIdentifier;
}
} else if (iosdevice) {
result = {
name: iosdevice == 'iphone' ? 'iPhone' : iosdevice == 'ipad' ? 'iPad' : 'iPod'
};
// WTF: version is not part of user agent in web apps
if (versionIdentifier) {
result.version = versionIdentifier;
}
} else if (/googlebot/i.test(ua)) {
result = {
name: 'Googlebot',
googlebot: t,
version: getFirstMatch(/googlebot\/(\d+(\.\d+))/i) || versionIdentifier
};
} else {
result = {
name: getFirstMatch(/^(.*)\/(.*) /),
version: getSecondMatch(/^(.*)\/(.*) /)
};
}
// set webkit or gecko flag for browsers based on these engines
if (!result.msedge && /(apple)?webkit/i.test(ua)) {
if (/(apple)?webkit\/537\.36/i.test(ua)) {
result.name = result.name || "Blink";
result.blink = t;
} else {
result.name = result.name || "Webkit";
result.webkit = t;
}
if (!result.version && versionIdentifier) {
result.version = versionIdentifier;
}
} else if (!result.opera && /gecko\//i.test(ua)) {
result.name = result.name || "Gecko";
result.gecko = t;
result.version = result.version || getFirstMatch(/gecko\/(\d+(\.\d+)?)/i);
}
// set OS flags for platforms that have multiple browsers
if (!result.windowsphone && !result.msedge && (android || result.silk)) {
result.android = t;
} else if (!result.windowsphone && !result.msedge && iosdevice) {
result[iosdevice] = t;
result.ios = t;
} else if (mac) {
result.mac = t;
} else if (xbox) {
result.xbox = t;
} else if (windows) {
result.windows = t;
} else if (linux) {
result.linux = t;
}
// OS version extraction
var osVersion = '';
if (result.windowsphone) {
osVersion = getFirstMatch(/windows phone (?:os)?\s?(\d+(\.\d+)*)/i);
} else if (iosdevice) {
osVersion = getFirstMatch(/os (\d+([_\s]\d+)*) like mac os x/i);
osVersion = osVersion.replace(/[_\s]/g, '.');
} else if (android) {
osVersion = getFirstMatch(/android[ \/-](\d+(\.\d+)*)/i);
} else if (result.webos) {
osVersion = getFirstMatch(/(?:web|hpw)os\/(\d+(\.\d+)*)/i);
} else if (result.blackberry) {
osVersion = getFirstMatch(/rim\stablet\sos\s(\d+(\.\d+)*)/i);
} else if (result.bada) {
osVersion = getFirstMatch(/bada\/(\d+(\.\d+)*)/i);
} else if (result.tizen) {
osVersion = getFirstMatch(/tizen[\/\s](\d+(\.\d+)*)/i);
}
if (osVersion) {
result.osversion = osVersion;
}
// device type extraction
var osMajorVersion = osVersion.split('.')[0];
if (tablet || nexusTablet || iosdevice == 'ipad' || android && (osMajorVersion == 3 || osMajorVersion >= 4 && !mobile) || result.silk) {
result.tablet = t;
} else if (mobile || iosdevice == 'iphone' || iosdevice == 'ipod' || android || nexusMobile || result.blackberry || result.webos || result.bada) {
result.mobile = t;
}
// Graded Browser Support
// http://developer.yahoo.com/yui/articles/gbs
if (result.msedge || result.msie && result.version >= 10 || result.yandexbrowser && result.version >= 15 || result.vivaldi && result.version >= 1.0 || result.chrome && result.version >= 20 || result.samsungBrowser && result.version >= 4 || result.firefox && result.version >= 20.0 || result.safari && result.version >= 6 || result.opera && result.version >= 10.0 || result.ios && result.osversion && result.osversion.split(".")[0] >= 6 || result.blackberry && result.version >= 10.1 || result.chromium && result.version >= 20) {
result.a = t;
} else if (result.msie && result.version < 10 || result.chrome && result.version < 20 || result.firefox && result.version < 20.0 || result.safari && result.version < 6 || result.opera && result.version < 10.0 || result.ios && result.osversion && result.osversion.split(".")[0] < 6 || result.chromium && result.version < 20) {
result.c = t;
} else result.x = t;
return result;
}
var bowser = detect(typeof navigator !== 'undefined' ? navigator.userAgent || '' : '');
bowser.test = function (browserList) {
for (var i = 0; i < browserList.length; ++i) {
var browserItem = browserList[i];
if (typeof browserItem === 'string') {
if (browserItem in bowser) {
return true;
}
}
}
return false;
};
/**
* Get version precisions count
*
* @example
* getVersionPrecision("1.10.3") // 3
*
* @param {string} version
* @return {number}
*/
function getVersionPrecision(version) {
return version.split(".").length;
}
/**
* Array::map polyfill
*
* @param {Array} arr
* @param {Function} iterator
* @return {Array}
*/
function map(arr, iterator) {
var result = [],
i;
if (Array.prototype.map) {
return Array.prototype.map.call(arr, iterator);
}
for (i = 0; i < arr.length; i++) {
result.push(iterator(arr[i]));
}
return result;
}
/**
* Calculate browser version weight
*
* @example
* compareVersions(['1.10.2.1', '1.8.2.1.90']) // 1
* compareVersions(['1.010.2.1', '1.09.2.1.90']); // 1
* compareVersions(['1.10.2.1', '1.10.2.1']); // 0
* compareVersions(['1.10.2.1', '1.0800.2']); // -1
*
* @param {Array<String>} versions versions to compare
* @return {Number} comparison result
*/
function compareVersions(versions) {
// 1) get common precision for both versions, for example for "10.0" and "9" it should be 2
var precision = Math.max(getVersionPrecision(versions[0]), getVersionPrecision(versions[1]));
var chunks = map(versions, function (version) {
var delta = precision - getVersionPrecision(version);
// 2) "9" -> "9.0" (for precision = 2)
version = version + new Array(delta + 1).join(".0");
// 3) "9.0" -> ["000000000"", "000000009"]
return map(version.split("."), function (chunk) {
return new Array(20 - chunk.length).join("0") + chunk;
}).reverse();
});
// iterate in reverse order by reversed chunks array
while (--precision >= 0) {
// 4) compare: "000000009" > "000000010" = false (but "9" > "10" = true)
if (chunks[0][precision] > chunks[1][precision]) {
return 1;
} else if (chunks[0][precision] === chunks[1][precision]) {
if (precision === 0) {
// all version chunks are same
return 0;
}
} else {
return -1;
}
}
}
/**
* Check if browser is unsupported
*
* @example
* bowser.isUnsupportedBrowser({
* msie: "10",
* firefox: "23",
* chrome: "29",
* safari: "5.1",
* opera: "16",
* phantom: "534"
* });
*
* @param {Object} minVersions map of minimal version to browser
* @param {Boolean} [strictMode = false] flag to return false if browser wasn't found in map
* @param {String} [ua] user agent string
* @return {Boolean}
*/
function isUnsupportedBrowser(minVersions, strictMode, ua) {
var _bowser = bowser;
// make strictMode param optional with ua param usage
if (typeof strictMode === 'string') {
ua = strictMode;
strictMode = void 0;
}
if (strictMode === void 0) {
strictMode = false;
}
if (ua) {
_bowser = detect(ua);
}
var version = "" + _bowser.version;
for (var browser in minVersions) {
if (minVersions.hasOwnProperty(browser)) {
if (_bowser[browser]) {
if (typeof minVersions[browser] !== 'string') {
throw new Error('Browser version in the minVersion map should be a string: ' + browser + ': ' + String(minVersions));
}
// browser version and min supported version.
return compareVersions([version, minVersions[browser]]) < 0;
}
}
}
return strictMode; // not found
}
/**
* Check if browser is supported
*
* @param {Object} minVersions map of minimal version to browser
* @param {Boolean} [strictMode = false] flag to return false if browser wasn't found in map
* @param {String} [ua] user agent string
* @return {Boolean}
*/
function check(minVersions, strictMode, ua) {
return !isUnsupportedBrowser(minVersions, strictMode, ua);
}
bowser.isUnsupportedBrowser = isUnsupportedBrowser;
bowser.compareVersions = compareVersions;
bowser.check = check;
/*
* Set our detect method to the main bowser object so we can
* reuse it to test other user agents.
* This is needed to implement future tests.
*/
bowser._detect = detect;
return bowser;
});
/***/ }),
/* 25 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
var __WEBPACK_AMD_DEFINE_RESULT__;
var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; };
/*!
Copyright (c) 2015 Jed Watson.
Based on code that is Copyright 2013-2015, Facebook, Inc.
All rights reserved.
*/
/* global define */
(function () {
'use strict';
var canUseDOM = !!(typeof window !== 'undefined' && window.document && window.document.createElement);
var ExecutionEnvironment = {
canUseDOM: canUseDOM,
canUseWorkers: typeof Worker !== 'undefined',
canUseEventListeners: canUseDOM && !!(window.addEventListener || window.attachEvent),
canUseViewport: canUseDOM && !!window.screen
};
if ("function" === 'function' && _typeof(__webpack_require__(20)) === 'object' && __webpack_require__(20)) {
!(__WEBPACK_AMD_DEFINE_RESULT__ = function () {
return ExecutionEnvironment;
}.call(exports, __webpack_require__, exports, module),
__WEBPACK_AMD_DEFINE_RESULT__ !== undefined && (module.exports = __WEBPACK_AMD_DEFINE_RESULT__));
} else if (typeof module !== 'undefined' && module.exports) {
module.exports = ExecutionEnvironment;
} else {
window.ExecutionEnvironment = ExecutionEnvironment;
}
})();
/***/ }),
/* 26 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _createClass = function () {
function defineProperties(target, props) {
for (var i = 0; i < props.length; i++) {
var descriptor = props[i];descriptor.enumerable = descriptor.enumerable || false;descriptor.configurable = true;if ("value" in descriptor) descriptor.writable = true;Object.defineProperty(target, descriptor.key, descriptor);
}
}return function (Constructor, protoProps, staticProps) {
if (protoProps) defineProperties(Constructor.prototype, protoProps);if (staticProps) defineProperties(Constructor, staticProps);return Constructor;
};
}();
// special flexbox specifications
var _prefixAll2 = __webpack_require__(47);
var _prefixAll3 = _interopRequireDefault(_prefixAll2);
var _getBrowserInformation = __webpack_require__(48);
var _getBrowserInformation2 = _interopRequireDefault(_getBrowserInformation);
var _getPrefixedKeyframes = __webpack_require__(49);
var _getPrefixedKeyframes2 = _interopRequireDefault(_getPrefixedKeyframes);
var _capitalizeString = __webpack_require__(4);
var _capitalizeString2 = _interopRequireDefault(_capitalizeString);
var _sortPrefixedStyle = __webpack_require__(15);
var _sortPrefixedStyle2 = _interopRequireDefault(_sortPrefixedStyle);
var _prefixProps = __webpack_require__(37);
var _prefixProps2 = _interopRequireDefault(_prefixProps);
var _position = __webpack_require__(33);
var _position2 = _interopRequireDefault(_position);
var _calc = __webpack_require__(27);
var _calc2 = _interopRequireDefault(_calc);
var _zoomCursor = __webpack_require__(36);
var _zoomCursor2 = _interopRequireDefault(_zoomCursor);
var _grabCursor = __webpack_require__(31);
var _grabCursor2 = _interopRequireDefault(_grabCursor);
var _flex = __webpack_require__(28);
var _flex2 = _interopRequireDefault(_flex);
var _sizing = __webpack_require__(34);
var _sizing2 = _interopRequireDefault(_sizing);
var _gradient = __webpack_require__(32);
var _gradient2 = _interopRequireDefault(_gradient);
var _transition = __webpack_require__(35);
var _transition2 = _interopRequireDefault(_transition);
var _flexboxIE = __webpack_require__(29);
var _flexboxIE2 = _interopRequireDefault(_flexboxIE);
var _flexboxOld = __webpack_require__(30);
var _flexboxOld2 = _interopRequireDefault(_flexboxOld);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
function _classCallCheck(instance, Constructor) {
if (!(instance instanceof Constructor)) {
throw new TypeError("Cannot call a class as a function");
}
}
var plugins = [_position2.default, _calc2.default, _zoomCursor2.default, _grabCursor2.default, _sizing2.default, _gradient2.default, _transition2.default, _flexboxIE2.default, _flexboxOld2.default,
// this must be run AFTER the flexbox specs
_flex2.default];
var Prefixer = function () {
/**
* Instantiante a new prefixer
* @param {string} userAgent - userAgent to gather prefix information according to caniuse.com
* @param {string} keepUnprefixed - keeps unprefixed properties and values
*/
function Prefixer() {
var _this = this;
var options = arguments.length <= 0 || arguments[0] === undefined ? {} : arguments[0];
_classCallCheck(this, Prefixer);
var defaultUserAgent = typeof navigator !== 'undefined' ? navigator.userAgent : undefined;
this._userAgent = options.userAgent || defaultUserAgent;
this._keepUnprefixed = options.keepUnprefixed || false;
this._browserInfo = (0, _getBrowserInformation2.default)(this._userAgent);
// Checks if the userAgent was resolved correctly
if (this._browserInfo && this._browserInfo.prefix) {
// set additional prefix information
this.cssPrefix = this._browserInfo.prefix.css;
this.jsPrefix = this._browserInfo.prefix.inline;
this.prefixedKeyframes = (0, _getPrefixedKeyframes2.default)(this._browserInfo);
} else {
this._usePrefixAllFallback = true;
return false;
}
var data = this._browserInfo.browser && _prefixProps2.default[this._browserInfo.browser];
if (data) {
this._requiresPrefix = Object.keys(data).filter(function (key) {
return data[key] >= _this._browserInfo.version;
}).reduce(function (result, name) {
result[name] = true;
return result;
}, {});
this._hasPropsRequiringPrefix = Object.keys(this._requiresPrefix).length > 0;
} else {
this._usePrefixAllFallback = true;
}
}
/**
* Returns a prefixed version of the style object
* @param {Object} styles - Style object that gets prefixed properties added
* @returns {Object} - Style object with prefixed properties and values
*/
_createClass(Prefixer, [{
key: 'prefix',
value: function prefix(styles) {
var _this2 = this;
// use prefixAll as fallback if userAgent can not be resolved
if (this._usePrefixAllFallback) {
return (0, _prefixAll3.default)(styles);
}
// only add prefixes if needed
if (!this._hasPropsRequiringPrefix) {
return styles;
}
Object.keys(styles).forEach(function (property) {
var value = styles[property];
if (value instanceof Object && !Array.isArray(value)) {
// recurse through nested style objects
styles[property] = _this2.prefix(value);
} else {
// add prefixes if needed
if (_this2._requiresPrefix[property]) {
styles[_this2.jsPrefix + (0, _capitalizeString2.default)(property)] = value;
if (!_this2._keepUnprefixed) {
delete styles[property];
}
}
}
});
Object.keys(styles).forEach(function (property) {
[].concat(styles[property]).forEach(function (value) {
// resolve plugins
plugins.forEach(function (plugin) {
// generates a new plugin interface with current data
assignStyles(styles, plugin({
property: property,
value: value,
styles: styles,
browserInfo: _this2._browserInfo,
prefix: {
js: _this2.jsPrefix,
css: _this2.cssPrefix,
keyframes: _this2.prefixedKeyframes
},
keepUnprefixed: _this2._keepUnprefixed,
requiresPrefix: _this2._requiresPrefix
}), value, _this2._keepUnprefixed);
});
});
});
return (0, _sortPrefixedStyle2.default)(styles);
}
/**
* Returns a prefixed version of the style object using all vendor prefixes
* @param {Object} styles - Style object that gets prefixed properties added
* @returns {Object} - Style object with prefixed properties and values
*/
}], [{
key: 'prefixAll',
value: function prefixAll(styles) {
return (0, _prefixAll3.default)(styles);
}
}]);
return Prefixer;
}();
exports.default = Prefixer;
function assignStyles(base) {
var extend = arguments.length <= 1 || arguments[1] === undefined ? {} : arguments[1];
var value = arguments[2];
var keepUnprefixed = arguments[3];
Object.keys(extend).forEach(function (property) {
var baseValue = base[property];
if (Array.isArray(baseValue)) {
[].concat(extend[property]).forEach(function (val) {
if (base[property].indexOf(val) === -1) {
base[property].splice(baseValue.indexOf(value), keepUnprefixed ? 0 : 1, val);
}
});
} else {
base[property] = extend[property];
}
});
}
module.exports = exports['default'];
/***/ }),
/* 27 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = calc;
var _getPrefixedValue = __webpack_require__(0);
var _getPrefixedValue2 = _interopRequireDefault(_getPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true });
} else {
obj[key] = value;
}return obj;
}
function calc(_ref) {
var property = _ref.property;
var value = _ref.value;
var _ref$browserInfo = _ref.browserInfo;
var browser = _ref$browserInfo.browser;
var version = _ref$browserInfo.version;
var css = _ref.prefix.css;
var keepUnprefixed = _ref.keepUnprefixed;
if (typeof value === 'string' && value.indexOf('calc(') > -1 && (browser === 'firefox' && version < 15 || browser === 'chrome' && version < 25 || browser === 'safari' && version < 6.1 || browser === 'ios_saf' && version < 7)) {
return _defineProperty({}, property, (0, _getPrefixedValue2.default)(value.replace(/calc\(/g, css + 'calc('), value, keepUnprefixed));
}
}
module.exports = exports['default'];
/***/ }),
/* 28 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = flex;
var _getPrefixedValue = __webpack_require__(0);
var _getPrefixedValue2 = _interopRequireDefault(_getPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
var values = { flex: true, 'inline-flex': true };
function flex(_ref) {
var property = _ref.property;
var value = _ref.value;
var _ref$browserInfo = _ref.browserInfo;
var browser = _ref$browserInfo.browser;
var version = _ref$browserInfo.version;
var css = _ref.prefix.css;
var keepUnprefixed = _ref.keepUnprefixed;
if (property === 'display' && values[value] && (browser === 'chrome' && version < 29 && version > 20 || (browser === 'safari' || browser === 'ios_saf') && version < 9 && version > 6 || browser === 'opera' && (version == 15 || version == 16))) {
return {
display: (0, _getPrefixedValue2.default)(css + value, value, keepUnprefixed)
};
}
}
module.exports = exports['default'];
/***/ }),
/* 29 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = flexboxIE;
var _getPrefixedValue = __webpack_require__(0);
var _getPrefixedValue2 = _interopRequireDefault(_getPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true });
} else {
obj[key] = value;
}return obj;
}
var alternativeValues = {
'space-around': 'distribute',
'space-between': 'justify',
'flex-start': 'start',
'flex-end': 'end',
flex: 'flexbox',
'inline-flex': 'inline-flexbox'
};
var alternativeProps = {
alignContent: 'msFlexLinePack',
alignSelf: 'msFlexItemAlign',
alignItems: 'msFlexAlign',
justifyContent: 'msFlexPack',
order: 'msFlexOrder',
flexGrow: 'msFlexPositive',
flexShrink: 'msFlexNegative',
flexBasis: 'msPreferredSize'
};
function flexboxIE(_ref) {
var property = _ref.property;
var value = _ref.value;
var styles = _ref.styles;
var _ref$browserInfo = _ref.browserInfo;
var browser = _ref$browserInfo.browser;
var version = _ref$browserInfo.version;
var css = _ref.prefix.css;
var keepUnprefixed = _ref.keepUnprefixed;
if ((alternativeProps[property] || property === 'display' && typeof value === 'string' && value.indexOf('flex') > -1) && (browser === 'ie_mob' || browser === 'ie') && version == 10) {
if (!keepUnprefixed && !Array.isArray(styles[property])) {
delete styles[property];
}
if (property === 'display' && alternativeValues[value]) {
return {
display: (0, _getPrefixedValue2.default)(css + alternativeValues[value], value, keepUnprefixed)
};
}
if (alternativeProps[property]) {
return _defineProperty({}, alternativeProps[property], alternativeValues[value] || value);
}
}
}
module.exports = exports['default'];
/***/ }),
/* 30 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = flexboxOld;
var _getPrefixedValue = __webpack_require__(0);
var _getPrefixedValue2 = _interopRequireDefault(_getPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true });
} else {
obj[key] = value;
}return obj;
}
var alternativeValues = {
'space-around': 'justify',
'space-between': 'justify',
'flex-start': 'start',
'flex-end': 'end',
'wrap-reverse': 'multiple',
wrap: 'multiple',
flex: 'box',
'inline-flex': 'inline-box'
};
var alternativeProps = {
alignItems: 'WebkitBoxAlign',
justifyContent: 'WebkitBoxPack',
flexWrap: 'WebkitBoxLines'
};
var otherProps = ['alignContent', 'alignSelf', 'order', 'flexGrow', 'flexShrink', 'flexBasis', 'flexDirection'];
var properties = Object.keys(alternativeProps).concat(otherProps);
function flexboxOld(_ref) {
var property = _ref.property;
var value = _ref.value;
var styles = _ref.styles;
var _ref$browserInfo = _ref.browserInfo;
var browser = _ref$browserInfo.browser;
var version = _ref$browserInfo.version;
var css = _ref.prefix.css;
var keepUnprefixed = _ref.keepUnprefixed;
if ((properties.indexOf(property) > -1 || property === 'display' && typeof value === 'string' && value.indexOf('flex') > -1) && (browser === 'firefox' && version < 22 || browser === 'chrome' && version < 21 || (browser === 'safari' || browser === 'ios_saf') && version <= 6.1 || browser === 'android' && version < 4.4 || browser === 'and_uc')) {
if (!keepUnprefixed && !Array.isArray(styles[property])) {
delete styles[property];
}
if (property === 'flexDirection' && typeof value === 'string') {
return {
WebkitBoxOrient: value.indexOf('column') > -1 ? 'vertical' : 'horizontal',
WebkitBoxDirection: value.indexOf('reverse') > -1 ? 'reverse' : 'normal'
};
}
if (property === 'display' && alternativeValues[value]) {
return {
display: (0, _getPrefixedValue2.default)(css + alternativeValues[value], value, keepUnprefixed)
};
}
if (alternativeProps[property]) {
return _defineProperty({}, alternativeProps[property], alternativeValues[value] || value);
}
}
}
module.exports = exports['default'];
/***/ }),
/* 31 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = grabCursor;
var _getPrefixedValue = __webpack_require__(0);
var _getPrefixedValue2 = _interopRequireDefault(_getPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
var values = { grab: true, grabbing: true };
function grabCursor(_ref) {
var property = _ref.property;
var value = _ref.value;
var browser = _ref.browserInfo.browser;
var css = _ref.prefix.css;
var keepUnprefixed = _ref.keepUnprefixed;
// adds prefixes for firefox, chrome, safari, and opera regardless of version until a reliable brwoser support info can be found (see: https://github.com/rofrischmann/inline-style-prefixer/issues/79)
if (property === 'cursor' && values[value] && (browser === 'firefox' || browser === 'chrome' || browser === 'safari' || browser === 'opera')) {
return {
cursor: (0, _getPrefixedValue2.default)(css + value, value, keepUnprefixed)
};
}
}
module.exports = exports['default'];
/***/ }),
/* 32 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = gradient;
var _getPrefixedValue = __webpack_require__(0);
var _getPrefixedValue2 = _interopRequireDefault(_getPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true });
} else {
obj[key] = value;
}return obj;
}
var values = /linear-gradient|radial-gradient|repeating-linear-gradient|repeating-radial-gradient/;
function gradient(_ref) {
var property = _ref.property;
var value = _ref.value;
var _ref$browserInfo = _ref.browserInfo;
var browser = _ref$browserInfo.browser;
var version = _ref$browserInfo.version;
var css = _ref.prefix.css;
var keepUnprefixed = _ref.keepUnprefixed;
if (typeof value === 'string' && value.match(values) !== null && (browser === 'firefox' && version < 16 || browser === 'chrome' && version < 26 || (browser === 'safari' || browser === 'ios_saf') && version < 7 || (browser === 'opera' || browser === 'op_mini') && version < 12.1 || browser === 'android' && version < 4.4 || browser === 'and_uc')) {
return _defineProperty({}, property, (0, _getPrefixedValue2.default)(css + value, value, keepUnprefixed));
}
}
module.exports = exports['default'];
/***/ }),
/* 33 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = position;
var _getPrefixedValue = __webpack_require__(0);
var _getPrefixedValue2 = _interopRequireDefault(_getPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true });
} else {
obj[key] = value;
}return obj;
}
function position(_ref) {
var property = _ref.property;
var value = _ref.value;
var browser = _ref.browserInfo.browser;
var css = _ref.prefix.css;
var keepUnprefixed = _ref.keepUnprefixed;
if (property === 'position' && value === 'sticky' && (browser === 'safari' || browser === 'ios_saf')) {
return _defineProperty({}, property, (0, _getPrefixedValue2.default)(css + value, value, keepUnprefixed));
}
}
module.exports = exports['default'];
/***/ }),
/* 34 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = sizing;
var _getPrefixedValue = __webpack_require__(0);
var _getPrefixedValue2 = _interopRequireDefault(_getPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true });
} else {
obj[key] = value;
}return obj;
}
var properties = {
maxHeight: true,
maxWidth: true,
width: true,
height: true,
columnWidth: true,
minWidth: true,
minHeight: true
};
var values = {
'min-content': true,
'max-content': true,
'fill-available': true,
'fit-content': true,
'contain-floats': true
};
function sizing(_ref) {
var property = _ref.property;
var value = _ref.value;
var css = _ref.prefix.css;
var keepUnprefixed = _ref.keepUnprefixed;
// This might change in the future
// Keep an eye on it
if (properties[property] && values[value]) {
return _defineProperty({}, property, (0, _getPrefixedValue2.default)(css + value, value, keepUnprefixed));
}
}
module.exports = exports['default'];
/***/ }),
/* 35 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
var _typeof2 = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; };
Object.defineProperty(exports, "__esModule", {
value: true
});
var _typeof = typeof Symbol === "function" && _typeof2(Symbol.iterator) === "symbol" ? function (obj) {
return typeof obj === "undefined" ? "undefined" : _typeof2(obj);
} : function (obj) {
return obj && typeof Symbol === "function" && obj.constructor === Symbol ? "symbol" : typeof obj === "undefined" ? "undefined" : _typeof2(obj);
};
exports.default = transition;
var _hyphenateStyleName = __webpack_require__(13);
var _hyphenateStyleName2 = _interopRequireDefault(_hyphenateStyleName);
var _unprefixProperty = __webpack_require__(51);
var _unprefixProperty2 = _interopRequireDefault(_unprefixProperty);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true });
} else {
obj[key] = value;
}return obj;
}
var properties = { transition: true, transitionProperty: true };
function transition(_ref) {
var property = _ref.property;
var value = _ref.value;
var css = _ref.prefix.css;
var requiresPrefix = _ref.requiresPrefix;
var keepUnprefixed = _ref.keepUnprefixed;
// also check for already prefixed transitions
var unprefixedProperty = (0, _unprefixProperty2.default)(property);
if (typeof value === 'string' && properties[unprefixedProperty]) {
var _ret = function () {
// TODO: memoize this array
var requiresPrefixDashCased = Object.keys(requiresPrefix).map(function (prop) {
return (0, _hyphenateStyleName2.default)(prop);
});
// only split multi values, not cubic beziers
var multipleValues = value.split(/,(?![^()]*(?:\([^()]*\))?\))/g);
requiresPrefixDashCased.forEach(function (prop) {
multipleValues.forEach(function (val, index) {
if (val.indexOf(prop) > -1 && prop !== 'order') {
multipleValues[index] = val.replace(prop, css + prop) + (keepUnprefixed ? ',' + val : '');
}
});
});
return {
v: _defineProperty({}, property, multipleValues.join(','))
};
}();
if ((typeof _ret === 'undefined' ? 'undefined' : _typeof(_ret)) === "object") return _ret.v;
}
}
module.exports = exports['default'];
/***/ }),
/* 36 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = zoomCursor;
var _getPrefixedValue = __webpack_require__(0);
var _getPrefixedValue2 = _interopRequireDefault(_getPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
var values = { 'zoom-in': true, 'zoom-out': true };
function zoomCursor(_ref) {
var property = _ref.property;
var value = _ref.value;
var _ref$browserInfo = _ref.browserInfo;
var browser = _ref$browserInfo.browser;
var version = _ref$browserInfo.version;
var css = _ref.prefix.css;
var keepUnprefixed = _ref.keepUnprefixed;
if (property === 'cursor' && values[value] && (browser === 'firefox' && version < 24 || browser === 'chrome' && version < 37 || browser === 'safari' && version < 9 || browser === 'opera' && version < 24)) {
return {
cursor: (0, _getPrefixedValue2.default)(css + value, value, keepUnprefixed)
};
}
}
module.exports = exports['default'];
/***/ }),
/* 37 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = { "chrome": { "transform": 35, "transformOrigin": 35, "transformOriginX": 35, "transformOriginY": 35, "backfaceVisibility": 35, "perspective": 35, "perspectiveOrigin": 35, "transformStyle": 35, "transformOriginZ": 35, "animation": 42, "animationDelay": 42, "animationDirection": 42, "animationFillMode": 42, "animationDuration": 42, "animationIterationCount": 42, "animationName": 42, "animationPlayState": 42, "animationTimingFunction": 42, "appearance": 55, "userSelect": 55, "fontKerning": 32, "textEmphasisPosition": 55, "textEmphasis": 55, "textEmphasisStyle": 55, "textEmphasisColor": 55, "boxDecorationBreak": 55, "clipPath": 55, "maskImage": 55, "maskMode": 55, "maskRepeat": 55, "maskPosition": 55, "maskClip": 55, "maskOrigin": 55, "maskSize": 55, "maskComposite": 55, "mask": 55, "maskBorderSource": 55, "maskBorderMode": 55, "maskBorderSlice": 55, "maskBorderWidth": 55, "maskBorderOutset": 55, "maskBorderRepeat": 55, "maskBorder": 55, "maskType": 55, "textDecorationStyle": 55, "textDecorationSkip": 55, "textDecorationLine": 55, "textDecorationColor": 55, "filter": 52, "fontFeatureSettings": 47, "breakAfter": 49, "breakBefore": 49, "breakInside": 49, "columnCount": 49, "columnFill": 49, "columnGap": 49, "columnRule": 49, "columnRuleColor": 49, "columnRuleStyle": 49, "columnRuleWidth": 49, "columns": 49, "columnSpan": 49, "columnWidth": 49 }, "safari": { "flex": 8, "flexBasis": 8, "flexDirection": 8, "flexGrow": 8, "flexFlow": 8, "flexShrink": 8, "flexWrap": 8, "alignContent": 8, "alignItems": 8, "alignSelf": 8, "justifyContent": 8, "order": 8, "transition": 6, "transitionDelay": 6, "transitionDuration": 6, "transitionProperty": 6, "transitionTimingFunction": 6, "transform": 8, "transformOrigin": 8, "transformOriginX": 8, "transformOriginY": 8, "backfaceVisibility": 8, "perspective": 8, "perspectiveOrigin": 8, "transformStyle": 8, "transformOriginZ": 8, "animation": 8, "animationDelay": 8, "animationDirection": 8, "animationFillMode": 8, "animationDuration": 8, "animationIterationCount": 8, "animationName": 8, "animationPlayState": 8, "animationTimingFunction": 8, "appearance": 10, "userSelect": 10, "backdropFilter": 10, "fontKerning": 9, "scrollSnapType": 10, "scrollSnapPointsX": 10, "scrollSnapPointsY": 10, "scrollSnapDestination": 10, "scrollSnapCoordinate": 10, "textEmphasisPosition": 7, "textEmphasis": 7, "textEmphasisStyle": 7, "textEmphasisColor": 7, "boxDecorationBreak": 10, "clipPath": 10, "maskImage": 10, "maskMode": 10, "maskRepeat": 10, "maskPosition": 10, "maskClip": 10, "maskOrigin": 10, "maskSize": 10, "maskComposite": 10, "mask": 10, "maskBorderSource": 10, "maskBorderMode": 10, "maskBorderSlice": 10, "maskBorderWidth": 10, "maskBorderOutset": 10, "maskBorderRepeat": 10, "maskBorder": 10, "maskType": 10, "textDecorationStyle": 10, "textDecorationSkip": 10, "textDecorationLine": 10, "textDecorationColor": 10, "shapeImageThreshold": 10, "shapeImageMargin": 10, "shapeImageOutside": 10, "filter": 9, "hyphens": 10, "flowInto": 10, "flowFrom": 10, "breakBefore": 8, "breakAfter": 8, "breakInside": 8, "regionFragment": 10, "columnCount": 8, "columnFill": 8, "columnGap": 8, "columnRule": 8, "columnRuleColor": 8, "columnRuleStyle": 8, "columnRuleWidth": 8, "columns": 8, "columnSpan": 8, "columnWidth": 8 }, "firefox": { "appearance": 51, "userSelect": 51, "boxSizing": 28, "textAlignLast": 48, "textDecorationStyle": 35, "textDecorationSkip": 35, "textDecorationLine": 35, "textDecorationColor": 35, "tabSize": 51, "hyphens": 42, "fontFeatureSettings": 33, "breakAfter": 51, "breakBefore": 51, "breakInside": 51, "columnCount": 51, "columnFill": 51, "columnGap": 51, "columnRule": 51, "columnRuleColor": 51, "columnRuleStyle": 51, "columnRuleWidth": 51, "columns": 51, "columnSpan": 51, "columnWidth": 51 }, "opera": { "flex": 16, "flexBasis": 16, "flexDirection": 16, "flexGrow": 16, "flexFlow": 16, "flexShrink": 16, "flexWrap": 16, "alignContent": 16, "alignItems": 16, "alignSelf": 16, "justifyContent": 16, "order": 16, "transform": 22, "transformOrigin": 22, "transformOriginX": 22, "transformOriginY": 22, "backfaceVisibility": 22, "perspective": 22, "perspectiveOrigin": 22, "transformStyle": 22, "transformOriginZ": 22, "animation": 29, "animationDelay": 29, "animationDirection": 29, "animationFillMode": 29, "animationDuration": 29, "animationIterationCount": 29, "animationName": 29, "animationPlayState": 29, "animationTimingFunction": 29, "appearance": 41, "userSelect": 41, "fontKerning": 19, "textEmphasisPosition": 41, "textEmphasis": 41, "textEmphasisStyle": 41, "textEmphasisColor": 41, "boxDecorationBreak": 41, "clipPath": 41, "maskImage": 41, "maskMode": 41, "maskRepeat": 41, "maskPosition": 41, "maskClip": 41, "maskOrigin": 41, "maskSize": 41, "maskComposite": 41, "mask": 41, "maskBorderSource": 41, "maskBorderMode": 41, "maskBorderSlice": 41, "maskBorderWidth": 41, "maskBorderOutset": 41, "maskBorderRepeat": 41, "maskBorder": 41, "maskType": 41, "textDecorationStyle": 41, "textDecorationSkip": 41, "textDecorationLine": 41, "textDecorationColor": 41, "filter": 39, "fontFeatureSettings": 34, "breakAfter": 36, "breakBefore": 36, "breakInside": 36, "columnCount": 36, "columnFill": 36, "columnGap": 36, "columnRule": 36, "columnRuleColor": 36, "columnRuleStyle": 36, "columnRuleWidth": 36, "columns": 36, "columnSpan": 36, "columnWidth": 36 }, "ie": { "flex": 10, "flexDirection": 10, "flexFlow": 10, "flexWrap": 10, "transform": 9, "transformOrigin": 9, "transformOriginX": 9, "transformOriginY": 9, "userSelect": 11, "wrapFlow": 11, "wrapThrough": 11, "wrapMargin": 11, "scrollSnapType": 11, "scrollSnapPointsX": 11, "scrollSnapPointsY": 11, "scrollSnapDestination": 11, "scrollSnapCoordinate": 11, "touchAction": 10, "hyphens": 11, "flowInto": 11, "flowFrom": 11, "breakBefore": 11, "breakAfter": 11, "breakInside": 11, "regionFragment": 11, "gridTemplateColumns": 11, "gridTemplateRows": 11, "gridTemplateAreas": 11, "gridTemplate": 11, "gridAutoColumns": 11, "gridAutoRows": 11, "gridAutoFlow": 11, "grid": 11, "gridRowStart": 11, "gridColumnStart": 11, "gridRowEnd": 11, "gridRow": 11, "gridColumn": 11, "gridColumnEnd": 11, "gridColumnGap": 11, "gridRowGap": 11, "gridArea": 11, "gridGap": 11, "textSizeAdjust": 11 }, "edge": { "userSelect": 14, "wrapFlow": 14, "wrapThrough": 14, "wrapMargin": 14, "scrollSnapType": 14, "scrollSnapPointsX": 14, "scrollSnapPointsY": 14, "scrollSnapDestination": 14, "scrollSnapCoordinate": 14, "hyphens": 14, "flowInto": 14, "flowFrom": 14, "breakBefore": 14, "breakAfter": 14, "breakInside": 14, "regionFragment": 14, "gridTemplateColumns": 14, "gridTemplateRows": 14, "gridTemplateAreas": 14, "gridTemplate": 14, "gridAutoColumns": 14, "gridAutoRows": 14, "gridAutoFlow": 14, "grid": 14, "gridRowStart": 14, "gridColumnStart": 14, "gridRowEnd": 14, "gridRow": 14, "gridColumn": 14, "gridColumnEnd": 14, "gridColumnGap": 14, "gridRowGap": 14, "gridArea": 14, "gridGap": 14 }, "ios_saf": { "flex": 8.1, "flexBasis": 8.1, "flexDirection": 8.1, "flexGrow": 8.1, "flexFlow": 8.1, "flexShrink": 8.1, "flexWrap": 8.1, "alignContent": 8.1, "alignItems": 8.1, "alignSelf": 8.1, "justifyContent": 8.1, "order": 8.1, "transition": 6, "transitionDelay": 6, "transitionDuration": 6, "transitionProperty": 6, "transitionTimingFunction": 6, "transform": 8.1, "transformOrigin": 8.1, "transformOriginX": 8.1, "transformOriginY": 8.1, "backfaceVisibility": 8.1, "perspective": 8.1, "perspectiveOrigin": 8.1, "transformStyle": 8.1, "transformOriginZ": 8.1, "animation": 8.1, "animationDelay": 8.1, "animationDirection": 8.1, "animationFillMode": 8.1, "animationDuration": 8.1, "animationIterationCount": 8.1, "animationName": 8.1, "animationPlayState": 8.1, "animationTimingFunction": 8.1, "appearance": 9.3, "userSelect": 9.3, "backdropFilter": 9.3, "fontKerning": 9.3, "scrollSnapType": 9.3, "scrollSnapPointsX": 9.3, "scrollSnapPointsY": 9.3, "scrollSnapDestination": 9.3, "scrollSnapCoordinate": 9.3, "boxDecorationBreak": 9.3, "clipPath": 9.3, "maskImage": 9.3, "maskMode": 9.3, "maskRepeat": 9.3, "maskPosition": 9.3, "maskClip": 9.3, "maskOrigin": 9.3, "maskSize": 9.3, "maskComposite": 9.3, "mask": 9.3, "maskBorderSource": 9.3, "maskBorderMode": 9.3, "maskBorderSlice": 9.3, "maskBorderWidth": 9.3, "maskBorderOutset": 9.3, "maskBorderRepeat": 9.3, "maskBorder": 9.3, "maskType": 9.3, "textSizeAdjust": 9.3, "textDecorationStyle": 9.3, "textDecorationSkip": 9.3, "textDecorationLine": 9.3, "textDecorationColor": 9.3, "shapeImageThreshold": 9.3, "shapeImageMargin": 9.3, "shapeImageOutside": 9.3, "filter": 9, "hyphens": 9.3, "flowInto": 9.3, "flowFrom": 9.3, "breakBefore": 8.1, "breakAfter": 8.1, "breakInside": 8.1, "regionFragment": 9.3, "columnCount": 8.1, "columnFill": 8.1, "columnGap": 8.1, "columnRule": 8.1, "columnRuleColor": 8.1, "columnRuleStyle": 8.1, "columnRuleWidth": 8.1, "columns": 8.1, "columnSpan": 8.1, "columnWidth": 8.1 }, "android": { "flex": 4.2, "flexBasis": 4.2, "flexDirection": 4.2, "flexGrow": 4.2, "flexFlow": 4.2, "flexShrink": 4.2, "flexWrap": 4.2, "alignContent": 4.2, "alignItems": 4.2, "alignSelf": 4.2, "justifyContent": 4.2, "order": 4.2, "transition": 4.2, "transitionDelay": 4.2, "transitionDuration": 4.2, "transitionProperty": 4.2, "transitionTimingFunction": 4.2, "transform": 4.4, "transformOrigin": 4.4, "transformOriginX": 4.4, "transformOriginY": 4.4, "backfaceVisibility": 4.4, "perspective": 4.4, "perspectiveOrigin": 4.4, "transformStyle": 4.4, "transformOriginZ": 4.4, "animation": 4.4, "animationDelay": 4.4, "animationDirection": 4.4, "animationFillMode": 4.4, "animationDuration": 4.4, "animationIterationCount": 4.4, "animationName": 4.4, "animationPlayState": 4.4, "animationTimingFunction": 4.4, "appearance": 51, "userSelect": 51, "fontKerning": 4.4, "textEmphasisPosition": 51, "textEmphasis": 51, "textEmphasisStyle": 51, "textEmphasisColor": 51, "boxDecorationBreak": 51, "clipPath": 51, "maskImage": 51, "maskMode": 51, "maskRepeat": 51, "maskPosition": 51, "maskClip": 51, "maskOrigin": 51, "maskSize": 51, "maskComposite": 51, "mask": 51, "maskBorderSource": 51, "maskBorderMode": 51, "maskBorderSlice": 51, "maskBorderWidth": 51, "maskBorderOutset": 51, "maskBorderRepeat": 51, "maskBorder": 51, "maskType": 51, "filter": 51, "fontFeatureSettings": 4.4, "breakAfter": 51, "breakBefore": 51, "breakInside": 51, "columnCount": 51, "columnFill": 51, "columnGap": 51, "columnRule": 51, "columnRuleColor": 51, "columnRuleStyle": 51, "columnRuleWidth": 51, "columns": 51, "columnSpan": 51, "columnWidth": 51 }, "and_chr": { "appearance": 51, "userSelect": 51, "textEmphasisPosition": 51, "textEmphasis": 51, "textEmphasisStyle": 51, "textEmphasisColor": 51, "boxDecorationBreak": 51, "clipPath": 51, "maskImage": 51, "maskMode": 51, "maskRepeat": 51, "maskPosition": 51, "maskClip": 51, "maskOrigin": 51, "maskSize": 51, "maskComposite": 51, "mask": 51, "maskBorderSource": 51, "maskBorderMode": 51, "maskBorderSlice": 51, "maskBorderWidth": 51, "maskBorderOutset": 51, "maskBorderRepeat": 51, "maskBorder": 51, "maskType": 51, "textDecorationStyle": 51, "textDecorationSkip": 51, "textDecorationLine": 51, "textDecorationColor": 51, "filter": 51 }, "and_uc": { "flex": 9.9, "flexBasis": 9.9, "flexDirection": 9.9, "flexGrow": 9.9, "flexFlow": 9.9, "flexShrink": 9.9, "flexWrap": 9.9, "alignContent": 9.9, "alignItems": 9.9, "alignSelf": 9.9, "justifyContent": 9.9, "order": 9.9, "transition": 9.9, "transitionDelay": 9.9, "transitionDuration": 9.9, "transitionProperty": 9.9, "transitionTimingFunction": 9.9, "transform": 9.9, "transformOrigin": 9.9, "transformOriginX": 9.9, "transformOriginY": 9.9, "backfaceVisibility": 9.9, "perspective": 9.9, "perspectiveOrigin": 9.9, "transformStyle": 9.9, "transformOriginZ": 9.9, "animation": 9.9, "animationDelay": 9.9, "animationDirection": 9.9, "animationFillMode": 9.9, "animationDuration": 9.9, "animationIterationCount": 9.9, "animationName": 9.9, "animationPlayState": 9.9, "animationTimingFunction": 9.9, "appearance": 9.9, "userSelect": 9.9, "fontKerning": 9.9, "textEmphasisPosition": 9.9, "textEmphasis": 9.9, "textEmphasisStyle": 9.9, "textEmphasisColor": 9.9, "maskImage": 9.9, "maskMode": 9.9, "maskRepeat": 9.9, "maskPosition": 9.9, "maskClip": 9.9, "maskOrigin": 9.9, "maskSize": 9.9, "maskComposite": 9.9, "mask": 9.9, "maskBorderSource": 9.9, "maskBorderMode": 9.9, "maskBorderSlice": 9.9, "maskBorderWidth": 9.9, "maskBorderOutset": 9.9, "maskBorderRepeat": 9.9, "maskBorder": 9.9, "maskType": 9.9, "textSizeAdjust": 9.9, "filter": 9.9, "hyphens": 9.9, "flowInto": 9.9, "flowFrom": 9.9, "breakBefore": 9.9, "breakAfter": 9.9, "breakInside": 9.9, "regionFragment": 9.9, "fontFeatureSettings": 9.9, "columnCount": 9.9, "columnFill": 9.9, "columnGap": 9.9, "columnRule": 9.9, "columnRuleColor": 9.9, "columnRuleStyle": 9.9, "columnRuleWidth": 9.9, "columns": 9.9, "columnSpan": 9.9, "columnWidth": 9.9 }, "op_mini": {} };
module.exports = exports["default"];
/***/ }),
/* 38 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = calc;
var _joinPrefixedValue = __webpack_require__(3);
var _joinPrefixedValue2 = _interopRequireDefault(_joinPrefixedValue);
var _isPrefixedValue = __webpack_require__(5);
var _isPrefixedValue2 = _interopRequireDefault(_isPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
function calc(property, value) {
if (typeof value === 'string' && !(0, _isPrefixedValue2.default)(value) && value.indexOf('calc(') > -1) {
return (0, _joinPrefixedValue2.default)(property, value, function (prefix, value) {
return value.replace(/calc\(/g, prefix + 'calc(');
});
}
}
module.exports = exports['default'];
/***/ }),
/* 39 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = cursor;
var _joinPrefixedValue = __webpack_require__(3);
var _joinPrefixedValue2 = _interopRequireDefault(_joinPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
var values = {
'zoom-in': true,
'zoom-out': true,
grab: true,
grabbing: true
};
function cursor(property, value) {
if (property === 'cursor' && values[value]) {
return (0, _joinPrefixedValue2.default)(property, value);
}
}
module.exports = exports['default'];
/***/ }),
/* 40 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = flex;
var values = { flex: true, 'inline-flex': true };
function flex(property, value) {
if (property === 'display' && values[value]) {
return {
display: ['-webkit-box', '-moz-box', '-ms-' + value + 'box', '-webkit-' + value, value]
};
}
}
module.exports = exports['default'];
/***/ }),
/* 41 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = flexboxIE;
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true });
} else {
obj[key] = value;
}return obj;
}
var alternativeValues = {
'space-around': 'distribute',
'space-between': 'justify',
'flex-start': 'start',
'flex-end': 'end'
};
var alternativeProps = {
alignContent: 'msFlexLinePack',
alignSelf: 'msFlexItemAlign',
alignItems: 'msFlexAlign',
justifyContent: 'msFlexPack',
order: 'msFlexOrder',
flexGrow: 'msFlexPositive',
flexShrink: 'msFlexNegative',
flexBasis: 'msPreferredSize'
};
function flexboxIE(property, value) {
if (alternativeProps[property]) {
return _defineProperty({}, alternativeProps[property], alternativeValues[value] || value);
}
}
module.exports = exports['default'];
/***/ }),
/* 42 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = flexboxOld;
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true });
} else {
obj[key] = value;
}return obj;
}
var alternativeValues = {
'space-around': 'justify',
'space-between': 'justify',
'flex-start': 'start',
'flex-end': 'end',
'wrap-reverse': 'multiple',
wrap: 'multiple'
};
var alternativeProps = {
alignItems: 'WebkitBoxAlign',
justifyContent: 'WebkitBoxPack',
flexWrap: 'WebkitBoxLines'
};
function flexboxOld(property, value) {
if (property === 'flexDirection' && typeof value === 'string') {
return {
WebkitBoxOrient: value.indexOf('column') > -1 ? 'vertical' : 'horizontal',
WebkitBoxDirection: value.indexOf('reverse') > -1 ? 'reverse' : 'normal'
};
}
if (alternativeProps[property]) {
return _defineProperty({}, alternativeProps[property], alternativeValues[value] || value);
}
}
module.exports = exports['default'];
/***/ }),
/* 43 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = gradient;
var _joinPrefixedValue = __webpack_require__(3);
var _joinPrefixedValue2 = _interopRequireDefault(_joinPrefixedValue);
var _isPrefixedValue = __webpack_require__(5);
var _isPrefixedValue2 = _interopRequireDefault(_isPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
var values = /linear-gradient|radial-gradient|repeating-linear-gradient|repeating-radial-gradient/;
function gradient(property, value) {
if (typeof value === 'string' && !(0, _isPrefixedValue2.default)(value) && value.match(values) !== null) {
return (0, _joinPrefixedValue2.default)(property, value);
}
}
module.exports = exports['default'];
/***/ }),
/* 44 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = position;
function position(property, value) {
if (property === 'position' && value === 'sticky') {
return { position: ['-webkit-sticky', 'sticky'] };
}
}
module.exports = exports['default'];
/***/ }),
/* 45 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = sizing;
var _joinPrefixedValue = __webpack_require__(3);
var _joinPrefixedValue2 = _interopRequireDefault(_joinPrefixedValue);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
var properties = {
maxHeight: true,
maxWidth: true,
width: true,
height: true,
columnWidth: true,
minWidth: true,
minHeight: true
};
var values = {
'min-content': true,
'max-content': true,
'fill-available': true,
'fit-content': true,
'contain-floats': true
};
function sizing(property, value) {
if (properties[property] && values[value]) {
return (0, _joinPrefixedValue2.default)(property, value);
}
}
module.exports = exports['default'];
/***/ }),
/* 46 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = transition;
var _hyphenateStyleName = __webpack_require__(13);
var _hyphenateStyleName2 = _interopRequireDefault(_hyphenateStyleName);
var _capitalizeString = __webpack_require__(4);
var _capitalizeString2 = _interopRequireDefault(_capitalizeString);
var _isPrefixedValue = __webpack_require__(5);
var _isPrefixedValue2 = _interopRequireDefault(_isPrefixedValue);
var _prefixProps = __webpack_require__(14);
var _prefixProps2 = _interopRequireDefault(_prefixProps);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, { value: value, enumerable: true, configurable: true, writable: true });
} else {
obj[key] = value;
}return obj;
}
var properties = {
transition: true,
transitionProperty: true,
WebkitTransition: true,
WebkitTransitionProperty: true
};
function transition(property, value) {
// also check for already prefixed transitions
if (typeof value === 'string' && properties[property]) {
var _ref2;
var outputValue = prefixValue(value);
var webkitOutput = outputValue.split(/,(?![^()]*(?:\([^()]*\))?\))/g).filter(function (value) {
return value.match(/-moz-|-ms-/) === null;
}).join(',');
// if the property is already prefixed
if (property.indexOf('Webkit') > -1) {
return _defineProperty({}, property, webkitOutput);
}
return _ref2 = {}, _defineProperty(_ref2, 'Webkit' + (0, _capitalizeString2.default)(property), webkitOutput), _defineProperty(_ref2, property, outputValue), _ref2;
}
}
function prefixValue(value) {
if ((0, _isPrefixedValue2.default)(value)) {
return value;
}
// only split multi values, not cubic beziers
var multipleValues = value.split(/,(?![^()]*(?:\([^()]*\))?\))/g);
// iterate each single value and check for transitioned properties
// that need to be prefixed as well
multipleValues.forEach(function (val, index) {
multipleValues[index] = Object.keys(_prefixProps2.default).reduce(function (out, prefix) {
var dashCasePrefix = '-' + prefix.toLowerCase() + '-';
Object.keys(_prefixProps2.default[prefix]).forEach(function (prop) {
var dashCaseProperty = (0, _hyphenateStyleName2.default)(prop);
if (val.indexOf(dashCaseProperty) > -1 && dashCaseProperty !== 'order') {
// join all prefixes and create a new value
out = val.replace(dashCaseProperty, dashCasePrefix + dashCaseProperty) + ',' + out;
}
});
return out;
}, val);
});
return multipleValues.join(',');
}
module.exports = exports['default'];
/***/ }),
/* 47 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = prefixAll;
var _prefixProps = __webpack_require__(14);
var _prefixProps2 = _interopRequireDefault(_prefixProps);
var _capitalizeString = __webpack_require__(4);
var _capitalizeString2 = _interopRequireDefault(_capitalizeString);
var _sortPrefixedStyle = __webpack_require__(15);
var _sortPrefixedStyle2 = _interopRequireDefault(_sortPrefixedStyle);
var _position = __webpack_require__(44);
var _position2 = _interopRequireDefault(_position);
var _calc = __webpack_require__(38);
var _calc2 = _interopRequireDefault(_calc);
var _cursor = __webpack_require__(39);
var _cursor2 = _interopRequireDefault(_cursor);
var _flex = __webpack_require__(40);
var _flex2 = _interopRequireDefault(_flex);
var _sizing = __webpack_require__(45);
var _sizing2 = _interopRequireDefault(_sizing);
var _gradient = __webpack_require__(43);
var _gradient2 = _interopRequireDefault(_gradient);
var _transition = __webpack_require__(46);
var _transition2 = _interopRequireDefault(_transition);
var _flexboxIE = __webpack_require__(41);
var _flexboxIE2 = _interopRequireDefault(_flexboxIE);
var _flexboxOld = __webpack_require__(42);
var _flexboxOld2 = _interopRequireDefault(_flexboxOld);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
// special flexbox specifications
var plugins = [_position2.default, _calc2.default, _cursor2.default, _sizing2.default, _gradient2.default, _transition2.default, _flexboxIE2.default, _flexboxOld2.default, _flex2.default];
/**
* Returns a prefixed version of the style object using all vendor prefixes
* @param {Object} styles - Style object that gets prefixed properties added
* @returns {Object} - Style object with prefixed properties and values
*/
function prefixAll(styles) {
Object.keys(styles).forEach(function (property) {
var value = styles[property];
if (value instanceof Object && !Array.isArray(value)) {
// recurse through nested style objects
styles[property] = prefixAll(value);
} else {
Object.keys(_prefixProps2.default).forEach(function (prefix) {
var properties = _prefixProps2.default[prefix];
// add prefixes if needed
if (properties[property]) {
styles[prefix + (0, _capitalizeString2.default)(property)] = value;
}
});
}
});
Object.keys(styles).forEach(function (property) {
[].concat(styles[property]).forEach(function (value, index) {
// resolve every special plugins
plugins.forEach(function (plugin) {
return assignStyles(styles, plugin(property, value));
});
});
});
return (0, _sortPrefixedStyle2.default)(styles);
}
function assignStyles(base) {
var extend = arguments.length <= 1 || arguments[1] === undefined ? {} : arguments[1];
Object.keys(extend).forEach(function (property) {
var baseValue = base[property];
if (Array.isArray(baseValue)) {
[].concat(extend[property]).forEach(function (value) {
var valueIndex = baseValue.indexOf(value);
if (valueIndex > -1) {
base[property].splice(valueIndex, 1);
}
base[property].push(value);
});
} else {
base[property] = extend[property];
}
});
}
module.exports = exports['default'];
/***/ }),
/* 48 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _bowser = __webpack_require__(24);
var _bowser2 = _interopRequireDefault(_bowser);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : { default: obj };
}
var vendorPrefixes = {
Webkit: ['chrome', 'safari', 'ios', 'android', 'phantom', 'opera', 'webos', 'blackberry', 'bada', 'tizen', 'chromium', 'vivaldi'],
Moz: ['firefox', 'seamonkey', 'sailfish'],
ms: ['msie', 'msedge']
};
var browsers = {
chrome: [['chrome'], ['chromium']],
safari: [['safari']],
firefox: [['firefox']],
edge: [['msedge']],
opera: [['opera'], ['vivaldi']],
ios_saf: [['ios', 'mobile'], ['ios', 'tablet']],
ie: [['msie']],
op_mini: [['opera', 'mobile'], ['opera', 'tablet']],
and_uc: [['android', 'mobile'], ['android', 'tablet']],
android: [['android', 'mobile'], ['android', 'tablet']]
};
var browserByInfo = function browserByInfo(info) {
if (info.firefox) {
return 'firefox';
}
var name = '';
Object.keys(browsers).forEach(function (browser) {
browsers[browser].forEach(function (condition) {
var match = 0;
condition.forEach(function (single) {
if (info[single]) {
match += 1;
}
});
if (condition.length === match) {
name = browser;
}
});
});
return name;
};
/**
* Uses bowser to get default browser information such as version and name
* Evaluates bowser info and adds vendorPrefix information
* @param {string} userAgent - userAgent that gets evaluated
*/
exports.default = function (userAgent) {
if (!userAgent) {
return false;
}
var info = _bowser2.default._detect(userAgent);
Object.keys(vendorPrefixes).forEach(function (prefix) {
vendorPrefixes[prefix].forEach(function (browser) {
if (info[browser]) {
info.prefix = {
inline: prefix,
css: '-' + prefix.toLowerCase() + '-'
};
}
});
});
info.browser = browserByInfo(info);
// For cordova IOS 8 the version is missing, set truncated osversion to prevent NaN
info.version = info.version ? parseFloat(info.version) : parseInt(parseFloat(info.osversion), 10);
info.osversion = parseFloat(info.osversion);
// iOS forces all browsers to use Safari under the hood
// as the Safari version seems to match the iOS version
// we just explicitely use the osversion instead
// https://github.com/rofrischmann/inline-style-prefixer/issues/72
if (info.browser === 'ios_saf' && info.version > info.osversion) {
info.version = info.osversion;
info.safari = true;
}
// seperate native android chrome
// https://github.com/rofrischmann/inline-style-prefixer/issues/45
if (info.browser === 'android' && info.chrome && info.version > 37) {
info.browser = 'and_chr';
}
// For android < 4.4 we want to check the osversion
// not the chrome version, see issue #26
// https://github.com/rofrischmann/inline-style-prefixer/issues/26
if (info.browser === 'android' && info.osversion < 5) {
info.version = info.osversion;
}
return info;
};
module.exports = exports['default'];
/***/ }),
/* 49 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = function (_ref) {
var browser = _ref.browser;
var version = _ref.version;
var prefix = _ref.prefix;
var prefixedKeyframes = 'keyframes';
if (browser === 'chrome' && version < 43 || (browser === 'safari' || browser === 'ios_saf') && version < 9 || browser === 'opera' && version < 30 || browser === 'android' && version <= 4.4 || browser === 'and_uc') {
prefixedKeyframes = prefix.css + prefixedKeyframes;
}
return prefixedKeyframes;
};
module.exports = exports['default'];
/***/ }),
/* 50 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = function (property) {
return property.match(/^(Webkit|Moz|O|ms)/) !== null;
};
module.exports = exports["default"];
/***/ }),
/* 51 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = function (property) {
var unprefixed = property.replace(/^(ms|Webkit|Moz|O)/, '');
return unprefixed.charAt(0).toLowerCase() + unprefixed.slice(1);
};
module.exports = exports['default'];
/***/ }),
/* 52 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; };
var g;
// This works in non-strict mode
g = function () {
return this;
}();
try {
// This works if eval is allowed (see CSP)
g = g || Function("return this")() || (1, eval)("this");
} catch (e) {
// This works if the window reference is available
if ((typeof window === "undefined" ? "undefined" : _typeof(window)) === "object") g = window;
}
// g can still be undefined, but nothing to do about it...
// We return undefined, instead of nothing here, so it's
// easier to handle this case. if(!global) { ...}
module.exports = g;
/***/ }),
/* 53 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = appendImportantToEachValue;
var _appendPxIfNeeded = __webpack_require__(16);
var _appendPxIfNeeded2 = _interopRequireDefault(_appendPxIfNeeded);
var _mapObject = __webpack_require__(19);
var _mapObject2 = _interopRequireDefault(_mapObject);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
function appendImportantToEachValue(style) {
return (0, _mapObject2.default)(style, function (result, key) {
return (0, _appendPxIfNeeded2.default)(key, style[key]) + ' !important';
});
}
module.exports = exports['default'];
/***/ }),
/* 54 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _camelCaseRegex = /([a-z])?([A-Z])/g;
var _camelCaseReplacer = function _camelCaseReplacer(match, p1, p2) {
return (p1 || '') + '-' + p2.toLowerCase();
};
var _camelCaseToDashCase = function _camelCaseToDashCase(s) {
return s.replace(_camelCaseRegex, _camelCaseReplacer);
};
var camelCasePropsToDashCase = function camelCasePropsToDashCase(prefixedStyle) {
// Since prefix is expected to work on inline style objects, we must
// translate the keys to dash case for rendering to CSS.
return Object.keys(prefixedStyle).reduce(function (result, key) {
var dashCaseKey = _camelCaseToDashCase(key);
// Fix IE vendor prefix
if (/^ms-/.test(dashCaseKey)) {
dashCaseKey = '-' + dashCaseKey;
}
result[dashCaseKey] = prefixedStyle[key];
return result;
}, {});
};
exports.default = camelCasePropsToDashCase;
module.exports = exports['default'];
/***/ }),
/* 55 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = undefined;
var _class, _temp;
var _react = __webpack_require__(2);
var _react2 = _interopRequireDefault(_react);
var _styleKeeper = __webpack_require__(8);
var _styleKeeper2 = _interopRequireDefault(_styleKeeper);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
var StyleSheet = (_temp = _class = function (_PureComponent) {
_inherits(StyleSheet, _PureComponent);
function StyleSheet() {
_classCallCheck(this, StyleSheet);
var _this = _possibleConstructorReturn(this, _PureComponent.apply(this, arguments));
_this._onChange = function () {
setTimeout(function () {
_this._isMounted && _this.setState(_this._getCSSState());
}, 0);
};
_this.state = _this._getCSSState();
return _this;
}
StyleSheet.prototype.componentDidMount = function componentDidMount() {
this._isMounted = true;
this._subscription = this.context._radiumStyleKeeper.subscribe(this._onChange);
this._onChange();
};
StyleSheet.prototype.componentWillUnmount = function componentWillUnmount() {
this._isMounted = false;
if (this._subscription) {
this._subscription.remove();
}
};
StyleSheet.prototype._getCSSState = function _getCSSState() {
return { css: this.context._radiumStyleKeeper.getCSS() };
};
StyleSheet.prototype.render = function render() {
return _react2.default.createElement('style', { dangerouslySetInnerHTML: { __html: this.state.css } });
};
return StyleSheet;
}(_react.PureComponent), _class.contextTypes = {
_radiumStyleKeeper: _react2.default.PropTypes.instanceOf(_styleKeeper2.default)
}, _temp);
exports.default = StyleSheet;
module.exports = exports['default'];
/***/ }),
/* 56 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; };
exports.isNestedStyle = isNestedStyle;
exports.mergeStyles = mergeStyles;
function isNestedStyle(value) {
// Don't merge objects overriding toString, since they should be converted
// to string values.
return value && value.constructor === Object && value.toString === Object.prototype.toString;
}
// Merge style objects. Deep merge plain object values.
function mergeStyles(styles) {
var result = {};
styles.forEach(function (style) {
if (!style || (typeof style === 'undefined' ? 'undefined' : _typeof(style)) !== 'object') {
return;
}
if (Array.isArray(style)) {
style = mergeStyles(style);
}
Object.keys(style).forEach(function (key) {
// Simple case, nothing nested
if (!isNestedStyle(style[key]) || !isNestedStyle(result[key])) {
result[key] = style[key];
return;
}
// If nested media, don't merge the nested styles, append a space to the
// end (benign when converted to CSS). This way we don't end up merging
// media queries that appear later in the chain with those that appear
// earlier.
if (key.indexOf('@media') === 0) {
var newKey = key;
// eslint-disable-next-line no-constant-condition
while (true) {
newKey += ' ';
if (!result[newKey]) {
result[newKey] = style[key];
return;
}
}
}
// Merge all other nested styles recursively
result[key] = mergeStyles([result[key], style[key]]);
});
});
return result;
}
/***/ }),
/* 57 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
/* WEBPACK VAR INJECTION */(function(process) {
Object.defineProperty(exports, "__esModule", {
value: true
});
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
var _typeof = typeof Symbol === "function" && typeof Symbol.iterator === "symbol" ? function (obj) { return typeof obj; } : function (obj) { return obj && typeof Symbol === "function" && obj.constructor === Symbol && obj !== Symbol.prototype ? "symbol" : typeof obj; };
var _checkProps = function checkProps() {};
if (process.env.NODE_ENV !== 'production') {
// Warn if you use longhand and shorthand properties in the same style
// object.
// https://developer.mozilla.org/en-US/docs/Web/CSS/Shorthand_properties
var shorthandPropertyExpansions = {
background: ['backgroundAttachment', 'backgroundBlendMode', 'backgroundClip', 'backgroundColor', 'backgroundImage', 'backgroundOrigin', 'backgroundPosition', 'backgroundPositionX', 'backgroundPositionY', 'backgroundRepeat', 'backgroundRepeatX', 'backgroundRepeatY', 'backgroundSize'],
border: ['borderBottom', 'borderBottomColor', 'borderBottomStyle', 'borderBottomWidth', 'borderColor', 'borderLeft', 'borderLeftColor', 'borderLeftStyle', 'borderLeftWidth', 'borderRight', 'borderRightColor', 'borderRightStyle', 'borderRightWidth', 'borderStyle', 'borderTop', 'borderTopColor', 'borderTopStyle', 'borderTopWidth', 'borderWidth'],
borderImage: ['borderImageOutset', 'borderImageRepeat', 'borderImageSlice', 'borderImageSource', 'borderImageWidth'],
borderRadius: ['borderBottomLeftRadius', 'borderBottomRightRadius', 'borderTopLeftRadius', 'borderTopRightRadius'],
font: ['fontFamily', 'fontKerning', 'fontSize', 'fontStretch', 'fontStyle', 'fontVariant', 'fontVariantLigatures', 'fontWeight', 'lineHeight'],
listStyle: ['listStyleImage', 'listStylePosition', 'listStyleType'],
margin: ['marginBottom', 'marginLeft', 'marginRight', 'marginTop'],
padding: ['paddingBottom', 'paddingLeft', 'paddingRight', 'paddingTop'],
transition: ['transitionDelay', 'transitionDuration', 'transitionProperty', 'transitionTimingFunction']
};
_checkProps = function checkProps(config) {
var componentName = config.componentName,
style = config.style;
if ((typeof style === 'undefined' ? 'undefined' : _typeof(style)) !== 'object' || !style) {
return;
}
var styleKeys = Object.keys(style);
styleKeys.forEach(function (styleKey) {
if (Array.isArray(shorthandPropertyExpansions[styleKey]) && shorthandPropertyExpansions[styleKey].some(function (sp) {
return styleKeys.indexOf(sp) !== -1;
})) {
if (process.env.NODE_ENV !== 'production') {
/* eslint-disable no-console */
console.warn('Radium: property "' + styleKey + '" in style object', style, ': do not mix longhand and ' + 'shorthand properties in the same style object. Check the render ' + 'method of ' + componentName + '.', 'See https://github.com/FormidableLabs/radium/issues/95 for more ' + 'information.');
/* eslint-enable no-console */
}
}
});
styleKeys.forEach(function (k) {
return _checkProps(_extends({}, config, { style: style[k] }));
});
return;
};
}
exports.default = _checkProps;
module.exports = exports['default'];
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(1)))
/***/ }),
/* 58 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = keyframesPlugin;
function keyframesPlugin(_ref) {
var addCSS = _ref.addCSS,
config = _ref.config,
style = _ref.style;
var newStyle = Object.keys(style).reduce(function (newStyleInProgress, key) {
var value = style[key];
if (key === 'animationName' && value && value.__radiumKeyframes) {
var keyframesValue = value;
var _keyframesValue$__pro = keyframesValue.__process(config.userAgent),
animationName = _keyframesValue$__pro.animationName,
css = _keyframesValue$__pro.css;
addCSS(css);
value = animationName;
}
newStyleInProgress[key] = value;
return newStyleInProgress;
}, {});
return { style: newStyle };
}
module.exports = exports['default'];
/***/ }),
/* 59 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
// Convenient syntax for multiple styles: `style={[style1, style2, etc]}`
// Ignores non-objects, so you can do `this.state.isCool && styles.cool`.
var mergeStyleArrayPlugin = function mergeStyleArrayPlugin(_ref) {
var style = _ref.style,
mergeStyles = _ref.mergeStyles;
// eslint-disable-line no-shadow
var newStyle = Array.isArray(style) ? mergeStyles(style) : style;
return { style: newStyle };
};
exports.default = mergeStyleArrayPlugin;
module.exports = exports['default'];
/***/ }),
/* 60 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _callbacks = [];
var _mouseUpListenerIsActive = false;
function _handleMouseUp() {
_callbacks.forEach(function (callback) {
callback();
});
}
var subscribe = function subscribe(callback) {
if (_callbacks.indexOf(callback) === -1) {
_callbacks.push(callback);
}
if (!_mouseUpListenerIsActive) {
window.addEventListener('mouseup', _handleMouseUp);
_mouseUpListenerIsActive = true;
}
return {
remove: function remove() {
var index = _callbacks.indexOf(callback);
_callbacks.splice(index, 1);
if (_callbacks.length === 0 && _mouseUpListenerIsActive) {
window.removeEventListener('mouseup', _handleMouseUp);
_mouseUpListenerIsActive = false;
}
}
};
};
exports.default = {
subscribe: subscribe,
__triggerForTests: _handleMouseUp
};
module.exports = exports['default'];
/***/ }),
/* 61 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = prefixPlugin;
var _prefixer = __webpack_require__(7);
function prefixPlugin(_ref) {
var config = _ref.config,
style = _ref.style;
var newStyle = (0, _prefixer.getPrefixedStyle)(style, config.userAgent);
return { style: newStyle };
}
module.exports = exports['default'];
/***/ }),
/* 62 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = removeNestedStyles;
function removeNestedStyles(_ref) {
var isNestedStyle = _ref.isNestedStyle,
style = _ref.style;
// eslint-disable-line no-shadow
var newStyle = Object.keys(style).reduce(function (newStyleInProgress, key) {
var value = style[key];
if (!isNestedStyle(value)) {
newStyleInProgress[key] = value;
}
return newStyleInProgress;
}, {});
return {
style: newStyle
};
}
module.exports = exports['default'];
/***/ }),
/* 63 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _mouseUpListener = __webpack_require__(60);
var _mouseUpListener2 = _interopRequireDefault(_mouseUpListener);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
var _isInteractiveStyleField = function _isInteractiveStyleField(styleFieldName) {
return styleFieldName === ':hover' || styleFieldName === ':active' || styleFieldName === ':focus';
};
var resolveInteractionStyles = function resolveInteractionStyles(config) {
var ExecutionEnvironment = config.ExecutionEnvironment,
getComponentField = config.getComponentField,
getState = config.getState,
mergeStyles = config.mergeStyles,
props = config.props,
setState = config.setState,
style = config.style;
var newComponentFields = {};
var newProps = {};
// Only add handlers if necessary
if (style[':hover']) {
// Always call the existing handler if one is already defined.
// This code, and the very similar ones below, could be abstracted a bit
// more, but it hurts readability IMO.
var existingOnMouseEnter = props.onMouseEnter;
newProps.onMouseEnter = function (e) {
existingOnMouseEnter && existingOnMouseEnter(e);
setState(':hover', true);
};
var existingOnMouseLeave = props.onMouseLeave;
newProps.onMouseLeave = function (e) {
existingOnMouseLeave && existingOnMouseLeave(e);
setState(':hover', false);
};
}
if (style[':active']) {
var existingOnMouseDown = props.onMouseDown;
newProps.onMouseDown = function (e) {
existingOnMouseDown && existingOnMouseDown(e);
newComponentFields._lastMouseDown = Date.now();
setState(':active', 'viamousedown');
};
var existingOnKeyDown = props.onKeyDown;
newProps.onKeyDown = function (e) {
existingOnKeyDown && existingOnKeyDown(e);
if (e.key === ' ' || e.key === 'Enter') {
setState(':active', 'viakeydown');
}
};
var existingOnKeyUp = props.onKeyUp;
newProps.onKeyUp = function (e) {
existingOnKeyUp && existingOnKeyUp(e);
if (e.key === ' ' || e.key === 'Enter') {
setState(':active', false);
}
};
}
if (style[':focus']) {
var existingOnFocus = props.onFocus;
newProps.onFocus = function (e) {
existingOnFocus && existingOnFocus(e);
setState(':focus', true);
};
var existingOnBlur = props.onBlur;
newProps.onBlur = function (e) {
existingOnBlur && existingOnBlur(e);
setState(':focus', false);
};
}
if (style[':active'] && !getComponentField('_radiumMouseUpListener') && ExecutionEnvironment.canUseEventListeners) {
newComponentFields._radiumMouseUpListener = _mouseUpListener2.default.subscribe(function () {
Object.keys(getComponentField('state')._radiumStyleState).forEach(function (key) {
if (getState(':active', key) === 'viamousedown') {
setState(':active', false, key);
}
});
});
}
// Merge the styles in the order they were defined
var interactionStyles = props.disabled ? [style[':disabled']] : Object.keys(style).filter(function (name) {
return _isInteractiveStyleField(name) && getState(name);
}).map(function (name) {
return style[name];
});
var newStyle = mergeStyles([style].concat(interactionStyles));
// Remove interactive styles
newStyle = Object.keys(newStyle).reduce(function (styleWithoutInteractions, name) {
if (!_isInteractiveStyleField(name) && name !== ':disabled') {
styleWithoutInteractions[name] = newStyle[name];
}
return styleWithoutInteractions;
}, {});
return {
componentFields: newComponentFields,
props: newProps,
style: newStyle
};
};
exports.default = resolveInteractionStyles;
module.exports = exports['default'];
/***/ }),
/* 64 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
exports.default = resolveMediaQueries;
var _windowMatchMedia = void 0;
function _getWindowMatchMedia(ExecutionEnvironment) {
if (_windowMatchMedia === undefined) {
_windowMatchMedia = !!ExecutionEnvironment.canUseDOM && !!window && !!window.matchMedia && function (mediaQueryString) {
return window.matchMedia(mediaQueryString);
} || null;
}
return _windowMatchMedia;
}
function _filterObject(obj, predicate) {
return Object.keys(obj).filter(function (key) {
return predicate(obj[key], key);
}).reduce(function (result, key) {
result[key] = obj[key];
return result;
}, {});
}
function _removeMediaQueries(style) {
return Object.keys(style).reduce(function (styleWithoutMedia, key) {
if (key.indexOf('@media') !== 0) {
styleWithoutMedia[key] = style[key];
}
return styleWithoutMedia;
}, {});
}
function _topLevelRulesToCSS(_ref) {
var addCSS = _ref.addCSS,
appendImportantToEachValue = _ref.appendImportantToEachValue,
cssRuleSetToString = _ref.cssRuleSetToString,
hash = _ref.hash,
isNestedStyle = _ref.isNestedStyle,
style = _ref.style,
userAgent = _ref.userAgent;
var className = '';
Object.keys(style).filter(function (name) {
return name.indexOf('@media') === 0;
}).map(function (query) {
var topLevelRules = appendImportantToEachValue(_filterObject(style[query], function (value) {
return !isNestedStyle(value);
}));
if (!Object.keys(topLevelRules).length) {
return;
}
var ruleCSS = cssRuleSetToString('', topLevelRules, userAgent);
// CSS classes cannot start with a number
var mediaQueryClassName = 'rmq-' + hash(query + ruleCSS);
var css = query + '{ .' + mediaQueryClassName + ruleCSS + '}';
addCSS(css);
className += (className ? ' ' : '') + mediaQueryClassName;
});
return className;
}
function _subscribeToMediaQuery(_ref2) {
var listener = _ref2.listener,
listenersByQuery = _ref2.listenersByQuery,
matchMedia = _ref2.matchMedia,
mediaQueryListsByQuery = _ref2.mediaQueryListsByQuery,
query = _ref2.query;
query = query.replace('@media ', '');
var mql = mediaQueryListsByQuery[query];
if (!mql && matchMedia) {
mediaQueryListsByQuery[query] = mql = matchMedia(query);
}
if (!listenersByQuery || !listenersByQuery[query]) {
mql.addListener(listener);
listenersByQuery[query] = {
remove: function remove() {
mql.removeListener(listener);
}
};
}
return mql;
}
function resolveMediaQueries(_ref3) {
var ExecutionEnvironment = _ref3.ExecutionEnvironment,
addCSS = _ref3.addCSS,
appendImportantToEachValue = _ref3.appendImportantToEachValue,
config = _ref3.config,
cssRuleSetToString = _ref3.cssRuleSetToString,
getComponentField = _ref3.getComponentField,
getGlobalState = _ref3.getGlobalState,
hash = _ref3.hash,
isNestedStyle = _ref3.isNestedStyle,
mergeStyles = _ref3.mergeStyles,
props = _ref3.props,
setState = _ref3.setState,
style = _ref3.style;
// eslint-disable-line no-shadow
var newStyle = _removeMediaQueries(style);
var mediaQueryClassNames = _topLevelRulesToCSS({
addCSS: addCSS,
appendImportantToEachValue: appendImportantToEachValue,
cssRuleSetToString: cssRuleSetToString,
hash: hash,
isNestedStyle: isNestedStyle,
style: style,
userAgent: config.userAgent
});
var newProps = mediaQueryClassNames ? {
className: mediaQueryClassNames + (props.className ? ' ' + props.className : '')
} : null;
var matchMedia = config.matchMedia || _getWindowMatchMedia(ExecutionEnvironment);
if (!matchMedia) {
return {
props: newProps,
style: newStyle
};
}
var listenersByQuery = _extends({}, getComponentField('_radiumMediaQueryListenersByQuery'));
var mediaQueryListsByQuery = getGlobalState('mediaQueryListsByQuery') || {};
Object.keys(style).filter(function (name) {
return name.indexOf('@media') === 0;
}).map(function (query) {
var nestedRules = _filterObject(style[query], isNestedStyle);
if (!Object.keys(nestedRules).length) {
return;
}
var mql = _subscribeToMediaQuery({
listener: function listener() {
return setState(query, mql.matches, '_all');
},
listenersByQuery: listenersByQuery,
matchMedia: matchMedia,
mediaQueryListsByQuery: mediaQueryListsByQuery,
query: query
});
// Apply media query states
if (mql.matches) {
newStyle = mergeStyles([newStyle, nestedRules]);
}
});
return {
componentFields: {
_radiumMediaQueryListenersByQuery: listenersByQuery
},
globalState: { mediaQueryListsByQuery: mediaQueryListsByQuery },
props: newProps,
style: newStyle
};
}
module.exports = exports['default'];
/***/ }),
/* 65 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.default = visited;
function visited(_ref) {
var addCSS = _ref.addCSS,
appendImportantToEachValue = _ref.appendImportantToEachValue,
config = _ref.config,
cssRuleSetToString = _ref.cssRuleSetToString,
hash = _ref.hash,
props = _ref.props,
style = _ref.style;
// eslint-disable-line no-shadow
var className = props.className;
var newStyle = Object.keys(style).reduce(function (newStyleInProgress, key) {
var value = style[key];
if (key === ':visited') {
value = appendImportantToEachValue(value);
var ruleCSS = cssRuleSetToString('', value, config.userAgent);
var visitedClassName = 'rad-' + hash(ruleCSS);
var css = '.' + visitedClassName + ':visited' + ruleCSS;
addCSS(css);
className = (className ? className + ' ' : '') + visitedClassName;
} else {
newStyleInProgress[key] = value;
}
return newStyleInProgress;
}, {});
return {
props: className === props.className ? null : { className: className },
style: newStyle
};
}
module.exports = exports['default'];
/***/ }),
/* 66 */
/***/ (function(module, exports) {
module.exports = function() {
throw new Error("define cannot be used indirect");
};
/***/ }),
/* 67 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
/* WEBPACK VAR INJECTION */(function(process) {
Object.defineProperty(exports, "__esModule", {
value: true
});
var _enhancer = __webpack_require__(9);
var _enhancer2 = _interopRequireDefault(_enhancer);
var _plugins = __webpack_require__(11);
var _plugins2 = _interopRequireDefault(_plugins);
var _style = __webpack_require__(22);
var _style2 = _interopRequireDefault(_style);
var _styleRoot = __webpack_require__(21);
var _styleRoot2 = _interopRequireDefault(_styleRoot);
var _getState = __webpack_require__(10);
var _getState2 = _interopRequireDefault(_getState);
var _keyframes = __webpack_require__(23);
var _keyframes2 = _interopRequireDefault(_keyframes);
var _resolveStyles = __webpack_require__(12);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
function Radium(ComposedComponent) {
return (0, _enhancer2.default)(ComposedComponent);
}
Radium.Plugins = _plugins2.default;
Radium.Style = _style2.default;
Radium.StyleRoot = _styleRoot2.default;
Radium.getState = _getState2.default;
Radium.keyframes = _keyframes2.default;
if (process.env.NODE_ENV !== 'production') {
Radium.TestMode = {
clearState: _resolveStyles.__clearStateForTests,
disable: _resolveStyles.__setTestMode.bind(null, false),
enable: _resolveStyles.__setTestMode.bind(null, true)
};
}
exports.default = Radium;
module.exports = exports['default'];
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(1)))
/***/ })
/******/ ]);
}); |
pathfinder/vtables/decommissioningcommissioningapplications/src/MultiSelectField.js | leanix/leanix-custom-reports | import React, { Component } from 'react';
import PropTypes from 'prop-types';
import Select from 'react-select';
import TableUtilities from './common/TableUtilities';
import Utilities from './common/Utilities';
class MultiSelectField extends Component {
constructor(props) {
super(props);
this._onChange = this._onChange.bind(this);
}
_onChange(val) {
const values = !val ? [] : val.map((e) => {
return e.label;
});
this.props.onChange(values);
}
_sortByLabel(a, b) {
return a.label.localeCompare(b.label);
}
render() {
if (Object.keys(this.props.items).length === 0) {
return null;
}
const options = [];
for (let key in this.props.items) {
options.push({
value: parseInt(key, 10),
label: this.props.items[key]
});
}
options.sort(this._sortByLabel);
const values = this.props.values.map((e) => {
const value = Utilities.getKeyToValue(this.props.items, e);
if (!value) {
return;
}
return {
value: parseInt(value, 10),
label: e
};
}).filter((e) => {
return e !== undefined;
});
return (
<div className='form-group'>
<label>{this.props.label}</label>
<Select multi
name='MultiSelectField'
placeholder={this.props.placeholder}
options={options}
matchPos='start'
matchProp='label'
ignoreCase={true}
value={values}
onChange={this._onChange} />
</div>
);
}
}
MultiSelectField.propTypes = {
label: PropTypes.string.isRequired,
placeholder: PropTypes.string.isRequired,
items: TableUtilities.PropTypes.options,
onChange: PropTypes.func.isRequired,
values: PropTypes.arrayOf(PropTypes.string.isRequired).isRequired
};
export default MultiSelectField; |
app/js/components/Rippler.js | raulalgo/button_rr | 'use strict';
import React from 'react';
class Rippler extends React.Component {
style;
constructor(props) {
super(props);
}
render() {
this.style = ({ top : this.props.y-50,
left : this.props.x-50})
return (
<div>
<div className={this.props.t_w_classes}>
<div className={this.props.tr_classes} style={this.style}> </div>
</div>
</div>
);
}
componentWillUpdate() {
}
}
export default Rippler;
/*
<div className={this.t_w_classes}>
<div className={'transitioner' + this.tr_classes } style={this.rippleStyle}> </div>
</div>
*/
|
src/components/Toggle.js | gj262/my-react-redux-starter-kit | import React from 'react'
import './Toggle.scss'
export default class Toggle extends React.Component {
static propTypes = {
toggle: React.PropTypes.func.isRequired,
assign: React.PropTypes.func.isRequired,
enabled: React.PropTypes.bool.isRequired
};
render () {
return (
<div className='container text-center'>
<div className='checkbox'>
<label>
<input type='checkbox' checked={this.props.enabled} onChange={this.props.toggle} />
Sample Enabled
</label>
</div>
<button type='submit' className='btn btn-default' onClick={this.props.assign.bind({}, true)}>Enable</button>
<button type='submit' className='btn btn-default' onClick={this.props.assign.bind({}, false)}>Disable</button>
</div>
)
}
}
|
modules/__tests__/serverRendering-test.js | cold-brew-coding/react-router | /*eslint-env mocha */
/*eslint react/prop-types: 0*/
import expect from 'expect'
import React from 'react'
import createLocation from 'history/lib/createLocation'
import RoutingContext from '../RoutingContext'
import match from '../match'
import Link from '../Link'
describe('server rendering', function () {
let App, Dashboard, About, RedirectRoute, AboutRoute, DashboardRoute, routes
beforeEach(function () {
App = React.createClass({
render() {
return (
<div className="App">
<h1>App</h1>
<Link to="/about" activeClassName="about-is-active">About</Link>{' '}
<Link to="/dashboard" activeClassName="dashboard-is-active">Dashboard</Link>
<div>
{this.props.children}
</div>
</div>
)
}
})
Dashboard = React.createClass({
render() {
return (
<div className="Dashboard">
<h1>The Dashboard</h1>
</div>
)
}
})
About = React.createClass({
render() {
return (
<div className="About">
<h1>About</h1>
</div>
)
}
})
DashboardRoute = {
path: '/dashboard',
component: Dashboard
}
AboutRoute = {
path: '/about',
component: About
}
RedirectRoute = {
path: '/company',
onEnter (nextState, replaceState) {
replaceState(null, '/about')
}
}
routes = {
path: '/',
component: App,
childRoutes: [ DashboardRoute, AboutRoute, RedirectRoute ]
}
})
it('works', function (done) {
const location = createLocation('/dashboard')
match({ routes, location }, function (error, redirectLocation, renderProps) {
const string = React.renderToString(
<RoutingContext {...renderProps} />
)
expect(string).toMatch(/The Dashboard/)
done()
})
})
it('renders active Links as active', function (done) {
const location = createLocation('/about')
match({ routes, location }, function (error, redirectLocation, renderProps) {
const string = React.renderToString(
<RoutingContext {...renderProps} />
)
expect(string).toMatch(/about-is-active/)
//expect(string).toNotMatch(/dashboard-is-active/) TODO add toNotMatch to expect
done()
})
})
it('sends the redirect location', function (done) {
const location = createLocation('/company')
match({ routes, location }, function (error, redirectLocation) {
expect(redirectLocation).toExist()
expect(redirectLocation.pathname).toEqual('/about')
expect(redirectLocation.search).toEqual('')
expect(redirectLocation.state).toEqual(null)
expect(redirectLocation.action).toEqual('REPLACE')
done()
})
})
it('sends null values when no routes match', function (done) {
const location = createLocation('/no-match')
match({ routes, location }, function (error, redirectLocation, state) {
expect(error).toBe(null)
expect(redirectLocation).toBe(null)
expect(state).toBe(null)
done()
})
})
})
|
src/js/containers/PictureGridContainer/PictureGridContainer.js | shane-arthur/react-redux-and-more-boilerplate | import React, { Component } from 'react';
import PictureIcon from '../../components/PictureIcon/PictureIcon';
import { styles } from './styles';
import { sendData } from '../../data/dataFetcher';
export default class PictureGridContainer extends Component {
_getPictureContainerItems() {
const pictureList = this.props.pictureList;
const pictureIds = this.props.items.map(item => {
return item.pictureId;
});
const doesPicExist = (pictureId) => {
return pictureIds.indexOf(Number(pictureId)) >= 0;
};
const translatePictureId = (pictureName) => {
return findObjectByValue(this.props.pictureMappings, pictureName, NaN);
};
const findObjectByValue = (collection, value, defaultValue = null) => {
let keyForValue = defaultValue;
Object.keys(collection).forEach(key => {
if (collection[key] === value)
keyForValue = key;
});
return keyForValue;
}
const formSelectedPicturePayload = (pictureName, pictureId, pageId) => {
return { content: pictureName, pictureId, pageId }
}
const onCheckboxClick = (payload) => {
sendData('/endpoint', payload).then(response => {
//do nothing
}).catch(error => {
//catch error
})
};
if (this.props.pictureList) {
const pageId = this.props.pageId;
return this.props.pictureList.map(picture => {
const pictureName = picture.split(".jpg")[0];
const pictureId = translatePictureId(pictureName);
const isPicSelected = doesPicExist(pictureId);
return <PictureIcon
selectedData={formSelectedPicturePayload(pictureName, pictureId, pageId)}
onClick={onCheckboxClick}
key={pictureName} selectedClass={'icon'}
pictureName={pictureName}
selected={isPicSelected}
onClick={this.props.onClick}
/>
}, this);
}
else {
return null
}
}
render() {
const picturesToDisplay = this._getPictureContainerItems();
return (
<div style={styles.wrapper}>
{picturesToDisplay}
</div>
);
}
} |
node_modules/react-router/modules/IndexLink.js | sauceSquatch/we_are_razorfish_experience | import React from 'react'
import Link from './Link'
/**
* An <IndexLink> is used to link to an <IndexRoute>.
*/
const IndexLink = React.createClass({
render() {
return <Link {...this.props} onlyActiveOnIndex={true} />
}
})
export default IndexLink
|
src/docs/components/tabs/TabsDoc.js | karatechops/grommet-docs | // (C) Copyright 2014-2016 Hewlett Packard Enterprise Development LP
import React, { Component } from 'react';
import Tabs from 'grommet/components/Tabs';
import Tab from 'grommet/components/Tab';
import Paragraph from 'grommet/components/Paragraph';
import Button from 'grommet/components/Button';
import DocsArticle from '../../../components/DocsArticle';
export default class TabsDoc extends Component {
render () {
return (
<DocsArticle title='Tab(s)' action={
<Button primary={true} path='/docs/tabs/examples' label='Examples' />
}>
<section>
<p>A tabular view component.</p>
<Tabs>
<Tab title='First Title'>
<Paragraph>First contents</Paragraph>
</Tab>
<Tab title='Second Title'>
<Paragraph>Second contents</Paragraph>
</Tab>
</Tabs>
</section>
<section>
<h2>Properties</h2>
<dl>
<dt><code>activeIndex {'{number}'}</code></dt>
<dd>Active tab index. Defaults to 0.</dd>
<dt><code>justify start|center|end</code></dt>
<dd>How to align the tabs along the main axis.
Defaults to <code>center</code>.</dd>
<dt><code>onActive {'{function}'}</code></dt>
<dd>Function that will be called with the active tab index when
the currently active tab changes.</dd>
<dt><code>responsive true|false</code></dt>
<dd>Whether the row of tabs should be
switched to a centered column layout when the display
area narrows.
Defaults to <code>true</code>.</dd>
</dl>
</section>
</DocsArticle>
);
}
};
|
node_modules/bower/node_modules/inquirer/node_modules/rx/dist/rx.lite.compat.js | jeanralphaviles/WhatsHappenin | // Copyright (c) Microsoft Open Technologies, Inc. All rights reserved. See License.txt in the project root for license information.
;(function (undefined) {
var objectTypes = {
'boolean': false,
'function': true,
'object': true,
'number': false,
'string': false,
'undefined': false
};
var root = (objectTypes[typeof window] && window) || this,
freeExports = objectTypes[typeof exports] && exports && !exports.nodeType && exports,
freeModule = objectTypes[typeof module] && module && !module.nodeType && module,
moduleExports = freeModule && freeModule.exports === freeExports && freeExports,
freeGlobal = objectTypes[typeof global] && global;
if (freeGlobal && (freeGlobal.global === freeGlobal || freeGlobal.window === freeGlobal)) {
root = freeGlobal;
}
var Rx = {
internals: {},
config: {
Promise: root.Promise
},
helpers: { }
};
// Defaults
var noop = Rx.helpers.noop = function () { },
notDefined = Rx.helpers.notDefined = function (x) { return typeof x === 'undefined'; },
isScheduler = Rx.helpers.isScheduler = function (x) { return x instanceof Rx.Scheduler; },
identity = Rx.helpers.identity = function (x) { return x; },
pluck = Rx.helpers.pluck = function (property) { return function (x) { return x[property]; }; },
just = Rx.helpers.just = function (value) { return function () { return value; }; },
defaultNow = Rx.helpers.defaultNow = (function () { return !!Date.now ? Date.now : function () { return +new Date; }; }()),
defaultComparer = Rx.helpers.defaultComparer = function (x, y) { return isEqual(x, y); },
defaultSubComparer = Rx.helpers.defaultSubComparer = function (x, y) { return x > y ? 1 : (x < y ? -1 : 0); },
defaultKeySerializer = Rx.helpers.defaultKeySerializer = function (x) { return x.toString(); },
defaultError = Rx.helpers.defaultError = function (err) { throw err; },
isPromise = Rx.helpers.isPromise = function (p) { return !!p && typeof p.then === 'function'; },
asArray = Rx.helpers.asArray = function () { return Array.prototype.slice.call(arguments); },
not = Rx.helpers.not = function (a) { return !a; },
isFunction = Rx.helpers.isFunction = (function () {
var isFn = function (value) {
return typeof value == 'function' || false;
}
// fallback for older versions of Chrome and Safari
if (isFn(/x/)) {
isFn = function(value) {
return typeof value == 'function' && toString.call(value) == '[object Function]';
};
}
return isFn;
}());
// Errors
var sequenceContainsNoElements = 'Sequence contains no elements.';
var argumentOutOfRange = 'Argument out of range';
var objectDisposed = 'Object has been disposed';
function checkDisposed(self) { if (self.isDisposed) { throw new Error(objectDisposed); } }
function cloneArray(arr) { for(var a = [], i = 0, len = arr.length; i < len; i++) { a.push(arr[i]); } return a;}
Rx.config.longStackSupport = false;
var hasStacks = false;
try {
throw new Error();
} catch (e) {
hasStacks = !!e.stack;
}
// All code after this point will be filtered from stack traces reported by RxJS
var rStartingLine = captureLine(), rFileName;
var STACK_JUMP_SEPARATOR = "From previous event:";
function makeStackTraceLong(error, observable) {
// If possible, transform the error stack trace by removing Node and RxJS
// cruft, then concatenating with the stack trace of `observable`.
if (hasStacks &&
observable.stack &&
typeof error === "object" &&
error !== null &&
error.stack &&
error.stack.indexOf(STACK_JUMP_SEPARATOR) === -1
) {
var stacks = [];
for (var o = observable; !!o; o = o.source) {
if (o.stack) {
stacks.unshift(o.stack);
}
}
stacks.unshift(error.stack);
var concatedStacks = stacks.join("\n" + STACK_JUMP_SEPARATOR + "\n");
error.stack = filterStackString(concatedStacks);
}
}
function filterStackString(stackString) {
var lines = stackString.split("\n"),
desiredLines = [];
for (var i = 0, len = lines.length; i < len; i++) {
var line = lines[i];
if (!isInternalFrame(line) && !isNodeFrame(line) && line) {
desiredLines.push(line);
}
}
return desiredLines.join("\n");
}
function isInternalFrame(stackLine) {
var fileNameAndLineNumber = getFileNameAndLineNumber(stackLine);
if (!fileNameAndLineNumber) {
return false;
}
var fileName = fileNameAndLineNumber[0], lineNumber = fileNameAndLineNumber[1];
return fileName === rFileName &&
lineNumber >= rStartingLine &&
lineNumber <= rEndingLine;
}
function isNodeFrame(stackLine) {
return stackLine.indexOf("(module.js:") !== -1 ||
stackLine.indexOf("(node.js:") !== -1;
}
function captureLine() {
if (!hasStacks) { return; }
try {
throw new Error();
} catch (e) {
var lines = e.stack.split("\n");
var firstLine = lines[0].indexOf("@") > 0 ? lines[1] : lines[2];
var fileNameAndLineNumber = getFileNameAndLineNumber(firstLine);
if (!fileNameAndLineNumber) { return; }
rFileName = fileNameAndLineNumber[0];
return fileNameAndLineNumber[1];
}
}
function getFileNameAndLineNumber(stackLine) {
// Named functions: "at functionName (filename:lineNumber:columnNumber)"
var attempt1 = /at .+ \((.+):(\d+):(?:\d+)\)$/.exec(stackLine);
if (attempt1) { return [attempt1[1], Number(attempt1[2])]; }
// Anonymous functions: "at filename:lineNumber:columnNumber"
var attempt2 = /at ([^ ]+):(\d+):(?:\d+)$/.exec(stackLine);
if (attempt2) { return [attempt2[1], Number(attempt2[2])]; }
// Firefox style: "function@filename:lineNumber or @filename:lineNumber"
var attempt3 = /.*@(.+):(\d+)$/.exec(stackLine);
if (attempt3) { return [attempt3[1], Number(attempt3[2])]; }
}
// Shim in iterator support
var $iterator$ = (typeof Symbol === 'function' && Symbol.iterator) ||
'_es6shim_iterator_';
// Bug for mozilla version
if (root.Set && typeof new root.Set()['@@iterator'] === 'function') {
$iterator$ = '@@iterator';
}
var doneEnumerator = Rx.doneEnumerator = { done: true, value: undefined };
var isIterable = Rx.helpers.isIterable = function (o) {
return o[$iterator$] !== undefined;
}
var isArrayLike = Rx.helpers.isArrayLike = function (o) {
return o && o.length !== undefined;
}
Rx.helpers.iterator = $iterator$;
var bindCallback = Rx.internals.bindCallback = function (func, thisArg, argCount) {
if (typeof thisArg === 'undefined') { return func; }
switch(argCount) {
case 0:
return function() {
return func.call(thisArg)
};
case 1:
return function(arg) {
return func.call(thisArg, arg);
}
case 2:
return function(value, index) {
return func.call(thisArg, value, index);
};
case 3:
return function(value, index, collection) {
return func.call(thisArg, value, index, collection);
};
}
return function() {
return func.apply(thisArg, arguments);
};
};
/** Used to determine if values are of the language type Object */
var dontEnums = ['toString',
'toLocaleString',
'valueOf',
'hasOwnProperty',
'isPrototypeOf',
'propertyIsEnumerable',
'constructor'],
dontEnumsLength = dontEnums.length;
/** `Object#toString` result shortcuts */
var argsClass = '[object Arguments]',
arrayClass = '[object Array]',
boolClass = '[object Boolean]',
dateClass = '[object Date]',
errorClass = '[object Error]',
funcClass = '[object Function]',
numberClass = '[object Number]',
objectClass = '[object Object]',
regexpClass = '[object RegExp]',
stringClass = '[object String]';
var toString = Object.prototype.toString,
hasOwnProperty = Object.prototype.hasOwnProperty,
supportsArgsClass = toString.call(arguments) == argsClass, // For less <IE9 && FF<4
supportNodeClass,
errorProto = Error.prototype,
objectProto = Object.prototype,
stringProto = String.prototype,
propertyIsEnumerable = objectProto.propertyIsEnumerable;
try {
supportNodeClass = !(toString.call(document) == objectClass && !({ 'toString': 0 } + ''));
} catch (e) {
supportNodeClass = true;
}
var nonEnumProps = {};
nonEnumProps[arrayClass] = nonEnumProps[dateClass] = nonEnumProps[numberClass] = { 'constructor': true, 'toLocaleString': true, 'toString': true, 'valueOf': true };
nonEnumProps[boolClass] = nonEnumProps[stringClass] = { 'constructor': true, 'toString': true, 'valueOf': true };
nonEnumProps[errorClass] = nonEnumProps[funcClass] = nonEnumProps[regexpClass] = { 'constructor': true, 'toString': true };
nonEnumProps[objectClass] = { 'constructor': true };
var support = {};
(function () {
var ctor = function() { this.x = 1; },
props = [];
ctor.prototype = { 'valueOf': 1, 'y': 1 };
for (var key in new ctor) { props.push(key); }
for (key in arguments) { }
// Detect if `name` or `message` properties of `Error.prototype` are enumerable by default.
support.enumErrorProps = propertyIsEnumerable.call(errorProto, 'message') || propertyIsEnumerable.call(errorProto, 'name');
// Detect if `prototype` properties are enumerable by default.
support.enumPrototypes = propertyIsEnumerable.call(ctor, 'prototype');
// Detect if `arguments` object indexes are non-enumerable
support.nonEnumArgs = key != 0;
// Detect if properties shadowing those on `Object.prototype` are non-enumerable.
support.nonEnumShadows = !/valueOf/.test(props);
}(1));
var isObject = Rx.internals.isObject = function(value) {
var type = typeof value;
return value && (type == 'function' || type == 'object') || false;
};
function keysIn(object) {
var result = [];
if (!isObject(object)) {
return result;
}
if (support.nonEnumArgs && object.length && isArguments(object)) {
object = slice.call(object);
}
var skipProto = support.enumPrototypes && typeof object == 'function',
skipErrorProps = support.enumErrorProps && (object === errorProto || object instanceof Error);
for (var key in object) {
if (!(skipProto && key == 'prototype') &&
!(skipErrorProps && (key == 'message' || key == 'name'))) {
result.push(key);
}
}
if (support.nonEnumShadows && object !== objectProto) {
var ctor = object.constructor,
index = -1,
length = dontEnumsLength;
if (object === (ctor && ctor.prototype)) {
var className = object === stringProto ? stringClass : object === errorProto ? errorClass : toString.call(object),
nonEnum = nonEnumProps[className];
}
while (++index < length) {
key = dontEnums[index];
if (!(nonEnum && nonEnum[key]) && hasOwnProperty.call(object, key)) {
result.push(key);
}
}
}
return result;
}
function internalFor(object, callback, keysFunc) {
var index = -1,
props = keysFunc(object),
length = props.length;
while (++index < length) {
var key = props[index];
if (callback(object[key], key, object) === false) {
break;
}
}
return object;
}
function internalForIn(object, callback) {
return internalFor(object, callback, keysIn);
}
function isNode(value) {
// IE < 9 presents DOM nodes as `Object` objects except they have `toString`
// methods that are `typeof` "string" and still can coerce nodes to strings
return typeof value.toString != 'function' && typeof (value + '') == 'string';
}
var isArguments = function(value) {
return (value && typeof value == 'object') ? toString.call(value) == argsClass : false;
}
// fallback for browsers that can't detect `arguments` objects by [[Class]]
if (!supportsArgsClass) {
isArguments = function(value) {
return (value && typeof value == 'object') ? hasOwnProperty.call(value, 'callee') : false;
};
}
var isEqual = Rx.internals.isEqual = function (x, y) {
return deepEquals(x, y, [], []);
};
/** @private
* Used for deep comparison
**/
function deepEquals(a, b, stackA, stackB) {
// exit early for identical values
if (a === b) {
// treat `+0` vs. `-0` as not equal
return a !== 0 || (1 / a == 1 / b);
}
var type = typeof a,
otherType = typeof b;
// exit early for unlike primitive values
if (a === a && (a == null || b == null ||
(type != 'function' && type != 'object' && otherType != 'function' && otherType != 'object'))) {
return false;
}
// compare [[Class]] names
var className = toString.call(a),
otherClass = toString.call(b);
if (className == argsClass) {
className = objectClass;
}
if (otherClass == argsClass) {
otherClass = objectClass;
}
if (className != otherClass) {
return false;
}
switch (className) {
case boolClass:
case dateClass:
// coerce dates and booleans to numbers, dates to milliseconds and booleans
// to `1` or `0` treating invalid dates coerced to `NaN` as not equal
return +a == +b;
case numberClass:
// treat `NaN` vs. `NaN` as equal
return (a != +a) ?
b != +b :
// but treat `-0` vs. `+0` as not equal
(a == 0 ? (1 / a == 1 / b) : a == +b);
case regexpClass:
case stringClass:
// coerce regexes to strings (http://es5.github.io/#x15.10.6.4)
// treat string primitives and their corresponding object instances as equal
return a == String(b);
}
var isArr = className == arrayClass;
if (!isArr) {
// exit for functions and DOM nodes
if (className != objectClass || (!support.nodeClass && (isNode(a) || isNode(b)))) {
return false;
}
// in older versions of Opera, `arguments` objects have `Array` constructors
var ctorA = !support.argsObject && isArguments(a) ? Object : a.constructor,
ctorB = !support.argsObject && isArguments(b) ? Object : b.constructor;
// non `Object` object instances with different constructors are not equal
if (ctorA != ctorB &&
!(hasOwnProperty.call(a, 'constructor') && hasOwnProperty.call(b, 'constructor')) &&
!(isFunction(ctorA) && ctorA instanceof ctorA && isFunction(ctorB) && ctorB instanceof ctorB) &&
('constructor' in a && 'constructor' in b)
) {
return false;
}
}
// assume cyclic structures are equal
// the algorithm for detecting cyclic structures is adapted from ES 5.1
// section 15.12.3, abstract operation `JO` (http://es5.github.io/#x15.12.3)
var initedStack = !stackA;
stackA || (stackA = []);
stackB || (stackB = []);
var length = stackA.length;
while (length--) {
if (stackA[length] == a) {
return stackB[length] == b;
}
}
var size = 0;
var result = true;
// add `a` and `b` to the stack of traversed objects
stackA.push(a);
stackB.push(b);
// recursively compare objects and arrays (susceptible to call stack limits)
if (isArr) {
// compare lengths to determine if a deep comparison is necessary
length = a.length;
size = b.length;
result = size == length;
if (result) {
// deep compare the contents, ignoring non-numeric properties
while (size--) {
var index = length,
value = b[size];
if (!(result = deepEquals(a[size], value, stackA, stackB))) {
break;
}
}
}
}
else {
// deep compare objects using `forIn`, instead of `forOwn`, to avoid `Object.keys`
// which, in this case, is more costly
internalForIn(b, function(value, key, b) {
if (hasOwnProperty.call(b, key)) {
// count the number of properties.
size++;
// deep compare each property value.
return (result = hasOwnProperty.call(a, key) && deepEquals(a[key], value, stackA, stackB));
}
});
if (result) {
// ensure both objects have the same number of properties
internalForIn(a, function(value, key, a) {
if (hasOwnProperty.call(a, key)) {
// `size` will be `-1` if `a` has more properties than `b`
return (result = --size > -1);
}
});
}
}
stackA.pop();
stackB.pop();
return result;
}
var errorObj = {e: {}};
var tryCatchTarget;
function tryCatcher() {
try {
return tryCatchTarget.apply(this, arguments);
} catch (e) {
errorObj.e = e;
return errorObj;
}
}
function tryCatch(fn) {
if (!isFunction(fn)) { throw new TypeError('fn must be a function'); }
tryCatchTarget = fn;
return tryCatcher;
}
function thrower(e) {
throw e;
}
var hasProp = {}.hasOwnProperty,
slice = Array.prototype.slice;
var inherits = this.inherits = Rx.internals.inherits = function (child, parent) {
function __() { this.constructor = child; }
__.prototype = parent.prototype;
child.prototype = new __();
};
var addProperties = Rx.internals.addProperties = function (obj) {
for(var sources = [], i = 1, len = arguments.length; i < len; i++) { sources.push(arguments[i]); }
for (var idx = 0, ln = sources.length; idx < ln; idx++) {
var source = sources[idx];
for (var prop in source) {
obj[prop] = source[prop];
}
}
};
// Rx Utils
var addRef = Rx.internals.addRef = function (xs, r) {
return new AnonymousObservable(function (observer) {
return new CompositeDisposable(r.getDisposable(), xs.subscribe(observer));
});
};
function arrayInitialize(count, factory) {
var a = new Array(count);
for (var i = 0; i < count; i++) {
a[i] = factory();
}
return a;
}
// Utilities
if (!Function.prototype.bind) {
Function.prototype.bind = function (that) {
var target = this,
args = slice.call(arguments, 1);
var bound = function () {
if (this instanceof bound) {
function F() { }
F.prototype = target.prototype;
var self = new F();
var result = target.apply(self, args.concat(slice.call(arguments)));
if (Object(result) === result) {
return result;
}
return self;
} else {
return target.apply(that, args.concat(slice.call(arguments)));
}
};
return bound;
};
}
if (!Array.prototype.forEach) {
Array.prototype.forEach = function (callback, thisArg) {
var T, k;
if (this == null) {
throw new TypeError(" this is null or not defined");
}
var O = Object(this);
var len = O.length >>> 0;
if (typeof callback !== "function") {
throw new TypeError(callback + " is not a function");
}
if (arguments.length > 1) {
T = thisArg;
}
k = 0;
while (k < len) {
var kValue;
if (k in O) {
kValue = O[k];
callback.call(T, kValue, k, O);
}
k++;
}
};
}
var boxedString = Object("a"),
splitString = boxedString[0] != "a" || !(0 in boxedString);
if (!Array.prototype.every) {
Array.prototype.every = function every(fun /*, thisp */) {
var object = Object(this),
self = splitString && {}.toString.call(this) == stringClass ?
this.split("") :
object,
length = self.length >>> 0,
thisp = arguments[1];
if ({}.toString.call(fun) != funcClass) {
throw new TypeError(fun + " is not a function");
}
for (var i = 0; i < length; i++) {
if (i in self && !fun.call(thisp, self[i], i, object)) {
return false;
}
}
return true;
};
}
if (!Array.prototype.map) {
Array.prototype.map = function map(fun /*, thisp*/) {
var object = Object(this),
self = splitString && {}.toString.call(this) == stringClass ?
this.split("") :
object,
length = self.length >>> 0,
result = Array(length),
thisp = arguments[1];
if ({}.toString.call(fun) != funcClass) {
throw new TypeError(fun + " is not a function");
}
for (var i = 0; i < length; i++) {
if (i in self) {
result[i] = fun.call(thisp, self[i], i, object);
}
}
return result;
};
}
if (!Array.prototype.filter) {
Array.prototype.filter = function (predicate) {
var results = [], item, t = new Object(this);
for (var i = 0, len = t.length >>> 0; i < len; i++) {
item = t[i];
if (i in t && predicate.call(arguments[1], item, i, t)) {
results.push(item);
}
}
return results;
};
}
if (!Array.isArray) {
Array.isArray = function (arg) {
return {}.toString.call(arg) == arrayClass;
};
}
if (!Array.prototype.indexOf) {
Array.prototype.indexOf = function indexOf(searchElement) {
var t = Object(this);
var len = t.length >>> 0;
if (len === 0) {
return -1;
}
var n = 0;
if (arguments.length > 1) {
n = Number(arguments[1]);
if (n !== n) {
n = 0;
} else if (n !== 0 && n != Infinity && n !== -Infinity) {
n = (n > 0 || -1) * Math.floor(Math.abs(n));
}
}
if (n >= len) {
return -1;
}
var k = n >= 0 ? n : Math.max(len - Math.abs(n), 0);
for (; k < len; k++) {
if (k in t && t[k] === searchElement) {
return k;
}
}
return -1;
};
}
// Fix for Tessel
if (!Object.prototype.propertyIsEnumerable) {
Object.prototype.propertyIsEnumerable = function (key) {
for (var k in this) { if (k === key) { return true; } }
return false;
};
}
if (!Object.keys) {
Object.keys = (function() {
'use strict';
var hasOwnProperty = Object.prototype.hasOwnProperty,
hasDontEnumBug = !({ toString: null }).propertyIsEnumerable('toString');
return function(obj) {
if (typeof obj !== 'object' && (typeof obj !== 'function' || obj === null)) {
throw new TypeError('Object.keys called on non-object');
}
var result = [], prop, i;
for (prop in obj) {
if (hasOwnProperty.call(obj, prop)) {
result.push(prop);
}
}
if (hasDontEnumBug) {
for (i = 0; i < dontEnumsLength; i++) {
if (hasOwnProperty.call(obj, dontEnums[i])) {
result.push(dontEnums[i]);
}
}
}
return result;
};
}());
}
// Collections
function IndexedItem(id, value) {
this.id = id;
this.value = value;
}
IndexedItem.prototype.compareTo = function (other) {
var c = this.value.compareTo(other.value);
c === 0 && (c = this.id - other.id);
return c;
};
// Priority Queue for Scheduling
var PriorityQueue = Rx.internals.PriorityQueue = function (capacity) {
this.items = new Array(capacity);
this.length = 0;
};
var priorityProto = PriorityQueue.prototype;
priorityProto.isHigherPriority = function (left, right) {
return this.items[left].compareTo(this.items[right]) < 0;
};
priorityProto.percolate = function (index) {
if (index >= this.length || index < 0) { return; }
var parent = index - 1 >> 1;
if (parent < 0 || parent === index) { return; }
if (this.isHigherPriority(index, parent)) {
var temp = this.items[index];
this.items[index] = this.items[parent];
this.items[parent] = temp;
this.percolate(parent);
}
};
priorityProto.heapify = function (index) {
+index || (index = 0);
if (index >= this.length || index < 0) { return; }
var left = 2 * index + 1,
right = 2 * index + 2,
first = index;
if (left < this.length && this.isHigherPriority(left, first)) {
first = left;
}
if (right < this.length && this.isHigherPriority(right, first)) {
first = right;
}
if (first !== index) {
var temp = this.items[index];
this.items[index] = this.items[first];
this.items[first] = temp;
this.heapify(first);
}
};
priorityProto.peek = function () { return this.items[0].value; };
priorityProto.removeAt = function (index) {
this.items[index] = this.items[--this.length];
this.items[this.length] = undefined;
this.heapify();
};
priorityProto.dequeue = function () {
var result = this.peek();
this.removeAt(0);
return result;
};
priorityProto.enqueue = function (item) {
var index = this.length++;
this.items[index] = new IndexedItem(PriorityQueue.count++, item);
this.percolate(index);
};
priorityProto.remove = function (item) {
for (var i = 0; i < this.length; i++) {
if (this.items[i].value === item) {
this.removeAt(i);
return true;
}
}
return false;
};
PriorityQueue.count = 0;
/**
* Represents a group of disposable resources that are disposed together.
* @constructor
*/
var CompositeDisposable = Rx.CompositeDisposable = function () {
var args = [], i, len;
if (Array.isArray(arguments[0])) {
args = arguments[0];
len = args.length;
} else {
len = arguments.length;
args = new Array(len);
for(i = 0; i < len; i++) { args[i] = arguments[i]; }
}
for(i = 0; i < len; i++) {
if (!isDisposable(args[i])) { throw new TypeError('Not a disposable'); }
}
this.disposables = args;
this.isDisposed = false;
this.length = args.length;
};
var CompositeDisposablePrototype = CompositeDisposable.prototype;
/**
* Adds a disposable to the CompositeDisposable or disposes the disposable if the CompositeDisposable is disposed.
* @param {Mixed} item Disposable to add.
*/
CompositeDisposablePrototype.add = function (item) {
if (this.isDisposed) {
item.dispose();
} else {
this.disposables.push(item);
this.length++;
}
};
/**
* Removes and disposes the first occurrence of a disposable from the CompositeDisposable.
* @param {Mixed} item Disposable to remove.
* @returns {Boolean} true if found; false otherwise.
*/
CompositeDisposablePrototype.remove = function (item) {
var shouldDispose = false;
if (!this.isDisposed) {
var idx = this.disposables.indexOf(item);
if (idx !== -1) {
shouldDispose = true;
this.disposables.splice(idx, 1);
this.length--;
item.dispose();
}
}
return shouldDispose;
};
/**
* Disposes all disposables in the group and removes them from the group.
*/
CompositeDisposablePrototype.dispose = function () {
if (!this.isDisposed) {
this.isDisposed = true;
var len = this.disposables.length, currentDisposables = new Array(len);
for(var i = 0; i < len; i++) { currentDisposables[i] = this.disposables[i]; }
this.disposables = [];
this.length = 0;
for (i = 0; i < len; i++) {
currentDisposables[i].dispose();
}
}
};
/**
* Provides a set of static methods for creating Disposables.
* @param {Function} dispose Action to run during the first call to dispose. The action is guaranteed to be run at most once.
*/
var Disposable = Rx.Disposable = function (action) {
this.isDisposed = false;
this.action = action || noop;
};
/** Performs the task of cleaning up resources. */
Disposable.prototype.dispose = function () {
if (!this.isDisposed) {
this.action();
this.isDisposed = true;
}
};
/**
* Creates a disposable object that invokes the specified action when disposed.
* @param {Function} dispose Action to run during the first call to dispose. The action is guaranteed to be run at most once.
* @return {Disposable} The disposable object that runs the given action upon disposal.
*/
var disposableCreate = Disposable.create = function (action) { return new Disposable(action); };
/**
* Gets the disposable that does nothing when disposed.
*/
var disposableEmpty = Disposable.empty = { dispose: noop };
/**
* Validates whether the given object is a disposable
* @param {Object} Object to test whether it has a dispose method
* @returns {Boolean} true if a disposable object, else false.
*/
var isDisposable = Disposable.isDisposable = function (d) {
return d && isFunction(d.dispose);
};
var SingleAssignmentDisposable = Rx.SingleAssignmentDisposable = (function () {
function BooleanDisposable () {
this.isDisposed = false;
this.current = null;
}
var booleanDisposablePrototype = BooleanDisposable.prototype;
/**
* Gets the underlying disposable.
* @return The underlying disposable.
*/
booleanDisposablePrototype.getDisposable = function () {
return this.current;
};
/**
* Sets the underlying disposable.
* @param {Disposable} value The new underlying disposable.
*/
booleanDisposablePrototype.setDisposable = function (value) {
var shouldDispose = this.isDisposed;
if (!shouldDispose) {
var old = this.current;
this.current = value;
}
old && old.dispose();
shouldDispose && value && value.dispose();
};
/**
* Disposes the underlying disposable as well as all future replacements.
*/
booleanDisposablePrototype.dispose = function () {
if (!this.isDisposed) {
this.isDisposed = true;
var old = this.current;
this.current = null;
}
old && old.dispose();
};
return BooleanDisposable;
}());
var SerialDisposable = Rx.SerialDisposable = SingleAssignmentDisposable;
/**
* Represents a disposable resource that only disposes its underlying disposable resource when all dependent disposable objects have been disposed.
*/
var RefCountDisposable = Rx.RefCountDisposable = (function () {
function InnerDisposable(disposable) {
this.disposable = disposable;
this.disposable.count++;
this.isInnerDisposed = false;
}
InnerDisposable.prototype.dispose = function () {
if (!this.disposable.isDisposed && !this.isInnerDisposed) {
this.isInnerDisposed = true;
this.disposable.count--;
if (this.disposable.count === 0 && this.disposable.isPrimaryDisposed) {
this.disposable.isDisposed = true;
this.disposable.underlyingDisposable.dispose();
}
}
};
/**
* Initializes a new instance of the RefCountDisposable with the specified disposable.
* @constructor
* @param {Disposable} disposable Underlying disposable.
*/
function RefCountDisposable(disposable) {
this.underlyingDisposable = disposable;
this.isDisposed = false;
this.isPrimaryDisposed = false;
this.count = 0;
}
/**
* Disposes the underlying disposable only when all dependent disposables have been disposed
*/
RefCountDisposable.prototype.dispose = function () {
if (!this.isDisposed && !this.isPrimaryDisposed) {
this.isPrimaryDisposed = true;
if (this.count === 0) {
this.isDisposed = true;
this.underlyingDisposable.dispose();
}
}
};
/**
* Returns a dependent disposable that when disposed decreases the refcount on the underlying disposable.
* @returns {Disposable} A dependent disposable contributing to the reference count that manages the underlying disposable's lifetime.
*/
RefCountDisposable.prototype.getDisposable = function () {
return this.isDisposed ? disposableEmpty : new InnerDisposable(this);
};
return RefCountDisposable;
})();
var ScheduledItem = Rx.internals.ScheduledItem = function (scheduler, state, action, dueTime, comparer) {
this.scheduler = scheduler;
this.state = state;
this.action = action;
this.dueTime = dueTime;
this.comparer = comparer || defaultSubComparer;
this.disposable = new SingleAssignmentDisposable();
}
ScheduledItem.prototype.invoke = function () {
this.disposable.setDisposable(this.invokeCore());
};
ScheduledItem.prototype.compareTo = function (other) {
return this.comparer(this.dueTime, other.dueTime);
};
ScheduledItem.prototype.isCancelled = function () {
return this.disposable.isDisposed;
};
ScheduledItem.prototype.invokeCore = function () {
return this.action(this.scheduler, this.state);
};
/** Provides a set of static properties to access commonly used schedulers. */
var Scheduler = Rx.Scheduler = (function () {
function Scheduler(now, schedule, scheduleRelative, scheduleAbsolute) {
this.now = now;
this._schedule = schedule;
this._scheduleRelative = scheduleRelative;
this._scheduleAbsolute = scheduleAbsolute;
}
function invokeAction(scheduler, action) {
action();
return disposableEmpty;
}
var schedulerProto = Scheduler.prototype;
/**
* Schedules an action to be executed.
* @param {Function} action Action to execute.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.schedule = function (action) {
return this._schedule(action, invokeAction);
};
/**
* Schedules an action to be executed.
* @param state State passed to the action to be executed.
* @param {Function} action Action to be executed.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithState = function (state, action) {
return this._schedule(state, action);
};
/**
* Schedules an action to be executed after the specified relative due time.
* @param {Function} action Action to execute.
* @param {Number} dueTime Relative time after which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithRelative = function (dueTime, action) {
return this._scheduleRelative(action, dueTime, invokeAction);
};
/**
* Schedules an action to be executed after dueTime.
* @param state State passed to the action to be executed.
* @param {Function} action Action to be executed.
* @param {Number} dueTime Relative time after which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithRelativeAndState = function (state, dueTime, action) {
return this._scheduleRelative(state, dueTime, action);
};
/**
* Schedules an action to be executed at the specified absolute due time.
* @param {Function} action Action to execute.
* @param {Number} dueTime Absolute time at which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithAbsolute = function (dueTime, action) {
return this._scheduleAbsolute(action, dueTime, invokeAction);
};
/**
* Schedules an action to be executed at dueTime.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to be executed.
* @param {Number}dueTime Absolute time at which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithAbsoluteAndState = function (state, dueTime, action) {
return this._scheduleAbsolute(state, dueTime, action);
};
/** Gets the current time according to the local machine's system clock. */
Scheduler.now = defaultNow;
/**
* Normalizes the specified TimeSpan value to a positive value.
* @param {Number} timeSpan The time span value to normalize.
* @returns {Number} The specified TimeSpan value if it is zero or positive; otherwise, 0
*/
Scheduler.normalize = function (timeSpan) {
timeSpan < 0 && (timeSpan = 0);
return timeSpan;
};
return Scheduler;
}());
var normalizeTime = Scheduler.normalize;
(function (schedulerProto) {
function invokeRecImmediate(scheduler, pair) {
var state = pair.first, action = pair.second, group = new CompositeDisposable(),
recursiveAction = function (state1) {
action(state1, function (state2) {
var isAdded = false, isDone = false,
d = scheduler.scheduleWithState(state2, function (scheduler1, state3) {
if (isAdded) {
group.remove(d);
} else {
isDone = true;
}
recursiveAction(state3);
return disposableEmpty;
});
if (!isDone) {
group.add(d);
isAdded = true;
}
});
};
recursiveAction(state);
return group;
}
function invokeRecDate(scheduler, pair, method) {
var state = pair.first, action = pair.second, group = new CompositeDisposable(),
recursiveAction = function (state1) {
action(state1, function (state2, dueTime1) {
var isAdded = false, isDone = false,
d = scheduler[method](state2, dueTime1, function (scheduler1, state3) {
if (isAdded) {
group.remove(d);
} else {
isDone = true;
}
recursiveAction(state3);
return disposableEmpty;
});
if (!isDone) {
group.add(d);
isAdded = true;
}
});
};
recursiveAction(state);
return group;
}
function scheduleInnerRecursive(action, self) {
action(function(dt) { self(action, dt); });
}
/**
* Schedules an action to be executed recursively.
* @param {Function} action Action to execute recursively. The parameter passed to the action is used to trigger recursive scheduling of the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursive = function (action) {
return this.scheduleRecursiveWithState(action, function (_action, self) {
_action(function () { self(_action); }); });
};
/**
* Schedules an action to be executed recursively.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to execute recursively. The last parameter passed to the action is used to trigger recursive scheduling of the action, passing in recursive invocation state.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithState = function (state, action) {
return this.scheduleWithState({ first: state, second: action }, invokeRecImmediate);
};
/**
* Schedules an action to be executed recursively after a specified relative due time.
* @param {Function} action Action to execute recursively. The parameter passed to the action is used to trigger recursive scheduling of the action at the specified relative time.
* @param {Number}dueTime Relative time after which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithRelative = function (dueTime, action) {
return this.scheduleRecursiveWithRelativeAndState(action, dueTime, scheduleInnerRecursive);
};
/**
* Schedules an action to be executed recursively after a specified relative due time.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to execute recursively. The last parameter passed to the action is used to trigger recursive scheduling of the action, passing in the recursive due time and invocation state.
* @param {Number}dueTime Relative time after which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithRelativeAndState = function (state, dueTime, action) {
return this._scheduleRelative({ first: state, second: action }, dueTime, function (s, p) {
return invokeRecDate(s, p, 'scheduleWithRelativeAndState');
});
};
/**
* Schedules an action to be executed recursively at a specified absolute due time.
* @param {Function} action Action to execute recursively. The parameter passed to the action is used to trigger recursive scheduling of the action at the specified absolute time.
* @param {Number}dueTime Absolute time at which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithAbsolute = function (dueTime, action) {
return this.scheduleRecursiveWithAbsoluteAndState(action, dueTime, scheduleInnerRecursive);
};
/**
* Schedules an action to be executed recursively at a specified absolute due time.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to execute recursively. The last parameter passed to the action is used to trigger recursive scheduling of the action, passing in the recursive due time and invocation state.
* @param {Number}dueTime Absolute time at which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithAbsoluteAndState = function (state, dueTime, action) {
return this._scheduleAbsolute({ first: state, second: action }, dueTime, function (s, p) {
return invokeRecDate(s, p, 'scheduleWithAbsoluteAndState');
});
};
}(Scheduler.prototype));
(function (schedulerProto) {
/**
* Schedules a periodic piece of work by dynamically discovering the scheduler's capabilities. The periodic task will be scheduled using window.setInterval for the base implementation.
* @param {Number} period Period for running the work periodically.
* @param {Function} action Action to be executed.
* @returns {Disposable} The disposable object used to cancel the scheduled recurring action (best effort).
*/
Scheduler.prototype.schedulePeriodic = function (period, action) {
return this.schedulePeriodicWithState(null, period, action);
};
/**
* Schedules a periodic piece of work by dynamically discovering the scheduler's capabilities. The periodic task will be scheduled using window.setInterval for the base implementation.
* @param {Mixed} state Initial state passed to the action upon the first iteration.
* @param {Number} period Period for running the work periodically.
* @param {Function} action Action to be executed, potentially updating the state.
* @returns {Disposable} The disposable object used to cancel the scheduled recurring action (best effort).
*/
Scheduler.prototype.schedulePeriodicWithState = function(state, period, action) {
if (typeof root.setInterval === 'undefined') { throw new Error('Periodic scheduling not supported.'); }
var s = state;
var id = root.setInterval(function () {
s = action(s);
}, period);
return disposableCreate(function () {
root.clearInterval(id);
});
};
}(Scheduler.prototype));
/** Gets a scheduler that schedules work immediately on the current thread. */
var immediateScheduler = Scheduler.immediate = (function () {
function scheduleNow(state, action) { return action(this, state); }
function notSupported() { throw new Error('Not supported'); }
return new Scheduler(defaultNow, scheduleNow, notSupported, notSupported);
}());
/**
* Gets a scheduler that schedules work as soon as possible on the current thread.
*/
var currentThreadScheduler = Scheduler.currentThread = (function () {
var queue;
function runTrampoline () {
while (queue.length > 0) {
var item = queue.dequeue();
if (!item.isCancelled()) {
!item.isCancelled() && item.invoke();
}
}
}
function scheduleNow(state, action) {
var si = new ScheduledItem(this, state, action, this.now());
if (!queue) {
queue = new PriorityQueue(4);
queue.enqueue(si);
var result = tryCatch(runTrampoline)();
queue = null;
if (result === errorObj) { return thrower(result.e); }
} else {
queue.enqueue(si);
}
return si.disposable;
}
function notSupported() { throw new Error('Not supported'); }
var currentScheduler = new Scheduler(defaultNow, scheduleNow, notSupported, notSupported);
currentScheduler.scheduleRequired = function () { return !queue; };
currentScheduler.ensureTrampoline = function (action) {
if (!queue) { this.schedule(action); } else { action(); }
};
return currentScheduler;
}());
var SchedulePeriodicRecursive = Rx.internals.SchedulePeriodicRecursive = (function () {
function tick(command, recurse) {
recurse(0, this._period);
try {
this._state = this._action(this._state);
} catch (e) {
this._cancel.dispose();
throw e;
}
}
function SchedulePeriodicRecursive(scheduler, state, period, action) {
this._scheduler = scheduler;
this._state = state;
this._period = period;
this._action = action;
}
SchedulePeriodicRecursive.prototype.start = function () {
var d = new SingleAssignmentDisposable();
this._cancel = d;
d.setDisposable(this._scheduler.scheduleRecursiveWithRelativeAndState(0, this._period, tick.bind(this)));
return d;
};
return SchedulePeriodicRecursive;
}());
var scheduleMethod, clearMethod = noop;
var localTimer = (function () {
var localSetTimeout, localClearTimeout = noop;
if ('WScript' in this) {
localSetTimeout = function (fn, time) {
WScript.Sleep(time);
fn();
};
} else if (!!root.setTimeout) {
localSetTimeout = root.setTimeout;
localClearTimeout = root.clearTimeout;
} else {
throw new Error('No concurrency detected!');
}
return {
setTimeout: localSetTimeout,
clearTimeout: localClearTimeout
};
}());
var localSetTimeout = localTimer.setTimeout,
localClearTimeout = localTimer.clearTimeout;
(function () {
var reNative = RegExp('^' +
String(toString)
.replace(/[.*+?^${}()|[\]\\]/g, '\\$&')
.replace(/toString| for [^\]]+/g, '.*?') + '$'
);
var setImmediate = typeof (setImmediate = freeGlobal && moduleExports && freeGlobal.setImmediate) == 'function' &&
!reNative.test(setImmediate) && setImmediate,
clearImmediate = typeof (clearImmediate = freeGlobal && moduleExports && freeGlobal.clearImmediate) == 'function' &&
!reNative.test(clearImmediate) && clearImmediate;
function postMessageSupported () {
// Ensure not in a worker
if (!root.postMessage || root.importScripts) { return false; }
var isAsync = false,
oldHandler = root.onmessage;
// Test for async
root.onmessage = function () { isAsync = true; };
root.postMessage('', '*');
root.onmessage = oldHandler;
return isAsync;
}
// Use in order, setImmediate, nextTick, postMessage, MessageChannel, script readystatechanged, setTimeout
if (typeof setImmediate === 'function') {
scheduleMethod = setImmediate;
clearMethod = clearImmediate;
} else if (typeof process !== 'undefined' && {}.toString.call(process) === '[object process]') {
scheduleMethod = process.nextTick;
} else if (postMessageSupported()) {
var MSG_PREFIX = 'ms.rx.schedule' + Math.random(),
tasks = {},
taskId = 0;
var onGlobalPostMessage = function (event) {
// Only if we're a match to avoid any other global events
if (typeof event.data === 'string' && event.data.substring(0, MSG_PREFIX.length) === MSG_PREFIX) {
var handleId = event.data.substring(MSG_PREFIX.length),
action = tasks[handleId];
action();
delete tasks[handleId];
}
}
if (root.addEventListener) {
root.addEventListener('message', onGlobalPostMessage, false);
} else {
root.attachEvent('onmessage', onGlobalPostMessage, false);
}
scheduleMethod = function (action) {
var currentId = taskId++;
tasks[currentId] = action;
root.postMessage(MSG_PREFIX + currentId, '*');
};
} else if (!!root.MessageChannel) {
var channel = new root.MessageChannel(),
channelTasks = {},
channelTaskId = 0;
channel.port1.onmessage = function (event) {
var id = event.data,
action = channelTasks[id];
action();
delete channelTasks[id];
};
scheduleMethod = function (action) {
var id = channelTaskId++;
channelTasks[id] = action;
channel.port2.postMessage(id);
};
} else if ('document' in root && 'onreadystatechange' in root.document.createElement('script')) {
scheduleMethod = function (action) {
var scriptElement = root.document.createElement('script');
scriptElement.onreadystatechange = function () {
action();
scriptElement.onreadystatechange = null;
scriptElement.parentNode.removeChild(scriptElement);
scriptElement = null;
};
root.document.documentElement.appendChild(scriptElement);
};
} else {
scheduleMethod = function (action) { return localSetTimeout(action, 0); };
clearMethod = localClearTimeout;
}
}());
/**
* Gets a scheduler that schedules work via a timed callback based upon platform.
*/
var timeoutScheduler = Scheduler.timeout = (function () {
function scheduleNow(state, action) {
var scheduler = this,
disposable = new SingleAssignmentDisposable();
var id = scheduleMethod(function () {
if (!disposable.isDisposed) {
disposable.setDisposable(action(scheduler, state));
}
});
return new CompositeDisposable(disposable, disposableCreate(function () {
clearMethod(id);
}));
}
function scheduleRelative(state, dueTime, action) {
var scheduler = this,
dt = Scheduler.normalize(dueTime);
if (dt === 0) {
return scheduler.scheduleWithState(state, action);
}
var disposable = new SingleAssignmentDisposable();
var id = localSetTimeout(function () {
if (!disposable.isDisposed) {
disposable.setDisposable(action(scheduler, state));
}
}, dt);
return new CompositeDisposable(disposable, disposableCreate(function () {
localClearTimeout(id);
}));
}
function scheduleAbsolute(state, dueTime, action) {
return this.scheduleWithRelativeAndState(state, dueTime - this.now(), action);
}
return new Scheduler(defaultNow, scheduleNow, scheduleRelative, scheduleAbsolute);
})();
/**
* Represents a notification to an observer.
*/
var Notification = Rx.Notification = (function () {
function Notification(kind, hasValue) {
this.hasValue = hasValue == null ? false : hasValue;
this.kind = kind;
}
/**
* Invokes the delegate corresponding to the notification or the observer's method corresponding to the notification and returns the produced result.
*
* @memberOf Notification
* @param {Any} observerOrOnNext Delegate to invoke for an OnNext notification or Observer to invoke the notification on..
* @param {Function} onError Delegate to invoke for an OnError notification.
* @param {Function} onCompleted Delegate to invoke for an OnCompleted notification.
* @returns {Any} Result produced by the observation.
*/
Notification.prototype.accept = function (observerOrOnNext, onError, onCompleted) {
return observerOrOnNext && typeof observerOrOnNext === 'object' ?
this._acceptObservable(observerOrOnNext) :
this._accept(observerOrOnNext, onError, onCompleted);
};
/**
* Returns an observable sequence with a single notification.
*
* @memberOf Notifications
* @param {Scheduler} [scheduler] Scheduler to send out the notification calls on.
* @returns {Observable} The observable sequence that surfaces the behavior of the notification upon subscription.
*/
Notification.prototype.toObservable = function (scheduler) {
var notification = this;
isScheduler(scheduler) || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.schedule(function () {
notification._acceptObservable(observer);
notification.kind === 'N' && observer.onCompleted();
});
});
};
return Notification;
})();
/**
* Creates an object that represents an OnNext notification to an observer.
* @param {Any} value The value contained in the notification.
* @returns {Notification} The OnNext notification containing the value.
*/
var notificationCreateOnNext = Notification.createOnNext = (function () {
function _accept (onNext) { return onNext(this.value); }
function _acceptObservable(observer) { return observer.onNext(this.value); }
function toString () { return 'OnNext(' + this.value + ')'; }
return function (value) {
var notification = new Notification('N', true);
notification.value = value;
notification._accept = _accept;
notification._acceptObservable = _acceptObservable;
notification.toString = toString;
return notification;
};
}());
/**
* Creates an object that represents an OnError notification to an observer.
* @param {Any} error The exception contained in the notification.
* @returns {Notification} The OnError notification containing the exception.
*/
var notificationCreateOnError = Notification.createOnError = (function () {
function _accept (onNext, onError) { return onError(this.exception); }
function _acceptObservable(observer) { return observer.onError(this.exception); }
function toString () { return 'OnError(' + this.exception + ')'; }
return function (e) {
var notification = new Notification('E');
notification.exception = e;
notification._accept = _accept;
notification._acceptObservable = _acceptObservable;
notification.toString = toString;
return notification;
};
}());
/**
* Creates an object that represents an OnCompleted notification to an observer.
* @returns {Notification} The OnCompleted notification.
*/
var notificationCreateOnCompleted = Notification.createOnCompleted = (function () {
function _accept (onNext, onError, onCompleted) { return onCompleted(); }
function _acceptObservable(observer) { return observer.onCompleted(); }
function toString () { return 'OnCompleted()'; }
return function () {
var notification = new Notification('C');
notification._accept = _accept;
notification._acceptObservable = _acceptObservable;
notification.toString = toString;
return notification;
};
}());
var Enumerator = Rx.internals.Enumerator = function (next) {
this._next = next;
};
Enumerator.prototype.next = function () {
return this._next();
};
Enumerator.prototype[$iterator$] = function () { return this; }
var Enumerable = Rx.internals.Enumerable = function (iterator) {
this._iterator = iterator;
};
Enumerable.prototype[$iterator$] = function () {
return this._iterator();
};
Enumerable.prototype.concat = function () {
var sources = this;
return new AnonymousObservable(function (o) {
var e = sources[$iterator$]();
var isDisposed, subscription = new SerialDisposable();
var cancelable = immediateScheduler.scheduleRecursive(function (self) {
if (isDisposed) { return; }
try {
var currentItem = e.next();
} catch (ex) {
return o.onError(ex);
}
if (currentItem.done) {
return o.onCompleted();
}
// Check if promise
var currentValue = currentItem.value;
isPromise(currentValue) && (currentValue = observableFromPromise(currentValue));
var d = new SingleAssignmentDisposable();
subscription.setDisposable(d);
d.setDisposable(currentValue.subscribe(
function(x) { o.onNext(x); },
function(err) { o.onError(err); },
self)
);
});
return new CompositeDisposable(subscription, cancelable, disposableCreate(function () {
isDisposed = true;
}));
});
};
Enumerable.prototype.catchError = function () {
var sources = this;
return new AnonymousObservable(function (o) {
var e = sources[$iterator$]();
var isDisposed, subscription = new SerialDisposable();
var cancelable = immediateScheduler.scheduleRecursiveWithState(null, function (lastException, self) {
if (isDisposed) { return; }
try {
var currentItem = e.next();
} catch (ex) {
return observer.onError(ex);
}
if (currentItem.done) {
if (lastException !== null) {
o.onError(lastException);
} else {
o.onCompleted();
}
return;
}
// Check if promise
var currentValue = currentItem.value;
isPromise(currentValue) && (currentValue = observableFromPromise(currentValue));
var d = new SingleAssignmentDisposable();
subscription.setDisposable(d);
d.setDisposable(currentValue.subscribe(
function(x) { o.onNext(x); },
self,
function() { o.onCompleted(); }));
});
return new CompositeDisposable(subscription, cancelable, disposableCreate(function () {
isDisposed = true;
}));
});
};
Enumerable.prototype.catchErrorWhen = function (notificationHandler) {
var sources = this;
return new AnonymousObservable(function (o) {
var exceptions = new Subject(),
notifier = new Subject(),
handled = notificationHandler(exceptions),
notificationDisposable = handled.subscribe(notifier);
var e = sources[$iterator$]();
var isDisposed,
lastException,
subscription = new SerialDisposable();
var cancelable = immediateScheduler.scheduleRecursive(function (self) {
if (isDisposed) { return; }
try {
var currentItem = e.next();
} catch (ex) {
return o.onError(ex);
}
if (currentItem.done) {
if (lastException) {
o.onError(lastException);
} else {
o.onCompleted();
}
return;
}
// Check if promise
var currentValue = currentItem.value;
isPromise(currentValue) && (currentValue = observableFromPromise(currentValue));
var outer = new SingleAssignmentDisposable();
var inner = new SingleAssignmentDisposable();
subscription.setDisposable(new CompositeDisposable(inner, outer));
outer.setDisposable(currentValue.subscribe(
function(x) { o.onNext(x); },
function (exn) {
inner.setDisposable(notifier.subscribe(self, function(ex) {
o.onError(ex);
}, function() {
o.onCompleted();
}));
exceptions.onNext(exn);
},
function() { o.onCompleted(); }));
});
return new CompositeDisposable(notificationDisposable, subscription, cancelable, disposableCreate(function () {
isDisposed = true;
}));
});
};
var enumerableRepeat = Enumerable.repeat = function (value, repeatCount) {
if (repeatCount == null) { repeatCount = -1; }
return new Enumerable(function () {
var left = repeatCount;
return new Enumerator(function () {
if (left === 0) { return doneEnumerator; }
if (left > 0) { left--; }
return { done: false, value: value };
});
});
};
var enumerableOf = Enumerable.of = function (source, selector, thisArg) {
if (selector) {
var selectorFn = bindCallback(selector, thisArg, 3);
}
return new Enumerable(function () {
var index = -1;
return new Enumerator(
function () {
return ++index < source.length ?
{ done: false, value: !selector ? source[index] : selectorFn(source[index], index, source) } :
doneEnumerator;
});
});
};
/**
* Supports push-style iteration over an observable sequence.
*/
var Observer = Rx.Observer = function () { };
/**
* Creates an observer from the specified OnNext, along with optional OnError, and OnCompleted actions.
* @param {Function} [onNext] Observer's OnNext action implementation.
* @param {Function} [onError] Observer's OnError action implementation.
* @param {Function} [onCompleted] Observer's OnCompleted action implementation.
* @returns {Observer} The observer object implemented using the given actions.
*/
var observerCreate = Observer.create = function (onNext, onError, onCompleted) {
onNext || (onNext = noop);
onError || (onError = defaultError);
onCompleted || (onCompleted = noop);
return new AnonymousObserver(onNext, onError, onCompleted);
};
/**
* Abstract base class for implementations of the Observer class.
* This base class enforces the grammar of observers where OnError and OnCompleted are terminal messages.
*/
var AbstractObserver = Rx.internals.AbstractObserver = (function (__super__) {
inherits(AbstractObserver, __super__);
/**
* Creates a new observer in a non-stopped state.
*/
function AbstractObserver() {
this.isStopped = false;
__super__.call(this);
}
function notImplemented() {
throw new Error('Method not implemented');
}
// Must be implemented by other observers
AbstractObserver.prototype.next = notImplemented;
AbstractObserver.prototype.error = notImplemented;
AbstractObserver.prototype.completed = notImplemented;
/**
* Notifies the observer of a new element in the sequence.
* @param {Any} value Next element in the sequence.
*/
AbstractObserver.prototype.onNext = function (value) {
if (!this.isStopped) { this.next(value); }
};
/**
* Notifies the observer that an exception has occurred.
* @param {Any} error The error that has occurred.
*/
AbstractObserver.prototype.onError = function (error) {
if (!this.isStopped) {
this.isStopped = true;
this.error(error);
}
};
/**
* Notifies the observer of the end of the sequence.
*/
AbstractObserver.prototype.onCompleted = function () {
if (!this.isStopped) {
this.isStopped = true;
this.completed();
}
};
/**
* Disposes the observer, causing it to transition to the stopped state.
*/
AbstractObserver.prototype.dispose = function () {
this.isStopped = true;
};
AbstractObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.error(e);
return true;
}
return false;
};
return AbstractObserver;
}(Observer));
/**
* Class to create an Observer instance from delegate-based implementations of the on* methods.
*/
var AnonymousObserver = Rx.AnonymousObserver = (function (__super__) {
inherits(AnonymousObserver, __super__);
/**
* Creates an observer from the specified OnNext, OnError, and OnCompleted actions.
* @param {Any} onNext Observer's OnNext action implementation.
* @param {Any} onError Observer's OnError action implementation.
* @param {Any} onCompleted Observer's OnCompleted action implementation.
*/
function AnonymousObserver(onNext, onError, onCompleted) {
__super__.call(this);
this._onNext = onNext;
this._onError = onError;
this._onCompleted = onCompleted;
}
/**
* Calls the onNext action.
* @param {Any} value Next element in the sequence.
*/
AnonymousObserver.prototype.next = function (value) {
this._onNext(value);
};
/**
* Calls the onError action.
* @param {Any} error The error that has occurred.
*/
AnonymousObserver.prototype.error = function (error) {
this._onError(error);
};
/**
* Calls the onCompleted action.
*/
AnonymousObserver.prototype.completed = function () {
this._onCompleted();
};
return AnonymousObserver;
}(AbstractObserver));
var observableProto;
/**
* Represents a push-style collection.
*/
var Observable = Rx.Observable = (function () {
function Observable(subscribe) {
if (Rx.config.longStackSupport && hasStacks) {
try {
throw new Error();
} catch (e) {
this.stack = e.stack.substring(e.stack.indexOf("\n") + 1);
}
var self = this;
this._subscribe = function (observer) {
var oldOnError = observer.onError.bind(observer);
observer.onError = function (err) {
makeStackTraceLong(err, self);
oldOnError(err);
};
return subscribe.call(self, observer);
};
} else {
this._subscribe = subscribe;
}
}
observableProto = Observable.prototype;
/**
* Subscribes an observer to the observable sequence.
* @param {Mixed} [observerOrOnNext] The object that is to receive notifications or an action to invoke for each element in the observable sequence.
* @param {Function} [onError] Action to invoke upon exceptional termination of the observable sequence.
* @param {Function} [onCompleted] Action to invoke upon graceful termination of the observable sequence.
* @returns {Diposable} A disposable handling the subscriptions and unsubscriptions.
*/
observableProto.subscribe = observableProto.forEach = function (observerOrOnNext, onError, onCompleted) {
return this._subscribe(typeof observerOrOnNext === 'object' ?
observerOrOnNext :
observerCreate(observerOrOnNext, onError, onCompleted));
};
/**
* Subscribes to the next value in the sequence with an optional "this" argument.
* @param {Function} onNext The function to invoke on each element in the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Disposable} A disposable handling the subscriptions and unsubscriptions.
*/
observableProto.subscribeOnNext = function (onNext, thisArg) {
return this._subscribe(observerCreate(typeof thisArg !== 'undefined' ? function(x) { onNext.call(thisArg, x); } : onNext));
};
/**
* Subscribes to an exceptional condition in the sequence with an optional "this" argument.
* @param {Function} onError The function to invoke upon exceptional termination of the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Disposable} A disposable handling the subscriptions and unsubscriptions.
*/
observableProto.subscribeOnError = function (onError, thisArg) {
return this._subscribe(observerCreate(null, typeof thisArg !== 'undefined' ? function(e) { onError.call(thisArg, e); } : onError));
};
/**
* Subscribes to the next value in the sequence with an optional "this" argument.
* @param {Function} onCompleted The function to invoke upon graceful termination of the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Disposable} A disposable handling the subscriptions and unsubscriptions.
*/
observableProto.subscribeOnCompleted = function (onCompleted, thisArg) {
return this._subscribe(observerCreate(null, null, typeof thisArg !== 'undefined' ? function() { onCompleted.call(thisArg); } : onCompleted));
};
return Observable;
})();
var ObservableBase = Rx.ObservableBase = (function (__super__) {
inherits(ObservableBase, __super__);
// Fix subscriber to check for undefined or function returned to decorate as Disposable
function fixSubscriber(subscriber) {
if (subscriber && typeof subscriber.dispose === 'function') { return subscriber; }
return typeof subscriber === 'function' ?
disposableCreate(subscriber) :
disposableEmpty;
}
function setDisposable(s, state) {
var ado = state[0], self = state[1];
var sub = tryCatch(self.subscribeCore).call(self, ado);
if (sub === errorObj) {
if(!ado.fail(errorObj.e)) { return thrower(errorObj.e); }
}
ado.setDisposable(fixSubscriber(sub));
}
function subscribe(observer) {
var ado = new AutoDetachObserver(observer), state = [ado, this];
if (currentThreadScheduler.scheduleRequired()) {
currentThreadScheduler.scheduleWithState(state, setDisposable);
} else {
setDisposable(null, state);
}
return ado;
}
function ObservableBase() {
__super__.call(this, subscribe);
}
ObservableBase.prototype.subscribeCore = function(observer) {
throw new Error('Not implemeneted');
}
return ObservableBase;
}(Observable));
var ScheduledObserver = Rx.internals.ScheduledObserver = (function (__super__) {
inherits(ScheduledObserver, __super__);
function ScheduledObserver(scheduler, observer) {
__super__.call(this);
this.scheduler = scheduler;
this.observer = observer;
this.isAcquired = false;
this.hasFaulted = false;
this.queue = [];
this.disposable = new SerialDisposable();
}
ScheduledObserver.prototype.next = function (value) {
var self = this;
this.queue.push(function () { self.observer.onNext(value); });
};
ScheduledObserver.prototype.error = function (e) {
var self = this;
this.queue.push(function () { self.observer.onError(e); });
};
ScheduledObserver.prototype.completed = function () {
var self = this;
this.queue.push(function () { self.observer.onCompleted(); });
};
ScheduledObserver.prototype.ensureActive = function () {
var isOwner = false, parent = this;
if (!this.hasFaulted && this.queue.length > 0) {
isOwner = !this.isAcquired;
this.isAcquired = true;
}
if (isOwner) {
this.disposable.setDisposable(this.scheduler.scheduleRecursive(function (self) {
var work;
if (parent.queue.length > 0) {
work = parent.queue.shift();
} else {
parent.isAcquired = false;
return;
}
try {
work();
} catch (ex) {
parent.queue = [];
parent.hasFaulted = true;
throw ex;
}
self();
}));
}
};
ScheduledObserver.prototype.dispose = function () {
__super__.prototype.dispose.call(this);
this.disposable.dispose();
};
return ScheduledObserver;
}(AbstractObserver));
var ToArrayObservable = (function(__super__) {
inherits(ToArrayObservable, __super__);
function ToArrayObservable(source) {
this.source = source;
__super__.call(this);
}
ToArrayObservable.prototype.subscribeCore = function(observer) {
return this.source.subscribe(new ToArrayObserver(observer));
};
return ToArrayObservable;
}(ObservableBase));
function ToArrayObserver(observer) {
this.observer = observer;
this.a = [];
this.isStopped = false;
}
ToArrayObserver.prototype.onNext = function (x) { if(!this.isStopped) { this.a.push(x); } };
ToArrayObserver.prototype.onError = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.observer.onError(e);
}
};
ToArrayObserver.prototype.onCompleted = function () {
if (!this.isStopped) {
this.isStopped = true;
this.observer.onNext(this.a);
this.observer.onCompleted();
}
};
ToArrayObserver.prototype.dispose = function () { this.isStopped = true; }
ToArrayObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.observer.onError(e);
return true;
}
return false;
};
/**
* Creates an array from an observable sequence.
* @returns {Observable} An observable sequence containing a single element with a list containing all the elements of the source sequence.
*/
observableProto.toArray = function () {
return new ToArrayObservable(this);
};
/**
* Creates an observable sequence from a specified subscribe method implementation.
* @example
* var res = Rx.Observable.create(function (observer) { return function () { } );
* var res = Rx.Observable.create(function (observer) { return Rx.Disposable.empty; } );
* var res = Rx.Observable.create(function (observer) { } );
* @param {Function} subscribe Implementation of the resulting observable sequence's subscribe method, returning a function that will be wrapped in a Disposable.
* @returns {Observable} The observable sequence with the specified implementation for the Subscribe method.
*/
Observable.create = Observable.createWithDisposable = function (subscribe, parent) {
return new AnonymousObservable(subscribe, parent);
};
/**
* Returns an observable sequence that invokes the specified factory function whenever a new observer subscribes.
*
* @example
* var res = Rx.Observable.defer(function () { return Rx.Observable.fromArray([1,2,3]); });
* @param {Function} observableFactory Observable factory function to invoke for each observer that subscribes to the resulting sequence or Promise.
* @returns {Observable} An observable sequence whose observers trigger an invocation of the given observable factory function.
*/
var observableDefer = Observable.defer = function (observableFactory) {
return new AnonymousObservable(function (observer) {
var result;
try {
result = observableFactory();
} catch (e) {
return observableThrow(e).subscribe(observer);
}
isPromise(result) && (result = observableFromPromise(result));
return result.subscribe(observer);
});
};
/**
* Returns an empty observable sequence, using the specified scheduler to send out the single OnCompleted message.
*
* @example
* var res = Rx.Observable.empty();
* var res = Rx.Observable.empty(Rx.Scheduler.timeout);
* @param {Scheduler} [scheduler] Scheduler to send the termination call on.
* @returns {Observable} An observable sequence with no elements.
*/
var observableEmpty = Observable.empty = function (scheduler) {
isScheduler(scheduler) || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.schedule(function () {
observer.onCompleted();
});
});
};
var FromObservable = (function(__super__) {
inherits(FromObservable, __super__);
function FromObservable(iterable, mapper, scheduler) {
this.iterable = iterable;
this.mapper = mapper;
this.scheduler = scheduler;
__super__.call(this);
}
FromObservable.prototype.subscribeCore = function (observer) {
var sink = new FromSink(observer, this);
return sink.run();
};
return FromObservable;
}(ObservableBase));
var FromSink = (function () {
function FromSink(observer, parent) {
this.observer = observer;
this.parent = parent;
}
FromSink.prototype.run = function () {
var list = Object(this.parent.iterable),
it = getIterable(list),
observer = this.observer,
mapper = this.parent.mapper;
function loopRecursive(i, recurse) {
try {
var next = it.next();
} catch (e) {
return observer.onError(e);
}
if (next.done) {
return observer.onCompleted();
}
var result = next.value;
if (mapper) {
try {
result = mapper(result, i);
} catch (e) {
return observer.onError(e);
}
}
observer.onNext(result);
recurse(i + 1);
}
return this.parent.scheduler.scheduleRecursiveWithState(0, loopRecursive);
};
return FromSink;
}());
var maxSafeInteger = Math.pow(2, 53) - 1;
function StringIterable(str) {
this._s = s;
}
StringIterable.prototype[$iterator$] = function () {
return new StringIterator(this._s);
};
function StringIterator(str) {
this._s = s;
this._l = s.length;
this._i = 0;
}
StringIterator.prototype[$iterator$] = function () {
return this;
};
StringIterator.prototype.next = function () {
return this._i < this._l ? { done: false, value: this._s.charAt(this._i++) } : doneEnumerator;
};
function ArrayIterable(a) {
this._a = a;
}
ArrayIterable.prototype[$iterator$] = function () {
return new ArrayIterator(this._a);
};
function ArrayIterator(a) {
this._a = a;
this._l = toLength(a);
this._i = 0;
}
ArrayIterator.prototype[$iterator$] = function () {
return this;
};
ArrayIterator.prototype.next = function () {
return this._i < this._l ? { done: false, value: this._a[this._i++] } : doneEnumerator;
};
function numberIsFinite(value) {
return typeof value === 'number' && root.isFinite(value);
}
function isNan(n) {
return n !== n;
}
function getIterable(o) {
var i = o[$iterator$], it;
if (!i && typeof o === 'string') {
it = new StringIterable(o);
return it[$iterator$]();
}
if (!i && o.length !== undefined) {
it = new ArrayIterable(o);
return it[$iterator$]();
}
if (!i) { throw new TypeError('Object is not iterable'); }
return o[$iterator$]();
}
function sign(value) {
var number = +value;
if (number === 0) { return number; }
if (isNaN(number)) { return number; }
return number < 0 ? -1 : 1;
}
function toLength(o) {
var len = +o.length;
if (isNaN(len)) { return 0; }
if (len === 0 || !numberIsFinite(len)) { return len; }
len = sign(len) * Math.floor(Math.abs(len));
if (len <= 0) { return 0; }
if (len > maxSafeInteger) { return maxSafeInteger; }
return len;
}
/**
* This method creates a new Observable sequence from an array-like or iterable object.
* @param {Any} arrayLike An array-like or iterable object to convert to an Observable sequence.
* @param {Function} [mapFn] Map function to call on every element of the array.
* @param {Any} [thisArg] The context to use calling the mapFn if provided.
* @param {Scheduler} [scheduler] Optional scheduler to use for scheduling. If not provided, defaults to Scheduler.currentThread.
*/
var observableFrom = Observable.from = function (iterable, mapFn, thisArg, scheduler) {
if (iterable == null) {
throw new Error('iterable cannot be null.')
}
if (mapFn && !isFunction(mapFn)) {
throw new Error('mapFn when provided must be a function');
}
if (mapFn) {
var mapper = bindCallback(mapFn, thisArg, 2);
}
isScheduler(scheduler) || (scheduler = currentThreadScheduler);
return new FromObservable(iterable, mapper, scheduler);
}
var FromArrayObservable = (function(__super__) {
inherits(FromArrayObservable, __super__);
function FromArrayObservable(args, scheduler) {
this.args = args;
this.scheduler = scheduler || currentThreadScheduler;
__super__.call(this);
}
FromArrayObservable.prototype.subscribeCore = function (observer) {
var sink = new FromArraySink(observer, this);
return sink.run();
};
return FromArrayObservable;
}(ObservableBase));
function FromArraySink(observer, parent) {
this.observer = observer;
this.parent = parent;
}
FromArraySink.prototype.run = function () {
var observer = this.observer, args = this.parent.args, len = args.length;
function loopRecursive(i, recurse) {
if (i < len) {
observer.onNext(args[i]);
recurse(i + 1);
} else {
observer.onCompleted();
}
}
return this.parent.scheduler.scheduleRecursiveWithState(0, loopRecursive);
};
/**
* Converts an array to an observable sequence, using an optional scheduler to enumerate the array.
* @deprecated use Observable.from or Observable.of
* @param {Scheduler} [scheduler] Scheduler to run the enumeration of the input sequence on.
* @returns {Observable} The observable sequence whose elements are pulled from the given enumerable sequence.
*/
var observableFromArray = Observable.fromArray = function (array, scheduler) {
return new FromArrayObservable(array, scheduler)
};
/**
* Returns a non-terminating observable sequence, which can be used to denote an infinite duration (e.g. when using reactive joins).
* @returns {Observable} An observable sequence whose observers will never get called.
*/
var observableNever = Observable.never = function () {
return new AnonymousObservable(function () {
return disposableEmpty;
});
};
function observableOf (scheduler, array) {
return new FromArrayObservable(array, scheduler);
}
/**
* This method creates a new Observable instance with a variable number of arguments, regardless of number or type of the arguments.
* @returns {Observable} The observable sequence whose elements are pulled from the given arguments.
*/
Observable.of = function () {
var len = arguments.length, args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
return new FromArrayObservable(args);
};
/**
* This method creates a new Observable instance with a variable number of arguments, regardless of number or type of the arguments.
* @param {Scheduler} scheduler A scheduler to use for scheduling the arguments.
* @returns {Observable} The observable sequence whose elements are pulled from the given arguments.
*/
Observable.ofWithScheduler = function (scheduler) {
var len = arguments.length, args = new Array(len - 1);
for(var i = 1; i < len; i++) { args[i - 1] = arguments[i]; }
return new FromArrayObservable(args, scheduler);
};
/**
* Convert an object into an observable sequence of [key, value] pairs.
* @param {Object} obj The object to inspect.
* @param {Scheduler} [scheduler] Scheduler to run the enumeration of the input sequence on.
* @returns {Observable} An observable sequence of [key, value] pairs from the object.
*/
Observable.pairs = function (obj, scheduler) {
scheduler || (scheduler = Rx.Scheduler.currentThread);
return new AnonymousObservable(function (observer) {
var keys = Object.keys(obj), len = keys.length;
return scheduler.scheduleRecursiveWithState(0, function (idx, self) {
if (idx < len) {
var key = keys[idx];
observer.onNext([key, obj[key]]);
self(idx + 1);
} else {
observer.onCompleted();
}
});
});
};
var RangeObservable = (function(__super__) {
inherits(RangeObservable, __super__);
function RangeObservable(start, count, scheduler) {
this.start = start;
this.count = count;
this.scheduler = scheduler;
__super__.call(this);
}
RangeObservable.prototype.subscribeCore = function (observer) {
var sink = new RangeSink(observer, this);
return sink.run();
};
return RangeObservable;
}(ObservableBase));
var RangeSink = (function () {
function RangeSink(observer, parent) {
this.observer = observer;
this.parent = parent;
}
RangeSink.prototype.run = function () {
var start = this.parent.start, count = this.parent.count, observer = this.observer;
function loopRecursive(i, recurse) {
if (i < count) {
observer.onNext(start + i);
recurse(i + 1);
} else {
observer.onCompleted();
}
}
return this.parent.scheduler.scheduleRecursiveWithState(0, loopRecursive);
};
return RangeSink;
}());
/**
* Generates an observable sequence of integral numbers within a specified range, using the specified scheduler to send out observer messages.
* @param {Number} start The value of the first integer in the sequence.
* @param {Number} count The number of sequential integers to generate.
* @param {Scheduler} [scheduler] Scheduler to run the generator loop on. If not specified, defaults to Scheduler.currentThread.
* @returns {Observable} An observable sequence that contains a range of sequential integral numbers.
*/
Observable.range = function (start, count, scheduler) {
isScheduler(scheduler) || (scheduler = currentThreadScheduler);
return new RangeObservable(start, count, scheduler);
};
/**
* Generates an observable sequence that repeats the given element the specified number of times, using the specified scheduler to send out observer messages.
*
* @example
* var res = Rx.Observable.repeat(42);
* var res = Rx.Observable.repeat(42, 4);
* 3 - res = Rx.Observable.repeat(42, 4, Rx.Scheduler.timeout);
* 4 - res = Rx.Observable.repeat(42, null, Rx.Scheduler.timeout);
* @param {Mixed} value Element to repeat.
* @param {Number} repeatCount [Optiona] Number of times to repeat the element. If not specified, repeats indefinitely.
* @param {Scheduler} scheduler Scheduler to run the producer loop on. If not specified, defaults to Scheduler.immediate.
* @returns {Observable} An observable sequence that repeats the given element the specified number of times.
*/
Observable.repeat = function (value, repeatCount, scheduler) {
isScheduler(scheduler) || (scheduler = currentThreadScheduler);
return observableReturn(value, scheduler).repeat(repeatCount == null ? -1 : repeatCount);
};
/**
* Returns an observable sequence that contains a single element, using the specified scheduler to send out observer messages.
* There is an alias called 'just', and 'returnValue' for browsers <IE9.
* @param {Mixed} value Single element in the resulting observable sequence.
* @param {Scheduler} scheduler Scheduler to send the single element on. If not specified, defaults to Scheduler.immediate.
* @returns {Observable} An observable sequence containing the single specified element.
*/
var observableReturn = Observable['return'] = Observable.just = function (value, scheduler) {
isScheduler(scheduler) || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.schedule(function () {
observer.onNext(value);
observer.onCompleted();
});
});
};
/** @deprecated use return or just */
Observable.returnValue = function () {
//deprecate('returnValue', 'return or just');
return observableReturn.apply(null, arguments);
};
/**
* Returns an observable sequence that terminates with an exception, using the specified scheduler to send out the single onError message.
* There is an alias to this method called 'throwError' for browsers <IE9.
* @param {Mixed} error An object used for the sequence's termination.
* @param {Scheduler} scheduler Scheduler to send the exceptional termination call on. If not specified, defaults to Scheduler.immediate.
* @returns {Observable} The observable sequence that terminates exceptionally with the specified exception object.
*/
var observableThrow = Observable['throw'] = Observable.throwError = function (error, scheduler) {
isScheduler(scheduler) || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.schedule(function () {
observer.onError(error);
});
});
};
/** @deprecated use #some instead */
Observable.throwException = function () {
//deprecate('throwException', 'throwError');
return Observable.throwError.apply(null, arguments);
};
function observableCatchHandler(source, handler) {
return new AnonymousObservable(function (o) {
var d1 = new SingleAssignmentDisposable(), subscription = new SerialDisposable();
subscription.setDisposable(d1);
d1.setDisposable(source.subscribe(function (x) { o.onNext(x); }, function (e) {
try {
var result = handler(e);
} catch (ex) {
return o.onError(ex);
}
isPromise(result) && (result = observableFromPromise(result));
var d = new SingleAssignmentDisposable();
subscription.setDisposable(d);
d.setDisposable(result.subscribe(o));
}, function (x) { o.onCompleted(x); }));
return subscription;
}, source);
}
/**
* Continues an observable sequence that is terminated by an exception with the next observable sequence.
* @example
* 1 - xs.catchException(ys)
* 2 - xs.catchException(function (ex) { return ys(ex); })
* @param {Mixed} handlerOrSecond Exception handler function that returns an observable sequence given the error that occurred in the first sequence, or a second observable sequence used to produce results when an error occurred in the first sequence.
* @returns {Observable} An observable sequence containing the first sequence's elements, followed by the elements of the handler sequence in case an exception occurred.
*/
observableProto['catch'] = observableProto.catchError = observableProto.catchException = function (handlerOrSecond) {
return typeof handlerOrSecond === 'function' ?
observableCatchHandler(this, handlerOrSecond) :
observableCatch([this, handlerOrSecond]);
};
/**
* Continues an observable sequence that is terminated by an exception with the next observable sequence.
* @param {Array | Arguments} args Arguments or an array to use as the next sequence if an error occurs.
* @returns {Observable} An observable sequence containing elements from consecutive source sequences until a source sequence terminates successfully.
*/
var observableCatch = Observable.catchError = Observable['catch'] = Observable.catchException = function () {
var items = [];
if (Array.isArray(arguments[0])) {
items = arguments[0];
} else {
for(var i = 0, len = arguments.length; i < len; i++) { items.push(arguments[i]); }
}
return enumerableOf(items).catchError();
};
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever any of the observable sequences or Promises produces an element.
* This can be in the form of an argument list of observables or an array.
*
* @example
* 1 - obs = observable.combineLatest(obs1, obs2, obs3, function (o1, o2, o3) { return o1 + o2 + o3; });
* 2 - obs = observable.combineLatest([obs1, obs2, obs3], function (o1, o2, o3) { return o1 + o2 + o3; });
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
observableProto.combineLatest = function () {
var len = arguments.length, args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
if (Array.isArray(args[0])) {
args[0].unshift(this);
} else {
args.unshift(this);
}
return combineLatest.apply(this, args);
};
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever any of the observable sequences or Promises produces an element.
*
* @example
* 1 - obs = Rx.Observable.combineLatest(obs1, obs2, obs3, function (o1, o2, o3) { return o1 + o2 + o3; });
* 2 - obs = Rx.Observable.combineLatest([obs1, obs2, obs3], function (o1, o2, o3) { return o1 + o2 + o3; });
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
var combineLatest = Observable.combineLatest = function () {
var len = arguments.length, args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
var resultSelector = args.pop();
len--;
Array.isArray(args[0]) && (args = args[0]);
return new AnonymousObservable(function (o) {
var falseFactory = function () { return false; },
hasValue = arrayInitialize(len, falseFactory),
hasValueAll = false,
isDone = arrayInitialize(len, falseFactory),
values = new Array(len);
function next(i) {
hasValue[i] = true;
if (hasValueAll || (hasValueAll = hasValue.every(identity))) {
try {
var res = resultSelector.apply(null, values);
} catch (ex) {
o.onError(ex);
return;
}
o.onNext(res);
} else if (isDone.filter(function (x, j) { return j !== i; }).every(identity)) {
o.onCompleted();
}
}
function done (i) {
isDone[i] = true;
isDone.every(identity) && o.onCompleted();
}
var subscriptions = new Array(len);
for (var idx = 0; idx < len; idx++) {
(function (i) {
var source = args[i], sad = new SingleAssignmentDisposable();
isPromise(source) && (source = observableFromPromise(source));
sad.setDisposable(source.subscribe(function (x) {
values[i] = x;
next(i);
},
function(e) { o.onError(e); },
function () { done(i); }
));
subscriptions[i] = sad;
}(idx));
}
return new CompositeDisposable(subscriptions);
}, this);
};
/**
* Concatenates all the observable sequences. This takes in either an array or variable arguments to concatenate.
* @returns {Observable} An observable sequence that contains the elements of each given sequence, in sequential order.
*/
observableProto.concat = function () {
for(var args = [], i = 0, len = arguments.length; i < len; i++) { args.push(arguments[i]); }
args.unshift(this);
return observableConcat.apply(null, args);
};
/**
* Concatenates all the observable sequences.
* @param {Array | Arguments} args Arguments or an array to concat to the observable sequence.
* @returns {Observable} An observable sequence that contains the elements of each given sequence, in sequential order.
*/
var observableConcat = Observable.concat = function () {
var args;
if (Array.isArray(arguments[0])) {
args = arguments[0];
} else {
args = new Array(arguments.length);
for(var i = 0, len = arguments.length; i < len; i++) { args[i] = arguments[i]; }
}
return enumerableOf(args).concat();
};
/**
* Concatenates an observable sequence of observable sequences.
* @returns {Observable} An observable sequence that contains the elements of each observed inner sequence, in sequential order.
*/
observableProto.concatAll = observableProto.concatObservable = function () {
return this.merge(1);
};
var MergeObservable = (function (__super__) {
inherits(MergeObservable, __super__);
function MergeObservable(source, maxConcurrent) {
this.source = source;
this.maxConcurrent = maxConcurrent;
__super__.call(this);
}
MergeObservable.prototype.subscribeCore = function(observer) {
var g = new CompositeDisposable();
g.add(this.source.subscribe(new MergeObserver(observer, this.maxConcurrent, g)));
return g;
};
return MergeObservable;
}(ObservableBase));
var MergeObserver = (function () {
function MergeObserver(o, max, g) {
this.o = o;
this.max = max;
this.g = g;
this.done = false;
this.q = [];
this.activeCount = 0;
this.isStopped = false;
}
MergeObserver.prototype.handleSubscribe = function (xs) {
var sad = new SingleAssignmentDisposable();
this.g.add(sad);
isPromise(xs) && (xs = observableFromPromise(xs));
sad.setDisposable(xs.subscribe(new InnerObserver(this, sad)));
};
MergeObserver.prototype.onNext = function (innerSource) {
if (this.isStopped) { return; }
if(this.activeCount < this.max) {
this.activeCount++;
this.handleSubscribe(innerSource);
} else {
this.q.push(innerSource);
}
};
MergeObserver.prototype.onError = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.o.onError(e);
}
};
MergeObserver.prototype.onCompleted = function () {
if (!this.isStopped) {
this.isStopped = true;
this.done = true;
this.activeCount === 0 && this.o.onCompleted();
}
};
MergeObserver.prototype.dispose = function() { this.isStopped = true; };
MergeObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.o.onError(e);
return true;
}
return false;
};
function InnerObserver(parent, sad) {
this.parent = parent;
this.sad = sad;
this.isStopped = false;
}
InnerObserver.prototype.onNext = function (x) { if(!this.isStopped) { this.parent.o.onNext(x); } };
InnerObserver.prototype.onError = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.parent.o.onError(e);
}
};
InnerObserver.prototype.onCompleted = function () {
if(!this.isStopped) {
var parent = this.parent;
parent.g.remove(this.sad);
if (parent.q.length > 0) {
parent.handleSubscribe(parent.q.shift());
} else {
parent.activeCount--;
parent.done && parent.activeCount === 0 && parent.o.onCompleted();
}
}
};
InnerObserver.prototype.dispose = function() { this.isStopped = true; };
InnerObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.parent.o.onError(e);
return true;
}
return false;
};
return MergeObserver;
}());
/**
* Merges an observable sequence of observable sequences into an observable sequence, limiting the number of concurrent subscriptions to inner sequences.
* Or merges two observable sequences into a single observable sequence.
*
* @example
* 1 - merged = sources.merge(1);
* 2 - merged = source.merge(otherSource);
* @param {Mixed} [maxConcurrentOrOther] Maximum number of inner observable sequences being subscribed to concurrently or the second observable sequence.
* @returns {Observable} The observable sequence that merges the elements of the inner sequences.
*/
observableProto.merge = function (maxConcurrentOrOther) {
return typeof maxConcurrentOrOther !== 'number' ?
observableMerge(this, maxConcurrentOrOther) :
new MergeObservable(this, maxConcurrentOrOther);
};
/**
* Merges all the observable sequences into a single observable sequence.
* The scheduler is optional and if not specified, the immediate scheduler is used.
* @returns {Observable} The observable sequence that merges the elements of the observable sequences.
*/
var observableMerge = Observable.merge = function () {
var scheduler, sources = [], i, len = arguments.length;
if (!arguments[0]) {
scheduler = immediateScheduler;
for(i = 1; i < len; i++) { sources.push(arguments[i]); }
} else if (isScheduler(arguments[0])) {
scheduler = arguments[0];
for(i = 1; i < len; i++) { sources.push(arguments[i]); }
} else {
scheduler = immediateScheduler;
for(i = 0; i < len; i++) { sources.push(arguments[i]); }
}
if (Array.isArray(sources[0])) {
sources = sources[0];
}
return observableOf(scheduler, sources).mergeAll();
};
var MergeAllObservable = (function (__super__) {
inherits(MergeAllObservable, __super__);
function MergeAllObservable(source) {
this.source = source;
__super__.call(this);
}
MergeAllObservable.prototype.subscribeCore = function (observer) {
var g = new CompositeDisposable(), m = new SingleAssignmentDisposable();
g.add(m);
m.setDisposable(this.source.subscribe(new MergeAllObserver(observer, g)));
return g;
};
return MergeAllObservable;
}(ObservableBase));
var MergeAllObserver = (function() {
function MergeAllObserver(o, g) {
this.o = o;
this.g = g;
this.isStopped = false;
this.done = false;
}
MergeAllObserver.prototype.onNext = function(innerSource) {
if(this.isStopped) { return; }
var sad = new SingleAssignmentDisposable();
this.g.add(sad);
isPromise(innerSource) && (innerSource = observableFromPromise(innerSource));
sad.setDisposable(innerSource.subscribe(new InnerObserver(this, this.g, sad)));
};
MergeAllObserver.prototype.onError = function (e) {
if(!this.isStopped) {
this.isStopped = true;
this.o.onError(e);
}
};
MergeAllObserver.prototype.onCompleted = function () {
if(!this.isStopped) {
this.isStopped = true;
this.done = true;
this.g.length === 1 && this.o.onCompleted();
}
};
MergeAllObserver.prototype.dispose = function() { this.isStopped = true; };
MergeAllObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.o.onError(e);
return true;
}
return false;
};
function InnerObserver(parent, g, sad) {
this.parent = parent;
this.g = g;
this.sad = sad;
this.isStopped = false;
}
InnerObserver.prototype.onNext = function (x) { if (!this.isStopped) { this.parent.o.onNext(x); } };
InnerObserver.prototype.onError = function (e) {
if(!this.isStopped) {
this.isStopped = true;
this.parent.o.onError(e);
}
};
InnerObserver.prototype.onCompleted = function () {
if(!this.isStopped) {
var parent = this.parent;
this.isStopped = true;
parent.g.remove(this.sad);
parent.done && parent.g.length === 1 && parent.o.onCompleted();
}
};
InnerObserver.prototype.dispose = function() { this.isStopped = true; };
InnerObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.parent.o.onError(e);
return true;
}
return false;
};
return MergeAllObserver;
}());
/**
* Merges an observable sequence of observable sequences into an observable sequence.
* @returns {Observable} The observable sequence that merges the elements of the inner sequences.
*/
observableProto.mergeAll = observableProto.mergeObservable = function () {
return new MergeAllObservable(this);
};
var CompositeError = Rx.CompositeError = function(errors) {
this.name = "NotImplementedError";
this.innerErrors = errors;
this.message = 'This contains multiple errors. Check the innerErrors';
Error.call(this);
}
CompositeError.prototype = Error.prototype;
/**
* Flattens an Observable that emits Observables into one Observable, in a way that allows an Observer to
* receive all successfully emitted items from all of the source Observables without being interrupted by
* an error notification from one of them.
*
* This behaves like Observable.prototype.mergeAll except that if any of the merged Observables notify of an
* error via the Observer's onError, mergeDelayError will refrain from propagating that
* error notification until all of the merged Observables have finished emitting items.
* @param {Array | Arguments} args Arguments or an array to merge.
* @returns {Observable} an Observable that emits all of the items emitted by the Observables emitted by the Observable
*/
Observable.mergeDelayError = function() {
var args;
if (Array.isArray(arguments[0])) {
args = arguments[0];
} else {
var len = arguments.length;
args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
}
var source = observableOf(null, args);
return new AnonymousObservable(function (o) {
var group = new CompositeDisposable(),
m = new SingleAssignmentDisposable(),
isStopped = false,
errors = [];
function setCompletion() {
if (errors.length === 0) {
o.onCompleted();
} else if (errors.length === 1) {
o.onError(errors[0]);
} else {
o.onError(new CompositeError(errors));
}
}
group.add(m);
m.setDisposable(source.subscribe(
function (innerSource) {
var innerSubscription = new SingleAssignmentDisposable();
group.add(innerSubscription);
// Check for promises support
isPromise(innerSource) && (innerSource = observableFromPromise(innerSource));
innerSubscription.setDisposable(innerSource.subscribe(
function (x) { o.onNext(x); },
function (e) {
errors.push(e);
group.remove(innerSubscription);
isStopped && group.length === 1 && setCompletion();
},
function () {
group.remove(innerSubscription);
isStopped && group.length === 1 && setCompletion();
}));
},
function (e) {
errors.push(e);
isStopped = true;
group.length === 1 && setCompletion();
},
function () {
isStopped = true;
group.length === 1 && setCompletion();
}));
return group;
});
};
/**
* Returns the values from the source observable sequence only after the other observable sequence produces a value.
* @param {Observable | Promise} other The observable sequence or Promise that triggers propagation of elements of the source sequence.
* @returns {Observable} An observable sequence containing the elements of the source sequence starting from the point the other sequence triggered propagation.
*/
observableProto.skipUntil = function (other) {
var source = this;
return new AnonymousObservable(function (o) {
var isOpen = false;
var disposables = new CompositeDisposable(source.subscribe(function (left) {
isOpen && o.onNext(left);
}, function (e) { o.onError(e); }, function () {
isOpen && o.onCompleted();
}));
isPromise(other) && (other = observableFromPromise(other));
var rightSubscription = new SingleAssignmentDisposable();
disposables.add(rightSubscription);
rightSubscription.setDisposable(other.subscribe(function () {
isOpen = true;
rightSubscription.dispose();
}, function (e) { o.onError(e); }, function () {
rightSubscription.dispose();
}));
return disposables;
}, source);
};
/**
* Transforms an observable sequence of observable sequences into an observable sequence producing values only from the most recent observable sequence.
* @returns {Observable} The observable sequence that at any point in time produces the elements of the most recent inner observable sequence that has been received.
*/
observableProto['switch'] = observableProto.switchLatest = function () {
var sources = this;
return new AnonymousObservable(function (observer) {
var hasLatest = false,
innerSubscription = new SerialDisposable(),
isStopped = false,
latest = 0,
subscription = sources.subscribe(
function (innerSource) {
var d = new SingleAssignmentDisposable(), id = ++latest;
hasLatest = true;
innerSubscription.setDisposable(d);
// Check if Promise or Observable
isPromise(innerSource) && (innerSource = observableFromPromise(innerSource));
d.setDisposable(innerSource.subscribe(
function (x) { latest === id && observer.onNext(x); },
function (e) { latest === id && observer.onError(e); },
function () {
if (latest === id) {
hasLatest = false;
isStopped && observer.onCompleted();
}
}));
},
function (e) { observer.onError(e); },
function () {
isStopped = true;
!hasLatest && observer.onCompleted();
});
return new CompositeDisposable(subscription, innerSubscription);
}, sources);
};
/**
* Returns the values from the source observable sequence until the other observable sequence produces a value.
* @param {Observable | Promise} other Observable sequence or Promise that terminates propagation of elements of the source sequence.
* @returns {Observable} An observable sequence containing the elements of the source sequence up to the point the other sequence interrupted further propagation.
*/
observableProto.takeUntil = function (other) {
var source = this;
return new AnonymousObservable(function (o) {
isPromise(other) && (other = observableFromPromise(other));
return new CompositeDisposable(
source.subscribe(o),
other.subscribe(function () { o.onCompleted(); }, function (e) { o.onError(e); }, noop)
);
}, source);
};
/**
* Merges the specified observable sequences into one observable sequence by using the selector function only when the (first) source observable sequence produces an element.
*
* @example
* 1 - obs = obs1.withLatestFrom(obs2, obs3, function (o1, o2, o3) { return o1 + o2 + o3; });
* 2 - obs = obs1.withLatestFrom([obs2, obs3], function (o1, o2, o3) { return o1 + o2 + o3; });
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
observableProto.withLatestFrom = function () {
for(var args = [], i = 0, len = arguments.length; i < len; i++) { args.push(arguments[i]); }
var resultSelector = args.pop(), source = this;
if (typeof source === 'undefined') {
throw new Error('Source observable not found for withLatestFrom().');
}
if (typeof resultSelector !== 'function') {
throw new Error('withLatestFrom() expects a resultSelector function.');
}
if (Array.isArray(args[0])) {
args = args[0];
}
return new AnonymousObservable(function (observer) {
var falseFactory = function () { return false; },
n = args.length,
hasValue = arrayInitialize(n, falseFactory),
hasValueAll = false,
values = new Array(n);
var subscriptions = new Array(n + 1);
for (var idx = 0; idx < n; idx++) {
(function (i) {
var other = args[i], sad = new SingleAssignmentDisposable();
isPromise(other) && (other = observableFromPromise(other));
sad.setDisposable(other.subscribe(function (x) {
values[i] = x;
hasValue[i] = true;
hasValueAll = hasValue.every(identity);
}, observer.onError.bind(observer), function () {}));
subscriptions[i] = sad;
}(idx));
}
var sad = new SingleAssignmentDisposable();
sad.setDisposable(source.subscribe(function (x) {
var res;
var allValues = [x].concat(values);
if (!hasValueAll) return;
try {
res = resultSelector.apply(null, allValues);
} catch (ex) {
observer.onError(ex);
return;
}
observer.onNext(res);
}, observer.onError.bind(observer), function () {
observer.onCompleted();
}));
subscriptions[n] = sad;
return new CompositeDisposable(subscriptions);
}, this);
};
function zipArray(second, resultSelector) {
var first = this;
return new AnonymousObservable(function (observer) {
var index = 0, len = second.length;
return first.subscribe(function (left) {
if (index < len) {
var right = second[index++], result;
try {
result = resultSelector(left, right);
} catch (e) {
return observer.onError(e);
}
observer.onNext(result);
} else {
observer.onCompleted();
}
}, function (e) { observer.onError(e); }, function () { observer.onCompleted(); });
}, first);
}
function falseFactory() { return false; }
function emptyArrayFactory() { return []; }
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever all of the observable sequences or an array have produced an element at a corresponding index.
* The last element in the arguments must be a function to invoke for each series of elements at corresponding indexes in the args.
*
* @example
* 1 - res = obs1.zip(obs2, fn);
* 1 - res = x1.zip([1,2,3], fn);
* @returns {Observable} An observable sequence containing the result of combining elements of the args using the specified result selector function.
*/
observableProto.zip = function () {
if (Array.isArray(arguments[0])) { return zipArray.apply(this, arguments); }
var len = arguments.length, args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
var parent = this, resultSelector = args.pop();
args.unshift(parent);
return new AnonymousObservable(function (observer) {
var n = args.length,
queues = arrayInitialize(n, emptyArrayFactory),
isDone = arrayInitialize(n, falseFactory);
function next(i) {
var res, queuedValues;
if (queues.every(function (x) { return x.length > 0; })) {
try {
queuedValues = queues.map(function (x) { return x.shift(); });
res = resultSelector.apply(parent, queuedValues);
} catch (ex) {
observer.onError(ex);
return;
}
observer.onNext(res);
} else if (isDone.filter(function (x, j) { return j !== i; }).every(identity)) {
observer.onCompleted();
}
};
function done(i) {
isDone[i] = true;
if (isDone.every(function (x) { return x; })) {
observer.onCompleted();
}
}
var subscriptions = new Array(n);
for (var idx = 0; idx < n; idx++) {
(function (i) {
var source = args[i], sad = new SingleAssignmentDisposable();
isPromise(source) && (source = observableFromPromise(source));
sad.setDisposable(source.subscribe(function (x) {
queues[i].push(x);
next(i);
}, function (e) { observer.onError(e); }, function () {
done(i);
}));
subscriptions[i] = sad;
})(idx);
}
return new CompositeDisposable(subscriptions);
}, parent);
};
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever all of the observable sequences have produced an element at a corresponding index.
* @param arguments Observable sources.
* @param {Function} resultSelector Function to invoke for each series of elements at corresponding indexes in the sources.
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
Observable.zip = function () {
var len = arguments.length, args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
var first = args.shift();
return first.zip.apply(first, args);
};
/**
* Merges the specified observable sequences into one observable sequence by emitting a list with the elements of the observable sequences at corresponding indexes.
* @param arguments Observable sources.
* @returns {Observable} An observable sequence containing lists of elements at corresponding indexes.
*/
Observable.zipArray = function () {
var sources;
if (Array.isArray(arguments[0])) {
sources = arguments[0];
} else {
var len = arguments.length;
sources = new Array(len);
for(var i = 0; i < len; i++) { sources[i] = arguments[i]; }
}
return new AnonymousObservable(function (observer) {
var n = sources.length,
queues = arrayInitialize(n, function () { return []; }),
isDone = arrayInitialize(n, function () { return false; });
function next(i) {
if (queues.every(function (x) { return x.length > 0; })) {
var res = queues.map(function (x) { return x.shift(); });
observer.onNext(res);
} else if (isDone.filter(function (x, j) { return j !== i; }).every(identity)) {
observer.onCompleted();
return;
}
};
function done(i) {
isDone[i] = true;
if (isDone.every(identity)) {
observer.onCompleted();
return;
}
}
var subscriptions = new Array(n);
for (var idx = 0; idx < n; idx++) {
(function (i) {
subscriptions[i] = new SingleAssignmentDisposable();
subscriptions[i].setDisposable(sources[i].subscribe(function (x) {
queues[i].push(x);
next(i);
}, function (e) { observer.onError(e); }, function () {
done(i);
}));
})(idx);
}
return new CompositeDisposable(subscriptions);
});
};
/**
* Hides the identity of an observable sequence.
* @returns {Observable} An observable sequence that hides the identity of the source sequence.
*/
observableProto.asObservable = function () {
var source = this;
return new AnonymousObservable(function (o) { return source.subscribe(o); }, this);
};
/**
* Dematerializes the explicit notification values of an observable sequence as implicit notifications.
* @returns {Observable} An observable sequence exhibiting the behavior corresponding to the source sequence's notification values.
*/
observableProto.dematerialize = function () {
var source = this;
return new AnonymousObservable(function (o) {
return source.subscribe(function (x) { return x.accept(o); }, function(e) { o.onError(e); }, function () { o.onCompleted(); });
}, this);
};
/**
* Returns an observable sequence that contains only distinct contiguous elements according to the keySelector and the comparer.
*
* var obs = observable.distinctUntilChanged();
* var obs = observable.distinctUntilChanged(function (x) { return x.id; });
* var obs = observable.distinctUntilChanged(function (x) { return x.id; }, function (x, y) { return x === y; });
*
* @param {Function} [keySelector] A function to compute the comparison key for each element. If not provided, it projects the value.
* @param {Function} [comparer] Equality comparer for computed key values. If not provided, defaults to an equality comparer function.
* @returns {Observable} An observable sequence only containing the distinct contiguous elements, based on a computed key value, from the source sequence.
*/
observableProto.distinctUntilChanged = function (keySelector, comparer) {
var source = this;
comparer || (comparer = defaultComparer);
return new AnonymousObservable(function (o) {
var hasCurrentKey = false, currentKey;
return source.subscribe(function (value) {
var key = value;
if (keySelector) {
try {
key = keySelector(value);
} catch (e) {
o.onError(e);
return;
}
}
if (hasCurrentKey) {
try {
var comparerEquals = comparer(currentKey, key);
} catch (e) {
o.onError(e);
return;
}
}
if (!hasCurrentKey || !comparerEquals) {
hasCurrentKey = true;
currentKey = key;
o.onNext(value);
}
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, this);
};
/**
* Invokes an action for each element in the observable sequence and invokes an action upon graceful or exceptional termination of the observable sequence.
* This method can be used for debugging, logging, etc. of query behavior by intercepting the message stream to run arbitrary actions for messages on the pipeline.
* @param {Function | Observer} observerOrOnNext Action to invoke for each element in the observable sequence or an observer.
* @param {Function} [onError] Action to invoke upon exceptional termination of the observable sequence. Used if only the observerOrOnNext parameter is also a function.
* @param {Function} [onCompleted] Action to invoke upon graceful termination of the observable sequence. Used if only the observerOrOnNext parameter is also a function.
* @returns {Observable} The source sequence with the side-effecting behavior applied.
*/
observableProto['do'] = observableProto.tap = observableProto.doAction = function (observerOrOnNext, onError, onCompleted) {
var source = this, tapObserver = typeof observerOrOnNext === 'function' || typeof observerOrOnNext === 'undefined'?
observerCreate(observerOrOnNext || noop, onError || noop, onCompleted || noop) :
observerOrOnNext;
return new AnonymousObservable(function (observer) {
return source.subscribe(function (x) {
try {
tapObserver.onNext(x);
} catch (e) {
observer.onError(e);
}
observer.onNext(x);
}, function (err) {
try {
tapObserver.onError(err);
} catch (e) {
observer.onError(e);
}
observer.onError(err);
}, function () {
try {
tapObserver.onCompleted();
} catch (e) {
observer.onError(e);
}
observer.onCompleted();
});
}, this);
};
/**
* Invokes an action for each element in the observable sequence.
* This method can be used for debugging, logging, etc. of query behavior by intercepting the message stream to run arbitrary actions for messages on the pipeline.
* @param {Function} onNext Action to invoke for each element in the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} The source sequence with the side-effecting behavior applied.
*/
observableProto.doOnNext = observableProto.tapOnNext = function (onNext, thisArg) {
return this.tap(typeof thisArg !== 'undefined' ? function (x) { onNext.call(thisArg, x); } : onNext);
};
/**
* Invokes an action upon exceptional termination of the observable sequence.
* This method can be used for debugging, logging, etc. of query behavior by intercepting the message stream to run arbitrary actions for messages on the pipeline.
* @param {Function} onError Action to invoke upon exceptional termination of the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} The source sequence with the side-effecting behavior applied.
*/
observableProto.doOnError = observableProto.tapOnError = function (onError, thisArg) {
return this.tap(noop, typeof thisArg !== 'undefined' ? function (e) { onError.call(thisArg, e); } : onError);
};
/**
* Invokes an action upon graceful termination of the observable sequence.
* This method can be used for debugging, logging, etc. of query behavior by intercepting the message stream to run arbitrary actions for messages on the pipeline.
* @param {Function} onCompleted Action to invoke upon graceful termination of the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} The source sequence with the side-effecting behavior applied.
*/
observableProto.doOnCompleted = observableProto.tapOnCompleted = function (onCompleted, thisArg) {
return this.tap(noop, null, typeof thisArg !== 'undefined' ? function () { onCompleted.call(thisArg); } : onCompleted);
};
/**
* Invokes a specified action after the source observable sequence terminates gracefully or exceptionally.
* @param {Function} finallyAction Action to invoke after the source observable sequence terminates.
* @returns {Observable} Source sequence with the action-invoking termination behavior applied.
*/
observableProto['finally'] = observableProto.ensure = function (action) {
var source = this;
return new AnonymousObservable(function (observer) {
var subscription;
try {
subscription = source.subscribe(observer);
} catch (e) {
action();
throw e;
}
return disposableCreate(function () {
try {
subscription.dispose();
} catch (e) {
throw e;
} finally {
action();
}
});
}, this);
};
/**
* @deprecated use #finally or #ensure instead.
*/
observableProto.finallyAction = function (action) {
//deprecate('finallyAction', 'finally or ensure');
return this.ensure(action);
};
/**
* Ignores all elements in an observable sequence leaving only the termination messages.
* @returns {Observable} An empty observable sequence that signals termination, successful or exceptional, of the source sequence.
*/
observableProto.ignoreElements = function () {
var source = this;
return new AnonymousObservable(function (o) {
return source.subscribe(noop, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
/**
* Materializes the implicit notifications of an observable sequence as explicit notification values.
* @returns {Observable} An observable sequence containing the materialized notification values from the source sequence.
*/
observableProto.materialize = function () {
var source = this;
return new AnonymousObservable(function (observer) {
return source.subscribe(function (value) {
observer.onNext(notificationCreateOnNext(value));
}, function (e) {
observer.onNext(notificationCreateOnError(e));
observer.onCompleted();
}, function () {
observer.onNext(notificationCreateOnCompleted());
observer.onCompleted();
});
}, source);
};
/**
* Repeats the observable sequence a specified number of times. If the repeat count is not specified, the sequence repeats indefinitely.
* @param {Number} [repeatCount] Number of times to repeat the sequence. If not provided, repeats the sequence indefinitely.
* @returns {Observable} The observable sequence producing the elements of the given sequence repeatedly.
*/
observableProto.repeat = function (repeatCount) {
return enumerableRepeat(this, repeatCount).concat();
};
/**
* Repeats the source observable sequence the specified number of times or until it successfully terminates. If the retry count is not specified, it retries indefinitely.
* Note if you encounter an error and want it to retry once, then you must use .retry(2);
*
* @example
* var res = retried = retry.repeat();
* var res = retried = retry.repeat(2);
* @param {Number} [retryCount] Number of times to retry the sequence. If not provided, retry the sequence indefinitely.
* @returns {Observable} An observable sequence producing the elements of the given sequence repeatedly until it terminates successfully.
*/
observableProto.retry = function (retryCount) {
return enumerableRepeat(this, retryCount).catchError();
};
/**
* Repeats the source observable sequence upon error each time the notifier emits or until it successfully terminates.
* if the notifier completes, the observable sequence completes.
*
* @example
* var timer = Observable.timer(500);
* var source = observable.retryWhen(timer);
* @param {Observable} [notifier] An observable that triggers the retries or completes the observable with onNext or onCompleted respectively.
* @returns {Observable} An observable sequence producing the elements of the given sequence repeatedly until it terminates successfully.
*/
observableProto.retryWhen = function (notifier) {
return enumerableRepeat(this).catchErrorWhen(notifier);
};
/**
* Applies an accumulator function over an observable sequence and returns each intermediate result. The optional seed value is used as the initial accumulator value.
* For aggregation behavior with no intermediate results, see Observable.aggregate.
* @example
* var res = source.scan(function (acc, x) { return acc + x; });
* var res = source.scan(0, function (acc, x) { return acc + x; });
* @param {Mixed} [seed] The initial accumulator value.
* @param {Function} accumulator An accumulator function to be invoked on each element.
* @returns {Observable} An observable sequence containing the accumulated values.
*/
observableProto.scan = function () {
var hasSeed = false, seed, accumulator, source = this;
if (arguments.length === 2) {
hasSeed = true;
seed = arguments[0];
accumulator = arguments[1];
} else {
accumulator = arguments[0];
}
return new AnonymousObservable(function (o) {
var hasAccumulation, accumulation, hasValue;
return source.subscribe (
function (x) {
!hasValue && (hasValue = true);
try {
if (hasAccumulation) {
accumulation = accumulator(accumulation, x);
} else {
accumulation = hasSeed ? accumulator(seed, x) : x;
hasAccumulation = true;
}
} catch (e) {
o.onError(e);
return;
}
o.onNext(accumulation);
},
function (e) { o.onError(e); },
function () {
!hasValue && hasSeed && o.onNext(seed);
o.onCompleted();
}
);
}, source);
};
/**
* Bypasses a specified number of elements at the end of an observable sequence.
* @description
* This operator accumulates a queue with a length enough to store the first `count` elements. As more elements are
* received, elements are taken from the front of the queue and produced on the result sequence. This causes elements to be delayed.
* @param count Number of elements to bypass at the end of the source sequence.
* @returns {Observable} An observable sequence containing the source sequence elements except for the bypassed ones at the end.
*/
observableProto.skipLast = function (count) {
var source = this;
return new AnonymousObservable(function (o) {
var q = [];
return source.subscribe(function (x) {
q.push(x);
q.length > count && o.onNext(q.shift());
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
/**
* Prepends a sequence of values to an observable sequence with an optional scheduler and an argument list of values to prepend.
* @example
* var res = source.startWith(1, 2, 3);
* var res = source.startWith(Rx.Scheduler.timeout, 1, 2, 3);
* @param {Arguments} args The specified values to prepend to the observable sequence
* @returns {Observable} The source sequence prepended with the specified values.
*/
observableProto.startWith = function () {
var values, scheduler, start = 0;
if (!!arguments.length && isScheduler(arguments[0])) {
scheduler = arguments[0];
start = 1;
} else {
scheduler = immediateScheduler;
}
for(var args = [], i = start, len = arguments.length; i < len; i++) { args.push(arguments[i]); }
return enumerableOf([observableFromArray(args, scheduler), this]).concat();
};
/**
* Returns a specified number of contiguous elements from the end of an observable sequence.
* @description
* This operator accumulates a buffer with a length enough to store elements count elements. Upon completion of
* the source sequence, this buffer is drained on the result sequence. This causes the elements to be delayed.
* @param {Number} count Number of elements to take from the end of the source sequence.
* @returns {Observable} An observable sequence containing the specified number of elements from the end of the source sequence.
*/
observableProto.takeLast = function (count) {
var source = this;
return new AnonymousObservable(function (o) {
var q = [];
return source.subscribe(function (x) {
q.push(x);
q.length > count && q.shift();
}, function (e) { o.onError(e); }, function () {
while (q.length > 0) { o.onNext(q.shift()); }
o.onCompleted();
});
}, source);
};
function concatMap(source, selector, thisArg) {
var selectorFunc = bindCallback(selector, thisArg, 3);
return source.map(function (x, i) {
var result = selectorFunc(x, i, source);
isPromise(result) && (result = observableFromPromise(result));
(isArrayLike(result) || isIterable(result)) && (result = observableFrom(result));
return result;
}).concatAll();
}
/**
* One of the Following:
* Projects each element of an observable sequence to an observable sequence and merges the resulting observable sequences into one observable sequence.
*
* @example
* var res = source.concatMap(function (x) { return Rx.Observable.range(0, x); });
* Or:
* Projects each element of an observable sequence to an observable sequence, invokes the result selector for the source element and each of the corresponding inner sequence's elements, and merges the results into one observable sequence.
*
* var res = source.concatMap(function (x) { return Rx.Observable.range(0, x); }, function (x, y) { return x + y; });
* Or:
* Projects each element of the source observable sequence to the other observable sequence and merges the resulting observable sequences into one observable sequence.
*
* var res = source.concatMap(Rx.Observable.fromArray([1,2,3]));
* @param {Function} selector A transform function to apply to each element or an observable sequence to project each element from the
* source sequence onto which could be either an observable or Promise.
* @param {Function} [resultSelector] A transform function to apply to each element of the intermediate sequence.
* @returns {Observable} An observable sequence whose elements are the result of invoking the one-to-many transform function collectionSelector on each element of the input sequence and then mapping each of those sequence elements and their corresponding source element to a result element.
*/
observableProto.selectConcat = observableProto.concatMap = function (selector, resultSelector, thisArg) {
if (isFunction(selector) && isFunction(resultSelector)) {
return this.concatMap(function (x, i) {
var selectorResult = selector(x, i);
isPromise(selectorResult) && (selectorResult = observableFromPromise(selectorResult));
(isArrayLike(selectorResult) || isIterable(selectorResult)) && (selectorResult = observableFrom(selectorResult));
return selectorResult.map(function (y, i2) {
return resultSelector(x, y, i, i2);
});
});
}
return isFunction(selector) ?
concatMap(this, selector, thisArg) :
concatMap(this, function () { return selector; });
};
var MapObservable = (function (__super__) {
inherits(MapObservable, __super__);
function MapObservable(source, selector, thisArg) {
this.source = source;
this.selector = bindCallback(selector, thisArg, 3);
__super__.call(this);
}
MapObservable.prototype.internalMap = function (selector, thisArg) {
var self = this;
return new MapObservable(this.source, function (x, i, o) { return selector(self.selector(x, i, o), i, o); }, thisArg)
};
MapObservable.prototype.subscribeCore = function (observer) {
return this.source.subscribe(new MapObserver(observer, this.selector, this));
};
return MapObservable;
}(ObservableBase));
function MapObserver(observer, selector, source) {
this.observer = observer;
this.selector = selector;
this.source = source;
this.i = 0;
this.isStopped = false;
}
MapObserver.prototype.onNext = function(x) {
if (this.isStopped) { return; }
var result = tryCatch(this.selector).call(this, x, this.i++, this.source);
if (result === errorObj) {
return this.observer.onError(result.e);
}
this.observer.onNext(result);
/*try {
var result = this.selector(x, this.i++, this.source);
} catch (e) {
return this.observer.onError(e);
}
this.observer.onNext(result);*/
};
MapObserver.prototype.onError = function (e) {
if(!this.isStopped) { this.isStopped = true; this.observer.onError(e); }
};
MapObserver.prototype.onCompleted = function () {
if(!this.isStopped) { this.isStopped = true; this.observer.onCompleted(); }
};
MapObserver.prototype.dispose = function() { this.isStopped = true; };
MapObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.observer.onError(e);
return true;
}
return false;
};
/**
* Projects each element of an observable sequence into a new form by incorporating the element's index.
* @param {Function} selector A transform function to apply to each source element; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence whose elements are the result of invoking the transform function on each element of source.
*/
observableProto.map = observableProto.select = function (selector, thisArg) {
var selectorFn = typeof selector === 'function' ? selector : function () { return selector; };
return this instanceof MapObservable ?
this.internalMap(selectorFn, thisArg) :
new MapObservable(this, selectorFn, thisArg);
};
/**
* Retrieves the value of a specified nested property from all elements in
* the Observable sequence.
* @param {Arguments} arguments The nested properties to pluck.
* @returns {Observable} Returns a new Observable sequence of property values.
*/
observableProto.pluck = function () {
var args = arguments, len = arguments.length;
if (len === 0) { throw new Error('List of properties cannot be empty.'); }
return this.map(function (x) {
var currentProp = x;
for (var i = 0; i < len; i++) {
var p = currentProp[args[i]];
if (typeof p !== 'undefined') {
currentProp = p;
} else {
return undefined;
}
}
return currentProp;
});
};
function flatMap(source, selector, thisArg) {
var selectorFunc = bindCallback(selector, thisArg, 3);
return source.map(function (x, i) {
var result = selectorFunc(x, i, source);
isPromise(result) && (result = observableFromPromise(result));
(isArrayLike(result) || isIterable(result)) && (result = observableFrom(result));
return result;
}).mergeAll();
}
/**
* One of the Following:
* Projects each element of an observable sequence to an observable sequence and merges the resulting observable sequences into one observable sequence.
*
* @example
* var res = source.selectMany(function (x) { return Rx.Observable.range(0, x); });
* Or:
* Projects each element of an observable sequence to an observable sequence, invokes the result selector for the source element and each of the corresponding inner sequence's elements, and merges the results into one observable sequence.
*
* var res = source.selectMany(function (x) { return Rx.Observable.range(0, x); }, function (x, y) { return x + y; });
* Or:
* Projects each element of the source observable sequence to the other observable sequence and merges the resulting observable sequences into one observable sequence.
*
* var res = source.selectMany(Rx.Observable.fromArray([1,2,3]));
* @param {Function} selector A transform function to apply to each element or an observable sequence to project each element from the source sequence onto which could be either an observable or Promise.
* @param {Function} [resultSelector] A transform function to apply to each element of the intermediate sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence whose elements are the result of invoking the one-to-many transform function collectionSelector on each element of the input sequence and then mapping each of those sequence elements and their corresponding source element to a result element.
*/
observableProto.selectMany = observableProto.flatMap = function (selector, resultSelector, thisArg) {
if (isFunction(selector) && isFunction(resultSelector)) {
return this.flatMap(function (x, i) {
var selectorResult = selector(x, i);
isPromise(selectorResult) && (selectorResult = observableFromPromise(selectorResult));
(isArrayLike(selectorResult) || isIterable(selectorResult)) && (selectorResult = observableFrom(selectorResult));
return selectorResult.map(function (y, i2) {
return resultSelector(x, y, i, i2);
});
}, thisArg);
}
return isFunction(selector) ?
flatMap(this, selector, thisArg) :
flatMap(this, function () { return selector; });
};
/**
* Projects each element of an observable sequence into a new sequence of observable sequences by incorporating the element's index and then
* transforms an observable sequence of observable sequences into an observable sequence producing values only from the most recent observable sequence.
* @param {Function} selector A transform function to apply to each source element; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence whose elements are the result of invoking the transform function on each element of source producing an Observable of Observable sequences
* and that at any point in time produces the elements of the most recent inner observable sequence that has been received.
*/
observableProto.selectSwitch = observableProto.flatMapLatest = observableProto.switchMap = function (selector, thisArg) {
return this.select(selector, thisArg).switchLatest();
};
/**
* Bypasses a specified number of elements in an observable sequence and then returns the remaining elements.
* @param {Number} count The number of elements to skip before returning the remaining elements.
* @returns {Observable} An observable sequence that contains the elements that occur after the specified index in the input sequence.
*/
observableProto.skip = function (count) {
if (count < 0) { throw new Error(argumentOutOfRange); }
var source = this;
return new AnonymousObservable(function (o) {
var remaining = count;
return source.subscribe(function (x) {
if (remaining <= 0) {
o.onNext(x);
} else {
remaining--;
}
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
/**
* Bypasses elements in an observable sequence as long as a specified condition is true and then returns the remaining elements.
* The element's index is used in the logic of the predicate function.
*
* var res = source.skipWhile(function (value) { return value < 10; });
* var res = source.skipWhile(function (value, index) { return value < 10 || index < 10; });
* @param {Function} predicate A function to test each element for a condition; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence that contains the elements from the input sequence starting at the first element in the linear series that does not pass the test specified by predicate.
*/
observableProto.skipWhile = function (predicate, thisArg) {
var source = this,
callback = bindCallback(predicate, thisArg, 3);
return new AnonymousObservable(function (o) {
var i = 0, running = false;
return source.subscribe(function (x) {
if (!running) {
try {
running = !callback(x, i++, source);
} catch (e) {
o.onError(e);
return;
}
}
running && o.onNext(x);
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
/**
* Returns a specified number of contiguous elements from the start of an observable sequence, using the specified scheduler for the edge case of take(0).
*
* var res = source.take(5);
* var res = source.take(0, Rx.Scheduler.timeout);
* @param {Number} count The number of elements to return.
* @param {Scheduler} [scheduler] Scheduler used to produce an OnCompleted message in case <paramref name="count count</paramref> is set to 0.
* @returns {Observable} An observable sequence that contains the specified number of elements from the start of the input sequence.
*/
observableProto.take = function (count, scheduler) {
if (count < 0) { throw new RangeError(argumentOutOfRange); }
if (count === 0) { return observableEmpty(scheduler); }
var source = this;
return new AnonymousObservable(function (o) {
var remaining = count;
return source.subscribe(function (x) {
if (remaining-- > 0) {
o.onNext(x);
remaining === 0 && o.onCompleted();
}
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
/**
* Returns elements from an observable sequence as long as a specified condition is true.
* The element's index is used in the logic of the predicate function.
* @param {Function} predicate A function to test each element for a condition; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence that contains the elements from the input sequence that occur before the element at which the test no longer passes.
*/
observableProto.takeWhile = function (predicate, thisArg) {
var source = this,
callback = bindCallback(predicate, thisArg, 3);
return new AnonymousObservable(function (o) {
var i = 0, running = true;
return source.subscribe(function (x) {
if (running) {
try {
running = callback(x, i++, source);
} catch (e) {
o.onError(e);
return;
}
if (running) {
o.onNext(x);
} else {
o.onCompleted();
}
}
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
var FilterObservable = (function (__super__) {
inherits(FilterObservable, __super__);
function FilterObservable(source, predicate, thisArg) {
this.source = source;
this.predicate = bindCallback(predicate, thisArg, 3);
__super__.call(this);
}
FilterObservable.prototype.subscribeCore = function (observer) {
return this.source.subscribe(new FilterObserver(observer, this.predicate, this));
};
FilterObservable.prototype.internalFilter = function(predicate, thisArg) {
var self = this;
return new FilterObservable(this.source, function(x, i, o) { return self.predicate(x, i, o) && predicate(x, i, o); }, thisArg);
};
return FilterObservable;
}(ObservableBase));
function FilterObserver(observer, predicate, source) {
this.observer = observer;
this.predicate = predicate;
this.source = source;
this.i = 0;
this.isStopped = false;
}
FilterObserver.prototype.onNext = function(x) {
if (this.isStopped) { return; }
var shouldYield = tryCatch(this.predicate).call(this, x, this.i++, this.source);
if (shouldYield === errorObj) {
return this.observer.onError(shouldYield.e);
}
shouldYield && this.observer.onNext(x);
};
FilterObserver.prototype.onError = function (e) {
if(!this.isStopped) { this.isStopped = true; this.observer.onError(e); }
};
FilterObserver.prototype.onCompleted = function () {
if(!this.isStopped) { this.isStopped = true; this.observer.onCompleted(); }
};
FilterObserver.prototype.dispose = function() { this.isStopped = true; };
FilterObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.observer.onError(e);
return true;
}
return false;
};
/**
* Filters the elements of an observable sequence based on a predicate by incorporating the element's index.
* @param {Function} predicate A function to test each source element for a condition; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence that contains elements from the input sequence that satisfy the condition.
*/
observableProto.filter = observableProto.where = function (predicate, thisArg) {
return this instanceof FilterObservable ? this.internalFilter(predicate, thisArg) :
new FilterObservable(this, predicate, thisArg);
};
/**
* Converts a callback function to an observable sequence.
*
* @param {Function} function Function with a callback as the last parameter to convert to an Observable sequence.
* @param {Mixed} [context] The context for the func parameter to be executed. If not specified, defaults to undefined.
* @param {Function} [selector] A selector which takes the arguments from the callback to produce a single item to yield on next.
* @returns {Function} A function, when executed with the required parameters minus the callback, produces an Observable sequence with a single value of the arguments to the callback as an array.
*/
Observable.fromCallback = function (func, context, selector) {
return function () {
for(var args = [], i = 0, len = arguments.length; i < len; i++) { args.push(arguments[i]); }
return new AnonymousObservable(function (observer) {
function handler() {
var results = arguments;
if (selector) {
try {
results = selector(results);
} catch (err) {
return observer.onError(err);
}
observer.onNext(results);
} else {
if (results.length <= 1) {
observer.onNext.apply(observer, results);
} else {
observer.onNext(results);
}
}
observer.onCompleted();
}
args.push(handler);
func.apply(context, args);
}).publishLast().refCount();
};
};
/**
* Converts a Node.js callback style function to an observable sequence. This must be in function (err, ...) format.
* @param {Function} func The function to call
* @param {Mixed} [context] The context for the func parameter to be executed. If not specified, defaults to undefined.
* @param {Function} [selector] A selector which takes the arguments from the callback minus the error to produce a single item to yield on next.
* @returns {Function} An async function which when applied, returns an observable sequence with the callback arguments as an array.
*/
Observable.fromNodeCallback = function (func, context, selector) {
return function () {
for(var args = [], i = 0, len = arguments.length; i < len; i++) { args.push(arguments[i]); }
return new AnonymousObservable(function (observer) {
function handler(err) {
if (err) {
observer.onError(err);
return;
}
for(var results = [], i = 1, len = arguments.length; i < len; i++) { results.push(arguments[i]); }
if (selector) {
try {
results = selector(results);
} catch (e) {
return observer.onError(e);
}
observer.onNext(results);
} else {
if (results.length <= 1) {
observer.onNext.apply(observer, results);
} else {
observer.onNext(results);
}
}
observer.onCompleted();
}
args.push(handler);
func.apply(context, args);
}).publishLast().refCount();
};
};
function fixEvent(event) {
var stopPropagation = function () {
this.cancelBubble = true;
};
var preventDefault = function () {
this.bubbledKeyCode = this.keyCode;
if (this.ctrlKey) {
try {
this.keyCode = 0;
} catch (e) { }
}
this.defaultPrevented = true;
this.returnValue = false;
this.modified = true;
};
event || (event = root.event);
if (!event.target) {
event.target = event.target || event.srcElement;
if (event.type == 'mouseover') {
event.relatedTarget = event.fromElement;
}
if (event.type == 'mouseout') {
event.relatedTarget = event.toElement;
}
// Adding stopPropogation and preventDefault to IE
if (!event.stopPropagation) {
event.stopPropagation = stopPropagation;
event.preventDefault = preventDefault;
}
// Normalize key events
switch (event.type) {
case 'keypress':
var c = ('charCode' in event ? event.charCode : event.keyCode);
if (c == 10) {
c = 0;
event.keyCode = 13;
} else if (c == 13 || c == 27) {
c = 0;
} else if (c == 3) {
c = 99;
}
event.charCode = c;
event.keyChar = event.charCode ? String.fromCharCode(event.charCode) : '';
break;
}
}
return event;
}
function createListener (element, name, handler) {
// Standards compliant
if (element.addEventListener) {
element.addEventListener(name, handler, false);
return disposableCreate(function () {
element.removeEventListener(name, handler, false);
});
}
if (element.attachEvent) {
// IE Specific
var innerHandler = function (event) {
handler(fixEvent(event));
};
element.attachEvent('on' + name, innerHandler);
return disposableCreate(function () {
element.detachEvent('on' + name, innerHandler);
});
}
// Level 1 DOM Events
element['on' + name] = handler;
return disposableCreate(function () {
element['on' + name] = null;
});
}
function createEventListener (el, eventName, handler) {
var disposables = new CompositeDisposable();
// Asume NodeList
if (Object.prototype.toString.call(el) === '[object NodeList]') {
for (var i = 0, len = el.length; i < len; i++) {
disposables.add(createEventListener(el.item(i), eventName, handler));
}
} else if (el) {
disposables.add(createListener(el, eventName, handler));
}
return disposables;
}
/**
* Configuration option to determine whether to use native events only
*/
Rx.config.useNativeEvents = false;
/**
* Creates an observable sequence by adding an event listener to the matching DOMElement or each item in the NodeList.
*
* @example
* var source = Rx.Observable.fromEvent(element, 'mouseup');
*
* @param {Object} element The DOMElement or NodeList to attach a listener.
* @param {String} eventName The event name to attach the observable sequence.
* @param {Function} [selector] A selector which takes the arguments from the event handler to produce a single item to yield on next.
* @returns {Observable} An observable sequence of events from the specified element and the specified event.
*/
Observable.fromEvent = function (element, eventName, selector) {
// Node.js specific
if (element.addListener) {
return fromEventPattern(
function (h) { element.addListener(eventName, h); },
function (h) { element.removeListener(eventName, h); },
selector);
}
// Use only if non-native events are allowed
if (!Rx.config.useNativeEvents) {
// Handles jq, Angular.js, Zepto, Marionette, Ember.js
if (typeof element.on === 'function' && typeof element.off === 'function') {
return fromEventPattern(
function (h) { element.on(eventName, h); },
function (h) { element.off(eventName, h); },
selector);
}
}
return new AnonymousObservable(function (observer) {
return createEventListener(
element,
eventName,
function handler (e) {
var results = e;
if (selector) {
try {
results = selector(arguments);
} catch (err) {
observer.onError(err);
return
}
}
observer.onNext(results);
});
}).publish().refCount();
};
/**
* Creates an observable sequence from an event emitter via an addHandler/removeHandler pair.
* @param {Function} addHandler The function to add a handler to the emitter.
* @param {Function} [removeHandler] The optional function to remove a handler from an emitter.
* @param {Function} [selector] A selector which takes the arguments from the event handler to produce a single item to yield on next.
* @returns {Observable} An observable sequence which wraps an event from an event emitter
*/
var fromEventPattern = Observable.fromEventPattern = function (addHandler, removeHandler, selector) {
return new AnonymousObservable(function (observer) {
function innerHandler (e) {
var result = e;
if (selector) {
try {
result = selector(arguments);
} catch (err) {
observer.onError(err);
return;
}
}
observer.onNext(result);
}
var returnValue = addHandler(innerHandler);
return disposableCreate(function () {
if (removeHandler) {
removeHandler(innerHandler, returnValue);
}
});
}).publish().refCount();
};
/**
* Converts a Promise to an Observable sequence
* @param {Promise} An ES6 Compliant promise.
* @returns {Observable} An Observable sequence which wraps the existing promise success and failure.
*/
var observableFromPromise = Observable.fromPromise = function (promise) {
return observableDefer(function () {
var subject = new Rx.AsyncSubject();
promise.then(
function (value) {
subject.onNext(value);
subject.onCompleted();
},
subject.onError.bind(subject));
return subject;
});
};
/*
* Converts an existing observable sequence to an ES6 Compatible Promise
* @example
* var promise = Rx.Observable.return(42).toPromise(RSVP.Promise);
*
* // With config
* Rx.config.Promise = RSVP.Promise;
* var promise = Rx.Observable.return(42).toPromise();
* @param {Function} [promiseCtor] The constructor of the promise. If not provided, it looks for it in Rx.config.Promise.
* @returns {Promise} An ES6 compatible promise with the last value from the observable sequence.
*/
observableProto.toPromise = function (promiseCtor) {
promiseCtor || (promiseCtor = Rx.config.Promise);
if (!promiseCtor) { throw new TypeError('Promise type not provided nor in Rx.config.Promise'); }
var source = this;
return new promiseCtor(function (resolve, reject) {
// No cancellation can be done
var value, hasValue = false;
source.subscribe(function (v) {
value = v;
hasValue = true;
}, reject, function () {
hasValue && resolve(value);
});
});
};
/**
* Invokes the asynchronous function, surfacing the result through an observable sequence.
* @param {Function} functionAsync Asynchronous function which returns a Promise to run.
* @returns {Observable} An observable sequence exposing the function's result value, or an exception.
*/
Observable.startAsync = function (functionAsync) {
var promise;
try {
promise = functionAsync();
} catch (e) {
return observableThrow(e);
}
return observableFromPromise(promise);
}
/**
* Multicasts the source sequence notifications through an instantiated subject into all uses of the sequence within a selector function. Each
* subscription to the resulting sequence causes a separate multicast invocation, exposing the sequence resulting from the selector function's
* invocation. For specializations with fixed subject types, see Publish, PublishLast, and Replay.
*
* @example
* 1 - res = source.multicast(observable);
* 2 - res = source.multicast(function () { return new Subject(); }, function (x) { return x; });
*
* @param {Function|Subject} subjectOrSubjectSelector
* Factory function to create an intermediate subject through which the source sequence's elements will be multicast to the selector function.
* Or:
* Subject to push source elements into.
*
* @param {Function} [selector] Optional selector function which can use the multicasted source sequence subject to the policies enforced by the created subject. Specified only if <paramref name="subjectOrSubjectSelector" is a factory function.
* @returns {Observable} An observable sequence that contains the elements of a sequence produced by multicasting the source sequence within a selector function.
*/
observableProto.multicast = function (subjectOrSubjectSelector, selector) {
var source = this;
return typeof subjectOrSubjectSelector === 'function' ?
new AnonymousObservable(function (observer) {
var connectable = source.multicast(subjectOrSubjectSelector());
return new CompositeDisposable(selector(connectable).subscribe(observer), connectable.connect());
}, source) :
new ConnectableObservable(source, subjectOrSubjectSelector);
};
/**
* Returns an observable sequence that is the result of invoking the selector on a connectable observable sequence that shares a single subscription to the underlying sequence.
* This operator is a specialization of Multicast using a regular Subject.
*
* @example
* var resres = source.publish();
* var res = source.publish(function (x) { return x; });
*
* @param {Function} [selector] Selector function which can use the multicasted source sequence as many times as needed, without causing multiple subscriptions to the source sequence. Subscribers to the given source will receive all notifications of the source from the time of the subscription on.
* @returns {Observable} An observable sequence that contains the elements of a sequence produced by multicasting the source sequence within a selector function.
*/
observableProto.publish = function (selector) {
return selector && isFunction(selector) ?
this.multicast(function () { return new Subject(); }, selector) :
this.multicast(new Subject());
};
/**
* Returns an observable sequence that shares a single subscription to the underlying sequence.
* This operator is a specialization of publish which creates a subscription when the number of observers goes from zero to one, then shares that subscription with all subsequent observers until the number of observers returns to zero, at which point the subscription is disposed.
* @returns {Observable} An observable sequence that contains the elements of a sequence produced by multicasting the source sequence.
*/
observableProto.share = function () {
return this.publish().refCount();
};
/**
* Returns an observable sequence that is the result of invoking the selector on a connectable observable sequence that shares a single subscription to the underlying sequence containing only the last notification.
* This operator is a specialization of Multicast using a AsyncSubject.
*
* @example
* var res = source.publishLast();
* var res = source.publishLast(function (x) { return x; });
*
* @param selector [Optional] Selector function which can use the multicasted source sequence as many times as needed, without causing multiple subscriptions to the source sequence. Subscribers to the given source will only receive the last notification of the source.
* @returns {Observable} An observable sequence that contains the elements of a sequence produced by multicasting the source sequence within a selector function.
*/
observableProto.publishLast = function (selector) {
return selector && isFunction(selector) ?
this.multicast(function () { return new AsyncSubject(); }, selector) :
this.multicast(new AsyncSubject());
};
/**
* Returns an observable sequence that is the result of invoking the selector on a connectable observable sequence that shares a single subscription to the underlying sequence and starts with initialValue.
* This operator is a specialization of Multicast using a BehaviorSubject.
*
* @example
* var res = source.publishValue(42);
* var res = source.publishValue(function (x) { return x.select(function (y) { return y * y; }) }, 42);
*
* @param {Function} [selector] Optional selector function which can use the multicasted source sequence as many times as needed, without causing multiple subscriptions to the source sequence. Subscribers to the given source will receive immediately receive the initial value, followed by all notifications of the source from the time of the subscription on.
* @param {Mixed} initialValue Initial value received by observers upon subscription.
* @returns {Observable} An observable sequence that contains the elements of a sequence produced by multicasting the source sequence within a selector function.
*/
observableProto.publishValue = function (initialValueOrSelector, initialValue) {
return arguments.length === 2 ?
this.multicast(function () {
return new BehaviorSubject(initialValue);
}, initialValueOrSelector) :
this.multicast(new BehaviorSubject(initialValueOrSelector));
};
/**
* Returns an observable sequence that shares a single subscription to the underlying sequence and starts with an initialValue.
* This operator is a specialization of publishValue which creates a subscription when the number of observers goes from zero to one, then shares that subscription with all subsequent observers until the number of observers returns to zero, at which point the subscription is disposed.
* @param {Mixed} initialValue Initial value received by observers upon subscription.
* @returns {Observable} An observable sequence that contains the elements of a sequence produced by multicasting the source sequence.
*/
observableProto.shareValue = function (initialValue) {
return this.publishValue(initialValue).refCount();
};
/**
* Returns an observable sequence that is the result of invoking the selector on a connectable observable sequence that shares a single subscription to the underlying sequence replaying notifications subject to a maximum time length for the replay buffer.
* This operator is a specialization of Multicast using a ReplaySubject.
*
* @example
* var res = source.replay(null, 3);
* var res = source.replay(null, 3, 500);
* var res = source.replay(null, 3, 500, scheduler);
* var res = source.replay(function (x) { return x.take(6).repeat(); }, 3, 500, scheduler);
*
* @param selector [Optional] Selector function which can use the multicasted source sequence as many times as needed, without causing multiple subscriptions to the source sequence. Subscribers to the given source will receive all the notifications of the source subject to the specified replay buffer trimming policy.
* @param bufferSize [Optional] Maximum element count of the replay buffer.
* @param window [Optional] Maximum time length of the replay buffer.
* @param scheduler [Optional] Scheduler where connected observers within the selector function will be invoked on.
* @returns {Observable} An observable sequence that contains the elements of a sequence produced by multicasting the source sequence within a selector function.
*/
observableProto.replay = function (selector, bufferSize, window, scheduler) {
return selector && isFunction(selector) ?
this.multicast(function () { return new ReplaySubject(bufferSize, window, scheduler); }, selector) :
this.multicast(new ReplaySubject(bufferSize, window, scheduler));
};
/**
* Returns an observable sequence that shares a single subscription to the underlying sequence replaying notifications subject to a maximum time length for the replay buffer.
* This operator is a specialization of replay which creates a subscription when the number of observers goes from zero to one, then shares that subscription with all subsequent observers until the number of observers returns to zero, at which point the subscription is disposed.
*
* @example
* var res = source.shareReplay(3);
* var res = source.shareReplay(3, 500);
* var res = source.shareReplay(3, 500, scheduler);
*
* @param bufferSize [Optional] Maximum element count of the replay buffer.
* @param window [Optional] Maximum time length of the replay buffer.
* @param scheduler [Optional] Scheduler where connected observers within the selector function will be invoked on.
* @returns {Observable} An observable sequence that contains the elements of a sequence produced by multicasting the source sequence.
*/
observableProto.shareReplay = function (bufferSize, window, scheduler) {
return this.replay(null, bufferSize, window, scheduler).refCount();
};
var ConnectableObservable = Rx.ConnectableObservable = (function (__super__) {
inherits(ConnectableObservable, __super__);
function ConnectableObservable(source, subject) {
var hasSubscription = false,
subscription,
sourceObservable = source.asObservable();
this.connect = function () {
if (!hasSubscription) {
hasSubscription = true;
subscription = new CompositeDisposable(sourceObservable.subscribe(subject), disposableCreate(function () {
hasSubscription = false;
}));
}
return subscription;
};
__super__.call(this, function (o) { return subject.subscribe(o); });
}
ConnectableObservable.prototype.refCount = function () {
var connectableSubscription, count = 0, source = this;
return new AnonymousObservable(function (observer) {
var shouldConnect = ++count === 1,
subscription = source.subscribe(observer);
shouldConnect && (connectableSubscription = source.connect());
return function () {
subscription.dispose();
--count === 0 && connectableSubscription.dispose();
};
});
};
return ConnectableObservable;
}(Observable));
function observableTimerDate(dueTime, scheduler) {
return new AnonymousObservable(function (observer) {
return scheduler.scheduleWithAbsolute(dueTime, function () {
observer.onNext(0);
observer.onCompleted();
});
});
}
function observableTimerDateAndPeriod(dueTime, period, scheduler) {
return new AnonymousObservable(function (observer) {
var d = dueTime, p = normalizeTime(period);
return scheduler.scheduleRecursiveWithAbsoluteAndState(0, d, function (count, self) {
if (p > 0) {
var now = scheduler.now();
d = d + p;
d <= now && (d = now + p);
}
observer.onNext(count);
self(count + 1, d);
});
});
}
function observableTimerTimeSpan(dueTime, scheduler) {
return new AnonymousObservable(function (observer) {
return scheduler.scheduleWithRelative(normalizeTime(dueTime), function () {
observer.onNext(0);
observer.onCompleted();
});
});
}
function observableTimerTimeSpanAndPeriod(dueTime, period, scheduler) {
return dueTime === period ?
new AnonymousObservable(function (observer) {
return scheduler.schedulePeriodicWithState(0, period, function (count) {
observer.onNext(count);
return count + 1;
});
}) :
observableDefer(function () {
return observableTimerDateAndPeriod(scheduler.now() + dueTime, period, scheduler);
});
}
/**
* Returns an observable sequence that produces a value after each period.
*
* @example
* 1 - res = Rx.Observable.interval(1000);
* 2 - res = Rx.Observable.interval(1000, Rx.Scheduler.timeout);
*
* @param {Number} period Period for producing the values in the resulting sequence (specified as an integer denoting milliseconds).
* @param {Scheduler} [scheduler] Scheduler to run the timer on. If not specified, Rx.Scheduler.timeout is used.
* @returns {Observable} An observable sequence that produces a value after each period.
*/
var observableinterval = Observable.interval = function (period, scheduler) {
return observableTimerTimeSpanAndPeriod(period, period, isScheduler(scheduler) ? scheduler : timeoutScheduler);
};
/**
* Returns an observable sequence that produces a value after dueTime has elapsed and then after each period.
* @param {Number} dueTime Absolute (specified as a Date object) or relative time (specified as an integer denoting milliseconds) at which to produce the first value.
* @param {Mixed} [periodOrScheduler] Period to produce subsequent values (specified as an integer denoting milliseconds), or the scheduler to run the timer on. If not specified, the resulting timer is not recurring.
* @param {Scheduler} [scheduler] Scheduler to run the timer on. If not specified, the timeout scheduler is used.
* @returns {Observable} An observable sequence that produces a value after due time has elapsed and then each period.
*/
var observableTimer = Observable.timer = function (dueTime, periodOrScheduler, scheduler) {
var period;
isScheduler(scheduler) || (scheduler = timeoutScheduler);
if (periodOrScheduler !== undefined && typeof periodOrScheduler === 'number') {
period = periodOrScheduler;
} else if (isScheduler(periodOrScheduler)) {
scheduler = periodOrScheduler;
}
if (dueTime instanceof Date && period === undefined) {
return observableTimerDate(dueTime.getTime(), scheduler);
}
if (dueTime instanceof Date && period !== undefined) {
period = periodOrScheduler;
return observableTimerDateAndPeriod(dueTime.getTime(), period, scheduler);
}
return period === undefined ?
observableTimerTimeSpan(dueTime, scheduler) :
observableTimerTimeSpanAndPeriod(dueTime, period, scheduler);
};
function observableDelayTimeSpan(source, dueTime, scheduler) {
return new AnonymousObservable(function (observer) {
var active = false,
cancelable = new SerialDisposable(),
exception = null,
q = [],
running = false,
subscription;
subscription = source.materialize().timestamp(scheduler).subscribe(function (notification) {
var d, shouldRun;
if (notification.value.kind === 'E') {
q = [];
q.push(notification);
exception = notification.value.exception;
shouldRun = !running;
} else {
q.push({ value: notification.value, timestamp: notification.timestamp + dueTime });
shouldRun = !active;
active = true;
}
if (shouldRun) {
if (exception !== null) {
observer.onError(exception);
} else {
d = new SingleAssignmentDisposable();
cancelable.setDisposable(d);
d.setDisposable(scheduler.scheduleRecursiveWithRelative(dueTime, function (self) {
var e, recurseDueTime, result, shouldRecurse;
if (exception !== null) {
return;
}
running = true;
do {
result = null;
if (q.length > 0 && q[0].timestamp - scheduler.now() <= 0) {
result = q.shift().value;
}
if (result !== null) {
result.accept(observer);
}
} while (result !== null);
shouldRecurse = false;
recurseDueTime = 0;
if (q.length > 0) {
shouldRecurse = true;
recurseDueTime = Math.max(0, q[0].timestamp - scheduler.now());
} else {
active = false;
}
e = exception;
running = false;
if (e !== null) {
observer.onError(e);
} else if (shouldRecurse) {
self(recurseDueTime);
}
}));
}
}
});
return new CompositeDisposable(subscription, cancelable);
}, source);
}
function observableDelayDate(source, dueTime, scheduler) {
return observableDefer(function () {
return observableDelayTimeSpan(source, dueTime - scheduler.now(), scheduler);
});
}
/**
* Time shifts the observable sequence by dueTime. The relative time intervals between the values are preserved.
*
* @example
* 1 - res = Rx.Observable.delay(new Date());
* 2 - res = Rx.Observable.delay(new Date(), Rx.Scheduler.timeout);
*
* 3 - res = Rx.Observable.delay(5000);
* 4 - res = Rx.Observable.delay(5000, 1000, Rx.Scheduler.timeout);
* @memberOf Observable#
* @param {Number} dueTime Absolute (specified as a Date object) or relative time (specified as an integer denoting milliseconds) by which to shift the observable sequence.
* @param {Scheduler} [scheduler] Scheduler to run the delay timers on. If not specified, the timeout scheduler is used.
* @returns {Observable} Time-shifted sequence.
*/
observableProto.delay = function (dueTime, scheduler) {
isScheduler(scheduler) || (scheduler = timeoutScheduler);
return dueTime instanceof Date ?
observableDelayDate(this, dueTime.getTime(), scheduler) :
observableDelayTimeSpan(this, dueTime, scheduler);
};
/**
* Ignores values from an observable sequence which are followed by another value before dueTime.
* @param {Number} dueTime Duration of the debounce period for each value (specified as an integer denoting milliseconds).
* @param {Scheduler} [scheduler] Scheduler to run the debounce timers on. If not specified, the timeout scheduler is used.
* @returns {Observable} The debounced sequence.
*/
observableProto.debounce = observableProto.throttleWithTimeout = function (dueTime, scheduler) {
isScheduler(scheduler) || (scheduler = timeoutScheduler);
var source = this;
return new AnonymousObservable(function (observer) {
var cancelable = new SerialDisposable(), hasvalue = false, value, id = 0;
var subscription = source.subscribe(
function (x) {
hasvalue = true;
value = x;
id++;
var currentId = id,
d = new SingleAssignmentDisposable();
cancelable.setDisposable(d);
d.setDisposable(scheduler.scheduleWithRelative(dueTime, function () {
hasvalue && id === currentId && observer.onNext(value);
hasvalue = false;
}));
},
function (e) {
cancelable.dispose();
observer.onError(e);
hasvalue = false;
id++;
},
function () {
cancelable.dispose();
hasvalue && observer.onNext(value);
observer.onCompleted();
hasvalue = false;
id++;
});
return new CompositeDisposable(subscription, cancelable);
}, this);
};
/**
* @deprecated use #debounce or #throttleWithTimeout instead.
*/
observableProto.throttle = function(dueTime, scheduler) {
//deprecate('throttle', 'debounce or throttleWithTimeout');
return this.debounce(dueTime, scheduler);
};
/**
* Records the timestamp for each value in an observable sequence.
*
* @example
* 1 - res = source.timestamp(); // produces { value: x, timestamp: ts }
* 2 - res = source.timestamp(Rx.Scheduler.timeout);
*
* @param {Scheduler} [scheduler] Scheduler used to compute timestamps. If not specified, the timeout scheduler is used.
* @returns {Observable} An observable sequence with timestamp information on values.
*/
observableProto.timestamp = function (scheduler) {
isScheduler(scheduler) || (scheduler = timeoutScheduler);
return this.map(function (x) {
return { value: x, timestamp: scheduler.now() };
});
};
function sampleObservable(source, sampler) {
return new AnonymousObservable(function (observer) {
var atEnd, value, hasValue;
function sampleSubscribe() {
if (hasValue) {
hasValue = false;
observer.onNext(value);
}
atEnd && observer.onCompleted();
}
return new CompositeDisposable(
source.subscribe(function (newValue) {
hasValue = true;
value = newValue;
}, observer.onError.bind(observer), function () {
atEnd = true;
}),
sampler.subscribe(sampleSubscribe, observer.onError.bind(observer), sampleSubscribe)
);
}, source);
}
/**
* Samples the observable sequence at each interval.
*
* @example
* 1 - res = source.sample(sampleObservable); // Sampler tick sequence
* 2 - res = source.sample(5000); // 5 seconds
* 2 - res = source.sample(5000, Rx.Scheduler.timeout); // 5 seconds
*
* @param {Mixed} intervalOrSampler Interval at which to sample (specified as an integer denoting milliseconds) or Sampler Observable.
* @param {Scheduler} [scheduler] Scheduler to run the sampling timer on. If not specified, the timeout scheduler is used.
* @returns {Observable} Sampled observable sequence.
*/
observableProto.sample = observableProto.throttleLatest = function (intervalOrSampler, scheduler) {
isScheduler(scheduler) || (scheduler = timeoutScheduler);
return typeof intervalOrSampler === 'number' ?
sampleObservable(this, observableinterval(intervalOrSampler, scheduler)) :
sampleObservable(this, intervalOrSampler);
};
/**
* Returns the source observable sequence or the other observable sequence if dueTime elapses.
* @param {Number} dueTime Absolute (specified as a Date object) or relative time (specified as an integer denoting milliseconds) when a timeout occurs.
* @param {Observable} [other] Sequence to return in case of a timeout. If not specified, a timeout error throwing sequence will be used.
* @param {Scheduler} [scheduler] Scheduler to run the timeout timers on. If not specified, the timeout scheduler is used.
* @returns {Observable} The source sequence switching to the other sequence in case of a timeout.
*/
observableProto.timeout = function (dueTime, other, scheduler) {
(other == null || typeof other === 'string') && (other = observableThrow(new Error(other || 'Timeout')));
isScheduler(scheduler) || (scheduler = timeoutScheduler);
var source = this, schedulerMethod = dueTime instanceof Date ?
'scheduleWithAbsolute' :
'scheduleWithRelative';
return new AnonymousObservable(function (observer) {
var id = 0,
original = new SingleAssignmentDisposable(),
subscription = new SerialDisposable(),
switched = false,
timer = new SerialDisposable();
subscription.setDisposable(original);
function createTimer() {
var myId = id;
timer.setDisposable(scheduler[schedulerMethod](dueTime, function () {
if (id === myId) {
isPromise(other) && (other = observableFromPromise(other));
subscription.setDisposable(other.subscribe(observer));
}
}));
}
createTimer();
original.setDisposable(source.subscribe(function (x) {
if (!switched) {
id++;
observer.onNext(x);
createTimer();
}
}, function (e) {
if (!switched) {
id++;
observer.onError(e);
}
}, function () {
if (!switched) {
id++;
observer.onCompleted();
}
}));
return new CompositeDisposable(subscription, timer);
}, source);
};
/**
* Returns an Observable that emits only the first item emitted by the source Observable during sequential time windows of a specified duration.
* @param {Number} windowDuration time to wait before emitting another item after emitting the last item
* @param {Scheduler} [scheduler] the Scheduler to use internally to manage the timers that handle timeout for each item. If not provided, defaults to Scheduler.timeout.
* @returns {Observable} An Observable that performs the throttle operation.
*/
observableProto.throttleFirst = function (windowDuration, scheduler) {
isScheduler(scheduler) || (scheduler = timeoutScheduler);
var duration = +windowDuration || 0;
if (duration <= 0) { throw new RangeError('windowDuration cannot be less or equal zero.'); }
var source = this;
return new AnonymousObservable(function (o) {
var lastOnNext = 0;
return source.subscribe(
function (x) {
var now = scheduler.now();
if (lastOnNext === 0 || now - lastOnNext >= duration) {
lastOnNext = now;
o.onNext(x);
}
},function (e) { o.onError(e); }, function () { o.onCompleted(); }
);
}, source);
};
var PausableObservable = (function (__super__) {
inherits(PausableObservable, __super__);
function subscribe(observer) {
var conn = this.source.publish(),
subscription = conn.subscribe(observer),
connection = disposableEmpty;
var pausable = this.pauser.distinctUntilChanged().subscribe(function (b) {
if (b) {
connection = conn.connect();
} else {
connection.dispose();
connection = disposableEmpty;
}
});
return new CompositeDisposable(subscription, connection, pausable);
}
function PausableObservable(source, pauser) {
this.source = source;
this.controller = new Subject();
if (pauser && pauser.subscribe) {
this.pauser = this.controller.merge(pauser);
} else {
this.pauser = this.controller;
}
__super__.call(this, subscribe, source);
}
PausableObservable.prototype.pause = function () {
this.controller.onNext(false);
};
PausableObservable.prototype.resume = function () {
this.controller.onNext(true);
};
return PausableObservable;
}(Observable));
/**
* Pauses the underlying observable sequence based upon the observable sequence which yields true/false.
* @example
* var pauser = new Rx.Subject();
* var source = Rx.Observable.interval(100).pausable(pauser);
* @param {Observable} pauser The observable sequence used to pause the underlying sequence.
* @returns {Observable} The observable sequence which is paused based upon the pauser.
*/
observableProto.pausable = function (pauser) {
return new PausableObservable(this, pauser);
};
function combineLatestSource(source, subject, resultSelector) {
return new AnonymousObservable(function (o) {
var hasValue = [false, false],
hasValueAll = false,
isDone = false,
values = new Array(2),
err;
function next(x, i) {
values[i] = x
var res;
hasValue[i] = true;
if (hasValueAll || (hasValueAll = hasValue.every(identity))) {
if (err) {
o.onError(err);
return;
}
try {
res = resultSelector.apply(null, values);
} catch (ex) {
o.onError(ex);
return;
}
o.onNext(res);
}
if (isDone && values[1]) {
o.onCompleted();
}
}
return new CompositeDisposable(
source.subscribe(
function (x) {
next(x, 0);
},
function (e) {
if (values[1]) {
o.onError(e);
} else {
err = e;
}
},
function () {
isDone = true;
values[1] && o.onCompleted();
}),
subject.subscribe(
function (x) {
next(x, 1);
},
function (e) { o.onError(e); },
function () {
isDone = true;
next(true, 1);
})
);
}, source);
}
var PausableBufferedObservable = (function (__super__) {
inherits(PausableBufferedObservable, __super__);
function subscribe(o) {
var q = [], previousShouldFire;
var subscription =
combineLatestSource(
this.source,
this.pauser.distinctUntilChanged().startWith(false),
function (data, shouldFire) {
return { data: data, shouldFire: shouldFire };
})
.subscribe(
function (results) {
if (previousShouldFire !== undefined && results.shouldFire != previousShouldFire) {
previousShouldFire = results.shouldFire;
// change in shouldFire
if (results.shouldFire) {
while (q.length > 0) {
o.onNext(q.shift());
}
}
} else {
previousShouldFire = results.shouldFire;
// new data
if (results.shouldFire) {
o.onNext(results.data);
} else {
q.push(results.data);
}
}
},
function (err) {
// Empty buffer before sending error
while (q.length > 0) {
o.onNext(q.shift());
}
o.onError(err);
},
function () {
// Empty buffer before sending completion
while (q.length > 0) {
o.onNext(q.shift());
}
o.onCompleted();
}
);
return subscription;
}
function PausableBufferedObservable(source, pauser) {
this.source = source;
this.controller = new Subject();
if (pauser && pauser.subscribe) {
this.pauser = this.controller.merge(pauser);
} else {
this.pauser = this.controller;
}
__super__.call(this, subscribe, source);
}
PausableBufferedObservable.prototype.pause = function () {
this.controller.onNext(false);
};
PausableBufferedObservable.prototype.resume = function () {
this.controller.onNext(true);
};
return PausableBufferedObservable;
}(Observable));
/**
* Pauses the underlying observable sequence based upon the observable sequence which yields true/false,
* and yields the values that were buffered while paused.
* @example
* var pauser = new Rx.Subject();
* var source = Rx.Observable.interval(100).pausableBuffered(pauser);
* @param {Observable} pauser The observable sequence used to pause the underlying sequence.
* @returns {Observable} The observable sequence which is paused based upon the pauser.
*/
observableProto.pausableBuffered = function (subject) {
return new PausableBufferedObservable(this, subject);
};
var ControlledObservable = (function (__super__) {
inherits(ControlledObservable, __super__);
function subscribe (observer) {
return this.source.subscribe(observer);
}
function ControlledObservable (source, enableQueue) {
__super__.call(this, subscribe, source);
this.subject = new ControlledSubject(enableQueue);
this.source = source.multicast(this.subject).refCount();
}
ControlledObservable.prototype.request = function (numberOfItems) {
if (numberOfItems == null) { numberOfItems = -1; }
return this.subject.request(numberOfItems);
};
return ControlledObservable;
}(Observable));
var ControlledSubject = (function (__super__) {
function subscribe (observer) {
return this.subject.subscribe(observer);
}
inherits(ControlledSubject, __super__);
function ControlledSubject(enableQueue) {
enableQueue == null && (enableQueue = true);
__super__.call(this, subscribe);
this.subject = new Subject();
this.enableQueue = enableQueue;
this.queue = enableQueue ? [] : null;
this.requestedCount = 0;
this.requestedDisposable = disposableEmpty;
this.error = null;
this.hasFailed = false;
this.hasCompleted = false;
this.controlledDisposable = disposableEmpty;
}
addProperties(ControlledSubject.prototype, Observer, {
onCompleted: function () {
this.hasCompleted = true;
(!this.enableQueue || this.queue.length === 0) && this.subject.onCompleted();
},
onError: function (error) {
this.hasFailed = true;
this.error = error;
(!this.enableQueue || this.queue.length === 0) && this.subject.onError(error);
},
onNext: function (value) {
var hasRequested = false;
if (this.requestedCount === 0) {
this.enableQueue && this.queue.push(value);
} else {
(this.requestedCount !== -1 && this.requestedCount-- === 0) && this.disposeCurrentRequest();
hasRequested = true;
}
hasRequested && this.subject.onNext(value);
},
_processRequest: function (numberOfItems) {
if (this.enableQueue) {
while (this.queue.length >= numberOfItems && numberOfItems > 0) {
this.subject.onNext(this.queue.shift());
numberOfItems--;
}
return this.queue.length !== 0 ?
{ numberOfItems: numberOfItems, returnValue: true } :
{ numberOfItems: numberOfItems, returnValue: false };
}
if (this.hasFailed) {
this.subject.onError(this.error);
this.controlledDisposable.dispose();
this.controlledDisposable = disposableEmpty;
} else if (this.hasCompleted) {
this.subject.onCompleted();
this.controlledDisposable.dispose();
this.controlledDisposable = disposableEmpty;
}
return { numberOfItems: numberOfItems, returnValue: false };
},
request: function (number) {
this.disposeCurrentRequest();
var self = this, r = this._processRequest(number);
var number = r.numberOfItems;
if (!r.returnValue) {
this.requestedCount = number;
this.requestedDisposable = disposableCreate(function () {
self.requestedCount = 0;
});
return this.requestedDisposable
} else {
return disposableEmpty;
}
},
disposeCurrentRequest: function () {
this.requestedDisposable.dispose();
this.requestedDisposable = disposableEmpty;
}
});
return ControlledSubject;
}(Observable));
/**
* Attaches a controller to the observable sequence with the ability to queue.
* @example
* var source = Rx.Observable.interval(100).controlled();
* source.request(3); // Reads 3 values
* @param {Observable} pauser The observable sequence used to pause the underlying sequence.
* @returns {Observable} The observable sequence which is paused based upon the pauser.
*/
observableProto.controlled = function (enableQueue) {
if (enableQueue == null) { enableQueue = true; }
return new ControlledObservable(this, enableQueue);
};
/**
* Executes a transducer to transform the observable sequence
* @param {Transducer} transducer A transducer to execute
* @returns {Observable} An Observable sequence containing the results from the transducer.
*/
observableProto.transduce = function(transducer) {
var source = this;
function transformForObserver(observer) {
return {
init: function() {
return observer;
},
step: function(obs, input) {
return obs.onNext(input);
},
result: function(obs) {
return obs.onCompleted();
}
};
}
return new AnonymousObservable(function(observer) {
var xform = transducer(transformForObserver(observer));
return source.subscribe(
function(v) {
try {
xform.step(observer, v);
} catch (e) {
observer.onError(e);
}
},
observer.onError.bind(observer),
function() { xform.result(observer); }
);
}, source);
};
var AnonymousObservable = Rx.AnonymousObservable = (function (__super__) {
inherits(AnonymousObservable, __super__);
// Fix subscriber to check for undefined or function returned to decorate as Disposable
function fixSubscriber(subscriber) {
if (subscriber && typeof subscriber.dispose === 'function') { return subscriber; }
return typeof subscriber === 'function' ?
disposableCreate(subscriber) :
disposableEmpty;
}
function setDisposable(s, state) {
var ado = state[0], subscribe = state[1];
try {
ado.setDisposable(fixSubscriber(subscribe(ado)));
} catch (e) {
if (!ado.fail(e)) { throw e; }
}
}
function AnonymousObservable(subscribe, parent) {
this.source = parent;
function s(observer) {
var ado = new AutoDetachObserver(observer), state = [ado, subscribe];
if (currentThreadScheduler.scheduleRequired()) {
currentThreadScheduler.scheduleWithState(state, setDisposable);
} else {
setDisposable(null, state);
}
return ado;
}
__super__.call(this, s);
}
return AnonymousObservable;
}(Observable));
var AutoDetachObserver = (function (__super__) {
inherits(AutoDetachObserver, __super__);
function AutoDetachObserver(observer) {
__super__.call(this);
this.observer = observer;
this.m = new SingleAssignmentDisposable();
}
var AutoDetachObserverPrototype = AutoDetachObserver.prototype;
AutoDetachObserverPrototype.next = function (value) {
var result = tryCatch(this.observer.onNext).call(this.observer, value);
if (result === errorObj) {
this.dispose();
return thrower(result.e);
}
};
AutoDetachObserverPrototype.error = function (err) {
var result = tryCatch(this.observer.onError).call(this.observer, err);
this.dispose();
if (result === errorObj) {
return thrower(result.e);
}
};
AutoDetachObserverPrototype.completed = function () {
var result = tryCatch(this.observer.onCompleted).call(this.observer);
this.dispose();
if (result === errorObj) {
return thrower(result.e);
}
};
AutoDetachObserverPrototype.setDisposable = function (value) { this.m.setDisposable(value); };
AutoDetachObserverPrototype.getDisposable = function () { return this.m.getDisposable(); };
AutoDetachObserverPrototype.dispose = function () {
__super__.prototype.dispose.call(this);
this.m.dispose();
};
return AutoDetachObserver;
}(AbstractObserver));
var InnerSubscription = function (subject, observer) {
this.subject = subject;
this.observer = observer;
};
InnerSubscription.prototype.dispose = function () {
if (!this.subject.isDisposed && this.observer !== null) {
var idx = this.subject.observers.indexOf(this.observer);
this.subject.observers.splice(idx, 1);
this.observer = null;
}
};
/**
* Represents an object that is both an observable sequence as well as an observer.
* Each notification is broadcasted to all subscribed observers.
*/
var Subject = Rx.Subject = (function (__super__) {
function subscribe(observer) {
checkDisposed(this);
if (!this.isStopped) {
this.observers.push(observer);
return new InnerSubscription(this, observer);
}
if (this.hasError) {
observer.onError(this.error);
return disposableEmpty;
}
observer.onCompleted();
return disposableEmpty;
}
inherits(Subject, __super__);
/**
* Creates a subject.
*/
function Subject() {
__super__.call(this, subscribe);
this.isDisposed = false,
this.isStopped = false,
this.observers = [];
this.hasError = false;
}
addProperties(Subject.prototype, Observer.prototype, {
/**
* Indicates whether the subject has observers subscribed to it.
* @returns {Boolean} Indicates whether the subject has observers subscribed to it.
*/
hasObservers: function () { return this.observers.length > 0; },
/**
* Notifies all subscribed observers about the end of the sequence.
*/
onCompleted: function () {
checkDisposed(this);
if (!this.isStopped) {
this.isStopped = true;
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
os[i].onCompleted();
}
this.observers.length = 0;
}
},
/**
* Notifies all subscribed observers about the exception.
* @param {Mixed} error The exception to send to all observers.
*/
onError: function (error) {
checkDisposed(this);
if (!this.isStopped) {
this.isStopped = true;
this.error = error;
this.hasError = true;
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
os[i].onError(error);
}
this.observers.length = 0;
}
},
/**
* Notifies all subscribed observers about the arrival of the specified element in the sequence.
* @param {Mixed} value The value to send to all observers.
*/
onNext: function (value) {
checkDisposed(this);
if (!this.isStopped) {
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
os[i].onNext(value);
}
}
},
/**
* Unsubscribe all observers and release resources.
*/
dispose: function () {
this.isDisposed = true;
this.observers = null;
}
});
/**
* Creates a subject from the specified observer and observable.
* @param {Observer} observer The observer used to send messages to the subject.
* @param {Observable} observable The observable used to subscribe to messages sent from the subject.
* @returns {Subject} Subject implemented using the given observer and observable.
*/
Subject.create = function (observer, observable) {
return new AnonymousSubject(observer, observable);
};
return Subject;
}(Observable));
/**
* Represents the result of an asynchronous operation.
* The last value before the OnCompleted notification, or the error received through OnError, is sent to all subscribed observers.
*/
var AsyncSubject = Rx.AsyncSubject = (function (__super__) {
function subscribe(observer) {
checkDisposed(this);
if (!this.isStopped) {
this.observers.push(observer);
return new InnerSubscription(this, observer);
}
if (this.hasError) {
observer.onError(this.error);
} else if (this.hasValue) {
observer.onNext(this.value);
observer.onCompleted();
} else {
observer.onCompleted();
}
return disposableEmpty;
}
inherits(AsyncSubject, __super__);
/**
* Creates a subject that can only receive one value and that value is cached for all future observations.
* @constructor
*/
function AsyncSubject() {
__super__.call(this, subscribe);
this.isDisposed = false;
this.isStopped = false;
this.hasValue = false;
this.observers = [];
this.hasError = false;
}
addProperties(AsyncSubject.prototype, Observer, {
/**
* Indicates whether the subject has observers subscribed to it.
* @returns {Boolean} Indicates whether the subject has observers subscribed to it.
*/
hasObservers: function () {
checkDisposed(this);
return this.observers.length > 0;
},
/**
* Notifies all subscribed observers about the end of the sequence, also causing the last received value to be sent out (if any).
*/
onCompleted: function () {
var i, len;
checkDisposed(this);
if (!this.isStopped) {
this.isStopped = true;
var os = cloneArray(this.observers), len = os.length;
if (this.hasValue) {
for (i = 0; i < len; i++) {
var o = os[i];
o.onNext(this.value);
o.onCompleted();
}
} else {
for (i = 0; i < len; i++) {
os[i].onCompleted();
}
}
this.observers.length = 0;
}
},
/**
* Notifies all subscribed observers about the error.
* @param {Mixed} error The Error to send to all observers.
*/
onError: function (error) {
checkDisposed(this);
if (!this.isStopped) {
this.isStopped = true;
this.hasError = true;
this.error = error;
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
os[i].onError(error);
}
this.observers.length = 0;
}
},
/**
* Sends a value to the subject. The last value received before successful termination will be sent to all subscribed and future observers.
* @param {Mixed} value The value to store in the subject.
*/
onNext: function (value) {
checkDisposed(this);
if (this.isStopped) { return; }
this.value = value;
this.hasValue = true;
},
/**
* Unsubscribe all observers and release resources.
*/
dispose: function () {
this.isDisposed = true;
this.observers = null;
this.exception = null;
this.value = null;
}
});
return AsyncSubject;
}(Observable));
var AnonymousSubject = Rx.AnonymousSubject = (function (__super__) {
inherits(AnonymousSubject, __super__);
function subscribe(observer) {
return this.observable.subscribe(observer);
}
function AnonymousSubject(observer, observable) {
this.observer = observer;
this.observable = observable;
__super__.call(this, subscribe);
}
addProperties(AnonymousSubject.prototype, Observer.prototype, {
onCompleted: function () {
this.observer.onCompleted();
},
onError: function (error) {
this.observer.onError(error);
},
onNext: function (value) {
this.observer.onNext(value);
}
});
return AnonymousSubject;
}(Observable));
/**
* Represents a value that changes over time.
* Observers can subscribe to the subject to receive the last (or initial) value and all subsequent notifications.
*/
var BehaviorSubject = Rx.BehaviorSubject = (function (__super__) {
function subscribe(observer) {
checkDisposed(this);
if (!this.isStopped) {
this.observers.push(observer);
observer.onNext(this.value);
return new InnerSubscription(this, observer);
}
if (this.hasError) {
observer.onError(this.error);
} else {
observer.onCompleted();
}
return disposableEmpty;
}
inherits(BehaviorSubject, __super__);
/**
* Initializes a new instance of the BehaviorSubject class which creates a subject that caches its last value and starts with the specified value.
* @param {Mixed} value Initial value sent to observers when no other value has been received by the subject yet.
*/
function BehaviorSubject(value) {
__super__.call(this, subscribe);
this.value = value,
this.observers = [],
this.isDisposed = false,
this.isStopped = false,
this.hasError = false;
}
addProperties(BehaviorSubject.prototype, Observer, {
/**
* Indicates whether the subject has observers subscribed to it.
* @returns {Boolean} Indicates whether the subject has observers subscribed to it.
*/
hasObservers: function () { return this.observers.length > 0; },
/**
* Notifies all subscribed observers about the end of the sequence.
*/
onCompleted: function () {
checkDisposed(this);
if (this.isStopped) { return; }
this.isStopped = true;
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
os[i].onCompleted();
}
this.observers.length = 0;
},
/**
* Notifies all subscribed observers about the exception.
* @param {Mixed} error The exception to send to all observers.
*/
onError: function (error) {
checkDisposed(this);
if (this.isStopped) { return; }
this.isStopped = true;
this.hasError = true;
this.error = error;
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
os[i].onError(error);
}
this.observers.length = 0;
},
/**
* Notifies all subscribed observers about the arrival of the specified element in the sequence.
* @param {Mixed} value The value to send to all observers.
*/
onNext: function (value) {
checkDisposed(this);
if (this.isStopped) { return; }
this.value = value;
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
os[i].onNext(value);
}
},
/**
* Unsubscribe all observers and release resources.
*/
dispose: function () {
this.isDisposed = true;
this.observers = null;
this.value = null;
this.exception = null;
}
});
return BehaviorSubject;
}(Observable));
/**
* Represents an object that is both an observable sequence as well as an observer.
* Each notification is broadcasted to all subscribed and future observers, subject to buffer trimming policies.
*/
var ReplaySubject = Rx.ReplaySubject = (function (__super__) {
function createRemovableDisposable(subject, observer) {
return disposableCreate(function () {
observer.dispose();
!subject.isDisposed && subject.observers.splice(subject.observers.indexOf(observer), 1);
});
}
function subscribe(observer) {
var so = new ScheduledObserver(this.scheduler, observer),
subscription = createRemovableDisposable(this, so);
checkDisposed(this);
this._trim(this.scheduler.now());
this.observers.push(so);
for (var i = 0, len = this.q.length; i < len; i++) {
so.onNext(this.q[i].value);
}
if (this.hasError) {
so.onError(this.error);
} else if (this.isStopped) {
so.onCompleted();
}
so.ensureActive();
return subscription;
}
inherits(ReplaySubject, __super__);
/**
* Initializes a new instance of the ReplaySubject class with the specified buffer size, window size and scheduler.
* @param {Number} [bufferSize] Maximum element count of the replay buffer.
* @param {Number} [windowSize] Maximum time length of the replay buffer.
* @param {Scheduler} [scheduler] Scheduler the observers are invoked on.
*/
function ReplaySubject(bufferSize, windowSize, scheduler) {
this.bufferSize = bufferSize == null ? Number.MAX_VALUE : bufferSize;
this.windowSize = windowSize == null ? Number.MAX_VALUE : windowSize;
this.scheduler = scheduler || currentThreadScheduler;
this.q = [];
this.observers = [];
this.isStopped = false;
this.isDisposed = false;
this.hasError = false;
this.error = null;
__super__.call(this, subscribe);
}
addProperties(ReplaySubject.prototype, Observer.prototype, {
/**
* Indicates whether the subject has observers subscribed to it.
* @returns {Boolean} Indicates whether the subject has observers subscribed to it.
*/
hasObservers: function () {
return this.observers.length > 0;
},
_trim: function (now) {
while (this.q.length > this.bufferSize) {
this.q.shift();
}
while (this.q.length > 0 && (now - this.q[0].interval) > this.windowSize) {
this.q.shift();
}
},
/**
* Notifies all subscribed observers about the arrival of the specified element in the sequence.
* @param {Mixed} value The value to send to all observers.
*/
onNext: function (value) {
checkDisposed(this);
if (this.isStopped) { return; }
var now = this.scheduler.now();
this.q.push({ interval: now, value: value });
this._trim(now);
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
var observer = os[i];
observer.onNext(value);
observer.ensureActive();
}
},
/**
* Notifies all subscribed observers about the exception.
* @param {Mixed} error The exception to send to all observers.
*/
onError: function (error) {
checkDisposed(this);
if (this.isStopped) { return; }
this.isStopped = true;
this.error = error;
this.hasError = true;
var now = this.scheduler.now();
this._trim(now);
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
var observer = os[i];
observer.onError(error);
observer.ensureActive();
}
this.observers.length = 0;
},
/**
* Notifies all subscribed observers about the end of the sequence.
*/
onCompleted: function () {
checkDisposed(this);
if (this.isStopped) { return; }
this.isStopped = true;
var now = this.scheduler.now();
this._trim(now);
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
var observer = os[i];
observer.onCompleted();
observer.ensureActive();
}
this.observers.length = 0;
},
/**
* Unsubscribe all observers and release resources.
*/
dispose: function () {
this.isDisposed = true;
this.observers = null;
}
});
return ReplaySubject;
}(Observable));
/**
* Used to pause and resume streams.
*/
Rx.Pauser = (function (__super__) {
inherits(Pauser, __super__);
function Pauser() {
__super__.call(this);
}
/**
* Pauses the underlying sequence.
*/
Pauser.prototype.pause = function () { this.onNext(false); };
/**
* Resumes the underlying sequence.
*/
Pauser.prototype.resume = function () { this.onNext(true); };
return Pauser;
}(Subject));
if (typeof define == 'function' && typeof define.amd == 'object' && define.amd) {
root.Rx = Rx;
define(function() {
return Rx;
});
} else if (freeExports && freeModule) {
// in Node.js or RingoJS
if (moduleExports) {
(freeModule.exports = Rx).Rx = Rx;
} else {
freeExports.Rx = Rx;
}
} else {
// in a browser or Rhino
root.Rx = Rx;
}
// All code before this point will be filtered from stack traces.
var rEndingLine = captureLine();
}.call(this));
|
node_modules/react-icons/md/control-point-duplicate.js | bairrada97/festival |
import React from 'react'
import Icon from 'react-icon-base'
const MdControlPointDuplicate = props => (
<Icon viewBox="0 0 40 40" {...props}>
<g><path d="m25 31.6q4.8 0 8.2-3.4t3.4-8.2-3.4-8.2-8.2-3.4-8.2 3.4-3.4 8.2 3.4 8.2 8.2 3.4z m0-26.6q6.3 0 10.6 4.4t4.4 10.6-4.4 10.6-10.6 4.4-10.6-4.4-4.4-10.6 4.4-10.6 10.6-4.4z m-21.6 15q0 3.4 1.8 6.3t4.8 4.2v3.6q-4.4-1.5-7.2-5.4t-2.8-8.7 2.8-8.7 7.2-5.4v3.5q-3 1.5-4.8 4.3t-1.8 6.3z m23.2-6.6v5h5v3.2h-5v5h-3.2v-5h-5v-3.2h5v-5h3.2z"/></g>
</Icon>
)
export default MdControlPointDuplicate
|
pages/api/step-button.js | cherniavskii/material-ui | import React from 'react';
import withRoot from 'docs/src/modules/components/withRoot';
import MarkdownDocs from 'docs/src/modules/components/MarkdownDocs';
import markdown from './step-button.md';
function Page() {
return <MarkdownDocs markdown={markdown} />;
}
export default withRoot(Page);
|
app/components/overview/index.js | wfa207/portfolio-page | 'use strict';
import React, { Component } from 'react';
import SocialBtn from '../social_button';
import { buttons } from '../../data';
export default class Overview extends Component {
constructor(props) {
super(props);
}
render() {
return (
<header>
<div className='container'>
<div className='row'>
<div className='col-lg-12'>
<img className='img-responsive' src={require('../../../assets/img/profile_icon.png')}/>
<div className='intro-text'>
<span className='name'>Wes Auyeung</span>
<hr className='star-light'/>
<span className='skills'>Web Developer - Software Engineer</span>
<ul className='list-inline'>
{ buttons.map(elem => <SocialBtn
key={elem.name}
link={elem.link}
icon={elem.name}/>) }
</ul>
</div>
</div>
</div>
</div>
</header>
);
}
}
|
app/javascript/mastodon/features/list_editor/components/edit_list_form.js | musashino205/mastodon | import React from 'react';
import { connect } from 'react-redux';
import PropTypes from 'prop-types';
import { changeListEditorTitle, submitListEditor } from '../../../actions/lists';
import IconButton from '../../../components/icon_button';
import { defineMessages, injectIntl } from 'react-intl';
const messages = defineMessages({
title: { id: 'lists.edit.submit', defaultMessage: 'Change title' },
});
const mapStateToProps = state => ({
value: state.getIn(['listEditor', 'title']),
disabled: !state.getIn(['listEditor', 'isChanged']) || !state.getIn(['listEditor', 'title']),
});
const mapDispatchToProps = dispatch => ({
onChange: value => dispatch(changeListEditorTitle(value)),
onSubmit: () => dispatch(submitListEditor(false)),
});
export default @connect(mapStateToProps, mapDispatchToProps)
@injectIntl
class ListForm extends React.PureComponent {
static propTypes = {
value: PropTypes.string.isRequired,
disabled: PropTypes.bool,
intl: PropTypes.object.isRequired,
onChange: PropTypes.func.isRequired,
onSubmit: PropTypes.func.isRequired,
};
handleChange = e => {
this.props.onChange(e.target.value);
}
handleSubmit = e => {
e.preventDefault();
this.props.onSubmit();
}
handleClick = () => {
this.props.onSubmit();
}
render () {
const { value, disabled, intl } = this.props;
const title = intl.formatMessage(messages.title);
return (
<form className='column-inline-form' onSubmit={this.handleSubmit}>
<input
className='setting-text'
value={value}
onChange={this.handleChange}
/>
<IconButton
disabled={disabled}
icon='check'
title={title}
onClick={this.handleClick}
/>
</form>
);
}
}
|
app/javascript/components/collections/list/ButtonCollectionListShowAll.js | avalonmediasystem/avalon | /*
* Copyright 2011-2022, The Trustees of Indiana University and Northwestern
* University. Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
*
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed
* under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
* --- END LICENSE_HEADER BLOCK ---
*/
import React from 'react';
import PropTypes from 'prop-types';
const ButtonCollectionListShowAll = ({
collectionsLength,
maxItems,
handleShowAll,
showAll
}) => {
if (collectionsLength > maxItems) {
return (
<button
aria-controls="collections-list-remaining-collections"
aria-expanded={showAll}
onClick={handleShowAll}
className="btn btn-link show-all"
role="button"
>
<i
className={`fa ${showAll ? 'fa-chevron-down' : 'fa-chevron-right'}`}
/>
{` Show ${showAll ? `less` : `${collectionsLength} items`}`}
</button>
);
}
return null;
};
ButtonCollectionListShowAll.propTypes = {
collectionsLength: PropTypes.number,
maxItems: PropTypes.number,
handleShowAll: PropTypes.func,
showAll: PropTypes.bool
};
export default ButtonCollectionListShowAll;
|
src/component/Name.js | munyrasirio/mss-chat | import React, { Component } from 'react';
import { StyleSheet, TouchableOpacity, Text } from 'react-native';
export default class Name extends Component {
render() {
return (
<TouchableOpacity style={styles.buttonName}>
<Text style={styles.button}>{this.props.name}</Text>
</TouchableOpacity>
);
}
}
const styles = StyleSheet.create({
buttonName: {
flex: 1,
justifyContent: 'center',
paddingLeft: 5
},
button: {
color: 'white',
fontSize: 20
}
})
Name.defaulProps = {
name: null
}
Name.propTypes = {
name: React.PropTypes.string.isRequired
} |
plugins/system/t3/base-bs3/js/jquery-1.11.2.min.js | Sunella/Restart | /*! jQuery v1.11.2 | (c) 2005, 2014 jQuery Foundation, Inc. | jquery.org/license */
!function(a,b){"object"==typeof module&&"object"==typeof module.exports?module.exports=a.document?b(a,!0):function(a){if(!a.document)throw new Error("jQuery requires a window with a document");return b(a)}:b(a)}("undefined"!=typeof window?window:this,function(a,b){var c=[],d=c.slice,e=c.concat,f=c.push,g=c.indexOf,h={},i=h.toString,j=h.hasOwnProperty,k={},l="1.11.2",m=function(a,b){return new m.fn.init(a,b)},n=/^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g,o=/^-ms-/,p=/-([\da-z])/gi,q=function(a,b){return b.toUpperCase()};m.fn=m.prototype={jquery:l,constructor:m,selector:"",length:0,toArray:function(){return d.call(this)},get:function(a){return null!=a?0>a?this[a+this.length]:this[a]:d.call(this)},pushStack:function(a){var b=m.merge(this.constructor(),a);return b.prevObject=this,b.context=this.context,b},each:function(a,b){return m.each(this,a,b)},map:function(a){return this.pushStack(m.map(this,function(b,c){return a.call(b,c,b)}))},slice:function(){return this.pushStack(d.apply(this,arguments))},first:function(){return this.eq(0)},last:function(){return this.eq(-1)},eq:function(a){var b=this.length,c=+a+(0>a?b:0);return this.pushStack(c>=0&&b>c?[this[c]]:[])},end:function(){return this.prevObject||this.constructor(null)},push:f,sort:c.sort,splice:c.splice},m.extend=m.fn.extend=function(){var a,b,c,d,e,f,g=arguments[0]||{},h=1,i=arguments.length,j=!1;for("boolean"==typeof g&&(j=g,g=arguments[h]||{},h++),"object"==typeof g||m.isFunction(g)||(g={}),h===i&&(g=this,h--);i>h;h++)if(null!=(e=arguments[h]))for(d in e)a=g[d],c=e[d],g!==c&&(j&&c&&(m.isPlainObject(c)||(b=m.isArray(c)))?(b?(b=!1,f=a&&m.isArray(a)?a:[]):f=a&&m.isPlainObject(a)?a:{},g[d]=m.extend(j,f,c)):void 0!==c&&(g[d]=c));return g},m.extend({expando:"jQuery"+(l+Math.random()).replace(/\D/g,""),isReady:!0,error:function(a){throw new Error(a)},noop:function(){},isFunction:function(a){return"function"===m.type(a)},isArray:Array.isArray||function(a){return"array"===m.type(a)},isWindow:function(a){return null!=a&&a==a.window},isNumeric:function(a){return!m.isArray(a)&&a-parseFloat(a)+1>=0},isEmptyObject:function(a){var b;for(b in a)return!1;return!0},isPlainObject:function(a){var b;if(!a||"object"!==m.type(a)||a.nodeType||m.isWindow(a))return!1;try{if(a.constructor&&!j.call(a,"constructor")&&!j.call(a.constructor.prototype,"isPrototypeOf"))return!1}catch(c){return!1}if(k.ownLast)for(b in a)return j.call(a,b);for(b in a);return void 0===b||j.call(a,b)},type:function(a){return null==a?a+"":"object"==typeof a||"function"==typeof a?h[i.call(a)]||"object":typeof a},globalEval:function(b){b&&m.trim(b)&&(a.execScript||function(b){a.eval.call(a,b)})(b)},camelCase:function(a){return a.replace(o,"ms-").replace(p,q)},nodeName:function(a,b){return a.nodeName&&a.nodeName.toLowerCase()===b.toLowerCase()},each:function(a,b,c){var d,e=0,f=a.length,g=r(a);if(c){if(g){for(;f>e;e++)if(d=b.apply(a[e],c),d===!1)break}else for(e in a)if(d=b.apply(a[e],c),d===!1)break}else if(g){for(;f>e;e++)if(d=b.call(a[e],e,a[e]),d===!1)break}else for(e in a)if(d=b.call(a[e],e,a[e]),d===!1)break;return a},trim:function(a){return null==a?"":(a+"").replace(n,"")},makeArray:function(a,b){var c=b||[];return null!=a&&(r(Object(a))?m.merge(c,"string"==typeof a?[a]:a):f.call(c,a)),c},inArray:function(a,b,c){var d;if(b){if(g)return g.call(b,a,c);for(d=b.length,c=c?0>c?Math.max(0,d+c):c:0;d>c;c++)if(c in b&&b[c]===a)return c}return-1},merge:function(a,b){var c=+b.length,d=0,e=a.length;while(c>d)a[e++]=b[d++];if(c!==c)while(void 0!==b[d])a[e++]=b[d++];return a.length=e,a},grep:function(a,b,c){for(var d,e=[],f=0,g=a.length,h=!c;g>f;f++)d=!b(a[f],f),d!==h&&e.push(a[f]);return e},map:function(a,b,c){var d,f=0,g=a.length,h=r(a),i=[];if(h)for(;g>f;f++)d=b(a[f],f,c),null!=d&&i.push(d);else for(f in a)d=b(a[f],f,c),null!=d&&i.push(d);return e.apply([],i)},guid:1,proxy:function(a,b){var c,e,f;return"string"==typeof b&&(f=a[b],b=a,a=f),m.isFunction(a)?(c=d.call(arguments,2),e=function(){return a.apply(b||this,c.concat(d.call(arguments)))},e.guid=a.guid=a.guid||m.guid++,e):void 0},now:function(){return+new Date},support:k}),m.each("Boolean Number String Function Array Date RegExp Object Error".split(" "),function(a,b){h["[object "+b+"]"]=b.toLowerCase()});function r(a){var b=a.length,c=m.type(a);return"function"===c||m.isWindow(a)?!1:1===a.nodeType&&b?!0:"array"===c||0===b||"number"==typeof b&&b>0&&b-1 in a}var s=function(a){var b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u="sizzle"+1*new Date,v=a.document,w=0,x=0,y=hb(),z=hb(),A=hb(),B=function(a,b){return a===b&&(l=!0),0},C=1<<31,D={}.hasOwnProperty,E=[],F=E.pop,G=E.push,H=E.push,I=E.slice,J=function(a,b){for(var c=0,d=a.length;d>c;c++)if(a[c]===b)return c;return-1},K="checked|selected|async|autofocus|autoplay|controls|defer|disabled|hidden|ismap|loop|multiple|open|readonly|required|scoped",L="[\\x20\\t\\r\\n\\f]",M="(?:\\\\.|[\\w-]|[^\\x00-\\xa0])+",N=M.replace("w","w#"),O="\\["+L+"*("+M+")(?:"+L+"*([*^$|!~]?=)"+L+"*(?:'((?:\\\\.|[^\\\\'])*)'|\"((?:\\\\.|[^\\\\\"])*)\"|("+N+"))|)"+L+"*\\]",P=":("+M+")(?:\\((('((?:\\\\.|[^\\\\'])*)'|\"((?:\\\\.|[^\\\\\"])*)\")|((?:\\\\.|[^\\\\()[\\]]|"+O+")*)|.*)\\)|)",Q=new RegExp(L+"+","g"),R=new RegExp("^"+L+"+|((?:^|[^\\\\])(?:\\\\.)*)"+L+"+$","g"),S=new RegExp("^"+L+"*,"+L+"*"),T=new RegExp("^"+L+"*([>+~]|"+L+")"+L+"*"),U=new RegExp("="+L+"*([^\\]'\"]*?)"+L+"*\\]","g"),V=new RegExp(P),W=new RegExp("^"+N+"$"),X={ID:new RegExp("^#("+M+")"),CLASS:new RegExp("^\\.("+M+")"),TAG:new RegExp("^("+M.replace("w","w*")+")"),ATTR:new RegExp("^"+O),PSEUDO:new RegExp("^"+P),CHILD:new RegExp("^:(only|first|last|nth|nth-last)-(child|of-type)(?:\\("+L+"*(even|odd|(([+-]|)(\\d*)n|)"+L+"*(?:([+-]|)"+L+"*(\\d+)|))"+L+"*\\)|)","i"),bool:new RegExp("^(?:"+K+")$","i"),needsContext:new RegExp("^"+L+"*[>+~]|:(even|odd|eq|gt|lt|nth|first|last)(?:\\("+L+"*((?:-\\d)?\\d*)"+L+"*\\)|)(?=[^-]|$)","i")},Y=/^(?:input|select|textarea|button)$/i,Z=/^h\d$/i,$=/^[^{]+\{\s*\[native \w/,_=/^(?:#([\w-]+)|(\w+)|\.([\w-]+))$/,ab=/[+~]/,bb=/'|\\/g,cb=new RegExp("\\\\([\\da-f]{1,6}"+L+"?|("+L+")|.)","ig"),db=function(a,b,c){var d="0x"+b-65536;return d!==d||c?b:0>d?String.fromCharCode(d+65536):String.fromCharCode(d>>10|55296,1023&d|56320)},eb=function(){m()};try{H.apply(E=I.call(v.childNodes),v.childNodes),E[v.childNodes.length].nodeType}catch(fb){H={apply:E.length?function(a,b){G.apply(a,I.call(b))}:function(a,b){var c=a.length,d=0;while(a[c++]=b[d++]);a.length=c-1}}}function gb(a,b,d,e){var f,h,j,k,l,o,r,s,w,x;if((b?b.ownerDocument||b:v)!==n&&m(b),b=b||n,d=d||[],k=b.nodeType,"string"!=typeof a||!a||1!==k&&9!==k&&11!==k)return d;if(!e&&p){if(11!==k&&(f=_.exec(a)))if(j=f[1]){if(9===k){if(h=b.getElementById(j),!h||!h.parentNode)return d;if(h.id===j)return d.push(h),d}else if(b.ownerDocument&&(h=b.ownerDocument.getElementById(j))&&t(b,h)&&h.id===j)return d.push(h),d}else{if(f[2])return H.apply(d,b.getElementsByTagName(a)),d;if((j=f[3])&&c.getElementsByClassName)return H.apply(d,b.getElementsByClassName(j)),d}if(c.qsa&&(!q||!q.test(a))){if(s=r=u,w=b,x=1!==k&&a,1===k&&"object"!==b.nodeName.toLowerCase()){o=g(a),(r=b.getAttribute("id"))?s=r.replace(bb,"\\$&"):b.setAttribute("id",s),s="[id='"+s+"'] ",l=o.length;while(l--)o[l]=s+rb(o[l]);w=ab.test(a)&&pb(b.parentNode)||b,x=o.join(",")}if(x)try{return H.apply(d,w.querySelectorAll(x)),d}catch(y){}finally{r||b.removeAttribute("id")}}}return i(a.replace(R,"$1"),b,d,e)}function hb(){var a=[];function b(c,e){return a.push(c+" ")>d.cacheLength&&delete b[a.shift()],b[c+" "]=e}return b}function ib(a){return a[u]=!0,a}function jb(a){var b=n.createElement("div");try{return!!a(b)}catch(c){return!1}finally{b.parentNode&&b.parentNode.removeChild(b),b=null}}function kb(a,b){var c=a.split("|"),e=a.length;while(e--)d.attrHandle[c[e]]=b}function lb(a,b){var c=b&&a,d=c&&1===a.nodeType&&1===b.nodeType&&(~b.sourceIndex||C)-(~a.sourceIndex||C);if(d)return d;if(c)while(c=c.nextSibling)if(c===b)return-1;return a?1:-1}function mb(a){return function(b){var c=b.nodeName.toLowerCase();return"input"===c&&b.type===a}}function nb(a){return function(b){var c=b.nodeName.toLowerCase();return("input"===c||"button"===c)&&b.type===a}}function ob(a){return ib(function(b){return b=+b,ib(function(c,d){var e,f=a([],c.length,b),g=f.length;while(g--)c[e=f[g]]&&(c[e]=!(d[e]=c[e]))})})}function pb(a){return a&&"undefined"!=typeof a.getElementsByTagName&&a}c=gb.support={},f=gb.isXML=function(a){var b=a&&(a.ownerDocument||a).documentElement;return b?"HTML"!==b.nodeName:!1},m=gb.setDocument=function(a){var b,e,g=a?a.ownerDocument||a:v;return g!==n&&9===g.nodeType&&g.documentElement?(n=g,o=g.documentElement,e=g.defaultView,e&&e!==e.top&&(e.addEventListener?e.addEventListener("unload",eb,!1):e.attachEvent&&e.attachEvent("onunload",eb)),p=!f(g),c.attributes=jb(function(a){return a.className="i",!a.getAttribute("className")}),c.getElementsByTagName=jb(function(a){return a.appendChild(g.createComment("")),!a.getElementsByTagName("*").length}),c.getElementsByClassName=$.test(g.getElementsByClassName),c.getById=jb(function(a){return o.appendChild(a).id=u,!g.getElementsByName||!g.getElementsByName(u).length}),c.getById?(d.find.ID=function(a,b){if("undefined"!=typeof b.getElementById&&p){var c=b.getElementById(a);return c&&c.parentNode?[c]:[]}},d.filter.ID=function(a){var b=a.replace(cb,db);return function(a){return a.getAttribute("id")===b}}):(delete d.find.ID,d.filter.ID=function(a){var b=a.replace(cb,db);return function(a){var c="undefined"!=typeof a.getAttributeNode&&a.getAttributeNode("id");return c&&c.value===b}}),d.find.TAG=c.getElementsByTagName?function(a,b){return"undefined"!=typeof b.getElementsByTagName?b.getElementsByTagName(a):c.qsa?b.querySelectorAll(a):void 0}:function(a,b){var c,d=[],e=0,f=b.getElementsByTagName(a);if("*"===a){while(c=f[e++])1===c.nodeType&&d.push(c);return d}return f},d.find.CLASS=c.getElementsByClassName&&function(a,b){return p?b.getElementsByClassName(a):void 0},r=[],q=[],(c.qsa=$.test(g.querySelectorAll))&&(jb(function(a){o.appendChild(a).innerHTML="<a id='"+u+"'></a><select id='"+u+"-\f]' msallowcapture=''><option selected=''></option></select>",a.querySelectorAll("[msallowcapture^='']").length&&q.push("[*^$]="+L+"*(?:''|\"\")"),a.querySelectorAll("[selected]").length||q.push("\\["+L+"*(?:value|"+K+")"),a.querySelectorAll("[id~="+u+"-]").length||q.push("~="),a.querySelectorAll(":checked").length||q.push(":checked"),a.querySelectorAll("a#"+u+"+*").length||q.push(".#.+[+~]")}),jb(function(a){var b=g.createElement("input");b.setAttribute("type","hidden"),a.appendChild(b).setAttribute("name","D"),a.querySelectorAll("[name=d]").length&&q.push("name"+L+"*[*^$|!~]?="),a.querySelectorAll(":enabled").length||q.push(":enabled",":disabled"),a.querySelectorAll("*,:x"),q.push(",.*:")})),(c.matchesSelector=$.test(s=o.matches||o.webkitMatchesSelector||o.mozMatchesSelector||o.oMatchesSelector||o.msMatchesSelector))&&jb(function(a){c.disconnectedMatch=s.call(a,"div"),s.call(a,"[s!='']:x"),r.push("!=",P)}),q=q.length&&new RegExp(q.join("|")),r=r.length&&new RegExp(r.join("|")),b=$.test(o.compareDocumentPosition),t=b||$.test(o.contains)?function(a,b){var c=9===a.nodeType?a.documentElement:a,d=b&&b.parentNode;return a===d||!(!d||1!==d.nodeType||!(c.contains?c.contains(d):a.compareDocumentPosition&&16&a.compareDocumentPosition(d)))}:function(a,b){if(b)while(b=b.parentNode)if(b===a)return!0;return!1},B=b?function(a,b){if(a===b)return l=!0,0;var d=!a.compareDocumentPosition-!b.compareDocumentPosition;return d?d:(d=(a.ownerDocument||a)===(b.ownerDocument||b)?a.compareDocumentPosition(b):1,1&d||!c.sortDetached&&b.compareDocumentPosition(a)===d?a===g||a.ownerDocument===v&&t(v,a)?-1:b===g||b.ownerDocument===v&&t(v,b)?1:k?J(k,a)-J(k,b):0:4&d?-1:1)}:function(a,b){if(a===b)return l=!0,0;var c,d=0,e=a.parentNode,f=b.parentNode,h=[a],i=[b];if(!e||!f)return a===g?-1:b===g?1:e?-1:f?1:k?J(k,a)-J(k,b):0;if(e===f)return lb(a,b);c=a;while(c=c.parentNode)h.unshift(c);c=b;while(c=c.parentNode)i.unshift(c);while(h[d]===i[d])d++;return d?lb(h[d],i[d]):h[d]===v?-1:i[d]===v?1:0},g):n},gb.matches=function(a,b){return gb(a,null,null,b)},gb.matchesSelector=function(a,b){if((a.ownerDocument||a)!==n&&m(a),b=b.replace(U,"='$1']"),!(!c.matchesSelector||!p||r&&r.test(b)||q&&q.test(b)))try{var d=s.call(a,b);if(d||c.disconnectedMatch||a.document&&11!==a.document.nodeType)return d}catch(e){}return gb(b,n,null,[a]).length>0},gb.contains=function(a,b){return(a.ownerDocument||a)!==n&&m(a),t(a,b)},gb.attr=function(a,b){(a.ownerDocument||a)!==n&&m(a);var e=d.attrHandle[b.toLowerCase()],f=e&&D.call(d.attrHandle,b.toLowerCase())?e(a,b,!p):void 0;return void 0!==f?f:c.attributes||!p?a.getAttribute(b):(f=a.getAttributeNode(b))&&f.specified?f.value:null},gb.error=function(a){throw new Error("Syntax error, unrecognized expression: "+a)},gb.uniqueSort=function(a){var b,d=[],e=0,f=0;if(l=!c.detectDuplicates,k=!c.sortStable&&a.slice(0),a.sort(B),l){while(b=a[f++])b===a[f]&&(e=d.push(f));while(e--)a.splice(d[e],1)}return k=null,a},e=gb.getText=function(a){var b,c="",d=0,f=a.nodeType;if(f){if(1===f||9===f||11===f){if("string"==typeof a.textContent)return a.textContent;for(a=a.firstChild;a;a=a.nextSibling)c+=e(a)}else if(3===f||4===f)return a.nodeValue}else while(b=a[d++])c+=e(b);return c},d=gb.selectors={cacheLength:50,createPseudo:ib,match:X,attrHandle:{},find:{},relative:{">":{dir:"parentNode",first:!0}," ":{dir:"parentNode"},"+":{dir:"previousSibling",first:!0},"~":{dir:"previousSibling"}},preFilter:{ATTR:function(a){return a[1]=a[1].replace(cb,db),a[3]=(a[3]||a[4]||a[5]||"").replace(cb,db),"~="===a[2]&&(a[3]=" "+a[3]+" "),a.slice(0,4)},CHILD:function(a){return a[1]=a[1].toLowerCase(),"nth"===a[1].slice(0,3)?(a[3]||gb.error(a[0]),a[4]=+(a[4]?a[5]+(a[6]||1):2*("even"===a[3]||"odd"===a[3])),a[5]=+(a[7]+a[8]||"odd"===a[3])):a[3]&&gb.error(a[0]),a},PSEUDO:function(a){var b,c=!a[6]&&a[2];return X.CHILD.test(a[0])?null:(a[3]?a[2]=a[4]||a[5]||"":c&&V.test(c)&&(b=g(c,!0))&&(b=c.indexOf(")",c.length-b)-c.length)&&(a[0]=a[0].slice(0,b),a[2]=c.slice(0,b)),a.slice(0,3))}},filter:{TAG:function(a){var b=a.replace(cb,db).toLowerCase();return"*"===a?function(){return!0}:function(a){return a.nodeName&&a.nodeName.toLowerCase()===b}},CLASS:function(a){var b=y[a+" "];return b||(b=new RegExp("(^|"+L+")"+a+"("+L+"|$)"))&&y(a,function(a){return b.test("string"==typeof a.className&&a.className||"undefined"!=typeof a.getAttribute&&a.getAttribute("class")||"")})},ATTR:function(a,b,c){return function(d){var e=gb.attr(d,a);return null==e?"!="===b:b?(e+="","="===b?e===c:"!="===b?e!==c:"^="===b?c&&0===e.indexOf(c):"*="===b?c&&e.indexOf(c)>-1:"$="===b?c&&e.slice(-c.length)===c:"~="===b?(" "+e.replace(Q," ")+" ").indexOf(c)>-1:"|="===b?e===c||e.slice(0,c.length+1)===c+"-":!1):!0}},CHILD:function(a,b,c,d,e){var f="nth"!==a.slice(0,3),g="last"!==a.slice(-4),h="of-type"===b;return 1===d&&0===e?function(a){return!!a.parentNode}:function(b,c,i){var j,k,l,m,n,o,p=f!==g?"nextSibling":"previousSibling",q=b.parentNode,r=h&&b.nodeName.toLowerCase(),s=!i&&!h;if(q){if(f){while(p){l=b;while(l=l[p])if(h?l.nodeName.toLowerCase()===r:1===l.nodeType)return!1;o=p="only"===a&&!o&&"nextSibling"}return!0}if(o=[g?q.firstChild:q.lastChild],g&&s){k=q[u]||(q[u]={}),j=k[a]||[],n=j[0]===w&&j[1],m=j[0]===w&&j[2],l=n&&q.childNodes[n];while(l=++n&&l&&l[p]||(m=n=0)||o.pop())if(1===l.nodeType&&++m&&l===b){k[a]=[w,n,m];break}}else if(s&&(j=(b[u]||(b[u]={}))[a])&&j[0]===w)m=j[1];else while(l=++n&&l&&l[p]||(m=n=0)||o.pop())if((h?l.nodeName.toLowerCase()===r:1===l.nodeType)&&++m&&(s&&((l[u]||(l[u]={}))[a]=[w,m]),l===b))break;return m-=e,m===d||m%d===0&&m/d>=0}}},PSEUDO:function(a,b){var c,e=d.pseudos[a]||d.setFilters[a.toLowerCase()]||gb.error("unsupported pseudo: "+a);return e[u]?e(b):e.length>1?(c=[a,a,"",b],d.setFilters.hasOwnProperty(a.toLowerCase())?ib(function(a,c){var d,f=e(a,b),g=f.length;while(g--)d=J(a,f[g]),a[d]=!(c[d]=f[g])}):function(a){return e(a,0,c)}):e}},pseudos:{not:ib(function(a){var b=[],c=[],d=h(a.replace(R,"$1"));return d[u]?ib(function(a,b,c,e){var f,g=d(a,null,e,[]),h=a.length;while(h--)(f=g[h])&&(a[h]=!(b[h]=f))}):function(a,e,f){return b[0]=a,d(b,null,f,c),b[0]=null,!c.pop()}}),has:ib(function(a){return function(b){return gb(a,b).length>0}}),contains:ib(function(a){return a=a.replace(cb,db),function(b){return(b.textContent||b.innerText||e(b)).indexOf(a)>-1}}),lang:ib(function(a){return W.test(a||"")||gb.error("unsupported lang: "+a),a=a.replace(cb,db).toLowerCase(),function(b){var c;do if(c=p?b.lang:b.getAttribute("xml:lang")||b.getAttribute("lang"))return c=c.toLowerCase(),c===a||0===c.indexOf(a+"-");while((b=b.parentNode)&&1===b.nodeType);return!1}}),target:function(b){var c=a.location&&a.location.hash;return c&&c.slice(1)===b.id},root:function(a){return a===o},focus:function(a){return a===n.activeElement&&(!n.hasFocus||n.hasFocus())&&!!(a.type||a.href||~a.tabIndex)},enabled:function(a){return a.disabled===!1},disabled:function(a){return a.disabled===!0},checked:function(a){var b=a.nodeName.toLowerCase();return"input"===b&&!!a.checked||"option"===b&&!!a.selected},selected:function(a){return a.parentNode&&a.parentNode.selectedIndex,a.selected===!0},empty:function(a){for(a=a.firstChild;a;a=a.nextSibling)if(a.nodeType<6)return!1;return!0},parent:function(a){return!d.pseudos.empty(a)},header:function(a){return Z.test(a.nodeName)},input:function(a){return Y.test(a.nodeName)},button:function(a){var b=a.nodeName.toLowerCase();return"input"===b&&"button"===a.type||"button"===b},text:function(a){var b;return"input"===a.nodeName.toLowerCase()&&"text"===a.type&&(null==(b=a.getAttribute("type"))||"text"===b.toLowerCase())},first:ob(function(){return[0]}),last:ob(function(a,b){return[b-1]}),eq:ob(function(a,b,c){return[0>c?c+b:c]}),even:ob(function(a,b){for(var c=0;b>c;c+=2)a.push(c);return a}),odd:ob(function(a,b){for(var c=1;b>c;c+=2)a.push(c);return a}),lt:ob(function(a,b,c){for(var d=0>c?c+b:c;--d>=0;)a.push(d);return a}),gt:ob(function(a,b,c){for(var d=0>c?c+b:c;++d<b;)a.push(d);return a})}},d.pseudos.nth=d.pseudos.eq;for(b in{radio:!0,checkbox:!0,file:!0,password:!0,image:!0})d.pseudos[b]=mb(b);for(b in{submit:!0,reset:!0})d.pseudos[b]=nb(b);function qb(){}qb.prototype=d.filters=d.pseudos,d.setFilters=new qb,g=gb.tokenize=function(a,b){var c,e,f,g,h,i,j,k=z[a+" "];if(k)return b?0:k.slice(0);h=a,i=[],j=d.preFilter;while(h){(!c||(e=S.exec(h)))&&(e&&(h=h.slice(e[0].length)||h),i.push(f=[])),c=!1,(e=T.exec(h))&&(c=e.shift(),f.push({value:c,type:e[0].replace(R," ")}),h=h.slice(c.length));for(g in d.filter)!(e=X[g].exec(h))||j[g]&&!(e=j[g](e))||(c=e.shift(),f.push({value:c,type:g,matches:e}),h=h.slice(c.length));if(!c)break}return b?h.length:h?gb.error(a):z(a,i).slice(0)};function rb(a){for(var b=0,c=a.length,d="";c>b;b++)d+=a[b].value;return d}function sb(a,b,c){var d=b.dir,e=c&&"parentNode"===d,f=x++;return b.first?function(b,c,f){while(b=b[d])if(1===b.nodeType||e)return a(b,c,f)}:function(b,c,g){var h,i,j=[w,f];if(g){while(b=b[d])if((1===b.nodeType||e)&&a(b,c,g))return!0}else while(b=b[d])if(1===b.nodeType||e){if(i=b[u]||(b[u]={}),(h=i[d])&&h[0]===w&&h[1]===f)return j[2]=h[2];if(i[d]=j,j[2]=a(b,c,g))return!0}}}function tb(a){return a.length>1?function(b,c,d){var e=a.length;while(e--)if(!a[e](b,c,d))return!1;return!0}:a[0]}function ub(a,b,c){for(var d=0,e=b.length;e>d;d++)gb(a,b[d],c);return c}function vb(a,b,c,d,e){for(var f,g=[],h=0,i=a.length,j=null!=b;i>h;h++)(f=a[h])&&(!c||c(f,d,e))&&(g.push(f),j&&b.push(h));return g}function wb(a,b,c,d,e,f){return d&&!d[u]&&(d=wb(d)),e&&!e[u]&&(e=wb(e,f)),ib(function(f,g,h,i){var j,k,l,m=[],n=[],o=g.length,p=f||ub(b||"*",h.nodeType?[h]:h,[]),q=!a||!f&&b?p:vb(p,m,a,h,i),r=c?e||(f?a:o||d)?[]:g:q;if(c&&c(q,r,h,i),d){j=vb(r,n),d(j,[],h,i),k=j.length;while(k--)(l=j[k])&&(r[n[k]]=!(q[n[k]]=l))}if(f){if(e||a){if(e){j=[],k=r.length;while(k--)(l=r[k])&&j.push(q[k]=l);e(null,r=[],j,i)}k=r.length;while(k--)(l=r[k])&&(j=e?J(f,l):m[k])>-1&&(f[j]=!(g[j]=l))}}else r=vb(r===g?r.splice(o,r.length):r),e?e(null,g,r,i):H.apply(g,r)})}function xb(a){for(var b,c,e,f=a.length,g=d.relative[a[0].type],h=g||d.relative[" "],i=g?1:0,k=sb(function(a){return a===b},h,!0),l=sb(function(a){return J(b,a)>-1},h,!0),m=[function(a,c,d){var e=!g&&(d||c!==j)||((b=c).nodeType?k(a,c,d):l(a,c,d));return b=null,e}];f>i;i++)if(c=d.relative[a[i].type])m=[sb(tb(m),c)];else{if(c=d.filter[a[i].type].apply(null,a[i].matches),c[u]){for(e=++i;f>e;e++)if(d.relative[a[e].type])break;return wb(i>1&&tb(m),i>1&&rb(a.slice(0,i-1).concat({value:" "===a[i-2].type?"*":""})).replace(R,"$1"),c,e>i&&xb(a.slice(i,e)),f>e&&xb(a=a.slice(e)),f>e&&rb(a))}m.push(c)}return tb(m)}function yb(a,b){var c=b.length>0,e=a.length>0,f=function(f,g,h,i,k){var l,m,o,p=0,q="0",r=f&&[],s=[],t=j,u=f||e&&d.find.TAG("*",k),v=w+=null==t?1:Math.random()||.1,x=u.length;for(k&&(j=g!==n&&g);q!==x&&null!=(l=u[q]);q++){if(e&&l){m=0;while(o=a[m++])if(o(l,g,h)){i.push(l);break}k&&(w=v)}c&&((l=!o&&l)&&p--,f&&r.push(l))}if(p+=q,c&&q!==p){m=0;while(o=b[m++])o(r,s,g,h);if(f){if(p>0)while(q--)r[q]||s[q]||(s[q]=F.call(i));s=vb(s)}H.apply(i,s),k&&!f&&s.length>0&&p+b.length>1&&gb.uniqueSort(i)}return k&&(w=v,j=t),r};return c?ib(f):f}return h=gb.compile=function(a,b){var c,d=[],e=[],f=A[a+" "];if(!f){b||(b=g(a)),c=b.length;while(c--)f=xb(b[c]),f[u]?d.push(f):e.push(f);f=A(a,yb(e,d)),f.selector=a}return f},i=gb.select=function(a,b,e,f){var i,j,k,l,m,n="function"==typeof a&&a,o=!f&&g(a=n.selector||a);if(e=e||[],1===o.length){if(j=o[0]=o[0].slice(0),j.length>2&&"ID"===(k=j[0]).type&&c.getById&&9===b.nodeType&&p&&d.relative[j[1].type]){if(b=(d.find.ID(k.matches[0].replace(cb,db),b)||[])[0],!b)return e;n&&(b=b.parentNode),a=a.slice(j.shift().value.length)}i=X.needsContext.test(a)?0:j.length;while(i--){if(k=j[i],d.relative[l=k.type])break;if((m=d.find[l])&&(f=m(k.matches[0].replace(cb,db),ab.test(j[0].type)&&pb(b.parentNode)||b))){if(j.splice(i,1),a=f.length&&rb(j),!a)return H.apply(e,f),e;break}}}return(n||h(a,o))(f,b,!p,e,ab.test(a)&&pb(b.parentNode)||b),e},c.sortStable=u.split("").sort(B).join("")===u,c.detectDuplicates=!!l,m(),c.sortDetached=jb(function(a){return 1&a.compareDocumentPosition(n.createElement("div"))}),jb(function(a){return a.innerHTML="<a href='#'></a>","#"===a.firstChild.getAttribute("href")})||kb("type|href|height|width",function(a,b,c){return c?void 0:a.getAttribute(b,"type"===b.toLowerCase()?1:2)}),c.attributes&&jb(function(a){return a.innerHTML="<input/>",a.firstChild.setAttribute("value",""),""===a.firstChild.getAttribute("value")})||kb("value",function(a,b,c){return c||"input"!==a.nodeName.toLowerCase()?void 0:a.defaultValue}),jb(function(a){return null==a.getAttribute("disabled")})||kb(K,function(a,b,c){var d;return c?void 0:a[b]===!0?b.toLowerCase():(d=a.getAttributeNode(b))&&d.specified?d.value:null}),gb}(a);m.find=s,m.expr=s.selectors,m.expr[":"]=m.expr.pseudos,m.unique=s.uniqueSort,m.text=s.getText,m.isXMLDoc=s.isXML,m.contains=s.contains;var t=m.expr.match.needsContext,u=/^<(\w+)\s*\/?>(?:<\/\1>|)$/,v=/^.[^:#\[\.,]*$/;function w(a,b,c){if(m.isFunction(b))return m.grep(a,function(a,d){return!!b.call(a,d,a)!==c});if(b.nodeType)return m.grep(a,function(a){return a===b!==c});if("string"==typeof b){if(v.test(b))return m.filter(b,a,c);b=m.filter(b,a)}return m.grep(a,function(a){return m.inArray(a,b)>=0!==c})}m.filter=function(a,b,c){var d=b[0];return c&&(a=":not("+a+")"),1===b.length&&1===d.nodeType?m.find.matchesSelector(d,a)?[d]:[]:m.find.matches(a,m.grep(b,function(a){return 1===a.nodeType}))},m.fn.extend({find:function(a){var b,c=[],d=this,e=d.length;if("string"!=typeof a)return this.pushStack(m(a).filter(function(){for(b=0;e>b;b++)if(m.contains(d[b],this))return!0}));for(b=0;e>b;b++)m.find(a,d[b],c);return c=this.pushStack(e>1?m.unique(c):c),c.selector=this.selector?this.selector+" "+a:a,c},filter:function(a){return this.pushStack(w(this,a||[],!1))},not:function(a){return this.pushStack(w(this,a||[],!0))},is:function(a){return!!w(this,"string"==typeof a&&t.test(a)?m(a):a||[],!1).length}});var x,y=a.document,z=/^(?:\s*(<[\w\W]+>)[^>]*|#([\w-]*))$/,A=m.fn.init=function(a,b){var c,d;if(!a)return this;if("string"==typeof a){if(c="<"===a.charAt(0)&&">"===a.charAt(a.length-1)&&a.length>=3?[null,a,null]:z.exec(a),!c||!c[1]&&b)return!b||b.jquery?(b||x).find(a):this.constructor(b).find(a);if(c[1]){if(b=b instanceof m?b[0]:b,m.merge(this,m.parseHTML(c[1],b&&b.nodeType?b.ownerDocument||b:y,!0)),u.test(c[1])&&m.isPlainObject(b))for(c in b)m.isFunction(this[c])?this[c](b[c]):this.attr(c,b[c]);return this}if(d=y.getElementById(c[2]),d&&d.parentNode){if(d.id!==c[2])return x.find(a);this.length=1,this[0]=d}return this.context=y,this.selector=a,this}return a.nodeType?(this.context=this[0]=a,this.length=1,this):m.isFunction(a)?"undefined"!=typeof x.ready?x.ready(a):a(m):(void 0!==a.selector&&(this.selector=a.selector,this.context=a.context),m.makeArray(a,this))};A.prototype=m.fn,x=m(y);var B=/^(?:parents|prev(?:Until|All))/,C={children:!0,contents:!0,next:!0,prev:!0};m.extend({dir:function(a,b,c){var d=[],e=a[b];while(e&&9!==e.nodeType&&(void 0===c||1!==e.nodeType||!m(e).is(c)))1===e.nodeType&&d.push(e),e=e[b];return d},sibling:function(a,b){for(var c=[];a;a=a.nextSibling)1===a.nodeType&&a!==b&&c.push(a);return c}}),m.fn.extend({has:function(a){var b,c=m(a,this),d=c.length;return this.filter(function(){for(b=0;d>b;b++)if(m.contains(this,c[b]))return!0})},closest:function(a,b){for(var c,d=0,e=this.length,f=[],g=t.test(a)||"string"!=typeof a?m(a,b||this.context):0;e>d;d++)for(c=this[d];c&&c!==b;c=c.parentNode)if(c.nodeType<11&&(g?g.index(c)>-1:1===c.nodeType&&m.find.matchesSelector(c,a))){f.push(c);break}return this.pushStack(f.length>1?m.unique(f):f)},index:function(a){return a?"string"==typeof a?m.inArray(this[0],m(a)):m.inArray(a.jquery?a[0]:a,this):this[0]&&this[0].parentNode?this.first().prevAll().length:-1},add:function(a,b){return this.pushStack(m.unique(m.merge(this.get(),m(a,b))))},addBack:function(a){return this.add(null==a?this.prevObject:this.prevObject.filter(a))}});function D(a,b){do a=a[b];while(a&&1!==a.nodeType);return a}m.each({parent:function(a){var b=a.parentNode;return b&&11!==b.nodeType?b:null},parents:function(a){return m.dir(a,"parentNode")},parentsUntil:function(a,b,c){return m.dir(a,"parentNode",c)},next:function(a){return D(a,"nextSibling")},prev:function(a){return D(a,"previousSibling")},nextAll:function(a){return m.dir(a,"nextSibling")},prevAll:function(a){return m.dir(a,"previousSibling")},nextUntil:function(a,b,c){return m.dir(a,"nextSibling",c)},prevUntil:function(a,b,c){return m.dir(a,"previousSibling",c)},siblings:function(a){return m.sibling((a.parentNode||{}).firstChild,a)},children:function(a){return m.sibling(a.firstChild)},contents:function(a){return m.nodeName(a,"iframe")?a.contentDocument||a.contentWindow.document:m.merge([],a.childNodes)}},function(a,b){m.fn[a]=function(c,d){var e=m.map(this,b,c);return"Until"!==a.slice(-5)&&(d=c),d&&"string"==typeof d&&(e=m.filter(d,e)),this.length>1&&(C[a]||(e=m.unique(e)),B.test(a)&&(e=e.reverse())),this.pushStack(e)}});var E=/\S+/g,F={};function G(a){var b=F[a]={};return m.each(a.match(E)||[],function(a,c){b[c]=!0}),b}m.Callbacks=function(a){a="string"==typeof a?F[a]||G(a):m.extend({},a);var b,c,d,e,f,g,h=[],i=!a.once&&[],j=function(l){for(c=a.memory&&l,d=!0,f=g||0,g=0,e=h.length,b=!0;h&&e>f;f++)if(h[f].apply(l[0],l[1])===!1&&a.stopOnFalse){c=!1;break}b=!1,h&&(i?i.length&&j(i.shift()):c?h=[]:k.disable())},k={add:function(){if(h){var d=h.length;!function f(b){m.each(b,function(b,c){var d=m.type(c);"function"===d?a.unique&&k.has(c)||h.push(c):c&&c.length&&"string"!==d&&f(c)})}(arguments),b?e=h.length:c&&(g=d,j(c))}return this},remove:function(){return h&&m.each(arguments,function(a,c){var d;while((d=m.inArray(c,h,d))>-1)h.splice(d,1),b&&(e>=d&&e--,f>=d&&f--)}),this},has:function(a){return a?m.inArray(a,h)>-1:!(!h||!h.length)},empty:function(){return h=[],e=0,this},disable:function(){return h=i=c=void 0,this},disabled:function(){return!h},lock:function(){return i=void 0,c||k.disable(),this},locked:function(){return!i},fireWith:function(a,c){return!h||d&&!i||(c=c||[],c=[a,c.slice?c.slice():c],b?i.push(c):j(c)),this},fire:function(){return k.fireWith(this,arguments),this},fired:function(){return!!d}};return k},m.extend({Deferred:function(a){var b=[["resolve","done",m.Callbacks("once memory"),"resolved"],["reject","fail",m.Callbacks("once memory"),"rejected"],["notify","progress",m.Callbacks("memory")]],c="pending",d={state:function(){return c},always:function(){return e.done(arguments).fail(arguments),this},then:function(){var a=arguments;return m.Deferred(function(c){m.each(b,function(b,f){var g=m.isFunction(a[b])&&a[b];e[f[1]](function(){var a=g&&g.apply(this,arguments);a&&m.isFunction(a.promise)?a.promise().done(c.resolve).fail(c.reject).progress(c.notify):c[f[0]+"With"](this===d?c.promise():this,g?[a]:arguments)})}),a=null}).promise()},promise:function(a){return null!=a?m.extend(a,d):d}},e={};return d.pipe=d.then,m.each(b,function(a,f){var g=f[2],h=f[3];d[f[1]]=g.add,h&&g.add(function(){c=h},b[1^a][2].disable,b[2][2].lock),e[f[0]]=function(){return e[f[0]+"With"](this===e?d:this,arguments),this},e[f[0]+"With"]=g.fireWith}),d.promise(e),a&&a.call(e,e),e},when:function(a){var b=0,c=d.call(arguments),e=c.length,f=1!==e||a&&m.isFunction(a.promise)?e:0,g=1===f?a:m.Deferred(),h=function(a,b,c){return function(e){b[a]=this,c[a]=arguments.length>1?d.call(arguments):e,c===i?g.notifyWith(b,c):--f||g.resolveWith(b,c)}},i,j,k;if(e>1)for(i=new Array(e),j=new Array(e),k=new Array(e);e>b;b++)c[b]&&m.isFunction(c[b].promise)?c[b].promise().done(h(b,k,c)).fail(g.reject).progress(h(b,j,i)):--f;return f||g.resolveWith(k,c),g.promise()}});var H;m.fn.ready=function(a){return m.ready.promise().done(a),this},m.extend({isReady:!1,readyWait:1,holdReady:function(a){a?m.readyWait++:m.ready(!0)},ready:function(a){if(a===!0?!--m.readyWait:!m.isReady){if(!y.body)return setTimeout(m.ready);m.isReady=!0,a!==!0&&--m.readyWait>0||(H.resolveWith(y,[m]),m.fn.triggerHandler&&(m(y).triggerHandler("ready"),m(y).off("ready")))}}});function I(){y.addEventListener?(y.removeEventListener("DOMContentLoaded",J,!1),a.removeEventListener("load",J,!1)):(y.detachEvent("onreadystatechange",J),a.detachEvent("onload",J))}function J(){(y.addEventListener||"load"===event.type||"complete"===y.readyState)&&(I(),m.ready())}m.ready.promise=function(b){if(!H)if(H=m.Deferred(),"complete"===y.readyState)setTimeout(m.ready);else if(y.addEventListener)y.addEventListener("DOMContentLoaded",J,!1),a.addEventListener("load",J,!1);else{y.attachEvent("onreadystatechange",J),a.attachEvent("onload",J);var c=!1;try{c=null==a.frameElement&&y.documentElement}catch(d){}c&&c.doScroll&&!function e(){if(!m.isReady){try{c.doScroll("left")}catch(a){return setTimeout(e,50)}I(),m.ready()}}()}return H.promise(b)};var K="undefined",L;for(L in m(k))break;k.ownLast="0"!==L,k.inlineBlockNeedsLayout=!1,m(function(){var a,b,c,d;c=y.getElementsByTagName("body")[0],c&&c.style&&(b=y.createElement("div"),d=y.createElement("div"),d.style.cssText="position:absolute;border:0;width:0;height:0;top:0;left:-9999px",c.appendChild(d).appendChild(b),typeof b.style.zoom!==K&&(b.style.cssText="display:inline;margin:0;border:0;padding:1px;width:1px;zoom:1",k.inlineBlockNeedsLayout=a=3===b.offsetWidth,a&&(c.style.zoom=1)),c.removeChild(d))}),function(){var a=y.createElement("div");if(null==k.deleteExpando){k.deleteExpando=!0;try{delete a.test}catch(b){k.deleteExpando=!1}}a=null}(),m.acceptData=function(a){var b=m.noData[(a.nodeName+" ").toLowerCase()],c=+a.nodeType||1;return 1!==c&&9!==c?!1:!b||b!==!0&&a.getAttribute("classid")===b};var M=/^(?:\{[\w\W]*\}|\[[\w\W]*\])$/,N=/([A-Z])/g;function O(a,b,c){if(void 0===c&&1===a.nodeType){var d="data-"+b.replace(N,"-$1").toLowerCase();if(c=a.getAttribute(d),"string"==typeof c){try{c="true"===c?!0:"false"===c?!1:"null"===c?null:+c+""===c?+c:M.test(c)?m.parseJSON(c):c}catch(e){}m.data(a,b,c)}else c=void 0}return c}function P(a){var b;for(b in a)if(("data"!==b||!m.isEmptyObject(a[b]))&&"toJSON"!==b)return!1;
return!0}function Q(a,b,d,e){if(m.acceptData(a)){var f,g,h=m.expando,i=a.nodeType,j=i?m.cache:a,k=i?a[h]:a[h]&&h;if(k&&j[k]&&(e||j[k].data)||void 0!==d||"string"!=typeof b)return k||(k=i?a[h]=c.pop()||m.guid++:h),j[k]||(j[k]=i?{}:{toJSON:m.noop}),("object"==typeof b||"function"==typeof b)&&(e?j[k]=m.extend(j[k],b):j[k].data=m.extend(j[k].data,b)),g=j[k],e||(g.data||(g.data={}),g=g.data),void 0!==d&&(g[m.camelCase(b)]=d),"string"==typeof b?(f=g[b],null==f&&(f=g[m.camelCase(b)])):f=g,f}}function R(a,b,c){if(m.acceptData(a)){var d,e,f=a.nodeType,g=f?m.cache:a,h=f?a[m.expando]:m.expando;if(g[h]){if(b&&(d=c?g[h]:g[h].data)){m.isArray(b)?b=b.concat(m.map(b,m.camelCase)):b in d?b=[b]:(b=m.camelCase(b),b=b in d?[b]:b.split(" ")),e=b.length;while(e--)delete d[b[e]];if(c?!P(d):!m.isEmptyObject(d))return}(c||(delete g[h].data,P(g[h])))&&(f?m.cleanData([a],!0):k.deleteExpando||g!=g.window?delete g[h]:g[h]=null)}}}m.extend({cache:{},noData:{"applet ":!0,"embed ":!0,"object ":"clsid:D27CDB6E-AE6D-11cf-96B8-444553540000"},hasData:function(a){return a=a.nodeType?m.cache[a[m.expando]]:a[m.expando],!!a&&!P(a)},data:function(a,b,c){return Q(a,b,c)},removeData:function(a,b){return R(a,b)},_data:function(a,b,c){return Q(a,b,c,!0)},_removeData:function(a,b){return R(a,b,!0)}}),m.fn.extend({data:function(a,b){var c,d,e,f=this[0],g=f&&f.attributes;if(void 0===a){if(this.length&&(e=m.data(f),1===f.nodeType&&!m._data(f,"parsedAttrs"))){c=g.length;while(c--)g[c]&&(d=g[c].name,0===d.indexOf("data-")&&(d=m.camelCase(d.slice(5)),O(f,d,e[d])));m._data(f,"parsedAttrs",!0)}return e}return"object"==typeof a?this.each(function(){m.data(this,a)}):arguments.length>1?this.each(function(){m.data(this,a,b)}):f?O(f,a,m.data(f,a)):void 0},removeData:function(a){return this.each(function(){m.removeData(this,a)})}}),m.extend({queue:function(a,b,c){var d;return a?(b=(b||"fx")+"queue",d=m._data(a,b),c&&(!d||m.isArray(c)?d=m._data(a,b,m.makeArray(c)):d.push(c)),d||[]):void 0},dequeue:function(a,b){b=b||"fx";var c=m.queue(a,b),d=c.length,e=c.shift(),f=m._queueHooks(a,b),g=function(){m.dequeue(a,b)};"inprogress"===e&&(e=c.shift(),d--),e&&("fx"===b&&c.unshift("inprogress"),delete f.stop,e.call(a,g,f)),!d&&f&&f.empty.fire()},_queueHooks:function(a,b){var c=b+"queueHooks";return m._data(a,c)||m._data(a,c,{empty:m.Callbacks("once memory").add(function(){m._removeData(a,b+"queue"),m._removeData(a,c)})})}}),m.fn.extend({queue:function(a,b){var c=2;return"string"!=typeof a&&(b=a,a="fx",c--),arguments.length<c?m.queue(this[0],a):void 0===b?this:this.each(function(){var c=m.queue(this,a,b);m._queueHooks(this,a),"fx"===a&&"inprogress"!==c[0]&&m.dequeue(this,a)})},dequeue:function(a){return this.each(function(){m.dequeue(this,a)})},clearQueue:function(a){return this.queue(a||"fx",[])},promise:function(a,b){var c,d=1,e=m.Deferred(),f=this,g=this.length,h=function(){--d||e.resolveWith(f,[f])};"string"!=typeof a&&(b=a,a=void 0),a=a||"fx";while(g--)c=m._data(f[g],a+"queueHooks"),c&&c.empty&&(d++,c.empty.add(h));return h(),e.promise(b)}});var S=/[+-]?(?:\d*\.|)\d+(?:[eE][+-]?\d+|)/.source,T=["Top","Right","Bottom","Left"],U=function(a,b){return a=b||a,"none"===m.css(a,"display")||!m.contains(a.ownerDocument,a)},V=m.access=function(a,b,c,d,e,f,g){var h=0,i=a.length,j=null==c;if("object"===m.type(c)){e=!0;for(h in c)m.access(a,b,h,c[h],!0,f,g)}else if(void 0!==d&&(e=!0,m.isFunction(d)||(g=!0),j&&(g?(b.call(a,d),b=null):(j=b,b=function(a,b,c){return j.call(m(a),c)})),b))for(;i>h;h++)b(a[h],c,g?d:d.call(a[h],h,b(a[h],c)));return e?a:j?b.call(a):i?b(a[0],c):f},W=/^(?:checkbox|radio)$/i;!function(){var a=y.createElement("input"),b=y.createElement("div"),c=y.createDocumentFragment();if(b.innerHTML=" <link/><table></table><a href='/a'>a</a><input type='checkbox'/>",k.leadingWhitespace=3===b.firstChild.nodeType,k.tbody=!b.getElementsByTagName("tbody").length,k.htmlSerialize=!!b.getElementsByTagName("link").length,k.html5Clone="<:nav></:nav>"!==y.createElement("nav").cloneNode(!0).outerHTML,a.type="checkbox",a.checked=!0,c.appendChild(a),k.appendChecked=a.checked,b.innerHTML="<textarea>x</textarea>",k.noCloneChecked=!!b.cloneNode(!0).lastChild.defaultValue,c.appendChild(b),b.innerHTML="<input type='radio' checked='checked' name='t'/>",k.checkClone=b.cloneNode(!0).cloneNode(!0).lastChild.checked,k.noCloneEvent=!0,b.attachEvent&&(b.attachEvent("onclick",function(){k.noCloneEvent=!1}),b.cloneNode(!0).click()),null==k.deleteExpando){k.deleteExpando=!0;try{delete b.test}catch(d){k.deleteExpando=!1}}}(),function(){var b,c,d=y.createElement("div");for(b in{submit:!0,change:!0,focusin:!0})c="on"+b,(k[b+"Bubbles"]=c in a)||(d.setAttribute(c,"t"),k[b+"Bubbles"]=d.attributes[c].expando===!1);d=null}();var X=/^(?:input|select|textarea)$/i,Y=/^key/,Z=/^(?:mouse|pointer|contextmenu)|click/,$=/^(?:focusinfocus|focusoutblur)$/,_=/^([^.]*)(?:\.(.+)|)$/;function ab(){return!0}function bb(){return!1}function cb(){try{return y.activeElement}catch(a){}}m.event={global:{},add:function(a,b,c,d,e){var f,g,h,i,j,k,l,n,o,p,q,r=m._data(a);if(r){c.handler&&(i=c,c=i.handler,e=i.selector),c.guid||(c.guid=m.guid++),(g=r.events)||(g=r.events={}),(k=r.handle)||(k=r.handle=function(a){return typeof m===K||a&&m.event.triggered===a.type?void 0:m.event.dispatch.apply(k.elem,arguments)},k.elem=a),b=(b||"").match(E)||[""],h=b.length;while(h--)f=_.exec(b[h])||[],o=q=f[1],p=(f[2]||"").split(".").sort(),o&&(j=m.event.special[o]||{},o=(e?j.delegateType:j.bindType)||o,j=m.event.special[o]||{},l=m.extend({type:o,origType:q,data:d,handler:c,guid:c.guid,selector:e,needsContext:e&&m.expr.match.needsContext.test(e),namespace:p.join(".")},i),(n=g[o])||(n=g[o]=[],n.delegateCount=0,j.setup&&j.setup.call(a,d,p,k)!==!1||(a.addEventListener?a.addEventListener(o,k,!1):a.attachEvent&&a.attachEvent("on"+o,k))),j.add&&(j.add.call(a,l),l.handler.guid||(l.handler.guid=c.guid)),e?n.splice(n.delegateCount++,0,l):n.push(l),m.event.global[o]=!0);a=null}},remove:function(a,b,c,d,e){var f,g,h,i,j,k,l,n,o,p,q,r=m.hasData(a)&&m._data(a);if(r&&(k=r.events)){b=(b||"").match(E)||[""],j=b.length;while(j--)if(h=_.exec(b[j])||[],o=q=h[1],p=(h[2]||"").split(".").sort(),o){l=m.event.special[o]||{},o=(d?l.delegateType:l.bindType)||o,n=k[o]||[],h=h[2]&&new RegExp("(^|\\.)"+p.join("\\.(?:.*\\.|)")+"(\\.|$)"),i=f=n.length;while(f--)g=n[f],!e&&q!==g.origType||c&&c.guid!==g.guid||h&&!h.test(g.namespace)||d&&d!==g.selector&&("**"!==d||!g.selector)||(n.splice(f,1),g.selector&&n.delegateCount--,l.remove&&l.remove.call(a,g));i&&!n.length&&(l.teardown&&l.teardown.call(a,p,r.handle)!==!1||m.removeEvent(a,o,r.handle),delete k[o])}else for(o in k)m.event.remove(a,o+b[j],c,d,!0);m.isEmptyObject(k)&&(delete r.handle,m._removeData(a,"events"))}},trigger:function(b,c,d,e){var f,g,h,i,k,l,n,o=[d||y],p=j.call(b,"type")?b.type:b,q=j.call(b,"namespace")?b.namespace.split("."):[];if(h=l=d=d||y,3!==d.nodeType&&8!==d.nodeType&&!$.test(p+m.event.triggered)&&(p.indexOf(".")>=0&&(q=p.split("."),p=q.shift(),q.sort()),g=p.indexOf(":")<0&&"on"+p,b=b[m.expando]?b:new m.Event(p,"object"==typeof b&&b),b.isTrigger=e?2:3,b.namespace=q.join("."),b.namespace_re=b.namespace?new RegExp("(^|\\.)"+q.join("\\.(?:.*\\.|)")+"(\\.|$)"):null,b.result=void 0,b.target||(b.target=d),c=null==c?[b]:m.makeArray(c,[b]),k=m.event.special[p]||{},e||!k.trigger||k.trigger.apply(d,c)!==!1)){if(!e&&!k.noBubble&&!m.isWindow(d)){for(i=k.delegateType||p,$.test(i+p)||(h=h.parentNode);h;h=h.parentNode)o.push(h),l=h;l===(d.ownerDocument||y)&&o.push(l.defaultView||l.parentWindow||a)}n=0;while((h=o[n++])&&!b.isPropagationStopped())b.type=n>1?i:k.bindType||p,f=(m._data(h,"events")||{})[b.type]&&m._data(h,"handle"),f&&f.apply(h,c),f=g&&h[g],f&&f.apply&&m.acceptData(h)&&(b.result=f.apply(h,c),b.result===!1&&b.preventDefault());if(b.type=p,!e&&!b.isDefaultPrevented()&&(!k._default||k._default.apply(o.pop(),c)===!1)&&m.acceptData(d)&&g&&d[p]&&!m.isWindow(d)){l=d[g],l&&(d[g]=null),m.event.triggered=p;try{d[p]()}catch(r){}m.event.triggered=void 0,l&&(d[g]=l)}return b.result}},dispatch:function(a){a=m.event.fix(a);var b,c,e,f,g,h=[],i=d.call(arguments),j=(m._data(this,"events")||{})[a.type]||[],k=m.event.special[a.type]||{};if(i[0]=a,a.delegateTarget=this,!k.preDispatch||k.preDispatch.call(this,a)!==!1){h=m.event.handlers.call(this,a,j),b=0;while((f=h[b++])&&!a.isPropagationStopped()){a.currentTarget=f.elem,g=0;while((e=f.handlers[g++])&&!a.isImmediatePropagationStopped())(!a.namespace_re||a.namespace_re.test(e.namespace))&&(a.handleObj=e,a.data=e.data,c=((m.event.special[e.origType]||{}).handle||e.handler).apply(f.elem,i),void 0!==c&&(a.result=c)===!1&&(a.preventDefault(),a.stopPropagation()))}return k.postDispatch&&k.postDispatch.call(this,a),a.result}},handlers:function(a,b){var c,d,e,f,g=[],h=b.delegateCount,i=a.target;if(h&&i.nodeType&&(!a.button||"click"!==a.type))for(;i!=this;i=i.parentNode||this)if(1===i.nodeType&&(i.disabled!==!0||"click"!==a.type)){for(e=[],f=0;h>f;f++)d=b[f],c=d.selector+" ",void 0===e[c]&&(e[c]=d.needsContext?m(c,this).index(i)>=0:m.find(c,this,null,[i]).length),e[c]&&e.push(d);e.length&&g.push({elem:i,handlers:e})}return h<b.length&&g.push({elem:this,handlers:b.slice(h)}),g},fix:function(a){if(a[m.expando])return a;var b,c,d,e=a.type,f=a,g=this.fixHooks[e];g||(this.fixHooks[e]=g=Z.test(e)?this.mouseHooks:Y.test(e)?this.keyHooks:{}),d=g.props?this.props.concat(g.props):this.props,a=new m.Event(f),b=d.length;while(b--)c=d[b],a[c]=f[c];return a.target||(a.target=f.srcElement||y),3===a.target.nodeType&&(a.target=a.target.parentNode),a.metaKey=!!a.metaKey,g.filter?g.filter(a,f):a},props:"altKey bubbles cancelable ctrlKey currentTarget eventPhase metaKey relatedTarget shiftKey target timeStamp view which".split(" "),fixHooks:{},keyHooks:{props:"char charCode key keyCode".split(" "),filter:function(a,b){return null==a.which&&(a.which=null!=b.charCode?b.charCode:b.keyCode),a}},mouseHooks:{props:"button buttons clientX clientY fromElement offsetX offsetY pageX pageY screenX screenY toElement".split(" "),filter:function(a,b){var c,d,e,f=b.button,g=b.fromElement;return null==a.pageX&&null!=b.clientX&&(d=a.target.ownerDocument||y,e=d.documentElement,c=d.body,a.pageX=b.clientX+(e&&e.scrollLeft||c&&c.scrollLeft||0)-(e&&e.clientLeft||c&&c.clientLeft||0),a.pageY=b.clientY+(e&&e.scrollTop||c&&c.scrollTop||0)-(e&&e.clientTop||c&&c.clientTop||0)),!a.relatedTarget&&g&&(a.relatedTarget=g===a.target?b.toElement:g),a.which||void 0===f||(a.which=1&f?1:2&f?3:4&f?2:0),a}},special:{load:{noBubble:!0},focus:{trigger:function(){if(this!==cb()&&this.focus)try{return this.focus(),!1}catch(a){}},delegateType:"focusin"},blur:{trigger:function(){return this===cb()&&this.blur?(this.blur(),!1):void 0},delegateType:"focusout"},click:{trigger:function(){return m.nodeName(this,"input")&&"checkbox"===this.type&&this.click?(this.click(),!1):void 0},_default:function(a){return m.nodeName(a.target,"a")}},beforeunload:{postDispatch:function(a){void 0!==a.result&&a.originalEvent&&(a.originalEvent.returnValue=a.result)}}},simulate:function(a,b,c,d){var e=m.extend(new m.Event,c,{type:a,isSimulated:!0,originalEvent:{}});d?m.event.trigger(e,null,b):m.event.dispatch.call(b,e),e.isDefaultPrevented()&&c.preventDefault()}},m.removeEvent=y.removeEventListener?function(a,b,c){a.removeEventListener&&a.removeEventListener(b,c,!1)}:function(a,b,c){var d="on"+b;a.detachEvent&&(typeof a[d]===K&&(a[d]=null),a.detachEvent(d,c))},m.Event=function(a,b){return this instanceof m.Event?(a&&a.type?(this.originalEvent=a,this.type=a.type,this.isDefaultPrevented=a.defaultPrevented||void 0===a.defaultPrevented&&a.returnValue===!1?ab:bb):this.type=a,b&&m.extend(this,b),this.timeStamp=a&&a.timeStamp||m.now(),void(this[m.expando]=!0)):new m.Event(a,b)},m.Event.prototype={isDefaultPrevented:bb,isPropagationStopped:bb,isImmediatePropagationStopped:bb,preventDefault:function(){var a=this.originalEvent;this.isDefaultPrevented=ab,a&&(a.preventDefault?a.preventDefault():a.returnValue=!1)},stopPropagation:function(){var a=this.originalEvent;this.isPropagationStopped=ab,a&&(a.stopPropagation&&a.stopPropagation(),a.cancelBubble=!0)},stopImmediatePropagation:function(){var a=this.originalEvent;this.isImmediatePropagationStopped=ab,a&&a.stopImmediatePropagation&&a.stopImmediatePropagation(),this.stopPropagation()}},m.each({mouseenter:"mouseover",mouseleave:"mouseout",pointerenter:"pointerover",pointerleave:"pointerout"},function(a,b){m.event.special[a]={delegateType:b,bindType:b,handle:function(a){var c,d=this,e=a.relatedTarget,f=a.handleObj;return(!e||e!==d&&!m.contains(d,e))&&(a.type=f.origType,c=f.handler.apply(this,arguments),a.type=b),c}}}),k.submitBubbles||(m.event.special.submit={setup:function(){return m.nodeName(this,"form")?!1:void m.event.add(this,"click._submit keypress._submit",function(a){var b=a.target,c=m.nodeName(b,"input")||m.nodeName(b,"button")?b.form:void 0;c&&!m._data(c,"submitBubbles")&&(m.event.add(c,"submit._submit",function(a){a._submit_bubble=!0}),m._data(c,"submitBubbles",!0))})},postDispatch:function(a){a._submit_bubble&&(delete a._submit_bubble,this.parentNode&&!a.isTrigger&&m.event.simulate("submit",this.parentNode,a,!0))},teardown:function(){return m.nodeName(this,"form")?!1:void m.event.remove(this,"._submit")}}),k.changeBubbles||(m.event.special.change={setup:function(){return X.test(this.nodeName)?(("checkbox"===this.type||"radio"===this.type)&&(m.event.add(this,"propertychange._change",function(a){"checked"===a.originalEvent.propertyName&&(this._just_changed=!0)}),m.event.add(this,"click._change",function(a){this._just_changed&&!a.isTrigger&&(this._just_changed=!1),m.event.simulate("change",this,a,!0)})),!1):void m.event.add(this,"beforeactivate._change",function(a){var b=a.target;X.test(b.nodeName)&&!m._data(b,"changeBubbles")&&(m.event.add(b,"change._change",function(a){!this.parentNode||a.isSimulated||a.isTrigger||m.event.simulate("change",this.parentNode,a,!0)}),m._data(b,"changeBubbles",!0))})},handle:function(a){var b=a.target;return this!==b||a.isSimulated||a.isTrigger||"radio"!==b.type&&"checkbox"!==b.type?a.handleObj.handler.apply(this,arguments):void 0},teardown:function(){return m.event.remove(this,"._change"),!X.test(this.nodeName)}}),k.focusinBubbles||m.each({focus:"focusin",blur:"focusout"},function(a,b){var c=function(a){m.event.simulate(b,a.target,m.event.fix(a),!0)};m.event.special[b]={setup:function(){var d=this.ownerDocument||this,e=m._data(d,b);e||d.addEventListener(a,c,!0),m._data(d,b,(e||0)+1)},teardown:function(){var d=this.ownerDocument||this,e=m._data(d,b)-1;e?m._data(d,b,e):(d.removeEventListener(a,c,!0),m._removeData(d,b))}}}),m.fn.extend({on:function(a,b,c,d,e){var f,g;if("object"==typeof a){"string"!=typeof b&&(c=c||b,b=void 0);for(f in a)this.on(f,b,c,a[f],e);return this}if(null==c&&null==d?(d=b,c=b=void 0):null==d&&("string"==typeof b?(d=c,c=void 0):(d=c,c=b,b=void 0)),d===!1)d=bb;else if(!d)return this;return 1===e&&(g=d,d=function(a){return m().off(a),g.apply(this,arguments)},d.guid=g.guid||(g.guid=m.guid++)),this.each(function(){m.event.add(this,a,d,c,b)})},one:function(a,b,c,d){return this.on(a,b,c,d,1)},off:function(a,b,c){var d,e;if(a&&a.preventDefault&&a.handleObj)return d=a.handleObj,m(a.delegateTarget).off(d.namespace?d.origType+"."+d.namespace:d.origType,d.selector,d.handler),this;if("object"==typeof a){for(e in a)this.off(e,b,a[e]);return this}return(b===!1||"function"==typeof b)&&(c=b,b=void 0),c===!1&&(c=bb),this.each(function(){m.event.remove(this,a,c,b)})},trigger:function(a,b){return this.each(function(){m.event.trigger(a,b,this)})},triggerHandler:function(a,b){var c=this[0];return c?m.event.trigger(a,b,c,!0):void 0}});function db(a){var b=eb.split("|"),c=a.createDocumentFragment();if(c.createElement)while(b.length)c.createElement(b.pop());return c}var eb="abbr|article|aside|audio|bdi|canvas|data|datalist|details|figcaption|figure|footer|header|hgroup|mark|meter|nav|output|progress|section|summary|time|video",fb=/ jQuery\d+="(?:null|\d+)"/g,gb=new RegExp("<(?:"+eb+")[\\s/>]","i"),hb=/^\s+/,ib=/<(?!area|br|col|embed|hr|img|input|link|meta|param)(([\w:]+)[^>]*)\/>/gi,jb=/<([\w:]+)/,kb=/<tbody/i,lb=/<|&#?\w+;/,mb=/<(?:script|style|link)/i,nb=/checked\s*(?:[^=]|=\s*.checked.)/i,ob=/^$|\/(?:java|ecma)script/i,pb=/^true\/(.*)/,qb=/^\s*<!(?:\[CDATA\[|--)|(?:\]\]|--)>\s*$/g,rb={option:[1,"<select multiple='multiple'>","</select>"],legend:[1,"<fieldset>","</fieldset>"],area:[1,"<map>","</map>"],param:[1,"<object>","</object>"],thead:[1,"<table>","</table>"],tr:[2,"<table><tbody>","</tbody></table>"],col:[2,"<table><tbody></tbody><colgroup>","</colgroup></table>"],td:[3,"<table><tbody><tr>","</tr></tbody></table>"],_default:k.htmlSerialize?[0,"",""]:[1,"X<div>","</div>"]},sb=db(y),tb=sb.appendChild(y.createElement("div"));rb.optgroup=rb.option,rb.tbody=rb.tfoot=rb.colgroup=rb.caption=rb.thead,rb.th=rb.td;function ub(a,b){var c,d,e=0,f=typeof a.getElementsByTagName!==K?a.getElementsByTagName(b||"*"):typeof a.querySelectorAll!==K?a.querySelectorAll(b||"*"):void 0;if(!f)for(f=[],c=a.childNodes||a;null!=(d=c[e]);e++)!b||m.nodeName(d,b)?f.push(d):m.merge(f,ub(d,b));return void 0===b||b&&m.nodeName(a,b)?m.merge([a],f):f}function vb(a){W.test(a.type)&&(a.defaultChecked=a.checked)}function wb(a,b){return m.nodeName(a,"table")&&m.nodeName(11!==b.nodeType?b:b.firstChild,"tr")?a.getElementsByTagName("tbody")[0]||a.appendChild(a.ownerDocument.createElement("tbody")):a}function xb(a){return a.type=(null!==m.find.attr(a,"type"))+"/"+a.type,a}function yb(a){var b=pb.exec(a.type);return b?a.type=b[1]:a.removeAttribute("type"),a}function zb(a,b){for(var c,d=0;null!=(c=a[d]);d++)m._data(c,"globalEval",!b||m._data(b[d],"globalEval"))}function Ab(a,b){if(1===b.nodeType&&m.hasData(a)){var c,d,e,f=m._data(a),g=m._data(b,f),h=f.events;if(h){delete g.handle,g.events={};for(c in h)for(d=0,e=h[c].length;e>d;d++)m.event.add(b,c,h[c][d])}g.data&&(g.data=m.extend({},g.data))}}function Bb(a,b){var c,d,e;if(1===b.nodeType){if(c=b.nodeName.toLowerCase(),!k.noCloneEvent&&b[m.expando]){e=m._data(b);for(d in e.events)m.removeEvent(b,d,e.handle);b.removeAttribute(m.expando)}"script"===c&&b.text!==a.text?(xb(b).text=a.text,yb(b)):"object"===c?(b.parentNode&&(b.outerHTML=a.outerHTML),k.html5Clone&&a.innerHTML&&!m.trim(b.innerHTML)&&(b.innerHTML=a.innerHTML)):"input"===c&&W.test(a.type)?(b.defaultChecked=b.checked=a.checked,b.value!==a.value&&(b.value=a.value)):"option"===c?b.defaultSelected=b.selected=a.defaultSelected:("input"===c||"textarea"===c)&&(b.defaultValue=a.defaultValue)}}m.extend({clone:function(a,b,c){var d,e,f,g,h,i=m.contains(a.ownerDocument,a);if(k.html5Clone||m.isXMLDoc(a)||!gb.test("<"+a.nodeName+">")?f=a.cloneNode(!0):(tb.innerHTML=a.outerHTML,tb.removeChild(f=tb.firstChild)),!(k.noCloneEvent&&k.noCloneChecked||1!==a.nodeType&&11!==a.nodeType||m.isXMLDoc(a)))for(d=ub(f),h=ub(a),g=0;null!=(e=h[g]);++g)d[g]&&Bb(e,d[g]);if(b)if(c)for(h=h||ub(a),d=d||ub(f),g=0;null!=(e=h[g]);g++)Ab(e,d[g]);else Ab(a,f);return d=ub(f,"script"),d.length>0&&zb(d,!i&&ub(a,"script")),d=h=e=null,f},buildFragment:function(a,b,c,d){for(var e,f,g,h,i,j,l,n=a.length,o=db(b),p=[],q=0;n>q;q++)if(f=a[q],f||0===f)if("object"===m.type(f))m.merge(p,f.nodeType?[f]:f);else if(lb.test(f)){h=h||o.appendChild(b.createElement("div")),i=(jb.exec(f)||["",""])[1].toLowerCase(),l=rb[i]||rb._default,h.innerHTML=l[1]+f.replace(ib,"<$1></$2>")+l[2],e=l[0];while(e--)h=h.lastChild;if(!k.leadingWhitespace&&hb.test(f)&&p.push(b.createTextNode(hb.exec(f)[0])),!k.tbody){f="table"!==i||kb.test(f)?"<table>"!==l[1]||kb.test(f)?0:h:h.firstChild,e=f&&f.childNodes.length;while(e--)m.nodeName(j=f.childNodes[e],"tbody")&&!j.childNodes.length&&f.removeChild(j)}m.merge(p,h.childNodes),h.textContent="";while(h.firstChild)h.removeChild(h.firstChild);h=o.lastChild}else p.push(b.createTextNode(f));h&&o.removeChild(h),k.appendChecked||m.grep(ub(p,"input"),vb),q=0;while(f=p[q++])if((!d||-1===m.inArray(f,d))&&(g=m.contains(f.ownerDocument,f),h=ub(o.appendChild(f),"script"),g&&zb(h),c)){e=0;while(f=h[e++])ob.test(f.type||"")&&c.push(f)}return h=null,o},cleanData:function(a,b){for(var d,e,f,g,h=0,i=m.expando,j=m.cache,l=k.deleteExpando,n=m.event.special;null!=(d=a[h]);h++)if((b||m.acceptData(d))&&(f=d[i],g=f&&j[f])){if(g.events)for(e in g.events)n[e]?m.event.remove(d,e):m.removeEvent(d,e,g.handle);j[f]&&(delete j[f],l?delete d[i]:typeof d.removeAttribute!==K?d.removeAttribute(i):d[i]=null,c.push(f))}}}),m.fn.extend({text:function(a){return V(this,function(a){return void 0===a?m.text(this):this.empty().append((this[0]&&this[0].ownerDocument||y).createTextNode(a))},null,a,arguments.length)},append:function(){return this.domManip(arguments,function(a){if(1===this.nodeType||11===this.nodeType||9===this.nodeType){var b=wb(this,a);b.appendChild(a)}})},prepend:function(){return this.domManip(arguments,function(a){if(1===this.nodeType||11===this.nodeType||9===this.nodeType){var b=wb(this,a);b.insertBefore(a,b.firstChild)}})},before:function(){return this.domManip(arguments,function(a){this.parentNode&&this.parentNode.insertBefore(a,this)})},after:function(){return this.domManip(arguments,function(a){this.parentNode&&this.parentNode.insertBefore(a,this.nextSibling)})},remove:function(a,b){for(var c,d=a?m.filter(a,this):this,e=0;null!=(c=d[e]);e++)b||1!==c.nodeType||m.cleanData(ub(c)),c.parentNode&&(b&&m.contains(c.ownerDocument,c)&&zb(ub(c,"script")),c.parentNode.removeChild(c));return this},empty:function(){for(var a,b=0;null!=(a=this[b]);b++){1===a.nodeType&&m.cleanData(ub(a,!1));while(a.firstChild)a.removeChild(a.firstChild);a.options&&m.nodeName(a,"select")&&(a.options.length=0)}return this},clone:function(a,b){return a=null==a?!1:a,b=null==b?a:b,this.map(function(){return m.clone(this,a,b)})},html:function(a){return V(this,function(a){var b=this[0]||{},c=0,d=this.length;if(void 0===a)return 1===b.nodeType?b.innerHTML.replace(fb,""):void 0;if(!("string"!=typeof a||mb.test(a)||!k.htmlSerialize&&gb.test(a)||!k.leadingWhitespace&&hb.test(a)||rb[(jb.exec(a)||["",""])[1].toLowerCase()])){a=a.replace(ib,"<$1></$2>");try{for(;d>c;c++)b=this[c]||{},1===b.nodeType&&(m.cleanData(ub(b,!1)),b.innerHTML=a);b=0}catch(e){}}b&&this.empty().append(a)},null,a,arguments.length)},replaceWith:function(){var a=arguments[0];return this.domManip(arguments,function(b){a=this.parentNode,m.cleanData(ub(this)),a&&a.replaceChild(b,this)}),a&&(a.length||a.nodeType)?this:this.remove()},detach:function(a){return this.remove(a,!0)},domManip:function(a,b){a=e.apply([],a);var c,d,f,g,h,i,j=0,l=this.length,n=this,o=l-1,p=a[0],q=m.isFunction(p);if(q||l>1&&"string"==typeof p&&!k.checkClone&&nb.test(p))return this.each(function(c){var d=n.eq(c);q&&(a[0]=p.call(this,c,d.html())),d.domManip(a,b)});if(l&&(i=m.buildFragment(a,this[0].ownerDocument,!1,this),c=i.firstChild,1===i.childNodes.length&&(i=c),c)){for(g=m.map(ub(i,"script"),xb),f=g.length;l>j;j++)d=i,j!==o&&(d=m.clone(d,!0,!0),f&&m.merge(g,ub(d,"script"))),b.call(this[j],d,j);if(f)for(h=g[g.length-1].ownerDocument,m.map(g,yb),j=0;f>j;j++)d=g[j],ob.test(d.type||"")&&!m._data(d,"globalEval")&&m.contains(h,d)&&(d.src?m._evalUrl&&m._evalUrl(d.src):m.globalEval((d.text||d.textContent||d.innerHTML||"").replace(qb,"")));i=c=null}return this}}),m.each({appendTo:"append",prependTo:"prepend",insertBefore:"before",insertAfter:"after",replaceAll:"replaceWith"},function(a,b){m.fn[a]=function(a){for(var c,d=0,e=[],g=m(a),h=g.length-1;h>=d;d++)c=d===h?this:this.clone(!0),m(g[d])[b](c),f.apply(e,c.get());return this.pushStack(e)}});var Cb,Db={};function Eb(b,c){var d,e=m(c.createElement(b)).appendTo(c.body),f=a.getDefaultComputedStyle&&(d=a.getDefaultComputedStyle(e[0]))?d.display:m.css(e[0],"display");return e.detach(),f}function Fb(a){var b=y,c=Db[a];return c||(c=Eb(a,b),"none"!==c&&c||(Cb=(Cb||m("<iframe frameborder='0' width='0' height='0'/>")).appendTo(b.documentElement),b=(Cb[0].contentWindow||Cb[0].contentDocument).document,b.write(),b.close(),c=Eb(a,b),Cb.detach()),Db[a]=c),c}!function(){var a;k.shrinkWrapBlocks=function(){if(null!=a)return a;a=!1;var b,c,d;return c=y.getElementsByTagName("body")[0],c&&c.style?(b=y.createElement("div"),d=y.createElement("div"),d.style.cssText="position:absolute;border:0;width:0;height:0;top:0;left:-9999px",c.appendChild(d).appendChild(b),typeof b.style.zoom!==K&&(b.style.cssText="-webkit-box-sizing:content-box;-moz-box-sizing:content-box;box-sizing:content-box;display:block;margin:0;border:0;padding:1px;width:1px;zoom:1",b.appendChild(y.createElement("div")).style.width="5px",a=3!==b.offsetWidth),c.removeChild(d),a):void 0}}();var Gb=/^margin/,Hb=new RegExp("^("+S+")(?!px)[a-z%]+$","i"),Ib,Jb,Kb=/^(top|right|bottom|left)$/;a.getComputedStyle?(Ib=function(b){return b.ownerDocument.defaultView.opener?b.ownerDocument.defaultView.getComputedStyle(b,null):a.getComputedStyle(b,null)},Jb=function(a,b,c){var d,e,f,g,h=a.style;return c=c||Ib(a),g=c?c.getPropertyValue(b)||c[b]:void 0,c&&(""!==g||m.contains(a.ownerDocument,a)||(g=m.style(a,b)),Hb.test(g)&&Gb.test(b)&&(d=h.width,e=h.minWidth,f=h.maxWidth,h.minWidth=h.maxWidth=h.width=g,g=c.width,h.width=d,h.minWidth=e,h.maxWidth=f)),void 0===g?g:g+""}):y.documentElement.currentStyle&&(Ib=function(a){return a.currentStyle},Jb=function(a,b,c){var d,e,f,g,h=a.style;return c=c||Ib(a),g=c?c[b]:void 0,null==g&&h&&h[b]&&(g=h[b]),Hb.test(g)&&!Kb.test(b)&&(d=h.left,e=a.runtimeStyle,f=e&&e.left,f&&(e.left=a.currentStyle.left),h.left="fontSize"===b?"1em":g,g=h.pixelLeft+"px",h.left=d,f&&(e.left=f)),void 0===g?g:g+""||"auto"});function Lb(a,b){return{get:function(){var c=a();if(null!=c)return c?void delete this.get:(this.get=b).apply(this,arguments)}}}!function(){var b,c,d,e,f,g,h;if(b=y.createElement("div"),b.innerHTML=" <link/><table></table><a href='/a'>a</a><input type='checkbox'/>",d=b.getElementsByTagName("a")[0],c=d&&d.style){c.cssText="float:left;opacity:.5",k.opacity="0.5"===c.opacity,k.cssFloat=!!c.cssFloat,b.style.backgroundClip="content-box",b.cloneNode(!0).style.backgroundClip="",k.clearCloneStyle="content-box"===b.style.backgroundClip,k.boxSizing=""===c.boxSizing||""===c.MozBoxSizing||""===c.WebkitBoxSizing,m.extend(k,{reliableHiddenOffsets:function(){return null==g&&i(),g},boxSizingReliable:function(){return null==f&&i(),f},pixelPosition:function(){return null==e&&i(),e},reliableMarginRight:function(){return null==h&&i(),h}});function i(){var b,c,d,i;c=y.getElementsByTagName("body")[0],c&&c.style&&(b=y.createElement("div"),d=y.createElement("div"),d.style.cssText="position:absolute;border:0;width:0;height:0;top:0;left:-9999px",c.appendChild(d).appendChild(b),b.style.cssText="-webkit-box-sizing:border-box;-moz-box-sizing:border-box;box-sizing:border-box;display:block;margin-top:1%;top:1%;border:1px;padding:1px;width:4px;position:absolute",e=f=!1,h=!0,a.getComputedStyle&&(e="1%"!==(a.getComputedStyle(b,null)||{}).top,f="4px"===(a.getComputedStyle(b,null)||{width:"4px"}).width,i=b.appendChild(y.createElement("div")),i.style.cssText=b.style.cssText="-webkit-box-sizing:content-box;-moz-box-sizing:content-box;box-sizing:content-box;display:block;margin:0;border:0;padding:0",i.style.marginRight=i.style.width="0",b.style.width="1px",h=!parseFloat((a.getComputedStyle(i,null)||{}).marginRight),b.removeChild(i)),b.innerHTML="<table><tr><td></td><td>t</td></tr></table>",i=b.getElementsByTagName("td"),i[0].style.cssText="margin:0;border:0;padding:0;display:none",g=0===i[0].offsetHeight,g&&(i[0].style.display="",i[1].style.display="none",g=0===i[0].offsetHeight),c.removeChild(d))}}}(),m.swap=function(a,b,c,d){var e,f,g={};for(f in b)g[f]=a.style[f],a.style[f]=b[f];e=c.apply(a,d||[]);for(f in b)a.style[f]=g[f];return e};var Mb=/alpha\([^)]*\)/i,Nb=/opacity\s*=\s*([^)]*)/,Ob=/^(none|table(?!-c[ea]).+)/,Pb=new RegExp("^("+S+")(.*)$","i"),Qb=new RegExp("^([+-])=("+S+")","i"),Rb={position:"absolute",visibility:"hidden",display:"block"},Sb={letterSpacing:"0",fontWeight:"400"},Tb=["Webkit","O","Moz","ms"];function Ub(a,b){if(b in a)return b;var c=b.charAt(0).toUpperCase()+b.slice(1),d=b,e=Tb.length;while(e--)if(b=Tb[e]+c,b in a)return b;return d}function Vb(a,b){for(var c,d,e,f=[],g=0,h=a.length;h>g;g++)d=a[g],d.style&&(f[g]=m._data(d,"olddisplay"),c=d.style.display,b?(f[g]||"none"!==c||(d.style.display=""),""===d.style.display&&U(d)&&(f[g]=m._data(d,"olddisplay",Fb(d.nodeName)))):(e=U(d),(c&&"none"!==c||!e)&&m._data(d,"olddisplay",e?c:m.css(d,"display"))));for(g=0;h>g;g++)d=a[g],d.style&&(b&&"none"!==d.style.display&&""!==d.style.display||(d.style.display=b?f[g]||"":"none"));return a}function Wb(a,b,c){var d=Pb.exec(b);return d?Math.max(0,d[1]-(c||0))+(d[2]||"px"):b}function Xb(a,b,c,d,e){for(var f=c===(d?"border":"content")?4:"width"===b?1:0,g=0;4>f;f+=2)"margin"===c&&(g+=m.css(a,c+T[f],!0,e)),d?("content"===c&&(g-=m.css(a,"padding"+T[f],!0,e)),"margin"!==c&&(g-=m.css(a,"border"+T[f]+"Width",!0,e))):(g+=m.css(a,"padding"+T[f],!0,e),"padding"!==c&&(g+=m.css(a,"border"+T[f]+"Width",!0,e)));return g}function Yb(a,b,c){var d=!0,e="width"===b?a.offsetWidth:a.offsetHeight,f=Ib(a),g=k.boxSizing&&"border-box"===m.css(a,"boxSizing",!1,f);if(0>=e||null==e){if(e=Jb(a,b,f),(0>e||null==e)&&(e=a.style[b]),Hb.test(e))return e;d=g&&(k.boxSizingReliable()||e===a.style[b]),e=parseFloat(e)||0}return e+Xb(a,b,c||(g?"border":"content"),d,f)+"px"}m.extend({cssHooks:{opacity:{get:function(a,b){if(b){var c=Jb(a,"opacity");return""===c?"1":c}}}},cssNumber:{columnCount:!0,fillOpacity:!0,flexGrow:!0,flexShrink:!0,fontWeight:!0,lineHeight:!0,opacity:!0,order:!0,orphans:!0,widows:!0,zIndex:!0,zoom:!0},cssProps:{"float":k.cssFloat?"cssFloat":"styleFloat"},style:function(a,b,c,d){if(a&&3!==a.nodeType&&8!==a.nodeType&&a.style){var e,f,g,h=m.camelCase(b),i=a.style;if(b=m.cssProps[h]||(m.cssProps[h]=Ub(i,h)),g=m.cssHooks[b]||m.cssHooks[h],void 0===c)return g&&"get"in g&&void 0!==(e=g.get(a,!1,d))?e:i[b];if(f=typeof c,"string"===f&&(e=Qb.exec(c))&&(c=(e[1]+1)*e[2]+parseFloat(m.css(a,b)),f="number"),null!=c&&c===c&&("number"!==f||m.cssNumber[h]||(c+="px"),k.clearCloneStyle||""!==c||0!==b.indexOf("background")||(i[b]="inherit"),!(g&&"set"in g&&void 0===(c=g.set(a,c,d)))))try{i[b]=c}catch(j){}}},css:function(a,b,c,d){var e,f,g,h=m.camelCase(b);return b=m.cssProps[h]||(m.cssProps[h]=Ub(a.style,h)),g=m.cssHooks[b]||m.cssHooks[h],g&&"get"in g&&(f=g.get(a,!0,c)),void 0===f&&(f=Jb(a,b,d)),"normal"===f&&b in Sb&&(f=Sb[b]),""===c||c?(e=parseFloat(f),c===!0||m.isNumeric(e)?e||0:f):f}}),m.each(["height","width"],function(a,b){m.cssHooks[b]={get:function(a,c,d){return c?Ob.test(m.css(a,"display"))&&0===a.offsetWidth?m.swap(a,Rb,function(){return Yb(a,b,d)}):Yb(a,b,d):void 0},set:function(a,c,d){var e=d&&Ib(a);return Wb(a,c,d?Xb(a,b,d,k.boxSizing&&"border-box"===m.css(a,"boxSizing",!1,e),e):0)}}}),k.opacity||(m.cssHooks.opacity={get:function(a,b){return Nb.test((b&&a.currentStyle?a.currentStyle.filter:a.style.filter)||"")?.01*parseFloat(RegExp.$1)+"":b?"1":""},set:function(a,b){var c=a.style,d=a.currentStyle,e=m.isNumeric(b)?"alpha(opacity="+100*b+")":"",f=d&&d.filter||c.filter||"";c.zoom=1,(b>=1||""===b)&&""===m.trim(f.replace(Mb,""))&&c.removeAttribute&&(c.removeAttribute("filter"),""===b||d&&!d.filter)||(c.filter=Mb.test(f)?f.replace(Mb,e):f+" "+e)}}),m.cssHooks.marginRight=Lb(k.reliableMarginRight,function(a,b){return b?m.swap(a,{display:"inline-block"},Jb,[a,"marginRight"]):void 0}),m.each({margin:"",padding:"",border:"Width"},function(a,b){m.cssHooks[a+b]={expand:function(c){for(var d=0,e={},f="string"==typeof c?c.split(" "):[c];4>d;d++)e[a+T[d]+b]=f[d]||f[d-2]||f[0];return e}},Gb.test(a)||(m.cssHooks[a+b].set=Wb)}),m.fn.extend({css:function(a,b){return V(this,function(a,b,c){var d,e,f={},g=0;if(m.isArray(b)){for(d=Ib(a),e=b.length;e>g;g++)f[b[g]]=m.css(a,b[g],!1,d);return f}return void 0!==c?m.style(a,b,c):m.css(a,b)},a,b,arguments.length>1)},show:function(){return Vb(this,!0)},hide:function(){return Vb(this)},toggle:function(a){return"boolean"==typeof a?a?this.show():this.hide():this.each(function(){U(this)?m(this).show():m(this).hide()})}});function Zb(a,b,c,d,e){return new Zb.prototype.init(a,b,c,d,e)
}m.Tween=Zb,Zb.prototype={constructor:Zb,init:function(a,b,c,d,e,f){this.elem=a,this.prop=c,this.easing=e||"swing",this.options=b,this.start=this.now=this.cur(),this.end=d,this.unit=f||(m.cssNumber[c]?"":"px")},cur:function(){var a=Zb.propHooks[this.prop];return a&&a.get?a.get(this):Zb.propHooks._default.get(this)},run:function(a){var b,c=Zb.propHooks[this.prop];return this.pos=b=this.options.duration?m.easing[this.easing](a,this.options.duration*a,0,1,this.options.duration):a,this.now=(this.end-this.start)*b+this.start,this.options.step&&this.options.step.call(this.elem,this.now,this),c&&c.set?c.set(this):Zb.propHooks._default.set(this),this}},Zb.prototype.init.prototype=Zb.prototype,Zb.propHooks={_default:{get:function(a){var b;return null==a.elem[a.prop]||a.elem.style&&null!=a.elem.style[a.prop]?(b=m.css(a.elem,a.prop,""),b&&"auto"!==b?b:0):a.elem[a.prop]},set:function(a){m.fx.step[a.prop]?m.fx.step[a.prop](a):a.elem.style&&(null!=a.elem.style[m.cssProps[a.prop]]||m.cssHooks[a.prop])?m.style(a.elem,a.prop,a.now+a.unit):a.elem[a.prop]=a.now}}},Zb.propHooks.scrollTop=Zb.propHooks.scrollLeft={set:function(a){a.elem.nodeType&&a.elem.parentNode&&(a.elem[a.prop]=a.now)}},m.easing={linear:function(a){return a},swing:function(a){return.5-Math.cos(a*Math.PI)/2}},m.fx=Zb.prototype.init,m.fx.step={};var $b,_b,ac=/^(?:toggle|show|hide)$/,bc=new RegExp("^(?:([+-])=|)("+S+")([a-z%]*)$","i"),cc=/queueHooks$/,dc=[ic],ec={"*":[function(a,b){var c=this.createTween(a,b),d=c.cur(),e=bc.exec(b),f=e&&e[3]||(m.cssNumber[a]?"":"px"),g=(m.cssNumber[a]||"px"!==f&&+d)&&bc.exec(m.css(c.elem,a)),h=1,i=20;if(g&&g[3]!==f){f=f||g[3],e=e||[],g=+d||1;do h=h||".5",g/=h,m.style(c.elem,a,g+f);while(h!==(h=c.cur()/d)&&1!==h&&--i)}return e&&(g=c.start=+g||+d||0,c.unit=f,c.end=e[1]?g+(e[1]+1)*e[2]:+e[2]),c}]};function fc(){return setTimeout(function(){$b=void 0}),$b=m.now()}function gc(a,b){var c,d={height:a},e=0;for(b=b?1:0;4>e;e+=2-b)c=T[e],d["margin"+c]=d["padding"+c]=a;return b&&(d.opacity=d.width=a),d}function hc(a,b,c){for(var d,e=(ec[b]||[]).concat(ec["*"]),f=0,g=e.length;g>f;f++)if(d=e[f].call(c,b,a))return d}function ic(a,b,c){var d,e,f,g,h,i,j,l,n=this,o={},p=a.style,q=a.nodeType&&U(a),r=m._data(a,"fxshow");c.queue||(h=m._queueHooks(a,"fx"),null==h.unqueued&&(h.unqueued=0,i=h.empty.fire,h.empty.fire=function(){h.unqueued||i()}),h.unqueued++,n.always(function(){n.always(function(){h.unqueued--,m.queue(a,"fx").length||h.empty.fire()})})),1===a.nodeType&&("height"in b||"width"in b)&&(c.overflow=[p.overflow,p.overflowX,p.overflowY],j=m.css(a,"display"),l="none"===j?m._data(a,"olddisplay")||Fb(a.nodeName):j,"inline"===l&&"none"===m.css(a,"float")&&(k.inlineBlockNeedsLayout&&"inline"!==Fb(a.nodeName)?p.zoom=1:p.display="inline-block")),c.overflow&&(p.overflow="hidden",k.shrinkWrapBlocks()||n.always(function(){p.overflow=c.overflow[0],p.overflowX=c.overflow[1],p.overflowY=c.overflow[2]}));for(d in b)if(e=b[d],ac.exec(e)){if(delete b[d],f=f||"toggle"===e,e===(q?"hide":"show")){if("show"!==e||!r||void 0===r[d])continue;q=!0}o[d]=r&&r[d]||m.style(a,d)}else j=void 0;if(m.isEmptyObject(o))"inline"===("none"===j?Fb(a.nodeName):j)&&(p.display=j);else{r?"hidden"in r&&(q=r.hidden):r=m._data(a,"fxshow",{}),f&&(r.hidden=!q),q?m(a).show():n.done(function(){m(a).hide()}),n.done(function(){var b;m._removeData(a,"fxshow");for(b in o)m.style(a,b,o[b])});for(d in o)g=hc(q?r[d]:0,d,n),d in r||(r[d]=g.start,q&&(g.end=g.start,g.start="width"===d||"height"===d?1:0))}}function jc(a,b){var c,d,e,f,g;for(c in a)if(d=m.camelCase(c),e=b[d],f=a[c],m.isArray(f)&&(e=f[1],f=a[c]=f[0]),c!==d&&(a[d]=f,delete a[c]),g=m.cssHooks[d],g&&"expand"in g){f=g.expand(f),delete a[d];for(c in f)c in a||(a[c]=f[c],b[c]=e)}else b[d]=e}function kc(a,b,c){var d,e,f=0,g=dc.length,h=m.Deferred().always(function(){delete i.elem}),i=function(){if(e)return!1;for(var b=$b||fc(),c=Math.max(0,j.startTime+j.duration-b),d=c/j.duration||0,f=1-d,g=0,i=j.tweens.length;i>g;g++)j.tweens[g].run(f);return h.notifyWith(a,[j,f,c]),1>f&&i?c:(h.resolveWith(a,[j]),!1)},j=h.promise({elem:a,props:m.extend({},b),opts:m.extend(!0,{specialEasing:{}},c),originalProperties:b,originalOptions:c,startTime:$b||fc(),duration:c.duration,tweens:[],createTween:function(b,c){var d=m.Tween(a,j.opts,b,c,j.opts.specialEasing[b]||j.opts.easing);return j.tweens.push(d),d},stop:function(b){var c=0,d=b?j.tweens.length:0;if(e)return this;for(e=!0;d>c;c++)j.tweens[c].run(1);return b?h.resolveWith(a,[j,b]):h.rejectWith(a,[j,b]),this}}),k=j.props;for(jc(k,j.opts.specialEasing);g>f;f++)if(d=dc[f].call(j,a,k,j.opts))return d;return m.map(k,hc,j),m.isFunction(j.opts.start)&&j.opts.start.call(a,j),m.fx.timer(m.extend(i,{elem:a,anim:j,queue:j.opts.queue})),j.progress(j.opts.progress).done(j.opts.done,j.opts.complete).fail(j.opts.fail).always(j.opts.always)}m.Animation=m.extend(kc,{tweener:function(a,b){m.isFunction(a)?(b=a,a=["*"]):a=a.split(" ");for(var c,d=0,e=a.length;e>d;d++)c=a[d],ec[c]=ec[c]||[],ec[c].unshift(b)},prefilter:function(a,b){b?dc.unshift(a):dc.push(a)}}),m.speed=function(a,b,c){var d=a&&"object"==typeof a?m.extend({},a):{complete:c||!c&&b||m.isFunction(a)&&a,duration:a,easing:c&&b||b&&!m.isFunction(b)&&b};return d.duration=m.fx.off?0:"number"==typeof d.duration?d.duration:d.duration in m.fx.speeds?m.fx.speeds[d.duration]:m.fx.speeds._default,(null==d.queue||d.queue===!0)&&(d.queue="fx"),d.old=d.complete,d.complete=function(){m.isFunction(d.old)&&d.old.call(this),d.queue&&m.dequeue(this,d.queue)},d},m.fn.extend({fadeTo:function(a,b,c,d){return this.filter(U).css("opacity",0).show().end().animate({opacity:b},a,c,d)},animate:function(a,b,c,d){var e=m.isEmptyObject(a),f=m.speed(b,c,d),g=function(){var b=kc(this,m.extend({},a),f);(e||m._data(this,"finish"))&&b.stop(!0)};return g.finish=g,e||f.queue===!1?this.each(g):this.queue(f.queue,g)},stop:function(a,b,c){var d=function(a){var b=a.stop;delete a.stop,b(c)};return"string"!=typeof a&&(c=b,b=a,a=void 0),b&&a!==!1&&this.queue(a||"fx",[]),this.each(function(){var b=!0,e=null!=a&&a+"queueHooks",f=m.timers,g=m._data(this);if(e)g[e]&&g[e].stop&&d(g[e]);else for(e in g)g[e]&&g[e].stop&&cc.test(e)&&d(g[e]);for(e=f.length;e--;)f[e].elem!==this||null!=a&&f[e].queue!==a||(f[e].anim.stop(c),b=!1,f.splice(e,1));(b||!c)&&m.dequeue(this,a)})},finish:function(a){return a!==!1&&(a=a||"fx"),this.each(function(){var b,c=m._data(this),d=c[a+"queue"],e=c[a+"queueHooks"],f=m.timers,g=d?d.length:0;for(c.finish=!0,m.queue(this,a,[]),e&&e.stop&&e.stop.call(this,!0),b=f.length;b--;)f[b].elem===this&&f[b].queue===a&&(f[b].anim.stop(!0),f.splice(b,1));for(b=0;g>b;b++)d[b]&&d[b].finish&&d[b].finish.call(this);delete c.finish})}}),m.each(["toggle","show","hide"],function(a,b){var c=m.fn[b];m.fn[b]=function(a,d,e){return null==a||"boolean"==typeof a?c.apply(this,arguments):this.animate(gc(b,!0),a,d,e)}}),m.each({slideDown:gc("show"),slideUp:gc("hide"),slideToggle:gc("toggle"),fadeIn:{opacity:"show"},fadeOut:{opacity:"hide"},fadeToggle:{opacity:"toggle"}},function(a,b){m.fn[a]=function(a,c,d){return this.animate(b,a,c,d)}}),m.timers=[],m.fx.tick=function(){var a,b=m.timers,c=0;for($b=m.now();c<b.length;c++)a=b[c],a()||b[c]!==a||b.splice(c--,1);b.length||m.fx.stop(),$b=void 0},m.fx.timer=function(a){m.timers.push(a),a()?m.fx.start():m.timers.pop()},m.fx.interval=13,m.fx.start=function(){_b||(_b=setInterval(m.fx.tick,m.fx.interval))},m.fx.stop=function(){clearInterval(_b),_b=null},m.fx.speeds={slow:600,fast:200,_default:400},m.fn.delay=function(a,b){return a=m.fx?m.fx.speeds[a]||a:a,b=b||"fx",this.queue(b,function(b,c){var d=setTimeout(b,a);c.stop=function(){clearTimeout(d)}})},function(){var a,b,c,d,e;b=y.createElement("div"),b.setAttribute("className","t"),b.innerHTML=" <link/><table></table><a href='/a'>a</a><input type='checkbox'/>",d=b.getElementsByTagName("a")[0],c=y.createElement("select"),e=c.appendChild(y.createElement("option")),a=b.getElementsByTagName("input")[0],d.style.cssText="top:1px",k.getSetAttribute="t"!==b.className,k.style=/top/.test(d.getAttribute("style")),k.hrefNormalized="/a"===d.getAttribute("href"),k.checkOn=!!a.value,k.optSelected=e.selected,k.enctype=!!y.createElement("form").enctype,c.disabled=!0,k.optDisabled=!e.disabled,a=y.createElement("input"),a.setAttribute("value",""),k.input=""===a.getAttribute("value"),a.value="t",a.setAttribute("type","radio"),k.radioValue="t"===a.value}();var lc=/\r/g;m.fn.extend({val:function(a){var b,c,d,e=this[0];{if(arguments.length)return d=m.isFunction(a),this.each(function(c){var e;1===this.nodeType&&(e=d?a.call(this,c,m(this).val()):a,null==e?e="":"number"==typeof e?e+="":m.isArray(e)&&(e=m.map(e,function(a){return null==a?"":a+""})),b=m.valHooks[this.type]||m.valHooks[this.nodeName.toLowerCase()],b&&"set"in b&&void 0!==b.set(this,e,"value")||(this.value=e))});if(e)return b=m.valHooks[e.type]||m.valHooks[e.nodeName.toLowerCase()],b&&"get"in b&&void 0!==(c=b.get(e,"value"))?c:(c=e.value,"string"==typeof c?c.replace(lc,""):null==c?"":c)}}}),m.extend({valHooks:{option:{get:function(a){var b=m.find.attr(a,"value");return null!=b?b:m.trim(m.text(a))}},select:{get:function(a){for(var b,c,d=a.options,e=a.selectedIndex,f="select-one"===a.type||0>e,g=f?null:[],h=f?e+1:d.length,i=0>e?h:f?e:0;h>i;i++)if(c=d[i],!(!c.selected&&i!==e||(k.optDisabled?c.disabled:null!==c.getAttribute("disabled"))||c.parentNode.disabled&&m.nodeName(c.parentNode,"optgroup"))){if(b=m(c).val(),f)return b;g.push(b)}return g},set:function(a,b){var c,d,e=a.options,f=m.makeArray(b),g=e.length;while(g--)if(d=e[g],m.inArray(m.valHooks.option.get(d),f)>=0)try{d.selected=c=!0}catch(h){d.scrollHeight}else d.selected=!1;return c||(a.selectedIndex=-1),e}}}}),m.each(["radio","checkbox"],function(){m.valHooks[this]={set:function(a,b){return m.isArray(b)?a.checked=m.inArray(m(a).val(),b)>=0:void 0}},k.checkOn||(m.valHooks[this].get=function(a){return null===a.getAttribute("value")?"on":a.value})});var mc,nc,oc=m.expr.attrHandle,pc=/^(?:checked|selected)$/i,qc=k.getSetAttribute,rc=k.input;m.fn.extend({attr:function(a,b){return V(this,m.attr,a,b,arguments.length>1)},removeAttr:function(a){return this.each(function(){m.removeAttr(this,a)})}}),m.extend({attr:function(a,b,c){var d,e,f=a.nodeType;if(a&&3!==f&&8!==f&&2!==f)return typeof a.getAttribute===K?m.prop(a,b,c):(1===f&&m.isXMLDoc(a)||(b=b.toLowerCase(),d=m.attrHooks[b]||(m.expr.match.bool.test(b)?nc:mc)),void 0===c?d&&"get"in d&&null!==(e=d.get(a,b))?e:(e=m.find.attr(a,b),null==e?void 0:e):null!==c?d&&"set"in d&&void 0!==(e=d.set(a,c,b))?e:(a.setAttribute(b,c+""),c):void m.removeAttr(a,b))},removeAttr:function(a,b){var c,d,e=0,f=b&&b.match(E);if(f&&1===a.nodeType)while(c=f[e++])d=m.propFix[c]||c,m.expr.match.bool.test(c)?rc&&qc||!pc.test(c)?a[d]=!1:a[m.camelCase("default-"+c)]=a[d]=!1:m.attr(a,c,""),a.removeAttribute(qc?c:d)},attrHooks:{type:{set:function(a,b){if(!k.radioValue&&"radio"===b&&m.nodeName(a,"input")){var c=a.value;return a.setAttribute("type",b),c&&(a.value=c),b}}}}}),nc={set:function(a,b,c){return b===!1?m.removeAttr(a,c):rc&&qc||!pc.test(c)?a.setAttribute(!qc&&m.propFix[c]||c,c):a[m.camelCase("default-"+c)]=a[c]=!0,c}},m.each(m.expr.match.bool.source.match(/\w+/g),function(a,b){var c=oc[b]||m.find.attr;oc[b]=rc&&qc||!pc.test(b)?function(a,b,d){var e,f;return d||(f=oc[b],oc[b]=e,e=null!=c(a,b,d)?b.toLowerCase():null,oc[b]=f),e}:function(a,b,c){return c?void 0:a[m.camelCase("default-"+b)]?b.toLowerCase():null}}),rc&&qc||(m.attrHooks.value={set:function(a,b,c){return m.nodeName(a,"input")?void(a.defaultValue=b):mc&&mc.set(a,b,c)}}),qc||(mc={set:function(a,b,c){var d=a.getAttributeNode(c);return d||a.setAttributeNode(d=a.ownerDocument.createAttribute(c)),d.value=b+="","value"===c||b===a.getAttribute(c)?b:void 0}},oc.id=oc.name=oc.coords=function(a,b,c){var d;return c?void 0:(d=a.getAttributeNode(b))&&""!==d.value?d.value:null},m.valHooks.button={get:function(a,b){var c=a.getAttributeNode(b);return c&&c.specified?c.value:void 0},set:mc.set},m.attrHooks.contenteditable={set:function(a,b,c){mc.set(a,""===b?!1:b,c)}},m.each(["width","height"],function(a,b){m.attrHooks[b]={set:function(a,c){return""===c?(a.setAttribute(b,"auto"),c):void 0}}})),k.style||(m.attrHooks.style={get:function(a){return a.style.cssText||void 0},set:function(a,b){return a.style.cssText=b+""}});var sc=/^(?:input|select|textarea|button|object)$/i,tc=/^(?:a|area)$/i;m.fn.extend({prop:function(a,b){return V(this,m.prop,a,b,arguments.length>1)},removeProp:function(a){return a=m.propFix[a]||a,this.each(function(){try{this[a]=void 0,delete this[a]}catch(b){}})}}),m.extend({propFix:{"for":"htmlFor","class":"className"},prop:function(a,b,c){var d,e,f,g=a.nodeType;if(a&&3!==g&&8!==g&&2!==g)return f=1!==g||!m.isXMLDoc(a),f&&(b=m.propFix[b]||b,e=m.propHooks[b]),void 0!==c?e&&"set"in e&&void 0!==(d=e.set(a,c,b))?d:a[b]=c:e&&"get"in e&&null!==(d=e.get(a,b))?d:a[b]},propHooks:{tabIndex:{get:function(a){var b=m.find.attr(a,"tabindex");return b?parseInt(b,10):sc.test(a.nodeName)||tc.test(a.nodeName)&&a.href?0:-1}}}}),k.hrefNormalized||m.each(["href","src"],function(a,b){m.propHooks[b]={get:function(a){return a.getAttribute(b,4)}}}),k.optSelected||(m.propHooks.selected={get:function(a){var b=a.parentNode;return b&&(b.selectedIndex,b.parentNode&&b.parentNode.selectedIndex),null}}),m.each(["tabIndex","readOnly","maxLength","cellSpacing","cellPadding","rowSpan","colSpan","useMap","frameBorder","contentEditable"],function(){m.propFix[this.toLowerCase()]=this}),k.enctype||(m.propFix.enctype="encoding");var uc=/[\t\r\n\f]/g;m.fn.extend({addClass:function(a){var b,c,d,e,f,g,h=0,i=this.length,j="string"==typeof a&&a;if(m.isFunction(a))return this.each(function(b){m(this).addClass(a.call(this,b,this.className))});if(j)for(b=(a||"").match(E)||[];i>h;h++)if(c=this[h],d=1===c.nodeType&&(c.className?(" "+c.className+" ").replace(uc," "):" ")){f=0;while(e=b[f++])d.indexOf(" "+e+" ")<0&&(d+=e+" ");g=m.trim(d),c.className!==g&&(c.className=g)}return this},removeClass:function(a){var b,c,d,e,f,g,h=0,i=this.length,j=0===arguments.length||"string"==typeof a&&a;if(m.isFunction(a))return this.each(function(b){m(this).removeClass(a.call(this,b,this.className))});if(j)for(b=(a||"").match(E)||[];i>h;h++)if(c=this[h],d=1===c.nodeType&&(c.className?(" "+c.className+" ").replace(uc," "):"")){f=0;while(e=b[f++])while(d.indexOf(" "+e+" ")>=0)d=d.replace(" "+e+" "," ");g=a?m.trim(d):"",c.className!==g&&(c.className=g)}return this},toggleClass:function(a,b){var c=typeof a;return"boolean"==typeof b&&"string"===c?b?this.addClass(a):this.removeClass(a):this.each(m.isFunction(a)?function(c){m(this).toggleClass(a.call(this,c,this.className,b),b)}:function(){if("string"===c){var b,d=0,e=m(this),f=a.match(E)||[];while(b=f[d++])e.hasClass(b)?e.removeClass(b):e.addClass(b)}else(c===K||"boolean"===c)&&(this.className&&m._data(this,"__className__",this.className),this.className=this.className||a===!1?"":m._data(this,"__className__")||"")})},hasClass:function(a){for(var b=" "+a+" ",c=0,d=this.length;d>c;c++)if(1===this[c].nodeType&&(" "+this[c].className+" ").replace(uc," ").indexOf(b)>=0)return!0;return!1}}),m.each("blur focus focusin focusout load resize scroll unload click dblclick mousedown mouseup mousemove mouseover mouseout mouseenter mouseleave change select submit keydown keypress keyup error contextmenu".split(" "),function(a,b){m.fn[b]=function(a,c){return arguments.length>0?this.on(b,null,a,c):this.trigger(b)}}),m.fn.extend({hover:function(a,b){return this.mouseenter(a).mouseleave(b||a)},bind:function(a,b,c){return this.on(a,null,b,c)},unbind:function(a,b){return this.off(a,null,b)},delegate:function(a,b,c,d){return this.on(b,a,c,d)},undelegate:function(a,b,c){return 1===arguments.length?this.off(a,"**"):this.off(b,a||"**",c)}});var vc=m.now(),wc=/\?/,xc=/(,)|(\[|{)|(}|])|"(?:[^"\\\r\n]|\\["\\\/bfnrt]|\\u[\da-fA-F]{4})*"\s*:?|true|false|null|-?(?!0\d)\d+(?:\.\d+|)(?:[eE][+-]?\d+|)/g;m.parseJSON=function(b){if(a.JSON&&a.JSON.parse)return a.JSON.parse(b+"");var c,d=null,e=m.trim(b+"");return e&&!m.trim(e.replace(xc,function(a,b,e,f){return c&&b&&(d=0),0===d?a:(c=e||b,d+=!f-!e,"")}))?Function("return "+e)():m.error("Invalid JSON: "+b)},m.parseXML=function(b){var c,d;if(!b||"string"!=typeof b)return null;try{a.DOMParser?(d=new DOMParser,c=d.parseFromString(b,"text/xml")):(c=new ActiveXObject("Microsoft.XMLDOM"),c.async="false",c.loadXML(b))}catch(e){c=void 0}return c&&c.documentElement&&!c.getElementsByTagName("parsererror").length||m.error("Invalid XML: "+b),c};var yc,zc,Ac=/#.*$/,Bc=/([?&])_=[^&]*/,Cc=/^(.*?):[ \t]*([^\r\n]*)\r?$/gm,Dc=/^(?:about|app|app-storage|.+-extension|file|res|widget):$/,Ec=/^(?:GET|HEAD)$/,Fc=/^\/\//,Gc=/^([\w.+-]+:)(?:\/\/(?:[^\/?#]*@|)([^\/?#:]*)(?::(\d+)|)|)/,Hc={},Ic={},Jc="*/".concat("*");try{zc=location.href}catch(Kc){zc=y.createElement("a"),zc.href="",zc=zc.href}yc=Gc.exec(zc.toLowerCase())||[];function Lc(a){return function(b,c){"string"!=typeof b&&(c=b,b="*");var d,e=0,f=b.toLowerCase().match(E)||[];if(m.isFunction(c))while(d=f[e++])"+"===d.charAt(0)?(d=d.slice(1)||"*",(a[d]=a[d]||[]).unshift(c)):(a[d]=a[d]||[]).push(c)}}function Mc(a,b,c,d){var e={},f=a===Ic;function g(h){var i;return e[h]=!0,m.each(a[h]||[],function(a,h){var j=h(b,c,d);return"string"!=typeof j||f||e[j]?f?!(i=j):void 0:(b.dataTypes.unshift(j),g(j),!1)}),i}return g(b.dataTypes[0])||!e["*"]&&g("*")}function Nc(a,b){var c,d,e=m.ajaxSettings.flatOptions||{};for(d in b)void 0!==b[d]&&((e[d]?a:c||(c={}))[d]=b[d]);return c&&m.extend(!0,a,c),a}function Oc(a,b,c){var d,e,f,g,h=a.contents,i=a.dataTypes;while("*"===i[0])i.shift(),void 0===e&&(e=a.mimeType||b.getResponseHeader("Content-Type"));if(e)for(g in h)if(h[g]&&h[g].test(e)){i.unshift(g);break}if(i[0]in c)f=i[0];else{for(g in c){if(!i[0]||a.converters[g+" "+i[0]]){f=g;break}d||(d=g)}f=f||d}return f?(f!==i[0]&&i.unshift(f),c[f]):void 0}function Pc(a,b,c,d){var e,f,g,h,i,j={},k=a.dataTypes.slice();if(k[1])for(g in a.converters)j[g.toLowerCase()]=a.converters[g];f=k.shift();while(f)if(a.responseFields[f]&&(c[a.responseFields[f]]=b),!i&&d&&a.dataFilter&&(b=a.dataFilter(b,a.dataType)),i=f,f=k.shift())if("*"===f)f=i;else if("*"!==i&&i!==f){if(g=j[i+" "+f]||j["* "+f],!g)for(e in j)if(h=e.split(" "),h[1]===f&&(g=j[i+" "+h[0]]||j["* "+h[0]])){g===!0?g=j[e]:j[e]!==!0&&(f=h[0],k.unshift(h[1]));break}if(g!==!0)if(g&&a["throws"])b=g(b);else try{b=g(b)}catch(l){return{state:"parsererror",error:g?l:"No conversion from "+i+" to "+f}}}return{state:"success",data:b}}m.extend({active:0,lastModified:{},etag:{},ajaxSettings:{url:zc,type:"GET",isLocal:Dc.test(yc[1]),global:!0,processData:!0,async:!0,contentType:"application/x-www-form-urlencoded; charset=UTF-8",accepts:{"*":Jc,text:"text/plain",html:"text/html",xml:"application/xml, text/xml",json:"application/json, text/javascript"},contents:{xml:/xml/,html:/html/,json:/json/},responseFields:{xml:"responseXML",text:"responseText",json:"responseJSON"},converters:{"* text":String,"text html":!0,"text json":m.parseJSON,"text xml":m.parseXML},flatOptions:{url:!0,context:!0}},ajaxSetup:function(a,b){return b?Nc(Nc(a,m.ajaxSettings),b):Nc(m.ajaxSettings,a)},ajaxPrefilter:Lc(Hc),ajaxTransport:Lc(Ic),ajax:function(a,b){"object"==typeof a&&(b=a,a=void 0),b=b||{};var c,d,e,f,g,h,i,j,k=m.ajaxSetup({},b),l=k.context||k,n=k.context&&(l.nodeType||l.jquery)?m(l):m.event,o=m.Deferred(),p=m.Callbacks("once memory"),q=k.statusCode||{},r={},s={},t=0,u="canceled",v={readyState:0,getResponseHeader:function(a){var b;if(2===t){if(!j){j={};while(b=Cc.exec(f))j[b[1].toLowerCase()]=b[2]}b=j[a.toLowerCase()]}return null==b?null:b},getAllResponseHeaders:function(){return 2===t?f:null},setRequestHeader:function(a,b){var c=a.toLowerCase();return t||(a=s[c]=s[c]||a,r[a]=b),this},overrideMimeType:function(a){return t||(k.mimeType=a),this},statusCode:function(a){var b;if(a)if(2>t)for(b in a)q[b]=[q[b],a[b]];else v.always(a[v.status]);return this},abort:function(a){var b=a||u;return i&&i.abort(b),x(0,b),this}};if(o.promise(v).complete=p.add,v.success=v.done,v.error=v.fail,k.url=((a||k.url||zc)+"").replace(Ac,"").replace(Fc,yc[1]+"//"),k.type=b.method||b.type||k.method||k.type,k.dataTypes=m.trim(k.dataType||"*").toLowerCase().match(E)||[""],null==k.crossDomain&&(c=Gc.exec(k.url.toLowerCase()),k.crossDomain=!(!c||c[1]===yc[1]&&c[2]===yc[2]&&(c[3]||("http:"===c[1]?"80":"443"))===(yc[3]||("http:"===yc[1]?"80":"443")))),k.data&&k.processData&&"string"!=typeof k.data&&(k.data=m.param(k.data,k.traditional)),Mc(Hc,k,b,v),2===t)return v;h=m.event&&k.global,h&&0===m.active++&&m.event.trigger("ajaxStart"),k.type=k.type.toUpperCase(),k.hasContent=!Ec.test(k.type),e=k.url,k.hasContent||(k.data&&(e=k.url+=(wc.test(e)?"&":"?")+k.data,delete k.data),k.cache===!1&&(k.url=Bc.test(e)?e.replace(Bc,"$1_="+vc++):e+(wc.test(e)?"&":"?")+"_="+vc++)),k.ifModified&&(m.lastModified[e]&&v.setRequestHeader("If-Modified-Since",m.lastModified[e]),m.etag[e]&&v.setRequestHeader("If-None-Match",m.etag[e])),(k.data&&k.hasContent&&k.contentType!==!1||b.contentType)&&v.setRequestHeader("Content-Type",k.contentType),v.setRequestHeader("Accept",k.dataTypes[0]&&k.accepts[k.dataTypes[0]]?k.accepts[k.dataTypes[0]]+("*"!==k.dataTypes[0]?", "+Jc+"; q=0.01":""):k.accepts["*"]);for(d in k.headers)v.setRequestHeader(d,k.headers[d]);if(k.beforeSend&&(k.beforeSend.call(l,v,k)===!1||2===t))return v.abort();u="abort";for(d in{success:1,error:1,complete:1})v[d](k[d]);if(i=Mc(Ic,k,b,v)){v.readyState=1,h&&n.trigger("ajaxSend",[v,k]),k.async&&k.timeout>0&&(g=setTimeout(function(){v.abort("timeout")},k.timeout));try{t=1,i.send(r,x)}catch(w){if(!(2>t))throw w;x(-1,w)}}else x(-1,"No Transport");function x(a,b,c,d){var j,r,s,u,w,x=b;2!==t&&(t=2,g&&clearTimeout(g),i=void 0,f=d||"",v.readyState=a>0?4:0,j=a>=200&&300>a||304===a,c&&(u=Oc(k,v,c)),u=Pc(k,u,v,j),j?(k.ifModified&&(w=v.getResponseHeader("Last-Modified"),w&&(m.lastModified[e]=w),w=v.getResponseHeader("etag"),w&&(m.etag[e]=w)),204===a||"HEAD"===k.type?x="nocontent":304===a?x="notmodified":(x=u.state,r=u.data,s=u.error,j=!s)):(s=x,(a||!x)&&(x="error",0>a&&(a=0))),v.status=a,v.statusText=(b||x)+"",j?o.resolveWith(l,[r,x,v]):o.rejectWith(l,[v,x,s]),v.statusCode(q),q=void 0,h&&n.trigger(j?"ajaxSuccess":"ajaxError",[v,k,j?r:s]),p.fireWith(l,[v,x]),h&&(n.trigger("ajaxComplete",[v,k]),--m.active||m.event.trigger("ajaxStop")))}return v},getJSON:function(a,b,c){return m.get(a,b,c,"json")},getScript:function(a,b){return m.get(a,void 0,b,"script")}}),m.each(["get","post"],function(a,b){m[b]=function(a,c,d,e){return m.isFunction(c)&&(e=e||d,d=c,c=void 0),m.ajax({url:a,type:b,dataType:e,data:c,success:d})}}),m._evalUrl=function(a){return m.ajax({url:a,type:"GET",dataType:"script",async:!1,global:!1,"throws":!0})},m.fn.extend({wrapAll:function(a){if(m.isFunction(a))return this.each(function(b){m(this).wrapAll(a.call(this,b))});if(this[0]){var b=m(a,this[0].ownerDocument).eq(0).clone(!0);this[0].parentNode&&b.insertBefore(this[0]),b.map(function(){var a=this;while(a.firstChild&&1===a.firstChild.nodeType)a=a.firstChild;return a}).append(this)}return this},wrapInner:function(a){return this.each(m.isFunction(a)?function(b){m(this).wrapInner(a.call(this,b))}:function(){var b=m(this),c=b.contents();c.length?c.wrapAll(a):b.append(a)})},wrap:function(a){var b=m.isFunction(a);return this.each(function(c){m(this).wrapAll(b?a.call(this,c):a)})},unwrap:function(){return this.parent().each(function(){m.nodeName(this,"body")||m(this).replaceWith(this.childNodes)}).end()}}),m.expr.filters.hidden=function(a){return a.offsetWidth<=0&&a.offsetHeight<=0||!k.reliableHiddenOffsets()&&"none"===(a.style&&a.style.display||m.css(a,"display"))},m.expr.filters.visible=function(a){return!m.expr.filters.hidden(a)};var Qc=/%20/g,Rc=/\[\]$/,Sc=/\r?\n/g,Tc=/^(?:submit|button|image|reset|file)$/i,Uc=/^(?:input|select|textarea|keygen)/i;function Vc(a,b,c,d){var e;if(m.isArray(b))m.each(b,function(b,e){c||Rc.test(a)?d(a,e):Vc(a+"["+("object"==typeof e?b:"")+"]",e,c,d)});else if(c||"object"!==m.type(b))d(a,b);else for(e in b)Vc(a+"["+e+"]",b[e],c,d)}m.param=function(a,b){var c,d=[],e=function(a,b){b=m.isFunction(b)?b():null==b?"":b,d[d.length]=encodeURIComponent(a)+"="+encodeURIComponent(b)};if(void 0===b&&(b=m.ajaxSettings&&m.ajaxSettings.traditional),m.isArray(a)||a.jquery&&!m.isPlainObject(a))m.each(a,function(){e(this.name,this.value)});else for(c in a)Vc(c,a[c],b,e);return d.join("&").replace(Qc,"+")},m.fn.extend({serialize:function(){return m.param(this.serializeArray())},serializeArray:function(){return this.map(function(){var a=m.prop(this,"elements");return a?m.makeArray(a):this}).filter(function(){var a=this.type;return this.name&&!m(this).is(":disabled")&&Uc.test(this.nodeName)&&!Tc.test(a)&&(this.checked||!W.test(a))}).map(function(a,b){var c=m(this).val();return null==c?null:m.isArray(c)?m.map(c,function(a){return{name:b.name,value:a.replace(Sc,"\r\n")}}):{name:b.name,value:c.replace(Sc,"\r\n")}}).get()}}),m.ajaxSettings.xhr=void 0!==a.ActiveXObject?function(){return!this.isLocal&&/^(get|post|head|put|delete|options)$/i.test(this.type)&&Zc()||$c()}:Zc;var Wc=0,Xc={},Yc=m.ajaxSettings.xhr();a.attachEvent&&a.attachEvent("onunload",function(){for(var a in Xc)Xc[a](void 0,!0)}),k.cors=!!Yc&&"withCredentials"in Yc,Yc=k.ajax=!!Yc,Yc&&m.ajaxTransport(function(a){if(!a.crossDomain||k.cors){var b;return{send:function(c,d){var e,f=a.xhr(),g=++Wc;if(f.open(a.type,a.url,a.async,a.username,a.password),a.xhrFields)for(e in a.xhrFields)f[e]=a.xhrFields[e];a.mimeType&&f.overrideMimeType&&f.overrideMimeType(a.mimeType),a.crossDomain||c["X-Requested-With"]||(c["X-Requested-With"]="XMLHttpRequest");for(e in c)void 0!==c[e]&&f.setRequestHeader(e,c[e]+"");f.send(a.hasContent&&a.data||null),b=function(c,e){var h,i,j;if(b&&(e||4===f.readyState))if(delete Xc[g],b=void 0,f.onreadystatechange=m.noop,e)4!==f.readyState&&f.abort();else{j={},h=f.status,"string"==typeof f.responseText&&(j.text=f.responseText);try{i=f.statusText}catch(k){i=""}h||!a.isLocal||a.crossDomain?1223===h&&(h=204):h=j.text?200:404}j&&d(h,i,j,f.getAllResponseHeaders())},a.async?4===f.readyState?setTimeout(b):f.onreadystatechange=Xc[g]=b:b()},abort:function(){b&&b(void 0,!0)}}}});function Zc(){try{return new a.XMLHttpRequest}catch(b){}}function $c(){try{return new a.ActiveXObject("Microsoft.XMLHTTP")}catch(b){}}m.ajaxSetup({accepts:{script:"text/javascript, application/javascript, application/ecmascript, application/x-ecmascript"},contents:{script:/(?:java|ecma)script/},converters:{"text script":function(a){return m.globalEval(a),a}}}),m.ajaxPrefilter("script",function(a){void 0===a.cache&&(a.cache=!1),a.crossDomain&&(a.type="GET",a.global=!1)}),m.ajaxTransport("script",function(a){if(a.crossDomain){var b,c=y.head||m("head")[0]||y.documentElement;return{send:function(d,e){b=y.createElement("script"),b.async=!0,a.scriptCharset&&(b.charset=a.scriptCharset),b.src=a.url,b.onload=b.onreadystatechange=function(a,c){(c||!b.readyState||/loaded|complete/.test(b.readyState))&&(b.onload=b.onreadystatechange=null,b.parentNode&&b.parentNode.removeChild(b),b=null,c||e(200,"success"))},c.insertBefore(b,c.firstChild)},abort:function(){b&&b.onload(void 0,!0)}}}});var _c=[],ad=/(=)\?(?=&|$)|\?\?/;m.ajaxSetup({jsonp:"callback",jsonpCallback:function(){var a=_c.pop()||m.expando+"_"+vc++;return this[a]=!0,a}}),m.ajaxPrefilter("json jsonp",function(b,c,d){var e,f,g,h=b.jsonp!==!1&&(ad.test(b.url)?"url":"string"==typeof b.data&&!(b.contentType||"").indexOf("application/x-www-form-urlencoded")&&ad.test(b.data)&&"data");return h||"jsonp"===b.dataTypes[0]?(e=b.jsonpCallback=m.isFunction(b.jsonpCallback)?b.jsonpCallback():b.jsonpCallback,h?b[h]=b[h].replace(ad,"$1"+e):b.jsonp!==!1&&(b.url+=(wc.test(b.url)?"&":"?")+b.jsonp+"="+e),b.converters["script json"]=function(){return g||m.error(e+" was not called"),g[0]},b.dataTypes[0]="json",f=a[e],a[e]=function(){g=arguments},d.always(function(){a[e]=f,b[e]&&(b.jsonpCallback=c.jsonpCallback,_c.push(e)),g&&m.isFunction(f)&&f(g[0]),g=f=void 0}),"script"):void 0}),m.parseHTML=function(a,b,c){if(!a||"string"!=typeof a)return null;"boolean"==typeof b&&(c=b,b=!1),b=b||y;var d=u.exec(a),e=!c&&[];return d?[b.createElement(d[1])]:(d=m.buildFragment([a],b,e),e&&e.length&&m(e).remove(),m.merge([],d.childNodes))};var bd=m.fn.load;m.fn.load=function(a,b,c){if("string"!=typeof a&&bd)return bd.apply(this,arguments);var d,e,f,g=this,h=a.indexOf(" ");return h>=0&&(d=m.trim(a.slice(h,a.length)),a=a.slice(0,h)),m.isFunction(b)?(c=b,b=void 0):b&&"object"==typeof b&&(f="POST"),g.length>0&&m.ajax({url:a,type:f,dataType:"html",data:b}).done(function(a){e=arguments,g.html(d?m("<div>").append(m.parseHTML(a)).find(d):a)}).complete(c&&function(a,b){g.each(c,e||[a.responseText,b,a])}),this},m.each(["ajaxStart","ajaxStop","ajaxComplete","ajaxError","ajaxSuccess","ajaxSend"],function(a,b){m.fn[b]=function(a){return this.on(b,a)}}),m.expr.filters.animated=function(a){return m.grep(m.timers,function(b){return a===b.elem}).length};var cd=a.document.documentElement;function dd(a){return m.isWindow(a)?a:9===a.nodeType?a.defaultView||a.parentWindow:!1}m.offset={setOffset:function(a,b,c){var d,e,f,g,h,i,j,k=m.css(a,"position"),l=m(a),n={};"static"===k&&(a.style.position="relative"),h=l.offset(),f=m.css(a,"top"),i=m.css(a,"left"),j=("absolute"===k||"fixed"===k)&&m.inArray("auto",[f,i])>-1,j?(d=l.position(),g=d.top,e=d.left):(g=parseFloat(f)||0,e=parseFloat(i)||0),m.isFunction(b)&&(b=b.call(a,c,h)),null!=b.top&&(n.top=b.top-h.top+g),null!=b.left&&(n.left=b.left-h.left+e),"using"in b?b.using.call(a,n):l.css(n)}},m.fn.extend({offset:function(a){if(arguments.length)return void 0===a?this:this.each(function(b){m.offset.setOffset(this,a,b)});var b,c,d={top:0,left:0},e=this[0],f=e&&e.ownerDocument;if(f)return b=f.documentElement,m.contains(b,e)?(typeof e.getBoundingClientRect!==K&&(d=e.getBoundingClientRect()),c=dd(f),{top:d.top+(c.pageYOffset||b.scrollTop)-(b.clientTop||0),left:d.left+(c.pageXOffset||b.scrollLeft)-(b.clientLeft||0)}):d},position:function(){if(this[0]){var a,b,c={top:0,left:0},d=this[0];return"fixed"===m.css(d,"position")?b=d.getBoundingClientRect():(a=this.offsetParent(),b=this.offset(),m.nodeName(a[0],"html")||(c=a.offset()),c.top+=m.css(a[0],"borderTopWidth",!0),c.left+=m.css(a[0],"borderLeftWidth",!0)),{top:b.top-c.top-m.css(d,"marginTop",!0),left:b.left-c.left-m.css(d,"marginLeft",!0)}}},offsetParent:function(){return this.map(function(){var a=this.offsetParent||cd;while(a&&!m.nodeName(a,"html")&&"static"===m.css(a,"position"))a=a.offsetParent;return a||cd})}}),m.each({scrollLeft:"pageXOffset",scrollTop:"pageYOffset"},function(a,b){var c=/Y/.test(b);m.fn[a]=function(d){return V(this,function(a,d,e){var f=dd(a);return void 0===e?f?b in f?f[b]:f.document.documentElement[d]:a[d]:void(f?f.scrollTo(c?m(f).scrollLeft():e,c?e:m(f).scrollTop()):a[d]=e)},a,d,arguments.length,null)}}),m.each(["top","left"],function(a,b){m.cssHooks[b]=Lb(k.pixelPosition,function(a,c){return c?(c=Jb(a,b),Hb.test(c)?m(a).position()[b]+"px":c):void 0})}),m.each({Height:"height",Width:"width"},function(a,b){m.each({padding:"inner"+a,content:b,"":"outer"+a},function(c,d){m.fn[d]=function(d,e){var f=arguments.length&&(c||"boolean"!=typeof d),g=c||(d===!0||e===!0?"margin":"border");return V(this,function(b,c,d){var e;return m.isWindow(b)?b.document.documentElement["client"+a]:9===b.nodeType?(e=b.documentElement,Math.max(b.body["scroll"+a],e["scroll"+a],b.body["offset"+a],e["offset"+a],e["client"+a])):void 0===d?m.css(b,c,g):m.style(b,c,d,g)},b,f?d:void 0,f,null)}})}),m.fn.size=function(){return this.length},m.fn.andSelf=m.fn.addBack,"function"==typeof define&&define.amd&&define("jquery",[],function(){return m});var ed=a.jQuery,fd=a.$;return m.noConflict=function(b){return a.$===m&&(a.$=fd),b&&a.jQuery===m&&(a.jQuery=ed),m},typeof b===K&&(a.jQuery=a.$=m),m});
|
src/thirdparty/react.js | petetnt/brackets | /**
* React (with addons) v0.14.7
*/
(function(f){if(typeof exports==="object"&&typeof module!=="undefined"){module.exports=f()}else if(typeof define==="function"&&define.amd){define([],f)}else{var g;if(typeof window!=="undefined"){g=window}else if(typeof global!=="undefined"){g=global}else if(typeof self!=="undefined"){g=self}else{g=this}g.React = f()}})(function(){var define,module,exports;return (function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s})({1:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactWithAddons
*/
/**
* This module exists purely in the open source project, and is meant as a way
* to create a separate standalone build of React. This build has "addons", or
* functionality we've built and think might be useful but doesn't have a good
* place to live inside React core.
*/
'use strict';
var LinkedStateMixin = _dereq_(22);
var React = _dereq_(26);
var ReactComponentWithPureRenderMixin = _dereq_(37);
var ReactCSSTransitionGroup = _dereq_(29);
var ReactFragment = _dereq_(64);
var ReactTransitionGroup = _dereq_(94);
var ReactUpdates = _dereq_(96);
var cloneWithProps = _dereq_(118);
var shallowCompare = _dereq_(140);
var update = _dereq_(143);
var warning = _dereq_(173);
var warnedAboutBatchedUpdates = false;
React.addons = {
CSSTransitionGroup: ReactCSSTransitionGroup,
LinkedStateMixin: LinkedStateMixin,
PureRenderMixin: ReactComponentWithPureRenderMixin,
TransitionGroup: ReactTransitionGroup,
batchedUpdates: function () {
if ("development" !== 'production') {
"development" !== 'production' ? warning(warnedAboutBatchedUpdates, 'React.addons.batchedUpdates is deprecated. Use ' + 'ReactDOM.unstable_batchedUpdates instead.') : undefined;
warnedAboutBatchedUpdates = true;
}
return ReactUpdates.batchedUpdates.apply(this, arguments);
},
cloneWithProps: cloneWithProps,
createFragment: ReactFragment.create,
shallowCompare: shallowCompare,
update: update
};
if ("development" !== 'production') {
React.addons.Perf = _dereq_(55);
React.addons.TestUtils = _dereq_(91);
}
module.exports = React;
},{"118":118,"140":140,"143":143,"173":173,"22":22,"26":26,"29":29,"37":37,"55":55,"64":64,"91":91,"94":94,"96":96}],2:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule AutoFocusUtils
* @typechecks static-only
*/
'use strict';
var ReactMount = _dereq_(72);
var findDOMNode = _dereq_(122);
var focusNode = _dereq_(155);
var Mixin = {
componentDidMount: function () {
if (this.props.autoFocus) {
focusNode(findDOMNode(this));
}
}
};
var AutoFocusUtils = {
Mixin: Mixin,
focusDOMComponent: function () {
focusNode(ReactMount.getNode(this._rootNodeID));
}
};
module.exports = AutoFocusUtils;
},{"122":122,"155":155,"72":72}],3:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015 Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule BeforeInputEventPlugin
* @typechecks static-only
*/
'use strict';
var EventConstants = _dereq_(15);
var EventPropagators = _dereq_(19);
var ExecutionEnvironment = _dereq_(147);
var FallbackCompositionState = _dereq_(20);
var SyntheticCompositionEvent = _dereq_(103);
var SyntheticInputEvent = _dereq_(107);
var keyOf = _dereq_(166);
var END_KEYCODES = [9, 13, 27, 32]; // Tab, Return, Esc, Space
var START_KEYCODE = 229;
var canUseCompositionEvent = ExecutionEnvironment.canUseDOM && 'CompositionEvent' in window;
var documentMode = null;
if (ExecutionEnvironment.canUseDOM && 'documentMode' in document) {
documentMode = document.documentMode;
}
// Webkit offers a very useful `textInput` event that can be used to
// directly represent `beforeInput`. The IE `textinput` event is not as
// useful, so we don't use it.
var canUseTextInputEvent = ExecutionEnvironment.canUseDOM && 'TextEvent' in window && !documentMode && !isPresto();
// In IE9+, we have access to composition events, but the data supplied
// by the native compositionend event may be incorrect. Japanese ideographic
// spaces, for instance (\u3000) are not recorded correctly.
var useFallbackCompositionData = ExecutionEnvironment.canUseDOM && (!canUseCompositionEvent || documentMode && documentMode > 8 && documentMode <= 11);
/**
* Opera <= 12 includes TextEvent in window, but does not fire
* text input events. Rely on keypress instead.
*/
function isPresto() {
var opera = window.opera;
return typeof opera === 'object' && typeof opera.version === 'function' && parseInt(opera.version(), 10) <= 12;
}
var SPACEBAR_CODE = 32;
var SPACEBAR_CHAR = String.fromCharCode(SPACEBAR_CODE);
var topLevelTypes = EventConstants.topLevelTypes;
// Events and their corresponding property names.
var eventTypes = {
beforeInput: {
phasedRegistrationNames: {
bubbled: keyOf({ onBeforeInput: null }),
captured: keyOf({ onBeforeInputCapture: null })
},
dependencies: [topLevelTypes.topCompositionEnd, topLevelTypes.topKeyPress, topLevelTypes.topTextInput, topLevelTypes.topPaste]
},
compositionEnd: {
phasedRegistrationNames: {
bubbled: keyOf({ onCompositionEnd: null }),
captured: keyOf({ onCompositionEndCapture: null })
},
dependencies: [topLevelTypes.topBlur, topLevelTypes.topCompositionEnd, topLevelTypes.topKeyDown, topLevelTypes.topKeyPress, topLevelTypes.topKeyUp, topLevelTypes.topMouseDown]
},
compositionStart: {
phasedRegistrationNames: {
bubbled: keyOf({ onCompositionStart: null }),
captured: keyOf({ onCompositionStartCapture: null })
},
dependencies: [topLevelTypes.topBlur, topLevelTypes.topCompositionStart, topLevelTypes.topKeyDown, topLevelTypes.topKeyPress, topLevelTypes.topKeyUp, topLevelTypes.topMouseDown]
},
compositionUpdate: {
phasedRegistrationNames: {
bubbled: keyOf({ onCompositionUpdate: null }),
captured: keyOf({ onCompositionUpdateCapture: null })
},
dependencies: [topLevelTypes.topBlur, topLevelTypes.topCompositionUpdate, topLevelTypes.topKeyDown, topLevelTypes.topKeyPress, topLevelTypes.topKeyUp, topLevelTypes.topMouseDown]
}
};
// Track whether we've ever handled a keypress on the space key.
var hasSpaceKeypress = false;
/**
* Return whether a native keypress event is assumed to be a command.
* This is required because Firefox fires `keypress` events for key commands
* (cut, copy, select-all, etc.) even though no character is inserted.
*/
function isKeypressCommand(nativeEvent) {
return (nativeEvent.ctrlKey || nativeEvent.altKey || nativeEvent.metaKey) &&
// ctrlKey && altKey is equivalent to AltGr, and is not a command.
!(nativeEvent.ctrlKey && nativeEvent.altKey);
}
/**
* Translate native top level events into event types.
*
* @param {string} topLevelType
* @return {object}
*/
function getCompositionEventType(topLevelType) {
switch (topLevelType) {
case topLevelTypes.topCompositionStart:
return eventTypes.compositionStart;
case topLevelTypes.topCompositionEnd:
return eventTypes.compositionEnd;
case topLevelTypes.topCompositionUpdate:
return eventTypes.compositionUpdate;
}
}
/**
* Does our fallback best-guess model think this event signifies that
* composition has begun?
*
* @param {string} topLevelType
* @param {object} nativeEvent
* @return {boolean}
*/
function isFallbackCompositionStart(topLevelType, nativeEvent) {
return topLevelType === topLevelTypes.topKeyDown && nativeEvent.keyCode === START_KEYCODE;
}
/**
* Does our fallback mode think that this event is the end of composition?
*
* @param {string} topLevelType
* @param {object} nativeEvent
* @return {boolean}
*/
function isFallbackCompositionEnd(topLevelType, nativeEvent) {
switch (topLevelType) {
case topLevelTypes.topKeyUp:
// Command keys insert or clear IME input.
return END_KEYCODES.indexOf(nativeEvent.keyCode) !== -1;
case topLevelTypes.topKeyDown:
// Expect IME keyCode on each keydown. If we get any other
// code we must have exited earlier.
return nativeEvent.keyCode !== START_KEYCODE;
case topLevelTypes.topKeyPress:
case topLevelTypes.topMouseDown:
case topLevelTypes.topBlur:
// Events are not possible without cancelling IME.
return true;
default:
return false;
}
}
/**
* Google Input Tools provides composition data via a CustomEvent,
* with the `data` property populated in the `detail` object. If this
* is available on the event object, use it. If not, this is a plain
* composition event and we have nothing special to extract.
*
* @param {object} nativeEvent
* @return {?string}
*/
function getDataFromCustomEvent(nativeEvent) {
var detail = nativeEvent.detail;
if (typeof detail === 'object' && 'data' in detail) {
return detail.data;
}
return null;
}
// Track the current IME composition fallback object, if any.
var currentComposition = null;
/**
* @param {string} topLevelType Record from `EventConstants`.
* @param {DOMEventTarget} topLevelTarget The listening component root node.
* @param {string} topLevelTargetID ID of `topLevelTarget`.
* @param {object} nativeEvent Native browser event.
* @return {?object} A SyntheticCompositionEvent.
*/
function extractCompositionEvent(topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget) {
var eventType;
var fallbackData;
if (canUseCompositionEvent) {
eventType = getCompositionEventType(topLevelType);
} else if (!currentComposition) {
if (isFallbackCompositionStart(topLevelType, nativeEvent)) {
eventType = eventTypes.compositionStart;
}
} else if (isFallbackCompositionEnd(topLevelType, nativeEvent)) {
eventType = eventTypes.compositionEnd;
}
if (!eventType) {
return null;
}
if (useFallbackCompositionData) {
// The current composition is stored statically and must not be
// overwritten while composition continues.
if (!currentComposition && eventType === eventTypes.compositionStart) {
currentComposition = FallbackCompositionState.getPooled(topLevelTarget);
} else if (eventType === eventTypes.compositionEnd) {
if (currentComposition) {
fallbackData = currentComposition.getData();
}
}
}
var event = SyntheticCompositionEvent.getPooled(eventType, topLevelTargetID, nativeEvent, nativeEventTarget);
if (fallbackData) {
// Inject data generated from fallback path into the synthetic event.
// This matches the property of native CompositionEventInterface.
event.data = fallbackData;
} else {
var customData = getDataFromCustomEvent(nativeEvent);
if (customData !== null) {
event.data = customData;
}
}
EventPropagators.accumulateTwoPhaseDispatches(event);
return event;
}
/**
* @param {string} topLevelType Record from `EventConstants`.
* @param {object} nativeEvent Native browser event.
* @return {?string} The string corresponding to this `beforeInput` event.
*/
function getNativeBeforeInputChars(topLevelType, nativeEvent) {
switch (topLevelType) {
case topLevelTypes.topCompositionEnd:
return getDataFromCustomEvent(nativeEvent);
case topLevelTypes.topKeyPress:
/**
* If native `textInput` events are available, our goal is to make
* use of them. However, there is a special case: the spacebar key.
* In Webkit, preventing default on a spacebar `textInput` event
* cancels character insertion, but it *also* causes the browser
* to fall back to its default spacebar behavior of scrolling the
* page.
*
* Tracking at:
* https://code.google.com/p/chromium/issues/detail?id=355103
*
* To avoid this issue, use the keypress event as if no `textInput`
* event is available.
*/
var which = nativeEvent.which;
if (which !== SPACEBAR_CODE) {
return null;
}
hasSpaceKeypress = true;
return SPACEBAR_CHAR;
case topLevelTypes.topTextInput:
// Record the characters to be added to the DOM.
var chars = nativeEvent.data;
// If it's a spacebar character, assume that we have already handled
// it at the keypress level and bail immediately. Android Chrome
// doesn't give us keycodes, so we need to blacklist it.
if (chars === SPACEBAR_CHAR && hasSpaceKeypress) {
return null;
}
return chars;
default:
// For other native event types, do nothing.
return null;
}
}
/**
* For browsers that do not provide the `textInput` event, extract the
* appropriate string to use for SyntheticInputEvent.
*
* @param {string} topLevelType Record from `EventConstants`.
* @param {object} nativeEvent Native browser event.
* @return {?string} The fallback string for this `beforeInput` event.
*/
function getFallbackBeforeInputChars(topLevelType, nativeEvent) {
// If we are currently composing (IME) and using a fallback to do so,
// try to extract the composed characters from the fallback object.
if (currentComposition) {
if (topLevelType === topLevelTypes.topCompositionEnd || isFallbackCompositionEnd(topLevelType, nativeEvent)) {
var chars = currentComposition.getData();
FallbackCompositionState.release(currentComposition);
currentComposition = null;
return chars;
}
return null;
}
switch (topLevelType) {
case topLevelTypes.topPaste:
// If a paste event occurs after a keypress, throw out the input
// chars. Paste events should not lead to BeforeInput events.
return null;
case topLevelTypes.topKeyPress:
/**
* As of v27, Firefox may fire keypress events even when no character
* will be inserted. A few possibilities:
*
* - `which` is `0`. Arrow keys, Esc key, etc.
*
* - `which` is the pressed key code, but no char is available.
* Ex: 'AltGr + d` in Polish. There is no modified character for
* this key combination and no character is inserted into the
* document, but FF fires the keypress for char code `100` anyway.
* No `input` event will occur.
*
* - `which` is the pressed key code, but a command combination is
* being used. Ex: `Cmd+C`. No character is inserted, and no
* `input` event will occur.
*/
if (nativeEvent.which && !isKeypressCommand(nativeEvent)) {
return String.fromCharCode(nativeEvent.which);
}
return null;
case topLevelTypes.topCompositionEnd:
return useFallbackCompositionData ? null : nativeEvent.data;
default:
return null;
}
}
/**
* Extract a SyntheticInputEvent for `beforeInput`, based on either native
* `textInput` or fallback behavior.
*
* @param {string} topLevelType Record from `EventConstants`.
* @param {DOMEventTarget} topLevelTarget The listening component root node.
* @param {string} topLevelTargetID ID of `topLevelTarget`.
* @param {object} nativeEvent Native browser event.
* @return {?object} A SyntheticInputEvent.
*/
function extractBeforeInputEvent(topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget) {
var chars;
if (canUseTextInputEvent) {
chars = getNativeBeforeInputChars(topLevelType, nativeEvent);
} else {
chars = getFallbackBeforeInputChars(topLevelType, nativeEvent);
}
// If no characters are being inserted, no BeforeInput event should
// be fired.
if (!chars) {
return null;
}
var event = SyntheticInputEvent.getPooled(eventTypes.beforeInput, topLevelTargetID, nativeEvent, nativeEventTarget);
event.data = chars;
EventPropagators.accumulateTwoPhaseDispatches(event);
return event;
}
/**
* Create an `onBeforeInput` event to match
* http://www.w3.org/TR/2013/WD-DOM-Level-3-Events-20131105/#events-inputevents.
*
* This event plugin is based on the native `textInput` event
* available in Chrome, Safari, Opera, and IE. This event fires after
* `onKeyPress` and `onCompositionEnd`, but before `onInput`.
*
* `beforeInput` is spec'd but not implemented in any browsers, and
* the `input` event does not provide any useful information about what has
* actually been added, contrary to the spec. Thus, `textInput` is the best
* available event to identify the characters that have actually been inserted
* into the target node.
*
* This plugin is also responsible for emitting `composition` events, thus
* allowing us to share composition fallback code for both `beforeInput` and
* `composition` event types.
*/
var BeforeInputEventPlugin = {
eventTypes: eventTypes,
/**
* @param {string} topLevelType Record from `EventConstants`.
* @param {DOMEventTarget} topLevelTarget The listening component root node.
* @param {string} topLevelTargetID ID of `topLevelTarget`.
* @param {object} nativeEvent Native browser event.
* @return {*} An accumulation of synthetic events.
* @see {EventPluginHub.extractEvents}
*/
extractEvents: function (topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget) {
return [extractCompositionEvent(topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget), extractBeforeInputEvent(topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget)];
}
};
module.exports = BeforeInputEventPlugin;
},{"103":103,"107":107,"147":147,"15":15,"166":166,"19":19,"20":20}],4:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule CSSProperty
*/
'use strict';
/**
* CSS properties which accept numbers but are not in units of "px".
*/
var isUnitlessNumber = {
animationIterationCount: true,
boxFlex: true,
boxFlexGroup: true,
boxOrdinalGroup: true,
columnCount: true,
flex: true,
flexGrow: true,
flexPositive: true,
flexShrink: true,
flexNegative: true,
flexOrder: true,
fontWeight: true,
lineClamp: true,
lineHeight: true,
opacity: true,
order: true,
orphans: true,
tabSize: true,
widows: true,
zIndex: true,
zoom: true,
// SVG-related properties
fillOpacity: true,
stopOpacity: true,
strokeDashoffset: true,
strokeOpacity: true,
strokeWidth: true
};
/**
* @param {string} prefix vendor-specific prefix, eg: Webkit
* @param {string} key style name, eg: transitionDuration
* @return {string} style name prefixed with `prefix`, properly camelCased, eg:
* WebkitTransitionDuration
*/
function prefixKey(prefix, key) {
return prefix + key.charAt(0).toUpperCase() + key.substring(1);
}
/**
* Support style names that may come passed in prefixed by adding permutations
* of vendor prefixes.
*/
var prefixes = ['Webkit', 'ms', 'Moz', 'O'];
// Using Object.keys here, or else the vanilla for-in loop makes IE8 go into an
// infinite loop, because it iterates over the newly added props too.
Object.keys(isUnitlessNumber).forEach(function (prop) {
prefixes.forEach(function (prefix) {
isUnitlessNumber[prefixKey(prefix, prop)] = isUnitlessNumber[prop];
});
});
/**
* Most style properties can be unset by doing .style[prop] = '' but IE8
* doesn't like doing that with shorthand properties so for the properties that
* IE8 breaks on, which are listed here, we instead unset each of the
* individual properties. See http://bugs.jquery.com/ticket/12385.
* The 4-value 'clock' properties like margin, padding, border-width seem to
* behave without any problems. Curiously, list-style works too without any
* special prodding.
*/
var shorthandPropertyExpansions = {
background: {
backgroundAttachment: true,
backgroundColor: true,
backgroundImage: true,
backgroundPositionX: true,
backgroundPositionY: true,
backgroundRepeat: true
},
backgroundPosition: {
backgroundPositionX: true,
backgroundPositionY: true
},
border: {
borderWidth: true,
borderStyle: true,
borderColor: true
},
borderBottom: {
borderBottomWidth: true,
borderBottomStyle: true,
borderBottomColor: true
},
borderLeft: {
borderLeftWidth: true,
borderLeftStyle: true,
borderLeftColor: true
},
borderRight: {
borderRightWidth: true,
borderRightStyle: true,
borderRightColor: true
},
borderTop: {
borderTopWidth: true,
borderTopStyle: true,
borderTopColor: true
},
font: {
fontStyle: true,
fontVariant: true,
fontWeight: true,
fontSize: true,
lineHeight: true,
fontFamily: true
},
outline: {
outlineWidth: true,
outlineStyle: true,
outlineColor: true
}
};
var CSSProperty = {
isUnitlessNumber: isUnitlessNumber,
shorthandPropertyExpansions: shorthandPropertyExpansions
};
module.exports = CSSProperty;
},{}],5:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule CSSPropertyOperations
* @typechecks static-only
*/
'use strict';
var CSSProperty = _dereq_(4);
var ExecutionEnvironment = _dereq_(147);
var ReactPerf = _dereq_(78);
var camelizeStyleName = _dereq_(149);
var dangerousStyleValue = _dereq_(119);
var hyphenateStyleName = _dereq_(160);
var memoizeStringOnly = _dereq_(168);
var warning = _dereq_(173);
var processStyleName = memoizeStringOnly(function (styleName) {
return hyphenateStyleName(styleName);
});
var hasShorthandPropertyBug = false;
var styleFloatAccessor = 'cssFloat';
if (ExecutionEnvironment.canUseDOM) {
var tempStyle = document.createElement('div').style;
try {
// IE8 throws "Invalid argument." if resetting shorthand style properties.
tempStyle.font = '';
} catch (e) {
hasShorthandPropertyBug = true;
}
// IE8 only supports accessing cssFloat (standard) as styleFloat
if (document.documentElement.style.cssFloat === undefined) {
styleFloatAccessor = 'styleFloat';
}
}
if ("development" !== 'production') {
// 'msTransform' is correct, but the other prefixes should be capitalized
var badVendoredStyleNamePattern = /^(?:webkit|moz|o)[A-Z]/;
// style values shouldn't contain a semicolon
var badStyleValueWithSemicolonPattern = /;\s*$/;
var warnedStyleNames = {};
var warnedStyleValues = {};
var warnHyphenatedStyleName = function (name) {
if (warnedStyleNames.hasOwnProperty(name) && warnedStyleNames[name]) {
return;
}
warnedStyleNames[name] = true;
"development" !== 'production' ? warning(false, 'Unsupported style property %s. Did you mean %s?', name, camelizeStyleName(name)) : undefined;
};
var warnBadVendoredStyleName = function (name) {
if (warnedStyleNames.hasOwnProperty(name) && warnedStyleNames[name]) {
return;
}
warnedStyleNames[name] = true;
"development" !== 'production' ? warning(false, 'Unsupported vendor-prefixed style property %s. Did you mean %s?', name, name.charAt(0).toUpperCase() + name.slice(1)) : undefined;
};
var warnStyleValueWithSemicolon = function (name, value) {
if (warnedStyleValues.hasOwnProperty(value) && warnedStyleValues[value]) {
return;
}
warnedStyleValues[value] = true;
"development" !== 'production' ? warning(false, 'Style property values shouldn\'t contain a semicolon. ' + 'Try "%s: %s" instead.', name, value.replace(badStyleValueWithSemicolonPattern, '')) : undefined;
};
/**
* @param {string} name
* @param {*} value
*/
var warnValidStyle = function (name, value) {
if (name.indexOf('-') > -1) {
warnHyphenatedStyleName(name);
} else if (badVendoredStyleNamePattern.test(name)) {
warnBadVendoredStyleName(name);
} else if (badStyleValueWithSemicolonPattern.test(value)) {
warnStyleValueWithSemicolon(name, value);
}
};
}
/**
* Operations for dealing with CSS properties.
*/
var CSSPropertyOperations = {
/**
* Serializes a mapping of style properties for use as inline styles:
*
* > createMarkupForStyles({width: '200px', height: 0})
* "width:200px;height:0;"
*
* Undefined values are ignored so that declarative programming is easier.
* The result should be HTML-escaped before insertion into the DOM.
*
* @param {object} styles
* @return {?string}
*/
createMarkupForStyles: function (styles) {
var serialized = '';
for (var styleName in styles) {
if (!styles.hasOwnProperty(styleName)) {
continue;
}
var styleValue = styles[styleName];
if ("development" !== 'production') {
warnValidStyle(styleName, styleValue);
}
if (styleValue != null) {
serialized += processStyleName(styleName) + ':';
serialized += dangerousStyleValue(styleName, styleValue) + ';';
}
}
return serialized || null;
},
/**
* Sets the value for multiple styles on a node. If a value is specified as
* '' (empty string), the corresponding style property will be unset.
*
* @param {DOMElement} node
* @param {object} styles
*/
setValueForStyles: function (node, styles) {
var style = node.style;
for (var styleName in styles) {
if (!styles.hasOwnProperty(styleName)) {
continue;
}
if ("development" !== 'production') {
warnValidStyle(styleName, styles[styleName]);
}
var styleValue = dangerousStyleValue(styleName, styles[styleName]);
if (styleName === 'float') {
styleName = styleFloatAccessor;
}
if (styleValue) {
style[styleName] = styleValue;
} else {
var expansion = hasShorthandPropertyBug && CSSProperty.shorthandPropertyExpansions[styleName];
if (expansion) {
// Shorthand property that IE8 won't like unsetting, so unset each
// component to placate it
for (var individualStyleName in expansion) {
style[individualStyleName] = '';
}
} else {
style[styleName] = '';
}
}
}
}
};
ReactPerf.measureMethods(CSSPropertyOperations, 'CSSPropertyOperations', {
setValueForStyles: 'setValueForStyles'
});
module.exports = CSSPropertyOperations;
},{"119":119,"147":147,"149":149,"160":160,"168":168,"173":173,"4":4,"78":78}],6:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule CallbackQueue
*/
'use strict';
var PooledClass = _dereq_(25);
var assign = _dereq_(24);
var invariant = _dereq_(161);
/**
* A specialized pseudo-event module to help keep track of components waiting to
* be notified when their DOM representations are available for use.
*
* This implements `PooledClass`, so you should never need to instantiate this.
* Instead, use `CallbackQueue.getPooled()`.
*
* @class ReactMountReady
* @implements PooledClass
* @internal
*/
function CallbackQueue() {
this._callbacks = null;
this._contexts = null;
}
assign(CallbackQueue.prototype, {
/**
* Enqueues a callback to be invoked when `notifyAll` is invoked.
*
* @param {function} callback Invoked when `notifyAll` is invoked.
* @param {?object} context Context to call `callback` with.
* @internal
*/
enqueue: function (callback, context) {
this._callbacks = this._callbacks || [];
this._contexts = this._contexts || [];
this._callbacks.push(callback);
this._contexts.push(context);
},
/**
* Invokes all enqueued callbacks and clears the queue. This is invoked after
* the DOM representation of a component has been created or updated.
*
* @internal
*/
notifyAll: function () {
var callbacks = this._callbacks;
var contexts = this._contexts;
if (callbacks) {
!(callbacks.length === contexts.length) ? "development" !== 'production' ? invariant(false, 'Mismatched list of contexts in callback queue') : invariant(false) : undefined;
this._callbacks = null;
this._contexts = null;
for (var i = 0; i < callbacks.length; i++) {
callbacks[i].call(contexts[i]);
}
callbacks.length = 0;
contexts.length = 0;
}
},
/**
* Resets the internal queue.
*
* @internal
*/
reset: function () {
this._callbacks = null;
this._contexts = null;
},
/**
* `PooledClass` looks for this.
*/
destructor: function () {
this.reset();
}
});
PooledClass.addPoolingTo(CallbackQueue);
module.exports = CallbackQueue;
},{"161":161,"24":24,"25":25}],7:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ChangeEventPlugin
*/
'use strict';
var EventConstants = _dereq_(15);
var EventPluginHub = _dereq_(16);
var EventPropagators = _dereq_(19);
var ExecutionEnvironment = _dereq_(147);
var ReactUpdates = _dereq_(96);
var SyntheticEvent = _dereq_(105);
var getEventTarget = _dereq_(128);
var isEventSupported = _dereq_(133);
var isTextInputElement = _dereq_(134);
var keyOf = _dereq_(166);
var topLevelTypes = EventConstants.topLevelTypes;
var eventTypes = {
change: {
phasedRegistrationNames: {
bubbled: keyOf({ onChange: null }),
captured: keyOf({ onChangeCapture: null })
},
dependencies: [topLevelTypes.topBlur, topLevelTypes.topChange, topLevelTypes.topClick, topLevelTypes.topFocus, topLevelTypes.topInput, topLevelTypes.topKeyDown, topLevelTypes.topKeyUp, topLevelTypes.topSelectionChange]
}
};
/**
* For IE shims
*/
var activeElement = null;
var activeElementID = null;
var activeElementValue = null;
var activeElementValueProp = null;
/**
* SECTION: handle `change` event
*/
function shouldUseChangeEvent(elem) {
var nodeName = elem.nodeName && elem.nodeName.toLowerCase();
return nodeName === 'select' || nodeName === 'input' && elem.type === 'file';
}
var doesChangeEventBubble = false;
if (ExecutionEnvironment.canUseDOM) {
// See `handleChange` comment below
doesChangeEventBubble = isEventSupported('change') && (!('documentMode' in document) || document.documentMode > 8);
}
function manualDispatchChangeEvent(nativeEvent) {
var event = SyntheticEvent.getPooled(eventTypes.change, activeElementID, nativeEvent, getEventTarget(nativeEvent));
EventPropagators.accumulateTwoPhaseDispatches(event);
// If change and propertychange bubbled, we'd just bind to it like all the
// other events and have it go through ReactBrowserEventEmitter. Since it
// doesn't, we manually listen for the events and so we have to enqueue and
// process the abstract event manually.
//
// Batching is necessary here in order to ensure that all event handlers run
// before the next rerender (including event handlers attached to ancestor
// elements instead of directly on the input). Without this, controlled
// components don't work properly in conjunction with event bubbling because
// the component is rerendered and the value reverted before all the event
// handlers can run. See https://github.com/facebook/react/issues/708.
ReactUpdates.batchedUpdates(runEventInBatch, event);
}
function runEventInBatch(event) {
EventPluginHub.enqueueEvents(event);
EventPluginHub.processEventQueue(false);
}
function startWatchingForChangeEventIE8(target, targetID) {
activeElement = target;
activeElementID = targetID;
activeElement.attachEvent('onchange', manualDispatchChangeEvent);
}
function stopWatchingForChangeEventIE8() {
if (!activeElement) {
return;
}
activeElement.detachEvent('onchange', manualDispatchChangeEvent);
activeElement = null;
activeElementID = null;
}
function getTargetIDForChangeEvent(topLevelType, topLevelTarget, topLevelTargetID) {
if (topLevelType === topLevelTypes.topChange) {
return topLevelTargetID;
}
}
function handleEventsForChangeEventIE8(topLevelType, topLevelTarget, topLevelTargetID) {
if (topLevelType === topLevelTypes.topFocus) {
// stopWatching() should be a noop here but we call it just in case we
// missed a blur event somehow.
stopWatchingForChangeEventIE8();
startWatchingForChangeEventIE8(topLevelTarget, topLevelTargetID);
} else if (topLevelType === topLevelTypes.topBlur) {
stopWatchingForChangeEventIE8();
}
}
/**
* SECTION: handle `input` event
*/
var isInputEventSupported = false;
if (ExecutionEnvironment.canUseDOM) {
// IE9 claims to support the input event but fails to trigger it when
// deleting text, so we ignore its input events
isInputEventSupported = isEventSupported('input') && (!('documentMode' in document) || document.documentMode > 9);
}
/**
* (For old IE.) Replacement getter/setter for the `value` property that gets
* set on the active element.
*/
var newValueProp = {
get: function () {
return activeElementValueProp.get.call(this);
},
set: function (val) {
// Cast to a string so we can do equality checks.
activeElementValue = '' + val;
activeElementValueProp.set.call(this, val);
}
};
/**
* (For old IE.) Starts tracking propertychange events on the passed-in element
* and override the value property so that we can distinguish user events from
* value changes in JS.
*/
function startWatchingForValueChange(target, targetID) {
activeElement = target;
activeElementID = targetID;
activeElementValue = target.value;
activeElementValueProp = Object.getOwnPropertyDescriptor(target.constructor.prototype, 'value');
// Not guarded in a canDefineProperty check: IE8 supports defineProperty only
// on DOM elements
Object.defineProperty(activeElement, 'value', newValueProp);
activeElement.attachEvent('onpropertychange', handlePropertyChange);
}
/**
* (For old IE.) Removes the event listeners from the currently-tracked element,
* if any exists.
*/
function stopWatchingForValueChange() {
if (!activeElement) {
return;
}
// delete restores the original property definition
delete activeElement.value;
activeElement.detachEvent('onpropertychange', handlePropertyChange);
activeElement = null;
activeElementID = null;
activeElementValue = null;
activeElementValueProp = null;
}
/**
* (For old IE.) Handles a propertychange event, sending a `change` event if
* the value of the active element has changed.
*/
function handlePropertyChange(nativeEvent) {
if (nativeEvent.propertyName !== 'value') {
return;
}
var value = nativeEvent.srcElement.value;
if (value === activeElementValue) {
return;
}
activeElementValue = value;
manualDispatchChangeEvent(nativeEvent);
}
/**
* If a `change` event should be fired, returns the target's ID.
*/
function getTargetIDForInputEvent(topLevelType, topLevelTarget, topLevelTargetID) {
if (topLevelType === topLevelTypes.topInput) {
// In modern browsers (i.e., not IE8 or IE9), the input event is exactly
// what we want so fall through here and trigger an abstract event
return topLevelTargetID;
}
}
// For IE8 and IE9.
function handleEventsForInputEventIE(topLevelType, topLevelTarget, topLevelTargetID) {
if (topLevelType === topLevelTypes.topFocus) {
// In IE8, we can capture almost all .value changes by adding a
// propertychange handler and looking for events with propertyName
// equal to 'value'
// In IE9, propertychange fires for most input events but is buggy and
// doesn't fire when text is deleted, but conveniently, selectionchange
// appears to fire in all of the remaining cases so we catch those and
// forward the event if the value has changed
// In either case, we don't want to call the event handler if the value
// is changed from JS so we redefine a setter for `.value` that updates
// our activeElementValue variable, allowing us to ignore those changes
//
// stopWatching() should be a noop here but we call it just in case we
// missed a blur event somehow.
stopWatchingForValueChange();
startWatchingForValueChange(topLevelTarget, topLevelTargetID);
} else if (topLevelType === topLevelTypes.topBlur) {
stopWatchingForValueChange();
}
}
// For IE8 and IE9.
function getTargetIDForInputEventIE(topLevelType, topLevelTarget, topLevelTargetID) {
if (topLevelType === topLevelTypes.topSelectionChange || topLevelType === topLevelTypes.topKeyUp || topLevelType === topLevelTypes.topKeyDown) {
// On the selectionchange event, the target is just document which isn't
// helpful for us so just check activeElement instead.
//
// 99% of the time, keydown and keyup aren't necessary. IE8 fails to fire
// propertychange on the first input event after setting `value` from a
// script and fires only keydown, keypress, keyup. Catching keyup usually
// gets it and catching keydown lets us fire an event for the first
// keystroke if user does a key repeat (it'll be a little delayed: right
// before the second keystroke). Other input methods (e.g., paste) seem to
// fire selectionchange normally.
if (activeElement && activeElement.value !== activeElementValue) {
activeElementValue = activeElement.value;
return activeElementID;
}
}
}
/**
* SECTION: handle `click` event
*/
function shouldUseClickEvent(elem) {
// Use the `click` event to detect changes to checkbox and radio inputs.
// This approach works across all browsers, whereas `change` does not fire
// until `blur` in IE8.
return elem.nodeName && elem.nodeName.toLowerCase() === 'input' && (elem.type === 'checkbox' || elem.type === 'radio');
}
function getTargetIDForClickEvent(topLevelType, topLevelTarget, topLevelTargetID) {
if (topLevelType === topLevelTypes.topClick) {
return topLevelTargetID;
}
}
/**
* This plugin creates an `onChange` event that normalizes change events
* across form elements. This event fires at a time when it's possible to
* change the element's value without seeing a flicker.
*
* Supported elements are:
* - input (see `isTextInputElement`)
* - textarea
* - select
*/
var ChangeEventPlugin = {
eventTypes: eventTypes,
/**
* @param {string} topLevelType Record from `EventConstants`.
* @param {DOMEventTarget} topLevelTarget The listening component root node.
* @param {string} topLevelTargetID ID of `topLevelTarget`.
* @param {object} nativeEvent Native browser event.
* @return {*} An accumulation of synthetic events.
* @see {EventPluginHub.extractEvents}
*/
extractEvents: function (topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget) {
var getTargetIDFunc, handleEventFunc;
if (shouldUseChangeEvent(topLevelTarget)) {
if (doesChangeEventBubble) {
getTargetIDFunc = getTargetIDForChangeEvent;
} else {
handleEventFunc = handleEventsForChangeEventIE8;
}
} else if (isTextInputElement(topLevelTarget)) {
if (isInputEventSupported) {
getTargetIDFunc = getTargetIDForInputEvent;
} else {
getTargetIDFunc = getTargetIDForInputEventIE;
handleEventFunc = handleEventsForInputEventIE;
}
} else if (shouldUseClickEvent(topLevelTarget)) {
getTargetIDFunc = getTargetIDForClickEvent;
}
if (getTargetIDFunc) {
var targetID = getTargetIDFunc(topLevelType, topLevelTarget, topLevelTargetID);
if (targetID) {
var event = SyntheticEvent.getPooled(eventTypes.change, targetID, nativeEvent, nativeEventTarget);
event.type = 'change';
EventPropagators.accumulateTwoPhaseDispatches(event);
return event;
}
}
if (handleEventFunc) {
handleEventFunc(topLevelType, topLevelTarget, topLevelTargetID);
}
}
};
module.exports = ChangeEventPlugin;
},{"105":105,"128":128,"133":133,"134":134,"147":147,"15":15,"16":16,"166":166,"19":19,"96":96}],8:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ClientReactRootIndex
* @typechecks
*/
'use strict';
var nextReactRootIndex = 0;
var ClientReactRootIndex = {
createReactRootIndex: function () {
return nextReactRootIndex++;
}
};
module.exports = ClientReactRootIndex;
},{}],9:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule DOMChildrenOperations
* @typechecks static-only
*/
'use strict';
var Danger = _dereq_(12);
var ReactMultiChildUpdateTypes = _dereq_(74);
var ReactPerf = _dereq_(78);
var setInnerHTML = _dereq_(138);
var setTextContent = _dereq_(139);
var invariant = _dereq_(161);
/**
* Inserts `childNode` as a child of `parentNode` at the `index`.
*
* @param {DOMElement} parentNode Parent node in which to insert.
* @param {DOMElement} childNode Child node to insert.
* @param {number} index Index at which to insert the child.
* @internal
*/
function insertChildAt(parentNode, childNode, index) {
// By exploiting arrays returning `undefined` for an undefined index, we can
// rely exclusively on `insertBefore(node, null)` instead of also using
// `appendChild(node)`. However, using `undefined` is not allowed by all
// browsers so we must replace it with `null`.
// fix render order error in safari
// IE8 will throw error when index out of list size.
var beforeChild = index >= parentNode.childNodes.length ? null : parentNode.childNodes.item(index);
parentNode.insertBefore(childNode, beforeChild);
}
/**
* Operations for updating with DOM children.
*/
var DOMChildrenOperations = {
dangerouslyReplaceNodeWithMarkup: Danger.dangerouslyReplaceNodeWithMarkup,
updateTextContent: setTextContent,
/**
* Updates a component's children by processing a series of updates. The
* update configurations are each expected to have a `parentNode` property.
*
* @param {array<object>} updates List of update configurations.
* @param {array<string>} markupList List of markup strings.
* @internal
*/
processUpdates: function (updates, markupList) {
var update;
// Mapping from parent IDs to initial child orderings.
var initialChildren = null;
// List of children that will be moved or removed.
var updatedChildren = null;
for (var i = 0; i < updates.length; i++) {
update = updates[i];
if (update.type === ReactMultiChildUpdateTypes.MOVE_EXISTING || update.type === ReactMultiChildUpdateTypes.REMOVE_NODE) {
var updatedIndex = update.fromIndex;
var updatedChild = update.parentNode.childNodes[updatedIndex];
var parentID = update.parentID;
!updatedChild ? "development" !== 'production' ? invariant(false, 'processUpdates(): Unable to find child %s of element. This ' + 'probably means the DOM was unexpectedly mutated (e.g., by the ' + 'browser), usually due to forgetting a <tbody> when using tables, ' + 'nesting tags like <form>, <p>, or <a>, or using non-SVG elements ' + 'in an <svg> parent. Try inspecting the child nodes of the element ' + 'with React ID `%s`.', updatedIndex, parentID) : invariant(false) : undefined;
initialChildren = initialChildren || {};
initialChildren[parentID] = initialChildren[parentID] || [];
initialChildren[parentID][updatedIndex] = updatedChild;
updatedChildren = updatedChildren || [];
updatedChildren.push(updatedChild);
}
}
var renderedMarkup;
// markupList is either a list of markup or just a list of elements
if (markupList.length && typeof markupList[0] === 'string') {
renderedMarkup = Danger.dangerouslyRenderMarkup(markupList);
} else {
renderedMarkup = markupList;
}
// Remove updated children first so that `toIndex` is consistent.
if (updatedChildren) {
for (var j = 0; j < updatedChildren.length; j++) {
updatedChildren[j].parentNode.removeChild(updatedChildren[j]);
}
}
for (var k = 0; k < updates.length; k++) {
update = updates[k];
switch (update.type) {
case ReactMultiChildUpdateTypes.INSERT_MARKUP:
insertChildAt(update.parentNode, renderedMarkup[update.markupIndex], update.toIndex);
break;
case ReactMultiChildUpdateTypes.MOVE_EXISTING:
insertChildAt(update.parentNode, initialChildren[update.parentID][update.fromIndex], update.toIndex);
break;
case ReactMultiChildUpdateTypes.SET_MARKUP:
setInnerHTML(update.parentNode, update.content);
break;
case ReactMultiChildUpdateTypes.TEXT_CONTENT:
setTextContent(update.parentNode, update.content);
break;
case ReactMultiChildUpdateTypes.REMOVE_NODE:
// Already removed by the for-loop above.
break;
}
}
}
};
ReactPerf.measureMethods(DOMChildrenOperations, 'DOMChildrenOperations', {
updateTextContent: 'updateTextContent'
});
module.exports = DOMChildrenOperations;
},{"12":12,"138":138,"139":139,"161":161,"74":74,"78":78}],10:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule DOMProperty
* @typechecks static-only
*/
'use strict';
var invariant = _dereq_(161);
function checkMask(value, bitmask) {
return (value & bitmask) === bitmask;
}
var DOMPropertyInjection = {
/**
* Mapping from normalized, camelcased property names to a configuration that
* specifies how the associated DOM property should be accessed or rendered.
*/
MUST_USE_ATTRIBUTE: 0x1,
MUST_USE_PROPERTY: 0x2,
HAS_SIDE_EFFECTS: 0x4,
HAS_BOOLEAN_VALUE: 0x8,
HAS_NUMERIC_VALUE: 0x10,
HAS_POSITIVE_NUMERIC_VALUE: 0x20 | 0x10,
HAS_OVERLOADED_BOOLEAN_VALUE: 0x40,
/**
* Inject some specialized knowledge about the DOM. This takes a config object
* with the following properties:
*
* isCustomAttribute: function that given an attribute name will return true
* if it can be inserted into the DOM verbatim. Useful for data-* or aria-*
* attributes where it's impossible to enumerate all of the possible
* attribute names,
*
* Properties: object mapping DOM property name to one of the
* DOMPropertyInjection constants or null. If your attribute isn't in here,
* it won't get written to the DOM.
*
* DOMAttributeNames: object mapping React attribute name to the DOM
* attribute name. Attribute names not specified use the **lowercase**
* normalized name.
*
* DOMAttributeNamespaces: object mapping React attribute name to the DOM
* attribute namespace URL. (Attribute names not specified use no namespace.)
*
* DOMPropertyNames: similar to DOMAttributeNames but for DOM properties.
* Property names not specified use the normalized name.
*
* DOMMutationMethods: Properties that require special mutation methods. If
* `value` is undefined, the mutation method should unset the property.
*
* @param {object} domPropertyConfig the config as described above.
*/
injectDOMPropertyConfig: function (domPropertyConfig) {
var Injection = DOMPropertyInjection;
var Properties = domPropertyConfig.Properties || {};
var DOMAttributeNamespaces = domPropertyConfig.DOMAttributeNamespaces || {};
var DOMAttributeNames = domPropertyConfig.DOMAttributeNames || {};
var DOMPropertyNames = domPropertyConfig.DOMPropertyNames || {};
var DOMMutationMethods = domPropertyConfig.DOMMutationMethods || {};
if (domPropertyConfig.isCustomAttribute) {
DOMProperty._isCustomAttributeFunctions.push(domPropertyConfig.isCustomAttribute);
}
for (var propName in Properties) {
!!DOMProperty.properties.hasOwnProperty(propName) ? "development" !== 'production' ? invariant(false, 'injectDOMPropertyConfig(...): You\'re trying to inject DOM property ' + '\'%s\' which has already been injected. You may be accidentally ' + 'injecting the same DOM property config twice, or you may be ' + 'injecting two configs that have conflicting property names.', propName) : invariant(false) : undefined;
var lowerCased = propName.toLowerCase();
var propConfig = Properties[propName];
var propertyInfo = {
attributeName: lowerCased,
attributeNamespace: null,
propertyName: propName,
mutationMethod: null,
mustUseAttribute: checkMask(propConfig, Injection.MUST_USE_ATTRIBUTE),
mustUseProperty: checkMask(propConfig, Injection.MUST_USE_PROPERTY),
hasSideEffects: checkMask(propConfig, Injection.HAS_SIDE_EFFECTS),
hasBooleanValue: checkMask(propConfig, Injection.HAS_BOOLEAN_VALUE),
hasNumericValue: checkMask(propConfig, Injection.HAS_NUMERIC_VALUE),
hasPositiveNumericValue: checkMask(propConfig, Injection.HAS_POSITIVE_NUMERIC_VALUE),
hasOverloadedBooleanValue: checkMask(propConfig, Injection.HAS_OVERLOADED_BOOLEAN_VALUE)
};
!(!propertyInfo.mustUseAttribute || !propertyInfo.mustUseProperty) ? "development" !== 'production' ? invariant(false, 'DOMProperty: Cannot require using both attribute and property: %s', propName) : invariant(false) : undefined;
!(propertyInfo.mustUseProperty || !propertyInfo.hasSideEffects) ? "development" !== 'production' ? invariant(false, 'DOMProperty: Properties that have side effects must use property: %s', propName) : invariant(false) : undefined;
!(propertyInfo.hasBooleanValue + propertyInfo.hasNumericValue + propertyInfo.hasOverloadedBooleanValue <= 1) ? "development" !== 'production' ? invariant(false, 'DOMProperty: Value can be one of boolean, overloaded boolean, or ' + 'numeric value, but not a combination: %s', propName) : invariant(false) : undefined;
if ("development" !== 'production') {
DOMProperty.getPossibleStandardName[lowerCased] = propName;
}
if (DOMAttributeNames.hasOwnProperty(propName)) {
var attributeName = DOMAttributeNames[propName];
propertyInfo.attributeName = attributeName;
if ("development" !== 'production') {
DOMProperty.getPossibleStandardName[attributeName] = propName;
}
}
if (DOMAttributeNamespaces.hasOwnProperty(propName)) {
propertyInfo.attributeNamespace = DOMAttributeNamespaces[propName];
}
if (DOMPropertyNames.hasOwnProperty(propName)) {
propertyInfo.propertyName = DOMPropertyNames[propName];
}
if (DOMMutationMethods.hasOwnProperty(propName)) {
propertyInfo.mutationMethod = DOMMutationMethods[propName];
}
DOMProperty.properties[propName] = propertyInfo;
}
}
};
var defaultValueCache = {};
/**
* DOMProperty exports lookup objects that can be used like functions:
*
* > DOMProperty.isValid['id']
* true
* > DOMProperty.isValid['foobar']
* undefined
*
* Although this may be confusing, it performs better in general.
*
* @see http://jsperf.com/key-exists
* @see http://jsperf.com/key-missing
*/
var DOMProperty = {
ID_ATTRIBUTE_NAME: 'data-reactid',
/**
* Map from property "standard name" to an object with info about how to set
* the property in the DOM. Each object contains:
*
* attributeName:
* Used when rendering markup or with `*Attribute()`.
* attributeNamespace
* propertyName:
* Used on DOM node instances. (This includes properties that mutate due to
* external factors.)
* mutationMethod:
* If non-null, used instead of the property or `setAttribute()` after
* initial render.
* mustUseAttribute:
* Whether the property must be accessed and mutated using `*Attribute()`.
* (This includes anything that fails `<propName> in <element>`.)
* mustUseProperty:
* Whether the property must be accessed and mutated as an object property.
* hasSideEffects:
* Whether or not setting a value causes side effects such as triggering
* resources to be loaded or text selection changes. If true, we read from
* the DOM before updating to ensure that the value is only set if it has
* changed.
* hasBooleanValue:
* Whether the property should be removed when set to a falsey value.
* hasNumericValue:
* Whether the property must be numeric or parse as a numeric and should be
* removed when set to a falsey value.
* hasPositiveNumericValue:
* Whether the property must be positive numeric or parse as a positive
* numeric and should be removed when set to a falsey value.
* hasOverloadedBooleanValue:
* Whether the property can be used as a flag as well as with a value.
* Removed when strictly equal to false; present without a value when
* strictly equal to true; present with a value otherwise.
*/
properties: {},
/**
* Mapping from lowercase property names to the properly cased version, used
* to warn in the case of missing properties. Available only in __DEV__.
* @type {Object}
*/
getPossibleStandardName: "development" !== 'production' ? {} : null,
/**
* All of the isCustomAttribute() functions that have been injected.
*/
_isCustomAttributeFunctions: [],
/**
* Checks whether a property name is a custom attribute.
* @method
*/
isCustomAttribute: function (attributeName) {
for (var i = 0; i < DOMProperty._isCustomAttributeFunctions.length; i++) {
var isCustomAttributeFn = DOMProperty._isCustomAttributeFunctions[i];
if (isCustomAttributeFn(attributeName)) {
return true;
}
}
return false;
},
/**
* Returns the default property value for a DOM property (i.e., not an
* attribute). Most default values are '' or false, but not all. Worse yet,
* some (in particular, `type`) vary depending on the type of element.
*
* TODO: Is it better to grab all the possible properties when creating an
* element to avoid having to create the same element twice?
*/
getDefaultValueForProperty: function (nodeName, prop) {
var nodeDefaults = defaultValueCache[nodeName];
var testElement;
if (!nodeDefaults) {
defaultValueCache[nodeName] = nodeDefaults = {};
}
if (!(prop in nodeDefaults)) {
testElement = document.createElement(nodeName);
nodeDefaults[prop] = testElement[prop];
}
return nodeDefaults[prop];
},
injection: DOMPropertyInjection
};
module.exports = DOMProperty;
},{"161":161}],11:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule DOMPropertyOperations
* @typechecks static-only
*/
'use strict';
var DOMProperty = _dereq_(10);
var ReactPerf = _dereq_(78);
var quoteAttributeValueForBrowser = _dereq_(136);
var warning = _dereq_(173);
// Simplified subset
var VALID_ATTRIBUTE_NAME_REGEX = /^[a-zA-Z_][\w\.\-]*$/;
var illegalAttributeNameCache = {};
var validatedAttributeNameCache = {};
function isAttributeNameSafe(attributeName) {
if (validatedAttributeNameCache.hasOwnProperty(attributeName)) {
return true;
}
if (illegalAttributeNameCache.hasOwnProperty(attributeName)) {
return false;
}
if (VALID_ATTRIBUTE_NAME_REGEX.test(attributeName)) {
validatedAttributeNameCache[attributeName] = true;
return true;
}
illegalAttributeNameCache[attributeName] = true;
"development" !== 'production' ? warning(false, 'Invalid attribute name: `%s`', attributeName) : undefined;
return false;
}
function shouldIgnoreValue(propertyInfo, value) {
return value == null || propertyInfo.hasBooleanValue && !value || propertyInfo.hasNumericValue && isNaN(value) || propertyInfo.hasPositiveNumericValue && value < 1 || propertyInfo.hasOverloadedBooleanValue && value === false;
}
if ("development" !== 'production') {
var reactProps = {
children: true,
dangerouslySetInnerHTML: true,
key: true,
ref: true
};
var warnedProperties = {};
var warnUnknownProperty = function (name) {
if (reactProps.hasOwnProperty(name) && reactProps[name] || warnedProperties.hasOwnProperty(name) && warnedProperties[name]) {
return;
}
warnedProperties[name] = true;
var lowerCasedName = name.toLowerCase();
// data-* attributes should be lowercase; suggest the lowercase version
var standardName = DOMProperty.isCustomAttribute(lowerCasedName) ? lowerCasedName : DOMProperty.getPossibleStandardName.hasOwnProperty(lowerCasedName) ? DOMProperty.getPossibleStandardName[lowerCasedName] : null;
// For now, only warn when we have a suggested correction. This prevents
// logging too much when using transferPropsTo.
"development" !== 'production' ? warning(standardName == null, 'Unknown DOM property %s. Did you mean %s?', name, standardName) : undefined;
};
}
/**
* Operations for dealing with DOM properties.
*/
var DOMPropertyOperations = {
/**
* Creates markup for the ID property.
*
* @param {string} id Unescaped ID.
* @return {string} Markup string.
*/
createMarkupForID: function (id) {
return DOMProperty.ID_ATTRIBUTE_NAME + '=' + quoteAttributeValueForBrowser(id);
},
setAttributeForID: function (node, id) {
node.setAttribute(DOMProperty.ID_ATTRIBUTE_NAME, id);
},
/**
* Creates markup for a property.
*
* @param {string} name
* @param {*} value
* @return {?string} Markup string, or null if the property was invalid.
*/
createMarkupForProperty: function (name, value) {
var propertyInfo = DOMProperty.properties.hasOwnProperty(name) ? DOMProperty.properties[name] : null;
if (propertyInfo) {
if (shouldIgnoreValue(propertyInfo, value)) {
return '';
}
var attributeName = propertyInfo.attributeName;
if (propertyInfo.hasBooleanValue || propertyInfo.hasOverloadedBooleanValue && value === true) {
return attributeName + '=""';
}
return attributeName + '=' + quoteAttributeValueForBrowser(value);
} else if (DOMProperty.isCustomAttribute(name)) {
if (value == null) {
return '';
}
return name + '=' + quoteAttributeValueForBrowser(value);
} else if ("development" !== 'production') {
warnUnknownProperty(name);
}
return null;
},
/**
* Creates markup for a custom property.
*
* @param {string} name
* @param {*} value
* @return {string} Markup string, or empty string if the property was invalid.
*/
createMarkupForCustomAttribute: function (name, value) {
if (!isAttributeNameSafe(name) || value == null) {
return '';
}
return name + '=' + quoteAttributeValueForBrowser(value);
},
/**
* Sets the value for a property on a node.
*
* @param {DOMElement} node
* @param {string} name
* @param {*} value
*/
setValueForProperty: function (node, name, value) {
var propertyInfo = DOMProperty.properties.hasOwnProperty(name) ? DOMProperty.properties[name] : null;
if (propertyInfo) {
var mutationMethod = propertyInfo.mutationMethod;
if (mutationMethod) {
mutationMethod(node, value);
} else if (shouldIgnoreValue(propertyInfo, value)) {
this.deleteValueForProperty(node, name);
} else if (propertyInfo.mustUseAttribute) {
var attributeName = propertyInfo.attributeName;
var namespace = propertyInfo.attributeNamespace;
// `setAttribute` with objects becomes only `[object]` in IE8/9,
// ('' + value) makes it output the correct toString()-value.
if (namespace) {
node.setAttributeNS(namespace, attributeName, '' + value);
} else if (propertyInfo.hasBooleanValue || propertyInfo.hasOverloadedBooleanValue && value === true) {
node.setAttribute(attributeName, '');
} else {
node.setAttribute(attributeName, '' + value);
}
} else {
var propName = propertyInfo.propertyName;
// Must explicitly cast values for HAS_SIDE_EFFECTS-properties to the
// property type before comparing; only `value` does and is string.
if (!propertyInfo.hasSideEffects || '' + node[propName] !== '' + value) {
// Contrary to `setAttribute`, object properties are properly
// `toString`ed by IE8/9.
node[propName] = value;
}
}
} else if (DOMProperty.isCustomAttribute(name)) {
DOMPropertyOperations.setValueForAttribute(node, name, value);
} else if ("development" !== 'production') {
warnUnknownProperty(name);
}
},
setValueForAttribute: function (node, name, value) {
if (!isAttributeNameSafe(name)) {
return;
}
if (value == null) {
node.removeAttribute(name);
} else {
node.setAttribute(name, '' + value);
}
},
/**
* Deletes the value for a property on a node.
*
* @param {DOMElement} node
* @param {string} name
*/
deleteValueForProperty: function (node, name) {
var propertyInfo = DOMProperty.properties.hasOwnProperty(name) ? DOMProperty.properties[name] : null;
if (propertyInfo) {
var mutationMethod = propertyInfo.mutationMethod;
if (mutationMethod) {
mutationMethod(node, undefined);
} else if (propertyInfo.mustUseAttribute) {
node.removeAttribute(propertyInfo.attributeName);
} else {
var propName = propertyInfo.propertyName;
var defaultValue = DOMProperty.getDefaultValueForProperty(node.nodeName, propName);
if (!propertyInfo.hasSideEffects || '' + node[propName] !== defaultValue) {
node[propName] = defaultValue;
}
}
} else if (DOMProperty.isCustomAttribute(name)) {
node.removeAttribute(name);
} else if ("development" !== 'production') {
warnUnknownProperty(name);
}
}
};
ReactPerf.measureMethods(DOMPropertyOperations, 'DOMPropertyOperations', {
setValueForProperty: 'setValueForProperty',
setValueForAttribute: 'setValueForAttribute',
deleteValueForProperty: 'deleteValueForProperty'
});
module.exports = DOMPropertyOperations;
},{"10":10,"136":136,"173":173,"78":78}],12:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule Danger
* @typechecks static-only
*/
'use strict';
var ExecutionEnvironment = _dereq_(147);
var createNodesFromMarkup = _dereq_(152);
var emptyFunction = _dereq_(153);
var getMarkupWrap = _dereq_(157);
var invariant = _dereq_(161);
var OPEN_TAG_NAME_EXP = /^(<[^ \/>]+)/;
var RESULT_INDEX_ATTR = 'data-danger-index';
/**
* Extracts the `nodeName` from a string of markup.
*
* NOTE: Extracting the `nodeName` does not require a regular expression match
* because we make assumptions about React-generated markup (i.e. there are no
* spaces surrounding the opening tag and there is at least one attribute).
*
* @param {string} markup String of markup.
* @return {string} Node name of the supplied markup.
* @see http://jsperf.com/extract-nodename
*/
function getNodeName(markup) {
return markup.substring(1, markup.indexOf(' '));
}
var Danger = {
/**
* Renders markup into an array of nodes. The markup is expected to render
* into a list of root nodes. Also, the length of `resultList` and
* `markupList` should be the same.
*
* @param {array<string>} markupList List of markup strings to render.
* @return {array<DOMElement>} List of rendered nodes.
* @internal
*/
dangerouslyRenderMarkup: function (markupList) {
!ExecutionEnvironment.canUseDOM ? "development" !== 'production' ? invariant(false, 'dangerouslyRenderMarkup(...): Cannot render markup in a worker ' + 'thread. Make sure `window` and `document` are available globally ' + 'before requiring React when unit testing or use ' + 'ReactDOMServer.renderToString for server rendering.') : invariant(false) : undefined;
var nodeName;
var markupByNodeName = {};
// Group markup by `nodeName` if a wrap is necessary, else by '*'.
for (var i = 0; i < markupList.length; i++) {
!markupList[i] ? "development" !== 'production' ? invariant(false, 'dangerouslyRenderMarkup(...): Missing markup.') : invariant(false) : undefined;
nodeName = getNodeName(markupList[i]);
nodeName = getMarkupWrap(nodeName) ? nodeName : '*';
markupByNodeName[nodeName] = markupByNodeName[nodeName] || [];
markupByNodeName[nodeName][i] = markupList[i];
}
var resultList = [];
var resultListAssignmentCount = 0;
for (nodeName in markupByNodeName) {
if (!markupByNodeName.hasOwnProperty(nodeName)) {
continue;
}
var markupListByNodeName = markupByNodeName[nodeName];
// This for-in loop skips the holes of the sparse array. The order of
// iteration should follow the order of assignment, which happens to match
// numerical index order, but we don't rely on that.
var resultIndex;
for (resultIndex in markupListByNodeName) {
if (markupListByNodeName.hasOwnProperty(resultIndex)) {
var markup = markupListByNodeName[resultIndex];
// Push the requested markup with an additional RESULT_INDEX_ATTR
// attribute. If the markup does not start with a < character, it
// will be discarded below (with an appropriate console.error).
markupListByNodeName[resultIndex] = markup.replace(OPEN_TAG_NAME_EXP,
// This index will be parsed back out below.
'$1 ' + RESULT_INDEX_ATTR + '="' + resultIndex + '" ');
}
}
// Render each group of markup with similar wrapping `nodeName`.
var renderNodes = createNodesFromMarkup(markupListByNodeName.join(''), emptyFunction // Do nothing special with <script> tags.
);
for (var j = 0; j < renderNodes.length; ++j) {
var renderNode = renderNodes[j];
if (renderNode.hasAttribute && renderNode.hasAttribute(RESULT_INDEX_ATTR)) {
resultIndex = +renderNode.getAttribute(RESULT_INDEX_ATTR);
renderNode.removeAttribute(RESULT_INDEX_ATTR);
!!resultList.hasOwnProperty(resultIndex) ? "development" !== 'production' ? invariant(false, 'Danger: Assigning to an already-occupied result index.') : invariant(false) : undefined;
resultList[resultIndex] = renderNode;
// This should match resultList.length and markupList.length when
// we're done.
resultListAssignmentCount += 1;
} else if ("development" !== 'production') {
console.error('Danger: Discarding unexpected node:', renderNode);
}
}
}
// Although resultList was populated out of order, it should now be a dense
// array.
!(resultListAssignmentCount === resultList.length) ? "development" !== 'production' ? invariant(false, 'Danger: Did not assign to every index of resultList.') : invariant(false) : undefined;
!(resultList.length === markupList.length) ? "development" !== 'production' ? invariant(false, 'Danger: Expected markup to render %s nodes, but rendered %s.', markupList.length, resultList.length) : invariant(false) : undefined;
return resultList;
},
/**
* Replaces a node with a string of markup at its current position within its
* parent. The markup must render into a single root node.
*
* @param {DOMElement} oldChild Child node to replace.
* @param {string} markup Markup to render in place of the child node.
* @internal
*/
dangerouslyReplaceNodeWithMarkup: function (oldChild, markup) {
!ExecutionEnvironment.canUseDOM ? "development" !== 'production' ? invariant(false, 'dangerouslyReplaceNodeWithMarkup(...): Cannot render markup in a ' + 'worker thread. Make sure `window` and `document` are available ' + 'globally before requiring React when unit testing or use ' + 'ReactDOMServer.renderToString() for server rendering.') : invariant(false) : undefined;
!markup ? "development" !== 'production' ? invariant(false, 'dangerouslyReplaceNodeWithMarkup(...): Missing markup.') : invariant(false) : undefined;
!(oldChild.tagName.toLowerCase() !== 'html') ? "development" !== 'production' ? invariant(false, 'dangerouslyReplaceNodeWithMarkup(...): Cannot replace markup of the ' + '<html> node. This is because browser quirks make this unreliable ' + 'and/or slow. If you want to render to the root you must use ' + 'server rendering. See ReactDOMServer.renderToString().') : invariant(false) : undefined;
var newChild;
if (typeof markup === 'string') {
newChild = createNodesFromMarkup(markup, emptyFunction)[0];
} else {
newChild = markup;
}
oldChild.parentNode.replaceChild(newChild, oldChild);
}
};
module.exports = Danger;
},{"147":147,"152":152,"153":153,"157":157,"161":161}],13:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule DefaultEventPluginOrder
*/
'use strict';
var keyOf = _dereq_(166);
/**
* Module that is injectable into `EventPluginHub`, that specifies a
* deterministic ordering of `EventPlugin`s. A convenient way to reason about
* plugins, without having to package every one of them. This is better than
* having plugins be ordered in the same order that they are injected because
* that ordering would be influenced by the packaging order.
* `ResponderEventPlugin` must occur before `SimpleEventPlugin` so that
* preventing default on events is convenient in `SimpleEventPlugin` handlers.
*/
var DefaultEventPluginOrder = [keyOf({ ResponderEventPlugin: null }), keyOf({ SimpleEventPlugin: null }), keyOf({ TapEventPlugin: null }), keyOf({ EnterLeaveEventPlugin: null }), keyOf({ ChangeEventPlugin: null }), keyOf({ SelectEventPlugin: null }), keyOf({ BeforeInputEventPlugin: null })];
module.exports = DefaultEventPluginOrder;
},{"166":166}],14:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule EnterLeaveEventPlugin
* @typechecks static-only
*/
'use strict';
var EventConstants = _dereq_(15);
var EventPropagators = _dereq_(19);
var SyntheticMouseEvent = _dereq_(109);
var ReactMount = _dereq_(72);
var keyOf = _dereq_(166);
var topLevelTypes = EventConstants.topLevelTypes;
var getFirstReactDOM = ReactMount.getFirstReactDOM;
var eventTypes = {
mouseEnter: {
registrationName: keyOf({ onMouseEnter: null }),
dependencies: [topLevelTypes.topMouseOut, topLevelTypes.topMouseOver]
},
mouseLeave: {
registrationName: keyOf({ onMouseLeave: null }),
dependencies: [topLevelTypes.topMouseOut, topLevelTypes.topMouseOver]
}
};
var extractedEvents = [null, null];
var EnterLeaveEventPlugin = {
eventTypes: eventTypes,
/**
* For almost every interaction we care about, there will be both a top-level
* `mouseover` and `mouseout` event that occurs. Only use `mouseout` so that
* we do not extract duplicate events. However, moving the mouse into the
* browser from outside will not fire a `mouseout` event. In this case, we use
* the `mouseover` top-level event.
*
* @param {string} topLevelType Record from `EventConstants`.
* @param {DOMEventTarget} topLevelTarget The listening component root node.
* @param {string} topLevelTargetID ID of `topLevelTarget`.
* @param {object} nativeEvent Native browser event.
* @return {*} An accumulation of synthetic events.
* @see {EventPluginHub.extractEvents}
*/
extractEvents: function (topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget) {
if (topLevelType === topLevelTypes.topMouseOver && (nativeEvent.relatedTarget || nativeEvent.fromElement)) {
return null;
}
if (topLevelType !== topLevelTypes.topMouseOut && topLevelType !== topLevelTypes.topMouseOver) {
// Must not be a mouse in or mouse out - ignoring.
return null;
}
var win;
if (topLevelTarget.window === topLevelTarget) {
// `topLevelTarget` is probably a window object.
win = topLevelTarget;
} else {
// TODO: Figure out why `ownerDocument` is sometimes undefined in IE8.
var doc = topLevelTarget.ownerDocument;
if (doc) {
win = doc.defaultView || doc.parentWindow;
} else {
win = window;
}
}
var from;
var to;
var fromID = '';
var toID = '';
if (topLevelType === topLevelTypes.topMouseOut) {
from = topLevelTarget;
fromID = topLevelTargetID;
to = getFirstReactDOM(nativeEvent.relatedTarget || nativeEvent.toElement);
if (to) {
toID = ReactMount.getID(to);
} else {
to = win;
}
to = to || win;
} else {
from = win;
to = topLevelTarget;
toID = topLevelTargetID;
}
if (from === to) {
// Nothing pertains to our managed components.
return null;
}
var leave = SyntheticMouseEvent.getPooled(eventTypes.mouseLeave, fromID, nativeEvent, nativeEventTarget);
leave.type = 'mouseleave';
leave.target = from;
leave.relatedTarget = to;
var enter = SyntheticMouseEvent.getPooled(eventTypes.mouseEnter, toID, nativeEvent, nativeEventTarget);
enter.type = 'mouseenter';
enter.target = to;
enter.relatedTarget = from;
EventPropagators.accumulateEnterLeaveDispatches(leave, enter, fromID, toID);
extractedEvents[0] = leave;
extractedEvents[1] = enter;
return extractedEvents;
}
};
module.exports = EnterLeaveEventPlugin;
},{"109":109,"15":15,"166":166,"19":19,"72":72}],15:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule EventConstants
*/
'use strict';
var keyMirror = _dereq_(165);
var PropagationPhases = keyMirror({ bubbled: null, captured: null });
/**
* Types of raw signals from the browser caught at the top level.
*/
var topLevelTypes = keyMirror({
topAbort: null,
topBlur: null,
topCanPlay: null,
topCanPlayThrough: null,
topChange: null,
topClick: null,
topCompositionEnd: null,
topCompositionStart: null,
topCompositionUpdate: null,
topContextMenu: null,
topCopy: null,
topCut: null,
topDoubleClick: null,
topDrag: null,
topDragEnd: null,
topDragEnter: null,
topDragExit: null,
topDragLeave: null,
topDragOver: null,
topDragStart: null,
topDrop: null,
topDurationChange: null,
topEmptied: null,
topEncrypted: null,
topEnded: null,
topError: null,
topFocus: null,
topInput: null,
topKeyDown: null,
topKeyPress: null,
topKeyUp: null,
topLoad: null,
topLoadedData: null,
topLoadedMetadata: null,
topLoadStart: null,
topMouseDown: null,
topMouseMove: null,
topMouseOut: null,
topMouseOver: null,
topMouseUp: null,
topPaste: null,
topPause: null,
topPlay: null,
topPlaying: null,
topProgress: null,
topRateChange: null,
topReset: null,
topScroll: null,
topSeeked: null,
topSeeking: null,
topSelectionChange: null,
topStalled: null,
topSubmit: null,
topSuspend: null,
topTextInput: null,
topTimeUpdate: null,
topTouchCancel: null,
topTouchEnd: null,
topTouchMove: null,
topTouchStart: null,
topVolumeChange: null,
topWaiting: null,
topWheel: null
});
var EventConstants = {
topLevelTypes: topLevelTypes,
PropagationPhases: PropagationPhases
};
module.exports = EventConstants;
},{"165":165}],16:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule EventPluginHub
*/
'use strict';
var EventPluginRegistry = _dereq_(17);
var EventPluginUtils = _dereq_(18);
var ReactErrorUtils = _dereq_(61);
var accumulateInto = _dereq_(115);
var forEachAccumulated = _dereq_(124);
var invariant = _dereq_(161);
var warning = _dereq_(173);
/**
* Internal store for event listeners
*/
var listenerBank = {};
/**
* Internal queue of events that have accumulated their dispatches and are
* waiting to have their dispatches executed.
*/
var eventQueue = null;
/**
* Dispatches an event and releases it back into the pool, unless persistent.
*
* @param {?object} event Synthetic event to be dispatched.
* @param {boolean} simulated If the event is simulated (changes exn behavior)
* @private
*/
var executeDispatchesAndRelease = function (event, simulated) {
if (event) {
EventPluginUtils.executeDispatchesInOrder(event, simulated);
if (!event.isPersistent()) {
event.constructor.release(event);
}
}
};
var executeDispatchesAndReleaseSimulated = function (e) {
return executeDispatchesAndRelease(e, true);
};
var executeDispatchesAndReleaseTopLevel = function (e) {
return executeDispatchesAndRelease(e, false);
};
/**
* - `InstanceHandle`: [required] Module that performs logical traversals of DOM
* hierarchy given ids of the logical DOM elements involved.
*/
var InstanceHandle = null;
function validateInstanceHandle() {
var valid = InstanceHandle && InstanceHandle.traverseTwoPhase && InstanceHandle.traverseEnterLeave;
"development" !== 'production' ? warning(valid, 'InstanceHandle not injected before use!') : undefined;
}
/**
* This is a unified interface for event plugins to be installed and configured.
*
* Event plugins can implement the following properties:
*
* `extractEvents` {function(string, DOMEventTarget, string, object): *}
* Required. When a top-level event is fired, this method is expected to
* extract synthetic events that will in turn be queued and dispatched.
*
* `eventTypes` {object}
* Optional, plugins that fire events must publish a mapping of registration
* names that are used to register listeners. Values of this mapping must
* be objects that contain `registrationName` or `phasedRegistrationNames`.
*
* `executeDispatch` {function(object, function, string)}
* Optional, allows plugins to override how an event gets dispatched. By
* default, the listener is simply invoked.
*
* Each plugin that is injected into `EventsPluginHub` is immediately operable.
*
* @public
*/
var EventPluginHub = {
/**
* Methods for injecting dependencies.
*/
injection: {
/**
* @param {object} InjectedMount
* @public
*/
injectMount: EventPluginUtils.injection.injectMount,
/**
* @param {object} InjectedInstanceHandle
* @public
*/
injectInstanceHandle: function (InjectedInstanceHandle) {
InstanceHandle = InjectedInstanceHandle;
if ("development" !== 'production') {
validateInstanceHandle();
}
},
getInstanceHandle: function () {
if ("development" !== 'production') {
validateInstanceHandle();
}
return InstanceHandle;
},
/**
* @param {array} InjectedEventPluginOrder
* @public
*/
injectEventPluginOrder: EventPluginRegistry.injectEventPluginOrder,
/**
* @param {object} injectedNamesToPlugins Map from names to plugin modules.
*/
injectEventPluginsByName: EventPluginRegistry.injectEventPluginsByName
},
eventNameDispatchConfigs: EventPluginRegistry.eventNameDispatchConfigs,
registrationNameModules: EventPluginRegistry.registrationNameModules,
/**
* Stores `listener` at `listenerBank[registrationName][id]`. Is idempotent.
*
* @param {string} id ID of the DOM element.
* @param {string} registrationName Name of listener (e.g. `onClick`).
* @param {?function} listener The callback to store.
*/
putListener: function (id, registrationName, listener) {
!(typeof listener === 'function') ? "development" !== 'production' ? invariant(false, 'Expected %s listener to be a function, instead got type %s', registrationName, typeof listener) : invariant(false) : undefined;
var bankForRegistrationName = listenerBank[registrationName] || (listenerBank[registrationName] = {});
bankForRegistrationName[id] = listener;
var PluginModule = EventPluginRegistry.registrationNameModules[registrationName];
if (PluginModule && PluginModule.didPutListener) {
PluginModule.didPutListener(id, registrationName, listener);
}
},
/**
* @param {string} id ID of the DOM element.
* @param {string} registrationName Name of listener (e.g. `onClick`).
* @return {?function} The stored callback.
*/
getListener: function (id, registrationName) {
var bankForRegistrationName = listenerBank[registrationName];
return bankForRegistrationName && bankForRegistrationName[id];
},
/**
* Deletes a listener from the registration bank.
*
* @param {string} id ID of the DOM element.
* @param {string} registrationName Name of listener (e.g. `onClick`).
*/
deleteListener: function (id, registrationName) {
var PluginModule = EventPluginRegistry.registrationNameModules[registrationName];
if (PluginModule && PluginModule.willDeleteListener) {
PluginModule.willDeleteListener(id, registrationName);
}
var bankForRegistrationName = listenerBank[registrationName];
// TODO: This should never be null -- when is it?
if (bankForRegistrationName) {
delete bankForRegistrationName[id];
}
},
/**
* Deletes all listeners for the DOM element with the supplied ID.
*
* @param {string} id ID of the DOM element.
*/
deleteAllListeners: function (id) {
for (var registrationName in listenerBank) {
if (!listenerBank[registrationName][id]) {
continue;
}
var PluginModule = EventPluginRegistry.registrationNameModules[registrationName];
if (PluginModule && PluginModule.willDeleteListener) {
PluginModule.willDeleteListener(id, registrationName);
}
delete listenerBank[registrationName][id];
}
},
/**
* Allows registered plugins an opportunity to extract events from top-level
* native browser events.
*
* @param {string} topLevelType Record from `EventConstants`.
* @param {DOMEventTarget} topLevelTarget The listening component root node.
* @param {string} topLevelTargetID ID of `topLevelTarget`.
* @param {object} nativeEvent Native browser event.
* @return {*} An accumulation of synthetic events.
* @internal
*/
extractEvents: function (topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget) {
var events;
var plugins = EventPluginRegistry.plugins;
for (var i = 0; i < plugins.length; i++) {
// Not every plugin in the ordering may be loaded at runtime.
var possiblePlugin = plugins[i];
if (possiblePlugin) {
var extractedEvents = possiblePlugin.extractEvents(topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget);
if (extractedEvents) {
events = accumulateInto(events, extractedEvents);
}
}
}
return events;
},
/**
* Enqueues a synthetic event that should be dispatched when
* `processEventQueue` is invoked.
*
* @param {*} events An accumulation of synthetic events.
* @internal
*/
enqueueEvents: function (events) {
if (events) {
eventQueue = accumulateInto(eventQueue, events);
}
},
/**
* Dispatches all synthetic events on the event queue.
*
* @internal
*/
processEventQueue: function (simulated) {
// Set `eventQueue` to null before processing it so that we can tell if more
// events get enqueued while processing.
var processingEventQueue = eventQueue;
eventQueue = null;
if (simulated) {
forEachAccumulated(processingEventQueue, executeDispatchesAndReleaseSimulated);
} else {
forEachAccumulated(processingEventQueue, executeDispatchesAndReleaseTopLevel);
}
!!eventQueue ? "development" !== 'production' ? invariant(false, 'processEventQueue(): Additional events were enqueued while processing ' + 'an event queue. Support for this has not yet been implemented.') : invariant(false) : undefined;
// This would be a good time to rethrow if any of the event handlers threw.
ReactErrorUtils.rethrowCaughtError();
},
/**
* These are needed for tests only. Do not use!
*/
__purge: function () {
listenerBank = {};
},
__getListenerBank: function () {
return listenerBank;
}
};
module.exports = EventPluginHub;
},{"115":115,"124":124,"161":161,"17":17,"173":173,"18":18,"61":61}],17:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule EventPluginRegistry
* @typechecks static-only
*/
'use strict';
var invariant = _dereq_(161);
/**
* Injectable ordering of event plugins.
*/
var EventPluginOrder = null;
/**
* Injectable mapping from names to event plugin modules.
*/
var namesToPlugins = {};
/**
* Recomputes the plugin list using the injected plugins and plugin ordering.
*
* @private
*/
function recomputePluginOrdering() {
if (!EventPluginOrder) {
// Wait until an `EventPluginOrder` is injected.
return;
}
for (var pluginName in namesToPlugins) {
var PluginModule = namesToPlugins[pluginName];
var pluginIndex = EventPluginOrder.indexOf(pluginName);
!(pluginIndex > -1) ? "development" !== 'production' ? invariant(false, 'EventPluginRegistry: Cannot inject event plugins that do not exist in ' + 'the plugin ordering, `%s`.', pluginName) : invariant(false) : undefined;
if (EventPluginRegistry.plugins[pluginIndex]) {
continue;
}
!PluginModule.extractEvents ? "development" !== 'production' ? invariant(false, 'EventPluginRegistry: Event plugins must implement an `extractEvents` ' + 'method, but `%s` does not.', pluginName) : invariant(false) : undefined;
EventPluginRegistry.plugins[pluginIndex] = PluginModule;
var publishedEvents = PluginModule.eventTypes;
for (var eventName in publishedEvents) {
!publishEventForPlugin(publishedEvents[eventName], PluginModule, eventName) ? "development" !== 'production' ? invariant(false, 'EventPluginRegistry: Failed to publish event `%s` for plugin `%s`.', eventName, pluginName) : invariant(false) : undefined;
}
}
}
/**
* Publishes an event so that it can be dispatched by the supplied plugin.
*
* @param {object} dispatchConfig Dispatch configuration for the event.
* @param {object} PluginModule Plugin publishing the event.
* @return {boolean} True if the event was successfully published.
* @private
*/
function publishEventForPlugin(dispatchConfig, PluginModule, eventName) {
!!EventPluginRegistry.eventNameDispatchConfigs.hasOwnProperty(eventName) ? "development" !== 'production' ? invariant(false, 'EventPluginHub: More than one plugin attempted to publish the same ' + 'event name, `%s`.', eventName) : invariant(false) : undefined;
EventPluginRegistry.eventNameDispatchConfigs[eventName] = dispatchConfig;
var phasedRegistrationNames = dispatchConfig.phasedRegistrationNames;
if (phasedRegistrationNames) {
for (var phaseName in phasedRegistrationNames) {
if (phasedRegistrationNames.hasOwnProperty(phaseName)) {
var phasedRegistrationName = phasedRegistrationNames[phaseName];
publishRegistrationName(phasedRegistrationName, PluginModule, eventName);
}
}
return true;
} else if (dispatchConfig.registrationName) {
publishRegistrationName(dispatchConfig.registrationName, PluginModule, eventName);
return true;
}
return false;
}
/**
* Publishes a registration name that is used to identify dispatched events and
* can be used with `EventPluginHub.putListener` to register listeners.
*
* @param {string} registrationName Registration name to add.
* @param {object} PluginModule Plugin publishing the event.
* @private
*/
function publishRegistrationName(registrationName, PluginModule, eventName) {
!!EventPluginRegistry.registrationNameModules[registrationName] ? "development" !== 'production' ? invariant(false, 'EventPluginHub: More than one plugin attempted to publish the same ' + 'registration name, `%s`.', registrationName) : invariant(false) : undefined;
EventPluginRegistry.registrationNameModules[registrationName] = PluginModule;
EventPluginRegistry.registrationNameDependencies[registrationName] = PluginModule.eventTypes[eventName].dependencies;
}
/**
* Registers plugins so that they can extract and dispatch events.
*
* @see {EventPluginHub}
*/
var EventPluginRegistry = {
/**
* Ordered list of injected plugins.
*/
plugins: [],
/**
* Mapping from event name to dispatch config
*/
eventNameDispatchConfigs: {},
/**
* Mapping from registration name to plugin module
*/
registrationNameModules: {},
/**
* Mapping from registration name to event name
*/
registrationNameDependencies: {},
/**
* Injects an ordering of plugins (by plugin name). This allows the ordering
* to be decoupled from injection of the actual plugins so that ordering is
* always deterministic regardless of packaging, on-the-fly injection, etc.
*
* @param {array} InjectedEventPluginOrder
* @internal
* @see {EventPluginHub.injection.injectEventPluginOrder}
*/
injectEventPluginOrder: function (InjectedEventPluginOrder) {
!!EventPluginOrder ? "development" !== 'production' ? invariant(false, 'EventPluginRegistry: Cannot inject event plugin ordering more than ' + 'once. You are likely trying to load more than one copy of React.') : invariant(false) : undefined;
// Clone the ordering so it cannot be dynamically mutated.
EventPluginOrder = Array.prototype.slice.call(InjectedEventPluginOrder);
recomputePluginOrdering();
},
/**
* Injects plugins to be used by `EventPluginHub`. The plugin names must be
* in the ordering injected by `injectEventPluginOrder`.
*
* Plugins can be injected as part of page initialization or on-the-fly.
*
* @param {object} injectedNamesToPlugins Map from names to plugin modules.
* @internal
* @see {EventPluginHub.injection.injectEventPluginsByName}
*/
injectEventPluginsByName: function (injectedNamesToPlugins) {
var isOrderingDirty = false;
for (var pluginName in injectedNamesToPlugins) {
if (!injectedNamesToPlugins.hasOwnProperty(pluginName)) {
continue;
}
var PluginModule = injectedNamesToPlugins[pluginName];
if (!namesToPlugins.hasOwnProperty(pluginName) || namesToPlugins[pluginName] !== PluginModule) {
!!namesToPlugins[pluginName] ? "development" !== 'production' ? invariant(false, 'EventPluginRegistry: Cannot inject two different event plugins ' + 'using the same name, `%s`.', pluginName) : invariant(false) : undefined;
namesToPlugins[pluginName] = PluginModule;
isOrderingDirty = true;
}
}
if (isOrderingDirty) {
recomputePluginOrdering();
}
},
/**
* Looks up the plugin for the supplied event.
*
* @param {object} event A synthetic event.
* @return {?object} The plugin that created the supplied event.
* @internal
*/
getPluginModuleForEvent: function (event) {
var dispatchConfig = event.dispatchConfig;
if (dispatchConfig.registrationName) {
return EventPluginRegistry.registrationNameModules[dispatchConfig.registrationName] || null;
}
for (var phase in dispatchConfig.phasedRegistrationNames) {
if (!dispatchConfig.phasedRegistrationNames.hasOwnProperty(phase)) {
continue;
}
var PluginModule = EventPluginRegistry.registrationNameModules[dispatchConfig.phasedRegistrationNames[phase]];
if (PluginModule) {
return PluginModule;
}
}
return null;
},
/**
* Exposed for unit testing.
* @private
*/
_resetEventPlugins: function () {
EventPluginOrder = null;
for (var pluginName in namesToPlugins) {
if (namesToPlugins.hasOwnProperty(pluginName)) {
delete namesToPlugins[pluginName];
}
}
EventPluginRegistry.plugins.length = 0;
var eventNameDispatchConfigs = EventPluginRegistry.eventNameDispatchConfigs;
for (var eventName in eventNameDispatchConfigs) {
if (eventNameDispatchConfigs.hasOwnProperty(eventName)) {
delete eventNameDispatchConfigs[eventName];
}
}
var registrationNameModules = EventPluginRegistry.registrationNameModules;
for (var registrationName in registrationNameModules) {
if (registrationNameModules.hasOwnProperty(registrationName)) {
delete registrationNameModules[registrationName];
}
}
}
};
module.exports = EventPluginRegistry;
},{"161":161}],18:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule EventPluginUtils
*/
'use strict';
var EventConstants = _dereq_(15);
var ReactErrorUtils = _dereq_(61);
var invariant = _dereq_(161);
var warning = _dereq_(173);
/**
* Injected dependencies:
*/
/**
* - `Mount`: [required] Module that can convert between React dom IDs and
* actual node references.
*/
var injection = {
Mount: null,
injectMount: function (InjectedMount) {
injection.Mount = InjectedMount;
if ("development" !== 'production') {
"development" !== 'production' ? warning(InjectedMount && InjectedMount.getNode && InjectedMount.getID, 'EventPluginUtils.injection.injectMount(...): Injected Mount ' + 'module is missing getNode or getID.') : undefined;
}
}
};
var topLevelTypes = EventConstants.topLevelTypes;
function isEndish(topLevelType) {
return topLevelType === topLevelTypes.topMouseUp || topLevelType === topLevelTypes.topTouchEnd || topLevelType === topLevelTypes.topTouchCancel;
}
function isMoveish(topLevelType) {
return topLevelType === topLevelTypes.topMouseMove || topLevelType === topLevelTypes.topTouchMove;
}
function isStartish(topLevelType) {
return topLevelType === topLevelTypes.topMouseDown || topLevelType === topLevelTypes.topTouchStart;
}
var validateEventDispatches;
if ("development" !== 'production') {
validateEventDispatches = function (event) {
var dispatchListeners = event._dispatchListeners;
var dispatchIDs = event._dispatchIDs;
var listenersIsArr = Array.isArray(dispatchListeners);
var idsIsArr = Array.isArray(dispatchIDs);
var IDsLen = idsIsArr ? dispatchIDs.length : dispatchIDs ? 1 : 0;
var listenersLen = listenersIsArr ? dispatchListeners.length : dispatchListeners ? 1 : 0;
"development" !== 'production' ? warning(idsIsArr === listenersIsArr && IDsLen === listenersLen, 'EventPluginUtils: Invalid `event`.') : undefined;
};
}
/**
* Dispatch the event to the listener.
* @param {SyntheticEvent} event SyntheticEvent to handle
* @param {boolean} simulated If the event is simulated (changes exn behavior)
* @param {function} listener Application-level callback
* @param {string} domID DOM id to pass to the callback.
*/
function executeDispatch(event, simulated, listener, domID) {
var type = event.type || 'unknown-event';
event.currentTarget = injection.Mount.getNode(domID);
if (simulated) {
ReactErrorUtils.invokeGuardedCallbackWithCatch(type, listener, event, domID);
} else {
ReactErrorUtils.invokeGuardedCallback(type, listener, event, domID);
}
event.currentTarget = null;
}
/**
* Standard/simple iteration through an event's collected dispatches.
*/
function executeDispatchesInOrder(event, simulated) {
var dispatchListeners = event._dispatchListeners;
var dispatchIDs = event._dispatchIDs;
if ("development" !== 'production') {
validateEventDispatches(event);
}
if (Array.isArray(dispatchListeners)) {
for (var i = 0; i < dispatchListeners.length; i++) {
if (event.isPropagationStopped()) {
break;
}
// Listeners and IDs are two parallel arrays that are always in sync.
executeDispatch(event, simulated, dispatchListeners[i], dispatchIDs[i]);
}
} else if (dispatchListeners) {
executeDispatch(event, simulated, dispatchListeners, dispatchIDs);
}
event._dispatchListeners = null;
event._dispatchIDs = null;
}
/**
* Standard/simple iteration through an event's collected dispatches, but stops
* at the first dispatch execution returning true, and returns that id.
*
* @return {?string} id of the first dispatch execution who's listener returns
* true, or null if no listener returned true.
*/
function executeDispatchesInOrderStopAtTrueImpl(event) {
var dispatchListeners = event._dispatchListeners;
var dispatchIDs = event._dispatchIDs;
if ("development" !== 'production') {
validateEventDispatches(event);
}
if (Array.isArray(dispatchListeners)) {
for (var i = 0; i < dispatchListeners.length; i++) {
if (event.isPropagationStopped()) {
break;
}
// Listeners and IDs are two parallel arrays that are always in sync.
if (dispatchListeners[i](event, dispatchIDs[i])) {
return dispatchIDs[i];
}
}
} else if (dispatchListeners) {
if (dispatchListeners(event, dispatchIDs)) {
return dispatchIDs;
}
}
return null;
}
/**
* @see executeDispatchesInOrderStopAtTrueImpl
*/
function executeDispatchesInOrderStopAtTrue(event) {
var ret = executeDispatchesInOrderStopAtTrueImpl(event);
event._dispatchIDs = null;
event._dispatchListeners = null;
return ret;
}
/**
* Execution of a "direct" dispatch - there must be at most one dispatch
* accumulated on the event or it is considered an error. It doesn't really make
* sense for an event with multiple dispatches (bubbled) to keep track of the
* return values at each dispatch execution, but it does tend to make sense when
* dealing with "direct" dispatches.
*
* @return {*} The return value of executing the single dispatch.
*/
function executeDirectDispatch(event) {
if ("development" !== 'production') {
validateEventDispatches(event);
}
var dispatchListener = event._dispatchListeners;
var dispatchID = event._dispatchIDs;
!!Array.isArray(dispatchListener) ? "development" !== 'production' ? invariant(false, 'executeDirectDispatch(...): Invalid `event`.') : invariant(false) : undefined;
var res = dispatchListener ? dispatchListener(event, dispatchID) : null;
event._dispatchListeners = null;
event._dispatchIDs = null;
return res;
}
/**
* @param {SyntheticEvent} event
* @return {boolean} True iff number of dispatches accumulated is greater than 0.
*/
function hasDispatches(event) {
return !!event._dispatchListeners;
}
/**
* General utilities that are useful in creating custom Event Plugins.
*/
var EventPluginUtils = {
isEndish: isEndish,
isMoveish: isMoveish,
isStartish: isStartish,
executeDirectDispatch: executeDirectDispatch,
executeDispatchesInOrder: executeDispatchesInOrder,
executeDispatchesInOrderStopAtTrue: executeDispatchesInOrderStopAtTrue,
hasDispatches: hasDispatches,
getNode: function (id) {
return injection.Mount.getNode(id);
},
getID: function (node) {
return injection.Mount.getID(node);
},
injection: injection
};
module.exports = EventPluginUtils;
},{"15":15,"161":161,"173":173,"61":61}],19:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule EventPropagators
*/
'use strict';
var EventConstants = _dereq_(15);
var EventPluginHub = _dereq_(16);
var warning = _dereq_(173);
var accumulateInto = _dereq_(115);
var forEachAccumulated = _dereq_(124);
var PropagationPhases = EventConstants.PropagationPhases;
var getListener = EventPluginHub.getListener;
/**
* Some event types have a notion of different registration names for different
* "phases" of propagation. This finds listeners by a given phase.
*/
function listenerAtPhase(id, event, propagationPhase) {
var registrationName = event.dispatchConfig.phasedRegistrationNames[propagationPhase];
return getListener(id, registrationName);
}
/**
* Tags a `SyntheticEvent` with dispatched listeners. Creating this function
* here, allows us to not have to bind or create functions for each event.
* Mutating the event's members allows us to not have to create a wrapping
* "dispatch" object that pairs the event with the listener.
*/
function accumulateDirectionalDispatches(domID, upwards, event) {
if ("development" !== 'production') {
"development" !== 'production' ? warning(domID, 'Dispatching id must not be null') : undefined;
}
var phase = upwards ? PropagationPhases.bubbled : PropagationPhases.captured;
var listener = listenerAtPhase(domID, event, phase);
if (listener) {
event._dispatchListeners = accumulateInto(event._dispatchListeners, listener);
event._dispatchIDs = accumulateInto(event._dispatchIDs, domID);
}
}
/**
* Collect dispatches (must be entirely collected before dispatching - see unit
* tests). Lazily allocate the array to conserve memory. We must loop through
* each event and perform the traversal for each one. We cannot perform a
* single traversal for the entire collection of events because each event may
* have a different target.
*/
function accumulateTwoPhaseDispatchesSingle(event) {
if (event && event.dispatchConfig.phasedRegistrationNames) {
EventPluginHub.injection.getInstanceHandle().traverseTwoPhase(event.dispatchMarker, accumulateDirectionalDispatches, event);
}
}
/**
* Same as `accumulateTwoPhaseDispatchesSingle`, but skips over the targetID.
*/
function accumulateTwoPhaseDispatchesSingleSkipTarget(event) {
if (event && event.dispatchConfig.phasedRegistrationNames) {
EventPluginHub.injection.getInstanceHandle().traverseTwoPhaseSkipTarget(event.dispatchMarker, accumulateDirectionalDispatches, event);
}
}
/**
* Accumulates without regard to direction, does not look for phased
* registration names. Same as `accumulateDirectDispatchesSingle` but without
* requiring that the `dispatchMarker` be the same as the dispatched ID.
*/
function accumulateDispatches(id, ignoredDirection, event) {
if (event && event.dispatchConfig.registrationName) {
var registrationName = event.dispatchConfig.registrationName;
var listener = getListener(id, registrationName);
if (listener) {
event._dispatchListeners = accumulateInto(event._dispatchListeners, listener);
event._dispatchIDs = accumulateInto(event._dispatchIDs, id);
}
}
}
/**
* Accumulates dispatches on an `SyntheticEvent`, but only for the
* `dispatchMarker`.
* @param {SyntheticEvent} event
*/
function accumulateDirectDispatchesSingle(event) {
if (event && event.dispatchConfig.registrationName) {
accumulateDispatches(event.dispatchMarker, null, event);
}
}
function accumulateTwoPhaseDispatches(events) {
forEachAccumulated(events, accumulateTwoPhaseDispatchesSingle);
}
function accumulateTwoPhaseDispatchesSkipTarget(events) {
forEachAccumulated(events, accumulateTwoPhaseDispatchesSingleSkipTarget);
}
function accumulateEnterLeaveDispatches(leave, enter, fromID, toID) {
EventPluginHub.injection.getInstanceHandle().traverseEnterLeave(fromID, toID, accumulateDispatches, leave, enter);
}
function accumulateDirectDispatches(events) {
forEachAccumulated(events, accumulateDirectDispatchesSingle);
}
/**
* A small set of propagation patterns, each of which will accept a small amount
* of information, and generate a set of "dispatch ready event objects" - which
* are sets of events that have already been annotated with a set of dispatched
* listener functions/ids. The API is designed this way to discourage these
* propagation strategies from actually executing the dispatches, since we
* always want to collect the entire set of dispatches before executing event a
* single one.
*
* @constructor EventPropagators
*/
var EventPropagators = {
accumulateTwoPhaseDispatches: accumulateTwoPhaseDispatches,
accumulateTwoPhaseDispatchesSkipTarget: accumulateTwoPhaseDispatchesSkipTarget,
accumulateDirectDispatches: accumulateDirectDispatches,
accumulateEnterLeaveDispatches: accumulateEnterLeaveDispatches
};
module.exports = EventPropagators;
},{"115":115,"124":124,"15":15,"16":16,"173":173}],20:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule FallbackCompositionState
* @typechecks static-only
*/
'use strict';
var PooledClass = _dereq_(25);
var assign = _dereq_(24);
var getTextContentAccessor = _dereq_(131);
/**
* This helper class stores information about text content of a target node,
* allowing comparison of content before and after a given event.
*
* Identify the node where selection currently begins, then observe
* both its text content and its current position in the DOM. Since the
* browser may natively replace the target node during composition, we can
* use its position to find its replacement.
*
* @param {DOMEventTarget} root
*/
function FallbackCompositionState(root) {
this._root = root;
this._startText = this.getText();
this._fallbackText = null;
}
assign(FallbackCompositionState.prototype, {
destructor: function () {
this._root = null;
this._startText = null;
this._fallbackText = null;
},
/**
* Get current text of input.
*
* @return {string}
*/
getText: function () {
if ('value' in this._root) {
return this._root.value;
}
return this._root[getTextContentAccessor()];
},
/**
* Determine the differing substring between the initially stored
* text content and the current content.
*
* @return {string}
*/
getData: function () {
if (this._fallbackText) {
return this._fallbackText;
}
var start;
var startValue = this._startText;
var startLength = startValue.length;
var end;
var endValue = this.getText();
var endLength = endValue.length;
for (start = 0; start < startLength; start++) {
if (startValue[start] !== endValue[start]) {
break;
}
}
var minEnd = startLength - start;
for (end = 1; end <= minEnd; end++) {
if (startValue[startLength - end] !== endValue[endLength - end]) {
break;
}
}
var sliceTail = end > 1 ? 1 - end : undefined;
this._fallbackText = endValue.slice(start, sliceTail);
return this._fallbackText;
}
});
PooledClass.addPoolingTo(FallbackCompositionState);
module.exports = FallbackCompositionState;
},{"131":131,"24":24,"25":25}],21:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule HTMLDOMPropertyConfig
*/
'use strict';
var DOMProperty = _dereq_(10);
var ExecutionEnvironment = _dereq_(147);
var MUST_USE_ATTRIBUTE = DOMProperty.injection.MUST_USE_ATTRIBUTE;
var MUST_USE_PROPERTY = DOMProperty.injection.MUST_USE_PROPERTY;
var HAS_BOOLEAN_VALUE = DOMProperty.injection.HAS_BOOLEAN_VALUE;
var HAS_SIDE_EFFECTS = DOMProperty.injection.HAS_SIDE_EFFECTS;
var HAS_NUMERIC_VALUE = DOMProperty.injection.HAS_NUMERIC_VALUE;
var HAS_POSITIVE_NUMERIC_VALUE = DOMProperty.injection.HAS_POSITIVE_NUMERIC_VALUE;
var HAS_OVERLOADED_BOOLEAN_VALUE = DOMProperty.injection.HAS_OVERLOADED_BOOLEAN_VALUE;
var hasSVG;
if (ExecutionEnvironment.canUseDOM) {
var implementation = document.implementation;
hasSVG = implementation && implementation.hasFeature && implementation.hasFeature('http://www.w3.org/TR/SVG11/feature#BasicStructure', '1.1');
}
var HTMLDOMPropertyConfig = {
isCustomAttribute: RegExp.prototype.test.bind(/^(data|aria)-[a-z_][a-z\d_.\-]*$/),
Properties: {
/**
* Standard Properties
*/
accept: null,
acceptCharset: null,
accessKey: null,
action: null,
allowFullScreen: MUST_USE_ATTRIBUTE | HAS_BOOLEAN_VALUE,
allowTransparency: MUST_USE_ATTRIBUTE,
alt: null,
async: HAS_BOOLEAN_VALUE,
autoComplete: null,
// autoFocus is polyfilled/normalized by AutoFocusUtils
// autoFocus: HAS_BOOLEAN_VALUE,
autoPlay: HAS_BOOLEAN_VALUE,
capture: MUST_USE_ATTRIBUTE | HAS_BOOLEAN_VALUE,
cellPadding: null,
cellSpacing: null,
charSet: MUST_USE_ATTRIBUTE,
challenge: MUST_USE_ATTRIBUTE,
checked: MUST_USE_PROPERTY | HAS_BOOLEAN_VALUE,
classID: MUST_USE_ATTRIBUTE,
// To set className on SVG elements, it's necessary to use .setAttribute;
// this works on HTML elements too in all browsers except IE8. Conveniently,
// IE8 doesn't support SVG and so we can simply use the attribute in
// browsers that support SVG and the property in browsers that don't,
// regardless of whether the element is HTML or SVG.
className: hasSVG ? MUST_USE_ATTRIBUTE : MUST_USE_PROPERTY,
cols: MUST_USE_ATTRIBUTE | HAS_POSITIVE_NUMERIC_VALUE,
colSpan: null,
content: null,
contentEditable: null,
contextMenu: MUST_USE_ATTRIBUTE,
controls: MUST_USE_PROPERTY | HAS_BOOLEAN_VALUE,
coords: null,
crossOrigin: null,
data: null, // For `<object />` acts as `src`.
dateTime: MUST_USE_ATTRIBUTE,
'default': HAS_BOOLEAN_VALUE,
defer: HAS_BOOLEAN_VALUE,
dir: null,
disabled: MUST_USE_ATTRIBUTE | HAS_BOOLEAN_VALUE,
download: HAS_OVERLOADED_BOOLEAN_VALUE,
draggable: null,
encType: null,
form: MUST_USE_ATTRIBUTE,
formAction: MUST_USE_ATTRIBUTE,
formEncType: MUST_USE_ATTRIBUTE,
formMethod: MUST_USE_ATTRIBUTE,
formNoValidate: HAS_BOOLEAN_VALUE,
formTarget: MUST_USE_ATTRIBUTE,
frameBorder: MUST_USE_ATTRIBUTE,
headers: null,
height: MUST_USE_ATTRIBUTE,
hidden: MUST_USE_ATTRIBUTE | HAS_BOOLEAN_VALUE,
high: null,
href: null,
hrefLang: null,
htmlFor: null,
httpEquiv: null,
icon: null,
id: MUST_USE_PROPERTY,
inputMode: MUST_USE_ATTRIBUTE,
integrity: null,
is: MUST_USE_ATTRIBUTE,
keyParams: MUST_USE_ATTRIBUTE,
keyType: MUST_USE_ATTRIBUTE,
kind: null,
label: null,
lang: null,
list: MUST_USE_ATTRIBUTE,
loop: MUST_USE_PROPERTY | HAS_BOOLEAN_VALUE,
low: null,
manifest: MUST_USE_ATTRIBUTE,
marginHeight: null,
marginWidth: null,
max: null,
maxLength: MUST_USE_ATTRIBUTE,
media: MUST_USE_ATTRIBUTE,
mediaGroup: null,
method: null,
min: null,
minLength: MUST_USE_ATTRIBUTE,
multiple: MUST_USE_PROPERTY | HAS_BOOLEAN_VALUE,
muted: MUST_USE_PROPERTY | HAS_BOOLEAN_VALUE,
name: null,
nonce: MUST_USE_ATTRIBUTE,
noValidate: HAS_BOOLEAN_VALUE,
open: HAS_BOOLEAN_VALUE,
optimum: null,
pattern: null,
placeholder: null,
poster: null,
preload: null,
radioGroup: null,
readOnly: MUST_USE_PROPERTY | HAS_BOOLEAN_VALUE,
rel: null,
required: HAS_BOOLEAN_VALUE,
reversed: HAS_BOOLEAN_VALUE,
role: MUST_USE_ATTRIBUTE,
rows: MUST_USE_ATTRIBUTE | HAS_POSITIVE_NUMERIC_VALUE,
rowSpan: null,
sandbox: null,
scope: null,
scoped: HAS_BOOLEAN_VALUE,
scrolling: null,
seamless: MUST_USE_ATTRIBUTE | HAS_BOOLEAN_VALUE,
selected: MUST_USE_PROPERTY | HAS_BOOLEAN_VALUE,
shape: null,
size: MUST_USE_ATTRIBUTE | HAS_POSITIVE_NUMERIC_VALUE,
sizes: MUST_USE_ATTRIBUTE,
span: HAS_POSITIVE_NUMERIC_VALUE,
spellCheck: null,
src: null,
srcDoc: MUST_USE_PROPERTY,
srcLang: null,
srcSet: MUST_USE_ATTRIBUTE,
start: HAS_NUMERIC_VALUE,
step: null,
style: null,
summary: null,
tabIndex: null,
target: null,
title: null,
type: null,
useMap: null,
value: MUST_USE_PROPERTY | HAS_SIDE_EFFECTS,
width: MUST_USE_ATTRIBUTE,
wmode: MUST_USE_ATTRIBUTE,
wrap: null,
/**
* RDFa Properties
*/
about: MUST_USE_ATTRIBUTE,
datatype: MUST_USE_ATTRIBUTE,
inlist: MUST_USE_ATTRIBUTE,
prefix: MUST_USE_ATTRIBUTE,
// property is also supported for OpenGraph in meta tags.
property: MUST_USE_ATTRIBUTE,
resource: MUST_USE_ATTRIBUTE,
'typeof': MUST_USE_ATTRIBUTE,
vocab: MUST_USE_ATTRIBUTE,
/**
* Non-standard Properties
*/
// autoCapitalize and autoCorrect are supported in Mobile Safari for
// keyboard hints.
autoCapitalize: MUST_USE_ATTRIBUTE,
autoCorrect: MUST_USE_ATTRIBUTE,
// autoSave allows WebKit/Blink to persist values of input fields on page reloads
autoSave: null,
// color is for Safari mask-icon link
color: null,
// itemProp, itemScope, itemType are for
// Microdata support. See http://schema.org/docs/gs.html
itemProp: MUST_USE_ATTRIBUTE,
itemScope: MUST_USE_ATTRIBUTE | HAS_BOOLEAN_VALUE,
itemType: MUST_USE_ATTRIBUTE,
// itemID and itemRef are for Microdata support as well but
// only specified in the the WHATWG spec document. See
// https://html.spec.whatwg.org/multipage/microdata.html#microdata-dom-api
itemID: MUST_USE_ATTRIBUTE,
itemRef: MUST_USE_ATTRIBUTE,
// results show looking glass icon and recent searches on input
// search fields in WebKit/Blink
results: null,
// IE-only attribute that specifies security restrictions on an iframe
// as an alternative to the sandbox attribute on IE<10
security: MUST_USE_ATTRIBUTE,
// IE-only attribute that controls focus behavior
unselectable: MUST_USE_ATTRIBUTE
},
DOMAttributeNames: {
acceptCharset: 'accept-charset',
className: 'class',
htmlFor: 'for',
httpEquiv: 'http-equiv'
},
DOMPropertyNames: {
autoComplete: 'autocomplete',
autoFocus: 'autofocus',
autoPlay: 'autoplay',
autoSave: 'autosave',
// `encoding` is equivalent to `enctype`, IE8 lacks an `enctype` setter.
// http://www.w3.org/TR/html5/forms.html#dom-fs-encoding
encType: 'encoding',
hrefLang: 'hreflang',
radioGroup: 'radiogroup',
spellCheck: 'spellcheck',
srcDoc: 'srcdoc',
srcSet: 'srcset'
}
};
module.exports = HTMLDOMPropertyConfig;
},{"10":10,"147":147}],22:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule LinkedStateMixin
* @typechecks static-only
*/
'use strict';
var ReactLink = _dereq_(70);
var ReactStateSetters = _dereq_(90);
/**
* A simple mixin around ReactLink.forState().
*/
var LinkedStateMixin = {
/**
* Create a ReactLink that's linked to part of this component's state. The
* ReactLink will have the current value of this.state[key] and will call
* setState() when a change is requested.
*
* @param {string} key state key to update. Note: you may want to use keyOf()
* if you're using Google Closure Compiler advanced mode.
* @return {ReactLink} ReactLink instance linking to the state.
*/
linkState: function (key) {
return new ReactLink(this.state[key], ReactStateSetters.createStateKeySetter(this, key));
}
};
module.exports = LinkedStateMixin;
},{"70":70,"90":90}],23:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule LinkedValueUtils
* @typechecks static-only
*/
'use strict';
var ReactPropTypes = _dereq_(82);
var ReactPropTypeLocations = _dereq_(81);
var invariant = _dereq_(161);
var warning = _dereq_(173);
var hasReadOnlyValue = {
'button': true,
'checkbox': true,
'image': true,
'hidden': true,
'radio': true,
'reset': true,
'submit': true
};
function _assertSingleLink(inputProps) {
!(inputProps.checkedLink == null || inputProps.valueLink == null) ? "development" !== 'production' ? invariant(false, 'Cannot provide a checkedLink and a valueLink. If you want to use ' + 'checkedLink, you probably don\'t want to use valueLink and vice versa.') : invariant(false) : undefined;
}
function _assertValueLink(inputProps) {
_assertSingleLink(inputProps);
!(inputProps.value == null && inputProps.onChange == null) ? "development" !== 'production' ? invariant(false, 'Cannot provide a valueLink and a value or onChange event. If you want ' + 'to use value or onChange, you probably don\'t want to use valueLink.') : invariant(false) : undefined;
}
function _assertCheckedLink(inputProps) {
_assertSingleLink(inputProps);
!(inputProps.checked == null && inputProps.onChange == null) ? "development" !== 'production' ? invariant(false, 'Cannot provide a checkedLink and a checked property or onChange event. ' + 'If you want to use checked or onChange, you probably don\'t want to ' + 'use checkedLink') : invariant(false) : undefined;
}
var propTypes = {
value: function (props, propName, componentName) {
if (!props[propName] || hasReadOnlyValue[props.type] || props.onChange || props.readOnly || props.disabled) {
return null;
}
return new Error('You provided a `value` prop to a form field without an ' + '`onChange` handler. This will render a read-only field. If ' + 'the field should be mutable use `defaultValue`. Otherwise, ' + 'set either `onChange` or `readOnly`.');
},
checked: function (props, propName, componentName) {
if (!props[propName] || props.onChange || props.readOnly || props.disabled) {
return null;
}
return new Error('You provided a `checked` prop to a form field without an ' + '`onChange` handler. This will render a read-only field. If ' + 'the field should be mutable use `defaultChecked`. Otherwise, ' + 'set either `onChange` or `readOnly`.');
},
onChange: ReactPropTypes.func
};
var loggedTypeFailures = {};
function getDeclarationErrorAddendum(owner) {
if (owner) {
var name = owner.getName();
if (name) {
return ' Check the render method of `' + name + '`.';
}
}
return '';
}
/**
* Provide a linked `value` attribute for controlled forms. You should not use
* this outside of the ReactDOM controlled form components.
*/
var LinkedValueUtils = {
checkPropTypes: function (tagName, props, owner) {
for (var propName in propTypes) {
if (propTypes.hasOwnProperty(propName)) {
var error = propTypes[propName](props, propName, tagName, ReactPropTypeLocations.prop);
}
if (error instanceof Error && !(error.message in loggedTypeFailures)) {
// Only monitor this failure once because there tends to be a lot of the
// same error.
loggedTypeFailures[error.message] = true;
var addendum = getDeclarationErrorAddendum(owner);
"development" !== 'production' ? warning(false, 'Failed form propType: %s%s', error.message, addendum) : undefined;
}
}
},
/**
* @param {object} inputProps Props for form component
* @return {*} current value of the input either from value prop or link.
*/
getValue: function (inputProps) {
if (inputProps.valueLink) {
_assertValueLink(inputProps);
return inputProps.valueLink.value;
}
return inputProps.value;
},
/**
* @param {object} inputProps Props for form component
* @return {*} current checked status of the input either from checked prop
* or link.
*/
getChecked: function (inputProps) {
if (inputProps.checkedLink) {
_assertCheckedLink(inputProps);
return inputProps.checkedLink.value;
}
return inputProps.checked;
},
/**
* @param {object} inputProps Props for form component
* @param {SyntheticEvent} event change event to handle
*/
executeOnChange: function (inputProps, event) {
if (inputProps.valueLink) {
_assertValueLink(inputProps);
return inputProps.valueLink.requestChange(event.target.value);
} else if (inputProps.checkedLink) {
_assertCheckedLink(inputProps);
return inputProps.checkedLink.requestChange(event.target.checked);
} else if (inputProps.onChange) {
return inputProps.onChange.call(undefined, event);
}
}
};
module.exports = LinkedValueUtils;
},{"161":161,"173":173,"81":81,"82":82}],24:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule Object.assign
*/
// https://people.mozilla.org/~jorendorff/es6-draft.html#sec-object.assign
'use strict';
function assign(target, sources) {
if (target == null) {
throw new TypeError('Object.assign target cannot be null or undefined');
}
var to = Object(target);
var hasOwnProperty = Object.prototype.hasOwnProperty;
for (var nextIndex = 1; nextIndex < arguments.length; nextIndex++) {
var nextSource = arguments[nextIndex];
if (nextSource == null) {
continue;
}
var from = Object(nextSource);
// We don't currently support accessors nor proxies. Therefore this
// copy cannot throw. If we ever supported this then we must handle
// exceptions and side-effects. We don't support symbols so they won't
// be transferred.
for (var key in from) {
if (hasOwnProperty.call(from, key)) {
to[key] = from[key];
}
}
}
return to;
}
module.exports = assign;
},{}],25:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule PooledClass
*/
'use strict';
var invariant = _dereq_(161);
/**
* Static poolers. Several custom versions for each potential number of
* arguments. A completely generic pooler is easy to implement, but would
* require accessing the `arguments` object. In each of these, `this` refers to
* the Class itself, not an instance. If any others are needed, simply add them
* here, or in their own files.
*/
var oneArgumentPooler = function (copyFieldsFrom) {
var Klass = this;
if (Klass.instancePool.length) {
var instance = Klass.instancePool.pop();
Klass.call(instance, copyFieldsFrom);
return instance;
} else {
return new Klass(copyFieldsFrom);
}
};
var twoArgumentPooler = function (a1, a2) {
var Klass = this;
if (Klass.instancePool.length) {
var instance = Klass.instancePool.pop();
Klass.call(instance, a1, a2);
return instance;
} else {
return new Klass(a1, a2);
}
};
var threeArgumentPooler = function (a1, a2, a3) {
var Klass = this;
if (Klass.instancePool.length) {
var instance = Klass.instancePool.pop();
Klass.call(instance, a1, a2, a3);
return instance;
} else {
return new Klass(a1, a2, a3);
}
};
var fourArgumentPooler = function (a1, a2, a3, a4) {
var Klass = this;
if (Klass.instancePool.length) {
var instance = Klass.instancePool.pop();
Klass.call(instance, a1, a2, a3, a4);
return instance;
} else {
return new Klass(a1, a2, a3, a4);
}
};
var fiveArgumentPooler = function (a1, a2, a3, a4, a5) {
var Klass = this;
if (Klass.instancePool.length) {
var instance = Klass.instancePool.pop();
Klass.call(instance, a1, a2, a3, a4, a5);
return instance;
} else {
return new Klass(a1, a2, a3, a4, a5);
}
};
var standardReleaser = function (instance) {
var Klass = this;
!(instance instanceof Klass) ? "development" !== 'production' ? invariant(false, 'Trying to release an instance into a pool of a different type.') : invariant(false) : undefined;
instance.destructor();
if (Klass.instancePool.length < Klass.poolSize) {
Klass.instancePool.push(instance);
}
};
var DEFAULT_POOL_SIZE = 10;
var DEFAULT_POOLER = oneArgumentPooler;
/**
* Augments `CopyConstructor` to be a poolable class, augmenting only the class
* itself (statically) not adding any prototypical fields. Any CopyConstructor
* you give this may have a `poolSize` property, and will look for a
* prototypical `destructor` on instances (optional).
*
* @param {Function} CopyConstructor Constructor that can be used to reset.
* @param {Function} pooler Customizable pooler.
*/
var addPoolingTo = function (CopyConstructor, pooler) {
var NewKlass = CopyConstructor;
NewKlass.instancePool = [];
NewKlass.getPooled = pooler || DEFAULT_POOLER;
if (!NewKlass.poolSize) {
NewKlass.poolSize = DEFAULT_POOL_SIZE;
}
NewKlass.release = standardReleaser;
return NewKlass;
};
var PooledClass = {
addPoolingTo: addPoolingTo,
oneArgumentPooler: oneArgumentPooler,
twoArgumentPooler: twoArgumentPooler,
threeArgumentPooler: threeArgumentPooler,
fourArgumentPooler: fourArgumentPooler,
fiveArgumentPooler: fiveArgumentPooler
};
module.exports = PooledClass;
},{"161":161}],26:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule React
*/
'use strict';
var ReactDOM = _dereq_(40);
var ReactDOMServer = _dereq_(50);
var ReactIsomorphic = _dereq_(69);
var assign = _dereq_(24);
var deprecated = _dereq_(120);
// `version` will be added here by ReactIsomorphic.
var React = {};
assign(React, ReactIsomorphic);
assign(React, {
// ReactDOM
findDOMNode: deprecated('findDOMNode', 'ReactDOM', 'react-dom', ReactDOM, ReactDOM.findDOMNode),
render: deprecated('render', 'ReactDOM', 'react-dom', ReactDOM, ReactDOM.render),
unmountComponentAtNode: deprecated('unmountComponentAtNode', 'ReactDOM', 'react-dom', ReactDOM, ReactDOM.unmountComponentAtNode),
// ReactDOMServer
renderToString: deprecated('renderToString', 'ReactDOMServer', 'react-dom/server', ReactDOMServer, ReactDOMServer.renderToString),
renderToStaticMarkup: deprecated('renderToStaticMarkup', 'ReactDOMServer', 'react-dom/server', ReactDOMServer, ReactDOMServer.renderToStaticMarkup)
});
React.__SECRET_DOM_DO_NOT_USE_OR_YOU_WILL_BE_FIRED = ReactDOM;
React.__SECRET_DOM_SERVER_DO_NOT_USE_OR_YOU_WILL_BE_FIRED = ReactDOMServer;
module.exports = React;
},{"120":120,"24":24,"40":40,"50":50,"69":69}],27:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactBrowserComponentMixin
*/
'use strict';
var ReactInstanceMap = _dereq_(68);
var findDOMNode = _dereq_(122);
var warning = _dereq_(173);
var didWarnKey = '_getDOMNodeDidWarn';
var ReactBrowserComponentMixin = {
/**
* Returns the DOM node rendered by this component.
*
* @return {DOMElement} The root node of this component.
* @final
* @protected
*/
getDOMNode: function () {
"development" !== 'production' ? warning(this.constructor[didWarnKey], '%s.getDOMNode(...) is deprecated. Please use ' + 'ReactDOM.findDOMNode(instance) instead.', ReactInstanceMap.get(this).getName() || this.tagName || 'Unknown') : undefined;
this.constructor[didWarnKey] = true;
return findDOMNode(this);
}
};
module.exports = ReactBrowserComponentMixin;
},{"122":122,"173":173,"68":68}],28:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactBrowserEventEmitter
* @typechecks static-only
*/
'use strict';
var EventConstants = _dereq_(15);
var EventPluginHub = _dereq_(16);
var EventPluginRegistry = _dereq_(17);
var ReactEventEmitterMixin = _dereq_(62);
var ReactPerf = _dereq_(78);
var ViewportMetrics = _dereq_(114);
var assign = _dereq_(24);
var isEventSupported = _dereq_(133);
/**
* Summary of `ReactBrowserEventEmitter` event handling:
*
* - Top-level delegation is used to trap most native browser events. This
* may only occur in the main thread and is the responsibility of
* ReactEventListener, which is injected and can therefore support pluggable
* event sources. This is the only work that occurs in the main thread.
*
* - We normalize and de-duplicate events to account for browser quirks. This
* may be done in the worker thread.
*
* - Forward these native events (with the associated top-level type used to
* trap it) to `EventPluginHub`, which in turn will ask plugins if they want
* to extract any synthetic events.
*
* - The `EventPluginHub` will then process each event by annotating them with
* "dispatches", a sequence of listeners and IDs that care about that event.
*
* - The `EventPluginHub` then dispatches the events.
*
* Overview of React and the event system:
*
* +------------+ .
* | DOM | .
* +------------+ .
* | .
* v .
* +------------+ .
* | ReactEvent | .
* | Listener | .
* +------------+ . +-----------+
* | . +--------+|SimpleEvent|
* | . | |Plugin |
* +-----|------+ . v +-----------+
* | | | . +--------------+ +------------+
* | +-----------.--->|EventPluginHub| | Event |
* | | . | | +-----------+ | Propagators|
* | ReactEvent | . | | |TapEvent | |------------|
* | Emitter | . | |<---+|Plugin | |other plugin|
* | | . | | +-----------+ | utilities |
* | +-----------.--->| | +------------+
* | | | . +--------------+
* +-----|------+ . ^ +-----------+
* | . | |Enter/Leave|
* + . +-------+|Plugin |
* +-------------+ . +-----------+
* | application | .
* |-------------| .
* | | .
* | | .
* +-------------+ .
* .
* React Core . General Purpose Event Plugin System
*/
var alreadyListeningTo = {};
var isMonitoringScrollValue = false;
var reactTopListenersCounter = 0;
// For events like 'submit' which don't consistently bubble (which we trap at a
// lower node than `document`), binding at `document` would cause duplicate
// events so we don't include them here
var topEventMapping = {
topAbort: 'abort',
topBlur: 'blur',
topCanPlay: 'canplay',
topCanPlayThrough: 'canplaythrough',
topChange: 'change',
topClick: 'click',
topCompositionEnd: 'compositionend',
topCompositionStart: 'compositionstart',
topCompositionUpdate: 'compositionupdate',
topContextMenu: 'contextmenu',
topCopy: 'copy',
topCut: 'cut',
topDoubleClick: 'dblclick',
topDrag: 'drag',
topDragEnd: 'dragend',
topDragEnter: 'dragenter',
topDragExit: 'dragexit',
topDragLeave: 'dragleave',
topDragOver: 'dragover',
topDragStart: 'dragstart',
topDrop: 'drop',
topDurationChange: 'durationchange',
topEmptied: 'emptied',
topEncrypted: 'encrypted',
topEnded: 'ended',
topError: 'error',
topFocus: 'focus',
topInput: 'input',
topKeyDown: 'keydown',
topKeyPress: 'keypress',
topKeyUp: 'keyup',
topLoadedData: 'loadeddata',
topLoadedMetadata: 'loadedmetadata',
topLoadStart: 'loadstart',
topMouseDown: 'mousedown',
topMouseMove: 'mousemove',
topMouseOut: 'mouseout',
topMouseOver: 'mouseover',
topMouseUp: 'mouseup',
topPaste: 'paste',
topPause: 'pause',
topPlay: 'play',
topPlaying: 'playing',
topProgress: 'progress',
topRateChange: 'ratechange',
topScroll: 'scroll',
topSeeked: 'seeked',
topSeeking: 'seeking',
topSelectionChange: 'selectionchange',
topStalled: 'stalled',
topSuspend: 'suspend',
topTextInput: 'textInput',
topTimeUpdate: 'timeupdate',
topTouchCancel: 'touchcancel',
topTouchEnd: 'touchend',
topTouchMove: 'touchmove',
topTouchStart: 'touchstart',
topVolumeChange: 'volumechange',
topWaiting: 'waiting',
topWheel: 'wheel'
};
/**
* To ensure no conflicts with other potential React instances on the page
*/
var topListenersIDKey = '_reactListenersID' + String(Math.random()).slice(2);
function getListeningForDocument(mountAt) {
// In IE8, `mountAt` is a host object and doesn't have `hasOwnProperty`
// directly.
if (!Object.prototype.hasOwnProperty.call(mountAt, topListenersIDKey)) {
mountAt[topListenersIDKey] = reactTopListenersCounter++;
alreadyListeningTo[mountAt[topListenersIDKey]] = {};
}
return alreadyListeningTo[mountAt[topListenersIDKey]];
}
/**
* `ReactBrowserEventEmitter` is used to attach top-level event listeners. For
* example:
*
* ReactBrowserEventEmitter.putListener('myID', 'onClick', myFunction);
*
* This would allocate a "registration" of `('onClick', myFunction)` on 'myID'.
*
* @internal
*/
var ReactBrowserEventEmitter = assign({}, ReactEventEmitterMixin, {
/**
* Injectable event backend
*/
ReactEventListener: null,
injection: {
/**
* @param {object} ReactEventListener
*/
injectReactEventListener: function (ReactEventListener) {
ReactEventListener.setHandleTopLevel(ReactBrowserEventEmitter.handleTopLevel);
ReactBrowserEventEmitter.ReactEventListener = ReactEventListener;
}
},
/**
* Sets whether or not any created callbacks should be enabled.
*
* @param {boolean} enabled True if callbacks should be enabled.
*/
setEnabled: function (enabled) {
if (ReactBrowserEventEmitter.ReactEventListener) {
ReactBrowserEventEmitter.ReactEventListener.setEnabled(enabled);
}
},
/**
* @return {boolean} True if callbacks are enabled.
*/
isEnabled: function () {
return !!(ReactBrowserEventEmitter.ReactEventListener && ReactBrowserEventEmitter.ReactEventListener.isEnabled());
},
/**
* We listen for bubbled touch events on the document object.
*
* Firefox v8.01 (and possibly others) exhibited strange behavior when
* mounting `onmousemove` events at some node that was not the document
* element. The symptoms were that if your mouse is not moving over something
* contained within that mount point (for example on the background) the
* top-level listeners for `onmousemove` won't be called. However, if you
* register the `mousemove` on the document object, then it will of course
* catch all `mousemove`s. This along with iOS quirks, justifies restricting
* top-level listeners to the document object only, at least for these
* movement types of events and possibly all events.
*
* @see http://www.quirksmode.org/blog/archives/2010/09/click_event_del.html
*
* Also, `keyup`/`keypress`/`keydown` do not bubble to the window on IE, but
* they bubble to document.
*
* @param {string} registrationName Name of listener (e.g. `onClick`).
* @param {object} contentDocumentHandle Document which owns the container
*/
listenTo: function (registrationName, contentDocumentHandle) {
var mountAt = contentDocumentHandle;
var isListening = getListeningForDocument(mountAt);
var dependencies = EventPluginRegistry.registrationNameDependencies[registrationName];
var topLevelTypes = EventConstants.topLevelTypes;
for (var i = 0; i < dependencies.length; i++) {
var dependency = dependencies[i];
if (!(isListening.hasOwnProperty(dependency) && isListening[dependency])) {
if (dependency === topLevelTypes.topWheel) {
if (isEventSupported('wheel')) {
ReactBrowserEventEmitter.ReactEventListener.trapBubbledEvent(topLevelTypes.topWheel, 'wheel', mountAt);
} else if (isEventSupported('mousewheel')) {
ReactBrowserEventEmitter.ReactEventListener.trapBubbledEvent(topLevelTypes.topWheel, 'mousewheel', mountAt);
} else {
// Firefox needs to capture a different mouse scroll event.
// @see http://www.quirksmode.org/dom/events/tests/scroll.html
ReactBrowserEventEmitter.ReactEventListener.trapBubbledEvent(topLevelTypes.topWheel, 'DOMMouseScroll', mountAt);
}
} else if (dependency === topLevelTypes.topScroll) {
if (isEventSupported('scroll', true)) {
ReactBrowserEventEmitter.ReactEventListener.trapCapturedEvent(topLevelTypes.topScroll, 'scroll', mountAt);
} else {
ReactBrowserEventEmitter.ReactEventListener.trapBubbledEvent(topLevelTypes.topScroll, 'scroll', ReactBrowserEventEmitter.ReactEventListener.WINDOW_HANDLE);
}
} else if (dependency === topLevelTypes.topFocus || dependency === topLevelTypes.topBlur) {
if (isEventSupported('focus', true)) {
ReactBrowserEventEmitter.ReactEventListener.trapCapturedEvent(topLevelTypes.topFocus, 'focus', mountAt);
ReactBrowserEventEmitter.ReactEventListener.trapCapturedEvent(topLevelTypes.topBlur, 'blur', mountAt);
} else if (isEventSupported('focusin')) {
// IE has `focusin` and `focusout` events which bubble.
// @see http://www.quirksmode.org/blog/archives/2008/04/delegating_the.html
ReactBrowserEventEmitter.ReactEventListener.trapBubbledEvent(topLevelTypes.topFocus, 'focusin', mountAt);
ReactBrowserEventEmitter.ReactEventListener.trapBubbledEvent(topLevelTypes.topBlur, 'focusout', mountAt);
}
// to make sure blur and focus event listeners are only attached once
isListening[topLevelTypes.topBlur] = true;
isListening[topLevelTypes.topFocus] = true;
} else if (topEventMapping.hasOwnProperty(dependency)) {
ReactBrowserEventEmitter.ReactEventListener.trapBubbledEvent(dependency, topEventMapping[dependency], mountAt);
}
isListening[dependency] = true;
}
}
},
trapBubbledEvent: function (topLevelType, handlerBaseName, handle) {
return ReactBrowserEventEmitter.ReactEventListener.trapBubbledEvent(topLevelType, handlerBaseName, handle);
},
trapCapturedEvent: function (topLevelType, handlerBaseName, handle) {
return ReactBrowserEventEmitter.ReactEventListener.trapCapturedEvent(topLevelType, handlerBaseName, handle);
},
/**
* Listens to window scroll and resize events. We cache scroll values so that
* application code can access them without triggering reflows.
*
* NOTE: Scroll events do not bubble.
*
* @see http://www.quirksmode.org/dom/events/scroll.html
*/
ensureScrollValueMonitoring: function () {
if (!isMonitoringScrollValue) {
var refresh = ViewportMetrics.refreshScrollValues;
ReactBrowserEventEmitter.ReactEventListener.monitorScrollValue(refresh);
isMonitoringScrollValue = true;
}
},
eventNameDispatchConfigs: EventPluginHub.eventNameDispatchConfigs,
registrationNameModules: EventPluginHub.registrationNameModules,
putListener: EventPluginHub.putListener,
getListener: EventPluginHub.getListener,
deleteListener: EventPluginHub.deleteListener,
deleteAllListeners: EventPluginHub.deleteAllListeners
});
ReactPerf.measureMethods(ReactBrowserEventEmitter, 'ReactBrowserEventEmitter', {
putListener: 'putListener',
deleteListener: 'deleteListener'
});
module.exports = ReactBrowserEventEmitter;
},{"114":114,"133":133,"15":15,"16":16,"17":17,"24":24,"62":62,"78":78}],29:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @typechecks
* @providesModule ReactCSSTransitionGroup
*/
'use strict';
var React = _dereq_(26);
var assign = _dereq_(24);
var ReactTransitionGroup = _dereq_(94);
var ReactCSSTransitionGroupChild = _dereq_(30);
function createTransitionTimeoutPropValidator(transitionType) {
var timeoutPropName = 'transition' + transitionType + 'Timeout';
var enabledPropName = 'transition' + transitionType;
return function (props) {
// If the transition is enabled
if (props[enabledPropName]) {
// If no timeout duration is provided
if (props[timeoutPropName] == null) {
return new Error(timeoutPropName + ' wasn\'t supplied to ReactCSSTransitionGroup: ' + 'this can cause unreliable animations and won\'t be supported in ' + 'a future version of React. See ' + 'https://fb.me/react-animation-transition-group-timeout for more ' + 'information.');
// If the duration isn't a number
} else if (typeof props[timeoutPropName] !== 'number') {
return new Error(timeoutPropName + ' must be a number (in milliseconds)');
}
}
};
}
var ReactCSSTransitionGroup = React.createClass({
displayName: 'ReactCSSTransitionGroup',
propTypes: {
transitionName: ReactCSSTransitionGroupChild.propTypes.name,
transitionAppear: React.PropTypes.bool,
transitionEnter: React.PropTypes.bool,
transitionLeave: React.PropTypes.bool,
transitionAppearTimeout: createTransitionTimeoutPropValidator('Appear'),
transitionEnterTimeout: createTransitionTimeoutPropValidator('Enter'),
transitionLeaveTimeout: createTransitionTimeoutPropValidator('Leave')
},
getDefaultProps: function () {
return {
transitionAppear: false,
transitionEnter: true,
transitionLeave: true
};
},
_wrapChild: function (child) {
// We need to provide this childFactory so that
// ReactCSSTransitionGroupChild can receive updates to name, enter, and
// leave while it is leaving.
return React.createElement(ReactCSSTransitionGroupChild, {
name: this.props.transitionName,
appear: this.props.transitionAppear,
enter: this.props.transitionEnter,
leave: this.props.transitionLeave,
appearTimeout: this.props.transitionAppearTimeout,
enterTimeout: this.props.transitionEnterTimeout,
leaveTimeout: this.props.transitionLeaveTimeout
}, child);
},
render: function () {
return React.createElement(ReactTransitionGroup, assign({}, this.props, { childFactory: this._wrapChild }));
}
});
module.exports = ReactCSSTransitionGroup;
},{"24":24,"26":26,"30":30,"94":94}],30:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @typechecks
* @providesModule ReactCSSTransitionGroupChild
*/
'use strict';
var React = _dereq_(26);
var ReactDOM = _dereq_(40);
var CSSCore = _dereq_(145);
var ReactTransitionEvents = _dereq_(93);
var onlyChild = _dereq_(135);
// We don't remove the element from the DOM until we receive an animationend or
// transitionend event. If the user screws up and forgets to add an animation
// their node will be stuck in the DOM forever, so we detect if an animation
// does not start and if it doesn't, we just call the end listener immediately.
var TICK = 17;
var ReactCSSTransitionGroupChild = React.createClass({
displayName: 'ReactCSSTransitionGroupChild',
propTypes: {
name: React.PropTypes.oneOfType([React.PropTypes.string, React.PropTypes.shape({
enter: React.PropTypes.string,
leave: React.PropTypes.string,
active: React.PropTypes.string
}), React.PropTypes.shape({
enter: React.PropTypes.string,
enterActive: React.PropTypes.string,
leave: React.PropTypes.string,
leaveActive: React.PropTypes.string,
appear: React.PropTypes.string,
appearActive: React.PropTypes.string
})]).isRequired,
// Once we require timeouts to be specified, we can remove the
// boolean flags (appear etc.) and just accept a number
// or a bool for the timeout flags (appearTimeout etc.)
appear: React.PropTypes.bool,
enter: React.PropTypes.bool,
leave: React.PropTypes.bool,
appearTimeout: React.PropTypes.number,
enterTimeout: React.PropTypes.number,
leaveTimeout: React.PropTypes.number
},
transition: function (animationType, finishCallback, userSpecifiedDelay) {
var node = ReactDOM.findDOMNode(this);
if (!node) {
if (finishCallback) {
finishCallback();
}
return;
}
var className = this.props.name[animationType] || this.props.name + '-' + animationType;
var activeClassName = this.props.name[animationType + 'Active'] || className + '-active';
var timeout = null;
var endListener = function (e) {
if (e && e.target !== node) {
return;
}
clearTimeout(timeout);
CSSCore.removeClass(node, className);
CSSCore.removeClass(node, activeClassName);
ReactTransitionEvents.removeEndEventListener(node, endListener);
// Usually this optional callback is used for informing an owner of
// a leave animation and telling it to remove the child.
if (finishCallback) {
finishCallback();
}
};
CSSCore.addClass(node, className);
// Need to do this to actually trigger a transition.
this.queueClass(activeClassName);
// If the user specified a timeout delay.
if (userSpecifiedDelay) {
// Clean-up the animation after the specified delay
timeout = setTimeout(endListener, userSpecifiedDelay);
this.transitionTimeouts.push(timeout);
} else {
// DEPRECATED: this listener will be removed in a future version of react
ReactTransitionEvents.addEndEventListener(node, endListener);
}
},
queueClass: function (className) {
this.classNameQueue.push(className);
if (!this.timeout) {
this.timeout = setTimeout(this.flushClassNameQueue, TICK);
}
},
flushClassNameQueue: function () {
if (this.isMounted()) {
this.classNameQueue.forEach(CSSCore.addClass.bind(CSSCore, ReactDOM.findDOMNode(this)));
}
this.classNameQueue.length = 0;
this.timeout = null;
},
componentWillMount: function () {
this.classNameQueue = [];
this.transitionTimeouts = [];
},
componentWillUnmount: function () {
if (this.timeout) {
clearTimeout(this.timeout);
}
this.transitionTimeouts.forEach(function (timeout) {
clearTimeout(timeout);
});
},
componentWillAppear: function (done) {
if (this.props.appear) {
this.transition('appear', done, this.props.appearTimeout);
} else {
done();
}
},
componentWillEnter: function (done) {
if (this.props.enter) {
this.transition('enter', done, this.props.enterTimeout);
} else {
done();
}
},
componentWillLeave: function (done) {
if (this.props.leave) {
this.transition('leave', done, this.props.leaveTimeout);
} else {
done();
}
},
render: function () {
return onlyChild(this.props.children);
}
});
module.exports = ReactCSSTransitionGroupChild;
},{"135":135,"145":145,"26":26,"40":40,"93":93}],31:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactChildReconciler
* @typechecks static-only
*/
'use strict';
var ReactReconciler = _dereq_(84);
var instantiateReactComponent = _dereq_(132);
var shouldUpdateReactComponent = _dereq_(141);
var traverseAllChildren = _dereq_(142);
var warning = _dereq_(173);
function instantiateChild(childInstances, child, name) {
// We found a component instance.
var keyUnique = childInstances[name] === undefined;
if ("development" !== 'production') {
"development" !== 'production' ? warning(keyUnique, 'flattenChildren(...): Encountered two children with the same key, ' + '`%s`. Child keys must be unique; when two children share a key, only ' + 'the first child will be used.', name) : undefined;
}
if (child != null && keyUnique) {
childInstances[name] = instantiateReactComponent(child, null);
}
}
/**
* ReactChildReconciler provides helpers for initializing or updating a set of
* children. Its output is suitable for passing it onto ReactMultiChild which
* does diffed reordering and insertion.
*/
var ReactChildReconciler = {
/**
* Generates a "mount image" for each of the supplied children. In the case
* of `ReactDOMComponent`, a mount image is a string of markup.
*
* @param {?object} nestedChildNodes Nested child maps.
* @return {?object} A set of child instances.
* @internal
*/
instantiateChildren: function (nestedChildNodes, transaction, context) {
if (nestedChildNodes == null) {
return null;
}
var childInstances = {};
traverseAllChildren(nestedChildNodes, instantiateChild, childInstances);
return childInstances;
},
/**
* Updates the rendered children and returns a new set of children.
*
* @param {?object} prevChildren Previously initialized set of children.
* @param {?object} nextChildren Flat child element maps.
* @param {ReactReconcileTransaction} transaction
* @param {object} context
* @return {?object} A new set of child instances.
* @internal
*/
updateChildren: function (prevChildren, nextChildren, transaction, context) {
// We currently don't have a way to track moves here but if we use iterators
// instead of for..in we can zip the iterators and check if an item has
// moved.
// TODO: If nothing has changed, return the prevChildren object so that we
// can quickly bailout if nothing has changed.
if (!nextChildren && !prevChildren) {
return null;
}
var name;
for (name in nextChildren) {
if (!nextChildren.hasOwnProperty(name)) {
continue;
}
var prevChild = prevChildren && prevChildren[name];
var prevElement = prevChild && prevChild._currentElement;
var nextElement = nextChildren[name];
if (prevChild != null && shouldUpdateReactComponent(prevElement, nextElement)) {
ReactReconciler.receiveComponent(prevChild, nextElement, transaction, context);
nextChildren[name] = prevChild;
} else {
if (prevChild) {
ReactReconciler.unmountComponent(prevChild, name);
}
// The child must be instantiated before it's mounted.
var nextChildInstance = instantiateReactComponent(nextElement, null);
nextChildren[name] = nextChildInstance;
}
}
// Unmount children that are no longer present.
for (name in prevChildren) {
if (prevChildren.hasOwnProperty(name) && !(nextChildren && nextChildren.hasOwnProperty(name))) {
ReactReconciler.unmountComponent(prevChildren[name]);
}
}
return nextChildren;
},
/**
* Unmounts all rendered children. This should be used to clean up children
* when this component is unmounted.
*
* @param {?object} renderedChildren Previously initialized set of children.
* @internal
*/
unmountChildren: function (renderedChildren) {
for (var name in renderedChildren) {
if (renderedChildren.hasOwnProperty(name)) {
var renderedChild = renderedChildren[name];
ReactReconciler.unmountComponent(renderedChild);
}
}
}
};
module.exports = ReactChildReconciler;
},{"132":132,"141":141,"142":142,"173":173,"84":84}],32:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactChildren
*/
'use strict';
var PooledClass = _dereq_(25);
var ReactElement = _dereq_(57);
var emptyFunction = _dereq_(153);
var traverseAllChildren = _dereq_(142);
var twoArgumentPooler = PooledClass.twoArgumentPooler;
var fourArgumentPooler = PooledClass.fourArgumentPooler;
var userProvidedKeyEscapeRegex = /\/(?!\/)/g;
function escapeUserProvidedKey(text) {
return ('' + text).replace(userProvidedKeyEscapeRegex, '//');
}
/**
* PooledClass representing the bookkeeping associated with performing a child
* traversal. Allows avoiding binding callbacks.
*
* @constructor ForEachBookKeeping
* @param {!function} forEachFunction Function to perform traversal with.
* @param {?*} forEachContext Context to perform context with.
*/
function ForEachBookKeeping(forEachFunction, forEachContext) {
this.func = forEachFunction;
this.context = forEachContext;
this.count = 0;
}
ForEachBookKeeping.prototype.destructor = function () {
this.func = null;
this.context = null;
this.count = 0;
};
PooledClass.addPoolingTo(ForEachBookKeeping, twoArgumentPooler);
function forEachSingleChild(bookKeeping, child, name) {
var func = bookKeeping.func;
var context = bookKeeping.context;
func.call(context, child, bookKeeping.count++);
}
/**
* Iterates through children that are typically specified as `props.children`.
*
* The provided forEachFunc(child, index) will be called for each
* leaf child.
*
* @param {?*} children Children tree container.
* @param {function(*, int)} forEachFunc
* @param {*} forEachContext Context for forEachContext.
*/
function forEachChildren(children, forEachFunc, forEachContext) {
if (children == null) {
return children;
}
var traverseContext = ForEachBookKeeping.getPooled(forEachFunc, forEachContext);
traverseAllChildren(children, forEachSingleChild, traverseContext);
ForEachBookKeeping.release(traverseContext);
}
/**
* PooledClass representing the bookkeeping associated with performing a child
* mapping. Allows avoiding binding callbacks.
*
* @constructor MapBookKeeping
* @param {!*} mapResult Object containing the ordered map of results.
* @param {!function} mapFunction Function to perform mapping with.
* @param {?*} mapContext Context to perform mapping with.
*/
function MapBookKeeping(mapResult, keyPrefix, mapFunction, mapContext) {
this.result = mapResult;
this.keyPrefix = keyPrefix;
this.func = mapFunction;
this.context = mapContext;
this.count = 0;
}
MapBookKeeping.prototype.destructor = function () {
this.result = null;
this.keyPrefix = null;
this.func = null;
this.context = null;
this.count = 0;
};
PooledClass.addPoolingTo(MapBookKeeping, fourArgumentPooler);
function mapSingleChildIntoContext(bookKeeping, child, childKey) {
var result = bookKeeping.result;
var keyPrefix = bookKeeping.keyPrefix;
var func = bookKeeping.func;
var context = bookKeeping.context;
var mappedChild = func.call(context, child, bookKeeping.count++);
if (Array.isArray(mappedChild)) {
mapIntoWithKeyPrefixInternal(mappedChild, result, childKey, emptyFunction.thatReturnsArgument);
} else if (mappedChild != null) {
if (ReactElement.isValidElement(mappedChild)) {
mappedChild = ReactElement.cloneAndReplaceKey(mappedChild,
// Keep both the (mapped) and old keys if they differ, just as
// traverseAllChildren used to do for objects as children
keyPrefix + (mappedChild !== child ? escapeUserProvidedKey(mappedChild.key || '') + '/' : '') + childKey);
}
result.push(mappedChild);
}
}
function mapIntoWithKeyPrefixInternal(children, array, prefix, func, context) {
var escapedPrefix = '';
if (prefix != null) {
escapedPrefix = escapeUserProvidedKey(prefix) + '/';
}
var traverseContext = MapBookKeeping.getPooled(array, escapedPrefix, func, context);
traverseAllChildren(children, mapSingleChildIntoContext, traverseContext);
MapBookKeeping.release(traverseContext);
}
/**
* Maps children that are typically specified as `props.children`.
*
* The provided mapFunction(child, key, index) will be called for each
* leaf child.
*
* @param {?*} children Children tree container.
* @param {function(*, int)} func The map function.
* @param {*} context Context for mapFunction.
* @return {object} Object containing the ordered map of results.
*/
function mapChildren(children, func, context) {
if (children == null) {
return children;
}
var result = [];
mapIntoWithKeyPrefixInternal(children, result, null, func, context);
return result;
}
function forEachSingleChildDummy(traverseContext, child, name) {
return null;
}
/**
* Count the number of children that are typically specified as
* `props.children`.
*
* @param {?*} children Children tree container.
* @return {number} The number of children.
*/
function countChildren(children, context) {
return traverseAllChildren(children, forEachSingleChildDummy, null);
}
/**
* Flatten a children object (typically specified as `props.children`) and
* return an array with appropriately re-keyed children.
*/
function toArray(children) {
var result = [];
mapIntoWithKeyPrefixInternal(children, result, null, emptyFunction.thatReturnsArgument);
return result;
}
var ReactChildren = {
forEach: forEachChildren,
map: mapChildren,
mapIntoWithKeyPrefixInternal: mapIntoWithKeyPrefixInternal,
count: countChildren,
toArray: toArray
};
module.exports = ReactChildren;
},{"142":142,"153":153,"25":25,"57":57}],33:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactClass
*/
'use strict';
var ReactComponent = _dereq_(34);
var ReactElement = _dereq_(57);
var ReactPropTypeLocations = _dereq_(81);
var ReactPropTypeLocationNames = _dereq_(80);
var ReactNoopUpdateQueue = _dereq_(76);
var assign = _dereq_(24);
var emptyObject = _dereq_(154);
var invariant = _dereq_(161);
var keyMirror = _dereq_(165);
var keyOf = _dereq_(166);
var warning = _dereq_(173);
var MIXINS_KEY = keyOf({ mixins: null });
/**
* Policies that describe methods in `ReactClassInterface`.
*/
var SpecPolicy = keyMirror({
/**
* These methods may be defined only once by the class specification or mixin.
*/
DEFINE_ONCE: null,
/**
* These methods may be defined by both the class specification and mixins.
* Subsequent definitions will be chained. These methods must return void.
*/
DEFINE_MANY: null,
/**
* These methods are overriding the base class.
*/
OVERRIDE_BASE: null,
/**
* These methods are similar to DEFINE_MANY, except we assume they return
* objects. We try to merge the keys of the return values of all the mixed in
* functions. If there is a key conflict we throw.
*/
DEFINE_MANY_MERGED: null
});
var injectedMixins = [];
var warnedSetProps = false;
function warnSetProps() {
if (!warnedSetProps) {
warnedSetProps = true;
"development" !== 'production' ? warning(false, 'setProps(...) and replaceProps(...) are deprecated. ' + 'Instead, call render again at the top level.') : undefined;
}
}
/**
* Composite components are higher-level components that compose other composite
* or native components.
*
* To create a new type of `ReactClass`, pass a specification of
* your new class to `React.createClass`. The only requirement of your class
* specification is that you implement a `render` method.
*
* var MyComponent = React.createClass({
* render: function() {
* return <div>Hello World</div>;
* }
* });
*
* The class specification supports a specific protocol of methods that have
* special meaning (e.g. `render`). See `ReactClassInterface` for
* more the comprehensive protocol. Any other properties and methods in the
* class specification will be available on the prototype.
*
* @interface ReactClassInterface
* @internal
*/
var ReactClassInterface = {
/**
* An array of Mixin objects to include when defining your component.
*
* @type {array}
* @optional
*/
mixins: SpecPolicy.DEFINE_MANY,
/**
* An object containing properties and methods that should be defined on
* the component's constructor instead of its prototype (static methods).
*
* @type {object}
* @optional
*/
statics: SpecPolicy.DEFINE_MANY,
/**
* Definition of prop types for this component.
*
* @type {object}
* @optional
*/
propTypes: SpecPolicy.DEFINE_MANY,
/**
* Definition of context types for this component.
*
* @type {object}
* @optional
*/
contextTypes: SpecPolicy.DEFINE_MANY,
/**
* Definition of context types this component sets for its children.
*
* @type {object}
* @optional
*/
childContextTypes: SpecPolicy.DEFINE_MANY,
// ==== Definition methods ====
/**
* Invoked when the component is mounted. Values in the mapping will be set on
* `this.props` if that prop is not specified (i.e. using an `in` check).
*
* This method is invoked before `getInitialState` and therefore cannot rely
* on `this.state` or use `this.setState`.
*
* @return {object}
* @optional
*/
getDefaultProps: SpecPolicy.DEFINE_MANY_MERGED,
/**
* Invoked once before the component is mounted. The return value will be used
* as the initial value of `this.state`.
*
* getInitialState: function() {
* return {
* isOn: false,
* fooBaz: new BazFoo()
* }
* }
*
* @return {object}
* @optional
*/
getInitialState: SpecPolicy.DEFINE_MANY_MERGED,
/**
* @return {object}
* @optional
*/
getChildContext: SpecPolicy.DEFINE_MANY_MERGED,
/**
* Uses props from `this.props` and state from `this.state` to render the
* structure of the component.
*
* No guarantees are made about when or how often this method is invoked, so
* it must not have side effects.
*
* render: function() {
* var name = this.props.name;
* return <div>Hello, {name}!</div>;
* }
*
* @return {ReactComponent}
* @nosideeffects
* @required
*/
render: SpecPolicy.DEFINE_ONCE,
// ==== Delegate methods ====
/**
* Invoked when the component is initially created and about to be mounted.
* This may have side effects, but any external subscriptions or data created
* by this method must be cleaned up in `componentWillUnmount`.
*
* @optional
*/
componentWillMount: SpecPolicy.DEFINE_MANY,
/**
* Invoked when the component has been mounted and has a DOM representation.
* However, there is no guarantee that the DOM node is in the document.
*
* Use this as an opportunity to operate on the DOM when the component has
* been mounted (initialized and rendered) for the first time.
*
* @param {DOMElement} rootNode DOM element representing the component.
* @optional
*/
componentDidMount: SpecPolicy.DEFINE_MANY,
/**
* Invoked before the component receives new props.
*
* Use this as an opportunity to react to a prop transition by updating the
* state using `this.setState`. Current props are accessed via `this.props`.
*
* componentWillReceiveProps: function(nextProps, nextContext) {
* this.setState({
* likesIncreasing: nextProps.likeCount > this.props.likeCount
* });
* }
*
* NOTE: There is no equivalent `componentWillReceiveState`. An incoming prop
* transition may cause a state change, but the opposite is not true. If you
* need it, you are probably looking for `componentWillUpdate`.
*
* @param {object} nextProps
* @optional
*/
componentWillReceiveProps: SpecPolicy.DEFINE_MANY,
/**
* Invoked while deciding if the component should be updated as a result of
* receiving new props, state and/or context.
*
* Use this as an opportunity to `return false` when you're certain that the
* transition to the new props/state/context will not require a component
* update.
*
* shouldComponentUpdate: function(nextProps, nextState, nextContext) {
* return !equal(nextProps, this.props) ||
* !equal(nextState, this.state) ||
* !equal(nextContext, this.context);
* }
*
* @param {object} nextProps
* @param {?object} nextState
* @param {?object} nextContext
* @return {boolean} True if the component should update.
* @optional
*/
shouldComponentUpdate: SpecPolicy.DEFINE_ONCE,
/**
* Invoked when the component is about to update due to a transition from
* `this.props`, `this.state` and `this.context` to `nextProps`, `nextState`
* and `nextContext`.
*
* Use this as an opportunity to perform preparation before an update occurs.
*
* NOTE: You **cannot** use `this.setState()` in this method.
*
* @param {object} nextProps
* @param {?object} nextState
* @param {?object} nextContext
* @param {ReactReconcileTransaction} transaction
* @optional
*/
componentWillUpdate: SpecPolicy.DEFINE_MANY,
/**
* Invoked when the component's DOM representation has been updated.
*
* Use this as an opportunity to operate on the DOM when the component has
* been updated.
*
* @param {object} prevProps
* @param {?object} prevState
* @param {?object} prevContext
* @param {DOMElement} rootNode DOM element representing the component.
* @optional
*/
componentDidUpdate: SpecPolicy.DEFINE_MANY,
/**
* Invoked when the component is about to be removed from its parent and have
* its DOM representation destroyed.
*
* Use this as an opportunity to deallocate any external resources.
*
* NOTE: There is no `componentDidUnmount` since your component will have been
* destroyed by that point.
*
* @optional
*/
componentWillUnmount: SpecPolicy.DEFINE_MANY,
// ==== Advanced methods ====
/**
* Updates the component's currently mounted DOM representation.
*
* By default, this implements React's rendering and reconciliation algorithm.
* Sophisticated clients may wish to override this.
*
* @param {ReactReconcileTransaction} transaction
* @internal
* @overridable
*/
updateComponent: SpecPolicy.OVERRIDE_BASE
};
/**
* Mapping from class specification keys to special processing functions.
*
* Although these are declared like instance properties in the specification
* when defining classes using `React.createClass`, they are actually static
* and are accessible on the constructor instead of the prototype. Despite
* being static, they must be defined outside of the "statics" key under
* which all other static methods are defined.
*/
var RESERVED_SPEC_KEYS = {
displayName: function (Constructor, displayName) {
Constructor.displayName = displayName;
},
mixins: function (Constructor, mixins) {
if (mixins) {
for (var i = 0; i < mixins.length; i++) {
mixSpecIntoComponent(Constructor, mixins[i]);
}
}
},
childContextTypes: function (Constructor, childContextTypes) {
if ("development" !== 'production') {
validateTypeDef(Constructor, childContextTypes, ReactPropTypeLocations.childContext);
}
Constructor.childContextTypes = assign({}, Constructor.childContextTypes, childContextTypes);
},
contextTypes: function (Constructor, contextTypes) {
if ("development" !== 'production') {
validateTypeDef(Constructor, contextTypes, ReactPropTypeLocations.context);
}
Constructor.contextTypes = assign({}, Constructor.contextTypes, contextTypes);
},
/**
* Special case getDefaultProps which should move into statics but requires
* automatic merging.
*/
getDefaultProps: function (Constructor, getDefaultProps) {
if (Constructor.getDefaultProps) {
Constructor.getDefaultProps = createMergedResultFunction(Constructor.getDefaultProps, getDefaultProps);
} else {
Constructor.getDefaultProps = getDefaultProps;
}
},
propTypes: function (Constructor, propTypes) {
if ("development" !== 'production') {
validateTypeDef(Constructor, propTypes, ReactPropTypeLocations.prop);
}
Constructor.propTypes = assign({}, Constructor.propTypes, propTypes);
},
statics: function (Constructor, statics) {
mixStaticSpecIntoComponent(Constructor, statics);
},
autobind: function () {} };
// noop
function validateTypeDef(Constructor, typeDef, location) {
for (var propName in typeDef) {
if (typeDef.hasOwnProperty(propName)) {
// use a warning instead of an invariant so components
// don't show up in prod but not in __DEV__
"development" !== 'production' ? warning(typeof typeDef[propName] === 'function', '%s: %s type `%s` is invalid; it must be a function, usually from ' + 'React.PropTypes.', Constructor.displayName || 'ReactClass', ReactPropTypeLocationNames[location], propName) : undefined;
}
}
}
function validateMethodOverride(proto, name) {
var specPolicy = ReactClassInterface.hasOwnProperty(name) ? ReactClassInterface[name] : null;
// Disallow overriding of base class methods unless explicitly allowed.
if (ReactClassMixin.hasOwnProperty(name)) {
!(specPolicy === SpecPolicy.OVERRIDE_BASE) ? "development" !== 'production' ? invariant(false, 'ReactClassInterface: You are attempting to override ' + '`%s` from your class specification. Ensure that your method names ' + 'do not overlap with React methods.', name) : invariant(false) : undefined;
}
// Disallow defining methods more than once unless explicitly allowed.
if (proto.hasOwnProperty(name)) {
!(specPolicy === SpecPolicy.DEFINE_MANY || specPolicy === SpecPolicy.DEFINE_MANY_MERGED) ? "development" !== 'production' ? invariant(false, 'ReactClassInterface: You are attempting to define ' + '`%s` on your component more than once. This conflict may be due ' + 'to a mixin.', name) : invariant(false) : undefined;
}
}
/**
* Mixin helper which handles policy validation and reserved
* specification keys when building React classses.
*/
function mixSpecIntoComponent(Constructor, spec) {
if (!spec) {
return;
}
!(typeof spec !== 'function') ? "development" !== 'production' ? invariant(false, 'ReactClass: You\'re attempting to ' + 'use a component class as a mixin. Instead, just use a regular object.') : invariant(false) : undefined;
!!ReactElement.isValidElement(spec) ? "development" !== 'production' ? invariant(false, 'ReactClass: You\'re attempting to ' + 'use a component as a mixin. Instead, just use a regular object.') : invariant(false) : undefined;
var proto = Constructor.prototype;
// By handling mixins before any other properties, we ensure the same
// chaining order is applied to methods with DEFINE_MANY policy, whether
// mixins are listed before or after these methods in the spec.
if (spec.hasOwnProperty(MIXINS_KEY)) {
RESERVED_SPEC_KEYS.mixins(Constructor, spec.mixins);
}
for (var name in spec) {
if (!spec.hasOwnProperty(name)) {
continue;
}
if (name === MIXINS_KEY) {
// We have already handled mixins in a special case above.
continue;
}
var property = spec[name];
validateMethodOverride(proto, name);
if (RESERVED_SPEC_KEYS.hasOwnProperty(name)) {
RESERVED_SPEC_KEYS[name](Constructor, property);
} else {
// Setup methods on prototype:
// The following member methods should not be automatically bound:
// 1. Expected ReactClass methods (in the "interface").
// 2. Overridden methods (that were mixed in).
var isReactClassMethod = ReactClassInterface.hasOwnProperty(name);
var isAlreadyDefined = proto.hasOwnProperty(name);
var isFunction = typeof property === 'function';
var shouldAutoBind = isFunction && !isReactClassMethod && !isAlreadyDefined && spec.autobind !== false;
if (shouldAutoBind) {
if (!proto.__reactAutoBindMap) {
proto.__reactAutoBindMap = {};
}
proto.__reactAutoBindMap[name] = property;
proto[name] = property;
} else {
if (isAlreadyDefined) {
var specPolicy = ReactClassInterface[name];
// These cases should already be caught by validateMethodOverride.
!(isReactClassMethod && (specPolicy === SpecPolicy.DEFINE_MANY_MERGED || specPolicy === SpecPolicy.DEFINE_MANY)) ? "development" !== 'production' ? invariant(false, 'ReactClass: Unexpected spec policy %s for key %s ' + 'when mixing in component specs.', specPolicy, name) : invariant(false) : undefined;
// For methods which are defined more than once, call the existing
// methods before calling the new property, merging if appropriate.
if (specPolicy === SpecPolicy.DEFINE_MANY_MERGED) {
proto[name] = createMergedResultFunction(proto[name], property);
} else if (specPolicy === SpecPolicy.DEFINE_MANY) {
proto[name] = createChainedFunction(proto[name], property);
}
} else {
proto[name] = property;
if ("development" !== 'production') {
// Add verbose displayName to the function, which helps when looking
// at profiling tools.
if (typeof property === 'function' && spec.displayName) {
proto[name].displayName = spec.displayName + '_' + name;
}
}
}
}
}
}
}
function mixStaticSpecIntoComponent(Constructor, statics) {
if (!statics) {
return;
}
for (var name in statics) {
var property = statics[name];
if (!statics.hasOwnProperty(name)) {
continue;
}
var isReserved = (name in RESERVED_SPEC_KEYS);
!!isReserved ? "development" !== 'production' ? invariant(false, 'ReactClass: You are attempting to define a reserved ' + 'property, `%s`, that shouldn\'t be on the "statics" key. Define it ' + 'as an instance property instead; it will still be accessible on the ' + 'constructor.', name) : invariant(false) : undefined;
var isInherited = (name in Constructor);
!!isInherited ? "development" !== 'production' ? invariant(false, 'ReactClass: You are attempting to define ' + '`%s` on your component more than once. This conflict may be ' + 'due to a mixin.', name) : invariant(false) : undefined;
Constructor[name] = property;
}
}
/**
* Merge two objects, but throw if both contain the same key.
*
* @param {object} one The first object, which is mutated.
* @param {object} two The second object
* @return {object} one after it has been mutated to contain everything in two.
*/
function mergeIntoWithNoDuplicateKeys(one, two) {
!(one && two && typeof one === 'object' && typeof two === 'object') ? "development" !== 'production' ? invariant(false, 'mergeIntoWithNoDuplicateKeys(): Cannot merge non-objects.') : invariant(false) : undefined;
for (var key in two) {
if (two.hasOwnProperty(key)) {
!(one[key] === undefined) ? "development" !== 'production' ? invariant(false, 'mergeIntoWithNoDuplicateKeys(): ' + 'Tried to merge two objects with the same key: `%s`. This conflict ' + 'may be due to a mixin; in particular, this may be caused by two ' + 'getInitialState() or getDefaultProps() methods returning objects ' + 'with clashing keys.', key) : invariant(false) : undefined;
one[key] = two[key];
}
}
return one;
}
/**
* Creates a function that invokes two functions and merges their return values.
*
* @param {function} one Function to invoke first.
* @param {function} two Function to invoke second.
* @return {function} Function that invokes the two argument functions.
* @private
*/
function createMergedResultFunction(one, two) {
return function mergedResult() {
var a = one.apply(this, arguments);
var b = two.apply(this, arguments);
if (a == null) {
return b;
} else if (b == null) {
return a;
}
var c = {};
mergeIntoWithNoDuplicateKeys(c, a);
mergeIntoWithNoDuplicateKeys(c, b);
return c;
};
}
/**
* Creates a function that invokes two functions and ignores their return vales.
*
* @param {function} one Function to invoke first.
* @param {function} two Function to invoke second.
* @return {function} Function that invokes the two argument functions.
* @private
*/
function createChainedFunction(one, two) {
return function chainedFunction() {
one.apply(this, arguments);
two.apply(this, arguments);
};
}
/**
* Binds a method to the component.
*
* @param {object} component Component whose method is going to be bound.
* @param {function} method Method to be bound.
* @return {function} The bound method.
*/
function bindAutoBindMethod(component, method) {
var boundMethod = method.bind(component);
if ("development" !== 'production') {
boundMethod.__reactBoundContext = component;
boundMethod.__reactBoundMethod = method;
boundMethod.__reactBoundArguments = null;
var componentName = component.constructor.displayName;
var _bind = boundMethod.bind;
/* eslint-disable block-scoped-var, no-undef */
boundMethod.bind = function (newThis) {
for (var _len = arguments.length, args = Array(_len > 1 ? _len - 1 : 0), _key = 1; _key < _len; _key++) {
args[_key - 1] = arguments[_key];
}
// User is trying to bind() an autobound method; we effectively will
// ignore the value of "this" that the user is trying to use, so
// let's warn.
if (newThis !== component && newThis !== null) {
"development" !== 'production' ? warning(false, 'bind(): React component methods may only be bound to the ' + 'component instance. See %s', componentName) : undefined;
} else if (!args.length) {
"development" !== 'production' ? warning(false, 'bind(): You are binding a component method to the component. ' + 'React does this for you automatically in a high-performance ' + 'way, so you can safely remove this call. See %s', componentName) : undefined;
return boundMethod;
}
var reboundMethod = _bind.apply(boundMethod, arguments);
reboundMethod.__reactBoundContext = component;
reboundMethod.__reactBoundMethod = method;
reboundMethod.__reactBoundArguments = args;
return reboundMethod;
/* eslint-enable */
};
}
return boundMethod;
}
/**
* Binds all auto-bound methods in a component.
*
* @param {object} component Component whose method is going to be bound.
*/
function bindAutoBindMethods(component) {
for (var autoBindKey in component.__reactAutoBindMap) {
if (component.__reactAutoBindMap.hasOwnProperty(autoBindKey)) {
var method = component.__reactAutoBindMap[autoBindKey];
component[autoBindKey] = bindAutoBindMethod(component, method);
}
}
}
/**
* Add more to the ReactClass base class. These are all legacy features and
* therefore not already part of the modern ReactComponent.
*/
var ReactClassMixin = {
/**
* TODO: This will be deprecated because state should always keep a consistent
* type signature and the only use case for this, is to avoid that.
*/
replaceState: function (newState, callback) {
this.updater.enqueueReplaceState(this, newState);
if (callback) {
this.updater.enqueueCallback(this, callback);
}
},
/**
* Checks whether or not this composite component is mounted.
* @return {boolean} True if mounted, false otherwise.
* @protected
* @final
*/
isMounted: function () {
return this.updater.isMounted(this);
},
/**
* Sets a subset of the props.
*
* @param {object} partialProps Subset of the next props.
* @param {?function} callback Called after props are updated.
* @final
* @public
* @deprecated
*/
setProps: function (partialProps, callback) {
if ("development" !== 'production') {
warnSetProps();
}
this.updater.enqueueSetProps(this, partialProps);
if (callback) {
this.updater.enqueueCallback(this, callback);
}
},
/**
* Replace all the props.
*
* @param {object} newProps Subset of the next props.
* @param {?function} callback Called after props are updated.
* @final
* @public
* @deprecated
*/
replaceProps: function (newProps, callback) {
if ("development" !== 'production') {
warnSetProps();
}
this.updater.enqueueReplaceProps(this, newProps);
if (callback) {
this.updater.enqueueCallback(this, callback);
}
}
};
var ReactClassComponent = function () {};
assign(ReactClassComponent.prototype, ReactComponent.prototype, ReactClassMixin);
/**
* Module for creating composite components.
*
* @class ReactClass
*/
var ReactClass = {
/**
* Creates a composite component class given a class specification.
*
* @param {object} spec Class specification (which must define `render`).
* @return {function} Component constructor function.
* @public
*/
createClass: function (spec) {
var Constructor = function (props, context, updater) {
// This constructor is overridden by mocks. The argument is used
// by mocks to assert on what gets mounted.
if ("development" !== 'production') {
"development" !== 'production' ? warning(this instanceof Constructor, 'Something is calling a React component directly. Use a factory or ' + 'JSX instead. See: https://fb.me/react-legacyfactory') : undefined;
}
// Wire up auto-binding
if (this.__reactAutoBindMap) {
bindAutoBindMethods(this);
}
this.props = props;
this.context = context;
this.refs = emptyObject;
this.updater = updater || ReactNoopUpdateQueue;
this.state = null;
// ReactClasses doesn't have constructors. Instead, they use the
// getInitialState and componentWillMount methods for initialization.
var initialState = this.getInitialState ? this.getInitialState() : null;
if ("development" !== 'production') {
// We allow auto-mocks to proceed as if they're returning null.
if (typeof initialState === 'undefined' && this.getInitialState._isMockFunction) {
// This is probably bad practice. Consider warning here and
// deprecating this convenience.
initialState = null;
}
}
!(typeof initialState === 'object' && !Array.isArray(initialState)) ? "development" !== 'production' ? invariant(false, '%s.getInitialState(): must return an object or null', Constructor.displayName || 'ReactCompositeComponent') : invariant(false) : undefined;
this.state = initialState;
};
Constructor.prototype = new ReactClassComponent();
Constructor.prototype.constructor = Constructor;
injectedMixins.forEach(mixSpecIntoComponent.bind(null, Constructor));
mixSpecIntoComponent(Constructor, spec);
// Initialize the defaultProps property after all mixins have been merged.
if (Constructor.getDefaultProps) {
Constructor.defaultProps = Constructor.getDefaultProps();
}
if ("development" !== 'production') {
// This is a tag to indicate that the use of these method names is ok,
// since it's used with createClass. If it's not, then it's likely a
// mistake so we'll warn you to use the static property, property
// initializer or constructor respectively.
if (Constructor.getDefaultProps) {
Constructor.getDefaultProps.isReactClassApproved = {};
}
if (Constructor.prototype.getInitialState) {
Constructor.prototype.getInitialState.isReactClassApproved = {};
}
}
!Constructor.prototype.render ? "development" !== 'production' ? invariant(false, 'createClass(...): Class specification must implement a `render` method.') : invariant(false) : undefined;
if ("development" !== 'production') {
"development" !== 'production' ? warning(!Constructor.prototype.componentShouldUpdate, '%s has a method called ' + 'componentShouldUpdate(). Did you mean shouldComponentUpdate()? ' + 'The name is phrased as a question because the function is ' + 'expected to return a value.', spec.displayName || 'A component') : undefined;
"development" !== 'production' ? warning(!Constructor.prototype.componentWillRecieveProps, '%s has a method called ' + 'componentWillRecieveProps(). Did you mean componentWillReceiveProps()?', spec.displayName || 'A component') : undefined;
}
// Reduce time spent doing lookups by setting these on the prototype.
for (var methodName in ReactClassInterface) {
if (!Constructor.prototype[methodName]) {
Constructor.prototype[methodName] = null;
}
}
return Constructor;
},
injection: {
injectMixin: function (mixin) {
injectedMixins.push(mixin);
}
}
};
module.exports = ReactClass;
},{"154":154,"161":161,"165":165,"166":166,"173":173,"24":24,"34":34,"57":57,"76":76,"80":80,"81":81}],34:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactComponent
*/
'use strict';
var ReactNoopUpdateQueue = _dereq_(76);
var canDefineProperty = _dereq_(117);
var emptyObject = _dereq_(154);
var invariant = _dereq_(161);
var warning = _dereq_(173);
/**
* Base class helpers for the updating state of a component.
*/
function ReactComponent(props, context, updater) {
this.props = props;
this.context = context;
this.refs = emptyObject;
// We initialize the default updater but the real one gets injected by the
// renderer.
this.updater = updater || ReactNoopUpdateQueue;
}
ReactComponent.prototype.isReactComponent = {};
/**
* Sets a subset of the state. Always use this to mutate
* state. You should treat `this.state` as immutable.
*
* There is no guarantee that `this.state` will be immediately updated, so
* accessing `this.state` after calling this method may return the old value.
*
* There is no guarantee that calls to `setState` will run synchronously,
* as they may eventually be batched together. You can provide an optional
* callback that will be executed when the call to setState is actually
* completed.
*
* When a function is provided to setState, it will be called at some point in
* the future (not synchronously). It will be called with the up to date
* component arguments (state, props, context). These values can be different
* from this.* because your function may be called after receiveProps but before
* shouldComponentUpdate, and this new state, props, and context will not yet be
* assigned to this.
*
* @param {object|function} partialState Next partial state or function to
* produce next partial state to be merged with current state.
* @param {?function} callback Called after state is updated.
* @final
* @protected
*/
ReactComponent.prototype.setState = function (partialState, callback) {
!(typeof partialState === 'object' || typeof partialState === 'function' || partialState == null) ? "development" !== 'production' ? invariant(false, 'setState(...): takes an object of state variables to update or a ' + 'function which returns an object of state variables.') : invariant(false) : undefined;
if ("development" !== 'production') {
"development" !== 'production' ? warning(partialState != null, 'setState(...): You passed an undefined or null state object; ' + 'instead, use forceUpdate().') : undefined;
}
this.updater.enqueueSetState(this, partialState);
if (callback) {
this.updater.enqueueCallback(this, callback);
}
};
/**
* Forces an update. This should only be invoked when it is known with
* certainty that we are **not** in a DOM transaction.
*
* You may want to call this when you know that some deeper aspect of the
* component's state has changed but `setState` was not called.
*
* This will not invoke `shouldComponentUpdate`, but it will invoke
* `componentWillUpdate` and `componentDidUpdate`.
*
* @param {?function} callback Called after update is complete.
* @final
* @protected
*/
ReactComponent.prototype.forceUpdate = function (callback) {
this.updater.enqueueForceUpdate(this);
if (callback) {
this.updater.enqueueCallback(this, callback);
}
};
/**
* Deprecated APIs. These APIs used to exist on classic React classes but since
* we would like to deprecate them, we're not going to move them over to this
* modern base class. Instead, we define a getter that warns if it's accessed.
*/
if ("development" !== 'production') {
var deprecatedAPIs = {
getDOMNode: ['getDOMNode', 'Use ReactDOM.findDOMNode(component) instead.'],
isMounted: ['isMounted', 'Instead, make sure to clean up subscriptions and pending requests in ' + 'componentWillUnmount to prevent memory leaks.'],
replaceProps: ['replaceProps', 'Instead, call render again at the top level.'],
replaceState: ['replaceState', 'Refactor your code to use setState instead (see ' + 'https://github.com/facebook/react/issues/3236).'],
setProps: ['setProps', 'Instead, call render again at the top level.']
};
var defineDeprecationWarning = function (methodName, info) {
if (canDefineProperty) {
Object.defineProperty(ReactComponent.prototype, methodName, {
get: function () {
"development" !== 'production' ? warning(false, '%s(...) is deprecated in plain JavaScript React classes. %s', info[0], info[1]) : undefined;
return undefined;
}
});
}
};
for (var fnName in deprecatedAPIs) {
if (deprecatedAPIs.hasOwnProperty(fnName)) {
defineDeprecationWarning(fnName, deprecatedAPIs[fnName]);
}
}
}
module.exports = ReactComponent;
},{"117":117,"154":154,"161":161,"173":173,"76":76}],35:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactComponentBrowserEnvironment
*/
'use strict';
var ReactDOMIDOperations = _dereq_(45);
var ReactMount = _dereq_(72);
/**
* Abstracts away all functionality of the reconciler that requires knowledge of
* the browser context. TODO: These callers should be refactored to avoid the
* need for this injection.
*/
var ReactComponentBrowserEnvironment = {
processChildrenUpdates: ReactDOMIDOperations.dangerouslyProcessChildrenUpdates,
replaceNodeWithMarkupByID: ReactDOMIDOperations.dangerouslyReplaceNodeWithMarkupByID,
/**
* If a particular environment requires that some resources be cleaned up,
* specify this in the injected Mixin. In the DOM, we would likely want to
* purge any cached node ID lookups.
*
* @private
*/
unmountIDFromEnvironment: function (rootNodeID) {
ReactMount.purgeID(rootNodeID);
}
};
module.exports = ReactComponentBrowserEnvironment;
},{"45":45,"72":72}],36:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactComponentEnvironment
*/
'use strict';
var invariant = _dereq_(161);
var injected = false;
var ReactComponentEnvironment = {
/**
* Optionally injectable environment dependent cleanup hook. (server vs.
* browser etc). Example: A browser system caches DOM nodes based on component
* ID and must remove that cache entry when this instance is unmounted.
*/
unmountIDFromEnvironment: null,
/**
* Optionally injectable hook for swapping out mount images in the middle of
* the tree.
*/
replaceNodeWithMarkupByID: null,
/**
* Optionally injectable hook for processing a queue of child updates. Will
* later move into MultiChildComponents.
*/
processChildrenUpdates: null,
injection: {
injectEnvironment: function (environment) {
!!injected ? "development" !== 'production' ? invariant(false, 'ReactCompositeComponent: injectEnvironment() can only be called once.') : invariant(false) : undefined;
ReactComponentEnvironment.unmountIDFromEnvironment = environment.unmountIDFromEnvironment;
ReactComponentEnvironment.replaceNodeWithMarkupByID = environment.replaceNodeWithMarkupByID;
ReactComponentEnvironment.processChildrenUpdates = environment.processChildrenUpdates;
injected = true;
}
}
};
module.exports = ReactComponentEnvironment;
},{"161":161}],37:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactComponentWithPureRenderMixin
*/
'use strict';
var shallowCompare = _dereq_(140);
/**
* If your React component's render function is "pure", e.g. it will render the
* same result given the same props and state, provide this Mixin for a
* considerable performance boost.
*
* Most React components have pure render functions.
*
* Example:
*
* var ReactComponentWithPureRenderMixin =
* require('ReactComponentWithPureRenderMixin');
* React.createClass({
* mixins: [ReactComponentWithPureRenderMixin],
*
* render: function() {
* return <div className={this.props.className}>foo</div>;
* }
* });
*
* Note: This only checks shallow equality for props and state. If these contain
* complex data structures this mixin may have false-negatives for deeper
* differences. Only mixin to components which have simple props and state, or
* use `forceUpdate()` when you know deep data structures have changed.
*/
var ReactComponentWithPureRenderMixin = {
shouldComponentUpdate: function (nextProps, nextState) {
return shallowCompare(this, nextProps, nextState);
}
};
module.exports = ReactComponentWithPureRenderMixin;
},{"140":140}],38:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactCompositeComponent
*/
'use strict';
var ReactComponentEnvironment = _dereq_(36);
var ReactCurrentOwner = _dereq_(39);
var ReactElement = _dereq_(57);
var ReactInstanceMap = _dereq_(68);
var ReactPerf = _dereq_(78);
var ReactPropTypeLocations = _dereq_(81);
var ReactPropTypeLocationNames = _dereq_(80);
var ReactReconciler = _dereq_(84);
var ReactUpdateQueue = _dereq_(95);
var assign = _dereq_(24);
var emptyObject = _dereq_(154);
var invariant = _dereq_(161);
var shouldUpdateReactComponent = _dereq_(141);
var warning = _dereq_(173);
function getDeclarationErrorAddendum(component) {
var owner = component._currentElement._owner || null;
if (owner) {
var name = owner.getName();
if (name) {
return ' Check the render method of `' + name + '`.';
}
}
return '';
}
function StatelessComponent(Component) {}
StatelessComponent.prototype.render = function () {
var Component = ReactInstanceMap.get(this)._currentElement.type;
return Component(this.props, this.context, this.updater);
};
/**
* ------------------ The Life-Cycle of a Composite Component ------------------
*
* - constructor: Initialization of state. The instance is now retained.
* - componentWillMount
* - render
* - [children's constructors]
* - [children's componentWillMount and render]
* - [children's componentDidMount]
* - componentDidMount
*
* Update Phases:
* - componentWillReceiveProps (only called if parent updated)
* - shouldComponentUpdate
* - componentWillUpdate
* - render
* - [children's constructors or receive props phases]
* - componentDidUpdate
*
* - componentWillUnmount
* - [children's componentWillUnmount]
* - [children destroyed]
* - (destroyed): The instance is now blank, released by React and ready for GC.
*
* -----------------------------------------------------------------------------
*/
/**
* An incrementing ID assigned to each component when it is mounted. This is
* used to enforce the order in which `ReactUpdates` updates dirty components.
*
* @private
*/
var nextMountID = 1;
/**
* @lends {ReactCompositeComponent.prototype}
*/
var ReactCompositeComponentMixin = {
/**
* Base constructor for all composite component.
*
* @param {ReactElement} element
* @final
* @internal
*/
construct: function (element) {
this._currentElement = element;
this._rootNodeID = null;
this._instance = null;
// See ReactUpdateQueue
this._pendingElement = null;
this._pendingStateQueue = null;
this._pendingReplaceState = false;
this._pendingForceUpdate = false;
this._renderedComponent = null;
this._context = null;
this._mountOrder = 0;
this._topLevelWrapper = null;
// See ReactUpdates and ReactUpdateQueue.
this._pendingCallbacks = null;
},
/**
* Initializes the component, renders markup, and registers event listeners.
*
* @param {string} rootID DOM ID of the root node.
* @param {ReactReconcileTransaction|ReactServerRenderingTransaction} transaction
* @return {?string} Rendered markup to be inserted into the DOM.
* @final
* @internal
*/
mountComponent: function (rootID, transaction, context) {
this._context = context;
this._mountOrder = nextMountID++;
this._rootNodeID = rootID;
var publicProps = this._processProps(this._currentElement.props);
var publicContext = this._processContext(context);
var Component = this._currentElement.type;
// Initialize the public class
var inst;
var renderedElement;
// This is a way to detect if Component is a stateless arrow function
// component, which is not newable. It might not be 100% reliable but is
// something we can do until we start detecting that Component extends
// React.Component. We already assume that typeof Component === 'function'.
var canInstantiate = ('prototype' in Component);
if (canInstantiate) {
if ("development" !== 'production') {
ReactCurrentOwner.current = this;
try {
inst = new Component(publicProps, publicContext, ReactUpdateQueue);
} finally {
ReactCurrentOwner.current = null;
}
} else {
inst = new Component(publicProps, publicContext, ReactUpdateQueue);
}
}
if (!canInstantiate || inst === null || inst === false || ReactElement.isValidElement(inst)) {
renderedElement = inst;
inst = new StatelessComponent(Component);
}
if ("development" !== 'production') {
// This will throw later in _renderValidatedComponent, but add an early
// warning now to help debugging
if (inst.render == null) {
"development" !== 'production' ? warning(false, '%s(...): No `render` method found on the returned component ' + 'instance: you may have forgotten to define `render`, returned ' + 'null/false from a stateless component, or tried to render an ' + 'element whose type is a function that isn\'t a React component.', Component.displayName || Component.name || 'Component') : undefined;
} else {
// We support ES6 inheriting from React.Component, the module pattern,
// and stateless components, but not ES6 classes that don't extend
"development" !== 'production' ? warning(Component.prototype && Component.prototype.isReactComponent || !canInstantiate || !(inst instanceof Component), '%s(...): React component classes must extend React.Component.', Component.displayName || Component.name || 'Component') : undefined;
}
}
// These should be set up in the constructor, but as a convenience for
// simpler class abstractions, we set them up after the fact.
inst.props = publicProps;
inst.context = publicContext;
inst.refs = emptyObject;
inst.updater = ReactUpdateQueue;
this._instance = inst;
// Store a reference from the instance back to the internal representation
ReactInstanceMap.set(inst, this);
if ("development" !== 'production') {
// Since plain JS classes are defined without any special initialization
// logic, we can not catch common errors early. Therefore, we have to
// catch them here, at initialization time, instead.
"development" !== 'production' ? warning(!inst.getInitialState || inst.getInitialState.isReactClassApproved, 'getInitialState was defined on %s, a plain JavaScript class. ' + 'This is only supported for classes created using React.createClass. ' + 'Did you mean to define a state property instead?', this.getName() || 'a component') : undefined;
"development" !== 'production' ? warning(!inst.getDefaultProps || inst.getDefaultProps.isReactClassApproved, 'getDefaultProps was defined on %s, a plain JavaScript class. ' + 'This is only supported for classes created using React.createClass. ' + 'Use a static property to define defaultProps instead.', this.getName() || 'a component') : undefined;
"development" !== 'production' ? warning(!inst.propTypes, 'propTypes was defined as an instance property on %s. Use a static ' + 'property to define propTypes instead.', this.getName() || 'a component') : undefined;
"development" !== 'production' ? warning(!inst.contextTypes, 'contextTypes was defined as an instance property on %s. Use a ' + 'static property to define contextTypes instead.', this.getName() || 'a component') : undefined;
"development" !== 'production' ? warning(typeof inst.componentShouldUpdate !== 'function', '%s has a method called ' + 'componentShouldUpdate(). Did you mean shouldComponentUpdate()? ' + 'The name is phrased as a question because the function is ' + 'expected to return a value.', this.getName() || 'A component') : undefined;
"development" !== 'production' ? warning(typeof inst.componentDidUnmount !== 'function', '%s has a method called ' + 'componentDidUnmount(). But there is no such lifecycle method. ' + 'Did you mean componentWillUnmount()?', this.getName() || 'A component') : undefined;
"development" !== 'production' ? warning(typeof inst.componentWillRecieveProps !== 'function', '%s has a method called ' + 'componentWillRecieveProps(). Did you mean componentWillReceiveProps()?', this.getName() || 'A component') : undefined;
}
var initialState = inst.state;
if (initialState === undefined) {
inst.state = initialState = null;
}
!(typeof initialState === 'object' && !Array.isArray(initialState)) ? "development" !== 'production' ? invariant(false, '%s.state: must be set to an object or null', this.getName() || 'ReactCompositeComponent') : invariant(false) : undefined;
this._pendingStateQueue = null;
this._pendingReplaceState = false;
this._pendingForceUpdate = false;
if (inst.componentWillMount) {
inst.componentWillMount();
// When mounting, calls to `setState` by `componentWillMount` will set
// `this._pendingStateQueue` without triggering a re-render.
if (this._pendingStateQueue) {
inst.state = this._processPendingState(inst.props, inst.context);
}
}
// If not a stateless component, we now render
if (renderedElement === undefined) {
renderedElement = this._renderValidatedComponent();
}
this._renderedComponent = this._instantiateReactComponent(renderedElement);
var markup = ReactReconciler.mountComponent(this._renderedComponent, rootID, transaction, this._processChildContext(context));
if (inst.componentDidMount) {
transaction.getReactMountReady().enqueue(inst.componentDidMount, inst);
}
return markup;
},
/**
* Releases any resources allocated by `mountComponent`.
*
* @final
* @internal
*/
unmountComponent: function () {
var inst = this._instance;
if (inst.componentWillUnmount) {
inst.componentWillUnmount();
}
ReactReconciler.unmountComponent(this._renderedComponent);
this._renderedComponent = null;
this._instance = null;
// Reset pending fields
// Even if this component is scheduled for another update in ReactUpdates,
// it would still be ignored because these fields are reset.
this._pendingStateQueue = null;
this._pendingReplaceState = false;
this._pendingForceUpdate = false;
this._pendingCallbacks = null;
this._pendingElement = null;
// These fields do not really need to be reset since this object is no
// longer accessible.
this._context = null;
this._rootNodeID = null;
this._topLevelWrapper = null;
// Delete the reference from the instance to this internal representation
// which allow the internals to be properly cleaned up even if the user
// leaks a reference to the public instance.
ReactInstanceMap.remove(inst);
// Some existing components rely on inst.props even after they've been
// destroyed (in event handlers).
// TODO: inst.props = null;
// TODO: inst.state = null;
// TODO: inst.context = null;
},
/**
* Filters the context object to only contain keys specified in
* `contextTypes`
*
* @param {object} context
* @return {?object}
* @private
*/
_maskContext: function (context) {
var maskedContext = null;
var Component = this._currentElement.type;
var contextTypes = Component.contextTypes;
if (!contextTypes) {
return emptyObject;
}
maskedContext = {};
for (var contextName in contextTypes) {
maskedContext[contextName] = context[contextName];
}
return maskedContext;
},
/**
* Filters the context object to only contain keys specified in
* `contextTypes`, and asserts that they are valid.
*
* @param {object} context
* @return {?object}
* @private
*/
_processContext: function (context) {
var maskedContext = this._maskContext(context);
if ("development" !== 'production') {
var Component = this._currentElement.type;
if (Component.contextTypes) {
this._checkPropTypes(Component.contextTypes, maskedContext, ReactPropTypeLocations.context);
}
}
return maskedContext;
},
/**
* @param {object} currentContext
* @return {object}
* @private
*/
_processChildContext: function (currentContext) {
var Component = this._currentElement.type;
var inst = this._instance;
var childContext = inst.getChildContext && inst.getChildContext();
if (childContext) {
!(typeof Component.childContextTypes === 'object') ? "development" !== 'production' ? invariant(false, '%s.getChildContext(): childContextTypes must be defined in order to ' + 'use getChildContext().', this.getName() || 'ReactCompositeComponent') : invariant(false) : undefined;
if ("development" !== 'production') {
this._checkPropTypes(Component.childContextTypes, childContext, ReactPropTypeLocations.childContext);
}
for (var name in childContext) {
!(name in Component.childContextTypes) ? "development" !== 'production' ? invariant(false, '%s.getChildContext(): key "%s" is not defined in childContextTypes.', this.getName() || 'ReactCompositeComponent', name) : invariant(false) : undefined;
}
return assign({}, currentContext, childContext);
}
return currentContext;
},
/**
* Processes props by setting default values for unspecified props and
* asserting that the props are valid. Does not mutate its argument; returns
* a new props object with defaults merged in.
*
* @param {object} newProps
* @return {object}
* @private
*/
_processProps: function (newProps) {
if ("development" !== 'production') {
var Component = this._currentElement.type;
if (Component.propTypes) {
this._checkPropTypes(Component.propTypes, newProps, ReactPropTypeLocations.prop);
}
}
return newProps;
},
/**
* Assert that the props are valid
*
* @param {object} propTypes Map of prop name to a ReactPropType
* @param {object} props
* @param {string} location e.g. "prop", "context", "child context"
* @private
*/
_checkPropTypes: function (propTypes, props, location) {
// TODO: Stop validating prop types here and only use the element
// validation.
var componentName = this.getName();
for (var propName in propTypes) {
if (propTypes.hasOwnProperty(propName)) {
var error;
try {
// This is intentionally an invariant that gets caught. It's the same
// behavior as without this statement except with a better message.
!(typeof propTypes[propName] === 'function') ? "development" !== 'production' ? invariant(false, '%s: %s type `%s` is invalid; it must be a function, usually ' + 'from React.PropTypes.', componentName || 'React class', ReactPropTypeLocationNames[location], propName) : invariant(false) : undefined;
error = propTypes[propName](props, propName, componentName, location);
} catch (ex) {
error = ex;
}
if (error instanceof Error) {
// We may want to extend this logic for similar errors in
// top-level render calls, so I'm abstracting it away into
// a function to minimize refactoring in the future
var addendum = getDeclarationErrorAddendum(this);
if (location === ReactPropTypeLocations.prop) {
// Preface gives us something to blacklist in warning module
"development" !== 'production' ? warning(false, 'Failed Composite propType: %s%s', error.message, addendum) : undefined;
} else {
"development" !== 'production' ? warning(false, 'Failed Context Types: %s%s', error.message, addendum) : undefined;
}
}
}
}
},
receiveComponent: function (nextElement, transaction, nextContext) {
var prevElement = this._currentElement;
var prevContext = this._context;
this._pendingElement = null;
this.updateComponent(transaction, prevElement, nextElement, prevContext, nextContext);
},
/**
* If any of `_pendingElement`, `_pendingStateQueue`, or `_pendingForceUpdate`
* is set, update the component.
*
* @param {ReactReconcileTransaction} transaction
* @internal
*/
performUpdateIfNecessary: function (transaction) {
if (this._pendingElement != null) {
ReactReconciler.receiveComponent(this, this._pendingElement || this._currentElement, transaction, this._context);
}
if (this._pendingStateQueue !== null || this._pendingForceUpdate) {
this.updateComponent(transaction, this._currentElement, this._currentElement, this._context, this._context);
}
},
/**
* Perform an update to a mounted component. The componentWillReceiveProps and
* shouldComponentUpdate methods are called, then (assuming the update isn't
* skipped) the remaining update lifecycle methods are called and the DOM
* representation is updated.
*
* By default, this implements React's rendering and reconciliation algorithm.
* Sophisticated clients may wish to override this.
*
* @param {ReactReconcileTransaction} transaction
* @param {ReactElement} prevParentElement
* @param {ReactElement} nextParentElement
* @internal
* @overridable
*/
updateComponent: function (transaction, prevParentElement, nextParentElement, prevUnmaskedContext, nextUnmaskedContext) {
var inst = this._instance;
var nextContext = this._context === nextUnmaskedContext ? inst.context : this._processContext(nextUnmaskedContext);
var nextProps;
// Distinguish between a props update versus a simple state update
if (prevParentElement === nextParentElement) {
// Skip checking prop types again -- we don't read inst.props to avoid
// warning for DOM component props in this upgrade
nextProps = nextParentElement.props;
} else {
nextProps = this._processProps(nextParentElement.props);
// An update here will schedule an update but immediately set
// _pendingStateQueue which will ensure that any state updates gets
// immediately reconciled instead of waiting for the next batch.
if (inst.componentWillReceiveProps) {
inst.componentWillReceiveProps(nextProps, nextContext);
}
}
var nextState = this._processPendingState(nextProps, nextContext);
var shouldUpdate = this._pendingForceUpdate || !inst.shouldComponentUpdate || inst.shouldComponentUpdate(nextProps, nextState, nextContext);
if ("development" !== 'production') {
"development" !== 'production' ? warning(typeof shouldUpdate !== 'undefined', '%s.shouldComponentUpdate(): Returned undefined instead of a ' + 'boolean value. Make sure to return true or false.', this.getName() || 'ReactCompositeComponent') : undefined;
}
if (shouldUpdate) {
this._pendingForceUpdate = false;
// Will set `this.props`, `this.state` and `this.context`.
this._performComponentUpdate(nextParentElement, nextProps, nextState, nextContext, transaction, nextUnmaskedContext);
} else {
// If it's determined that a component should not update, we still want
// to set props and state but we shortcut the rest of the update.
this._currentElement = nextParentElement;
this._context = nextUnmaskedContext;
inst.props = nextProps;
inst.state = nextState;
inst.context = nextContext;
}
},
_processPendingState: function (props, context) {
var inst = this._instance;
var queue = this._pendingStateQueue;
var replace = this._pendingReplaceState;
this._pendingReplaceState = false;
this._pendingStateQueue = null;
if (!queue) {
return inst.state;
}
if (replace && queue.length === 1) {
return queue[0];
}
var nextState = assign({}, replace ? queue[0] : inst.state);
for (var i = replace ? 1 : 0; i < queue.length; i++) {
var partial = queue[i];
assign(nextState, typeof partial === 'function' ? partial.call(inst, nextState, props, context) : partial);
}
return nextState;
},
/**
* Merges new props and state, notifies delegate methods of update and
* performs update.
*
* @param {ReactElement} nextElement Next element
* @param {object} nextProps Next public object to set as properties.
* @param {?object} nextState Next object to set as state.
* @param {?object} nextContext Next public object to set as context.
* @param {ReactReconcileTransaction} transaction
* @param {?object} unmaskedContext
* @private
*/
_performComponentUpdate: function (nextElement, nextProps, nextState, nextContext, transaction, unmaskedContext) {
var inst = this._instance;
var hasComponentDidUpdate = Boolean(inst.componentDidUpdate);
var prevProps;
var prevState;
var prevContext;
if (hasComponentDidUpdate) {
prevProps = inst.props;
prevState = inst.state;
prevContext = inst.context;
}
if (inst.componentWillUpdate) {
inst.componentWillUpdate(nextProps, nextState, nextContext);
}
this._currentElement = nextElement;
this._context = unmaskedContext;
inst.props = nextProps;
inst.state = nextState;
inst.context = nextContext;
this._updateRenderedComponent(transaction, unmaskedContext);
if (hasComponentDidUpdate) {
transaction.getReactMountReady().enqueue(inst.componentDidUpdate.bind(inst, prevProps, prevState, prevContext), inst);
}
},
/**
* Call the component's `render` method and update the DOM accordingly.
*
* @param {ReactReconcileTransaction} transaction
* @internal
*/
_updateRenderedComponent: function (transaction, context) {
var prevComponentInstance = this._renderedComponent;
var prevRenderedElement = prevComponentInstance._currentElement;
var nextRenderedElement = this._renderValidatedComponent();
if (shouldUpdateReactComponent(prevRenderedElement, nextRenderedElement)) {
ReactReconciler.receiveComponent(prevComponentInstance, nextRenderedElement, transaction, this._processChildContext(context));
} else {
// These two IDs are actually the same! But nothing should rely on that.
var thisID = this._rootNodeID;
var prevComponentID = prevComponentInstance._rootNodeID;
ReactReconciler.unmountComponent(prevComponentInstance);
this._renderedComponent = this._instantiateReactComponent(nextRenderedElement);
var nextMarkup = ReactReconciler.mountComponent(this._renderedComponent, thisID, transaction, this._processChildContext(context));
this._replaceNodeWithMarkupByID(prevComponentID, nextMarkup);
}
},
/**
* @protected
*/
_replaceNodeWithMarkupByID: function (prevComponentID, nextMarkup) {
ReactComponentEnvironment.replaceNodeWithMarkupByID(prevComponentID, nextMarkup);
},
/**
* @protected
*/
_renderValidatedComponentWithoutOwnerOrContext: function () {
var inst = this._instance;
var renderedComponent = inst.render();
if ("development" !== 'production') {
// We allow auto-mocks to proceed as if they're returning null.
if (typeof renderedComponent === 'undefined' && inst.render._isMockFunction) {
// This is probably bad practice. Consider warning here and
// deprecating this convenience.
renderedComponent = null;
}
}
return renderedComponent;
},
/**
* @private
*/
_renderValidatedComponent: function () {
var renderedComponent;
ReactCurrentOwner.current = this;
try {
renderedComponent = this._renderValidatedComponentWithoutOwnerOrContext();
} finally {
ReactCurrentOwner.current = null;
}
!(
// TODO: An `isValidNode` function would probably be more appropriate
renderedComponent === null || renderedComponent === false || ReactElement.isValidElement(renderedComponent)) ? "development" !== 'production' ? invariant(false, '%s.render(): A valid ReactComponent must be returned. You may have ' + 'returned undefined, an array or some other invalid object.', this.getName() || 'ReactCompositeComponent') : invariant(false) : undefined;
return renderedComponent;
},
/**
* Lazily allocates the refs object and stores `component` as `ref`.
*
* @param {string} ref Reference name.
* @param {component} component Component to store as `ref`.
* @final
* @private
*/
attachRef: function (ref, component) {
var inst = this.getPublicInstance();
!(inst != null) ? "development" !== 'production' ? invariant(false, 'Stateless function components cannot have refs.') : invariant(false) : undefined;
var publicComponentInstance = component.getPublicInstance();
if ("development" !== 'production') {
var componentName = component && component.getName ? component.getName() : 'a component';
"development" !== 'production' ? warning(publicComponentInstance != null, 'Stateless function components cannot be given refs ' + '(See ref "%s" in %s created by %s). ' + 'Attempts to access this ref will fail.', ref, componentName, this.getName()) : undefined;
}
var refs = inst.refs === emptyObject ? inst.refs = {} : inst.refs;
refs[ref] = publicComponentInstance;
},
/**
* Detaches a reference name.
*
* @param {string} ref Name to dereference.
* @final
* @private
*/
detachRef: function (ref) {
var refs = this.getPublicInstance().refs;
delete refs[ref];
},
/**
* Get a text description of the component that can be used to identify it
* in error messages.
* @return {string} The name or null.
* @internal
*/
getName: function () {
var type = this._currentElement.type;
var constructor = this._instance && this._instance.constructor;
return type.displayName || constructor && constructor.displayName || type.name || constructor && constructor.name || null;
},
/**
* Get the publicly accessible representation of this component - i.e. what
* is exposed by refs and returned by render. Can be null for stateless
* components.
*
* @return {ReactComponent} the public component instance.
* @internal
*/
getPublicInstance: function () {
var inst = this._instance;
if (inst instanceof StatelessComponent) {
return null;
}
return inst;
},
// Stub
_instantiateReactComponent: null
};
ReactPerf.measureMethods(ReactCompositeComponentMixin, 'ReactCompositeComponent', {
mountComponent: 'mountComponent',
updateComponent: 'updateComponent',
_renderValidatedComponent: '_renderValidatedComponent'
});
var ReactCompositeComponent = {
Mixin: ReactCompositeComponentMixin
};
module.exports = ReactCompositeComponent;
},{"141":141,"154":154,"161":161,"173":173,"24":24,"36":36,"39":39,"57":57,"68":68,"78":78,"80":80,"81":81,"84":84,"95":95}],39:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactCurrentOwner
*/
'use strict';
/**
* Keeps track of the current owner.
*
* The current owner is the component who should own any components that are
* currently being constructed.
*/
var ReactCurrentOwner = {
/**
* @internal
* @type {ReactComponent}
*/
current: null
};
module.exports = ReactCurrentOwner;
},{}],40:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOM
*/
/* globals __REACT_DEVTOOLS_GLOBAL_HOOK__*/
'use strict';
var ReactCurrentOwner = _dereq_(39);
var ReactDOMTextComponent = _dereq_(51);
var ReactDefaultInjection = _dereq_(54);
var ReactInstanceHandles = _dereq_(67);
var ReactMount = _dereq_(72);
var ReactPerf = _dereq_(78);
var ReactReconciler = _dereq_(84);
var ReactUpdates = _dereq_(96);
var ReactVersion = _dereq_(97);
var findDOMNode = _dereq_(122);
var renderSubtreeIntoContainer = _dereq_(137);
var warning = _dereq_(173);
ReactDefaultInjection.inject();
var render = ReactPerf.measure('React', 'render', ReactMount.render);
var React = {
findDOMNode: findDOMNode,
render: render,
unmountComponentAtNode: ReactMount.unmountComponentAtNode,
version: ReactVersion,
/* eslint-disable camelcase */
unstable_batchedUpdates: ReactUpdates.batchedUpdates,
unstable_renderSubtreeIntoContainer: renderSubtreeIntoContainer
};
// Inject the runtime into a devtools global hook regardless of browser.
// Allows for debugging when the hook is injected on the page.
/* eslint-enable camelcase */
if (typeof __REACT_DEVTOOLS_GLOBAL_HOOK__ !== 'undefined' && typeof __REACT_DEVTOOLS_GLOBAL_HOOK__.inject === 'function') {
__REACT_DEVTOOLS_GLOBAL_HOOK__.inject({
CurrentOwner: ReactCurrentOwner,
InstanceHandles: ReactInstanceHandles,
Mount: ReactMount,
Reconciler: ReactReconciler,
TextComponent: ReactDOMTextComponent
});
}
if ("development" !== 'production') {
var ExecutionEnvironment = _dereq_(147);
if (ExecutionEnvironment.canUseDOM && window.top === window.self) {
// First check if devtools is not installed
if (typeof __REACT_DEVTOOLS_GLOBAL_HOOK__ === 'undefined') {
// If we're in Chrome or Firefox, provide a download link if not installed.
if (navigator.userAgent.indexOf('Chrome') > -1 && navigator.userAgent.indexOf('Edge') === -1 || navigator.userAgent.indexOf('Firefox') > -1) {
console.debug('Download the React DevTools for a better development experience: ' + 'https://fb.me/react-devtools');
}
}
// If we're in IE8, check to see if we are in compatibility mode and provide
// information on preventing compatibility mode
var ieCompatibilityMode = document.documentMode && document.documentMode < 8;
"development" !== 'production' ? warning(!ieCompatibilityMode, 'Internet Explorer is running in compatibility mode; please add the ' + 'following tag to your HTML to prevent this from happening: ' + '<meta http-equiv="X-UA-Compatible" content="IE=edge" />') : undefined;
var expectedFeatures = [
// shims
Array.isArray, Array.prototype.every, Array.prototype.forEach, Array.prototype.indexOf, Array.prototype.map, Date.now, Function.prototype.bind, Object.keys, String.prototype.split, String.prototype.trim,
// shams
Object.create, Object.freeze];
for (var i = 0; i < expectedFeatures.length; i++) {
if (!expectedFeatures[i]) {
console.error('One or more ES5 shim/shams expected by React are not available: ' + 'https://fb.me/react-warning-polyfills');
break;
}
}
}
}
module.exports = React;
},{"122":122,"137":137,"147":147,"173":173,"39":39,"51":51,"54":54,"67":67,"72":72,"78":78,"84":84,"96":96,"97":97}],41:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMButton
*/
'use strict';
var mouseListenerNames = {
onClick: true,
onDoubleClick: true,
onMouseDown: true,
onMouseMove: true,
onMouseUp: true,
onClickCapture: true,
onDoubleClickCapture: true,
onMouseDownCapture: true,
onMouseMoveCapture: true,
onMouseUpCapture: true
};
/**
* Implements a <button> native component that does not receive mouse events
* when `disabled` is set.
*/
var ReactDOMButton = {
getNativeProps: function (inst, props, context) {
if (!props.disabled) {
return props;
}
// Copy the props, except the mouse listeners
var nativeProps = {};
for (var key in props) {
if (props.hasOwnProperty(key) && !mouseListenerNames[key]) {
nativeProps[key] = props[key];
}
}
return nativeProps;
}
};
module.exports = ReactDOMButton;
},{}],42:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMComponent
* @typechecks static-only
*/
/* global hasOwnProperty:true */
'use strict';
var AutoFocusUtils = _dereq_(2);
var CSSPropertyOperations = _dereq_(5);
var DOMProperty = _dereq_(10);
var DOMPropertyOperations = _dereq_(11);
var EventConstants = _dereq_(15);
var ReactBrowserEventEmitter = _dereq_(28);
var ReactComponentBrowserEnvironment = _dereq_(35);
var ReactDOMButton = _dereq_(41);
var ReactDOMInput = _dereq_(46);
var ReactDOMOption = _dereq_(47);
var ReactDOMSelect = _dereq_(48);
var ReactDOMTextarea = _dereq_(52);
var ReactMount = _dereq_(72);
var ReactMultiChild = _dereq_(73);
var ReactPerf = _dereq_(78);
var ReactUpdateQueue = _dereq_(95);
var assign = _dereq_(24);
var canDefineProperty = _dereq_(117);
var escapeTextContentForBrowser = _dereq_(121);
var invariant = _dereq_(161);
var isEventSupported = _dereq_(133);
var keyOf = _dereq_(166);
var setInnerHTML = _dereq_(138);
var setTextContent = _dereq_(139);
var shallowEqual = _dereq_(171);
var validateDOMNesting = _dereq_(144);
var warning = _dereq_(173);
var deleteListener = ReactBrowserEventEmitter.deleteListener;
var listenTo = ReactBrowserEventEmitter.listenTo;
var registrationNameModules = ReactBrowserEventEmitter.registrationNameModules;
// For quickly matching children type, to test if can be treated as content.
var CONTENT_TYPES = { 'string': true, 'number': true };
var CHILDREN = keyOf({ children: null });
var STYLE = keyOf({ style: null });
var HTML = keyOf({ __html: null });
var ELEMENT_NODE_TYPE = 1;
function getDeclarationErrorAddendum(internalInstance) {
if (internalInstance) {
var owner = internalInstance._currentElement._owner || null;
if (owner) {
var name = owner.getName();
if (name) {
return ' This DOM node was rendered by `' + name + '`.';
}
}
}
return '';
}
var legacyPropsDescriptor;
if ("development" !== 'production') {
legacyPropsDescriptor = {
props: {
enumerable: false,
get: function () {
var component = this._reactInternalComponent;
"development" !== 'production' ? warning(false, 'ReactDOMComponent: Do not access .props of a DOM node; instead, ' + 'recreate the props as `render` did originally or read the DOM ' + 'properties/attributes directly from this node (e.g., ' + 'this.refs.box.className).%s', getDeclarationErrorAddendum(component)) : undefined;
return component._currentElement.props;
}
}
};
}
function legacyGetDOMNode() {
if ("development" !== 'production') {
var component = this._reactInternalComponent;
"development" !== 'production' ? warning(false, 'ReactDOMComponent: Do not access .getDOMNode() of a DOM node; ' + 'instead, use the node directly.%s', getDeclarationErrorAddendum(component)) : undefined;
}
return this;
}
function legacyIsMounted() {
var component = this._reactInternalComponent;
if ("development" !== 'production') {
"development" !== 'production' ? warning(false, 'ReactDOMComponent: Do not access .isMounted() of a DOM node.%s', getDeclarationErrorAddendum(component)) : undefined;
}
return !!component;
}
function legacySetStateEtc() {
if ("development" !== 'production') {
var component = this._reactInternalComponent;
"development" !== 'production' ? warning(false, 'ReactDOMComponent: Do not access .setState(), .replaceState(), or ' + '.forceUpdate() of a DOM node. This is a no-op.%s', getDeclarationErrorAddendum(component)) : undefined;
}
}
function legacySetProps(partialProps, callback) {
var component = this._reactInternalComponent;
if ("development" !== 'production') {
"development" !== 'production' ? warning(false, 'ReactDOMComponent: Do not access .setProps() of a DOM node. ' + 'Instead, call ReactDOM.render again at the top level.%s', getDeclarationErrorAddendum(component)) : undefined;
}
if (!component) {
return;
}
ReactUpdateQueue.enqueueSetPropsInternal(component, partialProps);
if (callback) {
ReactUpdateQueue.enqueueCallbackInternal(component, callback);
}
}
function legacyReplaceProps(partialProps, callback) {
var component = this._reactInternalComponent;
if ("development" !== 'production') {
"development" !== 'production' ? warning(false, 'ReactDOMComponent: Do not access .replaceProps() of a DOM node. ' + 'Instead, call ReactDOM.render again at the top level.%s', getDeclarationErrorAddendum(component)) : undefined;
}
if (!component) {
return;
}
ReactUpdateQueue.enqueueReplacePropsInternal(component, partialProps);
if (callback) {
ReactUpdateQueue.enqueueCallbackInternal(component, callback);
}
}
function friendlyStringify(obj) {
if (typeof obj === 'object') {
if (Array.isArray(obj)) {
return '[' + obj.map(friendlyStringify).join(', ') + ']';
} else {
var pairs = [];
for (var key in obj) {
if (Object.prototype.hasOwnProperty.call(obj, key)) {
var keyEscaped = /^[a-z$_][\w$_]*$/i.test(key) ? key : JSON.stringify(key);
pairs.push(keyEscaped + ': ' + friendlyStringify(obj[key]));
}
}
return '{' + pairs.join(', ') + '}';
}
} else if (typeof obj === 'string') {
return JSON.stringify(obj);
} else if (typeof obj === 'function') {
return '[function object]';
}
// Differs from JSON.stringify in that undefined becauses undefined and that
// inf and nan don't become null
return String(obj);
}
var styleMutationWarning = {};
function checkAndWarnForMutatedStyle(style1, style2, component) {
if (style1 == null || style2 == null) {
return;
}
if (shallowEqual(style1, style2)) {
return;
}
var componentName = component._tag;
var owner = component._currentElement._owner;
var ownerName;
if (owner) {
ownerName = owner.getName();
}
var hash = ownerName + '|' + componentName;
if (styleMutationWarning.hasOwnProperty(hash)) {
return;
}
styleMutationWarning[hash] = true;
"development" !== 'production' ? warning(false, '`%s` was passed a style object that has previously been mutated. ' + 'Mutating `style` is deprecated. Consider cloning it beforehand. Check ' + 'the `render` %s. Previous style: %s. Mutated style: %s.', componentName, owner ? 'of `' + ownerName + '`' : 'using <' + componentName + '>', friendlyStringify(style1), friendlyStringify(style2)) : undefined;
}
/**
* @param {object} component
* @param {?object} props
*/
function assertValidProps(component, props) {
if (!props) {
return;
}
// Note the use of `==` which checks for null or undefined.
if ("development" !== 'production') {
if (voidElementTags[component._tag]) {
"development" !== 'production' ? warning(props.children == null && props.dangerouslySetInnerHTML == null, '%s is a void element tag and must not have `children` or ' + 'use `props.dangerouslySetInnerHTML`.%s', component._tag, component._currentElement._owner ? ' Check the render method of ' + component._currentElement._owner.getName() + '.' : '') : undefined;
}
}
if (props.dangerouslySetInnerHTML != null) {
!(props.children == null) ? "development" !== 'production' ? invariant(false, 'Can only set one of `children` or `props.dangerouslySetInnerHTML`.') : invariant(false) : undefined;
!(typeof props.dangerouslySetInnerHTML === 'object' && HTML in props.dangerouslySetInnerHTML) ? "development" !== 'production' ? invariant(false, '`props.dangerouslySetInnerHTML` must be in the form `{__html: ...}`. ' + 'Please visit https://fb.me/react-invariant-dangerously-set-inner-html ' + 'for more information.') : invariant(false) : undefined;
}
if ("development" !== 'production') {
"development" !== 'production' ? warning(props.innerHTML == null, 'Directly setting property `innerHTML` is not permitted. ' + 'For more information, lookup documentation on `dangerouslySetInnerHTML`.') : undefined;
"development" !== 'production' ? warning(!props.contentEditable || props.children == null, 'A component is `contentEditable` and contains `children` managed by ' + 'React. It is now your responsibility to guarantee that none of ' + 'those nodes are unexpectedly modified or duplicated. This is ' + 'probably not intentional.') : undefined;
}
!(props.style == null || typeof props.style === 'object') ? "development" !== 'production' ? invariant(false, 'The `style` prop expects a mapping from style properties to values, ' + 'not a string. For example, style={{marginRight: spacing + \'em\'}} when ' + 'using JSX.%s', getDeclarationErrorAddendum(component)) : invariant(false) : undefined;
}
function enqueuePutListener(id, registrationName, listener, transaction) {
if ("development" !== 'production') {
// IE8 has no API for event capturing and the `onScroll` event doesn't
// bubble.
"development" !== 'production' ? warning(registrationName !== 'onScroll' || isEventSupported('scroll', true), 'This browser doesn\'t support the `onScroll` event') : undefined;
}
var container = ReactMount.findReactContainerForID(id);
if (container) {
var doc = container.nodeType === ELEMENT_NODE_TYPE ? container.ownerDocument : container;
listenTo(registrationName, doc);
}
transaction.getReactMountReady().enqueue(putListener, {
id: id,
registrationName: registrationName,
listener: listener
});
}
function putListener() {
var listenerToPut = this;
ReactBrowserEventEmitter.putListener(listenerToPut.id, listenerToPut.registrationName, listenerToPut.listener);
}
// There are so many media events, it makes sense to just
// maintain a list rather than create a `trapBubbledEvent` for each
var mediaEvents = {
topAbort: 'abort',
topCanPlay: 'canplay',
topCanPlayThrough: 'canplaythrough',
topDurationChange: 'durationchange',
topEmptied: 'emptied',
topEncrypted: 'encrypted',
topEnded: 'ended',
topError: 'error',
topLoadedData: 'loadeddata',
topLoadedMetadata: 'loadedmetadata',
topLoadStart: 'loadstart',
topPause: 'pause',
topPlay: 'play',
topPlaying: 'playing',
topProgress: 'progress',
topRateChange: 'ratechange',
topSeeked: 'seeked',
topSeeking: 'seeking',
topStalled: 'stalled',
topSuspend: 'suspend',
topTimeUpdate: 'timeupdate',
topVolumeChange: 'volumechange',
topWaiting: 'waiting'
};
function trapBubbledEventsLocal() {
var inst = this;
// If a component renders to null or if another component fatals and causes
// the state of the tree to be corrupted, `node` here can be null.
!inst._rootNodeID ? "development" !== 'production' ? invariant(false, 'Must be mounted to trap events') : invariant(false) : undefined;
var node = ReactMount.getNode(inst._rootNodeID);
!node ? "development" !== 'production' ? invariant(false, 'trapBubbledEvent(...): Requires node to be rendered.') : invariant(false) : undefined;
switch (inst._tag) {
case 'iframe':
inst._wrapperState.listeners = [ReactBrowserEventEmitter.trapBubbledEvent(EventConstants.topLevelTypes.topLoad, 'load', node)];
break;
case 'video':
case 'audio':
inst._wrapperState.listeners = [];
// create listener for each media event
for (var event in mediaEvents) {
if (mediaEvents.hasOwnProperty(event)) {
inst._wrapperState.listeners.push(ReactBrowserEventEmitter.trapBubbledEvent(EventConstants.topLevelTypes[event], mediaEvents[event], node));
}
}
break;
case 'img':
inst._wrapperState.listeners = [ReactBrowserEventEmitter.trapBubbledEvent(EventConstants.topLevelTypes.topError, 'error', node), ReactBrowserEventEmitter.trapBubbledEvent(EventConstants.topLevelTypes.topLoad, 'load', node)];
break;
case 'form':
inst._wrapperState.listeners = [ReactBrowserEventEmitter.trapBubbledEvent(EventConstants.topLevelTypes.topReset, 'reset', node), ReactBrowserEventEmitter.trapBubbledEvent(EventConstants.topLevelTypes.topSubmit, 'submit', node)];
break;
}
}
function mountReadyInputWrapper() {
ReactDOMInput.mountReadyWrapper(this);
}
function postUpdateSelectWrapper() {
ReactDOMSelect.postUpdateWrapper(this);
}
// For HTML, certain tags should omit their close tag. We keep a whitelist for
// those special cased tags.
var omittedCloseTags = {
'area': true,
'base': true,
'br': true,
'col': true,
'embed': true,
'hr': true,
'img': true,
'input': true,
'keygen': true,
'link': true,
'meta': true,
'param': true,
'source': true,
'track': true,
'wbr': true
};
// NOTE: menuitem's close tag should be omitted, but that causes problems.
var newlineEatingTags = {
'listing': true,
'pre': true,
'textarea': true
};
// For HTML, certain tags cannot have children. This has the same purpose as
// `omittedCloseTags` except that `menuitem` should still have its closing tag.
var voidElementTags = assign({
'menuitem': true
}, omittedCloseTags);
// We accept any tag to be rendered but since this gets injected into arbitrary
// HTML, we want to make sure that it's a safe tag.
// http://www.w3.org/TR/REC-xml/#NT-Name
var VALID_TAG_REGEX = /^[a-zA-Z][a-zA-Z:_\.\-\d]*$/; // Simplified subset
var validatedTagCache = {};
var hasOwnProperty = ({}).hasOwnProperty;
function validateDangerousTag(tag) {
if (!hasOwnProperty.call(validatedTagCache, tag)) {
!VALID_TAG_REGEX.test(tag) ? "development" !== 'production' ? invariant(false, 'Invalid tag: %s', tag) : invariant(false) : undefined;
validatedTagCache[tag] = true;
}
}
function processChildContextDev(context, inst) {
// Pass down our tag name to child components for validation purposes
context = assign({}, context);
var info = context[validateDOMNesting.ancestorInfoContextKey];
context[validateDOMNesting.ancestorInfoContextKey] = validateDOMNesting.updatedAncestorInfo(info, inst._tag, inst);
return context;
}
function isCustomComponent(tagName, props) {
return tagName.indexOf('-') >= 0 || props.is != null;
}
/**
* Creates a new React class that is idempotent and capable of containing other
* React components. It accepts event listeners and DOM properties that are
* valid according to `DOMProperty`.
*
* - Event listeners: `onClick`, `onMouseDown`, etc.
* - DOM properties: `className`, `name`, `title`, etc.
*
* The `style` property functions differently from the DOM API. It accepts an
* object mapping of style properties to values.
*
* @constructor ReactDOMComponent
* @extends ReactMultiChild
*/
function ReactDOMComponent(tag) {
validateDangerousTag(tag);
this._tag = tag.toLowerCase();
this._renderedChildren = null;
this._previousStyle = null;
this._previousStyleCopy = null;
this._rootNodeID = null;
this._wrapperState = null;
this._topLevelWrapper = null;
this._nodeWithLegacyProperties = null;
if ("development" !== 'production') {
this._unprocessedContextDev = null;
this._processedContextDev = null;
}
}
ReactDOMComponent.displayName = 'ReactDOMComponent';
ReactDOMComponent.Mixin = {
construct: function (element) {
this._currentElement = element;
},
/**
* Generates root tag markup then recurses. This method has side effects and
* is not idempotent.
*
* @internal
* @param {string} rootID The root DOM ID for this node.
* @param {ReactReconcileTransaction|ReactServerRenderingTransaction} transaction
* @param {object} context
* @return {string} The computed markup.
*/
mountComponent: function (rootID, transaction, context) {
this._rootNodeID = rootID;
var props = this._currentElement.props;
switch (this._tag) {
case 'iframe':
case 'img':
case 'form':
case 'video':
case 'audio':
this._wrapperState = {
listeners: null
};
transaction.getReactMountReady().enqueue(trapBubbledEventsLocal, this);
break;
case 'button':
props = ReactDOMButton.getNativeProps(this, props, context);
break;
case 'input':
ReactDOMInput.mountWrapper(this, props, context);
props = ReactDOMInput.getNativeProps(this, props, context);
break;
case 'option':
ReactDOMOption.mountWrapper(this, props, context);
props = ReactDOMOption.getNativeProps(this, props, context);
break;
case 'select':
ReactDOMSelect.mountWrapper(this, props, context);
props = ReactDOMSelect.getNativeProps(this, props, context);
context = ReactDOMSelect.processChildContext(this, props, context);
break;
case 'textarea':
ReactDOMTextarea.mountWrapper(this, props, context);
props = ReactDOMTextarea.getNativeProps(this, props, context);
break;
}
assertValidProps(this, props);
if ("development" !== 'production') {
if (context[validateDOMNesting.ancestorInfoContextKey]) {
validateDOMNesting(this._tag, this, context[validateDOMNesting.ancestorInfoContextKey]);
}
}
if ("development" !== 'production') {
this._unprocessedContextDev = context;
this._processedContextDev = processChildContextDev(context, this);
context = this._processedContextDev;
}
var mountImage;
if (transaction.useCreateElement) {
var ownerDocument = context[ReactMount.ownerDocumentContextKey];
var el = ownerDocument.createElement(this._currentElement.type);
DOMPropertyOperations.setAttributeForID(el, this._rootNodeID);
// Populate node cache
ReactMount.getID(el);
this._updateDOMProperties({}, props, transaction, el);
this._createInitialChildren(transaction, props, context, el);
mountImage = el;
} else {
var tagOpen = this._createOpenTagMarkupAndPutListeners(transaction, props);
var tagContent = this._createContentMarkup(transaction, props, context);
if (!tagContent && omittedCloseTags[this._tag]) {
mountImage = tagOpen + '/>';
} else {
mountImage = tagOpen + '>' + tagContent + '</' + this._currentElement.type + '>';
}
}
switch (this._tag) {
case 'input':
transaction.getReactMountReady().enqueue(mountReadyInputWrapper, this);
// falls through
case 'button':
case 'select':
case 'textarea':
if (props.autoFocus) {
transaction.getReactMountReady().enqueue(AutoFocusUtils.focusDOMComponent, this);
}
break;
}
return mountImage;
},
/**
* Creates markup for the open tag and all attributes.
*
* This method has side effects because events get registered.
*
* Iterating over object properties is faster than iterating over arrays.
* @see http://jsperf.com/obj-vs-arr-iteration
*
* @private
* @param {ReactReconcileTransaction|ReactServerRenderingTransaction} transaction
* @param {object} props
* @return {string} Markup of opening tag.
*/
_createOpenTagMarkupAndPutListeners: function (transaction, props) {
var ret = '<' + this._currentElement.type;
for (var propKey in props) {
if (!props.hasOwnProperty(propKey)) {
continue;
}
var propValue = props[propKey];
if (propValue == null) {
continue;
}
if (registrationNameModules.hasOwnProperty(propKey)) {
if (propValue) {
enqueuePutListener(this._rootNodeID, propKey, propValue, transaction);
}
} else {
if (propKey === STYLE) {
if (propValue) {
if ("development" !== 'production') {
// See `_updateDOMProperties`. style block
this._previousStyle = propValue;
}
propValue = this._previousStyleCopy = assign({}, props.style);
}
propValue = CSSPropertyOperations.createMarkupForStyles(propValue);
}
var markup = null;
if (this._tag != null && isCustomComponent(this._tag, props)) {
if (propKey !== CHILDREN) {
markup = DOMPropertyOperations.createMarkupForCustomAttribute(propKey, propValue);
}
} else {
markup = DOMPropertyOperations.createMarkupForProperty(propKey, propValue);
}
if (markup) {
ret += ' ' + markup;
}
}
}
// For static pages, no need to put React ID and checksum. Saves lots of
// bytes.
if (transaction.renderToStaticMarkup) {
return ret;
}
var markupForID = DOMPropertyOperations.createMarkupForID(this._rootNodeID);
return ret + ' ' + markupForID;
},
/**
* Creates markup for the content between the tags.
*
* @private
* @param {ReactReconcileTransaction|ReactServerRenderingTransaction} transaction
* @param {object} props
* @param {object} context
* @return {string} Content markup.
*/
_createContentMarkup: function (transaction, props, context) {
var ret = '';
// Intentional use of != to avoid catching zero/false.
var innerHTML = props.dangerouslySetInnerHTML;
if (innerHTML != null) {
if (innerHTML.__html != null) {
ret = innerHTML.__html;
}
} else {
var contentToUse = CONTENT_TYPES[typeof props.children] ? props.children : null;
var childrenToUse = contentToUse != null ? null : props.children;
if (contentToUse != null) {
// TODO: Validate that text is allowed as a child of this node
ret = escapeTextContentForBrowser(contentToUse);
} else if (childrenToUse != null) {
var mountImages = this.mountChildren(childrenToUse, transaction, context);
ret = mountImages.join('');
}
}
if (newlineEatingTags[this._tag] && ret.charAt(0) === '\n') {
// text/html ignores the first character in these tags if it's a newline
// Prefer to break application/xml over text/html (for now) by adding
// a newline specifically to get eaten by the parser. (Alternately for
// textareas, replacing "^\n" with "\r\n" doesn't get eaten, and the first
// \r is normalized out by HTMLTextAreaElement#value.)
// See: <http://www.w3.org/TR/html-polyglot/#newlines-in-textarea-and-pre>
// See: <http://www.w3.org/TR/html5/syntax.html#element-restrictions>
// See: <http://www.w3.org/TR/html5/syntax.html#newlines>
// See: Parsing of "textarea" "listing" and "pre" elements
// from <http://www.w3.org/TR/html5/syntax.html#parsing-main-inbody>
return '\n' + ret;
} else {
return ret;
}
},
_createInitialChildren: function (transaction, props, context, el) {
// Intentional use of != to avoid catching zero/false.
var innerHTML = props.dangerouslySetInnerHTML;
if (innerHTML != null) {
if (innerHTML.__html != null) {
setInnerHTML(el, innerHTML.__html);
}
} else {
var contentToUse = CONTENT_TYPES[typeof props.children] ? props.children : null;
var childrenToUse = contentToUse != null ? null : props.children;
if (contentToUse != null) {
// TODO: Validate that text is allowed as a child of this node
setTextContent(el, contentToUse);
} else if (childrenToUse != null) {
var mountImages = this.mountChildren(childrenToUse, transaction, context);
for (var i = 0; i < mountImages.length; i++) {
el.appendChild(mountImages[i]);
}
}
}
},
/**
* Receives a next element and updates the component.
*
* @internal
* @param {ReactElement} nextElement
* @param {ReactReconcileTransaction|ReactServerRenderingTransaction} transaction
* @param {object} context
*/
receiveComponent: function (nextElement, transaction, context) {
var prevElement = this._currentElement;
this._currentElement = nextElement;
this.updateComponent(transaction, prevElement, nextElement, context);
},
/**
* Updates a native DOM component after it has already been allocated and
* attached to the DOM. Reconciles the root DOM node, then recurses.
*
* @param {ReactReconcileTransaction} transaction
* @param {ReactElement} prevElement
* @param {ReactElement} nextElement
* @internal
* @overridable
*/
updateComponent: function (transaction, prevElement, nextElement, context) {
var lastProps = prevElement.props;
var nextProps = this._currentElement.props;
switch (this._tag) {
case 'button':
lastProps = ReactDOMButton.getNativeProps(this, lastProps);
nextProps = ReactDOMButton.getNativeProps(this, nextProps);
break;
case 'input':
ReactDOMInput.updateWrapper(this);
lastProps = ReactDOMInput.getNativeProps(this, lastProps);
nextProps = ReactDOMInput.getNativeProps(this, nextProps);
break;
case 'option':
lastProps = ReactDOMOption.getNativeProps(this, lastProps);
nextProps = ReactDOMOption.getNativeProps(this, nextProps);
break;
case 'select':
lastProps = ReactDOMSelect.getNativeProps(this, lastProps);
nextProps = ReactDOMSelect.getNativeProps(this, nextProps);
break;
case 'textarea':
ReactDOMTextarea.updateWrapper(this);
lastProps = ReactDOMTextarea.getNativeProps(this, lastProps);
nextProps = ReactDOMTextarea.getNativeProps(this, nextProps);
break;
}
if ("development" !== 'production') {
// If the context is reference-equal to the old one, pass down the same
// processed object so the update bailout in ReactReconciler behaves
// correctly (and identically in dev and prod). See #5005.
if (this._unprocessedContextDev !== context) {
this._unprocessedContextDev = context;
this._processedContextDev = processChildContextDev(context, this);
}
context = this._processedContextDev;
}
assertValidProps(this, nextProps);
this._updateDOMProperties(lastProps, nextProps, transaction, null);
this._updateDOMChildren(lastProps, nextProps, transaction, context);
if (!canDefineProperty && this._nodeWithLegacyProperties) {
this._nodeWithLegacyProperties.props = nextProps;
}
if (this._tag === 'select') {
// <select> value update needs to occur after <option> children
// reconciliation
transaction.getReactMountReady().enqueue(postUpdateSelectWrapper, this);
}
},
/**
* Reconciles the properties by detecting differences in property values and
* updating the DOM as necessary. This function is probably the single most
* critical path for performance optimization.
*
* TODO: Benchmark whether checking for changed values in memory actually
* improves performance (especially statically positioned elements).
* TODO: Benchmark the effects of putting this at the top since 99% of props
* do not change for a given reconciliation.
* TODO: Benchmark areas that can be improved with caching.
*
* @private
* @param {object} lastProps
* @param {object} nextProps
* @param {ReactReconcileTransaction} transaction
* @param {?DOMElement} node
*/
_updateDOMProperties: function (lastProps, nextProps, transaction, node) {
var propKey;
var styleName;
var styleUpdates;
for (propKey in lastProps) {
if (nextProps.hasOwnProperty(propKey) || !lastProps.hasOwnProperty(propKey)) {
continue;
}
if (propKey === STYLE) {
var lastStyle = this._previousStyleCopy;
for (styleName in lastStyle) {
if (lastStyle.hasOwnProperty(styleName)) {
styleUpdates = styleUpdates || {};
styleUpdates[styleName] = '';
}
}
this._previousStyleCopy = null;
} else if (registrationNameModules.hasOwnProperty(propKey)) {
if (lastProps[propKey]) {
// Only call deleteListener if there was a listener previously or
// else willDeleteListener gets called when there wasn't actually a
// listener (e.g., onClick={null})
deleteListener(this._rootNodeID, propKey);
}
} else if (DOMProperty.properties[propKey] || DOMProperty.isCustomAttribute(propKey)) {
if (!node) {
node = ReactMount.getNode(this._rootNodeID);
}
DOMPropertyOperations.deleteValueForProperty(node, propKey);
}
}
for (propKey in nextProps) {
var nextProp = nextProps[propKey];
var lastProp = propKey === STYLE ? this._previousStyleCopy : lastProps[propKey];
if (!nextProps.hasOwnProperty(propKey) || nextProp === lastProp) {
continue;
}
if (propKey === STYLE) {
if (nextProp) {
if ("development" !== 'production') {
checkAndWarnForMutatedStyle(this._previousStyleCopy, this._previousStyle, this);
this._previousStyle = nextProp;
}
nextProp = this._previousStyleCopy = assign({}, nextProp);
} else {
this._previousStyleCopy = null;
}
if (lastProp) {
// Unset styles on `lastProp` but not on `nextProp`.
for (styleName in lastProp) {
if (lastProp.hasOwnProperty(styleName) && (!nextProp || !nextProp.hasOwnProperty(styleName))) {
styleUpdates = styleUpdates || {};
styleUpdates[styleName] = '';
}
}
// Update styles that changed since `lastProp`.
for (styleName in nextProp) {
if (nextProp.hasOwnProperty(styleName) && lastProp[styleName] !== nextProp[styleName]) {
styleUpdates = styleUpdates || {};
styleUpdates[styleName] = nextProp[styleName];
}
}
} else {
// Relies on `updateStylesByID` not mutating `styleUpdates`.
styleUpdates = nextProp;
}
} else if (registrationNameModules.hasOwnProperty(propKey)) {
if (nextProp) {
enqueuePutListener(this._rootNodeID, propKey, nextProp, transaction);
} else if (lastProp) {
deleteListener(this._rootNodeID, propKey);
}
} else if (isCustomComponent(this._tag, nextProps)) {
if (!node) {
node = ReactMount.getNode(this._rootNodeID);
}
if (propKey === CHILDREN) {
nextProp = null;
}
DOMPropertyOperations.setValueForAttribute(node, propKey, nextProp);
} else if (DOMProperty.properties[propKey] || DOMProperty.isCustomAttribute(propKey)) {
if (!node) {
node = ReactMount.getNode(this._rootNodeID);
}
// If we're updating to null or undefined, we should remove the property
// from the DOM node instead of inadvertantly setting to a string. This
// brings us in line with the same behavior we have on initial render.
if (nextProp != null) {
DOMPropertyOperations.setValueForProperty(node, propKey, nextProp);
} else {
DOMPropertyOperations.deleteValueForProperty(node, propKey);
}
}
}
if (styleUpdates) {
if (!node) {
node = ReactMount.getNode(this._rootNodeID);
}
CSSPropertyOperations.setValueForStyles(node, styleUpdates);
}
},
/**
* Reconciles the children with the various properties that affect the
* children content.
*
* @param {object} lastProps
* @param {object} nextProps
* @param {ReactReconcileTransaction} transaction
* @param {object} context
*/
_updateDOMChildren: function (lastProps, nextProps, transaction, context) {
var lastContent = CONTENT_TYPES[typeof lastProps.children] ? lastProps.children : null;
var nextContent = CONTENT_TYPES[typeof nextProps.children] ? nextProps.children : null;
var lastHtml = lastProps.dangerouslySetInnerHTML && lastProps.dangerouslySetInnerHTML.__html;
var nextHtml = nextProps.dangerouslySetInnerHTML && nextProps.dangerouslySetInnerHTML.__html;
// Note the use of `!=` which checks for null or undefined.
var lastChildren = lastContent != null ? null : lastProps.children;
var nextChildren = nextContent != null ? null : nextProps.children;
// If we're switching from children to content/html or vice versa, remove
// the old content
var lastHasContentOrHtml = lastContent != null || lastHtml != null;
var nextHasContentOrHtml = nextContent != null || nextHtml != null;
if (lastChildren != null && nextChildren == null) {
this.updateChildren(null, transaction, context);
} else if (lastHasContentOrHtml && !nextHasContentOrHtml) {
this.updateTextContent('');
}
if (nextContent != null) {
if (lastContent !== nextContent) {
this.updateTextContent('' + nextContent);
}
} else if (nextHtml != null) {
if (lastHtml !== nextHtml) {
this.updateMarkup('' + nextHtml);
}
} else if (nextChildren != null) {
this.updateChildren(nextChildren, transaction, context);
}
},
/**
* Destroys all event registrations for this instance. Does not remove from
* the DOM. That must be done by the parent.
*
* @internal
*/
unmountComponent: function () {
switch (this._tag) {
case 'iframe':
case 'img':
case 'form':
case 'video':
case 'audio':
var listeners = this._wrapperState.listeners;
if (listeners) {
for (var i = 0; i < listeners.length; i++) {
listeners[i].remove();
}
}
break;
case 'input':
ReactDOMInput.unmountWrapper(this);
break;
case 'html':
case 'head':
case 'body':
/**
* Components like <html> <head> and <body> can't be removed or added
* easily in a cross-browser way, however it's valuable to be able to
* take advantage of React's reconciliation for styling and <title>
* management. So we just document it and throw in dangerous cases.
*/
!false ? "development" !== 'production' ? invariant(false, '<%s> tried to unmount. Because of cross-browser quirks it is ' + 'impossible to unmount some top-level components (eg <html>, ' + '<head>, and <body>) reliably and efficiently. To fix this, have a ' + 'single top-level component that never unmounts render these ' + 'elements.', this._tag) : invariant(false) : undefined;
break;
}
this.unmountChildren();
ReactBrowserEventEmitter.deleteAllListeners(this._rootNodeID);
ReactComponentBrowserEnvironment.unmountIDFromEnvironment(this._rootNodeID);
this._rootNodeID = null;
this._wrapperState = null;
if (this._nodeWithLegacyProperties) {
var node = this._nodeWithLegacyProperties;
node._reactInternalComponent = null;
this._nodeWithLegacyProperties = null;
}
},
getPublicInstance: function () {
if (!this._nodeWithLegacyProperties) {
var node = ReactMount.getNode(this._rootNodeID);
node._reactInternalComponent = this;
node.getDOMNode = legacyGetDOMNode;
node.isMounted = legacyIsMounted;
node.setState = legacySetStateEtc;
node.replaceState = legacySetStateEtc;
node.forceUpdate = legacySetStateEtc;
node.setProps = legacySetProps;
node.replaceProps = legacyReplaceProps;
if ("development" !== 'production') {
if (canDefineProperty) {
Object.defineProperties(node, legacyPropsDescriptor);
} else {
// updateComponent will update this property on subsequent renders
node.props = this._currentElement.props;
}
} else {
// updateComponent will update this property on subsequent renders
node.props = this._currentElement.props;
}
this._nodeWithLegacyProperties = node;
}
return this._nodeWithLegacyProperties;
}
};
ReactPerf.measureMethods(ReactDOMComponent, 'ReactDOMComponent', {
mountComponent: 'mountComponent',
updateComponent: 'updateComponent'
});
assign(ReactDOMComponent.prototype, ReactDOMComponent.Mixin, ReactMultiChild.Mixin);
module.exports = ReactDOMComponent;
},{"10":10,"11":11,"117":117,"121":121,"133":133,"138":138,"139":139,"144":144,"15":15,"161":161,"166":166,"171":171,"173":173,"2":2,"24":24,"28":28,"35":35,"41":41,"46":46,"47":47,"48":48,"5":5,"52":52,"72":72,"73":73,"78":78,"95":95}],43:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMFactories
* @typechecks static-only
*/
'use strict';
var ReactElement = _dereq_(57);
var ReactElementValidator = _dereq_(58);
var mapObject = _dereq_(167);
/**
* Create a factory that creates HTML tag elements.
*
* @param {string} tag Tag name (e.g. `div`).
* @private
*/
function createDOMFactory(tag) {
if ("development" !== 'production') {
return ReactElementValidator.createFactory(tag);
}
return ReactElement.createFactory(tag);
}
/**
* Creates a mapping from supported HTML tags to `ReactDOMComponent` classes.
* This is also accessible via `React.DOM`.
*
* @public
*/
var ReactDOMFactories = mapObject({
a: 'a',
abbr: 'abbr',
address: 'address',
area: 'area',
article: 'article',
aside: 'aside',
audio: 'audio',
b: 'b',
base: 'base',
bdi: 'bdi',
bdo: 'bdo',
big: 'big',
blockquote: 'blockquote',
body: 'body',
br: 'br',
button: 'button',
canvas: 'canvas',
caption: 'caption',
cite: 'cite',
code: 'code',
col: 'col',
colgroup: 'colgroup',
data: 'data',
datalist: 'datalist',
dd: 'dd',
del: 'del',
details: 'details',
dfn: 'dfn',
dialog: 'dialog',
div: 'div',
dl: 'dl',
dt: 'dt',
em: 'em',
embed: 'embed',
fieldset: 'fieldset',
figcaption: 'figcaption',
figure: 'figure',
footer: 'footer',
form: 'form',
h1: 'h1',
h2: 'h2',
h3: 'h3',
h4: 'h4',
h5: 'h5',
h6: 'h6',
head: 'head',
header: 'header',
hgroup: 'hgroup',
hr: 'hr',
html: 'html',
i: 'i',
iframe: 'iframe',
img: 'img',
input: 'input',
ins: 'ins',
kbd: 'kbd',
keygen: 'keygen',
label: 'label',
legend: 'legend',
li: 'li',
link: 'link',
main: 'main',
map: 'map',
mark: 'mark',
menu: 'menu',
menuitem: 'menuitem',
meta: 'meta',
meter: 'meter',
nav: 'nav',
noscript: 'noscript',
object: 'object',
ol: 'ol',
optgroup: 'optgroup',
option: 'option',
output: 'output',
p: 'p',
param: 'param',
picture: 'picture',
pre: 'pre',
progress: 'progress',
q: 'q',
rp: 'rp',
rt: 'rt',
ruby: 'ruby',
s: 's',
samp: 'samp',
script: 'script',
section: 'section',
select: 'select',
small: 'small',
source: 'source',
span: 'span',
strong: 'strong',
style: 'style',
sub: 'sub',
summary: 'summary',
sup: 'sup',
table: 'table',
tbody: 'tbody',
td: 'td',
textarea: 'textarea',
tfoot: 'tfoot',
th: 'th',
thead: 'thead',
time: 'time',
title: 'title',
tr: 'tr',
track: 'track',
u: 'u',
ul: 'ul',
'var': 'var',
video: 'video',
wbr: 'wbr',
// SVG
circle: 'circle',
clipPath: 'clipPath',
defs: 'defs',
ellipse: 'ellipse',
g: 'g',
image: 'image',
line: 'line',
linearGradient: 'linearGradient',
mask: 'mask',
path: 'path',
pattern: 'pattern',
polygon: 'polygon',
polyline: 'polyline',
radialGradient: 'radialGradient',
rect: 'rect',
stop: 'stop',
svg: 'svg',
text: 'text',
tspan: 'tspan'
}, createDOMFactory);
module.exports = ReactDOMFactories;
},{"167":167,"57":57,"58":58}],44:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMFeatureFlags
*/
'use strict';
var ReactDOMFeatureFlags = {
useCreateElement: false
};
module.exports = ReactDOMFeatureFlags;
},{}],45:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMIDOperations
* @typechecks static-only
*/
'use strict';
var DOMChildrenOperations = _dereq_(9);
var DOMPropertyOperations = _dereq_(11);
var ReactMount = _dereq_(72);
var ReactPerf = _dereq_(78);
var invariant = _dereq_(161);
/**
* Errors for properties that should not be updated with `updatePropertyByID()`.
*
* @type {object}
* @private
*/
var INVALID_PROPERTY_ERRORS = {
dangerouslySetInnerHTML: '`dangerouslySetInnerHTML` must be set using `updateInnerHTMLByID()`.',
style: '`style` must be set using `updateStylesByID()`.'
};
/**
* Operations used to process updates to DOM nodes.
*/
var ReactDOMIDOperations = {
/**
* Updates a DOM node with new property values. This should only be used to
* update DOM properties in `DOMProperty`.
*
* @param {string} id ID of the node to update.
* @param {string} name A valid property name, see `DOMProperty`.
* @param {*} value New value of the property.
* @internal
*/
updatePropertyByID: function (id, name, value) {
var node = ReactMount.getNode(id);
!!INVALID_PROPERTY_ERRORS.hasOwnProperty(name) ? "development" !== 'production' ? invariant(false, 'updatePropertyByID(...): %s', INVALID_PROPERTY_ERRORS[name]) : invariant(false) : undefined;
// If we're updating to null or undefined, we should remove the property
// from the DOM node instead of inadvertantly setting to a string. This
// brings us in line with the same behavior we have on initial render.
if (value != null) {
DOMPropertyOperations.setValueForProperty(node, name, value);
} else {
DOMPropertyOperations.deleteValueForProperty(node, name);
}
},
/**
* Replaces a DOM node that exists in the document with markup.
*
* @param {string} id ID of child to be replaced.
* @param {string} markup Dangerous markup to inject in place of child.
* @internal
* @see {Danger.dangerouslyReplaceNodeWithMarkup}
*/
dangerouslyReplaceNodeWithMarkupByID: function (id, markup) {
var node = ReactMount.getNode(id);
DOMChildrenOperations.dangerouslyReplaceNodeWithMarkup(node, markup);
},
/**
* Updates a component's children by processing a series of updates.
*
* @param {array<object>} updates List of update configurations.
* @param {array<string>} markup List of markup strings.
* @internal
*/
dangerouslyProcessChildrenUpdates: function (updates, markup) {
for (var i = 0; i < updates.length; i++) {
updates[i].parentNode = ReactMount.getNode(updates[i].parentID);
}
DOMChildrenOperations.processUpdates(updates, markup);
}
};
ReactPerf.measureMethods(ReactDOMIDOperations, 'ReactDOMIDOperations', {
dangerouslyReplaceNodeWithMarkupByID: 'dangerouslyReplaceNodeWithMarkupByID',
dangerouslyProcessChildrenUpdates: 'dangerouslyProcessChildrenUpdates'
});
module.exports = ReactDOMIDOperations;
},{"11":11,"161":161,"72":72,"78":78,"9":9}],46:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMInput
*/
'use strict';
var ReactDOMIDOperations = _dereq_(45);
var LinkedValueUtils = _dereq_(23);
var ReactMount = _dereq_(72);
var ReactUpdates = _dereq_(96);
var assign = _dereq_(24);
var invariant = _dereq_(161);
var instancesByReactID = {};
function forceUpdateIfMounted() {
if (this._rootNodeID) {
// DOM component is still mounted; update
ReactDOMInput.updateWrapper(this);
}
}
/**
* Implements an <input> native component that allows setting these optional
* props: `checked`, `value`, `defaultChecked`, and `defaultValue`.
*
* If `checked` or `value` are not supplied (or null/undefined), user actions
* that affect the checked state or value will trigger updates to the element.
*
* If they are supplied (and not null/undefined), the rendered element will not
* trigger updates to the element. Instead, the props must change in order for
* the rendered element to be updated.
*
* The rendered element will be initialized as unchecked (or `defaultChecked`)
* with an empty value (or `defaultValue`).
*
* @see http://www.w3.org/TR/2012/WD-html5-20121025/the-input-element.html
*/
var ReactDOMInput = {
getNativeProps: function (inst, props, context) {
var value = LinkedValueUtils.getValue(props);
var checked = LinkedValueUtils.getChecked(props);
var nativeProps = assign({}, props, {
defaultChecked: undefined,
defaultValue: undefined,
value: value != null ? value : inst._wrapperState.initialValue,
checked: checked != null ? checked : inst._wrapperState.initialChecked,
onChange: inst._wrapperState.onChange
});
return nativeProps;
},
mountWrapper: function (inst, props) {
if ("development" !== 'production') {
LinkedValueUtils.checkPropTypes('input', props, inst._currentElement._owner);
}
var defaultValue = props.defaultValue;
inst._wrapperState = {
initialChecked: props.defaultChecked || false,
initialValue: defaultValue != null ? defaultValue : null,
onChange: _handleChange.bind(inst)
};
},
mountReadyWrapper: function (inst) {
// Can't be in mountWrapper or else server rendering leaks.
instancesByReactID[inst._rootNodeID] = inst;
},
unmountWrapper: function (inst) {
delete instancesByReactID[inst._rootNodeID];
},
updateWrapper: function (inst) {
var props = inst._currentElement.props;
// TODO: Shouldn't this be getChecked(props)?
var checked = props.checked;
if (checked != null) {
ReactDOMIDOperations.updatePropertyByID(inst._rootNodeID, 'checked', checked || false);
}
var value = LinkedValueUtils.getValue(props);
if (value != null) {
// Cast `value` to a string to ensure the value is set correctly. While
// browsers typically do this as necessary, jsdom doesn't.
ReactDOMIDOperations.updatePropertyByID(inst._rootNodeID, 'value', '' + value);
}
}
};
function _handleChange(event) {
var props = this._currentElement.props;
var returnValue = LinkedValueUtils.executeOnChange(props, event);
// Here we use asap to wait until all updates have propagated, which
// is important when using controlled components within layers:
// https://github.com/facebook/react/issues/1698
ReactUpdates.asap(forceUpdateIfMounted, this);
var name = props.name;
if (props.type === 'radio' && name != null) {
var rootNode = ReactMount.getNode(this._rootNodeID);
var queryRoot = rootNode;
while (queryRoot.parentNode) {
queryRoot = queryRoot.parentNode;
}
// If `rootNode.form` was non-null, then we could try `form.elements`,
// but that sometimes behaves strangely in IE8. We could also try using
// `form.getElementsByName`, but that will only return direct children
// and won't include inputs that use the HTML5 `form=` attribute. Since
// the input might not even be in a form, let's just use the global
// `querySelectorAll` to ensure we don't miss anything.
var group = queryRoot.querySelectorAll('input[name=' + JSON.stringify('' + name) + '][type="radio"]');
for (var i = 0; i < group.length; i++) {
var otherNode = group[i];
if (otherNode === rootNode || otherNode.form !== rootNode.form) {
continue;
}
// This will throw if radio buttons rendered by different copies of React
// and the same name are rendered into the same form (same as #1939).
// That's probably okay; we don't support it just as we don't support
// mixing React with non-React.
var otherID = ReactMount.getID(otherNode);
!otherID ? "development" !== 'production' ? invariant(false, 'ReactDOMInput: Mixing React and non-React radio inputs with the ' + 'same `name` is not supported.') : invariant(false) : undefined;
var otherInstance = instancesByReactID[otherID];
!otherInstance ? "development" !== 'production' ? invariant(false, 'ReactDOMInput: Unknown radio button ID %s.', otherID) : invariant(false) : undefined;
// If this is a controlled radio button group, forcing the input that
// was previously checked to update will cause it to be come re-checked
// as appropriate.
ReactUpdates.asap(forceUpdateIfMounted, otherInstance);
}
}
return returnValue;
}
module.exports = ReactDOMInput;
},{"161":161,"23":23,"24":24,"45":45,"72":72,"96":96}],47:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMOption
*/
'use strict';
var ReactChildren = _dereq_(32);
var ReactDOMSelect = _dereq_(48);
var assign = _dereq_(24);
var warning = _dereq_(173);
var valueContextKey = ReactDOMSelect.valueContextKey;
/**
* Implements an <option> native component that warns when `selected` is set.
*/
var ReactDOMOption = {
mountWrapper: function (inst, props, context) {
// TODO (yungsters): Remove support for `selected` in <option>.
if ("development" !== 'production') {
"development" !== 'production' ? warning(props.selected == null, 'Use the `defaultValue` or `value` props on <select> instead of ' + 'setting `selected` on <option>.') : undefined;
}
// Look up whether this option is 'selected' via context
var selectValue = context[valueContextKey];
// If context key is null (e.g., no specified value or after initial mount)
// or missing (e.g., for <datalist>), we don't change props.selected
var selected = null;
if (selectValue != null) {
selected = false;
if (Array.isArray(selectValue)) {
// multiple
for (var i = 0; i < selectValue.length; i++) {
if ('' + selectValue[i] === '' + props.value) {
selected = true;
break;
}
}
} else {
selected = '' + selectValue === '' + props.value;
}
}
inst._wrapperState = { selected: selected };
},
getNativeProps: function (inst, props, context) {
var nativeProps = assign({ selected: undefined, children: undefined }, props);
// Read state only from initial mount because <select> updates value
// manually; we need the initial state only for server rendering
if (inst._wrapperState.selected != null) {
nativeProps.selected = inst._wrapperState.selected;
}
var content = '';
// Flatten children and warn if they aren't strings or numbers;
// invalid types are ignored.
ReactChildren.forEach(props.children, function (child) {
if (child == null) {
return;
}
if (typeof child === 'string' || typeof child === 'number') {
content += child;
} else {
"development" !== 'production' ? warning(false, 'Only strings and numbers are supported as <option> children.') : undefined;
}
});
if (content) {
nativeProps.children = content;
}
return nativeProps;
}
};
module.exports = ReactDOMOption;
},{"173":173,"24":24,"32":32,"48":48}],48:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMSelect
*/
'use strict';
var LinkedValueUtils = _dereq_(23);
var ReactMount = _dereq_(72);
var ReactUpdates = _dereq_(96);
var assign = _dereq_(24);
var warning = _dereq_(173);
var valueContextKey = '__ReactDOMSelect_value$' + Math.random().toString(36).slice(2);
function updateOptionsIfPendingUpdateAndMounted() {
if (this._rootNodeID && this._wrapperState.pendingUpdate) {
this._wrapperState.pendingUpdate = false;
var props = this._currentElement.props;
var value = LinkedValueUtils.getValue(props);
if (value != null) {
updateOptions(this, Boolean(props.multiple), value);
}
}
}
function getDeclarationErrorAddendum(owner) {
if (owner) {
var name = owner.getName();
if (name) {
return ' Check the render method of `' + name + '`.';
}
}
return '';
}
var valuePropNames = ['value', 'defaultValue'];
/**
* Validation function for `value` and `defaultValue`.
* @private
*/
function checkSelectPropTypes(inst, props) {
var owner = inst._currentElement._owner;
LinkedValueUtils.checkPropTypes('select', props, owner);
for (var i = 0; i < valuePropNames.length; i++) {
var propName = valuePropNames[i];
if (props[propName] == null) {
continue;
}
if (props.multiple) {
"development" !== 'production' ? warning(Array.isArray(props[propName]), 'The `%s` prop supplied to <select> must be an array if ' + '`multiple` is true.%s', propName, getDeclarationErrorAddendum(owner)) : undefined;
} else {
"development" !== 'production' ? warning(!Array.isArray(props[propName]), 'The `%s` prop supplied to <select> must be a scalar ' + 'value if `multiple` is false.%s', propName, getDeclarationErrorAddendum(owner)) : undefined;
}
}
}
/**
* @param {ReactDOMComponent} inst
* @param {boolean} multiple
* @param {*} propValue A stringable (with `multiple`, a list of stringables).
* @private
*/
function updateOptions(inst, multiple, propValue) {
var selectedValue, i;
var options = ReactMount.getNode(inst._rootNodeID).options;
if (multiple) {
selectedValue = {};
for (i = 0; i < propValue.length; i++) {
selectedValue['' + propValue[i]] = true;
}
for (i = 0; i < options.length; i++) {
var selected = selectedValue.hasOwnProperty(options[i].value);
if (options[i].selected !== selected) {
options[i].selected = selected;
}
}
} else {
// Do not set `select.value` as exact behavior isn't consistent across all
// browsers for all cases.
selectedValue = '' + propValue;
for (i = 0; i < options.length; i++) {
if (options[i].value === selectedValue) {
options[i].selected = true;
return;
}
}
if (options.length) {
options[0].selected = true;
}
}
}
/**
* Implements a <select> native component that allows optionally setting the
* props `value` and `defaultValue`. If `multiple` is false, the prop must be a
* stringable. If `multiple` is true, the prop must be an array of stringables.
*
* If `value` is not supplied (or null/undefined), user actions that change the
* selected option will trigger updates to the rendered options.
*
* If it is supplied (and not null/undefined), the rendered options will not
* update in response to user actions. Instead, the `value` prop must change in
* order for the rendered options to update.
*
* If `defaultValue` is provided, any options with the supplied values will be
* selected.
*/
var ReactDOMSelect = {
valueContextKey: valueContextKey,
getNativeProps: function (inst, props, context) {
return assign({}, props, {
onChange: inst._wrapperState.onChange,
value: undefined
});
},
mountWrapper: function (inst, props) {
if ("development" !== 'production') {
checkSelectPropTypes(inst, props);
}
var value = LinkedValueUtils.getValue(props);
inst._wrapperState = {
pendingUpdate: false,
initialValue: value != null ? value : props.defaultValue,
onChange: _handleChange.bind(inst),
wasMultiple: Boolean(props.multiple)
};
},
processChildContext: function (inst, props, context) {
// Pass down initial value so initial generated markup has correct
// `selected` attributes
var childContext = assign({}, context);
childContext[valueContextKey] = inst._wrapperState.initialValue;
return childContext;
},
postUpdateWrapper: function (inst) {
var props = inst._currentElement.props;
// After the initial mount, we control selected-ness manually so don't pass
// the context value down
inst._wrapperState.initialValue = undefined;
var wasMultiple = inst._wrapperState.wasMultiple;
inst._wrapperState.wasMultiple = Boolean(props.multiple);
var value = LinkedValueUtils.getValue(props);
if (value != null) {
inst._wrapperState.pendingUpdate = false;
updateOptions(inst, Boolean(props.multiple), value);
} else if (wasMultiple !== Boolean(props.multiple)) {
// For simplicity, reapply `defaultValue` if `multiple` is toggled.
if (props.defaultValue != null) {
updateOptions(inst, Boolean(props.multiple), props.defaultValue);
} else {
// Revert the select back to its default unselected state.
updateOptions(inst, Boolean(props.multiple), props.multiple ? [] : '');
}
}
}
};
function _handleChange(event) {
var props = this._currentElement.props;
var returnValue = LinkedValueUtils.executeOnChange(props, event);
this._wrapperState.pendingUpdate = true;
ReactUpdates.asap(updateOptionsIfPendingUpdateAndMounted, this);
return returnValue;
}
module.exports = ReactDOMSelect;
},{"173":173,"23":23,"24":24,"72":72,"96":96}],49:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMSelection
*/
'use strict';
var ExecutionEnvironment = _dereq_(147);
var getNodeForCharacterOffset = _dereq_(130);
var getTextContentAccessor = _dereq_(131);
/**
* While `isCollapsed` is available on the Selection object and `collapsed`
* is available on the Range object, IE11 sometimes gets them wrong.
* If the anchor/focus nodes and offsets are the same, the range is collapsed.
*/
function isCollapsed(anchorNode, anchorOffset, focusNode, focusOffset) {
return anchorNode === focusNode && anchorOffset === focusOffset;
}
/**
* Get the appropriate anchor and focus node/offset pairs for IE.
*
* The catch here is that IE's selection API doesn't provide information
* about whether the selection is forward or backward, so we have to
* behave as though it's always forward.
*
* IE text differs from modern selection in that it behaves as though
* block elements end with a new line. This means character offsets will
* differ between the two APIs.
*
* @param {DOMElement} node
* @return {object}
*/
function getIEOffsets(node) {
var selection = document.selection;
var selectedRange = selection.createRange();
var selectedLength = selectedRange.text.length;
// Duplicate selection so we can move range without breaking user selection.
var fromStart = selectedRange.duplicate();
fromStart.moveToElementText(node);
fromStart.setEndPoint('EndToStart', selectedRange);
var startOffset = fromStart.text.length;
var endOffset = startOffset + selectedLength;
return {
start: startOffset,
end: endOffset
};
}
/**
* @param {DOMElement} node
* @return {?object}
*/
function getModernOffsets(node) {
var selection = window.getSelection && window.getSelection();
if (!selection || selection.rangeCount === 0) {
return null;
}
var anchorNode = selection.anchorNode;
var anchorOffset = selection.anchorOffset;
var focusNode = selection.focusNode;
var focusOffset = selection.focusOffset;
var currentRange = selection.getRangeAt(0);
// In Firefox, range.startContainer and range.endContainer can be "anonymous
// divs", e.g. the up/down buttons on an <input type="number">. Anonymous
// divs do not seem to expose properties, triggering a "Permission denied
// error" if any of its properties are accessed. The only seemingly possible
// way to avoid erroring is to access a property that typically works for
// non-anonymous divs and catch any error that may otherwise arise. See
// https://bugzilla.mozilla.org/show_bug.cgi?id=208427
try {
/* eslint-disable no-unused-expressions */
currentRange.startContainer.nodeType;
currentRange.endContainer.nodeType;
/* eslint-enable no-unused-expressions */
} catch (e) {
return null;
}
// If the node and offset values are the same, the selection is collapsed.
// `Selection.isCollapsed` is available natively, but IE sometimes gets
// this value wrong.
var isSelectionCollapsed = isCollapsed(selection.anchorNode, selection.anchorOffset, selection.focusNode, selection.focusOffset);
var rangeLength = isSelectionCollapsed ? 0 : currentRange.toString().length;
var tempRange = currentRange.cloneRange();
tempRange.selectNodeContents(node);
tempRange.setEnd(currentRange.startContainer, currentRange.startOffset);
var isTempRangeCollapsed = isCollapsed(tempRange.startContainer, tempRange.startOffset, tempRange.endContainer, tempRange.endOffset);
var start = isTempRangeCollapsed ? 0 : tempRange.toString().length;
var end = start + rangeLength;
// Detect whether the selection is backward.
var detectionRange = document.createRange();
detectionRange.setStart(anchorNode, anchorOffset);
detectionRange.setEnd(focusNode, focusOffset);
var isBackward = detectionRange.collapsed;
return {
start: isBackward ? end : start,
end: isBackward ? start : end
};
}
/**
* @param {DOMElement|DOMTextNode} node
* @param {object} offsets
*/
function setIEOffsets(node, offsets) {
var range = document.selection.createRange().duplicate();
var start, end;
if (typeof offsets.end === 'undefined') {
start = offsets.start;
end = start;
} else if (offsets.start > offsets.end) {
start = offsets.end;
end = offsets.start;
} else {
start = offsets.start;
end = offsets.end;
}
range.moveToElementText(node);
range.moveStart('character', start);
range.setEndPoint('EndToStart', range);
range.moveEnd('character', end - start);
range.select();
}
/**
* In modern non-IE browsers, we can support both forward and backward
* selections.
*
* Note: IE10+ supports the Selection object, but it does not support
* the `extend` method, which means that even in modern IE, it's not possible
* to programatically create a backward selection. Thus, for all IE
* versions, we use the old IE API to create our selections.
*
* @param {DOMElement|DOMTextNode} node
* @param {object} offsets
*/
function setModernOffsets(node, offsets) {
if (!window.getSelection) {
return;
}
var selection = window.getSelection();
var length = node[getTextContentAccessor()].length;
var start = Math.min(offsets.start, length);
var end = typeof offsets.end === 'undefined' ? start : Math.min(offsets.end, length);
// IE 11 uses modern selection, but doesn't support the extend method.
// Flip backward selections, so we can set with a single range.
if (!selection.extend && start > end) {
var temp = end;
end = start;
start = temp;
}
var startMarker = getNodeForCharacterOffset(node, start);
var endMarker = getNodeForCharacterOffset(node, end);
if (startMarker && endMarker) {
var range = document.createRange();
range.setStart(startMarker.node, startMarker.offset);
selection.removeAllRanges();
if (start > end) {
selection.addRange(range);
selection.extend(endMarker.node, endMarker.offset);
} else {
range.setEnd(endMarker.node, endMarker.offset);
selection.addRange(range);
}
}
}
var useIEOffsets = ExecutionEnvironment.canUseDOM && 'selection' in document && !('getSelection' in window);
var ReactDOMSelection = {
/**
* @param {DOMElement} node
*/
getOffsets: useIEOffsets ? getIEOffsets : getModernOffsets,
/**
* @param {DOMElement|DOMTextNode} node
* @param {object} offsets
*/
setOffsets: useIEOffsets ? setIEOffsets : setModernOffsets
};
module.exports = ReactDOMSelection;
},{"130":130,"131":131,"147":147}],50:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMServer
*/
'use strict';
var ReactDefaultInjection = _dereq_(54);
var ReactServerRendering = _dereq_(88);
var ReactVersion = _dereq_(97);
ReactDefaultInjection.inject();
var ReactDOMServer = {
renderToString: ReactServerRendering.renderToString,
renderToStaticMarkup: ReactServerRendering.renderToStaticMarkup,
version: ReactVersion
};
module.exports = ReactDOMServer;
},{"54":54,"88":88,"97":97}],51:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMTextComponent
* @typechecks static-only
*/
'use strict';
var DOMChildrenOperations = _dereq_(9);
var DOMPropertyOperations = _dereq_(11);
var ReactComponentBrowserEnvironment = _dereq_(35);
var ReactMount = _dereq_(72);
var assign = _dereq_(24);
var escapeTextContentForBrowser = _dereq_(121);
var setTextContent = _dereq_(139);
var validateDOMNesting = _dereq_(144);
/**
* Text nodes violate a couple assumptions that React makes about components:
*
* - When mounting text into the DOM, adjacent text nodes are merged.
* - Text nodes cannot be assigned a React root ID.
*
* This component is used to wrap strings in elements so that they can undergo
* the same reconciliation that is applied to elements.
*
* TODO: Investigate representing React components in the DOM with text nodes.
*
* @class ReactDOMTextComponent
* @extends ReactComponent
* @internal
*/
var ReactDOMTextComponent = function (props) {
// This constructor and its argument is currently used by mocks.
};
assign(ReactDOMTextComponent.prototype, {
/**
* @param {ReactText} text
* @internal
*/
construct: function (text) {
// TODO: This is really a ReactText (ReactNode), not a ReactElement
this._currentElement = text;
this._stringText = '' + text;
// Properties
this._rootNodeID = null;
this._mountIndex = 0;
},
/**
* Creates the markup for this text node. This node is not intended to have
* any features besides containing text content.
*
* @param {string} rootID DOM ID of the root node.
* @param {ReactReconcileTransaction|ReactServerRenderingTransaction} transaction
* @return {string} Markup for this text node.
* @internal
*/
mountComponent: function (rootID, transaction, context) {
if ("development" !== 'production') {
if (context[validateDOMNesting.ancestorInfoContextKey]) {
validateDOMNesting('span', null, context[validateDOMNesting.ancestorInfoContextKey]);
}
}
this._rootNodeID = rootID;
if (transaction.useCreateElement) {
var ownerDocument = context[ReactMount.ownerDocumentContextKey];
var el = ownerDocument.createElement('span');
DOMPropertyOperations.setAttributeForID(el, rootID);
// Populate node cache
ReactMount.getID(el);
setTextContent(el, this._stringText);
return el;
} else {
var escapedText = escapeTextContentForBrowser(this._stringText);
if (transaction.renderToStaticMarkup) {
// Normally we'd wrap this in a `span` for the reasons stated above, but
// since this is a situation where React won't take over (static pages),
// we can simply return the text as it is.
return escapedText;
}
return '<span ' + DOMPropertyOperations.createMarkupForID(rootID) + '>' + escapedText + '</span>';
}
},
/**
* Updates this component by updating the text content.
*
* @param {ReactText} nextText The next text content
* @param {ReactReconcileTransaction} transaction
* @internal
*/
receiveComponent: function (nextText, transaction) {
if (nextText !== this._currentElement) {
this._currentElement = nextText;
var nextStringText = '' + nextText;
if (nextStringText !== this._stringText) {
// TODO: Save this as pending props and use performUpdateIfNecessary
// and/or updateComponent to do the actual update for consistency with
// other component types?
this._stringText = nextStringText;
var node = ReactMount.getNode(this._rootNodeID);
DOMChildrenOperations.updateTextContent(node, nextStringText);
}
}
},
unmountComponent: function () {
ReactComponentBrowserEnvironment.unmountIDFromEnvironment(this._rootNodeID);
}
});
module.exports = ReactDOMTextComponent;
},{"11":11,"121":121,"139":139,"144":144,"24":24,"35":35,"72":72,"9":9}],52:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDOMTextarea
*/
'use strict';
var LinkedValueUtils = _dereq_(23);
var ReactDOMIDOperations = _dereq_(45);
var ReactUpdates = _dereq_(96);
var assign = _dereq_(24);
var invariant = _dereq_(161);
var warning = _dereq_(173);
function forceUpdateIfMounted() {
if (this._rootNodeID) {
// DOM component is still mounted; update
ReactDOMTextarea.updateWrapper(this);
}
}
/**
* Implements a <textarea> native component that allows setting `value`, and
* `defaultValue`. This differs from the traditional DOM API because value is
* usually set as PCDATA children.
*
* If `value` is not supplied (or null/undefined), user actions that affect the
* value will trigger updates to the element.
*
* If `value` is supplied (and not null/undefined), the rendered element will
* not trigger updates to the element. Instead, the `value` prop must change in
* order for the rendered element to be updated.
*
* The rendered element will be initialized with an empty value, the prop
* `defaultValue` if specified, or the children content (deprecated).
*/
var ReactDOMTextarea = {
getNativeProps: function (inst, props, context) {
!(props.dangerouslySetInnerHTML == null) ? "development" !== 'production' ? invariant(false, '`dangerouslySetInnerHTML` does not make sense on <textarea>.') : invariant(false) : undefined;
// Always set children to the same thing. In IE9, the selection range will
// get reset if `textContent` is mutated.
var nativeProps = assign({}, props, {
defaultValue: undefined,
value: undefined,
children: inst._wrapperState.initialValue,
onChange: inst._wrapperState.onChange
});
return nativeProps;
},
mountWrapper: function (inst, props) {
if ("development" !== 'production') {
LinkedValueUtils.checkPropTypes('textarea', props, inst._currentElement._owner);
}
var defaultValue = props.defaultValue;
// TODO (yungsters): Remove support for children content in <textarea>.
var children = props.children;
if (children != null) {
if ("development" !== 'production') {
"development" !== 'production' ? warning(false, 'Use the `defaultValue` or `value` props instead of setting ' + 'children on <textarea>.') : undefined;
}
!(defaultValue == null) ? "development" !== 'production' ? invariant(false, 'If you supply `defaultValue` on a <textarea>, do not pass children.') : invariant(false) : undefined;
if (Array.isArray(children)) {
!(children.length <= 1) ? "development" !== 'production' ? invariant(false, '<textarea> can only have at most one child.') : invariant(false) : undefined;
children = children[0];
}
defaultValue = '' + children;
}
if (defaultValue == null) {
defaultValue = '';
}
var value = LinkedValueUtils.getValue(props);
inst._wrapperState = {
// We save the initial value so that `ReactDOMComponent` doesn't update
// `textContent` (unnecessary since we update value).
// The initial value can be a boolean or object so that's why it's
// forced to be a string.
initialValue: '' + (value != null ? value : defaultValue),
onChange: _handleChange.bind(inst)
};
},
updateWrapper: function (inst) {
var props = inst._currentElement.props;
var value = LinkedValueUtils.getValue(props);
if (value != null) {
// Cast `value` to a string to ensure the value is set correctly. While
// browsers typically do this as necessary, jsdom doesn't.
ReactDOMIDOperations.updatePropertyByID(inst._rootNodeID, 'value', '' + value);
}
}
};
function _handleChange(event) {
var props = this._currentElement.props;
var returnValue = LinkedValueUtils.executeOnChange(props, event);
ReactUpdates.asap(forceUpdateIfMounted, this);
return returnValue;
}
module.exports = ReactDOMTextarea;
},{"161":161,"173":173,"23":23,"24":24,"45":45,"96":96}],53:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDefaultBatchingStrategy
*/
'use strict';
var ReactUpdates = _dereq_(96);
var Transaction = _dereq_(113);
var assign = _dereq_(24);
var emptyFunction = _dereq_(153);
var RESET_BATCHED_UPDATES = {
initialize: emptyFunction,
close: function () {
ReactDefaultBatchingStrategy.isBatchingUpdates = false;
}
};
var FLUSH_BATCHED_UPDATES = {
initialize: emptyFunction,
close: ReactUpdates.flushBatchedUpdates.bind(ReactUpdates)
};
var TRANSACTION_WRAPPERS = [FLUSH_BATCHED_UPDATES, RESET_BATCHED_UPDATES];
function ReactDefaultBatchingStrategyTransaction() {
this.reinitializeTransaction();
}
assign(ReactDefaultBatchingStrategyTransaction.prototype, Transaction.Mixin, {
getTransactionWrappers: function () {
return TRANSACTION_WRAPPERS;
}
});
var transaction = new ReactDefaultBatchingStrategyTransaction();
var ReactDefaultBatchingStrategy = {
isBatchingUpdates: false,
/**
* Call the provided function in a context within which calls to `setState`
* and friends are batched such that components aren't updated unnecessarily.
*/
batchedUpdates: function (callback, a, b, c, d, e) {
var alreadyBatchingUpdates = ReactDefaultBatchingStrategy.isBatchingUpdates;
ReactDefaultBatchingStrategy.isBatchingUpdates = true;
// The code is written this way to avoid extra allocations
if (alreadyBatchingUpdates) {
callback(a, b, c, d, e);
} else {
transaction.perform(callback, null, a, b, c, d, e);
}
}
};
module.exports = ReactDefaultBatchingStrategy;
},{"113":113,"153":153,"24":24,"96":96}],54:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDefaultInjection
*/
'use strict';
var BeforeInputEventPlugin = _dereq_(3);
var ChangeEventPlugin = _dereq_(7);
var ClientReactRootIndex = _dereq_(8);
var DefaultEventPluginOrder = _dereq_(13);
var EnterLeaveEventPlugin = _dereq_(14);
var ExecutionEnvironment = _dereq_(147);
var HTMLDOMPropertyConfig = _dereq_(21);
var ReactBrowserComponentMixin = _dereq_(27);
var ReactComponentBrowserEnvironment = _dereq_(35);
var ReactDefaultBatchingStrategy = _dereq_(53);
var ReactDOMComponent = _dereq_(42);
var ReactDOMTextComponent = _dereq_(51);
var ReactEventListener = _dereq_(63);
var ReactInjection = _dereq_(65);
var ReactInstanceHandles = _dereq_(67);
var ReactMount = _dereq_(72);
var ReactReconcileTransaction = _dereq_(83);
var SelectEventPlugin = _dereq_(99);
var ServerReactRootIndex = _dereq_(100);
var SimpleEventPlugin = _dereq_(101);
var SVGDOMPropertyConfig = _dereq_(98);
var alreadyInjected = false;
function inject() {
if (alreadyInjected) {
// TODO: This is currently true because these injections are shared between
// the client and the server package. They should be built independently
// and not share any injection state. Then this problem will be solved.
return;
}
alreadyInjected = true;
ReactInjection.EventEmitter.injectReactEventListener(ReactEventListener);
/**
* Inject modules for resolving DOM hierarchy and plugin ordering.
*/
ReactInjection.EventPluginHub.injectEventPluginOrder(DefaultEventPluginOrder);
ReactInjection.EventPluginHub.injectInstanceHandle(ReactInstanceHandles);
ReactInjection.EventPluginHub.injectMount(ReactMount);
/**
* Some important event plugins included by default (without having to require
* them).
*/
ReactInjection.EventPluginHub.injectEventPluginsByName({
SimpleEventPlugin: SimpleEventPlugin,
EnterLeaveEventPlugin: EnterLeaveEventPlugin,
ChangeEventPlugin: ChangeEventPlugin,
SelectEventPlugin: SelectEventPlugin,
BeforeInputEventPlugin: BeforeInputEventPlugin
});
ReactInjection.NativeComponent.injectGenericComponentClass(ReactDOMComponent);
ReactInjection.NativeComponent.injectTextComponentClass(ReactDOMTextComponent);
ReactInjection.Class.injectMixin(ReactBrowserComponentMixin);
ReactInjection.DOMProperty.injectDOMPropertyConfig(HTMLDOMPropertyConfig);
ReactInjection.DOMProperty.injectDOMPropertyConfig(SVGDOMPropertyConfig);
ReactInjection.EmptyComponent.injectEmptyComponent('noscript');
ReactInjection.Updates.injectReconcileTransaction(ReactReconcileTransaction);
ReactInjection.Updates.injectBatchingStrategy(ReactDefaultBatchingStrategy);
ReactInjection.RootIndex.injectCreateReactRootIndex(ExecutionEnvironment.canUseDOM ? ClientReactRootIndex.createReactRootIndex : ServerReactRootIndex.createReactRootIndex);
ReactInjection.Component.injectEnvironment(ReactComponentBrowserEnvironment);
if ("development" !== 'production') {
var url = ExecutionEnvironment.canUseDOM && window.location.href || '';
if (/[?&]react_perf\b/.test(url)) {
var ReactDefaultPerf = _dereq_(55);
ReactDefaultPerf.start();
}
}
}
module.exports = {
inject: inject
};
},{"100":100,"101":101,"13":13,"14":14,"147":147,"21":21,"27":27,"3":3,"35":35,"42":42,"51":51,"53":53,"55":55,"63":63,"65":65,"67":67,"7":7,"72":72,"8":8,"83":83,"98":98,"99":99}],55:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDefaultPerf
* @typechecks static-only
*/
'use strict';
var DOMProperty = _dereq_(10);
var ReactDefaultPerfAnalysis = _dereq_(56);
var ReactMount = _dereq_(72);
var ReactPerf = _dereq_(78);
var performanceNow = _dereq_(170);
function roundFloat(val) {
return Math.floor(val * 100) / 100;
}
function addValue(obj, key, val) {
obj[key] = (obj[key] || 0) + val;
}
var ReactDefaultPerf = {
_allMeasurements: [], // last item in the list is the current one
_mountStack: [0],
_injected: false,
start: function () {
if (!ReactDefaultPerf._injected) {
ReactPerf.injection.injectMeasure(ReactDefaultPerf.measure);
}
ReactDefaultPerf._allMeasurements.length = 0;
ReactPerf.enableMeasure = true;
},
stop: function () {
ReactPerf.enableMeasure = false;
},
getLastMeasurements: function () {
return ReactDefaultPerf._allMeasurements;
},
printExclusive: function (measurements) {
measurements = measurements || ReactDefaultPerf._allMeasurements;
var summary = ReactDefaultPerfAnalysis.getExclusiveSummary(measurements);
console.table(summary.map(function (item) {
return {
'Component class name': item.componentName,
'Total inclusive time (ms)': roundFloat(item.inclusive),
'Exclusive mount time (ms)': roundFloat(item.exclusive),
'Exclusive render time (ms)': roundFloat(item.render),
'Mount time per instance (ms)': roundFloat(item.exclusive / item.count),
'Render time per instance (ms)': roundFloat(item.render / item.count),
'Instances': item.count
};
}));
// TODO: ReactDefaultPerfAnalysis.getTotalTime() does not return the correct
// number.
},
printInclusive: function (measurements) {
measurements = measurements || ReactDefaultPerf._allMeasurements;
var summary = ReactDefaultPerfAnalysis.getInclusiveSummary(measurements);
console.table(summary.map(function (item) {
return {
'Owner > component': item.componentName,
'Inclusive time (ms)': roundFloat(item.time),
'Instances': item.count
};
}));
console.log('Total time:', ReactDefaultPerfAnalysis.getTotalTime(measurements).toFixed(2) + ' ms');
},
getMeasurementsSummaryMap: function (measurements) {
var summary = ReactDefaultPerfAnalysis.getInclusiveSummary(measurements, true);
return summary.map(function (item) {
return {
'Owner > component': item.componentName,
'Wasted time (ms)': item.time,
'Instances': item.count
};
});
},
printWasted: function (measurements) {
measurements = measurements || ReactDefaultPerf._allMeasurements;
console.table(ReactDefaultPerf.getMeasurementsSummaryMap(measurements));
console.log('Total time:', ReactDefaultPerfAnalysis.getTotalTime(measurements).toFixed(2) + ' ms');
},
printDOM: function (measurements) {
measurements = measurements || ReactDefaultPerf._allMeasurements;
var summary = ReactDefaultPerfAnalysis.getDOMSummary(measurements);
console.table(summary.map(function (item) {
var result = {};
result[DOMProperty.ID_ATTRIBUTE_NAME] = item.id;
result.type = item.type;
result.args = JSON.stringify(item.args);
return result;
}));
console.log('Total time:', ReactDefaultPerfAnalysis.getTotalTime(measurements).toFixed(2) + ' ms');
},
_recordWrite: function (id, fnName, totalTime, args) {
// TODO: totalTime isn't that useful since it doesn't count paints/reflows
var writes = ReactDefaultPerf._allMeasurements[ReactDefaultPerf._allMeasurements.length - 1].writes;
writes[id] = writes[id] || [];
writes[id].push({
type: fnName,
time: totalTime,
args: args
});
},
measure: function (moduleName, fnName, func) {
return function () {
for (var _len = arguments.length, args = Array(_len), _key = 0; _key < _len; _key++) {
args[_key] = arguments[_key];
}
var totalTime;
var rv;
var start;
if (fnName === '_renderNewRootComponent' || fnName === 'flushBatchedUpdates') {
// A "measurement" is a set of metrics recorded for each flush. We want
// to group the metrics for a given flush together so we can look at the
// components that rendered and the DOM operations that actually
// happened to determine the amount of "wasted work" performed.
ReactDefaultPerf._allMeasurements.push({
exclusive: {},
inclusive: {},
render: {},
counts: {},
writes: {},
displayNames: {},
totalTime: 0,
created: {}
});
start = performanceNow();
rv = func.apply(this, args);
ReactDefaultPerf._allMeasurements[ReactDefaultPerf._allMeasurements.length - 1].totalTime = performanceNow() - start;
return rv;
} else if (fnName === '_mountImageIntoNode' || moduleName === 'ReactBrowserEventEmitter' || moduleName === 'ReactDOMIDOperations' || moduleName === 'CSSPropertyOperations' || moduleName === 'DOMChildrenOperations' || moduleName === 'DOMPropertyOperations') {
start = performanceNow();
rv = func.apply(this, args);
totalTime = performanceNow() - start;
if (fnName === '_mountImageIntoNode') {
var mountID = ReactMount.getID(args[1]);
ReactDefaultPerf._recordWrite(mountID, fnName, totalTime, args[0]);
} else if (fnName === 'dangerouslyProcessChildrenUpdates') {
// special format
args[0].forEach(function (update) {
var writeArgs = {};
if (update.fromIndex !== null) {
writeArgs.fromIndex = update.fromIndex;
}
if (update.toIndex !== null) {
writeArgs.toIndex = update.toIndex;
}
if (update.textContent !== null) {
writeArgs.textContent = update.textContent;
}
if (update.markupIndex !== null) {
writeArgs.markup = args[1][update.markupIndex];
}
ReactDefaultPerf._recordWrite(update.parentID, update.type, totalTime, writeArgs);
});
} else {
// basic format
var id = args[0];
if (typeof id === 'object') {
id = ReactMount.getID(args[0]);
}
ReactDefaultPerf._recordWrite(id, fnName, totalTime, Array.prototype.slice.call(args, 1));
}
return rv;
} else if (moduleName === 'ReactCompositeComponent' && (fnName === 'mountComponent' || fnName === 'updateComponent' || // TODO: receiveComponent()?
fnName === '_renderValidatedComponent')) {
if (this._currentElement.type === ReactMount.TopLevelWrapper) {
return func.apply(this, args);
}
var rootNodeID = fnName === 'mountComponent' ? args[0] : this._rootNodeID;
var isRender = fnName === '_renderValidatedComponent';
var isMount = fnName === 'mountComponent';
var mountStack = ReactDefaultPerf._mountStack;
var entry = ReactDefaultPerf._allMeasurements[ReactDefaultPerf._allMeasurements.length - 1];
if (isRender) {
addValue(entry.counts, rootNodeID, 1);
} else if (isMount) {
entry.created[rootNodeID] = true;
mountStack.push(0);
}
start = performanceNow();
rv = func.apply(this, args);
totalTime = performanceNow() - start;
if (isRender) {
addValue(entry.render, rootNodeID, totalTime);
} else if (isMount) {
var subMountTime = mountStack.pop();
mountStack[mountStack.length - 1] += totalTime;
addValue(entry.exclusive, rootNodeID, totalTime - subMountTime);
addValue(entry.inclusive, rootNodeID, totalTime);
} else {
addValue(entry.inclusive, rootNodeID, totalTime);
}
entry.displayNames[rootNodeID] = {
current: this.getName(),
owner: this._currentElement._owner ? this._currentElement._owner.getName() : '<root>'
};
return rv;
} else {
return func.apply(this, args);
}
};
}
};
module.exports = ReactDefaultPerf;
},{"10":10,"170":170,"56":56,"72":72,"78":78}],56:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactDefaultPerfAnalysis
*/
'use strict';
var assign = _dereq_(24);
// Don't try to save users less than 1.2ms (a number I made up)
var DONT_CARE_THRESHOLD = 1.2;
var DOM_OPERATION_TYPES = {
'_mountImageIntoNode': 'set innerHTML',
INSERT_MARKUP: 'set innerHTML',
MOVE_EXISTING: 'move',
REMOVE_NODE: 'remove',
SET_MARKUP: 'set innerHTML',
TEXT_CONTENT: 'set textContent',
'setValueForProperty': 'update attribute',
'setValueForAttribute': 'update attribute',
'deleteValueForProperty': 'remove attribute',
'setValueForStyles': 'update styles',
'replaceNodeWithMarkup': 'replace',
'updateTextContent': 'set textContent'
};
function getTotalTime(measurements) {
// TODO: return number of DOM ops? could be misleading.
// TODO: measure dropped frames after reconcile?
// TODO: log total time of each reconcile and the top-level component
// class that triggered it.
var totalTime = 0;
for (var i = 0; i < measurements.length; i++) {
var measurement = measurements[i];
totalTime += measurement.totalTime;
}
return totalTime;
}
function getDOMSummary(measurements) {
var items = [];
measurements.forEach(function (measurement) {
Object.keys(measurement.writes).forEach(function (id) {
measurement.writes[id].forEach(function (write) {
items.push({
id: id,
type: DOM_OPERATION_TYPES[write.type] || write.type,
args: write.args
});
});
});
});
return items;
}
function getExclusiveSummary(measurements) {
var candidates = {};
var displayName;
for (var i = 0; i < measurements.length; i++) {
var measurement = measurements[i];
var allIDs = assign({}, measurement.exclusive, measurement.inclusive);
for (var id in allIDs) {
displayName = measurement.displayNames[id].current;
candidates[displayName] = candidates[displayName] || {
componentName: displayName,
inclusive: 0,
exclusive: 0,
render: 0,
count: 0
};
if (measurement.render[id]) {
candidates[displayName].render += measurement.render[id];
}
if (measurement.exclusive[id]) {
candidates[displayName].exclusive += measurement.exclusive[id];
}
if (measurement.inclusive[id]) {
candidates[displayName].inclusive += measurement.inclusive[id];
}
if (measurement.counts[id]) {
candidates[displayName].count += measurement.counts[id];
}
}
}
// Now make a sorted array with the results.
var arr = [];
for (displayName in candidates) {
if (candidates[displayName].exclusive >= DONT_CARE_THRESHOLD) {
arr.push(candidates[displayName]);
}
}
arr.sort(function (a, b) {
return b.exclusive - a.exclusive;
});
return arr;
}
function getInclusiveSummary(measurements, onlyClean) {
var candidates = {};
var inclusiveKey;
for (var i = 0; i < measurements.length; i++) {
var measurement = measurements[i];
var allIDs = assign({}, measurement.exclusive, measurement.inclusive);
var cleanComponents;
if (onlyClean) {
cleanComponents = getUnchangedComponents(measurement);
}
for (var id in allIDs) {
if (onlyClean && !cleanComponents[id]) {
continue;
}
var displayName = measurement.displayNames[id];
// Inclusive time is not useful for many components without knowing where
// they are instantiated. So we aggregate inclusive time with both the
// owner and current displayName as the key.
inclusiveKey = displayName.owner + ' > ' + displayName.current;
candidates[inclusiveKey] = candidates[inclusiveKey] || {
componentName: inclusiveKey,
time: 0,
count: 0
};
if (measurement.inclusive[id]) {
candidates[inclusiveKey].time += measurement.inclusive[id];
}
if (measurement.counts[id]) {
candidates[inclusiveKey].count += measurement.counts[id];
}
}
}
// Now make a sorted array with the results.
var arr = [];
for (inclusiveKey in candidates) {
if (candidates[inclusiveKey].time >= DONT_CARE_THRESHOLD) {
arr.push(candidates[inclusiveKey]);
}
}
arr.sort(function (a, b) {
return b.time - a.time;
});
return arr;
}
function getUnchangedComponents(measurement) {
// For a given reconcile, look at which components did not actually
// render anything to the DOM and return a mapping of their ID to
// the amount of time it took to render the entire subtree.
var cleanComponents = {};
var dirtyLeafIDs = Object.keys(measurement.writes);
var allIDs = assign({}, measurement.exclusive, measurement.inclusive);
for (var id in allIDs) {
var isDirty = false;
// For each component that rendered, see if a component that triggered
// a DOM op is in its subtree.
for (var i = 0; i < dirtyLeafIDs.length; i++) {
if (dirtyLeafIDs[i].indexOf(id) === 0) {
isDirty = true;
break;
}
}
// check if component newly created
if (measurement.created[id]) {
isDirty = true;
}
if (!isDirty && measurement.counts[id] > 0) {
cleanComponents[id] = true;
}
}
return cleanComponents;
}
var ReactDefaultPerfAnalysis = {
getExclusiveSummary: getExclusiveSummary,
getInclusiveSummary: getInclusiveSummary,
getDOMSummary: getDOMSummary,
getTotalTime: getTotalTime
};
module.exports = ReactDefaultPerfAnalysis;
},{"24":24}],57:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactElement
*/
'use strict';
var ReactCurrentOwner = _dereq_(39);
var assign = _dereq_(24);
var canDefineProperty = _dereq_(117);
// The Symbol used to tag the ReactElement type. If there is no native Symbol
// nor polyfill, then a plain number is used for performance.
var REACT_ELEMENT_TYPE = typeof Symbol === 'function' && Symbol['for'] && Symbol['for']('react.element') || 0xeac7;
var RESERVED_PROPS = {
key: true,
ref: true,
__self: true,
__source: true
};
/**
* Base constructor for all React elements. This is only used to make this
* work with a dynamic instanceof check. Nothing should live on this prototype.
*
* @param {*} type
* @param {*} key
* @param {string|object} ref
* @param {*} self A *temporary* helper to detect places where `this` is
* different from the `owner` when React.createElement is called, so that we
* can warn. We want to get rid of owner and replace string `ref`s with arrow
* functions, and as long as `this` and owner are the same, there will be no
* change in behavior.
* @param {*} source An annotation object (added by a transpiler or otherwise)
* indicating filename, line number, and/or other information.
* @param {*} owner
* @param {*} props
* @internal
*/
var ReactElement = function (type, key, ref, self, source, owner, props) {
var element = {
// This tag allow us to uniquely identify this as a React Element
$$typeof: REACT_ELEMENT_TYPE,
// Built-in properties that belong on the element
type: type,
key: key,
ref: ref,
props: props,
// Record the component responsible for creating this element.
_owner: owner
};
if ("development" !== 'production') {
// The validation flag is currently mutative. We put it on
// an external backing store so that we can freeze the whole object.
// This can be replaced with a WeakMap once they are implemented in
// commonly used development environments.
element._store = {};
// To make comparing ReactElements easier for testing purposes, we make
// the validation flag non-enumerable (where possible, which should
// include every environment we run tests in), so the test framework
// ignores it.
if (canDefineProperty) {
Object.defineProperty(element._store, 'validated', {
configurable: false,
enumerable: false,
writable: true,
value: false
});
// self and source are DEV only properties.
Object.defineProperty(element, '_self', {
configurable: false,
enumerable: false,
writable: false,
value: self
});
// Two elements created in two different places should be considered
// equal for testing purposes and therefore we hide it from enumeration.
Object.defineProperty(element, '_source', {
configurable: false,
enumerable: false,
writable: false,
value: source
});
} else {
element._store.validated = false;
element._self = self;
element._source = source;
}
Object.freeze(element.props);
Object.freeze(element);
}
return element;
};
ReactElement.createElement = function (type, config, children) {
var propName;
// Reserved names are extracted
var props = {};
var key = null;
var ref = null;
var self = null;
var source = null;
if (config != null) {
ref = config.ref === undefined ? null : config.ref;
key = config.key === undefined ? null : '' + config.key;
self = config.__self === undefined ? null : config.__self;
source = config.__source === undefined ? null : config.__source;
// Remaining properties are added to a new props object
for (propName in config) {
if (config.hasOwnProperty(propName) && !RESERVED_PROPS.hasOwnProperty(propName)) {
props[propName] = config[propName];
}
}
}
// Children can be more than one argument, and those are transferred onto
// the newly allocated props object.
var childrenLength = arguments.length - 2;
if (childrenLength === 1) {
props.children = children;
} else if (childrenLength > 1) {
var childArray = Array(childrenLength);
for (var i = 0; i < childrenLength; i++) {
childArray[i] = arguments[i + 2];
}
props.children = childArray;
}
// Resolve default props
if (type && type.defaultProps) {
var defaultProps = type.defaultProps;
for (propName in defaultProps) {
if (typeof props[propName] === 'undefined') {
props[propName] = defaultProps[propName];
}
}
}
return ReactElement(type, key, ref, self, source, ReactCurrentOwner.current, props);
};
ReactElement.createFactory = function (type) {
var factory = ReactElement.createElement.bind(null, type);
// Expose the type on the factory and the prototype so that it can be
// easily accessed on elements. E.g. `<Foo />.type === Foo`.
// This should not be named `constructor` since this may not be the function
// that created the element, and it may not even be a constructor.
// Legacy hook TODO: Warn if this is accessed
factory.type = type;
return factory;
};
ReactElement.cloneAndReplaceKey = function (oldElement, newKey) {
var newElement = ReactElement(oldElement.type, newKey, oldElement.ref, oldElement._self, oldElement._source, oldElement._owner, oldElement.props);
return newElement;
};
ReactElement.cloneAndReplaceProps = function (oldElement, newProps) {
var newElement = ReactElement(oldElement.type, oldElement.key, oldElement.ref, oldElement._self, oldElement._source, oldElement._owner, newProps);
if ("development" !== 'production') {
// If the key on the original is valid, then the clone is valid
newElement._store.validated = oldElement._store.validated;
}
return newElement;
};
ReactElement.cloneElement = function (element, config, children) {
var propName;
// Original props are copied
var props = assign({}, element.props);
// Reserved names are extracted
var key = element.key;
var ref = element.ref;
// Self is preserved since the owner is preserved.
var self = element._self;
// Source is preserved since cloneElement is unlikely to be targeted by a
// transpiler, and the original source is probably a better indicator of the
// true owner.
var source = element._source;
// Owner will be preserved, unless ref is overridden
var owner = element._owner;
if (config != null) {
if (config.ref !== undefined) {
// Silently steal the ref from the parent.
ref = config.ref;
owner = ReactCurrentOwner.current;
}
if (config.key !== undefined) {
key = '' + config.key;
}
// Remaining properties override existing props
for (propName in config) {
if (config.hasOwnProperty(propName) && !RESERVED_PROPS.hasOwnProperty(propName)) {
props[propName] = config[propName];
}
}
}
// Children can be more than one argument, and those are transferred onto
// the newly allocated props object.
var childrenLength = arguments.length - 2;
if (childrenLength === 1) {
props.children = children;
} else if (childrenLength > 1) {
var childArray = Array(childrenLength);
for (var i = 0; i < childrenLength; i++) {
childArray[i] = arguments[i + 2];
}
props.children = childArray;
}
return ReactElement(element.type, key, ref, self, source, owner, props);
};
/**
* @param {?object} object
* @return {boolean} True if `object` is a valid component.
* @final
*/
ReactElement.isValidElement = function (object) {
return typeof object === 'object' && object !== null && object.$$typeof === REACT_ELEMENT_TYPE;
};
module.exports = ReactElement;
},{"117":117,"24":24,"39":39}],58:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactElementValidator
*/
/**
* ReactElementValidator provides a wrapper around a element factory
* which validates the props passed to the element. This is intended to be
* used only in DEV and could be replaced by a static type checker for languages
* that support it.
*/
'use strict';
var ReactElement = _dereq_(57);
var ReactPropTypeLocations = _dereq_(81);
var ReactPropTypeLocationNames = _dereq_(80);
var ReactCurrentOwner = _dereq_(39);
var canDefineProperty = _dereq_(117);
var getIteratorFn = _dereq_(129);
var invariant = _dereq_(161);
var warning = _dereq_(173);
function getDeclarationErrorAddendum() {
if (ReactCurrentOwner.current) {
var name = ReactCurrentOwner.current.getName();
if (name) {
return ' Check the render method of `' + name + '`.';
}
}
return '';
}
/**
* Warn if there's no key explicitly set on dynamic arrays of children or
* object keys are not valid. This allows us to keep track of children between
* updates.
*/
var ownerHasKeyUseWarning = {};
var loggedTypeFailures = {};
/**
* Warn if the element doesn't have an explicit key assigned to it.
* This element is in an array. The array could grow and shrink or be
* reordered. All children that haven't already been validated are required to
* have a "key" property assigned to it.
*
* @internal
* @param {ReactElement} element Element that requires a key.
* @param {*} parentType element's parent's type.
*/
function validateExplicitKey(element, parentType) {
if (!element._store || element._store.validated || element.key != null) {
return;
}
element._store.validated = true;
var addenda = getAddendaForKeyUse('uniqueKey', element, parentType);
if (addenda === null) {
// we already showed the warning
return;
}
"development" !== 'production' ? warning(false, 'Each child in an array or iterator should have a unique "key" prop.' + '%s%s%s', addenda.parentOrOwner || '', addenda.childOwner || '', addenda.url || '') : undefined;
}
/**
* Shared warning and monitoring code for the key warnings.
*
* @internal
* @param {string} messageType A key used for de-duping warnings.
* @param {ReactElement} element Component that requires a key.
* @param {*} parentType element's parent's type.
* @returns {?object} A set of addenda to use in the warning message, or null
* if the warning has already been shown before (and shouldn't be shown again).
*/
function getAddendaForKeyUse(messageType, element, parentType) {
var addendum = getDeclarationErrorAddendum();
if (!addendum) {
var parentName = typeof parentType === 'string' ? parentType : parentType.displayName || parentType.name;
if (parentName) {
addendum = ' Check the top-level render call using <' + parentName + '>.';
}
}
var memoizer = ownerHasKeyUseWarning[messageType] || (ownerHasKeyUseWarning[messageType] = {});
if (memoizer[addendum]) {
return null;
}
memoizer[addendum] = true;
var addenda = {
parentOrOwner: addendum,
url: ' See https://fb.me/react-warning-keys for more information.',
childOwner: null
};
// Usually the current owner is the offender, but if it accepts children as a
// property, it may be the creator of the child that's responsible for
// assigning it a key.
if (element && element._owner && element._owner !== ReactCurrentOwner.current) {
// Give the component that originally created this child.
addenda.childOwner = ' It was passed a child from ' + element._owner.getName() + '.';
}
return addenda;
}
/**
* Ensure that every element either is passed in a static location, in an
* array with an explicit keys property defined, or in an object literal
* with valid key property.
*
* @internal
* @param {ReactNode} node Statically passed child of any type.
* @param {*} parentType node's parent's type.
*/
function validateChildKeys(node, parentType) {
if (typeof node !== 'object') {
return;
}
if (Array.isArray(node)) {
for (var i = 0; i < node.length; i++) {
var child = node[i];
if (ReactElement.isValidElement(child)) {
validateExplicitKey(child, parentType);
}
}
} else if (ReactElement.isValidElement(node)) {
// This element was passed in a valid location.
if (node._store) {
node._store.validated = true;
}
} else if (node) {
var iteratorFn = getIteratorFn(node);
// Entry iterators provide implicit keys.
if (iteratorFn) {
if (iteratorFn !== node.entries) {
var iterator = iteratorFn.call(node);
var step;
while (!(step = iterator.next()).done) {
if (ReactElement.isValidElement(step.value)) {
validateExplicitKey(step.value, parentType);
}
}
}
}
}
}
/**
* Assert that the props are valid
*
* @param {string} componentName Name of the component for error messages.
* @param {object} propTypes Map of prop name to a ReactPropType
* @param {object} props
* @param {string} location e.g. "prop", "context", "child context"
* @private
*/
function checkPropTypes(componentName, propTypes, props, location) {
for (var propName in propTypes) {
if (propTypes.hasOwnProperty(propName)) {
var error;
// Prop type validation may throw. In case they do, we don't want to
// fail the render phase where it didn't fail before. So we log it.
// After these have been cleaned up, we'll let them throw.
try {
// This is intentionally an invariant that gets caught. It's the same
// behavior as without this statement except with a better message.
!(typeof propTypes[propName] === 'function') ? "development" !== 'production' ? invariant(false, '%s: %s type `%s` is invalid; it must be a function, usually from ' + 'React.PropTypes.', componentName || 'React class', ReactPropTypeLocationNames[location], propName) : invariant(false) : undefined;
error = propTypes[propName](props, propName, componentName, location);
} catch (ex) {
error = ex;
}
"development" !== 'production' ? warning(!error || error instanceof Error, '%s: type specification of %s `%s` is invalid; the type checker ' + 'function must return `null` or an `Error` but returned a %s. ' + 'You may have forgotten to pass an argument to the type checker ' + 'creator (arrayOf, instanceOf, objectOf, oneOf, oneOfType, and ' + 'shape all require an argument).', componentName || 'React class', ReactPropTypeLocationNames[location], propName, typeof error) : undefined;
if (error instanceof Error && !(error.message in loggedTypeFailures)) {
// Only monitor this failure once because there tends to be a lot of the
// same error.
loggedTypeFailures[error.message] = true;
var addendum = getDeclarationErrorAddendum();
"development" !== 'production' ? warning(false, 'Failed propType: %s%s', error.message, addendum) : undefined;
}
}
}
}
/**
* Given an element, validate that its props follow the propTypes definition,
* provided by the type.
*
* @param {ReactElement} element
*/
function validatePropTypes(element) {
var componentClass = element.type;
if (typeof componentClass !== 'function') {
return;
}
var name = componentClass.displayName || componentClass.name;
if (componentClass.propTypes) {
checkPropTypes(name, componentClass.propTypes, element.props, ReactPropTypeLocations.prop);
}
if (typeof componentClass.getDefaultProps === 'function') {
"development" !== 'production' ? warning(componentClass.getDefaultProps.isReactClassApproved, 'getDefaultProps is only used on classic React.createClass ' + 'definitions. Use a static property named `defaultProps` instead.') : undefined;
}
}
var ReactElementValidator = {
createElement: function (type, props, children) {
var validType = typeof type === 'string' || typeof type === 'function';
// We warn in this case but don't throw. We expect the element creation to
// succeed and there will likely be errors in render.
"development" !== 'production' ? warning(validType, 'React.createElement: type should not be null, undefined, boolean, or ' + 'number. It should be a string (for DOM elements) or a ReactClass ' + '(for composite components).%s', getDeclarationErrorAddendum()) : undefined;
var element = ReactElement.createElement.apply(this, arguments);
// The result can be nullish if a mock or a custom function is used.
// TODO: Drop this when these are no longer allowed as the type argument.
if (element == null) {
return element;
}
// Skip key warning if the type isn't valid since our key validation logic
// doesn't expect a non-string/function type and can throw confusing errors.
// We don't want exception behavior to differ between dev and prod.
// (Rendering will throw with a helpful message and as soon as the type is
// fixed, the key warnings will appear.)
if (validType) {
for (var i = 2; i < arguments.length; i++) {
validateChildKeys(arguments[i], type);
}
}
validatePropTypes(element);
return element;
},
createFactory: function (type) {
var validatedFactory = ReactElementValidator.createElement.bind(null, type);
// Legacy hook TODO: Warn if this is accessed
validatedFactory.type = type;
if ("development" !== 'production') {
if (canDefineProperty) {
Object.defineProperty(validatedFactory, 'type', {
enumerable: false,
get: function () {
"development" !== 'production' ? warning(false, 'Factory.type is deprecated. Access the class directly ' + 'before passing it to createFactory.') : undefined;
Object.defineProperty(this, 'type', {
value: type
});
return type;
}
});
}
}
return validatedFactory;
},
cloneElement: function (element, props, children) {
var newElement = ReactElement.cloneElement.apply(this, arguments);
for (var i = 2; i < arguments.length; i++) {
validateChildKeys(arguments[i], newElement.type);
}
validatePropTypes(newElement);
return newElement;
}
};
module.exports = ReactElementValidator;
},{"117":117,"129":129,"161":161,"173":173,"39":39,"57":57,"80":80,"81":81}],59:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactEmptyComponent
*/
'use strict';
var ReactElement = _dereq_(57);
var ReactEmptyComponentRegistry = _dereq_(60);
var ReactReconciler = _dereq_(84);
var assign = _dereq_(24);
var placeholderElement;
var ReactEmptyComponentInjection = {
injectEmptyComponent: function (component) {
placeholderElement = ReactElement.createElement(component);
}
};
var ReactEmptyComponent = function (instantiate) {
this._currentElement = null;
this._rootNodeID = null;
this._renderedComponent = instantiate(placeholderElement);
};
assign(ReactEmptyComponent.prototype, {
construct: function (element) {},
mountComponent: function (rootID, transaction, context) {
ReactEmptyComponentRegistry.registerNullComponentID(rootID);
this._rootNodeID = rootID;
return ReactReconciler.mountComponent(this._renderedComponent, rootID, transaction, context);
},
receiveComponent: function () {},
unmountComponent: function (rootID, transaction, context) {
ReactReconciler.unmountComponent(this._renderedComponent);
ReactEmptyComponentRegistry.deregisterNullComponentID(this._rootNodeID);
this._rootNodeID = null;
this._renderedComponent = null;
}
});
ReactEmptyComponent.injection = ReactEmptyComponentInjection;
module.exports = ReactEmptyComponent;
},{"24":24,"57":57,"60":60,"84":84}],60:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactEmptyComponentRegistry
*/
'use strict';
// This registry keeps track of the React IDs of the components that rendered to
// `null` (in reality a placeholder such as `noscript`)
var nullComponentIDsRegistry = {};
/**
* @param {string} id Component's `_rootNodeID`.
* @return {boolean} True if the component is rendered to null.
*/
function isNullComponentID(id) {
return !!nullComponentIDsRegistry[id];
}
/**
* Mark the component as having rendered to null.
* @param {string} id Component's `_rootNodeID`.
*/
function registerNullComponentID(id) {
nullComponentIDsRegistry[id] = true;
}
/**
* Unmark the component as having rendered to null: it renders to something now.
* @param {string} id Component's `_rootNodeID`.
*/
function deregisterNullComponentID(id) {
delete nullComponentIDsRegistry[id];
}
var ReactEmptyComponentRegistry = {
isNullComponentID: isNullComponentID,
registerNullComponentID: registerNullComponentID,
deregisterNullComponentID: deregisterNullComponentID
};
module.exports = ReactEmptyComponentRegistry;
},{}],61:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactErrorUtils
* @typechecks
*/
'use strict';
var caughtError = null;
/**
* Call a function while guarding against errors that happens within it.
*
* @param {?String} name of the guard to use for logging or debugging
* @param {Function} func The function to invoke
* @param {*} a First argument
* @param {*} b Second argument
*/
function invokeGuardedCallback(name, func, a, b) {
try {
return func(a, b);
} catch (x) {
if (caughtError === null) {
caughtError = x;
}
return undefined;
}
}
var ReactErrorUtils = {
invokeGuardedCallback: invokeGuardedCallback,
/**
* Invoked by ReactTestUtils.Simulate so that any errors thrown by the event
* handler are sure to be rethrown by rethrowCaughtError.
*/
invokeGuardedCallbackWithCatch: invokeGuardedCallback,
/**
* During execution of guarded functions we will capture the first error which
* we will rethrow to be handled by the top level error handler.
*/
rethrowCaughtError: function () {
if (caughtError) {
var error = caughtError;
caughtError = null;
throw error;
}
}
};
if ("development" !== 'production') {
/**
* To help development we can get better devtools integration by simulating a
* real browser event.
*/
if (typeof window !== 'undefined' && typeof window.dispatchEvent === 'function' && typeof document !== 'undefined' && typeof document.createEvent === 'function') {
var fakeNode = document.createElement('react');
ReactErrorUtils.invokeGuardedCallback = function (name, func, a, b) {
var boundFunc = func.bind(null, a, b);
var evtType = 'react-' + name;
fakeNode.addEventListener(evtType, boundFunc, false);
var evt = document.createEvent('Event');
evt.initEvent(evtType, false, false);
fakeNode.dispatchEvent(evt);
fakeNode.removeEventListener(evtType, boundFunc, false);
};
}
}
module.exports = ReactErrorUtils;
},{}],62:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactEventEmitterMixin
*/
'use strict';
var EventPluginHub = _dereq_(16);
function runEventQueueInBatch(events) {
EventPluginHub.enqueueEvents(events);
EventPluginHub.processEventQueue(false);
}
var ReactEventEmitterMixin = {
/**
* Streams a fired top-level event to `EventPluginHub` where plugins have the
* opportunity to create `ReactEvent`s to be dispatched.
*
* @param {string} topLevelType Record from `EventConstants`.
* @param {object} topLevelTarget The listening component root node.
* @param {string} topLevelTargetID ID of `topLevelTarget`.
* @param {object} nativeEvent Native environment event.
*/
handleTopLevel: function (topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget) {
var events = EventPluginHub.extractEvents(topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget);
runEventQueueInBatch(events);
}
};
module.exports = ReactEventEmitterMixin;
},{"16":16}],63:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactEventListener
* @typechecks static-only
*/
'use strict';
var EventListener = _dereq_(146);
var ExecutionEnvironment = _dereq_(147);
var PooledClass = _dereq_(25);
var ReactInstanceHandles = _dereq_(67);
var ReactMount = _dereq_(72);
var ReactUpdates = _dereq_(96);
var assign = _dereq_(24);
var getEventTarget = _dereq_(128);
var getUnboundedScrollPosition = _dereq_(158);
var DOCUMENT_FRAGMENT_NODE_TYPE = 11;
/**
* Finds the parent React component of `node`.
*
* @param {*} node
* @return {?DOMEventTarget} Parent container, or `null` if the specified node
* is not nested.
*/
function findParent(node) {
// TODO: It may be a good idea to cache this to prevent unnecessary DOM
// traversal, but caching is difficult to do correctly without using a
// mutation observer to listen for all DOM changes.
var nodeID = ReactMount.getID(node);
var rootID = ReactInstanceHandles.getReactRootIDFromNodeID(nodeID);
var container = ReactMount.findReactContainerForID(rootID);
var parent = ReactMount.getFirstReactDOM(container);
return parent;
}
// Used to store ancestor hierarchy in top level callback
function TopLevelCallbackBookKeeping(topLevelType, nativeEvent) {
this.topLevelType = topLevelType;
this.nativeEvent = nativeEvent;
this.ancestors = [];
}
assign(TopLevelCallbackBookKeeping.prototype, {
destructor: function () {
this.topLevelType = null;
this.nativeEvent = null;
this.ancestors.length = 0;
}
});
PooledClass.addPoolingTo(TopLevelCallbackBookKeeping, PooledClass.twoArgumentPooler);
function handleTopLevelImpl(bookKeeping) {
// TODO: Re-enable event.path handling
//
// if (bookKeeping.nativeEvent.path && bookKeeping.nativeEvent.path.length > 1) {
// // New browsers have a path attribute on native events
// handleTopLevelWithPath(bookKeeping);
// } else {
// // Legacy browsers don't have a path attribute on native events
// handleTopLevelWithoutPath(bookKeeping);
// }
void handleTopLevelWithPath; // temporarily unused
handleTopLevelWithoutPath(bookKeeping);
}
// Legacy browsers don't have a path attribute on native events
function handleTopLevelWithoutPath(bookKeeping) {
var topLevelTarget = ReactMount.getFirstReactDOM(getEventTarget(bookKeeping.nativeEvent)) || window;
// Loop through the hierarchy, in case there's any nested components.
// It's important that we build the array of ancestors before calling any
// event handlers, because event handlers can modify the DOM, leading to
// inconsistencies with ReactMount's node cache. See #1105.
var ancestor = topLevelTarget;
while (ancestor) {
bookKeeping.ancestors.push(ancestor);
ancestor = findParent(ancestor);
}
for (var i = 0; i < bookKeeping.ancestors.length; i++) {
topLevelTarget = bookKeeping.ancestors[i];
var topLevelTargetID = ReactMount.getID(topLevelTarget) || '';
ReactEventListener._handleTopLevel(bookKeeping.topLevelType, topLevelTarget, topLevelTargetID, bookKeeping.nativeEvent, getEventTarget(bookKeeping.nativeEvent));
}
}
// New browsers have a path attribute on native events
function handleTopLevelWithPath(bookKeeping) {
var path = bookKeeping.nativeEvent.path;
var currentNativeTarget = path[0];
var eventsFired = 0;
for (var i = 0; i < path.length; i++) {
var currentPathElement = path[i];
if (currentPathElement.nodeType === DOCUMENT_FRAGMENT_NODE_TYPE) {
currentNativeTarget = path[i + 1];
}
// TODO: slow
var reactParent = ReactMount.getFirstReactDOM(currentPathElement);
if (reactParent === currentPathElement) {
var currentPathElementID = ReactMount.getID(currentPathElement);
var newRootID = ReactInstanceHandles.getReactRootIDFromNodeID(currentPathElementID);
bookKeeping.ancestors.push(currentPathElement);
var topLevelTargetID = ReactMount.getID(currentPathElement) || '';
eventsFired++;
ReactEventListener._handleTopLevel(bookKeeping.topLevelType, currentPathElement, topLevelTargetID, bookKeeping.nativeEvent, currentNativeTarget);
// Jump to the root of this React render tree
while (currentPathElementID !== newRootID) {
i++;
currentPathElement = path[i];
currentPathElementID = ReactMount.getID(currentPathElement);
}
}
}
if (eventsFired === 0) {
ReactEventListener._handleTopLevel(bookKeeping.topLevelType, window, '', bookKeeping.nativeEvent, getEventTarget(bookKeeping.nativeEvent));
}
}
function scrollValueMonitor(cb) {
var scrollPosition = getUnboundedScrollPosition(window);
cb(scrollPosition);
}
var ReactEventListener = {
_enabled: true,
_handleTopLevel: null,
WINDOW_HANDLE: ExecutionEnvironment.canUseDOM ? window : null,
setHandleTopLevel: function (handleTopLevel) {
ReactEventListener._handleTopLevel = handleTopLevel;
},
setEnabled: function (enabled) {
ReactEventListener._enabled = !!enabled;
},
isEnabled: function () {
return ReactEventListener._enabled;
},
/**
* Traps top-level events by using event bubbling.
*
* @param {string} topLevelType Record from `EventConstants`.
* @param {string} handlerBaseName Event name (e.g. "click").
* @param {object} handle Element on which to attach listener.
* @return {?object} An object with a remove function which will forcefully
* remove the listener.
* @internal
*/
trapBubbledEvent: function (topLevelType, handlerBaseName, handle) {
var element = handle;
if (!element) {
return null;
}
return EventListener.listen(element, handlerBaseName, ReactEventListener.dispatchEvent.bind(null, topLevelType));
},
/**
* Traps a top-level event by using event capturing.
*
* @param {string} topLevelType Record from `EventConstants`.
* @param {string} handlerBaseName Event name (e.g. "click").
* @param {object} handle Element on which to attach listener.
* @return {?object} An object with a remove function which will forcefully
* remove the listener.
* @internal
*/
trapCapturedEvent: function (topLevelType, handlerBaseName, handle) {
var element = handle;
if (!element) {
return null;
}
return EventListener.capture(element, handlerBaseName, ReactEventListener.dispatchEvent.bind(null, topLevelType));
},
monitorScrollValue: function (refresh) {
var callback = scrollValueMonitor.bind(null, refresh);
EventListener.listen(window, 'scroll', callback);
},
dispatchEvent: function (topLevelType, nativeEvent) {
if (!ReactEventListener._enabled) {
return;
}
var bookKeeping = TopLevelCallbackBookKeeping.getPooled(topLevelType, nativeEvent);
try {
// Event queue being processed in the same cycle allows
// `preventDefault`.
ReactUpdates.batchedUpdates(handleTopLevelImpl, bookKeeping);
} finally {
TopLevelCallbackBookKeeping.release(bookKeeping);
}
}
};
module.exports = ReactEventListener;
},{"128":128,"146":146,"147":147,"158":158,"24":24,"25":25,"67":67,"72":72,"96":96}],64:[function(_dereq_,module,exports){
/**
* Copyright 2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactFragment
*/
'use strict';
var ReactChildren = _dereq_(32);
var ReactElement = _dereq_(57);
var emptyFunction = _dereq_(153);
var invariant = _dereq_(161);
var warning = _dereq_(173);
/**
* We used to allow keyed objects to serve as a collection of ReactElements,
* or nested sets. This allowed us a way to explicitly key a set a fragment of
* components. This is now being replaced with an opaque data structure.
* The upgrade path is to call React.addons.createFragment({ key: value }) to
* create a keyed fragment. The resulting data structure is an array.
*/
var numericPropertyRegex = /^\d+$/;
var warnedAboutNumeric = false;
var ReactFragment = {
// Wrap a keyed object in an opaque proxy that warns you if you access any
// of its properties.
create: function (object) {
if (typeof object !== 'object' || !object || Array.isArray(object)) {
"development" !== 'production' ? warning(false, 'React.addons.createFragment only accepts a single object. Got: %s', object) : undefined;
return object;
}
if (ReactElement.isValidElement(object)) {
"development" !== 'production' ? warning(false, 'React.addons.createFragment does not accept a ReactElement ' + 'without a wrapper object.') : undefined;
return object;
}
!(object.nodeType !== 1) ? "development" !== 'production' ? invariant(false, 'React.addons.createFragment(...): Encountered an invalid child; DOM ' + 'elements are not valid children of React components.') : invariant(false) : undefined;
var result = [];
for (var key in object) {
if ("development" !== 'production') {
if (!warnedAboutNumeric && numericPropertyRegex.test(key)) {
"development" !== 'production' ? warning(false, 'React.addons.createFragment(...): Child objects should have ' + 'non-numeric keys so ordering is preserved.') : undefined;
warnedAboutNumeric = true;
}
}
ReactChildren.mapIntoWithKeyPrefixInternal(object[key], result, key, emptyFunction.thatReturnsArgument);
}
return result;
}
};
module.exports = ReactFragment;
},{"153":153,"161":161,"173":173,"32":32,"57":57}],65:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactInjection
*/
'use strict';
var DOMProperty = _dereq_(10);
var EventPluginHub = _dereq_(16);
var ReactComponentEnvironment = _dereq_(36);
var ReactClass = _dereq_(33);
var ReactEmptyComponent = _dereq_(59);
var ReactBrowserEventEmitter = _dereq_(28);
var ReactNativeComponent = _dereq_(75);
var ReactPerf = _dereq_(78);
var ReactRootIndex = _dereq_(86);
var ReactUpdates = _dereq_(96);
var ReactInjection = {
Component: ReactComponentEnvironment.injection,
Class: ReactClass.injection,
DOMProperty: DOMProperty.injection,
EmptyComponent: ReactEmptyComponent.injection,
EventPluginHub: EventPluginHub.injection,
EventEmitter: ReactBrowserEventEmitter.injection,
NativeComponent: ReactNativeComponent.injection,
Perf: ReactPerf.injection,
RootIndex: ReactRootIndex.injection,
Updates: ReactUpdates.injection
};
module.exports = ReactInjection;
},{"10":10,"16":16,"28":28,"33":33,"36":36,"59":59,"75":75,"78":78,"86":86,"96":96}],66:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactInputSelection
*/
'use strict';
var ReactDOMSelection = _dereq_(49);
var containsNode = _dereq_(150);
var focusNode = _dereq_(155);
var getActiveElement = _dereq_(156);
function isInDocument(node) {
return containsNode(document.documentElement, node);
}
/**
* @ReactInputSelection: React input selection module. Based on Selection.js,
* but modified to be suitable for react and has a couple of bug fixes (doesn't
* assume buttons have range selections allowed).
* Input selection module for React.
*/
var ReactInputSelection = {
hasSelectionCapabilities: function (elem) {
var nodeName = elem && elem.nodeName && elem.nodeName.toLowerCase();
return nodeName && (nodeName === 'input' && elem.type === 'text' || nodeName === 'textarea' || elem.contentEditable === 'true');
},
getSelectionInformation: function () {
var focusedElem = getActiveElement();
return {
focusedElem: focusedElem,
selectionRange: ReactInputSelection.hasSelectionCapabilities(focusedElem) ? ReactInputSelection.getSelection(focusedElem) : null
};
},
/**
* @restoreSelection: If any selection information was potentially lost,
* restore it. This is useful when performing operations that could remove dom
* nodes and place them back in, resulting in focus being lost.
*/
restoreSelection: function (priorSelectionInformation) {
var curFocusedElem = getActiveElement();
var priorFocusedElem = priorSelectionInformation.focusedElem;
var priorSelectionRange = priorSelectionInformation.selectionRange;
if (curFocusedElem !== priorFocusedElem && isInDocument(priorFocusedElem)) {
if (ReactInputSelection.hasSelectionCapabilities(priorFocusedElem)) {
ReactInputSelection.setSelection(priorFocusedElem, priorSelectionRange);
}
focusNode(priorFocusedElem);
}
},
/**
* @getSelection: Gets the selection bounds of a focused textarea, input or
* contentEditable node.
* -@input: Look up selection bounds of this input
* -@return {start: selectionStart, end: selectionEnd}
*/
getSelection: function (input) {
var selection;
if ('selectionStart' in input) {
// Modern browser with input or textarea.
selection = {
start: input.selectionStart,
end: input.selectionEnd
};
} else if (document.selection && (input.nodeName && input.nodeName.toLowerCase() === 'input')) {
// IE8 input.
var range = document.selection.createRange();
// There can only be one selection per document in IE, so it must
// be in our element.
if (range.parentElement() === input) {
selection = {
start: -range.moveStart('character', -input.value.length),
end: -range.moveEnd('character', -input.value.length)
};
}
} else {
// Content editable or old IE textarea.
selection = ReactDOMSelection.getOffsets(input);
}
return selection || { start: 0, end: 0 };
},
/**
* @setSelection: Sets the selection bounds of a textarea or input and focuses
* the input.
* -@input Set selection bounds of this input or textarea
* -@offsets Object of same form that is returned from get*
*/
setSelection: function (input, offsets) {
var start = offsets.start;
var end = offsets.end;
if (typeof end === 'undefined') {
end = start;
}
if ('selectionStart' in input) {
input.selectionStart = start;
input.selectionEnd = Math.min(end, input.value.length);
} else if (document.selection && (input.nodeName && input.nodeName.toLowerCase() === 'input')) {
var range = input.createTextRange();
range.collapse(true);
range.moveStart('character', start);
range.moveEnd('character', end - start);
range.select();
} else {
ReactDOMSelection.setOffsets(input, offsets);
}
}
};
module.exports = ReactInputSelection;
},{"150":150,"155":155,"156":156,"49":49}],67:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactInstanceHandles
* @typechecks static-only
*/
'use strict';
var ReactRootIndex = _dereq_(86);
var invariant = _dereq_(161);
var SEPARATOR = '.';
var SEPARATOR_LENGTH = SEPARATOR.length;
/**
* Maximum depth of traversals before we consider the possibility of a bad ID.
*/
var MAX_TREE_DEPTH = 10000;
/**
* Creates a DOM ID prefix to use when mounting React components.
*
* @param {number} index A unique integer
* @return {string} React root ID.
* @internal
*/
function getReactRootIDString(index) {
return SEPARATOR + index.toString(36);
}
/**
* Checks if a character in the supplied ID is a separator or the end.
*
* @param {string} id A React DOM ID.
* @param {number} index Index of the character to check.
* @return {boolean} True if the character is a separator or end of the ID.
* @private
*/
function isBoundary(id, index) {
return id.charAt(index) === SEPARATOR || index === id.length;
}
/**
* Checks if the supplied string is a valid React DOM ID.
*
* @param {string} id A React DOM ID, maybe.
* @return {boolean} True if the string is a valid React DOM ID.
* @private
*/
function isValidID(id) {
return id === '' || id.charAt(0) === SEPARATOR && id.charAt(id.length - 1) !== SEPARATOR;
}
/**
* Checks if the first ID is an ancestor of or equal to the second ID.
*
* @param {string} ancestorID
* @param {string} descendantID
* @return {boolean} True if `ancestorID` is an ancestor of `descendantID`.
* @internal
*/
function isAncestorIDOf(ancestorID, descendantID) {
return descendantID.indexOf(ancestorID) === 0 && isBoundary(descendantID, ancestorID.length);
}
/**
* Gets the parent ID of the supplied React DOM ID, `id`.
*
* @param {string} id ID of a component.
* @return {string} ID of the parent, or an empty string.
* @private
*/
function getParentID(id) {
return id ? id.substr(0, id.lastIndexOf(SEPARATOR)) : '';
}
/**
* Gets the next DOM ID on the tree path from the supplied `ancestorID` to the
* supplied `destinationID`. If they are equal, the ID is returned.
*
* @param {string} ancestorID ID of an ancestor node of `destinationID`.
* @param {string} destinationID ID of the destination node.
* @return {string} Next ID on the path from `ancestorID` to `destinationID`.
* @private
*/
function getNextDescendantID(ancestorID, destinationID) {
!(isValidID(ancestorID) && isValidID(destinationID)) ? "development" !== 'production' ? invariant(false, 'getNextDescendantID(%s, %s): Received an invalid React DOM ID.', ancestorID, destinationID) : invariant(false) : undefined;
!isAncestorIDOf(ancestorID, destinationID) ? "development" !== 'production' ? invariant(false, 'getNextDescendantID(...): React has made an invalid assumption about ' + 'the DOM hierarchy. Expected `%s` to be an ancestor of `%s`.', ancestorID, destinationID) : invariant(false) : undefined;
if (ancestorID === destinationID) {
return ancestorID;
}
// Skip over the ancestor and the immediate separator. Traverse until we hit
// another separator or we reach the end of `destinationID`.
var start = ancestorID.length + SEPARATOR_LENGTH;
var i;
for (i = start; i < destinationID.length; i++) {
if (isBoundary(destinationID, i)) {
break;
}
}
return destinationID.substr(0, i);
}
/**
* Gets the nearest common ancestor ID of two IDs.
*
* Using this ID scheme, the nearest common ancestor ID is the longest common
* prefix of the two IDs that immediately preceded a "marker" in both strings.
*
* @param {string} oneID
* @param {string} twoID
* @return {string} Nearest common ancestor ID, or the empty string if none.
* @private
*/
function getFirstCommonAncestorID(oneID, twoID) {
var minLength = Math.min(oneID.length, twoID.length);
if (minLength === 0) {
return '';
}
var lastCommonMarkerIndex = 0;
// Use `<=` to traverse until the "EOL" of the shorter string.
for (var i = 0; i <= minLength; i++) {
if (isBoundary(oneID, i) && isBoundary(twoID, i)) {
lastCommonMarkerIndex = i;
} else if (oneID.charAt(i) !== twoID.charAt(i)) {
break;
}
}
var longestCommonID = oneID.substr(0, lastCommonMarkerIndex);
!isValidID(longestCommonID) ? "development" !== 'production' ? invariant(false, 'getFirstCommonAncestorID(%s, %s): Expected a valid React DOM ID: %s', oneID, twoID, longestCommonID) : invariant(false) : undefined;
return longestCommonID;
}
/**
* Traverses the parent path between two IDs (either up or down). The IDs must
* not be the same, and there must exist a parent path between them. If the
* callback returns `false`, traversal is stopped.
*
* @param {?string} start ID at which to start traversal.
* @param {?string} stop ID at which to end traversal.
* @param {function} cb Callback to invoke each ID with.
* @param {*} arg Argument to invoke the callback with.
* @param {?boolean} skipFirst Whether or not to skip the first node.
* @param {?boolean} skipLast Whether or not to skip the last node.
* @private
*/
function traverseParentPath(start, stop, cb, arg, skipFirst, skipLast) {
start = start || '';
stop = stop || '';
!(start !== stop) ? "development" !== 'production' ? invariant(false, 'traverseParentPath(...): Cannot traverse from and to the same ID, `%s`.', start) : invariant(false) : undefined;
var traverseUp = isAncestorIDOf(stop, start);
!(traverseUp || isAncestorIDOf(start, stop)) ? "development" !== 'production' ? invariant(false, 'traverseParentPath(%s, %s, ...): Cannot traverse from two IDs that do ' + 'not have a parent path.', start, stop) : invariant(false) : undefined;
// Traverse from `start` to `stop` one depth at a time.
var depth = 0;
var traverse = traverseUp ? getParentID : getNextDescendantID;
for (var id = start;; /* until break */id = traverse(id, stop)) {
var ret;
if ((!skipFirst || id !== start) && (!skipLast || id !== stop)) {
ret = cb(id, traverseUp, arg);
}
if (ret === false || id === stop) {
// Only break //after// visiting `stop`.
break;
}
!(depth++ < MAX_TREE_DEPTH) ? "development" !== 'production' ? invariant(false, 'traverseParentPath(%s, %s, ...): Detected an infinite loop while ' + 'traversing the React DOM ID tree. This may be due to malformed IDs: %s', start, stop, id) : invariant(false) : undefined;
}
}
/**
* Manages the IDs assigned to DOM representations of React components. This
* uses a specific scheme in order to traverse the DOM efficiently (e.g. in
* order to simulate events).
*
* @internal
*/
var ReactInstanceHandles = {
/**
* Constructs a React root ID
* @return {string} A React root ID.
*/
createReactRootID: function () {
return getReactRootIDString(ReactRootIndex.createReactRootIndex());
},
/**
* Constructs a React ID by joining a root ID with a name.
*
* @param {string} rootID Root ID of a parent component.
* @param {string} name A component's name (as flattened children).
* @return {string} A React ID.
* @internal
*/
createReactID: function (rootID, name) {
return rootID + name;
},
/**
* Gets the DOM ID of the React component that is the root of the tree that
* contains the React component with the supplied DOM ID.
*
* @param {string} id DOM ID of a React component.
* @return {?string} DOM ID of the React component that is the root.
* @internal
*/
getReactRootIDFromNodeID: function (id) {
if (id && id.charAt(0) === SEPARATOR && id.length > 1) {
var index = id.indexOf(SEPARATOR, 1);
return index > -1 ? id.substr(0, index) : id;
}
return null;
},
/**
* Traverses the ID hierarchy and invokes the supplied `cb` on any IDs that
* should would receive a `mouseEnter` or `mouseLeave` event.
*
* NOTE: Does not invoke the callback on the nearest common ancestor because
* nothing "entered" or "left" that element.
*
* @param {string} leaveID ID being left.
* @param {string} enterID ID being entered.
* @param {function} cb Callback to invoke on each entered/left ID.
* @param {*} upArg Argument to invoke the callback with on left IDs.
* @param {*} downArg Argument to invoke the callback with on entered IDs.
* @internal
*/
traverseEnterLeave: function (leaveID, enterID, cb, upArg, downArg) {
var ancestorID = getFirstCommonAncestorID(leaveID, enterID);
if (ancestorID !== leaveID) {
traverseParentPath(leaveID, ancestorID, cb, upArg, false, true);
}
if (ancestorID !== enterID) {
traverseParentPath(ancestorID, enterID, cb, downArg, true, false);
}
},
/**
* Simulates the traversal of a two-phase, capture/bubble event dispatch.
*
* NOTE: This traversal happens on IDs without touching the DOM.
*
* @param {string} targetID ID of the target node.
* @param {function} cb Callback to invoke.
* @param {*} arg Argument to invoke the callback with.
* @internal
*/
traverseTwoPhase: function (targetID, cb, arg) {
if (targetID) {
traverseParentPath('', targetID, cb, arg, true, false);
traverseParentPath(targetID, '', cb, arg, false, true);
}
},
/**
* Same as `traverseTwoPhase` but skips the `targetID`.
*/
traverseTwoPhaseSkipTarget: function (targetID, cb, arg) {
if (targetID) {
traverseParentPath('', targetID, cb, arg, true, true);
traverseParentPath(targetID, '', cb, arg, true, true);
}
},
/**
* Traverse a node ID, calling the supplied `cb` for each ancestor ID. For
* example, passing `.0.$row-0.1` would result in `cb` getting called
* with `.0`, `.0.$row-0`, and `.0.$row-0.1`.
*
* NOTE: This traversal happens on IDs without touching the DOM.
*
* @param {string} targetID ID of the target node.
* @param {function} cb Callback to invoke.
* @param {*} arg Argument to invoke the callback with.
* @internal
*/
traverseAncestors: function (targetID, cb, arg) {
traverseParentPath('', targetID, cb, arg, true, false);
},
getFirstCommonAncestorID: getFirstCommonAncestorID,
/**
* Exposed for unit testing.
* @private
*/
_getNextDescendantID: getNextDescendantID,
isAncestorIDOf: isAncestorIDOf,
SEPARATOR: SEPARATOR
};
module.exports = ReactInstanceHandles;
},{"161":161,"86":86}],68:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactInstanceMap
*/
'use strict';
/**
* `ReactInstanceMap` maintains a mapping from a public facing stateful
* instance (key) and the internal representation (value). This allows public
* methods to accept the user facing instance as an argument and map them back
* to internal methods.
*/
// TODO: Replace this with ES6: var ReactInstanceMap = new Map();
var ReactInstanceMap = {
/**
* This API should be called `delete` but we'd have to make sure to always
* transform these to strings for IE support. When this transform is fully
* supported we can rename it.
*/
remove: function (key) {
key._reactInternalInstance = undefined;
},
get: function (key) {
return key._reactInternalInstance;
},
has: function (key) {
return key._reactInternalInstance !== undefined;
},
set: function (key, value) {
key._reactInternalInstance = value;
}
};
module.exports = ReactInstanceMap;
},{}],69:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactIsomorphic
*/
'use strict';
var ReactChildren = _dereq_(32);
var ReactComponent = _dereq_(34);
var ReactClass = _dereq_(33);
var ReactDOMFactories = _dereq_(43);
var ReactElement = _dereq_(57);
var ReactElementValidator = _dereq_(58);
var ReactPropTypes = _dereq_(82);
var ReactVersion = _dereq_(97);
var assign = _dereq_(24);
var onlyChild = _dereq_(135);
var createElement = ReactElement.createElement;
var createFactory = ReactElement.createFactory;
var cloneElement = ReactElement.cloneElement;
if ("development" !== 'production') {
createElement = ReactElementValidator.createElement;
createFactory = ReactElementValidator.createFactory;
cloneElement = ReactElementValidator.cloneElement;
}
var React = {
// Modern
Children: {
map: ReactChildren.map,
forEach: ReactChildren.forEach,
count: ReactChildren.count,
toArray: ReactChildren.toArray,
only: onlyChild
},
Component: ReactComponent,
createElement: createElement,
cloneElement: cloneElement,
isValidElement: ReactElement.isValidElement,
// Classic
PropTypes: ReactPropTypes,
createClass: ReactClass.createClass,
createFactory: createFactory,
createMixin: function (mixin) {
// Currently a noop. Will be used to validate and trace mixins.
return mixin;
},
// This looks DOM specific but these are actually isomorphic helpers
// since they are just generating DOM strings.
DOM: ReactDOMFactories,
version: ReactVersion,
// Hook for JSX spread, don't use this for anything else.
__spread: assign
};
module.exports = React;
},{"135":135,"24":24,"32":32,"33":33,"34":34,"43":43,"57":57,"58":58,"82":82,"97":97}],70:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactLink
* @typechecks static-only
*/
'use strict';
/**
* ReactLink encapsulates a common pattern in which a component wants to modify
* a prop received from its parent. ReactLink allows the parent to pass down a
* value coupled with a callback that, when invoked, expresses an intent to
* modify that value. For example:
*
* React.createClass({
* getInitialState: function() {
* return {value: ''};
* },
* render: function() {
* var valueLink = new ReactLink(this.state.value, this._handleValueChange);
* return <input valueLink={valueLink} />;
* },
* _handleValueChange: function(newValue) {
* this.setState({value: newValue});
* }
* });
*
* We have provided some sugary mixins to make the creation and
* consumption of ReactLink easier; see LinkedValueUtils and LinkedStateMixin.
*/
var React = _dereq_(26);
/**
* @param {*} value current value of the link
* @param {function} requestChange callback to request a change
*/
function ReactLink(value, requestChange) {
this.value = value;
this.requestChange = requestChange;
}
/**
* Creates a PropType that enforces the ReactLink API and optionally checks the
* type of the value being passed inside the link. Example:
*
* MyComponent.propTypes = {
* tabIndexLink: ReactLink.PropTypes.link(React.PropTypes.number)
* }
*/
function createLinkTypeChecker(linkType) {
var shapes = {
value: typeof linkType === 'undefined' ? React.PropTypes.any.isRequired : linkType.isRequired,
requestChange: React.PropTypes.func.isRequired
};
return React.PropTypes.shape(shapes);
}
ReactLink.PropTypes = {
link: createLinkTypeChecker
};
module.exports = ReactLink;
},{"26":26}],71:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactMarkupChecksum
*/
'use strict';
var adler32 = _dereq_(116);
var TAG_END = /\/?>/;
var ReactMarkupChecksum = {
CHECKSUM_ATTR_NAME: 'data-react-checksum',
/**
* @param {string} markup Markup string
* @return {string} Markup string with checksum attribute attached
*/
addChecksumToMarkup: function (markup) {
var checksum = adler32(markup);
// Add checksum (handle both parent tags and self-closing tags)
return markup.replace(TAG_END, ' ' + ReactMarkupChecksum.CHECKSUM_ATTR_NAME + '="' + checksum + '"$&');
},
/**
* @param {string} markup to use
* @param {DOMElement} element root React element
* @returns {boolean} whether or not the markup is the same
*/
canReuseMarkup: function (markup, element) {
var existingChecksum = element.getAttribute(ReactMarkupChecksum.CHECKSUM_ATTR_NAME);
existingChecksum = existingChecksum && parseInt(existingChecksum, 10);
var markupChecksum = adler32(markup);
return markupChecksum === existingChecksum;
}
};
module.exports = ReactMarkupChecksum;
},{"116":116}],72:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactMount
*/
'use strict';
var DOMProperty = _dereq_(10);
var ReactBrowserEventEmitter = _dereq_(28);
var ReactCurrentOwner = _dereq_(39);
var ReactDOMFeatureFlags = _dereq_(44);
var ReactElement = _dereq_(57);
var ReactEmptyComponentRegistry = _dereq_(60);
var ReactInstanceHandles = _dereq_(67);
var ReactInstanceMap = _dereq_(68);
var ReactMarkupChecksum = _dereq_(71);
var ReactPerf = _dereq_(78);
var ReactReconciler = _dereq_(84);
var ReactUpdateQueue = _dereq_(95);
var ReactUpdates = _dereq_(96);
var assign = _dereq_(24);
var emptyObject = _dereq_(154);
var containsNode = _dereq_(150);
var instantiateReactComponent = _dereq_(132);
var invariant = _dereq_(161);
var setInnerHTML = _dereq_(138);
var shouldUpdateReactComponent = _dereq_(141);
var validateDOMNesting = _dereq_(144);
var warning = _dereq_(173);
var ATTR_NAME = DOMProperty.ID_ATTRIBUTE_NAME;
var nodeCache = {};
var ELEMENT_NODE_TYPE = 1;
var DOC_NODE_TYPE = 9;
var DOCUMENT_FRAGMENT_NODE_TYPE = 11;
var ownerDocumentContextKey = '__ReactMount_ownerDocument$' + Math.random().toString(36).slice(2);
/** Mapping from reactRootID to React component instance. */
var instancesByReactRootID = {};
/** Mapping from reactRootID to `container` nodes. */
var containersByReactRootID = {};
if ("development" !== 'production') {
/** __DEV__-only mapping from reactRootID to root elements. */
var rootElementsByReactRootID = {};
}
// Used to store breadth-first search state in findComponentRoot.
var findComponentRootReusableArray = [];
/**
* Finds the index of the first character
* that's not common between the two given strings.
*
* @return {number} the index of the character where the strings diverge
*/
function firstDifferenceIndex(string1, string2) {
var minLen = Math.min(string1.length, string2.length);
for (var i = 0; i < minLen; i++) {
if (string1.charAt(i) !== string2.charAt(i)) {
return i;
}
}
return string1.length === string2.length ? -1 : minLen;
}
/**
* @param {DOMElement|DOMDocument} container DOM element that may contain
* a React component
* @return {?*} DOM element that may have the reactRoot ID, or null.
*/
function getReactRootElementInContainer(container) {
if (!container) {
return null;
}
if (container.nodeType === DOC_NODE_TYPE) {
return container.documentElement;
} else {
return container.firstChild;
}
}
/**
* @param {DOMElement} container DOM element that may contain a React component.
* @return {?string} A "reactRoot" ID, if a React component is rendered.
*/
function getReactRootID(container) {
var rootElement = getReactRootElementInContainer(container);
return rootElement && ReactMount.getID(rootElement);
}
/**
* Accessing node[ATTR_NAME] or calling getAttribute(ATTR_NAME) on a form
* element can return its control whose name or ID equals ATTR_NAME. All
* DOM nodes support `getAttributeNode` but this can also get called on
* other objects so just return '' if we're given something other than a
* DOM node (such as window).
*
* @param {?DOMElement|DOMWindow|DOMDocument|DOMTextNode} node DOM node.
* @return {string} ID of the supplied `domNode`.
*/
function getID(node) {
var id = internalGetID(node);
if (id) {
if (nodeCache.hasOwnProperty(id)) {
var cached = nodeCache[id];
if (cached !== node) {
!!isValid(cached, id) ? "development" !== 'production' ? invariant(false, 'ReactMount: Two valid but unequal nodes with the same `%s`: %s', ATTR_NAME, id) : invariant(false) : undefined;
nodeCache[id] = node;
}
} else {
nodeCache[id] = node;
}
}
return id;
}
function internalGetID(node) {
// If node is something like a window, document, or text node, none of
// which support attributes or a .getAttribute method, gracefully return
// the empty string, as if the attribute were missing.
return node && node.getAttribute && node.getAttribute(ATTR_NAME) || '';
}
/**
* Sets the React-specific ID of the given node.
*
* @param {DOMElement} node The DOM node whose ID will be set.
* @param {string} id The value of the ID attribute.
*/
function setID(node, id) {
var oldID = internalGetID(node);
if (oldID !== id) {
delete nodeCache[oldID];
}
node.setAttribute(ATTR_NAME, id);
nodeCache[id] = node;
}
/**
* Finds the node with the supplied React-generated DOM ID.
*
* @param {string} id A React-generated DOM ID.
* @return {DOMElement} DOM node with the suppled `id`.
* @internal
*/
function getNode(id) {
if (!nodeCache.hasOwnProperty(id) || !isValid(nodeCache[id], id)) {
nodeCache[id] = ReactMount.findReactNodeByID(id);
}
return nodeCache[id];
}
/**
* Finds the node with the supplied public React instance.
*
* @param {*} instance A public React instance.
* @return {?DOMElement} DOM node with the suppled `id`.
* @internal
*/
function getNodeFromInstance(instance) {
var id = ReactInstanceMap.get(instance)._rootNodeID;
if (ReactEmptyComponentRegistry.isNullComponentID(id)) {
return null;
}
if (!nodeCache.hasOwnProperty(id) || !isValid(nodeCache[id], id)) {
nodeCache[id] = ReactMount.findReactNodeByID(id);
}
return nodeCache[id];
}
/**
* A node is "valid" if it is contained by a currently mounted container.
*
* This means that the node does not have to be contained by a document in
* order to be considered valid.
*
* @param {?DOMElement} node The candidate DOM node.
* @param {string} id The expected ID of the node.
* @return {boolean} Whether the node is contained by a mounted container.
*/
function isValid(node, id) {
if (node) {
!(internalGetID(node) === id) ? "development" !== 'production' ? invariant(false, 'ReactMount: Unexpected modification of `%s`', ATTR_NAME) : invariant(false) : undefined;
var container = ReactMount.findReactContainerForID(id);
if (container && containsNode(container, node)) {
return true;
}
}
return false;
}
/**
* Causes the cache to forget about one React-specific ID.
*
* @param {string} id The ID to forget.
*/
function purgeID(id) {
delete nodeCache[id];
}
var deepestNodeSoFar = null;
function findDeepestCachedAncestorImpl(ancestorID) {
var ancestor = nodeCache[ancestorID];
if (ancestor && isValid(ancestor, ancestorID)) {
deepestNodeSoFar = ancestor;
} else {
// This node isn't populated in the cache, so presumably none of its
// descendants are. Break out of the loop.
return false;
}
}
/**
* Return the deepest cached node whose ID is a prefix of `targetID`.
*/
function findDeepestCachedAncestor(targetID) {
deepestNodeSoFar = null;
ReactInstanceHandles.traverseAncestors(targetID, findDeepestCachedAncestorImpl);
var foundNode = deepestNodeSoFar;
deepestNodeSoFar = null;
return foundNode;
}
/**
* Mounts this component and inserts it into the DOM.
*
* @param {ReactComponent} componentInstance The instance to mount.
* @param {string} rootID DOM ID of the root node.
* @param {DOMElement} container DOM element to mount into.
* @param {ReactReconcileTransaction} transaction
* @param {boolean} shouldReuseMarkup If true, do not insert markup
*/
function mountComponentIntoNode(componentInstance, rootID, container, transaction, shouldReuseMarkup, context) {
if (ReactDOMFeatureFlags.useCreateElement) {
context = assign({}, context);
if (container.nodeType === DOC_NODE_TYPE) {
context[ownerDocumentContextKey] = container;
} else {
context[ownerDocumentContextKey] = container.ownerDocument;
}
}
if ("development" !== 'production') {
if (context === emptyObject) {
context = {};
}
var tag = container.nodeName.toLowerCase();
context[validateDOMNesting.ancestorInfoContextKey] = validateDOMNesting.updatedAncestorInfo(null, tag, null);
}
var markup = ReactReconciler.mountComponent(componentInstance, rootID, transaction, context);
componentInstance._renderedComponent._topLevelWrapper = componentInstance;
ReactMount._mountImageIntoNode(markup, container, shouldReuseMarkup, transaction);
}
/**
* Batched mount.
*
* @param {ReactComponent} componentInstance The instance to mount.
* @param {string} rootID DOM ID of the root node.
* @param {DOMElement} container DOM element to mount into.
* @param {boolean} shouldReuseMarkup If true, do not insert markup
*/
function batchedMountComponentIntoNode(componentInstance, rootID, container, shouldReuseMarkup, context) {
var transaction = ReactUpdates.ReactReconcileTransaction.getPooled(
/* forceHTML */shouldReuseMarkup);
transaction.perform(mountComponentIntoNode, null, componentInstance, rootID, container, transaction, shouldReuseMarkup, context);
ReactUpdates.ReactReconcileTransaction.release(transaction);
}
/**
* Unmounts a component and removes it from the DOM.
*
* @param {ReactComponent} instance React component instance.
* @param {DOMElement} container DOM element to unmount from.
* @final
* @internal
* @see {ReactMount.unmountComponentAtNode}
*/
function unmountComponentFromNode(instance, container) {
ReactReconciler.unmountComponent(instance);
if (container.nodeType === DOC_NODE_TYPE) {
container = container.documentElement;
}
// http://jsperf.com/emptying-a-node
while (container.lastChild) {
container.removeChild(container.lastChild);
}
}
/**
* True if the supplied DOM node has a direct React-rendered child that is
* not a React root element. Useful for warning in `render`,
* `unmountComponentAtNode`, etc.
*
* @param {?DOMElement} node The candidate DOM node.
* @return {boolean} True if the DOM element contains a direct child that was
* rendered by React but is not a root element.
* @internal
*/
function hasNonRootReactChild(node) {
var reactRootID = getReactRootID(node);
return reactRootID ? reactRootID !== ReactInstanceHandles.getReactRootIDFromNodeID(reactRootID) : false;
}
/**
* Returns the first (deepest) ancestor of a node which is rendered by this copy
* of React.
*/
function findFirstReactDOMImpl(node) {
// This node might be from another React instance, so we make sure not to
// examine the node cache here
for (; node && node.parentNode !== node; node = node.parentNode) {
if (node.nodeType !== 1) {
// Not a DOMElement, therefore not a React component
continue;
}
var nodeID = internalGetID(node);
if (!nodeID) {
continue;
}
var reactRootID = ReactInstanceHandles.getReactRootIDFromNodeID(nodeID);
// If containersByReactRootID contains the container we find by crawling up
// the tree, we know that this instance of React rendered the node.
// nb. isValid's strategy (with containsNode) does not work because render
// trees may be nested and we don't want a false positive in that case.
var current = node;
var lastID;
do {
lastID = internalGetID(current);
current = current.parentNode;
if (current == null) {
// The passed-in node has been detached from the container it was
// originally rendered into.
return null;
}
} while (lastID !== reactRootID);
if (current === containersByReactRootID[reactRootID]) {
return node;
}
}
return null;
}
/**
* Temporary (?) hack so that we can store all top-level pending updates on
* composites instead of having to worry about different types of components
* here.
*/
var TopLevelWrapper = function () {};
TopLevelWrapper.prototype.isReactComponent = {};
if ("development" !== 'production') {
TopLevelWrapper.displayName = 'TopLevelWrapper';
}
TopLevelWrapper.prototype.render = function () {
// this.props is actually a ReactElement
return this.props;
};
/**
* Mounting is the process of initializing a React component by creating its
* representative DOM elements and inserting them into a supplied `container`.
* Any prior content inside `container` is destroyed in the process.
*
* ReactMount.render(
* component,
* document.getElementById('container')
* );
*
* <div id="container"> <-- Supplied `container`.
* <div data-reactid=".3"> <-- Rendered reactRoot of React
* // ... component.
* </div>
* </div>
*
* Inside of `container`, the first element rendered is the "reactRoot".
*/
var ReactMount = {
TopLevelWrapper: TopLevelWrapper,
/** Exposed for debugging purposes **/
_instancesByReactRootID: instancesByReactRootID,
/**
* This is a hook provided to support rendering React components while
* ensuring that the apparent scroll position of its `container` does not
* change.
*
* @param {DOMElement} container The `container` being rendered into.
* @param {function} renderCallback This must be called once to do the render.
*/
scrollMonitor: function (container, renderCallback) {
renderCallback();
},
/**
* Take a component that's already mounted into the DOM and replace its props
* @param {ReactComponent} prevComponent component instance already in the DOM
* @param {ReactElement} nextElement component instance to render
* @param {DOMElement} container container to render into
* @param {?function} callback function triggered on completion
*/
_updateRootComponent: function (prevComponent, nextElement, container, callback) {
ReactMount.scrollMonitor(container, function () {
ReactUpdateQueue.enqueueElementInternal(prevComponent, nextElement);
if (callback) {
ReactUpdateQueue.enqueueCallbackInternal(prevComponent, callback);
}
});
if ("development" !== 'production') {
// Record the root element in case it later gets transplanted.
rootElementsByReactRootID[getReactRootID(container)] = getReactRootElementInContainer(container);
}
return prevComponent;
},
/**
* Register a component into the instance map and starts scroll value
* monitoring
* @param {ReactComponent} nextComponent component instance to render
* @param {DOMElement} container container to render into
* @return {string} reactRoot ID prefix
*/
_registerComponent: function (nextComponent, container) {
!(container && (container.nodeType === ELEMENT_NODE_TYPE || container.nodeType === DOC_NODE_TYPE || container.nodeType === DOCUMENT_FRAGMENT_NODE_TYPE)) ? "development" !== 'production' ? invariant(false, '_registerComponent(...): Target container is not a DOM element.') : invariant(false) : undefined;
ReactBrowserEventEmitter.ensureScrollValueMonitoring();
var reactRootID = ReactMount.registerContainer(container);
instancesByReactRootID[reactRootID] = nextComponent;
return reactRootID;
},
/**
* Render a new component into the DOM.
* @param {ReactElement} nextElement element to render
* @param {DOMElement} container container to render into
* @param {boolean} shouldReuseMarkup if we should skip the markup insertion
* @return {ReactComponent} nextComponent
*/
_renderNewRootComponent: function (nextElement, container, shouldReuseMarkup, context) {
// Various parts of our code (such as ReactCompositeComponent's
// _renderValidatedComponent) assume that calls to render aren't nested;
// verify that that's the case.
"development" !== 'production' ? warning(ReactCurrentOwner.current == null, '_renderNewRootComponent(): Render methods should be a pure function ' + 'of props and state; triggering nested component updates from ' + 'render is not allowed. If necessary, trigger nested updates in ' + 'componentDidUpdate. Check the render method of %s.', ReactCurrentOwner.current && ReactCurrentOwner.current.getName() || 'ReactCompositeComponent') : undefined;
var componentInstance = instantiateReactComponent(nextElement, null);
var reactRootID = ReactMount._registerComponent(componentInstance, container);
// The initial render is synchronous but any updates that happen during
// rendering, in componentWillMount or componentDidMount, will be batched
// according to the current batching strategy.
ReactUpdates.batchedUpdates(batchedMountComponentIntoNode, componentInstance, reactRootID, container, shouldReuseMarkup, context);
if ("development" !== 'production') {
// Record the root element in case it later gets transplanted.
rootElementsByReactRootID[reactRootID] = getReactRootElementInContainer(container);
}
return componentInstance;
},
/**
* Renders a React component into the DOM in the supplied `container`.
*
* If the React component was previously rendered into `container`, this will
* perform an update on it and only mutate the DOM as necessary to reflect the
* latest React component.
*
* @param {ReactComponent} parentComponent The conceptual parent of this render tree.
* @param {ReactElement} nextElement Component element to render.
* @param {DOMElement} container DOM element to render into.
* @param {?function} callback function triggered on completion
* @return {ReactComponent} Component instance rendered in `container`.
*/
renderSubtreeIntoContainer: function (parentComponent, nextElement, container, callback) {
!(parentComponent != null && parentComponent._reactInternalInstance != null) ? "development" !== 'production' ? invariant(false, 'parentComponent must be a valid React Component') : invariant(false) : undefined;
return ReactMount._renderSubtreeIntoContainer(parentComponent, nextElement, container, callback);
},
_renderSubtreeIntoContainer: function (parentComponent, nextElement, container, callback) {
!ReactElement.isValidElement(nextElement) ? "development" !== 'production' ? invariant(false, 'ReactDOM.render(): Invalid component element.%s', typeof nextElement === 'string' ? ' Instead of passing an element string, make sure to instantiate ' + 'it by passing it to React.createElement.' : typeof nextElement === 'function' ? ' Instead of passing a component class, make sure to instantiate ' + 'it by passing it to React.createElement.' :
// Check if it quacks like an element
nextElement != null && nextElement.props !== undefined ? ' This may be caused by unintentionally loading two independent ' + 'copies of React.' : '') : invariant(false) : undefined;
"development" !== 'production' ? warning(!container || !container.tagName || container.tagName.toUpperCase() !== 'BODY', 'render(): Rendering components directly into document.body is ' + 'discouraged, since its children are often manipulated by third-party ' + 'scripts and browser extensions. This may lead to subtle ' + 'reconciliation issues. Try rendering into a container element created ' + 'for your app.') : undefined;
var nextWrappedElement = new ReactElement(TopLevelWrapper, null, null, null, null, null, nextElement);
var prevComponent = instancesByReactRootID[getReactRootID(container)];
if (prevComponent) {
var prevWrappedElement = prevComponent._currentElement;
var prevElement = prevWrappedElement.props;
if (shouldUpdateReactComponent(prevElement, nextElement)) {
var publicInst = prevComponent._renderedComponent.getPublicInstance();
var updatedCallback = callback && function () {
callback.call(publicInst);
};
ReactMount._updateRootComponent(prevComponent, nextWrappedElement, container, updatedCallback);
return publicInst;
} else {
ReactMount.unmountComponentAtNode(container);
}
}
var reactRootElement = getReactRootElementInContainer(container);
var containerHasReactMarkup = reactRootElement && !!internalGetID(reactRootElement);
var containerHasNonRootReactChild = hasNonRootReactChild(container);
if ("development" !== 'production') {
"development" !== 'production' ? warning(!containerHasNonRootReactChild, 'render(...): Replacing React-rendered children with a new root ' + 'component. If you intended to update the children of this node, ' + 'you should instead have the existing children update their state ' + 'and render the new components instead of calling ReactDOM.render.') : undefined;
if (!containerHasReactMarkup || reactRootElement.nextSibling) {
var rootElementSibling = reactRootElement;
while (rootElementSibling) {
if (internalGetID(rootElementSibling)) {
"development" !== 'production' ? warning(false, 'render(): Target node has markup rendered by React, but there ' + 'are unrelated nodes as well. This is most commonly caused by ' + 'white-space inserted around server-rendered markup.') : undefined;
break;
}
rootElementSibling = rootElementSibling.nextSibling;
}
}
}
var shouldReuseMarkup = containerHasReactMarkup && !prevComponent && !containerHasNonRootReactChild;
var component = ReactMount._renderNewRootComponent(nextWrappedElement, container, shouldReuseMarkup, parentComponent != null ? parentComponent._reactInternalInstance._processChildContext(parentComponent._reactInternalInstance._context) : emptyObject)._renderedComponent.getPublicInstance();
if (callback) {
callback.call(component);
}
return component;
},
/**
* Renders a React component into the DOM in the supplied `container`.
*
* If the React component was previously rendered into `container`, this will
* perform an update on it and only mutate the DOM as necessary to reflect the
* latest React component.
*
* @param {ReactElement} nextElement Component element to render.
* @param {DOMElement} container DOM element to render into.
* @param {?function} callback function triggered on completion
* @return {ReactComponent} Component instance rendered in `container`.
*/
render: function (nextElement, container, callback) {
return ReactMount._renderSubtreeIntoContainer(null, nextElement, container, callback);
},
/**
* Registers a container node into which React components will be rendered.
* This also creates the "reactRoot" ID that will be assigned to the element
* rendered within.
*
* @param {DOMElement} container DOM element to register as a container.
* @return {string} The "reactRoot" ID of elements rendered within.
*/
registerContainer: function (container) {
var reactRootID = getReactRootID(container);
if (reactRootID) {
// If one exists, make sure it is a valid "reactRoot" ID.
reactRootID = ReactInstanceHandles.getReactRootIDFromNodeID(reactRootID);
}
if (!reactRootID) {
// No valid "reactRoot" ID found, create one.
reactRootID = ReactInstanceHandles.createReactRootID();
}
containersByReactRootID[reactRootID] = container;
return reactRootID;
},
/**
* Unmounts and destroys the React component rendered in the `container`.
*
* @param {DOMElement} container DOM element containing a React component.
* @return {boolean} True if a component was found in and unmounted from
* `container`
*/
unmountComponentAtNode: function (container) {
// Various parts of our code (such as ReactCompositeComponent's
// _renderValidatedComponent) assume that calls to render aren't nested;
// verify that that's the case. (Strictly speaking, unmounting won't cause a
// render but we still don't expect to be in a render call here.)
"development" !== 'production' ? warning(ReactCurrentOwner.current == null, 'unmountComponentAtNode(): Render methods should be a pure function ' + 'of props and state; triggering nested component updates from render ' + 'is not allowed. If necessary, trigger nested updates in ' + 'componentDidUpdate. Check the render method of %s.', ReactCurrentOwner.current && ReactCurrentOwner.current.getName() || 'ReactCompositeComponent') : undefined;
!(container && (container.nodeType === ELEMENT_NODE_TYPE || container.nodeType === DOC_NODE_TYPE || container.nodeType === DOCUMENT_FRAGMENT_NODE_TYPE)) ? "development" !== 'production' ? invariant(false, 'unmountComponentAtNode(...): Target container is not a DOM element.') : invariant(false) : undefined;
var reactRootID = getReactRootID(container);
var component = instancesByReactRootID[reactRootID];
if (!component) {
// Check if the node being unmounted was rendered by React, but isn't a
// root node.
var containerHasNonRootReactChild = hasNonRootReactChild(container);
// Check if the container itself is a React root node.
var containerID = internalGetID(container);
var isContainerReactRoot = containerID && containerID === ReactInstanceHandles.getReactRootIDFromNodeID(containerID);
if ("development" !== 'production') {
"development" !== 'production' ? warning(!containerHasNonRootReactChild, 'unmountComponentAtNode(): The node you\'re attempting to unmount ' + 'was rendered by React and is not a top-level container. %s', isContainerReactRoot ? 'You may have accidentally passed in a React root node instead ' + 'of its container.' : 'Instead, have the parent component update its state and ' + 'rerender in order to remove this component.') : undefined;
}
return false;
}
ReactUpdates.batchedUpdates(unmountComponentFromNode, component, container);
delete instancesByReactRootID[reactRootID];
delete containersByReactRootID[reactRootID];
if ("development" !== 'production') {
delete rootElementsByReactRootID[reactRootID];
}
return true;
},
/**
* Finds the container DOM element that contains React component to which the
* supplied DOM `id` belongs.
*
* @param {string} id The ID of an element rendered by a React component.
* @return {?DOMElement} DOM element that contains the `id`.
*/
findReactContainerForID: function (id) {
var reactRootID = ReactInstanceHandles.getReactRootIDFromNodeID(id);
var container = containersByReactRootID[reactRootID];
if ("development" !== 'production') {
var rootElement = rootElementsByReactRootID[reactRootID];
if (rootElement && rootElement.parentNode !== container) {
"development" !== 'production' ? warning(
// Call internalGetID here because getID calls isValid which calls
// findReactContainerForID (this function).
internalGetID(rootElement) === reactRootID, 'ReactMount: Root element ID differed from reactRootID.') : undefined;
var containerChild = container.firstChild;
if (containerChild && reactRootID === internalGetID(containerChild)) {
// If the container has a new child with the same ID as the old
// root element, then rootElementsByReactRootID[reactRootID] is
// just stale and needs to be updated. The case that deserves a
// warning is when the container is empty.
rootElementsByReactRootID[reactRootID] = containerChild;
} else {
"development" !== 'production' ? warning(false, 'ReactMount: Root element has been removed from its original ' + 'container. New container: %s', rootElement.parentNode) : undefined;
}
}
}
return container;
},
/**
* Finds an element rendered by React with the supplied ID.
*
* @param {string} id ID of a DOM node in the React component.
* @return {DOMElement} Root DOM node of the React component.
*/
findReactNodeByID: function (id) {
var reactRoot = ReactMount.findReactContainerForID(id);
return ReactMount.findComponentRoot(reactRoot, id);
},
/**
* Traverses up the ancestors of the supplied node to find a node that is a
* DOM representation of a React component rendered by this copy of React.
*
* @param {*} node
* @return {?DOMEventTarget}
* @internal
*/
getFirstReactDOM: function (node) {
return findFirstReactDOMImpl(node);
},
/**
* Finds a node with the supplied `targetID` inside of the supplied
* `ancestorNode`. Exploits the ID naming scheme to perform the search
* quickly.
*
* @param {DOMEventTarget} ancestorNode Search from this root.
* @pararm {string} targetID ID of the DOM representation of the component.
* @return {DOMEventTarget} DOM node with the supplied `targetID`.
* @internal
*/
findComponentRoot: function (ancestorNode, targetID) {
var firstChildren = findComponentRootReusableArray;
var childIndex = 0;
var deepestAncestor = findDeepestCachedAncestor(targetID) || ancestorNode;
if ("development" !== 'production') {
// This will throw on the next line; give an early warning
"development" !== 'production' ? warning(deepestAncestor != null, 'React can\'t find the root component node for data-reactid value ' + '`%s`. If you\'re seeing this message, it probably means that ' + 'you\'ve loaded two copies of React on the page. At this time, only ' + 'a single copy of React can be loaded at a time.', targetID) : undefined;
}
firstChildren[0] = deepestAncestor.firstChild;
firstChildren.length = 1;
while (childIndex < firstChildren.length) {
var child = firstChildren[childIndex++];
var targetChild;
while (child) {
var childID = ReactMount.getID(child);
if (childID) {
// Even if we find the node we're looking for, we finish looping
// through its siblings to ensure they're cached so that we don't have
// to revisit this node again. Otherwise, we make n^2 calls to getID
// when visiting the many children of a single node in order.
if (targetID === childID) {
targetChild = child;
} else if (ReactInstanceHandles.isAncestorIDOf(childID, targetID)) {
// If we find a child whose ID is an ancestor of the given ID,
// then we can be sure that we only want to search the subtree
// rooted at this child, so we can throw out the rest of the
// search state.
firstChildren.length = childIndex = 0;
firstChildren.push(child.firstChild);
}
} else {
// If this child had no ID, then there's a chance that it was
// injected automatically by the browser, as when a `<table>`
// element sprouts an extra `<tbody>` child as a side effect of
// `.innerHTML` parsing. Optimistically continue down this
// branch, but not before examining the other siblings.
firstChildren.push(child.firstChild);
}
child = child.nextSibling;
}
if (targetChild) {
// Emptying firstChildren/findComponentRootReusableArray is
// not necessary for correctness, but it helps the GC reclaim
// any nodes that were left at the end of the search.
firstChildren.length = 0;
return targetChild;
}
}
firstChildren.length = 0;
!false ? "development" !== 'production' ? invariant(false, 'findComponentRoot(..., %s): Unable to find element. This probably ' + 'means the DOM was unexpectedly mutated (e.g., by the browser), ' + 'usually due to forgetting a <tbody> when using tables, nesting tags ' + 'like <form>, <p>, or <a>, or using non-SVG elements in an <svg> ' + 'parent. ' + 'Try inspecting the child nodes of the element with React ID `%s`.', targetID, ReactMount.getID(ancestorNode)) : invariant(false) : undefined;
},
_mountImageIntoNode: function (markup, container, shouldReuseMarkup, transaction) {
!(container && (container.nodeType === ELEMENT_NODE_TYPE || container.nodeType === DOC_NODE_TYPE || container.nodeType === DOCUMENT_FRAGMENT_NODE_TYPE)) ? "development" !== 'production' ? invariant(false, 'mountComponentIntoNode(...): Target container is not valid.') : invariant(false) : undefined;
if (shouldReuseMarkup) {
var rootElement = getReactRootElementInContainer(container);
if (ReactMarkupChecksum.canReuseMarkup(markup, rootElement)) {
return;
} else {
var checksum = rootElement.getAttribute(ReactMarkupChecksum.CHECKSUM_ATTR_NAME);
rootElement.removeAttribute(ReactMarkupChecksum.CHECKSUM_ATTR_NAME);
var rootMarkup = rootElement.outerHTML;
rootElement.setAttribute(ReactMarkupChecksum.CHECKSUM_ATTR_NAME, checksum);
var normalizedMarkup = markup;
if ("development" !== 'production') {
// because rootMarkup is retrieved from the DOM, various normalizations
// will have occurred which will not be present in `markup`. Here,
// insert markup into a <div> or <iframe> depending on the container
// type to perform the same normalizations before comparing.
var normalizer;
if (container.nodeType === ELEMENT_NODE_TYPE) {
normalizer = document.createElement('div');
normalizer.innerHTML = markup;
normalizedMarkup = normalizer.innerHTML;
} else {
normalizer = document.createElement('iframe');
document.body.appendChild(normalizer);
normalizer.contentDocument.write(markup);
normalizedMarkup = normalizer.contentDocument.documentElement.outerHTML;
document.body.removeChild(normalizer);
}
}
var diffIndex = firstDifferenceIndex(normalizedMarkup, rootMarkup);
var difference = ' (client) ' + normalizedMarkup.substring(diffIndex - 20, diffIndex + 20) + '\n (server) ' + rootMarkup.substring(diffIndex - 20, diffIndex + 20);
!(container.nodeType !== DOC_NODE_TYPE) ? "development" !== 'production' ? invariant(false, 'You\'re trying to render a component to the document using ' + 'server rendering but the checksum was invalid. This usually ' + 'means you rendered a different component type or props on ' + 'the client from the one on the server, or your render() ' + 'methods are impure. React cannot handle this case due to ' + 'cross-browser quirks by rendering at the document root. You ' + 'should look for environment dependent code in your components ' + 'and ensure the props are the same client and server side:\n%s', difference) : invariant(false) : undefined;
if ("development" !== 'production') {
"development" !== 'production' ? warning(false, 'React attempted to reuse markup in a container but the ' + 'checksum was invalid. This generally means that you are ' + 'using server rendering and the markup generated on the ' + 'server was not what the client was expecting. React injected ' + 'new markup to compensate which works but you have lost many ' + 'of the benefits of server rendering. Instead, figure out ' + 'why the markup being generated is different on the client ' + 'or server:\n%s', difference) : undefined;
}
}
}
!(container.nodeType !== DOC_NODE_TYPE) ? "development" !== 'production' ? invariant(false, 'You\'re trying to render a component to the document but ' + 'you didn\'t use server rendering. We can\'t do this ' + 'without using server rendering due to cross-browser quirks. ' + 'See ReactDOMServer.renderToString() for server rendering.') : invariant(false) : undefined;
if (transaction.useCreateElement) {
while (container.lastChild) {
container.removeChild(container.lastChild);
}
container.appendChild(markup);
} else {
setInnerHTML(container, markup);
}
},
ownerDocumentContextKey: ownerDocumentContextKey,
/**
* React ID utilities.
*/
getReactRootID: getReactRootID,
getID: getID,
setID: setID,
getNode: getNode,
getNodeFromInstance: getNodeFromInstance,
isValid: isValid,
purgeID: purgeID
};
ReactPerf.measureMethods(ReactMount, 'ReactMount', {
_renderNewRootComponent: '_renderNewRootComponent',
_mountImageIntoNode: '_mountImageIntoNode'
});
module.exports = ReactMount;
},{"10":10,"132":132,"138":138,"141":141,"144":144,"150":150,"154":154,"161":161,"173":173,"24":24,"28":28,"39":39,"44":44,"57":57,"60":60,"67":67,"68":68,"71":71,"78":78,"84":84,"95":95,"96":96}],73:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactMultiChild
* @typechecks static-only
*/
'use strict';
var ReactComponentEnvironment = _dereq_(36);
var ReactMultiChildUpdateTypes = _dereq_(74);
var ReactCurrentOwner = _dereq_(39);
var ReactReconciler = _dereq_(84);
var ReactChildReconciler = _dereq_(31);
var flattenChildren = _dereq_(123);
/**
* Updating children of a component may trigger recursive updates. The depth is
* used to batch recursive updates to render markup more efficiently.
*
* @type {number}
* @private
*/
var updateDepth = 0;
/**
* Queue of update configuration objects.
*
* Each object has a `type` property that is in `ReactMultiChildUpdateTypes`.
*
* @type {array<object>}
* @private
*/
var updateQueue = [];
/**
* Queue of markup to be rendered.
*
* @type {array<string>}
* @private
*/
var markupQueue = [];
/**
* Enqueues markup to be rendered and inserted at a supplied index.
*
* @param {string} parentID ID of the parent component.
* @param {string} markup Markup that renders into an element.
* @param {number} toIndex Destination index.
* @private
*/
function enqueueInsertMarkup(parentID, markup, toIndex) {
// NOTE: Null values reduce hidden classes.
updateQueue.push({
parentID: parentID,
parentNode: null,
type: ReactMultiChildUpdateTypes.INSERT_MARKUP,
markupIndex: markupQueue.push(markup) - 1,
content: null,
fromIndex: null,
toIndex: toIndex
});
}
/**
* Enqueues moving an existing element to another index.
*
* @param {string} parentID ID of the parent component.
* @param {number} fromIndex Source index of the existing element.
* @param {number} toIndex Destination index of the element.
* @private
*/
function enqueueMove(parentID, fromIndex, toIndex) {
// NOTE: Null values reduce hidden classes.
updateQueue.push({
parentID: parentID,
parentNode: null,
type: ReactMultiChildUpdateTypes.MOVE_EXISTING,
markupIndex: null,
content: null,
fromIndex: fromIndex,
toIndex: toIndex
});
}
/**
* Enqueues removing an element at an index.
*
* @param {string} parentID ID of the parent component.
* @param {number} fromIndex Index of the element to remove.
* @private
*/
function enqueueRemove(parentID, fromIndex) {
// NOTE: Null values reduce hidden classes.
updateQueue.push({
parentID: parentID,
parentNode: null,
type: ReactMultiChildUpdateTypes.REMOVE_NODE,
markupIndex: null,
content: null,
fromIndex: fromIndex,
toIndex: null
});
}
/**
* Enqueues setting the markup of a node.
*
* @param {string} parentID ID of the parent component.
* @param {string} markup Markup that renders into an element.
* @private
*/
function enqueueSetMarkup(parentID, markup) {
// NOTE: Null values reduce hidden classes.
updateQueue.push({
parentID: parentID,
parentNode: null,
type: ReactMultiChildUpdateTypes.SET_MARKUP,
markupIndex: null,
content: markup,
fromIndex: null,
toIndex: null
});
}
/**
* Enqueues setting the text content.
*
* @param {string} parentID ID of the parent component.
* @param {string} textContent Text content to set.
* @private
*/
function enqueueTextContent(parentID, textContent) {
// NOTE: Null values reduce hidden classes.
updateQueue.push({
parentID: parentID,
parentNode: null,
type: ReactMultiChildUpdateTypes.TEXT_CONTENT,
markupIndex: null,
content: textContent,
fromIndex: null,
toIndex: null
});
}
/**
* Processes any enqueued updates.
*
* @private
*/
function processQueue() {
if (updateQueue.length) {
ReactComponentEnvironment.processChildrenUpdates(updateQueue, markupQueue);
clearQueue();
}
}
/**
* Clears any enqueued updates.
*
* @private
*/
function clearQueue() {
updateQueue.length = 0;
markupQueue.length = 0;
}
/**
* ReactMultiChild are capable of reconciling multiple children.
*
* @class ReactMultiChild
* @internal
*/
var ReactMultiChild = {
/**
* Provides common functionality for components that must reconcile multiple
* children. This is used by `ReactDOMComponent` to mount, update, and
* unmount child components.
*
* @lends {ReactMultiChild.prototype}
*/
Mixin: {
_reconcilerInstantiateChildren: function (nestedChildren, transaction, context) {
if ("development" !== 'production') {
if (this._currentElement) {
try {
ReactCurrentOwner.current = this._currentElement._owner;
return ReactChildReconciler.instantiateChildren(nestedChildren, transaction, context);
} finally {
ReactCurrentOwner.current = null;
}
}
}
return ReactChildReconciler.instantiateChildren(nestedChildren, transaction, context);
},
_reconcilerUpdateChildren: function (prevChildren, nextNestedChildrenElements, transaction, context) {
var nextChildren;
if ("development" !== 'production') {
if (this._currentElement) {
try {
ReactCurrentOwner.current = this._currentElement._owner;
nextChildren = flattenChildren(nextNestedChildrenElements);
} finally {
ReactCurrentOwner.current = null;
}
return ReactChildReconciler.updateChildren(prevChildren, nextChildren, transaction, context);
}
}
nextChildren = flattenChildren(nextNestedChildrenElements);
return ReactChildReconciler.updateChildren(prevChildren, nextChildren, transaction, context);
},
/**
* Generates a "mount image" for each of the supplied children. In the case
* of `ReactDOMComponent`, a mount image is a string of markup.
*
* @param {?object} nestedChildren Nested child maps.
* @return {array} An array of mounted representations.
* @internal
*/
mountChildren: function (nestedChildren, transaction, context) {
var children = this._reconcilerInstantiateChildren(nestedChildren, transaction, context);
this._renderedChildren = children;
var mountImages = [];
var index = 0;
for (var name in children) {
if (children.hasOwnProperty(name)) {
var child = children[name];
// Inlined for performance, see `ReactInstanceHandles.createReactID`.
var rootID = this._rootNodeID + name;
var mountImage = ReactReconciler.mountComponent(child, rootID, transaction, context);
child._mountIndex = index++;
mountImages.push(mountImage);
}
}
return mountImages;
},
/**
* Replaces any rendered children with a text content string.
*
* @param {string} nextContent String of content.
* @internal
*/
updateTextContent: function (nextContent) {
updateDepth++;
var errorThrown = true;
try {
var prevChildren = this._renderedChildren;
// Remove any rendered children.
ReactChildReconciler.unmountChildren(prevChildren);
// TODO: The setTextContent operation should be enough
for (var name in prevChildren) {
if (prevChildren.hasOwnProperty(name)) {
this._unmountChild(prevChildren[name]);
}
}
// Set new text content.
this.setTextContent(nextContent);
errorThrown = false;
} finally {
updateDepth--;
if (!updateDepth) {
if (errorThrown) {
clearQueue();
} else {
processQueue();
}
}
}
},
/**
* Replaces any rendered children with a markup string.
*
* @param {string} nextMarkup String of markup.
* @internal
*/
updateMarkup: function (nextMarkup) {
updateDepth++;
var errorThrown = true;
try {
var prevChildren = this._renderedChildren;
// Remove any rendered children.
ReactChildReconciler.unmountChildren(prevChildren);
for (var name in prevChildren) {
if (prevChildren.hasOwnProperty(name)) {
this._unmountChildByName(prevChildren[name], name);
}
}
this.setMarkup(nextMarkup);
errorThrown = false;
} finally {
updateDepth--;
if (!updateDepth) {
if (errorThrown) {
clearQueue();
} else {
processQueue();
}
}
}
},
/**
* Updates the rendered children with new children.
*
* @param {?object} nextNestedChildrenElements Nested child element maps.
* @param {ReactReconcileTransaction} transaction
* @internal
*/
updateChildren: function (nextNestedChildrenElements, transaction, context) {
updateDepth++;
var errorThrown = true;
try {
this._updateChildren(nextNestedChildrenElements, transaction, context);
errorThrown = false;
} finally {
updateDepth--;
if (!updateDepth) {
if (errorThrown) {
clearQueue();
} else {
processQueue();
}
}
}
},
/**
* Improve performance by isolating this hot code path from the try/catch
* block in `updateChildren`.
*
* @param {?object} nextNestedChildrenElements Nested child element maps.
* @param {ReactReconcileTransaction} transaction
* @final
* @protected
*/
_updateChildren: function (nextNestedChildrenElements, transaction, context) {
var prevChildren = this._renderedChildren;
var nextChildren = this._reconcilerUpdateChildren(prevChildren, nextNestedChildrenElements, transaction, context);
this._renderedChildren = nextChildren;
if (!nextChildren && !prevChildren) {
return;
}
var name;
// `nextIndex` will increment for each child in `nextChildren`, but
// `lastIndex` will be the last index visited in `prevChildren`.
var lastIndex = 0;
var nextIndex = 0;
for (name in nextChildren) {
if (!nextChildren.hasOwnProperty(name)) {
continue;
}
var prevChild = prevChildren && prevChildren[name];
var nextChild = nextChildren[name];
if (prevChild === nextChild) {
this.moveChild(prevChild, nextIndex, lastIndex);
lastIndex = Math.max(prevChild._mountIndex, lastIndex);
prevChild._mountIndex = nextIndex;
} else {
if (prevChild) {
// Update `lastIndex` before `_mountIndex` gets unset by unmounting.
lastIndex = Math.max(prevChild._mountIndex, lastIndex);
this._unmountChild(prevChild);
}
// The child must be instantiated before it's mounted.
this._mountChildByNameAtIndex(nextChild, name, nextIndex, transaction, context);
}
nextIndex++;
}
// Remove children that are no longer present.
for (name in prevChildren) {
if (prevChildren.hasOwnProperty(name) && !(nextChildren && nextChildren.hasOwnProperty(name))) {
this._unmountChild(prevChildren[name]);
}
}
},
/**
* Unmounts all rendered children. This should be used to clean up children
* when this component is unmounted.
*
* @internal
*/
unmountChildren: function () {
var renderedChildren = this._renderedChildren;
ReactChildReconciler.unmountChildren(renderedChildren);
this._renderedChildren = null;
},
/**
* Moves a child component to the supplied index.
*
* @param {ReactComponent} child Component to move.
* @param {number} toIndex Destination index of the element.
* @param {number} lastIndex Last index visited of the siblings of `child`.
* @protected
*/
moveChild: function (child, toIndex, lastIndex) {
// If the index of `child` is less than `lastIndex`, then it needs to
// be moved. Otherwise, we do not need to move it because a child will be
// inserted or moved before `child`.
if (child._mountIndex < lastIndex) {
enqueueMove(this._rootNodeID, child._mountIndex, toIndex);
}
},
/**
* Creates a child component.
*
* @param {ReactComponent} child Component to create.
* @param {string} mountImage Markup to insert.
* @protected
*/
createChild: function (child, mountImage) {
enqueueInsertMarkup(this._rootNodeID, mountImage, child._mountIndex);
},
/**
* Removes a child component.
*
* @param {ReactComponent} child Child to remove.
* @protected
*/
removeChild: function (child) {
enqueueRemove(this._rootNodeID, child._mountIndex);
},
/**
* Sets this text content string.
*
* @param {string} textContent Text content to set.
* @protected
*/
setTextContent: function (textContent) {
enqueueTextContent(this._rootNodeID, textContent);
},
/**
* Sets this markup string.
*
* @param {string} markup Markup to set.
* @protected
*/
setMarkup: function (markup) {
enqueueSetMarkup(this._rootNodeID, markup);
},
/**
* Mounts a child with the supplied name.
*
* NOTE: This is part of `updateChildren` and is here for readability.
*
* @param {ReactComponent} child Component to mount.
* @param {string} name Name of the child.
* @param {number} index Index at which to insert the child.
* @param {ReactReconcileTransaction} transaction
* @private
*/
_mountChildByNameAtIndex: function (child, name, index, transaction, context) {
// Inlined for performance, see `ReactInstanceHandles.createReactID`.
var rootID = this._rootNodeID + name;
var mountImage = ReactReconciler.mountComponent(child, rootID, transaction, context);
child._mountIndex = index;
this.createChild(child, mountImage);
},
/**
* Unmounts a rendered child.
*
* NOTE: This is part of `updateChildren` and is here for readability.
*
* @param {ReactComponent} child Component to unmount.
* @private
*/
_unmountChild: function (child) {
this.removeChild(child);
child._mountIndex = null;
}
}
};
module.exports = ReactMultiChild;
},{"123":123,"31":31,"36":36,"39":39,"74":74,"84":84}],74:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactMultiChildUpdateTypes
*/
'use strict';
var keyMirror = _dereq_(165);
/**
* When a component's children are updated, a series of update configuration
* objects are created in order to batch and serialize the required changes.
*
* Enumerates all the possible types of update configurations.
*
* @internal
*/
var ReactMultiChildUpdateTypes = keyMirror({
INSERT_MARKUP: null,
MOVE_EXISTING: null,
REMOVE_NODE: null,
SET_MARKUP: null,
TEXT_CONTENT: null
});
module.exports = ReactMultiChildUpdateTypes;
},{"165":165}],75:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactNativeComponent
*/
'use strict';
var assign = _dereq_(24);
var invariant = _dereq_(161);
var autoGenerateWrapperClass = null;
var genericComponentClass = null;
// This registry keeps track of wrapper classes around native tags.
var tagToComponentClass = {};
var textComponentClass = null;
var ReactNativeComponentInjection = {
// This accepts a class that receives the tag string. This is a catch all
// that can render any kind of tag.
injectGenericComponentClass: function (componentClass) {
genericComponentClass = componentClass;
},
// This accepts a text component class that takes the text string to be
// rendered as props.
injectTextComponentClass: function (componentClass) {
textComponentClass = componentClass;
},
// This accepts a keyed object with classes as values. Each key represents a
// tag. That particular tag will use this class instead of the generic one.
injectComponentClasses: function (componentClasses) {
assign(tagToComponentClass, componentClasses);
}
};
/**
* Get a composite component wrapper class for a specific tag.
*
* @param {ReactElement} element The tag for which to get the class.
* @return {function} The React class constructor function.
*/
function getComponentClassForElement(element) {
if (typeof element.type === 'function') {
return element.type;
}
var tag = element.type;
var componentClass = tagToComponentClass[tag];
if (componentClass == null) {
tagToComponentClass[tag] = componentClass = autoGenerateWrapperClass(tag);
}
return componentClass;
}
/**
* Get a native internal component class for a specific tag.
*
* @param {ReactElement} element The element to create.
* @return {function} The internal class constructor function.
*/
function createInternalComponent(element) {
!genericComponentClass ? "development" !== 'production' ? invariant(false, 'There is no registered component for the tag %s', element.type) : invariant(false) : undefined;
return new genericComponentClass(element.type, element.props);
}
/**
* @param {ReactText} text
* @return {ReactComponent}
*/
function createInstanceForText(text) {
return new textComponentClass(text);
}
/**
* @param {ReactComponent} component
* @return {boolean}
*/
function isTextComponent(component) {
return component instanceof textComponentClass;
}
var ReactNativeComponent = {
getComponentClassForElement: getComponentClassForElement,
createInternalComponent: createInternalComponent,
createInstanceForText: createInstanceForText,
isTextComponent: isTextComponent,
injection: ReactNativeComponentInjection
};
module.exports = ReactNativeComponent;
},{"161":161,"24":24}],76:[function(_dereq_,module,exports){
/**
* Copyright 2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactNoopUpdateQueue
*/
'use strict';
var warning = _dereq_(173);
function warnTDZ(publicInstance, callerName) {
if ("development" !== 'production') {
"development" !== 'production' ? warning(false, '%s(...): Can only update a mounted or mounting component. ' + 'This usually means you called %s() on an unmounted component. ' + 'This is a no-op. Please check the code for the %s component.', callerName, callerName, publicInstance.constructor && publicInstance.constructor.displayName || '') : undefined;
}
}
/**
* This is the abstract API for an update queue.
*/
var ReactNoopUpdateQueue = {
/**
* Checks whether or not this composite component is mounted.
* @param {ReactClass} publicInstance The instance we want to test.
* @return {boolean} True if mounted, false otherwise.
* @protected
* @final
*/
isMounted: function (publicInstance) {
return false;
},
/**
* Enqueue a callback that will be executed after all the pending updates
* have processed.
*
* @param {ReactClass} publicInstance The instance to use as `this` context.
* @param {?function} callback Called after state is updated.
* @internal
*/
enqueueCallback: function (publicInstance, callback) {},
/**
* Forces an update. This should only be invoked when it is known with
* certainty that we are **not** in a DOM transaction.
*
* You may want to call this when you know that some deeper aspect of the
* component's state has changed but `setState` was not called.
*
* This will not invoke `shouldComponentUpdate`, but it will invoke
* `componentWillUpdate` and `componentDidUpdate`.
*
* @param {ReactClass} publicInstance The instance that should rerender.
* @internal
*/
enqueueForceUpdate: function (publicInstance) {
warnTDZ(publicInstance, 'forceUpdate');
},
/**
* Replaces all of the state. Always use this or `setState` to mutate state.
* You should treat `this.state` as immutable.
*
* There is no guarantee that `this.state` will be immediately updated, so
* accessing `this.state` after calling this method may return the old value.
*
* @param {ReactClass} publicInstance The instance that should rerender.
* @param {object} completeState Next state.
* @internal
*/
enqueueReplaceState: function (publicInstance, completeState) {
warnTDZ(publicInstance, 'replaceState');
},
/**
* Sets a subset of the state. This only exists because _pendingState is
* internal. This provides a merging strategy that is not available to deep
* properties which is confusing. TODO: Expose pendingState or don't use it
* during the merge.
*
* @param {ReactClass} publicInstance The instance that should rerender.
* @param {object} partialState Next partial state to be merged with state.
* @internal
*/
enqueueSetState: function (publicInstance, partialState) {
warnTDZ(publicInstance, 'setState');
},
/**
* Sets a subset of the props.
*
* @param {ReactClass} publicInstance The instance that should rerender.
* @param {object} partialProps Subset of the next props.
* @internal
*/
enqueueSetProps: function (publicInstance, partialProps) {
warnTDZ(publicInstance, 'setProps');
},
/**
* Replaces all of the props.
*
* @param {ReactClass} publicInstance The instance that should rerender.
* @param {object} props New props.
* @internal
*/
enqueueReplaceProps: function (publicInstance, props) {
warnTDZ(publicInstance, 'replaceProps');
}
};
module.exports = ReactNoopUpdateQueue;
},{"173":173}],77:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactOwner
*/
'use strict';
var invariant = _dereq_(161);
/**
* ReactOwners are capable of storing references to owned components.
*
* All components are capable of //being// referenced by owner components, but
* only ReactOwner components are capable of //referencing// owned components.
* The named reference is known as a "ref".
*
* Refs are available when mounted and updated during reconciliation.
*
* var MyComponent = React.createClass({
* render: function() {
* return (
* <div onClick={this.handleClick}>
* <CustomComponent ref="custom" />
* </div>
* );
* },
* handleClick: function() {
* this.refs.custom.handleClick();
* },
* componentDidMount: function() {
* this.refs.custom.initialize();
* }
* });
*
* Refs should rarely be used. When refs are used, they should only be done to
* control data that is not handled by React's data flow.
*
* @class ReactOwner
*/
var ReactOwner = {
/**
* @param {?object} object
* @return {boolean} True if `object` is a valid owner.
* @final
*/
isValidOwner: function (object) {
return !!(object && typeof object.attachRef === 'function' && typeof object.detachRef === 'function');
},
/**
* Adds a component by ref to an owner component.
*
* @param {ReactComponent} component Component to reference.
* @param {string} ref Name by which to refer to the component.
* @param {ReactOwner} owner Component on which to record the ref.
* @final
* @internal
*/
addComponentAsRefTo: function (component, ref, owner) {
!ReactOwner.isValidOwner(owner) ? "development" !== 'production' ? invariant(false, 'addComponentAsRefTo(...): Only a ReactOwner can have refs. You might ' + 'be adding a ref to a component that was not created inside a component\'s ' + '`render` method, or you have multiple copies of React loaded ' + '(details: https://fb.me/react-refs-must-have-owner).') : invariant(false) : undefined;
owner.attachRef(ref, component);
},
/**
* Removes a component by ref from an owner component.
*
* @param {ReactComponent} component Component to dereference.
* @param {string} ref Name of the ref to remove.
* @param {ReactOwner} owner Component on which the ref is recorded.
* @final
* @internal
*/
removeComponentAsRefFrom: function (component, ref, owner) {
!ReactOwner.isValidOwner(owner) ? "development" !== 'production' ? invariant(false, 'removeComponentAsRefFrom(...): Only a ReactOwner can have refs. You might ' + 'be removing a ref to a component that was not created inside a component\'s ' + '`render` method, or you have multiple copies of React loaded ' + '(details: https://fb.me/react-refs-must-have-owner).') : invariant(false) : undefined;
// Check that `component` is still the current ref because we do not want to
// detach the ref if another component stole it.
if (owner.getPublicInstance().refs[ref] === component.getPublicInstance()) {
owner.detachRef(ref);
}
}
};
module.exports = ReactOwner;
},{"161":161}],78:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactPerf
* @typechecks static-only
*/
'use strict';
/**
* ReactPerf is a general AOP system designed to measure performance. This
* module only has the hooks: see ReactDefaultPerf for the analysis tool.
*/
var ReactPerf = {
/**
* Boolean to enable/disable measurement. Set to false by default to prevent
* accidental logging and perf loss.
*/
enableMeasure: false,
/**
* Holds onto the measure function in use. By default, don't measure
* anything, but we'll override this if we inject a measure function.
*/
storedMeasure: _noMeasure,
/**
* @param {object} object
* @param {string} objectName
* @param {object<string>} methodNames
*/
measureMethods: function (object, objectName, methodNames) {
if ("development" !== 'production') {
for (var key in methodNames) {
if (!methodNames.hasOwnProperty(key)) {
continue;
}
object[key] = ReactPerf.measure(objectName, methodNames[key], object[key]);
}
}
},
/**
* Use this to wrap methods you want to measure. Zero overhead in production.
*
* @param {string} objName
* @param {string} fnName
* @param {function} func
* @return {function}
*/
measure: function (objName, fnName, func) {
if ("development" !== 'production') {
var measuredFunc = null;
var wrapper = function () {
if (ReactPerf.enableMeasure) {
if (!measuredFunc) {
measuredFunc = ReactPerf.storedMeasure(objName, fnName, func);
}
return measuredFunc.apply(this, arguments);
}
return func.apply(this, arguments);
};
wrapper.displayName = objName + '_' + fnName;
return wrapper;
}
return func;
},
injection: {
/**
* @param {function} measure
*/
injectMeasure: function (measure) {
ReactPerf.storedMeasure = measure;
}
}
};
/**
* Simply passes through the measured function, without measuring it.
*
* @param {string} objName
* @param {string} fnName
* @param {function} func
* @return {function}
*/
function _noMeasure(objName, fnName, func) {
return func;
}
module.exports = ReactPerf;
},{}],79:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactPropTransferer
*/
'use strict';
var assign = _dereq_(24);
var emptyFunction = _dereq_(153);
var joinClasses = _dereq_(164);
/**
* Creates a transfer strategy that will merge prop values using the supplied
* `mergeStrategy`. If a prop was previously unset, this just sets it.
*
* @param {function} mergeStrategy
* @return {function}
*/
function createTransferStrategy(mergeStrategy) {
return function (props, key, value) {
if (!props.hasOwnProperty(key)) {
props[key] = value;
} else {
props[key] = mergeStrategy(props[key], value);
}
};
}
var transferStrategyMerge = createTransferStrategy(function (a, b) {
// `merge` overrides the first object's (`props[key]` above) keys using the
// second object's (`value`) keys. An object's style's existing `propA` would
// get overridden. Flip the order here.
return assign({}, b, a);
});
/**
* Transfer strategies dictate how props are transferred by `transferPropsTo`.
* NOTE: if you add any more exceptions to this list you should be sure to
* update `cloneWithProps()` accordingly.
*/
var TransferStrategies = {
/**
* Never transfer `children`.
*/
children: emptyFunction,
/**
* Transfer the `className` prop by merging them.
*/
className: createTransferStrategy(joinClasses),
/**
* Transfer the `style` prop (which is an object) by merging them.
*/
style: transferStrategyMerge
};
/**
* Mutates the first argument by transferring the properties from the second
* argument.
*
* @param {object} props
* @param {object} newProps
* @return {object}
*/
function transferInto(props, newProps) {
for (var thisKey in newProps) {
if (!newProps.hasOwnProperty(thisKey)) {
continue;
}
var transferStrategy = TransferStrategies[thisKey];
if (transferStrategy && TransferStrategies.hasOwnProperty(thisKey)) {
transferStrategy(props, thisKey, newProps[thisKey]);
} else if (!props.hasOwnProperty(thisKey)) {
props[thisKey] = newProps[thisKey];
}
}
return props;
}
/**
* ReactPropTransferer are capable of transferring props to another component
* using a `transferPropsTo` method.
*
* @class ReactPropTransferer
*/
var ReactPropTransferer = {
/**
* Merge two props objects using TransferStrategies.
*
* @param {object} oldProps original props (they take precedence)
* @param {object} newProps new props to merge in
* @return {object} a new object containing both sets of props merged.
*/
mergeProps: function (oldProps, newProps) {
return transferInto(assign({}, oldProps), newProps);
}
};
module.exports = ReactPropTransferer;
},{"153":153,"164":164,"24":24}],80:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactPropTypeLocationNames
*/
'use strict';
var ReactPropTypeLocationNames = {};
if ("development" !== 'production') {
ReactPropTypeLocationNames = {
prop: 'prop',
context: 'context',
childContext: 'child context'
};
}
module.exports = ReactPropTypeLocationNames;
},{}],81:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactPropTypeLocations
*/
'use strict';
var keyMirror = _dereq_(165);
var ReactPropTypeLocations = keyMirror({
prop: null,
context: null,
childContext: null
});
module.exports = ReactPropTypeLocations;
},{"165":165}],82:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactPropTypes
*/
'use strict';
var ReactElement = _dereq_(57);
var ReactPropTypeLocationNames = _dereq_(80);
var emptyFunction = _dereq_(153);
var getIteratorFn = _dereq_(129);
/**
* Collection of methods that allow declaration and validation of props that are
* supplied to React components. Example usage:
*
* var Props = require('ReactPropTypes');
* var MyArticle = React.createClass({
* propTypes: {
* // An optional string prop named "description".
* description: Props.string,
*
* // A required enum prop named "category".
* category: Props.oneOf(['News','Photos']).isRequired,
*
* // A prop named "dialog" that requires an instance of Dialog.
* dialog: Props.instanceOf(Dialog).isRequired
* },
* render: function() { ... }
* });
*
* A more formal specification of how these methods are used:
*
* type := array|bool|func|object|number|string|oneOf([...])|instanceOf(...)
* decl := ReactPropTypes.{type}(.isRequired)?
*
* Each and every declaration produces a function with the same signature. This
* allows the creation of custom validation functions. For example:
*
* var MyLink = React.createClass({
* propTypes: {
* // An optional string or URI prop named "href".
* href: function(props, propName, componentName) {
* var propValue = props[propName];
* if (propValue != null && typeof propValue !== 'string' &&
* !(propValue instanceof URI)) {
* return new Error(
* 'Expected a string or an URI for ' + propName + ' in ' +
* componentName
* );
* }
* }
* },
* render: function() {...}
* });
*
* @internal
*/
var ANONYMOUS = '<<anonymous>>';
var ReactPropTypes = {
array: createPrimitiveTypeChecker('array'),
bool: createPrimitiveTypeChecker('boolean'),
func: createPrimitiveTypeChecker('function'),
number: createPrimitiveTypeChecker('number'),
object: createPrimitiveTypeChecker('object'),
string: createPrimitiveTypeChecker('string'),
any: createAnyTypeChecker(),
arrayOf: createArrayOfTypeChecker,
element: createElementTypeChecker(),
instanceOf: createInstanceTypeChecker,
node: createNodeChecker(),
objectOf: createObjectOfTypeChecker,
oneOf: createEnumTypeChecker,
oneOfType: createUnionTypeChecker,
shape: createShapeTypeChecker
};
function createChainableTypeChecker(validate) {
function checkType(isRequired, props, propName, componentName, location, propFullName) {
componentName = componentName || ANONYMOUS;
propFullName = propFullName || propName;
if (props[propName] == null) {
var locationName = ReactPropTypeLocationNames[location];
if (isRequired) {
return new Error('Required ' + locationName + ' `' + propFullName + '` was not specified in ' + ('`' + componentName + '`.'));
}
return null;
} else {
return validate(props, propName, componentName, location, propFullName);
}
}
var chainedCheckType = checkType.bind(null, false);
chainedCheckType.isRequired = checkType.bind(null, true);
return chainedCheckType;
}
function createPrimitiveTypeChecker(expectedType) {
function validate(props, propName, componentName, location, propFullName) {
var propValue = props[propName];
var propType = getPropType(propValue);
if (propType !== expectedType) {
var locationName = ReactPropTypeLocationNames[location];
// `propValue` being instance of, say, date/regexp, pass the 'object'
// check, but we can offer a more precise error message here rather than
// 'of type `object`'.
var preciseType = getPreciseType(propValue);
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of type ' + ('`' + preciseType + '` supplied to `' + componentName + '`, expected ') + ('`' + expectedType + '`.'));
}
return null;
}
return createChainableTypeChecker(validate);
}
function createAnyTypeChecker() {
return createChainableTypeChecker(emptyFunction.thatReturns(null));
}
function createArrayOfTypeChecker(typeChecker) {
function validate(props, propName, componentName, location, propFullName) {
var propValue = props[propName];
if (!Array.isArray(propValue)) {
var locationName = ReactPropTypeLocationNames[location];
var propType = getPropType(propValue);
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of type ' + ('`' + propType + '` supplied to `' + componentName + '`, expected an array.'));
}
for (var i = 0; i < propValue.length; i++) {
var error = typeChecker(propValue, i, componentName, location, propFullName + '[' + i + ']');
if (error instanceof Error) {
return error;
}
}
return null;
}
return createChainableTypeChecker(validate);
}
function createElementTypeChecker() {
function validate(props, propName, componentName, location, propFullName) {
if (!ReactElement.isValidElement(props[propName])) {
var locationName = ReactPropTypeLocationNames[location];
return new Error('Invalid ' + locationName + ' `' + propFullName + '` supplied to ' + ('`' + componentName + '`, expected a single ReactElement.'));
}
return null;
}
return createChainableTypeChecker(validate);
}
function createInstanceTypeChecker(expectedClass) {
function validate(props, propName, componentName, location, propFullName) {
if (!(props[propName] instanceof expectedClass)) {
var locationName = ReactPropTypeLocationNames[location];
var expectedClassName = expectedClass.name || ANONYMOUS;
var actualClassName = getClassName(props[propName]);
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of type ' + ('`' + actualClassName + '` supplied to `' + componentName + '`, expected ') + ('instance of `' + expectedClassName + '`.'));
}
return null;
}
return createChainableTypeChecker(validate);
}
function createEnumTypeChecker(expectedValues) {
if (!Array.isArray(expectedValues)) {
return createChainableTypeChecker(function () {
return new Error('Invalid argument supplied to oneOf, expected an instance of array.');
});
}
function validate(props, propName, componentName, location, propFullName) {
var propValue = props[propName];
for (var i = 0; i < expectedValues.length; i++) {
if (propValue === expectedValues[i]) {
return null;
}
}
var locationName = ReactPropTypeLocationNames[location];
var valuesString = JSON.stringify(expectedValues);
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of value `' + propValue + '` ' + ('supplied to `' + componentName + '`, expected one of ' + valuesString + '.'));
}
return createChainableTypeChecker(validate);
}
function createObjectOfTypeChecker(typeChecker) {
function validate(props, propName, componentName, location, propFullName) {
var propValue = props[propName];
var propType = getPropType(propValue);
if (propType !== 'object') {
var locationName = ReactPropTypeLocationNames[location];
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of type ' + ('`' + propType + '` supplied to `' + componentName + '`, expected an object.'));
}
for (var key in propValue) {
if (propValue.hasOwnProperty(key)) {
var error = typeChecker(propValue, key, componentName, location, propFullName + '.' + key);
if (error instanceof Error) {
return error;
}
}
}
return null;
}
return createChainableTypeChecker(validate);
}
function createUnionTypeChecker(arrayOfTypeCheckers) {
if (!Array.isArray(arrayOfTypeCheckers)) {
return createChainableTypeChecker(function () {
return new Error('Invalid argument supplied to oneOfType, expected an instance of array.');
});
}
function validate(props, propName, componentName, location, propFullName) {
for (var i = 0; i < arrayOfTypeCheckers.length; i++) {
var checker = arrayOfTypeCheckers[i];
if (checker(props, propName, componentName, location, propFullName) == null) {
return null;
}
}
var locationName = ReactPropTypeLocationNames[location];
return new Error('Invalid ' + locationName + ' `' + propFullName + '` supplied to ' + ('`' + componentName + '`.'));
}
return createChainableTypeChecker(validate);
}
function createNodeChecker() {
function validate(props, propName, componentName, location, propFullName) {
if (!isNode(props[propName])) {
var locationName = ReactPropTypeLocationNames[location];
return new Error('Invalid ' + locationName + ' `' + propFullName + '` supplied to ' + ('`' + componentName + '`, expected a ReactNode.'));
}
return null;
}
return createChainableTypeChecker(validate);
}
function createShapeTypeChecker(shapeTypes) {
function validate(props, propName, componentName, location, propFullName) {
var propValue = props[propName];
var propType = getPropType(propValue);
if (propType !== 'object') {
var locationName = ReactPropTypeLocationNames[location];
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of type `' + propType + '` ' + ('supplied to `' + componentName + '`, expected `object`.'));
}
for (var key in shapeTypes) {
var checker = shapeTypes[key];
if (!checker) {
continue;
}
var error = checker(propValue, key, componentName, location, propFullName + '.' + key);
if (error) {
return error;
}
}
return null;
}
return createChainableTypeChecker(validate);
}
function isNode(propValue) {
switch (typeof propValue) {
case 'number':
case 'string':
case 'undefined':
return true;
case 'boolean':
return !propValue;
case 'object':
if (Array.isArray(propValue)) {
return propValue.every(isNode);
}
if (propValue === null || ReactElement.isValidElement(propValue)) {
return true;
}
var iteratorFn = getIteratorFn(propValue);
if (iteratorFn) {
var iterator = iteratorFn.call(propValue);
var step;
if (iteratorFn !== propValue.entries) {
while (!(step = iterator.next()).done) {
if (!isNode(step.value)) {
return false;
}
}
} else {
// Iterator will provide entry [k,v] tuples rather than values.
while (!(step = iterator.next()).done) {
var entry = step.value;
if (entry) {
if (!isNode(entry[1])) {
return false;
}
}
}
}
} else {
return false;
}
return true;
default:
return false;
}
}
// Equivalent of `typeof` but with special handling for array and regexp.
function getPropType(propValue) {
var propType = typeof propValue;
if (Array.isArray(propValue)) {
return 'array';
}
if (propValue instanceof RegExp) {
// Old webkits (at least until Android 4.0) return 'function' rather than
// 'object' for typeof a RegExp. We'll normalize this here so that /bla/
// passes PropTypes.object.
return 'object';
}
return propType;
}
// This handles more types than `getPropType`. Only used for error messages.
// See `createPrimitiveTypeChecker`.
function getPreciseType(propValue) {
var propType = getPropType(propValue);
if (propType === 'object') {
if (propValue instanceof Date) {
return 'date';
} else if (propValue instanceof RegExp) {
return 'regexp';
}
}
return propType;
}
// Returns class name of the object, if any.
function getClassName(propValue) {
if (!propValue.constructor || !propValue.constructor.name) {
return '<<anonymous>>';
}
return propValue.constructor.name;
}
module.exports = ReactPropTypes;
},{"129":129,"153":153,"57":57,"80":80}],83:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactReconcileTransaction
* @typechecks static-only
*/
'use strict';
var CallbackQueue = _dereq_(6);
var PooledClass = _dereq_(25);
var ReactBrowserEventEmitter = _dereq_(28);
var ReactDOMFeatureFlags = _dereq_(44);
var ReactInputSelection = _dereq_(66);
var Transaction = _dereq_(113);
var assign = _dereq_(24);
/**
* Ensures that, when possible, the selection range (currently selected text
* input) is not disturbed by performing the transaction.
*/
var SELECTION_RESTORATION = {
/**
* @return {Selection} Selection information.
*/
initialize: ReactInputSelection.getSelectionInformation,
/**
* @param {Selection} sel Selection information returned from `initialize`.
*/
close: ReactInputSelection.restoreSelection
};
/**
* Suppresses events (blur/focus) that could be inadvertently dispatched due to
* high level DOM manipulations (like temporarily removing a text input from the
* DOM).
*/
var EVENT_SUPPRESSION = {
/**
* @return {boolean} The enabled status of `ReactBrowserEventEmitter` before
* the reconciliation.
*/
initialize: function () {
var currentlyEnabled = ReactBrowserEventEmitter.isEnabled();
ReactBrowserEventEmitter.setEnabled(false);
return currentlyEnabled;
},
/**
* @param {boolean} previouslyEnabled Enabled status of
* `ReactBrowserEventEmitter` before the reconciliation occurred. `close`
* restores the previous value.
*/
close: function (previouslyEnabled) {
ReactBrowserEventEmitter.setEnabled(previouslyEnabled);
}
};
/**
* Provides a queue for collecting `componentDidMount` and
* `componentDidUpdate` callbacks during the the transaction.
*/
var ON_DOM_READY_QUEUEING = {
/**
* Initializes the internal `onDOMReady` queue.
*/
initialize: function () {
this.reactMountReady.reset();
},
/**
* After DOM is flushed, invoke all registered `onDOMReady` callbacks.
*/
close: function () {
this.reactMountReady.notifyAll();
}
};
/**
* Executed within the scope of the `Transaction` instance. Consider these as
* being member methods, but with an implied ordering while being isolated from
* each other.
*/
var TRANSACTION_WRAPPERS = [SELECTION_RESTORATION, EVENT_SUPPRESSION, ON_DOM_READY_QUEUEING];
/**
* Currently:
* - The order that these are listed in the transaction is critical:
* - Suppresses events.
* - Restores selection range.
*
* Future:
* - Restore document/overflow scroll positions that were unintentionally
* modified via DOM insertions above the top viewport boundary.
* - Implement/integrate with customized constraint based layout system and keep
* track of which dimensions must be remeasured.
*
* @class ReactReconcileTransaction
*/
function ReactReconcileTransaction(forceHTML) {
this.reinitializeTransaction();
// Only server-side rendering really needs this option (see
// `ReactServerRendering`), but server-side uses
// `ReactServerRenderingTransaction` instead. This option is here so that it's
// accessible and defaults to false when `ReactDOMComponent` and
// `ReactTextComponent` checks it in `mountComponent`.`
this.renderToStaticMarkup = false;
this.reactMountReady = CallbackQueue.getPooled(null);
this.useCreateElement = !forceHTML && ReactDOMFeatureFlags.useCreateElement;
}
var Mixin = {
/**
* @see Transaction
* @abstract
* @final
* @return {array<object>} List of operation wrap procedures.
* TODO: convert to array<TransactionWrapper>
*/
getTransactionWrappers: function () {
return TRANSACTION_WRAPPERS;
},
/**
* @return {object} The queue to collect `onDOMReady` callbacks with.
*/
getReactMountReady: function () {
return this.reactMountReady;
},
/**
* `PooledClass` looks for this, and will invoke this before allowing this
* instance to be reused.
*/
destructor: function () {
CallbackQueue.release(this.reactMountReady);
this.reactMountReady = null;
}
};
assign(ReactReconcileTransaction.prototype, Transaction.Mixin, Mixin);
PooledClass.addPoolingTo(ReactReconcileTransaction);
module.exports = ReactReconcileTransaction;
},{"113":113,"24":24,"25":25,"28":28,"44":44,"6":6,"66":66}],84:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactReconciler
*/
'use strict';
var ReactRef = _dereq_(85);
/**
* Helper to call ReactRef.attachRefs with this composite component, split out
* to avoid allocations in the transaction mount-ready queue.
*/
function attachRefs() {
ReactRef.attachRefs(this, this._currentElement);
}
var ReactReconciler = {
/**
* Initializes the component, renders markup, and registers event listeners.
*
* @param {ReactComponent} internalInstance
* @param {string} rootID DOM ID of the root node.
* @param {ReactReconcileTransaction|ReactServerRenderingTransaction} transaction
* @return {?string} Rendered markup to be inserted into the DOM.
* @final
* @internal
*/
mountComponent: function (internalInstance, rootID, transaction, context) {
var markup = internalInstance.mountComponent(rootID, transaction, context);
if (internalInstance._currentElement && internalInstance._currentElement.ref != null) {
transaction.getReactMountReady().enqueue(attachRefs, internalInstance);
}
return markup;
},
/**
* Releases any resources allocated by `mountComponent`.
*
* @final
* @internal
*/
unmountComponent: function (internalInstance) {
ReactRef.detachRefs(internalInstance, internalInstance._currentElement);
internalInstance.unmountComponent();
},
/**
* Update a component using a new element.
*
* @param {ReactComponent} internalInstance
* @param {ReactElement} nextElement
* @param {ReactReconcileTransaction} transaction
* @param {object} context
* @internal
*/
receiveComponent: function (internalInstance, nextElement, transaction, context) {
var prevElement = internalInstance._currentElement;
if (nextElement === prevElement && context === internalInstance._context) {
// Since elements are immutable after the owner is rendered,
// we can do a cheap identity compare here to determine if this is a
// superfluous reconcile. It's possible for state to be mutable but such
// change should trigger an update of the owner which would recreate
// the element. We explicitly check for the existence of an owner since
// it's possible for an element created outside a composite to be
// deeply mutated and reused.
// TODO: Bailing out early is just a perf optimization right?
// TODO: Removing the return statement should affect correctness?
return;
}
var refsChanged = ReactRef.shouldUpdateRefs(prevElement, nextElement);
if (refsChanged) {
ReactRef.detachRefs(internalInstance, prevElement);
}
internalInstance.receiveComponent(nextElement, transaction, context);
if (refsChanged && internalInstance._currentElement && internalInstance._currentElement.ref != null) {
transaction.getReactMountReady().enqueue(attachRefs, internalInstance);
}
},
/**
* Flush any dirty changes in a component.
*
* @param {ReactComponent} internalInstance
* @param {ReactReconcileTransaction} transaction
* @internal
*/
performUpdateIfNecessary: function (internalInstance, transaction) {
internalInstance.performUpdateIfNecessary(transaction);
}
};
module.exports = ReactReconciler;
},{"85":85}],85:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactRef
*/
'use strict';
var ReactOwner = _dereq_(77);
var ReactRef = {};
function attachRef(ref, component, owner) {
if (typeof ref === 'function') {
ref(component.getPublicInstance());
} else {
// Legacy ref
ReactOwner.addComponentAsRefTo(component, ref, owner);
}
}
function detachRef(ref, component, owner) {
if (typeof ref === 'function') {
ref(null);
} else {
// Legacy ref
ReactOwner.removeComponentAsRefFrom(component, ref, owner);
}
}
ReactRef.attachRefs = function (instance, element) {
if (element === null || element === false) {
return;
}
var ref = element.ref;
if (ref != null) {
attachRef(ref, instance, element._owner);
}
};
ReactRef.shouldUpdateRefs = function (prevElement, nextElement) {
// If either the owner or a `ref` has changed, make sure the newest owner
// has stored a reference to `this`, and the previous owner (if different)
// has forgotten the reference to `this`. We use the element instead
// of the public this.props because the post processing cannot determine
// a ref. The ref conceptually lives on the element.
// TODO: Should this even be possible? The owner cannot change because
// it's forbidden by shouldUpdateReactComponent. The ref can change
// if you swap the keys of but not the refs. Reconsider where this check
// is made. It probably belongs where the key checking and
// instantiateReactComponent is done.
var prevEmpty = prevElement === null || prevElement === false;
var nextEmpty = nextElement === null || nextElement === false;
return(
// This has a few false positives w/r/t empty components.
prevEmpty || nextEmpty || nextElement._owner !== prevElement._owner || nextElement.ref !== prevElement.ref
);
};
ReactRef.detachRefs = function (instance, element) {
if (element === null || element === false) {
return;
}
var ref = element.ref;
if (ref != null) {
detachRef(ref, instance, element._owner);
}
};
module.exports = ReactRef;
},{"77":77}],86:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactRootIndex
* @typechecks
*/
'use strict';
var ReactRootIndexInjection = {
/**
* @param {function} _createReactRootIndex
*/
injectCreateReactRootIndex: function (_createReactRootIndex) {
ReactRootIndex.createReactRootIndex = _createReactRootIndex;
}
};
var ReactRootIndex = {
createReactRootIndex: null,
injection: ReactRootIndexInjection
};
module.exports = ReactRootIndex;
},{}],87:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactServerBatchingStrategy
* @typechecks
*/
'use strict';
var ReactServerBatchingStrategy = {
isBatchingUpdates: false,
batchedUpdates: function (callback) {
// Don't do anything here. During the server rendering we don't want to
// schedule any updates. We will simply ignore them.
}
};
module.exports = ReactServerBatchingStrategy;
},{}],88:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @typechecks static-only
* @providesModule ReactServerRendering
*/
'use strict';
var ReactDefaultBatchingStrategy = _dereq_(53);
var ReactElement = _dereq_(57);
var ReactInstanceHandles = _dereq_(67);
var ReactMarkupChecksum = _dereq_(71);
var ReactServerBatchingStrategy = _dereq_(87);
var ReactServerRenderingTransaction = _dereq_(89);
var ReactUpdates = _dereq_(96);
var emptyObject = _dereq_(154);
var instantiateReactComponent = _dereq_(132);
var invariant = _dereq_(161);
/**
* @param {ReactElement} element
* @return {string} the HTML markup
*/
function renderToString(element) {
!ReactElement.isValidElement(element) ? "development" !== 'production' ? invariant(false, 'renderToString(): You must pass a valid ReactElement.') : invariant(false) : undefined;
var transaction;
try {
ReactUpdates.injection.injectBatchingStrategy(ReactServerBatchingStrategy);
var id = ReactInstanceHandles.createReactRootID();
transaction = ReactServerRenderingTransaction.getPooled(false);
return transaction.perform(function () {
var componentInstance = instantiateReactComponent(element, null);
var markup = componentInstance.mountComponent(id, transaction, emptyObject);
return ReactMarkupChecksum.addChecksumToMarkup(markup);
}, null);
} finally {
ReactServerRenderingTransaction.release(transaction);
// Revert to the DOM batching strategy since these two renderers
// currently share these stateful modules.
ReactUpdates.injection.injectBatchingStrategy(ReactDefaultBatchingStrategy);
}
}
/**
* @param {ReactElement} element
* @return {string} the HTML markup, without the extra React ID and checksum
* (for generating static pages)
*/
function renderToStaticMarkup(element) {
!ReactElement.isValidElement(element) ? "development" !== 'production' ? invariant(false, 'renderToStaticMarkup(): You must pass a valid ReactElement.') : invariant(false) : undefined;
var transaction;
try {
ReactUpdates.injection.injectBatchingStrategy(ReactServerBatchingStrategy);
var id = ReactInstanceHandles.createReactRootID();
transaction = ReactServerRenderingTransaction.getPooled(true);
return transaction.perform(function () {
var componentInstance = instantiateReactComponent(element, null);
return componentInstance.mountComponent(id, transaction, emptyObject);
}, null);
} finally {
ReactServerRenderingTransaction.release(transaction);
// Revert to the DOM batching strategy since these two renderers
// currently share these stateful modules.
ReactUpdates.injection.injectBatchingStrategy(ReactDefaultBatchingStrategy);
}
}
module.exports = {
renderToString: renderToString,
renderToStaticMarkup: renderToStaticMarkup
};
},{"132":132,"154":154,"161":161,"53":53,"57":57,"67":67,"71":71,"87":87,"89":89,"96":96}],89:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactServerRenderingTransaction
* @typechecks
*/
'use strict';
var PooledClass = _dereq_(25);
var CallbackQueue = _dereq_(6);
var Transaction = _dereq_(113);
var assign = _dereq_(24);
var emptyFunction = _dereq_(153);
/**
* Provides a `CallbackQueue` queue for collecting `onDOMReady` callbacks
* during the performing of the transaction.
*/
var ON_DOM_READY_QUEUEING = {
/**
* Initializes the internal `onDOMReady` queue.
*/
initialize: function () {
this.reactMountReady.reset();
},
close: emptyFunction
};
/**
* Executed within the scope of the `Transaction` instance. Consider these as
* being member methods, but with an implied ordering while being isolated from
* each other.
*/
var TRANSACTION_WRAPPERS = [ON_DOM_READY_QUEUEING];
/**
* @class ReactServerRenderingTransaction
* @param {boolean} renderToStaticMarkup
*/
function ReactServerRenderingTransaction(renderToStaticMarkup) {
this.reinitializeTransaction();
this.renderToStaticMarkup = renderToStaticMarkup;
this.reactMountReady = CallbackQueue.getPooled(null);
this.useCreateElement = false;
}
var Mixin = {
/**
* @see Transaction
* @abstract
* @final
* @return {array} Empty list of operation wrap procedures.
*/
getTransactionWrappers: function () {
return TRANSACTION_WRAPPERS;
},
/**
* @return {object} The queue to collect `onDOMReady` callbacks with.
*/
getReactMountReady: function () {
return this.reactMountReady;
},
/**
* `PooledClass` looks for this, and will invoke this before allowing this
* instance to be reused.
*/
destructor: function () {
CallbackQueue.release(this.reactMountReady);
this.reactMountReady = null;
}
};
assign(ReactServerRenderingTransaction.prototype, Transaction.Mixin, Mixin);
PooledClass.addPoolingTo(ReactServerRenderingTransaction);
module.exports = ReactServerRenderingTransaction;
},{"113":113,"153":153,"24":24,"25":25,"6":6}],90:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactStateSetters
*/
'use strict';
var ReactStateSetters = {
/**
* Returns a function that calls the provided function, and uses the result
* of that to set the component's state.
*
* @param {ReactCompositeComponent} component
* @param {function} funcReturningState Returned callback uses this to
* determine how to update state.
* @return {function} callback that when invoked uses funcReturningState to
* determined the object literal to setState.
*/
createStateSetter: function (component, funcReturningState) {
return function (a, b, c, d, e, f) {
var partialState = funcReturningState.call(component, a, b, c, d, e, f);
if (partialState) {
component.setState(partialState);
}
};
},
/**
* Returns a single-argument callback that can be used to update a single
* key in the component's state.
*
* Note: this is memoized function, which makes it inexpensive to call.
*
* @param {ReactCompositeComponent} component
* @param {string} key The key in the state that you should update.
* @return {function} callback of 1 argument which calls setState() with
* the provided keyName and callback argument.
*/
createStateKeySetter: function (component, key) {
// Memoize the setters.
var cache = component.__keySetters || (component.__keySetters = {});
return cache[key] || (cache[key] = createStateKeySetter(component, key));
}
};
function createStateKeySetter(component, key) {
// Partial state is allocated outside of the function closure so it can be
// reused with every call, avoiding memory allocation when this function
// is called.
var partialState = {};
return function stateKeySetter(value) {
partialState[key] = value;
component.setState(partialState);
};
}
ReactStateSetters.Mixin = {
/**
* Returns a function that calls the provided function, and uses the result
* of that to set the component's state.
*
* For example, these statements are equivalent:
*
* this.setState({x: 1});
* this.createStateSetter(function(xValue) {
* return {x: xValue};
* })(1);
*
* @param {function} funcReturningState Returned callback uses this to
* determine how to update state.
* @return {function} callback that when invoked uses funcReturningState to
* determined the object literal to setState.
*/
createStateSetter: function (funcReturningState) {
return ReactStateSetters.createStateSetter(this, funcReturningState);
},
/**
* Returns a single-argument callback that can be used to update a single
* key in the component's state.
*
* For example, these statements are equivalent:
*
* this.setState({x: 1});
* this.createStateKeySetter('x')(1);
*
* Note: this is memoized function, which makes it inexpensive to call.
*
* @param {string} key The key in the state that you should update.
* @return {function} callback of 1 argument which calls setState() with
* the provided keyName and callback argument.
*/
createStateKeySetter: function (key) {
return ReactStateSetters.createStateKeySetter(this, key);
}
};
module.exports = ReactStateSetters;
},{}],91:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactTestUtils
*/
'use strict';
var EventConstants = _dereq_(15);
var EventPluginHub = _dereq_(16);
var EventPropagators = _dereq_(19);
var React = _dereq_(26);
var ReactDOM = _dereq_(40);
var ReactElement = _dereq_(57);
var ReactBrowserEventEmitter = _dereq_(28);
var ReactCompositeComponent = _dereq_(38);
var ReactInstanceHandles = _dereq_(67);
var ReactInstanceMap = _dereq_(68);
var ReactMount = _dereq_(72);
var ReactUpdates = _dereq_(96);
var SyntheticEvent = _dereq_(105);
var assign = _dereq_(24);
var emptyObject = _dereq_(154);
var findDOMNode = _dereq_(122);
var invariant = _dereq_(161);
var topLevelTypes = EventConstants.topLevelTypes;
function Event(suffix) {}
/**
* @class ReactTestUtils
*/
function findAllInRenderedTreeInternal(inst, test) {
if (!inst || !inst.getPublicInstance) {
return [];
}
var publicInst = inst.getPublicInstance();
var ret = test(publicInst) ? [publicInst] : [];
var currentElement = inst._currentElement;
if (ReactTestUtils.isDOMComponent(publicInst)) {
var renderedChildren = inst._renderedChildren;
var key;
for (key in renderedChildren) {
if (!renderedChildren.hasOwnProperty(key)) {
continue;
}
ret = ret.concat(findAllInRenderedTreeInternal(renderedChildren[key], test));
}
} else if (ReactElement.isValidElement(currentElement) && typeof currentElement.type === 'function') {
ret = ret.concat(findAllInRenderedTreeInternal(inst._renderedComponent, test));
}
return ret;
}
/**
* Todo: Support the entire DOM.scry query syntax. For now, these simple
* utilities will suffice for testing purposes.
* @lends ReactTestUtils
*/
var ReactTestUtils = {
renderIntoDocument: function (instance) {
var div = document.createElement('div');
// None of our tests actually require attaching the container to the
// DOM, and doing so creates a mess that we rely on test isolation to
// clean up, so we're going to stop honoring the name of this method
// (and probably rename it eventually) if no problems arise.
// document.documentElement.appendChild(div);
return ReactDOM.render(instance, div);
},
isElement: function (element) {
return ReactElement.isValidElement(element);
},
isElementOfType: function (inst, convenienceConstructor) {
return ReactElement.isValidElement(inst) && inst.type === convenienceConstructor;
},
isDOMComponent: function (inst) {
return !!(inst && inst.nodeType === 1 && inst.tagName);
},
isDOMComponentElement: function (inst) {
return !!(inst && ReactElement.isValidElement(inst) && !!inst.tagName);
},
isCompositeComponent: function (inst) {
if (ReactTestUtils.isDOMComponent(inst)) {
// Accessing inst.setState warns; just return false as that'll be what
// this returns when we have DOM nodes as refs directly
return false;
}
return inst != null && typeof inst.render === 'function' && typeof inst.setState === 'function';
},
isCompositeComponentWithType: function (inst, type) {
if (!ReactTestUtils.isCompositeComponent(inst)) {
return false;
}
var internalInstance = ReactInstanceMap.get(inst);
var constructor = internalInstance._currentElement.type;
return constructor === type;
},
isCompositeComponentElement: function (inst) {
if (!ReactElement.isValidElement(inst)) {
return false;
}
// We check the prototype of the type that will get mounted, not the
// instance itself. This is a future proof way of duck typing.
var prototype = inst.type.prototype;
return typeof prototype.render === 'function' && typeof prototype.setState === 'function';
},
isCompositeComponentElementWithType: function (inst, type) {
var internalInstance = ReactInstanceMap.get(inst);
var constructor = internalInstance._currentElement.type;
return !!(ReactTestUtils.isCompositeComponentElement(inst) && constructor === type);
},
getRenderedChildOfCompositeComponent: function (inst) {
if (!ReactTestUtils.isCompositeComponent(inst)) {
return null;
}
var internalInstance = ReactInstanceMap.get(inst);
return internalInstance._renderedComponent.getPublicInstance();
},
findAllInRenderedTree: function (inst, test) {
if (!inst) {
return [];
}
!ReactTestUtils.isCompositeComponent(inst) ? "development" !== 'production' ? invariant(false, 'findAllInRenderedTree(...): instance must be a composite component') : invariant(false) : undefined;
return findAllInRenderedTreeInternal(ReactInstanceMap.get(inst), test);
},
/**
* Finds all instance of components in the rendered tree that are DOM
* components with the class name matching `className`.
* @return {array} an array of all the matches.
*/
scryRenderedDOMComponentsWithClass: function (root, classNames) {
if (!Array.isArray(classNames)) {
classNames = classNames.split(/\s+/);
}
return ReactTestUtils.findAllInRenderedTree(root, function (inst) {
if (ReactTestUtils.isDOMComponent(inst)) {
var className = inst.className;
if (typeof className !== 'string') {
// SVG, probably.
className = inst.getAttribute('class') || '';
}
var classList = className.split(/\s+/);
return classNames.every(function (name) {
return classList.indexOf(name) !== -1;
});
}
return false;
});
},
/**
* Like scryRenderedDOMComponentsWithClass but expects there to be one result,
* and returns that one result, or throws exception if there is any other
* number of matches besides one.
* @return {!ReactDOMComponent} The one match.
*/
findRenderedDOMComponentWithClass: function (root, className) {
var all = ReactTestUtils.scryRenderedDOMComponentsWithClass(root, className);
if (all.length !== 1) {
throw new Error('Did not find exactly one match ' + '(found: ' + all.length + ') for class:' + className);
}
return all[0];
},
/**
* Finds all instance of components in the rendered tree that are DOM
* components with the tag name matching `tagName`.
* @return {array} an array of all the matches.
*/
scryRenderedDOMComponentsWithTag: function (root, tagName) {
return ReactTestUtils.findAllInRenderedTree(root, function (inst) {
return ReactTestUtils.isDOMComponent(inst) && inst.tagName.toUpperCase() === tagName.toUpperCase();
});
},
/**
* Like scryRenderedDOMComponentsWithTag but expects there to be one result,
* and returns that one result, or throws exception if there is any other
* number of matches besides one.
* @return {!ReactDOMComponent} The one match.
*/
findRenderedDOMComponentWithTag: function (root, tagName) {
var all = ReactTestUtils.scryRenderedDOMComponentsWithTag(root, tagName);
if (all.length !== 1) {
throw new Error('Did not find exactly one match for tag:' + tagName);
}
return all[0];
},
/**
* Finds all instances of components with type equal to `componentType`.
* @return {array} an array of all the matches.
*/
scryRenderedComponentsWithType: function (root, componentType) {
return ReactTestUtils.findAllInRenderedTree(root, function (inst) {
return ReactTestUtils.isCompositeComponentWithType(inst, componentType);
});
},
/**
* Same as `scryRenderedComponentsWithType` but expects there to be one result
* and returns that one result, or throws exception if there is any other
* number of matches besides one.
* @return {!ReactComponent} The one match.
*/
findRenderedComponentWithType: function (root, componentType) {
var all = ReactTestUtils.scryRenderedComponentsWithType(root, componentType);
if (all.length !== 1) {
throw new Error('Did not find exactly one match for componentType:' + componentType + ' (found ' + all.length + ')');
}
return all[0];
},
/**
* Pass a mocked component module to this method to augment it with
* useful methods that allow it to be used as a dummy React component.
* Instead of rendering as usual, the component will become a simple
* <div> containing any provided children.
*
* @param {object} module the mock function object exported from a
* module that defines the component to be mocked
* @param {?string} mockTagName optional dummy root tag name to return
* from render method (overrides
* module.mockTagName if provided)
* @return {object} the ReactTestUtils object (for chaining)
*/
mockComponent: function (module, mockTagName) {
mockTagName = mockTagName || module.mockTagName || 'div';
module.prototype.render.mockImplementation(function () {
return React.createElement(mockTagName, null, this.props.children);
});
return this;
},
/**
* Simulates a top level event being dispatched from a raw event that occurred
* on an `Element` node.
* @param {Object} topLevelType A type from `EventConstants.topLevelTypes`
* @param {!Element} node The dom to simulate an event occurring on.
* @param {?Event} fakeNativeEvent Fake native event to use in SyntheticEvent.
*/
simulateNativeEventOnNode: function (topLevelType, node, fakeNativeEvent) {
fakeNativeEvent.target = node;
ReactBrowserEventEmitter.ReactEventListener.dispatchEvent(topLevelType, fakeNativeEvent);
},
/**
* Simulates a top level event being dispatched from a raw event that occurred
* on the `ReactDOMComponent` `comp`.
* @param {Object} topLevelType A type from `EventConstants.topLevelTypes`.
* @param {!ReactDOMComponent} comp
* @param {?Event} fakeNativeEvent Fake native event to use in SyntheticEvent.
*/
simulateNativeEventOnDOMComponent: function (topLevelType, comp, fakeNativeEvent) {
ReactTestUtils.simulateNativeEventOnNode(topLevelType, findDOMNode(comp), fakeNativeEvent);
},
nativeTouchData: function (x, y) {
return {
touches: [{ pageX: x, pageY: y }]
};
},
createRenderer: function () {
return new ReactShallowRenderer();
},
Simulate: null,
SimulateNative: {}
};
/**
* @class ReactShallowRenderer
*/
var ReactShallowRenderer = function () {
this._instance = null;
};
ReactShallowRenderer.prototype.getRenderOutput = function () {
return this._instance && this._instance._renderedComponent && this._instance._renderedComponent._renderedOutput || null;
};
var NoopInternalComponent = function (element) {
this._renderedOutput = element;
this._currentElement = element;
};
NoopInternalComponent.prototype = {
mountComponent: function () {},
receiveComponent: function (element) {
this._renderedOutput = element;
this._currentElement = element;
},
unmountComponent: function () {},
getPublicInstance: function () {
return null;
}
};
var ShallowComponentWrapper = function () {};
assign(ShallowComponentWrapper.prototype, ReactCompositeComponent.Mixin, {
_instantiateReactComponent: function (element) {
return new NoopInternalComponent(element);
},
_replaceNodeWithMarkupByID: function () {},
_renderValidatedComponent: ReactCompositeComponent.Mixin._renderValidatedComponentWithoutOwnerOrContext
});
ReactShallowRenderer.prototype.render = function (element, context) {
!ReactElement.isValidElement(element) ? "development" !== 'production' ? invariant(false, 'ReactShallowRenderer render(): Invalid component element.%s', typeof element === 'function' ? ' Instead of passing a component class, make sure to instantiate ' + 'it by passing it to React.createElement.' : '') : invariant(false) : undefined;
!(typeof element.type !== 'string') ? "development" !== 'production' ? invariant(false, 'ReactShallowRenderer render(): Shallow rendering works only with custom ' + 'components, not primitives (%s). Instead of calling `.render(el)` and ' + 'inspecting the rendered output, look at `el.props` directly instead.', element.type) : invariant(false) : undefined;
if (!context) {
context = emptyObject;
}
ReactUpdates.batchedUpdates(_batchedRender, this, element, context);
};
function _batchedRender(renderer, element, context) {
var transaction = ReactUpdates.ReactReconcileTransaction.getPooled(false);
renderer._render(element, transaction, context);
ReactUpdates.ReactReconcileTransaction.release(transaction);
}
ReactShallowRenderer.prototype.unmount = function () {
if (this._instance) {
this._instance.unmountComponent();
}
};
ReactShallowRenderer.prototype._render = function (element, transaction, context) {
if (this._instance) {
this._instance.receiveComponent(element, transaction, context);
} else {
var rootID = ReactInstanceHandles.createReactRootID();
var instance = new ShallowComponentWrapper(element.type);
instance.construct(element);
instance.mountComponent(rootID, transaction, context);
this._instance = instance;
}
};
/**
* Exports:
*
* - `ReactTestUtils.Simulate.click(Element/ReactDOMComponent)`
* - `ReactTestUtils.Simulate.mouseMove(Element/ReactDOMComponent)`
* - `ReactTestUtils.Simulate.change(Element/ReactDOMComponent)`
* - ... (All keys from event plugin `eventTypes` objects)
*/
function makeSimulator(eventType) {
return function (domComponentOrNode, eventData) {
var node;
if (ReactTestUtils.isDOMComponent(domComponentOrNode)) {
node = findDOMNode(domComponentOrNode);
} else if (domComponentOrNode.tagName) {
node = domComponentOrNode;
}
var dispatchConfig = ReactBrowserEventEmitter.eventNameDispatchConfigs[eventType];
var fakeNativeEvent = new Event();
fakeNativeEvent.target = node;
// We don't use SyntheticEvent.getPooled in order to not have to worry about
// properly destroying any properties assigned from `eventData` upon release
var event = new SyntheticEvent(dispatchConfig, ReactMount.getID(node), fakeNativeEvent, node);
assign(event, eventData);
if (dispatchConfig.phasedRegistrationNames) {
EventPropagators.accumulateTwoPhaseDispatches(event);
} else {
EventPropagators.accumulateDirectDispatches(event);
}
ReactUpdates.batchedUpdates(function () {
EventPluginHub.enqueueEvents(event);
EventPluginHub.processEventQueue(true);
});
};
}
function buildSimulators() {
ReactTestUtils.Simulate = {};
var eventType;
for (eventType in ReactBrowserEventEmitter.eventNameDispatchConfigs) {
/**
* @param {!Element|ReactDOMComponent} domComponentOrNode
* @param {?object} eventData Fake event data to use in SyntheticEvent.
*/
ReactTestUtils.Simulate[eventType] = makeSimulator(eventType);
}
}
// Rebuild ReactTestUtils.Simulate whenever event plugins are injected
var oldInjectEventPluginOrder = EventPluginHub.injection.injectEventPluginOrder;
EventPluginHub.injection.injectEventPluginOrder = function () {
oldInjectEventPluginOrder.apply(this, arguments);
buildSimulators();
};
var oldInjectEventPlugins = EventPluginHub.injection.injectEventPluginsByName;
EventPluginHub.injection.injectEventPluginsByName = function () {
oldInjectEventPlugins.apply(this, arguments);
buildSimulators();
};
buildSimulators();
/**
* Exports:
*
* - `ReactTestUtils.SimulateNative.click(Element/ReactDOMComponent)`
* - `ReactTestUtils.SimulateNative.mouseMove(Element/ReactDOMComponent)`
* - `ReactTestUtils.SimulateNative.mouseIn/ReactDOMComponent)`
* - `ReactTestUtils.SimulateNative.mouseOut(Element/ReactDOMComponent)`
* - ... (All keys from `EventConstants.topLevelTypes`)
*
* Note: Top level event types are a subset of the entire set of handler types
* (which include a broader set of "synthetic" events). For example, onDragDone
* is a synthetic event. Except when testing an event plugin or React's event
* handling code specifically, you probably want to use ReactTestUtils.Simulate
* to dispatch synthetic events.
*/
function makeNativeSimulator(eventType) {
return function (domComponentOrNode, nativeEventData) {
var fakeNativeEvent = new Event(eventType);
assign(fakeNativeEvent, nativeEventData);
if (ReactTestUtils.isDOMComponent(domComponentOrNode)) {
ReactTestUtils.simulateNativeEventOnDOMComponent(eventType, domComponentOrNode, fakeNativeEvent);
} else if (domComponentOrNode.tagName) {
// Will allow on actual dom nodes.
ReactTestUtils.simulateNativeEventOnNode(eventType, domComponentOrNode, fakeNativeEvent);
}
};
}
Object.keys(topLevelTypes).forEach(function (eventType) {
// Event type is stored as 'topClick' - we transform that to 'click'
var convenienceName = eventType.indexOf('top') === 0 ? eventType.charAt(3).toLowerCase() + eventType.substr(4) : eventType;
/**
* @param {!Element|ReactDOMComponent} domComponentOrNode
* @param {?Event} nativeEventData Fake native event to use in SyntheticEvent.
*/
ReactTestUtils.SimulateNative[convenienceName] = makeNativeSimulator(eventType);
});
module.exports = ReactTestUtils;
},{"105":105,"122":122,"15":15,"154":154,"16":16,"161":161,"19":19,"24":24,"26":26,"28":28,"38":38,"40":40,"57":57,"67":67,"68":68,"72":72,"96":96}],92:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @typechecks static-only
* @providesModule ReactTransitionChildMapping
*/
'use strict';
var flattenChildren = _dereq_(123);
var ReactTransitionChildMapping = {
/**
* Given `this.props.children`, return an object mapping key to child. Just
* simple syntactic sugar around flattenChildren().
*
* @param {*} children `this.props.children`
* @return {object} Mapping of key to child
*/
getChildMapping: function (children) {
if (!children) {
return children;
}
return flattenChildren(children);
},
/**
* When you're adding or removing children some may be added or removed in the
* same render pass. We want to show *both* since we want to simultaneously
* animate elements in and out. This function takes a previous set of keys
* and a new set of keys and merges them with its best guess of the correct
* ordering. In the future we may expose some of the utilities in
* ReactMultiChild to make this easy, but for now React itself does not
* directly have this concept of the union of prevChildren and nextChildren
* so we implement it here.
*
* @param {object} prev prev children as returned from
* `ReactTransitionChildMapping.getChildMapping()`.
* @param {object} next next children as returned from
* `ReactTransitionChildMapping.getChildMapping()`.
* @return {object} a key set that contains all keys in `prev` and all keys
* in `next` in a reasonable order.
*/
mergeChildMappings: function (prev, next) {
prev = prev || {};
next = next || {};
function getValueForKey(key) {
if (next.hasOwnProperty(key)) {
return next[key];
} else {
return prev[key];
}
}
// For each key of `next`, the list of keys to insert before that key in
// the combined list
var nextKeysPending = {};
var pendingKeys = [];
for (var prevKey in prev) {
if (next.hasOwnProperty(prevKey)) {
if (pendingKeys.length) {
nextKeysPending[prevKey] = pendingKeys;
pendingKeys = [];
}
} else {
pendingKeys.push(prevKey);
}
}
var i;
var childMapping = {};
for (var nextKey in next) {
if (nextKeysPending.hasOwnProperty(nextKey)) {
for (i = 0; i < nextKeysPending[nextKey].length; i++) {
var pendingNextKey = nextKeysPending[nextKey][i];
childMapping[nextKeysPending[nextKey][i]] = getValueForKey(pendingNextKey);
}
}
childMapping[nextKey] = getValueForKey(nextKey);
}
// Finally, add the keys which didn't appear before any key in `next`
for (i = 0; i < pendingKeys.length; i++) {
childMapping[pendingKeys[i]] = getValueForKey(pendingKeys[i]);
}
return childMapping;
}
};
module.exports = ReactTransitionChildMapping;
},{"123":123}],93:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactTransitionEvents
*/
'use strict';
var ExecutionEnvironment = _dereq_(147);
/**
* EVENT_NAME_MAP is used to determine which event fired when a
* transition/animation ends, based on the style property used to
* define that event.
*/
var EVENT_NAME_MAP = {
transitionend: {
'transition': 'transitionend',
'WebkitTransition': 'webkitTransitionEnd',
'MozTransition': 'mozTransitionEnd',
'OTransition': 'oTransitionEnd',
'msTransition': 'MSTransitionEnd'
},
animationend: {
'animation': 'animationend',
'WebkitAnimation': 'webkitAnimationEnd',
'MozAnimation': 'mozAnimationEnd',
'OAnimation': 'oAnimationEnd',
'msAnimation': 'MSAnimationEnd'
}
};
var endEvents = [];
function detectEvents() {
var testEl = document.createElement('div');
var style = testEl.style;
// On some platforms, in particular some releases of Android 4.x,
// the un-prefixed "animation" and "transition" properties are defined on the
// style object but the events that fire will still be prefixed, so we need
// to check if the un-prefixed events are useable, and if not remove them
// from the map
if (!('AnimationEvent' in window)) {
delete EVENT_NAME_MAP.animationend.animation;
}
if (!('TransitionEvent' in window)) {
delete EVENT_NAME_MAP.transitionend.transition;
}
for (var baseEventName in EVENT_NAME_MAP) {
var baseEvents = EVENT_NAME_MAP[baseEventName];
for (var styleName in baseEvents) {
if (styleName in style) {
endEvents.push(baseEvents[styleName]);
break;
}
}
}
}
if (ExecutionEnvironment.canUseDOM) {
detectEvents();
}
// We use the raw {add|remove}EventListener() call because EventListener
// does not know how to remove event listeners and we really should
// clean up. Also, these events are not triggered in older browsers
// so we should be A-OK here.
function addEventListener(node, eventName, eventListener) {
node.addEventListener(eventName, eventListener, false);
}
function removeEventListener(node, eventName, eventListener) {
node.removeEventListener(eventName, eventListener, false);
}
var ReactTransitionEvents = {
addEndEventListener: function (node, eventListener) {
if (endEvents.length === 0) {
// If CSS transitions are not supported, trigger an "end animation"
// event immediately.
window.setTimeout(eventListener, 0);
return;
}
endEvents.forEach(function (endEvent) {
addEventListener(node, endEvent, eventListener);
});
},
removeEndEventListener: function (node, eventListener) {
if (endEvents.length === 0) {
return;
}
endEvents.forEach(function (endEvent) {
removeEventListener(node, endEvent, eventListener);
});
}
};
module.exports = ReactTransitionEvents;
},{"147":147}],94:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactTransitionGroup
*/
'use strict';
var React = _dereq_(26);
var ReactTransitionChildMapping = _dereq_(92);
var assign = _dereq_(24);
var emptyFunction = _dereq_(153);
var ReactTransitionGroup = React.createClass({
displayName: 'ReactTransitionGroup',
propTypes: {
component: React.PropTypes.any,
childFactory: React.PropTypes.func
},
getDefaultProps: function () {
return {
component: 'span',
childFactory: emptyFunction.thatReturnsArgument
};
},
getInitialState: function () {
return {
children: ReactTransitionChildMapping.getChildMapping(this.props.children)
};
},
componentWillMount: function () {
this.currentlyTransitioningKeys = {};
this.keysToEnter = [];
this.keysToLeave = [];
},
componentDidMount: function () {
var initialChildMapping = this.state.children;
for (var key in initialChildMapping) {
if (initialChildMapping[key]) {
this.performAppear(key);
}
}
},
componentWillReceiveProps: function (nextProps) {
var nextChildMapping = ReactTransitionChildMapping.getChildMapping(nextProps.children);
var prevChildMapping = this.state.children;
this.setState({
children: ReactTransitionChildMapping.mergeChildMappings(prevChildMapping, nextChildMapping)
});
var key;
for (key in nextChildMapping) {
var hasPrev = prevChildMapping && prevChildMapping.hasOwnProperty(key);
if (nextChildMapping[key] && !hasPrev && !this.currentlyTransitioningKeys[key]) {
this.keysToEnter.push(key);
}
}
for (key in prevChildMapping) {
var hasNext = nextChildMapping && nextChildMapping.hasOwnProperty(key);
if (prevChildMapping[key] && !hasNext && !this.currentlyTransitioningKeys[key]) {
this.keysToLeave.push(key);
}
}
// If we want to someday check for reordering, we could do it here.
},
componentDidUpdate: function () {
var keysToEnter = this.keysToEnter;
this.keysToEnter = [];
keysToEnter.forEach(this.performEnter);
var keysToLeave = this.keysToLeave;
this.keysToLeave = [];
keysToLeave.forEach(this.performLeave);
},
performAppear: function (key) {
this.currentlyTransitioningKeys[key] = true;
var component = this.refs[key];
if (component.componentWillAppear) {
component.componentWillAppear(this._handleDoneAppearing.bind(this, key));
} else {
this._handleDoneAppearing(key);
}
},
_handleDoneAppearing: function (key) {
var component = this.refs[key];
if (component.componentDidAppear) {
component.componentDidAppear();
}
delete this.currentlyTransitioningKeys[key];
var currentChildMapping = ReactTransitionChildMapping.getChildMapping(this.props.children);
if (!currentChildMapping || !currentChildMapping.hasOwnProperty(key)) {
// This was removed before it had fully appeared. Remove it.
this.performLeave(key);
}
},
performEnter: function (key) {
this.currentlyTransitioningKeys[key] = true;
var component = this.refs[key];
if (component.componentWillEnter) {
component.componentWillEnter(this._handleDoneEntering.bind(this, key));
} else {
this._handleDoneEntering(key);
}
},
_handleDoneEntering: function (key) {
var component = this.refs[key];
if (component.componentDidEnter) {
component.componentDidEnter();
}
delete this.currentlyTransitioningKeys[key];
var currentChildMapping = ReactTransitionChildMapping.getChildMapping(this.props.children);
if (!currentChildMapping || !currentChildMapping.hasOwnProperty(key)) {
// This was removed before it had fully entered. Remove it.
this.performLeave(key);
}
},
performLeave: function (key) {
this.currentlyTransitioningKeys[key] = true;
var component = this.refs[key];
if (component.componentWillLeave) {
component.componentWillLeave(this._handleDoneLeaving.bind(this, key));
} else {
// Note that this is somewhat dangerous b/c it calls setState()
// again, effectively mutating the component before all the work
// is done.
this._handleDoneLeaving(key);
}
},
_handleDoneLeaving: function (key) {
var component = this.refs[key];
if (component.componentDidLeave) {
component.componentDidLeave();
}
delete this.currentlyTransitioningKeys[key];
var currentChildMapping = ReactTransitionChildMapping.getChildMapping(this.props.children);
if (currentChildMapping && currentChildMapping.hasOwnProperty(key)) {
// This entered again before it fully left. Add it again.
this.performEnter(key);
} else {
this.setState(function (state) {
var newChildren = assign({}, state.children);
delete newChildren[key];
return { children: newChildren };
});
}
},
render: function () {
// TODO: we could get rid of the need for the wrapper node
// by cloning a single child
var childrenToRender = [];
for (var key in this.state.children) {
var child = this.state.children[key];
if (child) {
// You may need to apply reactive updates to a child as it is leaving.
// The normal React way to do it won't work since the child will have
// already been removed. In case you need this behavior you can provide
// a childFactory function to wrap every child, even the ones that are
// leaving.
childrenToRender.push(React.cloneElement(this.props.childFactory(child), { ref: key, key: key }));
}
}
return React.createElement(this.props.component, this.props, childrenToRender);
}
});
module.exports = ReactTransitionGroup;
},{"153":153,"24":24,"26":26,"92":92}],95:[function(_dereq_,module,exports){
/**
* Copyright 2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactUpdateQueue
*/
'use strict';
var ReactCurrentOwner = _dereq_(39);
var ReactElement = _dereq_(57);
var ReactInstanceMap = _dereq_(68);
var ReactUpdates = _dereq_(96);
var assign = _dereq_(24);
var invariant = _dereq_(161);
var warning = _dereq_(173);
function enqueueUpdate(internalInstance) {
ReactUpdates.enqueueUpdate(internalInstance);
}
function getInternalInstanceReadyForUpdate(publicInstance, callerName) {
var internalInstance = ReactInstanceMap.get(publicInstance);
if (!internalInstance) {
if ("development" !== 'production') {
// Only warn when we have a callerName. Otherwise we should be silent.
// We're probably calling from enqueueCallback. We don't want to warn
// there because we already warned for the corresponding lifecycle method.
"development" !== 'production' ? warning(!callerName, '%s(...): Can only update a mounted or mounting component. ' + 'This usually means you called %s() on an unmounted component. ' + 'This is a no-op. Please check the code for the %s component.', callerName, callerName, publicInstance.constructor.displayName) : undefined;
}
return null;
}
if ("development" !== 'production') {
"development" !== 'production' ? warning(ReactCurrentOwner.current == null, '%s(...): Cannot update during an existing state transition ' + '(such as within `render`). Render methods should be a pure function ' + 'of props and state.', callerName) : undefined;
}
return internalInstance;
}
/**
* ReactUpdateQueue allows for state updates to be scheduled into a later
* reconciliation step.
*/
var ReactUpdateQueue = {
/**
* Checks whether or not this composite component is mounted.
* @param {ReactClass} publicInstance The instance we want to test.
* @return {boolean} True if mounted, false otherwise.
* @protected
* @final
*/
isMounted: function (publicInstance) {
if ("development" !== 'production') {
var owner = ReactCurrentOwner.current;
if (owner !== null) {
"development" !== 'production' ? warning(owner._warnedAboutRefsInRender, '%s is accessing isMounted inside its render() function. ' + 'render() should be a pure function of props and state. It should ' + 'never access something that requires stale data from the previous ' + 'render, such as refs. Move this logic to componentDidMount and ' + 'componentDidUpdate instead.', owner.getName() || 'A component') : undefined;
owner._warnedAboutRefsInRender = true;
}
}
var internalInstance = ReactInstanceMap.get(publicInstance);
if (internalInstance) {
// During componentWillMount and render this will still be null but after
// that will always render to something. At least for now. So we can use
// this hack.
return !!internalInstance._renderedComponent;
} else {
return false;
}
},
/**
* Enqueue a callback that will be executed after all the pending updates
* have processed.
*
* @param {ReactClass} publicInstance The instance to use as `this` context.
* @param {?function} callback Called after state is updated.
* @internal
*/
enqueueCallback: function (publicInstance, callback) {
!(typeof callback === 'function') ? "development" !== 'production' ? invariant(false, 'enqueueCallback(...): You called `setProps`, `replaceProps`, ' + '`setState`, `replaceState`, or `forceUpdate` with a callback that ' + 'isn\'t callable.') : invariant(false) : undefined;
var internalInstance = getInternalInstanceReadyForUpdate(publicInstance);
// Previously we would throw an error if we didn't have an internal
// instance. Since we want to make it a no-op instead, we mirror the same
// behavior we have in other enqueue* methods.
// We also need to ignore callbacks in componentWillMount. See
// enqueueUpdates.
if (!internalInstance) {
return null;
}
if (internalInstance._pendingCallbacks) {
internalInstance._pendingCallbacks.push(callback);
} else {
internalInstance._pendingCallbacks = [callback];
}
// TODO: The callback here is ignored when setState is called from
// componentWillMount. Either fix it or disallow doing so completely in
// favor of getInitialState. Alternatively, we can disallow
// componentWillMount during server-side rendering.
enqueueUpdate(internalInstance);
},
enqueueCallbackInternal: function (internalInstance, callback) {
!(typeof callback === 'function') ? "development" !== 'production' ? invariant(false, 'enqueueCallback(...): You called `setProps`, `replaceProps`, ' + '`setState`, `replaceState`, or `forceUpdate` with a callback that ' + 'isn\'t callable.') : invariant(false) : undefined;
if (internalInstance._pendingCallbacks) {
internalInstance._pendingCallbacks.push(callback);
} else {
internalInstance._pendingCallbacks = [callback];
}
enqueueUpdate(internalInstance);
},
/**
* Forces an update. This should only be invoked when it is known with
* certainty that we are **not** in a DOM transaction.
*
* You may want to call this when you know that some deeper aspect of the
* component's state has changed but `setState` was not called.
*
* This will not invoke `shouldComponentUpdate`, but it will invoke
* `componentWillUpdate` and `componentDidUpdate`.
*
* @param {ReactClass} publicInstance The instance that should rerender.
* @internal
*/
enqueueForceUpdate: function (publicInstance) {
var internalInstance = getInternalInstanceReadyForUpdate(publicInstance, 'forceUpdate');
if (!internalInstance) {
return;
}
internalInstance._pendingForceUpdate = true;
enqueueUpdate(internalInstance);
},
/**
* Replaces all of the state. Always use this or `setState` to mutate state.
* You should treat `this.state` as immutable.
*
* There is no guarantee that `this.state` will be immediately updated, so
* accessing `this.state` after calling this method may return the old value.
*
* @param {ReactClass} publicInstance The instance that should rerender.
* @param {object} completeState Next state.
* @internal
*/
enqueueReplaceState: function (publicInstance, completeState) {
var internalInstance = getInternalInstanceReadyForUpdate(publicInstance, 'replaceState');
if (!internalInstance) {
return;
}
internalInstance._pendingStateQueue = [completeState];
internalInstance._pendingReplaceState = true;
enqueueUpdate(internalInstance);
},
/**
* Sets a subset of the state. This only exists because _pendingState is
* internal. This provides a merging strategy that is not available to deep
* properties which is confusing. TODO: Expose pendingState or don't use it
* during the merge.
*
* @param {ReactClass} publicInstance The instance that should rerender.
* @param {object} partialState Next partial state to be merged with state.
* @internal
*/
enqueueSetState: function (publicInstance, partialState) {
var internalInstance = getInternalInstanceReadyForUpdate(publicInstance, 'setState');
if (!internalInstance) {
return;
}
var queue = internalInstance._pendingStateQueue || (internalInstance._pendingStateQueue = []);
queue.push(partialState);
enqueueUpdate(internalInstance);
},
/**
* Sets a subset of the props.
*
* @param {ReactClass} publicInstance The instance that should rerender.
* @param {object} partialProps Subset of the next props.
* @internal
*/
enqueueSetProps: function (publicInstance, partialProps) {
var internalInstance = getInternalInstanceReadyForUpdate(publicInstance, 'setProps');
if (!internalInstance) {
return;
}
ReactUpdateQueue.enqueueSetPropsInternal(internalInstance, partialProps);
},
enqueueSetPropsInternal: function (internalInstance, partialProps) {
var topLevelWrapper = internalInstance._topLevelWrapper;
!topLevelWrapper ? "development" !== 'production' ? invariant(false, 'setProps(...): You called `setProps` on a ' + 'component with a parent. This is an anti-pattern since props will ' + 'get reactively updated when rendered. Instead, change the owner\'s ' + '`render` method to pass the correct value as props to the component ' + 'where it is created.') : invariant(false) : undefined;
// Merge with the pending element if it exists, otherwise with existing
// element props.
var wrapElement = topLevelWrapper._pendingElement || topLevelWrapper._currentElement;
var element = wrapElement.props;
var props = assign({}, element.props, partialProps);
topLevelWrapper._pendingElement = ReactElement.cloneAndReplaceProps(wrapElement, ReactElement.cloneAndReplaceProps(element, props));
enqueueUpdate(topLevelWrapper);
},
/**
* Replaces all of the props.
*
* @param {ReactClass} publicInstance The instance that should rerender.
* @param {object} props New props.
* @internal
*/
enqueueReplaceProps: function (publicInstance, props) {
var internalInstance = getInternalInstanceReadyForUpdate(publicInstance, 'replaceProps');
if (!internalInstance) {
return;
}
ReactUpdateQueue.enqueueReplacePropsInternal(internalInstance, props);
},
enqueueReplacePropsInternal: function (internalInstance, props) {
var topLevelWrapper = internalInstance._topLevelWrapper;
!topLevelWrapper ? "development" !== 'production' ? invariant(false, 'replaceProps(...): You called `replaceProps` on a ' + 'component with a parent. This is an anti-pattern since props will ' + 'get reactively updated when rendered. Instead, change the owner\'s ' + '`render` method to pass the correct value as props to the component ' + 'where it is created.') : invariant(false) : undefined;
// Merge with the pending element if it exists, otherwise with existing
// element props.
var wrapElement = topLevelWrapper._pendingElement || topLevelWrapper._currentElement;
var element = wrapElement.props;
topLevelWrapper._pendingElement = ReactElement.cloneAndReplaceProps(wrapElement, ReactElement.cloneAndReplaceProps(element, props));
enqueueUpdate(topLevelWrapper);
},
enqueueElementInternal: function (internalInstance, newElement) {
internalInstance._pendingElement = newElement;
enqueueUpdate(internalInstance);
}
};
module.exports = ReactUpdateQueue;
},{"161":161,"173":173,"24":24,"39":39,"57":57,"68":68,"96":96}],96:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactUpdates
*/
'use strict';
var CallbackQueue = _dereq_(6);
var PooledClass = _dereq_(25);
var ReactPerf = _dereq_(78);
var ReactReconciler = _dereq_(84);
var Transaction = _dereq_(113);
var assign = _dereq_(24);
var invariant = _dereq_(161);
var dirtyComponents = [];
var asapCallbackQueue = CallbackQueue.getPooled();
var asapEnqueued = false;
var batchingStrategy = null;
function ensureInjected() {
!(ReactUpdates.ReactReconcileTransaction && batchingStrategy) ? "development" !== 'production' ? invariant(false, 'ReactUpdates: must inject a reconcile transaction class and batching ' + 'strategy') : invariant(false) : undefined;
}
var NESTED_UPDATES = {
initialize: function () {
this.dirtyComponentsLength = dirtyComponents.length;
},
close: function () {
if (this.dirtyComponentsLength !== dirtyComponents.length) {
// Additional updates were enqueued by componentDidUpdate handlers or
// similar; before our own UPDATE_QUEUEING wrapper closes, we want to run
// these new updates so that if A's componentDidUpdate calls setState on
// B, B will update before the callback A's updater provided when calling
// setState.
dirtyComponents.splice(0, this.dirtyComponentsLength);
flushBatchedUpdates();
} else {
dirtyComponents.length = 0;
}
}
};
var UPDATE_QUEUEING = {
initialize: function () {
this.callbackQueue.reset();
},
close: function () {
this.callbackQueue.notifyAll();
}
};
var TRANSACTION_WRAPPERS = [NESTED_UPDATES, UPDATE_QUEUEING];
function ReactUpdatesFlushTransaction() {
this.reinitializeTransaction();
this.dirtyComponentsLength = null;
this.callbackQueue = CallbackQueue.getPooled();
this.reconcileTransaction = ReactUpdates.ReactReconcileTransaction.getPooled( /* forceHTML */false);
}
assign(ReactUpdatesFlushTransaction.prototype, Transaction.Mixin, {
getTransactionWrappers: function () {
return TRANSACTION_WRAPPERS;
},
destructor: function () {
this.dirtyComponentsLength = null;
CallbackQueue.release(this.callbackQueue);
this.callbackQueue = null;
ReactUpdates.ReactReconcileTransaction.release(this.reconcileTransaction);
this.reconcileTransaction = null;
},
perform: function (method, scope, a) {
// Essentially calls `this.reconcileTransaction.perform(method, scope, a)`
// with this transaction's wrappers around it.
return Transaction.Mixin.perform.call(this, this.reconcileTransaction.perform, this.reconcileTransaction, method, scope, a);
}
});
PooledClass.addPoolingTo(ReactUpdatesFlushTransaction);
function batchedUpdates(callback, a, b, c, d, e) {
ensureInjected();
batchingStrategy.batchedUpdates(callback, a, b, c, d, e);
}
/**
* Array comparator for ReactComponents by mount ordering.
*
* @param {ReactComponent} c1 first component you're comparing
* @param {ReactComponent} c2 second component you're comparing
* @return {number} Return value usable by Array.prototype.sort().
*/
function mountOrderComparator(c1, c2) {
return c1._mountOrder - c2._mountOrder;
}
function runBatchedUpdates(transaction) {
var len = transaction.dirtyComponentsLength;
!(len === dirtyComponents.length) ? "development" !== 'production' ? invariant(false, 'Expected flush transaction\'s stored dirty-components length (%s) to ' + 'match dirty-components array length (%s).', len, dirtyComponents.length) : invariant(false) : undefined;
// Since reconciling a component higher in the owner hierarchy usually (not
// always -- see shouldComponentUpdate()) will reconcile children, reconcile
// them before their children by sorting the array.
dirtyComponents.sort(mountOrderComparator);
for (var i = 0; i < len; i++) {
// If a component is unmounted before pending changes apply, it will still
// be here, but we assume that it has cleared its _pendingCallbacks and
// that performUpdateIfNecessary is a noop.
var component = dirtyComponents[i];
// If performUpdateIfNecessary happens to enqueue any new updates, we
// shouldn't execute the callbacks until the next render happens, so
// stash the callbacks first
var callbacks = component._pendingCallbacks;
component._pendingCallbacks = null;
ReactReconciler.performUpdateIfNecessary(component, transaction.reconcileTransaction);
if (callbacks) {
for (var j = 0; j < callbacks.length; j++) {
transaction.callbackQueue.enqueue(callbacks[j], component.getPublicInstance());
}
}
}
}
var flushBatchedUpdates = function () {
// ReactUpdatesFlushTransaction's wrappers will clear the dirtyComponents
// array and perform any updates enqueued by mount-ready handlers (i.e.,
// componentDidUpdate) but we need to check here too in order to catch
// updates enqueued by setState callbacks and asap calls.
while (dirtyComponents.length || asapEnqueued) {
if (dirtyComponents.length) {
var transaction = ReactUpdatesFlushTransaction.getPooled();
transaction.perform(runBatchedUpdates, null, transaction);
ReactUpdatesFlushTransaction.release(transaction);
}
if (asapEnqueued) {
asapEnqueued = false;
var queue = asapCallbackQueue;
asapCallbackQueue = CallbackQueue.getPooled();
queue.notifyAll();
CallbackQueue.release(queue);
}
}
};
flushBatchedUpdates = ReactPerf.measure('ReactUpdates', 'flushBatchedUpdates', flushBatchedUpdates);
/**
* Mark a component as needing a rerender, adding an optional callback to a
* list of functions which will be executed once the rerender occurs.
*/
function enqueueUpdate(component) {
ensureInjected();
// Various parts of our code (such as ReactCompositeComponent's
// _renderValidatedComponent) assume that calls to render aren't nested;
// verify that that's the case. (This is called by each top-level update
// function, like setProps, setState, forceUpdate, etc.; creation and
// destruction of top-level components is guarded in ReactMount.)
if (!batchingStrategy.isBatchingUpdates) {
batchingStrategy.batchedUpdates(enqueueUpdate, component);
return;
}
dirtyComponents.push(component);
}
/**
* Enqueue a callback to be run at the end of the current batching cycle. Throws
* if no updates are currently being performed.
*/
function asap(callback, context) {
!batchingStrategy.isBatchingUpdates ? "development" !== 'production' ? invariant(false, 'ReactUpdates.asap: Can\'t enqueue an asap callback in a context where' + 'updates are not being batched.') : invariant(false) : undefined;
asapCallbackQueue.enqueue(callback, context);
asapEnqueued = true;
}
var ReactUpdatesInjection = {
injectReconcileTransaction: function (ReconcileTransaction) {
!ReconcileTransaction ? "development" !== 'production' ? invariant(false, 'ReactUpdates: must provide a reconcile transaction class') : invariant(false) : undefined;
ReactUpdates.ReactReconcileTransaction = ReconcileTransaction;
},
injectBatchingStrategy: function (_batchingStrategy) {
!_batchingStrategy ? "development" !== 'production' ? invariant(false, 'ReactUpdates: must provide a batching strategy') : invariant(false) : undefined;
!(typeof _batchingStrategy.batchedUpdates === 'function') ? "development" !== 'production' ? invariant(false, 'ReactUpdates: must provide a batchedUpdates() function') : invariant(false) : undefined;
!(typeof _batchingStrategy.isBatchingUpdates === 'boolean') ? "development" !== 'production' ? invariant(false, 'ReactUpdates: must provide an isBatchingUpdates boolean attribute') : invariant(false) : undefined;
batchingStrategy = _batchingStrategy;
}
};
var ReactUpdates = {
/**
* React references `ReactReconcileTransaction` using this property in order
* to allow dependency injection.
*
* @internal
*/
ReactReconcileTransaction: null,
batchedUpdates: batchedUpdates,
enqueueUpdate: enqueueUpdate,
flushBatchedUpdates: flushBatchedUpdates,
injection: ReactUpdatesInjection,
asap: asap
};
module.exports = ReactUpdates;
},{"113":113,"161":161,"24":24,"25":25,"6":6,"78":78,"84":84}],97:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ReactVersion
*/
'use strict';
module.exports = '0.14.7';
},{}],98:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SVGDOMPropertyConfig
*/
'use strict';
var DOMProperty = _dereq_(10);
var MUST_USE_ATTRIBUTE = DOMProperty.injection.MUST_USE_ATTRIBUTE;
var NS = {
xlink: 'http://www.w3.org/1999/xlink',
xml: 'http://www.w3.org/XML/1998/namespace'
};
var SVGDOMPropertyConfig = {
Properties: {
clipPath: MUST_USE_ATTRIBUTE,
cx: MUST_USE_ATTRIBUTE,
cy: MUST_USE_ATTRIBUTE,
d: MUST_USE_ATTRIBUTE,
dx: MUST_USE_ATTRIBUTE,
dy: MUST_USE_ATTRIBUTE,
fill: MUST_USE_ATTRIBUTE,
fillOpacity: MUST_USE_ATTRIBUTE,
fontFamily: MUST_USE_ATTRIBUTE,
fontSize: MUST_USE_ATTRIBUTE,
fx: MUST_USE_ATTRIBUTE,
fy: MUST_USE_ATTRIBUTE,
gradientTransform: MUST_USE_ATTRIBUTE,
gradientUnits: MUST_USE_ATTRIBUTE,
markerEnd: MUST_USE_ATTRIBUTE,
markerMid: MUST_USE_ATTRIBUTE,
markerStart: MUST_USE_ATTRIBUTE,
offset: MUST_USE_ATTRIBUTE,
opacity: MUST_USE_ATTRIBUTE,
patternContentUnits: MUST_USE_ATTRIBUTE,
patternUnits: MUST_USE_ATTRIBUTE,
points: MUST_USE_ATTRIBUTE,
preserveAspectRatio: MUST_USE_ATTRIBUTE,
r: MUST_USE_ATTRIBUTE,
rx: MUST_USE_ATTRIBUTE,
ry: MUST_USE_ATTRIBUTE,
spreadMethod: MUST_USE_ATTRIBUTE,
stopColor: MUST_USE_ATTRIBUTE,
stopOpacity: MUST_USE_ATTRIBUTE,
stroke: MUST_USE_ATTRIBUTE,
strokeDasharray: MUST_USE_ATTRIBUTE,
strokeLinecap: MUST_USE_ATTRIBUTE,
strokeOpacity: MUST_USE_ATTRIBUTE,
strokeWidth: MUST_USE_ATTRIBUTE,
textAnchor: MUST_USE_ATTRIBUTE,
transform: MUST_USE_ATTRIBUTE,
version: MUST_USE_ATTRIBUTE,
viewBox: MUST_USE_ATTRIBUTE,
x1: MUST_USE_ATTRIBUTE,
x2: MUST_USE_ATTRIBUTE,
x: MUST_USE_ATTRIBUTE,
xlinkActuate: MUST_USE_ATTRIBUTE,
xlinkArcrole: MUST_USE_ATTRIBUTE,
xlinkHref: MUST_USE_ATTRIBUTE,
xlinkRole: MUST_USE_ATTRIBUTE,
xlinkShow: MUST_USE_ATTRIBUTE,
xlinkTitle: MUST_USE_ATTRIBUTE,
xlinkType: MUST_USE_ATTRIBUTE,
xmlBase: MUST_USE_ATTRIBUTE,
xmlLang: MUST_USE_ATTRIBUTE,
xmlSpace: MUST_USE_ATTRIBUTE,
y1: MUST_USE_ATTRIBUTE,
y2: MUST_USE_ATTRIBUTE,
y: MUST_USE_ATTRIBUTE
},
DOMAttributeNamespaces: {
xlinkActuate: NS.xlink,
xlinkArcrole: NS.xlink,
xlinkHref: NS.xlink,
xlinkRole: NS.xlink,
xlinkShow: NS.xlink,
xlinkTitle: NS.xlink,
xlinkType: NS.xlink,
xmlBase: NS.xml,
xmlLang: NS.xml,
xmlSpace: NS.xml
},
DOMAttributeNames: {
clipPath: 'clip-path',
fillOpacity: 'fill-opacity',
fontFamily: 'font-family',
fontSize: 'font-size',
gradientTransform: 'gradientTransform',
gradientUnits: 'gradientUnits',
markerEnd: 'marker-end',
markerMid: 'marker-mid',
markerStart: 'marker-start',
patternContentUnits: 'patternContentUnits',
patternUnits: 'patternUnits',
preserveAspectRatio: 'preserveAspectRatio',
spreadMethod: 'spreadMethod',
stopColor: 'stop-color',
stopOpacity: 'stop-opacity',
strokeDasharray: 'stroke-dasharray',
strokeLinecap: 'stroke-linecap',
strokeOpacity: 'stroke-opacity',
strokeWidth: 'stroke-width',
textAnchor: 'text-anchor',
viewBox: 'viewBox',
xlinkActuate: 'xlink:actuate',
xlinkArcrole: 'xlink:arcrole',
xlinkHref: 'xlink:href',
xlinkRole: 'xlink:role',
xlinkShow: 'xlink:show',
xlinkTitle: 'xlink:title',
xlinkType: 'xlink:type',
xmlBase: 'xml:base',
xmlLang: 'xml:lang',
xmlSpace: 'xml:space'
}
};
module.exports = SVGDOMPropertyConfig;
},{"10":10}],99:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SelectEventPlugin
*/
'use strict';
var EventConstants = _dereq_(15);
var EventPropagators = _dereq_(19);
var ExecutionEnvironment = _dereq_(147);
var ReactInputSelection = _dereq_(66);
var SyntheticEvent = _dereq_(105);
var getActiveElement = _dereq_(156);
var isTextInputElement = _dereq_(134);
var keyOf = _dereq_(166);
var shallowEqual = _dereq_(171);
var topLevelTypes = EventConstants.topLevelTypes;
var skipSelectionChangeEvent = ExecutionEnvironment.canUseDOM && 'documentMode' in document && document.documentMode <= 11;
var eventTypes = {
select: {
phasedRegistrationNames: {
bubbled: keyOf({ onSelect: null }),
captured: keyOf({ onSelectCapture: null })
},
dependencies: [topLevelTypes.topBlur, topLevelTypes.topContextMenu, topLevelTypes.topFocus, topLevelTypes.topKeyDown, topLevelTypes.topMouseDown, topLevelTypes.topMouseUp, topLevelTypes.topSelectionChange]
}
};
var activeElement = null;
var activeElementID = null;
var lastSelection = null;
var mouseDown = false;
// Track whether a listener exists for this plugin. If none exist, we do
// not extract events.
var hasListener = false;
var ON_SELECT_KEY = keyOf({ onSelect: null });
/**
* Get an object which is a unique representation of the current selection.
*
* The return value will not be consistent across nodes or browsers, but
* two identical selections on the same node will return identical objects.
*
* @param {DOMElement} node
* @return {object}
*/
function getSelection(node) {
if ('selectionStart' in node && ReactInputSelection.hasSelectionCapabilities(node)) {
return {
start: node.selectionStart,
end: node.selectionEnd
};
} else if (window.getSelection) {
var selection = window.getSelection();
return {
anchorNode: selection.anchorNode,
anchorOffset: selection.anchorOffset,
focusNode: selection.focusNode,
focusOffset: selection.focusOffset
};
} else if (document.selection) {
var range = document.selection.createRange();
return {
parentElement: range.parentElement(),
text: range.text,
top: range.boundingTop,
left: range.boundingLeft
};
}
}
/**
* Poll selection to see whether it's changed.
*
* @param {object} nativeEvent
* @return {?SyntheticEvent}
*/
function constructSelectEvent(nativeEvent, nativeEventTarget) {
// Ensure we have the right element, and that the user is not dragging a
// selection (this matches native `select` event behavior). In HTML5, select
// fires only on input and textarea thus if there's no focused element we
// won't dispatch.
if (mouseDown || activeElement == null || activeElement !== getActiveElement()) {
return null;
}
// Only fire when selection has actually changed.
var currentSelection = getSelection(activeElement);
if (!lastSelection || !shallowEqual(lastSelection, currentSelection)) {
lastSelection = currentSelection;
var syntheticEvent = SyntheticEvent.getPooled(eventTypes.select, activeElementID, nativeEvent, nativeEventTarget);
syntheticEvent.type = 'select';
syntheticEvent.target = activeElement;
EventPropagators.accumulateTwoPhaseDispatches(syntheticEvent);
return syntheticEvent;
}
return null;
}
/**
* This plugin creates an `onSelect` event that normalizes select events
* across form elements.
*
* Supported elements are:
* - input (see `isTextInputElement`)
* - textarea
* - contentEditable
*
* This differs from native browser implementations in the following ways:
* - Fires on contentEditable fields as well as inputs.
* - Fires for collapsed selection.
* - Fires after user input.
*/
var SelectEventPlugin = {
eventTypes: eventTypes,
/**
* @param {string} topLevelType Record from `EventConstants`.
* @param {DOMEventTarget} topLevelTarget The listening component root node.
* @param {string} topLevelTargetID ID of `topLevelTarget`.
* @param {object} nativeEvent Native browser event.
* @return {*} An accumulation of synthetic events.
* @see {EventPluginHub.extractEvents}
*/
extractEvents: function (topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget) {
if (!hasListener) {
return null;
}
switch (topLevelType) {
// Track the input node that has focus.
case topLevelTypes.topFocus:
if (isTextInputElement(topLevelTarget) || topLevelTarget.contentEditable === 'true') {
activeElement = topLevelTarget;
activeElementID = topLevelTargetID;
lastSelection = null;
}
break;
case topLevelTypes.topBlur:
activeElement = null;
activeElementID = null;
lastSelection = null;
break;
// Don't fire the event while the user is dragging. This matches the
// semantics of the native select event.
case topLevelTypes.topMouseDown:
mouseDown = true;
break;
case topLevelTypes.topContextMenu:
case topLevelTypes.topMouseUp:
mouseDown = false;
return constructSelectEvent(nativeEvent, nativeEventTarget);
// Chrome and IE fire non-standard event when selection is changed (and
// sometimes when it hasn't). IE's event fires out of order with respect
// to key and input events on deletion, so we discard it.
//
// Firefox doesn't support selectionchange, so check selection status
// after each key entry. The selection changes after keydown and before
// keyup, but we check on keydown as well in the case of holding down a
// key, when multiple keydown events are fired but only one keyup is.
// This is also our approach for IE handling, for the reason above.
case topLevelTypes.topSelectionChange:
if (skipSelectionChangeEvent) {
break;
}
// falls through
case topLevelTypes.topKeyDown:
case topLevelTypes.topKeyUp:
return constructSelectEvent(nativeEvent, nativeEventTarget);
}
return null;
},
didPutListener: function (id, registrationName, listener) {
if (registrationName === ON_SELECT_KEY) {
hasListener = true;
}
}
};
module.exports = SelectEventPlugin;
},{"105":105,"134":134,"147":147,"15":15,"156":156,"166":166,"171":171,"19":19,"66":66}],100:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ServerReactRootIndex
* @typechecks
*/
'use strict';
/**
* Size of the reactRoot ID space. We generate random numbers for React root
* IDs and if there's a collision the events and DOM update system will
* get confused. In the future we need a way to generate GUIDs but for
* now this will work on a smaller scale.
*/
var GLOBAL_MOUNT_POINT_MAX = Math.pow(2, 53);
var ServerReactRootIndex = {
createReactRootIndex: function () {
return Math.ceil(Math.random() * GLOBAL_MOUNT_POINT_MAX);
}
};
module.exports = ServerReactRootIndex;
},{}],101:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SimpleEventPlugin
*/
'use strict';
var EventConstants = _dereq_(15);
var EventListener = _dereq_(146);
var EventPropagators = _dereq_(19);
var ReactMount = _dereq_(72);
var SyntheticClipboardEvent = _dereq_(102);
var SyntheticEvent = _dereq_(105);
var SyntheticFocusEvent = _dereq_(106);
var SyntheticKeyboardEvent = _dereq_(108);
var SyntheticMouseEvent = _dereq_(109);
var SyntheticDragEvent = _dereq_(104);
var SyntheticTouchEvent = _dereq_(110);
var SyntheticUIEvent = _dereq_(111);
var SyntheticWheelEvent = _dereq_(112);
var emptyFunction = _dereq_(153);
var getEventCharCode = _dereq_(125);
var invariant = _dereq_(161);
var keyOf = _dereq_(166);
var topLevelTypes = EventConstants.topLevelTypes;
var eventTypes = {
abort: {
phasedRegistrationNames: {
bubbled: keyOf({ onAbort: true }),
captured: keyOf({ onAbortCapture: true })
}
},
blur: {
phasedRegistrationNames: {
bubbled: keyOf({ onBlur: true }),
captured: keyOf({ onBlurCapture: true })
}
},
canPlay: {
phasedRegistrationNames: {
bubbled: keyOf({ onCanPlay: true }),
captured: keyOf({ onCanPlayCapture: true })
}
},
canPlayThrough: {
phasedRegistrationNames: {
bubbled: keyOf({ onCanPlayThrough: true }),
captured: keyOf({ onCanPlayThroughCapture: true })
}
},
click: {
phasedRegistrationNames: {
bubbled: keyOf({ onClick: true }),
captured: keyOf({ onClickCapture: true })
}
},
contextMenu: {
phasedRegistrationNames: {
bubbled: keyOf({ onContextMenu: true }),
captured: keyOf({ onContextMenuCapture: true })
}
},
copy: {
phasedRegistrationNames: {
bubbled: keyOf({ onCopy: true }),
captured: keyOf({ onCopyCapture: true })
}
},
cut: {
phasedRegistrationNames: {
bubbled: keyOf({ onCut: true }),
captured: keyOf({ onCutCapture: true })
}
},
doubleClick: {
phasedRegistrationNames: {
bubbled: keyOf({ onDoubleClick: true }),
captured: keyOf({ onDoubleClickCapture: true })
}
},
drag: {
phasedRegistrationNames: {
bubbled: keyOf({ onDrag: true }),
captured: keyOf({ onDragCapture: true })
}
},
dragEnd: {
phasedRegistrationNames: {
bubbled: keyOf({ onDragEnd: true }),
captured: keyOf({ onDragEndCapture: true })
}
},
dragEnter: {
phasedRegistrationNames: {
bubbled: keyOf({ onDragEnter: true }),
captured: keyOf({ onDragEnterCapture: true })
}
},
dragExit: {
phasedRegistrationNames: {
bubbled: keyOf({ onDragExit: true }),
captured: keyOf({ onDragExitCapture: true })
}
},
dragLeave: {
phasedRegistrationNames: {
bubbled: keyOf({ onDragLeave: true }),
captured: keyOf({ onDragLeaveCapture: true })
}
},
dragOver: {
phasedRegistrationNames: {
bubbled: keyOf({ onDragOver: true }),
captured: keyOf({ onDragOverCapture: true })
}
},
dragStart: {
phasedRegistrationNames: {
bubbled: keyOf({ onDragStart: true }),
captured: keyOf({ onDragStartCapture: true })
}
},
drop: {
phasedRegistrationNames: {
bubbled: keyOf({ onDrop: true }),
captured: keyOf({ onDropCapture: true })
}
},
durationChange: {
phasedRegistrationNames: {
bubbled: keyOf({ onDurationChange: true }),
captured: keyOf({ onDurationChangeCapture: true })
}
},
emptied: {
phasedRegistrationNames: {
bubbled: keyOf({ onEmptied: true }),
captured: keyOf({ onEmptiedCapture: true })
}
},
encrypted: {
phasedRegistrationNames: {
bubbled: keyOf({ onEncrypted: true }),
captured: keyOf({ onEncryptedCapture: true })
}
},
ended: {
phasedRegistrationNames: {
bubbled: keyOf({ onEnded: true }),
captured: keyOf({ onEndedCapture: true })
}
},
error: {
phasedRegistrationNames: {
bubbled: keyOf({ onError: true }),
captured: keyOf({ onErrorCapture: true })
}
},
focus: {
phasedRegistrationNames: {
bubbled: keyOf({ onFocus: true }),
captured: keyOf({ onFocusCapture: true })
}
},
input: {
phasedRegistrationNames: {
bubbled: keyOf({ onInput: true }),
captured: keyOf({ onInputCapture: true })
}
},
keyDown: {
phasedRegistrationNames: {
bubbled: keyOf({ onKeyDown: true }),
captured: keyOf({ onKeyDownCapture: true })
}
},
keyPress: {
phasedRegistrationNames: {
bubbled: keyOf({ onKeyPress: true }),
captured: keyOf({ onKeyPressCapture: true })
}
},
keyUp: {
phasedRegistrationNames: {
bubbled: keyOf({ onKeyUp: true }),
captured: keyOf({ onKeyUpCapture: true })
}
},
load: {
phasedRegistrationNames: {
bubbled: keyOf({ onLoad: true }),
captured: keyOf({ onLoadCapture: true })
}
},
loadedData: {
phasedRegistrationNames: {
bubbled: keyOf({ onLoadedData: true }),
captured: keyOf({ onLoadedDataCapture: true })
}
},
loadedMetadata: {
phasedRegistrationNames: {
bubbled: keyOf({ onLoadedMetadata: true }),
captured: keyOf({ onLoadedMetadataCapture: true })
}
},
loadStart: {
phasedRegistrationNames: {
bubbled: keyOf({ onLoadStart: true }),
captured: keyOf({ onLoadStartCapture: true })
}
},
// Note: We do not allow listening to mouseOver events. Instead, use the
// onMouseEnter/onMouseLeave created by `EnterLeaveEventPlugin`.
mouseDown: {
phasedRegistrationNames: {
bubbled: keyOf({ onMouseDown: true }),
captured: keyOf({ onMouseDownCapture: true })
}
},
mouseMove: {
phasedRegistrationNames: {
bubbled: keyOf({ onMouseMove: true }),
captured: keyOf({ onMouseMoveCapture: true })
}
},
mouseOut: {
phasedRegistrationNames: {
bubbled: keyOf({ onMouseOut: true }),
captured: keyOf({ onMouseOutCapture: true })
}
},
mouseOver: {
phasedRegistrationNames: {
bubbled: keyOf({ onMouseOver: true }),
captured: keyOf({ onMouseOverCapture: true })
}
},
mouseUp: {
phasedRegistrationNames: {
bubbled: keyOf({ onMouseUp: true }),
captured: keyOf({ onMouseUpCapture: true })
}
},
paste: {
phasedRegistrationNames: {
bubbled: keyOf({ onPaste: true }),
captured: keyOf({ onPasteCapture: true })
}
},
pause: {
phasedRegistrationNames: {
bubbled: keyOf({ onPause: true }),
captured: keyOf({ onPauseCapture: true })
}
},
play: {
phasedRegistrationNames: {
bubbled: keyOf({ onPlay: true }),
captured: keyOf({ onPlayCapture: true })
}
},
playing: {
phasedRegistrationNames: {
bubbled: keyOf({ onPlaying: true }),
captured: keyOf({ onPlayingCapture: true })
}
},
progress: {
phasedRegistrationNames: {
bubbled: keyOf({ onProgress: true }),
captured: keyOf({ onProgressCapture: true })
}
},
rateChange: {
phasedRegistrationNames: {
bubbled: keyOf({ onRateChange: true }),
captured: keyOf({ onRateChangeCapture: true })
}
},
reset: {
phasedRegistrationNames: {
bubbled: keyOf({ onReset: true }),
captured: keyOf({ onResetCapture: true })
}
},
scroll: {
phasedRegistrationNames: {
bubbled: keyOf({ onScroll: true }),
captured: keyOf({ onScrollCapture: true })
}
},
seeked: {
phasedRegistrationNames: {
bubbled: keyOf({ onSeeked: true }),
captured: keyOf({ onSeekedCapture: true })
}
},
seeking: {
phasedRegistrationNames: {
bubbled: keyOf({ onSeeking: true }),
captured: keyOf({ onSeekingCapture: true })
}
},
stalled: {
phasedRegistrationNames: {
bubbled: keyOf({ onStalled: true }),
captured: keyOf({ onStalledCapture: true })
}
},
submit: {
phasedRegistrationNames: {
bubbled: keyOf({ onSubmit: true }),
captured: keyOf({ onSubmitCapture: true })
}
},
suspend: {
phasedRegistrationNames: {
bubbled: keyOf({ onSuspend: true }),
captured: keyOf({ onSuspendCapture: true })
}
},
timeUpdate: {
phasedRegistrationNames: {
bubbled: keyOf({ onTimeUpdate: true }),
captured: keyOf({ onTimeUpdateCapture: true })
}
},
touchCancel: {
phasedRegistrationNames: {
bubbled: keyOf({ onTouchCancel: true }),
captured: keyOf({ onTouchCancelCapture: true })
}
},
touchEnd: {
phasedRegistrationNames: {
bubbled: keyOf({ onTouchEnd: true }),
captured: keyOf({ onTouchEndCapture: true })
}
},
touchMove: {
phasedRegistrationNames: {
bubbled: keyOf({ onTouchMove: true }),
captured: keyOf({ onTouchMoveCapture: true })
}
},
touchStart: {
phasedRegistrationNames: {
bubbled: keyOf({ onTouchStart: true }),
captured: keyOf({ onTouchStartCapture: true })
}
},
volumeChange: {
phasedRegistrationNames: {
bubbled: keyOf({ onVolumeChange: true }),
captured: keyOf({ onVolumeChangeCapture: true })
}
},
waiting: {
phasedRegistrationNames: {
bubbled: keyOf({ onWaiting: true }),
captured: keyOf({ onWaitingCapture: true })
}
},
wheel: {
phasedRegistrationNames: {
bubbled: keyOf({ onWheel: true }),
captured: keyOf({ onWheelCapture: true })
}
}
};
var topLevelEventsToDispatchConfig = {
topAbort: eventTypes.abort,
topBlur: eventTypes.blur,
topCanPlay: eventTypes.canPlay,
topCanPlayThrough: eventTypes.canPlayThrough,
topClick: eventTypes.click,
topContextMenu: eventTypes.contextMenu,
topCopy: eventTypes.copy,
topCut: eventTypes.cut,
topDoubleClick: eventTypes.doubleClick,
topDrag: eventTypes.drag,
topDragEnd: eventTypes.dragEnd,
topDragEnter: eventTypes.dragEnter,
topDragExit: eventTypes.dragExit,
topDragLeave: eventTypes.dragLeave,
topDragOver: eventTypes.dragOver,
topDragStart: eventTypes.dragStart,
topDrop: eventTypes.drop,
topDurationChange: eventTypes.durationChange,
topEmptied: eventTypes.emptied,
topEncrypted: eventTypes.encrypted,
topEnded: eventTypes.ended,
topError: eventTypes.error,
topFocus: eventTypes.focus,
topInput: eventTypes.input,
topKeyDown: eventTypes.keyDown,
topKeyPress: eventTypes.keyPress,
topKeyUp: eventTypes.keyUp,
topLoad: eventTypes.load,
topLoadedData: eventTypes.loadedData,
topLoadedMetadata: eventTypes.loadedMetadata,
topLoadStart: eventTypes.loadStart,
topMouseDown: eventTypes.mouseDown,
topMouseMove: eventTypes.mouseMove,
topMouseOut: eventTypes.mouseOut,
topMouseOver: eventTypes.mouseOver,
topMouseUp: eventTypes.mouseUp,
topPaste: eventTypes.paste,
topPause: eventTypes.pause,
topPlay: eventTypes.play,
topPlaying: eventTypes.playing,
topProgress: eventTypes.progress,
topRateChange: eventTypes.rateChange,
topReset: eventTypes.reset,
topScroll: eventTypes.scroll,
topSeeked: eventTypes.seeked,
topSeeking: eventTypes.seeking,
topStalled: eventTypes.stalled,
topSubmit: eventTypes.submit,
topSuspend: eventTypes.suspend,
topTimeUpdate: eventTypes.timeUpdate,
topTouchCancel: eventTypes.touchCancel,
topTouchEnd: eventTypes.touchEnd,
topTouchMove: eventTypes.touchMove,
topTouchStart: eventTypes.touchStart,
topVolumeChange: eventTypes.volumeChange,
topWaiting: eventTypes.waiting,
topWheel: eventTypes.wheel
};
for (var type in topLevelEventsToDispatchConfig) {
topLevelEventsToDispatchConfig[type].dependencies = [type];
}
var ON_CLICK_KEY = keyOf({ onClick: null });
var onClickListeners = {};
var SimpleEventPlugin = {
eventTypes: eventTypes,
/**
* @param {string} topLevelType Record from `EventConstants`.
* @param {DOMEventTarget} topLevelTarget The listening component root node.
* @param {string} topLevelTargetID ID of `topLevelTarget`.
* @param {object} nativeEvent Native browser event.
* @return {*} An accumulation of synthetic events.
* @see {EventPluginHub.extractEvents}
*/
extractEvents: function (topLevelType, topLevelTarget, topLevelTargetID, nativeEvent, nativeEventTarget) {
var dispatchConfig = topLevelEventsToDispatchConfig[topLevelType];
if (!dispatchConfig) {
return null;
}
var EventConstructor;
switch (topLevelType) {
case topLevelTypes.topAbort:
case topLevelTypes.topCanPlay:
case topLevelTypes.topCanPlayThrough:
case topLevelTypes.topDurationChange:
case topLevelTypes.topEmptied:
case topLevelTypes.topEncrypted:
case topLevelTypes.topEnded:
case topLevelTypes.topError:
case topLevelTypes.topInput:
case topLevelTypes.topLoad:
case topLevelTypes.topLoadedData:
case topLevelTypes.topLoadedMetadata:
case topLevelTypes.topLoadStart:
case topLevelTypes.topPause:
case topLevelTypes.topPlay:
case topLevelTypes.topPlaying:
case topLevelTypes.topProgress:
case topLevelTypes.topRateChange:
case topLevelTypes.topReset:
case topLevelTypes.topSeeked:
case topLevelTypes.topSeeking:
case topLevelTypes.topStalled:
case topLevelTypes.topSubmit:
case topLevelTypes.topSuspend:
case topLevelTypes.topTimeUpdate:
case topLevelTypes.topVolumeChange:
case topLevelTypes.topWaiting:
// HTML Events
// @see http://www.w3.org/TR/html5/index.html#events-0
EventConstructor = SyntheticEvent;
break;
case topLevelTypes.topKeyPress:
// FireFox creates a keypress event for function keys too. This removes
// the unwanted keypress events. Enter is however both printable and
// non-printable. One would expect Tab to be as well (but it isn't).
if (getEventCharCode(nativeEvent) === 0) {
return null;
}
/* falls through */
case topLevelTypes.topKeyDown:
case topLevelTypes.topKeyUp:
EventConstructor = SyntheticKeyboardEvent;
break;
case topLevelTypes.topBlur:
case topLevelTypes.topFocus:
EventConstructor = SyntheticFocusEvent;
break;
case topLevelTypes.topClick:
// Firefox creates a click event on right mouse clicks. This removes the
// unwanted click events.
if (nativeEvent.button === 2) {
return null;
}
/* falls through */
case topLevelTypes.topContextMenu:
case topLevelTypes.topDoubleClick:
case topLevelTypes.topMouseDown:
case topLevelTypes.topMouseMove:
case topLevelTypes.topMouseOut:
case topLevelTypes.topMouseOver:
case topLevelTypes.topMouseUp:
EventConstructor = SyntheticMouseEvent;
break;
case topLevelTypes.topDrag:
case topLevelTypes.topDragEnd:
case topLevelTypes.topDragEnter:
case topLevelTypes.topDragExit:
case topLevelTypes.topDragLeave:
case topLevelTypes.topDragOver:
case topLevelTypes.topDragStart:
case topLevelTypes.topDrop:
EventConstructor = SyntheticDragEvent;
break;
case topLevelTypes.topTouchCancel:
case topLevelTypes.topTouchEnd:
case topLevelTypes.topTouchMove:
case topLevelTypes.topTouchStart:
EventConstructor = SyntheticTouchEvent;
break;
case topLevelTypes.topScroll:
EventConstructor = SyntheticUIEvent;
break;
case topLevelTypes.topWheel:
EventConstructor = SyntheticWheelEvent;
break;
case topLevelTypes.topCopy:
case topLevelTypes.topCut:
case topLevelTypes.topPaste:
EventConstructor = SyntheticClipboardEvent;
break;
}
!EventConstructor ? "development" !== 'production' ? invariant(false, 'SimpleEventPlugin: Unhandled event type, `%s`.', topLevelType) : invariant(false) : undefined;
var event = EventConstructor.getPooled(dispatchConfig, topLevelTargetID, nativeEvent, nativeEventTarget);
EventPropagators.accumulateTwoPhaseDispatches(event);
return event;
},
didPutListener: function (id, registrationName, listener) {
// Mobile Safari does not fire properly bubble click events on
// non-interactive elements, which means delegated click listeners do not
// fire. The workaround for this bug involves attaching an empty click
// listener on the target node.
if (registrationName === ON_CLICK_KEY) {
var node = ReactMount.getNode(id);
if (!onClickListeners[id]) {
onClickListeners[id] = EventListener.listen(node, 'click', emptyFunction);
}
}
},
willDeleteListener: function (id, registrationName) {
if (registrationName === ON_CLICK_KEY) {
onClickListeners[id].remove();
delete onClickListeners[id];
}
}
};
module.exports = SimpleEventPlugin;
},{"102":102,"104":104,"105":105,"106":106,"108":108,"109":109,"110":110,"111":111,"112":112,"125":125,"146":146,"15":15,"153":153,"161":161,"166":166,"19":19,"72":72}],102:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SyntheticClipboardEvent
* @typechecks static-only
*/
'use strict';
var SyntheticEvent = _dereq_(105);
/**
* @interface Event
* @see http://www.w3.org/TR/clipboard-apis/
*/
var ClipboardEventInterface = {
clipboardData: function (event) {
return 'clipboardData' in event ? event.clipboardData : window.clipboardData;
}
};
/**
* @param {object} dispatchConfig Configuration used to dispatch this event.
* @param {string} dispatchMarker Marker identifying the event target.
* @param {object} nativeEvent Native browser event.
* @extends {SyntheticUIEvent}
*/
function SyntheticClipboardEvent(dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget) {
SyntheticEvent.call(this, dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget);
}
SyntheticEvent.augmentClass(SyntheticClipboardEvent, ClipboardEventInterface);
module.exports = SyntheticClipboardEvent;
},{"105":105}],103:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SyntheticCompositionEvent
* @typechecks static-only
*/
'use strict';
var SyntheticEvent = _dereq_(105);
/**
* @interface Event
* @see http://www.w3.org/TR/DOM-Level-3-Events/#events-compositionevents
*/
var CompositionEventInterface = {
data: null
};
/**
* @param {object} dispatchConfig Configuration used to dispatch this event.
* @param {string} dispatchMarker Marker identifying the event target.
* @param {object} nativeEvent Native browser event.
* @extends {SyntheticUIEvent}
*/
function SyntheticCompositionEvent(dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget) {
SyntheticEvent.call(this, dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget);
}
SyntheticEvent.augmentClass(SyntheticCompositionEvent, CompositionEventInterface);
module.exports = SyntheticCompositionEvent;
},{"105":105}],104:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SyntheticDragEvent
* @typechecks static-only
*/
'use strict';
var SyntheticMouseEvent = _dereq_(109);
/**
* @interface DragEvent
* @see http://www.w3.org/TR/DOM-Level-3-Events/
*/
var DragEventInterface = {
dataTransfer: null
};
/**
* @param {object} dispatchConfig Configuration used to dispatch this event.
* @param {string} dispatchMarker Marker identifying the event target.
* @param {object} nativeEvent Native browser event.
* @extends {SyntheticUIEvent}
*/
function SyntheticDragEvent(dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget) {
SyntheticMouseEvent.call(this, dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget);
}
SyntheticMouseEvent.augmentClass(SyntheticDragEvent, DragEventInterface);
module.exports = SyntheticDragEvent;
},{"109":109}],105:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SyntheticEvent
* @typechecks static-only
*/
'use strict';
var PooledClass = _dereq_(25);
var assign = _dereq_(24);
var emptyFunction = _dereq_(153);
var warning = _dereq_(173);
/**
* @interface Event
* @see http://www.w3.org/TR/DOM-Level-3-Events/
*/
var EventInterface = {
type: null,
target: null,
// currentTarget is set when dispatching; no use in copying it here
currentTarget: emptyFunction.thatReturnsNull,
eventPhase: null,
bubbles: null,
cancelable: null,
timeStamp: function (event) {
return event.timeStamp || Date.now();
},
defaultPrevented: null,
isTrusted: null
};
/**
* Synthetic events are dispatched by event plugins, typically in response to a
* top-level event delegation handler.
*
* These systems should generally use pooling to reduce the frequency of garbage
* collection. The system should check `isPersistent` to determine whether the
* event should be released into the pool after being dispatched. Users that
* need a persisted event should invoke `persist`.
*
* Synthetic events (and subclasses) implement the DOM Level 3 Events API by
* normalizing browser quirks. Subclasses do not necessarily have to implement a
* DOM interface; custom application-specific events can also subclass this.
*
* @param {object} dispatchConfig Configuration used to dispatch this event.
* @param {string} dispatchMarker Marker identifying the event target.
* @param {object} nativeEvent Native browser event.
*/
function SyntheticEvent(dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget) {
this.dispatchConfig = dispatchConfig;
this.dispatchMarker = dispatchMarker;
this.nativeEvent = nativeEvent;
var Interface = this.constructor.Interface;
for (var propName in Interface) {
if (!Interface.hasOwnProperty(propName)) {
continue;
}
var normalize = Interface[propName];
if (normalize) {
this[propName] = normalize(nativeEvent);
} else {
if (propName === 'target') {
this.target = nativeEventTarget;
} else {
this[propName] = nativeEvent[propName];
}
}
}
var defaultPrevented = nativeEvent.defaultPrevented != null ? nativeEvent.defaultPrevented : nativeEvent.returnValue === false;
if (defaultPrevented) {
this.isDefaultPrevented = emptyFunction.thatReturnsTrue;
} else {
this.isDefaultPrevented = emptyFunction.thatReturnsFalse;
}
this.isPropagationStopped = emptyFunction.thatReturnsFalse;
}
assign(SyntheticEvent.prototype, {
preventDefault: function () {
this.defaultPrevented = true;
var event = this.nativeEvent;
if ("development" !== 'production') {
"development" !== 'production' ? warning(event, 'This synthetic event is reused for performance reasons. If you\'re ' + 'seeing this, you\'re calling `preventDefault` on a ' + 'released/nullified synthetic event. This is a no-op. See ' + 'https://fb.me/react-event-pooling for more information.') : undefined;
}
if (!event) {
return;
}
if (event.preventDefault) {
event.preventDefault();
} else {
event.returnValue = false;
}
this.isDefaultPrevented = emptyFunction.thatReturnsTrue;
},
stopPropagation: function () {
var event = this.nativeEvent;
if ("development" !== 'production') {
"development" !== 'production' ? warning(event, 'This synthetic event is reused for performance reasons. If you\'re ' + 'seeing this, you\'re calling `stopPropagation` on a ' + 'released/nullified synthetic event. This is a no-op. See ' + 'https://fb.me/react-event-pooling for more information.') : undefined;
}
if (!event) {
return;
}
if (event.stopPropagation) {
event.stopPropagation();
} else {
event.cancelBubble = true;
}
this.isPropagationStopped = emptyFunction.thatReturnsTrue;
},
/**
* We release all dispatched `SyntheticEvent`s after each event loop, adding
* them back into the pool. This allows a way to hold onto a reference that
* won't be added back into the pool.
*/
persist: function () {
this.isPersistent = emptyFunction.thatReturnsTrue;
},
/**
* Checks if this event should be released back into the pool.
*
* @return {boolean} True if this should not be released, false otherwise.
*/
isPersistent: emptyFunction.thatReturnsFalse,
/**
* `PooledClass` looks for `destructor` on each instance it releases.
*/
destructor: function () {
var Interface = this.constructor.Interface;
for (var propName in Interface) {
this[propName] = null;
}
this.dispatchConfig = null;
this.dispatchMarker = null;
this.nativeEvent = null;
}
});
SyntheticEvent.Interface = EventInterface;
/**
* Helper to reduce boilerplate when creating subclasses.
*
* @param {function} Class
* @param {?object} Interface
*/
SyntheticEvent.augmentClass = function (Class, Interface) {
var Super = this;
var prototype = Object.create(Super.prototype);
assign(prototype, Class.prototype);
Class.prototype = prototype;
Class.prototype.constructor = Class;
Class.Interface = assign({}, Super.Interface, Interface);
Class.augmentClass = Super.augmentClass;
PooledClass.addPoolingTo(Class, PooledClass.fourArgumentPooler);
};
PooledClass.addPoolingTo(SyntheticEvent, PooledClass.fourArgumentPooler);
module.exports = SyntheticEvent;
},{"153":153,"173":173,"24":24,"25":25}],106:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SyntheticFocusEvent
* @typechecks static-only
*/
'use strict';
var SyntheticUIEvent = _dereq_(111);
/**
* @interface FocusEvent
* @see http://www.w3.org/TR/DOM-Level-3-Events/
*/
var FocusEventInterface = {
relatedTarget: null
};
/**
* @param {object} dispatchConfig Configuration used to dispatch this event.
* @param {string} dispatchMarker Marker identifying the event target.
* @param {object} nativeEvent Native browser event.
* @extends {SyntheticUIEvent}
*/
function SyntheticFocusEvent(dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget) {
SyntheticUIEvent.call(this, dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget);
}
SyntheticUIEvent.augmentClass(SyntheticFocusEvent, FocusEventInterface);
module.exports = SyntheticFocusEvent;
},{"111":111}],107:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SyntheticInputEvent
* @typechecks static-only
*/
'use strict';
var SyntheticEvent = _dereq_(105);
/**
* @interface Event
* @see http://www.w3.org/TR/2013/WD-DOM-Level-3-Events-20131105
* /#events-inputevents
*/
var InputEventInterface = {
data: null
};
/**
* @param {object} dispatchConfig Configuration used to dispatch this event.
* @param {string} dispatchMarker Marker identifying the event target.
* @param {object} nativeEvent Native browser event.
* @extends {SyntheticUIEvent}
*/
function SyntheticInputEvent(dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget) {
SyntheticEvent.call(this, dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget);
}
SyntheticEvent.augmentClass(SyntheticInputEvent, InputEventInterface);
module.exports = SyntheticInputEvent;
},{"105":105}],108:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SyntheticKeyboardEvent
* @typechecks static-only
*/
'use strict';
var SyntheticUIEvent = _dereq_(111);
var getEventCharCode = _dereq_(125);
var getEventKey = _dereq_(126);
var getEventModifierState = _dereq_(127);
/**
* @interface KeyboardEvent
* @see http://www.w3.org/TR/DOM-Level-3-Events/
*/
var KeyboardEventInterface = {
key: getEventKey,
location: null,
ctrlKey: null,
shiftKey: null,
altKey: null,
metaKey: null,
repeat: null,
locale: null,
getModifierState: getEventModifierState,
// Legacy Interface
charCode: function (event) {
// `charCode` is the result of a KeyPress event and represents the value of
// the actual printable character.
// KeyPress is deprecated, but its replacement is not yet final and not
// implemented in any major browser. Only KeyPress has charCode.
if (event.type === 'keypress') {
return getEventCharCode(event);
}
return 0;
},
keyCode: function (event) {
// `keyCode` is the result of a KeyDown/Up event and represents the value of
// physical keyboard key.
// The actual meaning of the value depends on the users' keyboard layout
// which cannot be detected. Assuming that it is a US keyboard layout
// provides a surprisingly accurate mapping for US and European users.
// Due to this, it is left to the user to implement at this time.
if (event.type === 'keydown' || event.type === 'keyup') {
return event.keyCode;
}
return 0;
},
which: function (event) {
// `which` is an alias for either `keyCode` or `charCode` depending on the
// type of the event.
if (event.type === 'keypress') {
return getEventCharCode(event);
}
if (event.type === 'keydown' || event.type === 'keyup') {
return event.keyCode;
}
return 0;
}
};
/**
* @param {object} dispatchConfig Configuration used to dispatch this event.
* @param {string} dispatchMarker Marker identifying the event target.
* @param {object} nativeEvent Native browser event.
* @extends {SyntheticUIEvent}
*/
function SyntheticKeyboardEvent(dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget) {
SyntheticUIEvent.call(this, dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget);
}
SyntheticUIEvent.augmentClass(SyntheticKeyboardEvent, KeyboardEventInterface);
module.exports = SyntheticKeyboardEvent;
},{"111":111,"125":125,"126":126,"127":127}],109:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SyntheticMouseEvent
* @typechecks static-only
*/
'use strict';
var SyntheticUIEvent = _dereq_(111);
var ViewportMetrics = _dereq_(114);
var getEventModifierState = _dereq_(127);
/**
* @interface MouseEvent
* @see http://www.w3.org/TR/DOM-Level-3-Events/
*/
var MouseEventInterface = {
screenX: null,
screenY: null,
clientX: null,
clientY: null,
ctrlKey: null,
shiftKey: null,
altKey: null,
metaKey: null,
getModifierState: getEventModifierState,
button: function (event) {
// Webkit, Firefox, IE9+
// which: 1 2 3
// button: 0 1 2 (standard)
var button = event.button;
if ('which' in event) {
return button;
}
// IE<9
// which: undefined
// button: 0 0 0
// button: 1 4 2 (onmouseup)
return button === 2 ? 2 : button === 4 ? 1 : 0;
},
buttons: null,
relatedTarget: function (event) {
return event.relatedTarget || (event.fromElement === event.srcElement ? event.toElement : event.fromElement);
},
// "Proprietary" Interface.
pageX: function (event) {
return 'pageX' in event ? event.pageX : event.clientX + ViewportMetrics.currentScrollLeft;
},
pageY: function (event) {
return 'pageY' in event ? event.pageY : event.clientY + ViewportMetrics.currentScrollTop;
}
};
/**
* @param {object} dispatchConfig Configuration used to dispatch this event.
* @param {string} dispatchMarker Marker identifying the event target.
* @param {object} nativeEvent Native browser event.
* @extends {SyntheticUIEvent}
*/
function SyntheticMouseEvent(dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget) {
SyntheticUIEvent.call(this, dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget);
}
SyntheticUIEvent.augmentClass(SyntheticMouseEvent, MouseEventInterface);
module.exports = SyntheticMouseEvent;
},{"111":111,"114":114,"127":127}],110:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SyntheticTouchEvent
* @typechecks static-only
*/
'use strict';
var SyntheticUIEvent = _dereq_(111);
var getEventModifierState = _dereq_(127);
/**
* @interface TouchEvent
* @see http://www.w3.org/TR/touch-events/
*/
var TouchEventInterface = {
touches: null,
targetTouches: null,
changedTouches: null,
altKey: null,
metaKey: null,
ctrlKey: null,
shiftKey: null,
getModifierState: getEventModifierState
};
/**
* @param {object} dispatchConfig Configuration used to dispatch this event.
* @param {string} dispatchMarker Marker identifying the event target.
* @param {object} nativeEvent Native browser event.
* @extends {SyntheticUIEvent}
*/
function SyntheticTouchEvent(dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget) {
SyntheticUIEvent.call(this, dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget);
}
SyntheticUIEvent.augmentClass(SyntheticTouchEvent, TouchEventInterface);
module.exports = SyntheticTouchEvent;
},{"111":111,"127":127}],111:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SyntheticUIEvent
* @typechecks static-only
*/
'use strict';
var SyntheticEvent = _dereq_(105);
var getEventTarget = _dereq_(128);
/**
* @interface UIEvent
* @see http://www.w3.org/TR/DOM-Level-3-Events/
*/
var UIEventInterface = {
view: function (event) {
if (event.view) {
return event.view;
}
var target = getEventTarget(event);
if (target != null && target.window === target) {
// target is a window object
return target;
}
var doc = target.ownerDocument;
// TODO: Figure out why `ownerDocument` is sometimes undefined in IE8.
if (doc) {
return doc.defaultView || doc.parentWindow;
} else {
return window;
}
},
detail: function (event) {
return event.detail || 0;
}
};
/**
* @param {object} dispatchConfig Configuration used to dispatch this event.
* @param {string} dispatchMarker Marker identifying the event target.
* @param {object} nativeEvent Native browser event.
* @extends {SyntheticEvent}
*/
function SyntheticUIEvent(dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget) {
SyntheticEvent.call(this, dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget);
}
SyntheticEvent.augmentClass(SyntheticUIEvent, UIEventInterface);
module.exports = SyntheticUIEvent;
},{"105":105,"128":128}],112:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule SyntheticWheelEvent
* @typechecks static-only
*/
'use strict';
var SyntheticMouseEvent = _dereq_(109);
/**
* @interface WheelEvent
* @see http://www.w3.org/TR/DOM-Level-3-Events/
*/
var WheelEventInterface = {
deltaX: function (event) {
return 'deltaX' in event ? event.deltaX :
// Fallback to `wheelDeltaX` for Webkit and normalize (right is positive).
'wheelDeltaX' in event ? -event.wheelDeltaX : 0;
},
deltaY: function (event) {
return 'deltaY' in event ? event.deltaY :
// Fallback to `wheelDeltaY` for Webkit and normalize (down is positive).
'wheelDeltaY' in event ? -event.wheelDeltaY :
// Fallback to `wheelDelta` for IE<9 and normalize (down is positive).
'wheelDelta' in event ? -event.wheelDelta : 0;
},
deltaZ: null,
// Browsers without "deltaMode" is reporting in raw wheel delta where one
// notch on the scroll is always +/- 120, roughly equivalent to pixels.
// A good approximation of DOM_DELTA_LINE (1) is 5% of viewport size or
// ~40 pixels, for DOM_DELTA_SCREEN (2) it is 87.5% of viewport size.
deltaMode: null
};
/**
* @param {object} dispatchConfig Configuration used to dispatch this event.
* @param {string} dispatchMarker Marker identifying the event target.
* @param {object} nativeEvent Native browser event.
* @extends {SyntheticMouseEvent}
*/
function SyntheticWheelEvent(dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget) {
SyntheticMouseEvent.call(this, dispatchConfig, dispatchMarker, nativeEvent, nativeEventTarget);
}
SyntheticMouseEvent.augmentClass(SyntheticWheelEvent, WheelEventInterface);
module.exports = SyntheticWheelEvent;
},{"109":109}],113:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule Transaction
*/
'use strict';
var invariant = _dereq_(161);
/**
* `Transaction` creates a black box that is able to wrap any method such that
* certain invariants are maintained before and after the method is invoked
* (Even if an exception is thrown while invoking the wrapped method). Whoever
* instantiates a transaction can provide enforcers of the invariants at
* creation time. The `Transaction` class itself will supply one additional
* automatic invariant for you - the invariant that any transaction instance
* should not be run while it is already being run. You would typically create a
* single instance of a `Transaction` for reuse multiple times, that potentially
* is used to wrap several different methods. Wrappers are extremely simple -
* they only require implementing two methods.
*
* <pre>
* wrappers (injected at creation time)
* + +
* | |
* +-----------------|--------|--------------+
* | v | |
* | +---------------+ | |
* | +--| wrapper1 |---|----+ |
* | | +---------------+ v | |
* | | +-------------+ | |
* | | +----| wrapper2 |--------+ |
* | | | +-------------+ | | |
* | | | | | |
* | v v v v | wrapper
* | +---+ +---+ +---------+ +---+ +---+ | invariants
* perform(anyMethod) | | | | | | | | | | | | maintained
* +----------------->|-|---|-|---|-->|anyMethod|---|---|-|---|-|-------->
* | | | | | | | | | | | |
* | | | | | | | | | | | |
* | | | | | | | | | | | |
* | +---+ +---+ +---------+ +---+ +---+ |
* | initialize close |
* +-----------------------------------------+
* </pre>
*
* Use cases:
* - Preserving the input selection ranges before/after reconciliation.
* Restoring selection even in the event of an unexpected error.
* - Deactivating events while rearranging the DOM, preventing blurs/focuses,
* while guaranteeing that afterwards, the event system is reactivated.
* - Flushing a queue of collected DOM mutations to the main UI thread after a
* reconciliation takes place in a worker thread.
* - Invoking any collected `componentDidUpdate` callbacks after rendering new
* content.
* - (Future use case): Wrapping particular flushes of the `ReactWorker` queue
* to preserve the `scrollTop` (an automatic scroll aware DOM).
* - (Future use case): Layout calculations before and after DOM updates.
*
* Transactional plugin API:
* - A module that has an `initialize` method that returns any precomputation.
* - and a `close` method that accepts the precomputation. `close` is invoked
* when the wrapped process is completed, or has failed.
*
* @param {Array<TransactionalWrapper>} transactionWrapper Wrapper modules
* that implement `initialize` and `close`.
* @return {Transaction} Single transaction for reuse in thread.
*
* @class Transaction
*/
var Mixin = {
/**
* Sets up this instance so that it is prepared for collecting metrics. Does
* so such that this setup method may be used on an instance that is already
* initialized, in a way that does not consume additional memory upon reuse.
* That can be useful if you decide to make your subclass of this mixin a
* "PooledClass".
*/
reinitializeTransaction: function () {
this.transactionWrappers = this.getTransactionWrappers();
if (this.wrapperInitData) {
this.wrapperInitData.length = 0;
} else {
this.wrapperInitData = [];
}
this._isInTransaction = false;
},
_isInTransaction: false,
/**
* @abstract
* @return {Array<TransactionWrapper>} Array of transaction wrappers.
*/
getTransactionWrappers: null,
isInTransaction: function () {
return !!this._isInTransaction;
},
/**
* Executes the function within a safety window. Use this for the top level
* methods that result in large amounts of computation/mutations that would
* need to be safety checked. The optional arguments helps prevent the need
* to bind in many cases.
*
* @param {function} method Member of scope to call.
* @param {Object} scope Scope to invoke from.
* @param {Object?=} a Argument to pass to the method.
* @param {Object?=} b Argument to pass to the method.
* @param {Object?=} c Argument to pass to the method.
* @param {Object?=} d Argument to pass to the method.
* @param {Object?=} e Argument to pass to the method.
* @param {Object?=} f Argument to pass to the method.
*
* @return {*} Return value from `method`.
*/
perform: function (method, scope, a, b, c, d, e, f) {
!!this.isInTransaction() ? "development" !== 'production' ? invariant(false, 'Transaction.perform(...): Cannot initialize a transaction when there ' + 'is already an outstanding transaction.') : invariant(false) : undefined;
var errorThrown;
var ret;
try {
this._isInTransaction = true;
// Catching errors makes debugging more difficult, so we start with
// errorThrown set to true before setting it to false after calling
// close -- if it's still set to true in the finally block, it means
// one of these calls threw.
errorThrown = true;
this.initializeAll(0);
ret = method.call(scope, a, b, c, d, e, f);
errorThrown = false;
} finally {
try {
if (errorThrown) {
// If `method` throws, prefer to show that stack trace over any thrown
// by invoking `closeAll`.
try {
this.closeAll(0);
} catch (err) {}
} else {
// Since `method` didn't throw, we don't want to silence the exception
// here.
this.closeAll(0);
}
} finally {
this._isInTransaction = false;
}
}
return ret;
},
initializeAll: function (startIndex) {
var transactionWrappers = this.transactionWrappers;
for (var i = startIndex; i < transactionWrappers.length; i++) {
var wrapper = transactionWrappers[i];
try {
// Catching errors makes debugging more difficult, so we start with the
// OBSERVED_ERROR state before overwriting it with the real return value
// of initialize -- if it's still set to OBSERVED_ERROR in the finally
// block, it means wrapper.initialize threw.
this.wrapperInitData[i] = Transaction.OBSERVED_ERROR;
this.wrapperInitData[i] = wrapper.initialize ? wrapper.initialize.call(this) : null;
} finally {
if (this.wrapperInitData[i] === Transaction.OBSERVED_ERROR) {
// The initializer for wrapper i threw an error; initialize the
// remaining wrappers but silence any exceptions from them to ensure
// that the first error is the one to bubble up.
try {
this.initializeAll(i + 1);
} catch (err) {}
}
}
}
},
/**
* Invokes each of `this.transactionWrappers.close[i]` functions, passing into
* them the respective return values of `this.transactionWrappers.init[i]`
* (`close`rs that correspond to initializers that failed will not be
* invoked).
*/
closeAll: function (startIndex) {
!this.isInTransaction() ? "development" !== 'production' ? invariant(false, 'Transaction.closeAll(): Cannot close transaction when none are open.') : invariant(false) : undefined;
var transactionWrappers = this.transactionWrappers;
for (var i = startIndex; i < transactionWrappers.length; i++) {
var wrapper = transactionWrappers[i];
var initData = this.wrapperInitData[i];
var errorThrown;
try {
// Catching errors makes debugging more difficult, so we start with
// errorThrown set to true before setting it to false after calling
// close -- if it's still set to true in the finally block, it means
// wrapper.close threw.
errorThrown = true;
if (initData !== Transaction.OBSERVED_ERROR && wrapper.close) {
wrapper.close.call(this, initData);
}
errorThrown = false;
} finally {
if (errorThrown) {
// The closer for wrapper i threw an error; close the remaining
// wrappers but silence any exceptions from them to ensure that the
// first error is the one to bubble up.
try {
this.closeAll(i + 1);
} catch (e) {}
}
}
}
this.wrapperInitData.length = 0;
}
};
var Transaction = {
Mixin: Mixin,
/**
* Token to look for to determine if an error occurred.
*/
OBSERVED_ERROR: {}
};
module.exports = Transaction;
},{"161":161}],114:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ViewportMetrics
*/
'use strict';
var ViewportMetrics = {
currentScrollLeft: 0,
currentScrollTop: 0,
refreshScrollValues: function (scrollPosition) {
ViewportMetrics.currentScrollLeft = scrollPosition.x;
ViewportMetrics.currentScrollTop = scrollPosition.y;
}
};
module.exports = ViewportMetrics;
},{}],115:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule accumulateInto
*/
'use strict';
var invariant = _dereq_(161);
/**
*
* Accumulates items that must not be null or undefined into the first one. This
* is used to conserve memory by avoiding array allocations, and thus sacrifices
* API cleanness. Since `current` can be null before being passed in and not
* null after this function, make sure to assign it back to `current`:
*
* `a = accumulateInto(a, b);`
*
* This API should be sparingly used. Try `accumulate` for something cleaner.
*
* @return {*|array<*>} An accumulation of items.
*/
function accumulateInto(current, next) {
!(next != null) ? "development" !== 'production' ? invariant(false, 'accumulateInto(...): Accumulated items must not be null or undefined.') : invariant(false) : undefined;
if (current == null) {
return next;
}
// Both are not empty. Warning: Never call x.concat(y) when you are not
// certain that x is an Array (x could be a string with concat method).
var currentIsArray = Array.isArray(current);
var nextIsArray = Array.isArray(next);
if (currentIsArray && nextIsArray) {
current.push.apply(current, next);
return current;
}
if (currentIsArray) {
current.push(next);
return current;
}
if (nextIsArray) {
// A bit too dangerous to mutate `next`.
return [current].concat(next);
}
return [current, next];
}
module.exports = accumulateInto;
},{"161":161}],116:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule adler32
*/
'use strict';
var MOD = 65521;
// adler32 is not cryptographically strong, and is only used to sanity check that
// markup generated on the server matches the markup generated on the client.
// This implementation (a modified version of the SheetJS version) has been optimized
// for our use case, at the expense of conforming to the adler32 specification
// for non-ascii inputs.
function adler32(data) {
var a = 1;
var b = 0;
var i = 0;
var l = data.length;
var m = l & ~0x3;
while (i < m) {
for (; i < Math.min(i + 4096, m); i += 4) {
b += (a += data.charCodeAt(i)) + (a += data.charCodeAt(i + 1)) + (a += data.charCodeAt(i + 2)) + (a += data.charCodeAt(i + 3));
}
a %= MOD;
b %= MOD;
}
for (; i < l; i++) {
b += a += data.charCodeAt(i);
}
a %= MOD;
b %= MOD;
return a | b << 16;
}
module.exports = adler32;
},{}],117:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule canDefineProperty
*/
'use strict';
var canDefineProperty = false;
if ("development" !== 'production') {
try {
Object.defineProperty({}, 'x', { get: function () {} });
canDefineProperty = true;
} catch (x) {
// IE will fail on defineProperty
}
}
module.exports = canDefineProperty;
},{}],118:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @typechecks static-only
* @providesModule cloneWithProps
*/
'use strict';
var ReactElement = _dereq_(57);
var ReactPropTransferer = _dereq_(79);
var keyOf = _dereq_(166);
var warning = _dereq_(173);
var CHILDREN_PROP = keyOf({ children: null });
var didDeprecatedWarn = false;
/**
* Sometimes you want to change the props of a child passed to you. Usually
* this is to add a CSS class.
*
* @param {ReactElement} child child element you'd like to clone
* @param {object} props props you'd like to modify. className and style will be
* merged automatically.
* @return {ReactElement} a clone of child with props merged in.
* @deprecated
*/
function cloneWithProps(child, props) {
if ("development" !== 'production') {
"development" !== 'production' ? warning(didDeprecatedWarn, 'cloneWithProps(...) is deprecated. ' + 'Please use React.cloneElement instead.') : undefined;
didDeprecatedWarn = true;
"development" !== 'production' ? warning(!child.ref, 'You are calling cloneWithProps() on a child with a ref. This is ' + 'dangerous because you\'re creating a new child which will not be ' + 'added as a ref to its parent.') : undefined;
}
var newProps = ReactPropTransferer.mergeProps(props, child.props);
// Use `child.props.children` if it is provided.
if (!newProps.hasOwnProperty(CHILDREN_PROP) && child.props.hasOwnProperty(CHILDREN_PROP)) {
newProps.children = child.props.children;
}
// The current API doesn't retain _owner, which is why this
// doesn't use ReactElement.cloneAndReplaceProps.
return ReactElement.createElement(child.type, newProps);
}
module.exports = cloneWithProps;
},{"166":166,"173":173,"57":57,"79":79}],119:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule dangerousStyleValue
* @typechecks static-only
*/
'use strict';
var CSSProperty = _dereq_(4);
var isUnitlessNumber = CSSProperty.isUnitlessNumber;
/**
* Convert a value into the proper css writable value. The style name `name`
* should be logical (no hyphens), as specified
* in `CSSProperty.isUnitlessNumber`.
*
* @param {string} name CSS property name such as `topMargin`.
* @param {*} value CSS property value such as `10px`.
* @return {string} Normalized style value with dimensions applied.
*/
function dangerousStyleValue(name, value) {
// Note that we've removed escapeTextForBrowser() calls here since the
// whole string will be escaped when the attribute is injected into
// the markup. If you provide unsafe user data here they can inject
// arbitrary CSS which may be problematic (I couldn't repro this):
// https://www.owasp.org/index.php/XSS_Filter_Evasion_Cheat_Sheet
// http://www.thespanner.co.uk/2007/11/26/ultimate-xss-css-injection/
// This is not an XSS hole but instead a potential CSS injection issue
// which has lead to a greater discussion about how we're going to
// trust URLs moving forward. See #2115901
var isEmpty = value == null || typeof value === 'boolean' || value === '';
if (isEmpty) {
return '';
}
var isNonNumeric = isNaN(value);
if (isNonNumeric || value === 0 || isUnitlessNumber.hasOwnProperty(name) && isUnitlessNumber[name]) {
return '' + value; // cast to string
}
if (typeof value === 'string') {
value = value.trim();
}
return value + 'px';
}
module.exports = dangerousStyleValue;
},{"4":4}],120:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule deprecated
*/
'use strict';
var assign = _dereq_(24);
var warning = _dereq_(173);
/**
* This will log a single deprecation notice per function and forward the call
* on to the new API.
*
* @param {string} fnName The name of the function
* @param {string} newModule The module that fn will exist in
* @param {string} newPackage The module that fn will exist in
* @param {*} ctx The context this forwarded call should run in
* @param {function} fn The function to forward on to
* @return {function} The function that will warn once and then call fn
*/
function deprecated(fnName, newModule, newPackage, ctx, fn) {
var warned = false;
if ("development" !== 'production') {
var newFn = function () {
"development" !== 'production' ? warning(warned,
// Require examples in this string must be split to prevent React's
// build tools from mistaking them for real requires.
// Otherwise the build tools will attempt to build a '%s' module.
'React.%s is deprecated. Please use %s.%s from require' + '(\'%s\') ' + 'instead.', fnName, newModule, fnName, newPackage) : undefined;
warned = true;
return fn.apply(ctx, arguments);
};
// We need to make sure all properties of the original fn are copied over.
// In particular, this is needed to support PropTypes
return assign(newFn, fn);
}
return fn;
}
module.exports = deprecated;
},{"173":173,"24":24}],121:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule escapeTextContentForBrowser
*/
'use strict';
var ESCAPE_LOOKUP = {
'&': '&',
'>': '>',
'<': '<',
'"': '"',
'\'': '''
};
var ESCAPE_REGEX = /[&><"']/g;
function escaper(match) {
return ESCAPE_LOOKUP[match];
}
/**
* Escapes text to prevent scripting attacks.
*
* @param {*} text Text value to escape.
* @return {string} An escaped string.
*/
function escapeTextContentForBrowser(text) {
return ('' + text).replace(ESCAPE_REGEX, escaper);
}
module.exports = escapeTextContentForBrowser;
},{}],122:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule findDOMNode
* @typechecks static-only
*/
'use strict';
var ReactCurrentOwner = _dereq_(39);
var ReactInstanceMap = _dereq_(68);
var ReactMount = _dereq_(72);
var invariant = _dereq_(161);
var warning = _dereq_(173);
/**
* Returns the DOM node rendered by this element.
*
* @param {ReactComponent|DOMElement} componentOrElement
* @return {?DOMElement} The root node of this element.
*/
function findDOMNode(componentOrElement) {
if ("development" !== 'production') {
var owner = ReactCurrentOwner.current;
if (owner !== null) {
"development" !== 'production' ? warning(owner._warnedAboutRefsInRender, '%s is accessing getDOMNode or findDOMNode inside its render(). ' + 'render() should be a pure function of props and state. It should ' + 'never access something that requires stale data from the previous ' + 'render, such as refs. Move this logic to componentDidMount and ' + 'componentDidUpdate instead.', owner.getName() || 'A component') : undefined;
owner._warnedAboutRefsInRender = true;
}
}
if (componentOrElement == null) {
return null;
}
if (componentOrElement.nodeType === 1) {
return componentOrElement;
}
if (ReactInstanceMap.has(componentOrElement)) {
return ReactMount.getNodeFromInstance(componentOrElement);
}
!(componentOrElement.render == null || typeof componentOrElement.render !== 'function') ? "development" !== 'production' ? invariant(false, 'findDOMNode was called on an unmounted component.') : invariant(false) : undefined;
!false ? "development" !== 'production' ? invariant(false, 'Element appears to be neither ReactComponent nor DOMNode (keys: %s)', Object.keys(componentOrElement)) : invariant(false) : undefined;
}
module.exports = findDOMNode;
},{"161":161,"173":173,"39":39,"68":68,"72":72}],123:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule flattenChildren
*/
'use strict';
var traverseAllChildren = _dereq_(142);
var warning = _dereq_(173);
/**
* @param {function} traverseContext Context passed through traversal.
* @param {?ReactComponent} child React child component.
* @param {!string} name String name of key path to child.
*/
function flattenSingleChildIntoContext(traverseContext, child, name) {
// We found a component instance.
var result = traverseContext;
var keyUnique = result[name] === undefined;
if ("development" !== 'production') {
"development" !== 'production' ? warning(keyUnique, 'flattenChildren(...): Encountered two children with the same key, ' + '`%s`. Child keys must be unique; when two children share a key, only ' + 'the first child will be used.', name) : undefined;
}
if (keyUnique && child != null) {
result[name] = child;
}
}
/**
* Flattens children that are typically specified as `props.children`. Any null
* children will not be included in the resulting object.
* @return {!object} flattened children keyed by name.
*/
function flattenChildren(children) {
if (children == null) {
return children;
}
var result = {};
traverseAllChildren(children, flattenSingleChildIntoContext, result);
return result;
}
module.exports = flattenChildren;
},{"142":142,"173":173}],124:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule forEachAccumulated
*/
'use strict';
/**
* @param {array} arr an "accumulation" of items which is either an Array or
* a single item. Useful when paired with the `accumulate` module. This is a
* simple utility that allows us to reason about a collection of items, but
* handling the case when there is exactly one item (and we do not need to
* allocate an array).
*/
var forEachAccumulated = function (arr, cb, scope) {
if (Array.isArray(arr)) {
arr.forEach(cb, scope);
} else if (arr) {
cb.call(scope, arr);
}
};
module.exports = forEachAccumulated;
},{}],125:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule getEventCharCode
* @typechecks static-only
*/
'use strict';
/**
* `charCode` represents the actual "character code" and is safe to use with
* `String.fromCharCode`. As such, only keys that correspond to printable
* characters produce a valid `charCode`, the only exception to this is Enter.
* The Tab-key is considered non-printable and does not have a `charCode`,
* presumably because it does not produce a tab-character in browsers.
*
* @param {object} nativeEvent Native browser event.
* @return {number} Normalized `charCode` property.
*/
function getEventCharCode(nativeEvent) {
var charCode;
var keyCode = nativeEvent.keyCode;
if ('charCode' in nativeEvent) {
charCode = nativeEvent.charCode;
// FF does not set `charCode` for the Enter-key, check against `keyCode`.
if (charCode === 0 && keyCode === 13) {
charCode = 13;
}
} else {
// IE8 does not implement `charCode`, but `keyCode` has the correct value.
charCode = keyCode;
}
// Some non-printable keys are reported in `charCode`/`keyCode`, discard them.
// Must not discard the (non-)printable Enter-key.
if (charCode >= 32 || charCode === 13) {
return charCode;
}
return 0;
}
module.exports = getEventCharCode;
},{}],126:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule getEventKey
* @typechecks static-only
*/
'use strict';
var getEventCharCode = _dereq_(125);
/**
* Normalization of deprecated HTML5 `key` values
* @see https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent#Key_names
*/
var normalizeKey = {
'Esc': 'Escape',
'Spacebar': ' ',
'Left': 'ArrowLeft',
'Up': 'ArrowUp',
'Right': 'ArrowRight',
'Down': 'ArrowDown',
'Del': 'Delete',
'Win': 'OS',
'Menu': 'ContextMenu',
'Apps': 'ContextMenu',
'Scroll': 'ScrollLock',
'MozPrintableKey': 'Unidentified'
};
/**
* Translation from legacy `keyCode` to HTML5 `key`
* Only special keys supported, all others depend on keyboard layout or browser
* @see https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent#Key_names
*/
var translateToKey = {
8: 'Backspace',
9: 'Tab',
12: 'Clear',
13: 'Enter',
16: 'Shift',
17: 'Control',
18: 'Alt',
19: 'Pause',
20: 'CapsLock',
27: 'Escape',
32: ' ',
33: 'PageUp',
34: 'PageDown',
35: 'End',
36: 'Home',
37: 'ArrowLeft',
38: 'ArrowUp',
39: 'ArrowRight',
40: 'ArrowDown',
45: 'Insert',
46: 'Delete',
112: 'F1', 113: 'F2', 114: 'F3', 115: 'F4', 116: 'F5', 117: 'F6',
118: 'F7', 119: 'F8', 120: 'F9', 121: 'F10', 122: 'F11', 123: 'F12',
144: 'NumLock',
145: 'ScrollLock',
224: 'Meta'
};
/**
* @param {object} nativeEvent Native browser event.
* @return {string} Normalized `key` property.
*/
function getEventKey(nativeEvent) {
if (nativeEvent.key) {
// Normalize inconsistent values reported by browsers due to
// implementations of a working draft specification.
// FireFox implements `key` but returns `MozPrintableKey` for all
// printable characters (normalized to `Unidentified`), ignore it.
var key = normalizeKey[nativeEvent.key] || nativeEvent.key;
if (key !== 'Unidentified') {
return key;
}
}
// Browser does not implement `key`, polyfill as much of it as we can.
if (nativeEvent.type === 'keypress') {
var charCode = getEventCharCode(nativeEvent);
// The enter-key is technically both printable and non-printable and can
// thus be captured by `keypress`, no other non-printable key should.
return charCode === 13 ? 'Enter' : String.fromCharCode(charCode);
}
if (nativeEvent.type === 'keydown' || nativeEvent.type === 'keyup') {
// While user keyboard layout determines the actual meaning of each
// `keyCode` value, almost all function keys have a universal value.
return translateToKey[nativeEvent.keyCode] || 'Unidentified';
}
return '';
}
module.exports = getEventKey;
},{"125":125}],127:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule getEventModifierState
* @typechecks static-only
*/
'use strict';
/**
* Translation from modifier key to the associated property in the event.
* @see http://www.w3.org/TR/DOM-Level-3-Events/#keys-Modifiers
*/
var modifierKeyToProp = {
'Alt': 'altKey',
'Control': 'ctrlKey',
'Meta': 'metaKey',
'Shift': 'shiftKey'
};
// IE8 does not implement getModifierState so we simply map it to the only
// modifier keys exposed by the event itself, does not support Lock-keys.
// Currently, all major browsers except Chrome seems to support Lock-keys.
function modifierStateGetter(keyArg) {
var syntheticEvent = this;
var nativeEvent = syntheticEvent.nativeEvent;
if (nativeEvent.getModifierState) {
return nativeEvent.getModifierState(keyArg);
}
var keyProp = modifierKeyToProp[keyArg];
return keyProp ? !!nativeEvent[keyProp] : false;
}
function getEventModifierState(nativeEvent) {
return modifierStateGetter;
}
module.exports = getEventModifierState;
},{}],128:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule getEventTarget
* @typechecks static-only
*/
'use strict';
/**
* Gets the target node from a native browser event by accounting for
* inconsistencies in browser DOM APIs.
*
* @param {object} nativeEvent Native browser event.
* @return {DOMEventTarget} Target node.
*/
function getEventTarget(nativeEvent) {
var target = nativeEvent.target || nativeEvent.srcElement || window;
// Safari may fire events on text nodes (Node.TEXT_NODE is 3).
// @see http://www.quirksmode.org/js/events_properties.html
return target.nodeType === 3 ? target.parentNode : target;
}
module.exports = getEventTarget;
},{}],129:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule getIteratorFn
* @typechecks static-only
*/
'use strict';
/* global Symbol */
var ITERATOR_SYMBOL = typeof Symbol === 'function' && Symbol.iterator;
var FAUX_ITERATOR_SYMBOL = '@@iterator'; // Before Symbol spec.
/**
* Returns the iterator method function contained on the iterable object.
*
* Be sure to invoke the function with the iterable as context:
*
* var iteratorFn = getIteratorFn(myIterable);
* if (iteratorFn) {
* var iterator = iteratorFn.call(myIterable);
* ...
* }
*
* @param {?object} maybeIterable
* @return {?function}
*/
function getIteratorFn(maybeIterable) {
var iteratorFn = maybeIterable && (ITERATOR_SYMBOL && maybeIterable[ITERATOR_SYMBOL] || maybeIterable[FAUX_ITERATOR_SYMBOL]);
if (typeof iteratorFn === 'function') {
return iteratorFn;
}
}
module.exports = getIteratorFn;
},{}],130:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule getNodeForCharacterOffset
*/
'use strict';
/**
* Given any node return the first leaf node without children.
*
* @param {DOMElement|DOMTextNode} node
* @return {DOMElement|DOMTextNode}
*/
function getLeafNode(node) {
while (node && node.firstChild) {
node = node.firstChild;
}
return node;
}
/**
* Get the next sibling within a container. This will walk up the
* DOM if a node's siblings have been exhausted.
*
* @param {DOMElement|DOMTextNode} node
* @return {?DOMElement|DOMTextNode}
*/
function getSiblingNode(node) {
while (node) {
if (node.nextSibling) {
return node.nextSibling;
}
node = node.parentNode;
}
}
/**
* Get object describing the nodes which contain characters at offset.
*
* @param {DOMElement|DOMTextNode} root
* @param {number} offset
* @return {?object}
*/
function getNodeForCharacterOffset(root, offset) {
var node = getLeafNode(root);
var nodeStart = 0;
var nodeEnd = 0;
while (node) {
if (node.nodeType === 3) {
nodeEnd = nodeStart + node.textContent.length;
if (nodeStart <= offset && nodeEnd >= offset) {
return {
node: node,
offset: offset - nodeStart
};
}
nodeStart = nodeEnd;
}
node = getLeafNode(getSiblingNode(node));
}
}
module.exports = getNodeForCharacterOffset;
},{}],131:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule getTextContentAccessor
*/
'use strict';
var ExecutionEnvironment = _dereq_(147);
var contentKey = null;
/**
* Gets the key used to access text content on a DOM node.
*
* @return {?string} Key used to access text content.
* @internal
*/
function getTextContentAccessor() {
if (!contentKey && ExecutionEnvironment.canUseDOM) {
// Prefer textContent to innerText because many browsers support both but
// SVG <text> elements don't support innerText even when <div> does.
contentKey = 'textContent' in document.documentElement ? 'textContent' : 'innerText';
}
return contentKey;
}
module.exports = getTextContentAccessor;
},{"147":147}],132:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule instantiateReactComponent
* @typechecks static-only
*/
'use strict';
var ReactCompositeComponent = _dereq_(38);
var ReactEmptyComponent = _dereq_(59);
var ReactNativeComponent = _dereq_(75);
var assign = _dereq_(24);
var invariant = _dereq_(161);
var warning = _dereq_(173);
// To avoid a cyclic dependency, we create the final class in this module
var ReactCompositeComponentWrapper = function () {};
assign(ReactCompositeComponentWrapper.prototype, ReactCompositeComponent.Mixin, {
_instantiateReactComponent: instantiateReactComponent
});
function getDeclarationErrorAddendum(owner) {
if (owner) {
var name = owner.getName();
if (name) {
return ' Check the render method of `' + name + '`.';
}
}
return '';
}
/**
* Check if the type reference is a known internal type. I.e. not a user
* provided composite type.
*
* @param {function} type
* @return {boolean} Returns true if this is a valid internal type.
*/
function isInternalComponentType(type) {
return typeof type === 'function' && typeof type.prototype !== 'undefined' && typeof type.prototype.mountComponent === 'function' && typeof type.prototype.receiveComponent === 'function';
}
/**
* Given a ReactNode, create an instance that will actually be mounted.
*
* @param {ReactNode} node
* @return {object} A new instance of the element's constructor.
* @protected
*/
function instantiateReactComponent(node) {
var instance;
if (node === null || node === false) {
instance = new ReactEmptyComponent(instantiateReactComponent);
} else if (typeof node === 'object') {
var element = node;
!(element && (typeof element.type === 'function' || typeof element.type === 'string')) ? "development" !== 'production' ? invariant(false, 'Element type is invalid: expected a string (for built-in components) ' + 'or a class/function (for composite components) but got: %s.%s', element.type == null ? element.type : typeof element.type, getDeclarationErrorAddendum(element._owner)) : invariant(false) : undefined;
// Special case string values
if (typeof element.type === 'string') {
instance = ReactNativeComponent.createInternalComponent(element);
} else if (isInternalComponentType(element.type)) {
// This is temporarily available for custom components that are not string
// representations. I.e. ART. Once those are updated to use the string
// representation, we can drop this code path.
instance = new element.type(element);
} else {
instance = new ReactCompositeComponentWrapper();
}
} else if (typeof node === 'string' || typeof node === 'number') {
instance = ReactNativeComponent.createInstanceForText(node);
} else {
!false ? "development" !== 'production' ? invariant(false, 'Encountered invalid React node of type %s', typeof node) : invariant(false) : undefined;
}
if ("development" !== 'production') {
"development" !== 'production' ? warning(typeof instance.construct === 'function' && typeof instance.mountComponent === 'function' && typeof instance.receiveComponent === 'function' && typeof instance.unmountComponent === 'function', 'Only React Components can be mounted.') : undefined;
}
// Sets up the instance. This can probably just move into the constructor now.
instance.construct(node);
// These two fields are used by the DOM and ART diffing algorithms
// respectively. Instead of using expandos on components, we should be
// storing the state needed by the diffing algorithms elsewhere.
instance._mountIndex = 0;
instance._mountImage = null;
if ("development" !== 'production') {
instance._isOwnerNecessary = false;
instance._warnedAboutRefsInRender = false;
}
// Internal instances should fully constructed at this point, so they should
// not get any new fields added to them at this point.
if ("development" !== 'production') {
if (Object.preventExtensions) {
Object.preventExtensions(instance);
}
}
return instance;
}
module.exports = instantiateReactComponent;
},{"161":161,"173":173,"24":24,"38":38,"59":59,"75":75}],133:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule isEventSupported
*/
'use strict';
var ExecutionEnvironment = _dereq_(147);
var useHasFeature;
if (ExecutionEnvironment.canUseDOM) {
useHasFeature = document.implementation && document.implementation.hasFeature &&
// always returns true in newer browsers as per the standard.
// @see http://dom.spec.whatwg.org/#dom-domimplementation-hasfeature
document.implementation.hasFeature('', '') !== true;
}
/**
* Checks if an event is supported in the current execution environment.
*
* NOTE: This will not work correctly for non-generic events such as `change`,
* `reset`, `load`, `error`, and `select`.
*
* Borrows from Modernizr.
*
* @param {string} eventNameSuffix Event name, e.g. "click".
* @param {?boolean} capture Check if the capture phase is supported.
* @return {boolean} True if the event is supported.
* @internal
* @license Modernizr 3.0.0pre (Custom Build) | MIT
*/
function isEventSupported(eventNameSuffix, capture) {
if (!ExecutionEnvironment.canUseDOM || capture && !('addEventListener' in document)) {
return false;
}
var eventName = 'on' + eventNameSuffix;
var isSupported = (eventName in document);
if (!isSupported) {
var element = document.createElement('div');
element.setAttribute(eventName, 'return;');
isSupported = typeof element[eventName] === 'function';
}
if (!isSupported && useHasFeature && eventNameSuffix === 'wheel') {
// This is the only way to test support for the `wheel` event in IE9+.
isSupported = document.implementation.hasFeature('Events.wheel', '3.0');
}
return isSupported;
}
module.exports = isEventSupported;
},{"147":147}],134:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule isTextInputElement
*/
'use strict';
/**
* @see http://www.whatwg.org/specs/web-apps/current-work/multipage/the-input-element.html#input-type-attr-summary
*/
var supportedInputTypes = {
'color': true,
'date': true,
'datetime': true,
'datetime-local': true,
'email': true,
'month': true,
'number': true,
'password': true,
'range': true,
'search': true,
'tel': true,
'text': true,
'time': true,
'url': true,
'week': true
};
function isTextInputElement(elem) {
var nodeName = elem && elem.nodeName && elem.nodeName.toLowerCase();
return nodeName && (nodeName === 'input' && supportedInputTypes[elem.type] || nodeName === 'textarea');
}
module.exports = isTextInputElement;
},{}],135:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule onlyChild
*/
'use strict';
var ReactElement = _dereq_(57);
var invariant = _dereq_(161);
/**
* Returns the first child in a collection of children and verifies that there
* is only one child in the collection. The current implementation of this
* function assumes that a single child gets passed without a wrapper, but the
* purpose of this helper function is to abstract away the particular structure
* of children.
*
* @param {?object} children Child collection structure.
* @return {ReactComponent} The first and only `ReactComponent` contained in the
* structure.
*/
function onlyChild(children) {
!ReactElement.isValidElement(children) ? "development" !== 'production' ? invariant(false, 'onlyChild must be passed a children with exactly one child.') : invariant(false) : undefined;
return children;
}
module.exports = onlyChild;
},{"161":161,"57":57}],136:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule quoteAttributeValueForBrowser
*/
'use strict';
var escapeTextContentForBrowser = _dereq_(121);
/**
* Escapes attribute value to prevent scripting attacks.
*
* @param {*} value Value to escape.
* @return {string} An escaped string.
*/
function quoteAttributeValueForBrowser(value) {
return '"' + escapeTextContentForBrowser(value) + '"';
}
module.exports = quoteAttributeValueForBrowser;
},{"121":121}],137:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule renderSubtreeIntoContainer
*/
'use strict';
var ReactMount = _dereq_(72);
module.exports = ReactMount.renderSubtreeIntoContainer;
},{"72":72}],138:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule setInnerHTML
*/
/* globals MSApp */
'use strict';
var ExecutionEnvironment = _dereq_(147);
var WHITESPACE_TEST = /^[ \r\n\t\f]/;
var NONVISIBLE_TEST = /<(!--|link|noscript|meta|script|style)[ \r\n\t\f\/>]/;
/**
* Set the innerHTML property of a node, ensuring that whitespace is preserved
* even in IE8.
*
* @param {DOMElement} node
* @param {string} html
* @internal
*/
var setInnerHTML = function (node, html) {
node.innerHTML = html;
};
// Win8 apps: Allow all html to be inserted
if (typeof MSApp !== 'undefined' && MSApp.execUnsafeLocalFunction) {
setInnerHTML = function (node, html) {
MSApp.execUnsafeLocalFunction(function () {
node.innerHTML = html;
});
};
}
if (ExecutionEnvironment.canUseDOM) {
// IE8: When updating a just created node with innerHTML only leading
// whitespace is removed. When updating an existing node with innerHTML
// whitespace in root TextNodes is also collapsed.
// @see quirksmode.org/bugreports/archives/2004/11/innerhtml_and_t.html
// Feature detection; only IE8 is known to behave improperly like this.
var testElement = document.createElement('div');
testElement.innerHTML = ' ';
if (testElement.innerHTML === '') {
setInnerHTML = function (node, html) {
// Magic theory: IE8 supposedly differentiates between added and updated
// nodes when processing innerHTML, innerHTML on updated nodes suffers
// from worse whitespace behavior. Re-adding a node like this triggers
// the initial and more favorable whitespace behavior.
// TODO: What to do on a detached node?
if (node.parentNode) {
node.parentNode.replaceChild(node, node);
}
// We also implement a workaround for non-visible tags disappearing into
// thin air on IE8, this only happens if there is no visible text
// in-front of the non-visible tags. Piggyback on the whitespace fix
// and simply check if any non-visible tags appear in the source.
if (WHITESPACE_TEST.test(html) || html[0] === '<' && NONVISIBLE_TEST.test(html)) {
// Recover leading whitespace by temporarily prepending any character.
// \uFEFF has the potential advantage of being zero-width/invisible.
// UglifyJS drops U+FEFF chars when parsing, so use String.fromCharCode
// in hopes that this is preserved even if "\uFEFF" is transformed to
// the actual Unicode character (by Babel, for example).
// https://github.com/mishoo/UglifyJS2/blob/v2.4.20/lib/parse.js#L216
node.innerHTML = String.fromCharCode(0xFEFF) + html;
// deleteData leaves an empty `TextNode` which offsets the index of all
// children. Definitely want to avoid this.
var textNode = node.firstChild;
if (textNode.data.length === 1) {
node.removeChild(textNode);
} else {
textNode.deleteData(0, 1);
}
} else {
node.innerHTML = html;
}
};
}
}
module.exports = setInnerHTML;
},{"147":147}],139:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule setTextContent
*/
'use strict';
var ExecutionEnvironment = _dereq_(147);
var escapeTextContentForBrowser = _dereq_(121);
var setInnerHTML = _dereq_(138);
/**
* Set the textContent property of a node, ensuring that whitespace is preserved
* even in IE8. innerText is a poor substitute for textContent and, among many
* issues, inserts <br> instead of the literal newline chars. innerHTML behaves
* as it should.
*
* @param {DOMElement} node
* @param {string} text
* @internal
*/
var setTextContent = function (node, text) {
node.textContent = text;
};
if (ExecutionEnvironment.canUseDOM) {
if (!('textContent' in document.documentElement)) {
setTextContent = function (node, text) {
setInnerHTML(node, escapeTextContentForBrowser(text));
};
}
}
module.exports = setTextContent;
},{"121":121,"138":138,"147":147}],140:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule shallowCompare
*/
'use strict';
var shallowEqual = _dereq_(171);
/**
* Does a shallow comparison for props and state.
* See ReactComponentWithPureRenderMixin
*/
function shallowCompare(instance, nextProps, nextState) {
return !shallowEqual(instance.props, nextProps) || !shallowEqual(instance.state, nextState);
}
module.exports = shallowCompare;
},{"171":171}],141:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule shouldUpdateReactComponent
* @typechecks static-only
*/
'use strict';
/**
* Given a `prevElement` and `nextElement`, determines if the existing
* instance should be updated as opposed to being destroyed or replaced by a new
* instance. Both arguments are elements. This ensures that this logic can
* operate on stateless trees without any backing instance.
*
* @param {?object} prevElement
* @param {?object} nextElement
* @return {boolean} True if the existing instance should be updated.
* @protected
*/
function shouldUpdateReactComponent(prevElement, nextElement) {
var prevEmpty = prevElement === null || prevElement === false;
var nextEmpty = nextElement === null || nextElement === false;
if (prevEmpty || nextEmpty) {
return prevEmpty === nextEmpty;
}
var prevType = typeof prevElement;
var nextType = typeof nextElement;
if (prevType === 'string' || prevType === 'number') {
return nextType === 'string' || nextType === 'number';
} else {
return nextType === 'object' && prevElement.type === nextElement.type && prevElement.key === nextElement.key;
}
return false;
}
module.exports = shouldUpdateReactComponent;
},{}],142:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule traverseAllChildren
*/
'use strict';
var ReactCurrentOwner = _dereq_(39);
var ReactElement = _dereq_(57);
var ReactInstanceHandles = _dereq_(67);
var getIteratorFn = _dereq_(129);
var invariant = _dereq_(161);
var warning = _dereq_(173);
var SEPARATOR = ReactInstanceHandles.SEPARATOR;
var SUBSEPARATOR = ':';
/**
* TODO: Test that a single child and an array with one item have the same key
* pattern.
*/
var userProvidedKeyEscaperLookup = {
'=': '=0',
'.': '=1',
':': '=2'
};
var userProvidedKeyEscapeRegex = /[=.:]/g;
var didWarnAboutMaps = false;
function userProvidedKeyEscaper(match) {
return userProvidedKeyEscaperLookup[match];
}
/**
* Generate a key string that identifies a component within a set.
*
* @param {*} component A component that could contain a manual key.
* @param {number} index Index that is used if a manual key is not provided.
* @return {string}
*/
function getComponentKey(component, index) {
if (component && component.key != null) {
// Explicit key
return wrapUserProvidedKey(component.key);
}
// Implicit key determined by the index in the set
return index.toString(36);
}
/**
* Escape a component key so that it is safe to use in a reactid.
*
* @param {*} text Component key to be escaped.
* @return {string} An escaped string.
*/
function escapeUserProvidedKey(text) {
return ('' + text).replace(userProvidedKeyEscapeRegex, userProvidedKeyEscaper);
}
/**
* Wrap a `key` value explicitly provided by the user to distinguish it from
* implicitly-generated keys generated by a component's index in its parent.
*
* @param {string} key Value of a user-provided `key` attribute
* @return {string}
*/
function wrapUserProvidedKey(key) {
return '$' + escapeUserProvidedKey(key);
}
/**
* @param {?*} children Children tree container.
* @param {!string} nameSoFar Name of the key path so far.
* @param {!function} callback Callback to invoke with each child found.
* @param {?*} traverseContext Used to pass information throughout the traversal
* process.
* @return {!number} The number of children in this subtree.
*/
function traverseAllChildrenImpl(children, nameSoFar, callback, traverseContext) {
var type = typeof children;
if (type === 'undefined' || type === 'boolean') {
// All of the above are perceived as null.
children = null;
}
if (children === null || type === 'string' || type === 'number' || ReactElement.isValidElement(children)) {
callback(traverseContext, children,
// If it's the only child, treat the name as if it was wrapped in an array
// so that it's consistent if the number of children grows.
nameSoFar === '' ? SEPARATOR + getComponentKey(children, 0) : nameSoFar);
return 1;
}
var child;
var nextName;
var subtreeCount = 0; // Count of children found in the current subtree.
var nextNamePrefix = nameSoFar === '' ? SEPARATOR : nameSoFar + SUBSEPARATOR;
if (Array.isArray(children)) {
for (var i = 0; i < children.length; i++) {
child = children[i];
nextName = nextNamePrefix + getComponentKey(child, i);
subtreeCount += traverseAllChildrenImpl(child, nextName, callback, traverseContext);
}
} else {
var iteratorFn = getIteratorFn(children);
if (iteratorFn) {
var iterator = iteratorFn.call(children);
var step;
if (iteratorFn !== children.entries) {
var ii = 0;
while (!(step = iterator.next()).done) {
child = step.value;
nextName = nextNamePrefix + getComponentKey(child, ii++);
subtreeCount += traverseAllChildrenImpl(child, nextName, callback, traverseContext);
}
} else {
if ("development" !== 'production') {
"development" !== 'production' ? warning(didWarnAboutMaps, 'Using Maps as children is not yet fully supported. It is an ' + 'experimental feature that might be removed. Convert it to a ' + 'sequence / iterable of keyed ReactElements instead.') : undefined;
didWarnAboutMaps = true;
}
// Iterator will provide entry [k,v] tuples rather than values.
while (!(step = iterator.next()).done) {
var entry = step.value;
if (entry) {
child = entry[1];
nextName = nextNamePrefix + wrapUserProvidedKey(entry[0]) + SUBSEPARATOR + getComponentKey(child, 0);
subtreeCount += traverseAllChildrenImpl(child, nextName, callback, traverseContext);
}
}
}
} else if (type === 'object') {
var addendum = '';
if ("development" !== 'production') {
addendum = ' If you meant to render a collection of children, use an array ' + 'instead or wrap the object using createFragment(object) from the ' + 'React add-ons.';
if (children._isReactElement) {
addendum = ' It looks like you\'re using an element created by a different ' + 'version of React. Make sure to use only one copy of React.';
}
if (ReactCurrentOwner.current) {
var name = ReactCurrentOwner.current.getName();
if (name) {
addendum += ' Check the render method of `' + name + '`.';
}
}
}
var childrenString = String(children);
!false ? "development" !== 'production' ? invariant(false, 'Objects are not valid as a React child (found: %s).%s', childrenString === '[object Object]' ? 'object with keys {' + Object.keys(children).join(', ') + '}' : childrenString, addendum) : invariant(false) : undefined;
}
}
return subtreeCount;
}
/**
* Traverses children that are typically specified as `props.children`, but
* might also be specified through attributes:
*
* - `traverseAllChildren(this.props.children, ...)`
* - `traverseAllChildren(this.props.leftPanelChildren, ...)`
*
* The `traverseContext` is an optional argument that is passed through the
* entire traversal. It can be used to store accumulations or anything else that
* the callback might find relevant.
*
* @param {?*} children Children tree object.
* @param {!function} callback To invoke upon traversing each child.
* @param {?*} traverseContext Context for traversal.
* @return {!number} The number of children in this subtree.
*/
function traverseAllChildren(children, callback, traverseContext) {
if (children == null) {
return 0;
}
return traverseAllChildrenImpl(children, '', callback, traverseContext);
}
module.exports = traverseAllChildren;
},{"129":129,"161":161,"173":173,"39":39,"57":57,"67":67}],143:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule update
*/
/* global hasOwnProperty:true */
'use strict';
var assign = _dereq_(24);
var keyOf = _dereq_(166);
var invariant = _dereq_(161);
var hasOwnProperty = ({}).hasOwnProperty;
function shallowCopy(x) {
if (Array.isArray(x)) {
return x.concat();
} else if (x && typeof x === 'object') {
return assign(new x.constructor(), x);
} else {
return x;
}
}
var COMMAND_PUSH = keyOf({ $push: null });
var COMMAND_UNSHIFT = keyOf({ $unshift: null });
var COMMAND_SPLICE = keyOf({ $splice: null });
var COMMAND_SET = keyOf({ $set: null });
var COMMAND_MERGE = keyOf({ $merge: null });
var COMMAND_APPLY = keyOf({ $apply: null });
var ALL_COMMANDS_LIST = [COMMAND_PUSH, COMMAND_UNSHIFT, COMMAND_SPLICE, COMMAND_SET, COMMAND_MERGE, COMMAND_APPLY];
var ALL_COMMANDS_SET = {};
ALL_COMMANDS_LIST.forEach(function (command) {
ALL_COMMANDS_SET[command] = true;
});
function invariantArrayCase(value, spec, command) {
!Array.isArray(value) ? "development" !== 'production' ? invariant(false, 'update(): expected target of %s to be an array; got %s.', command, value) : invariant(false) : undefined;
var specValue = spec[command];
!Array.isArray(specValue) ? "development" !== 'production' ? invariant(false, 'update(): expected spec of %s to be an array; got %s. ' + 'Did you forget to wrap your parameter in an array?', command, specValue) : invariant(false) : undefined;
}
function update(value, spec) {
!(typeof spec === 'object') ? "development" !== 'production' ? invariant(false, 'update(): You provided a key path to update() that did not contain one ' + 'of %s. Did you forget to include {%s: ...}?', ALL_COMMANDS_LIST.join(', '), COMMAND_SET) : invariant(false) : undefined;
if (hasOwnProperty.call(spec, COMMAND_SET)) {
!(Object.keys(spec).length === 1) ? "development" !== 'production' ? invariant(false, 'Cannot have more than one key in an object with %s', COMMAND_SET) : invariant(false) : undefined;
return spec[COMMAND_SET];
}
var nextValue = shallowCopy(value);
if (hasOwnProperty.call(spec, COMMAND_MERGE)) {
var mergeObj = spec[COMMAND_MERGE];
!(mergeObj && typeof mergeObj === 'object') ? "development" !== 'production' ? invariant(false, 'update(): %s expects a spec of type \'object\'; got %s', COMMAND_MERGE, mergeObj) : invariant(false) : undefined;
!(nextValue && typeof nextValue === 'object') ? "development" !== 'production' ? invariant(false, 'update(): %s expects a target of type \'object\'; got %s', COMMAND_MERGE, nextValue) : invariant(false) : undefined;
assign(nextValue, spec[COMMAND_MERGE]);
}
if (hasOwnProperty.call(spec, COMMAND_PUSH)) {
invariantArrayCase(value, spec, COMMAND_PUSH);
spec[COMMAND_PUSH].forEach(function (item) {
nextValue.push(item);
});
}
if (hasOwnProperty.call(spec, COMMAND_UNSHIFT)) {
invariantArrayCase(value, spec, COMMAND_UNSHIFT);
spec[COMMAND_UNSHIFT].forEach(function (item) {
nextValue.unshift(item);
});
}
if (hasOwnProperty.call(spec, COMMAND_SPLICE)) {
!Array.isArray(value) ? "development" !== 'production' ? invariant(false, 'Expected %s target to be an array; got %s', COMMAND_SPLICE, value) : invariant(false) : undefined;
!Array.isArray(spec[COMMAND_SPLICE]) ? "development" !== 'production' ? invariant(false, 'update(): expected spec of %s to be an array of arrays; got %s. ' + 'Did you forget to wrap your parameters in an array?', COMMAND_SPLICE, spec[COMMAND_SPLICE]) : invariant(false) : undefined;
spec[COMMAND_SPLICE].forEach(function (args) {
!Array.isArray(args) ? "development" !== 'production' ? invariant(false, 'update(): expected spec of %s to be an array of arrays; got %s. ' + 'Did you forget to wrap your parameters in an array?', COMMAND_SPLICE, spec[COMMAND_SPLICE]) : invariant(false) : undefined;
nextValue.splice.apply(nextValue, args);
});
}
if (hasOwnProperty.call(spec, COMMAND_APPLY)) {
!(typeof spec[COMMAND_APPLY] === 'function') ? "development" !== 'production' ? invariant(false, 'update(): expected spec of %s to be a function; got %s.', COMMAND_APPLY, spec[COMMAND_APPLY]) : invariant(false) : undefined;
nextValue = spec[COMMAND_APPLY](nextValue);
}
for (var k in spec) {
if (!(ALL_COMMANDS_SET.hasOwnProperty(k) && ALL_COMMANDS_SET[k])) {
nextValue[k] = update(value[k], spec[k]);
}
}
return nextValue;
}
module.exports = update;
},{"161":161,"166":166,"24":24}],144:[function(_dereq_,module,exports){
/**
* Copyright 2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule validateDOMNesting
*/
'use strict';
var assign = _dereq_(24);
var emptyFunction = _dereq_(153);
var warning = _dereq_(173);
var validateDOMNesting = emptyFunction;
if ("development" !== 'production') {
// This validation code was written based on the HTML5 parsing spec:
// https://html.spec.whatwg.org/multipage/syntax.html#has-an-element-in-scope
//
// Note: this does not catch all invalid nesting, nor does it try to (as it's
// not clear what practical benefit doing so provides); instead, we warn only
// for cases where the parser will give a parse tree differing from what React
// intended. For example, <b><div></div></b> is invalid but we don't warn
// because it still parses correctly; we do warn for other cases like nested
// <p> tags where the beginning of the second element implicitly closes the
// first, causing a confusing mess.
// https://html.spec.whatwg.org/multipage/syntax.html#special
var specialTags = ['address', 'applet', 'area', 'article', 'aside', 'base', 'basefont', 'bgsound', 'blockquote', 'body', 'br', 'button', 'caption', 'center', 'col', 'colgroup', 'dd', 'details', 'dir', 'div', 'dl', 'dt', 'embed', 'fieldset', 'figcaption', 'figure', 'footer', 'form', 'frame', 'frameset', 'h1', 'h2', 'h3', 'h4', 'h5', 'h6', 'head', 'header', 'hgroup', 'hr', 'html', 'iframe', 'img', 'input', 'isindex', 'li', 'link', 'listing', 'main', 'marquee', 'menu', 'menuitem', 'meta', 'nav', 'noembed', 'noframes', 'noscript', 'object', 'ol', 'p', 'param', 'plaintext', 'pre', 'script', 'section', 'select', 'source', 'style', 'summary', 'table', 'tbody', 'td', 'template', 'textarea', 'tfoot', 'th', 'thead', 'title', 'tr', 'track', 'ul', 'wbr', 'xmp'];
// https://html.spec.whatwg.org/multipage/syntax.html#has-an-element-in-scope
var inScopeTags = ['applet', 'caption', 'html', 'table', 'td', 'th', 'marquee', 'object', 'template',
// https://html.spec.whatwg.org/multipage/syntax.html#html-integration-point
// TODO: Distinguish by namespace here -- for <title>, including it here
// errs on the side of fewer warnings
'foreignObject', 'desc', 'title'];
// https://html.spec.whatwg.org/multipage/syntax.html#has-an-element-in-button-scope
var buttonScopeTags = inScopeTags.concat(['button']);
// https://html.spec.whatwg.org/multipage/syntax.html#generate-implied-end-tags
var impliedEndTags = ['dd', 'dt', 'li', 'option', 'optgroup', 'p', 'rp', 'rt'];
var emptyAncestorInfo = {
parentTag: null,
formTag: null,
aTagInScope: null,
buttonTagInScope: null,
nobrTagInScope: null,
pTagInButtonScope: null,
listItemTagAutoclosing: null,
dlItemTagAutoclosing: null
};
var updatedAncestorInfo = function (oldInfo, tag, instance) {
var ancestorInfo = assign({}, oldInfo || emptyAncestorInfo);
var info = { tag: tag, instance: instance };
if (inScopeTags.indexOf(tag) !== -1) {
ancestorInfo.aTagInScope = null;
ancestorInfo.buttonTagInScope = null;
ancestorInfo.nobrTagInScope = null;
}
if (buttonScopeTags.indexOf(tag) !== -1) {
ancestorInfo.pTagInButtonScope = null;
}
// See rules for 'li', 'dd', 'dt' start tags in
// https://html.spec.whatwg.org/multipage/syntax.html#parsing-main-inbody
if (specialTags.indexOf(tag) !== -1 && tag !== 'address' && tag !== 'div' && tag !== 'p') {
ancestorInfo.listItemTagAutoclosing = null;
ancestorInfo.dlItemTagAutoclosing = null;
}
ancestorInfo.parentTag = info;
if (tag === 'form') {
ancestorInfo.formTag = info;
}
if (tag === 'a') {
ancestorInfo.aTagInScope = info;
}
if (tag === 'button') {
ancestorInfo.buttonTagInScope = info;
}
if (tag === 'nobr') {
ancestorInfo.nobrTagInScope = info;
}
if (tag === 'p') {
ancestorInfo.pTagInButtonScope = info;
}
if (tag === 'li') {
ancestorInfo.listItemTagAutoclosing = info;
}
if (tag === 'dd' || tag === 'dt') {
ancestorInfo.dlItemTagAutoclosing = info;
}
return ancestorInfo;
};
/**
* Returns whether
*/
var isTagValidWithParent = function (tag, parentTag) {
// First, let's check if we're in an unusual parsing mode...
switch (parentTag) {
// https://html.spec.whatwg.org/multipage/syntax.html#parsing-main-inselect
case 'select':
return tag === 'option' || tag === 'optgroup' || tag === '#text';
case 'optgroup':
return tag === 'option' || tag === '#text';
// Strictly speaking, seeing an <option> doesn't mean we're in a <select>
// but
case 'option':
return tag === '#text';
// https://html.spec.whatwg.org/multipage/syntax.html#parsing-main-intd
// https://html.spec.whatwg.org/multipage/syntax.html#parsing-main-incaption
// No special behavior since these rules fall back to "in body" mode for
// all except special table nodes which cause bad parsing behavior anyway.
// https://html.spec.whatwg.org/multipage/syntax.html#parsing-main-intr
case 'tr':
return tag === 'th' || tag === 'td' || tag === 'style' || tag === 'script' || tag === 'template';
// https://html.spec.whatwg.org/multipage/syntax.html#parsing-main-intbody
case 'tbody':
case 'thead':
case 'tfoot':
return tag === 'tr' || tag === 'style' || tag === 'script' || tag === 'template';
// https://html.spec.whatwg.org/multipage/syntax.html#parsing-main-incolgroup
case 'colgroup':
return tag === 'col' || tag === 'template';
// https://html.spec.whatwg.org/multipage/syntax.html#parsing-main-intable
case 'table':
return tag === 'caption' || tag === 'colgroup' || tag === 'tbody' || tag === 'tfoot' || tag === 'thead' || tag === 'style' || tag === 'script' || tag === 'template';
// https://html.spec.whatwg.org/multipage/syntax.html#parsing-main-inhead
case 'head':
return tag === 'base' || tag === 'basefont' || tag === 'bgsound' || tag === 'link' || tag === 'meta' || tag === 'title' || tag === 'noscript' || tag === 'noframes' || tag === 'style' || tag === 'script' || tag === 'template';
// https://html.spec.whatwg.org/multipage/semantics.html#the-html-element
case 'html':
return tag === 'head' || tag === 'body';
}
// Probably in the "in body" parsing mode, so we outlaw only tag combos
// where the parsing rules cause implicit opens or closes to be added.
// https://html.spec.whatwg.org/multipage/syntax.html#parsing-main-inbody
switch (tag) {
case 'h1':
case 'h2':
case 'h3':
case 'h4':
case 'h5':
case 'h6':
return parentTag !== 'h1' && parentTag !== 'h2' && parentTag !== 'h3' && parentTag !== 'h4' && parentTag !== 'h5' && parentTag !== 'h6';
case 'rp':
case 'rt':
return impliedEndTags.indexOf(parentTag) === -1;
case 'caption':
case 'col':
case 'colgroup':
case 'frame':
case 'head':
case 'tbody':
case 'td':
case 'tfoot':
case 'th':
case 'thead':
case 'tr':
// These tags are only valid with a few parents that have special child
// parsing rules -- if we're down here, then none of those matched and
// so we allow it only if we don't know what the parent is, as all other
// cases are invalid.
return parentTag == null;
}
return true;
};
/**
* Returns whether
*/
var findInvalidAncestorForTag = function (tag, ancestorInfo) {
switch (tag) {
case 'address':
case 'article':
case 'aside':
case 'blockquote':
case 'center':
case 'details':
case 'dialog':
case 'dir':
case 'div':
case 'dl':
case 'fieldset':
case 'figcaption':
case 'figure':
case 'footer':
case 'header':
case 'hgroup':
case 'main':
case 'menu':
case 'nav':
case 'ol':
case 'p':
case 'section':
case 'summary':
case 'ul':
case 'pre':
case 'listing':
case 'table':
case 'hr':
case 'xmp':
case 'h1':
case 'h2':
case 'h3':
case 'h4':
case 'h5':
case 'h6':
return ancestorInfo.pTagInButtonScope;
case 'form':
return ancestorInfo.formTag || ancestorInfo.pTagInButtonScope;
case 'li':
return ancestorInfo.listItemTagAutoclosing;
case 'dd':
case 'dt':
return ancestorInfo.dlItemTagAutoclosing;
case 'button':
return ancestorInfo.buttonTagInScope;
case 'a':
// Spec says something about storing a list of markers, but it sounds
// equivalent to this check.
return ancestorInfo.aTagInScope;
case 'nobr':
return ancestorInfo.nobrTagInScope;
}
return null;
};
/**
* Given a ReactCompositeComponent instance, return a list of its recursive
* owners, starting at the root and ending with the instance itself.
*/
var findOwnerStack = function (instance) {
if (!instance) {
return [];
}
var stack = [];
/*eslint-disable space-after-keywords */
do {
/*eslint-enable space-after-keywords */
stack.push(instance);
} while (instance = instance._currentElement._owner);
stack.reverse();
return stack;
};
var didWarn = {};
validateDOMNesting = function (childTag, childInstance, ancestorInfo) {
ancestorInfo = ancestorInfo || emptyAncestorInfo;
var parentInfo = ancestorInfo.parentTag;
var parentTag = parentInfo && parentInfo.tag;
var invalidParent = isTagValidWithParent(childTag, parentTag) ? null : parentInfo;
var invalidAncestor = invalidParent ? null : findInvalidAncestorForTag(childTag, ancestorInfo);
var problematic = invalidParent || invalidAncestor;
if (problematic) {
var ancestorTag = problematic.tag;
var ancestorInstance = problematic.instance;
var childOwner = childInstance && childInstance._currentElement._owner;
var ancestorOwner = ancestorInstance && ancestorInstance._currentElement._owner;
var childOwners = findOwnerStack(childOwner);
var ancestorOwners = findOwnerStack(ancestorOwner);
var minStackLen = Math.min(childOwners.length, ancestorOwners.length);
var i;
var deepestCommon = -1;
for (i = 0; i < minStackLen; i++) {
if (childOwners[i] === ancestorOwners[i]) {
deepestCommon = i;
} else {
break;
}
}
var UNKNOWN = '(unknown)';
var childOwnerNames = childOwners.slice(deepestCommon + 1).map(function (inst) {
return inst.getName() || UNKNOWN;
});
var ancestorOwnerNames = ancestorOwners.slice(deepestCommon + 1).map(function (inst) {
return inst.getName() || UNKNOWN;
});
var ownerInfo = [].concat(
// If the parent and child instances have a common owner ancestor, start
// with that -- otherwise we just start with the parent's owners.
deepestCommon !== -1 ? childOwners[deepestCommon].getName() || UNKNOWN : [], ancestorOwnerNames, ancestorTag,
// If we're warning about an invalid (non-parent) ancestry, add '...'
invalidAncestor ? ['...'] : [], childOwnerNames, childTag).join(' > ');
var warnKey = !!invalidParent + '|' + childTag + '|' + ancestorTag + '|' + ownerInfo;
if (didWarn[warnKey]) {
return;
}
didWarn[warnKey] = true;
if (invalidParent) {
var info = '';
if (ancestorTag === 'table' && childTag === 'tr') {
info += ' Add a <tbody> to your code to match the DOM tree generated by ' + 'the browser.';
}
"development" !== 'production' ? warning(false, 'validateDOMNesting(...): <%s> cannot appear as a child of <%s>. ' + 'See %s.%s', childTag, ancestorTag, ownerInfo, info) : undefined;
} else {
"development" !== 'production' ? warning(false, 'validateDOMNesting(...): <%s> cannot appear as a descendant of ' + '<%s>. See %s.', childTag, ancestorTag, ownerInfo) : undefined;
}
}
};
validateDOMNesting.ancestorInfoContextKey = '__validateDOMNesting_ancestorInfo$' + Math.random().toString(36).slice(2);
validateDOMNesting.updatedAncestorInfo = updatedAncestorInfo;
// For testing
validateDOMNesting.isTagValidInContext = function (tag, ancestorInfo) {
ancestorInfo = ancestorInfo || emptyAncestorInfo;
var parentInfo = ancestorInfo.parentTag;
var parentTag = parentInfo && parentInfo.tag;
return isTagValidWithParent(tag, parentTag) && !findInvalidAncestorForTag(tag, ancestorInfo);
};
}
module.exports = validateDOMNesting;
},{"153":153,"173":173,"24":24}],145:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule CSSCore
* @typechecks
*/
'use strict';
var invariant = _dereq_(161);
/**
* The CSSCore module specifies the API (and implements most of the methods)
* that should be used when dealing with the display of elements (via their
* CSS classes and visibility on screen. It is an API focused on mutating the
* display and not reading it as no logical state should be encoded in the
* display of elements.
*/
var CSSCore = {
/**
* Adds the class passed in to the element if it doesn't already have it.
*
* @param {DOMElement} element the element to set the class on
* @param {string} className the CSS className
* @return {DOMElement} the element passed in
*/
addClass: function (element, className) {
!!/\s/.test(className) ? "development" !== 'production' ? invariant(false, 'CSSCore.addClass takes only a single class name. "%s" contains ' + 'multiple classes.', className) : invariant(false) : undefined;
if (className) {
if (element.classList) {
element.classList.add(className);
} else if (!CSSCore.hasClass(element, className)) {
element.className = element.className + ' ' + className;
}
}
return element;
},
/**
* Removes the class passed in from the element
*
* @param {DOMElement} element the element to set the class on
* @param {string} className the CSS className
* @return {DOMElement} the element passed in
*/
removeClass: function (element, className) {
!!/\s/.test(className) ? "development" !== 'production' ? invariant(false, 'CSSCore.removeClass takes only a single class name. "%s" contains ' + 'multiple classes.', className) : invariant(false) : undefined;
if (className) {
if (element.classList) {
element.classList.remove(className);
} else if (CSSCore.hasClass(element, className)) {
element.className = element.className.replace(new RegExp('(^|\\s)' + className + '(?:\\s|$)', 'g'), '$1').replace(/\s+/g, ' ') // multiple spaces to one
.replace(/^\s*|\s*$/g, ''); // trim the ends
}
}
return element;
},
/**
* Helper to add or remove a class from an element based on a condition.
*
* @param {DOMElement} element the element to set the class on
* @param {string} className the CSS className
* @param {*} bool condition to whether to add or remove the class
* @return {DOMElement} the element passed in
*/
conditionClass: function (element, className, bool) {
return (bool ? CSSCore.addClass : CSSCore.removeClass)(element, className);
},
/**
* Tests whether the element has the class specified.
*
* @param {DOMNode|DOMWindow} element the element to set the class on
* @param {string} className the CSS className
* @return {boolean} true if the element has the class, false if not
*/
hasClass: function (element, className) {
!!/\s/.test(className) ? "development" !== 'production' ? invariant(false, 'CSS.hasClass takes only a single class name.') : invariant(false) : undefined;
if (element.classList) {
return !!className && element.classList.contains(className);
}
return (' ' + element.className + ' ').indexOf(' ' + className + ' ') > -1;
}
};
module.exports = CSSCore;
},{"161":161}],146:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* @providesModule EventListener
* @typechecks
*/
'use strict';
var emptyFunction = _dereq_(153);
/**
* Upstream version of event listener. Does not take into account specific
* nature of platform.
*/
var EventListener = {
/**
* Listen to DOM events during the bubble phase.
*
* @param {DOMEventTarget} target DOM element to register listener on.
* @param {string} eventType Event type, e.g. 'click' or 'mouseover'.
* @param {function} callback Callback function.
* @return {object} Object with a `remove` method.
*/
listen: function (target, eventType, callback) {
if (target.addEventListener) {
target.addEventListener(eventType, callback, false);
return {
remove: function () {
target.removeEventListener(eventType, callback, false);
}
};
} else if (target.attachEvent) {
target.attachEvent('on' + eventType, callback);
return {
remove: function () {
target.detachEvent('on' + eventType, callback);
}
};
}
},
/**
* Listen to DOM events during the capture phase.
*
* @param {DOMEventTarget} target DOM element to register listener on.
* @param {string} eventType Event type, e.g. 'click' or 'mouseover'.
* @param {function} callback Callback function.
* @return {object} Object with a `remove` method.
*/
capture: function (target, eventType, callback) {
if (target.addEventListener) {
target.addEventListener(eventType, callback, true);
return {
remove: function () {
target.removeEventListener(eventType, callback, true);
}
};
} else {
if ("development" !== 'production') {
console.error('Attempted to listen to events during the capture phase on a ' + 'browser that does not support the capture phase. Your application ' + 'will not receive some events.');
}
return {
remove: emptyFunction
};
}
},
registerDefault: function () {}
};
module.exports = EventListener;
},{"153":153}],147:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule ExecutionEnvironment
*/
'use strict';
var canUseDOM = !!(typeof window !== 'undefined' && window.document && window.document.createElement);
/**
* Simple, lightweight module assisting with the detection and context of
* Worker. Helps avoid circular dependencies and allows code to reason about
* whether or not they are in a Worker, even if they never include the main
* `ReactWorker` dependency.
*/
var ExecutionEnvironment = {
canUseDOM: canUseDOM,
canUseWorkers: typeof Worker !== 'undefined',
canUseEventListeners: canUseDOM && !!(window.addEventListener || window.attachEvent),
canUseViewport: canUseDOM && !!window.screen,
isInWorker: !canUseDOM // For now, this is true - might change in the future.
};
module.exports = ExecutionEnvironment;
},{}],148:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule camelize
* @typechecks
*/
"use strict";
var _hyphenPattern = /-(.)/g;
/**
* Camelcases a hyphenated string, for example:
*
* > camelize('background-color')
* < "backgroundColor"
*
* @param {string} string
* @return {string}
*/
function camelize(string) {
return string.replace(_hyphenPattern, function (_, character) {
return character.toUpperCase();
});
}
module.exports = camelize;
},{}],149:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule camelizeStyleName
* @typechecks
*/
'use strict';
var camelize = _dereq_(148);
var msPattern = /^-ms-/;
/**
* Camelcases a hyphenated CSS property name, for example:
*
* > camelizeStyleName('background-color')
* < "backgroundColor"
* > camelizeStyleName('-moz-transition')
* < "MozTransition"
* > camelizeStyleName('-ms-transition')
* < "msTransition"
*
* As Andi Smith suggests
* (http://www.andismith.com/blog/2012/02/modernizr-prefixed/), an `-ms` prefix
* is converted to lowercase `ms`.
*
* @param {string} string
* @return {string}
*/
function camelizeStyleName(string) {
return camelize(string.replace(msPattern, 'ms-'));
}
module.exports = camelizeStyleName;
},{"148":148}],150:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule containsNode
* @typechecks
*/
'use strict';
var isTextNode = _dereq_(163);
/*eslint-disable no-bitwise */
/**
* Checks if a given DOM node contains or is another DOM node.
*
* @param {?DOMNode} outerNode Outer DOM node.
* @param {?DOMNode} innerNode Inner DOM node.
* @return {boolean} True if `outerNode` contains or is `innerNode`.
*/
function containsNode(_x, _x2) {
var _again = true;
_function: while (_again) {
var outerNode = _x,
innerNode = _x2;
_again = false;
if (!outerNode || !innerNode) {
return false;
} else if (outerNode === innerNode) {
return true;
} else if (isTextNode(outerNode)) {
return false;
} else if (isTextNode(innerNode)) {
_x = outerNode;
_x2 = innerNode.parentNode;
_again = true;
continue _function;
} else if (outerNode.contains) {
return outerNode.contains(innerNode);
} else if (outerNode.compareDocumentPosition) {
return !!(outerNode.compareDocumentPosition(innerNode) & 16);
} else {
return false;
}
}
}
module.exports = containsNode;
},{"163":163}],151:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule createArrayFromMixed
* @typechecks
*/
'use strict';
var toArray = _dereq_(172);
/**
* Perform a heuristic test to determine if an object is "array-like".
*
* A monk asked Joshu, a Zen master, "Has a dog Buddha nature?"
* Joshu replied: "Mu."
*
* This function determines if its argument has "array nature": it returns
* true if the argument is an actual array, an `arguments' object, or an
* HTMLCollection (e.g. node.childNodes or node.getElementsByTagName()).
*
* It will return false for other array-like objects like Filelist.
*
* @param {*} obj
* @return {boolean}
*/
function hasArrayNature(obj) {
return(
// not null/false
!!obj && (
// arrays are objects, NodeLists are functions in Safari
typeof obj == 'object' || typeof obj == 'function') &&
// quacks like an array
'length' in obj &&
// not window
!('setInterval' in obj) &&
// no DOM node should be considered an array-like
// a 'select' element has 'length' and 'item' properties on IE8
typeof obj.nodeType != 'number' && (
// a real array
Array.isArray(obj) ||
// arguments
'callee' in obj ||
// HTMLCollection/NodeList
'item' in obj)
);
}
/**
* Ensure that the argument is an array by wrapping it in an array if it is not.
* Creates a copy of the argument if it is already an array.
*
* This is mostly useful idiomatically:
*
* var createArrayFromMixed = require('createArrayFromMixed');
*
* function takesOneOrMoreThings(things) {
* things = createArrayFromMixed(things);
* ...
* }
*
* This allows you to treat `things' as an array, but accept scalars in the API.
*
* If you need to convert an array-like object, like `arguments`, into an array
* use toArray instead.
*
* @param {*} obj
* @return {array}
*/
function createArrayFromMixed(obj) {
if (!hasArrayNature(obj)) {
return [obj];
} else if (Array.isArray(obj)) {
return obj.slice();
} else {
return toArray(obj);
}
}
module.exports = createArrayFromMixed;
},{"172":172}],152:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule createNodesFromMarkup
* @typechecks
*/
/*eslint-disable fb-www/unsafe-html*/
'use strict';
var ExecutionEnvironment = _dereq_(147);
var createArrayFromMixed = _dereq_(151);
var getMarkupWrap = _dereq_(157);
var invariant = _dereq_(161);
/**
* Dummy container used to render all markup.
*/
var dummyNode = ExecutionEnvironment.canUseDOM ? document.createElement('div') : null;
/**
* Pattern used by `getNodeName`.
*/
var nodeNamePattern = /^\s*<(\w+)/;
/**
* Extracts the `nodeName` of the first element in a string of markup.
*
* @param {string} markup String of markup.
* @return {?string} Node name of the supplied markup.
*/
function getNodeName(markup) {
var nodeNameMatch = markup.match(nodeNamePattern);
return nodeNameMatch && nodeNameMatch[1].toLowerCase();
}
/**
* Creates an array containing the nodes rendered from the supplied markup. The
* optionally supplied `handleScript` function will be invoked once for each
* <script> element that is rendered. If no `handleScript` function is supplied,
* an exception is thrown if any <script> elements are rendered.
*
* @param {string} markup A string of valid HTML markup.
* @param {?function} handleScript Invoked once for each rendered <script>.
* @return {array<DOMElement|DOMTextNode>} An array of rendered nodes.
*/
function createNodesFromMarkup(markup, handleScript) {
var node = dummyNode;
!!!dummyNode ? "development" !== 'production' ? invariant(false, 'createNodesFromMarkup dummy not initialized') : invariant(false) : undefined;
var nodeName = getNodeName(markup);
var wrap = nodeName && getMarkupWrap(nodeName);
if (wrap) {
node.innerHTML = wrap[1] + markup + wrap[2];
var wrapDepth = wrap[0];
while (wrapDepth--) {
node = node.lastChild;
}
} else {
node.innerHTML = markup;
}
var scripts = node.getElementsByTagName('script');
if (scripts.length) {
!handleScript ? "development" !== 'production' ? invariant(false, 'createNodesFromMarkup(...): Unexpected <script> element rendered.') : invariant(false) : undefined;
createArrayFromMixed(scripts).forEach(handleScript);
}
var nodes = createArrayFromMixed(node.childNodes);
while (node.lastChild) {
node.removeChild(node.lastChild);
}
return nodes;
}
module.exports = createNodesFromMarkup;
},{"147":147,"151":151,"157":157,"161":161}],153:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule emptyFunction
*/
"use strict";
function makeEmptyFunction(arg) {
return function () {
return arg;
};
}
/**
* This function accepts and discards inputs; it has no side effects. This is
* primarily useful idiomatically for overridable function endpoints which
* always need to be callable, since JS lacks a null-call idiom ala Cocoa.
*/
function emptyFunction() {}
emptyFunction.thatReturns = makeEmptyFunction;
emptyFunction.thatReturnsFalse = makeEmptyFunction(false);
emptyFunction.thatReturnsTrue = makeEmptyFunction(true);
emptyFunction.thatReturnsNull = makeEmptyFunction(null);
emptyFunction.thatReturnsThis = function () {
return this;
};
emptyFunction.thatReturnsArgument = function (arg) {
return arg;
};
module.exports = emptyFunction;
},{}],154:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule emptyObject
*/
'use strict';
var emptyObject = {};
if ("development" !== 'production') {
Object.freeze(emptyObject);
}
module.exports = emptyObject;
},{}],155:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule focusNode
*/
'use strict';
/**
* @param {DOMElement} node input/textarea to focus
*/
function focusNode(node) {
// IE8 can throw "Can't move focus to the control because it is invisible,
// not enabled, or of a type that does not accept the focus." for all kinds of
// reasons that are too expensive and fragile to test.
try {
node.focus();
} catch (e) {}
}
module.exports = focusNode;
},{}],156:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule getActiveElement
* @typechecks
*/
/* eslint-disable fb-www/typeof-undefined */
/**
* Same as document.activeElement but wraps in a try-catch block. In IE it is
* not safe to call document.activeElement if there is nothing focused.
*
* The activeElement will be null only if the document or document body is not
* yet defined.
*/
'use strict';
function getActiveElement() /*?DOMElement*/{
if (typeof document === 'undefined') {
return null;
}
try {
return document.activeElement || document.body;
} catch (e) {
return document.body;
}
}
module.exports = getActiveElement;
},{}],157:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule getMarkupWrap
*/
/*eslint-disable fb-www/unsafe-html */
'use strict';
var ExecutionEnvironment = _dereq_(147);
var invariant = _dereq_(161);
/**
* Dummy container used to detect which wraps are necessary.
*/
var dummyNode = ExecutionEnvironment.canUseDOM ? document.createElement('div') : null;
/**
* Some browsers cannot use `innerHTML` to render certain elements standalone,
* so we wrap them, render the wrapped nodes, then extract the desired node.
*
* In IE8, certain elements cannot render alone, so wrap all elements ('*').
*/
var shouldWrap = {};
var selectWrap = [1, '<select multiple="true">', '</select>'];
var tableWrap = [1, '<table>', '</table>'];
var trWrap = [3, '<table><tbody><tr>', '</tr></tbody></table>'];
var svgWrap = [1, '<svg xmlns="http://www.w3.org/2000/svg">', '</svg>'];
var markupWrap = {
'*': [1, '?<div>', '</div>'],
'area': [1, '<map>', '</map>'],
'col': [2, '<table><tbody></tbody><colgroup>', '</colgroup></table>'],
'legend': [1, '<fieldset>', '</fieldset>'],
'param': [1, '<object>', '</object>'],
'tr': [2, '<table><tbody>', '</tbody></table>'],
'optgroup': selectWrap,
'option': selectWrap,
'caption': tableWrap,
'colgroup': tableWrap,
'tbody': tableWrap,
'tfoot': tableWrap,
'thead': tableWrap,
'td': trWrap,
'th': trWrap
};
// Initialize the SVG elements since we know they'll always need to be wrapped
// consistently. If they are created inside a <div> they will be initialized in
// the wrong namespace (and will not display).
var svgElements = ['circle', 'clipPath', 'defs', 'ellipse', 'g', 'image', 'line', 'linearGradient', 'mask', 'path', 'pattern', 'polygon', 'polyline', 'radialGradient', 'rect', 'stop', 'text', 'tspan'];
svgElements.forEach(function (nodeName) {
markupWrap[nodeName] = svgWrap;
shouldWrap[nodeName] = true;
});
/**
* Gets the markup wrap configuration for the supplied `nodeName`.
*
* NOTE: This lazily detects which wraps are necessary for the current browser.
*
* @param {string} nodeName Lowercase `nodeName`.
* @return {?array} Markup wrap configuration, if applicable.
*/
function getMarkupWrap(nodeName) {
!!!dummyNode ? "development" !== 'production' ? invariant(false, 'Markup wrapping node not initialized') : invariant(false) : undefined;
if (!markupWrap.hasOwnProperty(nodeName)) {
nodeName = '*';
}
if (!shouldWrap.hasOwnProperty(nodeName)) {
if (nodeName === '*') {
dummyNode.innerHTML = '<link />';
} else {
dummyNode.innerHTML = '<' + nodeName + '></' + nodeName + '>';
}
shouldWrap[nodeName] = !dummyNode.firstChild;
}
return shouldWrap[nodeName] ? markupWrap[nodeName] : null;
}
module.exports = getMarkupWrap;
},{"147":147,"161":161}],158:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule getUnboundedScrollPosition
* @typechecks
*/
'use strict';
/**
* Gets the scroll position of the supplied element or window.
*
* The return values are unbounded, unlike `getScrollPosition`. This means they
* may be negative or exceed the element boundaries (which is possible using
* inertial scrolling).
*
* @param {DOMWindow|DOMElement} scrollable
* @return {object} Map with `x` and `y` keys.
*/
function getUnboundedScrollPosition(scrollable) {
if (scrollable === window) {
return {
x: window.pageXOffset || document.documentElement.scrollLeft,
y: window.pageYOffset || document.documentElement.scrollTop
};
}
return {
x: scrollable.scrollLeft,
y: scrollable.scrollTop
};
}
module.exports = getUnboundedScrollPosition;
},{}],159:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule hyphenate
* @typechecks
*/
'use strict';
var _uppercasePattern = /([A-Z])/g;
/**
* Hyphenates a camelcased string, for example:
*
* > hyphenate('backgroundColor')
* < "background-color"
*
* For CSS style names, use `hyphenateStyleName` instead which works properly
* with all vendor prefixes, including `ms`.
*
* @param {string} string
* @return {string}
*/
function hyphenate(string) {
return string.replace(_uppercasePattern, '-$1').toLowerCase();
}
module.exports = hyphenate;
},{}],160:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule hyphenateStyleName
* @typechecks
*/
'use strict';
var hyphenate = _dereq_(159);
var msPattern = /^ms-/;
/**
* Hyphenates a camelcased CSS property name, for example:
*
* > hyphenateStyleName('backgroundColor')
* < "background-color"
* > hyphenateStyleName('MozTransition')
* < "-moz-transition"
* > hyphenateStyleName('msTransition')
* < "-ms-transition"
*
* As Modernizr suggests (http://modernizr.com/docs/#prefixed), an `ms` prefix
* is converted to `-ms-`.
*
* @param {string} string
* @return {string}
*/
function hyphenateStyleName(string) {
return hyphenate(string).replace(msPattern, '-ms-');
}
module.exports = hyphenateStyleName;
},{"159":159}],161:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule invariant
*/
'use strict';
/**
* Use invariant() to assert state which your program assumes to be true.
*
* Provide sprintf-style format (only %s is supported) and arguments
* to provide information about what broke and what you were
* expecting.
*
* The invariant message will be stripped in production, but the invariant
* will remain to ensure logic does not differ in production.
*/
function invariant(condition, format, a, b, c, d, e, f) {
if ("development" !== 'production') {
if (format === undefined) {
throw new Error('invariant requires an error message argument');
}
}
if (!condition) {
var error;
if (format === undefined) {
error = new Error('Minified exception occurred; use the non-minified dev environment ' + 'for the full error message and additional helpful warnings.');
} else {
var args = [a, b, c, d, e, f];
var argIndex = 0;
error = new Error(format.replace(/%s/g, function () {
return args[argIndex++];
}));
error.name = 'Invariant Violation';
}
error.framesToPop = 1; // we don't care about invariant's own frame
throw error;
}
}
module.exports = invariant;
},{}],162:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule isNode
* @typechecks
*/
/**
* @param {*} object The object to check.
* @return {boolean} Whether or not the object is a DOM node.
*/
'use strict';
function isNode(object) {
return !!(object && (typeof Node === 'function' ? object instanceof Node : typeof object === 'object' && typeof object.nodeType === 'number' && typeof object.nodeName === 'string'));
}
module.exports = isNode;
},{}],163:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule isTextNode
* @typechecks
*/
'use strict';
var isNode = _dereq_(162);
/**
* @param {*} object The object to check.
* @return {boolean} Whether or not the object is a DOM text node.
*/
function isTextNode(object) {
return isNode(object) && object.nodeType == 3;
}
module.exports = isTextNode;
},{"162":162}],164:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule joinClasses
* @typechecks static-only
*/
'use strict';
/**
* Combines multiple className strings into one.
* http://jsperf.com/joinclasses-args-vs-array
*
* @param {...?string} className
* @return {string}
*/
function joinClasses(className /*, ... */) {
if (!className) {
className = '';
}
var nextClass;
var argLength = arguments.length;
if (argLength > 1) {
for (var ii = 1; ii < argLength; ii++) {
nextClass = arguments[ii];
if (nextClass) {
className = (className ? className + ' ' : '') + nextClass;
}
}
}
return className;
}
module.exports = joinClasses;
},{}],165:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule keyMirror
* @typechecks static-only
*/
'use strict';
var invariant = _dereq_(161);
/**
* Constructs an enumeration with keys equal to their value.
*
* For example:
*
* var COLORS = keyMirror({blue: null, red: null});
* var myColor = COLORS.blue;
* var isColorValid = !!COLORS[myColor];
*
* The last line could not be performed if the values of the generated enum were
* not equal to their keys.
*
* Input: {key1: val1, key2: val2}
* Output: {key1: key1, key2: key2}
*
* @param {object} obj
* @return {object}
*/
var keyMirror = function (obj) {
var ret = {};
var key;
!(obj instanceof Object && !Array.isArray(obj)) ? "development" !== 'production' ? invariant(false, 'keyMirror(...): Argument must be an object.') : invariant(false) : undefined;
for (key in obj) {
if (!obj.hasOwnProperty(key)) {
continue;
}
ret[key] = key;
}
return ret;
};
module.exports = keyMirror;
},{"161":161}],166:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule keyOf
*/
/**
* Allows extraction of a minified key. Let's the build system minify keys
* without losing the ability to dynamically use key strings as values
* themselves. Pass in an object with a single key/val pair and it will return
* you the string key of that single record. Suppose you want to grab the
* value for a key 'className' inside of an object. Key/val minification may
* have aliased that key to be 'xa12'. keyOf({className: null}) will return
* 'xa12' in that case. Resolve keys you want to use once at startup time, then
* reuse those resolutions.
*/
"use strict";
var keyOf = function (oneKeyObj) {
var key;
for (key in oneKeyObj) {
if (!oneKeyObj.hasOwnProperty(key)) {
continue;
}
return key;
}
return null;
};
module.exports = keyOf;
},{}],167:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule mapObject
*/
'use strict';
var hasOwnProperty = Object.prototype.hasOwnProperty;
/**
* Executes the provided `callback` once for each enumerable own property in the
* object and constructs a new object from the results. The `callback` is
* invoked with three arguments:
*
* - the property value
* - the property name
* - the object being traversed
*
* Properties that are added after the call to `mapObject` will not be visited
* by `callback`. If the values of existing properties are changed, the value
* passed to `callback` will be the value at the time `mapObject` visits them.
* Properties that are deleted before being visited are not visited.
*
* @grep function objectMap()
* @grep function objMap()
*
* @param {?object} object
* @param {function} callback
* @param {*} context
* @return {?object}
*/
function mapObject(object, callback, context) {
if (!object) {
return null;
}
var result = {};
for (var name in object) {
if (hasOwnProperty.call(object, name)) {
result[name] = callback.call(context, object[name], name, object);
}
}
return result;
}
module.exports = mapObject;
},{}],168:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule memoizeStringOnly
* @typechecks static-only
*/
'use strict';
/**
* Memoizes the return value of a function that accepts one string argument.
*
* @param {function} callback
* @return {function}
*/
function memoizeStringOnly(callback) {
var cache = {};
return function (string) {
if (!cache.hasOwnProperty(string)) {
cache[string] = callback.call(this, string);
}
return cache[string];
};
}
module.exports = memoizeStringOnly;
},{}],169:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule performance
* @typechecks
*/
'use strict';
var ExecutionEnvironment = _dereq_(147);
var performance;
if (ExecutionEnvironment.canUseDOM) {
performance = window.performance || window.msPerformance || window.webkitPerformance;
}
module.exports = performance || {};
},{"147":147}],170:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule performanceNow
* @typechecks
*/
'use strict';
var performance = _dereq_(169);
var performanceNow;
/**
* Detect if we can use `window.performance.now()` and gracefully fallback to
* `Date.now()` if it doesn't exist. We need to support Firefox < 15 for now
* because of Facebook's testing infrastructure.
*/
if (performance.now) {
performanceNow = function () {
return performance.now();
};
} else {
performanceNow = function () {
return Date.now();
};
}
module.exports = performanceNow;
},{"169":169}],171:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule shallowEqual
* @typechecks
*
*/
'use strict';
var hasOwnProperty = Object.prototype.hasOwnProperty;
/**
* Performs equality by iterating through keys on an object and returning false
* when any key has values which are not strictly equal between the arguments.
* Returns true when the values of all keys are strictly equal.
*/
function shallowEqual(objA, objB) {
if (objA === objB) {
return true;
}
if (typeof objA !== 'object' || objA === null || typeof objB !== 'object' || objB === null) {
return false;
}
var keysA = Object.keys(objA);
var keysB = Object.keys(objB);
if (keysA.length !== keysB.length) {
return false;
}
// Test for A's keys different from B.
var bHasOwnProperty = hasOwnProperty.bind(objB);
for (var i = 0; i < keysA.length; i++) {
if (!bHasOwnProperty(keysA[i]) || objA[keysA[i]] !== objB[keysA[i]]) {
return false;
}
}
return true;
}
module.exports = shallowEqual;
},{}],172:[function(_dereq_,module,exports){
/**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule toArray
* @typechecks
*/
'use strict';
var invariant = _dereq_(161);
/**
* Convert array-like objects to arrays.
*
* This API assumes the caller knows the contents of the data type. For less
* well defined inputs use createArrayFromMixed.
*
* @param {object|function|filelist} obj
* @return {array}
*/
function toArray(obj) {
var length = obj.length;
// Some browse builtin objects can report typeof 'function' (e.g. NodeList in
// old versions of Safari).
!(!Array.isArray(obj) && (typeof obj === 'object' || typeof obj === 'function')) ? "development" !== 'production' ? invariant(false, 'toArray: Array-like object expected') : invariant(false) : undefined;
!(typeof length === 'number') ? "development" !== 'production' ? invariant(false, 'toArray: Object needs a length property') : invariant(false) : undefined;
!(length === 0 || length - 1 in obj) ? "development" !== 'production' ? invariant(false, 'toArray: Object should have keys for indices') : invariant(false) : undefined;
// Old IE doesn't give collections access to hasOwnProperty. Assume inputs
// without method will throw during the slice call and skip straight to the
// fallback.
if (obj.hasOwnProperty) {
try {
return Array.prototype.slice.call(obj);
} catch (e) {
// IE < 9 does not support Array#slice on collections objects
}
}
// Fall back to copying key by key. This assumes all keys have a value,
// so will not preserve sparsely populated inputs.
var ret = Array(length);
for (var ii = 0; ii < length; ii++) {
ret[ii] = obj[ii];
}
return ret;
}
module.exports = toArray;
},{"161":161}],173:[function(_dereq_,module,exports){
/**
* Copyright 2014-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @providesModule warning
*/
'use strict';
var emptyFunction = _dereq_(153);
/**
* Similar to invariant but only logs a warning if the condition is not met.
* This can be used to log issues in development environments in critical
* paths. Removing the logging code for production environments will keep the
* same logic and follow the same code paths.
*/
var warning = emptyFunction;
if ("development" !== 'production') {
warning = function (condition, format) {
for (var _len = arguments.length, args = Array(_len > 2 ? _len - 2 : 0), _key = 2; _key < _len; _key++) {
args[_key - 2] = arguments[_key];
}
if (format === undefined) {
throw new Error('`warning(condition, format, ...args)` requires a warning ' + 'message argument');
}
if (format.indexOf('Failed Composite propType: ') === 0) {
return; // Ignore CompositeComponent proptype check.
}
if (!condition) {
var argIndex = 0;
var message = 'Warning: ' + format.replace(/%s/g, function () {
return args[argIndex++];
});
if (typeof console !== 'undefined') {
console.error(message);
}
try {
// --- Welcome to debugging React ---
// This error was thrown as a convenience so that you can use this stack
// to find the callsite that caused this warning to fire.
throw new Error(message);
} catch (x) {}
}
};
}
module.exports = warning;
},{"153":153}]},{},[1])(1)
}); |
fixtures/output/webpack-message-formatting/src/AppLintWarning.js | IamJoseph/create-react-app | import React, { Component } from 'react';
function foo() {}
class App extends Component {
render() {
return <div />;
}
}
export default App;
|
src/routes/contact/index.js | Jose4gg/GSW-P2 | /**
* React Starter Kit (https://www.reactstarterkit.com/)
*
* Copyright © 2014-2016 Kriasoft, LLC. All rights reserved.
*
* This source code is licensed under the MIT license found in the
* LICENSE.txt file in the root directory of this source tree.
*/
import React from 'react';
import Contact from './Contact';
const title = 'Contact Us';
export default {
path: '/contact',
action() {
return {
title,
component: <Contact title={title} />,
};
},
};
|
public/assets/publify_admin-cf16b40390492b41111b6ea26a9f5ce5.js | burakicel/Burakicel.com | /*!
* jQuery JavaScript Library v1.10.2
* http://jquery.com/
*
* Includes Sizzle.js
* http://sizzlejs.com/
*
* Copyright 2005, 2013 jQuery Foundation, Inc. and other contributors
* Released under the MIT license
* http://jquery.org/license
*
* Date: 2013-07-03T13:48Z
*/
(function( window, undefined ) {
// Can't do this because several apps including ASP.NET trace
// the stack via arguments.caller.callee and Firefox dies if
// you try to trace through "use strict" call chains. (#13335)
// Support: Firefox 18+
//"use strict";
var
// The deferred used on DOM ready
readyList,
// A central reference to the root jQuery(document)
rootjQuery,
// Support: IE<10
// For `typeof xmlNode.method` instead of `xmlNode.method !== undefined`
core_strundefined = typeof undefined,
// Use the correct document accordingly with window argument (sandbox)
location = window.location,
document = window.document,
docElem = document.documentElement,
// Map over jQuery in case of overwrite
_jQuery = window.jQuery,
// Map over the $ in case of overwrite
_$ = window.$,
// [[Class]] -> type pairs
class2type = {},
// List of deleted data cache ids, so we can reuse them
core_deletedIds = [],
core_version = "1.10.2",
// Save a reference to some core methods
core_concat = core_deletedIds.concat,
core_push = core_deletedIds.push,
core_slice = core_deletedIds.slice,
core_indexOf = core_deletedIds.indexOf,
core_toString = class2type.toString,
core_hasOwn = class2type.hasOwnProperty,
core_trim = core_version.trim,
// Define a local copy of jQuery
jQuery = function( selector, context ) {
// The jQuery object is actually just the init constructor 'enhanced'
return new jQuery.fn.init( selector, context, rootjQuery );
},
// Used for matching numbers
core_pnum = /[+-]?(?:\d*\.|)\d+(?:[eE][+-]?\d+|)/.source,
// Used for splitting on whitespace
core_rnotwhite = /\S+/g,
// Make sure we trim BOM and NBSP (here's looking at you, Safari 5.0 and IE)
rtrim = /^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g,
// A simple way to check for HTML strings
// Prioritize #id over <tag> to avoid XSS via location.hash (#9521)
// Strict HTML recognition (#11290: must start with <)
rquickExpr = /^(?:\s*(<[\w\W]+>)[^>]*|#([\w-]*))$/,
// Match a standalone tag
rsingleTag = /^<(\w+)\s*\/?>(?:<\/\1>|)$/,
// JSON RegExp
rvalidchars = /^[\],:{}\s]*$/,
rvalidbraces = /(?:^|:|,)(?:\s*\[)+/g,
rvalidescape = /\\(?:["\\\/bfnrt]|u[\da-fA-F]{4})/g,
rvalidtokens = /"[^"\\\r\n]*"|true|false|null|-?(?:\d+\.|)\d+(?:[eE][+-]?\d+|)/g,
// Matches dashed string for camelizing
rmsPrefix = /^-ms-/,
rdashAlpha = /-([\da-z])/gi,
// Used by jQuery.camelCase as callback to replace()
fcamelCase = function( all, letter ) {
return letter.toUpperCase();
},
// The ready event handler
completed = function( event ) {
// readyState === "complete" is good enough for us to call the dom ready in oldIE
if ( document.addEventListener || event.type === "load" || document.readyState === "complete" ) {
detach();
jQuery.ready();
}
},
// Clean-up method for dom ready events
detach = function() {
if ( document.addEventListener ) {
document.removeEventListener( "DOMContentLoaded", completed, false );
window.removeEventListener( "load", completed, false );
} else {
document.detachEvent( "onreadystatechange", completed );
window.detachEvent( "onload", completed );
}
};
jQuery.fn = jQuery.prototype = {
// The current version of jQuery being used
jquery: core_version,
constructor: jQuery,
init: function( selector, context, rootjQuery ) {
var match, elem;
// HANDLE: $(""), $(null), $(undefined), $(false)
if ( !selector ) {
return this;
}
// Handle HTML strings
if ( typeof selector === "string" ) {
if ( selector.charAt(0) === "<" && selector.charAt( selector.length - 1 ) === ">" && selector.length >= 3 ) {
// Assume that strings that start and end with <> are HTML and skip the regex check
match = [ null, selector, null ];
} else {
match = rquickExpr.exec( selector );
}
// Match html or make sure no context is specified for #id
if ( match && (match[1] || !context) ) {
// HANDLE: $(html) -> $(array)
if ( match[1] ) {
context = context instanceof jQuery ? context[0] : context;
// scripts is true for back-compat
jQuery.merge( this, jQuery.parseHTML(
match[1],
context && context.nodeType ? context.ownerDocument || context : document,
true
) );
// HANDLE: $(html, props)
if ( rsingleTag.test( match[1] ) && jQuery.isPlainObject( context ) ) {
for ( match in context ) {
// Properties of context are called as methods if possible
if ( jQuery.isFunction( this[ match ] ) ) {
this[ match ]( context[ match ] );
// ...and otherwise set as attributes
} else {
this.attr( match, context[ match ] );
}
}
}
return this;
// HANDLE: $(#id)
} else {
elem = document.getElementById( match[2] );
// Check parentNode to catch when Blackberry 4.6 returns
// nodes that are no longer in the document #6963
if ( elem && elem.parentNode ) {
// Handle the case where IE and Opera return items
// by name instead of ID
if ( elem.id !== match[2] ) {
return rootjQuery.find( selector );
}
// Otherwise, we inject the element directly into the jQuery object
this.length = 1;
this[0] = elem;
}
this.context = document;
this.selector = selector;
return this;
}
// HANDLE: $(expr, $(...))
} else if ( !context || context.jquery ) {
return ( context || rootjQuery ).find( selector );
// HANDLE: $(expr, context)
// (which is just equivalent to: $(context).find(expr)
} else {
return this.constructor( context ).find( selector );
}
// HANDLE: $(DOMElement)
} else if ( selector.nodeType ) {
this.context = this[0] = selector;
this.length = 1;
return this;
// HANDLE: $(function)
// Shortcut for document ready
} else if ( jQuery.isFunction( selector ) ) {
return rootjQuery.ready( selector );
}
if ( selector.selector !== undefined ) {
this.selector = selector.selector;
this.context = selector.context;
}
return jQuery.makeArray( selector, this );
},
// Start with an empty selector
selector: "",
// The default length of a jQuery object is 0
length: 0,
toArray: function() {
return core_slice.call( this );
},
// Get the Nth element in the matched element set OR
// Get the whole matched element set as a clean array
get: function( num ) {
return num == null ?
// Return a 'clean' array
this.toArray() :
// Return just the object
( num < 0 ? this[ this.length + num ] : this[ num ] );
},
// Take an array of elements and push it onto the stack
// (returning the new matched element set)
pushStack: function( elems ) {
// Build a new jQuery matched element set
var ret = jQuery.merge( this.constructor(), elems );
// Add the old object onto the stack (as a reference)
ret.prevObject = this;
ret.context = this.context;
// Return the newly-formed element set
return ret;
},
// Execute a callback for every element in the matched set.
// (You can seed the arguments with an array of args, but this is
// only used internally.)
each: function( callback, args ) {
return jQuery.each( this, callback, args );
},
ready: function( fn ) {
// Add the callback
jQuery.ready.promise().done( fn );
return this;
},
slice: function() {
return this.pushStack( core_slice.apply( this, arguments ) );
},
first: function() {
return this.eq( 0 );
},
last: function() {
return this.eq( -1 );
},
eq: function( i ) {
var len = this.length,
j = +i + ( i < 0 ? len : 0 );
return this.pushStack( j >= 0 && j < len ? [ this[j] ] : [] );
},
map: function( callback ) {
return this.pushStack( jQuery.map(this, function( elem, i ) {
return callback.call( elem, i, elem );
}));
},
end: function() {
return this.prevObject || this.constructor(null);
},
// For internal use only.
// Behaves like an Array's method, not like a jQuery method.
push: core_push,
sort: [].sort,
splice: [].splice
};
// Give the init function the jQuery prototype for later instantiation
jQuery.fn.init.prototype = jQuery.fn;
jQuery.extend = jQuery.fn.extend = function() {
var src, copyIsArray, copy, name, options, clone,
target = arguments[0] || {},
i = 1,
length = arguments.length,
deep = false;
// Handle a deep copy situation
if ( typeof target === "boolean" ) {
deep = target;
target = arguments[1] || {};
// skip the boolean and the target
i = 2;
}
// Handle case when target is a string or something (possible in deep copy)
if ( typeof target !== "object" && !jQuery.isFunction(target) ) {
target = {};
}
// extend jQuery itself if only one argument is passed
if ( length === i ) {
target = this;
--i;
}
for ( ; i < length; i++ ) {
// Only deal with non-null/undefined values
if ( (options = arguments[ i ]) != null ) {
// Extend the base object
for ( name in options ) {
src = target[ name ];
copy = options[ name ];
// Prevent never-ending loop
if ( target === copy ) {
continue;
}
// Recurse if we're merging plain objects or arrays
if ( deep && copy && ( jQuery.isPlainObject(copy) || (copyIsArray = jQuery.isArray(copy)) ) ) {
if ( copyIsArray ) {
copyIsArray = false;
clone = src && jQuery.isArray(src) ? src : [];
} else {
clone = src && jQuery.isPlainObject(src) ? src : {};
}
// Never move original objects, clone them
target[ name ] = jQuery.extend( deep, clone, copy );
// Don't bring in undefined values
} else if ( copy !== undefined ) {
target[ name ] = copy;
}
}
}
}
// Return the modified object
return target;
};
jQuery.extend({
// Unique for each copy of jQuery on the page
// Non-digits removed to match rinlinejQuery
expando: "jQuery" + ( core_version + Math.random() ).replace( /\D/g, "" ),
noConflict: function( deep ) {
if ( window.$ === jQuery ) {
window.$ = _$;
}
if ( deep && window.jQuery === jQuery ) {
window.jQuery = _jQuery;
}
return jQuery;
},
// Is the DOM ready to be used? Set to true once it occurs.
isReady: false,
// A counter to track how many items to wait for before
// the ready event fires. See #6781
readyWait: 1,
// Hold (or release) the ready event
holdReady: function( hold ) {
if ( hold ) {
jQuery.readyWait++;
} else {
jQuery.ready( true );
}
},
// Handle when the DOM is ready
ready: function( wait ) {
// Abort if there are pending holds or we're already ready
if ( wait === true ? --jQuery.readyWait : jQuery.isReady ) {
return;
}
// Make sure body exists, at least, in case IE gets a little overzealous (ticket #5443).
if ( !document.body ) {
return setTimeout( jQuery.ready );
}
// Remember that the DOM is ready
jQuery.isReady = true;
// If a normal DOM Ready event fired, decrement, and wait if need be
if ( wait !== true && --jQuery.readyWait > 0 ) {
return;
}
// If there are functions bound, to execute
readyList.resolveWith( document, [ jQuery ] );
// Trigger any bound ready events
if ( jQuery.fn.trigger ) {
jQuery( document ).trigger("ready").off("ready");
}
},
// See test/unit/core.js for details concerning isFunction.
// Since version 1.3, DOM methods and functions like alert
// aren't supported. They return false on IE (#2968).
isFunction: function( obj ) {
return jQuery.type(obj) === "function";
},
isArray: Array.isArray || function( obj ) {
return jQuery.type(obj) === "array";
},
isWindow: function( obj ) {
/* jshint eqeqeq: false */
return obj != null && obj == obj.window;
},
isNumeric: function( obj ) {
return !isNaN( parseFloat(obj) ) && isFinite( obj );
},
type: function( obj ) {
if ( obj == null ) {
return String( obj );
}
return typeof obj === "object" || typeof obj === "function" ?
class2type[ core_toString.call(obj) ] || "object" :
typeof obj;
},
isPlainObject: function( obj ) {
var key;
// Must be an Object.
// Because of IE, we also have to check the presence of the constructor property.
// Make sure that DOM nodes and window objects don't pass through, as well
if ( !obj || jQuery.type(obj) !== "object" || obj.nodeType || jQuery.isWindow( obj ) ) {
return false;
}
try {
// Not own constructor property must be Object
if ( obj.constructor &&
!core_hasOwn.call(obj, "constructor") &&
!core_hasOwn.call(obj.constructor.prototype, "isPrototypeOf") ) {
return false;
}
} catch ( e ) {
// IE8,9 Will throw exceptions on certain host objects #9897
return false;
}
// Support: IE<9
// Handle iteration over inherited properties before own properties.
if ( jQuery.support.ownLast ) {
for ( key in obj ) {
return core_hasOwn.call( obj, key );
}
}
// Own properties are enumerated firstly, so to speed up,
// if last one is own, then all properties are own.
for ( key in obj ) {}
return key === undefined || core_hasOwn.call( obj, key );
},
isEmptyObject: function( obj ) {
var name;
for ( name in obj ) {
return false;
}
return true;
},
error: function( msg ) {
throw new Error( msg );
},
// data: string of html
// context (optional): If specified, the fragment will be created in this context, defaults to document
// keepScripts (optional): If true, will include scripts passed in the html string
parseHTML: function( data, context, keepScripts ) {
if ( !data || typeof data !== "string" ) {
return null;
}
if ( typeof context === "boolean" ) {
keepScripts = context;
context = false;
}
context = context || document;
var parsed = rsingleTag.exec( data ),
scripts = !keepScripts && [];
// Single tag
if ( parsed ) {
return [ context.createElement( parsed[1] ) ];
}
parsed = jQuery.buildFragment( [ data ], context, scripts );
if ( scripts ) {
jQuery( scripts ).remove();
}
return jQuery.merge( [], parsed.childNodes );
},
parseJSON: function( data ) {
// Attempt to parse using the native JSON parser first
if ( window.JSON && window.JSON.parse ) {
return window.JSON.parse( data );
}
if ( data === null ) {
return data;
}
if ( typeof data === "string" ) {
// Make sure leading/trailing whitespace is removed (IE can't handle it)
data = jQuery.trim( data );
if ( data ) {
// Make sure the incoming data is actual JSON
// Logic borrowed from http://json.org/json2.js
if ( rvalidchars.test( data.replace( rvalidescape, "@" )
.replace( rvalidtokens, "]" )
.replace( rvalidbraces, "")) ) {
return ( new Function( "return " + data ) )();
}
}
}
jQuery.error( "Invalid JSON: " + data );
},
// Cross-browser xml parsing
parseXML: function( data ) {
var xml, tmp;
if ( !data || typeof data !== "string" ) {
return null;
}
try {
if ( window.DOMParser ) { // Standard
tmp = new DOMParser();
xml = tmp.parseFromString( data , "text/xml" );
} else { // IE
xml = new ActiveXObject( "Microsoft.XMLDOM" );
xml.async = "false";
xml.loadXML( data );
}
} catch( e ) {
xml = undefined;
}
if ( !xml || !xml.documentElement || xml.getElementsByTagName( "parsererror" ).length ) {
jQuery.error( "Invalid XML: " + data );
}
return xml;
},
noop: function() {},
// Evaluates a script in a global context
// Workarounds based on findings by Jim Driscoll
// http://weblogs.java.net/blog/driscoll/archive/2009/09/08/eval-javascript-global-context
globalEval: function( data ) {
if ( data && jQuery.trim( data ) ) {
// We use execScript on Internet Explorer
// We use an anonymous function so that context is window
// rather than jQuery in Firefox
( window.execScript || function( data ) {
window[ "eval" ].call( window, data );
} )( data );
}
},
// Convert dashed to camelCase; used by the css and data modules
// Microsoft forgot to hump their vendor prefix (#9572)
camelCase: function( string ) {
return string.replace( rmsPrefix, "ms-" ).replace( rdashAlpha, fcamelCase );
},
nodeName: function( elem, name ) {
return elem.nodeName && elem.nodeName.toLowerCase() === name.toLowerCase();
},
// args is for internal usage only
each: function( obj, callback, args ) {
var value,
i = 0,
length = obj.length,
isArray = isArraylike( obj );
if ( args ) {
if ( isArray ) {
for ( ; i < length; i++ ) {
value = callback.apply( obj[ i ], args );
if ( value === false ) {
break;
}
}
} else {
for ( i in obj ) {
value = callback.apply( obj[ i ], args );
if ( value === false ) {
break;
}
}
}
// A special, fast, case for the most common use of each
} else {
if ( isArray ) {
for ( ; i < length; i++ ) {
value = callback.call( obj[ i ], i, obj[ i ] );
if ( value === false ) {
break;
}
}
} else {
for ( i in obj ) {
value = callback.call( obj[ i ], i, obj[ i ] );
if ( value === false ) {
break;
}
}
}
}
return obj;
},
// Use native String.trim function wherever possible
trim: core_trim && !core_trim.call("\uFEFF\xA0") ?
function( text ) {
return text == null ?
"" :
core_trim.call( text );
} :
// Otherwise use our own trimming functionality
function( text ) {
return text == null ?
"" :
( text + "" ).replace( rtrim, "" );
},
// results is for internal usage only
makeArray: function( arr, results ) {
var ret = results || [];
if ( arr != null ) {
if ( isArraylike( Object(arr) ) ) {
jQuery.merge( ret,
typeof arr === "string" ?
[ arr ] : arr
);
} else {
core_push.call( ret, arr );
}
}
return ret;
},
inArray: function( elem, arr, i ) {
var len;
if ( arr ) {
if ( core_indexOf ) {
return core_indexOf.call( arr, elem, i );
}
len = arr.length;
i = i ? i < 0 ? Math.max( 0, len + i ) : i : 0;
for ( ; i < len; i++ ) {
// Skip accessing in sparse arrays
if ( i in arr && arr[ i ] === elem ) {
return i;
}
}
}
return -1;
},
merge: function( first, second ) {
var l = second.length,
i = first.length,
j = 0;
if ( typeof l === "number" ) {
for ( ; j < l; j++ ) {
first[ i++ ] = second[ j ];
}
} else {
while ( second[j] !== undefined ) {
first[ i++ ] = second[ j++ ];
}
}
first.length = i;
return first;
},
grep: function( elems, callback, inv ) {
var retVal,
ret = [],
i = 0,
length = elems.length;
inv = !!inv;
// Go through the array, only saving the items
// that pass the validator function
for ( ; i < length; i++ ) {
retVal = !!callback( elems[ i ], i );
if ( inv !== retVal ) {
ret.push( elems[ i ] );
}
}
return ret;
},
// arg is for internal usage only
map: function( elems, callback, arg ) {
var value,
i = 0,
length = elems.length,
isArray = isArraylike( elems ),
ret = [];
// Go through the array, translating each of the items to their
if ( isArray ) {
for ( ; i < length; i++ ) {
value = callback( elems[ i ], i, arg );
if ( value != null ) {
ret[ ret.length ] = value;
}
}
// Go through every key on the object,
} else {
for ( i in elems ) {
value = callback( elems[ i ], i, arg );
if ( value != null ) {
ret[ ret.length ] = value;
}
}
}
// Flatten any nested arrays
return core_concat.apply( [], ret );
},
// A global GUID counter for objects
guid: 1,
// Bind a function to a context, optionally partially applying any
// arguments.
proxy: function( fn, context ) {
var args, proxy, tmp;
if ( typeof context === "string" ) {
tmp = fn[ context ];
context = fn;
fn = tmp;
}
// Quick check to determine if target is callable, in the spec
// this throws a TypeError, but we will just return undefined.
if ( !jQuery.isFunction( fn ) ) {
return undefined;
}
// Simulated bind
args = core_slice.call( arguments, 2 );
proxy = function() {
return fn.apply( context || this, args.concat( core_slice.call( arguments ) ) );
};
// Set the guid of unique handler to the same of original handler, so it can be removed
proxy.guid = fn.guid = fn.guid || jQuery.guid++;
return proxy;
},
// Multifunctional method to get and set values of a collection
// The value/s can optionally be executed if it's a function
access: function( elems, fn, key, value, chainable, emptyGet, raw ) {
var i = 0,
length = elems.length,
bulk = key == null;
// Sets many values
if ( jQuery.type( key ) === "object" ) {
chainable = true;
for ( i in key ) {
jQuery.access( elems, fn, i, key[i], true, emptyGet, raw );
}
// Sets one value
} else if ( value !== undefined ) {
chainable = true;
if ( !jQuery.isFunction( value ) ) {
raw = true;
}
if ( bulk ) {
// Bulk operations run against the entire set
if ( raw ) {
fn.call( elems, value );
fn = null;
// ...except when executing function values
} else {
bulk = fn;
fn = function( elem, key, value ) {
return bulk.call( jQuery( elem ), value );
};
}
}
if ( fn ) {
for ( ; i < length; i++ ) {
fn( elems[i], key, raw ? value : value.call( elems[i], i, fn( elems[i], key ) ) );
}
}
}
return chainable ?
elems :
// Gets
bulk ?
fn.call( elems ) :
length ? fn( elems[0], key ) : emptyGet;
},
now: function() {
return ( new Date() ).getTime();
},
// A method for quickly swapping in/out CSS properties to get correct calculations.
// Note: this method belongs to the css module but it's needed here for the support module.
// If support gets modularized, this method should be moved back to the css module.
swap: function( elem, options, callback, args ) {
var ret, name,
old = {};
// Remember the old values, and insert the new ones
for ( name in options ) {
old[ name ] = elem.style[ name ];
elem.style[ name ] = options[ name ];
}
ret = callback.apply( elem, args || [] );
// Revert the old values
for ( name in options ) {
elem.style[ name ] = old[ name ];
}
return ret;
}
});
jQuery.ready.promise = function( obj ) {
if ( !readyList ) {
readyList = jQuery.Deferred();
// Catch cases where $(document).ready() is called after the browser event has already occurred.
// we once tried to use readyState "interactive" here, but it caused issues like the one
// discovered by ChrisS here: http://bugs.jquery.com/ticket/12282#comment:15
if ( document.readyState === "complete" ) {
// Handle it asynchronously to allow scripts the opportunity to delay ready
setTimeout( jQuery.ready );
// Standards-based browsers support DOMContentLoaded
} else if ( document.addEventListener ) {
// Use the handy event callback
document.addEventListener( "DOMContentLoaded", completed, false );
// A fallback to window.onload, that will always work
window.addEventListener( "load", completed, false );
// If IE event model is used
} else {
// Ensure firing before onload, maybe late but safe also for iframes
document.attachEvent( "onreadystatechange", completed );
// A fallback to window.onload, that will always work
window.attachEvent( "onload", completed );
// If IE and not a frame
// continually check to see if the document is ready
var top = false;
try {
top = window.frameElement == null && document.documentElement;
} catch(e) {}
if ( top && top.doScroll ) {
(function doScrollCheck() {
if ( !jQuery.isReady ) {
try {
// Use the trick by Diego Perini
// http://javascript.nwbox.com/IEContentLoaded/
top.doScroll("left");
} catch(e) {
return setTimeout( doScrollCheck, 50 );
}
// detach all dom ready events
detach();
// and execute any waiting functions
jQuery.ready();
}
})();
}
}
}
return readyList.promise( obj );
};
// Populate the class2type map
jQuery.each("Boolean Number String Function Array Date RegExp Object Error".split(" "), function(i, name) {
class2type[ "[object " + name + "]" ] = name.toLowerCase();
});
function isArraylike( obj ) {
var length = obj.length,
type = jQuery.type( obj );
if ( jQuery.isWindow( obj ) ) {
return false;
}
if ( obj.nodeType === 1 && length ) {
return true;
}
return type === "array" || type !== "function" &&
( length === 0 ||
typeof length === "number" && length > 0 && ( length - 1 ) in obj );
}
// All jQuery objects should point back to these
rootjQuery = jQuery(document);
/*!
* Sizzle CSS Selector Engine v1.10.2
* http://sizzlejs.com/
*
* Copyright 2013 jQuery Foundation, Inc. and other contributors
* Released under the MIT license
* http://jquery.org/license
*
* Date: 2013-07-03
*/
(function( window, undefined ) {
var i,
support,
cachedruns,
Expr,
getText,
isXML,
compile,
outermostContext,
sortInput,
// Local document vars
setDocument,
document,
docElem,
documentIsHTML,
rbuggyQSA,
rbuggyMatches,
matches,
contains,
// Instance-specific data
expando = "sizzle" + -(new Date()),
preferredDoc = window.document,
dirruns = 0,
done = 0,
classCache = createCache(),
tokenCache = createCache(),
compilerCache = createCache(),
hasDuplicate = false,
sortOrder = function( a, b ) {
if ( a === b ) {
hasDuplicate = true;
return 0;
}
return 0;
},
// General-purpose constants
strundefined = typeof undefined,
MAX_NEGATIVE = 1 << 31,
// Instance methods
hasOwn = ({}).hasOwnProperty,
arr = [],
pop = arr.pop,
push_native = arr.push,
push = arr.push,
slice = arr.slice,
// Use a stripped-down indexOf if we can't use a native one
indexOf = arr.indexOf || function( elem ) {
var i = 0,
len = this.length;
for ( ; i < len; i++ ) {
if ( this[i] === elem ) {
return i;
}
}
return -1;
},
booleans = "checked|selected|async|autofocus|autoplay|controls|defer|disabled|hidden|ismap|loop|multiple|open|readonly|required|scoped",
// Regular expressions
// Whitespace characters http://www.w3.org/TR/css3-selectors/#whitespace
whitespace = "[\\x20\\t\\r\\n\\f]",
// http://www.w3.org/TR/css3-syntax/#characters
characterEncoding = "(?:\\\\.|[\\w-]|[^\\x00-\\xa0])+",
// Loosely modeled on CSS identifier characters
// An unquoted value should be a CSS identifier http://www.w3.org/TR/css3-selectors/#attribute-selectors
// Proper syntax: http://www.w3.org/TR/CSS21/syndata.html#value-def-identifier
identifier = characterEncoding.replace( "w", "w#" ),
// Acceptable operators http://www.w3.org/TR/selectors/#attribute-selectors
attributes = "\\[" + whitespace + "*(" + characterEncoding + ")" + whitespace +
"*(?:([*^$|!~]?=)" + whitespace + "*(?:(['\"])((?:\\\\.|[^\\\\])*?)\\3|(" + identifier + ")|)|)" + whitespace + "*\\]",
// Prefer arguments quoted,
// then not containing pseudos/brackets,
// then attribute selectors/non-parenthetical expressions,
// then anything else
// These preferences are here to reduce the number of selectors
// needing tokenize in the PSEUDO preFilter
pseudos = ":(" + characterEncoding + ")(?:\\(((['\"])((?:\\\\.|[^\\\\])*?)\\3|((?:\\\\.|[^\\\\()[\\]]|" + attributes.replace( 3, 8 ) + ")*)|.*)\\)|)",
// Leading and non-escaped trailing whitespace, capturing some non-whitespace characters preceding the latter
rtrim = new RegExp( "^" + whitespace + "+|((?:^|[^\\\\])(?:\\\\.)*)" + whitespace + "+$", "g" ),
rcomma = new RegExp( "^" + whitespace + "*," + whitespace + "*" ),
rcombinators = new RegExp( "^" + whitespace + "*([>+~]|" + whitespace + ")" + whitespace + "*" ),
rsibling = new RegExp( whitespace + "*[+~]" ),
rattributeQuotes = new RegExp( "=" + whitespace + "*([^\\]'\"]*)" + whitespace + "*\\]", "g" ),
rpseudo = new RegExp( pseudos ),
ridentifier = new RegExp( "^" + identifier + "$" ),
matchExpr = {
"ID": new RegExp( "^#(" + characterEncoding + ")" ),
"CLASS": new RegExp( "^\\.(" + characterEncoding + ")" ),
"TAG": new RegExp( "^(" + characterEncoding.replace( "w", "w*" ) + ")" ),
"ATTR": new RegExp( "^" + attributes ),
"PSEUDO": new RegExp( "^" + pseudos ),
"CHILD": new RegExp( "^:(only|first|last|nth|nth-last)-(child|of-type)(?:\\(" + whitespace +
"*(even|odd|(([+-]|)(\\d*)n|)" + whitespace + "*(?:([+-]|)" + whitespace +
"*(\\d+)|))" + whitespace + "*\\)|)", "i" ),
"bool": new RegExp( "^(?:" + booleans + ")$", "i" ),
// For use in libraries implementing .is()
// We use this for POS matching in `select`
"needsContext": new RegExp( "^" + whitespace + "*[>+~]|:(even|odd|eq|gt|lt|nth|first|last)(?:\\(" +
whitespace + "*((?:-\\d)?\\d*)" + whitespace + "*\\)|)(?=[^-]|$)", "i" )
},
rnative = /^[^{]+\{\s*\[native \w/,
// Easily-parseable/retrievable ID or TAG or CLASS selectors
rquickExpr = /^(?:#([\w-]+)|(\w+)|\.([\w-]+))$/,
rinputs = /^(?:input|select|textarea|button)$/i,
rheader = /^h\d$/i,
rescape = /'|\\/g,
// CSS escapes http://www.w3.org/TR/CSS21/syndata.html#escaped-characters
runescape = new RegExp( "\\\\([\\da-f]{1,6}" + whitespace + "?|(" + whitespace + ")|.)", "ig" ),
funescape = function( _, escaped, escapedWhitespace ) {
var high = "0x" + escaped - 0x10000;
// NaN means non-codepoint
// Support: Firefox
// Workaround erroneous numeric interpretation of +"0x"
return high !== high || escapedWhitespace ?
escaped :
// BMP codepoint
high < 0 ?
String.fromCharCode( high + 0x10000 ) :
// Supplemental Plane codepoint (surrogate pair)
String.fromCharCode( high >> 10 | 0xD800, high & 0x3FF | 0xDC00 );
};
// Optimize for push.apply( _, NodeList )
try {
push.apply(
(arr = slice.call( preferredDoc.childNodes )),
preferredDoc.childNodes
);
// Support: Android<4.0
// Detect silently failing push.apply
arr[ preferredDoc.childNodes.length ].nodeType;
} catch ( e ) {
push = { apply: arr.length ?
// Leverage slice if possible
function( target, els ) {
push_native.apply( target, slice.call(els) );
} :
// Support: IE<9
// Otherwise append directly
function( target, els ) {
var j = target.length,
i = 0;
// Can't trust NodeList.length
while ( (target[j++] = els[i++]) ) {}
target.length = j - 1;
}
};
}
function Sizzle( selector, context, results, seed ) {
var match, elem, m, nodeType,
// QSA vars
i, groups, old, nid, newContext, newSelector;
if ( ( context ? context.ownerDocument || context : preferredDoc ) !== document ) {
setDocument( context );
}
context = context || document;
results = results || [];
if ( !selector || typeof selector !== "string" ) {
return results;
}
if ( (nodeType = context.nodeType) !== 1 && nodeType !== 9 ) {
return [];
}
if ( documentIsHTML && !seed ) {
// Shortcuts
if ( (match = rquickExpr.exec( selector )) ) {
// Speed-up: Sizzle("#ID")
if ( (m = match[1]) ) {
if ( nodeType === 9 ) {
elem = context.getElementById( m );
// Check parentNode to catch when Blackberry 4.6 returns
// nodes that are no longer in the document #6963
if ( elem && elem.parentNode ) {
// Handle the case where IE, Opera, and Webkit return items
// by name instead of ID
if ( elem.id === m ) {
results.push( elem );
return results;
}
} else {
return results;
}
} else {
// Context is not a document
if ( context.ownerDocument && (elem = context.ownerDocument.getElementById( m )) &&
contains( context, elem ) && elem.id === m ) {
results.push( elem );
return results;
}
}
// Speed-up: Sizzle("TAG")
} else if ( match[2] ) {
push.apply( results, context.getElementsByTagName( selector ) );
return results;
// Speed-up: Sizzle(".CLASS")
} else if ( (m = match[3]) && support.getElementsByClassName && context.getElementsByClassName ) {
push.apply( results, context.getElementsByClassName( m ) );
return results;
}
}
// QSA path
if ( support.qsa && (!rbuggyQSA || !rbuggyQSA.test( selector )) ) {
nid = old = expando;
newContext = context;
newSelector = nodeType === 9 && selector;
// qSA works strangely on Element-rooted queries
// We can work around this by specifying an extra ID on the root
// and working up from there (Thanks to Andrew Dupont for the technique)
// IE 8 doesn't work on object elements
if ( nodeType === 1 && context.nodeName.toLowerCase() !== "object" ) {
groups = tokenize( selector );
if ( (old = context.getAttribute("id")) ) {
nid = old.replace( rescape, "\\$&" );
} else {
context.setAttribute( "id", nid );
}
nid = "[id='" + nid + "'] ";
i = groups.length;
while ( i-- ) {
groups[i] = nid + toSelector( groups[i] );
}
newContext = rsibling.test( selector ) && context.parentNode || context;
newSelector = groups.join(",");
}
if ( newSelector ) {
try {
push.apply( results,
newContext.querySelectorAll( newSelector )
);
return results;
} catch(qsaError) {
} finally {
if ( !old ) {
context.removeAttribute("id");
}
}
}
}
}
// All others
return select( selector.replace( rtrim, "$1" ), context, results, seed );
}
/**
* Create key-value caches of limited size
* @returns {Function(string, Object)} Returns the Object data after storing it on itself with
* property name the (space-suffixed) string and (if the cache is larger than Expr.cacheLength)
* deleting the oldest entry
*/
function createCache() {
var keys = [];
function cache( key, value ) {
// Use (key + " ") to avoid collision with native prototype properties (see Issue #157)
if ( keys.push( key += " " ) > Expr.cacheLength ) {
// Only keep the most recent entries
delete cache[ keys.shift() ];
}
return (cache[ key ] = value);
}
return cache;
}
/**
* Mark a function for special use by Sizzle
* @param {Function} fn The function to mark
*/
function markFunction( fn ) {
fn[ expando ] = true;
return fn;
}
/**
* Support testing using an element
* @param {Function} fn Passed the created div and expects a boolean result
*/
function assert( fn ) {
var div = document.createElement("div");
try {
return !!fn( div );
} catch (e) {
return false;
} finally {
// Remove from its parent by default
if ( div.parentNode ) {
div.parentNode.removeChild( div );
}
// release memory in IE
div = null;
}
}
/**
* Adds the same handler for all of the specified attrs
* @param {String} attrs Pipe-separated list of attributes
* @param {Function} handler The method that will be applied
*/
function addHandle( attrs, handler ) {
var arr = attrs.split("|"),
i = attrs.length;
while ( i-- ) {
Expr.attrHandle[ arr[i] ] = handler;
}
}
/**
* Checks document order of two siblings
* @param {Element} a
* @param {Element} b
* @returns {Number} Returns less than 0 if a precedes b, greater than 0 if a follows b
*/
function siblingCheck( a, b ) {
var cur = b && a,
diff = cur && a.nodeType === 1 && b.nodeType === 1 &&
( ~b.sourceIndex || MAX_NEGATIVE ) -
( ~a.sourceIndex || MAX_NEGATIVE );
// Use IE sourceIndex if available on both nodes
if ( diff ) {
return diff;
}
// Check if b follows a
if ( cur ) {
while ( (cur = cur.nextSibling) ) {
if ( cur === b ) {
return -1;
}
}
}
return a ? 1 : -1;
}
/**
* Returns a function to use in pseudos for input types
* @param {String} type
*/
function createInputPseudo( type ) {
return function( elem ) {
var name = elem.nodeName.toLowerCase();
return name === "input" && elem.type === type;
};
}
/**
* Returns a function to use in pseudos for buttons
* @param {String} type
*/
function createButtonPseudo( type ) {
return function( elem ) {
var name = elem.nodeName.toLowerCase();
return (name === "input" || name === "button") && elem.type === type;
};
}
/**
* Returns a function to use in pseudos for positionals
* @param {Function} fn
*/
function createPositionalPseudo( fn ) {
return markFunction(function( argument ) {
argument = +argument;
return markFunction(function( seed, matches ) {
var j,
matchIndexes = fn( [], seed.length, argument ),
i = matchIndexes.length;
// Match elements found at the specified indexes
while ( i-- ) {
if ( seed[ (j = matchIndexes[i]) ] ) {
seed[j] = !(matches[j] = seed[j]);
}
}
});
});
}
/**
* Detect xml
* @param {Element|Object} elem An element or a document
*/
isXML = Sizzle.isXML = function( elem ) {
// documentElement is verified for cases where it doesn't yet exist
// (such as loading iframes in IE - #4833)
var documentElement = elem && (elem.ownerDocument || elem).documentElement;
return documentElement ? documentElement.nodeName !== "HTML" : false;
};
// Expose support vars for convenience
support = Sizzle.support = {};
/**
* Sets document-related variables once based on the current document
* @param {Element|Object} [doc] An element or document object to use to set the document
* @returns {Object} Returns the current document
*/
setDocument = Sizzle.setDocument = function( node ) {
var doc = node ? node.ownerDocument || node : preferredDoc,
parent = doc.defaultView;
// If no document and documentElement is available, return
if ( doc === document || doc.nodeType !== 9 || !doc.documentElement ) {
return document;
}
// Set our document
document = doc;
docElem = doc.documentElement;
// Support tests
documentIsHTML = !isXML( doc );
// Support: IE>8
// If iframe document is assigned to "document" variable and if iframe has been reloaded,
// IE will throw "permission denied" error when accessing "document" variable, see jQuery #13936
// IE6-8 do not support the defaultView property so parent will be undefined
if ( parent && parent.attachEvent && parent !== parent.top ) {
parent.attachEvent( "onbeforeunload", function() {
setDocument();
});
}
/* Attributes
---------------------------------------------------------------------- */
// Support: IE<8
// Verify that getAttribute really returns attributes and not properties (excepting IE8 booleans)
support.attributes = assert(function( div ) {
div.className = "i";
return !div.getAttribute("className");
});
/* getElement(s)By*
---------------------------------------------------------------------- */
// Check if getElementsByTagName("*") returns only elements
support.getElementsByTagName = assert(function( div ) {
div.appendChild( doc.createComment("") );
return !div.getElementsByTagName("*").length;
});
// Check if getElementsByClassName can be trusted
support.getElementsByClassName = assert(function( div ) {
div.innerHTML = "<div class='a'></div><div class='a i'></div>";
// Support: Safari<4
// Catch class over-caching
div.firstChild.className = "i";
// Support: Opera<10
// Catch gEBCN failure to find non-leading classes
return div.getElementsByClassName("i").length === 2;
});
// Support: IE<10
// Check if getElementById returns elements by name
// The broken getElementById methods don't pick up programatically-set names,
// so use a roundabout getElementsByName test
support.getById = assert(function( div ) {
docElem.appendChild( div ).id = expando;
return !doc.getElementsByName || !doc.getElementsByName( expando ).length;
});
// ID find and filter
if ( support.getById ) {
Expr.find["ID"] = function( id, context ) {
if ( typeof context.getElementById !== strundefined && documentIsHTML ) {
var m = context.getElementById( id );
// Check parentNode to catch when Blackberry 4.6 returns
// nodes that are no longer in the document #6963
return m && m.parentNode ? [m] : [];
}
};
Expr.filter["ID"] = function( id ) {
var attrId = id.replace( runescape, funescape );
return function( elem ) {
return elem.getAttribute("id") === attrId;
};
};
} else {
// Support: IE6/7
// getElementById is not reliable as a find shortcut
delete Expr.find["ID"];
Expr.filter["ID"] = function( id ) {
var attrId = id.replace( runescape, funescape );
return function( elem ) {
var node = typeof elem.getAttributeNode !== strundefined && elem.getAttributeNode("id");
return node && node.value === attrId;
};
};
}
// Tag
Expr.find["TAG"] = support.getElementsByTagName ?
function( tag, context ) {
if ( typeof context.getElementsByTagName !== strundefined ) {
return context.getElementsByTagName( tag );
}
} :
function( tag, context ) {
var elem,
tmp = [],
i = 0,
results = context.getElementsByTagName( tag );
// Filter out possible comments
if ( tag === "*" ) {
while ( (elem = results[i++]) ) {
if ( elem.nodeType === 1 ) {
tmp.push( elem );
}
}
return tmp;
}
return results;
};
// Class
Expr.find["CLASS"] = support.getElementsByClassName && function( className, context ) {
if ( typeof context.getElementsByClassName !== strundefined && documentIsHTML ) {
return context.getElementsByClassName( className );
}
};
/* QSA/matchesSelector
---------------------------------------------------------------------- */
// QSA and matchesSelector support
// matchesSelector(:active) reports false when true (IE9/Opera 11.5)
rbuggyMatches = [];
// qSa(:focus) reports false when true (Chrome 21)
// We allow this because of a bug in IE8/9 that throws an error
// whenever `document.activeElement` is accessed on an iframe
// So, we allow :focus to pass through QSA all the time to avoid the IE error
// See http://bugs.jquery.com/ticket/13378
rbuggyQSA = [];
if ( (support.qsa = rnative.test( doc.querySelectorAll )) ) {
// Build QSA regex
// Regex strategy adopted from Diego Perini
assert(function( div ) {
// Select is set to empty string on purpose
// This is to test IE's treatment of not explicitly
// setting a boolean content attribute,
// since its presence should be enough
// http://bugs.jquery.com/ticket/12359
div.innerHTML = "<select><option selected=''></option></select>";
// Support: IE8
// Boolean attributes and "value" are not treated correctly
if ( !div.querySelectorAll("[selected]").length ) {
rbuggyQSA.push( "\\[" + whitespace + "*(?:value|" + booleans + ")" );
}
// Webkit/Opera - :checked should return selected option elements
// http://www.w3.org/TR/2011/REC-css3-selectors-20110929/#checked
// IE8 throws error here and will not see later tests
if ( !div.querySelectorAll(":checked").length ) {
rbuggyQSA.push(":checked");
}
});
assert(function( div ) {
// Support: Opera 10-12/IE8
// ^= $= *= and empty values
// Should not select anything
// Support: Windows 8 Native Apps
// The type attribute is restricted during .innerHTML assignment
var input = doc.createElement("input");
input.setAttribute( "type", "hidden" );
div.appendChild( input ).setAttribute( "t", "" );
if ( div.querySelectorAll("[t^='']").length ) {
rbuggyQSA.push( "[*^$]=" + whitespace + "*(?:''|\"\")" );
}
// FF 3.5 - :enabled/:disabled and hidden elements (hidden elements are still enabled)
// IE8 throws error here and will not see later tests
if ( !div.querySelectorAll(":enabled").length ) {
rbuggyQSA.push( ":enabled", ":disabled" );
}
// Opera 10-11 does not throw on post-comma invalid pseudos
div.querySelectorAll("*,:x");
rbuggyQSA.push(",.*:");
});
}
if ( (support.matchesSelector = rnative.test( (matches = docElem.webkitMatchesSelector ||
docElem.mozMatchesSelector ||
docElem.oMatchesSelector ||
docElem.msMatchesSelector) )) ) {
assert(function( div ) {
// Check to see if it's possible to do matchesSelector
// on a disconnected node (IE 9)
support.disconnectedMatch = matches.call( div, "div" );
// This should fail with an exception
// Gecko does not error, returns false instead
matches.call( div, "[s!='']:x" );
rbuggyMatches.push( "!=", pseudos );
});
}
rbuggyQSA = rbuggyQSA.length && new RegExp( rbuggyQSA.join("|") );
rbuggyMatches = rbuggyMatches.length && new RegExp( rbuggyMatches.join("|") );
/* Contains
---------------------------------------------------------------------- */
// Element contains another
// Purposefully does not implement inclusive descendent
// As in, an element does not contain itself
contains = rnative.test( docElem.contains ) || docElem.compareDocumentPosition ?
function( a, b ) {
var adown = a.nodeType === 9 ? a.documentElement : a,
bup = b && b.parentNode;
return a === bup || !!( bup && bup.nodeType === 1 && (
adown.contains ?
adown.contains( bup ) :
a.compareDocumentPosition && a.compareDocumentPosition( bup ) & 16
));
} :
function( a, b ) {
if ( b ) {
while ( (b = b.parentNode) ) {
if ( b === a ) {
return true;
}
}
}
return false;
};
/* Sorting
---------------------------------------------------------------------- */
// Document order sorting
sortOrder = docElem.compareDocumentPosition ?
function( a, b ) {
// Flag for duplicate removal
if ( a === b ) {
hasDuplicate = true;
return 0;
}
var compare = b.compareDocumentPosition && a.compareDocumentPosition && a.compareDocumentPosition( b );
if ( compare ) {
// Disconnected nodes
if ( compare & 1 ||
(!support.sortDetached && b.compareDocumentPosition( a ) === compare) ) {
// Choose the first element that is related to our preferred document
if ( a === doc || contains(preferredDoc, a) ) {
return -1;
}
if ( b === doc || contains(preferredDoc, b) ) {
return 1;
}
// Maintain original order
return sortInput ?
( indexOf.call( sortInput, a ) - indexOf.call( sortInput, b ) ) :
0;
}
return compare & 4 ? -1 : 1;
}
// Not directly comparable, sort on existence of method
return a.compareDocumentPosition ? -1 : 1;
} :
function( a, b ) {
var cur,
i = 0,
aup = a.parentNode,
bup = b.parentNode,
ap = [ a ],
bp = [ b ];
// Exit early if the nodes are identical
if ( a === b ) {
hasDuplicate = true;
return 0;
// Parentless nodes are either documents or disconnected
} else if ( !aup || !bup ) {
return a === doc ? -1 :
b === doc ? 1 :
aup ? -1 :
bup ? 1 :
sortInput ?
( indexOf.call( sortInput, a ) - indexOf.call( sortInput, b ) ) :
0;
// If the nodes are siblings, we can do a quick check
} else if ( aup === bup ) {
return siblingCheck( a, b );
}
// Otherwise we need full lists of their ancestors for comparison
cur = a;
while ( (cur = cur.parentNode) ) {
ap.unshift( cur );
}
cur = b;
while ( (cur = cur.parentNode) ) {
bp.unshift( cur );
}
// Walk down the tree looking for a discrepancy
while ( ap[i] === bp[i] ) {
i++;
}
return i ?
// Do a sibling check if the nodes have a common ancestor
siblingCheck( ap[i], bp[i] ) :
// Otherwise nodes in our document sort first
ap[i] === preferredDoc ? -1 :
bp[i] === preferredDoc ? 1 :
0;
};
return doc;
};
Sizzle.matches = function( expr, elements ) {
return Sizzle( expr, null, null, elements );
};
Sizzle.matchesSelector = function( elem, expr ) {
// Set document vars if needed
if ( ( elem.ownerDocument || elem ) !== document ) {
setDocument( elem );
}
// Make sure that attribute selectors are quoted
expr = expr.replace( rattributeQuotes, "='$1']" );
if ( support.matchesSelector && documentIsHTML &&
( !rbuggyMatches || !rbuggyMatches.test( expr ) ) &&
( !rbuggyQSA || !rbuggyQSA.test( expr ) ) ) {
try {
var ret = matches.call( elem, expr );
// IE 9's matchesSelector returns false on disconnected nodes
if ( ret || support.disconnectedMatch ||
// As well, disconnected nodes are said to be in a document
// fragment in IE 9
elem.document && elem.document.nodeType !== 11 ) {
return ret;
}
} catch(e) {}
}
return Sizzle( expr, document, null, [elem] ).length > 0;
};
Sizzle.contains = function( context, elem ) {
// Set document vars if needed
if ( ( context.ownerDocument || context ) !== document ) {
setDocument( context );
}
return contains( context, elem );
};
Sizzle.attr = function( elem, name ) {
// Set document vars if needed
if ( ( elem.ownerDocument || elem ) !== document ) {
setDocument( elem );
}
var fn = Expr.attrHandle[ name.toLowerCase() ],
// Don't get fooled by Object.prototype properties (jQuery #13807)
val = fn && hasOwn.call( Expr.attrHandle, name.toLowerCase() ) ?
fn( elem, name, !documentIsHTML ) :
undefined;
return val === undefined ?
support.attributes || !documentIsHTML ?
elem.getAttribute( name ) :
(val = elem.getAttributeNode(name)) && val.specified ?
val.value :
null :
val;
};
Sizzle.error = function( msg ) {
throw new Error( "Syntax error, unrecognized expression: " + msg );
};
/**
* Document sorting and removing duplicates
* @param {ArrayLike} results
*/
Sizzle.uniqueSort = function( results ) {
var elem,
duplicates = [],
j = 0,
i = 0;
// Unless we *know* we can detect duplicates, assume their presence
hasDuplicate = !support.detectDuplicates;
sortInput = !support.sortStable && results.slice( 0 );
results.sort( sortOrder );
if ( hasDuplicate ) {
while ( (elem = results[i++]) ) {
if ( elem === results[ i ] ) {
j = duplicates.push( i );
}
}
while ( j-- ) {
results.splice( duplicates[ j ], 1 );
}
}
return results;
};
/**
* Utility function for retrieving the text value of an array of DOM nodes
* @param {Array|Element} elem
*/
getText = Sizzle.getText = function( elem ) {
var node,
ret = "",
i = 0,
nodeType = elem.nodeType;
if ( !nodeType ) {
// If no nodeType, this is expected to be an array
for ( ; (node = elem[i]); i++ ) {
// Do not traverse comment nodes
ret += getText( node );
}
} else if ( nodeType === 1 || nodeType === 9 || nodeType === 11 ) {
// Use textContent for elements
// innerText usage removed for consistency of new lines (see #11153)
if ( typeof elem.textContent === "string" ) {
return elem.textContent;
} else {
// Traverse its children
for ( elem = elem.firstChild; elem; elem = elem.nextSibling ) {
ret += getText( elem );
}
}
} else if ( nodeType === 3 || nodeType === 4 ) {
return elem.nodeValue;
}
// Do not include comment or processing instruction nodes
return ret;
};
Expr = Sizzle.selectors = {
// Can be adjusted by the user
cacheLength: 50,
createPseudo: markFunction,
match: matchExpr,
attrHandle: {},
find: {},
relative: {
">": { dir: "parentNode", first: true },
" ": { dir: "parentNode" },
"+": { dir: "previousSibling", first: true },
"~": { dir: "previousSibling" }
},
preFilter: {
"ATTR": function( match ) {
match[1] = match[1].replace( runescape, funescape );
// Move the given value to match[3] whether quoted or unquoted
match[3] = ( match[4] || match[5] || "" ).replace( runescape, funescape );
if ( match[2] === "~=" ) {
match[3] = " " + match[3] + " ";
}
return match.slice( 0, 4 );
},
"CHILD": function( match ) {
/* matches from matchExpr["CHILD"]
1 type (only|nth|...)
2 what (child|of-type)
3 argument (even|odd|\d*|\d*n([+-]\d+)?|...)
4 xn-component of xn+y argument ([+-]?\d*n|)
5 sign of xn-component
6 x of xn-component
7 sign of y-component
8 y of y-component
*/
match[1] = match[1].toLowerCase();
if ( match[1].slice( 0, 3 ) === "nth" ) {
// nth-* requires argument
if ( !match[3] ) {
Sizzle.error( match[0] );
}
// numeric x and y parameters for Expr.filter.CHILD
// remember that false/true cast respectively to 0/1
match[4] = +( match[4] ? match[5] + (match[6] || 1) : 2 * ( match[3] === "even" || match[3] === "odd" ) );
match[5] = +( ( match[7] + match[8] ) || match[3] === "odd" );
// other types prohibit arguments
} else if ( match[3] ) {
Sizzle.error( match[0] );
}
return match;
},
"PSEUDO": function( match ) {
var excess,
unquoted = !match[5] && match[2];
if ( matchExpr["CHILD"].test( match[0] ) ) {
return null;
}
// Accept quoted arguments as-is
if ( match[3] && match[4] !== undefined ) {
match[2] = match[4];
// Strip excess characters from unquoted arguments
} else if ( unquoted && rpseudo.test( unquoted ) &&
// Get excess from tokenize (recursively)
(excess = tokenize( unquoted, true )) &&
// advance to the next closing parenthesis
(excess = unquoted.indexOf( ")", unquoted.length - excess ) - unquoted.length) ) {
// excess is a negative index
match[0] = match[0].slice( 0, excess );
match[2] = unquoted.slice( 0, excess );
}
// Return only captures needed by the pseudo filter method (type and argument)
return match.slice( 0, 3 );
}
},
filter: {
"TAG": function( nodeNameSelector ) {
var nodeName = nodeNameSelector.replace( runescape, funescape ).toLowerCase();
return nodeNameSelector === "*" ?
function() { return true; } :
function( elem ) {
return elem.nodeName && elem.nodeName.toLowerCase() === nodeName;
};
},
"CLASS": function( className ) {
var pattern = classCache[ className + " " ];
return pattern ||
(pattern = new RegExp( "(^|" + whitespace + ")" + className + "(" + whitespace + "|$)" )) &&
classCache( className, function( elem ) {
return pattern.test( typeof elem.className === "string" && elem.className || typeof elem.getAttribute !== strundefined && elem.getAttribute("class") || "" );
});
},
"ATTR": function( name, operator, check ) {
return function( elem ) {
var result = Sizzle.attr( elem, name );
if ( result == null ) {
return operator === "!=";
}
if ( !operator ) {
return true;
}
result += "";
return operator === "=" ? result === check :
operator === "!=" ? result !== check :
operator === "^=" ? check && result.indexOf( check ) === 0 :
operator === "*=" ? check && result.indexOf( check ) > -1 :
operator === "$=" ? check && result.slice( -check.length ) === check :
operator === "~=" ? ( " " + result + " " ).indexOf( check ) > -1 :
operator === "|=" ? result === check || result.slice( 0, check.length + 1 ) === check + "-" :
false;
};
},
"CHILD": function( type, what, argument, first, last ) {
var simple = type.slice( 0, 3 ) !== "nth",
forward = type.slice( -4 ) !== "last",
ofType = what === "of-type";
return first === 1 && last === 0 ?
// Shortcut for :nth-*(n)
function( elem ) {
return !!elem.parentNode;
} :
function( elem, context, xml ) {
var cache, outerCache, node, diff, nodeIndex, start,
dir = simple !== forward ? "nextSibling" : "previousSibling",
parent = elem.parentNode,
name = ofType && elem.nodeName.toLowerCase(),
useCache = !xml && !ofType;
if ( parent ) {
// :(first|last|only)-(child|of-type)
if ( simple ) {
while ( dir ) {
node = elem;
while ( (node = node[ dir ]) ) {
if ( ofType ? node.nodeName.toLowerCase() === name : node.nodeType === 1 ) {
return false;
}
}
// Reverse direction for :only-* (if we haven't yet done so)
start = dir = type === "only" && !start && "nextSibling";
}
return true;
}
start = [ forward ? parent.firstChild : parent.lastChild ];
// non-xml :nth-child(...) stores cache data on `parent`
if ( forward && useCache ) {
// Seek `elem` from a previously-cached index
outerCache = parent[ expando ] || (parent[ expando ] = {});
cache = outerCache[ type ] || [];
nodeIndex = cache[0] === dirruns && cache[1];
diff = cache[0] === dirruns && cache[2];
node = nodeIndex && parent.childNodes[ nodeIndex ];
while ( (node = ++nodeIndex && node && node[ dir ] ||
// Fallback to seeking `elem` from the start
(diff = nodeIndex = 0) || start.pop()) ) {
// When found, cache indexes on `parent` and break
if ( node.nodeType === 1 && ++diff && node === elem ) {
outerCache[ type ] = [ dirruns, nodeIndex, diff ];
break;
}
}
// Use previously-cached element index if available
} else if ( useCache && (cache = (elem[ expando ] || (elem[ expando ] = {}))[ type ]) && cache[0] === dirruns ) {
diff = cache[1];
// xml :nth-child(...) or :nth-last-child(...) or :nth(-last)?-of-type(...)
} else {
// Use the same loop as above to seek `elem` from the start
while ( (node = ++nodeIndex && node && node[ dir ] ||
(diff = nodeIndex = 0) || start.pop()) ) {
if ( ( ofType ? node.nodeName.toLowerCase() === name : node.nodeType === 1 ) && ++diff ) {
// Cache the index of each encountered element
if ( useCache ) {
(node[ expando ] || (node[ expando ] = {}))[ type ] = [ dirruns, diff ];
}
if ( node === elem ) {
break;
}
}
}
}
// Incorporate the offset, then check against cycle size
diff -= last;
return diff === first || ( diff % first === 0 && diff / first >= 0 );
}
};
},
"PSEUDO": function( pseudo, argument ) {
// pseudo-class names are case-insensitive
// http://www.w3.org/TR/selectors/#pseudo-classes
// Prioritize by case sensitivity in case custom pseudos are added with uppercase letters
// Remember that setFilters inherits from pseudos
var args,
fn = Expr.pseudos[ pseudo ] || Expr.setFilters[ pseudo.toLowerCase() ] ||
Sizzle.error( "unsupported pseudo: " + pseudo );
// The user may use createPseudo to indicate that
// arguments are needed to create the filter function
// just as Sizzle does
if ( fn[ expando ] ) {
return fn( argument );
}
// But maintain support for old signatures
if ( fn.length > 1 ) {
args = [ pseudo, pseudo, "", argument ];
return Expr.setFilters.hasOwnProperty( pseudo.toLowerCase() ) ?
markFunction(function( seed, matches ) {
var idx,
matched = fn( seed, argument ),
i = matched.length;
while ( i-- ) {
idx = indexOf.call( seed, matched[i] );
seed[ idx ] = !( matches[ idx ] = matched[i] );
}
}) :
function( elem ) {
return fn( elem, 0, args );
};
}
return fn;
}
},
pseudos: {
// Potentially complex pseudos
"not": markFunction(function( selector ) {
// Trim the selector passed to compile
// to avoid treating leading and trailing
// spaces as combinators
var input = [],
results = [],
matcher = compile( selector.replace( rtrim, "$1" ) );
return matcher[ expando ] ?
markFunction(function( seed, matches, context, xml ) {
var elem,
unmatched = matcher( seed, null, xml, [] ),
i = seed.length;
// Match elements unmatched by `matcher`
while ( i-- ) {
if ( (elem = unmatched[i]) ) {
seed[i] = !(matches[i] = elem);
}
}
}) :
function( elem, context, xml ) {
input[0] = elem;
matcher( input, null, xml, results );
return !results.pop();
};
}),
"has": markFunction(function( selector ) {
return function( elem ) {
return Sizzle( selector, elem ).length > 0;
};
}),
"contains": markFunction(function( text ) {
return function( elem ) {
return ( elem.textContent || elem.innerText || getText( elem ) ).indexOf( text ) > -1;
};
}),
// "Whether an element is represented by a :lang() selector
// is based solely on the element's language value
// being equal to the identifier C,
// or beginning with the identifier C immediately followed by "-".
// The matching of C against the element's language value is performed case-insensitively.
// The identifier C does not have to be a valid language name."
// http://www.w3.org/TR/selectors/#lang-pseudo
"lang": markFunction( function( lang ) {
// lang value must be a valid identifier
if ( !ridentifier.test(lang || "") ) {
Sizzle.error( "unsupported lang: " + lang );
}
lang = lang.replace( runescape, funescape ).toLowerCase();
return function( elem ) {
var elemLang;
do {
if ( (elemLang = documentIsHTML ?
elem.lang :
elem.getAttribute("xml:lang") || elem.getAttribute("lang")) ) {
elemLang = elemLang.toLowerCase();
return elemLang === lang || elemLang.indexOf( lang + "-" ) === 0;
}
} while ( (elem = elem.parentNode) && elem.nodeType === 1 );
return false;
};
}),
// Miscellaneous
"target": function( elem ) {
var hash = window.location && window.location.hash;
return hash && hash.slice( 1 ) === elem.id;
},
"root": function( elem ) {
return elem === docElem;
},
"focus": function( elem ) {
return elem === document.activeElement && (!document.hasFocus || document.hasFocus()) && !!(elem.type || elem.href || ~elem.tabIndex);
},
// Boolean properties
"enabled": function( elem ) {
return elem.disabled === false;
},
"disabled": function( elem ) {
return elem.disabled === true;
},
"checked": function( elem ) {
// In CSS3, :checked should return both checked and selected elements
// http://www.w3.org/TR/2011/REC-css3-selectors-20110929/#checked
var nodeName = elem.nodeName.toLowerCase();
return (nodeName === "input" && !!elem.checked) || (nodeName === "option" && !!elem.selected);
},
"selected": function( elem ) {
// Accessing this property makes selected-by-default
// options in Safari work properly
if ( elem.parentNode ) {
elem.parentNode.selectedIndex;
}
return elem.selected === true;
},
// Contents
"empty": function( elem ) {
// http://www.w3.org/TR/selectors/#empty-pseudo
// :empty is only affected by element nodes and content nodes(including text(3), cdata(4)),
// not comment, processing instructions, or others
// Thanks to Diego Perini for the nodeName shortcut
// Greater than "@" means alpha characters (specifically not starting with "#" or "?")
for ( elem = elem.firstChild; elem; elem = elem.nextSibling ) {
if ( elem.nodeName > "@" || elem.nodeType === 3 || elem.nodeType === 4 ) {
return false;
}
}
return true;
},
"parent": function( elem ) {
return !Expr.pseudos["empty"]( elem );
},
// Element/input types
"header": function( elem ) {
return rheader.test( elem.nodeName );
},
"input": function( elem ) {
return rinputs.test( elem.nodeName );
},
"button": function( elem ) {
var name = elem.nodeName.toLowerCase();
return name === "input" && elem.type === "button" || name === "button";
},
"text": function( elem ) {
var attr;
// IE6 and 7 will map elem.type to 'text' for new HTML5 types (search, etc)
// use getAttribute instead to test this case
return elem.nodeName.toLowerCase() === "input" &&
elem.type === "text" &&
( (attr = elem.getAttribute("type")) == null || attr.toLowerCase() === elem.type );
},
// Position-in-collection
"first": createPositionalPseudo(function() {
return [ 0 ];
}),
"last": createPositionalPseudo(function( matchIndexes, length ) {
return [ length - 1 ];
}),
"eq": createPositionalPseudo(function( matchIndexes, length, argument ) {
return [ argument < 0 ? argument + length : argument ];
}),
"even": createPositionalPseudo(function( matchIndexes, length ) {
var i = 0;
for ( ; i < length; i += 2 ) {
matchIndexes.push( i );
}
return matchIndexes;
}),
"odd": createPositionalPseudo(function( matchIndexes, length ) {
var i = 1;
for ( ; i < length; i += 2 ) {
matchIndexes.push( i );
}
return matchIndexes;
}),
"lt": createPositionalPseudo(function( matchIndexes, length, argument ) {
var i = argument < 0 ? argument + length : argument;
for ( ; --i >= 0; ) {
matchIndexes.push( i );
}
return matchIndexes;
}),
"gt": createPositionalPseudo(function( matchIndexes, length, argument ) {
var i = argument < 0 ? argument + length : argument;
for ( ; ++i < length; ) {
matchIndexes.push( i );
}
return matchIndexes;
})
}
};
Expr.pseudos["nth"] = Expr.pseudos["eq"];
// Add button/input type pseudos
for ( i in { radio: true, checkbox: true, file: true, password: true, image: true } ) {
Expr.pseudos[ i ] = createInputPseudo( i );
}
for ( i in { submit: true, reset: true } ) {
Expr.pseudos[ i ] = createButtonPseudo( i );
}
// Easy API for creating new setFilters
function setFilters() {}
setFilters.prototype = Expr.filters = Expr.pseudos;
Expr.setFilters = new setFilters();
function tokenize( selector, parseOnly ) {
var matched, match, tokens, type,
soFar, groups, preFilters,
cached = tokenCache[ selector + " " ];
if ( cached ) {
return parseOnly ? 0 : cached.slice( 0 );
}
soFar = selector;
groups = [];
preFilters = Expr.preFilter;
while ( soFar ) {
// Comma and first run
if ( !matched || (match = rcomma.exec( soFar )) ) {
if ( match ) {
// Don't consume trailing commas as valid
soFar = soFar.slice( match[0].length ) || soFar;
}
groups.push( tokens = [] );
}
matched = false;
// Combinators
if ( (match = rcombinators.exec( soFar )) ) {
matched = match.shift();
tokens.push({
value: matched,
// Cast descendant combinators to space
type: match[0].replace( rtrim, " " )
});
soFar = soFar.slice( matched.length );
}
// Filters
for ( type in Expr.filter ) {
if ( (match = matchExpr[ type ].exec( soFar )) && (!preFilters[ type ] ||
(match = preFilters[ type ]( match ))) ) {
matched = match.shift();
tokens.push({
value: matched,
type: type,
matches: match
});
soFar = soFar.slice( matched.length );
}
}
if ( !matched ) {
break;
}
}
// Return the length of the invalid excess
// if we're just parsing
// Otherwise, throw an error or return tokens
return parseOnly ?
soFar.length :
soFar ?
Sizzle.error( selector ) :
// Cache the tokens
tokenCache( selector, groups ).slice( 0 );
}
function toSelector( tokens ) {
var i = 0,
len = tokens.length,
selector = "";
for ( ; i < len; i++ ) {
selector += tokens[i].value;
}
return selector;
}
function addCombinator( matcher, combinator, base ) {
var dir = combinator.dir,
checkNonElements = base && dir === "parentNode",
doneName = done++;
return combinator.first ?
// Check against closest ancestor/preceding element
function( elem, context, xml ) {
while ( (elem = elem[ dir ]) ) {
if ( elem.nodeType === 1 || checkNonElements ) {
return matcher( elem, context, xml );
}
}
} :
// Check against all ancestor/preceding elements
function( elem, context, xml ) {
var data, cache, outerCache,
dirkey = dirruns + " " + doneName;
// We can't set arbitrary data on XML nodes, so they don't benefit from dir caching
if ( xml ) {
while ( (elem = elem[ dir ]) ) {
if ( elem.nodeType === 1 || checkNonElements ) {
if ( matcher( elem, context, xml ) ) {
return true;
}
}
}
} else {
while ( (elem = elem[ dir ]) ) {
if ( elem.nodeType === 1 || checkNonElements ) {
outerCache = elem[ expando ] || (elem[ expando ] = {});
if ( (cache = outerCache[ dir ]) && cache[0] === dirkey ) {
if ( (data = cache[1]) === true || data === cachedruns ) {
return data === true;
}
} else {
cache = outerCache[ dir ] = [ dirkey ];
cache[1] = matcher( elem, context, xml ) || cachedruns;
if ( cache[1] === true ) {
return true;
}
}
}
}
}
};
}
function elementMatcher( matchers ) {
return matchers.length > 1 ?
function( elem, context, xml ) {
var i = matchers.length;
while ( i-- ) {
if ( !matchers[i]( elem, context, xml ) ) {
return false;
}
}
return true;
} :
matchers[0];
}
function condense( unmatched, map, filter, context, xml ) {
var elem,
newUnmatched = [],
i = 0,
len = unmatched.length,
mapped = map != null;
for ( ; i < len; i++ ) {
if ( (elem = unmatched[i]) ) {
if ( !filter || filter( elem, context, xml ) ) {
newUnmatched.push( elem );
if ( mapped ) {
map.push( i );
}
}
}
}
return newUnmatched;
}
function setMatcher( preFilter, selector, matcher, postFilter, postFinder, postSelector ) {
if ( postFilter && !postFilter[ expando ] ) {
postFilter = setMatcher( postFilter );
}
if ( postFinder && !postFinder[ expando ] ) {
postFinder = setMatcher( postFinder, postSelector );
}
return markFunction(function( seed, results, context, xml ) {
var temp, i, elem,
preMap = [],
postMap = [],
preexisting = results.length,
// Get initial elements from seed or context
elems = seed || multipleContexts( selector || "*", context.nodeType ? [ context ] : context, [] ),
// Prefilter to get matcher input, preserving a map for seed-results synchronization
matcherIn = preFilter && ( seed || !selector ) ?
condense( elems, preMap, preFilter, context, xml ) :
elems,
matcherOut = matcher ?
// If we have a postFinder, or filtered seed, or non-seed postFilter or preexisting results,
postFinder || ( seed ? preFilter : preexisting || postFilter ) ?
// ...intermediate processing is necessary
[] :
// ...otherwise use results directly
results :
matcherIn;
// Find primary matches
if ( matcher ) {
matcher( matcherIn, matcherOut, context, xml );
}
// Apply postFilter
if ( postFilter ) {
temp = condense( matcherOut, postMap );
postFilter( temp, [], context, xml );
// Un-match failing elements by moving them back to matcherIn
i = temp.length;
while ( i-- ) {
if ( (elem = temp[i]) ) {
matcherOut[ postMap[i] ] = !(matcherIn[ postMap[i] ] = elem);
}
}
}
if ( seed ) {
if ( postFinder || preFilter ) {
if ( postFinder ) {
// Get the final matcherOut by condensing this intermediate into postFinder contexts
temp = [];
i = matcherOut.length;
while ( i-- ) {
if ( (elem = matcherOut[i]) ) {
// Restore matcherIn since elem is not yet a final match
temp.push( (matcherIn[i] = elem) );
}
}
postFinder( null, (matcherOut = []), temp, xml );
}
// Move matched elements from seed to results to keep them synchronized
i = matcherOut.length;
while ( i-- ) {
if ( (elem = matcherOut[i]) &&
(temp = postFinder ? indexOf.call( seed, elem ) : preMap[i]) > -1 ) {
seed[temp] = !(results[temp] = elem);
}
}
}
// Add elements to results, through postFinder if defined
} else {
matcherOut = condense(
matcherOut === results ?
matcherOut.splice( preexisting, matcherOut.length ) :
matcherOut
);
if ( postFinder ) {
postFinder( null, results, matcherOut, xml );
} else {
push.apply( results, matcherOut );
}
}
});
}
function matcherFromTokens( tokens ) {
var checkContext, matcher, j,
len = tokens.length,
leadingRelative = Expr.relative[ tokens[0].type ],
implicitRelative = leadingRelative || Expr.relative[" "],
i = leadingRelative ? 1 : 0,
// The foundational matcher ensures that elements are reachable from top-level context(s)
matchContext = addCombinator( function( elem ) {
return elem === checkContext;
}, implicitRelative, true ),
matchAnyContext = addCombinator( function( elem ) {
return indexOf.call( checkContext, elem ) > -1;
}, implicitRelative, true ),
matchers = [ function( elem, context, xml ) {
return ( !leadingRelative && ( xml || context !== outermostContext ) ) || (
(checkContext = context).nodeType ?
matchContext( elem, context, xml ) :
matchAnyContext( elem, context, xml ) );
} ];
for ( ; i < len; i++ ) {
if ( (matcher = Expr.relative[ tokens[i].type ]) ) {
matchers = [ addCombinator(elementMatcher( matchers ), matcher) ];
} else {
matcher = Expr.filter[ tokens[i].type ].apply( null, tokens[i].matches );
// Return special upon seeing a positional matcher
if ( matcher[ expando ] ) {
// Find the next relative operator (if any) for proper handling
j = ++i;
for ( ; j < len; j++ ) {
if ( Expr.relative[ tokens[j].type ] ) {
break;
}
}
return setMatcher(
i > 1 && elementMatcher( matchers ),
i > 1 && toSelector(
// If the preceding token was a descendant combinator, insert an implicit any-element `*`
tokens.slice( 0, i - 1 ).concat({ value: tokens[ i - 2 ].type === " " ? "*" : "" })
).replace( rtrim, "$1" ),
matcher,
i < j && matcherFromTokens( tokens.slice( i, j ) ),
j < len && matcherFromTokens( (tokens = tokens.slice( j )) ),
j < len && toSelector( tokens )
);
}
matchers.push( matcher );
}
}
return elementMatcher( matchers );
}
function matcherFromGroupMatchers( elementMatchers, setMatchers ) {
// A counter to specify which element is currently being matched
var matcherCachedRuns = 0,
bySet = setMatchers.length > 0,
byElement = elementMatchers.length > 0,
superMatcher = function( seed, context, xml, results, expandContext ) {
var elem, j, matcher,
setMatched = [],
matchedCount = 0,
i = "0",
unmatched = seed && [],
outermost = expandContext != null,
contextBackup = outermostContext,
// We must always have either seed elements or context
elems = seed || byElement && Expr.find["TAG"]( "*", expandContext && context.parentNode || context ),
// Use integer dirruns iff this is the outermost matcher
dirrunsUnique = (dirruns += contextBackup == null ? 1 : Math.random() || 0.1);
if ( outermost ) {
outermostContext = context !== document && context;
cachedruns = matcherCachedRuns;
}
// Add elements passing elementMatchers directly to results
// Keep `i` a string if there are no elements so `matchedCount` will be "00" below
for ( ; (elem = elems[i]) != null; i++ ) {
if ( byElement && elem ) {
j = 0;
while ( (matcher = elementMatchers[j++]) ) {
if ( matcher( elem, context, xml ) ) {
results.push( elem );
break;
}
}
if ( outermost ) {
dirruns = dirrunsUnique;
cachedruns = ++matcherCachedRuns;
}
}
// Track unmatched elements for set filters
if ( bySet ) {
// They will have gone through all possible matchers
if ( (elem = !matcher && elem) ) {
matchedCount--;
}
// Lengthen the array for every element, matched or not
if ( seed ) {
unmatched.push( elem );
}
}
}
// Apply set filters to unmatched elements
matchedCount += i;
if ( bySet && i !== matchedCount ) {
j = 0;
while ( (matcher = setMatchers[j++]) ) {
matcher( unmatched, setMatched, context, xml );
}
if ( seed ) {
// Reintegrate element matches to eliminate the need for sorting
if ( matchedCount > 0 ) {
while ( i-- ) {
if ( !(unmatched[i] || setMatched[i]) ) {
setMatched[i] = pop.call( results );
}
}
}
// Discard index placeholder values to get only actual matches
setMatched = condense( setMatched );
}
// Add matches to results
push.apply( results, setMatched );
// Seedless set matches succeeding multiple successful matchers stipulate sorting
if ( outermost && !seed && setMatched.length > 0 &&
( matchedCount + setMatchers.length ) > 1 ) {
Sizzle.uniqueSort( results );
}
}
// Override manipulation of globals by nested matchers
if ( outermost ) {
dirruns = dirrunsUnique;
outermostContext = contextBackup;
}
return unmatched;
};
return bySet ?
markFunction( superMatcher ) :
superMatcher;
}
compile = Sizzle.compile = function( selector, group /* Internal Use Only */ ) {
var i,
setMatchers = [],
elementMatchers = [],
cached = compilerCache[ selector + " " ];
if ( !cached ) {
// Generate a function of recursive functions that can be used to check each element
if ( !group ) {
group = tokenize( selector );
}
i = group.length;
while ( i-- ) {
cached = matcherFromTokens( group[i] );
if ( cached[ expando ] ) {
setMatchers.push( cached );
} else {
elementMatchers.push( cached );
}
}
// Cache the compiled function
cached = compilerCache( selector, matcherFromGroupMatchers( elementMatchers, setMatchers ) );
}
return cached;
};
function multipleContexts( selector, contexts, results ) {
var i = 0,
len = contexts.length;
for ( ; i < len; i++ ) {
Sizzle( selector, contexts[i], results );
}
return results;
}
function select( selector, context, results, seed ) {
var i, tokens, token, type, find,
match = tokenize( selector );
if ( !seed ) {
// Try to minimize operations if there is only one group
if ( match.length === 1 ) {
// Take a shortcut and set the context if the root selector is an ID
tokens = match[0] = match[0].slice( 0 );
if ( tokens.length > 2 && (token = tokens[0]).type === "ID" &&
support.getById && context.nodeType === 9 && documentIsHTML &&
Expr.relative[ tokens[1].type ] ) {
context = ( Expr.find["ID"]( token.matches[0].replace(runescape, funescape), context ) || [] )[0];
if ( !context ) {
return results;
}
selector = selector.slice( tokens.shift().value.length );
}
// Fetch a seed set for right-to-left matching
i = matchExpr["needsContext"].test( selector ) ? 0 : tokens.length;
while ( i-- ) {
token = tokens[i];
// Abort if we hit a combinator
if ( Expr.relative[ (type = token.type) ] ) {
break;
}
if ( (find = Expr.find[ type ]) ) {
// Search, expanding context for leading sibling combinators
if ( (seed = find(
token.matches[0].replace( runescape, funescape ),
rsibling.test( tokens[0].type ) && context.parentNode || context
)) ) {
// If seed is empty or no tokens remain, we can return early
tokens.splice( i, 1 );
selector = seed.length && toSelector( tokens );
if ( !selector ) {
push.apply( results, seed );
return results;
}
break;
}
}
}
}
}
// Compile and execute a filtering function
// Provide `match` to avoid retokenization if we modified the selector above
compile( selector, match )(
seed,
context,
!documentIsHTML,
results,
rsibling.test( selector )
);
return results;
}
// One-time assignments
// Sort stability
support.sortStable = expando.split("").sort( sortOrder ).join("") === expando;
// Support: Chrome<14
// Always assume duplicates if they aren't passed to the comparison function
support.detectDuplicates = hasDuplicate;
// Initialize against the default document
setDocument();
// Support: Webkit<537.32 - Safari 6.0.3/Chrome 25 (fixed in Chrome 27)
// Detached nodes confoundingly follow *each other*
support.sortDetached = assert(function( div1 ) {
// Should return 1, but returns 4 (following)
return div1.compareDocumentPosition( document.createElement("div") ) & 1;
});
// Support: IE<8
// Prevent attribute/property "interpolation"
// http://msdn.microsoft.com/en-us/library/ms536429%28VS.85%29.aspx
if ( !assert(function( div ) {
div.innerHTML = "<a href='#'></a>";
return div.firstChild.getAttribute("href") === "#" ;
}) ) {
addHandle( "type|href|height|width", function( elem, name, isXML ) {
if ( !isXML ) {
return elem.getAttribute( name, name.toLowerCase() === "type" ? 1 : 2 );
}
});
}
// Support: IE<9
// Use defaultValue in place of getAttribute("value")
if ( !support.attributes || !assert(function( div ) {
div.innerHTML = "<input/>";
div.firstChild.setAttribute( "value", "" );
return div.firstChild.getAttribute( "value" ) === "";
}) ) {
addHandle( "value", function( elem, name, isXML ) {
if ( !isXML && elem.nodeName.toLowerCase() === "input" ) {
return elem.defaultValue;
}
});
}
// Support: IE<9
// Use getAttributeNode to fetch booleans when getAttribute lies
if ( !assert(function( div ) {
return div.getAttribute("disabled") == null;
}) ) {
addHandle( booleans, function( elem, name, isXML ) {
var val;
if ( !isXML ) {
return (val = elem.getAttributeNode( name )) && val.specified ?
val.value :
elem[ name ] === true ? name.toLowerCase() : null;
}
});
}
jQuery.find = Sizzle;
jQuery.expr = Sizzle.selectors;
jQuery.expr[":"] = jQuery.expr.pseudos;
jQuery.unique = Sizzle.uniqueSort;
jQuery.text = Sizzle.getText;
jQuery.isXMLDoc = Sizzle.isXML;
jQuery.contains = Sizzle.contains;
})( window );
// String to Object options format cache
var optionsCache = {};
// Convert String-formatted options into Object-formatted ones and store in cache
function createOptions( options ) {
var object = optionsCache[ options ] = {};
jQuery.each( options.match( core_rnotwhite ) || [], function( _, flag ) {
object[ flag ] = true;
});
return object;
}
/*
* Create a callback list using the following parameters:
*
* options: an optional list of space-separated options that will change how
* the callback list behaves or a more traditional option object
*
* By default a callback list will act like an event callback list and can be
* "fired" multiple times.
*
* Possible options:
*
* once: will ensure the callback list can only be fired once (like a Deferred)
*
* memory: will keep track of previous values and will call any callback added
* after the list has been fired right away with the latest "memorized"
* values (like a Deferred)
*
* unique: will ensure a callback can only be added once (no duplicate in the list)
*
* stopOnFalse: interrupt callings when a callback returns false
*
*/
jQuery.Callbacks = function( options ) {
// Convert options from String-formatted to Object-formatted if needed
// (we check in cache first)
options = typeof options === "string" ?
( optionsCache[ options ] || createOptions( options ) ) :
jQuery.extend( {}, options );
var // Flag to know if list is currently firing
firing,
// Last fire value (for non-forgettable lists)
memory,
// Flag to know if list was already fired
fired,
// End of the loop when firing
firingLength,
// Index of currently firing callback (modified by remove if needed)
firingIndex,
// First callback to fire (used internally by add and fireWith)
firingStart,
// Actual callback list
list = [],
// Stack of fire calls for repeatable lists
stack = !options.once && [],
// Fire callbacks
fire = function( data ) {
memory = options.memory && data;
fired = true;
firingIndex = firingStart || 0;
firingStart = 0;
firingLength = list.length;
firing = true;
for ( ; list && firingIndex < firingLength; firingIndex++ ) {
if ( list[ firingIndex ].apply( data[ 0 ], data[ 1 ] ) === false && options.stopOnFalse ) {
memory = false; // To prevent further calls using add
break;
}
}
firing = false;
if ( list ) {
if ( stack ) {
if ( stack.length ) {
fire( stack.shift() );
}
} else if ( memory ) {
list = [];
} else {
self.disable();
}
}
},
// Actual Callbacks object
self = {
// Add a callback or a collection of callbacks to the list
add: function() {
if ( list ) {
// First, we save the current length
var start = list.length;
(function add( args ) {
jQuery.each( args, function( _, arg ) {
var type = jQuery.type( arg );
if ( type === "function" ) {
if ( !options.unique || !self.has( arg ) ) {
list.push( arg );
}
} else if ( arg && arg.length && type !== "string" ) {
// Inspect recursively
add( arg );
}
});
})( arguments );
// Do we need to add the callbacks to the
// current firing batch?
if ( firing ) {
firingLength = list.length;
// With memory, if we're not firing then
// we should call right away
} else if ( memory ) {
firingStart = start;
fire( memory );
}
}
return this;
},
// Remove a callback from the list
remove: function() {
if ( list ) {
jQuery.each( arguments, function( _, arg ) {
var index;
while( ( index = jQuery.inArray( arg, list, index ) ) > -1 ) {
list.splice( index, 1 );
// Handle firing indexes
if ( firing ) {
if ( index <= firingLength ) {
firingLength--;
}
if ( index <= firingIndex ) {
firingIndex--;
}
}
}
});
}
return this;
},
// Check if a given callback is in the list.
// If no argument is given, return whether or not list has callbacks attached.
has: function( fn ) {
return fn ? jQuery.inArray( fn, list ) > -1 : !!( list && list.length );
},
// Remove all callbacks from the list
empty: function() {
list = [];
firingLength = 0;
return this;
},
// Have the list do nothing anymore
disable: function() {
list = stack = memory = undefined;
return this;
},
// Is it disabled?
disabled: function() {
return !list;
},
// Lock the list in its current state
lock: function() {
stack = undefined;
if ( !memory ) {
self.disable();
}
return this;
},
// Is it locked?
locked: function() {
return !stack;
},
// Call all callbacks with the given context and arguments
fireWith: function( context, args ) {
if ( list && ( !fired || stack ) ) {
args = args || [];
args = [ context, args.slice ? args.slice() : args ];
if ( firing ) {
stack.push( args );
} else {
fire( args );
}
}
return this;
},
// Call all the callbacks with the given arguments
fire: function() {
self.fireWith( this, arguments );
return this;
},
// To know if the callbacks have already been called at least once
fired: function() {
return !!fired;
}
};
return self;
};
jQuery.extend({
Deferred: function( func ) {
var tuples = [
// action, add listener, listener list, final state
[ "resolve", "done", jQuery.Callbacks("once memory"), "resolved" ],
[ "reject", "fail", jQuery.Callbacks("once memory"), "rejected" ],
[ "notify", "progress", jQuery.Callbacks("memory") ]
],
state = "pending",
promise = {
state: function() {
return state;
},
always: function() {
deferred.done( arguments ).fail( arguments );
return this;
},
then: function( /* fnDone, fnFail, fnProgress */ ) {
var fns = arguments;
return jQuery.Deferred(function( newDefer ) {
jQuery.each( tuples, function( i, tuple ) {
var action = tuple[ 0 ],
fn = jQuery.isFunction( fns[ i ] ) && fns[ i ];
// deferred[ done | fail | progress ] for forwarding actions to newDefer
deferred[ tuple[1] ](function() {
var returned = fn && fn.apply( this, arguments );
if ( returned && jQuery.isFunction( returned.promise ) ) {
returned.promise()
.done( newDefer.resolve )
.fail( newDefer.reject )
.progress( newDefer.notify );
} else {
newDefer[ action + "With" ]( this === promise ? newDefer.promise() : this, fn ? [ returned ] : arguments );
}
});
});
fns = null;
}).promise();
},
// Get a promise for this deferred
// If obj is provided, the promise aspect is added to the object
promise: function( obj ) {
return obj != null ? jQuery.extend( obj, promise ) : promise;
}
},
deferred = {};
// Keep pipe for back-compat
promise.pipe = promise.then;
// Add list-specific methods
jQuery.each( tuples, function( i, tuple ) {
var list = tuple[ 2 ],
stateString = tuple[ 3 ];
// promise[ done | fail | progress ] = list.add
promise[ tuple[1] ] = list.add;
// Handle state
if ( stateString ) {
list.add(function() {
// state = [ resolved | rejected ]
state = stateString;
// [ reject_list | resolve_list ].disable; progress_list.lock
}, tuples[ i ^ 1 ][ 2 ].disable, tuples[ 2 ][ 2 ].lock );
}
// deferred[ resolve | reject | notify ]
deferred[ tuple[0] ] = function() {
deferred[ tuple[0] + "With" ]( this === deferred ? promise : this, arguments );
return this;
};
deferred[ tuple[0] + "With" ] = list.fireWith;
});
// Make the deferred a promise
promise.promise( deferred );
// Call given func if any
if ( func ) {
func.call( deferred, deferred );
}
// All done!
return deferred;
},
// Deferred helper
when: function( subordinate /* , ..., subordinateN */ ) {
var i = 0,
resolveValues = core_slice.call( arguments ),
length = resolveValues.length,
// the count of uncompleted subordinates
remaining = length !== 1 || ( subordinate && jQuery.isFunction( subordinate.promise ) ) ? length : 0,
// the master Deferred. If resolveValues consist of only a single Deferred, just use that.
deferred = remaining === 1 ? subordinate : jQuery.Deferred(),
// Update function for both resolve and progress values
updateFunc = function( i, contexts, values ) {
return function( value ) {
contexts[ i ] = this;
values[ i ] = arguments.length > 1 ? core_slice.call( arguments ) : value;
if( values === progressValues ) {
deferred.notifyWith( contexts, values );
} else if ( !( --remaining ) ) {
deferred.resolveWith( contexts, values );
}
};
},
progressValues, progressContexts, resolveContexts;
// add listeners to Deferred subordinates; treat others as resolved
if ( length > 1 ) {
progressValues = new Array( length );
progressContexts = new Array( length );
resolveContexts = new Array( length );
for ( ; i < length; i++ ) {
if ( resolveValues[ i ] && jQuery.isFunction( resolveValues[ i ].promise ) ) {
resolveValues[ i ].promise()
.done( updateFunc( i, resolveContexts, resolveValues ) )
.fail( deferred.reject )
.progress( updateFunc( i, progressContexts, progressValues ) );
} else {
--remaining;
}
}
}
// if we're not waiting on anything, resolve the master
if ( !remaining ) {
deferred.resolveWith( resolveContexts, resolveValues );
}
return deferred.promise();
}
});
jQuery.support = (function( support ) {
var all, a, input, select, fragment, opt, eventName, isSupported, i,
div = document.createElement("div");
// Setup
div.setAttribute( "className", "t" );
div.innerHTML = " <link/><table></table><a href='/a'>a</a><input type='checkbox'/>";
// Finish early in limited (non-browser) environments
all = div.getElementsByTagName("*") || [];
a = div.getElementsByTagName("a")[ 0 ];
if ( !a || !a.style || !all.length ) {
return support;
}
// First batch of tests
select = document.createElement("select");
opt = select.appendChild( document.createElement("option") );
input = div.getElementsByTagName("input")[ 0 ];
a.style.cssText = "top:1px;float:left;opacity:.5";
// Test setAttribute on camelCase class. If it works, we need attrFixes when doing get/setAttribute (ie6/7)
support.getSetAttribute = div.className !== "t";
// IE strips leading whitespace when .innerHTML is used
support.leadingWhitespace = div.firstChild.nodeType === 3;
// Make sure that tbody elements aren't automatically inserted
// IE will insert them into empty tables
support.tbody = !div.getElementsByTagName("tbody").length;
// Make sure that link elements get serialized correctly by innerHTML
// This requires a wrapper element in IE
support.htmlSerialize = !!div.getElementsByTagName("link").length;
// Get the style information from getAttribute
// (IE uses .cssText instead)
support.style = /top/.test( a.getAttribute("style") );
// Make sure that URLs aren't manipulated
// (IE normalizes it by default)
support.hrefNormalized = a.getAttribute("href") === "/a";
// Make sure that element opacity exists
// (IE uses filter instead)
// Use a regex to work around a WebKit issue. See #5145
support.opacity = /^0.5/.test( a.style.opacity );
// Verify style float existence
// (IE uses styleFloat instead of cssFloat)
support.cssFloat = !!a.style.cssFloat;
// Check the default checkbox/radio value ("" on WebKit; "on" elsewhere)
support.checkOn = !!input.value;
// Make sure that a selected-by-default option has a working selected property.
// (WebKit defaults to false instead of true, IE too, if it's in an optgroup)
support.optSelected = opt.selected;
// Tests for enctype support on a form (#6743)
support.enctype = !!document.createElement("form").enctype;
// Makes sure cloning an html5 element does not cause problems
// Where outerHTML is undefined, this still works
support.html5Clone = document.createElement("nav").cloneNode( true ).outerHTML !== "<:nav></:nav>";
// Will be defined later
support.inlineBlockNeedsLayout = false;
support.shrinkWrapBlocks = false;
support.pixelPosition = false;
support.deleteExpando = true;
support.noCloneEvent = true;
support.reliableMarginRight = true;
support.boxSizingReliable = true;
// Make sure checked status is properly cloned
input.checked = true;
support.noCloneChecked = input.cloneNode( true ).checked;
// Make sure that the options inside disabled selects aren't marked as disabled
// (WebKit marks them as disabled)
select.disabled = true;
support.optDisabled = !opt.disabled;
// Support: IE<9
try {
delete div.test;
} catch( e ) {
support.deleteExpando = false;
}
// Check if we can trust getAttribute("value")
input = document.createElement("input");
input.setAttribute( "value", "" );
support.input = input.getAttribute( "value" ) === "";
// Check if an input maintains its value after becoming a radio
input.value = "t";
input.setAttribute( "type", "radio" );
support.radioValue = input.value === "t";
// #11217 - WebKit loses check when the name is after the checked attribute
input.setAttribute( "checked", "t" );
input.setAttribute( "name", "t" );
fragment = document.createDocumentFragment();
fragment.appendChild( input );
// Check if a disconnected checkbox will retain its checked
// value of true after appended to the DOM (IE6/7)
support.appendChecked = input.checked;
// WebKit doesn't clone checked state correctly in fragments
support.checkClone = fragment.cloneNode( true ).cloneNode( true ).lastChild.checked;
// Support: IE<9
// Opera does not clone events (and typeof div.attachEvent === undefined).
// IE9-10 clones events bound via attachEvent, but they don't trigger with .click()
if ( div.attachEvent ) {
div.attachEvent( "onclick", function() {
support.noCloneEvent = false;
});
div.cloneNode( true ).click();
}
// Support: IE<9 (lack submit/change bubble), Firefox 17+ (lack focusin event)
// Beware of CSP restrictions (https://developer.mozilla.org/en/Security/CSP)
for ( i in { submit: true, change: true, focusin: true }) {
div.setAttribute( eventName = "on" + i, "t" );
support[ i + "Bubbles" ] = eventName in window || div.attributes[ eventName ].expando === false;
}
div.style.backgroundClip = "content-box";
div.cloneNode( true ).style.backgroundClip = "";
support.clearCloneStyle = div.style.backgroundClip === "content-box";
// Support: IE<9
// Iteration over object's inherited properties before its own.
for ( i in jQuery( support ) ) {
break;
}
support.ownLast = i !== "0";
// Run tests that need a body at doc ready
jQuery(function() {
var container, marginDiv, tds,
divReset = "padding:0;margin:0;border:0;display:block;box-sizing:content-box;-moz-box-sizing:content-box;-webkit-box-sizing:content-box;",
body = document.getElementsByTagName("body")[0];
if ( !body ) {
// Return for frameset docs that don't have a body
return;
}
container = document.createElement("div");
container.style.cssText = "border:0;width:0;height:0;position:absolute;top:0;left:-9999px;margin-top:1px";
body.appendChild( container ).appendChild( div );
// Support: IE8
// Check if table cells still have offsetWidth/Height when they are set
// to display:none and there are still other visible table cells in a
// table row; if so, offsetWidth/Height are not reliable for use when
// determining if an element has been hidden directly using
// display:none (it is still safe to use offsets if a parent element is
// hidden; don safety goggles and see bug #4512 for more information).
div.innerHTML = "<table><tr><td></td><td>t</td></tr></table>";
tds = div.getElementsByTagName("td");
tds[ 0 ].style.cssText = "padding:0;margin:0;border:0;display:none";
isSupported = ( tds[ 0 ].offsetHeight === 0 );
tds[ 0 ].style.display = "";
tds[ 1 ].style.display = "none";
// Support: IE8
// Check if empty table cells still have offsetWidth/Height
support.reliableHiddenOffsets = isSupported && ( tds[ 0 ].offsetHeight === 0 );
// Check box-sizing and margin behavior.
div.innerHTML = "";
div.style.cssText = "box-sizing:border-box;-moz-box-sizing:border-box;-webkit-box-sizing:border-box;padding:1px;border:1px;display:block;width:4px;margin-top:1%;position:absolute;top:1%;";
// Workaround failing boxSizing test due to offsetWidth returning wrong value
// with some non-1 values of body zoom, ticket #13543
jQuery.swap( body, body.style.zoom != null ? { zoom: 1 } : {}, function() {
support.boxSizing = div.offsetWidth === 4;
});
// Use window.getComputedStyle because jsdom on node.js will break without it.
if ( window.getComputedStyle ) {
support.pixelPosition = ( window.getComputedStyle( div, null ) || {} ).top !== "1%";
support.boxSizingReliable = ( window.getComputedStyle( div, null ) || { width: "4px" } ).width === "4px";
// Check if div with explicit width and no margin-right incorrectly
// gets computed margin-right based on width of container. (#3333)
// Fails in WebKit before Feb 2011 nightlies
// WebKit Bug 13343 - getComputedStyle returns wrong value for margin-right
marginDiv = div.appendChild( document.createElement("div") );
marginDiv.style.cssText = div.style.cssText = divReset;
marginDiv.style.marginRight = marginDiv.style.width = "0";
div.style.width = "1px";
support.reliableMarginRight =
!parseFloat( ( window.getComputedStyle( marginDiv, null ) || {} ).marginRight );
}
if ( typeof div.style.zoom !== core_strundefined ) {
// Support: IE<8
// Check if natively block-level elements act like inline-block
// elements when setting their display to 'inline' and giving
// them layout
div.innerHTML = "";
div.style.cssText = divReset + "width:1px;padding:1px;display:inline;zoom:1";
support.inlineBlockNeedsLayout = ( div.offsetWidth === 3 );
// Support: IE6
// Check if elements with layout shrink-wrap their children
div.style.display = "block";
div.innerHTML = "<div></div>";
div.firstChild.style.width = "5px";
support.shrinkWrapBlocks = ( div.offsetWidth !== 3 );
if ( support.inlineBlockNeedsLayout ) {
// Prevent IE 6 from affecting layout for positioned elements #11048
// Prevent IE from shrinking the body in IE 7 mode #12869
// Support: IE<8
body.style.zoom = 1;
}
}
body.removeChild( container );
// Null elements to avoid leaks in IE
container = div = tds = marginDiv = null;
});
// Null elements to avoid leaks in IE
all = select = fragment = opt = a = input = null;
return support;
})({});
var rbrace = /(?:\{[\s\S]*\}|\[[\s\S]*\])$/,
rmultiDash = /([A-Z])/g;
function internalData( elem, name, data, pvt /* Internal Use Only */ ){
if ( !jQuery.acceptData( elem ) ) {
return;
}
var ret, thisCache,
internalKey = jQuery.expando,
// We have to handle DOM nodes and JS objects differently because IE6-7
// can't GC object references properly across the DOM-JS boundary
isNode = elem.nodeType,
// Only DOM nodes need the global jQuery cache; JS object data is
// attached directly to the object so GC can occur automatically
cache = isNode ? jQuery.cache : elem,
// Only defining an ID for JS objects if its cache already exists allows
// the code to shortcut on the same path as a DOM node with no cache
id = isNode ? elem[ internalKey ] : elem[ internalKey ] && internalKey;
// Avoid doing any more work than we need to when trying to get data on an
// object that has no data at all
if ( (!id || !cache[id] || (!pvt && !cache[id].data)) && data === undefined && typeof name === "string" ) {
return;
}
if ( !id ) {
// Only DOM nodes need a new unique ID for each element since their data
// ends up in the global cache
if ( isNode ) {
id = elem[ internalKey ] = core_deletedIds.pop() || jQuery.guid++;
} else {
id = internalKey;
}
}
if ( !cache[ id ] ) {
// Avoid exposing jQuery metadata on plain JS objects when the object
// is serialized using JSON.stringify
cache[ id ] = isNode ? {} : { toJSON: jQuery.noop };
}
// An object can be passed to jQuery.data instead of a key/value pair; this gets
// shallow copied over onto the existing cache
if ( typeof name === "object" || typeof name === "function" ) {
if ( pvt ) {
cache[ id ] = jQuery.extend( cache[ id ], name );
} else {
cache[ id ].data = jQuery.extend( cache[ id ].data, name );
}
}
thisCache = cache[ id ];
// jQuery data() is stored in a separate object inside the object's internal data
// cache in order to avoid key collisions between internal data and user-defined
// data.
if ( !pvt ) {
if ( !thisCache.data ) {
thisCache.data = {};
}
thisCache = thisCache.data;
}
if ( data !== undefined ) {
thisCache[ jQuery.camelCase( name ) ] = data;
}
// Check for both converted-to-camel and non-converted data property names
// If a data property was specified
if ( typeof name === "string" ) {
// First Try to find as-is property data
ret = thisCache[ name ];
// Test for null|undefined property data
if ( ret == null ) {
// Try to find the camelCased property
ret = thisCache[ jQuery.camelCase( name ) ];
}
} else {
ret = thisCache;
}
return ret;
}
function internalRemoveData( elem, name, pvt ) {
if ( !jQuery.acceptData( elem ) ) {
return;
}
var thisCache, i,
isNode = elem.nodeType,
// See jQuery.data for more information
cache = isNode ? jQuery.cache : elem,
id = isNode ? elem[ jQuery.expando ] : jQuery.expando;
// If there is already no cache entry for this object, there is no
// purpose in continuing
if ( !cache[ id ] ) {
return;
}
if ( name ) {
thisCache = pvt ? cache[ id ] : cache[ id ].data;
if ( thisCache ) {
// Support array or space separated string names for data keys
if ( !jQuery.isArray( name ) ) {
// try the string as a key before any manipulation
if ( name in thisCache ) {
name = [ name ];
} else {
// split the camel cased version by spaces unless a key with the spaces exists
name = jQuery.camelCase( name );
if ( name in thisCache ) {
name = [ name ];
} else {
name = name.split(" ");
}
}
} else {
// If "name" is an array of keys...
// When data is initially created, via ("key", "val") signature,
// keys will be converted to camelCase.
// Since there is no way to tell _how_ a key was added, remove
// both plain key and camelCase key. #12786
// This will only penalize the array argument path.
name = name.concat( jQuery.map( name, jQuery.camelCase ) );
}
i = name.length;
while ( i-- ) {
delete thisCache[ name[i] ];
}
// If there is no data left in the cache, we want to continue
// and let the cache object itself get destroyed
if ( pvt ? !isEmptyDataObject(thisCache) : !jQuery.isEmptyObject(thisCache) ) {
return;
}
}
}
// See jQuery.data for more information
if ( !pvt ) {
delete cache[ id ].data;
// Don't destroy the parent cache unless the internal data object
// had been the only thing left in it
if ( !isEmptyDataObject( cache[ id ] ) ) {
return;
}
}
// Destroy the cache
if ( isNode ) {
jQuery.cleanData( [ elem ], true );
// Use delete when supported for expandos or `cache` is not a window per isWindow (#10080)
/* jshint eqeqeq: false */
} else if ( jQuery.support.deleteExpando || cache != cache.window ) {
/* jshint eqeqeq: true */
delete cache[ id ];
// When all else fails, null
} else {
cache[ id ] = null;
}
}
jQuery.extend({
cache: {},
// The following elements throw uncatchable exceptions if you
// attempt to add expando properties to them.
noData: {
"applet": true,
"embed": true,
// Ban all objects except for Flash (which handle expandos)
"object": "clsid:D27CDB6E-AE6D-11cf-96B8-444553540000"
},
hasData: function( elem ) {
elem = elem.nodeType ? jQuery.cache[ elem[jQuery.expando] ] : elem[ jQuery.expando ];
return !!elem && !isEmptyDataObject( elem );
},
data: function( elem, name, data ) {
return internalData( elem, name, data );
},
removeData: function( elem, name ) {
return internalRemoveData( elem, name );
},
// For internal use only.
_data: function( elem, name, data ) {
return internalData( elem, name, data, true );
},
_removeData: function( elem, name ) {
return internalRemoveData( elem, name, true );
},
// A method for determining if a DOM node can handle the data expando
acceptData: function( elem ) {
// Do not set data on non-element because it will not be cleared (#8335).
if ( elem.nodeType && elem.nodeType !== 1 && elem.nodeType !== 9 ) {
return false;
}
var noData = elem.nodeName && jQuery.noData[ elem.nodeName.toLowerCase() ];
// nodes accept data unless otherwise specified; rejection can be conditional
return !noData || noData !== true && elem.getAttribute("classid") === noData;
}
});
jQuery.fn.extend({
data: function( key, value ) {
var attrs, name,
data = null,
i = 0,
elem = this[0];
// Special expections of .data basically thwart jQuery.access,
// so implement the relevant behavior ourselves
// Gets all values
if ( key === undefined ) {
if ( this.length ) {
data = jQuery.data( elem );
if ( elem.nodeType === 1 && !jQuery._data( elem, "parsedAttrs" ) ) {
attrs = elem.attributes;
for ( ; i < attrs.length; i++ ) {
name = attrs[i].name;
if ( name.indexOf("data-") === 0 ) {
name = jQuery.camelCase( name.slice(5) );
dataAttr( elem, name, data[ name ] );
}
}
jQuery._data( elem, "parsedAttrs", true );
}
}
return data;
}
// Sets multiple values
if ( typeof key === "object" ) {
return this.each(function() {
jQuery.data( this, key );
});
}
return arguments.length > 1 ?
// Sets one value
this.each(function() {
jQuery.data( this, key, value );
}) :
// Gets one value
// Try to fetch any internally stored data first
elem ? dataAttr( elem, key, jQuery.data( elem, key ) ) : null;
},
removeData: function( key ) {
return this.each(function() {
jQuery.removeData( this, key );
});
}
});
function dataAttr( elem, key, data ) {
// If nothing was found internally, try to fetch any
// data from the HTML5 data-* attribute
if ( data === undefined && elem.nodeType === 1 ) {
var name = "data-" + key.replace( rmultiDash, "-$1" ).toLowerCase();
data = elem.getAttribute( name );
if ( typeof data === "string" ) {
try {
data = data === "true" ? true :
data === "false" ? false :
data === "null" ? null :
// Only convert to a number if it doesn't change the string
+data + "" === data ? +data :
rbrace.test( data ) ? jQuery.parseJSON( data ) :
data;
} catch( e ) {}
// Make sure we set the data so it isn't changed later
jQuery.data( elem, key, data );
} else {
data = undefined;
}
}
return data;
}
// checks a cache object for emptiness
function isEmptyDataObject( obj ) {
var name;
for ( name in obj ) {
// if the public data object is empty, the private is still empty
if ( name === "data" && jQuery.isEmptyObject( obj[name] ) ) {
continue;
}
if ( name !== "toJSON" ) {
return false;
}
}
return true;
}
jQuery.extend({
queue: function( elem, type, data ) {
var queue;
if ( elem ) {
type = ( type || "fx" ) + "queue";
queue = jQuery._data( elem, type );
// Speed up dequeue by getting out quickly if this is just a lookup
if ( data ) {
if ( !queue || jQuery.isArray(data) ) {
queue = jQuery._data( elem, type, jQuery.makeArray(data) );
} else {
queue.push( data );
}
}
return queue || [];
}
},
dequeue: function( elem, type ) {
type = type || "fx";
var queue = jQuery.queue( elem, type ),
startLength = queue.length,
fn = queue.shift(),
hooks = jQuery._queueHooks( elem, type ),
next = function() {
jQuery.dequeue( elem, type );
};
// If the fx queue is dequeued, always remove the progress sentinel
if ( fn === "inprogress" ) {
fn = queue.shift();
startLength--;
}
if ( fn ) {
// Add a progress sentinel to prevent the fx queue from being
// automatically dequeued
if ( type === "fx" ) {
queue.unshift( "inprogress" );
}
// clear up the last queue stop function
delete hooks.stop;
fn.call( elem, next, hooks );
}
if ( !startLength && hooks ) {
hooks.empty.fire();
}
},
// not intended for public consumption - generates a queueHooks object, or returns the current one
_queueHooks: function( elem, type ) {
var key = type + "queueHooks";
return jQuery._data( elem, key ) || jQuery._data( elem, key, {
empty: jQuery.Callbacks("once memory").add(function() {
jQuery._removeData( elem, type + "queue" );
jQuery._removeData( elem, key );
})
});
}
});
jQuery.fn.extend({
queue: function( type, data ) {
var setter = 2;
if ( typeof type !== "string" ) {
data = type;
type = "fx";
setter--;
}
if ( arguments.length < setter ) {
return jQuery.queue( this[0], type );
}
return data === undefined ?
this :
this.each(function() {
var queue = jQuery.queue( this, type, data );
// ensure a hooks for this queue
jQuery._queueHooks( this, type );
if ( type === "fx" && queue[0] !== "inprogress" ) {
jQuery.dequeue( this, type );
}
});
},
dequeue: function( type ) {
return this.each(function() {
jQuery.dequeue( this, type );
});
},
// Based off of the plugin by Clint Helfers, with permission.
// http://blindsignals.com/index.php/2009/07/jquery-delay/
delay: function( time, type ) {
time = jQuery.fx ? jQuery.fx.speeds[ time ] || time : time;
type = type || "fx";
return this.queue( type, function( next, hooks ) {
var timeout = setTimeout( next, time );
hooks.stop = function() {
clearTimeout( timeout );
};
});
},
clearQueue: function( type ) {
return this.queue( type || "fx", [] );
},
// Get a promise resolved when queues of a certain type
// are emptied (fx is the type by default)
promise: function( type, obj ) {
var tmp,
count = 1,
defer = jQuery.Deferred(),
elements = this,
i = this.length,
resolve = function() {
if ( !( --count ) ) {
defer.resolveWith( elements, [ elements ] );
}
};
if ( typeof type !== "string" ) {
obj = type;
type = undefined;
}
type = type || "fx";
while( i-- ) {
tmp = jQuery._data( elements[ i ], type + "queueHooks" );
if ( tmp && tmp.empty ) {
count++;
tmp.empty.add( resolve );
}
}
resolve();
return defer.promise( obj );
}
});
var nodeHook, boolHook,
rclass = /[\t\r\n\f]/g,
rreturn = /\r/g,
rfocusable = /^(?:input|select|textarea|button|object)$/i,
rclickable = /^(?:a|area)$/i,
ruseDefault = /^(?:checked|selected)$/i,
getSetAttribute = jQuery.support.getSetAttribute,
getSetInput = jQuery.support.input;
jQuery.fn.extend({
attr: function( name, value ) {
return jQuery.access( this, jQuery.attr, name, value, arguments.length > 1 );
},
removeAttr: function( name ) {
return this.each(function() {
jQuery.removeAttr( this, name );
});
},
prop: function( name, value ) {
return jQuery.access( this, jQuery.prop, name, value, arguments.length > 1 );
},
removeProp: function( name ) {
name = jQuery.propFix[ name ] || name;
return this.each(function() {
// try/catch handles cases where IE balks (such as removing a property on window)
try {
this[ name ] = undefined;
delete this[ name ];
} catch( e ) {}
});
},
addClass: function( value ) {
var classes, elem, cur, clazz, j,
i = 0,
len = this.length,
proceed = typeof value === "string" && value;
if ( jQuery.isFunction( value ) ) {
return this.each(function( j ) {
jQuery( this ).addClass( value.call( this, j, this.className ) );
});
}
if ( proceed ) {
// The disjunction here is for better compressibility (see removeClass)
classes = ( value || "" ).match( core_rnotwhite ) || [];
for ( ; i < len; i++ ) {
elem = this[ i ];
cur = elem.nodeType === 1 && ( elem.className ?
( " " + elem.className + " " ).replace( rclass, " " ) :
" "
);
if ( cur ) {
j = 0;
while ( (clazz = classes[j++]) ) {
if ( cur.indexOf( " " + clazz + " " ) < 0 ) {
cur += clazz + " ";
}
}
elem.className = jQuery.trim( cur );
}
}
}
return this;
},
removeClass: function( value ) {
var classes, elem, cur, clazz, j,
i = 0,
len = this.length,
proceed = arguments.length === 0 || typeof value === "string" && value;
if ( jQuery.isFunction( value ) ) {
return this.each(function( j ) {
jQuery( this ).removeClass( value.call( this, j, this.className ) );
});
}
if ( proceed ) {
classes = ( value || "" ).match( core_rnotwhite ) || [];
for ( ; i < len; i++ ) {
elem = this[ i ];
// This expression is here for better compressibility (see addClass)
cur = elem.nodeType === 1 && ( elem.className ?
( " " + elem.className + " " ).replace( rclass, " " ) :
""
);
if ( cur ) {
j = 0;
while ( (clazz = classes[j++]) ) {
// Remove *all* instances
while ( cur.indexOf( " " + clazz + " " ) >= 0 ) {
cur = cur.replace( " " + clazz + " ", " " );
}
}
elem.className = value ? jQuery.trim( cur ) : "";
}
}
}
return this;
},
toggleClass: function( value, stateVal ) {
var type = typeof value;
if ( typeof stateVal === "boolean" && type === "string" ) {
return stateVal ? this.addClass( value ) : this.removeClass( value );
}
if ( jQuery.isFunction( value ) ) {
return this.each(function( i ) {
jQuery( this ).toggleClass( value.call(this, i, this.className, stateVal), stateVal );
});
}
return this.each(function() {
if ( type === "string" ) {
// toggle individual class names
var className,
i = 0,
self = jQuery( this ),
classNames = value.match( core_rnotwhite ) || [];
while ( (className = classNames[ i++ ]) ) {
// check each className given, space separated list
if ( self.hasClass( className ) ) {
self.removeClass( className );
} else {
self.addClass( className );
}
}
// Toggle whole class name
} else if ( type === core_strundefined || type === "boolean" ) {
if ( this.className ) {
// store className if set
jQuery._data( this, "__className__", this.className );
}
// If the element has a class name or if we're passed "false",
// then remove the whole classname (if there was one, the above saved it).
// Otherwise bring back whatever was previously saved (if anything),
// falling back to the empty string if nothing was stored.
this.className = this.className || value === false ? "" : jQuery._data( this, "__className__" ) || "";
}
});
},
hasClass: function( selector ) {
var className = " " + selector + " ",
i = 0,
l = this.length;
for ( ; i < l; i++ ) {
if ( this[i].nodeType === 1 && (" " + this[i].className + " ").replace(rclass, " ").indexOf( className ) >= 0 ) {
return true;
}
}
return false;
},
val: function( value ) {
var ret, hooks, isFunction,
elem = this[0];
if ( !arguments.length ) {
if ( elem ) {
hooks = jQuery.valHooks[ elem.type ] || jQuery.valHooks[ elem.nodeName.toLowerCase() ];
if ( hooks && "get" in hooks && (ret = hooks.get( elem, "value" )) !== undefined ) {
return ret;
}
ret = elem.value;
return typeof ret === "string" ?
// handle most common string cases
ret.replace(rreturn, "") :
// handle cases where value is null/undef or number
ret == null ? "" : ret;
}
return;
}
isFunction = jQuery.isFunction( value );
return this.each(function( i ) {
var val;
if ( this.nodeType !== 1 ) {
return;
}
if ( isFunction ) {
val = value.call( this, i, jQuery( this ).val() );
} else {
val = value;
}
// Treat null/undefined as ""; convert numbers to string
if ( val == null ) {
val = "";
} else if ( typeof val === "number" ) {
val += "";
} else if ( jQuery.isArray( val ) ) {
val = jQuery.map(val, function ( value ) {
return value == null ? "" : value + "";
});
}
hooks = jQuery.valHooks[ this.type ] || jQuery.valHooks[ this.nodeName.toLowerCase() ];
// If set returns undefined, fall back to normal setting
if ( !hooks || !("set" in hooks) || hooks.set( this, val, "value" ) === undefined ) {
this.value = val;
}
});
}
});
jQuery.extend({
valHooks: {
option: {
get: function( elem ) {
// Use proper attribute retrieval(#6932, #12072)
var val = jQuery.find.attr( elem, "value" );
return val != null ?
val :
elem.text;
}
},
select: {
get: function( elem ) {
var value, option,
options = elem.options,
index = elem.selectedIndex,
one = elem.type === "select-one" || index < 0,
values = one ? null : [],
max = one ? index + 1 : options.length,
i = index < 0 ?
max :
one ? index : 0;
// Loop through all the selected options
for ( ; i < max; i++ ) {
option = options[ i ];
// oldIE doesn't update selected after form reset (#2551)
if ( ( option.selected || i === index ) &&
// Don't return options that are disabled or in a disabled optgroup
( jQuery.support.optDisabled ? !option.disabled : option.getAttribute("disabled") === null ) &&
( !option.parentNode.disabled || !jQuery.nodeName( option.parentNode, "optgroup" ) ) ) {
// Get the specific value for the option
value = jQuery( option ).val();
// We don't need an array for one selects
if ( one ) {
return value;
}
// Multi-Selects return an array
values.push( value );
}
}
return values;
},
set: function( elem, value ) {
var optionSet, option,
options = elem.options,
values = jQuery.makeArray( value ),
i = options.length;
while ( i-- ) {
option = options[ i ];
if ( (option.selected = jQuery.inArray( jQuery(option).val(), values ) >= 0) ) {
optionSet = true;
}
}
// force browsers to behave consistently when non-matching value is set
if ( !optionSet ) {
elem.selectedIndex = -1;
}
return values;
}
}
},
attr: function( elem, name, value ) {
var hooks, ret,
nType = elem.nodeType;
// don't get/set attributes on text, comment and attribute nodes
if ( !elem || nType === 3 || nType === 8 || nType === 2 ) {
return;
}
// Fallback to prop when attributes are not supported
if ( typeof elem.getAttribute === core_strundefined ) {
return jQuery.prop( elem, name, value );
}
// All attributes are lowercase
// Grab necessary hook if one is defined
if ( nType !== 1 || !jQuery.isXMLDoc( elem ) ) {
name = name.toLowerCase();
hooks = jQuery.attrHooks[ name ] ||
( jQuery.expr.match.bool.test( name ) ? boolHook : nodeHook );
}
if ( value !== undefined ) {
if ( value === null ) {
jQuery.removeAttr( elem, name );
} else if ( hooks && "set" in hooks && (ret = hooks.set( elem, value, name )) !== undefined ) {
return ret;
} else {
elem.setAttribute( name, value + "" );
return value;
}
} else if ( hooks && "get" in hooks && (ret = hooks.get( elem, name )) !== null ) {
return ret;
} else {
ret = jQuery.find.attr( elem, name );
// Non-existent attributes return null, we normalize to undefined
return ret == null ?
undefined :
ret;
}
},
removeAttr: function( elem, value ) {
var name, propName,
i = 0,
attrNames = value && value.match( core_rnotwhite );
if ( attrNames && elem.nodeType === 1 ) {
while ( (name = attrNames[i++]) ) {
propName = jQuery.propFix[ name ] || name;
// Boolean attributes get special treatment (#10870)
if ( jQuery.expr.match.bool.test( name ) ) {
// Set corresponding property to false
if ( getSetInput && getSetAttribute || !ruseDefault.test( name ) ) {
elem[ propName ] = false;
// Support: IE<9
// Also clear defaultChecked/defaultSelected (if appropriate)
} else {
elem[ jQuery.camelCase( "default-" + name ) ] =
elem[ propName ] = false;
}
// See #9699 for explanation of this approach (setting first, then removal)
} else {
jQuery.attr( elem, name, "" );
}
elem.removeAttribute( getSetAttribute ? name : propName );
}
}
},
attrHooks: {
type: {
set: function( elem, value ) {
if ( !jQuery.support.radioValue && value === "radio" && jQuery.nodeName(elem, "input") ) {
// Setting the type on a radio button after the value resets the value in IE6-9
// Reset value to default in case type is set after value during creation
var val = elem.value;
elem.setAttribute( "type", value );
if ( val ) {
elem.value = val;
}
return value;
}
}
}
},
propFix: {
"for": "htmlFor",
"class": "className"
},
prop: function( elem, name, value ) {
var ret, hooks, notxml,
nType = elem.nodeType;
// don't get/set properties on text, comment and attribute nodes
if ( !elem || nType === 3 || nType === 8 || nType === 2 ) {
return;
}
notxml = nType !== 1 || !jQuery.isXMLDoc( elem );
if ( notxml ) {
// Fix name and attach hooks
name = jQuery.propFix[ name ] || name;
hooks = jQuery.propHooks[ name ];
}
if ( value !== undefined ) {
return hooks && "set" in hooks && (ret = hooks.set( elem, value, name )) !== undefined ?
ret :
( elem[ name ] = value );
} else {
return hooks && "get" in hooks && (ret = hooks.get( elem, name )) !== null ?
ret :
elem[ name ];
}
},
propHooks: {
tabIndex: {
get: function( elem ) {
// elem.tabIndex doesn't always return the correct value when it hasn't been explicitly set
// http://fluidproject.org/blog/2008/01/09/getting-setting-and-removing-tabindex-values-with-javascript/
// Use proper attribute retrieval(#12072)
var tabindex = jQuery.find.attr( elem, "tabindex" );
return tabindex ?
parseInt( tabindex, 10 ) :
rfocusable.test( elem.nodeName ) || rclickable.test( elem.nodeName ) && elem.href ?
0 :
-1;
}
}
}
});
// Hooks for boolean attributes
boolHook = {
set: function( elem, value, name ) {
if ( value === false ) {
// Remove boolean attributes when set to false
jQuery.removeAttr( elem, name );
} else if ( getSetInput && getSetAttribute || !ruseDefault.test( name ) ) {
// IE<8 needs the *property* name
elem.setAttribute( !getSetAttribute && jQuery.propFix[ name ] || name, name );
// Use defaultChecked and defaultSelected for oldIE
} else {
elem[ jQuery.camelCase( "default-" + name ) ] = elem[ name ] = true;
}
return name;
}
};
jQuery.each( jQuery.expr.match.bool.source.match( /\w+/g ), function( i, name ) {
var getter = jQuery.expr.attrHandle[ name ] || jQuery.find.attr;
jQuery.expr.attrHandle[ name ] = getSetInput && getSetAttribute || !ruseDefault.test( name ) ?
function( elem, name, isXML ) {
var fn = jQuery.expr.attrHandle[ name ],
ret = isXML ?
undefined :
/* jshint eqeqeq: false */
(jQuery.expr.attrHandle[ name ] = undefined) !=
getter( elem, name, isXML ) ?
name.toLowerCase() :
null;
jQuery.expr.attrHandle[ name ] = fn;
return ret;
} :
function( elem, name, isXML ) {
return isXML ?
undefined :
elem[ jQuery.camelCase( "default-" + name ) ] ?
name.toLowerCase() :
null;
};
});
// fix oldIE attroperties
if ( !getSetInput || !getSetAttribute ) {
jQuery.attrHooks.value = {
set: function( elem, value, name ) {
if ( jQuery.nodeName( elem, "input" ) ) {
// Does not return so that setAttribute is also used
elem.defaultValue = value;
} else {
// Use nodeHook if defined (#1954); otherwise setAttribute is fine
return nodeHook && nodeHook.set( elem, value, name );
}
}
};
}
// IE6/7 do not support getting/setting some attributes with get/setAttribute
if ( !getSetAttribute ) {
// Use this for any attribute in IE6/7
// This fixes almost every IE6/7 issue
nodeHook = {
set: function( elem, value, name ) {
// Set the existing or create a new attribute node
var ret = elem.getAttributeNode( name );
if ( !ret ) {
elem.setAttributeNode(
(ret = elem.ownerDocument.createAttribute( name ))
);
}
ret.value = value += "";
// Break association with cloned elements by also using setAttribute (#9646)
return name === "value" || value === elem.getAttribute( name ) ?
value :
undefined;
}
};
jQuery.expr.attrHandle.id = jQuery.expr.attrHandle.name = jQuery.expr.attrHandle.coords =
// Some attributes are constructed with empty-string values when not defined
function( elem, name, isXML ) {
var ret;
return isXML ?
undefined :
(ret = elem.getAttributeNode( name )) && ret.value !== "" ?
ret.value :
null;
};
jQuery.valHooks.button = {
get: function( elem, name ) {
var ret = elem.getAttributeNode( name );
return ret && ret.specified ?
ret.value :
undefined;
},
set: nodeHook.set
};
// Set contenteditable to false on removals(#10429)
// Setting to empty string throws an error as an invalid value
jQuery.attrHooks.contenteditable = {
set: function( elem, value, name ) {
nodeHook.set( elem, value === "" ? false : value, name );
}
};
// Set width and height to auto instead of 0 on empty string( Bug #8150 )
// This is for removals
jQuery.each([ "width", "height" ], function( i, name ) {
jQuery.attrHooks[ name ] = {
set: function( elem, value ) {
if ( value === "" ) {
elem.setAttribute( name, "auto" );
return value;
}
}
};
});
}
// Some attributes require a special call on IE
// http://msdn.microsoft.com/en-us/library/ms536429%28VS.85%29.aspx
if ( !jQuery.support.hrefNormalized ) {
// href/src property should get the full normalized URL (#10299/#12915)
jQuery.each([ "href", "src" ], function( i, name ) {
jQuery.propHooks[ name ] = {
get: function( elem ) {
return elem.getAttribute( name, 4 );
}
};
});
}
if ( !jQuery.support.style ) {
jQuery.attrHooks.style = {
get: function( elem ) {
// Return undefined in the case of empty string
// Note: IE uppercases css property names, but if we were to .toLowerCase()
// .cssText, that would destroy case senstitivity in URL's, like in "background"
return elem.style.cssText || undefined;
},
set: function( elem, value ) {
return ( elem.style.cssText = value + "" );
}
};
}
// Safari mis-reports the default selected property of an option
// Accessing the parent's selectedIndex property fixes it
if ( !jQuery.support.optSelected ) {
jQuery.propHooks.selected = {
get: function( elem ) {
var parent = elem.parentNode;
if ( parent ) {
parent.selectedIndex;
// Make sure that it also works with optgroups, see #5701
if ( parent.parentNode ) {
parent.parentNode.selectedIndex;
}
}
return null;
}
};
}
jQuery.each([
"tabIndex",
"readOnly",
"maxLength",
"cellSpacing",
"cellPadding",
"rowSpan",
"colSpan",
"useMap",
"frameBorder",
"contentEditable"
], function() {
jQuery.propFix[ this.toLowerCase() ] = this;
});
// IE6/7 call enctype encoding
if ( !jQuery.support.enctype ) {
jQuery.propFix.enctype = "encoding";
}
// Radios and checkboxes getter/setter
jQuery.each([ "radio", "checkbox" ], function() {
jQuery.valHooks[ this ] = {
set: function( elem, value ) {
if ( jQuery.isArray( value ) ) {
return ( elem.checked = jQuery.inArray( jQuery(elem).val(), value ) >= 0 );
}
}
};
if ( !jQuery.support.checkOn ) {
jQuery.valHooks[ this ].get = function( elem ) {
// Support: Webkit
// "" is returned instead of "on" if a value isn't specified
return elem.getAttribute("value") === null ? "on" : elem.value;
};
}
});
var rformElems = /^(?:input|select|textarea)$/i,
rkeyEvent = /^key/,
rmouseEvent = /^(?:mouse|contextmenu)|click/,
rfocusMorph = /^(?:focusinfocus|focusoutblur)$/,
rtypenamespace = /^([^.]*)(?:\.(.+)|)$/;
function returnTrue() {
return true;
}
function returnFalse() {
return false;
}
function safeActiveElement() {
try {
return document.activeElement;
} catch ( err ) { }
}
/*
* Helper functions for managing events -- not part of the public interface.
* Props to Dean Edwards' addEvent library for many of the ideas.
*/
jQuery.event = {
global: {},
add: function( elem, types, handler, data, selector ) {
var tmp, events, t, handleObjIn,
special, eventHandle, handleObj,
handlers, type, namespaces, origType,
elemData = jQuery._data( elem );
// Don't attach events to noData or text/comment nodes (but allow plain objects)
if ( !elemData ) {
return;
}
// Caller can pass in an object of custom data in lieu of the handler
if ( handler.handler ) {
handleObjIn = handler;
handler = handleObjIn.handler;
selector = handleObjIn.selector;
}
// Make sure that the handler has a unique ID, used to find/remove it later
if ( !handler.guid ) {
handler.guid = jQuery.guid++;
}
// Init the element's event structure and main handler, if this is the first
if ( !(events = elemData.events) ) {
events = elemData.events = {};
}
if ( !(eventHandle = elemData.handle) ) {
eventHandle = elemData.handle = function( e ) {
// Discard the second event of a jQuery.event.trigger() and
// when an event is called after a page has unloaded
return typeof jQuery !== core_strundefined && (!e || jQuery.event.triggered !== e.type) ?
jQuery.event.dispatch.apply( eventHandle.elem, arguments ) :
undefined;
};
// Add elem as a property of the handle fn to prevent a memory leak with IE non-native events
eventHandle.elem = elem;
}
// Handle multiple events separated by a space
types = ( types || "" ).match( core_rnotwhite ) || [""];
t = types.length;
while ( t-- ) {
tmp = rtypenamespace.exec( types[t] ) || [];
type = origType = tmp[1];
namespaces = ( tmp[2] || "" ).split( "." ).sort();
// There *must* be a type, no attaching namespace-only handlers
if ( !type ) {
continue;
}
// If event changes its type, use the special event handlers for the changed type
special = jQuery.event.special[ type ] || {};
// If selector defined, determine special event api type, otherwise given type
type = ( selector ? special.delegateType : special.bindType ) || type;
// Update special based on newly reset type
special = jQuery.event.special[ type ] || {};
// handleObj is passed to all event handlers
handleObj = jQuery.extend({
type: type,
origType: origType,
data: data,
handler: handler,
guid: handler.guid,
selector: selector,
needsContext: selector && jQuery.expr.match.needsContext.test( selector ),
namespace: namespaces.join(".")
}, handleObjIn );
// Init the event handler queue if we're the first
if ( !(handlers = events[ type ]) ) {
handlers = events[ type ] = [];
handlers.delegateCount = 0;
// Only use addEventListener/attachEvent if the special events handler returns false
if ( !special.setup || special.setup.call( elem, data, namespaces, eventHandle ) === false ) {
// Bind the global event handler to the element
if ( elem.addEventListener ) {
elem.addEventListener( type, eventHandle, false );
} else if ( elem.attachEvent ) {
elem.attachEvent( "on" + type, eventHandle );
}
}
}
if ( special.add ) {
special.add.call( elem, handleObj );
if ( !handleObj.handler.guid ) {
handleObj.handler.guid = handler.guid;
}
}
// Add to the element's handler list, delegates in front
if ( selector ) {
handlers.splice( handlers.delegateCount++, 0, handleObj );
} else {
handlers.push( handleObj );
}
// Keep track of which events have ever been used, for event optimization
jQuery.event.global[ type ] = true;
}
// Nullify elem to prevent memory leaks in IE
elem = null;
},
// Detach an event or set of events from an element
remove: function( elem, types, handler, selector, mappedTypes ) {
var j, handleObj, tmp,
origCount, t, events,
special, handlers, type,
namespaces, origType,
elemData = jQuery.hasData( elem ) && jQuery._data( elem );
if ( !elemData || !(events = elemData.events) ) {
return;
}
// Once for each type.namespace in types; type may be omitted
types = ( types || "" ).match( core_rnotwhite ) || [""];
t = types.length;
while ( t-- ) {
tmp = rtypenamespace.exec( types[t] ) || [];
type = origType = tmp[1];
namespaces = ( tmp[2] || "" ).split( "." ).sort();
// Unbind all events (on this namespace, if provided) for the element
if ( !type ) {
for ( type in events ) {
jQuery.event.remove( elem, type + types[ t ], handler, selector, true );
}
continue;
}
special = jQuery.event.special[ type ] || {};
type = ( selector ? special.delegateType : special.bindType ) || type;
handlers = events[ type ] || [];
tmp = tmp[2] && new RegExp( "(^|\\.)" + namespaces.join("\\.(?:.*\\.|)") + "(\\.|$)" );
// Remove matching events
origCount = j = handlers.length;
while ( j-- ) {
handleObj = handlers[ j ];
if ( ( mappedTypes || origType === handleObj.origType ) &&
( !handler || handler.guid === handleObj.guid ) &&
( !tmp || tmp.test( handleObj.namespace ) ) &&
( !selector || selector === handleObj.selector || selector === "**" && handleObj.selector ) ) {
handlers.splice( j, 1 );
if ( handleObj.selector ) {
handlers.delegateCount--;
}
if ( special.remove ) {
special.remove.call( elem, handleObj );
}
}
}
// Remove generic event handler if we removed something and no more handlers exist
// (avoids potential for endless recursion during removal of special event handlers)
if ( origCount && !handlers.length ) {
if ( !special.teardown || special.teardown.call( elem, namespaces, elemData.handle ) === false ) {
jQuery.removeEvent( elem, type, elemData.handle );
}
delete events[ type ];
}
}
// Remove the expando if it's no longer used
if ( jQuery.isEmptyObject( events ) ) {
delete elemData.handle;
// removeData also checks for emptiness and clears the expando if empty
// so use it instead of delete
jQuery._removeData( elem, "events" );
}
},
trigger: function( event, data, elem, onlyHandlers ) {
var handle, ontype, cur,
bubbleType, special, tmp, i,
eventPath = [ elem || document ],
type = core_hasOwn.call( event, "type" ) ? event.type : event,
namespaces = core_hasOwn.call( event, "namespace" ) ? event.namespace.split(".") : [];
cur = tmp = elem = elem || document;
// Don't do events on text and comment nodes
if ( elem.nodeType === 3 || elem.nodeType === 8 ) {
return;
}
// focus/blur morphs to focusin/out; ensure we're not firing them right now
if ( rfocusMorph.test( type + jQuery.event.triggered ) ) {
return;
}
if ( type.indexOf(".") >= 0 ) {
// Namespaced trigger; create a regexp to match event type in handle()
namespaces = type.split(".");
type = namespaces.shift();
namespaces.sort();
}
ontype = type.indexOf(":") < 0 && "on" + type;
// Caller can pass in a jQuery.Event object, Object, or just an event type string
event = event[ jQuery.expando ] ?
event :
new jQuery.Event( type, typeof event === "object" && event );
// Trigger bitmask: & 1 for native handlers; & 2 for jQuery (always true)
event.isTrigger = onlyHandlers ? 2 : 3;
event.namespace = namespaces.join(".");
event.namespace_re = event.namespace ?
new RegExp( "(^|\\.)" + namespaces.join("\\.(?:.*\\.|)") + "(\\.|$)" ) :
null;
// Clean up the event in case it is being reused
event.result = undefined;
if ( !event.target ) {
event.target = elem;
}
// Clone any incoming data and prepend the event, creating the handler arg list
data = data == null ?
[ event ] :
jQuery.makeArray( data, [ event ] );
// Allow special events to draw outside the lines
special = jQuery.event.special[ type ] || {};
if ( !onlyHandlers && special.trigger && special.trigger.apply( elem, data ) === false ) {
return;
}
// Determine event propagation path in advance, per W3C events spec (#9951)
// Bubble up to document, then to window; watch for a global ownerDocument var (#9724)
if ( !onlyHandlers && !special.noBubble && !jQuery.isWindow( elem ) ) {
bubbleType = special.delegateType || type;
if ( !rfocusMorph.test( bubbleType + type ) ) {
cur = cur.parentNode;
}
for ( ; cur; cur = cur.parentNode ) {
eventPath.push( cur );
tmp = cur;
}
// Only add window if we got to document (e.g., not plain obj or detached DOM)
if ( tmp === (elem.ownerDocument || document) ) {
eventPath.push( tmp.defaultView || tmp.parentWindow || window );
}
}
// Fire handlers on the event path
i = 0;
while ( (cur = eventPath[i++]) && !event.isPropagationStopped() ) {
event.type = i > 1 ?
bubbleType :
special.bindType || type;
// jQuery handler
handle = ( jQuery._data( cur, "events" ) || {} )[ event.type ] && jQuery._data( cur, "handle" );
if ( handle ) {
handle.apply( cur, data );
}
// Native handler
handle = ontype && cur[ ontype ];
if ( handle && jQuery.acceptData( cur ) && handle.apply && handle.apply( cur, data ) === false ) {
event.preventDefault();
}
}
event.type = type;
// If nobody prevented the default action, do it now
if ( !onlyHandlers && !event.isDefaultPrevented() ) {
if ( (!special._default || special._default.apply( eventPath.pop(), data ) === false) &&
jQuery.acceptData( elem ) ) {
// Call a native DOM method on the target with the same name name as the event.
// Can't use an .isFunction() check here because IE6/7 fails that test.
// Don't do default actions on window, that's where global variables be (#6170)
if ( ontype && elem[ type ] && !jQuery.isWindow( elem ) ) {
// Don't re-trigger an onFOO event when we call its FOO() method
tmp = elem[ ontype ];
if ( tmp ) {
elem[ ontype ] = null;
}
// Prevent re-triggering of the same event, since we already bubbled it above
jQuery.event.triggered = type;
try {
elem[ type ]();
} catch ( e ) {
// IE<9 dies on focus/blur to hidden element (#1486,#12518)
// only reproducible on winXP IE8 native, not IE9 in IE8 mode
}
jQuery.event.triggered = undefined;
if ( tmp ) {
elem[ ontype ] = tmp;
}
}
}
}
return event.result;
},
dispatch: function( event ) {
// Make a writable jQuery.Event from the native event object
event = jQuery.event.fix( event );
var i, ret, handleObj, matched, j,
handlerQueue = [],
args = core_slice.call( arguments ),
handlers = ( jQuery._data( this, "events" ) || {} )[ event.type ] || [],
special = jQuery.event.special[ event.type ] || {};
// Use the fix-ed jQuery.Event rather than the (read-only) native event
args[0] = event;
event.delegateTarget = this;
// Call the preDispatch hook for the mapped type, and let it bail if desired
if ( special.preDispatch && special.preDispatch.call( this, event ) === false ) {
return;
}
// Determine handlers
handlerQueue = jQuery.event.handlers.call( this, event, handlers );
// Run delegates first; they may want to stop propagation beneath us
i = 0;
while ( (matched = handlerQueue[ i++ ]) && !event.isPropagationStopped() ) {
event.currentTarget = matched.elem;
j = 0;
while ( (handleObj = matched.handlers[ j++ ]) && !event.isImmediatePropagationStopped() ) {
// Triggered event must either 1) have no namespace, or
// 2) have namespace(s) a subset or equal to those in the bound event (both can have no namespace).
if ( !event.namespace_re || event.namespace_re.test( handleObj.namespace ) ) {
event.handleObj = handleObj;
event.data = handleObj.data;
ret = ( (jQuery.event.special[ handleObj.origType ] || {}).handle || handleObj.handler )
.apply( matched.elem, args );
if ( ret !== undefined ) {
if ( (event.result = ret) === false ) {
event.preventDefault();
event.stopPropagation();
}
}
}
}
}
// Call the postDispatch hook for the mapped type
if ( special.postDispatch ) {
special.postDispatch.call( this, event );
}
return event.result;
},
handlers: function( event, handlers ) {
var sel, handleObj, matches, i,
handlerQueue = [],
delegateCount = handlers.delegateCount,
cur = event.target;
// Find delegate handlers
// Black-hole SVG <use> instance trees (#13180)
// Avoid non-left-click bubbling in Firefox (#3861)
if ( delegateCount && cur.nodeType && (!event.button || event.type !== "click") ) {
/* jshint eqeqeq: false */
for ( ; cur != this; cur = cur.parentNode || this ) {
/* jshint eqeqeq: true */
// Don't check non-elements (#13208)
// Don't process clicks on disabled elements (#6911, #8165, #11382, #11764)
if ( cur.nodeType === 1 && (cur.disabled !== true || event.type !== "click") ) {
matches = [];
for ( i = 0; i < delegateCount; i++ ) {
handleObj = handlers[ i ];
// Don't conflict with Object.prototype properties (#13203)
sel = handleObj.selector + " ";
if ( matches[ sel ] === undefined ) {
matches[ sel ] = handleObj.needsContext ?
jQuery( sel, this ).index( cur ) >= 0 :
jQuery.find( sel, this, null, [ cur ] ).length;
}
if ( matches[ sel ] ) {
matches.push( handleObj );
}
}
if ( matches.length ) {
handlerQueue.push({ elem: cur, handlers: matches });
}
}
}
}
// Add the remaining (directly-bound) handlers
if ( delegateCount < handlers.length ) {
handlerQueue.push({ elem: this, handlers: handlers.slice( delegateCount ) });
}
return handlerQueue;
},
fix: function( event ) {
if ( event[ jQuery.expando ] ) {
return event;
}
// Create a writable copy of the event object and normalize some properties
var i, prop, copy,
type = event.type,
originalEvent = event,
fixHook = this.fixHooks[ type ];
if ( !fixHook ) {
this.fixHooks[ type ] = fixHook =
rmouseEvent.test( type ) ? this.mouseHooks :
rkeyEvent.test( type ) ? this.keyHooks :
{};
}
copy = fixHook.props ? this.props.concat( fixHook.props ) : this.props;
event = new jQuery.Event( originalEvent );
i = copy.length;
while ( i-- ) {
prop = copy[ i ];
event[ prop ] = originalEvent[ prop ];
}
// Support: IE<9
// Fix target property (#1925)
if ( !event.target ) {
event.target = originalEvent.srcElement || document;
}
// Support: Chrome 23+, Safari?
// Target should not be a text node (#504, #13143)
if ( event.target.nodeType === 3 ) {
event.target = event.target.parentNode;
}
// Support: IE<9
// For mouse/key events, metaKey==false if it's undefined (#3368, #11328)
event.metaKey = !!event.metaKey;
return fixHook.filter ? fixHook.filter( event, originalEvent ) : event;
},
// Includes some event props shared by KeyEvent and MouseEvent
props: "altKey bubbles cancelable ctrlKey currentTarget eventPhase metaKey relatedTarget shiftKey target timeStamp view which".split(" "),
fixHooks: {},
keyHooks: {
props: "char charCode key keyCode".split(" "),
filter: function( event, original ) {
// Add which for key events
if ( event.which == null ) {
event.which = original.charCode != null ? original.charCode : original.keyCode;
}
return event;
}
},
mouseHooks: {
props: "button buttons clientX clientY fromElement offsetX offsetY pageX pageY screenX screenY toElement".split(" "),
filter: function( event, original ) {
var body, eventDoc, doc,
button = original.button,
fromElement = original.fromElement;
// Calculate pageX/Y if missing and clientX/Y available
if ( event.pageX == null && original.clientX != null ) {
eventDoc = event.target.ownerDocument || document;
doc = eventDoc.documentElement;
body = eventDoc.body;
event.pageX = original.clientX + ( doc && doc.scrollLeft || body && body.scrollLeft || 0 ) - ( doc && doc.clientLeft || body && body.clientLeft || 0 );
event.pageY = original.clientY + ( doc && doc.scrollTop || body && body.scrollTop || 0 ) - ( doc && doc.clientTop || body && body.clientTop || 0 );
}
// Add relatedTarget, if necessary
if ( !event.relatedTarget && fromElement ) {
event.relatedTarget = fromElement === event.target ? original.toElement : fromElement;
}
// Add which for click: 1 === left; 2 === middle; 3 === right
// Note: button is not normalized, so don't use it
if ( !event.which && button !== undefined ) {
event.which = ( button & 1 ? 1 : ( button & 2 ? 3 : ( button & 4 ? 2 : 0 ) ) );
}
return event;
}
},
special: {
load: {
// Prevent triggered image.load events from bubbling to window.load
noBubble: true
},
focus: {
// Fire native event if possible so blur/focus sequence is correct
trigger: function() {
if ( this !== safeActiveElement() && this.focus ) {
try {
this.focus();
return false;
} catch ( e ) {
// Support: IE<9
// If we error on focus to hidden element (#1486, #12518),
// let .trigger() run the handlers
}
}
},
delegateType: "focusin"
},
blur: {
trigger: function() {
if ( this === safeActiveElement() && this.blur ) {
this.blur();
return false;
}
},
delegateType: "focusout"
},
click: {
// For checkbox, fire native event so checked state will be right
trigger: function() {
if ( jQuery.nodeName( this, "input" ) && this.type === "checkbox" && this.click ) {
this.click();
return false;
}
},
// For cross-browser consistency, don't fire native .click() on links
_default: function( event ) {
return jQuery.nodeName( event.target, "a" );
}
},
beforeunload: {
postDispatch: function( event ) {
// Even when returnValue equals to undefined Firefox will still show alert
if ( event.result !== undefined ) {
event.originalEvent.returnValue = event.result;
}
}
}
},
simulate: function( type, elem, event, bubble ) {
// Piggyback on a donor event to simulate a different one.
// Fake originalEvent to avoid donor's stopPropagation, but if the
// simulated event prevents default then we do the same on the donor.
var e = jQuery.extend(
new jQuery.Event(),
event,
{
type: type,
isSimulated: true,
originalEvent: {}
}
);
if ( bubble ) {
jQuery.event.trigger( e, null, elem );
} else {
jQuery.event.dispatch.call( elem, e );
}
if ( e.isDefaultPrevented() ) {
event.preventDefault();
}
}
};
jQuery.removeEvent = document.removeEventListener ?
function( elem, type, handle ) {
if ( elem.removeEventListener ) {
elem.removeEventListener( type, handle, false );
}
} :
function( elem, type, handle ) {
var name = "on" + type;
if ( elem.detachEvent ) {
// #8545, #7054, preventing memory leaks for custom events in IE6-8
// detachEvent needed property on element, by name of that event, to properly expose it to GC
if ( typeof elem[ name ] === core_strundefined ) {
elem[ name ] = null;
}
elem.detachEvent( name, handle );
}
};
jQuery.Event = function( src, props ) {
// Allow instantiation without the 'new' keyword
if ( !(this instanceof jQuery.Event) ) {
return new jQuery.Event( src, props );
}
// Event object
if ( src && src.type ) {
this.originalEvent = src;
this.type = src.type;
// Events bubbling up the document may have been marked as prevented
// by a handler lower down the tree; reflect the correct value.
this.isDefaultPrevented = ( src.defaultPrevented || src.returnValue === false ||
src.getPreventDefault && src.getPreventDefault() ) ? returnTrue : returnFalse;
// Event type
} else {
this.type = src;
}
// Put explicitly provided properties onto the event object
if ( props ) {
jQuery.extend( this, props );
}
// Create a timestamp if incoming event doesn't have one
this.timeStamp = src && src.timeStamp || jQuery.now();
// Mark it as fixed
this[ jQuery.expando ] = true;
};
// jQuery.Event is based on DOM3 Events as specified by the ECMAScript Language Binding
// http://www.w3.org/TR/2003/WD-DOM-Level-3-Events-20030331/ecma-script-binding.html
jQuery.Event.prototype = {
isDefaultPrevented: returnFalse,
isPropagationStopped: returnFalse,
isImmediatePropagationStopped: returnFalse,
preventDefault: function() {
var e = this.originalEvent;
this.isDefaultPrevented = returnTrue;
if ( !e ) {
return;
}
// If preventDefault exists, run it on the original event
if ( e.preventDefault ) {
e.preventDefault();
// Support: IE
// Otherwise set the returnValue property of the original event to false
} else {
e.returnValue = false;
}
},
stopPropagation: function() {
var e = this.originalEvent;
this.isPropagationStopped = returnTrue;
if ( !e ) {
return;
}
// If stopPropagation exists, run it on the original event
if ( e.stopPropagation ) {
e.stopPropagation();
}
// Support: IE
// Set the cancelBubble property of the original event to true
e.cancelBubble = true;
},
stopImmediatePropagation: function() {
this.isImmediatePropagationStopped = returnTrue;
this.stopPropagation();
}
};
// Create mouseenter/leave events using mouseover/out and event-time checks
jQuery.each({
mouseenter: "mouseover",
mouseleave: "mouseout"
}, function( orig, fix ) {
jQuery.event.special[ orig ] = {
delegateType: fix,
bindType: fix,
handle: function( event ) {
var ret,
target = this,
related = event.relatedTarget,
handleObj = event.handleObj;
// For mousenter/leave call the handler if related is outside the target.
// NB: No relatedTarget if the mouse left/entered the browser window
if ( !related || (related !== target && !jQuery.contains( target, related )) ) {
event.type = handleObj.origType;
ret = handleObj.handler.apply( this, arguments );
event.type = fix;
}
return ret;
}
};
});
// IE submit delegation
if ( !jQuery.support.submitBubbles ) {
jQuery.event.special.submit = {
setup: function() {
// Only need this for delegated form submit events
if ( jQuery.nodeName( this, "form" ) ) {
return false;
}
// Lazy-add a submit handler when a descendant form may potentially be submitted
jQuery.event.add( this, "click._submit keypress._submit", function( e ) {
// Node name check avoids a VML-related crash in IE (#9807)
var elem = e.target,
form = jQuery.nodeName( elem, "input" ) || jQuery.nodeName( elem, "button" ) ? elem.form : undefined;
if ( form && !jQuery._data( form, "submitBubbles" ) ) {
jQuery.event.add( form, "submit._submit", function( event ) {
event._submit_bubble = true;
});
jQuery._data( form, "submitBubbles", true );
}
});
// return undefined since we don't need an event listener
},
postDispatch: function( event ) {
// If form was submitted by the user, bubble the event up the tree
if ( event._submit_bubble ) {
delete event._submit_bubble;
if ( this.parentNode && !event.isTrigger ) {
jQuery.event.simulate( "submit", this.parentNode, event, true );
}
}
},
teardown: function() {
// Only need this for delegated form submit events
if ( jQuery.nodeName( this, "form" ) ) {
return false;
}
// Remove delegated handlers; cleanData eventually reaps submit handlers attached above
jQuery.event.remove( this, "._submit" );
}
};
}
// IE change delegation and checkbox/radio fix
if ( !jQuery.support.changeBubbles ) {
jQuery.event.special.change = {
setup: function() {
if ( rformElems.test( this.nodeName ) ) {
// IE doesn't fire change on a check/radio until blur; trigger it on click
// after a propertychange. Eat the blur-change in special.change.handle.
// This still fires onchange a second time for check/radio after blur.
if ( this.type === "checkbox" || this.type === "radio" ) {
jQuery.event.add( this, "propertychange._change", function( event ) {
if ( event.originalEvent.propertyName === "checked" ) {
this._just_changed = true;
}
});
jQuery.event.add( this, "click._change", function( event ) {
if ( this._just_changed && !event.isTrigger ) {
this._just_changed = false;
}
// Allow triggered, simulated change events (#11500)
jQuery.event.simulate( "change", this, event, true );
});
}
return false;
}
// Delegated event; lazy-add a change handler on descendant inputs
jQuery.event.add( this, "beforeactivate._change", function( e ) {
var elem = e.target;
if ( rformElems.test( elem.nodeName ) && !jQuery._data( elem, "changeBubbles" ) ) {
jQuery.event.add( elem, "change._change", function( event ) {
if ( this.parentNode && !event.isSimulated && !event.isTrigger ) {
jQuery.event.simulate( "change", this.parentNode, event, true );
}
});
jQuery._data( elem, "changeBubbles", true );
}
});
},
handle: function( event ) {
var elem = event.target;
// Swallow native change events from checkbox/radio, we already triggered them above
if ( this !== elem || event.isSimulated || event.isTrigger || (elem.type !== "radio" && elem.type !== "checkbox") ) {
return event.handleObj.handler.apply( this, arguments );
}
},
teardown: function() {
jQuery.event.remove( this, "._change" );
return !rformElems.test( this.nodeName );
}
};
}
// Create "bubbling" focus and blur events
if ( !jQuery.support.focusinBubbles ) {
jQuery.each({ focus: "focusin", blur: "focusout" }, function( orig, fix ) {
// Attach a single capturing handler while someone wants focusin/focusout
var attaches = 0,
handler = function( event ) {
jQuery.event.simulate( fix, event.target, jQuery.event.fix( event ), true );
};
jQuery.event.special[ fix ] = {
setup: function() {
if ( attaches++ === 0 ) {
document.addEventListener( orig, handler, true );
}
},
teardown: function() {
if ( --attaches === 0 ) {
document.removeEventListener( orig, handler, true );
}
}
};
});
}
jQuery.fn.extend({
on: function( types, selector, data, fn, /*INTERNAL*/ one ) {
var type, origFn;
// Types can be a map of types/handlers
if ( typeof types === "object" ) {
// ( types-Object, selector, data )
if ( typeof selector !== "string" ) {
// ( types-Object, data )
data = data || selector;
selector = undefined;
}
for ( type in types ) {
this.on( type, selector, data, types[ type ], one );
}
return this;
}
if ( data == null && fn == null ) {
// ( types, fn )
fn = selector;
data = selector = undefined;
} else if ( fn == null ) {
if ( typeof selector === "string" ) {
// ( types, selector, fn )
fn = data;
data = undefined;
} else {
// ( types, data, fn )
fn = data;
data = selector;
selector = undefined;
}
}
if ( fn === false ) {
fn = returnFalse;
} else if ( !fn ) {
return this;
}
if ( one === 1 ) {
origFn = fn;
fn = function( event ) {
// Can use an empty set, since event contains the info
jQuery().off( event );
return origFn.apply( this, arguments );
};
// Use same guid so caller can remove using origFn
fn.guid = origFn.guid || ( origFn.guid = jQuery.guid++ );
}
return this.each( function() {
jQuery.event.add( this, types, fn, data, selector );
});
},
one: function( types, selector, data, fn ) {
return this.on( types, selector, data, fn, 1 );
},
off: function( types, selector, fn ) {
var handleObj, type;
if ( types && types.preventDefault && types.handleObj ) {
// ( event ) dispatched jQuery.Event
handleObj = types.handleObj;
jQuery( types.delegateTarget ).off(
handleObj.namespace ? handleObj.origType + "." + handleObj.namespace : handleObj.origType,
handleObj.selector,
handleObj.handler
);
return this;
}
if ( typeof types === "object" ) {
// ( types-object [, selector] )
for ( type in types ) {
this.off( type, selector, types[ type ] );
}
return this;
}
if ( selector === false || typeof selector === "function" ) {
// ( types [, fn] )
fn = selector;
selector = undefined;
}
if ( fn === false ) {
fn = returnFalse;
}
return this.each(function() {
jQuery.event.remove( this, types, fn, selector );
});
},
trigger: function( type, data ) {
return this.each(function() {
jQuery.event.trigger( type, data, this );
});
},
triggerHandler: function( type, data ) {
var elem = this[0];
if ( elem ) {
return jQuery.event.trigger( type, data, elem, true );
}
}
});
var isSimple = /^.[^:#\[\.,]*$/,
rparentsprev = /^(?:parents|prev(?:Until|All))/,
rneedsContext = jQuery.expr.match.needsContext,
// methods guaranteed to produce a unique set when starting from a unique set
guaranteedUnique = {
children: true,
contents: true,
next: true,
prev: true
};
jQuery.fn.extend({
find: function( selector ) {
var i,
ret = [],
self = this,
len = self.length;
if ( typeof selector !== "string" ) {
return this.pushStack( jQuery( selector ).filter(function() {
for ( i = 0; i < len; i++ ) {
if ( jQuery.contains( self[ i ], this ) ) {
return true;
}
}
}) );
}
for ( i = 0; i < len; i++ ) {
jQuery.find( selector, self[ i ], ret );
}
// Needed because $( selector, context ) becomes $( context ).find( selector )
ret = this.pushStack( len > 1 ? jQuery.unique( ret ) : ret );
ret.selector = this.selector ? this.selector + " " + selector : selector;
return ret;
},
has: function( target ) {
var i,
targets = jQuery( target, this ),
len = targets.length;
return this.filter(function() {
for ( i = 0; i < len; i++ ) {
if ( jQuery.contains( this, targets[i] ) ) {
return true;
}
}
});
},
not: function( selector ) {
return this.pushStack( winnow(this, selector || [], true) );
},
filter: function( selector ) {
return this.pushStack( winnow(this, selector || [], false) );
},
is: function( selector ) {
return !!winnow(
this,
// If this is a positional/relative selector, check membership in the returned set
// so $("p:first").is("p:last") won't return true for a doc with two "p".
typeof selector === "string" && rneedsContext.test( selector ) ?
jQuery( selector ) :
selector || [],
false
).length;
},
closest: function( selectors, context ) {
var cur,
i = 0,
l = this.length,
ret = [],
pos = rneedsContext.test( selectors ) || typeof selectors !== "string" ?
jQuery( selectors, context || this.context ) :
0;
for ( ; i < l; i++ ) {
for ( cur = this[i]; cur && cur !== context; cur = cur.parentNode ) {
// Always skip document fragments
if ( cur.nodeType < 11 && (pos ?
pos.index(cur) > -1 :
// Don't pass non-elements to Sizzle
cur.nodeType === 1 &&
jQuery.find.matchesSelector(cur, selectors)) ) {
cur = ret.push( cur );
break;
}
}
}
return this.pushStack( ret.length > 1 ? jQuery.unique( ret ) : ret );
},
// Determine the position of an element within
// the matched set of elements
index: function( elem ) {
// No argument, return index in parent
if ( !elem ) {
return ( this[0] && this[0].parentNode ) ? this.first().prevAll().length : -1;
}
// index in selector
if ( typeof elem === "string" ) {
return jQuery.inArray( this[0], jQuery( elem ) );
}
// Locate the position of the desired element
return jQuery.inArray(
// If it receives a jQuery object, the first element is used
elem.jquery ? elem[0] : elem, this );
},
add: function( selector, context ) {
var set = typeof selector === "string" ?
jQuery( selector, context ) :
jQuery.makeArray( selector && selector.nodeType ? [ selector ] : selector ),
all = jQuery.merge( this.get(), set );
return this.pushStack( jQuery.unique(all) );
},
addBack: function( selector ) {
return this.add( selector == null ?
this.prevObject : this.prevObject.filter(selector)
);
}
});
function sibling( cur, dir ) {
do {
cur = cur[ dir ];
} while ( cur && cur.nodeType !== 1 );
return cur;
}
jQuery.each({
parent: function( elem ) {
var parent = elem.parentNode;
return parent && parent.nodeType !== 11 ? parent : null;
},
parents: function( elem ) {
return jQuery.dir( elem, "parentNode" );
},
parentsUntil: function( elem, i, until ) {
return jQuery.dir( elem, "parentNode", until );
},
next: function( elem ) {
return sibling( elem, "nextSibling" );
},
prev: function( elem ) {
return sibling( elem, "previousSibling" );
},
nextAll: function( elem ) {
return jQuery.dir( elem, "nextSibling" );
},
prevAll: function( elem ) {
return jQuery.dir( elem, "previousSibling" );
},
nextUntil: function( elem, i, until ) {
return jQuery.dir( elem, "nextSibling", until );
},
prevUntil: function( elem, i, until ) {
return jQuery.dir( elem, "previousSibling", until );
},
siblings: function( elem ) {
return jQuery.sibling( ( elem.parentNode || {} ).firstChild, elem );
},
children: function( elem ) {
return jQuery.sibling( elem.firstChild );
},
contents: function( elem ) {
return jQuery.nodeName( elem, "iframe" ) ?
elem.contentDocument || elem.contentWindow.document :
jQuery.merge( [], elem.childNodes );
}
}, function( name, fn ) {
jQuery.fn[ name ] = function( until, selector ) {
var ret = jQuery.map( this, fn, until );
if ( name.slice( -5 ) !== "Until" ) {
selector = until;
}
if ( selector && typeof selector === "string" ) {
ret = jQuery.filter( selector, ret );
}
if ( this.length > 1 ) {
// Remove duplicates
if ( !guaranteedUnique[ name ] ) {
ret = jQuery.unique( ret );
}
// Reverse order for parents* and prev-derivatives
if ( rparentsprev.test( name ) ) {
ret = ret.reverse();
}
}
return this.pushStack( ret );
};
});
jQuery.extend({
filter: function( expr, elems, not ) {
var elem = elems[ 0 ];
if ( not ) {
expr = ":not(" + expr + ")";
}
return elems.length === 1 && elem.nodeType === 1 ?
jQuery.find.matchesSelector( elem, expr ) ? [ elem ] : [] :
jQuery.find.matches( expr, jQuery.grep( elems, function( elem ) {
return elem.nodeType === 1;
}));
},
dir: function( elem, dir, until ) {
var matched = [],
cur = elem[ dir ];
while ( cur && cur.nodeType !== 9 && (until === undefined || cur.nodeType !== 1 || !jQuery( cur ).is( until )) ) {
if ( cur.nodeType === 1 ) {
matched.push( cur );
}
cur = cur[dir];
}
return matched;
},
sibling: function( n, elem ) {
var r = [];
for ( ; n; n = n.nextSibling ) {
if ( n.nodeType === 1 && n !== elem ) {
r.push( n );
}
}
return r;
}
});
// Implement the identical functionality for filter and not
function winnow( elements, qualifier, not ) {
if ( jQuery.isFunction( qualifier ) ) {
return jQuery.grep( elements, function( elem, i ) {
/* jshint -W018 */
return !!qualifier.call( elem, i, elem ) !== not;
});
}
if ( qualifier.nodeType ) {
return jQuery.grep( elements, function( elem ) {
return ( elem === qualifier ) !== not;
});
}
if ( typeof qualifier === "string" ) {
if ( isSimple.test( qualifier ) ) {
return jQuery.filter( qualifier, elements, not );
}
qualifier = jQuery.filter( qualifier, elements );
}
return jQuery.grep( elements, function( elem ) {
return ( jQuery.inArray( elem, qualifier ) >= 0 ) !== not;
});
}
function createSafeFragment( document ) {
var list = nodeNames.split( "|" ),
safeFrag = document.createDocumentFragment();
if ( safeFrag.createElement ) {
while ( list.length ) {
safeFrag.createElement(
list.pop()
);
}
}
return safeFrag;
}
var nodeNames = "abbr|article|aside|audio|bdi|canvas|data|datalist|details|figcaption|figure|footer|" +
"header|hgroup|mark|meter|nav|output|progress|section|summary|time|video",
rinlinejQuery = / jQuery\d+="(?:null|\d+)"/g,
rnoshimcache = new RegExp("<(?:" + nodeNames + ")[\\s/>]", "i"),
rleadingWhitespace = /^\s+/,
rxhtmlTag = /<(?!area|br|col|embed|hr|img|input|link|meta|param)(([\w:]+)[^>]*)\/>/gi,
rtagName = /<([\w:]+)/,
rtbody = /<tbody/i,
rhtml = /<|&#?\w+;/,
rnoInnerhtml = /<(?:script|style|link)/i,
manipulation_rcheckableType = /^(?:checkbox|radio)$/i,
// checked="checked" or checked
rchecked = /checked\s*(?:[^=]|=\s*.checked.)/i,
rscriptType = /^$|\/(?:java|ecma)script/i,
rscriptTypeMasked = /^true\/(.*)/,
rcleanScript = /^\s*<!(?:\[CDATA\[|--)|(?:\]\]|--)>\s*$/g,
// We have to close these tags to support XHTML (#13200)
wrapMap = {
option: [ 1, "<select multiple='multiple'>", "</select>" ],
legend: [ 1, "<fieldset>", "</fieldset>" ],
area: [ 1, "<map>", "</map>" ],
param: [ 1, "<object>", "</object>" ],
thead: [ 1, "<table>", "</table>" ],
tr: [ 2, "<table><tbody>", "</tbody></table>" ],
col: [ 2, "<table><tbody></tbody><colgroup>", "</colgroup></table>" ],
td: [ 3, "<table><tbody><tr>", "</tr></tbody></table>" ],
// IE6-8 can't serialize link, script, style, or any html5 (NoScope) tags,
// unless wrapped in a div with non-breaking characters in front of it.
_default: jQuery.support.htmlSerialize ? [ 0, "", "" ] : [ 1, "X<div>", "</div>" ]
},
safeFragment = createSafeFragment( document ),
fragmentDiv = safeFragment.appendChild( document.createElement("div") );
wrapMap.optgroup = wrapMap.option;
wrapMap.tbody = wrapMap.tfoot = wrapMap.colgroup = wrapMap.caption = wrapMap.thead;
wrapMap.th = wrapMap.td;
jQuery.fn.extend({
text: function( value ) {
return jQuery.access( this, function( value ) {
return value === undefined ?
jQuery.text( this ) :
this.empty().append( ( this[0] && this[0].ownerDocument || document ).createTextNode( value ) );
}, null, value, arguments.length );
},
append: function() {
return this.domManip( arguments, function( elem ) {
if ( this.nodeType === 1 || this.nodeType === 11 || this.nodeType === 9 ) {
var target = manipulationTarget( this, elem );
target.appendChild( elem );
}
});
},
prepend: function() {
return this.domManip( arguments, function( elem ) {
if ( this.nodeType === 1 || this.nodeType === 11 || this.nodeType === 9 ) {
var target = manipulationTarget( this, elem );
target.insertBefore( elem, target.firstChild );
}
});
},
before: function() {
return this.domManip( arguments, function( elem ) {
if ( this.parentNode ) {
this.parentNode.insertBefore( elem, this );
}
});
},
after: function() {
return this.domManip( arguments, function( elem ) {
if ( this.parentNode ) {
this.parentNode.insertBefore( elem, this.nextSibling );
}
});
},
// keepData is for internal use only--do not document
remove: function( selector, keepData ) {
var elem,
elems = selector ? jQuery.filter( selector, this ) : this,
i = 0;
for ( ; (elem = elems[i]) != null; i++ ) {
if ( !keepData && elem.nodeType === 1 ) {
jQuery.cleanData( getAll( elem ) );
}
if ( elem.parentNode ) {
if ( keepData && jQuery.contains( elem.ownerDocument, elem ) ) {
setGlobalEval( getAll( elem, "script" ) );
}
elem.parentNode.removeChild( elem );
}
}
return this;
},
empty: function() {
var elem,
i = 0;
for ( ; (elem = this[i]) != null; i++ ) {
// Remove element nodes and prevent memory leaks
if ( elem.nodeType === 1 ) {
jQuery.cleanData( getAll( elem, false ) );
}
// Remove any remaining nodes
while ( elem.firstChild ) {
elem.removeChild( elem.firstChild );
}
// If this is a select, ensure that it displays empty (#12336)
// Support: IE<9
if ( elem.options && jQuery.nodeName( elem, "select" ) ) {
elem.options.length = 0;
}
}
return this;
},
clone: function( dataAndEvents, deepDataAndEvents ) {
dataAndEvents = dataAndEvents == null ? false : dataAndEvents;
deepDataAndEvents = deepDataAndEvents == null ? dataAndEvents : deepDataAndEvents;
return this.map( function () {
return jQuery.clone( this, dataAndEvents, deepDataAndEvents );
});
},
html: function( value ) {
return jQuery.access( this, function( value ) {
var elem = this[0] || {},
i = 0,
l = this.length;
if ( value === undefined ) {
return elem.nodeType === 1 ?
elem.innerHTML.replace( rinlinejQuery, "" ) :
undefined;
}
// See if we can take a shortcut and just use innerHTML
if ( typeof value === "string" && !rnoInnerhtml.test( value ) &&
( jQuery.support.htmlSerialize || !rnoshimcache.test( value ) ) &&
( jQuery.support.leadingWhitespace || !rleadingWhitespace.test( value ) ) &&
!wrapMap[ ( rtagName.exec( value ) || ["", ""] )[1].toLowerCase() ] ) {
value = value.replace( rxhtmlTag, "<$1></$2>" );
try {
for (; i < l; i++ ) {
// Remove element nodes and prevent memory leaks
elem = this[i] || {};
if ( elem.nodeType === 1 ) {
jQuery.cleanData( getAll( elem, false ) );
elem.innerHTML = value;
}
}
elem = 0;
// If using innerHTML throws an exception, use the fallback method
} catch(e) {}
}
if ( elem ) {
this.empty().append( value );
}
}, null, value, arguments.length );
},
replaceWith: function() {
var
// Snapshot the DOM in case .domManip sweeps something relevant into its fragment
args = jQuery.map( this, function( elem ) {
return [ elem.nextSibling, elem.parentNode ];
}),
i = 0;
// Make the changes, replacing each context element with the new content
this.domManip( arguments, function( elem ) {
var next = args[ i++ ],
parent = args[ i++ ];
if ( parent ) {
// Don't use the snapshot next if it has moved (#13810)
if ( next && next.parentNode !== parent ) {
next = this.nextSibling;
}
jQuery( this ).remove();
parent.insertBefore( elem, next );
}
// Allow new content to include elements from the context set
}, true );
// Force removal if there was no new content (e.g., from empty arguments)
return i ? this : this.remove();
},
detach: function( selector ) {
return this.remove( selector, true );
},
domManip: function( args, callback, allowIntersection ) {
// Flatten any nested arrays
args = core_concat.apply( [], args );
var first, node, hasScripts,
scripts, doc, fragment,
i = 0,
l = this.length,
set = this,
iNoClone = l - 1,
value = args[0],
isFunction = jQuery.isFunction( value );
// We can't cloneNode fragments that contain checked, in WebKit
if ( isFunction || !( l <= 1 || typeof value !== "string" || jQuery.support.checkClone || !rchecked.test( value ) ) ) {
return this.each(function( index ) {
var self = set.eq( index );
if ( isFunction ) {
args[0] = value.call( this, index, self.html() );
}
self.domManip( args, callback, allowIntersection );
});
}
if ( l ) {
fragment = jQuery.buildFragment( args, this[ 0 ].ownerDocument, false, !allowIntersection && this );
first = fragment.firstChild;
if ( fragment.childNodes.length === 1 ) {
fragment = first;
}
if ( first ) {
scripts = jQuery.map( getAll( fragment, "script" ), disableScript );
hasScripts = scripts.length;
// Use the original fragment for the last item instead of the first because it can end up
// being emptied incorrectly in certain situations (#8070).
for ( ; i < l; i++ ) {
node = fragment;
if ( i !== iNoClone ) {
node = jQuery.clone( node, true, true );
// Keep references to cloned scripts for later restoration
if ( hasScripts ) {
jQuery.merge( scripts, getAll( node, "script" ) );
}
}
callback.call( this[i], node, i );
}
if ( hasScripts ) {
doc = scripts[ scripts.length - 1 ].ownerDocument;
// Reenable scripts
jQuery.map( scripts, restoreScript );
// Evaluate executable scripts on first document insertion
for ( i = 0; i < hasScripts; i++ ) {
node = scripts[ i ];
if ( rscriptType.test( node.type || "" ) &&
!jQuery._data( node, "globalEval" ) && jQuery.contains( doc, node ) ) {
if ( node.src ) {
// Hope ajax is available...
jQuery._evalUrl( node.src );
} else {
jQuery.globalEval( ( node.text || node.textContent || node.innerHTML || "" ).replace( rcleanScript, "" ) );
}
}
}
}
// Fix #11809: Avoid leaking memory
fragment = first = null;
}
}
return this;
}
});
// Support: IE<8
// Manipulating tables requires a tbody
function manipulationTarget( elem, content ) {
return jQuery.nodeName( elem, "table" ) &&
jQuery.nodeName( content.nodeType === 1 ? content : content.firstChild, "tr" ) ?
elem.getElementsByTagName("tbody")[0] ||
elem.appendChild( elem.ownerDocument.createElement("tbody") ) :
elem;
}
// Replace/restore the type attribute of script elements for safe DOM manipulation
function disableScript( elem ) {
elem.type = (jQuery.find.attr( elem, "type" ) !== null) + "/" + elem.type;
return elem;
}
function restoreScript( elem ) {
var match = rscriptTypeMasked.exec( elem.type );
if ( match ) {
elem.type = match[1];
} else {
elem.removeAttribute("type");
}
return elem;
}
// Mark scripts as having already been evaluated
function setGlobalEval( elems, refElements ) {
var elem,
i = 0;
for ( ; (elem = elems[i]) != null; i++ ) {
jQuery._data( elem, "globalEval", !refElements || jQuery._data( refElements[i], "globalEval" ) );
}
}
function cloneCopyEvent( src, dest ) {
if ( dest.nodeType !== 1 || !jQuery.hasData( src ) ) {
return;
}
var type, i, l,
oldData = jQuery._data( src ),
curData = jQuery._data( dest, oldData ),
events = oldData.events;
if ( events ) {
delete curData.handle;
curData.events = {};
for ( type in events ) {
for ( i = 0, l = events[ type ].length; i < l; i++ ) {
jQuery.event.add( dest, type, events[ type ][ i ] );
}
}
}
// make the cloned public data object a copy from the original
if ( curData.data ) {
curData.data = jQuery.extend( {}, curData.data );
}
}
function fixCloneNodeIssues( src, dest ) {
var nodeName, e, data;
// We do not need to do anything for non-Elements
if ( dest.nodeType !== 1 ) {
return;
}
nodeName = dest.nodeName.toLowerCase();
// IE6-8 copies events bound via attachEvent when using cloneNode.
if ( !jQuery.support.noCloneEvent && dest[ jQuery.expando ] ) {
data = jQuery._data( dest );
for ( e in data.events ) {
jQuery.removeEvent( dest, e, data.handle );
}
// Event data gets referenced instead of copied if the expando gets copied too
dest.removeAttribute( jQuery.expando );
}
// IE blanks contents when cloning scripts, and tries to evaluate newly-set text
if ( nodeName === "script" && dest.text !== src.text ) {
disableScript( dest ).text = src.text;
restoreScript( dest );
// IE6-10 improperly clones children of object elements using classid.
// IE10 throws NoModificationAllowedError if parent is null, #12132.
} else if ( nodeName === "object" ) {
if ( dest.parentNode ) {
dest.outerHTML = src.outerHTML;
}
// This path appears unavoidable for IE9. When cloning an object
// element in IE9, the outerHTML strategy above is not sufficient.
// If the src has innerHTML and the destination does not,
// copy the src.innerHTML into the dest.innerHTML. #10324
if ( jQuery.support.html5Clone && ( src.innerHTML && !jQuery.trim(dest.innerHTML) ) ) {
dest.innerHTML = src.innerHTML;
}
} else if ( nodeName === "input" && manipulation_rcheckableType.test( src.type ) ) {
// IE6-8 fails to persist the checked state of a cloned checkbox
// or radio button. Worse, IE6-7 fail to give the cloned element
// a checked appearance if the defaultChecked value isn't also set
dest.defaultChecked = dest.checked = src.checked;
// IE6-7 get confused and end up setting the value of a cloned
// checkbox/radio button to an empty string instead of "on"
if ( dest.value !== src.value ) {
dest.value = src.value;
}
// IE6-8 fails to return the selected option to the default selected
// state when cloning options
} else if ( nodeName === "option" ) {
dest.defaultSelected = dest.selected = src.defaultSelected;
// IE6-8 fails to set the defaultValue to the correct value when
// cloning other types of input fields
} else if ( nodeName === "input" || nodeName === "textarea" ) {
dest.defaultValue = src.defaultValue;
}
}
jQuery.each({
appendTo: "append",
prependTo: "prepend",
insertBefore: "before",
insertAfter: "after",
replaceAll: "replaceWith"
}, function( name, original ) {
jQuery.fn[ name ] = function( selector ) {
var elems,
i = 0,
ret = [],
insert = jQuery( selector ),
last = insert.length - 1;
for ( ; i <= last; i++ ) {
elems = i === last ? this : this.clone(true);
jQuery( insert[i] )[ original ]( elems );
// Modern browsers can apply jQuery collections as arrays, but oldIE needs a .get()
core_push.apply( ret, elems.get() );
}
return this.pushStack( ret );
};
});
function getAll( context, tag ) {
var elems, elem,
i = 0,
found = typeof context.getElementsByTagName !== core_strundefined ? context.getElementsByTagName( tag || "*" ) :
typeof context.querySelectorAll !== core_strundefined ? context.querySelectorAll( tag || "*" ) :
undefined;
if ( !found ) {
for ( found = [], elems = context.childNodes || context; (elem = elems[i]) != null; i++ ) {
if ( !tag || jQuery.nodeName( elem, tag ) ) {
found.push( elem );
} else {
jQuery.merge( found, getAll( elem, tag ) );
}
}
}
return tag === undefined || tag && jQuery.nodeName( context, tag ) ?
jQuery.merge( [ context ], found ) :
found;
}
// Used in buildFragment, fixes the defaultChecked property
function fixDefaultChecked( elem ) {
if ( manipulation_rcheckableType.test( elem.type ) ) {
elem.defaultChecked = elem.checked;
}
}
jQuery.extend({
clone: function( elem, dataAndEvents, deepDataAndEvents ) {
var destElements, node, clone, i, srcElements,
inPage = jQuery.contains( elem.ownerDocument, elem );
if ( jQuery.support.html5Clone || jQuery.isXMLDoc(elem) || !rnoshimcache.test( "<" + elem.nodeName + ">" ) ) {
clone = elem.cloneNode( true );
// IE<=8 does not properly clone detached, unknown element nodes
} else {
fragmentDiv.innerHTML = elem.outerHTML;
fragmentDiv.removeChild( clone = fragmentDiv.firstChild );
}
if ( (!jQuery.support.noCloneEvent || !jQuery.support.noCloneChecked) &&
(elem.nodeType === 1 || elem.nodeType === 11) && !jQuery.isXMLDoc(elem) ) {
// We eschew Sizzle here for performance reasons: http://jsperf.com/getall-vs-sizzle/2
destElements = getAll( clone );
srcElements = getAll( elem );
// Fix all IE cloning issues
for ( i = 0; (node = srcElements[i]) != null; ++i ) {
// Ensure that the destination node is not null; Fixes #9587
if ( destElements[i] ) {
fixCloneNodeIssues( node, destElements[i] );
}
}
}
// Copy the events from the original to the clone
if ( dataAndEvents ) {
if ( deepDataAndEvents ) {
srcElements = srcElements || getAll( elem );
destElements = destElements || getAll( clone );
for ( i = 0; (node = srcElements[i]) != null; i++ ) {
cloneCopyEvent( node, destElements[i] );
}
} else {
cloneCopyEvent( elem, clone );
}
}
// Preserve script evaluation history
destElements = getAll( clone, "script" );
if ( destElements.length > 0 ) {
setGlobalEval( destElements, !inPage && getAll( elem, "script" ) );
}
destElements = srcElements = node = null;
// Return the cloned set
return clone;
},
buildFragment: function( elems, context, scripts, selection ) {
var j, elem, contains,
tmp, tag, tbody, wrap,
l = elems.length,
// Ensure a safe fragment
safe = createSafeFragment( context ),
nodes = [],
i = 0;
for ( ; i < l; i++ ) {
elem = elems[ i ];
if ( elem || elem === 0 ) {
// Add nodes directly
if ( jQuery.type( elem ) === "object" ) {
jQuery.merge( nodes, elem.nodeType ? [ elem ] : elem );
// Convert non-html into a text node
} else if ( !rhtml.test( elem ) ) {
nodes.push( context.createTextNode( elem ) );
// Convert html into DOM nodes
} else {
tmp = tmp || safe.appendChild( context.createElement("div") );
// Deserialize a standard representation
tag = ( rtagName.exec( elem ) || ["", ""] )[1].toLowerCase();
wrap = wrapMap[ tag ] || wrapMap._default;
tmp.innerHTML = wrap[1] + elem.replace( rxhtmlTag, "<$1></$2>" ) + wrap[2];
// Descend through wrappers to the right content
j = wrap[0];
while ( j-- ) {
tmp = tmp.lastChild;
}
// Manually add leading whitespace removed by IE
if ( !jQuery.support.leadingWhitespace && rleadingWhitespace.test( elem ) ) {
nodes.push( context.createTextNode( rleadingWhitespace.exec( elem )[0] ) );
}
// Remove IE's autoinserted <tbody> from table fragments
if ( !jQuery.support.tbody ) {
// String was a <table>, *may* have spurious <tbody>
elem = tag === "table" && !rtbody.test( elem ) ?
tmp.firstChild :
// String was a bare <thead> or <tfoot>
wrap[1] === "<table>" && !rtbody.test( elem ) ?
tmp :
0;
j = elem && elem.childNodes.length;
while ( j-- ) {
if ( jQuery.nodeName( (tbody = elem.childNodes[j]), "tbody" ) && !tbody.childNodes.length ) {
elem.removeChild( tbody );
}
}
}
jQuery.merge( nodes, tmp.childNodes );
// Fix #12392 for WebKit and IE > 9
tmp.textContent = "";
// Fix #12392 for oldIE
while ( tmp.firstChild ) {
tmp.removeChild( tmp.firstChild );
}
// Remember the top-level container for proper cleanup
tmp = safe.lastChild;
}
}
}
// Fix #11356: Clear elements from fragment
if ( tmp ) {
safe.removeChild( tmp );
}
// Reset defaultChecked for any radios and checkboxes
// about to be appended to the DOM in IE 6/7 (#8060)
if ( !jQuery.support.appendChecked ) {
jQuery.grep( getAll( nodes, "input" ), fixDefaultChecked );
}
i = 0;
while ( (elem = nodes[ i++ ]) ) {
// #4087 - If origin and destination elements are the same, and this is
// that element, do not do anything
if ( selection && jQuery.inArray( elem, selection ) !== -1 ) {
continue;
}
contains = jQuery.contains( elem.ownerDocument, elem );
// Append to fragment
tmp = getAll( safe.appendChild( elem ), "script" );
// Preserve script evaluation history
if ( contains ) {
setGlobalEval( tmp );
}
// Capture executables
if ( scripts ) {
j = 0;
while ( (elem = tmp[ j++ ]) ) {
if ( rscriptType.test( elem.type || "" ) ) {
scripts.push( elem );
}
}
}
}
tmp = null;
return safe;
},
cleanData: function( elems, /* internal */ acceptData ) {
var elem, type, id, data,
i = 0,
internalKey = jQuery.expando,
cache = jQuery.cache,
deleteExpando = jQuery.support.deleteExpando,
special = jQuery.event.special;
for ( ; (elem = elems[i]) != null; i++ ) {
if ( acceptData || jQuery.acceptData( elem ) ) {
id = elem[ internalKey ];
data = id && cache[ id ];
if ( data ) {
if ( data.events ) {
for ( type in data.events ) {
if ( special[ type ] ) {
jQuery.event.remove( elem, type );
// This is a shortcut to avoid jQuery.event.remove's overhead
} else {
jQuery.removeEvent( elem, type, data.handle );
}
}
}
// Remove cache only if it was not already removed by jQuery.event.remove
if ( cache[ id ] ) {
delete cache[ id ];
// IE does not allow us to delete expando properties from nodes,
// nor does it have a removeAttribute function on Document nodes;
// we must handle all of these cases
if ( deleteExpando ) {
delete elem[ internalKey ];
} else if ( typeof elem.removeAttribute !== core_strundefined ) {
elem.removeAttribute( internalKey );
} else {
elem[ internalKey ] = null;
}
core_deletedIds.push( id );
}
}
}
}
},
_evalUrl: function( url ) {
return jQuery.ajax({
url: url,
type: "GET",
dataType: "script",
async: false,
global: false,
"throws": true
});
}
});
jQuery.fn.extend({
wrapAll: function( html ) {
if ( jQuery.isFunction( html ) ) {
return this.each(function(i) {
jQuery(this).wrapAll( html.call(this, i) );
});
}
if ( this[0] ) {
// The elements to wrap the target around
var wrap = jQuery( html, this[0].ownerDocument ).eq(0).clone(true);
if ( this[0].parentNode ) {
wrap.insertBefore( this[0] );
}
wrap.map(function() {
var elem = this;
while ( elem.firstChild && elem.firstChild.nodeType === 1 ) {
elem = elem.firstChild;
}
return elem;
}).append( this );
}
return this;
},
wrapInner: function( html ) {
if ( jQuery.isFunction( html ) ) {
return this.each(function(i) {
jQuery(this).wrapInner( html.call(this, i) );
});
}
return this.each(function() {
var self = jQuery( this ),
contents = self.contents();
if ( contents.length ) {
contents.wrapAll( html );
} else {
self.append( html );
}
});
},
wrap: function( html ) {
var isFunction = jQuery.isFunction( html );
return this.each(function(i) {
jQuery( this ).wrapAll( isFunction ? html.call(this, i) : html );
});
},
unwrap: function() {
return this.parent().each(function() {
if ( !jQuery.nodeName( this, "body" ) ) {
jQuery( this ).replaceWith( this.childNodes );
}
}).end();
}
});
var iframe, getStyles, curCSS,
ralpha = /alpha\([^)]*\)/i,
ropacity = /opacity\s*=\s*([^)]*)/,
rposition = /^(top|right|bottom|left)$/,
// swappable if display is none or starts with table except "table", "table-cell", or "table-caption"
// see here for display values: https://developer.mozilla.org/en-US/docs/CSS/display
rdisplayswap = /^(none|table(?!-c[ea]).+)/,
rmargin = /^margin/,
rnumsplit = new RegExp( "^(" + core_pnum + ")(.*)$", "i" ),
rnumnonpx = new RegExp( "^(" + core_pnum + ")(?!px)[a-z%]+$", "i" ),
rrelNum = new RegExp( "^([+-])=(" + core_pnum + ")", "i" ),
elemdisplay = { BODY: "block" },
cssShow = { position: "absolute", visibility: "hidden", display: "block" },
cssNormalTransform = {
letterSpacing: 0,
fontWeight: 400
},
cssExpand = [ "Top", "Right", "Bottom", "Left" ],
cssPrefixes = [ "Webkit", "O", "Moz", "ms" ];
// return a css property mapped to a potentially vendor prefixed property
function vendorPropName( style, name ) {
// shortcut for names that are not vendor prefixed
if ( name in style ) {
return name;
}
// check for vendor prefixed names
var capName = name.charAt(0).toUpperCase() + name.slice(1),
origName = name,
i = cssPrefixes.length;
while ( i-- ) {
name = cssPrefixes[ i ] + capName;
if ( name in style ) {
return name;
}
}
return origName;
}
function isHidden( elem, el ) {
// isHidden might be called from jQuery#filter function;
// in that case, element will be second argument
elem = el || elem;
return jQuery.css( elem, "display" ) === "none" || !jQuery.contains( elem.ownerDocument, elem );
}
function showHide( elements, show ) {
var display, elem, hidden,
values = [],
index = 0,
length = elements.length;
for ( ; index < length; index++ ) {
elem = elements[ index ];
if ( !elem.style ) {
continue;
}
values[ index ] = jQuery._data( elem, "olddisplay" );
display = elem.style.display;
if ( show ) {
// Reset the inline display of this element to learn if it is
// being hidden by cascaded rules or not
if ( !values[ index ] && display === "none" ) {
elem.style.display = "";
}
// Set elements which have been overridden with display: none
// in a stylesheet to whatever the default browser style is
// for such an element
if ( elem.style.display === "" && isHidden( elem ) ) {
values[ index ] = jQuery._data( elem, "olddisplay", css_defaultDisplay(elem.nodeName) );
}
} else {
if ( !values[ index ] ) {
hidden = isHidden( elem );
if ( display && display !== "none" || !hidden ) {
jQuery._data( elem, "olddisplay", hidden ? display : jQuery.css( elem, "display" ) );
}
}
}
}
// Set the display of most of the elements in a second loop
// to avoid the constant reflow
for ( index = 0; index < length; index++ ) {
elem = elements[ index ];
if ( !elem.style ) {
continue;
}
if ( !show || elem.style.display === "none" || elem.style.display === "" ) {
elem.style.display = show ? values[ index ] || "" : "none";
}
}
return elements;
}
jQuery.fn.extend({
css: function( name, value ) {
return jQuery.access( this, function( elem, name, value ) {
var len, styles,
map = {},
i = 0;
if ( jQuery.isArray( name ) ) {
styles = getStyles( elem );
len = name.length;
for ( ; i < len; i++ ) {
map[ name[ i ] ] = jQuery.css( elem, name[ i ], false, styles );
}
return map;
}
return value !== undefined ?
jQuery.style( elem, name, value ) :
jQuery.css( elem, name );
}, name, value, arguments.length > 1 );
},
show: function() {
return showHide( this, true );
},
hide: function() {
return showHide( this );
},
toggle: function( state ) {
if ( typeof state === "boolean" ) {
return state ? this.show() : this.hide();
}
return this.each(function() {
if ( isHidden( this ) ) {
jQuery( this ).show();
} else {
jQuery( this ).hide();
}
});
}
});
jQuery.extend({
// Add in style property hooks for overriding the default
// behavior of getting and setting a style property
cssHooks: {
opacity: {
get: function( elem, computed ) {
if ( computed ) {
// We should always get a number back from opacity
var ret = curCSS( elem, "opacity" );
return ret === "" ? "1" : ret;
}
}
}
},
// Don't automatically add "px" to these possibly-unitless properties
cssNumber: {
"columnCount": true,
"fillOpacity": true,
"fontWeight": true,
"lineHeight": true,
"opacity": true,
"order": true,
"orphans": true,
"widows": true,
"zIndex": true,
"zoom": true
},
// Add in properties whose names you wish to fix before
// setting or getting the value
cssProps: {
// normalize float css property
"float": jQuery.support.cssFloat ? "cssFloat" : "styleFloat"
},
// Get and set the style property on a DOM Node
style: function( elem, name, value, extra ) {
// Don't set styles on text and comment nodes
if ( !elem || elem.nodeType === 3 || elem.nodeType === 8 || !elem.style ) {
return;
}
// Make sure that we're working with the right name
var ret, type, hooks,
origName = jQuery.camelCase( name ),
style = elem.style;
name = jQuery.cssProps[ origName ] || ( jQuery.cssProps[ origName ] = vendorPropName( style, origName ) );
// gets hook for the prefixed version
// followed by the unprefixed version
hooks = jQuery.cssHooks[ name ] || jQuery.cssHooks[ origName ];
// Check if we're setting a value
if ( value !== undefined ) {
type = typeof value;
// convert relative number strings (+= or -=) to relative numbers. #7345
if ( type === "string" && (ret = rrelNum.exec( value )) ) {
value = ( ret[1] + 1 ) * ret[2] + parseFloat( jQuery.css( elem, name ) );
// Fixes bug #9237
type = "number";
}
// Make sure that NaN and null values aren't set. See: #7116
if ( value == null || type === "number" && isNaN( value ) ) {
return;
}
// If a number was passed in, add 'px' to the (except for certain CSS properties)
if ( type === "number" && !jQuery.cssNumber[ origName ] ) {
value += "px";
}
// Fixes #8908, it can be done more correctly by specifing setters in cssHooks,
// but it would mean to define eight (for every problematic property) identical functions
if ( !jQuery.support.clearCloneStyle && value === "" && name.indexOf("background") === 0 ) {
style[ name ] = "inherit";
}
// If a hook was provided, use that value, otherwise just set the specified value
if ( !hooks || !("set" in hooks) || (value = hooks.set( elem, value, extra )) !== undefined ) {
// Wrapped to prevent IE from throwing errors when 'invalid' values are provided
// Fixes bug #5509
try {
style[ name ] = value;
} catch(e) {}
}
} else {
// If a hook was provided get the non-computed value from there
if ( hooks && "get" in hooks && (ret = hooks.get( elem, false, extra )) !== undefined ) {
return ret;
}
// Otherwise just get the value from the style object
return style[ name ];
}
},
css: function( elem, name, extra, styles ) {
var num, val, hooks,
origName = jQuery.camelCase( name );
// Make sure that we're working with the right name
name = jQuery.cssProps[ origName ] || ( jQuery.cssProps[ origName ] = vendorPropName( elem.style, origName ) );
// gets hook for the prefixed version
// followed by the unprefixed version
hooks = jQuery.cssHooks[ name ] || jQuery.cssHooks[ origName ];
// If a hook was provided get the computed value from there
if ( hooks && "get" in hooks ) {
val = hooks.get( elem, true, extra );
}
// Otherwise, if a way to get the computed value exists, use that
if ( val === undefined ) {
val = curCSS( elem, name, styles );
}
//convert "normal" to computed value
if ( val === "normal" && name in cssNormalTransform ) {
val = cssNormalTransform[ name ];
}
// Return, converting to number if forced or a qualifier was provided and val looks numeric
if ( extra === "" || extra ) {
num = parseFloat( val );
return extra === true || jQuery.isNumeric( num ) ? num || 0 : val;
}
return val;
}
});
// NOTE: we've included the "window" in window.getComputedStyle
// because jsdom on node.js will break without it.
if ( window.getComputedStyle ) {
getStyles = function( elem ) {
return window.getComputedStyle( elem, null );
};
curCSS = function( elem, name, _computed ) {
var width, minWidth, maxWidth,
computed = _computed || getStyles( elem ),
// getPropertyValue is only needed for .css('filter') in IE9, see #12537
ret = computed ? computed.getPropertyValue( name ) || computed[ name ] : undefined,
style = elem.style;
if ( computed ) {
if ( ret === "" && !jQuery.contains( elem.ownerDocument, elem ) ) {
ret = jQuery.style( elem, name );
}
// A tribute to the "awesome hack by Dean Edwards"
// Chrome < 17 and Safari 5.0 uses "computed value" instead of "used value" for margin-right
// Safari 5.1.7 (at least) returns percentage for a larger set of values, but width seems to be reliably pixels
// this is against the CSSOM draft spec: http://dev.w3.org/csswg/cssom/#resolved-values
if ( rnumnonpx.test( ret ) && rmargin.test( name ) ) {
// Remember the original values
width = style.width;
minWidth = style.minWidth;
maxWidth = style.maxWidth;
// Put in the new values to get a computed value out
style.minWidth = style.maxWidth = style.width = ret;
ret = computed.width;
// Revert the changed values
style.width = width;
style.minWidth = minWidth;
style.maxWidth = maxWidth;
}
}
return ret;
};
} else if ( document.documentElement.currentStyle ) {
getStyles = function( elem ) {
return elem.currentStyle;
};
curCSS = function( elem, name, _computed ) {
var left, rs, rsLeft,
computed = _computed || getStyles( elem ),
ret = computed ? computed[ name ] : undefined,
style = elem.style;
// Avoid setting ret to empty string here
// so we don't default to auto
if ( ret == null && style && style[ name ] ) {
ret = style[ name ];
}
// From the awesome hack by Dean Edwards
// http://erik.eae.net/archives/2007/07/27/18.54.15/#comment-102291
// If we're not dealing with a regular pixel number
// but a number that has a weird ending, we need to convert it to pixels
// but not position css attributes, as those are proportional to the parent element instead
// and we can't measure the parent instead because it might trigger a "stacking dolls" problem
if ( rnumnonpx.test( ret ) && !rposition.test( name ) ) {
// Remember the original values
left = style.left;
rs = elem.runtimeStyle;
rsLeft = rs && rs.left;
// Put in the new values to get a computed value out
if ( rsLeft ) {
rs.left = elem.currentStyle.left;
}
style.left = name === "fontSize" ? "1em" : ret;
ret = style.pixelLeft + "px";
// Revert the changed values
style.left = left;
if ( rsLeft ) {
rs.left = rsLeft;
}
}
return ret === "" ? "auto" : ret;
};
}
function setPositiveNumber( elem, value, subtract ) {
var matches = rnumsplit.exec( value );
return matches ?
// Guard against undefined "subtract", e.g., when used as in cssHooks
Math.max( 0, matches[ 1 ] - ( subtract || 0 ) ) + ( matches[ 2 ] || "px" ) :
value;
}
function augmentWidthOrHeight( elem, name, extra, isBorderBox, styles ) {
var i = extra === ( isBorderBox ? "border" : "content" ) ?
// If we already have the right measurement, avoid augmentation
4 :
// Otherwise initialize for horizontal or vertical properties
name === "width" ? 1 : 0,
val = 0;
for ( ; i < 4; i += 2 ) {
// both box models exclude margin, so add it if we want it
if ( extra === "margin" ) {
val += jQuery.css( elem, extra + cssExpand[ i ], true, styles );
}
if ( isBorderBox ) {
// border-box includes padding, so remove it if we want content
if ( extra === "content" ) {
val -= jQuery.css( elem, "padding" + cssExpand[ i ], true, styles );
}
// at this point, extra isn't border nor margin, so remove border
if ( extra !== "margin" ) {
val -= jQuery.css( elem, "border" + cssExpand[ i ] + "Width", true, styles );
}
} else {
// at this point, extra isn't content, so add padding
val += jQuery.css( elem, "padding" + cssExpand[ i ], true, styles );
// at this point, extra isn't content nor padding, so add border
if ( extra !== "padding" ) {
val += jQuery.css( elem, "border" + cssExpand[ i ] + "Width", true, styles );
}
}
}
return val;
}
function getWidthOrHeight( elem, name, extra ) {
// Start with offset property, which is equivalent to the border-box value
var valueIsBorderBox = true,
val = name === "width" ? elem.offsetWidth : elem.offsetHeight,
styles = getStyles( elem ),
isBorderBox = jQuery.support.boxSizing && jQuery.css( elem, "boxSizing", false, styles ) === "border-box";
// some non-html elements return undefined for offsetWidth, so check for null/undefined
// svg - https://bugzilla.mozilla.org/show_bug.cgi?id=649285
// MathML - https://bugzilla.mozilla.org/show_bug.cgi?id=491668
if ( val <= 0 || val == null ) {
// Fall back to computed then uncomputed css if necessary
val = curCSS( elem, name, styles );
if ( val < 0 || val == null ) {
val = elem.style[ name ];
}
// Computed unit is not pixels. Stop here and return.
if ( rnumnonpx.test(val) ) {
return val;
}
// we need the check for style in case a browser which returns unreliable values
// for getComputedStyle silently falls back to the reliable elem.style
valueIsBorderBox = isBorderBox && ( jQuery.support.boxSizingReliable || val === elem.style[ name ] );
// Normalize "", auto, and prepare for extra
val = parseFloat( val ) || 0;
}
// use the active box-sizing model to add/subtract irrelevant styles
return ( val +
augmentWidthOrHeight(
elem,
name,
extra || ( isBorderBox ? "border" : "content" ),
valueIsBorderBox,
styles
)
) + "px";
}
// Try to determine the default display value of an element
function css_defaultDisplay( nodeName ) {
var doc = document,
display = elemdisplay[ nodeName ];
if ( !display ) {
display = actualDisplay( nodeName, doc );
// If the simple way fails, read from inside an iframe
if ( display === "none" || !display ) {
// Use the already-created iframe if possible
iframe = ( iframe ||
jQuery("<iframe frameborder='0' width='0' height='0'/>")
.css( "cssText", "display:block !important" )
).appendTo( doc.documentElement );
// Always write a new HTML skeleton so Webkit and Firefox don't choke on reuse
doc = ( iframe[0].contentWindow || iframe[0].contentDocument ).document;
doc.write("<!doctype html><html><body>");
doc.close();
display = actualDisplay( nodeName, doc );
iframe.detach();
}
// Store the correct default display
elemdisplay[ nodeName ] = display;
}
return display;
}
// Called ONLY from within css_defaultDisplay
function actualDisplay( name, doc ) {
var elem = jQuery( doc.createElement( name ) ).appendTo( doc.body ),
display = jQuery.css( elem[0], "display" );
elem.remove();
return display;
}
jQuery.each([ "height", "width" ], function( i, name ) {
jQuery.cssHooks[ name ] = {
get: function( elem, computed, extra ) {
if ( computed ) {
// certain elements can have dimension info if we invisibly show them
// however, it must have a current display style that would benefit from this
return elem.offsetWidth === 0 && rdisplayswap.test( jQuery.css( elem, "display" ) ) ?
jQuery.swap( elem, cssShow, function() {
return getWidthOrHeight( elem, name, extra );
}) :
getWidthOrHeight( elem, name, extra );
}
},
set: function( elem, value, extra ) {
var styles = extra && getStyles( elem );
return setPositiveNumber( elem, value, extra ?
augmentWidthOrHeight(
elem,
name,
extra,
jQuery.support.boxSizing && jQuery.css( elem, "boxSizing", false, styles ) === "border-box",
styles
) : 0
);
}
};
});
if ( !jQuery.support.opacity ) {
jQuery.cssHooks.opacity = {
get: function( elem, computed ) {
// IE uses filters for opacity
return ropacity.test( (computed && elem.currentStyle ? elem.currentStyle.filter : elem.style.filter) || "" ) ?
( 0.01 * parseFloat( RegExp.$1 ) ) + "" :
computed ? "1" : "";
},
set: function( elem, value ) {
var style = elem.style,
currentStyle = elem.currentStyle,
opacity = jQuery.isNumeric( value ) ? "alpha(opacity=" + value * 100 + ")" : "",
filter = currentStyle && currentStyle.filter || style.filter || "";
// IE has trouble with opacity if it does not have layout
// Force it by setting the zoom level
style.zoom = 1;
// if setting opacity to 1, and no other filters exist - attempt to remove filter attribute #6652
// if value === "", then remove inline opacity #12685
if ( ( value >= 1 || value === "" ) &&
jQuery.trim( filter.replace( ralpha, "" ) ) === "" &&
style.removeAttribute ) {
// Setting style.filter to null, "" & " " still leave "filter:" in the cssText
// if "filter:" is present at all, clearType is disabled, we want to avoid this
// style.removeAttribute is IE Only, but so apparently is this code path...
style.removeAttribute( "filter" );
// if there is no filter style applied in a css rule or unset inline opacity, we are done
if ( value === "" || currentStyle && !currentStyle.filter ) {
return;
}
}
// otherwise, set new filter values
style.filter = ralpha.test( filter ) ?
filter.replace( ralpha, opacity ) :
filter + " " + opacity;
}
};
}
// These hooks cannot be added until DOM ready because the support test
// for it is not run until after DOM ready
jQuery(function() {
if ( !jQuery.support.reliableMarginRight ) {
jQuery.cssHooks.marginRight = {
get: function( elem, computed ) {
if ( computed ) {
// WebKit Bug 13343 - getComputedStyle returns wrong value for margin-right
// Work around by temporarily setting element display to inline-block
return jQuery.swap( elem, { "display": "inline-block" },
curCSS, [ elem, "marginRight" ] );
}
}
};
}
// Webkit bug: https://bugs.webkit.org/show_bug.cgi?id=29084
// getComputedStyle returns percent when specified for top/left/bottom/right
// rather than make the css module depend on the offset module, we just check for it here
if ( !jQuery.support.pixelPosition && jQuery.fn.position ) {
jQuery.each( [ "top", "left" ], function( i, prop ) {
jQuery.cssHooks[ prop ] = {
get: function( elem, computed ) {
if ( computed ) {
computed = curCSS( elem, prop );
// if curCSS returns percentage, fallback to offset
return rnumnonpx.test( computed ) ?
jQuery( elem ).position()[ prop ] + "px" :
computed;
}
}
};
});
}
});
if ( jQuery.expr && jQuery.expr.filters ) {
jQuery.expr.filters.hidden = function( elem ) {
// Support: Opera <= 12.12
// Opera reports offsetWidths and offsetHeights less than zero on some elements
return elem.offsetWidth <= 0 && elem.offsetHeight <= 0 ||
(!jQuery.support.reliableHiddenOffsets && ((elem.style && elem.style.display) || jQuery.css( elem, "display" )) === "none");
};
jQuery.expr.filters.visible = function( elem ) {
return !jQuery.expr.filters.hidden( elem );
};
}
// These hooks are used by animate to expand properties
jQuery.each({
margin: "",
padding: "",
border: "Width"
}, function( prefix, suffix ) {
jQuery.cssHooks[ prefix + suffix ] = {
expand: function( value ) {
var i = 0,
expanded = {},
// assumes a single number if not a string
parts = typeof value === "string" ? value.split(" ") : [ value ];
for ( ; i < 4; i++ ) {
expanded[ prefix + cssExpand[ i ] + suffix ] =
parts[ i ] || parts[ i - 2 ] || parts[ 0 ];
}
return expanded;
}
};
if ( !rmargin.test( prefix ) ) {
jQuery.cssHooks[ prefix + suffix ].set = setPositiveNumber;
}
});
var r20 = /%20/g,
rbracket = /\[\]$/,
rCRLF = /\r?\n/g,
rsubmitterTypes = /^(?:submit|button|image|reset|file)$/i,
rsubmittable = /^(?:input|select|textarea|keygen)/i;
jQuery.fn.extend({
serialize: function() {
return jQuery.param( this.serializeArray() );
},
serializeArray: function() {
return this.map(function(){
// Can add propHook for "elements" to filter or add form elements
var elements = jQuery.prop( this, "elements" );
return elements ? jQuery.makeArray( elements ) : this;
})
.filter(function(){
var type = this.type;
// Use .is(":disabled") so that fieldset[disabled] works
return this.name && !jQuery( this ).is( ":disabled" ) &&
rsubmittable.test( this.nodeName ) && !rsubmitterTypes.test( type ) &&
( this.checked || !manipulation_rcheckableType.test( type ) );
})
.map(function( i, elem ){
var val = jQuery( this ).val();
return val == null ?
null :
jQuery.isArray( val ) ?
jQuery.map( val, function( val ){
return { name: elem.name, value: val.replace( rCRLF, "\r\n" ) };
}) :
{ name: elem.name, value: val.replace( rCRLF, "\r\n" ) };
}).get();
}
});
//Serialize an array of form elements or a set of
//key/values into a query string
jQuery.param = function( a, traditional ) {
var prefix,
s = [],
add = function( key, value ) {
// If value is a function, invoke it and return its value
value = jQuery.isFunction( value ) ? value() : ( value == null ? "" : value );
s[ s.length ] = encodeURIComponent( key ) + "=" + encodeURIComponent( value );
};
// Set traditional to true for jQuery <= 1.3.2 behavior.
if ( traditional === undefined ) {
traditional = jQuery.ajaxSettings && jQuery.ajaxSettings.traditional;
}
// If an array was passed in, assume that it is an array of form elements.
if ( jQuery.isArray( a ) || ( a.jquery && !jQuery.isPlainObject( a ) ) ) {
// Serialize the form elements
jQuery.each( a, function() {
add( this.name, this.value );
});
} else {
// If traditional, encode the "old" way (the way 1.3.2 or older
// did it), otherwise encode params recursively.
for ( prefix in a ) {
buildParams( prefix, a[ prefix ], traditional, add );
}
}
// Return the resulting serialization
return s.join( "&" ).replace( r20, "+" );
};
function buildParams( prefix, obj, traditional, add ) {
var name;
if ( jQuery.isArray( obj ) ) {
// Serialize array item.
jQuery.each( obj, function( i, v ) {
if ( traditional || rbracket.test( prefix ) ) {
// Treat each array item as a scalar.
add( prefix, v );
} else {
// Item is non-scalar (array or object), encode its numeric index.
buildParams( prefix + "[" + ( typeof v === "object" ? i : "" ) + "]", v, traditional, add );
}
});
} else if ( !traditional && jQuery.type( obj ) === "object" ) {
// Serialize object item.
for ( name in obj ) {
buildParams( prefix + "[" + name + "]", obj[ name ], traditional, add );
}
} else {
// Serialize scalar item.
add( prefix, obj );
}
}
jQuery.each( ("blur focus focusin focusout load resize scroll unload click dblclick " +
"mousedown mouseup mousemove mouseover mouseout mouseenter mouseleave " +
"change select submit keydown keypress keyup error contextmenu").split(" "), function( i, name ) {
// Handle event binding
jQuery.fn[ name ] = function( data, fn ) {
return arguments.length > 0 ?
this.on( name, null, data, fn ) :
this.trigger( name );
};
});
jQuery.fn.extend({
hover: function( fnOver, fnOut ) {
return this.mouseenter( fnOver ).mouseleave( fnOut || fnOver );
},
bind: function( types, data, fn ) {
return this.on( types, null, data, fn );
},
unbind: function( types, fn ) {
return this.off( types, null, fn );
},
delegate: function( selector, types, data, fn ) {
return this.on( types, selector, data, fn );
},
undelegate: function( selector, types, fn ) {
// ( namespace ) or ( selector, types [, fn] )
return arguments.length === 1 ? this.off( selector, "**" ) : this.off( types, selector || "**", fn );
}
});
var
// Document location
ajaxLocParts,
ajaxLocation,
ajax_nonce = jQuery.now(),
ajax_rquery = /\?/,
rhash = /#.*$/,
rts = /([?&])_=[^&]*/,
rheaders = /^(.*?):[ \t]*([^\r\n]*)\r?$/mg, // IE leaves an \r character at EOL
// #7653, #8125, #8152: local protocol detection
rlocalProtocol = /^(?:about|app|app-storage|.+-extension|file|res|widget):$/,
rnoContent = /^(?:GET|HEAD)$/,
rprotocol = /^\/\//,
rurl = /^([\w.+-]+:)(?:\/\/([^\/?#:]*)(?::(\d+)|)|)/,
// Keep a copy of the old load method
_load = jQuery.fn.load,
/* Prefilters
* 1) They are useful to introduce custom dataTypes (see ajax/jsonp.js for an example)
* 2) These are called:
* - BEFORE asking for a transport
* - AFTER param serialization (s.data is a string if s.processData is true)
* 3) key is the dataType
* 4) the catchall symbol "*" can be used
* 5) execution will start with transport dataType and THEN continue down to "*" if needed
*/
prefilters = {},
/* Transports bindings
* 1) key is the dataType
* 2) the catchall symbol "*" can be used
* 3) selection will start with transport dataType and THEN go to "*" if needed
*/
transports = {},
// Avoid comment-prolog char sequence (#10098); must appease lint and evade compression
allTypes = "*/".concat("*");
// #8138, IE may throw an exception when accessing
// a field from window.location if document.domain has been set
try {
ajaxLocation = location.href;
} catch( e ) {
// Use the href attribute of an A element
// since IE will modify it given document.location
ajaxLocation = document.createElement( "a" );
ajaxLocation.href = "";
ajaxLocation = ajaxLocation.href;
}
// Segment location into parts
ajaxLocParts = rurl.exec( ajaxLocation.toLowerCase() ) || [];
// Base "constructor" for jQuery.ajaxPrefilter and jQuery.ajaxTransport
function addToPrefiltersOrTransports( structure ) {
// dataTypeExpression is optional and defaults to "*"
return function( dataTypeExpression, func ) {
if ( typeof dataTypeExpression !== "string" ) {
func = dataTypeExpression;
dataTypeExpression = "*";
}
var dataType,
i = 0,
dataTypes = dataTypeExpression.toLowerCase().match( core_rnotwhite ) || [];
if ( jQuery.isFunction( func ) ) {
// For each dataType in the dataTypeExpression
while ( (dataType = dataTypes[i++]) ) {
// Prepend if requested
if ( dataType[0] === "+" ) {
dataType = dataType.slice( 1 ) || "*";
(structure[ dataType ] = structure[ dataType ] || []).unshift( func );
// Otherwise append
} else {
(structure[ dataType ] = structure[ dataType ] || []).push( func );
}
}
}
};
}
// Base inspection function for prefilters and transports
function inspectPrefiltersOrTransports( structure, options, originalOptions, jqXHR ) {
var inspected = {},
seekingTransport = ( structure === transports );
function inspect( dataType ) {
var selected;
inspected[ dataType ] = true;
jQuery.each( structure[ dataType ] || [], function( _, prefilterOrFactory ) {
var dataTypeOrTransport = prefilterOrFactory( options, originalOptions, jqXHR );
if( typeof dataTypeOrTransport === "string" && !seekingTransport && !inspected[ dataTypeOrTransport ] ) {
options.dataTypes.unshift( dataTypeOrTransport );
inspect( dataTypeOrTransport );
return false;
} else if ( seekingTransport ) {
return !( selected = dataTypeOrTransport );
}
});
return selected;
}
return inspect( options.dataTypes[ 0 ] ) || !inspected[ "*" ] && inspect( "*" );
}
// A special extend for ajax options
// that takes "flat" options (not to be deep extended)
// Fixes #9887
function ajaxExtend( target, src ) {
var deep, key,
flatOptions = jQuery.ajaxSettings.flatOptions || {};
for ( key in src ) {
if ( src[ key ] !== undefined ) {
( flatOptions[ key ] ? target : ( deep || (deep = {}) ) )[ key ] = src[ key ];
}
}
if ( deep ) {
jQuery.extend( true, target, deep );
}
return target;
}
jQuery.fn.load = function( url, params, callback ) {
if ( typeof url !== "string" && _load ) {
return _load.apply( this, arguments );
}
var selector, response, type,
self = this,
off = url.indexOf(" ");
if ( off >= 0 ) {
selector = url.slice( off, url.length );
url = url.slice( 0, off );
}
// If it's a function
if ( jQuery.isFunction( params ) ) {
// We assume that it's the callback
callback = params;
params = undefined;
// Otherwise, build a param string
} else if ( params && typeof params === "object" ) {
type = "POST";
}
// If we have elements to modify, make the request
if ( self.length > 0 ) {
jQuery.ajax({
url: url,
// if "type" variable is undefined, then "GET" method will be used
type: type,
dataType: "html",
data: params
}).done(function( responseText ) {
// Save response for use in complete callback
response = arguments;
self.html( selector ?
// If a selector was specified, locate the right elements in a dummy div
// Exclude scripts to avoid IE 'Permission Denied' errors
jQuery("<div>").append( jQuery.parseHTML( responseText ) ).find( selector ) :
// Otherwise use the full result
responseText );
}).complete( callback && function( jqXHR, status ) {
self.each( callback, response || [ jqXHR.responseText, status, jqXHR ] );
});
}
return this;
};
// Attach a bunch of functions for handling common AJAX events
jQuery.each( [ "ajaxStart", "ajaxStop", "ajaxComplete", "ajaxError", "ajaxSuccess", "ajaxSend" ], function( i, type ){
jQuery.fn[ type ] = function( fn ){
return this.on( type, fn );
};
});
jQuery.extend({
// Counter for holding the number of active queries
active: 0,
// Last-Modified header cache for next request
lastModified: {},
etag: {},
ajaxSettings: {
url: ajaxLocation,
type: "GET",
isLocal: rlocalProtocol.test( ajaxLocParts[ 1 ] ),
global: true,
processData: true,
async: true,
contentType: "application/x-www-form-urlencoded; charset=UTF-8",
/*
timeout: 0,
data: null,
dataType: null,
username: null,
password: null,
cache: null,
throws: false,
traditional: false,
headers: {},
*/
accepts: {
"*": allTypes,
text: "text/plain",
html: "text/html",
xml: "application/xml, text/xml",
json: "application/json, text/javascript"
},
contents: {
xml: /xml/,
html: /html/,
json: /json/
},
responseFields: {
xml: "responseXML",
text: "responseText",
json: "responseJSON"
},
// Data converters
// Keys separate source (or catchall "*") and destination types with a single space
converters: {
// Convert anything to text
"* text": String,
// Text to html (true = no transformation)
"text html": true,
// Evaluate text as a json expression
"text json": jQuery.parseJSON,
// Parse text as xml
"text xml": jQuery.parseXML
},
// For options that shouldn't be deep extended:
// you can add your own custom options here if
// and when you create one that shouldn't be
// deep extended (see ajaxExtend)
flatOptions: {
url: true,
context: true
}
},
// Creates a full fledged settings object into target
// with both ajaxSettings and settings fields.
// If target is omitted, writes into ajaxSettings.
ajaxSetup: function( target, settings ) {
return settings ?
// Building a settings object
ajaxExtend( ajaxExtend( target, jQuery.ajaxSettings ), settings ) :
// Extending ajaxSettings
ajaxExtend( jQuery.ajaxSettings, target );
},
ajaxPrefilter: addToPrefiltersOrTransports( prefilters ),
ajaxTransport: addToPrefiltersOrTransports( transports ),
// Main method
ajax: function( url, options ) {
// If url is an object, simulate pre-1.5 signature
if ( typeof url === "object" ) {
options = url;
url = undefined;
}
// Force options to be an object
options = options || {};
var // Cross-domain detection vars
parts,
// Loop variable
i,
// URL without anti-cache param
cacheURL,
// Response headers as string
responseHeadersString,
// timeout handle
timeoutTimer,
// To know if global events are to be dispatched
fireGlobals,
transport,
// Response headers
responseHeaders,
// Create the final options object
s = jQuery.ajaxSetup( {}, options ),
// Callbacks context
callbackContext = s.context || s,
// Context for global events is callbackContext if it is a DOM node or jQuery collection
globalEventContext = s.context && ( callbackContext.nodeType || callbackContext.jquery ) ?
jQuery( callbackContext ) :
jQuery.event,
// Deferreds
deferred = jQuery.Deferred(),
completeDeferred = jQuery.Callbacks("once memory"),
// Status-dependent callbacks
statusCode = s.statusCode || {},
// Headers (they are sent all at once)
requestHeaders = {},
requestHeadersNames = {},
// The jqXHR state
state = 0,
// Default abort message
strAbort = "canceled",
// Fake xhr
jqXHR = {
readyState: 0,
// Builds headers hashtable if needed
getResponseHeader: function( key ) {
var match;
if ( state === 2 ) {
if ( !responseHeaders ) {
responseHeaders = {};
while ( (match = rheaders.exec( responseHeadersString )) ) {
responseHeaders[ match[1].toLowerCase() ] = match[ 2 ];
}
}
match = responseHeaders[ key.toLowerCase() ];
}
return match == null ? null : match;
},
// Raw string
getAllResponseHeaders: function() {
return state === 2 ? responseHeadersString : null;
},
// Caches the header
setRequestHeader: function( name, value ) {
var lname = name.toLowerCase();
if ( !state ) {
name = requestHeadersNames[ lname ] = requestHeadersNames[ lname ] || name;
requestHeaders[ name ] = value;
}
return this;
},
// Overrides response content-type header
overrideMimeType: function( type ) {
if ( !state ) {
s.mimeType = type;
}
return this;
},
// Status-dependent callbacks
statusCode: function( map ) {
var code;
if ( map ) {
if ( state < 2 ) {
for ( code in map ) {
// Lazy-add the new callback in a way that preserves old ones
statusCode[ code ] = [ statusCode[ code ], map[ code ] ];
}
} else {
// Execute the appropriate callbacks
jqXHR.always( map[ jqXHR.status ] );
}
}
return this;
},
// Cancel the request
abort: function( statusText ) {
var finalText = statusText || strAbort;
if ( transport ) {
transport.abort( finalText );
}
done( 0, finalText );
return this;
}
};
// Attach deferreds
deferred.promise( jqXHR ).complete = completeDeferred.add;
jqXHR.success = jqXHR.done;
jqXHR.error = jqXHR.fail;
// Remove hash character (#7531: and string promotion)
// Add protocol if not provided (#5866: IE7 issue with protocol-less urls)
// Handle falsy url in the settings object (#10093: consistency with old signature)
// We also use the url parameter if available
s.url = ( ( url || s.url || ajaxLocation ) + "" ).replace( rhash, "" ).replace( rprotocol, ajaxLocParts[ 1 ] + "//" );
// Alias method option to type as per ticket #12004
s.type = options.method || options.type || s.method || s.type;
// Extract dataTypes list
s.dataTypes = jQuery.trim( s.dataType || "*" ).toLowerCase().match( core_rnotwhite ) || [""];
// A cross-domain request is in order when we have a protocol:host:port mismatch
if ( s.crossDomain == null ) {
parts = rurl.exec( s.url.toLowerCase() );
s.crossDomain = !!( parts &&
( parts[ 1 ] !== ajaxLocParts[ 1 ] || parts[ 2 ] !== ajaxLocParts[ 2 ] ||
( parts[ 3 ] || ( parts[ 1 ] === "http:" ? "80" : "443" ) ) !==
( ajaxLocParts[ 3 ] || ( ajaxLocParts[ 1 ] === "http:" ? "80" : "443" ) ) )
);
}
// Convert data if not already a string
if ( s.data && s.processData && typeof s.data !== "string" ) {
s.data = jQuery.param( s.data, s.traditional );
}
// Apply prefilters
inspectPrefiltersOrTransports( prefilters, s, options, jqXHR );
// If request was aborted inside a prefilter, stop there
if ( state === 2 ) {
return jqXHR;
}
// We can fire global events as of now if asked to
fireGlobals = s.global;
// Watch for a new set of requests
if ( fireGlobals && jQuery.active++ === 0 ) {
jQuery.event.trigger("ajaxStart");
}
// Uppercase the type
s.type = s.type.toUpperCase();
// Determine if request has content
s.hasContent = !rnoContent.test( s.type );
// Save the URL in case we're toying with the If-Modified-Since
// and/or If-None-Match header later on
cacheURL = s.url;
// More options handling for requests with no content
if ( !s.hasContent ) {
// If data is available, append data to url
if ( s.data ) {
cacheURL = ( s.url += ( ajax_rquery.test( cacheURL ) ? "&" : "?" ) + s.data );
// #9682: remove data so that it's not used in an eventual retry
delete s.data;
}
// Add anti-cache in url if needed
if ( s.cache === false ) {
s.url = rts.test( cacheURL ) ?
// If there is already a '_' parameter, set its value
cacheURL.replace( rts, "$1_=" + ajax_nonce++ ) :
// Otherwise add one to the end
cacheURL + ( ajax_rquery.test( cacheURL ) ? "&" : "?" ) + "_=" + ajax_nonce++;
}
}
// Set the If-Modified-Since and/or If-None-Match header, if in ifModified mode.
if ( s.ifModified ) {
if ( jQuery.lastModified[ cacheURL ] ) {
jqXHR.setRequestHeader( "If-Modified-Since", jQuery.lastModified[ cacheURL ] );
}
if ( jQuery.etag[ cacheURL ] ) {
jqXHR.setRequestHeader( "If-None-Match", jQuery.etag[ cacheURL ] );
}
}
// Set the correct header, if data is being sent
if ( s.data && s.hasContent && s.contentType !== false || options.contentType ) {
jqXHR.setRequestHeader( "Content-Type", s.contentType );
}
// Set the Accepts header for the server, depending on the dataType
jqXHR.setRequestHeader(
"Accept",
s.dataTypes[ 0 ] && s.accepts[ s.dataTypes[0] ] ?
s.accepts[ s.dataTypes[0] ] + ( s.dataTypes[ 0 ] !== "*" ? ", " + allTypes + "; q=0.01" : "" ) :
s.accepts[ "*" ]
);
// Check for headers option
for ( i in s.headers ) {
jqXHR.setRequestHeader( i, s.headers[ i ] );
}
// Allow custom headers/mimetypes and early abort
if ( s.beforeSend && ( s.beforeSend.call( callbackContext, jqXHR, s ) === false || state === 2 ) ) {
// Abort if not done already and return
return jqXHR.abort();
}
// aborting is no longer a cancellation
strAbort = "abort";
// Install callbacks on deferreds
for ( i in { success: 1, error: 1, complete: 1 } ) {
jqXHR[ i ]( s[ i ] );
}
// Get transport
transport = inspectPrefiltersOrTransports( transports, s, options, jqXHR );
// If no transport, we auto-abort
if ( !transport ) {
done( -1, "No Transport" );
} else {
jqXHR.readyState = 1;
// Send global event
if ( fireGlobals ) {
globalEventContext.trigger( "ajaxSend", [ jqXHR, s ] );
}
// Timeout
if ( s.async && s.timeout > 0 ) {
timeoutTimer = setTimeout(function() {
jqXHR.abort("timeout");
}, s.timeout );
}
try {
state = 1;
transport.send( requestHeaders, done );
} catch ( e ) {
// Propagate exception as error if not done
if ( state < 2 ) {
done( -1, e );
// Simply rethrow otherwise
} else {
throw e;
}
}
}
// Callback for when everything is done
function done( status, nativeStatusText, responses, headers ) {
var isSuccess, success, error, response, modified,
statusText = nativeStatusText;
// Called once
if ( state === 2 ) {
return;
}
// State is "done" now
state = 2;
// Clear timeout if it exists
if ( timeoutTimer ) {
clearTimeout( timeoutTimer );
}
// Dereference transport for early garbage collection
// (no matter how long the jqXHR object will be used)
transport = undefined;
// Cache response headers
responseHeadersString = headers || "";
// Set readyState
jqXHR.readyState = status > 0 ? 4 : 0;
// Determine if successful
isSuccess = status >= 200 && status < 300 || status === 304;
// Get response data
if ( responses ) {
response = ajaxHandleResponses( s, jqXHR, responses );
}
// Convert no matter what (that way responseXXX fields are always set)
response = ajaxConvert( s, response, jqXHR, isSuccess );
// If successful, handle type chaining
if ( isSuccess ) {
// Set the If-Modified-Since and/or If-None-Match header, if in ifModified mode.
if ( s.ifModified ) {
modified = jqXHR.getResponseHeader("Last-Modified");
if ( modified ) {
jQuery.lastModified[ cacheURL ] = modified;
}
modified = jqXHR.getResponseHeader("etag");
if ( modified ) {
jQuery.etag[ cacheURL ] = modified;
}
}
// if no content
if ( status === 204 || s.type === "HEAD" ) {
statusText = "nocontent";
// if not modified
} else if ( status === 304 ) {
statusText = "notmodified";
// If we have data, let's convert it
} else {
statusText = response.state;
success = response.data;
error = response.error;
isSuccess = !error;
}
} else {
// We extract error from statusText
// then normalize statusText and status for non-aborts
error = statusText;
if ( status || !statusText ) {
statusText = "error";
if ( status < 0 ) {
status = 0;
}
}
}
// Set data for the fake xhr object
jqXHR.status = status;
jqXHR.statusText = ( nativeStatusText || statusText ) + "";
// Success/Error
if ( isSuccess ) {
deferred.resolveWith( callbackContext, [ success, statusText, jqXHR ] );
} else {
deferred.rejectWith( callbackContext, [ jqXHR, statusText, error ] );
}
// Status-dependent callbacks
jqXHR.statusCode( statusCode );
statusCode = undefined;
if ( fireGlobals ) {
globalEventContext.trigger( isSuccess ? "ajaxSuccess" : "ajaxError",
[ jqXHR, s, isSuccess ? success : error ] );
}
// Complete
completeDeferred.fireWith( callbackContext, [ jqXHR, statusText ] );
if ( fireGlobals ) {
globalEventContext.trigger( "ajaxComplete", [ jqXHR, s ] );
// Handle the global AJAX counter
if ( !( --jQuery.active ) ) {
jQuery.event.trigger("ajaxStop");
}
}
}
return jqXHR;
},
getJSON: function( url, data, callback ) {
return jQuery.get( url, data, callback, "json" );
},
getScript: function( url, callback ) {
return jQuery.get( url, undefined, callback, "script" );
}
});
jQuery.each( [ "get", "post" ], function( i, method ) {
jQuery[ method ] = function( url, data, callback, type ) {
// shift arguments if data argument was omitted
if ( jQuery.isFunction( data ) ) {
type = type || callback;
callback = data;
data = undefined;
}
return jQuery.ajax({
url: url,
type: method,
dataType: type,
data: data,
success: callback
});
};
});
/* Handles responses to an ajax request:
* - finds the right dataType (mediates between content-type and expected dataType)
* - returns the corresponding response
*/
function ajaxHandleResponses( s, jqXHR, responses ) {
var firstDataType, ct, finalDataType, type,
contents = s.contents,
dataTypes = s.dataTypes;
// Remove auto dataType and get content-type in the process
while( dataTypes[ 0 ] === "*" ) {
dataTypes.shift();
if ( ct === undefined ) {
ct = s.mimeType || jqXHR.getResponseHeader("Content-Type");
}
}
// Check if we're dealing with a known content-type
if ( ct ) {
for ( type in contents ) {
if ( contents[ type ] && contents[ type ].test( ct ) ) {
dataTypes.unshift( type );
break;
}
}
}
// Check to see if we have a response for the expected dataType
if ( dataTypes[ 0 ] in responses ) {
finalDataType = dataTypes[ 0 ];
} else {
// Try convertible dataTypes
for ( type in responses ) {
if ( !dataTypes[ 0 ] || s.converters[ type + " " + dataTypes[0] ] ) {
finalDataType = type;
break;
}
if ( !firstDataType ) {
firstDataType = type;
}
}
// Or just use first one
finalDataType = finalDataType || firstDataType;
}
// If we found a dataType
// We add the dataType to the list if needed
// and return the corresponding response
if ( finalDataType ) {
if ( finalDataType !== dataTypes[ 0 ] ) {
dataTypes.unshift( finalDataType );
}
return responses[ finalDataType ];
}
}
/* Chain conversions given the request and the original response
* Also sets the responseXXX fields on the jqXHR instance
*/
function ajaxConvert( s, response, jqXHR, isSuccess ) {
var conv2, current, conv, tmp, prev,
converters = {},
// Work with a copy of dataTypes in case we need to modify it for conversion
dataTypes = s.dataTypes.slice();
// Create converters map with lowercased keys
if ( dataTypes[ 1 ] ) {
for ( conv in s.converters ) {
converters[ conv.toLowerCase() ] = s.converters[ conv ];
}
}
current = dataTypes.shift();
// Convert to each sequential dataType
while ( current ) {
if ( s.responseFields[ current ] ) {
jqXHR[ s.responseFields[ current ] ] = response;
}
// Apply the dataFilter if provided
if ( !prev && isSuccess && s.dataFilter ) {
response = s.dataFilter( response, s.dataType );
}
prev = current;
current = dataTypes.shift();
if ( current ) {
// There's only work to do if current dataType is non-auto
if ( current === "*" ) {
current = prev;
// Convert response if prev dataType is non-auto and differs from current
} else if ( prev !== "*" && prev !== current ) {
// Seek a direct converter
conv = converters[ prev + " " + current ] || converters[ "* " + current ];
// If none found, seek a pair
if ( !conv ) {
for ( conv2 in converters ) {
// If conv2 outputs current
tmp = conv2.split( " " );
if ( tmp[ 1 ] === current ) {
// If prev can be converted to accepted input
conv = converters[ prev + " " + tmp[ 0 ] ] ||
converters[ "* " + tmp[ 0 ] ];
if ( conv ) {
// Condense equivalence converters
if ( conv === true ) {
conv = converters[ conv2 ];
// Otherwise, insert the intermediate dataType
} else if ( converters[ conv2 ] !== true ) {
current = tmp[ 0 ];
dataTypes.unshift( tmp[ 1 ] );
}
break;
}
}
}
}
// Apply converter (if not an equivalence)
if ( conv !== true ) {
// Unless errors are allowed to bubble, catch and return them
if ( conv && s[ "throws" ] ) {
response = conv( response );
} else {
try {
response = conv( response );
} catch ( e ) {
return { state: "parsererror", error: conv ? e : "No conversion from " + prev + " to " + current };
}
}
}
}
}
}
return { state: "success", data: response };
}
// Install script dataType
jQuery.ajaxSetup({
accepts: {
script: "text/javascript, application/javascript, application/ecmascript, application/x-ecmascript"
},
contents: {
script: /(?:java|ecma)script/
},
converters: {
"text script": function( text ) {
jQuery.globalEval( text );
return text;
}
}
});
// Handle cache's special case and global
jQuery.ajaxPrefilter( "script", function( s ) {
if ( s.cache === undefined ) {
s.cache = false;
}
if ( s.crossDomain ) {
s.type = "GET";
s.global = false;
}
});
// Bind script tag hack transport
jQuery.ajaxTransport( "script", function(s) {
// This transport only deals with cross domain requests
if ( s.crossDomain ) {
var script,
head = document.head || jQuery("head")[0] || document.documentElement;
return {
send: function( _, callback ) {
script = document.createElement("script");
script.async = true;
if ( s.scriptCharset ) {
script.charset = s.scriptCharset;
}
script.src = s.url;
// Attach handlers for all browsers
script.onload = script.onreadystatechange = function( _, isAbort ) {
if ( isAbort || !script.readyState || /loaded|complete/.test( script.readyState ) ) {
// Handle memory leak in IE
script.onload = script.onreadystatechange = null;
// Remove the script
if ( script.parentNode ) {
script.parentNode.removeChild( script );
}
// Dereference the script
script = null;
// Callback if not abort
if ( !isAbort ) {
callback( 200, "success" );
}
}
};
// Circumvent IE6 bugs with base elements (#2709 and #4378) by prepending
// Use native DOM manipulation to avoid our domManip AJAX trickery
head.insertBefore( script, head.firstChild );
},
abort: function() {
if ( script ) {
script.onload( undefined, true );
}
}
};
}
});
var oldCallbacks = [],
rjsonp = /(=)\?(?=&|$)|\?\?/;
// Default jsonp settings
jQuery.ajaxSetup({
jsonp: "callback",
jsonpCallback: function() {
var callback = oldCallbacks.pop() || ( jQuery.expando + "_" + ( ajax_nonce++ ) );
this[ callback ] = true;
return callback;
}
});
// Detect, normalize options and install callbacks for jsonp requests
jQuery.ajaxPrefilter( "json jsonp", function( s, originalSettings, jqXHR ) {
var callbackName, overwritten, responseContainer,
jsonProp = s.jsonp !== false && ( rjsonp.test( s.url ) ?
"url" :
typeof s.data === "string" && !( s.contentType || "" ).indexOf("application/x-www-form-urlencoded") && rjsonp.test( s.data ) && "data"
);
// Handle iff the expected data type is "jsonp" or we have a parameter to set
if ( jsonProp || s.dataTypes[ 0 ] === "jsonp" ) {
// Get callback name, remembering preexisting value associated with it
callbackName = s.jsonpCallback = jQuery.isFunction( s.jsonpCallback ) ?
s.jsonpCallback() :
s.jsonpCallback;
// Insert callback into url or form data
if ( jsonProp ) {
s[ jsonProp ] = s[ jsonProp ].replace( rjsonp, "$1" + callbackName );
} else if ( s.jsonp !== false ) {
s.url += ( ajax_rquery.test( s.url ) ? "&" : "?" ) + s.jsonp + "=" + callbackName;
}
// Use data converter to retrieve json after script execution
s.converters["script json"] = function() {
if ( !responseContainer ) {
jQuery.error( callbackName + " was not called" );
}
return responseContainer[ 0 ];
};
// force json dataType
s.dataTypes[ 0 ] = "json";
// Install callback
overwritten = window[ callbackName ];
window[ callbackName ] = function() {
responseContainer = arguments;
};
// Clean-up function (fires after converters)
jqXHR.always(function() {
// Restore preexisting value
window[ callbackName ] = overwritten;
// Save back as free
if ( s[ callbackName ] ) {
// make sure that re-using the options doesn't screw things around
s.jsonpCallback = originalSettings.jsonpCallback;
// save the callback name for future use
oldCallbacks.push( callbackName );
}
// Call if it was a function and we have a response
if ( responseContainer && jQuery.isFunction( overwritten ) ) {
overwritten( responseContainer[ 0 ] );
}
responseContainer = overwritten = undefined;
});
// Delegate to script
return "script";
}
});
var xhrCallbacks, xhrSupported,
xhrId = 0,
// #5280: Internet Explorer will keep connections alive if we don't abort on unload
xhrOnUnloadAbort = window.ActiveXObject && function() {
// Abort all pending requests
var key;
for ( key in xhrCallbacks ) {
xhrCallbacks[ key ]( undefined, true );
}
};
// Functions to create xhrs
function createStandardXHR() {
try {
return new window.XMLHttpRequest();
} catch( e ) {}
}
function createActiveXHR() {
try {
return new window.ActiveXObject("Microsoft.XMLHTTP");
} catch( e ) {}
}
// Create the request object
// (This is still attached to ajaxSettings for backward compatibility)
jQuery.ajaxSettings.xhr = window.ActiveXObject ?
/* Microsoft failed to properly
* implement the XMLHttpRequest in IE7 (can't request local files),
* so we use the ActiveXObject when it is available
* Additionally XMLHttpRequest can be disabled in IE7/IE8 so
* we need a fallback.
*/
function() {
return !this.isLocal && createStandardXHR() || createActiveXHR();
} :
// For all other browsers, use the standard XMLHttpRequest object
createStandardXHR;
// Determine support properties
xhrSupported = jQuery.ajaxSettings.xhr();
jQuery.support.cors = !!xhrSupported && ( "withCredentials" in xhrSupported );
xhrSupported = jQuery.support.ajax = !!xhrSupported;
// Create transport if the browser can provide an xhr
if ( xhrSupported ) {
jQuery.ajaxTransport(function( s ) {
// Cross domain only allowed if supported through XMLHttpRequest
if ( !s.crossDomain || jQuery.support.cors ) {
var callback;
return {
send: function( headers, complete ) {
// Get a new xhr
var handle, i,
xhr = s.xhr();
// Open the socket
// Passing null username, generates a login popup on Opera (#2865)
if ( s.username ) {
xhr.open( s.type, s.url, s.async, s.username, s.password );
} else {
xhr.open( s.type, s.url, s.async );
}
// Apply custom fields if provided
if ( s.xhrFields ) {
for ( i in s.xhrFields ) {
xhr[ i ] = s.xhrFields[ i ];
}
}
// Override mime type if needed
if ( s.mimeType && xhr.overrideMimeType ) {
xhr.overrideMimeType( s.mimeType );
}
// X-Requested-With header
// For cross-domain requests, seeing as conditions for a preflight are
// akin to a jigsaw puzzle, we simply never set it to be sure.
// (it can always be set on a per-request basis or even using ajaxSetup)
// For same-domain requests, won't change header if already provided.
if ( !s.crossDomain && !headers["X-Requested-With"] ) {
headers["X-Requested-With"] = "XMLHttpRequest";
}
// Need an extra try/catch for cross domain requests in Firefox 3
try {
for ( i in headers ) {
xhr.setRequestHeader( i, headers[ i ] );
}
} catch( err ) {}
// Do send the request
// This may raise an exception which is actually
// handled in jQuery.ajax (so no try/catch here)
xhr.send( ( s.hasContent && s.data ) || null );
// Listener
callback = function( _, isAbort ) {
var status, responseHeaders, statusText, responses;
// Firefox throws exceptions when accessing properties
// of an xhr when a network error occurred
// http://helpful.knobs-dials.com/index.php/Component_returned_failure_code:_0x80040111_(NS_ERROR_NOT_AVAILABLE)
try {
// Was never called and is aborted or complete
if ( callback && ( isAbort || xhr.readyState === 4 ) ) {
// Only called once
callback = undefined;
// Do not keep as active anymore
if ( handle ) {
xhr.onreadystatechange = jQuery.noop;
if ( xhrOnUnloadAbort ) {
delete xhrCallbacks[ handle ];
}
}
// If it's an abort
if ( isAbort ) {
// Abort it manually if needed
if ( xhr.readyState !== 4 ) {
xhr.abort();
}
} else {
responses = {};
status = xhr.status;
responseHeaders = xhr.getAllResponseHeaders();
// When requesting binary data, IE6-9 will throw an exception
// on any attempt to access responseText (#11426)
if ( typeof xhr.responseText === "string" ) {
responses.text = xhr.responseText;
}
// Firefox throws an exception when accessing
// statusText for faulty cross-domain requests
try {
statusText = xhr.statusText;
} catch( e ) {
// We normalize with Webkit giving an empty statusText
statusText = "";
}
// Filter status for non standard behaviors
// If the request is local and we have data: assume a success
// (success with no data won't get notified, that's the best we
// can do given current implementations)
if ( !status && s.isLocal && !s.crossDomain ) {
status = responses.text ? 200 : 404;
// IE - #1450: sometimes returns 1223 when it should be 204
} else if ( status === 1223 ) {
status = 204;
}
}
}
} catch( firefoxAccessException ) {
if ( !isAbort ) {
complete( -1, firefoxAccessException );
}
}
// Call complete if needed
if ( responses ) {
complete( status, statusText, responses, responseHeaders );
}
};
if ( !s.async ) {
// if we're in sync mode we fire the callback
callback();
} else if ( xhr.readyState === 4 ) {
// (IE6 & IE7) if it's in cache and has been
// retrieved directly we need to fire the callback
setTimeout( callback );
} else {
handle = ++xhrId;
if ( xhrOnUnloadAbort ) {
// Create the active xhrs callbacks list if needed
// and attach the unload handler
if ( !xhrCallbacks ) {
xhrCallbacks = {};
jQuery( window ).unload( xhrOnUnloadAbort );
}
// Add to list of active xhrs callbacks
xhrCallbacks[ handle ] = callback;
}
xhr.onreadystatechange = callback;
}
},
abort: function() {
if ( callback ) {
callback( undefined, true );
}
}
};
}
});
}
var fxNow, timerId,
rfxtypes = /^(?:toggle|show|hide)$/,
rfxnum = new RegExp( "^(?:([+-])=|)(" + core_pnum + ")([a-z%]*)$", "i" ),
rrun = /queueHooks$/,
animationPrefilters = [ defaultPrefilter ],
tweeners = {
"*": [function( prop, value ) {
var tween = this.createTween( prop, value ),
target = tween.cur(),
parts = rfxnum.exec( value ),
unit = parts && parts[ 3 ] || ( jQuery.cssNumber[ prop ] ? "" : "px" ),
// Starting value computation is required for potential unit mismatches
start = ( jQuery.cssNumber[ prop ] || unit !== "px" && +target ) &&
rfxnum.exec( jQuery.css( tween.elem, prop ) ),
scale = 1,
maxIterations = 20;
if ( start && start[ 3 ] !== unit ) {
// Trust units reported by jQuery.css
unit = unit || start[ 3 ];
// Make sure we update the tween properties later on
parts = parts || [];
// Iteratively approximate from a nonzero starting point
start = +target || 1;
do {
// If previous iteration zeroed out, double until we get *something*
// Use a string for doubling factor so we don't accidentally see scale as unchanged below
scale = scale || ".5";
// Adjust and apply
start = start / scale;
jQuery.style( tween.elem, prop, start + unit );
// Update scale, tolerating zero or NaN from tween.cur()
// And breaking the loop if scale is unchanged or perfect, or if we've just had enough
} while ( scale !== (scale = tween.cur() / target) && scale !== 1 && --maxIterations );
}
// Update tween properties
if ( parts ) {
start = tween.start = +start || +target || 0;
tween.unit = unit;
// If a +=/-= token was provided, we're doing a relative animation
tween.end = parts[ 1 ] ?
start + ( parts[ 1 ] + 1 ) * parts[ 2 ] :
+parts[ 2 ];
}
return tween;
}]
};
// Animations created synchronously will run synchronously
function createFxNow() {
setTimeout(function() {
fxNow = undefined;
});
return ( fxNow = jQuery.now() );
}
function createTween( value, prop, animation ) {
var tween,
collection = ( tweeners[ prop ] || [] ).concat( tweeners[ "*" ] ),
index = 0,
length = collection.length;
for ( ; index < length; index++ ) {
if ( (tween = collection[ index ].call( animation, prop, value )) ) {
// we're done with this property
return tween;
}
}
}
function Animation( elem, properties, options ) {
var result,
stopped,
index = 0,
length = animationPrefilters.length,
deferred = jQuery.Deferred().always( function() {
// don't match elem in the :animated selector
delete tick.elem;
}),
tick = function() {
if ( stopped ) {
return false;
}
var currentTime = fxNow || createFxNow(),
remaining = Math.max( 0, animation.startTime + animation.duration - currentTime ),
// archaic crash bug won't allow us to use 1 - ( 0.5 || 0 ) (#12497)
temp = remaining / animation.duration || 0,
percent = 1 - temp,
index = 0,
length = animation.tweens.length;
for ( ; index < length ; index++ ) {
animation.tweens[ index ].run( percent );
}
deferred.notifyWith( elem, [ animation, percent, remaining ]);
if ( percent < 1 && length ) {
return remaining;
} else {
deferred.resolveWith( elem, [ animation ] );
return false;
}
},
animation = deferred.promise({
elem: elem,
props: jQuery.extend( {}, properties ),
opts: jQuery.extend( true, { specialEasing: {} }, options ),
originalProperties: properties,
originalOptions: options,
startTime: fxNow || createFxNow(),
duration: options.duration,
tweens: [],
createTween: function( prop, end ) {
var tween = jQuery.Tween( elem, animation.opts, prop, end,
animation.opts.specialEasing[ prop ] || animation.opts.easing );
animation.tweens.push( tween );
return tween;
},
stop: function( gotoEnd ) {
var index = 0,
// if we are going to the end, we want to run all the tweens
// otherwise we skip this part
length = gotoEnd ? animation.tweens.length : 0;
if ( stopped ) {
return this;
}
stopped = true;
for ( ; index < length ; index++ ) {
animation.tweens[ index ].run( 1 );
}
// resolve when we played the last frame
// otherwise, reject
if ( gotoEnd ) {
deferred.resolveWith( elem, [ animation, gotoEnd ] );
} else {
deferred.rejectWith( elem, [ animation, gotoEnd ] );
}
return this;
}
}),
props = animation.props;
propFilter( props, animation.opts.specialEasing );
for ( ; index < length ; index++ ) {
result = animationPrefilters[ index ].call( animation, elem, props, animation.opts );
if ( result ) {
return result;
}
}
jQuery.map( props, createTween, animation );
if ( jQuery.isFunction( animation.opts.start ) ) {
animation.opts.start.call( elem, animation );
}
jQuery.fx.timer(
jQuery.extend( tick, {
elem: elem,
anim: animation,
queue: animation.opts.queue
})
);
// attach callbacks from options
return animation.progress( animation.opts.progress )
.done( animation.opts.done, animation.opts.complete )
.fail( animation.opts.fail )
.always( animation.opts.always );
}
function propFilter( props, specialEasing ) {
var index, name, easing, value, hooks;
// camelCase, specialEasing and expand cssHook pass
for ( index in props ) {
name = jQuery.camelCase( index );
easing = specialEasing[ name ];
value = props[ index ];
if ( jQuery.isArray( value ) ) {
easing = value[ 1 ];
value = props[ index ] = value[ 0 ];
}
if ( index !== name ) {
props[ name ] = value;
delete props[ index ];
}
hooks = jQuery.cssHooks[ name ];
if ( hooks && "expand" in hooks ) {
value = hooks.expand( value );
delete props[ name ];
// not quite $.extend, this wont overwrite keys already present.
// also - reusing 'index' from above because we have the correct "name"
for ( index in value ) {
if ( !( index in props ) ) {
props[ index ] = value[ index ];
specialEasing[ index ] = easing;
}
}
} else {
specialEasing[ name ] = easing;
}
}
}
jQuery.Animation = jQuery.extend( Animation, {
tweener: function( props, callback ) {
if ( jQuery.isFunction( props ) ) {
callback = props;
props = [ "*" ];
} else {
props = props.split(" ");
}
var prop,
index = 0,
length = props.length;
for ( ; index < length ; index++ ) {
prop = props[ index ];
tweeners[ prop ] = tweeners[ prop ] || [];
tweeners[ prop ].unshift( callback );
}
},
prefilter: function( callback, prepend ) {
if ( prepend ) {
animationPrefilters.unshift( callback );
} else {
animationPrefilters.push( callback );
}
}
});
function defaultPrefilter( elem, props, opts ) {
/* jshint validthis: true */
var prop, value, toggle, tween, hooks, oldfire,
anim = this,
orig = {},
style = elem.style,
hidden = elem.nodeType && isHidden( elem ),
dataShow = jQuery._data( elem, "fxshow" );
// handle queue: false promises
if ( !opts.queue ) {
hooks = jQuery._queueHooks( elem, "fx" );
if ( hooks.unqueued == null ) {
hooks.unqueued = 0;
oldfire = hooks.empty.fire;
hooks.empty.fire = function() {
if ( !hooks.unqueued ) {
oldfire();
}
};
}
hooks.unqueued++;
anim.always(function() {
// doing this makes sure that the complete handler will be called
// before this completes
anim.always(function() {
hooks.unqueued--;
if ( !jQuery.queue( elem, "fx" ).length ) {
hooks.empty.fire();
}
});
});
}
// height/width overflow pass
if ( elem.nodeType === 1 && ( "height" in props || "width" in props ) ) {
// Make sure that nothing sneaks out
// Record all 3 overflow attributes because IE does not
// change the overflow attribute when overflowX and
// overflowY are set to the same value
opts.overflow = [ style.overflow, style.overflowX, style.overflowY ];
// Set display property to inline-block for height/width
// animations on inline elements that are having width/height animated
if ( jQuery.css( elem, "display" ) === "inline" &&
jQuery.css( elem, "float" ) === "none" ) {
// inline-level elements accept inline-block;
// block-level elements need to be inline with layout
if ( !jQuery.support.inlineBlockNeedsLayout || css_defaultDisplay( elem.nodeName ) === "inline" ) {
style.display = "inline-block";
} else {
style.zoom = 1;
}
}
}
if ( opts.overflow ) {
style.overflow = "hidden";
if ( !jQuery.support.shrinkWrapBlocks ) {
anim.always(function() {
style.overflow = opts.overflow[ 0 ];
style.overflowX = opts.overflow[ 1 ];
style.overflowY = opts.overflow[ 2 ];
});
}
}
// show/hide pass
for ( prop in props ) {
value = props[ prop ];
if ( rfxtypes.exec( value ) ) {
delete props[ prop ];
toggle = toggle || value === "toggle";
if ( value === ( hidden ? "hide" : "show" ) ) {
continue;
}
orig[ prop ] = dataShow && dataShow[ prop ] || jQuery.style( elem, prop );
}
}
if ( !jQuery.isEmptyObject( orig ) ) {
if ( dataShow ) {
if ( "hidden" in dataShow ) {
hidden = dataShow.hidden;
}
} else {
dataShow = jQuery._data( elem, "fxshow", {} );
}
// store state if its toggle - enables .stop().toggle() to "reverse"
if ( toggle ) {
dataShow.hidden = !hidden;
}
if ( hidden ) {
jQuery( elem ).show();
} else {
anim.done(function() {
jQuery( elem ).hide();
});
}
anim.done(function() {
var prop;
jQuery._removeData( elem, "fxshow" );
for ( prop in orig ) {
jQuery.style( elem, prop, orig[ prop ] );
}
});
for ( prop in orig ) {
tween = createTween( hidden ? dataShow[ prop ] : 0, prop, anim );
if ( !( prop in dataShow ) ) {
dataShow[ prop ] = tween.start;
if ( hidden ) {
tween.end = tween.start;
tween.start = prop === "width" || prop === "height" ? 1 : 0;
}
}
}
}
}
function Tween( elem, options, prop, end, easing ) {
return new Tween.prototype.init( elem, options, prop, end, easing );
}
jQuery.Tween = Tween;
Tween.prototype = {
constructor: Tween,
init: function( elem, options, prop, end, easing, unit ) {
this.elem = elem;
this.prop = prop;
this.easing = easing || "swing";
this.options = options;
this.start = this.now = this.cur();
this.end = end;
this.unit = unit || ( jQuery.cssNumber[ prop ] ? "" : "px" );
},
cur: function() {
var hooks = Tween.propHooks[ this.prop ];
return hooks && hooks.get ?
hooks.get( this ) :
Tween.propHooks._default.get( this );
},
run: function( percent ) {
var eased,
hooks = Tween.propHooks[ this.prop ];
if ( this.options.duration ) {
this.pos = eased = jQuery.easing[ this.easing ](
percent, this.options.duration * percent, 0, 1, this.options.duration
);
} else {
this.pos = eased = percent;
}
this.now = ( this.end - this.start ) * eased + this.start;
if ( this.options.step ) {
this.options.step.call( this.elem, this.now, this );
}
if ( hooks && hooks.set ) {
hooks.set( this );
} else {
Tween.propHooks._default.set( this );
}
return this;
}
};
Tween.prototype.init.prototype = Tween.prototype;
Tween.propHooks = {
_default: {
get: function( tween ) {
var result;
if ( tween.elem[ tween.prop ] != null &&
(!tween.elem.style || tween.elem.style[ tween.prop ] == null) ) {
return tween.elem[ tween.prop ];
}
// passing an empty string as a 3rd parameter to .css will automatically
// attempt a parseFloat and fallback to a string if the parse fails
// so, simple values such as "10px" are parsed to Float.
// complex values such as "rotate(1rad)" are returned as is.
result = jQuery.css( tween.elem, tween.prop, "" );
// Empty strings, null, undefined and "auto" are converted to 0.
return !result || result === "auto" ? 0 : result;
},
set: function( tween ) {
// use step hook for back compat - use cssHook if its there - use .style if its
// available and use plain properties where available
if ( jQuery.fx.step[ tween.prop ] ) {
jQuery.fx.step[ tween.prop ]( tween );
} else if ( tween.elem.style && ( tween.elem.style[ jQuery.cssProps[ tween.prop ] ] != null || jQuery.cssHooks[ tween.prop ] ) ) {
jQuery.style( tween.elem, tween.prop, tween.now + tween.unit );
} else {
tween.elem[ tween.prop ] = tween.now;
}
}
}
};
// Support: IE <=9
// Panic based approach to setting things on disconnected nodes
Tween.propHooks.scrollTop = Tween.propHooks.scrollLeft = {
set: function( tween ) {
if ( tween.elem.nodeType && tween.elem.parentNode ) {
tween.elem[ tween.prop ] = tween.now;
}
}
};
jQuery.each([ "toggle", "show", "hide" ], function( i, name ) {
var cssFn = jQuery.fn[ name ];
jQuery.fn[ name ] = function( speed, easing, callback ) {
return speed == null || typeof speed === "boolean" ?
cssFn.apply( this, arguments ) :
this.animate( genFx( name, true ), speed, easing, callback );
};
});
jQuery.fn.extend({
fadeTo: function( speed, to, easing, callback ) {
// show any hidden elements after setting opacity to 0
return this.filter( isHidden ).css( "opacity", 0 ).show()
// animate to the value specified
.end().animate({ opacity: to }, speed, easing, callback );
},
animate: function( prop, speed, easing, callback ) {
var empty = jQuery.isEmptyObject( prop ),
optall = jQuery.speed( speed, easing, callback ),
doAnimation = function() {
// Operate on a copy of prop so per-property easing won't be lost
var anim = Animation( this, jQuery.extend( {}, prop ), optall );
// Empty animations, or finishing resolves immediately
if ( empty || jQuery._data( this, "finish" ) ) {
anim.stop( true );
}
};
doAnimation.finish = doAnimation;
return empty || optall.queue === false ?
this.each( doAnimation ) :
this.queue( optall.queue, doAnimation );
},
stop: function( type, clearQueue, gotoEnd ) {
var stopQueue = function( hooks ) {
var stop = hooks.stop;
delete hooks.stop;
stop( gotoEnd );
};
if ( typeof type !== "string" ) {
gotoEnd = clearQueue;
clearQueue = type;
type = undefined;
}
if ( clearQueue && type !== false ) {
this.queue( type || "fx", [] );
}
return this.each(function() {
var dequeue = true,
index = type != null && type + "queueHooks",
timers = jQuery.timers,
data = jQuery._data( this );
if ( index ) {
if ( data[ index ] && data[ index ].stop ) {
stopQueue( data[ index ] );
}
} else {
for ( index in data ) {
if ( data[ index ] && data[ index ].stop && rrun.test( index ) ) {
stopQueue( data[ index ] );
}
}
}
for ( index = timers.length; index--; ) {
if ( timers[ index ].elem === this && (type == null || timers[ index ].queue === type) ) {
timers[ index ].anim.stop( gotoEnd );
dequeue = false;
timers.splice( index, 1 );
}
}
// start the next in the queue if the last step wasn't forced
// timers currently will call their complete callbacks, which will dequeue
// but only if they were gotoEnd
if ( dequeue || !gotoEnd ) {
jQuery.dequeue( this, type );
}
});
},
finish: function( type ) {
if ( type !== false ) {
type = type || "fx";
}
return this.each(function() {
var index,
data = jQuery._data( this ),
queue = data[ type + "queue" ],
hooks = data[ type + "queueHooks" ],
timers = jQuery.timers,
length = queue ? queue.length : 0;
// enable finishing flag on private data
data.finish = true;
// empty the queue first
jQuery.queue( this, type, [] );
if ( hooks && hooks.stop ) {
hooks.stop.call( this, true );
}
// look for any active animations, and finish them
for ( index = timers.length; index--; ) {
if ( timers[ index ].elem === this && timers[ index ].queue === type ) {
timers[ index ].anim.stop( true );
timers.splice( index, 1 );
}
}
// look for any animations in the old queue and finish them
for ( index = 0; index < length; index++ ) {
if ( queue[ index ] && queue[ index ].finish ) {
queue[ index ].finish.call( this );
}
}
// turn off finishing flag
delete data.finish;
});
}
});
// Generate parameters to create a standard animation
function genFx( type, includeWidth ) {
var which,
attrs = { height: type },
i = 0;
// if we include width, step value is 1 to do all cssExpand values,
// if we don't include width, step value is 2 to skip over Left and Right
includeWidth = includeWidth? 1 : 0;
for( ; i < 4 ; i += 2 - includeWidth ) {
which = cssExpand[ i ];
attrs[ "margin" + which ] = attrs[ "padding" + which ] = type;
}
if ( includeWidth ) {
attrs.opacity = attrs.width = type;
}
return attrs;
}
// Generate shortcuts for custom animations
jQuery.each({
slideDown: genFx("show"),
slideUp: genFx("hide"),
slideToggle: genFx("toggle"),
fadeIn: { opacity: "show" },
fadeOut: { opacity: "hide" },
fadeToggle: { opacity: "toggle" }
}, function( name, props ) {
jQuery.fn[ name ] = function( speed, easing, callback ) {
return this.animate( props, speed, easing, callback );
};
});
jQuery.speed = function( speed, easing, fn ) {
var opt = speed && typeof speed === "object" ? jQuery.extend( {}, speed ) : {
complete: fn || !fn && easing ||
jQuery.isFunction( speed ) && speed,
duration: speed,
easing: fn && easing || easing && !jQuery.isFunction( easing ) && easing
};
opt.duration = jQuery.fx.off ? 0 : typeof opt.duration === "number" ? opt.duration :
opt.duration in jQuery.fx.speeds ? jQuery.fx.speeds[ opt.duration ] : jQuery.fx.speeds._default;
// normalize opt.queue - true/undefined/null -> "fx"
if ( opt.queue == null || opt.queue === true ) {
opt.queue = "fx";
}
// Queueing
opt.old = opt.complete;
opt.complete = function() {
if ( jQuery.isFunction( opt.old ) ) {
opt.old.call( this );
}
if ( opt.queue ) {
jQuery.dequeue( this, opt.queue );
}
};
return opt;
};
jQuery.easing = {
linear: function( p ) {
return p;
},
swing: function( p ) {
return 0.5 - Math.cos( p*Math.PI ) / 2;
}
};
jQuery.timers = [];
jQuery.fx = Tween.prototype.init;
jQuery.fx.tick = function() {
var timer,
timers = jQuery.timers,
i = 0;
fxNow = jQuery.now();
for ( ; i < timers.length; i++ ) {
timer = timers[ i ];
// Checks the timer has not already been removed
if ( !timer() && timers[ i ] === timer ) {
timers.splice( i--, 1 );
}
}
if ( !timers.length ) {
jQuery.fx.stop();
}
fxNow = undefined;
};
jQuery.fx.timer = function( timer ) {
if ( timer() && jQuery.timers.push( timer ) ) {
jQuery.fx.start();
}
};
jQuery.fx.interval = 13;
jQuery.fx.start = function() {
if ( !timerId ) {
timerId = setInterval( jQuery.fx.tick, jQuery.fx.interval );
}
};
jQuery.fx.stop = function() {
clearInterval( timerId );
timerId = null;
};
jQuery.fx.speeds = {
slow: 600,
fast: 200,
// Default speed
_default: 400
};
// Back Compat <1.8 extension point
jQuery.fx.step = {};
if ( jQuery.expr && jQuery.expr.filters ) {
jQuery.expr.filters.animated = function( elem ) {
return jQuery.grep(jQuery.timers, function( fn ) {
return elem === fn.elem;
}).length;
};
}
jQuery.fn.offset = function( options ) {
if ( arguments.length ) {
return options === undefined ?
this :
this.each(function( i ) {
jQuery.offset.setOffset( this, options, i );
});
}
var docElem, win,
box = { top: 0, left: 0 },
elem = this[ 0 ],
doc = elem && elem.ownerDocument;
if ( !doc ) {
return;
}
docElem = doc.documentElement;
// Make sure it's not a disconnected DOM node
if ( !jQuery.contains( docElem, elem ) ) {
return box;
}
// If we don't have gBCR, just use 0,0 rather than error
// BlackBerry 5, iOS 3 (original iPhone)
if ( typeof elem.getBoundingClientRect !== core_strundefined ) {
box = elem.getBoundingClientRect();
}
win = getWindow( doc );
return {
top: box.top + ( win.pageYOffset || docElem.scrollTop ) - ( docElem.clientTop || 0 ),
left: box.left + ( win.pageXOffset || docElem.scrollLeft ) - ( docElem.clientLeft || 0 )
};
};
jQuery.offset = {
setOffset: function( elem, options, i ) {
var position = jQuery.css( elem, "position" );
// set position first, in-case top/left are set even on static elem
if ( position === "static" ) {
elem.style.position = "relative";
}
var curElem = jQuery( elem ),
curOffset = curElem.offset(),
curCSSTop = jQuery.css( elem, "top" ),
curCSSLeft = jQuery.css( elem, "left" ),
calculatePosition = ( position === "absolute" || position === "fixed" ) && jQuery.inArray("auto", [curCSSTop, curCSSLeft]) > -1,
props = {}, curPosition = {}, curTop, curLeft;
// need to be able to calculate position if either top or left is auto and position is either absolute or fixed
if ( calculatePosition ) {
curPosition = curElem.position();
curTop = curPosition.top;
curLeft = curPosition.left;
} else {
curTop = parseFloat( curCSSTop ) || 0;
curLeft = parseFloat( curCSSLeft ) || 0;
}
if ( jQuery.isFunction( options ) ) {
options = options.call( elem, i, curOffset );
}
if ( options.top != null ) {
props.top = ( options.top - curOffset.top ) + curTop;
}
if ( options.left != null ) {
props.left = ( options.left - curOffset.left ) + curLeft;
}
if ( "using" in options ) {
options.using.call( elem, props );
} else {
curElem.css( props );
}
}
};
jQuery.fn.extend({
position: function() {
if ( !this[ 0 ] ) {
return;
}
var offsetParent, offset,
parentOffset = { top: 0, left: 0 },
elem = this[ 0 ];
// fixed elements are offset from window (parentOffset = {top:0, left: 0}, because it is it's only offset parent
if ( jQuery.css( elem, "position" ) === "fixed" ) {
// we assume that getBoundingClientRect is available when computed position is fixed
offset = elem.getBoundingClientRect();
} else {
// Get *real* offsetParent
offsetParent = this.offsetParent();
// Get correct offsets
offset = this.offset();
if ( !jQuery.nodeName( offsetParent[ 0 ], "html" ) ) {
parentOffset = offsetParent.offset();
}
// Add offsetParent borders
parentOffset.top += jQuery.css( offsetParent[ 0 ], "borderTopWidth", true );
parentOffset.left += jQuery.css( offsetParent[ 0 ], "borderLeftWidth", true );
}
// Subtract parent offsets and element margins
// note: when an element has margin: auto the offsetLeft and marginLeft
// are the same in Safari causing offset.left to incorrectly be 0
return {
top: offset.top - parentOffset.top - jQuery.css( elem, "marginTop", true ),
left: offset.left - parentOffset.left - jQuery.css( elem, "marginLeft", true)
};
},
offsetParent: function() {
return this.map(function() {
var offsetParent = this.offsetParent || docElem;
while ( offsetParent && ( !jQuery.nodeName( offsetParent, "html" ) && jQuery.css( offsetParent, "position") === "static" ) ) {
offsetParent = offsetParent.offsetParent;
}
return offsetParent || docElem;
});
}
});
// Create scrollLeft and scrollTop methods
jQuery.each( {scrollLeft: "pageXOffset", scrollTop: "pageYOffset"}, function( method, prop ) {
var top = /Y/.test( prop );
jQuery.fn[ method ] = function( val ) {
return jQuery.access( this, function( elem, method, val ) {
var win = getWindow( elem );
if ( val === undefined ) {
return win ? (prop in win) ? win[ prop ] :
win.document.documentElement[ method ] :
elem[ method ];
}
if ( win ) {
win.scrollTo(
!top ? val : jQuery( win ).scrollLeft(),
top ? val : jQuery( win ).scrollTop()
);
} else {
elem[ method ] = val;
}
}, method, val, arguments.length, null );
};
});
function getWindow( elem ) {
return jQuery.isWindow( elem ) ?
elem :
elem.nodeType === 9 ?
elem.defaultView || elem.parentWindow :
false;
}
// Create innerHeight, innerWidth, height, width, outerHeight and outerWidth methods
jQuery.each( { Height: "height", Width: "width" }, function( name, type ) {
jQuery.each( { padding: "inner" + name, content: type, "": "outer" + name }, function( defaultExtra, funcName ) {
// margin is only for outerHeight, outerWidth
jQuery.fn[ funcName ] = function( margin, value ) {
var chainable = arguments.length && ( defaultExtra || typeof margin !== "boolean" ),
extra = defaultExtra || ( margin === true || value === true ? "margin" : "border" );
return jQuery.access( this, function( elem, type, value ) {
var doc;
if ( jQuery.isWindow( elem ) ) {
// As of 5/8/2012 this will yield incorrect results for Mobile Safari, but there
// isn't a whole lot we can do. See pull request at this URL for discussion:
// https://github.com/jquery/jquery/pull/764
return elem.document.documentElement[ "client" + name ];
}
// Get document width or height
if ( elem.nodeType === 9 ) {
doc = elem.documentElement;
// Either scroll[Width/Height] or offset[Width/Height] or client[Width/Height], whichever is greatest
// unfortunately, this causes bug #3838 in IE6/8 only, but there is currently no good, small way to fix it.
return Math.max(
elem.body[ "scroll" + name ], doc[ "scroll" + name ],
elem.body[ "offset" + name ], doc[ "offset" + name ],
doc[ "client" + name ]
);
}
return value === undefined ?
// Get width or height on the element, requesting but not forcing parseFloat
jQuery.css( elem, type, extra ) :
// Set width or height on the element
jQuery.style( elem, type, value, extra );
}, type, chainable ? margin : undefined, chainable, null );
};
});
});
// Limit scope pollution from any deprecated API
// (function() {
// The number of elements contained in the matched element set
jQuery.fn.size = function() {
return this.length;
};
jQuery.fn.andSelf = jQuery.fn.addBack;
// })();
if ( typeof module === "object" && module && typeof module.exports === "object" ) {
// Expose jQuery as module.exports in loaders that implement the Node
// module pattern (including browserify). Do not create the global, since
// the user will be storing it themselves locally, and globals are frowned
// upon in the Node module world.
module.exports = jQuery;
} else {
// Otherwise expose jQuery to the global object as usual
window.jQuery = window.$ = jQuery;
// Register as a named AMD module, since jQuery can be concatenated with other
// files that may use define, but not via a proper concatenation script that
// understands anonymous AMD modules. A named AMD is safest and most robust
// way to register. Lowercase jquery is used because AMD module names are
// derived from file names, and jQuery is normally delivered in a lowercase
// file name. Do this after creating the global so that if an AMD module wants
// to call noConflict to hide this version of jQuery, it will work.
if ( typeof define === "function" && define.amd ) {
define( "jquery", [], function () { return jQuery; } );
}
}
})( window );
(function($, undefined) {
/**
* Unobtrusive scripting adapter for jQuery
* https://github.com/rails/jquery-ujs
*
* Requires jQuery 1.7.0 or later.
*
* Released under the MIT license
*
*/
// Cut down on the number of issues from people inadvertently including jquery_ujs twice
// by detecting and raising an error when it happens.
if ( $.rails !== undefined ) {
$.error('jquery-ujs has already been loaded!');
}
// Shorthand to make it a little easier to call public rails functions from within rails.js
var rails;
var $document = $(document);
$.rails = rails = {
// Link elements bound by jquery-ujs
linkClickSelector: 'a[data-confirm], a[data-method], a[data-remote], a[data-disable-with]',
// Button elements boud jquery-ujs
buttonClickSelector: 'button[data-remote]',
// Select elements bound by jquery-ujs
inputChangeSelector: 'select[data-remote], input[data-remote], textarea[data-remote]',
// Form elements bound by jquery-ujs
formSubmitSelector: 'form',
// Form input elements bound by jquery-ujs
formInputClickSelector: 'form input[type=submit], form input[type=image], form button[type=submit], form button:not([type])',
// Form input elements disabled during form submission
disableSelector: 'input[data-disable-with], button[data-disable-with], textarea[data-disable-with]',
// Form input elements re-enabled after form submission
enableSelector: 'input[data-disable-with]:disabled, button[data-disable-with]:disabled, textarea[data-disable-with]:disabled',
// Form required input elements
requiredInputSelector: 'input[name][required]:not([disabled]),textarea[name][required]:not([disabled])',
// Form file input elements
fileInputSelector: 'input[type=file]',
// Link onClick disable selector with possible reenable after remote submission
linkDisableSelector: 'a[data-disable-with]',
// Make sure that every Ajax request sends the CSRF token
CSRFProtection: function(xhr) {
var token = $('meta[name="csrf-token"]').attr('content');
if (token) xhr.setRequestHeader('X-CSRF-Token', token);
},
// Triggers an event on an element and returns false if the event result is false
fire: function(obj, name, data) {
var event = $.Event(name);
obj.trigger(event, data);
return event.result !== false;
},
// Default confirm dialog, may be overridden with custom confirm dialog in $.rails.confirm
confirm: function(message) {
return confirm(message);
},
// Default ajax function, may be overridden with custom function in $.rails.ajax
ajax: function(options) {
return $.ajax(options);
},
// Default way to get an element's href. May be overridden at $.rails.href.
href: function(element) {
return element.attr('href');
},
// Submits "remote" forms and links with ajax
handleRemote: function(element) {
var method, url, data, elCrossDomain, crossDomain, withCredentials, dataType, options;
if (rails.fire(element, 'ajax:before')) {
elCrossDomain = element.data('cross-domain');
crossDomain = elCrossDomain === undefined ? null : elCrossDomain;
withCredentials = element.data('with-credentials') || null;
dataType = element.data('type') || ($.ajaxSettings && $.ajaxSettings.dataType);
if (element.is('form')) {
method = element.attr('method');
url = element.attr('action');
data = element.serializeArray();
// memoized value from clicked submit button
var button = element.data('ujs:submit-button');
if (button) {
data.push(button);
element.data('ujs:submit-button', null);
}
} else if (element.is(rails.inputChangeSelector)) {
method = element.data('method');
url = element.data('url');
data = element.serialize();
if (element.data('params')) data = data + "&" + element.data('params');
} else if (element.is(rails.buttonClickSelector)) {
method = element.data('method') || 'get';
url = element.data('url');
data = element.serialize();
if (element.data('params')) data = data + "&" + element.data('params');
} else {
method = element.data('method');
url = rails.href(element);
data = element.data('params') || null;
}
options = {
type: method || 'GET', data: data, dataType: dataType,
// stopping the "ajax:beforeSend" event will cancel the ajax request
beforeSend: function(xhr, settings) {
if (settings.dataType === undefined) {
xhr.setRequestHeader('accept', '*/*;q=0.5, ' + settings.accepts.script);
}
return rails.fire(element, 'ajax:beforeSend', [xhr, settings]);
},
success: function(data, status, xhr) {
element.trigger('ajax:success', [data, status, xhr]);
},
complete: function(xhr, status) {
element.trigger('ajax:complete', [xhr, status]);
},
error: function(xhr, status, error) {
element.trigger('ajax:error', [xhr, status, error]);
},
crossDomain: crossDomain
};
// There is no withCredentials for IE6-8 when
// "Enable native XMLHTTP support" is disabled
if (withCredentials) {
options.xhrFields = {
withCredentials: withCredentials
};
}
// Only pass url to `ajax` options if not blank
if (url) { options.url = url; }
var jqxhr = rails.ajax(options);
element.trigger('ajax:send', jqxhr);
return jqxhr;
} else {
return false;
}
},
// Handles "data-method" on links such as:
// <a href="/users/5" data-method="delete" rel="nofollow" data-confirm="Are you sure?">Delete</a>
handleMethod: function(link) {
var href = rails.href(link),
method = link.data('method'),
target = link.attr('target'),
csrf_token = $('meta[name=csrf-token]').attr('content'),
csrf_param = $('meta[name=csrf-param]').attr('content'),
form = $('<form method="post" action="' + href + '"></form>'),
metadata_input = '<input name="_method" value="' + method + '" type="hidden" />';
if (csrf_param !== undefined && csrf_token !== undefined) {
metadata_input += '<input name="' + csrf_param + '" value="' + csrf_token + '" type="hidden" />';
}
if (target) { form.attr('target', target); }
form.hide().append(metadata_input).appendTo('body');
form.submit();
},
/* Disables form elements:
- Caches element value in 'ujs:enable-with' data store
- Replaces element text with value of 'data-disable-with' attribute
- Sets disabled property to true
*/
disableFormElements: function(form) {
form.find(rails.disableSelector).each(function() {
var element = $(this), method = element.is('button') ? 'html' : 'val';
element.data('ujs:enable-with', element[method]());
element[method](element.data('disable-with'));
element.prop('disabled', true);
});
},
/* Re-enables disabled form elements:
- Replaces element text with cached value from 'ujs:enable-with' data store (created in `disableFormElements`)
- Sets disabled property to false
*/
enableFormElements: function(form) {
form.find(rails.enableSelector).each(function() {
var element = $(this), method = element.is('button') ? 'html' : 'val';
if (element.data('ujs:enable-with')) element[method](element.data('ujs:enable-with'));
element.prop('disabled', false);
});
},
/* For 'data-confirm' attribute:
- Fires `confirm` event
- Shows the confirmation dialog
- Fires the `confirm:complete` event
Returns `true` if no function stops the chain and user chose yes; `false` otherwise.
Attaching a handler to the element's `confirm` event that returns a `falsy` value cancels the confirmation dialog.
Attaching a handler to the element's `confirm:complete` event that returns a `falsy` value makes this function
return false. The `confirm:complete` event is fired whether or not the user answered true or false to the dialog.
*/
allowAction: function(element) {
var message = element.data('confirm'),
answer = false, callback;
if (!message) { return true; }
if (rails.fire(element, 'confirm')) {
answer = rails.confirm(message);
callback = rails.fire(element, 'confirm:complete', [answer]);
}
return answer && callback;
},
// Helper function which checks for blank inputs in a form that match the specified CSS selector
blankInputs: function(form, specifiedSelector, nonBlank) {
var inputs = $(), input, valueToCheck,
selector = specifiedSelector || 'input,textarea',
allInputs = form.find(selector);
allInputs.each(function() {
input = $(this);
valueToCheck = input.is('input[type=checkbox],input[type=radio]') ? input.is(':checked') : input.val();
// If nonBlank and valueToCheck are both truthy, or nonBlank and valueToCheck are both falsey
if (!valueToCheck === !nonBlank) {
// Don't count unchecked required radio if other radio with same name is checked
if (input.is('input[type=radio]') && allInputs.filter('input[type=radio]:checked[name="' + input.attr('name') + '"]').length) {
return true; // Skip to next input
}
inputs = inputs.add(input);
}
});
return inputs.length ? inputs : false;
},
// Helper function which checks for non-blank inputs in a form that match the specified CSS selector
nonBlankInputs: function(form, specifiedSelector) {
return rails.blankInputs(form, specifiedSelector, true); // true specifies nonBlank
},
// Helper function, needed to provide consistent behavior in IE
stopEverything: function(e) {
$(e.target).trigger('ujs:everythingStopped');
e.stopImmediatePropagation();
return false;
},
// replace element's html with the 'data-disable-with' after storing original html
// and prevent clicking on it
disableElement: function(element) {
element.data('ujs:enable-with', element.html()); // store enabled state
element.html(element.data('disable-with')); // set to disabled state
element.bind('click.railsDisable', function(e) { // prevent further clicking
return rails.stopEverything(e);
});
},
// restore element to its original state which was disabled by 'disableElement' above
enableElement: function(element) {
if (element.data('ujs:enable-with') !== undefined) {
element.html(element.data('ujs:enable-with')); // set to old enabled state
element.removeData('ujs:enable-with'); // clean up cache
}
element.unbind('click.railsDisable'); // enable element
}
};
if (rails.fire($document, 'rails:attachBindings')) {
$.ajaxPrefilter(function(options, originalOptions, xhr){ if ( !options.crossDomain ) { rails.CSRFProtection(xhr); }});
$document.delegate(rails.linkDisableSelector, 'ajax:complete', function() {
rails.enableElement($(this));
});
$document.delegate(rails.linkClickSelector, 'click.rails', function(e) {
var link = $(this), method = link.data('method'), data = link.data('params');
if (!rails.allowAction(link)) return rails.stopEverything(e);
if (link.is(rails.linkDisableSelector)) rails.disableElement(link);
if (link.data('remote') !== undefined) {
if ( (e.metaKey || e.ctrlKey) && (!method || method === 'GET') && !data ) { return true; }
var handleRemote = rails.handleRemote(link);
// response from rails.handleRemote() will either be false or a deferred object promise.
if (handleRemote === false) {
rails.enableElement(link);
} else {
handleRemote.error( function() { rails.enableElement(link); } );
}
return false;
} else if (link.data('method')) {
rails.handleMethod(link);
return false;
}
});
$document.delegate(rails.buttonClickSelector, 'click.rails', function(e) {
var button = $(this);
if (!rails.allowAction(button)) return rails.stopEverything(e);
rails.handleRemote(button);
return false;
});
$document.delegate(rails.inputChangeSelector, 'change.rails', function(e) {
var link = $(this);
if (!rails.allowAction(link)) return rails.stopEverything(e);
rails.handleRemote(link);
return false;
});
$document.delegate(rails.formSubmitSelector, 'submit.rails', function(e) {
var form = $(this),
remote = form.data('remote') !== undefined,
blankRequiredInputs = rails.blankInputs(form, rails.requiredInputSelector),
nonBlankFileInputs = rails.nonBlankInputs(form, rails.fileInputSelector);
if (!rails.allowAction(form)) return rails.stopEverything(e);
// skip other logic when required values are missing or file upload is present
if (blankRequiredInputs && form.attr("novalidate") == undefined && rails.fire(form, 'ajax:aborted:required', [blankRequiredInputs])) {
return rails.stopEverything(e);
}
if (remote) {
if (nonBlankFileInputs) {
// slight timeout so that the submit button gets properly serialized
// (make it easy for event handler to serialize form without disabled values)
setTimeout(function(){ rails.disableFormElements(form); }, 13);
var aborted = rails.fire(form, 'ajax:aborted:file', [nonBlankFileInputs]);
// re-enable form elements if event bindings return false (canceling normal form submission)
if (!aborted) { setTimeout(function(){ rails.enableFormElements(form); }, 13); }
return aborted;
}
rails.handleRemote(form);
return false;
} else {
// slight timeout so that the submit button gets properly serialized
setTimeout(function(){ rails.disableFormElements(form); }, 13);
}
});
$document.delegate(rails.formInputClickSelector, 'click.rails', function(event) {
var button = $(this);
if (!rails.allowAction(button)) return rails.stopEverything(event);
// register the pressed submit button
var name = button.attr('name'),
data = name ? {name:name, value:button.val()} : null;
button.closest('form').data('ujs:submit-button', data);
});
$document.delegate(rails.formSubmitSelector, 'ajax:beforeSend.rails', function(event) {
if (this == event.target) rails.disableFormElements($(this));
});
$document.delegate(rails.formSubmitSelector, 'ajax:complete.rails', function(event) {
if (this == event.target) rails.enableFormElements($(this));
});
$(function(){
// making sure that all forms have actual up-to-date token(cached forms contain old one)
var csrf_token = $('meta[name=csrf-token]').attr('content');
var csrf_param = $('meta[name=csrf-param]').attr('content');
$('form input[name="' + csrf_param + '"]').val(csrf_token);
});
}
})( jQuery );
/*! jQuery UI - v1.10.3 - 2013-05-03
* http://jqueryui.com
* Includes: jquery.ui.core.js, jquery.ui.widget.js, jquery.ui.mouse.js, jquery.ui.draggable.js, jquery.ui.droppable.js, jquery.ui.resizable.js, jquery.ui.selectable.js, jquery.ui.sortable.js, jquery.ui.effect.js, jquery.ui.accordion.js, jquery.ui.autocomplete.js, jquery.ui.button.js, jquery.ui.datepicker.js, jquery.ui.dialog.js, jquery.ui.effect-blind.js, jquery.ui.effect-bounce.js, jquery.ui.effect-clip.js, jquery.ui.effect-drop.js, jquery.ui.effect-explode.js, jquery.ui.effect-fade.js, jquery.ui.effect-fold.js, jquery.ui.effect-highlight.js, jquery.ui.effect-pulsate.js, jquery.ui.effect-scale.js, jquery.ui.effect-shake.js, jquery.ui.effect-slide.js, jquery.ui.effect-transfer.js, jquery.ui.menu.js, jquery.ui.position.js, jquery.ui.progressbar.js, jquery.ui.slider.js, jquery.ui.spinner.js, jquery.ui.tabs.js, jquery.ui.tooltip.js
* Copyright 2013 jQuery Foundation and other contributors; Licensed MIT */
(function( $, undefined ) {
var uuid = 0,
runiqueId = /^ui-id-\d+$/;
// $.ui might exist from components with no dependencies, e.g., $.ui.position
$.ui = $.ui || {};
$.extend( $.ui, {
version: "1.10.3",
keyCode: {
BACKSPACE: 8,
COMMA: 188,
DELETE: 46,
DOWN: 40,
END: 35,
ENTER: 13,
ESCAPE: 27,
HOME: 36,
LEFT: 37,
NUMPAD_ADD: 107,
NUMPAD_DECIMAL: 110,
NUMPAD_DIVIDE: 111,
NUMPAD_ENTER: 108,
NUMPAD_MULTIPLY: 106,
NUMPAD_SUBTRACT: 109,
PAGE_DOWN: 34,
PAGE_UP: 33,
PERIOD: 190,
RIGHT: 39,
SPACE: 32,
TAB: 9,
UP: 38
}
});
// plugins
$.fn.extend({
focus: (function( orig ) {
return function( delay, fn ) {
return typeof delay === "number" ?
this.each(function() {
var elem = this;
setTimeout(function() {
$( elem ).focus();
if ( fn ) {
fn.call( elem );
}
}, delay );
}) :
orig.apply( this, arguments );
};
})( $.fn.focus ),
scrollParent: function() {
var scrollParent;
if (($.ui.ie && (/(static|relative)/).test(this.css("position"))) || (/absolute/).test(this.css("position"))) {
scrollParent = this.parents().filter(function() {
return (/(relative|absolute|fixed)/).test($.css(this,"position")) && (/(auto|scroll)/).test($.css(this,"overflow")+$.css(this,"overflow-y")+$.css(this,"overflow-x"));
}).eq(0);
} else {
scrollParent = this.parents().filter(function() {
return (/(auto|scroll)/).test($.css(this,"overflow")+$.css(this,"overflow-y")+$.css(this,"overflow-x"));
}).eq(0);
}
return (/fixed/).test(this.css("position")) || !scrollParent.length ? $(document) : scrollParent;
},
zIndex: function( zIndex ) {
if ( zIndex !== undefined ) {
return this.css( "zIndex", zIndex );
}
if ( this.length ) {
var elem = $( this[ 0 ] ), position, value;
while ( elem.length && elem[ 0 ] !== document ) {
// Ignore z-index if position is set to a value where z-index is ignored by the browser
// This makes behavior of this function consistent across browsers
// WebKit always returns auto if the element is positioned
position = elem.css( "position" );
if ( position === "absolute" || position === "relative" || position === "fixed" ) {
// IE returns 0 when zIndex is not specified
// other browsers return a string
// we ignore the case of nested elements with an explicit value of 0
// <div style="z-index: -10;"><div style="z-index: 0;"></div></div>
value = parseInt( elem.css( "zIndex" ), 10 );
if ( !isNaN( value ) && value !== 0 ) {
return value;
}
}
elem = elem.parent();
}
}
return 0;
},
uniqueId: function() {
return this.each(function() {
if ( !this.id ) {
this.id = "ui-id-" + (++uuid);
}
});
},
removeUniqueId: function() {
return this.each(function() {
if ( runiqueId.test( this.id ) ) {
$( this ).removeAttr( "id" );
}
});
}
});
// selectors
function focusable( element, isTabIndexNotNaN ) {
var map, mapName, img,
nodeName = element.nodeName.toLowerCase();
if ( "area" === nodeName ) {
map = element.parentNode;
mapName = map.name;
if ( !element.href || !mapName || map.nodeName.toLowerCase() !== "map" ) {
return false;
}
img = $( "img[usemap=#" + mapName + "]" )[0];
return !!img && visible( img );
}
return ( /input|select|textarea|button|object/.test( nodeName ) ?
!element.disabled :
"a" === nodeName ?
element.href || isTabIndexNotNaN :
isTabIndexNotNaN) &&
// the element and all of its ancestors must be visible
visible( element );
}
function visible( element ) {
return $.expr.filters.visible( element ) &&
!$( element ).parents().addBack().filter(function() {
return $.css( this, "visibility" ) === "hidden";
}).length;
}
$.extend( $.expr[ ":" ], {
data: $.expr.createPseudo ?
$.expr.createPseudo(function( dataName ) {
return function( elem ) {
return !!$.data( elem, dataName );
};
}) :
// support: jQuery <1.8
function( elem, i, match ) {
return !!$.data( elem, match[ 3 ] );
},
focusable: function( element ) {
return focusable( element, !isNaN( $.attr( element, "tabindex" ) ) );
},
tabbable: function( element ) {
var tabIndex = $.attr( element, "tabindex" ),
isTabIndexNaN = isNaN( tabIndex );
return ( isTabIndexNaN || tabIndex >= 0 ) && focusable( element, !isTabIndexNaN );
}
});
// support: jQuery <1.8
if ( !$( "<a>" ).outerWidth( 1 ).jquery ) {
$.each( [ "Width", "Height" ], function( i, name ) {
var side = name === "Width" ? [ "Left", "Right" ] : [ "Top", "Bottom" ],
type = name.toLowerCase(),
orig = {
innerWidth: $.fn.innerWidth,
innerHeight: $.fn.innerHeight,
outerWidth: $.fn.outerWidth,
outerHeight: $.fn.outerHeight
};
function reduce( elem, size, border, margin ) {
$.each( side, function() {
size -= parseFloat( $.css( elem, "padding" + this ) ) || 0;
if ( border ) {
size -= parseFloat( $.css( elem, "border" + this + "Width" ) ) || 0;
}
if ( margin ) {
size -= parseFloat( $.css( elem, "margin" + this ) ) || 0;
}
});
return size;
}
$.fn[ "inner" + name ] = function( size ) {
if ( size === undefined ) {
return orig[ "inner" + name ].call( this );
}
return this.each(function() {
$( this ).css( type, reduce( this, size ) + "px" );
});
};
$.fn[ "outer" + name] = function( size, margin ) {
if ( typeof size !== "number" ) {
return orig[ "outer" + name ].call( this, size );
}
return this.each(function() {
$( this).css( type, reduce( this, size, true, margin ) + "px" );
});
};
});
}
// support: jQuery <1.8
if ( !$.fn.addBack ) {
$.fn.addBack = function( selector ) {
return this.add( selector == null ?
this.prevObject : this.prevObject.filter( selector )
);
};
}
// support: jQuery 1.6.1, 1.6.2 (http://bugs.jquery.com/ticket/9413)
if ( $( "<a>" ).data( "a-b", "a" ).removeData( "a-b" ).data( "a-b" ) ) {
$.fn.removeData = (function( removeData ) {
return function( key ) {
if ( arguments.length ) {
return removeData.call( this, $.camelCase( key ) );
} else {
return removeData.call( this );
}
};
})( $.fn.removeData );
}
// deprecated
$.ui.ie = !!/msie [\w.]+/.exec( navigator.userAgent.toLowerCase() );
$.support.selectstart = "onselectstart" in document.createElement( "div" );
$.fn.extend({
disableSelection: function() {
return this.bind( ( $.support.selectstart ? "selectstart" : "mousedown" ) +
".ui-disableSelection", function( event ) {
event.preventDefault();
});
},
enableSelection: function() {
return this.unbind( ".ui-disableSelection" );
}
});
$.extend( $.ui, {
// $.ui.plugin is deprecated. Use $.widget() extensions instead.
plugin: {
add: function( module, option, set ) {
var i,
proto = $.ui[ module ].prototype;
for ( i in set ) {
proto.plugins[ i ] = proto.plugins[ i ] || [];
proto.plugins[ i ].push( [ option, set[ i ] ] );
}
},
call: function( instance, name, args ) {
var i,
set = instance.plugins[ name ];
if ( !set || !instance.element[ 0 ].parentNode || instance.element[ 0 ].parentNode.nodeType === 11 ) {
return;
}
for ( i = 0; i < set.length; i++ ) {
if ( instance.options[ set[ i ][ 0 ] ] ) {
set[ i ][ 1 ].apply( instance.element, args );
}
}
}
},
// only used by resizable
hasScroll: function( el, a ) {
//If overflow is hidden, the element might have extra content, but the user wants to hide it
if ( $( el ).css( "overflow" ) === "hidden") {
return false;
}
var scroll = ( a && a === "left" ) ? "scrollLeft" : "scrollTop",
has = false;
if ( el[ scroll ] > 0 ) {
return true;
}
// TODO: determine which cases actually cause this to happen
// if the element doesn't have the scroll set, see if it's possible to
// set the scroll
el[ scroll ] = 1;
has = ( el[ scroll ] > 0 );
el[ scroll ] = 0;
return has;
}
});
})( jQuery );
(function( $, undefined ) {
var uuid = 0,
slice = Array.prototype.slice,
_cleanData = $.cleanData;
$.cleanData = function( elems ) {
for ( var i = 0, elem; (elem = elems[i]) != null; i++ ) {
try {
$( elem ).triggerHandler( "remove" );
// http://bugs.jquery.com/ticket/8235
} catch( e ) {}
}
_cleanData( elems );
};
$.widget = function( name, base, prototype ) {
var fullName, existingConstructor, constructor, basePrototype,
// proxiedPrototype allows the provided prototype to remain unmodified
// so that it can be used as a mixin for multiple widgets (#8876)
proxiedPrototype = {},
namespace = name.split( "." )[ 0 ];
name = name.split( "." )[ 1 ];
fullName = namespace + "-" + name;
if ( !prototype ) {
prototype = base;
base = $.Widget;
}
// create selector for plugin
$.expr[ ":" ][ fullName.toLowerCase() ] = function( elem ) {
return !!$.data( elem, fullName );
};
$[ namespace ] = $[ namespace ] || {};
existingConstructor = $[ namespace ][ name ];
constructor = $[ namespace ][ name ] = function( options, element ) {
// allow instantiation without "new" keyword
if ( !this._createWidget ) {
return new constructor( options, element );
}
// allow instantiation without initializing for simple inheritance
// must use "new" keyword (the code above always passes args)
if ( arguments.length ) {
this._createWidget( options, element );
}
};
// extend with the existing constructor to carry over any static properties
$.extend( constructor, existingConstructor, {
version: prototype.version,
// copy the object used to create the prototype in case we need to
// redefine the widget later
_proto: $.extend( {}, prototype ),
// track widgets that inherit from this widget in case this widget is
// redefined after a widget inherits from it
_childConstructors: []
});
basePrototype = new base();
// we need to make the options hash a property directly on the new instance
// otherwise we'll modify the options hash on the prototype that we're
// inheriting from
basePrototype.options = $.widget.extend( {}, basePrototype.options );
$.each( prototype, function( prop, value ) {
if ( !$.isFunction( value ) ) {
proxiedPrototype[ prop ] = value;
return;
}
proxiedPrototype[ prop ] = (function() {
var _super = function() {
return base.prototype[ prop ].apply( this, arguments );
},
_superApply = function( args ) {
return base.prototype[ prop ].apply( this, args );
};
return function() {
var __super = this._super,
__superApply = this._superApply,
returnValue;
this._super = _super;
this._superApply = _superApply;
returnValue = value.apply( this, arguments );
this._super = __super;
this._superApply = __superApply;
return returnValue;
};
})();
});
constructor.prototype = $.widget.extend( basePrototype, {
// TODO: remove support for widgetEventPrefix
// always use the name + a colon as the prefix, e.g., draggable:start
// don't prefix for widgets that aren't DOM-based
widgetEventPrefix: existingConstructor ? basePrototype.widgetEventPrefix : name
}, proxiedPrototype, {
constructor: constructor,
namespace: namespace,
widgetName: name,
widgetFullName: fullName
});
// If this widget is being redefined then we need to find all widgets that
// are inheriting from it and redefine all of them so that they inherit from
// the new version of this widget. We're essentially trying to replace one
// level in the prototype chain.
if ( existingConstructor ) {
$.each( existingConstructor._childConstructors, function( i, child ) {
var childPrototype = child.prototype;
// redefine the child widget using the same prototype that was
// originally used, but inherit from the new version of the base
$.widget( childPrototype.namespace + "." + childPrototype.widgetName, constructor, child._proto );
});
// remove the list of existing child constructors from the old constructor
// so the old child constructors can be garbage collected
delete existingConstructor._childConstructors;
} else {
base._childConstructors.push( constructor );
}
$.widget.bridge( name, constructor );
};
$.widget.extend = function( target ) {
var input = slice.call( arguments, 1 ),
inputIndex = 0,
inputLength = input.length,
key,
value;
for ( ; inputIndex < inputLength; inputIndex++ ) {
for ( key in input[ inputIndex ] ) {
value = input[ inputIndex ][ key ];
if ( input[ inputIndex ].hasOwnProperty( key ) && value !== undefined ) {
// Clone objects
if ( $.isPlainObject( value ) ) {
target[ key ] = $.isPlainObject( target[ key ] ) ?
$.widget.extend( {}, target[ key ], value ) :
// Don't extend strings, arrays, etc. with objects
$.widget.extend( {}, value );
// Copy everything else by reference
} else {
target[ key ] = value;
}
}
}
}
return target;
};
$.widget.bridge = function( name, object ) {
var fullName = object.prototype.widgetFullName || name;
$.fn[ name ] = function( options ) {
var isMethodCall = typeof options === "string",
args = slice.call( arguments, 1 ),
returnValue = this;
// allow multiple hashes to be passed on init
options = !isMethodCall && args.length ?
$.widget.extend.apply( null, [ options ].concat(args) ) :
options;
if ( isMethodCall ) {
this.each(function() {
var methodValue,
instance = $.data( this, fullName );
if ( !instance ) {
return $.error( "cannot call methods on " + name + " prior to initialization; " +
"attempted to call method '" + options + "'" );
}
if ( !$.isFunction( instance[options] ) || options.charAt( 0 ) === "_" ) {
return $.error( "no such method '" + options + "' for " + name + " widget instance" );
}
methodValue = instance[ options ].apply( instance, args );
if ( methodValue !== instance && methodValue !== undefined ) {
returnValue = methodValue && methodValue.jquery ?
returnValue.pushStack( methodValue.get() ) :
methodValue;
return false;
}
});
} else {
this.each(function() {
var instance = $.data( this, fullName );
if ( instance ) {
instance.option( options || {} )._init();
} else {
$.data( this, fullName, new object( options, this ) );
}
});
}
return returnValue;
};
};
$.Widget = function( /* options, element */ ) {};
$.Widget._childConstructors = [];
$.Widget.prototype = {
widgetName: "widget",
widgetEventPrefix: "",
defaultElement: "<div>",
options: {
disabled: false,
// callbacks
create: null
},
_createWidget: function( options, element ) {
element = $( element || this.defaultElement || this )[ 0 ];
this.element = $( element );
this.uuid = uuid++;
this.eventNamespace = "." + this.widgetName + this.uuid;
this.options = $.widget.extend( {},
this.options,
this._getCreateOptions(),
options );
this.bindings = $();
this.hoverable = $();
this.focusable = $();
if ( element !== this ) {
$.data( element, this.widgetFullName, this );
this._on( true, this.element, {
remove: function( event ) {
if ( event.target === element ) {
this.destroy();
}
}
});
this.document = $( element.style ?
// element within the document
element.ownerDocument :
// element is window or document
element.document || element );
this.window = $( this.document[0].defaultView || this.document[0].parentWindow );
}
this._create();
this._trigger( "create", null, this._getCreateEventData() );
this._init();
},
_getCreateOptions: $.noop,
_getCreateEventData: $.noop,
_create: $.noop,
_init: $.noop,
destroy: function() {
this._destroy();
// we can probably remove the unbind calls in 2.0
// all event bindings should go through this._on()
this.element
.unbind( this.eventNamespace )
// 1.9 BC for #7810
// TODO remove dual storage
.removeData( this.widgetName )
.removeData( this.widgetFullName )
// support: jquery <1.6.3
// http://bugs.jquery.com/ticket/9413
.removeData( $.camelCase( this.widgetFullName ) );
this.widget()
.unbind( this.eventNamespace )
.removeAttr( "aria-disabled" )
.removeClass(
this.widgetFullName + "-disabled " +
"ui-state-disabled" );
// clean up events and states
this.bindings.unbind( this.eventNamespace );
this.hoverable.removeClass( "ui-state-hover" );
this.focusable.removeClass( "ui-state-focus" );
},
_destroy: $.noop,
widget: function() {
return this.element;
},
option: function( key, value ) {
var options = key,
parts,
curOption,
i;
if ( arguments.length === 0 ) {
// don't return a reference to the internal hash
return $.widget.extend( {}, this.options );
}
if ( typeof key === "string" ) {
// handle nested keys, e.g., "foo.bar" => { foo: { bar: ___ } }
options = {};
parts = key.split( "." );
key = parts.shift();
if ( parts.length ) {
curOption = options[ key ] = $.widget.extend( {}, this.options[ key ] );
for ( i = 0; i < parts.length - 1; i++ ) {
curOption[ parts[ i ] ] = curOption[ parts[ i ] ] || {};
curOption = curOption[ parts[ i ] ];
}
key = parts.pop();
if ( value === undefined ) {
return curOption[ key ] === undefined ? null : curOption[ key ];
}
curOption[ key ] = value;
} else {
if ( value === undefined ) {
return this.options[ key ] === undefined ? null : this.options[ key ];
}
options[ key ] = value;
}
}
this._setOptions( options );
return this;
},
_setOptions: function( options ) {
var key;
for ( key in options ) {
this._setOption( key, options[ key ] );
}
return this;
},
_setOption: function( key, value ) {
this.options[ key ] = value;
if ( key === "disabled" ) {
this.widget()
.toggleClass( this.widgetFullName + "-disabled ui-state-disabled", !!value )
.attr( "aria-disabled", value );
this.hoverable.removeClass( "ui-state-hover" );
this.focusable.removeClass( "ui-state-focus" );
}
return this;
},
enable: function() {
return this._setOption( "disabled", false );
},
disable: function() {
return this._setOption( "disabled", true );
},
_on: function( suppressDisabledCheck, element, handlers ) {
var delegateElement,
instance = this;
// no suppressDisabledCheck flag, shuffle arguments
if ( typeof suppressDisabledCheck !== "boolean" ) {
handlers = element;
element = suppressDisabledCheck;
suppressDisabledCheck = false;
}
// no element argument, shuffle and use this.element
if ( !handlers ) {
handlers = element;
element = this.element;
delegateElement = this.widget();
} else {
// accept selectors, DOM elements
element = delegateElement = $( element );
this.bindings = this.bindings.add( element );
}
$.each( handlers, function( event, handler ) {
function handlerProxy() {
// allow widgets to customize the disabled handling
// - disabled as an array instead of boolean
// - disabled class as method for disabling individual parts
if ( !suppressDisabledCheck &&
( instance.options.disabled === true ||
$( this ).hasClass( "ui-state-disabled" ) ) ) {
return;
}
return ( typeof handler === "string" ? instance[ handler ] : handler )
.apply( instance, arguments );
}
// copy the guid so direct unbinding works
if ( typeof handler !== "string" ) {
handlerProxy.guid = handler.guid =
handler.guid || handlerProxy.guid || $.guid++;
}
var match = event.match( /^(\w+)\s*(.*)$/ ),
eventName = match[1] + instance.eventNamespace,
selector = match[2];
if ( selector ) {
delegateElement.delegate( selector, eventName, handlerProxy );
} else {
element.bind( eventName, handlerProxy );
}
});
},
_off: function( element, eventName ) {
eventName = (eventName || "").split( " " ).join( this.eventNamespace + " " ) + this.eventNamespace;
element.unbind( eventName ).undelegate( eventName );
},
_delay: function( handler, delay ) {
function handlerProxy() {
return ( typeof handler === "string" ? instance[ handler ] : handler )
.apply( instance, arguments );
}
var instance = this;
return setTimeout( handlerProxy, delay || 0 );
},
_hoverable: function( element ) {
this.hoverable = this.hoverable.add( element );
this._on( element, {
mouseenter: function( event ) {
$( event.currentTarget ).addClass( "ui-state-hover" );
},
mouseleave: function( event ) {
$( event.currentTarget ).removeClass( "ui-state-hover" );
}
});
},
_focusable: function( element ) {
this.focusable = this.focusable.add( element );
this._on( element, {
focusin: function( event ) {
$( event.currentTarget ).addClass( "ui-state-focus" );
},
focusout: function( event ) {
$( event.currentTarget ).removeClass( "ui-state-focus" );
}
});
},
_trigger: function( type, event, data ) {
var prop, orig,
callback = this.options[ type ];
data = data || {};
event = $.Event( event );
event.type = ( type === this.widgetEventPrefix ?
type :
this.widgetEventPrefix + type ).toLowerCase();
// the original event may come from any element
// so we need to reset the target on the new event
event.target = this.element[ 0 ];
// copy original event properties over to the new event
orig = event.originalEvent;
if ( orig ) {
for ( prop in orig ) {
if ( !( prop in event ) ) {
event[ prop ] = orig[ prop ];
}
}
}
this.element.trigger( event, data );
return !( $.isFunction( callback ) &&
callback.apply( this.element[0], [ event ].concat( data ) ) === false ||
event.isDefaultPrevented() );
}
};
$.each( { show: "fadeIn", hide: "fadeOut" }, function( method, defaultEffect ) {
$.Widget.prototype[ "_" + method ] = function( element, options, callback ) {
if ( typeof options === "string" ) {
options = { effect: options };
}
var hasOptions,
effectName = !options ?
method :
options === true || typeof options === "number" ?
defaultEffect :
options.effect || defaultEffect;
options = options || {};
if ( typeof options === "number" ) {
options = { duration: options };
}
hasOptions = !$.isEmptyObject( options );
options.complete = callback;
if ( options.delay ) {
element.delay( options.delay );
}
if ( hasOptions && $.effects && $.effects.effect[ effectName ] ) {
element[ method ]( options );
} else if ( effectName !== method && element[ effectName ] ) {
element[ effectName ]( options.duration, options.easing, callback );
} else {
element.queue(function( next ) {
$( this )[ method ]();
if ( callback ) {
callback.call( element[ 0 ] );
}
next();
});
}
};
});
})( jQuery );
(function( $, undefined ) {
var mouseHandled = false;
$( document ).mouseup( function() {
mouseHandled = false;
});
$.widget("ui.mouse", {
version: "1.10.3",
options: {
cancel: "input,textarea,button,select,option",
distance: 1,
delay: 0
},
_mouseInit: function() {
var that = this;
this.element
.bind("mousedown."+this.widgetName, function(event) {
return that._mouseDown(event);
})
.bind("click."+this.widgetName, function(event) {
if (true === $.data(event.target, that.widgetName + ".preventClickEvent")) {
$.removeData(event.target, that.widgetName + ".preventClickEvent");
event.stopImmediatePropagation();
return false;
}
});
this.started = false;
},
// TODO: make sure destroying one instance of mouse doesn't mess with
// other instances of mouse
_mouseDestroy: function() {
this.element.unbind("."+this.widgetName);
if ( this._mouseMoveDelegate ) {
$(document)
.unbind("mousemove."+this.widgetName, this._mouseMoveDelegate)
.unbind("mouseup."+this.widgetName, this._mouseUpDelegate);
}
},
_mouseDown: function(event) {
// don't let more than one widget handle mouseStart
if( mouseHandled ) { return; }
// we may have missed mouseup (out of window)
(this._mouseStarted && this._mouseUp(event));
this._mouseDownEvent = event;
var that = this,
btnIsLeft = (event.which === 1),
// event.target.nodeName works around a bug in IE 8 with
// disabled inputs (#7620)
elIsCancel = (typeof this.options.cancel === "string" && event.target.nodeName ? $(event.target).closest(this.options.cancel).length : false);
if (!btnIsLeft || elIsCancel || !this._mouseCapture(event)) {
return true;
}
this.mouseDelayMet = !this.options.delay;
if (!this.mouseDelayMet) {
this._mouseDelayTimer = setTimeout(function() {
that.mouseDelayMet = true;
}, this.options.delay);
}
if (this._mouseDistanceMet(event) && this._mouseDelayMet(event)) {
this._mouseStarted = (this._mouseStart(event) !== false);
if (!this._mouseStarted) {
event.preventDefault();
return true;
}
}
// Click event may never have fired (Gecko & Opera)
if (true === $.data(event.target, this.widgetName + ".preventClickEvent")) {
$.removeData(event.target, this.widgetName + ".preventClickEvent");
}
// these delegates are required to keep context
this._mouseMoveDelegate = function(event) {
return that._mouseMove(event);
};
this._mouseUpDelegate = function(event) {
return that._mouseUp(event);
};
$(document)
.bind("mousemove."+this.widgetName, this._mouseMoveDelegate)
.bind("mouseup."+this.widgetName, this._mouseUpDelegate);
event.preventDefault();
mouseHandled = true;
return true;
},
_mouseMove: function(event) {
// IE mouseup check - mouseup happened when mouse was out of window
if ($.ui.ie && ( !document.documentMode || document.documentMode < 9 ) && !event.button) {
return this._mouseUp(event);
}
if (this._mouseStarted) {
this._mouseDrag(event);
return event.preventDefault();
}
if (this._mouseDistanceMet(event) && this._mouseDelayMet(event)) {
this._mouseStarted =
(this._mouseStart(this._mouseDownEvent, event) !== false);
(this._mouseStarted ? this._mouseDrag(event) : this._mouseUp(event));
}
return !this._mouseStarted;
},
_mouseUp: function(event) {
$(document)
.unbind("mousemove."+this.widgetName, this._mouseMoveDelegate)
.unbind("mouseup."+this.widgetName, this._mouseUpDelegate);
if (this._mouseStarted) {
this._mouseStarted = false;
if (event.target === this._mouseDownEvent.target) {
$.data(event.target, this.widgetName + ".preventClickEvent", true);
}
this._mouseStop(event);
}
return false;
},
_mouseDistanceMet: function(event) {
return (Math.max(
Math.abs(this._mouseDownEvent.pageX - event.pageX),
Math.abs(this._mouseDownEvent.pageY - event.pageY)
) >= this.options.distance
);
},
_mouseDelayMet: function(/* event */) {
return this.mouseDelayMet;
},
// These are placeholder methods, to be overriden by extending plugin
_mouseStart: function(/* event */) {},
_mouseDrag: function(/* event */) {},
_mouseStop: function(/* event */) {},
_mouseCapture: function(/* event */) { return true; }
});
})(jQuery);
(function( $, undefined ) {
$.widget("ui.draggable", $.ui.mouse, {
version: "1.10.3",
widgetEventPrefix: "drag",
options: {
addClasses: true,
appendTo: "parent",
axis: false,
connectToSortable: false,
containment: false,
cursor: "auto",
cursorAt: false,
grid: false,
handle: false,
helper: "original",
iframeFix: false,
opacity: false,
refreshPositions: false,
revert: false,
revertDuration: 500,
scope: "default",
scroll: true,
scrollSensitivity: 20,
scrollSpeed: 20,
snap: false,
snapMode: "both",
snapTolerance: 20,
stack: false,
zIndex: false,
// callbacks
drag: null,
start: null,
stop: null
},
_create: function() {
if (this.options.helper === "original" && !(/^(?:r|a|f)/).test(this.element.css("position"))) {
this.element[0].style.position = "relative";
}
if (this.options.addClasses){
this.element.addClass("ui-draggable");
}
if (this.options.disabled){
this.element.addClass("ui-draggable-disabled");
}
this._mouseInit();
},
_destroy: function() {
this.element.removeClass( "ui-draggable ui-draggable-dragging ui-draggable-disabled" );
this._mouseDestroy();
},
_mouseCapture: function(event) {
var o = this.options;
// among others, prevent a drag on a resizable-handle
if (this.helper || o.disabled || $(event.target).closest(".ui-resizable-handle").length > 0) {
return false;
}
//Quit if we're not on a valid handle
this.handle = this._getHandle(event);
if (!this.handle) {
return false;
}
$(o.iframeFix === true ? "iframe" : o.iframeFix).each(function() {
$("<div class='ui-draggable-iframeFix' style='background: #fff;'></div>")
.css({
width: this.offsetWidth+"px", height: this.offsetHeight+"px",
position: "absolute", opacity: "0.001", zIndex: 1000
})
.css($(this).offset())
.appendTo("body");
});
return true;
},
_mouseStart: function(event) {
var o = this.options;
//Create and append the visible helper
this.helper = this._createHelper(event);
this.helper.addClass("ui-draggable-dragging");
//Cache the helper size
this._cacheHelperProportions();
//If ddmanager is used for droppables, set the global draggable
if($.ui.ddmanager) {
$.ui.ddmanager.current = this;
}
/*
* - Position generation -
* This block generates everything position related - it's the core of draggables.
*/
//Cache the margins of the original element
this._cacheMargins();
//Store the helper's css position
this.cssPosition = this.helper.css( "position" );
this.scrollParent = this.helper.scrollParent();
this.offsetParent = this.helper.offsetParent();
this.offsetParentCssPosition = this.offsetParent.css( "position" );
//The element's absolute position on the page minus margins
this.offset = this.positionAbs = this.element.offset();
this.offset = {
top: this.offset.top - this.margins.top,
left: this.offset.left - this.margins.left
};
//Reset scroll cache
this.offset.scroll = false;
$.extend(this.offset, {
click: { //Where the click happened, relative to the element
left: event.pageX - this.offset.left,
top: event.pageY - this.offset.top
},
parent: this._getParentOffset(),
relative: this._getRelativeOffset() //This is a relative to absolute position minus the actual position calculation - only used for relative positioned helper
});
//Generate the original position
this.originalPosition = this.position = this._generatePosition(event);
this.originalPageX = event.pageX;
this.originalPageY = event.pageY;
//Adjust the mouse offset relative to the helper if "cursorAt" is supplied
(o.cursorAt && this._adjustOffsetFromHelper(o.cursorAt));
//Set a containment if given in the options
this._setContainment();
//Trigger event + callbacks
if(this._trigger("start", event) === false) {
this._clear();
return false;
}
//Recache the helper size
this._cacheHelperProportions();
//Prepare the droppable offsets
if ($.ui.ddmanager && !o.dropBehaviour) {
$.ui.ddmanager.prepareOffsets(this, event);
}
this._mouseDrag(event, true); //Execute the drag once - this causes the helper not to be visible before getting its correct position
//If the ddmanager is used for droppables, inform the manager that dragging has started (see #5003)
if ( $.ui.ddmanager ) {
$.ui.ddmanager.dragStart(this, event);
}
return true;
},
_mouseDrag: function(event, noPropagation) {
// reset any necessary cached properties (see #5009)
if ( this.offsetParentCssPosition === "fixed" ) {
this.offset.parent = this._getParentOffset();
}
//Compute the helpers position
this.position = this._generatePosition(event);
this.positionAbs = this._convertPositionTo("absolute");
//Call plugins and callbacks and use the resulting position if something is returned
if (!noPropagation) {
var ui = this._uiHash();
if(this._trigger("drag", event, ui) === false) {
this._mouseUp({});
return false;
}
this.position = ui.position;
}
if(!this.options.axis || this.options.axis !== "y") {
this.helper[0].style.left = this.position.left+"px";
}
if(!this.options.axis || this.options.axis !== "x") {
this.helper[0].style.top = this.position.top+"px";
}
if($.ui.ddmanager) {
$.ui.ddmanager.drag(this, event);
}
return false;
},
_mouseStop: function(event) {
//If we are using droppables, inform the manager about the drop
var that = this,
dropped = false;
if ($.ui.ddmanager && !this.options.dropBehaviour) {
dropped = $.ui.ddmanager.drop(this, event);
}
//if a drop comes from outside (a sortable)
if(this.dropped) {
dropped = this.dropped;
this.dropped = false;
}
//if the original element is no longer in the DOM don't bother to continue (see #8269)
if ( this.options.helper === "original" && !$.contains( this.element[ 0 ].ownerDocument, this.element[ 0 ] ) ) {
return false;
}
if((this.options.revert === "invalid" && !dropped) || (this.options.revert === "valid" && dropped) || this.options.revert === true || ($.isFunction(this.options.revert) && this.options.revert.call(this.element, dropped))) {
$(this.helper).animate(this.originalPosition, parseInt(this.options.revertDuration, 10), function() {
if(that._trigger("stop", event) !== false) {
that._clear();
}
});
} else {
if(this._trigger("stop", event) !== false) {
this._clear();
}
}
return false;
},
_mouseUp: function(event) {
//Remove frame helpers
$("div.ui-draggable-iframeFix").each(function() {
this.parentNode.removeChild(this);
});
//If the ddmanager is used for droppables, inform the manager that dragging has stopped (see #5003)
if( $.ui.ddmanager ) {
$.ui.ddmanager.dragStop(this, event);
}
return $.ui.mouse.prototype._mouseUp.call(this, event);
},
cancel: function() {
if(this.helper.is(".ui-draggable-dragging")) {
this._mouseUp({});
} else {
this._clear();
}
return this;
},
_getHandle: function(event) {
return this.options.handle ?
!!$( event.target ).closest( this.element.find( this.options.handle ) ).length :
true;
},
_createHelper: function(event) {
var o = this.options,
helper = $.isFunction(o.helper) ? $(o.helper.apply(this.element[0], [event])) : (o.helper === "clone" ? this.element.clone().removeAttr("id") : this.element);
if(!helper.parents("body").length) {
helper.appendTo((o.appendTo === "parent" ? this.element[0].parentNode : o.appendTo));
}
if(helper[0] !== this.element[0] && !(/(fixed|absolute)/).test(helper.css("position"))) {
helper.css("position", "absolute");
}
return helper;
},
_adjustOffsetFromHelper: function(obj) {
if (typeof obj === "string") {
obj = obj.split(" ");
}
if ($.isArray(obj)) {
obj = {left: +obj[0], top: +obj[1] || 0};
}
if ("left" in obj) {
this.offset.click.left = obj.left + this.margins.left;
}
if ("right" in obj) {
this.offset.click.left = this.helperProportions.width - obj.right + this.margins.left;
}
if ("top" in obj) {
this.offset.click.top = obj.top + this.margins.top;
}
if ("bottom" in obj) {
this.offset.click.top = this.helperProportions.height - obj.bottom + this.margins.top;
}
},
_getParentOffset: function() {
//Get the offsetParent and cache its position
var po = this.offsetParent.offset();
// This is a special case where we need to modify a offset calculated on start, since the following happened:
// 1. The position of the helper is absolute, so it's position is calculated based on the next positioned parent
// 2. The actual offset parent is a child of the scroll parent, and the scroll parent isn't the document, which means that
// the scroll is included in the initial calculation of the offset of the parent, and never recalculated upon drag
if(this.cssPosition === "absolute" && this.scrollParent[0] !== document && $.contains(this.scrollParent[0], this.offsetParent[0])) {
po.left += this.scrollParent.scrollLeft();
po.top += this.scrollParent.scrollTop();
}
//This needs to be actually done for all browsers, since pageX/pageY includes this information
//Ugly IE fix
if((this.offsetParent[0] === document.body) ||
(this.offsetParent[0].tagName && this.offsetParent[0].tagName.toLowerCase() === "html" && $.ui.ie)) {
po = { top: 0, left: 0 };
}
return {
top: po.top + (parseInt(this.offsetParent.css("borderTopWidth"),10) || 0),
left: po.left + (parseInt(this.offsetParent.css("borderLeftWidth"),10) || 0)
};
},
_getRelativeOffset: function() {
if(this.cssPosition === "relative") {
var p = this.element.position();
return {
top: p.top - (parseInt(this.helper.css("top"),10) || 0) + this.scrollParent.scrollTop(),
left: p.left - (parseInt(this.helper.css("left"),10) || 0) + this.scrollParent.scrollLeft()
};
} else {
return { top: 0, left: 0 };
}
},
_cacheMargins: function() {
this.margins = {
left: (parseInt(this.element.css("marginLeft"),10) || 0),
top: (parseInt(this.element.css("marginTop"),10) || 0),
right: (parseInt(this.element.css("marginRight"),10) || 0),
bottom: (parseInt(this.element.css("marginBottom"),10) || 0)
};
},
_cacheHelperProportions: function() {
this.helperProportions = {
width: this.helper.outerWidth(),
height: this.helper.outerHeight()
};
},
_setContainment: function() {
var over, c, ce,
o = this.options;
if ( !o.containment ) {
this.containment = null;
return;
}
if ( o.containment === "window" ) {
this.containment = [
$( window ).scrollLeft() - this.offset.relative.left - this.offset.parent.left,
$( window ).scrollTop() - this.offset.relative.top - this.offset.parent.top,
$( window ).scrollLeft() + $( window ).width() - this.helperProportions.width - this.margins.left,
$( window ).scrollTop() + ( $( window ).height() || document.body.parentNode.scrollHeight ) - this.helperProportions.height - this.margins.top
];
return;
}
if ( o.containment === "document") {
this.containment = [
0,
0,
$( document ).width() - this.helperProportions.width - this.margins.left,
( $( document ).height() || document.body.parentNode.scrollHeight ) - this.helperProportions.height - this.margins.top
];
return;
}
if ( o.containment.constructor === Array ) {
this.containment = o.containment;
return;
}
if ( o.containment === "parent" ) {
o.containment = this.helper[ 0 ].parentNode;
}
c = $( o.containment );
ce = c[ 0 ];
if( !ce ) {
return;
}
over = c.css( "overflow" ) !== "hidden";
this.containment = [
( parseInt( c.css( "borderLeftWidth" ), 10 ) || 0 ) + ( parseInt( c.css( "paddingLeft" ), 10 ) || 0 ),
( parseInt( c.css( "borderTopWidth" ), 10 ) || 0 ) + ( parseInt( c.css( "paddingTop" ), 10 ) || 0 ) ,
( over ? Math.max( ce.scrollWidth, ce.offsetWidth ) : ce.offsetWidth ) - ( parseInt( c.css( "borderRightWidth" ), 10 ) || 0 ) - ( parseInt( c.css( "paddingRight" ), 10 ) || 0 ) - this.helperProportions.width - this.margins.left - this.margins.right,
( over ? Math.max( ce.scrollHeight, ce.offsetHeight ) : ce.offsetHeight ) - ( parseInt( c.css( "borderBottomWidth" ), 10 ) || 0 ) - ( parseInt( c.css( "paddingBottom" ), 10 ) || 0 ) - this.helperProportions.height - this.margins.top - this.margins.bottom
];
this.relative_container = c;
},
_convertPositionTo: function(d, pos) {
if(!pos) {
pos = this.position;
}
var mod = d === "absolute" ? 1 : -1,
scroll = this.cssPosition === "absolute" && !( this.scrollParent[ 0 ] !== document && $.contains( this.scrollParent[ 0 ], this.offsetParent[ 0 ] ) ) ? this.offsetParent : this.scrollParent;
//Cache the scroll
if (!this.offset.scroll) {
this.offset.scroll = {top : scroll.scrollTop(), left : scroll.scrollLeft()};
}
return {
top: (
pos.top + // The absolute mouse position
this.offset.relative.top * mod + // Only for relative positioned nodes: Relative offset from element to offset parent
this.offset.parent.top * mod - // The offsetParent's offset without borders (offset + border)
( ( this.cssPosition === "fixed" ? -this.scrollParent.scrollTop() : this.offset.scroll.top ) * mod )
),
left: (
pos.left + // The absolute mouse position
this.offset.relative.left * mod + // Only for relative positioned nodes: Relative offset from element to offset parent
this.offset.parent.left * mod - // The offsetParent's offset without borders (offset + border)
( ( this.cssPosition === "fixed" ? -this.scrollParent.scrollLeft() : this.offset.scroll.left ) * mod )
)
};
},
_generatePosition: function(event) {
var containment, co, top, left,
o = this.options,
scroll = this.cssPosition === "absolute" && !( this.scrollParent[ 0 ] !== document && $.contains( this.scrollParent[ 0 ], this.offsetParent[ 0 ] ) ) ? this.offsetParent : this.scrollParent,
pageX = event.pageX,
pageY = event.pageY;
//Cache the scroll
if (!this.offset.scroll) {
this.offset.scroll = {top : scroll.scrollTop(), left : scroll.scrollLeft()};
}
/*
* - Position constraining -
* Constrain the position to a mix of grid, containment.
*/
// If we are not dragging yet, we won't check for options
if ( this.originalPosition ) {
if ( this.containment ) {
if ( this.relative_container ){
co = this.relative_container.offset();
containment = [
this.containment[ 0 ] + co.left,
this.containment[ 1 ] + co.top,
this.containment[ 2 ] + co.left,
this.containment[ 3 ] + co.top
];
}
else {
containment = this.containment;
}
if(event.pageX - this.offset.click.left < containment[0]) {
pageX = containment[0] + this.offset.click.left;
}
if(event.pageY - this.offset.click.top < containment[1]) {
pageY = containment[1] + this.offset.click.top;
}
if(event.pageX - this.offset.click.left > containment[2]) {
pageX = containment[2] + this.offset.click.left;
}
if(event.pageY - this.offset.click.top > containment[3]) {
pageY = containment[3] + this.offset.click.top;
}
}
if(o.grid) {
//Check for grid elements set to 0 to prevent divide by 0 error causing invalid argument errors in IE (see ticket #6950)
top = o.grid[1] ? this.originalPageY + Math.round((pageY - this.originalPageY) / o.grid[1]) * o.grid[1] : this.originalPageY;
pageY = containment ? ((top - this.offset.click.top >= containment[1] || top - this.offset.click.top > containment[3]) ? top : ((top - this.offset.click.top >= containment[1]) ? top - o.grid[1] : top + o.grid[1])) : top;
left = o.grid[0] ? this.originalPageX + Math.round((pageX - this.originalPageX) / o.grid[0]) * o.grid[0] : this.originalPageX;
pageX = containment ? ((left - this.offset.click.left >= containment[0] || left - this.offset.click.left > containment[2]) ? left : ((left - this.offset.click.left >= containment[0]) ? left - o.grid[0] : left + o.grid[0])) : left;
}
}
return {
top: (
pageY - // The absolute mouse position
this.offset.click.top - // Click offset (relative to the element)
this.offset.relative.top - // Only for relative positioned nodes: Relative offset from element to offset parent
this.offset.parent.top + // The offsetParent's offset without borders (offset + border)
( this.cssPosition === "fixed" ? -this.scrollParent.scrollTop() : this.offset.scroll.top )
),
left: (
pageX - // The absolute mouse position
this.offset.click.left - // Click offset (relative to the element)
this.offset.relative.left - // Only for relative positioned nodes: Relative offset from element to offset parent
this.offset.parent.left + // The offsetParent's offset without borders (offset + border)
( this.cssPosition === "fixed" ? -this.scrollParent.scrollLeft() : this.offset.scroll.left )
)
};
},
_clear: function() {
this.helper.removeClass("ui-draggable-dragging");
if(this.helper[0] !== this.element[0] && !this.cancelHelperRemoval) {
this.helper.remove();
}
this.helper = null;
this.cancelHelperRemoval = false;
},
// From now on bulk stuff - mainly helpers
_trigger: function(type, event, ui) {
ui = ui || this._uiHash();
$.ui.plugin.call(this, type, [event, ui]);
//The absolute position has to be recalculated after plugins
if(type === "drag") {
this.positionAbs = this._convertPositionTo("absolute");
}
return $.Widget.prototype._trigger.call(this, type, event, ui);
},
plugins: {},
_uiHash: function() {
return {
helper: this.helper,
position: this.position,
originalPosition: this.originalPosition,
offset: this.positionAbs
};
}
});
$.ui.plugin.add("draggable", "connectToSortable", {
start: function(event, ui) {
var inst = $(this).data("ui-draggable"), o = inst.options,
uiSortable = $.extend({}, ui, { item: inst.element });
inst.sortables = [];
$(o.connectToSortable).each(function() {
var sortable = $.data(this, "ui-sortable");
if (sortable && !sortable.options.disabled) {
inst.sortables.push({
instance: sortable,
shouldRevert: sortable.options.revert
});
sortable.refreshPositions(); // Call the sortable's refreshPositions at drag start to refresh the containerCache since the sortable container cache is used in drag and needs to be up to date (this will ensure it's initialised as well as being kept in step with any changes that might have happened on the page).
sortable._trigger("activate", event, uiSortable);
}
});
},
stop: function(event, ui) {
//If we are still over the sortable, we fake the stop event of the sortable, but also remove helper
var inst = $(this).data("ui-draggable"),
uiSortable = $.extend({}, ui, { item: inst.element });
$.each(inst.sortables, function() {
if(this.instance.isOver) {
this.instance.isOver = 0;
inst.cancelHelperRemoval = true; //Don't remove the helper in the draggable instance
this.instance.cancelHelperRemoval = false; //Remove it in the sortable instance (so sortable plugins like revert still work)
//The sortable revert is supported, and we have to set a temporary dropped variable on the draggable to support revert: "valid/invalid"
if(this.shouldRevert) {
this.instance.options.revert = this.shouldRevert;
}
//Trigger the stop of the sortable
this.instance._mouseStop(event);
this.instance.options.helper = this.instance.options._helper;
//If the helper has been the original item, restore properties in the sortable
if(inst.options.helper === "original") {
this.instance.currentItem.css({ top: "auto", left: "auto" });
}
} else {
this.instance.cancelHelperRemoval = false; //Remove the helper in the sortable instance
this.instance._trigger("deactivate", event, uiSortable);
}
});
},
drag: function(event, ui) {
var inst = $(this).data("ui-draggable"), that = this;
$.each(inst.sortables, function() {
var innermostIntersecting = false,
thisSortable = this;
//Copy over some variables to allow calling the sortable's native _intersectsWith
this.instance.positionAbs = inst.positionAbs;
this.instance.helperProportions = inst.helperProportions;
this.instance.offset.click = inst.offset.click;
if(this.instance._intersectsWith(this.instance.containerCache)) {
innermostIntersecting = true;
$.each(inst.sortables, function () {
this.instance.positionAbs = inst.positionAbs;
this.instance.helperProportions = inst.helperProportions;
this.instance.offset.click = inst.offset.click;
if (this !== thisSortable &&
this.instance._intersectsWith(this.instance.containerCache) &&
$.contains(thisSortable.instance.element[0], this.instance.element[0])
) {
innermostIntersecting = false;
}
return innermostIntersecting;
});
}
if(innermostIntersecting) {
//If it intersects, we use a little isOver variable and set it once, so our move-in stuff gets fired only once
if(!this.instance.isOver) {
this.instance.isOver = 1;
//Now we fake the start of dragging for the sortable instance,
//by cloning the list group item, appending it to the sortable and using it as inst.currentItem
//We can then fire the start event of the sortable with our passed browser event, and our own helper (so it doesn't create a new one)
this.instance.currentItem = $(that).clone().removeAttr("id").appendTo(this.instance.element).data("ui-sortable-item", true);
this.instance.options._helper = this.instance.options.helper; //Store helper option to later restore it
this.instance.options.helper = function() { return ui.helper[0]; };
event.target = this.instance.currentItem[0];
this.instance._mouseCapture(event, true);
this.instance._mouseStart(event, true, true);
//Because the browser event is way off the new appended portlet, we modify a couple of variables to reflect the changes
this.instance.offset.click.top = inst.offset.click.top;
this.instance.offset.click.left = inst.offset.click.left;
this.instance.offset.parent.left -= inst.offset.parent.left - this.instance.offset.parent.left;
this.instance.offset.parent.top -= inst.offset.parent.top - this.instance.offset.parent.top;
inst._trigger("toSortable", event);
inst.dropped = this.instance.element; //draggable revert needs that
//hack so receive/update callbacks work (mostly)
inst.currentItem = inst.element;
this.instance.fromOutside = inst;
}
//Provided we did all the previous steps, we can fire the drag event of the sortable on every draggable drag, when it intersects with the sortable
if(this.instance.currentItem) {
this.instance._mouseDrag(event);
}
} else {
//If it doesn't intersect with the sortable, and it intersected before,
//we fake the drag stop of the sortable, but make sure it doesn't remove the helper by using cancelHelperRemoval
if(this.instance.isOver) {
this.instance.isOver = 0;
this.instance.cancelHelperRemoval = true;
//Prevent reverting on this forced stop
this.instance.options.revert = false;
// The out event needs to be triggered independently
this.instance._trigger("out", event, this.instance._uiHash(this.instance));
this.instance._mouseStop(event, true);
this.instance.options.helper = this.instance.options._helper;
//Now we remove our currentItem, the list group clone again, and the placeholder, and animate the helper back to it's original size
this.instance.currentItem.remove();
if(this.instance.placeholder) {
this.instance.placeholder.remove();
}
inst._trigger("fromSortable", event);
inst.dropped = false; //draggable revert needs that
}
}
});
}
});
$.ui.plugin.add("draggable", "cursor", {
start: function() {
var t = $("body"), o = $(this).data("ui-draggable").options;
if (t.css("cursor")) {
o._cursor = t.css("cursor");
}
t.css("cursor", o.cursor);
},
stop: function() {
var o = $(this).data("ui-draggable").options;
if (o._cursor) {
$("body").css("cursor", o._cursor);
}
}
});
$.ui.plugin.add("draggable", "opacity", {
start: function(event, ui) {
var t = $(ui.helper), o = $(this).data("ui-draggable").options;
if(t.css("opacity")) {
o._opacity = t.css("opacity");
}
t.css("opacity", o.opacity);
},
stop: function(event, ui) {
var o = $(this).data("ui-draggable").options;
if(o._opacity) {
$(ui.helper).css("opacity", o._opacity);
}
}
});
$.ui.plugin.add("draggable", "scroll", {
start: function() {
var i = $(this).data("ui-draggable");
if(i.scrollParent[0] !== document && i.scrollParent[0].tagName !== "HTML") {
i.overflowOffset = i.scrollParent.offset();
}
},
drag: function( event ) {
var i = $(this).data("ui-draggable"), o = i.options, scrolled = false;
if(i.scrollParent[0] !== document && i.scrollParent[0].tagName !== "HTML") {
if(!o.axis || o.axis !== "x") {
if((i.overflowOffset.top + i.scrollParent[0].offsetHeight) - event.pageY < o.scrollSensitivity) {
i.scrollParent[0].scrollTop = scrolled = i.scrollParent[0].scrollTop + o.scrollSpeed;
} else if(event.pageY - i.overflowOffset.top < o.scrollSensitivity) {
i.scrollParent[0].scrollTop = scrolled = i.scrollParent[0].scrollTop - o.scrollSpeed;
}
}
if(!o.axis || o.axis !== "y") {
if((i.overflowOffset.left + i.scrollParent[0].offsetWidth) - event.pageX < o.scrollSensitivity) {
i.scrollParent[0].scrollLeft = scrolled = i.scrollParent[0].scrollLeft + o.scrollSpeed;
} else if(event.pageX - i.overflowOffset.left < o.scrollSensitivity) {
i.scrollParent[0].scrollLeft = scrolled = i.scrollParent[0].scrollLeft - o.scrollSpeed;
}
}
} else {
if(!o.axis || o.axis !== "x") {
if(event.pageY - $(document).scrollTop() < o.scrollSensitivity) {
scrolled = $(document).scrollTop($(document).scrollTop() - o.scrollSpeed);
} else if($(window).height() - (event.pageY - $(document).scrollTop()) < o.scrollSensitivity) {
scrolled = $(document).scrollTop($(document).scrollTop() + o.scrollSpeed);
}
}
if(!o.axis || o.axis !== "y") {
if(event.pageX - $(document).scrollLeft() < o.scrollSensitivity) {
scrolled = $(document).scrollLeft($(document).scrollLeft() - o.scrollSpeed);
} else if($(window).width() - (event.pageX - $(document).scrollLeft()) < o.scrollSensitivity) {
scrolled = $(document).scrollLeft($(document).scrollLeft() + o.scrollSpeed);
}
}
}
if(scrolled !== false && $.ui.ddmanager && !o.dropBehaviour) {
$.ui.ddmanager.prepareOffsets(i, event);
}
}
});
$.ui.plugin.add("draggable", "snap", {
start: function() {
var i = $(this).data("ui-draggable"),
o = i.options;
i.snapElements = [];
$(o.snap.constructor !== String ? ( o.snap.items || ":data(ui-draggable)" ) : o.snap).each(function() {
var $t = $(this),
$o = $t.offset();
if(this !== i.element[0]) {
i.snapElements.push({
item: this,
width: $t.outerWidth(), height: $t.outerHeight(),
top: $o.top, left: $o.left
});
}
});
},
drag: function(event, ui) {
var ts, bs, ls, rs, l, r, t, b, i, first,
inst = $(this).data("ui-draggable"),
o = inst.options,
d = o.snapTolerance,
x1 = ui.offset.left, x2 = x1 + inst.helperProportions.width,
y1 = ui.offset.top, y2 = y1 + inst.helperProportions.height;
for (i = inst.snapElements.length - 1; i >= 0; i--){
l = inst.snapElements[i].left;
r = l + inst.snapElements[i].width;
t = inst.snapElements[i].top;
b = t + inst.snapElements[i].height;
if ( x2 < l - d || x1 > r + d || y2 < t - d || y1 > b + d || !$.contains( inst.snapElements[ i ].item.ownerDocument, inst.snapElements[ i ].item ) ) {
if(inst.snapElements[i].snapping) {
(inst.options.snap.release && inst.options.snap.release.call(inst.element, event, $.extend(inst._uiHash(), { snapItem: inst.snapElements[i].item })));
}
inst.snapElements[i].snapping = false;
continue;
}
if(o.snapMode !== "inner") {
ts = Math.abs(t - y2) <= d;
bs = Math.abs(b - y1) <= d;
ls = Math.abs(l - x2) <= d;
rs = Math.abs(r - x1) <= d;
if(ts) {
ui.position.top = inst._convertPositionTo("relative", { top: t - inst.helperProportions.height, left: 0 }).top - inst.margins.top;
}
if(bs) {
ui.position.top = inst._convertPositionTo("relative", { top: b, left: 0 }).top - inst.margins.top;
}
if(ls) {
ui.position.left = inst._convertPositionTo("relative", { top: 0, left: l - inst.helperProportions.width }).left - inst.margins.left;
}
if(rs) {
ui.position.left = inst._convertPositionTo("relative", { top: 0, left: r }).left - inst.margins.left;
}
}
first = (ts || bs || ls || rs);
if(o.snapMode !== "outer") {
ts = Math.abs(t - y1) <= d;
bs = Math.abs(b - y2) <= d;
ls = Math.abs(l - x1) <= d;
rs = Math.abs(r - x2) <= d;
if(ts) {
ui.position.top = inst._convertPositionTo("relative", { top: t, left: 0 }).top - inst.margins.top;
}
if(bs) {
ui.position.top = inst._convertPositionTo("relative", { top: b - inst.helperProportions.height, left: 0 }).top - inst.margins.top;
}
if(ls) {
ui.position.left = inst._convertPositionTo("relative", { top: 0, left: l }).left - inst.margins.left;
}
if(rs) {
ui.position.left = inst._convertPositionTo("relative", { top: 0, left: r - inst.helperProportions.width }).left - inst.margins.left;
}
}
if(!inst.snapElements[i].snapping && (ts || bs || ls || rs || first)) {
(inst.options.snap.snap && inst.options.snap.snap.call(inst.element, event, $.extend(inst._uiHash(), { snapItem: inst.snapElements[i].item })));
}
inst.snapElements[i].snapping = (ts || bs || ls || rs || first);
}
}
});
$.ui.plugin.add("draggable", "stack", {
start: function() {
var min,
o = this.data("ui-draggable").options,
group = $.makeArray($(o.stack)).sort(function(a,b) {
return (parseInt($(a).css("zIndex"),10) || 0) - (parseInt($(b).css("zIndex"),10) || 0);
});
if (!group.length) { return; }
min = parseInt($(group[0]).css("zIndex"), 10) || 0;
$(group).each(function(i) {
$(this).css("zIndex", min + i);
});
this.css("zIndex", (min + group.length));
}
});
$.ui.plugin.add("draggable", "zIndex", {
start: function(event, ui) {
var t = $(ui.helper), o = $(this).data("ui-draggable").options;
if(t.css("zIndex")) {
o._zIndex = t.css("zIndex");
}
t.css("zIndex", o.zIndex);
},
stop: function(event, ui) {
var o = $(this).data("ui-draggable").options;
if(o._zIndex) {
$(ui.helper).css("zIndex", o._zIndex);
}
}
});
})(jQuery);
(function( $, undefined ) {
function isOverAxis( x, reference, size ) {
return ( x > reference ) && ( x < ( reference + size ) );
}
$.widget("ui.droppable", {
version: "1.10.3",
widgetEventPrefix: "drop",
options: {
accept: "*",
activeClass: false,
addClasses: true,
greedy: false,
hoverClass: false,
scope: "default",
tolerance: "intersect",
// callbacks
activate: null,
deactivate: null,
drop: null,
out: null,
over: null
},
_create: function() {
var o = this.options,
accept = o.accept;
this.isover = false;
this.isout = true;
this.accept = $.isFunction(accept) ? accept : function(d) {
return d.is(accept);
};
//Store the droppable's proportions
this.proportions = { width: this.element[0].offsetWidth, height: this.element[0].offsetHeight };
// Add the reference and positions to the manager
$.ui.ddmanager.droppables[o.scope] = $.ui.ddmanager.droppables[o.scope] || [];
$.ui.ddmanager.droppables[o.scope].push(this);
(o.addClasses && this.element.addClass("ui-droppable"));
},
_destroy: function() {
var i = 0,
drop = $.ui.ddmanager.droppables[this.options.scope];
for ( ; i < drop.length; i++ ) {
if ( drop[i] === this ) {
drop.splice(i, 1);
}
}
this.element.removeClass("ui-droppable ui-droppable-disabled");
},
_setOption: function(key, value) {
if(key === "accept") {
this.accept = $.isFunction(value) ? value : function(d) {
return d.is(value);
};
}
$.Widget.prototype._setOption.apply(this, arguments);
},
_activate: function(event) {
var draggable = $.ui.ddmanager.current;
if(this.options.activeClass) {
this.element.addClass(this.options.activeClass);
}
if(draggable){
this._trigger("activate", event, this.ui(draggable));
}
},
_deactivate: function(event) {
var draggable = $.ui.ddmanager.current;
if(this.options.activeClass) {
this.element.removeClass(this.options.activeClass);
}
if(draggable){
this._trigger("deactivate", event, this.ui(draggable));
}
},
_over: function(event) {
var draggable = $.ui.ddmanager.current;
// Bail if draggable and droppable are same element
if (!draggable || (draggable.currentItem || draggable.element)[0] === this.element[0]) {
return;
}
if (this.accept.call(this.element[0],(draggable.currentItem || draggable.element))) {
if(this.options.hoverClass) {
this.element.addClass(this.options.hoverClass);
}
this._trigger("over", event, this.ui(draggable));
}
},
_out: function(event) {
var draggable = $.ui.ddmanager.current;
// Bail if draggable and droppable are same element
if (!draggable || (draggable.currentItem || draggable.element)[0] === this.element[0]) {
return;
}
if (this.accept.call(this.element[0],(draggable.currentItem || draggable.element))) {
if(this.options.hoverClass) {
this.element.removeClass(this.options.hoverClass);
}
this._trigger("out", event, this.ui(draggable));
}
},
_drop: function(event,custom) {
var draggable = custom || $.ui.ddmanager.current,
childrenIntersection = false;
// Bail if draggable and droppable are same element
if (!draggable || (draggable.currentItem || draggable.element)[0] === this.element[0]) {
return false;
}
this.element.find(":data(ui-droppable)").not(".ui-draggable-dragging").each(function() {
var inst = $.data(this, "ui-droppable");
if(
inst.options.greedy &&
!inst.options.disabled &&
inst.options.scope === draggable.options.scope &&
inst.accept.call(inst.element[0], (draggable.currentItem || draggable.element)) &&
$.ui.intersect(draggable, $.extend(inst, { offset: inst.element.offset() }), inst.options.tolerance)
) { childrenIntersection = true; return false; }
});
if(childrenIntersection) {
return false;
}
if(this.accept.call(this.element[0],(draggable.currentItem || draggable.element))) {
if(this.options.activeClass) {
this.element.removeClass(this.options.activeClass);
}
if(this.options.hoverClass) {
this.element.removeClass(this.options.hoverClass);
}
this._trigger("drop", event, this.ui(draggable));
return this.element;
}
return false;
},
ui: function(c) {
return {
draggable: (c.currentItem || c.element),
helper: c.helper,
position: c.position,
offset: c.positionAbs
};
}
});
$.ui.intersect = function(draggable, droppable, toleranceMode) {
if (!droppable.offset) {
return false;
}
var draggableLeft, draggableTop,
x1 = (draggable.positionAbs || draggable.position.absolute).left, x2 = x1 + draggable.helperProportions.width,
y1 = (draggable.positionAbs || draggable.position.absolute).top, y2 = y1 + draggable.helperProportions.height,
l = droppable.offset.left, r = l + droppable.proportions.width,
t = droppable.offset.top, b = t + droppable.proportions.height;
switch (toleranceMode) {
case "fit":
return (l <= x1 && x2 <= r && t <= y1 && y2 <= b);
case "intersect":
return (l < x1 + (draggable.helperProportions.width / 2) && // Right Half
x2 - (draggable.helperProportions.width / 2) < r && // Left Half
t < y1 + (draggable.helperProportions.height / 2) && // Bottom Half
y2 - (draggable.helperProportions.height / 2) < b ); // Top Half
case "pointer":
draggableLeft = ((draggable.positionAbs || draggable.position.absolute).left + (draggable.clickOffset || draggable.offset.click).left);
draggableTop = ((draggable.positionAbs || draggable.position.absolute).top + (draggable.clickOffset || draggable.offset.click).top);
return isOverAxis( draggableTop, t, droppable.proportions.height ) && isOverAxis( draggableLeft, l, droppable.proportions.width );
case "touch":
return (
(y1 >= t && y1 <= b) || // Top edge touching
(y2 >= t && y2 <= b) || // Bottom edge touching
(y1 < t && y2 > b) // Surrounded vertically
) && (
(x1 >= l && x1 <= r) || // Left edge touching
(x2 >= l && x2 <= r) || // Right edge touching
(x1 < l && x2 > r) // Surrounded horizontally
);
default:
return false;
}
};
/*
This manager tracks offsets of draggables and droppables
*/
$.ui.ddmanager = {
current: null,
droppables: { "default": [] },
prepareOffsets: function(t, event) {
var i, j,
m = $.ui.ddmanager.droppables[t.options.scope] || [],
type = event ? event.type : null, // workaround for #2317
list = (t.currentItem || t.element).find(":data(ui-droppable)").addBack();
droppablesLoop: for (i = 0; i < m.length; i++) {
//No disabled and non-accepted
if(m[i].options.disabled || (t && !m[i].accept.call(m[i].element[0],(t.currentItem || t.element)))) {
continue;
}
// Filter out elements in the current dragged item
for (j=0; j < list.length; j++) {
if(list[j] === m[i].element[0]) {
m[i].proportions.height = 0;
continue droppablesLoop;
}
}
m[i].visible = m[i].element.css("display") !== "none";
if(!m[i].visible) {
continue;
}
//Activate the droppable if used directly from draggables
if(type === "mousedown") {
m[i]._activate.call(m[i], event);
}
m[i].offset = m[i].element.offset();
m[i].proportions = { width: m[i].element[0].offsetWidth, height: m[i].element[0].offsetHeight };
}
},
drop: function(draggable, event) {
var dropped = false;
// Create a copy of the droppables in case the list changes during the drop (#9116)
$.each(($.ui.ddmanager.droppables[draggable.options.scope] || []).slice(), function() {
if(!this.options) {
return;
}
if (!this.options.disabled && this.visible && $.ui.intersect(draggable, this, this.options.tolerance)) {
dropped = this._drop.call(this, event) || dropped;
}
if (!this.options.disabled && this.visible && this.accept.call(this.element[0],(draggable.currentItem || draggable.element))) {
this.isout = true;
this.isover = false;
this._deactivate.call(this, event);
}
});
return dropped;
},
dragStart: function( draggable, event ) {
//Listen for scrolling so that if the dragging causes scrolling the position of the droppables can be recalculated (see #5003)
draggable.element.parentsUntil( "body" ).bind( "scroll.droppable", function() {
if( !draggable.options.refreshPositions ) {
$.ui.ddmanager.prepareOffsets( draggable, event );
}
});
},
drag: function(draggable, event) {
//If you have a highly dynamic page, you might try this option. It renders positions every time you move the mouse.
if(draggable.options.refreshPositions) {
$.ui.ddmanager.prepareOffsets(draggable, event);
}
//Run through all droppables and check their positions based on specific tolerance options
$.each($.ui.ddmanager.droppables[draggable.options.scope] || [], function() {
if(this.options.disabled || this.greedyChild || !this.visible) {
return;
}
var parentInstance, scope, parent,
intersects = $.ui.intersect(draggable, this, this.options.tolerance),
c = !intersects && this.isover ? "isout" : (intersects && !this.isover ? "isover" : null);
if(!c) {
return;
}
if (this.options.greedy) {
// find droppable parents with same scope
scope = this.options.scope;
parent = this.element.parents(":data(ui-droppable)").filter(function () {
return $.data(this, "ui-droppable").options.scope === scope;
});
if (parent.length) {
parentInstance = $.data(parent[0], "ui-droppable");
parentInstance.greedyChild = (c === "isover");
}
}
// we just moved into a greedy child
if (parentInstance && c === "isover") {
parentInstance.isover = false;
parentInstance.isout = true;
parentInstance._out.call(parentInstance, event);
}
this[c] = true;
this[c === "isout" ? "isover" : "isout"] = false;
this[c === "isover" ? "_over" : "_out"].call(this, event);
// we just moved out of a greedy child
if (parentInstance && c === "isout") {
parentInstance.isout = false;
parentInstance.isover = true;
parentInstance._over.call(parentInstance, event);
}
});
},
dragStop: function( draggable, event ) {
draggable.element.parentsUntil( "body" ).unbind( "scroll.droppable" );
//Call prepareOffsets one final time since IE does not fire return scroll events when overflow was caused by drag (see #5003)
if( !draggable.options.refreshPositions ) {
$.ui.ddmanager.prepareOffsets( draggable, event );
}
}
};
})(jQuery);
(function( $, undefined ) {
function num(v) {
return parseInt(v, 10) || 0;
}
function isNumber(value) {
return !isNaN(parseInt(value, 10));
}
$.widget("ui.resizable", $.ui.mouse, {
version: "1.10.3",
widgetEventPrefix: "resize",
options: {
alsoResize: false,
animate: false,
animateDuration: "slow",
animateEasing: "swing",
aspectRatio: false,
autoHide: false,
containment: false,
ghost: false,
grid: false,
handles: "e,s,se",
helper: false,
maxHeight: null,
maxWidth: null,
minHeight: 10,
minWidth: 10,
// See #7960
zIndex: 90,
// callbacks
resize: null,
start: null,
stop: null
},
_create: function() {
var n, i, handle, axis, hname,
that = this,
o = this.options;
this.element.addClass("ui-resizable");
$.extend(this, {
_aspectRatio: !!(o.aspectRatio),
aspectRatio: o.aspectRatio,
originalElement: this.element,
_proportionallyResizeElements: [],
_helper: o.helper || o.ghost || o.animate ? o.helper || "ui-resizable-helper" : null
});
//Wrap the element if it cannot hold child nodes
if(this.element[0].nodeName.match(/canvas|textarea|input|select|button|img/i)) {
//Create a wrapper element and set the wrapper to the new current internal element
this.element.wrap(
$("<div class='ui-wrapper' style='overflow: hidden;'></div>").css({
position: this.element.css("position"),
width: this.element.outerWidth(),
height: this.element.outerHeight(),
top: this.element.css("top"),
left: this.element.css("left")
})
);
//Overwrite the original this.element
this.element = this.element.parent().data(
"ui-resizable", this.element.data("ui-resizable")
);
this.elementIsWrapper = true;
//Move margins to the wrapper
this.element.css({ marginLeft: this.originalElement.css("marginLeft"), marginTop: this.originalElement.css("marginTop"), marginRight: this.originalElement.css("marginRight"), marginBottom: this.originalElement.css("marginBottom") });
this.originalElement.css({ marginLeft: 0, marginTop: 0, marginRight: 0, marginBottom: 0});
//Prevent Safari textarea resize
this.originalResizeStyle = this.originalElement.css("resize");
this.originalElement.css("resize", "none");
//Push the actual element to our proportionallyResize internal array
this._proportionallyResizeElements.push(this.originalElement.css({ position: "static", zoom: 1, display: "block" }));
// avoid IE jump (hard set the margin)
this.originalElement.css({ margin: this.originalElement.css("margin") });
// fix handlers offset
this._proportionallyResize();
}
this.handles = o.handles || (!$(".ui-resizable-handle", this.element).length ? "e,s,se" : { n: ".ui-resizable-n", e: ".ui-resizable-e", s: ".ui-resizable-s", w: ".ui-resizable-w", se: ".ui-resizable-se", sw: ".ui-resizable-sw", ne: ".ui-resizable-ne", nw: ".ui-resizable-nw" });
if(this.handles.constructor === String) {
if ( this.handles === "all") {
this.handles = "n,e,s,w,se,sw,ne,nw";
}
n = this.handles.split(",");
this.handles = {};
for(i = 0; i < n.length; i++) {
handle = $.trim(n[i]);
hname = "ui-resizable-"+handle;
axis = $("<div class='ui-resizable-handle " + hname + "'></div>");
// Apply zIndex to all handles - see #7960
axis.css({ zIndex: o.zIndex });
//TODO : What's going on here?
if ("se" === handle) {
axis.addClass("ui-icon ui-icon-gripsmall-diagonal-se");
}
//Insert into internal handles object and append to element
this.handles[handle] = ".ui-resizable-"+handle;
this.element.append(axis);
}
}
this._renderAxis = function(target) {
var i, axis, padPos, padWrapper;
target = target || this.element;
for(i in this.handles) {
if(this.handles[i].constructor === String) {
this.handles[i] = $(this.handles[i], this.element).show();
}
//Apply pad to wrapper element, needed to fix axis position (textarea, inputs, scrolls)
if (this.elementIsWrapper && this.originalElement[0].nodeName.match(/textarea|input|select|button/i)) {
axis = $(this.handles[i], this.element);
//Checking the correct pad and border
padWrapper = /sw|ne|nw|se|n|s/.test(i) ? axis.outerHeight() : axis.outerWidth();
//The padding type i have to apply...
padPos = [ "padding",
/ne|nw|n/.test(i) ? "Top" :
/se|sw|s/.test(i) ? "Bottom" :
/^e$/.test(i) ? "Right" : "Left" ].join("");
target.css(padPos, padWrapper);
this._proportionallyResize();
}
//TODO: What's that good for? There's not anything to be executed left
if(!$(this.handles[i]).length) {
continue;
}
}
};
//TODO: make renderAxis a prototype function
this._renderAxis(this.element);
this._handles = $(".ui-resizable-handle", this.element)
.disableSelection();
//Matching axis name
this._handles.mouseover(function() {
if (!that.resizing) {
if (this.className) {
axis = this.className.match(/ui-resizable-(se|sw|ne|nw|n|e|s|w)/i);
}
//Axis, default = se
that.axis = axis && axis[1] ? axis[1] : "se";
}
});
//If we want to auto hide the elements
if (o.autoHide) {
this._handles.hide();
$(this.element)
.addClass("ui-resizable-autohide")
.mouseenter(function() {
if (o.disabled) {
return;
}
$(this).removeClass("ui-resizable-autohide");
that._handles.show();
})
.mouseleave(function(){
if (o.disabled) {
return;
}
if (!that.resizing) {
$(this).addClass("ui-resizable-autohide");
that._handles.hide();
}
});
}
//Initialize the mouse interaction
this._mouseInit();
},
_destroy: function() {
this._mouseDestroy();
var wrapper,
_destroy = function(exp) {
$(exp).removeClass("ui-resizable ui-resizable-disabled ui-resizable-resizing")
.removeData("resizable").removeData("ui-resizable").unbind(".resizable").find(".ui-resizable-handle").remove();
};
//TODO: Unwrap at same DOM position
if (this.elementIsWrapper) {
_destroy(this.element);
wrapper = this.element;
this.originalElement.css({
position: wrapper.css("position"),
width: wrapper.outerWidth(),
height: wrapper.outerHeight(),
top: wrapper.css("top"),
left: wrapper.css("left")
}).insertAfter( wrapper );
wrapper.remove();
}
this.originalElement.css("resize", this.originalResizeStyle);
_destroy(this.originalElement);
return this;
},
_mouseCapture: function(event) {
var i, handle,
capture = false;
for (i in this.handles) {
handle = $(this.handles[i])[0];
if (handle === event.target || $.contains(handle, event.target)) {
capture = true;
}
}
return !this.options.disabled && capture;
},
_mouseStart: function(event) {
var curleft, curtop, cursor,
o = this.options,
iniPos = this.element.position(),
el = this.element;
this.resizing = true;
// bugfix for http://dev.jquery.com/ticket/1749
if ( (/absolute/).test( el.css("position") ) ) {
el.css({ position: "absolute", top: el.css("top"), left: el.css("left") });
} else if (el.is(".ui-draggable")) {
el.css({ position: "absolute", top: iniPos.top, left: iniPos.left });
}
this._renderProxy();
curleft = num(this.helper.css("left"));
curtop = num(this.helper.css("top"));
if (o.containment) {
curleft += $(o.containment).scrollLeft() || 0;
curtop += $(o.containment).scrollTop() || 0;
}
//Store needed variables
this.offset = this.helper.offset();
this.position = { left: curleft, top: curtop };
this.size = this._helper ? { width: el.outerWidth(), height: el.outerHeight() } : { width: el.width(), height: el.height() };
this.originalSize = this._helper ? { width: el.outerWidth(), height: el.outerHeight() } : { width: el.width(), height: el.height() };
this.originalPosition = { left: curleft, top: curtop };
this.sizeDiff = { width: el.outerWidth() - el.width(), height: el.outerHeight() - el.height() };
this.originalMousePosition = { left: event.pageX, top: event.pageY };
//Aspect Ratio
this.aspectRatio = (typeof o.aspectRatio === "number") ? o.aspectRatio : ((this.originalSize.width / this.originalSize.height) || 1);
cursor = $(".ui-resizable-" + this.axis).css("cursor");
$("body").css("cursor", cursor === "auto" ? this.axis + "-resize" : cursor);
el.addClass("ui-resizable-resizing");
this._propagate("start", event);
return true;
},
_mouseDrag: function(event) {
//Increase performance, avoid regex
var data,
el = this.helper, props = {},
smp = this.originalMousePosition,
a = this.axis,
prevTop = this.position.top,
prevLeft = this.position.left,
prevWidth = this.size.width,
prevHeight = this.size.height,
dx = (event.pageX-smp.left)||0,
dy = (event.pageY-smp.top)||0,
trigger = this._change[a];
if (!trigger) {
return false;
}
// Calculate the attrs that will be change
data = trigger.apply(this, [event, dx, dy]);
// Put this in the mouseDrag handler since the user can start pressing shift while resizing
this._updateVirtualBoundaries(event.shiftKey);
if (this._aspectRatio || event.shiftKey) {
data = this._updateRatio(data, event);
}
data = this._respectSize(data, event);
this._updateCache(data);
// plugins callbacks need to be called first
this._propagate("resize", event);
if (this.position.top !== prevTop) {
props.top = this.position.top + "px";
}
if (this.position.left !== prevLeft) {
props.left = this.position.left + "px";
}
if (this.size.width !== prevWidth) {
props.width = this.size.width + "px";
}
if (this.size.height !== prevHeight) {
props.height = this.size.height + "px";
}
el.css(props);
if (!this._helper && this._proportionallyResizeElements.length) {
this._proportionallyResize();
}
// Call the user callback if the element was resized
if ( ! $.isEmptyObject(props) ) {
this._trigger("resize", event, this.ui());
}
return false;
},
_mouseStop: function(event) {
this.resizing = false;
var pr, ista, soffseth, soffsetw, s, left, top,
o = this.options, that = this;
if(this._helper) {
pr = this._proportionallyResizeElements;
ista = pr.length && (/textarea/i).test(pr[0].nodeName);
soffseth = ista && $.ui.hasScroll(pr[0], "left") /* TODO - jump height */ ? 0 : that.sizeDiff.height;
soffsetw = ista ? 0 : that.sizeDiff.width;
s = { width: (that.helper.width() - soffsetw), height: (that.helper.height() - soffseth) };
left = (parseInt(that.element.css("left"), 10) + (that.position.left - that.originalPosition.left)) || null;
top = (parseInt(that.element.css("top"), 10) + (that.position.top - that.originalPosition.top)) || null;
if (!o.animate) {
this.element.css($.extend(s, { top: top, left: left }));
}
that.helper.height(that.size.height);
that.helper.width(that.size.width);
if (this._helper && !o.animate) {
this._proportionallyResize();
}
}
$("body").css("cursor", "auto");
this.element.removeClass("ui-resizable-resizing");
this._propagate("stop", event);
if (this._helper) {
this.helper.remove();
}
return false;
},
_updateVirtualBoundaries: function(forceAspectRatio) {
var pMinWidth, pMaxWidth, pMinHeight, pMaxHeight, b,
o = this.options;
b = {
minWidth: isNumber(o.minWidth) ? o.minWidth : 0,
maxWidth: isNumber(o.maxWidth) ? o.maxWidth : Infinity,
minHeight: isNumber(o.minHeight) ? o.minHeight : 0,
maxHeight: isNumber(o.maxHeight) ? o.maxHeight : Infinity
};
if(this._aspectRatio || forceAspectRatio) {
// We want to create an enclosing box whose aspect ration is the requested one
// First, compute the "projected" size for each dimension based on the aspect ratio and other dimension
pMinWidth = b.minHeight * this.aspectRatio;
pMinHeight = b.minWidth / this.aspectRatio;
pMaxWidth = b.maxHeight * this.aspectRatio;
pMaxHeight = b.maxWidth / this.aspectRatio;
if(pMinWidth > b.minWidth) {
b.minWidth = pMinWidth;
}
if(pMinHeight > b.minHeight) {
b.minHeight = pMinHeight;
}
if(pMaxWidth < b.maxWidth) {
b.maxWidth = pMaxWidth;
}
if(pMaxHeight < b.maxHeight) {
b.maxHeight = pMaxHeight;
}
}
this._vBoundaries = b;
},
_updateCache: function(data) {
this.offset = this.helper.offset();
if (isNumber(data.left)) {
this.position.left = data.left;
}
if (isNumber(data.top)) {
this.position.top = data.top;
}
if (isNumber(data.height)) {
this.size.height = data.height;
}
if (isNumber(data.width)) {
this.size.width = data.width;
}
},
_updateRatio: function( data ) {
var cpos = this.position,
csize = this.size,
a = this.axis;
if (isNumber(data.height)) {
data.width = (data.height * this.aspectRatio);
} else if (isNumber(data.width)) {
data.height = (data.width / this.aspectRatio);
}
if (a === "sw") {
data.left = cpos.left + (csize.width - data.width);
data.top = null;
}
if (a === "nw") {
data.top = cpos.top + (csize.height - data.height);
data.left = cpos.left + (csize.width - data.width);
}
return data;
},
_respectSize: function( data ) {
var o = this._vBoundaries,
a = this.axis,
ismaxw = isNumber(data.width) && o.maxWidth && (o.maxWidth < data.width), ismaxh = isNumber(data.height) && o.maxHeight && (o.maxHeight < data.height),
isminw = isNumber(data.width) && o.minWidth && (o.minWidth > data.width), isminh = isNumber(data.height) && o.minHeight && (o.minHeight > data.height),
dw = this.originalPosition.left + this.originalSize.width,
dh = this.position.top + this.size.height,
cw = /sw|nw|w/.test(a), ch = /nw|ne|n/.test(a);
if (isminw) {
data.width = o.minWidth;
}
if (isminh) {
data.height = o.minHeight;
}
if (ismaxw) {
data.width = o.maxWidth;
}
if (ismaxh) {
data.height = o.maxHeight;
}
if (isminw && cw) {
data.left = dw - o.minWidth;
}
if (ismaxw && cw) {
data.left = dw - o.maxWidth;
}
if (isminh && ch) {
data.top = dh - o.minHeight;
}
if (ismaxh && ch) {
data.top = dh - o.maxHeight;
}
// fixing jump error on top/left - bug #2330
if (!data.width && !data.height && !data.left && data.top) {
data.top = null;
} else if (!data.width && !data.height && !data.top && data.left) {
data.left = null;
}
return data;
},
_proportionallyResize: function() {
if (!this._proportionallyResizeElements.length) {
return;
}
var i, j, borders, paddings, prel,
element = this.helper || this.element;
for ( i=0; i < this._proportionallyResizeElements.length; i++) {
prel = this._proportionallyResizeElements[i];
if (!this.borderDif) {
this.borderDif = [];
borders = [prel.css("borderTopWidth"), prel.css("borderRightWidth"), prel.css("borderBottomWidth"), prel.css("borderLeftWidth")];
paddings = [prel.css("paddingTop"), prel.css("paddingRight"), prel.css("paddingBottom"), prel.css("paddingLeft")];
for ( j = 0; j < borders.length; j++ ) {
this.borderDif[ j ] = ( parseInt( borders[ j ], 10 ) || 0 ) + ( parseInt( paddings[ j ], 10 ) || 0 );
}
}
prel.css({
height: (element.height() - this.borderDif[0] - this.borderDif[2]) || 0,
width: (element.width() - this.borderDif[1] - this.borderDif[3]) || 0
});
}
},
_renderProxy: function() {
var el = this.element, o = this.options;
this.elementOffset = el.offset();
if(this._helper) {
this.helper = this.helper || $("<div style='overflow:hidden;'></div>");
this.helper.addClass(this._helper).css({
width: this.element.outerWidth() - 1,
height: this.element.outerHeight() - 1,
position: "absolute",
left: this.elementOffset.left +"px",
top: this.elementOffset.top +"px",
zIndex: ++o.zIndex //TODO: Don't modify option
});
this.helper
.appendTo("body")
.disableSelection();
} else {
this.helper = this.element;
}
},
_change: {
e: function(event, dx) {
return { width: this.originalSize.width + dx };
},
w: function(event, dx) {
var cs = this.originalSize, sp = this.originalPosition;
return { left: sp.left + dx, width: cs.width - dx };
},
n: function(event, dx, dy) {
var cs = this.originalSize, sp = this.originalPosition;
return { top: sp.top + dy, height: cs.height - dy };
},
s: function(event, dx, dy) {
return { height: this.originalSize.height + dy };
},
se: function(event, dx, dy) {
return $.extend(this._change.s.apply(this, arguments), this._change.e.apply(this, [event, dx, dy]));
},
sw: function(event, dx, dy) {
return $.extend(this._change.s.apply(this, arguments), this._change.w.apply(this, [event, dx, dy]));
},
ne: function(event, dx, dy) {
return $.extend(this._change.n.apply(this, arguments), this._change.e.apply(this, [event, dx, dy]));
},
nw: function(event, dx, dy) {
return $.extend(this._change.n.apply(this, arguments), this._change.w.apply(this, [event, dx, dy]));
}
},
_propagate: function(n, event) {
$.ui.plugin.call(this, n, [event, this.ui()]);
(n !== "resize" && this._trigger(n, event, this.ui()));
},
plugins: {},
ui: function() {
return {
originalElement: this.originalElement,
element: this.element,
helper: this.helper,
position: this.position,
size: this.size,
originalSize: this.originalSize,
originalPosition: this.originalPosition
};
}
});
/*
* Resizable Extensions
*/
$.ui.plugin.add("resizable", "animate", {
stop: function( event ) {
var that = $(this).data("ui-resizable"),
o = that.options,
pr = that._proportionallyResizeElements,
ista = pr.length && (/textarea/i).test(pr[0].nodeName),
soffseth = ista && $.ui.hasScroll(pr[0], "left") /* TODO - jump height */ ? 0 : that.sizeDiff.height,
soffsetw = ista ? 0 : that.sizeDiff.width,
style = { width: (that.size.width - soffsetw), height: (that.size.height - soffseth) },
left = (parseInt(that.element.css("left"), 10) + (that.position.left - that.originalPosition.left)) || null,
top = (parseInt(that.element.css("top"), 10) + (that.position.top - that.originalPosition.top)) || null;
that.element.animate(
$.extend(style, top && left ? { top: top, left: left } : {}), {
duration: o.animateDuration,
easing: o.animateEasing,
step: function() {
var data = {
width: parseInt(that.element.css("width"), 10),
height: parseInt(that.element.css("height"), 10),
top: parseInt(that.element.css("top"), 10),
left: parseInt(that.element.css("left"), 10)
};
if (pr && pr.length) {
$(pr[0]).css({ width: data.width, height: data.height });
}
// propagating resize, and updating values for each animation step
that._updateCache(data);
that._propagate("resize", event);
}
}
);
}
});
$.ui.plugin.add("resizable", "containment", {
start: function() {
var element, p, co, ch, cw, width, height,
that = $(this).data("ui-resizable"),
o = that.options,
el = that.element,
oc = o.containment,
ce = (oc instanceof $) ? oc.get(0) : (/parent/.test(oc)) ? el.parent().get(0) : oc;
if (!ce) {
return;
}
that.containerElement = $(ce);
if (/document/.test(oc) || oc === document) {
that.containerOffset = { left: 0, top: 0 };
that.containerPosition = { left: 0, top: 0 };
that.parentData = {
element: $(document), left: 0, top: 0,
width: $(document).width(), height: $(document).height() || document.body.parentNode.scrollHeight
};
}
// i'm a node, so compute top, left, right, bottom
else {
element = $(ce);
p = [];
$([ "Top", "Right", "Left", "Bottom" ]).each(function(i, name) { p[i] = num(element.css("padding" + name)); });
that.containerOffset = element.offset();
that.containerPosition = element.position();
that.containerSize = { height: (element.innerHeight() - p[3]), width: (element.innerWidth() - p[1]) };
co = that.containerOffset;
ch = that.containerSize.height;
cw = that.containerSize.width;
width = ($.ui.hasScroll(ce, "left") ? ce.scrollWidth : cw );
height = ($.ui.hasScroll(ce) ? ce.scrollHeight : ch);
that.parentData = {
element: ce, left: co.left, top: co.top, width: width, height: height
};
}
},
resize: function( event ) {
var woset, hoset, isParent, isOffsetRelative,
that = $(this).data("ui-resizable"),
o = that.options,
co = that.containerOffset, cp = that.position,
pRatio = that._aspectRatio || event.shiftKey,
cop = { top:0, left:0 }, ce = that.containerElement;
if (ce[0] !== document && (/static/).test(ce.css("position"))) {
cop = co;
}
if (cp.left < (that._helper ? co.left : 0)) {
that.size.width = that.size.width + (that._helper ? (that.position.left - co.left) : (that.position.left - cop.left));
if (pRatio) {
that.size.height = that.size.width / that.aspectRatio;
}
that.position.left = o.helper ? co.left : 0;
}
if (cp.top < (that._helper ? co.top : 0)) {
that.size.height = that.size.height + (that._helper ? (that.position.top - co.top) : that.position.top);
if (pRatio) {
that.size.width = that.size.height * that.aspectRatio;
}
that.position.top = that._helper ? co.top : 0;
}
that.offset.left = that.parentData.left+that.position.left;
that.offset.top = that.parentData.top+that.position.top;
woset = Math.abs( (that._helper ? that.offset.left - cop.left : (that.offset.left - cop.left)) + that.sizeDiff.width );
hoset = Math.abs( (that._helper ? that.offset.top - cop.top : (that.offset.top - co.top)) + that.sizeDiff.height );
isParent = that.containerElement.get(0) === that.element.parent().get(0);
isOffsetRelative = /relative|absolute/.test(that.containerElement.css("position"));
if(isParent && isOffsetRelative) {
woset -= that.parentData.left;
}
if (woset + that.size.width >= that.parentData.width) {
that.size.width = that.parentData.width - woset;
if (pRatio) {
that.size.height = that.size.width / that.aspectRatio;
}
}
if (hoset + that.size.height >= that.parentData.height) {
that.size.height = that.parentData.height - hoset;
if (pRatio) {
that.size.width = that.size.height * that.aspectRatio;
}
}
},
stop: function(){
var that = $(this).data("ui-resizable"),
o = that.options,
co = that.containerOffset,
cop = that.containerPosition,
ce = that.containerElement,
helper = $(that.helper),
ho = helper.offset(),
w = helper.outerWidth() - that.sizeDiff.width,
h = helper.outerHeight() - that.sizeDiff.height;
if (that._helper && !o.animate && (/relative/).test(ce.css("position"))) {
$(this).css({ left: ho.left - cop.left - co.left, width: w, height: h });
}
if (that._helper && !o.animate && (/static/).test(ce.css("position"))) {
$(this).css({ left: ho.left - cop.left - co.left, width: w, height: h });
}
}
});
$.ui.plugin.add("resizable", "alsoResize", {
start: function () {
var that = $(this).data("ui-resizable"),
o = that.options,
_store = function (exp) {
$(exp).each(function() {
var el = $(this);
el.data("ui-resizable-alsoresize", {
width: parseInt(el.width(), 10), height: parseInt(el.height(), 10),
left: parseInt(el.css("left"), 10), top: parseInt(el.css("top"), 10)
});
});
};
if (typeof(o.alsoResize) === "object" && !o.alsoResize.parentNode) {
if (o.alsoResize.length) { o.alsoResize = o.alsoResize[0]; _store(o.alsoResize); }
else { $.each(o.alsoResize, function (exp) { _store(exp); }); }
}else{
_store(o.alsoResize);
}
},
resize: function (event, ui) {
var that = $(this).data("ui-resizable"),
o = that.options,
os = that.originalSize,
op = that.originalPosition,
delta = {
height: (that.size.height - os.height) || 0, width: (that.size.width - os.width) || 0,
top: (that.position.top - op.top) || 0, left: (that.position.left - op.left) || 0
},
_alsoResize = function (exp, c) {
$(exp).each(function() {
var el = $(this), start = $(this).data("ui-resizable-alsoresize"), style = {},
css = c && c.length ? c : el.parents(ui.originalElement[0]).length ? ["width", "height"] : ["width", "height", "top", "left"];
$.each(css, function (i, prop) {
var sum = (start[prop]||0) + (delta[prop]||0);
if (sum && sum >= 0) {
style[prop] = sum || null;
}
});
el.css(style);
});
};
if (typeof(o.alsoResize) === "object" && !o.alsoResize.nodeType) {
$.each(o.alsoResize, function (exp, c) { _alsoResize(exp, c); });
}else{
_alsoResize(o.alsoResize);
}
},
stop: function () {
$(this).removeData("resizable-alsoresize");
}
});
$.ui.plugin.add("resizable", "ghost", {
start: function() {
var that = $(this).data("ui-resizable"), o = that.options, cs = that.size;
that.ghost = that.originalElement.clone();
that.ghost
.css({ opacity: 0.25, display: "block", position: "relative", height: cs.height, width: cs.width, margin: 0, left: 0, top: 0 })
.addClass("ui-resizable-ghost")
.addClass(typeof o.ghost === "string" ? o.ghost : "");
that.ghost.appendTo(that.helper);
},
resize: function(){
var that = $(this).data("ui-resizable");
if (that.ghost) {
that.ghost.css({ position: "relative", height: that.size.height, width: that.size.width });
}
},
stop: function() {
var that = $(this).data("ui-resizable");
if (that.ghost && that.helper) {
that.helper.get(0).removeChild(that.ghost.get(0));
}
}
});
$.ui.plugin.add("resizable", "grid", {
resize: function() {
var that = $(this).data("ui-resizable"),
o = that.options,
cs = that.size,
os = that.originalSize,
op = that.originalPosition,
a = that.axis,
grid = typeof o.grid === "number" ? [o.grid, o.grid] : o.grid,
gridX = (grid[0]||1),
gridY = (grid[1]||1),
ox = Math.round((cs.width - os.width) / gridX) * gridX,
oy = Math.round((cs.height - os.height) / gridY) * gridY,
newWidth = os.width + ox,
newHeight = os.height + oy,
isMaxWidth = o.maxWidth && (o.maxWidth < newWidth),
isMaxHeight = o.maxHeight && (o.maxHeight < newHeight),
isMinWidth = o.minWidth && (o.minWidth > newWidth),
isMinHeight = o.minHeight && (o.minHeight > newHeight);
o.grid = grid;
if (isMinWidth) {
newWidth = newWidth + gridX;
}
if (isMinHeight) {
newHeight = newHeight + gridY;
}
if (isMaxWidth) {
newWidth = newWidth - gridX;
}
if (isMaxHeight) {
newHeight = newHeight - gridY;
}
if (/^(se|s|e)$/.test(a)) {
that.size.width = newWidth;
that.size.height = newHeight;
} else if (/^(ne)$/.test(a)) {
that.size.width = newWidth;
that.size.height = newHeight;
that.position.top = op.top - oy;
} else if (/^(sw)$/.test(a)) {
that.size.width = newWidth;
that.size.height = newHeight;
that.position.left = op.left - ox;
} else {
that.size.width = newWidth;
that.size.height = newHeight;
that.position.top = op.top - oy;
that.position.left = op.left - ox;
}
}
});
})(jQuery);
(function( $, undefined ) {
$.widget("ui.selectable", $.ui.mouse, {
version: "1.10.3",
options: {
appendTo: "body",
autoRefresh: true,
distance: 0,
filter: "*",
tolerance: "touch",
// callbacks
selected: null,
selecting: null,
start: null,
stop: null,
unselected: null,
unselecting: null
},
_create: function() {
var selectees,
that = this;
this.element.addClass("ui-selectable");
this.dragged = false;
// cache selectee children based on filter
this.refresh = function() {
selectees = $(that.options.filter, that.element[0]);
selectees.addClass("ui-selectee");
selectees.each(function() {
var $this = $(this),
pos = $this.offset();
$.data(this, "selectable-item", {
element: this,
$element: $this,
left: pos.left,
top: pos.top,
right: pos.left + $this.outerWidth(),
bottom: pos.top + $this.outerHeight(),
startselected: false,
selected: $this.hasClass("ui-selected"),
selecting: $this.hasClass("ui-selecting"),
unselecting: $this.hasClass("ui-unselecting")
});
});
};
this.refresh();
this.selectees = selectees.addClass("ui-selectee");
this._mouseInit();
this.helper = $("<div class='ui-selectable-helper'></div>");
},
_destroy: function() {
this.selectees
.removeClass("ui-selectee")
.removeData("selectable-item");
this.element
.removeClass("ui-selectable ui-selectable-disabled");
this._mouseDestroy();
},
_mouseStart: function(event) {
var that = this,
options = this.options;
this.opos = [event.pageX, event.pageY];
if (this.options.disabled) {
return;
}
this.selectees = $(options.filter, this.element[0]);
this._trigger("start", event);
$(options.appendTo).append(this.helper);
// position helper (lasso)
this.helper.css({
"left": event.pageX,
"top": event.pageY,
"width": 0,
"height": 0
});
if (options.autoRefresh) {
this.refresh();
}
this.selectees.filter(".ui-selected").each(function() {
var selectee = $.data(this, "selectable-item");
selectee.startselected = true;
if (!event.metaKey && !event.ctrlKey) {
selectee.$element.removeClass("ui-selected");
selectee.selected = false;
selectee.$element.addClass("ui-unselecting");
selectee.unselecting = true;
// selectable UNSELECTING callback
that._trigger("unselecting", event, {
unselecting: selectee.element
});
}
});
$(event.target).parents().addBack().each(function() {
var doSelect,
selectee = $.data(this, "selectable-item");
if (selectee) {
doSelect = (!event.metaKey && !event.ctrlKey) || !selectee.$element.hasClass("ui-selected");
selectee.$element
.removeClass(doSelect ? "ui-unselecting" : "ui-selected")
.addClass(doSelect ? "ui-selecting" : "ui-unselecting");
selectee.unselecting = !doSelect;
selectee.selecting = doSelect;
selectee.selected = doSelect;
// selectable (UN)SELECTING callback
if (doSelect) {
that._trigger("selecting", event, {
selecting: selectee.element
});
} else {
that._trigger("unselecting", event, {
unselecting: selectee.element
});
}
return false;
}
});
},
_mouseDrag: function(event) {
this.dragged = true;
if (this.options.disabled) {
return;
}
var tmp,
that = this,
options = this.options,
x1 = this.opos[0],
y1 = this.opos[1],
x2 = event.pageX,
y2 = event.pageY;
if (x1 > x2) { tmp = x2; x2 = x1; x1 = tmp; }
if (y1 > y2) { tmp = y2; y2 = y1; y1 = tmp; }
this.helper.css({left: x1, top: y1, width: x2-x1, height: y2-y1});
this.selectees.each(function() {
var selectee = $.data(this, "selectable-item"),
hit = false;
//prevent helper from being selected if appendTo: selectable
if (!selectee || selectee.element === that.element[0]) {
return;
}
if (options.tolerance === "touch") {
hit = ( !(selectee.left > x2 || selectee.right < x1 || selectee.top > y2 || selectee.bottom < y1) );
} else if (options.tolerance === "fit") {
hit = (selectee.left > x1 && selectee.right < x2 && selectee.top > y1 && selectee.bottom < y2);
}
if (hit) {
// SELECT
if (selectee.selected) {
selectee.$element.removeClass("ui-selected");
selectee.selected = false;
}
if (selectee.unselecting) {
selectee.$element.removeClass("ui-unselecting");
selectee.unselecting = false;
}
if (!selectee.selecting) {
selectee.$element.addClass("ui-selecting");
selectee.selecting = true;
// selectable SELECTING callback
that._trigger("selecting", event, {
selecting: selectee.element
});
}
} else {
// UNSELECT
if (selectee.selecting) {
if ((event.metaKey || event.ctrlKey) && selectee.startselected) {
selectee.$element.removeClass("ui-selecting");
selectee.selecting = false;
selectee.$element.addClass("ui-selected");
selectee.selected = true;
} else {
selectee.$element.removeClass("ui-selecting");
selectee.selecting = false;
if (selectee.startselected) {
selectee.$element.addClass("ui-unselecting");
selectee.unselecting = true;
}
// selectable UNSELECTING callback
that._trigger("unselecting", event, {
unselecting: selectee.element
});
}
}
if (selectee.selected) {
if (!event.metaKey && !event.ctrlKey && !selectee.startselected) {
selectee.$element.removeClass("ui-selected");
selectee.selected = false;
selectee.$element.addClass("ui-unselecting");
selectee.unselecting = true;
// selectable UNSELECTING callback
that._trigger("unselecting", event, {
unselecting: selectee.element
});
}
}
}
});
return false;
},
_mouseStop: function(event) {
var that = this;
this.dragged = false;
$(".ui-unselecting", this.element[0]).each(function() {
var selectee = $.data(this, "selectable-item");
selectee.$element.removeClass("ui-unselecting");
selectee.unselecting = false;
selectee.startselected = false;
that._trigger("unselected", event, {
unselected: selectee.element
});
});
$(".ui-selecting", this.element[0]).each(function() {
var selectee = $.data(this, "selectable-item");
selectee.$element.removeClass("ui-selecting").addClass("ui-selected");
selectee.selecting = false;
selectee.selected = true;
selectee.startselected = true;
that._trigger("selected", event, {
selected: selectee.element
});
});
this._trigger("stop", event);
this.helper.remove();
return false;
}
});
})(jQuery);
(function( $, undefined ) {
/*jshint loopfunc: true */
function isOverAxis( x, reference, size ) {
return ( x > reference ) && ( x < ( reference + size ) );
}
function isFloating(item) {
return (/left|right/).test(item.css("float")) || (/inline|table-cell/).test(item.css("display"));
}
$.widget("ui.sortable", $.ui.mouse, {
version: "1.10.3",
widgetEventPrefix: "sort",
ready: false,
options: {
appendTo: "parent",
axis: false,
connectWith: false,
containment: false,
cursor: "auto",
cursorAt: false,
dropOnEmpty: true,
forcePlaceholderSize: false,
forceHelperSize: false,
grid: false,
handle: false,
helper: "original",
items: "> *",
opacity: false,
placeholder: false,
revert: false,
scroll: true,
scrollSensitivity: 20,
scrollSpeed: 20,
scope: "default",
tolerance: "intersect",
zIndex: 1000,
// callbacks
activate: null,
beforeStop: null,
change: null,
deactivate: null,
out: null,
over: null,
receive: null,
remove: null,
sort: null,
start: null,
stop: null,
update: null
},
_create: function() {
var o = this.options;
this.containerCache = {};
this.element.addClass("ui-sortable");
//Get the items
this.refresh();
//Let's determine if the items are being displayed horizontally
this.floating = this.items.length ? o.axis === "x" || isFloating(this.items[0].item) : false;
//Let's determine the parent's offset
this.offset = this.element.offset();
//Initialize mouse events for interaction
this._mouseInit();
//We're ready to go
this.ready = true;
},
_destroy: function() {
this.element
.removeClass("ui-sortable ui-sortable-disabled");
this._mouseDestroy();
for ( var i = this.items.length - 1; i >= 0; i-- ) {
this.items[i].item.removeData(this.widgetName + "-item");
}
return this;
},
_setOption: function(key, value){
if ( key === "disabled" ) {
this.options[ key ] = value;
this.widget().toggleClass( "ui-sortable-disabled", !!value );
} else {
// Don't call widget base _setOption for disable as it adds ui-state-disabled class
$.Widget.prototype._setOption.apply(this, arguments);
}
},
_mouseCapture: function(event, overrideHandle) {
var currentItem = null,
validHandle = false,
that = this;
if (this.reverting) {
return false;
}
if(this.options.disabled || this.options.type === "static") {
return false;
}
//We have to refresh the items data once first
this._refreshItems(event);
//Find out if the clicked node (or one of its parents) is a actual item in this.items
$(event.target).parents().each(function() {
if($.data(this, that.widgetName + "-item") === that) {
currentItem = $(this);
return false;
}
});
if($.data(event.target, that.widgetName + "-item") === that) {
currentItem = $(event.target);
}
if(!currentItem) {
return false;
}
if(this.options.handle && !overrideHandle) {
$(this.options.handle, currentItem).find("*").addBack().each(function() {
if(this === event.target) {
validHandle = true;
}
});
if(!validHandle) {
return false;
}
}
this.currentItem = currentItem;
this._removeCurrentsFromItems();
return true;
},
_mouseStart: function(event, overrideHandle, noActivation) {
var i, body,
o = this.options;
this.currentContainer = this;
//We only need to call refreshPositions, because the refreshItems call has been moved to mouseCapture
this.refreshPositions();
//Create and append the visible helper
this.helper = this._createHelper(event);
//Cache the helper size
this._cacheHelperProportions();
/*
* - Position generation -
* This block generates everything position related - it's the core of draggables.
*/
//Cache the margins of the original element
this._cacheMargins();
//Get the next scrolling parent
this.scrollParent = this.helper.scrollParent();
//The element's absolute position on the page minus margins
this.offset = this.currentItem.offset();
this.offset = {
top: this.offset.top - this.margins.top,
left: this.offset.left - this.margins.left
};
$.extend(this.offset, {
click: { //Where the click happened, relative to the element
left: event.pageX - this.offset.left,
top: event.pageY - this.offset.top
},
parent: this._getParentOffset(),
relative: this._getRelativeOffset() //This is a relative to absolute position minus the actual position calculation - only used for relative positioned helper
});
// Only after we got the offset, we can change the helper's position to absolute
// TODO: Still need to figure out a way to make relative sorting possible
this.helper.css("position", "absolute");
this.cssPosition = this.helper.css("position");
//Generate the original position
this.originalPosition = this._generatePosition(event);
this.originalPageX = event.pageX;
this.originalPageY = event.pageY;
//Adjust the mouse offset relative to the helper if "cursorAt" is supplied
(o.cursorAt && this._adjustOffsetFromHelper(o.cursorAt));
//Cache the former DOM position
this.domPosition = { prev: this.currentItem.prev()[0], parent: this.currentItem.parent()[0] };
//If the helper is not the original, hide the original so it's not playing any role during the drag, won't cause anything bad this way
if(this.helper[0] !== this.currentItem[0]) {
this.currentItem.hide();
}
//Create the placeholder
this._createPlaceholder();
//Set a containment if given in the options
if(o.containment) {
this._setContainment();
}
if( o.cursor && o.cursor !== "auto" ) { // cursor option
body = this.document.find( "body" );
// support: IE
this.storedCursor = body.css( "cursor" );
body.css( "cursor", o.cursor );
this.storedStylesheet = $( "<style>*{ cursor: "+o.cursor+" !important; }</style>" ).appendTo( body );
}
if(o.opacity) { // opacity option
if (this.helper.css("opacity")) {
this._storedOpacity = this.helper.css("opacity");
}
this.helper.css("opacity", o.opacity);
}
if(o.zIndex) { // zIndex option
if (this.helper.css("zIndex")) {
this._storedZIndex = this.helper.css("zIndex");
}
this.helper.css("zIndex", o.zIndex);
}
//Prepare scrolling
if(this.scrollParent[0] !== document && this.scrollParent[0].tagName !== "HTML") {
this.overflowOffset = this.scrollParent.offset();
}
//Call callbacks
this._trigger("start", event, this._uiHash());
//Recache the helper size
if(!this._preserveHelperProportions) {
this._cacheHelperProportions();
}
//Post "activate" events to possible containers
if( !noActivation ) {
for ( i = this.containers.length - 1; i >= 0; i-- ) {
this.containers[ i ]._trigger( "activate", event, this._uiHash( this ) );
}
}
//Prepare possible droppables
if($.ui.ddmanager) {
$.ui.ddmanager.current = this;
}
if ($.ui.ddmanager && !o.dropBehaviour) {
$.ui.ddmanager.prepareOffsets(this, event);
}
this.dragging = true;
this.helper.addClass("ui-sortable-helper");
this._mouseDrag(event); //Execute the drag once - this causes the helper not to be visible before getting its correct position
return true;
},
_mouseDrag: function(event) {
var i, item, itemElement, intersection,
o = this.options,
scrolled = false;
//Compute the helpers position
this.position = this._generatePosition(event);
this.positionAbs = this._convertPositionTo("absolute");
if (!this.lastPositionAbs) {
this.lastPositionAbs = this.positionAbs;
}
//Do scrolling
if(this.options.scroll) {
if(this.scrollParent[0] !== document && this.scrollParent[0].tagName !== "HTML") {
if((this.overflowOffset.top + this.scrollParent[0].offsetHeight) - event.pageY < o.scrollSensitivity) {
this.scrollParent[0].scrollTop = scrolled = this.scrollParent[0].scrollTop + o.scrollSpeed;
} else if(event.pageY - this.overflowOffset.top < o.scrollSensitivity) {
this.scrollParent[0].scrollTop = scrolled = this.scrollParent[0].scrollTop - o.scrollSpeed;
}
if((this.overflowOffset.left + this.scrollParent[0].offsetWidth) - event.pageX < o.scrollSensitivity) {
this.scrollParent[0].scrollLeft = scrolled = this.scrollParent[0].scrollLeft + o.scrollSpeed;
} else if(event.pageX - this.overflowOffset.left < o.scrollSensitivity) {
this.scrollParent[0].scrollLeft = scrolled = this.scrollParent[0].scrollLeft - o.scrollSpeed;
}
} else {
if(event.pageY - $(document).scrollTop() < o.scrollSensitivity) {
scrolled = $(document).scrollTop($(document).scrollTop() - o.scrollSpeed);
} else if($(window).height() - (event.pageY - $(document).scrollTop()) < o.scrollSensitivity) {
scrolled = $(document).scrollTop($(document).scrollTop() + o.scrollSpeed);
}
if(event.pageX - $(document).scrollLeft() < o.scrollSensitivity) {
scrolled = $(document).scrollLeft($(document).scrollLeft() - o.scrollSpeed);
} else if($(window).width() - (event.pageX - $(document).scrollLeft()) < o.scrollSensitivity) {
scrolled = $(document).scrollLeft($(document).scrollLeft() + o.scrollSpeed);
}
}
if(scrolled !== false && $.ui.ddmanager && !o.dropBehaviour) {
$.ui.ddmanager.prepareOffsets(this, event);
}
}
//Regenerate the absolute position used for position checks
this.positionAbs = this._convertPositionTo("absolute");
//Set the helper position
if(!this.options.axis || this.options.axis !== "y") {
this.helper[0].style.left = this.position.left+"px";
}
if(!this.options.axis || this.options.axis !== "x") {
this.helper[0].style.top = this.position.top+"px";
}
//Rearrange
for (i = this.items.length - 1; i >= 0; i--) {
//Cache variables and intersection, continue if no intersection
item = this.items[i];
itemElement = item.item[0];
intersection = this._intersectsWithPointer(item);
if (!intersection) {
continue;
}
// Only put the placeholder inside the current Container, skip all
// items form other containers. This works because when moving
// an item from one container to another the
// currentContainer is switched before the placeholder is moved.
//
// Without this moving items in "sub-sortables" can cause the placeholder to jitter
// beetween the outer and inner container.
if (item.instance !== this.currentContainer) {
continue;
}
// cannot intersect with itself
// no useless actions that have been done before
// no action if the item moved is the parent of the item checked
if (itemElement !== this.currentItem[0] &&
this.placeholder[intersection === 1 ? "next" : "prev"]()[0] !== itemElement &&
!$.contains(this.placeholder[0], itemElement) &&
(this.options.type === "semi-dynamic" ? !$.contains(this.element[0], itemElement) : true)
) {
this.direction = intersection === 1 ? "down" : "up";
if (this.options.tolerance === "pointer" || this._intersectsWithSides(item)) {
this._rearrange(event, item);
} else {
break;
}
this._trigger("change", event, this._uiHash());
break;
}
}
//Post events to containers
this._contactContainers(event);
//Interconnect with droppables
if($.ui.ddmanager) {
$.ui.ddmanager.drag(this, event);
}
//Call callbacks
this._trigger("sort", event, this._uiHash());
this.lastPositionAbs = this.positionAbs;
return false;
},
_mouseStop: function(event, noPropagation) {
if(!event) {
return;
}
//If we are using droppables, inform the manager about the drop
if ($.ui.ddmanager && !this.options.dropBehaviour) {
$.ui.ddmanager.drop(this, event);
}
if(this.options.revert) {
var that = this,
cur = this.placeholder.offset(),
axis = this.options.axis,
animation = {};
if ( !axis || axis === "x" ) {
animation.left = cur.left - this.offset.parent.left - this.margins.left + (this.offsetParent[0] === document.body ? 0 : this.offsetParent[0].scrollLeft);
}
if ( !axis || axis === "y" ) {
animation.top = cur.top - this.offset.parent.top - this.margins.top + (this.offsetParent[0] === document.body ? 0 : this.offsetParent[0].scrollTop);
}
this.reverting = true;
$(this.helper).animate( animation, parseInt(this.options.revert, 10) || 500, function() {
that._clear(event);
});
} else {
this._clear(event, noPropagation);
}
return false;
},
cancel: function() {
if(this.dragging) {
this._mouseUp({ target: null });
if(this.options.helper === "original") {
this.currentItem.css(this._storedCSS).removeClass("ui-sortable-helper");
} else {
this.currentItem.show();
}
//Post deactivating events to containers
for (var i = this.containers.length - 1; i >= 0; i--){
this.containers[i]._trigger("deactivate", null, this._uiHash(this));
if(this.containers[i].containerCache.over) {
this.containers[i]._trigger("out", null, this._uiHash(this));
this.containers[i].containerCache.over = 0;
}
}
}
if (this.placeholder) {
//$(this.placeholder[0]).remove(); would have been the jQuery way - unfortunately, it unbinds ALL events from the original node!
if(this.placeholder[0].parentNode) {
this.placeholder[0].parentNode.removeChild(this.placeholder[0]);
}
if(this.options.helper !== "original" && this.helper && this.helper[0].parentNode) {
this.helper.remove();
}
$.extend(this, {
helper: null,
dragging: false,
reverting: false,
_noFinalSort: null
});
if(this.domPosition.prev) {
$(this.domPosition.prev).after(this.currentItem);
} else {
$(this.domPosition.parent).prepend(this.currentItem);
}
}
return this;
},
serialize: function(o) {
var items = this._getItemsAsjQuery(o && o.connected),
str = [];
o = o || {};
$(items).each(function() {
var res = ($(o.item || this).attr(o.attribute || "id") || "").match(o.expression || (/(.+)[\-=_](.+)/));
if (res) {
str.push((o.key || res[1]+"[]")+"="+(o.key && o.expression ? res[1] : res[2]));
}
});
if(!str.length && o.key) {
str.push(o.key + "=");
}
return str.join("&");
},
toArray: function(o) {
var items = this._getItemsAsjQuery(o && o.connected),
ret = [];
o = o || {};
items.each(function() { ret.push($(o.item || this).attr(o.attribute || "id") || ""); });
return ret;
},
/* Be careful with the following core functions */
_intersectsWith: function(item) {
var x1 = this.positionAbs.left,
x2 = x1 + this.helperProportions.width,
y1 = this.positionAbs.top,
y2 = y1 + this.helperProportions.height,
l = item.left,
r = l + item.width,
t = item.top,
b = t + item.height,
dyClick = this.offset.click.top,
dxClick = this.offset.click.left,
isOverElementHeight = ( this.options.axis === "x" ) || ( ( y1 + dyClick ) > t && ( y1 + dyClick ) < b ),
isOverElementWidth = ( this.options.axis === "y" ) || ( ( x1 + dxClick ) > l && ( x1 + dxClick ) < r ),
isOverElement = isOverElementHeight && isOverElementWidth;
if ( this.options.tolerance === "pointer" ||
this.options.forcePointerForContainers ||
(this.options.tolerance !== "pointer" && this.helperProportions[this.floating ? "width" : "height"] > item[this.floating ? "width" : "height"])
) {
return isOverElement;
} else {
return (l < x1 + (this.helperProportions.width / 2) && // Right Half
x2 - (this.helperProportions.width / 2) < r && // Left Half
t < y1 + (this.helperProportions.height / 2) && // Bottom Half
y2 - (this.helperProportions.height / 2) < b ); // Top Half
}
},
_intersectsWithPointer: function(item) {
var isOverElementHeight = (this.options.axis === "x") || isOverAxis(this.positionAbs.top + this.offset.click.top, item.top, item.height),
isOverElementWidth = (this.options.axis === "y") || isOverAxis(this.positionAbs.left + this.offset.click.left, item.left, item.width),
isOverElement = isOverElementHeight && isOverElementWidth,
verticalDirection = this._getDragVerticalDirection(),
horizontalDirection = this._getDragHorizontalDirection();
if (!isOverElement) {
return false;
}
return this.floating ?
( ((horizontalDirection && horizontalDirection === "right") || verticalDirection === "down") ? 2 : 1 )
: ( verticalDirection && (verticalDirection === "down" ? 2 : 1) );
},
_intersectsWithSides: function(item) {
var isOverBottomHalf = isOverAxis(this.positionAbs.top + this.offset.click.top, item.top + (item.height/2), item.height),
isOverRightHalf = isOverAxis(this.positionAbs.left + this.offset.click.left, item.left + (item.width/2), item.width),
verticalDirection = this._getDragVerticalDirection(),
horizontalDirection = this._getDragHorizontalDirection();
if (this.floating && horizontalDirection) {
return ((horizontalDirection === "right" && isOverRightHalf) || (horizontalDirection === "left" && !isOverRightHalf));
} else {
return verticalDirection && ((verticalDirection === "down" && isOverBottomHalf) || (verticalDirection === "up" && !isOverBottomHalf));
}
},
_getDragVerticalDirection: function() {
var delta = this.positionAbs.top - this.lastPositionAbs.top;
return delta !== 0 && (delta > 0 ? "down" : "up");
},
_getDragHorizontalDirection: function() {
var delta = this.positionAbs.left - this.lastPositionAbs.left;
return delta !== 0 && (delta > 0 ? "right" : "left");
},
refresh: function(event) {
this._refreshItems(event);
this.refreshPositions();
return this;
},
_connectWith: function() {
var options = this.options;
return options.connectWith.constructor === String ? [options.connectWith] : options.connectWith;
},
_getItemsAsjQuery: function(connected) {
var i, j, cur, inst,
items = [],
queries = [],
connectWith = this._connectWith();
if(connectWith && connected) {
for (i = connectWith.length - 1; i >= 0; i--){
cur = $(connectWith[i]);
for ( j = cur.length - 1; j >= 0; j--){
inst = $.data(cur[j], this.widgetFullName);
if(inst && inst !== this && !inst.options.disabled) {
queries.push([$.isFunction(inst.options.items) ? inst.options.items.call(inst.element) : $(inst.options.items, inst.element).not(".ui-sortable-helper").not(".ui-sortable-placeholder"), inst]);
}
}
}
}
queries.push([$.isFunction(this.options.items) ? this.options.items.call(this.element, null, { options: this.options, item: this.currentItem }) : $(this.options.items, this.element).not(".ui-sortable-helper").not(".ui-sortable-placeholder"), this]);
for (i = queries.length - 1; i >= 0; i--){
queries[i][0].each(function() {
items.push(this);
});
}
return $(items);
},
_removeCurrentsFromItems: function() {
var list = this.currentItem.find(":data(" + this.widgetName + "-item)");
this.items = $.grep(this.items, function (item) {
for (var j=0; j < list.length; j++) {
if(list[j] === item.item[0]) {
return false;
}
}
return true;
});
},
_refreshItems: function(event) {
this.items = [];
this.containers = [this];
var i, j, cur, inst, targetData, _queries, item, queriesLength,
items = this.items,
queries = [[$.isFunction(this.options.items) ? this.options.items.call(this.element[0], event, { item: this.currentItem }) : $(this.options.items, this.element), this]],
connectWith = this._connectWith();
if(connectWith && this.ready) { //Shouldn't be run the first time through due to massive slow-down
for (i = connectWith.length - 1; i >= 0; i--){
cur = $(connectWith[i]);
for (j = cur.length - 1; j >= 0; j--){
inst = $.data(cur[j], this.widgetFullName);
if(inst && inst !== this && !inst.options.disabled) {
queries.push([$.isFunction(inst.options.items) ? inst.options.items.call(inst.element[0], event, { item: this.currentItem }) : $(inst.options.items, inst.element), inst]);
this.containers.push(inst);
}
}
}
}
for (i = queries.length - 1; i >= 0; i--) {
targetData = queries[i][1];
_queries = queries[i][0];
for (j=0, queriesLength = _queries.length; j < queriesLength; j++) {
item = $(_queries[j]);
item.data(this.widgetName + "-item", targetData); // Data for target checking (mouse manager)
items.push({
item: item,
instance: targetData,
width: 0, height: 0,
left: 0, top: 0
});
}
}
},
refreshPositions: function(fast) {
//This has to be redone because due to the item being moved out/into the offsetParent, the offsetParent's position will change
if(this.offsetParent && this.helper) {
this.offset.parent = this._getParentOffset();
}
var i, item, t, p;
for (i = this.items.length - 1; i >= 0; i--){
item = this.items[i];
//We ignore calculating positions of all connected containers when we're not over them
if(item.instance !== this.currentContainer && this.currentContainer && item.item[0] !== this.currentItem[0]) {
continue;
}
t = this.options.toleranceElement ? $(this.options.toleranceElement, item.item) : item.item;
if (!fast) {
item.width = t.outerWidth();
item.height = t.outerHeight();
}
p = t.offset();
item.left = p.left;
item.top = p.top;
}
if(this.options.custom && this.options.custom.refreshContainers) {
this.options.custom.refreshContainers.call(this);
} else {
for (i = this.containers.length - 1; i >= 0; i--){
p = this.containers[i].element.offset();
this.containers[i].containerCache.left = p.left;
this.containers[i].containerCache.top = p.top;
this.containers[i].containerCache.width = this.containers[i].element.outerWidth();
this.containers[i].containerCache.height = this.containers[i].element.outerHeight();
}
}
return this;
},
_createPlaceholder: function(that) {
that = that || this;
var className,
o = that.options;
if(!o.placeholder || o.placeholder.constructor === String) {
className = o.placeholder;
o.placeholder = {
element: function() {
var nodeName = that.currentItem[0].nodeName.toLowerCase(),
element = $( "<" + nodeName + ">", that.document[0] )
.addClass(className || that.currentItem[0].className+" ui-sortable-placeholder")
.removeClass("ui-sortable-helper");
if ( nodeName === "tr" ) {
that.currentItem.children().each(function() {
$( "<td> </td>", that.document[0] )
.attr( "colspan", $( this ).attr( "colspan" ) || 1 )
.appendTo( element );
});
} else if ( nodeName === "img" ) {
element.attr( "src", that.currentItem.attr( "src" ) );
}
if ( !className ) {
element.css( "visibility", "hidden" );
}
return element;
},
update: function(container, p) {
// 1. If a className is set as 'placeholder option, we don't force sizes - the class is responsible for that
// 2. The option 'forcePlaceholderSize can be enabled to force it even if a class name is specified
if(className && !o.forcePlaceholderSize) {
return;
}
//If the element doesn't have a actual height by itself (without styles coming from a stylesheet), it receives the inline height from the dragged item
if(!p.height()) { p.height(that.currentItem.innerHeight() - parseInt(that.currentItem.css("paddingTop")||0, 10) - parseInt(that.currentItem.css("paddingBottom")||0, 10)); }
if(!p.width()) { p.width(that.currentItem.innerWidth() - parseInt(that.currentItem.css("paddingLeft")||0, 10) - parseInt(that.currentItem.css("paddingRight")||0, 10)); }
}
};
}
//Create the placeholder
that.placeholder = $(o.placeholder.element.call(that.element, that.currentItem));
//Append it after the actual current item
that.currentItem.after(that.placeholder);
//Update the size of the placeholder (TODO: Logic to fuzzy, see line 316/317)
o.placeholder.update(that, that.placeholder);
},
_contactContainers: function(event) {
var i, j, dist, itemWithLeastDistance, posProperty, sizeProperty, base, cur, nearBottom, floating,
innermostContainer = null,
innermostIndex = null;
// get innermost container that intersects with item
for (i = this.containers.length - 1; i >= 0; i--) {
// never consider a container that's located within the item itself
if($.contains(this.currentItem[0], this.containers[i].element[0])) {
continue;
}
if(this._intersectsWith(this.containers[i].containerCache)) {
// if we've already found a container and it's more "inner" than this, then continue
if(innermostContainer && $.contains(this.containers[i].element[0], innermostContainer.element[0])) {
continue;
}
innermostContainer = this.containers[i];
innermostIndex = i;
} else {
// container doesn't intersect. trigger "out" event if necessary
if(this.containers[i].containerCache.over) {
this.containers[i]._trigger("out", event, this._uiHash(this));
this.containers[i].containerCache.over = 0;
}
}
}
// if no intersecting containers found, return
if(!innermostContainer) {
return;
}
// move the item into the container if it's not there already
if(this.containers.length === 1) {
if (!this.containers[innermostIndex].containerCache.over) {
this.containers[innermostIndex]._trigger("over", event, this._uiHash(this));
this.containers[innermostIndex].containerCache.over = 1;
}
} else {
//When entering a new container, we will find the item with the least distance and append our item near it
dist = 10000;
itemWithLeastDistance = null;
floating = innermostContainer.floating || isFloating(this.currentItem);
posProperty = floating ? "left" : "top";
sizeProperty = floating ? "width" : "height";
base = this.positionAbs[posProperty] + this.offset.click[posProperty];
for (j = this.items.length - 1; j >= 0; j--) {
if(!$.contains(this.containers[innermostIndex].element[0], this.items[j].item[0])) {
continue;
}
if(this.items[j].item[0] === this.currentItem[0]) {
continue;
}
if (floating && !isOverAxis(this.positionAbs.top + this.offset.click.top, this.items[j].top, this.items[j].height)) {
continue;
}
cur = this.items[j].item.offset()[posProperty];
nearBottom = false;
if(Math.abs(cur - base) > Math.abs(cur + this.items[j][sizeProperty] - base)){
nearBottom = true;
cur += this.items[j][sizeProperty];
}
if(Math.abs(cur - base) < dist) {
dist = Math.abs(cur - base); itemWithLeastDistance = this.items[j];
this.direction = nearBottom ? "up": "down";
}
}
//Check if dropOnEmpty is enabled
if(!itemWithLeastDistance && !this.options.dropOnEmpty) {
return;
}
if(this.currentContainer === this.containers[innermostIndex]) {
return;
}
itemWithLeastDistance ? this._rearrange(event, itemWithLeastDistance, null, true) : this._rearrange(event, null, this.containers[innermostIndex].element, true);
this._trigger("change", event, this._uiHash());
this.containers[innermostIndex]._trigger("change", event, this._uiHash(this));
this.currentContainer = this.containers[innermostIndex];
//Update the placeholder
this.options.placeholder.update(this.currentContainer, this.placeholder);
this.containers[innermostIndex]._trigger("over", event, this._uiHash(this));
this.containers[innermostIndex].containerCache.over = 1;
}
},
_createHelper: function(event) {
var o = this.options,
helper = $.isFunction(o.helper) ? $(o.helper.apply(this.element[0], [event, this.currentItem])) : (o.helper === "clone" ? this.currentItem.clone() : this.currentItem);
//Add the helper to the DOM if that didn't happen already
if(!helper.parents("body").length) {
$(o.appendTo !== "parent" ? o.appendTo : this.currentItem[0].parentNode)[0].appendChild(helper[0]);
}
if(helper[0] === this.currentItem[0]) {
this._storedCSS = { width: this.currentItem[0].style.width, height: this.currentItem[0].style.height, position: this.currentItem.css("position"), top: this.currentItem.css("top"), left: this.currentItem.css("left") };
}
if(!helper[0].style.width || o.forceHelperSize) {
helper.width(this.currentItem.width());
}
if(!helper[0].style.height || o.forceHelperSize) {
helper.height(this.currentItem.height());
}
return helper;
},
_adjustOffsetFromHelper: function(obj) {
if (typeof obj === "string") {
obj = obj.split(" ");
}
if ($.isArray(obj)) {
obj = {left: +obj[0], top: +obj[1] || 0};
}
if ("left" in obj) {
this.offset.click.left = obj.left + this.margins.left;
}
if ("right" in obj) {
this.offset.click.left = this.helperProportions.width - obj.right + this.margins.left;
}
if ("top" in obj) {
this.offset.click.top = obj.top + this.margins.top;
}
if ("bottom" in obj) {
this.offset.click.top = this.helperProportions.height - obj.bottom + this.margins.top;
}
},
_getParentOffset: function() {
//Get the offsetParent and cache its position
this.offsetParent = this.helper.offsetParent();
var po = this.offsetParent.offset();
// This is a special case where we need to modify a offset calculated on start, since the following happened:
// 1. The position of the helper is absolute, so it's position is calculated based on the next positioned parent
// 2. The actual offset parent is a child of the scroll parent, and the scroll parent isn't the document, which means that
// the scroll is included in the initial calculation of the offset of the parent, and never recalculated upon drag
if(this.cssPosition === "absolute" && this.scrollParent[0] !== document && $.contains(this.scrollParent[0], this.offsetParent[0])) {
po.left += this.scrollParent.scrollLeft();
po.top += this.scrollParent.scrollTop();
}
// This needs to be actually done for all browsers, since pageX/pageY includes this information
// with an ugly IE fix
if( this.offsetParent[0] === document.body || (this.offsetParent[0].tagName && this.offsetParent[0].tagName.toLowerCase() === "html" && $.ui.ie)) {
po = { top: 0, left: 0 };
}
return {
top: po.top + (parseInt(this.offsetParent.css("borderTopWidth"),10) || 0),
left: po.left + (parseInt(this.offsetParent.css("borderLeftWidth"),10) || 0)
};
},
_getRelativeOffset: function() {
if(this.cssPosition === "relative") {
var p = this.currentItem.position();
return {
top: p.top - (parseInt(this.helper.css("top"),10) || 0) + this.scrollParent.scrollTop(),
left: p.left - (parseInt(this.helper.css("left"),10) || 0) + this.scrollParent.scrollLeft()
};
} else {
return { top: 0, left: 0 };
}
},
_cacheMargins: function() {
this.margins = {
left: (parseInt(this.currentItem.css("marginLeft"),10) || 0),
top: (parseInt(this.currentItem.css("marginTop"),10) || 0)
};
},
_cacheHelperProportions: function() {
this.helperProportions = {
width: this.helper.outerWidth(),
height: this.helper.outerHeight()
};
},
_setContainment: function() {
var ce, co, over,
o = this.options;
if(o.containment === "parent") {
o.containment = this.helper[0].parentNode;
}
if(o.containment === "document" || o.containment === "window") {
this.containment = [
0 - this.offset.relative.left - this.offset.parent.left,
0 - this.offset.relative.top - this.offset.parent.top,
$(o.containment === "document" ? document : window).width() - this.helperProportions.width - this.margins.left,
($(o.containment === "document" ? document : window).height() || document.body.parentNode.scrollHeight) - this.helperProportions.height - this.margins.top
];
}
if(!(/^(document|window|parent)$/).test(o.containment)) {
ce = $(o.containment)[0];
co = $(o.containment).offset();
over = ($(ce).css("overflow") !== "hidden");
this.containment = [
co.left + (parseInt($(ce).css("borderLeftWidth"),10) || 0) + (parseInt($(ce).css("paddingLeft"),10) || 0) - this.margins.left,
co.top + (parseInt($(ce).css("borderTopWidth"),10) || 0) + (parseInt($(ce).css("paddingTop"),10) || 0) - this.margins.top,
co.left+(over ? Math.max(ce.scrollWidth,ce.offsetWidth) : ce.offsetWidth) - (parseInt($(ce).css("borderLeftWidth"),10) || 0) - (parseInt($(ce).css("paddingRight"),10) || 0) - this.helperProportions.width - this.margins.left,
co.top+(over ? Math.max(ce.scrollHeight,ce.offsetHeight) : ce.offsetHeight) - (parseInt($(ce).css("borderTopWidth"),10) || 0) - (parseInt($(ce).css("paddingBottom"),10) || 0) - this.helperProportions.height - this.margins.top
];
}
},
_convertPositionTo: function(d, pos) {
if(!pos) {
pos = this.position;
}
var mod = d === "absolute" ? 1 : -1,
scroll = this.cssPosition === "absolute" && !(this.scrollParent[0] !== document && $.contains(this.scrollParent[0], this.offsetParent[0])) ? this.offsetParent : this.scrollParent,
scrollIsRootNode = (/(html|body)/i).test(scroll[0].tagName);
return {
top: (
pos.top + // The absolute mouse position
this.offset.relative.top * mod + // Only for relative positioned nodes: Relative offset from element to offset parent
this.offset.parent.top * mod - // The offsetParent's offset without borders (offset + border)
( ( this.cssPosition === "fixed" ? -this.scrollParent.scrollTop() : ( scrollIsRootNode ? 0 : scroll.scrollTop() ) ) * mod)
),
left: (
pos.left + // The absolute mouse position
this.offset.relative.left * mod + // Only for relative positioned nodes: Relative offset from element to offset parent
this.offset.parent.left * mod - // The offsetParent's offset without borders (offset + border)
( ( this.cssPosition === "fixed" ? -this.scrollParent.scrollLeft() : scrollIsRootNode ? 0 : scroll.scrollLeft() ) * mod)
)
};
},
_generatePosition: function(event) {
var top, left,
o = this.options,
pageX = event.pageX,
pageY = event.pageY,
scroll = this.cssPosition === "absolute" && !(this.scrollParent[0] !== document && $.contains(this.scrollParent[0], this.offsetParent[0])) ? this.offsetParent : this.scrollParent, scrollIsRootNode = (/(html|body)/i).test(scroll[0].tagName);
// This is another very weird special case that only happens for relative elements:
// 1. If the css position is relative
// 2. and the scroll parent is the document or similar to the offset parent
// we have to refresh the relative offset during the scroll so there are no jumps
if(this.cssPosition === "relative" && !(this.scrollParent[0] !== document && this.scrollParent[0] !== this.offsetParent[0])) {
this.offset.relative = this._getRelativeOffset();
}
/*
* - Position constraining -
* Constrain the position to a mix of grid, containment.
*/
if(this.originalPosition) { //If we are not dragging yet, we won't check for options
if(this.containment) {
if(event.pageX - this.offset.click.left < this.containment[0]) {
pageX = this.containment[0] + this.offset.click.left;
}
if(event.pageY - this.offset.click.top < this.containment[1]) {
pageY = this.containment[1] + this.offset.click.top;
}
if(event.pageX - this.offset.click.left > this.containment[2]) {
pageX = this.containment[2] + this.offset.click.left;
}
if(event.pageY - this.offset.click.top > this.containment[3]) {
pageY = this.containment[3] + this.offset.click.top;
}
}
if(o.grid) {
top = this.originalPageY + Math.round((pageY - this.originalPageY) / o.grid[1]) * o.grid[1];
pageY = this.containment ? ( (top - this.offset.click.top >= this.containment[1] && top - this.offset.click.top <= this.containment[3]) ? top : ((top - this.offset.click.top >= this.containment[1]) ? top - o.grid[1] : top + o.grid[1])) : top;
left = this.originalPageX + Math.round((pageX - this.originalPageX) / o.grid[0]) * o.grid[0];
pageX = this.containment ? ( (left - this.offset.click.left >= this.containment[0] && left - this.offset.click.left <= this.containment[2]) ? left : ((left - this.offset.click.left >= this.containment[0]) ? left - o.grid[0] : left + o.grid[0])) : left;
}
}
return {
top: (
pageY - // The absolute mouse position
this.offset.click.top - // Click offset (relative to the element)
this.offset.relative.top - // Only for relative positioned nodes: Relative offset from element to offset parent
this.offset.parent.top + // The offsetParent's offset without borders (offset + border)
( ( this.cssPosition === "fixed" ? -this.scrollParent.scrollTop() : ( scrollIsRootNode ? 0 : scroll.scrollTop() ) ))
),
left: (
pageX - // The absolute mouse position
this.offset.click.left - // Click offset (relative to the element)
this.offset.relative.left - // Only for relative positioned nodes: Relative offset from element to offset parent
this.offset.parent.left + // The offsetParent's offset without borders (offset + border)
( ( this.cssPosition === "fixed" ? -this.scrollParent.scrollLeft() : scrollIsRootNode ? 0 : scroll.scrollLeft() ))
)
};
},
_rearrange: function(event, i, a, hardRefresh) {
a ? a[0].appendChild(this.placeholder[0]) : i.item[0].parentNode.insertBefore(this.placeholder[0], (this.direction === "down" ? i.item[0] : i.item[0].nextSibling));
//Various things done here to improve the performance:
// 1. we create a setTimeout, that calls refreshPositions
// 2. on the instance, we have a counter variable, that get's higher after every append
// 3. on the local scope, we copy the counter variable, and check in the timeout, if it's still the same
// 4. this lets only the last addition to the timeout stack through
this.counter = this.counter ? ++this.counter : 1;
var counter = this.counter;
this._delay(function() {
if(counter === this.counter) {
this.refreshPositions(!hardRefresh); //Precompute after each DOM insertion, NOT on mousemove
}
});
},
_clear: function(event, noPropagation) {
this.reverting = false;
// We delay all events that have to be triggered to after the point where the placeholder has been removed and
// everything else normalized again
var i,
delayedTriggers = [];
// We first have to update the dom position of the actual currentItem
// Note: don't do it if the current item is already removed (by a user), or it gets reappended (see #4088)
if(!this._noFinalSort && this.currentItem.parent().length) {
this.placeholder.before(this.currentItem);
}
this._noFinalSort = null;
if(this.helper[0] === this.currentItem[0]) {
for(i in this._storedCSS) {
if(this._storedCSS[i] === "auto" || this._storedCSS[i] === "static") {
this._storedCSS[i] = "";
}
}
this.currentItem.css(this._storedCSS).removeClass("ui-sortable-helper");
} else {
this.currentItem.show();
}
if(this.fromOutside && !noPropagation) {
delayedTriggers.push(function(event) { this._trigger("receive", event, this._uiHash(this.fromOutside)); });
}
if((this.fromOutside || this.domPosition.prev !== this.currentItem.prev().not(".ui-sortable-helper")[0] || this.domPosition.parent !== this.currentItem.parent()[0]) && !noPropagation) {
delayedTriggers.push(function(event) { this._trigger("update", event, this._uiHash()); }); //Trigger update callback if the DOM position has changed
}
// Check if the items Container has Changed and trigger appropriate
// events.
if (this !== this.currentContainer) {
if(!noPropagation) {
delayedTriggers.push(function(event) { this._trigger("remove", event, this._uiHash()); });
delayedTriggers.push((function(c) { return function(event) { c._trigger("receive", event, this._uiHash(this)); }; }).call(this, this.currentContainer));
delayedTriggers.push((function(c) { return function(event) { c._trigger("update", event, this._uiHash(this)); }; }).call(this, this.currentContainer));
}
}
//Post events to containers
for (i = this.containers.length - 1; i >= 0; i--){
if(!noPropagation) {
delayedTriggers.push((function(c) { return function(event) { c._trigger("deactivate", event, this._uiHash(this)); }; }).call(this, this.containers[i]));
}
if(this.containers[i].containerCache.over) {
delayedTriggers.push((function(c) { return function(event) { c._trigger("out", event, this._uiHash(this)); }; }).call(this, this.containers[i]));
this.containers[i].containerCache.over = 0;
}
}
//Do what was originally in plugins
if ( this.storedCursor ) {
this.document.find( "body" ).css( "cursor", this.storedCursor );
this.storedStylesheet.remove();
}
if(this._storedOpacity) {
this.helper.css("opacity", this._storedOpacity);
}
if(this._storedZIndex) {
this.helper.css("zIndex", this._storedZIndex === "auto" ? "" : this._storedZIndex);
}
this.dragging = false;
if(this.cancelHelperRemoval) {
if(!noPropagation) {
this._trigger("beforeStop", event, this._uiHash());
for (i=0; i < delayedTriggers.length; i++) {
delayedTriggers[i].call(this, event);
} //Trigger all delayed events
this._trigger("stop", event, this._uiHash());
}
this.fromOutside = false;
return false;
}
if(!noPropagation) {
this._trigger("beforeStop", event, this._uiHash());
}
//$(this.placeholder[0]).remove(); would have been the jQuery way - unfortunately, it unbinds ALL events from the original node!
this.placeholder[0].parentNode.removeChild(this.placeholder[0]);
if(this.helper[0] !== this.currentItem[0]) {
this.helper.remove();
}
this.helper = null;
if(!noPropagation) {
for (i=0; i < delayedTriggers.length; i++) {
delayedTriggers[i].call(this, event);
} //Trigger all delayed events
this._trigger("stop", event, this._uiHash());
}
this.fromOutside = false;
return true;
},
_trigger: function() {
if ($.Widget.prototype._trigger.apply(this, arguments) === false) {
this.cancel();
}
},
_uiHash: function(_inst) {
var inst = _inst || this;
return {
helper: inst.helper,
placeholder: inst.placeholder || $([]),
position: inst.position,
originalPosition: inst.originalPosition,
offset: inst.positionAbs,
item: inst.currentItem,
sender: _inst ? _inst.element : null
};
}
});
})(jQuery);
(function($, undefined) {
var dataSpace = "ui-effects-";
$.effects = {
effect: {}
};
/*!
* jQuery Color Animations v2.1.2
* https://github.com/jquery/jquery-color
*
* Copyright 2013 jQuery Foundation and other contributors
* Released under the MIT license.
* http://jquery.org/license
*
* Date: Wed Jan 16 08:47:09 2013 -0600
*/
(function( jQuery, undefined ) {
var stepHooks = "backgroundColor borderBottomColor borderLeftColor borderRightColor borderTopColor color columnRuleColor outlineColor textDecorationColor textEmphasisColor",
// plusequals test for += 100 -= 100
rplusequals = /^([\-+])=\s*(\d+\.?\d*)/,
// a set of RE's that can match strings and generate color tuples.
stringParsers = [{
re: /rgba?\(\s*(\d{1,3})\s*,\s*(\d{1,3})\s*,\s*(\d{1,3})\s*(?:,\s*(\d?(?:\.\d+)?)\s*)?\)/,
parse: function( execResult ) {
return [
execResult[ 1 ],
execResult[ 2 ],
execResult[ 3 ],
execResult[ 4 ]
];
}
}, {
re: /rgba?\(\s*(\d+(?:\.\d+)?)\%\s*,\s*(\d+(?:\.\d+)?)\%\s*,\s*(\d+(?:\.\d+)?)\%\s*(?:,\s*(\d?(?:\.\d+)?)\s*)?\)/,
parse: function( execResult ) {
return [
execResult[ 1 ] * 2.55,
execResult[ 2 ] * 2.55,
execResult[ 3 ] * 2.55,
execResult[ 4 ]
];
}
}, {
// this regex ignores A-F because it's compared against an already lowercased string
re: /#([a-f0-9]{2})([a-f0-9]{2})([a-f0-9]{2})/,
parse: function( execResult ) {
return [
parseInt( execResult[ 1 ], 16 ),
parseInt( execResult[ 2 ], 16 ),
parseInt( execResult[ 3 ], 16 )
];
}
}, {
// this regex ignores A-F because it's compared against an already lowercased string
re: /#([a-f0-9])([a-f0-9])([a-f0-9])/,
parse: function( execResult ) {
return [
parseInt( execResult[ 1 ] + execResult[ 1 ], 16 ),
parseInt( execResult[ 2 ] + execResult[ 2 ], 16 ),
parseInt( execResult[ 3 ] + execResult[ 3 ], 16 )
];
}
}, {
re: /hsla?\(\s*(\d+(?:\.\d+)?)\s*,\s*(\d+(?:\.\d+)?)\%\s*,\s*(\d+(?:\.\d+)?)\%\s*(?:,\s*(\d?(?:\.\d+)?)\s*)?\)/,
space: "hsla",
parse: function( execResult ) {
return [
execResult[ 1 ],
execResult[ 2 ] / 100,
execResult[ 3 ] / 100,
execResult[ 4 ]
];
}
}],
// jQuery.Color( )
color = jQuery.Color = function( color, green, blue, alpha ) {
return new jQuery.Color.fn.parse( color, green, blue, alpha );
},
spaces = {
rgba: {
props: {
red: {
idx: 0,
type: "byte"
},
green: {
idx: 1,
type: "byte"
},
blue: {
idx: 2,
type: "byte"
}
}
},
hsla: {
props: {
hue: {
idx: 0,
type: "degrees"
},
saturation: {
idx: 1,
type: "percent"
},
lightness: {
idx: 2,
type: "percent"
}
}
}
},
propTypes = {
"byte": {
floor: true,
max: 255
},
"percent": {
max: 1
},
"degrees": {
mod: 360,
floor: true
}
},
support = color.support = {},
// element for support tests
supportElem = jQuery( "<p>" )[ 0 ],
// colors = jQuery.Color.names
colors,
// local aliases of functions called often
each = jQuery.each;
// determine rgba support immediately
supportElem.style.cssText = "background-color:rgba(1,1,1,.5)";
support.rgba = supportElem.style.backgroundColor.indexOf( "rgba" ) > -1;
// define cache name and alpha properties
// for rgba and hsla spaces
each( spaces, function( spaceName, space ) {
space.cache = "_" + spaceName;
space.props.alpha = {
idx: 3,
type: "percent",
def: 1
};
});
function clamp( value, prop, allowEmpty ) {
var type = propTypes[ prop.type ] || {};
if ( value == null ) {
return (allowEmpty || !prop.def) ? null : prop.def;
}
// ~~ is an short way of doing floor for positive numbers
value = type.floor ? ~~value : parseFloat( value );
// IE will pass in empty strings as value for alpha,
// which will hit this case
if ( isNaN( value ) ) {
return prop.def;
}
if ( type.mod ) {
// we add mod before modding to make sure that negatives values
// get converted properly: -10 -> 350
return (value + type.mod) % type.mod;
}
// for now all property types without mod have min and max
return 0 > value ? 0 : type.max < value ? type.max : value;
}
function stringParse( string ) {
var inst = color(),
rgba = inst._rgba = [];
string = string.toLowerCase();
each( stringParsers, function( i, parser ) {
var parsed,
match = parser.re.exec( string ),
values = match && parser.parse( match ),
spaceName = parser.space || "rgba";
if ( values ) {
parsed = inst[ spaceName ]( values );
// if this was an rgba parse the assignment might happen twice
// oh well....
inst[ spaces[ spaceName ].cache ] = parsed[ spaces[ spaceName ].cache ];
rgba = inst._rgba = parsed._rgba;
// exit each( stringParsers ) here because we matched
return false;
}
});
// Found a stringParser that handled it
if ( rgba.length ) {
// if this came from a parsed string, force "transparent" when alpha is 0
// chrome, (and maybe others) return "transparent" as rgba(0,0,0,0)
if ( rgba.join() === "0,0,0,0" ) {
jQuery.extend( rgba, colors.transparent );
}
return inst;
}
// named colors
return colors[ string ];
}
color.fn = jQuery.extend( color.prototype, {
parse: function( red, green, blue, alpha ) {
if ( red === undefined ) {
this._rgba = [ null, null, null, null ];
return this;
}
if ( red.jquery || red.nodeType ) {
red = jQuery( red ).css( green );
green = undefined;
}
var inst = this,
type = jQuery.type( red ),
rgba = this._rgba = [];
// more than 1 argument specified - assume ( red, green, blue, alpha )
if ( green !== undefined ) {
red = [ red, green, blue, alpha ];
type = "array";
}
if ( type === "string" ) {
return this.parse( stringParse( red ) || colors._default );
}
if ( type === "array" ) {
each( spaces.rgba.props, function( key, prop ) {
rgba[ prop.idx ] = clamp( red[ prop.idx ], prop );
});
return this;
}
if ( type === "object" ) {
if ( red instanceof color ) {
each( spaces, function( spaceName, space ) {
if ( red[ space.cache ] ) {
inst[ space.cache ] = red[ space.cache ].slice();
}
});
} else {
each( spaces, function( spaceName, space ) {
var cache = space.cache;
each( space.props, function( key, prop ) {
// if the cache doesn't exist, and we know how to convert
if ( !inst[ cache ] && space.to ) {
// if the value was null, we don't need to copy it
// if the key was alpha, we don't need to copy it either
if ( key === "alpha" || red[ key ] == null ) {
return;
}
inst[ cache ] = space.to( inst._rgba );
}
// this is the only case where we allow nulls for ALL properties.
// call clamp with alwaysAllowEmpty
inst[ cache ][ prop.idx ] = clamp( red[ key ], prop, true );
});
// everything defined but alpha?
if ( inst[ cache ] && jQuery.inArray( null, inst[ cache ].slice( 0, 3 ) ) < 0 ) {
// use the default of 1
inst[ cache ][ 3 ] = 1;
if ( space.from ) {
inst._rgba = space.from( inst[ cache ] );
}
}
});
}
return this;
}
},
is: function( compare ) {
var is = color( compare ),
same = true,
inst = this;
each( spaces, function( _, space ) {
var localCache,
isCache = is[ space.cache ];
if (isCache) {
localCache = inst[ space.cache ] || space.to && space.to( inst._rgba ) || [];
each( space.props, function( _, prop ) {
if ( isCache[ prop.idx ] != null ) {
same = ( isCache[ prop.idx ] === localCache[ prop.idx ] );
return same;
}
});
}
return same;
});
return same;
},
_space: function() {
var used = [],
inst = this;
each( spaces, function( spaceName, space ) {
if ( inst[ space.cache ] ) {
used.push( spaceName );
}
});
return used.pop();
},
transition: function( other, distance ) {
var end = color( other ),
spaceName = end._space(),
space = spaces[ spaceName ],
startColor = this.alpha() === 0 ? color( "transparent" ) : this,
start = startColor[ space.cache ] || space.to( startColor._rgba ),
result = start.slice();
end = end[ space.cache ];
each( space.props, function( key, prop ) {
var index = prop.idx,
startValue = start[ index ],
endValue = end[ index ],
type = propTypes[ prop.type ] || {};
// if null, don't override start value
if ( endValue === null ) {
return;
}
// if null - use end
if ( startValue === null ) {
result[ index ] = endValue;
} else {
if ( type.mod ) {
if ( endValue - startValue > type.mod / 2 ) {
startValue += type.mod;
} else if ( startValue - endValue > type.mod / 2 ) {
startValue -= type.mod;
}
}
result[ index ] = clamp( ( endValue - startValue ) * distance + startValue, prop );
}
});
return this[ spaceName ]( result );
},
blend: function( opaque ) {
// if we are already opaque - return ourself
if ( this._rgba[ 3 ] === 1 ) {
return this;
}
var rgb = this._rgba.slice(),
a = rgb.pop(),
blend = color( opaque )._rgba;
return color( jQuery.map( rgb, function( v, i ) {
return ( 1 - a ) * blend[ i ] + a * v;
}));
},
toRgbaString: function() {
var prefix = "rgba(",
rgba = jQuery.map( this._rgba, function( v, i ) {
return v == null ? ( i > 2 ? 1 : 0 ) : v;
});
if ( rgba[ 3 ] === 1 ) {
rgba.pop();
prefix = "rgb(";
}
return prefix + rgba.join() + ")";
},
toHslaString: function() {
var prefix = "hsla(",
hsla = jQuery.map( this.hsla(), function( v, i ) {
if ( v == null ) {
v = i > 2 ? 1 : 0;
}
// catch 1 and 2
if ( i && i < 3 ) {
v = Math.round( v * 100 ) + "%";
}
return v;
});
if ( hsla[ 3 ] === 1 ) {
hsla.pop();
prefix = "hsl(";
}
return prefix + hsla.join() + ")";
},
toHexString: function( includeAlpha ) {
var rgba = this._rgba.slice(),
alpha = rgba.pop();
if ( includeAlpha ) {
rgba.push( ~~( alpha * 255 ) );
}
return "#" + jQuery.map( rgba, function( v ) {
// default to 0 when nulls exist
v = ( v || 0 ).toString( 16 );
return v.length === 1 ? "0" + v : v;
}).join("");
},
toString: function() {
return this._rgba[ 3 ] === 0 ? "transparent" : this.toRgbaString();
}
});
color.fn.parse.prototype = color.fn;
// hsla conversions adapted from:
// https://code.google.com/p/maashaack/source/browse/packages/graphics/trunk/src/graphics/colors/HUE2RGB.as?r=5021
function hue2rgb( p, q, h ) {
h = ( h + 1 ) % 1;
if ( h * 6 < 1 ) {
return p + (q - p) * h * 6;
}
if ( h * 2 < 1) {
return q;
}
if ( h * 3 < 2 ) {
return p + (q - p) * ((2/3) - h) * 6;
}
return p;
}
spaces.hsla.to = function ( rgba ) {
if ( rgba[ 0 ] == null || rgba[ 1 ] == null || rgba[ 2 ] == null ) {
return [ null, null, null, rgba[ 3 ] ];
}
var r = rgba[ 0 ] / 255,
g = rgba[ 1 ] / 255,
b = rgba[ 2 ] / 255,
a = rgba[ 3 ],
max = Math.max( r, g, b ),
min = Math.min( r, g, b ),
diff = max - min,
add = max + min,
l = add * 0.5,
h, s;
if ( min === max ) {
h = 0;
} else if ( r === max ) {
h = ( 60 * ( g - b ) / diff ) + 360;
} else if ( g === max ) {
h = ( 60 * ( b - r ) / diff ) + 120;
} else {
h = ( 60 * ( r - g ) / diff ) + 240;
}
// chroma (diff) == 0 means greyscale which, by definition, saturation = 0%
// otherwise, saturation is based on the ratio of chroma (diff) to lightness (add)
if ( diff === 0 ) {
s = 0;
} else if ( l <= 0.5 ) {
s = diff / add;
} else {
s = diff / ( 2 - add );
}
return [ Math.round(h) % 360, s, l, a == null ? 1 : a ];
};
spaces.hsla.from = function ( hsla ) {
if ( hsla[ 0 ] == null || hsla[ 1 ] == null || hsla[ 2 ] == null ) {
return [ null, null, null, hsla[ 3 ] ];
}
var h = hsla[ 0 ] / 360,
s = hsla[ 1 ],
l = hsla[ 2 ],
a = hsla[ 3 ],
q = l <= 0.5 ? l * ( 1 + s ) : l + s - l * s,
p = 2 * l - q;
return [
Math.round( hue2rgb( p, q, h + ( 1 / 3 ) ) * 255 ),
Math.round( hue2rgb( p, q, h ) * 255 ),
Math.round( hue2rgb( p, q, h - ( 1 / 3 ) ) * 255 ),
a
];
};
each( spaces, function( spaceName, space ) {
var props = space.props,
cache = space.cache,
to = space.to,
from = space.from;
// makes rgba() and hsla()
color.fn[ spaceName ] = function( value ) {
// generate a cache for this space if it doesn't exist
if ( to && !this[ cache ] ) {
this[ cache ] = to( this._rgba );
}
if ( value === undefined ) {
return this[ cache ].slice();
}
var ret,
type = jQuery.type( value ),
arr = ( type === "array" || type === "object" ) ? value : arguments,
local = this[ cache ].slice();
each( props, function( key, prop ) {
var val = arr[ type === "object" ? key : prop.idx ];
if ( val == null ) {
val = local[ prop.idx ];
}
local[ prop.idx ] = clamp( val, prop );
});
if ( from ) {
ret = color( from( local ) );
ret[ cache ] = local;
return ret;
} else {
return color( local );
}
};
// makes red() green() blue() alpha() hue() saturation() lightness()
each( props, function( key, prop ) {
// alpha is included in more than one space
if ( color.fn[ key ] ) {
return;
}
color.fn[ key ] = function( value ) {
var vtype = jQuery.type( value ),
fn = ( key === "alpha" ? ( this._hsla ? "hsla" : "rgba" ) : spaceName ),
local = this[ fn ](),
cur = local[ prop.idx ],
match;
if ( vtype === "undefined" ) {
return cur;
}
if ( vtype === "function" ) {
value = value.call( this, cur );
vtype = jQuery.type( value );
}
if ( value == null && prop.empty ) {
return this;
}
if ( vtype === "string" ) {
match = rplusequals.exec( value );
if ( match ) {
value = cur + parseFloat( match[ 2 ] ) * ( match[ 1 ] === "+" ? 1 : -1 );
}
}
local[ prop.idx ] = value;
return this[ fn ]( local );
};
});
});
// add cssHook and .fx.step function for each named hook.
// accept a space separated string of properties
color.hook = function( hook ) {
var hooks = hook.split( " " );
each( hooks, function( i, hook ) {
jQuery.cssHooks[ hook ] = {
set: function( elem, value ) {
var parsed, curElem,
backgroundColor = "";
if ( value !== "transparent" && ( jQuery.type( value ) !== "string" || ( parsed = stringParse( value ) ) ) ) {
value = color( parsed || value );
if ( !support.rgba && value._rgba[ 3 ] !== 1 ) {
curElem = hook === "backgroundColor" ? elem.parentNode : elem;
while (
(backgroundColor === "" || backgroundColor === "transparent") &&
curElem && curElem.style
) {
try {
backgroundColor = jQuery.css( curElem, "backgroundColor" );
curElem = curElem.parentNode;
} catch ( e ) {
}
}
value = value.blend( backgroundColor && backgroundColor !== "transparent" ?
backgroundColor :
"_default" );
}
value = value.toRgbaString();
}
try {
elem.style[ hook ] = value;
} catch( e ) {
// wrapped to prevent IE from throwing errors on "invalid" values like 'auto' or 'inherit'
}
}
};
jQuery.fx.step[ hook ] = function( fx ) {
if ( !fx.colorInit ) {
fx.start = color( fx.elem, hook );
fx.end = color( fx.end );
fx.colorInit = true;
}
jQuery.cssHooks[ hook ].set( fx.elem, fx.start.transition( fx.end, fx.pos ) );
};
});
};
color.hook( stepHooks );
jQuery.cssHooks.borderColor = {
expand: function( value ) {
var expanded = {};
each( [ "Top", "Right", "Bottom", "Left" ], function( i, part ) {
expanded[ "border" + part + "Color" ] = value;
});
return expanded;
}
};
// Basic color names only.
// Usage of any of the other color names requires adding yourself or including
// jquery.color.svg-names.js.
colors = jQuery.Color.names = {
// 4.1. Basic color keywords
aqua: "#00ffff",
black: "#000000",
blue: "#0000ff",
fuchsia: "#ff00ff",
gray: "#808080",
green: "#008000",
lime: "#00ff00",
maroon: "#800000",
navy: "#000080",
olive: "#808000",
purple: "#800080",
red: "#ff0000",
silver: "#c0c0c0",
teal: "#008080",
white: "#ffffff",
yellow: "#ffff00",
// 4.2.3. "transparent" color keyword
transparent: [ null, null, null, 0 ],
_default: "#ffffff"
};
})( jQuery );
/******************************************************************************/
/****************************** CLASS ANIMATIONS ******************************/
/******************************************************************************/
(function() {
var classAnimationActions = [ "add", "remove", "toggle" ],
shorthandStyles = {
border: 1,
borderBottom: 1,
borderColor: 1,
borderLeft: 1,
borderRight: 1,
borderTop: 1,
borderWidth: 1,
margin: 1,
padding: 1
};
$.each([ "borderLeftStyle", "borderRightStyle", "borderBottomStyle", "borderTopStyle" ], function( _, prop ) {
$.fx.step[ prop ] = function( fx ) {
if ( fx.end !== "none" && !fx.setAttr || fx.pos === 1 && !fx.setAttr ) {
jQuery.style( fx.elem, prop, fx.end );
fx.setAttr = true;
}
};
});
function getElementStyles( elem ) {
var key, len,
style = elem.ownerDocument.defaultView ?
elem.ownerDocument.defaultView.getComputedStyle( elem, null ) :
elem.currentStyle,
styles = {};
if ( style && style.length && style[ 0 ] && style[ style[ 0 ] ] ) {
len = style.length;
while ( len-- ) {
key = style[ len ];
if ( typeof style[ key ] === "string" ) {
styles[ $.camelCase( key ) ] = style[ key ];
}
}
// support: Opera, IE <9
} else {
for ( key in style ) {
if ( typeof style[ key ] === "string" ) {
styles[ key ] = style[ key ];
}
}
}
return styles;
}
function styleDifference( oldStyle, newStyle ) {
var diff = {},
name, value;
for ( name in newStyle ) {
value = newStyle[ name ];
if ( oldStyle[ name ] !== value ) {
if ( !shorthandStyles[ name ] ) {
if ( $.fx.step[ name ] || !isNaN( parseFloat( value ) ) ) {
diff[ name ] = value;
}
}
}
}
return diff;
}
// support: jQuery <1.8
if ( !$.fn.addBack ) {
$.fn.addBack = function( selector ) {
return this.add( selector == null ?
this.prevObject : this.prevObject.filter( selector )
);
};
}
$.effects.animateClass = function( value, duration, easing, callback ) {
var o = $.speed( duration, easing, callback );
return this.queue( function() {
var animated = $( this ),
baseClass = animated.attr( "class" ) || "",
applyClassChange,
allAnimations = o.children ? animated.find( "*" ).addBack() : animated;
// map the animated objects to store the original styles.
allAnimations = allAnimations.map(function() {
var el = $( this );
return {
el: el,
start: getElementStyles( this )
};
});
// apply class change
applyClassChange = function() {
$.each( classAnimationActions, function(i, action) {
if ( value[ action ] ) {
animated[ action + "Class" ]( value[ action ] );
}
});
};
applyClassChange();
// map all animated objects again - calculate new styles and diff
allAnimations = allAnimations.map(function() {
this.end = getElementStyles( this.el[ 0 ] );
this.diff = styleDifference( this.start, this.end );
return this;
});
// apply original class
animated.attr( "class", baseClass );
// map all animated objects again - this time collecting a promise
allAnimations = allAnimations.map(function() {
var styleInfo = this,
dfd = $.Deferred(),
opts = $.extend({}, o, {
queue: false,
complete: function() {
dfd.resolve( styleInfo );
}
});
this.el.animate( this.diff, opts );
return dfd.promise();
});
// once all animations have completed:
$.when.apply( $, allAnimations.get() ).done(function() {
// set the final class
applyClassChange();
// for each animated element,
// clear all css properties that were animated
$.each( arguments, function() {
var el = this.el;
$.each( this.diff, function(key) {
el.css( key, "" );
});
});
// this is guarnteed to be there if you use jQuery.speed()
// it also handles dequeuing the next anim...
o.complete.call( animated[ 0 ] );
});
});
};
$.fn.extend({
addClass: (function( orig ) {
return function( classNames, speed, easing, callback ) {
return speed ?
$.effects.animateClass.call( this,
{ add: classNames }, speed, easing, callback ) :
orig.apply( this, arguments );
};
})( $.fn.addClass ),
removeClass: (function( orig ) {
return function( classNames, speed, easing, callback ) {
return arguments.length > 1 ?
$.effects.animateClass.call( this,
{ remove: classNames }, speed, easing, callback ) :
orig.apply( this, arguments );
};
})( $.fn.removeClass ),
toggleClass: (function( orig ) {
return function( classNames, force, speed, easing, callback ) {
if ( typeof force === "boolean" || force === undefined ) {
if ( !speed ) {
// without speed parameter
return orig.apply( this, arguments );
} else {
return $.effects.animateClass.call( this,
(force ? { add: classNames } : { remove: classNames }),
speed, easing, callback );
}
} else {
// without force parameter
return $.effects.animateClass.call( this,
{ toggle: classNames }, force, speed, easing );
}
};
})( $.fn.toggleClass ),
switchClass: function( remove, add, speed, easing, callback) {
return $.effects.animateClass.call( this, {
add: add,
remove: remove
}, speed, easing, callback );
}
});
})();
/******************************************************************************/
/*********************************** EFFECTS **********************************/
/******************************************************************************/
(function() {
$.extend( $.effects, {
version: "1.10.3",
// Saves a set of properties in a data storage
save: function( element, set ) {
for( var i=0; i < set.length; i++ ) {
if ( set[ i ] !== null ) {
element.data( dataSpace + set[ i ], element[ 0 ].style[ set[ i ] ] );
}
}
},
// Restores a set of previously saved properties from a data storage
restore: function( element, set ) {
var val, i;
for( i=0; i < set.length; i++ ) {
if ( set[ i ] !== null ) {
val = element.data( dataSpace + set[ i ] );
// support: jQuery 1.6.2
// http://bugs.jquery.com/ticket/9917
// jQuery 1.6.2 incorrectly returns undefined for any falsy value.
// We can't differentiate between "" and 0 here, so we just assume
// empty string since it's likely to be a more common value...
if ( val === undefined ) {
val = "";
}
element.css( set[ i ], val );
}
}
},
setMode: function( el, mode ) {
if (mode === "toggle") {
mode = el.is( ":hidden" ) ? "show" : "hide";
}
return mode;
},
// Translates a [top,left] array into a baseline value
// this should be a little more flexible in the future to handle a string & hash
getBaseline: function( origin, original ) {
var y, x;
switch ( origin[ 0 ] ) {
case "top": y = 0; break;
case "middle": y = 0.5; break;
case "bottom": y = 1; break;
default: y = origin[ 0 ] / original.height;
}
switch ( origin[ 1 ] ) {
case "left": x = 0; break;
case "center": x = 0.5; break;
case "right": x = 1; break;
default: x = origin[ 1 ] / original.width;
}
return {
x: x,
y: y
};
},
// Wraps the element around a wrapper that copies position properties
createWrapper: function( element ) {
// if the element is already wrapped, return it
if ( element.parent().is( ".ui-effects-wrapper" )) {
return element.parent();
}
// wrap the element
var props = {
width: element.outerWidth(true),
height: element.outerHeight(true),
"float": element.css( "float" )
},
wrapper = $( "<div></div>" )
.addClass( "ui-effects-wrapper" )
.css({
fontSize: "100%",
background: "transparent",
border: "none",
margin: 0,
padding: 0
}),
// Store the size in case width/height are defined in % - Fixes #5245
size = {
width: element.width(),
height: element.height()
},
active = document.activeElement;
// support: Firefox
// Firefox incorrectly exposes anonymous content
// https://bugzilla.mozilla.org/show_bug.cgi?id=561664
try {
active.id;
} catch( e ) {
active = document.body;
}
element.wrap( wrapper );
// Fixes #7595 - Elements lose focus when wrapped.
if ( element[ 0 ] === active || $.contains( element[ 0 ], active ) ) {
$( active ).focus();
}
wrapper = element.parent(); //Hotfix for jQuery 1.4 since some change in wrap() seems to actually lose the reference to the wrapped element
// transfer positioning properties to the wrapper
if ( element.css( "position" ) === "static" ) {
wrapper.css({ position: "relative" });
element.css({ position: "relative" });
} else {
$.extend( props, {
position: element.css( "position" ),
zIndex: element.css( "z-index" )
});
$.each([ "top", "left", "bottom", "right" ], function(i, pos) {
props[ pos ] = element.css( pos );
if ( isNaN( parseInt( props[ pos ], 10 ) ) ) {
props[ pos ] = "auto";
}
});
element.css({
position: "relative",
top: 0,
left: 0,
right: "auto",
bottom: "auto"
});
}
element.css(size);
return wrapper.css( props ).show();
},
removeWrapper: function( element ) {
var active = document.activeElement;
if ( element.parent().is( ".ui-effects-wrapper" ) ) {
element.parent().replaceWith( element );
// Fixes #7595 - Elements lose focus when wrapped.
if ( element[ 0 ] === active || $.contains( element[ 0 ], active ) ) {
$( active ).focus();
}
}
return element;
},
setTransition: function( element, list, factor, value ) {
value = value || {};
$.each( list, function( i, x ) {
var unit = element.cssUnit( x );
if ( unit[ 0 ] > 0 ) {
value[ x ] = unit[ 0 ] * factor + unit[ 1 ];
}
});
return value;
}
});
// return an effect options object for the given parameters:
function _normalizeArguments( effect, options, speed, callback ) {
// allow passing all options as the first parameter
if ( $.isPlainObject( effect ) ) {
options = effect;
effect = effect.effect;
}
// convert to an object
effect = { effect: effect };
// catch (effect, null, ...)
if ( options == null ) {
options = {};
}
// catch (effect, callback)
if ( $.isFunction( options ) ) {
callback = options;
speed = null;
options = {};
}
// catch (effect, speed, ?)
if ( typeof options === "number" || $.fx.speeds[ options ] ) {
callback = speed;
speed = options;
options = {};
}
// catch (effect, options, callback)
if ( $.isFunction( speed ) ) {
callback = speed;
speed = null;
}
// add options to effect
if ( options ) {
$.extend( effect, options );
}
speed = speed || options.duration;
effect.duration = $.fx.off ? 0 :
typeof speed === "number" ? speed :
speed in $.fx.speeds ? $.fx.speeds[ speed ] :
$.fx.speeds._default;
effect.complete = callback || options.complete;
return effect;
}
function standardAnimationOption( option ) {
// Valid standard speeds (nothing, number, named speed)
if ( !option || typeof option === "number" || $.fx.speeds[ option ] ) {
return true;
}
// Invalid strings - treat as "normal" speed
if ( typeof option === "string" && !$.effects.effect[ option ] ) {
return true;
}
// Complete callback
if ( $.isFunction( option ) ) {
return true;
}
// Options hash (but not naming an effect)
if ( typeof option === "object" && !option.effect ) {
return true;
}
// Didn't match any standard API
return false;
}
$.fn.extend({
effect: function( /* effect, options, speed, callback */ ) {
var args = _normalizeArguments.apply( this, arguments ),
mode = args.mode,
queue = args.queue,
effectMethod = $.effects.effect[ args.effect ];
if ( $.fx.off || !effectMethod ) {
// delegate to the original method (e.g., .show()) if possible
if ( mode ) {
return this[ mode ]( args.duration, args.complete );
} else {
return this.each( function() {
if ( args.complete ) {
args.complete.call( this );
}
});
}
}
function run( next ) {
var elem = $( this ),
complete = args.complete,
mode = args.mode;
function done() {
if ( $.isFunction( complete ) ) {
complete.call( elem[0] );
}
if ( $.isFunction( next ) ) {
next();
}
}
// If the element already has the correct final state, delegate to
// the core methods so the internal tracking of "olddisplay" works.
if ( elem.is( ":hidden" ) ? mode === "hide" : mode === "show" ) {
elem[ mode ]();
done();
} else {
effectMethod.call( elem[0], args, done );
}
}
return queue === false ? this.each( run ) : this.queue( queue || "fx", run );
},
show: (function( orig ) {
return function( option ) {
if ( standardAnimationOption( option ) ) {
return orig.apply( this, arguments );
} else {
var args = _normalizeArguments.apply( this, arguments );
args.mode = "show";
return this.effect.call( this, args );
}
};
})( $.fn.show ),
hide: (function( orig ) {
return function( option ) {
if ( standardAnimationOption( option ) ) {
return orig.apply( this, arguments );
} else {
var args = _normalizeArguments.apply( this, arguments );
args.mode = "hide";
return this.effect.call( this, args );
}
};
})( $.fn.hide ),
toggle: (function( orig ) {
return function( option ) {
if ( standardAnimationOption( option ) || typeof option === "boolean" ) {
return orig.apply( this, arguments );
} else {
var args = _normalizeArguments.apply( this, arguments );
args.mode = "toggle";
return this.effect.call( this, args );
}
};
})( $.fn.toggle ),
// helper functions
cssUnit: function(key) {
var style = this.css( key ),
val = [];
$.each( [ "em", "px", "%", "pt" ], function( i, unit ) {
if ( style.indexOf( unit ) > 0 ) {
val = [ parseFloat( style ), unit ];
}
});
return val;
}
});
})();
/******************************************************************************/
/*********************************** EASING ***********************************/
/******************************************************************************/
(function() {
// based on easing equations from Robert Penner (http://www.robertpenner.com/easing)
var baseEasings = {};
$.each( [ "Quad", "Cubic", "Quart", "Quint", "Expo" ], function( i, name ) {
baseEasings[ name ] = function( p ) {
return Math.pow( p, i + 2 );
};
});
$.extend( baseEasings, {
Sine: function ( p ) {
return 1 - Math.cos( p * Math.PI / 2 );
},
Circ: function ( p ) {
return 1 - Math.sqrt( 1 - p * p );
},
Elastic: function( p ) {
return p === 0 || p === 1 ? p :
-Math.pow( 2, 8 * (p - 1) ) * Math.sin( ( (p - 1) * 80 - 7.5 ) * Math.PI / 15 );
},
Back: function( p ) {
return p * p * ( 3 * p - 2 );
},
Bounce: function ( p ) {
var pow2,
bounce = 4;
while ( p < ( ( pow2 = Math.pow( 2, --bounce ) ) - 1 ) / 11 ) {}
return 1 / Math.pow( 4, 3 - bounce ) - 7.5625 * Math.pow( ( pow2 * 3 - 2 ) / 22 - p, 2 );
}
});
$.each( baseEasings, function( name, easeIn ) {
$.easing[ "easeIn" + name ] = easeIn;
$.easing[ "easeOut" + name ] = function( p ) {
return 1 - easeIn( 1 - p );
};
$.easing[ "easeInOut" + name ] = function( p ) {
return p < 0.5 ?
easeIn( p * 2 ) / 2 :
1 - easeIn( p * -2 + 2 ) / 2;
};
});
})();
})(jQuery);
(function( $, undefined ) {
var uid = 0,
hideProps = {},
showProps = {};
hideProps.height = hideProps.paddingTop = hideProps.paddingBottom =
hideProps.borderTopWidth = hideProps.borderBottomWidth = "hide";
showProps.height = showProps.paddingTop = showProps.paddingBottom =
showProps.borderTopWidth = showProps.borderBottomWidth = "show";
$.widget( "ui.accordion", {
version: "1.10.3",
options: {
active: 0,
animate: {},
collapsible: false,
event: "click",
header: "> li > :first-child,> :not(li):even",
heightStyle: "auto",
icons: {
activeHeader: "ui-icon-triangle-1-s",
header: "ui-icon-triangle-1-e"
},
// callbacks
activate: null,
beforeActivate: null
},
_create: function() {
var options = this.options;
this.prevShow = this.prevHide = $();
this.element.addClass( "ui-accordion ui-widget ui-helper-reset" )
// ARIA
.attr( "role", "tablist" );
// don't allow collapsible: false and active: false / null
if ( !options.collapsible && (options.active === false || options.active == null) ) {
options.active = 0;
}
this._processPanels();
// handle negative values
if ( options.active < 0 ) {
options.active += this.headers.length;
}
this._refresh();
},
_getCreateEventData: function() {
return {
header: this.active,
panel: !this.active.length ? $() : this.active.next(),
content: !this.active.length ? $() : this.active.next()
};
},
_createIcons: function() {
var icons = this.options.icons;
if ( icons ) {
$( "<span>" )
.addClass( "ui-accordion-header-icon ui-icon " + icons.header )
.prependTo( this.headers );
this.active.children( ".ui-accordion-header-icon" )
.removeClass( icons.header )
.addClass( icons.activeHeader );
this.headers.addClass( "ui-accordion-icons" );
}
},
_destroyIcons: function() {
this.headers
.removeClass( "ui-accordion-icons" )
.children( ".ui-accordion-header-icon" )
.remove();
},
_destroy: function() {
var contents;
// clean up main element
this.element
.removeClass( "ui-accordion ui-widget ui-helper-reset" )
.removeAttr( "role" );
// clean up headers
this.headers
.removeClass( "ui-accordion-header ui-accordion-header-active ui-helper-reset ui-state-default ui-corner-all ui-state-active ui-state-disabled ui-corner-top" )
.removeAttr( "role" )
.removeAttr( "aria-selected" )
.removeAttr( "aria-controls" )
.removeAttr( "tabIndex" )
.each(function() {
if ( /^ui-accordion/.test( this.id ) ) {
this.removeAttribute( "id" );
}
});
this._destroyIcons();
// clean up content panels
contents = this.headers.next()
.css( "display", "" )
.removeAttr( "role" )
.removeAttr( "aria-expanded" )
.removeAttr( "aria-hidden" )
.removeAttr( "aria-labelledby" )
.removeClass( "ui-helper-reset ui-widget-content ui-corner-bottom ui-accordion-content ui-accordion-content-active ui-state-disabled" )
.each(function() {
if ( /^ui-accordion/.test( this.id ) ) {
this.removeAttribute( "id" );
}
});
if ( this.options.heightStyle !== "content" ) {
contents.css( "height", "" );
}
},
_setOption: function( key, value ) {
if ( key === "active" ) {
// _activate() will handle invalid values and update this.options
this._activate( value );
return;
}
if ( key === "event" ) {
if ( this.options.event ) {
this._off( this.headers, this.options.event );
}
this._setupEvents( value );
}
this._super( key, value );
// setting collapsible: false while collapsed; open first panel
if ( key === "collapsible" && !value && this.options.active === false ) {
this._activate( 0 );
}
if ( key === "icons" ) {
this._destroyIcons();
if ( value ) {
this._createIcons();
}
}
// #5332 - opacity doesn't cascade to positioned elements in IE
// so we need to add the disabled class to the headers and panels
if ( key === "disabled" ) {
this.headers.add( this.headers.next() )
.toggleClass( "ui-state-disabled", !!value );
}
},
_keydown: function( event ) {
/*jshint maxcomplexity:15*/
if ( event.altKey || event.ctrlKey ) {
return;
}
var keyCode = $.ui.keyCode,
length = this.headers.length,
currentIndex = this.headers.index( event.target ),
toFocus = false;
switch ( event.keyCode ) {
case keyCode.RIGHT:
case keyCode.DOWN:
toFocus = this.headers[ ( currentIndex + 1 ) % length ];
break;
case keyCode.LEFT:
case keyCode.UP:
toFocus = this.headers[ ( currentIndex - 1 + length ) % length ];
break;
case keyCode.SPACE:
case keyCode.ENTER:
this._eventHandler( event );
break;
case keyCode.HOME:
toFocus = this.headers[ 0 ];
break;
case keyCode.END:
toFocus = this.headers[ length - 1 ];
break;
}
if ( toFocus ) {
$( event.target ).attr( "tabIndex", -1 );
$( toFocus ).attr( "tabIndex", 0 );
toFocus.focus();
event.preventDefault();
}
},
_panelKeyDown : function( event ) {
if ( event.keyCode === $.ui.keyCode.UP && event.ctrlKey ) {
$( event.currentTarget ).prev().focus();
}
},
refresh: function() {
var options = this.options;
this._processPanels();
// was collapsed or no panel
if ( ( options.active === false && options.collapsible === true ) || !this.headers.length ) {
options.active = false;
this.active = $();
// active false only when collapsible is true
} else if ( options.active === false ) {
this._activate( 0 );
// was active, but active panel is gone
} else if ( this.active.length && !$.contains( this.element[ 0 ], this.active[ 0 ] ) ) {
// all remaining panel are disabled
if ( this.headers.length === this.headers.find(".ui-state-disabled").length ) {
options.active = false;
this.active = $();
// activate previous panel
} else {
this._activate( Math.max( 0, options.active - 1 ) );
}
// was active, active panel still exists
} else {
// make sure active index is correct
options.active = this.headers.index( this.active );
}
this._destroyIcons();
this._refresh();
},
_processPanels: function() {
this.headers = this.element.find( this.options.header )
.addClass( "ui-accordion-header ui-helper-reset ui-state-default ui-corner-all" );
this.headers.next()
.addClass( "ui-accordion-content ui-helper-reset ui-widget-content ui-corner-bottom" )
.filter(":not(.ui-accordion-content-active)")
.hide();
},
_refresh: function() {
var maxHeight,
options = this.options,
heightStyle = options.heightStyle,
parent = this.element.parent(),
accordionId = this.accordionId = "ui-accordion-" +
(this.element.attr( "id" ) || ++uid);
this.active = this._findActive( options.active )
.addClass( "ui-accordion-header-active ui-state-active ui-corner-top" )
.removeClass( "ui-corner-all" );
this.active.next()
.addClass( "ui-accordion-content-active" )
.show();
this.headers
.attr( "role", "tab" )
.each(function( i ) {
var header = $( this ),
headerId = header.attr( "id" ),
panel = header.next(),
panelId = panel.attr( "id" );
if ( !headerId ) {
headerId = accordionId + "-header-" + i;
header.attr( "id", headerId );
}
if ( !panelId ) {
panelId = accordionId + "-panel-" + i;
panel.attr( "id", panelId );
}
header.attr( "aria-controls", panelId );
panel.attr( "aria-labelledby", headerId );
})
.next()
.attr( "role", "tabpanel" );
this.headers
.not( this.active )
.attr({
"aria-selected": "false",
tabIndex: -1
})
.next()
.attr({
"aria-expanded": "false",
"aria-hidden": "true"
})
.hide();
// make sure at least one header is in the tab order
if ( !this.active.length ) {
this.headers.eq( 0 ).attr( "tabIndex", 0 );
} else {
this.active.attr({
"aria-selected": "true",
tabIndex: 0
})
.next()
.attr({
"aria-expanded": "true",
"aria-hidden": "false"
});
}
this._createIcons();
this._setupEvents( options.event );
if ( heightStyle === "fill" ) {
maxHeight = parent.height();
this.element.siblings( ":visible" ).each(function() {
var elem = $( this ),
position = elem.css( "position" );
if ( position === "absolute" || position === "fixed" ) {
return;
}
maxHeight -= elem.outerHeight( true );
});
this.headers.each(function() {
maxHeight -= $( this ).outerHeight( true );
});
this.headers.next()
.each(function() {
$( this ).height( Math.max( 0, maxHeight -
$( this ).innerHeight() + $( this ).height() ) );
})
.css( "overflow", "auto" );
} else if ( heightStyle === "auto" ) {
maxHeight = 0;
this.headers.next()
.each(function() {
maxHeight = Math.max( maxHeight, $( this ).css( "height", "" ).height() );
})
.height( maxHeight );
}
},
_activate: function( index ) {
var active = this._findActive( index )[ 0 ];
// trying to activate the already active panel
if ( active === this.active[ 0 ] ) {
return;
}
// trying to collapse, simulate a click on the currently active header
active = active || this.active[ 0 ];
this._eventHandler({
target: active,
currentTarget: active,
preventDefault: $.noop
});
},
_findActive: function( selector ) {
return typeof selector === "number" ? this.headers.eq( selector ) : $();
},
_setupEvents: function( event ) {
var events = {
keydown: "_keydown"
};
if ( event ) {
$.each( event.split(" "), function( index, eventName ) {
events[ eventName ] = "_eventHandler";
});
}
this._off( this.headers.add( this.headers.next() ) );
this._on( this.headers, events );
this._on( this.headers.next(), { keydown: "_panelKeyDown" });
this._hoverable( this.headers );
this._focusable( this.headers );
},
_eventHandler: function( event ) {
var options = this.options,
active = this.active,
clicked = $( event.currentTarget ),
clickedIsActive = clicked[ 0 ] === active[ 0 ],
collapsing = clickedIsActive && options.collapsible,
toShow = collapsing ? $() : clicked.next(),
toHide = active.next(),
eventData = {
oldHeader: active,
oldPanel: toHide,
newHeader: collapsing ? $() : clicked,
newPanel: toShow
};
event.preventDefault();
if (
// click on active header, but not collapsible
( clickedIsActive && !options.collapsible ) ||
// allow canceling activation
( this._trigger( "beforeActivate", event, eventData ) === false ) ) {
return;
}
options.active = collapsing ? false : this.headers.index( clicked );
// when the call to ._toggle() comes after the class changes
// it causes a very odd bug in IE 8 (see #6720)
this.active = clickedIsActive ? $() : clicked;
this._toggle( eventData );
// switch classes
// corner classes on the previously active header stay after the animation
active.removeClass( "ui-accordion-header-active ui-state-active" );
if ( options.icons ) {
active.children( ".ui-accordion-header-icon" )
.removeClass( options.icons.activeHeader )
.addClass( options.icons.header );
}
if ( !clickedIsActive ) {
clicked
.removeClass( "ui-corner-all" )
.addClass( "ui-accordion-header-active ui-state-active ui-corner-top" );
if ( options.icons ) {
clicked.children( ".ui-accordion-header-icon" )
.removeClass( options.icons.header )
.addClass( options.icons.activeHeader );
}
clicked
.next()
.addClass( "ui-accordion-content-active" );
}
},
_toggle: function( data ) {
var toShow = data.newPanel,
toHide = this.prevShow.length ? this.prevShow : data.oldPanel;
// handle activating a panel during the animation for another activation
this.prevShow.add( this.prevHide ).stop( true, true );
this.prevShow = toShow;
this.prevHide = toHide;
if ( this.options.animate ) {
this._animate( toShow, toHide, data );
} else {
toHide.hide();
toShow.show();
this._toggleComplete( data );
}
toHide.attr({
"aria-expanded": "false",
"aria-hidden": "true"
});
toHide.prev().attr( "aria-selected", "false" );
// if we're switching panels, remove the old header from the tab order
// if we're opening from collapsed state, remove the previous header from the tab order
// if we're collapsing, then keep the collapsing header in the tab order
if ( toShow.length && toHide.length ) {
toHide.prev().attr( "tabIndex", -1 );
} else if ( toShow.length ) {
this.headers.filter(function() {
return $( this ).attr( "tabIndex" ) === 0;
})
.attr( "tabIndex", -1 );
}
toShow
.attr({
"aria-expanded": "true",
"aria-hidden": "false"
})
.prev()
.attr({
"aria-selected": "true",
tabIndex: 0
});
},
_animate: function( toShow, toHide, data ) {
var total, easing, duration,
that = this,
adjust = 0,
down = toShow.length &&
( !toHide.length || ( toShow.index() < toHide.index() ) ),
animate = this.options.animate || {},
options = down && animate.down || animate,
complete = function() {
that._toggleComplete( data );
};
if ( typeof options === "number" ) {
duration = options;
}
if ( typeof options === "string" ) {
easing = options;
}
// fall back from options to animation in case of partial down settings
easing = easing || options.easing || animate.easing;
duration = duration || options.duration || animate.duration;
if ( !toHide.length ) {
return toShow.animate( showProps, duration, easing, complete );
}
if ( !toShow.length ) {
return toHide.animate( hideProps, duration, easing, complete );
}
total = toShow.show().outerHeight();
toHide.animate( hideProps, {
duration: duration,
easing: easing,
step: function( now, fx ) {
fx.now = Math.round( now );
}
});
toShow
.hide()
.animate( showProps, {
duration: duration,
easing: easing,
complete: complete,
step: function( now, fx ) {
fx.now = Math.round( now );
if ( fx.prop !== "height" ) {
adjust += fx.now;
} else if ( that.options.heightStyle !== "content" ) {
fx.now = Math.round( total - toHide.outerHeight() - adjust );
adjust = 0;
}
}
});
},
_toggleComplete: function( data ) {
var toHide = data.oldPanel;
toHide
.removeClass( "ui-accordion-content-active" )
.prev()
.removeClass( "ui-corner-top" )
.addClass( "ui-corner-all" );
// Work around for rendering bug in IE (#5421)
if ( toHide.length ) {
toHide.parent()[0].className = toHide.parent()[0].className;
}
this._trigger( "activate", null, data );
}
});
})( jQuery );
(function( $, undefined ) {
// used to prevent race conditions with remote data sources
var requestIndex = 0;
$.widget( "ui.autocomplete", {
version: "1.10.3",
defaultElement: "<input>",
options: {
appendTo: null,
autoFocus: false,
delay: 300,
minLength: 1,
position: {
my: "left top",
at: "left bottom",
collision: "none"
},
source: null,
// callbacks
change: null,
close: null,
focus: null,
open: null,
response: null,
search: null,
select: null
},
pending: 0,
_create: function() {
// Some browsers only repeat keydown events, not keypress events,
// so we use the suppressKeyPress flag to determine if we've already
// handled the keydown event. #7269
// Unfortunately the code for & in keypress is the same as the up arrow,
// so we use the suppressKeyPressRepeat flag to avoid handling keypress
// events when we know the keydown event was used to modify the
// search term. #7799
var suppressKeyPress, suppressKeyPressRepeat, suppressInput,
nodeName = this.element[0].nodeName.toLowerCase(),
isTextarea = nodeName === "textarea",
isInput = nodeName === "input";
this.isMultiLine =
// Textareas are always multi-line
isTextarea ? true :
// Inputs are always single-line, even if inside a contentEditable element
// IE also treats inputs as contentEditable
isInput ? false :
// All other element types are determined by whether or not they're contentEditable
this.element.prop( "isContentEditable" );
this.valueMethod = this.element[ isTextarea || isInput ? "val" : "text" ];
this.isNewMenu = true;
this.element
.addClass( "ui-autocomplete-input" )
.attr( "autocomplete", "off" );
this._on( this.element, {
keydown: function( event ) {
/*jshint maxcomplexity:15*/
if ( this.element.prop( "readOnly" ) ) {
suppressKeyPress = true;
suppressInput = true;
suppressKeyPressRepeat = true;
return;
}
suppressKeyPress = false;
suppressInput = false;
suppressKeyPressRepeat = false;
var keyCode = $.ui.keyCode;
switch( event.keyCode ) {
case keyCode.PAGE_UP:
suppressKeyPress = true;
this._move( "previousPage", event );
break;
case keyCode.PAGE_DOWN:
suppressKeyPress = true;
this._move( "nextPage", event );
break;
case keyCode.UP:
suppressKeyPress = true;
this._keyEvent( "previous", event );
break;
case keyCode.DOWN:
suppressKeyPress = true;
this._keyEvent( "next", event );
break;
case keyCode.ENTER:
case keyCode.NUMPAD_ENTER:
// when menu is open and has focus
if ( this.menu.active ) {
// #6055 - Opera still allows the keypress to occur
// which causes forms to submit
suppressKeyPress = true;
event.preventDefault();
this.menu.select( event );
}
break;
case keyCode.TAB:
if ( this.menu.active ) {
this.menu.select( event );
}
break;
case keyCode.ESCAPE:
if ( this.menu.element.is( ":visible" ) ) {
this._value( this.term );
this.close( event );
// Different browsers have different default behavior for escape
// Single press can mean undo or clear
// Double press in IE means clear the whole form
event.preventDefault();
}
break;
default:
suppressKeyPressRepeat = true;
// search timeout should be triggered before the input value is changed
this._searchTimeout( event );
break;
}
},
keypress: function( event ) {
if ( suppressKeyPress ) {
suppressKeyPress = false;
if ( !this.isMultiLine || this.menu.element.is( ":visible" ) ) {
event.preventDefault();
}
return;
}
if ( suppressKeyPressRepeat ) {
return;
}
// replicate some key handlers to allow them to repeat in Firefox and Opera
var keyCode = $.ui.keyCode;
switch( event.keyCode ) {
case keyCode.PAGE_UP:
this._move( "previousPage", event );
break;
case keyCode.PAGE_DOWN:
this._move( "nextPage", event );
break;
case keyCode.UP:
this._keyEvent( "previous", event );
break;
case keyCode.DOWN:
this._keyEvent( "next", event );
break;
}
},
input: function( event ) {
if ( suppressInput ) {
suppressInput = false;
event.preventDefault();
return;
}
this._searchTimeout( event );
},
focus: function() {
this.selectedItem = null;
this.previous = this._value();
},
blur: function( event ) {
if ( this.cancelBlur ) {
delete this.cancelBlur;
return;
}
clearTimeout( this.searching );
this.close( event );
this._change( event );
}
});
this._initSource();
this.menu = $( "<ul>" )
.addClass( "ui-autocomplete ui-front" )
.appendTo( this._appendTo() )
.menu({
// disable ARIA support, the live region takes care of that
role: null
})
.hide()
.data( "ui-menu" );
this._on( this.menu.element, {
mousedown: function( event ) {
// prevent moving focus out of the text field
event.preventDefault();
// IE doesn't prevent moving focus even with event.preventDefault()
// so we set a flag to know when we should ignore the blur event
this.cancelBlur = true;
this._delay(function() {
delete this.cancelBlur;
});
// clicking on the scrollbar causes focus to shift to the body
// but we can't detect a mouseup or a click immediately afterward
// so we have to track the next mousedown and close the menu if
// the user clicks somewhere outside of the autocomplete
var menuElement = this.menu.element[ 0 ];
if ( !$( event.target ).closest( ".ui-menu-item" ).length ) {
this._delay(function() {
var that = this;
this.document.one( "mousedown", function( event ) {
if ( event.target !== that.element[ 0 ] &&
event.target !== menuElement &&
!$.contains( menuElement, event.target ) ) {
that.close();
}
});
});
}
},
menufocus: function( event, ui ) {
// support: Firefox
// Prevent accidental activation of menu items in Firefox (#7024 #9118)
if ( this.isNewMenu ) {
this.isNewMenu = false;
if ( event.originalEvent && /^mouse/.test( event.originalEvent.type ) ) {
this.menu.blur();
this.document.one( "mousemove", function() {
$( event.target ).trigger( event.originalEvent );
});
return;
}
}
var item = ui.item.data( "ui-autocomplete-item" );
if ( false !== this._trigger( "focus", event, { item: item } ) ) {
// use value to match what will end up in the input, if it was a key event
if ( event.originalEvent && /^key/.test( event.originalEvent.type ) ) {
this._value( item.value );
}
} else {
// Normally the input is populated with the item's value as the
// menu is navigated, causing screen readers to notice a change and
// announce the item. Since the focus event was canceled, this doesn't
// happen, so we update the live region so that screen readers can
// still notice the change and announce it.
this.liveRegion.text( item.value );
}
},
menuselect: function( event, ui ) {
var item = ui.item.data( "ui-autocomplete-item" ),
previous = this.previous;
// only trigger when focus was lost (click on menu)
if ( this.element[0] !== this.document[0].activeElement ) {
this.element.focus();
this.previous = previous;
// #6109 - IE triggers two focus events and the second
// is asynchronous, so we need to reset the previous
// term synchronously and asynchronously :-(
this._delay(function() {
this.previous = previous;
this.selectedItem = item;
});
}
if ( false !== this._trigger( "select", event, { item: item } ) ) {
this._value( item.value );
}
// reset the term after the select event
// this allows custom select handling to work properly
this.term = this._value();
this.close( event );
this.selectedItem = item;
}
});
this.liveRegion = $( "<span>", {
role: "status",
"aria-live": "polite"
})
.addClass( "ui-helper-hidden-accessible" )
.insertBefore( this.element );
// turning off autocomplete prevents the browser from remembering the
// value when navigating through history, so we re-enable autocomplete
// if the page is unloaded before the widget is destroyed. #7790
this._on( this.window, {
beforeunload: function() {
this.element.removeAttr( "autocomplete" );
}
});
},
_destroy: function() {
clearTimeout( this.searching );
this.element
.removeClass( "ui-autocomplete-input" )
.removeAttr( "autocomplete" );
this.menu.element.remove();
this.liveRegion.remove();
},
_setOption: function( key, value ) {
this._super( key, value );
if ( key === "source" ) {
this._initSource();
}
if ( key === "appendTo" ) {
this.menu.element.appendTo( this._appendTo() );
}
if ( key === "disabled" && value && this.xhr ) {
this.xhr.abort();
}
},
_appendTo: function() {
var element = this.options.appendTo;
if ( element ) {
element = element.jquery || element.nodeType ?
$( element ) :
this.document.find( element ).eq( 0 );
}
if ( !element ) {
element = this.element.closest( ".ui-front" );
}
if ( !element.length ) {
element = this.document[0].body;
}
return element;
},
_initSource: function() {
var array, url,
that = this;
if ( $.isArray(this.options.source) ) {
array = this.options.source;
this.source = function( request, response ) {
response( $.ui.autocomplete.filter( array, request.term ) );
};
} else if ( typeof this.options.source === "string" ) {
url = this.options.source;
this.source = function( request, response ) {
if ( that.xhr ) {
that.xhr.abort();
}
that.xhr = $.ajax({
url: url,
data: request,
dataType: "json",
success: function( data ) {
response( data );
},
error: function() {
response( [] );
}
});
};
} else {
this.source = this.options.source;
}
},
_searchTimeout: function( event ) {
clearTimeout( this.searching );
this.searching = this._delay(function() {
// only search if the value has changed
if ( this.term !== this._value() ) {
this.selectedItem = null;
this.search( null, event );
}
}, this.options.delay );
},
search: function( value, event ) {
value = value != null ? value : this._value();
// always save the actual value, not the one passed as an argument
this.term = this._value();
if ( value.length < this.options.minLength ) {
return this.close( event );
}
if ( this._trigger( "search", event ) === false ) {
return;
}
return this._search( value );
},
_search: function( value ) {
this.pending++;
this.element.addClass( "ui-autocomplete-loading" );
this.cancelSearch = false;
this.source( { term: value }, this._response() );
},
_response: function() {
var that = this,
index = ++requestIndex;
return function( content ) {
if ( index === requestIndex ) {
that.__response( content );
}
that.pending--;
if ( !that.pending ) {
that.element.removeClass( "ui-autocomplete-loading" );
}
};
},
__response: function( content ) {
if ( content ) {
content = this._normalize( content );
}
this._trigger( "response", null, { content: content } );
if ( !this.options.disabled && content && content.length && !this.cancelSearch ) {
this._suggest( content );
this._trigger( "open" );
} else {
// use ._close() instead of .close() so we don't cancel future searches
this._close();
}
},
close: function( event ) {
this.cancelSearch = true;
this._close( event );
},
_close: function( event ) {
if ( this.menu.element.is( ":visible" ) ) {
this.menu.element.hide();
this.menu.blur();
this.isNewMenu = true;
this._trigger( "close", event );
}
},
_change: function( event ) {
if ( this.previous !== this._value() ) {
this._trigger( "change", event, { item: this.selectedItem } );
}
},
_normalize: function( items ) {
// assume all items have the right format when the first item is complete
if ( items.length && items[0].label && items[0].value ) {
return items;
}
return $.map( items, function( item ) {
if ( typeof item === "string" ) {
return {
label: item,
value: item
};
}
return $.extend({
label: item.label || item.value,
value: item.value || item.label
}, item );
});
},
_suggest: function( items ) {
var ul = this.menu.element.empty();
this._renderMenu( ul, items );
this.isNewMenu = true;
this.menu.refresh();
// size and position menu
ul.show();
this._resizeMenu();
ul.position( $.extend({
of: this.element
}, this.options.position ));
if ( this.options.autoFocus ) {
this.menu.next();
}
},
_resizeMenu: function() {
var ul = this.menu.element;
ul.outerWidth( Math.max(
// Firefox wraps long text (possibly a rounding bug)
// so we add 1px to avoid the wrapping (#7513)
ul.width( "" ).outerWidth() + 1,
this.element.outerWidth()
) );
},
_renderMenu: function( ul, items ) {
var that = this;
$.each( items, function( index, item ) {
that._renderItemData( ul, item );
});
},
_renderItemData: function( ul, item ) {
return this._renderItem( ul, item ).data( "ui-autocomplete-item", item );
},
_renderItem: function( ul, item ) {
return $( "<li>" )
.append( $( "<a>" ).text( item.label ) )
.appendTo( ul );
},
_move: function( direction, event ) {
if ( !this.menu.element.is( ":visible" ) ) {
this.search( null, event );
return;
}
if ( this.menu.isFirstItem() && /^previous/.test( direction ) ||
this.menu.isLastItem() && /^next/.test( direction ) ) {
this._value( this.term );
this.menu.blur();
return;
}
this.menu[ direction ]( event );
},
widget: function() {
return this.menu.element;
},
_value: function() {
return this.valueMethod.apply( this.element, arguments );
},
_keyEvent: function( keyEvent, event ) {
if ( !this.isMultiLine || this.menu.element.is( ":visible" ) ) {
this._move( keyEvent, event );
// prevents moving cursor to beginning/end of the text field in some browsers
event.preventDefault();
}
}
});
$.extend( $.ui.autocomplete, {
escapeRegex: function( value ) {
return value.replace(/[\-\[\]{}()*+?.,\\\^$|#\s]/g, "\\$&");
},
filter: function(array, term) {
var matcher = new RegExp( $.ui.autocomplete.escapeRegex(term), "i" );
return $.grep( array, function(value) {
return matcher.test( value.label || value.value || value );
});
}
});
// live region extension, adding a `messages` option
// NOTE: This is an experimental API. We are still investigating
// a full solution for string manipulation and internationalization.
$.widget( "ui.autocomplete", $.ui.autocomplete, {
options: {
messages: {
noResults: "No search results.",
results: function( amount ) {
return amount + ( amount > 1 ? " results are" : " result is" ) +
" available, use up and down arrow keys to navigate.";
}
}
},
__response: function( content ) {
var message;
this._superApply( arguments );
if ( this.options.disabled || this.cancelSearch ) {
return;
}
if ( content && content.length ) {
message = this.options.messages.results( content.length );
} else {
message = this.options.messages.noResults;
}
this.liveRegion.text( message );
}
});
}( jQuery ));
(function( $, undefined ) {
var lastActive, startXPos, startYPos, clickDragged,
baseClasses = "ui-button ui-widget ui-state-default ui-corner-all",
stateClasses = "ui-state-hover ui-state-active ",
typeClasses = "ui-button-icons-only ui-button-icon-only ui-button-text-icons ui-button-text-icon-primary ui-button-text-icon-secondary ui-button-text-only",
formResetHandler = function() {
var form = $( this );
setTimeout(function() {
form.find( ":ui-button" ).button( "refresh" );
}, 1 );
},
radioGroup = function( radio ) {
var name = radio.name,
form = radio.form,
radios = $( [] );
if ( name ) {
name = name.replace( /'/g, "\\'" );
if ( form ) {
radios = $( form ).find( "[name='" + name + "']" );
} else {
radios = $( "[name='" + name + "']", radio.ownerDocument )
.filter(function() {
return !this.form;
});
}
}
return radios;
};
$.widget( "ui.button", {
version: "1.10.3",
defaultElement: "<button>",
options: {
disabled: null,
text: true,
label: null,
icons: {
primary: null,
secondary: null
}
},
_create: function() {
this.element.closest( "form" )
.unbind( "reset" + this.eventNamespace )
.bind( "reset" + this.eventNamespace, formResetHandler );
if ( typeof this.options.disabled !== "boolean" ) {
this.options.disabled = !!this.element.prop( "disabled" );
} else {
this.element.prop( "disabled", this.options.disabled );
}
this._determineButtonType();
this.hasTitle = !!this.buttonElement.attr( "title" );
var that = this,
options = this.options,
toggleButton = this.type === "checkbox" || this.type === "radio",
activeClass = !toggleButton ? "ui-state-active" : "",
focusClass = "ui-state-focus";
if ( options.label === null ) {
options.label = (this.type === "input" ? this.buttonElement.val() : this.buttonElement.html());
}
this._hoverable( this.buttonElement );
this.buttonElement
.addClass( baseClasses )
.attr( "role", "button" )
.bind( "mouseenter" + this.eventNamespace, function() {
if ( options.disabled ) {
return;
}
if ( this === lastActive ) {
$( this ).addClass( "ui-state-active" );
}
})
.bind( "mouseleave" + this.eventNamespace, function() {
if ( options.disabled ) {
return;
}
$( this ).removeClass( activeClass );
})
.bind( "click" + this.eventNamespace, function( event ) {
if ( options.disabled ) {
event.preventDefault();
event.stopImmediatePropagation();
}
});
this.element
.bind( "focus" + this.eventNamespace, function() {
// no need to check disabled, focus won't be triggered anyway
that.buttonElement.addClass( focusClass );
})
.bind( "blur" + this.eventNamespace, function() {
that.buttonElement.removeClass( focusClass );
});
if ( toggleButton ) {
this.element.bind( "change" + this.eventNamespace, function() {
if ( clickDragged ) {
return;
}
that.refresh();
});
// if mouse moves between mousedown and mouseup (drag) set clickDragged flag
// prevents issue where button state changes but checkbox/radio checked state
// does not in Firefox (see ticket #6970)
this.buttonElement
.bind( "mousedown" + this.eventNamespace, function( event ) {
if ( options.disabled ) {
return;
}
clickDragged = false;
startXPos = event.pageX;
startYPos = event.pageY;
})
.bind( "mouseup" + this.eventNamespace, function( event ) {
if ( options.disabled ) {
return;
}
if ( startXPos !== event.pageX || startYPos !== event.pageY ) {
clickDragged = true;
}
});
}
if ( this.type === "checkbox" ) {
this.buttonElement.bind( "click" + this.eventNamespace, function() {
if ( options.disabled || clickDragged ) {
return false;
}
});
} else if ( this.type === "radio" ) {
this.buttonElement.bind( "click" + this.eventNamespace, function() {
if ( options.disabled || clickDragged ) {
return false;
}
$( this ).addClass( "ui-state-active" );
that.buttonElement.attr( "aria-pressed", "true" );
var radio = that.element[ 0 ];
radioGroup( radio )
.not( radio )
.map(function() {
return $( this ).button( "widget" )[ 0 ];
})
.removeClass( "ui-state-active" )
.attr( "aria-pressed", "false" );
});
} else {
this.buttonElement
.bind( "mousedown" + this.eventNamespace, function() {
if ( options.disabled ) {
return false;
}
$( this ).addClass( "ui-state-active" );
lastActive = this;
that.document.one( "mouseup", function() {
lastActive = null;
});
})
.bind( "mouseup" + this.eventNamespace, function() {
if ( options.disabled ) {
return false;
}
$( this ).removeClass( "ui-state-active" );
})
.bind( "keydown" + this.eventNamespace, function(event) {
if ( options.disabled ) {
return false;
}
if ( event.keyCode === $.ui.keyCode.SPACE || event.keyCode === $.ui.keyCode.ENTER ) {
$( this ).addClass( "ui-state-active" );
}
})
// see #8559, we bind to blur here in case the button element loses
// focus between keydown and keyup, it would be left in an "active" state
.bind( "keyup" + this.eventNamespace + " blur" + this.eventNamespace, function() {
$( this ).removeClass( "ui-state-active" );
});
if ( this.buttonElement.is("a") ) {
this.buttonElement.keyup(function(event) {
if ( event.keyCode === $.ui.keyCode.SPACE ) {
// TODO pass through original event correctly (just as 2nd argument doesn't work)
$( this ).click();
}
});
}
}
// TODO: pull out $.Widget's handling for the disabled option into
// $.Widget.prototype._setOptionDisabled so it's easy to proxy and can
// be overridden by individual plugins
this._setOption( "disabled", options.disabled );
this._resetButton();
},
_determineButtonType: function() {
var ancestor, labelSelector, checked;
if ( this.element.is("[type=checkbox]") ) {
this.type = "checkbox";
} else if ( this.element.is("[type=radio]") ) {
this.type = "radio";
} else if ( this.element.is("input") ) {
this.type = "input";
} else {
this.type = "button";
}
if ( this.type === "checkbox" || this.type === "radio" ) {
// we don't search against the document in case the element
// is disconnected from the DOM
ancestor = this.element.parents().last();
labelSelector = "label[for='" + this.element.attr("id") + "']";
this.buttonElement = ancestor.find( labelSelector );
if ( !this.buttonElement.length ) {
ancestor = ancestor.length ? ancestor.siblings() : this.element.siblings();
this.buttonElement = ancestor.filter( labelSelector );
if ( !this.buttonElement.length ) {
this.buttonElement = ancestor.find( labelSelector );
}
}
this.element.addClass( "ui-helper-hidden-accessible" );
checked = this.element.is( ":checked" );
if ( checked ) {
this.buttonElement.addClass( "ui-state-active" );
}
this.buttonElement.prop( "aria-pressed", checked );
} else {
this.buttonElement = this.element;
}
},
widget: function() {
return this.buttonElement;
},
_destroy: function() {
this.element
.removeClass( "ui-helper-hidden-accessible" );
this.buttonElement
.removeClass( baseClasses + " " + stateClasses + " " + typeClasses )
.removeAttr( "role" )
.removeAttr( "aria-pressed" )
.html( this.buttonElement.find(".ui-button-text").html() );
if ( !this.hasTitle ) {
this.buttonElement.removeAttr( "title" );
}
},
_setOption: function( key, value ) {
this._super( key, value );
if ( key === "disabled" ) {
if ( value ) {
this.element.prop( "disabled", true );
} else {
this.element.prop( "disabled", false );
}
return;
}
this._resetButton();
},
refresh: function() {
//See #8237 & #8828
var isDisabled = this.element.is( "input, button" ) ? this.element.is( ":disabled" ) : this.element.hasClass( "ui-button-disabled" );
if ( isDisabled !== this.options.disabled ) {
this._setOption( "disabled", isDisabled );
}
if ( this.type === "radio" ) {
radioGroup( this.element[0] ).each(function() {
if ( $( this ).is( ":checked" ) ) {
$( this ).button( "widget" )
.addClass( "ui-state-active" )
.attr( "aria-pressed", "true" );
} else {
$( this ).button( "widget" )
.removeClass( "ui-state-active" )
.attr( "aria-pressed", "false" );
}
});
} else if ( this.type === "checkbox" ) {
if ( this.element.is( ":checked" ) ) {
this.buttonElement
.addClass( "ui-state-active" )
.attr( "aria-pressed", "true" );
} else {
this.buttonElement
.removeClass( "ui-state-active" )
.attr( "aria-pressed", "false" );
}
}
},
_resetButton: function() {
if ( this.type === "input" ) {
if ( this.options.label ) {
this.element.val( this.options.label );
}
return;
}
var buttonElement = this.buttonElement.removeClass( typeClasses ),
buttonText = $( "<span></span>", this.document[0] )
.addClass( "ui-button-text" )
.html( this.options.label )
.appendTo( buttonElement.empty() )
.text(),
icons = this.options.icons,
multipleIcons = icons.primary && icons.secondary,
buttonClasses = [];
if ( icons.primary || icons.secondary ) {
if ( this.options.text ) {
buttonClasses.push( "ui-button-text-icon" + ( multipleIcons ? "s" : ( icons.primary ? "-primary" : "-secondary" ) ) );
}
if ( icons.primary ) {
buttonElement.prepend( "<span class='ui-button-icon-primary ui-icon " + icons.primary + "'></span>" );
}
if ( icons.secondary ) {
buttonElement.append( "<span class='ui-button-icon-secondary ui-icon " + icons.secondary + "'></span>" );
}
if ( !this.options.text ) {
buttonClasses.push( multipleIcons ? "ui-button-icons-only" : "ui-button-icon-only" );
if ( !this.hasTitle ) {
buttonElement.attr( "title", $.trim( buttonText ) );
}
}
} else {
buttonClasses.push( "ui-button-text-only" );
}
buttonElement.addClass( buttonClasses.join( " " ) );
}
});
$.widget( "ui.buttonset", {
version: "1.10.3",
options: {
items: "button, input[type=button], input[type=submit], input[type=reset], input[type=checkbox], input[type=radio], a, :data(ui-button)"
},
_create: function() {
this.element.addClass( "ui-buttonset" );
},
_init: function() {
this.refresh();
},
_setOption: function( key, value ) {
if ( key === "disabled" ) {
this.buttons.button( "option", key, value );
}
this._super( key, value );
},
refresh: function() {
var rtl = this.element.css( "direction" ) === "rtl";
this.buttons = this.element.find( this.options.items )
.filter( ":ui-button" )
.button( "refresh" )
.end()
.not( ":ui-button" )
.button()
.end()
.map(function() {
return $( this ).button( "widget" )[ 0 ];
})
.removeClass( "ui-corner-all ui-corner-left ui-corner-right" )
.filter( ":first" )
.addClass( rtl ? "ui-corner-right" : "ui-corner-left" )
.end()
.filter( ":last" )
.addClass( rtl ? "ui-corner-left" : "ui-corner-right" )
.end()
.end();
},
_destroy: function() {
this.element.removeClass( "ui-buttonset" );
this.buttons
.map(function() {
return $( this ).button( "widget" )[ 0 ];
})
.removeClass( "ui-corner-left ui-corner-right" )
.end()
.button( "destroy" );
}
});
}( jQuery ) );
(function( $, undefined ) {
$.extend($.ui, { datepicker: { version: "1.10.3" } });
var PROP_NAME = "datepicker",
instActive;
/* Date picker manager.
Use the singleton instance of this class, $.datepicker, to interact with the date picker.
Settings for (groups of) date pickers are maintained in an instance object,
allowing multiple different settings on the same page. */
function Datepicker() {
this._curInst = null; // The current instance in use
this._keyEvent = false; // If the last event was a key event
this._disabledInputs = []; // List of date picker inputs that have been disabled
this._datepickerShowing = false; // True if the popup picker is showing , false if not
this._inDialog = false; // True if showing within a "dialog", false if not
this._mainDivId = "ui-datepicker-div"; // The ID of the main datepicker division
this._inlineClass = "ui-datepicker-inline"; // The name of the inline marker class
this._appendClass = "ui-datepicker-append"; // The name of the append marker class
this._triggerClass = "ui-datepicker-trigger"; // The name of the trigger marker class
this._dialogClass = "ui-datepicker-dialog"; // The name of the dialog marker class
this._disableClass = "ui-datepicker-disabled"; // The name of the disabled covering marker class
this._unselectableClass = "ui-datepicker-unselectable"; // The name of the unselectable cell marker class
this._currentClass = "ui-datepicker-current-day"; // The name of the current day marker class
this._dayOverClass = "ui-datepicker-days-cell-over"; // The name of the day hover marker class
this.regional = []; // Available regional settings, indexed by language code
this.regional[""] = { // Default regional settings
closeText: "Done", // Display text for close link
prevText: "Prev", // Display text for previous month link
nextText: "Next", // Display text for next month link
currentText: "Today", // Display text for current month link
monthNames: ["January","February","March","April","May","June",
"July","August","September","October","November","December"], // Names of months for drop-down and formatting
monthNamesShort: ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"], // For formatting
dayNames: ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"], // For formatting
dayNamesShort: ["Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"], // For formatting
dayNamesMin: ["Su","Mo","Tu","We","Th","Fr","Sa"], // Column headings for days starting at Sunday
weekHeader: "Wk", // Column header for week of the year
dateFormat: "mm/dd/yy", // See format options on parseDate
firstDay: 0, // The first day of the week, Sun = 0, Mon = 1, ...
isRTL: false, // True if right-to-left language, false if left-to-right
showMonthAfterYear: false, // True if the year select precedes month, false for month then year
yearSuffix: "" // Additional text to append to the year in the month headers
};
this._defaults = { // Global defaults for all the date picker instances
showOn: "focus", // "focus" for popup on focus,
// "button" for trigger button, or "both" for either
showAnim: "fadeIn", // Name of jQuery animation for popup
showOptions: {}, // Options for enhanced animations
defaultDate: null, // Used when field is blank: actual date,
// +/-number for offset from today, null for today
appendText: "", // Display text following the input box, e.g. showing the format
buttonText: "...", // Text for trigger button
buttonImage: "", // URL for trigger button image
buttonImageOnly: false, // True if the image appears alone, false if it appears on a button
hideIfNoPrevNext: false, // True to hide next/previous month links
// if not applicable, false to just disable them
navigationAsDateFormat: false, // True if date formatting applied to prev/today/next links
gotoCurrent: false, // True if today link goes back to current selection instead
changeMonth: false, // True if month can be selected directly, false if only prev/next
changeYear: false, // True if year can be selected directly, false if only prev/next
yearRange: "c-10:c+10", // Range of years to display in drop-down,
// either relative to today's year (-nn:+nn), relative to currently displayed year
// (c-nn:c+nn), absolute (nnnn:nnnn), or a combination of the above (nnnn:-n)
showOtherMonths: false, // True to show dates in other months, false to leave blank
selectOtherMonths: false, // True to allow selection of dates in other months, false for unselectable
showWeek: false, // True to show week of the year, false to not show it
calculateWeek: this.iso8601Week, // How to calculate the week of the year,
// takes a Date and returns the number of the week for it
shortYearCutoff: "+10", // Short year values < this are in the current century,
// > this are in the previous century,
// string value starting with "+" for current year + value
minDate: null, // The earliest selectable date, or null for no limit
maxDate: null, // The latest selectable date, or null for no limit
duration: "fast", // Duration of display/closure
beforeShowDay: null, // Function that takes a date and returns an array with
// [0] = true if selectable, false if not, [1] = custom CSS class name(s) or "",
// [2] = cell title (optional), e.g. $.datepicker.noWeekends
beforeShow: null, // Function that takes an input field and
// returns a set of custom settings for the date picker
onSelect: null, // Define a callback function when a date is selected
onChangeMonthYear: null, // Define a callback function when the month or year is changed
onClose: null, // Define a callback function when the datepicker is closed
numberOfMonths: 1, // Number of months to show at a time
showCurrentAtPos: 0, // The position in multipe months at which to show the current month (starting at 0)
stepMonths: 1, // Number of months to step back/forward
stepBigMonths: 12, // Number of months to step back/forward for the big links
altField: "", // Selector for an alternate field to store selected dates into
altFormat: "", // The date format to use for the alternate field
constrainInput: true, // The input is constrained by the current date format
showButtonPanel: false, // True to show button panel, false to not show it
autoSize: false, // True to size the input for the date format, false to leave as is
disabled: false // The initial disabled state
};
$.extend(this._defaults, this.regional[""]);
this.dpDiv = bindHover($("<div id='" + this._mainDivId + "' class='ui-datepicker ui-widget ui-widget-content ui-helper-clearfix ui-corner-all'></div>"));
}
$.extend(Datepicker.prototype, {
/* Class name added to elements to indicate already configured with a date picker. */
markerClassName: "hasDatepicker",
//Keep track of the maximum number of rows displayed (see #7043)
maxRows: 4,
// TODO rename to "widget" when switching to widget factory
_widgetDatepicker: function() {
return this.dpDiv;
},
/* Override the default settings for all instances of the date picker.
* @param settings object - the new settings to use as defaults (anonymous object)
* @return the manager object
*/
setDefaults: function(settings) {
extendRemove(this._defaults, settings || {});
return this;
},
/* Attach the date picker to a jQuery selection.
* @param target element - the target input field or division or span
* @param settings object - the new settings to use for this date picker instance (anonymous)
*/
_attachDatepicker: function(target, settings) {
var nodeName, inline, inst;
nodeName = target.nodeName.toLowerCase();
inline = (nodeName === "div" || nodeName === "span");
if (!target.id) {
this.uuid += 1;
target.id = "dp" + this.uuid;
}
inst = this._newInst($(target), inline);
inst.settings = $.extend({}, settings || {});
if (nodeName === "input") {
this._connectDatepicker(target, inst);
} else if (inline) {
this._inlineDatepicker(target, inst);
}
},
/* Create a new instance object. */
_newInst: function(target, inline) {
var id = target[0].id.replace(/([^A-Za-z0-9_\-])/g, "\\\\$1"); // escape jQuery meta chars
return {id: id, input: target, // associated target
selectedDay: 0, selectedMonth: 0, selectedYear: 0, // current selection
drawMonth: 0, drawYear: 0, // month being drawn
inline: inline, // is datepicker inline or not
dpDiv: (!inline ? this.dpDiv : // presentation div
bindHover($("<div class='" + this._inlineClass + " ui-datepicker ui-widget ui-widget-content ui-helper-clearfix ui-corner-all'></div>")))};
},
/* Attach the date picker to an input field. */
_connectDatepicker: function(target, inst) {
var input = $(target);
inst.append = $([]);
inst.trigger = $([]);
if (input.hasClass(this.markerClassName)) {
return;
}
this._attachments(input, inst);
input.addClass(this.markerClassName).keydown(this._doKeyDown).
keypress(this._doKeyPress).keyup(this._doKeyUp);
this._autoSize(inst);
$.data(target, PROP_NAME, inst);
//If disabled option is true, disable the datepicker once it has been attached to the input (see ticket #5665)
if( inst.settings.disabled ) {
this._disableDatepicker( target );
}
},
/* Make attachments based on settings. */
_attachments: function(input, inst) {
var showOn, buttonText, buttonImage,
appendText = this._get(inst, "appendText"),
isRTL = this._get(inst, "isRTL");
if (inst.append) {
inst.append.remove();
}
if (appendText) {
inst.append = $("<span class='" + this._appendClass + "'>" + appendText + "</span>");
input[isRTL ? "before" : "after"](inst.append);
}
input.unbind("focus", this._showDatepicker);
if (inst.trigger) {
inst.trigger.remove();
}
showOn = this._get(inst, "showOn");
if (showOn === "focus" || showOn === "both") { // pop-up date picker when in the marked field
input.focus(this._showDatepicker);
}
if (showOn === "button" || showOn === "both") { // pop-up date picker when button clicked
buttonText = this._get(inst, "buttonText");
buttonImage = this._get(inst, "buttonImage");
inst.trigger = $(this._get(inst, "buttonImageOnly") ?
$("<img/>").addClass(this._triggerClass).
attr({ src: buttonImage, alt: buttonText, title: buttonText }) :
$("<button type='button'></button>").addClass(this._triggerClass).
html(!buttonImage ? buttonText : $("<img/>").attr(
{ src:buttonImage, alt:buttonText, title:buttonText })));
input[isRTL ? "before" : "after"](inst.trigger);
inst.trigger.click(function() {
if ($.datepicker._datepickerShowing && $.datepicker._lastInput === input[0]) {
$.datepicker._hideDatepicker();
} else if ($.datepicker._datepickerShowing && $.datepicker._lastInput !== input[0]) {
$.datepicker._hideDatepicker();
$.datepicker._showDatepicker(input[0]);
} else {
$.datepicker._showDatepicker(input[0]);
}
return false;
});
}
},
/* Apply the maximum length for the date format. */
_autoSize: function(inst) {
if (this._get(inst, "autoSize") && !inst.inline) {
var findMax, max, maxI, i,
date = new Date(2009, 12 - 1, 20), // Ensure double digits
dateFormat = this._get(inst, "dateFormat");
if (dateFormat.match(/[DM]/)) {
findMax = function(names) {
max = 0;
maxI = 0;
for (i = 0; i < names.length; i++) {
if (names[i].length > max) {
max = names[i].length;
maxI = i;
}
}
return maxI;
};
date.setMonth(findMax(this._get(inst, (dateFormat.match(/MM/) ?
"monthNames" : "monthNamesShort"))));
date.setDate(findMax(this._get(inst, (dateFormat.match(/DD/) ?
"dayNames" : "dayNamesShort"))) + 20 - date.getDay());
}
inst.input.attr("size", this._formatDate(inst, date).length);
}
},
/* Attach an inline date picker to a div. */
_inlineDatepicker: function(target, inst) {
var divSpan = $(target);
if (divSpan.hasClass(this.markerClassName)) {
return;
}
divSpan.addClass(this.markerClassName).append(inst.dpDiv);
$.data(target, PROP_NAME, inst);
this._setDate(inst, this._getDefaultDate(inst), true);
this._updateDatepicker(inst);
this._updateAlternate(inst);
//If disabled option is true, disable the datepicker before showing it (see ticket #5665)
if( inst.settings.disabled ) {
this._disableDatepicker( target );
}
// Set display:block in place of inst.dpDiv.show() which won't work on disconnected elements
// http://bugs.jqueryui.com/ticket/7552 - A Datepicker created on a detached div has zero height
inst.dpDiv.css( "display", "block" );
},
/* Pop-up the date picker in a "dialog" box.
* @param input element - ignored
* @param date string or Date - the initial date to display
* @param onSelect function - the function to call when a date is selected
* @param settings object - update the dialog date picker instance's settings (anonymous object)
* @param pos int[2] - coordinates for the dialog's position within the screen or
* event - with x/y coordinates or
* leave empty for default (screen centre)
* @return the manager object
*/
_dialogDatepicker: function(input, date, onSelect, settings, pos) {
var id, browserWidth, browserHeight, scrollX, scrollY,
inst = this._dialogInst; // internal instance
if (!inst) {
this.uuid += 1;
id = "dp" + this.uuid;
this._dialogInput = $("<input type='text' id='" + id +
"' style='position: absolute; top: -100px; width: 0px;'/>");
this._dialogInput.keydown(this._doKeyDown);
$("body").append(this._dialogInput);
inst = this._dialogInst = this._newInst(this._dialogInput, false);
inst.settings = {};
$.data(this._dialogInput[0], PROP_NAME, inst);
}
extendRemove(inst.settings, settings || {});
date = (date && date.constructor === Date ? this._formatDate(inst, date) : date);
this._dialogInput.val(date);
this._pos = (pos ? (pos.length ? pos : [pos.pageX, pos.pageY]) : null);
if (!this._pos) {
browserWidth = document.documentElement.clientWidth;
browserHeight = document.documentElement.clientHeight;
scrollX = document.documentElement.scrollLeft || document.body.scrollLeft;
scrollY = document.documentElement.scrollTop || document.body.scrollTop;
this._pos = // should use actual width/height below
[(browserWidth / 2) - 100 + scrollX, (browserHeight / 2) - 150 + scrollY];
}
// move input on screen for focus, but hidden behind dialog
this._dialogInput.css("left", (this._pos[0] + 20) + "px").css("top", this._pos[1] + "px");
inst.settings.onSelect = onSelect;
this._inDialog = true;
this.dpDiv.addClass(this._dialogClass);
this._showDatepicker(this._dialogInput[0]);
if ($.blockUI) {
$.blockUI(this.dpDiv);
}
$.data(this._dialogInput[0], PROP_NAME, inst);
return this;
},
/* Detach a datepicker from its control.
* @param target element - the target input field or division or span
*/
_destroyDatepicker: function(target) {
var nodeName,
$target = $(target),
inst = $.data(target, PROP_NAME);
if (!$target.hasClass(this.markerClassName)) {
return;
}
nodeName = target.nodeName.toLowerCase();
$.removeData(target, PROP_NAME);
if (nodeName === "input") {
inst.append.remove();
inst.trigger.remove();
$target.removeClass(this.markerClassName).
unbind("focus", this._showDatepicker).
unbind("keydown", this._doKeyDown).
unbind("keypress", this._doKeyPress).
unbind("keyup", this._doKeyUp);
} else if (nodeName === "div" || nodeName === "span") {
$target.removeClass(this.markerClassName).empty();
}
},
/* Enable the date picker to a jQuery selection.
* @param target element - the target input field or division or span
*/
_enableDatepicker: function(target) {
var nodeName, inline,
$target = $(target),
inst = $.data(target, PROP_NAME);
if (!$target.hasClass(this.markerClassName)) {
return;
}
nodeName = target.nodeName.toLowerCase();
if (nodeName === "input") {
target.disabled = false;
inst.trigger.filter("button").
each(function() { this.disabled = false; }).end().
filter("img").css({opacity: "1.0", cursor: ""});
} else if (nodeName === "div" || nodeName === "span") {
inline = $target.children("." + this._inlineClass);
inline.children().removeClass("ui-state-disabled");
inline.find("select.ui-datepicker-month, select.ui-datepicker-year").
prop("disabled", false);
}
this._disabledInputs = $.map(this._disabledInputs,
function(value) { return (value === target ? null : value); }); // delete entry
},
/* Disable the date picker to a jQuery selection.
* @param target element - the target input field or division or span
*/
_disableDatepicker: function(target) {
var nodeName, inline,
$target = $(target),
inst = $.data(target, PROP_NAME);
if (!$target.hasClass(this.markerClassName)) {
return;
}
nodeName = target.nodeName.toLowerCase();
if (nodeName === "input") {
target.disabled = true;
inst.trigger.filter("button").
each(function() { this.disabled = true; }).end().
filter("img").css({opacity: "0.5", cursor: "default"});
} else if (nodeName === "div" || nodeName === "span") {
inline = $target.children("." + this._inlineClass);
inline.children().addClass("ui-state-disabled");
inline.find("select.ui-datepicker-month, select.ui-datepicker-year").
prop("disabled", true);
}
this._disabledInputs = $.map(this._disabledInputs,
function(value) { return (value === target ? null : value); }); // delete entry
this._disabledInputs[this._disabledInputs.length] = target;
},
/* Is the first field in a jQuery collection disabled as a datepicker?
* @param target element - the target input field or division or span
* @return boolean - true if disabled, false if enabled
*/
_isDisabledDatepicker: function(target) {
if (!target) {
return false;
}
for (var i = 0; i < this._disabledInputs.length; i++) {
if (this._disabledInputs[i] === target) {
return true;
}
}
return false;
},
/* Retrieve the instance data for the target control.
* @param target element - the target input field or division or span
* @return object - the associated instance data
* @throws error if a jQuery problem getting data
*/
_getInst: function(target) {
try {
return $.data(target, PROP_NAME);
}
catch (err) {
throw "Missing instance data for this datepicker";
}
},
/* Update or retrieve the settings for a date picker attached to an input field or division.
* @param target element - the target input field or division or span
* @param name object - the new settings to update or
* string - the name of the setting to change or retrieve,
* when retrieving also "all" for all instance settings or
* "defaults" for all global defaults
* @param value any - the new value for the setting
* (omit if above is an object or to retrieve a value)
*/
_optionDatepicker: function(target, name, value) {
var settings, date, minDate, maxDate,
inst = this._getInst(target);
if (arguments.length === 2 && typeof name === "string") {
return (name === "defaults" ? $.extend({}, $.datepicker._defaults) :
(inst ? (name === "all" ? $.extend({}, inst.settings) :
this._get(inst, name)) : null));
}
settings = name || {};
if (typeof name === "string") {
settings = {};
settings[name] = value;
}
if (inst) {
if (this._curInst === inst) {
this._hideDatepicker();
}
date = this._getDateDatepicker(target, true);
minDate = this._getMinMaxDate(inst, "min");
maxDate = this._getMinMaxDate(inst, "max");
extendRemove(inst.settings, settings);
// reformat the old minDate/maxDate values if dateFormat changes and a new minDate/maxDate isn't provided
if (minDate !== null && settings.dateFormat !== undefined && settings.minDate === undefined) {
inst.settings.minDate = this._formatDate(inst, minDate);
}
if (maxDate !== null && settings.dateFormat !== undefined && settings.maxDate === undefined) {
inst.settings.maxDate = this._formatDate(inst, maxDate);
}
if ( "disabled" in settings ) {
if ( settings.disabled ) {
this._disableDatepicker(target);
} else {
this._enableDatepicker(target);
}
}
this._attachments($(target), inst);
this._autoSize(inst);
this._setDate(inst, date);
this._updateAlternate(inst);
this._updateDatepicker(inst);
}
},
// change method deprecated
_changeDatepicker: function(target, name, value) {
this._optionDatepicker(target, name, value);
},
/* Redraw the date picker attached to an input field or division.
* @param target element - the target input field or division or span
*/
_refreshDatepicker: function(target) {
var inst = this._getInst(target);
if (inst) {
this._updateDatepicker(inst);
}
},
/* Set the dates for a jQuery selection.
* @param target element - the target input field or division or span
* @param date Date - the new date
*/
_setDateDatepicker: function(target, date) {
var inst = this._getInst(target);
if (inst) {
this._setDate(inst, date);
this._updateDatepicker(inst);
this._updateAlternate(inst);
}
},
/* Get the date(s) for the first entry in a jQuery selection.
* @param target element - the target input field or division or span
* @param noDefault boolean - true if no default date is to be used
* @return Date - the current date
*/
_getDateDatepicker: function(target, noDefault) {
var inst = this._getInst(target);
if (inst && !inst.inline) {
this._setDateFromField(inst, noDefault);
}
return (inst ? this._getDate(inst) : null);
},
/* Handle keystrokes. */
_doKeyDown: function(event) {
var onSelect, dateStr, sel,
inst = $.datepicker._getInst(event.target),
handled = true,
isRTL = inst.dpDiv.is(".ui-datepicker-rtl");
inst._keyEvent = true;
if ($.datepicker._datepickerShowing) {
switch (event.keyCode) {
case 9: $.datepicker._hideDatepicker();
handled = false;
break; // hide on tab out
case 13: sel = $("td." + $.datepicker._dayOverClass + ":not(." +
$.datepicker._currentClass + ")", inst.dpDiv);
if (sel[0]) {
$.datepicker._selectDay(event.target, inst.selectedMonth, inst.selectedYear, sel[0]);
}
onSelect = $.datepicker._get(inst, "onSelect");
if (onSelect) {
dateStr = $.datepicker._formatDate(inst);
// trigger custom callback
onSelect.apply((inst.input ? inst.input[0] : null), [dateStr, inst]);
} else {
$.datepicker._hideDatepicker();
}
return false; // don't submit the form
case 27: $.datepicker._hideDatepicker();
break; // hide on escape
case 33: $.datepicker._adjustDate(event.target, (event.ctrlKey ?
-$.datepicker._get(inst, "stepBigMonths") :
-$.datepicker._get(inst, "stepMonths")), "M");
break; // previous month/year on page up/+ ctrl
case 34: $.datepicker._adjustDate(event.target, (event.ctrlKey ?
+$.datepicker._get(inst, "stepBigMonths") :
+$.datepicker._get(inst, "stepMonths")), "M");
break; // next month/year on page down/+ ctrl
case 35: if (event.ctrlKey || event.metaKey) {
$.datepicker._clearDate(event.target);
}
handled = event.ctrlKey || event.metaKey;
break; // clear on ctrl or command +end
case 36: if (event.ctrlKey || event.metaKey) {
$.datepicker._gotoToday(event.target);
}
handled = event.ctrlKey || event.metaKey;
break; // current on ctrl or command +home
case 37: if (event.ctrlKey || event.metaKey) {
$.datepicker._adjustDate(event.target, (isRTL ? +1 : -1), "D");
}
handled = event.ctrlKey || event.metaKey;
// -1 day on ctrl or command +left
if (event.originalEvent.altKey) {
$.datepicker._adjustDate(event.target, (event.ctrlKey ?
-$.datepicker._get(inst, "stepBigMonths") :
-$.datepicker._get(inst, "stepMonths")), "M");
}
// next month/year on alt +left on Mac
break;
case 38: if (event.ctrlKey || event.metaKey) {
$.datepicker._adjustDate(event.target, -7, "D");
}
handled = event.ctrlKey || event.metaKey;
break; // -1 week on ctrl or command +up
case 39: if (event.ctrlKey || event.metaKey) {
$.datepicker._adjustDate(event.target, (isRTL ? -1 : +1), "D");
}
handled = event.ctrlKey || event.metaKey;
// +1 day on ctrl or command +right
if (event.originalEvent.altKey) {
$.datepicker._adjustDate(event.target, (event.ctrlKey ?
+$.datepicker._get(inst, "stepBigMonths") :
+$.datepicker._get(inst, "stepMonths")), "M");
}
// next month/year on alt +right
break;
case 40: if (event.ctrlKey || event.metaKey) {
$.datepicker._adjustDate(event.target, +7, "D");
}
handled = event.ctrlKey || event.metaKey;
break; // +1 week on ctrl or command +down
default: handled = false;
}
} else if (event.keyCode === 36 && event.ctrlKey) { // display the date picker on ctrl+home
$.datepicker._showDatepicker(this);
} else {
handled = false;
}
if (handled) {
event.preventDefault();
event.stopPropagation();
}
},
/* Filter entered characters - based on date format. */
_doKeyPress: function(event) {
var chars, chr,
inst = $.datepicker._getInst(event.target);
if ($.datepicker._get(inst, "constrainInput")) {
chars = $.datepicker._possibleChars($.datepicker._get(inst, "dateFormat"));
chr = String.fromCharCode(event.charCode == null ? event.keyCode : event.charCode);
return event.ctrlKey || event.metaKey || (chr < " " || !chars || chars.indexOf(chr) > -1);
}
},
/* Synchronise manual entry and field/alternate field. */
_doKeyUp: function(event) {
var date,
inst = $.datepicker._getInst(event.target);
if (inst.input.val() !== inst.lastVal) {
try {
date = $.datepicker.parseDate($.datepicker._get(inst, "dateFormat"),
(inst.input ? inst.input.val() : null),
$.datepicker._getFormatConfig(inst));
if (date) { // only if valid
$.datepicker._setDateFromField(inst);
$.datepicker._updateAlternate(inst);
$.datepicker._updateDatepicker(inst);
}
}
catch (err) {
}
}
return true;
},
/* Pop-up the date picker for a given input field.
* If false returned from beforeShow event handler do not show.
* @param input element - the input field attached to the date picker or
* event - if triggered by focus
*/
_showDatepicker: function(input) {
input = input.target || input;
if (input.nodeName.toLowerCase() !== "input") { // find from button/image trigger
input = $("input", input.parentNode)[0];
}
if ($.datepicker._isDisabledDatepicker(input) || $.datepicker._lastInput === input) { // already here
return;
}
var inst, beforeShow, beforeShowSettings, isFixed,
offset, showAnim, duration;
inst = $.datepicker._getInst(input);
if ($.datepicker._curInst && $.datepicker._curInst !== inst) {
$.datepicker._curInst.dpDiv.stop(true, true);
if ( inst && $.datepicker._datepickerShowing ) {
$.datepicker._hideDatepicker( $.datepicker._curInst.input[0] );
}
}
beforeShow = $.datepicker._get(inst, "beforeShow");
beforeShowSettings = beforeShow ? beforeShow.apply(input, [input, inst]) : {};
if(beforeShowSettings === false){
return;
}
extendRemove(inst.settings, beforeShowSettings);
inst.lastVal = null;
$.datepicker._lastInput = input;
$.datepicker._setDateFromField(inst);
if ($.datepicker._inDialog) { // hide cursor
input.value = "";
}
if (!$.datepicker._pos) { // position below input
$.datepicker._pos = $.datepicker._findPos(input);
$.datepicker._pos[1] += input.offsetHeight; // add the height
}
isFixed = false;
$(input).parents().each(function() {
isFixed |= $(this).css("position") === "fixed";
return !isFixed;
});
offset = {left: $.datepicker._pos[0], top: $.datepicker._pos[1]};
$.datepicker._pos = null;
//to avoid flashes on Firefox
inst.dpDiv.empty();
// determine sizing offscreen
inst.dpDiv.css({position: "absolute", display: "block", top: "-1000px"});
$.datepicker._updateDatepicker(inst);
// fix width for dynamic number of date pickers
// and adjust position before showing
offset = $.datepicker._checkOffset(inst, offset, isFixed);
inst.dpDiv.css({position: ($.datepicker._inDialog && $.blockUI ?
"static" : (isFixed ? "fixed" : "absolute")), display: "none",
left: offset.left + "px", top: offset.top + "px"});
if (!inst.inline) {
showAnim = $.datepicker._get(inst, "showAnim");
duration = $.datepicker._get(inst, "duration");
inst.dpDiv.zIndex($(input).zIndex()+1);
$.datepicker._datepickerShowing = true;
if ( $.effects && $.effects.effect[ showAnim ] ) {
inst.dpDiv.show(showAnim, $.datepicker._get(inst, "showOptions"), duration);
} else {
inst.dpDiv[showAnim || "show"](showAnim ? duration : null);
}
if ( $.datepicker._shouldFocusInput( inst ) ) {
inst.input.focus();
}
$.datepicker._curInst = inst;
}
},
/* Generate the date picker content. */
_updateDatepicker: function(inst) {
this.maxRows = 4; //Reset the max number of rows being displayed (see #7043)
instActive = inst; // for delegate hover events
inst.dpDiv.empty().append(this._generateHTML(inst));
this._attachHandlers(inst);
inst.dpDiv.find("." + this._dayOverClass + " a").mouseover();
var origyearshtml,
numMonths = this._getNumberOfMonths(inst),
cols = numMonths[1],
width = 17;
inst.dpDiv.removeClass("ui-datepicker-multi-2 ui-datepicker-multi-3 ui-datepicker-multi-4").width("");
if (cols > 1) {
inst.dpDiv.addClass("ui-datepicker-multi-" + cols).css("width", (width * cols) + "em");
}
inst.dpDiv[(numMonths[0] !== 1 || numMonths[1] !== 1 ? "add" : "remove") +
"Class"]("ui-datepicker-multi");
inst.dpDiv[(this._get(inst, "isRTL") ? "add" : "remove") +
"Class"]("ui-datepicker-rtl");
if (inst === $.datepicker._curInst && $.datepicker._datepickerShowing && $.datepicker._shouldFocusInput( inst ) ) {
inst.input.focus();
}
// deffered render of the years select (to avoid flashes on Firefox)
if( inst.yearshtml ){
origyearshtml = inst.yearshtml;
setTimeout(function(){
//assure that inst.yearshtml didn't change.
if( origyearshtml === inst.yearshtml && inst.yearshtml ){
inst.dpDiv.find("select.ui-datepicker-year:first").replaceWith(inst.yearshtml);
}
origyearshtml = inst.yearshtml = null;
}, 0);
}
},
// #6694 - don't focus the input if it's already focused
// this breaks the change event in IE
// Support: IE and jQuery <1.9
_shouldFocusInput: function( inst ) {
return inst.input && inst.input.is( ":visible" ) && !inst.input.is( ":disabled" ) && !inst.input.is( ":focus" );
},
/* Check positioning to remain on screen. */
_checkOffset: function(inst, offset, isFixed) {
var dpWidth = inst.dpDiv.outerWidth(),
dpHeight = inst.dpDiv.outerHeight(),
inputWidth = inst.input ? inst.input.outerWidth() : 0,
inputHeight = inst.input ? inst.input.outerHeight() : 0,
viewWidth = document.documentElement.clientWidth + (isFixed ? 0 : $(document).scrollLeft()),
viewHeight = document.documentElement.clientHeight + (isFixed ? 0 : $(document).scrollTop());
offset.left -= (this._get(inst, "isRTL") ? (dpWidth - inputWidth) : 0);
offset.left -= (isFixed && offset.left === inst.input.offset().left) ? $(document).scrollLeft() : 0;
offset.top -= (isFixed && offset.top === (inst.input.offset().top + inputHeight)) ? $(document).scrollTop() : 0;
// now check if datepicker is showing outside window viewport - move to a better place if so.
offset.left -= Math.min(offset.left, (offset.left + dpWidth > viewWidth && viewWidth > dpWidth) ?
Math.abs(offset.left + dpWidth - viewWidth) : 0);
offset.top -= Math.min(offset.top, (offset.top + dpHeight > viewHeight && viewHeight > dpHeight) ?
Math.abs(dpHeight + inputHeight) : 0);
return offset;
},
/* Find an object's position on the screen. */
_findPos: function(obj) {
var position,
inst = this._getInst(obj),
isRTL = this._get(inst, "isRTL");
while (obj && (obj.type === "hidden" || obj.nodeType !== 1 || $.expr.filters.hidden(obj))) {
obj = obj[isRTL ? "previousSibling" : "nextSibling"];
}
position = $(obj).offset();
return [position.left, position.top];
},
/* Hide the date picker from view.
* @param input element - the input field attached to the date picker
*/
_hideDatepicker: function(input) {
var showAnim, duration, postProcess, onClose,
inst = this._curInst;
if (!inst || (input && inst !== $.data(input, PROP_NAME))) {
return;
}
if (this._datepickerShowing) {
showAnim = this._get(inst, "showAnim");
duration = this._get(inst, "duration");
postProcess = function() {
$.datepicker._tidyDialog(inst);
};
// DEPRECATED: after BC for 1.8.x $.effects[ showAnim ] is not needed
if ( $.effects && ( $.effects.effect[ showAnim ] || $.effects[ showAnim ] ) ) {
inst.dpDiv.hide(showAnim, $.datepicker._get(inst, "showOptions"), duration, postProcess);
} else {
inst.dpDiv[(showAnim === "slideDown" ? "slideUp" :
(showAnim === "fadeIn" ? "fadeOut" : "hide"))]((showAnim ? duration : null), postProcess);
}
if (!showAnim) {
postProcess();
}
this._datepickerShowing = false;
onClose = this._get(inst, "onClose");
if (onClose) {
onClose.apply((inst.input ? inst.input[0] : null), [(inst.input ? inst.input.val() : ""), inst]);
}
this._lastInput = null;
if (this._inDialog) {
this._dialogInput.css({ position: "absolute", left: "0", top: "-100px" });
if ($.blockUI) {
$.unblockUI();
$("body").append(this.dpDiv);
}
}
this._inDialog = false;
}
},
/* Tidy up after a dialog display. */
_tidyDialog: function(inst) {
inst.dpDiv.removeClass(this._dialogClass).unbind(".ui-datepicker-calendar");
},
/* Close date picker if clicked elsewhere. */
_checkExternalClick: function(event) {
if (!$.datepicker._curInst) {
return;
}
var $target = $(event.target),
inst = $.datepicker._getInst($target[0]);
if ( ( ( $target[0].id !== $.datepicker._mainDivId &&
$target.parents("#" + $.datepicker._mainDivId).length === 0 &&
!$target.hasClass($.datepicker.markerClassName) &&
!$target.closest("." + $.datepicker._triggerClass).length &&
$.datepicker._datepickerShowing && !($.datepicker._inDialog && $.blockUI) ) ) ||
( $target.hasClass($.datepicker.markerClassName) && $.datepicker._curInst !== inst ) ) {
$.datepicker._hideDatepicker();
}
},
/* Adjust one of the date sub-fields. */
_adjustDate: function(id, offset, period) {
var target = $(id),
inst = this._getInst(target[0]);
if (this._isDisabledDatepicker(target[0])) {
return;
}
this._adjustInstDate(inst, offset +
(period === "M" ? this._get(inst, "showCurrentAtPos") : 0), // undo positioning
period);
this._updateDatepicker(inst);
},
/* Action for current link. */
_gotoToday: function(id) {
var date,
target = $(id),
inst = this._getInst(target[0]);
if (this._get(inst, "gotoCurrent") && inst.currentDay) {
inst.selectedDay = inst.currentDay;
inst.drawMonth = inst.selectedMonth = inst.currentMonth;
inst.drawYear = inst.selectedYear = inst.currentYear;
} else {
date = new Date();
inst.selectedDay = date.getDate();
inst.drawMonth = inst.selectedMonth = date.getMonth();
inst.drawYear = inst.selectedYear = date.getFullYear();
}
this._notifyChange(inst);
this._adjustDate(target);
},
/* Action for selecting a new month/year. */
_selectMonthYear: function(id, select, period) {
var target = $(id),
inst = this._getInst(target[0]);
inst["selected" + (period === "M" ? "Month" : "Year")] =
inst["draw" + (period === "M" ? "Month" : "Year")] =
parseInt(select.options[select.selectedIndex].value,10);
this._notifyChange(inst);
this._adjustDate(target);
},
/* Action for selecting a day. */
_selectDay: function(id, month, year, td) {
var inst,
target = $(id);
if ($(td).hasClass(this._unselectableClass) || this._isDisabledDatepicker(target[0])) {
return;
}
inst = this._getInst(target[0]);
inst.selectedDay = inst.currentDay = $("a", td).html();
inst.selectedMonth = inst.currentMonth = month;
inst.selectedYear = inst.currentYear = year;
this._selectDate(id, this._formatDate(inst,
inst.currentDay, inst.currentMonth, inst.currentYear));
},
/* Erase the input field and hide the date picker. */
_clearDate: function(id) {
var target = $(id);
this._selectDate(target, "");
},
/* Update the input field with the selected date. */
_selectDate: function(id, dateStr) {
var onSelect,
target = $(id),
inst = this._getInst(target[0]);
dateStr = (dateStr != null ? dateStr : this._formatDate(inst));
if (inst.input) {
inst.input.val(dateStr);
}
this._updateAlternate(inst);
onSelect = this._get(inst, "onSelect");
if (onSelect) {
onSelect.apply((inst.input ? inst.input[0] : null), [dateStr, inst]); // trigger custom callback
} else if (inst.input) {
inst.input.trigger("change"); // fire the change event
}
if (inst.inline){
this._updateDatepicker(inst);
} else {
this._hideDatepicker();
this._lastInput = inst.input[0];
if (typeof(inst.input[0]) !== "object") {
inst.input.focus(); // restore focus
}
this._lastInput = null;
}
},
/* Update any alternate field to synchronise with the main field. */
_updateAlternate: function(inst) {
var altFormat, date, dateStr,
altField = this._get(inst, "altField");
if (altField) { // update alternate field too
altFormat = this._get(inst, "altFormat") || this._get(inst, "dateFormat");
date = this._getDate(inst);
dateStr = this.formatDate(altFormat, date, this._getFormatConfig(inst));
$(altField).each(function() { $(this).val(dateStr); });
}
},
/* Set as beforeShowDay function to prevent selection of weekends.
* @param date Date - the date to customise
* @return [boolean, string] - is this date selectable?, what is its CSS class?
*/
noWeekends: function(date) {
var day = date.getDay();
return [(day > 0 && day < 6), ""];
},
/* Set as calculateWeek to determine the week of the year based on the ISO 8601 definition.
* @param date Date - the date to get the week for
* @return number - the number of the week within the year that contains this date
*/
iso8601Week: function(date) {
var time,
checkDate = new Date(date.getTime());
// Find Thursday of this week starting on Monday
checkDate.setDate(checkDate.getDate() + 4 - (checkDate.getDay() || 7));
time = checkDate.getTime();
checkDate.setMonth(0); // Compare with Jan 1
checkDate.setDate(1);
return Math.floor(Math.round((time - checkDate) / 86400000) / 7) + 1;
},
/* Parse a string value into a date object.
* See formatDate below for the possible formats.
*
* @param format string - the expected format of the date
* @param value string - the date in the above format
* @param settings Object - attributes include:
* shortYearCutoff number - the cutoff year for determining the century (optional)
* dayNamesShort string[7] - abbreviated names of the days from Sunday (optional)
* dayNames string[7] - names of the days from Sunday (optional)
* monthNamesShort string[12] - abbreviated names of the months (optional)
* monthNames string[12] - names of the months (optional)
* @return Date - the extracted date value or null if value is blank
*/
parseDate: function (format, value, settings) {
if (format == null || value == null) {
throw "Invalid arguments";
}
value = (typeof value === "object" ? value.toString() : value + "");
if (value === "") {
return null;
}
var iFormat, dim, extra,
iValue = 0,
shortYearCutoffTemp = (settings ? settings.shortYearCutoff : null) || this._defaults.shortYearCutoff,
shortYearCutoff = (typeof shortYearCutoffTemp !== "string" ? shortYearCutoffTemp :
new Date().getFullYear() % 100 + parseInt(shortYearCutoffTemp, 10)),
dayNamesShort = (settings ? settings.dayNamesShort : null) || this._defaults.dayNamesShort,
dayNames = (settings ? settings.dayNames : null) || this._defaults.dayNames,
monthNamesShort = (settings ? settings.monthNamesShort : null) || this._defaults.monthNamesShort,
monthNames = (settings ? settings.monthNames : null) || this._defaults.monthNames,
year = -1,
month = -1,
day = -1,
doy = -1,
literal = false,
date,
// Check whether a format character is doubled
lookAhead = function(match) {
var matches = (iFormat + 1 < format.length && format.charAt(iFormat + 1) === match);
if (matches) {
iFormat++;
}
return matches;
},
// Extract a number from the string value
getNumber = function(match) {
var isDoubled = lookAhead(match),
size = (match === "@" ? 14 : (match === "!" ? 20 :
(match === "y" && isDoubled ? 4 : (match === "o" ? 3 : 2)))),
digits = new RegExp("^\\d{1," + size + "}"),
num = value.substring(iValue).match(digits);
if (!num) {
throw "Missing number at position " + iValue;
}
iValue += num[0].length;
return parseInt(num[0], 10);
},
// Extract a name from the string value and convert to an index
getName = function(match, shortNames, longNames) {
var index = -1,
names = $.map(lookAhead(match) ? longNames : shortNames, function (v, k) {
return [ [k, v] ];
}).sort(function (a, b) {
return -(a[1].length - b[1].length);
});
$.each(names, function (i, pair) {
var name = pair[1];
if (value.substr(iValue, name.length).toLowerCase() === name.toLowerCase()) {
index = pair[0];
iValue += name.length;
return false;
}
});
if (index !== -1) {
return index + 1;
} else {
throw "Unknown name at position " + iValue;
}
},
// Confirm that a literal character matches the string value
checkLiteral = function() {
if (value.charAt(iValue) !== format.charAt(iFormat)) {
throw "Unexpected literal at position " + iValue;
}
iValue++;
};
for (iFormat = 0; iFormat < format.length; iFormat++) {
if (literal) {
if (format.charAt(iFormat) === "'" && !lookAhead("'")) {
literal = false;
} else {
checkLiteral();
}
} else {
switch (format.charAt(iFormat)) {
case "d":
day = getNumber("d");
break;
case "D":
getName("D", dayNamesShort, dayNames);
break;
case "o":
doy = getNumber("o");
break;
case "m":
month = getNumber("m");
break;
case "M":
month = getName("M", monthNamesShort, monthNames);
break;
case "y":
year = getNumber("y");
break;
case "@":
date = new Date(getNumber("@"));
year = date.getFullYear();
month = date.getMonth() + 1;
day = date.getDate();
break;
case "!":
date = new Date((getNumber("!") - this._ticksTo1970) / 10000);
year = date.getFullYear();
month = date.getMonth() + 1;
day = date.getDate();
break;
case "'":
if (lookAhead("'")){
checkLiteral();
} else {
literal = true;
}
break;
default:
checkLiteral();
}
}
}
if (iValue < value.length){
extra = value.substr(iValue);
if (!/^\s+/.test(extra)) {
throw "Extra/unparsed characters found in date: " + extra;
}
}
if (year === -1) {
year = new Date().getFullYear();
} else if (year < 100) {
year += new Date().getFullYear() - new Date().getFullYear() % 100 +
(year <= shortYearCutoff ? 0 : -100);
}
if (doy > -1) {
month = 1;
day = doy;
do {
dim = this._getDaysInMonth(year, month - 1);
if (day <= dim) {
break;
}
month++;
day -= dim;
} while (true);
}
date = this._daylightSavingAdjust(new Date(year, month - 1, day));
if (date.getFullYear() !== year || date.getMonth() + 1 !== month || date.getDate() !== day) {
throw "Invalid date"; // E.g. 31/02/00
}
return date;
},
/* Standard date formats. */
ATOM: "yy-mm-dd", // RFC 3339 (ISO 8601)
COOKIE: "D, dd M yy",
ISO_8601: "yy-mm-dd",
RFC_822: "D, d M y",
RFC_850: "DD, dd-M-y",
RFC_1036: "D, d M y",
RFC_1123: "D, d M yy",
RFC_2822: "D, d M yy",
RSS: "D, d M y", // RFC 822
TICKS: "!",
TIMESTAMP: "@",
W3C: "yy-mm-dd", // ISO 8601
_ticksTo1970: (((1970 - 1) * 365 + Math.floor(1970 / 4) - Math.floor(1970 / 100) +
Math.floor(1970 / 400)) * 24 * 60 * 60 * 10000000),
/* Format a date object into a string value.
* The format can be combinations of the following:
* d - day of month (no leading zero)
* dd - day of month (two digit)
* o - day of year (no leading zeros)
* oo - day of year (three digit)
* D - day name short
* DD - day name long
* m - month of year (no leading zero)
* mm - month of year (two digit)
* M - month name short
* MM - month name long
* y - year (two digit)
* yy - year (four digit)
* @ - Unix timestamp (ms since 01/01/1970)
* ! - Windows ticks (100ns since 01/01/0001)
* "..." - literal text
* '' - single quote
*
* @param format string - the desired format of the date
* @param date Date - the date value to format
* @param settings Object - attributes include:
* dayNamesShort string[7] - abbreviated names of the days from Sunday (optional)
* dayNames string[7] - names of the days from Sunday (optional)
* monthNamesShort string[12] - abbreviated names of the months (optional)
* monthNames string[12] - names of the months (optional)
* @return string - the date in the above format
*/
formatDate: function (format, date, settings) {
if (!date) {
return "";
}
var iFormat,
dayNamesShort = (settings ? settings.dayNamesShort : null) || this._defaults.dayNamesShort,
dayNames = (settings ? settings.dayNames : null) || this._defaults.dayNames,
monthNamesShort = (settings ? settings.monthNamesShort : null) || this._defaults.monthNamesShort,
monthNames = (settings ? settings.monthNames : null) || this._defaults.monthNames,
// Check whether a format character is doubled
lookAhead = function(match) {
var matches = (iFormat + 1 < format.length && format.charAt(iFormat + 1) === match);
if (matches) {
iFormat++;
}
return matches;
},
// Format a number, with leading zero if necessary
formatNumber = function(match, value, len) {
var num = "" + value;
if (lookAhead(match)) {
while (num.length < len) {
num = "0" + num;
}
}
return num;
},
// Format a name, short or long as requested
formatName = function(match, value, shortNames, longNames) {
return (lookAhead(match) ? longNames[value] : shortNames[value]);
},
output = "",
literal = false;
if (date) {
for (iFormat = 0; iFormat < format.length; iFormat++) {
if (literal) {
if (format.charAt(iFormat) === "'" && !lookAhead("'")) {
literal = false;
} else {
output += format.charAt(iFormat);
}
} else {
switch (format.charAt(iFormat)) {
case "d":
output += formatNumber("d", date.getDate(), 2);
break;
case "D":
output += formatName("D", date.getDay(), dayNamesShort, dayNames);
break;
case "o":
output += formatNumber("o",
Math.round((new Date(date.getFullYear(), date.getMonth(), date.getDate()).getTime() - new Date(date.getFullYear(), 0, 0).getTime()) / 86400000), 3);
break;
case "m":
output += formatNumber("m", date.getMonth() + 1, 2);
break;
case "M":
output += formatName("M", date.getMonth(), monthNamesShort, monthNames);
break;
case "y":
output += (lookAhead("y") ? date.getFullYear() :
(date.getYear() % 100 < 10 ? "0" : "") + date.getYear() % 100);
break;
case "@":
output += date.getTime();
break;
case "!":
output += date.getTime() * 10000 + this._ticksTo1970;
break;
case "'":
if (lookAhead("'")) {
output += "'";
} else {
literal = true;
}
break;
default:
output += format.charAt(iFormat);
}
}
}
}
return output;
},
/* Extract all possible characters from the date format. */
_possibleChars: function (format) {
var iFormat,
chars = "",
literal = false,
// Check whether a format character is doubled
lookAhead = function(match) {
var matches = (iFormat + 1 < format.length && format.charAt(iFormat + 1) === match);
if (matches) {
iFormat++;
}
return matches;
};
for (iFormat = 0; iFormat < format.length; iFormat++) {
if (literal) {
if (format.charAt(iFormat) === "'" && !lookAhead("'")) {
literal = false;
} else {
chars += format.charAt(iFormat);
}
} else {
switch (format.charAt(iFormat)) {
case "d": case "m": case "y": case "@":
chars += "0123456789";
break;
case "D": case "M":
return null; // Accept anything
case "'":
if (lookAhead("'")) {
chars += "'";
} else {
literal = true;
}
break;
default:
chars += format.charAt(iFormat);
}
}
}
return chars;
},
/* Get a setting value, defaulting if necessary. */
_get: function(inst, name) {
return inst.settings[name] !== undefined ?
inst.settings[name] : this._defaults[name];
},
/* Parse existing date and initialise date picker. */
_setDateFromField: function(inst, noDefault) {
if (inst.input.val() === inst.lastVal) {
return;
}
var dateFormat = this._get(inst, "dateFormat"),
dates = inst.lastVal = inst.input ? inst.input.val() : null,
defaultDate = this._getDefaultDate(inst),
date = defaultDate,
settings = this._getFormatConfig(inst);
try {
date = this.parseDate(dateFormat, dates, settings) || defaultDate;
} catch (event) {
dates = (noDefault ? "" : dates);
}
inst.selectedDay = date.getDate();
inst.drawMonth = inst.selectedMonth = date.getMonth();
inst.drawYear = inst.selectedYear = date.getFullYear();
inst.currentDay = (dates ? date.getDate() : 0);
inst.currentMonth = (dates ? date.getMonth() : 0);
inst.currentYear = (dates ? date.getFullYear() : 0);
this._adjustInstDate(inst);
},
/* Retrieve the default date shown on opening. */
_getDefaultDate: function(inst) {
return this._restrictMinMax(inst,
this._determineDate(inst, this._get(inst, "defaultDate"), new Date()));
},
/* A date may be specified as an exact value or a relative one. */
_determineDate: function(inst, date, defaultDate) {
var offsetNumeric = function(offset) {
var date = new Date();
date.setDate(date.getDate() + offset);
return date;
},
offsetString = function(offset) {
try {
return $.datepicker.parseDate($.datepicker._get(inst, "dateFormat"),
offset, $.datepicker._getFormatConfig(inst));
}
catch (e) {
// Ignore
}
var date = (offset.toLowerCase().match(/^c/) ?
$.datepicker._getDate(inst) : null) || new Date(),
year = date.getFullYear(),
month = date.getMonth(),
day = date.getDate(),
pattern = /([+\-]?[0-9]+)\s*(d|D|w|W|m|M|y|Y)?/g,
matches = pattern.exec(offset);
while (matches) {
switch (matches[2] || "d") {
case "d" : case "D" :
day += parseInt(matches[1],10); break;
case "w" : case "W" :
day += parseInt(matches[1],10) * 7; break;
case "m" : case "M" :
month += parseInt(matches[1],10);
day = Math.min(day, $.datepicker._getDaysInMonth(year, month));
break;
case "y": case "Y" :
year += parseInt(matches[1],10);
day = Math.min(day, $.datepicker._getDaysInMonth(year, month));
break;
}
matches = pattern.exec(offset);
}
return new Date(year, month, day);
},
newDate = (date == null || date === "" ? defaultDate : (typeof date === "string" ? offsetString(date) :
(typeof date === "number" ? (isNaN(date) ? defaultDate : offsetNumeric(date)) : new Date(date.getTime()))));
newDate = (newDate && newDate.toString() === "Invalid Date" ? defaultDate : newDate);
if (newDate) {
newDate.setHours(0);
newDate.setMinutes(0);
newDate.setSeconds(0);
newDate.setMilliseconds(0);
}
return this._daylightSavingAdjust(newDate);
},
/* Handle switch to/from daylight saving.
* Hours may be non-zero on daylight saving cut-over:
* > 12 when midnight changeover, but then cannot generate
* midnight datetime, so jump to 1AM, otherwise reset.
* @param date (Date) the date to check
* @return (Date) the corrected date
*/
_daylightSavingAdjust: function(date) {
if (!date) {
return null;
}
date.setHours(date.getHours() > 12 ? date.getHours() + 2 : 0);
return date;
},
/* Set the date(s) directly. */
_setDate: function(inst, date, noChange) {
var clear = !date,
origMonth = inst.selectedMonth,
origYear = inst.selectedYear,
newDate = this._restrictMinMax(inst, this._determineDate(inst, date, new Date()));
inst.selectedDay = inst.currentDay = newDate.getDate();
inst.drawMonth = inst.selectedMonth = inst.currentMonth = newDate.getMonth();
inst.drawYear = inst.selectedYear = inst.currentYear = newDate.getFullYear();
if ((origMonth !== inst.selectedMonth || origYear !== inst.selectedYear) && !noChange) {
this._notifyChange(inst);
}
this._adjustInstDate(inst);
if (inst.input) {
inst.input.val(clear ? "" : this._formatDate(inst));
}
},
/* Retrieve the date(s) directly. */
_getDate: function(inst) {
var startDate = (!inst.currentYear || (inst.input && inst.input.val() === "") ? null :
this._daylightSavingAdjust(new Date(
inst.currentYear, inst.currentMonth, inst.currentDay)));
return startDate;
},
/* Attach the onxxx handlers. These are declared statically so
* they work with static code transformers like Caja.
*/
_attachHandlers: function(inst) {
var stepMonths = this._get(inst, "stepMonths"),
id = "#" + inst.id.replace( /\\\\/g, "\\" );
inst.dpDiv.find("[data-handler]").map(function () {
var handler = {
prev: function () {
$.datepicker._adjustDate(id, -stepMonths, "M");
},
next: function () {
$.datepicker._adjustDate(id, +stepMonths, "M");
},
hide: function () {
$.datepicker._hideDatepicker();
},
today: function () {
$.datepicker._gotoToday(id);
},
selectDay: function () {
$.datepicker._selectDay(id, +this.getAttribute("data-month"), +this.getAttribute("data-year"), this);
return false;
},
selectMonth: function () {
$.datepicker._selectMonthYear(id, this, "M");
return false;
},
selectYear: function () {
$.datepicker._selectMonthYear(id, this, "Y");
return false;
}
};
$(this).bind(this.getAttribute("data-event"), handler[this.getAttribute("data-handler")]);
});
},
/* Generate the HTML for the current state of the date picker. */
_generateHTML: function(inst) {
var maxDraw, prevText, prev, nextText, next, currentText, gotoDate,
controls, buttonPanel, firstDay, showWeek, dayNames, dayNamesMin,
monthNames, monthNamesShort, beforeShowDay, showOtherMonths,
selectOtherMonths, defaultDate, html, dow, row, group, col, selectedDate,
cornerClass, calender, thead, day, daysInMonth, leadDays, curRows, numRows,
printDate, dRow, tbody, daySettings, otherMonth, unselectable,
tempDate = new Date(),
today = this._daylightSavingAdjust(
new Date(tempDate.getFullYear(), tempDate.getMonth(), tempDate.getDate())), // clear time
isRTL = this._get(inst, "isRTL"),
showButtonPanel = this._get(inst, "showButtonPanel"),
hideIfNoPrevNext = this._get(inst, "hideIfNoPrevNext"),
navigationAsDateFormat = this._get(inst, "navigationAsDateFormat"),
numMonths = this._getNumberOfMonths(inst),
showCurrentAtPos = this._get(inst, "showCurrentAtPos"),
stepMonths = this._get(inst, "stepMonths"),
isMultiMonth = (numMonths[0] !== 1 || numMonths[1] !== 1),
currentDate = this._daylightSavingAdjust((!inst.currentDay ? new Date(9999, 9, 9) :
new Date(inst.currentYear, inst.currentMonth, inst.currentDay))),
minDate = this._getMinMaxDate(inst, "min"),
maxDate = this._getMinMaxDate(inst, "max"),
drawMonth = inst.drawMonth - showCurrentAtPos,
drawYear = inst.drawYear;
if (drawMonth < 0) {
drawMonth += 12;
drawYear--;
}
if (maxDate) {
maxDraw = this._daylightSavingAdjust(new Date(maxDate.getFullYear(),
maxDate.getMonth() - (numMonths[0] * numMonths[1]) + 1, maxDate.getDate()));
maxDraw = (minDate && maxDraw < minDate ? minDate : maxDraw);
while (this._daylightSavingAdjust(new Date(drawYear, drawMonth, 1)) > maxDraw) {
drawMonth--;
if (drawMonth < 0) {
drawMonth = 11;
drawYear--;
}
}
}
inst.drawMonth = drawMonth;
inst.drawYear = drawYear;
prevText = this._get(inst, "prevText");
prevText = (!navigationAsDateFormat ? prevText : this.formatDate(prevText,
this._daylightSavingAdjust(new Date(drawYear, drawMonth - stepMonths, 1)),
this._getFormatConfig(inst)));
prev = (this._canAdjustMonth(inst, -1, drawYear, drawMonth) ?
"<a class='ui-datepicker-prev ui-corner-all' data-handler='prev' data-event='click'" +
" title='" + prevText + "'><span class='ui-icon ui-icon-circle-triangle-" + ( isRTL ? "e" : "w") + "'>" + prevText + "</span></a>" :
(hideIfNoPrevNext ? "" : "<a class='ui-datepicker-prev ui-corner-all ui-state-disabled' title='"+ prevText +"'><span class='ui-icon ui-icon-circle-triangle-" + ( isRTL ? "e" : "w") + "'>" + prevText + "</span></a>"));
nextText = this._get(inst, "nextText");
nextText = (!navigationAsDateFormat ? nextText : this.formatDate(nextText,
this._daylightSavingAdjust(new Date(drawYear, drawMonth + stepMonths, 1)),
this._getFormatConfig(inst)));
next = (this._canAdjustMonth(inst, +1, drawYear, drawMonth) ?
"<a class='ui-datepicker-next ui-corner-all' data-handler='next' data-event='click'" +
" title='" + nextText + "'><span class='ui-icon ui-icon-circle-triangle-" + ( isRTL ? "w" : "e") + "'>" + nextText + "</span></a>" :
(hideIfNoPrevNext ? "" : "<a class='ui-datepicker-next ui-corner-all ui-state-disabled' title='"+ nextText + "'><span class='ui-icon ui-icon-circle-triangle-" + ( isRTL ? "w" : "e") + "'>" + nextText + "</span></a>"));
currentText = this._get(inst, "currentText");
gotoDate = (this._get(inst, "gotoCurrent") && inst.currentDay ? currentDate : today);
currentText = (!navigationAsDateFormat ? currentText :
this.formatDate(currentText, gotoDate, this._getFormatConfig(inst)));
controls = (!inst.inline ? "<button type='button' class='ui-datepicker-close ui-state-default ui-priority-primary ui-corner-all' data-handler='hide' data-event='click'>" +
this._get(inst, "closeText") + "</button>" : "");
buttonPanel = (showButtonPanel) ? "<div class='ui-datepicker-buttonpane ui-widget-content'>" + (isRTL ? controls : "") +
(this._isInRange(inst, gotoDate) ? "<button type='button' class='ui-datepicker-current ui-state-default ui-priority-secondary ui-corner-all' data-handler='today' data-event='click'" +
">" + currentText + "</button>" : "") + (isRTL ? "" : controls) + "</div>" : "";
firstDay = parseInt(this._get(inst, "firstDay"),10);
firstDay = (isNaN(firstDay) ? 0 : firstDay);
showWeek = this._get(inst, "showWeek");
dayNames = this._get(inst, "dayNames");
dayNamesMin = this._get(inst, "dayNamesMin");
monthNames = this._get(inst, "monthNames");
monthNamesShort = this._get(inst, "monthNamesShort");
beforeShowDay = this._get(inst, "beforeShowDay");
showOtherMonths = this._get(inst, "showOtherMonths");
selectOtherMonths = this._get(inst, "selectOtherMonths");
defaultDate = this._getDefaultDate(inst);
html = "";
dow;
for (row = 0; row < numMonths[0]; row++) {
group = "";
this.maxRows = 4;
for (col = 0; col < numMonths[1]; col++) {
selectedDate = this._daylightSavingAdjust(new Date(drawYear, drawMonth, inst.selectedDay));
cornerClass = " ui-corner-all";
calender = "";
if (isMultiMonth) {
calender += "<div class='ui-datepicker-group";
if (numMonths[1] > 1) {
switch (col) {
case 0: calender += " ui-datepicker-group-first";
cornerClass = " ui-corner-" + (isRTL ? "right" : "left"); break;
case numMonths[1]-1: calender += " ui-datepicker-group-last";
cornerClass = " ui-corner-" + (isRTL ? "left" : "right"); break;
default: calender += " ui-datepicker-group-middle"; cornerClass = ""; break;
}
}
calender += "'>";
}
calender += "<div class='ui-datepicker-header ui-widget-header ui-helper-clearfix" + cornerClass + "'>" +
(/all|left/.test(cornerClass) && row === 0 ? (isRTL ? next : prev) : "") +
(/all|right/.test(cornerClass) && row === 0 ? (isRTL ? prev : next) : "") +
this._generateMonthYearHeader(inst, drawMonth, drawYear, minDate, maxDate,
row > 0 || col > 0, monthNames, monthNamesShort) + // draw month headers
"</div><table class='ui-datepicker-calendar'><thead>" +
"<tr>";
thead = (showWeek ? "<th class='ui-datepicker-week-col'>" + this._get(inst, "weekHeader") + "</th>" : "");
for (dow = 0; dow < 7; dow++) { // days of the week
day = (dow + firstDay) % 7;
thead += "<th" + ((dow + firstDay + 6) % 7 >= 5 ? " class='ui-datepicker-week-end'" : "") + ">" +
"<span title='" + dayNames[day] + "'>" + dayNamesMin[day] + "</span></th>";
}
calender += thead + "</tr></thead><tbody>";
daysInMonth = this._getDaysInMonth(drawYear, drawMonth);
if (drawYear === inst.selectedYear && drawMonth === inst.selectedMonth) {
inst.selectedDay = Math.min(inst.selectedDay, daysInMonth);
}
leadDays = (this._getFirstDayOfMonth(drawYear, drawMonth) - firstDay + 7) % 7;
curRows = Math.ceil((leadDays + daysInMonth) / 7); // calculate the number of rows to generate
numRows = (isMultiMonth ? this.maxRows > curRows ? this.maxRows : curRows : curRows); //If multiple months, use the higher number of rows (see #7043)
this.maxRows = numRows;
printDate = this._daylightSavingAdjust(new Date(drawYear, drawMonth, 1 - leadDays));
for (dRow = 0; dRow < numRows; dRow++) { // create date picker rows
calender += "<tr>";
tbody = (!showWeek ? "" : "<td class='ui-datepicker-week-col'>" +
this._get(inst, "calculateWeek")(printDate) + "</td>");
for (dow = 0; dow < 7; dow++) { // create date picker days
daySettings = (beforeShowDay ?
beforeShowDay.apply((inst.input ? inst.input[0] : null), [printDate]) : [true, ""]);
otherMonth = (printDate.getMonth() !== drawMonth);
unselectable = (otherMonth && !selectOtherMonths) || !daySettings[0] ||
(minDate && printDate < minDate) || (maxDate && printDate > maxDate);
tbody += "<td class='" +
((dow + firstDay + 6) % 7 >= 5 ? " ui-datepicker-week-end" : "") + // highlight weekends
(otherMonth ? " ui-datepicker-other-month" : "") + // highlight days from other months
((printDate.getTime() === selectedDate.getTime() && drawMonth === inst.selectedMonth && inst._keyEvent) || // user pressed key
(defaultDate.getTime() === printDate.getTime() && defaultDate.getTime() === selectedDate.getTime()) ?
// or defaultDate is current printedDate and defaultDate is selectedDate
" " + this._dayOverClass : "") + // highlight selected day
(unselectable ? " " + this._unselectableClass + " ui-state-disabled": "") + // highlight unselectable days
(otherMonth && !showOtherMonths ? "" : " " + daySettings[1] + // highlight custom dates
(printDate.getTime() === currentDate.getTime() ? " " + this._currentClass : "") + // highlight selected day
(printDate.getTime() === today.getTime() ? " ui-datepicker-today" : "")) + "'" + // highlight today (if different)
((!otherMonth || showOtherMonths) && daySettings[2] ? " title='" + daySettings[2].replace(/'/g, "'") + "'" : "") + // cell title
(unselectable ? "" : " data-handler='selectDay' data-event='click' data-month='" + printDate.getMonth() + "' data-year='" + printDate.getFullYear() + "'") + ">" + // actions
(otherMonth && !showOtherMonths ? " " : // display for other months
(unselectable ? "<span class='ui-state-default'>" + printDate.getDate() + "</span>" : "<a class='ui-state-default" +
(printDate.getTime() === today.getTime() ? " ui-state-highlight" : "") +
(printDate.getTime() === currentDate.getTime() ? " ui-state-active" : "") + // highlight selected day
(otherMonth ? " ui-priority-secondary" : "") + // distinguish dates from other months
"' href='#'>" + printDate.getDate() + "</a>")) + "</td>"; // display selectable date
printDate.setDate(printDate.getDate() + 1);
printDate = this._daylightSavingAdjust(printDate);
}
calender += tbody + "</tr>";
}
drawMonth++;
if (drawMonth > 11) {
drawMonth = 0;
drawYear++;
}
calender += "</tbody></table>" + (isMultiMonth ? "</div>" +
((numMonths[0] > 0 && col === numMonths[1]-1) ? "<div class='ui-datepicker-row-break'></div>" : "") : "");
group += calender;
}
html += group;
}
html += buttonPanel;
inst._keyEvent = false;
return html;
},
/* Generate the month and year header. */
_generateMonthYearHeader: function(inst, drawMonth, drawYear, minDate, maxDate,
secondary, monthNames, monthNamesShort) {
var inMinYear, inMaxYear, month, years, thisYear, determineYear, year, endYear,
changeMonth = this._get(inst, "changeMonth"),
changeYear = this._get(inst, "changeYear"),
showMonthAfterYear = this._get(inst, "showMonthAfterYear"),
html = "<div class='ui-datepicker-title'>",
monthHtml = "";
// month selection
if (secondary || !changeMonth) {
monthHtml += "<span class='ui-datepicker-month'>" + monthNames[drawMonth] + "</span>";
} else {
inMinYear = (minDate && minDate.getFullYear() === drawYear);
inMaxYear = (maxDate && maxDate.getFullYear() === drawYear);
monthHtml += "<select class='ui-datepicker-month' data-handler='selectMonth' data-event='change'>";
for ( month = 0; month < 12; month++) {
if ((!inMinYear || month >= minDate.getMonth()) && (!inMaxYear || month <= maxDate.getMonth())) {
monthHtml += "<option value='" + month + "'" +
(month === drawMonth ? " selected='selected'" : "") +
">" + monthNamesShort[month] + "</option>";
}
}
monthHtml += "</select>";
}
if (!showMonthAfterYear) {
html += monthHtml + (secondary || !(changeMonth && changeYear) ? " " : "");
}
// year selection
if ( !inst.yearshtml ) {
inst.yearshtml = "";
if (secondary || !changeYear) {
html += "<span class='ui-datepicker-year'>" + drawYear + "</span>";
} else {
// determine range of years to display
years = this._get(inst, "yearRange").split(":");
thisYear = new Date().getFullYear();
determineYear = function(value) {
var year = (value.match(/c[+\-].*/) ? drawYear + parseInt(value.substring(1), 10) :
(value.match(/[+\-].*/) ? thisYear + parseInt(value, 10) :
parseInt(value, 10)));
return (isNaN(year) ? thisYear : year);
};
year = determineYear(years[0]);
endYear = Math.max(year, determineYear(years[1] || ""));
year = (minDate ? Math.max(year, minDate.getFullYear()) : year);
endYear = (maxDate ? Math.min(endYear, maxDate.getFullYear()) : endYear);
inst.yearshtml += "<select class='ui-datepicker-year' data-handler='selectYear' data-event='change'>";
for (; year <= endYear; year++) {
inst.yearshtml += "<option value='" + year + "'" +
(year === drawYear ? " selected='selected'" : "") +
">" + year + "</option>";
}
inst.yearshtml += "</select>";
html += inst.yearshtml;
inst.yearshtml = null;
}
}
html += this._get(inst, "yearSuffix");
if (showMonthAfterYear) {
html += (secondary || !(changeMonth && changeYear) ? " " : "") + monthHtml;
}
html += "</div>"; // Close datepicker_header
return html;
},
/* Adjust one of the date sub-fields. */
_adjustInstDate: function(inst, offset, period) {
var year = inst.drawYear + (period === "Y" ? offset : 0),
month = inst.drawMonth + (period === "M" ? offset : 0),
day = Math.min(inst.selectedDay, this._getDaysInMonth(year, month)) + (period === "D" ? offset : 0),
date = this._restrictMinMax(inst, this._daylightSavingAdjust(new Date(year, month, day)));
inst.selectedDay = date.getDate();
inst.drawMonth = inst.selectedMonth = date.getMonth();
inst.drawYear = inst.selectedYear = date.getFullYear();
if (period === "M" || period === "Y") {
this._notifyChange(inst);
}
},
/* Ensure a date is within any min/max bounds. */
_restrictMinMax: function(inst, date) {
var minDate = this._getMinMaxDate(inst, "min"),
maxDate = this._getMinMaxDate(inst, "max"),
newDate = (minDate && date < minDate ? minDate : date);
return (maxDate && newDate > maxDate ? maxDate : newDate);
},
/* Notify change of month/year. */
_notifyChange: function(inst) {
var onChange = this._get(inst, "onChangeMonthYear");
if (onChange) {
onChange.apply((inst.input ? inst.input[0] : null),
[inst.selectedYear, inst.selectedMonth + 1, inst]);
}
},
/* Determine the number of months to show. */
_getNumberOfMonths: function(inst) {
var numMonths = this._get(inst, "numberOfMonths");
return (numMonths == null ? [1, 1] : (typeof numMonths === "number" ? [1, numMonths] : numMonths));
},
/* Determine the current maximum date - ensure no time components are set. */
_getMinMaxDate: function(inst, minMax) {
return this._determineDate(inst, this._get(inst, minMax + "Date"), null);
},
/* Find the number of days in a given month. */
_getDaysInMonth: function(year, month) {
return 32 - this._daylightSavingAdjust(new Date(year, month, 32)).getDate();
},
/* Find the day of the week of the first of a month. */
_getFirstDayOfMonth: function(year, month) {
return new Date(year, month, 1).getDay();
},
/* Determines if we should allow a "next/prev" month display change. */
_canAdjustMonth: function(inst, offset, curYear, curMonth) {
var numMonths = this._getNumberOfMonths(inst),
date = this._daylightSavingAdjust(new Date(curYear,
curMonth + (offset < 0 ? offset : numMonths[0] * numMonths[1]), 1));
if (offset < 0) {
date.setDate(this._getDaysInMonth(date.getFullYear(), date.getMonth()));
}
return this._isInRange(inst, date);
},
/* Is the given date in the accepted range? */
_isInRange: function(inst, date) {
var yearSplit, currentYear,
minDate = this._getMinMaxDate(inst, "min"),
maxDate = this._getMinMaxDate(inst, "max"),
minYear = null,
maxYear = null,
years = this._get(inst, "yearRange");
if (years){
yearSplit = years.split(":");
currentYear = new Date().getFullYear();
minYear = parseInt(yearSplit[0], 10);
maxYear = parseInt(yearSplit[1], 10);
if ( yearSplit[0].match(/[+\-].*/) ) {
minYear += currentYear;
}
if ( yearSplit[1].match(/[+\-].*/) ) {
maxYear += currentYear;
}
}
return ((!minDate || date.getTime() >= minDate.getTime()) &&
(!maxDate || date.getTime() <= maxDate.getTime()) &&
(!minYear || date.getFullYear() >= minYear) &&
(!maxYear || date.getFullYear() <= maxYear));
},
/* Provide the configuration settings for formatting/parsing. */
_getFormatConfig: function(inst) {
var shortYearCutoff = this._get(inst, "shortYearCutoff");
shortYearCutoff = (typeof shortYearCutoff !== "string" ? shortYearCutoff :
new Date().getFullYear() % 100 + parseInt(shortYearCutoff, 10));
return {shortYearCutoff: shortYearCutoff,
dayNamesShort: this._get(inst, "dayNamesShort"), dayNames: this._get(inst, "dayNames"),
monthNamesShort: this._get(inst, "monthNamesShort"), monthNames: this._get(inst, "monthNames")};
},
/* Format the given date for display. */
_formatDate: function(inst, day, month, year) {
if (!day) {
inst.currentDay = inst.selectedDay;
inst.currentMonth = inst.selectedMonth;
inst.currentYear = inst.selectedYear;
}
var date = (day ? (typeof day === "object" ? day :
this._daylightSavingAdjust(new Date(year, month, day))) :
this._daylightSavingAdjust(new Date(inst.currentYear, inst.currentMonth, inst.currentDay)));
return this.formatDate(this._get(inst, "dateFormat"), date, this._getFormatConfig(inst));
}
});
/*
* Bind hover events for datepicker elements.
* Done via delegate so the binding only occurs once in the lifetime of the parent div.
* Global instActive, set by _updateDatepicker allows the handlers to find their way back to the active picker.
*/
function bindHover(dpDiv) {
var selector = "button, .ui-datepicker-prev, .ui-datepicker-next, .ui-datepicker-calendar td a";
return dpDiv.delegate(selector, "mouseout", function() {
$(this).removeClass("ui-state-hover");
if (this.className.indexOf("ui-datepicker-prev") !== -1) {
$(this).removeClass("ui-datepicker-prev-hover");
}
if (this.className.indexOf("ui-datepicker-next") !== -1) {
$(this).removeClass("ui-datepicker-next-hover");
}
})
.delegate(selector, "mouseover", function(){
if (!$.datepicker._isDisabledDatepicker( instActive.inline ? dpDiv.parent()[0] : instActive.input[0])) {
$(this).parents(".ui-datepicker-calendar").find("a").removeClass("ui-state-hover");
$(this).addClass("ui-state-hover");
if (this.className.indexOf("ui-datepicker-prev") !== -1) {
$(this).addClass("ui-datepicker-prev-hover");
}
if (this.className.indexOf("ui-datepicker-next") !== -1) {
$(this).addClass("ui-datepicker-next-hover");
}
}
});
}
/* jQuery extend now ignores nulls! */
function extendRemove(target, props) {
$.extend(target, props);
for (var name in props) {
if (props[name] == null) {
target[name] = props[name];
}
}
return target;
}
/* Invoke the datepicker functionality.
@param options string - a command, optionally followed by additional parameters or
Object - settings for attaching new datepicker functionality
@return jQuery object */
$.fn.datepicker = function(options){
/* Verify an empty collection wasn't passed - Fixes #6976 */
if ( !this.length ) {
return this;
}
/* Initialise the date picker. */
if (!$.datepicker.initialized) {
$(document).mousedown($.datepicker._checkExternalClick);
$.datepicker.initialized = true;
}
/* Append datepicker main container to body if not exist. */
if ($("#"+$.datepicker._mainDivId).length === 0) {
$("body").append($.datepicker.dpDiv);
}
var otherArgs = Array.prototype.slice.call(arguments, 1);
if (typeof options === "string" && (options === "isDisabled" || options === "getDate" || options === "widget")) {
return $.datepicker["_" + options + "Datepicker"].
apply($.datepicker, [this[0]].concat(otherArgs));
}
if (options === "option" && arguments.length === 2 && typeof arguments[1] === "string") {
return $.datepicker["_" + options + "Datepicker"].
apply($.datepicker, [this[0]].concat(otherArgs));
}
return this.each(function() {
typeof options === "string" ?
$.datepicker["_" + options + "Datepicker"].
apply($.datepicker, [this].concat(otherArgs)) :
$.datepicker._attachDatepicker(this, options);
});
};
$.datepicker = new Datepicker(); // singleton instance
$.datepicker.initialized = false;
$.datepicker.uuid = new Date().getTime();
$.datepicker.version = "1.10.3";
})(jQuery);
(function( $, undefined ) {
var sizeRelatedOptions = {
buttons: true,
height: true,
maxHeight: true,
maxWidth: true,
minHeight: true,
minWidth: true,
width: true
},
resizableRelatedOptions = {
maxHeight: true,
maxWidth: true,
minHeight: true,
minWidth: true
};
$.widget( "ui.dialog", {
version: "1.10.3",
options: {
appendTo: "body",
autoOpen: true,
buttons: [],
closeOnEscape: true,
closeText: "close",
dialogClass: "",
draggable: true,
hide: null,
height: "auto",
maxHeight: null,
maxWidth: null,
minHeight: 150,
minWidth: 150,
modal: false,
position: {
my: "center",
at: "center",
of: window,
collision: "fit",
// Ensure the titlebar is always visible
using: function( pos ) {
var topOffset = $( this ).css( pos ).offset().top;
if ( topOffset < 0 ) {
$( this ).css( "top", pos.top - topOffset );
}
}
},
resizable: true,
show: null,
title: null,
width: 300,
// callbacks
beforeClose: null,
close: null,
drag: null,
dragStart: null,
dragStop: null,
focus: null,
open: null,
resize: null,
resizeStart: null,
resizeStop: null
},
_create: function() {
this.originalCss = {
display: this.element[0].style.display,
width: this.element[0].style.width,
minHeight: this.element[0].style.minHeight,
maxHeight: this.element[0].style.maxHeight,
height: this.element[0].style.height
};
this.originalPosition = {
parent: this.element.parent(),
index: this.element.parent().children().index( this.element )
};
this.originalTitle = this.element.attr("title");
this.options.title = this.options.title || this.originalTitle;
this._createWrapper();
this.element
.show()
.removeAttr("title")
.addClass("ui-dialog-content ui-widget-content")
.appendTo( this.uiDialog );
this._createTitlebar();
this._createButtonPane();
if ( this.options.draggable && $.fn.draggable ) {
this._makeDraggable();
}
if ( this.options.resizable && $.fn.resizable ) {
this._makeResizable();
}
this._isOpen = false;
},
_init: function() {
if ( this.options.autoOpen ) {
this.open();
}
},
_appendTo: function() {
var element = this.options.appendTo;
if ( element && (element.jquery || element.nodeType) ) {
return $( element );
}
return this.document.find( element || "body" ).eq( 0 );
},
_destroy: function() {
var next,
originalPosition = this.originalPosition;
this._destroyOverlay();
this.element
.removeUniqueId()
.removeClass("ui-dialog-content ui-widget-content")
.css( this.originalCss )
// Without detaching first, the following becomes really slow
.detach();
this.uiDialog.stop( true, true ).remove();
if ( this.originalTitle ) {
this.element.attr( "title", this.originalTitle );
}
next = originalPosition.parent.children().eq( originalPosition.index );
// Don't try to place the dialog next to itself (#8613)
if ( next.length && next[0] !== this.element[0] ) {
next.before( this.element );
} else {
originalPosition.parent.append( this.element );
}
},
widget: function() {
return this.uiDialog;
},
disable: $.noop,
enable: $.noop,
close: function( event ) {
var that = this;
if ( !this._isOpen || this._trigger( "beforeClose", event ) === false ) {
return;
}
this._isOpen = false;
this._destroyOverlay();
if ( !this.opener.filter(":focusable").focus().length ) {
// Hiding a focused element doesn't trigger blur in WebKit
// so in case we have nothing to focus on, explicitly blur the active element
// https://bugs.webkit.org/show_bug.cgi?id=47182
$( this.document[0].activeElement ).blur();
}
this._hide( this.uiDialog, this.options.hide, function() {
that._trigger( "close", event );
});
},
isOpen: function() {
return this._isOpen;
},
moveToTop: function() {
this._moveToTop();
},
_moveToTop: function( event, silent ) {
var moved = !!this.uiDialog.nextAll(":visible").insertBefore( this.uiDialog ).length;
if ( moved && !silent ) {
this._trigger( "focus", event );
}
return moved;
},
open: function() {
var that = this;
if ( this._isOpen ) {
if ( this._moveToTop() ) {
this._focusTabbable();
}
return;
}
this._isOpen = true;
this.opener = $( this.document[0].activeElement );
this._size();
this._position();
this._createOverlay();
this._moveToTop( null, true );
this._show( this.uiDialog, this.options.show, function() {
that._focusTabbable();
that._trigger("focus");
});
this._trigger("open");
},
_focusTabbable: function() {
// Set focus to the first match:
// 1. First element inside the dialog matching [autofocus]
// 2. Tabbable element inside the content element
// 3. Tabbable element inside the buttonpane
// 4. The close button
// 5. The dialog itself
var hasFocus = this.element.find("[autofocus]");
if ( !hasFocus.length ) {
hasFocus = this.element.find(":tabbable");
}
if ( !hasFocus.length ) {
hasFocus = this.uiDialogButtonPane.find(":tabbable");
}
if ( !hasFocus.length ) {
hasFocus = this.uiDialogTitlebarClose.filter(":tabbable");
}
if ( !hasFocus.length ) {
hasFocus = this.uiDialog;
}
hasFocus.eq( 0 ).focus();
},
_keepFocus: function( event ) {
function checkFocus() {
var activeElement = this.document[0].activeElement,
isActive = this.uiDialog[0] === activeElement ||
$.contains( this.uiDialog[0], activeElement );
if ( !isActive ) {
this._focusTabbable();
}
}
event.preventDefault();
checkFocus.call( this );
// support: IE
// IE <= 8 doesn't prevent moving focus even with event.preventDefault()
// so we check again later
this._delay( checkFocus );
},
_createWrapper: function() {
this.uiDialog = $("<div>")
.addClass( "ui-dialog ui-widget ui-widget-content ui-corner-all ui-front " +
this.options.dialogClass )
.hide()
.attr({
// Setting tabIndex makes the div focusable
tabIndex: -1,
role: "dialog"
})
.appendTo( this._appendTo() );
this._on( this.uiDialog, {
keydown: function( event ) {
if ( this.options.closeOnEscape && !event.isDefaultPrevented() && event.keyCode &&
event.keyCode === $.ui.keyCode.ESCAPE ) {
event.preventDefault();
this.close( event );
return;
}
// prevent tabbing out of dialogs
if ( event.keyCode !== $.ui.keyCode.TAB ) {
return;
}
var tabbables = this.uiDialog.find(":tabbable"),
first = tabbables.filter(":first"),
last = tabbables.filter(":last");
if ( ( event.target === last[0] || event.target === this.uiDialog[0] ) && !event.shiftKey ) {
first.focus( 1 );
event.preventDefault();
} else if ( ( event.target === first[0] || event.target === this.uiDialog[0] ) && event.shiftKey ) {
last.focus( 1 );
event.preventDefault();
}
},
mousedown: function( event ) {
if ( this._moveToTop( event ) ) {
this._focusTabbable();
}
}
});
// We assume that any existing aria-describedby attribute means
// that the dialog content is marked up properly
// otherwise we brute force the content as the description
if ( !this.element.find("[aria-describedby]").length ) {
this.uiDialog.attr({
"aria-describedby": this.element.uniqueId().attr("id")
});
}
},
_createTitlebar: function() {
var uiDialogTitle;
this.uiDialogTitlebar = $("<div>")
.addClass("ui-dialog-titlebar ui-widget-header ui-corner-all ui-helper-clearfix")
.prependTo( this.uiDialog );
this._on( this.uiDialogTitlebar, {
mousedown: function( event ) {
// Don't prevent click on close button (#8838)
// Focusing a dialog that is partially scrolled out of view
// causes the browser to scroll it into view, preventing the click event
if ( !$( event.target ).closest(".ui-dialog-titlebar-close") ) {
// Dialog isn't getting focus when dragging (#8063)
this.uiDialog.focus();
}
}
});
this.uiDialogTitlebarClose = $("<button></button>")
.button({
label: this.options.closeText,
icons: {
primary: "ui-icon-closethick"
},
text: false
})
.addClass("ui-dialog-titlebar-close")
.appendTo( this.uiDialogTitlebar );
this._on( this.uiDialogTitlebarClose, {
click: function( event ) {
event.preventDefault();
this.close( event );
}
});
uiDialogTitle = $("<span>")
.uniqueId()
.addClass("ui-dialog-title")
.prependTo( this.uiDialogTitlebar );
this._title( uiDialogTitle );
this.uiDialog.attr({
"aria-labelledby": uiDialogTitle.attr("id")
});
},
_title: function( title ) {
if ( !this.options.title ) {
title.html(" ");
}
title.text( this.options.title );
},
_createButtonPane: function() {
this.uiDialogButtonPane = $("<div>")
.addClass("ui-dialog-buttonpane ui-widget-content ui-helper-clearfix");
this.uiButtonSet = $("<div>")
.addClass("ui-dialog-buttonset")
.appendTo( this.uiDialogButtonPane );
this._createButtons();
},
_createButtons: function() {
var that = this,
buttons = this.options.buttons;
// if we already have a button pane, remove it
this.uiDialogButtonPane.remove();
this.uiButtonSet.empty();
if ( $.isEmptyObject( buttons ) || ($.isArray( buttons ) && !buttons.length) ) {
this.uiDialog.removeClass("ui-dialog-buttons");
return;
}
$.each( buttons, function( name, props ) {
var click, buttonOptions;
props = $.isFunction( props ) ?
{ click: props, text: name } :
props;
// Default to a non-submitting button
props = $.extend( { type: "button" }, props );
// Change the context for the click callback to be the main element
click = props.click;
props.click = function() {
click.apply( that.element[0], arguments );
};
buttonOptions = {
icons: props.icons,
text: props.showText
};
delete props.icons;
delete props.showText;
$( "<button></button>", props )
.button( buttonOptions )
.appendTo( that.uiButtonSet );
});
this.uiDialog.addClass("ui-dialog-buttons");
this.uiDialogButtonPane.appendTo( this.uiDialog );
},
_makeDraggable: function() {
var that = this,
options = this.options;
function filteredUi( ui ) {
return {
position: ui.position,
offset: ui.offset
};
}
this.uiDialog.draggable({
cancel: ".ui-dialog-content, .ui-dialog-titlebar-close",
handle: ".ui-dialog-titlebar",
containment: "document",
start: function( event, ui ) {
$( this ).addClass("ui-dialog-dragging");
that._blockFrames();
that._trigger( "dragStart", event, filteredUi( ui ) );
},
drag: function( event, ui ) {
that._trigger( "drag", event, filteredUi( ui ) );
},
stop: function( event, ui ) {
options.position = [
ui.position.left - that.document.scrollLeft(),
ui.position.top - that.document.scrollTop()
];
$( this ).removeClass("ui-dialog-dragging");
that._unblockFrames();
that._trigger( "dragStop", event, filteredUi( ui ) );
}
});
},
_makeResizable: function() {
var that = this,
options = this.options,
handles = options.resizable,
// .ui-resizable has position: relative defined in the stylesheet
// but dialogs have to use absolute or fixed positioning
position = this.uiDialog.css("position"),
resizeHandles = typeof handles === "string" ?
handles :
"n,e,s,w,se,sw,ne,nw";
function filteredUi( ui ) {
return {
originalPosition: ui.originalPosition,
originalSize: ui.originalSize,
position: ui.position,
size: ui.size
};
}
this.uiDialog.resizable({
cancel: ".ui-dialog-content",
containment: "document",
alsoResize: this.element,
maxWidth: options.maxWidth,
maxHeight: options.maxHeight,
minWidth: options.minWidth,
minHeight: this._minHeight(),
handles: resizeHandles,
start: function( event, ui ) {
$( this ).addClass("ui-dialog-resizing");
that._blockFrames();
that._trigger( "resizeStart", event, filteredUi( ui ) );
},
resize: function( event, ui ) {
that._trigger( "resize", event, filteredUi( ui ) );
},
stop: function( event, ui ) {
options.height = $( this ).height();
options.width = $( this ).width();
$( this ).removeClass("ui-dialog-resizing");
that._unblockFrames();
that._trigger( "resizeStop", event, filteredUi( ui ) );
}
})
.css( "position", position );
},
_minHeight: function() {
var options = this.options;
return options.height === "auto" ?
options.minHeight :
Math.min( options.minHeight, options.height );
},
_position: function() {
// Need to show the dialog to get the actual offset in the position plugin
var isVisible = this.uiDialog.is(":visible");
if ( !isVisible ) {
this.uiDialog.show();
}
this.uiDialog.position( this.options.position );
if ( !isVisible ) {
this.uiDialog.hide();
}
},
_setOptions: function( options ) {
var that = this,
resize = false,
resizableOptions = {};
$.each( options, function( key, value ) {
that._setOption( key, value );
if ( key in sizeRelatedOptions ) {
resize = true;
}
if ( key in resizableRelatedOptions ) {
resizableOptions[ key ] = value;
}
});
if ( resize ) {
this._size();
this._position();
}
if ( this.uiDialog.is(":data(ui-resizable)") ) {
this.uiDialog.resizable( "option", resizableOptions );
}
},
_setOption: function( key, value ) {
/*jshint maxcomplexity:15*/
var isDraggable, isResizable,
uiDialog = this.uiDialog;
if ( key === "dialogClass" ) {
uiDialog
.removeClass( this.options.dialogClass )
.addClass( value );
}
if ( key === "disabled" ) {
return;
}
this._super( key, value );
if ( key === "appendTo" ) {
this.uiDialog.appendTo( this._appendTo() );
}
if ( key === "buttons" ) {
this._createButtons();
}
if ( key === "closeText" ) {
this.uiDialogTitlebarClose.button({
// Ensure that we always pass a string
label: "" + value
});
}
if ( key === "draggable" ) {
isDraggable = uiDialog.is(":data(ui-draggable)");
if ( isDraggable && !value ) {
uiDialog.draggable("destroy");
}
if ( !isDraggable && value ) {
this._makeDraggable();
}
}
if ( key === "position" ) {
this._position();
}
if ( key === "resizable" ) {
// currently resizable, becoming non-resizable
isResizable = uiDialog.is(":data(ui-resizable)");
if ( isResizable && !value ) {
uiDialog.resizable("destroy");
}
// currently resizable, changing handles
if ( isResizable && typeof value === "string" ) {
uiDialog.resizable( "option", "handles", value );
}
// currently non-resizable, becoming resizable
if ( !isResizable && value !== false ) {
this._makeResizable();
}
}
if ( key === "title" ) {
this._title( this.uiDialogTitlebar.find(".ui-dialog-title") );
}
},
_size: function() {
// If the user has resized the dialog, the .ui-dialog and .ui-dialog-content
// divs will both have width and height set, so we need to reset them
var nonContentHeight, minContentHeight, maxContentHeight,
options = this.options;
// Reset content sizing
this.element.show().css({
width: "auto",
minHeight: 0,
maxHeight: "none",
height: 0
});
if ( options.minWidth > options.width ) {
options.width = options.minWidth;
}
// reset wrapper sizing
// determine the height of all the non-content elements
nonContentHeight = this.uiDialog.css({
height: "auto",
width: options.width
})
.outerHeight();
minContentHeight = Math.max( 0, options.minHeight - nonContentHeight );
maxContentHeight = typeof options.maxHeight === "number" ?
Math.max( 0, options.maxHeight - nonContentHeight ) :
"none";
if ( options.height === "auto" ) {
this.element.css({
minHeight: minContentHeight,
maxHeight: maxContentHeight,
height: "auto"
});
} else {
this.element.height( Math.max( 0, options.height - nonContentHeight ) );
}
if (this.uiDialog.is(":data(ui-resizable)") ) {
this.uiDialog.resizable( "option", "minHeight", this._minHeight() );
}
},
_blockFrames: function() {
this.iframeBlocks = this.document.find( "iframe" ).map(function() {
var iframe = $( this );
return $( "<div>" )
.css({
position: "absolute",
width: iframe.outerWidth(),
height: iframe.outerHeight()
})
.appendTo( iframe.parent() )
.offset( iframe.offset() )[0];
});
},
_unblockFrames: function() {
if ( this.iframeBlocks ) {
this.iframeBlocks.remove();
delete this.iframeBlocks;
}
},
_allowInteraction: function( event ) {
if ( $( event.target ).closest(".ui-dialog").length ) {
return true;
}
// TODO: Remove hack when datepicker implements
// the .ui-front logic (#8989)
return !!$( event.target ).closest(".ui-datepicker").length;
},
_createOverlay: function() {
if ( !this.options.modal ) {
return;
}
var that = this,
widgetFullName = this.widgetFullName;
if ( !$.ui.dialog.overlayInstances ) {
// Prevent use of anchors and inputs.
// We use a delay in case the overlay is created from an
// event that we're going to be cancelling. (#2804)
this._delay(function() {
// Handle .dialog().dialog("close") (#4065)
if ( $.ui.dialog.overlayInstances ) {
this.document.bind( "focusin.dialog", function( event ) {
if ( !that._allowInteraction( event ) ) {
event.preventDefault();
$(".ui-dialog:visible:last .ui-dialog-content")
.data( widgetFullName )._focusTabbable();
}
});
}
});
}
this.overlay = $("<div>")
.addClass("ui-widget-overlay ui-front")
.appendTo( this._appendTo() );
this._on( this.overlay, {
mousedown: "_keepFocus"
});
$.ui.dialog.overlayInstances++;
},
_destroyOverlay: function() {
if ( !this.options.modal ) {
return;
}
if ( this.overlay ) {
$.ui.dialog.overlayInstances--;
if ( !$.ui.dialog.overlayInstances ) {
this.document.unbind( "focusin.dialog" );
}
this.overlay.remove();
this.overlay = null;
}
}
});
$.ui.dialog.overlayInstances = 0;
// DEPRECATED
if ( $.uiBackCompat !== false ) {
// position option with array notation
// just override with old implementation
$.widget( "ui.dialog", $.ui.dialog, {
_position: function() {
var position = this.options.position,
myAt = [],
offset = [ 0, 0 ],
isVisible;
if ( position ) {
if ( typeof position === "string" || (typeof position === "object" && "0" in position ) ) {
myAt = position.split ? position.split(" ") : [ position[0], position[1] ];
if ( myAt.length === 1 ) {
myAt[1] = myAt[0];
}
$.each( [ "left", "top" ], function( i, offsetPosition ) {
if ( +myAt[ i ] === myAt[ i ] ) {
offset[ i ] = myAt[ i ];
myAt[ i ] = offsetPosition;
}
});
position = {
my: myAt[0] + (offset[0] < 0 ? offset[0] : "+" + offset[0]) + " " +
myAt[1] + (offset[1] < 0 ? offset[1] : "+" + offset[1]),
at: myAt.join(" ")
};
}
position = $.extend( {}, $.ui.dialog.prototype.options.position, position );
} else {
position = $.ui.dialog.prototype.options.position;
}
// need to show the dialog to get the actual offset in the position plugin
isVisible = this.uiDialog.is(":visible");
if ( !isVisible ) {
this.uiDialog.show();
}
this.uiDialog.position( position );
if ( !isVisible ) {
this.uiDialog.hide();
}
}
});
}
}( jQuery ) );
(function( $, undefined ) {
var rvertical = /up|down|vertical/,
rpositivemotion = /up|left|vertical|horizontal/;
$.effects.effect.blind = function( o, done ) {
// Create element
var el = $( this ),
props = [ "position", "top", "bottom", "left", "right", "height", "width" ],
mode = $.effects.setMode( el, o.mode || "hide" ),
direction = o.direction || "up",
vertical = rvertical.test( direction ),
ref = vertical ? "height" : "width",
ref2 = vertical ? "top" : "left",
motion = rpositivemotion.test( direction ),
animation = {},
show = mode === "show",
wrapper, distance, margin;
// if already wrapped, the wrapper's properties are my property. #6245
if ( el.parent().is( ".ui-effects-wrapper" ) ) {
$.effects.save( el.parent(), props );
} else {
$.effects.save( el, props );
}
el.show();
wrapper = $.effects.createWrapper( el ).css({
overflow: "hidden"
});
distance = wrapper[ ref ]();
margin = parseFloat( wrapper.css( ref2 ) ) || 0;
animation[ ref ] = show ? distance : 0;
if ( !motion ) {
el
.css( vertical ? "bottom" : "right", 0 )
.css( vertical ? "top" : "left", "auto" )
.css({ position: "absolute" });
animation[ ref2 ] = show ? margin : distance + margin;
}
// start at 0 if we are showing
if ( show ) {
wrapper.css( ref, 0 );
if ( ! motion ) {
wrapper.css( ref2, margin + distance );
}
}
// Animate
wrapper.animate( animation, {
duration: o.duration,
easing: o.easing,
queue: false,
complete: function() {
if ( mode === "hide" ) {
el.hide();
}
$.effects.restore( el, props );
$.effects.removeWrapper( el );
done();
}
});
};
})(jQuery);
(function( $, undefined ) {
$.effects.effect.bounce = function( o, done ) {
var el = $( this ),
props = [ "position", "top", "bottom", "left", "right", "height", "width" ],
// defaults:
mode = $.effects.setMode( el, o.mode || "effect" ),
hide = mode === "hide",
show = mode === "show",
direction = o.direction || "up",
distance = o.distance,
times = o.times || 5,
// number of internal animations
anims = times * 2 + ( show || hide ? 1 : 0 ),
speed = o.duration / anims,
easing = o.easing,
// utility:
ref = ( direction === "up" || direction === "down" ) ? "top" : "left",
motion = ( direction === "up" || direction === "left" ),
i,
upAnim,
downAnim,
// we will need to re-assemble the queue to stack our animations in place
queue = el.queue(),
queuelen = queue.length;
// Avoid touching opacity to prevent clearType and PNG issues in IE
if ( show || hide ) {
props.push( "opacity" );
}
$.effects.save( el, props );
el.show();
$.effects.createWrapper( el ); // Create Wrapper
// default distance for the BIGGEST bounce is the outer Distance / 3
if ( !distance ) {
distance = el[ ref === "top" ? "outerHeight" : "outerWidth" ]() / 3;
}
if ( show ) {
downAnim = { opacity: 1 };
downAnim[ ref ] = 0;
// if we are showing, force opacity 0 and set the initial position
// then do the "first" animation
el.css( "opacity", 0 )
.css( ref, motion ? -distance * 2 : distance * 2 )
.animate( downAnim, speed, easing );
}
// start at the smallest distance if we are hiding
if ( hide ) {
distance = distance / Math.pow( 2, times - 1 );
}
downAnim = {};
downAnim[ ref ] = 0;
// Bounces up/down/left/right then back to 0 -- times * 2 animations happen here
for ( i = 0; i < times; i++ ) {
upAnim = {};
upAnim[ ref ] = ( motion ? "-=" : "+=" ) + distance;
el.animate( upAnim, speed, easing )
.animate( downAnim, speed, easing );
distance = hide ? distance * 2 : distance / 2;
}
// Last Bounce when Hiding
if ( hide ) {
upAnim = { opacity: 0 };
upAnim[ ref ] = ( motion ? "-=" : "+=" ) + distance;
el.animate( upAnim, speed, easing );
}
el.queue(function() {
if ( hide ) {
el.hide();
}
$.effects.restore( el, props );
$.effects.removeWrapper( el );
done();
});
// inject all the animations we just queued to be first in line (after "inprogress")
if ( queuelen > 1) {
queue.splice.apply( queue,
[ 1, 0 ].concat( queue.splice( queuelen, anims + 1 ) ) );
}
el.dequeue();
};
})(jQuery);
(function( $, undefined ) {
$.effects.effect.clip = function( o, done ) {
// Create element
var el = $( this ),
props = [ "position", "top", "bottom", "left", "right", "height", "width" ],
mode = $.effects.setMode( el, o.mode || "hide" ),
show = mode === "show",
direction = o.direction || "vertical",
vert = direction === "vertical",
size = vert ? "height" : "width",
position = vert ? "top" : "left",
animation = {},
wrapper, animate, distance;
// Save & Show
$.effects.save( el, props );
el.show();
// Create Wrapper
wrapper = $.effects.createWrapper( el ).css({
overflow: "hidden"
});
animate = ( el[0].tagName === "IMG" ) ? wrapper : el;
distance = animate[ size ]();
// Shift
if ( show ) {
animate.css( size, 0 );
animate.css( position, distance / 2 );
}
// Create Animation Object:
animation[ size ] = show ? distance : 0;
animation[ position ] = show ? 0 : distance / 2;
// Animate
animate.animate( animation, {
queue: false,
duration: o.duration,
easing: o.easing,
complete: function() {
if ( !show ) {
el.hide();
}
$.effects.restore( el, props );
$.effects.removeWrapper( el );
done();
}
});
};
})(jQuery);
(function( $, undefined ) {
$.effects.effect.drop = function( o, done ) {
var el = $( this ),
props = [ "position", "top", "bottom", "left", "right", "opacity", "height", "width" ],
mode = $.effects.setMode( el, o.mode || "hide" ),
show = mode === "show",
direction = o.direction || "left",
ref = ( direction === "up" || direction === "down" ) ? "top" : "left",
motion = ( direction === "up" || direction === "left" ) ? "pos" : "neg",
animation = {
opacity: show ? 1 : 0
},
distance;
// Adjust
$.effects.save( el, props );
el.show();
$.effects.createWrapper( el );
distance = o.distance || el[ ref === "top" ? "outerHeight": "outerWidth" ]( true ) / 2;
if ( show ) {
el
.css( "opacity", 0 )
.css( ref, motion === "pos" ? -distance : distance );
}
// Animation
animation[ ref ] = ( show ?
( motion === "pos" ? "+=" : "-=" ) :
( motion === "pos" ? "-=" : "+=" ) ) +
distance;
// Animate
el.animate( animation, {
queue: false,
duration: o.duration,
easing: o.easing,
complete: function() {
if ( mode === "hide" ) {
el.hide();
}
$.effects.restore( el, props );
$.effects.removeWrapper( el );
done();
}
});
};
})(jQuery);
(function( $, undefined ) {
$.effects.effect.explode = function( o, done ) {
var rows = o.pieces ? Math.round( Math.sqrt( o.pieces ) ) : 3,
cells = rows,
el = $( this ),
mode = $.effects.setMode( el, o.mode || "hide" ),
show = mode === "show",
// show and then visibility:hidden the element before calculating offset
offset = el.show().css( "visibility", "hidden" ).offset(),
// width and height of a piece
width = Math.ceil( el.outerWidth() / cells ),
height = Math.ceil( el.outerHeight() / rows ),
pieces = [],
// loop
i, j, left, top, mx, my;
// children animate complete:
function childComplete() {
pieces.push( this );
if ( pieces.length === rows * cells ) {
animComplete();
}
}
// clone the element for each row and cell.
for( i = 0; i < rows ; i++ ) { // ===>
top = offset.top + i * height;
my = i - ( rows - 1 ) / 2 ;
for( j = 0; j < cells ; j++ ) { // |||
left = offset.left + j * width;
mx = j - ( cells - 1 ) / 2 ;
// Create a clone of the now hidden main element that will be absolute positioned
// within a wrapper div off the -left and -top equal to size of our pieces
el
.clone()
.appendTo( "body" )
.wrap( "<div></div>" )
.css({
position: "absolute",
visibility: "visible",
left: -j * width,
top: -i * height
})
// select the wrapper - make it overflow: hidden and absolute positioned based on
// where the original was located +left and +top equal to the size of pieces
.parent()
.addClass( "ui-effects-explode" )
.css({
position: "absolute",
overflow: "hidden",
width: width,
height: height,
left: left + ( show ? mx * width : 0 ),
top: top + ( show ? my * height : 0 ),
opacity: show ? 0 : 1
}).animate({
left: left + ( show ? 0 : mx * width ),
top: top + ( show ? 0 : my * height ),
opacity: show ? 1 : 0
}, o.duration || 500, o.easing, childComplete );
}
}
function animComplete() {
el.css({
visibility: "visible"
});
$( pieces ).remove();
if ( !show ) {
el.hide();
}
done();
}
};
})(jQuery);
(function( $, undefined ) {
$.effects.effect.fade = function( o, done ) {
var el = $( this ),
mode = $.effects.setMode( el, o.mode || "toggle" );
el.animate({
opacity: mode
}, {
queue: false,
duration: o.duration,
easing: o.easing,
complete: done
});
};
})( jQuery );
(function( $, undefined ) {
$.effects.effect.fold = function( o, done ) {
// Create element
var el = $( this ),
props = [ "position", "top", "bottom", "left", "right", "height", "width" ],
mode = $.effects.setMode( el, o.mode || "hide" ),
show = mode === "show",
hide = mode === "hide",
size = o.size || 15,
percent = /([0-9]+)%/.exec( size ),
horizFirst = !!o.horizFirst,
widthFirst = show !== horizFirst,
ref = widthFirst ? [ "width", "height" ] : [ "height", "width" ],
duration = o.duration / 2,
wrapper, distance,
animation1 = {},
animation2 = {};
$.effects.save( el, props );
el.show();
// Create Wrapper
wrapper = $.effects.createWrapper( el ).css({
overflow: "hidden"
});
distance = widthFirst ?
[ wrapper.width(), wrapper.height() ] :
[ wrapper.height(), wrapper.width() ];
if ( percent ) {
size = parseInt( percent[ 1 ], 10 ) / 100 * distance[ hide ? 0 : 1 ];
}
if ( show ) {
wrapper.css( horizFirst ? {
height: 0,
width: size
} : {
height: size,
width: 0
});
}
// Animation
animation1[ ref[ 0 ] ] = show ? distance[ 0 ] : size;
animation2[ ref[ 1 ] ] = show ? distance[ 1 ] : 0;
// Animate
wrapper
.animate( animation1, duration, o.easing )
.animate( animation2, duration, o.easing, function() {
if ( hide ) {
el.hide();
}
$.effects.restore( el, props );
$.effects.removeWrapper( el );
done();
});
};
})(jQuery);
(function( $, undefined ) {
$.effects.effect.highlight = function( o, done ) {
var elem = $( this ),
props = [ "backgroundImage", "backgroundColor", "opacity" ],
mode = $.effects.setMode( elem, o.mode || "show" ),
animation = {
backgroundColor: elem.css( "backgroundColor" )
};
if (mode === "hide") {
animation.opacity = 0;
}
$.effects.save( elem, props );
elem
.show()
.css({
backgroundImage: "none",
backgroundColor: o.color || "#ffff99"
})
.animate( animation, {
queue: false,
duration: o.duration,
easing: o.easing,
complete: function() {
if ( mode === "hide" ) {
elem.hide();
}
$.effects.restore( elem, props );
done();
}
});
};
})(jQuery);
(function( $, undefined ) {
$.effects.effect.pulsate = function( o, done ) {
var elem = $( this ),
mode = $.effects.setMode( elem, o.mode || "show" ),
show = mode === "show",
hide = mode === "hide",
showhide = ( show || mode === "hide" ),
// showing or hiding leaves of the "last" animation
anims = ( ( o.times || 5 ) * 2 ) + ( showhide ? 1 : 0 ),
duration = o.duration / anims,
animateTo = 0,
queue = elem.queue(),
queuelen = queue.length,
i;
if ( show || !elem.is(":visible")) {
elem.css( "opacity", 0 ).show();
animateTo = 1;
}
// anims - 1 opacity "toggles"
for ( i = 1; i < anims; i++ ) {
elem.animate({
opacity: animateTo
}, duration, o.easing );
animateTo = 1 - animateTo;
}
elem.animate({
opacity: animateTo
}, duration, o.easing);
elem.queue(function() {
if ( hide ) {
elem.hide();
}
done();
});
// We just queued up "anims" animations, we need to put them next in the queue
if ( queuelen > 1 ) {
queue.splice.apply( queue,
[ 1, 0 ].concat( queue.splice( queuelen, anims + 1 ) ) );
}
elem.dequeue();
};
})(jQuery);
(function( $, undefined ) {
$.effects.effect.puff = function( o, done ) {
var elem = $( this ),
mode = $.effects.setMode( elem, o.mode || "hide" ),
hide = mode === "hide",
percent = parseInt( o.percent, 10 ) || 150,
factor = percent / 100,
original = {
height: elem.height(),
width: elem.width(),
outerHeight: elem.outerHeight(),
outerWidth: elem.outerWidth()
};
$.extend( o, {
effect: "scale",
queue: false,
fade: true,
mode: mode,
complete: done,
percent: hide ? percent : 100,
from: hide ?
original :
{
height: original.height * factor,
width: original.width * factor,
outerHeight: original.outerHeight * factor,
outerWidth: original.outerWidth * factor
}
});
elem.effect( o );
};
$.effects.effect.scale = function( o, done ) {
// Create element
var el = $( this ),
options = $.extend( true, {}, o ),
mode = $.effects.setMode( el, o.mode || "effect" ),
percent = parseInt( o.percent, 10 ) ||
( parseInt( o.percent, 10 ) === 0 ? 0 : ( mode === "hide" ? 0 : 100 ) ),
direction = o.direction || "both",
origin = o.origin,
original = {
height: el.height(),
width: el.width(),
outerHeight: el.outerHeight(),
outerWidth: el.outerWidth()
},
factor = {
y: direction !== "horizontal" ? (percent / 100) : 1,
x: direction !== "vertical" ? (percent / 100) : 1
};
// We are going to pass this effect to the size effect:
options.effect = "size";
options.queue = false;
options.complete = done;
// Set default origin and restore for show/hide
if ( mode !== "effect" ) {
options.origin = origin || ["middle","center"];
options.restore = true;
}
options.from = o.from || ( mode === "show" ? {
height: 0,
width: 0,
outerHeight: 0,
outerWidth: 0
} : original );
options.to = {
height: original.height * factor.y,
width: original.width * factor.x,
outerHeight: original.outerHeight * factor.y,
outerWidth: original.outerWidth * factor.x
};
// Fade option to support puff
if ( options.fade ) {
if ( mode === "show" ) {
options.from.opacity = 0;
options.to.opacity = 1;
}
if ( mode === "hide" ) {
options.from.opacity = 1;
options.to.opacity = 0;
}
}
// Animate
el.effect( options );
};
$.effects.effect.size = function( o, done ) {
// Create element
var original, baseline, factor,
el = $( this ),
props0 = [ "position", "top", "bottom", "left", "right", "width", "height", "overflow", "opacity" ],
// Always restore
props1 = [ "position", "top", "bottom", "left", "right", "overflow", "opacity" ],
// Copy for children
props2 = [ "width", "height", "overflow" ],
cProps = [ "fontSize" ],
vProps = [ "borderTopWidth", "borderBottomWidth", "paddingTop", "paddingBottom" ],
hProps = [ "borderLeftWidth", "borderRightWidth", "paddingLeft", "paddingRight" ],
// Set options
mode = $.effects.setMode( el, o.mode || "effect" ),
restore = o.restore || mode !== "effect",
scale = o.scale || "both",
origin = o.origin || [ "middle", "center" ],
position = el.css( "position" ),
props = restore ? props0 : props1,
zero = {
height: 0,
width: 0,
outerHeight: 0,
outerWidth: 0
};
if ( mode === "show" ) {
el.show();
}
original = {
height: el.height(),
width: el.width(),
outerHeight: el.outerHeight(),
outerWidth: el.outerWidth()
};
if ( o.mode === "toggle" && mode === "show" ) {
el.from = o.to || zero;
el.to = o.from || original;
} else {
el.from = o.from || ( mode === "show" ? zero : original );
el.to = o.to || ( mode === "hide" ? zero : original );
}
// Set scaling factor
factor = {
from: {
y: el.from.height / original.height,
x: el.from.width / original.width
},
to: {
y: el.to.height / original.height,
x: el.to.width / original.width
}
};
// Scale the css box
if ( scale === "box" || scale === "both" ) {
// Vertical props scaling
if ( factor.from.y !== factor.to.y ) {
props = props.concat( vProps );
el.from = $.effects.setTransition( el, vProps, factor.from.y, el.from );
el.to = $.effects.setTransition( el, vProps, factor.to.y, el.to );
}
// Horizontal props scaling
if ( factor.from.x !== factor.to.x ) {
props = props.concat( hProps );
el.from = $.effects.setTransition( el, hProps, factor.from.x, el.from );
el.to = $.effects.setTransition( el, hProps, factor.to.x, el.to );
}
}
// Scale the content
if ( scale === "content" || scale === "both" ) {
// Vertical props scaling
if ( factor.from.y !== factor.to.y ) {
props = props.concat( cProps ).concat( props2 );
el.from = $.effects.setTransition( el, cProps, factor.from.y, el.from );
el.to = $.effects.setTransition( el, cProps, factor.to.y, el.to );
}
}
$.effects.save( el, props );
el.show();
$.effects.createWrapper( el );
el.css( "overflow", "hidden" ).css( el.from );
// Adjust
if (origin) { // Calculate baseline shifts
baseline = $.effects.getBaseline( origin, original );
el.from.top = ( original.outerHeight - el.outerHeight() ) * baseline.y;
el.from.left = ( original.outerWidth - el.outerWidth() ) * baseline.x;
el.to.top = ( original.outerHeight - el.to.outerHeight ) * baseline.y;
el.to.left = ( original.outerWidth - el.to.outerWidth ) * baseline.x;
}
el.css( el.from ); // set top & left
// Animate
if ( scale === "content" || scale === "both" ) { // Scale the children
// Add margins/font-size
vProps = vProps.concat([ "marginTop", "marginBottom" ]).concat(cProps);
hProps = hProps.concat([ "marginLeft", "marginRight" ]);
props2 = props0.concat(vProps).concat(hProps);
el.find( "*[width]" ).each( function(){
var child = $( this ),
c_original = {
height: child.height(),
width: child.width(),
outerHeight: child.outerHeight(),
outerWidth: child.outerWidth()
};
if (restore) {
$.effects.save(child, props2);
}
child.from = {
height: c_original.height * factor.from.y,
width: c_original.width * factor.from.x,
outerHeight: c_original.outerHeight * factor.from.y,
outerWidth: c_original.outerWidth * factor.from.x
};
child.to = {
height: c_original.height * factor.to.y,
width: c_original.width * factor.to.x,
outerHeight: c_original.height * factor.to.y,
outerWidth: c_original.width * factor.to.x
};
// Vertical props scaling
if ( factor.from.y !== factor.to.y ) {
child.from = $.effects.setTransition( child, vProps, factor.from.y, child.from );
child.to = $.effects.setTransition( child, vProps, factor.to.y, child.to );
}
// Horizontal props scaling
if ( factor.from.x !== factor.to.x ) {
child.from = $.effects.setTransition( child, hProps, factor.from.x, child.from );
child.to = $.effects.setTransition( child, hProps, factor.to.x, child.to );
}
// Animate children
child.css( child.from );
child.animate( child.to, o.duration, o.easing, function() {
// Restore children
if ( restore ) {
$.effects.restore( child, props2 );
}
});
});
}
// Animate
el.animate( el.to, {
queue: false,
duration: o.duration,
easing: o.easing,
complete: function() {
if ( el.to.opacity === 0 ) {
el.css( "opacity", el.from.opacity );
}
if( mode === "hide" ) {
el.hide();
}
$.effects.restore( el, props );
if ( !restore ) {
// we need to calculate our new positioning based on the scaling
if ( position === "static" ) {
el.css({
position: "relative",
top: el.to.top,
left: el.to.left
});
} else {
$.each([ "top", "left" ], function( idx, pos ) {
el.css( pos, function( _, str ) {
var val = parseInt( str, 10 ),
toRef = idx ? el.to.left : el.to.top;
// if original was "auto", recalculate the new value from wrapper
if ( str === "auto" ) {
return toRef + "px";
}
return val + toRef + "px";
});
});
}
}
$.effects.removeWrapper( el );
done();
}
});
};
})(jQuery);
(function( $, undefined ) {
$.effects.effect.shake = function( o, done ) {
var el = $( this ),
props = [ "position", "top", "bottom", "left", "right", "height", "width" ],
mode = $.effects.setMode( el, o.mode || "effect" ),
direction = o.direction || "left",
distance = o.distance || 20,
times = o.times || 3,
anims = times * 2 + 1,
speed = Math.round(o.duration/anims),
ref = (direction === "up" || direction === "down") ? "top" : "left",
positiveMotion = (direction === "up" || direction === "left"),
animation = {},
animation1 = {},
animation2 = {},
i,
// we will need to re-assemble the queue to stack our animations in place
queue = el.queue(),
queuelen = queue.length;
$.effects.save( el, props );
el.show();
$.effects.createWrapper( el );
// Animation
animation[ ref ] = ( positiveMotion ? "-=" : "+=" ) + distance;
animation1[ ref ] = ( positiveMotion ? "+=" : "-=" ) + distance * 2;
animation2[ ref ] = ( positiveMotion ? "-=" : "+=" ) + distance * 2;
// Animate
el.animate( animation, speed, o.easing );
// Shakes
for ( i = 1; i < times; i++ ) {
el.animate( animation1, speed, o.easing ).animate( animation2, speed, o.easing );
}
el
.animate( animation1, speed, o.easing )
.animate( animation, speed / 2, o.easing )
.queue(function() {
if ( mode === "hide" ) {
el.hide();
}
$.effects.restore( el, props );
$.effects.removeWrapper( el );
done();
});
// inject all the animations we just queued to be first in line (after "inprogress")
if ( queuelen > 1) {
queue.splice.apply( queue,
[ 1, 0 ].concat( queue.splice( queuelen, anims + 1 ) ) );
}
el.dequeue();
};
})(jQuery);
(function( $, undefined ) {
$.effects.effect.slide = function( o, done ) {
// Create element
var el = $( this ),
props = [ "position", "top", "bottom", "left", "right", "width", "height" ],
mode = $.effects.setMode( el, o.mode || "show" ),
show = mode === "show",
direction = o.direction || "left",
ref = (direction === "up" || direction === "down") ? "top" : "left",
positiveMotion = (direction === "up" || direction === "left"),
distance,
animation = {};
// Adjust
$.effects.save( el, props );
el.show();
distance = o.distance || el[ ref === "top" ? "outerHeight" : "outerWidth" ]( true );
$.effects.createWrapper( el ).css({
overflow: "hidden"
});
if ( show ) {
el.css( ref, positiveMotion ? (isNaN(distance) ? "-" + distance : -distance) : distance );
}
// Animation
animation[ ref ] = ( show ?
( positiveMotion ? "+=" : "-=") :
( positiveMotion ? "-=" : "+=")) +
distance;
// Animate
el.animate( animation, {
queue: false,
duration: o.duration,
easing: o.easing,
complete: function() {
if ( mode === "hide" ) {
el.hide();
}
$.effects.restore( el, props );
$.effects.removeWrapper( el );
done();
}
});
};
})(jQuery);
(function( $, undefined ) {
$.effects.effect.transfer = function( o, done ) {
var elem = $( this ),
target = $( o.to ),
targetFixed = target.css( "position" ) === "fixed",
body = $("body"),
fixTop = targetFixed ? body.scrollTop() : 0,
fixLeft = targetFixed ? body.scrollLeft() : 0,
endPosition = target.offset(),
animation = {
top: endPosition.top - fixTop ,
left: endPosition.left - fixLeft ,
height: target.innerHeight(),
width: target.innerWidth()
},
startPosition = elem.offset(),
transfer = $( "<div class='ui-effects-transfer'></div>" )
.appendTo( document.body )
.addClass( o.className )
.css({
top: startPosition.top - fixTop ,
left: startPosition.left - fixLeft ,
height: elem.innerHeight(),
width: elem.innerWidth(),
position: targetFixed ? "fixed" : "absolute"
})
.animate( animation, o.duration, o.easing, function() {
transfer.remove();
done();
});
};
})(jQuery);
(function( $, undefined ) {
$.widget( "ui.menu", {
version: "1.10.3",
defaultElement: "<ul>",
delay: 300,
options: {
icons: {
submenu: "ui-icon-carat-1-e"
},
menus: "ul",
position: {
my: "left top",
at: "right top"
},
role: "menu",
// callbacks
blur: null,
focus: null,
select: null
},
_create: function() {
this.activeMenu = this.element;
// flag used to prevent firing of the click handler
// as the event bubbles up through nested menus
this.mouseHandled = false;
this.element
.uniqueId()
.addClass( "ui-menu ui-widget ui-widget-content ui-corner-all" )
.toggleClass( "ui-menu-icons", !!this.element.find( ".ui-icon" ).length )
.attr({
role: this.options.role,
tabIndex: 0
})
// need to catch all clicks on disabled menu
// not possible through _on
.bind( "click" + this.eventNamespace, $.proxy(function( event ) {
if ( this.options.disabled ) {
event.preventDefault();
}
}, this ));
if ( this.options.disabled ) {
this.element
.addClass( "ui-state-disabled" )
.attr( "aria-disabled", "true" );
}
this._on({
// Prevent focus from sticking to links inside menu after clicking
// them (focus should always stay on UL during navigation).
"mousedown .ui-menu-item > a": function( event ) {
event.preventDefault();
},
"click .ui-state-disabled > a": function( event ) {
event.preventDefault();
},
"click .ui-menu-item:has(a)": function( event ) {
var target = $( event.target ).closest( ".ui-menu-item" );
if ( !this.mouseHandled && target.not( ".ui-state-disabled" ).length ) {
this.mouseHandled = true;
this.select( event );
// Open submenu on click
if ( target.has( ".ui-menu" ).length ) {
this.expand( event );
} else if ( !this.element.is( ":focus" ) ) {
// Redirect focus to the menu
this.element.trigger( "focus", [ true ] );
// If the active item is on the top level, let it stay active.
// Otherwise, blur the active item since it is no longer visible.
if ( this.active && this.active.parents( ".ui-menu" ).length === 1 ) {
clearTimeout( this.timer );
}
}
}
},
"mouseenter .ui-menu-item": function( event ) {
var target = $( event.currentTarget );
// Remove ui-state-active class from siblings of the newly focused menu item
// to avoid a jump caused by adjacent elements both having a class with a border
target.siblings().children( ".ui-state-active" ).removeClass( "ui-state-active" );
this.focus( event, target );
},
mouseleave: "collapseAll",
"mouseleave .ui-menu": "collapseAll",
focus: function( event, keepActiveItem ) {
// If there's already an active item, keep it active
// If not, activate the first item
var item = this.active || this.element.children( ".ui-menu-item" ).eq( 0 );
if ( !keepActiveItem ) {
this.focus( event, item );
}
},
blur: function( event ) {
this._delay(function() {
if ( !$.contains( this.element[0], this.document[0].activeElement ) ) {
this.collapseAll( event );
}
});
},
keydown: "_keydown"
});
this.refresh();
// Clicks outside of a menu collapse any open menus
this._on( this.document, {
click: function( event ) {
if ( !$( event.target ).closest( ".ui-menu" ).length ) {
this.collapseAll( event );
}
// Reset the mouseHandled flag
this.mouseHandled = false;
}
});
},
_destroy: function() {
// Destroy (sub)menus
this.element
.removeAttr( "aria-activedescendant" )
.find( ".ui-menu" ).addBack()
.removeClass( "ui-menu ui-widget ui-widget-content ui-corner-all ui-menu-icons" )
.removeAttr( "role" )
.removeAttr( "tabIndex" )
.removeAttr( "aria-labelledby" )
.removeAttr( "aria-expanded" )
.removeAttr( "aria-hidden" )
.removeAttr( "aria-disabled" )
.removeUniqueId()
.show();
// Destroy menu items
this.element.find( ".ui-menu-item" )
.removeClass( "ui-menu-item" )
.removeAttr( "role" )
.removeAttr( "aria-disabled" )
.children( "a" )
.removeUniqueId()
.removeClass( "ui-corner-all ui-state-hover" )
.removeAttr( "tabIndex" )
.removeAttr( "role" )
.removeAttr( "aria-haspopup" )
.children().each( function() {
var elem = $( this );
if ( elem.data( "ui-menu-submenu-carat" ) ) {
elem.remove();
}
});
// Destroy menu dividers
this.element.find( ".ui-menu-divider" ).removeClass( "ui-menu-divider ui-widget-content" );
},
_keydown: function( event ) {
/*jshint maxcomplexity:20*/
var match, prev, character, skip, regex,
preventDefault = true;
function escape( value ) {
return value.replace( /[\-\[\]{}()*+?.,\\\^$|#\s]/g, "\\$&" );
}
switch ( event.keyCode ) {
case $.ui.keyCode.PAGE_UP:
this.previousPage( event );
break;
case $.ui.keyCode.PAGE_DOWN:
this.nextPage( event );
break;
case $.ui.keyCode.HOME:
this._move( "first", "first", event );
break;
case $.ui.keyCode.END:
this._move( "last", "last", event );
break;
case $.ui.keyCode.UP:
this.previous( event );
break;
case $.ui.keyCode.DOWN:
this.next( event );
break;
case $.ui.keyCode.LEFT:
this.collapse( event );
break;
case $.ui.keyCode.RIGHT:
if ( this.active && !this.active.is( ".ui-state-disabled" ) ) {
this.expand( event );
}
break;
case $.ui.keyCode.ENTER:
case $.ui.keyCode.SPACE:
this._activate( event );
break;
case $.ui.keyCode.ESCAPE:
this.collapse( event );
break;
default:
preventDefault = false;
prev = this.previousFilter || "";
character = String.fromCharCode( event.keyCode );
skip = false;
clearTimeout( this.filterTimer );
if ( character === prev ) {
skip = true;
} else {
character = prev + character;
}
regex = new RegExp( "^" + escape( character ), "i" );
match = this.activeMenu.children( ".ui-menu-item" ).filter(function() {
return regex.test( $( this ).children( "a" ).text() );
});
match = skip && match.index( this.active.next() ) !== -1 ?
this.active.nextAll( ".ui-menu-item" ) :
match;
// If no matches on the current filter, reset to the last character pressed
// to move down the menu to the first item that starts with that character
if ( !match.length ) {
character = String.fromCharCode( event.keyCode );
regex = new RegExp( "^" + escape( character ), "i" );
match = this.activeMenu.children( ".ui-menu-item" ).filter(function() {
return regex.test( $( this ).children( "a" ).text() );
});
}
if ( match.length ) {
this.focus( event, match );
if ( match.length > 1 ) {
this.previousFilter = character;
this.filterTimer = this._delay(function() {
delete this.previousFilter;
}, 1000 );
} else {
delete this.previousFilter;
}
} else {
delete this.previousFilter;
}
}
if ( preventDefault ) {
event.preventDefault();
}
},
_activate: function( event ) {
if ( !this.active.is( ".ui-state-disabled" ) ) {
if ( this.active.children( "a[aria-haspopup='true']" ).length ) {
this.expand( event );
} else {
this.select( event );
}
}
},
refresh: function() {
var menus,
icon = this.options.icons.submenu,
submenus = this.element.find( this.options.menus );
// Initialize nested menus
submenus.filter( ":not(.ui-menu)" )
.addClass( "ui-menu ui-widget ui-widget-content ui-corner-all" )
.hide()
.attr({
role: this.options.role,
"aria-hidden": "true",
"aria-expanded": "false"
})
.each(function() {
var menu = $( this ),
item = menu.prev( "a" ),
submenuCarat = $( "<span>" )
.addClass( "ui-menu-icon ui-icon " + icon )
.data( "ui-menu-submenu-carat", true );
item
.attr( "aria-haspopup", "true" )
.prepend( submenuCarat );
menu.attr( "aria-labelledby", item.attr( "id" ) );
});
menus = submenus.add( this.element );
// Don't refresh list items that are already adapted
menus.children( ":not(.ui-menu-item):has(a)" )
.addClass( "ui-menu-item" )
.attr( "role", "presentation" )
.children( "a" )
.uniqueId()
.addClass( "ui-corner-all" )
.attr({
tabIndex: -1,
role: this._itemRole()
});
// Initialize unlinked menu-items containing spaces and/or dashes only as dividers
menus.children( ":not(.ui-menu-item)" ).each(function() {
var item = $( this );
// hyphen, em dash, en dash
if ( !/[^\-\u2014\u2013\s]/.test( item.text() ) ) {
item.addClass( "ui-widget-content ui-menu-divider" );
}
});
// Add aria-disabled attribute to any disabled menu item
menus.children( ".ui-state-disabled" ).attr( "aria-disabled", "true" );
// If the active item has been removed, blur the menu
if ( this.active && !$.contains( this.element[ 0 ], this.active[ 0 ] ) ) {
this.blur();
}
},
_itemRole: function() {
return {
menu: "menuitem",
listbox: "option"
}[ this.options.role ];
},
_setOption: function( key, value ) {
if ( key === "icons" ) {
this.element.find( ".ui-menu-icon" )
.removeClass( this.options.icons.submenu )
.addClass( value.submenu );
}
this._super( key, value );
},
focus: function( event, item ) {
var nested, focused;
this.blur( event, event && event.type === "focus" );
this._scrollIntoView( item );
this.active = item.first();
focused = this.active.children( "a" ).addClass( "ui-state-focus" );
// Only update aria-activedescendant if there's a role
// otherwise we assume focus is managed elsewhere
if ( this.options.role ) {
this.element.attr( "aria-activedescendant", focused.attr( "id" ) );
}
// Highlight active parent menu item, if any
this.active
.parent()
.closest( ".ui-menu-item" )
.children( "a:first" )
.addClass( "ui-state-active" );
if ( event && event.type === "keydown" ) {
this._close();
} else {
this.timer = this._delay(function() {
this._close();
}, this.delay );
}
nested = item.children( ".ui-menu" );
if ( nested.length && ( /^mouse/.test( event.type ) ) ) {
this._startOpening(nested);
}
this.activeMenu = item.parent();
this._trigger( "focus", event, { item: item } );
},
_scrollIntoView: function( item ) {
var borderTop, paddingTop, offset, scroll, elementHeight, itemHeight;
if ( this._hasScroll() ) {
borderTop = parseFloat( $.css( this.activeMenu[0], "borderTopWidth" ) ) || 0;
paddingTop = parseFloat( $.css( this.activeMenu[0], "paddingTop" ) ) || 0;
offset = item.offset().top - this.activeMenu.offset().top - borderTop - paddingTop;
scroll = this.activeMenu.scrollTop();
elementHeight = this.activeMenu.height();
itemHeight = item.height();
if ( offset < 0 ) {
this.activeMenu.scrollTop( scroll + offset );
} else if ( offset + itemHeight > elementHeight ) {
this.activeMenu.scrollTop( scroll + offset - elementHeight + itemHeight );
}
}
},
blur: function( event, fromFocus ) {
if ( !fromFocus ) {
clearTimeout( this.timer );
}
if ( !this.active ) {
return;
}
this.active.children( "a" ).removeClass( "ui-state-focus" );
this.active = null;
this._trigger( "blur", event, { item: this.active } );
},
_startOpening: function( submenu ) {
clearTimeout( this.timer );
// Don't open if already open fixes a Firefox bug that caused a .5 pixel
// shift in the submenu position when mousing over the carat icon
if ( submenu.attr( "aria-hidden" ) !== "true" ) {
return;
}
this.timer = this._delay(function() {
this._close();
this._open( submenu );
}, this.delay );
},
_open: function( submenu ) {
var position = $.extend({
of: this.active
}, this.options.position );
clearTimeout( this.timer );
this.element.find( ".ui-menu" ).not( submenu.parents( ".ui-menu" ) )
.hide()
.attr( "aria-hidden", "true" );
submenu
.show()
.removeAttr( "aria-hidden" )
.attr( "aria-expanded", "true" )
.position( position );
},
collapseAll: function( event, all ) {
clearTimeout( this.timer );
this.timer = this._delay(function() {
// If we were passed an event, look for the submenu that contains the event
var currentMenu = all ? this.element :
$( event && event.target ).closest( this.element.find( ".ui-menu" ) );
// If we found no valid submenu ancestor, use the main menu to close all sub menus anyway
if ( !currentMenu.length ) {
currentMenu = this.element;
}
this._close( currentMenu );
this.blur( event );
this.activeMenu = currentMenu;
}, this.delay );
},
// With no arguments, closes the currently active menu - if nothing is active
// it closes all menus. If passed an argument, it will search for menus BELOW
_close: function( startMenu ) {
if ( !startMenu ) {
startMenu = this.active ? this.active.parent() : this.element;
}
startMenu
.find( ".ui-menu" )
.hide()
.attr( "aria-hidden", "true" )
.attr( "aria-expanded", "false" )
.end()
.find( "a.ui-state-active" )
.removeClass( "ui-state-active" );
},
collapse: function( event ) {
var newItem = this.active &&
this.active.parent().closest( ".ui-menu-item", this.element );
if ( newItem && newItem.length ) {
this._close();
this.focus( event, newItem );
}
},
expand: function( event ) {
var newItem = this.active &&
this.active
.children( ".ui-menu " )
.children( ".ui-menu-item" )
.first();
if ( newItem && newItem.length ) {
this._open( newItem.parent() );
// Delay so Firefox will not hide activedescendant change in expanding submenu from AT
this._delay(function() {
this.focus( event, newItem );
});
}
},
next: function( event ) {
this._move( "next", "first", event );
},
previous: function( event ) {
this._move( "prev", "last", event );
},
isFirstItem: function() {
return this.active && !this.active.prevAll( ".ui-menu-item" ).length;
},
isLastItem: function() {
return this.active && !this.active.nextAll( ".ui-menu-item" ).length;
},
_move: function( direction, filter, event ) {
var next;
if ( this.active ) {
if ( direction === "first" || direction === "last" ) {
next = this.active
[ direction === "first" ? "prevAll" : "nextAll" ]( ".ui-menu-item" )
.eq( -1 );
} else {
next = this.active
[ direction + "All" ]( ".ui-menu-item" )
.eq( 0 );
}
}
if ( !next || !next.length || !this.active ) {
next = this.activeMenu.children( ".ui-menu-item" )[ filter ]();
}
this.focus( event, next );
},
nextPage: function( event ) {
var item, base, height;
if ( !this.active ) {
this.next( event );
return;
}
if ( this.isLastItem() ) {
return;
}
if ( this._hasScroll() ) {
base = this.active.offset().top;
height = this.element.height();
this.active.nextAll( ".ui-menu-item" ).each(function() {
item = $( this );
return item.offset().top - base - height < 0;
});
this.focus( event, item );
} else {
this.focus( event, this.activeMenu.children( ".ui-menu-item" )
[ !this.active ? "first" : "last" ]() );
}
},
previousPage: function( event ) {
var item, base, height;
if ( !this.active ) {
this.next( event );
return;
}
if ( this.isFirstItem() ) {
return;
}
if ( this._hasScroll() ) {
base = this.active.offset().top;
height = this.element.height();
this.active.prevAll( ".ui-menu-item" ).each(function() {
item = $( this );
return item.offset().top - base + height > 0;
});
this.focus( event, item );
} else {
this.focus( event, this.activeMenu.children( ".ui-menu-item" ).first() );
}
},
_hasScroll: function() {
return this.element.outerHeight() < this.element.prop( "scrollHeight" );
},
select: function( event ) {
// TODO: It should never be possible to not have an active item at this
// point, but the tests don't trigger mouseenter before click.
this.active = this.active || $( event.target ).closest( ".ui-menu-item" );
var ui = { item: this.active };
if ( !this.active.has( ".ui-menu" ).length ) {
this.collapseAll( event, true );
}
this._trigger( "select", event, ui );
}
});
}( jQuery ));
(function( $, undefined ) {
$.ui = $.ui || {};
var cachedScrollbarWidth,
max = Math.max,
abs = Math.abs,
round = Math.round,
rhorizontal = /left|center|right/,
rvertical = /top|center|bottom/,
roffset = /[\+\-]\d+(\.[\d]+)?%?/,
rposition = /^\w+/,
rpercent = /%$/,
_position = $.fn.position;
function getOffsets( offsets, width, height ) {
return [
parseFloat( offsets[ 0 ] ) * ( rpercent.test( offsets[ 0 ] ) ? width / 100 : 1 ),
parseFloat( offsets[ 1 ] ) * ( rpercent.test( offsets[ 1 ] ) ? height / 100 : 1 )
];
}
function parseCss( element, property ) {
return parseInt( $.css( element, property ), 10 ) || 0;
}
function getDimensions( elem ) {
var raw = elem[0];
if ( raw.nodeType === 9 ) {
return {
width: elem.width(),
height: elem.height(),
offset: { top: 0, left: 0 }
};
}
if ( $.isWindow( raw ) ) {
return {
width: elem.width(),
height: elem.height(),
offset: { top: elem.scrollTop(), left: elem.scrollLeft() }
};
}
if ( raw.preventDefault ) {
return {
width: 0,
height: 0,
offset: { top: raw.pageY, left: raw.pageX }
};
}
return {
width: elem.outerWidth(),
height: elem.outerHeight(),
offset: elem.offset()
};
}
$.position = {
scrollbarWidth: function() {
if ( cachedScrollbarWidth !== undefined ) {
return cachedScrollbarWidth;
}
var w1, w2,
div = $( "<div style='display:block;width:50px;height:50px;overflow:hidden;'><div style='height:100px;width:auto;'></div></div>" ),
innerDiv = div.children()[0];
$( "body" ).append( div );
w1 = innerDiv.offsetWidth;
div.css( "overflow", "scroll" );
w2 = innerDiv.offsetWidth;
if ( w1 === w2 ) {
w2 = div[0].clientWidth;
}
div.remove();
return (cachedScrollbarWidth = w1 - w2);
},
getScrollInfo: function( within ) {
var overflowX = within.isWindow ? "" : within.element.css( "overflow-x" ),
overflowY = within.isWindow ? "" : within.element.css( "overflow-y" ),
hasOverflowX = overflowX === "scroll" ||
( overflowX === "auto" && within.width < within.element[0].scrollWidth ),
hasOverflowY = overflowY === "scroll" ||
( overflowY === "auto" && within.height < within.element[0].scrollHeight );
return {
width: hasOverflowY ? $.position.scrollbarWidth() : 0,
height: hasOverflowX ? $.position.scrollbarWidth() : 0
};
},
getWithinInfo: function( element ) {
var withinElement = $( element || window ),
isWindow = $.isWindow( withinElement[0] );
return {
element: withinElement,
isWindow: isWindow,
offset: withinElement.offset() || { left: 0, top: 0 },
scrollLeft: withinElement.scrollLeft(),
scrollTop: withinElement.scrollTop(),
width: isWindow ? withinElement.width() : withinElement.outerWidth(),
height: isWindow ? withinElement.height() : withinElement.outerHeight()
};
}
};
$.fn.position = function( options ) {
if ( !options || !options.of ) {
return _position.apply( this, arguments );
}
// make a copy, we don't want to modify arguments
options = $.extend( {}, options );
var atOffset, targetWidth, targetHeight, targetOffset, basePosition, dimensions,
target = $( options.of ),
within = $.position.getWithinInfo( options.within ),
scrollInfo = $.position.getScrollInfo( within ),
collision = ( options.collision || "flip" ).split( " " ),
offsets = {};
dimensions = getDimensions( target );
if ( target[0].preventDefault ) {
// force left top to allow flipping
options.at = "left top";
}
targetWidth = dimensions.width;
targetHeight = dimensions.height;
targetOffset = dimensions.offset;
// clone to reuse original targetOffset later
basePosition = $.extend( {}, targetOffset );
// force my and at to have valid horizontal and vertical positions
// if a value is missing or invalid, it will be converted to center
$.each( [ "my", "at" ], function() {
var pos = ( options[ this ] || "" ).split( " " ),
horizontalOffset,
verticalOffset;
if ( pos.length === 1) {
pos = rhorizontal.test( pos[ 0 ] ) ?
pos.concat( [ "center" ] ) :
rvertical.test( pos[ 0 ] ) ?
[ "center" ].concat( pos ) :
[ "center", "center" ];
}
pos[ 0 ] = rhorizontal.test( pos[ 0 ] ) ? pos[ 0 ] : "center";
pos[ 1 ] = rvertical.test( pos[ 1 ] ) ? pos[ 1 ] : "center";
// calculate offsets
horizontalOffset = roffset.exec( pos[ 0 ] );
verticalOffset = roffset.exec( pos[ 1 ] );
offsets[ this ] = [
horizontalOffset ? horizontalOffset[ 0 ] : 0,
verticalOffset ? verticalOffset[ 0 ] : 0
];
// reduce to just the positions without the offsets
options[ this ] = [
rposition.exec( pos[ 0 ] )[ 0 ],
rposition.exec( pos[ 1 ] )[ 0 ]
];
});
// normalize collision option
if ( collision.length === 1 ) {
collision[ 1 ] = collision[ 0 ];
}
if ( options.at[ 0 ] === "right" ) {
basePosition.left += targetWidth;
} else if ( options.at[ 0 ] === "center" ) {
basePosition.left += targetWidth / 2;
}
if ( options.at[ 1 ] === "bottom" ) {
basePosition.top += targetHeight;
} else if ( options.at[ 1 ] === "center" ) {
basePosition.top += targetHeight / 2;
}
atOffset = getOffsets( offsets.at, targetWidth, targetHeight );
basePosition.left += atOffset[ 0 ];
basePosition.top += atOffset[ 1 ];
return this.each(function() {
var collisionPosition, using,
elem = $( this ),
elemWidth = elem.outerWidth(),
elemHeight = elem.outerHeight(),
marginLeft = parseCss( this, "marginLeft" ),
marginTop = parseCss( this, "marginTop" ),
collisionWidth = elemWidth + marginLeft + parseCss( this, "marginRight" ) + scrollInfo.width,
collisionHeight = elemHeight + marginTop + parseCss( this, "marginBottom" ) + scrollInfo.height,
position = $.extend( {}, basePosition ),
myOffset = getOffsets( offsets.my, elem.outerWidth(), elem.outerHeight() );
if ( options.my[ 0 ] === "right" ) {
position.left -= elemWidth;
} else if ( options.my[ 0 ] === "center" ) {
position.left -= elemWidth / 2;
}
if ( options.my[ 1 ] === "bottom" ) {
position.top -= elemHeight;
} else if ( options.my[ 1 ] === "center" ) {
position.top -= elemHeight / 2;
}
position.left += myOffset[ 0 ];
position.top += myOffset[ 1 ];
// if the browser doesn't support fractions, then round for consistent results
if ( !$.support.offsetFractions ) {
position.left = round( position.left );
position.top = round( position.top );
}
collisionPosition = {
marginLeft: marginLeft,
marginTop: marginTop
};
$.each( [ "left", "top" ], function( i, dir ) {
if ( $.ui.position[ collision[ i ] ] ) {
$.ui.position[ collision[ i ] ][ dir ]( position, {
targetWidth: targetWidth,
targetHeight: targetHeight,
elemWidth: elemWidth,
elemHeight: elemHeight,
collisionPosition: collisionPosition,
collisionWidth: collisionWidth,
collisionHeight: collisionHeight,
offset: [ atOffset[ 0 ] + myOffset[ 0 ], atOffset [ 1 ] + myOffset[ 1 ] ],
my: options.my,
at: options.at,
within: within,
elem : elem
});
}
});
if ( options.using ) {
// adds feedback as second argument to using callback, if present
using = function( props ) {
var left = targetOffset.left - position.left,
right = left + targetWidth - elemWidth,
top = targetOffset.top - position.top,
bottom = top + targetHeight - elemHeight,
feedback = {
target: {
element: target,
left: targetOffset.left,
top: targetOffset.top,
width: targetWidth,
height: targetHeight
},
element: {
element: elem,
left: position.left,
top: position.top,
width: elemWidth,
height: elemHeight
},
horizontal: right < 0 ? "left" : left > 0 ? "right" : "center",
vertical: bottom < 0 ? "top" : top > 0 ? "bottom" : "middle"
};
if ( targetWidth < elemWidth && abs( left + right ) < targetWidth ) {
feedback.horizontal = "center";
}
if ( targetHeight < elemHeight && abs( top + bottom ) < targetHeight ) {
feedback.vertical = "middle";
}
if ( max( abs( left ), abs( right ) ) > max( abs( top ), abs( bottom ) ) ) {
feedback.important = "horizontal";
} else {
feedback.important = "vertical";
}
options.using.call( this, props, feedback );
};
}
elem.offset( $.extend( position, { using: using } ) );
});
};
$.ui.position = {
fit: {
left: function( position, data ) {
var within = data.within,
withinOffset = within.isWindow ? within.scrollLeft : within.offset.left,
outerWidth = within.width,
collisionPosLeft = position.left - data.collisionPosition.marginLeft,
overLeft = withinOffset - collisionPosLeft,
overRight = collisionPosLeft + data.collisionWidth - outerWidth - withinOffset,
newOverRight;
// element is wider than within
if ( data.collisionWidth > outerWidth ) {
// element is initially over the left side of within
if ( overLeft > 0 && overRight <= 0 ) {
newOverRight = position.left + overLeft + data.collisionWidth - outerWidth - withinOffset;
position.left += overLeft - newOverRight;
// element is initially over right side of within
} else if ( overRight > 0 && overLeft <= 0 ) {
position.left = withinOffset;
// element is initially over both left and right sides of within
} else {
if ( overLeft > overRight ) {
position.left = withinOffset + outerWidth - data.collisionWidth;
} else {
position.left = withinOffset;
}
}
// too far left -> align with left edge
} else if ( overLeft > 0 ) {
position.left += overLeft;
// too far right -> align with right edge
} else if ( overRight > 0 ) {
position.left -= overRight;
// adjust based on position and margin
} else {
position.left = max( position.left - collisionPosLeft, position.left );
}
},
top: function( position, data ) {
var within = data.within,
withinOffset = within.isWindow ? within.scrollTop : within.offset.top,
outerHeight = data.within.height,
collisionPosTop = position.top - data.collisionPosition.marginTop,
overTop = withinOffset - collisionPosTop,
overBottom = collisionPosTop + data.collisionHeight - outerHeight - withinOffset,
newOverBottom;
// element is taller than within
if ( data.collisionHeight > outerHeight ) {
// element is initially over the top of within
if ( overTop > 0 && overBottom <= 0 ) {
newOverBottom = position.top + overTop + data.collisionHeight - outerHeight - withinOffset;
position.top += overTop - newOverBottom;
// element is initially over bottom of within
} else if ( overBottom > 0 && overTop <= 0 ) {
position.top = withinOffset;
// element is initially over both top and bottom of within
} else {
if ( overTop > overBottom ) {
position.top = withinOffset + outerHeight - data.collisionHeight;
} else {
position.top = withinOffset;
}
}
// too far up -> align with top
} else if ( overTop > 0 ) {
position.top += overTop;
// too far down -> align with bottom edge
} else if ( overBottom > 0 ) {
position.top -= overBottom;
// adjust based on position and margin
} else {
position.top = max( position.top - collisionPosTop, position.top );
}
}
},
flip: {
left: function( position, data ) {
var within = data.within,
withinOffset = within.offset.left + within.scrollLeft,
outerWidth = within.width,
offsetLeft = within.isWindow ? within.scrollLeft : within.offset.left,
collisionPosLeft = position.left - data.collisionPosition.marginLeft,
overLeft = collisionPosLeft - offsetLeft,
overRight = collisionPosLeft + data.collisionWidth - outerWidth - offsetLeft,
myOffset = data.my[ 0 ] === "left" ?
-data.elemWidth :
data.my[ 0 ] === "right" ?
data.elemWidth :
0,
atOffset = data.at[ 0 ] === "left" ?
data.targetWidth :
data.at[ 0 ] === "right" ?
-data.targetWidth :
0,
offset = -2 * data.offset[ 0 ],
newOverRight,
newOverLeft;
if ( overLeft < 0 ) {
newOverRight = position.left + myOffset + atOffset + offset + data.collisionWidth - outerWidth - withinOffset;
if ( newOverRight < 0 || newOverRight < abs( overLeft ) ) {
position.left += myOffset + atOffset + offset;
}
}
else if ( overRight > 0 ) {
newOverLeft = position.left - data.collisionPosition.marginLeft + myOffset + atOffset + offset - offsetLeft;
if ( newOverLeft > 0 || abs( newOverLeft ) < overRight ) {
position.left += myOffset + atOffset + offset;
}
}
},
top: function( position, data ) {
var within = data.within,
withinOffset = within.offset.top + within.scrollTop,
outerHeight = within.height,
offsetTop = within.isWindow ? within.scrollTop : within.offset.top,
collisionPosTop = position.top - data.collisionPosition.marginTop,
overTop = collisionPosTop - offsetTop,
overBottom = collisionPosTop + data.collisionHeight - outerHeight - offsetTop,
top = data.my[ 1 ] === "top",
myOffset = top ?
-data.elemHeight :
data.my[ 1 ] === "bottom" ?
data.elemHeight :
0,
atOffset = data.at[ 1 ] === "top" ?
data.targetHeight :
data.at[ 1 ] === "bottom" ?
-data.targetHeight :
0,
offset = -2 * data.offset[ 1 ],
newOverTop,
newOverBottom;
if ( overTop < 0 ) {
newOverBottom = position.top + myOffset + atOffset + offset + data.collisionHeight - outerHeight - withinOffset;
if ( ( position.top + myOffset + atOffset + offset) > overTop && ( newOverBottom < 0 || newOverBottom < abs( overTop ) ) ) {
position.top += myOffset + atOffset + offset;
}
}
else if ( overBottom > 0 ) {
newOverTop = position.top - data.collisionPosition.marginTop + myOffset + atOffset + offset - offsetTop;
if ( ( position.top + myOffset + atOffset + offset) > overBottom && ( newOverTop > 0 || abs( newOverTop ) < overBottom ) ) {
position.top += myOffset + atOffset + offset;
}
}
}
},
flipfit: {
left: function() {
$.ui.position.flip.left.apply( this, arguments );
$.ui.position.fit.left.apply( this, arguments );
},
top: function() {
$.ui.position.flip.top.apply( this, arguments );
$.ui.position.fit.top.apply( this, arguments );
}
}
};
// fraction support test
(function () {
var testElement, testElementParent, testElementStyle, offsetLeft, i,
body = document.getElementsByTagName( "body" )[ 0 ],
div = document.createElement( "div" );
//Create a "fake body" for testing based on method used in jQuery.support
testElement = document.createElement( body ? "div" : "body" );
testElementStyle = {
visibility: "hidden",
width: 0,
height: 0,
border: 0,
margin: 0,
background: "none"
};
if ( body ) {
$.extend( testElementStyle, {
position: "absolute",
left: "-1000px",
top: "-1000px"
});
}
for ( i in testElementStyle ) {
testElement.style[ i ] = testElementStyle[ i ];
}
testElement.appendChild( div );
testElementParent = body || document.documentElement;
testElementParent.insertBefore( testElement, testElementParent.firstChild );
div.style.cssText = "position: absolute; left: 10.7432222px;";
offsetLeft = $( div ).offset().left;
$.support.offsetFractions = offsetLeft > 10 && offsetLeft < 11;
testElement.innerHTML = "";
testElementParent.removeChild( testElement );
})();
}( jQuery ) );
(function( $, undefined ) {
$.widget( "ui.progressbar", {
version: "1.10.3",
options: {
max: 100,
value: 0,
change: null,
complete: null
},
min: 0,
_create: function() {
// Constrain initial value
this.oldValue = this.options.value = this._constrainedValue();
this.element
.addClass( "ui-progressbar ui-widget ui-widget-content ui-corner-all" )
.attr({
// Only set static values, aria-valuenow and aria-valuemax are
// set inside _refreshValue()
role: "progressbar",
"aria-valuemin": this.min
});
this.valueDiv = $( "<div class='ui-progressbar-value ui-widget-header ui-corner-left'></div>" )
.appendTo( this.element );
this._refreshValue();
},
_destroy: function() {
this.element
.removeClass( "ui-progressbar ui-widget ui-widget-content ui-corner-all" )
.removeAttr( "role" )
.removeAttr( "aria-valuemin" )
.removeAttr( "aria-valuemax" )
.removeAttr( "aria-valuenow" );
this.valueDiv.remove();
},
value: function( newValue ) {
if ( newValue === undefined ) {
return this.options.value;
}
this.options.value = this._constrainedValue( newValue );
this._refreshValue();
},
_constrainedValue: function( newValue ) {
if ( newValue === undefined ) {
newValue = this.options.value;
}
this.indeterminate = newValue === false;
// sanitize value
if ( typeof newValue !== "number" ) {
newValue = 0;
}
return this.indeterminate ? false :
Math.min( this.options.max, Math.max( this.min, newValue ) );
},
_setOptions: function( options ) {
// Ensure "value" option is set after other values (like max)
var value = options.value;
delete options.value;
this._super( options );
this.options.value = this._constrainedValue( value );
this._refreshValue();
},
_setOption: function( key, value ) {
if ( key === "max" ) {
// Don't allow a max less than min
value = Math.max( this.min, value );
}
this._super( key, value );
},
_percentage: function() {
return this.indeterminate ? 100 : 100 * ( this.options.value - this.min ) / ( this.options.max - this.min );
},
_refreshValue: function() {
var value = this.options.value,
percentage = this._percentage();
this.valueDiv
.toggle( this.indeterminate || value > this.min )
.toggleClass( "ui-corner-right", value === this.options.max )
.width( percentage.toFixed(0) + "%" );
this.element.toggleClass( "ui-progressbar-indeterminate", this.indeterminate );
if ( this.indeterminate ) {
this.element.removeAttr( "aria-valuenow" );
if ( !this.overlayDiv ) {
this.overlayDiv = $( "<div class='ui-progressbar-overlay'></div>" ).appendTo( this.valueDiv );
}
} else {
this.element.attr({
"aria-valuemax": this.options.max,
"aria-valuenow": value
});
if ( this.overlayDiv ) {
this.overlayDiv.remove();
this.overlayDiv = null;
}
}
if ( this.oldValue !== value ) {
this.oldValue = value;
this._trigger( "change" );
}
if ( value === this.options.max ) {
this._trigger( "complete" );
}
}
});
})( jQuery );
(function( $, undefined ) {
// number of pages in a slider
// (how many times can you page up/down to go through the whole range)
var numPages = 5;
$.widget( "ui.slider", $.ui.mouse, {
version: "1.10.3",
widgetEventPrefix: "slide",
options: {
animate: false,
distance: 0,
max: 100,
min: 0,
orientation: "horizontal",
range: false,
step: 1,
value: 0,
values: null,
// callbacks
change: null,
slide: null,
start: null,
stop: null
},
_create: function() {
this._keySliding = false;
this._mouseSliding = false;
this._animateOff = true;
this._handleIndex = null;
this._detectOrientation();
this._mouseInit();
this.element
.addClass( "ui-slider" +
" ui-slider-" + this.orientation +
" ui-widget" +
" ui-widget-content" +
" ui-corner-all");
this._refresh();
this._setOption( "disabled", this.options.disabled );
this._animateOff = false;
},
_refresh: function() {
this._createRange();
this._createHandles();
this._setupEvents();
this._refreshValue();
},
_createHandles: function() {
var i, handleCount,
options = this.options,
existingHandles = this.element.find( ".ui-slider-handle" ).addClass( "ui-state-default ui-corner-all" ),
handle = "<a class='ui-slider-handle ui-state-default ui-corner-all' href='#'></a>",
handles = [];
handleCount = ( options.values && options.values.length ) || 1;
if ( existingHandles.length > handleCount ) {
existingHandles.slice( handleCount ).remove();
existingHandles = existingHandles.slice( 0, handleCount );
}
for ( i = existingHandles.length; i < handleCount; i++ ) {
handles.push( handle );
}
this.handles = existingHandles.add( $( handles.join( "" ) ).appendTo( this.element ) );
this.handle = this.handles.eq( 0 );
this.handles.each(function( i ) {
$( this ).data( "ui-slider-handle-index", i );
});
},
_createRange: function() {
var options = this.options,
classes = "";
if ( options.range ) {
if ( options.range === true ) {
if ( !options.values ) {
options.values = [ this._valueMin(), this._valueMin() ];
} else if ( options.values.length && options.values.length !== 2 ) {
options.values = [ options.values[0], options.values[0] ];
} else if ( $.isArray( options.values ) ) {
options.values = options.values.slice(0);
}
}
if ( !this.range || !this.range.length ) {
this.range = $( "<div></div>" )
.appendTo( this.element );
classes = "ui-slider-range" +
// note: this isn't the most fittingly semantic framework class for this element,
// but worked best visually with a variety of themes
" ui-widget-header ui-corner-all";
} else {
this.range.removeClass( "ui-slider-range-min ui-slider-range-max" )
// Handle range switching from true to min/max
.css({
"left": "",
"bottom": ""
});
}
this.range.addClass( classes +
( ( options.range === "min" || options.range === "max" ) ? " ui-slider-range-" + options.range : "" ) );
} else {
this.range = $([]);
}
},
_setupEvents: function() {
var elements = this.handles.add( this.range ).filter( "a" );
this._off( elements );
this._on( elements, this._handleEvents );
this._hoverable( elements );
this._focusable( elements );
},
_destroy: function() {
this.handles.remove();
this.range.remove();
this.element
.removeClass( "ui-slider" +
" ui-slider-horizontal" +
" ui-slider-vertical" +
" ui-widget" +
" ui-widget-content" +
" ui-corner-all" );
this._mouseDestroy();
},
_mouseCapture: function( event ) {
var position, normValue, distance, closestHandle, index, allowed, offset, mouseOverHandle,
that = this,
o = this.options;
if ( o.disabled ) {
return false;
}
this.elementSize = {
width: this.element.outerWidth(),
height: this.element.outerHeight()
};
this.elementOffset = this.element.offset();
position = { x: event.pageX, y: event.pageY };
normValue = this._normValueFromMouse( position );
distance = this._valueMax() - this._valueMin() + 1;
this.handles.each(function( i ) {
var thisDistance = Math.abs( normValue - that.values(i) );
if (( distance > thisDistance ) ||
( distance === thisDistance &&
(i === that._lastChangedValue || that.values(i) === o.min ))) {
distance = thisDistance;
closestHandle = $( this );
index = i;
}
});
allowed = this._start( event, index );
if ( allowed === false ) {
return false;
}
this._mouseSliding = true;
this._handleIndex = index;
closestHandle
.addClass( "ui-state-active" )
.focus();
offset = closestHandle.offset();
mouseOverHandle = !$( event.target ).parents().addBack().is( ".ui-slider-handle" );
this._clickOffset = mouseOverHandle ? { left: 0, top: 0 } : {
left: event.pageX - offset.left - ( closestHandle.width() / 2 ),
top: event.pageY - offset.top -
( closestHandle.height() / 2 ) -
( parseInt( closestHandle.css("borderTopWidth"), 10 ) || 0 ) -
( parseInt( closestHandle.css("borderBottomWidth"), 10 ) || 0) +
( parseInt( closestHandle.css("marginTop"), 10 ) || 0)
};
if ( !this.handles.hasClass( "ui-state-hover" ) ) {
this._slide( event, index, normValue );
}
this._animateOff = true;
return true;
},
_mouseStart: function() {
return true;
},
_mouseDrag: function( event ) {
var position = { x: event.pageX, y: event.pageY },
normValue = this._normValueFromMouse( position );
this._slide( event, this._handleIndex, normValue );
return false;
},
_mouseStop: function( event ) {
this.handles.removeClass( "ui-state-active" );
this._mouseSliding = false;
this._stop( event, this._handleIndex );
this._change( event, this._handleIndex );
this._handleIndex = null;
this._clickOffset = null;
this._animateOff = false;
return false;
},
_detectOrientation: function() {
this.orientation = ( this.options.orientation === "vertical" ) ? "vertical" : "horizontal";
},
_normValueFromMouse: function( position ) {
var pixelTotal,
pixelMouse,
percentMouse,
valueTotal,
valueMouse;
if ( this.orientation === "horizontal" ) {
pixelTotal = this.elementSize.width;
pixelMouse = position.x - this.elementOffset.left - ( this._clickOffset ? this._clickOffset.left : 0 );
} else {
pixelTotal = this.elementSize.height;
pixelMouse = position.y - this.elementOffset.top - ( this._clickOffset ? this._clickOffset.top : 0 );
}
percentMouse = ( pixelMouse / pixelTotal );
if ( percentMouse > 1 ) {
percentMouse = 1;
}
if ( percentMouse < 0 ) {
percentMouse = 0;
}
if ( this.orientation === "vertical" ) {
percentMouse = 1 - percentMouse;
}
valueTotal = this._valueMax() - this._valueMin();
valueMouse = this._valueMin() + percentMouse * valueTotal;
return this._trimAlignValue( valueMouse );
},
_start: function( event, index ) {
var uiHash = {
handle: this.handles[ index ],
value: this.value()
};
if ( this.options.values && this.options.values.length ) {
uiHash.value = this.values( index );
uiHash.values = this.values();
}
return this._trigger( "start", event, uiHash );
},
_slide: function( event, index, newVal ) {
var otherVal,
newValues,
allowed;
if ( this.options.values && this.options.values.length ) {
otherVal = this.values( index ? 0 : 1 );
if ( ( this.options.values.length === 2 && this.options.range === true ) &&
( ( index === 0 && newVal > otherVal) || ( index === 1 && newVal < otherVal ) )
) {
newVal = otherVal;
}
if ( newVal !== this.values( index ) ) {
newValues = this.values();
newValues[ index ] = newVal;
// A slide can be canceled by returning false from the slide callback
allowed = this._trigger( "slide", event, {
handle: this.handles[ index ],
value: newVal,
values: newValues
} );
otherVal = this.values( index ? 0 : 1 );
if ( allowed !== false ) {
this.values( index, newVal, true );
}
}
} else {
if ( newVal !== this.value() ) {
// A slide can be canceled by returning false from the slide callback
allowed = this._trigger( "slide", event, {
handle: this.handles[ index ],
value: newVal
} );
if ( allowed !== false ) {
this.value( newVal );
}
}
}
},
_stop: function( event, index ) {
var uiHash = {
handle: this.handles[ index ],
value: this.value()
};
if ( this.options.values && this.options.values.length ) {
uiHash.value = this.values( index );
uiHash.values = this.values();
}
this._trigger( "stop", event, uiHash );
},
_change: function( event, index ) {
if ( !this._keySliding && !this._mouseSliding ) {
var uiHash = {
handle: this.handles[ index ],
value: this.value()
};
if ( this.options.values && this.options.values.length ) {
uiHash.value = this.values( index );
uiHash.values = this.values();
}
//store the last changed value index for reference when handles overlap
this._lastChangedValue = index;
this._trigger( "change", event, uiHash );
}
},
value: function( newValue ) {
if ( arguments.length ) {
this.options.value = this._trimAlignValue( newValue );
this._refreshValue();
this._change( null, 0 );
return;
}
return this._value();
},
values: function( index, newValue ) {
var vals,
newValues,
i;
if ( arguments.length > 1 ) {
this.options.values[ index ] = this._trimAlignValue( newValue );
this._refreshValue();
this._change( null, index );
return;
}
if ( arguments.length ) {
if ( $.isArray( arguments[ 0 ] ) ) {
vals = this.options.values;
newValues = arguments[ 0 ];
for ( i = 0; i < vals.length; i += 1 ) {
vals[ i ] = this._trimAlignValue( newValues[ i ] );
this._change( null, i );
}
this._refreshValue();
} else {
if ( this.options.values && this.options.values.length ) {
return this._values( index );
} else {
return this.value();
}
}
} else {
return this._values();
}
},
_setOption: function( key, value ) {
var i,
valsLength = 0;
if ( key === "range" && this.options.range === true ) {
if ( value === "min" ) {
this.options.value = this._values( 0 );
this.options.values = null;
} else if ( value === "max" ) {
this.options.value = this._values( this.options.values.length-1 );
this.options.values = null;
}
}
if ( $.isArray( this.options.values ) ) {
valsLength = this.options.values.length;
}
$.Widget.prototype._setOption.apply( this, arguments );
switch ( key ) {
case "orientation":
this._detectOrientation();
this.element
.removeClass( "ui-slider-horizontal ui-slider-vertical" )
.addClass( "ui-slider-" + this.orientation );
this._refreshValue();
break;
case "value":
this._animateOff = true;
this._refreshValue();
this._change( null, 0 );
this._animateOff = false;
break;
case "values":
this._animateOff = true;
this._refreshValue();
for ( i = 0; i < valsLength; i += 1 ) {
this._change( null, i );
}
this._animateOff = false;
break;
case "min":
case "max":
this._animateOff = true;
this._refreshValue();
this._animateOff = false;
break;
case "range":
this._animateOff = true;
this._refresh();
this._animateOff = false;
break;
}
},
//internal value getter
// _value() returns value trimmed by min and max, aligned by step
_value: function() {
var val = this.options.value;
val = this._trimAlignValue( val );
return val;
},
//internal values getter
// _values() returns array of values trimmed by min and max, aligned by step
// _values( index ) returns single value trimmed by min and max, aligned by step
_values: function( index ) {
var val,
vals,
i;
if ( arguments.length ) {
val = this.options.values[ index ];
val = this._trimAlignValue( val );
return val;
} else if ( this.options.values && this.options.values.length ) {
// .slice() creates a copy of the array
// this copy gets trimmed by min and max and then returned
vals = this.options.values.slice();
for ( i = 0; i < vals.length; i+= 1) {
vals[ i ] = this._trimAlignValue( vals[ i ] );
}
return vals;
} else {
return [];
}
},
// returns the step-aligned value that val is closest to, between (inclusive) min and max
_trimAlignValue: function( val ) {
if ( val <= this._valueMin() ) {
return this._valueMin();
}
if ( val >= this._valueMax() ) {
return this._valueMax();
}
var step = ( this.options.step > 0 ) ? this.options.step : 1,
valModStep = (val - this._valueMin()) % step,
alignValue = val - valModStep;
if ( Math.abs(valModStep) * 2 >= step ) {
alignValue += ( valModStep > 0 ) ? step : ( -step );
}
// Since JavaScript has problems with large floats, round
// the final value to 5 digits after the decimal point (see #4124)
return parseFloat( alignValue.toFixed(5) );
},
_valueMin: function() {
return this.options.min;
},
_valueMax: function() {
return this.options.max;
},
_refreshValue: function() {
var lastValPercent, valPercent, value, valueMin, valueMax,
oRange = this.options.range,
o = this.options,
that = this,
animate = ( !this._animateOff ) ? o.animate : false,
_set = {};
if ( this.options.values && this.options.values.length ) {
this.handles.each(function( i ) {
valPercent = ( that.values(i) - that._valueMin() ) / ( that._valueMax() - that._valueMin() ) * 100;
_set[ that.orientation === "horizontal" ? "left" : "bottom" ] = valPercent + "%";
$( this ).stop( 1, 1 )[ animate ? "animate" : "css" ]( _set, o.animate );
if ( that.options.range === true ) {
if ( that.orientation === "horizontal" ) {
if ( i === 0 ) {
that.range.stop( 1, 1 )[ animate ? "animate" : "css" ]( { left: valPercent + "%" }, o.animate );
}
if ( i === 1 ) {
that.range[ animate ? "animate" : "css" ]( { width: ( valPercent - lastValPercent ) + "%" }, { queue: false, duration: o.animate } );
}
} else {
if ( i === 0 ) {
that.range.stop( 1, 1 )[ animate ? "animate" : "css" ]( { bottom: ( valPercent ) + "%" }, o.animate );
}
if ( i === 1 ) {
that.range[ animate ? "animate" : "css" ]( { height: ( valPercent - lastValPercent ) + "%" }, { queue: false, duration: o.animate } );
}
}
}
lastValPercent = valPercent;
});
} else {
value = this.value();
valueMin = this._valueMin();
valueMax = this._valueMax();
valPercent = ( valueMax !== valueMin ) ?
( value - valueMin ) / ( valueMax - valueMin ) * 100 :
0;
_set[ this.orientation === "horizontal" ? "left" : "bottom" ] = valPercent + "%";
this.handle.stop( 1, 1 )[ animate ? "animate" : "css" ]( _set, o.animate );
if ( oRange === "min" && this.orientation === "horizontal" ) {
this.range.stop( 1, 1 )[ animate ? "animate" : "css" ]( { width: valPercent + "%" }, o.animate );
}
if ( oRange === "max" && this.orientation === "horizontal" ) {
this.range[ animate ? "animate" : "css" ]( { width: ( 100 - valPercent ) + "%" }, { queue: false, duration: o.animate } );
}
if ( oRange === "min" && this.orientation === "vertical" ) {
this.range.stop( 1, 1 )[ animate ? "animate" : "css" ]( { height: valPercent + "%" }, o.animate );
}
if ( oRange === "max" && this.orientation === "vertical" ) {
this.range[ animate ? "animate" : "css" ]( { height: ( 100 - valPercent ) + "%" }, { queue: false, duration: o.animate } );
}
}
},
_handleEvents: {
keydown: function( event ) {
/*jshint maxcomplexity:25*/
var allowed, curVal, newVal, step,
index = $( event.target ).data( "ui-slider-handle-index" );
switch ( event.keyCode ) {
case $.ui.keyCode.HOME:
case $.ui.keyCode.END:
case $.ui.keyCode.PAGE_UP:
case $.ui.keyCode.PAGE_DOWN:
case $.ui.keyCode.UP:
case $.ui.keyCode.RIGHT:
case $.ui.keyCode.DOWN:
case $.ui.keyCode.LEFT:
event.preventDefault();
if ( !this._keySliding ) {
this._keySliding = true;
$( event.target ).addClass( "ui-state-active" );
allowed = this._start( event, index );
if ( allowed === false ) {
return;
}
}
break;
}
step = this.options.step;
if ( this.options.values && this.options.values.length ) {
curVal = newVal = this.values( index );
} else {
curVal = newVal = this.value();
}
switch ( event.keyCode ) {
case $.ui.keyCode.HOME:
newVal = this._valueMin();
break;
case $.ui.keyCode.END:
newVal = this._valueMax();
break;
case $.ui.keyCode.PAGE_UP:
newVal = this._trimAlignValue( curVal + ( (this._valueMax() - this._valueMin()) / numPages ) );
break;
case $.ui.keyCode.PAGE_DOWN:
newVal = this._trimAlignValue( curVal - ( (this._valueMax() - this._valueMin()) / numPages ) );
break;
case $.ui.keyCode.UP:
case $.ui.keyCode.RIGHT:
if ( curVal === this._valueMax() ) {
return;
}
newVal = this._trimAlignValue( curVal + step );
break;
case $.ui.keyCode.DOWN:
case $.ui.keyCode.LEFT:
if ( curVal === this._valueMin() ) {
return;
}
newVal = this._trimAlignValue( curVal - step );
break;
}
this._slide( event, index, newVal );
},
click: function( event ) {
event.preventDefault();
},
keyup: function( event ) {
var index = $( event.target ).data( "ui-slider-handle-index" );
if ( this._keySliding ) {
this._keySliding = false;
this._stop( event, index );
this._change( event, index );
$( event.target ).removeClass( "ui-state-active" );
}
}
}
});
}(jQuery));
(function( $ ) {
function modifier( fn ) {
return function() {
var previous = this.element.val();
fn.apply( this, arguments );
this._refresh();
if ( previous !== this.element.val() ) {
this._trigger( "change" );
}
};
}
$.widget( "ui.spinner", {
version: "1.10.3",
defaultElement: "<input>",
widgetEventPrefix: "spin",
options: {
culture: null,
icons: {
down: "ui-icon-triangle-1-s",
up: "ui-icon-triangle-1-n"
},
incremental: true,
max: null,
min: null,
numberFormat: null,
page: 10,
step: 1,
change: null,
spin: null,
start: null,
stop: null
},
_create: function() {
// handle string values that need to be parsed
this._setOption( "max", this.options.max );
this._setOption( "min", this.options.min );
this._setOption( "step", this.options.step );
// format the value, but don't constrain
this._value( this.element.val(), true );
this._draw();
this._on( this._events );
this._refresh();
// turning off autocomplete prevents the browser from remembering the
// value when navigating through history, so we re-enable autocomplete
// if the page is unloaded before the widget is destroyed. #7790
this._on( this.window, {
beforeunload: function() {
this.element.removeAttr( "autocomplete" );
}
});
},
_getCreateOptions: function() {
var options = {},
element = this.element;
$.each( [ "min", "max", "step" ], function( i, option ) {
var value = element.attr( option );
if ( value !== undefined && value.length ) {
options[ option ] = value;
}
});
return options;
},
_events: {
keydown: function( event ) {
if ( this._start( event ) && this._keydown( event ) ) {
event.preventDefault();
}
},
keyup: "_stop",
focus: function() {
this.previous = this.element.val();
},
blur: function( event ) {
if ( this.cancelBlur ) {
delete this.cancelBlur;
return;
}
this._stop();
this._refresh();
if ( this.previous !== this.element.val() ) {
this._trigger( "change", event );
}
},
mousewheel: function( event, delta ) {
if ( !delta ) {
return;
}
if ( !this.spinning && !this._start( event ) ) {
return false;
}
this._spin( (delta > 0 ? 1 : -1) * this.options.step, event );
clearTimeout( this.mousewheelTimer );
this.mousewheelTimer = this._delay(function() {
if ( this.spinning ) {
this._stop( event );
}
}, 100 );
event.preventDefault();
},
"mousedown .ui-spinner-button": function( event ) {
var previous;
// We never want the buttons to have focus; whenever the user is
// interacting with the spinner, the focus should be on the input.
// If the input is focused then this.previous is properly set from
// when the input first received focus. If the input is not focused
// then we need to set this.previous based on the value before spinning.
previous = this.element[0] === this.document[0].activeElement ?
this.previous : this.element.val();
function checkFocus() {
var isActive = this.element[0] === this.document[0].activeElement;
if ( !isActive ) {
this.element.focus();
this.previous = previous;
// support: IE
// IE sets focus asynchronously, so we need to check if focus
// moved off of the input because the user clicked on the button.
this._delay(function() {
this.previous = previous;
});
}
}
// ensure focus is on (or stays on) the text field
event.preventDefault();
checkFocus.call( this );
// support: IE
// IE doesn't prevent moving focus even with event.preventDefault()
// so we set a flag to know when we should ignore the blur event
// and check (again) if focus moved off of the input.
this.cancelBlur = true;
this._delay(function() {
delete this.cancelBlur;
checkFocus.call( this );
});
if ( this._start( event ) === false ) {
return;
}
this._repeat( null, $( event.currentTarget ).hasClass( "ui-spinner-up" ) ? 1 : -1, event );
},
"mouseup .ui-spinner-button": "_stop",
"mouseenter .ui-spinner-button": function( event ) {
// button will add ui-state-active if mouse was down while mouseleave and kept down
if ( !$( event.currentTarget ).hasClass( "ui-state-active" ) ) {
return;
}
if ( this._start( event ) === false ) {
return false;
}
this._repeat( null, $( event.currentTarget ).hasClass( "ui-spinner-up" ) ? 1 : -1, event );
},
// TODO: do we really want to consider this a stop?
// shouldn't we just stop the repeater and wait until mouseup before
// we trigger the stop event?
"mouseleave .ui-spinner-button": "_stop"
},
_draw: function() {
var uiSpinner = this.uiSpinner = this.element
.addClass( "ui-spinner-input" )
.attr( "autocomplete", "off" )
.wrap( this._uiSpinnerHtml() )
.parent()
// add buttons
.append( this._buttonHtml() );
this.element.attr( "role", "spinbutton" );
// button bindings
this.buttons = uiSpinner.find( ".ui-spinner-button" )
.attr( "tabIndex", -1 )
.button()
.removeClass( "ui-corner-all" );
// IE 6 doesn't understand height: 50% for the buttons
// unless the wrapper has an explicit height
if ( this.buttons.height() > Math.ceil( uiSpinner.height() * 0.5 ) &&
uiSpinner.height() > 0 ) {
uiSpinner.height( uiSpinner.height() );
}
// disable spinner if element was already disabled
if ( this.options.disabled ) {
this.disable();
}
},
_keydown: function( event ) {
var options = this.options,
keyCode = $.ui.keyCode;
switch ( event.keyCode ) {
case keyCode.UP:
this._repeat( null, 1, event );
return true;
case keyCode.DOWN:
this._repeat( null, -1, event );
return true;
case keyCode.PAGE_UP:
this._repeat( null, options.page, event );
return true;
case keyCode.PAGE_DOWN:
this._repeat( null, -options.page, event );
return true;
}
return false;
},
_uiSpinnerHtml: function() {
return "<span class='ui-spinner ui-widget ui-widget-content ui-corner-all'></span>";
},
_buttonHtml: function() {
return "" +
"<a class='ui-spinner-button ui-spinner-up ui-corner-tr'>" +
"<span class='ui-icon " + this.options.icons.up + "'>▲</span>" +
"</a>" +
"<a class='ui-spinner-button ui-spinner-down ui-corner-br'>" +
"<span class='ui-icon " + this.options.icons.down + "'>▼</span>" +
"</a>";
},
_start: function( event ) {
if ( !this.spinning && this._trigger( "start", event ) === false ) {
return false;
}
if ( !this.counter ) {
this.counter = 1;
}
this.spinning = true;
return true;
},
_repeat: function( i, steps, event ) {
i = i || 500;
clearTimeout( this.timer );
this.timer = this._delay(function() {
this._repeat( 40, steps, event );
}, i );
this._spin( steps * this.options.step, event );
},
_spin: function( step, event ) {
var value = this.value() || 0;
if ( !this.counter ) {
this.counter = 1;
}
value = this._adjustValue( value + step * this._increment( this.counter ) );
if ( !this.spinning || this._trigger( "spin", event, { value: value } ) !== false) {
this._value( value );
this.counter++;
}
},
_increment: function( i ) {
var incremental = this.options.incremental;
if ( incremental ) {
return $.isFunction( incremental ) ?
incremental( i ) :
Math.floor( i*i*i/50000 - i*i/500 + 17*i/200 + 1 );
}
return 1;
},
_precision: function() {
var precision = this._precisionOf( this.options.step );
if ( this.options.min !== null ) {
precision = Math.max( precision, this._precisionOf( this.options.min ) );
}
return precision;
},
_precisionOf: function( num ) {
var str = num.toString(),
decimal = str.indexOf( "." );
return decimal === -1 ? 0 : str.length - decimal - 1;
},
_adjustValue: function( value ) {
var base, aboveMin,
options = this.options;
// make sure we're at a valid step
// - find out where we are relative to the base (min or 0)
base = options.min !== null ? options.min : 0;
aboveMin = value - base;
// - round to the nearest step
aboveMin = Math.round(aboveMin / options.step) * options.step;
// - rounding is based on 0, so adjust back to our base
value = base + aboveMin;
// fix precision from bad JS floating point math
value = parseFloat( value.toFixed( this._precision() ) );
// clamp the value
if ( options.max !== null && value > options.max) {
return options.max;
}
if ( options.min !== null && value < options.min ) {
return options.min;
}
return value;
},
_stop: function( event ) {
if ( !this.spinning ) {
return;
}
clearTimeout( this.timer );
clearTimeout( this.mousewheelTimer );
this.counter = 0;
this.spinning = false;
this._trigger( "stop", event );
},
_setOption: function( key, value ) {
if ( key === "culture" || key === "numberFormat" ) {
var prevValue = this._parse( this.element.val() );
this.options[ key ] = value;
this.element.val( this._format( prevValue ) );
return;
}
if ( key === "max" || key === "min" || key === "step" ) {
if ( typeof value === "string" ) {
value = this._parse( value );
}
}
if ( key === "icons" ) {
this.buttons.first().find( ".ui-icon" )
.removeClass( this.options.icons.up )
.addClass( value.up );
this.buttons.last().find( ".ui-icon" )
.removeClass( this.options.icons.down )
.addClass( value.down );
}
this._super( key, value );
if ( key === "disabled" ) {
if ( value ) {
this.element.prop( "disabled", true );
this.buttons.button( "disable" );
} else {
this.element.prop( "disabled", false );
this.buttons.button( "enable" );
}
}
},
_setOptions: modifier(function( options ) {
this._super( options );
this._value( this.element.val() );
}),
_parse: function( val ) {
if ( typeof val === "string" && val !== "" ) {
val = window.Globalize && this.options.numberFormat ?
Globalize.parseFloat( val, 10, this.options.culture ) : +val;
}
return val === "" || isNaN( val ) ? null : val;
},
_format: function( value ) {
if ( value === "" ) {
return "";
}
return window.Globalize && this.options.numberFormat ?
Globalize.format( value, this.options.numberFormat, this.options.culture ) :
value;
},
_refresh: function() {
this.element.attr({
"aria-valuemin": this.options.min,
"aria-valuemax": this.options.max,
// TODO: what should we do with values that can't be parsed?
"aria-valuenow": this._parse( this.element.val() )
});
},
// update the value without triggering change
_value: function( value, allowAny ) {
var parsed;
if ( value !== "" ) {
parsed = this._parse( value );
if ( parsed !== null ) {
if ( !allowAny ) {
parsed = this._adjustValue( parsed );
}
value = this._format( parsed );
}
}
this.element.val( value );
this._refresh();
},
_destroy: function() {
this.element
.removeClass( "ui-spinner-input" )
.prop( "disabled", false )
.removeAttr( "autocomplete" )
.removeAttr( "role" )
.removeAttr( "aria-valuemin" )
.removeAttr( "aria-valuemax" )
.removeAttr( "aria-valuenow" );
this.uiSpinner.replaceWith( this.element );
},
stepUp: modifier(function( steps ) {
this._stepUp( steps );
}),
_stepUp: function( steps ) {
if ( this._start() ) {
this._spin( (steps || 1) * this.options.step );
this._stop();
}
},
stepDown: modifier(function( steps ) {
this._stepDown( steps );
}),
_stepDown: function( steps ) {
if ( this._start() ) {
this._spin( (steps || 1) * -this.options.step );
this._stop();
}
},
pageUp: modifier(function( pages ) {
this._stepUp( (pages || 1) * this.options.page );
}),
pageDown: modifier(function( pages ) {
this._stepDown( (pages || 1) * this.options.page );
}),
value: function( newVal ) {
if ( !arguments.length ) {
return this._parse( this.element.val() );
}
modifier( this._value ).call( this, newVal );
},
widget: function() {
return this.uiSpinner;
}
});
}( jQuery ) );
(function( $, undefined ) {
var tabId = 0,
rhash = /#.*$/;
function getNextTabId() {
return ++tabId;
}
function isLocal( anchor ) {
return anchor.hash.length > 1 &&
decodeURIComponent( anchor.href.replace( rhash, "" ) ) ===
decodeURIComponent( location.href.replace( rhash, "" ) );
}
$.widget( "ui.tabs", {
version: "1.10.3",
delay: 300,
options: {
active: null,
collapsible: false,
event: "click",
heightStyle: "content",
hide: null,
show: null,
// callbacks
activate: null,
beforeActivate: null,
beforeLoad: null,
load: null
},
_create: function() {
var that = this,
options = this.options;
this.running = false;
this.element
.addClass( "ui-tabs ui-widget ui-widget-content ui-corner-all" )
.toggleClass( "ui-tabs-collapsible", options.collapsible )
// Prevent users from focusing disabled tabs via click
.delegate( ".ui-tabs-nav > li", "mousedown" + this.eventNamespace, function( event ) {
if ( $( this ).is( ".ui-state-disabled" ) ) {
event.preventDefault();
}
})
// support: IE <9
// Preventing the default action in mousedown doesn't prevent IE
// from focusing the element, so if the anchor gets focused, blur.
// We don't have to worry about focusing the previously focused
// element since clicking on a non-focusable element should focus
// the body anyway.
.delegate( ".ui-tabs-anchor", "focus" + this.eventNamespace, function() {
if ( $( this ).closest( "li" ).is( ".ui-state-disabled" ) ) {
this.blur();
}
});
this._processTabs();
options.active = this._initialActive();
// Take disabling tabs via class attribute from HTML
// into account and update option properly.
if ( $.isArray( options.disabled ) ) {
options.disabled = $.unique( options.disabled.concat(
$.map( this.tabs.filter( ".ui-state-disabled" ), function( li ) {
return that.tabs.index( li );
})
) ).sort();
}
// check for length avoids error when initializing empty list
if ( this.options.active !== false && this.anchors.length ) {
this.active = this._findActive( options.active );
} else {
this.active = $();
}
this._refresh();
if ( this.active.length ) {
this.load( options.active );
}
},
_initialActive: function() {
var active = this.options.active,
collapsible = this.options.collapsible,
locationHash = location.hash.substring( 1 );
if ( active === null ) {
// check the fragment identifier in the URL
if ( locationHash ) {
this.tabs.each(function( i, tab ) {
if ( $( tab ).attr( "aria-controls" ) === locationHash ) {
active = i;
return false;
}
});
}
// check for a tab marked active via a class
if ( active === null ) {
active = this.tabs.index( this.tabs.filter( ".ui-tabs-active" ) );
}
// no active tab, set to false
if ( active === null || active === -1 ) {
active = this.tabs.length ? 0 : false;
}
}
// handle numbers: negative, out of range
if ( active !== false ) {
active = this.tabs.index( this.tabs.eq( active ) );
if ( active === -1 ) {
active = collapsible ? false : 0;
}
}
// don't allow collapsible: false and active: false
if ( !collapsible && active === false && this.anchors.length ) {
active = 0;
}
return active;
},
_getCreateEventData: function() {
return {
tab: this.active,
panel: !this.active.length ? $() : this._getPanelForTab( this.active )
};
},
_tabKeydown: function( event ) {
/*jshint maxcomplexity:15*/
var focusedTab = $( this.document[0].activeElement ).closest( "li" ),
selectedIndex = this.tabs.index( focusedTab ),
goingForward = true;
if ( this._handlePageNav( event ) ) {
return;
}
switch ( event.keyCode ) {
case $.ui.keyCode.RIGHT:
case $.ui.keyCode.DOWN:
selectedIndex++;
break;
case $.ui.keyCode.UP:
case $.ui.keyCode.LEFT:
goingForward = false;
selectedIndex--;
break;
case $.ui.keyCode.END:
selectedIndex = this.anchors.length - 1;
break;
case $.ui.keyCode.HOME:
selectedIndex = 0;
break;
case $.ui.keyCode.SPACE:
// Activate only, no collapsing
event.preventDefault();
clearTimeout( this.activating );
this._activate( selectedIndex );
return;
case $.ui.keyCode.ENTER:
// Toggle (cancel delayed activation, allow collapsing)
event.preventDefault();
clearTimeout( this.activating );
// Determine if we should collapse or activate
this._activate( selectedIndex === this.options.active ? false : selectedIndex );
return;
default:
return;
}
// Focus the appropriate tab, based on which key was pressed
event.preventDefault();
clearTimeout( this.activating );
selectedIndex = this._focusNextTab( selectedIndex, goingForward );
// Navigating with control key will prevent automatic activation
if ( !event.ctrlKey ) {
// Update aria-selected immediately so that AT think the tab is already selected.
// Otherwise AT may confuse the user by stating that they need to activate the tab,
// but the tab will already be activated by the time the announcement finishes.
focusedTab.attr( "aria-selected", "false" );
this.tabs.eq( selectedIndex ).attr( "aria-selected", "true" );
this.activating = this._delay(function() {
this.option( "active", selectedIndex );
}, this.delay );
}
},
_panelKeydown: function( event ) {
if ( this._handlePageNav( event ) ) {
return;
}
// Ctrl+up moves focus to the current tab
if ( event.ctrlKey && event.keyCode === $.ui.keyCode.UP ) {
event.preventDefault();
this.active.focus();
}
},
// Alt+page up/down moves focus to the previous/next tab (and activates)
_handlePageNav: function( event ) {
if ( event.altKey && event.keyCode === $.ui.keyCode.PAGE_UP ) {
this._activate( this._focusNextTab( this.options.active - 1, false ) );
return true;
}
if ( event.altKey && event.keyCode === $.ui.keyCode.PAGE_DOWN ) {
this._activate( this._focusNextTab( this.options.active + 1, true ) );
return true;
}
},
_findNextTab: function( index, goingForward ) {
var lastTabIndex = this.tabs.length - 1;
function constrain() {
if ( index > lastTabIndex ) {
index = 0;
}
if ( index < 0 ) {
index = lastTabIndex;
}
return index;
}
while ( $.inArray( constrain(), this.options.disabled ) !== -1 ) {
index = goingForward ? index + 1 : index - 1;
}
return index;
},
_focusNextTab: function( index, goingForward ) {
index = this._findNextTab( index, goingForward );
this.tabs.eq( index ).focus();
return index;
},
_setOption: function( key, value ) {
if ( key === "active" ) {
// _activate() will handle invalid values and update this.options
this._activate( value );
return;
}
if ( key === "disabled" ) {
// don't use the widget factory's disabled handling
this._setupDisabled( value );
return;
}
this._super( key, value);
if ( key === "collapsible" ) {
this.element.toggleClass( "ui-tabs-collapsible", value );
// Setting collapsible: false while collapsed; open first panel
if ( !value && this.options.active === false ) {
this._activate( 0 );
}
}
if ( key === "event" ) {
this._setupEvents( value );
}
if ( key === "heightStyle" ) {
this._setupHeightStyle( value );
}
},
_tabId: function( tab ) {
return tab.attr( "aria-controls" ) || "ui-tabs-" + getNextTabId();
},
_sanitizeSelector: function( hash ) {
return hash ? hash.replace( /[!"$%&'()*+,.\/:;<=>?@\[\]\^`{|}~]/g, "\\$&" ) : "";
},
refresh: function() {
var options = this.options,
lis = this.tablist.children( ":has(a[href])" );
// get disabled tabs from class attribute from HTML
// this will get converted to a boolean if needed in _refresh()
options.disabled = $.map( lis.filter( ".ui-state-disabled" ), function( tab ) {
return lis.index( tab );
});
this._processTabs();
// was collapsed or no tabs
if ( options.active === false || !this.anchors.length ) {
options.active = false;
this.active = $();
// was active, but active tab is gone
} else if ( this.active.length && !$.contains( this.tablist[ 0 ], this.active[ 0 ] ) ) {
// all remaining tabs are disabled
if ( this.tabs.length === options.disabled.length ) {
options.active = false;
this.active = $();
// activate previous tab
} else {
this._activate( this._findNextTab( Math.max( 0, options.active - 1 ), false ) );
}
// was active, active tab still exists
} else {
// make sure active index is correct
options.active = this.tabs.index( this.active );
}
this._refresh();
},
_refresh: function() {
this._setupDisabled( this.options.disabled );
this._setupEvents( this.options.event );
this._setupHeightStyle( this.options.heightStyle );
this.tabs.not( this.active ).attr({
"aria-selected": "false",
tabIndex: -1
});
this.panels.not( this._getPanelForTab( this.active ) )
.hide()
.attr({
"aria-expanded": "false",
"aria-hidden": "true"
});
// Make sure one tab is in the tab order
if ( !this.active.length ) {
this.tabs.eq( 0 ).attr( "tabIndex", 0 );
} else {
this.active
.addClass( "ui-tabs-active ui-state-active" )
.attr({
"aria-selected": "true",
tabIndex: 0
});
this._getPanelForTab( this.active )
.show()
.attr({
"aria-expanded": "true",
"aria-hidden": "false"
});
}
},
_processTabs: function() {
var that = this;
this.tablist = this._getList()
.addClass( "ui-tabs-nav ui-helper-reset ui-helper-clearfix ui-widget-header ui-corner-all" )
.attr( "role", "tablist" );
this.tabs = this.tablist.find( "> li:has(a[href])" )
.addClass( "ui-state-default ui-corner-top" )
.attr({
role: "tab",
tabIndex: -1
});
this.anchors = this.tabs.map(function() {
return $( "a", this )[ 0 ];
})
.addClass( "ui-tabs-anchor" )
.attr({
role: "presentation",
tabIndex: -1
});
this.panels = $();
this.anchors.each(function( i, anchor ) {
var selector, panel, panelId,
anchorId = $( anchor ).uniqueId().attr( "id" ),
tab = $( anchor ).closest( "li" ),
originalAriaControls = tab.attr( "aria-controls" );
// inline tab
if ( isLocal( anchor ) ) {
selector = anchor.hash;
panel = that.element.find( that._sanitizeSelector( selector ) );
// remote tab
} else {
panelId = that._tabId( tab );
selector = "#" + panelId;
panel = that.element.find( selector );
if ( !panel.length ) {
panel = that._createPanel( panelId );
panel.insertAfter( that.panels[ i - 1 ] || that.tablist );
}
panel.attr( "aria-live", "polite" );
}
if ( panel.length) {
that.panels = that.panels.add( panel );
}
if ( originalAriaControls ) {
tab.data( "ui-tabs-aria-controls", originalAriaControls );
}
tab.attr({
"aria-controls": selector.substring( 1 ),
"aria-labelledby": anchorId
});
panel.attr( "aria-labelledby", anchorId );
});
this.panels
.addClass( "ui-tabs-panel ui-widget-content ui-corner-bottom" )
.attr( "role", "tabpanel" );
},
// allow overriding how to find the list for rare usage scenarios (#7715)
_getList: function() {
return this.element.find( "ol,ul" ).eq( 0 );
},
_createPanel: function( id ) {
return $( "<div>" )
.attr( "id", id )
.addClass( "ui-tabs-panel ui-widget-content ui-corner-bottom" )
.data( "ui-tabs-destroy", true );
},
_setupDisabled: function( disabled ) {
if ( $.isArray( disabled ) ) {
if ( !disabled.length ) {
disabled = false;
} else if ( disabled.length === this.anchors.length ) {
disabled = true;
}
}
// disable tabs
for ( var i = 0, li; ( li = this.tabs[ i ] ); i++ ) {
if ( disabled === true || $.inArray( i, disabled ) !== -1 ) {
$( li )
.addClass( "ui-state-disabled" )
.attr( "aria-disabled", "true" );
} else {
$( li )
.removeClass( "ui-state-disabled" )
.removeAttr( "aria-disabled" );
}
}
this.options.disabled = disabled;
},
_setupEvents: function( event ) {
var events = {
click: function( event ) {
event.preventDefault();
}
};
if ( event ) {
$.each( event.split(" "), function( index, eventName ) {
events[ eventName ] = "_eventHandler";
});
}
this._off( this.anchors.add( this.tabs ).add( this.panels ) );
this._on( this.anchors, events );
this._on( this.tabs, { keydown: "_tabKeydown" } );
this._on( this.panels, { keydown: "_panelKeydown" } );
this._focusable( this.tabs );
this._hoverable( this.tabs );
},
_setupHeightStyle: function( heightStyle ) {
var maxHeight,
parent = this.element.parent();
if ( heightStyle === "fill" ) {
maxHeight = parent.height();
maxHeight -= this.element.outerHeight() - this.element.height();
this.element.siblings( ":visible" ).each(function() {
var elem = $( this ),
position = elem.css( "position" );
if ( position === "absolute" || position === "fixed" ) {
return;
}
maxHeight -= elem.outerHeight( true );
});
this.element.children().not( this.panels ).each(function() {
maxHeight -= $( this ).outerHeight( true );
});
this.panels.each(function() {
$( this ).height( Math.max( 0, maxHeight -
$( this ).innerHeight() + $( this ).height() ) );
})
.css( "overflow", "auto" );
} else if ( heightStyle === "auto" ) {
maxHeight = 0;
this.panels.each(function() {
maxHeight = Math.max( maxHeight, $( this ).height( "" ).height() );
}).height( maxHeight );
}
},
_eventHandler: function( event ) {
var options = this.options,
active = this.active,
anchor = $( event.currentTarget ),
tab = anchor.closest( "li" ),
clickedIsActive = tab[ 0 ] === active[ 0 ],
collapsing = clickedIsActive && options.collapsible,
toShow = collapsing ? $() : this._getPanelForTab( tab ),
toHide = !active.length ? $() : this._getPanelForTab( active ),
eventData = {
oldTab: active,
oldPanel: toHide,
newTab: collapsing ? $() : tab,
newPanel: toShow
};
event.preventDefault();
if ( tab.hasClass( "ui-state-disabled" ) ||
// tab is already loading
tab.hasClass( "ui-tabs-loading" ) ||
// can't switch durning an animation
this.running ||
// click on active header, but not collapsible
( clickedIsActive && !options.collapsible ) ||
// allow canceling activation
( this._trigger( "beforeActivate", event, eventData ) === false ) ) {
return;
}
options.active = collapsing ? false : this.tabs.index( tab );
this.active = clickedIsActive ? $() : tab;
if ( this.xhr ) {
this.xhr.abort();
}
if ( !toHide.length && !toShow.length ) {
$.error( "jQuery UI Tabs: Mismatching fragment identifier." );
}
if ( toShow.length ) {
this.load( this.tabs.index( tab ), event );
}
this._toggle( event, eventData );
},
// handles show/hide for selecting tabs
_toggle: function( event, eventData ) {
var that = this,
toShow = eventData.newPanel,
toHide = eventData.oldPanel;
this.running = true;
function complete() {
that.running = false;
that._trigger( "activate", event, eventData );
}
function show() {
eventData.newTab.closest( "li" ).addClass( "ui-tabs-active ui-state-active" );
if ( toShow.length && that.options.show ) {
that._show( toShow, that.options.show, complete );
} else {
toShow.show();
complete();
}
}
// start out by hiding, then showing, then completing
if ( toHide.length && this.options.hide ) {
this._hide( toHide, this.options.hide, function() {
eventData.oldTab.closest( "li" ).removeClass( "ui-tabs-active ui-state-active" );
show();
});
} else {
eventData.oldTab.closest( "li" ).removeClass( "ui-tabs-active ui-state-active" );
toHide.hide();
show();
}
toHide.attr({
"aria-expanded": "false",
"aria-hidden": "true"
});
eventData.oldTab.attr( "aria-selected", "false" );
// If we're switching tabs, remove the old tab from the tab order.
// If we're opening from collapsed state, remove the previous tab from the tab order.
// If we're collapsing, then keep the collapsing tab in the tab order.
if ( toShow.length && toHide.length ) {
eventData.oldTab.attr( "tabIndex", -1 );
} else if ( toShow.length ) {
this.tabs.filter(function() {
return $( this ).attr( "tabIndex" ) === 0;
})
.attr( "tabIndex", -1 );
}
toShow.attr({
"aria-expanded": "true",
"aria-hidden": "false"
});
eventData.newTab.attr({
"aria-selected": "true",
tabIndex: 0
});
},
_activate: function( index ) {
var anchor,
active = this._findActive( index );
// trying to activate the already active panel
if ( active[ 0 ] === this.active[ 0 ] ) {
return;
}
// trying to collapse, simulate a click on the current active header
if ( !active.length ) {
active = this.active;
}
anchor = active.find( ".ui-tabs-anchor" )[ 0 ];
this._eventHandler({
target: anchor,
currentTarget: anchor,
preventDefault: $.noop
});
},
_findActive: function( index ) {
return index === false ? $() : this.tabs.eq( index );
},
_getIndex: function( index ) {
// meta-function to give users option to provide a href string instead of a numerical index.
if ( typeof index === "string" ) {
index = this.anchors.index( this.anchors.filter( "[href$='" + index + "']" ) );
}
return index;
},
_destroy: function() {
if ( this.xhr ) {
this.xhr.abort();
}
this.element.removeClass( "ui-tabs ui-widget ui-widget-content ui-corner-all ui-tabs-collapsible" );
this.tablist
.removeClass( "ui-tabs-nav ui-helper-reset ui-helper-clearfix ui-widget-header ui-corner-all" )
.removeAttr( "role" );
this.anchors
.removeClass( "ui-tabs-anchor" )
.removeAttr( "role" )
.removeAttr( "tabIndex" )
.removeUniqueId();
this.tabs.add( this.panels ).each(function() {
if ( $.data( this, "ui-tabs-destroy" ) ) {
$( this ).remove();
} else {
$( this )
.removeClass( "ui-state-default ui-state-active ui-state-disabled " +
"ui-corner-top ui-corner-bottom ui-widget-content ui-tabs-active ui-tabs-panel" )
.removeAttr( "tabIndex" )
.removeAttr( "aria-live" )
.removeAttr( "aria-busy" )
.removeAttr( "aria-selected" )
.removeAttr( "aria-labelledby" )
.removeAttr( "aria-hidden" )
.removeAttr( "aria-expanded" )
.removeAttr( "role" );
}
});
this.tabs.each(function() {
var li = $( this ),
prev = li.data( "ui-tabs-aria-controls" );
if ( prev ) {
li
.attr( "aria-controls", prev )
.removeData( "ui-tabs-aria-controls" );
} else {
li.removeAttr( "aria-controls" );
}
});
this.panels.show();
if ( this.options.heightStyle !== "content" ) {
this.panels.css( "height", "" );
}
},
enable: function( index ) {
var disabled = this.options.disabled;
if ( disabled === false ) {
return;
}
if ( index === undefined ) {
disabled = false;
} else {
index = this._getIndex( index );
if ( $.isArray( disabled ) ) {
disabled = $.map( disabled, function( num ) {
return num !== index ? num : null;
});
} else {
disabled = $.map( this.tabs, function( li, num ) {
return num !== index ? num : null;
});
}
}
this._setupDisabled( disabled );
},
disable: function( index ) {
var disabled = this.options.disabled;
if ( disabled === true ) {
return;
}
if ( index === undefined ) {
disabled = true;
} else {
index = this._getIndex( index );
if ( $.inArray( index, disabled ) !== -1 ) {
return;
}
if ( $.isArray( disabled ) ) {
disabled = $.merge( [ index ], disabled ).sort();
} else {
disabled = [ index ];
}
}
this._setupDisabled( disabled );
},
load: function( index, event ) {
index = this._getIndex( index );
var that = this,
tab = this.tabs.eq( index ),
anchor = tab.find( ".ui-tabs-anchor" ),
panel = this._getPanelForTab( tab ),
eventData = {
tab: tab,
panel: panel
};
// not remote
if ( isLocal( anchor[ 0 ] ) ) {
return;
}
this.xhr = $.ajax( this._ajaxSettings( anchor, event, eventData ) );
// support: jQuery <1.8
// jQuery <1.8 returns false if the request is canceled in beforeSend,
// but as of 1.8, $.ajax() always returns a jqXHR object.
if ( this.xhr && this.xhr.statusText !== "canceled" ) {
tab.addClass( "ui-tabs-loading" );
panel.attr( "aria-busy", "true" );
this.xhr
.success(function( response ) {
// support: jQuery <1.8
// http://bugs.jquery.com/ticket/11778
setTimeout(function() {
panel.html( response );
that._trigger( "load", event, eventData );
}, 1 );
})
.complete(function( jqXHR, status ) {
// support: jQuery <1.8
// http://bugs.jquery.com/ticket/11778
setTimeout(function() {
if ( status === "abort" ) {
that.panels.stop( false, true );
}
tab.removeClass( "ui-tabs-loading" );
panel.removeAttr( "aria-busy" );
if ( jqXHR === that.xhr ) {
delete that.xhr;
}
}, 1 );
});
}
},
_ajaxSettings: function( anchor, event, eventData ) {
var that = this;
return {
url: anchor.attr( "href" ),
beforeSend: function( jqXHR, settings ) {
return that._trigger( "beforeLoad", event,
$.extend( { jqXHR : jqXHR, ajaxSettings: settings }, eventData ) );
}
};
},
_getPanelForTab: function( tab ) {
var id = $( tab ).attr( "aria-controls" );
return this.element.find( this._sanitizeSelector( "#" + id ) );
}
});
})( jQuery );
(function( $ ) {
var increments = 0;
function addDescribedBy( elem, id ) {
var describedby = (elem.attr( "aria-describedby" ) || "").split( /\s+/ );
describedby.push( id );
elem
.data( "ui-tooltip-id", id )
.attr( "aria-describedby", $.trim( describedby.join( " " ) ) );
}
function removeDescribedBy( elem ) {
var id = elem.data( "ui-tooltip-id" ),
describedby = (elem.attr( "aria-describedby" ) || "").split( /\s+/ ),
index = $.inArray( id, describedby );
if ( index !== -1 ) {
describedby.splice( index, 1 );
}
elem.removeData( "ui-tooltip-id" );
describedby = $.trim( describedby.join( " " ) );
if ( describedby ) {
elem.attr( "aria-describedby", describedby );
} else {
elem.removeAttr( "aria-describedby" );
}
}
$.widget( "ui.tooltip", {
version: "1.10.3",
options: {
content: function() {
// support: IE<9, Opera in jQuery <1.7
// .text() can't accept undefined, so coerce to a string
var title = $( this ).attr( "title" ) || "";
// Escape title, since we're going from an attribute to raw HTML
return $( "<a>" ).text( title ).html();
},
hide: true,
// Disabled elements have inconsistent behavior across browsers (#8661)
items: "[title]:not([disabled])",
position: {
my: "left top+15",
at: "left bottom",
collision: "flipfit flip"
},
show: true,
tooltipClass: null,
track: false,
// callbacks
close: null,
open: null
},
_create: function() {
this._on({
mouseover: "open",
focusin: "open"
});
// IDs of generated tooltips, needed for destroy
this.tooltips = {};
// IDs of parent tooltips where we removed the title attribute
this.parents = {};
if ( this.options.disabled ) {
this._disable();
}
},
_setOption: function( key, value ) {
var that = this;
if ( key === "disabled" ) {
this[ value ? "_disable" : "_enable" ]();
this.options[ key ] = value;
// disable element style changes
return;
}
this._super( key, value );
if ( key === "content" ) {
$.each( this.tooltips, function( id, element ) {
that._updateContent( element );
});
}
},
_disable: function() {
var that = this;
// close open tooltips
$.each( this.tooltips, function( id, element ) {
var event = $.Event( "blur" );
event.target = event.currentTarget = element[0];
that.close( event, true );
});
// remove title attributes to prevent native tooltips
this.element.find( this.options.items ).addBack().each(function() {
var element = $( this );
if ( element.is( "[title]" ) ) {
element
.data( "ui-tooltip-title", element.attr( "title" ) )
.attr( "title", "" );
}
});
},
_enable: function() {
// restore title attributes
this.element.find( this.options.items ).addBack().each(function() {
var element = $( this );
if ( element.data( "ui-tooltip-title" ) ) {
element.attr( "title", element.data( "ui-tooltip-title" ) );
}
});
},
open: function( event ) {
var that = this,
target = $( event ? event.target : this.element )
// we need closest here due to mouseover bubbling,
// but always pointing at the same event target
.closest( this.options.items );
// No element to show a tooltip for or the tooltip is already open
if ( !target.length || target.data( "ui-tooltip-id" ) ) {
return;
}
if ( target.attr( "title" ) ) {
target.data( "ui-tooltip-title", target.attr( "title" ) );
}
target.data( "ui-tooltip-open", true );
// kill parent tooltips, custom or native, for hover
if ( event && event.type === "mouseover" ) {
target.parents().each(function() {
var parent = $( this ),
blurEvent;
if ( parent.data( "ui-tooltip-open" ) ) {
blurEvent = $.Event( "blur" );
blurEvent.target = blurEvent.currentTarget = this;
that.close( blurEvent, true );
}
if ( parent.attr( "title" ) ) {
parent.uniqueId();
that.parents[ this.id ] = {
element: this,
title: parent.attr( "title" )
};
parent.attr( "title", "" );
}
});
}
this._updateContent( target, event );
},
_updateContent: function( target, event ) {
var content,
contentOption = this.options.content,
that = this,
eventType = event ? event.type : null;
if ( typeof contentOption === "string" ) {
return this._open( event, target, contentOption );
}
content = contentOption.call( target[0], function( response ) {
// ignore async response if tooltip was closed already
if ( !target.data( "ui-tooltip-open" ) ) {
return;
}
// IE may instantly serve a cached response for ajax requests
// delay this call to _open so the other call to _open runs first
that._delay(function() {
// jQuery creates a special event for focusin when it doesn't
// exist natively. To improve performance, the native event
// object is reused and the type is changed. Therefore, we can't
// rely on the type being correct after the event finished
// bubbling, so we set it back to the previous value. (#8740)
if ( event ) {
event.type = eventType;
}
this._open( event, target, response );
});
});
if ( content ) {
this._open( event, target, content );
}
},
_open: function( event, target, content ) {
var tooltip, events, delayedShow,
positionOption = $.extend( {}, this.options.position );
if ( !content ) {
return;
}
// Content can be updated multiple times. If the tooltip already
// exists, then just update the content and bail.
tooltip = this._find( target );
if ( tooltip.length ) {
tooltip.find( ".ui-tooltip-content" ).html( content );
return;
}
// if we have a title, clear it to prevent the native tooltip
// we have to check first to avoid defining a title if none exists
// (we don't want to cause an element to start matching [title])
//
// We use removeAttr only for key events, to allow IE to export the correct
// accessible attributes. For mouse events, set to empty string to avoid
// native tooltip showing up (happens only when removing inside mouseover).
if ( target.is( "[title]" ) ) {
if ( event && event.type === "mouseover" ) {
target.attr( "title", "" );
} else {
target.removeAttr( "title" );
}
}
tooltip = this._tooltip( target );
addDescribedBy( target, tooltip.attr( "id" ) );
tooltip.find( ".ui-tooltip-content" ).html( content );
function position( event ) {
positionOption.of = event;
if ( tooltip.is( ":hidden" ) ) {
return;
}
tooltip.position( positionOption );
}
if ( this.options.track && event && /^mouse/.test( event.type ) ) {
this._on( this.document, {
mousemove: position
});
// trigger once to override element-relative positioning
position( event );
} else {
tooltip.position( $.extend({
of: target
}, this.options.position ) );
}
tooltip.hide();
this._show( tooltip, this.options.show );
// Handle tracking tooltips that are shown with a delay (#8644). As soon
// as the tooltip is visible, position the tooltip using the most recent
// event.
if ( this.options.show && this.options.show.delay ) {
delayedShow = this.delayedShow = setInterval(function() {
if ( tooltip.is( ":visible" ) ) {
position( positionOption.of );
clearInterval( delayedShow );
}
}, $.fx.interval );
}
this._trigger( "open", event, { tooltip: tooltip } );
events = {
keyup: function( event ) {
if ( event.keyCode === $.ui.keyCode.ESCAPE ) {
var fakeEvent = $.Event(event);
fakeEvent.currentTarget = target[0];
this.close( fakeEvent, true );
}
},
remove: function() {
this._removeTooltip( tooltip );
}
};
if ( !event || event.type === "mouseover" ) {
events.mouseleave = "close";
}
if ( !event || event.type === "focusin" ) {
events.focusout = "close";
}
this._on( true, target, events );
},
close: function( event ) {
var that = this,
target = $( event ? event.currentTarget : this.element ),
tooltip = this._find( target );
// disabling closes the tooltip, so we need to track when we're closing
// to avoid an infinite loop in case the tooltip becomes disabled on close
if ( this.closing ) {
return;
}
// Clear the interval for delayed tracking tooltips
clearInterval( this.delayedShow );
// only set title if we had one before (see comment in _open())
if ( target.data( "ui-tooltip-title" ) ) {
target.attr( "title", target.data( "ui-tooltip-title" ) );
}
removeDescribedBy( target );
tooltip.stop( true );
this._hide( tooltip, this.options.hide, function() {
that._removeTooltip( $( this ) );
});
target.removeData( "ui-tooltip-open" );
this._off( target, "mouseleave focusout keyup" );
// Remove 'remove' binding only on delegated targets
if ( target[0] !== this.element[0] ) {
this._off( target, "remove" );
}
this._off( this.document, "mousemove" );
if ( event && event.type === "mouseleave" ) {
$.each( this.parents, function( id, parent ) {
$( parent.element ).attr( "title", parent.title );
delete that.parents[ id ];
});
}
this.closing = true;
this._trigger( "close", event, { tooltip: tooltip } );
this.closing = false;
},
_tooltip: function( element ) {
var id = "ui-tooltip-" + increments++,
tooltip = $( "<div>" )
.attr({
id: id,
role: "tooltip"
})
.addClass( "ui-tooltip ui-widget ui-corner-all ui-widget-content " +
( this.options.tooltipClass || "" ) );
$( "<div>" )
.addClass( "ui-tooltip-content" )
.appendTo( tooltip );
tooltip.appendTo( this.document[0].body );
this.tooltips[ id ] = element;
return tooltip;
},
_find: function( target ) {
var id = target.data( "ui-tooltip-id" );
return id ? $( "#" + id ) : $();
},
_removeTooltip: function( tooltip ) {
tooltip.remove();
delete this.tooltips[ tooltip.attr( "id" ) ];
},
_destroy: function() {
var that = this;
// close open tooltips
$.each( this.tooltips, function( id, element ) {
// Delegate to close method to handle common cleanup
var event = $.Event( "blur" );
event.target = event.currentTarget = element[0];
that.close( event, true );
// Remove immediately; destroying an open tooltip doesn't use the
// hide animation
$( "#" + id ).remove();
// Restore the title
if ( element.data( "ui-tooltip-title" ) ) {
element.attr( "title", element.data( "ui-tooltip-title" ) );
element.removeData( "ui-tooltip-title" );
}
});
}
});
}( jQuery ) );
/**
* @preserve jQuery DateTimePicker plugin v2.3.4
* @homepage http://xdsoft.net/jqplugins/datetimepicker/
* (c) 2014, Chupurnov Valeriy.
*/
(function( $ ) {
'use strict';
var default_options = {
i18n:{
bg:{ // Bulgarian
months:[
"Януари", "Февруари", "Март", "Април", "Май", "Юни", "Юли", "Август", "Септември", "Октомври", "Ноември", "Декември"
],
dayOfWeek:[
"Нд", "Пн", "Вт", "Ср", "Чт", "Пт", "Сб"
]
},
fa:{ // Persian/Farsi
months:[
'فروردین', 'اردیبهشت', 'خرداد', 'تیر', 'مرداد', 'شهریور', 'مهر', 'آبان', 'آذر', 'دی', 'بهمن', 'اسفند'
],
dayOfWeek:[
'یکشنبه', 'دوشنبه', 'سه شنبه', 'چهارشنبه', 'پنجشنبه', 'جمعه', 'شنبه'
]
},
ru:{ // Russian
months:[
'Январь','Февраль','Март','Апрель','Май','Июнь','Июль','Август','Сентябрь','Октябрь','Ноябрь','Декабрь'
],
dayOfWeek:[
"Вск", "Пн", "Вт", "Ср", "Чт", "Пт", "Сб"
]
},
uk:{ // Ukrainian
months:[
'Січень','Лютий','Березень','Квітень','Травень','Червень','Липень','Серпень','Вересень','Жовтень','Листопад','Грудень'
],
dayOfWeek:[
"Ндл", "Пнд", "Втр", "Срд", "Чтв", "Птн", "Сбт"
]
},
en:{ // English
months: [
"January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"
],
dayOfWeek: [
"Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"
]
},
el:{ // Ελληνικά
months: [
"Ιανουάριος", "Φεβρουάριος", "Μάρτιος", "Απρίλιος", "Μάιος", "Ιούνιος", "Ιούλιος", "Αύγουστος", "Σεπτέμβριος", "Οκτώβριος", "Νοέμβριος", "Δεκέμβριος"
],
dayOfWeek: [
"Κυρ", "Δευ", "Τρι", "Τετ", "Πεμ", "Παρ", "Σαβ"
]
},
de:{ // German
months:[
'Januar','Februar','März','April','Mai','Juni','Juli','August','September','Oktober','November','Dezember'
],
dayOfWeek:[
"So", "Mo", "Di", "Mi", "Do", "Fr", "Sa"
]
},
nl:{ // Dutch
months:[
"januari", "februari", "maart", "april", "mei", "juni", "juli", "augustus", "september", "oktober", "november", "december"
],
dayOfWeek:[
"zo", "ma", "di", "wo", "do", "vr", "za"
]
},
tr:{ // Turkish
months:[
"Ocak", "Şubat", "Mart", "Nisan", "Mayıs", "Haziran", "Temmuz", "Ağustos", "Eylül", "Ekim", "Kasım", "Aralık"
],
dayOfWeek:[
"Paz", "Pts", "Sal", "Çar", "Per", "Cum", "Cts"
]
},
fr:{ //French
months:[
"Janvier", "Février", "Mars", "Avril", "Mai", "Juin", "Juillet", "Août", "Septembre", "Octobre", "Novembre", "Décembre"
],
dayOfWeek:[
"Dim", "Lun", "Mar", "Mer", "Jeu", "Ven", "Sam"
]
},
es:{ // Spanish
months: [
"Enero", "Febrero", "Marzo", "Abril", "Mayo", "Junio", "Julio", "Agosto", "Septiembre", "Octubre", "Noviembre", "Diciembre"
],
dayOfWeek: [
"Dom", "Lun", "Mar", "Mié", "Jue", "Vie", "Sáb"
]
},
th:{ // Thai
months:[
'มกราคม','กุมภาพันธ์','มีนาคม','เมษายน','พฤษภาคม','มิถุนายน','กรกฎาคม','สิงหาคม','กันยายน','ตุลาคม','พฤศจิกายน','ธันวาคม'
],
dayOfWeek:[
'อา.','จ.','อ.','พ.','พฤ.','ศ.','ส.'
]
},
pl:{ // Polish
months: [
"styczeń", "luty", "marzec", "kwiecień", "maj", "czerwiec", "lipiec", "sierpień", "wrzesień", "październik", "listopad", "grudzień"
],
dayOfWeek: [
"nd", "pn", "wt", "śr", "cz", "pt", "sb"
]
},
pt:{ // Portuguese
months: [
"Janeiro", "Fevereiro", "Março", "Abril", "Maio", "Junho", "Julho", "Agosto", "Setembro", "Outubro", "Novembro", "Dezembro"
],
dayOfWeek: [
"Dom", "Seg", "Ter", "Qua", "Qui", "Sex", "Sab"
]
},
ch:{ // Simplified Chinese
months: [
"一月","二月","三月","四月","五月","六月","七月","八月","九月","十月","十一月","十二月"
],
dayOfWeek: [
"日", "一","二","三","四","五","六"
]
},
se:{ // Swedish
months: [
"Januari", "Februari", "Mars", "April", "Maj", "Juni", "Juli", "Augusti", "September","Oktober", "November", "December"
],
dayOfWeek: [
"Sön", "Mån", "Tis", "Ons", "Tor", "Fre", "Lör"
]
},
kr:{ // Korean
months: [
"1월", "2월", "3월", "4월", "5월", "6월", "7월", "8월", "9월", "10월", "11월", "12월"
],
dayOfWeek: [
"일", "월", "화", "수", "목", "금", "토"
]
},
it:{ // Italian
months: [
"Gennaio", "Febbraio", "Marzo", "Aprile", "Maggio", "Giugno", "Luglio", "Agosto", "Settembre", "Ottobre", "Novembre", "Dicembre"
],
dayOfWeek: [
"Dom", "Lun", "Mar", "Mer", "Gio", "Ven", "Sab"
]
},
da:{ // Dansk
months: [
"January", "Februar", "Marts", "April", "Maj", "Juni", "July", "August", "September", "Oktober", "November", "December"
],
dayOfWeek: [
"Søn", "Man", "Tir", "Ons", "Tor", "Fre", "Lør"
]
},
no:{ // Norwegian
months: [
"Januar", "Februar", "Mars", "April", "Mai", "Juni", "Juli", "August", "September", "Oktober", "November", "Desember"
],
dayOfWeek: [
"Søn", "Man", "Tir", "Ons", "Tor", "Fre", "Lør"
]
},
ja:{ // Japanese
months: [
"1月", "2月", "3月", "4月", "5月", "6月", "7月", "8月", "9月", "10月", "11月", "12月"
],
dayOfWeek: [
"日", "月", "火", "水", "木", "金", "土"
]
},
vi:{ // Vietnamese
months: [
"Tháng 1", "Tháng 2", "Tháng 3", "Tháng 4", "Tháng 5", "Tháng 6", "Tháng 7", "Tháng 8", "Tháng 9", "Tháng 10", "Tháng 11", "Tháng 12"
],
dayOfWeek: [
"CN", "T2", "T3", "T4", "T5", "T6", "T7"
]
},
sl:{ // Slovenščina
months: [
"Januar", "Februar", "Marec", "April", "Maj", "Junij", "Julij", "Avgust", "September", "Oktober", "November", "December"
],
dayOfWeek: [
"Ned", "Pon", "Tor", "Sre", "Čet", "Pet", "Sob"
]
},
cs:{ // Čeština
months: [
"Leden", "Únor", "Březen", "Duben", "Květen", "Červen", "Červenec", "Srpen", "Září", "Říjen", "Listopad", "Prosinec"
],
dayOfWeek: [
"Ne", "Po", "Út", "St", "Čt", "Pá", "So"
]
},
hu:{ // Hungarian
months: [
"Január", "Február", "Március", "Április", "Május", "Június", "Július", "Augusztus", "Szeptember", "Október", "November", "December"
],
dayOfWeek: [
"Va", "Hé", "Ke", "Sze", "Cs", "Pé", "Szo"
]
}
},
value:'',
lang:'en',
format: 'Y/m/d H:i',
formatTime: 'H:i',
formatDate: 'Y/m/d',
startDate: false, // new Date(), '1986/12/08', '-1970/01/05','-1970/01/05',
step:60,
monthChangeSpinner:true,
closeOnDateSelect:false,
closeOnWithoutClick:true,
closeOnInputClick: true,
timepicker:true,
datepicker:true,
weeks:false,
defaultTime:false, // use formatTime format (ex. '10:00' for formatTime: 'H:i')
defaultDate:false, // use formatDate format (ex new Date() or '1986/12/08' or '-1970/01/05' or '-1970/01/05')
minDate:false,
maxDate:false,
minTime:false,
maxTime:false,
allowTimes:[],
opened:false,
initTime:true,
inline:false,
onSelectDate:function() {},
onSelectTime:function() {},
onChangeMonth:function() {},
onChangeDateTime:function() {},
onShow:function() {},
onClose:function() {},
onGenerate:function() {},
withoutCopyright:true,
inverseButton:false,
hours12:false,
next: 'xdsoft_next',
prev : 'xdsoft_prev',
dayOfWeekStart:0,
timeHeightInTimePicker:25,
timepickerScrollbar:true,
todayButton:true, // 2.1.0
defaultSelect:true, // 2.1.0
scrollMonth:true,
scrollTime:true,
scrollInput:true,
lazyInit:false,
mask:false,
validateOnBlur:true,
allowBlank:true,
yearStart:1950,
yearEnd:2050,
style:'',
id:'',
fixed: false,
roundTime:'round', // ceil, floor
className:'',
weekends : [],
yearOffset:0,
beforeShowDay: null
};
// fix for ie8
if ( !Array.prototype.indexOf ) {
Array.prototype.indexOf = function(obj, start) {
for (var i = (start || 0), j = this.length; i < j; i++) {
if (this[i] === obj) { return i; }
}
return -1;
}
}
Date.prototype.countDaysInMonth = function(){
return new Date(this.getFullYear(), this.getMonth()+1, 0).getDate();
};
$.fn.xdsoftScroller = function( _percent ) {
return this.each(function() {
var timeboxparent = $(this);
if( !$(this).hasClass('xdsoft_scroller_box') ) {
var pointerEventToXY = function( e ) {
var out = {x:0, y:0};
if( e.type == 'touchstart' || e.type == 'touchmove' || e.type == 'touchend' || e.type == 'touchcancel' ) {
var touch = e.originalEvent.touches[0] || e.originalEvent.changedTouches[0];
out.x = touch.pageX;
out.y = touch.pageY;
}else if (e.type == 'mousedown' || e.type == 'mouseup' || e.type == 'mousemove' || e.type == 'mouseover'|| e.type=='mouseout' || e.type=='mouseenter' || e.type=='mouseleave') {
out.x = e.pageX;
out.y = e.pageY;
}
return out;
},
move = 0,
timebox = timeboxparent.children().eq(0),
parentHeight = timeboxparent[0].clientHeight,
height = timebox[0].offsetHeight,
scrollbar = $('<div class="xdsoft_scrollbar"></div>'),
scroller = $('<div class="xdsoft_scroller"></div>'),
maximumOffset = 100,
start = false;
scrollbar.append(scroller);
timeboxparent.addClass('xdsoft_scroller_box').append(scrollbar);
scroller.on('mousedown.xdsoft_scroller',function ( event ) {
if( !parentHeight )
timeboxparent.trigger('resize_scroll.xdsoft_scroller',[_percent]);
var pageY = event.pageY,
top = parseInt(scroller.css('margin-top')),
h1 = scrollbar[0].offsetHeight;
$(document.body).addClass('xdsoft_noselect');
$([document.body,window]).on('mouseup.xdsoft_scroller',function arguments_callee() {
$([document.body,window]).off('mouseup.xdsoft_scroller',arguments_callee)
.off('mousemove.xdsoft_scroller',move)
.removeClass('xdsoft_noselect');
});
$(document.body).on('mousemove.xdsoft_scroller',move = function(event) {
var offset = event.pageY-pageY+top;
if( offset<0 )
offset = 0;
if( offset+scroller[0].offsetHeight>h1 )
offset = h1-scroller[0].offsetHeight;
timeboxparent.trigger('scroll_element.xdsoft_scroller',[maximumOffset?offset/maximumOffset:0]);
});
});
timeboxparent
.on('scroll_element.xdsoft_scroller',function( event,percent ) {
if( !parentHeight )
timeboxparent.trigger('resize_scroll.xdsoft_scroller',[percent,true]);
percent = percent>1?1:(percent<0||isNaN(percent))?0:percent;
scroller.css('margin-top',maximumOffset*percent);
timebox.css('marginTop',-parseInt((height-parentHeight)*percent))
})
.on('resize_scroll.xdsoft_scroller',function( event,_percent,noTriggerScroll ) {
parentHeight = timeboxparent[0].clientHeight;
height = timebox[0].offsetHeight;
var percent = parentHeight/height,
sh = percent*scrollbar[0].offsetHeight;
if( percent>1 )
scroller.hide();
else{
scroller.show();
scroller.css('height',parseInt(sh>10?sh:10));
maximumOffset = scrollbar[0].offsetHeight-scroller[0].offsetHeight;
if( noTriggerScroll!==true )
timeboxparent.trigger('scroll_element.xdsoft_scroller',[_percent?_percent:Math.abs(parseInt(timebox.css('marginTop')))/(height-parentHeight)]);
}
});
timeboxparent.mousewheel&&timeboxparent.mousewheel(function(event, delta, deltaX, deltaY) {
var top = Math.abs(parseInt(timebox.css('marginTop')));
timeboxparent.trigger('scroll_element.xdsoft_scroller',[(top-delta*20)/(height-parentHeight)]);
event.stopPropagation();
return false;
});
timeboxparent.on('touchstart',function( event ) {
start = pointerEventToXY(event);
});
timeboxparent.on('touchmove',function( event ) {
if( start ) {
var coord = pointerEventToXY(event), top = Math.abs(parseInt(timebox.css('marginTop')));
timeboxparent.trigger('scroll_element.xdsoft_scroller',[(top-(coord.y-start.y))/(height-parentHeight)]);
event.stopPropagation();
event.preventDefault();
start = pointerEventToXY(event);
}
});
timeboxparent.on('touchend touchcancel',function( event ) {
start = false;
});
}
timeboxparent.trigger('resize_scroll.xdsoft_scroller',[_percent]);
});
};
$.fn.datetimepicker = function( opt ) {
var KEY0 = 48,
KEY9 = 57,
_KEY0 = 96,
_KEY9 = 105,
CTRLKEY = 17,
DEL = 46,
ENTER = 13,
ESC = 27,
BACKSPACE = 8,
ARROWLEFT = 37,
ARROWUP = 38,
ARROWRIGHT = 39,
ARROWDOWN = 40,
TAB = 9,
F5 = 116,
AKEY = 65,
CKEY = 67,
VKEY = 86,
ZKEY = 90,
YKEY = 89,
ctrlDown = false,
options = ($.isPlainObject(opt)||!opt)?$.extend(true,{},default_options,opt):$.extend({},default_options),
lazyInitTimer = 0,
lazyInit = function( input ){
input
.on('open.xdsoft focusin.xdsoft mousedown.xdsoft',function initOnActionCallback(event) {
if( input.is(':disabled')||input.is(':hidden')||!input.is(':visible')||input.data( 'xdsoft_datetimepicker') )
return;
clearTimeout(lazyInitTimer);
lazyInitTimer = setTimeout(function() {
if( !input.data( 'xdsoft_datetimepicker') )
createDateTimePicker(input);
input
.off('open.xdsoft focusin.xdsoft mousedown.xdsoft',initOnActionCallback)
.trigger('open.xdsoft');
},100);
});
},
createDateTimePicker = function( input ) {
var datetimepicker = $('<div '+(options.id?'id="'+options.id+'"':'')+' '+(options.style?'style="'+options.style+'"':'')+' class="xdsoft_datetimepicker xdsoft_noselect '+(options.weeks?' xdsoft_showweeks':'')+options.className+'"></div>'),
xdsoft_copyright = $('<div class="xdsoft_copyright"><a target="_blank" href="http://xdsoft.net/jqplugins/datetimepicker/">xdsoft.net</a></div>'),
datepicker = $('<div class="xdsoft_datepicker active"></div>'),
mounth_picker = $('<div class="xdsoft_mounthpicker"><button type="button" class="xdsoft_prev"></button><button type="button" class="xdsoft_today_button"></button><div class="xdsoft_label xdsoft_month"><span></span></div><div class="xdsoft_label xdsoft_year"><span></span></div><button type="button" class="xdsoft_next"></button></div>'),
calendar = $('<div class="xdsoft_calendar"></div>'),
timepicker = $('<div class="xdsoft_timepicker active"><button type="button" class="xdsoft_prev"></button><div class="xdsoft_time_box"></div><button type="button" class="xdsoft_next"></button></div>'),
timeboxparent = timepicker.find('.xdsoft_time_box').eq(0),
timebox = $('<div class="xdsoft_time_variant"></div>'),
scrollbar = $('<div class="xdsoft_scrollbar"></div>'),
scroller = $('<div class="xdsoft_scroller"></div>'),
monthselect =$('<div class="xdsoft_select xdsoft_monthselect"><div></div></div>'),
yearselect =$('<div class="xdsoft_select xdsoft_yearselect"><div></div></div>');
//constructor lego
mounth_picker
.find('.xdsoft_month span')
.after(monthselect);
mounth_picker
.find('.xdsoft_year span')
.after(yearselect);
mounth_picker
.find('.xdsoft_month,.xdsoft_year')
.on('mousedown.xdsoft',function(event) {
mounth_picker
.find('.xdsoft_select')
.hide();
var select = $(this).find('.xdsoft_select').eq(0),
val = 0,
top = 0;
if( _xdsoft_datetime.currentTime )
val = _xdsoft_datetime.currentTime[$(this).hasClass('xdsoft_month')?'getMonth':'getFullYear']();
select.show();
for(var items = select.find('div.xdsoft_option'),i = 0;i<items.length;i++) {
if( items.eq(i).data('value')==val ) {
break;
}else top+=items[0].offsetHeight;
}
select.xdsoftScroller(top/(select.children()[0].offsetHeight-(select[0].clientHeight)));
event.stopPropagation();
return false;
});
mounth_picker
.find('.xdsoft_select')
.xdsoftScroller()
.on('mousedown.xdsoft',function( event ) {
event.stopPropagation();
event.preventDefault();
})
.on('mousedown.xdsoft','.xdsoft_option',function( event ) {
if( _xdsoft_datetime&&_xdsoft_datetime.currentTime )
_xdsoft_datetime.currentTime[$(this).parent().parent().hasClass('xdsoft_monthselect')?'setMonth':'setFullYear']($(this).data('value'));
$(this).parent().parent().hide();
datetimepicker.trigger('xchange.xdsoft');
options.onChangeMonth&&options.onChangeMonth.call&&options.onChangeMonth.call(datetimepicker,_xdsoft_datetime.currentTime,datetimepicker.data('input'));
});
// set options
datetimepicker.setOptions = function( _options ) {
options = $.extend(true,{},options,_options);
if( _options.allowTimes && $.isArray(_options.allowTimes) && _options.allowTimes.length ){
options['allowTimes'] = $.extend(true,[],_options.allowTimes);
}
if( _options.weekends && $.isArray(_options.weekends) && _options.weekends.length ){
options['weekends'] = $.extend(true,[],_options.weekends);
}
if( (options.open||options.opened)&&(!options.inline) ) {
input.trigger('open.xdsoft');
}
if( options.inline ) {
triggerAfterOpen = true;
datetimepicker.addClass('xdsoft_inline');
input.after(datetimepicker).hide();
}
if( options.inverseButton ) {
options.next = 'xdsoft_prev';
options.prev = 'xdsoft_next';
}
if( options.datepicker )
datepicker.addClass('active');
else
datepicker.removeClass('active');
if( options.timepicker )
timepicker.addClass('active');
else
timepicker.removeClass('active');
if( options.value ){
input&&input.val&&input.val(options.value);
_xdsoft_datetime.setCurrentTime(options.value);
}
if( isNaN(options.dayOfWeekStart) )
options.dayOfWeekStart = 0;
else
options.dayOfWeekStart = parseInt(options.dayOfWeekStart)%7;
if( !options.timepickerScrollbar )
scrollbar.hide();
if( options.minDate && /^-(.*)$/.test(options.minDate) ){
options.minDate = _xdsoft_datetime.strToDateTime(options.minDate).dateFormat( options.formatDate );
}
if( options.maxDate && /^\+(.*)$/.test(options.maxDate) ) {
options.maxDate = _xdsoft_datetime.strToDateTime(options.maxDate).dateFormat( options.formatDate );
}
mounth_picker
.find('.xdsoft_today_button')
.css('visibility',!options.todayButton?'hidden':'visible');
if( options.mask ) {
var e,
getCaretPos = function ( input ) {
try{
if ( document.selection && document.selection.createRange ) {
var range = document.selection.createRange();
return range.getBookmark().charCodeAt(2) - 2;
}else
if ( input.setSelectionRange )
return input.selectionStart;
}catch(e) {
return 0;
}
},
setCaretPos = function ( node,pos ) {
node = (typeof node == "string" || node instanceof String) ? document.getElementById(node) : node;
if(!node) {
return false;
}else if(node.createTextRange) {
var textRange = node.createTextRange();
textRange.collapse(true);
textRange.moveEnd(pos);
textRange.moveStart(pos);
textRange.select();
return true;
}else if(node.setSelectionRange) {
node.setSelectionRange(pos,pos);
return true;
}
return false;
},
isValidValue = function ( mask,value ) {
var reg = mask
.replace(/([\[\]\/\{\}\(\)\-\.\+]{1})/g,'\\$1')
.replace(/_/g,'{digit+}')
.replace(/([0-9]{1})/g,'{digit$1}')
.replace(/\{digit([0-9]{1})\}/g,'[0-$1_]{1}')
.replace(/\{digit[\+]\}/g,'[0-9_]{1}');
return RegExp(reg).test(value);
};
input.off('keydown.xdsoft');
switch(true) {
case ( options.mask===true ):
options.mask = options.format
.replace(/Y/g,'9999')
.replace(/F/g,'9999')
.replace(/m/g,'19')
.replace(/d/g,'39')
.replace(/H/g,'29')
.replace(/i/g,'59')
.replace(/s/g,'59');
case ( $.type(options.mask) == 'string' ):
if( !isValidValue( options.mask,input.val() ) )
input.val(options.mask.replace(/[0-9]/g,'_'));
input.on('keydown.xdsoft',function( event ) {
var val = this.value,
key = event.which;
switch(true) {
case (( key>=KEY0&&key<=KEY9 )||( key>=_KEY0&&key<=_KEY9 ))||(key==BACKSPACE||key==DEL):
var pos = getCaretPos(this),
digit = ( key!=BACKSPACE&&key!=DEL )?String.fromCharCode((_KEY0 <= key && key <= _KEY9)? key-KEY0 : key):'_';
if( (key==BACKSPACE||key==DEL)&&pos ) {
pos--;
digit='_';
}
while( /[^0-9_]/.test(options.mask.substr(pos,1))&&pos<options.mask.length&&pos>0 )
pos+=( key==BACKSPACE||key==DEL )?-1:1;
val = val.substr(0,pos)+digit+val.substr(pos+1);
if( $.trim(val)=='' ){
val = options.mask.replace(/[0-9]/g,'_');
}else{
if( pos==options.mask.length )
break;
}
pos+=(key==BACKSPACE||key==DEL)?0:1;
while( /[^0-9_]/.test(options.mask.substr(pos,1))&&pos<options.mask.length&&pos>0 )
pos+=(key==BACKSPACE||key==DEL)?-1:1;
if( isValidValue( options.mask,val ) ) {
this.value = val;
setCaretPos(this,pos);
}else if( $.trim(val)=='' )
this.value = options.mask.replace(/[0-9]/g,'_');
else{
input.trigger('error_input.xdsoft');
}
break;
case ( !!~([AKEY,CKEY,VKEY,ZKEY,YKEY].indexOf(key))&&ctrlDown ):
case !!~([ESC,ARROWUP,ARROWDOWN,ARROWLEFT,ARROWRIGHT,F5,CTRLKEY,TAB,ENTER].indexOf(key)):
return true;
}
event.preventDefault();
return false;
});
break;
}
}
if( options.validateOnBlur ) {
input
.off('blur.xdsoft')
.on('blur.xdsoft', function() {
if( options.allowBlank && !$.trim($(this).val()).length ) {
$(this).val(null);
datetimepicker.data('xdsoft_datetime').empty();
}else if( !Date.parseDate( $(this).val(), options.format ) ) {
$(this).val((_xdsoft_datetime.now()).dateFormat( options.format ));
datetimepicker.data('xdsoft_datetime').setCurrentTime($(this).val());
}
else{
datetimepicker.data('xdsoft_datetime').setCurrentTime($(this).val());
}
datetimepicker.trigger('changedatetime.xdsoft');
});
}
options.dayOfWeekStartPrev = (options.dayOfWeekStart==0)?6:options.dayOfWeekStart-1;
datetimepicker
.trigger('xchange.xdsoft')
.trigger('afterOpen.xdsoft')
};
datetimepicker
.data('options',options)
.on('mousedown.xdsoft',function( event ) {
event.stopPropagation();
event.preventDefault();
yearselect.hide();
monthselect.hide();
return false;
});
var scroll_element = timepicker.find('.xdsoft_time_box');
scroll_element.append(timebox);
scroll_element.xdsoftScroller();
datetimepicker.on('afterOpen.xdsoft',function() {
scroll_element.xdsoftScroller();
});
datetimepicker
.append(datepicker)
.append(timepicker);
if( options.withoutCopyright!==true )
datetimepicker
.append(xdsoft_copyright);
datepicker
.append(mounth_picker)
.append(calendar);
$('body').append(datetimepicker);
var _xdsoft_datetime = new function() {
var _this = this;
_this.now = function( norecursion ) {
var d = new Date();
if( !norecursion && options.defaultDate ){
var date = _this.strtodate(options.defaultDate);
d.setFullYear( date.getFullYear() );
d.setMonth( date.getMonth() );
d.setDate( date.getDate() );
}
if( options.yearOffset ){
d.setFullYear(d.getFullYear()+options.yearOffset);
}
if( !norecursion && options.defaultTime ){
var time = _this.strtotime(options.defaultTime);
d.setHours( time.getHours() );
d.setMinutes( time.getMinutes() );
}
return d;
};
_this.isValidDate = function (d) {
if ( Object.prototype.toString.call(d) !== "[object Date]" )
return false;
return !isNaN(d.getTime());
};
_this.setCurrentTime = function( dTime ) {
_this.currentTime = (typeof dTime == 'string')? _this.strToDateTime(dTime) : _this.isValidDate(dTime) ? dTime: _this.now();
datetimepicker.trigger('xchange.xdsoft');
};
_this.empty = function() {
_this.currentTime = null;
};
_this.getCurrentTime = function( dTime) {
return _this.currentTime;
};
_this.nextMonth = function() {
var month = _this.currentTime.getMonth()+1;
if( month==12 ) {
_this.currentTime.setFullYear(_this.currentTime.getFullYear()+1);
month = 0;
}
_this.currentTime.setDate(
Math.min(
Date.daysInMonth[month],
_this.currentTime.getDate()
)
);
_this.currentTime.setMonth(month);
options.onChangeMonth&&options.onChangeMonth.call&&options.onChangeMonth.call(datetimepicker,_xdsoft_datetime.currentTime,datetimepicker.data('input'));
datetimepicker.trigger('xchange.xdsoft');
return month;
};
_this.prevMonth = function() {
var month = _this.currentTime.getMonth()-1;
if( month==-1 ) {
_this.currentTime.setFullYear(_this.currentTime.getFullYear()-1);
month = 11;
}
_this.currentTime.setDate(
Math.min(
Date.daysInMonth[month],
_this.currentTime.getDate()
)
);
_this.currentTime.setMonth(month);
options.onChangeMonth&&options.onChangeMonth.call&&options.onChangeMonth.call(datetimepicker,_xdsoft_datetime.currentTime,datetimepicker.data('input'));
datetimepicker.trigger('xchange.xdsoft');
return month;
};
_this.strToDateTime = function( sDateTime ) {
if( sDateTime && sDateTime instanceof Date && _this.isValidDate(sDateTime) )
return sDateTime;
var tmpDate = [],timeOffset,currentTime;
if( ( tmpDate = /^(\+|\-)(.*)$/.exec(sDateTime) ) && ( tmpDate[2]=Date.parseDate(tmpDate[2], options.formatDate) ) ) {
timeOffset = tmpDate[2].getTime()-(tmpDate[2].getTimezoneOffset())*60000;
currentTime = new Date((_xdsoft_datetime.now()).getTime()+parseInt(tmpDate[1]+'1')*timeOffset);
}else
currentTime = sDateTime?Date.parseDate(sDateTime, options.format):_this.now();
if( !_this.isValidDate(currentTime) )
currentTime = _this.now();
return currentTime;
};
_this.strtodate = function( sDate ) {
if( sDate && sDate instanceof Date && _this.isValidDate(sDate) )
return sDate;
var currentTime = sDate?Date.parseDate(sDate, options.formatDate):_this.now(true);
if( !_this.isValidDate(currentTime) )
currentTime = _this.now(true);
return currentTime;
};
_this.strtotime = function( sTime ) {
if( sTime && sTime instanceof Date && _this.isValidDate(sTime) )
return sTime;
var currentTime = sTime?Date.parseDate(sTime, options.formatTime):_this.now();
if( !_this.isValidDate(currentTime) )
currentTime = _this.now(true);
return currentTime;
};
_this.str = function() {
return _this.currentTime.dateFormat(options.format);
};
_this.currentTime = this.now();
};
mounth_picker
.find('.xdsoft_today_button')
.on('mousedown.xdsoft',function() {
datetimepicker.data('changed',true);
_xdsoft_datetime.setCurrentTime(0);
datetimepicker.trigger('afterOpen.xdsoft');
}).on('dblclick.xdsoft',function(){
input.val( _xdsoft_datetime.str() );
datetimepicker.trigger('close.xdsoft');
});
mounth_picker
.find('.xdsoft_prev,.xdsoft_next')
.on('mousedown.xdsoft',function() {
var $this = $(this),
timer = 0,
stop = false;
(function arguments_callee1(v) {
var month = _xdsoft_datetime.currentTime.getMonth();
if( $this.hasClass( options.next ) ) {
_xdsoft_datetime.nextMonth();
}else if( $this.hasClass( options.prev ) ) {
_xdsoft_datetime.prevMonth();
}
if (options.monthChangeSpinner) {
!stop&&(timer = setTimeout(arguments_callee1,v?v:100));
}
})(500);
$([document.body,window]).on('mouseup.xdsoft',function arguments_callee2() {
clearTimeout(timer);
stop = true;
$([document.body,window]).off('mouseup.xdsoft',arguments_callee2);
});
});
timepicker
.find('.xdsoft_prev,.xdsoft_next')
.on('mousedown.xdsoft',function() {
var $this = $(this),
timer = 0,
stop = false,
period = 110;
(function arguments_callee4(v) {
var pheight = timeboxparent[0].clientHeight,
height = timebox[0].offsetHeight,
top = Math.abs(parseInt(timebox.css('marginTop')));
if( $this.hasClass(options.next) && (height-pheight)- options.timeHeightInTimePicker>=top ) {
timebox.css('marginTop','-'+(top+options.timeHeightInTimePicker)+'px')
}else if( $this.hasClass(options.prev) && top-options.timeHeightInTimePicker>=0 ) {
timebox.css('marginTop','-'+(top-options.timeHeightInTimePicker)+'px')
}
timeboxparent.trigger('scroll_element.xdsoft_scroller',[Math.abs(parseInt(timebox.css('marginTop'))/(height-pheight))]);
period= ( period>10 )?10:period-10;
!stop&&(timer = setTimeout(arguments_callee4,v?v:period));
})(500);
$([document.body,window]).on('mouseup.xdsoft',function arguments_callee5() {
clearTimeout(timer);
stop = true;
$([document.body,window])
.off('mouseup.xdsoft',arguments_callee5);
});
});
var xchangeTimer = 0;
// base handler - generating a calendar and timepicker
datetimepicker
.on('xchange.xdsoft',function( event ) {
clearTimeout(xchangeTimer);
xchangeTimer = setTimeout(function(){
var table = '',
start = new Date(_xdsoft_datetime.currentTime.getFullYear(),_xdsoft_datetime.currentTime.getMonth(),1, 12, 0, 0),
i = 0,
today = _xdsoft_datetime.now();
while( start.getDay()!=options.dayOfWeekStart )
start.setDate(start.getDate()-1);
//generate calendar
table+='<table><thead><tr>';
if(options.weeks) {
table+='<th></th>';
}
// days
for(var j = 0; j<7; j++) {
table+='<th>'+options.i18n[options.lang].dayOfWeek[(j+options.dayOfWeekStart)%7]+'</th>';
}
table+='</tr></thead>';
table+='<tbody>';
var maxDate = false, minDate = false;
if( options.maxDate!==false ) {
maxDate = _xdsoft_datetime.strtodate(options.maxDate);
maxDate = new Date(maxDate.getFullYear(),maxDate.getMonth(),maxDate.getDate(),23,59,59,999);
}
if( options.minDate!==false ) {
minDate = _xdsoft_datetime.strtodate(options.minDate);
minDate = new Date(minDate.getFullYear(),minDate.getMonth(),minDate.getDate());
}
var d,y,m,w,classes = [],customDateSettings,newRow=true;
while( i<_xdsoft_datetime.currentTime.countDaysInMonth()||start.getDay()!=options.dayOfWeekStart||_xdsoft_datetime.currentTime.getMonth()==start.getMonth() ) {
classes = [];
i++;
d = start.getDate(); y = start.getFullYear(); m = start.getMonth(); w = start.getWeekOfYear();
classes.push('xdsoft_date');
if ( options.beforeShowDay && options.beforeShowDay.call ) {
customDateSettings = options.beforeShowDay.call(datetimepicker, start);
} else {
customDateSettings = null;
}
if( ( maxDate!==false && start > maxDate )||( minDate!==false && start < minDate )||(customDateSettings && customDateSettings[0] === false) ){
classes.push('xdsoft_disabled');
}
if ( customDateSettings && customDateSettings[1] != "" ) {
classes.push(customDateSettings[1]);
}
if( _xdsoft_datetime.currentTime.getMonth()!=m ) classes.push('xdsoft_other_month');
if( (options.defaultSelect||datetimepicker.data('changed')) && _xdsoft_datetime.currentTime.dateFormat( options.formatDate )==start.dateFormat( options.formatDate ) ) {
classes.push('xdsoft_current');
}
if( today.dateFormat( options.formatDate )==start.dateFormat( options.formatDate ) ) {
classes.push('xdsoft_today');
}
if( start.getDay()==0||start.getDay()==6||~options.weekends.indexOf(start.dateFormat( options.formatDate )) ) {
classes.push('xdsoft_weekend');
}
if(options.beforeShowDay && typeof options.beforeShowDay == 'function') {
classes.push(options.beforeShowDay(start))
}
if(newRow) {
table+='<tr>';
newRow = false;
if(options.weeks) {
table+='<th>'+w+'</th>';
}
}
table+='<td data-date="'+d+'" data-month="'+m+'" data-year="'+y+'"'+' class="xdsoft_date xdsoft_day_of_week'+start.getDay()+' '+ classes.join(' ')+'">'+
'<div>'+d+'</div>'+
'</td>';
if( start.getDay()==options.dayOfWeekStartPrev ) {
table+='</tr>';
newRow = true;
}
start.setDate(d+1);
}
table+='</tbody></table>';
calendar.html(table);
mounth_picker.find('.xdsoft_label span').eq(0).text(options.i18n[options.lang].months[_xdsoft_datetime.currentTime.getMonth()]);
mounth_picker.find('.xdsoft_label span').eq(1).text(_xdsoft_datetime.currentTime.getFullYear());
// generate timebox
var time = '',
h = '',
m ='',
line_time = function line_time( h,m ) {
var now = _xdsoft_datetime.now();
now.setHours(h);
h = parseInt(now.getHours());
now.setMinutes(m);
m = parseInt(now.getMinutes());
classes = [];
if( (options.maxTime!==false&&_xdsoft_datetime.strtotime(options.maxTime).getTime()<now.getTime())||(options.minTime!==false&&_xdsoft_datetime.strtotime(options.minTime).getTime()>now.getTime()))
classes.push('xdsoft_disabled');
if( (options.initTime||options.defaultSelect||datetimepicker.data('changed')) && parseInt(_xdsoft_datetime.currentTime.getHours())==parseInt(h)&&(options.step>59||Math[options.roundTime](_xdsoft_datetime.currentTime.getMinutes()/options.step)*options.step==parseInt(m))) {
if( options.defaultSelect||datetimepicker.data('changed')) {
classes.push('xdsoft_current');
} else if( options.initTime ) {
classes.push('xdsoft_init_time');
}
}
if( parseInt(today.getHours())==parseInt(h)&&parseInt(today.getMinutes())==parseInt(m))
classes.push('xdsoft_today');
time+= '<div class="xdsoft_time '+classes.join(' ')+'" data-hour="'+h+'" data-minute="'+m+'">'+now.dateFormat(options.formatTime)+'</div>';
};
if( !options.allowTimes || !$.isArray(options.allowTimes) || !options.allowTimes.length ) {
for( var i=0,j=0;i<(options.hours12?12:24);i++ ) {
for( j=0;j<60;j+=options.step ) {
h = (i<10?'0':'')+i;
m = (j<10?'0':'')+j;
line_time( h,m );
}
}
}else{
for( var i=0;i<options.allowTimes.length;i++ ) {
h = _xdsoft_datetime.strtotime(options.allowTimes[i]).getHours();
m = _xdsoft_datetime.strtotime(options.allowTimes[i]).getMinutes();
line_time( h,m );
}
}
timebox.html(time);
var opt = '',
i = 0;
for( i = parseInt(options.yearStart,10)+options.yearOffset;i<= parseInt(options.yearEnd,10)+options.yearOffset;i++ ) {
opt+='<div class="xdsoft_option '+(_xdsoft_datetime.currentTime.getFullYear()==i?'xdsoft_current':'')+'" data-value="'+i+'">'+i+'</div>';
}
yearselect.children().eq(0)
.html(opt);
for( i = 0,opt = '';i<= 11;i++ ) {
opt+='<div class="xdsoft_option '+(_xdsoft_datetime.currentTime.getMonth()==i?'xdsoft_current':'')+'" data-value="'+i+'">'+options.i18n[options.lang].months[i]+'</div>';
}
monthselect.children().eq(0).html(opt);
$(datetimepicker)
.trigger('generate.xdsoft');
},10);
event.stopPropagation();
})
.on('afterOpen.xdsoft',function() {
if( options.timepicker ) {
var classType;
if( timebox.find('.xdsoft_current').length ) {
classType = '.xdsoft_current';
} else if( timebox.find('.xdsoft_init_time').length ) {
classType = '.xdsoft_init_time';
}
if( classType ) {
var pheight = timeboxparent[0].clientHeight,
height = timebox[0].offsetHeight,
top = timebox.find(classType).index()*options.timeHeightInTimePicker+1;
if( (height-pheight)<top )
top = height-pheight;
timeboxparent.trigger('scroll_element.xdsoft_scroller',[parseInt(top)/(height-pheight)]);
}else{
timeboxparent.trigger('scroll_element.xdsoft_scroller',[0]);
}
}
});
var timerclick = 0;
calendar
.on('click.xdsoft', 'td', function (xdevent) {
xdevent.stopPropagation(); // Prevents closing of Pop-ups, Modals and Flyouts in Bootstrap
timerclick++;
var $this = $(this),
currentTime = _xdsoft_datetime.currentTime;
if( currentTime===undefined||currentTime===null ){
_xdsoft_datetime.currentTime = _xdsoft_datetime.now();
currentTime = _xdsoft_datetime.currentTime;
}
if( $this.hasClass('xdsoft_disabled') )
return false;
currentTime.setDate( 1 );
currentTime.setFullYear( $this.data('year') );
currentTime.setMonth( $this.data('month') );
currentTime.setDate( $this.data('date') );
datetimepicker.trigger('select.xdsoft',[currentTime]);
input.val( _xdsoft_datetime.str() );
if( (timerclick>1||(options.closeOnDateSelect===true||( options.closeOnDateSelect===0&&!options.timepicker )))&&!options.inline ) {
datetimepicker.trigger('close.xdsoft');
}
if( options.onSelectDate && options.onSelectDate.call ) {
options.onSelectDate.call(datetimepicker,_xdsoft_datetime.currentTime,datetimepicker.data('input'));
}
datetimepicker.data('changed',true);
datetimepicker.trigger('xchange.xdsoft');
datetimepicker.trigger('changedatetime.xdsoft');
setTimeout(function(){
timerclick = 0;
},200);
});
timebox
.on('click.xdsoft', 'div', function (xdevent) {
xdevent.stopPropagation(); // NAJ: Prevents closing of Pop-ups, Modals and Flyouts
var $this = $(this),
currentTime = _xdsoft_datetime.currentTime;
if( currentTime===undefined||currentTime===null ){
_xdsoft_datetime.currentTime = _xdsoft_datetime.now();
currentTime = _xdsoft_datetime.currentTime;
}
if( $this.hasClass('xdsoft_disabled') )
return false;
currentTime.setHours($this.data('hour'));
currentTime.setMinutes($this.data('minute'));
datetimepicker.trigger('select.xdsoft',[currentTime]);
datetimepicker.data('input').val( _xdsoft_datetime.str() );
!options.inline&&datetimepicker.trigger('close.xdsoft');
if( options.onSelectTime&&options.onSelectTime.call ) {
options.onSelectTime.call(datetimepicker,_xdsoft_datetime.currentTime,datetimepicker.data('input'));
}
datetimepicker.data('changed',true);
datetimepicker.trigger('xchange.xdsoft');
datetimepicker.trigger('changedatetime.xdsoft');
});
datetimepicker.mousewheel&&datepicker.mousewheel(function(event, delta, deltaX, deltaY) {
if( !options.scrollMonth )
return true;
if( delta<0 )
_xdsoft_datetime.nextMonth();
else
_xdsoft_datetime.prevMonth();
return false;
});
datetimepicker.mousewheel&&timeboxparent.unmousewheel().mousewheel(function(event, delta, deltaX, deltaY) {
if( !options.scrollTime )
return true;
var pheight = timeboxparent[0].clientHeight,
height = timebox[0].offsetHeight,
top = Math.abs(parseInt(timebox.css('marginTop'))),
fl = true;
if( delta<0 && (height-pheight)-options.timeHeightInTimePicker>=top ) {
timebox.css('marginTop','-'+(top+options.timeHeightInTimePicker)+'px');
fl = false;
}else if( delta>0&&top-options.timeHeightInTimePicker>=0 ) {
timebox.css('marginTop','-'+(top-options.timeHeightInTimePicker)+'px');
fl = false;
}
timeboxparent.trigger('scroll_element.xdsoft_scroller',[Math.abs(parseInt(timebox.css('marginTop'))/(height-pheight))]);
event.stopPropagation();
return fl;
});
var triggerAfterOpen = false;
datetimepicker
.on('changedatetime.xdsoft',function() {
if( options.onChangeDateTime&&options.onChangeDateTime.call ) {
var $input = datetimepicker.data('input');
options.onChangeDateTime.call(datetimepicker, _xdsoft_datetime.currentTime, $input);
delete options.value;
$input.trigger('change');
}
})
.on('generate.xdsoft',function() {
if( options.onGenerate&&options.onGenerate.call )
options.onGenerate.call(datetimepicker,_xdsoft_datetime.currentTime,datetimepicker.data('input'));
if( triggerAfterOpen ){
datetimepicker.trigger('afterOpen.xdsoft');
triggerAfterOpen = false;
}
})
.on( 'click.xdsoft', function( xdevent )
{
xdevent.stopPropagation(); // Prevents closing of Pop-ups, Modals and Flyouts in Bootstrap
});
var current_time_index = 0;
input.mousewheel&&input.mousewheel(function( event, delta, deltaX, deltaY ) {
if( !options.scrollInput )
return true;
if( !options.datepicker && options.timepicker ) {
current_time_index = timebox.find('.xdsoft_current').length?timebox.find('.xdsoft_current').eq(0).index():0;
if( current_time_index+delta>=0&¤t_time_index+delta<timebox.children().length )
current_time_index+=delta;
timebox.children().eq(current_time_index).length&&timebox.children().eq(current_time_index).trigger('mousedown');
return false;
}else if( options.datepicker && !options.timepicker ) {
datepicker.trigger( event, [delta, deltaX, deltaY]);
input.val&&input.val( _xdsoft_datetime.str() );
datetimepicker.trigger('changedatetime.xdsoft');
return false;
}
});
var setPos = function() {
var offset = datetimepicker.data('input').offset(), top = offset.top+datetimepicker.data('input')[0].offsetHeight-1, left = offset.left, position = "absolute";
if (options.fixed) {
top -= $(window).scrollTop();
left -= $(window).scrollLeft();
position = "fixed";
}else {
if( top+datetimepicker[0].offsetHeight>$(window).height()+$(window).scrollTop() )
top = offset.top-datetimepicker[0].offsetHeight+1;
if (top < 0)
top = 0;
if( left+datetimepicker[0].offsetWidth>$(window).width() )
left = offset.left-datetimepicker[0].offsetWidth+datetimepicker.data('input')[0].offsetWidth;
}
datetimepicker.css({
left:left,
top:top,
position: position
});
};
datetimepicker
.on('open.xdsoft', function() {
var onShow = true;
if( options.onShow&&options.onShow.call) {
onShow = options.onShow.call(datetimepicker,_xdsoft_datetime.currentTime,datetimepicker.data('input'));
}
if( onShow!==false ) {
datetimepicker.show();
setPos();
$(window)
.off('resize.xdsoft',setPos)
.on('resize.xdsoft',setPos);
if( options.closeOnWithoutClick ) {
$([document.body,window]).on('mousedown.xdsoft',function arguments_callee6() {
datetimepicker.trigger('close.xdsoft');
$([document.body,window]).off('mousedown.xdsoft',arguments_callee6);
});
}
}
})
.on('close.xdsoft', function( event ) {
var onClose = true;
if( options.onClose&&options.onClose.call ) {
onClose=options.onClose.call(datetimepicker,_xdsoft_datetime.currentTime,datetimepicker.data('input'));
}
if( onClose!==false&&!options.opened&&!options.inline ) {
datetimepicker.hide();
}
event.stopPropagation();
})
.data('input',input);
var timer = 0,
timer1 = 0;
datetimepicker.data('xdsoft_datetime',_xdsoft_datetime);
datetimepicker.setOptions(options);
function getCurrentValue(){
var ct = false;
if ( options.startDate ) {
ct = _xdsoft_datetime.strToDateTime(options.startDate);
} else {
ct = options.value?options.value:(input&&input.val&&input.val())?input.val():'';
ct = Date.parseDate(ct, options.format);
}
if ( ct && _xdsoft_datetime.isValidDate(ct) ) {
datetimepicker.data('changed',true);
} else {
ct = '';
}
return ct?ct:0;
}
//debugger
_xdsoft_datetime.setCurrentTime( getCurrentValue() );
input
.data( 'xdsoft_datetimepicker',datetimepicker )
.on('open.xdsoft focusin.xdsoft mousedown.xdsoft',function(event) {
if( input.is(':disabled')||input.is(':hidden')||!input.is(':visible')||(input.data('xdsoft_datetimepicker').is(':visible') && options.closeOnInputClick) )
return;
clearTimeout(timer);
timer = setTimeout(function() {
if( input.is(':disabled')||input.is(':hidden')||!input.is(':visible') )
return;
triggerAfterOpen = true;
_xdsoft_datetime.setCurrentTime(getCurrentValue());
datetimepicker.trigger('open.xdsoft');
},100);
})
.on('keydown.xdsoft',function( event ) {
var val = this.value,
key = event.which;
switch(true) {
case !!~([ENTER].indexOf(key)):
var elementSelector = $("input:visible,textarea:visible");
datetimepicker.trigger('close.xdsoft');
elementSelector.eq(elementSelector.index(this) + 1).focus();
return false;
case !!~[TAB].indexOf(key):
datetimepicker.trigger('close.xdsoft');
return true;
}
});
},
destroyDateTimePicker = function( input ) {
var datetimepicker = input.data('xdsoft_datetimepicker');
if( datetimepicker ) {
datetimepicker.data('xdsoft_datetime',null);
datetimepicker.remove();
input
.data( 'xdsoft_datetimepicker',null )
.off( 'open.xdsoft focusin.xdsoft focusout.xdsoft mousedown.xdsoft blur.xdsoft keydown.xdsoft' );
$(window).off('resize.xdsoft');
$([window,document.body]).off('mousedown.xdsoft');
input.unmousewheel&&input.unmousewheel();
}
};
$(document)
.off('keydown.xdsoftctrl keyup.xdsoftctrl')
.on('keydown.xdsoftctrl',function(e) {
if ( e.keyCode == CTRLKEY )
ctrlDown = true;
})
.on('keyup.xdsoftctrl',function(e) {
if ( e.keyCode == CTRLKEY )
ctrlDown = false;
});
return this.each(function() {
var datetimepicker;
if( datetimepicker = $(this).data('xdsoft_datetimepicker') ) {
if( $.type(opt) === 'string' ) {
switch(opt) {
case 'show':
$(this).select().focus();
datetimepicker.trigger( 'open.xdsoft' );
break;
case 'hide':
datetimepicker.trigger('close.xdsoft');
break;
case 'destroy':
destroyDateTimePicker($(this));
break;
case 'reset':
this.value = this.defaultValue;
if(!this.value || !datetimepicker.data('xdsoft_datetime').isValidDate(Date.parseDate(this.value, options.format)))
datetimepicker.data('changed',false);
datetimepicker.data('xdsoft_datetime').setCurrentTime(this.value);
break;
}
}else{
datetimepicker
.setOptions(opt);
}
return 0;
}else
if( ($.type(opt) !== 'string') ){
if( !options.lazyInit||options.open||options.inline ){
createDateTimePicker($(this));
}else
lazyInit($(this));
}
});
};
$.fn.datetimepicker.defaults = default_options;
})( jQuery );
/*
* Copyright (c) 2013 Brandon Aaron (http://brandonaaron.net)
*
* Licensed under the MIT License (LICENSE.txt).
*
* Thanks to: http://adomas.org/javascript-mouse-wheel/ for some pointers.
* Thanks to: Mathias Bank(http://www.mathias-bank.de) for a scope bug fix.
* Thanks to: Seamus Leahy for adding deltaX and deltaY
*
* Version: 3.1.3
*
* Requires: 1.2.2+
*/
(function(factory) {if(typeof define==='function'&&define.amd) {define(['jquery'],factory)}else if(typeof exports==='object') {module.exports=factory}else{factory(jQuery)}}(function($) {var toFix=['wheel','mousewheel','DOMMouseScroll','MozMousePixelScroll'];var toBind='onwheel'in document||document.documentMode>=9?['wheel']:['mousewheel','DomMouseScroll','MozMousePixelScroll'];var lowestDelta,lowestDeltaXY;if($.event.fixHooks) {for(var i=toFix.length;i;) {$.event.fixHooks[toFix[--i]]=$.event.mouseHooks}}$.event.special.mousewheel={setup:function() {if(this.addEventListener) {for(var i=toBind.length;i;) {this.addEventListener(toBind[--i],handler,false)}}else{this.onmousewheel=handler}},teardown:function() {if(this.removeEventListener) {for(var i=toBind.length;i;) {this.removeEventListener(toBind[--i],handler,false)}}else{this.onmousewheel=null}}};$.fn.extend({mousewheel:function(fn) {return fn?this.bind("mousewheel",fn):this.trigger("mousewheel")},unmousewheel:function(fn) {return this.unbind("mousewheel",fn)}});function handler(event) {var orgEvent=event||window.event,args=[].slice.call(arguments,1),delta=0,deltaX=0,deltaY=0,absDelta=0,absDeltaXY=0,fn;event=$.event.fix(orgEvent);event.type="mousewheel";if(orgEvent.wheelDelta) {delta=orgEvent.wheelDelta}if(orgEvent.detail) {delta=orgEvent.detail*-1}if(orgEvent.deltaY) {deltaY=orgEvent.deltaY*-1;delta=deltaY}if(orgEvent.deltaX) {deltaX=orgEvent.deltaX;delta=deltaX*-1}if(orgEvent.wheelDeltaY!==undefined) {deltaY=orgEvent.wheelDeltaY}if(orgEvent.wheelDeltaX!==undefined) {deltaX=orgEvent.wheelDeltaX*-1}absDelta=Math.abs(delta);if(!lowestDelta||absDelta<lowestDelta) {lowestDelta=absDelta}absDeltaXY=Math.max(Math.abs(deltaY),Math.abs(deltaX));if(!lowestDeltaXY||absDeltaXY<lowestDeltaXY) {lowestDeltaXY=absDeltaXY}fn=delta>0?'floor':'ceil';delta=Math[fn](delta/lowestDelta);deltaX=Math[fn](deltaX/lowestDeltaXY);deltaY=Math[fn](deltaY/lowestDeltaXY);args.unshift(event,delta,deltaX,deltaY);return($.event.dispatch||$.event.handle).apply(this,args)}}));
// Parse and Format Library
//http://www.xaprb.com/blog/2005/12/12/javascript-closures-for-runtime-efficiency/
/*
* Copyright (C) 2004 Baron Schwartz <baron at sequent dot org>
*
* This program is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by the
* Free Software Foundation, version 2.1.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*/
Date.parseFunctions={count:0};Date.parseRegexes=[];Date.formatFunctions={count:0};Date.prototype.dateFormat=function(b){if(b=="unixtime"){return parseInt(this.getTime()/1000);}if(Date.formatFunctions[b]==null){Date.createNewFormat(b);}var a=Date.formatFunctions[b];return this[a]();};Date.createNewFormat=function(format){var funcName="format"+Date.formatFunctions.count++;Date.formatFunctions[format]=funcName;var code="Date.prototype."+funcName+" = function() {return ";var special=false;var ch="";for(var i=0;i<format.length;++i){ch=format.charAt(i);if(!special&&ch=="\\"){special=true;}else{if(special){special=false;code+="'"+String.escape(ch)+"' + ";}else{code+=Date.getFormatCode(ch);}}}eval(code.substring(0,code.length-3)+";}");};Date.getFormatCode=function(a){switch(a){case"d":return"String.leftPad(this.getDate(), 2, '0') + ";case"D":return"Date.dayNames[this.getDay()].substring(0, 3) + ";case"j":return"this.getDate() + ";case"l":return"Date.dayNames[this.getDay()] + ";case"S":return"this.getSuffix() + ";case"w":return"this.getDay() + ";case"z":return"this.getDayOfYear() + ";case"W":return"this.getWeekOfYear() + ";case"F":return"Date.monthNames[this.getMonth()] + ";case"m":return"String.leftPad(this.getMonth() + 1, 2, '0') + ";case"M":return"Date.monthNames[this.getMonth()].substring(0, 3) + ";case"n":return"(this.getMonth() + 1) + ";case"t":return"this.getDaysInMonth() + ";case"L":return"(this.isLeapYear() ? 1 : 0) + ";case"Y":return"this.getFullYear() + ";case"y":return"('' + this.getFullYear()).substring(2, 4) + ";case"a":return"(this.getHours() < 12 ? 'am' : 'pm') + ";case"A":return"(this.getHours() < 12 ? 'AM' : 'PM') + ";case"g":return"((this.getHours() %12) ? this.getHours() % 12 : 12) + ";case"G":return"this.getHours() + ";case"h":return"String.leftPad((this.getHours() %12) ? this.getHours() % 12 : 12, 2, '0') + ";case"H":return"String.leftPad(this.getHours(), 2, '0') + ";case"i":return"String.leftPad(this.getMinutes(), 2, '0') + ";case"s":return"String.leftPad(this.getSeconds(), 2, '0') + ";case"O":return"this.getGMTOffset() + ";case"T":return"this.getTimezone() + ";case"Z":return"(this.getTimezoneOffset() * -60) + ";default:return"'"+String.escape(a)+"' + ";}};Date.parseDate=function(a,c){if(c=="unixtime"){return new Date(!isNaN(parseInt(a))?parseInt(a)*1000:0);}if(Date.parseFunctions[c]==null){Date.createParser(c);}var b=Date.parseFunctions[c];return Date[b](a);};Date.createParser=function(format){var funcName="parse"+Date.parseFunctions.count++;var regexNum=Date.parseRegexes.length;var currentGroup=1;Date.parseFunctions[format]=funcName;var code="Date."+funcName+" = function(input) {\nvar y = -1, m = -1, d = -1, h = -1, i = -1, s = -1, z = -1;\nvar d = new Date();\ny = d.getFullYear();\nm = d.getMonth();\nd = d.getDate();\nvar results = input.match(Date.parseRegexes["+regexNum+"]);\nif (results && results.length > 0) {";var regex="";var special=false;var ch="";for(var i=0;i<format.length;++i){ch=format.charAt(i);if(!special&&ch=="\\"){special=true;}else{if(special){special=false;regex+=String.escape(ch);}else{obj=Date.formatCodeToRegex(ch,currentGroup);currentGroup+=obj.g;regex+=obj.s;if(obj.g&&obj.c){code+=obj.c;}}}}code+="if (y > 0 && z > 0){\nvar doyDate = new Date(y,0);\ndoyDate.setDate(z);\nm = doyDate.getMonth();\nd = doyDate.getDate();\n}";code+="if (y > 0 && m >= 0 && d > 0 && h >= 0 && i >= 0 && s >= 0)\n{return new Date(y, m, d, h, i, s);}\nelse if (y > 0 && m >= 0 && d > 0 && h >= 0 && i >= 0)\n{return new Date(y, m, d, h, i);}\nelse if (y > 0 && m >= 0 && d > 0 && h >= 0)\n{return new Date(y, m, d, h);}\nelse if (y > 0 && m >= 0 && d > 0)\n{return new Date(y, m, d);}\nelse if (y > 0 && m >= 0)\n{return new Date(y, m);}\nelse if (y > 0)\n{return new Date(y);}\n}return null;}";Date.parseRegexes[regexNum]=new RegExp("^"+regex+"$");eval(code);};Date.formatCodeToRegex=function(b,a){switch(b){case"D":return{g:0,c:null,s:"(?:Sun|Mon|Tue|Wed|Thu|Fri|Sat)"};case"j":case"d":return{g:1,c:"d = parseInt(results["+a+"], 10);\n",s:"(\\d{1,2})"};case"l":return{g:0,c:null,s:"(?:"+Date.dayNames.join("|")+")"};case"S":return{g:0,c:null,s:"(?:st|nd|rd|th)"};case"w":return{g:0,c:null,s:"\\d"};case"z":return{g:1,c:"z = parseInt(results["+a+"], 10);\n",s:"(\\d{1,3})"};case"W":return{g:0,c:null,s:"(?:\\d{2})"};case"F":return{g:1,c:"m = parseInt(Date.monthNumbers[results["+a+"].substring(0, 3)], 10);\n",s:"("+Date.monthNames.join("|")+")"};case"M":return{g:1,c:"m = parseInt(Date.monthNumbers[results["+a+"]], 10);\n",s:"(Jan|Feb|Mar|Apr|May|Jun|Jul|Aug|Sep|Oct|Nov|Dec)"};case"n":case"m":return{g:1,c:"m = parseInt(results["+a+"], 10) - 1;\n",s:"(\\d{1,2})"};case"t":return{g:0,c:null,s:"\\d{1,2}"};case"L":return{g:0,c:null,s:"(?:1|0)"};case"Y":return{g:1,c:"y = parseInt(results["+a+"], 10);\n",s:"(\\d{4})"};case"y":return{g:1,c:"var ty = parseInt(results["+a+"], 10);\ny = ty > Date.y2kYear ? 1900 + ty : 2000 + ty;\n",s:"(\\d{1,2})"};case"a":return{g:1,c:"if (results["+a+"] == 'am') {\nif (h == 12) { h = 0; }\n} else { if (h < 12) { h += 12; }}",s:"(am|pm)"};case"A":return{g:1,c:"if (results["+a+"] == 'AM') {\nif (h == 12) { h = 0; }\n} else { if (h < 12) { h += 12; }}",s:"(AM|PM)"};case"g":case"G":case"h":case"H":return{g:1,c:"h = parseInt(results["+a+"], 10);\n",s:"(\\d{1,2})"};case"i":return{g:1,c:"i = parseInt(results["+a+"], 10);\n",s:"(\\d{2})"};case"s":return{g:1,c:"s = parseInt(results["+a+"], 10);\n",s:"(\\d{2})"};case"O":return{g:0,c:null,s:"[+-]\\d{4}"};case"T":return{g:0,c:null,s:"[A-Z]{3}"};case"Z":return{g:0,c:null,s:"[+-]\\d{1,5}"};default:return{g:0,c:null,s:String.escape(b)};}};Date.prototype.getTimezone=function(){return this.toString().replace(/^.*? ([A-Z]{3}) [0-9]{4}.*$/,"$1").replace(/^.*?\(([A-Z])[a-z]+ ([A-Z])[a-z]+ ([A-Z])[a-z]+\)$/,"$1$2$3");};Date.prototype.getGMTOffset=function(){return(this.getTimezoneOffset()>0?"-":"+")+String.leftPad(Math.floor(Math.abs(this.getTimezoneOffset())/60),2,"0")+String.leftPad(Math.abs(this.getTimezoneOffset())%60,2,"0");};Date.prototype.getDayOfYear=function(){var a=0;Date.daysInMonth[1]=this.isLeapYear()?29:28;for(var b=0;b<this.getMonth();++b){a+=Date.daysInMonth[b];}return a+this.getDate();};Date.prototype.getWeekOfYear=function(){var b=this.getDayOfYear()+(4-this.getDay());var a=new Date(this.getFullYear(),0,1);var c=(7-a.getDay()+4);return String.leftPad(Math.ceil((b-c)/7)+1,2,"0");};Date.prototype.isLeapYear=function(){var a=this.getFullYear();return((a&3)==0&&(a%100||(a%400==0&&a)));};Date.prototype.getFirstDayOfMonth=function(){var a=(this.getDay()-(this.getDate()-1))%7;return(a<0)?(a+7):a;};Date.prototype.getLastDayOfMonth=function(){var a=(this.getDay()+(Date.daysInMonth[this.getMonth()]-this.getDate()))%7;return(a<0)?(a+7):a;};Date.prototype.getDaysInMonth=function(){Date.daysInMonth[1]=this.isLeapYear()?29:28;return Date.daysInMonth[this.getMonth()];};Date.prototype.getSuffix=function(){switch(this.getDate()){case 1:case 21:case 31:return"st";case 2:case 22:return"nd";case 3:case 23:return"rd";default:return"th";}};String.escape=function(a){return a.replace(/('|\\)/g,"\\$1");};String.leftPad=function(d,b,c){var a=new String(d);if(c==null){c=" ";}while(a.length<b){a=c+a;}return a;};Date.daysInMonth=[31,28,31,30,31,30,31,31,30,31,30,31];Date.monthNames=["January","February","March","April","May","June","July","August","September","October","November","December"];Date.dayNames=["Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday"];Date.y2kYear=50;Date.monthNumbers={Jan:0,Feb:1,Mar:2,Apr:3,May:4,Jun:5,Jul:6,Aug:7,Sep:8,Oct:9,Nov:10,Dec:11};Date.patterns={ISO8601LongPattern:"Y-m-d H:i:s",ISO8601ShortPattern:"Y-m-d",ShortDatePattern:"n/j/Y",LongDatePattern:"l, F d, Y",FullDateTimePattern:"l, F d, Y g:i:s A",MonthDayPattern:"F d",ShortTimePattern:"g:i A",LongTimePattern:"g:i:s A",SortableDateTimePattern:"Y-m-d\\TH:i:s",UniversalSortableDateTimePattern:"Y-m-d H:i:sO",YearMonthPattern:"F, Y"};
/*!
* Bootstrap v3.0.2 by @fat and @mdo
* Copyright 2013 Twitter, Inc.
* Licensed under http://www.apache.org/licenses/LICENSE-2.0
*
* Designed and built with all the love in the world by @mdo and @fat.
*/
if (typeof jQuery === "undefined") { throw new Error("Bootstrap requires jQuery") }
/* ========================================================================
* Bootstrap: transition.js v3.0.2
* http://getbootstrap.com/javascript/#transitions
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// CSS TRANSITION SUPPORT (Shoutout: http://www.modernizr.com/)
// ============================================================
function transitionEnd() {
var el = document.createElement('bootstrap')
var transEndEventNames = {
'WebkitTransition' : 'webkitTransitionEnd'
, 'MozTransition' : 'transitionend'
, 'OTransition' : 'oTransitionEnd otransitionend'
, 'transition' : 'transitionend'
}
for (var name in transEndEventNames) {
if (el.style[name] !== undefined) {
return { end: transEndEventNames[name] }
}
}
}
// http://blog.alexmaccaw.com/css-transitions
$.fn.emulateTransitionEnd = function (duration) {
var called = false, $el = this
$(this).one($.support.transition.end, function () { called = true })
var callback = function () { if (!called) $($el).trigger($.support.transition.end) }
setTimeout(callback, duration)
return this
}
$(function () {
$.support.transition = transitionEnd()
})
}(jQuery);
/* ========================================================================
* Bootstrap: alert.js v3.0.2
* http://getbootstrap.com/javascript/#alerts
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// ALERT CLASS DEFINITION
// ======================
var dismiss = '[data-dismiss="alert"]'
var Alert = function (el) {
$(el).on('click', dismiss, this.close)
}
Alert.prototype.close = function (e) {
var $this = $(this)
var selector = $this.attr('data-target')
if (!selector) {
selector = $this.attr('href')
selector = selector && selector.replace(/.*(?=#[^\s]*$)/, '') // strip for ie7
}
var $parent = $(selector)
if (e) e.preventDefault()
if (!$parent.length) {
$parent = $this.hasClass('alert') ? $this : $this.parent()
}
$parent.trigger(e = $.Event('close.bs.alert'))
if (e.isDefaultPrevented()) return
$parent.removeClass('in')
function removeElement() {
$parent.trigger('closed.bs.alert').remove()
}
$.support.transition && $parent.hasClass('fade') ?
$parent
.one($.support.transition.end, removeElement)
.emulateTransitionEnd(150) :
removeElement()
}
// ALERT PLUGIN DEFINITION
// =======================
var old = $.fn.alert
$.fn.alert = function (option) {
return this.each(function () {
var $this = $(this)
var data = $this.data('bs.alert')
if (!data) $this.data('bs.alert', (data = new Alert(this)))
if (typeof option == 'string') data[option].call($this)
})
}
$.fn.alert.Constructor = Alert
// ALERT NO CONFLICT
// =================
$.fn.alert.noConflict = function () {
$.fn.alert = old
return this
}
// ALERT DATA-API
// ==============
$(document).on('click.bs.alert.data-api', dismiss, Alert.prototype.close)
}(jQuery);
/* ========================================================================
* Bootstrap: button.js v3.0.2
* http://getbootstrap.com/javascript/#buttons
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// BUTTON PUBLIC CLASS DEFINITION
// ==============================
var Button = function (element, options) {
this.$element = $(element)
this.options = $.extend({}, Button.DEFAULTS, options)
}
Button.DEFAULTS = {
loadingText: 'loading...'
}
Button.prototype.setState = function (state) {
var d = 'disabled'
var $el = this.$element
var val = $el.is('input') ? 'val' : 'html'
var data = $el.data()
state = state + 'Text'
if (!data.resetText) $el.data('resetText', $el[val]())
$el[val](data[state] || this.options[state])
// push to event loop to allow forms to submit
setTimeout(function () {
state == 'loadingText' ?
$el.addClass(d).attr(d, d) :
$el.removeClass(d).removeAttr(d);
}, 0)
}
Button.prototype.toggle = function () {
var $parent = this.$element.closest('[data-toggle="buttons"]')
if ($parent.length) {
var $input = this.$element.find('input')
.prop('checked', !this.$element.hasClass('active'))
.trigger('change')
if ($input.prop('type') === 'radio') $parent.find('.active').removeClass('active')
}
this.$element.toggleClass('active')
}
// BUTTON PLUGIN DEFINITION
// ========================
var old = $.fn.button
$.fn.button = function (option) {
return this.each(function () {
var $this = $(this)
var data = $this.data('bs.button')
var options = typeof option == 'object' && option
if (!data) $this.data('bs.button', (data = new Button(this, options)))
if (option == 'toggle') data.toggle()
else if (option) data.setState(option)
})
}
$.fn.button.Constructor = Button
// BUTTON NO CONFLICT
// ==================
$.fn.button.noConflict = function () {
$.fn.button = old
return this
}
// BUTTON DATA-API
// ===============
$(document).on('click.bs.button.data-api', '[data-toggle^=button]', function (e) {
var $btn = $(e.target)
if (!$btn.hasClass('btn')) $btn = $btn.closest('.btn')
$btn.button('toggle')
e.preventDefault()
})
}(jQuery);
/* ========================================================================
* Bootstrap: carousel.js v3.0.2
* http://getbootstrap.com/javascript/#carousel
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// CAROUSEL CLASS DEFINITION
// =========================
var Carousel = function (element, options) {
this.$element = $(element)
this.$indicators = this.$element.find('.carousel-indicators')
this.options = options
this.paused =
this.sliding =
this.interval =
this.$active =
this.$items = null
this.options.pause == 'hover' && this.$element
.on('mouseenter', $.proxy(this.pause, this))
.on('mouseleave', $.proxy(this.cycle, this))
}
Carousel.DEFAULTS = {
interval: 5000
, pause: 'hover'
, wrap: true
}
Carousel.prototype.cycle = function (e) {
e || (this.paused = false)
this.interval && clearInterval(this.interval)
this.options.interval
&& !this.paused
&& (this.interval = setInterval($.proxy(this.next, this), this.options.interval))
return this
}
Carousel.prototype.getActiveIndex = function () {
this.$active = this.$element.find('.item.active')
this.$items = this.$active.parent().children()
return this.$items.index(this.$active)
}
Carousel.prototype.to = function (pos) {
var that = this
var activeIndex = this.getActiveIndex()
if (pos > (this.$items.length - 1) || pos < 0) return
if (this.sliding) return this.$element.one('slid', function () { that.to(pos) })
if (activeIndex == pos) return this.pause().cycle()
return this.slide(pos > activeIndex ? 'next' : 'prev', $(this.$items[pos]))
}
Carousel.prototype.pause = function (e) {
e || (this.paused = true)
if (this.$element.find('.next, .prev').length && $.support.transition.end) {
this.$element.trigger($.support.transition.end)
this.cycle(true)
}
this.interval = clearInterval(this.interval)
return this
}
Carousel.prototype.next = function () {
if (this.sliding) return
return this.slide('next')
}
Carousel.prototype.prev = function () {
if (this.sliding) return
return this.slide('prev')
}
Carousel.prototype.slide = function (type, next) {
var $active = this.$element.find('.item.active')
var $next = next || $active[type]()
var isCycling = this.interval
var direction = type == 'next' ? 'left' : 'right'
var fallback = type == 'next' ? 'first' : 'last'
var that = this
if (!$next.length) {
if (!this.options.wrap) return
$next = this.$element.find('.item')[fallback]()
}
this.sliding = true
isCycling && this.pause()
var e = $.Event('slide.bs.carousel', { relatedTarget: $next[0], direction: direction })
if ($next.hasClass('active')) return
if (this.$indicators.length) {
this.$indicators.find('.active').removeClass('active')
this.$element.one('slid', function () {
var $nextIndicator = $(that.$indicators.children()[that.getActiveIndex()])
$nextIndicator && $nextIndicator.addClass('active')
})
}
if ($.support.transition && this.$element.hasClass('slide')) {
this.$element.trigger(e)
if (e.isDefaultPrevented()) return
$next.addClass(type)
$next[0].offsetWidth // force reflow
$active.addClass(direction)
$next.addClass(direction)
$active
.one($.support.transition.end, function () {
$next.removeClass([type, direction].join(' ')).addClass('active')
$active.removeClass(['active', direction].join(' '))
that.sliding = false
setTimeout(function () { that.$element.trigger('slid') }, 0)
})
.emulateTransitionEnd(600)
} else {
this.$element.trigger(e)
if (e.isDefaultPrevented()) return
$active.removeClass('active')
$next.addClass('active')
this.sliding = false
this.$element.trigger('slid')
}
isCycling && this.cycle()
return this
}
// CAROUSEL PLUGIN DEFINITION
// ==========================
var old = $.fn.carousel
$.fn.carousel = function (option) {
return this.each(function () {
var $this = $(this)
var data = $this.data('bs.carousel')
var options = $.extend({}, Carousel.DEFAULTS, $this.data(), typeof option == 'object' && option)
var action = typeof option == 'string' ? option : options.slide
if (!data) $this.data('bs.carousel', (data = new Carousel(this, options)))
if (typeof option == 'number') data.to(option)
else if (action) data[action]()
else if (options.interval) data.pause().cycle()
})
}
$.fn.carousel.Constructor = Carousel
// CAROUSEL NO CONFLICT
// ====================
$.fn.carousel.noConflict = function () {
$.fn.carousel = old
return this
}
// CAROUSEL DATA-API
// =================
$(document).on('click.bs.carousel.data-api', '[data-slide], [data-slide-to]', function (e) {
var $this = $(this), href
var $target = $($this.attr('data-target') || (href = $this.attr('href')) && href.replace(/.*(?=#[^\s]+$)/, '')) //strip for ie7
var options = $.extend({}, $target.data(), $this.data())
var slideIndex = $this.attr('data-slide-to')
if (slideIndex) options.interval = false
$target.carousel(options)
if (slideIndex = $this.attr('data-slide-to')) {
$target.data('bs.carousel').to(slideIndex)
}
e.preventDefault()
})
$(window).on('load', function () {
$('[data-ride="carousel"]').each(function () {
var $carousel = $(this)
$carousel.carousel($carousel.data())
})
})
}(jQuery);
/* ========================================================================
* Bootstrap: collapse.js v3.0.2
* http://getbootstrap.com/javascript/#collapse
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// COLLAPSE PUBLIC CLASS DEFINITION
// ================================
var Collapse = function (element, options) {
this.$element = $(element)
this.options = $.extend({}, Collapse.DEFAULTS, options)
this.transitioning = null
if (this.options.parent) this.$parent = $(this.options.parent)
if (this.options.toggle) this.toggle()
}
Collapse.DEFAULTS = {
toggle: true
}
Collapse.prototype.dimension = function () {
var hasWidth = this.$element.hasClass('width')
return hasWidth ? 'width' : 'height'
}
Collapse.prototype.show = function () {
if (this.transitioning || this.$element.hasClass('in')) return
var startEvent = $.Event('show.bs.collapse')
this.$element.trigger(startEvent)
if (startEvent.isDefaultPrevented()) return
var actives = this.$parent && this.$parent.find('> .panel > .in')
if (actives && actives.length) {
var hasData = actives.data('bs.collapse')
if (hasData && hasData.transitioning) return
actives.collapse('hide')
hasData || actives.data('bs.collapse', null)
}
var dimension = this.dimension()
this.$element
.removeClass('collapse')
.addClass('collapsing')
[dimension](0)
this.transitioning = 1
var complete = function () {
this.$element
.removeClass('collapsing')
.addClass('in')
[dimension]('auto')
this.transitioning = 0
this.$element.trigger('shown.bs.collapse')
}
if (!$.support.transition) return complete.call(this)
var scrollSize = $.camelCase(['scroll', dimension].join('-'))
this.$element
.one($.support.transition.end, $.proxy(complete, this))
.emulateTransitionEnd(350)
[dimension](this.$element[0][scrollSize])
}
Collapse.prototype.hide = function () {
if (this.transitioning || !this.$element.hasClass('in')) return
var startEvent = $.Event('hide.bs.collapse')
this.$element.trigger(startEvent)
if (startEvent.isDefaultPrevented()) return
var dimension = this.dimension()
this.$element
[dimension](this.$element[dimension]())
[0].offsetHeight
this.$element
.addClass('collapsing')
.removeClass('collapse')
.removeClass('in')
this.transitioning = 1
var complete = function () {
this.transitioning = 0
this.$element
.trigger('hidden.bs.collapse')
.removeClass('collapsing')
.addClass('collapse')
}
if (!$.support.transition) return complete.call(this)
this.$element
[dimension](0)
.one($.support.transition.end, $.proxy(complete, this))
.emulateTransitionEnd(350)
}
Collapse.prototype.toggle = function () {
this[this.$element.hasClass('in') ? 'hide' : 'show']()
}
// COLLAPSE PLUGIN DEFINITION
// ==========================
var old = $.fn.collapse
$.fn.collapse = function (option) {
return this.each(function () {
var $this = $(this)
var data = $this.data('bs.collapse')
var options = $.extend({}, Collapse.DEFAULTS, $this.data(), typeof option == 'object' && option)
if (!data) $this.data('bs.collapse', (data = new Collapse(this, options)))
if (typeof option == 'string') data[option]()
})
}
$.fn.collapse.Constructor = Collapse
// COLLAPSE NO CONFLICT
// ====================
$.fn.collapse.noConflict = function () {
$.fn.collapse = old
return this
}
// COLLAPSE DATA-API
// =================
$(document).on('click.bs.collapse.data-api', '[data-toggle=collapse]', function (e) {
var $this = $(this), href
var target = $this.attr('data-target')
|| e.preventDefault()
|| (href = $this.attr('href')) && href.replace(/.*(?=#[^\s]+$)/, '') //strip for ie7
var $target = $(target)
var data = $target.data('bs.collapse')
var option = data ? 'toggle' : $this.data()
var parent = $this.attr('data-parent')
var $parent = parent && $(parent)
if (!data || !data.transitioning) {
if ($parent) $parent.find('[data-toggle=collapse][data-parent="' + parent + '"]').not($this).addClass('collapsed')
$this[$target.hasClass('in') ? 'addClass' : 'removeClass']('collapsed')
}
$target.collapse(option)
})
}(jQuery);
/* ========================================================================
* Bootstrap: dropdown.js v3.0.2
* http://getbootstrap.com/javascript/#dropdowns
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// DROPDOWN CLASS DEFINITION
// =========================
var backdrop = '.dropdown-backdrop'
var toggle = '[data-toggle=dropdown]'
var Dropdown = function (element) {
var $el = $(element).on('click.bs.dropdown', this.toggle)
}
Dropdown.prototype.toggle = function (e) {
var $this = $(this)
if ($this.is('.disabled, :disabled')) return
var $parent = getParent($this)
var isActive = $parent.hasClass('open')
clearMenus()
if (!isActive) {
if ('ontouchstart' in document.documentElement && !$parent.closest('.navbar-nav').length) {
// if mobile we we use a backdrop because click events don't delegate
$('<div class="dropdown-backdrop"/>').insertAfter($(this)).on('click', clearMenus)
}
$parent.trigger(e = $.Event('show.bs.dropdown'))
if (e.isDefaultPrevented()) return
$parent
.toggleClass('open')
.trigger('shown.bs.dropdown')
$this.focus()
}
return false
}
Dropdown.prototype.keydown = function (e) {
if (!/(38|40|27)/.test(e.keyCode)) return
var $this = $(this)
e.preventDefault()
e.stopPropagation()
if ($this.is('.disabled, :disabled')) return
var $parent = getParent($this)
var isActive = $parent.hasClass('open')
if (!isActive || (isActive && e.keyCode == 27)) {
if (e.which == 27) $parent.find(toggle).focus()
return $this.click()
}
var $items = $('[role=menu] li:not(.divider):visible a', $parent)
if (!$items.length) return
var index = $items.index($items.filter(':focus'))
if (e.keyCode == 38 && index > 0) index-- // up
if (e.keyCode == 40 && index < $items.length - 1) index++ // down
if (!~index) index=0
$items.eq(index).focus()
}
function clearMenus() {
$(backdrop).remove()
$(toggle).each(function (e) {
var $parent = getParent($(this))
if (!$parent.hasClass('open')) return
$parent.trigger(e = $.Event('hide.bs.dropdown'))
if (e.isDefaultPrevented()) return
$parent.removeClass('open').trigger('hidden.bs.dropdown')
})
}
function getParent($this) {
var selector = $this.attr('data-target')
if (!selector) {
selector = $this.attr('href')
selector = selector && /#/.test(selector) && selector.replace(/.*(?=#[^\s]*$)/, '') //strip for ie7
}
var $parent = selector && $(selector)
return $parent && $parent.length ? $parent : $this.parent()
}
// DROPDOWN PLUGIN DEFINITION
// ==========================
var old = $.fn.dropdown
$.fn.dropdown = function (option) {
return this.each(function () {
var $this = $(this)
var data = $this.data('dropdown')
if (!data) $this.data('dropdown', (data = new Dropdown(this)))
if (typeof option == 'string') data[option].call($this)
})
}
$.fn.dropdown.Constructor = Dropdown
// DROPDOWN NO CONFLICT
// ====================
$.fn.dropdown.noConflict = function () {
$.fn.dropdown = old
return this
}
// APPLY TO STANDARD DROPDOWN ELEMENTS
// ===================================
$(document)
.on('click.bs.dropdown.data-api', clearMenus)
.on('click.bs.dropdown.data-api', '.dropdown form', function (e) { e.stopPropagation() })
.on('click.bs.dropdown.data-api' , toggle, Dropdown.prototype.toggle)
.on('keydown.bs.dropdown.data-api', toggle + ', [role=menu]' , Dropdown.prototype.keydown)
}(jQuery);
/* ========================================================================
* Bootstrap: modal.js v3.0.2
* http://getbootstrap.com/javascript/#modals
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// MODAL CLASS DEFINITION
// ======================
var Modal = function (element, options) {
this.options = options
this.$element = $(element)
this.$backdrop =
this.isShown = null
if (this.options.remote) this.$element.load(this.options.remote)
}
Modal.DEFAULTS = {
backdrop: true
, keyboard: true
, show: true
}
Modal.prototype.toggle = function (_relatedTarget) {
return this[!this.isShown ? 'show' : 'hide'](_relatedTarget)
}
Modal.prototype.show = function (_relatedTarget) {
var that = this
var e = $.Event('show.bs.modal', { relatedTarget: _relatedTarget })
this.$element.trigger(e)
if (this.isShown || e.isDefaultPrevented()) return
this.isShown = true
this.escape()
this.$element.on('click.dismiss.modal', '[data-dismiss="modal"]', $.proxy(this.hide, this))
this.backdrop(function () {
var transition = $.support.transition && that.$element.hasClass('fade')
if (!that.$element.parent().length) {
that.$element.appendTo(document.body) // don't move modals dom position
}
that.$element.show()
if (transition) {
that.$element[0].offsetWidth // force reflow
}
that.$element
.addClass('in')
.attr('aria-hidden', false)
that.enforceFocus()
var e = $.Event('shown.bs.modal', { relatedTarget: _relatedTarget })
transition ?
that.$element.find('.modal-dialog') // wait for modal to slide in
.one($.support.transition.end, function () {
that.$element.focus().trigger(e)
})
.emulateTransitionEnd(300) :
that.$element.focus().trigger(e)
})
}
Modal.prototype.hide = function (e) {
if (e) e.preventDefault()
e = $.Event('hide.bs.modal')
this.$element.trigger(e)
if (!this.isShown || e.isDefaultPrevented()) return
this.isShown = false
this.escape()
$(document).off('focusin.bs.modal')
this.$element
.removeClass('in')
.attr('aria-hidden', true)
.off('click.dismiss.modal')
$.support.transition && this.$element.hasClass('fade') ?
this.$element
.one($.support.transition.end, $.proxy(this.hideModal, this))
.emulateTransitionEnd(300) :
this.hideModal()
}
Modal.prototype.enforceFocus = function () {
$(document)
.off('focusin.bs.modal') // guard against infinite focus loop
.on('focusin.bs.modal', $.proxy(function (e) {
if (this.$element[0] !== e.target && !this.$element.has(e.target).length) {
this.$element.focus()
}
}, this))
}
Modal.prototype.escape = function () {
if (this.isShown && this.options.keyboard) {
this.$element.on('keyup.dismiss.bs.modal', $.proxy(function (e) {
e.which == 27 && this.hide()
}, this))
} else if (!this.isShown) {
this.$element.off('keyup.dismiss.bs.modal')
}
}
Modal.prototype.hideModal = function () {
var that = this
this.$element.hide()
this.backdrop(function () {
that.removeBackdrop()
that.$element.trigger('hidden.bs.modal')
})
}
Modal.prototype.removeBackdrop = function () {
this.$backdrop && this.$backdrop.remove()
this.$backdrop = null
}
Modal.prototype.backdrop = function (callback) {
var that = this
var animate = this.$element.hasClass('fade') ? 'fade' : ''
if (this.isShown && this.options.backdrop) {
var doAnimate = $.support.transition && animate
this.$backdrop = $('<div class="modal-backdrop ' + animate + '" />')
.appendTo(document.body)
this.$element.on('click.dismiss.modal', $.proxy(function (e) {
if (e.target !== e.currentTarget) return
this.options.backdrop == 'static'
? this.$element[0].focus.call(this.$element[0])
: this.hide.call(this)
}, this))
if (doAnimate) this.$backdrop[0].offsetWidth // force reflow
this.$backdrop.addClass('in')
if (!callback) return
doAnimate ?
this.$backdrop
.one($.support.transition.end, callback)
.emulateTransitionEnd(150) :
callback()
} else if (!this.isShown && this.$backdrop) {
this.$backdrop.removeClass('in')
$.support.transition && this.$element.hasClass('fade')?
this.$backdrop
.one($.support.transition.end, callback)
.emulateTransitionEnd(150) :
callback()
} else if (callback) {
callback()
}
}
// MODAL PLUGIN DEFINITION
// =======================
var old = $.fn.modal
$.fn.modal = function (option, _relatedTarget) {
return this.each(function () {
var $this = $(this)
var data = $this.data('bs.modal')
var options = $.extend({}, Modal.DEFAULTS, $this.data(), typeof option == 'object' && option)
if (!data) $this.data('bs.modal', (data = new Modal(this, options)))
if (typeof option == 'string') data[option](_relatedTarget)
else if (options.show) data.show(_relatedTarget)
})
}
$.fn.modal.Constructor = Modal
// MODAL NO CONFLICT
// =================
$.fn.modal.noConflict = function () {
$.fn.modal = old
return this
}
// MODAL DATA-API
// ==============
$(document).on('click.bs.modal.data-api', '[data-toggle="modal"]', function (e) {
var $this = $(this)
var href = $this.attr('href')
var $target = $($this.attr('data-target') || (href && href.replace(/.*(?=#[^\s]+$)/, ''))) //strip for ie7
var option = $target.data('modal') ? 'toggle' : $.extend({ remote: !/#/.test(href) && href }, $target.data(), $this.data())
e.preventDefault()
$target
.modal(option, this)
.one('hide', function () {
$this.is(':visible') && $this.focus()
})
})
$(document)
.on('show.bs.modal', '.modal', function () { $(document.body).addClass('modal-open') })
.on('hidden.bs.modal', '.modal', function () { $(document.body).removeClass('modal-open') })
}(jQuery);
/* ========================================================================
* Bootstrap: tooltip.js v3.0.2
* http://getbootstrap.com/javascript/#tooltip
* Inspired by the original jQuery.tipsy by Jason Frame
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// TOOLTIP PUBLIC CLASS DEFINITION
// ===============================
var Tooltip = function (element, options) {
this.type =
this.options =
this.enabled =
this.timeout =
this.hoverState =
this.$element = null
this.init('tooltip', element, options)
}
Tooltip.DEFAULTS = {
animation: true
, placement: 'top'
, selector: false
, template: '<div class="tooltip"><div class="tooltip-arrow"></div><div class="tooltip-inner"></div></div>'
, trigger: 'hover focus'
, title: ''
, delay: 0
, html: false
, container: false
}
Tooltip.prototype.init = function (type, element, options) {
this.enabled = true
this.type = type
this.$element = $(element)
this.options = this.getOptions(options)
var triggers = this.options.trigger.split(' ')
for (var i = triggers.length; i--;) {
var trigger = triggers[i]
if (trigger == 'click') {
this.$element.on('click.' + this.type, this.options.selector, $.proxy(this.toggle, this))
} else if (trigger != 'manual') {
var eventIn = trigger == 'hover' ? 'mouseenter' : 'focus'
var eventOut = trigger == 'hover' ? 'mouseleave' : 'blur'
this.$element.on(eventIn + '.' + this.type, this.options.selector, $.proxy(this.enter, this))
this.$element.on(eventOut + '.' + this.type, this.options.selector, $.proxy(this.leave, this))
}
}
this.options.selector ?
(this._options = $.extend({}, this.options, { trigger: 'manual', selector: '' })) :
this.fixTitle()
}
Tooltip.prototype.getDefaults = function () {
return Tooltip.DEFAULTS
}
Tooltip.prototype.getOptions = function (options) {
options = $.extend({}, this.getDefaults(), this.$element.data(), options)
if (options.delay && typeof options.delay == 'number') {
options.delay = {
show: options.delay
, hide: options.delay
}
}
return options
}
Tooltip.prototype.getDelegateOptions = function () {
var options = {}
var defaults = this.getDefaults()
this._options && $.each(this._options, function (key, value) {
if (defaults[key] != value) options[key] = value
})
return options
}
Tooltip.prototype.enter = function (obj) {
var self = obj instanceof this.constructor ?
obj : $(obj.currentTarget)[this.type](this.getDelegateOptions()).data('bs.' + this.type)
clearTimeout(self.timeout)
self.hoverState = 'in'
if (!self.options.delay || !self.options.delay.show) return self.show()
self.timeout = setTimeout(function () {
if (self.hoverState == 'in') self.show()
}, self.options.delay.show)
}
Tooltip.prototype.leave = function (obj) {
var self = obj instanceof this.constructor ?
obj : $(obj.currentTarget)[this.type](this.getDelegateOptions()).data('bs.' + this.type)
clearTimeout(self.timeout)
self.hoverState = 'out'
if (!self.options.delay || !self.options.delay.hide) return self.hide()
self.timeout = setTimeout(function () {
if (self.hoverState == 'out') self.hide()
}, self.options.delay.hide)
}
Tooltip.prototype.show = function () {
var e = $.Event('show.bs.'+ this.type)
if (this.hasContent() && this.enabled) {
this.$element.trigger(e)
if (e.isDefaultPrevented()) return
var $tip = this.tip()
this.setContent()
if (this.options.animation) $tip.addClass('fade')
var placement = typeof this.options.placement == 'function' ?
this.options.placement.call(this, $tip[0], this.$element[0]) :
this.options.placement
var autoToken = /\s?auto?\s?/i
var autoPlace = autoToken.test(placement)
if (autoPlace) placement = placement.replace(autoToken, '') || 'top'
$tip
.detach()
.css({ top: 0, left: 0, display: 'block' })
.addClass(placement)
this.options.container ? $tip.appendTo(this.options.container) : $tip.insertAfter(this.$element)
var pos = this.getPosition()
var actualWidth = $tip[0].offsetWidth
var actualHeight = $tip[0].offsetHeight
if (autoPlace) {
var $parent = this.$element.parent()
var orgPlacement = placement
var docScroll = document.documentElement.scrollTop || document.body.scrollTop
var parentWidth = this.options.container == 'body' ? window.innerWidth : $parent.outerWidth()
var parentHeight = this.options.container == 'body' ? window.innerHeight : $parent.outerHeight()
var parentLeft = this.options.container == 'body' ? 0 : $parent.offset().left
placement = placement == 'bottom' && pos.top + pos.height + actualHeight - docScroll > parentHeight ? 'top' :
placement == 'top' && pos.top - docScroll - actualHeight < 0 ? 'bottom' :
placement == 'right' && pos.right + actualWidth > parentWidth ? 'left' :
placement == 'left' && pos.left - actualWidth < parentLeft ? 'right' :
placement
$tip
.removeClass(orgPlacement)
.addClass(placement)
}
var calculatedOffset = this.getCalculatedOffset(placement, pos, actualWidth, actualHeight)
this.applyPlacement(calculatedOffset, placement)
this.$element.trigger('shown.bs.' + this.type)
}
}
Tooltip.prototype.applyPlacement = function(offset, placement) {
var replace
var $tip = this.tip()
var width = $tip[0].offsetWidth
var height = $tip[0].offsetHeight
// manually read margins because getBoundingClientRect includes difference
var marginTop = parseInt($tip.css('margin-top'), 10)
var marginLeft = parseInt($tip.css('margin-left'), 10)
// we must check for NaN for ie 8/9
if (isNaN(marginTop)) marginTop = 0
if (isNaN(marginLeft)) marginLeft = 0
offset.top = offset.top + marginTop
offset.left = offset.left + marginLeft
$tip
.offset(offset)
.addClass('in')
// check to see if placing tip in new offset caused the tip to resize itself
var actualWidth = $tip[0].offsetWidth
var actualHeight = $tip[0].offsetHeight
if (placement == 'top' && actualHeight != height) {
replace = true
offset.top = offset.top + height - actualHeight
}
if (/bottom|top/.test(placement)) {
var delta = 0
if (offset.left < 0) {
delta = offset.left * -2
offset.left = 0
$tip.offset(offset)
actualWidth = $tip[0].offsetWidth
actualHeight = $tip[0].offsetHeight
}
this.replaceArrow(delta - width + actualWidth, actualWidth, 'left')
} else {
this.replaceArrow(actualHeight - height, actualHeight, 'top')
}
if (replace) $tip.offset(offset)
}
Tooltip.prototype.replaceArrow = function(delta, dimension, position) {
this.arrow().css(position, delta ? (50 * (1 - delta / dimension) + "%") : '')
}
Tooltip.prototype.setContent = function () {
var $tip = this.tip()
var title = this.getTitle()
$tip.find('.tooltip-inner')[this.options.html ? 'html' : 'text'](title)
$tip.removeClass('fade in top bottom left right')
}
Tooltip.prototype.hide = function () {
var that = this
var $tip = this.tip()
var e = $.Event('hide.bs.' + this.type)
function complete() {
if (that.hoverState != 'in') $tip.detach()
}
this.$element.trigger(e)
if (e.isDefaultPrevented()) return
$tip.removeClass('in')
$.support.transition && this.$tip.hasClass('fade') ?
$tip
.one($.support.transition.end, complete)
.emulateTransitionEnd(150) :
complete()
this.$element.trigger('hidden.bs.' + this.type)
return this
}
Tooltip.prototype.fixTitle = function () {
var $e = this.$element
if ($e.attr('title') || typeof($e.attr('data-original-title')) != 'string') {
$e.attr('data-original-title', $e.attr('title') || '').attr('title', '')
}
}
Tooltip.prototype.hasContent = function () {
return this.getTitle()
}
Tooltip.prototype.getPosition = function () {
var el = this.$element[0]
return $.extend({}, (typeof el.getBoundingClientRect == 'function') ? el.getBoundingClientRect() : {
width: el.offsetWidth
, height: el.offsetHeight
}, this.$element.offset())
}
Tooltip.prototype.getCalculatedOffset = function (placement, pos, actualWidth, actualHeight) {
return placement == 'bottom' ? { top: pos.top + pos.height, left: pos.left + pos.width / 2 - actualWidth / 2 } :
placement == 'top' ? { top: pos.top - actualHeight, left: pos.left + pos.width / 2 - actualWidth / 2 } :
placement == 'left' ? { top: pos.top + pos.height / 2 - actualHeight / 2, left: pos.left - actualWidth } :
/* placement == 'right' */ { top: pos.top + pos.height / 2 - actualHeight / 2, left: pos.left + pos.width }
}
Tooltip.prototype.getTitle = function () {
var title
var $e = this.$element
var o = this.options
title = $e.attr('data-original-title')
|| (typeof o.title == 'function' ? o.title.call($e[0]) : o.title)
return title
}
Tooltip.prototype.tip = function () {
return this.$tip = this.$tip || $(this.options.template)
}
Tooltip.prototype.arrow = function () {
return this.$arrow = this.$arrow || this.tip().find('.tooltip-arrow')
}
Tooltip.prototype.validate = function () {
if (!this.$element[0].parentNode) {
this.hide()
this.$element = null
this.options = null
}
}
Tooltip.prototype.enable = function () {
this.enabled = true
}
Tooltip.prototype.disable = function () {
this.enabled = false
}
Tooltip.prototype.toggleEnabled = function () {
this.enabled = !this.enabled
}
Tooltip.prototype.toggle = function (e) {
var self = e ? $(e.currentTarget)[this.type](this.getDelegateOptions()).data('bs.' + this.type) : this
self.tip().hasClass('in') ? self.leave(self) : self.enter(self)
}
Tooltip.prototype.destroy = function () {
this.hide().$element.off('.' + this.type).removeData('bs.' + this.type)
}
// TOOLTIP PLUGIN DEFINITION
// =========================
var old = $.fn.tooltip
$.fn.tooltip = function (option) {
return this.each(function () {
var $this = $(this)
var data = $this.data('bs.tooltip')
var options = typeof option == 'object' && option
if (!data) $this.data('bs.tooltip', (data = new Tooltip(this, options)))
if (typeof option == 'string') data[option]()
})
}
$.fn.tooltip.Constructor = Tooltip
// TOOLTIP NO CONFLICT
// ===================
$.fn.tooltip.noConflict = function () {
$.fn.tooltip = old
return this
}
}(jQuery);
/* ========================================================================
* Bootstrap: popover.js v3.0.2
* http://getbootstrap.com/javascript/#popovers
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// POPOVER PUBLIC CLASS DEFINITION
// ===============================
var Popover = function (element, options) {
this.init('popover', element, options)
}
if (!$.fn.tooltip) throw new Error('Popover requires tooltip.js')
Popover.DEFAULTS = $.extend({} , $.fn.tooltip.Constructor.DEFAULTS, {
placement: 'right'
, trigger: 'click'
, content: ''
, template: '<div class="popover"><div class="arrow"></div><h3 class="popover-title"></h3><div class="popover-content"></div></div>'
})
// NOTE: POPOVER EXTENDS tooltip.js
// ================================
Popover.prototype = $.extend({}, $.fn.tooltip.Constructor.prototype)
Popover.prototype.constructor = Popover
Popover.prototype.getDefaults = function () {
return Popover.DEFAULTS
}
Popover.prototype.setContent = function () {
var $tip = this.tip()
var title = this.getTitle()
var content = this.getContent()
$tip.find('.popover-title')[this.options.html ? 'html' : 'text'](title)
$tip.find('.popover-content')[this.options.html ? 'html' : 'text'](content)
$tip.removeClass('fade top bottom left right in')
// IE8 doesn't accept hiding via the `:empty` pseudo selector, we have to do
// this manually by checking the contents.
if (!$tip.find('.popover-title').html()) $tip.find('.popover-title').hide()
}
Popover.prototype.hasContent = function () {
return this.getTitle() || this.getContent()
}
Popover.prototype.getContent = function () {
var $e = this.$element
var o = this.options
return $e.attr('data-content')
|| (typeof o.content == 'function' ?
o.content.call($e[0]) :
o.content)
}
Popover.prototype.arrow = function () {
return this.$arrow = this.$arrow || this.tip().find('.arrow')
}
Popover.prototype.tip = function () {
if (!this.$tip) this.$tip = $(this.options.template)
return this.$tip
}
// POPOVER PLUGIN DEFINITION
// =========================
var old = $.fn.popover
$.fn.popover = function (option) {
return this.each(function () {
var $this = $(this)
var data = $this.data('bs.popover')
var options = typeof option == 'object' && option
if (!data) $this.data('bs.popover', (data = new Popover(this, options)))
if (typeof option == 'string') data[option]()
})
}
$.fn.popover.Constructor = Popover
// POPOVER NO CONFLICT
// ===================
$.fn.popover.noConflict = function () {
$.fn.popover = old
return this
}
}(jQuery);
/* ========================================================================
* Bootstrap: scrollspy.js v3.0.2
* http://getbootstrap.com/javascript/#scrollspy
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// SCROLLSPY CLASS DEFINITION
// ==========================
function ScrollSpy(element, options) {
var href
var process = $.proxy(this.process, this)
this.$element = $(element).is('body') ? $(window) : $(element)
this.$body = $('body')
this.$scrollElement = this.$element.on('scroll.bs.scroll-spy.data-api', process)
this.options = $.extend({}, ScrollSpy.DEFAULTS, options)
this.selector = (this.options.target
|| ((href = $(element).attr('href')) && href.replace(/.*(?=#[^\s]+$)/, '')) //strip for ie7
|| '') + ' .nav li > a'
this.offsets = $([])
this.targets = $([])
this.activeTarget = null
this.refresh()
this.process()
}
ScrollSpy.DEFAULTS = {
offset: 10
}
ScrollSpy.prototype.refresh = function () {
var offsetMethod = this.$element[0] == window ? 'offset' : 'position'
this.offsets = $([])
this.targets = $([])
var self = this
var $targets = this.$body
.find(this.selector)
.map(function () {
var $el = $(this)
var href = $el.data('target') || $el.attr('href')
var $href = /^#\w/.test(href) && $(href)
return ($href
&& $href.length
&& [[ $href[offsetMethod]().top + (!$.isWindow(self.$scrollElement.get(0)) && self.$scrollElement.scrollTop()), href ]]) || null
})
.sort(function (a, b) { return a[0] - b[0] })
.each(function () {
self.offsets.push(this[0])
self.targets.push(this[1])
})
}
ScrollSpy.prototype.process = function () {
var scrollTop = this.$scrollElement.scrollTop() + this.options.offset
var scrollHeight = this.$scrollElement[0].scrollHeight || this.$body[0].scrollHeight
var maxScroll = scrollHeight - this.$scrollElement.height()
var offsets = this.offsets
var targets = this.targets
var activeTarget = this.activeTarget
var i
if (scrollTop >= maxScroll) {
return activeTarget != (i = targets.last()[0]) && this.activate(i)
}
for (i = offsets.length; i--;) {
activeTarget != targets[i]
&& scrollTop >= offsets[i]
&& (!offsets[i + 1] || scrollTop <= offsets[i + 1])
&& this.activate( targets[i] )
}
}
ScrollSpy.prototype.activate = function (target) {
this.activeTarget = target
$(this.selector)
.parents('.active')
.removeClass('active')
var selector = this.selector
+ '[data-target="' + target + '"],'
+ this.selector + '[href="' + target + '"]'
var active = $(selector)
.parents('li')
.addClass('active')
if (active.parent('.dropdown-menu').length) {
active = active
.closest('li.dropdown')
.addClass('active')
}
active.trigger('activate')
}
// SCROLLSPY PLUGIN DEFINITION
// ===========================
var old = $.fn.scrollspy
$.fn.scrollspy = function (option) {
return this.each(function () {
var $this = $(this)
var data = $this.data('bs.scrollspy')
var options = typeof option == 'object' && option
if (!data) $this.data('bs.scrollspy', (data = new ScrollSpy(this, options)))
if (typeof option == 'string') data[option]()
})
}
$.fn.scrollspy.Constructor = ScrollSpy
// SCROLLSPY NO CONFLICT
// =====================
$.fn.scrollspy.noConflict = function () {
$.fn.scrollspy = old
return this
}
// SCROLLSPY DATA-API
// ==================
$(window).on('load', function () {
$('[data-spy="scroll"]').each(function () {
var $spy = $(this)
$spy.scrollspy($spy.data())
})
})
}(jQuery);
/* ========================================================================
* Bootstrap: tab.js v3.0.2
* http://getbootstrap.com/javascript/#tabs
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// TAB CLASS DEFINITION
// ====================
var Tab = function (element) {
this.element = $(element)
}
Tab.prototype.show = function () {
var $this = this.element
var $ul = $this.closest('ul:not(.dropdown-menu)')
var selector = $this.data('target')
if (!selector) {
selector = $this.attr('href')
selector = selector && selector.replace(/.*(?=#[^\s]*$)/, '') //strip for ie7
}
if ($this.parent('li').hasClass('active')) return
var previous = $ul.find('.active:last a')[0]
var e = $.Event('show.bs.tab', {
relatedTarget: previous
})
$this.trigger(e)
if (e.isDefaultPrevented()) return
var $target = $(selector)
this.activate($this.parent('li'), $ul)
this.activate($target, $target.parent(), function () {
$this.trigger({
type: 'shown.bs.tab'
, relatedTarget: previous
})
})
}
Tab.prototype.activate = function (element, container, callback) {
var $active = container.find('> .active')
var transition = callback
&& $.support.transition
&& $active.hasClass('fade')
function next() {
$active
.removeClass('active')
.find('> .dropdown-menu > .active')
.removeClass('active')
element.addClass('active')
if (transition) {
element[0].offsetWidth // reflow for transition
element.addClass('in')
} else {
element.removeClass('fade')
}
if (element.parent('.dropdown-menu')) {
element.closest('li.dropdown').addClass('active')
}
callback && callback()
}
transition ?
$active
.one($.support.transition.end, next)
.emulateTransitionEnd(150) :
next()
$active.removeClass('in')
}
// TAB PLUGIN DEFINITION
// =====================
var old = $.fn.tab
$.fn.tab = function ( option ) {
return this.each(function () {
var $this = $(this)
var data = $this.data('bs.tab')
if (!data) $this.data('bs.tab', (data = new Tab(this)))
if (typeof option == 'string') data[option]()
})
}
$.fn.tab.Constructor = Tab
// TAB NO CONFLICT
// ===============
$.fn.tab.noConflict = function () {
$.fn.tab = old
return this
}
// TAB DATA-API
// ============
$(document).on('click.bs.tab.data-api', '[data-toggle="tab"], [data-toggle="pill"]', function (e) {
e.preventDefault()
$(this).tab('show')
})
}(jQuery);
/* ========================================================================
* Bootstrap: affix.js v3.0.2
* http://getbootstrap.com/javascript/#affix
* ========================================================================
* Copyright 2013 Twitter, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ======================================================================== */
+function ($) { "use strict";
// AFFIX CLASS DEFINITION
// ======================
var Affix = function (element, options) {
this.options = $.extend({}, Affix.DEFAULTS, options)
this.$window = $(window)
.on('scroll.bs.affix.data-api', $.proxy(this.checkPosition, this))
.on('click.bs.affix.data-api', $.proxy(this.checkPositionWithEventLoop, this))
this.$element = $(element)
this.affixed =
this.unpin = null
this.checkPosition()
}
Affix.RESET = 'affix affix-top affix-bottom'
Affix.DEFAULTS = {
offset: 0
}
Affix.prototype.checkPositionWithEventLoop = function () {
setTimeout($.proxy(this.checkPosition, this), 1)
}
Affix.prototype.checkPosition = function () {
if (!this.$element.is(':visible')) return
var scrollHeight = $(document).height()
var scrollTop = this.$window.scrollTop()
var position = this.$element.offset()
var offset = this.options.offset
var offsetTop = offset.top
var offsetBottom = offset.bottom
if (typeof offset != 'object') offsetBottom = offsetTop = offset
if (typeof offsetTop == 'function') offsetTop = offset.top()
if (typeof offsetBottom == 'function') offsetBottom = offset.bottom()
var affix = this.unpin != null && (scrollTop + this.unpin <= position.top) ? false :
offsetBottom != null && (position.top + this.$element.height() >= scrollHeight - offsetBottom) ? 'bottom' :
offsetTop != null && (scrollTop <= offsetTop) ? 'top' : false
if (this.affixed === affix) return
if (this.unpin) this.$element.css('top', '')
this.affixed = affix
this.unpin = affix == 'bottom' ? position.top - scrollTop : null
this.$element.removeClass(Affix.RESET).addClass('affix' + (affix ? '-' + affix : ''))
if (affix == 'bottom') {
this.$element.offset({ top: document.body.offsetHeight - offsetBottom - this.$element.height() })
}
}
// AFFIX PLUGIN DEFINITION
// =======================
var old = $.fn.affix
$.fn.affix = function (option) {
return this.each(function () {
var $this = $(this)
var data = $this.data('bs.affix')
var options = typeof option == 'object' && option
if (!data) $this.data('bs.affix', (data = new Affix(this, options)))
if (typeof option == 'string') data[option]()
})
}
$.fn.affix.Constructor = Affix
// AFFIX NO CONFLICT
// =================
$.fn.affix.noConflict = function () {
$.fn.affix = old
return this
}
// AFFIX DATA-API
// ==============
$(window).on('load', function () {
$('[data-spy="affix"]').each(function () {
var $spy = $(this)
var data = $spy.data()
data.offset = data.offset || {}
if (data.offsetBottom) data.offset.bottom = data.offsetBottom
if (data.offsetTop) data.offset.top = data.offsetTop
$spy.affix(data)
})
})
}(jQuery);
$(document).ready(function() {
$(function() {
$( "#article_published_at" ).datetimepicker();
});
});
$(document).ready(function(){
// Spinners
$('form.spinnable').on('ajax:before', function(evt, xhr, status){ $('#spinner').show();})
$('form.spinnable').on('ajax:after', function(evt, xhr, status){ $('#spinner').hide();})
// DatePickers
$('.datepicker').datepicker();
});
// Front javascript manifest
;
// JS QuickTags version 1.3.1
//
// Copyright (c) 2002-2008 Alex King
// http://alexking.org/projects/js-quicktags
//
// Thanks to Greg Heo <greg@node79.com> for his changes
// to support multiple toolbars per page.
//
// Licensed under the LGPL license
// http://www.gnu.org/copyleft/lesser.html
//
// **********************************************************************
// This program is distributed in the hope that it will be useful, but
// WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
// **********************************************************************
var dictionaryUrl = 'http://www.ninjawords.com/';
var edButtons = new Array();
var edLinks = new Array();
var edOpenTags = new Array();
function edButton(id, display, tagStart, tagEnd, access, open) {
this.id = id; // used to name the toolbar button
this.display = display; // label on button
this.tagStart = tagStart; // open tag
this.tagEnd = tagEnd; // close tag
this.access = access; // set to -1 if tag does not need to be closed
this.open = open; // set to -1 if tag does not need to be closed
}
function get_buttons(textfilter) {
switch (textfilter) {
case "textile":
edButtons.push(new edButton('ed_bold', 'b', '**', '**', 'b'));
edButtons.push(new edButton('ed_italic', 'i', '_', '_', 'i'));
edButtons.push(new edButton('ed_link', 'link', '', '</a>', 'a'));
edButtons.push(new edButton('ed_img', 'img', '!', '!', 'm' ,-1));
edButtons.push(new edButton('ed_li', 'li', '* ', '', 'l', -1));
edButtons.push(new edButton('ed_block', 'b-quote', '>', '', 'q', -1));
edButtons.push(new edButton('ed_del', 'del', '<del>', '</del>'));
edButtons.push(new edButton('ed_code', 'code', '@', '@', 'c'));
edButtons.push(new edButton('ed_more', 'more', '\n<!--more-->\n', '', ''));
edButtons.push(new edButton('ed_publifycode', 'publify:code', '', '\n</publify:code>\n\n', 'publify:code'));
break;
case "markdown":
case "markdown smartypants":
edButtons.push(new edButton('ed_bold', 'b', '**', '**', 'b'));
edButtons.push(new edButton('ed_italic', 'i', '_', '_', 'i'));
edButtons.push(new edButton('ed_link', 'link', '', '</a>', 'a'));
edButtons.push(new edButton('ed_img', 'img', '', '', 'm' ,-1));
edButtons.push(new edButton('ed_li', 'li', '* ', '', 'l', -1));
edButtons.push(new edButton('ed_ol', 'ol', '#', '', 'o', -1));
edButtons.push(new edButton('ed_block', 'b-quote', '>', '', 'q', -1));
edButtons.push(new edButton('ed_del', 'del', '<del>', '</del>'));
edButtons.push(new edButton('ed_code', 'code', '`', '`', 'c'));
edButtons.push(new edButton('ed_more', 'more', '\n<!--more-->\n', '', ''));
edButtons.push(new edButton('ed_publifycode', 'publify:code', '', '\n</publify:code>\n\n', 'publify:code'));
break;
default:
edButtons.push(new edButton('ed_bold', 'b', '<strong>', '</strong>', 'b'));
edButtons.push(new edButton('ed_italic', 'i', '<em>', '</em>', 'i'));
edButtons.push(new edButton('ed_link', 'link', '', '</a>', 'a'));
edButtons.push(new edButton('ed_img', 'img', '', '', 'm' ,-1));
edButtons.push(new edButton('ed_ul', 'ul', '<ul>\n', '</ul>\n\n', 'u'));
edButtons.push(new edButton('ed_ol', 'ol', '<ol>\n', '</ol>\n\n', 'o'));
edButtons.push(new edButton('ed_li', 'li', '\t<li>', '</li>\n', 'l'));
edButtons.push(new edButton('ed_block', 'b-quote', '<blockquote>', '</blockquote>', 'q'));
edButtons.push(new edButton('ed_del', 'del', '<del>', '</del>'));
edButtons.push(new edButton('ed_code', 'code', '<code>', '</code>', 'c'));
edButtons.push(new edButton('ed_more', 'more', '\n<!--more-->\n', '', ''));
edButtons.push(new edButton('ed_publifycode', 'publify:code', '', '\n</publify:code>\n\n', 'publify:code'));
break;
}
}
var extendedStart = edButtons.length;
function edLink(display, URL, newWin) {
this.display = display;
this.URL = URL;
if (!newWin) {
newWin = 0;
}
this.newWin = newWin;
}
edLinks[edLinks.length] = new edLink('alexking.org'
,'http://www.alexking.org/'
);
function edShowButton(which, button, i) {
if (button.access) {
var accesskey = ' accesskey = "' + button.access + '"'
}
else {
var accesskey = '';
}
switch (button.id) {
case 'ed_img':
document.write('<input type="button" id="' + button.id + '_' + which + '" ' + accesskey + ' class="btn btn-default ' + button.id + '" onclick="edInsertImage(\'' + which + '\');" value="' + button.display + '" />');
break;
case 'ed_link':
document.write('<input type="button" id="' + button.id + '_' + which + '" ' + accesskey + ' class="btn btn-default ' + button.id + '" onclick="edInsertLink(\'' + which + '\', ' + i + ');" value="' + button.display + '" />');
break;
case 'ed_publifycode':
document.write('<input type="button" id="' + button.id + '_' + which + '" ' + accesskey + ' class="btn btn-default ' + button.id + '" onclick="edInsertPublifyCode(\'' + which + '\', ' + i + ');" value="' + button.display + '" />');
break;
default:
document.write('<input type="button" id="' + button.id + '_' + which + '" ' + accesskey + ' class="btn btn-default ' + button.id + '" onclick="edInsertTag(\'' + which + '\', ' + i + ');" value="' + button.display + '" />');
break;
}
}
function edShowLinks() {
var tempStr = '<select onchange="edQuickLink(this.options[this.selectedIndex].value, this);"><option value="-1" selected>(Quick Links)</option>';
for (i = 0; i < edLinks.length; i++) {
tempStr += '<option value="' + i + '">' + edLinks[i].display + '</option>';
}
tempStr += '</select>';
document.write(tempStr);
}
function edAddTag(which, button) {
if (edButtons[button].tagEnd != '') {
edOpenTags[which][edOpenTags[which].length] = button;
document.getElementById(edButtons[button].id + '_' + which).value = '/' + document.getElementById(edButtons[button].id + '_' + which).value;
}
}
function edRemoveTag(which, button) {
for (i = 0; i < edOpenTags[which].length; i++) {
if (edOpenTags[which][i] == button) {
edOpenTags[which].splice(i, 1);
document.getElementById(edButtons[button].id + '_' + which).value = document.getElementById(edButtons[button].id + '_' + which).value.replace('/', '');
}
}
}
function edCheckOpenTags(which, button) {
var tag = 0;
for (i = 0; i < edOpenTags[which].length; i++) {
if (edOpenTags[which][i] == button) {
tag++;
}
}
if (tag > 0) {
return true; // tag found
}
else {
return false; // tag not found
}
}
function edCloseAllTags(which) {
var count = edOpenTags[which].length;
for (o = 0; o < count; o++) {
edInsertTag(which, edOpenTags[which][edOpenTags[which].length - 1]);
}
}
function edQuickLink(i, thisSelect) {
if (i > -1) {
var newWin = '';
if (edLinks[i].newWin == 1) {
newWin = ' target="_blank"';
}
var tempStr = '<a href="' + edLinks[i].URL + '"' + newWin + '>'
+ edLinks[i].display
+ '</a>';
thisSelect.selectedIndex = 0;
edInsertContent(edCanvas, tempStr);
}
else {
thisSelect.selectedIndex = 0;
}
}
function edSpell(which) {
myField = document.getElementById(which);
var word = '';
if (document.selection) {
myField.focus();
var sel = document.selection.createRange();
if (sel.text.length > 0) {
word = sel.text;
}
}
else if (myField.selectionStart || myField.selectionStart == '0') {
var startPos = myField.selectionStart;
var endPos = myField.selectionEnd;
if (startPos != endPos) {
word = myField.value.substring(startPos, endPos);
}
}
if (word == '') {
word = prompt('Enter a word to look up:', '');
}
if (word != '') {
window.open(dictionaryUrl + escape(word));
}
}
function edToolbar(which, textfilter) {
get_buttons(textfilter);
document.write('<div id="ed_toolbar_' + which + '" class="btn-toolbar"><div class="btn-group-vertical">');
for (i = 0; i < extendedStart; i++) {
edShowButton(which, edButtons[i], i);
}
for (i = extendedStart; i < edButtons.length; i++) {
edShowButton(which, edButtons[i], i);
}
if (edShowExtraCookie()) {
document.write(
'<input type="button" id="ed_close_' + which + '" class="btn btn-default" onclick="edCloseAllTags(\'' + which + '\');" value="Close Tags" />'
+ '<input type="button" id="ed_spell_' + which + '" class="btn btn-default" onclick="edSpell(\'' + which + '\');" value="Dict" />'
);
}
else {
document.write(
'<input type="button" id="ed_close_' + which + '" class="btn btn-default" onclick="edCloseAllTags(\'' + which + '\');" value="Close Tags" />'
+ '<input type="button" id="ed_spell_' + which + '" class="btn btn-default" onclick="edSpell(\'' + which + '\');" value="Dict" />'
);
}
// edShowLinks();
document.write('</div></div>');
edOpenTags[which] = new Array();
}
// insertion code
function edInsertTag(which, i) {
myField = document.getElementById(which);
//IE support
if (document.selection) {
myField.focus();
sel = document.selection.createRange();
if (sel.text.length > 0) {
sel.text = edButtons[i].tagStart + sel.text + edButtons[i].tagEnd;
}
else {
if (!edCheckOpenTags(which, i) || edButtons[i].tagEnd == '') {
sel.text = edButtons[i].tagStart;
edAddTag(which, i);
}
else {
sel.text = edButtons[i].tagEnd;
edRemoveTag(which, i);
}
}
myField.focus();
}
//MOZILLA/NETSCAPE support
else if (myField.selectionStart || myField.selectionStart == '0') {
var startPos = myField.selectionStart;
var endPos = myField.selectionEnd;
var cursorPos = endPos;
var scrollTop = myField.scrollTop;
if (startPos != endPos) {
myField.value = myField.value.substring(0, startPos)
+ edButtons[i].tagStart
+ myField.value.substring(startPos, endPos)
+ edButtons[i].tagEnd
+ myField.value.substring(endPos, myField.value.length);
cursorPos += edButtons[i].tagStart.length + edButtons[i].tagEnd.length;
}
else {
if (!edCheckOpenTags(which, i) || edButtons[i].tagEnd == '') {
myField.value = myField.value.substring(0, startPos)
+ edButtons[i].tagStart
+ myField.value.substring(endPos, myField.value.length);
edAddTag(which, i);
cursorPos = startPos + edButtons[i].tagStart.length;
}
else {
myField.value = myField.value.substring(0, startPos)
+ edButtons[i].tagEnd
+ myField.value.substring(endPos, myField.value.length);
edRemoveTag(which, i);
cursorPos = startPos + edButtons[i].tagEnd.length;
}
}
myField.focus();
myField.selectionStart = cursorPos;
myField.selectionEnd = cursorPos;
myField.scrollTop = scrollTop;
}
else {
if (!edCheckOpenTags(which, i) || edButtons[i].tagEnd == '') {
myField.value += edButtons[i].tagStart;
edAddTag(which, i);
}
else {
myField.value += edButtons[i].tagEnd;
edRemoveTag(which, i);
}
myField.focus();
}
}
function edInsertContent(which, myValue) {
myField = document.getElementById(which);
//IE support
if (document.selection) {
myField.focus();
sel = document.selection.createRange();
sel.text = myValue;
myField.focus();
}
//MOZILLA/NETSCAPE support
else if (myField.selectionStart || myField.selectionStart == '0') {
var startPos = myField.selectionStart;
var endPos = myField.selectionEnd;
var scrollTop = myField.scrollTop;
myField.value = myField.value.substring(0, startPos)
+ myValue
+ myField.value.substring(endPos, myField.value.length);
myField.focus();
myField.selectionStart = startPos + myValue.length;
myField.selectionEnd = startPos + myValue.length;
myField.scrollTop = scrollTop;
} else {
myField.value += myValue;
myField.focus();
}
}
function edInsertLink(which, i, defaultValue) {
myField = document.getElementById(which);
if (!defaultValue) {
defaultValue = 'http://';
}
if (!edCheckOpenTags(which, i)) {
var URL = prompt('Enter the URL' ,defaultValue);
if (URL) {
edButtons[i].tagStart = '<a href="' + URL + '">';
edInsertTag(which, i);
}
}
else {
edInsertTag(which, i);
}
}
function edInsertPublifyCode(which, i, defaultValue) {
myField = document.getElementById(which);
if (!defaultValue) {
defaultValue = '';
}
if (!edCheckOpenTags(which, i)) {
var code = prompt('Choose language' ,defaultValue);
if (code) {
edButtons[i].tagStart = '<publify:code lang="' + code + '">\n';
edInsertTag(which, i);
}
}
else {
edInsertTag(which, i);
}
}
function edInsertImage(which) {
myField = document.getElementById(which);
var myValue = prompt('Enter the URL of the image', 'http://');
if (myValue && myValue.length > 0) {
myValue = '<img src="'
+ myValue
+ '" alt="' + prompt('Enter a description of the image', '')
+ '" />';
edInsertContent(which, myValue);
}
}
function edInsertImageFromCarousel(which, image) {
myField = document.getElementById(which);
if (image) {
myValue = '<img src="'
+ image
+ '" alt="' + prompt('Enter a description of the image', '')
+ '" />';
edInsertContent(which, myValue);
}
}
function countInstances(string, substr) {
var count = string.split(substr);
return count.length - 1;
}
function edInsertVia(which) {
myField = document.getElementById(which);
var myValue = prompt('Enter the URL of the source link', 'http://');
if (myValue) {
myValue = '(Thanks <a href="' + myValue + '" rel="external">'
+ prompt('Enter the name of the source', '')
+ '</a>)';
edInsertContent(which, myValue);
}
}
function edSetCookie(name, value, expires, path, domain) {
document.cookie= name + "=" + escape(value) +
((expires) ? "; expires=" + expires.toGMTString() : "") +
((path) ? "; path=" + path : "") +
((domain) ? "; domain=" + domain : "");
}
function edShowExtraCookie() {
var cookies = document.cookie.split(';');
for (var i=0;i < cookies.length; i++) {
var cookieData = cookies[i];
while (cookieData.charAt(0) ==' ') {
cookieData = cookieData.substring(1, cookieData.length);
}
if (cookieData.indexOf('js_quicktags_extra') == 0) {
if (cookieData.substring(19, cookieData.length) == 'show') {
return true;
}
else {
return false;
}
}
}
return false;
}
;
/**
* WideArea v0.3.0
* https://github.com/usablica/widearea
* MIT licensed
*
* Copyright (C) 2013 usabli.ca - By Afshin Mehrabani (@afshinmeh)
*/
(function (root, factory) {
if (typeof exports === 'object') {
// CommonJS
factory(exports);
} else if (typeof define === 'function' && define.amd) {
// AMD. Register as an anonymous module.
define(['exports'], factory);
} else {
// Browser globals
factory(root);
}
} (this, function (exports) {
//Default config/variables
var VERSION = '0.3.0';
/**
* WideArea main class
*
* @class WideArea
*/
function WideArea(obj) {
this._targetElement = obj;
//starts with 1
this._wideAreaId = 1;
this._options = {
wideAreaAttr: 'data-widearea',
exitOnEsc: true,
defaultColorScheme: 'light',
closeIconLabel: 'Close WideArea',
changeThemeIconLabel: 'Toggle Color Scheme',
fullScreenIconLabel: 'WideArea Mode',
autoSaveKeyPrefix: 'WDATA-'
};
_enable.call(this);
}
/**
* Save textarea data to storage
*
* @api private
* @method _saveToStorage
*/
function _saveToStorage(id, value) {
//save textarea data in localStorage
//localStorage.setItem(this._options.autoSaveKeyPrefix + id, value);
}
/**
* Get data from storage
*
* @api private
* @method _getFromStorage
*/
function _getFromStorage(id) {
// return localStorage.getItem(this._options.autoSaveKeyPrefix + id);
}
/**
* Clear specific textarea data from storage
*
* @api private
* @method _clearStorage
*/
function _clearStorage(value) {
var currentElement, id;
if (typeof(value) == "string") {
//with css selector
currentElement = this._targetElement.querySelector(value);
if (currentElement) {
id = parseInt(currentElement.getAttribute("data-widearea-id"));
}
} else if (typeof(value) == "number") {
//first, clear it from storage
currentElement = this._targetElement.querySelector('textarea[data-widearea-id="' + value + '"]');
id = value;
} else if (typeof(value) == "object") {
//with DOM
currentElement = value;
if (currentElement) {
id = parseInt(currentElement.attr("data-widearea-id"));
}
}
//and then, clear the textarea
if (currentElement && id) {
currentElement.val('');
localStorage.removeItem(this._options.autoSaveKeyPrefix + id);
}
}
/**
* Enable the WideArea
*
* @api private
* @method _enable
*/
function _enable() {
var self = this,
//select all textareas in the target element
textAreaList = this._targetElement.querySelectorAll('textarea[' + this._options.wideAreaAttr + '=\'enable\']'),
// don't make functions within a loop.
fullscreenIconClickHandler = function() {
_enableFullScreen.call(self, this);
};
//to hold all textareas in the page
this._textareas = [];
//then, change all textareas to widearea
for (var i = textAreaList.length - 1; i >= 0; i--) {
var currentTextArea = textAreaList[i];
//create widearea wrapper element
var wideAreaWrapper = document.createElement('div'),
wideAreaIcons = document.createElement('div'),
fullscreenIcon = document.createElement('a');
wideAreaWrapper.className = 'widearea-wrapper';
wideAreaIcons.className = 'widearea-icons';
fullscreenIcon.className = 'widearea-icon fullscreen';
fullscreenIcon.title = this._options.fullScreenIconLabel;
//hack!
fullscreenIcon.href = 'javascript:void(0);';
fullscreenIcon.draggable = false;
//bind to click event
fullscreenIcon.onclick = fullscreenIconClickHandler;
//add widearea class to textarea
currentTextArea.className += (currentTextArea.className + " widearea").replace(/^\s+|\s+$/g, "");
//set wideArea id and increase the stepper
currentTextArea.setAttribute("data-widearea-id", this._wideAreaId);
wideAreaIcons.setAttribute("id", "widearea-" + this._wideAreaId);
//Autosaving
if (_getFromStorage.call(this, this._wideAreaId)) {
currentTextArea.value = _getFromStorage.call(this, this._wideAreaId);
}
var onTextChanged = function () {
_saveToStorage.call(self, this.getAttribute('data-widearea-id'), this.value);
};
//add textchange listener
if (currentTextArea.addEventListener) {
currentTextArea.addEventListener('input', onTextChanged, false);
} else if (currentTextArea.attachEvent) { //IE hack
currentTextArea.attachEvent('onpropertychange', onTextChanged);
}
//go to next wideArea
++this._wideAreaId;
//set icons panel position
_renewIconsPosition(currentTextArea, wideAreaIcons);
//append all prepared div(s)
wideAreaIcons.appendChild(fullscreenIcon);
wideAreaWrapper.appendChild(wideAreaIcons);
//and append it to the page
document.body.appendChild(wideAreaWrapper);
//add the textarea to internal variable
this._textareas.push(currentTextArea);
}
//set a timer to re-calculate the position of textareas, I don't know whether this is a good approach or not
this._timer = setInterval(function() {
for (var i = self._textareas.length - 1; i >= 0; i--) {
var currentTextArea = self._textareas[i];
//get the related icon panel. Using `getElementById` for better performance
var wideAreaIcons = document.getElementById("widearea-" + currentTextArea.getAttribute("data-widearea-id"));
//get old position
var oldPosition = _getOffset(wideAreaIcons);
//get the new element's position
var currentTextareaPosition = _getOffset(currentTextArea);
//only set the new position of old positions changed
if((oldPosition.left - currentTextareaPosition.width + 21) != currentTextareaPosition.left || oldPosition.top != currentTextareaPosition.top) {
//set icons panel position
_renewIconsPosition(currentTextArea, wideAreaIcons, currentTextareaPosition);
}
};
}, 200);
}
/**
* Set new position to icons panel
*
* @api private
* @method _renewIconsPosition
* @param {Object} textarea
* @param {Object} iconPanel
*/
function _renewIconsPosition(textarea, iconPanel, textAreaPosition) {
var currentTextareaPosition = textAreaPosition || _getOffset(textarea);
//set icon panel position
iconPanel.style.left = currentTextareaPosition.left + currentTextareaPosition.width - 21 + "px";
iconPanel.style.top = currentTextareaPosition.top + "px";
}
/**
* Get an element position on the page
* Thanks to `meouw`: http://stackoverflow.com/a/442474/375966
*
* @api private
* @method _getOffset
* @param {Object} element
* @returns Element's position info
*/
function _getOffset(element) {
var elementPosition = {};
//set width
elementPosition.width = element.offsetWidth;
//set height
elementPosition.height = element.offsetHeight;
//calculate element top and left
var _x = 0;
var _y = 0;
while(element && !isNaN(element.offsetLeft) && !isNaN(element.offsetTop)) {
_x += element.offsetLeft;
_y += element.offsetTop;
element = element.offsetParent;
}
//set top
elementPosition.top = _y;
//set left
elementPosition.left = _x;
return elementPosition;
}
/**
* Get an element CSS property on the page
* Thanks to JavaScript Kit: http://www.javascriptkit.com/dhtmltutors/dhtmlcascade4.shtml
*
* @api private
* @method _getPropValue
* @param {Object} element
* @param {String} propName
* @returns Element's property value
*/
function _getPropValue (element, propName) {
var propValue = '';
if (element.currentStyle) { //IE
propValue = element.currentStyle[propName];
} else if (document.defaultView && document.defaultView.getComputedStyle) { //Others
propValue = document.defaultView.getComputedStyle(element, null).getPropertyValue(propName);
}
//Prevent exception in IE
if(propValue.toLowerCase) {
return propValue.toLowerCase();
} else {
return propValue;
}
}
/**
* FullScreen the textarea
*
* @api private
* @method _enableFullScreen
* @param {Object} link
*/
function _enableFullScreen(link) {
var self = this;
//first of all, get the textarea id
var wideAreaId = parseInt(link.parentNode.id.replace(/widearea\-/, ""));
//I don't know whether is this correct or not, but I think it's not a bad way
var targetTextarea = document.querySelector("textarea[data-widearea-id='" + wideAreaId + "']");
//clone current textarea
var currentTextArea = targetTextarea.cloneNode();
//add proper css class names
currentTextArea.className = ('widearea-fullscreen ' + targetTextarea.className).replace(/^\s+|\s+$/g, "");
targetTextarea.className = ('widearea-fullscreened ' + targetTextarea.className).replace(/^\s+|\s+$/g, "");
var controlPanel = document.createElement('div');
controlPanel.className = 'widearea-controlPanel';
//create close icon
var closeIcon = document.createElement('a');
closeIcon.href = 'javascript:void(0);';
closeIcon.className = 'widearea-icon close';
closeIcon.title = this._options.closeIconLabel;
closeIcon.onclick = function(){
_disableFullScreen.call(self);
};
//disable dragging
closeIcon.draggable = false;
//create close icon
var changeThemeIcon = document.createElement('a');
changeThemeIcon.href = 'javascript:void(0);';
changeThemeIcon.className = 'widearea-icon changeTheme';
changeThemeIcon.title = this._options.changeThemeIconLabel;
changeThemeIcon.onclick = function() {
_toggleColorScheme.call(self);
};
//disable dragging
changeThemeIcon.draggable = false;
controlPanel.appendChild(closeIcon);
controlPanel.appendChild(changeThemeIcon);
//create overlay layer
var overlayLayer = document.createElement('div');
overlayLayer.className = 'widearea-overlayLayer ' + this._options.defaultColorScheme;
//add controls to overlay layer
overlayLayer.appendChild(currentTextArea);
overlayLayer.appendChild(controlPanel);
//finally add it to the body
document.body.appendChild(overlayLayer);
//set the focus to textarea
currentTextArea.focus();
//set the value of small textarea to fullscreen one
currentTextArea.value = targetTextarea.value;
var onTextChanged = function () {
_saveToStorage.call(self, this.getAttribute('data-widearea-id'), this.value);
};
//add textchange listener
if (currentTextArea.addEventListener) {
currentTextArea.addEventListener('input', onTextChanged, false);
} else if (currentTextArea.attachEvent) { //IE hack
currentTextArea.attachEvent('onpropertychange', onTextChanged);
}
//bind to keydown event
this._onKeyDown = function(e) {
if (e.keyCode === 27 && self._options.exitOnEsc) {
//escape key pressed
_disableFullScreen.call(self);
}
if (e.keyCode == 9) {
// tab key pressed
e.preventDefault();
var selectionStart = currentTextArea.selectionStart;
currentTextArea.value = currentTextArea.value.substring(0, selectionStart) + "\t" + currentTextArea.value.substring(currentTextArea.selectionEnd);
currentTextArea.selectionEnd = selectionStart + 1;
}
};
if (window.addEventListener) {
window.addEventListener('keydown', self._onKeyDown, true);
} else if (document.attachEvent) { //IE
document.attachEvent('onkeydown', self._onKeyDown);
}
}
/**
* Change/Toggle color scheme of WideArea
*
* @api private
* @method _toggleColorScheme
*/
function _toggleColorScheme() {
var overlayLayer = document.querySelector(".widearea-overlayLayer");
if(/dark/gi.test(overlayLayer.className)) {
overlayLayer.className = overlayLayer.className.replace('dark', 'light');
} else {
overlayLayer.className = overlayLayer.className.replace('light', 'dark');
}
}
/**
* Close FullScreen
*
* @api private
* @method _disableFullScreen
*/
function _disableFullScreen() {
var smallTextArea = document.querySelector("textarea.widearea-fullscreened");
var overlayLayer = document.querySelector(".widearea-overlayLayer");
var fullscreenTextArea = overlayLayer.querySelector("textarea");
//change the focus
smallTextArea.focus();
//set fullscreen textarea to small one
smallTextArea.value = fullscreenTextArea.value;
//reset class for targeted text
smallTextArea.className = smallTextArea.className.replace(/widearea-fullscreened/gi, "").replace(/^\s+|\s+$/g, "");
//and then remove the overlay layer
overlayLayer.parentNode.removeChild(overlayLayer);
//clean listeners
if (window.removeEventListener) {
window.removeEventListener('keydown', this._onKeyDown, true);
} else if (document.detachEvent) { //IE
document.detachEvent('onkeydown', this._onKeyDown);
}
adaptiveheight(smallTextArea);
}
/**
* Overwrites obj1's values with obj2's and adds obj2's if non existent in obj1
*
* @param obj1
* @param obj2
* @returns obj3 a new object based on obj1 and obj2
*/
function _mergeOptions(obj1, obj2) {
var obj3 = {}, attrname;
for (attrname in obj1) { obj3[attrname] = obj1[attrname]; }
for (attrname in obj2) { obj3[attrname] = obj2[attrname]; }
return obj3;
}
var wideArea = function (selector) {
if (typeof (selector) === 'string') {
//select the target element with query selector
var targetElement = document.querySelector(selector);
if (targetElement) {
return new WideArea(targetElement);
} else {
throw new Error('There is no element with given selector.');
}
} else {
return new WideArea(document.body);
}
};
/**
* Current WideArea version
*
* @property version
* @type String
*/
wideArea.version = VERSION;
//Prototype
wideArea.fn = WideArea.prototype = {
clone: function () {
return new WideArea(this);
},
setOption: function(option, value) {
this._options[option] = value;
return this;
},
setOptions: function(options) {
this._options = _mergeOptions(this._options, options);
return this;
},
clearData: function(value) {
_clearStorage.call(this, value);
return this;
}
};
exports.wideArea = wideArea;
return wideArea;
}));
/* ===================================================
* tagmanager.js v3.0.1
* http://welldonethings.com/tags/manager
* ===================================================
* Copyright 2012 Max Favilli
*
* Licensed under the Mozilla Public License, Version 2.0 You may not use this work except in compliance with the License.
*
* http://www.mozilla.org/MPL/2.0/
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ========================================================== */
(function($) {
"use strict";
var defaults = {
prefilled: null,
CapitalizeFirstLetter: false,
preventSubmitOnEnter: true, // deprecated
isClearInputOnEsc: true, // deprecated
AjaxPush: null,
AjaxPushAllTags: null,
AjaxPushParameters: null,
delimiters: [9, 13, 44], // tab, enter, comma
backspace: [8],
maxTags: 0,
hiddenTagListName: null, // deprecated
hiddenTagListId: null, // deprecated
replace: true,
output: null,
deleteTagsOnBackspace: true, // deprecated
tagsContainer: null,
tagCloseIcon: 'x',
tagClass: '',
validator: null,
onlyTagList: false,
tagList: null,
},
publicMethods = {
pushTag : function (tag, ignoreEvents) {
var $self = $(this), opts = $self.data('opts'), alreadyInList, tlisLowerCase, max, tagId,
tlis = $self.data("tlis"), tlid = $self.data("tlid"), idx, newTagId, newTagRemoveId, escaped,
html, $el, lastTagId, lastTagObj;
tag = privateMethods.trimTag(tag, opts.delimiterChars);
if (!tag || tag.length <= 0) { return; }
// check if restricted only to the tagList suggestions
if (opts.onlyTagList && undefined !== opts.tagList ){
//if the list has been updated by look pushed tag in the tagList. if not found return
if (opts.tagList){
var $tagList = opts.tagList;
// change each array item to lower case
$.each($tagList, function(index, item) {
$tagList[index] = item.toLowerCase();
});
var suggestion = $.inArray(tag.toLowerCase(), $tagList);
if ( -1 === suggestion ) {
//console.log("tag:" + tag + " not in tagList, not adding it");
return;
}
}
}
if (opts.CapitalizeFirstLetter && tag.length > 1) {
tag = tag.charAt(0).toUpperCase() + tag.slice(1).toLowerCase();
}
// call the validator (if any) and do not let the tag pass if invalid
if (opts.validator && !opts.validator(tag)) { return; }
// dont accept new tags beyond the defined maximum
if (opts.maxTags > 0 && tlis.length >= opts.maxTags) { return; }
alreadyInList = false;
//use jQuery.map to make this work in IE8 (pure JS map is JS 1.6 but IE8 only supports JS 1.5)
tlisLowerCase = jQuery.map(tlis, function(elem) {
return elem.toLowerCase();
});
idx = $.inArray(tag.toLowerCase(), tlisLowerCase);
if (-1 !== idx) {
// console.log("tag:" + tag + " !!already in list!!");
alreadyInList = true;
}
if (alreadyInList) {
$self.trigger('tm:duplicated', tag);
$("#" + $self.data("tm_rndid") + "_" + tlid[idx]).stop()
.animate({backgroundColor: opts.blinkBGColor_1}, 100)
.animate({backgroundColor: opts.blinkBGColor_2}, 100)
.animate({backgroundColor: opts.blinkBGColor_1}, 100)
.animate({backgroundColor: opts.blinkBGColor_2}, 100)
.animate({backgroundColor: opts.blinkBGColor_1}, 100)
.animate({backgroundColor: opts.blinkBGColor_2}, 100);
} else {
if (!ignoreEvents) { $self.trigger('tm:pushing', tag); }
max = Math.max.apply(null, tlid);
max = max === -Infinity ? 0 : max;
tagId = ++max;
tlis.push(tag);
tlid.push(tagId);
if (!ignoreEvents)
if (opts.AjaxPush !== null && opts.AjaxPushAllTags == null) {
if ($.inArray(tag, opts.prefilled) === -1) {
$.post(opts.AjaxPush, $.extend({tag: tag}, opts.AjaxPushParameters));
}
}
// console.log("tagList: " + tlis);
newTagId = $self.data("tm_rndid") + '_' + tagId;
newTagRemoveId = $self.data("tm_rndid") + '_Remover_' + tagId;
escaped = $("<span/>").text(tag).html();
html = '<span class="' + privateMethods.tagClasses.call($self) + '" id="' + newTagId + '">';
html+= '<span>' + escaped + '</span>';
html+= '<a href="#" class="tm-tag-remove" id="' + newTagRemoveId + '" TagIdToRemove="' + tagId + '">';
html+= opts.tagCloseIcon + '</a></span> ';
$el = $(html);
if (opts.tagsContainer !== null) {
$(opts.tagsContainer).append($el);
} else {
if (tagId > 1) {
lastTagId = tagId - 1;
lastTagObj = $("#" + $self.data("tm_rndid") + "_" + lastTagId);
lastTagObj.after($el);
} else {
$self.before($el);
}
}
$el.find("#" + newTagRemoveId).on("click", $self, function(e) {
e.preventDefault();
var TagIdToRemove = parseInt($(this).attr("TagIdToRemove"));
privateMethods.spliceTag.call($self, TagIdToRemove, e.data);
});
privateMethods.refreshHiddenTagList.call($self);
if (!ignoreEvents) { $self.trigger('tm:pushed', tag); }
privateMethods.showOrHide.call($self);
//if (tagManagerOptions.maxTags > 0 && tlis.length >= tagManagerOptions.maxTags) {
// obj.hide();
//}
}
$self.val("");
},
popTag : function () {
var $self = $(this), tagId, tagBeingRemoved,
tlis = $self.data("tlis"),
tlid = $self.data("tlid");
if (tlid.length > 0) {
tagId = tlid.pop();
tagBeingRemoved = tlis[tlis.length - 1];
$self.trigger('tm:popping', tagBeingRemoved);
tlis.pop();
// console.log("TagIdToRemove: " + tagId);
$("#" + $self.data("tm_rndid") + "_" + tagId).remove();
privateMethods.refreshHiddenTagList.call($self);
$self.trigger('tm:popped', tagBeingRemoved);
// console.log(tlis);
}
},
empty : function() {
var $self = $(this), tlis = $self.data("tlis"), tlid = $self.data("tlid"), tagId;
while (tlid.length > 0) {
tagId = tlid.pop();
tlis.pop();
// console.log("TagIdToRemove: " + tagId);
$("#" + $self.data("tm_rndid") + "_" + tagId).remove();
privateMethods.refreshHiddenTagList.call($self);
// console.log(tlis);
}
$self.trigger('tm:emptied', null);
privateMethods.showOrHide.call($self);
//if (tagManagerOptions.maxTags > 0 && tlis.length < tagManagerOptions.maxTags) {
// obj.show();
//}
},
tags : function() {
var $self = this, tlis = $self.data("tlis");
return tlis;
}
},
privateMethods = {
showOrHide : function () {
var $self = this, opts = $self.data('opts'), tlis = $self.data("tlis");
if (opts.maxTags > 0 && tlis.length < opts.maxTags) {
$self.show();
$self.trigger('tm:show');
}
if (opts.maxTags > 0 && tlis.length >= opts.maxTags) {
$self.hide();
$self.trigger('tm:hide');
}
},
tagClasses : function () {
var $self = $(this), opts = $self.data('opts'), tagBaseClass = opts.tagBaseClass,
inputBaseClass = opts.inputBaseClass, cl;
// 1) default class (tm-tag)
cl = tagBaseClass;
// 2) interpolate from input class: tm-input-xxx --> tm-tag-xxx
if ($self.attr('class')) {
$.each($self.attr('class').split(' '), function (index, value) {
if (value.indexOf(inputBaseClass + '-') !== -1) {
cl += ' ' + tagBaseClass + value.substring(inputBaseClass.length);
}
});
}
// 3) tags from tagClass option
cl += (opts.tagClass ? ' ' + opts.tagClass : '');
return cl;
},
trimTag : function (tag, delimiterChars) {
var i;
tag = $.trim(tag);
// truncate at the first delimiter char
i = 0;
for (i; i < tag.length; i++) {
if ($.inArray(tag.charCodeAt(i), delimiterChars) !== -1) { break; }
}
return tag.substring(0, i);
},
refreshHiddenTagList : function () {
var $self = $(this), tlis = $self.data("tlis"), lhiddenTagList = $self.data("lhiddenTagList");
if (lhiddenTagList) {
$(lhiddenTagList).val(tlis.join($self.data('opts').baseDelimiter)).change();
}
$self.trigger('tm:refresh', tlis.join($self.data('opts').baseDelimiter));
},
killEvent : function (e) {
e.cancelBubble = true;
e.returnValue = false;
e.stopPropagation();
e.preventDefault();
},
keyInArray : function (e, ary) {
return $.inArray(e.which, ary) !== -1;
},
applyDelimiter : function (e) {
var $self = $(this);
publicMethods.pushTag.call($self,$(this).val());
e.preventDefault();
},
prefill : function (pta) {
var $self = $(this);
$.each(pta, function (key, val) {
publicMethods.pushTag.call($self, val, true);
});
},
pushAllTags : function (e, tag) {
var $self = $(this), opts = $self.data('opts'), tlis = $self.data("tlis");
if (opts.AjaxPushAllTags) {
if (e.type !== 'tm:pushed' || $.inArray(tag, opts.prefilled) === -1) {
$.post(opts.AjaxPush, $.extend({ tags: tlis.join(opts.baseDelimiter) }, opts.AjaxPushParameters));
}
}
},
spliceTag : function (tagId) {
var $self = this, tlis = $self.data("tlis"), tlid = $self.data("tlid"), idx = $.inArray(tagId, tlid),
tagBeingRemoved;
// console.log("TagIdToRemove: " + tagId);
// console.log("position: " + idx);
if (-1 !== idx) {
tagBeingRemoved = tlis[idx];
$self.trigger('tm:splicing', tagBeingRemoved);
$("#" + $self.data("tm_rndid") + "_" + tagId).remove();
tlis.splice(idx, 1);
tlid.splice(idx, 1);
privateMethods.refreshHiddenTagList.call($self);
$self.trigger('tm:spliced', tagBeingRemoved);
// console.log(tlis);
}
privateMethods.showOrHide.call($self);
//if (tagManagerOptions.maxTags > 0 && tlis.length < tagManagerOptions.maxTags) {
// obj.show();
//}
},
init : function (options) {
var opts = $.extend({}, defaults, options), delimiters, keyNums;
opts.hiddenTagListName = (opts.hiddenTagListName === null)
? 'hidden-' + this.attr('name')
: opts.hiddenTagListName;
delimiters = opts.delimeters || opts.delimiters; // 'delimeter' is deprecated
keyNums = [9, 13, 17, 18, 19, 37, 38, 39, 40]; // delimiter values to be handled as key codes
opts.delimiterChars = [];
opts.delimiterKeys = [];
$.each(delimiters, function (i, v) {
if ($.inArray(v, keyNums) !== -1) {
opts.delimiterKeys.push(v);
} else {
opts.delimiterChars.push(v);
}
});
opts.baseDelimiter = String.fromCharCode(opts.delimiterChars[0] || 44);
opts.tagBaseClass = 'tm-tag';
opts.inputBaseClass = 'tm-input';
if (!$.isFunction(opts.validator)) { opts.validator = null; }
this.each(function() {
var $self = $(this), hiddenObj ='', rndid ='', albet = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz";
// prevent double-initialization of TagManager
if ($self.data('tagManager')) { return false; }
$self.data('tagManager', true);
for (var i = 0; i < 5; i++) {
rndid += albet.charAt(Math.floor(Math.random() * albet.length));
}
$self.data("tm_rndid", rndid);
// store instance-specific data in the DOM object
$self.data('opts',opts)
.data('tlis', []) //list of string tags
.data('tlid', []); //list of ID of the string tags
if (opts.output === null) {
hiddenObj = $('<input/>', {
type: 'hidden',
name: opts.hiddenTagListName
});
$self.after(hiddenObj);
$self.data("lhiddenTagList", hiddenObj);
} else {
$self.data("lhiddenTagList", $(opts.output));
}
if (opts.AjaxPushAllTags) {
$self.on('tm:spliced', privateMethods.pushAllTags);
$self.on('tm:popped', privateMethods.pushAllTags);
$self.on('tm:pushed', privateMethods.pushAllTags);
}
// hide popovers on focus and keypress events
$self.on('focus keypress', function(e) {
if ($(this).popover) { $(this).popover('hide'); }
});
// handle ESC (keyup used for browser compatibility)
if (opts.isClearInputOnEsc) {
$self.on('keyup', function(e) {
if (e.which === 27) {
// console.log('esc detected');
$(this).val('');
privateMethods.killEvent(e);
}
});
}
$self.on('keypress', function(e) {
// push ASCII-based delimiters
if (privateMethods.keyInArray(e, opts.delimiterChars)) {
privateMethods.applyDelimiter.call($self, e);
}
});
$self.on('keydown', function(e) {
// disable ENTER
if (e.which === 13) {
if (opts.preventSubmitOnEnter) {
privateMethods.killEvent(e);
}
}
// push key-based delimiters (includes <enter> by default)
if (privateMethods.keyInArray(e, opts.delimiterKeys)) {
privateMethods.applyDelimiter.call($self, e);
}
});
// BACKSPACE (keydown used for browser compatibility)
if (opts.deleteTagsOnBackspace) {
$self.on('keydown', function(e) {
if (privateMethods.keyInArray(e, opts.backspace)) {
// console.log("backspace detected");
if ($(this).val().length <= 0) {
publicMethods.popTag.call($self);
privateMethods.killEvent(e);
}
}
});
}
$self.change(function(e) {
if (!/webkit/.test(navigator.userAgent.toLowerCase())) {
$self.focus();
} // why?
/* unimplemented mode to push tag on blur
else if (tagManagerOptions.pushTagOnBlur) {
console.log('change: pushTagOnBlur ' + tag);
pushTag($(this).val());
} */
privateMethods.killEvent(e);
});
if (opts.prefilled !== null) {
if (typeof (opts.prefilled) === "object") {
privateMethods.prefill.call($self, opts.prefilled);
} else if (typeof (opts.prefilled) === "string") {
privateMethods.prefill.call($self, opts.prefilled.split(opts.baseDelimiter));
} else if (typeof (opts.prefilled) === "function") {
privateMethods.prefill.call($self, opts.prefilled());
}
} else if (opts.output !== null) {
if ($(opts.output) && $(opts.output).val()) { var existing_tags = $(opts.output); }
privateMethods.prefill.call($self,$(opts.output).val().split(opts.baseDelimiter));
}
});
return this;
}
};
$.fn.tagsManager = function(method) {
var $self = $(this);
if (!(0 in this)) { return this; }
if ( publicMethods[method] ) {
return publicMethods[method].apply( $self, Array.prototype.slice.call(arguments, 1) );
} else if ( typeof method === 'object' || ! method ) {
return privateMethods.init.apply( this, arguments );
} else {
$.error( 'Method ' + method + ' does not exist.' );
return false;
}
};
}(jQuery));
/*!
* typeahead.js 0.9.3
* https://github.com/twitter/typeahead
* Copyright 2013 Twitter, Inc. and other contributors; Licensed MIT
*/
(function($) {
var VERSION = "0.9.3";
var utils = {
isMsie: function() {
var match = /(msie) ([\w.]+)/i.exec(navigator.userAgent);
return match ? parseInt(match[2], 10) : false;
},
isBlankString: function(str) {
return !str || /^\s*$/.test(str);
},
escapeRegExChars: function(str) {
return str.replace(/[\-\[\]\/\{\}\(\)\*\+\?\.\\\^\$\|]/g, "\\$&");
},
isString: function(obj) {
return typeof obj === "string";
},
isNumber: function(obj) {
return typeof obj === "number";
},
isArray: $.isArray,
isFunction: $.isFunction,
isObject: $.isPlainObject,
isUndefined: function(obj) {
return typeof obj === "undefined";
},
bind: $.proxy,
bindAll: function(obj) {
var val;
for (var key in obj) {
$.isFunction(val = obj[key]) && (obj[key] = $.proxy(val, obj));
}
},
indexOf: function(haystack, needle) {
for (var i = 0; i < haystack.length; i++) {
if (haystack[i] === needle) {
return i;
}
}
return -1;
},
each: $.each,
map: $.map,
filter: $.grep,
every: function(obj, test) {
var result = true;
if (!obj) {
return result;
}
$.each(obj, function(key, val) {
if (!(result = test.call(null, val, key, obj))) {
return false;
}
});
return !!result;
},
some: function(obj, test) {
var result = false;
if (!obj) {
return result;
}
$.each(obj, function(key, val) {
if (result = test.call(null, val, key, obj)) {
return false;
}
});
return !!result;
},
mixin: $.extend,
getUniqueId: function() {
var counter = 0;
return function() {
return counter++;
};
}(),
defer: function(fn) {
setTimeout(fn, 0);
},
debounce: function(func, wait, immediate) {
var timeout, result;
return function() {
var context = this, args = arguments, later, callNow;
later = function() {
timeout = null;
if (!immediate) {
result = func.apply(context, args);
}
};
callNow = immediate && !timeout;
clearTimeout(timeout);
timeout = setTimeout(later, wait);
if (callNow) {
result = func.apply(context, args);
}
return result;
};
},
throttle: function(func, wait) {
var context, args, timeout, result, previous, later;
previous = 0;
later = function() {
previous = new Date();
timeout = null;
result = func.apply(context, args);
};
return function() {
var now = new Date(), remaining = wait - (now - previous);
context = this;
args = arguments;
if (remaining <= 0) {
clearTimeout(timeout);
timeout = null;
previous = now;
result = func.apply(context, args);
} else if (!timeout) {
timeout = setTimeout(later, remaining);
}
return result;
};
},
tokenizeQuery: function(str) {
return $.trim(str).toLowerCase().split(/[\s]+/);
},
tokenizeText: function(str) {
return $.trim(str).toLowerCase().split(/[\s\-_]+/);
},
getProtocol: function() {
return location.protocol;
},
noop: function() {}
};
var EventTarget = function() {
var eventSplitter = /\s+/;
return {
on: function(events, callback) {
var event;
if (!callback) {
return this;
}
this._callbacks = this._callbacks || {};
events = events.split(eventSplitter);
while (event = events.shift()) {
this._callbacks[event] = this._callbacks[event] || [];
this._callbacks[event].push(callback);
}
return this;
},
trigger: function(events, data) {
var event, callbacks;
if (!this._callbacks) {
return this;
}
events = events.split(eventSplitter);
while (event = events.shift()) {
if (callbacks = this._callbacks[event]) {
for (var i = 0; i < callbacks.length; i += 1) {
callbacks[i].call(this, {
type: event,
data: data
});
}
}
}
return this;
}
};
}();
var EventBus = function() {
var namespace = "typeahead:";
function EventBus(o) {
if (!o || !o.el) {
$.error("EventBus initialized without el");
}
this.$el = $(o.el);
}
utils.mixin(EventBus.prototype, {
trigger: function(type) {
var args = [].slice.call(arguments, 1);
this.$el.trigger(namespace + type, args);
}
});
return EventBus;
}();
var PersistentStorage = function() {
var ls, methods;
try {
ls = window.localStorage;
ls.setItem("~~~", "!");
ls.removeItem("~~~");
} catch (err) {
ls = null;
}
function PersistentStorage(namespace) {
this.prefix = [ "__", namespace, "__" ].join("");
this.ttlKey = "__ttl__";
this.keyMatcher = new RegExp("^" + this.prefix);
}
if (ls && window.JSON) {
methods = {
_prefix: function(key) {
return this.prefix + key;
},
_ttlKey: function(key) {
return this._prefix(key) + this.ttlKey;
},
get: function(key) {
if (this.isExpired(key)) {
this.remove(key);
}
return decode(ls.getItem(this._prefix(key)));
},
set: function(key, val, ttl) {
if (utils.isNumber(ttl)) {
ls.setItem(this._ttlKey(key), encode(now() + ttl));
} else {
ls.removeItem(this._ttlKey(key));
}
return ls.setItem(this._prefix(key), encode(val));
},
remove: function(key) {
ls.removeItem(this._ttlKey(key));
ls.removeItem(this._prefix(key));
return this;
},
clear: function() {
var i, key, keys = [], len = ls.length;
for (i = 0; i < len; i++) {
if ((key = ls.key(i)).match(this.keyMatcher)) {
keys.push(key.replace(this.keyMatcher, ""));
}
}
for (i = keys.length; i--; ) {
this.remove(keys[i]);
}
return this;
},
isExpired: function(key) {
var ttl = decode(ls.getItem(this._ttlKey(key)));
return utils.isNumber(ttl) && now() > ttl ? true : false;
}
};
} else {
methods = {
get: utils.noop,
set: utils.noop,
remove: utils.noop,
clear: utils.noop,
isExpired: utils.noop
};
}
utils.mixin(PersistentStorage.prototype, methods);
return PersistentStorage;
function now() {
return new Date().getTime();
}
function encode(val) {
return JSON.stringify(utils.isUndefined(val) ? null : val);
}
function decode(val) {
return JSON.parse(val);
}
}();
var RequestCache = function() {
function RequestCache(o) {
utils.bindAll(this);
o = o || {};
this.sizeLimit = o.sizeLimit || 10;
this.cache = {};
this.cachedKeysByAge = [];
}
utils.mixin(RequestCache.prototype, {
get: function(url) {
return this.cache[url];
},
set: function(url, resp) {
var requestToEvict;
if (this.cachedKeysByAge.length === this.sizeLimit) {
requestToEvict = this.cachedKeysByAge.shift();
delete this.cache[requestToEvict];
}
this.cache[url] = resp;
this.cachedKeysByAge.push(url);
}
});
return RequestCache;
}();
var Transport = function() {
var pendingRequestsCount = 0, pendingRequests = {}, maxPendingRequests, requestCache;
function Transport(o) {
utils.bindAll(this);
o = utils.isString(o) ? {
url: o
} : o;
requestCache = requestCache || new RequestCache();
maxPendingRequests = utils.isNumber(o.maxParallelRequests) ? o.maxParallelRequests : maxPendingRequests || 6;
this.url = o.url;
this.wildcard = o.wildcard || "%QUERY";
this.filter = o.filter;
this.replace = o.replace;
this.ajaxSettings = {
type: "get",
cache: o.cache,
timeout: o.timeout,
dataType: o.dataType || "json",
beforeSend: o.beforeSend
};
this._get = (/^throttle$/i.test(o.rateLimitFn) ? utils.throttle : utils.debounce)(this._get, o.rateLimitWait || 300);
}
utils.mixin(Transport.prototype, {
_get: function(url, cb) {
var that = this;
if (belowPendingRequestsThreshold()) {
this._sendRequest(url).done(done);
} else {
this.onDeckRequestArgs = [].slice.call(arguments, 0);
}
function done(resp) {
var data = that.filter ? that.filter(resp) : resp;
cb && cb(data);
requestCache.set(url, resp);
}
},
_sendRequest: function(url) {
var that = this, jqXhr = pendingRequests[url];
if (!jqXhr) {
incrementPendingRequests();
jqXhr = pendingRequests[url] = $.ajax(url, this.ajaxSettings).always(always);
}
return jqXhr;
function always() {
decrementPendingRequests();
pendingRequests[url] = null;
if (that.onDeckRequestArgs) {
that._get.apply(that, that.onDeckRequestArgs);
that.onDeckRequestArgs = null;
}
}
},
get: function(query, cb) {
var that = this, encodedQuery = encodeURIComponent(query || ""), url, resp;
cb = cb || utils.noop;
url = this.replace ? this.replace(this.url, encodedQuery) : this.url.replace(this.wildcard, encodedQuery);
if (resp = requestCache.get(url)) {
utils.defer(function() {
cb(that.filter ? that.filter(resp) : resp);
});
} else {
this._get(url, cb);
}
return !!resp;
}
});
return Transport;
function incrementPendingRequests() {
pendingRequestsCount++;
}
function decrementPendingRequests() {
pendingRequestsCount--;
}
function belowPendingRequestsThreshold() {
return pendingRequestsCount < maxPendingRequests;
}
}();
var Dataset = function() {
var keys = {
thumbprint: "thumbprint",
protocol: "protocol",
itemHash: "itemHash",
adjacencyList: "adjacencyList"
};
function Dataset(o) {
utils.bindAll(this);
if (utils.isString(o.template) && !o.engine) {
$.error("no template engine specified");
}
if (!o.local && !o.prefetch && !o.remote) {
$.error("one of local, prefetch, or remote is required");
}
this.name = o.name || utils.getUniqueId();
this.limit = o.limit || 5;
this.minLength = o.minLength || 1;
this.header = o.header;
this.footer = o.footer;
this.valueKey = o.valueKey || "value";
this.template = compileTemplate(o.template, o.engine, this.valueKey);
this.local = o.local;
this.prefetch = o.prefetch;
this.remote = o.remote;
this.itemHash = {};
this.adjacencyList = {};
this.storage = o.name ? new PersistentStorage(o.name) : null;
}
utils.mixin(Dataset.prototype, {
_processLocalData: function(data) {
this._mergeProcessedData(this._processData(data));
},
_loadPrefetchData: function(o) {
var that = this, thumbprint = VERSION + (o.thumbprint || ""), storedThumbprint, storedProtocol, storedItemHash, storedAdjacencyList, isExpired, deferred;
if (this.storage) {
storedThumbprint = this.storage.get(keys.thumbprint);
storedProtocol = this.storage.get(keys.protocol);
storedItemHash = this.storage.get(keys.itemHash);
storedAdjacencyList = this.storage.get(keys.adjacencyList);
}
isExpired = storedThumbprint !== thumbprint || storedProtocol !== utils.getProtocol();
o = utils.isString(o) ? {
url: o
} : o;
o.ttl = utils.isNumber(o.ttl) ? o.ttl : 24 * 60 * 60 * 1e3;
if (storedItemHash && storedAdjacencyList && !isExpired) {
this._mergeProcessedData({
itemHash: storedItemHash,
adjacencyList: storedAdjacencyList
});
deferred = $.Deferred().resolve();
} else {
deferred = $.getJSON(o.url).done(processPrefetchData);
}
return deferred;
function processPrefetchData(data) {
var filteredData = o.filter ? o.filter(data) : data, processedData = that._processData(filteredData), itemHash = processedData.itemHash, adjacencyList = processedData.adjacencyList;
if (that.storage) {
that.storage.set(keys.itemHash, itemHash, o.ttl);
that.storage.set(keys.adjacencyList, adjacencyList, o.ttl);
that.storage.set(keys.thumbprint, thumbprint, o.ttl);
that.storage.set(keys.protocol, utils.getProtocol(), o.ttl);
}
that._mergeProcessedData(processedData);
}
},
_transformDatum: function(datum) {
var value = utils.isString(datum) ? datum : datum[this.valueKey], tokens = datum.tokens || utils.tokenizeText(value), item = {
value: value,
tokens: tokens
};
if (utils.isString(datum)) {
item.datum = {};
item.datum[this.valueKey] = datum;
} else {
item.datum = datum;
}
item.tokens = utils.filter(item.tokens, function(token) {
return !utils.isBlankString(token);
});
item.tokens = utils.map(item.tokens, function(token) {
return token.toLowerCase();
});
return item;
},
_processData: function(data) {
var that = this, itemHash = {}, adjacencyList = {};
utils.each(data, function(i, datum) {
var item = that._transformDatum(datum), id = utils.getUniqueId(item.value);
itemHash[id] = item;
utils.each(item.tokens, function(i, token) {
var character = token.charAt(0), adjacency = adjacencyList[character] || (adjacencyList[character] = [ id ]);
!~utils.indexOf(adjacency, id) && adjacency.push(id);
});
});
return {
itemHash: itemHash,
adjacencyList: adjacencyList
};
},
_mergeProcessedData: function(processedData) {
var that = this;
utils.mixin(this.itemHash, processedData.itemHash);
utils.each(processedData.adjacencyList, function(character, adjacency) {
var masterAdjacency = that.adjacencyList[character];
that.adjacencyList[character] = masterAdjacency ? masterAdjacency.concat(adjacency) : adjacency;
});
},
_getLocalSuggestions: function(terms) {
var that = this, firstChars = [], lists = [], shortestList, suggestions = [];
utils.each(terms, function(i, term) {
var firstChar = term.charAt(0);
!~utils.indexOf(firstChars, firstChar) && firstChars.push(firstChar);
});
utils.each(firstChars, function(i, firstChar) {
var list = that.adjacencyList[firstChar];
if (!list) {
return false;
}
lists.push(list);
if (!shortestList || list.length < shortestList.length) {
shortestList = list;
}
});
if (lists.length < firstChars.length) {
return [];
}
utils.each(shortestList, function(i, id) {
var item = that.itemHash[id], isCandidate, isMatch;
isCandidate = utils.every(lists, function(list) {
return ~utils.indexOf(list, id);
});
isMatch = isCandidate && utils.every(terms, function(term) {
return utils.some(item.tokens, function(token) {
return token.indexOf(term) === 0;
});
});
isMatch && suggestions.push(item);
});
return suggestions;
},
initialize: function() {
var deferred;
this.local && this._processLocalData(this.local);
this.transport = this.remote ? new Transport(this.remote) : null;
deferred = this.prefetch ? this._loadPrefetchData(this.prefetch) : $.Deferred().resolve();
this.local = this.prefetch = this.remote = null;
this.initialize = function() {
return deferred;
};
return deferred;
},
getSuggestions: function(query, cb) {
var that = this, terms, suggestions, cacheHit = false;
if (query.length < this.minLength) {
return;
}
terms = utils.tokenizeQuery(query);
suggestions = this._getLocalSuggestions(terms).slice(0, this.limit);
if (suggestions.length < this.limit && this.transport) {
cacheHit = this.transport.get(query, processRemoteData);
}
!cacheHit && cb && cb(suggestions);
function processRemoteData(data) {
suggestions = suggestions.slice(0);
utils.each(data, function(i, datum) {
var item = that._transformDatum(datum), isDuplicate;
isDuplicate = utils.some(suggestions, function(suggestion) {
return item.value === suggestion.value;
});
!isDuplicate && suggestions.push(item);
return suggestions.length < that.limit;
});
cb && cb(suggestions);
}
}
});
return Dataset;
function compileTemplate(template, engine, valueKey) {
var renderFn, compiledTemplate;
if (utils.isFunction(template)) {
renderFn = template;
} else if (utils.isString(template)) {
compiledTemplate = engine.compile(template);
renderFn = utils.bind(compiledTemplate.render, compiledTemplate);
} else {
renderFn = function(context) {
return "<p>" + context[valueKey] + "</p>";
};
}
return renderFn;
}
}();
var InputView = function() {
function InputView(o) {
var that = this;
utils.bindAll(this);
this.specialKeyCodeMap = {
9: "tab",
27: "esc",
37: "left",
39: "right",
13: "enter",
38: "up",
40: "down"
};
this.$hint = $(o.hint);
this.$input = $(o.input).on("blur.tt", this._handleBlur).on("focus.tt", this._handleFocus).on("keydown.tt", this._handleSpecialKeyEvent);
if (!utils.isMsie()) {
this.$input.on("input.tt", this._compareQueryToInputValue);
} else {
this.$input.on("keydown.tt keypress.tt cut.tt paste.tt", function($e) {
if (that.specialKeyCodeMap[$e.which || $e.keyCode]) {
return;
}
utils.defer(that._compareQueryToInputValue);
});
}
this.query = this.$input.val();
this.$overflowHelper = buildOverflowHelper(this.$input);
}
utils.mixin(InputView.prototype, EventTarget, {
_handleFocus: function() {
this.trigger("focused");
},
_handleBlur: function() {
this.trigger("blured");
},
_handleSpecialKeyEvent: function($e) {
var keyName = this.specialKeyCodeMap[$e.which || $e.keyCode];
keyName && this.trigger(keyName + "Keyed", $e);
},
_compareQueryToInputValue: function() {
var inputValue = this.getInputValue(), isSameQuery = compareQueries(this.query, inputValue), isSameQueryExceptWhitespace = isSameQuery ? this.query.length !== inputValue.length : false;
if (isSameQueryExceptWhitespace) {
this.trigger("whitespaceChanged", {
value: this.query
});
} else if (!isSameQuery) {
this.trigger("queryChanged", {
value: this.query = inputValue
});
}
},
destroy: function() {
this.$hint.off(".tt");
this.$input.off(".tt");
this.$hint = this.$input = this.$overflowHelper = null;
},
focus: function() {
this.$input.focus();
},
blur: function() {
this.$input.blur();
},
getQuery: function() {
return this.query;
},
setQuery: function(query) {
this.query = query;
},
getInputValue: function() {
return this.$input.val();
},
setInputValue: function(value, silent) {
this.$input.val(value);
!silent && this._compareQueryToInputValue();
},
getHintValue: function() {
return this.$hint.val();
},
setHintValue: function(value) {
this.$hint.val(value);
},
getLanguageDirection: function() {
return (this.$input.css("direction") || "ltr").toLowerCase();
},
isOverflow: function() {
this.$overflowHelper.text(this.getInputValue());
return this.$overflowHelper.width() > this.$input.width();
},
isCursorAtEnd: function() {
var valueLength = this.$input.val().length, selectionStart = this.$input[0].selectionStart, range;
if (utils.isNumber(selectionStart)) {
return selectionStart === valueLength;
} else if (document.selection) {
range = document.selection.createRange();
range.moveStart("character", -valueLength);
return valueLength === range.text.length;
}
return true;
}
});
return InputView;
function buildOverflowHelper($input) {
return $("<span></span>").css({
position: "absolute",
left: "-9999px",
visibility: "hidden",
whiteSpace: "nowrap",
fontFamily: $input.css("font-family"),
fontSize: $input.css("font-size"),
fontStyle: $input.css("font-style"),
fontVariant: $input.css("font-variant"),
fontWeight: $input.css("font-weight"),
wordSpacing: $input.css("word-spacing"),
letterSpacing: $input.css("letter-spacing"),
textIndent: $input.css("text-indent"),
textRendering: $input.css("text-rendering"),
textTransform: $input.css("text-transform")
}).insertAfter($input);
}
function compareQueries(a, b) {
a = (a || "").replace(/^\s*/g, "").replace(/\s{2,}/g, " ");
b = (b || "").replace(/^\s*/g, "").replace(/\s{2,}/g, " ");
return a === b;
}
}();
var DropdownView = function() {
var html = {
suggestionsList: '<span class="tt-suggestions"></span>'
}, css = {
suggestionsList: {
display: "block"
},
suggestion: {
whiteSpace: "nowrap",
cursor: "pointer"
},
suggestionChild: {
whiteSpace: "normal"
}
};
function DropdownView(o) {
utils.bindAll(this);
this.isOpen = false;
this.isEmpty = true;
this.isMouseOverDropdown = false;
this.$menu = $(o.menu).on("mouseenter.tt", this._handleMouseenter).on("mouseleave.tt", this._handleMouseleave).on("click.tt", ".tt-suggestion", this._handleSelection).on("mouseover.tt", ".tt-suggestion", this._handleMouseover);
}
utils.mixin(DropdownView.prototype, EventTarget, {
_handleMouseenter: function() {
this.isMouseOverDropdown = true;
},
_handleMouseleave: function() {
this.isMouseOverDropdown = false;
},
_handleMouseover: function($e) {
var $suggestion = $($e.currentTarget);
this._getSuggestions().removeClass("tt-is-under-cursor");
$suggestion.addClass("tt-is-under-cursor");
},
_handleSelection: function($e) {
var $suggestion = $($e.currentTarget);
this.trigger("suggestionSelected", extractSuggestion($suggestion));
},
_show: function() {
this.$menu.css("display", "block");
},
_hide: function() {
this.$menu.hide();
},
_moveCursor: function(increment) {
var $suggestions, $cur, nextIndex, $underCursor;
if (!this.isVisible()) {
return;
}
$suggestions = this._getSuggestions();
$cur = $suggestions.filter(".tt-is-under-cursor");
$cur.removeClass("tt-is-under-cursor");
nextIndex = $suggestions.index($cur) + increment;
nextIndex = (nextIndex + 1) % ($suggestions.length + 1) - 1;
if (nextIndex === -1) {
this.trigger("cursorRemoved");
return;
} else if (nextIndex < -1) {
nextIndex = $suggestions.length - 1;
}
$underCursor = $suggestions.eq(nextIndex).addClass("tt-is-under-cursor");
this._ensureVisibility($underCursor);
this.trigger("cursorMoved", extractSuggestion($underCursor));
},
_getSuggestions: function() {
return this.$menu.find(".tt-suggestions > .tt-suggestion");
},
_ensureVisibility: function($el) {
var menuHeight = this.$menu.height() + parseInt(this.$menu.css("paddingTop"), 10) + parseInt(this.$menu.css("paddingBottom"), 10), menuScrollTop = this.$menu.scrollTop(), elTop = $el.position().top, elBottom = elTop + $el.outerHeight(true);
if (elTop < 0) {
this.$menu.scrollTop(menuScrollTop + elTop);
} else if (menuHeight < elBottom) {
this.$menu.scrollTop(menuScrollTop + (elBottom - menuHeight));
}
},
destroy: function() {
this.$menu.off(".tt");
this.$menu = null;
},
isVisible: function() {
return this.isOpen && !this.isEmpty;
},
closeUnlessMouseIsOverDropdown: function() {
if (!this.isMouseOverDropdown) {
this.close();
}
},
close: function() {
if (this.isOpen) {
this.isOpen = false;
this.isMouseOverDropdown = false;
this._hide();
this.$menu.find(".tt-suggestions > .tt-suggestion").removeClass("tt-is-under-cursor");
this.trigger("closed");
}
},
open: function() {
if (!this.isOpen) {
this.isOpen = true;
!this.isEmpty && this._show();
this.trigger("opened");
}
},
setLanguageDirection: function(dir) {
var ltrCss = {
left: "0",
right: "auto"
}, rtlCss = {
left: "auto",
right: " 0"
};
dir === "ltr" ? this.$menu.css(ltrCss) : this.$menu.css(rtlCss);
},
moveCursorUp: function() {
this._moveCursor(-1);
},
moveCursorDown: function() {
this._moveCursor(+1);
},
getSuggestionUnderCursor: function() {
var $suggestion = this._getSuggestions().filter(".tt-is-under-cursor").first();
return $suggestion.length > 0 ? extractSuggestion($suggestion) : null;
},
getFirstSuggestion: function() {
var $suggestion = this._getSuggestions().first();
return $suggestion.length > 0 ? extractSuggestion($suggestion) : null;
},
renderSuggestions: function(dataset, suggestions) {
var datasetClassName = "tt-dataset-" + dataset.name, wrapper = '<div class="tt-suggestion">%body</div>', compiledHtml, $suggestionsList, $dataset = this.$menu.find("." + datasetClassName), elBuilder, fragment, $el;
if ($dataset.length === 0) {
$suggestionsList = $(html.suggestionsList).css(css.suggestionsList);
$dataset = $("<div></div>").addClass(datasetClassName).append(dataset.header).append($suggestionsList).append(dataset.footer).appendTo(this.$menu);
}
if (suggestions.length > 0) {
this.isEmpty = false;
this.isOpen && this._show();
elBuilder = document.createElement("div");
fragment = document.createDocumentFragment();
utils.each(suggestions, function(i, suggestion) {
suggestion.dataset = dataset.name;
compiledHtml = dataset.template(suggestion.datum);
elBuilder.innerHTML = wrapper.replace("%body", compiledHtml);
$el = $(elBuilder.firstChild).css(css.suggestion).data("suggestion", suggestion);
$el.children().each(function() {
$(this).css(css.suggestionChild);
});
fragment.appendChild($el[0]);
});
$dataset.show().find(".tt-suggestions").html(fragment);
} else {
this.clearSuggestions(dataset.name);
}
this.trigger("suggestionsRendered");
},
clearSuggestions: function(datasetName) {
var $datasets = datasetName ? this.$menu.find(".tt-dataset-" + datasetName) : this.$menu.find('[class^="tt-dataset-"]'), $suggestions = $datasets.find(".tt-suggestions");
$datasets.hide();
$suggestions.empty();
if (this._getSuggestions().length === 0) {
this.isEmpty = true;
this._hide();
}
}
});
return DropdownView;
function extractSuggestion($el) {
return $el.data("suggestion");
}
}();
var TypeaheadView = function() {
var html = {
wrapper: '<span class="twitter-typeahead"></span>',
hint: '<input class="tt-hint" type="text" autocomplete="off" spellcheck="off" disabled>',
dropdown: '<span class="tt-dropdown-menu"></span>'
}, css = {
wrapper: {
position: "relative",
display: "block"
},
hint: {
position: "absolute",
top: "0",
left: "0",
borderColor: "transparent",
boxShadow: "none"
},
query: {
position: "relative",
verticalAlign: "top",
backgroundColor: "transparent"
},
dropdown: {
position: "absolute",
top: "100%",
left: "0",
zIndex: "100",
display: "none"
}
};
if (utils.isMsie()) {
utils.mixin(css.query, {
backgroundImage: "url(data:image/gif;base64,R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7)"
});
}
if (utils.isMsie() && utils.isMsie() <= 7) {
utils.mixin(css.wrapper, {
display: "inline",
zoom: "1"
});
utils.mixin(css.query, {
marginTop: "-1px"
});
}
function TypeaheadView(o) {
var $menu, $input, $hint;
utils.bindAll(this);
this.$node = buildDomStructure(o.input);
this.datasets = o.datasets;
this.dir = null;
this.eventBus = o.eventBus;
$menu = this.$node.find(".tt-dropdown-menu");
$input = this.$node.find(".tt-query");
$hint = this.$node.find(".tt-hint");
this.dropdownView = new DropdownView({
menu: $menu
}).on("suggestionSelected", this._handleSelection).on("cursorMoved", this._clearHint).on("cursorMoved", this._setInputValueToSuggestionUnderCursor).on("cursorRemoved", this._setInputValueToQuery).on("cursorRemoved", this._updateHint).on("suggestionsRendered", this._updateHint).on("opened", this._updateHint).on("closed", this._clearHint).on("opened closed", this._propagateEvent);
this.inputView = new InputView({
input: $input,
hint: $hint
}).on("focused", this._openDropdown).on("blured", this._closeDropdown).on("blured", this._setInputValueToQuery).on("enterKeyed tabKeyed", this._handleSelection).on("queryChanged", this._clearHint).on("queryChanged", this._clearSuggestions).on("queryChanged", this._getSuggestions).on("whitespaceChanged", this._updateHint).on("queryChanged whitespaceChanged", this._openDropdown).on("queryChanged whitespaceChanged", this._setLanguageDirection).on("escKeyed", this._closeDropdown).on("escKeyed", this._setInputValueToQuery).on("tabKeyed upKeyed downKeyed", this._managePreventDefault).on("upKeyed downKeyed", this._moveDropdownCursor).on("upKeyed downKeyed", this._openDropdown).on("tabKeyed leftKeyed rightKeyed", this._autocomplete);
}
utils.mixin(TypeaheadView.prototype, EventTarget, {
_managePreventDefault: function(e) {
var $e = e.data, hint, inputValue, preventDefault = false;
switch (e.type) {
case "tabKeyed":
hint = this.inputView.getHintValue();
inputValue = this.inputView.getInputValue();
preventDefault = hint && hint !== inputValue;
break;
case "upKeyed":
case "downKeyed":
preventDefault = !$e.shiftKey && !$e.ctrlKey && !$e.metaKey;
break;
}
preventDefault && $e.preventDefault();
},
_setLanguageDirection: function() {
var dir = this.inputView.getLanguageDirection();
if (dir !== this.dir) {
this.dir = dir;
this.$node.css("direction", dir);
this.dropdownView.setLanguageDirection(dir);
}
},
_updateHint: function() {
var suggestion = this.dropdownView.getFirstSuggestion(), hint = suggestion ? suggestion.value : null, dropdownIsVisible = this.dropdownView.isVisible(), inputHasOverflow = this.inputView.isOverflow(), inputValue, query, escapedQuery, beginsWithQuery, match;
if (hint && dropdownIsVisible && !inputHasOverflow) {
inputValue = this.inputView.getInputValue();
query = inputValue.replace(/\s{2,}/g, " ").replace(/^\s+/g, "");
escapedQuery = utils.escapeRegExChars(query);
beginsWithQuery = new RegExp("^(?:" + escapedQuery + ")(.*$)", "i");
match = beginsWithQuery.exec(hint);
this.inputView.setHintValue(inputValue + (match ? match[1] : ""));
}
},
_clearHint: function() {
this.inputView.setHintValue("");
},
_clearSuggestions: function() {
this.dropdownView.clearSuggestions();
},
_setInputValueToQuery: function() {
this.inputView.setInputValue(this.inputView.getQuery());
},
_setInputValueToSuggestionUnderCursor: function(e) {
var suggestion = e.data;
this.inputView.setInputValue(suggestion.value, true);
},
_openDropdown: function() {
this.dropdownView.open();
},
_closeDropdown: function(e) {
this.dropdownView[e.type === "blured" ? "closeUnlessMouseIsOverDropdown" : "close"]();
},
_moveDropdownCursor: function(e) {
var $e = e.data;
if (!$e.shiftKey && !$e.ctrlKey && !$e.metaKey) {
this.dropdownView[e.type === "upKeyed" ? "moveCursorUp" : "moveCursorDown"]();
}
},
_handleSelection: function(e) {
var byClick = e.type === "suggestionSelected", suggestion = byClick ? e.data : this.dropdownView.getSuggestionUnderCursor();
if (suggestion) {
this.inputView.setInputValue(suggestion.value);
byClick ? this.inputView.focus() : e.data.preventDefault();
byClick && utils.isMsie() ? utils.defer(this.dropdownView.close) : this.dropdownView.close();
this.eventBus.trigger("selected", suggestion.datum, suggestion.dataset);
}
},
_getSuggestions: function() {
var that = this, query = this.inputView.getQuery();
if (utils.isBlankString(query)) {
return;
}
utils.each(this.datasets, function(i, dataset) {
dataset.getSuggestions(query, function(suggestions) {
if (query === that.inputView.getQuery()) {
that.dropdownView.renderSuggestions(dataset, suggestions);
}
});
});
},
_autocomplete: function(e) {
var isCursorAtEnd, ignoreEvent, query, hint, suggestion;
if (e.type === "rightKeyed" || e.type === "leftKeyed") {
isCursorAtEnd = this.inputView.isCursorAtEnd();
ignoreEvent = this.inputView.getLanguageDirection() === "ltr" ? e.type === "leftKeyed" : e.type === "rightKeyed";
if (!isCursorAtEnd || ignoreEvent) {
return;
}
}
query = this.inputView.getQuery();
hint = this.inputView.getHintValue();
if (hint !== "" && query !== hint) {
suggestion = this.dropdownView.getFirstSuggestion();
this.inputView.setInputValue(suggestion.value);
this.eventBus.trigger("autocompleted", suggestion.datum, suggestion.dataset);
}
},
_propagateEvent: function(e) {
this.eventBus.trigger(e.type);
},
destroy: function() {
this.inputView.destroy();
this.dropdownView.destroy();
destroyDomStructure(this.$node);
this.$node = null;
},
setQuery: function(query) {
this.inputView.setQuery(query);
this.inputView.setInputValue(query);
this._clearHint();
this._clearSuggestions();
this._getSuggestions();
}
});
return TypeaheadView;
function buildDomStructure(input) {
var $wrapper = $(html.wrapper), $dropdown = $(html.dropdown), $input = $(input), $hint = $(html.hint);
$wrapper = $wrapper.css(css.wrapper);
$dropdown = $dropdown.css(css.dropdown);
$hint.css(css.hint).css({
backgroundAttachment: $input.css("background-attachment"),
backgroundClip: $input.css("background-clip"),
backgroundColor: $input.css("background-color"),
backgroundImage: $input.css("background-image"),
backgroundOrigin: $input.css("background-origin"),
backgroundPosition: $input.css("background-position"),
backgroundRepeat: $input.css("background-repeat"),
backgroundSize: $input.css("background-size")
});
$input.data("ttAttrs", {
dir: $input.attr("dir"),
autocomplete: $input.attr("autocomplete"),
spellcheck: $input.attr("spellcheck"),
style: $input.attr("style")
});
$input.addClass("tt-query").attr({
autocomplete: "off",
spellcheck: false
}).css(css.query);
try {
!$input.attr("dir") && $input.attr("dir", "auto");
} catch (e) {}
return $input.wrap($wrapper).parent().prepend($hint).append($dropdown);
}
function destroyDomStructure($node) {
var $input = $node.find(".tt-query");
utils.each($input.data("ttAttrs"), function(key, val) {
utils.isUndefined(val) ? $input.removeAttr(key) : $input.attr(key, val);
});
$input.detach().removeData("ttAttrs").removeClass("tt-query").insertAfter($node);
$node.remove();
}
}();
(function() {
var cache = {}, viewKey = "ttView", methods;
methods = {
initialize: function(datasetDefs) {
var datasets;
datasetDefs = utils.isArray(datasetDefs) ? datasetDefs : [ datasetDefs ];
if (datasetDefs.length === 0) {
$.error("no datasets provided");
}
datasets = utils.map(datasetDefs, function(o) {
var dataset = cache[o.name] ? cache[o.name] : new Dataset(o);
if (o.name) {
cache[o.name] = dataset;
}
return dataset;
});
return this.each(initialize);
function initialize() {
var $input = $(this), deferreds, eventBus = new EventBus({
el: $input
});
deferreds = utils.map(datasets, function(dataset) {
return dataset.initialize();
});
$input.data(viewKey, new TypeaheadView({
input: $input,
eventBus: eventBus = new EventBus({
el: $input
}),
datasets: datasets
}));
$.when.apply($, deferreds).always(function() {
utils.defer(function() {
eventBus.trigger("initialized");
});
});
}
},
destroy: function() {
return this.each(destroy);
function destroy() {
var $this = $(this), view = $this.data(viewKey);
if (view) {
view.destroy();
$this.removeData(viewKey);
}
}
},
setQuery: function(query) {
return this.each(setQuery);
function setQuery() {
var view = $(this).data(viewKey);
view && view.setQuery(query);
}
}
};
jQuery.fn.typeahead = function(method) {
if (methods[method]) {
return methods[method].apply(this, [].slice.call(arguments, 1));
} else {
return methods.initialize.apply(this, arguments);
}
};
})();
})(window.jQuery);
/* typewatch() borrowed from http://stackoverflow.com/questions/2219924/idiomatic-jquery-delayed-event-only-after-a-short-pause-in-typing-e-g-timew */
var typewatch = (function(){
var timer = 0;
return function(callback, ms){
clearTimeout (timer);
timer = setTimeout(callback, ms);
}
})();
function autosave_request(e) {
$('#article_form').keyup(function() {
typewatch(function() {
$.ajax({
type: "POST",
url: '/admin/content/autosave',
data: $("#article_form").serialize()});
}, 5000)
});
}
function set_widerea(element) {
if ($("#article_id").val() == "") {
wideArea().clearData(element);
}
wideArea();
}
function tag_manager() {
var tagApi = jQuery("#article_keywords").tagsManager({
prefilled: $('#article_keywords').val()
});
jQuery("#article_keywords").typeahead({
name: 'tags',
limit: 15,
prefetch: '/admin/content/auto_complete_for_article_keywords'
}).on('typeahead:selected', function (e, d) {
tagApi.tagsManager("pushTag", d.value);
});
}
function save_article_tags() {
$('#article_keywords').val($('#article_form').find('input[name="hidden-article[keywords]"]').val());
}
function doneTyping () {
$( "#save-bar").fadeIn(2000, function() {
});
}
function set_savebar() {
var typingTimer;
var doneTypingInterval = 3000;
$( "#article_body_and_extended" ).keydown(function() {
$( "#save-bar").fadeOut(2000, function() {
});
clearTimeout(typingTimer);
});
$('#article_body_and_extended').keyup(function(){
typingTimer = setTimeout(doneTyping, doneTypingInterval);
});
}
$(document).ready(function() {
$('#article_form').each(function(e){autosave_request(e)});
$('#article_form').submit(function(e){save_article_tags()});
$('#article_form').each(function(e){tag_manager()});
$('#article_form').each(function(e){set_widerea($('#article_body_and_extended'))});
$('#article_body_and_extended').each(function(e){set_savebar()});
$('#page_form').each(function(e){set_widerea($('#page_body'))});
});
$(document).delegate('*[data-toggle="lightbox"]', 'click', function(event) {
event.preventDefault();
$(this).ekkoLightbox();
});
var bind_sortable = function() {
$('.sortable').sortable({
dropOnEmpty: true,
stop: function(evt, ui) {
var data = $(this).sortable('serialize', {attribute: 'data-sortable'});
$.ajax({
data: data,
type: 'PUT',
dataType: 'json',
url: '/admin/sidebar/sortable',
statusCode: {
200: function(data, textStatus, jqXHR) {
$('#sidebar-config').replaceWith(data.html);
bind_sortable();
},
500: function(jqXHR, textStatus, errorThrown) {
alert('Oups?');
}
}
});
},
});
$('.draggable').draggable({
connectToSortable: '.sortable',
helper: "clone",
revert: "invalid"
});
$('.sidebar_item').on('ajax:success', function(data, textStatus, xhr) {
$(this).parent().replaceWith(data);
}
);
$('.deletion_link').on('ajax:success', function(data, textStatus, xhr) {
$(this).parent().remove();
}
);
}
$(document).ready(function() {
bind_sortable();
});
/*
Lightbox for Bootstrap 3 by @ashleydw
https://github.com/ashleydw/lightbox
License: https://github.com/ashleydw/lightbox/blob/master/LICENSE
*/
(function() {
"use strict";
var $, EkkoLightbox;
$ = jQuery;
EkkoLightbox = function(element, options) {
var content, footer, header,
_this = this;
this.options = $.extend({
title: null,
footer: null,
remote: null
}, $.fn.ekkoLightbox.defaults, options || {});
this.$element = $(element);
content = '';
this.modal_id = this.options.modal_id ? this.options.modal_id : 'ekkoLightbox-' + Math.floor((Math.random() * 1000) + 1);
header = '<div class="modal-header"' + (this.options.title || this.options.always_show_close ? '' : ' style="display:none"') + '><button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button><h4 class="modal-title">' + (this.options.title || " ") + '</h4></div>';
footer = '<div class="modal-footer"' + (this.options.footer ? '' : ' style="display:none"') + '>' + this.options.footer + '</div>';
$(document.body).append('<div id="' + this.modal_id + '" class="ekko-lightbox modal fade" tabindex="-1"><div class="modal-dialog"><div class="modal-content">' + header + '<div class="modal-body"><div class="ekko-lightbox-container"><div></div></div></div>' + footer + '</div></div></div>');
this.modal = $('#' + this.modal_id);
this.modal_dialog = this.modal.find('.modal-dialog').first();
this.modal_content = this.modal.find('.modal-content').first();
this.modal_body = this.modal.find('.modal-body').first();
this.lightbox_container = this.modal_body.find('.ekko-lightbox-container').first();
this.lightbox_body = this.lightbox_container.find('> div:first-child').first();
this.showLoading();
this.modal_arrows = null;
this.border = {
top: parseFloat(this.modal_dialog.css('border-top-width')) + parseFloat(this.modal_content.css('border-top-width')) + parseFloat(this.modal_body.css('border-top-width')),
right: parseFloat(this.modal_dialog.css('border-right-width')) + parseFloat(this.modal_content.css('border-right-width')) + parseFloat(this.modal_body.css('border-right-width')),
bottom: parseFloat(this.modal_dialog.css('border-bottom-width')) + parseFloat(this.modal_content.css('border-bottom-width')) + parseFloat(this.modal_body.css('border-bottom-width')),
left: parseFloat(this.modal_dialog.css('border-left-width')) + parseFloat(this.modal_content.css('border-left-width')) + parseFloat(this.modal_body.css('border-left-width'))
};
this.padding = {
top: parseFloat(this.modal_dialog.css('padding-top')) + parseFloat(this.modal_content.css('padding-top')) + parseFloat(this.modal_body.css('padding-top')),
right: parseFloat(this.modal_dialog.css('padding-right')) + parseFloat(this.modal_content.css('padding-right')) + parseFloat(this.modal_body.css('padding-right')),
bottom: parseFloat(this.modal_dialog.css('padding-bottom')) + parseFloat(this.modal_content.css('padding-bottom')) + parseFloat(this.modal_body.css('padding-bottom')),
left: parseFloat(this.modal_dialog.css('padding-left')) + parseFloat(this.modal_content.css('padding-left')) + parseFloat(this.modal_body.css('padding-left'))
};
this.modal.on('show.bs.modal', this.options.onShow.bind(this)).on('shown.bs.modal', function() {
_this.modal_shown();
return _this.options.onShown.call(_this);
}).on('hide.bs.modal', this.options.onHide.bind(this)).on('hidden.bs.modal', function() {
if (_this.gallery) {
$(document).off('keydown.ekkoLightbox');
}
_this.modal.remove();
return _this.options.onHidden.call(_this);
}).modal('show', options);
return this.modal;
};
EkkoLightbox.prototype = {
modal_shown: function() {
var video_id,
_this = this;
if (!this.options.remote) {
return this.error('No remote target given');
} else {
this.gallery = this.$element.data('gallery');
if (this.gallery) {
if (this.options.gallery_parent_selector === 'document.body' || this.options.gallery_parent_selector === '') {
this.gallery_items = $(document.body).find('*[data-toggle="lightbox"][data-gallery="' + this.gallery + '"]');
} else {
this.gallery_items = this.$element.parents(this.options.gallery_parent_selector).first().find('*[data-toggle="lightbox"][data-gallery="' + this.gallery + '"]');
}
this.gallery_index = this.gallery_items.index(this.$element);
$(document).on('keydown.ekkoLightbox', this.navigate.bind(this));
if (this.options.directional_arrows && this.gallery_items.length > 1) {
this.lightbox_container.prepend('<div class="ekko-lightbox-nav-overlay"><a href="#" class="' + this.strip_stops(this.options.left_arrow_class) + '"></a><a href="#" class="' + this.strip_stops(this.options.right_arrow_class) + '"></a></div>');
this.modal_arrows = this.lightbox_container.find('div.ekko-lightbox-nav-overlay').first();
this.lightbox_container.find('a' + this.strip_spaces(this.options.left_arrow_class)).on('click', function(event) {
event.preventDefault();
return _this.navigate_left();
});
this.lightbox_container.find('a' + this.strip_spaces(this.options.right_arrow_class)).on('click', function(event) {
event.preventDefault();
return _this.navigate_right();
});
}
}
if (this.options.type) {
if (this.options.type === 'image') {
return this.preloadImage(this.options.remote, true);
} else if (this.options.type === 'youtube' && (video_id = this.getYoutubeId(this.options.remote))) {
return this.showYoutubeVideo(video_id);
} else if (this.options.type === 'vimeo') {
return this.showVimeoVideo(this.options.remote);
} else if (this.options.type === 'instagram') {
return this.showInstagramVideo(this.options.remote);
} else {
return this.error("Could not detect remote target type. Force the type using data-type=\"image|youtube|vimeo\"");
}
} else {
return this.detectRemoteType(this.options.remote);
}
}
},
strip_stops: function(str) {
return str.replace(/\./g, '');
},
strip_spaces: function(str) {
return str.replace(/\s/g, '');
},
isImage: function(str) {
return str.match(/(^data:image\/.*,)|(\.(jp(e|g|eg)|gif|png|bmp|webp|svg)((\?|#).*)?$)/i);
},
isSwf: function(str) {
return str.match(/\.(swf)((\?|#).*)?$/i);
},
getYoutubeId: function(str) {
var match;
match = str.match(/^.*(youtu.be\/|v\/|u\/\w\/|embed\/|watch\?v=|\&v=)([^#\&\?]*).*/);
if (match && match[2].length === 11) {
return match[2];
} else {
return false;
}
},
getVimeoId: function(str) {
if (str.indexOf('vimeo') > 0) {
return str;
} else {
return false;
}
},
getInstagramId: function(str) {
if (str.indexOf('instagram') > 0) {
return str;
} else {
return false;
}
},
navigate: function(event) {
event = event || window.event;
if (event.keyCode === 39 || event.keyCode === 37) {
if (event.keyCode === 39) {
return this.navigate_right();
} else if (event.keyCode === 37) {
return this.navigate_left();
}
}
},
navigate_left: function() {
var src;
this.showLoading();
if (this.gallery_items.length === 1) {
return;
}
if (this.gallery_index === 0) {
this.gallery_index = this.gallery_items.length - 1;
} else {
this.gallery_index--;
}
this.$element = $(this.gallery_items.get(this.gallery_index));
this.updateTitleAndFooter();
src = this.$element.attr('data-remote') || this.$element.attr('href');
return this.detectRemoteType(src, this.$element.attr('data-type'));
},
navigate_right: function() {
var next, src;
this.showLoading();
if (this.gallery_items.length === 1) {
return;
}
if (this.gallery_index === this.gallery_items.length - 1) {
this.gallery_index = 0;
} else {
this.gallery_index++;
}
this.$element = $(this.gallery_items.get(this.gallery_index));
src = this.$element.attr('data-remote') || this.$element.attr('href');
this.updateTitleAndFooter();
this.detectRemoteType(src, this.$element.attr('data-type'));
if (this.gallery_index + 1 < this.gallery_items.length) {
next = $(this.gallery_items.get(this.gallery_index + 1), false);
src = next.attr('data-remote') || next.attr('href');
if (next.attr('data-type') === 'image' || this.isImage(src)) {
return this.preloadImage(src, false);
}
}
},
detectRemoteType: function(src, type) {
var video_id;
if (type === 'image' || this.isImage(src)) {
this.options.type = 'image';
return this.preloadImage(src, true);
} else if (type === 'youtube' || (video_id = this.getYoutubeId(src))) {
this.options.type = 'youtube';
return this.showYoutubeVideo(video_id);
} else if (type === 'vimeo' || (video_id = this.getVimeoId(src))) {
this.options.type = 'vimeo';
return this.showVimeoVideo(video_id);
} else if (type === 'instagram' || (video_id = this.getInstagramId(src))) {
this.options.type = 'instagram';
return this.showInstagramVideo(video_id);
} else {
return this.error("Could not detect remote target type. Force the type using data-type=\"image|youtube|vimeo\"");
}
},
updateTitleAndFooter: function() {
var caption, footer, header, title;
header = this.modal_content.find('.modal-header');
footer = this.modal_content.find('.modal-footer');
title = this.$element.data('title') || "";
caption = this.$element.data('footer') || "";
if (title || this.options.always_show_close) {
header.css('display', '').find('.modal-title').html(title || " ");
} else {
header.css('display', 'none');
}
if (caption) {
footer.css('display', '').html(caption);
} else {
footer.css('display', 'none');
}
return this;
},
showLoading: function() {
this.lightbox_body.html('<div class="modal-loading">Loading..</div>');
return this;
},
showYoutubeVideo: function(id) {
var aspectRatio, height, width;
aspectRatio = 560 / 315;
width = this.$element.data('width') || 560;
width = this.checkDimensions(width);
height = width / aspectRatio;
this.resize(width);
this.lightbox_body.html('<iframe width="' + width + '" height="' + height + '" src="//www.youtube.com/embed/' + id + '?badge=0&autoplay=1&html5=1" frameborder="0" allowfullscreen></iframe>');
if (this.modal_arrows) {
return this.modal_arrows.css('display', 'none');
}
},
showVimeoVideo: function(id) {
var aspectRatio, height, width;
aspectRatio = 500 / 281;
width = this.$element.data('width') || 560;
width = this.checkDimensions(width);
height = width / aspectRatio;
this.resize(width);
this.lightbox_body.html('<iframe width="' + width + '" height="' + height + '" src="' + id + '?autoplay=1" frameborder="0" allowfullscreen></iframe>');
if (this.modal_arrows) {
return this.modal_arrows.css('display', 'none');
}
},
showInstagramVideo: function(id) {
var height, width;
width = this.$element.data('width') || 612;
width = this.checkDimensions(width);
height = width;
this.resize(width);
this.lightbox_body.html('<iframe width="' + width + '" height="' + height + '" src="' + this.addTrailingSlash(id) + 'embed/" frameborder="0" allowfullscreen></iframe>');
if (this.modal_arrows) {
return this.modal_arrows.css('display', 'none');
}
},
error: function(message) {
this.lightbox_body.html(message);
return this;
},
preloadImage: function(src, onLoadShowImage) {
var img,
_this = this;
img = new Image();
if ((onLoadShowImage == null) || onLoadShowImage === true) {
img.onload = function() {
var image;
image = $('<img />');
image.attr('src', img.src);
image.addClass('img-responsive');
_this.lightbox_body.html(image);
if (_this.modal_arrows) {
_this.modal_arrows.css('display', 'block');
}
return _this.resize(img.width);
};
img.onerror = function() {
return _this.error('Failed to load image: ' + src);
};
}
img.src = src;
return img;
},
resize: function(width) {
var width_total;
width_total = width + this.border.left + this.padding.left + this.padding.right + this.border.right;
this.modal_dialog.css('width', 'auto').css('max-width', width_total);
this.lightbox_container.find('a').css('padding-top', function() {
return $(this).parent().height() / 2;
});
return this;
},
checkDimensions: function(width) {
var body_width, width_total;
width_total = width + this.border.left + this.padding.left + this.padding.right + this.border.right;
body_width = document.body.clientWidth;
if (width_total > body_width) {
width = this.modal_body.width();
}
return width;
},
close: function() {
return this.modal.modal('hide');
},
addTrailingSlash: function(url) {
if (url.substr(-1) !== '/') {
url += '/';
}
return url;
}
};
$.fn.ekkoLightbox = function(options) {
return this.each(function() {
var $this;
$this = $(this);
options = $.extend({
remote: $this.attr('data-remote') || $this.attr('href'),
gallery_parent_selector: $this.attr('data-parent'),
type: $this.attr('data-type')
}, options, $this.data());
new EkkoLightbox(this, options);
return this;
});
};
$.fn.ekkoLightbox.defaults = {
gallery_parent_selector: '*:not(.row)',
left_arrow_class: '.glyphicon .glyphicon-chevron-left',
right_arrow_class: '.glyphicon .glyphicon-chevron-right',
directional_arrows: true,
type: null,
always_show_close: true,
onShow: function() {},
onShown: function() {},
onHide: function() {},
onHidden: function() {}
};
}).call(this);
|
vendor/ob/highcharts-bundle/Ob/HighchartsBundle/Resources/public/js/highcharts/highcharts.src.js | AmineBelhaj/GoldenCageWeb | // ==ClosureCompiler==
// @compilation_level SIMPLE_OPTIMIZATIONS
/**
* @license Highcharts JS v3.0.6 (2013-10-04)
*
* (c) 2009-2013 Torstein Hønsi
*
* License: www.highcharts.com/license
*/
// JSLint options:
/*global Highcharts, document, window, navigator, setInterval, clearInterval, clearTimeout, setTimeout, location, jQuery, $, console, each, grep */
(function () {
// encapsulated variables
var UNDEFINED,
doc = document,
win = window,
math = Math,
mathRound = math.round,
mathFloor = math.floor,
mathCeil = math.ceil,
mathMax = math.max,
mathMin = math.min,
mathAbs = math.abs,
mathCos = math.cos,
mathSin = math.sin,
mathPI = math.PI,
deg2rad = mathPI * 2 / 360,
// some variables
userAgent = navigator.userAgent,
isOpera = win.opera,
isIE = /msie/i.test(userAgent) && !isOpera,
docMode8 = doc.documentMode === 8,
isWebKit = /AppleWebKit/.test(userAgent),
isFirefox = /Firefox/.test(userAgent),
isTouchDevice = /(Mobile|Android|Windows Phone)/.test(userAgent),
SVG_NS = 'http://www.w3.org/2000/svg',
hasSVG = !!doc.createElementNS && !!doc.createElementNS(SVG_NS, 'svg').createSVGRect,
hasBidiBug = isFirefox && parseInt(userAgent.split('Firefox/')[1], 10) < 4, // issue #38
useCanVG = !hasSVG && !isIE && !!doc.createElement('canvas').getContext,
Renderer,
hasTouch = doc.documentElement.ontouchstart !== UNDEFINED,
symbolSizes = {},
idCounter = 0,
garbageBin,
defaultOptions,
dateFormat, // function
globalAnimation,
pathAnim,
timeUnits,
noop = function () {},
charts = [],
PRODUCT = 'Highcharts',
VERSION = '3.0.6',
// some constants for frequently used strings
DIV = 'div',
ABSOLUTE = 'absolute',
RELATIVE = 'relative',
HIDDEN = 'hidden',
PREFIX = 'highcharts-',
VISIBLE = 'visible',
PX = 'px',
NONE = 'none',
M = 'M',
L = 'L',
/*
* Empirical lowest possible opacities for TRACKER_FILL
* IE6: 0.002
* IE7: 0.002
* IE8: 0.002
* IE9: 0.00000000001 (unlimited)
* IE10: 0.0001 (exporting only)
* FF: 0.00000000001 (unlimited)
* Chrome: 0.000001
* Safari: 0.000001
* Opera: 0.00000000001 (unlimited)
*/
TRACKER_FILL = 'rgba(192,192,192,' + (hasSVG ? 0.0001 : 0.002) + ')', // invisible but clickable
//TRACKER_FILL = 'rgba(192,192,192,0.5)',
NORMAL_STATE = '',
HOVER_STATE = 'hover',
SELECT_STATE = 'select',
MILLISECOND = 'millisecond',
SECOND = 'second',
MINUTE = 'minute',
HOUR = 'hour',
DAY = 'day',
WEEK = 'week',
MONTH = 'month',
YEAR = 'year',
// constants for attributes
LINEAR_GRADIENT = 'linearGradient',
STOPS = 'stops',
STROKE_WIDTH = 'stroke-width',
// time methods, changed based on whether or not UTC is used
makeTime,
getMinutes,
getHours,
getDay,
getDate,
getMonth,
getFullYear,
setMinutes,
setHours,
setDate,
setMonth,
setFullYear,
// lookup over the types and the associated classes
seriesTypes = {};
// The Highcharts namespace
win.Highcharts = win.Highcharts ? error(16, true) : {};
/**
* Extend an object with the members of another
* @param {Object} a The object to be extended
* @param {Object} b The object to add to the first one
*/
function extend(a, b) {
var n;
if (!a) {
a = {};
}
for (n in b) {
a[n] = b[n];
}
return a;
}
/**
* Deep merge two or more objects and return a third object.
* Previously this function redirected to jQuery.extend(true), but this had two limitations.
* First, it deep merged arrays, which lead to workarounds in Highcharts. Second,
* it copied properties from extended prototypes.
*/
function merge() {
var i,
len = arguments.length,
ret = {},
doCopy = function (copy, original) {
var value, key;
// An object is replacing a primitive
if (typeof copy !== 'object') {
copy = {};
}
for (key in original) {
if (original.hasOwnProperty(key)) {
value = original[key];
// Copy the contents of objects, but not arrays or DOM nodes
if (value && typeof value === 'object' && Object.prototype.toString.call(value) !== '[object Array]'
&& typeof value.nodeType !== 'number') {
copy[key] = doCopy(copy[key] || {}, value);
// Primitives and arrays are copied over directly
} else {
copy[key] = original[key];
}
}
}
return copy;
};
// For each argument, extend the return
for (i = 0; i < len; i++) {
ret = doCopy(ret, arguments[i]);
}
return ret;
}
/**
* Take an array and turn into a hash with even number arguments as keys and odd numbers as
* values. Allows creating constants for commonly used style properties, attributes etc.
* Avoid it in performance critical situations like looping
*/
function hash() {
var i = 0,
args = arguments,
length = args.length,
obj = {};
for (; i < length; i++) {
obj[args[i++]] = args[i];
}
return obj;
}
/**
* Shortcut for parseInt
* @param {Object} s
* @param {Number} mag Magnitude
*/
function pInt(s, mag) {
return parseInt(s, mag || 10);
}
/**
* Check for string
* @param {Object} s
*/
function isString(s) {
return typeof s === 'string';
}
/**
* Check for object
* @param {Object} obj
*/
function isObject(obj) {
return typeof obj === 'object';
}
/**
* Check for array
* @param {Object} obj
*/
function isArray(obj) {
return Object.prototype.toString.call(obj) === '[object Array]';
}
/**
* Check for number
* @param {Object} n
*/
function isNumber(n) {
return typeof n === 'number';
}
function log2lin(num) {
return math.log(num) / math.LN10;
}
function lin2log(num) {
return math.pow(10, num);
}
/**
* Remove last occurence of an item from an array
* @param {Array} arr
* @param {Mixed} item
*/
function erase(arr, item) {
var i = arr.length;
while (i--) {
if (arr[i] === item) {
arr.splice(i, 1);
break;
}
}
//return arr;
}
/**
* Returns true if the object is not null or undefined. Like MooTools' $.defined.
* @param {Object} obj
*/
function defined(obj) {
return obj !== UNDEFINED && obj !== null;
}
/**
* Set or get an attribute or an object of attributes. Can't use jQuery attr because
* it attempts to set expando properties on the SVG element, which is not allowed.
*
* @param {Object} elem The DOM element to receive the attribute(s)
* @param {String|Object} prop The property or an abject of key-value pairs
* @param {String} value The value if a single property is set
*/
function attr(elem, prop, value) {
var key,
setAttribute = 'setAttribute',
ret;
// if the prop is a string
if (isString(prop)) {
// set the value
if (defined(value)) {
elem[setAttribute](prop, value);
// get the value
} else if (elem && elem.getAttribute) { // elem not defined when printing pie demo...
ret = elem.getAttribute(prop);
}
// else if prop is defined, it is a hash of key/value pairs
} else if (defined(prop) && isObject(prop)) {
for (key in prop) {
elem[setAttribute](key, prop[key]);
}
}
return ret;
}
/**
* Check if an element is an array, and if not, make it into an array. Like
* MooTools' $.splat.
*/
function splat(obj) {
return isArray(obj) ? obj : [obj];
}
/**
* Return the first value that is defined. Like MooTools' $.pick.
*/
function pick() {
var args = arguments,
i,
arg,
length = args.length;
for (i = 0; i < length; i++) {
arg = args[i];
if (typeof arg !== 'undefined' && arg !== null) {
return arg;
}
}
}
/**
* Set CSS on a given element
* @param {Object} el
* @param {Object} styles Style object with camel case property names
*/
function css(el, styles) {
if (isIE) {
if (styles && styles.opacity !== UNDEFINED) {
styles.filter = 'alpha(opacity=' + (styles.opacity * 100) + ')';
}
}
extend(el.style, styles);
}
/**
* Utility function to create element with attributes and styles
* @param {Object} tag
* @param {Object} attribs
* @param {Object} styles
* @param {Object} parent
* @param {Object} nopad
*/
function createElement(tag, attribs, styles, parent, nopad) {
var el = doc.createElement(tag);
if (attribs) {
extend(el, attribs);
}
if (nopad) {
css(el, {padding: 0, border: NONE, margin: 0});
}
if (styles) {
css(el, styles);
}
if (parent) {
parent.appendChild(el);
}
return el;
}
/**
* Extend a prototyped class by new members
* @param {Object} parent
* @param {Object} members
*/
function extendClass(parent, members) {
var object = function () {};
object.prototype = new parent();
extend(object.prototype, members);
return object;
}
/**
* Format a number and return a string based on input settings
* @param {Number} number The input number to format
* @param {Number} decimals The amount of decimals
* @param {String} decPoint The decimal point, defaults to the one given in the lang options
* @param {String} thousandsSep The thousands separator, defaults to the one given in the lang options
*/
function numberFormat(number, decimals, decPoint, thousandsSep) {
var lang = defaultOptions.lang,
// http://kevin.vanzonneveld.net/techblog/article/javascript_equivalent_for_phps_number_format/
n = +number || 0,
c = decimals === -1 ?
(n.toString().split('.')[1] || '').length : // preserve decimals
(isNaN(decimals = mathAbs(decimals)) ? 2 : decimals),
d = decPoint === undefined ? lang.decimalPoint : decPoint,
t = thousandsSep === undefined ? lang.thousandsSep : thousandsSep,
s = n < 0 ? "-" : "",
i = String(pInt(n = mathAbs(n).toFixed(c))),
j = i.length > 3 ? i.length % 3 : 0;
return s + (j ? i.substr(0, j) + t : "") + i.substr(j).replace(/(\d{3})(?=\d)/g, "$1" + t) +
(c ? d + mathAbs(n - i).toFixed(c).slice(2) : "");
}
/**
* Pad a string to a given length by adding 0 to the beginning
* @param {Number} number
* @param {Number} length
*/
function pad(number, length) {
// Create an array of the remaining length +1 and join it with 0's
return new Array((length || 2) + 1 - String(number).length).join(0) + number;
}
/**
* Wrap a method with extended functionality, preserving the original function
* @param {Object} obj The context object that the method belongs to
* @param {String} method The name of the method to extend
* @param {Function} func A wrapper function callback. This function is called with the same arguments
* as the original function, except that the original function is unshifted and passed as the first
* argument.
*/
function wrap(obj, method, func) {
var proceed = obj[method];
obj[method] = function () {
var args = Array.prototype.slice.call(arguments);
args.unshift(proceed);
return func.apply(this, args);
};
}
/**
* Based on http://www.php.net/manual/en/function.strftime.php
* @param {String} format
* @param {Number} timestamp
* @param {Boolean} capitalize
*/
dateFormat = function (format, timestamp, capitalize) {
if (!defined(timestamp) || isNaN(timestamp)) {
return 'Invalid date';
}
format = pick(format, '%Y-%m-%d %H:%M:%S');
var date = new Date(timestamp),
key, // used in for constuct below
// get the basic time values
hours = date[getHours](),
day = date[getDay](),
dayOfMonth = date[getDate](),
month = date[getMonth](),
fullYear = date[getFullYear](),
lang = defaultOptions.lang,
langWeekdays = lang.weekdays,
// List all format keys. Custom formats can be added from the outside.
replacements = extend({
// Day
'a': langWeekdays[day].substr(0, 3), // Short weekday, like 'Mon'
'A': langWeekdays[day], // Long weekday, like 'Monday'
'd': pad(dayOfMonth), // Two digit day of the month, 01 to 31
'e': dayOfMonth, // Day of the month, 1 through 31
// Week (none implemented)
//'W': weekNumber(),
// Month
'b': lang.shortMonths[month], // Short month, like 'Jan'
'B': lang.months[month], // Long month, like 'January'
'm': pad(month + 1), // Two digit month number, 01 through 12
// Year
'y': fullYear.toString().substr(2, 2), // Two digits year, like 09 for 2009
'Y': fullYear, // Four digits year, like 2009
// Time
'H': pad(hours), // Two digits hours in 24h format, 00 through 23
'I': pad((hours % 12) || 12), // Two digits hours in 12h format, 00 through 11
'l': (hours % 12) || 12, // Hours in 12h format, 1 through 12
'M': pad(date[getMinutes]()), // Two digits minutes, 00 through 59
'p': hours < 12 ? 'AM' : 'PM', // Upper case AM or PM
'P': hours < 12 ? 'am' : 'pm', // Lower case AM or PM
'S': pad(date.getSeconds()), // Two digits seconds, 00 through 59
'L': pad(mathRound(timestamp % 1000), 3) // Milliseconds (naming from Ruby)
}, Highcharts.dateFormats);
// do the replaces
for (key in replacements) {
while (format.indexOf('%' + key) !== -1) { // regex would do it in one line, but this is faster
format = format.replace('%' + key, typeof replacements[key] === 'function' ? replacements[key](timestamp) : replacements[key]);
}
}
// Optionally capitalize the string and return
return capitalize ? format.substr(0, 1).toUpperCase() + format.substr(1) : format;
};
/**
* Format a single variable. Similar to sprintf, without the % prefix.
*/
function formatSingle(format, val) {
var floatRegex = /f$/,
decRegex = /\.([0-9])/,
lang = defaultOptions.lang,
decimals;
if (floatRegex.test(format)) { // float
decimals = format.match(decRegex);
decimals = decimals ? decimals[1] : -1;
val = numberFormat(
val,
decimals,
lang.decimalPoint,
format.indexOf(',') > -1 ? lang.thousandsSep : ''
);
} else {
val = dateFormat(format, val);
}
return val;
}
/**
* Format a string according to a subset of the rules of Python's String.format method.
*/
function format(str, ctx) {
var splitter = '{',
isInside = false,
segment,
valueAndFormat,
path,
i,
len,
ret = [],
val,
index;
while ((index = str.indexOf(splitter)) !== -1) {
segment = str.slice(0, index);
if (isInside) { // we're on the closing bracket looking back
valueAndFormat = segment.split(':');
path = valueAndFormat.shift().split('.'); // get first and leave format
len = path.length;
val = ctx;
// Assign deeper paths
for (i = 0; i < len; i++) {
val = val[path[i]];
}
// Format the replacement
if (valueAndFormat.length) {
val = formatSingle(valueAndFormat.join(':'), val);
}
// Push the result and advance the cursor
ret.push(val);
} else {
ret.push(segment);
}
str = str.slice(index + 1); // the rest
isInside = !isInside; // toggle
splitter = isInside ? '}' : '{'; // now look for next matching bracket
}
ret.push(str);
return ret.join('');
}
/**
* Get the magnitude of a number
*/
function getMagnitude(num) {
return math.pow(10, mathFloor(math.log(num) / math.LN10));
}
/**
* Take an interval and normalize it to multiples of 1, 2, 2.5 and 5
* @param {Number} interval
* @param {Array} multiples
* @param {Number} magnitude
* @param {Object} options
*/
function normalizeTickInterval(interval, multiples, magnitude, options) {
var normalized, i;
// round to a tenfold of 1, 2, 2.5 or 5
magnitude = pick(magnitude, 1);
normalized = interval / magnitude;
// multiples for a linear scale
if (!multiples) {
multiples = [1, 2, 2.5, 5, 10];
// the allowDecimals option
if (options && options.allowDecimals === false) {
if (magnitude === 1) {
multiples = [1, 2, 5, 10];
} else if (magnitude <= 0.1) {
multiples = [1 / magnitude];
}
}
}
// normalize the interval to the nearest multiple
for (i = 0; i < multiples.length; i++) {
interval = multiples[i];
if (normalized <= (multiples[i] + (multiples[i + 1] || multiples[i])) / 2) {
break;
}
}
// multiply back to the correct magnitude
interval *= magnitude;
return interval;
}
/**
* Get a normalized tick interval for dates. Returns a configuration object with
* unit range (interval), count and name. Used to prepare data for getTimeTicks.
* Previously this logic was part of getTimeTicks, but as getTimeTicks now runs
* of segments in stock charts, the normalizing logic was extracted in order to
* prevent it for running over again for each segment having the same interval.
* #662, #697.
*/
function normalizeTimeTickInterval(tickInterval, unitsOption) {
var units = unitsOption || [[
MILLISECOND, // unit name
[1, 2, 5, 10, 20, 25, 50, 100, 200, 500] // allowed multiples
], [
SECOND,
[1, 2, 5, 10, 15, 30]
], [
MINUTE,
[1, 2, 5, 10, 15, 30]
], [
HOUR,
[1, 2, 3, 4, 6, 8, 12]
], [
DAY,
[1, 2]
], [
WEEK,
[1, 2]
], [
MONTH,
[1, 2, 3, 4, 6]
], [
YEAR,
null
]],
unit = units[units.length - 1], // default unit is years
interval = timeUnits[unit[0]],
multiples = unit[1],
count,
i;
// loop through the units to find the one that best fits the tickInterval
for (i = 0; i < units.length; i++) {
unit = units[i];
interval = timeUnits[unit[0]];
multiples = unit[1];
if (units[i + 1]) {
// lessThan is in the middle between the highest multiple and the next unit.
var lessThan = (interval * multiples[multiples.length - 1] +
timeUnits[units[i + 1][0]]) / 2;
// break and keep the current unit
if (tickInterval <= lessThan) {
break;
}
}
}
// prevent 2.5 years intervals, though 25, 250 etc. are allowed
if (interval === timeUnits[YEAR] && tickInterval < 5 * interval) {
multiples = [1, 2, 5];
}
// get the count
count = normalizeTickInterval(
tickInterval / interval,
multiples,
unit[0] === YEAR ? getMagnitude(tickInterval / interval) : 1 // #1913
);
return {
unitRange: interval,
count: count,
unitName: unit[0]
};
}
/**
* Set the tick positions to a time unit that makes sense, for example
* on the first of each month or on every Monday. Return an array
* with the time positions. Used in datetime axes as well as for grouping
* data on a datetime axis.
*
* @param {Object} normalizedInterval The interval in axis values (ms) and the count
* @param {Number} min The minimum in axis values
* @param {Number} max The maximum in axis values
* @param {Number} startOfWeek
*/
function getTimeTicks(normalizedInterval, min, max, startOfWeek) {
var tickPositions = [],
i,
higherRanks = {},
useUTC = defaultOptions.global.useUTC,
minYear, // used in months and years as a basis for Date.UTC()
minDate = new Date(min),
interval = normalizedInterval.unitRange,
count = normalizedInterval.count;
if (defined(min)) { // #1300
if (interval >= timeUnits[SECOND]) { // second
minDate.setMilliseconds(0);
minDate.setSeconds(interval >= timeUnits[MINUTE] ? 0 :
count * mathFloor(minDate.getSeconds() / count));
}
if (interval >= timeUnits[MINUTE]) { // minute
minDate[setMinutes](interval >= timeUnits[HOUR] ? 0 :
count * mathFloor(minDate[getMinutes]() / count));
}
if (interval >= timeUnits[HOUR]) { // hour
minDate[setHours](interval >= timeUnits[DAY] ? 0 :
count * mathFloor(minDate[getHours]() / count));
}
if (interval >= timeUnits[DAY]) { // day
minDate[setDate](interval >= timeUnits[MONTH] ? 1 :
count * mathFloor(minDate[getDate]() / count));
}
if (interval >= timeUnits[MONTH]) { // month
minDate[setMonth](interval >= timeUnits[YEAR] ? 0 :
count * mathFloor(minDate[getMonth]() / count));
minYear = minDate[getFullYear]();
}
if (interval >= timeUnits[YEAR]) { // year
minYear -= minYear % count;
minDate[setFullYear](minYear);
}
// week is a special case that runs outside the hierarchy
if (interval === timeUnits[WEEK]) {
// get start of current week, independent of count
minDate[setDate](minDate[getDate]() - minDate[getDay]() +
pick(startOfWeek, 1));
}
// get tick positions
i = 1;
minYear = minDate[getFullYear]();
var time = minDate.getTime(),
minMonth = minDate[getMonth](),
minDateDate = minDate[getDate](),
timezoneOffset = useUTC ?
0 :
(24 * 3600 * 1000 + minDate.getTimezoneOffset() * 60 * 1000) % (24 * 3600 * 1000); // #950
// iterate and add tick positions at appropriate values
while (time < max) {
tickPositions.push(time);
// if the interval is years, use Date.UTC to increase years
if (interval === timeUnits[YEAR]) {
time = makeTime(minYear + i * count, 0);
// if the interval is months, use Date.UTC to increase months
} else if (interval === timeUnits[MONTH]) {
time = makeTime(minYear, minMonth + i * count);
// if we're using global time, the interval is not fixed as it jumps
// one hour at the DST crossover
} else if (!useUTC && (interval === timeUnits[DAY] || interval === timeUnits[WEEK])) {
time = makeTime(minYear, minMonth, minDateDate +
i * count * (interval === timeUnits[DAY] ? 1 : 7));
// else, the interval is fixed and we use simple addition
} else {
time += interval * count;
}
i++;
}
// push the last time
tickPositions.push(time);
// mark new days if the time is dividible by day (#1649, #1760)
each(grep(tickPositions, function (time) {
return interval <= timeUnits[HOUR] && time % timeUnits[DAY] === timezoneOffset;
}), function (time) {
higherRanks[time] = DAY;
});
}
// record information on the chosen unit - for dynamic label formatter
tickPositions.info = extend(normalizedInterval, {
higherRanks: higherRanks,
totalRange: interval * count
});
return tickPositions;
}
/**
* Helper class that contains variuos counters that are local to the chart.
*/
function ChartCounters() {
this.color = 0;
this.symbol = 0;
}
ChartCounters.prototype = {
/**
* Wraps the color counter if it reaches the specified length.
*/
wrapColor: function (length) {
if (this.color >= length) {
this.color = 0;
}
},
/**
* Wraps the symbol counter if it reaches the specified length.
*/
wrapSymbol: function (length) {
if (this.symbol >= length) {
this.symbol = 0;
}
}
};
/**
* Utility method that sorts an object array and keeping the order of equal items.
* ECMA script standard does not specify the behaviour when items are equal.
*/
function stableSort(arr, sortFunction) {
var length = arr.length,
sortValue,
i;
// Add index to each item
for (i = 0; i < length; i++) {
arr[i].ss_i = i; // stable sort index
}
arr.sort(function (a, b) {
sortValue = sortFunction(a, b);
return sortValue === 0 ? a.ss_i - b.ss_i : sortValue;
});
// Remove index from items
for (i = 0; i < length; i++) {
delete arr[i].ss_i; // stable sort index
}
}
/**
* Non-recursive method to find the lowest member of an array. Math.min raises a maximum
* call stack size exceeded error in Chrome when trying to apply more than 150.000 points. This
* method is slightly slower, but safe.
*/
function arrayMin(data) {
var i = data.length,
min = data[0];
while (i--) {
if (data[i] < min) {
min = data[i];
}
}
return min;
}
/**
* Non-recursive method to find the lowest member of an array. Math.min raises a maximum
* call stack size exceeded error in Chrome when trying to apply more than 150.000 points. This
* method is slightly slower, but safe.
*/
function arrayMax(data) {
var i = data.length,
max = data[0];
while (i--) {
if (data[i] > max) {
max = data[i];
}
}
return max;
}
/**
* Utility method that destroys any SVGElement or VMLElement that are properties on the given object.
* It loops all properties and invokes destroy if there is a destroy method. The property is
* then delete'ed.
* @param {Object} The object to destroy properties on
* @param {Object} Exception, do not destroy this property, only delete it.
*/
function destroyObjectProperties(obj, except) {
var n;
for (n in obj) {
// If the object is non-null and destroy is defined
if (obj[n] && obj[n] !== except && obj[n].destroy) {
// Invoke the destroy
obj[n].destroy();
}
// Delete the property from the object.
delete obj[n];
}
}
/**
* Discard an element by moving it to the bin and delete
* @param {Object} The HTML node to discard
*/
function discardElement(element) {
// create a garbage bin element, not part of the DOM
if (!garbageBin) {
garbageBin = createElement(DIV);
}
// move the node and empty bin
if (element) {
garbageBin.appendChild(element);
}
garbageBin.innerHTML = '';
}
/**
* Provide error messages for debugging, with links to online explanation
*/
function error(code, stop) {
var msg = 'Highcharts error #' + code + ': www.highcharts.com/errors/' + code;
if (stop) {
throw msg;
} else if (win.console) {
console.log(msg);
}
}
/**
* Fix JS round off float errors
* @param {Number} num
*/
function correctFloat(num) {
return parseFloat(
num.toPrecision(14)
);
}
/**
* Set the global animation to either a given value, or fall back to the
* given chart's animation option
* @param {Object} animation
* @param {Object} chart
*/
function setAnimation(animation, chart) {
globalAnimation = pick(animation, chart.animation);
}
/**
* The time unit lookup
*/
/*jslint white: true*/
timeUnits = hash(
MILLISECOND, 1,
SECOND, 1000,
MINUTE, 60000,
HOUR, 3600000,
DAY, 24 * 3600000,
WEEK, 7 * 24 * 3600000,
MONTH, 31 * 24 * 3600000,
YEAR, 31556952000
);
/*jslint white: false*/
/**
* Path interpolation algorithm used across adapters
*/
pathAnim = {
/**
* Prepare start and end values so that the path can be animated one to one
*/
init: function (elem, fromD, toD) {
fromD = fromD || '';
var shift = elem.shift,
bezier = fromD.indexOf('C') > -1,
numParams = bezier ? 7 : 3,
endLength,
slice,
i,
start = fromD.split(' '),
end = [].concat(toD), // copy
startBaseLine,
endBaseLine,
sixify = function (arr) { // in splines make move points have six parameters like bezier curves
i = arr.length;
while (i--) {
if (arr[i] === M) {
arr.splice(i + 1, 0, arr[i + 1], arr[i + 2], arr[i + 1], arr[i + 2]);
}
}
};
if (bezier) {
sixify(start);
sixify(end);
}
// pull out the base lines before padding
if (elem.isArea) {
startBaseLine = start.splice(start.length - 6, 6);
endBaseLine = end.splice(end.length - 6, 6);
}
// if shifting points, prepend a dummy point to the end path
if (shift <= end.length / numParams && start.length === end.length) {
while (shift--) {
end = [].concat(end).splice(0, numParams).concat(end);
}
}
elem.shift = 0; // reset for following animations
// copy and append last point until the length matches the end length
if (start.length) {
endLength = end.length;
while (start.length < endLength) {
//bezier && sixify(start);
slice = [].concat(start).splice(start.length - numParams, numParams);
if (bezier) { // disable first control point
slice[numParams - 6] = slice[numParams - 2];
slice[numParams - 5] = slice[numParams - 1];
}
start = start.concat(slice);
}
}
if (startBaseLine) { // append the base lines for areas
start = start.concat(startBaseLine);
end = end.concat(endBaseLine);
}
return [start, end];
},
/**
* Interpolate each value of the path and return the array
*/
step: function (start, end, pos, complete) {
var ret = [],
i = start.length,
startVal;
if (pos === 1) { // land on the final path without adjustment points appended in the ends
ret = complete;
} else if (i === end.length && pos < 1) {
while (i--) {
startVal = parseFloat(start[i]);
ret[i] =
isNaN(startVal) ? // a letter instruction like M or L
start[i] :
pos * (parseFloat(end[i] - startVal)) + startVal;
}
} else { // if animation is finished or length not matching, land on right value
ret = end;
}
return ret;
}
};
(function ($) {
/**
* The default HighchartsAdapter for jQuery
*/
win.HighchartsAdapter = win.HighchartsAdapter || ($ && {
/**
* Initialize the adapter by applying some extensions to jQuery
*/
init: function (pathAnim) {
// extend the animate function to allow SVG animations
var Fx = $.fx,
Step = Fx.step,
dSetter,
Tween = $.Tween,
propHooks = Tween && Tween.propHooks,
opacityHook = $.cssHooks.opacity;
/*jslint unparam: true*//* allow unused param x in this function */
$.extend($.easing, {
easeOutQuad: function (x, t, b, c, d) {
return -c * (t /= d) * (t - 2) + b;
}
});
/*jslint unparam: false*/
// extend some methods to check for elem.attr, which means it is a Highcharts SVG object
$.each(['cur', '_default', 'width', 'height', 'opacity'], function (i, fn) {
var obj = Step,
base,
elem;
// Handle different parent objects
if (fn === 'cur') {
obj = Fx.prototype; // 'cur', the getter, relates to Fx.prototype
} else if (fn === '_default' && Tween) { // jQuery 1.8 model
obj = propHooks[fn];
fn = 'set';
}
// Overwrite the method
base = obj[fn];
if (base) { // step.width and step.height don't exist in jQuery < 1.7
// create the extended function replacement
obj[fn] = function (fx) {
// Fx.prototype.cur does not use fx argument
fx = i ? fx : this;
// Don't run animations on textual properties like align (#1821)
if (fx.prop === 'align') {
return;
}
// shortcut
elem = fx.elem;
// Fx.prototype.cur returns the current value. The other ones are setters
// and returning a value has no effect.
return elem.attr ? // is SVG element wrapper
elem.attr(fx.prop, fn === 'cur' ? UNDEFINED : fx.now) : // apply the SVG wrapper's method
base.apply(this, arguments); // use jQuery's built-in method
};
}
});
// Extend the opacity getter, needed for fading opacity with IE9 and jQuery 1.10+
wrap(opacityHook, 'get', function (proceed, elem, computed) {
return elem.attr ? (elem.opacity || 0) : proceed.call(this, elem, computed);
});
// Define the setter function for d (path definitions)
dSetter = function (fx) {
var elem = fx.elem,
ends;
// Normally start and end should be set in state == 0, but sometimes,
// for reasons unknown, this doesn't happen. Perhaps state == 0 is skipped
// in these cases
if (!fx.started) {
ends = pathAnim.init(elem, elem.d, elem.toD);
fx.start = ends[0];
fx.end = ends[1];
fx.started = true;
}
// interpolate each value of the path
elem.attr('d', pathAnim.step(fx.start, fx.end, fx.pos, elem.toD));
};
// jQuery 1.8 style
if (Tween) {
propHooks.d = {
set: dSetter
};
// pre 1.8
} else {
// animate paths
Step.d = dSetter;
}
/**
* Utility for iterating over an array. Parameters are reversed compared to jQuery.
* @param {Array} arr
* @param {Function} fn
*/
this.each = Array.prototype.forEach ?
function (arr, fn) { // modern browsers
return Array.prototype.forEach.call(arr, fn);
} :
function (arr, fn) { // legacy
var i = 0,
len = arr.length;
for (; i < len; i++) {
if (fn.call(arr[i], arr[i], i, arr) === false) {
return i;
}
}
};
/**
* Register Highcharts as a plugin in the respective framework
*/
$.fn.highcharts = function () {
var constr = 'Chart', // default constructor
args = arguments,
options,
ret,
chart;
if (isString(args[0])) {
constr = args[0];
args = Array.prototype.slice.call(args, 1);
}
options = args[0];
// Create the chart
if (options !== UNDEFINED) {
/*jslint unused:false*/
options.chart = options.chart || {};
options.chart.renderTo = this[0];
chart = new Highcharts[constr](options, args[1]);
ret = this;
/*jslint unused:true*/
}
// When called without parameters or with the return argument, get a predefined chart
if (options === UNDEFINED) {
ret = charts[attr(this[0], 'data-highcharts-chart')];
}
return ret;
};
},
/**
* Downloads a script and executes a callback when done.
* @param {String} scriptLocation
* @param {Function} callback
*/
getScript: $.getScript,
/**
* Return the index of an item in an array, or -1 if not found
*/
inArray: $.inArray,
/**
* A direct link to jQuery methods. MooTools and Prototype adapters must be implemented for each case of method.
* @param {Object} elem The HTML element
* @param {String} method Which method to run on the wrapped element
*/
adapterRun: function (elem, method) {
return $(elem)[method]();
},
/**
* Filter an array
*/
grep: $.grep,
/**
* Map an array
* @param {Array} arr
* @param {Function} fn
*/
map: function (arr, fn) {
//return jQuery.map(arr, fn);
var results = [],
i = 0,
len = arr.length;
for (; i < len; i++) {
results[i] = fn.call(arr[i], arr[i], i, arr);
}
return results;
},
/**
* Get the position of an element relative to the top left of the page
*/
offset: function (el) {
return $(el).offset();
},
/**
* Add an event listener
* @param {Object} el A HTML element or custom object
* @param {String} event The event type
* @param {Function} fn The event handler
*/
addEvent: function (el, event, fn) {
$(el).bind(event, fn);
},
/**
* Remove event added with addEvent
* @param {Object} el The object
* @param {String} eventType The event type. Leave blank to remove all events.
* @param {Function} handler The function to remove
*/
removeEvent: function (el, eventType, handler) {
// workaround for jQuery issue with unbinding custom events:
// http://forum.jQuery.com/topic/javascript-error-when-unbinding-a-custom-event-using-jQuery-1-4-2
var func = doc.removeEventListener ? 'removeEventListener' : 'detachEvent';
if (doc[func] && el && !el[func]) {
el[func] = function () {};
}
$(el).unbind(eventType, handler);
},
/**
* Fire an event on a custom object
* @param {Object} el
* @param {String} type
* @param {Object} eventArguments
* @param {Function} defaultFunction
*/
fireEvent: function (el, type, eventArguments, defaultFunction) {
var event = $.Event(type),
detachedType = 'detached' + type,
defaultPrevented;
// Remove warnings in Chrome when accessing layerX and layerY. Although Highcharts
// never uses these properties, Chrome includes them in the default click event and
// raises the warning when they are copied over in the extend statement below.
//
// To avoid problems in IE (see #1010) where we cannot delete the properties and avoid
// testing if they are there (warning in chrome) the only option is to test if running IE.
if (!isIE && eventArguments) {
delete eventArguments.layerX;
delete eventArguments.layerY;
}
extend(event, eventArguments);
// Prevent jQuery from triggering the object method that is named the
// same as the event. For example, if the event is 'select', jQuery
// attempts calling el.select and it goes into a loop.
if (el[type]) {
el[detachedType] = el[type];
el[type] = null;
}
// Wrap preventDefault and stopPropagation in try/catch blocks in
// order to prevent JS errors when cancelling events on non-DOM
// objects. #615.
/*jslint unparam: true*/
$.each(['preventDefault', 'stopPropagation'], function (i, fn) {
var base = event[fn];
event[fn] = function () {
try {
base.call(event);
} catch (e) {
if (fn === 'preventDefault') {
defaultPrevented = true;
}
}
};
});
/*jslint unparam: false*/
// trigger it
$(el).trigger(event);
// attach the method
if (el[detachedType]) {
el[type] = el[detachedType];
el[detachedType] = null;
}
if (defaultFunction && !event.isDefaultPrevented() && !defaultPrevented) {
defaultFunction(event);
}
},
/**
* Extension method needed for MooTools
*/
washMouseEvent: function (e) {
var ret = e.originalEvent || e;
// computed by jQuery, needed by IE8
if (ret.pageX === UNDEFINED) { // #1236
ret.pageX = e.pageX;
ret.pageY = e.pageY;
}
return ret;
},
/**
* Animate a HTML element or SVG element wrapper
* @param {Object} el
* @param {Object} params
* @param {Object} options jQuery-like animation options: duration, easing, callback
*/
animate: function (el, params, options) {
var $el = $(el);
if (!el.style) {
el.style = {}; // #1881
}
if (params.d) {
el.toD = params.d; // keep the array form for paths, used in $.fx.step.d
params.d = 1; // because in jQuery, animating to an array has a different meaning
}
$el.stop();
if (params.opacity !== UNDEFINED && el.attr) {
params.opacity += 'px'; // force jQuery to use same logic as width and height (#2161)
}
$el.animate(params, options);
},
/**
* Stop running animation
*/
stop: function (el) {
$(el).stop();
}
});
}(win.jQuery));
// check for a custom HighchartsAdapter defined prior to this file
var globalAdapter = win.HighchartsAdapter,
adapter = globalAdapter || {};
// Initialize the adapter
if (globalAdapter) {
globalAdapter.init.call(globalAdapter, pathAnim);
}
// Utility functions. If the HighchartsAdapter is not defined, adapter is an empty object
// and all the utility functions will be null. In that case they are populated by the
// default adapters below.
var adapterRun = adapter.adapterRun,
getScript = adapter.getScript,
inArray = adapter.inArray,
each = adapter.each,
grep = adapter.grep,
offset = adapter.offset,
map = adapter.map,
addEvent = adapter.addEvent,
removeEvent = adapter.removeEvent,
fireEvent = adapter.fireEvent,
washMouseEvent = adapter.washMouseEvent,
animate = adapter.animate,
stop = adapter.stop;
/* ****************************************************************************
* Handle the options *
*****************************************************************************/
var
defaultLabelOptions = {
enabled: true,
// rotation: 0,
// align: 'center',
x: 0,
y: 15,
/*formatter: function () {
return this.value;
},*/
style: {
color: '#666',
cursor: 'default',
fontSize: '11px',
lineHeight: '14px'
}
};
defaultOptions = {
colors: ['#2f7ed8', '#0d233a', '#8bbc21', '#910000', '#1aadce', '#492970',
'#f28f43', '#77a1e5', '#c42525', '#a6c96a'],
symbols: ['circle', 'diamond', 'square', 'triangle', 'triangle-down'],
lang: {
loading: 'Loading...',
months: ['January', 'February', 'March', 'April', 'May', 'June', 'July',
'August', 'September', 'October', 'November', 'December'],
shortMonths: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'],
weekdays: ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'],
decimalPoint: '.',
numericSymbols: ['k', 'M', 'G', 'T', 'P', 'E'], // SI prefixes used in axis labels
resetZoom: 'Reset zoom',
resetZoomTitle: 'Reset zoom level 1:1',
thousandsSep: ','
},
global: {
useUTC: true,
canvasToolsURL: 'http://code.highcharts.com/3.0.6/modules/canvas-tools.js',
VMLRadialGradientURL: 'http://code.highcharts.com/3.0.6/gfx/vml-radial-gradient.png'
},
chart: {
//animation: true,
//alignTicks: false,
//reflow: true,
//className: null,
//events: { load, selection },
//margin: [null],
//marginTop: null,
//marginRight: null,
//marginBottom: null,
//marginLeft: null,
borderColor: '#4572A7',
//borderWidth: 0,
borderRadius: 5,
defaultSeriesType: 'line',
ignoreHiddenSeries: true,
//inverted: false,
//shadow: false,
spacing: [10, 10, 15, 10],
//spacingTop: 10,
//spacingRight: 10,
//spacingBottom: 15,
//spacingLeft: 10,
style: {
fontFamily: '"Lucida Grande", "Lucida Sans Unicode", Verdana, Arial, Helvetica, sans-serif', // default font
fontSize: '12px'
},
backgroundColor: '#FFFFFF',
//plotBackgroundColor: null,
plotBorderColor: '#C0C0C0',
//plotBorderWidth: 0,
//plotShadow: false,
//zoomType: ''
resetZoomButton: {
theme: {
zIndex: 20
},
position: {
align: 'right',
x: -10,
//verticalAlign: 'top',
y: 10
}
// relativeTo: 'plot'
}
},
title: {
text: 'Chart title',
align: 'center',
// floating: false,
margin: 15,
// x: 0,
// verticalAlign: 'top',
// y: null,
style: {
color: '#274b6d',//#3E576F',
fontSize: '16px'
}
},
subtitle: {
text: '',
align: 'center',
// floating: false
// x: 0,
// verticalAlign: 'top',
// y: null,
style: {
color: '#4d759e'
}
},
plotOptions: {
line: { // base series options
allowPointSelect: false,
showCheckbox: false,
animation: {
duration: 1000
},
//connectNulls: false,
//cursor: 'default',
//clip: true,
//dashStyle: null,
//enableMouseTracking: true,
events: {},
//legendIndex: 0,
lineWidth: 2,
//shadow: false,
// stacking: null,
marker: {
enabled: true,
//symbol: null,
lineWidth: 0,
radius: 4,
lineColor: '#FFFFFF',
//fillColor: null,
states: { // states for a single point
hover: {
enabled: true
//radius: base + 2
},
select: {
fillColor: '#FFFFFF',
lineColor: '#000000',
lineWidth: 2
}
}
},
point: {
events: {}
},
dataLabels: merge(defaultLabelOptions, {
align: 'center',
enabled: false,
formatter: function () {
return this.y === null ? '' : numberFormat(this.y, -1);
},
verticalAlign: 'bottom', // above singular point
y: 0
// backgroundColor: undefined,
// borderColor: undefined,
// borderRadius: undefined,
// borderWidth: undefined,
// padding: 3,
// shadow: false
}),
cropThreshold: 300, // draw points outside the plot area when the number of points is less than this
pointRange: 0,
//pointStart: 0,
//pointInterval: 1,
showInLegend: true,
states: { // states for the entire series
hover: {
//enabled: false,
//lineWidth: base + 1,
marker: {
// lineWidth: base + 1,
// radius: base + 1
}
},
select: {
marker: {}
}
},
stickyTracking: true
//tooltip: {
//pointFormat: '<span style="color:{series.color}">{series.name}</span>: <b>{point.y}</b>'
//valueDecimals: null,
//xDateFormat: '%A, %b %e, %Y',
//valuePrefix: '',
//ySuffix: ''
//}
// turboThreshold: 1000
// zIndex: null
}
},
labels: {
//items: [],
style: {
//font: defaultFont,
position: ABSOLUTE,
color: '#3E576F'
}
},
legend: {
enabled: true,
align: 'center',
//floating: false,
layout: 'horizontal',
labelFormatter: function () {
return this.name;
},
borderWidth: 1,
borderColor: '#909090',
borderRadius: 5,
navigation: {
// animation: true,
activeColor: '#274b6d',
// arrowSize: 12
inactiveColor: '#CCC'
// style: {} // text styles
},
// margin: 10,
// reversed: false,
shadow: false,
// backgroundColor: null,
/*style: {
padding: '5px'
},*/
itemStyle: {
cursor: 'pointer',
color: '#274b6d',
fontSize: '12px'
},
itemHoverStyle: {
//cursor: 'pointer', removed as of #601
color: '#000'
},
itemHiddenStyle: {
color: '#CCC'
},
itemCheckboxStyle: {
position: ABSOLUTE,
width: '13px', // for IE precision
height: '13px'
},
// itemWidth: undefined,
symbolWidth: 16,
symbolPadding: 5,
verticalAlign: 'bottom',
// width: undefined,
x: 0,
y: 0,
title: {
//text: null,
style: {
fontWeight: 'bold'
}
}
},
loading: {
// hideDuration: 100,
labelStyle: {
fontWeight: 'bold',
position: RELATIVE,
top: '1em'
},
// showDuration: 0,
style: {
position: ABSOLUTE,
backgroundColor: 'white',
opacity: 0.5,
textAlign: 'center'
}
},
tooltip: {
enabled: true,
animation: hasSVG,
//crosshairs: null,
backgroundColor: 'rgba(255, 255, 255, .85)',
borderWidth: 1,
borderRadius: 3,
dateTimeLabelFormats: {
millisecond: '%A, %b %e, %H:%M:%S.%L',
second: '%A, %b %e, %H:%M:%S',
minute: '%A, %b %e, %H:%M',
hour: '%A, %b %e, %H:%M',
day: '%A, %b %e, %Y',
week: 'Week from %A, %b %e, %Y',
month: '%B %Y',
year: '%Y'
},
//formatter: defaultFormatter,
headerFormat: '<span style="font-size: 10px">{point.key}</span><br/>',
pointFormat: '<span style="color:{series.color}">{series.name}</span>: <b>{point.y}</b><br/>',
shadow: true,
//shared: false,
snap: isTouchDevice ? 25 : 10,
style: {
color: '#333333',
cursor: 'default',
fontSize: '12px',
padding: '8px',
whiteSpace: 'nowrap'
}
//xDateFormat: '%A, %b %e, %Y',
//valueDecimals: null,
//valuePrefix: '',
//valueSuffix: ''
},
credits: {
enabled: true,
text: 'Highcharts.com',
href: 'http://www.highcharts.com',
position: {
align: 'right',
x: -10,
verticalAlign: 'bottom',
y: -5
},
style: {
cursor: 'pointer',
color: '#909090',
fontSize: '9px'
}
}
};
// Series defaults
var defaultPlotOptions = defaultOptions.plotOptions,
defaultSeriesOptions = defaultPlotOptions.line;
// set the default time methods
setTimeMethods();
/**
* Set the time methods globally based on the useUTC option. Time method can be either
* local time or UTC (default).
*/
function setTimeMethods() {
var useUTC = defaultOptions.global.useUTC,
GET = useUTC ? 'getUTC' : 'get',
SET = useUTC ? 'setUTC' : 'set';
makeTime = useUTC ? Date.UTC : function (year, month, date, hours, minutes, seconds) {
return new Date(
year,
month,
pick(date, 1),
pick(hours, 0),
pick(minutes, 0),
pick(seconds, 0)
).getTime();
};
getMinutes = GET + 'Minutes';
getHours = GET + 'Hours';
getDay = GET + 'Day';
getDate = GET + 'Date';
getMonth = GET + 'Month';
getFullYear = GET + 'FullYear';
setMinutes = SET + 'Minutes';
setHours = SET + 'Hours';
setDate = SET + 'Date';
setMonth = SET + 'Month';
setFullYear = SET + 'FullYear';
}
/**
* Merge the default options with custom options and return the new options structure
* @param {Object} options The new custom options
*/
function setOptions(options) {
// Pull out axis options and apply them to the respective default axis options
/*defaultXAxisOptions = merge(defaultXAxisOptions, options.xAxis);
defaultYAxisOptions = merge(defaultYAxisOptions, options.yAxis);
options.xAxis = options.yAxis = UNDEFINED;*/
// Merge in the default options
defaultOptions = merge(defaultOptions, options);
// Apply UTC
setTimeMethods();
return defaultOptions;
}
/**
* Get the updated default options. Merely exposing defaultOptions for outside modules
* isn't enough because the setOptions method creates a new object.
*/
function getOptions() {
return defaultOptions;
}
/**
* Handle color operations. The object methods are chainable.
* @param {String} input The input color in either rbga or hex format
*/
var Color = function (input) {
// declare variables
var rgba = [], result, stops;
/**
* Parse the input color to rgba array
* @param {String} input
*/
function init(input) {
// Gradients
if (input && input.stops) {
stops = map(input.stops, function (stop) {
return Color(stop[1]);
});
// Solid colors
} else {
// rgba
result = /rgba\(\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*,\s*([0-9]?(?:\.[0-9]+)?)\s*\)/.exec(input);
if (result) {
rgba = [pInt(result[1]), pInt(result[2]), pInt(result[3]), parseFloat(result[4], 10)];
} else {
// hex
result = /#([a-fA-F0-9]{2})([a-fA-F0-9]{2})([a-fA-F0-9]{2})/.exec(input);
if (result) {
rgba = [pInt(result[1], 16), pInt(result[2], 16), pInt(result[3], 16), 1];
} else {
// rgb
result = /rgb\(\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*,\s*([0-9]{1,3})\s*\)/.exec(input);
if (result) {
rgba = [pInt(result[1]), pInt(result[2]), pInt(result[3]), 1];
}
}
}
}
}
/**
* Return the color a specified format
* @param {String} format
*/
function get(format) {
var ret;
if (stops) {
ret = merge(input);
ret.stops = [].concat(ret.stops);
each(stops, function (stop, i) {
ret.stops[i] = [ret.stops[i][0], stop.get(format)];
});
// it's NaN if gradient colors on a column chart
} else if (rgba && !isNaN(rgba[0])) {
if (format === 'rgb') {
ret = 'rgb(' + rgba[0] + ',' + rgba[1] + ',' + rgba[2] + ')';
} else if (format === 'a') {
ret = rgba[3];
} else {
ret = 'rgba(' + rgba.join(',') + ')';
}
} else {
ret = input;
}
return ret;
}
/**
* Brighten the color
* @param {Number} alpha
*/
function brighten(alpha) {
if (stops) {
each(stops, function (stop) {
stop.brighten(alpha);
});
} else if (isNumber(alpha) && alpha !== 0) {
var i;
for (i = 0; i < 3; i++) {
rgba[i] += pInt(alpha * 255);
if (rgba[i] < 0) {
rgba[i] = 0;
}
if (rgba[i] > 255) {
rgba[i] = 255;
}
}
}
return this;
}
/**
* Set the color's opacity to a given alpha value
* @param {Number} alpha
*/
function setOpacity(alpha) {
rgba[3] = alpha;
return this;
}
// initialize: parse the input
init(input);
// public methods
return {
get: get,
brighten: brighten,
rgba: rgba,
setOpacity: setOpacity
};
};
/**
* A wrapper object for SVG elements
*/
function SVGElement() {}
SVGElement.prototype = {
/**
* Initialize the SVG renderer
* @param {Object} renderer
* @param {String} nodeName
*/
init: function (renderer, nodeName) {
var wrapper = this;
wrapper.element = nodeName === 'span' ?
createElement(nodeName) :
doc.createElementNS(SVG_NS, nodeName);
wrapper.renderer = renderer;
/**
* A collection of attribute setters. These methods, if defined, are called right before a certain
* attribute is set on an element wrapper. Returning false prevents the default attribute
* setter to run. Returning a value causes the default setter to set that value. Used in
* Renderer.label.
*/
wrapper.attrSetters = {};
},
/**
* Default base for animation
*/
opacity: 1,
/**
* Animate a given attribute
* @param {Object} params
* @param {Number} options The same options as in jQuery animation
* @param {Function} complete Function to perform at the end of animation
*/
animate: function (params, options, complete) {
var animOptions = pick(options, globalAnimation, true);
stop(this); // stop regardless of animation actually running, or reverting to .attr (#607)
if (animOptions) {
animOptions = merge(animOptions);
if (complete) { // allows using a callback with the global animation without overwriting it
animOptions.complete = complete;
}
animate(this, params, animOptions);
} else {
this.attr(params);
if (complete) {
complete();
}
}
},
/**
* Set or get a given attribute
* @param {Object|String} hash
* @param {Mixed|Undefined} val
*/
attr: function (hash, val) {
var wrapper = this,
key,
value,
result,
i,
child,
element = wrapper.element,
nodeName = element.nodeName.toLowerCase(), // Android2 requires lower for "text"
renderer = wrapper.renderer,
skipAttr,
titleNode,
attrSetters = wrapper.attrSetters,
shadows = wrapper.shadows,
hasSetSymbolSize,
doTransform,
ret = wrapper;
// single key-value pair
if (isString(hash) && defined(val)) {
key = hash;
hash = {};
hash[key] = val;
}
// used as a getter: first argument is a string, second is undefined
if (isString(hash)) {
key = hash;
if (nodeName === 'circle') {
key = { x: 'cx', y: 'cy' }[key] || key;
} else if (key === 'strokeWidth') {
key = 'stroke-width';
}
ret = attr(element, key) || wrapper[key] || 0;
if (key !== 'd' && key !== 'visibility' && key !== 'fill') { // 'd' is string in animation step
ret = parseFloat(ret);
}
// setter
} else {
for (key in hash) {
skipAttr = false; // reset
value = hash[key];
// check for a specific attribute setter
result = attrSetters[key] && attrSetters[key].call(wrapper, value, key);
if (result !== false) {
if (result !== UNDEFINED) {
value = result; // the attribute setter has returned a new value to set
}
// paths
if (key === 'd') {
if (value && value.join) { // join path
value = value.join(' ');
}
if (/(NaN| {2}|^$)/.test(value)) {
value = 'M 0 0';
}
//wrapper.d = value; // shortcut for animations
// update child tspans x values
} else if (key === 'x' && nodeName === 'text') {
for (i = 0; i < element.childNodes.length; i++) {
child = element.childNodes[i];
// if the x values are equal, the tspan represents a linebreak
if (attr(child, 'x') === attr(element, 'x')) {
//child.setAttribute('x', value);
attr(child, 'x', value);
}
}
} else if (wrapper.rotation && (key === 'x' || key === 'y')) {
doTransform = true;
// apply gradients
} else if (key === 'fill') {
value = renderer.color(value, element, key);
// circle x and y
} else if (nodeName === 'circle' && (key === 'x' || key === 'y')) {
key = { x: 'cx', y: 'cy' }[key] || key;
// rectangle border radius
} else if (nodeName === 'rect' && key === 'r') {
attr(element, {
rx: value,
ry: value
});
skipAttr = true;
// translation and text rotation
} else if (key === 'translateX' || key === 'translateY' || key === 'rotation' ||
key === 'verticalAlign' || key === 'scaleX' || key === 'scaleY') {
doTransform = true;
skipAttr = true;
// apply opacity as subnode (required by legacy WebKit and Batik)
} else if (key === 'stroke') {
value = renderer.color(value, element, key);
// emulate VML's dashstyle implementation
} else if (key === 'dashstyle') {
key = 'stroke-dasharray';
value = value && value.toLowerCase();
if (value === 'solid') {
value = NONE;
} else if (value) {
value = value
.replace('shortdashdotdot', '3,1,1,1,1,1,')
.replace('shortdashdot', '3,1,1,1')
.replace('shortdot', '1,1,')
.replace('shortdash', '3,1,')
.replace('longdash', '8,3,')
.replace(/dot/g, '1,3,')
.replace('dash', '4,3,')
.replace(/,$/, '')
.split(','); // ending comma
i = value.length;
while (i--) {
value[i] = pInt(value[i]) * pick(hash['stroke-width'], wrapper['stroke-width']);
}
value = value.join(',');
}
// IE9/MooTools combo: MooTools returns objects instead of numbers and IE9 Beta 2
// is unable to cast them. Test again with final IE9.
} else if (key === 'width') {
value = pInt(value);
// Text alignment
} else if (key === 'align') {
key = 'text-anchor';
value = { left: 'start', center: 'middle', right: 'end' }[value];
// Title requires a subnode, #431
} else if (key === 'title') {
titleNode = element.getElementsByTagName('title')[0];
if (!titleNode) {
titleNode = doc.createElementNS(SVG_NS, 'title');
element.appendChild(titleNode);
}
titleNode.textContent = value;
}
// jQuery animate changes case
if (key === 'strokeWidth') {
key = 'stroke-width';
}
// In Chrome/Win < 6 as well as Batik, the stroke attribute can't be set when the stroke-
// width is 0. #1369
if (key === 'stroke-width' || key === 'stroke') {
wrapper[key] = value;
// Only apply the stroke attribute if the stroke width is defined and larger than 0
if (wrapper.stroke && wrapper['stroke-width']) {
attr(element, 'stroke', wrapper.stroke);
attr(element, 'stroke-width', wrapper['stroke-width']);
wrapper.hasStroke = true;
} else if (key === 'stroke-width' && value === 0 && wrapper.hasStroke) {
element.removeAttribute('stroke');
wrapper.hasStroke = false;
}
skipAttr = true;
}
// symbols
if (wrapper.symbolName && /^(x|y|width|height|r|start|end|innerR|anchorX|anchorY)/.test(key)) {
if (!hasSetSymbolSize) {
wrapper.symbolAttr(hash);
hasSetSymbolSize = true;
}
skipAttr = true;
}
// let the shadow follow the main element
if (shadows && /^(width|height|visibility|x|y|d|transform|cx|cy|r)$/.test(key)) {
i = shadows.length;
while (i--) {
attr(
shadows[i],
key,
key === 'height' ?
mathMax(value - (shadows[i].cutHeight || 0), 0) :
value
);
}
}
// validate heights
if ((key === 'width' || key === 'height') && nodeName === 'rect' && value < 0) {
value = 0;
}
// Record for animation and quick access without polling the DOM
wrapper[key] = value;
if (key === 'text') {
// Delete bBox memo when the text changes
if (value !== wrapper.textStr) {
delete wrapper.bBox;
}
wrapper.textStr = value;
if (wrapper.added) {
renderer.buildText(wrapper);
}
} else if (!skipAttr) {
attr(element, key, value);
}
}
}
// Update transform. Do this outside the loop to prevent redundant updating for batch setting
// of attributes.
if (doTransform) {
wrapper.updateTransform();
}
}
return ret;
},
/**
* Add a class name to an element
*/
addClass: function (className) {
var element = this.element,
currentClassName = attr(element, 'class') || '';
if (currentClassName.indexOf(className) === -1) {
attr(element, 'class', currentClassName + ' ' + className);
}
return this;
},
/* hasClass and removeClass are not (yet) needed
hasClass: function (className) {
return attr(this.element, 'class').indexOf(className) !== -1;
},
removeClass: function (className) {
attr(this.element, 'class', attr(this.element, 'class').replace(className, ''));
return this;
},
*/
/**
* If one of the symbol size affecting parameters are changed,
* check all the others only once for each call to an element's
* .attr() method
* @param {Object} hash
*/
symbolAttr: function (hash) {
var wrapper = this;
each(['x', 'y', 'r', 'start', 'end', 'width', 'height', 'innerR', 'anchorX', 'anchorY'], function (key) {
wrapper[key] = pick(hash[key], wrapper[key]);
});
wrapper.attr({
d: wrapper.renderer.symbols[wrapper.symbolName](
wrapper.x,
wrapper.y,
wrapper.width,
wrapper.height,
wrapper
)
});
},
/**
* Apply a clipping path to this object
* @param {String} id
*/
clip: function (clipRect) {
return this.attr('clip-path', clipRect ? 'url(' + this.renderer.url + '#' + clipRect.id + ')' : NONE);
},
/**
* Calculate the coordinates needed for drawing a rectangle crisply and return the
* calculated attributes
* @param {Number} strokeWidth
* @param {Number} x
* @param {Number} y
* @param {Number} width
* @param {Number} height
*/
crisp: function (strokeWidth, x, y, width, height) {
var wrapper = this,
key,
attribs = {},
values = {},
normalizer;
strokeWidth = strokeWidth || wrapper.strokeWidth || (wrapper.attr && wrapper.attr('stroke-width')) || 0;
normalizer = mathRound(strokeWidth) % 2 / 2; // mathRound because strokeWidth can sometimes have roundoff errors
// normalize for crisp edges
values.x = mathFloor(x || wrapper.x || 0) + normalizer;
values.y = mathFloor(y || wrapper.y || 0) + normalizer;
values.width = mathFloor((width || wrapper.width || 0) - 2 * normalizer);
values.height = mathFloor((height || wrapper.height || 0) - 2 * normalizer);
values.strokeWidth = strokeWidth;
for (key in values) {
if (wrapper[key] !== values[key]) { // only set attribute if changed
wrapper[key] = attribs[key] = values[key];
}
}
return attribs;
},
/**
* Set styles for the element
* @param {Object} styles
*/
css: function (styles) {
/*jslint unparam: true*//* allow unused param a in the regexp function below */
var elemWrapper = this,
elem = elemWrapper.element,
textWidth = styles && styles.width && elem.nodeName.toLowerCase() === 'text',
n,
serializedCss = '',
hyphenate = function (a, b) { return '-' + b.toLowerCase(); };
/*jslint unparam: false*/
// convert legacy
if (styles && styles.color) {
styles.fill = styles.color;
}
// Merge the new styles with the old ones
styles = extend(
elemWrapper.styles,
styles
);
// store object
elemWrapper.styles = styles;
// Don't handle line wrap on canvas
if (useCanVG && textWidth) {
delete styles.width;
}
// serialize and set style attribute
if (isIE && !hasSVG) { // legacy IE doesn't support setting style attribute
if (textWidth) {
delete styles.width;
}
css(elemWrapper.element, styles);
} else {
for (n in styles) {
serializedCss += n.replace(/([A-Z])/g, hyphenate) + ':' + styles[n] + ';';
}
attr(elem, 'style', serializedCss); // #1881
}
// re-build text
if (textWidth && elemWrapper.added) {
elemWrapper.renderer.buildText(elemWrapper);
}
return elemWrapper;
},
/**
* Add an event listener
* @param {String} eventType
* @param {Function} handler
*/
on: function (eventType, handler) {
var svgElement = this,
element = svgElement.element;
// touch
if (hasTouch && eventType === 'click') {
element.ontouchstart = function (e) {
svgElement.touchEventFired = Date.now();
e.preventDefault();
handler.call(element, e);
};
element.onclick = function (e) {
if (userAgent.indexOf('Android') === -1 || Date.now() - (svgElement.touchEventFired || 0) > 1100) { // #2269
handler.call(element, e);
}
};
} else {
// simplest possible event model for internal use
element['on' + eventType] = handler;
}
return this;
},
/**
* Set the coordinates needed to draw a consistent radial gradient across
* pie slices regardless of positioning inside the chart. The format is
* [centerX, centerY, diameter] in pixels.
*/
setRadialReference: function (coordinates) {
this.element.radialReference = coordinates;
return this;
},
/**
* Move an object and its children by x and y values
* @param {Number} x
* @param {Number} y
*/
translate: function (x, y) {
return this.attr({
translateX: x,
translateY: y
});
},
/**
* Invert a group, rotate and flip
*/
invert: function () {
var wrapper = this;
wrapper.inverted = true;
wrapper.updateTransform();
return wrapper;
},
/**
* Apply CSS to HTML elements. This is used in text within SVG rendering and
* by the VML renderer
*/
htmlCss: function (styles) {
var wrapper = this,
element = wrapper.element,
textWidth = styles && element.tagName === 'SPAN' && styles.width;
if (textWidth) {
delete styles.width;
wrapper.textWidth = textWidth;
wrapper.updateTransform();
}
wrapper.styles = extend(wrapper.styles, styles);
css(wrapper.element, styles);
return wrapper;
},
/**
* VML and useHTML method for calculating the bounding box based on offsets
* @param {Boolean} refresh Whether to force a fresh value from the DOM or to
* use the cached value
*
* @return {Object} A hash containing values for x, y, width and height
*/
htmlGetBBox: function () {
var wrapper = this,
element = wrapper.element,
bBox = wrapper.bBox;
// faking getBBox in exported SVG in legacy IE
if (!bBox) {
// faking getBBox in exported SVG in legacy IE (is this a duplicate of the fix for #1079?)
if (element.nodeName === 'text') {
element.style.position = ABSOLUTE;
}
bBox = wrapper.bBox = {
x: element.offsetLeft,
y: element.offsetTop,
width: element.offsetWidth,
height: element.offsetHeight
};
}
return bBox;
},
/**
* VML override private method to update elements based on internal
* properties based on SVG transform
*/
htmlUpdateTransform: function () {
// aligning non added elements is expensive
if (!this.added) {
this.alignOnAdd = true;
return;
}
var wrapper = this,
renderer = wrapper.renderer,
elem = wrapper.element,
translateX = wrapper.translateX || 0,
translateY = wrapper.translateY || 0,
x = wrapper.x || 0,
y = wrapper.y || 0,
align = wrapper.textAlign || 'left',
alignCorrection = { left: 0, center: 0.5, right: 1 }[align],
nonLeft = align && align !== 'left',
shadows = wrapper.shadows;
// apply translate
css(elem, {
marginLeft: translateX,
marginTop: translateY
});
if (shadows) { // used in labels/tooltip
each(shadows, function (shadow) {
css(shadow, {
marginLeft: translateX + 1,
marginTop: translateY + 1
});
});
}
// apply inversion
if (wrapper.inverted) { // wrapper is a group
each(elem.childNodes, function (child) {
renderer.invertChild(child, elem);
});
}
if (elem.tagName === 'SPAN') {
var width, height,
rotation = wrapper.rotation,
baseline,
radians = 0,
costheta = 1,
sintheta = 0,
quad,
textWidth = pInt(wrapper.textWidth),
xCorr = wrapper.xCorr || 0,
yCorr = wrapper.yCorr || 0,
currentTextTransform = [rotation, align, elem.innerHTML, wrapper.textWidth].join(',');
if (currentTextTransform !== wrapper.cTT) { // do the calculations and DOM access only if properties changed
if (defined(rotation)) {
radians = rotation * deg2rad; // deg to rad
costheta = mathCos(radians);
sintheta = mathSin(radians);
wrapper.setSpanRotation(rotation, sintheta, costheta);
}
width = pick(wrapper.elemWidth, elem.offsetWidth);
height = pick(wrapper.elemHeight, elem.offsetHeight);
// update textWidth
if (width > textWidth && /[ \-]/.test(elem.textContent || elem.innerText)) { // #983, #1254
css(elem, {
width: textWidth + PX,
display: 'block',
whiteSpace: 'normal'
});
width = textWidth;
}
// correct x and y
baseline = renderer.fontMetrics(elem.style.fontSize).b;
xCorr = costheta < 0 && -width;
yCorr = sintheta < 0 && -height;
// correct for baseline and corners spilling out after rotation
quad = costheta * sintheta < 0;
xCorr += sintheta * baseline * (quad ? 1 - alignCorrection : alignCorrection);
yCorr -= costheta * baseline * (rotation ? (quad ? alignCorrection : 1 - alignCorrection) : 1);
// correct for the length/height of the text
if (nonLeft) {
xCorr -= width * alignCorrection * (costheta < 0 ? -1 : 1);
if (rotation) {
yCorr -= height * alignCorrection * (sintheta < 0 ? -1 : 1);
}
css(elem, {
textAlign: align
});
}
// record correction
wrapper.xCorr = xCorr;
wrapper.yCorr = yCorr;
}
// apply position with correction
css(elem, {
left: (x + xCorr) + PX,
top: (y + yCorr) + PX
});
// force reflow in webkit to apply the left and top on useHTML element (#1249)
if (isWebKit) {
height = elem.offsetHeight; // assigned to height for JSLint purpose
}
// record current text transform
wrapper.cTT = currentTextTransform;
}
},
/**
* Set the rotation of an individual HTML span
*/
setSpanRotation: function (rotation) {
var rotationStyle = {},
cssTransformKey = isIE ? '-ms-transform' : isWebKit ? '-webkit-transform' : isFirefox ? 'MozTransform' : isOpera ? '-o-transform' : '';
rotationStyle[cssTransformKey] = rotationStyle.transform = 'rotate(' + rotation + 'deg)';
css(this.element, rotationStyle);
},
/**
* Private method to update the transform attribute based on internal
* properties
*/
updateTransform: function () {
var wrapper = this,
translateX = wrapper.translateX || 0,
translateY = wrapper.translateY || 0,
scaleX = wrapper.scaleX,
scaleY = wrapper.scaleY,
inverted = wrapper.inverted,
rotation = wrapper.rotation,
transform;
// flipping affects translate as adjustment for flipping around the group's axis
if (inverted) {
translateX += wrapper.attr('width');
translateY += wrapper.attr('height');
}
// Apply translate. Nearly all transformed elements have translation, so instead
// of checking for translate = 0, do it always (#1767, #1846).
transform = ['translate(' + translateX + ',' + translateY + ')'];
// apply rotation
if (inverted) {
transform.push('rotate(90) scale(-1,1)');
} else if (rotation) { // text rotation
transform.push('rotate(' + rotation + ' ' + (wrapper.x || 0) + ' ' + (wrapper.y || 0) + ')');
}
// apply scale
if (defined(scaleX) || defined(scaleY)) {
transform.push('scale(' + pick(scaleX, 1) + ' ' + pick(scaleY, 1) + ')');
}
if (transform.length) {
attr(wrapper.element, 'transform', transform.join(' '));
}
},
/**
* Bring the element to the front
*/
toFront: function () {
var element = this.element;
element.parentNode.appendChild(element);
return this;
},
/**
* Break down alignment options like align, verticalAlign, x and y
* to x and y relative to the chart.
*
* @param {Object} alignOptions
* @param {Boolean} alignByTranslate
* @param {String[Object} box The box to align to, needs a width and height. When the
* box is a string, it refers to an object in the Renderer. For example, when
* box is 'spacingBox', it refers to Renderer.spacingBox which holds width, height
* x and y properties.
*
*/
align: function (alignOptions, alignByTranslate, box) {
var align,
vAlign,
x,
y,
attribs = {},
alignTo,
renderer = this.renderer,
alignedObjects = renderer.alignedObjects;
// First call on instanciate
if (alignOptions) {
this.alignOptions = alignOptions;
this.alignByTranslate = alignByTranslate;
if (!box || isString(box)) { // boxes other than renderer handle this internally
this.alignTo = alignTo = box || 'renderer';
erase(alignedObjects, this); // prevent duplicates, like legendGroup after resize
alignedObjects.push(this);
box = null; // reassign it below
}
// When called on resize, no arguments are supplied
} else {
alignOptions = this.alignOptions;
alignByTranslate = this.alignByTranslate;
alignTo = this.alignTo;
}
box = pick(box, renderer[alignTo], renderer);
// Assign variables
align = alignOptions.align;
vAlign = alignOptions.verticalAlign;
x = (box.x || 0) + (alignOptions.x || 0); // default: left align
y = (box.y || 0) + (alignOptions.y || 0); // default: top align
// Align
if (align === 'right' || align === 'center') {
x += (box.width - (alignOptions.width || 0)) /
{ right: 1, center: 2 }[align];
}
attribs[alignByTranslate ? 'translateX' : 'x'] = mathRound(x);
// Vertical align
if (vAlign === 'bottom' || vAlign === 'middle') {
y += (box.height - (alignOptions.height || 0)) /
({ bottom: 1, middle: 2 }[vAlign] || 1);
}
attribs[alignByTranslate ? 'translateY' : 'y'] = mathRound(y);
// Animate only if already placed
this[this.placed ? 'animate' : 'attr'](attribs);
this.placed = true;
this.alignAttr = attribs;
return this;
},
/**
* Get the bounding box (width, height, x and y) for the element
*/
getBBox: function () {
var wrapper = this,
bBox = wrapper.bBox,
renderer = wrapper.renderer,
width,
height,
rotation = wrapper.rotation,
element = wrapper.element,
styles = wrapper.styles,
rad = rotation * deg2rad;
if (!bBox) {
// SVG elements
if (element.namespaceURI === SVG_NS || renderer.forExport) {
try { // Fails in Firefox if the container has display: none.
bBox = element.getBBox ?
// SVG: use extend because IE9 is not allowed to change width and height in case
// of rotation (below)
extend({}, element.getBBox()) :
// Canvas renderer and legacy IE in export mode
{
width: element.offsetWidth,
height: element.offsetHeight
};
} catch (e) {}
// If the bBox is not set, the try-catch block above failed. The other condition
// is for Opera that returns a width of -Infinity on hidden elements.
if (!bBox || bBox.width < 0) {
bBox = { width: 0, height: 0 };
}
// VML Renderer or useHTML within SVG
} else {
bBox = wrapper.htmlGetBBox();
}
// True SVG elements as well as HTML elements in modern browsers using the .useHTML option
// need to compensated for rotation
if (renderer.isSVG) {
width = bBox.width;
height = bBox.height;
// Workaround for wrong bounding box in IE9 and IE10 (#1101, #1505, #1669)
if (isIE && styles && styles.fontSize === '11px' && height.toPrecision(3) === '22.7') {
bBox.height = height = 14;
}
// Adjust for rotated text
if (rotation) {
bBox.width = mathAbs(height * mathSin(rad)) + mathAbs(width * mathCos(rad));
bBox.height = mathAbs(height * mathCos(rad)) + mathAbs(width * mathSin(rad));
}
}
wrapper.bBox = bBox;
}
return bBox;
},
/**
* Show the element
*/
show: function () {
return this.attr({ visibility: VISIBLE });
},
/**
* Hide the element
*/
hide: function () {
return this.attr({ visibility: HIDDEN });
},
fadeOut: function (duration) {
var elemWrapper = this;
elemWrapper.animate({
opacity: 0
}, {
duration: duration || 150,
complete: function () {
elemWrapper.hide();
}
});
},
/**
* Add the element
* @param {Object|Undefined} parent Can be an element, an element wrapper or undefined
* to append the element to the renderer.box.
*/
add: function (parent) {
var renderer = this.renderer,
parentWrapper = parent || renderer,
parentNode = parentWrapper.element || renderer.box,
childNodes = parentNode.childNodes,
element = this.element,
zIndex = attr(element, 'zIndex'),
otherElement,
otherZIndex,
i,
inserted;
if (parent) {
this.parentGroup = parent;
}
// mark as inverted
this.parentInverted = parent && parent.inverted;
// build formatted text
if (this.textStr !== undefined) {
renderer.buildText(this);
}
// mark the container as having z indexed children
if (zIndex) {
parentWrapper.handleZ = true;
zIndex = pInt(zIndex);
}
// insert according to this and other elements' zIndex
if (parentWrapper.handleZ) { // this element or any of its siblings has a z index
for (i = 0; i < childNodes.length; i++) {
otherElement = childNodes[i];
otherZIndex = attr(otherElement, 'zIndex');
if (otherElement !== element && (
// insert before the first element with a higher zIndex
pInt(otherZIndex) > zIndex ||
// if no zIndex given, insert before the first element with a zIndex
(!defined(zIndex) && defined(otherZIndex))
)) {
parentNode.insertBefore(element, otherElement);
inserted = true;
break;
}
}
}
// default: append at the end
if (!inserted) {
parentNode.appendChild(element);
}
// mark as added
this.added = true;
// fire an event for internal hooks
fireEvent(this, 'add');
return this;
},
/**
* Removes a child either by removeChild or move to garbageBin.
* Issue 490; in VML removeChild results in Orphaned nodes according to sIEve, discardElement does not.
*/
safeRemoveChild: function (element) {
var parentNode = element.parentNode;
if (parentNode) {
parentNode.removeChild(element);
}
},
/**
* Destroy the element and element wrapper
*/
destroy: function () {
var wrapper = this,
element = wrapper.element || {},
shadows = wrapper.shadows,
parentToClean = wrapper.renderer.isSVG && element.nodeName === 'SPAN' && element.parentNode,
grandParent,
key,
i;
// remove events
element.onclick = element.onmouseout = element.onmouseover = element.onmousemove = element.point = null;
stop(wrapper); // stop running animations
if (wrapper.clipPath) {
wrapper.clipPath = wrapper.clipPath.destroy();
}
// Destroy stops in case this is a gradient object
if (wrapper.stops) {
for (i = 0; i < wrapper.stops.length; i++) {
wrapper.stops[i] = wrapper.stops[i].destroy();
}
wrapper.stops = null;
}
// remove element
wrapper.safeRemoveChild(element);
// destroy shadows
if (shadows) {
each(shadows, function (shadow) {
wrapper.safeRemoveChild(shadow);
});
}
// In case of useHTML, clean up empty containers emulating SVG groups (#1960).
while (parentToClean && parentToClean.childNodes.length === 0) {
grandParent = parentToClean.parentNode;
wrapper.safeRemoveChild(parentToClean);
parentToClean = grandParent;
}
// remove from alignObjects
if (wrapper.alignTo) {
erase(wrapper.renderer.alignedObjects, wrapper);
}
for (key in wrapper) {
delete wrapper[key];
}
return null;
},
/**
* Add a shadow to the element. Must be done after the element is added to the DOM
* @param {Boolean|Object} shadowOptions
*/
shadow: function (shadowOptions, group, cutOff) {
var shadows = [],
i,
shadow,
element = this.element,
strokeWidth,
shadowWidth,
shadowElementOpacity,
// compensate for inverted plot area
transform;
if (shadowOptions) {
shadowWidth = pick(shadowOptions.width, 3);
shadowElementOpacity = (shadowOptions.opacity || 0.15) / shadowWidth;
transform = this.parentInverted ?
'(-1,-1)' :
'(' + pick(shadowOptions.offsetX, 1) + ', ' + pick(shadowOptions.offsetY, 1) + ')';
for (i = 1; i <= shadowWidth; i++) {
shadow = element.cloneNode(0);
strokeWidth = (shadowWidth * 2) + 1 - (2 * i);
attr(shadow, {
'isShadow': 'true',
'stroke': shadowOptions.color || 'black',
'stroke-opacity': shadowElementOpacity * i,
'stroke-width': strokeWidth,
'transform': 'translate' + transform,
'fill': NONE
});
if (cutOff) {
attr(shadow, 'height', mathMax(attr(shadow, 'height') - strokeWidth, 0));
shadow.cutHeight = strokeWidth;
}
if (group) {
group.element.appendChild(shadow);
} else {
element.parentNode.insertBefore(shadow, element);
}
shadows.push(shadow);
}
this.shadows = shadows;
}
return this;
}
};
/**
* The default SVG renderer
*/
var SVGRenderer = function () {
this.init.apply(this, arguments);
};
SVGRenderer.prototype = {
Element: SVGElement,
/**
* Initialize the SVGRenderer
* @param {Object} container
* @param {Number} width
* @param {Number} height
* @param {Boolean} forExport
*/
init: function (container, width, height, forExport) {
var renderer = this,
loc = location,
boxWrapper,
element,
desc;
boxWrapper = renderer.createElement('svg')
.attr({
version: '1.1'
});
element = boxWrapper.element;
container.appendChild(element);
// For browsers other than IE, add the namespace attribute (#1978)
if (container.innerHTML.indexOf('xmlns') === -1) {
attr(element, 'xmlns', SVG_NS);
}
// object properties
renderer.isSVG = true;
renderer.box = element;
renderer.boxWrapper = boxWrapper;
renderer.alignedObjects = [];
// Page url used for internal references. #24, #672, #1070
renderer.url = (isFirefox || isWebKit) && doc.getElementsByTagName('base').length ?
loc.href
.replace(/#.*?$/, '') // remove the hash
.replace(/([\('\)])/g, '\\$1') // escape parantheses and quotes
.replace(/ /g, '%20') : // replace spaces (needed for Safari only)
'';
// Add description
desc = this.createElement('desc').add();
desc.element.appendChild(doc.createTextNode('Created with ' + PRODUCT + ' ' + VERSION));
renderer.defs = this.createElement('defs').add();
renderer.forExport = forExport;
renderer.gradients = {}; // Object where gradient SvgElements are stored
renderer.setSize(width, height, false);
// Issue 110 workaround:
// In Firefox, if a div is positioned by percentage, its pixel position may land
// between pixels. The container itself doesn't display this, but an SVG element
// inside this container will be drawn at subpixel precision. In order to draw
// sharp lines, this must be compensated for. This doesn't seem to work inside
// iframes though (like in jsFiddle).
var subPixelFix, rect;
if (isFirefox && container.getBoundingClientRect) {
renderer.subPixelFix = subPixelFix = function () {
css(container, { left: 0, top: 0 });
rect = container.getBoundingClientRect();
css(container, {
left: (mathCeil(rect.left) - rect.left) + PX,
top: (mathCeil(rect.top) - rect.top) + PX
});
};
// run the fix now
subPixelFix();
// run it on resize
addEvent(win, 'resize', subPixelFix);
}
},
/**
* Detect whether the renderer is hidden. This happens when one of the parent elements
* has display: none. #608.
*/
isHidden: function () {
return !this.boxWrapper.getBBox().width;
},
/**
* Destroys the renderer and its allocated members.
*/
destroy: function () {
var renderer = this,
rendererDefs = renderer.defs;
renderer.box = null;
renderer.boxWrapper = renderer.boxWrapper.destroy();
// Call destroy on all gradient elements
destroyObjectProperties(renderer.gradients || {});
renderer.gradients = null;
// Defs are null in VMLRenderer
// Otherwise, destroy them here.
if (rendererDefs) {
renderer.defs = rendererDefs.destroy();
}
// Remove sub pixel fix handler
// We need to check that there is a handler, otherwise all functions that are registered for event 'resize' are removed
// See issue #982
if (renderer.subPixelFix) {
removeEvent(win, 'resize', renderer.subPixelFix);
}
renderer.alignedObjects = null;
return null;
},
/**
* Create a wrapper for an SVG element
* @param {Object} nodeName
*/
createElement: function (nodeName) {
var wrapper = new this.Element();
wrapper.init(this, nodeName);
return wrapper;
},
/**
* Dummy function for use in canvas renderer
*/
draw: function () {},
/**
* Parse a simple HTML string into SVG tspans
*
* @param {Object} textNode The parent text SVG node
*/
buildText: function (wrapper) {
var textNode = wrapper.element,
renderer = this,
forExport = renderer.forExport,
lines = pick(wrapper.textStr, '').toString()
.replace(/<(b|strong)>/g, '<span style="font-weight:bold">')
.replace(/<(i|em)>/g, '<span style="font-style:italic">')
.replace(/<a/g, '<span')
.replace(/<\/(b|strong|i|em|a)>/g, '</span>')
.split(/<br.*?>/g),
childNodes = textNode.childNodes,
styleRegex = /style="([^"]+)"/,
hrefRegex = /href="(http[^"]+)"/,
parentX = attr(textNode, 'x'),
textStyles = wrapper.styles,
width = textStyles && textStyles.width && pInt(textStyles.width),
textLineHeight = textStyles && textStyles.lineHeight,
i = childNodes.length;
/// remove old text
while (i--) {
textNode.removeChild(childNodes[i]);
}
if (width && !wrapper.added) {
this.box.appendChild(textNode); // attach it to the DOM to read offset width
}
// remove empty line at end
if (lines[lines.length - 1] === '') {
lines.pop();
}
// build the lines
each(lines, function (line, lineNo) {
var spans, spanNo = 0;
line = line.replace(/<span/g, '|||<span').replace(/<\/span>/g, '</span>|||');
spans = line.split('|||');
each(spans, function (span) {
if (span !== '' || spans.length === 1) {
var attributes = {},
tspan = doc.createElementNS(SVG_NS, 'tspan'),
spanStyle; // #390
if (styleRegex.test(span)) {
spanStyle = span.match(styleRegex)[1].replace(/(;| |^)color([ :])/, '$1fill$2');
attr(tspan, 'style', spanStyle);
}
if (hrefRegex.test(span) && !forExport) { // Not for export - #1529
attr(tspan, 'onclick', 'location.href=\"' + span.match(hrefRegex)[1] + '\"');
css(tspan, { cursor: 'pointer' });
}
span = (span.replace(/<(.|\n)*?>/g, '') || ' ')
.replace(/</g, '<')
.replace(/>/g, '>');
// Nested tags aren't supported, and cause crash in Safari (#1596)
if (span !== ' ') {
// add the text node
tspan.appendChild(doc.createTextNode(span));
if (!spanNo) { // first span in a line, align it to the left
attributes.x = parentX;
} else {
attributes.dx = 0; // #16
}
// add attributes
attr(tspan, attributes);
// first span on subsequent line, add the line height
if (!spanNo && lineNo) {
// allow getting the right offset height in exporting in IE
if (!hasSVG && forExport) {
css(tspan, { display: 'block' });
}
// Set the line height based on the font size of either
// the text element or the tspan element
attr(
tspan,
'dy',
textLineHeight || renderer.fontMetrics(
/px$/.test(tspan.style.fontSize) ?
tspan.style.fontSize :
textStyles.fontSize
).h,
// Safari 6.0.2 - too optimized for its own good (#1539)
// TODO: revisit this with future versions of Safari
isWebKit && tspan.offsetHeight
);
}
// Append it
textNode.appendChild(tspan);
spanNo++;
// check width and apply soft breaks
if (width) {
var words = span.replace(/([^\^])-/g, '$1- ').split(' '), // #1273
tooLong,
actualWidth,
clipHeight = wrapper._clipHeight,
rest = [],
dy = pInt(textLineHeight || 16),
softLineNo = 1,
bBox;
while (words.length || rest.length) {
delete wrapper.bBox; // delete cache
bBox = wrapper.getBBox();
actualWidth = bBox.width;
tooLong = actualWidth > width;
if (!tooLong || words.length === 1) { // new line needed
words = rest;
rest = [];
if (words.length) {
softLineNo++;
if (clipHeight && softLineNo * dy > clipHeight) {
words = ['...'];
wrapper.attr('title', wrapper.textStr);
} else {
tspan = doc.createElementNS(SVG_NS, 'tspan');
attr(tspan, {
dy: dy,
x: parentX
});
if (spanStyle) { // #390
attr(tspan, 'style', spanStyle);
}
textNode.appendChild(tspan);
if (actualWidth > width) { // a single word is pressing it out
width = actualWidth;
}
}
}
} else { // append to existing line tspan
tspan.removeChild(tspan.firstChild);
rest.unshift(words.pop());
}
if (words.length) {
tspan.appendChild(doc.createTextNode(words.join(' ').replace(/- /g, '-')));
}
}
}
}
}
});
});
},
/**
* Create a button with preset states
* @param {String} text
* @param {Number} x
* @param {Number} y
* @param {Function} callback
* @param {Object} normalState
* @param {Object} hoverState
* @param {Object} pressedState
*/
button: function (text, x, y, callback, normalState, hoverState, pressedState, disabledState) {
var label = this.label(text, x, y, null, null, null, null, null, 'button'),
curState = 0,
stateOptions,
stateStyle,
normalStyle,
hoverStyle,
pressedStyle,
disabledStyle,
STYLE = 'style',
verticalGradient = { x1: 0, y1: 0, x2: 0, y2: 1 };
// Normal state - prepare the attributes
normalState = merge({
'stroke-width': 1,
stroke: '#CCCCCC',
fill: {
linearGradient: verticalGradient,
stops: [
[0, '#FEFEFE'],
[1, '#F6F6F6']
]
},
r: 2,
padding: 5,
style: {
color: 'black'
}
}, normalState);
normalStyle = normalState[STYLE];
delete normalState[STYLE];
// Hover state
hoverState = merge(normalState, {
stroke: '#68A',
fill: {
linearGradient: verticalGradient,
stops: [
[0, '#FFF'],
[1, '#ACF']
]
}
}, hoverState);
hoverStyle = hoverState[STYLE];
delete hoverState[STYLE];
// Pressed state
pressedState = merge(normalState, {
stroke: '#68A',
fill: {
linearGradient: verticalGradient,
stops: [
[0, '#9BD'],
[1, '#CDF']
]
}
}, pressedState);
pressedStyle = pressedState[STYLE];
delete pressedState[STYLE];
// Disabled state
disabledState = merge(normalState, {
style: {
color: '#CCC'
}
}, disabledState);
disabledStyle = disabledState[STYLE];
delete disabledState[STYLE];
// Add the events. IE9 and IE10 need mouseover and mouseout to funciton (#667).
addEvent(label.element, isIE ? 'mouseover' : 'mouseenter', function () {
if (curState !== 3) {
label.attr(hoverState)
.css(hoverStyle);
}
});
addEvent(label.element, isIE ? 'mouseout' : 'mouseleave', function () {
if (curState !== 3) {
stateOptions = [normalState, hoverState, pressedState][curState];
stateStyle = [normalStyle, hoverStyle, pressedStyle][curState];
label.attr(stateOptions)
.css(stateStyle);
}
});
label.setState = function (state) {
label.state = curState = state;
if (!state) {
label.attr(normalState)
.css(normalStyle);
} else if (state === 2) {
label.attr(pressedState)
.css(pressedStyle);
} else if (state === 3) {
label.attr(disabledState)
.css(disabledStyle);
}
};
return label
.on('click', function () {
if (curState !== 3) {
callback.call(label);
}
})
.attr(normalState)
.css(extend({ cursor: 'default' }, normalStyle));
},
/**
* Make a straight line crisper by not spilling out to neighbour pixels
* @param {Array} points
* @param {Number} width
*/
crispLine: function (points, width) {
// points format: [M, 0, 0, L, 100, 0]
// normalize to a crisp line
if (points[1] === points[4]) {
// Substract due to #1129. Now bottom and left axis gridlines behave the same.
points[1] = points[4] = mathRound(points[1]) - (width % 2 / 2);
}
if (points[2] === points[5]) {
points[2] = points[5] = mathRound(points[2]) + (width % 2 / 2);
}
return points;
},
/**
* Draw a path
* @param {Array} path An SVG path in array form
*/
path: function (path) {
var attr = {
fill: NONE
};
if (isArray(path)) {
attr.d = path;
} else if (isObject(path)) { // attributes
extend(attr, path);
}
return this.createElement('path').attr(attr);
},
/**
* Draw and return an SVG circle
* @param {Number} x The x position
* @param {Number} y The y position
* @param {Number} r The radius
*/
circle: function (x, y, r) {
var attr = isObject(x) ?
x :
{
x: x,
y: y,
r: r
};
return this.createElement('circle').attr(attr);
},
/**
* Draw and return an arc
* @param {Number} x X position
* @param {Number} y Y position
* @param {Number} r Radius
* @param {Number} innerR Inner radius like used in donut charts
* @param {Number} start Starting angle
* @param {Number} end Ending angle
*/
arc: function (x, y, r, innerR, start, end) {
var arc;
if (isObject(x)) {
y = x.y;
r = x.r;
innerR = x.innerR;
start = x.start;
end = x.end;
x = x.x;
}
// Arcs are defined as symbols for the ability to set
// attributes in attr and animate
arc = this.symbol('arc', x || 0, y || 0, r || 0, r || 0, {
innerR: innerR || 0,
start: start || 0,
end: end || 0
});
arc.r = r; // #959
return arc;
},
/**
* Draw and return a rectangle
* @param {Number} x Left position
* @param {Number} y Top position
* @param {Number} width
* @param {Number} height
* @param {Number} r Border corner radius
* @param {Number} strokeWidth A stroke width can be supplied to allow crisp drawing
*/
rect: function (x, y, width, height, r, strokeWidth) {
r = isObject(x) ? x.r : r;
var wrapper = this.createElement('rect').attr({
rx: r,
ry: r,
fill: NONE
});
return wrapper.attr(
isObject(x) ?
x :
// do not crispify when an object is passed in (as in column charts)
wrapper.crisp(strokeWidth, x, y, mathMax(width, 0), mathMax(height, 0))
);
},
/**
* Resize the box and re-align all aligned elements
* @param {Object} width
* @param {Object} height
* @param {Boolean} animate
*
*/
setSize: function (width, height, animate) {
var renderer = this,
alignedObjects = renderer.alignedObjects,
i = alignedObjects.length;
renderer.width = width;
renderer.height = height;
renderer.boxWrapper[pick(animate, true) ? 'animate' : 'attr']({
width: width,
height: height
});
while (i--) {
alignedObjects[i].align();
}
},
/**
* Create a group
* @param {String} name The group will be given a class name of 'highcharts-{name}'.
* This can be used for styling and scripting.
*/
g: function (name) {
var elem = this.createElement('g');
return defined(name) ? elem.attr({ 'class': PREFIX + name }) : elem;
},
/**
* Display an image
* @param {String} src
* @param {Number} x
* @param {Number} y
* @param {Number} width
* @param {Number} height
*/
image: function (src, x, y, width, height) {
var attribs = {
preserveAspectRatio: NONE
},
elemWrapper;
// optional properties
if (arguments.length > 1) {
extend(attribs, {
x: x,
y: y,
width: width,
height: height
});
}
elemWrapper = this.createElement('image').attr(attribs);
// set the href in the xlink namespace
if (elemWrapper.element.setAttributeNS) {
elemWrapper.element.setAttributeNS('http://www.w3.org/1999/xlink',
'href', src);
} else {
// could be exporting in IE
// using href throws "not supported" in ie7 and under, requries regex shim to fix later
elemWrapper.element.setAttribute('hc-svg-href', src);
}
return elemWrapper;
},
/**
* Draw a symbol out of pre-defined shape paths from the namespace 'symbol' object.
*
* @param {Object} symbol
* @param {Object} x
* @param {Object} y
* @param {Object} radius
* @param {Object} options
*/
symbol: function (symbol, x, y, width, height, options) {
var obj,
// get the symbol definition function
symbolFn = this.symbols[symbol],
// check if there's a path defined for this symbol
path = symbolFn && symbolFn(
mathRound(x),
mathRound(y),
width,
height,
options
),
imageElement,
imageRegex = /^url\((.*?)\)$/,
imageSrc,
imageSize,
centerImage;
if (path) {
obj = this.path(path);
// expando properties for use in animate and attr
extend(obj, {
symbolName: symbol,
x: x,
y: y,
width: width,
height: height
});
if (options) {
extend(obj, options);
}
// image symbols
} else if (imageRegex.test(symbol)) {
// On image load, set the size and position
centerImage = function (img, size) {
if (img.element) { // it may be destroyed in the meantime (#1390)
img.attr({
width: size[0],
height: size[1]
});
if (!img.alignByTranslate) { // #185
img.translate(
mathRound((width - size[0]) / 2), // #1378
mathRound((height - size[1]) / 2)
);
}
}
};
imageSrc = symbol.match(imageRegex)[1];
imageSize = symbolSizes[imageSrc];
// Ireate the image synchronously, add attribs async
obj = this.image(imageSrc)
.attr({
x: x,
y: y
});
obj.isImg = true;
if (imageSize) {
centerImage(obj, imageSize);
} else {
// Initialize image to be 0 size so export will still function if there's no cached sizes.
//
obj.attr({ width: 0, height: 0 });
// Create a dummy JavaScript image to get the width and height. Due to a bug in IE < 8,
// the created element must be assigned to a variable in order to load (#292).
imageElement = createElement('img', {
onload: function () {
centerImage(obj, symbolSizes[imageSrc] = [this.width, this.height]);
},
src: imageSrc
});
}
}
return obj;
},
/**
* An extendable collection of functions for defining symbol paths.
*/
symbols: {
'circle': function (x, y, w, h) {
var cpw = 0.166 * w;
return [
M, x + w / 2, y,
'C', x + w + cpw, y, x + w + cpw, y + h, x + w / 2, y + h,
'C', x - cpw, y + h, x - cpw, y, x + w / 2, y,
'Z'
];
},
'square': function (x, y, w, h) {
return [
M, x, y,
L, x + w, y,
x + w, y + h,
x, y + h,
'Z'
];
},
'triangle': function (x, y, w, h) {
return [
M, x + w / 2, y,
L, x + w, y + h,
x, y + h,
'Z'
];
},
'triangle-down': function (x, y, w, h) {
return [
M, x, y,
L, x + w, y,
x + w / 2, y + h,
'Z'
];
},
'diamond': function (x, y, w, h) {
return [
M, x + w / 2, y,
L, x + w, y + h / 2,
x + w / 2, y + h,
x, y + h / 2,
'Z'
];
},
'arc': function (x, y, w, h, options) {
var start = options.start,
radius = options.r || w || h,
end = options.end - 0.001, // to prevent cos and sin of start and end from becoming equal on 360 arcs (related: #1561)
innerRadius = options.innerR,
open = options.open,
cosStart = mathCos(start),
sinStart = mathSin(start),
cosEnd = mathCos(end),
sinEnd = mathSin(end),
longArc = options.end - start < mathPI ? 0 : 1;
return [
M,
x + radius * cosStart,
y + radius * sinStart,
'A', // arcTo
radius, // x radius
radius, // y radius
0, // slanting
longArc, // long or short arc
1, // clockwise
x + radius * cosEnd,
y + radius * sinEnd,
open ? M : L,
x + innerRadius * cosEnd,
y + innerRadius * sinEnd,
'A', // arcTo
innerRadius, // x radius
innerRadius, // y radius
0, // slanting
longArc, // long or short arc
0, // clockwise
x + innerRadius * cosStart,
y + innerRadius * sinStart,
open ? '' : 'Z' // close
];
}
},
/**
* Define a clipping rectangle
* @param {String} id
* @param {Number} x
* @param {Number} y
* @param {Number} width
* @param {Number} height
*/
clipRect: function (x, y, width, height) {
var wrapper,
id = PREFIX + idCounter++,
clipPath = this.createElement('clipPath').attr({
id: id
}).add(this.defs);
wrapper = this.rect(x, y, width, height, 0).add(clipPath);
wrapper.id = id;
wrapper.clipPath = clipPath;
return wrapper;
},
/**
* Take a color and return it if it's a string, make it a gradient if it's a
* gradient configuration object. Prior to Highstock, an array was used to define
* a linear gradient with pixel positions relative to the SVG. In newer versions
* we change the coordinates to apply relative to the shape, using coordinates
* 0-1 within the shape. To preserve backwards compatibility, linearGradient
* in this definition is an object of x1, y1, x2 and y2.
*
* @param {Object} color The color or config object
*/
color: function (color, elem, prop) {
var renderer = this,
colorObject,
regexRgba = /^rgba/,
gradName,
gradAttr,
gradients,
gradientObject,
stops,
stopColor,
stopOpacity,
radialReference,
n,
id,
key = [];
// Apply linear or radial gradients
if (color && color.linearGradient) {
gradName = 'linearGradient';
} else if (color && color.radialGradient) {
gradName = 'radialGradient';
}
if (gradName) {
gradAttr = color[gradName];
gradients = renderer.gradients;
stops = color.stops;
radialReference = elem.radialReference;
// Keep < 2.2 kompatibility
if (isArray(gradAttr)) {
color[gradName] = gradAttr = {
x1: gradAttr[0],
y1: gradAttr[1],
x2: gradAttr[2],
y2: gradAttr[3],
gradientUnits: 'userSpaceOnUse'
};
}
// Correct the radial gradient for the radial reference system
if (gradName === 'radialGradient' && radialReference && !defined(gradAttr.gradientUnits)) {
gradAttr = merge(gradAttr, {
cx: (radialReference[0] - radialReference[2] / 2) + gradAttr.cx * radialReference[2],
cy: (radialReference[1] - radialReference[2] / 2) + gradAttr.cy * radialReference[2],
r: gradAttr.r * radialReference[2],
gradientUnits: 'userSpaceOnUse'
});
}
// Build the unique key to detect whether we need to create a new element (#1282)
for (n in gradAttr) {
if (n !== 'id') {
key.push(n, gradAttr[n]);
}
}
for (n in stops) {
key.push(stops[n]);
}
key = key.join(',');
// Check if a gradient object with the same config object is created within this renderer
if (gradients[key]) {
id = gradients[key].id;
} else {
// Set the id and create the element
gradAttr.id = id = PREFIX + idCounter++;
gradients[key] = gradientObject = renderer.createElement(gradName)
.attr(gradAttr)
.add(renderer.defs);
// The gradient needs to keep a list of stops to be able to destroy them
gradientObject.stops = [];
each(stops, function (stop) {
var stopObject;
if (regexRgba.test(stop[1])) {
colorObject = Color(stop[1]);
stopColor = colorObject.get('rgb');
stopOpacity = colorObject.get('a');
} else {
stopColor = stop[1];
stopOpacity = 1;
}
stopObject = renderer.createElement('stop').attr({
offset: stop[0],
'stop-color': stopColor,
'stop-opacity': stopOpacity
}).add(gradientObject);
// Add the stop element to the gradient
gradientObject.stops.push(stopObject);
});
}
// Return the reference to the gradient object
return 'url(' + renderer.url + '#' + id + ')';
// Webkit and Batik can't show rgba.
} else if (regexRgba.test(color)) {
colorObject = Color(color);
attr(elem, prop + '-opacity', colorObject.get('a'));
return colorObject.get('rgb');
} else {
// Remove the opacity attribute added above. Does not throw if the attribute is not there.
elem.removeAttribute(prop + '-opacity');
return color;
}
},
/**
* Add text to the SVG object
* @param {String} str
* @param {Number} x Left position
* @param {Number} y Top position
* @param {Boolean} useHTML Use HTML to render the text
*/
text: function (str, x, y, useHTML) {
// declare variables
var renderer = this,
defaultChartStyle = defaultOptions.chart.style,
fakeSVG = useCanVG || (!hasSVG && renderer.forExport),
wrapper;
if (useHTML && !renderer.forExport) {
return renderer.html(str, x, y);
}
x = mathRound(pick(x, 0));
y = mathRound(pick(y, 0));
wrapper = renderer.createElement('text')
.attr({
x: x,
y: y,
text: str
})
.css({
fontFamily: defaultChartStyle.fontFamily,
fontSize: defaultChartStyle.fontSize
});
// Prevent wrapping from creating false offsetWidths in export in legacy IE (#1079, #1063)
if (fakeSVG) {
wrapper.css({
position: ABSOLUTE
});
}
wrapper.x = x;
wrapper.y = y;
return wrapper;
},
/**
* Create HTML text node. This is used by the VML renderer as well as the SVG
* renderer through the useHTML option.
*
* @param {String} str
* @param {Number} x
* @param {Number} y
*/
html: function (str, x, y) {
var defaultChartStyle = defaultOptions.chart.style,
wrapper = this.createElement('span'),
attrSetters = wrapper.attrSetters,
element = wrapper.element,
renderer = wrapper.renderer;
// Text setter
attrSetters.text = function (value) {
if (value !== element.innerHTML) {
delete this.bBox;
}
element.innerHTML = value;
return false;
};
// Various setters which rely on update transform
attrSetters.x = attrSetters.y = attrSetters.align = function (value, key) {
if (key === 'align') {
key = 'textAlign'; // Do not overwrite the SVGElement.align method. Same as VML.
}
wrapper[key] = value;
wrapper.htmlUpdateTransform();
return false;
};
// Set the default attributes
wrapper.attr({
text: str,
x: mathRound(x),
y: mathRound(y)
})
.css({
position: ABSOLUTE,
whiteSpace: 'nowrap',
fontFamily: defaultChartStyle.fontFamily,
fontSize: defaultChartStyle.fontSize
});
// Use the HTML specific .css method
wrapper.css = wrapper.htmlCss;
// This is specific for HTML within SVG
if (renderer.isSVG) {
wrapper.add = function (svgGroupWrapper) {
var htmlGroup,
container = renderer.box.parentNode,
parentGroup,
parents = [];
// Create a mock group to hold the HTML elements
if (svgGroupWrapper) {
htmlGroup = svgGroupWrapper.div;
if (!htmlGroup) {
// Read the parent chain into an array and read from top down
parentGroup = svgGroupWrapper;
while (parentGroup) {
parents.push(parentGroup);
// Move up to the next parent group
parentGroup = parentGroup.parentGroup;
}
// Ensure dynamically updating position when any parent is translated
each(parents.reverse(), function (parentGroup) {
var htmlGroupStyle;
// Create a HTML div and append it to the parent div to emulate
// the SVG group structure
htmlGroup = parentGroup.div = parentGroup.div || createElement(DIV, {
className: attr(parentGroup.element, 'class')
}, {
position: ABSOLUTE,
left: (parentGroup.translateX || 0) + PX,
top: (parentGroup.translateY || 0) + PX
}, htmlGroup || container); // the top group is appended to container
// Shortcut
htmlGroupStyle = htmlGroup.style;
// Set listeners to update the HTML div's position whenever the SVG group
// position is changed
extend(parentGroup.attrSetters, {
translateX: function (value) {
htmlGroupStyle.left = value + PX;
},
translateY: function (value) {
htmlGroupStyle.top = value + PX;
},
visibility: function (value, key) {
htmlGroupStyle[key] = value;
}
});
});
}
} else {
htmlGroup = container;
}
htmlGroup.appendChild(element);
// Shared with VML:
wrapper.added = true;
if (wrapper.alignOnAdd) {
wrapper.htmlUpdateTransform();
}
return wrapper;
};
}
return wrapper;
},
/**
* Utility to return the baseline offset and total line height from the font size
*/
fontMetrics: function (fontSize) {
fontSize = pInt(fontSize || 11);
// Empirical values found by comparing font size and bounding box height.
// Applies to the default font family. http://jsfiddle.net/highcharts/7xvn7/
var lineHeight = fontSize < 24 ? fontSize + 4 : mathRound(fontSize * 1.2),
baseline = mathRound(lineHeight * 0.8);
return {
h: lineHeight,
b: baseline
};
},
/**
* Add a label, a text item that can hold a colored or gradient background
* as well as a border and shadow.
* @param {string} str
* @param {Number} x
* @param {Number} y
* @param {String} shape
* @param {Number} anchorX In case the shape has a pointer, like a flag, this is the
* coordinates it should be pinned to
* @param {Number} anchorY
* @param {Boolean} baseline Whether to position the label relative to the text baseline,
* like renderer.text, or to the upper border of the rectangle.
* @param {String} className Class name for the group
*/
label: function (str, x, y, shape, anchorX, anchorY, useHTML, baseline, className) {
var renderer = this,
wrapper = renderer.g(className),
text = renderer.text('', 0, 0, useHTML)
.attr({
zIndex: 1
}),
//.add(wrapper),
box,
bBox,
alignFactor = 0,
padding = 3,
paddingLeft = 0,
width,
height,
wrapperX,
wrapperY,
crispAdjust = 0,
deferredAttr = {},
baselineOffset,
attrSetters = wrapper.attrSetters,
needsBox;
/**
* This function runs after the label is added to the DOM (when the bounding box is
* available), and after the text of the label is updated to detect the new bounding
* box and reflect it in the border box.
*/
function updateBoxSize() {
var boxX,
boxY,
style = text.element.style;
bBox = (width === undefined || height === undefined || wrapper.styles.textAlign) &&
text.getBBox();
wrapper.width = (width || bBox.width || 0) + 2 * padding + paddingLeft;
wrapper.height = (height || bBox.height || 0) + 2 * padding;
// update the label-scoped y offset
baselineOffset = padding + renderer.fontMetrics(style && style.fontSize).b;
if (needsBox) {
// create the border box if it is not already present
if (!box) {
boxX = mathRound(-alignFactor * padding);
boxY = baseline ? -baselineOffset : 0;
wrapper.box = box = shape ?
renderer.symbol(shape, boxX, boxY, wrapper.width, wrapper.height) :
renderer.rect(boxX, boxY, wrapper.width, wrapper.height, 0, deferredAttr[STROKE_WIDTH]);
box.add(wrapper);
}
// apply the box attributes
if (!box.isImg) { // #1630
box.attr(merge({
width: wrapper.width,
height: wrapper.height
}, deferredAttr));
}
deferredAttr = null;
}
}
/**
* This function runs after setting text or padding, but only if padding is changed
*/
function updateTextPadding() {
var styles = wrapper.styles,
textAlign = styles && styles.textAlign,
x = paddingLeft + padding * (1 - alignFactor),
y;
// determin y based on the baseline
y = baseline ? 0 : baselineOffset;
// compensate for alignment
if (defined(width) && (textAlign === 'center' || textAlign === 'right')) {
x += { center: 0.5, right: 1 }[textAlign] * (width - bBox.width);
}
// update if anything changed
if (x !== text.x || y !== text.y) {
text.attr({
x: x,
y: y
});
}
// record current values
text.x = x;
text.y = y;
}
/**
* Set a box attribute, or defer it if the box is not yet created
* @param {Object} key
* @param {Object} value
*/
function boxAttr(key, value) {
if (box) {
box.attr(key, value);
} else {
deferredAttr[key] = value;
}
}
function getSizeAfterAdd() {
text.add(wrapper);
wrapper.attr({
text: str, // alignment is available now
x: x,
y: y
});
if (box && defined(anchorX)) {
wrapper.attr({
anchorX: anchorX,
anchorY: anchorY
});
}
}
/**
* After the text element is added, get the desired size of the border box
* and add it before the text in the DOM.
*/
addEvent(wrapper, 'add', getSizeAfterAdd);
/*
* Add specific attribute setters.
*/
// only change local variables
attrSetters.width = function (value) {
width = value;
return false;
};
attrSetters.height = function (value) {
height = value;
return false;
};
attrSetters.padding = function (value) {
if (defined(value) && value !== padding) {
padding = value;
updateTextPadding();
}
return false;
};
attrSetters.paddingLeft = function (value) {
if (defined(value) && value !== paddingLeft) {
paddingLeft = value;
updateTextPadding();
}
return false;
};
// change local variable and set attribue as well
attrSetters.align = function (value) {
alignFactor = { left: 0, center: 0.5, right: 1 }[value];
return false; // prevent setting text-anchor on the group
};
// apply these to the box and the text alike
attrSetters.text = function (value, key) {
text.attr(key, value);
updateBoxSize();
updateTextPadding();
return false;
};
// apply these to the box but not to the text
attrSetters[STROKE_WIDTH] = function (value, key) {
needsBox = true;
crispAdjust = value % 2 / 2;
boxAttr(key, value);
return false;
};
attrSetters.stroke = attrSetters.fill = attrSetters.r = function (value, key) {
if (key === 'fill') {
needsBox = true;
}
boxAttr(key, value);
return false;
};
attrSetters.anchorX = function (value, key) {
anchorX = value;
boxAttr(key, value + crispAdjust - wrapperX);
return false;
};
attrSetters.anchorY = function (value, key) {
anchorY = value;
boxAttr(key, value - wrapperY);
return false;
};
// rename attributes
attrSetters.x = function (value) {
wrapper.x = value; // for animation getter
value -= alignFactor * ((width || bBox.width) + padding);
wrapperX = mathRound(value);
wrapper.attr('translateX', wrapperX);
return false;
};
attrSetters.y = function (value) {
wrapperY = wrapper.y = mathRound(value);
wrapper.attr('translateY', wrapperY);
return false;
};
// Redirect certain methods to either the box or the text
var baseCss = wrapper.css;
return extend(wrapper, {
/**
* Pick up some properties and apply them to the text instead of the wrapper
*/
css: function (styles) {
if (styles) {
var textStyles = {};
styles = merge(styles); // create a copy to avoid altering the original object (#537)
each(['fontSize', 'fontWeight', 'fontFamily', 'color', 'lineHeight', 'width', 'textDecoration', 'textShadow'], function (prop) {
if (styles[prop] !== UNDEFINED) {
textStyles[prop] = styles[prop];
delete styles[prop];
}
});
text.css(textStyles);
}
return baseCss.call(wrapper, styles);
},
/**
* Return the bounding box of the box, not the group
*/
getBBox: function () {
return {
width: bBox.width + 2 * padding,
height: bBox.height + 2 * padding,
x: bBox.x - padding,
y: bBox.y - padding
};
},
/**
* Apply the shadow to the box
*/
shadow: function (b) {
if (box) {
box.shadow(b);
}
return wrapper;
},
/**
* Destroy and release memory.
*/
destroy: function () {
removeEvent(wrapper, 'add', getSizeAfterAdd);
// Added by button implementation
removeEvent(wrapper.element, 'mouseenter');
removeEvent(wrapper.element, 'mouseleave');
if (text) {
text = text.destroy();
}
if (box) {
box = box.destroy();
}
// Call base implementation to destroy the rest
SVGElement.prototype.destroy.call(wrapper);
// Release local pointers (#1298)
wrapper = renderer = updateBoxSize = updateTextPadding = boxAttr = getSizeAfterAdd = null;
}
});
}
}; // end SVGRenderer
// general renderer
Renderer = SVGRenderer;
/* ****************************************************************************
* *
* START OF INTERNET EXPLORER <= 8 SPECIFIC CODE *
* *
* For applications and websites that don't need IE support, like platform *
* targeted mobile apps and web apps, this code can be removed. *
* *
*****************************************************************************/
/**
* @constructor
*/
var VMLRenderer, VMLElement;
if (!hasSVG && !useCanVG) {
/**
* The VML element wrapper.
*/
Highcharts.VMLElement = VMLElement = {
/**
* Initialize a new VML element wrapper. It builds the markup as a string
* to minimize DOM traffic.
* @param {Object} renderer
* @param {Object} nodeName
*/
init: function (renderer, nodeName) {
var wrapper = this,
markup = ['<', nodeName, ' filled="f" stroked="f"'],
style = ['position: ', ABSOLUTE, ';'],
isDiv = nodeName === DIV;
// divs and shapes need size
if (nodeName === 'shape' || isDiv) {
style.push('left:0;top:0;width:1px;height:1px;');
}
style.push('visibility: ', isDiv ? HIDDEN : VISIBLE);
markup.push(' style="', style.join(''), '"/>');
// create element with default attributes and style
if (nodeName) {
markup = isDiv || nodeName === 'span' || nodeName === 'img' ?
markup.join('')
: renderer.prepVML(markup);
wrapper.element = createElement(markup);
}
wrapper.renderer = renderer;
wrapper.attrSetters = {};
},
/**
* Add the node to the given parent
* @param {Object} parent
*/
add: function (parent) {
var wrapper = this,
renderer = wrapper.renderer,
element = wrapper.element,
box = renderer.box,
inverted = parent && parent.inverted,
// get the parent node
parentNode = parent ?
parent.element || parent :
box;
// if the parent group is inverted, apply inversion on all children
if (inverted) { // only on groups
renderer.invertChild(element, parentNode);
}
// append it
parentNode.appendChild(element);
// align text after adding to be able to read offset
wrapper.added = true;
if (wrapper.alignOnAdd && !wrapper.deferUpdateTransform) {
wrapper.updateTransform();
}
// fire an event for internal hooks
fireEvent(wrapper, 'add');
return wrapper;
},
/**
* VML always uses htmlUpdateTransform
*/
updateTransform: SVGElement.prototype.htmlUpdateTransform,
/**
* Set the rotation of a span with oldIE's filter
*/
setSpanRotation: function (rotation, sintheta, costheta) {
// Adjust for alignment and rotation. Rotation of useHTML content is not yet implemented
// but it can probably be implemented for Firefox 3.5+ on user request. FF3.5+
// has support for CSS3 transform. The getBBox method also needs to be updated
// to compensate for the rotation, like it currently does for SVG.
// Test case: http://highcharts.com/tests/?file=text-rotation
css(this.element, {
filter: rotation ? ['progid:DXImageTransform.Microsoft.Matrix(M11=', costheta,
', M12=', -sintheta, ', M21=', sintheta, ', M22=', costheta,
', sizingMethod=\'auto expand\')'].join('') : NONE
});
},
/**
* Converts a subset of an SVG path definition to its VML counterpart. Takes an array
* as the parameter and returns a string.
*/
pathToVML: function (value) {
// convert paths
var i = value.length,
path = [],
clockwise;
while (i--) {
// Multiply by 10 to allow subpixel precision.
// Substracting half a pixel seems to make the coordinates
// align with SVG, but this hasn't been tested thoroughly
if (isNumber(value[i])) {
path[i] = mathRound(value[i] * 10) - 5;
} else if (value[i] === 'Z') { // close the path
path[i] = 'x';
} else {
path[i] = value[i];
// When the start X and end X coordinates of an arc are too close,
// they are rounded to the same value above. In this case, substract 1 from the end X
// position. #760, #1371.
if (value.isArc && (value[i] === 'wa' || value[i] === 'at')) {
clockwise = value[i] === 'wa' ? 1 : -1; // #1642
if (path[i + 5] === path[i + 7]) {
path[i + 7] -= clockwise;
}
// Start and end Y (#1410)
if (path[i + 6] === path[i + 8]) {
path[i + 8] -= clockwise;
}
}
}
}
// Loop up again to handle path shortcuts (#2132)
/*while (i++ < path.length) {
if (path[i] === 'H') { // horizontal line to
path[i] = 'L';
path.splice(i + 2, 0, path[i - 1]);
} else if (path[i] === 'V') { // vertical line to
path[i] = 'L';
path.splice(i + 1, 0, path[i - 2]);
}
}*/
return path.join(' ') || 'x';
},
/**
* Get or set attributes
*/
attr: function (hash, val) {
var wrapper = this,
key,
value,
i,
result,
element = wrapper.element || {},
elemStyle = element.style,
nodeName = element.nodeName,
renderer = wrapper.renderer,
symbolName = wrapper.symbolName,
hasSetSymbolSize,
shadows = wrapper.shadows,
skipAttr,
attrSetters = wrapper.attrSetters,
ret = wrapper;
// single key-value pair
if (isString(hash) && defined(val)) {
key = hash;
hash = {};
hash[key] = val;
}
// used as a getter, val is undefined
if (isString(hash)) {
key = hash;
if (key === 'strokeWidth' || key === 'stroke-width') {
ret = wrapper.strokeweight;
} else {
ret = wrapper[key];
}
// setter
} else {
for (key in hash) {
value = hash[key];
skipAttr = false;
// check for a specific attribute setter
result = attrSetters[key] && attrSetters[key].call(wrapper, value, key);
if (result !== false && value !== null) { // #620
if (result !== UNDEFINED) {
value = result; // the attribute setter has returned a new value to set
}
// prepare paths
// symbols
if (symbolName && /^(x|y|r|start|end|width|height|innerR|anchorX|anchorY)/.test(key)) {
// if one of the symbol size affecting parameters are changed,
// check all the others only once for each call to an element's
// .attr() method
if (!hasSetSymbolSize) {
wrapper.symbolAttr(hash);
hasSetSymbolSize = true;
}
skipAttr = true;
} else if (key === 'd') {
value = value || [];
wrapper.d = value.join(' '); // used in getter for animation
element.path = value = wrapper.pathToVML(value);
// update shadows
if (shadows) {
i = shadows.length;
while (i--) {
shadows[i].path = shadows[i].cutOff ? this.cutOffPath(value, shadows[i].cutOff) : value;
}
}
skipAttr = true;
// handle visibility
} else if (key === 'visibility') {
// let the shadow follow the main element
if (shadows) {
i = shadows.length;
while (i--) {
shadows[i].style[key] = value;
}
}
// Instead of toggling the visibility CSS property, move the div out of the viewport.
// This works around #61 and #586
if (nodeName === 'DIV') {
value = value === HIDDEN ? '-999em' : 0;
// In order to redraw, IE7 needs the div to be visible when tucked away
// outside the viewport. So the visibility is actually opposite of
// the expected value. This applies to the tooltip only.
if (!docMode8) {
elemStyle[key] = value ? VISIBLE : HIDDEN;
}
key = 'top';
}
elemStyle[key] = value;
skipAttr = true;
// directly mapped to css
} else if (key === 'zIndex') {
if (value) {
elemStyle[key] = value;
}
skipAttr = true;
// x, y, width, height
} else if (inArray(key, ['x', 'y', 'width', 'height']) !== -1) {
wrapper[key] = value; // used in getter
if (key === 'x' || key === 'y') {
key = { x: 'left', y: 'top' }[key];
} else {
value = mathMax(0, value); // don't set width or height below zero (#311)
}
// clipping rectangle special
if (wrapper.updateClipping) {
wrapper[key] = value; // the key is now 'left' or 'top' for 'x' and 'y'
wrapper.updateClipping();
} else {
// normal
elemStyle[key] = value;
}
skipAttr = true;
// class name
} else if (key === 'class' && nodeName === 'DIV') {
// IE8 Standards mode has problems retrieving the className
element.className = value;
// stroke
} else if (key === 'stroke') {
value = renderer.color(value, element, key);
key = 'strokecolor';
// stroke width
} else if (key === 'stroke-width' || key === 'strokeWidth') {
element.stroked = value ? true : false;
key = 'strokeweight';
wrapper[key] = value; // used in getter, issue #113
if (isNumber(value)) {
value += PX;
}
// dashStyle
} else if (key === 'dashstyle') {
var strokeElem = element.getElementsByTagName('stroke')[0] ||
createElement(renderer.prepVML(['<stroke/>']), null, null, element);
strokeElem[key] = value || 'solid';
wrapper.dashstyle = value; /* because changing stroke-width will change the dash length
and cause an epileptic effect */
skipAttr = true;
// fill
} else if (key === 'fill') {
if (nodeName === 'SPAN') { // text color
elemStyle.color = value;
} else if (nodeName !== 'IMG') { // #1336
element.filled = value !== NONE ? true : false;
value = renderer.color(value, element, key, wrapper);
key = 'fillcolor';
}
// opacity: don't bother - animation is too slow and filters introduce artifacts
} else if (key === 'opacity') {
/*css(element, {
opacity: value
});*/
skipAttr = true;
// rotation on VML elements
} else if (nodeName === 'shape' && key === 'rotation') {
wrapper[key] = element.style[key] = value; // style is for #1873
// Correction for the 1x1 size of the shape container. Used in gauge needles.
element.style.left = -mathRound(mathSin(value * deg2rad) + 1) + PX;
element.style.top = mathRound(mathCos(value * deg2rad)) + PX;
// translation for animation
} else if (key === 'translateX' || key === 'translateY' || key === 'rotation') {
wrapper[key] = value;
wrapper.updateTransform();
skipAttr = true;
// text for rotated and non-rotated elements
} else if (key === 'text') {
this.bBox = null;
element.innerHTML = value;
skipAttr = true;
}
if (!skipAttr) {
if (docMode8) { // IE8 setAttribute bug
element[key] = value;
} else {
attr(element, key, value);
}
}
}
}
}
return ret;
},
/**
* Set the element's clipping to a predefined rectangle
*
* @param {String} id The id of the clip rectangle
*/
clip: function (clipRect) {
var wrapper = this,
clipMembers,
cssRet;
if (clipRect) {
clipMembers = clipRect.members;
erase(clipMembers, wrapper); // Ensure unique list of elements (#1258)
clipMembers.push(wrapper);
wrapper.destroyClip = function () {
erase(clipMembers, wrapper);
};
cssRet = clipRect.getCSS(wrapper);
} else {
if (wrapper.destroyClip) {
wrapper.destroyClip();
}
cssRet = { clip: docMode8 ? 'inherit' : 'rect(auto)' }; // #1214
}
return wrapper.css(cssRet);
},
/**
* Set styles for the element
* @param {Object} styles
*/
css: SVGElement.prototype.htmlCss,
/**
* Removes a child either by removeChild or move to garbageBin.
* Issue 490; in VML removeChild results in Orphaned nodes according to sIEve, discardElement does not.
*/
safeRemoveChild: function (element) {
// discardElement will detach the node from its parent before attaching it
// to the garbage bin. Therefore it is important that the node is attached and have parent.
if (element.parentNode) {
discardElement(element);
}
},
/**
* Extend element.destroy by removing it from the clip members array
*/
destroy: function () {
if (this.destroyClip) {
this.destroyClip();
}
return SVGElement.prototype.destroy.apply(this);
},
/**
* Add an event listener. VML override for normalizing event parameters.
* @param {String} eventType
* @param {Function} handler
*/
on: function (eventType, handler) {
// simplest possible event model for internal use
this.element['on' + eventType] = function () {
var evt = win.event;
evt.target = evt.srcElement;
handler(evt);
};
return this;
},
/**
* In stacked columns, cut off the shadows so that they don't overlap
*/
cutOffPath: function (path, length) {
var len;
path = path.split(/[ ,]/);
len = path.length;
if (len === 9 || len === 11) {
path[len - 4] = path[len - 2] = pInt(path[len - 2]) - 10 * length;
}
return path.join(' ');
},
/**
* Apply a drop shadow by copying elements and giving them different strokes
* @param {Boolean|Object} shadowOptions
*/
shadow: function (shadowOptions, group, cutOff) {
var shadows = [],
i,
element = this.element,
renderer = this.renderer,
shadow,
elemStyle = element.style,
markup,
path = element.path,
strokeWidth,
modifiedPath,
shadowWidth,
shadowElementOpacity;
// some times empty paths are not strings
if (path && typeof path.value !== 'string') {
path = 'x';
}
modifiedPath = path;
if (shadowOptions) {
shadowWidth = pick(shadowOptions.width, 3);
shadowElementOpacity = (shadowOptions.opacity || 0.15) / shadowWidth;
for (i = 1; i <= 3; i++) {
strokeWidth = (shadowWidth * 2) + 1 - (2 * i);
// Cut off shadows for stacked column items
if (cutOff) {
modifiedPath = this.cutOffPath(path.value, strokeWidth + 0.5);
}
markup = ['<shape isShadow="true" strokeweight="', strokeWidth,
'" filled="false" path="', modifiedPath,
'" coordsize="10 10" style="', element.style.cssText, '" />'];
shadow = createElement(renderer.prepVML(markup),
null, {
left: pInt(elemStyle.left) + pick(shadowOptions.offsetX, 1),
top: pInt(elemStyle.top) + pick(shadowOptions.offsetY, 1)
}
);
if (cutOff) {
shadow.cutOff = strokeWidth + 1;
}
// apply the opacity
markup = ['<stroke color="', shadowOptions.color || 'black', '" opacity="', shadowElementOpacity * i, '"/>'];
createElement(renderer.prepVML(markup), null, null, shadow);
// insert it
if (group) {
group.element.appendChild(shadow);
} else {
element.parentNode.insertBefore(shadow, element);
}
// record it
shadows.push(shadow);
}
this.shadows = shadows;
}
return this;
}
};
VMLElement = extendClass(SVGElement, VMLElement);
/**
* The VML renderer
*/
var VMLRendererExtension = { // inherit SVGRenderer
Element: VMLElement,
isIE8: userAgent.indexOf('MSIE 8.0') > -1,
/**
* Initialize the VMLRenderer
* @param {Object} container
* @param {Number} width
* @param {Number} height
*/
init: function (container, width, height) {
var renderer = this,
boxWrapper,
box;
renderer.alignedObjects = [];
boxWrapper = renderer.createElement(DIV);
box = boxWrapper.element;
box.style.position = RELATIVE; // for freeform drawing using renderer directly
container.appendChild(boxWrapper.element);
// generate the containing box
renderer.isVML = true;
renderer.box = box;
renderer.boxWrapper = boxWrapper;
renderer.setSize(width, height, false);
// The only way to make IE6 and IE7 print is to use a global namespace. However,
// with IE8 the only way to make the dynamic shapes visible in screen and print mode
// seems to be to add the xmlns attribute and the behaviour style inline.
if (!doc.namespaces.hcv) {
doc.namespaces.add('hcv', 'urn:schemas-microsoft-com:vml');
// Setup default CSS (#2153)
(doc.styleSheets.length ? doc.styleSheets[0] : doc.createStyleSheet()).cssText +=
'hcv\\:fill, hcv\\:path, hcv\\:shape, hcv\\:stroke' +
'{ behavior:url(#default#VML); display: inline-block; } ';
}
},
/**
* Detect whether the renderer is hidden. This happens when one of the parent elements
* has display: none
*/
isHidden: function () {
return !this.box.offsetWidth;
},
/**
* Define a clipping rectangle. In VML it is accomplished by storing the values
* for setting the CSS style to all associated members.
*
* @param {Number} x
* @param {Number} y
* @param {Number} width
* @param {Number} height
*/
clipRect: function (x, y, width, height) {
// create a dummy element
var clipRect = this.createElement(),
isObj = isObject(x);
// mimic a rectangle with its style object for automatic updating in attr
return extend(clipRect, {
members: [],
left: (isObj ? x.x : x) + 1,
top: (isObj ? x.y : y) + 1,
width: (isObj ? x.width : width) - 1,
height: (isObj ? x.height : height) - 1,
getCSS: function (wrapper) {
var element = wrapper.element,
nodeName = element.nodeName,
isShape = nodeName === 'shape',
inverted = wrapper.inverted,
rect = this,
top = rect.top - (isShape ? element.offsetTop : 0),
left = rect.left,
right = left + rect.width,
bottom = top + rect.height,
ret = {
clip: 'rect(' +
mathRound(inverted ? left : top) + 'px,' +
mathRound(inverted ? bottom : right) + 'px,' +
mathRound(inverted ? right : bottom) + 'px,' +
mathRound(inverted ? top : left) + 'px)'
};
// issue 74 workaround
if (!inverted && docMode8 && nodeName === 'DIV') {
extend(ret, {
width: right + PX,
height: bottom + PX
});
}
return ret;
},
// used in attr and animation to update the clipping of all members
updateClipping: function () {
each(clipRect.members, function (member) {
member.css(clipRect.getCSS(member));
});
}
});
},
/**
* Take a color and return it if it's a string, make it a gradient if it's a
* gradient configuration object, and apply opacity.
*
* @param {Object} color The color or config object
*/
color: function (color, elem, prop, wrapper) {
var renderer = this,
colorObject,
regexRgba = /^rgba/,
markup,
fillType,
ret = NONE;
// Check for linear or radial gradient
if (color && color.linearGradient) {
fillType = 'gradient';
} else if (color && color.radialGradient) {
fillType = 'pattern';
}
if (fillType) {
var stopColor,
stopOpacity,
gradient = color.linearGradient || color.radialGradient,
x1,
y1,
x2,
y2,
opacity1,
opacity2,
color1,
color2,
fillAttr = '',
stops = color.stops,
firstStop,
lastStop,
colors = [],
addFillNode = function () {
// Add the fill subnode. When colors attribute is used, the meanings of opacity and o:opacity2
// are reversed.
markup = ['<fill colors="' + colors.join(',') + '" opacity="', opacity2, '" o:opacity2="', opacity1,
'" type="', fillType, '" ', fillAttr, 'focus="100%" method="any" />'];
createElement(renderer.prepVML(markup), null, null, elem);
};
// Extend from 0 to 1
firstStop = stops[0];
lastStop = stops[stops.length - 1];
if (firstStop[0] > 0) {
stops.unshift([
0,
firstStop[1]
]);
}
if (lastStop[0] < 1) {
stops.push([
1,
lastStop[1]
]);
}
// Compute the stops
each(stops, function (stop, i) {
if (regexRgba.test(stop[1])) {
colorObject = Color(stop[1]);
stopColor = colorObject.get('rgb');
stopOpacity = colorObject.get('a');
} else {
stopColor = stop[1];
stopOpacity = 1;
}
// Build the color attribute
colors.push((stop[0] * 100) + '% ' + stopColor);
// Only start and end opacities are allowed, so we use the first and the last
if (!i) {
opacity1 = stopOpacity;
color2 = stopColor;
} else {
opacity2 = stopOpacity;
color1 = stopColor;
}
});
// Apply the gradient to fills only.
if (prop === 'fill') {
// Handle linear gradient angle
if (fillType === 'gradient') {
x1 = gradient.x1 || gradient[0] || 0;
y1 = gradient.y1 || gradient[1] || 0;
x2 = gradient.x2 || gradient[2] || 0;
y2 = gradient.y2 || gradient[3] || 0;
fillAttr = 'angle="' + (90 - math.atan(
(y2 - y1) / // y vector
(x2 - x1) // x vector
) * 180 / mathPI) + '"';
addFillNode();
// Radial (circular) gradient
} else {
var r = gradient.r,
sizex = r * 2,
sizey = r * 2,
cx = gradient.cx,
cy = gradient.cy,
radialReference = elem.radialReference,
bBox,
applyRadialGradient = function () {
if (radialReference) {
bBox = wrapper.getBBox();
cx += (radialReference[0] - bBox.x) / bBox.width - 0.5;
cy += (radialReference[1] - bBox.y) / bBox.height - 0.5;
sizex *= radialReference[2] / bBox.width;
sizey *= radialReference[2] / bBox.height;
}
fillAttr = 'src="' + defaultOptions.global.VMLRadialGradientURL + '" ' +
'size="' + sizex + ',' + sizey + '" ' +
'origin="0.5,0.5" ' +
'position="' + cx + ',' + cy + '" ' +
'color2="' + color2 + '" ';
addFillNode();
};
// Apply radial gradient
if (wrapper.added) {
applyRadialGradient();
} else {
// We need to know the bounding box to get the size and position right
addEvent(wrapper, 'add', applyRadialGradient);
}
// The fill element's color attribute is broken in IE8 standards mode, so we
// need to set the parent shape's fillcolor attribute instead.
ret = color1;
}
// Gradients are not supported for VML stroke, return the first color. #722.
} else {
ret = stopColor;
}
// if the color is an rgba color, split it and add a fill node
// to hold the opacity component
} else if (regexRgba.test(color) && elem.tagName !== 'IMG') {
colorObject = Color(color);
markup = ['<', prop, ' opacity="', colorObject.get('a'), '"/>'];
createElement(this.prepVML(markup), null, null, elem);
ret = colorObject.get('rgb');
} else {
var propNodes = elem.getElementsByTagName(prop); // 'stroke' or 'fill' node
if (propNodes.length) {
propNodes[0].opacity = 1;
propNodes[0].type = 'solid';
}
ret = color;
}
return ret;
},
/**
* Take a VML string and prepare it for either IE8 or IE6/IE7.
* @param {Array} markup A string array of the VML markup to prepare
*/
prepVML: function (markup) {
var vmlStyle = 'display:inline-block;behavior:url(#default#VML);',
isIE8 = this.isIE8;
markup = markup.join('');
if (isIE8) { // add xmlns and style inline
markup = markup.replace('/>', ' xmlns="urn:schemas-microsoft-com:vml" />');
if (markup.indexOf('style="') === -1) {
markup = markup.replace('/>', ' style="' + vmlStyle + '" />');
} else {
markup = markup.replace('style="', 'style="' + vmlStyle);
}
} else { // add namespace
markup = markup.replace('<', '<hcv:');
}
return markup;
},
/**
* Create rotated and aligned text
* @param {String} str
* @param {Number} x
* @param {Number} y
*/
text: SVGRenderer.prototype.html,
/**
* Create and return a path element
* @param {Array} path
*/
path: function (path) {
var attr = {
// subpixel precision down to 0.1 (width and height = 1px)
coordsize: '10 10'
};
if (isArray(path)) {
attr.d = path;
} else if (isObject(path)) { // attributes
extend(attr, path);
}
// create the shape
return this.createElement('shape').attr(attr);
},
/**
* Create and return a circle element. In VML circles are implemented as
* shapes, which is faster than v:oval
* @param {Number} x
* @param {Number} y
* @param {Number} r
*/
circle: function (x, y, r) {
var circle = this.symbol('circle');
if (isObject(x)) {
r = x.r;
y = x.y;
x = x.x;
}
circle.isCircle = true; // Causes x and y to mean center (#1682)
circle.r = r;
return circle.attr({ x: x, y: y });
},
/**
* Create a group using an outer div and an inner v:group to allow rotating
* and flipping. A simple v:group would have problems with positioning
* child HTML elements and CSS clip.
*
* @param {String} name The name of the group
*/
g: function (name) {
var wrapper,
attribs;
// set the class name
if (name) {
attribs = { 'className': PREFIX + name, 'class': PREFIX + name };
}
// the div to hold HTML and clipping
wrapper = this.createElement(DIV).attr(attribs);
return wrapper;
},
/**
* VML override to create a regular HTML image
* @param {String} src
* @param {Number} x
* @param {Number} y
* @param {Number} width
* @param {Number} height
*/
image: function (src, x, y, width, height) {
var obj = this.createElement('img')
.attr({ src: src });
if (arguments.length > 1) {
obj.attr({
x: x,
y: y,
width: width,
height: height
});
}
return obj;
},
/**
* VML uses a shape for rect to overcome bugs and rotation problems
*/
rect: function (x, y, width, height, r, strokeWidth) {
var wrapper = this.symbol('rect');
wrapper.r = isObject(x) ? x.r : r;
//return wrapper.attr(wrapper.crisp(strokeWidth, x, y, mathMax(width, 0), mathMax(height, 0)));
return wrapper.attr(
isObject(x) ?
x :
// do not crispify when an object is passed in (as in column charts)
wrapper.crisp(strokeWidth, x, y, mathMax(width, 0), mathMax(height, 0))
);
},
/**
* In the VML renderer, each child of an inverted div (group) is inverted
* @param {Object} element
* @param {Object} parentNode
*/
invertChild: function (element, parentNode) {
var parentStyle = parentNode.style;
css(element, {
flip: 'x',
left: pInt(parentStyle.width) - 1,
top: pInt(parentStyle.height) - 1,
rotation: -90
});
},
/**
* Symbol definitions that override the parent SVG renderer's symbols
*
*/
symbols: {
// VML specific arc function
arc: function (x, y, w, h, options) {
var start = options.start,
end = options.end,
radius = options.r || w || h,
innerRadius = options.innerR,
cosStart = mathCos(start),
sinStart = mathSin(start),
cosEnd = mathCos(end),
sinEnd = mathSin(end),
ret;
if (end - start === 0) { // no angle, don't show it.
return ['x'];
}
ret = [
'wa', // clockwise arc to
x - radius, // left
y - radius, // top
x + radius, // right
y + radius, // bottom
x + radius * cosStart, // start x
y + radius * sinStart, // start y
x + radius * cosEnd, // end x
y + radius * sinEnd // end y
];
if (options.open && !innerRadius) {
ret.push(
'e',
M,
x,// - innerRadius,
y// - innerRadius
);
}
ret.push(
'at', // anti clockwise arc to
x - innerRadius, // left
y - innerRadius, // top
x + innerRadius, // right
y + innerRadius, // bottom
x + innerRadius * cosEnd, // start x
y + innerRadius * sinEnd, // start y
x + innerRadius * cosStart, // end x
y + innerRadius * sinStart, // end y
'x', // finish path
'e' // close
);
ret.isArc = true;
return ret;
},
// Add circle symbol path. This performs significantly faster than v:oval.
circle: function (x, y, w, h, wrapper) {
if (wrapper) {
w = h = 2 * wrapper.r;
}
// Center correction, #1682
if (wrapper && wrapper.isCircle) {
x -= w / 2;
y -= h / 2;
}
// Return the path
return [
'wa', // clockwisearcto
x, // left
y, // top
x + w, // right
y + h, // bottom
x + w, // start x
y + h / 2, // start y
x + w, // end x
y + h / 2, // end y
//'x', // finish path
'e' // close
];
},
/**
* Add rectangle symbol path which eases rotation and omits arcsize problems
* compared to the built-in VML roundrect shape
*
* @param {Number} left Left position
* @param {Number} top Top position
* @param {Number} r Border radius
* @param {Object} options Width and height
*/
rect: function (left, top, width, height, options) {
var right = left + width,
bottom = top + height,
ret,
r;
// No radius, return the more lightweight square
if (!defined(options) || !options.r) {
ret = SVGRenderer.prototype.symbols.square.apply(0, arguments);
// Has radius add arcs for the corners
} else {
r = mathMin(options.r, width, height);
ret = [
M,
left + r, top,
L,
right - r, top,
'wa',
right - 2 * r, top,
right, top + 2 * r,
right - r, top,
right, top + r,
L,
right, bottom - r,
'wa',
right - 2 * r, bottom - 2 * r,
right, bottom,
right, bottom - r,
right - r, bottom,
L,
left + r, bottom,
'wa',
left, bottom - 2 * r,
left + 2 * r, bottom,
left + r, bottom,
left, bottom - r,
L,
left, top + r,
'wa',
left, top,
left + 2 * r, top + 2 * r,
left, top + r,
left + r, top,
'x',
'e'
];
}
return ret;
}
}
};
Highcharts.VMLRenderer = VMLRenderer = function () {
this.init.apply(this, arguments);
};
VMLRenderer.prototype = merge(SVGRenderer.prototype, VMLRendererExtension);
// general renderer
Renderer = VMLRenderer;
}
/* ****************************************************************************
* *
* END OF INTERNET EXPLORER <= 8 SPECIFIC CODE *
* *
*****************************************************************************/
/* ****************************************************************************
* *
* START OF ANDROID < 3 SPECIFIC CODE. THIS CAN BE REMOVED IF YOU'RE NOT *
* TARGETING THAT SYSTEM. *
* *
*****************************************************************************/
var CanVGRenderer,
CanVGController;
if (useCanVG) {
/**
* The CanVGRenderer is empty from start to keep the source footprint small.
* When requested, the CanVGController downloads the rest of the source packaged
* together with the canvg library.
*/
Highcharts.CanVGRenderer = CanVGRenderer = function () {
// Override the global SVG namespace to fake SVG/HTML that accepts CSS
SVG_NS = 'http://www.w3.org/1999/xhtml';
};
/**
* Start with an empty symbols object. This is needed when exporting is used (exporting.src.js will add a few symbols), but
* the implementation from SvgRenderer will not be merged in until first render.
*/
CanVGRenderer.prototype.symbols = {};
/**
* Handles on demand download of canvg rendering support.
*/
CanVGController = (function () {
// List of renderering calls
var deferredRenderCalls = [];
/**
* When downloaded, we are ready to draw deferred charts.
*/
function drawDeferred() {
var callLength = deferredRenderCalls.length,
callIndex;
// Draw all pending render calls
for (callIndex = 0; callIndex < callLength; callIndex++) {
deferredRenderCalls[callIndex]();
}
// Clear the list
deferredRenderCalls = [];
}
return {
push: function (func, scriptLocation) {
// Only get the script once
if (deferredRenderCalls.length === 0) {
getScript(scriptLocation, drawDeferred);
}
// Register render call
deferredRenderCalls.push(func);
}
};
}());
Renderer = CanVGRenderer;
} // end CanVGRenderer
/* ****************************************************************************
* *
* END OF ANDROID < 3 SPECIFIC CODE *
* *
*****************************************************************************/
/**
* The Tick class
*/
function Tick(axis, pos, type, noLabel) {
this.axis = axis;
this.pos = pos;
this.type = type || '';
this.isNew = true;
if (!type && !noLabel) {
this.addLabel();
}
}
Tick.prototype = {
/**
* Write the tick label
*/
addLabel: function () {
var tick = this,
axis = tick.axis,
options = axis.options,
chart = axis.chart,
horiz = axis.horiz,
categories = axis.categories,
names = axis.series[0] && axis.series[0].names,
pos = tick.pos,
labelOptions = options.labels,
str,
tickPositions = axis.tickPositions,
width = (horiz && categories &&
!labelOptions.step && !labelOptions.staggerLines &&
!labelOptions.rotation &&
chart.plotWidth / tickPositions.length) ||
(!horiz && (chart.margin[3] || chart.chartWidth * 0.33)), // #1580, #1931
isFirst = pos === tickPositions[0],
isLast = pos === tickPositions[tickPositions.length - 1],
css,
attr,
value = categories ?
pick(categories[pos], names && names[pos], pos) :
pos,
label = tick.label,
tickPositionInfo = tickPositions.info,
dateTimeLabelFormat;
// Set the datetime label format. If a higher rank is set for this position, use that. If not,
// use the general format.
if (axis.isDatetimeAxis && tickPositionInfo) {
dateTimeLabelFormat = options.dateTimeLabelFormats[tickPositionInfo.higherRanks[pos] || tickPositionInfo.unitName];
}
// set properties for access in render method
tick.isFirst = isFirst;
tick.isLast = isLast;
// get the string
str = axis.labelFormatter.call({
axis: axis,
chart: chart,
isFirst: isFirst,
isLast: isLast,
dateTimeLabelFormat: dateTimeLabelFormat,
value: axis.isLog ? correctFloat(lin2log(value)) : value
});
// prepare CSS
css = width && { width: mathMax(1, mathRound(width - 2 * (labelOptions.padding || 10))) + PX };
css = extend(css, labelOptions.style);
// first call
if (!defined(label)) {
attr = {
align: axis.labelAlign
};
if (isNumber(labelOptions.rotation)) {
attr.rotation = labelOptions.rotation;
}
if (width && labelOptions.ellipsis) {
attr._clipHeight = axis.len / tickPositions.length;
}
tick.label =
defined(str) && labelOptions.enabled ?
chart.renderer.text(
str,
0,
0,
labelOptions.useHTML
)
.attr(attr)
// without position absolute, IE export sometimes is wrong
.css(css)
.add(axis.labelGroup) :
null;
// update
} else if (label) {
label.attr({
text: str
})
.css(css);
}
},
/**
* Get the offset height or width of the label
*/
getLabelSize: function () {
var label = this.label,
axis = this.axis;
return label ?
((this.labelBBox = label.getBBox()))[axis.horiz ? 'height' : 'width'] :
0;
},
/**
* Find how far the labels extend to the right and left of the tick's x position. Used for anti-collision
* detection with overflow logic.
*/
getLabelSides: function () {
var bBox = this.labelBBox, // assume getLabelSize has run at this point
axis = this.axis,
options = axis.options,
labelOptions = options.labels,
width = bBox.width,
leftSide = width * { left: 0, center: 0.5, right: 1 }[axis.labelAlign] - labelOptions.x;
return [-leftSide, width - leftSide];
},
/**
* Handle the label overflow by adjusting the labels to the left and right edge, or
* hide them if they collide into the neighbour label.
*/
handleOverflow: function (index, xy) {
var show = true,
axis = this.axis,
chart = axis.chart,
isFirst = this.isFirst,
isLast = this.isLast,
x = xy.x,
reversed = axis.reversed,
tickPositions = axis.tickPositions;
if (isFirst || isLast) {
var sides = this.getLabelSides(),
leftSide = sides[0],
rightSide = sides[1],
plotLeft = chart.plotLeft,
plotRight = plotLeft + axis.len,
neighbour = axis.ticks[tickPositions[index + (isFirst ? 1 : -1)]],
neighbourEdge = neighbour && neighbour.label.xy && neighbour.label.xy.x + neighbour.getLabelSides()[isFirst ? 0 : 1];
if ((isFirst && !reversed) || (isLast && reversed)) {
// Is the label spilling out to the left of the plot area?
if (x + leftSide < plotLeft) {
// Align it to plot left
x = plotLeft - leftSide;
// Hide it if it now overlaps the neighbour label
if (neighbour && x + rightSide > neighbourEdge) {
show = false;
}
}
} else {
// Is the label spilling out to the right of the plot area?
if (x + rightSide > plotRight) {
// Align it to plot right
x = plotRight - rightSide;
// Hide it if it now overlaps the neighbour label
if (neighbour && x + leftSide < neighbourEdge) {
show = false;
}
}
}
// Set the modified x position of the label
xy.x = x;
}
return show;
},
/**
* Get the x and y position for ticks and labels
*/
getPosition: function (horiz, pos, tickmarkOffset, old) {
var axis = this.axis,
chart = axis.chart,
cHeight = (old && chart.oldChartHeight) || chart.chartHeight;
return {
x: horiz ?
axis.translate(pos + tickmarkOffset, null, null, old) + axis.transB :
axis.left + axis.offset + (axis.opposite ? ((old && chart.oldChartWidth) || chart.chartWidth) - axis.right - axis.left : 0),
y: horiz ?
cHeight - axis.bottom + axis.offset - (axis.opposite ? axis.height : 0) :
cHeight - axis.translate(pos + tickmarkOffset, null, null, old) - axis.transB
};
},
/**
* Get the x, y position of the tick label
*/
getLabelPosition: function (x, y, label, horiz, labelOptions, tickmarkOffset, index, step) {
var axis = this.axis,
transA = axis.transA,
reversed = axis.reversed,
staggerLines = axis.staggerLines,
baseline = axis.chart.renderer.fontMetrics(labelOptions.style.fontSize).b,
rotation = labelOptions.rotation;
x = x + labelOptions.x - (tickmarkOffset && horiz ?
tickmarkOffset * transA * (reversed ? -1 : 1) : 0);
y = y + labelOptions.y - (tickmarkOffset && !horiz ?
tickmarkOffset * transA * (reversed ? 1 : -1) : 0);
// Correct for rotation (#1764)
if (rotation && axis.side === 2) {
y -= baseline - baseline * mathCos(rotation * deg2rad);
}
// Vertically centered
if (!defined(labelOptions.y) && !rotation) { // #1951
y += baseline - label.getBBox().height / 2;
}
// Correct for staggered labels
if (staggerLines) {
y += (index / (step || 1) % staggerLines) * (axis.labelOffset / staggerLines);
}
return {
x: x,
y: y
};
},
/**
* Extendible method to return the path of the marker
*/
getMarkPath: function (x, y, tickLength, tickWidth, horiz, renderer) {
return renderer.crispLine([
M,
x,
y,
L,
x + (horiz ? 0 : -tickLength),
y + (horiz ? tickLength : 0)
], tickWidth);
},
/**
* Put everything in place
*
* @param index {Number}
* @param old {Boolean} Use old coordinates to prepare an animation into new position
*/
render: function (index, old, opacity) {
var tick = this,
axis = tick.axis,
options = axis.options,
chart = axis.chart,
renderer = chart.renderer,
horiz = axis.horiz,
type = tick.type,
label = tick.label,
pos = tick.pos,
labelOptions = options.labels,
gridLine = tick.gridLine,
gridPrefix = type ? type + 'Grid' : 'grid',
tickPrefix = type ? type + 'Tick' : 'tick',
gridLineWidth = options[gridPrefix + 'LineWidth'],
gridLineColor = options[gridPrefix + 'LineColor'],
dashStyle = options[gridPrefix + 'LineDashStyle'],
tickLength = options[tickPrefix + 'Length'],
tickWidth = options[tickPrefix + 'Width'] || 0,
tickColor = options[tickPrefix + 'Color'],
tickPosition = options[tickPrefix + 'Position'],
gridLinePath,
mark = tick.mark,
markPath,
step = labelOptions.step,
attribs,
show = true,
tickmarkOffset = axis.tickmarkOffset,
xy = tick.getPosition(horiz, pos, tickmarkOffset, old),
x = xy.x,
y = xy.y,
reverseCrisp = ((horiz && x === axis.pos + axis.len) || (!horiz && y === axis.pos)) ? -1 : 1, // #1480, #1687
staggerLines = axis.staggerLines;
this.isActive = true;
// create the grid line
if (gridLineWidth) {
gridLinePath = axis.getPlotLinePath(pos + tickmarkOffset, gridLineWidth * reverseCrisp, old, true);
if (gridLine === UNDEFINED) {
attribs = {
stroke: gridLineColor,
'stroke-width': gridLineWidth
};
if (dashStyle) {
attribs.dashstyle = dashStyle;
}
if (!type) {
attribs.zIndex = 1;
}
if (old) {
attribs.opacity = 0;
}
tick.gridLine = gridLine =
gridLineWidth ?
renderer.path(gridLinePath)
.attr(attribs).add(axis.gridGroup) :
null;
}
// If the parameter 'old' is set, the current call will be followed
// by another call, therefore do not do any animations this time
if (!old && gridLine && gridLinePath) {
gridLine[tick.isNew ? 'attr' : 'animate']({
d: gridLinePath,
opacity: opacity
});
}
}
// create the tick mark
if (tickWidth && tickLength) {
// negate the length
if (tickPosition === 'inside') {
tickLength = -tickLength;
}
if (axis.opposite) {
tickLength = -tickLength;
}
markPath = tick.getMarkPath(x, y, tickLength, tickWidth * reverseCrisp, horiz, renderer);
if (mark) { // updating
mark.animate({
d: markPath,
opacity: opacity
});
} else { // first time
tick.mark = renderer.path(
markPath
).attr({
stroke: tickColor,
'stroke-width': tickWidth,
opacity: opacity
}).add(axis.axisGroup);
}
}
// the label is created on init - now move it into place
if (label && !isNaN(x)) {
label.xy = xy = tick.getLabelPosition(x, y, label, horiz, labelOptions, tickmarkOffset, index, step);
// Apply show first and show last. If the tick is both first and last, it is
// a single centered tick, in which case we show the label anyway (#2100).
if ((tick.isFirst && !tick.isLast && !pick(options.showFirstLabel, 1)) ||
(tick.isLast && !tick.isFirst && !pick(options.showLastLabel, 1))) {
show = false;
// Handle label overflow and show or hide accordingly
} else if (!staggerLines && horiz && labelOptions.overflow === 'justify' && !tick.handleOverflow(index, xy)) {
show = false;
}
// apply step
if (step && index % step) {
// show those indices dividable by step
show = false;
}
// Set the new position, and show or hide
if (show && !isNaN(xy.y)) {
xy.opacity = opacity;
label[tick.isNew ? 'attr' : 'animate'](xy);
tick.isNew = false;
} else {
label.attr('y', -9999); // #1338
}
}
},
/**
* Destructor for the tick prototype
*/
destroy: function () {
destroyObjectProperties(this, this.axis);
}
};
/**
* The object wrapper for plot lines and plot bands
* @param {Object} options
*/
function PlotLineOrBand(axis, options) {
this.axis = axis;
if (options) {
this.options = options;
this.id = options.id;
}
}
PlotLineOrBand.prototype = {
/**
* Render the plot line or plot band. If it is already existing,
* move it.
*/
render: function () {
var plotLine = this,
axis = plotLine.axis,
horiz = axis.horiz,
halfPointRange = (axis.pointRange || 0) / 2,
options = plotLine.options,
optionsLabel = options.label,
label = plotLine.label,
width = options.width,
to = options.to,
from = options.from,
isBand = defined(from) && defined(to),
value = options.value,
dashStyle = options.dashStyle,
svgElem = plotLine.svgElem,
path = [],
addEvent,
eventType,
xs,
ys,
x,
y,
color = options.color,
zIndex = options.zIndex,
events = options.events,
attribs,
renderer = axis.chart.renderer;
// logarithmic conversion
if (axis.isLog) {
from = log2lin(from);
to = log2lin(to);
value = log2lin(value);
}
// plot line
if (width) {
path = axis.getPlotLinePath(value, width);
attribs = {
stroke: color,
'stroke-width': width
};
if (dashStyle) {
attribs.dashstyle = dashStyle;
}
} else if (isBand) { // plot band
// keep within plot area
from = mathMax(from, axis.min - halfPointRange);
to = mathMin(to, axis.max + halfPointRange);
path = axis.getPlotBandPath(from, to, options);
attribs = {
fill: color
};
if (options.borderWidth) {
attribs.stroke = options.borderColor;
attribs['stroke-width'] = options.borderWidth;
}
} else {
return;
}
// zIndex
if (defined(zIndex)) {
attribs.zIndex = zIndex;
}
// common for lines and bands
if (svgElem) {
if (path) {
svgElem.animate({
d: path
}, null, svgElem.onGetPath);
} else {
svgElem.hide();
svgElem.onGetPath = function () {
svgElem.show();
};
}
} else if (path && path.length) {
plotLine.svgElem = svgElem = renderer.path(path)
.attr(attribs).add();
// events
if (events) {
addEvent = function (eventType) {
svgElem.on(eventType, function (e) {
events[eventType].apply(plotLine, [e]);
});
};
for (eventType in events) {
addEvent(eventType);
}
}
}
// the plot band/line label
if (optionsLabel && defined(optionsLabel.text) && path && path.length && axis.width > 0 && axis.height > 0) {
// apply defaults
optionsLabel = merge({
align: horiz && isBand && 'center',
x: horiz ? !isBand && 4 : 10,
verticalAlign : !horiz && isBand && 'middle',
y: horiz ? isBand ? 16 : 10 : isBand ? 6 : -4,
rotation: horiz && !isBand && 90
}, optionsLabel);
// add the SVG element
if (!label) {
plotLine.label = label = renderer.text(
optionsLabel.text,
0,
0,
optionsLabel.useHTML
)
.attr({
align: optionsLabel.textAlign || optionsLabel.align,
rotation: optionsLabel.rotation,
zIndex: zIndex
})
.css(optionsLabel.style)
.add();
}
// get the bounding box and align the label
xs = [path[1], path[4], pick(path[6], path[1])];
ys = [path[2], path[5], pick(path[7], path[2])];
x = arrayMin(xs);
y = arrayMin(ys);
label.align(optionsLabel, false, {
x: x,
y: y,
width: arrayMax(xs) - x,
height: arrayMax(ys) - y
});
label.show();
} else if (label) { // move out of sight
label.hide();
}
// chainable
return plotLine;
},
/**
* Remove the plot line or band
*/
destroy: function () {
// remove it from the lookup
erase(this.axis.plotLinesAndBands, this);
delete this.axis;
destroyObjectProperties(this);
}
};
/**
* The class for stack items
*/
function StackItem(axis, options, isNegative, x, stackOption, stacking) {
var inverted = axis.chart.inverted;
this.axis = axis;
// Tells if the stack is negative
this.isNegative = isNegative;
// Save the options to be able to style the label
this.options = options;
// Save the x value to be able to position the label later
this.x = x;
// Initialize total value
this.total = null;
// This will keep each points' extremes stored by series.index
this.points = {};
// Save the stack option on the series configuration object, and whether to treat it as percent
this.stack = stackOption;
this.percent = stacking === 'percent';
// The align options and text align varies on whether the stack is negative and
// if the chart is inverted or not.
// First test the user supplied value, then use the dynamic.
this.alignOptions = {
align: options.align || (inverted ? (isNegative ? 'left' : 'right') : 'center'),
verticalAlign: options.verticalAlign || (inverted ? 'middle' : (isNegative ? 'bottom' : 'top')),
y: pick(options.y, inverted ? 4 : (isNegative ? 14 : -6)),
x: pick(options.x, inverted ? (isNegative ? -6 : 6) : 0)
};
this.textAlign = options.textAlign || (inverted ? (isNegative ? 'right' : 'left') : 'center');
}
StackItem.prototype = {
destroy: function () {
destroyObjectProperties(this, this.axis);
},
/**
* Renders the stack total label and adds it to the stack label group.
*/
render: function (group) {
var options = this.options,
formatOption = options.format,
str = formatOption ?
format(formatOption, this) :
options.formatter.call(this); // format the text in the label
// Change the text to reflect the new total and set visibility to hidden in case the serie is hidden
if (this.label) {
this.label.attr({text: str, visibility: HIDDEN});
// Create new label
} else {
this.label =
this.axis.chart.renderer.text(str, 0, 0, options.useHTML) // dummy positions, actual position updated with setOffset method in columnseries
.css(options.style) // apply style
.attr({
align: this.textAlign, // fix the text-anchor
rotation: options.rotation, // rotation
visibility: HIDDEN // hidden until setOffset is called
})
.add(group); // add to the labels-group
}
},
/**
* Sets the offset that the stack has from the x value and repositions the label.
*/
setOffset: function (xOffset, xWidth) {
var stackItem = this,
axis = stackItem.axis,
chart = axis.chart,
inverted = chart.inverted,
neg = this.isNegative, // special treatment is needed for negative stacks
y = axis.translate(this.percent ? 100 : this.total, 0, 0, 0, 1), // stack value translated mapped to chart coordinates
yZero = axis.translate(0), // stack origin
h = mathAbs(y - yZero), // stack height
x = chart.xAxis[0].translate(this.x) + xOffset, // stack x position
plotHeight = chart.plotHeight,
stackBox = { // this is the box for the complete stack
x: inverted ? (neg ? y : y - h) : x,
y: inverted ? plotHeight - x - xWidth : (neg ? (plotHeight - y - h) : plotHeight - y),
width: inverted ? h : xWidth,
height: inverted ? xWidth : h
},
label = this.label,
alignAttr;
if (label) {
label.align(this.alignOptions, null, stackBox); // align the label to the box
// Set visibility (#678)
alignAttr = label.alignAttr;
label.attr({
visibility: this.options.crop === false || chart.isInsidePlot(alignAttr.x, alignAttr.y) ?
(hasSVG ? 'inherit' : VISIBLE) :
HIDDEN
});
}
}
};
/**
* Create a new axis object
* @param {Object} chart
* @param {Object} options
*/
function Axis() {
this.init.apply(this, arguments);
}
Axis.prototype = {
/**
* Default options for the X axis - the Y axis has extended defaults
*/
defaultOptions: {
// allowDecimals: null,
// alternateGridColor: null,
// categories: [],
dateTimeLabelFormats: {
millisecond: '%H:%M:%S.%L',
second: '%H:%M:%S',
minute: '%H:%M',
hour: '%H:%M',
day: '%e. %b',
week: '%e. %b',
month: '%b \'%y',
year: '%Y'
},
endOnTick: false,
gridLineColor: '#C0C0C0',
// gridLineDashStyle: 'solid',
// gridLineWidth: 0,
// reversed: false,
labels: defaultLabelOptions,
// { step: null },
lineColor: '#C0D0E0',
lineWidth: 1,
//linkedTo: null,
//max: undefined,
//min: undefined,
minPadding: 0.01,
maxPadding: 0.01,
//minRange: null,
minorGridLineColor: '#E0E0E0',
// minorGridLineDashStyle: null,
minorGridLineWidth: 1,
minorTickColor: '#A0A0A0',
//minorTickInterval: null,
minorTickLength: 2,
minorTickPosition: 'outside', // inside or outside
//minorTickWidth: 0,
//opposite: false,
//offset: 0,
//plotBands: [{
// events: {},
// zIndex: 1,
// labels: { align, x, verticalAlign, y, style, rotation, textAlign }
//}],
//plotLines: [{
// events: {}
// dashStyle: {}
// zIndex:
// labels: { align, x, verticalAlign, y, style, rotation, textAlign }
//}],
//reversed: false,
// showFirstLabel: true,
// showLastLabel: true,
startOfWeek: 1,
startOnTick: false,
tickColor: '#C0D0E0',
//tickInterval: null,
tickLength: 5,
tickmarkPlacement: 'between', // on or between
tickPixelInterval: 100,
tickPosition: 'outside',
tickWidth: 1,
title: {
//text: null,
align: 'middle', // low, middle or high
//margin: 0 for horizontal, 10 for vertical axes,
//rotation: 0,
//side: 'outside',
style: {
color: '#4d759e',
//font: defaultFont.replace('normal', 'bold')
fontWeight: 'bold'
}
//x: 0,
//y: 0
},
type: 'linear' // linear, logarithmic or datetime
},
/**
* This options set extends the defaultOptions for Y axes
*/
defaultYAxisOptions: {
endOnTick: true,
gridLineWidth: 1,
tickPixelInterval: 72,
showLastLabel: true,
labels: {
x: -8,
y: 3
},
lineWidth: 0,
maxPadding: 0.05,
minPadding: 0.05,
startOnTick: true,
tickWidth: 0,
title: {
rotation: 270,
text: 'Values'
},
stackLabels: {
enabled: false,
//align: dynamic,
//y: dynamic,
//x: dynamic,
//verticalAlign: dynamic,
//textAlign: dynamic,
//rotation: 0,
formatter: function () {
return numberFormat(this.total, -1);
},
style: defaultLabelOptions.style
}
},
/**
* These options extend the defaultOptions for left axes
*/
defaultLeftAxisOptions: {
labels: {
x: -8,
y: null
},
title: {
rotation: 270
}
},
/**
* These options extend the defaultOptions for right axes
*/
defaultRightAxisOptions: {
labels: {
x: 8,
y: null
},
title: {
rotation: 90
}
},
/**
* These options extend the defaultOptions for bottom axes
*/
defaultBottomAxisOptions: {
labels: {
x: 0,
y: 14
// overflow: undefined,
// staggerLines: null
},
title: {
rotation: 0
}
},
/**
* These options extend the defaultOptions for left axes
*/
defaultTopAxisOptions: {
labels: {
x: 0,
y: -5
// overflow: undefined
// staggerLines: null
},
title: {
rotation: 0
}
},
/**
* Initialize the axis
*/
init: function (chart, userOptions) {
var isXAxis = userOptions.isX,
axis = this;
// Flag, is the axis horizontal
axis.horiz = chart.inverted ? !isXAxis : isXAxis;
// Flag, isXAxis
axis.isXAxis = isXAxis;
axis.xOrY = isXAxis ? 'x' : 'y';
axis.opposite = userOptions.opposite; // needed in setOptions
axis.side = axis.horiz ?
(axis.opposite ? 0 : 2) : // top : bottom
(axis.opposite ? 1 : 3); // right : left
axis.setOptions(userOptions);
var options = this.options,
type = options.type,
isDatetimeAxis = type === 'datetime';
axis.labelFormatter = options.labels.formatter || axis.defaultLabelFormatter; // can be overwritten by dynamic format
// Flag, stagger lines or not
axis.userOptions = userOptions;
//axis.axisTitleMargin = UNDEFINED,// = options.title.margin,
axis.minPixelPadding = 0;
//axis.ignoreMinPadding = UNDEFINED; // can be set to true by a column or bar series
//axis.ignoreMaxPadding = UNDEFINED;
axis.chart = chart;
axis.reversed = options.reversed;
axis.zoomEnabled = options.zoomEnabled !== false;
// Initial categories
axis.categories = options.categories || type === 'category';
// Elements
//axis.axisGroup = UNDEFINED;
//axis.gridGroup = UNDEFINED;
//axis.axisTitle = UNDEFINED;
//axis.axisLine = UNDEFINED;
// Shorthand types
axis.isLog = type === 'logarithmic';
axis.isDatetimeAxis = isDatetimeAxis;
// Flag, if axis is linked to another axis
axis.isLinked = defined(options.linkedTo);
// Linked axis.
//axis.linkedParent = UNDEFINED;
// Tick positions
//axis.tickPositions = UNDEFINED; // array containing predefined positions
// Tick intervals
//axis.tickInterval = UNDEFINED;
//axis.minorTickInterval = UNDEFINED;
axis.tickmarkOffset = (axis.categories && options.tickmarkPlacement === 'between') ? 0.5 : 0;
// Major ticks
axis.ticks = {};
// Minor ticks
axis.minorTicks = {};
//axis.tickAmount = UNDEFINED;
// List of plotLines/Bands
axis.plotLinesAndBands = [];
// Alternate bands
axis.alternateBands = {};
// Axis metrics
//axis.left = UNDEFINED;
//axis.top = UNDEFINED;
//axis.width = UNDEFINED;
//axis.height = UNDEFINED;
//axis.bottom = UNDEFINED;
//axis.right = UNDEFINED;
//axis.transA = UNDEFINED;
//axis.transB = UNDEFINED;
//axis.oldTransA = UNDEFINED;
axis.len = 0;
//axis.oldMin = UNDEFINED;
//axis.oldMax = UNDEFINED;
//axis.oldUserMin = UNDEFINED;
//axis.oldUserMax = UNDEFINED;
//axis.oldAxisLength = UNDEFINED;
axis.minRange = axis.userMinRange = options.minRange || options.maxZoom;
axis.range = options.range;
axis.offset = options.offset || 0;
// Dictionary for stacks
axis.stacks = {};
axis.oldStacks = {};
// Dictionary for stacks max values
axis.stackExtremes = {};
// Min and max in the data
//axis.dataMin = UNDEFINED,
//axis.dataMax = UNDEFINED,
// The axis range
axis.max = null;
axis.min = null;
// User set min and max
//axis.userMin = UNDEFINED,
//axis.userMax = UNDEFINED,
// Run Axis
var eventType,
events = axis.options.events;
// Register
if (inArray(axis, chart.axes) === -1) { // don't add it again on Axis.update()
chart.axes.push(axis);
chart[isXAxis ? 'xAxis' : 'yAxis'].push(axis);
}
axis.series = axis.series || []; // populated by Series
// inverted charts have reversed xAxes as default
if (chart.inverted && isXAxis && axis.reversed === UNDEFINED) {
axis.reversed = true;
}
axis.removePlotBand = axis.removePlotBandOrLine;
axis.removePlotLine = axis.removePlotBandOrLine;
// register event listeners
for (eventType in events) {
addEvent(axis, eventType, events[eventType]);
}
// extend logarithmic axis
if (axis.isLog) {
axis.val2lin = log2lin;
axis.lin2val = lin2log;
}
},
/**
* Merge and set options
*/
setOptions: function (userOptions) {
this.options = merge(
this.defaultOptions,
this.isXAxis ? {} : this.defaultYAxisOptions,
[this.defaultTopAxisOptions, this.defaultRightAxisOptions,
this.defaultBottomAxisOptions, this.defaultLeftAxisOptions][this.side],
merge(
defaultOptions[this.isXAxis ? 'xAxis' : 'yAxis'], // if set in setOptions (#1053)
userOptions
)
);
},
/**
* Update the axis with a new options structure
*/
update: function (newOptions, redraw) {
var chart = this.chart;
newOptions = chart.options[this.xOrY + 'Axis'][this.options.index] = merge(this.userOptions, newOptions);
this.destroy(true);
this._addedPlotLB = this.userMin = this.userMax = UNDEFINED; // #1611, #2306
this.init(chart, extend(newOptions, { events: UNDEFINED }));
chart.isDirtyBox = true;
if (pick(redraw, true)) {
chart.redraw();
}
},
/**
* Remove the axis from the chart
*/
remove: function (redraw) {
var chart = this.chart,
key = this.xOrY + 'Axis'; // xAxis or yAxis
// Remove associated series
each(this.series, function (series) {
series.remove(false);
});
// Remove the axis
erase(chart.axes, this);
erase(chart[key], this);
chart.options[key].splice(this.options.index, 1);
each(chart[key], function (axis, i) { // Re-index, #1706
axis.options.index = i;
});
this.destroy();
chart.isDirtyBox = true;
if (pick(redraw, true)) {
chart.redraw();
}
},
/**
* The default label formatter. The context is a special config object for the label.
*/
defaultLabelFormatter: function () {
var axis = this.axis,
value = this.value,
categories = axis.categories,
dateTimeLabelFormat = this.dateTimeLabelFormat,
numericSymbols = defaultOptions.lang.numericSymbols,
i = numericSymbols && numericSymbols.length,
multi,
ret,
formatOption = axis.options.labels.format,
// make sure the same symbol is added for all labels on a linear axis
numericSymbolDetector = axis.isLog ? value : axis.tickInterval;
if (formatOption) {
ret = format(formatOption, this);
} else if (categories) {
ret = value;
} else if (dateTimeLabelFormat) { // datetime axis
ret = dateFormat(dateTimeLabelFormat, value);
} else if (i && numericSymbolDetector >= 1000) {
// Decide whether we should add a numeric symbol like k (thousands) or M (millions).
// If we are to enable this in tooltip or other places as well, we can move this
// logic to the numberFormatter and enable it by a parameter.
while (i-- && ret === UNDEFINED) {
multi = Math.pow(1000, i + 1);
if (numericSymbolDetector >= multi && numericSymbols[i] !== null) {
ret = numberFormat(value / multi, -1) + numericSymbols[i];
}
}
}
if (ret === UNDEFINED) {
if (value >= 1000) { // add thousands separators
ret = numberFormat(value, 0);
} else { // small numbers
ret = numberFormat(value, -1);
}
}
return ret;
},
/**
* Get the minimum and maximum for the series of each axis
*/
getSeriesExtremes: function () {
var axis = this,
chart = axis.chart;
axis.hasVisibleSeries = false;
// reset dataMin and dataMax in case we're redrawing
axis.dataMin = axis.dataMax = null;
// reset cached stacking extremes
axis.stackExtremes = {};
axis.buildStacks();
// loop through this axis' series
each(axis.series, function (series) {
if (series.visible || !chart.options.chart.ignoreHiddenSeries) {
var seriesOptions = series.options,
xData,
threshold = seriesOptions.threshold,
seriesDataMin,
seriesDataMax;
axis.hasVisibleSeries = true;
// Validate threshold in logarithmic axes
if (axis.isLog && threshold <= 0) {
threshold = null;
}
// Get dataMin and dataMax for X axes
if (axis.isXAxis) {
xData = series.xData;
if (xData.length) {
axis.dataMin = mathMin(pick(axis.dataMin, xData[0]), arrayMin(xData));
axis.dataMax = mathMax(pick(axis.dataMax, xData[0]), arrayMax(xData));
}
// Get dataMin and dataMax for Y axes, as well as handle stacking and processed data
} else {
// Get this particular series extremes
series.getExtremes();
seriesDataMax = series.dataMax;
seriesDataMin = series.dataMin;
// Get the dataMin and dataMax so far. If percentage is used, the min and max are
// always 0 and 100. If seriesDataMin and seriesDataMax is null, then series
// doesn't have active y data, we continue with nulls
if (defined(seriesDataMin) && defined(seriesDataMax)) {
axis.dataMin = mathMin(pick(axis.dataMin, seriesDataMin), seriesDataMin);
axis.dataMax = mathMax(pick(axis.dataMax, seriesDataMax), seriesDataMax);
}
// Adjust to threshold
if (defined(threshold)) {
if (axis.dataMin >= threshold) {
axis.dataMin = threshold;
axis.ignoreMinPadding = true;
} else if (axis.dataMax < threshold) {
axis.dataMax = threshold;
axis.ignoreMaxPadding = true;
}
}
}
}
});
},
/**
* Translate from axis value to pixel position on the chart, or back
*
*/
translate: function (val, backwards, cvsCoord, old, handleLog, pointPlacement) {
var axis = this,
axisLength = axis.len,
sign = 1,
cvsOffset = 0,
localA = old ? axis.oldTransA : axis.transA,
localMin = old ? axis.oldMin : axis.min,
returnValue,
minPixelPadding = axis.minPixelPadding,
postTranslate = (axis.options.ordinal || (axis.isLog && handleLog)) && axis.lin2val;
if (!localA) {
localA = axis.transA;
}
// In vertical axes, the canvas coordinates start from 0 at the top like in
// SVG.
if (cvsCoord) {
sign *= -1; // canvas coordinates inverts the value
cvsOffset = axisLength;
}
// Handle reversed axis
if (axis.reversed) {
sign *= -1;
cvsOffset -= sign * axisLength;
}
// From pixels to value
if (backwards) { // reverse translation
val = val * sign + cvsOffset;
val -= minPixelPadding;
returnValue = val / localA + localMin; // from chart pixel to value
if (postTranslate) { // log and ordinal axes
returnValue = axis.lin2val(returnValue);
}
// From value to pixels
} else {
if (postTranslate) { // log and ordinal axes
val = axis.val2lin(val);
}
if (pointPlacement === 'between') {
pointPlacement = 0.5;
}
returnValue = sign * (val - localMin) * localA + cvsOffset + (sign * minPixelPadding) +
(isNumber(pointPlacement) ? localA * pointPlacement * axis.pointRange : 0);
}
return returnValue;
},
/**
* Utility method to translate an axis value to pixel position.
* @param {Number} value A value in terms of axis units
* @param {Boolean} paneCoordinates Whether to return the pixel coordinate relative to the chart
* or just the axis/pane itself.
*/
toPixels: function (value, paneCoordinates) {
return this.translate(value, false, !this.horiz, null, true) + (paneCoordinates ? 0 : this.pos);
},
/*
* Utility method to translate a pixel position in to an axis value
* @param {Number} pixel The pixel value coordinate
* @param {Boolean} paneCoordiantes Whether the input pixel is relative to the chart or just the
* axis/pane itself.
*/
toValue: function (pixel, paneCoordinates) {
return this.translate(pixel - (paneCoordinates ? 0 : this.pos), true, !this.horiz, null, true);
},
/**
* Create the path for a plot line that goes from the given value on
* this axis, across the plot to the opposite side
* @param {Number} value
* @param {Number} lineWidth Used for calculation crisp line
* @param {Number] old Use old coordinates (for resizing and rescaling)
*/
getPlotLinePath: function (value, lineWidth, old, force) {
var axis = this,
chart = axis.chart,
axisLeft = axis.left,
axisTop = axis.top,
x1,
y1,
x2,
y2,
translatedValue = axis.translate(value, null, null, old),
cHeight = (old && chart.oldChartHeight) || chart.chartHeight,
cWidth = (old && chart.oldChartWidth) || chart.chartWidth,
skip,
transB = axis.transB;
x1 = x2 = mathRound(translatedValue + transB);
y1 = y2 = mathRound(cHeight - translatedValue - transB);
if (isNaN(translatedValue)) { // no min or max
skip = true;
} else if (axis.horiz) {
y1 = axisTop;
y2 = cHeight - axis.bottom;
if (x1 < axisLeft || x1 > axisLeft + axis.width) {
skip = true;
}
} else {
x1 = axisLeft;
x2 = cWidth - axis.right;
if (y1 < axisTop || y1 > axisTop + axis.height) {
skip = true;
}
}
return skip && !force ?
null :
chart.renderer.crispLine([M, x1, y1, L, x2, y2], lineWidth || 0);
},
/**
* Create the path for a plot band
*/
getPlotBandPath: function (from, to) {
var toPath = this.getPlotLinePath(to),
path = this.getPlotLinePath(from);
if (path && toPath) {
path.push(
toPath[4],
toPath[5],
toPath[1],
toPath[2]
);
} else { // outside the axis area
path = null;
}
return path;
},
/**
* Set the tick positions of a linear axis to round values like whole tens or every five.
*/
getLinearTickPositions: function (tickInterval, min, max) {
var pos,
lastPos,
roundedMin = correctFloat(mathFloor(min / tickInterval) * tickInterval),
roundedMax = correctFloat(mathCeil(max / tickInterval) * tickInterval),
tickPositions = [];
// Populate the intermediate values
pos = roundedMin;
while (pos <= roundedMax) {
// Place the tick on the rounded value
tickPositions.push(pos);
// Always add the raw tickInterval, not the corrected one.
pos = correctFloat(pos + tickInterval);
// If the interval is not big enough in the current min - max range to actually increase
// the loop variable, we need to break out to prevent endless loop. Issue #619
if (pos === lastPos) {
break;
}
// Record the last value
lastPos = pos;
}
return tickPositions;
},
/**
* Set the tick positions of a logarithmic axis
*/
getLogTickPositions: function (interval, min, max, minor) {
var axis = this,
options = axis.options,
axisLength = axis.len,
// Since we use this method for both major and minor ticks,
// use a local variable and return the result
positions = [];
// Reset
if (!minor) {
axis._minorAutoInterval = null;
}
// First case: All ticks fall on whole logarithms: 1, 10, 100 etc.
if (interval >= 0.5) {
interval = mathRound(interval);
positions = axis.getLinearTickPositions(interval, min, max);
// Second case: We need intermediary ticks. For example
// 1, 2, 4, 6, 8, 10, 20, 40 etc.
} else if (interval >= 0.08) {
var roundedMin = mathFloor(min),
intermediate,
i,
j,
len,
pos,
lastPos,
break2;
if (interval > 0.3) {
intermediate = [1, 2, 4];
} else if (interval > 0.15) { // 0.2 equals five minor ticks per 1, 10, 100 etc
intermediate = [1, 2, 4, 6, 8];
} else { // 0.1 equals ten minor ticks per 1, 10, 100 etc
intermediate = [1, 2, 3, 4, 5, 6, 7, 8, 9];
}
for (i = roundedMin; i < max + 1 && !break2; i++) {
len = intermediate.length;
for (j = 0; j < len && !break2; j++) {
pos = log2lin(lin2log(i) * intermediate[j]);
if (pos > min && (!minor || lastPos <= max)) { // #1670
positions.push(lastPos);
}
if (lastPos > max) {
break2 = true;
}
lastPos = pos;
}
}
// Third case: We are so deep in between whole logarithmic values that
// we might as well handle the tick positions like a linear axis. For
// example 1.01, 1.02, 1.03, 1.04.
} else {
var realMin = lin2log(min),
realMax = lin2log(max),
tickIntervalOption = options[minor ? 'minorTickInterval' : 'tickInterval'],
filteredTickIntervalOption = tickIntervalOption === 'auto' ? null : tickIntervalOption,
tickPixelIntervalOption = options.tickPixelInterval / (minor ? 5 : 1),
totalPixelLength = minor ? axisLength / axis.tickPositions.length : axisLength;
interval = pick(
filteredTickIntervalOption,
axis._minorAutoInterval,
(realMax - realMin) * tickPixelIntervalOption / (totalPixelLength || 1)
);
interval = normalizeTickInterval(
interval,
null,
getMagnitude(interval)
);
positions = map(axis.getLinearTickPositions(
interval,
realMin,
realMax
), log2lin);
if (!minor) {
axis._minorAutoInterval = interval / 5;
}
}
// Set the axis-level tickInterval variable
if (!minor) {
axis.tickInterval = interval;
}
return positions;
},
/**
* Return the minor tick positions. For logarithmic axes, reuse the same logic
* as for major ticks.
*/
getMinorTickPositions: function () {
var axis = this,
options = axis.options,
tickPositions = axis.tickPositions,
minorTickInterval = axis.minorTickInterval,
minorTickPositions = [],
pos,
i,
len;
if (axis.isLog) {
len = tickPositions.length;
for (i = 1; i < len; i++) {
minorTickPositions = minorTickPositions.concat(
axis.getLogTickPositions(minorTickInterval, tickPositions[i - 1], tickPositions[i], true)
);
}
} else if (axis.isDatetimeAxis && options.minorTickInterval === 'auto') { // #1314
minorTickPositions = minorTickPositions.concat(
getTimeTicks(
normalizeTimeTickInterval(minorTickInterval),
axis.min,
axis.max,
options.startOfWeek
)
);
if (minorTickPositions[0] < axis.min) {
minorTickPositions.shift();
}
} else {
for (pos = axis.min + (tickPositions[0] - axis.min) % minorTickInterval; pos <= axis.max; pos += minorTickInterval) {
minorTickPositions.push(pos);
}
}
return minorTickPositions;
},
/**
* Adjust the min and max for the minimum range. Keep in mind that the series data is
* not yet processed, so we don't have information on data cropping and grouping, or
* updated axis.pointRange or series.pointRange. The data can't be processed until
* we have finally established min and max.
*/
adjustForMinRange: function () {
var axis = this,
options = axis.options,
min = axis.min,
max = axis.max,
zoomOffset,
spaceAvailable = axis.dataMax - axis.dataMin >= axis.minRange,
closestDataRange,
i,
distance,
xData,
loopLength,
minArgs,
maxArgs;
// Set the automatic minimum range based on the closest point distance
if (axis.isXAxis && axis.minRange === UNDEFINED && !axis.isLog) {
if (defined(options.min) || defined(options.max)) {
axis.minRange = null; // don't do this again
} else {
// Find the closest distance between raw data points, as opposed to
// closestPointRange that applies to processed points (cropped and grouped)
each(axis.series, function (series) {
xData = series.xData;
loopLength = series.xIncrement ? 1 : xData.length - 1;
for (i = loopLength; i > 0; i--) {
distance = xData[i] - xData[i - 1];
if (closestDataRange === UNDEFINED || distance < closestDataRange) {
closestDataRange = distance;
}
}
});
axis.minRange = mathMin(closestDataRange * 5, axis.dataMax - axis.dataMin);
}
}
// if minRange is exceeded, adjust
if (max - min < axis.minRange) {
var minRange = axis.minRange;
zoomOffset = (minRange - max + min) / 2;
// if min and max options have been set, don't go beyond it
minArgs = [min - zoomOffset, pick(options.min, min - zoomOffset)];
if (spaceAvailable) { // if space is available, stay within the data range
minArgs[2] = axis.dataMin;
}
min = arrayMax(minArgs);
maxArgs = [min + minRange, pick(options.max, min + minRange)];
if (spaceAvailable) { // if space is availabe, stay within the data range
maxArgs[2] = axis.dataMax;
}
max = arrayMin(maxArgs);
// now if the max is adjusted, adjust the min back
if (max - min < minRange) {
minArgs[0] = max - minRange;
minArgs[1] = pick(options.min, max - minRange);
min = arrayMax(minArgs);
}
}
// Record modified extremes
axis.min = min;
axis.max = max;
},
/**
* Update translation information
*/
setAxisTranslation: function (saveOld) {
var axis = this,
range = axis.max - axis.min,
pointRange = 0,
closestPointRange,
minPointOffset = 0,
pointRangePadding = 0,
linkedParent = axis.linkedParent,
ordinalCorrection,
transA = axis.transA;
// adjust translation for padding
if (axis.isXAxis) {
if (linkedParent) {
minPointOffset = linkedParent.minPointOffset;
pointRangePadding = linkedParent.pointRangePadding;
} else {
each(axis.series, function (series) {
var seriesPointRange = series.pointRange,
pointPlacement = series.options.pointPlacement,
seriesClosestPointRange = series.closestPointRange;
if (seriesPointRange > range) { // #1446
seriesPointRange = 0;
}
pointRange = mathMax(pointRange, seriesPointRange);
// minPointOffset is the value padding to the left of the axis in order to make
// room for points with a pointRange, typically columns. When the pointPlacement option
// is 'between' or 'on', this padding does not apply.
minPointOffset = mathMax(
minPointOffset,
isString(pointPlacement) ? 0 : seriesPointRange / 2
);
// Determine the total padding needed to the length of the axis to make room for the
// pointRange. If the series' pointPlacement is 'on', no padding is added.
pointRangePadding = mathMax(
pointRangePadding,
pointPlacement === 'on' ? 0 : seriesPointRange
);
// Set the closestPointRange
if (!series.noSharedTooltip && defined(seriesClosestPointRange)) {
closestPointRange = defined(closestPointRange) ?
mathMin(closestPointRange, seriesClosestPointRange) :
seriesClosestPointRange;
}
});
}
// Record minPointOffset and pointRangePadding
ordinalCorrection = axis.ordinalSlope && closestPointRange ? axis.ordinalSlope / closestPointRange : 1; // #988, #1853
axis.minPointOffset = minPointOffset = minPointOffset * ordinalCorrection;
axis.pointRangePadding = pointRangePadding = pointRangePadding * ordinalCorrection;
// pointRange means the width reserved for each point, like in a column chart
axis.pointRange = mathMin(pointRange, range);
// closestPointRange means the closest distance between points. In columns
// it is mostly equal to pointRange, but in lines pointRange is 0 while closestPointRange
// is some other value
axis.closestPointRange = closestPointRange;
}
// Secondary values
if (saveOld) {
axis.oldTransA = transA;
}
axis.translationSlope = axis.transA = transA = axis.len / ((range + pointRangePadding) || 1);
axis.transB = axis.horiz ? axis.left : axis.bottom; // translation addend
axis.minPixelPadding = transA * minPointOffset;
},
/**
* Set the tick positions to round values and optionally extend the extremes
* to the nearest tick
*/
setTickPositions: function (secondPass) {
var axis = this,
chart = axis.chart,
options = axis.options,
isLog = axis.isLog,
isDatetimeAxis = axis.isDatetimeAxis,
isXAxis = axis.isXAxis,
isLinked = axis.isLinked,
tickPositioner = axis.options.tickPositioner,
maxPadding = options.maxPadding,
minPadding = options.minPadding,
length,
linkedParentExtremes,
tickIntervalOption = options.tickInterval,
minTickIntervalOption = options.minTickInterval,
tickPixelIntervalOption = options.tickPixelInterval,
tickPositions,
keepTwoTicksOnly,
categories = axis.categories;
// linked axis gets the extremes from the parent axis
if (isLinked) {
axis.linkedParent = chart[isXAxis ? 'xAxis' : 'yAxis'][options.linkedTo];
linkedParentExtremes = axis.linkedParent.getExtremes();
axis.min = pick(linkedParentExtremes.min, linkedParentExtremes.dataMin);
axis.max = pick(linkedParentExtremes.max, linkedParentExtremes.dataMax);
if (options.type !== axis.linkedParent.options.type) {
error(11, 1); // Can't link axes of different type
}
} else { // initial min and max from the extreme data values
axis.min = pick(axis.userMin, options.min, axis.dataMin);
axis.max = pick(axis.userMax, options.max, axis.dataMax);
}
if (isLog) {
if (!secondPass && mathMin(axis.min, pick(axis.dataMin, axis.min)) <= 0) { // #978
error(10, 1); // Can't plot negative values on log axis
}
axis.min = correctFloat(log2lin(axis.min)); // correctFloat cures #934
axis.max = correctFloat(log2lin(axis.max));
}
// handle zoomed range
if (axis.range) {
axis.userMin = axis.min = mathMax(axis.min, axis.max - axis.range); // #618
axis.userMax = axis.max;
if (secondPass) {
axis.range = null; // don't use it when running setExtremes
}
}
// Hook for adjusting this.min and this.max. Used by bubble series.
if (axis.beforePadding) {
axis.beforePadding();
}
// adjust min and max for the minimum range
axis.adjustForMinRange();
// Pad the values to get clear of the chart's edges. To avoid tickInterval taking the padding
// into account, we do this after computing tick interval (#1337).
if (!categories && !axis.usePercentage && !isLinked && defined(axis.min) && defined(axis.max)) {
length = axis.max - axis.min;
if (length) {
if (!defined(options.min) && !defined(axis.userMin) && minPadding && (axis.dataMin < 0 || !axis.ignoreMinPadding)) {
axis.min -= length * minPadding;
}
if (!defined(options.max) && !defined(axis.userMax) && maxPadding && (axis.dataMax > 0 || !axis.ignoreMaxPadding)) {
axis.max += length * maxPadding;
}
}
}
// get tickInterval
if (axis.min === axis.max || axis.min === undefined || axis.max === undefined) {
axis.tickInterval = 1;
} else if (isLinked && !tickIntervalOption &&
tickPixelIntervalOption === axis.linkedParent.options.tickPixelInterval) {
axis.tickInterval = axis.linkedParent.tickInterval;
} else {
axis.tickInterval = pick(
tickIntervalOption,
categories ? // for categoried axis, 1 is default, for linear axis use tickPix
1 :
// don't let it be more than the data range
(axis.max - axis.min) * tickPixelIntervalOption / mathMax(axis.len, tickPixelIntervalOption)
);
// For squished axes, set only two ticks
if (!defined(tickIntervalOption) && axis.len < tickPixelIntervalOption && !this.isRadial) {
keepTwoTicksOnly = true;
axis.tickInterval /= 4; // tick extremes closer to the real values
}
}
// Now we're finished detecting min and max, crop and group series data. This
// is in turn needed in order to find tick positions in ordinal axes.
if (isXAxis && !secondPass) {
each(axis.series, function (series) {
series.processData(axis.min !== axis.oldMin || axis.max !== axis.oldMax);
});
}
// set the translation factor used in translate function
axis.setAxisTranslation(true);
// hook for ordinal axes and radial axes
if (axis.beforeSetTickPositions) {
axis.beforeSetTickPositions();
}
// hook for extensions, used in Highstock ordinal axes
if (axis.postProcessTickInterval) {
axis.tickInterval = axis.postProcessTickInterval(axis.tickInterval);
}
// In column-like charts, don't cramp in more ticks than there are points (#1943)
if (axis.pointRange) {
axis.tickInterval = mathMax(axis.pointRange, axis.tickInterval);
}
// Before normalizing the tick interval, handle minimum tick interval. This applies only if tickInterval is not defined.
if (!tickIntervalOption && axis.tickInterval < minTickIntervalOption) {
axis.tickInterval = minTickIntervalOption;
}
// for linear axes, get magnitude and normalize the interval
if (!isDatetimeAxis && !isLog) { // linear
if (!tickIntervalOption) {
axis.tickInterval = normalizeTickInterval(axis.tickInterval, null, getMagnitude(axis.tickInterval), options);
}
}
// get minorTickInterval
axis.minorTickInterval = options.minorTickInterval === 'auto' && axis.tickInterval ?
axis.tickInterval / 5 : options.minorTickInterval;
// find the tick positions
axis.tickPositions = tickPositions = options.tickPositions ?
[].concat(options.tickPositions) : // Work on a copy (#1565)
(tickPositioner && tickPositioner.apply(axis, [axis.min, axis.max]));
if (!tickPositions) {
// Too many ticks
if (!axis.ordinalPositions && (axis.max - axis.min) / axis.tickInterval > mathMax(2 * axis.len, 200)) {
error(19, true);
}
if (isDatetimeAxis) {
tickPositions = (axis.getNonLinearTimeTicks || getTimeTicks)(
normalizeTimeTickInterval(axis.tickInterval, options.units),
axis.min,
axis.max,
options.startOfWeek,
axis.ordinalPositions,
axis.closestPointRange,
true
);
} else if (isLog) {
tickPositions = axis.getLogTickPositions(axis.tickInterval, axis.min, axis.max);
} else {
tickPositions = axis.getLinearTickPositions(axis.tickInterval, axis.min, axis.max);
}
if (keepTwoTicksOnly) {
tickPositions.splice(1, tickPositions.length - 2);
}
axis.tickPositions = tickPositions;
}
if (!isLinked) {
// reset min/max or remove extremes based on start/end on tick
var roundedMin = tickPositions[0],
roundedMax = tickPositions[tickPositions.length - 1],
minPointOffset = axis.minPointOffset || 0,
singlePad;
if (options.startOnTick) {
axis.min = roundedMin;
} else if (axis.min - minPointOffset > roundedMin) {
tickPositions.shift();
}
if (options.endOnTick) {
axis.max = roundedMax;
} else if (axis.max + minPointOffset < roundedMax) {
tickPositions.pop();
}
// When there is only one point, or all points have the same value on this axis, then min
// and max are equal and tickPositions.length is 1. In this case, add some padding
// in order to center the point, but leave it with one tick. #1337.
if (tickPositions.length === 1) {
singlePad = 0.001; // The lowest possible number to avoid extra padding on columns
axis.min -= singlePad;
axis.max += singlePad;
}
}
},
/**
* Set the max ticks of either the x and y axis collection
*/
setMaxTicks: function () {
var chart = this.chart,
maxTicks = chart.maxTicks || {},
tickPositions = this.tickPositions,
key = this._maxTicksKey = [this.xOrY, this.pos, this.len].join('-');
if (!this.isLinked && !this.isDatetimeAxis && tickPositions && tickPositions.length > (maxTicks[key] || 0) && this.options.alignTicks !== false) {
maxTicks[key] = tickPositions.length;
}
chart.maxTicks = maxTicks;
},
/**
* When using multiple axes, adjust the number of ticks to match the highest
* number of ticks in that group
*/
adjustTickAmount: function () {
var axis = this,
chart = axis.chart,
key = axis._maxTicksKey,
tickPositions = axis.tickPositions,
maxTicks = chart.maxTicks;
if (maxTicks && maxTicks[key] && !axis.isDatetimeAxis && !axis.categories && !axis.isLinked && axis.options.alignTicks !== false) { // only apply to linear scale
var oldTickAmount = axis.tickAmount,
calculatedTickAmount = tickPositions.length,
tickAmount;
// set the axis-level tickAmount to use below
axis.tickAmount = tickAmount = maxTicks[key];
if (calculatedTickAmount < tickAmount) {
while (tickPositions.length < tickAmount) {
tickPositions.push(correctFloat(
tickPositions[tickPositions.length - 1] + axis.tickInterval
));
}
axis.transA *= (calculatedTickAmount - 1) / (tickAmount - 1);
axis.max = tickPositions[tickPositions.length - 1];
}
if (defined(oldTickAmount) && tickAmount !== oldTickAmount) {
axis.isDirty = true;
}
}
},
/**
* Set the scale based on data min and max, user set min and max or options
*
*/
setScale: function () {
var axis = this,
stacks = axis.stacks,
type,
i,
isDirtyData,
isDirtyAxisLength;
axis.oldMin = axis.min;
axis.oldMax = axis.max;
axis.oldAxisLength = axis.len;
// set the new axisLength
axis.setAxisSize();
//axisLength = horiz ? axisWidth : axisHeight;
isDirtyAxisLength = axis.len !== axis.oldAxisLength;
// is there new data?
each(axis.series, function (series) {
if (series.isDirtyData || series.isDirty ||
series.xAxis.isDirty) { // when x axis is dirty, we need new data extremes for y as well
isDirtyData = true;
}
});
// do we really need to go through all this?
if (isDirtyAxisLength || isDirtyData || axis.isLinked || axis.forceRedraw ||
axis.userMin !== axis.oldUserMin || axis.userMax !== axis.oldUserMax) {
// reset stacks
if (!axis.isXAxis) {
for (type in stacks) {
delete stacks[type];
}
}
axis.forceRedraw = false;
// get data extremes if needed
axis.getSeriesExtremes();
// get fixed positions based on tickInterval
axis.setTickPositions();
// record old values to decide whether a rescale is necessary later on (#540)
axis.oldUserMin = axis.userMin;
axis.oldUserMax = axis.userMax;
// Mark as dirty if it is not already set to dirty and extremes have changed. #595.
if (!axis.isDirty) {
axis.isDirty = isDirtyAxisLength || axis.min !== axis.oldMin || axis.max !== axis.oldMax;
}
} else if (!axis.isXAxis) {
if (axis.oldStacks) {
stacks = axis.stacks = axis.oldStacks;
}
// reset stacks
for (type in stacks) {
for (i in stacks[type]) {
stacks[type][i].cum = stacks[type][i].total;
}
}
}
// Set the maximum tick amount
axis.setMaxTicks();
},
/**
* Set the extremes and optionally redraw
* @param {Number} newMin
* @param {Number} newMax
* @param {Boolean} redraw
* @param {Boolean|Object} animation Whether to apply animation, and optionally animation
* configuration
* @param {Object} eventArguments
*
*/
setExtremes: function (newMin, newMax, redraw, animation, eventArguments) {
var axis = this,
chart = axis.chart;
redraw = pick(redraw, true); // defaults to true
// Extend the arguments with min and max
eventArguments = extend(eventArguments, {
min: newMin,
max: newMax
});
// Fire the event
fireEvent(axis, 'setExtremes', eventArguments, function () { // the default event handler
axis.userMin = newMin;
axis.userMax = newMax;
axis.eventArgs = eventArguments;
// Mark for running afterSetExtremes
axis.isDirtyExtremes = true;
// redraw
if (redraw) {
chart.redraw(animation);
}
});
},
/**
* Overridable method for zooming chart. Pulled out in a separate method to allow overriding
* in stock charts.
*/
zoom: function (newMin, newMax) {
// Prevent pinch zooming out of range. Check for defined is for #1946.
if (!this.allowZoomOutside) {
if (defined(this.dataMin) && newMin <= this.dataMin) {
newMin = UNDEFINED;
}
if (defined(this.dataMax) && newMax >= this.dataMax) {
newMax = UNDEFINED;
}
}
// In full view, displaying the reset zoom button is not required
this.displayBtn = newMin !== UNDEFINED || newMax !== UNDEFINED;
// Do it
this.setExtremes(
newMin,
newMax,
false,
UNDEFINED,
{ trigger: 'zoom' }
);
return true;
},
/**
* Update the axis metrics
*/
setAxisSize: function () {
var chart = this.chart,
options = this.options,
offsetLeft = options.offsetLeft || 0,
offsetRight = options.offsetRight || 0,
horiz = this.horiz,
width,
height,
top,
left;
// Expose basic values to use in Series object and navigator
this.left = left = pick(options.left, chart.plotLeft + offsetLeft);
this.top = top = pick(options.top, chart.plotTop);
this.width = width = pick(options.width, chart.plotWidth - offsetLeft + offsetRight);
this.height = height = pick(options.height, chart.plotHeight);
this.bottom = chart.chartHeight - height - top;
this.right = chart.chartWidth - width - left;
// Direction agnostic properties
this.len = mathMax(horiz ? width : height, 0); // mathMax fixes #905
this.pos = horiz ? left : top; // distance from SVG origin
},
/**
* Get the actual axis extremes
*/
getExtremes: function () {
var axis = this,
isLog = axis.isLog;
return {
min: isLog ? correctFloat(lin2log(axis.min)) : axis.min,
max: isLog ? correctFloat(lin2log(axis.max)) : axis.max,
dataMin: axis.dataMin,
dataMax: axis.dataMax,
userMin: axis.userMin,
userMax: axis.userMax
};
},
/**
* Get the zero plane either based on zero or on the min or max value.
* Used in bar and area plots
*/
getThreshold: function (threshold) {
var axis = this,
isLog = axis.isLog;
var realMin = isLog ? lin2log(axis.min) : axis.min,
realMax = isLog ? lin2log(axis.max) : axis.max;
if (realMin > threshold || threshold === null) {
threshold = realMin;
} else if (realMax < threshold) {
threshold = realMax;
}
return axis.translate(threshold, 0, 1, 0, 1);
},
addPlotBand: function (options) {
this.addPlotBandOrLine(options, 'plotBands');
},
addPlotLine: function (options) {
this.addPlotBandOrLine(options, 'plotLines');
},
/**
* Add a plot band or plot line after render time
*
* @param options {Object} The plotBand or plotLine configuration object
*/
addPlotBandOrLine: function (options, coll) {
var obj = new PlotLineOrBand(this, options).render(),
userOptions = this.userOptions;
if (obj) { // #2189
// Add it to the user options for exporting and Axis.update
if (coll) {
userOptions[coll] = userOptions[coll] || [];
userOptions[coll].push(options);
}
this.plotLinesAndBands.push(obj);
}
return obj;
},
/**
* Compute auto alignment for the axis label based on which side the axis is on
* and the given rotation for the label
*/
autoLabelAlign: function (rotation) {
var ret,
angle = (pick(rotation, 0) - (this.side * 90) + 720) % 360;
if (angle > 15 && angle < 165) {
ret = 'right';
} else if (angle > 195 && angle < 345) {
ret = 'left';
} else {
ret = 'center';
}
return ret;
},
/**
* Render the tick labels to a preliminary position to get their sizes
*/
getOffset: function () {
var axis = this,
chart = axis.chart,
renderer = chart.renderer,
options = axis.options,
tickPositions = axis.tickPositions,
ticks = axis.ticks,
horiz = axis.horiz,
side = axis.side,
invertedSide = chart.inverted ? [1, 0, 3, 2][side] : side,
hasData,
showAxis,
titleOffset = 0,
titleOffsetOption,
titleMargin = 0,
axisTitleOptions = options.title,
labelOptions = options.labels,
labelOffset = 0, // reset
axisOffset = chart.axisOffset,
clipOffset = chart.clipOffset,
directionFactor = [-1, 1, 1, -1][side],
n,
i,
autoStaggerLines = 1,
maxStaggerLines = pick(labelOptions.maxStaggerLines, 5),
sortedPositions,
lastRight,
overlap,
pos,
bBox,
x,
w,
lineNo;
// For reuse in Axis.render
axis.hasData = hasData = (axis.hasVisibleSeries || (defined(axis.min) && defined(axis.max) && !!tickPositions));
axis.showAxis = showAxis = hasData || pick(options.showEmpty, true);
// Set/reset staggerLines
axis.staggerLines = axis.horiz && labelOptions.staggerLines;
// Create the axisGroup and gridGroup elements on first iteration
if (!axis.axisGroup) {
axis.gridGroup = renderer.g('grid')
.attr({ zIndex: options.gridZIndex || 1 })
.add();
axis.axisGroup = renderer.g('axis')
.attr({ zIndex: options.zIndex || 2 })
.add();
axis.labelGroup = renderer.g('axis-labels')
.attr({ zIndex: labelOptions.zIndex || 7 })
.add();
}
if (hasData || axis.isLinked) {
// Set the explicit or automatic label alignment
axis.labelAlign = pick(labelOptions.align || axis.autoLabelAlign(labelOptions.rotation));
each(tickPositions, function (pos) {
if (!ticks[pos]) {
ticks[pos] = new Tick(axis, pos);
} else {
ticks[pos].addLabel(); // update labels depending on tick interval
}
});
// Handle automatic stagger lines
if (axis.horiz && !axis.staggerLines && maxStaggerLines && !labelOptions.rotation) {
sortedPositions = axis.reversed ? [].concat(tickPositions).reverse() : tickPositions;
while (autoStaggerLines < maxStaggerLines) {
lastRight = [];
overlap = false;
for (i = 0; i < sortedPositions.length; i++) {
pos = sortedPositions[i];
bBox = ticks[pos].label && ticks[pos].label.getBBox();
w = bBox ? bBox.width : 0;
lineNo = i % autoStaggerLines;
if (w) {
x = axis.translate(pos); // don't handle log
if (lastRight[lineNo] !== UNDEFINED && x < lastRight[lineNo]) {
overlap = true;
}
lastRight[lineNo] = x + w;
}
}
if (overlap) {
autoStaggerLines++;
} else {
break;
}
}
if (autoStaggerLines > 1) {
axis.staggerLines = autoStaggerLines;
}
}
each(tickPositions, function (pos) {
// left side must be align: right and right side must have align: left for labels
if (side === 0 || side === 2 || { 1: 'left', 3: 'right' }[side] === axis.labelAlign) {
// get the highest offset
labelOffset = mathMax(
ticks[pos].getLabelSize(),
labelOffset
);
}
});
if (axis.staggerLines) {
labelOffset *= axis.staggerLines;
axis.labelOffset = labelOffset;
}
} else { // doesn't have data
for (n in ticks) {
ticks[n].destroy();
delete ticks[n];
}
}
if (axisTitleOptions && axisTitleOptions.text && axisTitleOptions.enabled !== false) {
if (!axis.axisTitle) {
axis.axisTitle = renderer.text(
axisTitleOptions.text,
0,
0,
axisTitleOptions.useHTML
)
.attr({
zIndex: 7,
rotation: axisTitleOptions.rotation || 0,
align:
axisTitleOptions.textAlign ||
{ low: 'left', middle: 'center', high: 'right' }[axisTitleOptions.align]
})
.css(axisTitleOptions.style)
.add(axis.axisGroup);
axis.axisTitle.isNew = true;
}
if (showAxis) {
titleOffset = axis.axisTitle.getBBox()[horiz ? 'height' : 'width'];
titleMargin = pick(axisTitleOptions.margin, horiz ? 5 : 10);
titleOffsetOption = axisTitleOptions.offset;
}
// hide or show the title depending on whether showEmpty is set
axis.axisTitle[showAxis ? 'show' : 'hide']();
}
// handle automatic or user set offset
axis.offset = directionFactor * pick(options.offset, axisOffset[side]);
axis.axisTitleMargin =
pick(titleOffsetOption,
labelOffset + titleMargin +
(side !== 2 && labelOffset && directionFactor * options.labels[horiz ? 'y' : 'x'])
);
axisOffset[side] = mathMax(
axisOffset[side],
axis.axisTitleMargin + titleOffset + directionFactor * axis.offset
);
clipOffset[invertedSide] = mathMax(clipOffset[invertedSide], mathFloor(options.lineWidth / 2) * 2);
},
/**
* Get the path for the axis line
*/
getLinePath: function (lineWidth) {
var chart = this.chart,
opposite = this.opposite,
offset = this.offset,
horiz = this.horiz,
lineLeft = this.left + (opposite ? this.width : 0) + offset,
lineTop = chart.chartHeight - this.bottom - (opposite ? this.height : 0) + offset;
if (opposite) {
lineWidth *= -1; // crispify the other way - #1480, #1687
}
return chart.renderer.crispLine([
M,
horiz ?
this.left :
lineLeft,
horiz ?
lineTop :
this.top,
L,
horiz ?
chart.chartWidth - this.right :
lineLeft,
horiz ?
lineTop :
chart.chartHeight - this.bottom
], lineWidth);
},
/**
* Position the title
*/
getTitlePosition: function () {
// compute anchor points for each of the title align options
var horiz = this.horiz,
axisLeft = this.left,
axisTop = this.top,
axisLength = this.len,
axisTitleOptions = this.options.title,
margin = horiz ? axisLeft : axisTop,
opposite = this.opposite,
offset = this.offset,
fontSize = pInt(axisTitleOptions.style.fontSize || 12),
// the position in the length direction of the axis
alongAxis = {
low: margin + (horiz ? 0 : axisLength),
middle: margin + axisLength / 2,
high: margin + (horiz ? axisLength : 0)
}[axisTitleOptions.align],
// the position in the perpendicular direction of the axis
offAxis = (horiz ? axisTop + this.height : axisLeft) +
(horiz ? 1 : -1) * // horizontal axis reverses the margin
(opposite ? -1 : 1) * // so does opposite axes
this.axisTitleMargin +
(this.side === 2 ? fontSize : 0);
return {
x: horiz ?
alongAxis :
offAxis + (opposite ? this.width : 0) + offset +
(axisTitleOptions.x || 0), // x
y: horiz ?
offAxis - (opposite ? this.height : 0) + offset :
alongAxis + (axisTitleOptions.y || 0) // y
};
},
/**
* Render the axis
*/
render: function () {
var axis = this,
chart = axis.chart,
renderer = chart.renderer,
options = axis.options,
isLog = axis.isLog,
isLinked = axis.isLinked,
tickPositions = axis.tickPositions,
axisTitle = axis.axisTitle,
stacks = axis.stacks,
ticks = axis.ticks,
minorTicks = axis.minorTicks,
alternateBands = axis.alternateBands,
stackLabelOptions = options.stackLabels,
alternateGridColor = options.alternateGridColor,
tickmarkOffset = axis.tickmarkOffset,
lineWidth = options.lineWidth,
linePath,
hasRendered = chart.hasRendered,
slideInTicks = hasRendered && defined(axis.oldMin) && !isNaN(axis.oldMin),
hasData = axis.hasData,
showAxis = axis.showAxis,
from,
to;
// Mark all elements inActive before we go over and mark the active ones
each([ticks, minorTicks, alternateBands], function (coll) {
var pos;
for (pos in coll) {
coll[pos].isActive = false;
}
});
// If the series has data draw the ticks. Else only the line and title
if (hasData || isLinked) {
// minor ticks
if (axis.minorTickInterval && !axis.categories) {
each(axis.getMinorTickPositions(), function (pos) {
if (!minorTicks[pos]) {
minorTicks[pos] = new Tick(axis, pos, 'minor');
}
// render new ticks in old position
if (slideInTicks && minorTicks[pos].isNew) {
minorTicks[pos].render(null, true);
}
minorTicks[pos].render(null, false, 1);
});
}
// Major ticks. Pull out the first item and render it last so that
// we can get the position of the neighbour label. #808.
if (tickPositions.length) { // #1300
each(tickPositions.slice(1).concat([tickPositions[0]]), function (pos, i) {
// Reorganize the indices
i = (i === tickPositions.length - 1) ? 0 : i + 1;
// linked axes need an extra check to find out if
if (!isLinked || (pos >= axis.min && pos <= axis.max)) {
if (!ticks[pos]) {
ticks[pos] = new Tick(axis, pos);
}
// render new ticks in old position
if (slideInTicks && ticks[pos].isNew) {
ticks[pos].render(i, true);
}
ticks[pos].render(i, false, 1);
}
});
// In a categorized axis, the tick marks are displayed between labels. So
// we need to add a tick mark and grid line at the left edge of the X axis.
if (tickmarkOffset && axis.min === 0) {
if (!ticks[-1]) {
ticks[-1] = new Tick(axis, -1, null, true);
}
ticks[-1].render(-1);
}
}
// alternate grid color
if (alternateGridColor) {
each(tickPositions, function (pos, i) {
if (i % 2 === 0 && pos < axis.max) {
if (!alternateBands[pos]) {
alternateBands[pos] = new PlotLineOrBand(axis);
}
from = pos + tickmarkOffset; // #949
to = tickPositions[i + 1] !== UNDEFINED ? tickPositions[i + 1] + tickmarkOffset : axis.max;
alternateBands[pos].options = {
from: isLog ? lin2log(from) : from,
to: isLog ? lin2log(to) : to,
color: alternateGridColor
};
alternateBands[pos].render();
alternateBands[pos].isActive = true;
}
});
}
// custom plot lines and bands
if (!axis._addedPlotLB) { // only first time
each((options.plotLines || []).concat(options.plotBands || []), function (plotLineOptions) {
axis.addPlotBandOrLine(plotLineOptions);
});
axis._addedPlotLB = true;
}
} // end if hasData
// Remove inactive ticks
each([ticks, minorTicks, alternateBands], function (coll) {
var pos,
i,
forDestruction = [],
delay = globalAnimation ? globalAnimation.duration || 500 : 0,
destroyInactiveItems = function () {
i = forDestruction.length;
while (i--) {
// When resizing rapidly, the same items may be destroyed in different timeouts,
// or the may be reactivated
if (coll[forDestruction[i]] && !coll[forDestruction[i]].isActive) {
coll[forDestruction[i]].destroy();
delete coll[forDestruction[i]];
}
}
};
for (pos in coll) {
if (!coll[pos].isActive) {
// Render to zero opacity
coll[pos].render(pos, false, 0);
coll[pos].isActive = false;
forDestruction.push(pos);
}
}
// When the objects are finished fading out, destroy them
if (coll === alternateBands || !chart.hasRendered || !delay) {
destroyInactiveItems();
} else if (delay) {
setTimeout(destroyInactiveItems, delay);
}
});
// Static items. As the axis group is cleared on subsequent calls
// to render, these items are added outside the group.
// axis line
if (lineWidth) {
linePath = axis.getLinePath(lineWidth);
if (!axis.axisLine) {
axis.axisLine = renderer.path(linePath)
.attr({
stroke: options.lineColor,
'stroke-width': lineWidth,
zIndex: 7
})
.add(axis.axisGroup);
} else {
axis.axisLine.animate({ d: linePath });
}
// show or hide the line depending on options.showEmpty
axis.axisLine[showAxis ? 'show' : 'hide']();
}
if (axisTitle && showAxis) {
axisTitle[axisTitle.isNew ? 'attr' : 'animate'](
axis.getTitlePosition()
);
axisTitle.isNew = false;
}
// Stacked totals:
if (stackLabelOptions && stackLabelOptions.enabled) {
var stackKey, oneStack, stackCategory,
stackTotalGroup = axis.stackTotalGroup;
// Create a separate group for the stack total labels
if (!stackTotalGroup) {
axis.stackTotalGroup = stackTotalGroup =
renderer.g('stack-labels')
.attr({
visibility: VISIBLE,
zIndex: 6
})
.add();
}
// plotLeft/Top will change when y axis gets wider so we need to translate the
// stackTotalGroup at every render call. See bug #506 and #516
stackTotalGroup.translate(chart.plotLeft, chart.plotTop);
// Render each stack total
for (stackKey in stacks) {
oneStack = stacks[stackKey];
for (stackCategory in oneStack) {
oneStack[stackCategory].render(stackTotalGroup);
}
}
}
// End stacked totals
axis.isDirty = false;
},
/**
* Remove a plot band or plot line from the chart by id
* @param {Object} id
*/
removePlotBandOrLine: function (id) {
var plotLinesAndBands = this.plotLinesAndBands,
options = this.options,
userOptions = this.userOptions,
i = plotLinesAndBands.length;
while (i--) {
if (plotLinesAndBands[i].id === id) {
plotLinesAndBands[i].destroy();
}
}
each([options.plotLines || [], userOptions.plotLines || [], options.plotBands || [], userOptions.plotBands || []], function (arr) {
i = arr.length;
while (i--) {
if (arr[i].id === id) {
erase(arr, arr[i]);
}
}
});
},
/**
* Update the axis title by options
*/
setTitle: function (newTitleOptions, redraw) {
this.update({ title: newTitleOptions }, redraw);
},
/**
* Redraw the axis to reflect changes in the data or axis extremes
*/
redraw: function () {
var axis = this,
chart = axis.chart,
pointer = chart.pointer;
// hide tooltip and hover states
if (pointer.reset) {
pointer.reset(true);
}
// render the axis
axis.render();
// move plot lines and bands
each(axis.plotLinesAndBands, function (plotLine) {
plotLine.render();
});
// mark associated series as dirty and ready for redraw
each(axis.series, function (series) {
series.isDirty = true;
});
},
/**
* Build the stacks from top down
*/
buildStacks: function () {
var series = this.series,
i = series.length;
if (!this.isXAxis) {
while (i--) {
series[i].setStackedPoints();
}
// Loop up again to compute percent stack
if (this.usePercentage) {
for (i = 0; i < series.length; i++) {
series[i].setPercentStacks();
}
}
}
},
/**
* Set new axis categories and optionally redraw
* @param {Array} categories
* @param {Boolean} redraw
*/
setCategories: function (categories, redraw) {
this.update({ categories: categories }, redraw);
},
/**
* Destroys an Axis instance.
*/
destroy: function (keepEvents) {
var axis = this,
stacks = axis.stacks,
stackKey,
plotLinesAndBands = axis.plotLinesAndBands,
i;
// Remove the events
if (!keepEvents) {
removeEvent(axis);
}
// Destroy each stack total
for (stackKey in stacks) {
destroyObjectProperties(stacks[stackKey]);
stacks[stackKey] = null;
}
// Destroy collections
each([axis.ticks, axis.minorTicks, axis.alternateBands], function (coll) {
destroyObjectProperties(coll);
});
i = plotLinesAndBands.length;
while (i--) { // #1975
plotLinesAndBands[i].destroy();
}
// Destroy local variables
each(['stackTotalGroup', 'axisLine', 'axisGroup', 'gridGroup', 'labelGroup', 'axisTitle'], function (prop) {
if (axis[prop]) {
axis[prop] = axis[prop].destroy();
}
});
}
}; // end Axis
/**
* The tooltip object
* @param {Object} chart The chart instance
* @param {Object} options Tooltip options
*/
function Tooltip() {
this.init.apply(this, arguments);
}
Tooltip.prototype = {
init: function (chart, options) {
var borderWidth = options.borderWidth,
style = options.style,
padding = pInt(style.padding);
// Save the chart and options
this.chart = chart;
this.options = options;
// Keep track of the current series
//this.currentSeries = UNDEFINED;
// List of crosshairs
this.crosshairs = [];
// Current values of x and y when animating
this.now = { x: 0, y: 0 };
// The tooltip is initially hidden
this.isHidden = true;
// create the label
this.label = chart.renderer.label('', 0, 0, options.shape, null, null, options.useHTML, null, 'tooltip')
.attr({
padding: padding,
fill: options.backgroundColor,
'stroke-width': borderWidth,
r: options.borderRadius,
zIndex: 8
})
.css(style)
.css({ padding: 0 }) // Remove it from VML, the padding is applied as an attribute instead (#1117)
.add()
.attr({ y: -999 }); // #2301
// When using canVG the shadow shows up as a gray circle
// even if the tooltip is hidden.
if (!useCanVG) {
this.label.shadow(options.shadow);
}
// Public property for getting the shared state.
this.shared = options.shared;
},
/**
* Destroy the tooltip and its elements.
*/
destroy: function () {
each(this.crosshairs, function (crosshair) {
if (crosshair) {
crosshair.destroy();
}
});
// Destroy and clear local variables
if (this.label) {
this.label = this.label.destroy();
}
clearTimeout(this.hideTimer);
clearTimeout(this.tooltipTimeout);
},
/**
* Provide a soft movement for the tooltip
*
* @param {Number} x
* @param {Number} y
* @private
*/
move: function (x, y, anchorX, anchorY) {
var tooltip = this,
now = tooltip.now,
animate = tooltip.options.animation !== false && !tooltip.isHidden;
// get intermediate values for animation
extend(now, {
x: animate ? (2 * now.x + x) / 3 : x,
y: animate ? (now.y + y) / 2 : y,
anchorX: animate ? (2 * now.anchorX + anchorX) / 3 : anchorX,
anchorY: animate ? (now.anchorY + anchorY) / 2 : anchorY
});
// move to the intermediate value
tooltip.label.attr(now);
// run on next tick of the mouse tracker
if (animate && (mathAbs(x - now.x) > 1 || mathAbs(y - now.y) > 1)) {
// never allow two timeouts
clearTimeout(this.tooltipTimeout);
// set the fixed interval ticking for the smooth tooltip
this.tooltipTimeout = setTimeout(function () {
// The interval function may still be running during destroy, so check that the chart is really there before calling.
if (tooltip) {
tooltip.move(x, y, anchorX, anchorY);
}
}, 32);
}
},
/**
* Hide the tooltip
*/
hide: function () {
var tooltip = this,
hoverPoints;
clearTimeout(this.hideTimer); // disallow duplicate timers (#1728, #1766)
if (!this.isHidden) {
hoverPoints = this.chart.hoverPoints;
this.hideTimer = setTimeout(function () {
tooltip.label.fadeOut();
tooltip.isHidden = true;
}, pick(this.options.hideDelay, 500));
// hide previous hoverPoints and set new
if (hoverPoints) {
each(hoverPoints, function (point) {
point.setState();
});
}
this.chart.hoverPoints = null;
}
},
/**
* Hide the crosshairs
*/
hideCrosshairs: function () {
each(this.crosshairs, function (crosshair) {
if (crosshair) {
crosshair.hide();
}
});
},
/**
* Extendable method to get the anchor position of the tooltip
* from a point or set of points
*/
getAnchor: function (points, mouseEvent) {
var ret,
chart = this.chart,
inverted = chart.inverted,
plotTop = chart.plotTop,
plotX = 0,
plotY = 0,
yAxis;
points = splat(points);
// Pie uses a special tooltipPos
ret = points[0].tooltipPos;
// When tooltip follows mouse, relate the position to the mouse
if (this.followPointer && mouseEvent) {
if (mouseEvent.chartX === UNDEFINED) {
mouseEvent = chart.pointer.normalize(mouseEvent);
}
ret = [
mouseEvent.chartX - chart.plotLeft,
mouseEvent.chartY - plotTop
];
}
// When shared, use the average position
if (!ret) {
each(points, function (point) {
yAxis = point.series.yAxis;
plotX += point.plotX;
plotY += (point.plotLow ? (point.plotLow + point.plotHigh) / 2 : point.plotY) +
(!inverted && yAxis ? yAxis.top - plotTop : 0); // #1151
});
plotX /= points.length;
plotY /= points.length;
ret = [
inverted ? chart.plotWidth - plotY : plotX,
this.shared && !inverted && points.length > 1 && mouseEvent ?
mouseEvent.chartY - plotTop : // place shared tooltip next to the mouse (#424)
inverted ? chart.plotHeight - plotX : plotY
];
}
return map(ret, mathRound);
},
/**
* Place the tooltip in a chart without spilling over
* and not covering the point it self.
*/
getPosition: function (boxWidth, boxHeight, point) {
// Set up the variables
var chart = this.chart,
plotLeft = chart.plotLeft,
plotTop = chart.plotTop,
plotWidth = chart.plotWidth,
plotHeight = chart.plotHeight,
distance = pick(this.options.distance, 12),
pointX = point.plotX,
pointY = point.plotY,
x = pointX + plotLeft + (chart.inverted ? distance : -boxWidth - distance),
y = pointY - boxHeight + plotTop + 15, // 15 means the point is 15 pixels up from the bottom of the tooltip
alignedRight;
// It is too far to the left, adjust it
if (x < 7) {
x = plotLeft + mathMax(pointX, 0) + distance;
}
// Test to see if the tooltip is too far to the right,
// if it is, move it back to be inside and then up to not cover the point.
if ((x + boxWidth) > (plotLeft + plotWidth)) {
x -= (x + boxWidth) - (plotLeft + plotWidth);
y = pointY - boxHeight + plotTop - distance;
alignedRight = true;
}
// If it is now above the plot area, align it to the top of the plot area
if (y < plotTop + 5) {
y = plotTop + 5;
// If the tooltip is still covering the point, move it below instead
if (alignedRight && pointY >= y && pointY <= (y + boxHeight)) {
y = pointY + plotTop + distance; // below
}
}
// Now if the tooltip is below the chart, move it up. It's better to cover the
// point than to disappear outside the chart. #834.
if (y + boxHeight > plotTop + plotHeight) {
y = mathMax(plotTop, plotTop + plotHeight - boxHeight - distance); // below
}
return {x: x, y: y};
},
/**
* In case no user defined formatter is given, this will be used. Note that the context
* here is an object holding point, series, x, y etc.
*/
defaultFormatter: function (tooltip) {
var items = this.points || splat(this),
series = items[0].series,
s;
// build the header
s = [series.tooltipHeaderFormatter(items[0])];
// build the values
each(items, function (item) {
series = item.series;
s.push((series.tooltipFormatter && series.tooltipFormatter(item)) ||
item.point.tooltipFormatter(series.tooltipOptions.pointFormat));
});
// footer
s.push(tooltip.options.footerFormat || '');
return s.join('');
},
/**
* Refresh the tooltip's text and position.
* @param {Object} point
*/
refresh: function (point, mouseEvent) {
var tooltip = this,
chart = tooltip.chart,
label = tooltip.label,
options = tooltip.options,
x,
y,
anchor,
textConfig = {},
text,
pointConfig = [],
formatter = options.formatter || tooltip.defaultFormatter,
hoverPoints = chart.hoverPoints,
borderColor,
crosshairsOptions = options.crosshairs,
shared = tooltip.shared,
currentSeries;
clearTimeout(this.hideTimer);
// get the reference point coordinates (pie charts use tooltipPos)
tooltip.followPointer = splat(point)[0].series.tooltipOptions.followPointer;
anchor = tooltip.getAnchor(point, mouseEvent);
x = anchor[0];
y = anchor[1];
// shared tooltip, array is sent over
if (shared && !(point.series && point.series.noSharedTooltip)) {
// hide previous hoverPoints and set new
chart.hoverPoints = point;
if (hoverPoints) {
each(hoverPoints, function (point) {
point.setState();
});
}
each(point, function (item) {
item.setState(HOVER_STATE);
pointConfig.push(item.getLabelConfig());
});
textConfig = {
x: point[0].category,
y: point[0].y
};
textConfig.points = pointConfig;
point = point[0];
// single point tooltip
} else {
textConfig = point.getLabelConfig();
}
text = formatter.call(textConfig, tooltip);
// register the current series
currentSeries = point.series;
// update the inner HTML
if (text === false) {
this.hide();
} else {
// show it
if (tooltip.isHidden) {
stop(label);
label.attr('opacity', 1).show();
}
// update text
label.attr({
text: text
});
// set the stroke color of the box
borderColor = options.borderColor || point.color || currentSeries.color || '#606060';
label.attr({
stroke: borderColor
});
tooltip.updatePosition({ plotX: x, plotY: y });
this.isHidden = false;
}
// crosshairs
if (crosshairsOptions) {
crosshairsOptions = splat(crosshairsOptions); // [x, y]
var path,
i = crosshairsOptions.length,
attribs,
axis,
val,
series;
while (i--) {
series = point.series;
axis = series[i ? 'yAxis' : 'xAxis'];
if (crosshairsOptions[i] && axis) {
val = i ? pick(point.stackY, point.y) : point.x; // #814
if (axis.isLog) { // #1671
val = log2lin(val);
}
if (i === 1 && series.modifyValue) { // #1205, #2316
val = series.modifyValue(val);
}
path = axis.getPlotLinePath(
val,
1
);
if (tooltip.crosshairs[i]) {
tooltip.crosshairs[i].attr({ d: path, visibility: VISIBLE });
} else {
attribs = {
'stroke-width': crosshairsOptions[i].width || 1,
stroke: crosshairsOptions[i].color || '#C0C0C0',
zIndex: crosshairsOptions[i].zIndex || 2
};
if (crosshairsOptions[i].dashStyle) {
attribs.dashstyle = crosshairsOptions[i].dashStyle;
}
tooltip.crosshairs[i] = chart.renderer.path(path)
.attr(attribs)
.add();
}
}
}
}
fireEvent(chart, 'tooltipRefresh', {
text: text,
x: x + chart.plotLeft,
y: y + chart.plotTop,
borderColor: borderColor
});
},
/**
* Find the new position and perform the move
*/
updatePosition: function (point) {
var chart = this.chart,
label = this.label,
pos = (this.options.positioner || this.getPosition).call(
this,
label.width,
label.height,
point
);
// do the move
this.move(
mathRound(pos.x),
mathRound(pos.y),
point.plotX + chart.plotLeft,
point.plotY + chart.plotTop
);
}
};
/**
* The mouse tracker object. All methods starting with "on" are primary DOM event handlers.
* Subsequent methods should be named differently from what they are doing.
* @param {Object} chart The Chart instance
* @param {Object} options The root options object
*/
function Pointer(chart, options) {
this.init(chart, options);
}
Pointer.prototype = {
/**
* Initialize Pointer
*/
init: function (chart, options) {
var chartOptions = options.chart,
chartEvents = chartOptions.events,
zoomType = useCanVG ? '' : chartOptions.zoomType,
inverted = chart.inverted,
zoomX,
zoomY;
// Store references
this.options = options;
this.chart = chart;
// Zoom status
this.zoomX = zoomX = /x/.test(zoomType);
this.zoomY = zoomY = /y/.test(zoomType);
this.zoomHor = (zoomX && !inverted) || (zoomY && inverted);
this.zoomVert = (zoomY && !inverted) || (zoomX && inverted);
// Do we need to handle click on a touch device?
this.runChartClick = chartEvents && !!chartEvents.click;
this.pinchDown = [];
this.lastValidTouch = {};
if (options.tooltip.enabled) {
chart.tooltip = new Tooltip(chart, options.tooltip);
}
this.setDOMEvents();
},
/**
* Add crossbrowser support for chartX and chartY
* @param {Object} e The event object in standard browsers
*/
normalize: function (e, chartPosition) {
var chartX,
chartY,
ePos;
// common IE normalizing
e = e || win.event;
if (!e.target) {
e.target = e.srcElement;
}
// Framework specific normalizing (#1165)
e = washMouseEvent(e);
// iOS
ePos = e.touches ? e.touches.item(0) : e;
// Get mouse position
if (!chartPosition) {
this.chartPosition = chartPosition = offset(this.chart.container);
}
// chartX and chartY
if (ePos.pageX === UNDEFINED) { // IE < 9. #886.
chartX = mathMax(e.x, e.clientX - chartPosition.left); // #2005, #2129: the second case is
// for IE10 quirks mode within framesets
chartY = e.y;
} else {
chartX = ePos.pageX - chartPosition.left;
chartY = ePos.pageY - chartPosition.top;
}
return extend(e, {
chartX: mathRound(chartX),
chartY: mathRound(chartY)
});
},
/**
* Get the click position in terms of axis values.
*
* @param {Object} e A pointer event
*/
getCoordinates: function (e) {
var coordinates = {
xAxis: [],
yAxis: []
};
each(this.chart.axes, function (axis) {
coordinates[axis.isXAxis ? 'xAxis' : 'yAxis'].push({
axis: axis,
value: axis.toValue(e[axis.horiz ? 'chartX' : 'chartY'])
});
});
return coordinates;
},
/**
* Return the index in the tooltipPoints array, corresponding to pixel position in
* the plot area.
*/
getIndex: function (e) {
var chart = this.chart;
return chart.inverted ?
chart.plotHeight + chart.plotTop - e.chartY :
e.chartX - chart.plotLeft;
},
/**
* With line type charts with a single tracker, get the point closest to the mouse.
* Run Point.onMouseOver and display tooltip for the point or points.
*/
runPointActions: function (e) {
var pointer = this,
chart = pointer.chart,
series = chart.series,
tooltip = chart.tooltip,
point,
points,
hoverPoint = chart.hoverPoint,
hoverSeries = chart.hoverSeries,
i,
j,
distance = chart.chartWidth,
index = pointer.getIndex(e),
anchor;
// shared tooltip
if (tooltip && pointer.options.tooltip.shared && !(hoverSeries && hoverSeries.noSharedTooltip)) {
points = [];
// loop over all series and find the ones with points closest to the mouse
i = series.length;
for (j = 0; j < i; j++) {
if (series[j].visible &&
series[j].options.enableMouseTracking !== false &&
!series[j].noSharedTooltip && series[j].tooltipPoints.length) {
point = series[j].tooltipPoints[index];
if (point && point.series) { // not a dummy point, #1544
point._dist = mathAbs(index - point.clientX);
distance = mathMin(distance, point._dist);
points.push(point);
}
}
}
// remove furthest points
i = points.length;
while (i--) {
if (points[i]._dist > distance) {
points.splice(i, 1);
}
}
// refresh the tooltip if necessary
if (points.length && (points[0].clientX !== pointer.hoverX)) {
tooltip.refresh(points, e);
pointer.hoverX = points[0].clientX;
}
}
// separate tooltip and general mouse events
if (hoverSeries && hoverSeries.tracker) { // only use for line-type series with common tracker
// get the point
point = hoverSeries.tooltipPoints[index];
// a new point is hovered, refresh the tooltip
if (point && point !== hoverPoint) {
// trigger the events
point.onMouseOver(e);
}
} else if (tooltip && tooltip.followPointer && !tooltip.isHidden) {
anchor = tooltip.getAnchor([{}], e);
tooltip.updatePosition({ plotX: anchor[0], plotY: anchor[1] });
}
},
/**
* Reset the tracking by hiding the tooltip, the hover series state and the hover point
*
* @param allowMove {Boolean} Instead of destroying the tooltip altogether, allow moving it if possible
*/
reset: function (allowMove) {
var pointer = this,
chart = pointer.chart,
hoverSeries = chart.hoverSeries,
hoverPoint = chart.hoverPoint,
tooltip = chart.tooltip,
tooltipPoints = tooltip && tooltip.shared ? chart.hoverPoints : hoverPoint;
// Narrow in allowMove
allowMove = allowMove && tooltip && tooltipPoints;
// Check if the points have moved outside the plot area, #1003
if (allowMove && splat(tooltipPoints)[0].plotX === UNDEFINED) {
allowMove = false;
}
// Just move the tooltip, #349
if (allowMove) {
tooltip.refresh(tooltipPoints);
// Full reset
} else {
if (hoverPoint) {
hoverPoint.onMouseOut();
}
if (hoverSeries) {
hoverSeries.onMouseOut();
}
if (tooltip) {
tooltip.hide();
tooltip.hideCrosshairs();
}
pointer.hoverX = null;
}
},
/**
* Scale series groups to a certain scale and translation
*/
scaleGroups: function (attribs, clip) {
var chart = this.chart,
seriesAttribs;
// Scale each series
each(chart.series, function (series) {
seriesAttribs = attribs || series.getPlotBox(); // #1701
if (series.xAxis && series.xAxis.zoomEnabled) {
series.group.attr(seriesAttribs);
if (series.markerGroup) {
series.markerGroup.attr(seriesAttribs);
series.markerGroup.clip(clip ? chart.clipRect : null);
}
if (series.dataLabelsGroup) {
series.dataLabelsGroup.attr(seriesAttribs);
}
}
});
// Clip
chart.clipRect.attr(clip || chart.clipBox);
},
/**
* Run translation operations for each direction (horizontal and vertical) independently
*/
pinchTranslateDirection: function (horiz, pinchDown, touches, transform, selectionMarker, clip, lastValidTouch) {
var chart = this.chart,
xy = horiz ? 'x' : 'y',
XY = horiz ? 'X' : 'Y',
sChartXY = 'chart' + XY,
wh = horiz ? 'width' : 'height',
plotLeftTop = chart['plot' + (horiz ? 'Left' : 'Top')],
selectionWH,
selectionXY,
clipXY,
scale = 1,
inverted = chart.inverted,
bounds = chart.bounds[horiz ? 'h' : 'v'],
singleTouch = pinchDown.length === 1,
touch0Start = pinchDown[0][sChartXY],
touch0Now = touches[0][sChartXY],
touch1Start = !singleTouch && pinchDown[1][sChartXY],
touch1Now = !singleTouch && touches[1][sChartXY],
outOfBounds,
transformScale,
scaleKey,
setScale = function () {
if (!singleTouch && mathAbs(touch0Start - touch1Start) > 20) { // Don't zoom if fingers are too close on this axis
scale = mathAbs(touch0Now - touch1Now) / mathAbs(touch0Start - touch1Start);
}
clipXY = ((plotLeftTop - touch0Now) / scale) + touch0Start;
selectionWH = chart['plot' + (horiz ? 'Width' : 'Height')] / scale;
};
// Set the scale, first pass
setScale();
selectionXY = clipXY; // the clip position (x or y) is altered if out of bounds, the selection position is not
// Out of bounds
if (selectionXY < bounds.min) {
selectionXY = bounds.min;
outOfBounds = true;
} else if (selectionXY + selectionWH > bounds.max) {
selectionXY = bounds.max - selectionWH;
outOfBounds = true;
}
// Is the chart dragged off its bounds, determined by dataMin and dataMax?
if (outOfBounds) {
// Modify the touchNow position in order to create an elastic drag movement. This indicates
// to the user that the chart is responsive but can't be dragged further.
touch0Now -= 0.8 * (touch0Now - lastValidTouch[xy][0]);
if (!singleTouch) {
touch1Now -= 0.8 * (touch1Now - lastValidTouch[xy][1]);
}
// Set the scale, second pass to adapt to the modified touchNow positions
setScale();
} else {
lastValidTouch[xy] = [touch0Now, touch1Now];
}
// Set geometry for clipping, selection and transformation
if (!inverted) { // TODO: implement clipping for inverted charts
clip[xy] = clipXY - plotLeftTop;
clip[wh] = selectionWH;
}
scaleKey = inverted ? (horiz ? 'scaleY' : 'scaleX') : 'scale' + XY;
transformScale = inverted ? 1 / scale : scale;
selectionMarker[wh] = selectionWH;
selectionMarker[xy] = selectionXY;
transform[scaleKey] = scale;
transform['translate' + XY] = (transformScale * plotLeftTop) + (touch0Now - (transformScale * touch0Start));
},
/**
* Handle touch events with two touches
*/
pinch: function (e) {
var self = this,
chart = self.chart,
pinchDown = self.pinchDown,
followTouchMove = chart.tooltip && chart.tooltip.options.followTouchMove,
touches = e.touches,
touchesLength = touches.length,
lastValidTouch = self.lastValidTouch,
zoomHor = self.zoomHor || self.pinchHor,
zoomVert = self.zoomVert || self.pinchVert,
hasZoom = zoomHor || zoomVert,
selectionMarker = self.selectionMarker,
transform = {},
fireClickEvent = touchesLength === 1 && ((self.inClass(e.target, PREFIX + 'tracker') &&
chart.runTrackerClick) || chart.runChartClick),
clip = {};
// On touch devices, only proceed to trigger click if a handler is defined
if ((hasZoom || followTouchMove) && !fireClickEvent) {
e.preventDefault();
}
// Normalize each touch
map(touches, function (e) {
return self.normalize(e);
});
// Register the touch start position
if (e.type === 'touchstart') {
each(touches, function (e, i) {
pinchDown[i] = { chartX: e.chartX, chartY: e.chartY };
});
lastValidTouch.x = [pinchDown[0].chartX, pinchDown[1] && pinchDown[1].chartX];
lastValidTouch.y = [pinchDown[0].chartY, pinchDown[1] && pinchDown[1].chartY];
// Identify the data bounds in pixels
each(chart.axes, function (axis) {
if (axis.zoomEnabled) {
var bounds = chart.bounds[axis.horiz ? 'h' : 'v'],
minPixelPadding = axis.minPixelPadding,
min = axis.toPixels(axis.dataMin),
max = axis.toPixels(axis.dataMax),
absMin = mathMin(min, max),
absMax = mathMax(min, max);
// Store the bounds for use in the touchmove handler
bounds.min = mathMin(axis.pos, absMin - minPixelPadding);
bounds.max = mathMax(axis.pos + axis.len, absMax + minPixelPadding);
}
});
// Event type is touchmove, handle panning and pinching
} else if (pinchDown.length) { // can be 0 when releasing, if touchend fires first
// Set the marker
if (!selectionMarker) {
self.selectionMarker = selectionMarker = extend({
destroy: noop
}, chart.plotBox);
}
if (zoomHor) {
self.pinchTranslateDirection(true, pinchDown, touches, transform, selectionMarker, clip, lastValidTouch);
}
if (zoomVert) {
self.pinchTranslateDirection(false, pinchDown, touches, transform, selectionMarker, clip, lastValidTouch);
}
self.hasPinched = hasZoom;
// Scale and translate the groups to provide visual feedback during pinching
self.scaleGroups(transform, clip);
// Optionally move the tooltip on touchmove
if (!hasZoom && followTouchMove && touchesLength === 1) {
this.runPointActions(self.normalize(e));
}
}
},
/**
* Start a drag operation
*/
dragStart: function (e) {
var chart = this.chart;
// Record the start position
chart.mouseIsDown = e.type;
chart.cancelClick = false;
chart.mouseDownX = this.mouseDownX = e.chartX;
chart.mouseDownY = this.mouseDownY = e.chartY;
},
/**
* Perform a drag operation in response to a mousemove event while the mouse is down
*/
drag: function (e) {
var chart = this.chart,
chartOptions = chart.options.chart,
chartX = e.chartX,
chartY = e.chartY,
zoomHor = this.zoomHor,
zoomVert = this.zoomVert,
plotLeft = chart.plotLeft,
plotTop = chart.plotTop,
plotWidth = chart.plotWidth,
plotHeight = chart.plotHeight,
clickedInside,
size,
mouseDownX = this.mouseDownX,
mouseDownY = this.mouseDownY;
// If the mouse is outside the plot area, adjust to cooordinates
// inside to prevent the selection marker from going outside
if (chartX < plotLeft) {
chartX = plotLeft;
} else if (chartX > plotLeft + plotWidth) {
chartX = plotLeft + plotWidth;
}
if (chartY < plotTop) {
chartY = plotTop;
} else if (chartY > plotTop + plotHeight) {
chartY = plotTop + plotHeight;
}
// determine if the mouse has moved more than 10px
this.hasDragged = Math.sqrt(
Math.pow(mouseDownX - chartX, 2) +
Math.pow(mouseDownY - chartY, 2)
);
if (this.hasDragged > 10) {
clickedInside = chart.isInsidePlot(mouseDownX - plotLeft, mouseDownY - plotTop);
// make a selection
if (chart.hasCartesianSeries && (this.zoomX || this.zoomY) && clickedInside) {
if (!this.selectionMarker) {
this.selectionMarker = chart.renderer.rect(
plotLeft,
plotTop,
zoomHor ? 1 : plotWidth,
zoomVert ? 1 : plotHeight,
0
)
.attr({
fill: chartOptions.selectionMarkerFill || 'rgba(69,114,167,0.25)',
zIndex: 7
})
.add();
}
}
// adjust the width of the selection marker
if (this.selectionMarker && zoomHor) {
size = chartX - mouseDownX;
this.selectionMarker.attr({
width: mathAbs(size),
x: (size > 0 ? 0 : size) + mouseDownX
});
}
// adjust the height of the selection marker
if (this.selectionMarker && zoomVert) {
size = chartY - mouseDownY;
this.selectionMarker.attr({
height: mathAbs(size),
y: (size > 0 ? 0 : size) + mouseDownY
});
}
// panning
if (clickedInside && !this.selectionMarker && chartOptions.panning) {
chart.pan(e, chartOptions.panning);
}
}
},
/**
* On mouse up or touch end across the entire document, drop the selection.
*/
drop: function (e) {
var chart = this.chart,
hasPinched = this.hasPinched;
if (this.selectionMarker) {
var selectionData = {
xAxis: [],
yAxis: [],
originalEvent: e.originalEvent || e
},
selectionBox = this.selectionMarker,
selectionLeft = selectionBox.x,
selectionTop = selectionBox.y,
runZoom;
// a selection has been made
if (this.hasDragged || hasPinched) {
// record each axis' min and max
each(chart.axes, function (axis) {
if (axis.zoomEnabled) {
var horiz = axis.horiz,
selectionMin = axis.toValue((horiz ? selectionLeft : selectionTop)),
selectionMax = axis.toValue((horiz ? selectionLeft + selectionBox.width : selectionTop + selectionBox.height));
if (!isNaN(selectionMin) && !isNaN(selectionMax)) { // #859
selectionData[axis.xOrY + 'Axis'].push({
axis: axis,
min: mathMin(selectionMin, selectionMax), // for reversed axes,
max: mathMax(selectionMin, selectionMax)
});
runZoom = true;
}
}
});
if (runZoom) {
fireEvent(chart, 'selection', selectionData, function (args) {
chart.zoom(extend(args, hasPinched ? { animation: false } : null));
});
}
}
this.selectionMarker = this.selectionMarker.destroy();
// Reset scaling preview
if (hasPinched) {
this.scaleGroups();
}
}
// Reset all
if (chart) { // it may be destroyed on mouse up - #877
css(chart.container, { cursor: chart._cursor });
chart.cancelClick = this.hasDragged > 10; // #370
chart.mouseIsDown = this.hasDragged = this.hasPinched = false;
this.pinchDown = [];
}
},
onContainerMouseDown: function (e) {
e = this.normalize(e);
// issue #295, dragging not always working in Firefox
if (e.preventDefault) {
e.preventDefault();
}
this.dragStart(e);
},
onDocumentMouseUp: function (e) {
this.drop(e);
},
/**
* Special handler for mouse move that will hide the tooltip when the mouse leaves the plotarea.
* Issue #149 workaround. The mouseleave event does not always fire.
*/
onDocumentMouseMove: function (e) {
var chart = this.chart,
chartPosition = this.chartPosition,
hoverSeries = chart.hoverSeries;
e = this.normalize(e, chartPosition);
// If we're outside, hide the tooltip
if (chartPosition && hoverSeries && !this.inClass(e.target, 'highcharts-tracker') &&
!chart.isInsidePlot(e.chartX - chart.plotLeft, e.chartY - chart.plotTop)) {
this.reset();
}
},
/**
* When mouse leaves the container, hide the tooltip.
*/
onContainerMouseLeave: function () {
this.reset();
this.chartPosition = null; // also reset the chart position, used in #149 fix
},
// The mousemove, touchmove and touchstart event handler
onContainerMouseMove: function (e) {
var chart = this.chart;
// normalize
e = this.normalize(e);
// #295
e.returnValue = false;
if (chart.mouseIsDown === 'mousedown') {
this.drag(e);
}
// Show the tooltip and run mouse over events (#977)
if ((this.inClass(e.target, 'highcharts-tracker') ||
chart.isInsidePlot(e.chartX - chart.plotLeft, e.chartY - chart.plotTop)) && !chart.openMenu) {
this.runPointActions(e);
}
},
/**
* Utility to detect whether an element has, or has a parent with, a specific
* class name. Used on detection of tracker objects and on deciding whether
* hovering the tooltip should cause the active series to mouse out.
*/
inClass: function (element, className) {
var elemClassName;
while (element) {
elemClassName = attr(element, 'class');
if (elemClassName) {
if (elemClassName.indexOf(className) !== -1) {
return true;
} else if (elemClassName.indexOf(PREFIX + 'container') !== -1) {
return false;
}
}
element = element.parentNode;
}
},
onTrackerMouseOut: function (e) {
var series = this.chart.hoverSeries;
if (series && !series.options.stickyTracking && !this.inClass(e.toElement || e.relatedTarget, PREFIX + 'tooltip')) {
series.onMouseOut();
}
},
onContainerClick: function (e) {
var chart = this.chart,
hoverPoint = chart.hoverPoint,
plotLeft = chart.plotLeft,
plotTop = chart.plotTop,
inverted = chart.inverted,
chartPosition,
plotX,
plotY;
e = this.normalize(e);
e.cancelBubble = true; // IE specific
if (!chart.cancelClick) {
// On tracker click, fire the series and point events. #783, #1583
if (hoverPoint && this.inClass(e.target, PREFIX + 'tracker')) {
chartPosition = this.chartPosition;
plotX = hoverPoint.plotX;
plotY = hoverPoint.plotY;
// add page position info
extend(hoverPoint, {
pageX: chartPosition.left + plotLeft +
(inverted ? chart.plotWidth - plotY : plotX),
pageY: chartPosition.top + plotTop +
(inverted ? chart.plotHeight - plotX : plotY)
});
// the series click event
fireEvent(hoverPoint.series, 'click', extend(e, {
point: hoverPoint
}));
// the point click event
if (chart.hoverPoint) { // it may be destroyed (#1844)
hoverPoint.firePointEvent('click', e);
}
// When clicking outside a tracker, fire a chart event
} else {
extend(e, this.getCoordinates(e));
// fire a click event in the chart
if (chart.isInsidePlot(e.chartX - plotLeft, e.chartY - plotTop)) {
fireEvent(chart, 'click', e);
}
}
}
},
onContainerTouchStart: function (e) {
var chart = this.chart;
if (e.touches.length === 1) {
e = this.normalize(e);
if (chart.isInsidePlot(e.chartX - chart.plotLeft, e.chartY - chart.plotTop)) {
// Prevent the click pseudo event from firing unless it is set in the options
/*if (!chart.runChartClick) {
e.preventDefault();
}*/
// Run mouse events and display tooltip etc
this.runPointActions(e);
this.pinch(e);
} else {
// Hide the tooltip on touching outside the plot area (#1203)
this.reset();
}
} else if (e.touches.length === 2) {
this.pinch(e);
}
},
onContainerTouchMove: function (e) {
if (e.touches.length === 1 || e.touches.length === 2) {
this.pinch(e);
}
},
onDocumentTouchEnd: function (e) {
this.drop(e);
},
/**
* Set the JS DOM events on the container and document. This method should contain
* a one-to-one assignment between methods and their handlers. Any advanced logic should
* be moved to the handler reflecting the event's name.
*/
setDOMEvents: function () {
var pointer = this,
container = pointer.chart.container,
events;
this._events = events = [
[container, 'onmousedown', 'onContainerMouseDown'],
[container, 'onmousemove', 'onContainerMouseMove'],
[container, 'onclick', 'onContainerClick'],
[container, 'mouseleave', 'onContainerMouseLeave'],
[doc, 'mousemove', 'onDocumentMouseMove'],
[doc, 'mouseup', 'onDocumentMouseUp']
];
if (hasTouch) {
events.push(
[container, 'ontouchstart', 'onContainerTouchStart'],
[container, 'ontouchmove', 'onContainerTouchMove'],
[doc, 'touchend', 'onDocumentTouchEnd']
);
}
each(events, function (eventConfig) {
// First, create the callback function that in turn calls the method on Pointer
pointer['_' + eventConfig[2]] = function (e) {
pointer[eventConfig[2]](e);
};
// Now attach the function, either as a direct property or through addEvent
if (eventConfig[1].indexOf('on') === 0) {
eventConfig[0][eventConfig[1]] = pointer['_' + eventConfig[2]];
} else {
addEvent(eventConfig[0], eventConfig[1], pointer['_' + eventConfig[2]]);
}
});
},
/**
* Destroys the Pointer object and disconnects DOM events.
*/
destroy: function () {
var pointer = this;
// Release all DOM events
each(pointer._events, function (eventConfig) {
if (eventConfig[1].indexOf('on') === 0) {
eventConfig[0][eventConfig[1]] = null; // delete breaks oldIE
} else {
removeEvent(eventConfig[0], eventConfig[1], pointer['_' + eventConfig[2]]);
}
});
delete pointer._events;
// memory and CPU leak
clearInterval(pointer.tooltipTimeout);
}
};
/**
* The overview of the chart's series
*/
function Legend(chart, options) {
this.init(chart, options);
}
Legend.prototype = {
/**
* Initialize the legend
*/
init: function (chart, options) {
var legend = this,
itemStyle = options.itemStyle,
padding = pick(options.padding, 8),
itemMarginTop = options.itemMarginTop || 0;
this.options = options;
if (!options.enabled) {
return;
}
legend.baseline = pInt(itemStyle.fontSize) + 3 + itemMarginTop; // used in Series prototype
legend.itemStyle = itemStyle;
legend.itemHiddenStyle = merge(itemStyle, options.itemHiddenStyle);
legend.itemMarginTop = itemMarginTop;
legend.padding = padding;
legend.initialItemX = padding;
legend.initialItemY = padding - 5; // 5 is the number of pixels above the text
legend.maxItemWidth = 0;
legend.chart = chart;
legend.itemHeight = 0;
legend.lastLineHeight = 0;
// Render it
legend.render();
// move checkboxes
addEvent(legend.chart, 'endResize', function () {
legend.positionCheckboxes();
});
},
/**
* Set the colors for the legend item
* @param {Object} item A Series or Point instance
* @param {Object} visible Dimmed or colored
*/
colorizeItem: function (item, visible) {
var legend = this,
options = legend.options,
legendItem = item.legendItem,
legendLine = item.legendLine,
legendSymbol = item.legendSymbol,
hiddenColor = legend.itemHiddenStyle.color,
textColor = visible ? options.itemStyle.color : hiddenColor,
symbolColor = visible ? item.color : hiddenColor,
markerOptions = item.options && item.options.marker,
symbolAttr = {
stroke: symbolColor,
fill: symbolColor
},
key,
val;
if (legendItem) {
legendItem.css({ fill: textColor, color: textColor }); // color for #1553, oldIE
}
if (legendLine) {
legendLine.attr({ stroke: symbolColor });
}
if (legendSymbol) {
// Apply marker options
if (markerOptions && legendSymbol.isMarker) { // #585
markerOptions = item.convertAttribs(markerOptions);
for (key in markerOptions) {
val = markerOptions[key];
if (val !== UNDEFINED) {
symbolAttr[key] = val;
}
}
}
legendSymbol.attr(symbolAttr);
}
},
/**
* Position the legend item
* @param {Object} item A Series or Point instance
*/
positionItem: function (item) {
var legend = this,
options = legend.options,
symbolPadding = options.symbolPadding,
ltr = !options.rtl,
legendItemPos = item._legendItemPos,
itemX = legendItemPos[0],
itemY = legendItemPos[1],
checkbox = item.checkbox;
if (item.legendGroup) {
item.legendGroup.translate(
ltr ? itemX : legend.legendWidth - itemX - 2 * symbolPadding - 4,
itemY
);
}
if (checkbox) {
checkbox.x = itemX;
checkbox.y = itemY;
}
},
/**
* Destroy a single legend item
* @param {Object} item The series or point
*/
destroyItem: function (item) {
var checkbox = item.checkbox;
// destroy SVG elements
each(['legendItem', 'legendLine', 'legendSymbol', 'legendGroup'], function (key) {
if (item[key]) {
item[key] = item[key].destroy();
}
});
if (checkbox) {
discardElement(item.checkbox);
}
},
/**
* Destroys the legend.
*/
destroy: function () {
var legend = this,
legendGroup = legend.group,
box = legend.box;
if (box) {
legend.box = box.destroy();
}
if (legendGroup) {
legend.group = legendGroup.destroy();
}
},
/**
* Position the checkboxes after the width is determined
*/
positionCheckboxes: function (scrollOffset) {
var alignAttr = this.group.alignAttr,
translateY,
clipHeight = this.clipHeight || this.legendHeight;
if (alignAttr) {
translateY = alignAttr.translateY;
each(this.allItems, function (item) {
var checkbox = item.checkbox,
top;
if (checkbox) {
top = (translateY + checkbox.y + (scrollOffset || 0) + 3);
css(checkbox, {
left: (alignAttr.translateX + item.legendItemWidth + checkbox.x - 20) + PX,
top: top + PX,
display: top > translateY - 6 && top < translateY + clipHeight - 6 ? '' : NONE
});
}
});
}
},
/**
* Render the legend title on top of the legend
*/
renderTitle: function () {
var options = this.options,
padding = this.padding,
titleOptions = options.title,
titleHeight = 0,
bBox;
if (titleOptions.text) {
if (!this.title) {
this.title = this.chart.renderer.label(titleOptions.text, padding - 3, padding - 4, null, null, null, null, null, 'legend-title')
.attr({ zIndex: 1 })
.css(titleOptions.style)
.add(this.group);
}
bBox = this.title.getBBox();
titleHeight = bBox.height;
this.offsetWidth = bBox.width; // #1717
this.contentGroup.attr({ translateY: titleHeight });
}
this.titleHeight = titleHeight;
},
/**
* Render a single specific legend item
* @param {Object} item A series or point
*/
renderItem: function (item) {
var legend = this,
chart = legend.chart,
renderer = chart.renderer,
options = legend.options,
horizontal = options.layout === 'horizontal',
symbolWidth = options.symbolWidth,
symbolPadding = options.symbolPadding,
itemStyle = legend.itemStyle,
itemHiddenStyle = legend.itemHiddenStyle,
padding = legend.padding,
itemDistance = horizontal ? pick(options.itemDistance, 8) : 0,
ltr = !options.rtl,
itemHeight,
widthOption = options.width,
itemMarginBottom = options.itemMarginBottom || 0,
itemMarginTop = legend.itemMarginTop,
initialItemX = legend.initialItemX,
bBox,
itemWidth,
li = item.legendItem,
series = item.series || item,
itemOptions = series.options,
showCheckbox = itemOptions.showCheckbox,
useHTML = options.useHTML;
if (!li) { // generate it once, later move it
// Generate the group box
// A group to hold the symbol and text. Text is to be appended in Legend class.
item.legendGroup = renderer.g('legend-item')
.attr({ zIndex: 1 })
.add(legend.scrollGroup);
// Draw the legend symbol inside the group box
series.drawLegendSymbol(legend, item);
// Generate the list item text and add it to the group
item.legendItem = li = renderer.text(
options.labelFormat ? format(options.labelFormat, item) : options.labelFormatter.call(item),
ltr ? symbolWidth + symbolPadding : -symbolPadding,
legend.baseline,
useHTML
)
.css(merge(item.visible ? itemStyle : itemHiddenStyle)) // merge to prevent modifying original (#1021)
.attr({
align: ltr ? 'left' : 'right',
zIndex: 2
})
.add(item.legendGroup);
// Set the events on the item group, or in case of useHTML, the item itself (#1249)
(useHTML ? li : item.legendGroup).on('mouseover', function () {
item.setState(HOVER_STATE);
li.css(legend.options.itemHoverStyle);
})
.on('mouseout', function () {
li.css(item.visible ? itemStyle : itemHiddenStyle);
item.setState();
})
.on('click', function (event) {
var strLegendItemClick = 'legendItemClick',
fnLegendItemClick = function () {
item.setVisible();
};
// Pass over the click/touch event. #4.
event = {
browserEvent: event
};
// click the name or symbol
if (item.firePointEvent) { // point
item.firePointEvent(strLegendItemClick, event, fnLegendItemClick);
} else {
fireEvent(item, strLegendItemClick, event, fnLegendItemClick);
}
});
// Colorize the items
legend.colorizeItem(item, item.visible);
// add the HTML checkbox on top
if (itemOptions && showCheckbox) {
item.checkbox = createElement('input', {
type: 'checkbox',
checked: item.selected,
defaultChecked: item.selected // required by IE7
}, options.itemCheckboxStyle, chart.container);
addEvent(item.checkbox, 'click', function (event) {
var target = event.target;
fireEvent(item, 'checkboxClick', {
checked: target.checked
},
function () {
item.select();
}
);
});
}
}
// calculate the positions for the next line
bBox = li.getBBox();
itemWidth = item.legendItemWidth =
options.itemWidth || symbolWidth + symbolPadding + bBox.width + itemDistance +
(showCheckbox ? 20 : 0);
legend.itemHeight = itemHeight = bBox.height;
// if the item exceeds the width, start a new line
if (horizontal && legend.itemX - initialItemX + itemWidth >
(widthOption || (chart.chartWidth - 2 * padding - initialItemX))) {
legend.itemX = initialItemX;
legend.itemY += itemMarginTop + legend.lastLineHeight + itemMarginBottom;
legend.lastLineHeight = 0; // reset for next line
}
// If the item exceeds the height, start a new column
/*if (!horizontal && legend.itemY + options.y + itemHeight > chart.chartHeight - spacingTop - spacingBottom) {
legend.itemY = legend.initialItemY;
legend.itemX += legend.maxItemWidth;
legend.maxItemWidth = 0;
}*/
// Set the edge positions
legend.maxItemWidth = mathMax(legend.maxItemWidth, itemWidth);
legend.lastItemY = itemMarginTop + legend.itemY + itemMarginBottom;
legend.lastLineHeight = mathMax(itemHeight, legend.lastLineHeight); // #915
// cache the position of the newly generated or reordered items
item._legendItemPos = [legend.itemX, legend.itemY];
// advance
if (horizontal) {
legend.itemX += itemWidth;
} else {
legend.itemY += itemMarginTop + itemHeight + itemMarginBottom;
legend.lastLineHeight = itemHeight;
}
// the width of the widest item
legend.offsetWidth = widthOption || mathMax(
(horizontal ? legend.itemX - initialItemX - itemDistance : itemWidth) + padding,
legend.offsetWidth
);
},
/**
* Render the legend. This method can be called both before and after
* chart.render. If called after, it will only rearrange items instead
* of creating new ones.
*/
render: function () {
var legend = this,
chart = legend.chart,
renderer = chart.renderer,
legendGroup = legend.group,
allItems,
display,
legendWidth,
legendHeight,
box = legend.box,
options = legend.options,
padding = legend.padding,
legendBorderWidth = options.borderWidth,
legendBackgroundColor = options.backgroundColor;
legend.itemX = legend.initialItemX;
legend.itemY = legend.initialItemY;
legend.offsetWidth = 0;
legend.lastItemY = 0;
if (!legendGroup) {
legend.group = legendGroup = renderer.g('legend')
.attr({ zIndex: 7 })
.add();
legend.contentGroup = renderer.g()
.attr({ zIndex: 1 }) // above background
.add(legendGroup);
legend.scrollGroup = renderer.g()
.add(legend.contentGroup);
}
legend.renderTitle();
// add each series or point
allItems = [];
each(chart.series, function (serie) {
var seriesOptions = serie.options;
if (!seriesOptions.showInLegend || defined(seriesOptions.linkedTo)) {
return;
}
// use points or series for the legend item depending on legendType
allItems = allItems.concat(
serie.legendItems ||
(seriesOptions.legendType === 'point' ?
serie.data :
serie)
);
});
// sort by legendIndex
stableSort(allItems, function (a, b) {
return ((a.options && a.options.legendIndex) || 0) - ((b.options && b.options.legendIndex) || 0);
});
// reversed legend
if (options.reversed) {
allItems.reverse();
}
legend.allItems = allItems;
legend.display = display = !!allItems.length;
// render the items
each(allItems, function (item) {
legend.renderItem(item);
});
// Draw the border
legendWidth = options.width || legend.offsetWidth;
legendHeight = legend.lastItemY + legend.lastLineHeight + legend.titleHeight;
legendHeight = legend.handleOverflow(legendHeight);
if (legendBorderWidth || legendBackgroundColor) {
legendWidth += padding;
legendHeight += padding;
if (!box) {
legend.box = box = renderer.rect(
0,
0,
legendWidth,
legendHeight,
options.borderRadius,
legendBorderWidth || 0
).attr({
stroke: options.borderColor,
'stroke-width': legendBorderWidth || 0,
fill: legendBackgroundColor || NONE
})
.add(legendGroup)
.shadow(options.shadow);
box.isNew = true;
} else if (legendWidth > 0 && legendHeight > 0) {
box[box.isNew ? 'attr' : 'animate'](
box.crisp(null, null, null, legendWidth, legendHeight)
);
box.isNew = false;
}
// hide the border if no items
box[display ? 'show' : 'hide']();
}
legend.legendWidth = legendWidth;
legend.legendHeight = legendHeight;
// Now that the legend width and height are established, put the items in the
// final position
each(allItems, function (item) {
legend.positionItem(item);
});
// 1.x compatibility: positioning based on style
/*var props = ['left', 'right', 'top', 'bottom'],
prop,
i = 4;
while (i--) {
prop = props[i];
if (options.style[prop] && options.style[prop] !== 'auto') {
options[i < 2 ? 'align' : 'verticalAlign'] = prop;
options[i < 2 ? 'x' : 'y'] = pInt(options.style[prop]) * (i % 2 ? -1 : 1);
}
}*/
if (display) {
legendGroup.align(extend({
width: legendWidth,
height: legendHeight
}, options), true, 'spacingBox');
}
if (!chart.isResizing) {
this.positionCheckboxes();
}
},
/**
* Set up the overflow handling by adding navigation with up and down arrows below the
* legend.
*/
handleOverflow: function (legendHeight) {
var legend = this,
chart = this.chart,
renderer = chart.renderer,
pageCount,
options = this.options,
optionsY = options.y,
alignTop = options.verticalAlign === 'top',
spaceHeight = chart.spacingBox.height + (alignTop ? -optionsY : optionsY) - this.padding,
maxHeight = options.maxHeight,
clipHeight,
clipRect = this.clipRect,
navOptions = options.navigation,
animation = pick(navOptions.animation, true),
arrowSize = navOptions.arrowSize || 12,
nav = this.nav;
// Adjust the height
if (options.layout === 'horizontal') {
spaceHeight /= 2;
}
if (maxHeight) {
spaceHeight = mathMin(spaceHeight, maxHeight);
}
// Reset the legend height and adjust the clipping rectangle
if (legendHeight > spaceHeight && !options.useHTML) {
this.clipHeight = clipHeight = spaceHeight - 20 - this.titleHeight;
this.pageCount = pageCount = mathCeil(legendHeight / clipHeight);
this.currentPage = pick(this.currentPage, 1);
this.fullHeight = legendHeight;
// Only apply clipping if needed. Clipping causes blurred legend in PDF export (#1787)
if (!clipRect) {
clipRect = legend.clipRect = renderer.clipRect(0, 0, 9999, 0);
legend.contentGroup.clip(clipRect);
}
clipRect.attr({
height: clipHeight
});
// Add navigation elements
if (!nav) {
this.nav = nav = renderer.g().attr({ zIndex: 1 }).add(this.group);
this.up = renderer.symbol('triangle', 0, 0, arrowSize, arrowSize)
.on('click', function () {
legend.scroll(-1, animation);
})
.add(nav);
this.pager = renderer.text('', 15, 10)
.css(navOptions.style)
.add(nav);
this.down = renderer.symbol('triangle-down', 0, 0, arrowSize, arrowSize)
.on('click', function () {
legend.scroll(1, animation);
})
.add(nav);
}
// Set initial position
legend.scroll(0);
legendHeight = spaceHeight;
} else if (nav) {
clipRect.attr({
height: chart.chartHeight
});
nav.hide();
this.scrollGroup.attr({
translateY: 1
});
this.clipHeight = 0; // #1379
}
return legendHeight;
},
/**
* Scroll the legend by a number of pages
* @param {Object} scrollBy
* @param {Object} animation
*/
scroll: function (scrollBy, animation) {
var pageCount = this.pageCount,
currentPage = this.currentPage + scrollBy,
clipHeight = this.clipHeight,
navOptions = this.options.navigation,
activeColor = navOptions.activeColor,
inactiveColor = navOptions.inactiveColor,
pager = this.pager,
padding = this.padding,
scrollOffset;
// When resizing while looking at the last page
if (currentPage > pageCount) {
currentPage = pageCount;
}
if (currentPage > 0) {
if (animation !== UNDEFINED) {
setAnimation(animation, this.chart);
}
this.nav.attr({
translateX: padding,
translateY: clipHeight + 7 + this.titleHeight,
visibility: VISIBLE
});
this.up.attr({
fill: currentPage === 1 ? inactiveColor : activeColor
})
.css({
cursor: currentPage === 1 ? 'default' : 'pointer'
});
pager.attr({
text: currentPage + '/' + this.pageCount
});
this.down.attr({
x: 18 + this.pager.getBBox().width, // adjust to text width
fill: currentPage === pageCount ? inactiveColor : activeColor
})
.css({
cursor: currentPage === pageCount ? 'default' : 'pointer'
});
scrollOffset = -mathMin(clipHeight * (currentPage - 1), this.fullHeight - clipHeight + padding) + 1;
this.scrollGroup.animate({
translateY: scrollOffset
});
pager.attr({
text: currentPage + '/' + pageCount
});
this.currentPage = currentPage;
this.positionCheckboxes(scrollOffset);
}
}
};
// Workaround for #2030, horizontal legend items not displaying in IE11 Preview.
// TODO: When IE11 is released, check again for this bug, and remove the fix
// or make a better one.
if (/Trident.*?11\.0/.test(userAgent)) {
wrap(Legend.prototype, 'positionItem', function (proceed, item) {
var legend = this;
setTimeout(function () {
proceed.call(legend, item);
});
});
}
/**
* The chart class
* @param {Object} options
* @param {Function} callback Function to run when the chart has loaded
*/
function Chart() {
this.init.apply(this, arguments);
}
Chart.prototype = {
/**
* Initialize the chart
*/
init: function (userOptions, callback) {
// Handle regular options
var options,
seriesOptions = userOptions.series; // skip merging data points to increase performance
userOptions.series = null;
options = merge(defaultOptions, userOptions); // do the merge
options.series = userOptions.series = seriesOptions; // set back the series data
var optionsChart = options.chart;
// Create margin & spacing array
this.margin = this.splashArray('margin', optionsChart);
this.spacing = this.splashArray('spacing', optionsChart);
var chartEvents = optionsChart.events;
//this.runChartClick = chartEvents && !!chartEvents.click;
this.bounds = { h: {}, v: {} }; // Pixel data bounds for touch zoom
this.callback = callback;
this.isResizing = 0;
this.options = options;
//chartTitleOptions = UNDEFINED;
//chartSubtitleOptions = UNDEFINED;
this.axes = [];
this.series = [];
this.hasCartesianSeries = optionsChart.showAxes;
//this.axisOffset = UNDEFINED;
//this.maxTicks = UNDEFINED; // handle the greatest amount of ticks on grouped axes
//this.inverted = UNDEFINED;
//this.loadingShown = UNDEFINED;
//this.container = UNDEFINED;
//this.chartWidth = UNDEFINED;
//this.chartHeight = UNDEFINED;
//this.marginRight = UNDEFINED;
//this.marginBottom = UNDEFINED;
//this.containerWidth = UNDEFINED;
//this.containerHeight = UNDEFINED;
//this.oldChartWidth = UNDEFINED;
//this.oldChartHeight = UNDEFINED;
//this.renderTo = UNDEFINED;
//this.renderToClone = UNDEFINED;
//this.spacingBox = UNDEFINED
//this.legend = UNDEFINED;
// Elements
//this.chartBackground = UNDEFINED;
//this.plotBackground = UNDEFINED;
//this.plotBGImage = UNDEFINED;
//this.plotBorder = UNDEFINED;
//this.loadingDiv = UNDEFINED;
//this.loadingSpan = UNDEFINED;
var chart = this,
eventType;
// Add the chart to the global lookup
chart.index = charts.length;
charts.push(chart);
// Set up auto resize
if (optionsChart.reflow !== false) {
addEvent(chart, 'load', function () {
chart.initReflow();
});
}
// Chart event handlers
if (chartEvents) {
for (eventType in chartEvents) {
addEvent(chart, eventType, chartEvents[eventType]);
}
}
chart.xAxis = [];
chart.yAxis = [];
// Expose methods and variables
chart.animation = useCanVG ? false : pick(optionsChart.animation, true);
chart.pointCount = 0;
chart.counters = new ChartCounters();
chart.firstRender();
},
/**
* Initialize an individual series, called internally before render time
*/
initSeries: function (options) {
var chart = this,
optionsChart = chart.options.chart,
type = options.type || optionsChart.type || optionsChart.defaultSeriesType,
series,
constr = seriesTypes[type];
// No such series type
if (!constr) {
error(17, true);
}
series = new constr();
series.init(this, options);
return series;
},
/**
* Add a series dynamically after time
*
* @param {Object} options The config options
* @param {Boolean} redraw Whether to redraw the chart after adding. Defaults to true.
* @param {Boolean|Object} animation Whether to apply animation, and optionally animation
* configuration
*
* @return {Object} series The newly created series object
*/
addSeries: function (options, redraw, animation) {
var series,
chart = this;
if (options) {
redraw = pick(redraw, true); // defaults to true
fireEvent(chart, 'addSeries', { options: options }, function () {
series = chart.initSeries(options);
chart.isDirtyLegend = true; // the series array is out of sync with the display
chart.linkSeries();
if (redraw) {
chart.redraw(animation);
}
});
}
return series;
},
/**
* Add an axis to the chart
* @param {Object} options The axis option
* @param {Boolean} isX Whether it is an X axis or a value axis
*/
addAxis: function (options, isX, redraw, animation) {
var key = isX ? 'xAxis' : 'yAxis',
chartOptions = this.options,
axis;
/*jslint unused: false*/
axis = new Axis(this, merge(options, {
index: this[key].length,
isX: isX
}));
/*jslint unused: true*/
// Push the new axis options to the chart options
chartOptions[key] = splat(chartOptions[key] || {});
chartOptions[key].push(options);
if (pick(redraw, true)) {
this.redraw(animation);
}
},
/**
* Check whether a given point is within the plot area
*
* @param {Number} plotX Pixel x relative to the plot area
* @param {Number} plotY Pixel y relative to the plot area
* @param {Boolean} inverted Whether the chart is inverted
*/
isInsidePlot: function (plotX, plotY, inverted) {
var x = inverted ? plotY : plotX,
y = inverted ? plotX : plotY;
return x >= 0 &&
x <= this.plotWidth &&
y >= 0 &&
y <= this.plotHeight;
},
/**
* Adjust all axes tick amounts
*/
adjustTickAmounts: function () {
if (this.options.chart.alignTicks !== false) {
each(this.axes, function (axis) {
axis.adjustTickAmount();
});
}
this.maxTicks = null;
},
/**
* Redraw legend, axes or series based on updated data
*
* @param {Boolean|Object} animation Whether to apply animation, and optionally animation
* configuration
*/
redraw: function (animation) {
var chart = this,
axes = chart.axes,
series = chart.series,
pointer = chart.pointer,
legend = chart.legend,
redrawLegend = chart.isDirtyLegend,
hasStackedSeries,
hasDirtyStacks,
isDirtyBox = chart.isDirtyBox, // todo: check if it has actually changed?
seriesLength = series.length,
i = seriesLength,
serie,
renderer = chart.renderer,
isHiddenChart = renderer.isHidden(),
afterRedraw = [];
setAnimation(animation, chart);
if (isHiddenChart) {
chart.cloneRenderTo();
}
// Adjust title layout (reflow multiline text)
chart.layOutTitles();
// link stacked series
while (i--) {
serie = series[i];
if (serie.options.stacking) {
hasStackedSeries = true;
if (serie.isDirty) {
hasDirtyStacks = true;
break;
}
}
}
if (hasDirtyStacks) { // mark others as dirty
i = seriesLength;
while (i--) {
serie = series[i];
if (serie.options.stacking) {
serie.isDirty = true;
}
}
}
// handle updated data in the series
each(series, function (serie) {
if (serie.isDirty) { // prepare the data so axis can read it
if (serie.options.legendType === 'point') {
redrawLegend = true;
}
}
});
// handle added or removed series
if (redrawLegend && legend.options.enabled) { // series or pie points are added or removed
// draw legend graphics
legend.render();
chart.isDirtyLegend = false;
}
// reset stacks
if (hasStackedSeries) {
chart.getStacks();
}
if (chart.hasCartesianSeries) {
if (!chart.isResizing) {
// reset maxTicks
chart.maxTicks = null;
// set axes scales
each(axes, function (axis) {
axis.setScale();
});
}
chart.adjustTickAmounts();
chart.getMargins();
// If one axis is dirty, all axes must be redrawn (#792, #2169)
each(axes, function (axis) {
if (axis.isDirty) {
isDirtyBox = true;
}
});
// redraw axes
each(axes, function (axis) {
// Fire 'afterSetExtremes' only if extremes are set
if (axis.isDirtyExtremes) { // #821
axis.isDirtyExtremes = false;
afterRedraw.push(function () { // prevent a recursive call to chart.redraw() (#1119)
fireEvent(axis, 'afterSetExtremes', extend(axis.eventArgs, axis.getExtremes())); // #747, #751
delete axis.eventArgs;
});
}
if (isDirtyBox || hasStackedSeries) {
axis.redraw();
}
});
}
// the plot areas size has changed
if (isDirtyBox) {
chart.drawChartBox();
}
// redraw affected series
each(series, function (serie) {
if (serie.isDirty && serie.visible &&
(!serie.isCartesian || serie.xAxis)) { // issue #153
serie.redraw();
}
});
// move tooltip or reset
if (pointer && pointer.reset) {
pointer.reset(true);
}
// redraw if canvas
renderer.draw();
// fire the event
fireEvent(chart, 'redraw'); // jQuery breaks this when calling it from addEvent. Overwrites chart.redraw
if (isHiddenChart) {
chart.cloneRenderTo(true);
}
// Fire callbacks that are put on hold until after the redraw
each(afterRedraw, function (callback) {
callback.call();
});
},
/**
* Dim the chart and show a loading text or symbol
* @param {String} str An optional text to show in the loading label instead of the default one
*/
showLoading: function (str) {
var chart = this,
options = chart.options,
loadingDiv = chart.loadingDiv;
var loadingOptions = options.loading;
// create the layer at the first call
if (!loadingDiv) {
chart.loadingDiv = loadingDiv = createElement(DIV, {
className: PREFIX + 'loading'
}, extend(loadingOptions.style, {
zIndex: 10,
display: NONE
}), chart.container);
chart.loadingSpan = createElement(
'span',
null,
loadingOptions.labelStyle,
loadingDiv
);
}
// update text
chart.loadingSpan.innerHTML = str || options.lang.loading;
// show it
if (!chart.loadingShown) {
css(loadingDiv, {
opacity: 0,
display: '',
left: chart.plotLeft + PX,
top: chart.plotTop + PX,
width: chart.plotWidth + PX,
height: chart.plotHeight + PX
});
animate(loadingDiv, {
opacity: loadingOptions.style.opacity
}, {
duration: loadingOptions.showDuration || 0
});
chart.loadingShown = true;
}
},
/**
* Hide the loading layer
*/
hideLoading: function () {
var options = this.options,
loadingDiv = this.loadingDiv;
if (loadingDiv) {
animate(loadingDiv, {
opacity: 0
}, {
duration: options.loading.hideDuration || 100,
complete: function () {
css(loadingDiv, { display: NONE });
}
});
}
this.loadingShown = false;
},
/**
* Get an axis, series or point object by id.
* @param id {String} The id as given in the configuration options
*/
get: function (id) {
var chart = this,
axes = chart.axes,
series = chart.series;
var i,
j,
points;
// search axes
for (i = 0; i < axes.length; i++) {
if (axes[i].options.id === id) {
return axes[i];
}
}
// search series
for (i = 0; i < series.length; i++) {
if (series[i].options.id === id) {
return series[i];
}
}
// search points
for (i = 0; i < series.length; i++) {
points = series[i].points || [];
for (j = 0; j < points.length; j++) {
if (points[j].id === id) {
return points[j];
}
}
}
return null;
},
/**
* Create the Axis instances based on the config options
*/
getAxes: function () {
var chart = this,
options = this.options,
xAxisOptions = options.xAxis = splat(options.xAxis || {}),
yAxisOptions = options.yAxis = splat(options.yAxis || {}),
optionsArray,
axis;
// make sure the options are arrays and add some members
each(xAxisOptions, function (axis, i) {
axis.index = i;
axis.isX = true;
});
each(yAxisOptions, function (axis, i) {
axis.index = i;
});
// concatenate all axis options into one array
optionsArray = xAxisOptions.concat(yAxisOptions);
each(optionsArray, function (axisOptions) {
axis = new Axis(chart, axisOptions);
});
chart.adjustTickAmounts();
},
/**
* Get the currently selected points from all series
*/
getSelectedPoints: function () {
var points = [];
each(this.series, function (serie) {
points = points.concat(grep(serie.points || [], function (point) {
return point.selected;
}));
});
return points;
},
/**
* Get the currently selected series
*/
getSelectedSeries: function () {
return grep(this.series, function (serie) {
return serie.selected;
});
},
/**
* Generate stacks for each series and calculate stacks total values
*/
getStacks: function () {
var chart = this;
// reset stacks for each yAxis
each(chart.yAxis, function (axis) {
if (axis.stacks && axis.hasVisibleSeries) {
axis.oldStacks = axis.stacks;
}
});
each(chart.series, function (series) {
if (series.options.stacking && (series.visible === true || chart.options.chart.ignoreHiddenSeries === false)) {
series.stackKey = series.type + pick(series.options.stack, '');
}
});
},
/**
* Display the zoom button
*/
showResetZoom: function () {
var chart = this,
lang = defaultOptions.lang,
btnOptions = chart.options.chart.resetZoomButton,
theme = btnOptions.theme,
states = theme.states,
alignTo = btnOptions.relativeTo === 'chart' ? null : 'plotBox';
this.resetZoomButton = chart.renderer.button(lang.resetZoom, null, null, function () { chart.zoomOut(); }, theme, states && states.hover)
.attr({
align: btnOptions.position.align,
title: lang.resetZoomTitle
})
.add()
.align(btnOptions.position, false, alignTo);
},
/**
* Zoom out to 1:1
*/
zoomOut: function () {
var chart = this;
fireEvent(chart, 'selection', { resetSelection: true }, function () {
chart.zoom();
});
},
/**
* Zoom into a given portion of the chart given by axis coordinates
* @param {Object} event
*/
zoom: function (event) {
var chart = this,
hasZoomed,
pointer = chart.pointer,
displayButton = false,
resetZoomButton;
// If zoom is called with no arguments, reset the axes
if (!event || event.resetSelection) {
each(chart.axes, function (axis) {
hasZoomed = axis.zoom();
});
} else { // else, zoom in on all axes
each(event.xAxis.concat(event.yAxis), function (axisData) {
var axis = axisData.axis,
isXAxis = axis.isXAxis;
// don't zoom more than minRange
if (pointer[isXAxis ? 'zoomX' : 'zoomY'] || pointer[isXAxis ? 'pinchX' : 'pinchY']) {
hasZoomed = axis.zoom(axisData.min, axisData.max);
if (axis.displayBtn) {
displayButton = true;
}
}
});
}
// Show or hide the Reset zoom button
resetZoomButton = chart.resetZoomButton;
if (displayButton && !resetZoomButton) {
chart.showResetZoom();
} else if (!displayButton && isObject(resetZoomButton)) {
chart.resetZoomButton = resetZoomButton.destroy();
}
// Redraw
if (hasZoomed) {
chart.redraw(
pick(chart.options.chart.animation, event && event.animation, chart.pointCount < 100) // animation
);
}
},
/**
* Pan the chart by dragging the mouse across the pane. This function is called
* on mouse move, and the distance to pan is computed from chartX compared to
* the first chartX position in the dragging operation.
*/
pan: function (e, panning) {
var chart = this,
hoverPoints = chart.hoverPoints,
doRedraw;
// remove active points for shared tooltip
if (hoverPoints) {
each(hoverPoints, function (point) {
point.setState();
});
}
each(panning === 'xy' ? [1, 0] : [1], function (isX) { // xy is used in maps
var mousePos = e[isX ? 'chartX' : 'chartY'],
axis = chart[isX ? 'xAxis' : 'yAxis'][0],
startPos = chart[isX ? 'mouseDownX' : 'mouseDownY'],
halfPointRange = (axis.pointRange || 0) / 2,
extremes = axis.getExtremes(),
newMin = axis.toValue(startPos - mousePos, true) + halfPointRange,
newMax = axis.toValue(startPos + chart[isX ? 'plotWidth' : 'plotHeight'] - mousePos, true) - halfPointRange;
if (axis.series.length && newMin > mathMin(extremes.dataMin, extremes.min) && newMax < mathMax(extremes.dataMax, extremes.max)) {
axis.setExtremes(newMin, newMax, false, false, { trigger: 'pan' });
doRedraw = true;
}
chart[isX ? 'mouseDownX' : 'mouseDownY'] = mousePos; // set new reference for next run
});
if (doRedraw) {
chart.redraw(false);
}
css(chart.container, { cursor: 'move' });
},
/**
* Show the title and subtitle of the chart
*
* @param titleOptions {Object} New title options
* @param subtitleOptions {Object} New subtitle options
*
*/
setTitle: function (titleOptions, subtitleOptions) {
var chart = this,
options = chart.options,
chartTitleOptions,
chartSubtitleOptions;
chartTitleOptions = options.title = merge(options.title, titleOptions);
chartSubtitleOptions = options.subtitle = merge(options.subtitle, subtitleOptions);
// add title and subtitle
each([
['title', titleOptions, chartTitleOptions],
['subtitle', subtitleOptions, chartSubtitleOptions]
], function (arr) {
var name = arr[0],
title = chart[name],
titleOptions = arr[1],
chartTitleOptions = arr[2];
if (title && titleOptions) {
chart[name] = title = title.destroy(); // remove old
}
if (chartTitleOptions && chartTitleOptions.text && !title) {
chart[name] = chart.renderer.text(
chartTitleOptions.text,
0,
0,
chartTitleOptions.useHTML
)
.attr({
align: chartTitleOptions.align,
'class': PREFIX + name,
zIndex: chartTitleOptions.zIndex || 4
})
.css(chartTitleOptions.style)
.add();
}
});
chart.layOutTitles();
},
/**
* Lay out the chart titles and cache the full offset height for use in getMargins
*/
layOutTitles: function () {
var titleOffset = 0,
title = this.title,
subtitle = this.subtitle,
options = this.options,
titleOptions = options.title,
subtitleOptions = options.subtitle,
autoWidth = this.spacingBox.width - 44; // 44 makes room for default context button
if (title) {
title
.css({ width: (titleOptions.width || autoWidth) + PX })
.align(extend({ y: 15 }, titleOptions), false, 'spacingBox');
if (!titleOptions.floating && !titleOptions.verticalAlign) {
titleOffset = title.getBBox().height;
// Adjust for browser consistency + backwards compat after #776 fix
if (titleOffset >= 18 && titleOffset <= 25) {
titleOffset = 15;
}
}
}
if (subtitle) {
subtitle
.css({ width: (subtitleOptions.width || autoWidth) + PX })
.align(extend({ y: titleOffset + titleOptions.margin }, subtitleOptions), false, 'spacingBox');
if (!subtitleOptions.floating && !subtitleOptions.verticalAlign) {
titleOffset = mathCeil(titleOffset + subtitle.getBBox().height);
}
}
this.titleOffset = titleOffset; // used in getMargins
},
/**
* Get chart width and height according to options and container size
*/
getChartSize: function () {
var chart = this,
optionsChart = chart.options.chart,
renderTo = chart.renderToClone || chart.renderTo;
// get inner width and height from jQuery (#824)
chart.containerWidth = adapterRun(renderTo, 'width');
chart.containerHeight = adapterRun(renderTo, 'height');
chart.chartWidth = mathMax(0, optionsChart.width || chart.containerWidth || 600); // #1393, 1460
chart.chartHeight = mathMax(0, pick(optionsChart.height,
// the offsetHeight of an empty container is 0 in standard browsers, but 19 in IE7:
chart.containerHeight > 19 ? chart.containerHeight : 400));
},
/**
* Create a clone of the chart's renderTo div and place it outside the viewport to allow
* size computation on chart.render and chart.redraw
*/
cloneRenderTo: function (revert) {
var clone = this.renderToClone,
container = this.container;
// Destroy the clone and bring the container back to the real renderTo div
if (revert) {
if (clone) {
this.renderTo.appendChild(container);
discardElement(clone);
delete this.renderToClone;
}
// Set up the clone
} else {
if (container && container.parentNode === this.renderTo) {
this.renderTo.removeChild(container); // do not clone this
}
this.renderToClone = clone = this.renderTo.cloneNode(0);
css(clone, {
position: ABSOLUTE,
top: '-9999px',
display: 'block' // #833
});
doc.body.appendChild(clone);
if (container) {
clone.appendChild(container);
}
}
},
/**
* Get the containing element, determine the size and create the inner container
* div to hold the chart
*/
getContainer: function () {
var chart = this,
container,
optionsChart = chart.options.chart,
chartWidth,
chartHeight,
renderTo,
indexAttrName = 'data-highcharts-chart',
oldChartIndex,
containerId;
chart.renderTo = renderTo = optionsChart.renderTo;
containerId = PREFIX + idCounter++;
if (isString(renderTo)) {
chart.renderTo = renderTo = doc.getElementById(renderTo);
}
// Display an error if the renderTo is wrong
if (!renderTo) {
error(13, true);
}
// If the container already holds a chart, destroy it
oldChartIndex = pInt(attr(renderTo, indexAttrName));
if (!isNaN(oldChartIndex) && charts[oldChartIndex]) {
charts[oldChartIndex].destroy();
}
// Make a reference to the chart from the div
attr(renderTo, indexAttrName, chart.index);
// remove previous chart
renderTo.innerHTML = '';
// If the container doesn't have an offsetWidth, it has or is a child of a node
// that has display:none. We need to temporarily move it out to a visible
// state to determine the size, else the legend and tooltips won't render
// properly
if (!renderTo.offsetWidth) {
chart.cloneRenderTo();
}
// get the width and height
chart.getChartSize();
chartWidth = chart.chartWidth;
chartHeight = chart.chartHeight;
// create the inner container
chart.container = container = createElement(DIV, {
className: PREFIX + 'container' +
(optionsChart.className ? ' ' + optionsChart.className : ''),
id: containerId
}, extend({
position: RELATIVE,
overflow: HIDDEN, // needed for context menu (avoid scrollbars) and
// content overflow in IE
width: chartWidth + PX,
height: chartHeight + PX,
textAlign: 'left',
lineHeight: 'normal', // #427
zIndex: 0, // #1072
'-webkit-tap-highlight-color': 'rgba(0,0,0,0)'
}, optionsChart.style),
chart.renderToClone || renderTo
);
// cache the cursor (#1650)
chart._cursor = container.style.cursor;
chart.renderer =
optionsChart.forExport ? // force SVG, used for SVG export
new SVGRenderer(container, chartWidth, chartHeight, true) :
new Renderer(container, chartWidth, chartHeight);
if (useCanVG) {
// If we need canvg library, extend and configure the renderer
// to get the tracker for translating mouse events
chart.renderer.create(chart, container, chartWidth, chartHeight);
}
},
/**
* Calculate margins by rendering axis labels in a preliminary position. Title,
* subtitle and legend have already been rendered at this stage, but will be
* moved into their final positions
*/
getMargins: function () {
var chart = this,
spacing = chart.spacing,
axisOffset,
legend = chart.legend,
margin = chart.margin,
legendOptions = chart.options.legend,
legendMargin = pick(legendOptions.margin, 10),
legendX = legendOptions.x,
legendY = legendOptions.y,
align = legendOptions.align,
verticalAlign = legendOptions.verticalAlign,
titleOffset = chart.titleOffset;
chart.resetMargins();
axisOffset = chart.axisOffset;
// Adjust for title and subtitle
if (titleOffset && !defined(margin[0])) {
chart.plotTop = mathMax(chart.plotTop, titleOffset + chart.options.title.margin + spacing[0]);
}
// Adjust for legend
if (legend.display && !legendOptions.floating) {
if (align === 'right') { // horizontal alignment handled first
if (!defined(margin[1])) {
chart.marginRight = mathMax(
chart.marginRight,
legend.legendWidth - legendX + legendMargin + spacing[1]
);
}
} else if (align === 'left') {
if (!defined(margin[3])) {
chart.plotLeft = mathMax(
chart.plotLeft,
legend.legendWidth + legendX + legendMargin + spacing[3]
);
}
} else if (verticalAlign === 'top') {
if (!defined(margin[0])) {
chart.plotTop = mathMax(
chart.plotTop,
legend.legendHeight + legendY + legendMargin + spacing[0]
);
}
} else if (verticalAlign === 'bottom') {
if (!defined(margin[2])) {
chart.marginBottom = mathMax(
chart.marginBottom,
legend.legendHeight - legendY + legendMargin + spacing[2]
);
}
}
}
// adjust for scroller
if (chart.extraBottomMargin) {
chart.marginBottom += chart.extraBottomMargin;
}
if (chart.extraTopMargin) {
chart.plotTop += chart.extraTopMargin;
}
// pre-render axes to get labels offset width
if (chart.hasCartesianSeries) {
each(chart.axes, function (axis) {
axis.getOffset();
});
}
if (!defined(margin[3])) {
chart.plotLeft += axisOffset[3];
}
if (!defined(margin[0])) {
chart.plotTop += axisOffset[0];
}
if (!defined(margin[2])) {
chart.marginBottom += axisOffset[2];
}
if (!defined(margin[1])) {
chart.marginRight += axisOffset[1];
}
chart.setChartSize();
},
/**
* Add the event handlers necessary for auto resizing
*
*/
initReflow: function () {
var chart = this,
optionsChart = chart.options.chart,
renderTo = chart.renderTo,
reflowTimeout;
function reflow(e) {
var width = optionsChart.width || adapterRun(renderTo, 'width'),
height = optionsChart.height || adapterRun(renderTo, 'height'),
target = e ? e.target : win; // #805 - MooTools doesn't supply e
// Width and height checks for display:none. Target is doc in IE8 and Opera,
// win in Firefox, Chrome and IE9.
if (!chart.hasUserSize && width && height && (target === win || target === doc)) {
if (width !== chart.containerWidth || height !== chart.containerHeight) {
clearTimeout(reflowTimeout);
chart.reflowTimeout = reflowTimeout = setTimeout(function () {
if (chart.container) { // It may have been destroyed in the meantime (#1257)
chart.setSize(width, height, false);
chart.hasUserSize = null;
}
}, 100);
}
chart.containerWidth = width;
chart.containerHeight = height;
}
}
chart.reflow = reflow;
addEvent(win, 'resize', reflow);
addEvent(chart, 'destroy', function () {
removeEvent(win, 'resize', reflow);
});
},
/**
* Resize the chart to a given width and height
* @param {Number} width
* @param {Number} height
* @param {Object|Boolean} animation
*/
setSize: function (width, height, animation) {
var chart = this,
chartWidth,
chartHeight,
fireEndResize;
// Handle the isResizing counter
chart.isResizing += 1;
fireEndResize = function () {
if (chart) {
fireEvent(chart, 'endResize', null, function () {
chart.isResizing -= 1;
});
}
};
// set the animation for the current process
setAnimation(animation, chart);
chart.oldChartHeight = chart.chartHeight;
chart.oldChartWidth = chart.chartWidth;
if (defined(width)) {
chart.chartWidth = chartWidth = mathMax(0, mathRound(width));
chart.hasUserSize = !!chartWidth;
}
if (defined(height)) {
chart.chartHeight = chartHeight = mathMax(0, mathRound(height));
}
css(chart.container, {
width: chartWidth + PX,
height: chartHeight + PX
});
chart.setChartSize(true);
chart.renderer.setSize(chartWidth, chartHeight, animation);
// handle axes
chart.maxTicks = null;
each(chart.axes, function (axis) {
axis.isDirty = true;
axis.setScale();
});
// make sure non-cartesian series are also handled
each(chart.series, function (serie) {
serie.isDirty = true;
});
chart.isDirtyLegend = true; // force legend redraw
chart.isDirtyBox = true; // force redraw of plot and chart border
chart.getMargins();
chart.redraw(animation);
chart.oldChartHeight = null;
fireEvent(chart, 'resize');
// fire endResize and set isResizing back
// If animation is disabled, fire without delay
if (globalAnimation === false) {
fireEndResize();
} else { // else set a timeout with the animation duration
setTimeout(fireEndResize, (globalAnimation && globalAnimation.duration) || 500);
}
},
/**
* Set the public chart properties. This is done before and after the pre-render
* to determine margin sizes
*/
setChartSize: function (skipAxes) {
var chart = this,
inverted = chart.inverted,
renderer = chart.renderer,
chartWidth = chart.chartWidth,
chartHeight = chart.chartHeight,
optionsChart = chart.options.chart,
spacing = chart.spacing,
clipOffset = chart.clipOffset,
clipX,
clipY,
plotLeft,
plotTop,
plotWidth,
plotHeight,
plotBorderWidth;
chart.plotLeft = plotLeft = mathRound(chart.plotLeft);
chart.plotTop = plotTop = mathRound(chart.plotTop);
chart.plotWidth = plotWidth = mathMax(0, mathRound(chartWidth - plotLeft - chart.marginRight));
chart.plotHeight = plotHeight = mathMax(0, mathRound(chartHeight - plotTop - chart.marginBottom));
chart.plotSizeX = inverted ? plotHeight : plotWidth;
chart.plotSizeY = inverted ? plotWidth : plotHeight;
chart.plotBorderWidth = optionsChart.plotBorderWidth || 0;
// Set boxes used for alignment
chart.spacingBox = renderer.spacingBox = {
x: spacing[3],
y: spacing[0],
width: chartWidth - spacing[3] - spacing[1],
height: chartHeight - spacing[0] - spacing[2]
};
chart.plotBox = renderer.plotBox = {
x: plotLeft,
y: plotTop,
width: plotWidth,
height: plotHeight
};
plotBorderWidth = 2 * mathFloor(chart.plotBorderWidth / 2);
clipX = mathCeil(mathMax(plotBorderWidth, clipOffset[3]) / 2);
clipY = mathCeil(mathMax(plotBorderWidth, clipOffset[0]) / 2);
chart.clipBox = {
x: clipX,
y: clipY,
width: mathFloor(chart.plotSizeX - mathMax(plotBorderWidth, clipOffset[1]) / 2 - clipX),
height: mathFloor(chart.plotSizeY - mathMax(plotBorderWidth, clipOffset[2]) / 2 - clipY)
};
if (!skipAxes) {
each(chart.axes, function (axis) {
axis.setAxisSize();
axis.setAxisTranslation();
});
}
},
/**
* Initial margins before auto size margins are applied
*/
resetMargins: function () {
var chart = this,
spacing = chart.spacing,
margin = chart.margin;
chart.plotTop = pick(margin[0], spacing[0]);
chart.marginRight = pick(margin[1], spacing[1]);
chart.marginBottom = pick(margin[2], spacing[2]);
chart.plotLeft = pick(margin[3], spacing[3]);
chart.axisOffset = [0, 0, 0, 0]; // top, right, bottom, left
chart.clipOffset = [0, 0, 0, 0];
},
/**
* Draw the borders and backgrounds for chart and plot area
*/
drawChartBox: function () {
var chart = this,
optionsChart = chart.options.chart,
renderer = chart.renderer,
chartWidth = chart.chartWidth,
chartHeight = chart.chartHeight,
chartBackground = chart.chartBackground,
plotBackground = chart.plotBackground,
plotBorder = chart.plotBorder,
plotBGImage = chart.plotBGImage,
chartBorderWidth = optionsChart.borderWidth || 0,
chartBackgroundColor = optionsChart.backgroundColor,
plotBackgroundColor = optionsChart.plotBackgroundColor,
plotBackgroundImage = optionsChart.plotBackgroundImage,
plotBorderWidth = optionsChart.plotBorderWidth || 0,
mgn,
bgAttr,
plotLeft = chart.plotLeft,
plotTop = chart.plotTop,
plotWidth = chart.plotWidth,
plotHeight = chart.plotHeight,
plotBox = chart.plotBox,
clipRect = chart.clipRect,
clipBox = chart.clipBox;
// Chart area
mgn = chartBorderWidth + (optionsChart.shadow ? 8 : 0);
if (chartBorderWidth || chartBackgroundColor) {
if (!chartBackground) {
bgAttr = {
fill: chartBackgroundColor || NONE
};
if (chartBorderWidth) { // #980
bgAttr.stroke = optionsChart.borderColor;
bgAttr['stroke-width'] = chartBorderWidth;
}
chart.chartBackground = renderer.rect(mgn / 2, mgn / 2, chartWidth - mgn, chartHeight - mgn,
optionsChart.borderRadius, chartBorderWidth)
.attr(bgAttr)
.add()
.shadow(optionsChart.shadow);
} else { // resize
chartBackground.animate(
chartBackground.crisp(null, null, null, chartWidth - mgn, chartHeight - mgn)
);
}
}
// Plot background
if (plotBackgroundColor) {
if (!plotBackground) {
chart.plotBackground = renderer.rect(plotLeft, plotTop, plotWidth, plotHeight, 0)
.attr({
fill: plotBackgroundColor
})
.add()
.shadow(optionsChart.plotShadow);
} else {
plotBackground.animate(plotBox);
}
}
if (plotBackgroundImage) {
if (!plotBGImage) {
chart.plotBGImage = renderer.image(plotBackgroundImage, plotLeft, plotTop, plotWidth, plotHeight)
.add();
} else {
plotBGImage.animate(plotBox);
}
}
// Plot clip
if (!clipRect) {
chart.clipRect = renderer.clipRect(clipBox);
} else {
clipRect.animate({
width: clipBox.width,
height: clipBox.height
});
}
// Plot area border
if (plotBorderWidth) {
if (!plotBorder) {
chart.plotBorder = renderer.rect(plotLeft, plotTop, plotWidth, plotHeight, 0, -plotBorderWidth)
.attr({
stroke: optionsChart.plotBorderColor,
'stroke-width': plotBorderWidth,
zIndex: 1
})
.add();
} else {
plotBorder.animate(
plotBorder.crisp(null, plotLeft, plotTop, plotWidth, plotHeight)
);
}
}
// reset
chart.isDirtyBox = false;
},
/**
* Detect whether a certain chart property is needed based on inspecting its options
* and series. This mainly applies to the chart.invert property, and in extensions to
* the chart.angular and chart.polar properties.
*/
propFromSeries: function () {
var chart = this,
optionsChart = chart.options.chart,
klass,
seriesOptions = chart.options.series,
i,
value;
each(['inverted', 'angular', 'polar'], function (key) {
// The default series type's class
klass = seriesTypes[optionsChart.type || optionsChart.defaultSeriesType];
// Get the value from available chart-wide properties
value = (
chart[key] || // 1. it is set before
optionsChart[key] || // 2. it is set in the options
(klass && klass.prototype[key]) // 3. it's default series class requires it
);
// 4. Check if any the chart's series require it
i = seriesOptions && seriesOptions.length;
while (!value && i--) {
klass = seriesTypes[seriesOptions[i].type];
if (klass && klass.prototype[key]) {
value = true;
}
}
// Set the chart property
chart[key] = value;
});
},
/**
* Link two or more series together. This is done initially from Chart.render,
* and after Chart.addSeries and Series.remove.
*/
linkSeries: function () {
var chart = this,
chartSeries = chart.series;
// Reset links
each(chartSeries, function (series) {
series.linkedSeries.length = 0;
});
// Apply new links
each(chartSeries, function (series) {
var linkedTo = series.options.linkedTo;
if (isString(linkedTo)) {
if (linkedTo === ':previous') {
linkedTo = chart.series[series.index - 1];
} else {
linkedTo = chart.get(linkedTo);
}
if (linkedTo) {
linkedTo.linkedSeries.push(series);
series.linkedParent = linkedTo;
}
}
});
},
/**
* Render all graphics for the chart
*/
render: function () {
var chart = this,
axes = chart.axes,
renderer = chart.renderer,
options = chart.options;
var labels = options.labels,
credits = options.credits,
creditsHref;
// Title
chart.setTitle();
// Legend
chart.legend = new Legend(chart, options.legend);
chart.getStacks(); // render stacks
// Get margins by pre-rendering axes
// set axes scales
each(axes, function (axis) {
axis.setScale();
});
chart.getMargins();
chart.maxTicks = null; // reset for second pass
each(axes, function (axis) {
axis.setTickPositions(true); // update to reflect the new margins
axis.setMaxTicks();
});
chart.adjustTickAmounts();
chart.getMargins(); // second pass to check for new labels
// Draw the borders and backgrounds
chart.drawChartBox();
// Axes
if (chart.hasCartesianSeries) {
each(axes, function (axis) {
axis.render();
});
}
// The series
if (!chart.seriesGroup) {
chart.seriesGroup = renderer.g('series-group')
.attr({ zIndex: 3 })
.add();
}
each(chart.series, function (serie) {
serie.translate();
serie.setTooltipPoints();
serie.render();
});
// Labels
if (labels.items) {
each(labels.items, function (label) {
var style = extend(labels.style, label.style),
x = pInt(style.left) + chart.plotLeft,
y = pInt(style.top) + chart.plotTop + 12;
// delete to prevent rewriting in IE
delete style.left;
delete style.top;
renderer.text(
label.html,
x,
y
)
.attr({ zIndex: 2 })
.css(style)
.add();
});
}
// Credits
if (credits.enabled && !chart.credits) {
creditsHref = credits.href;
chart.credits = renderer.text(
credits.text,
0,
0
)
.on('click', function () {
if (creditsHref) {
location.href = creditsHref;
}
})
.attr({
align: credits.position.align,
zIndex: 8
})
.css(credits.style)
.add()
.align(credits.position);
}
// Set flag
chart.hasRendered = true;
},
/**
* Clean up memory usage
*/
destroy: function () {
var chart = this,
axes = chart.axes,
series = chart.series,
container = chart.container,
i,
parentNode = container && container.parentNode;
// fire the chart.destoy event
fireEvent(chart, 'destroy');
// Delete the chart from charts lookup array
charts[chart.index] = UNDEFINED;
chart.renderTo.removeAttribute('data-highcharts-chart');
// remove events
removeEvent(chart);
// ==== Destroy collections:
// Destroy axes
i = axes.length;
while (i--) {
axes[i] = axes[i].destroy();
}
// Destroy each series
i = series.length;
while (i--) {
series[i] = series[i].destroy();
}
// ==== Destroy chart properties:
each(['title', 'subtitle', 'chartBackground', 'plotBackground', 'plotBGImage',
'plotBorder', 'seriesGroup', 'clipRect', 'credits', 'pointer', 'scroller',
'rangeSelector', 'legend', 'resetZoomButton', 'tooltip', 'renderer'], function (name) {
var prop = chart[name];
if (prop && prop.destroy) {
chart[name] = prop.destroy();
}
});
// remove container and all SVG
if (container) { // can break in IE when destroyed before finished loading
container.innerHTML = '';
removeEvent(container);
if (parentNode) {
discardElement(container);
}
}
// clean it all up
for (i in chart) {
delete chart[i];
}
},
/**
* VML namespaces can't be added until after complete. Listening
* for Perini's doScroll hack is not enough.
*/
isReadyToRender: function () {
var chart = this;
// Note: in spite of JSLint's complaints, win == win.top is required
/*jslint eqeq: true*/
if ((!hasSVG && (win == win.top && doc.readyState !== 'complete')) || (useCanVG && !win.canvg)) {
/*jslint eqeq: false*/
if (useCanVG) {
// Delay rendering until canvg library is downloaded and ready
CanVGController.push(function () { chart.firstRender(); }, chart.options.global.canvasToolsURL);
} else {
doc.attachEvent('onreadystatechange', function () {
doc.detachEvent('onreadystatechange', chart.firstRender);
if (doc.readyState === 'complete') {
chart.firstRender();
}
});
}
return false;
}
return true;
},
/**
* Prepare for first rendering after all data are loaded
*/
firstRender: function () {
var chart = this,
options = chart.options,
callback = chart.callback;
// Check whether the chart is ready to render
if (!chart.isReadyToRender()) {
return;
}
// Create the container
chart.getContainer();
// Run an early event after the container and renderer are established
fireEvent(chart, 'init');
chart.resetMargins();
chart.setChartSize();
// Set the common chart properties (mainly invert) from the given series
chart.propFromSeries();
// get axes
chart.getAxes();
// Initialize the series
each(options.series || [], function (serieOptions) {
chart.initSeries(serieOptions);
});
chart.linkSeries();
// Run an event after axes and series are initialized, but before render. At this stage,
// the series data is indexed and cached in the xData and yData arrays, so we can access
// those before rendering. Used in Highstock.
fireEvent(chart, 'beforeRender');
// depends on inverted and on margins being set
chart.pointer = new Pointer(chart, options);
chart.render();
// add canvas
chart.renderer.draw();
// run callbacks
if (callback) {
callback.apply(chart, [chart]);
}
each(chart.callbacks, function (fn) {
fn.apply(chart, [chart]);
});
// If the chart was rendered outside the top container, put it back in
chart.cloneRenderTo(true);
fireEvent(chart, 'load');
},
/**
* Creates arrays for spacing and margin from given options.
*/
splashArray: function (target, options) {
var oVar = options[target],
tArray = isObject(oVar) ? oVar : [oVar, oVar, oVar, oVar];
return [pick(options[target + 'Top'], tArray[0]),
pick(options[target + 'Right'], tArray[1]),
pick(options[target + 'Bottom'], tArray[2]),
pick(options[target + 'Left'], tArray[3])];
}
}; // end Chart
// Hook for exporting module
Chart.prototype.callbacks = [];
/**
* The Point object and prototype. Inheritable and used as base for PiePoint
*/
var Point = function () {};
Point.prototype = {
/**
* Initialize the point
* @param {Object} series The series object containing this point
* @param {Object} options The data in either number, array or object format
*/
init: function (series, options, x) {
var point = this,
colors;
point.series = series;
point.applyOptions(options, x);
point.pointAttr = {};
if (series.options.colorByPoint) {
colors = series.options.colors || series.chart.options.colors;
point.color = point.color || colors[series.colorCounter++];
// loop back to zero
if (series.colorCounter === colors.length) {
series.colorCounter = 0;
}
}
series.chart.pointCount++;
return point;
},
/**
* Apply the options containing the x and y data and possible some extra properties.
* This is called on point init or from point.update.
*
* @param {Object} options
*/
applyOptions: function (options, x) {
var point = this,
series = point.series,
pointValKey = series.pointValKey;
options = Point.prototype.optionsToObject.call(this, options);
// copy options directly to point
extend(point, options);
point.options = point.options ? extend(point.options, options) : options;
// For higher dimension series types. For instance, for ranges, point.y is mapped to point.low.
if (pointValKey) {
point.y = point[pointValKey];
}
// If no x is set by now, get auto incremented value. All points must have an
// x value, however the y value can be null to create a gap in the series
if (point.x === UNDEFINED && series) {
point.x = x === UNDEFINED ? series.autoIncrement() : x;
}
return point;
},
/**
* Transform number or array configs into objects
*/
optionsToObject: function (options) {
var ret,
series = this.series,
pointArrayMap = series.pointArrayMap || ['y'],
valueCount = pointArrayMap.length,
firstItemType,
i = 0,
j = 0;
if (typeof options === 'number' || options === null) {
ret = { y: options };
} else if (isArray(options)) {
ret = {};
// with leading x value
if (options.length > valueCount) {
firstItemType = typeof options[0];
if (firstItemType === 'string') {
ret.name = options[0];
} else if (firstItemType === 'number') {
ret.x = options[0];
}
i++;
}
while (j < valueCount) {
ret[pointArrayMap[j++]] = options[i++];
}
} else if (typeof options === 'object') {
ret = options;
// This is the fastest way to detect if there are individual point dataLabels that need
// to be considered in drawDataLabels. These can only occur in object configs.
if (options.dataLabels) {
series._hasPointLabels = true;
}
// Same approach as above for markers
if (options.marker) {
series._hasPointMarkers = true;
}
}
return ret;
},
/**
* Destroy a point to clear memory. Its reference still stays in series.data.
*/
destroy: function () {
var point = this,
series = point.series,
chart = series.chart,
hoverPoints = chart.hoverPoints,
prop;
chart.pointCount--;
if (hoverPoints) {
point.setState();
erase(hoverPoints, point);
if (!hoverPoints.length) {
chart.hoverPoints = null;
}
}
if (point === chart.hoverPoint) {
point.onMouseOut();
}
// remove all events
if (point.graphic || point.dataLabel) { // removeEvent and destroyElements are performance expensive
removeEvent(point);
point.destroyElements();
}
if (point.legendItem) { // pies have legend items
chart.legend.destroyItem(point);
}
for (prop in point) {
point[prop] = null;
}
},
/**
* Destroy SVG elements associated with the point
*/
destroyElements: function () {
var point = this,
props = ['graphic', 'dataLabel', 'dataLabelUpper', 'group', 'connector', 'shadowGroup'],
prop,
i = 6;
while (i--) {
prop = props[i];
if (point[prop]) {
point[prop] = point[prop].destroy();
}
}
},
/**
* Return the configuration hash needed for the data label and tooltip formatters
*/
getLabelConfig: function () {
var point = this;
return {
x: point.category,
y: point.y,
key: point.name || point.category,
series: point.series,
point: point,
percentage: point.percentage,
total: point.total || point.stackTotal
};
},
/**
* Toggle the selection status of a point
* @param {Boolean} selected Whether to select or unselect the point.
* @param {Boolean} accumulate Whether to add to the previous selection. By default,
* this happens if the control key (Cmd on Mac) was pressed during clicking.
*/
select: function (selected, accumulate) {
var point = this,
series = point.series,
chart = series.chart;
selected = pick(selected, !point.selected);
// fire the event with the defalut handler
point.firePointEvent(selected ? 'select' : 'unselect', { accumulate: accumulate }, function () {
point.selected = point.options.selected = selected;
series.options.data[inArray(point, series.data)] = point.options;
point.setState(selected && SELECT_STATE);
// unselect all other points unless Ctrl or Cmd + click
if (!accumulate) {
each(chart.getSelectedPoints(), function (loopPoint) {
if (loopPoint.selected && loopPoint !== point) {
loopPoint.selected = loopPoint.options.selected = false;
series.options.data[inArray(loopPoint, series.data)] = loopPoint.options;
loopPoint.setState(NORMAL_STATE);
loopPoint.firePointEvent('unselect');
}
});
}
});
},
/**
* Runs on mouse over the point
*/
onMouseOver: function (e) {
var point = this,
series = point.series,
chart = series.chart,
tooltip = chart.tooltip,
hoverPoint = chart.hoverPoint;
// set normal state to previous series
if (hoverPoint && hoverPoint !== point) {
hoverPoint.onMouseOut();
}
// trigger the event
point.firePointEvent('mouseOver');
// update the tooltip
if (tooltip && (!tooltip.shared || series.noSharedTooltip)) {
tooltip.refresh(point, e);
}
// hover this
point.setState(HOVER_STATE);
chart.hoverPoint = point;
},
/**
* Runs on mouse out from the point
*/
onMouseOut: function () {
var chart = this.series.chart,
hoverPoints = chart.hoverPoints;
if (!hoverPoints || inArray(this, hoverPoints) === -1) { // #887
this.firePointEvent('mouseOut');
this.setState();
chart.hoverPoint = null;
}
},
/**
* Extendable method for formatting each point's tooltip line
*
* @return {String} A string to be concatenated in to the common tooltip text
*/
tooltipFormatter: function (pointFormat) {
// Insert options for valueDecimals, valuePrefix, and valueSuffix
var series = this.series,
seriesTooltipOptions = series.tooltipOptions,
valueDecimals = pick(seriesTooltipOptions.valueDecimals, ''),
valuePrefix = seriesTooltipOptions.valuePrefix || '',
valueSuffix = seriesTooltipOptions.valueSuffix || '';
// Loop over the point array map and replace unformatted values with sprintf formatting markup
each(series.pointArrayMap || ['y'], function (key) {
key = '{point.' + key; // without the closing bracket
if (valuePrefix || valueSuffix) {
pointFormat = pointFormat.replace(key + '}', valuePrefix + key + '}' + valueSuffix);
}
pointFormat = pointFormat.replace(key + '}', key + ':,.' + valueDecimals + 'f}');
});
return format(pointFormat, {
point: this,
series: this.series
});
},
/**
* Update the point with new options (typically x/y data) and optionally redraw the series.
*
* @param {Object} options Point options as defined in the series.data array
* @param {Boolean} redraw Whether to redraw the chart or wait for an explicit call
* @param {Boolean|Object} animation Whether to apply animation, and optionally animation
* configuration
*
*/
update: function (options, redraw, animation) {
var point = this,
series = point.series,
graphic = point.graphic,
i,
data = series.data,
chart = series.chart,
seriesOptions = series.options;
redraw = pick(redraw, true);
// fire the event with a default handler of doing the update
point.firePointEvent('update', { options: options }, function () {
point.applyOptions(options);
// update visuals
if (isObject(options)) {
series.getAttribs();
if (graphic) {
if (options.marker && options.marker.symbol) {
point.graphic = graphic.destroy();
} else {
graphic.attr(point.pointAttr[point.state || '']);
}
}
}
// record changes in the parallel arrays
i = inArray(point, data);
series.xData[i] = point.x;
series.yData[i] = series.toYData ? series.toYData(point) : point.y;
series.zData[i] = point.z;
seriesOptions.data[i] = point.options;
// redraw
series.isDirty = series.isDirtyData = true;
if (!series.fixedBox && series.hasCartesianSeries) { // #1906, #2320
chart.isDirtyBox = true;
}
if (seriesOptions.legendType === 'point') { // #1831, #1885
chart.legend.destroyItem(point);
}
if (redraw) {
chart.redraw(animation);
}
});
},
/**
* Remove a point and optionally redraw the series and if necessary the axes
* @param {Boolean} redraw Whether to redraw the chart or wait for an explicit call
* @param {Boolean|Object} animation Whether to apply animation, and optionally animation
* configuration
*/
remove: function (redraw, animation) {
var point = this,
series = point.series,
points = series.points,
chart = series.chart,
i,
data = series.data;
setAnimation(animation, chart);
redraw = pick(redraw, true);
// fire the event with a default handler of removing the point
point.firePointEvent('remove', null, function () {
// splice all the parallel arrays
i = inArray(point, data);
if (data.length === points.length) {
points.splice(i, 1);
}
data.splice(i, 1);
series.options.data.splice(i, 1);
series.xData.splice(i, 1);
series.yData.splice(i, 1);
series.zData.splice(i, 1);
point.destroy();
// redraw
series.isDirty = true;
series.isDirtyData = true;
if (redraw) {
chart.redraw();
}
});
},
/**
* Fire an event on the Point object. Must not be renamed to fireEvent, as this
* causes a name clash in MooTools
* @param {String} eventType
* @param {Object} eventArgs Additional event arguments
* @param {Function} defaultFunction Default event handler
*/
firePointEvent: function (eventType, eventArgs, defaultFunction) {
var point = this,
series = this.series,
seriesOptions = series.options;
// load event handlers on demand to save time on mouseover/out
if (seriesOptions.point.events[eventType] || (point.options && point.options.events && point.options.events[eventType])) {
this.importEvents();
}
// add default handler if in selection mode
if (eventType === 'click' && seriesOptions.allowPointSelect) {
defaultFunction = function (event) {
// Control key is for Windows, meta (= Cmd key) for Mac, Shift for Opera
point.select(null, event.ctrlKey || event.metaKey || event.shiftKey);
};
}
fireEvent(this, eventType, eventArgs, defaultFunction);
},
/**
* Import events from the series' and point's options. Only do it on
* demand, to save processing time on hovering.
*/
importEvents: function () {
if (!this.hasImportedEvents) {
var point = this,
options = merge(point.series.options.point, point.options),
events = options.events,
eventType;
point.events = events;
for (eventType in events) {
addEvent(point, eventType, events[eventType]);
}
this.hasImportedEvents = true;
}
},
/**
* Set the point's state
* @param {String} state
*/
setState: function (state) {
var point = this,
plotX = point.plotX,
plotY = point.plotY,
series = point.series,
stateOptions = series.options.states,
markerOptions = defaultPlotOptions[series.type].marker && series.options.marker,
normalDisabled = markerOptions && !markerOptions.enabled,
markerStateOptions = markerOptions && markerOptions.states[state],
stateDisabled = markerStateOptions && markerStateOptions.enabled === false,
stateMarkerGraphic = series.stateMarkerGraphic,
pointMarker = point.marker || {},
chart = series.chart,
radius,
newSymbol,
pointAttr = point.pointAttr;
state = state || NORMAL_STATE; // empty string
if (
// already has this state
state === point.state ||
// selected points don't respond to hover
(point.selected && state !== SELECT_STATE) ||
// series' state options is disabled
(stateOptions[state] && stateOptions[state].enabled === false) ||
// point marker's state options is disabled
(state && (stateDisabled || (normalDisabled && !markerStateOptions.enabled)))
) {
return;
}
// apply hover styles to the existing point
if (point.graphic) {
radius = markerOptions && point.graphic.symbolName && pointAttr[state].r;
point.graphic.attr(merge(
pointAttr[state],
radius ? { // new symbol attributes (#507, #612)
x: plotX - radius,
y: plotY - radius,
width: 2 * radius,
height: 2 * radius
} : {}
));
} else {
// if a graphic is not applied to each point in the normal state, create a shared
// graphic for the hover state
if (state && markerStateOptions) {
radius = markerStateOptions.radius;
newSymbol = pointMarker.symbol || series.symbol;
// If the point has another symbol than the previous one, throw away the
// state marker graphic and force a new one (#1459)
if (stateMarkerGraphic && stateMarkerGraphic.currentSymbol !== newSymbol) {
stateMarkerGraphic = stateMarkerGraphic.destroy();
}
// Add a new state marker graphic
if (!stateMarkerGraphic) {
series.stateMarkerGraphic = stateMarkerGraphic = chart.renderer.symbol(
newSymbol,
plotX - radius,
plotY - radius,
2 * radius,
2 * radius
)
.attr(pointAttr[state])
.add(series.markerGroup);
stateMarkerGraphic.currentSymbol = newSymbol;
// Move the existing graphic
} else {
stateMarkerGraphic.attr({ // #1054
x: plotX - radius,
y: plotY - radius
});
}
}
if (stateMarkerGraphic) {
stateMarkerGraphic[state && chart.isInsidePlot(plotX, plotY) ? 'show' : 'hide']();
}
}
point.state = state;
}
};
/**
* @classDescription The base function which all other series types inherit from. The data in the series is stored
* in various arrays.
*
* - First, series.options.data contains all the original config options for
* each point whether added by options or methods like series.addPoint.
* - Next, series.data contains those values converted to points, but in case the series data length
* exceeds the cropThreshold, or if the data is grouped, series.data doesn't contain all the points. It
* only contains the points that have been created on demand.
* - Then there's series.points that contains all currently visible point objects. In case of cropping,
* the cropped-away points are not part of this array. The series.points array starts at series.cropStart
* compared to series.data and series.options.data. If however the series data is grouped, these can't
* be correlated one to one.
* - series.xData and series.processedXData contain clean x values, equivalent to series.data and series.points.
* - series.yData and series.processedYData contain clean x values, equivalent to series.data and series.points.
*
* @param {Object} chart
* @param {Object} options
*/
var Series = function () {};
Series.prototype = {
isCartesian: true,
type: 'line',
pointClass: Point,
sorted: true, // requires the data to be sorted
requireSorting: true,
pointAttrToOptions: { // mapping between SVG attributes and the corresponding options
stroke: 'lineColor',
'stroke-width': 'lineWidth',
fill: 'fillColor',
r: 'radius'
},
colorCounter: 0,
init: function (chart, options) {
var series = this,
eventType,
events,
chartSeries = chart.series;
series.chart = chart;
series.options = options = series.setOptions(options); // merge with plotOptions
series.linkedSeries = [];
// bind the axes
series.bindAxes();
// set some variables
extend(series, {
name: options.name,
state: NORMAL_STATE,
pointAttr: {},
visible: options.visible !== false, // true by default
selected: options.selected === true // false by default
});
// special
if (useCanVG) {
options.animation = false;
}
// register event listeners
events = options.events;
for (eventType in events) {
addEvent(series, eventType, events[eventType]);
}
if (
(events && events.click) ||
(options.point && options.point.events && options.point.events.click) ||
options.allowPointSelect
) {
chart.runTrackerClick = true;
}
series.getColor();
series.getSymbol();
// set the data
series.setData(options.data, false);
// Mark cartesian
if (series.isCartesian) {
chart.hasCartesianSeries = true;
}
// Register it in the chart
chartSeries.push(series);
series._i = chartSeries.length - 1;
// Sort series according to index option (#248, #1123)
stableSort(chartSeries, function (a, b) {
return pick(a.options.index, a._i) - pick(b.options.index, a._i);
});
each(chartSeries, function (series, i) {
series.index = i;
series.name = series.name || 'Series ' + (i + 1);
});
},
/**
* Set the xAxis and yAxis properties of cartesian series, and register the series
* in the axis.series array
*/
bindAxes: function () {
var series = this,
seriesOptions = series.options,
chart = series.chart,
axisOptions;
if (series.isCartesian) {
each(['xAxis', 'yAxis'], function (AXIS) { // repeat for xAxis and yAxis
each(chart[AXIS], function (axis) { // loop through the chart's axis objects
axisOptions = axis.options;
// apply if the series xAxis or yAxis option mathches the number of the
// axis, or if undefined, use the first axis
if ((seriesOptions[AXIS] === axisOptions.index) ||
(seriesOptions[AXIS] !== UNDEFINED && seriesOptions[AXIS] === axisOptions.id) ||
(seriesOptions[AXIS] === UNDEFINED && axisOptions.index === 0)) {
// register this series in the axis.series lookup
axis.series.push(series);
// set this series.xAxis or series.yAxis reference
series[AXIS] = axis;
// mark dirty for redraw
axis.isDirty = true;
}
});
// The series needs an X and an Y axis
if (!series[AXIS]) {
error(18, true);
}
});
}
},
/**
* Return an auto incremented x value based on the pointStart and pointInterval options.
* This is only used if an x value is not given for the point that calls autoIncrement.
*/
autoIncrement: function () {
var series = this,
options = series.options,
xIncrement = series.xIncrement;
xIncrement = pick(xIncrement, options.pointStart, 0);
series.pointInterval = pick(series.pointInterval, options.pointInterval, 1);
series.xIncrement = xIncrement + series.pointInterval;
return xIncrement;
},
/**
* Divide the series data into segments divided by null values.
*/
getSegments: function () {
var series = this,
lastNull = -1,
segments = [],
i,
points = series.points,
pointsLength = points.length;
if (pointsLength) { // no action required for []
// if connect nulls, just remove null points
if (series.options.connectNulls) {
i = pointsLength;
while (i--) {
if (points[i].y === null) {
points.splice(i, 1);
}
}
if (points.length) {
segments = [points];
}
// else, split on null points
} else {
each(points, function (point, i) {
if (point.y === null) {
if (i > lastNull + 1) {
segments.push(points.slice(lastNull + 1, i));
}
lastNull = i;
} else if (i === pointsLength - 1) { // last value
segments.push(points.slice(lastNull + 1, i + 1));
}
});
}
}
// register it
series.segments = segments;
},
/**
* Set the series options by merging from the options tree
* @param {Object} itemOptions
*/
setOptions: function (itemOptions) {
var chart = this.chart,
chartOptions = chart.options,
plotOptions = chartOptions.plotOptions,
typeOptions = plotOptions[this.type],
options;
this.userOptions = itemOptions;
options = merge(
typeOptions,
plotOptions.series,
itemOptions
);
// the tooltip options are merged between global and series specific options
this.tooltipOptions = merge(chartOptions.tooltip, options.tooltip);
// Delte marker object if not allowed (#1125)
if (typeOptions.marker === null) {
delete options.marker;
}
return options;
},
/**
* Get the series' color
*/
getColor: function () {
var options = this.options,
userOptions = this.userOptions,
defaultColors = this.chart.options.colors,
counters = this.chart.counters,
color,
colorIndex;
color = options.color || defaultPlotOptions[this.type].color;
if (!color && !options.colorByPoint) {
if (defined(userOptions._colorIndex)) { // after Series.update()
colorIndex = userOptions._colorIndex;
} else {
userOptions._colorIndex = counters.color;
colorIndex = counters.color++;
}
color = defaultColors[colorIndex];
}
this.color = color;
counters.wrapColor(defaultColors.length);
},
/**
* Get the series' symbol
*/
getSymbol: function () {
var series = this,
userOptions = series.userOptions,
seriesMarkerOption = series.options.marker,
chart = series.chart,
defaultSymbols = chart.options.symbols,
counters = chart.counters,
symbolIndex;
series.symbol = seriesMarkerOption.symbol;
if (!series.symbol) {
if (defined(userOptions._symbolIndex)) { // after Series.update()
symbolIndex = userOptions._symbolIndex;
} else {
userOptions._symbolIndex = counters.symbol;
symbolIndex = counters.symbol++;
}
series.symbol = defaultSymbols[symbolIndex];
}
// don't substract radius in image symbols (#604)
if (/^url/.test(series.symbol)) {
seriesMarkerOption.radius = 0;
}
counters.wrapSymbol(defaultSymbols.length);
},
/**
* Get the series' symbol in the legend. This method should be overridable to create custom
* symbols through Highcharts.seriesTypes[type].prototype.drawLegendSymbols.
*
* @param {Object} legend The legend object
*/
drawLegendSymbol: function (legend) {
var options = this.options,
markerOptions = options.marker,
radius,
legendOptions = legend.options,
legendSymbol,
symbolWidth = legendOptions.symbolWidth,
renderer = this.chart.renderer,
legendItemGroup = this.legendGroup,
verticalCenter = legend.baseline - mathRound(renderer.fontMetrics(legendOptions.itemStyle.fontSize).b * 0.3),
attr;
// Draw the line
if (options.lineWidth) {
attr = {
'stroke-width': options.lineWidth
};
if (options.dashStyle) {
attr.dashstyle = options.dashStyle;
}
this.legendLine = renderer.path([
M,
0,
verticalCenter,
L,
symbolWidth,
verticalCenter
])
.attr(attr)
.add(legendItemGroup);
}
// Draw the marker
if (markerOptions && markerOptions.enabled) {
radius = markerOptions.radius;
this.legendSymbol = legendSymbol = renderer.symbol(
this.symbol,
(symbolWidth / 2) - radius,
verticalCenter - radius,
2 * radius,
2 * radius
)
.add(legendItemGroup);
legendSymbol.isMarker = true;
}
},
/**
* Add a point dynamically after chart load time
* @param {Object} options Point options as given in series.data
* @param {Boolean} redraw Whether to redraw the chart or wait for an explicit call
* @param {Boolean} shift If shift is true, a point is shifted off the start
* of the series as one is appended to the end.
* @param {Boolean|Object} animation Whether to apply animation, and optionally animation
* configuration
*/
addPoint: function (options, redraw, shift, animation) {
var series = this,
seriesOptions = series.options,
data = series.data,
graph = series.graph,
area = series.area,
chart = series.chart,
xData = series.xData,
yData = series.yData,
zData = series.zData,
names = series.names,
currentShift = (graph && graph.shift) || 0,
dataOptions = seriesOptions.data,
point,
isInTheMiddle,
x,
i;
setAnimation(animation, chart);
// Make graph animate sideways
if (shift) {
each([graph, area, series.graphNeg, series.areaNeg], function (shape) {
if (shape) {
shape.shift = currentShift + 1;
}
});
}
if (area) {
area.isArea = true; // needed in animation, both with and without shift
}
// Optional redraw, defaults to true
redraw = pick(redraw, true);
// Get options and push the point to xData, yData and series.options. In series.generatePoints
// the Point instance will be created on demand and pushed to the series.data array.
point = { series: series };
series.pointClass.prototype.applyOptions.apply(point, [options]);
x = point.x;
// Get the insertion point
i = xData.length;
if (series.requireSorting && x < xData[i - 1]) {
isInTheMiddle = true;
while (i && xData[i - 1] > x) {
i--;
}
}
xData.splice(i, 0, x);
yData.splice(i, 0, series.toYData ? series.toYData(point) : point.y);
zData.splice(i, 0, point.z);
if (names) {
names[x] = point.name;
}
dataOptions.splice(i, 0, options);
if (isInTheMiddle) {
series.data.splice(i, 0, null);
series.processData();
}
// Generate points to be added to the legend (#1329)
if (seriesOptions.legendType === 'point') {
series.generatePoints();
}
// Shift the first point off the parallel arrays
// todo: consider series.removePoint(i) method
if (shift) {
if (data[0] && data[0].remove) {
data[0].remove(false);
} else {
data.shift();
xData.shift();
yData.shift();
zData.shift();
dataOptions.shift();
}
}
// redraw
series.isDirty = true;
series.isDirtyData = true;
if (redraw) {
series.getAttribs(); // #1937
chart.redraw();
}
},
/**
* Replace the series data with a new set of data
* @param {Object} data
* @param {Object} redraw
*/
setData: function (data, redraw) {
var series = this,
oldData = series.points,
options = series.options,
chart = series.chart,
firstPoint = null,
xAxis = series.xAxis,
names = xAxis && xAxis.categories && !xAxis.categories.length ? [] : null,
i;
// reset properties
series.xIncrement = null;
series.pointRange = xAxis && xAxis.categories ? 1 : options.pointRange;
series.colorCounter = 0; // for series with colorByPoint (#1547)
// parallel arrays
var xData = [],
yData = [],
zData = [],
dataLength = data ? data.length : [],
turboThreshold = pick(options.turboThreshold, 1000),
pt,
pointArrayMap = series.pointArrayMap,
valueCount = pointArrayMap && pointArrayMap.length,
hasToYData = !!series.toYData;
// In turbo mode, only one- or twodimensional arrays of numbers are allowed. The
// first value is tested, and we assume that all the rest are defined the same
// way. Although the 'for' loops are similar, they are repeated inside each
// if-else conditional for max performance.
if (turboThreshold && dataLength > turboThreshold) {
// find the first non-null point
i = 0;
while (firstPoint === null && i < dataLength) {
firstPoint = data[i];
i++;
}
if (isNumber(firstPoint)) { // assume all points are numbers
var x = pick(options.pointStart, 0),
pointInterval = pick(options.pointInterval, 1);
for (i = 0; i < dataLength; i++) {
xData[i] = x;
yData[i] = data[i];
x += pointInterval;
}
series.xIncrement = x;
} else if (isArray(firstPoint)) { // assume all points are arrays
if (valueCount) { // [x, low, high] or [x, o, h, l, c]
for (i = 0; i < dataLength; i++) {
pt = data[i];
xData[i] = pt[0];
yData[i] = pt.slice(1, valueCount + 1);
}
} else { // [x, y]
for (i = 0; i < dataLength; i++) {
pt = data[i];
xData[i] = pt[0];
yData[i] = pt[1];
}
}
} else {
error(12); // Highcharts expects configs to be numbers or arrays in turbo mode
}
} else {
for (i = 0; i < dataLength; i++) {
if (data[i] !== UNDEFINED) { // stray commas in oldIE
pt = { series: series };
series.pointClass.prototype.applyOptions.apply(pt, [data[i]]);
xData[i] = pt.x;
yData[i] = hasToYData ? series.toYData(pt) : pt.y;
zData[i] = pt.z;
if (names && pt.name) {
names[pt.x] = pt.name; // #2046
}
}
}
}
// Forgetting to cast strings to numbers is a common caveat when handling CSV or JSON
if (isString(yData[0])) {
error(14, true);
}
series.data = [];
series.options.data = data;
series.xData = xData;
series.yData = yData;
series.zData = zData;
series.names = names;
// destroy old points
i = (oldData && oldData.length) || 0;
while (i--) {
if (oldData[i] && oldData[i].destroy) {
oldData[i].destroy();
}
}
// reset minRange (#878)
if (xAxis) {
xAxis.minRange = xAxis.userMinRange;
}
// redraw
series.isDirty = series.isDirtyData = chart.isDirtyBox = true;
if (pick(redraw, true)) {
chart.redraw(false);
}
},
/**
* Remove a series and optionally redraw the chart
*
* @param {Boolean} redraw Whether to redraw the chart or wait for an explicit call
* @param {Boolean|Object} animation Whether to apply animation, and optionally animation
* configuration
*/
remove: function (redraw, animation) {
var series = this,
chart = series.chart;
redraw = pick(redraw, true);
if (!series.isRemoving) { /* prevent triggering native event in jQuery
(calling the remove function from the remove event) */
series.isRemoving = true;
// fire the event with a default handler of removing the point
fireEvent(series, 'remove', null, function () {
// destroy elements
series.destroy();
// redraw
chart.isDirtyLegend = chart.isDirtyBox = true;
chart.linkSeries();
if (redraw) {
chart.redraw(animation);
}
});
}
series.isRemoving = false;
},
/**
* Process the data by cropping away unused data points if the series is longer
* than the crop threshold. This saves computing time for lage series.
*/
processData: function (force) {
var series = this,
processedXData = series.xData, // copied during slice operation below
processedYData = series.yData,
dataLength = processedXData.length,
croppedData,
cropStart = 0,
cropped,
distance,
closestPointRange,
xAxis = series.xAxis,
i, // loop variable
options = series.options,
cropThreshold = options.cropThreshold,
isCartesian = series.isCartesian;
// If the series data or axes haven't changed, don't go through this. Return false to pass
// the message on to override methods like in data grouping.
if (isCartesian && !series.isDirty && !xAxis.isDirty && !series.yAxis.isDirty && !force) {
return false;
}
// optionally filter out points outside the plot area
if (isCartesian && series.sorted && (!cropThreshold || dataLength > cropThreshold || series.forceCrop)) {
var min = xAxis.min,
max = xAxis.max;
// it's outside current extremes
if (processedXData[dataLength - 1] < min || processedXData[0] > max) {
processedXData = [];
processedYData = [];
// only crop if it's actually spilling out
} else if (processedXData[0] < min || processedXData[dataLength - 1] > max) {
croppedData = this.cropData(series.xData, series.yData, min, max);
processedXData = croppedData.xData;
processedYData = croppedData.yData;
cropStart = croppedData.start;
cropped = true;
}
}
// Find the closest distance between processed points
for (i = processedXData.length - 1; i >= 0; i--) {
distance = processedXData[i] - processedXData[i - 1];
if (distance > 0 && (closestPointRange === UNDEFINED || distance < closestPointRange)) {
closestPointRange = distance;
// Unsorted data is not supported by the line tooltip, as well as data grouping and
// navigation in Stock charts (#725) and width calculation of columns (#1900)
} else if (distance < 0 && series.requireSorting) {
error(15);
}
}
// Record the properties
series.cropped = cropped; // undefined or true
series.cropStart = cropStart;
series.processedXData = processedXData;
series.processedYData = processedYData;
if (options.pointRange === null) { // null means auto, as for columns, candlesticks and OHLC
series.pointRange = closestPointRange || 1;
}
series.closestPointRange = closestPointRange;
},
/**
* Iterate over xData and crop values between min and max. Returns object containing crop start/end
* cropped xData with corresponding part of yData, dataMin and dataMax within the cropped range
*/
cropData: function (xData, yData, min, max) {
var dataLength = xData.length,
cropStart = 0,
cropEnd = dataLength,
cropShoulder = pick(this.cropShoulder, 1), // line-type series need one point outside
i;
// iterate up to find slice start
for (i = 0; i < dataLength; i++) {
if (xData[i] >= min) {
cropStart = mathMax(0, i - cropShoulder);
break;
}
}
// proceed to find slice end
for (; i < dataLength; i++) {
if (xData[i] > max) {
cropEnd = i + cropShoulder;
break;
}
}
return {
xData: xData.slice(cropStart, cropEnd),
yData: yData.slice(cropStart, cropEnd),
start: cropStart,
end: cropEnd
};
},
/**
* Generate the data point after the data has been processed by cropping away
* unused points and optionally grouped in Highcharts Stock.
*/
generatePoints: function () {
var series = this,
options = series.options,
dataOptions = options.data,
data = series.data,
dataLength,
processedXData = series.processedXData,
processedYData = series.processedYData,
pointClass = series.pointClass,
processedDataLength = processedXData.length,
cropStart = series.cropStart || 0,
cursor,
hasGroupedData = series.hasGroupedData,
point,
points = [],
i;
if (!data && !hasGroupedData) {
var arr = [];
arr.length = dataOptions.length;
data = series.data = arr;
}
for (i = 0; i < processedDataLength; i++) {
cursor = cropStart + i;
if (!hasGroupedData) {
if (data[cursor]) {
point = data[cursor];
} else if (dataOptions[cursor] !== UNDEFINED) { // #970
data[cursor] = point = (new pointClass()).init(series, dataOptions[cursor], processedXData[i]);
}
points[i] = point;
} else {
// splat the y data in case of ohlc data array
points[i] = (new pointClass()).init(series, [processedXData[i]].concat(splat(processedYData[i])));
}
}
// Hide cropped-away points - this only runs when the number of points is above cropThreshold, or when
// swithching view from non-grouped data to grouped data (#637)
if (data && (processedDataLength !== (dataLength = data.length) || hasGroupedData)) {
for (i = 0; i < dataLength; i++) {
if (i === cropStart && !hasGroupedData) { // when has grouped data, clear all points
i += processedDataLength;
}
if (data[i]) {
data[i].destroyElements();
data[i].plotX = UNDEFINED; // #1003
}
}
}
series.data = data;
series.points = points;
},
/**
* Adds series' points value to corresponding stack
*/
setStackedPoints: function () {
if (!this.options.stacking || (this.visible !== true && this.chart.options.chart.ignoreHiddenSeries !== false)) {
return;
}
var series = this,
xData = series.processedXData,
yData = series.processedYData,
stackedYData = [],
yDataLength = yData.length,
seriesOptions = series.options,
threshold = seriesOptions.threshold,
stackOption = seriesOptions.stack,
stacking = seriesOptions.stacking,
stackKey = series.stackKey,
negKey = '-' + stackKey,
negStacks = series.negStacks,
yAxis = series.yAxis,
stacks = yAxis.stacks,
oldStacks = yAxis.oldStacks,
isNegative,
stack,
other,
key,
i,
x,
y;
// loop over the non-null y values and read them into a local array
for (i = 0; i < yDataLength; i++) {
x = xData[i];
y = yData[i];
// Read stacked values into a stack based on the x value,
// the sign of y and the stack key. Stacking is also handled for null values (#739)
isNegative = negStacks && y < threshold;
key = isNegative ? negKey : stackKey;
// Create empty object for this stack if it doesn't exist yet
if (!stacks[key]) {
stacks[key] = {};
}
// Initialize StackItem for this x
if (!stacks[key][x]) {
if (oldStacks[key] && oldStacks[key][x]) {
stacks[key][x] = oldStacks[key][x];
stacks[key][x].total = null;
} else {
stacks[key][x] = new StackItem(yAxis, yAxis.options.stackLabels, isNegative, x, stackOption, stacking);
}
}
// If the StackItem doesn't exist, create it first
stack = stacks[key][x];
stack.points[series.index] = [stack.cum || 0];
// Add value to the stack total
if (stacking === 'percent') {
// Percent stacked column, totals are the same for the positive and negative stacks
other = isNegative ? stackKey : negKey;
if (negStacks && stacks[other] && stacks[other][x]) {
other = stacks[other][x];
stack.total = other.total = mathMax(other.total, stack.total) + mathAbs(y) || 0;
// Percent stacked areas
} else {
stack.total += mathAbs(y) || 0;
}
} else {
stack.total += y || 0;
}
stack.cum = (stack.cum || 0) + (y || 0);
stack.points[series.index].push(stack.cum);
stackedYData[i] = stack.cum;
}
if (stacking === 'percent') {
yAxis.usePercentage = true;
}
this.stackedYData = stackedYData; // To be used in getExtremes
// Reset old stacks
yAxis.oldStacks = {};
},
/**
* Iterate over all stacks and compute the absolute values to percent
*/
setPercentStacks: function () {
var series = this,
stackKey = series.stackKey,
stacks = series.yAxis.stacks;
each([stackKey, '-' + stackKey], function (key) {
var i = series.xData.length,
x,
stack,
pointExtremes,
totalFactor;
while (i--) {
x = series.xData[i];
stack = stacks[key] && stacks[key][x];
pointExtremes = stack && stack.points[series.index];
if (pointExtremes) {
totalFactor = stack.total ? 100 / stack.total : 0;
pointExtremes[0] = correctFloat(pointExtremes[0] * totalFactor); // Y bottom value
pointExtremes[1] = correctFloat(pointExtremes[1] * totalFactor); // Y value
series.stackedYData[i] = pointExtremes[1];
}
}
});
},
/**
* Calculate Y extremes for visible data
*/
getExtremes: function () {
var xAxis = this.xAxis,
yAxis = this.yAxis,
xData = this.processedXData,
yData = this.stackedYData || this.processedYData,
yDataLength = yData.length,
activeYData = [],
activeCounter = 0,
xExtremes = xAxis.getExtremes(), // #2117, need to compensate for log X axis
xMin = xExtremes.min,
xMax = xExtremes.max,
validValue,
withinRange,
dataMin,
dataMax,
x,
y,
i,
j;
for (i = 0; i < yDataLength; i++) {
x = xData[i];
y = yData[i];
// For points within the visible range, including the first point outside the
// visible range, consider y extremes
validValue = y !== null && y !== UNDEFINED && (!yAxis.isLog || (y.length || y > 0));
withinRange = this.getExtremesFromAll || this.cropped || ((xData[i + 1] || x) >= xMin &&
(xData[i - 1] || x) <= xMax);
if (validValue && withinRange) {
j = y.length;
if (j) { // array, like ohlc or range data
while (j--) {
if (y[j] !== null) {
activeYData[activeCounter++] = y[j];
}
}
} else {
activeYData[activeCounter++] = y;
}
}
}
this.dataMin = pick(dataMin, arrayMin(activeYData));
this.dataMax = pick(dataMax, arrayMax(activeYData));
},
/**
* Translate data points from raw data values to chart specific positioning data
* needed later in drawPoints, drawGraph and drawTracker.
*/
translate: function () {
if (!this.processedXData) { // hidden series
this.processData();
}
this.generatePoints();
var series = this,
options = series.options,
stacking = options.stacking,
xAxis = series.xAxis,
categories = xAxis.categories,
yAxis = series.yAxis,
points = series.points,
dataLength = points.length,
hasModifyValue = !!series.modifyValue,
i,
pointPlacement = options.pointPlacement,
dynamicallyPlaced = pointPlacement === 'between' || isNumber(pointPlacement),
threshold = options.threshold;
// Translate each point
for (i = 0; i < dataLength; i++) {
var point = points[i],
xValue = point.x,
yValue = point.y,
yBottom = point.low,
stack = yAxis.stacks[(series.negStacks && yValue < threshold ? '-' : '') + series.stackKey],
pointStack,
stackValues;
// Discard disallowed y values for log axes
if (yAxis.isLog && yValue <= 0) {
point.y = yValue = null;
}
// Get the plotX translation
point.plotX = xAxis.translate(xValue, 0, 0, 0, 1, pointPlacement, this.type === 'flags'); // Math.round fixes #591
// Calculate the bottom y value for stacked series
if (stacking && series.visible && stack && stack[xValue]) {
pointStack = stack[xValue];
stackValues = pointStack.points[series.index];
yBottom = stackValues[0];
yValue = stackValues[1];
if (yBottom === 0) {
yBottom = pick(threshold, yAxis.min);
}
if (yAxis.isLog && yBottom <= 0) { // #1200, #1232
yBottom = null;
}
point.percentage = stacking === 'percent' && yValue;
point.total = point.stackTotal = pointStack.total;
point.stackY = yValue;
// Place the stack label
pointStack.setOffset(series.pointXOffset || 0, series.barW || 0);
}
// Set translated yBottom or remove it
point.yBottom = defined(yBottom) ?
yAxis.translate(yBottom, 0, 1, 0, 1) :
null;
// general hook, used for Highstock compare mode
if (hasModifyValue) {
yValue = series.modifyValue(yValue, point);
}
// Set the the plotY value, reset it for redraws
point.plotY = (typeof yValue === 'number' && yValue !== Infinity) ?
//mathRound(yAxis.translate(yValue, 0, 1, 0, 1) * 10) / 10 : // Math.round fixes #591
yAxis.translate(yValue, 0, 1, 0, 1) :
UNDEFINED;
// Set client related positions for mouse tracking
point.clientX = dynamicallyPlaced ? xAxis.translate(xValue, 0, 0, 0, 1) : point.plotX; // #1514
point.negative = point.y < (threshold || 0);
// some API data
point.category = categories && categories[point.x] !== UNDEFINED ?
categories[point.x] : point.x;
}
// now that we have the cropped data, build the segments
series.getSegments();
},
/**
* Memoize tooltip texts and positions
*/
setTooltipPoints: function (renew) {
var series = this,
points = [],
pointsLength,
low,
high,
xAxis = series.xAxis,
xExtremes = xAxis && xAxis.getExtremes(),
axisLength = xAxis ? (xAxis.tooltipLen || xAxis.len) : series.chart.plotSizeX, // tooltipLen and tooltipPosName used in polar
point,
pointX,
nextPoint,
i,
tooltipPoints = []; // a lookup array for each pixel in the x dimension
// don't waste resources if tracker is disabled
if (series.options.enableMouseTracking === false) {
return;
}
// renew
if (renew) {
series.tooltipPoints = null;
}
// concat segments to overcome null values
each(series.segments || series.points, function (segment) {
points = points.concat(segment);
});
// Reverse the points in case the X axis is reversed
if (xAxis && xAxis.reversed) {
points = points.reverse();
}
// Polar needs additional shaping
if (series.orderTooltipPoints) {
series.orderTooltipPoints(points);
}
// Assign each pixel position to the nearest point
pointsLength = points.length;
for (i = 0; i < pointsLength; i++) {
point = points[i];
pointX = point.x;
if (pointX >= xExtremes.min && pointX <= xExtremes.max) { // #1149
nextPoint = points[i + 1];
// Set this range's low to the last range's high plus one
low = high === UNDEFINED ? 0 : high + 1;
// Now find the new high
high = points[i + 1] ?
mathMin(mathMax(0, mathFloor( // #2070
(point.clientX + (nextPoint ? (nextPoint.wrappedClientX || nextPoint.clientX) : axisLength)) / 2
)), axisLength) :
axisLength;
while (low >= 0 && low <= high) {
tooltipPoints[low++] = point;
}
}
}
series.tooltipPoints = tooltipPoints;
},
/**
* Format the header of the tooltip
*/
tooltipHeaderFormatter: function (point) {
var series = this,
tooltipOptions = series.tooltipOptions,
xDateFormat = tooltipOptions.xDateFormat,
dateTimeLabelFormats = tooltipOptions.dateTimeLabelFormats,
xAxis = series.xAxis,
isDateTime = xAxis && xAxis.options.type === 'datetime',
headerFormat = tooltipOptions.headerFormat,
closestPointRange = xAxis && xAxis.closestPointRange,
n;
// Guess the best date format based on the closest point distance (#568)
if (isDateTime && !xDateFormat) {
if (closestPointRange) {
for (n in timeUnits) {
if (timeUnits[n] >= closestPointRange) {
xDateFormat = dateTimeLabelFormats[n];
break;
}
}
} else {
xDateFormat = dateTimeLabelFormats.day;
}
}
// Insert the header date format if any
if (isDateTime && xDateFormat && isNumber(point.key)) {
headerFormat = headerFormat.replace('{point.key}', '{point.key:' + xDateFormat + '}');
}
return format(headerFormat, {
point: point,
series: series
});
},
/**
* Series mouse over handler
*/
onMouseOver: function () {
var series = this,
chart = series.chart,
hoverSeries = chart.hoverSeries;
// set normal state to previous series
if (hoverSeries && hoverSeries !== series) {
hoverSeries.onMouseOut();
}
// trigger the event, but to save processing time,
// only if defined
if (series.options.events.mouseOver) {
fireEvent(series, 'mouseOver');
}
// hover this
series.setState(HOVER_STATE);
chart.hoverSeries = series;
},
/**
* Series mouse out handler
*/
onMouseOut: function () {
// trigger the event only if listeners exist
var series = this,
options = series.options,
chart = series.chart,
tooltip = chart.tooltip,
hoverPoint = chart.hoverPoint;
// trigger mouse out on the point, which must be in this series
if (hoverPoint) {
hoverPoint.onMouseOut();
}
// fire the mouse out event
if (series && options.events.mouseOut) {
fireEvent(series, 'mouseOut');
}
// hide the tooltip
if (tooltip && !options.stickyTracking && (!tooltip.shared || series.noSharedTooltip)) {
tooltip.hide();
}
// set normal state
series.setState();
chart.hoverSeries = null;
},
/**
* Animate in the series
*/
animate: function (init) {
var series = this,
chart = series.chart,
renderer = chart.renderer,
clipRect,
markerClipRect,
animation = series.options.animation,
clipBox = chart.clipBox,
inverted = chart.inverted,
sharedClipKey;
// Animation option is set to true
if (animation && !isObject(animation)) {
animation = defaultPlotOptions[series.type].animation;
}
sharedClipKey = '_sharedClip' + animation.duration + animation.easing;
// Initialize the animation. Set up the clipping rectangle.
if (init) {
// If a clipping rectangle with the same properties is currently present in the chart, use that.
clipRect = chart[sharedClipKey];
markerClipRect = chart[sharedClipKey + 'm'];
if (!clipRect) {
chart[sharedClipKey] = clipRect = renderer.clipRect(
extend(clipBox, { width: 0 })
);
chart[sharedClipKey + 'm'] = markerClipRect = renderer.clipRect(
-99, // include the width of the first marker
inverted ? -chart.plotLeft : -chart.plotTop,
99,
inverted ? chart.chartWidth : chart.chartHeight
);
}
series.group.clip(clipRect);
series.markerGroup.clip(markerClipRect);
series.sharedClipKey = sharedClipKey;
// Run the animation
} else {
clipRect = chart[sharedClipKey];
if (clipRect) {
clipRect.animate({
width: chart.plotSizeX
}, animation);
chart[sharedClipKey + 'm'].animate({
width: chart.plotSizeX + 99
}, animation);
}
// Delete this function to allow it only once
series.animate = null;
// Call the afterAnimate function on animation complete (but don't overwrite the animation.complete option
// which should be available to the user).
series.animationTimeout = setTimeout(function () {
series.afterAnimate();
}, animation.duration);
}
},
/**
* This runs after animation to land on the final plot clipping
*/
afterAnimate: function () {
var chart = this.chart,
sharedClipKey = this.sharedClipKey,
group = this.group;
if (group && this.options.clip !== false) {
group.clip(chart.clipRect);
this.markerGroup.clip(); // no clip
}
// Remove the shared clipping rectancgle when all series are shown
setTimeout(function () {
if (sharedClipKey && chart[sharedClipKey]) {
chart[sharedClipKey] = chart[sharedClipKey].destroy();
chart[sharedClipKey + 'm'] = chart[sharedClipKey + 'm'].destroy();
}
}, 100);
},
/**
* Draw the markers
*/
drawPoints: function () {
var series = this,
pointAttr,
points = series.points,
chart = series.chart,
plotX,
plotY,
i,
point,
radius,
symbol,
isImage,
graphic,
options = series.options,
seriesMarkerOptions = options.marker,
pointMarkerOptions,
enabled,
isInside,
markerGroup = series.markerGroup;
if (seriesMarkerOptions.enabled || series._hasPointMarkers) {
i = points.length;
while (i--) {
point = points[i];
plotX = mathFloor(point.plotX); // #1843
plotY = point.plotY;
graphic = point.graphic;
pointMarkerOptions = point.marker || {};
enabled = (seriesMarkerOptions.enabled && pointMarkerOptions.enabled === UNDEFINED) || pointMarkerOptions.enabled;
isInside = chart.isInsidePlot(mathRound(plotX), plotY, chart.inverted); // #1858
// only draw the point if y is defined
if (enabled && plotY !== UNDEFINED && !isNaN(plotY) && point.y !== null) {
// shortcuts
pointAttr = point.pointAttr[point.selected ? SELECT_STATE : NORMAL_STATE];
radius = pointAttr.r;
symbol = pick(pointMarkerOptions.symbol, series.symbol);
isImage = symbol.indexOf('url') === 0;
if (graphic) { // update
graphic
.attr({ // Since the marker group isn't clipped, each individual marker must be toggled
visibility: isInside ? (hasSVG ? 'inherit' : VISIBLE) : HIDDEN
})
.animate(extend({
x: plotX - radius,
y: plotY - radius
}, graphic.symbolName ? { // don't apply to image symbols #507
width: 2 * radius,
height: 2 * radius
} : {}));
} else if (isInside && (radius > 0 || isImage)) {
point.graphic = graphic = chart.renderer.symbol(
symbol,
plotX - radius,
plotY - radius,
2 * radius,
2 * radius
)
.attr(pointAttr)
.add(markerGroup);
}
} else if (graphic) {
point.graphic = graphic.destroy(); // #1269
}
}
}
},
/**
* Convert state properties from API naming conventions to SVG attributes
*
* @param {Object} options API options object
* @param {Object} base1 SVG attribute object to inherit from
* @param {Object} base2 Second level SVG attribute object to inherit from
*/
convertAttribs: function (options, base1, base2, base3) {
var conversion = this.pointAttrToOptions,
attr,
option,
obj = {};
options = options || {};
base1 = base1 || {};
base2 = base2 || {};
base3 = base3 || {};
for (attr in conversion) {
option = conversion[attr];
obj[attr] = pick(options[option], base1[attr], base2[attr], base3[attr]);
}
return obj;
},
/**
* Get the state attributes. Each series type has its own set of attributes
* that are allowed to change on a point's state change. Series wide attributes are stored for
* all series, and additionally point specific attributes are stored for all
* points with individual marker options. If such options are not defined for the point,
* a reference to the series wide attributes is stored in point.pointAttr.
*/
getAttribs: function () {
var series = this,
seriesOptions = series.options,
normalOptions = defaultPlotOptions[series.type].marker ? seriesOptions.marker : seriesOptions,
stateOptions = normalOptions.states,
stateOptionsHover = stateOptions[HOVER_STATE],
pointStateOptionsHover,
seriesColor = series.color,
normalDefaults = {
stroke: seriesColor,
fill: seriesColor
},
points = series.points || [], // #927
i,
point,
seriesPointAttr = [],
pointAttr,
pointAttrToOptions = series.pointAttrToOptions,
hasPointSpecificOptions,
negativeColor = seriesOptions.negativeColor,
defaultLineColor = normalOptions.lineColor,
key;
// series type specific modifications
if (seriesOptions.marker) { // line, spline, area, areaspline, scatter
// if no hover radius is given, default to normal radius + 2
stateOptionsHover.radius = stateOptionsHover.radius || normalOptions.radius + 2;
stateOptionsHover.lineWidth = stateOptionsHover.lineWidth || normalOptions.lineWidth + 1;
} else { // column, bar, pie
// if no hover color is given, brighten the normal color
stateOptionsHover.color = stateOptionsHover.color ||
Color(stateOptionsHover.color || seriesColor)
.brighten(stateOptionsHover.brightness).get();
}
// general point attributes for the series normal state
seriesPointAttr[NORMAL_STATE] = series.convertAttribs(normalOptions, normalDefaults);
// HOVER_STATE and SELECT_STATE states inherit from normal state except the default radius
each([HOVER_STATE, SELECT_STATE], function (state) {
seriesPointAttr[state] =
series.convertAttribs(stateOptions[state], seriesPointAttr[NORMAL_STATE]);
});
// set it
series.pointAttr = seriesPointAttr;
// Generate the point-specific attribute collections if specific point
// options are given. If not, create a referance to the series wide point
// attributes
i = points.length;
while (i--) {
point = points[i];
normalOptions = (point.options && point.options.marker) || point.options;
if (normalOptions && normalOptions.enabled === false) {
normalOptions.radius = 0;
}
if (point.negative && negativeColor) {
point.color = point.fillColor = negativeColor;
}
hasPointSpecificOptions = seriesOptions.colorByPoint || point.color; // #868
// check if the point has specific visual options
if (point.options) {
for (key in pointAttrToOptions) {
if (defined(normalOptions[pointAttrToOptions[key]])) {
hasPointSpecificOptions = true;
}
}
}
// a specific marker config object is defined for the individual point:
// create it's own attribute collection
if (hasPointSpecificOptions) {
normalOptions = normalOptions || {};
pointAttr = [];
stateOptions = normalOptions.states || {}; // reassign for individual point
pointStateOptionsHover = stateOptions[HOVER_STATE] = stateOptions[HOVER_STATE] || {};
// Handle colors for column and pies
if (!seriesOptions.marker) { // column, bar, point
// if no hover color is given, brighten the normal color
pointStateOptionsHover.color =
Color(pointStateOptionsHover.color || point.color)
.brighten(pointStateOptionsHover.brightness ||
stateOptionsHover.brightness).get();
}
// normal point state inherits series wide normal state
pointAttr[NORMAL_STATE] = series.convertAttribs(extend({
color: point.color, // #868
fillColor: point.color, // Individual point color or negative color markers (#2219)
lineColor: defaultLineColor === null ? point.color : UNDEFINED // Bubbles take point color, line markers use white
}, normalOptions), seriesPointAttr[NORMAL_STATE]);
// inherit from point normal and series hover
pointAttr[HOVER_STATE] = series.convertAttribs(
stateOptions[HOVER_STATE],
seriesPointAttr[HOVER_STATE],
pointAttr[NORMAL_STATE]
);
// inherit from point normal and series hover
pointAttr[SELECT_STATE] = series.convertAttribs(
stateOptions[SELECT_STATE],
seriesPointAttr[SELECT_STATE],
pointAttr[NORMAL_STATE]
);
// no marker config object is created: copy a reference to the series-wide
// attribute collection
} else {
pointAttr = seriesPointAttr;
}
point.pointAttr = pointAttr;
}
},
/**
* Update the series with a new set of options
*/
update: function (newOptions, redraw) {
var chart = this.chart,
// must use user options when changing type because this.options is merged
// in with type specific plotOptions
oldOptions = this.userOptions,
oldType = this.type,
proto = seriesTypes[oldType].prototype,
n;
// Do the merge, with some forced options
newOptions = merge(oldOptions, {
animation: false,
index: this.index,
pointStart: this.xData[0] // when updating after addPoint
}, { data: this.options.data }, newOptions);
// Destroy the series and reinsert methods from the type prototype
this.remove(false);
for (n in proto) { // Overwrite series-type specific methods (#2270)
if (proto.hasOwnProperty(n)) {
this[n] = UNDEFINED;
}
}
extend(this, seriesTypes[newOptions.type || oldType].prototype);
this.init(chart, newOptions);
if (pick(redraw, true)) {
chart.redraw(false);
}
},
/**
* Clear DOM objects and free up memory
*/
destroy: function () {
var series = this,
chart = series.chart,
issue134 = /AppleWebKit\/533/.test(userAgent),
destroy,
i,
data = series.data || [],
point,
prop,
axis;
// add event hook
fireEvent(series, 'destroy');
// remove all events
removeEvent(series);
// erase from axes
each(['xAxis', 'yAxis'], function (AXIS) {
axis = series[AXIS];
if (axis) {
erase(axis.series, series);
axis.isDirty = axis.forceRedraw = true;
axis.stacks = {}; // Rebuild stacks when updating (#2229)
}
});
// remove legend items
if (series.legendItem) {
series.chart.legend.destroyItem(series);
}
// destroy all points with their elements
i = data.length;
while (i--) {
point = data[i];
if (point && point.destroy) {
point.destroy();
}
}
series.points = null;
// Clear the animation timeout if we are destroying the series during initial animation
clearTimeout(series.animationTimeout);
// destroy all SVGElements associated to the series
each(['area', 'graph', 'dataLabelsGroup', 'group', 'markerGroup', 'tracker',
'graphNeg', 'areaNeg', 'posClip', 'negClip'], function (prop) {
if (series[prop]) {
// issue 134 workaround
destroy = issue134 && prop === 'group' ?
'hide' :
'destroy';
series[prop][destroy]();
}
});
// remove from hoverSeries
if (chart.hoverSeries === series) {
chart.hoverSeries = null;
}
erase(chart.series, series);
// clear all members
for (prop in series) {
delete series[prop];
}
},
/**
* Draw the data labels
*/
drawDataLabels: function () {
var series = this,
seriesOptions = series.options,
options = seriesOptions.dataLabels,
points = series.points,
pointOptions,
generalOptions,
str,
dataLabelsGroup;
if (options.enabled || series._hasPointLabels) {
// Process default alignment of data labels for columns
if (series.dlProcessOptions) {
series.dlProcessOptions(options);
}
// Create a separate group for the data labels to avoid rotation
dataLabelsGroup = series.plotGroup(
'dataLabelsGroup',
'data-labels',
series.visible ? VISIBLE : HIDDEN,
options.zIndex || 6
);
// Make the labels for each point
generalOptions = options;
each(points, function (point) {
var enabled,
dataLabel = point.dataLabel,
labelConfig,
attr,
name,
rotation,
connector = point.connector,
isNew = true;
// Determine if each data label is enabled
pointOptions = point.options && point.options.dataLabels;
enabled = pick(pointOptions && pointOptions.enabled, generalOptions.enabled); // #2282
// If the point is outside the plot area, destroy it. #678, #820
if (dataLabel && !enabled) {
point.dataLabel = dataLabel.destroy();
// Individual labels are disabled if the are explicitly disabled
// in the point options, or if they fall outside the plot area.
} else if (enabled) {
// Create individual options structure that can be extended without
// affecting others
options = merge(generalOptions, pointOptions);
rotation = options.rotation;
// Get the string
labelConfig = point.getLabelConfig();
str = options.format ?
format(options.format, labelConfig) :
options.formatter.call(labelConfig, options);
// Determine the color
options.style.color = pick(options.color, options.style.color, series.color, 'black');
// update existing label
if (dataLabel) {
if (defined(str)) {
dataLabel
.attr({
text: str
});
isNew = false;
} else { // #1437 - the label is shown conditionally
point.dataLabel = dataLabel = dataLabel.destroy();
if (connector) {
point.connector = connector.destroy();
}
}
// create new label
} else if (defined(str)) {
attr = {
//align: align,
fill: options.backgroundColor,
stroke: options.borderColor,
'stroke-width': options.borderWidth,
r: options.borderRadius || 0,
rotation: rotation,
padding: options.padding,
zIndex: 1
};
// Remove unused attributes (#947)
for (name in attr) {
if (attr[name] === UNDEFINED) {
delete attr[name];
}
}
dataLabel = point.dataLabel = series.chart.renderer[rotation ? 'text' : 'label']( // labels don't support rotation
str,
0,
-999,
null,
null,
null,
options.useHTML
)
.attr(attr)
.css(options.style)
.add(dataLabelsGroup)
.shadow(options.shadow);
}
if (dataLabel) {
// Now the data label is created and placed at 0,0, so we need to align it
series.alignDataLabel(point, dataLabel, options, null, isNew);
}
}
});
}
},
/**
* Align each individual data label
*/
alignDataLabel: function (point, dataLabel, options, alignTo, isNew) {
var chart = this.chart,
inverted = chart.inverted,
plotX = pick(point.plotX, -999),
plotY = pick(point.plotY, -999),
bBox = dataLabel.getBBox(),
visible = this.visible && chart.isInsidePlot(point.plotX, point.plotY, inverted),
alignAttr; // the final position;
if (visible) {
// The alignment box is a singular point
alignTo = extend({
x: inverted ? chart.plotWidth - plotY : plotX,
y: mathRound(inverted ? chart.plotHeight - plotX : plotY),
width: 0,
height: 0
}, alignTo);
// Add the text size for alignment calculation
extend(options, {
width: bBox.width,
height: bBox.height
});
// Allow a hook for changing alignment in the last moment, then do the alignment
if (options.rotation) { // Fancy box alignment isn't supported for rotated text
alignAttr = {
align: options.align,
x: alignTo.x + options.x + alignTo.width / 2,
y: alignTo.y + options.y + alignTo.height / 2
};
dataLabel[isNew ? 'attr' : 'animate'](alignAttr);
} else {
dataLabel.align(options, null, alignTo);
alignAttr = dataLabel.alignAttr;
// Handle justify or crop
if (pick(options.overflow, 'justify') === 'justify') { // docs: overflow: justify, also crop only applies when not justify
this.justifyDataLabel(dataLabel, options, alignAttr, bBox, alignTo, isNew);
} else if (pick(options.crop, true)) {
// Now check that the data label is within the plot area
visible = chart.isInsidePlot(alignAttr.x, alignAttr.y) && chart.isInsidePlot(alignAttr.x + bBox.width, alignAttr.y + bBox.height);
}
}
}
// Show or hide based on the final aligned position
if (!visible) {
dataLabel.attr({ y: -999 });
}
},
/**
* If data labels fall partly outside the plot area, align them back in, in a way that
* doesn't hide the point.
*/
justifyDataLabel: function (dataLabel, options, alignAttr, bBox, alignTo, isNew) {
var chart = this.chart,
align = options.align,
verticalAlign = options.verticalAlign,
off,
justified;
// Off left
off = alignAttr.x;
if (off < 0) {
if (align === 'right') {
options.align = 'left';
} else {
options.x = -off;
}
justified = true;
}
// Off right
off = alignAttr.x + bBox.width;
if (off > chart.plotWidth) {
if (align === 'left') {
options.align = 'right';
} else {
options.x = chart.plotWidth - off;
}
justified = true;
}
// Off top
off = alignAttr.y;
if (off < 0) {
if (verticalAlign === 'bottom') {
options.verticalAlign = 'top';
} else {
options.y = -off;
}
justified = true;
}
// Off bottom
off = alignAttr.y + bBox.height;
if (off > chart.plotHeight) {
if (verticalAlign === 'top') {
options.verticalAlign = 'bottom';
} else {
options.y = chart.plotHeight - off;
}
justified = true;
}
if (justified) {
dataLabel.placed = !isNew;
dataLabel.align(options, null, alignTo);
}
},
/**
* Return the graph path of a segment
*/
getSegmentPath: function (segment) {
var series = this,
segmentPath = [],
step = series.options.step;
// build the segment line
each(segment, function (point, i) {
var plotX = point.plotX,
plotY = point.plotY,
lastPoint;
if (series.getPointSpline) { // generate the spline as defined in the SplineSeries object
segmentPath.push.apply(segmentPath, series.getPointSpline(segment, point, i));
} else {
// moveTo or lineTo
segmentPath.push(i ? L : M);
// step line?
if (step && i) {
lastPoint = segment[i - 1];
if (step === 'right') {
segmentPath.push(
lastPoint.plotX,
plotY
);
} else if (step === 'center') {
segmentPath.push(
(lastPoint.plotX + plotX) / 2,
lastPoint.plotY,
(lastPoint.plotX + plotX) / 2,
plotY
);
} else {
segmentPath.push(
plotX,
lastPoint.plotY
);
}
}
// normal line to next point
segmentPath.push(
point.plotX,
point.plotY
);
}
});
return segmentPath;
},
/**
* Get the graph path
*/
getGraphPath: function () {
var series = this,
graphPath = [],
segmentPath,
singlePoints = []; // used in drawTracker
// Divide into segments and build graph and area paths
each(series.segments, function (segment) {
segmentPath = series.getSegmentPath(segment);
// add the segment to the graph, or a single point for tracking
if (segment.length > 1) {
graphPath = graphPath.concat(segmentPath);
} else {
singlePoints.push(segment[0]);
}
});
// Record it for use in drawGraph and drawTracker, and return graphPath
series.singlePoints = singlePoints;
series.graphPath = graphPath;
return graphPath;
},
/**
* Draw the actual graph
*/
drawGraph: function () {
var series = this,
options = this.options,
props = [['graph', options.lineColor || this.color]],
lineWidth = options.lineWidth,
dashStyle = options.dashStyle,
graphPath = this.getGraphPath(),
negativeColor = options.negativeColor;
if (negativeColor) {
props.push(['graphNeg', negativeColor]);
}
// draw the graph
each(props, function (prop, i) {
var graphKey = prop[0],
graph = series[graphKey],
attribs;
if (graph) {
stop(graph); // cancel running animations, #459
graph.animate({ d: graphPath });
} else if (lineWidth && graphPath.length) { // #1487
attribs = {
stroke: prop[1],
'stroke-width': lineWidth,
zIndex: 1 // #1069
};
if (dashStyle) {
attribs.dashstyle = dashStyle;
} else {
attribs['stroke-linecap'] = attribs['stroke-linejoin'] = 'round';
}
series[graphKey] = series.chart.renderer.path(graphPath)
.attr(attribs)
.add(series.group)
.shadow(!i && options.shadow);
}
});
},
/**
* Clip the graphs into the positive and negative coloured graphs
*/
clipNeg: function () {
var options = this.options,
chart = this.chart,
renderer = chart.renderer,
negativeColor = options.negativeColor || options.negativeFillColor,
translatedThreshold,
posAttr,
negAttr,
graph = this.graph,
area = this.area,
posClip = this.posClip,
negClip = this.negClip,
chartWidth = chart.chartWidth,
chartHeight = chart.chartHeight,
chartSizeMax = mathMax(chartWidth, chartHeight),
yAxis = this.yAxis,
above,
below;
if (negativeColor && (graph || area)) {
translatedThreshold = mathRound(yAxis.toPixels(options.threshold || 0, true));
above = {
x: 0,
y: 0,
width: chartSizeMax,
height: translatedThreshold
};
below = {
x: 0,
y: translatedThreshold,
width: chartSizeMax,
height: chartSizeMax
};
if (chart.inverted) {
above.height = below.y = chart.plotWidth - translatedThreshold;
if (renderer.isVML) {
above = {
x: chart.plotWidth - translatedThreshold - chart.plotLeft,
y: 0,
width: chartWidth,
height: chartHeight
};
below = {
x: translatedThreshold + chart.plotLeft - chartWidth,
y: 0,
width: chart.plotLeft + translatedThreshold,
height: chartWidth
};
}
}
if (yAxis.reversed) {
posAttr = below;
negAttr = above;
} else {
posAttr = above;
negAttr = below;
}
if (posClip) { // update
posClip.animate(posAttr);
negClip.animate(negAttr);
} else {
this.posClip = posClip = renderer.clipRect(posAttr);
this.negClip = negClip = renderer.clipRect(negAttr);
if (graph && this.graphNeg) {
graph.clip(posClip);
this.graphNeg.clip(negClip);
}
if (area) {
area.clip(posClip);
this.areaNeg.clip(negClip);
}
}
}
},
/**
* Initialize and perform group inversion on series.group and series.markerGroup
*/
invertGroups: function () {
var series = this,
chart = series.chart;
// Pie, go away (#1736)
if (!series.xAxis) {
return;
}
// A fixed size is needed for inversion to work
function setInvert() {
var size = {
width: series.yAxis.len,
height: series.xAxis.len
};
each(['group', 'markerGroup'], function (groupName) {
if (series[groupName]) {
series[groupName].attr(size).invert();
}
});
}
addEvent(chart, 'resize', setInvert); // do it on resize
addEvent(series, 'destroy', function () {
removeEvent(chart, 'resize', setInvert);
});
// Do it now
setInvert(); // do it now
// On subsequent render and redraw, just do setInvert without setting up events again
series.invertGroups = setInvert;
},
/**
* General abstraction for creating plot groups like series.group, series.dataLabelsGroup and
* series.markerGroup. On subsequent calls, the group will only be adjusted to the updated plot size.
*/
plotGroup: function (prop, name, visibility, zIndex, parent) {
var group = this[prop],
isNew = !group;
// Generate it on first call
if (isNew) {
this[prop] = group = this.chart.renderer.g(name)
.attr({
visibility: visibility,
zIndex: zIndex || 0.1 // IE8 needs this
})
.add(parent);
}
// Place it on first and subsequent (redraw) calls
group[isNew ? 'attr' : 'animate'](this.getPlotBox());
return group;
},
/**
* Get the translation and scale for the plot area of this series
*/
getPlotBox: function () {
return {
translateX: this.xAxis ? this.xAxis.left : this.chart.plotLeft,
translateY: this.yAxis ? this.yAxis.top : this.chart.plotTop,
scaleX: 1, // #1623
scaleY: 1
};
},
/**
* Render the graph and markers
*/
render: function () {
var series = this,
chart = series.chart,
group,
options = series.options,
animation = options.animation,
doAnimation = animation && !!series.animate &&
chart.renderer.isSVG, // this animation doesn't work in IE8 quirks when the group div is hidden,
// and looks bad in other oldIE
visibility = series.visible ? VISIBLE : HIDDEN,
zIndex = options.zIndex,
hasRendered = series.hasRendered,
chartSeriesGroup = chart.seriesGroup;
// the group
group = series.plotGroup(
'group',
'series',
visibility,
zIndex,
chartSeriesGroup
);
series.markerGroup = series.plotGroup(
'markerGroup',
'markers',
visibility,
zIndex,
chartSeriesGroup
);
// initiate the animation
if (doAnimation) {
series.animate(true);
}
// cache attributes for shapes
series.getAttribs();
// SVGRenderer needs to know this before drawing elements (#1089, #1795)
group.inverted = series.isCartesian ? chart.inverted : false;
// draw the graph if any
if (series.drawGraph) {
series.drawGraph();
series.clipNeg();
}
// draw the data labels (inn pies they go before the points)
series.drawDataLabels();
// draw the points
series.drawPoints();
// draw the mouse tracking area
if (series.options.enableMouseTracking !== false) {
series.drawTracker();
}
// Handle inverted series and tracker groups
if (chart.inverted) {
series.invertGroups();
}
// Initial clipping, must be defined after inverting groups for VML
if (options.clip !== false && !series.sharedClipKey && !hasRendered) {
group.clip(chart.clipRect);
}
// Run the animation
if (doAnimation) {
series.animate();
} else if (!hasRendered) {
series.afterAnimate();
}
series.isDirty = series.isDirtyData = false; // means data is in accordance with what you see
// (See #322) series.isDirty = series.isDirtyData = false; // means data is in accordance with what you see
series.hasRendered = true;
},
/**
* Redraw the series after an update in the axes.
*/
redraw: function () {
var series = this,
chart = series.chart,
wasDirtyData = series.isDirtyData, // cache it here as it is set to false in render, but used after
group = series.group,
xAxis = series.xAxis,
yAxis = series.yAxis;
// reposition on resize
if (group) {
if (chart.inverted) {
group.attr({
width: chart.plotWidth,
height: chart.plotHeight
});
}
group.animate({
translateX: pick(xAxis && xAxis.left, chart.plotLeft),
translateY: pick(yAxis && yAxis.top, chart.plotTop)
});
}
series.translate();
series.setTooltipPoints(true);
series.render();
if (wasDirtyData) {
fireEvent(series, 'updatedData');
}
},
/**
* Set the state of the graph
*/
setState: function (state) {
var series = this,
options = series.options,
graph = series.graph,
graphNeg = series.graphNeg,
stateOptions = options.states,
lineWidth = options.lineWidth,
attribs;
state = state || NORMAL_STATE;
if (series.state !== state) {
series.state = state;
if (stateOptions[state] && stateOptions[state].enabled === false) {
return;
}
if (state) {
lineWidth = stateOptions[state].lineWidth || lineWidth + 1;
}
if (graph && !graph.dashstyle) { // hover is turned off for dashed lines in VML
attribs = {
'stroke-width': lineWidth
};
// use attr because animate will cause any other animation on the graph to stop
graph.attr(attribs);
if (graphNeg) {
graphNeg.attr(attribs);
}
}
}
},
/**
* Set the visibility of the graph
*
* @param vis {Boolean} True to show the series, false to hide. If UNDEFINED,
* the visibility is toggled.
*/
setVisible: function (vis, redraw) {
var series = this,
chart = series.chart,
legendItem = series.legendItem,
showOrHide,
ignoreHiddenSeries = chart.options.chart.ignoreHiddenSeries,
oldVisibility = series.visible;
// if called without an argument, toggle visibility
series.visible = vis = series.userOptions.visible = vis === UNDEFINED ? !oldVisibility : vis;
showOrHide = vis ? 'show' : 'hide';
// show or hide elements
each(['group', 'dataLabelsGroup', 'markerGroup', 'tracker'], function (key) {
if (series[key]) {
series[key][showOrHide]();
}
});
// hide tooltip (#1361)
if (chart.hoverSeries === series) {
series.onMouseOut();
}
if (legendItem) {
chart.legend.colorizeItem(series, vis);
}
// rescale or adapt to resized chart
series.isDirty = true;
// in a stack, all other series are affected
if (series.options.stacking) {
each(chart.series, function (otherSeries) {
if (otherSeries.options.stacking && otherSeries.visible) {
otherSeries.isDirty = true;
}
});
}
// show or hide linked series
each(series.linkedSeries, function (otherSeries) {
otherSeries.setVisible(vis, false);
});
if (ignoreHiddenSeries) {
chart.isDirtyBox = true;
}
if (redraw !== false) {
chart.redraw();
}
fireEvent(series, showOrHide);
},
/**
* Show the graph
*/
show: function () {
this.setVisible(true);
},
/**
* Hide the graph
*/
hide: function () {
this.setVisible(false);
},
/**
* Set the selected state of the graph
*
* @param selected {Boolean} True to select the series, false to unselect. If
* UNDEFINED, the selection state is toggled.
*/
select: function (selected) {
var series = this;
// if called without an argument, toggle
series.selected = selected = (selected === UNDEFINED) ? !series.selected : selected;
if (series.checkbox) {
series.checkbox.checked = selected;
}
fireEvent(series, selected ? 'select' : 'unselect');
},
/**
* Draw the tracker object that sits above all data labels and markers to
* track mouse events on the graph or points. For the line type charts
* the tracker uses the same graphPath, but with a greater stroke width
* for better control.
*/
drawTracker: function () {
var series = this,
options = series.options,
trackByArea = options.trackByArea,
trackerPath = [].concat(trackByArea ? series.areaPath : series.graphPath),
trackerPathLength = trackerPath.length,
chart = series.chart,
pointer = chart.pointer,
renderer = chart.renderer,
snap = chart.options.tooltip.snap,
tracker = series.tracker,
cursor = options.cursor,
css = cursor && { cursor: cursor },
singlePoints = series.singlePoints,
singlePoint,
i,
onMouseOver = function () {
if (chart.hoverSeries !== series) {
series.onMouseOver();
}
};
// Extend end points. A better way would be to use round linecaps,
// but those are not clickable in VML.
if (trackerPathLength && !trackByArea) {
i = trackerPathLength + 1;
while (i--) {
if (trackerPath[i] === M) { // extend left side
trackerPath.splice(i + 1, 0, trackerPath[i + 1] - snap, trackerPath[i + 2], L);
}
if ((i && trackerPath[i] === M) || i === trackerPathLength) { // extend right side
trackerPath.splice(i, 0, L, trackerPath[i - 2] + snap, trackerPath[i - 1]);
}
}
}
// handle single points
for (i = 0; i < singlePoints.length; i++) {
singlePoint = singlePoints[i];
trackerPath.push(M, singlePoint.plotX - snap, singlePoint.plotY,
L, singlePoint.plotX + snap, singlePoint.plotY);
}
// draw the tracker
if (tracker) {
tracker.attr({ d: trackerPath });
} else { // create
series.tracker = renderer.path(trackerPath)
.attr({
'stroke-linejoin': 'round', // #1225
visibility: series.visible ? VISIBLE : HIDDEN,
stroke: TRACKER_FILL,
fill: trackByArea ? TRACKER_FILL : NONE,
'stroke-width' : options.lineWidth + (trackByArea ? 0 : 2 * snap),
zIndex: 2
})
.add(series.group);
// The tracker is added to the series group, which is clipped, but is covered
// by the marker group. So the marker group also needs to capture events.
each([series.tracker, series.markerGroup], function (tracker) {
tracker.addClass(PREFIX + 'tracker')
.on('mouseover', onMouseOver)
.on('mouseout', function (e) { pointer.onTrackerMouseOut(e); })
.css(css);
if (hasTouch) {
tracker.on('touchstart', onMouseOver);
}
});
}
}
}; // end Series prototype
/**
* LineSeries object
*/
var LineSeries = extendClass(Series);
seriesTypes.line = LineSeries;
/**
* Set the default options for area
*/
defaultPlotOptions.area = merge(defaultSeriesOptions, {
threshold: 0
// trackByArea: false,
// lineColor: null, // overrides color, but lets fillColor be unaltered
// fillOpacity: 0.75,
// fillColor: null
});
/**
* AreaSeries object
*/
var AreaSeries = extendClass(Series, {
type: 'area',
/**
* For stacks, don't split segments on null values. Instead, draw null values with
* no marker. Also insert dummy points for any X position that exists in other series
* in the stack.
*/
getSegments: function () {
var segments = [],
segment = [],
keys = [],
xAxis = this.xAxis,
yAxis = this.yAxis,
stack = yAxis.stacks[this.stackKey],
pointMap = {},
plotX,
plotY,
points = this.points,
connectNulls = this.options.connectNulls,
val,
i,
x;
if (this.options.stacking && !this.cropped) { // cropped causes artefacts in Stock, and perf issue
// Create a map where we can quickly look up the points by their X value.
for (i = 0; i < points.length; i++) {
pointMap[points[i].x] = points[i];
}
// Sort the keys (#1651)
for (x in stack) {
keys.push(+x);
}
keys.sort(function (a, b) {
return a - b;
});
each(keys, function (x) {
if (connectNulls && (!pointMap[x] || pointMap[x].y === null)) { // #1836
return;
// The point exists, push it to the segment
} else if (pointMap[x]) {
segment.push(pointMap[x]);
// There is no point for this X value in this series, so we
// insert a dummy point in order for the areas to be drawn
// correctly.
} else {
plotX = xAxis.translate(x);
val = stack[x].percent ? (stack[x].total ? stack[x].cum * 100 / stack[x].total : 0) : stack[x].cum; // #1991
plotY = yAxis.toPixels(val, true);
segment.push({
y: null,
plotX: plotX,
clientX: plotX,
plotY: plotY,
yBottom: plotY,
onMouseOver: noop
});
}
});
if (segment.length) {
segments.push(segment);
}
} else {
Series.prototype.getSegments.call(this);
segments = this.segments;
}
this.segments = segments;
},
/**
* Extend the base Series getSegmentPath method by adding the path for the area.
* This path is pushed to the series.areaPath property.
*/
getSegmentPath: function (segment) {
var segmentPath = Series.prototype.getSegmentPath.call(this, segment), // call base method
areaSegmentPath = [].concat(segmentPath), // work on a copy for the area path
i,
options = this.options,
segLength = segmentPath.length,
translatedThreshold = this.yAxis.getThreshold(options.threshold), // #2181
yBottom;
if (segLength === 3) { // for animation from 1 to two points
areaSegmentPath.push(L, segmentPath[1], segmentPath[2]);
}
if (options.stacking && !this.closedStacks) {
// Follow stack back. Todo: implement areaspline. A general solution could be to
// reverse the entire graphPath of the previous series, though may be hard with
// splines and with series with different extremes
for (i = segment.length - 1; i >= 0; i--) {
yBottom = pick(segment[i].yBottom, translatedThreshold);
// step line?
if (i < segment.length - 1 && options.step) {
areaSegmentPath.push(segment[i + 1].plotX, yBottom);
}
areaSegmentPath.push(segment[i].plotX, yBottom);
}
} else { // follow zero line back
this.closeSegment(areaSegmentPath, segment, translatedThreshold);
}
this.areaPath = this.areaPath.concat(areaSegmentPath);
return segmentPath;
},
/**
* Extendable method to close the segment path of an area. This is overridden in polar
* charts.
*/
closeSegment: function (path, segment, translatedThreshold) {
path.push(
L,
segment[segment.length - 1].plotX,
translatedThreshold,
L,
segment[0].plotX,
translatedThreshold
);
},
/**
* Draw the graph and the underlying area. This method calls the Series base
* function and adds the area. The areaPath is calculated in the getSegmentPath
* method called from Series.prototype.drawGraph.
*/
drawGraph: function () {
// Define or reset areaPath
this.areaPath = [];
// Call the base method
Series.prototype.drawGraph.apply(this);
// Define local variables
var series = this,
areaPath = this.areaPath,
options = this.options,
negativeColor = options.negativeColor,
negativeFillColor = options.negativeFillColor,
props = [['area', this.color, options.fillColor]]; // area name, main color, fill color
if (negativeColor || negativeFillColor) {
props.push(['areaNeg', negativeColor, negativeFillColor]);
}
each(props, function (prop) {
var areaKey = prop[0],
area = series[areaKey];
// Create or update the area
if (area) { // update
area.animate({ d: areaPath });
} else { // create
series[areaKey] = series.chart.renderer.path(areaPath)
.attr({
fill: pick(
prop[2],
Color(prop[1]).setOpacity(pick(options.fillOpacity, 0.75)).get()
),
zIndex: 0 // #1069
}).add(series.group);
}
});
},
/**
* Get the series' symbol in the legend
*
* @param {Object} legend The legend object
* @param {Object} item The series (this) or point
*/
drawLegendSymbol: function (legend, item) {
item.legendSymbol = this.chart.renderer.rect(
0,
legend.baseline - 11,
legend.options.symbolWidth,
12,
2
).attr({
zIndex: 3
}).add(item.legendGroup);
}
});
seriesTypes.area = AreaSeries;/**
* Set the default options for spline
*/
defaultPlotOptions.spline = merge(defaultSeriesOptions);
/**
* SplineSeries object
*/
var SplineSeries = extendClass(Series, {
type: 'spline',
/**
* Get the spline segment from a given point's previous neighbour to the given point
*/
getPointSpline: function (segment, point, i) {
var smoothing = 1.5, // 1 means control points midway between points, 2 means 1/3 from the point, 3 is 1/4 etc
denom = smoothing + 1,
plotX = point.plotX,
plotY = point.plotY,
lastPoint = segment[i - 1],
nextPoint = segment[i + 1],
leftContX,
leftContY,
rightContX,
rightContY,
ret;
// find control points
if (lastPoint && nextPoint) {
var lastX = lastPoint.plotX,
lastY = lastPoint.plotY,
nextX = nextPoint.plotX,
nextY = nextPoint.plotY,
correction;
leftContX = (smoothing * plotX + lastX) / denom;
leftContY = (smoothing * plotY + lastY) / denom;
rightContX = (smoothing * plotX + nextX) / denom;
rightContY = (smoothing * plotY + nextY) / denom;
// have the two control points make a straight line through main point
correction = ((rightContY - leftContY) * (rightContX - plotX)) /
(rightContX - leftContX) + plotY - rightContY;
leftContY += correction;
rightContY += correction;
// to prevent false extremes, check that control points are between
// neighbouring points' y values
if (leftContY > lastY && leftContY > plotY) {
leftContY = mathMax(lastY, plotY);
rightContY = 2 * plotY - leftContY; // mirror of left control point
} else if (leftContY < lastY && leftContY < plotY) {
leftContY = mathMin(lastY, plotY);
rightContY = 2 * plotY - leftContY;
}
if (rightContY > nextY && rightContY > plotY) {
rightContY = mathMax(nextY, plotY);
leftContY = 2 * plotY - rightContY;
} else if (rightContY < nextY && rightContY < plotY) {
rightContY = mathMin(nextY, plotY);
leftContY = 2 * plotY - rightContY;
}
// record for drawing in next point
point.rightContX = rightContX;
point.rightContY = rightContY;
}
// Visualize control points for debugging
/*
if (leftContX) {
this.chart.renderer.circle(leftContX + this.chart.plotLeft, leftContY + this.chart.plotTop, 2)
.attr({
stroke: 'red',
'stroke-width': 1,
fill: 'none'
})
.add();
this.chart.renderer.path(['M', leftContX + this.chart.plotLeft, leftContY + this.chart.plotTop,
'L', plotX + this.chart.plotLeft, plotY + this.chart.plotTop])
.attr({
stroke: 'red',
'stroke-width': 1
})
.add();
this.chart.renderer.circle(rightContX + this.chart.plotLeft, rightContY + this.chart.plotTop, 2)
.attr({
stroke: 'green',
'stroke-width': 1,
fill: 'none'
})
.add();
this.chart.renderer.path(['M', rightContX + this.chart.plotLeft, rightContY + this.chart.plotTop,
'L', plotX + this.chart.plotLeft, plotY + this.chart.plotTop])
.attr({
stroke: 'green',
'stroke-width': 1
})
.add();
}
*/
// moveTo or lineTo
if (!i) {
ret = [M, plotX, plotY];
} else { // curve from last point to this
ret = [
'C',
lastPoint.rightContX || lastPoint.plotX,
lastPoint.rightContY || lastPoint.plotY,
leftContX || plotX,
leftContY || plotY,
plotX,
plotY
];
lastPoint.rightContX = lastPoint.rightContY = null; // reset for updating series later
}
return ret;
}
});
seriesTypes.spline = SplineSeries;
/**
* Set the default options for areaspline
*/
defaultPlotOptions.areaspline = merge(defaultPlotOptions.area);
/**
* AreaSplineSeries object
*/
var areaProto = AreaSeries.prototype,
AreaSplineSeries = extendClass(SplineSeries, {
type: 'areaspline',
closedStacks: true, // instead of following the previous graph back, follow the threshold back
// Mix in methods from the area series
getSegmentPath: areaProto.getSegmentPath,
closeSegment: areaProto.closeSegment,
drawGraph: areaProto.drawGraph,
drawLegendSymbol: areaProto.drawLegendSymbol
});
seriesTypes.areaspline = AreaSplineSeries;
/**
* Set the default options for column
*/
defaultPlotOptions.column = merge(defaultSeriesOptions, {
borderColor: '#FFFFFF',
borderWidth: 1,
borderRadius: 0,
//colorByPoint: undefined,
groupPadding: 0.2,
//grouping: true,
marker: null, // point options are specified in the base options
pointPadding: 0.1,
//pointWidth: null,
minPointLength: 0,
cropThreshold: 50, // when there are more points, they will not animate out of the chart on xAxis.setExtremes
pointRange: null, // null means auto, meaning 1 in a categorized axis and least distance between points if not categories
states: {
hover: {
brightness: 0.1,
shadow: false
},
select: {
color: '#C0C0C0',
borderColor: '#000000',
shadow: false
}
},
dataLabels: {
align: null, // auto
verticalAlign: null, // auto
y: null
},
stickyTracking: false,
threshold: 0
});
/**
* ColumnSeries object
*/
var ColumnSeries = extendClass(Series, {
type: 'column',
pointAttrToOptions: { // mapping between SVG attributes and the corresponding options
stroke: 'borderColor',
'stroke-width': 'borderWidth',
fill: 'color',
r: 'borderRadius'
},
cropShoulder: 0,
trackerGroups: ['group', 'dataLabelsGroup'],
negStacks: true, // use separate negative stacks, unlike area stacks where a negative
// point is substracted from previous (#1910)
/**
* Initialize the series
*/
init: function () {
Series.prototype.init.apply(this, arguments);
var series = this,
chart = series.chart;
// if the series is added dynamically, force redraw of other
// series affected by a new column
if (chart.hasRendered) {
each(chart.series, function (otherSeries) {
if (otherSeries.type === series.type) {
otherSeries.isDirty = true;
}
});
}
},
/**
* Return the width and x offset of the columns adjusted for grouping, groupPadding, pointPadding,
* pointWidth etc.
*/
getColumnMetrics: function () {
var series = this,
options = series.options,
xAxis = series.xAxis,
yAxis = series.yAxis,
reversedXAxis = xAxis.reversed,
stackKey,
stackGroups = {},
columnIndex,
columnCount = 0;
// Get the total number of column type series.
// This is called on every series. Consider moving this logic to a
// chart.orderStacks() function and call it on init, addSeries and removeSeries
if (options.grouping === false) {
columnCount = 1;
} else {
each(series.chart.series, function (otherSeries) {
var otherOptions = otherSeries.options,
otherYAxis = otherSeries.yAxis;
if (otherSeries.type === series.type && otherSeries.visible &&
yAxis.len === otherYAxis.len && yAxis.pos === otherYAxis.pos) { // #642, #2086
if (otherOptions.stacking) {
stackKey = otherSeries.stackKey;
if (stackGroups[stackKey] === UNDEFINED) {
stackGroups[stackKey] = columnCount++;
}
columnIndex = stackGroups[stackKey];
} else if (otherOptions.grouping !== false) { // #1162
columnIndex = columnCount++;
}
otherSeries.columnIndex = columnIndex;
}
});
}
var categoryWidth = mathMin(
mathAbs(xAxis.transA) * (xAxis.ordinalSlope || options.pointRange || xAxis.closestPointRange || 1),
xAxis.len // #1535
),
groupPadding = categoryWidth * options.groupPadding,
groupWidth = categoryWidth - 2 * groupPadding,
pointOffsetWidth = groupWidth / columnCount,
optionPointWidth = options.pointWidth,
pointPadding = defined(optionPointWidth) ? (pointOffsetWidth - optionPointWidth) / 2 :
pointOffsetWidth * options.pointPadding,
pointWidth = pick(optionPointWidth, pointOffsetWidth - 2 * pointPadding), // exact point width, used in polar charts
colIndex = (reversedXAxis ?
columnCount - (series.columnIndex || 0) : // #1251
series.columnIndex) || 0,
pointXOffset = pointPadding + (groupPadding + colIndex *
pointOffsetWidth - (categoryWidth / 2)) *
(reversedXAxis ? -1 : 1);
// Save it for reading in linked series (Error bars particularly)
return (series.columnMetrics = {
width: pointWidth,
offset: pointXOffset
});
},
/**
* Translate each point to the plot area coordinate system and find shape positions
*/
translate: function () {
var series = this,
chart = series.chart,
options = series.options,
borderWidth = options.borderWidth,
yAxis = series.yAxis,
threshold = options.threshold,
translatedThreshold = series.translatedThreshold = yAxis.getThreshold(threshold),
minPointLength = pick(options.minPointLength, 5),
metrics = series.getColumnMetrics(),
pointWidth = metrics.width,
seriesBarW = series.barW = mathCeil(mathMax(pointWidth, 1 + 2 * borderWidth)), // rounded and postprocessed for border width
pointXOffset = series.pointXOffset = metrics.offset,
xCrisp = -(borderWidth % 2 ? 0.5 : 0),
yCrisp = borderWidth % 2 ? 0.5 : 1;
if (chart.renderer.isVML && chart.inverted) {
yCrisp += 1;
}
Series.prototype.translate.apply(series);
// record the new values
each(series.points, function (point) {
var yBottom = pick(point.yBottom, translatedThreshold),
plotY = mathMin(mathMax(-999 - yBottom, point.plotY), yAxis.len + 999 + yBottom), // Don't draw too far outside plot area (#1303, #2241)
barX = point.plotX + pointXOffset,
barW = seriesBarW,
barY = mathMin(plotY, yBottom),
right,
bottom,
fromTop,
fromLeft,
barH = mathMax(plotY, yBottom) - barY;
// Handle options.minPointLength
if (mathAbs(barH) < minPointLength) {
if (minPointLength) {
barH = minPointLength;
barY =
mathRound(mathAbs(barY - translatedThreshold) > minPointLength ? // stacked
yBottom - minPointLength : // keep position
translatedThreshold - (yAxis.translate(point.y, 0, 1, 0, 1) <= translatedThreshold ? minPointLength : 0)); // use exact yAxis.translation (#1485)
}
}
// Cache for access in polar
point.barX = barX;
point.pointWidth = pointWidth;
// Round off to obtain crisp edges
fromLeft = mathAbs(barX) < 0.5;
right = mathRound(barX + barW) + xCrisp;
barX = mathRound(barX) + xCrisp;
barW = right - barX;
fromTop = mathAbs(barY) < 0.5;
bottom = mathRound(barY + barH) + yCrisp;
barY = mathRound(barY) + yCrisp;
barH = bottom - barY;
// Top and left edges are exceptions
if (fromLeft) {
barX += 1;
barW -= 1;
}
if (fromTop) {
barY -= 1;
barH += 1;
}
// Register shape type and arguments to be used in drawPoints
point.shapeType = 'rect';
point.shapeArgs = {
x: barX,
y: barY,
width: barW,
height: barH
};
});
},
getSymbol: noop,
/**
* Use a solid rectangle like the area series types
*/
drawLegendSymbol: AreaSeries.prototype.drawLegendSymbol,
/**
* Columns have no graph
*/
drawGraph: noop,
/**
* Draw the columns. For bars, the series.group is rotated, so the same coordinates
* apply for columns and bars. This method is inherited by scatter series.
*
*/
drawPoints: function () {
var series = this,
options = series.options,
renderer = series.chart.renderer,
shapeArgs;
// draw the columns
each(series.points, function (point) {
var plotY = point.plotY,
graphic = point.graphic;
if (plotY !== UNDEFINED && !isNaN(plotY) && point.y !== null) {
shapeArgs = point.shapeArgs;
if (graphic) { // update
stop(graphic);
graphic.animate(merge(shapeArgs));
} else {
point.graphic = graphic = renderer[point.shapeType](shapeArgs)
.attr(point.pointAttr[point.selected ? SELECT_STATE : NORMAL_STATE])
.add(series.group)
.shadow(options.shadow, null, options.stacking && !options.borderRadius);
}
} else if (graphic) {
point.graphic = graphic.destroy(); // #1269
}
});
},
/**
* Add tracking event listener to the series group, so the point graphics
* themselves act as trackers
*/
drawTracker: function () {
var series = this,
chart = series.chart,
pointer = chart.pointer,
cursor = series.options.cursor,
css = cursor && { cursor: cursor },
onMouseOver = function (e) {
var target = e.target,
point;
if (chart.hoverSeries !== series) {
series.onMouseOver();
}
while (target && !point) {
point = target.point;
target = target.parentNode;
}
if (point !== UNDEFINED && point !== chart.hoverPoint) { // undefined on graph in scatterchart
point.onMouseOver(e);
}
};
// Add reference to the point
each(series.points, function (point) {
if (point.graphic) {
point.graphic.element.point = point;
}
if (point.dataLabel) {
point.dataLabel.element.point = point;
}
});
// Add the event listeners, we need to do this only once
if (!series._hasTracking) {
each(series.trackerGroups, function (key) {
if (series[key]) { // we don't always have dataLabelsGroup
series[key]
.addClass(PREFIX + 'tracker')
.on('mouseover', onMouseOver)
.on('mouseout', function (e) { pointer.onTrackerMouseOut(e); })
.css(css);
if (hasTouch) {
series[key].on('touchstart', onMouseOver);
}
}
});
series._hasTracking = true;
}
},
/**
* Override the basic data label alignment by adjusting for the position of the column
*/
alignDataLabel: function (point, dataLabel, options, alignTo, isNew) {
var chart = this.chart,
inverted = chart.inverted,
dlBox = point.dlBox || point.shapeArgs, // data label box for alignment
below = point.below || (point.plotY > pick(this.translatedThreshold, chart.plotSizeY)),
inside = pick(options.inside, !!this.options.stacking); // draw it inside the box?
// Align to the column itself, or the top of it
if (dlBox) { // Area range uses this method but not alignTo
alignTo = merge(dlBox);
if (inverted) {
alignTo = {
x: chart.plotWidth - alignTo.y - alignTo.height,
y: chart.plotHeight - alignTo.x - alignTo.width,
width: alignTo.height,
height: alignTo.width
};
}
// Compute the alignment box
if (!inside) {
if (inverted) {
alignTo.x += below ? 0 : alignTo.width;
alignTo.width = 0;
} else {
alignTo.y += below ? alignTo.height : 0;
alignTo.height = 0;
}
}
}
// When alignment is undefined (typically columns and bars), display the individual
// point below or above the point depending on the threshold
options.align = pick(
options.align,
!inverted || inside ? 'center' : below ? 'right' : 'left'
);
options.verticalAlign = pick(
options.verticalAlign,
inverted || inside ? 'middle' : below ? 'top' : 'bottom'
);
// Call the parent method
Series.prototype.alignDataLabel.call(this, point, dataLabel, options, alignTo, isNew);
},
/**
* Animate the column heights one by one from zero
* @param {Boolean} init Whether to initialize the animation or run it
*/
animate: function (init) {
var series = this,
yAxis = this.yAxis,
options = series.options,
inverted = this.chart.inverted,
attr = {},
translatedThreshold;
if (hasSVG) { // VML is too slow anyway
if (init) {
attr.scaleY = 0.001;
translatedThreshold = mathMin(yAxis.pos + yAxis.len, mathMax(yAxis.pos, yAxis.toPixels(options.threshold)));
if (inverted) {
attr.translateX = translatedThreshold - yAxis.len;
} else {
attr.translateY = translatedThreshold;
}
series.group.attr(attr);
} else { // run the animation
attr.scaleY = 1;
attr[inverted ? 'translateX' : 'translateY'] = yAxis.pos;
series.group.animate(attr, series.options.animation);
// delete this function to allow it only once
series.animate = null;
}
}
},
/**
* Remove this series from the chart
*/
remove: function () {
var series = this,
chart = series.chart;
// column and bar series affects other series of the same type
// as they are either stacked or grouped
if (chart.hasRendered) {
each(chart.series, function (otherSeries) {
if (otherSeries.type === series.type) {
otherSeries.isDirty = true;
}
});
}
Series.prototype.remove.apply(series, arguments);
}
});
seriesTypes.column = ColumnSeries;
/**
* Set the default options for bar
*/
defaultPlotOptions.bar = merge(defaultPlotOptions.column);
/**
* The Bar series class
*/
var BarSeries = extendClass(ColumnSeries, {
type: 'bar',
inverted: true
});
seriesTypes.bar = BarSeries;
/**
* Set the default options for scatter
*/
defaultPlotOptions.scatter = merge(defaultSeriesOptions, {
lineWidth: 0,
tooltip: {
headerFormat: '<span style="font-size: 10px; color:{series.color}">{series.name}</span><br/>',
pointFormat: 'x: <b>{point.x}</b><br/>y: <b>{point.y}</b><br/>',
followPointer: true
},
stickyTracking: false
});
/**
* The scatter series class
*/
var ScatterSeries = extendClass(Series, {
type: 'scatter',
sorted: false,
requireSorting: false,
noSharedTooltip: true,
trackerGroups: ['markerGroup'],
drawTracker: ColumnSeries.prototype.drawTracker,
setTooltipPoints: noop
});
seriesTypes.scatter = ScatterSeries;
/**
* Set the default options for pie
*/
defaultPlotOptions.pie = merge(defaultSeriesOptions, {
borderColor: '#FFFFFF',
borderWidth: 1,
center: [null, null],
clip: false,
colorByPoint: true, // always true for pies
dataLabels: {
// align: null,
// connectorWidth: 1,
// connectorColor: point.color,
// connectorPadding: 5,
distance: 30,
enabled: true,
formatter: function () {
return this.point.name;
}
// softConnector: true,
//y: 0
},
ignoreHiddenPoint: true,
//innerSize: 0,
legendType: 'point',
marker: null, // point options are specified in the base options
size: null,
showInLegend: false,
slicedOffset: 10,
states: {
hover: {
brightness: 0.1,
shadow: false
}
},
stickyTracking: false,
tooltip: {
followPointer: true
}
});
/**
* Extended point object for pies
*/
var PiePoint = extendClass(Point, {
/**
* Initiate the pie slice
*/
init: function () {
Point.prototype.init.apply(this, arguments);
var point = this,
toggleSlice;
// Disallow negative values (#1530)
if (point.y < 0) {
point.y = null;
}
//visible: options.visible !== false,
extend(point, {
visible: point.visible !== false,
name: pick(point.name, 'Slice')
});
// add event listener for select
toggleSlice = function (e) {
point.slice(e.type === 'select');
};
addEvent(point, 'select', toggleSlice);
addEvent(point, 'unselect', toggleSlice);
return point;
},
/**
* Toggle the visibility of the pie slice
* @param {Boolean} vis Whether to show the slice or not. If undefined, the
* visibility is toggled
*/
setVisible: function (vis) {
var point = this,
series = point.series,
chart = series.chart,
method;
// if called without an argument, toggle visibility
point.visible = point.options.visible = vis = vis === UNDEFINED ? !point.visible : vis;
series.options.data[inArray(point, series.data)] = point.options; // update userOptions.data
method = vis ? 'show' : 'hide';
// Show and hide associated elements
each(['graphic', 'dataLabel', 'connector', 'shadowGroup'], function (key) {
if (point[key]) {
point[key][method]();
}
});
if (point.legendItem) {
chart.legend.colorizeItem(point, vis);
}
// Handle ignore hidden slices
if (!series.isDirty && series.options.ignoreHiddenPoint) {
series.isDirty = true;
chart.redraw();
}
},
/**
* Set or toggle whether the slice is cut out from the pie
* @param {Boolean} sliced When undefined, the slice state is toggled
* @param {Boolean} redraw Whether to redraw the chart. True by default.
*/
slice: function (sliced, redraw, animation) {
var point = this,
series = point.series,
chart = series.chart,
translation;
setAnimation(animation, chart);
// redraw is true by default
redraw = pick(redraw, true);
// if called without an argument, toggle
point.sliced = point.options.sliced = sliced = defined(sliced) ? sliced : !point.sliced;
series.options.data[inArray(point, series.data)] = point.options; // update userOptions.data
translation = sliced ? point.slicedTranslation : {
translateX: 0,
translateY: 0
};
point.graphic.animate(translation);
if (point.shadowGroup) {
point.shadowGroup.animate(translation);
}
}
});
/**
* The Pie series class
*/
var PieSeries = {
type: 'pie',
isCartesian: false,
pointClass: PiePoint,
requireSorting: false,
noSharedTooltip: true,
trackerGroups: ['group', 'dataLabelsGroup'],
pointAttrToOptions: { // mapping between SVG attributes and the corresponding options
stroke: 'borderColor',
'stroke-width': 'borderWidth',
fill: 'color'
},
/**
* Pies have one color each point
*/
getColor: noop,
/**
* Animate the pies in
*/
animate: function (init) {
var series = this,
points = series.points,
startAngleRad = series.startAngleRad;
if (!init) {
each(points, function (point) {
var graphic = point.graphic,
args = point.shapeArgs;
if (graphic) {
// start values
graphic.attr({
r: series.center[3] / 2, // animate from inner radius (#779)
start: startAngleRad,
end: startAngleRad
});
// animate
graphic.animate({
r: args.r,
start: args.start,
end: args.end
}, series.options.animation);
}
});
// delete this function to allow it only once
series.animate = null;
}
},
/**
* Extend the basic setData method by running processData and generatePoints immediately,
* in order to access the points from the legend.
*/
setData: function (data, redraw) {
Series.prototype.setData.call(this, data, false);
this.processData();
this.generatePoints();
if (pick(redraw, true)) {
this.chart.redraw();
}
},
/**
* Extend the generatePoints method by adding total and percentage properties to each point
*/
generatePoints: function () {
var i,
total = 0,
points,
len,
point,
ignoreHiddenPoint = this.options.ignoreHiddenPoint;
Series.prototype.generatePoints.call(this);
// Populate local vars
points = this.points;
len = points.length;
// Get the total sum
for (i = 0; i < len; i++) {
point = points[i];
total += (ignoreHiddenPoint && !point.visible) ? 0 : point.y;
}
this.total = total;
// Set each point's properties
for (i = 0; i < len; i++) {
point = points[i];
point.percentage = total > 0 ? (point.y / total) * 100 : 0;
point.total = total;
}
},
/**
* Get the center of the pie based on the size and center options relative to the
* plot area. Borrowed by the polar and gauge series types.
*/
getCenter: function () {
var options = this.options,
chart = this.chart,
slicingRoom = 2 * (options.slicedOffset || 0),
handleSlicingRoom,
plotWidth = chart.plotWidth - 2 * slicingRoom,
plotHeight = chart.plotHeight - 2 * slicingRoom,
centerOption = options.center,
positions = [pick(centerOption[0], '50%'), pick(centerOption[1], '50%'), options.size || '100%', options.innerSize || 0],
smallestSize = mathMin(plotWidth, plotHeight),
isPercent;
return map(positions, function (length, i) {
isPercent = /%$/.test(length);
handleSlicingRoom = i < 2 || (i === 2 && isPercent);
return (isPercent ?
// i == 0: centerX, relative to width
// i == 1: centerY, relative to height
// i == 2: size, relative to smallestSize
// i == 4: innerSize, relative to smallestSize
[plotWidth, plotHeight, smallestSize, smallestSize][i] *
pInt(length) / 100 :
length) + (handleSlicingRoom ? slicingRoom : 0);
});
},
/**
* Do translation for pie slices
*/
translate: function (positions) {
this.generatePoints();
var series = this,
cumulative = 0,
precision = 1000, // issue #172
options = series.options,
slicedOffset = options.slicedOffset,
connectorOffset = slicedOffset + options.borderWidth,
start,
end,
angle,
startAngle = options.startAngle || 0,
startAngleRad = series.startAngleRad = mathPI / 180 * (startAngle - 90),
endAngleRad = series.endAngleRad = mathPI / 180 * ((options.endAngle || (startAngle + 360)) - 90), // docs
circ = endAngleRad - startAngleRad, //2 * mathPI,
points = series.points,
radiusX, // the x component of the radius vector for a given point
radiusY,
labelDistance = options.dataLabels.distance,
ignoreHiddenPoint = options.ignoreHiddenPoint,
i,
len = points.length,
point;
// Get positions - either an integer or a percentage string must be given.
// If positions are passed as a parameter, we're in a recursive loop for adjusting
// space for data labels.
if (!positions) {
series.center = positions = series.getCenter();
}
// utility for getting the x value from a given y, used for anticollision logic in data labels
series.getX = function (y, left) {
angle = math.asin((y - positions[1]) / (positions[2] / 2 + labelDistance));
return positions[0] +
(left ? -1 : 1) *
(mathCos(angle) * (positions[2] / 2 + labelDistance));
};
// Calculate the geometry for each point
for (i = 0; i < len; i++) {
point = points[i];
// set start and end angle
start = startAngleRad + (cumulative * circ);
if (!ignoreHiddenPoint || point.visible) {
cumulative += point.percentage / 100;
}
end = startAngleRad + (cumulative * circ);
// set the shape
point.shapeType = 'arc';
point.shapeArgs = {
x: positions[0],
y: positions[1],
r: positions[2] / 2,
innerR: positions[3] / 2,
start: mathRound(start * precision) / precision,
end: mathRound(end * precision) / precision
};
// center for the sliced out slice
angle = (end + start) / 2;
if (angle > 0.75 * circ) {
angle -= 2 * mathPI;
}
point.slicedTranslation = {
translateX: mathRound(mathCos(angle) * slicedOffset),
translateY: mathRound(mathSin(angle) * slicedOffset)
};
// set the anchor point for tooltips
radiusX = mathCos(angle) * positions[2] / 2;
radiusY = mathSin(angle) * positions[2] / 2;
point.tooltipPos = [
positions[0] + radiusX * 0.7,
positions[1] + radiusY * 0.7
];
point.half = angle < -mathPI / 2 || angle > mathPI / 2 ? 1 : 0;
point.angle = angle;
// set the anchor point for data labels
connectorOffset = mathMin(connectorOffset, labelDistance / 2); // #1678
point.labelPos = [
positions[0] + radiusX + mathCos(angle) * labelDistance, // first break of connector
positions[1] + radiusY + mathSin(angle) * labelDistance, // a/a
positions[0] + radiusX + mathCos(angle) * connectorOffset, // second break, right outside pie
positions[1] + radiusY + mathSin(angle) * connectorOffset, // a/a
positions[0] + radiusX, // landing point for connector
positions[1] + radiusY, // a/a
labelDistance < 0 ? // alignment
'center' :
point.half ? 'right' : 'left', // alignment
angle // center angle
];
}
},
setTooltipPoints: noop,
drawGraph: null,
/**
* Draw the data points
*/
drawPoints: function () {
var series = this,
chart = series.chart,
renderer = chart.renderer,
groupTranslation,
//center,
graphic,
//group,
shadow = series.options.shadow,
shadowGroup,
shapeArgs;
if (shadow && !series.shadowGroup) {
series.shadowGroup = renderer.g('shadow')
.add(series.group);
}
// draw the slices
each(series.points, function (point) {
graphic = point.graphic;
shapeArgs = point.shapeArgs;
shadowGroup = point.shadowGroup;
// put the shadow behind all points
if (shadow && !shadowGroup) {
shadowGroup = point.shadowGroup = renderer.g('shadow')
.add(series.shadowGroup);
}
// if the point is sliced, use special translation, else use plot area traslation
groupTranslation = point.sliced ? point.slicedTranslation : {
translateX: 0,
translateY: 0
};
//group.translate(groupTranslation[0], groupTranslation[1]);
if (shadowGroup) {
shadowGroup.attr(groupTranslation);
}
// draw the slice
if (graphic) {
graphic.animate(extend(shapeArgs, groupTranslation));
} else {
point.graphic = graphic = renderer.arc(shapeArgs)
.setRadialReference(series.center)
.attr(
point.pointAttr[point.selected ? SELECT_STATE : NORMAL_STATE]
)
.attr({ 'stroke-linejoin': 'round' })
.attr(groupTranslation)
.add(series.group)
.shadow(shadow, shadowGroup);
}
// detect point specific visibility
if (point.visible === false) {
point.setVisible(false);
}
});
},
/**
* Utility for sorting data labels
*/
sortByAngle: function (points, sign) {
points.sort(function (a, b) {
return a.angle !== undefined && (b.angle - a.angle) * sign;
});
},
/**
* Override the base drawDataLabels method by pie specific functionality
*/
drawDataLabels: function () {
var series = this,
data = series.data,
point,
chart = series.chart,
options = series.options.dataLabels,
connectorPadding = pick(options.connectorPadding, 10),
connectorWidth = pick(options.connectorWidth, 1),
plotWidth = chart.plotWidth,
plotHeight = chart.plotHeight,
connector,
connectorPath,
softConnector = pick(options.softConnector, true),
distanceOption = options.distance,
seriesCenter = series.center,
radius = seriesCenter[2] / 2,
centerY = seriesCenter[1],
outside = distanceOption > 0,
dataLabel,
dataLabelWidth,
labelPos,
labelHeight,
halves = [// divide the points into right and left halves for anti collision
[], // right
[] // left
],
x,
y,
visibility,
rankArr,
i,
j,
overflow = [0, 0, 0, 0], // top, right, bottom, left
sort = function (a, b) {
return b.y - a.y;
};
// get out if not enabled
if (!series.visible || (!options.enabled && !series._hasPointLabels)) {
return;
}
// run parent method
Series.prototype.drawDataLabels.apply(series);
// arrange points for detection collision
each(data, function (point) {
if (point.dataLabel) { // it may have been cancelled in the base method (#407)
halves[point.half].push(point);
}
});
// assume equal label heights
i = 0;
while (!labelHeight && data[i]) { // #1569
labelHeight = data[i] && data[i].dataLabel && (data[i].dataLabel.getBBox().height || 21); // 21 is for #968
i++;
}
/* Loop over the points in each half, starting from the top and bottom
* of the pie to detect overlapping labels.
*/
i = 2;
while (i--) {
var slots = [],
slotsLength,
usedSlots = [],
points = halves[i],
pos,
length = points.length,
slotIndex;
// Sort by angle
series.sortByAngle(points, i - 0.5);
// Only do anti-collision when we are outside the pie and have connectors (#856)
if (distanceOption > 0) {
// build the slots
for (pos = centerY - radius - distanceOption; pos <= centerY + radius + distanceOption; pos += labelHeight) {
slots.push(pos);
// visualize the slot
/*
var slotX = series.getX(pos, i) + chart.plotLeft - (i ? 100 : 0),
slotY = pos + chart.plotTop;
if (!isNaN(slotX)) {
chart.renderer.rect(slotX, slotY - 7, 100, labelHeight, 1)
.attr({
'stroke-width': 1,
stroke: 'silver'
})
.add();
chart.renderer.text('Slot '+ (slots.length - 1), slotX, slotY + 4)
.attr({
fill: 'silver'
}).add();
}
*/
}
slotsLength = slots.length;
// if there are more values than available slots, remove lowest values
if (length > slotsLength) {
// create an array for sorting and ranking the points within each quarter
rankArr = [].concat(points);
rankArr.sort(sort);
j = length;
while (j--) {
rankArr[j].rank = j;
}
j = length;
while (j--) {
if (points[j].rank >= slotsLength) {
points.splice(j, 1);
}
}
length = points.length;
}
// The label goes to the nearest open slot, but not closer to the edge than
// the label's index.
for (j = 0; j < length; j++) {
point = points[j];
labelPos = point.labelPos;
var closest = 9999,
distance,
slotI;
// find the closest slot index
for (slotI = 0; slotI < slotsLength; slotI++) {
distance = mathAbs(slots[slotI] - labelPos[1]);
if (distance < closest) {
closest = distance;
slotIndex = slotI;
}
}
// if that slot index is closer to the edges of the slots, move it
// to the closest appropriate slot
if (slotIndex < j && slots[j] !== null) { // cluster at the top
slotIndex = j;
} else if (slotsLength < length - j + slotIndex && slots[j] !== null) { // cluster at the bottom
slotIndex = slotsLength - length + j;
while (slots[slotIndex] === null) { // make sure it is not taken
slotIndex++;
}
} else {
// Slot is taken, find next free slot below. In the next run, the next slice will find the
// slot above these, because it is the closest one
while (slots[slotIndex] === null) { // make sure it is not taken
slotIndex++;
}
}
usedSlots.push({ i: slotIndex, y: slots[slotIndex] });
slots[slotIndex] = null; // mark as taken
}
// sort them in order to fill in from the top
usedSlots.sort(sort);
}
// now the used slots are sorted, fill them up sequentially
for (j = 0; j < length; j++) {
var slot, naturalY;
point = points[j];
labelPos = point.labelPos;
dataLabel = point.dataLabel;
visibility = point.visible === false ? HIDDEN : VISIBLE;
naturalY = labelPos[1];
if (distanceOption > 0) {
slot = usedSlots.pop();
slotIndex = slot.i;
// if the slot next to currrent slot is free, the y value is allowed
// to fall back to the natural position
y = slot.y;
if ((naturalY > y && slots[slotIndex + 1] !== null) ||
(naturalY < y && slots[slotIndex - 1] !== null)) {
y = naturalY;
}
} else {
y = naturalY;
}
// get the x - use the natural x position for first and last slot, to prevent the top
// and botton slice connectors from touching each other on either side
x = options.justify ?
seriesCenter[0] + (i ? -1 : 1) * (radius + distanceOption) :
series.getX(slotIndex === 0 || slotIndex === slots.length - 1 ? naturalY : y, i);
// Record the placement and visibility
dataLabel._attr = {
visibility: visibility,
align: labelPos[6]
};
dataLabel._pos = {
x: x + options.x +
({ left: connectorPadding, right: -connectorPadding }[labelPos[6]] || 0),
y: y + options.y - 10 // 10 is for the baseline (label vs text)
};
dataLabel.connX = x;
dataLabel.connY = y;
// Detect overflowing data labels
if (this.options.size === null) {
dataLabelWidth = dataLabel.width;
// Overflow left
if (x - dataLabelWidth < connectorPadding) {
overflow[3] = mathMax(mathRound(dataLabelWidth - x + connectorPadding), overflow[3]);
// Overflow right
} else if (x + dataLabelWidth > plotWidth - connectorPadding) {
overflow[1] = mathMax(mathRound(x + dataLabelWidth - plotWidth + connectorPadding), overflow[1]);
}
// Overflow top
if (y - labelHeight / 2 < 0) {
overflow[0] = mathMax(mathRound(-y + labelHeight / 2), overflow[0]);
// Overflow left
} else if (y + labelHeight / 2 > plotHeight) {
overflow[2] = mathMax(mathRound(y + labelHeight / 2 - plotHeight), overflow[2]);
}
}
} // for each point
} // for each half
// Do not apply the final placement and draw the connectors until we have verified
// that labels are not spilling over.
if (arrayMax(overflow) === 0 || this.verifyDataLabelOverflow(overflow)) {
// Place the labels in the final position
this.placeDataLabels();
// Draw the connectors
if (outside && connectorWidth) {
each(this.points, function (point) {
connector = point.connector;
labelPos = point.labelPos;
dataLabel = point.dataLabel;
if (dataLabel && dataLabel._pos) {
visibility = dataLabel._attr.visibility;
x = dataLabel.connX;
y = dataLabel.connY;
connectorPath = softConnector ? [
M,
x + (labelPos[6] === 'left' ? 5 : -5), y, // end of the string at the label
'C',
x, y, // first break, next to the label
2 * labelPos[2] - labelPos[4], 2 * labelPos[3] - labelPos[5],
labelPos[2], labelPos[3], // second break
L,
labelPos[4], labelPos[5] // base
] : [
M,
x + (labelPos[6] === 'left' ? 5 : -5), y, // end of the string at the label
L,
labelPos[2], labelPos[3], // second break
L,
labelPos[4], labelPos[5] // base
];
if (connector) {
connector.animate({ d: connectorPath });
connector.attr('visibility', visibility);
} else {
point.connector = connector = series.chart.renderer.path(connectorPath).attr({
'stroke-width': connectorWidth,
stroke: options.connectorColor || point.color || '#606060',
visibility: visibility
})
.add(series.group);
}
} else if (connector) {
point.connector = connector.destroy();
}
});
}
}
},
/**
* Verify whether the data labels are allowed to draw, or we should run more translation and data
* label positioning to keep them inside the plot area. Returns true when data labels are ready
* to draw.
*/
verifyDataLabelOverflow: function (overflow) {
var center = this.center,
options = this.options,
centerOption = options.center,
minSize = options.minSize || 80,
newSize = minSize,
ret;
// Handle horizontal size and center
if (centerOption[0] !== null) { // Fixed center
newSize = mathMax(center[2] - mathMax(overflow[1], overflow[3]), minSize);
} else { // Auto center
newSize = mathMax(
center[2] - overflow[1] - overflow[3], // horizontal overflow
minSize
);
center[0] += (overflow[3] - overflow[1]) / 2; // horizontal center
}
// Handle vertical size and center
if (centerOption[1] !== null) { // Fixed center
newSize = mathMax(mathMin(newSize, center[2] - mathMax(overflow[0], overflow[2])), minSize);
} else { // Auto center
newSize = mathMax(
mathMin(
newSize,
center[2] - overflow[0] - overflow[2] // vertical overflow
),
minSize
);
center[1] += (overflow[0] - overflow[2]) / 2; // vertical center
}
// If the size must be decreased, we need to run translate and drawDataLabels again
if (newSize < center[2]) {
center[2] = newSize;
this.translate(center);
each(this.points, function (point) {
if (point.dataLabel) {
point.dataLabel._pos = null; // reset
}
});
this.drawDataLabels();
// Else, return true to indicate that the pie and its labels is within the plot area
} else {
ret = true;
}
return ret;
},
/**
* Perform the final placement of the data labels after we have verified that they
* fall within the plot area.
*/
placeDataLabels: function () {
each(this.points, function (point) {
var dataLabel = point.dataLabel,
_pos;
if (dataLabel) {
_pos = dataLabel._pos;
if (_pos) {
dataLabel.attr(dataLabel._attr);
dataLabel[dataLabel.moved ? 'animate' : 'attr'](_pos);
dataLabel.moved = true;
} else if (dataLabel) {
dataLabel.attr({ y: -999 });
}
}
});
},
alignDataLabel: noop,
/**
* Draw point specific tracker objects. Inherit directly from column series.
*/
drawTracker: ColumnSeries.prototype.drawTracker,
/**
* Use a simple symbol from column prototype
*/
drawLegendSymbol: AreaSeries.prototype.drawLegendSymbol,
/**
* Pies don't have point marker symbols
*/
getSymbol: noop
};
PieSeries = extendClass(Series, PieSeries);
seriesTypes.pie = PieSeries;
// global variables
extend(Highcharts, {
// Constructors
Axis: Axis,
Chart: Chart,
Color: Color,
Legend: Legend,
Pointer: Pointer,
Point: Point,
Tick: Tick,
Tooltip: Tooltip,
Renderer: Renderer,
Series: Series,
SVGElement: SVGElement,
SVGRenderer: SVGRenderer,
// Various
arrayMin: arrayMin,
arrayMax: arrayMax,
charts: charts,
dateFormat: dateFormat,
format: format,
pathAnim: pathAnim,
getOptions: getOptions,
hasBidiBug: hasBidiBug,
isTouchDevice: isTouchDevice,
numberFormat: numberFormat,
seriesTypes: seriesTypes,
setOptions: setOptions,
addEvent: addEvent,
removeEvent: removeEvent,
createElement: createElement,
discardElement: discardElement,
css: css,
each: each,
extend: extend,
map: map,
merge: merge,
pick: pick,
splat: splat,
extendClass: extendClass,
pInt: pInt,
wrap: wrap,
svg: hasSVG,
canvas: useCanVG,
vml: !hasSVG && !useCanVG,
product: PRODUCT,
version: VERSION
});
}());
|
packages/material-ui-icons/src/LocationDisabledSharp.js | Kagami/material-ui | import React from 'react';
import createSvgIcon from './utils/createSvgIcon';
export default createSvgIcon(
<React.Fragment><path fill="none" d="M0 0h24v24H0V0z" /><g><path d="M23 13v-2h-2.06c-.46-4.17-3.77-7.48-7.94-7.94V1h-2v2.06c-.98.11-1.91.38-2.77.78l1.53 1.53C10.46 5.13 11.22 5 12 5c3.87 0 7 3.13 7 7 0 .79-.13 1.54-.37 2.24l1.53 1.53c.4-.86.67-1.79.78-2.77H23zM4.41 2.86L3 4.27l2.04 2.04C3.97 7.62 3.26 9.23 3.06 11H1v2h2.06c.46 4.17 3.77 7.48 7.94 7.94V23h2v-2.06c1.77-.2 3.38-.91 4.69-1.98L19.73 21l1.41-1.41L4.41 2.86zM12 19c-3.87 0-7-3.13-7-7 0-1.61.55-3.09 1.46-4.27l9.81 9.81C15.09 18.45 13.61 19 12 19z" /></g></React.Fragment>
, 'LocationDisabledSharp');
|
ajax/libs/inferno/1.0.0-beta24/inferno-compat.js | wout/cdnjs | /*!
* inferno-compat v1.0.0-beta24
* (c) 2016 Dominic Gannaway
* Released under the MIT License.
*/
(function (global, factory) {
typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports, require('./inferno-component')) :
typeof define === 'function' && define.amd ? define(['exports', 'inferno-component'], factory) :
(factory((global.Inferno = global.Inferno || {}),global.Inferno.Component));
}(this, (function (exports,Component) { 'use strict';
Component = 'default' in Component ? Component['default'] : Component;
var commonjsGlobal = typeof window !== 'undefined' ? window : typeof global !== 'undefined' ? global : typeof self !== 'undefined' ? self : {};
function createCommonjsModule(fn, module) {
return module = { exports: {} }, fn(module, module.exports), module.exports;
}
var index$1 = createCommonjsModule(function (module, exports) {
(function (global, factory) {
if (typeof define === 'function' && define.amd) {
define('PropTypes', ['exports', 'module'], factory);
} else if (typeof exports !== 'undefined' && typeof module !== 'undefined') {
factory(exports, module);
} else {
var mod = {
exports: {}
};
factory(mod.exports, mod);
global.PropTypes = mod.exports;
}
})(commonjsGlobal, function (exports, module) {
'use strict';
var REACT_ELEMENT_TYPE = typeof Symbol === 'function' && Symbol['for'] && Symbol['for']('react.element') || 0xeac7;
var ReactElement = {};
ReactElement.isValidElement = function (object) {
return typeof object === 'object' && object !== null && object.$$typeof === REACT_ELEMENT_TYPE;
};
var ReactPropTypeLocationNames = {
prop: 'prop',
context: 'context',
childContext: 'child context'
};
var emptyFunction = {
thatReturns: function thatReturns(what) {
return function () {
return what;
};
}
};
var ITERATOR_SYMBOL = typeof Symbol === 'function' && Symbol.iterator;
var FAUX_ITERATOR_SYMBOL = '@@iterator';
function getIteratorFn(maybeIterable) {
var iteratorFn = maybeIterable && (ITERATOR_SYMBOL && maybeIterable[ITERATOR_SYMBOL] || maybeIterable[FAUX_ITERATOR_SYMBOL]);
if (typeof iteratorFn === 'function') {
return iteratorFn;
}
}
var ANONYMOUS = '<<anonymous>>';
var ReactPropTypes = {
array: createPrimitiveTypeChecker('array'),
bool: createPrimitiveTypeChecker('boolean'),
func: createPrimitiveTypeChecker('function'),
number: createPrimitiveTypeChecker('number'),
object: createPrimitiveTypeChecker('object'),
string: createPrimitiveTypeChecker('string'),
any: createAnyTypeChecker(),
arrayOf: createArrayOfTypeChecker,
element: createElementTypeChecker(),
instanceOf: createInstanceTypeChecker,
node: createNodeChecker(),
objectOf: createObjectOfTypeChecker,
oneOf: createEnumTypeChecker,
oneOfType: createUnionTypeChecker,
shape: createShapeTypeChecker
};
function createChainableTypeChecker(validate) {
function checkType(isRequired, props, propName, componentName, location, propFullName) {
componentName = componentName || ANONYMOUS;
propFullName = propFullName || propName;
if (props[propName] == null) {
var locationName = ReactPropTypeLocationNames[location];
if (isRequired) {
return new Error('Required ' + locationName + ' `' + propFullName + '` was not specified in ' + ('`' + componentName + '`.'));
}
return null;
} else {
return validate(props, propName, componentName, location, propFullName);
}
}
var chainedCheckType = checkType.bind(null, false);
chainedCheckType.isRequired = checkType.bind(null, true);
return chainedCheckType;
}
function createPrimitiveTypeChecker(expectedType) {
function validate(props, propName, componentName, location, propFullName) {
var propValue = props[propName];
var propType = getPropType(propValue);
if (propType !== expectedType) {
var locationName = ReactPropTypeLocationNames[location];
var preciseType = getPreciseType(propValue);
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of type ' + ('`' + preciseType + '` supplied to `' + componentName + '`, expected ') + ('`' + expectedType + '`.'));
}
return null;
}
return createChainableTypeChecker(validate);
}
function createAnyTypeChecker() {
return createChainableTypeChecker(emptyFunction.thatReturns(null));
}
function createArrayOfTypeChecker(typeChecker) {
function validate(props, propName, componentName, location, propFullName) {
var propValue = props[propName];
if (!Array.isArray(propValue)) {
var locationName = ReactPropTypeLocationNames[location];
var propType = getPropType(propValue);
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of type ' + ('`' + propType + '` supplied to `' + componentName + '`, expected an array.'));
}
for (var i = 0; i < propValue.length; i++) {
var error = typeChecker(propValue, i, componentName, location, propFullName + '[' + i + ']');
if (error instanceof Error) {
return error;
}
}
return null;
}
return createChainableTypeChecker(validate);
}
function createElementTypeChecker() {
function validate(props, propName, componentName, location, propFullName) {
if (!ReactElement.isValidElement(props[propName])) {
var locationName = ReactPropTypeLocationNames[location];
return new Error('Invalid ' + locationName + ' `' + propFullName + '` supplied to ' + ('`' + componentName + '`, expected a single ReactElement.'));
}
return null;
}
return createChainableTypeChecker(validate);
}
function createInstanceTypeChecker(expectedClass) {
function validate(props, propName, componentName, location, propFullName) {
if (!(props[propName] instanceof expectedClass)) {
var locationName = ReactPropTypeLocationNames[location];
var expectedClassName = expectedClass.name || ANONYMOUS;
var actualClassName = getClassName(props[propName]);
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of type ' + ('`' + actualClassName + '` supplied to `' + componentName + '`, expected ') + ('instance of `' + expectedClassName + '`.'));
}
return null;
}
return createChainableTypeChecker(validate);
}
function createEnumTypeChecker(expectedValues) {
if (!Array.isArray(expectedValues)) {
return createChainableTypeChecker(function () {
return new Error('Invalid argument supplied to oneOf, expected an instance of array.');
});
}
function validate(props, propName, componentName, location, propFullName) {
var propValue = props[propName];
for (var i = 0; i < expectedValues.length; i++) {
if (propValue === expectedValues[i]) {
return null;
}
}
var locationName = ReactPropTypeLocationNames[location];
var valuesString = JSON.stringify(expectedValues);
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of value `' + propValue + '` ' + ('supplied to `' + componentName + '`, expected one of ' + valuesString + '.'));
}
return createChainableTypeChecker(validate);
}
function createObjectOfTypeChecker(typeChecker) {
function validate(props, propName, componentName, location, propFullName) {
var propValue = props[propName];
var propType = getPropType(propValue);
if (propType !== 'object') {
var locationName = ReactPropTypeLocationNames[location];
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of type ' + ('`' + propType + '` supplied to `' + componentName + '`, expected an object.'));
}
for (var key in propValue) {
if (propValue.hasOwnProperty(key)) {
var error = typeChecker(propValue, key, componentName, location, propFullName + '.' + key);
if (error instanceof Error) {
return error;
}
}
}
return null;
}
return createChainableTypeChecker(validate);
}
function createUnionTypeChecker(arrayOfTypeCheckers) {
if (!Array.isArray(arrayOfTypeCheckers)) {
return createChainableTypeChecker(function () {
return new Error('Invalid argument supplied to oneOfType, expected an instance of array.');
});
}
function validate(props, propName, componentName, location, propFullName) {
for (var i = 0; i < arrayOfTypeCheckers.length; i++) {
var checker = arrayOfTypeCheckers[i];
if (checker(props, propName, componentName, location, propFullName) == null) {
return null;
}
}
var locationName = ReactPropTypeLocationNames[location];
return new Error('Invalid ' + locationName + ' `' + propFullName + '` supplied to ' + ('`' + componentName + '`.'));
}
return createChainableTypeChecker(validate);
}
function createNodeChecker() {
function validate(props, propName, componentName, location, propFullName) {
if (!isNode(props[propName])) {
var locationName = ReactPropTypeLocationNames[location];
return new Error('Invalid ' + locationName + ' `' + propFullName + '` supplied to ' + ('`' + componentName + '`, expected a ReactNode.'));
}
return null;
}
return createChainableTypeChecker(validate);
}
function createShapeTypeChecker(shapeTypes) {
function validate(props, propName, componentName, location, propFullName) {
var propValue = props[propName];
var propType = getPropType(propValue);
if (propType !== 'object') {
var locationName = ReactPropTypeLocationNames[location];
return new Error('Invalid ' + locationName + ' `' + propFullName + '` of type `' + propType + '` ' + ('supplied to `' + componentName + '`, expected `object`.'));
}
for (var key in shapeTypes) {
var checker = shapeTypes[key];
if (!checker) {
continue;
}
var error = checker(propValue, key, componentName, location, propFullName + '.' + key);
if (error) {
return error;
}
}
return null;
}
return createChainableTypeChecker(validate);
}
function isNode(propValue) {
switch (typeof propValue) {
case 'number':
case 'string':
case 'undefined':
return true;
case 'boolean':
return !propValue;
case 'object':
if (Array.isArray(propValue)) {
return propValue.every(isNode);
}
if (propValue === null || ReactElement.isValidElement(propValue)) {
return true;
}
var iteratorFn = getIteratorFn(propValue);
if (iteratorFn) {
var iterator = iteratorFn.call(propValue);
var step;
if (iteratorFn !== propValue.entries) {
while (!(step = iterator.next()).done) {
if (!isNode(step.value)) {
return false;
}
}
} else {
while (!(step = iterator.next()).done) {
var entry = step.value;
if (entry) {
if (!isNode(entry[1])) {
return false;
}
}
}
}
} else {
return false;
}
return true;
default:
return false;
}
}
function getPropType(propValue) {
var propType = typeof propValue;
if (Array.isArray(propValue)) {
return 'array';
}
if (propValue instanceof RegExp) {
return 'object';
}
return propType;
}
function getPreciseType(propValue) {
var propType = getPropType(propValue);
if (propType === 'object') {
if (propValue instanceof Date) {
return 'date';
} else if (propValue instanceof RegExp) {
return 'regexp';
}
}
return propType;
}
function getClassName(propValue) {
if (!propValue.constructor || !propValue.constructor.name) {
return ANONYMOUS;
}
return propValue.constructor.name;
}
module.exports = ReactPropTypes;
});
});
var NO_OP = '$NO_OP';
var ERROR_MSG = 'a runtime error occured! Use Inferno in development environment to find the error.';
var isBrowser = typeof window !== 'undefined' && window.document;
// this is MUCH faster than .constructor === Array and instanceof Array
// in Node 7 and the later versions of V8, slower in older versions though
var isArray = Array.isArray;
function isStatefulComponent(o) {
return !isUndefined(o.prototype) && !isUndefined(o.prototype.render);
}
function isStringOrNumber(obj) {
return isString(obj) || isNumber(obj);
}
function isNullOrUndef(obj) {
return isUndefined(obj) || isNull(obj);
}
function isInvalid(obj) {
return isNull(obj) || obj === false || isTrue(obj) || isUndefined(obj);
}
function isFunction(obj) {
return typeof obj === 'function';
}
function isAttrAnEvent(attr) {
return attr[0] === 'o' && attr[1] === 'n' && attr.length > 3;
}
function isString(obj) {
return typeof obj === 'string';
}
function isNumber(obj) {
return typeof obj === 'number';
}
function isNull(obj) {
return obj === null;
}
function isTrue(obj) {
return obj === true;
}
function isUndefined(obj) {
return obj === undefined;
}
function isObject(o) {
return typeof o === 'object';
}
function throwError(message) {
if (!message) {
message = ERROR_MSG;
}
throw new Error(("Inferno Error: " + message));
}
var EMPTY_OBJ = {};
function isValidElement(obj) {
var isNotANullObject = isObject(obj) && isNull(obj) === false;
if (isNotANullObject === false) {
return false;
}
var flags = obj.flags;
return !!(flags & (28 /* Component */ | 3970 /* Element */));
}
// don't autobind these methods since they already have guaranteed context.
var AUTOBIND_BLACKLIST = {
constructor: 1,
render: 1,
shouldComponentUpdate: 1,
componentWillRecieveProps: 1,
componentWillUpdate: 1,
componentDidUpdate: 1,
componentWillMount: 1,
componentDidMount: 1,
componentWillUnmount: 1,
componentDidUnmount: 1
};
function extend(base, props, all) {
for (var key in props) {
if (all === true || !isNullOrUndef(props[key])) {
base[key] = props[key];
}
}
return base;
}
function bindAll(ctx) {
for (var i in ctx) {
var v = ctx[i];
if (typeof v === 'function' && !v.__bound && !AUTOBIND_BLACKLIST[i]) {
(ctx[i] = v.bind(ctx)).__bound = true;
}
}
}
function collateMixins(mixins) {
var keyed = {};
for (var i = 0; i < mixins.length; i++) {
var mixin = mixins[i];
for (var key in mixin) {
if (mixin.hasOwnProperty(key) && typeof mixin[key] === 'function') {
(keyed[key] || (keyed[key] = [])).push(mixin[key]);
}
}
}
return keyed;
}
function applyMixin(key, inst, mixin) {
var original = inst[key];
inst[key] = function () {
var arguments$1 = arguments;
var ret;
for (var i = 0; i < mixin.length; i++) {
var method = mixin[i];
var _ret = method.apply(inst, arguments$1);
if (!isUndefined(_ret)) {
ret = _ret;
}
}
if (original) {
var _ret$1 = original.call(inst);
if (!isUndefined(_ret$1)) {
ret = _ret$1;
}
}
return ret;
};
}
function applyMixins(inst, mixins) {
for (var key in mixins) {
if (mixins.hasOwnProperty(key)) {
var mixin = mixins[key];
if (isFunction(mixin[0])) {
applyMixin(key, inst, mixin);
}
else {
inst[key] = mixin;
}
}
}
}
function createClass(obj) {
var Cl = (function (Component$$1) {
function Cl(props) {
Component$$1.call(this, props);
this.isMounted = function () { return !this._unmounted; };
extend(this, obj);
if (Cl.mixins) {
applyMixins(this, Cl.mixins);
}
bindAll(this);
if (obj.getInitialState) {
this.state = obj.getInitialState.call(this);
}
}
if ( Component$$1 ) Cl.__proto__ = Component$$1;
Cl.prototype = Object.create( Component$$1 && Component$$1.prototype );
Cl.prototype.constructor = Cl;
return Cl;
}(Component));
Cl.displayName = obj.displayName || 'Component';
Cl.propTypes = obj.propTypes;
Cl.defaultProps = obj.getDefaultProps ? obj.getDefaultProps() : undefined;
Cl.mixins = obj.mixins && collateMixins(obj.mixins);
if (obj.statics) {
extend(Cl, obj.statics);
}
return Cl;
}
function cloneVNode(vNodeToClone, props) {
var _children = [], len = arguments.length - 2;
while ( len-- > 0 ) _children[ len ] = arguments[ len + 2 ];
var children = _children;
if (_children.length > 0 && !isNull(_children[0])) {
if (!props) {
props = {};
}
if (_children.length === 1) {
children = _children[0];
}
if (isUndefined(props.children)) {
props.children = children;
}
else {
if (isArray(children)) {
if (isArray(props.children)) {
props.children = props.children.concat(children);
}
else {
props.children = [props.children].concat(children);
}
}
else {
if (isArray(props.children)) {
props.children.push(children);
}
else {
props.children = [props.children];
props.children.push(children);
}
}
}
}
children = null;
var flags = vNodeToClone.flags;
var newVNode;
if (isArray(vNodeToClone)) {
newVNode = vNodeToClone.map(function (vNode) { return cloneVNode(vNode); });
}
else if (isNullOrUndef(props) && isNullOrUndef(children)) {
newVNode = Object.assign({}, vNodeToClone);
}
else {
var key = !isNullOrUndef(vNodeToClone.key) ? vNodeToClone.key : props.key;
var ref = vNodeToClone.ref || props.ref;
if (flags & 28 /* Component */) {
newVNode = createVNode(flags, vNodeToClone.type, Object.assign({}, vNodeToClone.props, props), null, key, ref, true);
}
else if (flags & 3970 /* Element */) {
children = (props && props.children) || vNodeToClone.children;
newVNode = createVNode(flags, vNodeToClone.type, Object.assign({}, vNodeToClone.props, props), children, key, ref, !children);
}
}
if (flags & 28 /* Component */) {
var newProps = newVNode.props;
if (newProps) {
var newChildren = newProps.children;
// we need to also clone component children that are in props
// as the children may also have been hoisted
if (newChildren) {
if (isArray(newChildren)) {
for (var i = 0; i < newChildren.length; i++) {
var child = newChildren[i];
if (!isInvalid(child) && isVNode(child)) {
newProps.children[i] = cloneVNode(child);
}
}
}
else if (isVNode(newChildren)) {
newProps.children = cloneVNode(newChildren);
}
}
}
newVNode.children = null;
}
newVNode.dom = null;
return newVNode;
}
function _normalizeVNodes(nodes, result, i) {
for (; i < nodes.length; i++) {
var n = nodes[i];
if (!isInvalid(n)) {
if (Array.isArray(n)) {
_normalizeVNodes(n, result, 0);
}
else {
if (isStringOrNumber(n)) {
n = createTextVNode(n);
}
else if (isVNode(n) && n.dom) {
n = cloneVNode(n);
}
result.push(n);
}
}
}
}
function normalizeVNodes(nodes) {
var newNodes;
// we assign $ which basically means we've flagged this array for future note
// if it comes back again, we need to clone it, as people are using it
// in an immutable way
// tslint:disable
if (nodes['$']) {
nodes = nodes.slice();
}
else {
nodes['$'] = true;
}
// tslint:enable
for (var i = 0; i < nodes.length; i++) {
var n = nodes[i];
if (isInvalid(n)) {
if (!newNodes) {
newNodes = nodes.slice(0, i);
}
newNodes.push(n);
}
else if (Array.isArray(n)) {
var result = (newNodes || nodes).slice(0, i);
_normalizeVNodes(nodes, result, i);
return result;
}
else if (isStringOrNumber(n)) {
if (!newNodes) {
newNodes = nodes.slice(0, i);
}
newNodes.push(createTextVNode(n));
}
else if (isVNode(n) && n.dom) {
if (!newNodes) {
newNodes = nodes.slice(0, i);
}
newNodes.push(cloneVNode(n));
}
else if (newNodes) {
newNodes.push(cloneVNode(n));
}
}
return newNodes || nodes;
}
function normalize(vNode) {
var props = vNode.props;
var children = vNode.children;
if (props) {
if (!(vNode.flags & 28 /* Component */) && isNullOrUndef(children) && !isNullOrUndef(props.children)) {
vNode.children = props.children;
}
if (props.ref) {
vNode.ref = props.ref;
}
if (!isNullOrUndef(props.key)) {
vNode.key = props.key;
}
}
if (!isInvalid(children)) {
if (isArray(children)) {
vNode.children = normalizeVNodes(children);
}
else if (isVNode(children) && children.dom) {
vNode.children = cloneVNode(children);
}
}
}
function createVNode(flags, type, props, children, key, ref, noNormalise) {
if (flags & 16 /* ComponentUnknown */) {
flags = isStatefulComponent(type) ? 4 /* ComponentClass */ : 8 /* ComponentFunction */;
}
var vNode = {
children: isUndefined(children) ? null : children,
dom: null,
flags: flags || 0,
key: key === undefined ? null : key,
props: props || null,
ref: ref || null,
type: type
};
if (!noNormalise) {
normalize(vNode);
}
return vNode;
}
// when a components root VNode is also a component, we can run into issues
// this will recursively look for vNode.parentNode if the VNode is a component
function updateParentComponentVNodes(vNode, dom) {
if (vNode.flags & 28 /* Component */) {
var parentVNode = vNode.parentVNode;
if (parentVNode) {
parentVNode.dom = dom;
updateParentComponentVNodes(parentVNode, dom);
}
}
}
function createVoidVNode() {
return createVNode(4096 /* Void */);
}
function createTextVNode(text) {
return createVNode(1 /* Text */, null, null, text);
}
function isVNode(o) {
return !!o.flags;
}
var componentHooks = {
onComponentWillMount: true,
onComponentDidMount: true,
onComponentWillUnmount: true,
onComponentShouldUpdate: true,
onComponentWillUpdate: true,
onComponentDidUpdate: true
};
function createElement$1(name, props) {
var _children = [], len = arguments.length - 2;
while ( len-- > 0 ) _children[ len ] = arguments[ len + 2 ];
if (isInvalid(name) || isObject(name)) {
throw new Error('Inferno Error: createElement() name paramater cannot be undefined, null, false or true, It must be a string, class or function.');
}
var children = _children;
var vNode = createVNode(0);
var ref = null;
var key = null;
var flags = 0;
if (_children) {
if (_children.length === 1) {
children = _children[0];
}
else if (_children.length === 0) {
children = undefined;
}
}
if (isString(name)) {
flags = 2 /* HtmlElement */;
switch (name) {
case 'svg':
flags = 128 /* SvgElement */;
break;
case 'input':
flags = 512 /* InputElement */;
break;
case 'textarea':
flags = 1024 /* TextareaElement */;
break;
case 'select':
flags = 2048 /* SelectElement */;
break;
default:
}
for (var prop in props) {
if (prop === 'key') {
key = props.key;
delete props.key;
}
else if (prop === 'children' && isUndefined(children)) {
children = props.children; // always favour children args, default to props
}
else if (prop === 'ref') {
ref = props.ref;
}
else if (isAttrAnEvent(prop)) {
var lowerCase = prop.toLowerCase();
if (lowerCase !== prop) {
props[prop.toLowerCase()] = props[prop];
delete props[prop];
}
}
}
}
else {
flags = isStatefulComponent(name) ? 4 /* ComponentClass */ : 8 /* ComponentFunction */;
if (!isUndefined(children)) {
if (!props) {
props = {};
}
props.children = children;
}
for (var prop$1 in props) {
if (componentHooks[prop$1]) {
if (!ref) {
ref = {};
}
ref[prop$1] = props[prop$1];
}
else if (prop$1 === 'key') {
key = props.key;
delete props.key;
}
}
vNode.props = props;
}
return createVNode(flags, name, props, children, key, ref);
}
var devToolsStatus = {
connected: false
};
var internalIncrementer = {
id: 0
};
var componentIdMap = new Map();
function getIncrementalId() {
return internalIncrementer.id++;
}
function sendToDevTools(global, data) {
var event = new CustomEvent('inferno.client.message', {
detail: JSON.stringify(data, function (key, val) {
if (!isNull(val) && !isUndefined(val)) {
if (key === '_vComponent' || !isUndefined(val.nodeType)) {
return;
}
else if (isFunction(val)) {
return ("$$f:" + (val.name));
}
}
return val;
})
});
global.dispatchEvent(event);
}
function rerenderRoots() {
for (var i = 0; i < roots.length; i++) {
var root = roots[i];
render(root.input, root.dom);
}
}
function sendRoots(global) {
sendToDevTools(global, { type: 'roots', data: roots });
}
var Lifecycle = function Lifecycle() {
this.listeners = [];
this.fastUnmount = true;
};
Lifecycle.prototype.addListener = function addListener (callback) {
this.listeners.push(callback);
};
Lifecycle.prototype.trigger = function trigger () {
var this$1 = this;
for (var i = 0; i < this.listeners.length; i++) {
this$1.listeners[i]();
}
};
function constructDefaults(string, object, value) {
/* eslint no-return-assign: 0 */
string.split(',').forEach(function (i) { return object[i] = value; });
}
var xlinkNS = 'http://www.w3.org/1999/xlink';
var xmlNS = 'http://www.w3.org/XML/1998/namespace';
var svgNS = 'http://www.w3.org/2000/svg';
var strictProps = {};
var booleanProps = {};
var namespaces = {};
var isUnitlessNumber = {};
constructDefaults('xlink:href,xlink:arcrole,xlink:actuate,xlink:role,xlink:titlef,xlink:type', namespaces, xlinkNS);
constructDefaults('xml:base,xml:lang,xml:space', namespaces, xmlNS);
constructDefaults('volume,defaultValue,defaultChecked', strictProps, true);
constructDefaults('muted,scoped,loop,open,checked,default,capture,disabled,readonly,required,autoplay,controls,seamless,reversed,allowfullscreen,novalidate', booleanProps, true);
constructDefaults('animationIterationCount,borderImageOutset,borderImageSlice,borderImageWidth,boxFlex,boxFlexGroup,boxOrdinalGroup,columnCount,flex,flexGrow,flexPositive,flexShrink,flexNegative,flexOrder,gridRow,gridColumn,fontWeight,lineClamp,lineHeight,opacity,order,orphans,tabSize,widows,zIndex,zoom,fillOpacity,floodOpacity,stopOpacity,strokeDasharray,strokeDashoffset,strokeMiterlimit,strokeOpacity,strokeWidth,', isUnitlessNumber, true);
function isCheckedType(type) {
return type === 'checkbox' || type === 'radio';
}
function isControlled(props) {
var usesChecked = isCheckedType(props.type);
return usesChecked ? !isNullOrUndef(props.checked) : !isNullOrUndef(props.value);
}
function onTextInputChange(e) {
var vNode = this.vNode;
var props = vNode.props;
var dom = vNode.dom;
if (props.onInput) {
props.onInput(e);
}
else if (props.oninput) {
props.oninput(e);
}
// the user may have updated the vNode from the above onInput events
// so we need to get it from the context of `this` again
applyValue(this.vNode, dom);
}
function onCheckboxChange(e) {
var vNode = this.vNode;
var props = vNode.props;
var dom = vNode.dom;
if (props.onClick) {
props.onClick(e);
}
else if (props.onclick) {
props.onclick(e);
}
// the user may have updated the vNode from the above onClick events
// so we need to get it from the context of `this` again
applyValue(this.vNode, dom);
}
function handleAssociatedRadioInputs(name) {
var inputs = document.querySelectorAll(("input[type=\"radio\"][name=\"" + name + "\"]"));
[].forEach.call(inputs, function (dom) {
var inputWrapper = wrappers.get(dom);
if (inputWrapper) {
var props = inputWrapper.vNode.props;
if (props) {
dom.checked = inputWrapper.vNode.props.checked;
}
}
});
}
function processInput(vNode, dom) {
var props = vNode.props || EMPTY_OBJ;
applyValue(vNode, dom);
if (isControlled(props)) {
var inputWrapper = wrappers.get(dom);
if (!inputWrapper) {
inputWrapper = {
vNode: vNode
};
if (isCheckedType(props.type)) {
dom.onclick = onCheckboxChange.bind(inputWrapper);
dom.onclick.wrapped = true;
}
else {
dom.oninput = onTextInputChange.bind(inputWrapper);
dom.oninput.wrapped = true;
}
wrappers.set(dom, inputWrapper);
}
inputWrapper.vNode = vNode;
}
}
function applyValue(vNode, dom) {
var props = vNode.props || EMPTY_OBJ;
var type = props.type;
var value = props.value;
var checked = props.checked;
if (type !== dom.type && type) {
dom.type = type;
}
if (props.multiple !== dom.multiple) {
dom.multiple = props.multiple;
}
if (isCheckedType(type)) {
if (!isNullOrUndef(value)) {
dom.value = value;
}
dom.checked = checked;
if (type === 'radio' && props.name) {
handleAssociatedRadioInputs(props.name);
}
}
else {
if (!isNullOrUndef(value) && dom.value !== value) {
dom.value = value;
}
else if (!isNullOrUndef(checked)) {
dom.checked = checked;
}
}
}
function isControlled$1(props) {
return !isNullOrUndef(props.value);
}
function updateChildOption(vNode, value) {
var props = vNode.props || EMPTY_OBJ;
var dom = vNode.dom;
// we do this as multiple may have changed
dom.value = props.value;
if ((isArray(value) && value.indexOf(props.value) !== -1) || props.value === value) {
dom.selected = true;
}
else {
dom.selected = props.selected || false;
}
}
function onSelectChange(e) {
var vNode = this.vNode;
var props = vNode.props;
var dom = vNode.dom;
if (props.onChange) {
props.onChange(e);
}
else if (props.onchange) {
props.onchange(e);
}
// the user may have updated the vNode from the above onChange events
// so we need to get it from the context of `this` again
applyValue$1(this.vNode, dom);
}
function processSelect(vNode, dom) {
var props = vNode.props || EMPTY_OBJ;
applyValue$1(vNode, dom);
if (isControlled$1(props)) {
var selectWrapper = wrappers.get(dom);
if (!selectWrapper) {
selectWrapper = {
vNode: vNode
};
dom.onchange = onSelectChange.bind(selectWrapper);
dom.onchange.wrapped = true;
wrappers.set(dom, selectWrapper);
}
selectWrapper.vNode = vNode;
}
}
function applyValue$1(vNode, dom) {
var props = vNode.props || EMPTY_OBJ;
if (props.multiple !== dom.multiple) {
dom.multiple = props.multiple;
}
var children = vNode.children;
var value = props.value;
if (isArray(children)) {
for (var i = 0; i < children.length; i++) {
updateChildOption(children[i], value);
}
}
else if (isVNode(children)) {
updateChildOption(children, value);
}
}
// import { isVNode } from '../../core/shapes';
function isControlled$2(props) {
return !isNullOrUndef(props.value);
}
function onTextareaInputChange(e) {
var vNode = this.vNode;
var props = vNode.props;
var dom = vNode.dom;
if (props.onInput) {
props.onInput(e);
}
else if (props.oninput) {
props.oninput(e);
}
// the user may have updated the vNode from the above onInput events
// so we need to get it from the context of `this` again
applyValue$2(this.vNode, dom);
}
function processTextarea(vNode, dom) {
var props = vNode.props || EMPTY_OBJ;
applyValue$2(vNode, dom);
var textareaWrapper = wrappers.get(dom);
if (isControlled$2(props)) {
if (!textareaWrapper) {
textareaWrapper = {
vNode: vNode
};
dom.oninput = onTextareaInputChange.bind(textareaWrapper);
dom.oninput.wrapped = true;
wrappers.set(dom, textareaWrapper);
}
textareaWrapper.vNode = vNode;
}
}
function applyValue$2(vNode, dom) {
var props = vNode.props || EMPTY_OBJ;
var value = props.value;
if (dom.value !== value) {
dom.value = value;
}
}
var wrappers = new Map();
function processElement(flags, vNode, dom) {
if (flags & 512 /* InputElement */) {
processInput(vNode, dom);
}
else if (flags & 2048 /* SelectElement */) {
processSelect(vNode, dom);
}
else if (flags & 1024 /* TextareaElement */) {
processTextarea(vNode, dom);
}
}
function unmount(vNode, parentDom, lifecycle, canRecycle, shallowUnmount, isRecycling) {
var flags = vNode.flags;
if (flags & 28 /* Component */) {
unmountComponent(vNode, parentDom, lifecycle, canRecycle, shallowUnmount, isRecycling);
}
else if (flags & 3970 /* Element */) {
unmountElement(vNode, parentDom, lifecycle, canRecycle, shallowUnmount, isRecycling);
}
else if (flags & 1 /* Text */) {
unmountText(vNode, parentDom);
}
else if (flags & 4096 /* Void */) {
unmountVoid(vNode, parentDom);
}
}
function unmountVoid(vNode, parentDom) {
if (parentDom) {
removeChild(parentDom, vNode.dom);
}
}
function unmountText(vNode, parentDom) {
if (parentDom) {
removeChild(parentDom, vNode.dom);
}
}
function unmountComponent(vNode, parentDom, lifecycle, canRecycle, shallowUnmount, isRecycling) {
var instance = vNode.children;
var flags = vNode.flags;
var isStatefulComponent$$1 = flags & 4;
var ref = vNode.ref;
var dom = vNode.dom;
if (!isRecycling) {
if (!shallowUnmount) {
if (isStatefulComponent$$1) {
var subLifecycle = instance._lifecycle;
if (!subLifecycle.fastUnmount) {
unmount(instance._lastInput, null, lifecycle, false, shallowUnmount, isRecycling);
}
}
else {
if (!lifecycle.fastUnmount) {
unmount(instance, null, lifecycle, false, shallowUnmount, isRecycling);
}
}
}
if (isStatefulComponent$$1) {
instance._ignoreSetState = true;
instance.componentWillUnmount();
if (ref && !isRecycling) {
ref(null);
}
instance._unmounted = true;
componentToDOMNodeMap.delete(instance);
}
else if (!isNullOrUndef(ref)) {
if (!isNullOrUndef(ref.onComponentWillUnmount)) {
ref.onComponentWillUnmount(dom);
}
}
}
if (parentDom) {
var lastInput = instance._lastInput;
if (isNullOrUndef(lastInput)) {
lastInput = instance;
}
removeChild(parentDom, dom);
}
if (recyclingEnabled && (parentDom || canRecycle)) {
poolComponent(vNode);
}
}
function unmountElement(vNode, parentDom, lifecycle, canRecycle, shallowUnmount, isRecycling) {
var dom = vNode.dom;
var ref = vNode.ref;
if (!shallowUnmount && !lifecycle.fastUnmount) {
if (ref && !isRecycling) {
unmountRef(ref);
}
var children = vNode.children;
if (!isNullOrUndef(children)) {
unmountChildren$1(children, lifecycle, shallowUnmount, isRecycling);
}
}
if (parentDom) {
removeChild(parentDom, dom);
}
if (recyclingEnabled && (parentDom || canRecycle)) {
poolElement(vNode);
}
}
function unmountChildren$1(children, lifecycle, shallowUnmount, isRecycling) {
if (isArray(children)) {
for (var i = 0; i < children.length; i++) {
var child = children[i];
if (!isInvalid(child) && isObject(child)) {
unmount(child, null, lifecycle, false, shallowUnmount, isRecycling);
}
}
}
else if (isObject(children)) {
unmount(children, null, lifecycle, false, shallowUnmount, isRecycling);
}
}
function unmountRef(ref) {
if (isFunction(ref)) {
ref(null);
}
else {
if (isInvalid(ref)) {
return;
}
if (process.env.NODE_ENV !== 'production') {
throwError('string "refs" are not supported in Inferno 1.0. Use callback "refs" instead.');
}
throwError();
}
}
function patch(lastVNode, nextVNode, parentDom, lifecycle, context, isSVG, isRecycling) {
if (lastVNode !== nextVNode) {
var lastFlags = lastVNode.flags;
var nextFlags = nextVNode.flags;
if (nextFlags & 28 /* Component */) {
if (lastFlags & 28 /* Component */) {
patchComponent(lastVNode, nextVNode, parentDom, lifecycle, context, isSVG, nextFlags & 4 /* ComponentClass */, isRecycling);
}
else {
replaceVNode(parentDom, mountComponent(nextVNode, null, lifecycle, context, isSVG, nextFlags & 4 /* ComponentClass */), lastVNode, lifecycle, isRecycling);
}
}
else if (nextFlags & 3970 /* Element */) {
if (lastFlags & 3970 /* Element */) {
patchElement(lastVNode, nextVNode, parentDom, lifecycle, context, isSVG, isRecycling);
}
else {
replaceVNode(parentDom, mountElement(nextVNode, null, lifecycle, context, isSVG), lastVNode, lifecycle, isRecycling);
}
}
else if (nextFlags & 1 /* Text */) {
if (lastFlags & 1 /* Text */) {
patchText(lastVNode, nextVNode);
}
else {
replaceVNode(parentDom, mountText(nextVNode, null), lastVNode, lifecycle, isRecycling);
}
}
else if (nextFlags & 4096 /* Void */) {
if (lastFlags & 4096 /* Void */) {
patchVoid(lastVNode, nextVNode);
}
else {
replaceVNode(parentDom, mountVoid(nextVNode, null), lastVNode, lifecycle, isRecycling);
}
}
else {
// Error case: mount new one replacing old one
replaceLastChildAndUnmount(lastVNode, nextVNode, parentDom, lifecycle, context, isSVG, isRecycling);
}
}
}
function unmountChildren(children, dom, lifecycle, isRecycling) {
if (isVNode(children)) {
unmount(children, dom, lifecycle, true, false, isRecycling);
}
else if (isArray(children)) {
removeAllChildren(dom, children, lifecycle, false, isRecycling);
}
else {
dom.textContent = '';
}
}
function patchElement(lastVNode, nextVNode, parentDom, lifecycle, context, isSVG, isRecycling) {
var nextTag = nextVNode.type;
var lastTag = lastVNode.type;
if (lastTag !== nextTag) {
replaceWithNewNode(lastVNode, nextVNode, parentDom, lifecycle, context, isSVG, isRecycling);
}
else {
var dom = lastVNode.dom;
var lastProps = lastVNode.props;
var nextProps = nextVNode.props;
var lastChildren = lastVNode.children;
var nextChildren = nextVNode.children;
var lastFlags = lastVNode.flags;
var nextFlags = nextVNode.flags;
var lastRef = lastVNode.ref;
var nextRef = nextVNode.ref;
nextVNode.dom = dom;
if (isSVG || (nextFlags & 128 /* SvgElement */)) {
isSVG = true;
}
if (lastChildren !== nextChildren) {
patchChildren(lastFlags, nextFlags, lastChildren, nextChildren, dom, lifecycle, context, isSVG, isRecycling);
}
if (!(nextFlags & 2 /* HtmlElement */)) {
processElement(nextFlags, nextVNode, dom);
}
if (lastProps !== nextProps) {
patchProps(lastProps, nextProps, dom, lifecycle, context, isSVG);
}
if (nextRef) {
if (lastRef !== nextRef || isRecycling) {
mountRef(dom, nextRef, lifecycle);
}
}
}
}
function patchChildren(lastFlags, nextFlags, lastChildren, nextChildren, dom, lifecycle, context, isSVG, isRecycling) {
var patchArray = false;
var patchKeyed = false;
if (nextFlags & 64 /* HasNonKeyedChildren */) {
patchArray = true;
}
else if ((lastFlags & 32 /* HasKeyedChildren */) && (nextFlags & 32 /* HasKeyedChildren */)) {
patchKeyed = true;
patchArray = true;
}
else if (isInvalid(nextChildren)) {
unmountChildren(lastChildren, dom, lifecycle, isRecycling);
}
else if (isInvalid(lastChildren)) {
if (isStringOrNumber(nextChildren)) {
setTextContent(dom, nextChildren);
}
else {
if (isArray(nextChildren)) {
mountArrayChildren(nextChildren, dom, lifecycle, context, isSVG);
}
else {
mount(nextChildren, dom, lifecycle, context, isSVG);
}
}
}
else if (isStringOrNumber(nextChildren)) {
if (isStringOrNumber(lastChildren)) {
updateTextContent(dom, nextChildren);
}
else {
unmountChildren(lastChildren, dom, lifecycle, isRecycling);
setTextContent(dom, nextChildren);
}
}
else if (isArray(nextChildren)) {
if (isArray(lastChildren)) {
patchArray = true;
if (isKeyed(lastChildren, nextChildren)) {
patchKeyed = true;
}
}
else {
unmountChildren(lastChildren, dom, lifecycle, isRecycling);
mountArrayChildren(nextChildren, dom, lifecycle, context, isSVG);
}
}
else if (isArray(lastChildren)) {
removeAllChildren(dom, lastChildren, lifecycle, false, isRecycling);
mount(nextChildren, dom, lifecycle, context, isSVG);
}
else if (isVNode(nextChildren)) {
if (isVNode(lastChildren)) {
patch(lastChildren, nextChildren, dom, lifecycle, context, isSVG, isRecycling);
}
else {
unmountChildren(lastChildren, dom, lifecycle, isRecycling);
mount(nextChildren, dom, lifecycle, context, isSVG);
}
}
else if (isVNode(lastChildren)) {
}
else {
}
if (patchArray) {
if (patchKeyed) {
patchKeyedChildren(lastChildren, nextChildren, dom, lifecycle, context, isSVG, isRecycling);
}
else {
patchNonKeyedChildren(lastChildren, nextChildren, dom, lifecycle, context, isSVG, isRecycling);
}
}
}
function patchComponent(lastVNode, nextVNode, parentDom, lifecycle, context, isSVG, isClass, isRecycling) {
var lastType = lastVNode.type;
var nextType = nextVNode.type;
var nextProps = nextVNode.props || EMPTY_OBJ;
var lastKey = lastVNode.key;
var nextKey = nextVNode.key;
if (lastType !== nextType) {
if (isClass) {
replaceWithNewNode(lastVNode, nextVNode, parentDom, lifecycle, context, isSVG, isRecycling);
}
else {
var lastInput = lastVNode.children._lastInput || lastVNode.children;
var nextInput = createStatelessComponentInput(nextVNode, nextType, nextProps, context);
patch(lastInput, nextInput, parentDom, lifecycle, context, isSVG, isRecycling);
var dom = nextVNode.dom = nextInput.dom;
nextVNode.children = nextInput;
mountStatelessComponentCallbacks(nextVNode.ref, dom, lifecycle);
unmount(lastVNode, null, lifecycle, false, true, isRecycling);
}
}
else {
if (isClass) {
if (lastKey !== nextKey) {
replaceWithNewNode(lastVNode, nextVNode, parentDom, lifecycle, context, isSVG, isRecycling);
return false;
}
var instance = lastVNode.children;
if (instance._unmounted) {
if (isNull(parentDom)) {
return true;
}
replaceChild(parentDom, mountComponent(nextVNode, null, lifecycle, context, isSVG, nextVNode.flags & 4 /* ComponentClass */), lastVNode.dom);
}
else {
var defaultProps = nextType.defaultProps;
var lastProps = instance.props;
if (instance._devToolsStatus.connected && !instance._devToolsId) {
componentIdMap.set(instance._devToolsId = getIncrementalId(), instance);
}
lifecycle.fastUnmount = false;
if (!isUndefined(defaultProps)) {
copyPropsTo(lastProps, nextProps);
nextVNode.props = nextProps;
}
var lastState = instance.state;
var nextState = instance.state;
var childContext = instance.getChildContext();
nextVNode.children = instance;
instance._isSVG = isSVG;
if (!isNullOrUndef(childContext)) {
childContext = Object.assign({}, context, childContext);
}
else {
childContext = context;
}
var lastInput$1 = instance._lastInput;
var nextInput$1 = instance._updateComponent(lastState, nextState, lastProps, nextProps, context, false);
var didUpdate = true;
instance._childContext = childContext;
if (isInvalid(nextInput$1)) {
nextInput$1 = createVoidVNode();
}
else if (isArray(nextInput$1)) {
if (process.env.NODE_ENV !== 'production') {
throwError('a valid Inferno VNode (or null) must be returned from a component render. You may have returned an array or an invalid object.');
}
throwError();
}
else if (nextInput$1 === NO_OP) {
nextInput$1 = lastInput$1;
didUpdate = false;
}
else if (isObject(nextInput$1) && nextInput$1.dom) {
nextInput$1 = cloneVNode(nextInput$1);
}
if (nextInput$1.flags & 28 /* Component */) {
nextInput$1.parentVNode = nextVNode;
}
else if (lastInput$1.flags & 28 /* Component */) {
lastInput$1.parentVNode = nextVNode;
}
instance._lastInput = nextInput$1;
instance._vNode = nextVNode;
if (didUpdate) {
patch(lastInput$1, nextInput$1, parentDom, lifecycle, childContext, isSVG, isRecycling);
instance.componentDidUpdate(lastProps, lastState);
componentToDOMNodeMap.set(instance, nextInput$1.dom);
}
nextVNode.dom = nextInput$1.dom;
}
}
else {
var shouldUpdate = true;
var lastProps$1 = lastVNode.props;
var nextHooks = nextVNode.ref;
var nextHooksDefined = !isNullOrUndef(nextHooks);
var lastInput$2 = lastVNode.children;
var nextInput$2 = lastInput$2;
nextVNode.dom = lastVNode.dom;
nextVNode.children = lastInput$2;
if (lastKey !== nextKey) {
shouldUpdate = true;
}
else {
if (nextHooksDefined && !isNullOrUndef(nextHooks.onComponentShouldUpdate)) {
shouldUpdate = nextHooks.onComponentShouldUpdate(lastProps$1, nextProps);
}
}
if (shouldUpdate !== false) {
if (nextHooksDefined && !isNullOrUndef(nextHooks.onComponentWillUpdate)) {
lifecycle.fastUnmount = false;
nextHooks.onComponentWillUpdate(lastProps$1, nextProps);
}
nextInput$2 = nextType(nextProps, context);
if (isInvalid(nextInput$2)) {
nextInput$2 = createVoidVNode();
}
else if (isArray(nextInput$2)) {
if (process.env.NODE_ENV !== 'production') {
throwError('a valid Inferno VNode (or null) must be returned from a component render. You may have returned an array or an invalid object.');
}
throwError();
}
else if (isObject(nextInput$2) && nextInput$2.dom) {
nextInput$2 = cloneVNode(nextInput$2);
}
if (nextInput$2 !== NO_OP) {
patch(lastInput$2, nextInput$2, parentDom, lifecycle, context, isSVG, isRecycling);
nextVNode.children = nextInput$2;
if (nextHooksDefined && !isNullOrUndef(nextHooks.onComponentDidUpdate)) {
lifecycle.fastUnmount = false;
nextHooks.onComponentDidUpdate(lastProps$1, nextProps);
}
nextVNode.dom = nextInput$2.dom;
}
}
if (nextInput$2.flags & 28 /* Component */) {
nextInput$2.parentVNode = nextVNode;
}
else if (lastInput$2.flags & 28 /* Component */) {
lastInput$2.parentVNode = nextVNode;
}
}
}
return false;
}
function patchText(lastVNode, nextVNode) {
var nextText = nextVNode.children;
var dom = lastVNode.dom;
nextVNode.dom = dom;
if (lastVNode.children !== nextText) {
dom.nodeValue = nextText;
}
}
function patchVoid(lastVNode, nextVNode) {
nextVNode.dom = lastVNode.dom;
}
function patchNonKeyedChildren(lastChildren, nextChildren, dom, lifecycle, context, isSVG, isRecycling) {
var lastChildrenLength = lastChildren.length;
var nextChildrenLength = nextChildren.length;
var commonLength = lastChildrenLength > nextChildrenLength ? nextChildrenLength : lastChildrenLength;
var i;
var nextNode = null;
var newNode;
// Loop backwards so we can use insertBefore
if (lastChildrenLength < nextChildrenLength) {
for (i = nextChildrenLength - 1; i >= commonLength; i--) {
var child = nextChildren[i];
if (!isInvalid(child)) {
if (child.dom) {
nextChildren[i] = child = cloneVNode(child);
}
newNode = mount(child, null, lifecycle, context, isSVG);
insertOrAppend(dom, newNode, nextNode);
nextNode = newNode;
}
}
}
else if (nextChildrenLength === 0) {
removeAllChildren(dom, lastChildren, lifecycle, false, isRecycling);
}
else if (lastChildrenLength > nextChildrenLength) {
for (i = commonLength; i < lastChildrenLength; i++) {
var child$1 = lastChildren[i];
if (!isInvalid(child$1)) {
unmount(lastChildren[i], dom, lifecycle, false, false, isRecycling);
}
}
}
for (i = commonLength - 1; i >= 0; i--) {
var lastChild = lastChildren[i];
var nextChild = nextChildren[i];
if (isInvalid(nextChild)) {
if (!isInvalid(lastChild)) {
unmount(lastChild, dom, lifecycle, true, false, isRecycling);
}
}
else {
if (nextChild.dom) {
nextChildren[i] = nextChild = cloneVNode(nextChild);
}
if (isInvalid(lastChild)) {
newNode = mount(nextChild, null, lifecycle, context, isSVG);
insertOrAppend(dom, newNode, nextNode);
nextNode = newNode;
}
else {
patch(lastChild, nextChild, dom, lifecycle, context, isSVG, isRecycling);
nextNode = nextChild.dom;
}
}
}
}
function patchKeyedChildren(a, b, dom, lifecycle, context, isSVG, isRecycling) {
var aLength = a.length;
var bLength = b.length;
var aEnd = aLength - 1;
var bEnd = bLength - 1;
var aStart = 0;
var bStart = 0;
var i;
var j;
var aNode;
var bNode;
var nextNode;
var nextPos;
var node;
if (aLength === 0) {
if (bLength !== 0) {
mountArrayChildren(b, dom, lifecycle, context, isSVG);
}
return;
}
else if (bLength === 0) {
removeAllChildren(dom, a, lifecycle, false, isRecycling);
return;
}
var aStartNode = a[aStart];
var bStartNode = b[bStart];
var aEndNode = a[aEnd];
var bEndNode = b[bEnd];
if (bStartNode.dom) {
b[bStart] = bStartNode = cloneVNode(bStartNode);
}
if (bEndNode.dom) {
b[bEnd] = bEndNode = cloneVNode(bEndNode);
}
// Step 1
/* eslint no-constant-condition: 0 */
outer: while (true) {
// Sync nodes with the same key at the beginning.
while (aStartNode.key === bStartNode.key) {
patch(aStartNode, bStartNode, dom, lifecycle, context, isSVG, isRecycling);
aStart++;
bStart++;
if (aStart > aEnd || bStart > bEnd) {
break outer;
}
aStartNode = a[aStart];
bStartNode = b[bStart];
if (bStartNode.dom) {
b[bStart] = bStartNode = cloneVNode(bStartNode);
}
}
// Sync nodes with the same key at the end.
while (aEndNode.key === bEndNode.key) {
patch(aEndNode, bEndNode, dom, lifecycle, context, isSVG, isRecycling);
aEnd--;
bEnd--;
if (aStart > aEnd || bStart > bEnd) {
break outer;
}
aEndNode = a[aEnd];
bEndNode = b[bEnd];
if (bEndNode.dom) {
b[bEnd] = bEndNode = cloneVNode(bEndNode);
}
}
// Move and sync nodes from right to left.
if (aEndNode.key === bStartNode.key) {
patch(aEndNode, bStartNode, dom, lifecycle, context, isSVG, isRecycling);
insertOrAppend(dom, bStartNode.dom, aStartNode.dom);
aEnd--;
bStart++;
aEndNode = a[aEnd];
bStartNode = b[bStart];
if (bStartNode.dom) {
b[bStart] = bStartNode = cloneVNode(bStartNode);
}
continue;
}
// Move and sync nodes from left to right.
if (aStartNode.key === bEndNode.key) {
patch(aStartNode, bEndNode, dom, lifecycle, context, isSVG, isRecycling);
nextPos = bEnd + 1;
nextNode = nextPos < b.length ? b[nextPos].dom : null;
insertOrAppend(dom, bEndNode.dom, nextNode);
aStart++;
bEnd--;
aStartNode = a[aStart];
bEndNode = b[bEnd];
if (bEndNode.dom) {
b[bEnd] = bEndNode = cloneVNode(bEndNode);
}
continue;
}
break;
}
if (aStart > aEnd) {
if (bStart <= bEnd) {
nextPos = bEnd + 1;
nextNode = nextPos < b.length ? b[nextPos].dom : null;
while (bStart <= bEnd) {
node = b[bStart];
if (node.dom) {
b[bStart] = node = cloneVNode(node);
}
bStart++;
insertOrAppend(dom, mount(node, null, lifecycle, context, isSVG), nextNode);
}
}
}
else if (bStart > bEnd) {
while (aStart <= aEnd) {
unmount(a[aStart++], dom, lifecycle, false, false, isRecycling);
}
}
else {
aLength = aEnd - aStart + 1;
bLength = bEnd - bStart + 1;
var aNullable = a;
var sources = new Array(bLength);
// Mark all nodes as inserted.
for (i = 0; i < bLength; i++) {
sources[i] = -1;
}
var moved = false;
var pos = 0;
var patched = 0;
if ((bLength <= 4) || (aLength * bLength <= 16)) {
for (i = aStart; i <= aEnd; i++) {
aNode = a[i];
if (patched < bLength) {
for (j = bStart; j <= bEnd; j++) {
bNode = b[j];
if (aNode.key === bNode.key) {
sources[j - bStart] = i;
if (pos > j) {
moved = true;
}
else {
pos = j;
}
if (bNode.dom) {
b[j] = bNode = cloneVNode(bNode);
}
patch(aNode, bNode, dom, lifecycle, context, isSVG, isRecycling);
patched++;
aNullable[i] = null;
break;
}
}
}
}
}
else {
var keyIndex = new Map();
for (i = bStart; i <= bEnd; i++) {
node = b[i];
keyIndex.set(node.key, i);
}
for (i = aStart; i <= aEnd; i++) {
aNode = a[i];
if (patched < bLength) {
j = keyIndex.get(aNode.key);
if (!isUndefined(j)) {
bNode = b[j];
sources[j - bStart] = i;
if (pos > j) {
moved = true;
}
else {
pos = j;
}
if (bNode.dom) {
b[j] = bNode = cloneVNode(bNode);
}
patch(aNode, bNode, dom, lifecycle, context, isSVG, isRecycling);
patched++;
aNullable[i] = null;
}
}
}
}
if (aLength === a.length && patched === 0) {
removeAllChildren(dom, a, lifecycle, false, isRecycling);
while (bStart < bLength) {
node = b[bStart];
if (node.dom) {
b[bStart] = node = cloneVNode(node);
}
bStart++;
insertOrAppend(dom, mount(node, null, lifecycle, context, isSVG), null);
}
}
else {
i = aLength - patched;
while (i > 0) {
aNode = aNullable[aStart++];
if (!isNull(aNode)) {
unmount(aNode, dom, lifecycle, false, false, isRecycling);
i--;
}
}
if (moved) {
var seq = lis_algorithm(sources);
j = seq.length - 1;
for (i = bLength - 1; i >= 0; i--) {
if (sources[i] === -1) {
pos = i + bStart;
node = b[pos];
if (node.dom) {
b[pos] = node = cloneVNode(node);
}
nextPos = pos + 1;
nextNode = nextPos < b.length ? b[nextPos].dom : null;
insertOrAppend(dom, mount(node, dom, lifecycle, context, isSVG), nextNode);
}
else {
if (j < 0 || i !== seq[j]) {
pos = i + bStart;
node = b[pos];
nextPos = pos + 1;
nextNode = nextPos < b.length ? b[nextPos].dom : null;
insertOrAppend(dom, node.dom, nextNode);
}
else {
j--;
}
}
}
}
else if (patched !== bLength) {
for (i = bLength - 1; i >= 0; i--) {
if (sources[i] === -1) {
pos = i + bStart;
node = b[pos];
if (node.dom) {
b[pos] = node = cloneVNode(node);
}
nextPos = pos + 1;
nextNode = nextPos < b.length ? b[nextPos].dom : null;
insertOrAppend(dom, mount(node, null, lifecycle, context, isSVG), nextNode);
}
}
}
}
}
}
// // https://en.wikipedia.org/wiki/Longest_increasing_subsequence
function lis_algorithm(a) {
var p = a.slice(0);
var result = [];
result.push(0);
var i;
var j;
var u;
var v;
var c;
for (i = 0; i < a.length; i++) {
if (a[i] === -1) {
continue;
}
j = result[result.length - 1];
if (a[j] < a[i]) {
p[i] = j;
result.push(i);
continue;
}
u = 0;
v = result.length - 1;
while (u < v) {
c = ((u + v) / 2) | 0;
if (a[result[c]] < a[i]) {
u = c + 1;
}
else {
v = c;
}
}
if (a[i] < a[result[u]]) {
if (u > 0) {
p[i] = result[u - 1];
}
result[u] = i;
}
}
u = result.length;
v = result[u - 1];
while (u-- > 0) {
result[u] = v;
v = p[v];
}
return result;
}
// TODO we can shorten this code and put it in constants for smaller size bundles
// these are handled by other parts of Inferno, e.g. input wrappers
var skipProps = {
children: true,
ref: true,
key: true,
selected: true,
checked: true,
value: true,
multiple: true
};
var dehyphenProps = {
textAnchor: 'text-anchor'
};
function patchProp(prop, lastValue, nextValue, dom, isSVG) {
if (skipProps[prop]) {
return;
}
if (booleanProps[prop]) {
dom[prop] = nextValue ? true : false;
}
else if (strictProps[prop]) {
var value = isNullOrUndef(nextValue) ? '' : nextValue;
if (dom[prop] !== value) {
dom[prop] = value;
}
}
else if (lastValue !== nextValue) {
if (isNullOrUndef(nextValue)) {
dom.removeAttribute(prop);
}
else if (prop === 'className') {
if (isSVG) {
dom.setAttribute('class', nextValue);
}
else {
dom.className = nextValue;
}
}
else if (prop === 'style') {
patchStyle(lastValue, nextValue, dom);
}
else if (isAttrAnEvent(prop)) {
var eventName = prop.toLowerCase();
var event = dom[eventName];
if (!event || !event.wrapped) {
dom[eventName] = nextValue;
}
}
else if (prop === 'dangerouslySetInnerHTML') {
var lastHtml = lastValue && lastValue.__html;
var nextHtml = nextValue && nextValue.__html;
if (lastHtml !== nextHtml) {
if (!isNullOrUndef(nextHtml)) {
dom.innerHTML = nextHtml;
}
}
}
else if (prop !== 'childrenType' && prop !== 'ref' && prop !== 'key') {
var dehyphenProp = dehyphenProps[prop];
var ns = namespaces[prop];
if (ns) {
dom.setAttributeNS(ns, dehyphenProp || prop, nextValue);
}
else {
dom.setAttribute(dehyphenProp || prop, nextValue);
}
}
}
}
function patchProps(lastProps, nextProps, dom, lifecycle, context, isSVG) {
lastProps = lastProps || EMPTY_OBJ;
nextProps = nextProps || EMPTY_OBJ;
if (nextProps !== EMPTY_OBJ) {
for (var prop in nextProps) {
// do not add a hasOwnProperty check here, it affects performance
var nextValue = nextProps[prop];
var lastValue = lastProps[prop];
if (isNullOrUndef(nextValue)) {
removeProp(prop, dom);
}
else {
patchProp(prop, lastValue, nextValue, dom, isSVG);
}
}
}
if (lastProps !== EMPTY_OBJ) {
for (var prop$1 in lastProps) {
// do not add a hasOwnProperty check here, it affects performance
if (isNullOrUndef(nextProps[prop$1])) {
removeProp(prop$1, dom);
}
}
}
}
// We are assuming here that we come from patchProp routine
// -nextAttrValue cannot be null or undefined
function patchStyle(lastAttrValue, nextAttrValue, dom) {
if (isString(nextAttrValue)) {
dom.style.cssText = nextAttrValue;
}
else if (isNullOrUndef(lastAttrValue)) {
for (var style in nextAttrValue) {
// do not add a hasOwnProperty check here, it affects performance
var value = nextAttrValue[style];
if (isNumber(value) && !isUnitlessNumber[style]) {
dom.style[style] = value + 'px';
}
else {
dom.style[style] = value;
}
}
}
else {
for (var style$1 in nextAttrValue) {
// do not add a hasOwnProperty check here, it affects performance
var value$1 = nextAttrValue[style$1];
if (isNumber(value$1) && !isUnitlessNumber[style$1]) {
dom.style[style$1] = value$1 + 'px';
}
else {
dom.style[style$1] = value$1;
}
}
for (var style$2 in lastAttrValue) {
if (isNullOrUndef(nextAttrValue[style$2])) {
dom.style[style$2] = '';
}
}
}
}
function removeProp(prop, dom) {
if (prop === 'className') {
dom.removeAttribute('class');
}
else if (prop === 'value') {
dom.value = '';
}
else if (prop === 'style') {
dom.style.cssText = null;
dom.removeAttribute('style');
}
else {
dom.removeAttribute(prop);
}
}
var recyclingEnabled = true;
var componentPools = new Map();
var elementPools = new Map();
function recycleElement(vNode, lifecycle, context, isSVG) {
var tag = vNode.type;
var key = vNode.key;
var pools = elementPools.get(tag);
if (!isUndefined(pools)) {
var pool = key === null ? pools.nonKeyed : pools.keyed.get(key);
if (!isUndefined(pool)) {
var recycledVNode = pool.pop();
if (!isUndefined(recycledVNode)) {
patchElement(recycledVNode, vNode, null, lifecycle, context, isSVG, true);
return vNode.dom;
}
}
}
return null;
}
function poolElement(vNode) {
var tag = vNode.type;
var key = vNode.key;
var pools = elementPools.get(tag);
if (isUndefined(pools)) {
pools = {
nonKeyed: [],
keyed: new Map()
};
elementPools.set(tag, pools);
}
if (isNull(key)) {
pools.nonKeyed.push(vNode);
}
else {
var pool = pools.keyed.get(key);
if (isUndefined(pool)) {
pool = [];
pools.keyed.set(key, pool);
}
pool.push(vNode);
}
}
function recycleComponent(vNode, lifecycle, context, isSVG) {
var type = vNode.type;
var key = vNode.key;
var pools = componentPools.get(type);
if (!isUndefined(pools)) {
var pool = key === null ? pools.nonKeyed : pools.keyed.get(key);
if (!isUndefined(pool)) {
var recycledVNode = pool.pop();
if (!isUndefined(recycledVNode)) {
var flags = vNode.flags;
var failed = patchComponent(recycledVNode, vNode, null, lifecycle, context, isSVG, flags & 4 /* ComponentClass */, true);
if (!failed) {
return vNode.dom;
}
}
}
}
return null;
}
function poolComponent(vNode) {
var type = vNode.type;
var key = vNode.key;
var hooks = vNode.ref;
var nonRecycleHooks = hooks && (hooks.onComponentWillMount ||
hooks.onComponentWillUnmount ||
hooks.onComponentDidMount ||
hooks.onComponentWillUpdate ||
hooks.onComponentDidUpdate);
if (nonRecycleHooks) {
return;
}
var pools = componentPools.get(type);
if (isUndefined(pools)) {
pools = {
nonKeyed: [],
keyed: new Map()
};
componentPools.set(type, pools);
}
if (isNull(key)) {
pools.nonKeyed.push(vNode);
}
else {
var pool = pools.keyed.get(key);
if (isUndefined(pool)) {
pool = [];
pools.keyed.set(key, pool);
}
pool.push(vNode);
}
}
function mount(vNode, parentDom, lifecycle, context, isSVG) {
var flags = vNode.flags;
if (flags & 3970 /* Element */) {
return mountElement(vNode, parentDom, lifecycle, context, isSVG);
}
else if (flags & 28 /* Component */) {
return mountComponent(vNode, parentDom, lifecycle, context, isSVG, flags & 4 /* ComponentClass */);
}
else if (flags & 4096 /* Void */) {
return mountVoid(vNode, parentDom);
}
else if (flags & 1 /* Text */) {
return mountText(vNode, parentDom);
}
else {
if (process.env.NODE_ENV !== 'production') {
throwError(("mount() expects a valid VNode, instead it received an object with the type \"" + (typeof vNode) + "\"."));
}
throwError();
}
}
function mountText(vNode, parentDom) {
var dom = document.createTextNode(vNode.children);
vNode.dom = dom;
if (parentDom) {
appendChild(parentDom, dom);
}
return dom;
}
function mountVoid(vNode, parentDom) {
var dom = document.createTextNode('');
vNode.dom = dom;
if (parentDom) {
appendChild(parentDom, dom);
}
return dom;
}
function mountElement(vNode, parentDom, lifecycle, context, isSVG) {
if (recyclingEnabled) {
var dom$1 = recycleElement(vNode, lifecycle, context, isSVG);
if (!isNull(dom$1)) {
if (!isNull(parentDom)) {
appendChild(parentDom, dom$1);
}
return dom$1;
}
}
var tag = vNode.type;
var flags = vNode.flags;
if (isSVG || (flags & 128 /* SvgElement */)) {
isSVG = true;
}
var dom = documentCreateElement(tag, isSVG);
var children = vNode.children;
var props = vNode.props;
var ref = vNode.ref;
vNode.dom = dom;
if (!isNull(children)) {
if (isStringOrNumber(children)) {
setTextContent(dom, children);
}
else if (isArray(children)) {
mountArrayChildren(children, dom, lifecycle, context, isSVG);
}
else if (isVNode(children)) {
mount(children, dom, lifecycle, context, isSVG);
}
}
if (!(flags & 2 /* HtmlElement */)) {
processElement(flags, vNode, dom);
}
if (!isNull(props)) {
for (var prop in props) {
// do not add a hasOwnProperty check here, it affects performance
patchProp(prop, null, props[prop], dom, isSVG);
}
}
if (!isNull(ref)) {
mountRef(dom, ref, lifecycle);
}
if (!isNull(parentDom)) {
appendChild(parentDom, dom);
}
return dom;
}
function mountArrayChildren(children, dom, lifecycle, context, isSVG) {
for (var i = 0; i < children.length; i++) {
var child = children[i];
if (!isInvalid(child)) {
if (child.dom) {
children[i] = child = cloneVNode(child);
}
mount(children[i], dom, lifecycle, context, isSVG);
}
}
}
function mountComponent(vNode, parentDom, lifecycle, context, isSVG, isClass) {
if (recyclingEnabled) {
var dom$1 = recycleComponent(vNode, lifecycle, context, isSVG);
if (!isNull(dom$1)) {
if (!isNull(parentDom)) {
appendChild(parentDom, dom$1);
}
return dom$1;
}
}
var type = vNode.type;
var props = vNode.props || EMPTY_OBJ;
var ref = vNode.ref;
var dom;
if (isClass) {
var defaultProps = type.defaultProps;
lifecycle.fastUnmount = false;
if (!isUndefined(defaultProps)) {
copyPropsTo(defaultProps, props);
vNode.props = props;
}
var instance = createStatefulComponentInstance(vNode, type, props, context, isSVG, devToolsStatus);
var input = instance._lastInput;
var fastUnmount = lifecycle.fastUnmount;
// we store the fastUnmount value, but we set it back to true on the lifecycle
// we do this so we can determine if the component render has a fastUnmount or not
lifecycle.fastUnmount = true;
instance._vNode = vNode;
vNode.dom = dom = mount(input, null, lifecycle, instance._childContext, isSVG);
// we now create a lifecycle for this component and store the fastUnmount value
var subLifecycle = instance._lifecycle = new Lifecycle();
subLifecycle.fastUnmount = lifecycle.fastUnmount;
// we then set the lifecycle fastUnmount value back to what it was before the mount
lifecycle.fastUnmount = fastUnmount;
if (!isNull(parentDom)) {
appendChild(parentDom, dom);
}
mountStatefulComponentCallbacks(ref, instance, lifecycle);
componentToDOMNodeMap.set(instance, dom);
vNode.children = instance;
}
else {
var input$1 = createStatelessComponentInput(vNode, type, props, context);
vNode.dom = dom = mount(input$1, null, lifecycle, context, isSVG);
vNode.children = input$1;
mountStatelessComponentCallbacks(ref, dom, lifecycle);
if (!isNull(parentDom)) {
appendChild(parentDom, dom);
}
}
return dom;
}
function mountStatefulComponentCallbacks(ref, instance, lifecycle) {
if (ref) {
if (isFunction(ref)) {
ref(instance);
}
else {
if (process.env.NODE_ENV !== 'production') {
throwError('string "refs" are not supported in Inferno 1.0. Use callback "refs" instead.');
}
throwError();
}
}
if (!isNull(instance.componentDidMount)) {
lifecycle.addListener(function () {
instance.componentDidMount();
});
}
}
function mountStatelessComponentCallbacks(ref, dom, lifecycle) {
if (ref) {
if (!isNullOrUndef(ref.onComponentWillMount)) {
lifecycle.fastUnmount = false;
ref.onComponentWillMount();
}
if (!isNullOrUndef(ref.onComponentDidMount)) {
lifecycle.fastUnmount = false;
lifecycle.addListener(function () { return ref.onComponentDidMount(dom); });
}
}
}
function mountRef(dom, value, lifecycle) {
if (isFunction(value)) {
lifecycle.fastUnmount = false;
lifecycle.addListener(function () { return value(dom); });
}
else {
if (isInvalid(value)) {
return;
}
if (process.env.NODE_ENV !== 'production') {
throwError('string "refs" are not supported in Inferno 1.0. Use callback "refs" instead.');
}
throwError();
}
}
function copyPropsTo(copyFrom, copyTo) {
for (var prop in copyFrom) {
if (isUndefined(copyTo[prop])) {
copyTo[prop] = copyFrom[prop];
}
}
}
function createStatefulComponentInstance(vNode, Component$$1, props, context, isSVG, devToolsStatus) {
var instance = new Component$$1(props, context);
instance.context = context;
instance._patch = patch;
instance._devToolsStatus = devToolsStatus;
instance._componentToDOMNodeMap = componentToDOMNodeMap;
var childContext = instance.getChildContext();
if (!isNullOrUndef(childContext)) {
instance._childContext = Object.assign({}, context, childContext);
}
else {
instance._childContext = context;
}
instance._unmounted = false;
instance._pendingSetState = true;
instance._isSVG = isSVG;
instance.componentWillMount();
instance._beforeRender && instance._beforeRender();
var input = instance.render(props, instance.state, context);
instance._afterRender && instance._afterRender();
if (isArray(input)) {
if (process.env.NODE_ENV !== 'production') {
throwError('a valid Inferno VNode (or null) must be returned from a component render. You may have returned an array or an invalid object.');
}
throwError();
}
else if (isInvalid(input)) {
input = createVoidVNode();
}
else {
if (input.dom) {
input = cloneVNode(input);
}
if (input.flags & 28 /* Component */) {
// if we have an input that is also a component, we run into a tricky situation
// where the root vNode needs to always have the correct DOM entry
// so we break monomorphism on our input and supply it our vNode as parentVNode
// we can optimise this in the future, but this gets us out of a lot of issues
input.parentVNode = vNode;
}
}
instance._pendingSetState = false;
instance._lastInput = input;
return instance;
}
function replaceLastChildAndUnmount(lastInput, nextInput, parentDom, lifecycle, context, isSVG, isRecycling) {
replaceVNode(parentDom, mount(nextInput, null, lifecycle, context, isSVG), lastInput, lifecycle, isRecycling);
}
function replaceVNode(parentDom, dom, vNode, lifecycle, isRecycling) {
var shallowUnmount = false;
// we cannot cache nodeType here as vNode might be re-assigned below
if (vNode.flags & 28 /* Component */) {
// if we are accessing a stateful or stateless component, we want to access their last rendered input
// accessing their DOM node is not useful to us here
unmount(vNode, null, lifecycle, false, false, isRecycling);
vNode = vNode.children._lastInput || vNode.children;
shallowUnmount = true;
}
replaceChild(parentDom, dom, vNode.dom);
unmount(vNode, null, lifecycle, false, shallowUnmount, isRecycling);
}
function createStatelessComponentInput(vNode, component, props, context) {
var input = component(props, context);
if (isArray(input)) {
if (process.env.NODE_ENV !== 'production') {
throwError('a valid Inferno VNode (or null) must be returned from a component render. You may have returned an array or an invalid object.');
}
throwError();
}
else if (isInvalid(input)) {
input = createVoidVNode();
}
else {
if (input.dom) {
input = cloneVNode(input);
}
if (input.flags & 28 /* Component */) {
// if we have an input that is also a component, we run into a tricky situation
// where the root vNode needs to always have the correct DOM entry
// so we break monomorphism on our input and supply it our vNode as parentVNode
// we can optimise this in the future, but this gets us out of a lot of issues
input.parentVNode = vNode;
}
}
return input;
}
function setTextContent(dom, text) {
if (text !== '') {
dom.textContent = text;
}
else {
dom.appendChild(document.createTextNode(''));
}
}
function updateTextContent(dom, text) {
dom.firstChild.nodeValue = text;
}
function appendChild(parentDom, dom) {
parentDom.appendChild(dom);
}
function insertOrAppend(parentDom, newNode, nextNode) {
if (isNullOrUndef(nextNode)) {
appendChild(parentDom, newNode);
}
else {
parentDom.insertBefore(newNode, nextNode);
}
}
function documentCreateElement(tag, isSVG) {
if (isSVG === true) {
return document.createElementNS(svgNS, tag);
}
else {
return document.createElement(tag);
}
}
function replaceWithNewNode(lastNode, nextNode, parentDom, lifecycle, context, isSVG, isRecycling) {
var lastInstance = null;
var instanceLastNode = lastNode._lastInput;
if (!isNullOrUndef(instanceLastNode)) {
lastInstance = lastNode;
lastNode = instanceLastNode;
}
unmount(lastNode, null, lifecycle, false, false, isRecycling);
var dom = mount(nextNode, null, lifecycle, context, isSVG);
nextNode.dom = dom;
replaceChild(parentDom, dom, lastNode.dom);
if (lastInstance !== null) {
lastInstance._lasInput = nextNode;
}
}
function replaceChild(parentDom, nextDom, lastDom) {
if (!parentDom) {
parentDom = lastDom.parentNode;
}
parentDom.replaceChild(nextDom, lastDom);
}
function removeChild(parentDom, dom) {
parentDom.removeChild(dom);
}
function removeAllChildren(dom, children, lifecycle, shallowUnmount, isRecycling) {
dom.textContent = '';
if (!lifecycle.fastUnmount) {
removeChildren(null, children, lifecycle, shallowUnmount, isRecycling);
}
}
function removeChildren(dom, children, lifecycle, shallowUnmount, isRecycling) {
for (var i = 0; i < children.length; i++) {
var child = children[i];
if (!isInvalid(child)) {
unmount(child, dom, lifecycle, true, shallowUnmount, isRecycling);
}
}
}
function isKeyed(lastChildren, nextChildren) {
return nextChildren.length && !isNullOrUndef(nextChildren[0]) && !isNullOrUndef(nextChildren[0].key)
&& lastChildren.length && !isNullOrUndef(lastChildren[0]) && !isNullOrUndef(lastChildren[0].key);
}
function normaliseChildNodes(dom) {
var rawChildNodes = dom.childNodes;
var length = rawChildNodes.length;
var i = 0;
while (i < length) {
var rawChild = rawChildNodes[i];
if (rawChild.nodeType === 8) {
if (rawChild.data === '!') {
var placeholder = document.createTextNode('');
dom.replaceChild(placeholder, rawChild);
i++;
}
else {
dom.removeChild(rawChild);
length--;
}
}
else {
i++;
}
}
}
function hydrateComponent(vNode, dom, lifecycle, context, isSVG, isClass) {
var type = vNode.type;
var props = vNode.props;
var ref = vNode.ref;
vNode.dom = dom;
if (isClass) {
var _isSVG = dom.namespaceURI === svgNS;
var defaultProps = type.defaultProps;
lifecycle.fastUnmount = false;
if (!isUndefined(defaultProps)) {
copyPropsTo(defaultProps, props);
vNode.props = props;
}
var instance = createStatefulComponentInstance(vNode, type, props, context, _isSVG, devToolsStatus);
var input = instance._lastInput;
var fastUnmount = lifecycle.fastUnmount;
// we store the fastUnmount value, but we set it back to true on the lifecycle
// we do this so we can determine if the component render has a fastUnmount or not
lifecycle.fastUnmount = true;
instance._vComponent = vNode;
instance._vNode = vNode;
hydrate(input, dom, lifecycle, instance._childContext, _isSVG);
var subLifecycle = instance._lifecycle = new Lifecycle();
subLifecycle.fastUnmount = lifecycle.fastUnmount;
// we then set the lifecycle fastUnmount value back to what it was before the mount
lifecycle.fastUnmount = fastUnmount;
mountStatefulComponentCallbacks(ref, instance, lifecycle);
componentToDOMNodeMap.set(instance, dom);
vNode.children = instance;
}
else {
var input$1 = createStatelessComponentInput(vNode, type, props, context);
hydrate(input$1, dom, lifecycle, context, isSVG);
vNode.children = input$1;
vNode.dom = input$1.dom;
mountStatelessComponentCallbacks(ref, dom, lifecycle);
}
}
function hydrateElement(vNode, dom, lifecycle, context, isSVG) {
var tag = vNode.type;
var children = vNode.children;
var props = vNode.props;
var flags = vNode.flags;
if (isSVG || (flags & 128 /* SvgElement */)) {
isSVG = true;
}
if (dom.nodeType !== 1 || dom.tagName.toLowerCase() !== tag) {
var newDom = mountElement(vNode, null, lifecycle, context, isSVG);
vNode.dom = newDom;
replaceChild(dom.parentNode, newDom, dom);
}
else {
vNode.dom = dom;
if (children) {
hydrateChildren(children, dom, lifecycle, context, isSVG);
}
if (!(flags & 2 /* HtmlElement */)) {
processElement(flags, vNode, dom);
}
for (var prop in props) {
var value = props[prop];
patchProp(prop, null, value, dom, isSVG);
}
}
}
function hydrateChildren(children, dom, lifecycle, context, isSVG) {
normaliseChildNodes(dom);
var domNodes = Array.prototype.slice.call(dom.childNodes);
var childNodeIndex = 0;
if (isArray(children)) {
for (var i = 0; i < children.length; i++) {
var child = children[i];
if (isObject(child) && !isNull(child)) {
hydrate(child, domNodes[childNodeIndex++], lifecycle, context, isSVG);
}
}
}
else if (isObject(children)) {
hydrate(children, dom.firstChild, lifecycle, context, isSVG);
}
}
function hydrateText(vNode, dom) {
if (dom.nodeType === 3) {
var newDom = mountText(vNode, null);
vNode.dom = newDom;
replaceChild(dom.parentNode, newDom, dom);
}
else {
vNode.dom = dom;
}
}
function hydrateVoid(vNode, dom) {
vNode.dom = dom;
}
function hydrate(vNode, dom, lifecycle, context, isSVG) {
if (process.env.NODE_ENV !== 'production') {
if (isInvalid(dom)) {
throwError("failed to hydrate. The server-side render doesn't match client side.");
}
}
var flags = vNode.flags;
if (flags & 28 /* Component */) {
return hydrateComponent(vNode, dom, lifecycle, context, isSVG, flags & 4 /* ComponentClass */);
}
else if (flags & 3970 /* Element */) {
return hydrateElement(vNode, dom, lifecycle, context, isSVG);
}
else if (flags & 1 /* Text */) {
return hydrateText(vNode, dom);
}
else if (flags & 4096 /* Void */) {
return hydrateVoid(vNode, dom);
}
else {
if (process.env.NODE_ENV !== 'production') {
throwError(("hydrate() expects a valid VNode, instead it received an object with the type \"" + (typeof vNode) + "\"."));
}
throwError();
}
}
function hydrateRoot(input, parentDom, lifecycle) {
if (parentDom && parentDom.nodeType === 1 && parentDom.firstChild) {
hydrate(input, parentDom.firstChild, lifecycle, {}, false);
return true;
}
return false;
}
// rather than use a Map, like we did before, we can use an array here
// given there shouldn't be THAT many roots on the page, the difference
// in performance is huge: https://esbench.com/bench/5802a691330ab09900a1a2da
var roots = [];
var componentToDOMNodeMap = new Map();
function findDOMNode(ref) {
var dom = ref && ref.nodeType ? ref : null;
return componentToDOMNodeMap.get(ref) || dom;
}
function getRoot(dom) {
for (var i = 0; i < roots.length; i++) {
var root = roots[i];
if (root.dom === dom) {
return root;
}
}
return null;
}
function setRoot(dom, input, lifecycle) {
roots.push({
dom: dom,
input: input,
lifecycle: lifecycle
});
}
function removeRoot(root) {
for (var i = 0; i < roots.length; i++) {
if (roots[i] === root) {
roots.splice(i, 1);
return;
}
}
}
var documentBody = isBrowser ? document.body : null;
function render(input, parentDom) {
if (documentBody === parentDom) {
if (process.env.NODE_ENV !== 'production') {
throwError('you cannot render() to the "document.body". Use an empty element as a container instead.');
}
throwError();
}
if (input === NO_OP) {
return;
}
var root = getRoot(parentDom);
if (isNull(root)) {
var lifecycle = new Lifecycle();
if (!isInvalid(input)) {
if (input.dom) {
input = cloneVNode(input);
}
if (!hydrateRoot(input, parentDom, lifecycle)) {
mount(input, parentDom, lifecycle, {}, false);
}
lifecycle.trigger();
setRoot(parentDom, input, lifecycle);
}
}
else {
var lifecycle$1 = root.lifecycle;
lifecycle$1.listeners = [];
if (isNullOrUndef(input)) {
unmount(root.input, parentDom, lifecycle$1, false, false, false);
removeRoot(root);
}
else {
if (input.dom) {
input = cloneVNode(input);
}
patch(root.input, input, parentDom, lifecycle$1, {}, false, false);
}
lifecycle$1.trigger();
root.input = input;
}
if (devToolsStatus.connected) {
sendRoots(window);
}
}
function unmountComponentAtNode(container) {
render(null, container);
return true;
}
var ARR = [];
var Children = {
map: function map(children, fn, ctx) {
children = Children.toArray(children);
if (ctx && ctx!==children) { fn = fn.bind(ctx); }
return children.map(fn);
},
forEach: function forEach(children, fn, ctx) {
children = Children.toArray(children);
if (ctx && ctx!==children) { fn = fn.bind(ctx); }
children.forEach(fn);
},
count: function count(children) {
children = Children.toArray(children);
return children.length;
},
only: function only(children) {
children = Children.toArray(children);
if (children.length!==1) { throw new Error('Children.only() expects only one child.'); }
return children[0];
},
toArray: function toArray(children) {
return Array.isArray && Array.isArray(children) ? children : ARR.concat(children);
}
};
var currentComponent = null;
Component.prototype.isReactComponent = {};
Component.prototype._beforeRender = function() {
currentComponent = this;
};
Component.prototype._afterRender = function() {
currentComponent = null;
};
var cloneElement = cloneVNode;
var version = '15.4.1';
function normalizeProps(name, props) {
if ((name === 'input' || name === 'textarea') && props.onChange) {
var eventName = props.type === 'checkbox' ? 'onclick' : 'oninput';
if (!props[eventName]) {
props[eventName] = props.onChange;
delete props.onChange;
}
}
}
// we need to add persist() to Event (as React has it for synthetic events)
// this is a hack and we really shouldn't be modifying a global object this way,
// but there isn't a performant way of doing this apart from trying to proxy
// every prop event that starts with "on", i.e. onClick or onKeyPress
// but in reality devs use onSomething for many things, not only for
// input events
if (Event && !Event.prototype.persist) {
Event.prototype.persist = function () {};
}
var createElement = function (name, _props) {
var children = [], len = arguments.length - 2;
while ( len-- > 0 ) children[ len ] = arguments[ len + 2 ];
var props = _props || {};
var ref = props.ref;
if (typeof ref === 'string') {
props.ref = function (val) {
if (this && this.refs) {
this.refs[ref] = val;
}
}.bind(currentComponent || null);
}
if (typeof name === 'string') {
normalizeProps(name, props);
}
return createElement$1.apply(void 0, [ name, props ].concat( children ));
};
// Credit: preact-compat - https://github.com/developit/preact-compat :)
function shallowDiffers (a, b) {
for (var i in a) { if (!(i in b)) { return true; } }
for (var i$1 in b) { if (a[i$1] !== b[i$1]) { return true; } }
return false;
}
function PureComponent(props, context) {
Component.call(this, props, context);
}
PureComponent.prototype = new Component({});
PureComponent.prototype.shouldComponentUpdate = function (props, state) {
return shallowDiffers(this.props, props) || shallowDiffers(this.state, state);
};
var index = {
createVNode: createVNode,
render: render,
isValidElement: isValidElement,
createElement: createElement,
Component: Component,
PureComponent: PureComponent,
unmountComponentAtNode: unmountComponentAtNode,
cloneElement: cloneElement,
PropTypes: index$1,
createClass: createClass,
findDOMNode: findDOMNode,
Children: Children,
cloneVNode: cloneVNode,
NO_OP: NO_OP,
version: version
};
exports.createVNode = createVNode;
exports.render = render;
exports.isValidElement = isValidElement;
exports.createElement = createElement;
exports.Component = Component;
exports.PureComponent = PureComponent;
exports.unmountComponentAtNode = unmountComponentAtNode;
exports.cloneElement = cloneElement;
exports.PropTypes = index$1;
exports.createClass = createClass;
exports.findDOMNode = findDOMNode;
exports.Children = Children;
exports.cloneVNode = cloneVNode;
exports.NO_OP = NO_OP;
exports.version = version;
exports['default'] = index;
Object.defineProperty(exports, '__esModule', { value: true });
})));
|
test/PageItemSpec.js | egauci/react-bootstrap | import React from 'react';
import ReactTestUtils from 'react/lib/ReactTestUtils';
import ReactDOM from 'react-dom';
import PageItem from '../src/PageItem';
describe('PageItem', () => {
it('Should output a "list item" as root element, and an "anchor" as a child item', () => {
let instance = ReactTestUtils.renderIntoDocument(
<PageItem href="#">Text</PageItem>
);
let node = ReactDOM.findDOMNode(instance);
assert.equal(node.nodeName, 'LI');
assert.equal(node.children.length, 1);
assert.equal(node.children[0].nodeName, 'A');
});
it('Should output "disabled" attribute as a class', () => {
let instance = ReactTestUtils.renderIntoDocument(
<PageItem disabled href="#">Text</PageItem>
);
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(instance, 'disabled'));
});
it('Should output "next" attribute as a class', () => {
let instance = ReactTestUtils.renderIntoDocument(
<PageItem previous href="#">Previous</PageItem>
);
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(instance, 'previous'));
});
it('Should output "previous" attribute as a class', () => {
let instance = ReactTestUtils.renderIntoDocument(
<PageItem next href="#">Next</PageItem>
);
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(instance, 'next'));
});
it('Should call "onSelect" when item is clicked', (done) => {
function handleSelect(key, href) {
assert.equal(key, 1);
assert.equal(href, undefined);
done();
}
let instance = ReactTestUtils.renderIntoDocument(
<PageItem eventKey={1} onSelect={handleSelect}>Next</PageItem>
);
ReactTestUtils.Simulate.click(ReactTestUtils.findRenderedDOMComponentWithTag(instance, 'a'));
});
it('Should not call "onSelect" when item disabled and is clicked', () => {
function handleSelect() {
throw new Error('onSelect should not be called');
}
let instance = ReactTestUtils.renderIntoDocument(
<PageItem disabled onSelect={handleSelect}>Next</PageItem>
);
ReactTestUtils.Simulate.click(ReactTestUtils.findRenderedDOMComponentWithTag(instance, 'a'));
});
it('Should set target attribute on anchor', () => {
let instance = ReactTestUtils.renderIntoDocument(
<PageItem next href="#" target="_blank">Next</PageItem>
);
let anchor = ReactTestUtils.findRenderedDOMComponentWithTag(instance, 'a');
assert.equal(anchor.getAttribute('target'), '_blank');
});
it('Should call "onSelect" with target attribute', (done) => {
function handleSelect(key, href, target) {
assert.equal(target, '_blank');
done();
}
let instance = ReactTestUtils.renderIntoDocument(
<PageItem eventKey={1} onSelect={handleSelect} target="_blank">Next</PageItem>
);
ReactTestUtils.Simulate.click(ReactTestUtils.findRenderedDOMComponentWithTag(instance, 'a'));
});
});
|
ajax/libs/angular-google-maps/1.0.16/angular-google-maps.js | fbender/cdnjs | /*! angular-google-maps 1.0.16 2014-03-26
* AngularJS directives for Google Maps
* git: https://github.com/nlaplante/angular-google-maps.git
*/
(function() {
angular.element(document).ready(function() {
if (!(google || (typeof google !== "undefined" && google !== null ? google.maps : void 0) || (google.maps.InfoWindow != null))) {
return;
}
google.maps.InfoWindow.prototype._open = google.maps.InfoWindow.prototype.open;
google.maps.InfoWindow.prototype._close = google.maps.InfoWindow.prototype.close;
google.maps.InfoWindow.prototype._isOpen = false;
google.maps.InfoWindow.prototype.open = function(map, anchor) {
this._isOpen = true;
this._open(map, anchor);
};
google.maps.InfoWindow.prototype.close = function() {
this._isOpen = false;
this._close();
};
google.maps.InfoWindow.prototype.isOpen = function(val) {
if (val == null) {
val = void 0;
}
if (val == null) {
return this._isOpen;
} else {
return this._isOpen = val;
}
};
/*
Do the same for InfoBox
TODO: Clean this up so the logic is defined once, wait until develop becomes master as this will be easier
*/
if (!window.InfoBox) {
return;
}
window.InfoBox.prototype._open = window.InfoBox.prototype.open;
window.InfoBox.prototype._close = window.InfoBox.prototype.close;
window.InfoBox.prototype._isOpen = false;
window.InfoBox.prototype.open = function(map, anchor) {
this._isOpen = true;
this._open(map, anchor);
};
window.InfoBox.prototype.close = function() {
this._isOpen = false;
this._close();
};
return window.InfoBox.prototype.isOpen = function(val) {
if (val == null) {
val = void 0;
}
if (val == null) {
return this._isOpen;
} else {
return this._isOpen = val;
}
};
});
}).call(this);
/*
Author Nick McCready
Intersection of Objects if the arrays have something in common each intersecting object will be returned
in an new array.
*/
(function() {
_.intersectionObjects = function(array1, array2, comparison) {
var res,
_this = this;
if (comparison == null) {
comparison = void 0;
}
res = _.map(array1, function(obj1) {
return _.find(array2, function(obj2) {
if (comparison != null) {
return comparison(obj1, obj2);
} else {
return _.isEqual(obj1, obj2);
}
});
});
return _.filter(res, function(o) {
return o != null;
});
};
}).call(this);
/*
!
The MIT License
Copyright (c) 2010-2013 Google, Inc. http://angularjs.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
angular-google-maps
https://github.com/nlaplante/angular-google-maps
@authors
Nicolas Laplante - https://plus.google.com/108189012221374960701
Nicholas McCready - https://twitter.com/nmccready
*/
(function() {
(function() {
var app;
app = angular.module("google-maps", []);
return app.factory("debounce", [
"$timeout", function($timeout) {
return function(fn) {
var nthCall;
nthCall = 0;
return function() {
var argz, later, that;
that = this;
argz = arguments;
nthCall++;
later = (function(version) {
return function() {
if (version === nthCall) {
return fn.apply(that, argz);
}
};
})(nthCall);
return $timeout(later, 0, true);
};
};
}
]);
})();
}).call(this);
(function() {
this.ngGmapModule = function(names, fn) {
var space, _name;
if (fn == null) {
fn = function() {};
}
if (typeof names === 'string') {
names = names.split('.');
}
space = this[_name = names.shift()] || (this[_name] = {});
space.ngGmapModule || (space.ngGmapModule = this.ngGmapModule);
if (names.length) {
return space.ngGmapModule(names, fn);
} else {
return fn.call(space);
}
};
}).call(this);
(function() {
angular.module("google-maps").factory("array-sync", [
"add-events", function(mapEvents) {
var LatLngArraySync;
return LatLngArraySync = function(mapArray, scope, pathEval) {
var mapArrayListener, scopeArray, watchListener;
scopeArray = scope.$eval(pathEval);
mapArrayListener = mapEvents(mapArray, {
set_at: function(index) {
var value;
value = mapArray.getAt(index);
if (!value) {
return;
}
if (!value.lng || !value.lat) {
return;
}
scopeArray[index].latitude = value.lat();
return scopeArray[index].longitude = value.lng();
},
insert_at: function(index) {
var value;
value = mapArray.getAt(index);
if (!value) {
return;
}
if (!value.lng || !value.lat) {
return;
}
return scopeArray.splice(index, 0, {
latitude: value.lat(),
longitude: value.lng()
});
},
remove_at: function(index) {
return scopeArray.splice(index, 1);
}
});
watchListener = scope.$watch(pathEval, function(newArray) {
var i, l, newLength, newValue, oldArray, oldLength, oldValue, _results;
oldArray = mapArray;
if (newArray) {
i = 0;
oldLength = oldArray.getLength();
newLength = newArray.length;
l = Math.min(oldLength, newLength);
newValue = void 0;
while (i < l) {
oldValue = oldArray.getAt(i);
newValue = newArray[i];
if ((oldValue.lat() !== newValue.latitude) || (oldValue.lng() !== newValue.longitude)) {
oldArray.setAt(i, new google.maps.LatLng(newValue.latitude, newValue.longitude));
}
i++;
}
while (i < newLength) {
newValue = newArray[i];
oldArray.push(new google.maps.LatLng(newValue.latitude, newValue.longitude));
i++;
}
_results = [];
while (i < oldLength) {
oldArray.pop();
_results.push(i++);
}
return _results;
}
}, true);
return function() {
if (mapArrayListener) {
mapArrayListener();
mapArrayListener = null;
}
if (watchListener) {
watchListener();
return watchListener = null;
}
};
};
}
]);
}).call(this);
(function() {
angular.module("google-maps").factory("add-events", [
"$timeout", function($timeout) {
var addEvent, addEvents;
addEvent = function(target, eventName, handler) {
return google.maps.event.addListener(target, eventName, function() {
handler.apply(this, arguments);
return $timeout((function() {}), true);
});
};
addEvents = function(target, eventName, handler) {
var remove;
if (handler) {
return addEvent(target, eventName, handler);
}
remove = [];
angular.forEach(eventName, function(_handler, key) {
return remove.push(addEvent(target, key, _handler));
});
return function() {
angular.forEach(remove, function(fn) {
if (_.isFunction(fn)) {
fn();
}
if (fn.e !== null && _.isFunction(fn.e)) {
return fn.e();
}
});
return remove = null;
};
};
return addEvents;
}
]);
}).call(this);
(function() {
var __indexOf = [].indexOf || function(item) { for (var i = 0, l = this.length; i < l; i++) { if (i in this && this[i] === item) return i; } return -1; };
this.ngGmapModule("oo", function() {
var baseObjectKeywords;
baseObjectKeywords = ['extended', 'included'];
return this.BaseObject = (function() {
function BaseObject() {}
BaseObject.extend = function(obj) {
var key, value, _ref;
for (key in obj) {
value = obj[key];
if (__indexOf.call(baseObjectKeywords, key) < 0) {
this[key] = value;
}
}
if ((_ref = obj.extended) != null) {
_ref.apply(0);
}
return this;
};
BaseObject.include = function(obj) {
var key, value, _ref;
for (key in obj) {
value = obj[key];
if (__indexOf.call(baseObjectKeywords, key) < 0) {
this.prototype[key] = value;
}
}
if ((_ref = obj.included) != null) {
_ref.apply(0);
}
return this;
};
return BaseObject;
})();
});
}).call(this);
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.managers", function() {
return this.ClustererMarkerManager = (function(_super) {
__extends(ClustererMarkerManager, _super);
function ClustererMarkerManager(gMap, opt_markers, opt_options) {
this.clear = __bind(this.clear, this);
this.draw = __bind(this.draw, this);
this.removeMany = __bind(this.removeMany, this);
this.remove = __bind(this.remove, this);
this.addMany = __bind(this.addMany, this);
this.add = __bind(this.add, this);
var self;
ClustererMarkerManager.__super__.constructor.call(this);
self = this;
this.opt_options = opt_options;
if ((opt_options != null) && opt_markers === void 0) {
this.clusterer = new MarkerClusterer(gMap, void 0, opt_options);
} else if ((opt_options != null) && (opt_markers != null)) {
this.clusterer = new MarkerClusterer(gMap, opt_markers, opt_options);
} else {
this.clusterer = new MarkerClusterer(gMap);
}
this.clusterer.setIgnoreHidden(true);
this.$log = directives.api.utils.Logger;
this.noDrawOnSingleAddRemoves = true;
this.$log.info(this);
}
ClustererMarkerManager.prototype.add = function(gMarker) {
return this.clusterer.addMarker(gMarker, this.noDrawOnSingleAddRemoves);
};
ClustererMarkerManager.prototype.addMany = function(gMarkers) {
return this.clusterer.addMarkers(gMarkers);
};
ClustererMarkerManager.prototype.remove = function(gMarker) {
return this.clusterer.removeMarker(gMarker, this.noDrawOnSingleAddRemoves);
};
ClustererMarkerManager.prototype.removeMany = function(gMarkers) {
return this.clusterer.addMarkers(gMarkers);
};
ClustererMarkerManager.prototype.draw = function() {
return this.clusterer.repaint();
};
ClustererMarkerManager.prototype.clear = function() {
this.clusterer.clearMarkers();
return this.clusterer.repaint();
};
return ClustererMarkerManager;
})(oo.BaseObject);
});
}).call(this);
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.managers", function() {
return this.MarkerManager = (function(_super) {
__extends(MarkerManager, _super);
function MarkerManager(gMap, opt_markers, opt_options) {
this.handleOptDraw = __bind(this.handleOptDraw, this);
this.clear = __bind(this.clear, this);
this.draw = __bind(this.draw, this);
this.removeMany = __bind(this.removeMany, this);
this.remove = __bind(this.remove, this);
this.addMany = __bind(this.addMany, this);
this.add = __bind(this.add, this);
var self;
MarkerManager.__super__.constructor.call(this);
self = this;
this.gMap = gMap;
this.gMarkers = [];
this.$log = directives.api.utils.Logger;
this.$log.info(this);
}
MarkerManager.prototype.add = function(gMarker, optDraw) {
this.handleOptDraw(gMarker, optDraw, true);
return this.gMarkers.push(gMarker);
};
MarkerManager.prototype.addMany = function(gMarkers) {
var gMarker, _i, _len, _results;
_results = [];
for (_i = 0, _len = gMarkers.length; _i < _len; _i++) {
gMarker = gMarkers[_i];
_results.push(this.add(gMarker));
}
return _results;
};
MarkerManager.prototype.remove = function(gMarker, optDraw) {
var index, tempIndex;
this.handleOptDraw(gMarker, optDraw, false);
if (!optDraw) {
return;
}
index = void 0;
if (this.gMarkers.indexOf != null) {
index = this.gMarkers.indexOf(gMarker);
} else {
tempIndex = 0;
_.find(this.gMarkers, function(marker) {
tempIndex += 1;
if (marker === gMarker) {
index = tempIndex;
}
});
}
if (index != null) {
return this.gMarkers.splice(index, 1);
}
};
MarkerManager.prototype.removeMany = function(gMarkers) {
var marker, _i, _len, _ref, _results;
_ref = this.gMarkers;
_results = [];
for (_i = 0, _len = _ref.length; _i < _len; _i++) {
marker = _ref[_i];
_results.push(this.remove(marker));
}
return _results;
};
MarkerManager.prototype.draw = function() {
var deletes, gMarker, _fn, _i, _j, _len, _len1, _ref, _results,
_this = this;
deletes = [];
_ref = this.gMarkers;
_fn = function(gMarker) {
if (!gMarker.isDrawn) {
if (gMarker.doAdd) {
return gMarker.setMap(_this.gMap);
} else {
return deletes.push(gMarker);
}
}
};
for (_i = 0, _len = _ref.length; _i < _len; _i++) {
gMarker = _ref[_i];
_fn(gMarker);
}
_results = [];
for (_j = 0, _len1 = deletes.length; _j < _len1; _j++) {
gMarker = deletes[_j];
_results.push(this.remove(gMarker, true));
}
return _results;
};
MarkerManager.prototype.clear = function() {
var gMarker, _i, _len, _ref;
_ref = this.gMarkers;
for (_i = 0, _len = _ref.length; _i < _len; _i++) {
gMarker = _ref[_i];
gMarker.setMap(null);
}
delete this.gMarkers;
return this.gMarkers = [];
};
MarkerManager.prototype.handleOptDraw = function(gMarker, optDraw, doAdd) {
if (optDraw === true) {
if (doAdd) {
gMarker.setMap(this.gMap);
} else {
gMarker.setMap(null);
}
return gMarker.isDrawn = true;
} else {
gMarker.isDrawn = false;
return gMarker.doAdd = doAdd;
}
};
return MarkerManager;
})(oo.BaseObject);
});
}).call(this);
/*
Author: Nicholas McCready & jfriend00
AsyncProcessor handles things asynchronous-like :), to allow the UI to be free'd to do other things
Code taken from http://stackoverflow.com/questions/10344498/best-way-to-iterate-over-an-array-without-blocking-the-ui
*/
(function() {
this.ngGmapModule("directives.api.utils", function() {
return this.AsyncProcessor = {
handleLargeArray: function(array, callback, pausedCallBack, doneCallBack, chunk, index) {
var doChunk;
if (chunk == null) {
chunk = 100;
}
if (index == null) {
index = 0;
}
if (array === void 0 || array.length <= 0) {
doneCallBack();
return;
}
doChunk = function() {
var cnt, i;
cnt = chunk;
i = index;
while (cnt-- && i < array.length) {
callback(array[i]);
++i;
}
if (i < array.length) {
index = i;
if (pausedCallBack != null) {
pausedCallBack();
}
return setTimeout(doChunk, 1);
} else {
return doneCallBack();
}
};
return doChunk();
}
};
});
}).call(this);
/*
Useful function callbacks that should be defined at later time.
Mainly to be used for specs to verify creation / linking.
This is to lead a common design in notifying child stuff.
*/
(function() {
this.ngGmapModule("directives.api.utils", function() {
return this.ChildEvents = {
onChildCreation: function(child) {}
};
});
}).call(this);
(function() {
this.ngGmapModule("directives.api.utils", function() {
return this.GmapUtil = {
getLabelPositionPoint: function(anchor) {
var xPos, yPos;
if (anchor === void 0) {
return void 0;
}
anchor = /^([\d\.]+)\s([\d\.]+)$/.exec(anchor);
xPos = anchor[1];
yPos = anchor[2];
if (xPos && yPos) {
return new google.maps.Point(xPos, yPos);
}
},
createMarkerOptions: function(coords, icon, defaults, map) {
var opts;
if (map == null) {
map = void 0;
}
if (defaults == null) {
defaults = {};
}
opts = angular.extend({}, defaults, {
position: defaults.position != null ? defaults.position : new google.maps.LatLng(coords.latitude, coords.longitude),
icon: defaults.icon != null ? defaults.icon : icon,
visible: defaults.visible != null ? defaults.visible : (coords.latitude != null) && (coords.longitude != null)
});
if (map != null) {
opts.map = map;
}
return opts;
},
createWindowOptions: function(gMarker, scope, content, defaults) {
if ((content != null) && (defaults != null)) {
return angular.extend({}, defaults, {
content: defaults.content != null ? defaults.content : content,
position: defaults.position != null ? defaults.position : angular.isObject(gMarker) ? gMarker.getPosition() : new google.maps.LatLng(scope.coords.latitude, scope.coords.longitude)
});
} else {
if (!defaults) {
} else {
return defaults;
}
}
},
defaultDelay: 50
};
});
}).call(this);
(function() {
var __hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.utils", function() {
return this.Linked = (function(_super) {
__extends(Linked, _super);
function Linked(scope, element, attrs, ctrls) {
this.scope = scope;
this.element = element;
this.attrs = attrs;
this.ctrls = ctrls;
}
return Linked;
})(oo.BaseObject);
});
}).call(this);
(function() {
this.ngGmapModule("directives.api.utils", function() {
var logger;
this.Logger = {
logger: void 0,
doLog: false,
info: function(msg) {
if (logger.doLog) {
if (logger.logger != null) {
return logger.logger.info(msg);
} else {
return console.info(msg);
}
}
},
error: function(msg) {
if (logger.doLog) {
if (logger.logger != null) {
return logger.logger.error(msg);
} else {
return console.error(msg);
}
}
}
};
return logger = this.Logger;
});
}).call(this);
(function() {
this.ngGmapModule("directives.api.utils", function() {
return this.ModelsWatcher = {
didModelsChange: function(newValue, oldValue) {
var didModelsChange, hasIntersectionDiff;
if (!_.isArray(newValue)) {
directives.api.utils.Logger.error("models property must be an array newValue of: " + (newValue.toString()) + " is not!!");
return false;
}
if (newValue === oldValue) {
return false;
}
hasIntersectionDiff = _.intersectionObjects(newValue, oldValue).length !== oldValue.length;
didModelsChange = true;
if (!hasIntersectionDiff) {
didModelsChange = newValue.length !== oldValue.length;
}
return didModelsChange;
}
};
});
}).call(this);
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.models.child", function() {
return this.MarkerLabelChildModel = (function(_super) {
__extends(MarkerLabelChildModel, _super);
MarkerLabelChildModel.include(directives.api.utils.GmapUtil);
function MarkerLabelChildModel(gMarker, opt_options) {
this.destroy = __bind(this.destroy, this);
this.draw = __bind(this.draw, this);
this.setPosition = __bind(this.setPosition, this);
this.setZIndex = __bind(this.setZIndex, this);
this.setVisible = __bind(this.setVisible, this);
this.setAnchor = __bind(this.setAnchor, this);
this.setMandatoryStyles = __bind(this.setMandatoryStyles, this);
this.setStyles = __bind(this.setStyles, this);
this.setContent = __bind(this.setContent, this);
this.setTitle = __bind(this.setTitle, this);
this.getSharedCross = __bind(this.getSharedCross, this);
var self, _ref, _ref1;
MarkerLabelChildModel.__super__.constructor.call(this);
self = this;
this.marker = gMarker;
this.marker.set("labelContent", opt_options.labelContent);
this.marker.set("labelAnchor", this.getLabelPositionPoint(opt_options.labelAnchor));
this.marker.set("labelClass", opt_options.labelClass || 'labels');
this.marker.set("labelStyle", opt_options.labelStyle || {
opacity: 100
});
this.marker.set("labelInBackground", opt_options.labelInBackground || false);
if (!opt_options.labelVisible) {
this.marker.set("labelVisible", true);
}
if (!opt_options.raiseOnDrag) {
this.marker.set("raiseOnDrag", true);
}
if (!opt_options.clickable) {
this.marker.set("clickable", true);
}
if (!opt_options.draggable) {
this.marker.set("draggable", false);
}
if (!opt_options.optimized) {
this.marker.set("optimized", false);
}
opt_options.crossImage = (_ref = opt_options.crossImage) != null ? _ref : document.location.protocol + "//maps.gstatic.com/intl/en_us/mapfiles/drag_cross_67_16.png";
opt_options.handCursor = (_ref1 = opt_options.handCursor) != null ? _ref1 : document.location.protocol + "//maps.gstatic.com/intl/en_us/mapfiles/closedhand_8_8.cur";
this.markerLabel = new MarkerLabel_(this.marker, opt_options.crossImage, opt_options.handCursor);
this.marker.set("setMap", function(theMap) {
google.maps.Marker.prototype.setMap.apply(this, arguments);
return self.markerLabel.setMap(theMap);
});
this.marker.setMap(this.marker.getMap());
}
MarkerLabelChildModel.prototype.getSharedCross = function(crossUrl) {
return this.markerLabel.getSharedCross(crossUrl);
};
MarkerLabelChildModel.prototype.setTitle = function() {
return this.markerLabel.setTitle();
};
MarkerLabelChildModel.prototype.setContent = function() {
return this.markerLabel.setContent();
};
MarkerLabelChildModel.prototype.setStyles = function() {
return this.markerLabel.setStyles();
};
MarkerLabelChildModel.prototype.setMandatoryStyles = function() {
return this.markerLabel.setMandatoryStyles();
};
MarkerLabelChildModel.prototype.setAnchor = function() {
return this.markerLabel.setAnchor();
};
MarkerLabelChildModel.prototype.setVisible = function() {
return this.markerLabel.setVisible();
};
MarkerLabelChildModel.prototype.setZIndex = function() {
return this.markerLabel.setZIndex();
};
MarkerLabelChildModel.prototype.setPosition = function() {
return this.markerLabel.setPosition();
};
MarkerLabelChildModel.prototype.draw = function() {
return this.markerLabel.draw();
};
MarkerLabelChildModel.prototype.destroy = function() {
if ((this.markerLabel.labelDiv_.parentNode != null) && (this.markerLabel.eventDiv_.parentNode != null)) {
return this.markerLabel.onRemove();
}
};
return MarkerLabelChildModel;
})(oo.BaseObject);
});
}).call(this);
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.models.child", function() {
return this.MarkerChildModel = (function(_super) {
__extends(MarkerChildModel, _super);
MarkerChildModel.include(directives.api.utils.GmapUtil);
function MarkerChildModel(index, model, parentScope, gMap, $timeout, defaults, doClick, gMarkerManager) {
var self,
_this = this;
this.index = index;
this.model = model;
this.parentScope = parentScope;
this.gMap = gMap;
this.defaults = defaults;
this.doClick = doClick;
this.gMarkerManager = gMarkerManager;
this.watchDestroy = __bind(this.watchDestroy, this);
this.setLabelOptions = __bind(this.setLabelOptions, this);
this.isLabelDefined = __bind(this.isLabelDefined, this);
this.setOptions = __bind(this.setOptions, this);
this.setIcon = __bind(this.setIcon, this);
this.setCoords = __bind(this.setCoords, this);
this.destroy = __bind(this.destroy, this);
this.maybeSetScopeValue = __bind(this.maybeSetScopeValue, this);
this.createMarker = __bind(this.createMarker, this);
this.setMyScope = __bind(this.setMyScope, this);
self = this;
this.iconKey = this.parentScope.icon;
this.coordsKey = this.parentScope.coords;
this.clickKey = this.parentScope.click();
this.labelContentKey = this.parentScope.labelContent;
this.optionsKey = this.parentScope.options;
this.labelOptionsKey = this.parentScope.labelOptions;
this.myScope = this.parentScope.$new(false);
this.myScope.model = this.model;
this.setMyScope(this.model, void 0, true);
this.createMarker(this.model);
this.myScope.$watch('model', function(newValue, oldValue) {
if (newValue !== oldValue) {
return _this.setMyScope(newValue, oldValue);
}
}, true);
this.$log = directives.api.utils.Logger;
this.$log.info(self);
this.watchDestroy(this.myScope);
}
MarkerChildModel.prototype.setMyScope = function(model, oldModel, isInit) {
if (oldModel == null) {
oldModel = void 0;
}
if (isInit == null) {
isInit = false;
}
this.maybeSetScopeValue('icon', model, oldModel, this.iconKey, this.evalModelHandle, isInit, this.setIcon);
this.maybeSetScopeValue('coords', model, oldModel, this.coordsKey, this.evalModelHandle, isInit, this.setCoords);
this.maybeSetScopeValue('labelContent', model, oldModel, this.labelContentKey, this.evalModelHandle, isInit);
this.maybeSetScopeValue('click', model, oldModel, this.clickKey, this.evalModelHandle, isInit);
return this.createMarker(model, oldModel, isInit);
};
MarkerChildModel.prototype.createMarker = function(model, oldModel, isInit) {
var _this = this;
if (oldModel == null) {
oldModel = void 0;
}
if (isInit == null) {
isInit = false;
}
return this.maybeSetScopeValue('options', model, oldModel, this.optionsKey, function(lModel, lModelKey) {
var value;
if (lModel === void 0) {
return void 0;
}
value = lModelKey === 'self' ? lModel : lModel[lModelKey];
if (value === void 0) {
return value = lModelKey === void 0 ? _this.defaults : _this.myScope.options;
} else {
return value;
}
}, isInit, this.setOptions);
};
MarkerChildModel.prototype.evalModelHandle = function(model, modelKey) {
if (model === void 0) {
return void 0;
}
if (modelKey === 'self') {
return model;
} else {
return model[modelKey];
}
};
MarkerChildModel.prototype.maybeSetScopeValue = function(scopePropName, model, oldModel, modelKey, evaluate, isInit, gSetter) {
var newValue, oldVal;
if (gSetter == null) {
gSetter = void 0;
}
if (oldModel === void 0) {
this.myScope[scopePropName] = evaluate(model, modelKey);
if (!isInit) {
if (gSetter != null) {
gSetter(this.myScope);
}
}
return;
}
oldVal = evaluate(oldModel, modelKey);
newValue = evaluate(model, modelKey);
if (newValue !== oldVal && this.myScope[scopePropName] !== newValue) {
this.myScope[scopePropName] = newValue;
if (!isInit) {
if (gSetter != null) {
gSetter(this.myScope);
}
return this.gMarkerManager.draw();
}
}
};
MarkerChildModel.prototype.destroy = function() {
return this.myScope.$destroy();
};
MarkerChildModel.prototype.setCoords = function(scope) {
if (scope.$id !== this.myScope.$id || this.gMarker === void 0) {
return;
}
if ((scope.coords != null)) {
if ((this.scope.coords.latitude == null) || (this.scope.coords.longitude == null)) {
this.$log.error("MarkerChildMarker cannot render marker as scope.coords as no position on marker: " + (JSON.stringify(this.model)));
return;
}
this.gMarker.setPosition(new google.maps.LatLng(scope.coords.latitude, scope.coords.longitude));
this.gMarker.setVisible((scope.coords.latitude != null) && (scope.coords.longitude != null));
this.gMarkerManager.remove(this.gMarker);
return this.gMarkerManager.add(this.gMarker);
} else {
return this.gMarkerManager.remove(this.gMarker);
}
};
MarkerChildModel.prototype.setIcon = function(scope) {
if (scope.$id !== this.myScope.$id || this.gMarker === void 0) {
return;
}
this.gMarkerManager.remove(this.gMarker);
this.gMarker.setIcon(scope.icon);
this.gMarkerManager.add(this.gMarker);
this.gMarker.setPosition(new google.maps.LatLng(scope.coords.latitude, scope.coords.longitude));
return this.gMarker.setVisible(scope.coords.latitude && (scope.coords.longitude != null));
};
MarkerChildModel.prototype.setOptions = function(scope) {
var _ref,
_this = this;
if (scope.$id !== this.myScope.$id) {
return;
}
if (this.gMarker != null) {
this.gMarkerManager.remove(this.gMarker);
delete this.gMarker;
}
if (!((_ref = scope.coords) != null ? _ref : typeof scope.icon === "function" ? scope.icon(scope.options != null) : void 0)) {
return;
}
this.opts = this.createMarkerOptions(scope.coords, scope.icon, scope.options);
delete this.gMarker;
if (this.isLabelDefined(scope)) {
this.gMarker = new MarkerWithLabel(this.setLabelOptions(this.opts, scope));
} else {
this.gMarker = new google.maps.Marker(this.opts);
}
this.gMarkerManager.add(this.gMarker);
return google.maps.event.addListener(this.gMarker, 'click', function() {
if (_this.doClick && (_this.myScope.click != null)) {
return _this.myScope.click();
}
});
};
MarkerChildModel.prototype.isLabelDefined = function(scope) {
return scope.labelContent != null;
};
MarkerChildModel.prototype.setLabelOptions = function(opts, scope) {
opts.labelAnchor = this.getLabelPositionPoint(scope.labelAnchor);
opts.labelClass = scope.labelClass;
opts.labelContent = scope.labelContent;
return opts;
};
MarkerChildModel.prototype.watchDestroy = function(scope) {
var _this = this;
return scope.$on("$destroy", function() {
var self;
if (_this.gMarker != null) {
_this.gMarkerManager.remove(_this.gMarker);
delete _this.gMarker;
}
return self = void 0;
});
};
return MarkerChildModel;
})(oo.BaseObject);
});
}).call(this);
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.models.child", function() {
return this.WindowChildModel = (function(_super) {
__extends(WindowChildModel, _super);
WindowChildModel.include(directives.api.utils.GmapUtil);
function WindowChildModel(scope, opts, isIconVisibleOnClick, mapCtrl, markerCtrl, $http, $templateCache, $compile, element, needToManualDestroy) {
this.element = element;
if (needToManualDestroy == null) {
needToManualDestroy = false;
}
this.destroy = __bind(this.destroy, this);
this.hideWindow = __bind(this.hideWindow, this);
this.getLatestPosition = __bind(this.getLatestPosition, this);
this.showWindow = __bind(this.showWindow, this);
this.handleClick = __bind(this.handleClick, this);
this.watchCoords = __bind(this.watchCoords, this);
this.watchShow = __bind(this.watchShow, this);
this.createGWin = __bind(this.createGWin, this);
this.scope = scope;
this.googleMapsHandles = [];
this.opts = opts;
this.mapCtrl = mapCtrl;
this.markerCtrl = markerCtrl;
this.isIconVisibleOnClick = isIconVisibleOnClick;
this.initialMarkerVisibility = this.markerCtrl != null ? this.markerCtrl.getVisible() : false;
this.$log = directives.api.utils.Logger;
this.$http = $http;
this.$templateCache = $templateCache;
this.$compile = $compile;
this.createGWin();
if (this.markerCtrl != null) {
this.markerCtrl.setClickable(true);
}
this.handleClick();
this.watchShow();
this.watchCoords();
this.needToManualDestroy = needToManualDestroy;
this.$log.info(this);
}
WindowChildModel.prototype.createGWin = function() {
var defaults, html,
_this = this;
if ((this.gWin == null) && (this.markerCtrl != null)) {
defaults = this.opts != null ? this.opts : {};
html = (this.element != null) && _.isFunction(this.element.html) ? this.element.html() : this.element;
this.opts = this.markerCtrl != null ? this.createWindowOptions(this.markerCtrl, this.scope, html, defaults) : {};
}
if ((this.opts != null) && this.gWin === void 0) {
if (this.opts.boxClass && (window.InfoBox && typeof window.InfoBox === 'function')) {
this.gWin = new window.InfoBox(this.opts);
} else {
this.gWin = new google.maps.InfoWindow(this.opts);
}
return this.googleMapsHandles.push(google.maps.event.addListener(this.gWin, 'closeclick', function() {
if (_this.markerCtrl != null) {
_this.markerCtrl.setVisible(_this.initialMarkerVisibility);
}
_this.gWin.isOpen(false);
if (_this.scope.closeClick != null) {
return _this.scope.closeClick();
}
}));
}
};
WindowChildModel.prototype.watchShow = function() {
var _this = this;
return this.scope.$watch('show', function(newValue, oldValue) {
if (newValue) {
return _this.showWindow();
} else {
return _this.hideWindow();
}
});
};
WindowChildModel.prototype.watchCoords = function() {
var scope,
_this = this;
scope = this.markerCtrl != null ? this.scope.$parent : this.scope;
return scope.$watch('coords', function(newValue, oldValue) {
if (newValue !== oldValue) {
if (newValue == null) {
return _this.hideWindow();
} else {
if ((newValue.latitude == null) || (newValue.longitude == null)) {
_this.$log.error("WindowChildMarker cannot render marker as scope.coords as no position on marker: " + (JSON.stringify(_this.model)));
return;
}
return _this.gWin.setPosition(new google.maps.LatLng(newValue.latitude, newValue.longitude));
}
}
}, true);
};
WindowChildModel.prototype.handleClick = function() {
var _this = this;
if (this.markerCtrl != null) {
return this.googleMapsHandles.push(google.maps.event.addListener(this.markerCtrl, 'click', function() {
var pos;
if (_this.gWin == null) {
_this.createGWin();
}
pos = _this.markerCtrl.getPosition();
if (_this.gWin != null) {
_this.gWin.setPosition(pos);
_this.showWindow();
}
_this.initialMarkerVisibility = _this.markerCtrl.getVisible();
return _this.markerCtrl.setVisible(_this.isIconVisibleOnClick);
}));
}
};
WindowChildModel.prototype.showWindow = function() {
var show,
_this = this;
show = function() {
if (_this.gWin) {
if ((_this.scope.show || (_this.scope.show == null)) && !_this.gWin.isOpen()) {
return _this.gWin.open(_this.mapCtrl);
}
}
};
if (this.scope.templateUrl) {
if (this.gWin) {
return this.$http.get(this.scope.templateUrl, {
cache: this.$templateCache
}).then(function(content) {
var compiled, templateScope;
templateScope = _this.scope.$new();
if (angular.isDefined(_this.scope.templateParameter)) {
templateScope.parameter = _this.scope.templateParameter;
}
compiled = _this.$compile(content.data)(templateScope);
_this.gWin.setContent(compiled[0]);
return show();
});
}
} else {
return show();
}
};
WindowChildModel.prototype.getLatestPosition = function() {
if ((this.gWin != null) && (this.markerCtrl != null)) {
return this.gWin.setPosition(this.markerCtrl.getPosition());
}
};
WindowChildModel.prototype.hideWindow = function() {
if ((this.gWin != null) && this.gWin.isOpen()) {
return this.gWin.close();
}
};
WindowChildModel.prototype.destroy = function() {
var self;
this.hideWindow();
_.each(this.googleMapsHandles, function(h) {
return google.maps.event.removeListener(h);
});
this.googleMapsHandles.length = 0;
if ((this.scope != null) && this.needToManualDestroy) {
this.scope.$destroy();
}
delete this.gWin;
return self = void 0;
};
return WindowChildModel;
})(oo.BaseObject);
});
}).call(this);
/*
- interface for all markers to derrive from
- to enforce a minimum set of requirements
- attributes
- coords
- icon
- implementation needed on watches
*/
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.models.parent", function() {
return this.IMarkerParentModel = (function(_super) {
__extends(IMarkerParentModel, _super);
IMarkerParentModel.prototype.DEFAULTS = {};
IMarkerParentModel.prototype.isFalse = function(value) {
return ['false', 'FALSE', 0, 'n', 'N', 'no', 'NO'].indexOf(value) !== -1;
};
function IMarkerParentModel(scope, element, attrs, mapCtrl, $timeout) {
var self,
_this = this;
this.scope = scope;
this.element = element;
this.attrs = attrs;
this.mapCtrl = mapCtrl;
this.$timeout = $timeout;
this.linkInit = __bind(this.linkInit, this);
this.onDestroy = __bind(this.onDestroy, this);
this.onWatch = __bind(this.onWatch, this);
this.watch = __bind(this.watch, this);
this.validateScope = __bind(this.validateScope, this);
this.onTimeOut = __bind(this.onTimeOut, this);
self = this;
this.$log = directives.api.utils.Logger;
if (!this.validateScope(scope)) {
return;
}
this.doClick = angular.isDefined(attrs.click);
if (scope.options != null) {
this.DEFAULTS = scope.options;
}
this.$timeout(function() {
_this.watch('coords', scope);
_this.watch('icon', scope);
_this.watch('options', scope);
_this.onTimeOut(scope);
return scope.$on("$destroy", function() {
return _this.onDestroy(scope);
});
});
}
IMarkerParentModel.prototype.onTimeOut = function(scope) {};
IMarkerParentModel.prototype.validateScope = function(scope) {
var ret;
if (scope == null) {
return false;
}
ret = scope.coords != null;
if (!ret) {
this.$log.error(this.constructor.name + ": no valid coords attribute found");
}
return ret;
};
IMarkerParentModel.prototype.watch = function(propNameToWatch, scope) {
var _this = this;
return scope.$watch(propNameToWatch, function(newValue, oldValue) {
if (newValue !== oldValue) {
return _this.onWatch(propNameToWatch, scope, newValue, oldValue);
}
}, true);
};
IMarkerParentModel.prototype.onWatch = function(propNameToWatch, scope, newValue, oldValue) {
throw new Exception("Not Implemented!!");
};
IMarkerParentModel.prototype.onDestroy = function(scope) {
throw new Exception("Not Implemented!!");
};
IMarkerParentModel.prototype.linkInit = function(element, mapCtrl, scope, animate) {
throw new Exception("Not Implemented!!");
};
return IMarkerParentModel;
})(oo.BaseObject);
});
}).call(this);
/*
- interface directive for all window(s) to derrive from
*/
(function() {
var __hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.models.parent", function() {
return this.IWindowParentModel = (function(_super) {
__extends(IWindowParentModel, _super);
IWindowParentModel.include(directives.api.utils.GmapUtil);
IWindowParentModel.prototype.DEFAULTS = {};
function IWindowParentModel(scope, element, attrs, ctrls, $timeout, $compile, $http, $templateCache) {
var self;
self = this;
this.$log = directives.api.utils.Logger;
this.$timeout = $timeout;
this.$compile = $compile;
this.$http = $http;
this.$templateCache = $templateCache;
if (scope.options != null) {
this.DEFAULTS = scope.options;
}
}
return IWindowParentModel;
})(oo.BaseObject);
});
}).call(this);
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.models.parent", function() {
return this.LayerParentModel = (function(_super) {
__extends(LayerParentModel, _super);
function LayerParentModel(scope, element, attrs, mapCtrl, $timeout, onLayerCreated, $log) {
var _this = this;
this.scope = scope;
this.element = element;
this.attrs = attrs;
this.mapCtrl = mapCtrl;
this.$timeout = $timeout;
this.onLayerCreated = onLayerCreated != null ? onLayerCreated : void 0;
this.$log = $log != null ? $log : directives.api.utils.Logger;
this.createGoogleLayer = __bind(this.createGoogleLayer, this);
if (this.attrs.type == null) {
this.$log.info("type attribute for the layer directive is mandatory. Layer creation aborted!!");
return;
}
this.createGoogleLayer();
this.gMap = void 0;
this.doShow = true;
this.$timeout(function() {
_this.gMap = mapCtrl.getMap();
if (angular.isDefined(_this.attrs.show)) {
_this.doShow = _this.scope.show;
}
if (_this.doShow !== null && _this.doShow && _this.gMap !== null) {
_this.layer.setMap(_this.gMap);
}
_this.scope.$watch("show", function(newValue, oldValue) {
if (newValue !== oldValue) {
_this.doShow = newValue;
if (newValue) {
return _this.layer.setMap(_this.gMap);
} else {
return _this.layer.setMap(null);
}
}
}, true);
_this.scope.$watch("options", function(newValue, oldValue) {
if (newValue !== oldValue) {
_this.layer.setMap(null);
_this.layer = null;
return _this.createGoogleLayer();
}
}, true);
return _this.scope.$on("$destroy", function() {
return this.layer.setMap(null);
});
});
}
LayerParentModel.prototype.createGoogleLayer = function() {
var _this = this;
if (this.attrs.options == null) {
this.layer = this.attrs.namespace === void 0 ? new google.maps[this.attrs.type]() : new google.maps[this.attrs.namespace][this.attrs.type]();
} else {
this.layer = this.attrs.namespace === void 0 ? new google.maps[this.attrs.type](this.scope.options) : new google.maps[this.attrs.namespace][this.attrs.type](this.scope.options);
}
return this.$timeout(function() {
var fn;
if ((_this.layer != null) && (_this.onLayerCreated != null)) {
fn = _this.onLayerCreated(_this.scope, _this.layer);
if (fn) {
return fn(_this.layer);
}
}
});
};
return LayerParentModel;
})(oo.BaseObject);
});
}).call(this);
/*
Basic Directive api for a marker. Basic in the sense that this directive contains 1:1 on scope and model.
Thus there will be one html element per marker within the directive.
*/
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.models.parent", function() {
return this.MarkerParentModel = (function(_super) {
__extends(MarkerParentModel, _super);
MarkerParentModel.include(directives.api.utils.GmapUtil);
function MarkerParentModel(scope, element, attrs, mapCtrl, $timeout) {
this.onDestroy = __bind(this.onDestroy, this);
this.onWatch = __bind(this.onWatch, this);
this.onTimeOut = __bind(this.onTimeOut, this);
var self;
MarkerParentModel.__super__.constructor.call(this, scope, element, attrs, mapCtrl, $timeout);
self = this;
}
MarkerParentModel.prototype.onTimeOut = function(scope) {
var opts,
_this = this;
opts = this.createMarkerOptions(scope.coords, scope.icon, scope.options, this.mapCtrl.getMap());
this.scope.gMarker = new google.maps.Marker(opts);
google.maps.event.addListener(this.scope.gMarker, 'click', function() {
if (_this.doClick && (scope.click != null)) {
return _this.$timeout(function() {
return _this.scope.click();
});
}
});
this.setEvents(this.scope.gMarker, scope);
return this.$log.info(this);
};
MarkerParentModel.prototype.onWatch = function(propNameToWatch, scope) {
switch (propNameToWatch) {
case 'coords':
if ((scope.coords != null) && (this.scope.gMarker != null)) {
this.scope.gMarker.setMap(this.mapCtrl.getMap());
this.scope.gMarker.setPosition(new google.maps.LatLng(scope.coords.latitude, scope.coords.longitude));
this.scope.gMarker.setVisible((scope.coords.latitude != null) && (scope.coords.longitude != null));
return this.scope.gMarker.setOptions(scope.options);
} else {
return this.scope.gMarker.setMap(null);
}
break;
case 'icon':
if ((scope.icon != null) && (scope.coords != null) && (this.scope.gMarker != null)) {
this.scope.gMarker.setOptions(scope.options);
this.scope.gMarker.setIcon(scope.icon);
this.scope.gMarker.setMap(null);
this.scope.gMarker.setMap(this.mapCtrl.getMap());
this.scope.gMarker.setPosition(new google.maps.LatLng(scope.coords.latitude, scope.coords.longitude));
return this.scope.gMarker.setVisible(scope.coords.latitude && (scope.coords.longitude != null));
}
break;
case 'options':
if ((scope.coords != null) && (scope.icon != null) && scope.options) {
if (this.scope.gMarker != null) {
this.scope.gMarker.setMap(null);
}
delete this.scope.gMarker;
return this.scope.gMarker = new google.maps.Marker(this.createMarkerOptions(scope.coords, scope.icon, scope.options, this.mapCtrl.getMap()));
}
}
};
MarkerParentModel.prototype.onDestroy = function(scope) {
var self;
if (this.scope.gMarker === void 0) {
self = void 0;
return;
}
this.scope.gMarker.setMap(null);
delete this.scope.gMarker;
return self = void 0;
};
MarkerParentModel.prototype.setEvents = function(marker, scope) {
if (angular.isDefined(scope.events) && (scope.events != null) && angular.isObject(scope.events)) {
return _.compact(_.each(scope.events, function(eventHandler, eventName) {
if (scope.events.hasOwnProperty(eventName) && angular.isFunction(scope.events[eventName])) {
return google.maps.event.addListener(marker, eventName, function() {
return eventHandler.apply(scope, [marker, eventName, arguments]);
});
}
}));
}
};
return MarkerParentModel;
})(directives.api.models.parent.IMarkerParentModel);
});
}).call(this);
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.models.parent", function() {
return this.MarkersParentModel = (function(_super) {
__extends(MarkersParentModel, _super);
MarkersParentModel.include(directives.api.utils.ModelsWatcher);
function MarkersParentModel(scope, element, attrs, mapCtrl, $timeout) {
this.fit = __bind(this.fit, this);
this.onDestroy = __bind(this.onDestroy, this);
this.onWatch = __bind(this.onWatch, this);
this.reBuildMarkers = __bind(this.reBuildMarkers, this);
this.createMarkers = __bind(this.createMarkers, this);
this.validateScope = __bind(this.validateScope, this);
this.onTimeOut = __bind(this.onTimeOut, this);
var self;
MarkersParentModel.__super__.constructor.call(this, scope, element, attrs, mapCtrl, $timeout);
self = this;
this.markersIndex = 0;
this.gMarkerManager = void 0;
this.scope = scope;
this.scope.markerModels = [];
this.bigGulp = directives.api.utils.AsyncProcessor;
this.$timeout = $timeout;
this.$log.info(this);
}
MarkersParentModel.prototype.onTimeOut = function(scope) {
this.watch('models', scope);
this.watch('doCluster', scope);
this.watch('clusterOptions', scope);
this.watch('fit', scope);
return this.createMarkers(scope);
};
MarkersParentModel.prototype.validateScope = function(scope) {
var modelsNotDefined;
modelsNotDefined = angular.isUndefined(scope.models) || scope.models === void 0;
if (modelsNotDefined) {
this.$log.error(this.constructor.name + ": no valid models attribute found");
}
return MarkersParentModel.__super__.validateScope.call(this, scope) || modelsNotDefined;
};
MarkersParentModel.prototype.createMarkers = function(scope) {
var markers,
_this = this;
if ((scope.doCluster != null) && scope.doCluster === true) {
if (scope.clusterOptions != null) {
if (this.gMarkerManager === void 0) {
this.gMarkerManager = new directives.api.managers.ClustererMarkerManager(this.mapCtrl.getMap(), void 0, scope.clusterOptions);
} else {
if (this.gMarkerManager.opt_options !== scope.clusterOptions) {
this.gMarkerManager = new directives.api.managers.ClustererMarkerManager(this.mapCtrl.getMap(), void 0, scope.clusterOptions);
}
}
} else {
this.gMarkerManager = new directives.api.managers.ClustererMarkerManager(this.mapCtrl.getMap());
}
} else {
this.gMarkerManager = new directives.api.managers.MarkerManager(this.mapCtrl.getMap());
}
markers = [];
scope.isMarkerModelsReady = false;
return this.bigGulp.handleLargeArray(scope.models, function(model) {
var child;
scope.doRebuild = true;
child = new directives.api.models.child.MarkerChildModel(_this.markersIndex, model, scope, _this.mapCtrl, _this.$timeout, _this.DEFAULTS, _this.doClick, _this.gMarkerManager);
_this.$log.info('child', child, 'markers', markers);
markers.push(child);
return _this.markersIndex++;
}, (function() {}), function() {
_this.gMarkerManager.draw();
scope.markerModels = markers;
if (angular.isDefined(_this.attrs.fit) && (scope.fit != null) && scope.fit) {
_this.fit();
}
scope.isMarkerModelsReady = true;
if (scope.onMarkerModelsReady != null) {
return scope.onMarkerModelsReady(scope);
}
});
};
MarkersParentModel.prototype.reBuildMarkers = function(scope) {
var _this = this;
if (!scope.doRebuild && scope.doRebuild !== void 0) {
return;
}
_.each(scope.markerModels, function(oldM) {
return oldM.destroy();
});
this.markersIndex = 0;
if (this.gMarkerManager != null) {
this.gMarkerManager.clear();
}
return this.createMarkers(scope);
};
MarkersParentModel.prototype.onWatch = function(propNameToWatch, scope, newValue, oldValue) {
if (propNameToWatch === 'models') {
if (!this.didModelsChange(newValue, oldValue)) {
return;
}
}
if (propNameToWatch === 'options' && (newValue != null)) {
this.DEFAULTS = newValue;
return;
}
return this.reBuildMarkers(scope);
};
MarkersParentModel.prototype.onDestroy = function(scope) {
var model, _i, _len, _ref;
_ref = scope.markerModels;
for (_i = 0, _len = _ref.length; _i < _len; _i++) {
model = _ref[_i];
model.destroy();
}
if (this.gMarkerManager != null) {
return this.gMarkerManager.clear();
}
};
MarkersParentModel.prototype.fit = function() {
var bounds, everSet,
_this = this;
if (this.mapCtrl && (this.scope.markerModels != null) && this.scope.markerModels.length > 0) {
bounds = new google.maps.LatLngBounds();
everSet = false;
_.each(this.scope.markerModels, function(childModelMarker) {
if (childModelMarker.gMarker != null) {
if (!everSet) {
everSet = true;
}
return bounds.extend(childModelMarker.gMarker.getPosition());
}
});
if (everSet) {
return this.mapCtrl.getMap().fitBounds(bounds);
}
}
};
return MarkersParentModel;
})(directives.api.models.parent.IMarkerParentModel);
});
}).call(this);
/*
Windows directive where many windows map to the models property
*/
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api.models.parent", function() {
return this.WindowsParentModel = (function(_super) {
__extends(WindowsParentModel, _super);
WindowsParentModel.include(directives.api.utils.ModelsWatcher);
function WindowsParentModel(scope, element, attrs, ctrls, $timeout, $compile, $http, $templateCache, $interpolate) {
this.interpolateContent = __bind(this.interpolateContent, this);
this.setChildScope = __bind(this.setChildScope, this);
this.createWindow = __bind(this.createWindow, this);
this.setContentKeys = __bind(this.setContentKeys, this);
this.createChildScopesWindows = __bind(this.createChildScopesWindows, this);
this.onMarkerModelsReady = __bind(this.onMarkerModelsReady, this);
this.watchOurScope = __bind(this.watchOurScope, this);
this.destroy = __bind(this.destroy, this);
this.watchDestroy = __bind(this.watchDestroy, this);
this.watchModels = __bind(this.watchModels, this);
this.watch = __bind(this.watch, this);
var name, self, _i, _len, _ref,
_this = this;
WindowsParentModel.__super__.constructor.call(this, scope, element, attrs, ctrls, $timeout, $compile, $http, $templateCache, $interpolate);
self = this;
this.$interpolate = $interpolate;
this.windows = [];
this.windwsIndex = 0;
this.scopePropNames = ['show', 'coords', 'templateUrl', 'templateParameter', 'isIconVisibleOnClick', 'closeClick'];
_ref = this.scopePropNames;
for (_i = 0, _len = _ref.length; _i < _len; _i++) {
name = _ref[_i];
this[name + 'Key'] = void 0;
}
this.linked = new directives.api.utils.Linked(scope, element, attrs, ctrls);
this.models = void 0;
this.contentKeys = void 0;
this.isIconVisibleOnClick = void 0;
this.firstTime = true;
this.bigGulp = directives.api.utils.AsyncProcessor;
this.$log.info(self);
this.$timeout(function() {
_this.watchOurScope(scope);
return _this.createChildScopesWindows();
}, 50);
}
WindowsParentModel.prototype.watch = function(scope, name, nameKey) {
var _this = this;
return scope.$watch(name, function(newValue, oldValue) {
if (newValue !== oldValue) {
_this[nameKey] = typeof newValue === 'function' ? newValue() : newValue;
return _.each(_this.windows, function(model) {
return model.scope[name] = _this[nameKey] === 'self' ? model : model[_this[nameKey]];
});
}
}, true);
};
WindowsParentModel.prototype.watchModels = function(scope) {
var _this = this;
return scope.$watch('models', function(newValue, oldValue) {
if (_this.didModelsChange(newValue, oldValue)) {
_this.destroy();
return _this.createChildScopesWindows();
}
});
};
WindowsParentModel.prototype.watchDestroy = function(scope) {
var _this = this;
return scope.$on("$destroy", function() {
return _this.destroy();
});
};
WindowsParentModel.prototype.destroy = function() {
var _this = this;
_.each(this.windows, function(model) {
return model.destroy();
});
delete this.windows;
this.windows = [];
return this.windowsIndex = 0;
};
WindowsParentModel.prototype.watchOurScope = function(scope) {
var _this = this;
return _.each(this.scopePropNames, function(name) {
var nameKey;
nameKey = name + 'Key';
_this[nameKey] = typeof scope[name] === 'function' ? scope[name]() : scope[name];
return _this.watch(scope, name, nameKey);
});
};
WindowsParentModel.prototype.onMarkerModelsReady = function(scope) {
var _this = this;
this.destroy();
this.models = scope.models;
if (this.firstTime) {
this.watchDestroy(scope);
}
this.setContentKeys(scope.models);
return this.bigGulp.handleLargeArray(scope.markerModels, function(mm) {
return _this.createWindow(mm.model, mm.gMarker, _this.gMap);
}, (function() {}), function() {
return _this.firstTime = false;
});
};
WindowsParentModel.prototype.createChildScopesWindows = function() {
/*
being that we cannot tell the difference in Key String vs. a normal value string (TemplateUrl)
we will assume that all scope values are string expressions either pointing to a key (propName) or using
'self' to point the model as container/object of interest.
This may force redundant information into the model, but this appears to be the most flexible approach.
*/
var markersScope, modelsNotDefined,
_this = this;
this.isIconVisibleOnClick = true;
if (angular.isDefined(this.linked.attrs.isiconvisibleonclick)) {
this.isIconVisibleOnClick = this.linked.scope.isIconVisibleOnClick;
}
this.gMap = this.linked.ctrls[0].getMap();
markersScope = this.linked.ctrls.length > 1 && (this.linked.ctrls[1] != null) ? this.linked.ctrls[1].getMarkersScope() : void 0;
modelsNotDefined = angular.isUndefined(this.linked.scope.models);
if (modelsNotDefined && (markersScope === void 0 || (markersScope.markerModels === void 0 && markersScope.models === void 0))) {
this.$log.info("No models to create windows from! Need direct models or models derrived from markers!");
return;
}
if (this.gMap != null) {
if (this.linked.scope.models != null) {
this.models = this.linked.scope.models;
if (this.firstTime) {
this.watchModels(this.linked.scope);
this.watchDestroy(this.linked.scope);
}
this.setContentKeys(this.linked.scope.models);
return this.bigGulp.handleLargeArray(this.linked.scope.models, function(model) {
return _this.createWindow(model, void 0, _this.gMap);
}, (function() {}), function() {
return _this.firstTime = false;
});
} else {
markersScope.onMarkerModelsReady = this.onMarkerModelsReady;
if (markersScope.isMarkerModelsReady) {
return this.onMarkerModelsReady(markersScope);
}
}
}
};
WindowsParentModel.prototype.setContentKeys = function(models) {
if (models.length > 0) {
return this.contentKeys = Object.keys(models[0]);
}
};
WindowsParentModel.prototype.createWindow = function(model, gMarker, gMap) {
/*
Create ChildScope to Mimmick an ng-repeat created scope, must define the below scope
scope= {
coords: '=coords',
show: '&show',
templateUrl: '=templateurl',
templateParameter: '=templateparameter',
isIconVisibleOnClick: '=isiconvisibleonclick',
closeClick: '&closeclick'
}
*/
var childScope, opts, parsedContent,
_this = this;
childScope = this.linked.scope.$new(false);
this.setChildScope(childScope, model);
childScope.$watch('model', function(newValue, oldValue) {
if (newValue !== oldValue) {
return _this.setChildScope(childScope, newValue);
}
}, true);
parsedContent = this.interpolateContent(this.linked.element.html(), model);
opts = this.createWindowOptions(gMarker, childScope, parsedContent, this.DEFAULTS);
return this.windows.push(new directives.api.models.child.WindowChildModel(childScope, opts, this.isIconVisibleOnClick, gMap, gMarker, this.$http, this.$templateCache, this.$compile, void 0, true));
};
WindowsParentModel.prototype.setChildScope = function(childScope, model) {
var name, _fn, _i, _len, _ref,
_this = this;
_ref = this.scopePropNames;
_fn = function(name) {
var nameKey, newValue;
nameKey = name + 'Key';
newValue = _this[nameKey] === 'self' ? model : model[_this[nameKey]];
if (newValue !== childScope[name]) {
return childScope[name] = newValue;
}
};
for (_i = 0, _len = _ref.length; _i < _len; _i++) {
name = _ref[_i];
_fn(name);
}
return childScope.model = model;
};
WindowsParentModel.prototype.interpolateContent = function(content, model) {
var exp, interpModel, key, _i, _len, _ref;
if (this.contentKeys === void 0 || this.contentKeys.length === 0) {
return;
}
exp = this.$interpolate(content);
interpModel = {};
_ref = this.contentKeys;
for (_i = 0, _len = _ref.length; _i < _len; _i++) {
key = _ref[_i];
interpModel[key] = model[key];
}
return exp(interpModel);
};
return WindowsParentModel;
})(directives.api.models.parent.IWindowParentModel);
});
}).call(this);
/*
- interface for all labels to derrive from
- to enforce a minimum set of requirements
- attributes
- content
- anchor
- implementation needed on watches
*/
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api", function() {
return this.ILabel = (function(_super) {
__extends(ILabel, _super);
function ILabel($timeout) {
this.link = __bind(this.link, this);
var self;
self = this;
this.restrict = 'ECMA';
this.replace = true;
this.template = void 0;
this.require = void 0;
this.transclude = true;
this.priority = -100;
this.scope = {
labelContent: '=content',
labelAnchor: '@anchor',
labelClass: '@class',
labelStyle: '=style'
};
this.$log = directives.api.utils.Logger;
this.$timeout = $timeout;
}
ILabel.prototype.link = function(scope, element, attrs, ctrl) {
throw new Exception("Not Implemented!!");
};
return ILabel;
})(oo.BaseObject);
});
}).call(this);
/*
- interface for all markers to derrive from
- to enforce a minimum set of requirements
- attributes
- coords
- icon
- implementation needed on watches
*/
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api", function() {
return this.IMarker = (function(_super) {
__extends(IMarker, _super);
function IMarker($timeout) {
this.link = __bind(this.link, this);
var self;
self = this;
this.$log = directives.api.utils.Logger;
this.$timeout = $timeout;
this.restrict = 'ECMA';
this.require = '^googleMap';
this.priority = -1;
this.transclude = true;
this.replace = true;
this.scope = {
coords: '=coords',
icon: '=icon',
click: '&click',
options: '=options',
events: '=events'
};
}
IMarker.prototype.controller = [
'$scope', '$element', function($scope, $element) {
throw new Exception("Not Implemented!!");
}
];
IMarker.prototype.link = function(scope, element, attrs, ctrl) {
throw new Exception("Not implemented!!");
};
return IMarker;
})(oo.BaseObject);
});
}).call(this);
/*
- interface directive for all window(s) to derrive from
*/
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api", function() {
return this.IWindow = (function(_super) {
__extends(IWindow, _super);
IWindow.include(directives.api.utils.ChildEvents);
function IWindow($timeout, $compile, $http, $templateCache) {
var self;
this.$timeout = $timeout;
this.$compile = $compile;
this.$http = $http;
this.$templateCache = $templateCache;
this.link = __bind(this.link, this);
self = this;
this.restrict = 'ECMA';
this.template = void 0;
this.transclude = true;
this.priority = -100;
this.require = void 0;
this.replace = true;
this.scope = {
coords: '=coords',
show: '=show',
templateUrl: '=templateurl',
templateParameter: '=templateparameter',
isIconVisibleOnClick: '=isiconvisibleonclick',
closeClick: '&closeclick',
options: '=options'
};
this.$log = directives.api.utils.Logger;
}
IWindow.prototype.link = function(scope, element, attrs, ctrls) {
throw new Exception("Not Implemented!!");
};
return IWindow;
})(oo.BaseObject);
});
}).call(this);
/*
Basic Directive api for a label. Basic in the sense that this directive contains 1:1 on scope and model.
Thus there will be one html element per marker within the directive.
*/
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api", function() {
return this.Label = (function(_super) {
__extends(Label, _super);
function Label($timeout) {
this.link = __bind(this.link, this);
var self;
Label.__super__.constructor.call(this, $timeout);
self = this;
this.require = '^marker';
this.template = '<span class="angular-google-maps-marker-label" ng-transclude></span>';
this.$log.info(this);
}
Label.prototype.link = function(scope, element, attrs, ctrl) {
var _this = this;
return this.$timeout(function() {
var label, markerCtrl;
markerCtrl = ctrl.getMarkerScope().gMarker;
if (markerCtrl != null) {
label = new directives.api.models.child.MarkerLabelChildModel(markerCtrl, scope);
}
return scope.$on("$destroy", function() {
return label.destroy();
});
}, directives.api.utils.GmapUtil.defaultDelay + 25);
};
return Label;
})(directives.api.ILabel);
});
}).call(this);
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api", function() {
return this.Layer = (function(_super) {
__extends(Layer, _super);
function Layer($timeout) {
this.$timeout = $timeout;
this.link = __bind(this.link, this);
this.$log = directives.api.utils.Logger;
this.restrict = "ECMA";
this.require = "^googleMap";
this.priority = -1;
this.transclude = true;
this.template = '<span class=\"angular-google-map-layer\" ng-transclude></span>';
this.replace = true;
this.scope = {
show: "=show",
type: "=type",
namespace: "=namespace",
options: '=options',
onCreated: '&oncreated'
};
}
Layer.prototype.link = function(scope, element, attrs, mapCtrl) {
if (attrs.oncreated != null) {
return new directives.api.models.parent.LayerParentModel(scope, element, attrs, mapCtrl, this.$timeout, scope.onCreated);
} else {
return new directives.api.models.parent.LayerParentModel(scope, element, attrs, mapCtrl, this.$timeout);
}
};
return Layer;
})(oo.BaseObject);
});
}).call(this);
/*
Basic Directive api for a marker. Basic in the sense that this directive contains 1:1 on scope and model.
Thus there will be one html element per marker within the directive.
*/
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api", function() {
return this.Marker = (function(_super) {
__extends(Marker, _super);
function Marker($timeout) {
this.link = __bind(this.link, this);
var self;
Marker.__super__.constructor.call(this, $timeout);
self = this;
this.template = '<span class="angular-google-map-marker" ng-transclude></span>';
this.$log.info(this);
}
Marker.prototype.controller = [
'$scope', '$element', function($scope, $element) {
return {
getMarkerScope: function() {
return $scope;
}
};
}
];
Marker.prototype.link = function(scope, element, attrs, ctrl) {
return new directives.api.models.parent.MarkerParentModel(scope, element, attrs, ctrl, this.$timeout);
};
return Marker;
})(directives.api.IMarker);
});
}).call(this);
/*
Markers will map icon and coords differently than directibes.api.Marker. This is because Scope and the model marker are
not 1:1 in this setting.
- icon - will be the iconKey to the marker value ie: to get the icon marker[iconKey]
- coords - will be the coordsKey to the marker value ie: to get the icon marker[coordsKey]
- watches from IMarker reflect that the look up key for a value has changed and not the actual icon or coords itself
- actual changes to a model are tracked inside directives.api.model.MarkerModel
*/
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api", function() {
return this.Markers = (function(_super) {
__extends(Markers, _super);
function Markers($timeout) {
this.link = __bind(this.link, this);
var self;
Markers.__super__.constructor.call(this, $timeout);
self = this;
this.template = '<span class="angular-google-map-markers" ng-transclude></span>';
this.scope.models = '=models';
this.scope.doCluster = '=docluster';
this.scope.clusterOptions = '=clusteroptions';
this.scope.fit = '=fit';
this.scope.labelContent = '=labelcontent';
this.scope.labelAnchor = '@labelanchor';
this.scope.labelClass = '@labelclass';
this.$timeout = $timeout;
this.$log.info(this);
}
Markers.prototype.controller = [
'$scope', '$element', function($scope, $element) {
return {
getMarkersScope: function() {
return $scope;
}
};
}
];
Markers.prototype.link = function(scope, element, attrs, ctrl) {
return new directives.api.models.parent.MarkersParentModel(scope, element, attrs, ctrl, this.$timeout);
};
return Markers;
})(directives.api.IMarker);
});
}).call(this);
/*
Window directive for GoogleMap Info Windows, where ng-repeat is being used....
Where Html DOM element is 1:1 on Scope and a Model
*/
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api", function() {
return this.Window = (function(_super) {
__extends(Window, _super);
Window.include(directives.api.utils.GmapUtil);
function Window($timeout, $compile, $http, $templateCache) {
this.link = __bind(this.link, this);
var self;
Window.__super__.constructor.call(this, $timeout, $compile, $http, $templateCache);
self = this;
this.require = ['^googleMap', '^?marker'];
this.template = '<span class="angular-google-maps-window" ng-transclude></span>';
this.$log.info(self);
}
Window.prototype.link = function(scope, element, attrs, ctrls) {
var _this = this;
return this.$timeout(function() {
var defaults, hasScopeCoords, isIconVisibleOnClick, mapCtrl, markerCtrl, markerScope, opts, window;
isIconVisibleOnClick = true;
if (angular.isDefined(attrs.isiconvisibleonclick)) {
isIconVisibleOnClick = scope.isIconVisibleOnClick;
}
mapCtrl = ctrls[0].getMap();
markerCtrl = ctrls.length > 1 && (ctrls[1] != null) ? ctrls[1].getMarkerScope().gMarker : void 0;
defaults = scope.options != null ? scope.options : {};
hasScopeCoords = (scope != null) && (scope.coords != null) && (scope.coords.latitude != null) && (scope.coords.longitude != null);
opts = hasScopeCoords ? _this.createWindowOptions(markerCtrl, scope, element.html(), defaults) : defaults;
if (mapCtrl != null) {
window = new directives.api.models.child.WindowChildModel(scope, opts, isIconVisibleOnClick, mapCtrl, markerCtrl, _this.$http, _this.$templateCache, _this.$compile, element);
}
scope.$on("$destroy", function() {
return window.destroy();
});
if (ctrls[1] != null) {
markerScope = ctrls[1].getMarkerScope();
markerScope.$watch('coords', function(newValue, oldValue) {
if (newValue == null) {
return window.hideWindow();
}
});
markerScope.$watch('coords.latitude', function(newValue, oldValue) {
if (newValue !== oldValue) {
return window.getLatestPosition();
}
});
}
if ((_this.onChildCreation != null) && (window != null)) {
return _this.onChildCreation(window);
}
}, directives.api.utils.GmapUtil.defaultDelay + 25);
};
return Window;
})(directives.api.IWindow);
});
}).call(this);
/*
Windows directive where many windows map to the models property
*/
(function() {
var __bind = function(fn, me){ return function(){ return fn.apply(me, arguments); }; },
__hasProp = {}.hasOwnProperty,
__extends = function(child, parent) { for (var key in parent) { if (__hasProp.call(parent, key)) child[key] = parent[key]; } function ctor() { this.constructor = child; } ctor.prototype = parent.prototype; child.prototype = new ctor(); child.__super__ = parent.prototype; return child; };
this.ngGmapModule("directives.api", function() {
return this.Windows = (function(_super) {
__extends(Windows, _super);
function Windows($timeout, $compile, $http, $templateCache, $interpolate) {
this.link = __bind(this.link, this);
var self;
Windows.__super__.constructor.call(this, $timeout, $compile, $http, $templateCache);
self = this;
this.$interpolate = $interpolate;
this.require = ['^googleMap', '^?markers'];
this.template = '<span class="angular-google-maps-windows" ng-transclude></span>';
this.scope.models = '=models';
this.$log.info(self);
}
Windows.prototype.link = function(scope, element, attrs, ctrls) {
return new directives.api.models.parent.WindowsParentModel(scope, element, attrs, ctrls, this.$timeout, this.$compile, this.$http, this.$templateCache, this.$interpolate);
};
return Windows;
})(directives.api.IWindow);
});
}).call(this);
/*
!
The MIT License
Copyright (c) 2010-2013 Google, Inc. http://angularjs.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
angular-google-maps
https://github.com/nlaplante/angular-google-maps
@authors
Nicolas Laplante - https://plus.google.com/108189012221374960701
Nicholas McCready - https://twitter.com/nmccready
Nick Baugh - https://github.com/niftylettuce
*/
(function() {
angular.module("google-maps").directive("googleMap", [
"$log", "$timeout", function($log, $timeout) {
"use strict";
var DEFAULTS, getCoords, isTrue;
isTrue = function(val) {
return angular.isDefined(val) && val !== null && val === true || val === "1" || val === "y" || val === "true";
};
directives.api.utils.Logger.logger = $log;
DEFAULTS = {
mapTypeId: google.maps.MapTypeId.ROADMAP
};
getCoords = function(value) {
return new google.maps.LatLng(value.latitude, value.longitude);
};
return {
self: this,
restrict: "ECMA",
transclude: true,
replace: false,
template: "<div class=\"angular-google-map\"><div class=\"angular-google-map-container\"></div><div ng-transclude style=\"display: none\"></div></div>",
scope: {
center: "=center",
zoom: "=zoom",
dragging: "=dragging",
control: "=",
windows: "=windows",
options: "=options",
events: "=events",
styles: "=styles",
bounds: "=bounds"
},
controller: [
"$scope", function($scope) {
return {
getMap: function() {
return $scope.map;
}
};
}
],
/*
@param scope
@param element
@param attrs
*/
link: function(scope, element, attrs) {
var dragging, el, eventName, getEventHandler, opts, settingCenterFromScope, type, _m,
_this = this;
if (!angular.isDefined(scope.center) || (!angular.isDefined(scope.center.latitude) || !angular.isDefined(scope.center.longitude))) {
$log.error("angular-google-maps: could not find a valid center property");
return;
}
if (!angular.isDefined(scope.zoom)) {
$log.error("angular-google-maps: map zoom property not set");
return;
}
el = angular.element(element);
el.addClass("angular-google-map");
opts = {
options: {}
};
if (attrs.options) {
opts.options = scope.options;
}
if (attrs.styles) {
opts.styles = scope.styles;
}
if (attrs.type) {
type = attrs.type.toUpperCase();
if (google.maps.MapTypeId.hasOwnProperty(type)) {
opts.mapTypeId = google.maps.MapTypeId[attrs.type.toUpperCase()];
} else {
$log.error("angular-google-maps: invalid map type \"" + attrs.type + "\"");
}
}
_m = new google.maps.Map(el.find("div")[1], angular.extend({}, DEFAULTS, opts, {
center: new google.maps.LatLng(scope.center.latitude, scope.center.longitude),
draggable: isTrue(attrs.draggable),
zoom: scope.zoom,
bounds: scope.bounds
}));
dragging = false;
google.maps.event.addListener(_m, "dragstart", function() {
dragging = true;
return _.defer(function() {
return scope.$apply(function(s) {
if (s.dragging != null) {
return s.dragging = dragging;
}
});
});
});
google.maps.event.addListener(_m, "dragend", function() {
dragging = false;
return _.defer(function() {
return scope.$apply(function(s) {
if (s.dragging != null) {
return s.dragging = dragging;
}
});
});
});
google.maps.event.addListener(_m, "drag", function() {
var c;
c = _m.center;
return _.defer(function() {
return scope.$apply(function(s) {
s.center.latitude = c.lat();
return s.center.longitude = c.lng();
});
});
});
google.maps.event.addListener(_m, "zoom_changed", function() {
if (scope.zoom !== _m.zoom) {
return _.defer(function() {
return scope.$apply(function(s) {
return s.zoom = _m.zoom;
});
});
}
});
settingCenterFromScope = false;
google.maps.event.addListener(_m, "center_changed", function() {
var c;
c = _m.center;
if (settingCenterFromScope) {
return;
}
return _.defer(function() {
return scope.$apply(function(s) {
if (!_m.dragging) {
if (s.center.latitude !== c.lat()) {
s.center.latitude = c.lat();
}
if (s.center.longitude !== c.lng()) {
return s.center.longitude = c.lng();
}
}
});
});
});
google.maps.event.addListener(_m, "idle", function() {
var b, ne, sw;
b = _m.getBounds();
ne = b.getNorthEast();
sw = b.getSouthWest();
return _.defer(function() {
return scope.$apply(function(s) {
if (s.bounds !== null && s.bounds !== undefined && s.bounds !== void 0) {
s.bounds.northeast = {
latitude: ne.lat(),
longitude: ne.lng()
};
return s.bounds.southwest = {
latitude: sw.lat(),
longitude: sw.lng()
};
}
});
});
});
if (angular.isDefined(scope.events) && scope.events !== null && angular.isObject(scope.events)) {
getEventHandler = function(eventName) {
return function() {
return scope.events[eventName].apply(scope, [_m, eventName, arguments]);
};
};
for (eventName in scope.events) {
if (scope.events.hasOwnProperty(eventName) && angular.isFunction(scope.events[eventName])) {
google.maps.event.addListener(_m, eventName, getEventHandler(eventName));
}
}
}
scope.map = _m;
if ((attrs.control != null) && (scope.control != null)) {
scope.control.refresh = function(maybeCoords) {
var coords;
if (_m == null) {
return;
}
google.maps.event.trigger(_m, "resize");
if (((maybeCoords != null ? maybeCoords.latitude : void 0) != null) && ((maybeCoords != null ? maybeCoords.latitude : void 0) != null)) {
coords = getCoords(maybeCoords);
if (isTrue(attrs.pan)) {
return _m.panTo(coords);
} else {
return _m.setCenter(coords);
}
}
};
/*
I am sure you all will love this. You want the instance here you go.. BOOM!
*/
scope.control.getGMap = function() {
return _m;
};
}
scope.$watch("center", (function(newValue, oldValue) {
var coords;
coords = getCoords(newValue);
if (newValue === oldValue || (coords.lat() === _m.center.lat() && coords.lng() === _m.center.lng())) {
return;
}
settingCenterFromScope = true;
if (!dragging) {
if ((newValue.latitude == null) || (newValue.longitude == null)) {
$log.error("Invalid center for newValue: " + (JSON.stringify(newValue)));
}
if (isTrue(attrs.pan) && scope.zoom === _m.zoom) {
_m.panTo(coords);
} else {
_m.setCenter(coords);
}
}
return settingCenterFromScope = false;
}), true);
scope.$watch("zoom", function(newValue, oldValue) {
if (newValue === oldValue || newValue === _m.zoom) {
return;
}
return _.defer(function() {
return _m.setZoom(newValue);
});
});
scope.$watch("bounds", function(newValue, oldValue) {
var bounds, ne, sw;
if (newValue === oldValue) {
return;
}
if ((newValue.northeast.latitude == null) || (newValue.northeast.longitude == null) || (newValue.southwest.latitude == null) || (newValue.southwest.longitude == null)) {
$log.error("Invalid map bounds for new value: " + (JSON.stringify(newValue)));
return;
}
ne = new google.maps.LatLng(newValue.northeast.latitude, newValue.northeast.longitude);
sw = new google.maps.LatLng(newValue.southwest.latitude, newValue.southwest.longitude);
bounds = new google.maps.LatLngBounds(sw, ne);
return _m.fitBounds(bounds);
});
scope.$watch("options", function(newValue, oldValue) {
if (!_.isEqual(newValue, oldValue)) {
opts.options = newValue;
if (_m != null) {
return _m.setOptions(opts);
}
}
}, true);
return scope.$watch("styles", function(newValue, oldValue) {
if (!_.isEqual(newValue, oldValue)) {
opts.styles = newValue;
if (_m != null) {
return _m.setOptions(opts);
}
}
}, true);
}
};
}
]);
}).call(this);
/*
!
The MIT License
Copyright (c) 2010-2013 Google, Inc. http://angularjs.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
angular-google-maps
https://github.com/nlaplante/angular-google-maps
@authors
Nicolas Laplante - https://plus.google.com/108189012221374960701
Nicholas McCready - https://twitter.com/nmccready
*/
/*
Map marker directive
This directive is used to create a marker on an existing map.
This directive creates a new scope.
{attribute coords required} object containing latitude and longitude properties
{attribute icon optional} string url to image used for marker icon
{attribute animate optional} if set to false, the marker won't be animated (on by default)
*/
(function() {
angular.module("google-maps").directive("marker", [
"$timeout", function($timeout) {
return new directives.api.Marker($timeout);
}
]);
}).call(this);
/*
!
The MIT License
Copyright (c) 2010-2013 Google, Inc. http://angularjs.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
angular-google-maps
https://github.com/nlaplante/angular-google-maps
@authors
Nicolas Laplante - https://plus.google.com/108189012221374960701
Nicholas McCready - https://twitter.com/nmccready
*/
/*
Map marker directive
This directive is used to create a marker on an existing map.
This directive creates a new scope.
{attribute coords required} object containing latitude and longitude properties
{attribute icon optional} string url to image used for marker icon
{attribute animate optional} if set to false, the marker won't be animated (on by default)
*/
(function() {
angular.module("google-maps").directive("markers", [
"$timeout", function($timeout) {
return new directives.api.Markers($timeout);
}
]);
}).call(this);
/*
!
The MIT License
Copyright (c) 2010-2013 Google, Inc. http://angularjs.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
angular-google-maps
https://github.com/nlaplante/angular-google-maps
@authors Bruno Queiroz, creativelikeadog@gmail.com
*/
/*
Marker label directive
This directive is used to create a marker label on an existing map.
{attribute content required} content of the label
{attribute anchor required} string that contains the x and y point position of the label
{attribute class optional} class to DOM object
{attribute style optional} style for the label
*/
(function() {
angular.module("google-maps").directive("markerLabel", [
"$log", "$timeout", function($log, $timeout) {
return new directives.api.Label($timeout);
}
]);
}).call(this);
/*
!
The MIT License
Copyright (c) 2010-2013 Google, Inc. http://angularjs.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
angular-google-maps
https://github.com/nlaplante/angular-google-maps
@authors
Nicolas Laplante - https://plus.google.com/108189012221374960701
Nicholas McCready - https://twitter.com/nmccready
*/
(function() {
angular.module("google-maps").directive("polygon", [
"$log", "$timeout", function($log, $timeout) {
var DEFAULTS, convertPathPoints, extendMapBounds, isTrue, validatePathPoints;
validatePathPoints = function(path) {
var i;
i = 0;
while (i < path.length) {
if (angular.isUndefined(path[i].latitude) || angular.isUndefined(path[i].longitude)) {
return false;
}
i++;
}
return true;
};
convertPathPoints = function(path) {
var i, result;
result = new google.maps.MVCArray();
i = 0;
while (i < path.length) {
result.push(new google.maps.LatLng(path[i].latitude, path[i].longitude));
i++;
}
return result;
};
extendMapBounds = function(map, points) {
var bounds, i;
bounds = new google.maps.LatLngBounds();
i = 0;
while (i < points.length) {
bounds.extend(points.getAt(i));
i++;
}
return map.fitBounds(bounds);
};
/*
Check if a value is true
*/
isTrue = function(val) {
return angular.isDefined(val) && val !== null && val === true || val === "1" || val === "y" || val === "true";
};
"use strict";
DEFAULTS = {};
return {
restrict: "ECA",
require: "^googleMap",
replace: true,
scope: {
path: "=path",
stroke: "=stroke",
clickable: "=",
draggable: "=",
editable: "=",
geodesic: "=",
icons: "=icons",
visible: "="
},
link: function(scope, element, attrs, mapCtrl) {
if (angular.isUndefined(scope.path) || scope.path === null || scope.path.length < 2 || !validatePathPoints(scope.path)) {
$log.error("polyline: no valid path attribute found");
return;
}
return $timeout(function() {
var map, opts, pathInsertAtListener, pathPoints, pathRemoveAtListener, pathSetAtListener, polyPath, polyline;
map = mapCtrl.getMap();
pathPoints = convertPathPoints(scope.path);
opts = angular.extend({}, DEFAULTS, {
map: map,
path: pathPoints,
strokeColor: scope.stroke && scope.stroke.color,
strokeOpacity: scope.stroke && scope.stroke.opacity,
strokeWeight: scope.stroke && scope.stroke.weight
});
angular.forEach({
clickable: true,
draggable: false,
editable: false,
geodesic: false,
visible: true
}, function(defaultValue, key) {
if (angular.isUndefined(scope[key]) || scope[key] === null) {
return opts[key] = defaultValue;
} else {
return opts[key] = scope[key];
}
});
polyline = new google.maps.Polyline(opts);
if (isTrue(attrs.fit)) {
extendMapBounds(map, pathPoints);
}
if (angular.isDefined(scope.editable)) {
scope.$watch("editable", function(newValue, oldValue) {
return polyline.setEditable(newValue);
});
}
if (angular.isDefined(scope.draggable)) {
scope.$watch("draggable", function(newValue, oldValue) {
return polyline.setDraggable(newValue);
});
}
if (angular.isDefined(scope.visible)) {
scope.$watch("visible", function(newValue, oldValue) {
return polyline.setVisible(newValue);
});
}
pathSetAtListener = void 0;
pathInsertAtListener = void 0;
pathRemoveAtListener = void 0;
polyPath = polyline.getPath();
pathSetAtListener = google.maps.event.addListener(polyPath, "set_at", function(index) {
var value;
value = polyPath.getAt(index);
if (!value) {
return;
}
if (!value.lng || !value.lat) {
return;
}
scope.path[index].latitude = value.lat();
scope.path[index].longitude = value.lng();
return scope.$apply();
});
pathInsertAtListener = google.maps.event.addListener(polyPath, "insert_at", function(index) {
var value;
value = polyPath.getAt(index);
if (!value) {
return;
}
if (!value.lng || !value.lat) {
return;
}
scope.path.splice(index, 0, {
latitude: value.lat(),
longitude: value.lng()
});
return scope.$apply();
});
pathRemoveAtListener = google.maps.event.addListener(polyPath, "remove_at", function(index) {
scope.path.splice(index, 1);
return scope.$apply();
});
scope.$watch("path", (function(newArray) {
var i, l, newLength, newValue, oldArray, oldLength, oldValue;
oldArray = polyline.getPath();
if (newArray !== oldArray) {
if (newArray) {
polyline.setMap(map);
i = 0;
oldLength = oldArray.getLength();
newLength = newArray.length;
l = Math.min(oldLength, newLength);
while (i < l) {
oldValue = oldArray.getAt(i);
newValue = newArray[i];
if ((oldValue.lat() !== newValue.latitude) || (oldValue.lng() !== newValue.longitude)) {
oldArray.setAt(i, new google.maps.LatLng(newValue.latitude, newValue.longitude));
}
i++;
}
while (i < newLength) {
newValue = newArray[i];
oldArray.push(new google.maps.LatLng(newValue.latitude, newValue.longitude));
i++;
}
while (i < oldLength) {
oldArray.pop();
i++;
}
if (isTrue(attrs.fit)) {
return extendMapBounds(map, oldArray);
}
} else {
return polyline.setMap(null);
}
}
}), true);
return scope.$on("$destroy", function() {
polyline.setMap(null);
pathSetAtListener();
pathSetAtListener = null;
pathInsertAtListener();
pathInsertAtListener = null;
pathRemoveAtListener();
return pathRemoveAtListener = null;
});
});
}
};
}
]);
}).call(this);
/*
!
The MIT License
Copyright (c) 2010-2013 Google, Inc. http://angularjs.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
angular-google-maps
https://github.com/nlaplante/angular-google-maps
@authors
Nicolas Laplante - https://plus.google.com/108189012221374960701
Nicholas McCready - https://twitter.com/nmccready
*/
(function() {
angular.module("google-maps").directive("polyline", [
"$log", "$timeout", "array-sync", function($log, $timeout, arraySync) {
var DEFAULTS, convertPathPoints, extendMapBounds, isTrue, validatePathPoints;
validatePathPoints = function(path) {
var i;
i = 0;
while (i < path.length) {
if (angular.isUndefined(path[i].latitude) || angular.isUndefined(path[i].longitude)) {
return false;
}
i++;
}
return true;
};
convertPathPoints = function(path) {
var i, result;
result = new google.maps.MVCArray();
i = 0;
while (i < path.length) {
result.push(new google.maps.LatLng(path[i].latitude, path[i].longitude));
i++;
}
return result;
};
extendMapBounds = function(map, points) {
var bounds, i;
bounds = new google.maps.LatLngBounds();
i = 0;
while (i < points.length) {
bounds.extend(points.getAt(i));
i++;
}
return map.fitBounds(bounds);
};
/*
Check if a value is true
*/
isTrue = function(val) {
return angular.isDefined(val) && val !== null && val === true || val === "1" || val === "y" || val === "true";
};
"use strict";
DEFAULTS = {};
return {
restrict: "ECA",
replace: true,
require: "^googleMap",
scope: {
path: "=path",
stroke: "=stroke",
clickable: "=",
draggable: "=",
editable: "=",
geodesic: "=",
icons: "=icons",
visible: "="
},
link: function(scope, element, attrs, mapCtrl) {
if (angular.isUndefined(scope.path) || scope.path === null || scope.path.length < 2 || !validatePathPoints(scope.path)) {
$log.error("polyline: no valid path attribute found");
return;
}
return $timeout(function() {
var arraySyncer, buildOpts, map, polyline;
buildOpts = function(pathPoints) {
var opts;
opts = angular.extend({}, DEFAULTS, {
map: map,
path: pathPoints,
strokeColor: scope.stroke && scope.stroke.color,
strokeOpacity: scope.stroke && scope.stroke.opacity,
strokeWeight: scope.stroke && scope.stroke.weight
});
angular.forEach({
clickable: true,
draggable: false,
editable: false,
geodesic: false,
visible: true
}, function(defaultValue, key) {
if (angular.isUndefined(scope[key]) || scope[key] === null) {
return opts[key] = defaultValue;
} else {
return opts[key] = scope[key];
}
});
return opts;
};
map = mapCtrl.getMap();
polyline = new google.maps.Polyline(buildOpts(convertPathPoints(scope.path)));
if (isTrue(attrs.fit)) {
extendMapBounds(map, pathPoints);
}
if (angular.isDefined(scope.editable)) {
scope.$watch("editable", function(newValue, oldValue) {
return polyline.setEditable(newValue);
});
}
if (angular.isDefined(scope.draggable)) {
scope.$watch("draggable", function(newValue, oldValue) {
return polyline.setDraggable(newValue);
});
}
if (angular.isDefined(scope.visible)) {
scope.$watch("visible", function(newValue, oldValue) {
return polyline.setVisible(newValue);
});
}
if (angular.isDefined(scope.geodesic)) {
scope.$watch("geodesic", function(newValue, oldValue) {
return polyline.setOptions(buildOpts(polyline.getPath()));
});
}
if (angular.isDefined(scope.stroke) && angular.isDefined(scope.stroke.weight)) {
scope.$watch("stroke.weight", function(newValue, oldValue) {
return polyline.setOptions(buildOpts(polyline.getPath()));
});
}
if (angular.isDefined(scope.stroke) && angular.isDefined(scope.stroke.color)) {
scope.$watch("stroke.color", function(newValue, oldValue) {
return polyline.setOptions(buildOpts(polyline.getPath()));
});
}
arraySyncer = arraySync(polyline.getPath(), scope, "path");
return scope.$on("$destroy", function() {
polyline.setMap(null);
if (arraySyncer) {
arraySyncer();
return arraySyncer = null;
}
});
});
}
};
}
]);
}).call(this);
/*
!
The MIT License
Copyright (c) 2010-2013 Google, Inc. http://angularjs.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
angular-google-maps
https://github.com/nlaplante/angular-google-maps
@authors
Nicolas Laplante - https://plus.google.com/108189012221374960701
Nicholas McCready - https://twitter.com/nmccready
*/
/*
Map info window directive
This directive is used to create an info window on an existing map.
This directive creates a new scope.
{attribute coords required} object containing latitude and longitude properties
{attribute show optional} map will show when this expression returns true
*/
(function() {
angular.module("google-maps").directive("window", [
"$timeout", "$compile", "$http", "$templateCache", function($timeout, $compile, $http, $templateCache) {
return new directives.api.Window($timeout, $compile, $http, $templateCache);
}
]);
}).call(this);
/*
!
The MIT License
Copyright (c) 2010-2013 Google, Inc. http://angularjs.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
angular-google-maps
https://github.com/nlaplante/angular-google-maps
@authors
Nicolas Laplante - https://plus.google.com/108189012221374960701
Nicholas McCready - https://twitter.com/nmccready
*/
/*
Map info window directive
This directive is used to create an info window on an existing map.
This directive creates a new scope.
{attribute coords required} object containing latitude and longitude properties
{attribute show optional} map will show when this expression returns true
*/
(function() {
angular.module("google-maps").directive("windows", [
"$timeout", "$compile", "$http", "$templateCache", "$interpolate", function($timeout, $compile, $http, $templateCache, $interpolate) {
return new directives.api.Windows($timeout, $compile, $http, $templateCache, $interpolate);
}
]);
}).call(this);
/*
!
The MIT License
Copyright (c) 2010-2013 Google, Inc. http://angularjs.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
angular-google-maps
https://github.com/nlaplante/angular-google-maps
@authors:
- Nicolas Laplante https://plus.google.com/108189012221374960701
- Nicholas McCready - https://twitter.com/nmccready
*/
/*
Map Layer directive
This directive is used to create any type of Layer from the google maps sdk.
This directive creates a new scope.
{attribute show optional} true (default) shows the trafficlayer otherwise it is hidden
*/
(function() {
angular.module("google-maps").directive("layer", [
"$timeout", function($timeout) {
return new directives.api.Layer($timeout);
}
]);
}).call(this);
;/**
* @name InfoBox
* @version 1.1.12 [December 11, 2012]
* @author Gary Little (inspired by proof-of-concept code from Pamela Fox of Google)
* @copyright Copyright 2010 Gary Little [gary at luxcentral.com]
* @fileoverview InfoBox extends the Google Maps JavaScript API V3 <tt>OverlayView</tt> class.
* <p>
* An InfoBox behaves like a <tt>google.maps.InfoWindow</tt>, but it supports several
* additional properties for advanced styling. An InfoBox can also be used as a map label.
* <p>
* An InfoBox also fires the same events as a <tt>google.maps.InfoWindow</tt>.
*/
/*!
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/*jslint browser:true */
/*global google */
/**
* @name InfoBoxOptions
* @class This class represents the optional parameter passed to the {@link InfoBox} constructor.
* @property {string|Node} content The content of the InfoBox (plain text or an HTML DOM node).
* @property {boolean} [disableAutoPan=false] Disable auto-pan on <tt>open</tt>.
* @property {number} maxWidth The maximum width (in pixels) of the InfoBox. Set to 0 if no maximum.
* @property {Size} pixelOffset The offset (in pixels) from the top left corner of the InfoBox
* (or the bottom left corner if the <code>alignBottom</code> property is <code>true</code>)
* to the map pixel corresponding to <tt>position</tt>.
* @property {LatLng} position The geographic location at which to display the InfoBox.
* @property {number} zIndex The CSS z-index style value for the InfoBox.
* Note: This value overrides a zIndex setting specified in the <tt>boxStyle</tt> property.
* @property {string} [boxClass="infoBox"] The name of the CSS class defining the styles for the InfoBox container.
* @property {Object} [boxStyle] An object literal whose properties define specific CSS
* style values to be applied to the InfoBox. Style values defined here override those that may
* be defined in the <code>boxClass</code> style sheet. If this property is changed after the
* InfoBox has been created, all previously set styles (except those defined in the style sheet)
* are removed from the InfoBox before the new style values are applied.
* @property {string} closeBoxMargin The CSS margin style value for the close box.
* The default is "2px" (a 2-pixel margin on all sides).
* @property {string} closeBoxURL The URL of the image representing the close box.
* Note: The default is the URL for Google's standard close box.
* Set this property to "" if no close box is required.
* @property {Size} infoBoxClearance Minimum offset (in pixels) from the InfoBox to the
* map edge after an auto-pan.
* @property {boolean} [isHidden=false] Hide the InfoBox on <tt>open</tt>.
* [Deprecated in favor of the <tt>visible</tt> property.]
* @property {boolean} [visible=true] Show the InfoBox on <tt>open</tt>.
* @property {boolean} alignBottom Align the bottom left corner of the InfoBox to the <code>position</code>
* location (default is <tt>false</tt> which means that the top left corner of the InfoBox is aligned).
* @property {string} pane The pane where the InfoBox is to appear (default is "floatPane").
* Set the pane to "mapPane" if the InfoBox is being used as a map label.
* Valid pane names are the property names for the <tt>google.maps.MapPanes</tt> object.
* @property {boolean} enableEventPropagation Propagate mousedown, mousemove, mouseover, mouseout,
* mouseup, click, dblclick, touchstart, touchend, touchmove, and contextmenu events in the InfoBox
* (default is <tt>false</tt> to mimic the behavior of a <tt>google.maps.InfoWindow</tt>). Set
* this property to <tt>true</tt> if the InfoBox is being used as a map label.
*/
/**
* Creates an InfoBox with the options specified in {@link InfoBoxOptions}.
* Call <tt>InfoBox.open</tt> to add the box to the map.
* @constructor
* @param {InfoBoxOptions} [opt_opts]
*/
function InfoBox(opt_opts) {
opt_opts = opt_opts || {};
google.maps.OverlayView.apply(this, arguments);
// Standard options (in common with google.maps.InfoWindow):
//
this.content_ = opt_opts.content || "";
this.disableAutoPan_ = opt_opts.disableAutoPan || false;
this.maxWidth_ = opt_opts.maxWidth || 0;
this.pixelOffset_ = opt_opts.pixelOffset || new google.maps.Size(0, 0);
this.position_ = opt_opts.position || new google.maps.LatLng(0, 0);
this.zIndex_ = opt_opts.zIndex || null;
// Additional options (unique to InfoBox):
//
this.boxClass_ = opt_opts.boxClass || "infoBox";
this.boxStyle_ = opt_opts.boxStyle || {};
this.closeBoxMargin_ = opt_opts.closeBoxMargin || "2px";
this.closeBoxURL_ = opt_opts.closeBoxURL || "http://www.google.com/intl/en_us/mapfiles/close.gif";
if (opt_opts.closeBoxURL === "") {
this.closeBoxURL_ = "";
}
this.infoBoxClearance_ = opt_opts.infoBoxClearance || new google.maps.Size(1, 1);
if (typeof opt_opts.visible === "undefined") {
if (typeof opt_opts.isHidden === "undefined") {
opt_opts.visible = true;
} else {
opt_opts.visible = !opt_opts.isHidden;
}
}
this.isHidden_ = !opt_opts.visible;
this.alignBottom_ = opt_opts.alignBottom || false;
this.pane_ = opt_opts.pane || "floatPane";
this.enableEventPropagation_ = opt_opts.enableEventPropagation || false;
this.div_ = null;
this.closeListener_ = null;
this.moveListener_ = null;
this.contextListener_ = null;
this.eventListeners_ = null;
this.fixedWidthSet_ = null;
}
/* InfoBox extends OverlayView in the Google Maps API v3.
*/
InfoBox.prototype = new google.maps.OverlayView();
/**
* Creates the DIV representing the InfoBox.
* @private
*/
InfoBox.prototype.createInfoBoxDiv_ = function () {
var i;
var events;
var bw;
var me = this;
// This handler prevents an event in the InfoBox from being passed on to the map.
//
var cancelHandler = function (e) {
e.cancelBubble = true;
if (e.stopPropagation) {
e.stopPropagation();
}
};
// This handler ignores the current event in the InfoBox and conditionally prevents
// the event from being passed on to the map. It is used for the contextmenu event.
//
var ignoreHandler = function (e) {
e.returnValue = false;
if (e.preventDefault) {
e.preventDefault();
}
if (!me.enableEventPropagation_) {
cancelHandler(e);
}
};
if (!this.div_) {
this.div_ = document.createElement("div");
this.setBoxStyle_();
if (typeof this.content_.nodeType === "undefined") {
this.div_.innerHTML = this.getCloseBoxImg_() + this.content_;
} else {
this.div_.innerHTML = this.getCloseBoxImg_();
this.div_.appendChild(this.content_);
}
// Add the InfoBox DIV to the DOM
this.getPanes()[this.pane_].appendChild(this.div_);
this.addClickHandler_();
if (this.div_.style.width) {
this.fixedWidthSet_ = true;
} else {
if (this.maxWidth_ !== 0 && this.div_.offsetWidth > this.maxWidth_) {
this.div_.style.width = this.maxWidth_;
this.div_.style.overflow = "auto";
this.fixedWidthSet_ = true;
} else { // The following code is needed to overcome problems with MSIE
bw = this.getBoxWidths_();
this.div_.style.width = (this.div_.offsetWidth - bw.left - bw.right) + "px";
this.fixedWidthSet_ = false;
}
}
this.panBox_(this.disableAutoPan_);
if (!this.enableEventPropagation_) {
this.eventListeners_ = [];
// Cancel event propagation.
//
// Note: mousemove not included (to resolve Issue 152)
events = ["mousedown", "mouseover", "mouseout", "mouseup",
"click", "dblclick", "touchstart", "touchend", "touchmove"];
for (i = 0; i < events.length; i++) {
this.eventListeners_.push(google.maps.event.addDomListener(this.div_, events[i], cancelHandler));
}
// Workaround for Google bug that causes the cursor to change to a pointer
// when the mouse moves over a marker underneath InfoBox.
this.eventListeners_.push(google.maps.event.addDomListener(this.div_, "mouseover", function (e) {
this.style.cursor = "default";
}));
}
this.contextListener_ = google.maps.event.addDomListener(this.div_, "contextmenu", ignoreHandler);
/**
* This event is fired when the DIV containing the InfoBox's content is attached to the DOM.
* @name InfoBox#domready
* @event
*/
google.maps.event.trigger(this, "domready");
}
};
/**
* Returns the HTML <IMG> tag for the close box.
* @private
*/
InfoBox.prototype.getCloseBoxImg_ = function () {
var img = "";
if (this.closeBoxURL_ !== "") {
img = "<img";
img += " src='" + this.closeBoxURL_ + "'";
img += " align=right"; // Do this because Opera chokes on style='float: right;'
img += " style='";
img += " position: relative;"; // Required by MSIE
img += " cursor: pointer;";
img += " margin: " + this.closeBoxMargin_ + ";";
img += "'>";
}
return img;
};
/**
* Adds the click handler to the InfoBox close box.
* @private
*/
InfoBox.prototype.addClickHandler_ = function () {
var closeBox;
if (this.closeBoxURL_ !== "") {
closeBox = this.div_.firstChild;
this.closeListener_ = google.maps.event.addDomListener(closeBox, "click", this.getCloseClickHandler_());
} else {
this.closeListener_ = null;
}
};
/**
* Returns the function to call when the user clicks the close box of an InfoBox.
* @private
*/
InfoBox.prototype.getCloseClickHandler_ = function () {
var me = this;
return function (e) {
// 1.0.3 fix: Always prevent propagation of a close box click to the map:
e.cancelBubble = true;
if (e.stopPropagation) {
e.stopPropagation();
}
/**
* This event is fired when the InfoBox's close box is clicked.
* @name InfoBox#closeclick
* @event
*/
google.maps.event.trigger(me, "closeclick");
me.close();
};
};
/**
* Pans the map so that the InfoBox appears entirely within the map's visible area.
* @private
*/
InfoBox.prototype.panBox_ = function (disablePan) {
var map;
var bounds;
var xOffset = 0, yOffset = 0;
if (!disablePan) {
map = this.getMap();
if (map instanceof google.maps.Map) { // Only pan if attached to map, not panorama
if (!map.getBounds().contains(this.position_)) {
// Marker not in visible area of map, so set center
// of map to the marker position first.
map.setCenter(this.position_);
}
bounds = map.getBounds();
var mapDiv = map.getDiv();
var mapWidth = mapDiv.offsetWidth;
var mapHeight = mapDiv.offsetHeight;
var iwOffsetX = this.pixelOffset_.width;
var iwOffsetY = this.pixelOffset_.height;
var iwWidth = this.div_.offsetWidth;
var iwHeight = this.div_.offsetHeight;
var padX = this.infoBoxClearance_.width;
var padY = this.infoBoxClearance_.height;
var pixPosition = this.getProjection().fromLatLngToContainerPixel(this.position_);
if (pixPosition.x < (-iwOffsetX + padX)) {
xOffset = pixPosition.x + iwOffsetX - padX;
} else if ((pixPosition.x + iwWidth + iwOffsetX + padX) > mapWidth) {
xOffset = pixPosition.x + iwWidth + iwOffsetX + padX - mapWidth;
}
if (this.alignBottom_) {
if (pixPosition.y < (-iwOffsetY + padY + iwHeight)) {
yOffset = pixPosition.y + iwOffsetY - padY - iwHeight;
} else if ((pixPosition.y + iwOffsetY + padY) > mapHeight) {
yOffset = pixPosition.y + iwOffsetY + padY - mapHeight;
}
} else {
if (pixPosition.y < (-iwOffsetY + padY)) {
yOffset = pixPosition.y + iwOffsetY - padY;
} else if ((pixPosition.y + iwHeight + iwOffsetY + padY) > mapHeight) {
yOffset = pixPosition.y + iwHeight + iwOffsetY + padY - mapHeight;
}
}
if (!(xOffset === 0 && yOffset === 0)) {
// Move the map to the shifted center.
//
var c = map.getCenter();
map.panBy(xOffset, yOffset);
}
}
}
};
/**
* Sets the style of the InfoBox by setting the style sheet and applying
* other specific styles requested.
* @private
*/
InfoBox.prototype.setBoxStyle_ = function () {
var i, boxStyle;
if (this.div_) {
// Apply style values from the style sheet defined in the boxClass parameter:
this.div_.className = this.boxClass_;
// Clear existing inline style values:
this.div_.style.cssText = "";
// Apply style values defined in the boxStyle parameter:
boxStyle = this.boxStyle_;
for (i in boxStyle) {
if (boxStyle.hasOwnProperty(i)) {
this.div_.style[i] = boxStyle[i];
}
}
// Fix up opacity style for benefit of MSIE:
//
if (typeof this.div_.style.opacity !== "undefined" && this.div_.style.opacity !== "") {
this.div_.style.filter = "alpha(opacity=" + (this.div_.style.opacity * 100) + ")";
}
// Apply required styles:
//
this.div_.style.position = "absolute";
this.div_.style.visibility = 'hidden';
if (this.zIndex_ !== null) {
this.div_.style.zIndex = this.zIndex_;
}
}
};
/**
* Get the widths of the borders of the InfoBox.
* @private
* @return {Object} widths object (top, bottom left, right)
*/
InfoBox.prototype.getBoxWidths_ = function () {
var computedStyle;
var bw = {top: 0, bottom: 0, left: 0, right: 0};
var box = this.div_;
if (document.defaultView && document.defaultView.getComputedStyle) {
computedStyle = box.ownerDocument.defaultView.getComputedStyle(box, "");
if (computedStyle) {
// The computed styles are always in pixel units (good!)
bw.top = parseInt(computedStyle.borderTopWidth, 10) || 0;
bw.bottom = parseInt(computedStyle.borderBottomWidth, 10) || 0;
bw.left = parseInt(computedStyle.borderLeftWidth, 10) || 0;
bw.right = parseInt(computedStyle.borderRightWidth, 10) || 0;
}
} else if (document.documentElement.currentStyle) { // MSIE
if (box.currentStyle) {
// The current styles may not be in pixel units, but assume they are (bad!)
bw.top = parseInt(box.currentStyle.borderTopWidth, 10) || 0;
bw.bottom = parseInt(box.currentStyle.borderBottomWidth, 10) || 0;
bw.left = parseInt(box.currentStyle.borderLeftWidth, 10) || 0;
bw.right = parseInt(box.currentStyle.borderRightWidth, 10) || 0;
}
}
return bw;
};
/**
* Invoked when <tt>close</tt> is called. Do not call it directly.
*/
InfoBox.prototype.onRemove = function () {
if (this.div_) {
this.div_.parentNode.removeChild(this.div_);
this.div_ = null;
}
};
/**
* Draws the InfoBox based on the current map projection and zoom level.
*/
InfoBox.prototype.draw = function () {
this.createInfoBoxDiv_();
var pixPosition = this.getProjection().fromLatLngToDivPixel(this.position_);
this.div_.style.left = (pixPosition.x + this.pixelOffset_.width) + "px";
if (this.alignBottom_) {
this.div_.style.bottom = -(pixPosition.y + this.pixelOffset_.height) + "px";
} else {
this.div_.style.top = (pixPosition.y + this.pixelOffset_.height) + "px";
}
if (this.isHidden_) {
this.div_.style.visibility = 'hidden';
} else {
this.div_.style.visibility = "visible";
}
};
/**
* Sets the options for the InfoBox. Note that changes to the <tt>maxWidth</tt>,
* <tt>closeBoxMargin</tt>, <tt>closeBoxURL</tt>, and <tt>enableEventPropagation</tt>
* properties have no affect until the current InfoBox is <tt>close</tt>d and a new one
* is <tt>open</tt>ed.
* @param {InfoBoxOptions} opt_opts
*/
InfoBox.prototype.setOptions = function (opt_opts) {
if (typeof opt_opts.boxClass !== "undefined") { // Must be first
this.boxClass_ = opt_opts.boxClass;
this.setBoxStyle_();
}
if (typeof opt_opts.boxStyle !== "undefined") { // Must be second
this.boxStyle_ = opt_opts.boxStyle;
this.setBoxStyle_();
}
if (typeof opt_opts.content !== "undefined") {
this.setContent(opt_opts.content);
}
if (typeof opt_opts.disableAutoPan !== "undefined") {
this.disableAutoPan_ = opt_opts.disableAutoPan;
}
if (typeof opt_opts.maxWidth !== "undefined") {
this.maxWidth_ = opt_opts.maxWidth;
}
if (typeof opt_opts.pixelOffset !== "undefined") {
this.pixelOffset_ = opt_opts.pixelOffset;
}
if (typeof opt_opts.alignBottom !== "undefined") {
this.alignBottom_ = opt_opts.alignBottom;
}
if (typeof opt_opts.position !== "undefined") {
this.setPosition(opt_opts.position);
}
if (typeof opt_opts.zIndex !== "undefined") {
this.setZIndex(opt_opts.zIndex);
}
if (typeof opt_opts.closeBoxMargin !== "undefined") {
this.closeBoxMargin_ = opt_opts.closeBoxMargin;
}
if (typeof opt_opts.closeBoxURL !== "undefined") {
this.closeBoxURL_ = opt_opts.closeBoxURL;
}
if (typeof opt_opts.infoBoxClearance !== "undefined") {
this.infoBoxClearance_ = opt_opts.infoBoxClearance;
}
if (typeof opt_opts.isHidden !== "undefined") {
this.isHidden_ = opt_opts.isHidden;
}
if (typeof opt_opts.visible !== "undefined") {
this.isHidden_ = !opt_opts.visible;
}
if (typeof opt_opts.enableEventPropagation !== "undefined") {
this.enableEventPropagation_ = opt_opts.enableEventPropagation;
}
if (this.div_) {
this.draw();
}
};
/**
* Sets the content of the InfoBox.
* The content can be plain text or an HTML DOM node.
* @param {string|Node} content
*/
InfoBox.prototype.setContent = function (content) {
this.content_ = content;
if (this.div_) {
if (this.closeListener_) {
google.maps.event.removeListener(this.closeListener_);
this.closeListener_ = null;
}
// Odd code required to make things work with MSIE.
//
if (!this.fixedWidthSet_) {
this.div_.style.width = "";
}
if (typeof content.nodeType === "undefined") {
this.div_.innerHTML = this.getCloseBoxImg_() + content;
} else {
this.div_.innerHTML = this.getCloseBoxImg_();
this.div_.appendChild(content);
}
// Perverse code required to make things work with MSIE.
// (Ensures the close box does, in fact, float to the right.)
//
if (!this.fixedWidthSet_) {
this.div_.style.width = this.div_.offsetWidth + "px";
if (typeof content.nodeType === "undefined") {
this.div_.innerHTML = this.getCloseBoxImg_() + content;
} else {
this.div_.innerHTML = this.getCloseBoxImg_();
this.div_.appendChild(content);
}
}
this.addClickHandler_();
}
/**
* This event is fired when the content of the InfoBox changes.
* @name InfoBox#content_changed
* @event
*/
google.maps.event.trigger(this, "content_changed");
};
/**
* Sets the geographic location of the InfoBox.
* @param {LatLng} latlng
*/
InfoBox.prototype.setPosition = function (latlng) {
this.position_ = latlng;
if (this.div_) {
this.draw();
}
/**
* This event is fired when the position of the InfoBox changes.
* @name InfoBox#position_changed
* @event
*/
google.maps.event.trigger(this, "position_changed");
};
/**
* Sets the zIndex style for the InfoBox.
* @param {number} index
*/
InfoBox.prototype.setZIndex = function (index) {
this.zIndex_ = index;
if (this.div_) {
this.div_.style.zIndex = index;
}
/**
* This event is fired when the zIndex of the InfoBox changes.
* @name InfoBox#zindex_changed
* @event
*/
google.maps.event.trigger(this, "zindex_changed");
};
/**
* Sets the visibility of the InfoBox.
* @param {boolean} isVisible
*/
InfoBox.prototype.setVisible = function (isVisible) {
this.isHidden_ = !isVisible;
if (this.div_) {
this.div_.style.visibility = (this.isHidden_ ? "hidden" : "visible");
}
};
/**
* Returns the content of the InfoBox.
* @returns {string}
*/
InfoBox.prototype.getContent = function () {
return this.content_;
};
/**
* Returns the geographic location of the InfoBox.
* @returns {LatLng}
*/
InfoBox.prototype.getPosition = function () {
return this.position_;
};
/**
* Returns the zIndex for the InfoBox.
* @returns {number}
*/
InfoBox.prototype.getZIndex = function () {
return this.zIndex_;
};
/**
* Returns a flag indicating whether the InfoBox is visible.
* @returns {boolean}
*/
InfoBox.prototype.getVisible = function () {
var isVisible;
if ((typeof this.getMap() === "undefined") || (this.getMap() === null)) {
isVisible = false;
} else {
isVisible = !this.isHidden_;
}
return isVisible;
};
/**
* Shows the InfoBox. [Deprecated; use <tt>setVisible</tt> instead.]
*/
InfoBox.prototype.show = function () {
this.isHidden_ = false;
if (this.div_) {
this.div_.style.visibility = "visible";
}
};
/**
* Hides the InfoBox. [Deprecated; use <tt>setVisible</tt> instead.]
*/
InfoBox.prototype.hide = function () {
this.isHidden_ = true;
if (this.div_) {
this.div_.style.visibility = "hidden";
}
};
/**
* Adds the InfoBox to the specified map or Street View panorama. If <tt>anchor</tt>
* (usually a <tt>google.maps.Marker</tt>) is specified, the position
* of the InfoBox is set to the position of the <tt>anchor</tt>. If the
* anchor is dragged to a new location, the InfoBox moves as well.
* @param {Map|StreetViewPanorama} map
* @param {MVCObject} [anchor]
*/
InfoBox.prototype.open = function (map, anchor) {
var me = this;
if (anchor) {
this.position_ = anchor.getPosition();
this.moveListener_ = google.maps.event.addListener(anchor, "position_changed", function () {
me.setPosition(this.getPosition());
});
}
this.setMap(map);
if (this.div_) {
this.panBox_();
}
};
/**
* Removes the InfoBox from the map.
*/
InfoBox.prototype.close = function () {
var i;
if (this.closeListener_) {
google.maps.event.removeListener(this.closeListener_);
this.closeListener_ = null;
}
if (this.eventListeners_) {
for (i = 0; i < this.eventListeners_.length; i++) {
google.maps.event.removeListener(this.eventListeners_[i]);
}
this.eventListeners_ = null;
}
if (this.moveListener_) {
google.maps.event.removeListener(this.moveListener_);
this.moveListener_ = null;
}
if (this.contextListener_) {
google.maps.event.removeListener(this.contextListener_);
this.contextListener_ = null;
}
this.setMap(null);
};;/**
* @name MarkerClustererPlus for Google Maps V3
* @version 2.1.1 [November 4, 2013]
* @author Gary Little
* @fileoverview
* The library creates and manages per-zoom-level clusters for large amounts of markers.
* <p>
* This is an enhanced V3 implementation of the
* <a href="http://gmaps-utility-library-dev.googlecode.com/svn/tags/markerclusterer/"
* >V2 MarkerClusterer</a> by Xiaoxi Wu. It is based on the
* <a href="http://google-maps-utility-library-v3.googlecode.com/svn/tags/markerclusterer/"
* >V3 MarkerClusterer</a> port by Luke Mahe. MarkerClustererPlus was created by Gary Little.
* <p>
* v2.0 release: MarkerClustererPlus v2.0 is backward compatible with MarkerClusterer v1.0. It
* adds support for the <code>ignoreHidden</code>, <code>title</code>, <code>batchSizeIE</code>,
* and <code>calculator</code> properties as well as support for four more events. It also allows
* greater control over the styling of the text that appears on the cluster marker. The
* documentation has been significantly improved and the overall code has been simplified and
* polished. Very large numbers of markers can now be managed without causing Javascript timeout
* errors on Internet Explorer. Note that the name of the <code>clusterclick</code> event has been
* deprecated. The new name is <code>click</code>, so please change your application code now.
*/
/**
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* @name ClusterIconStyle
* @class This class represents the object for values in the <code>styles</code> array passed
* to the {@link MarkerClusterer} constructor. The element in this array that is used to
* style the cluster icon is determined by calling the <code>calculator</code> function.
*
* @property {string} url The URL of the cluster icon image file. Required.
* @property {number} height The display height (in pixels) of the cluster icon. Required.
* @property {number} width The display width (in pixels) of the cluster icon. Required.
* @property {Array} [anchorText] The position (in pixels) from the center of the cluster icon to
* where the text label is to be centered and drawn. The format is <code>[yoffset, xoffset]</code>
* where <code>yoffset</code> increases as you go down from center and <code>xoffset</code>
* increases to the right of center. The default is <code>[0, 0]</code>.
* @property {Array} [anchorIcon] The anchor position (in pixels) of the cluster icon. This is the
* spot on the cluster icon that is to be aligned with the cluster position. The format is
* <code>[yoffset, xoffset]</code> where <code>yoffset</code> increases as you go down and
* <code>xoffset</code> increases to the right of the top-left corner of the icon. The default
* anchor position is the center of the cluster icon.
* @property {string} [textColor="black"] The color of the label text shown on the
* cluster icon.
* @property {number} [textSize=11] The size (in pixels) of the label text shown on the
* cluster icon.
* @property {string} [textDecoration="none"] The value of the CSS <code>text-decoration</code>
* property for the label text shown on the cluster icon.
* @property {string} [fontWeight="bold"] The value of the CSS <code>font-weight</code>
* property for the label text shown on the cluster icon.
* @property {string} [fontStyle="normal"] The value of the CSS <code>font-style</code>
* property for the label text shown on the cluster icon.
* @property {string} [fontFamily="Arial,sans-serif"] The value of the CSS <code>font-family</code>
* property for the label text shown on the cluster icon.
* @property {string} [backgroundPosition="0 0"] The position of the cluster icon image
* within the image defined by <code>url</code>. The format is <code>"xpos ypos"</code>
* (the same format as for the CSS <code>background-position</code> property). You must set
* this property appropriately when the image defined by <code>url</code> represents a sprite
* containing multiple images. Note that the position <i>must</i> be specified in px units.
*/
/**
* @name ClusterIconInfo
* @class This class is an object containing general information about a cluster icon. This is
* the object that a <code>calculator</code> function returns.
*
* @property {string} text The text of the label to be shown on the cluster icon.
* @property {number} index The index plus 1 of the element in the <code>styles</code>
* array to be used to style the cluster icon.
* @property {string} title The tooltip to display when the mouse moves over the cluster icon.
* If this value is <code>undefined</code> or <code>""</code>, <code>title</code> is set to the
* value of the <code>title</code> property passed to the MarkerClusterer.
*/
/**
* A cluster icon.
*
* @constructor
* @extends google.maps.OverlayView
* @param {Cluster} cluster The cluster with which the icon is to be associated.
* @param {Array} [styles] An array of {@link ClusterIconStyle} defining the cluster icons
* to use for various cluster sizes.
* @private
*/
function ClusterIcon(cluster, styles) {
cluster.getMarkerClusterer().extend(ClusterIcon, google.maps.OverlayView);
this.cluster_ = cluster;
this.className_ = cluster.getMarkerClusterer().getClusterClass();
this.styles_ = styles;
this.center_ = null;
this.div_ = null;
this.sums_ = null;
this.visible_ = false;
this.setMap(cluster.getMap()); // Note: this causes onAdd to be called
}
/**
* Adds the icon to the DOM.
*/
ClusterIcon.prototype.onAdd = function () {
var cClusterIcon = this;
var cMouseDownInCluster;
var cDraggingMapByCluster;
this.div_ = document.createElement("div");
this.div_.className = this.className_;
if (this.visible_) {
this.show();
}
this.getPanes().overlayMouseTarget.appendChild(this.div_);
// Fix for Issue 157
this.boundsChangedListener_ = google.maps.event.addListener(this.getMap(), "bounds_changed", function () {
cDraggingMapByCluster = cMouseDownInCluster;
});
google.maps.event.addDomListener(this.div_, "mousedown", function () {
cMouseDownInCluster = true;
cDraggingMapByCluster = false;
});
google.maps.event.addDomListener(this.div_, "click", function (e) {
cMouseDownInCluster = false;
if (!cDraggingMapByCluster) {
var theBounds;
var mz;
var mc = cClusterIcon.cluster_.getMarkerClusterer();
/**
* This event is fired when a cluster marker is clicked.
* @name MarkerClusterer#click
* @param {Cluster} c The cluster that was clicked.
* @event
*/
google.maps.event.trigger(mc, "click", cClusterIcon.cluster_);
google.maps.event.trigger(mc, "clusterclick", cClusterIcon.cluster_); // deprecated name
// The default click handler follows. Disable it by setting
// the zoomOnClick property to false.
if (mc.getZoomOnClick()) {
// Zoom into the cluster.
mz = mc.getMaxZoom();
theBounds = cClusterIcon.cluster_.getBounds();
mc.getMap().fitBounds(theBounds);
// There is a fix for Issue 170 here:
setTimeout(function () {
mc.getMap().fitBounds(theBounds);
// Don't zoom beyond the max zoom level
if (mz !== null && (mc.getMap().getZoom() > mz)) {
mc.getMap().setZoom(mz + 1);
}
}, 100);
}
// Prevent event propagation to the map:
e.cancelBubble = true;
if (e.stopPropagation) {
e.stopPropagation();
}
}
});
google.maps.event.addDomListener(this.div_, "mouseover", function () {
var mc = cClusterIcon.cluster_.getMarkerClusterer();
/**
* This event is fired when the mouse moves over a cluster marker.
* @name MarkerClusterer#mouseover
* @param {Cluster} c The cluster that the mouse moved over.
* @event
*/
google.maps.event.trigger(mc, "mouseover", cClusterIcon.cluster_);
});
google.maps.event.addDomListener(this.div_, "mouseout", function () {
var mc = cClusterIcon.cluster_.getMarkerClusterer();
/**
* This event is fired when the mouse moves out of a cluster marker.
* @name MarkerClusterer#mouseout
* @param {Cluster} c The cluster that the mouse moved out of.
* @event
*/
google.maps.event.trigger(mc, "mouseout", cClusterIcon.cluster_);
});
};
/**
* Removes the icon from the DOM.
*/
ClusterIcon.prototype.onRemove = function () {
if (this.div_ && this.div_.parentNode) {
this.hide();
google.maps.event.removeListener(this.boundsChangedListener_);
google.maps.event.clearInstanceListeners(this.div_);
this.div_.parentNode.removeChild(this.div_);
this.div_ = null;
}
};
/**
* Draws the icon.
*/
ClusterIcon.prototype.draw = function () {
if (this.visible_) {
var pos = this.getPosFromLatLng_(this.center_);
this.div_.style.top = pos.y + "px";
this.div_.style.left = pos.x + "px";
}
};
/**
* Hides the icon.
*/
ClusterIcon.prototype.hide = function () {
if (this.div_) {
this.div_.style.display = "none";
}
this.visible_ = false;
};
/**
* Positions and shows the icon.
*/
ClusterIcon.prototype.show = function () {
if (this.div_) {
var img = "";
// NOTE: values must be specified in px units
var bp = this.backgroundPosition_.split(" ");
var spriteH = parseInt(bp[0].trim(), 10);
var spriteV = parseInt(bp[1].trim(), 10);
var pos = this.getPosFromLatLng_(this.center_);
this.div_.style.cssText = this.createCss(pos);
img = "<img src='" + this.url_ + "' style='position: absolute; top: " + spriteV + "px; left: " + spriteH + "px; ";
if (!this.cluster_.getMarkerClusterer().enableRetinaIcons_) {
img += "clip: rect(" + (-1 * spriteV) + "px, " + ((-1 * spriteH) + this.width_) + "px, " +
((-1 * spriteV) + this.height_) + "px, " + (-1 * spriteH) + "px);";
}
img += "'>";
this.div_.innerHTML = img + "<div style='" +
"position: absolute;" +
"top: " + this.anchorText_[0] + "px;" +
"left: " + this.anchorText_[1] + "px;" +
"color: " + this.textColor_ + ";" +
"font-size: " + this.textSize_ + "px;" +
"font-family: " + this.fontFamily_ + ";" +
"font-weight: " + this.fontWeight_ + ";" +
"font-style: " + this.fontStyle_ + ";" +
"text-decoration: " + this.textDecoration_ + ";" +
"text-align: center;" +
"width: " + this.width_ + "px;" +
"line-height:" + this.height_ + "px;" +
"'>" + this.sums_.text + "</div>";
if (typeof this.sums_.title === "undefined" || this.sums_.title === "") {
this.div_.title = this.cluster_.getMarkerClusterer().getTitle();
} else {
this.div_.title = this.sums_.title;
}
this.div_.style.display = "";
}
this.visible_ = true;
};
/**
* Sets the icon styles to the appropriate element in the styles array.
*
* @param {ClusterIconInfo} sums The icon label text and styles index.
*/
ClusterIcon.prototype.useStyle = function (sums) {
this.sums_ = sums;
var index = Math.max(0, sums.index - 1);
index = Math.min(this.styles_.length - 1, index);
var style = this.styles_[index];
this.url_ = style.url;
this.height_ = style.height;
this.width_ = style.width;
this.anchorText_ = style.anchorText || [0, 0];
this.anchorIcon_ = style.anchorIcon || [parseInt(this.height_ / 2, 10), parseInt(this.width_ / 2, 10)];
this.textColor_ = style.textColor || "black";
this.textSize_ = style.textSize || 11;
this.textDecoration_ = style.textDecoration || "none";
this.fontWeight_ = style.fontWeight || "bold";
this.fontStyle_ = style.fontStyle || "normal";
this.fontFamily_ = style.fontFamily || "Arial,sans-serif";
this.backgroundPosition_ = style.backgroundPosition || "0 0";
};
/**
* Sets the position at which to center the icon.
*
* @param {google.maps.LatLng} center The latlng to set as the center.
*/
ClusterIcon.prototype.setCenter = function (center) {
this.center_ = center;
};
/**
* Creates the cssText style parameter based on the position of the icon.
*
* @param {google.maps.Point} pos The position of the icon.
* @return {string} The CSS style text.
*/
ClusterIcon.prototype.createCss = function (pos) {
var style = [];
style.push("cursor: pointer;");
style.push("position: absolute; top: " + pos.y + "px; left: " + pos.x + "px;");
style.push("width: " + this.width_ + "px; height: " + this.height_ + "px;");
return style.join("");
};
/**
* Returns the position at which to place the DIV depending on the latlng.
*
* @param {google.maps.LatLng} latlng The position in latlng.
* @return {google.maps.Point} The position in pixels.
*/
ClusterIcon.prototype.getPosFromLatLng_ = function (latlng) {
var pos = this.getProjection().fromLatLngToDivPixel(latlng);
pos.x -= this.anchorIcon_[1];
pos.y -= this.anchorIcon_[0];
pos.x = parseInt(pos.x, 10);
pos.y = parseInt(pos.y, 10);
return pos;
};
/**
* Creates a single cluster that manages a group of proximate markers.
* Used internally, do not call this constructor directly.
* @constructor
* @param {MarkerClusterer} mc The <code>MarkerClusterer</code> object with which this
* cluster is associated.
*/
function Cluster(mc) {
this.markerClusterer_ = mc;
this.map_ = mc.getMap();
this.gridSize_ = mc.getGridSize();
this.minClusterSize_ = mc.getMinimumClusterSize();
this.averageCenter_ = mc.getAverageCenter();
this.markers_ = [];
this.center_ = null;
this.bounds_ = null;
this.clusterIcon_ = new ClusterIcon(this, mc.getStyles());
}
/**
* Returns the number of markers managed by the cluster. You can call this from
* a <code>click</code>, <code>mouseover</code>, or <code>mouseout</code> event handler
* for the <code>MarkerClusterer</code> object.
*
* @return {number} The number of markers in the cluster.
*/
Cluster.prototype.getSize = function () {
return this.markers_.length;
};
/**
* Returns the array of markers managed by the cluster. You can call this from
* a <code>click</code>, <code>mouseover</code>, or <code>mouseout</code> event handler
* for the <code>MarkerClusterer</code> object.
*
* @return {Array} The array of markers in the cluster.
*/
Cluster.prototype.getMarkers = function () {
return this.markers_;
};
/**
* Returns the center of the cluster. You can call this from
* a <code>click</code>, <code>mouseover</code>, or <code>mouseout</code> event handler
* for the <code>MarkerClusterer</code> object.
*
* @return {google.maps.LatLng} The center of the cluster.
*/
Cluster.prototype.getCenter = function () {
return this.center_;
};
/**
* Returns the map with which the cluster is associated.
*
* @return {google.maps.Map} The map.
* @ignore
*/
Cluster.prototype.getMap = function () {
return this.map_;
};
/**
* Returns the <code>MarkerClusterer</code> object with which the cluster is associated.
*
* @return {MarkerClusterer} The associated marker clusterer.
* @ignore
*/
Cluster.prototype.getMarkerClusterer = function () {
return this.markerClusterer_;
};
/**
* Returns the bounds of the cluster.
*
* @return {google.maps.LatLngBounds} the cluster bounds.
* @ignore
*/
Cluster.prototype.getBounds = function () {
var i;
var bounds = new google.maps.LatLngBounds(this.center_, this.center_);
var markers = this.getMarkers();
for (i = 0; i < markers.length; i++) {
bounds.extend(markers[i].getPosition());
}
return bounds;
};
/**
* Removes the cluster from the map.
*
* @ignore
*/
Cluster.prototype.remove = function () {
this.clusterIcon_.setMap(null);
this.markers_ = [];
delete this.markers_;
};
/**
* Adds a marker to the cluster.
*
* @param {google.maps.Marker} marker The marker to be added.
* @return {boolean} True if the marker was added.
* @ignore
*/
Cluster.prototype.addMarker = function (marker) {
var i;
var mCount;
var mz;
if (this.isMarkerAlreadyAdded_(marker)) {
return false;
}
if (!this.center_) {
this.center_ = marker.getPosition();
this.calculateBounds_();
} else {
if (this.averageCenter_) {
var l = this.markers_.length + 1;
var lat = (this.center_.lat() * (l - 1) + marker.getPosition().lat()) / l;
var lng = (this.center_.lng() * (l - 1) + marker.getPosition().lng()) / l;
this.center_ = new google.maps.LatLng(lat, lng);
this.calculateBounds_();
}
}
marker.isAdded = true;
this.markers_.push(marker);
mCount = this.markers_.length;
mz = this.markerClusterer_.getMaxZoom();
if (mz !== null && this.map_.getZoom() > mz) {
// Zoomed in past max zoom, so show the marker.
if (marker.getMap() !== this.map_) {
marker.setMap(this.map_);
}
} else if (mCount < this.minClusterSize_) {
// Min cluster size not reached so show the marker.
if (marker.getMap() !== this.map_) {
marker.setMap(this.map_);
}
} else if (mCount === this.minClusterSize_) {
// Hide the markers that were showing.
for (i = 0; i < mCount; i++) {
this.markers_[i].setMap(null);
}
} else {
marker.setMap(null);
}
this.updateIcon_();
return true;
};
/**
* Determines if a marker lies within the cluster's bounds.
*
* @param {google.maps.Marker} marker The marker to check.
* @return {boolean} True if the marker lies in the bounds.
* @ignore
*/
Cluster.prototype.isMarkerInClusterBounds = function (marker) {
return this.bounds_.contains(marker.getPosition());
};
/**
* Calculates the extended bounds of the cluster with the grid.
*/
Cluster.prototype.calculateBounds_ = function () {
var bounds = new google.maps.LatLngBounds(this.center_, this.center_);
this.bounds_ = this.markerClusterer_.getExtendedBounds(bounds);
};
/**
* Updates the cluster icon.
*/
Cluster.prototype.updateIcon_ = function () {
var mCount = this.markers_.length;
var mz = this.markerClusterer_.getMaxZoom();
if (mz !== null && this.map_.getZoom() > mz) {
this.clusterIcon_.hide();
return;
}
if (mCount < this.minClusterSize_) {
// Min cluster size not yet reached.
this.clusterIcon_.hide();
return;
}
var numStyles = this.markerClusterer_.getStyles().length;
var sums = this.markerClusterer_.getCalculator()(this.markers_, numStyles);
this.clusterIcon_.setCenter(this.center_);
this.clusterIcon_.useStyle(sums);
this.clusterIcon_.show();
};
/**
* Determines if a marker has already been added to the cluster.
*
* @param {google.maps.Marker} marker The marker to check.
* @return {boolean} True if the marker has already been added.
*/
Cluster.prototype.isMarkerAlreadyAdded_ = function (marker) {
var i;
if (this.markers_.indexOf) {
return this.markers_.indexOf(marker) !== -1;
} else {
for (i = 0; i < this.markers_.length; i++) {
if (marker === this.markers_[i]) {
return true;
}
}
}
return false;
};
/**
* @name MarkerClustererOptions
* @class This class represents the optional parameter passed to
* the {@link MarkerClusterer} constructor.
* @property {number} [gridSize=60] The grid size of a cluster in pixels. The grid is a square.
* @property {number} [maxZoom=null] The maximum zoom level at which clustering is enabled or
* <code>null</code> if clustering is to be enabled at all zoom levels.
* @property {boolean} [zoomOnClick=true] Whether to zoom the map when a cluster marker is
* clicked. You may want to set this to <code>false</code> if you have installed a handler
* for the <code>click</code> event and it deals with zooming on its own.
* @property {boolean} [averageCenter=false] Whether the position of a cluster marker should be
* the average position of all markers in the cluster. If set to <code>false</code>, the
* cluster marker is positioned at the location of the first marker added to the cluster.
* @property {number} [minimumClusterSize=2] The minimum number of markers needed in a cluster
* before the markers are hidden and a cluster marker appears.
* @property {boolean} [ignoreHidden=false] Whether to ignore hidden markers in clusters. You
* may want to set this to <code>true</code> to ensure that hidden markers are not included
* in the marker count that appears on a cluster marker (this count is the value of the
* <code>text</code> property of the result returned by the default <code>calculator</code>).
* If set to <code>true</code> and you change the visibility of a marker being clustered, be
* sure to also call <code>MarkerClusterer.repaint()</code>.
* @property {string} [title=""] The tooltip to display when the mouse moves over a cluster
* marker. (Alternatively, you can use a custom <code>calculator</code> function to specify a
* different tooltip for each cluster marker.)
* @property {function} [calculator=MarkerClusterer.CALCULATOR] The function used to determine
* the text to be displayed on a cluster marker and the index indicating which style to use
* for the cluster marker. The input parameters for the function are (1) the array of markers
* represented by a cluster marker and (2) the number of cluster icon styles. It returns a
* {@link ClusterIconInfo} object. The default <code>calculator</code> returns a
* <code>text</code> property which is the number of markers in the cluster and an
* <code>index</code> property which is one higher than the lowest integer such that
* <code>10^i</code> exceeds the number of markers in the cluster, or the size of the styles
* array, whichever is less. The <code>styles</code> array element used has an index of
* <code>index</code> minus 1. For example, the default <code>calculator</code> returns a
* <code>text</code> value of <code>"125"</code> and an <code>index</code> of <code>3</code>
* for a cluster icon representing 125 markers so the element used in the <code>styles</code>
* array is <code>2</code>. A <code>calculator</code> may also return a <code>title</code>
* property that contains the text of the tooltip to be used for the cluster marker. If
* <code>title</code> is not defined, the tooltip is set to the value of the <code>title</code>
* property for the MarkerClusterer.
* @property {string} [clusterClass="cluster"] The name of the CSS class defining general styles
* for the cluster markers. Use this class to define CSS styles that are not set up by the code
* that processes the <code>styles</code> array.
* @property {Array} [styles] An array of {@link ClusterIconStyle} elements defining the styles
* of the cluster markers to be used. The element to be used to style a given cluster marker
* is determined by the function defined by the <code>calculator</code> property.
* The default is an array of {@link ClusterIconStyle} elements whose properties are derived
* from the values for <code>imagePath</code>, <code>imageExtension</code>, and
* <code>imageSizes</code>.
* @property {boolean} [enableRetinaIcons=false] Whether to allow the use of cluster icons that
* have sizes that are some multiple (typically double) of their actual display size. Icons such
* as these look better when viewed on high-resolution monitors such as Apple's Retina displays.
* Note: if this property is <code>true</code>, sprites cannot be used as cluster icons.
* @property {number} [batchSize=MarkerClusterer.BATCH_SIZE] Set this property to the
* number of markers to be processed in a single batch when using a browser other than
* Internet Explorer (for Internet Explorer, use the batchSizeIE property instead).
* @property {number} [batchSizeIE=MarkerClusterer.BATCH_SIZE_IE] When Internet Explorer is
* being used, markers are processed in several batches with a small delay inserted between
* each batch in an attempt to avoid Javascript timeout errors. Set this property to the
* number of markers to be processed in a single batch; select as high a number as you can
* without causing a timeout error in the browser. This number might need to be as low as 100
* if 15,000 markers are being managed, for example.
* @property {string} [imagePath=MarkerClusterer.IMAGE_PATH]
* The full URL of the root name of the group of image files to use for cluster icons.
* The complete file name is of the form <code>imagePath</code>n.<code>imageExtension</code>
* where n is the image file number (1, 2, etc.).
* @property {string} [imageExtension=MarkerClusterer.IMAGE_EXTENSION]
* The extension name for the cluster icon image files (e.g., <code>"png"</code> or
* <code>"jpg"</code>).
* @property {Array} [imageSizes=MarkerClusterer.IMAGE_SIZES]
* An array of numbers containing the widths of the group of
* <code>imagePath</code>n.<code>imageExtension</code> image files.
* (The images are assumed to be square.)
*/
/**
* Creates a MarkerClusterer object with the options specified in {@link MarkerClustererOptions}.
* @constructor
* @extends google.maps.OverlayView
* @param {google.maps.Map} map The Google map to attach to.
* @param {Array.<google.maps.Marker>} [opt_markers] The markers to be added to the cluster.
* @param {MarkerClustererOptions} [opt_options] The optional parameters.
*/
function MarkerClusterer(map, opt_markers, opt_options) {
// MarkerClusterer implements google.maps.OverlayView interface. We use the
// extend function to extend MarkerClusterer with google.maps.OverlayView
// because it might not always be available when the code is defined so we
// look for it at the last possible moment. If it doesn't exist now then
// there is no point going ahead :)
this.extend(MarkerClusterer, google.maps.OverlayView);
opt_markers = opt_markers || [];
opt_options = opt_options || {};
this.markers_ = [];
this.clusters_ = [];
this.listeners_ = [];
this.activeMap_ = null;
this.ready_ = false;
this.gridSize_ = opt_options.gridSize || 60;
this.minClusterSize_ = opt_options.minimumClusterSize || 2;
this.maxZoom_ = opt_options.maxZoom || null;
this.styles_ = opt_options.styles || [];
this.title_ = opt_options.title || "";
this.zoomOnClick_ = true;
if (opt_options.zoomOnClick !== undefined) {
this.zoomOnClick_ = opt_options.zoomOnClick;
}
this.averageCenter_ = false;
if (opt_options.averageCenter !== undefined) {
this.averageCenter_ = opt_options.averageCenter;
}
this.ignoreHidden_ = false;
if (opt_options.ignoreHidden !== undefined) {
this.ignoreHidden_ = opt_options.ignoreHidden;
}
this.enableRetinaIcons_ = false;
if (opt_options.enableRetinaIcons !== undefined) {
this.enableRetinaIcons_ = opt_options.enableRetinaIcons;
}
this.imagePath_ = opt_options.imagePath || MarkerClusterer.IMAGE_PATH;
this.imageExtension_ = opt_options.imageExtension || MarkerClusterer.IMAGE_EXTENSION;
this.imageSizes_ = opt_options.imageSizes || MarkerClusterer.IMAGE_SIZES;
this.calculator_ = opt_options.calculator || MarkerClusterer.CALCULATOR;
this.batchSize_ = opt_options.batchSize || MarkerClusterer.BATCH_SIZE;
this.batchSizeIE_ = opt_options.batchSizeIE || MarkerClusterer.BATCH_SIZE_IE;
this.clusterClass_ = opt_options.clusterClass || "cluster";
if (navigator.userAgent.toLowerCase().indexOf("msie") !== -1) {
// Try to avoid IE timeout when processing a huge number of markers:
this.batchSize_ = this.batchSizeIE_;
}
this.setupStyles_();
this.addMarkers(opt_markers, true);
this.setMap(map); // Note: this causes onAdd to be called
}
/**
* Implementation of the onAdd interface method.
* @ignore
*/
MarkerClusterer.prototype.onAdd = function () {
var cMarkerClusterer = this;
this.activeMap_ = this.getMap();
this.ready_ = true;
this.repaint();
// Add the map event listeners
this.listeners_ = [
google.maps.event.addListener(this.getMap(), "zoom_changed", function () {
cMarkerClusterer.resetViewport_(false);
// Workaround for this Google bug: when map is at level 0 and "-" of
// zoom slider is clicked, a "zoom_changed" event is fired even though
// the map doesn't zoom out any further. In this situation, no "idle"
// event is triggered so the cluster markers that have been removed
// do not get redrawn. Same goes for a zoom in at maxZoom.
if (this.getZoom() === (this.get("minZoom") || 0) || this.getZoom() === this.get("maxZoom")) {
google.maps.event.trigger(this, "idle");
}
}),
google.maps.event.addListener(this.getMap(), "idle", function () {
cMarkerClusterer.redraw_();
})
];
};
/**
* Implementation of the onRemove interface method.
* Removes map event listeners and all cluster icons from the DOM.
* All managed markers are also put back on the map.
* @ignore
*/
MarkerClusterer.prototype.onRemove = function () {
var i;
// Put all the managed markers back on the map:
for (i = 0; i < this.markers_.length; i++) {
if (this.markers_[i].getMap() !== this.activeMap_) {
this.markers_[i].setMap(this.activeMap_);
}
}
// Remove all clusters:
for (i = 0; i < this.clusters_.length; i++) {
this.clusters_[i].remove();
}
this.clusters_ = [];
// Remove map event listeners:
for (i = 0; i < this.listeners_.length; i++) {
google.maps.event.removeListener(this.listeners_[i]);
}
this.listeners_ = [];
this.activeMap_ = null;
this.ready_ = false;
};
/**
* Implementation of the draw interface method.
* @ignore
*/
MarkerClusterer.prototype.draw = function () {};
/**
* Sets up the styles object.
*/
MarkerClusterer.prototype.setupStyles_ = function () {
var i, size;
if (this.styles_.length > 0) {
return;
}
for (i = 0; i < this.imageSizes_.length; i++) {
size = this.imageSizes_[i];
this.styles_.push({
url: this.imagePath_ + (i + 1) + "." + this.imageExtension_,
height: size,
width: size
});
}
};
/**
* Fits the map to the bounds of the markers managed by the clusterer.
*/
MarkerClusterer.prototype.fitMapToMarkers = function () {
var i;
var markers = this.getMarkers();
var bounds = new google.maps.LatLngBounds();
for (i = 0; i < markers.length; i++) {
bounds.extend(markers[i].getPosition());
}
this.getMap().fitBounds(bounds);
};
/**
* Returns the value of the <code>gridSize</code> property.
*
* @return {number} The grid size.
*/
MarkerClusterer.prototype.getGridSize = function () {
return this.gridSize_;
};
/**
* Sets the value of the <code>gridSize</code> property.
*
* @param {number} gridSize The grid size.
*/
MarkerClusterer.prototype.setGridSize = function (gridSize) {
this.gridSize_ = gridSize;
};
/**
* Returns the value of the <code>minimumClusterSize</code> property.
*
* @return {number} The minimum cluster size.
*/
MarkerClusterer.prototype.getMinimumClusterSize = function () {
return this.minClusterSize_;
};
/**
* Sets the value of the <code>minimumClusterSize</code> property.
*
* @param {number} minimumClusterSize The minimum cluster size.
*/
MarkerClusterer.prototype.setMinimumClusterSize = function (minimumClusterSize) {
this.minClusterSize_ = minimumClusterSize;
};
/**
* Returns the value of the <code>maxZoom</code> property.
*
* @return {number} The maximum zoom level.
*/
MarkerClusterer.prototype.getMaxZoom = function () {
return this.maxZoom_;
};
/**
* Sets the value of the <code>maxZoom</code> property.
*
* @param {number} maxZoom The maximum zoom level.
*/
MarkerClusterer.prototype.setMaxZoom = function (maxZoom) {
this.maxZoom_ = maxZoom;
};
/**
* Returns the value of the <code>styles</code> property.
*
* @return {Array} The array of styles defining the cluster markers to be used.
*/
MarkerClusterer.prototype.getStyles = function () {
return this.styles_;
};
/**
* Sets the value of the <code>styles</code> property.
*
* @param {Array.<ClusterIconStyle>} styles The array of styles to use.
*/
MarkerClusterer.prototype.setStyles = function (styles) {
this.styles_ = styles;
};
/**
* Returns the value of the <code>title</code> property.
*
* @return {string} The content of the title text.
*/
MarkerClusterer.prototype.getTitle = function () {
return this.title_;
};
/**
* Sets the value of the <code>title</code> property.
*
* @param {string} title The value of the title property.
*/
MarkerClusterer.prototype.setTitle = function (title) {
this.title_ = title;
};
/**
* Returns the value of the <code>zoomOnClick</code> property.
*
* @return {boolean} True if zoomOnClick property is set.
*/
MarkerClusterer.prototype.getZoomOnClick = function () {
return this.zoomOnClick_;
};
/**
* Sets the value of the <code>zoomOnClick</code> property.
*
* @param {boolean} zoomOnClick The value of the zoomOnClick property.
*/
MarkerClusterer.prototype.setZoomOnClick = function (zoomOnClick) {
this.zoomOnClick_ = zoomOnClick;
};
/**
* Returns the value of the <code>averageCenter</code> property.
*
* @return {boolean} True if averageCenter property is set.
*/
MarkerClusterer.prototype.getAverageCenter = function () {
return this.averageCenter_;
};
/**
* Sets the value of the <code>averageCenter</code> property.
*
* @param {boolean} averageCenter The value of the averageCenter property.
*/
MarkerClusterer.prototype.setAverageCenter = function (averageCenter) {
this.averageCenter_ = averageCenter;
};
/**
* Returns the value of the <code>ignoreHidden</code> property.
*
* @return {boolean} True if ignoreHidden property is set.
*/
MarkerClusterer.prototype.getIgnoreHidden = function () {
return this.ignoreHidden_;
};
/**
* Sets the value of the <code>ignoreHidden</code> property.
*
* @param {boolean} ignoreHidden The value of the ignoreHidden property.
*/
MarkerClusterer.prototype.setIgnoreHidden = function (ignoreHidden) {
this.ignoreHidden_ = ignoreHidden;
};
/**
* Returns the value of the <code>enableRetinaIcons</code> property.
*
* @return {boolean} True if enableRetinaIcons property is set.
*/
MarkerClusterer.prototype.getEnableRetinaIcons = function () {
return this.enableRetinaIcons_;
};
/**
* Sets the value of the <code>enableRetinaIcons</code> property.
*
* @param {boolean} enableRetinaIcons The value of the enableRetinaIcons property.
*/
MarkerClusterer.prototype.setEnableRetinaIcons = function (enableRetinaIcons) {
this.enableRetinaIcons_ = enableRetinaIcons;
};
/**
* Returns the value of the <code>imageExtension</code> property.
*
* @return {string} The value of the imageExtension property.
*/
MarkerClusterer.prototype.getImageExtension = function () {
return this.imageExtension_;
};
/**
* Sets the value of the <code>imageExtension</code> property.
*
* @param {string} imageExtension The value of the imageExtension property.
*/
MarkerClusterer.prototype.setImageExtension = function (imageExtension) {
this.imageExtension_ = imageExtension;
};
/**
* Returns the value of the <code>imagePath</code> property.
*
* @return {string} The value of the imagePath property.
*/
MarkerClusterer.prototype.getImagePath = function () {
return this.imagePath_;
};
/**
* Sets the value of the <code>imagePath</code> property.
*
* @param {string} imagePath The value of the imagePath property.
*/
MarkerClusterer.prototype.setImagePath = function (imagePath) {
this.imagePath_ = imagePath;
};
/**
* Returns the value of the <code>imageSizes</code> property.
*
* @return {Array} The value of the imageSizes property.
*/
MarkerClusterer.prototype.getImageSizes = function () {
return this.imageSizes_;
};
/**
* Sets the value of the <code>imageSizes</code> property.
*
* @param {Array} imageSizes The value of the imageSizes property.
*/
MarkerClusterer.prototype.setImageSizes = function (imageSizes) {
this.imageSizes_ = imageSizes;
};
/**
* Returns the value of the <code>calculator</code> property.
*
* @return {function} the value of the calculator property.
*/
MarkerClusterer.prototype.getCalculator = function () {
return this.calculator_;
};
/**
* Sets the value of the <code>calculator</code> property.
*
* @param {function(Array.<google.maps.Marker>, number)} calculator The value
* of the calculator property.
*/
MarkerClusterer.prototype.setCalculator = function (calculator) {
this.calculator_ = calculator;
};
/**
* Returns the value of the <code>batchSizeIE</code> property.
*
* @return {number} the value of the batchSizeIE property.
*/
MarkerClusterer.prototype.getBatchSizeIE = function () {
return this.batchSizeIE_;
};
/**
* Sets the value of the <code>batchSizeIE</code> property.
*
* @param {number} batchSizeIE The value of the batchSizeIE property.
*/
MarkerClusterer.prototype.setBatchSizeIE = function (batchSizeIE) {
this.batchSizeIE_ = batchSizeIE;
};
/**
* Returns the value of the <code>clusterClass</code> property.
*
* @return {string} the value of the clusterClass property.
*/
MarkerClusterer.prototype.getClusterClass = function () {
return this.clusterClass_;
};
/**
* Sets the value of the <code>clusterClass</code> property.
*
* @param {string} clusterClass The value of the clusterClass property.
*/
MarkerClusterer.prototype.setClusterClass = function (clusterClass) {
this.clusterClass_ = clusterClass;
};
/**
* Returns the array of markers managed by the clusterer.
*
* @return {Array} The array of markers managed by the clusterer.
*/
MarkerClusterer.prototype.getMarkers = function () {
return this.markers_;
};
/**
* Returns the number of markers managed by the clusterer.
*
* @return {number} The number of markers.
*/
MarkerClusterer.prototype.getTotalMarkers = function () {
return this.markers_.length;
};
/**
* Returns the current array of clusters formed by the clusterer.
*
* @return {Array} The array of clusters formed by the clusterer.
*/
MarkerClusterer.prototype.getClusters = function () {
return this.clusters_;
};
/**
* Returns the number of clusters formed by the clusterer.
*
* @return {number} The number of clusters formed by the clusterer.
*/
MarkerClusterer.prototype.getTotalClusters = function () {
return this.clusters_.length;
};
/**
* Adds a marker to the clusterer. The clusters are redrawn unless
* <code>opt_nodraw</code> is set to <code>true</code>.
*
* @param {google.maps.Marker} marker The marker to add.
* @param {boolean} [opt_nodraw] Set to <code>true</code> to prevent redrawing.
*/
MarkerClusterer.prototype.addMarker = function (marker, opt_nodraw) {
this.pushMarkerTo_(marker);
if (!opt_nodraw) {
this.redraw_();
}
};
/**
* Adds an array of markers to the clusterer. The clusters are redrawn unless
* <code>opt_nodraw</code> is set to <code>true</code>.
*
* @param {Array.<google.maps.Marker>} markers The markers to add.
* @param {boolean} [opt_nodraw] Set to <code>true</code> to prevent redrawing.
*/
MarkerClusterer.prototype.addMarkers = function (markers, opt_nodraw) {
var key;
for (key in markers) {
if (markers.hasOwnProperty(key)) {
this.pushMarkerTo_(markers[key]);
}
}
if (!opt_nodraw) {
this.redraw_();
}
};
/**
* Pushes a marker to the clusterer.
*
* @param {google.maps.Marker} marker The marker to add.
*/
MarkerClusterer.prototype.pushMarkerTo_ = function (marker) {
// If the marker is draggable add a listener so we can update the clusters on the dragend:
if (marker.getDraggable()) {
var cMarkerClusterer = this;
google.maps.event.addListener(marker, "dragend", function () {
if (cMarkerClusterer.ready_) {
this.isAdded = false;
cMarkerClusterer.repaint();
}
});
}
marker.isAdded = false;
this.markers_.push(marker);
};
/**
* Removes a marker from the cluster. The clusters are redrawn unless
* <code>opt_nodraw</code> is set to <code>true</code>. Returns <code>true</code> if the
* marker was removed from the clusterer.
*
* @param {google.maps.Marker} marker The marker to remove.
* @param {boolean} [opt_nodraw] Set to <code>true</code> to prevent redrawing.
* @return {boolean} True if the marker was removed from the clusterer.
*/
MarkerClusterer.prototype.removeMarker = function (marker, opt_nodraw) {
var removed = this.removeMarker_(marker);
if (!opt_nodraw && removed) {
this.repaint();
}
return removed;
};
/**
* Removes an array of markers from the cluster. The clusters are redrawn unless
* <code>opt_nodraw</code> is set to <code>true</code>. Returns <code>true</code> if markers
* were removed from the clusterer.
*
* @param {Array.<google.maps.Marker>} markers The markers to remove.
* @param {boolean} [opt_nodraw] Set to <code>true</code> to prevent redrawing.
* @return {boolean} True if markers were removed from the clusterer.
*/
MarkerClusterer.prototype.removeMarkers = function (markers, opt_nodraw) {
var i, r;
var removed = false;
for (i = 0; i < markers.length; i++) {
r = this.removeMarker_(markers[i]);
removed = removed || r;
}
if (!opt_nodraw && removed) {
this.repaint();
}
return removed;
};
/**
* Removes a marker and returns true if removed, false if not.
*
* @param {google.maps.Marker} marker The marker to remove
* @return {boolean} Whether the marker was removed or not
*/
MarkerClusterer.prototype.removeMarker_ = function (marker) {
var i;
var index = -1;
if (this.markers_.indexOf) {
index = this.markers_.indexOf(marker);
} else {
for (i = 0; i < this.markers_.length; i++) {
if (marker === this.markers_[i]) {
index = i;
break;
}
}
}
if (index === -1) {
// Marker is not in our list of markers, so do nothing:
return false;
}
marker.setMap(null);
this.markers_.splice(index, 1); // Remove the marker from the list of managed markers
return true;
};
/**
* Removes all clusters and markers from the map and also removes all markers
* managed by the clusterer.
*/
MarkerClusterer.prototype.clearMarkers = function () {
this.resetViewport_(true);
this.markers_ = [];
};
/**
* Recalculates and redraws all the marker clusters from scratch.
* Call this after changing any properties.
*/
MarkerClusterer.prototype.repaint = function () {
var oldClusters = this.clusters_.slice();
this.clusters_ = [];
this.resetViewport_(false);
this.redraw_();
// Remove the old clusters.
// Do it in a timeout to prevent blinking effect.
setTimeout(function () {
var i;
for (i = 0; i < oldClusters.length; i++) {
oldClusters[i].remove();
}
}, 0);
};
/**
* Returns the current bounds extended by the grid size.
*
* @param {google.maps.LatLngBounds} bounds The bounds to extend.
* @return {google.maps.LatLngBounds} The extended bounds.
* @ignore
*/
MarkerClusterer.prototype.getExtendedBounds = function (bounds) {
var projection = this.getProjection();
// Turn the bounds into latlng.
var tr = new google.maps.LatLng(bounds.getNorthEast().lat(),
bounds.getNorthEast().lng());
var bl = new google.maps.LatLng(bounds.getSouthWest().lat(),
bounds.getSouthWest().lng());
// Convert the points to pixels and the extend out by the grid size.
var trPix = projection.fromLatLngToDivPixel(tr);
trPix.x += this.gridSize_;
trPix.y -= this.gridSize_;
var blPix = projection.fromLatLngToDivPixel(bl);
blPix.x -= this.gridSize_;
blPix.y += this.gridSize_;
// Convert the pixel points back to LatLng
var ne = projection.fromDivPixelToLatLng(trPix);
var sw = projection.fromDivPixelToLatLng(blPix);
// Extend the bounds to contain the new bounds.
bounds.extend(ne);
bounds.extend(sw);
return bounds;
};
/**
* Redraws all the clusters.
*/
MarkerClusterer.prototype.redraw_ = function () {
this.createClusters_(0);
};
/**
* Removes all clusters from the map. The markers are also removed from the map
* if <code>opt_hide</code> is set to <code>true</code>.
*
* @param {boolean} [opt_hide] Set to <code>true</code> to also remove the markers
* from the map.
*/
MarkerClusterer.prototype.resetViewport_ = function (opt_hide) {
var i, marker;
// Remove all the clusters
for (i = 0; i < this.clusters_.length; i++) {
this.clusters_[i].remove();
}
this.clusters_ = [];
// Reset the markers to not be added and to be removed from the map.
for (i = 0; i < this.markers_.length; i++) {
marker = this.markers_[i];
marker.isAdded = false;
if (opt_hide) {
marker.setMap(null);
}
}
};
/**
* Calculates the distance between two latlng locations in km.
*
* @param {google.maps.LatLng} p1 The first lat lng point.
* @param {google.maps.LatLng} p2 The second lat lng point.
* @return {number} The distance between the two points in km.
* @see http://www.movable-type.co.uk/scripts/latlong.html
*/
MarkerClusterer.prototype.distanceBetweenPoints_ = function (p1, p2) {
var R = 6371; // Radius of the Earth in km
var dLat = (p2.lat() - p1.lat()) * Math.PI / 180;
var dLon = (p2.lng() - p1.lng()) * Math.PI / 180;
var a = Math.sin(dLat / 2) * Math.sin(dLat / 2) +
Math.cos(p1.lat() * Math.PI / 180) * Math.cos(p2.lat() * Math.PI / 180) *
Math.sin(dLon / 2) * Math.sin(dLon / 2);
var c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1 - a));
var d = R * c;
return d;
};
/**
* Determines if a marker is contained in a bounds.
*
* @param {google.maps.Marker} marker The marker to check.
* @param {google.maps.LatLngBounds} bounds The bounds to check against.
* @return {boolean} True if the marker is in the bounds.
*/
MarkerClusterer.prototype.isMarkerInBounds_ = function (marker, bounds) {
return bounds.contains(marker.getPosition());
};
/**
* Adds a marker to a cluster, or creates a new cluster.
*
* @param {google.maps.Marker} marker The marker to add.
*/
MarkerClusterer.prototype.addToClosestCluster_ = function (marker) {
var i, d, cluster, center;
var distance = 40000; // Some large number
var clusterToAddTo = null;
for (i = 0; i < this.clusters_.length; i++) {
cluster = this.clusters_[i];
center = cluster.getCenter();
if (center) {
d = this.distanceBetweenPoints_(center, marker.getPosition());
if (d < distance) {
distance = d;
clusterToAddTo = cluster;
}
}
}
if (clusterToAddTo && clusterToAddTo.isMarkerInClusterBounds(marker)) {
clusterToAddTo.addMarker(marker);
} else {
cluster = new Cluster(this);
cluster.addMarker(marker);
this.clusters_.push(cluster);
}
};
/**
* Creates the clusters. This is done in batches to avoid timeout errors
* in some browsers when there is a huge number of markers.
*
* @param {number} iFirst The index of the first marker in the batch of
* markers to be added to clusters.
*/
MarkerClusterer.prototype.createClusters_ = function (iFirst) {
var i, marker;
var mapBounds;
var cMarkerClusterer = this;
if (!this.ready_) {
return;
}
// Cancel previous batch processing if we're working on the first batch:
if (iFirst === 0) {
/**
* This event is fired when the <code>MarkerClusterer</code> begins
* clustering markers.
* @name MarkerClusterer#clusteringbegin
* @param {MarkerClusterer} mc The MarkerClusterer whose markers are being clustered.
* @event
*/
google.maps.event.trigger(this, "clusteringbegin", this);
if (typeof this.timerRefStatic !== "undefined") {
clearTimeout(this.timerRefStatic);
delete this.timerRefStatic;
}
}
// Get our current map view bounds.
// Create a new bounds object so we don't affect the map.
//
// See Comments 9 & 11 on Issue 3651 relating to this workaround for a Google Maps bug:
if (this.getMap().getZoom() > 3) {
mapBounds = new google.maps.LatLngBounds(this.getMap().getBounds().getSouthWest(),
this.getMap().getBounds().getNorthEast());
} else {
mapBounds = new google.maps.LatLngBounds(new google.maps.LatLng(85.02070771743472, -178.48388434375), new google.maps.LatLng(-85.08136444384544, 178.00048865625));
}
var bounds = this.getExtendedBounds(mapBounds);
var iLast = Math.min(iFirst + this.batchSize_, this.markers_.length);
for (i = iFirst; i < iLast; i++) {
marker = this.markers_[i];
if (!marker.isAdded && this.isMarkerInBounds_(marker, bounds)) {
if (!this.ignoreHidden_ || (this.ignoreHidden_ && marker.getVisible())) {
this.addToClosestCluster_(marker);
}
}
}
if (iLast < this.markers_.length) {
this.timerRefStatic = setTimeout(function () {
cMarkerClusterer.createClusters_(iLast);
}, 0);
} else {
delete this.timerRefStatic;
/**
* This event is fired when the <code>MarkerClusterer</code> stops
* clustering markers.
* @name MarkerClusterer#clusteringend
* @param {MarkerClusterer} mc The MarkerClusterer whose markers are being clustered.
* @event
*/
google.maps.event.trigger(this, "clusteringend", this);
}
};
/**
* Extends an object's prototype by another's.
*
* @param {Object} obj1 The object to be extended.
* @param {Object} obj2 The object to extend with.
* @return {Object} The new extended object.
* @ignore
*/
MarkerClusterer.prototype.extend = function (obj1, obj2) {
return (function (object) {
var property;
for (property in object.prototype) {
this.prototype[property] = object.prototype[property];
}
return this;
}).apply(obj1, [obj2]);
};
/**
* The default function for determining the label text and style
* for a cluster icon.
*
* @param {Array.<google.maps.Marker>} markers The array of markers represented by the cluster.
* @param {number} numStyles The number of marker styles available.
* @return {ClusterIconInfo} The information resource for the cluster.
* @constant
* @ignore
*/
MarkerClusterer.CALCULATOR = function (markers, numStyles) {
var index = 0;
var title = "";
var count = markers.length.toString();
var dv = count;
while (dv !== 0) {
dv = parseInt(dv / 10, 10);
index++;
}
index = Math.min(index, numStyles);
return {
text: count,
index: index,
title: title
};
};
/**
* The number of markers to process in one batch.
*
* @type {number}
* @constant
*/
MarkerClusterer.BATCH_SIZE = 2000;
/**
* The number of markers to process in one batch (IE only).
*
* @type {number}
* @constant
*/
MarkerClusterer.BATCH_SIZE_IE = 500;
/**
* The default root name for the marker cluster images.
*
* @type {string}
* @constant
*/
MarkerClusterer.IMAGE_PATH = "http://google-maps-utility-library-v3.googlecode.com/svn/trunk/markerclustererplus/images/m";
/**
* The default extension name for the marker cluster images.
*
* @type {string}
* @constant
*/
MarkerClusterer.IMAGE_EXTENSION = "png";
/**
* The default array of sizes for the marker cluster images.
*
* @type {Array.<number>}
* @constant
*/
MarkerClusterer.IMAGE_SIZES = [53, 56, 66, 78, 90];
if (typeof String.prototype.trim !== 'function') {
/**
* IE hack since trim() doesn't exist in all browsers
* @return {string} The string with removed whitespace
*/
String.prototype.trim = function() {
return this.replace(/^\s+|\s+$/g, '');
}
}
;/**
* 1.1.9-patched
* @name MarkerWithLabel for V3
* @version 1.1.8 [February 26, 2013]
* @author Gary Little (inspired by code from Marc Ridey of Google).
* @copyright Copyright 2012 Gary Little [gary at luxcentral.com]
* @fileoverview MarkerWithLabel extends the Google Maps JavaScript API V3
* <code>google.maps.Marker</code> class.
* <p>
* MarkerWithLabel allows you to define markers with associated labels. As you would expect,
* if the marker is draggable, so too will be the label. In addition, a marker with a label
* responds to all mouse events in the same manner as a regular marker. It also fires mouse
* events and "property changed" events just as a regular marker would. Version 1.1 adds
* support for the raiseOnDrag feature introduced in API V3.3.
* <p>
* If you drag a marker by its label, you can cancel the drag and return the marker to its
* original position by pressing the <code>Esc</code> key. This doesn't work if you drag the marker
* itself because this feature is not (yet) supported in the <code>google.maps.Marker</code> class.
*/
/*!
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/*jslint browser:true */
/*global document,google */
/**
* @param {Function} childCtor Child class.
* @param {Function} parentCtor Parent class.
*/
function inherits(childCtor, parentCtor) {
/** @constructor */
function tempCtor() {}
tempCtor.prototype = parentCtor.prototype;
childCtor.superClass_ = parentCtor.prototype;
childCtor.prototype = new tempCtor();
/** @override */
childCtor.prototype.constructor = childCtor;
}
/**
* This constructor creates a label and associates it with a marker.
* It is for the private use of the MarkerWithLabel class.
* @constructor
* @param {Marker} marker The marker with which the label is to be associated.
* @param {string} crossURL The URL of the cross image =.
* @param {string} handCursor The URL of the hand cursor.
* @private
*/
function MarkerLabel_(marker, crossURL, handCursorURL) {
this.marker_ = marker;
this.handCursorURL_ = marker.handCursorURL;
this.labelDiv_ = document.createElement("div");
this.labelDiv_.style.cssText = "position: absolute; overflow: hidden;";
// Set up the DIV for handling mouse events in the label. This DIV forms a transparent veil
// in the "overlayMouseTarget" pane, a veil that covers just the label. This is done so that
// events can be captured even if the label is in the shadow of a google.maps.InfoWindow.
// Code is included here to ensure the veil is always exactly the same size as the label.
this.eventDiv_ = document.createElement("div");
this.eventDiv_.style.cssText = this.labelDiv_.style.cssText;
// This is needed for proper behavior on MSIE:
this.eventDiv_.setAttribute("onselectstart", "return false;");
this.eventDiv_.setAttribute("ondragstart", "return false;");
// Get the DIV for the "X" to be displayed when the marker is raised.
this.crossDiv_ = MarkerLabel_.getSharedCross(crossURL);
}
inherits(MarkerLabel_, google.maps.OverlayView);
/**
* Returns the DIV for the cross used when dragging a marker when the
* raiseOnDrag parameter set to true. One cross is shared with all markers.
* @param {string} crossURL The URL of the cross image =.
* @private
*/
MarkerLabel_.getSharedCross = function (crossURL) {
var div;
if (typeof MarkerLabel_.getSharedCross.crossDiv === "undefined") {
div = document.createElement("img");
div.style.cssText = "position: absolute; z-index: 1000002; display: none;";
// Hopefully Google never changes the standard "X" attributes:
div.style.marginLeft = "-8px";
div.style.marginTop = "-9px";
div.src = crossURL;
MarkerLabel_.getSharedCross.crossDiv = div;
}
return MarkerLabel_.getSharedCross.crossDiv;
};
/**
* Adds the DIV representing the label to the DOM. This method is called
* automatically when the marker's <code>setMap</code> method is called.
* @private
*/
MarkerLabel_.prototype.onAdd = function () {
var me = this;
var cMouseIsDown = false;
var cDraggingLabel = false;
var cSavedZIndex;
var cLatOffset, cLngOffset;
var cIgnoreClick;
var cRaiseEnabled;
var cStartPosition;
var cStartCenter;
// Constants:
var cRaiseOffset = 20;
var cDraggingCursor = "url(" + this.handCursorURL_ + ")";
// Stops all processing of an event.
//
var cAbortEvent = function (e) {
if (e.preventDefault) {
e.preventDefault();
}
e.cancelBubble = true;
if (e.stopPropagation) {
e.stopPropagation();
}
};
var cStopBounce = function () {
me.marker_.setAnimation(null);
};
this.getPanes().overlayImage.appendChild(this.labelDiv_);
this.getPanes().overlayMouseTarget.appendChild(this.eventDiv_);
// One cross is shared with all markers, so only add it once:
if (typeof MarkerLabel_.getSharedCross.processed === "undefined") {
this.getPanes().overlayImage.appendChild(this.crossDiv_);
MarkerLabel_.getSharedCross.processed = true;
}
this.listeners_ = [
google.maps.event.addDomListener(this.eventDiv_, "mouseover", function (e) {
if (me.marker_.getDraggable() || me.marker_.getClickable()) {
this.style.cursor = "pointer";
google.maps.event.trigger(me.marker_, "mouseover", e);
}
}),
google.maps.event.addDomListener(this.eventDiv_, "mouseout", function (e) {
if ((me.marker_.getDraggable() || me.marker_.getClickable()) && !cDraggingLabel) {
this.style.cursor = me.marker_.getCursor();
google.maps.event.trigger(me.marker_, "mouseout", e);
}
}),
google.maps.event.addDomListener(this.eventDiv_, "mousedown", function (e) {
cDraggingLabel = false;
if (me.marker_.getDraggable()) {
cMouseIsDown = true;
this.style.cursor = cDraggingCursor;
}
if (me.marker_.getDraggable() || me.marker_.getClickable()) {
google.maps.event.trigger(me.marker_, "mousedown", e);
cAbortEvent(e); // Prevent map pan when starting a drag on a label
}
}),
google.maps.event.addDomListener(document, "mouseup", function (mEvent) {
var position;
if (cMouseIsDown) {
cMouseIsDown = false;
me.eventDiv_.style.cursor = "pointer";
google.maps.event.trigger(me.marker_, "mouseup", mEvent);
}
if (cDraggingLabel) {
if (cRaiseEnabled) { // Lower the marker & label
position = me.getProjection().fromLatLngToDivPixel(me.marker_.getPosition());
position.y += cRaiseOffset;
me.marker_.setPosition(me.getProjection().fromDivPixelToLatLng(position));
// This is not the same bouncing style as when the marker portion is dragged,
// but it will have to do:
try { // Will fail if running Google Maps API earlier than V3.3
me.marker_.setAnimation(google.maps.Animation.BOUNCE);
setTimeout(cStopBounce, 1406);
} catch (e) {}
}
me.crossDiv_.style.display = "none";
me.marker_.setZIndex(cSavedZIndex);
cIgnoreClick = true; // Set flag to ignore the click event reported after a label drag
cDraggingLabel = false;
mEvent.latLng = me.marker_.getPosition();
google.maps.event.trigger(me.marker_, "dragend", mEvent);
}
}),
google.maps.event.addListener(me.marker_.getMap(), "mousemove", function (mEvent) {
var position;
if (cMouseIsDown) {
if (cDraggingLabel) {
// Change the reported location from the mouse position to the marker position:
mEvent.latLng = new google.maps.LatLng(mEvent.latLng.lat() - cLatOffset, mEvent.latLng.lng() - cLngOffset);
position = me.getProjection().fromLatLngToDivPixel(mEvent.latLng);
if (cRaiseEnabled) {
me.crossDiv_.style.left = position.x + "px";
me.crossDiv_.style.top = position.y + "px";
me.crossDiv_.style.display = "";
position.y -= cRaiseOffset;
}
me.marker_.setPosition(me.getProjection().fromDivPixelToLatLng(position));
if (cRaiseEnabled) { // Don't raise the veil; this hack needed to make MSIE act properly
me.eventDiv_.style.top = (position.y + cRaiseOffset) + "px";
}
google.maps.event.trigger(me.marker_, "drag", mEvent);
} else {
// Calculate offsets from the click point to the marker position:
cLatOffset = mEvent.latLng.lat() - me.marker_.getPosition().lat();
cLngOffset = mEvent.latLng.lng() - me.marker_.getPosition().lng();
cSavedZIndex = me.marker_.getZIndex();
cStartPosition = me.marker_.getPosition();
cStartCenter = me.marker_.getMap().getCenter();
cRaiseEnabled = me.marker_.get("raiseOnDrag");
cDraggingLabel = true;
me.marker_.setZIndex(1000000); // Moves the marker & label to the foreground during a drag
mEvent.latLng = me.marker_.getPosition();
google.maps.event.trigger(me.marker_, "dragstart", mEvent);
}
}
}),
google.maps.event.addDomListener(document, "keydown", function (e) {
if (cDraggingLabel) {
if (e.keyCode === 27) { // Esc key
cRaiseEnabled = false;
me.marker_.setPosition(cStartPosition);
me.marker_.getMap().setCenter(cStartCenter);
google.maps.event.trigger(document, "mouseup", e);
}
}
}),
google.maps.event.addDomListener(this.eventDiv_, "click", function (e) {
if (me.marker_.getDraggable() || me.marker_.getClickable()) {
if (cIgnoreClick) { // Ignore the click reported when a label drag ends
cIgnoreClick = false;
} else {
google.maps.event.trigger(me.marker_, "click", e);
cAbortEvent(e); // Prevent click from being passed on to map
}
}
}),
google.maps.event.addDomListener(this.eventDiv_, "dblclick", function (e) {
if (me.marker_.getDraggable() || me.marker_.getClickable()) {
google.maps.event.trigger(me.marker_, "dblclick", e);
cAbortEvent(e); // Prevent map zoom when double-clicking on a label
}
}),
google.maps.event.addListener(this.marker_, "dragstart", function (mEvent) {
if (!cDraggingLabel) {
cRaiseEnabled = this.get("raiseOnDrag");
}
}),
google.maps.event.addListener(this.marker_, "drag", function (mEvent) {
if (!cDraggingLabel) {
if (cRaiseEnabled) {
me.setPosition(cRaiseOffset);
// During a drag, the marker's z-index is temporarily set to 1000000 to
// ensure it appears above all other markers. Also set the label's z-index
// to 1000000 (plus or minus 1 depending on whether the label is supposed
// to be above or below the marker).
me.labelDiv_.style.zIndex = 1000000 + (this.get("labelInBackground") ? -1 : +1);
}
}
}),
google.maps.event.addListener(this.marker_, "dragend", function (mEvent) {
if (!cDraggingLabel) {
if (cRaiseEnabled) {
me.setPosition(0); // Also restores z-index of label
}
}
}),
google.maps.event.addListener(this.marker_, "position_changed", function () {
me.setPosition();
}),
google.maps.event.addListener(this.marker_, "zindex_changed", function () {
me.setZIndex();
}),
google.maps.event.addListener(this.marker_, "visible_changed", function () {
me.setVisible();
}),
google.maps.event.addListener(this.marker_, "labelvisible_changed", function () {
me.setVisible();
}),
google.maps.event.addListener(this.marker_, "title_changed", function () {
me.setTitle();
}),
google.maps.event.addListener(this.marker_, "labelcontent_changed", function () {
me.setContent();
}),
google.maps.event.addListener(this.marker_, "labelanchor_changed", function () {
me.setAnchor();
}),
google.maps.event.addListener(this.marker_, "labelclass_changed", function () {
me.setStyles();
}),
google.maps.event.addListener(this.marker_, "labelstyle_changed", function () {
me.setStyles();
})
];
};
/**
* Removes the DIV for the label from the DOM. It also removes all event handlers.
* This method is called automatically when the marker's <code>setMap(null)</code>
* method is called.
* @private
*/
MarkerLabel_.prototype.onRemove = function () {
var i;
if (this.labelDiv_.parentNode !== null)
this.labelDiv_.parentNode.removeChild(this.labelDiv_);
if (this.eventDiv_.parentNode !== null)
this.eventDiv_.parentNode.removeChild(this.eventDiv_);
// Remove event listeners:
for (i = 0; i < this.listeners_.length; i++) {
google.maps.event.removeListener(this.listeners_[i]);
}
};
/**
* Draws the label on the map.
* @private
*/
MarkerLabel_.prototype.draw = function () {
this.setContent();
this.setTitle();
this.setStyles();
};
/**
* Sets the content of the label.
* The content can be plain text or an HTML DOM node.
* @private
*/
MarkerLabel_.prototype.setContent = function () {
var content = this.marker_.get("labelContent");
if (typeof content.nodeType === "undefined") {
this.labelDiv_.innerHTML = content;
this.eventDiv_.innerHTML = this.labelDiv_.innerHTML;
} else {
this.labelDiv_.innerHTML = ""; // Remove current content
this.labelDiv_.appendChild(content);
content = content.cloneNode(true);
this.eventDiv_.appendChild(content);
}
};
/**
* Sets the content of the tool tip for the label. It is
* always set to be the same as for the marker itself.
* @private
*/
MarkerLabel_.prototype.setTitle = function () {
this.eventDiv_.title = this.marker_.getTitle() || "";
};
/**
* Sets the style of the label by setting the style sheet and applying
* other specific styles requested.
* @private
*/
MarkerLabel_.prototype.setStyles = function () {
var i, labelStyle;
// Apply style values from the style sheet defined in the labelClass parameter:
this.labelDiv_.className = this.marker_.get("labelClass");
this.eventDiv_.className = this.labelDiv_.className;
// Clear existing inline style values:
this.labelDiv_.style.cssText = "";
this.eventDiv_.style.cssText = "";
// Apply style values defined in the labelStyle parameter:
labelStyle = this.marker_.get("labelStyle");
for (i in labelStyle) {
if (labelStyle.hasOwnProperty(i)) {
this.labelDiv_.style[i] = labelStyle[i];
this.eventDiv_.style[i] = labelStyle[i];
}
}
this.setMandatoryStyles();
};
/**
* Sets the mandatory styles to the DIV representing the label as well as to the
* associated event DIV. This includes setting the DIV position, z-index, and visibility.
* @private
*/
MarkerLabel_.prototype.setMandatoryStyles = function () {
this.labelDiv_.style.position = "absolute";
this.labelDiv_.style.overflow = "hidden";
// Make sure the opacity setting causes the desired effect on MSIE:
if (typeof this.labelDiv_.style.opacity !== "undefined" && this.labelDiv_.style.opacity !== "") {
this.labelDiv_.style.MsFilter = "\"progid:DXImageTransform.Microsoft.Alpha(opacity=" + (this.labelDiv_.style.opacity * 100) + ")\"";
this.labelDiv_.style.filter = "alpha(opacity=" + (this.labelDiv_.style.opacity * 100) + ")";
}
this.eventDiv_.style.position = this.labelDiv_.style.position;
this.eventDiv_.style.overflow = this.labelDiv_.style.overflow;
this.eventDiv_.style.opacity = 0.01; // Don't use 0; DIV won't be clickable on MSIE
this.eventDiv_.style.MsFilter = "\"progid:DXImageTransform.Microsoft.Alpha(opacity=1)\"";
this.eventDiv_.style.filter = "alpha(opacity=1)"; // For MSIE
this.setAnchor();
this.setPosition(); // This also updates z-index, if necessary.
this.setVisible();
};
/**
* Sets the anchor point of the label.
* @private
*/
MarkerLabel_.prototype.setAnchor = function () {
var anchor = this.marker_.get("labelAnchor");
this.labelDiv_.style.marginLeft = -anchor.x + "px";
this.labelDiv_.style.marginTop = -anchor.y + "px";
this.eventDiv_.style.marginLeft = -anchor.x + "px";
this.eventDiv_.style.marginTop = -anchor.y + "px";
};
/**
* Sets the position of the label. The z-index is also updated, if necessary.
* @private
*/
MarkerLabel_.prototype.setPosition = function (yOffset) {
var position = this.getProjection().fromLatLngToDivPixel(this.marker_.getPosition());
if (typeof yOffset === "undefined") {
yOffset = 0;
}
this.labelDiv_.style.left = Math.round(position.x) + "px";
this.labelDiv_.style.top = Math.round(position.y - yOffset) + "px";
this.eventDiv_.style.left = this.labelDiv_.style.left;
this.eventDiv_.style.top = this.labelDiv_.style.top;
this.setZIndex();
};
/**
* Sets the z-index of the label. If the marker's z-index property has not been defined, the z-index
* of the label is set to the vertical coordinate of the label. This is in keeping with the default
* stacking order for Google Maps: markers to the south are in front of markers to the north.
* @private
*/
MarkerLabel_.prototype.setZIndex = function () {
var zAdjust = (this.marker_.get("labelInBackground") ? -1 : +1);
if (typeof this.marker_.getZIndex() === "undefined") {
this.labelDiv_.style.zIndex = parseInt(this.labelDiv_.style.top, 10) + zAdjust;
this.eventDiv_.style.zIndex = this.labelDiv_.style.zIndex;
} else {
this.labelDiv_.style.zIndex = this.marker_.getZIndex() + zAdjust;
this.eventDiv_.style.zIndex = this.labelDiv_.style.zIndex;
}
};
/**
* Sets the visibility of the label. The label is visible only if the marker itself is
* visible (i.e., its visible property is true) and the labelVisible property is true.
* @private
*/
MarkerLabel_.prototype.setVisible = function () {
if (this.marker_.get("labelVisible")) {
this.labelDiv_.style.display = this.marker_.getVisible() ? "block" : "none";
} else {
this.labelDiv_.style.display = "none";
}
this.eventDiv_.style.display = this.labelDiv_.style.display;
};
/**
* @name MarkerWithLabelOptions
* @class This class represents the optional parameter passed to the {@link MarkerWithLabel} constructor.
* The properties available are the same as for <code>google.maps.Marker</code> with the addition
* of the properties listed below. To change any of these additional properties after the labeled
* marker has been created, call <code>google.maps.Marker.set(propertyName, propertyValue)</code>.
* <p>
* When any of these properties changes, a property changed event is fired. The names of these
* events are derived from the name of the property and are of the form <code>propertyname_changed</code>.
* For example, if the content of the label changes, a <code>labelcontent_changed</code> event
* is fired.
* <p>
* @property {string|Node} [labelContent] The content of the label (plain text or an HTML DOM node).
* @property {Point} [labelAnchor] By default, a label is drawn with its anchor point at (0,0) so
* that its top left corner is positioned at the anchor point of the associated marker. Use this
* property to change the anchor point of the label. For example, to center a 50px-wide label
* beneath a marker, specify a <code>labelAnchor</code> of <code>google.maps.Point(25, 0)</code>.
* (Note: x-values increase to the right and y-values increase to the top.)
* @property {string} [labelClass] The name of the CSS class defining the styles for the label.
* Note that style values for <code>position</code>, <code>overflow</code>, <code>top</code>,
* <code>left</code>, <code>zIndex</code>, <code>display</code>, <code>marginLeft</code>, and
* <code>marginTop</code> are ignored; these styles are for internal use only.
* @property {Object} [labelStyle] An object literal whose properties define specific CSS
* style values to be applied to the label. Style values defined here override those that may
* be defined in the <code>labelClass</code> style sheet. If this property is changed after the
* label has been created, all previously set styles (except those defined in the style sheet)
* are removed from the label before the new style values are applied.
* Note that style values for <code>position</code>, <code>overflow</code>, <code>top</code>,
* <code>left</code>, <code>zIndex</code>, <code>display</code>, <code>marginLeft</code>, and
* <code>marginTop</code> are ignored; these styles are for internal use only.
* @property {boolean} [labelInBackground] A flag indicating whether a label that overlaps its
* associated marker should appear in the background (i.e., in a plane below the marker).
* The default is <code>false</code>, which causes the label to appear in the foreground.
* @property {boolean} [labelVisible] A flag indicating whether the label is to be visible.
* The default is <code>true</code>. Note that even if <code>labelVisible</code> is
* <code>true</code>, the label will <i>not</i> be visible unless the associated marker is also
* visible (i.e., unless the marker's <code>visible</code> property is <code>true</code>).
* @property {boolean} [raiseOnDrag] A flag indicating whether the label and marker are to be
* raised when the marker is dragged. The default is <code>true</code>. If a draggable marker is
* being created and a version of Google Maps API earlier than V3.3 is being used, this property
* must be set to <code>false</code>.
* @property {boolean} [optimized] A flag indicating whether rendering is to be optimized for the
* marker. <b>Important: The optimized rendering technique is not supported by MarkerWithLabel,
* so the value of this parameter is always forced to <code>false</code>.
* @property {string} [crossImage="http://maps.gstatic.com/intl/en_us/mapfiles/drag_cross_67_16.png"]
* The URL of the cross image to be displayed while dragging a marker.
* @property {string} [handCursor="http://maps.gstatic.com/intl/en_us/mapfiles/closedhand_8_8.cur"]
* The URL of the cursor to be displayed while dragging a marker.
*/
/**
* Creates a MarkerWithLabel with the options specified in {@link MarkerWithLabelOptions}.
* @constructor
* @param {MarkerWithLabelOptions} [opt_options] The optional parameters.
*/
function MarkerWithLabel(opt_options) {
opt_options = opt_options || {};
opt_options.labelContent = opt_options.labelContent || "";
opt_options.labelAnchor = opt_options.labelAnchor || new google.maps.Point(0, 0);
opt_options.labelClass = opt_options.labelClass || "markerLabels";
opt_options.labelStyle = opt_options.labelStyle || {};
opt_options.labelInBackground = opt_options.labelInBackground || false;
if (typeof opt_options.labelVisible === "undefined") {
opt_options.labelVisible = true;
}
if (typeof opt_options.raiseOnDrag === "undefined") {
opt_options.raiseOnDrag = true;
}
if (typeof opt_options.clickable === "undefined") {
opt_options.clickable = true;
}
if (typeof opt_options.draggable === "undefined") {
opt_options.draggable = false;
}
if (typeof opt_options.optimized === "undefined") {
opt_options.optimized = false;
}
opt_options.crossImage = opt_options.crossImage || "http" + (document.location.protocol === "https:" ? "s" : "") + "://maps.gstatic.com/intl/en_us/mapfiles/drag_cross_67_16.png";
opt_options.handCursor = opt_options.handCursor || "http" + (document.location.protocol === "https:" ? "s" : "") + "://maps.gstatic.com/intl/en_us/mapfiles/closedhand_8_8.cur";
opt_options.optimized = false; // Optimized rendering is not supported
this.label = new MarkerLabel_(this, opt_options.crossImage, opt_options.handCursor); // Bind the label to the marker
// Call the parent constructor. It calls Marker.setValues to initialize, so all
// the new parameters are conveniently saved and can be accessed with get/set.
// Marker.set triggers a property changed event (called "propertyname_changed")
// that the marker label listens for in order to react to state changes.
google.maps.Marker.apply(this, arguments);
}
inherits(MarkerWithLabel, google.maps.Marker);
/**
* Overrides the standard Marker setMap function.
* @param {Map} theMap The map to which the marker is to be added.
* @private
*/
MarkerWithLabel.prototype.setMap = function (theMap) {
// Call the inherited function...
google.maps.Marker.prototype.setMap.apply(this, arguments);
// ... then deal with the label:
this.label.setMap(theMap);
}; |
src/component/PageWrapper/test.js | pittborndigital/the92club | import React from 'react'
import { shallow } from 'enzyme'
import PageWrapper from './component'
it('renders without crashing', () => {
const wrapper = shallow(<PageWrapper><h1>some content</h1></PageWrapper>)
expect(wrapper.exists()).toBe(true)
})
|
examples/decorators/router/NotFoundScreen.js | stackscz/re-app | import React from 'react';
export default class NotFoundScreen extends React.Component {
render() {
return (
<div className="NotFoundScreen">
<h1>404 - Not Found</h1>
</div>
);
}
}
|
sdncon/ui/static/js/thirdparty/jquery-1.9.1.js | mandeepdhami/netvirt-ctrl | /*!
* jQuery JavaScript Library v1.9.1
* http://jquery.com/
*
* Includes Sizzle.js
* http://sizzlejs.com/
*
* Copyright 2005, 2012 jQuery Foundation, Inc. and other contributors
* Released under the MIT license
* http://jquery.org/license
*
* Date: 2013-2-4
*/
(function( window, undefined ) {
// Can't do this because several apps including ASP.NET trace
// the stack via arguments.caller.callee and Firefox dies if
// you try to trace through "use strict" call chains. (#13335)
// Support: Firefox 18+
//"use strict";
var
// The deferred used on DOM ready
readyList,
// A central reference to the root jQuery(document)
rootjQuery,
// Support: IE<9
// For `typeof node.method` instead of `node.method !== undefined`
core_strundefined = typeof undefined,
// Use the correct document accordingly with window argument (sandbox)
document = window.document,
location = window.location,
// Map over jQuery in case of overwrite
_jQuery = window.jQuery,
// Map over the $ in case of overwrite
_$ = window.$,
// [[Class]] -> type pairs
class2type = {},
// List of deleted data cache ids, so we can reuse them
core_deletedIds = [],
core_version = "1.9.1",
// Save a reference to some core methods
core_concat = core_deletedIds.concat,
core_push = core_deletedIds.push,
core_slice = core_deletedIds.slice,
core_indexOf = core_deletedIds.indexOf,
core_toString = class2type.toString,
core_hasOwn = class2type.hasOwnProperty,
core_trim = core_version.trim,
// Define a local copy of jQuery
jQuery = function( selector, context ) {
// The jQuery object is actually just the init constructor 'enhanced'
return new jQuery.fn.init( selector, context, rootjQuery );
},
// Used for matching numbers
core_pnum = /[+-]?(?:\d*\.|)\d+(?:[eE][+-]?\d+|)/.source,
// Used for splitting on whitespace
core_rnotwhite = /\S+/g,
// Make sure we trim BOM and NBSP (here's looking at you, Safari 5.0 and IE)
rtrim = /^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g,
// A simple way to check for HTML strings
// Prioritize #id over <tag> to avoid XSS via location.hash (#9521)
// Strict HTML recognition (#11290: must start with <)
rquickExpr = /^(?:(<[\w\W]+>)[^>]*|#([\w-]*))$/,
// Match a standalone tag
rsingleTag = /^<(\w+)\s*\/?>(?:<\/\1>|)$/,
// JSON RegExp
rvalidchars = /^[\],:{}\s]*$/,
rvalidbraces = /(?:^|:|,)(?:\s*\[)+/g,
rvalidescape = /\\(?:["\\\/bfnrt]|u[\da-fA-F]{4})/g,
rvalidtokens = /"[^"\\\r\n]*"|true|false|null|-?(?:\d+\.|)\d+(?:[eE][+-]?\d+|)/g,
// Matches dashed string for camelizing
rmsPrefix = /^-ms-/,
rdashAlpha = /-([\da-z])/gi,
// Used by jQuery.camelCase as callback to replace()
fcamelCase = function( all, letter ) {
return letter.toUpperCase();
},
// The ready event handler
completed = function( event ) {
// readyState === "complete" is good enough for us to call the dom ready in oldIE
if ( document.addEventListener || event.type === "load" || document.readyState === "complete" ) {
detach();
jQuery.ready();
}
},
// Clean-up method for dom ready events
detach = function() {
if ( document.addEventListener ) {
document.removeEventListener( "DOMContentLoaded", completed, false );
window.removeEventListener( "load", completed, false );
} else {
document.detachEvent( "onreadystatechange", completed );
window.detachEvent( "onload", completed );
}
};
jQuery.fn = jQuery.prototype = {
// The current version of jQuery being used
jquery: core_version,
constructor: jQuery,
init: function( selector, context, rootjQuery ) {
var match, elem;
// HANDLE: $(""), $(null), $(undefined), $(false)
if ( !selector ) {
return this;
}
// Handle HTML strings
if ( typeof selector === "string" ) {
if ( selector.charAt(0) === "<" && selector.charAt( selector.length - 1 ) === ">" && selector.length >= 3 ) {
// Assume that strings that start and end with <> are HTML and skip the regex check
match = [ null, selector, null ];
} else {
match = rquickExpr.exec( selector );
}
// Match html or make sure no context is specified for #id
if ( match && (match[1] || !context) ) {
// HANDLE: $(html) -> $(array)
if ( match[1] ) {
context = context instanceof jQuery ? context[0] : context;
// scripts is true for back-compat
jQuery.merge( this, jQuery.parseHTML(
match[1],
context && context.nodeType ? context.ownerDocument || context : document,
true
) );
// HANDLE: $(html, props)
if ( rsingleTag.test( match[1] ) && jQuery.isPlainObject( context ) ) {
for ( match in context ) {
// Properties of context are called as methods if possible
if ( jQuery.isFunction( this[ match ] ) ) {
this[ match ]( context[ match ] );
// ...and otherwise set as attributes
} else {
this.attr( match, context[ match ] );
}
}
}
return this;
// HANDLE: $(#id)
} else {
elem = document.getElementById( match[2] );
// Check parentNode to catch when Blackberry 4.6 returns
// nodes that are no longer in the document #6963
if ( elem && elem.parentNode ) {
// Handle the case where IE and Opera return items
// by name instead of ID
if ( elem.id !== match[2] ) {
return rootjQuery.find( selector );
}
// Otherwise, we inject the element directly into the jQuery object
this.length = 1;
this[0] = elem;
}
this.context = document;
this.selector = selector;
return this;
}
// HANDLE: $(expr, $(...))
} else if ( !context || context.jquery ) {
return ( context || rootjQuery ).find( selector );
// HANDLE: $(expr, context)
// (which is just equivalent to: $(context).find(expr)
} else {
return this.constructor( context ).find( selector );
}
// HANDLE: $(DOMElement)
} else if ( selector.nodeType ) {
this.context = this[0] = selector;
this.length = 1;
return this;
// HANDLE: $(function)
// Shortcut for document ready
} else if ( jQuery.isFunction( selector ) ) {
return rootjQuery.ready( selector );
}
if ( selector.selector !== undefined ) {
this.selector = selector.selector;
this.context = selector.context;
}
return jQuery.makeArray( selector, this );
},
// Start with an empty selector
selector: "",
// The default length of a jQuery object is 0
length: 0,
// The number of elements contained in the matched element set
size: function() {
return this.length;
},
toArray: function() {
return core_slice.call( this );
},
// Get the Nth element in the matched element set OR
// Get the whole matched element set as a clean array
get: function( num ) {
return num == null ?
// Return a 'clean' array
this.toArray() :
// Return just the object
( num < 0 ? this[ this.length + num ] : this[ num ] );
},
// Take an array of elements and push it onto the stack
// (returning the new matched element set)
pushStack: function( elems ) {
// Build a new jQuery matched element set
var ret = jQuery.merge( this.constructor(), elems );
// Add the old object onto the stack (as a reference)
ret.prevObject = this;
ret.context = this.context;
// Return the newly-formed element set
return ret;
},
// Execute a callback for every element in the matched set.
// (You can seed the arguments with an array of args, but this is
// only used internally.)
each: function( callback, args ) {
return jQuery.each( this, callback, args );
},
ready: function( fn ) {
// Add the callback
jQuery.ready.promise().done( fn );
return this;
},
slice: function() {
return this.pushStack( core_slice.apply( this, arguments ) );
},
first: function() {
return this.eq( 0 );
},
last: function() {
return this.eq( -1 );
},
eq: function( i ) {
var len = this.length,
j = +i + ( i < 0 ? len : 0 );
return this.pushStack( j >= 0 && j < len ? [ this[j] ] : [] );
},
map: function( callback ) {
return this.pushStack( jQuery.map(this, function( elem, i ) {
return callback.call( elem, i, elem );
}));
},
end: function() {
return this.prevObject || this.constructor(null);
},
// For internal use only.
// Behaves like an Array's method, not like a jQuery method.
push: core_push,
sort: [].sort,
splice: [].splice
};
// Give the init function the jQuery prototype for later instantiation
jQuery.fn.init.prototype = jQuery.fn;
jQuery.extend = jQuery.fn.extend = function() {
var src, copyIsArray, copy, name, options, clone,
target = arguments[0] || {},
i = 1,
length = arguments.length,
deep = false;
// Handle a deep copy situation
if ( typeof target === "boolean" ) {
deep = target;
target = arguments[1] || {};
// skip the boolean and the target
i = 2;
}
// Handle case when target is a string or something (possible in deep copy)
if ( typeof target !== "object" && !jQuery.isFunction(target) ) {
target = {};
}
// extend jQuery itself if only one argument is passed
if ( length === i ) {
target = this;
--i;
}
for ( ; i < length; i++ ) {
// Only deal with non-null/undefined values
if ( (options = arguments[ i ]) != null ) {
// Extend the base object
for ( name in options ) {
src = target[ name ];
copy = options[ name ];
// Prevent never-ending loop
if ( target === copy ) {
continue;
}
// Recurse if we're merging plain objects or arrays
if ( deep && copy && ( jQuery.isPlainObject(copy) || (copyIsArray = jQuery.isArray(copy)) ) ) {
if ( copyIsArray ) {
copyIsArray = false;
clone = src && jQuery.isArray(src) ? src : [];
} else {
clone = src && jQuery.isPlainObject(src) ? src : {};
}
// Never move original objects, clone them
target[ name ] = jQuery.extend( deep, clone, copy );
// Don't bring in undefined values
} else if ( copy !== undefined ) {
target[ name ] = copy;
}
}
}
}
// Return the modified object
return target;
};
jQuery.extend({
noConflict: function( deep ) {
if ( window.$ === jQuery ) {
window.$ = _$;
}
if ( deep && window.jQuery === jQuery ) {
window.jQuery = _jQuery;
}
return jQuery;
},
// Is the DOM ready to be used? Set to true once it occurs.
isReady: false,
// A counter to track how many items to wait for before
// the ready event fires. See #6781
readyWait: 1,
// Hold (or release) the ready event
holdReady: function( hold ) {
if ( hold ) {
jQuery.readyWait++;
} else {
jQuery.ready( true );
}
},
// Handle when the DOM is ready
ready: function( wait ) {
// Abort if there are pending holds or we're already ready
if ( wait === true ? --jQuery.readyWait : jQuery.isReady ) {
return;
}
// Make sure body exists, at least, in case IE gets a little overzealous (ticket #5443).
if ( !document.body ) {
return setTimeout( jQuery.ready );
}
// Remember that the DOM is ready
jQuery.isReady = true;
// If a normal DOM Ready event fired, decrement, and wait if need be
if ( wait !== true && --jQuery.readyWait > 0 ) {
return;
}
// If there are functions bound, to execute
readyList.resolveWith( document, [ jQuery ] );
// Trigger any bound ready events
if ( jQuery.fn.trigger ) {
jQuery( document ).trigger("ready").off("ready");
}
},
// See test/unit/core.js for details concerning isFunction.
// Since version 1.3, DOM methods and functions like alert
// aren't supported. They return false on IE (#2968).
isFunction: function( obj ) {
return jQuery.type(obj) === "function";
},
isArray: Array.isArray || function( obj ) {
return jQuery.type(obj) === "array";
},
isWindow: function( obj ) {
return obj != null && obj == obj.window;
},
isNumeric: function( obj ) {
return !isNaN( parseFloat(obj) ) && isFinite( obj );
},
type: function( obj ) {
if ( obj == null ) {
return String( obj );
}
return typeof obj === "object" || typeof obj === "function" ?
class2type[ core_toString.call(obj) ] || "object" :
typeof obj;
},
isPlainObject: function( obj ) {
// Must be an Object.
// Because of IE, we also have to check the presence of the constructor property.
// Make sure that DOM nodes and window objects don't pass through, as well
if ( !obj || jQuery.type(obj) !== "object" || obj.nodeType || jQuery.isWindow( obj ) ) {
return false;
}
try {
// Not own constructor property must be Object
if ( obj.constructor &&
!core_hasOwn.call(obj, "constructor") &&
!core_hasOwn.call(obj.constructor.prototype, "isPrototypeOf") ) {
return false;
}
} catch ( e ) {
// IE8,9 Will throw exceptions on certain host objects #9897
return false;
}
// Own properties are enumerated firstly, so to speed up,
// if last one is own, then all properties are own.
var key;
for ( key in obj ) {}
return key === undefined || core_hasOwn.call( obj, key );
},
isEmptyObject: function( obj ) {
var name;
for ( name in obj ) {
return false;
}
return true;
},
error: function( msg ) {
throw new Error( msg );
},
// data: string of html
// context (optional): If specified, the fragment will be created in this context, defaults to document
// keepScripts (optional): If true, will include scripts passed in the html string
parseHTML: function( data, context, keepScripts ) {
if ( !data || typeof data !== "string" ) {
return null;
}
if ( typeof context === "boolean" ) {
keepScripts = context;
context = false;
}
context = context || document;
var parsed = rsingleTag.exec( data ),
scripts = !keepScripts && [];
// Single tag
if ( parsed ) {
return [ context.createElement( parsed[1] ) ];
}
parsed = jQuery.buildFragment( [ data ], context, scripts );
if ( scripts ) {
jQuery( scripts ).remove();
}
return jQuery.merge( [], parsed.childNodes );
},
parseJSON: function( data ) {
// Attempt to parse using the native JSON parser first
if ( window.JSON && window.JSON.parse ) {
return window.JSON.parse( data );
}
if ( data === null ) {
return data;
}
if ( typeof data === "string" ) {
// Make sure leading/trailing whitespace is removed (IE can't handle it)
data = jQuery.trim( data );
if ( data ) {
// Make sure the incoming data is actual JSON
// Logic borrowed from http://json.org/json2.js
if ( rvalidchars.test( data.replace( rvalidescape, "@" )
.replace( rvalidtokens, "]" )
.replace( rvalidbraces, "")) ) {
return ( new Function( "return " + data ) )();
}
}
}
jQuery.error( "Invalid JSON: " + data );
},
// Cross-browser xml parsing
parseXML: function( data ) {
var xml, tmp;
if ( !data || typeof data !== "string" ) {
return null;
}
try {
if ( window.DOMParser ) { // Standard
tmp = new DOMParser();
xml = tmp.parseFromString( data , "text/xml" );
} else { // IE
xml = new ActiveXObject( "Microsoft.XMLDOM" );
xml.async = "false";
xml.loadXML( data );
}
} catch( e ) {
xml = undefined;
}
if ( !xml || !xml.documentElement || xml.getElementsByTagName( "parsererror" ).length ) {
jQuery.error( "Invalid XML: " + data );
}
return xml;
},
noop: function() {},
// Evaluates a script in a global context
// Workarounds based on findings by Jim Driscoll
// http://weblogs.java.net/blog/driscoll/archive/2009/09/08/eval-javascript-global-context
globalEval: function( data ) {
if ( data && jQuery.trim( data ) ) {
// We use execScript on Internet Explorer
// We use an anonymous function so that context is window
// rather than jQuery in Firefox
( window.execScript || function( data ) {
window[ "eval" ].call( window, data );
} )( data );
}
},
// Convert dashed to camelCase; used by the css and data modules
// Microsoft forgot to hump their vendor prefix (#9572)
camelCase: function( string ) {
return string.replace( rmsPrefix, "ms-" ).replace( rdashAlpha, fcamelCase );
},
nodeName: function( elem, name ) {
return elem.nodeName && elem.nodeName.toLowerCase() === name.toLowerCase();
},
// args is for internal usage only
each: function( obj, callback, args ) {
var value,
i = 0,
length = obj.length,
isArray = isArraylike( obj );
if ( args ) {
if ( isArray ) {
for ( ; i < length; i++ ) {
value = callback.apply( obj[ i ], args );
if ( value === false ) {
break;
}
}
} else {
for ( i in obj ) {
value = callback.apply( obj[ i ], args );
if ( value === false ) {
break;
}
}
}
// A special, fast, case for the most common use of each
} else {
if ( isArray ) {
for ( ; i < length; i++ ) {
value = callback.call( obj[ i ], i, obj[ i ] );
if ( value === false ) {
break;
}
}
} else {
for ( i in obj ) {
value = callback.call( obj[ i ], i, obj[ i ] );
if ( value === false ) {
break;
}
}
}
}
return obj;
},
// Use native String.trim function wherever possible
trim: core_trim && !core_trim.call("\uFEFF\xA0") ?
function( text ) {
return text == null ?
"" :
core_trim.call( text );
} :
// Otherwise use our own trimming functionality
function( text ) {
return text == null ?
"" :
( text + "" ).replace( rtrim, "" );
},
// results is for internal usage only
makeArray: function( arr, results ) {
var ret = results || [];
if ( arr != null ) {
if ( isArraylike( Object(arr) ) ) {
jQuery.merge( ret,
typeof arr === "string" ?
[ arr ] : arr
);
} else {
core_push.call( ret, arr );
}
}
return ret;
},
inArray: function( elem, arr, i ) {
var len;
if ( arr ) {
if ( core_indexOf ) {
return core_indexOf.call( arr, elem, i );
}
len = arr.length;
i = i ? i < 0 ? Math.max( 0, len + i ) : i : 0;
for ( ; i < len; i++ ) {
// Skip accessing in sparse arrays
if ( i in arr && arr[ i ] === elem ) {
return i;
}
}
}
return -1;
},
merge: function( first, second ) {
var l = second.length,
i = first.length,
j = 0;
if ( typeof l === "number" ) {
for ( ; j < l; j++ ) {
first[ i++ ] = second[ j ];
}
} else {
while ( second[j] !== undefined ) {
first[ i++ ] = second[ j++ ];
}
}
first.length = i;
return first;
},
grep: function( elems, callback, inv ) {
var retVal,
ret = [],
i = 0,
length = elems.length;
inv = !!inv;
// Go through the array, only saving the items
// that pass the validator function
for ( ; i < length; i++ ) {
retVal = !!callback( elems[ i ], i );
if ( inv !== retVal ) {
ret.push( elems[ i ] );
}
}
return ret;
},
// arg is for internal usage only
map: function( elems, callback, arg ) {
var value,
i = 0,
length = elems.length,
isArray = isArraylike( elems ),
ret = [];
// Go through the array, translating each of the items to their
if ( isArray ) {
for ( ; i < length; i++ ) {
value = callback( elems[ i ], i, arg );
if ( value != null ) {
ret[ ret.length ] = value;
}
}
// Go through every key on the object,
} else {
for ( i in elems ) {
value = callback( elems[ i ], i, arg );
if ( value != null ) {
ret[ ret.length ] = value;
}
}
}
// Flatten any nested arrays
return core_concat.apply( [], ret );
},
// A global GUID counter for objects
guid: 1,
// Bind a function to a context, optionally partially applying any
// arguments.
proxy: function( fn, context ) {
var args, proxy, tmp;
if ( typeof context === "string" ) {
tmp = fn[ context ];
context = fn;
fn = tmp;
}
// Quick check to determine if target is callable, in the spec
// this throws a TypeError, but we will just return undefined.
if ( !jQuery.isFunction( fn ) ) {
return undefined;
}
// Simulated bind
args = core_slice.call( arguments, 2 );
proxy = function() {
return fn.apply( context || this, args.concat( core_slice.call( arguments ) ) );
};
// Set the guid of unique handler to the same of original handler, so it can be removed
proxy.guid = fn.guid = fn.guid || jQuery.guid++;
return proxy;
},
// Multifunctional method to get and set values of a collection
// The value/s can optionally be executed if it's a function
access: function( elems, fn, key, value, chainable, emptyGet, raw ) {
var i = 0,
length = elems.length,
bulk = key == null;
// Sets many values
if ( jQuery.type( key ) === "object" ) {
chainable = true;
for ( i in key ) {
jQuery.access( elems, fn, i, key[i], true, emptyGet, raw );
}
// Sets one value
} else if ( value !== undefined ) {
chainable = true;
if ( !jQuery.isFunction( value ) ) {
raw = true;
}
if ( bulk ) {
// Bulk operations run against the entire set
if ( raw ) {
fn.call( elems, value );
fn = null;
// ...except when executing function values
} else {
bulk = fn;
fn = function( elem, key, value ) {
return bulk.call( jQuery( elem ), value );
};
}
}
if ( fn ) {
for ( ; i < length; i++ ) {
fn( elems[i], key, raw ? value : value.call( elems[i], i, fn( elems[i], key ) ) );
}
}
}
return chainable ?
elems :
// Gets
bulk ?
fn.call( elems ) :
length ? fn( elems[0], key ) : emptyGet;
},
now: function() {
return ( new Date() ).getTime();
}
});
jQuery.ready.promise = function( obj ) {
if ( !readyList ) {
readyList = jQuery.Deferred();
// Catch cases where $(document).ready() is called after the browser event has already occurred.
// we once tried to use readyState "interactive" here, but it caused issues like the one
// discovered by ChrisS here: http://bugs.jquery.com/ticket/12282#comment:15
if ( document.readyState === "complete" ) {
// Handle it asynchronously to allow scripts the opportunity to delay ready
setTimeout( jQuery.ready );
// Standards-based browsers support DOMContentLoaded
} else if ( document.addEventListener ) {
// Use the handy event callback
document.addEventListener( "DOMContentLoaded", completed, false );
// A fallback to window.onload, that will always work
window.addEventListener( "load", completed, false );
// If IE event model is used
} else {
// Ensure firing before onload, maybe late but safe also for iframes
document.attachEvent( "onreadystatechange", completed );
// A fallback to window.onload, that will always work
window.attachEvent( "onload", completed );
// If IE and not a frame
// continually check to see if the document is ready
var top = false;
try {
top = window.frameElement == null && document.documentElement;
} catch(e) {}
if ( top && top.doScroll ) {
(function doScrollCheck() {
if ( !jQuery.isReady ) {
try {
// Use the trick by Diego Perini
// http://javascript.nwbox.com/IEContentLoaded/
top.doScroll("left");
} catch(e) {
return setTimeout( doScrollCheck, 50 );
}
// detach all dom ready events
detach();
// and execute any waiting functions
jQuery.ready();
}
})();
}
}
}
return readyList.promise( obj );
};
// Populate the class2type map
jQuery.each("Boolean Number String Function Array Date RegExp Object Error".split(" "), function(i, name) {
class2type[ "[object " + name + "]" ] = name.toLowerCase();
});
function isArraylike( obj ) {
var length = obj.length,
type = jQuery.type( obj );
if ( jQuery.isWindow( obj ) ) {
return false;
}
if ( obj.nodeType === 1 && length ) {
return true;
}
return type === "array" || type !== "function" &&
( length === 0 ||
typeof length === "number" && length > 0 && ( length - 1 ) in obj );
}
// All jQuery objects should point back to these
rootjQuery = jQuery(document);
// String to Object options format cache
var optionsCache = {};
// Convert String-formatted options into Object-formatted ones and store in cache
function createOptions( options ) {
var object = optionsCache[ options ] = {};
jQuery.each( options.match( core_rnotwhite ) || [], function( _, flag ) {
object[ flag ] = true;
});
return object;
}
/*
* Create a callback list using the following parameters:
*
* options: an optional list of space-separated options that will change how
* the callback list behaves or a more traditional option object
*
* By default a callback list will act like an event callback list and can be
* "fired" multiple times.
*
* Possible options:
*
* once: will ensure the callback list can only be fired once (like a Deferred)
*
* memory: will keep track of previous values and will call any callback added
* after the list has been fired right away with the latest "memorized"
* values (like a Deferred)
*
* unique: will ensure a callback can only be added once (no duplicate in the list)
*
* stopOnFalse: interrupt callings when a callback returns false
*
*/
jQuery.Callbacks = function( options ) {
// Convert options from String-formatted to Object-formatted if needed
// (we check in cache first)
options = typeof options === "string" ?
( optionsCache[ options ] || createOptions( options ) ) :
jQuery.extend( {}, options );
var // Flag to know if list is currently firing
firing,
// Last fire value (for non-forgettable lists)
memory,
// Flag to know if list was already fired
fired,
// End of the loop when firing
firingLength,
// Index of currently firing callback (modified by remove if needed)
firingIndex,
// First callback to fire (used internally by add and fireWith)
firingStart,
// Actual callback list
list = [],
// Stack of fire calls for repeatable lists
stack = !options.once && [],
// Fire callbacks
fire = function( data ) {
memory = options.memory && data;
fired = true;
firingIndex = firingStart || 0;
firingStart = 0;
firingLength = list.length;
firing = true;
for ( ; list && firingIndex < firingLength; firingIndex++ ) {
if ( list[ firingIndex ].apply( data[ 0 ], data[ 1 ] ) === false && options.stopOnFalse ) {
memory = false; // To prevent further calls using add
break;
}
}
firing = false;
if ( list ) {
if ( stack ) {
if ( stack.length ) {
fire( stack.shift() );
}
} else if ( memory ) {
list = [];
} else {
self.disable();
}
}
},
// Actual Callbacks object
self = {
// Add a callback or a collection of callbacks to the list
add: function() {
if ( list ) {
// First, we save the current length
var start = list.length;
(function add( args ) {
jQuery.each( args, function( _, arg ) {
var type = jQuery.type( arg );
if ( type === "function" ) {
if ( !options.unique || !self.has( arg ) ) {
list.push( arg );
}
} else if ( arg && arg.length && type !== "string" ) {
// Inspect recursively
add( arg );
}
});
})( arguments );
// Do we need to add the callbacks to the
// current firing batch?
if ( firing ) {
firingLength = list.length;
// With memory, if we're not firing then
// we should call right away
} else if ( memory ) {
firingStart = start;
fire( memory );
}
}
return this;
},
// Remove a callback from the list
remove: function() {
if ( list ) {
jQuery.each( arguments, function( _, arg ) {
var index;
while( ( index = jQuery.inArray( arg, list, index ) ) > -1 ) {
list.splice( index, 1 );
// Handle firing indexes
if ( firing ) {
if ( index <= firingLength ) {
firingLength--;
}
if ( index <= firingIndex ) {
firingIndex--;
}
}
}
});
}
return this;
},
// Check if a given callback is in the list.
// If no argument is given, return whether or not list has callbacks attached.
has: function( fn ) {
return fn ? jQuery.inArray( fn, list ) > -1 : !!( list && list.length );
},
// Remove all callbacks from the list
empty: function() {
list = [];
return this;
},
// Have the list do nothing anymore
disable: function() {
list = stack = memory = undefined;
return this;
},
// Is it disabled?
disabled: function() {
return !list;
},
// Lock the list in its current state
lock: function() {
stack = undefined;
if ( !memory ) {
self.disable();
}
return this;
},
// Is it locked?
locked: function() {
return !stack;
},
// Call all callbacks with the given context and arguments
fireWith: function( context, args ) {
args = args || [];
args = [ context, args.slice ? args.slice() : args ];
if ( list && ( !fired || stack ) ) {
if ( firing ) {
stack.push( args );
} else {
fire( args );
}
}
return this;
},
// Call all the callbacks with the given arguments
fire: function() {
self.fireWith( this, arguments );
return this;
},
// To know if the callbacks have already been called at least once
fired: function() {
return !!fired;
}
};
return self;
};
jQuery.extend({
Deferred: function( func ) {
var tuples = [
// action, add listener, listener list, final state
[ "resolve", "done", jQuery.Callbacks("once memory"), "resolved" ],
[ "reject", "fail", jQuery.Callbacks("once memory"), "rejected" ],
[ "notify", "progress", jQuery.Callbacks("memory") ]
],
state = "pending",
promise = {
state: function() {
return state;
},
always: function() {
deferred.done( arguments ).fail( arguments );
return this;
},
then: function( /* fnDone, fnFail, fnProgress */ ) {
var fns = arguments;
return jQuery.Deferred(function( newDefer ) {
jQuery.each( tuples, function( i, tuple ) {
var action = tuple[ 0 ],
fn = jQuery.isFunction( fns[ i ] ) && fns[ i ];
// deferred[ done | fail | progress ] for forwarding actions to newDefer
deferred[ tuple[1] ](function() {
var returned = fn && fn.apply( this, arguments );
if ( returned && jQuery.isFunction( returned.promise ) ) {
returned.promise()
.done( newDefer.resolve )
.fail( newDefer.reject )
.progress( newDefer.notify );
} else {
newDefer[ action + "With" ]( this === promise ? newDefer.promise() : this, fn ? [ returned ] : arguments );
}
});
});
fns = null;
}).promise();
},
// Get a promise for this deferred
// If obj is provided, the promise aspect is added to the object
promise: function( obj ) {
return obj != null ? jQuery.extend( obj, promise ) : promise;
}
},
deferred = {};
// Keep pipe for back-compat
promise.pipe = promise.then;
// Add list-specific methods
jQuery.each( tuples, function( i, tuple ) {
var list = tuple[ 2 ],
stateString = tuple[ 3 ];
// promise[ done | fail | progress ] = list.add
promise[ tuple[1] ] = list.add;
// Handle state
if ( stateString ) {
list.add(function() {
// state = [ resolved | rejected ]
state = stateString;
// [ reject_list | resolve_list ].disable; progress_list.lock
}, tuples[ i ^ 1 ][ 2 ].disable, tuples[ 2 ][ 2 ].lock );
}
// deferred[ resolve | reject | notify ]
deferred[ tuple[0] ] = function() {
deferred[ tuple[0] + "With" ]( this === deferred ? promise : this, arguments );
return this;
};
deferred[ tuple[0] + "With" ] = list.fireWith;
});
// Make the deferred a promise
promise.promise( deferred );
// Call given func if any
if ( func ) {
func.call( deferred, deferred );
}
// All done!
return deferred;
},
// Deferred helper
when: function( subordinate /* , ..., subordinateN */ ) {
var i = 0,
resolveValues = core_slice.call( arguments ),
length = resolveValues.length,
// the count of uncompleted subordinates
remaining = length !== 1 || ( subordinate && jQuery.isFunction( subordinate.promise ) ) ? length : 0,
// the master Deferred. If resolveValues consist of only a single Deferred, just use that.
deferred = remaining === 1 ? subordinate : jQuery.Deferred(),
// Update function for both resolve and progress values
updateFunc = function( i, contexts, values ) {
return function( value ) {
contexts[ i ] = this;
values[ i ] = arguments.length > 1 ? core_slice.call( arguments ) : value;
if( values === progressValues ) {
deferred.notifyWith( contexts, values );
} else if ( !( --remaining ) ) {
deferred.resolveWith( contexts, values );
}
};
},
progressValues, progressContexts, resolveContexts;
// add listeners to Deferred subordinates; treat others as resolved
if ( length > 1 ) {
progressValues = new Array( length );
progressContexts = new Array( length );
resolveContexts = new Array( length );
for ( ; i < length; i++ ) {
if ( resolveValues[ i ] && jQuery.isFunction( resolveValues[ i ].promise ) ) {
resolveValues[ i ].promise()
.done( updateFunc( i, resolveContexts, resolveValues ) )
.fail( deferred.reject )
.progress( updateFunc( i, progressContexts, progressValues ) );
} else {
--remaining;
}
}
}
// if we're not waiting on anything, resolve the master
if ( !remaining ) {
deferred.resolveWith( resolveContexts, resolveValues );
}
return deferred.promise();
}
});
jQuery.support = (function() {
var support, all, a,
input, select, fragment,
opt, eventName, isSupported, i,
div = document.createElement("div");
// Setup
div.setAttribute( "className", "t" );
div.innerHTML = " <link/><table></table><a href='/a'>a</a><input type='checkbox'/>";
// Support tests won't run in some limited or non-browser environments
all = div.getElementsByTagName("*");
a = div.getElementsByTagName("a")[ 0 ];
if ( !all || !a || !all.length ) {
return {};
}
// First batch of tests
select = document.createElement("select");
opt = select.appendChild( document.createElement("option") );
input = div.getElementsByTagName("input")[ 0 ];
a.style.cssText = "top:1px;float:left;opacity:.5";
support = {
// Test setAttribute on camelCase class. If it works, we need attrFixes when doing get/setAttribute (ie6/7)
getSetAttribute: div.className !== "t",
// IE strips leading whitespace when .innerHTML is used
leadingWhitespace: div.firstChild.nodeType === 3,
// Make sure that tbody elements aren't automatically inserted
// IE will insert them into empty tables
tbody: !div.getElementsByTagName("tbody").length,
// Make sure that link elements get serialized correctly by innerHTML
// This requires a wrapper element in IE
htmlSerialize: !!div.getElementsByTagName("link").length,
// Get the style information from getAttribute
// (IE uses .cssText instead)
style: /top/.test( a.getAttribute("style") ),
// Make sure that URLs aren't manipulated
// (IE normalizes it by default)
hrefNormalized: a.getAttribute("href") === "/a",
// Make sure that element opacity exists
// (IE uses filter instead)
// Use a regex to work around a WebKit issue. See #5145
opacity: /^0.5/.test( a.style.opacity ),
// Verify style float existence
// (IE uses styleFloat instead of cssFloat)
cssFloat: !!a.style.cssFloat,
// Check the default checkbox/radio value ("" on WebKit; "on" elsewhere)
checkOn: !!input.value,
// Make sure that a selected-by-default option has a working selected property.
// (WebKit defaults to false instead of true, IE too, if it's in an optgroup)
optSelected: opt.selected,
// Tests for enctype support on a form (#6743)
enctype: !!document.createElement("form").enctype,
// Makes sure cloning an html5 element does not cause problems
// Where outerHTML is undefined, this still works
html5Clone: document.createElement("nav").cloneNode( true ).outerHTML !== "<:nav></:nav>",
// jQuery.support.boxModel DEPRECATED in 1.8 since we don't support Quirks Mode
boxModel: document.compatMode === "CSS1Compat",
// Will be defined later
deleteExpando: true,
noCloneEvent: true,
inlineBlockNeedsLayout: false,
shrinkWrapBlocks: false,
reliableMarginRight: true,
boxSizingReliable: true,
pixelPosition: false
};
// Make sure checked status is properly cloned
input.checked = true;
support.noCloneChecked = input.cloneNode( true ).checked;
// Make sure that the options inside disabled selects aren't marked as disabled
// (WebKit marks them as disabled)
select.disabled = true;
support.optDisabled = !opt.disabled;
// Support: IE<9
try {
delete div.test;
} catch( e ) {
support.deleteExpando = false;
}
// Check if we can trust getAttribute("value")
input = document.createElement("input");
input.setAttribute( "value", "" );
support.input = input.getAttribute( "value" ) === "";
// Check if an input maintains its value after becoming a radio
input.value = "t";
input.setAttribute( "type", "radio" );
support.radioValue = input.value === "t";
// #11217 - WebKit loses check when the name is after the checked attribute
input.setAttribute( "checked", "t" );
input.setAttribute( "name", "t" );
fragment = document.createDocumentFragment();
fragment.appendChild( input );
// Check if a disconnected checkbox will retain its checked
// value of true after appended to the DOM (IE6/7)
support.appendChecked = input.checked;
// WebKit doesn't clone checked state correctly in fragments
support.checkClone = fragment.cloneNode( true ).cloneNode( true ).lastChild.checked;
// Support: IE<9
// Opera does not clone events (and typeof div.attachEvent === undefined).
// IE9-10 clones events bound via attachEvent, but they don't trigger with .click()
if ( div.attachEvent ) {
div.attachEvent( "onclick", function() {
support.noCloneEvent = false;
});
div.cloneNode( true ).click();
}
// Support: IE<9 (lack submit/change bubble), Firefox 17+ (lack focusin event)
// Beware of CSP restrictions (https://developer.mozilla.org/en/Security/CSP), test/csp.php
for ( i in { submit: true, change: true, focusin: true }) {
div.setAttribute( eventName = "on" + i, "t" );
support[ i + "Bubbles" ] = eventName in window || div.attributes[ eventName ].expando === false;
}
div.style.backgroundClip = "content-box";
div.cloneNode( true ).style.backgroundClip = "";
support.clearCloneStyle = div.style.backgroundClip === "content-box";
// Run tests that need a body at doc ready
jQuery(function() {
var container, marginDiv, tds,
divReset = "padding:0;margin:0;border:0;display:block;box-sizing:content-box;-moz-box-sizing:content-box;-webkit-box-sizing:content-box;",
body = document.getElementsByTagName("body")[0];
if ( !body ) {
// Return for frameset docs that don't have a body
return;
}
container = document.createElement("div");
container.style.cssText = "border:0;width:0;height:0;position:absolute;top:0;left:-9999px;margin-top:1px";
body.appendChild( container ).appendChild( div );
// Support: IE8
// Check if table cells still have offsetWidth/Height when they are set
// to display:none and there are still other visible table cells in a
// table row; if so, offsetWidth/Height are not reliable for use when
// determining if an element has been hidden directly using
// display:none (it is still safe to use offsets if a parent element is
// hidden; don safety goggles and see bug #4512 for more information).
div.innerHTML = "<table><tr><td></td><td>t</td></tr></table>";
tds = div.getElementsByTagName("td");
tds[ 0 ].style.cssText = "padding:0;margin:0;border:0;display:none";
isSupported = ( tds[ 0 ].offsetHeight === 0 );
tds[ 0 ].style.display = "";
tds[ 1 ].style.display = "none";
// Support: IE8
// Check if empty table cells still have offsetWidth/Height
support.reliableHiddenOffsets = isSupported && ( tds[ 0 ].offsetHeight === 0 );
// Check box-sizing and margin behavior
div.innerHTML = "";
div.style.cssText = "box-sizing:border-box;-moz-box-sizing:border-box;-webkit-box-sizing:border-box;padding:1px;border:1px;display:block;width:4px;margin-top:1%;position:absolute;top:1%;";
support.boxSizing = ( div.offsetWidth === 4 );
support.doesNotIncludeMarginInBodyOffset = ( body.offsetTop !== 1 );
// Use window.getComputedStyle because jsdom on node.js will break without it.
if ( window.getComputedStyle ) {
support.pixelPosition = ( window.getComputedStyle( div, null ) || {} ).top !== "1%";
support.boxSizingReliable = ( window.getComputedStyle( div, null ) || { width: "4px" } ).width === "4px";
// Check if div with explicit width and no margin-right incorrectly
// gets computed margin-right based on width of container. (#3333)
// Fails in WebKit before Feb 2011 nightlies
// WebKit Bug 13343 - getComputedStyle returns wrong value for margin-right
marginDiv = div.appendChild( document.createElement("div") );
marginDiv.style.cssText = div.style.cssText = divReset;
marginDiv.style.marginRight = marginDiv.style.width = "0";
div.style.width = "1px";
support.reliableMarginRight =
!parseFloat( ( window.getComputedStyle( marginDiv, null ) || {} ).marginRight );
}
if ( typeof div.style.zoom !== core_strundefined ) {
// Support: IE<8
// Check if natively block-level elements act like inline-block
// elements when setting their display to 'inline' and giving
// them layout
div.innerHTML = "";
div.style.cssText = divReset + "width:1px;padding:1px;display:inline;zoom:1";
support.inlineBlockNeedsLayout = ( div.offsetWidth === 3 );
// Support: IE6
// Check if elements with layout shrink-wrap their children
div.style.display = "block";
div.innerHTML = "<div></div>";
div.firstChild.style.width = "5px";
support.shrinkWrapBlocks = ( div.offsetWidth !== 3 );
if ( support.inlineBlockNeedsLayout ) {
// Prevent IE 6 from affecting layout for positioned elements #11048
// Prevent IE from shrinking the body in IE 7 mode #12869
// Support: IE<8
body.style.zoom = 1;
}
}
body.removeChild( container );
// Null elements to avoid leaks in IE
container = div = tds = marginDiv = null;
});
// Null elements to avoid leaks in IE
all = select = fragment = opt = a = input = null;
return support;
})();
var rbrace = /(?:\{[\s\S]*\}|\[[\s\S]*\])$/,
rmultiDash = /([A-Z])/g;
function internalData( elem, name, data, pvt /* Internal Use Only */ ){
if ( !jQuery.acceptData( elem ) ) {
return;
}
var thisCache, ret,
internalKey = jQuery.expando,
getByName = typeof name === "string",
// We have to handle DOM nodes and JS objects differently because IE6-7
// can't GC object references properly across the DOM-JS boundary
isNode = elem.nodeType,
// Only DOM nodes need the global jQuery cache; JS object data is
// attached directly to the object so GC can occur automatically
cache = isNode ? jQuery.cache : elem,
// Only defining an ID for JS objects if its cache already exists allows
// the code to shortcut on the same path as a DOM node with no cache
id = isNode ? elem[ internalKey ] : elem[ internalKey ] && internalKey;
// Avoid doing any more work than we need to when trying to get data on an
// object that has no data at all
if ( (!id || !cache[id] || (!pvt && !cache[id].data)) && getByName && data === undefined ) {
return;
}
if ( !id ) {
// Only DOM nodes need a new unique ID for each element since their data
// ends up in the global cache
if ( isNode ) {
elem[ internalKey ] = id = core_deletedIds.pop() || jQuery.guid++;
} else {
id = internalKey;
}
}
if ( !cache[ id ] ) {
cache[ id ] = {};
// Avoids exposing jQuery metadata on plain JS objects when the object
// is serialized using JSON.stringify
if ( !isNode ) {
cache[ id ].toJSON = jQuery.noop;
}
}
// An object can be passed to jQuery.data instead of a key/value pair; this gets
// shallow copied over onto the existing cache
if ( typeof name === "object" || typeof name === "function" ) {
if ( pvt ) {
cache[ id ] = jQuery.extend( cache[ id ], name );
} else {
cache[ id ].data = jQuery.extend( cache[ id ].data, name );
}
}
thisCache = cache[ id ];
// jQuery data() is stored in a separate object inside the object's internal data
// cache in order to avoid key collisions between internal data and user-defined
// data.
if ( !pvt ) {
if ( !thisCache.data ) {
thisCache.data = {};
}
thisCache = thisCache.data;
}
if ( data !== undefined ) {
thisCache[ jQuery.camelCase( name ) ] = data;
}
// Check for both converted-to-camel and non-converted data property names
// If a data property was specified
if ( getByName ) {
// First Try to find as-is property data
ret = thisCache[ name ];
// Test for null|undefined property data
if ( ret == null ) {
// Try to find the camelCased property
ret = thisCache[ jQuery.camelCase( name ) ];
}
} else {
ret = thisCache;
}
return ret;
}
function internalRemoveData( elem, name, pvt ) {
if ( !jQuery.acceptData( elem ) ) {
return;
}
var i, l, thisCache,
isNode = elem.nodeType,
// See jQuery.data for more information
cache = isNode ? jQuery.cache : elem,
id = isNode ? elem[ jQuery.expando ] : jQuery.expando;
// If there is already no cache entry for this object, there is no
// purpose in continuing
if ( !cache[ id ] ) {
return;
}
if ( name ) {
thisCache = pvt ? cache[ id ] : cache[ id ].data;
if ( thisCache ) {
// Support array or space separated string names for data keys
if ( !jQuery.isArray( name ) ) {
// try the string as a key before any manipulation
if ( name in thisCache ) {
name = [ name ];
} else {
// split the camel cased version by spaces unless a key with the spaces exists
name = jQuery.camelCase( name );
if ( name in thisCache ) {
name = [ name ];
} else {
name = name.split(" ");
}
}
} else {
// If "name" is an array of keys...
// When data is initially created, via ("key", "val") signature,
// keys will be converted to camelCase.
// Since there is no way to tell _how_ a key was added, remove
// both plain key and camelCase key. #12786
// This will only penalize the array argument path.
name = name.concat( jQuery.map( name, jQuery.camelCase ) );
}
for ( i = 0, l = name.length; i < l; i++ ) {
delete thisCache[ name[i] ];
}
// If there is no data left in the cache, we want to continue
// and let the cache object itself get destroyed
if ( !( pvt ? isEmptyDataObject : jQuery.isEmptyObject )( thisCache ) ) {
return;
}
}
}
// See jQuery.data for more information
if ( !pvt ) {
delete cache[ id ].data;
// Don't destroy the parent cache unless the internal data object
// had been the only thing left in it
if ( !isEmptyDataObject( cache[ id ] ) ) {
return;
}
}
// Destroy the cache
if ( isNode ) {
jQuery.cleanData( [ elem ], true );
// Use delete when supported for expandos or `cache` is not a window per isWindow (#10080)
} else if ( jQuery.support.deleteExpando || cache != cache.window ) {
delete cache[ id ];
// When all else fails, null
} else {
cache[ id ] = null;
}
}
jQuery.extend({
cache: {},
// Unique for each copy of jQuery on the page
// Non-digits removed to match rinlinejQuery
expando: "jQuery" + ( core_version + Math.random() ).replace( /\D/g, "" ),
// The following elements throw uncatchable exceptions if you
// attempt to add expando properties to them.
noData: {
"embed": true,
// Ban all objects except for Flash (which handle expandos)
"object": "clsid:D27CDB6E-AE6D-11cf-96B8-444553540000",
"applet": true
},
hasData: function( elem ) {
elem = elem.nodeType ? jQuery.cache[ elem[jQuery.expando] ] : elem[ jQuery.expando ];
return !!elem && !isEmptyDataObject( elem );
},
data: function( elem, name, data ) {
return internalData( elem, name, data );
},
removeData: function( elem, name ) {
return internalRemoveData( elem, name );
},
// For internal use only.
_data: function( elem, name, data ) {
return internalData( elem, name, data, true );
},
_removeData: function( elem, name ) {
return internalRemoveData( elem, name, true );
},
// A method for determining if a DOM node can handle the data expando
acceptData: function( elem ) {
// Do not set data on non-element because it will not be cleared (#8335).
if ( elem.nodeType && elem.nodeType !== 1 && elem.nodeType !== 9 ) {
return false;
}
var noData = elem.nodeName && jQuery.noData[ elem.nodeName.toLowerCase() ];
// nodes accept data unless otherwise specified; rejection can be conditional
return !noData || noData !== true && elem.getAttribute("classid") === noData;
}
});
jQuery.fn.extend({
data: function( key, value ) {
var attrs, name,
elem = this[0],
i = 0,
data = null;
// Gets all values
if ( key === undefined ) {
if ( this.length ) {
data = jQuery.data( elem );
if ( elem.nodeType === 1 && !jQuery._data( elem, "parsedAttrs" ) ) {
attrs = elem.attributes;
for ( ; i < attrs.length; i++ ) {
name = attrs[i].name;
if ( !name.indexOf( "data-" ) ) {
name = jQuery.camelCase( name.slice(5) );
dataAttr( elem, name, data[ name ] );
}
}
jQuery._data( elem, "parsedAttrs", true );
}
}
return data;
}
// Sets multiple values
if ( typeof key === "object" ) {
return this.each(function() {
jQuery.data( this, key );
});
}
return jQuery.access( this, function( value ) {
if ( value === undefined ) {
// Try to fetch any internally stored data first
return elem ? dataAttr( elem, key, jQuery.data( elem, key ) ) : null;
}
this.each(function() {
jQuery.data( this, key, value );
});
}, null, value, arguments.length > 1, null, true );
},
removeData: function( key ) {
return this.each(function() {
jQuery.removeData( this, key );
});
}
});
function dataAttr( elem, key, data ) {
// If nothing was found internally, try to fetch any
// data from the HTML5 data-* attribute
if ( data === undefined && elem.nodeType === 1 ) {
var name = "data-" + key.replace( rmultiDash, "-$1" ).toLowerCase();
data = elem.getAttribute( name );
if ( typeof data === "string" ) {
try {
data = data === "true" ? true :
data === "false" ? false :
data === "null" ? null :
// Only convert to a number if it doesn't change the string
+data + "" === data ? +data :
rbrace.test( data ) ? jQuery.parseJSON( data ) :
data;
} catch( e ) {}
// Make sure we set the data so it isn't changed later
jQuery.data( elem, key, data );
} else {
data = undefined;
}
}
return data;
}
// checks a cache object for emptiness
function isEmptyDataObject( obj ) {
var name;
for ( name in obj ) {
// if the public data object is empty, the private is still empty
if ( name === "data" && jQuery.isEmptyObject( obj[name] ) ) {
continue;
}
if ( name !== "toJSON" ) {
return false;
}
}
return true;
}
jQuery.extend({
queue: function( elem, type, data ) {
var queue;
if ( elem ) {
type = ( type || "fx" ) + "queue";
queue = jQuery._data( elem, type );
// Speed up dequeue by getting out quickly if this is just a lookup
if ( data ) {
if ( !queue || jQuery.isArray(data) ) {
queue = jQuery._data( elem, type, jQuery.makeArray(data) );
} else {
queue.push( data );
}
}
return queue || [];
}
},
dequeue: function( elem, type ) {
type = type || "fx";
var queue = jQuery.queue( elem, type ),
startLength = queue.length,
fn = queue.shift(),
hooks = jQuery._queueHooks( elem, type ),
next = function() {
jQuery.dequeue( elem, type );
};
// If the fx queue is dequeued, always remove the progress sentinel
if ( fn === "inprogress" ) {
fn = queue.shift();
startLength--;
}
hooks.cur = fn;
if ( fn ) {
// Add a progress sentinel to prevent the fx queue from being
// automatically dequeued
if ( type === "fx" ) {
queue.unshift( "inprogress" );
}
// clear up the last queue stop function
delete hooks.stop;
fn.call( elem, next, hooks );
}
if ( !startLength && hooks ) {
hooks.empty.fire();
}
},
// not intended for public consumption - generates a queueHooks object, or returns the current one
_queueHooks: function( elem, type ) {
var key = type + "queueHooks";
return jQuery._data( elem, key ) || jQuery._data( elem, key, {
empty: jQuery.Callbacks("once memory").add(function() {
jQuery._removeData( elem, type + "queue" );
jQuery._removeData( elem, key );
})
});
}
});
jQuery.fn.extend({
queue: function( type, data ) {
var setter = 2;
if ( typeof type !== "string" ) {
data = type;
type = "fx";
setter--;
}
if ( arguments.length < setter ) {
return jQuery.queue( this[0], type );
}
return data === undefined ?
this :
this.each(function() {
var queue = jQuery.queue( this, type, data );
// ensure a hooks for this queue
jQuery._queueHooks( this, type );
if ( type === "fx" && queue[0] !== "inprogress" ) {
jQuery.dequeue( this, type );
}
});
},
dequeue: function( type ) {
return this.each(function() {
jQuery.dequeue( this, type );
});
},
// Based off of the plugin by Clint Helfers, with permission.
// http://blindsignals.com/index.php/2009/07/jquery-delay/
delay: function( time, type ) {
time = jQuery.fx ? jQuery.fx.speeds[ time ] || time : time;
type = type || "fx";
return this.queue( type, function( next, hooks ) {
var timeout = setTimeout( next, time );
hooks.stop = function() {
clearTimeout( timeout );
};
});
},
clearQueue: function( type ) {
return this.queue( type || "fx", [] );
},
// Get a promise resolved when queues of a certain type
// are emptied (fx is the type by default)
promise: function( type, obj ) {
var tmp,
count = 1,
defer = jQuery.Deferred(),
elements = this,
i = this.length,
resolve = function() {
if ( !( --count ) ) {
defer.resolveWith( elements, [ elements ] );
}
};
if ( typeof type !== "string" ) {
obj = type;
type = undefined;
}
type = type || "fx";
while( i-- ) {
tmp = jQuery._data( elements[ i ], type + "queueHooks" );
if ( tmp && tmp.empty ) {
count++;
tmp.empty.add( resolve );
}
}
resolve();
return defer.promise( obj );
}
});
var nodeHook, boolHook,
rclass = /[\t\r\n]/g,
rreturn = /\r/g,
rfocusable = /^(?:input|select|textarea|button|object)$/i,
rclickable = /^(?:a|area)$/i,
rboolean = /^(?:checked|selected|autofocus|autoplay|async|controls|defer|disabled|hidden|loop|multiple|open|readonly|required|scoped)$/i,
ruseDefault = /^(?:checked|selected)$/i,
getSetAttribute = jQuery.support.getSetAttribute,
getSetInput = jQuery.support.input;
jQuery.fn.extend({
attr: function( name, value ) {
return jQuery.access( this, jQuery.attr, name, value, arguments.length > 1 );
},
removeAttr: function( name ) {
return this.each(function() {
jQuery.removeAttr( this, name );
});
},
prop: function( name, value ) {
return jQuery.access( this, jQuery.prop, name, value, arguments.length > 1 );
},
removeProp: function( name ) {
name = jQuery.propFix[ name ] || name;
return this.each(function() {
// try/catch handles cases where IE balks (such as removing a property on window)
try {
this[ name ] = undefined;
delete this[ name ];
} catch( e ) {}
});
},
addClass: function( value ) {
var classes, elem, cur, clazz, j,
i = 0,
len = this.length,
proceed = typeof value === "string" && value;
if ( jQuery.isFunction( value ) ) {
return this.each(function( j ) {
jQuery( this ).addClass( value.call( this, j, this.className ) );
});
}
if ( proceed ) {
// The disjunction here is for better compressibility (see removeClass)
classes = ( value || "" ).match( core_rnotwhite ) || [];
for ( ; i < len; i++ ) {
elem = this[ i ];
cur = elem.nodeType === 1 && ( elem.className ?
( " " + elem.className + " " ).replace( rclass, " " ) :
" "
);
if ( cur ) {
j = 0;
while ( (clazz = classes[j++]) ) {
if ( cur.indexOf( " " + clazz + " " ) < 0 ) {
cur += clazz + " ";
}
}
elem.className = jQuery.trim( cur );
}
}
}
return this;
},
removeClass: function( value ) {
var classes, elem, cur, clazz, j,
i = 0,
len = this.length,
proceed = arguments.length === 0 || typeof value === "string" && value;
if ( jQuery.isFunction( value ) ) {
return this.each(function( j ) {
jQuery( this ).removeClass( value.call( this, j, this.className ) );
});
}
if ( proceed ) {
classes = ( value || "" ).match( core_rnotwhite ) || [];
for ( ; i < len; i++ ) {
elem = this[ i ];
// This expression is here for better compressibility (see addClass)
cur = elem.nodeType === 1 && ( elem.className ?
( " " + elem.className + " " ).replace( rclass, " " ) :
""
);
if ( cur ) {
j = 0;
while ( (clazz = classes[j++]) ) {
// Remove *all* instances
while ( cur.indexOf( " " + clazz + " " ) >= 0 ) {
cur = cur.replace( " " + clazz + " ", " " );
}
}
elem.className = value ? jQuery.trim( cur ) : "";
}
}
}
return this;
},
toggleClass: function( value, stateVal ) {
var type = typeof value,
isBool = typeof stateVal === "boolean";
if ( jQuery.isFunction( value ) ) {
return this.each(function( i ) {
jQuery( this ).toggleClass( value.call(this, i, this.className, stateVal), stateVal );
});
}
return this.each(function() {
if ( type === "string" ) {
// toggle individual class names
var className,
i = 0,
self = jQuery( this ),
state = stateVal,
classNames = value.match( core_rnotwhite ) || [];
while ( (className = classNames[ i++ ]) ) {
// check each className given, space separated list
state = isBool ? state : !self.hasClass( className );
self[ state ? "addClass" : "removeClass" ]( className );
}
// Toggle whole class name
} else if ( type === core_strundefined || type === "boolean" ) {
if ( this.className ) {
// store className if set
jQuery._data( this, "__className__", this.className );
}
// If the element has a class name or if we're passed "false",
// then remove the whole classname (if there was one, the above saved it).
// Otherwise bring back whatever was previously saved (if anything),
// falling back to the empty string if nothing was stored.
this.className = this.className || value === false ? "" : jQuery._data( this, "__className__" ) || "";
}
});
},
hasClass: function( selector ) {
var className = " " + selector + " ",
i = 0,
l = this.length;
for ( ; i < l; i++ ) {
if ( this[i].nodeType === 1 && (" " + this[i].className + " ").replace(rclass, " ").indexOf( className ) >= 0 ) {
return true;
}
}
return false;
},
val: function( value ) {
var ret, hooks, isFunction,
elem = this[0];
if ( !arguments.length ) {
if ( elem ) {
hooks = jQuery.valHooks[ elem.type ] || jQuery.valHooks[ elem.nodeName.toLowerCase() ];
if ( hooks && "get" in hooks && (ret = hooks.get( elem, "value" )) !== undefined ) {
return ret;
}
ret = elem.value;
return typeof ret === "string" ?
// handle most common string cases
ret.replace(rreturn, "") :
// handle cases where value is null/undef or number
ret == null ? "" : ret;
}
return;
}
isFunction = jQuery.isFunction( value );
return this.each(function( i ) {
var val,
self = jQuery(this);
if ( this.nodeType !== 1 ) {
return;
}
if ( isFunction ) {
val = value.call( this, i, self.val() );
} else {
val = value;
}
// Treat null/undefined as ""; convert numbers to string
if ( val == null ) {
val = "";
} else if ( typeof val === "number" ) {
val += "";
} else if ( jQuery.isArray( val ) ) {
val = jQuery.map(val, function ( value ) {
return value == null ? "" : value + "";
});
}
hooks = jQuery.valHooks[ this.type ] || jQuery.valHooks[ this.nodeName.toLowerCase() ];
// If set returns undefined, fall back to normal setting
if ( !hooks || !("set" in hooks) || hooks.set( this, val, "value" ) === undefined ) {
this.value = val;
}
});
}
});
jQuery.extend({
valHooks: {
option: {
get: function( elem ) {
// attributes.value is undefined in Blackberry 4.7 but
// uses .value. See #6932
var val = elem.attributes.value;
return !val || val.specified ? elem.value : elem.text;
}
},
select: {
get: function( elem ) {
var value, option,
options = elem.options,
index = elem.selectedIndex,
one = elem.type === "select-one" || index < 0,
values = one ? null : [],
max = one ? index + 1 : options.length,
i = index < 0 ?
max :
one ? index : 0;
// Loop through all the selected options
for ( ; i < max; i++ ) {
option = options[ i ];
// oldIE doesn't update selected after form reset (#2551)
if ( ( option.selected || i === index ) &&
// Don't return options that are disabled or in a disabled optgroup
( jQuery.support.optDisabled ? !option.disabled : option.getAttribute("disabled") === null ) &&
( !option.parentNode.disabled || !jQuery.nodeName( option.parentNode, "optgroup" ) ) ) {
// Get the specific value for the option
value = jQuery( option ).val();
// We don't need an array for one selects
if ( one ) {
return value;
}
// Multi-Selects return an array
values.push( value );
}
}
return values;
},
set: function( elem, value ) {
var values = jQuery.makeArray( value );
jQuery(elem).find("option").each(function() {
this.selected = jQuery.inArray( jQuery(this).val(), values ) >= 0;
});
if ( !values.length ) {
elem.selectedIndex = -1;
}
return values;
}
}
},
attr: function( elem, name, value ) {
var hooks, notxml, ret,
nType = elem.nodeType;
// don't get/set attributes on text, comment and attribute nodes
if ( !elem || nType === 3 || nType === 8 || nType === 2 ) {
return;
}
// Fallback to prop when attributes are not supported
if ( typeof elem.getAttribute === core_strundefined ) {
return jQuery.prop( elem, name, value );
}
notxml = nType !== 1 || !jQuery.isXMLDoc( elem );
// All attributes are lowercase
// Grab necessary hook if one is defined
if ( notxml ) {
name = name.toLowerCase();
hooks = jQuery.attrHooks[ name ] || ( rboolean.test( name ) ? boolHook : nodeHook );
}
if ( value !== undefined ) {
if ( value === null ) {
jQuery.removeAttr( elem, name );
} else if ( hooks && notxml && "set" in hooks && (ret = hooks.set( elem, value, name )) !== undefined ) {
return ret;
} else {
elem.setAttribute( name, value + "" );
return value;
}
} else if ( hooks && notxml && "get" in hooks && (ret = hooks.get( elem, name )) !== null ) {
return ret;
} else {
// In IE9+, Flash objects don't have .getAttribute (#12945)
// Support: IE9+
if ( typeof elem.getAttribute !== core_strundefined ) {
ret = elem.getAttribute( name );
}
// Non-existent attributes return null, we normalize to undefined
return ret == null ?
undefined :
ret;
}
},
removeAttr: function( elem, value ) {
var name, propName,
i = 0,
attrNames = value && value.match( core_rnotwhite );
if ( attrNames && elem.nodeType === 1 ) {
while ( (name = attrNames[i++]) ) {
propName = jQuery.propFix[ name ] || name;
// Boolean attributes get special treatment (#10870)
if ( rboolean.test( name ) ) {
// Set corresponding property to false for boolean attributes
// Also clear defaultChecked/defaultSelected (if appropriate) for IE<8
if ( !getSetAttribute && ruseDefault.test( name ) ) {
elem[ jQuery.camelCase( "default-" + name ) ] =
elem[ propName ] = false;
} else {
elem[ propName ] = false;
}
// See #9699 for explanation of this approach (setting first, then removal)
} else {
jQuery.attr( elem, name, "" );
}
elem.removeAttribute( getSetAttribute ? name : propName );
}
}
},
attrHooks: {
type: {
set: function( elem, value ) {
if ( !jQuery.support.radioValue && value === "radio" && jQuery.nodeName(elem, "input") ) {
// Setting the type on a radio button after the value resets the value in IE6-9
// Reset value to default in case type is set after value during creation
var val = elem.value;
elem.setAttribute( "type", value );
if ( val ) {
elem.value = val;
}
return value;
}
}
}
},
propFix: {
tabindex: "tabIndex",
readonly: "readOnly",
"for": "htmlFor",
"class": "className",
maxlength: "maxLength",
cellspacing: "cellSpacing",
cellpadding: "cellPadding",
rowspan: "rowSpan",
colspan: "colSpan",
usemap: "useMap",
frameborder: "frameBorder",
contenteditable: "contentEditable"
},
prop: function( elem, name, value ) {
var ret, hooks, notxml,
nType = elem.nodeType;
// don't get/set properties on text, comment and attribute nodes
if ( !elem || nType === 3 || nType === 8 || nType === 2 ) {
return;
}
notxml = nType !== 1 || !jQuery.isXMLDoc( elem );
if ( notxml ) {
// Fix name and attach hooks
name = jQuery.propFix[ name ] || name;
hooks = jQuery.propHooks[ name ];
}
if ( value !== undefined ) {
if ( hooks && "set" in hooks && (ret = hooks.set( elem, value, name )) !== undefined ) {
return ret;
} else {
return ( elem[ name ] = value );
}
} else {
if ( hooks && "get" in hooks && (ret = hooks.get( elem, name )) !== null ) {
return ret;
} else {
return elem[ name ];
}
}
},
propHooks: {
tabIndex: {
get: function( elem ) {
// elem.tabIndex doesn't always return the correct value when it hasn't been explicitly set
// http://fluidproject.org/blog/2008/01/09/getting-setting-and-removing-tabindex-values-with-javascript/
var attributeNode = elem.getAttributeNode("tabindex");
return attributeNode && attributeNode.specified ?
parseInt( attributeNode.value, 10 ) :
rfocusable.test( elem.nodeName ) || rclickable.test( elem.nodeName ) && elem.href ?
0 :
undefined;
}
}
}
});
// Hook for boolean attributes
boolHook = {
get: function( elem, name ) {
var
// Use .prop to determine if this attribute is understood as boolean
prop = jQuery.prop( elem, name ),
// Fetch it accordingly
attr = typeof prop === "boolean" && elem.getAttribute( name ),
detail = typeof prop === "boolean" ?
getSetInput && getSetAttribute ?
attr != null :
// oldIE fabricates an empty string for missing boolean attributes
// and conflates checked/selected into attroperties
ruseDefault.test( name ) ?
elem[ jQuery.camelCase( "default-" + name ) ] :
!!attr :
// fetch an attribute node for properties not recognized as boolean
elem.getAttributeNode( name );
return detail && detail.value !== false ?
name.toLowerCase() :
undefined;
},
set: function( elem, value, name ) {
if ( value === false ) {
// Remove boolean attributes when set to false
jQuery.removeAttr( elem, name );
} else if ( getSetInput && getSetAttribute || !ruseDefault.test( name ) ) {
// IE<8 needs the *property* name
elem.setAttribute( !getSetAttribute && jQuery.propFix[ name ] || name, name );
// Use defaultChecked and defaultSelected for oldIE
} else {
elem[ jQuery.camelCase( "default-" + name ) ] = elem[ name ] = true;
}
return name;
}
};
// fix oldIE value attroperty
if ( !getSetInput || !getSetAttribute ) {
jQuery.attrHooks.value = {
get: function( elem, name ) {
var ret = elem.getAttributeNode( name );
return jQuery.nodeName( elem, "input" ) ?
// Ignore the value *property* by using defaultValue
elem.defaultValue :
ret && ret.specified ? ret.value : undefined;
},
set: function( elem, value, name ) {
if ( jQuery.nodeName( elem, "input" ) ) {
// Does not return so that setAttribute is also used
elem.defaultValue = value;
} else {
// Use nodeHook if defined (#1954); otherwise setAttribute is fine
return nodeHook && nodeHook.set( elem, value, name );
}
}
};
}
// IE6/7 do not support getting/setting some attributes with get/setAttribute
if ( !getSetAttribute ) {
// Use this for any attribute in IE6/7
// This fixes almost every IE6/7 issue
nodeHook = jQuery.valHooks.button = {
get: function( elem, name ) {
var ret = elem.getAttributeNode( name );
return ret && ( name === "id" || name === "name" || name === "coords" ? ret.value !== "" : ret.specified ) ?
ret.value :
undefined;
},
set: function( elem, value, name ) {
// Set the existing or create a new attribute node
var ret = elem.getAttributeNode( name );
if ( !ret ) {
elem.setAttributeNode(
(ret = elem.ownerDocument.createAttribute( name ))
);
}
ret.value = value += "";
// Break association with cloned elements by also using setAttribute (#9646)
return name === "value" || value === elem.getAttribute( name ) ?
value :
undefined;
}
};
// Set contenteditable to false on removals(#10429)
// Setting to empty string throws an error as an invalid value
jQuery.attrHooks.contenteditable = {
get: nodeHook.get,
set: function( elem, value, name ) {
nodeHook.set( elem, value === "" ? false : value, name );
}
};
// Set width and height to auto instead of 0 on empty string( Bug #8150 )
// This is for removals
jQuery.each([ "width", "height" ], function( i, name ) {
jQuery.attrHooks[ name ] = jQuery.extend( jQuery.attrHooks[ name ], {
set: function( elem, value ) {
if ( value === "" ) {
elem.setAttribute( name, "auto" );
return value;
}
}
});
});
}
// Some attributes require a special call on IE
// http://msdn.microsoft.com/en-us/library/ms536429%28VS.85%29.aspx
if ( !jQuery.support.hrefNormalized ) {
jQuery.each([ "href", "src", "width", "height" ], function( i, name ) {
jQuery.attrHooks[ name ] = jQuery.extend( jQuery.attrHooks[ name ], {
get: function( elem ) {
var ret = elem.getAttribute( name, 2 );
return ret == null ? undefined : ret;
}
});
});
// href/src property should get the full normalized URL (#10299/#12915)
jQuery.each([ "href", "src" ], function( i, name ) {
jQuery.propHooks[ name ] = {
get: function( elem ) {
return elem.getAttribute( name, 4 );
}
};
});
}
if ( !jQuery.support.style ) {
jQuery.attrHooks.style = {
get: function( elem ) {
// Return undefined in the case of empty string
// Note: IE uppercases css property names, but if we were to .toLowerCase()
// .cssText, that would destroy case senstitivity in URL's, like in "background"
return elem.style.cssText || undefined;
},
set: function( elem, value ) {
return ( elem.style.cssText = value + "" );
}
};
}
// Safari mis-reports the default selected property of an option
// Accessing the parent's selectedIndex property fixes it
if ( !jQuery.support.optSelected ) {
jQuery.propHooks.selected = jQuery.extend( jQuery.propHooks.selected, {
get: function( elem ) {
var parent = elem.parentNode;
if ( parent ) {
parent.selectedIndex;
// Make sure that it also works with optgroups, see #5701
if ( parent.parentNode ) {
parent.parentNode.selectedIndex;
}
}
return null;
}
});
}
// IE6/7 call enctype encoding
if ( !jQuery.support.enctype ) {
jQuery.propFix.enctype = "encoding";
}
// Radios and checkboxes getter/setter
if ( !jQuery.support.checkOn ) {
jQuery.each([ "radio", "checkbox" ], function() {
jQuery.valHooks[ this ] = {
get: function( elem ) {
// Handle the case where in Webkit "" is returned instead of "on" if a value isn't specified
return elem.getAttribute("value") === null ? "on" : elem.value;
}
};
});
}
jQuery.each([ "radio", "checkbox" ], function() {
jQuery.valHooks[ this ] = jQuery.extend( jQuery.valHooks[ this ], {
set: function( elem, value ) {
if ( jQuery.isArray( value ) ) {
return ( elem.checked = jQuery.inArray( jQuery(elem).val(), value ) >= 0 );
}
}
});
});
var rformElems = /^(?:input|select|textarea)$/i,
rkeyEvent = /^key/,
rmouseEvent = /^(?:mouse|contextmenu)|click/,
rfocusMorph = /^(?:focusinfocus|focusoutblur)$/,
rtypenamespace = /^([^.]*)(?:\.(.+)|)$/;
function returnTrue() {
return true;
}
function returnFalse() {
return false;
}
/*
* Helper functions for managing events -- not part of the public interface.
* Props to Dean Edwards' addEvent library for many of the ideas.
*/
jQuery.event = {
global: {},
add: function( elem, types, handler, data, selector ) {
var tmp, events, t, handleObjIn,
special, eventHandle, handleObj,
handlers, type, namespaces, origType,
elemData = jQuery._data( elem );
// Don't attach events to noData or text/comment nodes (but allow plain objects)
if ( !elemData ) {
return;
}
// Caller can pass in an object of custom data in lieu of the handler
if ( handler.handler ) {
handleObjIn = handler;
handler = handleObjIn.handler;
selector = handleObjIn.selector;
}
// Make sure that the handler has a unique ID, used to find/remove it later
if ( !handler.guid ) {
handler.guid = jQuery.guid++;
}
// Init the element's event structure and main handler, if this is the first
if ( !(events = elemData.events) ) {
events = elemData.events = {};
}
if ( !(eventHandle = elemData.handle) ) {
eventHandle = elemData.handle = function( e ) {
// Discard the second event of a jQuery.event.trigger() and
// when an event is called after a page has unloaded
return typeof jQuery !== core_strundefined && (!e || jQuery.event.triggered !== e.type) ?
jQuery.event.dispatch.apply( eventHandle.elem, arguments ) :
undefined;
};
// Add elem as a property of the handle fn to prevent a memory leak with IE non-native events
eventHandle.elem = elem;
}
// Handle multiple events separated by a space
// jQuery(...).bind("mouseover mouseout", fn);
types = ( types || "" ).match( core_rnotwhite ) || [""];
t = types.length;
while ( t-- ) {
tmp = rtypenamespace.exec( types[t] ) || [];
type = origType = tmp[1];
namespaces = ( tmp[2] || "" ).split( "." ).sort();
// If event changes its type, use the special event handlers for the changed type
special = jQuery.event.special[ type ] || {};
// If selector defined, determine special event api type, otherwise given type
type = ( selector ? special.delegateType : special.bindType ) || type;
// Update special based on newly reset type
special = jQuery.event.special[ type ] || {};
// handleObj is passed to all event handlers
handleObj = jQuery.extend({
type: type,
origType: origType,
data: data,
handler: handler,
guid: handler.guid,
selector: selector,
needsContext: selector && jQuery.expr.match.needsContext.test( selector ),
namespace: namespaces.join(".")
}, handleObjIn );
// Init the event handler queue if we're the first
if ( !(handlers = events[ type ]) ) {
handlers = events[ type ] = [];
handlers.delegateCount = 0;
// Only use addEventListener/attachEvent if the special events handler returns false
if ( !special.setup || special.setup.call( elem, data, namespaces, eventHandle ) === false ) {
// Bind the global event handler to the element
if ( elem.addEventListener ) {
elem.addEventListener( type, eventHandle, false );
} else if ( elem.attachEvent ) {
elem.attachEvent( "on" + type, eventHandle );
}
}
}
if ( special.add ) {
special.add.call( elem, handleObj );
if ( !handleObj.handler.guid ) {
handleObj.handler.guid = handler.guid;
}
}
// Add to the element's handler list, delegates in front
if ( selector ) {
handlers.splice( handlers.delegateCount++, 0, handleObj );
} else {
handlers.push( handleObj );
}
// Keep track of which events have ever been used, for event optimization
jQuery.event.global[ type ] = true;
}
// Nullify elem to prevent memory leaks in IE
elem = null;
},
// Detach an event or set of events from an element
remove: function( elem, types, handler, selector, mappedTypes ) {
var j, handleObj, tmp,
origCount, t, events,
special, handlers, type,
namespaces, origType,
elemData = jQuery.hasData( elem ) && jQuery._data( elem );
if ( !elemData || !(events = elemData.events) ) {
return;
}
// Once for each type.namespace in types; type may be omitted
types = ( types || "" ).match( core_rnotwhite ) || [""];
t = types.length;
while ( t-- ) {
tmp = rtypenamespace.exec( types[t] ) || [];
type = origType = tmp[1];
namespaces = ( tmp[2] || "" ).split( "." ).sort();
// Unbind all events (on this namespace, if provided) for the element
if ( !type ) {
for ( type in events ) {
jQuery.event.remove( elem, type + types[ t ], handler, selector, true );
}
continue;
}
special = jQuery.event.special[ type ] || {};
type = ( selector ? special.delegateType : special.bindType ) || type;
handlers = events[ type ] || [];
tmp = tmp[2] && new RegExp( "(^|\\.)" + namespaces.join("\\.(?:.*\\.|)") + "(\\.|$)" );
// Remove matching events
origCount = j = handlers.length;
while ( j-- ) {
handleObj = handlers[ j ];
if ( ( mappedTypes || origType === handleObj.origType ) &&
( !handler || handler.guid === handleObj.guid ) &&
( !tmp || tmp.test( handleObj.namespace ) ) &&
( !selector || selector === handleObj.selector || selector === "**" && handleObj.selector ) ) {
handlers.splice( j, 1 );
if ( handleObj.selector ) {
handlers.delegateCount--;
}
if ( special.remove ) {
special.remove.call( elem, handleObj );
}
}
}
// Remove generic event handler if we removed something and no more handlers exist
// (avoids potential for endless recursion during removal of special event handlers)
if ( origCount && !handlers.length ) {
if ( !special.teardown || special.teardown.call( elem, namespaces, elemData.handle ) === false ) {
jQuery.removeEvent( elem, type, elemData.handle );
}
delete events[ type ];
}
}
// Remove the expando if it's no longer used
if ( jQuery.isEmptyObject( events ) ) {
delete elemData.handle;
// removeData also checks for emptiness and clears the expando if empty
// so use it instead of delete
jQuery._removeData( elem, "events" );
}
},
trigger: function( event, data, elem, onlyHandlers ) {
var handle, ontype, cur,
bubbleType, special, tmp, i,
eventPath = [ elem || document ],
type = core_hasOwn.call( event, "type" ) ? event.type : event,
namespaces = core_hasOwn.call( event, "namespace" ) ? event.namespace.split(".") : [];
cur = tmp = elem = elem || document;
// Don't do events on text and comment nodes
if ( elem.nodeType === 3 || elem.nodeType === 8 ) {
return;
}
// focus/blur morphs to focusin/out; ensure we're not firing them right now
if ( rfocusMorph.test( type + jQuery.event.triggered ) ) {
return;
}
if ( type.indexOf(".") >= 0 ) {
// Namespaced trigger; create a regexp to match event type in handle()
namespaces = type.split(".");
type = namespaces.shift();
namespaces.sort();
}
ontype = type.indexOf(":") < 0 && "on" + type;
// Caller can pass in a jQuery.Event object, Object, or just an event type string
event = event[ jQuery.expando ] ?
event :
new jQuery.Event( type, typeof event === "object" && event );
event.isTrigger = true;
event.namespace = namespaces.join(".");
event.namespace_re = event.namespace ?
new RegExp( "(^|\\.)" + namespaces.join("\\.(?:.*\\.|)") + "(\\.|$)" ) :
null;
// Clean up the event in case it is being reused
event.result = undefined;
if ( !event.target ) {
event.target = elem;
}
// Clone any incoming data and prepend the event, creating the handler arg list
data = data == null ?
[ event ] :
jQuery.makeArray( data, [ event ] );
// Allow special events to draw outside the lines
special = jQuery.event.special[ type ] || {};
if ( !onlyHandlers && special.trigger && special.trigger.apply( elem, data ) === false ) {
return;
}
// Determine event propagation path in advance, per W3C events spec (#9951)
// Bubble up to document, then to window; watch for a global ownerDocument var (#9724)
if ( !onlyHandlers && !special.noBubble && !jQuery.isWindow( elem ) ) {
bubbleType = special.delegateType || type;
if ( !rfocusMorph.test( bubbleType + type ) ) {
cur = cur.parentNode;
}
for ( ; cur; cur = cur.parentNode ) {
eventPath.push( cur );
tmp = cur;
}
// Only add window if we got to document (e.g., not plain obj or detached DOM)
if ( tmp === (elem.ownerDocument || document) ) {
eventPath.push( tmp.defaultView || tmp.parentWindow || window );
}
}
// Fire handlers on the event path
i = 0;
while ( (cur = eventPath[i++]) && !event.isPropagationStopped() ) {
event.type = i > 1 ?
bubbleType :
special.bindType || type;
// jQuery handler
handle = ( jQuery._data( cur, "events" ) || {} )[ event.type ] && jQuery._data( cur, "handle" );
if ( handle ) {
handle.apply( cur, data );
}
// Native handler
handle = ontype && cur[ ontype ];
if ( handle && jQuery.acceptData( cur ) && handle.apply && handle.apply( cur, data ) === false ) {
event.preventDefault();
}
}
event.type = type;
// If nobody prevented the default action, do it now
if ( !onlyHandlers && !event.isDefaultPrevented() ) {
if ( (!special._default || special._default.apply( elem.ownerDocument, data ) === false) &&
!(type === "click" && jQuery.nodeName( elem, "a" )) && jQuery.acceptData( elem ) ) {
// Call a native DOM method on the target with the same name name as the event.
// Can't use an .isFunction() check here because IE6/7 fails that test.
// Don't do default actions on window, that's where global variables be (#6170)
if ( ontype && elem[ type ] && !jQuery.isWindow( elem ) ) {
// Don't re-trigger an onFOO event when we call its FOO() method
tmp = elem[ ontype ];
if ( tmp ) {
elem[ ontype ] = null;
}
// Prevent re-triggering of the same event, since we already bubbled it above
jQuery.event.triggered = type;
try {
elem[ type ]();
} catch ( e ) {
// IE<9 dies on focus/blur to hidden element (#1486,#12518)
// only reproducible on winXP IE8 native, not IE9 in IE8 mode
}
jQuery.event.triggered = undefined;
if ( tmp ) {
elem[ ontype ] = tmp;
}
}
}
}
return event.result;
},
dispatch: function( event ) {
// Make a writable jQuery.Event from the native event object
event = jQuery.event.fix( event );
var i, ret, handleObj, matched, j,
handlerQueue = [],
args = core_slice.call( arguments ),
handlers = ( jQuery._data( this, "events" ) || {} )[ event.type ] || [],
special = jQuery.event.special[ event.type ] || {};
// Use the fix-ed jQuery.Event rather than the (read-only) native event
args[0] = event;
event.delegateTarget = this;
// Call the preDispatch hook for the mapped type, and let it bail if desired
if ( special.preDispatch && special.preDispatch.call( this, event ) === false ) {
return;
}
// Determine handlers
handlerQueue = jQuery.event.handlers.call( this, event, handlers );
// Run delegates first; they may want to stop propagation beneath us
i = 0;
while ( (matched = handlerQueue[ i++ ]) && !event.isPropagationStopped() ) {
event.currentTarget = matched.elem;
j = 0;
while ( (handleObj = matched.handlers[ j++ ]) && !event.isImmediatePropagationStopped() ) {
// Triggered event must either 1) have no namespace, or
// 2) have namespace(s) a subset or equal to those in the bound event (both can have no namespace).
if ( !event.namespace_re || event.namespace_re.test( handleObj.namespace ) ) {
event.handleObj = handleObj;
event.data = handleObj.data;
ret = ( (jQuery.event.special[ handleObj.origType ] || {}).handle || handleObj.handler )
.apply( matched.elem, args );
if ( ret !== undefined ) {
if ( (event.result = ret) === false ) {
event.preventDefault();
event.stopPropagation();
}
}
}
}
}
// Call the postDispatch hook for the mapped type
if ( special.postDispatch ) {
special.postDispatch.call( this, event );
}
return event.result;
},
handlers: function( event, handlers ) {
var sel, handleObj, matches, i,
handlerQueue = [],
delegateCount = handlers.delegateCount,
cur = event.target;
// Find delegate handlers
// Black-hole SVG <use> instance trees (#13180)
// Avoid non-left-click bubbling in Firefox (#3861)
if ( delegateCount && cur.nodeType && (!event.button || event.type !== "click") ) {
for ( ; cur != this; cur = cur.parentNode || this ) {
// Don't check non-elements (#13208)
// Don't process clicks on disabled elements (#6911, #8165, #11382, #11764)
if ( cur.nodeType === 1 && (cur.disabled !== true || event.type !== "click") ) {
matches = [];
for ( i = 0; i < delegateCount; i++ ) {
handleObj = handlers[ i ];
// Don't conflict with Object.prototype properties (#13203)
sel = handleObj.selector + " ";
if ( matches[ sel ] === undefined ) {
matches[ sel ] = handleObj.needsContext ?
jQuery( sel, this ).index( cur ) >= 0 :
jQuery.find( sel, this, null, [ cur ] ).length;
}
if ( matches[ sel ] ) {
matches.push( handleObj );
}
}
if ( matches.length ) {
handlerQueue.push({ elem: cur, handlers: matches });
}
}
}
}
// Add the remaining (directly-bound) handlers
if ( delegateCount < handlers.length ) {
handlerQueue.push({ elem: this, handlers: handlers.slice( delegateCount ) });
}
return handlerQueue;
},
fix: function( event ) {
if ( event[ jQuery.expando ] ) {
return event;
}
// Create a writable copy of the event object and normalize some properties
var i, prop, copy,
type = event.type,
originalEvent = event,
fixHook = this.fixHooks[ type ];
if ( !fixHook ) {
this.fixHooks[ type ] = fixHook =
rmouseEvent.test( type ) ? this.mouseHooks :
rkeyEvent.test( type ) ? this.keyHooks :
{};
}
copy = fixHook.props ? this.props.concat( fixHook.props ) : this.props;
event = new jQuery.Event( originalEvent );
i = copy.length;
while ( i-- ) {
prop = copy[ i ];
event[ prop ] = originalEvent[ prop ];
}
// Support: IE<9
// Fix target property (#1925)
if ( !event.target ) {
event.target = originalEvent.srcElement || document;
}
// Support: Chrome 23+, Safari?
// Target should not be a text node (#504, #13143)
if ( event.target.nodeType === 3 ) {
event.target = event.target.parentNode;
}
// Support: IE<9
// For mouse/key events, metaKey==false if it's undefined (#3368, #11328)
event.metaKey = !!event.metaKey;
return fixHook.filter ? fixHook.filter( event, originalEvent ) : event;
},
// Includes some event props shared by KeyEvent and MouseEvent
props: "altKey bubbles cancelable ctrlKey currentTarget eventPhase metaKey relatedTarget shiftKey target timeStamp view which".split(" "),
fixHooks: {},
keyHooks: {
props: "char charCode key keyCode".split(" "),
filter: function( event, original ) {
// Add which for key events
if ( event.which == null ) {
event.which = original.charCode != null ? original.charCode : original.keyCode;
}
return event;
}
},
mouseHooks: {
props: "button buttons clientX clientY fromElement offsetX offsetY pageX pageY screenX screenY toElement".split(" "),
filter: function( event, original ) {
var body, eventDoc, doc,
button = original.button,
fromElement = original.fromElement;
// Calculate pageX/Y if missing and clientX/Y available
if ( event.pageX == null && original.clientX != null ) {
eventDoc = event.target.ownerDocument || document;
doc = eventDoc.documentElement;
body = eventDoc.body;
event.pageX = original.clientX + ( doc && doc.scrollLeft || body && body.scrollLeft || 0 ) - ( doc && doc.clientLeft || body && body.clientLeft || 0 );
event.pageY = original.clientY + ( doc && doc.scrollTop || body && body.scrollTop || 0 ) - ( doc && doc.clientTop || body && body.clientTop || 0 );
}
// Add relatedTarget, if necessary
if ( !event.relatedTarget && fromElement ) {
event.relatedTarget = fromElement === event.target ? original.toElement : fromElement;
}
// Add which for click: 1 === left; 2 === middle; 3 === right
// Note: button is not normalized, so don't use it
if ( !event.which && button !== undefined ) {
event.which = ( button & 1 ? 1 : ( button & 2 ? 3 : ( button & 4 ? 2 : 0 ) ) );
}
return event;
}
},
special: {
load: {
// Prevent triggered image.load events from bubbling to window.load
noBubble: true
},
click: {
// For checkbox, fire native event so checked state will be right
trigger: function() {
if ( jQuery.nodeName( this, "input" ) && this.type === "checkbox" && this.click ) {
this.click();
return false;
}
}
},
focus: {
// Fire native event if possible so blur/focus sequence is correct
trigger: function() {
if ( this !== document.activeElement && this.focus ) {
try {
this.focus();
return false;
} catch ( e ) {
// Support: IE<9
// If we error on focus to hidden element (#1486, #12518),
// let .trigger() run the handlers
}
}
},
delegateType: "focusin"
},
blur: {
trigger: function() {
if ( this === document.activeElement && this.blur ) {
this.blur();
return false;
}
},
delegateType: "focusout"
},
beforeunload: {
postDispatch: function( event ) {
// Even when returnValue equals to undefined Firefox will still show alert
if ( event.result !== undefined ) {
event.originalEvent.returnValue = event.result;
}
}
}
},
simulate: function( type, elem, event, bubble ) {
// Piggyback on a donor event to simulate a different one.
// Fake originalEvent to avoid donor's stopPropagation, but if the
// simulated event prevents default then we do the same on the donor.
var e = jQuery.extend(
new jQuery.Event(),
event,
{ type: type,
isSimulated: true,
originalEvent: {}
}
);
if ( bubble ) {
jQuery.event.trigger( e, null, elem );
} else {
jQuery.event.dispatch.call( elem, e );
}
if ( e.isDefaultPrevented() ) {
event.preventDefault();
}
}
};
jQuery.removeEvent = document.removeEventListener ?
function( elem, type, handle ) {
if ( elem.removeEventListener ) {
elem.removeEventListener( type, handle, false );
}
} :
function( elem, type, handle ) {
var name = "on" + type;
if ( elem.detachEvent ) {
// #8545, #7054, preventing memory leaks for custom events in IE6-8
// detachEvent needed property on element, by name of that event, to properly expose it to GC
if ( typeof elem[ name ] === core_strundefined ) {
elem[ name ] = null;
}
elem.detachEvent( name, handle );
}
};
jQuery.Event = function( src, props ) {
// Allow instantiation without the 'new' keyword
if ( !(this instanceof jQuery.Event) ) {
return new jQuery.Event( src, props );
}
// Event object
if ( src && src.type ) {
this.originalEvent = src;
this.type = src.type;
// Events bubbling up the document may have been marked as prevented
// by a handler lower down the tree; reflect the correct value.
this.isDefaultPrevented = ( src.defaultPrevented || src.returnValue === false ||
src.getPreventDefault && src.getPreventDefault() ) ? returnTrue : returnFalse;
// Event type
} else {
this.type = src;
}
// Put explicitly provided properties onto the event object
if ( props ) {
jQuery.extend( this, props );
}
// Create a timestamp if incoming event doesn't have one
this.timeStamp = src && src.timeStamp || jQuery.now();
// Mark it as fixed
this[ jQuery.expando ] = true;
};
// jQuery.Event is based on DOM3 Events as specified by the ECMAScript Language Binding
// http://www.w3.org/TR/2003/WD-DOM-Level-3-Events-20030331/ecma-script-binding.html
jQuery.Event.prototype = {
isDefaultPrevented: returnFalse,
isPropagationStopped: returnFalse,
isImmediatePropagationStopped: returnFalse,
preventDefault: function() {
var e = this.originalEvent;
this.isDefaultPrevented = returnTrue;
if ( !e ) {
return;
}
// If preventDefault exists, run it on the original event
if ( e.preventDefault ) {
e.preventDefault();
// Support: IE
// Otherwise set the returnValue property of the original event to false
} else {
e.returnValue = false;
}
},
stopPropagation: function() {
var e = this.originalEvent;
this.isPropagationStopped = returnTrue;
if ( !e ) {
return;
}
// If stopPropagation exists, run it on the original event
if ( e.stopPropagation ) {
e.stopPropagation();
}
// Support: IE
// Set the cancelBubble property of the original event to true
e.cancelBubble = true;
},
stopImmediatePropagation: function() {
this.isImmediatePropagationStopped = returnTrue;
this.stopPropagation();
}
};
// Create mouseenter/leave events using mouseover/out and event-time checks
jQuery.each({
mouseenter: "mouseover",
mouseleave: "mouseout"
}, function( orig, fix ) {
jQuery.event.special[ orig ] = {
delegateType: fix,
bindType: fix,
handle: function( event ) {
var ret,
target = this,
related = event.relatedTarget,
handleObj = event.handleObj;
// For mousenter/leave call the handler if related is outside the target.
// NB: No relatedTarget if the mouse left/entered the browser window
if ( !related || (related !== target && !jQuery.contains( target, related )) ) {
event.type = handleObj.origType;
ret = handleObj.handler.apply( this, arguments );
event.type = fix;
}
return ret;
}
};
});
// IE submit delegation
if ( !jQuery.support.submitBubbles ) {
jQuery.event.special.submit = {
setup: function() {
// Only need this for delegated form submit events
if ( jQuery.nodeName( this, "form" ) ) {
return false;
}
// Lazy-add a submit handler when a descendant form may potentially be submitted
jQuery.event.add( this, "click._submit keypress._submit", function( e ) {
// Node name check avoids a VML-related crash in IE (#9807)
var elem = e.target,
form = jQuery.nodeName( elem, "input" ) || jQuery.nodeName( elem, "button" ) ? elem.form : undefined;
if ( form && !jQuery._data( form, "submitBubbles" ) ) {
jQuery.event.add( form, "submit._submit", function( event ) {
event._submit_bubble = true;
});
jQuery._data( form, "submitBubbles", true );
}
});
// return undefined since we don't need an event listener
},
postDispatch: function( event ) {
// If form was submitted by the user, bubble the event up the tree
if ( event._submit_bubble ) {
delete event._submit_bubble;
if ( this.parentNode && !event.isTrigger ) {
jQuery.event.simulate( "submit", this.parentNode, event, true );
}
}
},
teardown: function() {
// Only need this for delegated form submit events
if ( jQuery.nodeName( this, "form" ) ) {
return false;
}
// Remove delegated handlers; cleanData eventually reaps submit handlers attached above
jQuery.event.remove( this, "._submit" );
}
};
}
// IE change delegation and checkbox/radio fix
if ( !jQuery.support.changeBubbles ) {
jQuery.event.special.change = {
setup: function() {
if ( rformElems.test( this.nodeName ) ) {
// IE doesn't fire change on a check/radio until blur; trigger it on click
// after a propertychange. Eat the blur-change in special.change.handle.
// This still fires onchange a second time for check/radio after blur.
if ( this.type === "checkbox" || this.type === "radio" ) {
jQuery.event.add( this, "propertychange._change", function( event ) {
if ( event.originalEvent.propertyName === "checked" ) {
this._just_changed = true;
}
});
jQuery.event.add( this, "click._change", function( event ) {
if ( this._just_changed && !event.isTrigger ) {
this._just_changed = false;
}
// Allow triggered, simulated change events (#11500)
jQuery.event.simulate( "change", this, event, true );
});
}
return false;
}
// Delegated event; lazy-add a change handler on descendant inputs
jQuery.event.add( this, "beforeactivate._change", function( e ) {
var elem = e.target;
if ( rformElems.test( elem.nodeName ) && !jQuery._data( elem, "changeBubbles" ) ) {
jQuery.event.add( elem, "change._change", function( event ) {
if ( this.parentNode && !event.isSimulated && !event.isTrigger ) {
jQuery.event.simulate( "change", this.parentNode, event, true );
}
});
jQuery._data( elem, "changeBubbles", true );
}
});
},
handle: function( event ) {
var elem = event.target;
// Swallow native change events from checkbox/radio, we already triggered them above
if ( this !== elem || event.isSimulated || event.isTrigger || (elem.type !== "radio" && elem.type !== "checkbox") ) {
return event.handleObj.handler.apply( this, arguments );
}
},
teardown: function() {
jQuery.event.remove( this, "._change" );
return !rformElems.test( this.nodeName );
}
};
}
// Create "bubbling" focus and blur events
if ( !jQuery.support.focusinBubbles ) {
jQuery.each({ focus: "focusin", blur: "focusout" }, function( orig, fix ) {
// Attach a single capturing handler while someone wants focusin/focusout
var attaches = 0,
handler = function( event ) {
jQuery.event.simulate( fix, event.target, jQuery.event.fix( event ), true );
};
jQuery.event.special[ fix ] = {
setup: function() {
if ( attaches++ === 0 ) {
document.addEventListener( orig, handler, true );
}
},
teardown: function() {
if ( --attaches === 0 ) {
document.removeEventListener( orig, handler, true );
}
}
};
});
}
jQuery.fn.extend({
on: function( types, selector, data, fn, /*INTERNAL*/ one ) {
var type, origFn;
// Types can be a map of types/handlers
if ( typeof types === "object" ) {
// ( types-Object, selector, data )
if ( typeof selector !== "string" ) {
// ( types-Object, data )
data = data || selector;
selector = undefined;
}
for ( type in types ) {
this.on( type, selector, data, types[ type ], one );
}
return this;
}
if ( data == null && fn == null ) {
// ( types, fn )
fn = selector;
data = selector = undefined;
} else if ( fn == null ) {
if ( typeof selector === "string" ) {
// ( types, selector, fn )
fn = data;
data = undefined;
} else {
// ( types, data, fn )
fn = data;
data = selector;
selector = undefined;
}
}
if ( fn === false ) {
fn = returnFalse;
} else if ( !fn ) {
return this;
}
if ( one === 1 ) {
origFn = fn;
fn = function( event ) {
// Can use an empty set, since event contains the info
jQuery().off( event );
return origFn.apply( this, arguments );
};
// Use same guid so caller can remove using origFn
fn.guid = origFn.guid || ( origFn.guid = jQuery.guid++ );
}
return this.each( function() {
jQuery.event.add( this, types, fn, data, selector );
});
},
one: function( types, selector, data, fn ) {
return this.on( types, selector, data, fn, 1 );
},
off: function( types, selector, fn ) {
var handleObj, type;
if ( types && types.preventDefault && types.handleObj ) {
// ( event ) dispatched jQuery.Event
handleObj = types.handleObj;
jQuery( types.delegateTarget ).off(
handleObj.namespace ? handleObj.origType + "." + handleObj.namespace : handleObj.origType,
handleObj.selector,
handleObj.handler
);
return this;
}
if ( typeof types === "object" ) {
// ( types-object [, selector] )
for ( type in types ) {
this.off( type, selector, types[ type ] );
}
return this;
}
if ( selector === false || typeof selector === "function" ) {
// ( types [, fn] )
fn = selector;
selector = undefined;
}
if ( fn === false ) {
fn = returnFalse;
}
return this.each(function() {
jQuery.event.remove( this, types, fn, selector );
});
},
bind: function( types, data, fn ) {
return this.on( types, null, data, fn );
},
unbind: function( types, fn ) {
return this.off( types, null, fn );
},
delegate: function( selector, types, data, fn ) {
return this.on( types, selector, data, fn );
},
undelegate: function( selector, types, fn ) {
// ( namespace ) or ( selector, types [, fn] )
return arguments.length === 1 ? this.off( selector, "**" ) : this.off( types, selector || "**", fn );
},
trigger: function( type, data ) {
return this.each(function() {
jQuery.event.trigger( type, data, this );
});
},
triggerHandler: function( type, data ) {
var elem = this[0];
if ( elem ) {
return jQuery.event.trigger( type, data, elem, true );
}
}
});
/*!
* Sizzle CSS Selector Engine
* Copyright 2012 jQuery Foundation and other contributors
* Released under the MIT license
* http://sizzlejs.com/
*/
(function( window, undefined ) {
var i,
cachedruns,
Expr,
getText,
isXML,
compile,
hasDuplicate,
outermostContext,
// Local document vars
setDocument,
document,
docElem,
documentIsXML,
rbuggyQSA,
rbuggyMatches,
matches,
contains,
sortOrder,
// Instance-specific data
expando = "sizzle" + -(new Date()),
preferredDoc = window.document,
support = {},
dirruns = 0,
done = 0,
classCache = createCache(),
tokenCache = createCache(),
compilerCache = createCache(),
// General-purpose constants
strundefined = typeof undefined,
MAX_NEGATIVE = 1 << 31,
// Array methods
arr = [],
pop = arr.pop,
push = arr.push,
slice = arr.slice,
// Use a stripped-down indexOf if we can't use a native one
indexOf = arr.indexOf || function( elem ) {
var i = 0,
len = this.length;
for ( ; i < len; i++ ) {
if ( this[i] === elem ) {
return i;
}
}
return -1;
},
// Regular expressions
// Whitespace characters http://www.w3.org/TR/css3-selectors/#whitespace
whitespace = "[\\x20\\t\\r\\n\\f]",
// http://www.w3.org/TR/css3-syntax/#characters
characterEncoding = "(?:\\\\.|[\\w-]|[^\\x00-\\xa0])+",
// Loosely modeled on CSS identifier characters
// An unquoted value should be a CSS identifier http://www.w3.org/TR/css3-selectors/#attribute-selectors
// Proper syntax: http://www.w3.org/TR/CSS21/syndata.html#value-def-identifier
identifier = characterEncoding.replace( "w", "w#" ),
// Acceptable operators http://www.w3.org/TR/selectors/#attribute-selectors
operators = "([*^$|!~]?=)",
attributes = "\\[" + whitespace + "*(" + characterEncoding + ")" + whitespace +
"*(?:" + operators + whitespace + "*(?:(['\"])((?:\\\\.|[^\\\\])*?)\\3|(" + identifier + ")|)|)" + whitespace + "*\\]",
// Prefer arguments quoted,
// then not containing pseudos/brackets,
// then attribute selectors/non-parenthetical expressions,
// then anything else
// These preferences are here to reduce the number of selectors
// needing tokenize in the PSEUDO preFilter
pseudos = ":(" + characterEncoding + ")(?:\\(((['\"])((?:\\\\.|[^\\\\])*?)\\3|((?:\\\\.|[^\\\\()[\\]]|" + attributes.replace( 3, 8 ) + ")*)|.*)\\)|)",
// Leading and non-escaped trailing whitespace, capturing some non-whitespace characters preceding the latter
rtrim = new RegExp( "^" + whitespace + "+|((?:^|[^\\\\])(?:\\\\.)*)" + whitespace + "+$", "g" ),
rcomma = new RegExp( "^" + whitespace + "*," + whitespace + "*" ),
rcombinators = new RegExp( "^" + whitespace + "*([\\x20\\t\\r\\n\\f>+~])" + whitespace + "*" ),
rpseudo = new RegExp( pseudos ),
ridentifier = new RegExp( "^" + identifier + "$" ),
matchExpr = {
"ID": new RegExp( "^#(" + characterEncoding + ")" ),
"CLASS": new RegExp( "^\\.(" + characterEncoding + ")" ),
"NAME": new RegExp( "^\\[name=['\"]?(" + characterEncoding + ")['\"]?\\]" ),
"TAG": new RegExp( "^(" + characterEncoding.replace( "w", "w*" ) + ")" ),
"ATTR": new RegExp( "^" + attributes ),
"PSEUDO": new RegExp( "^" + pseudos ),
"CHILD": new RegExp( "^:(only|first|last|nth|nth-last)-(child|of-type)(?:\\(" + whitespace +
"*(even|odd|(([+-]|)(\\d*)n|)" + whitespace + "*(?:([+-]|)" + whitespace +
"*(\\d+)|))" + whitespace + "*\\)|)", "i" ),
// For use in libraries implementing .is()
// We use this for POS matching in `select`
"needsContext": new RegExp( "^" + whitespace + "*[>+~]|:(even|odd|eq|gt|lt|nth|first|last)(?:\\(" +
whitespace + "*((?:-\\d)?\\d*)" + whitespace + "*\\)|)(?=[^-]|$)", "i" )
},
rsibling = /[\x20\t\r\n\f]*[+~]/,
rnative = /^[^{]+\{\s*\[native code/,
// Easily-parseable/retrievable ID or TAG or CLASS selectors
rquickExpr = /^(?:#([\w-]+)|(\w+)|\.([\w-]+))$/,
rinputs = /^(?:input|select|textarea|button)$/i,
rheader = /^h\d$/i,
rescape = /'|\\/g,
rattributeQuotes = /\=[\x20\t\r\n\f]*([^'"\]]*)[\x20\t\r\n\f]*\]/g,
// CSS escapes http://www.w3.org/TR/CSS21/syndata.html#escaped-characters
runescape = /\\([\da-fA-F]{1,6}[\x20\t\r\n\f]?|.)/g,
funescape = function( _, escaped ) {
var high = "0x" + escaped - 0x10000;
// NaN means non-codepoint
return high !== high ?
escaped :
// BMP codepoint
high < 0 ?
String.fromCharCode( high + 0x10000 ) :
// Supplemental Plane codepoint (surrogate pair)
String.fromCharCode( high >> 10 | 0xD800, high & 0x3FF | 0xDC00 );
};
// Use a stripped-down slice if we can't use a native one
try {
slice.call( preferredDoc.documentElement.childNodes, 0 )[0].nodeType;
} catch ( e ) {
slice = function( i ) {
var elem,
results = [];
while ( (elem = this[i++]) ) {
results.push( elem );
}
return results;
};
}
/**
* For feature detection
* @param {Function} fn The function to test for native support
*/
function isNative( fn ) {
return rnative.test( fn + "" );
}
/**
* Create key-value caches of limited size
* @returns {Function(string, Object)} Returns the Object data after storing it on itself with
* property name the (space-suffixed) string and (if the cache is larger than Expr.cacheLength)
* deleting the oldest entry
*/
function createCache() {
var cache,
keys = [];
return (cache = function( key, value ) {
// Use (key + " ") to avoid collision with native prototype properties (see Issue #157)
if ( keys.push( key += " " ) > Expr.cacheLength ) {
// Only keep the most recent entries
delete cache[ keys.shift() ];
}
return (cache[ key ] = value);
});
}
/**
* Mark a function for special use by Sizzle
* @param {Function} fn The function to mark
*/
function markFunction( fn ) {
fn[ expando ] = true;
return fn;
}
/**
* Support testing using an element
* @param {Function} fn Passed the created div and expects a boolean result
*/
function assert( fn ) {
var div = document.createElement("div");
try {
return fn( div );
} catch (e) {
return false;
} finally {
// release memory in IE
div = null;
}
}
function Sizzle( selector, context, results, seed ) {
var match, elem, m, nodeType,
// QSA vars
i, groups, old, nid, newContext, newSelector;
if ( ( context ? context.ownerDocument || context : preferredDoc ) !== document ) {
setDocument( context );
}
context = context || document;
results = results || [];
if ( !selector || typeof selector !== "string" ) {
return results;
}
if ( (nodeType = context.nodeType) !== 1 && nodeType !== 9 ) {
return [];
}
if ( !documentIsXML && !seed ) {
// Shortcuts
if ( (match = rquickExpr.exec( selector )) ) {
// Speed-up: Sizzle("#ID")
if ( (m = match[1]) ) {
if ( nodeType === 9 ) {
elem = context.getElementById( m );
// Check parentNode to catch when Blackberry 4.6 returns
// nodes that are no longer in the document #6963
if ( elem && elem.parentNode ) {
// Handle the case where IE, Opera, and Webkit return items
// by name instead of ID
if ( elem.id === m ) {
results.push( elem );
return results;
}
} else {
return results;
}
} else {
// Context is not a document
if ( context.ownerDocument && (elem = context.ownerDocument.getElementById( m )) &&
contains( context, elem ) && elem.id === m ) {
results.push( elem );
return results;
}
}
// Speed-up: Sizzle("TAG")
} else if ( match[2] ) {
push.apply( results, slice.call(context.getElementsByTagName( selector ), 0) );
return results;
// Speed-up: Sizzle(".CLASS")
} else if ( (m = match[3]) && support.getByClassName && context.getElementsByClassName ) {
push.apply( results, slice.call(context.getElementsByClassName( m ), 0) );
return results;
}
}
// QSA path
if ( support.qsa && !rbuggyQSA.test(selector) ) {
old = true;
nid = expando;
newContext = context;
newSelector = nodeType === 9 && selector;
// qSA works strangely on Element-rooted queries
// We can work around this by specifying an extra ID on the root
// and working up from there (Thanks to Andrew Dupont for the technique)
// IE 8 doesn't work on object elements
if ( nodeType === 1 && context.nodeName.toLowerCase() !== "object" ) {
groups = tokenize( selector );
if ( (old = context.getAttribute("id")) ) {
nid = old.replace( rescape, "\\$&" );
} else {
context.setAttribute( "id", nid );
}
nid = "[id='" + nid + "'] ";
i = groups.length;
while ( i-- ) {
groups[i] = nid + toSelector( groups[i] );
}
newContext = rsibling.test( selector ) && context.parentNode || context;
newSelector = groups.join(",");
}
if ( newSelector ) {
try {
push.apply( results, slice.call( newContext.querySelectorAll(
newSelector
), 0 ) );
return results;
} catch(qsaError) {
} finally {
if ( !old ) {
context.removeAttribute("id");
}
}
}
}
}
// All others
return select( selector.replace( rtrim, "$1" ), context, results, seed );
}
/**
* Detect xml
* @param {Element|Object} elem An element or a document
*/
isXML = Sizzle.isXML = function( elem ) {
// documentElement is verified for cases where it doesn't yet exist
// (such as loading iframes in IE - #4833)
var documentElement = elem && (elem.ownerDocument || elem).documentElement;
return documentElement ? documentElement.nodeName !== "HTML" : false;
};
/**
* Sets document-related variables once based on the current document
* @param {Element|Object} [doc] An element or document object to use to set the document
* @returns {Object} Returns the current document
*/
setDocument = Sizzle.setDocument = function( node ) {
var doc = node ? node.ownerDocument || node : preferredDoc;
// If no document and documentElement is available, return
if ( doc === document || doc.nodeType !== 9 || !doc.documentElement ) {
return document;
}
// Set our document
document = doc;
docElem = doc.documentElement;
// Support tests
documentIsXML = isXML( doc );
// Check if getElementsByTagName("*") returns only elements
support.tagNameNoComments = assert(function( div ) {
div.appendChild( doc.createComment("") );
return !div.getElementsByTagName("*").length;
});
// Check if attributes should be retrieved by attribute nodes
support.attributes = assert(function( div ) {
div.innerHTML = "<select></select>";
var type = typeof div.lastChild.getAttribute("multiple");
// IE8 returns a string for some attributes even when not present
return type !== "boolean" && type !== "string";
});
// Check if getElementsByClassName can be trusted
support.getByClassName = assert(function( div ) {
// Opera can't find a second classname (in 9.6)
div.innerHTML = "<div class='hidden e'></div><div class='hidden'></div>";
if ( !div.getElementsByClassName || !div.getElementsByClassName("e").length ) {
return false;
}
// Safari 3.2 caches class attributes and doesn't catch changes
div.lastChild.className = "e";
return div.getElementsByClassName("e").length === 2;
});
// Check if getElementById returns elements by name
// Check if getElementsByName privileges form controls or returns elements by ID
support.getByName = assert(function( div ) {
// Inject content
div.id = expando + 0;
div.innerHTML = "<a name='" + expando + "'></a><div name='" + expando + "'></div>";
docElem.insertBefore( div, docElem.firstChild );
// Test
var pass = doc.getElementsByName &&
// buggy browsers will return fewer than the correct 2
doc.getElementsByName( expando ).length === 2 +
// buggy browsers will return more than the correct 0
doc.getElementsByName( expando + 0 ).length;
support.getIdNotName = !doc.getElementById( expando );
// Cleanup
docElem.removeChild( div );
return pass;
});
// IE6/7 return modified attributes
Expr.attrHandle = assert(function( div ) {
div.innerHTML = "<a href='#'></a>";
return div.firstChild && typeof div.firstChild.getAttribute !== strundefined &&
div.firstChild.getAttribute("href") === "#";
}) ?
{} :
{
"href": function( elem ) {
return elem.getAttribute( "href", 2 );
},
"type": function( elem ) {
return elem.getAttribute("type");
}
};
// ID find and filter
if ( support.getIdNotName ) {
Expr.find["ID"] = function( id, context ) {
if ( typeof context.getElementById !== strundefined && !documentIsXML ) {
var m = context.getElementById( id );
// Check parentNode to catch when Blackberry 4.6 returns
// nodes that are no longer in the document #6963
return m && m.parentNode ? [m] : [];
}
};
Expr.filter["ID"] = function( id ) {
var attrId = id.replace( runescape, funescape );
return function( elem ) {
return elem.getAttribute("id") === attrId;
};
};
} else {
Expr.find["ID"] = function( id, context ) {
if ( typeof context.getElementById !== strundefined && !documentIsXML ) {
var m = context.getElementById( id );
return m ?
m.id === id || typeof m.getAttributeNode !== strundefined && m.getAttributeNode("id").value === id ?
[m] :
undefined :
[];
}
};
Expr.filter["ID"] = function( id ) {
var attrId = id.replace( runescape, funescape );
return function( elem ) {
var node = typeof elem.getAttributeNode !== strundefined && elem.getAttributeNode("id");
return node && node.value === attrId;
};
};
}
// Tag
Expr.find["TAG"] = support.tagNameNoComments ?
function( tag, context ) {
if ( typeof context.getElementsByTagName !== strundefined ) {
return context.getElementsByTagName( tag );
}
} :
function( tag, context ) {
var elem,
tmp = [],
i = 0,
results = context.getElementsByTagName( tag );
// Filter out possible comments
if ( tag === "*" ) {
while ( (elem = results[i++]) ) {
if ( elem.nodeType === 1 ) {
tmp.push( elem );
}
}
return tmp;
}
return results;
};
// Name
Expr.find["NAME"] = support.getByName && function( tag, context ) {
if ( typeof context.getElementsByName !== strundefined ) {
return context.getElementsByName( name );
}
};
// Class
Expr.find["CLASS"] = support.getByClassName && function( className, context ) {
if ( typeof context.getElementsByClassName !== strundefined && !documentIsXML ) {
return context.getElementsByClassName( className );
}
};
// QSA and matchesSelector support
// matchesSelector(:active) reports false when true (IE9/Opera 11.5)
rbuggyMatches = [];
// qSa(:focus) reports false when true (Chrome 21),
// no need to also add to buggyMatches since matches checks buggyQSA
// A support test would require too much code (would include document ready)
rbuggyQSA = [ ":focus" ];
if ( (support.qsa = isNative(doc.querySelectorAll)) ) {
// Build QSA regex
// Regex strategy adopted from Diego Perini
assert(function( div ) {
// Select is set to empty string on purpose
// This is to test IE's treatment of not explictly
// setting a boolean content attribute,
// since its presence should be enough
// http://bugs.jquery.com/ticket/12359
div.innerHTML = "<select><option selected=''></option></select>";
// IE8 - Some boolean attributes are not treated correctly
if ( !div.querySelectorAll("[selected]").length ) {
rbuggyQSA.push( "\\[" + whitespace + "*(?:checked|disabled|ismap|multiple|readonly|selected|value)" );
}
// Webkit/Opera - :checked should return selected option elements
// http://www.w3.org/TR/2011/REC-css3-selectors-20110929/#checked
// IE8 throws error here and will not see later tests
if ( !div.querySelectorAll(":checked").length ) {
rbuggyQSA.push(":checked");
}
});
assert(function( div ) {
// Opera 10-12/IE8 - ^= $= *= and empty values
// Should not select anything
div.innerHTML = "<input type='hidden' i=''/>";
if ( div.querySelectorAll("[i^='']").length ) {
rbuggyQSA.push( "[*^$]=" + whitespace + "*(?:\"\"|'')" );
}
// FF 3.5 - :enabled/:disabled and hidden elements (hidden elements are still enabled)
// IE8 throws error here and will not see later tests
if ( !div.querySelectorAll(":enabled").length ) {
rbuggyQSA.push( ":enabled", ":disabled" );
}
// Opera 10-11 does not throw on post-comma invalid pseudos
div.querySelectorAll("*,:x");
rbuggyQSA.push(",.*:");
});
}
if ( (support.matchesSelector = isNative( (matches = docElem.matchesSelector ||
docElem.mozMatchesSelector ||
docElem.webkitMatchesSelector ||
docElem.oMatchesSelector ||
docElem.msMatchesSelector) )) ) {
assert(function( div ) {
// Check to see if it's possible to do matchesSelector
// on a disconnected node (IE 9)
support.disconnectedMatch = matches.call( div, "div" );
// This should fail with an exception
// Gecko does not error, returns false instead
matches.call( div, "[s!='']:x" );
rbuggyMatches.push( "!=", pseudos );
});
}
rbuggyQSA = new RegExp( rbuggyQSA.join("|") );
rbuggyMatches = new RegExp( rbuggyMatches.join("|") );
// Element contains another
// Purposefully does not implement inclusive descendent
// As in, an element does not contain itself
contains = isNative(docElem.contains) || docElem.compareDocumentPosition ?
function( a, b ) {
var adown = a.nodeType === 9 ? a.documentElement : a,
bup = b && b.parentNode;
return a === bup || !!( bup && bup.nodeType === 1 && (
adown.contains ?
adown.contains( bup ) :
a.compareDocumentPosition && a.compareDocumentPosition( bup ) & 16
));
} :
function( a, b ) {
if ( b ) {
while ( (b = b.parentNode) ) {
if ( b === a ) {
return true;
}
}
}
return false;
};
// Document order sorting
sortOrder = docElem.compareDocumentPosition ?
function( a, b ) {
var compare;
if ( a === b ) {
hasDuplicate = true;
return 0;
}
if ( (compare = b.compareDocumentPosition && a.compareDocumentPosition && a.compareDocumentPosition( b )) ) {
if ( compare & 1 || a.parentNode && a.parentNode.nodeType === 11 ) {
if ( a === doc || contains( preferredDoc, a ) ) {
return -1;
}
if ( b === doc || contains( preferredDoc, b ) ) {
return 1;
}
return 0;
}
return compare & 4 ? -1 : 1;
}
return a.compareDocumentPosition ? -1 : 1;
} :
function( a, b ) {
var cur,
i = 0,
aup = a.parentNode,
bup = b.parentNode,
ap = [ a ],
bp = [ b ];
// Exit early if the nodes are identical
if ( a === b ) {
hasDuplicate = true;
return 0;
// Parentless nodes are either documents or disconnected
} else if ( !aup || !bup ) {
return a === doc ? -1 :
b === doc ? 1 :
aup ? -1 :
bup ? 1 :
0;
// If the nodes are siblings, we can do a quick check
} else if ( aup === bup ) {
return siblingCheck( a, b );
}
// Otherwise we need full lists of their ancestors for comparison
cur = a;
while ( (cur = cur.parentNode) ) {
ap.unshift( cur );
}
cur = b;
while ( (cur = cur.parentNode) ) {
bp.unshift( cur );
}
// Walk down the tree looking for a discrepancy
while ( ap[i] === bp[i] ) {
i++;
}
return i ?
// Do a sibling check if the nodes have a common ancestor
siblingCheck( ap[i], bp[i] ) :
// Otherwise nodes in our document sort first
ap[i] === preferredDoc ? -1 :
bp[i] === preferredDoc ? 1 :
0;
};
// Always assume the presence of duplicates if sort doesn't
// pass them to our comparison function (as in Google Chrome).
hasDuplicate = false;
[0, 0].sort( sortOrder );
support.detectDuplicates = hasDuplicate;
return document;
};
Sizzle.matches = function( expr, elements ) {
return Sizzle( expr, null, null, elements );
};
Sizzle.matchesSelector = function( elem, expr ) {
// Set document vars if needed
if ( ( elem.ownerDocument || elem ) !== document ) {
setDocument( elem );
}
// Make sure that attribute selectors are quoted
expr = expr.replace( rattributeQuotes, "='$1']" );
// rbuggyQSA always contains :focus, so no need for an existence check
if ( support.matchesSelector && !documentIsXML && (!rbuggyMatches || !rbuggyMatches.test(expr)) && !rbuggyQSA.test(expr) ) {
try {
var ret = matches.call( elem, expr );
// IE 9's matchesSelector returns false on disconnected nodes
if ( ret || support.disconnectedMatch ||
// As well, disconnected nodes are said to be in a document
// fragment in IE 9
elem.document && elem.document.nodeType !== 11 ) {
return ret;
}
} catch(e) {}
}
return Sizzle( expr, document, null, [elem] ).length > 0;
};
Sizzle.contains = function( context, elem ) {
// Set document vars if needed
if ( ( context.ownerDocument || context ) !== document ) {
setDocument( context );
}
return contains( context, elem );
};
Sizzle.attr = function( elem, name ) {
var val;
// Set document vars if needed
if ( ( elem.ownerDocument || elem ) !== document ) {
setDocument( elem );
}
if ( !documentIsXML ) {
name = name.toLowerCase();
}
if ( (val = Expr.attrHandle[ name ]) ) {
return val( elem );
}
if ( documentIsXML || support.attributes ) {
return elem.getAttribute( name );
}
return ( (val = elem.getAttributeNode( name )) || elem.getAttribute( name ) ) && elem[ name ] === true ?
name :
val && val.specified ? val.value : null;
};
Sizzle.error = function( msg ) {
throw new Error( "Syntax error, unrecognized expression: " + msg );
};
// Document sorting and removing duplicates
Sizzle.uniqueSort = function( results ) {
var elem,
duplicates = [],
i = 1,
j = 0;
// Unless we *know* we can detect duplicates, assume their presence
hasDuplicate = !support.detectDuplicates;
results.sort( sortOrder );
if ( hasDuplicate ) {
for ( ; (elem = results[i]); i++ ) {
if ( elem === results[ i - 1 ] ) {
j = duplicates.push( i );
}
}
while ( j-- ) {
results.splice( duplicates[ j ], 1 );
}
}
return results;
};
function siblingCheck( a, b ) {
var cur = b && a,
diff = cur && ( ~b.sourceIndex || MAX_NEGATIVE ) - ( ~a.sourceIndex || MAX_NEGATIVE );
// Use IE sourceIndex if available on both nodes
if ( diff ) {
return diff;
}
// Check if b follows a
if ( cur ) {
while ( (cur = cur.nextSibling) ) {
if ( cur === b ) {
return -1;
}
}
}
return a ? 1 : -1;
}
// Returns a function to use in pseudos for input types
function createInputPseudo( type ) {
return function( elem ) {
var name = elem.nodeName.toLowerCase();
return name === "input" && elem.type === type;
};
}
// Returns a function to use in pseudos for buttons
function createButtonPseudo( type ) {
return function( elem ) {
var name = elem.nodeName.toLowerCase();
return (name === "input" || name === "button") && elem.type === type;
};
}
// Returns a function to use in pseudos for positionals
function createPositionalPseudo( fn ) {
return markFunction(function( argument ) {
argument = +argument;
return markFunction(function( seed, matches ) {
var j,
matchIndexes = fn( [], seed.length, argument ),
i = matchIndexes.length;
// Match elements found at the specified indexes
while ( i-- ) {
if ( seed[ (j = matchIndexes[i]) ] ) {
seed[j] = !(matches[j] = seed[j]);
}
}
});
});
}
/**
* Utility function for retrieving the text value of an array of DOM nodes
* @param {Array|Element} elem
*/
getText = Sizzle.getText = function( elem ) {
var node,
ret = "",
i = 0,
nodeType = elem.nodeType;
if ( !nodeType ) {
// If no nodeType, this is expected to be an array
for ( ; (node = elem[i]); i++ ) {
// Do not traverse comment nodes
ret += getText( node );
}
} else if ( nodeType === 1 || nodeType === 9 || nodeType === 11 ) {
// Use textContent for elements
// innerText usage removed for consistency of new lines (see #11153)
if ( typeof elem.textContent === "string" ) {
return elem.textContent;
} else {
// Traverse its children
for ( elem = elem.firstChild; elem; elem = elem.nextSibling ) {
ret += getText( elem );
}
}
} else if ( nodeType === 3 || nodeType === 4 ) {
return elem.nodeValue;
}
// Do not include comment or processing instruction nodes
return ret;
};
Expr = Sizzle.selectors = {
// Can be adjusted by the user
cacheLength: 50,
createPseudo: markFunction,
match: matchExpr,
find: {},
relative: {
">": { dir: "parentNode", first: true },
" ": { dir: "parentNode" },
"+": { dir: "previousSibling", first: true },
"~": { dir: "previousSibling" }
},
preFilter: {
"ATTR": function( match ) {
match[1] = match[1].replace( runescape, funescape );
// Move the given value to match[3] whether quoted or unquoted
match[3] = ( match[4] || match[5] || "" ).replace( runescape, funescape );
if ( match[2] === "~=" ) {
match[3] = " " + match[3] + " ";
}
return match.slice( 0, 4 );
},
"CHILD": function( match ) {
/* matches from matchExpr["CHILD"]
1 type (only|nth|...)
2 what (child|of-type)
3 argument (even|odd|\d*|\d*n([+-]\d+)?|...)
4 xn-component of xn+y argument ([+-]?\d*n|)
5 sign of xn-component
6 x of xn-component
7 sign of y-component
8 y of y-component
*/
match[1] = match[1].toLowerCase();
if ( match[1].slice( 0, 3 ) === "nth" ) {
// nth-* requires argument
if ( !match[3] ) {
Sizzle.error( match[0] );
}
// numeric x and y parameters for Expr.filter.CHILD
// remember that false/true cast respectively to 0/1
match[4] = +( match[4] ? match[5] + (match[6] || 1) : 2 * ( match[3] === "even" || match[3] === "odd" ) );
match[5] = +( ( match[7] + match[8] ) || match[3] === "odd" );
// other types prohibit arguments
} else if ( match[3] ) {
Sizzle.error( match[0] );
}
return match;
},
"PSEUDO": function( match ) {
var excess,
unquoted = !match[5] && match[2];
if ( matchExpr["CHILD"].test( match[0] ) ) {
return null;
}
// Accept quoted arguments as-is
if ( match[4] ) {
match[2] = match[4];
// Strip excess characters from unquoted arguments
} else if ( unquoted && rpseudo.test( unquoted ) &&
// Get excess from tokenize (recursively)
(excess = tokenize( unquoted, true )) &&
// advance to the next closing parenthesis
(excess = unquoted.indexOf( ")", unquoted.length - excess ) - unquoted.length) ) {
// excess is a negative index
match[0] = match[0].slice( 0, excess );
match[2] = unquoted.slice( 0, excess );
}
// Return only captures needed by the pseudo filter method (type and argument)
return match.slice( 0, 3 );
}
},
filter: {
"TAG": function( nodeName ) {
if ( nodeName === "*" ) {
return function() { return true; };
}
nodeName = nodeName.replace( runescape, funescape ).toLowerCase();
return function( elem ) {
return elem.nodeName && elem.nodeName.toLowerCase() === nodeName;
};
},
"CLASS": function( className ) {
var pattern = classCache[ className + " " ];
return pattern ||
(pattern = new RegExp( "(^|" + whitespace + ")" + className + "(" + whitespace + "|$)" )) &&
classCache( className, function( elem ) {
return pattern.test( elem.className || (typeof elem.getAttribute !== strundefined && elem.getAttribute("class")) || "" );
});
},
"ATTR": function( name, operator, check ) {
return function( elem ) {
var result = Sizzle.attr( elem, name );
if ( result == null ) {
return operator === "!=";
}
if ( !operator ) {
return true;
}
result += "";
return operator === "=" ? result === check :
operator === "!=" ? result !== check :
operator === "^=" ? check && result.indexOf( check ) === 0 :
operator === "*=" ? check && result.indexOf( check ) > -1 :
operator === "$=" ? check && result.slice( -check.length ) === check :
operator === "~=" ? ( " " + result + " " ).indexOf( check ) > -1 :
operator === "|=" ? result === check || result.slice( 0, check.length + 1 ) === check + "-" :
false;
};
},
"CHILD": function( type, what, argument, first, last ) {
var simple = type.slice( 0, 3 ) !== "nth",
forward = type.slice( -4 ) !== "last",
ofType = what === "of-type";
return first === 1 && last === 0 ?
// Shortcut for :nth-*(n)
function( elem ) {
return !!elem.parentNode;
} :
function( elem, context, xml ) {
var cache, outerCache, node, diff, nodeIndex, start,
dir = simple !== forward ? "nextSibling" : "previousSibling",
parent = elem.parentNode,
name = ofType && elem.nodeName.toLowerCase(),
useCache = !xml && !ofType;
if ( parent ) {
// :(first|last|only)-(child|of-type)
if ( simple ) {
while ( dir ) {
node = elem;
while ( (node = node[ dir ]) ) {
if ( ofType ? node.nodeName.toLowerCase() === name : node.nodeType === 1 ) {
return false;
}
}
// Reverse direction for :only-* (if we haven't yet done so)
start = dir = type === "only" && !start && "nextSibling";
}
return true;
}
start = [ forward ? parent.firstChild : parent.lastChild ];
// non-xml :nth-child(...) stores cache data on `parent`
if ( forward && useCache ) {
// Seek `elem` from a previously-cached index
outerCache = parent[ expando ] || (parent[ expando ] = {});
cache = outerCache[ type ] || [];
nodeIndex = cache[0] === dirruns && cache[1];
diff = cache[0] === dirruns && cache[2];
node = nodeIndex && parent.childNodes[ nodeIndex ];
while ( (node = ++nodeIndex && node && node[ dir ] ||
// Fallback to seeking `elem` from the start
(diff = nodeIndex = 0) || start.pop()) ) {
// When found, cache indexes on `parent` and break
if ( node.nodeType === 1 && ++diff && node === elem ) {
outerCache[ type ] = [ dirruns, nodeIndex, diff ];
break;
}
}
// Use previously-cached element index if available
} else if ( useCache && (cache = (elem[ expando ] || (elem[ expando ] = {}))[ type ]) && cache[0] === dirruns ) {
diff = cache[1];
// xml :nth-child(...) or :nth-last-child(...) or :nth(-last)?-of-type(...)
} else {
// Use the same loop as above to seek `elem` from the start
while ( (node = ++nodeIndex && node && node[ dir ] ||
(diff = nodeIndex = 0) || start.pop()) ) {
if ( ( ofType ? node.nodeName.toLowerCase() === name : node.nodeType === 1 ) && ++diff ) {
// Cache the index of each encountered element
if ( useCache ) {
(node[ expando ] || (node[ expando ] = {}))[ type ] = [ dirruns, diff ];
}
if ( node === elem ) {
break;
}
}
}
}
// Incorporate the offset, then check against cycle size
diff -= last;
return diff === first || ( diff % first === 0 && diff / first >= 0 );
}
};
},
"PSEUDO": function( pseudo, argument ) {
// pseudo-class names are case-insensitive
// http://www.w3.org/TR/selectors/#pseudo-classes
// Prioritize by case sensitivity in case custom pseudos are added with uppercase letters
// Remember that setFilters inherits from pseudos
var args,
fn = Expr.pseudos[ pseudo ] || Expr.setFilters[ pseudo.toLowerCase() ] ||
Sizzle.error( "unsupported pseudo: " + pseudo );
// The user may use createPseudo to indicate that
// arguments are needed to create the filter function
// just as Sizzle does
if ( fn[ expando ] ) {
return fn( argument );
}
// But maintain support for old signatures
if ( fn.length > 1 ) {
args = [ pseudo, pseudo, "", argument ];
return Expr.setFilters.hasOwnProperty( pseudo.toLowerCase() ) ?
markFunction(function( seed, matches ) {
var idx,
matched = fn( seed, argument ),
i = matched.length;
while ( i-- ) {
idx = indexOf.call( seed, matched[i] );
seed[ idx ] = !( matches[ idx ] = matched[i] );
}
}) :
function( elem ) {
return fn( elem, 0, args );
};
}
return fn;
}
},
pseudos: {
// Potentially complex pseudos
"not": markFunction(function( selector ) {
// Trim the selector passed to compile
// to avoid treating leading and trailing
// spaces as combinators
var input = [],
results = [],
matcher = compile( selector.replace( rtrim, "$1" ) );
return matcher[ expando ] ?
markFunction(function( seed, matches, context, xml ) {
var elem,
unmatched = matcher( seed, null, xml, [] ),
i = seed.length;
// Match elements unmatched by `matcher`
while ( i-- ) {
if ( (elem = unmatched[i]) ) {
seed[i] = !(matches[i] = elem);
}
}
}) :
function( elem, context, xml ) {
input[0] = elem;
matcher( input, null, xml, results );
return !results.pop();
};
}),
"has": markFunction(function( selector ) {
return function( elem ) {
return Sizzle( selector, elem ).length > 0;
};
}),
"contains": markFunction(function( text ) {
return function( elem ) {
return ( elem.textContent || elem.innerText || getText( elem ) ).indexOf( text ) > -1;
};
}),
// "Whether an element is represented by a :lang() selector
// is based solely on the element's language value
// being equal to the identifier C,
// or beginning with the identifier C immediately followed by "-".
// The matching of C against the element's language value is performed case-insensitively.
// The identifier C does not have to be a valid language name."
// http://www.w3.org/TR/selectors/#lang-pseudo
"lang": markFunction( function( lang ) {
// lang value must be a valid identifider
if ( !ridentifier.test(lang || "") ) {
Sizzle.error( "unsupported lang: " + lang );
}
lang = lang.replace( runescape, funescape ).toLowerCase();
return function( elem ) {
var elemLang;
do {
if ( (elemLang = documentIsXML ?
elem.getAttribute("xml:lang") || elem.getAttribute("lang") :
elem.lang) ) {
elemLang = elemLang.toLowerCase();
return elemLang === lang || elemLang.indexOf( lang + "-" ) === 0;
}
} while ( (elem = elem.parentNode) && elem.nodeType === 1 );
return false;
};
}),
// Miscellaneous
"target": function( elem ) {
var hash = window.location && window.location.hash;
return hash && hash.slice( 1 ) === elem.id;
},
"root": function( elem ) {
return elem === docElem;
},
"focus": function( elem ) {
return elem === document.activeElement && (!document.hasFocus || document.hasFocus()) && !!(elem.type || elem.href || ~elem.tabIndex);
},
// Boolean properties
"enabled": function( elem ) {
return elem.disabled === false;
},
"disabled": function( elem ) {
return elem.disabled === true;
},
"checked": function( elem ) {
// In CSS3, :checked should return both checked and selected elements
// http://www.w3.org/TR/2011/REC-css3-selectors-20110929/#checked
var nodeName = elem.nodeName.toLowerCase();
return (nodeName === "input" && !!elem.checked) || (nodeName === "option" && !!elem.selected);
},
"selected": function( elem ) {
// Accessing this property makes selected-by-default
// options in Safari work properly
if ( elem.parentNode ) {
elem.parentNode.selectedIndex;
}
return elem.selected === true;
},
// Contents
"empty": function( elem ) {
// http://www.w3.org/TR/selectors/#empty-pseudo
// :empty is only affected by element nodes and content nodes(including text(3), cdata(4)),
// not comment, processing instructions, or others
// Thanks to Diego Perini for the nodeName shortcut
// Greater than "@" means alpha characters (specifically not starting with "#" or "?")
for ( elem = elem.firstChild; elem; elem = elem.nextSibling ) {
if ( elem.nodeName > "@" || elem.nodeType === 3 || elem.nodeType === 4 ) {
return false;
}
}
return true;
},
"parent": function( elem ) {
return !Expr.pseudos["empty"]( elem );
},
// Element/input types
"header": function( elem ) {
return rheader.test( elem.nodeName );
},
"input": function( elem ) {
return rinputs.test( elem.nodeName );
},
"button": function( elem ) {
var name = elem.nodeName.toLowerCase();
return name === "input" && elem.type === "button" || name === "button";
},
"text": function( elem ) {
var attr;
// IE6 and 7 will map elem.type to 'text' for new HTML5 types (search, etc)
// use getAttribute instead to test this case
return elem.nodeName.toLowerCase() === "input" &&
elem.type === "text" &&
( (attr = elem.getAttribute("type")) == null || attr.toLowerCase() === elem.type );
},
// Position-in-collection
"first": createPositionalPseudo(function() {
return [ 0 ];
}),
"last": createPositionalPseudo(function( matchIndexes, length ) {
return [ length - 1 ];
}),
"eq": createPositionalPseudo(function( matchIndexes, length, argument ) {
return [ argument < 0 ? argument + length : argument ];
}),
"even": createPositionalPseudo(function( matchIndexes, length ) {
var i = 0;
for ( ; i < length; i += 2 ) {
matchIndexes.push( i );
}
return matchIndexes;
}),
"odd": createPositionalPseudo(function( matchIndexes, length ) {
var i = 1;
for ( ; i < length; i += 2 ) {
matchIndexes.push( i );
}
return matchIndexes;
}),
"lt": createPositionalPseudo(function( matchIndexes, length, argument ) {
var i = argument < 0 ? argument + length : argument;
for ( ; --i >= 0; ) {
matchIndexes.push( i );
}
return matchIndexes;
}),
"gt": createPositionalPseudo(function( matchIndexes, length, argument ) {
var i = argument < 0 ? argument + length : argument;
for ( ; ++i < length; ) {
matchIndexes.push( i );
}
return matchIndexes;
})
}
};
// Add button/input type pseudos
for ( i in { radio: true, checkbox: true, file: true, password: true, image: true } ) {
Expr.pseudos[ i ] = createInputPseudo( i );
}
for ( i in { submit: true, reset: true } ) {
Expr.pseudos[ i ] = createButtonPseudo( i );
}
function tokenize( selector, parseOnly ) {
var matched, match, tokens, type,
soFar, groups, preFilters,
cached = tokenCache[ selector + " " ];
if ( cached ) {
return parseOnly ? 0 : cached.slice( 0 );
}
soFar = selector;
groups = [];
preFilters = Expr.preFilter;
while ( soFar ) {
// Comma and first run
if ( !matched || (match = rcomma.exec( soFar )) ) {
if ( match ) {
// Don't consume trailing commas as valid
soFar = soFar.slice( match[0].length ) || soFar;
}
groups.push( tokens = [] );
}
matched = false;
// Combinators
if ( (match = rcombinators.exec( soFar )) ) {
matched = match.shift();
tokens.push( {
value: matched,
// Cast descendant combinators to space
type: match[0].replace( rtrim, " " )
} );
soFar = soFar.slice( matched.length );
}
// Filters
for ( type in Expr.filter ) {
if ( (match = matchExpr[ type ].exec( soFar )) && (!preFilters[ type ] ||
(match = preFilters[ type ]( match ))) ) {
matched = match.shift();
tokens.push( {
value: matched,
type: type,
matches: match
} );
soFar = soFar.slice( matched.length );
}
}
if ( !matched ) {
break;
}
}
// Return the length of the invalid excess
// if we're just parsing
// Otherwise, throw an error or return tokens
return parseOnly ?
soFar.length :
soFar ?
Sizzle.error( selector ) :
// Cache the tokens
tokenCache( selector, groups ).slice( 0 );
}
function toSelector( tokens ) {
var i = 0,
len = tokens.length,
selector = "";
for ( ; i < len; i++ ) {
selector += tokens[i].value;
}
return selector;
}
function addCombinator( matcher, combinator, base ) {
var dir = combinator.dir,
checkNonElements = base && dir === "parentNode",
doneName = done++;
return combinator.first ?
// Check against closest ancestor/preceding element
function( elem, context, xml ) {
while ( (elem = elem[ dir ]) ) {
if ( elem.nodeType === 1 || checkNonElements ) {
return matcher( elem, context, xml );
}
}
} :
// Check against all ancestor/preceding elements
function( elem, context, xml ) {
var data, cache, outerCache,
dirkey = dirruns + " " + doneName;
// We can't set arbitrary data on XML nodes, so they don't benefit from dir caching
if ( xml ) {
while ( (elem = elem[ dir ]) ) {
if ( elem.nodeType === 1 || checkNonElements ) {
if ( matcher( elem, context, xml ) ) {
return true;
}
}
}
} else {
while ( (elem = elem[ dir ]) ) {
if ( elem.nodeType === 1 || checkNonElements ) {
outerCache = elem[ expando ] || (elem[ expando ] = {});
if ( (cache = outerCache[ dir ]) && cache[0] === dirkey ) {
if ( (data = cache[1]) === true || data === cachedruns ) {
return data === true;
}
} else {
cache = outerCache[ dir ] = [ dirkey ];
cache[1] = matcher( elem, context, xml ) || cachedruns;
if ( cache[1] === true ) {
return true;
}
}
}
}
}
};
}
function elementMatcher( matchers ) {
return matchers.length > 1 ?
function( elem, context, xml ) {
var i = matchers.length;
while ( i-- ) {
if ( !matchers[i]( elem, context, xml ) ) {
return false;
}
}
return true;
} :
matchers[0];
}
function condense( unmatched, map, filter, context, xml ) {
var elem,
newUnmatched = [],
i = 0,
len = unmatched.length,
mapped = map != null;
for ( ; i < len; i++ ) {
if ( (elem = unmatched[i]) ) {
if ( !filter || filter( elem, context, xml ) ) {
newUnmatched.push( elem );
if ( mapped ) {
map.push( i );
}
}
}
}
return newUnmatched;
}
function setMatcher( preFilter, selector, matcher, postFilter, postFinder, postSelector ) {
if ( postFilter && !postFilter[ expando ] ) {
postFilter = setMatcher( postFilter );
}
if ( postFinder && !postFinder[ expando ] ) {
postFinder = setMatcher( postFinder, postSelector );
}
return markFunction(function( seed, results, context, xml ) {
var temp, i, elem,
preMap = [],
postMap = [],
preexisting = results.length,
// Get initial elements from seed or context
elems = seed || multipleContexts( selector || "*", context.nodeType ? [ context ] : context, [] ),
// Prefilter to get matcher input, preserving a map for seed-results synchronization
matcherIn = preFilter && ( seed || !selector ) ?
condense( elems, preMap, preFilter, context, xml ) :
elems,
matcherOut = matcher ?
// If we have a postFinder, or filtered seed, or non-seed postFilter or preexisting results,
postFinder || ( seed ? preFilter : preexisting || postFilter ) ?
// ...intermediate processing is necessary
[] :
// ...otherwise use results directly
results :
matcherIn;
// Find primary matches
if ( matcher ) {
matcher( matcherIn, matcherOut, context, xml );
}
// Apply postFilter
if ( postFilter ) {
temp = condense( matcherOut, postMap );
postFilter( temp, [], context, xml );
// Un-match failing elements by moving them back to matcherIn
i = temp.length;
while ( i-- ) {
if ( (elem = temp[i]) ) {
matcherOut[ postMap[i] ] = !(matcherIn[ postMap[i] ] = elem);
}
}
}
if ( seed ) {
if ( postFinder || preFilter ) {
if ( postFinder ) {
// Get the final matcherOut by condensing this intermediate into postFinder contexts
temp = [];
i = matcherOut.length;
while ( i-- ) {
if ( (elem = matcherOut[i]) ) {
// Restore matcherIn since elem is not yet a final match
temp.push( (matcherIn[i] = elem) );
}
}
postFinder( null, (matcherOut = []), temp, xml );
}
// Move matched elements from seed to results to keep them synchronized
i = matcherOut.length;
while ( i-- ) {
if ( (elem = matcherOut[i]) &&
(temp = postFinder ? indexOf.call( seed, elem ) : preMap[i]) > -1 ) {
seed[temp] = !(results[temp] = elem);
}
}
}
// Add elements to results, through postFinder if defined
} else {
matcherOut = condense(
matcherOut === results ?
matcherOut.splice( preexisting, matcherOut.length ) :
matcherOut
);
if ( postFinder ) {
postFinder( null, results, matcherOut, xml );
} else {
push.apply( results, matcherOut );
}
}
});
}
function matcherFromTokens( tokens ) {
var checkContext, matcher, j,
len = tokens.length,
leadingRelative = Expr.relative[ tokens[0].type ],
implicitRelative = leadingRelative || Expr.relative[" "],
i = leadingRelative ? 1 : 0,
// The foundational matcher ensures that elements are reachable from top-level context(s)
matchContext = addCombinator( function( elem ) {
return elem === checkContext;
}, implicitRelative, true ),
matchAnyContext = addCombinator( function( elem ) {
return indexOf.call( checkContext, elem ) > -1;
}, implicitRelative, true ),
matchers = [ function( elem, context, xml ) {
return ( !leadingRelative && ( xml || context !== outermostContext ) ) || (
(checkContext = context).nodeType ?
matchContext( elem, context, xml ) :
matchAnyContext( elem, context, xml ) );
} ];
for ( ; i < len; i++ ) {
if ( (matcher = Expr.relative[ tokens[i].type ]) ) {
matchers = [ addCombinator(elementMatcher( matchers ), matcher) ];
} else {
matcher = Expr.filter[ tokens[i].type ].apply( null, tokens[i].matches );
// Return special upon seeing a positional matcher
if ( matcher[ expando ] ) {
// Find the next relative operator (if any) for proper handling
j = ++i;
for ( ; j < len; j++ ) {
if ( Expr.relative[ tokens[j].type ] ) {
break;
}
}
return setMatcher(
i > 1 && elementMatcher( matchers ),
i > 1 && toSelector( tokens.slice( 0, i - 1 ) ).replace( rtrim, "$1" ),
matcher,
i < j && matcherFromTokens( tokens.slice( i, j ) ),
j < len && matcherFromTokens( (tokens = tokens.slice( j )) ),
j < len && toSelector( tokens )
);
}
matchers.push( matcher );
}
}
return elementMatcher( matchers );
}
function matcherFromGroupMatchers( elementMatchers, setMatchers ) {
// A counter to specify which element is currently being matched
var matcherCachedRuns = 0,
bySet = setMatchers.length > 0,
byElement = elementMatchers.length > 0,
superMatcher = function( seed, context, xml, results, expandContext ) {
var elem, j, matcher,
setMatched = [],
matchedCount = 0,
i = "0",
unmatched = seed && [],
outermost = expandContext != null,
contextBackup = outermostContext,
// We must always have either seed elements or context
elems = seed || byElement && Expr.find["TAG"]( "*", expandContext && context.parentNode || context ),
// Use integer dirruns iff this is the outermost matcher
dirrunsUnique = (dirruns += contextBackup == null ? 1 : Math.random() || 0.1);
if ( outermost ) {
outermostContext = context !== document && context;
cachedruns = matcherCachedRuns;
}
// Add elements passing elementMatchers directly to results
// Keep `i` a string if there are no elements so `matchedCount` will be "00" below
for ( ; (elem = elems[i]) != null; i++ ) {
if ( byElement && elem ) {
j = 0;
while ( (matcher = elementMatchers[j++]) ) {
if ( matcher( elem, context, xml ) ) {
results.push( elem );
break;
}
}
if ( outermost ) {
dirruns = dirrunsUnique;
cachedruns = ++matcherCachedRuns;
}
}
// Track unmatched elements for set filters
if ( bySet ) {
// They will have gone through all possible matchers
if ( (elem = !matcher && elem) ) {
matchedCount--;
}
// Lengthen the array for every element, matched or not
if ( seed ) {
unmatched.push( elem );
}
}
}
// Apply set filters to unmatched elements
matchedCount += i;
if ( bySet && i !== matchedCount ) {
j = 0;
while ( (matcher = setMatchers[j++]) ) {
matcher( unmatched, setMatched, context, xml );
}
if ( seed ) {
// Reintegrate element matches to eliminate the need for sorting
if ( matchedCount > 0 ) {
while ( i-- ) {
if ( !(unmatched[i] || setMatched[i]) ) {
setMatched[i] = pop.call( results );
}
}
}
// Discard index placeholder values to get only actual matches
setMatched = condense( setMatched );
}
// Add matches to results
push.apply( results, setMatched );
// Seedless set matches succeeding multiple successful matchers stipulate sorting
if ( outermost && !seed && setMatched.length > 0 &&
( matchedCount + setMatchers.length ) > 1 ) {
Sizzle.uniqueSort( results );
}
}
// Override manipulation of globals by nested matchers
if ( outermost ) {
dirruns = dirrunsUnique;
outermostContext = contextBackup;
}
return unmatched;
};
return bySet ?
markFunction( superMatcher ) :
superMatcher;
}
compile = Sizzle.compile = function( selector, group /* Internal Use Only */ ) {
var i,
setMatchers = [],
elementMatchers = [],
cached = compilerCache[ selector + " " ];
if ( !cached ) {
// Generate a function of recursive functions that can be used to check each element
if ( !group ) {
group = tokenize( selector );
}
i = group.length;
while ( i-- ) {
cached = matcherFromTokens( group[i] );
if ( cached[ expando ] ) {
setMatchers.push( cached );
} else {
elementMatchers.push( cached );
}
}
// Cache the compiled function
cached = compilerCache( selector, matcherFromGroupMatchers( elementMatchers, setMatchers ) );
}
return cached;
};
function multipleContexts( selector, contexts, results ) {
var i = 0,
len = contexts.length;
for ( ; i < len; i++ ) {
Sizzle( selector, contexts[i], results );
}
return results;
}
function select( selector, context, results, seed ) {
var i, tokens, token, type, find,
match = tokenize( selector );
if ( !seed ) {
// Try to minimize operations if there is only one group
if ( match.length === 1 ) {
// Take a shortcut and set the context if the root selector is an ID
tokens = match[0] = match[0].slice( 0 );
if ( tokens.length > 2 && (token = tokens[0]).type === "ID" &&
context.nodeType === 9 && !documentIsXML &&
Expr.relative[ tokens[1].type ] ) {
context = Expr.find["ID"]( token.matches[0].replace( runescape, funescape ), context )[0];
if ( !context ) {
return results;
}
selector = selector.slice( tokens.shift().value.length );
}
// Fetch a seed set for right-to-left matching
i = matchExpr["needsContext"].test( selector ) ? 0 : tokens.length;
while ( i-- ) {
token = tokens[i];
// Abort if we hit a combinator
if ( Expr.relative[ (type = token.type) ] ) {
break;
}
if ( (find = Expr.find[ type ]) ) {
// Search, expanding context for leading sibling combinators
if ( (seed = find(
token.matches[0].replace( runescape, funescape ),
rsibling.test( tokens[0].type ) && context.parentNode || context
)) ) {
// If seed is empty or no tokens remain, we can return early
tokens.splice( i, 1 );
selector = seed.length && toSelector( tokens );
if ( !selector ) {
push.apply( results, slice.call( seed, 0 ) );
return results;
}
break;
}
}
}
}
}
// Compile and execute a filtering function
// Provide `match` to avoid retokenization if we modified the selector above
compile( selector, match )(
seed,
context,
documentIsXML,
results,
rsibling.test( selector )
);
return results;
}
// Deprecated
Expr.pseudos["nth"] = Expr.pseudos["eq"];
// Easy API for creating new setFilters
function setFilters() {}
Expr.filters = setFilters.prototype = Expr.pseudos;
Expr.setFilters = new setFilters();
// Initialize with the default document
setDocument();
// Override sizzle attribute retrieval
Sizzle.attr = jQuery.attr;
jQuery.find = Sizzle;
jQuery.expr = Sizzle.selectors;
jQuery.expr[":"] = jQuery.expr.pseudos;
jQuery.unique = Sizzle.uniqueSort;
jQuery.text = Sizzle.getText;
jQuery.isXMLDoc = Sizzle.isXML;
jQuery.contains = Sizzle.contains;
})( window );
var runtil = /Until$/,
rparentsprev = /^(?:parents|prev(?:Until|All))/,
isSimple = /^.[^:#\[\.,]*$/,
rneedsContext = jQuery.expr.match.needsContext,
// methods guaranteed to produce a unique set when starting from a unique set
guaranteedUnique = {
children: true,
contents: true,
next: true,
prev: true
};
jQuery.fn.extend({
find: function( selector ) {
var i, ret, self,
len = this.length;
if ( typeof selector !== "string" ) {
self = this;
return this.pushStack( jQuery( selector ).filter(function() {
for ( i = 0; i < len; i++ ) {
if ( jQuery.contains( self[ i ], this ) ) {
return true;
}
}
}) );
}
ret = [];
for ( i = 0; i < len; i++ ) {
jQuery.find( selector, this[ i ], ret );
}
// Needed because $( selector, context ) becomes $( context ).find( selector )
ret = this.pushStack( len > 1 ? jQuery.unique( ret ) : ret );
ret.selector = ( this.selector ? this.selector + " " : "" ) + selector;
return ret;
},
has: function( target ) {
var i,
targets = jQuery( target, this ),
len = targets.length;
return this.filter(function() {
for ( i = 0; i < len; i++ ) {
if ( jQuery.contains( this, targets[i] ) ) {
return true;
}
}
});
},
not: function( selector ) {
return this.pushStack( winnow(this, selector, false) );
},
filter: function( selector ) {
return this.pushStack( winnow(this, selector, true) );
},
is: function( selector ) {
return !!selector && (
typeof selector === "string" ?
// If this is a positional/relative selector, check membership in the returned set
// so $("p:first").is("p:last") won't return true for a doc with two "p".
rneedsContext.test( selector ) ?
jQuery( selector, this.context ).index( this[0] ) >= 0 :
jQuery.filter( selector, this ).length > 0 :
this.filter( selector ).length > 0 );
},
closest: function( selectors, context ) {
var cur,
i = 0,
l = this.length,
ret = [],
pos = rneedsContext.test( selectors ) || typeof selectors !== "string" ?
jQuery( selectors, context || this.context ) :
0;
for ( ; i < l; i++ ) {
cur = this[i];
while ( cur && cur.ownerDocument && cur !== context && cur.nodeType !== 11 ) {
if ( pos ? pos.index(cur) > -1 : jQuery.find.matchesSelector(cur, selectors) ) {
ret.push( cur );
break;
}
cur = cur.parentNode;
}
}
return this.pushStack( ret.length > 1 ? jQuery.unique( ret ) : ret );
},
// Determine the position of an element within
// the matched set of elements
index: function( elem ) {
// No argument, return index in parent
if ( !elem ) {
return ( this[0] && this[0].parentNode ) ? this.first().prevAll().length : -1;
}
// index in selector
if ( typeof elem === "string" ) {
return jQuery.inArray( this[0], jQuery( elem ) );
}
// Locate the position of the desired element
return jQuery.inArray(
// If it receives a jQuery object, the first element is used
elem.jquery ? elem[0] : elem, this );
},
add: function( selector, context ) {
var set = typeof selector === "string" ?
jQuery( selector, context ) :
jQuery.makeArray( selector && selector.nodeType ? [ selector ] : selector ),
all = jQuery.merge( this.get(), set );
return this.pushStack( jQuery.unique(all) );
},
addBack: function( selector ) {
return this.add( selector == null ?
this.prevObject : this.prevObject.filter(selector)
);
}
});
jQuery.fn.andSelf = jQuery.fn.addBack;
function sibling( cur, dir ) {
do {
cur = cur[ dir ];
} while ( cur && cur.nodeType !== 1 );
return cur;
}
jQuery.each({
parent: function( elem ) {
var parent = elem.parentNode;
return parent && parent.nodeType !== 11 ? parent : null;
},
parents: function( elem ) {
return jQuery.dir( elem, "parentNode" );
},
parentsUntil: function( elem, i, until ) {
return jQuery.dir( elem, "parentNode", until );
},
next: function( elem ) {
return sibling( elem, "nextSibling" );
},
prev: function( elem ) {
return sibling( elem, "previousSibling" );
},
nextAll: function( elem ) {
return jQuery.dir( elem, "nextSibling" );
},
prevAll: function( elem ) {
return jQuery.dir( elem, "previousSibling" );
},
nextUntil: function( elem, i, until ) {
return jQuery.dir( elem, "nextSibling", until );
},
prevUntil: function( elem, i, until ) {
return jQuery.dir( elem, "previousSibling", until );
},
siblings: function( elem ) {
return jQuery.sibling( ( elem.parentNode || {} ).firstChild, elem );
},
children: function( elem ) {
return jQuery.sibling( elem.firstChild );
},
contents: function( elem ) {
return jQuery.nodeName( elem, "iframe" ) ?
elem.contentDocument || elem.contentWindow.document :
jQuery.merge( [], elem.childNodes );
}
}, function( name, fn ) {
jQuery.fn[ name ] = function( until, selector ) {
var ret = jQuery.map( this, fn, until );
if ( !runtil.test( name ) ) {
selector = until;
}
if ( selector && typeof selector === "string" ) {
ret = jQuery.filter( selector, ret );
}
ret = this.length > 1 && !guaranteedUnique[ name ] ? jQuery.unique( ret ) : ret;
if ( this.length > 1 && rparentsprev.test( name ) ) {
ret = ret.reverse();
}
return this.pushStack( ret );
};
});
jQuery.extend({
filter: function( expr, elems, not ) {
if ( not ) {
expr = ":not(" + expr + ")";
}
return elems.length === 1 ?
jQuery.find.matchesSelector(elems[0], expr) ? [ elems[0] ] : [] :
jQuery.find.matches(expr, elems);
},
dir: function( elem, dir, until ) {
var matched = [],
cur = elem[ dir ];
while ( cur && cur.nodeType !== 9 && (until === undefined || cur.nodeType !== 1 || !jQuery( cur ).is( until )) ) {
if ( cur.nodeType === 1 ) {
matched.push( cur );
}
cur = cur[dir];
}
return matched;
},
sibling: function( n, elem ) {
var r = [];
for ( ; n; n = n.nextSibling ) {
if ( n.nodeType === 1 && n !== elem ) {
r.push( n );
}
}
return r;
}
});
// Implement the identical functionality for filter and not
function winnow( elements, qualifier, keep ) {
// Can't pass null or undefined to indexOf in Firefox 4
// Set to 0 to skip string check
qualifier = qualifier || 0;
if ( jQuery.isFunction( qualifier ) ) {
return jQuery.grep(elements, function( elem, i ) {
var retVal = !!qualifier.call( elem, i, elem );
return retVal === keep;
});
} else if ( qualifier.nodeType ) {
return jQuery.grep(elements, function( elem ) {
return ( elem === qualifier ) === keep;
});
} else if ( typeof qualifier === "string" ) {
var filtered = jQuery.grep(elements, function( elem ) {
return elem.nodeType === 1;
});
if ( isSimple.test( qualifier ) ) {
return jQuery.filter(qualifier, filtered, !keep);
} else {
qualifier = jQuery.filter( qualifier, filtered );
}
}
return jQuery.grep(elements, function( elem ) {
return ( jQuery.inArray( elem, qualifier ) >= 0 ) === keep;
});
}
function createSafeFragment( document ) {
var list = nodeNames.split( "|" ),
safeFrag = document.createDocumentFragment();
if ( safeFrag.createElement ) {
while ( list.length ) {
safeFrag.createElement(
list.pop()
);
}
}
return safeFrag;
}
var nodeNames = "abbr|article|aside|audio|bdi|canvas|data|datalist|details|figcaption|figure|footer|" +
"header|hgroup|mark|meter|nav|output|progress|section|summary|time|video",
rinlinejQuery = / jQuery\d+="(?:null|\d+)"/g,
rnoshimcache = new RegExp("<(?:" + nodeNames + ")[\\s/>]", "i"),
rleadingWhitespace = /^\s+/,
rxhtmlTag = /<(?!area|br|col|embed|hr|img|input|link|meta|param)(([\w:]+)[^>]*)\/>/gi,
rtagName = /<([\w:]+)/,
rtbody = /<tbody/i,
rhtml = /<|&#?\w+;/,
rnoInnerhtml = /<(?:script|style|link)/i,
manipulation_rcheckableType = /^(?:checkbox|radio)$/i,
// checked="checked" or checked
rchecked = /checked\s*(?:[^=]|=\s*.checked.)/i,
rscriptType = /^$|\/(?:java|ecma)script/i,
rscriptTypeMasked = /^true\/(.*)/,
rcleanScript = /^\s*<!(?:\[CDATA\[|--)|(?:\]\]|--)>\s*$/g,
// We have to close these tags to support XHTML (#13200)
wrapMap = {
option: [ 1, "<select multiple='multiple'>", "</select>" ],
legend: [ 1, "<fieldset>", "</fieldset>" ],
area: [ 1, "<map>", "</map>" ],
param: [ 1, "<object>", "</object>" ],
thead: [ 1, "<table>", "</table>" ],
tr: [ 2, "<table><tbody>", "</tbody></table>" ],
col: [ 2, "<table><tbody></tbody><colgroup>", "</colgroup></table>" ],
td: [ 3, "<table><tbody><tr>", "</tr></tbody></table>" ],
// IE6-8 can't serialize link, script, style, or any html5 (NoScope) tags,
// unless wrapped in a div with non-breaking characters in front of it.
_default: jQuery.support.htmlSerialize ? [ 0, "", "" ] : [ 1, "X<div>", "</div>" ]
},
safeFragment = createSafeFragment( document ),
fragmentDiv = safeFragment.appendChild( document.createElement("div") );
wrapMap.optgroup = wrapMap.option;
wrapMap.tbody = wrapMap.tfoot = wrapMap.colgroup = wrapMap.caption = wrapMap.thead;
wrapMap.th = wrapMap.td;
jQuery.fn.extend({
text: function( value ) {
return jQuery.access( this, function( value ) {
return value === undefined ?
jQuery.text( this ) :
this.empty().append( ( this[0] && this[0].ownerDocument || document ).createTextNode( value ) );
}, null, value, arguments.length );
},
wrapAll: function( html ) {
if ( jQuery.isFunction( html ) ) {
return this.each(function(i) {
jQuery(this).wrapAll( html.call(this, i) );
});
}
if ( this[0] ) {
// The elements to wrap the target around
var wrap = jQuery( html, this[0].ownerDocument ).eq(0).clone(true);
if ( this[0].parentNode ) {
wrap.insertBefore( this[0] );
}
wrap.map(function() {
var elem = this;
while ( elem.firstChild && elem.firstChild.nodeType === 1 ) {
elem = elem.firstChild;
}
return elem;
}).append( this );
}
return this;
},
wrapInner: function( html ) {
if ( jQuery.isFunction( html ) ) {
return this.each(function(i) {
jQuery(this).wrapInner( html.call(this, i) );
});
}
return this.each(function() {
var self = jQuery( this ),
contents = self.contents();
if ( contents.length ) {
contents.wrapAll( html );
} else {
self.append( html );
}
});
},
wrap: function( html ) {
var isFunction = jQuery.isFunction( html );
return this.each(function(i) {
jQuery( this ).wrapAll( isFunction ? html.call(this, i) : html );
});
},
unwrap: function() {
return this.parent().each(function() {
if ( !jQuery.nodeName( this, "body" ) ) {
jQuery( this ).replaceWith( this.childNodes );
}
}).end();
},
append: function() {
return this.domManip(arguments, true, function( elem ) {
if ( this.nodeType === 1 || this.nodeType === 11 || this.nodeType === 9 ) {
this.appendChild( elem );
}
});
},
prepend: function() {
return this.domManip(arguments, true, function( elem ) {
if ( this.nodeType === 1 || this.nodeType === 11 || this.nodeType === 9 ) {
this.insertBefore( elem, this.firstChild );
}
});
},
before: function() {
return this.domManip( arguments, false, function( elem ) {
if ( this.parentNode ) {
this.parentNode.insertBefore( elem, this );
}
});
},
after: function() {
return this.domManip( arguments, false, function( elem ) {
if ( this.parentNode ) {
this.parentNode.insertBefore( elem, this.nextSibling );
}
});
},
// keepData is for internal use only--do not document
remove: function( selector, keepData ) {
var elem,
i = 0;
for ( ; (elem = this[i]) != null; i++ ) {
if ( !selector || jQuery.filter( selector, [ elem ] ).length > 0 ) {
if ( !keepData && elem.nodeType === 1 ) {
jQuery.cleanData( getAll( elem ) );
}
if ( elem.parentNode ) {
if ( keepData && jQuery.contains( elem.ownerDocument, elem ) ) {
setGlobalEval( getAll( elem, "script" ) );
}
elem.parentNode.removeChild( elem );
}
}
}
return this;
},
empty: function() {
var elem,
i = 0;
for ( ; (elem = this[i]) != null; i++ ) {
// Remove element nodes and prevent memory leaks
if ( elem.nodeType === 1 ) {
jQuery.cleanData( getAll( elem, false ) );
}
// Remove any remaining nodes
while ( elem.firstChild ) {
elem.removeChild( elem.firstChild );
}
// If this is a select, ensure that it displays empty (#12336)
// Support: IE<9
if ( elem.options && jQuery.nodeName( elem, "select" ) ) {
elem.options.length = 0;
}
}
return this;
},
clone: function( dataAndEvents, deepDataAndEvents ) {
dataAndEvents = dataAndEvents == null ? false : dataAndEvents;
deepDataAndEvents = deepDataAndEvents == null ? dataAndEvents : deepDataAndEvents;
return this.map( function () {
return jQuery.clone( this, dataAndEvents, deepDataAndEvents );
});
},
html: function( value ) {
return jQuery.access( this, function( value ) {
var elem = this[0] || {},
i = 0,
l = this.length;
if ( value === undefined ) {
return elem.nodeType === 1 ?
elem.innerHTML.replace( rinlinejQuery, "" ) :
undefined;
}
// See if we can take a shortcut and just use innerHTML
if ( typeof value === "string" && !rnoInnerhtml.test( value ) &&
( jQuery.support.htmlSerialize || !rnoshimcache.test( value ) ) &&
( jQuery.support.leadingWhitespace || !rleadingWhitespace.test( value ) ) &&
!wrapMap[ ( rtagName.exec( value ) || ["", ""] )[1].toLowerCase() ] ) {
value = value.replace( rxhtmlTag, "<$1></$2>" );
try {
for (; i < l; i++ ) {
// Remove element nodes and prevent memory leaks
elem = this[i] || {};
if ( elem.nodeType === 1 ) {
jQuery.cleanData( getAll( elem, false ) );
elem.innerHTML = value;
}
}
elem = 0;
// If using innerHTML throws an exception, use the fallback method
} catch(e) {}
}
if ( elem ) {
this.empty().append( value );
}
}, null, value, arguments.length );
},
replaceWith: function( value ) {
var isFunc = jQuery.isFunction( value );
// Make sure that the elements are removed from the DOM before they are inserted
// this can help fix replacing a parent with child elements
if ( !isFunc && typeof value !== "string" ) {
value = jQuery( value ).not( this ).detach();
}
return this.domManip( [ value ], true, function( elem ) {
var next = this.nextSibling,
parent = this.parentNode;
if ( parent ) {
jQuery( this ).remove();
parent.insertBefore( elem, next );
}
});
},
detach: function( selector ) {
return this.remove( selector, true );
},
domManip: function( args, table, callback ) {
// Flatten any nested arrays
args = core_concat.apply( [], args );
var first, node, hasScripts,
scripts, doc, fragment,
i = 0,
l = this.length,
set = this,
iNoClone = l - 1,
value = args[0],
isFunction = jQuery.isFunction( value );
// We can't cloneNode fragments that contain checked, in WebKit
if ( isFunction || !( l <= 1 || typeof value !== "string" || jQuery.support.checkClone || !rchecked.test( value ) ) ) {
return this.each(function( index ) {
var self = set.eq( index );
if ( isFunction ) {
args[0] = value.call( this, index, table ? self.html() : undefined );
}
self.domManip( args, table, callback );
});
}
if ( l ) {
fragment = jQuery.buildFragment( args, this[ 0 ].ownerDocument, false, this );
first = fragment.firstChild;
if ( fragment.childNodes.length === 1 ) {
fragment = first;
}
if ( first ) {
table = table && jQuery.nodeName( first, "tr" );
scripts = jQuery.map( getAll( fragment, "script" ), disableScript );
hasScripts = scripts.length;
// Use the original fragment for the last item instead of the first because it can end up
// being emptied incorrectly in certain situations (#8070).
for ( ; i < l; i++ ) {
node = fragment;
if ( i !== iNoClone ) {
node = jQuery.clone( node, true, true );
// Keep references to cloned scripts for later restoration
if ( hasScripts ) {
jQuery.merge( scripts, getAll( node, "script" ) );
}
}
callback.call(
table && jQuery.nodeName( this[i], "table" ) ?
findOrAppend( this[i], "tbody" ) :
this[i],
node,
i
);
}
if ( hasScripts ) {
doc = scripts[ scripts.length - 1 ].ownerDocument;
// Reenable scripts
jQuery.map( scripts, restoreScript );
// Evaluate executable scripts on first document insertion
for ( i = 0; i < hasScripts; i++ ) {
node = scripts[ i ];
if ( rscriptType.test( node.type || "" ) &&
!jQuery._data( node, "globalEval" ) && jQuery.contains( doc, node ) ) {
if ( node.src ) {
// Hope ajax is available...
jQuery.ajax({
url: node.src,
type: "GET",
dataType: "script",
async: false,
global: false,
"throws": true
});
} else {
jQuery.globalEval( ( node.text || node.textContent || node.innerHTML || "" ).replace( rcleanScript, "" ) );
}
}
}
}
// Fix #11809: Avoid leaking memory
fragment = first = null;
}
}
return this;
}
});
function findOrAppend( elem, tag ) {
return elem.getElementsByTagName( tag )[0] || elem.appendChild( elem.ownerDocument.createElement( tag ) );
}
// Replace/restore the type attribute of script elements for safe DOM manipulation
function disableScript( elem ) {
var attr = elem.getAttributeNode("type");
elem.type = ( attr && attr.specified ) + "/" + elem.type;
return elem;
}
function restoreScript( elem ) {
var match = rscriptTypeMasked.exec( elem.type );
if ( match ) {
elem.type = match[1];
} else {
elem.removeAttribute("type");
}
return elem;
}
// Mark scripts as having already been evaluated
function setGlobalEval( elems, refElements ) {
var elem,
i = 0;
for ( ; (elem = elems[i]) != null; i++ ) {
jQuery._data( elem, "globalEval", !refElements || jQuery._data( refElements[i], "globalEval" ) );
}
}
function cloneCopyEvent( src, dest ) {
if ( dest.nodeType !== 1 || !jQuery.hasData( src ) ) {
return;
}
var type, i, l,
oldData = jQuery._data( src ),
curData = jQuery._data( dest, oldData ),
events = oldData.events;
if ( events ) {
delete curData.handle;
curData.events = {};
for ( type in events ) {
for ( i = 0, l = events[ type ].length; i < l; i++ ) {
jQuery.event.add( dest, type, events[ type ][ i ] );
}
}
}
// make the cloned public data object a copy from the original
if ( curData.data ) {
curData.data = jQuery.extend( {}, curData.data );
}
}
function fixCloneNodeIssues( src, dest ) {
var nodeName, e, data;
// We do not need to do anything for non-Elements
if ( dest.nodeType !== 1 ) {
return;
}
nodeName = dest.nodeName.toLowerCase();
// IE6-8 copies events bound via attachEvent when using cloneNode.
if ( !jQuery.support.noCloneEvent && dest[ jQuery.expando ] ) {
data = jQuery._data( dest );
for ( e in data.events ) {
jQuery.removeEvent( dest, e, data.handle );
}
// Event data gets referenced instead of copied if the expando gets copied too
dest.removeAttribute( jQuery.expando );
}
// IE blanks contents when cloning scripts, and tries to evaluate newly-set text
if ( nodeName === "script" && dest.text !== src.text ) {
disableScript( dest ).text = src.text;
restoreScript( dest );
// IE6-10 improperly clones children of object elements using classid.
// IE10 throws NoModificationAllowedError if parent is null, #12132.
} else if ( nodeName === "object" ) {
if ( dest.parentNode ) {
dest.outerHTML = src.outerHTML;
}
// This path appears unavoidable for IE9. When cloning an object
// element in IE9, the outerHTML strategy above is not sufficient.
// If the src has innerHTML and the destination does not,
// copy the src.innerHTML into the dest.innerHTML. #10324
if ( jQuery.support.html5Clone && ( src.innerHTML && !jQuery.trim(dest.innerHTML) ) ) {
dest.innerHTML = src.innerHTML;
}
} else if ( nodeName === "input" && manipulation_rcheckableType.test( src.type ) ) {
// IE6-8 fails to persist the checked state of a cloned checkbox
// or radio button. Worse, IE6-7 fail to give the cloned element
// a checked appearance if the defaultChecked value isn't also set
dest.defaultChecked = dest.checked = src.checked;
// IE6-7 get confused and end up setting the value of a cloned
// checkbox/radio button to an empty string instead of "on"
if ( dest.value !== src.value ) {
dest.value = src.value;
}
// IE6-8 fails to return the selected option to the default selected
// state when cloning options
} else if ( nodeName === "option" ) {
dest.defaultSelected = dest.selected = src.defaultSelected;
// IE6-8 fails to set the defaultValue to the correct value when
// cloning other types of input fields
} else if ( nodeName === "input" || nodeName === "textarea" ) {
dest.defaultValue = src.defaultValue;
}
}
jQuery.each({
appendTo: "append",
prependTo: "prepend",
insertBefore: "before",
insertAfter: "after",
replaceAll: "replaceWith"
}, function( name, original ) {
jQuery.fn[ name ] = function( selector ) {
var elems,
i = 0,
ret = [],
insert = jQuery( selector ),
last = insert.length - 1;
for ( ; i <= last; i++ ) {
elems = i === last ? this : this.clone(true);
jQuery( insert[i] )[ original ]( elems );
// Modern browsers can apply jQuery collections as arrays, but oldIE needs a .get()
core_push.apply( ret, elems.get() );
}
return this.pushStack( ret );
};
});
function getAll( context, tag ) {
var elems, elem,
i = 0,
found = typeof context.getElementsByTagName !== core_strundefined ? context.getElementsByTagName( tag || "*" ) :
typeof context.querySelectorAll !== core_strundefined ? context.querySelectorAll( tag || "*" ) :
undefined;
if ( !found ) {
for ( found = [], elems = context.childNodes || context; (elem = elems[i]) != null; i++ ) {
if ( !tag || jQuery.nodeName( elem, tag ) ) {
found.push( elem );
} else {
jQuery.merge( found, getAll( elem, tag ) );
}
}
}
return tag === undefined || tag && jQuery.nodeName( context, tag ) ?
jQuery.merge( [ context ], found ) :
found;
}
// Used in buildFragment, fixes the defaultChecked property
function fixDefaultChecked( elem ) {
if ( manipulation_rcheckableType.test( elem.type ) ) {
elem.defaultChecked = elem.checked;
}
}
jQuery.extend({
clone: function( elem, dataAndEvents, deepDataAndEvents ) {
var destElements, node, clone, i, srcElements,
inPage = jQuery.contains( elem.ownerDocument, elem );
if ( jQuery.support.html5Clone || jQuery.isXMLDoc(elem) || !rnoshimcache.test( "<" + elem.nodeName + ">" ) ) {
clone = elem.cloneNode( true );
// IE<=8 does not properly clone detached, unknown element nodes
} else {
fragmentDiv.innerHTML = elem.outerHTML;
fragmentDiv.removeChild( clone = fragmentDiv.firstChild );
}
if ( (!jQuery.support.noCloneEvent || !jQuery.support.noCloneChecked) &&
(elem.nodeType === 1 || elem.nodeType === 11) && !jQuery.isXMLDoc(elem) ) {
// We eschew Sizzle here for performance reasons: http://jsperf.com/getall-vs-sizzle/2
destElements = getAll( clone );
srcElements = getAll( elem );
// Fix all IE cloning issues
for ( i = 0; (node = srcElements[i]) != null; ++i ) {
// Ensure that the destination node is not null; Fixes #9587
if ( destElements[i] ) {
fixCloneNodeIssues( node, destElements[i] );
}
}
}
// Copy the events from the original to the clone
if ( dataAndEvents ) {
if ( deepDataAndEvents ) {
srcElements = srcElements || getAll( elem );
destElements = destElements || getAll( clone );
for ( i = 0; (node = srcElements[i]) != null; i++ ) {
cloneCopyEvent( node, destElements[i] );
}
} else {
cloneCopyEvent( elem, clone );
}
}
// Preserve script evaluation history
destElements = getAll( clone, "script" );
if ( destElements.length > 0 ) {
setGlobalEval( destElements, !inPage && getAll( elem, "script" ) );
}
destElements = srcElements = node = null;
// Return the cloned set
return clone;
},
buildFragment: function( elems, context, scripts, selection ) {
var j, elem, contains,
tmp, tag, tbody, wrap,
l = elems.length,
// Ensure a safe fragment
safe = createSafeFragment( context ),
nodes = [],
i = 0;
for ( ; i < l; i++ ) {
elem = elems[ i ];
if ( elem || elem === 0 ) {
// Add nodes directly
if ( jQuery.type( elem ) === "object" ) {
jQuery.merge( nodes, elem.nodeType ? [ elem ] : elem );
// Convert non-html into a text node
} else if ( !rhtml.test( elem ) ) {
nodes.push( context.createTextNode( elem ) );
// Convert html into DOM nodes
} else {
tmp = tmp || safe.appendChild( context.createElement("div") );
// Deserialize a standard representation
tag = ( rtagName.exec( elem ) || ["", ""] )[1].toLowerCase();
wrap = wrapMap[ tag ] || wrapMap._default;
tmp.innerHTML = wrap[1] + elem.replace( rxhtmlTag, "<$1></$2>" ) + wrap[2];
// Descend through wrappers to the right content
j = wrap[0];
while ( j-- ) {
tmp = tmp.lastChild;
}
// Manually add leading whitespace removed by IE
if ( !jQuery.support.leadingWhitespace && rleadingWhitespace.test( elem ) ) {
nodes.push( context.createTextNode( rleadingWhitespace.exec( elem )[0] ) );
}
// Remove IE's autoinserted <tbody> from table fragments
if ( !jQuery.support.tbody ) {
// String was a <table>, *may* have spurious <tbody>
elem = tag === "table" && !rtbody.test( elem ) ?
tmp.firstChild :
// String was a bare <thead> or <tfoot>
wrap[1] === "<table>" && !rtbody.test( elem ) ?
tmp :
0;
j = elem && elem.childNodes.length;
while ( j-- ) {
if ( jQuery.nodeName( (tbody = elem.childNodes[j]), "tbody" ) && !tbody.childNodes.length ) {
elem.removeChild( tbody );
}
}
}
jQuery.merge( nodes, tmp.childNodes );
// Fix #12392 for WebKit and IE > 9
tmp.textContent = "";
// Fix #12392 for oldIE
while ( tmp.firstChild ) {
tmp.removeChild( tmp.firstChild );
}
// Remember the top-level container for proper cleanup
tmp = safe.lastChild;
}
}
}
// Fix #11356: Clear elements from fragment
if ( tmp ) {
safe.removeChild( tmp );
}
// Reset defaultChecked for any radios and checkboxes
// about to be appended to the DOM in IE 6/7 (#8060)
if ( !jQuery.support.appendChecked ) {
jQuery.grep( getAll( nodes, "input" ), fixDefaultChecked );
}
i = 0;
while ( (elem = nodes[ i++ ]) ) {
// #4087 - If origin and destination elements are the same, and this is
// that element, do not do anything
if ( selection && jQuery.inArray( elem, selection ) !== -1 ) {
continue;
}
contains = jQuery.contains( elem.ownerDocument, elem );
// Append to fragment
tmp = getAll( safe.appendChild( elem ), "script" );
// Preserve script evaluation history
if ( contains ) {
setGlobalEval( tmp );
}
// Capture executables
if ( scripts ) {
j = 0;
while ( (elem = tmp[ j++ ]) ) {
if ( rscriptType.test( elem.type || "" ) ) {
scripts.push( elem );
}
}
}
}
tmp = null;
return safe;
},
cleanData: function( elems, /* internal */ acceptData ) {
var elem, type, id, data,
i = 0,
internalKey = jQuery.expando,
cache = jQuery.cache,
deleteExpando = jQuery.support.deleteExpando,
special = jQuery.event.special;
for ( ; (elem = elems[i]) != null; i++ ) {
if ( acceptData || jQuery.acceptData( elem ) ) {
id = elem[ internalKey ];
data = id && cache[ id ];
if ( data ) {
if ( data.events ) {
for ( type in data.events ) {
if ( special[ type ] ) {
jQuery.event.remove( elem, type );
// This is a shortcut to avoid jQuery.event.remove's overhead
} else {
jQuery.removeEvent( elem, type, data.handle );
}
}
}
// Remove cache only if it was not already removed by jQuery.event.remove
if ( cache[ id ] ) {
delete cache[ id ];
// IE does not allow us to delete expando properties from nodes,
// nor does it have a removeAttribute function on Document nodes;
// we must handle all of these cases
if ( deleteExpando ) {
delete elem[ internalKey ];
} else if ( typeof elem.removeAttribute !== core_strundefined ) {
elem.removeAttribute( internalKey );
} else {
elem[ internalKey ] = null;
}
core_deletedIds.push( id );
}
}
}
}
}
});
var iframe, getStyles, curCSS,
ralpha = /alpha\([^)]*\)/i,
ropacity = /opacity\s*=\s*([^)]*)/,
rposition = /^(top|right|bottom|left)$/,
// swappable if display is none or starts with table except "table", "table-cell", or "table-caption"
// see here for display values: https://developer.mozilla.org/en-US/docs/CSS/display
rdisplayswap = /^(none|table(?!-c[ea]).+)/,
rmargin = /^margin/,
rnumsplit = new RegExp( "^(" + core_pnum + ")(.*)$", "i" ),
rnumnonpx = new RegExp( "^(" + core_pnum + ")(?!px)[a-z%]+$", "i" ),
rrelNum = new RegExp( "^([+-])=(" + core_pnum + ")", "i" ),
elemdisplay = { BODY: "block" },
cssShow = { position: "absolute", visibility: "hidden", display: "block" },
cssNormalTransform = {
letterSpacing: 0,
fontWeight: 400
},
cssExpand = [ "Top", "Right", "Bottom", "Left" ],
cssPrefixes = [ "Webkit", "O", "Moz", "ms" ];
// return a css property mapped to a potentially vendor prefixed property
function vendorPropName( style, name ) {
// shortcut for names that are not vendor prefixed
if ( name in style ) {
return name;
}
// check for vendor prefixed names
var capName = name.charAt(0).toUpperCase() + name.slice(1),
origName = name,
i = cssPrefixes.length;
while ( i-- ) {
name = cssPrefixes[ i ] + capName;
if ( name in style ) {
return name;
}
}
return origName;
}
function isHidden( elem, el ) {
// isHidden might be called from jQuery#filter function;
// in that case, element will be second argument
elem = el || elem;
return jQuery.css( elem, "display" ) === "none" || !jQuery.contains( elem.ownerDocument, elem );
}
function showHide( elements, show ) {
var display, elem, hidden,
values = [],
index = 0,
length = elements.length;
for ( ; index < length; index++ ) {
elem = elements[ index ];
if ( !elem.style ) {
continue;
}
values[ index ] = jQuery._data( elem, "olddisplay" );
display = elem.style.display;
if ( show ) {
// Reset the inline display of this element to learn if it is
// being hidden by cascaded rules or not
if ( !values[ index ] && display === "none" ) {
elem.style.display = "";
}
// Set elements which have been overridden with display: none
// in a stylesheet to whatever the default browser style is
// for such an element
if ( elem.style.display === "" && isHidden( elem ) ) {
values[ index ] = jQuery._data( elem, "olddisplay", css_defaultDisplay(elem.nodeName) );
}
} else {
if ( !values[ index ] ) {
hidden = isHidden( elem );
if ( display && display !== "none" || !hidden ) {
jQuery._data( elem, "olddisplay", hidden ? display : jQuery.css( elem, "display" ) );
}
}
}
}
// Set the display of most of the elements in a second loop
// to avoid the constant reflow
for ( index = 0; index < length; index++ ) {
elem = elements[ index ];
if ( !elem.style ) {
continue;
}
if ( !show || elem.style.display === "none" || elem.style.display === "" ) {
elem.style.display = show ? values[ index ] || "" : "none";
}
}
return elements;
}
jQuery.fn.extend({
css: function( name, value ) {
return jQuery.access( this, function( elem, name, value ) {
var len, styles,
map = {},
i = 0;
if ( jQuery.isArray( name ) ) {
styles = getStyles( elem );
len = name.length;
for ( ; i < len; i++ ) {
map[ name[ i ] ] = jQuery.css( elem, name[ i ], false, styles );
}
return map;
}
return value !== undefined ?
jQuery.style( elem, name, value ) :
jQuery.css( elem, name );
}, name, value, arguments.length > 1 );
},
show: function() {
return showHide( this, true );
},
hide: function() {
return showHide( this );
},
toggle: function( state ) {
var bool = typeof state === "boolean";
return this.each(function() {
if ( bool ? state : isHidden( this ) ) {
jQuery( this ).show();
} else {
jQuery( this ).hide();
}
});
}
});
jQuery.extend({
// Add in style property hooks for overriding the default
// behavior of getting and setting a style property
cssHooks: {
opacity: {
get: function( elem, computed ) {
if ( computed ) {
// We should always get a number back from opacity
var ret = curCSS( elem, "opacity" );
return ret === "" ? "1" : ret;
}
}
}
},
// Exclude the following css properties to add px
cssNumber: {
"columnCount": true,
"fillOpacity": true,
"fontWeight": true,
"lineHeight": true,
"opacity": true,
"orphans": true,
"widows": true,
"zIndex": true,
"zoom": true
},
// Add in properties whose names you wish to fix before
// setting or getting the value
cssProps: {
// normalize float css property
"float": jQuery.support.cssFloat ? "cssFloat" : "styleFloat"
},
// Get and set the style property on a DOM Node
style: function( elem, name, value, extra ) {
// Don't set styles on text and comment nodes
if ( !elem || elem.nodeType === 3 || elem.nodeType === 8 || !elem.style ) {
return;
}
// Make sure that we're working with the right name
var ret, type, hooks,
origName = jQuery.camelCase( name ),
style = elem.style;
name = jQuery.cssProps[ origName ] || ( jQuery.cssProps[ origName ] = vendorPropName( style, origName ) );
// gets hook for the prefixed version
// followed by the unprefixed version
hooks = jQuery.cssHooks[ name ] || jQuery.cssHooks[ origName ];
// Check if we're setting a value
if ( value !== undefined ) {
type = typeof value;
// convert relative number strings (+= or -=) to relative numbers. #7345
if ( type === "string" && (ret = rrelNum.exec( value )) ) {
value = ( ret[1] + 1 ) * ret[2] + parseFloat( jQuery.css( elem, name ) );
// Fixes bug #9237
type = "number";
}
// Make sure that NaN and null values aren't set. See: #7116
if ( value == null || type === "number" && isNaN( value ) ) {
return;
}
// If a number was passed in, add 'px' to the (except for certain CSS properties)
if ( type === "number" && !jQuery.cssNumber[ origName ] ) {
value += "px";
}
// Fixes #8908, it can be done more correctly by specifing setters in cssHooks,
// but it would mean to define eight (for every problematic property) identical functions
if ( !jQuery.support.clearCloneStyle && value === "" && name.indexOf("background") === 0 ) {
style[ name ] = "inherit";
}
// If a hook was provided, use that value, otherwise just set the specified value
if ( !hooks || !("set" in hooks) || (value = hooks.set( elem, value, extra )) !== undefined ) {
// Wrapped to prevent IE from throwing errors when 'invalid' values are provided
// Fixes bug #5509
try {
style[ name ] = value;
} catch(e) {}
}
} else {
// If a hook was provided get the non-computed value from there
if ( hooks && "get" in hooks && (ret = hooks.get( elem, false, extra )) !== undefined ) {
return ret;
}
// Otherwise just get the value from the style object
return style[ name ];
}
},
css: function( elem, name, extra, styles ) {
var num, val, hooks,
origName = jQuery.camelCase( name );
// Make sure that we're working with the right name
name = jQuery.cssProps[ origName ] || ( jQuery.cssProps[ origName ] = vendorPropName( elem.style, origName ) );
// gets hook for the prefixed version
// followed by the unprefixed version
hooks = jQuery.cssHooks[ name ] || jQuery.cssHooks[ origName ];
// If a hook was provided get the computed value from there
if ( hooks && "get" in hooks ) {
val = hooks.get( elem, true, extra );
}
// Otherwise, if a way to get the computed value exists, use that
if ( val === undefined ) {
val = curCSS( elem, name, styles );
}
//convert "normal" to computed value
if ( val === "normal" && name in cssNormalTransform ) {
val = cssNormalTransform[ name ];
}
// Return, converting to number if forced or a qualifier was provided and val looks numeric
if ( extra === "" || extra ) {
num = parseFloat( val );
return extra === true || jQuery.isNumeric( num ) ? num || 0 : val;
}
return val;
},
// A method for quickly swapping in/out CSS properties to get correct calculations
swap: function( elem, options, callback, args ) {
var ret, name,
old = {};
// Remember the old values, and insert the new ones
for ( name in options ) {
old[ name ] = elem.style[ name ];
elem.style[ name ] = options[ name ];
}
ret = callback.apply( elem, args || [] );
// Revert the old values
for ( name in options ) {
elem.style[ name ] = old[ name ];
}
return ret;
}
});
// NOTE: we've included the "window" in window.getComputedStyle
// because jsdom on node.js will break without it.
if ( window.getComputedStyle ) {
getStyles = function( elem ) {
return window.getComputedStyle( elem, null );
};
curCSS = function( elem, name, _computed ) {
var width, minWidth, maxWidth,
computed = _computed || getStyles( elem ),
// getPropertyValue is only needed for .css('filter') in IE9, see #12537
ret = computed ? computed.getPropertyValue( name ) || computed[ name ] : undefined,
style = elem.style;
if ( computed ) {
if ( ret === "" && !jQuery.contains( elem.ownerDocument, elem ) ) {
ret = jQuery.style( elem, name );
}
// A tribute to the "awesome hack by Dean Edwards"
// Chrome < 17 and Safari 5.0 uses "computed value" instead of "used value" for margin-right
// Safari 5.1.7 (at least) returns percentage for a larger set of values, but width seems to be reliably pixels
// this is against the CSSOM draft spec: http://dev.w3.org/csswg/cssom/#resolved-values
if ( rnumnonpx.test( ret ) && rmargin.test( name ) ) {
// Remember the original values
width = style.width;
minWidth = style.minWidth;
maxWidth = style.maxWidth;
// Put in the new values to get a computed value out
style.minWidth = style.maxWidth = style.width = ret;
ret = computed.width;
// Revert the changed values
style.width = width;
style.minWidth = minWidth;
style.maxWidth = maxWidth;
}
}
return ret;
};
} else if ( document.documentElement.currentStyle ) {
getStyles = function( elem ) {
return elem.currentStyle;
};
curCSS = function( elem, name, _computed ) {
var left, rs, rsLeft,
computed = _computed || getStyles( elem ),
ret = computed ? computed[ name ] : undefined,
style = elem.style;
// Avoid setting ret to empty string here
// so we don't default to auto
if ( ret == null && style && style[ name ] ) {
ret = style[ name ];
}
// From the awesome hack by Dean Edwards
// http://erik.eae.net/archives/2007/07/27/18.54.15/#comment-102291
// If we're not dealing with a regular pixel number
// but a number that has a weird ending, we need to convert it to pixels
// but not position css attributes, as those are proportional to the parent element instead
// and we can't measure the parent instead because it might trigger a "stacking dolls" problem
if ( rnumnonpx.test( ret ) && !rposition.test( name ) ) {
// Remember the original values
left = style.left;
rs = elem.runtimeStyle;
rsLeft = rs && rs.left;
// Put in the new values to get a computed value out
if ( rsLeft ) {
rs.left = elem.currentStyle.left;
}
style.left = name === "fontSize" ? "1em" : ret;
ret = style.pixelLeft + "px";
// Revert the changed values
style.left = left;
if ( rsLeft ) {
rs.left = rsLeft;
}
}
return ret === "" ? "auto" : ret;
};
}
function setPositiveNumber( elem, value, subtract ) {
var matches = rnumsplit.exec( value );
return matches ?
// Guard against undefined "subtract", e.g., when used as in cssHooks
Math.max( 0, matches[ 1 ] - ( subtract || 0 ) ) + ( matches[ 2 ] || "px" ) :
value;
}
function augmentWidthOrHeight( elem, name, extra, isBorderBox, styles ) {
var i = extra === ( isBorderBox ? "border" : "content" ) ?
// If we already have the right measurement, avoid augmentation
4 :
// Otherwise initialize for horizontal or vertical properties
name === "width" ? 1 : 0,
val = 0;
for ( ; i < 4; i += 2 ) {
// both box models exclude margin, so add it if we want it
if ( extra === "margin" ) {
val += jQuery.css( elem, extra + cssExpand[ i ], true, styles );
}
if ( isBorderBox ) {
// border-box includes padding, so remove it if we want content
if ( extra === "content" ) {
val -= jQuery.css( elem, "padding" + cssExpand[ i ], true, styles );
}
// at this point, extra isn't border nor margin, so remove border
if ( extra !== "margin" ) {
val -= jQuery.css( elem, "border" + cssExpand[ i ] + "Width", true, styles );
}
} else {
// at this point, extra isn't content, so add padding
val += jQuery.css( elem, "padding" + cssExpand[ i ], true, styles );
// at this point, extra isn't content nor padding, so add border
if ( extra !== "padding" ) {
val += jQuery.css( elem, "border" + cssExpand[ i ] + "Width", true, styles );
}
}
}
return val;
}
function getWidthOrHeight( elem, name, extra ) {
// Start with offset property, which is equivalent to the border-box value
var valueIsBorderBox = true,
val = name === "width" ? elem.offsetWidth : elem.offsetHeight,
styles = getStyles( elem ),
isBorderBox = jQuery.support.boxSizing && jQuery.css( elem, "boxSizing", false, styles ) === "border-box";
// some non-html elements return undefined for offsetWidth, so check for null/undefined
// svg - https://bugzilla.mozilla.org/show_bug.cgi?id=649285
// MathML - https://bugzilla.mozilla.org/show_bug.cgi?id=491668
if ( val <= 0 || val == null ) {
// Fall back to computed then uncomputed css if necessary
val = curCSS( elem, name, styles );
if ( val < 0 || val == null ) {
val = elem.style[ name ];
}
// Computed unit is not pixels. Stop here and return.
if ( rnumnonpx.test(val) ) {
return val;
}
// we need the check for style in case a browser which returns unreliable values
// for getComputedStyle silently falls back to the reliable elem.style
valueIsBorderBox = isBorderBox && ( jQuery.support.boxSizingReliable || val === elem.style[ name ] );
// Normalize "", auto, and prepare for extra
val = parseFloat( val ) || 0;
}
// use the active box-sizing model to add/subtract irrelevant styles
return ( val +
augmentWidthOrHeight(
elem,
name,
extra || ( isBorderBox ? "border" : "content" ),
valueIsBorderBox,
styles
)
) + "px";
}
// Try to determine the default display value of an element
function css_defaultDisplay( nodeName ) {
var doc = document,
display = elemdisplay[ nodeName ];
if ( !display ) {
display = actualDisplay( nodeName, doc );
// If the simple way fails, read from inside an iframe
if ( display === "none" || !display ) {
// Use the already-created iframe if possible
iframe = ( iframe ||
jQuery("<iframe frameborder='0' width='0' height='0'/>")
.css( "cssText", "display:block !important" )
).appendTo( doc.documentElement );
// Always write a new HTML skeleton so Webkit and Firefox don't choke on reuse
doc = ( iframe[0].contentWindow || iframe[0].contentDocument ).document;
doc.write("<!doctype html><html><body>");
doc.close();
display = actualDisplay( nodeName, doc );
iframe.detach();
}
// Store the correct default display
elemdisplay[ nodeName ] = display;
}
return display;
}
// Called ONLY from within css_defaultDisplay
function actualDisplay( name, doc ) {
var elem = jQuery( doc.createElement( name ) ).appendTo( doc.body ),
display = jQuery.css( elem[0], "display" );
elem.remove();
return display;
}
jQuery.each([ "height", "width" ], function( i, name ) {
jQuery.cssHooks[ name ] = {
get: function( elem, computed, extra ) {
if ( computed ) {
// certain elements can have dimension info if we invisibly show them
// however, it must have a current display style that would benefit from this
return elem.offsetWidth === 0 && rdisplayswap.test( jQuery.css( elem, "display" ) ) ?
jQuery.swap( elem, cssShow, function() {
return getWidthOrHeight( elem, name, extra );
}) :
getWidthOrHeight( elem, name, extra );
}
},
set: function( elem, value, extra ) {
var styles = extra && getStyles( elem );
return setPositiveNumber( elem, value, extra ?
augmentWidthOrHeight(
elem,
name,
extra,
jQuery.support.boxSizing && jQuery.css( elem, "boxSizing", false, styles ) === "border-box",
styles
) : 0
);
}
};
});
if ( !jQuery.support.opacity ) {
jQuery.cssHooks.opacity = {
get: function( elem, computed ) {
// IE uses filters for opacity
return ropacity.test( (computed && elem.currentStyle ? elem.currentStyle.filter : elem.style.filter) || "" ) ?
( 0.01 * parseFloat( RegExp.$1 ) ) + "" :
computed ? "1" : "";
},
set: function( elem, value ) {
var style = elem.style,
currentStyle = elem.currentStyle,
opacity = jQuery.isNumeric( value ) ? "alpha(opacity=" + value * 100 + ")" : "",
filter = currentStyle && currentStyle.filter || style.filter || "";
// IE has trouble with opacity if it does not have layout
// Force it by setting the zoom level
style.zoom = 1;
// if setting opacity to 1, and no other filters exist - attempt to remove filter attribute #6652
// if value === "", then remove inline opacity #12685
if ( ( value >= 1 || value === "" ) &&
jQuery.trim( filter.replace( ralpha, "" ) ) === "" &&
style.removeAttribute ) {
// Setting style.filter to null, "" & " " still leave "filter:" in the cssText
// if "filter:" is present at all, clearType is disabled, we want to avoid this
// style.removeAttribute is IE Only, but so apparently is this code path...
style.removeAttribute( "filter" );
// if there is no filter style applied in a css rule or unset inline opacity, we are done
if ( value === "" || currentStyle && !currentStyle.filter ) {
return;
}
}
// otherwise, set new filter values
style.filter = ralpha.test( filter ) ?
filter.replace( ralpha, opacity ) :
filter + " " + opacity;
}
};
}
// These hooks cannot be added until DOM ready because the support test
// for it is not run until after DOM ready
jQuery(function() {
if ( !jQuery.support.reliableMarginRight ) {
jQuery.cssHooks.marginRight = {
get: function( elem, computed ) {
if ( computed ) {
// WebKit Bug 13343 - getComputedStyle returns wrong value for margin-right
// Work around by temporarily setting element display to inline-block
return jQuery.swap( elem, { "display": "inline-block" },
curCSS, [ elem, "marginRight" ] );
}
}
};
}
// Webkit bug: https://bugs.webkit.org/show_bug.cgi?id=29084
// getComputedStyle returns percent when specified for top/left/bottom/right
// rather than make the css module depend on the offset module, we just check for it here
if ( !jQuery.support.pixelPosition && jQuery.fn.position ) {
jQuery.each( [ "top", "left" ], function( i, prop ) {
jQuery.cssHooks[ prop ] = {
get: function( elem, computed ) {
if ( computed ) {
computed = curCSS( elem, prop );
// if curCSS returns percentage, fallback to offset
return rnumnonpx.test( computed ) ?
jQuery( elem ).position()[ prop ] + "px" :
computed;
}
}
};
});
}
});
if ( jQuery.expr && jQuery.expr.filters ) {
jQuery.expr.filters.hidden = function( elem ) {
// Support: Opera <= 12.12
// Opera reports offsetWidths and offsetHeights less than zero on some elements
return elem.offsetWidth <= 0 && elem.offsetHeight <= 0 ||
(!jQuery.support.reliableHiddenOffsets && ((elem.style && elem.style.display) || jQuery.css( elem, "display" )) === "none");
};
jQuery.expr.filters.visible = function( elem ) {
return !jQuery.expr.filters.hidden( elem );
};
}
// These hooks are used by animate to expand properties
jQuery.each({
margin: "",
padding: "",
border: "Width"
}, function( prefix, suffix ) {
jQuery.cssHooks[ prefix + suffix ] = {
expand: function( value ) {
var i = 0,
expanded = {},
// assumes a single number if not a string
parts = typeof value === "string" ? value.split(" ") : [ value ];
for ( ; i < 4; i++ ) {
expanded[ prefix + cssExpand[ i ] + suffix ] =
parts[ i ] || parts[ i - 2 ] || parts[ 0 ];
}
return expanded;
}
};
if ( !rmargin.test( prefix ) ) {
jQuery.cssHooks[ prefix + suffix ].set = setPositiveNumber;
}
});
var r20 = /%20/g,
rbracket = /\[\]$/,
rCRLF = /\r?\n/g,
rsubmitterTypes = /^(?:submit|button|image|reset|file)$/i,
rsubmittable = /^(?:input|select|textarea|keygen)/i;
jQuery.fn.extend({
serialize: function() {
return jQuery.param( this.serializeArray() );
},
serializeArray: function() {
return this.map(function(){
// Can add propHook for "elements" to filter or add form elements
var elements = jQuery.prop( this, "elements" );
return elements ? jQuery.makeArray( elements ) : this;
})
.filter(function(){
var type = this.type;
// Use .is(":disabled") so that fieldset[disabled] works
return this.name && !jQuery( this ).is( ":disabled" ) &&
rsubmittable.test( this.nodeName ) && !rsubmitterTypes.test( type ) &&
( this.checked || !manipulation_rcheckableType.test( type ) );
})
.map(function( i, elem ){
var val = jQuery( this ).val();
return val == null ?
null :
jQuery.isArray( val ) ?
jQuery.map( val, function( val ){
return { name: elem.name, value: val.replace( rCRLF, "\r\n" ) };
}) :
{ name: elem.name, value: val.replace( rCRLF, "\r\n" ) };
}).get();
}
});
//Serialize an array of form elements or a set of
//key/values into a query string
jQuery.param = function( a, traditional ) {
var prefix,
s = [],
add = function( key, value ) {
// If value is a function, invoke it and return its value
value = jQuery.isFunction( value ) ? value() : ( value == null ? "" : value );
s[ s.length ] = encodeURIComponent( key ) + "=" + encodeURIComponent( value );
};
// Set traditional to true for jQuery <= 1.3.2 behavior.
if ( traditional === undefined ) {
traditional = jQuery.ajaxSettings && jQuery.ajaxSettings.traditional;
}
// If an array was passed in, assume that it is an array of form elements.
if ( jQuery.isArray( a ) || ( a.jquery && !jQuery.isPlainObject( a ) ) ) {
// Serialize the form elements
jQuery.each( a, function() {
add( this.name, this.value );
});
} else {
// If traditional, encode the "old" way (the way 1.3.2 or older
// did it), otherwise encode params recursively.
for ( prefix in a ) {
buildParams( prefix, a[ prefix ], traditional, add );
}
}
// Return the resulting serialization
return s.join( "&" ).replace( r20, "+" );
};
function buildParams( prefix, obj, traditional, add ) {
var name;
if ( jQuery.isArray( obj ) ) {
// Serialize array item.
jQuery.each( obj, function( i, v ) {
if ( traditional || rbracket.test( prefix ) ) {
// Treat each array item as a scalar.
add( prefix, v );
} else {
// Item is non-scalar (array or object), encode its numeric index.
buildParams( prefix + "[" + ( typeof v === "object" ? i : "" ) + "]", v, traditional, add );
}
});
} else if ( !traditional && jQuery.type( obj ) === "object" ) {
// Serialize object item.
for ( name in obj ) {
buildParams( prefix + "[" + name + "]", obj[ name ], traditional, add );
}
} else {
// Serialize scalar item.
add( prefix, obj );
}
}
jQuery.each( ("blur focus focusin focusout load resize scroll unload click dblclick " +
"mousedown mouseup mousemove mouseover mouseout mouseenter mouseleave " +
"change select submit keydown keypress keyup error contextmenu").split(" "), function( i, name ) {
// Handle event binding
jQuery.fn[ name ] = function( data, fn ) {
return arguments.length > 0 ?
this.on( name, null, data, fn ) :
this.trigger( name );
};
});
jQuery.fn.hover = function( fnOver, fnOut ) {
return this.mouseenter( fnOver ).mouseleave( fnOut || fnOver );
};
var
// Document location
ajaxLocParts,
ajaxLocation,
ajax_nonce = jQuery.now(),
ajax_rquery = /\?/,
rhash = /#.*$/,
rts = /([?&])_=[^&]*/,
rheaders = /^(.*?):[ \t]*([^\r\n]*)\r?$/mg, // IE leaves an \r character at EOL
// #7653, #8125, #8152: local protocol detection
rlocalProtocol = /^(?:about|app|app-storage|.+-extension|file|res|widget):$/,
rnoContent = /^(?:GET|HEAD)$/,
rprotocol = /^\/\//,
rurl = /^([\w.+-]+:)(?:\/\/([^\/?#:]*)(?::(\d+)|)|)/,
// Keep a copy of the old load method
_load = jQuery.fn.load,
/* Prefilters
* 1) They are useful to introduce custom dataTypes (see ajax/jsonp.js for an example)
* 2) These are called:
* - BEFORE asking for a transport
* - AFTER param serialization (s.data is a string if s.processData is true)
* 3) key is the dataType
* 4) the catchall symbol "*" can be used
* 5) execution will start with transport dataType and THEN continue down to "*" if needed
*/
prefilters = {},
/* Transports bindings
* 1) key is the dataType
* 2) the catchall symbol "*" can be used
* 3) selection will start with transport dataType and THEN go to "*" if needed
*/
transports = {},
// Avoid comment-prolog char sequence (#10098); must appease lint and evade compression
allTypes = "*/".concat("*");
// #8138, IE may throw an exception when accessing
// a field from window.location if document.domain has been set
try {
ajaxLocation = location.href;
} catch( e ) {
// Use the href attribute of an A element
// since IE will modify it given document.location
ajaxLocation = document.createElement( "a" );
ajaxLocation.href = "";
ajaxLocation = ajaxLocation.href;
}
// Segment location into parts
ajaxLocParts = rurl.exec( ajaxLocation.toLowerCase() ) || [];
// Base "constructor" for jQuery.ajaxPrefilter and jQuery.ajaxTransport
function addToPrefiltersOrTransports( structure ) {
// dataTypeExpression is optional and defaults to "*"
return function( dataTypeExpression, func ) {
if ( typeof dataTypeExpression !== "string" ) {
func = dataTypeExpression;
dataTypeExpression = "*";
}
var dataType,
i = 0,
dataTypes = dataTypeExpression.toLowerCase().match( core_rnotwhite ) || [];
if ( jQuery.isFunction( func ) ) {
// For each dataType in the dataTypeExpression
while ( (dataType = dataTypes[i++]) ) {
// Prepend if requested
if ( dataType[0] === "+" ) {
dataType = dataType.slice( 1 ) || "*";
(structure[ dataType ] = structure[ dataType ] || []).unshift( func );
// Otherwise append
} else {
(structure[ dataType ] = structure[ dataType ] || []).push( func );
}
}
}
};
}
// Base inspection function for prefilters and transports
function inspectPrefiltersOrTransports( structure, options, originalOptions, jqXHR ) {
var inspected = {},
seekingTransport = ( structure === transports );
function inspect( dataType ) {
var selected;
inspected[ dataType ] = true;
jQuery.each( structure[ dataType ] || [], function( _, prefilterOrFactory ) {
var dataTypeOrTransport = prefilterOrFactory( options, originalOptions, jqXHR );
if( typeof dataTypeOrTransport === "string" && !seekingTransport && !inspected[ dataTypeOrTransport ] ) {
options.dataTypes.unshift( dataTypeOrTransport );
inspect( dataTypeOrTransport );
return false;
} else if ( seekingTransport ) {
return !( selected = dataTypeOrTransport );
}
});
return selected;
}
return inspect( options.dataTypes[ 0 ] ) || !inspected[ "*" ] && inspect( "*" );
}
// A special extend for ajax options
// that takes "flat" options (not to be deep extended)
// Fixes #9887
function ajaxExtend( target, src ) {
var deep, key,
flatOptions = jQuery.ajaxSettings.flatOptions || {};
for ( key in src ) {
if ( src[ key ] !== undefined ) {
( flatOptions[ key ] ? target : ( deep || (deep = {}) ) )[ key ] = src[ key ];
}
}
if ( deep ) {
jQuery.extend( true, target, deep );
}
return target;
}
jQuery.fn.load = function( url, params, callback ) {
if ( typeof url !== "string" && _load ) {
return _load.apply( this, arguments );
}
var selector, response, type,
self = this,
off = url.indexOf(" ");
if ( off >= 0 ) {
selector = url.slice( off, url.length );
url = url.slice( 0, off );
}
// If it's a function
if ( jQuery.isFunction( params ) ) {
// We assume that it's the callback
callback = params;
params = undefined;
// Otherwise, build a param string
} else if ( params && typeof params === "object" ) {
type = "POST";
}
// If we have elements to modify, make the request
if ( self.length > 0 ) {
jQuery.ajax({
url: url,
// if "type" variable is undefined, then "GET" method will be used
type: type,
dataType: "html",
data: params
}).done(function( responseText ) {
// Save response for use in complete callback
response = arguments;
self.html( selector ?
// If a selector was specified, locate the right elements in a dummy div
// Exclude scripts to avoid IE 'Permission Denied' errors
jQuery("<div>").append( jQuery.parseHTML( responseText ) ).find( selector ) :
// Otherwise use the full result
responseText );
}).complete( callback && function( jqXHR, status ) {
self.each( callback, response || [ jqXHR.responseText, status, jqXHR ] );
});
}
return this;
};
// Attach a bunch of functions for handling common AJAX events
jQuery.each( [ "ajaxStart", "ajaxStop", "ajaxComplete", "ajaxError", "ajaxSuccess", "ajaxSend" ], function( i, type ){
jQuery.fn[ type ] = function( fn ){
return this.on( type, fn );
};
});
jQuery.each( [ "get", "post" ], function( i, method ) {
jQuery[ method ] = function( url, data, callback, type ) {
// shift arguments if data argument was omitted
if ( jQuery.isFunction( data ) ) {
type = type || callback;
callback = data;
data = undefined;
}
return jQuery.ajax({
url: url,
type: method,
dataType: type,
data: data,
success: callback
});
};
});
jQuery.extend({
// Counter for holding the number of active queries
active: 0,
// Last-Modified header cache for next request
lastModified: {},
etag: {},
ajaxSettings: {
url: ajaxLocation,
type: "GET",
isLocal: rlocalProtocol.test( ajaxLocParts[ 1 ] ),
global: true,
processData: true,
async: true,
contentType: "application/x-www-form-urlencoded; charset=UTF-8",
/*
timeout: 0,
data: null,
dataType: null,
username: null,
password: null,
cache: null,
throws: false,
traditional: false,
headers: {},
*/
accepts: {
"*": allTypes,
text: "text/plain",
html: "text/html",
xml: "application/xml, text/xml",
json: "application/json, text/javascript"
},
contents: {
xml: /xml/,
html: /html/,
json: /json/
},
responseFields: {
xml: "responseXML",
text: "responseText"
},
// Data converters
// Keys separate source (or catchall "*") and destination types with a single space
converters: {
// Convert anything to text
"* text": window.String,
// Text to html (true = no transformation)
"text html": true,
// Evaluate text as a json expression
"text json": jQuery.parseJSON,
// Parse text as xml
"text xml": jQuery.parseXML
},
// For options that shouldn't be deep extended:
// you can add your own custom options here if
// and when you create one that shouldn't be
// deep extended (see ajaxExtend)
flatOptions: {
url: true,
context: true
}
},
// Creates a full fledged settings object into target
// with both ajaxSettings and settings fields.
// If target is omitted, writes into ajaxSettings.
ajaxSetup: function( target, settings ) {
return settings ?
// Building a settings object
ajaxExtend( ajaxExtend( target, jQuery.ajaxSettings ), settings ) :
// Extending ajaxSettings
ajaxExtend( jQuery.ajaxSettings, target );
},
ajaxPrefilter: addToPrefiltersOrTransports( prefilters ),
ajaxTransport: addToPrefiltersOrTransports( transports ),
// Main method
ajax: function( url, options ) {
// If url is an object, simulate pre-1.5 signature
if ( typeof url === "object" ) {
options = url;
url = undefined;
}
// Force options to be an object
options = options || {};
var // Cross-domain detection vars
parts,
// Loop variable
i,
// URL without anti-cache param
cacheURL,
// Response headers as string
responseHeadersString,
// timeout handle
timeoutTimer,
// To know if global events are to be dispatched
fireGlobals,
transport,
// Response headers
responseHeaders,
// Create the final options object
s = jQuery.ajaxSetup( {}, options ),
// Callbacks context
callbackContext = s.context || s,
// Context for global events is callbackContext if it is a DOM node or jQuery collection
globalEventContext = s.context && ( callbackContext.nodeType || callbackContext.jquery ) ?
jQuery( callbackContext ) :
jQuery.event,
// Deferreds
deferred = jQuery.Deferred(),
completeDeferred = jQuery.Callbacks("once memory"),
// Status-dependent callbacks
statusCode = s.statusCode || {},
// Headers (they are sent all at once)
requestHeaders = {},
requestHeadersNames = {},
// The jqXHR state
state = 0,
// Default abort message
strAbort = "canceled",
// Fake xhr
jqXHR = {
readyState: 0,
// Builds headers hashtable if needed
getResponseHeader: function( key ) {
var match;
if ( state === 2 ) {
if ( !responseHeaders ) {
responseHeaders = {};
while ( (match = rheaders.exec( responseHeadersString )) ) {
responseHeaders[ match[1].toLowerCase() ] = match[ 2 ];
}
}
match = responseHeaders[ key.toLowerCase() ];
}
return match == null ? null : match;
},
// Raw string
getAllResponseHeaders: function() {
return state === 2 ? responseHeadersString : null;
},
// Caches the header
setRequestHeader: function( name, value ) {
var lname = name.toLowerCase();
if ( !state ) {
name = requestHeadersNames[ lname ] = requestHeadersNames[ lname ] || name;
requestHeaders[ name ] = value;
}
return this;
},
// Overrides response content-type header
overrideMimeType: function( type ) {
if ( !state ) {
s.mimeType = type;
}
return this;
},
// Status-dependent callbacks
statusCode: function( map ) {
var code;
if ( map ) {
if ( state < 2 ) {
for ( code in map ) {
// Lazy-add the new callback in a way that preserves old ones
statusCode[ code ] = [ statusCode[ code ], map[ code ] ];
}
} else {
// Execute the appropriate callbacks
jqXHR.always( map[ jqXHR.status ] );
}
}
return this;
},
// Cancel the request
abort: function( statusText ) {
var finalText = statusText || strAbort;
if ( transport ) {
transport.abort( finalText );
}
done( 0, finalText );
return this;
}
};
// Attach deferreds
deferred.promise( jqXHR ).complete = completeDeferred.add;
jqXHR.success = jqXHR.done;
jqXHR.error = jqXHR.fail;
// Remove hash character (#7531: and string promotion)
// Add protocol if not provided (#5866: IE7 issue with protocol-less urls)
// Handle falsy url in the settings object (#10093: consistency with old signature)
// We also use the url parameter if available
s.url = ( ( url || s.url || ajaxLocation ) + "" ).replace( rhash, "" ).replace( rprotocol, ajaxLocParts[ 1 ] + "//" );
// Alias method option to type as per ticket #12004
s.type = options.method || options.type || s.method || s.type;
// Extract dataTypes list
s.dataTypes = jQuery.trim( s.dataType || "*" ).toLowerCase().match( core_rnotwhite ) || [""];
// A cross-domain request is in order when we have a protocol:host:port mismatch
if ( s.crossDomain == null ) {
parts = rurl.exec( s.url.toLowerCase() );
s.crossDomain = !!( parts &&
( parts[ 1 ] !== ajaxLocParts[ 1 ] || parts[ 2 ] !== ajaxLocParts[ 2 ] ||
( parts[ 3 ] || ( parts[ 1 ] === "http:" ? 80 : 443 ) ) !=
( ajaxLocParts[ 3 ] || ( ajaxLocParts[ 1 ] === "http:" ? 80 : 443 ) ) )
);
}
// Convert data if not already a string
if ( s.data && s.processData && typeof s.data !== "string" ) {
s.data = jQuery.param( s.data, s.traditional );
}
// Apply prefilters
inspectPrefiltersOrTransports( prefilters, s, options, jqXHR );
// If request was aborted inside a prefilter, stop there
if ( state === 2 ) {
return jqXHR;
}
// We can fire global events as of now if asked to
fireGlobals = s.global;
// Watch for a new set of requests
if ( fireGlobals && jQuery.active++ === 0 ) {
jQuery.event.trigger("ajaxStart");
}
// Uppercase the type
s.type = s.type.toUpperCase();
// Determine if request has content
s.hasContent = !rnoContent.test( s.type );
// Save the URL in case we're toying with the If-Modified-Since
// and/or If-None-Match header later on
cacheURL = s.url;
// More options handling for requests with no content
if ( !s.hasContent ) {
// If data is available, append data to url
if ( s.data ) {
cacheURL = ( s.url += ( ajax_rquery.test( cacheURL ) ? "&" : "?" ) + s.data );
// #9682: remove data so that it's not used in an eventual retry
delete s.data;
}
// Add anti-cache in url if needed
if ( s.cache === false ) {
s.url = rts.test( cacheURL ) ?
// If there is already a '_' parameter, set its value
cacheURL.replace( rts, "$1_=" + ajax_nonce++ ) :
// Otherwise add one to the end
cacheURL + ( ajax_rquery.test( cacheURL ) ? "&" : "?" ) + "_=" + ajax_nonce++;
}
}
// Set the If-Modified-Since and/or If-None-Match header, if in ifModified mode.
if ( s.ifModified ) {
if ( jQuery.lastModified[ cacheURL ] ) {
jqXHR.setRequestHeader( "If-Modified-Since", jQuery.lastModified[ cacheURL ] );
}
if ( jQuery.etag[ cacheURL ] ) {
jqXHR.setRequestHeader( "If-None-Match", jQuery.etag[ cacheURL ] );
}
}
// Set the correct header, if data is being sent
if ( s.data && s.hasContent && s.contentType !== false || options.contentType ) {
jqXHR.setRequestHeader( "Content-Type", s.contentType );
}
// Set the Accepts header for the server, depending on the dataType
jqXHR.setRequestHeader(
"Accept",
s.dataTypes[ 0 ] && s.accepts[ s.dataTypes[0] ] ?
s.accepts[ s.dataTypes[0] ] + ( s.dataTypes[ 0 ] !== "*" ? ", " + allTypes + "; q=0.01" : "" ) :
s.accepts[ "*" ]
);
// Check for headers option
for ( i in s.headers ) {
jqXHR.setRequestHeader( i, s.headers[ i ] );
}
// Allow custom headers/mimetypes and early abort
if ( s.beforeSend && ( s.beforeSend.call( callbackContext, jqXHR, s ) === false || state === 2 ) ) {
// Abort if not done already and return
return jqXHR.abort();
}
// aborting is no longer a cancellation
strAbort = "abort";
// Install callbacks on deferreds
for ( i in { success: 1, error: 1, complete: 1 } ) {
jqXHR[ i ]( s[ i ] );
}
// Get transport
transport = inspectPrefiltersOrTransports( transports, s, options, jqXHR );
// If no transport, we auto-abort
if ( !transport ) {
done( -1, "No Transport" );
} else {
jqXHR.readyState = 1;
// Send global event
if ( fireGlobals ) {
globalEventContext.trigger( "ajaxSend", [ jqXHR, s ] );
}
// Timeout
if ( s.async && s.timeout > 0 ) {
timeoutTimer = setTimeout(function() {
jqXHR.abort("timeout");
}, s.timeout );
}
try {
state = 1;
transport.send( requestHeaders, done );
} catch ( e ) {
// Propagate exception as error if not done
if ( state < 2 ) {
done( -1, e );
// Simply rethrow otherwise
} else {
throw e;
}
}
}
// Callback for when everything is done
function done( status, nativeStatusText, responses, headers ) {
var isSuccess, success, error, response, modified,
statusText = nativeStatusText;
// Called once
if ( state === 2 ) {
return;
}
// State is "done" now
state = 2;
// Clear timeout if it exists
if ( timeoutTimer ) {
clearTimeout( timeoutTimer );
}
// Dereference transport for early garbage collection
// (no matter how long the jqXHR object will be used)
transport = undefined;
// Cache response headers
responseHeadersString = headers || "";
// Set readyState
jqXHR.readyState = status > 0 ? 4 : 0;
// Get response data
if ( responses ) {
response = ajaxHandleResponses( s, jqXHR, responses );
}
// If successful, handle type chaining
if ( status >= 200 && status < 300 || status === 304 ) {
// Set the If-Modified-Since and/or If-None-Match header, if in ifModified mode.
if ( s.ifModified ) {
modified = jqXHR.getResponseHeader("Last-Modified");
if ( modified ) {
jQuery.lastModified[ cacheURL ] = modified;
}
modified = jqXHR.getResponseHeader("etag");
if ( modified ) {
jQuery.etag[ cacheURL ] = modified;
}
}
// if no content
if ( status === 204 ) {
isSuccess = true;
statusText = "nocontent";
// if not modified
} else if ( status === 304 ) {
isSuccess = true;
statusText = "notmodified";
// If we have data, let's convert it
} else {
isSuccess = ajaxConvert( s, response );
statusText = isSuccess.state;
success = isSuccess.data;
error = isSuccess.error;
isSuccess = !error;
}
} else {
// We extract error from statusText
// then normalize statusText and status for non-aborts
error = statusText;
if ( status || !statusText ) {
statusText = "error";
if ( status < 0 ) {
status = 0;
}
}
}
// Set data for the fake xhr object
jqXHR.status = status;
jqXHR.statusText = ( nativeStatusText || statusText ) + "";
// Success/Error
if ( isSuccess ) {
deferred.resolveWith( callbackContext, [ success, statusText, jqXHR ] );
} else {
deferred.rejectWith( callbackContext, [ jqXHR, statusText, error ] );
}
// Status-dependent callbacks
jqXHR.statusCode( statusCode );
statusCode = undefined;
if ( fireGlobals ) {
globalEventContext.trigger( isSuccess ? "ajaxSuccess" : "ajaxError",
[ jqXHR, s, isSuccess ? success : error ] );
}
// Complete
completeDeferred.fireWith( callbackContext, [ jqXHR, statusText ] );
if ( fireGlobals ) {
globalEventContext.trigger( "ajaxComplete", [ jqXHR, s ] );
// Handle the global AJAX counter
if ( !( --jQuery.active ) ) {
jQuery.event.trigger("ajaxStop");
}
}
}
return jqXHR;
},
getScript: function( url, callback ) {
return jQuery.get( url, undefined, callback, "script" );
},
getJSON: function( url, data, callback ) {
return jQuery.get( url, data, callback, "json" );
}
});
/* Handles responses to an ajax request:
* - sets all responseXXX fields accordingly
* - finds the right dataType (mediates between content-type and expected dataType)
* - returns the corresponding response
*/
function ajaxHandleResponses( s, jqXHR, responses ) {
var firstDataType, ct, finalDataType, type,
contents = s.contents,
dataTypes = s.dataTypes,
responseFields = s.responseFields;
// Fill responseXXX fields
for ( type in responseFields ) {
if ( type in responses ) {
jqXHR[ responseFields[type] ] = responses[ type ];
}
}
// Remove auto dataType and get content-type in the process
while( dataTypes[ 0 ] === "*" ) {
dataTypes.shift();
if ( ct === undefined ) {
ct = s.mimeType || jqXHR.getResponseHeader("Content-Type");
}
}
// Check if we're dealing with a known content-type
if ( ct ) {
for ( type in contents ) {
if ( contents[ type ] && contents[ type ].test( ct ) ) {
dataTypes.unshift( type );
break;
}
}
}
// Check to see if we have a response for the expected dataType
if ( dataTypes[ 0 ] in responses ) {
finalDataType = dataTypes[ 0 ];
} else {
// Try convertible dataTypes
for ( type in responses ) {
if ( !dataTypes[ 0 ] || s.converters[ type + " " + dataTypes[0] ] ) {
finalDataType = type;
break;
}
if ( !firstDataType ) {
firstDataType = type;
}
}
// Or just use first one
finalDataType = finalDataType || firstDataType;
}
// If we found a dataType
// We add the dataType to the list if needed
// and return the corresponding response
if ( finalDataType ) {
if ( finalDataType !== dataTypes[ 0 ] ) {
dataTypes.unshift( finalDataType );
}
return responses[ finalDataType ];
}
}
// Chain conversions given the request and the original response
function ajaxConvert( s, response ) {
var conv2, current, conv, tmp,
converters = {},
i = 0,
// Work with a copy of dataTypes in case we need to modify it for conversion
dataTypes = s.dataTypes.slice(),
prev = dataTypes[ 0 ];
// Apply the dataFilter if provided
if ( s.dataFilter ) {
response = s.dataFilter( response, s.dataType );
}
// Create converters map with lowercased keys
if ( dataTypes[ 1 ] ) {
for ( conv in s.converters ) {
converters[ conv.toLowerCase() ] = s.converters[ conv ];
}
}
// Convert to each sequential dataType, tolerating list modification
for ( ; (current = dataTypes[++i]); ) {
// There's only work to do if current dataType is non-auto
if ( current !== "*" ) {
// Convert response if prev dataType is non-auto and differs from current
if ( prev !== "*" && prev !== current ) {
// Seek a direct converter
conv = converters[ prev + " " + current ] || converters[ "* " + current ];
// If none found, seek a pair
if ( !conv ) {
for ( conv2 in converters ) {
// If conv2 outputs current
tmp = conv2.split(" ");
if ( tmp[ 1 ] === current ) {
// If prev can be converted to accepted input
conv = converters[ prev + " " + tmp[ 0 ] ] ||
converters[ "* " + tmp[ 0 ] ];
if ( conv ) {
// Condense equivalence converters
if ( conv === true ) {
conv = converters[ conv2 ];
// Otherwise, insert the intermediate dataType
} else if ( converters[ conv2 ] !== true ) {
current = tmp[ 0 ];
dataTypes.splice( i--, 0, current );
}
break;
}
}
}
}
// Apply converter (if not an equivalence)
if ( conv !== true ) {
// Unless errors are allowed to bubble, catch and return them
if ( conv && s["throws"] ) {
response = conv( response );
} else {
try {
response = conv( response );
} catch ( e ) {
return { state: "parsererror", error: conv ? e : "No conversion from " + prev + " to " + current };
}
}
}
}
// Update prev for next iteration
prev = current;
}
}
return { state: "success", data: response };
}
// Install script dataType
jQuery.ajaxSetup({
accepts: {
script: "text/javascript, application/javascript, application/ecmascript, application/x-ecmascript"
},
contents: {
script: /(?:java|ecma)script/
},
converters: {
"text script": function( text ) {
jQuery.globalEval( text );
return text;
}
}
});
// Handle cache's special case and global
jQuery.ajaxPrefilter( "script", function( s ) {
if ( s.cache === undefined ) {
s.cache = false;
}
if ( s.crossDomain ) {
s.type = "GET";
s.global = false;
}
});
// Bind script tag hack transport
jQuery.ajaxTransport( "script", function(s) {
// This transport only deals with cross domain requests
if ( s.crossDomain ) {
var script,
head = document.head || jQuery("head")[0] || document.documentElement;
return {
send: function( _, callback ) {
script = document.createElement("script");
script.async = true;
if ( s.scriptCharset ) {
script.charset = s.scriptCharset;
}
script.src = s.url;
// Attach handlers for all browsers
script.onload = script.onreadystatechange = function( _, isAbort ) {
if ( isAbort || !script.readyState || /loaded|complete/.test( script.readyState ) ) {
// Handle memory leak in IE
script.onload = script.onreadystatechange = null;
// Remove the script
if ( script.parentNode ) {
script.parentNode.removeChild( script );
}
// Dereference the script
script = null;
// Callback if not abort
if ( !isAbort ) {
callback( 200, "success" );
}
}
};
// Circumvent IE6 bugs with base elements (#2709 and #4378) by prepending
// Use native DOM manipulation to avoid our domManip AJAX trickery
head.insertBefore( script, head.firstChild );
},
abort: function() {
if ( script ) {
script.onload( undefined, true );
}
}
};
}
});
var oldCallbacks = [],
rjsonp = /(=)\?(?=&|$)|\?\?/;
// Default jsonp settings
jQuery.ajaxSetup({
jsonp: "callback",
jsonpCallback: function() {
var callback = oldCallbacks.pop() || ( jQuery.expando + "_" + ( ajax_nonce++ ) );
this[ callback ] = true;
return callback;
}
});
// Detect, normalize options and install callbacks for jsonp requests
jQuery.ajaxPrefilter( "json jsonp", function( s, originalSettings, jqXHR ) {
var callbackName, overwritten, responseContainer,
jsonProp = s.jsonp !== false && ( rjsonp.test( s.url ) ?
"url" :
typeof s.data === "string" && !( s.contentType || "" ).indexOf("application/x-www-form-urlencoded") && rjsonp.test( s.data ) && "data"
);
// Handle iff the expected data type is "jsonp" or we have a parameter to set
if ( jsonProp || s.dataTypes[ 0 ] === "jsonp" ) {
// Get callback name, remembering preexisting value associated with it
callbackName = s.jsonpCallback = jQuery.isFunction( s.jsonpCallback ) ?
s.jsonpCallback() :
s.jsonpCallback;
// Insert callback into url or form data
if ( jsonProp ) {
s[ jsonProp ] = s[ jsonProp ].replace( rjsonp, "$1" + callbackName );
} else if ( s.jsonp !== false ) {
s.url += ( ajax_rquery.test( s.url ) ? "&" : "?" ) + s.jsonp + "=" + callbackName;
}
// Use data converter to retrieve json after script execution
s.converters["script json"] = function() {
if ( !responseContainer ) {
jQuery.error( callbackName + " was not called" );
}
return responseContainer[ 0 ];
};
// force json dataType
s.dataTypes[ 0 ] = "json";
// Install callback
overwritten = window[ callbackName ];
window[ callbackName ] = function() {
responseContainer = arguments;
};
// Clean-up function (fires after converters)
jqXHR.always(function() {
// Restore preexisting value
window[ callbackName ] = overwritten;
// Save back as free
if ( s[ callbackName ] ) {
// make sure that re-using the options doesn't screw things around
s.jsonpCallback = originalSettings.jsonpCallback;
// save the callback name for future use
oldCallbacks.push( callbackName );
}
// Call if it was a function and we have a response
if ( responseContainer && jQuery.isFunction( overwritten ) ) {
overwritten( responseContainer[ 0 ] );
}
responseContainer = overwritten = undefined;
});
// Delegate to script
return "script";
}
});
var xhrCallbacks, xhrSupported,
xhrId = 0,
// #5280: Internet Explorer will keep connections alive if we don't abort on unload
xhrOnUnloadAbort = window.ActiveXObject && function() {
// Abort all pending requests
var key;
for ( key in xhrCallbacks ) {
xhrCallbacks[ key ]( undefined, true );
}
};
// Functions to create xhrs
function createStandardXHR() {
try {
return new window.XMLHttpRequest();
} catch( e ) {}
}
function createActiveXHR() {
try {
return new window.ActiveXObject("Microsoft.XMLHTTP");
} catch( e ) {}
}
// Create the request object
// (This is still attached to ajaxSettings for backward compatibility)
jQuery.ajaxSettings.xhr = window.ActiveXObject ?
/* Microsoft failed to properly
* implement the XMLHttpRequest in IE7 (can't request local files),
* so we use the ActiveXObject when it is available
* Additionally XMLHttpRequest can be disabled in IE7/IE8 so
* we need a fallback.
*/
function() {
return !this.isLocal && createStandardXHR() || createActiveXHR();
} :
// For all other browsers, use the standard XMLHttpRequest object
createStandardXHR;
// Determine support properties
xhrSupported = jQuery.ajaxSettings.xhr();
jQuery.support.cors = !!xhrSupported && ( "withCredentials" in xhrSupported );
xhrSupported = jQuery.support.ajax = !!xhrSupported;
// Create transport if the browser can provide an xhr
if ( xhrSupported ) {
jQuery.ajaxTransport(function( s ) {
// Cross domain only allowed if supported through XMLHttpRequest
if ( !s.crossDomain || jQuery.support.cors ) {
var callback;
return {
send: function( headers, complete ) {
// Get a new xhr
var handle, i,
xhr = s.xhr();
// Open the socket
// Passing null username, generates a login popup on Opera (#2865)
if ( s.username ) {
xhr.open( s.type, s.url, s.async, s.username, s.password );
} else {
xhr.open( s.type, s.url, s.async );
}
// Apply custom fields if provided
if ( s.xhrFields ) {
for ( i in s.xhrFields ) {
xhr[ i ] = s.xhrFields[ i ];
}
}
// Override mime type if needed
if ( s.mimeType && xhr.overrideMimeType ) {
xhr.overrideMimeType( s.mimeType );
}
// X-Requested-With header
// For cross-domain requests, seeing as conditions for a preflight are
// akin to a jigsaw puzzle, we simply never set it to be sure.
// (it can always be set on a per-request basis or even using ajaxSetup)
// For same-domain requests, won't change header if already provided.
if ( !s.crossDomain && !headers["X-Requested-With"] ) {
headers["X-Requested-With"] = "XMLHttpRequest";
}
// Need an extra try/catch for cross domain requests in Firefox 3
try {
for ( i in headers ) {
xhr.setRequestHeader( i, headers[ i ] );
}
} catch( err ) {}
// Do send the request
// This may raise an exception which is actually
// handled in jQuery.ajax (so no try/catch here)
xhr.send( ( s.hasContent && s.data ) || null );
// Listener
callback = function( _, isAbort ) {
var status, responseHeaders, statusText, responses;
// Firefox throws exceptions when accessing properties
// of an xhr when a network error occurred
// http://helpful.knobs-dials.com/index.php/Component_returned_failure_code:_0x80040111_(NS_ERROR_NOT_AVAILABLE)
try {
// Was never called and is aborted or complete
if ( callback && ( isAbort || xhr.readyState === 4 ) ) {
// Only called once
callback = undefined;
// Do not keep as active anymore
if ( handle ) {
xhr.onreadystatechange = jQuery.noop;
if ( xhrOnUnloadAbort ) {
delete xhrCallbacks[ handle ];
}
}
// If it's an abort
if ( isAbort ) {
// Abort it manually if needed
if ( xhr.readyState !== 4 ) {
xhr.abort();
}
} else {
responses = {};
status = xhr.status;
responseHeaders = xhr.getAllResponseHeaders();
// When requesting binary data, IE6-9 will throw an exception
// on any attempt to access responseText (#11426)
if ( typeof xhr.responseText === "string" ) {
responses.text = xhr.responseText;
}
// Firefox throws an exception when accessing
// statusText for faulty cross-domain requests
try {
statusText = xhr.statusText;
} catch( e ) {
// We normalize with Webkit giving an empty statusText
statusText = "";
}
// Filter status for non standard behaviors
// If the request is local and we have data: assume a success
// (success with no data won't get notified, that's the best we
// can do given current implementations)
if ( !status && s.isLocal && !s.crossDomain ) {
status = responses.text ? 200 : 404;
// IE - #1450: sometimes returns 1223 when it should be 204
} else if ( status === 1223 ) {
status = 204;
}
}
}
} catch( firefoxAccessException ) {
if ( !isAbort ) {
complete( -1, firefoxAccessException );
}
}
// Call complete if needed
if ( responses ) {
complete( status, statusText, responses, responseHeaders );
}
};
if ( !s.async ) {
// if we're in sync mode we fire the callback
callback();
} else if ( xhr.readyState === 4 ) {
// (IE6 & IE7) if it's in cache and has been
// retrieved directly we need to fire the callback
setTimeout( callback );
} else {
handle = ++xhrId;
if ( xhrOnUnloadAbort ) {
// Create the active xhrs callbacks list if needed
// and attach the unload handler
if ( !xhrCallbacks ) {
xhrCallbacks = {};
jQuery( window ).unload( xhrOnUnloadAbort );
}
// Add to list of active xhrs callbacks
xhrCallbacks[ handle ] = callback;
}
xhr.onreadystatechange = callback;
}
},
abort: function() {
if ( callback ) {
callback( undefined, true );
}
}
};
}
});
}
var fxNow, timerId,
rfxtypes = /^(?:toggle|show|hide)$/,
rfxnum = new RegExp( "^(?:([+-])=|)(" + core_pnum + ")([a-z%]*)$", "i" ),
rrun = /queueHooks$/,
animationPrefilters = [ defaultPrefilter ],
tweeners = {
"*": [function( prop, value ) {
var end, unit,
tween = this.createTween( prop, value ),
parts = rfxnum.exec( value ),
target = tween.cur(),
start = +target || 0,
scale = 1,
maxIterations = 20;
if ( parts ) {
end = +parts[2];
unit = parts[3] || ( jQuery.cssNumber[ prop ] ? "" : "px" );
// We need to compute starting value
if ( unit !== "px" && start ) {
// Iteratively approximate from a nonzero starting point
// Prefer the current property, because this process will be trivial if it uses the same units
// Fallback to end or a simple constant
start = jQuery.css( tween.elem, prop, true ) || end || 1;
do {
// If previous iteration zeroed out, double until we get *something*
// Use a string for doubling factor so we don't accidentally see scale as unchanged below
scale = scale || ".5";
// Adjust and apply
start = start / scale;
jQuery.style( tween.elem, prop, start + unit );
// Update scale, tolerating zero or NaN from tween.cur()
// And breaking the loop if scale is unchanged or perfect, or if we've just had enough
} while ( scale !== (scale = tween.cur() / target) && scale !== 1 && --maxIterations );
}
tween.unit = unit;
tween.start = start;
// If a +=/-= token was provided, we're doing a relative animation
tween.end = parts[1] ? start + ( parts[1] + 1 ) * end : end;
}
return tween;
}]
};
// Animations created synchronously will run synchronously
function createFxNow() {
setTimeout(function() {
fxNow = undefined;
});
return ( fxNow = jQuery.now() );
}
function createTweens( animation, props ) {
jQuery.each( props, function( prop, value ) {
var collection = ( tweeners[ prop ] || [] ).concat( tweeners[ "*" ] ),
index = 0,
length = collection.length;
for ( ; index < length; index++ ) {
if ( collection[ index ].call( animation, prop, value ) ) {
// we're done with this property
return;
}
}
});
}
function Animation( elem, properties, options ) {
var result,
stopped,
index = 0,
length = animationPrefilters.length,
deferred = jQuery.Deferred().always( function() {
// don't match elem in the :animated selector
delete tick.elem;
}),
tick = function() {
if ( stopped ) {
return false;
}
var currentTime = fxNow || createFxNow(),
remaining = Math.max( 0, animation.startTime + animation.duration - currentTime ),
// archaic crash bug won't allow us to use 1 - ( 0.5 || 0 ) (#12497)
temp = remaining / animation.duration || 0,
percent = 1 - temp,
index = 0,
length = animation.tweens.length;
for ( ; index < length ; index++ ) {
animation.tweens[ index ].run( percent );
}
deferred.notifyWith( elem, [ animation, percent, remaining ]);
if ( percent < 1 && length ) {
return remaining;
} else {
deferred.resolveWith( elem, [ animation ] );
return false;
}
},
animation = deferred.promise({
elem: elem,
props: jQuery.extend( {}, properties ),
opts: jQuery.extend( true, { specialEasing: {} }, options ),
originalProperties: properties,
originalOptions: options,
startTime: fxNow || createFxNow(),
duration: options.duration,
tweens: [],
createTween: function( prop, end ) {
var tween = jQuery.Tween( elem, animation.opts, prop, end,
animation.opts.specialEasing[ prop ] || animation.opts.easing );
animation.tweens.push( tween );
return tween;
},
stop: function( gotoEnd ) {
var index = 0,
// if we are going to the end, we want to run all the tweens
// otherwise we skip this part
length = gotoEnd ? animation.tweens.length : 0;
if ( stopped ) {
return this;
}
stopped = true;
for ( ; index < length ; index++ ) {
animation.tweens[ index ].run( 1 );
}
// resolve when we played the last frame
// otherwise, reject
if ( gotoEnd ) {
deferred.resolveWith( elem, [ animation, gotoEnd ] );
} else {
deferred.rejectWith( elem, [ animation, gotoEnd ] );
}
return this;
}
}),
props = animation.props;
propFilter( props, animation.opts.specialEasing );
for ( ; index < length ; index++ ) {
result = animationPrefilters[ index ].call( animation, elem, props, animation.opts );
if ( result ) {
return result;
}
}
createTweens( animation, props );
if ( jQuery.isFunction( animation.opts.start ) ) {
animation.opts.start.call( elem, animation );
}
jQuery.fx.timer(
jQuery.extend( tick, {
elem: elem,
anim: animation,
queue: animation.opts.queue
})
);
// attach callbacks from options
return animation.progress( animation.opts.progress )
.done( animation.opts.done, animation.opts.complete )
.fail( animation.opts.fail )
.always( animation.opts.always );
}
function propFilter( props, specialEasing ) {
var value, name, index, easing, hooks;
// camelCase, specialEasing and expand cssHook pass
for ( index in props ) {
name = jQuery.camelCase( index );
easing = specialEasing[ name ];
value = props[ index ];
if ( jQuery.isArray( value ) ) {
easing = value[ 1 ];
value = props[ index ] = value[ 0 ];
}
if ( index !== name ) {
props[ name ] = value;
delete props[ index ];
}
hooks = jQuery.cssHooks[ name ];
if ( hooks && "expand" in hooks ) {
value = hooks.expand( value );
delete props[ name ];
// not quite $.extend, this wont overwrite keys already present.
// also - reusing 'index' from above because we have the correct "name"
for ( index in value ) {
if ( !( index in props ) ) {
props[ index ] = value[ index ];
specialEasing[ index ] = easing;
}
}
} else {
specialEasing[ name ] = easing;
}
}
}
jQuery.Animation = jQuery.extend( Animation, {
tweener: function( props, callback ) {
if ( jQuery.isFunction( props ) ) {
callback = props;
props = [ "*" ];
} else {
props = props.split(" ");
}
var prop,
index = 0,
length = props.length;
for ( ; index < length ; index++ ) {
prop = props[ index ];
tweeners[ prop ] = tweeners[ prop ] || [];
tweeners[ prop ].unshift( callback );
}
},
prefilter: function( callback, prepend ) {
if ( prepend ) {
animationPrefilters.unshift( callback );
} else {
animationPrefilters.push( callback );
}
}
});
function defaultPrefilter( elem, props, opts ) {
/*jshint validthis:true */
var prop, index, length,
value, dataShow, toggle,
tween, hooks, oldfire,
anim = this,
style = elem.style,
orig = {},
handled = [],
hidden = elem.nodeType && isHidden( elem );
// handle queue: false promises
if ( !opts.queue ) {
hooks = jQuery._queueHooks( elem, "fx" );
if ( hooks.unqueued == null ) {
hooks.unqueued = 0;
oldfire = hooks.empty.fire;
hooks.empty.fire = function() {
if ( !hooks.unqueued ) {
oldfire();
}
};
}
hooks.unqueued++;
anim.always(function() {
// doing this makes sure that the complete handler will be called
// before this completes
anim.always(function() {
hooks.unqueued--;
if ( !jQuery.queue( elem, "fx" ).length ) {
hooks.empty.fire();
}
});
});
}
// height/width overflow pass
if ( elem.nodeType === 1 && ( "height" in props || "width" in props ) ) {
// Make sure that nothing sneaks out
// Record all 3 overflow attributes because IE does not
// change the overflow attribute when overflowX and
// overflowY are set to the same value
opts.overflow = [ style.overflow, style.overflowX, style.overflowY ];
// Set display property to inline-block for height/width
// animations on inline elements that are having width/height animated
if ( jQuery.css( elem, "display" ) === "inline" &&
jQuery.css( elem, "float" ) === "none" ) {
// inline-level elements accept inline-block;
// block-level elements need to be inline with layout
if ( !jQuery.support.inlineBlockNeedsLayout || css_defaultDisplay( elem.nodeName ) === "inline" ) {
style.display = "inline-block";
} else {
style.zoom = 1;
}
}
}
if ( opts.overflow ) {
style.overflow = "hidden";
if ( !jQuery.support.shrinkWrapBlocks ) {
anim.always(function() {
style.overflow = opts.overflow[ 0 ];
style.overflowX = opts.overflow[ 1 ];
style.overflowY = opts.overflow[ 2 ];
});
}
}
// show/hide pass
for ( index in props ) {
value = props[ index ];
if ( rfxtypes.exec( value ) ) {
delete props[ index ];
toggle = toggle || value === "toggle";
if ( value === ( hidden ? "hide" : "show" ) ) {
continue;
}
handled.push( index );
}
}
length = handled.length;
if ( length ) {
dataShow = jQuery._data( elem, "fxshow" ) || jQuery._data( elem, "fxshow", {} );
if ( "hidden" in dataShow ) {
hidden = dataShow.hidden;
}
// store state if its toggle - enables .stop().toggle() to "reverse"
if ( toggle ) {
dataShow.hidden = !hidden;
}
if ( hidden ) {
jQuery( elem ).show();
} else {
anim.done(function() {
jQuery( elem ).hide();
});
}
anim.done(function() {
var prop;
jQuery._removeData( elem, "fxshow" );
for ( prop in orig ) {
jQuery.style( elem, prop, orig[ prop ] );
}
});
for ( index = 0 ; index < length ; index++ ) {
prop = handled[ index ];
tween = anim.createTween( prop, hidden ? dataShow[ prop ] : 0 );
orig[ prop ] = dataShow[ prop ] || jQuery.style( elem, prop );
if ( !( prop in dataShow ) ) {
dataShow[ prop ] = tween.start;
if ( hidden ) {
tween.end = tween.start;
tween.start = prop === "width" || prop === "height" ? 1 : 0;
}
}
}
}
}
function Tween( elem, options, prop, end, easing ) {
return new Tween.prototype.init( elem, options, prop, end, easing );
}
jQuery.Tween = Tween;
Tween.prototype = {
constructor: Tween,
init: function( elem, options, prop, end, easing, unit ) {
this.elem = elem;
this.prop = prop;
this.easing = easing || "swing";
this.options = options;
this.start = this.now = this.cur();
this.end = end;
this.unit = unit || ( jQuery.cssNumber[ prop ] ? "" : "px" );
},
cur: function() {
var hooks = Tween.propHooks[ this.prop ];
return hooks && hooks.get ?
hooks.get( this ) :
Tween.propHooks._default.get( this );
},
run: function( percent ) {
var eased,
hooks = Tween.propHooks[ this.prop ];
if ( this.options.duration ) {
this.pos = eased = jQuery.easing[ this.easing ](
percent, this.options.duration * percent, 0, 1, this.options.duration
);
} else {
this.pos = eased = percent;
}
this.now = ( this.end - this.start ) * eased + this.start;
if ( this.options.step ) {
this.options.step.call( this.elem, this.now, this );
}
if ( hooks && hooks.set ) {
hooks.set( this );
} else {
Tween.propHooks._default.set( this );
}
return this;
}
};
Tween.prototype.init.prototype = Tween.prototype;
Tween.propHooks = {
_default: {
get: function( tween ) {
var result;
if ( tween.elem[ tween.prop ] != null &&
(!tween.elem.style || tween.elem.style[ tween.prop ] == null) ) {
return tween.elem[ tween.prop ];
}
// passing an empty string as a 3rd parameter to .css will automatically
// attempt a parseFloat and fallback to a string if the parse fails
// so, simple values such as "10px" are parsed to Float.
// complex values such as "rotate(1rad)" are returned as is.
result = jQuery.css( tween.elem, tween.prop, "" );
// Empty strings, null, undefined and "auto" are converted to 0.
return !result || result === "auto" ? 0 : result;
},
set: function( tween ) {
// use step hook for back compat - use cssHook if its there - use .style if its
// available and use plain properties where available
if ( jQuery.fx.step[ tween.prop ] ) {
jQuery.fx.step[ tween.prop ]( tween );
} else if ( tween.elem.style && ( tween.elem.style[ jQuery.cssProps[ tween.prop ] ] != null || jQuery.cssHooks[ tween.prop ] ) ) {
jQuery.style( tween.elem, tween.prop, tween.now + tween.unit );
} else {
tween.elem[ tween.prop ] = tween.now;
}
}
}
};
// Remove in 2.0 - this supports IE8's panic based approach
// to setting things on disconnected nodes
Tween.propHooks.scrollTop = Tween.propHooks.scrollLeft = {
set: function( tween ) {
if ( tween.elem.nodeType && tween.elem.parentNode ) {
tween.elem[ tween.prop ] = tween.now;
}
}
};
jQuery.each([ "toggle", "show", "hide" ], function( i, name ) {
var cssFn = jQuery.fn[ name ];
jQuery.fn[ name ] = function( speed, easing, callback ) {
return speed == null || typeof speed === "boolean" ?
cssFn.apply( this, arguments ) :
this.animate( genFx( name, true ), speed, easing, callback );
};
});
jQuery.fn.extend({
fadeTo: function( speed, to, easing, callback ) {
// show any hidden elements after setting opacity to 0
return this.filter( isHidden ).css( "opacity", 0 ).show()
// animate to the value specified
.end().animate({ opacity: to }, speed, easing, callback );
},
animate: function( prop, speed, easing, callback ) {
var empty = jQuery.isEmptyObject( prop ),
optall = jQuery.speed( speed, easing, callback ),
doAnimation = function() {
// Operate on a copy of prop so per-property easing won't be lost
var anim = Animation( this, jQuery.extend( {}, prop ), optall );
doAnimation.finish = function() {
anim.stop( true );
};
// Empty animations, or finishing resolves immediately
if ( empty || jQuery._data( this, "finish" ) ) {
anim.stop( true );
}
};
doAnimation.finish = doAnimation;
return empty || optall.queue === false ?
this.each( doAnimation ) :
this.queue( optall.queue, doAnimation );
},
stop: function( type, clearQueue, gotoEnd ) {
var stopQueue = function( hooks ) {
var stop = hooks.stop;
delete hooks.stop;
stop( gotoEnd );
};
if ( typeof type !== "string" ) {
gotoEnd = clearQueue;
clearQueue = type;
type = undefined;
}
if ( clearQueue && type !== false ) {
this.queue( type || "fx", [] );
}
return this.each(function() {
var dequeue = true,
index = type != null && type + "queueHooks",
timers = jQuery.timers,
data = jQuery._data( this );
if ( index ) {
if ( data[ index ] && data[ index ].stop ) {
stopQueue( data[ index ] );
}
} else {
for ( index in data ) {
if ( data[ index ] && data[ index ].stop && rrun.test( index ) ) {
stopQueue( data[ index ] );
}
}
}
for ( index = timers.length; index--; ) {
if ( timers[ index ].elem === this && (type == null || timers[ index ].queue === type) ) {
timers[ index ].anim.stop( gotoEnd );
dequeue = false;
timers.splice( index, 1 );
}
}
// start the next in the queue if the last step wasn't forced
// timers currently will call their complete callbacks, which will dequeue
// but only if they were gotoEnd
if ( dequeue || !gotoEnd ) {
jQuery.dequeue( this, type );
}
});
},
finish: function( type ) {
if ( type !== false ) {
type = type || "fx";
}
return this.each(function() {
var index,
data = jQuery._data( this ),
queue = data[ type + "queue" ],
hooks = data[ type + "queueHooks" ],
timers = jQuery.timers,
length = queue ? queue.length : 0;
// enable finishing flag on private data
data.finish = true;
// empty the queue first
jQuery.queue( this, type, [] );
if ( hooks && hooks.cur && hooks.cur.finish ) {
hooks.cur.finish.call( this );
}
// look for any active animations, and finish them
for ( index = timers.length; index--; ) {
if ( timers[ index ].elem === this && timers[ index ].queue === type ) {
timers[ index ].anim.stop( true );
timers.splice( index, 1 );
}
}
// look for any animations in the old queue and finish them
for ( index = 0; index < length; index++ ) {
if ( queue[ index ] && queue[ index ].finish ) {
queue[ index ].finish.call( this );
}
}
// turn off finishing flag
delete data.finish;
});
}
});
// Generate parameters to create a standard animation
function genFx( type, includeWidth ) {
var which,
attrs = { height: type },
i = 0;
// if we include width, step value is 1 to do all cssExpand values,
// if we don't include width, step value is 2 to skip over Left and Right
includeWidth = includeWidth? 1 : 0;
for( ; i < 4 ; i += 2 - includeWidth ) {
which = cssExpand[ i ];
attrs[ "margin" + which ] = attrs[ "padding" + which ] = type;
}
if ( includeWidth ) {
attrs.opacity = attrs.width = type;
}
return attrs;
}
// Generate shortcuts for custom animations
jQuery.each({
slideDown: genFx("show"),
slideUp: genFx("hide"),
slideToggle: genFx("toggle"),
fadeIn: { opacity: "show" },
fadeOut: { opacity: "hide" },
fadeToggle: { opacity: "toggle" }
}, function( name, props ) {
jQuery.fn[ name ] = function( speed, easing, callback ) {
return this.animate( props, speed, easing, callback );
};
});
jQuery.speed = function( speed, easing, fn ) {
var opt = speed && typeof speed === "object" ? jQuery.extend( {}, speed ) : {
complete: fn || !fn && easing ||
jQuery.isFunction( speed ) && speed,
duration: speed,
easing: fn && easing || easing && !jQuery.isFunction( easing ) && easing
};
opt.duration = jQuery.fx.off ? 0 : typeof opt.duration === "number" ? opt.duration :
opt.duration in jQuery.fx.speeds ? jQuery.fx.speeds[ opt.duration ] : jQuery.fx.speeds._default;
// normalize opt.queue - true/undefined/null -> "fx"
if ( opt.queue == null || opt.queue === true ) {
opt.queue = "fx";
}
// Queueing
opt.old = opt.complete;
opt.complete = function() {
if ( jQuery.isFunction( opt.old ) ) {
opt.old.call( this );
}
if ( opt.queue ) {
jQuery.dequeue( this, opt.queue );
}
};
return opt;
};
jQuery.easing = {
linear: function( p ) {
return p;
},
swing: function( p ) {
return 0.5 - Math.cos( p*Math.PI ) / 2;
}
};
jQuery.timers = [];
jQuery.fx = Tween.prototype.init;
jQuery.fx.tick = function() {
var timer,
timers = jQuery.timers,
i = 0;
fxNow = jQuery.now();
for ( ; i < timers.length; i++ ) {
timer = timers[ i ];
// Checks the timer has not already been removed
if ( !timer() && timers[ i ] === timer ) {
timers.splice( i--, 1 );
}
}
if ( !timers.length ) {
jQuery.fx.stop();
}
fxNow = undefined;
};
jQuery.fx.timer = function( timer ) {
if ( timer() && jQuery.timers.push( timer ) ) {
jQuery.fx.start();
}
};
jQuery.fx.interval = 13;
jQuery.fx.start = function() {
if ( !timerId ) {
timerId = setInterval( jQuery.fx.tick, jQuery.fx.interval );
}
};
jQuery.fx.stop = function() {
clearInterval( timerId );
timerId = null;
};
jQuery.fx.speeds = {
slow: 600,
fast: 200,
// Default speed
_default: 400
};
// Back Compat <1.8 extension point
jQuery.fx.step = {};
if ( jQuery.expr && jQuery.expr.filters ) {
jQuery.expr.filters.animated = function( elem ) {
return jQuery.grep(jQuery.timers, function( fn ) {
return elem === fn.elem;
}).length;
};
}
jQuery.fn.offset = function( options ) {
if ( arguments.length ) {
return options === undefined ?
this :
this.each(function( i ) {
jQuery.offset.setOffset( this, options, i );
});
}
var docElem, win,
box = { top: 0, left: 0 },
elem = this[ 0 ],
doc = elem && elem.ownerDocument;
if ( !doc ) {
return;
}
docElem = doc.documentElement;
// Make sure it's not a disconnected DOM node
if ( !jQuery.contains( docElem, elem ) ) {
return box;
}
// If we don't have gBCR, just use 0,0 rather than error
// BlackBerry 5, iOS 3 (original iPhone)
if ( typeof elem.getBoundingClientRect !== core_strundefined ) {
box = elem.getBoundingClientRect();
}
win = getWindow( doc );
return {
top: box.top + ( win.pageYOffset || docElem.scrollTop ) - ( docElem.clientTop || 0 ),
left: box.left + ( win.pageXOffset || docElem.scrollLeft ) - ( docElem.clientLeft || 0 )
};
};
jQuery.offset = {
setOffset: function( elem, options, i ) {
var position = jQuery.css( elem, "position" );
// set position first, in-case top/left are set even on static elem
if ( position === "static" ) {
elem.style.position = "relative";
}
var curElem = jQuery( elem ),
curOffset = curElem.offset(),
curCSSTop = jQuery.css( elem, "top" ),
curCSSLeft = jQuery.css( elem, "left" ),
calculatePosition = ( position === "absolute" || position === "fixed" ) && jQuery.inArray("auto", [curCSSTop, curCSSLeft]) > -1,
props = {}, curPosition = {}, curTop, curLeft;
// need to be able to calculate position if either top or left is auto and position is either absolute or fixed
if ( calculatePosition ) {
curPosition = curElem.position();
curTop = curPosition.top;
curLeft = curPosition.left;
} else {
curTop = parseFloat( curCSSTop ) || 0;
curLeft = parseFloat( curCSSLeft ) || 0;
}
if ( jQuery.isFunction( options ) ) {
options = options.call( elem, i, curOffset );
}
if ( options.top != null ) {
props.top = ( options.top - curOffset.top ) + curTop;
}
if ( options.left != null ) {
props.left = ( options.left - curOffset.left ) + curLeft;
}
if ( "using" in options ) {
options.using.call( elem, props );
} else {
curElem.css( props );
}
}
};
jQuery.fn.extend({
position: function() {
if ( !this[ 0 ] ) {
return;
}
var offsetParent, offset,
parentOffset = { top: 0, left: 0 },
elem = this[ 0 ];
// fixed elements are offset from window (parentOffset = {top:0, left: 0}, because it is it's only offset parent
if ( jQuery.css( elem, "position" ) === "fixed" ) {
// we assume that getBoundingClientRect is available when computed position is fixed
offset = elem.getBoundingClientRect();
} else {
// Get *real* offsetParent
offsetParent = this.offsetParent();
// Get correct offsets
offset = this.offset();
if ( !jQuery.nodeName( offsetParent[ 0 ], "html" ) ) {
parentOffset = offsetParent.offset();
}
// Add offsetParent borders
parentOffset.top += jQuery.css( offsetParent[ 0 ], "borderTopWidth", true );
parentOffset.left += jQuery.css( offsetParent[ 0 ], "borderLeftWidth", true );
}
// Subtract parent offsets and element margins
// note: when an element has margin: auto the offsetLeft and marginLeft
// are the same in Safari causing offset.left to incorrectly be 0
return {
top: offset.top - parentOffset.top - jQuery.css( elem, "marginTop", true ),
left: offset.left - parentOffset.left - jQuery.css( elem, "marginLeft", true)
};
},
offsetParent: function() {
return this.map(function() {
var offsetParent = this.offsetParent || document.documentElement;
while ( offsetParent && ( !jQuery.nodeName( offsetParent, "html" ) && jQuery.css( offsetParent, "position") === "static" ) ) {
offsetParent = offsetParent.offsetParent;
}
return offsetParent || document.documentElement;
});
}
});
// Create scrollLeft and scrollTop methods
jQuery.each( {scrollLeft: "pageXOffset", scrollTop: "pageYOffset"}, function( method, prop ) {
var top = /Y/.test( prop );
jQuery.fn[ method ] = function( val ) {
return jQuery.access( this, function( elem, method, val ) {
var win = getWindow( elem );
if ( val === undefined ) {
return win ? (prop in win) ? win[ prop ] :
win.document.documentElement[ method ] :
elem[ method ];
}
if ( win ) {
win.scrollTo(
!top ? val : jQuery( win ).scrollLeft(),
top ? val : jQuery( win ).scrollTop()
);
} else {
elem[ method ] = val;
}
}, method, val, arguments.length, null );
};
});
function getWindow( elem ) {
return jQuery.isWindow( elem ) ?
elem :
elem.nodeType === 9 ?
elem.defaultView || elem.parentWindow :
false;
}
// Create innerHeight, innerWidth, height, width, outerHeight and outerWidth methods
jQuery.each( { Height: "height", Width: "width" }, function( name, type ) {
jQuery.each( { padding: "inner" + name, content: type, "": "outer" + name }, function( defaultExtra, funcName ) {
// margin is only for outerHeight, outerWidth
jQuery.fn[ funcName ] = function( margin, value ) {
var chainable = arguments.length && ( defaultExtra || typeof margin !== "boolean" ),
extra = defaultExtra || ( margin === true || value === true ? "margin" : "border" );
return jQuery.access( this, function( elem, type, value ) {
var doc;
if ( jQuery.isWindow( elem ) ) {
// As of 5/8/2012 this will yield incorrect results for Mobile Safari, but there
// isn't a whole lot we can do. See pull request at this URL for discussion:
// https://github.com/jquery/jquery/pull/764
return elem.document.documentElement[ "client" + name ];
}
// Get document width or height
if ( elem.nodeType === 9 ) {
doc = elem.documentElement;
// Either scroll[Width/Height] or offset[Width/Height] or client[Width/Height], whichever is greatest
// unfortunately, this causes bug #3838 in IE6/8 only, but there is currently no good, small way to fix it.
return Math.max(
elem.body[ "scroll" + name ], doc[ "scroll" + name ],
elem.body[ "offset" + name ], doc[ "offset" + name ],
doc[ "client" + name ]
);
}
return value === undefined ?
// Get width or height on the element, requesting but not forcing parseFloat
jQuery.css( elem, type, extra ) :
// Set width or height on the element
jQuery.style( elem, type, value, extra );
}, type, chainable ? margin : undefined, chainable, null );
};
});
});
// Limit scope pollution from any deprecated API
// (function() {
// })();
// Expose jQuery to the global object
window.jQuery = window.$ = jQuery;
// Expose jQuery as an AMD module, but only for AMD loaders that
// understand the issues with loading multiple versions of jQuery
// in a page that all might call define(). The loader will indicate
// they have special allowances for multiple jQuery versions by
// specifying define.amd.jQuery = true. Register as a named module,
// since jQuery can be concatenated with other files that may use define,
// but not use a proper concatenation script that understands anonymous
// AMD modules. A named AMD is safest and most robust way to register.
// Lowercase jquery is used because AMD module names are derived from
// file names, and jQuery is normally delivered in a lowercase file name.
// Do this after creating the global so that if an AMD module wants to call
// noConflict to hide this version of jQuery, it will work.
if ( typeof define === "function" && define.amd && define.amd.jQuery ) {
define( "jquery", [], function () { return jQuery; } );
}
})( window );
|
web/getstarted/js/jquery.js | phongnhung/CnitSymfony | /*! jQuery v1.11.1 | (c) 2005, 2014 jQuery Foundation, Inc. | jquery.org/license */
!function(a,b){"object"==typeof module&&"object"==typeof module.exports?module.exports=a.document?b(a,!0):function(a){if(!a.document)throw new Error("jQuery requires a window with a document");return b(a)}:b(a)}("undefined"!=typeof window?window:this,function(a,b){var c=[],d=c.slice,e=c.concat,f=c.push,g=c.indexOf,h={},i=h.toString,j=h.hasOwnProperty,k={},l="1.11.1",m=function(a,b){return new m.fn.init(a,b)},n=/^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g,o=/^-ms-/,p=/-([\da-z])/gi,q=function(a,b){return b.toUpperCase()};m.fn=m.prototype={jquery:l,constructor:m,selector:"",length:0,toArray:function(){return d.call(this)},get:function(a){return null!=a?0>a?this[a+this.length]:this[a]:d.call(this)},pushStack:function(a){var b=m.merge(this.constructor(),a);return b.prevObject=this,b.context=this.context,b},each:function(a,b){return m.each(this,a,b)},map:function(a){return this.pushStack(m.map(this,function(b,c){return a.call(b,c,b)}))},slice:function(){return this.pushStack(d.apply(this,arguments))},first:function(){return this.eq(0)},last:function(){return this.eq(-1)},eq:function(a){var b=this.length,c=+a+(0>a?b:0);return this.pushStack(c>=0&&b>c?[this[c]]:[])},end:function(){return this.prevObject||this.constructor(null)},push:f,sort:c.sort,splice:c.splice},m.extend=m.fn.extend=function(){var a,b,c,d,e,f,g=arguments[0]||{},h=1,i=arguments.length,j=!1;for("boolean"==typeof g&&(j=g,g=arguments[h]||{},h++),"object"==typeof g||m.isFunction(g)||(g={}),h===i&&(g=this,h--);i>h;h++)if(null!=(e=arguments[h]))for(d in e)a=g[d],c=e[d],g!==c&&(j&&c&&(m.isPlainObject(c)||(b=m.isArray(c)))?(b?(b=!1,f=a&&m.isArray(a)?a:[]):f=a&&m.isPlainObject(a)?a:{},g[d]=m.extend(j,f,c)):void 0!==c&&(g[d]=c));return g},m.extend({expando:"jQuery"+(l+Math.random()).replace(/\D/g,""),isReady:!0,error:function(a){throw new Error(a)},noop:function(){},isFunction:function(a){return"function"===m.type(a)},isArray:Array.isArray||function(a){return"array"===m.type(a)},isWindow:function(a){return null!=a&&a==a.window},isNumeric:function(a){return!m.isArray(a)&&a-parseFloat(a)>=0},isEmptyObject:function(a){var b;for(b in a)return!1;return!0},isPlainObject:function(a){var b;if(!a||"object"!==m.type(a)||a.nodeType||m.isWindow(a))return!1;try{if(a.constructor&&!j.call(a,"constructor")&&!j.call(a.constructor.prototype,"isPrototypeOf"))return!1}catch(c){return!1}if(k.ownLast)for(b in a)return j.call(a,b);for(b in a);return void 0===b||j.call(a,b)},type:function(a){return null==a?a+"":"object"==typeof a||"function"==typeof a?h[i.call(a)]||"object":typeof a},globalEval:function(b){b&&m.trim(b)&&(a.execScript||function(b){a.eval.call(a,b)})(b)},camelCase:function(a){return a.replace(o,"ms-").replace(p,q)},nodeName:function(a,b){return a.nodeName&&a.nodeName.toLowerCase()===b.toLowerCase()},each:function(a,b,c){var d,e=0,f=a.length,g=r(a);if(c){if(g){for(;f>e;e++)if(d=b.apply(a[e],c),d===!1)break}else for(e in a)if(d=b.apply(a[e],c),d===!1)break}else if(g){for(;f>e;e++)if(d=b.call(a[e],e,a[e]),d===!1)break}else for(e in a)if(d=b.call(a[e],e,a[e]),d===!1)break;return a},trim:function(a){return null==a?"":(a+"").replace(n,"")},makeArray:function(a,b){var c=b||[];return null!=a&&(r(Object(a))?m.merge(c,"string"==typeof a?[a]:a):f.call(c,a)),c},inArray:function(a,b,c){var d;if(b){if(g)return g.call(b,a,c);for(d=b.length,c=c?0>c?Math.max(0,d+c):c:0;d>c;c++)if(c in b&&b[c]===a)return c}return-1},merge:function(a,b){var c=+b.length,d=0,e=a.length;while(c>d)a[e++]=b[d++];if(c!==c)while(void 0!==b[d])a[e++]=b[d++];return a.length=e,a},grep:function(a,b,c){for(var d,e=[],f=0,g=a.length,h=!c;g>f;f++)d=!b(a[f],f),d!==h&&e.push(a[f]);return e},map:function(a,b,c){var d,f=0,g=a.length,h=r(a),i=[];if(h)for(;g>f;f++)d=b(a[f],f,c),null!=d&&i.push(d);else for(f in a)d=b(a[f],f,c),null!=d&&i.push(d);return e.apply([],i)},guid:1,proxy:function(a,b){var c,e,f;return"string"==typeof b&&(f=a[b],b=a,a=f),m.isFunction(a)?(c=d.call(arguments,2),e=function(){return a.apply(b||this,c.concat(d.call(arguments)))},e.guid=a.guid=a.guid||m.guid++,e):void 0},now:function(){return+new Date},support:k}),m.each("Boolean Number String Function Array Date RegExp Object Error".split(" "),function(a,b){h["[object "+b+"]"]=b.toLowerCase()});function r(a){var b=a.length,c=m.type(a);return"function"===c||m.isWindow(a)?!1:1===a.nodeType&&b?!0:"array"===c||0===b||"number"==typeof b&&b>0&&b-1 in a}var s=function(a){var b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u="sizzle"+-new Date,v=a.document,w=0,x=0,y=gb(),z=gb(),A=gb(),B=function(a,b){return a===b&&(l=!0),0},C="undefined",D=1<<31,E={}.hasOwnProperty,F=[],G=F.pop,H=F.push,I=F.push,J=F.slice,K=F.indexOf||function(a){for(var b=0,c=this.length;c>b;b++)if(this[b]===a)return b;return-1},L="checked|selected|async|autofocus|autoplay|controls|defer|disabled|hidden|ismap|loop|multiple|open|readonly|required|scoped",M="[\\x20\\t\\r\\n\\f]",N="(?:\\\\.|[\\w-]|[^\\x00-\\xa0])+",O=N.replace("w","w#"),P="\\["+M+"*("+N+")(?:"+M+"*([*^$|!~]?=)"+M+"*(?:'((?:\\\\.|[^\\\\'])*)'|\"((?:\\\\.|[^\\\\\"])*)\"|("+O+"))|)"+M+"*\\]",Q=":("+N+")(?:\\((('((?:\\\\.|[^\\\\'])*)'|\"((?:\\\\.|[^\\\\\"])*)\")|((?:\\\\.|[^\\\\()[\\]]|"+P+")*)|.*)\\)|)",R=new RegExp("^"+M+"+|((?:^|[^\\\\])(?:\\\\.)*)"+M+"+$","g"),S=new RegExp("^"+M+"*,"+M+"*"),T=new RegExp("^"+M+"*([>+~]|"+M+")"+M+"*"),U=new RegExp("="+M+"*([^\\]'\"]*?)"+M+"*\\]","g"),V=new RegExp(Q),W=new RegExp("^"+O+"$"),X={ID:new RegExp("^#("+N+")"),CLASS:new RegExp("^\\.("+N+")"),TAG:new RegExp("^("+N.replace("w","w*")+")"),ATTR:new RegExp("^"+P),PSEUDO:new RegExp("^"+Q),CHILD:new RegExp("^:(only|first|last|nth|nth-last)-(child|of-type)(?:\\("+M+"*(even|odd|(([+-]|)(\\d*)n|)"+M+"*(?:([+-]|)"+M+"*(\\d+)|))"+M+"*\\)|)","i"),bool:new RegExp("^(?:"+L+")$","i"),needsContext:new RegExp("^"+M+"*[>+~]|:(even|odd|eq|gt|lt|nth|first|last)(?:\\("+M+"*((?:-\\d)?\\d*)"+M+"*\\)|)(?=[^-]|$)","i")},Y=/^(?:input|select|textarea|button)$/i,Z=/^h\d$/i,$=/^[^{]+\{\s*\[native \w/,_=/^(?:#([\w-]+)|(\w+)|\.([\w-]+))$/,ab=/[+~]/,bb=/'|\\/g,cb=new RegExp("\\\\([\\da-f]{1,6}"+M+"?|("+M+")|.)","ig"),db=function(a,b,c){var d="0x"+b-65536;return d!==d||c?b:0>d?String.fromCharCode(d+65536):String.fromCharCode(d>>10|55296,1023&d|56320)};try{I.apply(F=J.call(v.childNodes),v.childNodes),F[v.childNodes.length].nodeType}catch(eb){I={apply:F.length?function(a,b){H.apply(a,J.call(b))}:function(a,b){var c=a.length,d=0;while(a[c++]=b[d++]);a.length=c-1}}}function fb(a,b,d,e){var f,h,j,k,l,o,r,s,w,x;if((b?b.ownerDocument||b:v)!==n&&m(b),b=b||n,d=d||[],!a||"string"!=typeof a)return d;if(1!==(k=b.nodeType)&&9!==k)return[];if(p&&!e){if(f=_.exec(a))if(j=f[1]){if(9===k){if(h=b.getElementById(j),!h||!h.parentNode)return d;if(h.id===j)return d.push(h),d}else if(b.ownerDocument&&(h=b.ownerDocument.getElementById(j))&&t(b,h)&&h.id===j)return d.push(h),d}else{if(f[2])return I.apply(d,b.getElementsByTagName(a)),d;if((j=f[3])&&c.getElementsByClassName&&b.getElementsByClassName)return I.apply(d,b.getElementsByClassName(j)),d}if(c.qsa&&(!q||!q.test(a))){if(s=r=u,w=b,x=9===k&&a,1===k&&"object"!==b.nodeName.toLowerCase()){o=g(a),(r=b.getAttribute("id"))?s=r.replace(bb,"\\$&"):b.setAttribute("id",s),s="[id='"+s+"'] ",l=o.length;while(l--)o[l]=s+qb(o[l]);w=ab.test(a)&&ob(b.parentNode)||b,x=o.join(",")}if(x)try{return I.apply(d,w.querySelectorAll(x)),d}catch(y){}finally{r||b.removeAttribute("id")}}}return i(a.replace(R,"$1"),b,d,e)}function gb(){var a=[];function b(c,e){return a.push(c+" ")>d.cacheLength&&delete b[a.shift()],b[c+" "]=e}return b}function hb(a){return a[u]=!0,a}function ib(a){var b=n.createElement("div");try{return!!a(b)}catch(c){return!1}finally{b.parentNode&&b.parentNode.removeChild(b),b=null}}function jb(a,b){var c=a.split("|"),e=a.length;while(e--)d.attrHandle[c[e]]=b}function kb(a,b){var c=b&&a,d=c&&1===a.nodeType&&1===b.nodeType&&(~b.sourceIndex||D)-(~a.sourceIndex||D);if(d)return d;if(c)while(c=c.nextSibling)if(c===b)return-1;return a?1:-1}function lb(a){return function(b){var c=b.nodeName.toLowerCase();return"input"===c&&b.type===a}}function mb(a){return function(b){var c=b.nodeName.toLowerCase();return("input"===c||"button"===c)&&b.type===a}}function nb(a){return hb(function(b){return b=+b,hb(function(c,d){var e,f=a([],c.length,b),g=f.length;while(g--)c[e=f[g]]&&(c[e]=!(d[e]=c[e]))})})}function ob(a){return a&&typeof a.getElementsByTagName!==C&&a}c=fb.support={},f=fb.isXML=function(a){var b=a&&(a.ownerDocument||a).documentElement;return b?"HTML"!==b.nodeName:!1},m=fb.setDocument=function(a){var b,e=a?a.ownerDocument||a:v,g=e.defaultView;return e!==n&&9===e.nodeType&&e.documentElement?(n=e,o=e.documentElement,p=!f(e),g&&g!==g.top&&(g.addEventListener?g.addEventListener("unload",function(){m()},!1):g.attachEvent&&g.attachEvent("onunload",function(){m()})),c.attributes=ib(function(a){return a.className="i",!a.getAttribute("className")}),c.getElementsByTagName=ib(function(a){return a.appendChild(e.createComment("")),!a.getElementsByTagName("*").length}),c.getElementsByClassName=$.test(e.getElementsByClassName)&&ib(function(a){return a.innerHTML="<div class='a'></div><div class='a i'></div>",a.firstChild.className="i",2===a.getElementsByClassName("i").length}),c.getById=ib(function(a){return o.appendChild(a).id=u,!e.getElementsByName||!e.getElementsByName(u).length}),c.getById?(d.find.ID=function(a,b){if(typeof b.getElementById!==C&&p){var c=b.getElementById(a);return c&&c.parentNode?[c]:[]}},d.filter.ID=function(a){var b=a.replace(cb,db);return function(a){return a.getAttribute("id")===b}}):(delete d.find.ID,d.filter.ID=function(a){var b=a.replace(cb,db);return function(a){var c=typeof a.getAttributeNode!==C&&a.getAttributeNode("id");return c&&c.value===b}}),d.find.TAG=c.getElementsByTagName?function(a,b){return typeof b.getElementsByTagName!==C?b.getElementsByTagName(a):void 0}:function(a,b){var c,d=[],e=0,f=b.getElementsByTagName(a);if("*"===a){while(c=f[e++])1===c.nodeType&&d.push(c);return d}return f},d.find.CLASS=c.getElementsByClassName&&function(a,b){return typeof b.getElementsByClassName!==C&&p?b.getElementsByClassName(a):void 0},r=[],q=[],(c.qsa=$.test(e.querySelectorAll))&&(ib(function(a){a.innerHTML="<select msallowclip=''><option selected=''></option></select>",a.querySelectorAll("[msallowclip^='']").length&&q.push("[*^$]="+M+"*(?:''|\"\")"),a.querySelectorAll("[selected]").length||q.push("\\["+M+"*(?:value|"+L+")"),a.querySelectorAll(":checked").length||q.push(":checked")}),ib(function(a){var b=e.createElement("input");b.setAttribute("type","hidden"),a.appendChild(b).setAttribute("name","D"),a.querySelectorAll("[name=d]").length&&q.push("name"+M+"*[*^$|!~]?="),a.querySelectorAll(":enabled").length||q.push(":enabled",":disabled"),a.querySelectorAll("*,:x"),q.push(",.*:")})),(c.matchesSelector=$.test(s=o.matches||o.webkitMatchesSelector||o.mozMatchesSelector||o.oMatchesSelector||o.msMatchesSelector))&&ib(function(a){c.disconnectedMatch=s.call(a,"div"),s.call(a,"[s!='']:x"),r.push("!=",Q)}),q=q.length&&new RegExp(q.join("|")),r=r.length&&new RegExp(r.join("|")),b=$.test(o.compareDocumentPosition),t=b||$.test(o.contains)?function(a,b){var c=9===a.nodeType?a.documentElement:a,d=b&&b.parentNode;return a===d||!(!d||1!==d.nodeType||!(c.contains?c.contains(d):a.compareDocumentPosition&&16&a.compareDocumentPosition(d)))}:function(a,b){if(b)while(b=b.parentNode)if(b===a)return!0;return!1},B=b?function(a,b){if(a===b)return l=!0,0;var d=!a.compareDocumentPosition-!b.compareDocumentPosition;return d?d:(d=(a.ownerDocument||a)===(b.ownerDocument||b)?a.compareDocumentPosition(b):1,1&d||!c.sortDetached&&b.compareDocumentPosition(a)===d?a===e||a.ownerDocument===v&&t(v,a)?-1:b===e||b.ownerDocument===v&&t(v,b)?1:k?K.call(k,a)-K.call(k,b):0:4&d?-1:1)}:function(a,b){if(a===b)return l=!0,0;var c,d=0,f=a.parentNode,g=b.parentNode,h=[a],i=[b];if(!f||!g)return a===e?-1:b===e?1:f?-1:g?1:k?K.call(k,a)-K.call(k,b):0;if(f===g)return kb(a,b);c=a;while(c=c.parentNode)h.unshift(c);c=b;while(c=c.parentNode)i.unshift(c);while(h[d]===i[d])d++;return d?kb(h[d],i[d]):h[d]===v?-1:i[d]===v?1:0},e):n},fb.matches=function(a,b){return fb(a,null,null,b)},fb.matchesSelector=function(a,b){if((a.ownerDocument||a)!==n&&m(a),b=b.replace(U,"='$1']"),!(!c.matchesSelector||!p||r&&r.test(b)||q&&q.test(b)))try{var d=s.call(a,b);if(d||c.disconnectedMatch||a.document&&11!==a.document.nodeType)return d}catch(e){}return fb(b,n,null,[a]).length>0},fb.contains=function(a,b){return(a.ownerDocument||a)!==n&&m(a),t(a,b)},fb.attr=function(a,b){(a.ownerDocument||a)!==n&&m(a);var e=d.attrHandle[b.toLowerCase()],f=e&&E.call(d.attrHandle,b.toLowerCase())?e(a,b,!p):void 0;return void 0!==f?f:c.attributes||!p?a.getAttribute(b):(f=a.getAttributeNode(b))&&f.specified?f.value:null},fb.error=function(a){throw new Error("Syntax error, unrecognized expression: "+a)},fb.uniqueSort=function(a){var b,d=[],e=0,f=0;if(l=!c.detectDuplicates,k=!c.sortStable&&a.slice(0),a.sort(B),l){while(b=a[f++])b===a[f]&&(e=d.push(f));while(e--)a.splice(d[e],1)}return k=null,a},e=fb.getText=function(a){var b,c="",d=0,f=a.nodeType;if(f){if(1===f||9===f||11===f){if("string"==typeof a.textContent)return a.textContent;for(a=a.firstChild;a;a=a.nextSibling)c+=e(a)}else if(3===f||4===f)return a.nodeValue}else while(b=a[d++])c+=e(b);return c},d=fb.selectors={cacheLength:50,createPseudo:hb,match:X,attrHandle:{},find:{},relative:{">":{dir:"parentNode",first:!0}," ":{dir:"parentNode"},"+":{dir:"previousSibling",first:!0},"~":{dir:"previousSibling"}},preFilter:{ATTR:function(a){return a[1]=a[1].replace(cb,db),a[3]=(a[3]||a[4]||a[5]||"").replace(cb,db),"~="===a[2]&&(a[3]=" "+a[3]+" "),a.slice(0,4)},CHILD:function(a){return a[1]=a[1].toLowerCase(),"nth"===a[1].slice(0,3)?(a[3]||fb.error(a[0]),a[4]=+(a[4]?a[5]+(a[6]||1):2*("even"===a[3]||"odd"===a[3])),a[5]=+(a[7]+a[8]||"odd"===a[3])):a[3]&&fb.error(a[0]),a},PSEUDO:function(a){var b,c=!a[6]&&a[2];return X.CHILD.test(a[0])?null:(a[3]?a[2]=a[4]||a[5]||"":c&&V.test(c)&&(b=g(c,!0))&&(b=c.indexOf(")",c.length-b)-c.length)&&(a[0]=a[0].slice(0,b),a[2]=c.slice(0,b)),a.slice(0,3))}},filter:{TAG:function(a){var b=a.replace(cb,db).toLowerCase();return"*"===a?function(){return!0}:function(a){return a.nodeName&&a.nodeName.toLowerCase()===b}},CLASS:function(a){var b=y[a+" "];return b||(b=new RegExp("(^|"+M+")"+a+"("+M+"|$)"))&&y(a,function(a){return b.test("string"==typeof a.className&&a.className||typeof a.getAttribute!==C&&a.getAttribute("class")||"")})},ATTR:function(a,b,c){return function(d){var e=fb.attr(d,a);return null==e?"!="===b:b?(e+="","="===b?e===c:"!="===b?e!==c:"^="===b?c&&0===e.indexOf(c):"*="===b?c&&e.indexOf(c)>-1:"$="===b?c&&e.slice(-c.length)===c:"~="===b?(" "+e+" ").indexOf(c)>-1:"|="===b?e===c||e.slice(0,c.length+1)===c+"-":!1):!0}},CHILD:function(a,b,c,d,e){var f="nth"!==a.slice(0,3),g="last"!==a.slice(-4),h="of-type"===b;return 1===d&&0===e?function(a){return!!a.parentNode}:function(b,c,i){var j,k,l,m,n,o,p=f!==g?"nextSibling":"previousSibling",q=b.parentNode,r=h&&b.nodeName.toLowerCase(),s=!i&&!h;if(q){if(f){while(p){l=b;while(l=l[p])if(h?l.nodeName.toLowerCase()===r:1===l.nodeType)return!1;o=p="only"===a&&!o&&"nextSibling"}return!0}if(o=[g?q.firstChild:q.lastChild],g&&s){k=q[u]||(q[u]={}),j=k[a]||[],n=j[0]===w&&j[1],m=j[0]===w&&j[2],l=n&&q.childNodes[n];while(l=++n&&l&&l[p]||(m=n=0)||o.pop())if(1===l.nodeType&&++m&&l===b){k[a]=[w,n,m];break}}else if(s&&(j=(b[u]||(b[u]={}))[a])&&j[0]===w)m=j[1];else while(l=++n&&l&&l[p]||(m=n=0)||o.pop())if((h?l.nodeName.toLowerCase()===r:1===l.nodeType)&&++m&&(s&&((l[u]||(l[u]={}))[a]=[w,m]),l===b))break;return m-=e,m===d||m%d===0&&m/d>=0}}},PSEUDO:function(a,b){var c,e=d.pseudos[a]||d.setFilters[a.toLowerCase()]||fb.error("unsupported pseudo: "+a);return e[u]?e(b):e.length>1?(c=[a,a,"",b],d.setFilters.hasOwnProperty(a.toLowerCase())?hb(function(a,c){var d,f=e(a,b),g=f.length;while(g--)d=K.call(a,f[g]),a[d]=!(c[d]=f[g])}):function(a){return e(a,0,c)}):e}},pseudos:{not:hb(function(a){var b=[],c=[],d=h(a.replace(R,"$1"));return d[u]?hb(function(a,b,c,e){var f,g=d(a,null,e,[]),h=a.length;while(h--)(f=g[h])&&(a[h]=!(b[h]=f))}):function(a,e,f){return b[0]=a,d(b,null,f,c),!c.pop()}}),has:hb(function(a){return function(b){return fb(a,b).length>0}}),contains:hb(function(a){return function(b){return(b.textContent||b.innerText||e(b)).indexOf(a)>-1}}),lang:hb(function(a){return W.test(a||"")||fb.error("unsupported lang: "+a),a=a.replace(cb,db).toLowerCase(),function(b){var c;do if(c=p?b.lang:b.getAttribute("xml:lang")||b.getAttribute("lang"))return c=c.toLowerCase(),c===a||0===c.indexOf(a+"-");while((b=b.parentNode)&&1===b.nodeType);return!1}}),target:function(b){var c=a.location&&a.location.hash;return c&&c.slice(1)===b.id},root:function(a){return a===o},focus:function(a){return a===n.activeElement&&(!n.hasFocus||n.hasFocus())&&!!(a.type||a.href||~a.tabIndex)},enabled:function(a){return a.disabled===!1},disabled:function(a){return a.disabled===!0},checked:function(a){var b=a.nodeName.toLowerCase();return"input"===b&&!!a.checked||"option"===b&&!!a.selected},selected:function(a){return a.parentNode&&a.parentNode.selectedIndex,a.selected===!0},empty:function(a){for(a=a.firstChild;a;a=a.nextSibling)if(a.nodeType<6)return!1;return!0},parent:function(a){return!d.pseudos.empty(a)},header:function(a){return Z.test(a.nodeName)},input:function(a){return Y.test(a.nodeName)},button:function(a){var b=a.nodeName.toLowerCase();return"input"===b&&"button"===a.type||"button"===b},text:function(a){var b;return"input"===a.nodeName.toLowerCase()&&"text"===a.type&&(null==(b=a.getAttribute("type"))||"text"===b.toLowerCase())},first:nb(function(){return[0]}),last:nb(function(a,b){return[b-1]}),eq:nb(function(a,b,c){return[0>c?c+b:c]}),even:nb(function(a,b){for(var c=0;b>c;c+=2)a.push(c);return a}),odd:nb(function(a,b){for(var c=1;b>c;c+=2)a.push(c);return a}),lt:nb(function(a,b,c){for(var d=0>c?c+b:c;--d>=0;)a.push(d);return a}),gt:nb(function(a,b,c){for(var d=0>c?c+b:c;++d<b;)a.push(d);return a})}},d.pseudos.nth=d.pseudos.eq;for(b in{radio:!0,checkbox:!0,file:!0,password:!0,image:!0})d.pseudos[b]=lb(b);for(b in{submit:!0,reset:!0})d.pseudos[b]=mb(b);function pb(){}pb.prototype=d.filters=d.pseudos,d.setFilters=new pb,g=fb.tokenize=function(a,b){var c,e,f,g,h,i,j,k=z[a+" "];if(k)return b?0:k.slice(0);h=a,i=[],j=d.preFilter;while(h){(!c||(e=S.exec(h)))&&(e&&(h=h.slice(e[0].length)||h),i.push(f=[])),c=!1,(e=T.exec(h))&&(c=e.shift(),f.push({value:c,type:e[0].replace(R," ")}),h=h.slice(c.length));for(g in d.filter)!(e=X[g].exec(h))||j[g]&&!(e=j[g](e))||(c=e.shift(),f.push({value:c,type:g,matches:e}),h=h.slice(c.length));if(!c)break}return b?h.length:h?fb.error(a):z(a,i).slice(0)};function qb(a){for(var b=0,c=a.length,d="";c>b;b++)d+=a[b].value;return d}function rb(a,b,c){var d=b.dir,e=c&&"parentNode"===d,f=x++;return b.first?function(b,c,f){while(b=b[d])if(1===b.nodeType||e)return a(b,c,f)}:function(b,c,g){var h,i,j=[w,f];if(g){while(b=b[d])if((1===b.nodeType||e)&&a(b,c,g))return!0}else while(b=b[d])if(1===b.nodeType||e){if(i=b[u]||(b[u]={}),(h=i[d])&&h[0]===w&&h[1]===f)return j[2]=h[2];if(i[d]=j,j[2]=a(b,c,g))return!0}}}function sb(a){return a.length>1?function(b,c,d){var e=a.length;while(e--)if(!a[e](b,c,d))return!1;return!0}:a[0]}function tb(a,b,c){for(var d=0,e=b.length;e>d;d++)fb(a,b[d],c);return c}function ub(a,b,c,d,e){for(var f,g=[],h=0,i=a.length,j=null!=b;i>h;h++)(f=a[h])&&(!c||c(f,d,e))&&(g.push(f),j&&b.push(h));return g}function vb(a,b,c,d,e,f){return d&&!d[u]&&(d=vb(d)),e&&!e[u]&&(e=vb(e,f)),hb(function(f,g,h,i){var j,k,l,m=[],n=[],o=g.length,p=f||tb(b||"*",h.nodeType?[h]:h,[]),q=!a||!f&&b?p:ub(p,m,a,h,i),r=c?e||(f?a:o||d)?[]:g:q;if(c&&c(q,r,h,i),d){j=ub(r,n),d(j,[],h,i),k=j.length;while(k--)(l=j[k])&&(r[n[k]]=!(q[n[k]]=l))}if(f){if(e||a){if(e){j=[],k=r.length;while(k--)(l=r[k])&&j.push(q[k]=l);e(null,r=[],j,i)}k=r.length;while(k--)(l=r[k])&&(j=e?K.call(f,l):m[k])>-1&&(f[j]=!(g[j]=l))}}else r=ub(r===g?r.splice(o,r.length):r),e?e(null,g,r,i):I.apply(g,r)})}function wb(a){for(var b,c,e,f=a.length,g=d.relative[a[0].type],h=g||d.relative[" "],i=g?1:0,k=rb(function(a){return a===b},h,!0),l=rb(function(a){return K.call(b,a)>-1},h,!0),m=[function(a,c,d){return!g&&(d||c!==j)||((b=c).nodeType?k(a,c,d):l(a,c,d))}];f>i;i++)if(c=d.relative[a[i].type])m=[rb(sb(m),c)];else{if(c=d.filter[a[i].type].apply(null,a[i].matches),c[u]){for(e=++i;f>e;e++)if(d.relative[a[e].type])break;return vb(i>1&&sb(m),i>1&&qb(a.slice(0,i-1).concat({value:" "===a[i-2].type?"*":""})).replace(R,"$1"),c,e>i&&wb(a.slice(i,e)),f>e&&wb(a=a.slice(e)),f>e&&qb(a))}m.push(c)}return sb(m)}function xb(a,b){var c=b.length>0,e=a.length>0,f=function(f,g,h,i,k){var l,m,o,p=0,q="0",r=f&&[],s=[],t=j,u=f||e&&d.find.TAG("*",k),v=w+=null==t?1:Math.random()||.1,x=u.length;for(k&&(j=g!==n&&g);q!==x&&null!=(l=u[q]);q++){if(e&&l){m=0;while(o=a[m++])if(o(l,g,h)){i.push(l);break}k&&(w=v)}c&&((l=!o&&l)&&p--,f&&r.push(l))}if(p+=q,c&&q!==p){m=0;while(o=b[m++])o(r,s,g,h);if(f){if(p>0)while(q--)r[q]||s[q]||(s[q]=G.call(i));s=ub(s)}I.apply(i,s),k&&!f&&s.length>0&&p+b.length>1&&fb.uniqueSort(i)}return k&&(w=v,j=t),r};return c?hb(f):f}return h=fb.compile=function(a,b){var c,d=[],e=[],f=A[a+" "];if(!f){b||(b=g(a)),c=b.length;while(c--)f=wb(b[c]),f[u]?d.push(f):e.push(f);f=A(a,xb(e,d)),f.selector=a}return f},i=fb.select=function(a,b,e,f){var i,j,k,l,m,n="function"==typeof a&&a,o=!f&&g(a=n.selector||a);if(e=e||[],1===o.length){if(j=o[0]=o[0].slice(0),j.length>2&&"ID"===(k=j[0]).type&&c.getById&&9===b.nodeType&&p&&d.relative[j[1].type]){if(b=(d.find.ID(k.matches[0].replace(cb,db),b)||[])[0],!b)return e;n&&(b=b.parentNode),a=a.slice(j.shift().value.length)}i=X.needsContext.test(a)?0:j.length;while(i--){if(k=j[i],d.relative[l=k.type])break;if((m=d.find[l])&&(f=m(k.matches[0].replace(cb,db),ab.test(j[0].type)&&ob(b.parentNode)||b))){if(j.splice(i,1),a=f.length&&qb(j),!a)return I.apply(e,f),e;break}}}return(n||h(a,o))(f,b,!p,e,ab.test(a)&&ob(b.parentNode)||b),e},c.sortStable=u.split("").sort(B).join("")===u,c.detectDuplicates=!!l,m(),c.sortDetached=ib(function(a){return 1&a.compareDocumentPosition(n.createElement("div"))}),ib(function(a){return a.innerHTML="<a href='#'></a>","#"===a.firstChild.getAttribute("href")})||jb("type|href|height|width",function(a,b,c){return c?void 0:a.getAttribute(b,"type"===b.toLowerCase()?1:2)}),c.attributes&&ib(function(a){return a.innerHTML="<input/>",a.firstChild.setAttribute("value",""),""===a.firstChild.getAttribute("value")})||jb("value",function(a,b,c){return c||"input"!==a.nodeName.toLowerCase()?void 0:a.defaultValue}),ib(function(a){return null==a.getAttribute("disabled")})||jb(L,function(a,b,c){var d;return c?void 0:a[b]===!0?b.toLowerCase():(d=a.getAttributeNode(b))&&d.specified?d.value:null}),fb}(a);m.find=s,m.expr=s.selectors,m.expr[":"]=m.expr.pseudos,m.unique=s.uniqueSort,m.text=s.getText,m.isXMLDoc=s.isXML,m.contains=s.contains;var t=m.expr.match.needsContext,u=/^<(\w+)\s*\/?>(?:<\/\1>|)$/,v=/^.[^:#\[\.,]*$/;function w(a,b,c){if(m.isFunction(b))return m.grep(a,function(a,d){return!!b.call(a,d,a)!==c});if(b.nodeType)return m.grep(a,function(a){return a===b!==c});if("string"==typeof b){if(v.test(b))return m.filter(b,a,c);b=m.filter(b,a)}return m.grep(a,function(a){return m.inArray(a,b)>=0!==c})}m.filter=function(a,b,c){var d=b[0];return c&&(a=":not("+a+")"),1===b.length&&1===d.nodeType?m.find.matchesSelector(d,a)?[d]:[]:m.find.matches(a,m.grep(b,function(a){return 1===a.nodeType}))},m.fn.extend({find:function(a){var b,c=[],d=this,e=d.length;if("string"!=typeof a)return this.pushStack(m(a).filter(function(){for(b=0;e>b;b++)if(m.contains(d[b],this))return!0}));for(b=0;e>b;b++)m.find(a,d[b],c);return c=this.pushStack(e>1?m.unique(c):c),c.selector=this.selector?this.selector+" "+a:a,c},filter:function(a){return this.pushStack(w(this,a||[],!1))},not:function(a){return this.pushStack(w(this,a||[],!0))},is:function(a){return!!w(this,"string"==typeof a&&t.test(a)?m(a):a||[],!1).length}});var x,y=a.document,z=/^(?:\s*(<[\w\W]+>)[^>]*|#([\w-]*))$/,A=m.fn.init=function(a,b){var c,d;if(!a)return this;if("string"==typeof a){if(c="<"===a.charAt(0)&&">"===a.charAt(a.length-1)&&a.length>=3?[null,a,null]:z.exec(a),!c||!c[1]&&b)return!b||b.jquery?(b||x).find(a):this.constructor(b).find(a);if(c[1]){if(b=b instanceof m?b[0]:b,m.merge(this,m.parseHTML(c[1],b&&b.nodeType?b.ownerDocument||b:y,!0)),u.test(c[1])&&m.isPlainObject(b))for(c in b)m.isFunction(this[c])?this[c](b[c]):this.attr(c,b[c]);return this}if(d=y.getElementById(c[2]),d&&d.parentNode){if(d.id!==c[2])return x.find(a);this.length=1,this[0]=d}return this.context=y,this.selector=a,this}return a.nodeType?(this.context=this[0]=a,this.length=1,this):m.isFunction(a)?"undefined"!=typeof x.ready?x.ready(a):a(m):(void 0!==a.selector&&(this.selector=a.selector,this.context=a.context),m.makeArray(a,this))};A.prototype=m.fn,x=m(y);var B=/^(?:parents|prev(?:Until|All))/,C={children:!0,contents:!0,next:!0,prev:!0};m.extend({dir:function(a,b,c){var d=[],e=a[b];while(e&&9!==e.nodeType&&(void 0===c||1!==e.nodeType||!m(e).is(c)))1===e.nodeType&&d.push(e),e=e[b];return d},sibling:function(a,b){for(var c=[];a;a=a.nextSibling)1===a.nodeType&&a!==b&&c.push(a);return c}}),m.fn.extend({has:function(a){var b,c=m(a,this),d=c.length;return this.filter(function(){for(b=0;d>b;b++)if(m.contains(this,c[b]))return!0})},closest:function(a,b){for(var c,d=0,e=this.length,f=[],g=t.test(a)||"string"!=typeof a?m(a,b||this.context):0;e>d;d++)for(c=this[d];c&&c!==b;c=c.parentNode)if(c.nodeType<11&&(g?g.index(c)>-1:1===c.nodeType&&m.find.matchesSelector(c,a))){f.push(c);break}return this.pushStack(f.length>1?m.unique(f):f)},index:function(a){return a?"string"==typeof a?m.inArray(this[0],m(a)):m.inArray(a.jquery?a[0]:a,this):this[0]&&this[0].parentNode?this.first().prevAll().length:-1},add:function(a,b){return this.pushStack(m.unique(m.merge(this.get(),m(a,b))))},addBack:function(a){return this.add(null==a?this.prevObject:this.prevObject.filter(a))}});function D(a,b){do a=a[b];while(a&&1!==a.nodeType);return a}m.each({parent:function(a){var b=a.parentNode;return b&&11!==b.nodeType?b:null},parents:function(a){return m.dir(a,"parentNode")},parentsUntil:function(a,b,c){return m.dir(a,"parentNode",c)},next:function(a){return D(a,"nextSibling")},prev:function(a){return D(a,"previousSibling")},nextAll:function(a){return m.dir(a,"nextSibling")},prevAll:function(a){return m.dir(a,"previousSibling")},nextUntil:function(a,b,c){return m.dir(a,"nextSibling",c)},prevUntil:function(a,b,c){return m.dir(a,"previousSibling",c)},siblings:function(a){return m.sibling((a.parentNode||{}).firstChild,a)},children:function(a){return m.sibling(a.firstChild)},contents:function(a){return m.nodeName(a,"iframe")?a.contentDocument||a.contentWindow.document:m.merge([],a.childNodes)}},function(a,b){m.fn[a]=function(c,d){var e=m.map(this,b,c);return"Until"!==a.slice(-5)&&(d=c),d&&"string"==typeof d&&(e=m.filter(d,e)),this.length>1&&(C[a]||(e=m.unique(e)),B.test(a)&&(e=e.reverse())),this.pushStack(e)}});var E=/\S+/g,F={};function G(a){var b=F[a]={};return m.each(a.match(E)||[],function(a,c){b[c]=!0}),b}m.Callbacks=function(a){a="string"==typeof a?F[a]||G(a):m.extend({},a);var b,c,d,e,f,g,h=[],i=!a.once&&[],j=function(l){for(c=a.memory&&l,d=!0,f=g||0,g=0,e=h.length,b=!0;h&&e>f;f++)if(h[f].apply(l[0],l[1])===!1&&a.stopOnFalse){c=!1;break}b=!1,h&&(i?i.length&&j(i.shift()):c?h=[]:k.disable())},k={add:function(){if(h){var d=h.length;!function f(b){m.each(b,function(b,c){var d=m.type(c);"function"===d?a.unique&&k.has(c)||h.push(c):c&&c.length&&"string"!==d&&f(c)})}(arguments),b?e=h.length:c&&(g=d,j(c))}return this},remove:function(){return h&&m.each(arguments,function(a,c){var d;while((d=m.inArray(c,h,d))>-1)h.splice(d,1),b&&(e>=d&&e--,f>=d&&f--)}),this},has:function(a){return a?m.inArray(a,h)>-1:!(!h||!h.length)},empty:function(){return h=[],e=0,this},disable:function(){return h=i=c=void 0,this},disabled:function(){return!h},lock:function(){return i=void 0,c||k.disable(),this},locked:function(){return!i},fireWith:function(a,c){return!h||d&&!i||(c=c||[],c=[a,c.slice?c.slice():c],b?i.push(c):j(c)),this},fire:function(){return k.fireWith(this,arguments),this},fired:function(){return!!d}};return k},m.extend({Deferred:function(a){var b=[["resolve","done",m.Callbacks("once memory"),"resolved"],["reject","fail",m.Callbacks("once memory"),"rejected"],["notify","progress",m.Callbacks("memory")]],c="pending",d={state:function(){return c},always:function(){return e.done(arguments).fail(arguments),this},then:function(){var a=arguments;return m.Deferred(function(c){m.each(b,function(b,f){var g=m.isFunction(a[b])&&a[b];e[f[1]](function(){var a=g&&g.apply(this,arguments);a&&m.isFunction(a.promise)?a.promise().done(c.resolve).fail(c.reject).progress(c.notify):c[f[0]+"With"](this===d?c.promise():this,g?[a]:arguments)})}),a=null}).promise()},promise:function(a){return null!=a?m.extend(a,d):d}},e={};return d.pipe=d.then,m.each(b,function(a,f){var g=f[2],h=f[3];d[f[1]]=g.add,h&&g.add(function(){c=h},b[1^a][2].disable,b[2][2].lock),e[f[0]]=function(){return e[f[0]+"With"](this===e?d:this,arguments),this},e[f[0]+"With"]=g.fireWith}),d.promise(e),a&&a.call(e,e),e},when:function(a){var b=0,c=d.call(arguments),e=c.length,f=1!==e||a&&m.isFunction(a.promise)?e:0,g=1===f?a:m.Deferred(),h=function(a,b,c){return function(e){b[a]=this,c[a]=arguments.length>1?d.call(arguments):e,c===i?g.notifyWith(b,c):--f||g.resolveWith(b,c)}},i,j,k;if(e>1)for(i=new Array(e),j=new Array(e),k=new Array(e);e>b;b++)c[b]&&m.isFunction(c[b].promise)?c[b].promise().done(h(b,k,c)).fail(g.reject).progress(h(b,j,i)):--f;return f||g.resolveWith(k,c),g.promise()}});var H;m.fn.ready=function(a){return m.ready.promise().done(a),this},m.extend({isReady:!1,readyWait:1,holdReady:function(a){a?m.readyWait++:m.ready(!0)},ready:function(a){if(a===!0?!--m.readyWait:!m.isReady){if(!y.body)return setTimeout(m.ready);m.isReady=!0,a!==!0&&--m.readyWait>0||(H.resolveWith(y,[m]),m.fn.triggerHandler&&(m(y).triggerHandler("ready"),m(y).off("ready")))}}});function I(){y.addEventListener?(y.removeEventListener("DOMContentLoaded",J,!1),a.removeEventListener("load",J,!1)):(y.detachEvent("onreadystatechange",J),a.detachEvent("onload",J))}function J(){(y.addEventListener||"load"===event.type||"complete"===y.readyState)&&(I(),m.ready())}m.ready.promise=function(b){if(!H)if(H=m.Deferred(),"complete"===y.readyState)setTimeout(m.ready);else if(y.addEventListener)y.addEventListener("DOMContentLoaded",J,!1),a.addEventListener("load",J,!1);else{y.attachEvent("onreadystatechange",J),a.attachEvent("onload",J);var c=!1;try{c=null==a.frameElement&&y.documentElement}catch(d){}c&&c.doScroll&&!function e(){if(!m.isReady){try{c.doScroll("left")}catch(a){return setTimeout(e,50)}I(),m.ready()}}()}return H.promise(b)};var K="undefined",L;for(L in m(k))break;k.ownLast="0"!==L,k.inlineBlockNeedsLayout=!1,m(function(){var a,b,c,d;c=y.getElementsByTagName("body")[0],c&&c.style&&(b=y.createElement("div"),d=y.createElement("div"),d.style.cssText="position:absolute;border:0;width:0;height:0;top:0;left:-9999px",c.appendChild(d).appendChild(b),typeof b.style.zoom!==K&&(b.style.cssText="display:inline;margin:0;border:0;padding:1px;width:1px;zoom:1",k.inlineBlockNeedsLayout=a=3===b.offsetWidth,a&&(c.style.zoom=1)),c.removeChild(d))}),function(){var a=y.createElement("div");if(null==k.deleteExpando){k.deleteExpando=!0;try{delete a.test}catch(b){k.deleteExpando=!1}}a=null}(),m.acceptData=function(a){var b=m.noData[(a.nodeName+" ").toLowerCase()],c=+a.nodeType||1;return 1!==c&&9!==c?!1:!b||b!==!0&&a.getAttribute("classid")===b};var M=/^(?:\{[\w\W]*\}|\[[\w\W]*\])$/,N=/([A-Z])/g;function O(a,b,c){if(void 0===c&&1===a.nodeType){var d="data-"+b.replace(N,"-$1").toLowerCase();if(c=a.getAttribute(d),"string"==typeof c){try{c="true"===c?!0:"false"===c?!1:"null"===c?null:+c+""===c?+c:M.test(c)?m.parseJSON(c):c}catch(e){}m.data(a,b,c)}else c=void 0}return c}function P(a){var b;for(b in a)if(("data"!==b||!m.isEmptyObject(a[b]))&&"toJSON"!==b)return!1;return!0}function Q(a,b,d,e){if(m.acceptData(a)){var f,g,h=m.expando,i=a.nodeType,j=i?m.cache:a,k=i?a[h]:a[h]&&h;
if(k&&j[k]&&(e||j[k].data)||void 0!==d||"string"!=typeof b)return k||(k=i?a[h]=c.pop()||m.guid++:h),j[k]||(j[k]=i?{}:{toJSON:m.noop}),("object"==typeof b||"function"==typeof b)&&(e?j[k]=m.extend(j[k],b):j[k].data=m.extend(j[k].data,b)),g=j[k],e||(g.data||(g.data={}),g=g.data),void 0!==d&&(g[m.camelCase(b)]=d),"string"==typeof b?(f=g[b],null==f&&(f=g[m.camelCase(b)])):f=g,f}}function R(a,b,c){if(m.acceptData(a)){var d,e,f=a.nodeType,g=f?m.cache:a,h=f?a[m.expando]:m.expando;if(g[h]){if(b&&(d=c?g[h]:g[h].data)){m.isArray(b)?b=b.concat(m.map(b,m.camelCase)):b in d?b=[b]:(b=m.camelCase(b),b=b in d?[b]:b.split(" ")),e=b.length;while(e--)delete d[b[e]];if(c?!P(d):!m.isEmptyObject(d))return}(c||(delete g[h].data,P(g[h])))&&(f?m.cleanData([a],!0):k.deleteExpando||g!=g.window?delete g[h]:g[h]=null)}}}m.extend({cache:{},noData:{"applet ":!0,"embed ":!0,"object ":"clsid:D27CDB6E-AE6D-11cf-96B8-444553540000"},hasData:function(a){return a=a.nodeType?m.cache[a[m.expando]]:a[m.expando],!!a&&!P(a)},data:function(a,b,c){return Q(a,b,c)},removeData:function(a,b){return R(a,b)},_data:function(a,b,c){return Q(a,b,c,!0)},_removeData:function(a,b){return R(a,b,!0)}}),m.fn.extend({data:function(a,b){var c,d,e,f=this[0],g=f&&f.attributes;if(void 0===a){if(this.length&&(e=m.data(f),1===f.nodeType&&!m._data(f,"parsedAttrs"))){c=g.length;while(c--)g[c]&&(d=g[c].name,0===d.indexOf("data-")&&(d=m.camelCase(d.slice(5)),O(f,d,e[d])));m._data(f,"parsedAttrs",!0)}return e}return"object"==typeof a?this.each(function(){m.data(this,a)}):arguments.length>1?this.each(function(){m.data(this,a,b)}):f?O(f,a,m.data(f,a)):void 0},removeData:function(a){return this.each(function(){m.removeData(this,a)})}}),m.extend({queue:function(a,b,c){var d;return a?(b=(b||"fx")+"queue",d=m._data(a,b),c&&(!d||m.isArray(c)?d=m._data(a,b,m.makeArray(c)):d.push(c)),d||[]):void 0},dequeue:function(a,b){b=b||"fx";var c=m.queue(a,b),d=c.length,e=c.shift(),f=m._queueHooks(a,b),g=function(){m.dequeue(a,b)};"inprogress"===e&&(e=c.shift(),d--),e&&("fx"===b&&c.unshift("inprogress"),delete f.stop,e.call(a,g,f)),!d&&f&&f.empty.fire()},_queueHooks:function(a,b){var c=b+"queueHooks";return m._data(a,c)||m._data(a,c,{empty:m.Callbacks("once memory").add(function(){m._removeData(a,b+"queue"),m._removeData(a,c)})})}}),m.fn.extend({queue:function(a,b){var c=2;return"string"!=typeof a&&(b=a,a="fx",c--),arguments.length<c?m.queue(this[0],a):void 0===b?this:this.each(function(){var c=m.queue(this,a,b);m._queueHooks(this,a),"fx"===a&&"inprogress"!==c[0]&&m.dequeue(this,a)})},dequeue:function(a){return this.each(function(){m.dequeue(this,a)})},clearQueue:function(a){return this.queue(a||"fx",[])},promise:function(a,b){var c,d=1,e=m.Deferred(),f=this,g=this.length,h=function(){--d||e.resolveWith(f,[f])};"string"!=typeof a&&(b=a,a=void 0),a=a||"fx";while(g--)c=m._data(f[g],a+"queueHooks"),c&&c.empty&&(d++,c.empty.add(h));return h(),e.promise(b)}});var S=/[+-]?(?:\d*\.|)\d+(?:[eE][+-]?\d+|)/.source,T=["Top","Right","Bottom","Left"],U=function(a,b){return a=b||a,"none"===m.css(a,"display")||!m.contains(a.ownerDocument,a)},V=m.access=function(a,b,c,d,e,f,g){var h=0,i=a.length,j=null==c;if("object"===m.type(c)){e=!0;for(h in c)m.access(a,b,h,c[h],!0,f,g)}else if(void 0!==d&&(e=!0,m.isFunction(d)||(g=!0),j&&(g?(b.call(a,d),b=null):(j=b,b=function(a,b,c){return j.call(m(a),c)})),b))for(;i>h;h++)b(a[h],c,g?d:d.call(a[h],h,b(a[h],c)));return e?a:j?b.call(a):i?b(a[0],c):f},W=/^(?:checkbox|radio)$/i;!function(){var a=y.createElement("input"),b=y.createElement("div"),c=y.createDocumentFragment();if(b.innerHTML=" <link/><table></table><a href='/a'>a</a><input type='checkbox'/>",k.leadingWhitespace=3===b.firstChild.nodeType,k.tbody=!b.getElementsByTagName("tbody").length,k.htmlSerialize=!!b.getElementsByTagName("link").length,k.html5Clone="<:nav></:nav>"!==y.createElement("nav").cloneNode(!0).outerHTML,a.type="checkbox",a.checked=!0,c.appendChild(a),k.appendChecked=a.checked,b.innerHTML="<textarea>x</textarea>",k.noCloneChecked=!!b.cloneNode(!0).lastChild.defaultValue,c.appendChild(b),b.innerHTML="<input type='radio' checked='checked' name='t'/>",k.checkClone=b.cloneNode(!0).cloneNode(!0).lastChild.checked,k.noCloneEvent=!0,b.attachEvent&&(b.attachEvent("onclick",function(){k.noCloneEvent=!1}),b.cloneNode(!0).click()),null==k.deleteExpando){k.deleteExpando=!0;try{delete b.test}catch(d){k.deleteExpando=!1}}}(),function(){var b,c,d=y.createElement("div");for(b in{submit:!0,change:!0,focusin:!0})c="on"+b,(k[b+"Bubbles"]=c in a)||(d.setAttribute(c,"t"),k[b+"Bubbles"]=d.attributes[c].expando===!1);d=null}();var X=/^(?:input|select|textarea)$/i,Y=/^key/,Z=/^(?:mouse|pointer|contextmenu)|click/,$=/^(?:focusinfocus|focusoutblur)$/,_=/^([^.]*)(?:\.(.+)|)$/;function ab(){return!0}function bb(){return!1}function cb(){try{return y.activeElement}catch(a){}}m.event={global:{},add:function(a,b,c,d,e){var f,g,h,i,j,k,l,n,o,p,q,r=m._data(a);if(r){c.handler&&(i=c,c=i.handler,e=i.selector),c.guid||(c.guid=m.guid++),(g=r.events)||(g=r.events={}),(k=r.handle)||(k=r.handle=function(a){return typeof m===K||a&&m.event.triggered===a.type?void 0:m.event.dispatch.apply(k.elem,arguments)},k.elem=a),b=(b||"").match(E)||[""],h=b.length;while(h--)f=_.exec(b[h])||[],o=q=f[1],p=(f[2]||"").split(".").sort(),o&&(j=m.event.special[o]||{},o=(e?j.delegateType:j.bindType)||o,j=m.event.special[o]||{},l=m.extend({type:o,origType:q,data:d,handler:c,guid:c.guid,selector:e,needsContext:e&&m.expr.match.needsContext.test(e),namespace:p.join(".")},i),(n=g[o])||(n=g[o]=[],n.delegateCount=0,j.setup&&j.setup.call(a,d,p,k)!==!1||(a.addEventListener?a.addEventListener(o,k,!1):a.attachEvent&&a.attachEvent("on"+o,k))),j.add&&(j.add.call(a,l),l.handler.guid||(l.handler.guid=c.guid)),e?n.splice(n.delegateCount++,0,l):n.push(l),m.event.global[o]=!0);a=null}},remove:function(a,b,c,d,e){var f,g,h,i,j,k,l,n,o,p,q,r=m.hasData(a)&&m._data(a);if(r&&(k=r.events)){b=(b||"").match(E)||[""],j=b.length;while(j--)if(h=_.exec(b[j])||[],o=q=h[1],p=(h[2]||"").split(".").sort(),o){l=m.event.special[o]||{},o=(d?l.delegateType:l.bindType)||o,n=k[o]||[],h=h[2]&&new RegExp("(^|\\.)"+p.join("\\.(?:.*\\.|)")+"(\\.|$)"),i=f=n.length;while(f--)g=n[f],!e&&q!==g.origType||c&&c.guid!==g.guid||h&&!h.test(g.namespace)||d&&d!==g.selector&&("**"!==d||!g.selector)||(n.splice(f,1),g.selector&&n.delegateCount--,l.remove&&l.remove.call(a,g));i&&!n.length&&(l.teardown&&l.teardown.call(a,p,r.handle)!==!1||m.removeEvent(a,o,r.handle),delete k[o])}else for(o in k)m.event.remove(a,o+b[j],c,d,!0);m.isEmptyObject(k)&&(delete r.handle,m._removeData(a,"events"))}},trigger:function(b,c,d,e){var f,g,h,i,k,l,n,o=[d||y],p=j.call(b,"type")?b.type:b,q=j.call(b,"namespace")?b.namespace.split("."):[];if(h=l=d=d||y,3!==d.nodeType&&8!==d.nodeType&&!$.test(p+m.event.triggered)&&(p.indexOf(".")>=0&&(q=p.split("."),p=q.shift(),q.sort()),g=p.indexOf(":")<0&&"on"+p,b=b[m.expando]?b:new m.Event(p,"object"==typeof b&&b),b.isTrigger=e?2:3,b.namespace=q.join("."),b.namespace_re=b.namespace?new RegExp("(^|\\.)"+q.join("\\.(?:.*\\.|)")+"(\\.|$)"):null,b.result=void 0,b.target||(b.target=d),c=null==c?[b]:m.makeArray(c,[b]),k=m.event.special[p]||{},e||!k.trigger||k.trigger.apply(d,c)!==!1)){if(!e&&!k.noBubble&&!m.isWindow(d)){for(i=k.delegateType||p,$.test(i+p)||(h=h.parentNode);h;h=h.parentNode)o.push(h),l=h;l===(d.ownerDocument||y)&&o.push(l.defaultView||l.parentWindow||a)}n=0;while((h=o[n++])&&!b.isPropagationStopped())b.type=n>1?i:k.bindType||p,f=(m._data(h,"events")||{})[b.type]&&m._data(h,"handle"),f&&f.apply(h,c),f=g&&h[g],f&&f.apply&&m.acceptData(h)&&(b.result=f.apply(h,c),b.result===!1&&b.preventDefault());if(b.type=p,!e&&!b.isDefaultPrevented()&&(!k._default||k._default.apply(o.pop(),c)===!1)&&m.acceptData(d)&&g&&d[p]&&!m.isWindow(d)){l=d[g],l&&(d[g]=null),m.event.triggered=p;try{d[p]()}catch(r){}m.event.triggered=void 0,l&&(d[g]=l)}return b.result}},dispatch:function(a){a=m.event.fix(a);var b,c,e,f,g,h=[],i=d.call(arguments),j=(m._data(this,"events")||{})[a.type]||[],k=m.event.special[a.type]||{};if(i[0]=a,a.delegateTarget=this,!k.preDispatch||k.preDispatch.call(this,a)!==!1){h=m.event.handlers.call(this,a,j),b=0;while((f=h[b++])&&!a.isPropagationStopped()){a.currentTarget=f.elem,g=0;while((e=f.handlers[g++])&&!a.isImmediatePropagationStopped())(!a.namespace_re||a.namespace_re.test(e.namespace))&&(a.handleObj=e,a.data=e.data,c=((m.event.special[e.origType]||{}).handle||e.handler).apply(f.elem,i),void 0!==c&&(a.result=c)===!1&&(a.preventDefault(),a.stopPropagation()))}return k.postDispatch&&k.postDispatch.call(this,a),a.result}},handlers:function(a,b){var c,d,e,f,g=[],h=b.delegateCount,i=a.target;if(h&&i.nodeType&&(!a.button||"click"!==a.type))for(;i!=this;i=i.parentNode||this)if(1===i.nodeType&&(i.disabled!==!0||"click"!==a.type)){for(e=[],f=0;h>f;f++)d=b[f],c=d.selector+" ",void 0===e[c]&&(e[c]=d.needsContext?m(c,this).index(i)>=0:m.find(c,this,null,[i]).length),e[c]&&e.push(d);e.length&&g.push({elem:i,handlers:e})}return h<b.length&&g.push({elem:this,handlers:b.slice(h)}),g},fix:function(a){if(a[m.expando])return a;var b,c,d,e=a.type,f=a,g=this.fixHooks[e];g||(this.fixHooks[e]=g=Z.test(e)?this.mouseHooks:Y.test(e)?this.keyHooks:{}),d=g.props?this.props.concat(g.props):this.props,a=new m.Event(f),b=d.length;while(b--)c=d[b],a[c]=f[c];return a.target||(a.target=f.srcElement||y),3===a.target.nodeType&&(a.target=a.target.parentNode),a.metaKey=!!a.metaKey,g.filter?g.filter(a,f):a},props:"altKey bubbles cancelable ctrlKey currentTarget eventPhase metaKey relatedTarget shiftKey target timeStamp view which".split(" "),fixHooks:{},keyHooks:{props:"char charCode key keyCode".split(" "),filter:function(a,b){return null==a.which&&(a.which=null!=b.charCode?b.charCode:b.keyCode),a}},mouseHooks:{props:"button buttons clientX clientY fromElement offsetX offsetY pageX pageY screenX screenY toElement".split(" "),filter:function(a,b){var c,d,e,f=b.button,g=b.fromElement;return null==a.pageX&&null!=b.clientX&&(d=a.target.ownerDocument||y,e=d.documentElement,c=d.body,a.pageX=b.clientX+(e&&e.scrollLeft||c&&c.scrollLeft||0)-(e&&e.clientLeft||c&&c.clientLeft||0),a.pageY=b.clientY+(e&&e.scrollTop||c&&c.scrollTop||0)-(e&&e.clientTop||c&&c.clientTop||0)),!a.relatedTarget&&g&&(a.relatedTarget=g===a.target?b.toElement:g),a.which||void 0===f||(a.which=1&f?1:2&f?3:4&f?2:0),a}},special:{load:{noBubble:!0},focus:{trigger:function(){if(this!==cb()&&this.focus)try{return this.focus(),!1}catch(a){}},delegateType:"focusin"},blur:{trigger:function(){return this===cb()&&this.blur?(this.blur(),!1):void 0},delegateType:"focusout"},click:{trigger:function(){return m.nodeName(this,"input")&&"checkbox"===this.type&&this.click?(this.click(),!1):void 0},_default:function(a){return m.nodeName(a.target,"a")}},beforeunload:{postDispatch:function(a){void 0!==a.result&&a.originalEvent&&(a.originalEvent.returnValue=a.result)}}},simulate:function(a,b,c,d){var e=m.extend(new m.Event,c,{type:a,isSimulated:!0,originalEvent:{}});d?m.event.trigger(e,null,b):m.event.dispatch.call(b,e),e.isDefaultPrevented()&&c.preventDefault()}},m.removeEvent=y.removeEventListener?function(a,b,c){a.removeEventListener&&a.removeEventListener(b,c,!1)}:function(a,b,c){var d="on"+b;a.detachEvent&&(typeof a[d]===K&&(a[d]=null),a.detachEvent(d,c))},m.Event=function(a,b){return this instanceof m.Event?(a&&a.type?(this.originalEvent=a,this.type=a.type,this.isDefaultPrevented=a.defaultPrevented||void 0===a.defaultPrevented&&a.returnValue===!1?ab:bb):this.type=a,b&&m.extend(this,b),this.timeStamp=a&&a.timeStamp||m.now(),void(this[m.expando]=!0)):new m.Event(a,b)},m.Event.prototype={isDefaultPrevented:bb,isPropagationStopped:bb,isImmediatePropagationStopped:bb,preventDefault:function(){var a=this.originalEvent;this.isDefaultPrevented=ab,a&&(a.preventDefault?a.preventDefault():a.returnValue=!1)},stopPropagation:function(){var a=this.originalEvent;this.isPropagationStopped=ab,a&&(a.stopPropagation&&a.stopPropagation(),a.cancelBubble=!0)},stopImmediatePropagation:function(){var a=this.originalEvent;this.isImmediatePropagationStopped=ab,a&&a.stopImmediatePropagation&&a.stopImmediatePropagation(),this.stopPropagation()}},m.each({mouseenter:"mouseover",mouseleave:"mouseout",pointerenter:"pointerover",pointerleave:"pointerout"},function(a,b){m.event.special[a]={delegateType:b,bindType:b,handle:function(a){var c,d=this,e=a.relatedTarget,f=a.handleObj;return(!e||e!==d&&!m.contains(d,e))&&(a.type=f.origType,c=f.handler.apply(this,arguments),a.type=b),c}}}),k.submitBubbles||(m.event.special.submit={setup:function(){return m.nodeName(this,"form")?!1:void m.event.add(this,"click._submit keypress._submit",function(a){var b=a.target,c=m.nodeName(b,"input")||m.nodeName(b,"button")?b.form:void 0;c&&!m._data(c,"submitBubbles")&&(m.event.add(c,"submit._submit",function(a){a._submit_bubble=!0}),m._data(c,"submitBubbles",!0))})},postDispatch:function(a){a._submit_bubble&&(delete a._submit_bubble,this.parentNode&&!a.isTrigger&&m.event.simulate("submit",this.parentNode,a,!0))},teardown:function(){return m.nodeName(this,"form")?!1:void m.event.remove(this,"._submit")}}),k.changeBubbles||(m.event.special.change={setup:function(){return X.test(this.nodeName)?(("checkbox"===this.type||"radio"===this.type)&&(m.event.add(this,"propertychange._change",function(a){"checked"===a.originalEvent.propertyName&&(this._just_changed=!0)}),m.event.add(this,"click._change",function(a){this._just_changed&&!a.isTrigger&&(this._just_changed=!1),m.event.simulate("change",this,a,!0)})),!1):void m.event.add(this,"beforeactivate._change",function(a){var b=a.target;X.test(b.nodeName)&&!m._data(b,"changeBubbles")&&(m.event.add(b,"change._change",function(a){!this.parentNode||a.isSimulated||a.isTrigger||m.event.simulate("change",this.parentNode,a,!0)}),m._data(b,"changeBubbles",!0))})},handle:function(a){var b=a.target;return this!==b||a.isSimulated||a.isTrigger||"radio"!==b.type&&"checkbox"!==b.type?a.handleObj.handler.apply(this,arguments):void 0},teardown:function(){return m.event.remove(this,"._change"),!X.test(this.nodeName)}}),k.focusinBubbles||m.each({focus:"focusin",blur:"focusout"},function(a,b){var c=function(a){m.event.simulate(b,a.target,m.event.fix(a),!0)};m.event.special[b]={setup:function(){var d=this.ownerDocument||this,e=m._data(d,b);e||d.addEventListener(a,c,!0),m._data(d,b,(e||0)+1)},teardown:function(){var d=this.ownerDocument||this,e=m._data(d,b)-1;e?m._data(d,b,e):(d.removeEventListener(a,c,!0),m._removeData(d,b))}}}),m.fn.extend({on:function(a,b,c,d,e){var f,g;if("object"==typeof a){"string"!=typeof b&&(c=c||b,b=void 0);for(f in a)this.on(f,b,c,a[f],e);return this}if(null==c&&null==d?(d=b,c=b=void 0):null==d&&("string"==typeof b?(d=c,c=void 0):(d=c,c=b,b=void 0)),d===!1)d=bb;else if(!d)return this;return 1===e&&(g=d,d=function(a){return m().off(a),g.apply(this,arguments)},d.guid=g.guid||(g.guid=m.guid++)),this.each(function(){m.event.add(this,a,d,c,b)})},one:function(a,b,c,d){return this.on(a,b,c,d,1)},off:function(a,b,c){var d,e;if(a&&a.preventDefault&&a.handleObj)return d=a.handleObj,m(a.delegateTarget).off(d.namespace?d.origType+"."+d.namespace:d.origType,d.selector,d.handler),this;if("object"==typeof a){for(e in a)this.off(e,b,a[e]);return this}return(b===!1||"function"==typeof b)&&(c=b,b=void 0),c===!1&&(c=bb),this.each(function(){m.event.remove(this,a,c,b)})},trigger:function(a,b){return this.each(function(){m.event.trigger(a,b,this)})},triggerHandler:function(a,b){var c=this[0];return c?m.event.trigger(a,b,c,!0):void 0}});function db(a){var b=eb.split("|"),c=a.createDocumentFragment();if(c.createElement)while(b.length)c.createElement(b.pop());return c}var eb="abbr|article|aside|audio|bdi|canvas|data|datalist|details|figcaption|figure|footer|header|hgroup|mark|meter|nav|output|progress|section|summary|time|video",fb=/ jQuery\d+="(?:null|\d+)"/g,gb=new RegExp("<(?:"+eb+")[\\s/>]","i"),hb=/^\s+/,ib=/<(?!area|br|col|embed|hr|img|input|link|meta|param)(([\w:]+)[^>]*)\/>/gi,jb=/<([\w:]+)/,kb=/<tbody/i,lb=/<|&#?\w+;/,mb=/<(?:script|style|link)/i,nb=/checked\s*(?:[^=]|=\s*.checked.)/i,ob=/^$|\/(?:java|ecma)script/i,pb=/^true\/(.*)/,qb=/^\s*<!(?:\[CDATA\[|--)|(?:\]\]|--)>\s*$/g,rb={option:[1,"<select multiple='multiple'>","</select>"],legend:[1,"<fieldset>","</fieldset>"],area:[1,"<map>","</map>"],param:[1,"<object>","</object>"],thead:[1,"<table>","</table>"],tr:[2,"<table><tbody>","</tbody></table>"],col:[2,"<table><tbody></tbody><colgroup>","</colgroup></table>"],td:[3,"<table><tbody><tr>","</tr></tbody></table>"],_default:k.htmlSerialize?[0,"",""]:[1,"X<div>","</div>"]},sb=db(y),tb=sb.appendChild(y.createElement("div"));rb.optgroup=rb.option,rb.tbody=rb.tfoot=rb.colgroup=rb.caption=rb.thead,rb.th=rb.td;function ub(a,b){var c,d,e=0,f=typeof a.getElementsByTagName!==K?a.getElementsByTagName(b||"*"):typeof a.querySelectorAll!==K?a.querySelectorAll(b||"*"):void 0;if(!f)for(f=[],c=a.childNodes||a;null!=(d=c[e]);e++)!b||m.nodeName(d,b)?f.push(d):m.merge(f,ub(d,b));return void 0===b||b&&m.nodeName(a,b)?m.merge([a],f):f}function vb(a){W.test(a.type)&&(a.defaultChecked=a.checked)}function wb(a,b){return m.nodeName(a,"table")&&m.nodeName(11!==b.nodeType?b:b.firstChild,"tr")?a.getElementsByTagName("tbody")[0]||a.appendChild(a.ownerDocument.createElement("tbody")):a}function xb(a){return a.type=(null!==m.find.attr(a,"type"))+"/"+a.type,a}function yb(a){var b=pb.exec(a.type);return b?a.type=b[1]:a.removeAttribute("type"),a}function zb(a,b){for(var c,d=0;null!=(c=a[d]);d++)m._data(c,"globalEval",!b||m._data(b[d],"globalEval"))}function Ab(a,b){if(1===b.nodeType&&m.hasData(a)){var c,d,e,f=m._data(a),g=m._data(b,f),h=f.events;if(h){delete g.handle,g.events={};for(c in h)for(d=0,e=h[c].length;e>d;d++)m.event.add(b,c,h[c][d])}g.data&&(g.data=m.extend({},g.data))}}function Bb(a,b){var c,d,e;if(1===b.nodeType){if(c=b.nodeName.toLowerCase(),!k.noCloneEvent&&b[m.expando]){e=m._data(b);for(d in e.events)m.removeEvent(b,d,e.handle);b.removeAttribute(m.expando)}"script"===c&&b.text!==a.text?(xb(b).text=a.text,yb(b)):"object"===c?(b.parentNode&&(b.outerHTML=a.outerHTML),k.html5Clone&&a.innerHTML&&!m.trim(b.innerHTML)&&(b.innerHTML=a.innerHTML)):"input"===c&&W.test(a.type)?(b.defaultChecked=b.checked=a.checked,b.value!==a.value&&(b.value=a.value)):"option"===c?b.defaultSelected=b.selected=a.defaultSelected:("input"===c||"textarea"===c)&&(b.defaultValue=a.defaultValue)}}m.extend({clone:function(a,b,c){var d,e,f,g,h,i=m.contains(a.ownerDocument,a);if(k.html5Clone||m.isXMLDoc(a)||!gb.test("<"+a.nodeName+">")?f=a.cloneNode(!0):(tb.innerHTML=a.outerHTML,tb.removeChild(f=tb.firstChild)),!(k.noCloneEvent&&k.noCloneChecked||1!==a.nodeType&&11!==a.nodeType||m.isXMLDoc(a)))for(d=ub(f),h=ub(a),g=0;null!=(e=h[g]);++g)d[g]&&Bb(e,d[g]);if(b)if(c)for(h=h||ub(a),d=d||ub(f),g=0;null!=(e=h[g]);g++)Ab(e,d[g]);else Ab(a,f);return d=ub(f,"script"),d.length>0&&zb(d,!i&&ub(a,"script")),d=h=e=null,f},buildFragment:function(a,b,c,d){for(var e,f,g,h,i,j,l,n=a.length,o=db(b),p=[],q=0;n>q;q++)if(f=a[q],f||0===f)if("object"===m.type(f))m.merge(p,f.nodeType?[f]:f);else if(lb.test(f)){h=h||o.appendChild(b.createElement("div")),i=(jb.exec(f)||["",""])[1].toLowerCase(),l=rb[i]||rb._default,h.innerHTML=l[1]+f.replace(ib,"<$1></$2>")+l[2],e=l[0];while(e--)h=h.lastChild;if(!k.leadingWhitespace&&hb.test(f)&&p.push(b.createTextNode(hb.exec(f)[0])),!k.tbody){f="table"!==i||kb.test(f)?"<table>"!==l[1]||kb.test(f)?0:h:h.firstChild,e=f&&f.childNodes.length;while(e--)m.nodeName(j=f.childNodes[e],"tbody")&&!j.childNodes.length&&f.removeChild(j)}m.merge(p,h.childNodes),h.textContent="";while(h.firstChild)h.removeChild(h.firstChild);h=o.lastChild}else p.push(b.createTextNode(f));h&&o.removeChild(h),k.appendChecked||m.grep(ub(p,"input"),vb),q=0;while(f=p[q++])if((!d||-1===m.inArray(f,d))&&(g=m.contains(f.ownerDocument,f),h=ub(o.appendChild(f),"script"),g&&zb(h),c)){e=0;while(f=h[e++])ob.test(f.type||"")&&c.push(f)}return h=null,o},cleanData:function(a,b){for(var d,e,f,g,h=0,i=m.expando,j=m.cache,l=k.deleteExpando,n=m.event.special;null!=(d=a[h]);h++)if((b||m.acceptData(d))&&(f=d[i],g=f&&j[f])){if(g.events)for(e in g.events)n[e]?m.event.remove(d,e):m.removeEvent(d,e,g.handle);j[f]&&(delete j[f],l?delete d[i]:typeof d.removeAttribute!==K?d.removeAttribute(i):d[i]=null,c.push(f))}}}),m.fn.extend({text:function(a){return V(this,function(a){return void 0===a?m.text(this):this.empty().append((this[0]&&this[0].ownerDocument||y).createTextNode(a))},null,a,arguments.length)},append:function(){return this.domManip(arguments,function(a){if(1===this.nodeType||11===this.nodeType||9===this.nodeType){var b=wb(this,a);b.appendChild(a)}})},prepend:function(){return this.domManip(arguments,function(a){if(1===this.nodeType||11===this.nodeType||9===this.nodeType){var b=wb(this,a);b.insertBefore(a,b.firstChild)}})},before:function(){return this.domManip(arguments,function(a){this.parentNode&&this.parentNode.insertBefore(a,this)})},after:function(){return this.domManip(arguments,function(a){this.parentNode&&this.parentNode.insertBefore(a,this.nextSibling)})},remove:function(a,b){for(var c,d=a?m.filter(a,this):this,e=0;null!=(c=d[e]);e++)b||1!==c.nodeType||m.cleanData(ub(c)),c.parentNode&&(b&&m.contains(c.ownerDocument,c)&&zb(ub(c,"script")),c.parentNode.removeChild(c));return this},empty:function(){for(var a,b=0;null!=(a=this[b]);b++){1===a.nodeType&&m.cleanData(ub(a,!1));while(a.firstChild)a.removeChild(a.firstChild);a.options&&m.nodeName(a,"select")&&(a.options.length=0)}return this},clone:function(a,b){return a=null==a?!1:a,b=null==b?a:b,this.map(function(){return m.clone(this,a,b)})},html:function(a){return V(this,function(a){var b=this[0]||{},c=0,d=this.length;if(void 0===a)return 1===b.nodeType?b.innerHTML.replace(fb,""):void 0;if(!("string"!=typeof a||mb.test(a)||!k.htmlSerialize&&gb.test(a)||!k.leadingWhitespace&&hb.test(a)||rb[(jb.exec(a)||["",""])[1].toLowerCase()])){a=a.replace(ib,"<$1></$2>");try{for(;d>c;c++)b=this[c]||{},1===b.nodeType&&(m.cleanData(ub(b,!1)),b.innerHTML=a);b=0}catch(e){}}b&&this.empty().append(a)},null,a,arguments.length)},replaceWith:function(){var a=arguments[0];return this.domManip(arguments,function(b){a=this.parentNode,m.cleanData(ub(this)),a&&a.replaceChild(b,this)}),a&&(a.length||a.nodeType)?this:this.remove()},detach:function(a){return this.remove(a,!0)},domManip:function(a,b){a=e.apply([],a);var c,d,f,g,h,i,j=0,l=this.length,n=this,o=l-1,p=a[0],q=m.isFunction(p);if(q||l>1&&"string"==typeof p&&!k.checkClone&&nb.test(p))return this.each(function(c){var d=n.eq(c);q&&(a[0]=p.call(this,c,d.html())),d.domManip(a,b)});if(l&&(i=m.buildFragment(a,this[0].ownerDocument,!1,this),c=i.firstChild,1===i.childNodes.length&&(i=c),c)){for(g=m.map(ub(i,"script"),xb),f=g.length;l>j;j++)d=i,j!==o&&(d=m.clone(d,!0,!0),f&&m.merge(g,ub(d,"script"))),b.call(this[j],d,j);if(f)for(h=g[g.length-1].ownerDocument,m.map(g,yb),j=0;f>j;j++)d=g[j],ob.test(d.type||"")&&!m._data(d,"globalEval")&&m.contains(h,d)&&(d.src?m._evalUrl&&m._evalUrl(d.src):m.globalEval((d.text||d.textContent||d.innerHTML||"").replace(qb,"")));i=c=null}return this}}),m.each({appendTo:"append",prependTo:"prepend",insertBefore:"before",insertAfter:"after",replaceAll:"replaceWith"},function(a,b){m.fn[a]=function(a){for(var c,d=0,e=[],g=m(a),h=g.length-1;h>=d;d++)c=d===h?this:this.clone(!0),m(g[d])[b](c),f.apply(e,c.get());return this.pushStack(e)}});var Cb,Db={};function Eb(b,c){var d,e=m(c.createElement(b)).appendTo(c.body),f=a.getDefaultComputedStyle&&(d=a.getDefaultComputedStyle(e[0]))?d.display:m.css(e[0],"display");return e.detach(),f}function Fb(a){var b=y,c=Db[a];return c||(c=Eb(a,b),"none"!==c&&c||(Cb=(Cb||m("<iframe frameborder='0' width='0' height='0'/>")).appendTo(b.documentElement),b=(Cb[0].contentWindow||Cb[0].contentDocument).document,b.write(),b.close(),c=Eb(a,b),Cb.detach()),Db[a]=c),c}!function(){var a;k.shrinkWrapBlocks=function(){if(null!=a)return a;a=!1;var b,c,d;return c=y.getElementsByTagName("body")[0],c&&c.style?(b=y.createElement("div"),d=y.createElement("div"),d.style.cssText="position:absolute;border:0;width:0;height:0;top:0;left:-9999px",c.appendChild(d).appendChild(b),typeof b.style.zoom!==K&&(b.style.cssText="-webkit-box-sizing:content-box;-moz-box-sizing:content-box;box-sizing:content-box;display:block;margin:0;border:0;padding:1px;width:1px;zoom:1",b.appendChild(y.createElement("div")).style.width="5px",a=3!==b.offsetWidth),c.removeChild(d),a):void 0}}();var Gb=/^margin/,Hb=new RegExp("^("+S+")(?!px)[a-z%]+$","i"),Ib,Jb,Kb=/^(top|right|bottom|left)$/;a.getComputedStyle?(Ib=function(a){return a.ownerDocument.defaultView.getComputedStyle(a,null)},Jb=function(a,b,c){var d,e,f,g,h=a.style;return c=c||Ib(a),g=c?c.getPropertyValue(b)||c[b]:void 0,c&&(""!==g||m.contains(a.ownerDocument,a)||(g=m.style(a,b)),Hb.test(g)&&Gb.test(b)&&(d=h.width,e=h.minWidth,f=h.maxWidth,h.minWidth=h.maxWidth=h.width=g,g=c.width,h.width=d,h.minWidth=e,h.maxWidth=f)),void 0===g?g:g+""}):y.documentElement.currentStyle&&(Ib=function(a){return a.currentStyle},Jb=function(a,b,c){var d,e,f,g,h=a.style;return c=c||Ib(a),g=c?c[b]:void 0,null==g&&h&&h[b]&&(g=h[b]),Hb.test(g)&&!Kb.test(b)&&(d=h.left,e=a.runtimeStyle,f=e&&e.left,f&&(e.left=a.currentStyle.left),h.left="fontSize"===b?"1em":g,g=h.pixelLeft+"px",h.left=d,f&&(e.left=f)),void 0===g?g:g+""||"auto"});function Lb(a,b){return{get:function(){var c=a();if(null!=c)return c?void delete this.get:(this.get=b).apply(this,arguments)}}}!function(){var b,c,d,e,f,g,h;if(b=y.createElement("div"),b.innerHTML=" <link/><table></table><a href='/a'>a</a><input type='checkbox'/>",d=b.getElementsByTagName("a")[0],c=d&&d.style){c.cssText="float:left;opacity:.5",k.opacity="0.5"===c.opacity,k.cssFloat=!!c.cssFloat,b.style.backgroundClip="content-box",b.cloneNode(!0).style.backgroundClip="",k.clearCloneStyle="content-box"===b.style.backgroundClip,k.boxSizing=""===c.boxSizing||""===c.MozBoxSizing||""===c.WebkitBoxSizing,m.extend(k,{reliableHiddenOffsets:function(){return null==g&&i(),g},boxSizingReliable:function(){return null==f&&i(),f},pixelPosition:function(){return null==e&&i(),e},reliableMarginRight:function(){return null==h&&i(),h}});function i(){var b,c,d,i;c=y.getElementsByTagName("body")[0],c&&c.style&&(b=y.createElement("div"),d=y.createElement("div"),d.style.cssText="position:absolute;border:0;width:0;height:0;top:0;left:-9999px",c.appendChild(d).appendChild(b),b.style.cssText="-webkit-box-sizing:border-box;-moz-box-sizing:border-box;box-sizing:border-box;display:block;margin-top:1%;top:1%;border:1px;padding:1px;width:4px;position:absolute",e=f=!1,h=!0,a.getComputedStyle&&(e="1%"!==(a.getComputedStyle(b,null)||{}).top,f="4px"===(a.getComputedStyle(b,null)||{width:"4px"}).width,i=b.appendChild(y.createElement("div")),i.style.cssText=b.style.cssText="-webkit-box-sizing:content-box;-moz-box-sizing:content-box;box-sizing:content-box;display:block;margin:0;border:0;padding:0",i.style.marginRight=i.style.width="0",b.style.width="1px",h=!parseFloat((a.getComputedStyle(i,null)||{}).marginRight)),b.innerHTML="<table><tr><td></td><td>t</td></tr></table>",i=b.getElementsByTagName("td"),i[0].style.cssText="margin:0;border:0;padding:0;display:none",g=0===i[0].offsetHeight,g&&(i[0].style.display="",i[1].style.display="none",g=0===i[0].offsetHeight),c.removeChild(d))}}}(),m.swap=function(a,b,c,d){var e,f,g={};for(f in b)g[f]=a.style[f],a.style[f]=b[f];e=c.apply(a,d||[]);for(f in b)a.style[f]=g[f];return e};var Mb=/alpha\([^)]*\)/i,Nb=/opacity\s*=\s*([^)]*)/,Ob=/^(none|table(?!-c[ea]).+)/,Pb=new RegExp("^("+S+")(.*)$","i"),Qb=new RegExp("^([+-])=("+S+")","i"),Rb={position:"absolute",visibility:"hidden",display:"block"},Sb={letterSpacing:"0",fontWeight:"400"},Tb=["Webkit","O","Moz","ms"];function Ub(a,b){if(b in a)return b;var c=b.charAt(0).toUpperCase()+b.slice(1),d=b,e=Tb.length;while(e--)if(b=Tb[e]+c,b in a)return b;return d}function Vb(a,b){for(var c,d,e,f=[],g=0,h=a.length;h>g;g++)d=a[g],d.style&&(f[g]=m._data(d,"olddisplay"),c=d.style.display,b?(f[g]||"none"!==c||(d.style.display=""),""===d.style.display&&U(d)&&(f[g]=m._data(d,"olddisplay",Fb(d.nodeName)))):(e=U(d),(c&&"none"!==c||!e)&&m._data(d,"olddisplay",e?c:m.css(d,"display"))));for(g=0;h>g;g++)d=a[g],d.style&&(b&&"none"!==d.style.display&&""!==d.style.display||(d.style.display=b?f[g]||"":"none"));return a}function Wb(a,b,c){var d=Pb.exec(b);return d?Math.max(0,d[1]-(c||0))+(d[2]||"px"):b}function Xb(a,b,c,d,e){for(var f=c===(d?"border":"content")?4:"width"===b?1:0,g=0;4>f;f+=2)"margin"===c&&(g+=m.css(a,c+T[f],!0,e)),d?("content"===c&&(g-=m.css(a,"padding"+T[f],!0,e)),"margin"!==c&&(g-=m.css(a,"border"+T[f]+"Width",!0,e))):(g+=m.css(a,"padding"+T[f],!0,e),"padding"!==c&&(g+=m.css(a,"border"+T[f]+"Width",!0,e)));return g}function Yb(a,b,c){var d=!0,e="width"===b?a.offsetWidth:a.offsetHeight,f=Ib(a),g=k.boxSizing&&"border-box"===m.css(a,"boxSizing",!1,f);if(0>=e||null==e){if(e=Jb(a,b,f),(0>e||null==e)&&(e=a.style[b]),Hb.test(e))return e;d=g&&(k.boxSizingReliable()||e===a.style[b]),e=parseFloat(e)||0}return e+Xb(a,b,c||(g?"border":"content"),d,f)+"px"}m.extend({cssHooks:{opacity:{get:function(a,b){if(b){var c=Jb(a,"opacity");return""===c?"1":c}}}},cssNumber:{columnCount:!0,fillOpacity:!0,flexGrow:!0,flexShrink:!0,fontWeight:!0,lineHeight:!0,opacity:!0,order:!0,orphans:!0,widows:!0,zIndex:!0,zoom:!0},cssProps:{"float":k.cssFloat?"cssFloat":"styleFloat"},style:function(a,b,c,d){if(a&&3!==a.nodeType&&8!==a.nodeType&&a.style){var e,f,g,h=m.camelCase(b),i=a.style;if(b=m.cssProps[h]||(m.cssProps[h]=Ub(i,h)),g=m.cssHooks[b]||m.cssHooks[h],void 0===c)return g&&"get"in g&&void 0!==(e=g.get(a,!1,d))?e:i[b];if(f=typeof c,"string"===f&&(e=Qb.exec(c))&&(c=(e[1]+1)*e[2]+parseFloat(m.css(a,b)),f="number"),null!=c&&c===c&&("number"!==f||m.cssNumber[h]||(c+="px"),k.clearCloneStyle||""!==c||0!==b.indexOf("background")||(i[b]="inherit"),!(g&&"set"in g&&void 0===(c=g.set(a,c,d)))))try{i[b]=c}catch(j){}}},css:function(a,b,c,d){var e,f,g,h=m.camelCase(b);return b=m.cssProps[h]||(m.cssProps[h]=Ub(a.style,h)),g=m.cssHooks[b]||m.cssHooks[h],g&&"get"in g&&(f=g.get(a,!0,c)),void 0===f&&(f=Jb(a,b,d)),"normal"===f&&b in Sb&&(f=Sb[b]),""===c||c?(e=parseFloat(f),c===!0||m.isNumeric(e)?e||0:f):f}}),m.each(["height","width"],function(a,b){m.cssHooks[b]={get:function(a,c,d){return c?Ob.test(m.css(a,"display"))&&0===a.offsetWidth?m.swap(a,Rb,function(){return Yb(a,b,d)}):Yb(a,b,d):void 0},set:function(a,c,d){var e=d&&Ib(a);return Wb(a,c,d?Xb(a,b,d,k.boxSizing&&"border-box"===m.css(a,"boxSizing",!1,e),e):0)}}}),k.opacity||(m.cssHooks.opacity={get:function(a,b){return Nb.test((b&&a.currentStyle?a.currentStyle.filter:a.style.filter)||"")?.01*parseFloat(RegExp.$1)+"":b?"1":""},set:function(a,b){var c=a.style,d=a.currentStyle,e=m.isNumeric(b)?"alpha(opacity="+100*b+")":"",f=d&&d.filter||c.filter||"";c.zoom=1,(b>=1||""===b)&&""===m.trim(f.replace(Mb,""))&&c.removeAttribute&&(c.removeAttribute("filter"),""===b||d&&!d.filter)||(c.filter=Mb.test(f)?f.replace(Mb,e):f+" "+e)}}),m.cssHooks.marginRight=Lb(k.reliableMarginRight,function(a,b){return b?m.swap(a,{display:"inline-block"},Jb,[a,"marginRight"]):void 0}),m.each({margin:"",padding:"",border:"Width"},function(a,b){m.cssHooks[a+b]={expand:function(c){for(var d=0,e={},f="string"==typeof c?c.split(" "):[c];4>d;d++)e[a+T[d]+b]=f[d]||f[d-2]||f[0];return e}},Gb.test(a)||(m.cssHooks[a+b].set=Wb)}),m.fn.extend({css:function(a,b){return V(this,function(a,b,c){var d,e,f={},g=0;if(m.isArray(b)){for(d=Ib(a),e=b.length;e>g;g++)f[b[g]]=m.css(a,b[g],!1,d);return f}return void 0!==c?m.style(a,b,c):m.css(a,b)},a,b,arguments.length>1)},show:function(){return Vb(this,!0)},hide:function(){return Vb(this)},toggle:function(a){return"boolean"==typeof a?a?this.show():this.hide():this.each(function(){U(this)?m(this).show():m(this).hide()})}});function Zb(a,b,c,d,e){return new Zb.prototype.init(a,b,c,d,e)}m.Tween=Zb,Zb.prototype={constructor:Zb,init:function(a,b,c,d,e,f){this.elem=a,this.prop=c,this.easing=e||"swing",this.options=b,this.start=this.now=this.cur(),this.end=d,this.unit=f||(m.cssNumber[c]?"":"px")
},cur:function(){var a=Zb.propHooks[this.prop];return a&&a.get?a.get(this):Zb.propHooks._default.get(this)},run:function(a){var b,c=Zb.propHooks[this.prop];return this.pos=b=this.options.duration?m.easing[this.easing](a,this.options.duration*a,0,1,this.options.duration):a,this.now=(this.end-this.start)*b+this.start,this.options.step&&this.options.step.call(this.elem,this.now,this),c&&c.set?c.set(this):Zb.propHooks._default.set(this),this}},Zb.prototype.init.prototype=Zb.prototype,Zb.propHooks={_default:{get:function(a){var b;return null==a.elem[a.prop]||a.elem.style&&null!=a.elem.style[a.prop]?(b=m.css(a.elem,a.prop,""),b&&"auto"!==b?b:0):a.elem[a.prop]},set:function(a){m.fx.step[a.prop]?m.fx.step[a.prop](a):a.elem.style&&(null!=a.elem.style[m.cssProps[a.prop]]||m.cssHooks[a.prop])?m.style(a.elem,a.prop,a.now+a.unit):a.elem[a.prop]=a.now}}},Zb.propHooks.scrollTop=Zb.propHooks.scrollLeft={set:function(a){a.elem.nodeType&&a.elem.parentNode&&(a.elem[a.prop]=a.now)}},m.easing={linear:function(a){return a},swing:function(a){return.5-Math.cos(a*Math.PI)/2}},m.fx=Zb.prototype.init,m.fx.step={};var $b,_b,ac=/^(?:toggle|show|hide)$/,bc=new RegExp("^(?:([+-])=|)("+S+")([a-z%]*)$","i"),cc=/queueHooks$/,dc=[ic],ec={"*":[function(a,b){var c=this.createTween(a,b),d=c.cur(),e=bc.exec(b),f=e&&e[3]||(m.cssNumber[a]?"":"px"),g=(m.cssNumber[a]||"px"!==f&&+d)&&bc.exec(m.css(c.elem,a)),h=1,i=20;if(g&&g[3]!==f){f=f||g[3],e=e||[],g=+d||1;do h=h||".5",g/=h,m.style(c.elem,a,g+f);while(h!==(h=c.cur()/d)&&1!==h&&--i)}return e&&(g=c.start=+g||+d||0,c.unit=f,c.end=e[1]?g+(e[1]+1)*e[2]:+e[2]),c}]};function fc(){return setTimeout(function(){$b=void 0}),$b=m.now()}function gc(a,b){var c,d={height:a},e=0;for(b=b?1:0;4>e;e+=2-b)c=T[e],d["margin"+c]=d["padding"+c]=a;return b&&(d.opacity=d.width=a),d}function hc(a,b,c){for(var d,e=(ec[b]||[]).concat(ec["*"]),f=0,g=e.length;g>f;f++)if(d=e[f].call(c,b,a))return d}function ic(a,b,c){var d,e,f,g,h,i,j,l,n=this,o={},p=a.style,q=a.nodeType&&U(a),r=m._data(a,"fxshow");c.queue||(h=m._queueHooks(a,"fx"),null==h.unqueued&&(h.unqueued=0,i=h.empty.fire,h.empty.fire=function(){h.unqueued||i()}),h.unqueued++,n.always(function(){n.always(function(){h.unqueued--,m.queue(a,"fx").length||h.empty.fire()})})),1===a.nodeType&&("height"in b||"width"in b)&&(c.overflow=[p.overflow,p.overflowX,p.overflowY],j=m.css(a,"display"),l="none"===j?m._data(a,"olddisplay")||Fb(a.nodeName):j,"inline"===l&&"none"===m.css(a,"float")&&(k.inlineBlockNeedsLayout&&"inline"!==Fb(a.nodeName)?p.zoom=1:p.display="inline-block")),c.overflow&&(p.overflow="hidden",k.shrinkWrapBlocks()||n.always(function(){p.overflow=c.overflow[0],p.overflowX=c.overflow[1],p.overflowY=c.overflow[2]}));for(d in b)if(e=b[d],ac.exec(e)){if(delete b[d],f=f||"toggle"===e,e===(q?"hide":"show")){if("show"!==e||!r||void 0===r[d])continue;q=!0}o[d]=r&&r[d]||m.style(a,d)}else j=void 0;if(m.isEmptyObject(o))"inline"===("none"===j?Fb(a.nodeName):j)&&(p.display=j);else{r?"hidden"in r&&(q=r.hidden):r=m._data(a,"fxshow",{}),f&&(r.hidden=!q),q?m(a).show():n.done(function(){m(a).hide()}),n.done(function(){var b;m._removeData(a,"fxshow");for(b in o)m.style(a,b,o[b])});for(d in o)g=hc(q?r[d]:0,d,n),d in r||(r[d]=g.start,q&&(g.end=g.start,g.start="width"===d||"height"===d?1:0))}}function jc(a,b){var c,d,e,f,g;for(c in a)if(d=m.camelCase(c),e=b[d],f=a[c],m.isArray(f)&&(e=f[1],f=a[c]=f[0]),c!==d&&(a[d]=f,delete a[c]),g=m.cssHooks[d],g&&"expand"in g){f=g.expand(f),delete a[d];for(c in f)c in a||(a[c]=f[c],b[c]=e)}else b[d]=e}function kc(a,b,c){var d,e,f=0,g=dc.length,h=m.Deferred().always(function(){delete i.elem}),i=function(){if(e)return!1;for(var b=$b||fc(),c=Math.max(0,j.startTime+j.duration-b),d=c/j.duration||0,f=1-d,g=0,i=j.tweens.length;i>g;g++)j.tweens[g].run(f);return h.notifyWith(a,[j,f,c]),1>f&&i?c:(h.resolveWith(a,[j]),!1)},j=h.promise({elem:a,props:m.extend({},b),opts:m.extend(!0,{specialEasing:{}},c),originalProperties:b,originalOptions:c,startTime:$b||fc(),duration:c.duration,tweens:[],createTween:function(b,c){var d=m.Tween(a,j.opts,b,c,j.opts.specialEasing[b]||j.opts.easing);return j.tweens.push(d),d},stop:function(b){var c=0,d=b?j.tweens.length:0;if(e)return this;for(e=!0;d>c;c++)j.tweens[c].run(1);return b?h.resolveWith(a,[j,b]):h.rejectWith(a,[j,b]),this}}),k=j.props;for(jc(k,j.opts.specialEasing);g>f;f++)if(d=dc[f].call(j,a,k,j.opts))return d;return m.map(k,hc,j),m.isFunction(j.opts.start)&&j.opts.start.call(a,j),m.fx.timer(m.extend(i,{elem:a,anim:j,queue:j.opts.queue})),j.progress(j.opts.progress).done(j.opts.done,j.opts.complete).fail(j.opts.fail).always(j.opts.always)}m.Animation=m.extend(kc,{tweener:function(a,b){m.isFunction(a)?(b=a,a=["*"]):a=a.split(" ");for(var c,d=0,e=a.length;e>d;d++)c=a[d],ec[c]=ec[c]||[],ec[c].unshift(b)},prefilter:function(a,b){b?dc.unshift(a):dc.push(a)}}),m.speed=function(a,b,c){var d=a&&"object"==typeof a?m.extend({},a):{complete:c||!c&&b||m.isFunction(a)&&a,duration:a,easing:c&&b||b&&!m.isFunction(b)&&b};return d.duration=m.fx.off?0:"number"==typeof d.duration?d.duration:d.duration in m.fx.speeds?m.fx.speeds[d.duration]:m.fx.speeds._default,(null==d.queue||d.queue===!0)&&(d.queue="fx"),d.old=d.complete,d.complete=function(){m.isFunction(d.old)&&d.old.call(this),d.queue&&m.dequeue(this,d.queue)},d},m.fn.extend({fadeTo:function(a,b,c,d){return this.filter(U).css("opacity",0).show().end().animate({opacity:b},a,c,d)},animate:function(a,b,c,d){var e=m.isEmptyObject(a),f=m.speed(b,c,d),g=function(){var b=kc(this,m.extend({},a),f);(e||m._data(this,"finish"))&&b.stop(!0)};return g.finish=g,e||f.queue===!1?this.each(g):this.queue(f.queue,g)},stop:function(a,b,c){var d=function(a){var b=a.stop;delete a.stop,b(c)};return"string"!=typeof a&&(c=b,b=a,a=void 0),b&&a!==!1&&this.queue(a||"fx",[]),this.each(function(){var b=!0,e=null!=a&&a+"queueHooks",f=m.timers,g=m._data(this);if(e)g[e]&&g[e].stop&&d(g[e]);else for(e in g)g[e]&&g[e].stop&&cc.test(e)&&d(g[e]);for(e=f.length;e--;)f[e].elem!==this||null!=a&&f[e].queue!==a||(f[e].anim.stop(c),b=!1,f.splice(e,1));(b||!c)&&m.dequeue(this,a)})},finish:function(a){return a!==!1&&(a=a||"fx"),this.each(function(){var b,c=m._data(this),d=c[a+"queue"],e=c[a+"queueHooks"],f=m.timers,g=d?d.length:0;for(c.finish=!0,m.queue(this,a,[]),e&&e.stop&&e.stop.call(this,!0),b=f.length;b--;)f[b].elem===this&&f[b].queue===a&&(f[b].anim.stop(!0),f.splice(b,1));for(b=0;g>b;b++)d[b]&&d[b].finish&&d[b].finish.call(this);delete c.finish})}}),m.each(["toggle","show","hide"],function(a,b){var c=m.fn[b];m.fn[b]=function(a,d,e){return null==a||"boolean"==typeof a?c.apply(this,arguments):this.animate(gc(b,!0),a,d,e)}}),m.each({slideDown:gc("show"),slideUp:gc("hide"),slideToggle:gc("toggle"),fadeIn:{opacity:"show"},fadeOut:{opacity:"hide"},fadeToggle:{opacity:"toggle"}},function(a,b){m.fn[a]=function(a,c,d){return this.animate(b,a,c,d)}}),m.timers=[],m.fx.tick=function(){var a,b=m.timers,c=0;for($b=m.now();c<b.length;c++)a=b[c],a()||b[c]!==a||b.splice(c--,1);b.length||m.fx.stop(),$b=void 0},m.fx.timer=function(a){m.timers.push(a),a()?m.fx.start():m.timers.pop()},m.fx.interval=13,m.fx.start=function(){_b||(_b=setInterval(m.fx.tick,m.fx.interval))},m.fx.stop=function(){clearInterval(_b),_b=null},m.fx.speeds={slow:600,fast:200,_default:400},m.fn.delay=function(a,b){return a=m.fx?m.fx.speeds[a]||a:a,b=b||"fx",this.queue(b,function(b,c){var d=setTimeout(b,a);c.stop=function(){clearTimeout(d)}})},function(){var a,b,c,d,e;b=y.createElement("div"),b.setAttribute("className","t"),b.innerHTML=" <link/><table></table><a href='/a'>a</a><input type='checkbox'/>",d=b.getElementsByTagName("a")[0],c=y.createElement("select"),e=c.appendChild(y.createElement("option")),a=b.getElementsByTagName("input")[0],d.style.cssText="top:1px",k.getSetAttribute="t"!==b.className,k.style=/top/.test(d.getAttribute("style")),k.hrefNormalized="/a"===d.getAttribute("href"),k.checkOn=!!a.value,k.optSelected=e.selected,k.enctype=!!y.createElement("form").enctype,c.disabled=!0,k.optDisabled=!e.disabled,a=y.createElement("input"),a.setAttribute("value",""),k.input=""===a.getAttribute("value"),a.value="t",a.setAttribute("type","radio"),k.radioValue="t"===a.value}();var lc=/\r/g;m.fn.extend({val:function(a){var b,c,d,e=this[0];{if(arguments.length)return d=m.isFunction(a),this.each(function(c){var e;1===this.nodeType&&(e=d?a.call(this,c,m(this).val()):a,null==e?e="":"number"==typeof e?e+="":m.isArray(e)&&(e=m.map(e,function(a){return null==a?"":a+""})),b=m.valHooks[this.type]||m.valHooks[this.nodeName.toLowerCase()],b&&"set"in b&&void 0!==b.set(this,e,"value")||(this.value=e))});if(e)return b=m.valHooks[e.type]||m.valHooks[e.nodeName.toLowerCase()],b&&"get"in b&&void 0!==(c=b.get(e,"value"))?c:(c=e.value,"string"==typeof c?c.replace(lc,""):null==c?"":c)}}}),m.extend({valHooks:{option:{get:function(a){var b=m.find.attr(a,"value");return null!=b?b:m.trim(m.text(a))}},select:{get:function(a){for(var b,c,d=a.options,e=a.selectedIndex,f="select-one"===a.type||0>e,g=f?null:[],h=f?e+1:d.length,i=0>e?h:f?e:0;h>i;i++)if(c=d[i],!(!c.selected&&i!==e||(k.optDisabled?c.disabled:null!==c.getAttribute("disabled"))||c.parentNode.disabled&&m.nodeName(c.parentNode,"optgroup"))){if(b=m(c).val(),f)return b;g.push(b)}return g},set:function(a,b){var c,d,e=a.options,f=m.makeArray(b),g=e.length;while(g--)if(d=e[g],m.inArray(m.valHooks.option.get(d),f)>=0)try{d.selected=c=!0}catch(h){d.scrollHeight}else d.selected=!1;return c||(a.selectedIndex=-1),e}}}}),m.each(["radio","checkbox"],function(){m.valHooks[this]={set:function(a,b){return m.isArray(b)?a.checked=m.inArray(m(a).val(),b)>=0:void 0}},k.checkOn||(m.valHooks[this].get=function(a){return null===a.getAttribute("value")?"on":a.value})});var mc,nc,oc=m.expr.attrHandle,pc=/^(?:checked|selected)$/i,qc=k.getSetAttribute,rc=k.input;m.fn.extend({attr:function(a,b){return V(this,m.attr,a,b,arguments.length>1)},removeAttr:function(a){return this.each(function(){m.removeAttr(this,a)})}}),m.extend({attr:function(a,b,c){var d,e,f=a.nodeType;if(a&&3!==f&&8!==f&&2!==f)return typeof a.getAttribute===K?m.prop(a,b,c):(1===f&&m.isXMLDoc(a)||(b=b.toLowerCase(),d=m.attrHooks[b]||(m.expr.match.bool.test(b)?nc:mc)),void 0===c?d&&"get"in d&&null!==(e=d.get(a,b))?e:(e=m.find.attr(a,b),null==e?void 0:e):null!==c?d&&"set"in d&&void 0!==(e=d.set(a,c,b))?e:(a.setAttribute(b,c+""),c):void m.removeAttr(a,b))},removeAttr:function(a,b){var c,d,e=0,f=b&&b.match(E);if(f&&1===a.nodeType)while(c=f[e++])d=m.propFix[c]||c,m.expr.match.bool.test(c)?rc&&qc||!pc.test(c)?a[d]=!1:a[m.camelCase("default-"+c)]=a[d]=!1:m.attr(a,c,""),a.removeAttribute(qc?c:d)},attrHooks:{type:{set:function(a,b){if(!k.radioValue&&"radio"===b&&m.nodeName(a,"input")){var c=a.value;return a.setAttribute("type",b),c&&(a.value=c),b}}}}}),nc={set:function(a,b,c){return b===!1?m.removeAttr(a,c):rc&&qc||!pc.test(c)?a.setAttribute(!qc&&m.propFix[c]||c,c):a[m.camelCase("default-"+c)]=a[c]=!0,c}},m.each(m.expr.match.bool.source.match(/\w+/g),function(a,b){var c=oc[b]||m.find.attr;oc[b]=rc&&qc||!pc.test(b)?function(a,b,d){var e,f;return d||(f=oc[b],oc[b]=e,e=null!=c(a,b,d)?b.toLowerCase():null,oc[b]=f),e}:function(a,b,c){return c?void 0:a[m.camelCase("default-"+b)]?b.toLowerCase():null}}),rc&&qc||(m.attrHooks.value={set:function(a,b,c){return m.nodeName(a,"input")?void(a.defaultValue=b):mc&&mc.set(a,b,c)}}),qc||(mc={set:function(a,b,c){var d=a.getAttributeNode(c);return d||a.setAttributeNode(d=a.ownerDocument.createAttribute(c)),d.value=b+="","value"===c||b===a.getAttribute(c)?b:void 0}},oc.id=oc.name=oc.coords=function(a,b,c){var d;return c?void 0:(d=a.getAttributeNode(b))&&""!==d.value?d.value:null},m.valHooks.button={get:function(a,b){var c=a.getAttributeNode(b);return c&&c.specified?c.value:void 0},set:mc.set},m.attrHooks.contenteditable={set:function(a,b,c){mc.set(a,""===b?!1:b,c)}},m.each(["width","height"],function(a,b){m.attrHooks[b]={set:function(a,c){return""===c?(a.setAttribute(b,"auto"),c):void 0}}})),k.style||(m.attrHooks.style={get:function(a){return a.style.cssText||void 0},set:function(a,b){return a.style.cssText=b+""}});var sc=/^(?:input|select|textarea|button|object)$/i,tc=/^(?:a|area)$/i;m.fn.extend({prop:function(a,b){return V(this,m.prop,a,b,arguments.length>1)},removeProp:function(a){return a=m.propFix[a]||a,this.each(function(){try{this[a]=void 0,delete this[a]}catch(b){}})}}),m.extend({propFix:{"for":"htmlFor","class":"className"},prop:function(a,b,c){var d,e,f,g=a.nodeType;if(a&&3!==g&&8!==g&&2!==g)return f=1!==g||!m.isXMLDoc(a),f&&(b=m.propFix[b]||b,e=m.propHooks[b]),void 0!==c?e&&"set"in e&&void 0!==(d=e.set(a,c,b))?d:a[b]=c:e&&"get"in e&&null!==(d=e.get(a,b))?d:a[b]},propHooks:{tabIndex:{get:function(a){var b=m.find.attr(a,"tabindex");return b?parseInt(b,10):sc.test(a.nodeName)||tc.test(a.nodeName)&&a.href?0:-1}}}}),k.hrefNormalized||m.each(["href","src"],function(a,b){m.propHooks[b]={get:function(a){return a.getAttribute(b,4)}}}),k.optSelected||(m.propHooks.selected={get:function(a){var b=a.parentNode;return b&&(b.selectedIndex,b.parentNode&&b.parentNode.selectedIndex),null}}),m.each(["tabIndex","readOnly","maxLength","cellSpacing","cellPadding","rowSpan","colSpan","useMap","frameBorder","contentEditable"],function(){m.propFix[this.toLowerCase()]=this}),k.enctype||(m.propFix.enctype="encoding");var uc=/[\t\r\n\f]/g;m.fn.extend({addClass:function(a){var b,c,d,e,f,g,h=0,i=this.length,j="string"==typeof a&&a;if(m.isFunction(a))return this.each(function(b){m(this).addClass(a.call(this,b,this.className))});if(j)for(b=(a||"").match(E)||[];i>h;h++)if(c=this[h],d=1===c.nodeType&&(c.className?(" "+c.className+" ").replace(uc," "):" ")){f=0;while(e=b[f++])d.indexOf(" "+e+" ")<0&&(d+=e+" ");g=m.trim(d),c.className!==g&&(c.className=g)}return this},removeClass:function(a){var b,c,d,e,f,g,h=0,i=this.length,j=0===arguments.length||"string"==typeof a&&a;if(m.isFunction(a))return this.each(function(b){m(this).removeClass(a.call(this,b,this.className))});if(j)for(b=(a||"").match(E)||[];i>h;h++)if(c=this[h],d=1===c.nodeType&&(c.className?(" "+c.className+" ").replace(uc," "):"")){f=0;while(e=b[f++])while(d.indexOf(" "+e+" ")>=0)d=d.replace(" "+e+" "," ");g=a?m.trim(d):"",c.className!==g&&(c.className=g)}return this},toggleClass:function(a,b){var c=typeof a;return"boolean"==typeof b&&"string"===c?b?this.addClass(a):this.removeClass(a):this.each(m.isFunction(a)?function(c){m(this).toggleClass(a.call(this,c,this.className,b),b)}:function(){if("string"===c){var b,d=0,e=m(this),f=a.match(E)||[];while(b=f[d++])e.hasClass(b)?e.removeClass(b):e.addClass(b)}else(c===K||"boolean"===c)&&(this.className&&m._data(this,"__className__",this.className),this.className=this.className||a===!1?"":m._data(this,"__className__")||"")})},hasClass:function(a){for(var b=" "+a+" ",c=0,d=this.length;d>c;c++)if(1===this[c].nodeType&&(" "+this[c].className+" ").replace(uc," ").indexOf(b)>=0)return!0;return!1}}),m.each("blur focus focusin focusout load resize scroll unload click dblclick mousedown mouseup mousemove mouseover mouseout mouseenter mouseleave change select submit keydown keypress keyup error contextmenu".split(" "),function(a,b){m.fn[b]=function(a,c){return arguments.length>0?this.on(b,null,a,c):this.trigger(b)}}),m.fn.extend({hover:function(a,b){return this.mouseenter(a).mouseleave(b||a)},bind:function(a,b,c){return this.on(a,null,b,c)},unbind:function(a,b){return this.off(a,null,b)},delegate:function(a,b,c,d){return this.on(b,a,c,d)},undelegate:function(a,b,c){return 1===arguments.length?this.off(a,"**"):this.off(b,a||"**",c)}});var vc=m.now(),wc=/\?/,xc=/(,)|(\[|{)|(}|])|"(?:[^"\\\r\n]|\\["\\\/bfnrt]|\\u[\da-fA-F]{4})*"\s*:?|true|false|null|-?(?!0\d)\d+(?:\.\d+|)(?:[eE][+-]?\d+|)/g;m.parseJSON=function(b){if(a.JSON&&a.JSON.parse)return a.JSON.parse(b+"");var c,d=null,e=m.trim(b+"");return e&&!m.trim(e.replace(xc,function(a,b,e,f){return c&&b&&(d=0),0===d?a:(c=e||b,d+=!f-!e,"")}))?Function("return "+e)():m.error("Invalid JSON: "+b)},m.parseXML=function(b){var c,d;if(!b||"string"!=typeof b)return null;try{a.DOMParser?(d=new DOMParser,c=d.parseFromString(b,"text/xml")):(c=new ActiveXObject("Microsoft.XMLDOM"),c.async="false",c.loadXML(b))}catch(e){c=void 0}return c&&c.documentElement&&!c.getElementsByTagName("parsererror").length||m.error("Invalid XML: "+b),c};var yc,zc,Ac=/#.*$/,Bc=/([?&])_=[^&]*/,Cc=/^(.*?):[ \t]*([^\r\n]*)\r?$/gm,Dc=/^(?:about|app|app-storage|.+-extension|file|res|widget):$/,Ec=/^(?:GET|HEAD)$/,Fc=/^\/\//,Gc=/^([\w.+-]+:)(?:\/\/(?:[^\/?#]*@|)([^\/?#:]*)(?::(\d+)|)|)/,Hc={},Ic={},Jc="*/".concat("*");try{zc=location.href}catch(Kc){zc=y.createElement("a"),zc.href="",zc=zc.href}yc=Gc.exec(zc.toLowerCase())||[];function Lc(a){return function(b,c){"string"!=typeof b&&(c=b,b="*");var d,e=0,f=b.toLowerCase().match(E)||[];if(m.isFunction(c))while(d=f[e++])"+"===d.charAt(0)?(d=d.slice(1)||"*",(a[d]=a[d]||[]).unshift(c)):(a[d]=a[d]||[]).push(c)}}function Mc(a,b,c,d){var e={},f=a===Ic;function g(h){var i;return e[h]=!0,m.each(a[h]||[],function(a,h){var j=h(b,c,d);return"string"!=typeof j||f||e[j]?f?!(i=j):void 0:(b.dataTypes.unshift(j),g(j),!1)}),i}return g(b.dataTypes[0])||!e["*"]&&g("*")}function Nc(a,b){var c,d,e=m.ajaxSettings.flatOptions||{};for(d in b)void 0!==b[d]&&((e[d]?a:c||(c={}))[d]=b[d]);return c&&m.extend(!0,a,c),a}function Oc(a,b,c){var d,e,f,g,h=a.contents,i=a.dataTypes;while("*"===i[0])i.shift(),void 0===e&&(e=a.mimeType||b.getResponseHeader("Content-Type"));if(e)for(g in h)if(h[g]&&h[g].test(e)){i.unshift(g);break}if(i[0]in c)f=i[0];else{for(g in c){if(!i[0]||a.converters[g+" "+i[0]]){f=g;break}d||(d=g)}f=f||d}return f?(f!==i[0]&&i.unshift(f),c[f]):void 0}function Pc(a,b,c,d){var e,f,g,h,i,j={},k=a.dataTypes.slice();if(k[1])for(g in a.converters)j[g.toLowerCase()]=a.converters[g];f=k.shift();while(f)if(a.responseFields[f]&&(c[a.responseFields[f]]=b),!i&&d&&a.dataFilter&&(b=a.dataFilter(b,a.dataType)),i=f,f=k.shift())if("*"===f)f=i;else if("*"!==i&&i!==f){if(g=j[i+" "+f]||j["* "+f],!g)for(e in j)if(h=e.split(" "),h[1]===f&&(g=j[i+" "+h[0]]||j["* "+h[0]])){g===!0?g=j[e]:j[e]!==!0&&(f=h[0],k.unshift(h[1]));break}if(g!==!0)if(g&&a["throws"])b=g(b);else try{b=g(b)}catch(l){return{state:"parsererror",error:g?l:"No conversion from "+i+" to "+f}}}return{state:"success",data:b}}m.extend({active:0,lastModified:{},etag:{},ajaxSettings:{url:zc,type:"GET",isLocal:Dc.test(yc[1]),global:!0,processData:!0,async:!0,contentType:"application/x-www-form-urlencoded; charset=UTF-8",accepts:{"*":Jc,text:"text/plain",html:"text/html",xml:"application/xml, text/xml",json:"application/json, text/javascript"},contents:{xml:/xml/,html:/html/,json:/json/},responseFields:{xml:"responseXML",text:"responseText",json:"responseJSON"},converters:{"* text":String,"text html":!0,"text json":m.parseJSON,"text xml":m.parseXML},flatOptions:{url:!0,context:!0}},ajaxSetup:function(a,b){return b?Nc(Nc(a,m.ajaxSettings),b):Nc(m.ajaxSettings,a)},ajaxPrefilter:Lc(Hc),ajaxTransport:Lc(Ic),ajax:function(a,b){"object"==typeof a&&(b=a,a=void 0),b=b||{};var c,d,e,f,g,h,i,j,k=m.ajaxSetup({},b),l=k.context||k,n=k.context&&(l.nodeType||l.jquery)?m(l):m.event,o=m.Deferred(),p=m.Callbacks("once memory"),q=k.statusCode||{},r={},s={},t=0,u="canceled",v={readyState:0,getResponseHeader:function(a){var b;if(2===t){if(!j){j={};while(b=Cc.exec(f))j[b[1].toLowerCase()]=b[2]}b=j[a.toLowerCase()]}return null==b?null:b},getAllResponseHeaders:function(){return 2===t?f:null},setRequestHeader:function(a,b){var c=a.toLowerCase();return t||(a=s[c]=s[c]||a,r[a]=b),this},overrideMimeType:function(a){return t||(k.mimeType=a),this},statusCode:function(a){var b;if(a)if(2>t)for(b in a)q[b]=[q[b],a[b]];else v.always(a[v.status]);return this},abort:function(a){var b=a||u;return i&&i.abort(b),x(0,b),this}};if(o.promise(v).complete=p.add,v.success=v.done,v.error=v.fail,k.url=((a||k.url||zc)+"").replace(Ac,"").replace(Fc,yc[1]+"//"),k.type=b.method||b.type||k.method||k.type,k.dataTypes=m.trim(k.dataType||"*").toLowerCase().match(E)||[""],null==k.crossDomain&&(c=Gc.exec(k.url.toLowerCase()),k.crossDomain=!(!c||c[1]===yc[1]&&c[2]===yc[2]&&(c[3]||("http:"===c[1]?"80":"443"))===(yc[3]||("http:"===yc[1]?"80":"443")))),k.data&&k.processData&&"string"!=typeof k.data&&(k.data=m.param(k.data,k.traditional)),Mc(Hc,k,b,v),2===t)return v;h=k.global,h&&0===m.active++&&m.event.trigger("ajaxStart"),k.type=k.type.toUpperCase(),k.hasContent=!Ec.test(k.type),e=k.url,k.hasContent||(k.data&&(e=k.url+=(wc.test(e)?"&":"?")+k.data,delete k.data),k.cache===!1&&(k.url=Bc.test(e)?e.replace(Bc,"$1_="+vc++):e+(wc.test(e)?"&":"?")+"_="+vc++)),k.ifModified&&(m.lastModified[e]&&v.setRequestHeader("If-Modified-Since",m.lastModified[e]),m.etag[e]&&v.setRequestHeader("If-None-Match",m.etag[e])),(k.data&&k.hasContent&&k.contentType!==!1||b.contentType)&&v.setRequestHeader("Content-Type",k.contentType),v.setRequestHeader("Accept",k.dataTypes[0]&&k.accepts[k.dataTypes[0]]?k.accepts[k.dataTypes[0]]+("*"!==k.dataTypes[0]?", "+Jc+"; q=0.01":""):k.accepts["*"]);for(d in k.headers)v.setRequestHeader(d,k.headers[d]);if(k.beforeSend&&(k.beforeSend.call(l,v,k)===!1||2===t))return v.abort();u="abort";for(d in{success:1,error:1,complete:1})v[d](k[d]);if(i=Mc(Ic,k,b,v)){v.readyState=1,h&&n.trigger("ajaxSend",[v,k]),k.async&&k.timeout>0&&(g=setTimeout(function(){v.abort("timeout")},k.timeout));try{t=1,i.send(r,x)}catch(w){if(!(2>t))throw w;x(-1,w)}}else x(-1,"No Transport");function x(a,b,c,d){var j,r,s,u,w,x=b;2!==t&&(t=2,g&&clearTimeout(g),i=void 0,f=d||"",v.readyState=a>0?4:0,j=a>=200&&300>a||304===a,c&&(u=Oc(k,v,c)),u=Pc(k,u,v,j),j?(k.ifModified&&(w=v.getResponseHeader("Last-Modified"),w&&(m.lastModified[e]=w),w=v.getResponseHeader("etag"),w&&(m.etag[e]=w)),204===a||"HEAD"===k.type?x="nocontent":304===a?x="notmodified":(x=u.state,r=u.data,s=u.error,j=!s)):(s=x,(a||!x)&&(x="error",0>a&&(a=0))),v.status=a,v.statusText=(b||x)+"",j?o.resolveWith(l,[r,x,v]):o.rejectWith(l,[v,x,s]),v.statusCode(q),q=void 0,h&&n.trigger(j?"ajaxSuccess":"ajaxError",[v,k,j?r:s]),p.fireWith(l,[v,x]),h&&(n.trigger("ajaxComplete",[v,k]),--m.active||m.event.trigger("ajaxStop")))}return v},getJSON:function(a,b,c){return m.get(a,b,c,"json")},getScript:function(a,b){return m.get(a,void 0,b,"script")}}),m.each(["get","post"],function(a,b){m[b]=function(a,c,d,e){return m.isFunction(c)&&(e=e||d,d=c,c=void 0),m.ajax({url:a,type:b,dataType:e,data:c,success:d})}}),m.each(["ajaxStart","ajaxStop","ajaxComplete","ajaxError","ajaxSuccess","ajaxSend"],function(a,b){m.fn[b]=function(a){return this.on(b,a)}}),m._evalUrl=function(a){return m.ajax({url:a,type:"GET",dataType:"script",async:!1,global:!1,"throws":!0})},m.fn.extend({wrapAll:function(a){if(m.isFunction(a))return this.each(function(b){m(this).wrapAll(a.call(this,b))});if(this[0]){var b=m(a,this[0].ownerDocument).eq(0).clone(!0);this[0].parentNode&&b.insertBefore(this[0]),b.map(function(){var a=this;while(a.firstChild&&1===a.firstChild.nodeType)a=a.firstChild;return a}).append(this)}return this},wrapInner:function(a){return this.each(m.isFunction(a)?function(b){m(this).wrapInner(a.call(this,b))}:function(){var b=m(this),c=b.contents();c.length?c.wrapAll(a):b.append(a)})},wrap:function(a){var b=m.isFunction(a);return this.each(function(c){m(this).wrapAll(b?a.call(this,c):a)})},unwrap:function(){return this.parent().each(function(){m.nodeName(this,"body")||m(this).replaceWith(this.childNodes)}).end()}}),m.expr.filters.hidden=function(a){return a.offsetWidth<=0&&a.offsetHeight<=0||!k.reliableHiddenOffsets()&&"none"===(a.style&&a.style.display||m.css(a,"display"))},m.expr.filters.visible=function(a){return!m.expr.filters.hidden(a)};var Qc=/%20/g,Rc=/\[\]$/,Sc=/\r?\n/g,Tc=/^(?:submit|button|image|reset|file)$/i,Uc=/^(?:input|select|textarea|keygen)/i;function Vc(a,b,c,d){var e;if(m.isArray(b))m.each(b,function(b,e){c||Rc.test(a)?d(a,e):Vc(a+"["+("object"==typeof e?b:"")+"]",e,c,d)});else if(c||"object"!==m.type(b))d(a,b);else for(e in b)Vc(a+"["+e+"]",b[e],c,d)}m.param=function(a,b){var c,d=[],e=function(a,b){b=m.isFunction(b)?b():null==b?"":b,d[d.length]=encodeURIComponent(a)+"="+encodeURIComponent(b)};if(void 0===b&&(b=m.ajaxSettings&&m.ajaxSettings.traditional),m.isArray(a)||a.jquery&&!m.isPlainObject(a))m.each(a,function(){e(this.name,this.value)});else for(c in a)Vc(c,a[c],b,e);return d.join("&").replace(Qc,"+")},m.fn.extend({serialize:function(){return m.param(this.serializeArray())},serializeArray:function(){return this.map(function(){var a=m.prop(this,"elements");return a?m.makeArray(a):this}).filter(function(){var a=this.type;return this.name&&!m(this).is(":disabled")&&Uc.test(this.nodeName)&&!Tc.test(a)&&(this.checked||!W.test(a))}).map(function(a,b){var c=m(this).val();return null==c?null:m.isArray(c)?m.map(c,function(a){return{name:b.name,value:a.replace(Sc,"\r\n")}}):{name:b.name,value:c.replace(Sc,"\r\n")}}).get()}}),m.ajaxSettings.xhr=void 0!==a.ActiveXObject?function(){return!this.isLocal&&/^(get|post|head|put|delete|options)$/i.test(this.type)&&Zc()||$c()}:Zc;var Wc=0,Xc={},Yc=m.ajaxSettings.xhr();a.ActiveXObject&&m(a).on("unload",function(){for(var a in Xc)Xc[a](void 0,!0)}),k.cors=!!Yc&&"withCredentials"in Yc,Yc=k.ajax=!!Yc,Yc&&m.ajaxTransport(function(a){if(!a.crossDomain||k.cors){var b;return{send:function(c,d){var e,f=a.xhr(),g=++Wc;if(f.open(a.type,a.url,a.async,a.username,a.password),a.xhrFields)for(e in a.xhrFields)f[e]=a.xhrFields[e];a.mimeType&&f.overrideMimeType&&f.overrideMimeType(a.mimeType),a.crossDomain||c["X-Requested-With"]||(c["X-Requested-With"]="XMLHttpRequest");for(e in c)void 0!==c[e]&&f.setRequestHeader(e,c[e]+"");f.send(a.hasContent&&a.data||null),b=function(c,e){var h,i,j;if(b&&(e||4===f.readyState))if(delete Xc[g],b=void 0,f.onreadystatechange=m.noop,e)4!==f.readyState&&f.abort();else{j={},h=f.status,"string"==typeof f.responseText&&(j.text=f.responseText);try{i=f.statusText}catch(k){i=""}h||!a.isLocal||a.crossDomain?1223===h&&(h=204):h=j.text?200:404}j&&d(h,i,j,f.getAllResponseHeaders())},a.async?4===f.readyState?setTimeout(b):f.onreadystatechange=Xc[g]=b:b()},abort:function(){b&&b(void 0,!0)}}}});function Zc(){try{return new a.XMLHttpRequest}catch(b){}}function $c(){try{return new a.ActiveXObject("Microsoft.XMLHTTP")}catch(b){}}m.ajaxSetup({accepts:{script:"text/javascript, application/javascript, application/ecmascript, application/x-ecmascript"},contents:{script:/(?:java|ecma)script/},converters:{"text script":function(a){return m.globalEval(a),a}}}),m.ajaxPrefilter("script",function(a){void 0===a.cache&&(a.cache=!1),a.crossDomain&&(a.type="GET",a.global=!1)}),m.ajaxTransport("script",function(a){if(a.crossDomain){var b,c=y.head||m("head")[0]||y.documentElement;return{send:function(d,e){b=y.createElement("script"),b.async=!0,a.scriptCharset&&(b.charset=a.scriptCharset),b.src=a.url,b.onload=b.onreadystatechange=function(a,c){(c||!b.readyState||/loaded|complete/.test(b.readyState))&&(b.onload=b.onreadystatechange=null,b.parentNode&&b.parentNode.removeChild(b),b=null,c||e(200,"success"))},c.insertBefore(b,c.firstChild)},abort:function(){b&&b.onload(void 0,!0)}}}});var _c=[],ad=/(=)\?(?=&|$)|\?\?/;m.ajaxSetup({jsonp:"callback",jsonpCallback:function(){var a=_c.pop()||m.expando+"_"+vc++;return this[a]=!0,a}}),m.ajaxPrefilter("json jsonp",function(b,c,d){var e,f,g,h=b.jsonp!==!1&&(ad.test(b.url)?"url":"string"==typeof b.data&&!(b.contentType||"").indexOf("application/x-www-form-urlencoded")&&ad.test(b.data)&&"data");return h||"jsonp"===b.dataTypes[0]?(e=b.jsonpCallback=m.isFunction(b.jsonpCallback)?b.jsonpCallback():b.jsonpCallback,h?b[h]=b[h].replace(ad,"$1"+e):b.jsonp!==!1&&(b.url+=(wc.test(b.url)?"&":"?")+b.jsonp+"="+e),b.converters["script json"]=function(){return g||m.error(e+" was not called"),g[0]},b.dataTypes[0]="json",f=a[e],a[e]=function(){g=arguments},d.always(function(){a[e]=f,b[e]&&(b.jsonpCallback=c.jsonpCallback,_c.push(e)),g&&m.isFunction(f)&&f(g[0]),g=f=void 0}),"script"):void 0}),m.parseHTML=function(a,b,c){if(!a||"string"!=typeof a)return null;"boolean"==typeof b&&(c=b,b=!1),b=b||y;var d=u.exec(a),e=!c&&[];return d?[b.createElement(d[1])]:(d=m.buildFragment([a],b,e),e&&e.length&&m(e).remove(),m.merge([],d.childNodes))};var bd=m.fn.load;m.fn.load=function(a,b,c){if("string"!=typeof a&&bd)return bd.apply(this,arguments);var d,e,f,g=this,h=a.indexOf(" ");return h>=0&&(d=m.trim(a.slice(h,a.length)),a=a.slice(0,h)),m.isFunction(b)?(c=b,b=void 0):b&&"object"==typeof b&&(f="POST"),g.length>0&&m.ajax({url:a,type:f,dataType:"html",data:b}).done(function(a){e=arguments,g.html(d?m("<div>").append(m.parseHTML(a)).find(d):a)}).complete(c&&function(a,b){g.each(c,e||[a.responseText,b,a])}),this},m.expr.filters.animated=function(a){return m.grep(m.timers,function(b){return a===b.elem}).length};var cd=a.document.documentElement;function dd(a){return m.isWindow(a)?a:9===a.nodeType?a.defaultView||a.parentWindow:!1}m.offset={setOffset:function(a,b,c){var d,e,f,g,h,i,j,k=m.css(a,"position"),l=m(a),n={};"static"===k&&(a.style.position="relative"),h=l.offset(),f=m.css(a,"top"),i=m.css(a,"left"),j=("absolute"===k||"fixed"===k)&&m.inArray("auto",[f,i])>-1,j?(d=l.position(),g=d.top,e=d.left):(g=parseFloat(f)||0,e=parseFloat(i)||0),m.isFunction(b)&&(b=b.call(a,c,h)),null!=b.top&&(n.top=b.top-h.top+g),null!=b.left&&(n.left=b.left-h.left+e),"using"in b?b.using.call(a,n):l.css(n)}},m.fn.extend({offset:function(a){if(arguments.length)return void 0===a?this:this.each(function(b){m.offset.setOffset(this,a,b)});var b,c,d={top:0,left:0},e=this[0],f=e&&e.ownerDocument;if(f)return b=f.documentElement,m.contains(b,e)?(typeof e.getBoundingClientRect!==K&&(d=e.getBoundingClientRect()),c=dd(f),{top:d.top+(c.pageYOffset||b.scrollTop)-(b.clientTop||0),left:d.left+(c.pageXOffset||b.scrollLeft)-(b.clientLeft||0)}):d},position:function(){if(this[0]){var a,b,c={top:0,left:0},d=this[0];return"fixed"===m.css(d,"position")?b=d.getBoundingClientRect():(a=this.offsetParent(),b=this.offset(),m.nodeName(a[0],"html")||(c=a.offset()),c.top+=m.css(a[0],"borderTopWidth",!0),c.left+=m.css(a[0],"borderLeftWidth",!0)),{top:b.top-c.top-m.css(d,"marginTop",!0),left:b.left-c.left-m.css(d,"marginLeft",!0)}}},offsetParent:function(){return this.map(function(){var a=this.offsetParent||cd;while(a&&!m.nodeName(a,"html")&&"static"===m.css(a,"position"))a=a.offsetParent;return a||cd})}}),m.each({scrollLeft:"pageXOffset",scrollTop:"pageYOffset"},function(a,b){var c=/Y/.test(b);m.fn[a]=function(d){return V(this,function(a,d,e){var f=dd(a);return void 0===e?f?b in f?f[b]:f.document.documentElement[d]:a[d]:void(f?f.scrollTo(c?m(f).scrollLeft():e,c?e:m(f).scrollTop()):a[d]=e)},a,d,arguments.length,null)}}),m.each(["top","left"],function(a,b){m.cssHooks[b]=Lb(k.pixelPosition,function(a,c){return c?(c=Jb(a,b),Hb.test(c)?m(a).position()[b]+"px":c):void 0})}),m.each({Height:"height",Width:"width"},function(a,b){m.each({padding:"inner"+a,content:b,"":"outer"+a},function(c,d){m.fn[d]=function(d,e){var f=arguments.length&&(c||"boolean"!=typeof d),g=c||(d===!0||e===!0?"margin":"border");return V(this,function(b,c,d){var e;return m.isWindow(b)?b.document.documentElement["client"+a]:9===b.nodeType?(e=b.documentElement,Math.max(b.body["scroll"+a],e["scroll"+a],b.body["offset"+a],e["offset"+a],e["client"+a])):void 0===d?m.css(b,c,g):m.style(b,c,d,g)},b,f?d:void 0,f,null)}})}),m.fn.size=function(){return this.length},m.fn.andSelf=m.fn.addBack,"function"==typeof define&&define.amd&&define("jquery",[],function(){return m});var ed=a.jQuery,fd=a.$;return m.noConflict=function(b){return a.$===m&&(a.$=fd),b&&a.jQuery===m&&(a.jQuery=ed),m},typeof b===K&&(a.jQuery=a.$=m),m}); |
app/containers/BootStrap/BSFooterSitemap/index.js | perry-ugroop/ugroop-react-dup2 | /**
* Created by Ber on 25/11/16.
*/
import React from 'react';
const BSFooterSitemap = (props) => <div className={`${props.className}`}>
{props.children}
</div>;
BSFooterSitemap.propTypes = {
className: React.PropTypes.any,
children: React.PropTypes.any,
};
export default BSFooterSitemap;
|
tests/Rules-minLength-spec.js | bitgaming/formsy-react | import React from 'react';
import TestUtils from 'react-addons-test-utils';
import Formsy from './..';
import { InputFactory } from './utils/TestInput';
const TestInput = InputFactory({
render() {
return <input value={this.getValue()} readOnly/>;
}
});
const TestForm = React.createClass({
render() {
return (
<Formsy.Form>
<TestInput name="foo" validations={this.props.rule} value={this.props.inputValue}/>
</Formsy.Form>
);
}
});
export default {
'minLength:3': {
'should pass with a default value': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:3"/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), true);
test.done();
},
'should pass when a string\'s length is bigger': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:3" inputValue="myValue"/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), true);
test.done();
},
'should fail when a string\'s length is smaller': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:3" inputValue="my"/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), false);
test.done();
},
'should pass with empty string': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:3" inputValue=""/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), true);
test.done();
},
'should pass with an undefined': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:3" inputValue={undefined}/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), true);
test.done();
},
'should pass with a null': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:3" inputValue={null}/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), true);
test.done();
},
'should fail with a number': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:3" inputValue={42}/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), false);
test.done();
}
},
'minLength:0': {
'should pass with a default value': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:0"/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), true);
test.done();
},
'should pass when a string\'s length is bigger': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:0" inputValue="myValue"/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), true);
test.done();
},
'should pass with empty string': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:0" inputValue=""/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), true);
test.done();
},
'should pass with an undefined': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:0" inputValue={undefined}/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), true);
test.done();
},
'should pass with a null': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:0" inputValue={null}/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), true);
test.done();
},
'should fail with a number': function (test) {
const form = TestUtils.renderIntoDocument(<TestForm rule="minLength:0" inputValue={42}/>);
const inputComponent = TestUtils.findRenderedComponentWithType(form, TestInput);
test.equal(inputComponent.isValid(), false);
test.done();
}
}
};
|
src/js/components/InfiniteScroll/stories/Grid.js | HewlettPackard/grommet | import React from 'react';
import { Grid, Grommet, Box, Image, InfiniteScroll, Text } from 'grommet';
import { grommet } from 'grommet/themes';
const allItems = Array(2000)
.fill()
.map((_, i) => `item ${i + 1}`);
export const GridInfiniteScroll = () => (
<Grommet theme={grommet}>
<Grid columns="xsmall" rows="small">
<InfiniteScroll items={allItems} step={12}>
{item => (
<Box key={item} as="article" pad="xsmall">
<Image src="https://via.placeholder.com/350x150" />
<Text>{item}</Text>
</Box>
)}
</InfiniteScroll>
</Grid>
</Grommet>
);
GridInfiniteScroll.storyName = 'Grid';
export default {
title: 'Utilities/InfiniteScroll/Grid',
};
|
packages/react-router-dom/modules/Link.js | d-oliveros/react-router | import React from 'react'
import PropTypes from 'prop-types'
import invariant from 'invariant'
const isModifiedEvent = (event) =>
!!(event.metaKey || event.altKey || event.ctrlKey || event.shiftKey)
/**
* The public API for rendering a history-aware <a>.
*/
class Link extends React.Component {
static propTypes = {
onClick: PropTypes.func,
target: PropTypes.string,
replace: PropTypes.bool,
to: PropTypes.oneOfType([
PropTypes.string,
PropTypes.object
]).isRequired,
innerRef: PropTypes.oneOfType([
PropTypes.string,
PropTypes.func
])
}
static defaultProps = {
replace: false
}
static contextTypes = {
router: PropTypes.shape({
history: PropTypes.shape({
push: PropTypes.func.isRequired,
replace: PropTypes.func.isRequired,
createHref: PropTypes.func.isRequired
}).isRequired
}).isRequired
}
handleClick = (event) => {
if (this.props.onClick)
this.props.onClick(event)
if (
!event.defaultPrevented && // onClick prevented default
event.button === 0 && // ignore everything but left clicks
!this.props.target && // let browser handle "target=_blank" etc.
!isModifiedEvent(event) // ignore clicks with modifier keys
) {
event.preventDefault()
const { history } = this.context.router
const { replace, to } = this.props
if (replace) {
history.replace(to)
} else {
history.push(to)
}
}
}
render() {
const { replace, to, innerRef, ...props } = this.props // eslint-disable-line no-unused-vars
invariant(
this.context.router,
'You should not use <Link> outside a <Router>'
)
const href = this.context.router.history.createHref(
typeof to === 'string' ? { pathname: to } : to
)
return <a {...props} onClick={this.handleClick} href={href} ref={innerRef}/>
}
}
export default Link
|
ajax/libs/rxjs/2.2.22/rx.js | r3x/cdnjs | // Copyright (c) Microsoft Open Technologies, Inc. All rights reserved. See License.txt in the project root for license information.
;(function (undefined) {
var objectTypes = {
'boolean': false,
'function': true,
'object': true,
'number': false,
'string': false,
'undefined': false
};
var root = (objectTypes[typeof window] && window) || this,
freeExports = objectTypes[typeof exports] && exports && !exports.nodeType && exports,
freeModule = objectTypes[typeof module] && module && !module.nodeType && module,
moduleExports = freeModule && freeModule.exports === freeExports && freeExports,
freeGlobal = objectTypes[typeof global] && global;
if (freeGlobal && (freeGlobal.global === freeGlobal || freeGlobal.window === freeGlobal)) {
root = freeGlobal;
}
var Rx = {
internals: {},
config: {
Promise: root.Promise // Detect if promise exists
},
helpers: { }
};
// Defaults
var noop = Rx.helpers.noop = function () { },
identity = Rx.helpers.identity = function (x) { return x; },
defaultNow = Rx.helpers.defaultNow = Date.now,
defaultComparer = Rx.helpers.defaultComparer = function (x, y) { return isEqual(x, y); },
defaultSubComparer = Rx.helpers.defaultSubComparer = function (x, y) { return x > y ? 1 : (x < y ? -1 : 0); },
defaultKeySerializer = Rx.helpers.defaultKeySerializer = function (x) { return x.toString(); },
defaultError = Rx.helpers.defaultError = function (err) { throw err; },
isPromise = Rx.helpers.isPromise = function (p) { return !!p && typeof p.then === 'function' && p.then !== Rx.Observable.prototype.then; },
asArray = Rx.helpers.asArray = function () { return Array.prototype.slice.call(arguments); },
not = Rx.helpers.not = function (a) { return !a; };
// Errors
var sequenceContainsNoElements = 'Sequence contains no elements.';
var argumentOutOfRange = 'Argument out of range';
var objectDisposed = 'Object has been disposed';
function checkDisposed() { if (this.isDisposed) { throw new Error(objectDisposed); } }
// Shim in iterator support
var $iterator$ = (typeof Symbol === 'object' && Symbol.iterator) ||
'_es6shim_iterator_';
// Firefox ships a partial implementation using the name @@iterator.
// https://bugzilla.mozilla.org/show_bug.cgi?id=907077#c14
// So use that name if we detect it.
if (root.Set && typeof new root.Set()['@@iterator'] === 'function') {
$iterator$ = '@@iterator';
}
var doneEnumerator = { done: true, value: undefined };
/** `Object#toString` result shortcuts */
var argsClass = '[object Arguments]',
arrayClass = '[object Array]',
boolClass = '[object Boolean]',
dateClass = '[object Date]',
errorClass = '[object Error]',
funcClass = '[object Function]',
numberClass = '[object Number]',
objectClass = '[object Object]',
regexpClass = '[object RegExp]',
stringClass = '[object String]';
var toString = Object.prototype.toString,
hasOwnProperty = Object.prototype.hasOwnProperty,
supportsArgsClass = toString.call(arguments) == argsClass, // For less <IE9 && FF<4
suportNodeClass,
errorProto = Error.prototype,
objectProto = Object.prototype,
propertyIsEnumerable = objectProto.propertyIsEnumerable;
try {
suportNodeClass = !(toString.call(document) == objectClass && !({ 'toString': 0 } + ''));
} catch(e) {
suportNodeClass = true;
}
var shadowedProps = [
'constructor', 'hasOwnProperty', 'isPrototypeOf', 'propertyIsEnumerable', 'toLocaleString', 'toString', 'valueOf'
];
var nonEnumProps = {};
nonEnumProps[arrayClass] = nonEnumProps[dateClass] = nonEnumProps[numberClass] = { 'constructor': true, 'toLocaleString': true, 'toString': true, 'valueOf': true };
nonEnumProps[boolClass] = nonEnumProps[stringClass] = { 'constructor': true, 'toString': true, 'valueOf': true };
nonEnumProps[errorClass] = nonEnumProps[funcClass] = nonEnumProps[regexpClass] = { 'constructor': true, 'toString': true };
nonEnumProps[objectClass] = { 'constructor': true };
var support = {};
(function () {
var ctor = function() { this.x = 1; },
props = [];
ctor.prototype = { 'valueOf': 1, 'y': 1 };
for (var key in new ctor) { props.push(key); }
for (key in arguments) { }
// Detect if `name` or `message` properties of `Error.prototype` are enumerable by default.
support.enumErrorProps = propertyIsEnumerable.call(errorProto, 'message') || propertyIsEnumerable.call(errorProto, 'name');
// Detect if `prototype` properties are enumerable by default.
support.enumPrototypes = propertyIsEnumerable.call(ctor, 'prototype');
// Detect if `arguments` object indexes are non-enumerable
support.nonEnumArgs = key != 0;
// Detect if properties shadowing those on `Object.prototype` are non-enumerable.
support.nonEnumShadows = !/valueOf/.test(props);
}(1));
function isObject(value) {
// check if the value is the ECMAScript language type of Object
// http://es5.github.io/#x8
// and avoid a V8 bug
// https://code.google.com/p/v8/issues/detail?id=2291
var type = typeof value;
return value && (type == 'function' || type == 'object') || false;
}
function keysIn(object) {
var result = [];
if (!isObject(object)) {
return result;
}
if (support.nonEnumArgs && object.length && isArguments(object)) {
object = slice.call(object);
}
var skipProto = support.enumPrototypes && typeof object == 'function',
skipErrorProps = support.enumErrorProps && (object === errorProto || object instanceof Error);
for (var key in object) {
if (!(skipProto && key == 'prototype') &&
!(skipErrorProps && (key == 'message' || key == 'name'))) {
result.push(key);
}
}
if (support.nonEnumShadows && object !== objectProto) {
var ctor = object.constructor,
index = -1,
length = shadowedProps.length;
if (object === (ctor && ctor.prototype)) {
var className = object === stringProto ? stringClass : object === errorProto ? errorClass : toString.call(object),
nonEnum = nonEnumProps[className];
}
while (++index < length) {
key = shadowedProps[index];
if (!(nonEnum && nonEnum[key]) && hasOwnProperty.call(object, key)) {
result.push(key);
}
}
}
return result;
}
function internalFor(object, callback, keysFunc) {
var index = -1,
props = keysFunc(object),
length = props.length;
while (++index < length) {
var key = props[index];
if (callback(object[key], key, object) === false) {
break;
}
}
return object;
}
function internalForIn(object, callback) {
return internalFor(object, callback, keysIn);
}
function isNode(value) {
// IE < 9 presents DOM nodes as `Object` objects except they have `toString`
// methods that are `typeof` "string" and still can coerce nodes to strings
return typeof value.toString != 'function' && typeof (value + '') == 'string';
}
function isArguments(value) {
return (value && typeof value == 'object') ? toString.call(value) == argsClass : false;
}
// fallback for browsers that can't detect `arguments` objects by [[Class]]
if (!supportsArgsClass) {
isArguments = function(value) {
return (value && typeof value == 'object') ? hasOwnProperty.call(value, 'callee') : false;
};
}
function isFunction(value) {
return typeof value == 'function';
}
// fallback for older versions of Chrome and Safari
if (isFunction(/x/)) {
isFunction = function(value) {
return typeof value == 'function' && toString.call(value) == funcClass;
};
}
var isEqual = Rx.internals.isEqual = function (x, y) {
return deepEquals(x, y, [], []);
};
/** @private
* Used for deep comparison
**/
function deepEquals(a, b, stackA, stackB) {
// exit early for identical values
if (a === b) {
// treat `+0` vs. `-0` as not equal
return a !== 0 || (1 / a == 1 / b);
}
var type = typeof a,
otherType = typeof b;
// exit early for unlike primitive values
if (a === a && (a == null || b == null ||
(type != 'function' && type != 'object' && otherType != 'function' && otherType != 'object'))) {
return false;
}
// compare [[Class]] names
var className = toString.call(a),
otherClass = toString.call(b);
if (className == argsClass) {
className = objectClass;
}
if (otherClass == argsClass) {
otherClass = objectClass;
}
if (className != otherClass) {
return false;
}
switch (className) {
case boolClass:
case dateClass:
// coerce dates and booleans to numbers, dates to milliseconds and booleans
// to `1` or `0` treating invalid dates coerced to `NaN` as not equal
return +a == +b;
case numberClass:
// treat `NaN` vs. `NaN` as equal
return (a != +a)
? b != +b
// but treat `-0` vs. `+0` as not equal
: (a == 0 ? (1 / a == 1 / b) : a == +b);
case regexpClass:
case stringClass:
// coerce regexes to strings (http://es5.github.io/#x15.10.6.4)
// treat string primitives and their corresponding object instances as equal
return a == String(b);
}
var isArr = className == arrayClass;
if (!isArr) {
// exit for functions and DOM nodes
if (className != objectClass || (!support.nodeClass && (isNode(a) || isNode(b)))) {
return false;
}
// in older versions of Opera, `arguments` objects have `Array` constructors
var ctorA = !support.argsObject && isArguments(a) ? Object : a.constructor,
ctorB = !support.argsObject && isArguments(b) ? Object : b.constructor;
// non `Object` object instances with different constructors are not equal
if (ctorA != ctorB &&
!(hasOwnProperty.call(a, 'constructor') && hasOwnProperty.call(b, 'constructor')) &&
!(isFunction(ctorA) && ctorA instanceof ctorA && isFunction(ctorB) && ctorB instanceof ctorB) &&
('constructor' in a && 'constructor' in b)
) {
return false;
}
}
// assume cyclic structures are equal
// the algorithm for detecting cyclic structures is adapted from ES 5.1
// section 15.12.3, abstract operation `JO` (http://es5.github.io/#x15.12.3)
var initedStack = !stackA;
stackA || (stackA = []);
stackB || (stackB = []);
var length = stackA.length;
while (length--) {
if (stackA[length] == a) {
return stackB[length] == b;
}
}
var size = 0;
result = true;
// add `a` and `b` to the stack of traversed objects
stackA.push(a);
stackB.push(b);
// recursively compare objects and arrays (susceptible to call stack limits)
if (isArr) {
// compare lengths to determine if a deep comparison is necessary
length = a.length;
size = b.length;
result = size == length;
if (result) {
// deep compare the contents, ignoring non-numeric properties
while (size--) {
var index = length,
value = b[size];
if (!(result = deepEquals(a[size], value, stackA, stackB))) {
break;
}
}
}
}
else {
// deep compare objects using `forIn`, instead of `forOwn`, to avoid `Object.keys`
// which, in this case, is more costly
internalForIn(b, function(value, key, b) {
if (hasOwnProperty.call(b, key)) {
// count the number of properties.
size++;
// deep compare each property value.
return (result = hasOwnProperty.call(a, key) && deepEquals(a[key], value, stackA, stackB));
}
});
if (result) {
// ensure both objects have the same number of properties
internalForIn(a, function(value, key, a) {
if (hasOwnProperty.call(a, key)) {
// `size` will be `-1` if `a` has more properties than `b`
return (result = --size > -1);
}
});
}
}
stackA.pop();
stackB.pop();
return result;
}
var slice = Array.prototype.slice;
function argsOrArray(args, idx) {
return args.length === 1 && Array.isArray(args[idx]) ?
args[idx] :
slice.call(args);
}
var hasProp = {}.hasOwnProperty;
/** @private */
var inherits = this.inherits = Rx.internals.inherits = function (child, parent) {
function __() { this.constructor = child; }
__.prototype = parent.prototype;
child.prototype = new __();
};
/** @private */
var addProperties = Rx.internals.addProperties = function (obj) {
var sources = slice.call(arguments, 1);
for (var i = 0, len = sources.length; i < len; i++) {
var source = sources[i];
for (var prop in source) {
obj[prop] = source[prop];
}
}
};
// Rx Utils
var addRef = Rx.internals.addRef = function (xs, r) {
return new AnonymousObservable(function (observer) {
return new CompositeDisposable(r.getDisposable(), xs.subscribe(observer));
});
};
// Collection polyfills
function arrayInitialize(count, factory) {
var a = new Array(count);
for (var i = 0; i < count; i++) {
a[i] = factory();
}
return a;
}
// Collections
var IndexedItem = function (id, value) {
this.id = id;
this.value = value;
};
IndexedItem.prototype.compareTo = function (other) {
var c = this.value.compareTo(other.value);
if (c === 0) {
c = this.id - other.id;
}
return c;
};
// Priority Queue for Scheduling
var PriorityQueue = Rx.internals.PriorityQueue = function (capacity) {
this.items = new Array(capacity);
this.length = 0;
};
var priorityProto = PriorityQueue.prototype;
priorityProto.isHigherPriority = function (left, right) {
return this.items[left].compareTo(this.items[right]) < 0;
};
priorityProto.percolate = function (index) {
if (index >= this.length || index < 0) {
return;
}
var parent = index - 1 >> 1;
if (parent < 0 || parent === index) {
return;
}
if (this.isHigherPriority(index, parent)) {
var temp = this.items[index];
this.items[index] = this.items[parent];
this.items[parent] = temp;
this.percolate(parent);
}
};
priorityProto.heapify = function (index) {
if (index === undefined) {
index = 0;
}
if (index >= this.length || index < 0) {
return;
}
var left = 2 * index + 1,
right = 2 * index + 2,
first = index;
if (left < this.length && this.isHigherPriority(left, first)) {
first = left;
}
if (right < this.length && this.isHigherPriority(right, first)) {
first = right;
}
if (first !== index) {
var temp = this.items[index];
this.items[index] = this.items[first];
this.items[first] = temp;
this.heapify(first);
}
};
priorityProto.peek = function () { return this.items[0].value; };
priorityProto.removeAt = function (index) {
this.items[index] = this.items[--this.length];
delete this.items[this.length];
this.heapify();
};
priorityProto.dequeue = function () {
var result = this.peek();
this.removeAt(0);
return result;
};
priorityProto.enqueue = function (item) {
var index = this.length++;
this.items[index] = new IndexedItem(PriorityQueue.count++, item);
this.percolate(index);
};
priorityProto.remove = function (item) {
for (var i = 0; i < this.length; i++) {
if (this.items[i].value === item) {
this.removeAt(i);
return true;
}
}
return false;
};
PriorityQueue.count = 0;
/**
* Represents a group of disposable resources that are disposed together.
* @constructor
*/
var CompositeDisposable = Rx.CompositeDisposable = function () {
this.disposables = argsOrArray(arguments, 0);
this.isDisposed = false;
this.length = this.disposables.length;
};
var CompositeDisposablePrototype = CompositeDisposable.prototype;
/**
* Adds a disposable to the CompositeDisposable or disposes the disposable if the CompositeDisposable is disposed.
* @param {Mixed} item Disposable to add.
*/
CompositeDisposablePrototype.add = function (item) {
if (this.isDisposed) {
item.dispose();
} else {
this.disposables.push(item);
this.length++;
}
};
/**
* Removes and disposes the first occurrence of a disposable from the CompositeDisposable.
* @param {Mixed} item Disposable to remove.
* @returns {Boolean} true if found; false otherwise.
*/
CompositeDisposablePrototype.remove = function (item) {
var shouldDispose = false;
if (!this.isDisposed) {
var idx = this.disposables.indexOf(item);
if (idx !== -1) {
shouldDispose = true;
this.disposables.splice(idx, 1);
this.length--;
item.dispose();
}
}
return shouldDispose;
};
/**
* Disposes all disposables in the group and removes them from the group.
*/
CompositeDisposablePrototype.dispose = function () {
if (!this.isDisposed) {
this.isDisposed = true;
var currentDisposables = this.disposables.slice(0);
this.disposables = [];
this.length = 0;
for (var i = 0, len = currentDisposables.length; i < len; i++) {
currentDisposables[i].dispose();
}
}
};
/**
* Removes and disposes all disposables from the CompositeDisposable, but does not dispose the CompositeDisposable.
*/
CompositeDisposablePrototype.clear = function () {
var currentDisposables = this.disposables.slice(0);
this.disposables = [];
this.length = 0;
for (var i = 0, len = currentDisposables.length; i < len; i++) {
currentDisposables[i].dispose();
}
};
/**
* Determines whether the CompositeDisposable contains a specific disposable.
* @param {Mixed} item Disposable to search for.
* @returns {Boolean} true if the disposable was found; otherwise, false.
*/
CompositeDisposablePrototype.contains = function (item) {
return this.disposables.indexOf(item) !== -1;
};
/**
* Converts the existing CompositeDisposable to an array of disposables
* @returns {Array} An array of disposable objects.
*/
CompositeDisposablePrototype.toArray = function () {
return this.disposables.slice(0);
};
/**
* Provides a set of static methods for creating Disposables.
*
* @constructor
* @param {Function} dispose Action to run during the first call to dispose. The action is guaranteed to be run at most once.
*/
var Disposable = Rx.Disposable = function (action) {
this.isDisposed = false;
this.action = action || noop;
};
/** Performs the task of cleaning up resources. */
Disposable.prototype.dispose = function () {
if (!this.isDisposed) {
this.action();
this.isDisposed = true;
}
};
/**
* Creates a disposable object that invokes the specified action when disposed.
* @param {Function} dispose Action to run during the first call to dispose. The action is guaranteed to be run at most once.
* @return {Disposable} The disposable object that runs the given action upon disposal.
*/
var disposableCreate = Disposable.create = function (action) { return new Disposable(action); };
/**
* Gets the disposable that does nothing when disposed.
*/
var disposableEmpty = Disposable.empty = { dispose: noop };
var BooleanDisposable = (function () {
function BooleanDisposable (isSingle) {
this.isSingle = isSingle;
this.isDisposed = false;
this.current = null;
}
var booleanDisposablePrototype = BooleanDisposable.prototype;
/**
* Gets the underlying disposable.
* @return The underlying disposable.
*/
booleanDisposablePrototype.getDisposable = function () {
return this.current;
};
/**
* Sets the underlying disposable.
* @param {Disposable} value The new underlying disposable.
*/
booleanDisposablePrototype.setDisposable = function (value) {
if (this.current && this.isSingle) {
throw new Error('Disposable has already been assigned');
}
var shouldDispose = this.isDisposed, old;
if (!shouldDispose) {
old = this.current;
this.current = value;
}
if (old) {
old.dispose();
}
if (shouldDispose && value) {
value.dispose();
}
};
/**
* Disposes the underlying disposable as well as all future replacements.
*/
booleanDisposablePrototype.dispose = function () {
var old;
if (!this.isDisposed) {
this.isDisposed = true;
old = this.current;
this.current = null;
}
if (old) {
old.dispose();
}
};
return BooleanDisposable;
}());
/**
* Represents a disposable resource which only allows a single assignment of its underlying disposable resource.
* If an underlying disposable resource has already been set, future attempts to set the underlying disposable resource will throw an Error.
*/
var SingleAssignmentDisposable = Rx.SingleAssignmentDisposable = (function (super_) {
inherits(SingleAssignmentDisposable, super_);
function SingleAssignmentDisposable() {
super_.call(this, true);
}
return SingleAssignmentDisposable;
}(BooleanDisposable));
/**
* Represents a disposable resource whose underlying disposable resource can be replaced by another disposable resource, causing automatic disposal of the previous underlying disposable resource.
*/
var SerialDisposable = Rx.SerialDisposable = (function (super_) {
inherits(SerialDisposable, super_);
function SerialDisposable() {
super_.call(this, false);
}
return SerialDisposable;
}(BooleanDisposable));
/**
* Represents a disposable resource that only disposes its underlying disposable resource when all dependent disposable objects have been disposed.
*/
var RefCountDisposable = Rx.RefCountDisposable = (function () {
function InnerDisposable(disposable) {
this.disposable = disposable;
this.disposable.count++;
this.isInnerDisposed = false;
}
InnerDisposable.prototype.dispose = function () {
if (!this.disposable.isDisposed) {
if (!this.isInnerDisposed) {
this.isInnerDisposed = true;
this.disposable.count--;
if (this.disposable.count === 0 && this.disposable.isPrimaryDisposed) {
this.disposable.isDisposed = true;
this.disposable.underlyingDisposable.dispose();
}
}
}
};
/**
* Initializes a new instance of the RefCountDisposable with the specified disposable.
* @constructor
* @param {Disposable} disposable Underlying disposable.
*/
function RefCountDisposable(disposable) {
this.underlyingDisposable = disposable;
this.isDisposed = false;
this.isPrimaryDisposed = false;
this.count = 0;
}
/**
* Disposes the underlying disposable only when all dependent disposables have been disposed
*/
RefCountDisposable.prototype.dispose = function () {
if (!this.isDisposed) {
if (!this.isPrimaryDisposed) {
this.isPrimaryDisposed = true;
if (this.count === 0) {
this.isDisposed = true;
this.underlyingDisposable.dispose();
}
}
}
};
/**
* Returns a dependent disposable that when disposed decreases the refcount on the underlying disposable.
* @returns {Disposable} A dependent disposable contributing to the reference count that manages the underlying disposable's lifetime.
*/
RefCountDisposable.prototype.getDisposable = function () {
return this.isDisposed ? disposableEmpty : new InnerDisposable(this);
};
return RefCountDisposable;
})();
function ScheduledDisposable(scheduler, disposable) {
this.scheduler = scheduler;
this.disposable = disposable;
this.isDisposed = false;
}
ScheduledDisposable.prototype.dispose = function () {
var parent = this;
this.scheduler.schedule(function () {
if (!parent.isDisposed) {
parent.isDisposed = true;
parent.disposable.dispose();
}
});
};
var ScheduledItem = Rx.internals.ScheduledItem = function (scheduler, state, action, dueTime, comparer) {
this.scheduler = scheduler;
this.state = state;
this.action = action;
this.dueTime = dueTime;
this.comparer = comparer || defaultSubComparer;
this.disposable = new SingleAssignmentDisposable();
}
ScheduledItem.prototype.invoke = function () {
this.disposable.setDisposable(this.invokeCore());
};
ScheduledItem.prototype.compareTo = function (other) {
return this.comparer(this.dueTime, other.dueTime);
};
ScheduledItem.prototype.isCancelled = function () {
return this.disposable.isDisposed;
};
ScheduledItem.prototype.invokeCore = function () {
return this.action(this.scheduler, this.state);
};
/** Provides a set of static properties to access commonly used schedulers. */
var Scheduler = Rx.Scheduler = (function () {
/**
* @constructor
* @private
*/
function Scheduler(now, schedule, scheduleRelative, scheduleAbsolute) {
this.now = now;
this._schedule = schedule;
this._scheduleRelative = scheduleRelative;
this._scheduleAbsolute = scheduleAbsolute;
}
function invokeRecImmediate(scheduler, pair) {
var state = pair.first, action = pair.second, group = new CompositeDisposable(),
recursiveAction = function (state1) {
action(state1, function (state2) {
var isAdded = false, isDone = false,
d = scheduler.scheduleWithState(state2, function (scheduler1, state3) {
if (isAdded) {
group.remove(d);
} else {
isDone = true;
}
recursiveAction(state3);
return disposableEmpty;
});
if (!isDone) {
group.add(d);
isAdded = true;
}
});
};
recursiveAction(state);
return group;
}
function invokeRecDate(scheduler, pair, method) {
var state = pair.first, action = pair.second, group = new CompositeDisposable(),
recursiveAction = function (state1) {
action(state1, function (state2, dueTime1) {
var isAdded = false, isDone = false,
d = scheduler[method].call(scheduler, state2, dueTime1, function (scheduler1, state3) {
if (isAdded) {
group.remove(d);
} else {
isDone = true;
}
recursiveAction(state3);
return disposableEmpty;
});
if (!isDone) {
group.add(d);
isAdded = true;
}
});
};
recursiveAction(state);
return group;
}
function invokeAction(scheduler, action) {
action();
return disposableEmpty;
}
var schedulerProto = Scheduler.prototype;
/**
* Returns a scheduler that wraps the original scheduler, adding exception handling for scheduled actions.
* @param {Function} handler Handler that's run if an exception is caught. The exception will be rethrown if the handler returns false.
* @returns {Scheduler} Wrapper around the original scheduler, enforcing exception handling.
*/
schedulerProto.catchException = schedulerProto['catch'] = function (handler) {
return new CatchScheduler(this, handler);
};
/**
* Schedules a periodic piece of work by dynamically discovering the scheduler's capabilities. The periodic task will be scheduled using window.setInterval for the base implementation.
* @param {Number} period Period for running the work periodically.
* @param {Function} action Action to be executed.
* @returns {Disposable} The disposable object used to cancel the scheduled recurring action (best effort).
*/
schedulerProto.schedulePeriodic = function (period, action) {
return this.schedulePeriodicWithState(null, period, function () {
action();
});
};
/**
* Schedules a periodic piece of work by dynamically discovering the scheduler's capabilities. The periodic task will be scheduled using window.setInterval for the base implementation.
* @param {Mixed} state Initial state passed to the action upon the first iteration.
* @param {Number} period Period for running the work periodically.
* @param {Function} action Action to be executed, potentially updating the state.
* @returns {Disposable} The disposable object used to cancel the scheduled recurring action (best effort).
*/
schedulerProto.schedulePeriodicWithState = function (state, period, action) {
var s = state, id = setInterval(function () {
s = action(s);
}, period);
return disposableCreate(function () {
clearInterval(id);
});
};
/**
* Schedules an action to be executed.
* @param {Function} action Action to execute.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.schedule = function (action) {
return this._schedule(action, invokeAction);
};
/**
* Schedules an action to be executed.
* @param state State passed to the action to be executed.
* @param {Function} action Action to be executed.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithState = function (state, action) {
return this._schedule(state, action);
};
/**
* Schedules an action to be executed after the specified relative due time.
* @param {Function} action Action to execute.
* @param {Number} dueTime Relative time after which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithRelative = function (dueTime, action) {
return this._scheduleRelative(action, dueTime, invokeAction);
};
/**
* Schedules an action to be executed after dueTime.
* @param state State passed to the action to be executed.
* @param {Function} action Action to be executed.
* @param {Number} dueTime Relative time after which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithRelativeAndState = function (state, dueTime, action) {
return this._scheduleRelative(state, dueTime, action);
};
/**
* Schedules an action to be executed at the specified absolute due time.
* @param {Function} action Action to execute.
* @param {Number} dueTime Absolute time at which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithAbsolute = function (dueTime, action) {
return this._scheduleAbsolute(action, dueTime, invokeAction);
};
/**
* Schedules an action to be executed at dueTime.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to be executed.
* @param {Number}dueTime Absolute time at which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithAbsoluteAndState = function (state, dueTime, action) {
return this._scheduleAbsolute(state, dueTime, action);
};
/**
* Schedules an action to be executed recursively.
* @param {Function} action Action to execute recursively. The parameter passed to the action is used to trigger recursive scheduling of the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursive = function (action) {
return this.scheduleRecursiveWithState(action, function (_action, self) {
_action(function () {
self(_action);
});
});
};
/**
* Schedules an action to be executed recursively.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to execute recursively. The last parameter passed to the action is used to trigger recursive scheduling of the action, passing in recursive invocation state.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithState = function (state, action) {
return this.scheduleWithState({ first: state, second: action }, function (s, p) {
return invokeRecImmediate(s, p);
});
};
/**
* Schedules an action to be executed recursively after a specified relative due time.
* @param {Function} action Action to execute recursively. The parameter passed to the action is used to trigger recursive scheduling of the action at the specified relative time.
* @param {Number}dueTime Relative time after which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithRelative = function (dueTime, action) {
return this.scheduleRecursiveWithRelativeAndState(action, dueTime, function (_action, self) {
_action(function (dt) {
self(_action, dt);
});
});
};
/**
* Schedules an action to be executed recursively after a specified relative due time.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to execute recursively. The last parameter passed to the action is used to trigger recursive scheduling of the action, passing in the recursive due time and invocation state.
* @param {Number}dueTime Relative time after which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithRelativeAndState = function (state, dueTime, action) {
return this._scheduleRelative({ first: state, second: action }, dueTime, function (s, p) {
return invokeRecDate(s, p, 'scheduleWithRelativeAndState');
});
};
/**
* Schedules an action to be executed recursively at a specified absolute due time.
* @param {Function} action Action to execute recursively. The parameter passed to the action is used to trigger recursive scheduling of the action at the specified absolute time.
* @param {Number}dueTime Absolute time at which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithAbsolute = function (dueTime, action) {
return this.scheduleRecursiveWithAbsoluteAndState(action, dueTime, function (_action, self) {
_action(function (dt) {
self(_action, dt);
});
});
};
/**
* Schedules an action to be executed recursively at a specified absolute due time.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to execute recursively. The last parameter passed to the action is used to trigger recursive scheduling of the action, passing in the recursive due time and invocation state.
* @param {Number}dueTime Absolute time at which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithAbsoluteAndState = function (state, dueTime, action) {
return this._scheduleAbsolute({ first: state, second: action }, dueTime, function (s, p) {
return invokeRecDate(s, p, 'scheduleWithAbsoluteAndState');
});
};
/** Gets the current time according to the local machine's system clock. */
Scheduler.now = defaultNow;
/**
* Normalizes the specified TimeSpan value to a positive value.
* @param {Number} timeSpan The time span value to normalize.
* @returns {Number} The specified TimeSpan value if it is zero or positive; otherwise, 0
*/
Scheduler.normalize = function (timeSpan) {
if (timeSpan < 0) {
timeSpan = 0;
}
return timeSpan;
};
return Scheduler;
}());
var normalizeTime = Scheduler.normalize;
var SchedulePeriodicRecursive = Rx.internals.SchedulePeriodicRecursive = (function () {
function tick(command, recurse) {
recurse(0, this._period);
try {
this._state = this._action(this._state);
} catch (e) {
this._cancel.dispose();
throw e;
}
}
function SchedulePeriodicRecursive(scheduler, state, period, action) {
this._scheduler = scheduler;
this._state = state;
this._period = period;
this._action = action;
}
SchedulePeriodicRecursive.prototype.start = function () {
var d = new SingleAssignmentDisposable();
this._cancel = d;
d.setDisposable(this._scheduler.scheduleRecursiveWithRelativeAndState(0, this._period, tick.bind(this)));
return d;
};
return SchedulePeriodicRecursive;
}());
/**
* Gets a scheduler that schedules work immediately on the current thread.
*/
var immediateScheduler = Scheduler.immediate = (function () {
function scheduleNow(state, action) { return action(this, state); }
function scheduleRelative(state, dueTime, action) {
var dt = normalizeTime(dt);
while (dt - this.now() > 0) { }
return action(this, state);
}
function scheduleAbsolute(state, dueTime, action) {
return this.scheduleWithRelativeAndState(state, dueTime - this.now(), action);
}
return new Scheduler(defaultNow, scheduleNow, scheduleRelative, scheduleAbsolute);
}());
/**
* Gets a scheduler that schedules work as soon as possible on the current thread.
*/
var currentThreadScheduler = Scheduler.currentThread = (function () {
var queue;
function runTrampoline (q) {
var item;
while (q.length > 0) {
item = q.dequeue();
if (!item.isCancelled()) {
// Note, do not schedule blocking work!
while (item.dueTime - Scheduler.now() > 0) {
}
if (!item.isCancelled()) {
item.invoke();
}
}
}
}
function scheduleNow(state, action) {
return this.scheduleWithRelativeAndState(state, 0, action);
}
function scheduleRelative(state, dueTime, action) {
var dt = this.now() + Scheduler.normalize(dueTime),
si = new ScheduledItem(this, state, action, dt),
t;
if (!queue) {
queue = new PriorityQueue(4);
queue.enqueue(si);
try {
runTrampoline(queue);
} catch (e) {
throw e;
} finally {
queue = null;
}
} else {
queue.enqueue(si);
}
return si.disposable;
}
function scheduleAbsolute(state, dueTime, action) {
return this.scheduleWithRelativeAndState(state, dueTime - this.now(), action);
}
var currentScheduler = new Scheduler(defaultNow, scheduleNow, scheduleRelative, scheduleAbsolute);
currentScheduler.scheduleRequired = function () { return queue === null; };
currentScheduler.ensureTrampoline = function (action) {
if (queue === null) {
return this.schedule(action);
} else {
return action();
}
};
return currentScheduler;
}());
var scheduleMethod, clearMethod = noop;
(function () {
var reNative = RegExp('^' +
String(toString)
.replace(/[.*+?^${}()|[\]\\]/g, '\\$&')
.replace(/toString| for [^\]]+/g, '.*?') + '$'
);
var setImmediate = typeof (setImmediate = freeGlobal && moduleExports && freeGlobal.setImmediate) == 'function' &&
!reNative.test(setImmediate) && setImmediate,
clearImmediate = typeof (clearImmediate = freeGlobal && moduleExports && freeGlobal.clearImmediate) == 'function' &&
!reNative.test(clearImmediate) && clearImmediate;
function postMessageSupported () {
// Ensure not in a worker
if (!root.postMessage || root.importScripts) { return false; }
var isAsync = false,
oldHandler = root.onmessage;
// Test for async
root.onmessage = function () { isAsync = true; };
root.postMessage('','*');
root.onmessage = oldHandler;
return isAsync;
}
// Use in order, nextTick, setImmediate, postMessage, MessageChannel, script readystatechanged, setTimeout
if (typeof process !== 'undefined' && {}.toString.call(process) === '[object process]') {
scheduleMethod = process.nextTick;
} else if (typeof setImmediate === 'function') {
scheduleMethod = setImmediate;
clearMethod = clearImmediate;
} else if (postMessageSupported()) {
var MSG_PREFIX = 'ms.rx.schedule' + Math.random(),
tasks = {},
taskId = 0;
function onGlobalPostMessage(event) {
// Only if we're a match to avoid any other global events
if (typeof event.data === 'string' && event.data.substring(0, MSG_PREFIX.length) === MSG_PREFIX) {
var handleId = event.data.substring(MSG_PREFIX.length),
action = tasks[handleId];
action();
delete tasks[handleId];
}
}
if (root.addEventListener) {
root.addEventListener('message', onGlobalPostMessage, false);
} else {
root.attachEvent('onmessage', onGlobalPostMessage, false);
}
scheduleMethod = function (action) {
var currentId = taskId++;
tasks[currentId] = action;
root.postMessage(MSG_PREFIX + currentId, '*');
};
} else if (!!root.MessageChannel) {
var channel = new root.MessageChannel(),
channelTasks = {},
channelTaskId = 0;
channel.port1.onmessage = function (event) {
var id = event.data,
action = channelTasks[id];
action();
delete channelTasks[id];
};
scheduleMethod = function (action) {
var id = channelTaskId++;
channelTasks[id] = action;
channel.port2.postMessage(id);
};
} else if ('document' in root && 'onreadystatechange' in root.document.createElement('script')) {
scheduleMethod = function (action) {
var scriptElement = root.document.createElement('script');
scriptElement.onreadystatechange = function () {
action();
scriptElement.onreadystatechange = null;
scriptElement.parentNode.removeChild(scriptElement);
scriptElement = null;
};
root.document.documentElement.appendChild(scriptElement);
};
} else {
scheduleMethod = function (action) { return setTimeout(action, 0); };
clearMethod = clearTimeout;
}
}());
/**
* Gets a scheduler that schedules work via a timed callback based upon platform.
*/
var timeoutScheduler = Scheduler.timeout = (function () {
function scheduleNow(state, action) {
var scheduler = this,
disposable = new SingleAssignmentDisposable();
var id = scheduleMethod(function () {
if (!disposable.isDisposed) {
disposable.setDisposable(action(scheduler, state));
}
});
return new CompositeDisposable(disposable, disposableCreate(function () {
clearMethod(id);
}));
}
function scheduleRelative(state, dueTime, action) {
var scheduler = this,
dt = Scheduler.normalize(dueTime);
if (dt === 0) {
return scheduler.scheduleWithState(state, action);
}
var disposable = new SingleAssignmentDisposable();
var id = setTimeout(function () {
if (!disposable.isDisposed) {
disposable.setDisposable(action(scheduler, state));
}
}, dt);
return new CompositeDisposable(disposable, disposableCreate(function () {
clearTimeout(id);
}));
}
function scheduleAbsolute(state, dueTime, action) {
return this.scheduleWithRelativeAndState(state, dueTime - this.now(), action);
}
return new Scheduler(defaultNow, scheduleNow, scheduleRelative, scheduleAbsolute);
})();
/** @private */
var CatchScheduler = (function (_super) {
function localNow() {
return this._scheduler.now();
}
function scheduleNow(state, action) {
return this._scheduler.scheduleWithState(state, this._wrap(action));
}
function scheduleRelative(state, dueTime, action) {
return this._scheduler.scheduleWithRelativeAndState(state, dueTime, this._wrap(action));
}
function scheduleAbsolute(state, dueTime, action) {
return this._scheduler.scheduleWithAbsoluteAndState(state, dueTime, this._wrap(action));
}
inherits(CatchScheduler, _super);
/** @private */
function CatchScheduler(scheduler, handler) {
this._scheduler = scheduler;
this._handler = handler;
this._recursiveOriginal = null;
this._recursiveWrapper = null;
_super.call(this, localNow, scheduleNow, scheduleRelative, scheduleAbsolute);
}
/** @private */
CatchScheduler.prototype._clone = function (scheduler) {
return new CatchScheduler(scheduler, this._handler);
};
/** @private */
CatchScheduler.prototype._wrap = function (action) {
var parent = this;
return function (self, state) {
try {
return action(parent._getRecursiveWrapper(self), state);
} catch (e) {
if (!parent._handler(e)) { throw e; }
return disposableEmpty;
}
};
};
/** @private */
CatchScheduler.prototype._getRecursiveWrapper = function (scheduler) {
if (this._recursiveOriginal !== scheduler) {
this._recursiveOriginal = scheduler;
var wrapper = this._clone(scheduler);
wrapper._recursiveOriginal = scheduler;
wrapper._recursiveWrapper = wrapper;
this._recursiveWrapper = wrapper;
}
return this._recursiveWrapper;
};
/** @private */
CatchScheduler.prototype.schedulePeriodicWithState = function (state, period, action) {
var self = this, failed = false, d = new SingleAssignmentDisposable();
d.setDisposable(this._scheduler.schedulePeriodicWithState(state, period, function (state1) {
if (failed) { return null; }
try {
return action(state1);
} catch (e) {
failed = true;
if (!self._handler(e)) { throw e; }
d.dispose();
return null;
}
}));
return d;
};
return CatchScheduler;
}(Scheduler));
/**
* Represents a notification to an observer.
*/
var Notification = Rx.Notification = (function () {
function Notification(kind, hasValue) {
this.hasValue = hasValue == null ? false : hasValue;
this.kind = kind;
}
var NotificationPrototype = Notification.prototype;
/**
* Invokes the delegate corresponding to the notification or the observer's method corresponding to the notification and returns the produced result.
*
* @memberOf Notification
* @param {Any} observerOrOnNext Delegate to invoke for an OnNext notification or Observer to invoke the notification on..
* @param {Function} onError Delegate to invoke for an OnError notification.
* @param {Function} onCompleted Delegate to invoke for an OnCompleted notification.
* @returns {Any} Result produced by the observation.
*/
NotificationPrototype.accept = function (observerOrOnNext, onError, onCompleted) {
if (arguments.length === 1 && typeof observerOrOnNext === 'object') {
return this._acceptObservable(observerOrOnNext);
}
return this._accept(observerOrOnNext, onError, onCompleted);
};
/**
* Returns an observable sequence with a single notification.
*
* @memberOf Notification
* @param {Scheduler} [scheduler] Scheduler to send out the notification calls on.
* @returns {Observable} The observable sequence that surfaces the behavior of the notification upon subscription.
*/
NotificationPrototype.toObservable = function (scheduler) {
var notification = this;
scheduler || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.schedule(function () {
notification._acceptObservable(observer);
if (notification.kind === 'N') {
observer.onCompleted();
}
});
});
};
return Notification;
})();
/**
* Creates an object that represents an OnNext notification to an observer.
* @param {Any} value The value contained in the notification.
* @returns {Notification} The OnNext notification containing the value.
*/
var notificationCreateOnNext = Notification.createOnNext = (function () {
function _accept (onNext) {
return onNext(this.value);
}
function _acceptObservable(observer) {
return observer.onNext(this.value);
}
function toString () {
return 'OnNext(' + this.value + ')';
}
return function (value) {
var notification = new Notification('N', true);
notification.value = value;
notification._accept = _accept;
notification._acceptObservable = _acceptObservable;
notification.toString = toString;
return notification;
};
}());
/**
* Creates an object that represents an OnError notification to an observer.
* @param {Any} error The exception contained in the notification.
* @returns {Notification} The OnError notification containing the exception.
*/
var notificationCreateOnError = Notification.createOnError = (function () {
function _accept (onNext, onError) {
return onError(this.exception);
}
function _acceptObservable(observer) {
return observer.onError(this.exception);
}
function toString () {
return 'OnError(' + this.exception + ')';
}
return function (exception) {
var notification = new Notification('E');
notification.exception = exception;
notification._accept = _accept;
notification._acceptObservable = _acceptObservable;
notification.toString = toString;
return notification;
};
}());
/**
* Creates an object that represents an OnCompleted notification to an observer.
* @returns {Notification} The OnCompleted notification.
*/
var notificationCreateOnCompleted = Notification.createOnCompleted = (function () {
function _accept (onNext, onError, onCompleted) {
return onCompleted();
}
function _acceptObservable(observer) {
return observer.onCompleted();
}
function toString () {
return 'OnCompleted()';
}
return function () {
var notification = new Notification('C');
notification._accept = _accept;
notification._acceptObservable = _acceptObservable;
notification.toString = toString;
return notification;
};
}());
var Enumerator = Rx.internals.Enumerator = function (next) {
this._next = next;
};
Enumerator.prototype.next = function () {
return this._next();
};
Enumerator.prototype[$iterator$] = function () { return this; }
var Enumerable = Rx.internals.Enumerable = function (iterator) {
this._iterator = iterator;
};
Enumerable.prototype[$iterator$] = function () {
return this._iterator();
};
Enumerable.prototype.concat = function () {
var sources = this;
return new AnonymousObservable(function (observer) {
var e;
try {
e = sources[$iterator$]();
} catch(err) {
observer.onError();
return;
}
var isDisposed,
subscription = new SerialDisposable();
var cancelable = immediateScheduler.scheduleRecursive(function (self) {
var currentItem;
if (isDisposed) { return; }
try {
currentItem = e.next();
} catch (ex) {
observer.onError(ex);
return;
}
if (currentItem.done) {
observer.onCompleted();
return;
}
// Check if promise
var currentValue = currentItem.value;
isPromise(currentValue) && (currentValue = observableFromPromise(currentValue));
var d = new SingleAssignmentDisposable();
subscription.setDisposable(d);
d.setDisposable(currentValue.subscribe(
observer.onNext.bind(observer),
observer.onError.bind(observer),
function () { self(); })
);
});
return new CompositeDisposable(subscription, cancelable, disposableCreate(function () {
isDisposed = true;
}));
});
};
Enumerable.prototype.catchException = function () {
var sources = this;
return new AnonymousObservable(function (observer) {
var e;
try {
e = sources[$iterator$]();
} catch(err) {
observer.onError();
return;
}
var isDisposed,
lastException,
subscription = new SerialDisposable();
var cancelable = immediateScheduler.scheduleRecursive(function (self) {
if (isDisposed) { return; }
var currentItem;
try {
currentItem = e.next();
} catch (ex) {
observer.onError(ex);
return;
}
if (currentItem.done) {
if (lastException) {
observer.onError(lastException);
} else {
observer.onCompleted();
}
return;
}
// Check if promise
var currentValue = currentItem.value;
isPromise(currentValue) && (currentValue = observableFromPromise(currentValue));
var d = new SingleAssignmentDisposable();
subscription.setDisposable(d);
d.setDisposable(currentValue.subscribe(
observer.onNext.bind(observer),
function (exn) {
lastException = exn;
self();
},
observer.onCompleted.bind(observer)));
});
return new CompositeDisposable(subscription, cancelable, disposableCreate(function () {
isDisposed = true;
}));
});
};
var enumerableRepeat = Enumerable.repeat = function (value, repeatCount) {
if (repeatCount == null) { repeatCount = -1; }
return new Enumerable(function () {
var left = repeatCount;
return new Enumerator(function () {
if (left === 0) { return doneEnumerator; }
if (left > 0) { left--; }
return { done: false, value: value };
});
});
};
var enumerableFor = Enumerable.forEach = function (source, selector, thisArg) {
selector || (selector = identity);
return new Enumerable(function () {
var index = -1;
return new Enumerator(
function () {
return ++index < source.length ?
{ done: false, value: selector.call(thisArg, source[index], index, source) } :
doneEnumerator;
});
});
};
/**
* Supports push-style iteration over an observable sequence.
*/
var Observer = Rx.Observer = function () { };
/**
* Creates a notification callback from an observer.
*
* @param observer Observer object.
* @returns The action that forwards its input notification to the underlying observer.
*/
Observer.prototype.toNotifier = function () {
var observer = this;
return function (n) {
return n.accept(observer);
};
};
/**
* Hides the identity of an observer.
* @returns An observer that hides the identity of the specified observer.
*/
Observer.prototype.asObserver = function () {
return new AnonymousObserver(this.onNext.bind(this), this.onError.bind(this), this.onCompleted.bind(this));
};
/**
* Checks access to the observer for grammar violations. This includes checking for multiple OnError or OnCompleted calls, as well as reentrancy in any of the observer methods.
* If a violation is detected, an Error is thrown from the offending observer method call.
*
* @returns An observer that checks callbacks invocations against the observer grammar and, if the checks pass, forwards those to the specified observer.
*/
Observer.prototype.checked = function () { return new CheckedObserver(this); };
/**
* Creates an observer from the specified OnNext, along with optional OnError, and OnCompleted actions.
*
* @static
* @memberOf Observer
* @param {Function} [onNext] Observer's OnNext action implementation.
* @param {Function} [onError] Observer's OnError action implementation.
* @param {Function} [onCompleted] Observer's OnCompleted action implementation.
* @returns {Observer} The observer object implemented using the given actions.
*/
var observerCreate = Observer.create = function (onNext, onError, onCompleted) {
onNext || (onNext = noop);
onError || (onError = defaultError);
onCompleted || (onCompleted = noop);
return new AnonymousObserver(onNext, onError, onCompleted);
};
/**
* Creates an observer from a notification callback.
*
* @static
* @memberOf Observer
* @param {Function} handler Action that handles a notification.
* @returns The observer object that invokes the specified handler using a notification corresponding to each message it receives.
*/
Observer.fromNotifier = function (handler) {
return new AnonymousObserver(function (x) {
return handler(notificationCreateOnNext(x));
}, function (exception) {
return handler(notificationCreateOnError(exception));
}, function () {
return handler(notificationCreateOnCompleted());
});
};
/**
* Schedules the invocation of observer methods on the given scheduler.
* @param {Scheduler} scheduler Scheduler to schedule observer messages on.
* @returns {Observer} Observer whose messages are scheduled on the given scheduler.
*/
Observer.notifyOn = function (scheduler) {
return new ObserveOnObserver(scheduler, this);
};
/**
* Abstract base class for implementations of the Observer class.
* This base class enforces the grammar of observers where OnError and OnCompleted are terminal messages.
*/
var AbstractObserver = Rx.internals.AbstractObserver = (function (_super) {
inherits(AbstractObserver, _super);
/**
* Creates a new observer in a non-stopped state.
*
* @constructor
*/
function AbstractObserver() {
this.isStopped = false;
_super.call(this);
}
/**
* Notifies the observer of a new element in the sequence.
*
* @memberOf AbstractObserver
* @param {Any} value Next element in the sequence.
*/
AbstractObserver.prototype.onNext = function (value) {
if (!this.isStopped) {
this.next(value);
}
};
/**
* Notifies the observer that an exception has occurred.
*
* @memberOf AbstractObserver
* @param {Any} error The error that has occurred.
*/
AbstractObserver.prototype.onError = function (error) {
if (!this.isStopped) {
this.isStopped = true;
this.error(error);
}
};
/**
* Notifies the observer of the end of the sequence.
*/
AbstractObserver.prototype.onCompleted = function () {
if (!this.isStopped) {
this.isStopped = true;
this.completed();
}
};
/**
* Disposes the observer, causing it to transition to the stopped state.
*/
AbstractObserver.prototype.dispose = function () {
this.isStopped = true;
};
AbstractObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.error(e);
return true;
}
return false;
};
return AbstractObserver;
}(Observer));
/**
* Class to create an Observer instance from delegate-based implementations of the on* methods.
*/
var AnonymousObserver = Rx.AnonymousObserver = (function (_super) {
inherits(AnonymousObserver, _super);
/**
* Creates an observer from the specified OnNext, OnError, and OnCompleted actions.
* @param {Any} onNext Observer's OnNext action implementation.
* @param {Any} onError Observer's OnError action implementation.
* @param {Any} onCompleted Observer's OnCompleted action implementation.
*/
function AnonymousObserver(onNext, onError, onCompleted) {
_super.call(this);
this._onNext = onNext;
this._onError = onError;
this._onCompleted = onCompleted;
}
/**
* Calls the onNext action.
* @param {Any} value Next element in the sequence.
*/
AnonymousObserver.prototype.next = function (value) {
this._onNext(value);
};
/**
* Calls the onError action.
* @param {Any} error The error that has occurred.
*/
AnonymousObserver.prototype.error = function (exception) {
this._onError(exception);
};
/**
* Calls the onCompleted action.
*/
AnonymousObserver.prototype.completed = function () {
this._onCompleted();
};
return AnonymousObserver;
}(AbstractObserver));
var CheckedObserver = (function (_super) {
inherits(CheckedObserver, _super);
function CheckedObserver(observer) {
_super.call(this);
this._observer = observer;
this._state = 0; // 0 - idle, 1 - busy, 2 - done
}
var CheckedObserverPrototype = CheckedObserver.prototype;
CheckedObserverPrototype.onNext = function (value) {
this.checkAccess();
try {
this._observer.onNext(value);
} catch (e) {
throw e;
} finally {
this._state = 0;
}
};
CheckedObserverPrototype.onError = function (err) {
this.checkAccess();
try {
this._observer.onError(err);
} catch (e) {
throw e;
} finally {
this._state = 2;
}
};
CheckedObserverPrototype.onCompleted = function () {
this.checkAccess();
try {
this._observer.onCompleted();
} catch (e) {
throw e;
} finally {
this._state = 2;
}
};
CheckedObserverPrototype.checkAccess = function () {
if (this._state === 1) { throw new Error('Re-entrancy detected'); }
if (this._state === 2) { throw new Error('Observer completed'); }
if (this._state === 0) { this._state = 1; }
};
return CheckedObserver;
}(Observer));
var ScheduledObserver = Rx.internals.ScheduledObserver = (function (_super) {
inherits(ScheduledObserver, _super);
function ScheduledObserver(scheduler, observer) {
_super.call(this);
this.scheduler = scheduler;
this.observer = observer;
this.isAcquired = false;
this.hasFaulted = false;
this.queue = [];
this.disposable = new SerialDisposable();
}
ScheduledObserver.prototype.next = function (value) {
var self = this;
this.queue.push(function () {
self.observer.onNext(value);
});
};
ScheduledObserver.prototype.error = function (exception) {
var self = this;
this.queue.push(function () {
self.observer.onError(exception);
});
};
ScheduledObserver.prototype.completed = function () {
var self = this;
this.queue.push(function () {
self.observer.onCompleted();
});
};
ScheduledObserver.prototype.ensureActive = function () {
var isOwner = false, parent = this;
if (!this.hasFaulted && this.queue.length > 0) {
isOwner = !this.isAcquired;
this.isAcquired = true;
}
if (isOwner) {
this.disposable.setDisposable(this.scheduler.scheduleRecursive(function (self) {
var work;
if (parent.queue.length > 0) {
work = parent.queue.shift();
} else {
parent.isAcquired = false;
return;
}
try {
work();
} catch (ex) {
parent.queue = [];
parent.hasFaulted = true;
throw ex;
}
self();
}));
}
};
ScheduledObserver.prototype.dispose = function () {
_super.prototype.dispose.call(this);
this.disposable.dispose();
};
return ScheduledObserver;
}(AbstractObserver));
/** @private */
var ObserveOnObserver = (function (_super) {
inherits(ObserveOnObserver, _super);
/** @private */
function ObserveOnObserver() {
_super.apply(this, arguments);
}
/** @private */
ObserveOnObserver.prototype.next = function (value) {
_super.prototype.next.call(this, value);
this.ensureActive();
};
/** @private */
ObserveOnObserver.prototype.error = function (e) {
_super.prototype.error.call(this, e);
this.ensureActive();
};
/** @private */
ObserveOnObserver.prototype.completed = function () {
_super.prototype.completed.call(this);
this.ensureActive();
};
return ObserveOnObserver;
})(ScheduledObserver);
var observableProto;
/**
* Represents a push-style collection.
*/
var Observable = Rx.Observable = (function () {
function Observable(subscribe) {
this._subscribe = subscribe;
}
observableProto = Observable.prototype;
/**
* Subscribes an observer to the observable sequence.
*
* @example
* 1 - source.subscribe();
* 2 - source.subscribe(observer);
* 3 - source.subscribe(function (x) { console.log(x); });
* 4 - source.subscribe(function (x) { console.log(x); }, function (err) { console.log(err); });
* 5 - source.subscribe(function (x) { console.log(x); }, function (err) { console.log(err); }, function () { console.log('done'); });
* @param {Mixed} [observerOrOnNext] The object that is to receive notifications or an action to invoke for each element in the observable sequence.
* @param {Function} [onError] Action to invoke upon exceptional termination of the observable sequence.
* @param {Function} [onCompleted] Action to invoke upon graceful termination of the observable sequence.
* @returns {Diposable} The source sequence whose subscriptions and unsubscriptions happen on the specified scheduler.
*/
observableProto.subscribe = observableProto.forEach = function (observerOrOnNext, onError, onCompleted) {
var subscriber = typeof observerOrOnNext === 'object' ?
observerOrOnNext :
observerCreate(observerOrOnNext, onError, onCompleted);
return this._subscribe(subscriber);
};
return Observable;
})();
/**
* Wraps the source sequence in order to run its observer callbacks on the specified scheduler.
*
* This only invokes observer callbacks on a scheduler. In case the subscription and/or unsubscription actions have side-effects
* that require to be run on a scheduler, use subscribeOn.
*
* @param {Scheduler} scheduler Scheduler to notify observers on.
* @returns {Observable} The source sequence whose observations happen on the specified scheduler.
*/
observableProto.observeOn = function (scheduler) {
var source = this;
return new AnonymousObservable(function (observer) {
return source.subscribe(new ObserveOnObserver(scheduler, observer));
});
};
/**
* Wraps the source sequence in order to run its subscription and unsubscription logic on the specified scheduler. This operation is not commonly used;
* see the remarks section for more information on the distinction between subscribeOn and observeOn.
* This only performs the side-effects of subscription and unsubscription on the specified scheduler. In order to invoke observer
* callbacks on a scheduler, use observeOn.
* @param {Scheduler} scheduler Scheduler to perform subscription and unsubscription actions on.
* @returns {Observable} The source sequence whose subscriptions and unsubscriptions happen on the specified scheduler.
*/
observableProto.subscribeOn = function (scheduler) {
var source = this;
return new AnonymousObservable(function (observer) {
var m = new SingleAssignmentDisposable(), d = new SerialDisposable();
d.setDisposable(m);
m.setDisposable(scheduler.schedule(function () {
d.setDisposable(new ScheduledDisposable(scheduler, source.subscribe(observer)));
}));
return d;
});
};
/**
* Converts a Promise to an Observable sequence
* @param {Promise} An ES6 Compliant promise.
* @returns {Observable} An Observable sequence which wraps the existing promise success and failure.
*/
var observableFromPromise = Observable.fromPromise = function (promise) {
return new AnonymousObservable(function (observer) {
promise.then(
function (value) {
observer.onNext(value);
observer.onCompleted();
},
function (reason) {
observer.onError(reason);
});
return function () {
if (promise && promise.abort) {
promise.abort();
}
}
});
};
/*
* Converts an existing observable sequence to an ES6 Compatible Promise
* @example
* var promise = Rx.Observable.return(42).toPromise(RSVP.Promise);
*
* // With config
* Rx.config.Promise = RSVP.Promise;
* var promise = Rx.Observable.return(42).toPromise();
* @param {Function} [promiseCtor] The constructor of the promise. If not provided, it looks for it in Rx.config.Promise.
* @returns {Promise} An ES6 compatible promise with the last value from the observable sequence.
*/
observableProto.toPromise = function (promiseCtor) {
promiseCtor || (promiseCtor = Rx.config.Promise);
if (!promiseCtor) {
throw new Error('Promise type not provided nor in Rx.config.Promise');
}
var source = this;
return new promiseCtor(function (resolve, reject) {
// No cancellation can be done
var value, hasValue = false;
source.subscribe(function (v) {
value = v;
hasValue = true;
}, function (err) {
reject(err);
}, function () {
if (hasValue) {
resolve(value);
}
});
});
};
/**
* Creates a list from an observable sequence.
* @returns An observable sequence containing a single element with a list containing all the elements of the source sequence.
*/
observableProto.toArray = function () {
var self = this;
return new AnonymousObservable(function(observer) {
var arr = [];
return self.subscribe(
arr.push.bind(arr),
observer.onError.bind(observer),
function () {
observer.onNext(arr);
observer.onCompleted();
});
});
};
/**
* Creates an observable sequence from a specified subscribe method implementation.
*
* @example
* var res = Rx.Observable.create(function (observer) { return function () { } );
* var res = Rx.Observable.create(function (observer) { return Rx.Disposable.empty; } );
* var res = Rx.Observable.create(function (observer) { } );
*
* @param {Function} subscribe Implementation of the resulting observable sequence's subscribe method, returning a function that will be wrapped in a Disposable.
* @returns {Observable} The observable sequence with the specified implementation for the Subscribe method.
*/
Observable.create = Observable.createWithDisposable = function (subscribe) {
return new AnonymousObservable(subscribe);
};
/**
* Returns an observable sequence that invokes the specified factory function whenever a new observer subscribes.
*
* @example
* var res = Rx.Observable.defer(function () { return Rx.Observable.fromArray([1,2,3]); });
* @param {Function} observableFactory Observable factory function to invoke for each observer that subscribes to the resulting sequence or Promise.
* @returns {Observable} An observable sequence whose observers trigger an invocation of the given observable factory function.
*/
var observableDefer = Observable.defer = function (observableFactory) {
return new AnonymousObservable(function (observer) {
var result;
try {
result = observableFactory();
} catch (e) {
return observableThrow(e).subscribe(observer);
}
isPromise(result) && (result = observableFromPromise(result));
return result.subscribe(observer);
});
};
/**
* Returns an empty observable sequence, using the specified scheduler to send out the single OnCompleted message.
*
* @example
* var res = Rx.Observable.empty();
* var res = Rx.Observable.empty(Rx.Scheduler.timeout);
* @param {Scheduler} [scheduler] Scheduler to send the termination call on.
* @returns {Observable} An observable sequence with no elements.
*/
var observableEmpty = Observable.empty = function (scheduler) {
scheduler || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.schedule(function () {
observer.onCompleted();
});
});
};
/**
* Converts an array to an observable sequence, using an optional scheduler to enumerate the array.
*
* @example
* var res = Rx.Observable.fromArray([1,2,3]);
* var res = Rx.Observable.fromArray([1,2,3], Rx.Scheduler.timeout);
* @param {Scheduler} [scheduler] Scheduler to run the enumeration of the input sequence on.
* @returns {Observable} The observable sequence whose elements are pulled from the given enumerable sequence.
*/
var observableFromArray = Observable.fromArray = function (array, scheduler) {
scheduler || (scheduler = currentThreadScheduler);
return new AnonymousObservable(function (observer) {
var count = 0;
return scheduler.scheduleRecursive(function (self) {
if (count < array.length) {
observer.onNext(array[count++]);
self();
} else {
observer.onCompleted();
}
});
});
};
/**
* Converts an iterable into an Observable sequence
*
* @example
* var res = Rx.Observable.fromIterable(new Map());
* var res = Rx.Observable.fromIterable(new Set(), Rx.Scheduler.timeout);
* @param {Scheduler} [scheduler] Scheduler to run the enumeration of the input sequence on.
* @returns {Observable} The observable sequence whose elements are pulled from the given generator sequence.
*/
Observable.fromIterable = function (iterable, scheduler) {
scheduler || (scheduler = currentThreadScheduler);
return new AnonymousObservable(function (observer) {
var iterator;
try {
iterator = iterable[$iterator$]();
} catch (e) {
observer.onError(e);
return;
}
return scheduler.scheduleRecursive(function (self) {
var next;
try {
next = iterator.next();
} catch (err) {
observer.onError(err);
return;
}
if (next.done) {
observer.onCompleted();
} else {
observer.onNext(next.value);
self();
}
});
});
};
/**
* Generates an observable sequence by running a state-driven loop producing the sequence's elements, using the specified scheduler to send out observer messages.
*
* @example
* var res = Rx.Observable.generate(0, function (x) { return x < 10; }, function (x) { return x + 1; }, function (x) { return x; });
* var res = Rx.Observable.generate(0, function (x) { return x < 10; }, function (x) { return x + 1; }, function (x) { return x; }, Rx.Scheduler.timeout);
* @param {Mixed} initialState Initial state.
* @param {Function} condition Condition to terminate generation (upon returning false).
* @param {Function} iterate Iteration step function.
* @param {Function} resultSelector Selector function for results produced in the sequence.
* @param {Scheduler} [scheduler] Scheduler on which to run the generator loop. If not provided, defaults to Scheduler.currentThread.
* @returns {Observable} The generated sequence.
*/
Observable.generate = function (initialState, condition, iterate, resultSelector, scheduler) {
scheduler || (scheduler = currentThreadScheduler);
return new AnonymousObservable(function (observer) {
var first = true, state = initialState;
return scheduler.scheduleRecursive(function (self) {
var hasResult, result;
try {
if (first) {
first = false;
} else {
state = iterate(state);
}
hasResult = condition(state);
if (hasResult) {
result = resultSelector(state);
}
} catch (exception) {
observer.onError(exception);
return;
}
if (hasResult) {
observer.onNext(result);
self();
} else {
observer.onCompleted();
}
});
});
};
/**
* Returns a non-terminating observable sequence, which can be used to denote an infinite duration (e.g. when using reactive joins).
* @returns {Observable} An observable sequence whose observers will never get called.
*/
var observableNever = Observable.never = function () {
return new AnonymousObservable(function () {
return disposableEmpty;
});
};
/**
* Generates an observable sequence of integral numbers within a specified range, using the specified scheduler to send out observer messages.
*
* @example
* var res = Rx.Observable.range(0, 10);
* var res = Rx.Observable.range(0, 10, Rx.Scheduler.timeout);
* @param {Number} start The value of the first integer in the sequence.
* @param {Number} count The number of sequential integers to generate.
* @param {Scheduler} [scheduler] Scheduler to run the generator loop on. If not specified, defaults to Scheduler.currentThread.
* @returns {Observable} An observable sequence that contains a range of sequential integral numbers.
*/
Observable.range = function (start, count, scheduler) {
scheduler || (scheduler = currentThreadScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.scheduleRecursiveWithState(0, function (i, self) {
if (i < count) {
observer.onNext(start + i);
self(i + 1);
} else {
observer.onCompleted();
}
});
});
};
/**
* Generates an observable sequence that repeats the given element the specified number of times, using the specified scheduler to send out observer messages.
*
* @example
* var res = Rx.Observable.repeat(42);
* var res = Rx.Observable.repeat(42, 4);
* 3 - res = Rx.Observable.repeat(42, 4, Rx.Scheduler.timeout);
* 4 - res = Rx.Observable.repeat(42, null, Rx.Scheduler.timeout);
* @param {Mixed} value Element to repeat.
* @param {Number} repeatCount [Optiona] Number of times to repeat the element. If not specified, repeats indefinitely.
* @param {Scheduler} scheduler Scheduler to run the producer loop on. If not specified, defaults to Scheduler.immediate.
* @returns {Observable} An observable sequence that repeats the given element the specified number of times.
*/
Observable.repeat = function (value, repeatCount, scheduler) {
scheduler || (scheduler = currentThreadScheduler);
if (repeatCount == null) {
repeatCount = -1;
}
return observableReturn(value, scheduler).repeat(repeatCount);
};
/**
* Returns an observable sequence that contains a single element, using the specified scheduler to send out observer messages.
* There is an alias called 'returnValue' for browsers <IE9.
*
* @example
* var res = Rx.Observable.return(42);
* var res = Rx.Observable.return(42, Rx.Scheduler.timeout);
* @param {Mixed} value Single element in the resulting observable sequence.
* @param {Scheduler} scheduler Scheduler to send the single element on. If not specified, defaults to Scheduler.immediate.
* @returns {Observable} An observable sequence containing the single specified element.
*/
var observableReturn = Observable['return'] = Observable.returnValue = function (value, scheduler) {
scheduler || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.schedule(function () {
observer.onNext(value);
observer.onCompleted();
});
});
};
/**
* Returns an observable sequence that terminates with an exception, using the specified scheduler to send out the single onError message.
* There is an alias to this method called 'throwException' for browsers <IE9.
*
* @example
* var res = Rx.Observable.throwException(new Error('Error'));
* var res = Rx.Observable.throwException(new Error('Error'), Rx.Scheduler.timeout);
* @param {Mixed} exception An object used for the sequence's termination.
* @param {Scheduler} scheduler Scheduler to send the exceptional termination call on. If not specified, defaults to Scheduler.immediate.
* @returns {Observable} The observable sequence that terminates exceptionally with the specified exception object.
*/
var observableThrow = Observable['throw'] = Observable.throwException = function (exception, scheduler) {
scheduler || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.schedule(function () {
observer.onError(exception);
});
});
};
/**
* Constructs an observable sequence that depends on a resource object, whose lifetime is tied to the resulting observable sequence's lifetime.
*
* @example
* var res = Rx.Observable.using(function () { return new AsyncSubject(); }, function (s) { return s; });
* @param {Function} resourceFactory Factory function to obtain a resource object.
* @param {Function} observableFactory Factory function to obtain an observable sequence that depends on the obtained resource.
* @returns {Observable} An observable sequence whose lifetime controls the lifetime of the dependent resource object.
*/
Observable.using = function (resourceFactory, observableFactory) {
return new AnonymousObservable(function (observer) {
var disposable = disposableEmpty, resource, source;
try {
resource = resourceFactory();
if (resource) {
disposable = resource;
}
source = observableFactory(resource);
} catch (exception) {
return new CompositeDisposable(observableThrow(exception).subscribe(observer), disposable);
}
return new CompositeDisposable(source.subscribe(observer), disposable);
});
};
/**
* Propagates the observable sequence or Promise that reacts first.
* @param {Observable} rightSource Second observable sequence or Promise.
* @returns {Observable} {Observable} An observable sequence that surfaces either of the given sequences, whichever reacted first.
*/
observableProto.amb = function (rightSource) {
var leftSource = this;
return new AnonymousObservable(function (observer) {
var choice,
leftChoice = 'L', rightChoice = 'R',
leftSubscription = new SingleAssignmentDisposable(),
rightSubscription = new SingleAssignmentDisposable();
isPromise(rightSource) && (rightSource = observableFromPromise(rightSource));
function choiceL() {
if (!choice) {
choice = leftChoice;
rightSubscription.dispose();
}
}
function choiceR() {
if (!choice) {
choice = rightChoice;
leftSubscription.dispose();
}
}
leftSubscription.setDisposable(leftSource.subscribe(function (left) {
choiceL();
if (choice === leftChoice) {
observer.onNext(left);
}
}, function (err) {
choiceL();
if (choice === leftChoice) {
observer.onError(err);
}
}, function () {
choiceL();
if (choice === leftChoice) {
observer.onCompleted();
}
}));
rightSubscription.setDisposable(rightSource.subscribe(function (right) {
choiceR();
if (choice === rightChoice) {
observer.onNext(right);
}
}, function (err) {
choiceR();
if (choice === rightChoice) {
observer.onError(err);
}
}, function () {
choiceR();
if (choice === rightChoice) {
observer.onCompleted();
}
}));
return new CompositeDisposable(leftSubscription, rightSubscription);
});
};
/**
* Propagates the observable sequence or Promise that reacts first.
*
* @example
* var = Rx.Observable.amb(xs, ys, zs);
* @returns {Observable} An observable sequence that surfaces any of the given sequences, whichever reacted first.
*/
Observable.amb = function () {
var acc = observableNever(),
items = argsOrArray(arguments, 0);
function func(previous, current) {
return previous.amb(current);
}
for (var i = 0, len = items.length; i < len; i++) {
acc = func(acc, items[i]);
}
return acc;
};
function observableCatchHandler(source, handler) {
return new AnonymousObservable(function (observer) {
var d1 = new SingleAssignmentDisposable(), subscription = new SerialDisposable();
subscription.setDisposable(d1);
d1.setDisposable(source.subscribe(observer.onNext.bind(observer), function (exception) {
var d, result;
try {
result = handler(exception);
} catch (ex) {
observer.onError(ex);
return;
}
isPromise(result) && (result = observableFromPromise(result));
d = new SingleAssignmentDisposable();
subscription.setDisposable(d);
d.setDisposable(result.subscribe(observer));
}, observer.onCompleted.bind(observer)));
return subscription;
});
}
/**
* Continues an observable sequence that is terminated by an exception with the next observable sequence.
* @example
* 1 - xs.catchException(ys)
* 2 - xs.catchException(function (ex) { return ys(ex); })
* @param {Mixed} handlerOrSecond Exception handler function that returns an observable sequence given the error that occurred in the first sequence, or a second observable sequence used to produce results when an error occurred in the first sequence.
* @returns {Observable} An observable sequence containing the first sequence's elements, followed by the elements of the handler sequence in case an exception occurred.
*/
observableProto['catch'] = observableProto.catchException = function (handlerOrSecond) {
return typeof handlerOrSecond === 'function' ?
observableCatchHandler(this, handlerOrSecond) :
observableCatch([this, handlerOrSecond]);
};
/**
* Continues an observable sequence that is terminated by an exception with the next observable sequence.
*
* @example
* 1 - res = Rx.Observable.catchException(xs, ys, zs);
* 2 - res = Rx.Observable.catchException([xs, ys, zs]);
* @returns {Observable} An observable sequence containing elements from consecutive source sequences until a source sequence terminates successfully.
*/
var observableCatch = Observable.catchException = Observable['catch'] = function () {
var items = argsOrArray(arguments, 0);
return enumerableFor(items).catchException();
};
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever any of the observable sequences or Promises produces an element.
* This can be in the form of an argument list of observables or an array.
*
* @example
* 1 - obs = observable.combineLatest(obs1, obs2, obs3, function (o1, o2, o3) { return o1 + o2 + o3; });
* 2 - obs = observable.combineLatest([obs1, obs2, obs3], function (o1, o2, o3) { return o1 + o2 + o3; });
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
observableProto.combineLatest = function () {
var args = slice.call(arguments);
if (Array.isArray(args[0])) {
args[0].unshift(this);
} else {
args.unshift(this);
}
return combineLatest.apply(this, args);
};
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever any of the observable sequences or Promises produces an element.
*
* @example
* 1 - obs = Rx.Observable.combineLatest(obs1, obs2, obs3, function (o1, o2, o3) { return o1 + o2 + o3; });
* 2 - obs = Rx.Observable.combineLatest([obs1, obs2, obs3], function (o1, o2, o3) { return o1 + o2 + o3; });
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
var combineLatest = Observable.combineLatest = function () {
var args = slice.call(arguments), resultSelector = args.pop();
if (Array.isArray(args[0])) {
args = args[0];
}
return new AnonymousObservable(function (observer) {
var falseFactory = function () { return false; },
n = args.length,
hasValue = arrayInitialize(n, falseFactory),
hasValueAll = false,
isDone = arrayInitialize(n, falseFactory),
values = new Array(n);
function next(i) {
var res;
hasValue[i] = true;
if (hasValueAll || (hasValueAll = hasValue.every(identity))) {
try {
res = resultSelector.apply(null, values);
} catch (ex) {
observer.onError(ex);
return;
}
observer.onNext(res);
} else if (isDone.filter(function (x, j) { return j !== i; }).every(identity)) {
observer.onCompleted();
}
}
function done (i) {
isDone[i] = true;
if (isDone.every(identity)) {
observer.onCompleted();
}
}
var subscriptions = new Array(n);
for (var idx = 0; idx < n; idx++) {
(function (i) {
var source = args[i], sad = new SingleAssignmentDisposable();
isPromise(source) && (source = observableFromPromise(source));
sad.setDisposable(source.subscribe(function (x) {
values[i] = x;
next(i);
}, observer.onError.bind(observer), function () {
done(i);
}));
subscriptions[i] = sad;
}(idx));
}
return new CompositeDisposable(subscriptions);
});
};
/**
* Concatenates all the observable sequences. This takes in either an array or variable arguments to concatenate.
*
* @example
* 1 - concatenated = xs.concat(ys, zs);
* 2 - concatenated = xs.concat([ys, zs]);
* @returns {Observable} An observable sequence that contains the elements of each given sequence, in sequential order.
*/
observableProto.concat = function () {
var items = slice.call(arguments, 0);
items.unshift(this);
return observableConcat.apply(this, items);
};
/**
* Concatenates all the observable sequences.
*
* @example
* 1 - res = Rx.Observable.concat(xs, ys, zs);
* 2 - res = Rx.Observable.concat([xs, ys, zs]);
* @returns {Observable} An observable sequence that contains the elements of each given sequence, in sequential order.
*/
var observableConcat = Observable.concat = function () {
var sources = argsOrArray(arguments, 0);
return enumerableFor(sources).concat();
};
/**
* Concatenates an observable sequence of observable sequences.
* @returns {Observable} An observable sequence that contains the elements of each observed inner sequence, in sequential order.
*/
observableProto.concatObservable = observableProto.concatAll =function () {
return this.merge(1);
};
/**
* Merges an observable sequence of observable sequences into an observable sequence, limiting the number of concurrent subscriptions to inner sequences.
* Or merges two observable sequences into a single observable sequence.
*
* @example
* 1 - merged = sources.merge(1);
* 2 - merged = source.merge(otherSource);
* @param {Mixed} [maxConcurrentOrOther] Maximum number of inner observable sequences being subscribed to concurrently or the second observable sequence.
* @returns {Observable} The observable sequence that merges the elements of the inner sequences.
*/
observableProto.merge = function (maxConcurrentOrOther) {
if (typeof maxConcurrentOrOther !== 'number') {
return observableMerge(this, maxConcurrentOrOther);
}
var sources = this;
return new AnonymousObservable(function (observer) {
var activeCount = 0,
group = new CompositeDisposable(),
isStopped = false,
q = [],
subscribe = function (xs) {
var subscription = new SingleAssignmentDisposable();
group.add(subscription);
// Check for promises support
if (isPromise(xs)) { xs = observableFromPromise(xs); }
subscription.setDisposable(xs.subscribe(observer.onNext.bind(observer), observer.onError.bind(observer), function () {
var s;
group.remove(subscription);
if (q.length > 0) {
s = q.shift();
subscribe(s);
} else {
activeCount--;
if (isStopped && activeCount === 0) {
observer.onCompleted();
}
}
}));
};
group.add(sources.subscribe(function (innerSource) {
if (activeCount < maxConcurrentOrOther) {
activeCount++;
subscribe(innerSource);
} else {
q.push(innerSource);
}
}, observer.onError.bind(observer), function () {
isStopped = true;
if (activeCount === 0) {
observer.onCompleted();
}
}));
return group;
});
};
/**
* Merges all the observable sequences into a single observable sequence.
* The scheduler is optional and if not specified, the immediate scheduler is used.
*
* @example
* 1 - merged = Rx.Observable.merge(xs, ys, zs);
* 2 - merged = Rx.Observable.merge([xs, ys, zs]);
* 3 - merged = Rx.Observable.merge(scheduler, xs, ys, zs);
* 4 - merged = Rx.Observable.merge(scheduler, [xs, ys, zs]);
* @returns {Observable} The observable sequence that merges the elements of the observable sequences.
*/
var observableMerge = Observable.merge = function () {
var scheduler, sources;
if (!arguments[0]) {
scheduler = immediateScheduler;
sources = slice.call(arguments, 1);
} else if (arguments[0].now) {
scheduler = arguments[0];
sources = slice.call(arguments, 1);
} else {
scheduler = immediateScheduler;
sources = slice.call(arguments, 0);
}
if (Array.isArray(sources[0])) {
sources = sources[0];
}
return observableFromArray(sources, scheduler).mergeObservable();
};
/**
* Merges an observable sequence of observable sequences into an observable sequence.
* @returns {Observable} The observable sequence that merges the elements of the inner sequences.
*/
observableProto.mergeObservable = observableProto.mergeAll =function () {
var sources = this;
return new AnonymousObservable(function (observer) {
var group = new CompositeDisposable(),
isStopped = false,
m = new SingleAssignmentDisposable();
group.add(m);
m.setDisposable(sources.subscribe(function (innerSource) {
var innerSubscription = new SingleAssignmentDisposable();
group.add(innerSubscription);
// Check if Promise or Observable
if (isPromise(innerSource)) {
innerSource = observableFromPromise(innerSource);
}
innerSubscription.setDisposable(innerSource.subscribe(function (x) {
observer.onNext(x);
}, observer.onError.bind(observer), function () {
group.remove(innerSubscription);
if (isStopped && group.length === 1) { observer.onCompleted(); }
}));
}, observer.onError.bind(observer), function () {
isStopped = true;
if (group.length === 1) { observer.onCompleted(); }
}));
return group;
});
};
/**
* Continues an observable sequence that is terminated normally or by an exception with the next observable sequence.
* @param {Observable} second Second observable sequence used to produce results after the first sequence terminates.
* @returns {Observable} An observable sequence that concatenates the first and second sequence, even if the first sequence terminates exceptionally.
*/
observableProto.onErrorResumeNext = function (second) {
if (!second) {
throw new Error('Second observable is required');
}
return onErrorResumeNext([this, second]);
};
/**
* Continues an observable sequence that is terminated normally or by an exception with the next observable sequence.
*
* @example
* 1 - res = Rx.Observable.onErrorResumeNext(xs, ys, zs);
* 1 - res = Rx.Observable.onErrorResumeNext([xs, ys, zs]);
* @returns {Observable} An observable sequence that concatenates the source sequences, even if a sequence terminates exceptionally.
*/
var onErrorResumeNext = Observable.onErrorResumeNext = function () {
var sources = argsOrArray(arguments, 0);
return new AnonymousObservable(function (observer) {
var pos = 0, subscription = new SerialDisposable(),
cancelable = immediateScheduler.scheduleRecursive(function (self) {
var current, d;
if (pos < sources.length) {
current = sources[pos++];
isPromise(current) && (current = observableFromPromise(current));
d = new SingleAssignmentDisposable();
subscription.setDisposable(d);
d.setDisposable(current.subscribe(observer.onNext.bind(observer), function () {
self();
}, function () {
self();
}));
} else {
observer.onCompleted();
}
});
return new CompositeDisposable(subscription, cancelable);
});
};
/**
* Returns the values from the source observable sequence only after the other observable sequence produces a value.
* @param {Observable | Promise} other The observable sequence or Promise that triggers propagation of elements of the source sequence.
* @returns {Observable} An observable sequence containing the elements of the source sequence starting from the point the other sequence triggered propagation.
*/
observableProto.skipUntil = function (other) {
var source = this;
return new AnonymousObservable(function (observer) {
var isOpen = false;
var disposables = new CompositeDisposable(source.subscribe(function (left) {
isOpen && observer.onNext(left);
}, observer.onError.bind(observer), function () {
isOpen && observer.onCompleted();
}));
isPromise(other) && (other = observableFromPromise(other));
var rightSubscription = new SingleAssignmentDisposable();
disposables.add(rightSubscription);
rightSubscription.setDisposable(other.subscribe(function () {
isOpen = true;
rightSubscription.dispose();
}, observer.onError.bind(observer), function () {
rightSubscription.dispose();
}));
return disposables;
});
};
/**
* Transforms an observable sequence of observable sequences into an observable sequence producing values only from the most recent observable sequence.
* @returns {Observable} The observable sequence that at any point in time produces the elements of the most recent inner observable sequence that has been received.
*/
observableProto['switch'] = observableProto.switchLatest = function () {
var sources = this;
return new AnonymousObservable(function (observer) {
var hasLatest = false,
innerSubscription = new SerialDisposable(),
isStopped = false,
latest = 0,
subscription = sources.subscribe(function (innerSource) {
var d = new SingleAssignmentDisposable(), id = ++latest;
hasLatest = true;
innerSubscription.setDisposable(d);
// Check if Promise or Observable
if (isPromise(innerSource)) {
innerSource = observableFromPromise(innerSource);
}
d.setDisposable(innerSource.subscribe(function (x) {
if (latest === id) {
observer.onNext(x);
}
}, function (e) {
if (latest === id) {
observer.onError(e);
}
}, function () {
if (latest === id) {
hasLatest = false;
if (isStopped) {
observer.onCompleted();
}
}
}));
}, observer.onError.bind(observer), function () {
isStopped = true;
if (!hasLatest) {
observer.onCompleted();
}
});
return new CompositeDisposable(subscription, innerSubscription);
});
};
/**
* Returns the values from the source observable sequence until the other observable sequence produces a value.
* @param {Observable | Promise} other Observable sequence or Promise that terminates propagation of elements of the source sequence.
* @returns {Observable} An observable sequence containing the elements of the source sequence up to the point the other sequence interrupted further propagation.
*/
observableProto.takeUntil = function (other) {
var source = this;
return new AnonymousObservable(function (observer) {
isPromise(other) && (other = observableFromPromise(other));
return new CompositeDisposable(
source.subscribe(observer),
other.subscribe(observer.onCompleted.bind(observer), observer.onError.bind(observer), noop)
);
});
};
function zipArray(second, resultSelector) {
var first = this;
return new AnonymousObservable(function (observer) {
var index = 0, len = second.length;
return first.subscribe(function (left) {
if (index < len) {
var right = second[index++], result;
try {
result = resultSelector(left, right);
} catch (e) {
observer.onError(e);
return;
}
observer.onNext(result);
} else {
observer.onCompleted();
}
}, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
}
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever all of the observable sequences or an array have produced an element at a corresponding index.
* The last element in the arguments must be a function to invoke for each series of elements at corresponding indexes in the sources.
*
* @example
* 1 - res = obs1.zip(obs2, fn);
* 1 - res = x1.zip([1,2,3], fn);
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
observableProto.zip = function () {
if (Array.isArray(arguments[0])) {
return zipArray.apply(this, arguments);
}
var parent = this, sources = slice.call(arguments), resultSelector = sources.pop();
sources.unshift(parent);
return new AnonymousObservable(function (observer) {
var n = sources.length,
queues = arrayInitialize(n, function () { return []; }),
isDone = arrayInitialize(n, function () { return false; });
function next(i) {
var res, queuedValues;
if (queues.every(function (x) { return x.length > 0; })) {
try {
queuedValues = queues.map(function (x) { return x.shift(); });
res = resultSelector.apply(parent, queuedValues);
} catch (ex) {
observer.onError(ex);
return;
}
observer.onNext(res);
} else if (isDone.filter(function (x, j) { return j !== i; }).every(identity)) {
observer.onCompleted();
}
};
function done(i) {
isDone[i] = true;
if (isDone.every(function (x) { return x; })) {
observer.onCompleted();
}
}
var subscriptions = new Array(n);
for (var idx = 0; idx < n; idx++) {
(function (i) {
var source = sources[i], sad = new SingleAssignmentDisposable();
isPromise(source) && (source = observableFromPromise(source));
sad.setDisposable(source.subscribe(function (x) {
queues[i].push(x);
next(i);
}, observer.onError.bind(observer), function () {
done(i);
}));
subscriptions[i] = sad;
})(idx);
}
return new CompositeDisposable(subscriptions);
});
};
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever all of the observable sequences have produced an element at a corresponding index.
* @param arguments Observable sources.
* @param {Function} resultSelector Function to invoke for each series of elements at corresponding indexes in the sources.
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
Observable.zip = function () {
var args = slice.call(arguments, 0),
first = args.shift();
return first.zip.apply(first, args);
};
/**
* Merges the specified observable sequences into one observable sequence by emitting a list with the elements of the observable sequences at corresponding indexes.
* @param arguments Observable sources.
* @returns {Observable} An observable sequence containing lists of elements at corresponding indexes.
*/
Observable.zipArray = function () {
var sources = argsOrArray(arguments, 0);
return new AnonymousObservable(function (observer) {
var n = sources.length,
queues = arrayInitialize(n, function () { return []; }),
isDone = arrayInitialize(n, function () { return false; });
function next(i) {
if (queues.every(function (x) { return x.length > 0; })) {
var res = queues.map(function (x) { return x.shift(); });
observer.onNext(res);
} else if (isDone.filter(function (x, j) { return j !== i; }).every(identity)) {
observer.onCompleted();
return;
}
};
function done(i) {
isDone[i] = true;
if (isDone.every(identity)) {
observer.onCompleted();
return;
}
}
var subscriptions = new Array(n);
for (var idx = 0; idx < n; idx++) {
(function (i) {
subscriptions[i] = new SingleAssignmentDisposable();
subscriptions[i].setDisposable(sources[i].subscribe(function (x) {
queues[i].push(x);
next(i);
}, observer.onError.bind(observer), function () {
done(i);
}));
})(idx);
}
var compositeDisposable = new CompositeDisposable(subscriptions);
compositeDisposable.add(disposableCreate(function () {
for (var qIdx = 0, qLen = queues.length; qIdx < qLen; qIdx++) {
queues[qIdx] = [];
}
}));
return compositeDisposable;
});
};
/**
* Hides the identity of an observable sequence.
* @returns {Observable} An observable sequence that hides the identity of the source sequence.
*/
observableProto.asObservable = function () {
var source = this;
return new AnonymousObservable(function (observer) {
return source.subscribe(observer);
});
};
/**
* Projects each element of an observable sequence into zero or more buffers which are produced based on element count information.
*
* @example
* var res = xs.bufferWithCount(10);
* var res = xs.bufferWithCount(10, 1);
* @param {Number} count Length of each buffer.
* @param {Number} [skip] Number of elements to skip between creation of consecutive buffers. If not provided, defaults to the count.
* @returns {Observable} An observable sequence of buffers.
*/
observableProto.bufferWithCount = function (count, skip) {
if (typeof skip !== 'number') {
skip = count;
}
return this.windowWithCount(count, skip).selectMany(function (x) {
return x.toArray();
}).where(function (x) {
return x.length > 0;
});
};
/**
* Dematerializes the explicit notification values of an observable sequence as implicit notifications.
* @returns {Observable} An observable sequence exhibiting the behavior corresponding to the source sequence's notification values.
*/
observableProto.dematerialize = function () {
var source = this;
return new AnonymousObservable(function (observer) {
return source.subscribe(function (x) {
return x.accept(observer);
}, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
};
/**
* Returns an observable sequence that contains only distinct contiguous elements according to the keySelector and the comparer.
*
* var obs = observable.distinctUntilChanged();
* var obs = observable.distinctUntilChanged(function (x) { return x.id; });
* var obs = observable.distinctUntilChanged(function (x) { return x.id; }, function (x, y) { return x === y; });
*
* @param {Function} [keySelector] A function to compute the comparison key for each element. If not provided, it projects the value.
* @param {Function} [comparer] Equality comparer for computed key values. If not provided, defaults to an equality comparer function.
* @returns {Observable} An observable sequence only containing the distinct contiguous elements, based on a computed key value, from the source sequence.
*/
observableProto.distinctUntilChanged = function (keySelector, comparer) {
var source = this;
keySelector || (keySelector = identity);
comparer || (comparer = defaultComparer);
return new AnonymousObservable(function (observer) {
var hasCurrentKey = false, currentKey;
return source.subscribe(function (value) {
var comparerEquals = false, key;
try {
key = keySelector(value);
} catch (exception) {
observer.onError(exception);
return;
}
if (hasCurrentKey) {
try {
comparerEquals = comparer(currentKey, key);
} catch (exception) {
observer.onError(exception);
return;
}
}
if (!hasCurrentKey || !comparerEquals) {
hasCurrentKey = true;
currentKey = key;
observer.onNext(value);
}
}, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
};
/**
* Invokes an action for each element in the observable sequence and invokes an action upon graceful or exceptional termination of the observable sequence.
* This method can be used for debugging, logging, etc. of query behavior by intercepting the message stream to run arbitrary actions for messages on the pipeline.
*
* @example
* var res = observable.doAction(observer);
* var res = observable.doAction(onNext);
* var res = observable.doAction(onNext, onError);
* var res = observable.doAction(onNext, onError, onCompleted);
* @param {Mixed} observerOrOnNext Action to invoke for each element in the observable sequence or an observer.
* @param {Function} [onError] Action to invoke upon exceptional termination of the observable sequence. Used if only the observerOrOnNext parameter is also a function.
* @param {Function} [onCompleted] Action to invoke upon graceful termination of the observable sequence. Used if only the observerOrOnNext parameter is also a function.
* @returns {Observable} The source sequence with the side-effecting behavior applied.
*/
observableProto['do'] = observableProto.doAction = function (observerOrOnNext, onError, onCompleted) {
var source = this, onNextFunc;
if (typeof observerOrOnNext === 'function') {
onNextFunc = observerOrOnNext;
} else {
onNextFunc = observerOrOnNext.onNext.bind(observerOrOnNext);
onError = observerOrOnNext.onError.bind(observerOrOnNext);
onCompleted = observerOrOnNext.onCompleted.bind(observerOrOnNext);
}
return new AnonymousObservable(function (observer) {
return source.subscribe(function (x) {
try {
onNextFunc(x);
} catch (e) {
observer.onError(e);
}
observer.onNext(x);
}, function (exception) {
if (!onError) {
observer.onError(exception);
} else {
try {
onError(exception);
} catch (e) {
observer.onError(e);
}
observer.onError(exception);
}
}, function () {
if (!onCompleted) {
observer.onCompleted();
} else {
try {
onCompleted();
} catch (e) {
observer.onError(e);
}
observer.onCompleted();
}
});
});
};
/**
* Invokes a specified action after the source observable sequence terminates gracefully or exceptionally.
*
* @example
* var res = observable.finallyAction(function () { console.log('sequence ended'; });
* @param {Function} finallyAction Action to invoke after the source observable sequence terminates.
* @returns {Observable} Source sequence with the action-invoking termination behavior applied.
*/
observableProto['finally'] = observableProto.finallyAction = function (action) {
var source = this;
return new AnonymousObservable(function (observer) {
var subscription;
try {
subscription = source.subscribe(observer);
} catch (e) {
action();
throw e;
}
return disposableCreate(function () {
try {
subscription.dispose();
} catch (e) {
throw e;
} finally {
action();
}
});
});
};
/**
* Ignores all elements in an observable sequence leaving only the termination messages.
* @returns {Observable} An empty observable sequence that signals termination, successful or exceptional, of the source sequence.
*/
observableProto.ignoreElements = function () {
var source = this;
return new AnonymousObservable(function (observer) {
return source.subscribe(noop, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
};
/**
* Materializes the implicit notifications of an observable sequence as explicit notification values.
* @returns {Observable} An observable sequence containing the materialized notification values from the source sequence.
*/
observableProto.materialize = function () {
var source = this;
return new AnonymousObservable(function (observer) {
return source.subscribe(function (value) {
observer.onNext(notificationCreateOnNext(value));
}, function (e) {
observer.onNext(notificationCreateOnError(e));
observer.onCompleted();
}, function () {
observer.onNext(notificationCreateOnCompleted());
observer.onCompleted();
});
});
};
/**
* Repeats the observable sequence a specified number of times. If the repeat count is not specified, the sequence repeats indefinitely.
*
* @example
* var res = repeated = source.repeat();
* var res = repeated = source.repeat(42);
* @param {Number} [repeatCount] Number of times to repeat the sequence. If not provided, repeats the sequence indefinitely.
* @returns {Observable} The observable sequence producing the elements of the given sequence repeatedly.
*/
observableProto.repeat = function (repeatCount) {
return enumerableRepeat(this, repeatCount).concat();
};
/**
* Repeats the source observable sequence the specified number of times or until it successfully terminates. If the retry count is not specified, it retries indefinitely.
*
* @example
* var res = retried = retry.repeat();
* var res = retried = retry.repeat(42);
* @param {Number} [retryCount] Number of times to retry the sequence. If not provided, retry the sequence indefinitely.
* @returns {Observable} An observable sequence producing the elements of the given sequence repeatedly until it terminates successfully.
*/
observableProto.retry = function (retryCount) {
return enumerableRepeat(this, retryCount).catchException();
};
/**
* Applies an accumulator function over an observable sequence and returns each intermediate result. The optional seed value is used as the initial accumulator value.
* For aggregation behavior with no intermediate results, see Observable.aggregate.
* @example
* var res = source.scan(function (acc, x) { return acc + x; });
* var res = source.scan(0, function (acc, x) { return acc + x; });
* @param {Mixed} [seed] The initial accumulator value.
* @param {Function} accumulator An accumulator function to be invoked on each element.
* @returns {Observable} An observable sequence containing the accumulated values.
*/
observableProto.scan = function () {
var hasSeed = false, seed, accumulator, source = this;
if (arguments.length === 2) {
hasSeed = true;
seed = arguments[0];
accumulator = arguments[1];
} else {
accumulator = arguments[0];
}
return new AnonymousObservable(function (observer) {
var hasAccumulation, accumulation, hasValue;
return source.subscribe (
function (x) {
try {
if (!hasValue) {
hasValue = true;
}
if (hasAccumulation) {
accumulation = accumulator(accumulation, x);
} else {
accumulation = hasSeed ? accumulator(seed, x) : x;
hasAccumulation = true;
}
} catch (e) {
observer.onError(e);
return;
}
observer.onNext(accumulation);
},
observer.onError.bind(observer),
function () {
if (!hasValue && hasSeed) {
observer.onNext(seed);
}
observer.onCompleted();
}
);
});
};
/**
* Bypasses a specified number of elements at the end of an observable sequence.
* @description
* This operator accumulates a queue with a length enough to store the first `count` elements. As more elements are
* received, elements are taken from the front of the queue and produced on the result sequence. This causes elements to be delayed.
* @param count Number of elements to bypass at the end of the source sequence.
* @returns {Observable} An observable sequence containing the source sequence elements except for the bypassed ones at the end.
*/
observableProto.skipLast = function (count) {
var source = this;
return new AnonymousObservable(function (observer) {
var q = [];
return source.subscribe(function (x) {
q.push(x);
if (q.length > count) {
observer.onNext(q.shift());
}
}, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
};
/**
* Prepends a sequence of values to an observable sequence with an optional scheduler and an argument list of values to prepend.
*
* var res = source.startWith(1, 2, 3);
* var res = source.startWith(Rx.Scheduler.timeout, 1, 2, 3);
*
* @memberOf Observable#
* @returns {Observable} The source sequence prepended with the specified values.
*/
observableProto.startWith = function () {
var values, scheduler, start = 0;
if (!!arguments.length && 'now' in Object(arguments[0])) {
scheduler = arguments[0];
start = 1;
} else {
scheduler = immediateScheduler;
}
values = slice.call(arguments, start);
return enumerableFor([observableFromArray(values, scheduler), this]).concat();
};
/**
* Returns a specified number of contiguous elements from the end of an observable sequence, using an optional scheduler to drain the queue.
*
* @example
* var res = source.takeLast(5);
* var res = source.takeLast(5, Rx.Scheduler.timeout);
*
* @description
* This operator accumulates a buffer with a length enough to store elements count elements. Upon completion of
* the source sequence, this buffer is drained on the result sequence. This causes the elements to be delayed.
* @param {Number} count Number of elements to take from the end of the source sequence.
* @param {Scheduler} [scheduler] Scheduler used to drain the queue upon completion of the source sequence.
* @returns {Observable} An observable sequence containing the specified number of elements from the end of the source sequence.
*/
observableProto.takeLast = function (count, scheduler) {
return this.takeLastBuffer(count).selectMany(function (xs) { return observableFromArray(xs, scheduler); });
};
/**
* Returns an array with the specified number of contiguous elements from the end of an observable sequence.
*
* @description
* This operator accumulates a buffer with a length enough to store count elements. Upon completion of the
* source sequence, this buffer is produced on the result sequence.
* @param {Number} count Number of elements to take from the end of the source sequence.
* @returns {Observable} An observable sequence containing a single array with the specified number of elements from the end of the source sequence.
*/
observableProto.takeLastBuffer = function (count) {
var source = this;
return new AnonymousObservable(function (observer) {
var q = [];
return source.subscribe(function (x) {
q.push(x);
if (q.length > count) {
q.shift();
}
}, observer.onError.bind(observer), function () {
observer.onNext(q);
observer.onCompleted();
});
});
};
/**
* Projects each element of an observable sequence into zero or more windows which are produced based on element count information.
*
* var res = xs.windowWithCount(10);
* var res = xs.windowWithCount(10, 1);
* @param {Number} count Length of each window.
* @param {Number} [skip] Number of elements to skip between creation of consecutive windows. If not specified, defaults to the count.
* @returns {Observable} An observable sequence of windows.
*/
observableProto.windowWithCount = function (count, skip) {
var source = this;
if (count <= 0) {
throw new Error(argumentOutOfRange);
}
if (arguments.length === 1) {
skip = count;
}
if (skip <= 0) {
throw new Error(argumentOutOfRange);
}
return new AnonymousObservable(function (observer) {
var m = new SingleAssignmentDisposable(),
refCountDisposable = new RefCountDisposable(m),
n = 0,
q = [],
createWindow = function () {
var s = new Subject();
q.push(s);
observer.onNext(addRef(s, refCountDisposable));
};
createWindow();
m.setDisposable(source.subscribe(function (x) {
var s;
for (var i = 0, len = q.length; i < len; i++) {
q[i].onNext(x);
}
var c = n - count + 1;
if (c >= 0 && c % skip === 0) {
s = q.shift();
s.onCompleted();
}
n++;
if (n % skip === 0) {
createWindow();
}
}, function (exception) {
while (q.length > 0) {
q.shift().onError(exception);
}
observer.onError(exception);
}, function () {
while (q.length > 0) {
q.shift().onCompleted();
}
observer.onCompleted();
}));
return refCountDisposable;
});
};
/**
* Returns the elements of the specified sequence or the specified value in a singleton sequence if the sequence is empty.
*
* var res = obs = xs.defaultIfEmpty();
* 2 - obs = xs.defaultIfEmpty(false);
*
* @memberOf Observable#
* @param defaultValue The value to return if the sequence is empty. If not provided, this defaults to null.
* @returns {Observable} An observable sequence that contains the specified default value if the source is empty; otherwise, the elements of the source itself.
*/
observableProto.defaultIfEmpty = function (defaultValue) {
var source = this;
if (defaultValue === undefined) {
defaultValue = null;
}
return new AnonymousObservable(function (observer) {
var found = false;
return source.subscribe(function (x) {
found = true;
observer.onNext(x);
}, observer.onError.bind(observer), function () {
if (!found) {
observer.onNext(defaultValue);
}
observer.onCompleted();
});
});
};
/**
* Returns an observable sequence that contains only distinct elements according to the keySelector and the comparer.
* Usage of this operator should be considered carefully due to the maintenance of an internal lookup structure which can grow large.
*
* @example
* var res = obs = xs.distinct();
* 2 - obs = xs.distinct(function (x) { return x.id; });
* 2 - obs = xs.distinct(function (x) { return x.id; }, function (x) { return x.toString(); });
* @param {Function} [keySelector] A function to compute the comparison key for each element.
* @param {Function} [keySerializer] Used to serialize the given object into a string for object comparison.
* @returns {Observable} An observable sequence only containing the distinct elements, based on a computed key value, from the source sequence.
*/
observableProto.distinct = function (keySelector, keySerializer) {
var source = this;
keySelector || (keySelector = identity);
keySerializer || (keySerializer = defaultKeySerializer);
return new AnonymousObservable(function (observer) {
var hashSet = {};
return source.subscribe(function (x) {
var key, serializedKey, otherKey, hasMatch = false;
try {
key = keySelector(x);
serializedKey = keySerializer(key);
} catch (exception) {
observer.onError(exception);
return;
}
for (otherKey in hashSet) {
if (serializedKey === otherKey) {
hasMatch = true;
break;
}
}
if (!hasMatch) {
hashSet[serializedKey] = null;
observer.onNext(x);
}
}, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
};
/**
* Groups the elements of an observable sequence according to a specified key selector function and comparer and selects the resulting elements by using a specified function.
*
* @example
* var res = observable.groupBy(function (x) { return x.id; });
* 2 - observable.groupBy(function (x) { return x.id; }), function (x) { return x.name; });
* 3 - observable.groupBy(function (x) { return x.id; }), function (x) { return x.name; }, function (x) { return x.toString(); });
* @param {Function} keySelector A function to extract the key for each element.
* @param {Function} [elementSelector] A function to map each source element to an element in an observable group.
* @param {Function} [keySerializer] Used to serialize the given object into a string for object comparison.
* @returns {Observable} A sequence of observable groups, each of which corresponds to a unique key value, containing all elements that share that same key value.
*/
observableProto.groupBy = function (keySelector, elementSelector, keySerializer) {
return this.groupByUntil(keySelector, elementSelector, function () {
return observableNever();
}, keySerializer);
};
/**
* Groups the elements of an observable sequence according to a specified key selector function.
* A duration selector function is used to control the lifetime of groups. When a group expires, it receives an OnCompleted notification. When a new element with the same
* key value as a reclaimed group occurs, the group will be reborn with a new lifetime request.
*
* @example
* var res = observable.groupByUntil(function (x) { return x.id; }, null, function () { return Rx.Observable.never(); });
* 2 - observable.groupBy(function (x) { return x.id; }), function (x) { return x.name; }, function () { return Rx.Observable.never(); });
* 3 - observable.groupBy(function (x) { return x.id; }), function (x) { return x.name; }, function () { return Rx.Observable.never(); }, function (x) { return x.toString(); });
* @param {Function} keySelector A function to extract the key for each element.
* @param {Function} durationSelector A function to signal the expiration of a group.
* @param {Function} [keySerializer] Used to serialize the given object into a string for object comparison.
* @returns {Observable}
* A sequence of observable groups, each of which corresponds to a unique key value, containing all elements that share that same key value.
* If a group's lifetime expires, a new group with the same key value can be created once an element with such a key value is encoutered.
*
*/
observableProto.groupByUntil = function (keySelector, elementSelector, durationSelector, keySerializer) {
var source = this;
elementSelector || (elementSelector = identity);
keySerializer || (keySerializer = defaultKeySerializer);
return new AnonymousObservable(function (observer) {
var map = {},
groupDisposable = new CompositeDisposable(),
refCountDisposable = new RefCountDisposable(groupDisposable);
groupDisposable.add(source.subscribe(function (x) {
var duration, durationGroup, element, fireNewMapEntry, group, key, serializedKey, md, writer, w;
try {
key = keySelector(x);
serializedKey = keySerializer(key);
} catch (e) {
for (w in map) {
map[w].onError(e);
}
observer.onError(e);
return;
}
fireNewMapEntry = false;
try {
writer = map[serializedKey];
if (!writer) {
writer = new Subject();
map[serializedKey] = writer;
fireNewMapEntry = true;
}
} catch (e) {
for (w in map) {
map[w].onError(e);
}
observer.onError(e);
return;
}
if (fireNewMapEntry) {
group = new GroupedObservable(key, writer, refCountDisposable);
durationGroup = new GroupedObservable(key, writer);
try {
duration = durationSelector(durationGroup);
} catch (e) {
for (w in map) {
map[w].onError(e);
}
observer.onError(e);
return;
}
observer.onNext(group);
md = new SingleAssignmentDisposable();
groupDisposable.add(md);
var expire = function () {
if (serializedKey in map) {
delete map[serializedKey];
writer.onCompleted();
}
groupDisposable.remove(md);
};
md.setDisposable(duration.take(1).subscribe(noop, function (exn) {
for (w in map) {
map[w].onError(exn);
}
observer.onError(exn);
}, function () {
expire();
}));
}
try {
element = elementSelector(x);
} catch (e) {
for (w in map) {
map[w].onError(e);
}
observer.onError(e);
return;
}
writer.onNext(element);
}, function (ex) {
for (var w in map) {
map[w].onError(ex);
}
observer.onError(ex);
}, function () {
for (var w in map) {
map[w].onCompleted();
}
observer.onCompleted();
}));
return refCountDisposable;
});
};
/**
* Projects each element of an observable sequence into a new form by incorporating the element's index.
* @param {Function} selector A transform function to apply to each source element; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence whose elements are the result of invoking the transform function on each element of source.
*/
observableProto.select = observableProto.map = function (selector, thisArg) {
var parent = this;
return new AnonymousObservable(function (observer) {
var count = 0;
return parent.subscribe(function (value) {
var result;
try {
result = selector.call(thisArg, value, count++, parent);
} catch (exception) {
observer.onError(exception);
return;
}
observer.onNext(result);
}, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
};
/**
* Retrieves the value of a specified property from all elements in the Observable sequence.
* @param {String} property The property to pluck.
* @returns {Observable} Returns a new Observable sequence of property values.
*/
observableProto.pluck = function (property) {
return this.select(function (x) { return x[property]; });
};
function selectMany(selector) {
return this.select(function (x, i) {
var result = selector(x, i);
return isPromise(result) ? observableFromPromise(result) : result;
}).mergeObservable();
}
/**
* One of the Following:
* Projects each element of an observable sequence to an observable sequence and merges the resulting observable sequences into one observable sequence.
*
* @example
* var res = source.selectMany(function (x) { return Rx.Observable.range(0, x); });
* Or:
* Projects each element of an observable sequence to an observable sequence, invokes the result selector for the source element and each of the corresponding inner sequence's elements, and merges the results into one observable sequence.
*
* var res = source.selectMany(function (x) { return Rx.Observable.range(0, x); }, function (x, y) { return x + y; });
* Or:
* Projects each element of the source observable sequence to the other observable sequence and merges the resulting observable sequences into one observable sequence.
*
* var res = source.selectMany(Rx.Observable.fromArray([1,2,3]));
* @param selector A transform function to apply to each element or an observable sequence to project each element from the
* source sequence onto which could be either an observable or Promise.
* @param {Function} [resultSelector] A transform function to apply to each element of the intermediate sequence.
* @returns {Observable} An observable sequence whose elements are the result of invoking the one-to-many transform function collectionSelector on each element of the input sequence and then mapping each of those sequence elements and their corresponding source element to a result element.
*/
observableProto.selectMany = observableProto.flatMap = function (selector, resultSelector) {
if (resultSelector) {
return this.selectMany(function (x, i) {
var selectorResult = selector(x, i),
result = isPromise(selectorResult) ? observableFromPromise(selectorResult) : selectorResult;
return result.select(function (y) {
return resultSelector(x, y, i);
});
});
}
if (typeof selector === 'function') {
return selectMany.call(this, selector);
}
return selectMany.call(this, function () {
return selector;
});
};
/**
* Projects each element of an observable sequence into a new sequence of observable sequences by incorporating the element's index and then
* transforms an observable sequence of observable sequences into an observable sequence producing values only from the most recent observable sequence.
* @param {Function} selector A transform function to apply to each source element; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence whose elements are the result of invoking the transform function on each element of source producing an Observable of Observable sequences
* and that at any point in time produces the elements of the most recent inner observable sequence that has been received.
*/
observableProto.selectSwitch = observableProto.flatMapLatest = function (selector, thisArg) {
return this.select(selector, thisArg).switchLatest();
};
/**
* Bypasses a specified number of elements in an observable sequence and then returns the remaining elements.
* @param {Number} count The number of elements to skip before returning the remaining elements.
* @returns {Observable} An observable sequence that contains the elements that occur after the specified index in the input sequence.
*/
observableProto.skip = function (count) {
if (count < 0) {
throw new Error(argumentOutOfRange);
}
var observable = this;
return new AnonymousObservable(function (observer) {
var remaining = count;
return observable.subscribe(function (x) {
if (remaining <= 0) {
observer.onNext(x);
} else {
remaining--;
}
}, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
};
/**
* Bypasses elements in an observable sequence as long as a specified condition is true and then returns the remaining elements.
* The element's index is used in the logic of the predicate function.
*
* var res = source.skipWhile(function (value) { return value < 10; });
* var res = source.skipWhile(function (value, index) { return value < 10 || index < 10; });
* @param {Function} predicate A function to test each element for a condition; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence that contains the elements from the input sequence starting at the first element in the linear series that does not pass the test specified by predicate.
*/
observableProto.skipWhile = function (predicate, thisArg) {
var source = this;
return new AnonymousObservable(function (observer) {
var i = 0, running = false;
return source.subscribe(function (x) {
if (!running) {
try {
running = !predicate.call(thisArg, x, i++, source);
} catch (e) {
observer.onError(e);
return;
}
}
if (running) {
observer.onNext(x);
}
}, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
};
/**
* Returns a specified number of contiguous elements from the start of an observable sequence, using the specified scheduler for the edge case of take(0).
*
* var res = source.take(5);
* var res = source.take(0, Rx.Scheduler.timeout);
* @param {Number} count The number of elements to return.
* @param {Scheduler} [scheduler] Scheduler used to produce an OnCompleted message in case <paramref name="count count</paramref> is set to 0.
* @returns {Observable} An observable sequence that contains the specified number of elements from the start of the input sequence.
*/
observableProto.take = function (count, scheduler) {
if (count < 0) {
throw new Error(argumentOutOfRange);
}
if (count === 0) {
return observableEmpty(scheduler);
}
var observable = this;
return new AnonymousObservable(function (observer) {
var remaining = count;
return observable.subscribe(function (x) {
if (remaining > 0) {
remaining--;
observer.onNext(x);
if (remaining === 0) {
observer.onCompleted();
}
}
}, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
};
/**
* Returns elements from an observable sequence as long as a specified condition is true.
* The element's index is used in the logic of the predicate function.
*
* @example
* var res = source.takeWhile(function (value) { return value < 10; });
* var res = source.takeWhile(function (value, index) { return value < 10 || index < 10; });
* @param {Function} predicate A function to test each element for a condition; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence that contains the elements from the input sequence that occur before the element at which the test no longer passes.
*/
observableProto.takeWhile = function (predicate, thisArg) {
var observable = this;
return new AnonymousObservable(function (observer) {
var i = 0, running = true;
return observable.subscribe(function (x) {
if (running) {
try {
running = predicate.call(thisArg, x, i++, observable);
} catch (e) {
observer.onError(e);
return;
}
if (running) {
observer.onNext(x);
} else {
observer.onCompleted();
}
}
}, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
};
/**
* Filters the elements of an observable sequence based on a predicate by incorporating the element's index.
*
* @example
* var res = source.where(function (value) { return value < 10; });
* var res = source.where(function (value, index) { return value < 10 || index < 10; });
* @param {Function} predicate A function to test each source element for a condition; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence that contains elements from the input sequence that satisfy the condition.
*/
observableProto.where = observableProto.filter = function (predicate, thisArg) {
var parent = this;
return new AnonymousObservable(function (observer) {
var count = 0;
return parent.subscribe(function (value) {
var shouldRun;
try {
shouldRun = predicate.call(thisArg, value, count++, parent);
} catch (exception) {
observer.onError(exception);
return;
}
if (shouldRun) {
observer.onNext(value);
}
}, observer.onError.bind(observer), observer.onCompleted.bind(observer));
});
};
var AnonymousObservable = Rx.AnonymousObservable = (function (_super) {
inherits(AnonymousObservable, _super);
// Fix subscriber to check for undefined or function returned to decorate as Disposable
function fixSubscriber(subscriber) {
if (typeof subscriber === 'undefined') {
subscriber = disposableEmpty;
} else if (typeof subscriber === 'function') {
subscriber = disposableCreate(subscriber);
}
return subscriber;
}
function AnonymousObservable(subscribe) {
if (!(this instanceof AnonymousObservable)) {
return new AnonymousObservable(subscribe);
}
function s(observer) {
var autoDetachObserver = new AutoDetachObserver(observer);
if (currentThreadScheduler.scheduleRequired()) {
currentThreadScheduler.schedule(function () {
try {
autoDetachObserver.setDisposable(fixSubscriber(subscribe(autoDetachObserver)));
} catch (e) {
if (!autoDetachObserver.fail(e)) {
throw e;
}
}
});
} else {
try {
autoDetachObserver.setDisposable(fixSubscriber(subscribe(autoDetachObserver)));
} catch (e) {
if (!autoDetachObserver.fail(e)) {
throw e;
}
}
}
return autoDetachObserver;
}
_super.call(this, s);
}
return AnonymousObservable;
}(Observable));
/** @private */
var AutoDetachObserver = (function (_super) {
inherits(AutoDetachObserver, _super);
function AutoDetachObserver(observer) {
_super.call(this);
this.observer = observer;
this.m = new SingleAssignmentDisposable();
}
var AutoDetachObserverPrototype = AutoDetachObserver.prototype;
AutoDetachObserverPrototype.next = function (value) {
var noError = false;
try {
this.observer.onNext(value);
noError = true;
} catch (e) {
throw e;
} finally {
if (!noError) {
this.dispose();
}
}
};
AutoDetachObserverPrototype.error = function (exn) {
try {
this.observer.onError(exn);
} catch (e) {
throw e;
} finally {
this.dispose();
}
};
AutoDetachObserverPrototype.completed = function () {
try {
this.observer.onCompleted();
} catch (e) {
throw e;
} finally {
this.dispose();
}
};
AutoDetachObserverPrototype.setDisposable = function (value) { this.m.setDisposable(value); };
AutoDetachObserverPrototype.getDisposable = function (value) { return this.m.getDisposable(); };
/* @private */
AutoDetachObserverPrototype.disposable = function (value) {
return arguments.length ? this.getDisposable() : setDisposable(value);
};
AutoDetachObserverPrototype.dispose = function () {
_super.prototype.dispose.call(this);
this.m.dispose();
};
return AutoDetachObserver;
}(AbstractObserver));
/** @private */
var GroupedObservable = (function (_super) {
inherits(GroupedObservable, _super);
function subscribe(observer) {
return this.underlyingObservable.subscribe(observer);
}
/**
* @constructor
* @private
*/
function GroupedObservable(key, underlyingObservable, mergedDisposable) {
_super.call(this, subscribe);
this.key = key;
this.underlyingObservable = !mergedDisposable ?
underlyingObservable :
new AnonymousObservable(function (observer) {
return new CompositeDisposable(mergedDisposable.getDisposable(), underlyingObservable.subscribe(observer));
});
}
return GroupedObservable;
}(Observable));
/** @private */
var InnerSubscription = function (subject, observer) {
this.subject = subject;
this.observer = observer;
};
/**
* @private
* @memberOf InnerSubscription
*/
InnerSubscription.prototype.dispose = function () {
if (!this.subject.isDisposed && this.observer !== null) {
var idx = this.subject.observers.indexOf(this.observer);
this.subject.observers.splice(idx, 1);
this.observer = null;
}
};
/**
* Represents an object that is both an observable sequence as well as an observer.
* Each notification is broadcasted to all subscribed observers.
*/
var Subject = Rx.Subject = (function (_super) {
function subscribe(observer) {
checkDisposed.call(this);
if (!this.isStopped) {
this.observers.push(observer);
return new InnerSubscription(this, observer);
}
if (this.exception) {
observer.onError(this.exception);
return disposableEmpty;
}
observer.onCompleted();
return disposableEmpty;
}
inherits(Subject, _super);
/**
* Creates a subject.
* @constructor
*/
function Subject() {
_super.call(this, subscribe);
this.isDisposed = false,
this.isStopped = false,
this.observers = [];
}
addProperties(Subject.prototype, Observer, {
/**
* Indicates whether the subject has observers subscribed to it.
* @returns {Boolean} Indicates whether the subject has observers subscribed to it.
*/
hasObservers: function () {
return this.observers.length > 0;
},
/**
* Notifies all subscribed observers about the end of the sequence.
*/
onCompleted: function () {
checkDisposed.call(this);
if (!this.isStopped) {
var os = this.observers.slice(0);
this.isStopped = true;
for (var i = 0, len = os.length; i < len; i++) {
os[i].onCompleted();
}
this.observers = [];
}
},
/**
* Notifies all subscribed observers about the exception.
* @param {Mixed} error The exception to send to all observers.
*/
onError: function (exception) {
checkDisposed.call(this);
if (!this.isStopped) {
var os = this.observers.slice(0);
this.isStopped = true;
this.exception = exception;
for (var i = 0, len = os.length; i < len; i++) {
os[i].onError(exception);
}
this.observers = [];
}
},
/**
* Notifies all subscribed observers about the arrival of the specified element in the sequence.
* @param {Mixed} value The value to send to all observers.
*/
onNext: function (value) {
checkDisposed.call(this);
if (!this.isStopped) {
var os = this.observers.slice(0);
for (var i = 0, len = os.length; i < len; i++) {
os[i].onNext(value);
}
}
},
/**
* Unsubscribe all observers and release resources.
*/
dispose: function () {
this.isDisposed = true;
this.observers = null;
}
});
/**
* Creates a subject from the specified observer and observable.
* @param {Observer} observer The observer used to send messages to the subject.
* @param {Observable} observable The observable used to subscribe to messages sent from the subject.
* @returns {Subject} Subject implemented using the given observer and observable.
*/
Subject.create = function (observer, observable) {
return new AnonymousSubject(observer, observable);
};
return Subject;
}(Observable));
/**
* Represents the result of an asynchronous operation.
* The last value before the OnCompleted notification, or the error received through OnError, is sent to all subscribed observers.
*/
var AsyncSubject = Rx.AsyncSubject = (function (_super) {
function subscribe(observer) {
checkDisposed.call(this);
if (!this.isStopped) {
this.observers.push(observer);
return new InnerSubscription(this, observer);
}
var ex = this.exception,
hv = this.hasValue,
v = this.value;
if (ex) {
observer.onError(ex);
} else if (hv) {
observer.onNext(v);
observer.onCompleted();
} else {
observer.onCompleted();
}
return disposableEmpty;
}
inherits(AsyncSubject, _super);
/**
* Creates a subject that can only receive one value and that value is cached for all future observations.
* @constructor
*/
function AsyncSubject() {
_super.call(this, subscribe);
this.isDisposed = false;
this.isStopped = false;
this.value = null;
this.hasValue = false;
this.observers = [];
this.exception = null;
}
addProperties(AsyncSubject.prototype, Observer, {
/**
* Indicates whether the subject has observers subscribed to it.
* @returns {Boolean} Indicates whether the subject has observers subscribed to it.
*/
hasObservers: function () {
checkDisposed.call(this);
return this.observers.length > 0;
},
/**
* Notifies all subscribed observers about the end of the sequence, also causing the last received value to be sent out (if any).
*/
onCompleted: function () {
var o, i, len;
checkDisposed.call(this);
if (!this.isStopped) {
this.isStopped = true;
var os = this.observers.slice(0),
v = this.value,
hv = this.hasValue;
if (hv) {
for (i = 0, len = os.length; i < len; i++) {
o = os[i];
o.onNext(v);
o.onCompleted();
}
} else {
for (i = 0, len = os.length; i < len; i++) {
os[i].onCompleted();
}
}
this.observers = [];
}
},
/**
* Notifies all subscribed observers about the exception.
* @param {Mixed} error The exception to send to all observers.
*/
onError: function (exception) {
checkDisposed.call(this);
if (!this.isStopped) {
var os = this.observers.slice(0);
this.isStopped = true;
this.exception = exception;
for (var i = 0, len = os.length; i < len; i++) {
os[i].onError(exception);
}
this.observers = [];
}
},
/**
* Sends a value to the subject. The last value received before successful termination will be sent to all subscribed and future observers.
* @param {Mixed} value The value to store in the subject.
*/
onNext: function (value) {
checkDisposed.call(this);
if (!this.isStopped) {
this.value = value;
this.hasValue = true;
}
},
/**
* Unsubscribe all observers and release resources.
*/
dispose: function () {
this.isDisposed = true;
this.observers = null;
this.exception = null;
this.value = null;
}
});
return AsyncSubject;
}(Observable));
/** @private */
var AnonymousSubject = (function (_super) {
inherits(AnonymousSubject, _super);
function subscribe(observer) {
return this.observable.subscribe(observer);
}
/**
* @private
* @constructor
*/
function AnonymousSubject(observer, observable) {
_super.call(this, subscribe);
this.observer = observer;
this.observable = observable;
}
addProperties(AnonymousSubject.prototype, Observer, {
/**
* @private
* @memberOf AnonymousSubject#
*/
onCompleted: function () {
this.observer.onCompleted();
},
/**
* @private
* @memberOf AnonymousSubject#
*/
onError: function (exception) {
this.observer.onError(exception);
},
/**
* @private
* @memberOf AnonymousSubject#
*/
onNext: function (value) {
this.observer.onNext(value);
}
});
return AnonymousSubject;
}(Observable));
if (typeof define == 'function' && typeof define.amd == 'object' && define.amd) {
root.Rx = Rx;
define(function() {
return Rx;
});
} else if (freeExports && freeModule) {
// in Node.js or RingoJS
if (moduleExports) {
(freeModule.exports = Rx).Rx = Rx;
} else {
freeExports.Rx = Rx;
}
} else {
// in a browser or Rhino
root.Rx = Rx;
}
}.call(this)); |
src/FontIcon/FontIcon.js | skystebnicki/chamel | import React from 'react';
import PropTypes from 'prop-types';
import classnames from 'classnames';
import ThemeService from '../styles/ChamelThemeService';
/**
* Functional component for any button
*
* @param props
* @param context
* @returns {ReactDOM}
* @constructor
*/
const FontIcon = (props, context) => {
let theme = null,
themeName = null;
if (context.chamelTheme && context.chamelTheme.fontIcon) {
theme = context.chamelTheme.fontIcon;
themeName = context.chamelTheme.name;
} else {
theme = ThemeService.defaultTheme.fontIcon;
themeName = ThemeService.defaultTheme.name;
}
let { className, ...other } = props;
const classes = classnames(
theme.fontIcon,
{
[theme.iconSize18]: props.size == 18,
[theme.iconSize24]: props.size == 24,
[theme.iconSize36]: props.size == 36,
[theme.iconSize48]: props.size == 48,
},
className,
);
const content = themeName === 'material' ? props.children : null;
return (
<span className={classes} {...other}>
{content}
</span>
);
};
/**
* Set accepted properties
*/
FontIcon.propTypes = {
className: PropTypes.string,
size: PropTypes.oneOf([18, 24, 36, 48]),
};
/**
* Set property defaults
*/
FontIcon.defaultProps = {
className: '',
size: 24,
};
/**
* An alternate theme may be passed down by a provider
*/
FontIcon.contextTypes = {
chamelTheme: PropTypes.object,
};
export default FontIcon;
|
src/app/components/Diagram/Guitar.js | vinodronold/fivefrets-next | import React from 'react'
import glamorous from 'glamorous'
import Theme from '../../constants/Theme'
import { GetChordName, GetGuitarChordShape } from './chordMeta'
const Container = glamorous.div({
display: 'flex',
flexWrap: 'wrap',
justifyContent: 'center',
margin: '1rem'
})
const SVGText = ({ x, y, fontSize = 12, fill = Theme.color.primary(), children }) => (
<text x={x} y={y} stroke={'none'} fill={fill} fontSize={`${fontSize}px`}>
{children}
</text>
)
const Barre = ({ stroke, fret = 1 }) =>
fret > 0 ? <ellipse cx="100" cy={50 * fret} fill={stroke} strokeWidth="1.5" ry="3" rx="80" /> : null
const FretBoard = ({ children, fill, stroke, height = '15rem', r, q, x, style, ...rest }) => {
let ChordShape = GetGuitarChordShape(r, q, x)
let start = ChordShape.s ? ChordShape.s : 1
return (
<svg
xmlns="http://www.w3.org/2000/svg"
viewBox="0 0 200 250"
style={{
position: 'relative',
height: height,
width: 'auto',
...style
}}
{...rest}>
<path fill={fill} d="M-1-1h202v252H-1z" />
<g stroke={stroke}>
<path fill={fill} strokeWidth="1.5" d="M25 25h150v200H25z" />
<path
fill="none"
strokeOpacity="null"
strokeWidth="1.5"
d="M26 75h150M26 125h150M26 175h150M56 26v199M86 26v199m30-199v199m30-199v199"
strokeLinecap="null"
strokeLinejoin="null"
/>
<SVGText x="95" y="20" fontSize="16">
{GetChordName(r, q, x)}
</SVGText>
<SVGText x="9.5" y="50">
{start}
</SVGText>
<SVGText x="9.5" y="100">
{start + 1}
</SVGText>
<SVGText x="9.5" y="150">
{start + 2}
</SVGText>
<SVGText x="9.5" y="200">
{start + 3}
</SVGText>
<Barre fret={ChordShape.b} stroke={stroke} />
{ChordShape.p.map(
(f, i) =>
f > 0 && f <= 4 ? (
<ellipse
key={i}
cx={26 + 30 * i}
cy={50 * f}
fill={stroke}
strokeOpacity="null"
strokeWidth="1.5"
ry="5"
rx="5"
/>
) : null
)}
</g>
</svg>
)
}
export default ({ chordsToDraw, x = 0 }) =>
chordsToDraw ? (
<Container>
{chordsToDraw.map(({ r, q }, i) => (
<FretBoard key={i} r={r} q={q} x={x} fill={Theme.color.bg(0)} stroke={Theme.color.primary()} />
))}
</Container>
) : null
|
fields/types/boolean/BooleanFilter.js | ratecity/keystone | import React from 'react';
import { SegmentedControl } from '../../../admin/client/App/elemental';
const VALUE_OPTIONS = [
{ label: 'Is Checked', value: true },
{ label: 'Is NOT Checked', value: false },
];
function getDefaultValue () {
return {
value: true,
};
}
var BooleanFilter = React.createClass({
propTypes: {
filter: React.PropTypes.shape({
value: React.PropTypes.bool,
}),
},
statics: {
getDefaultValue: getDefaultValue,
},
getDefaultProps () {
return {
filter: getDefaultValue(),
};
},
updateValue (value) {
this.props.onChange({ value });
},
render () {
return <SegmentedControl equalWidthSegments options={VALUE_OPTIONS} value={this.props.filter.value} onChange={this.updateValue} />;
},
});
module.exports = BooleanFilter;
|
files/rxjs/2.4.1/rx.compat.js | ajkj/jsdelivr | // Copyright (c) Microsoft Open Technologies, Inc. All rights reserved. See License.txt in the project root for license information.
;(function (undefined) {
var objectTypes = {
'boolean': false,
'function': true,
'object': true,
'number': false,
'string': false,
'undefined': false
};
var root = (objectTypes[typeof window] && window) || this,
freeExports = objectTypes[typeof exports] && exports && !exports.nodeType && exports,
freeModule = objectTypes[typeof module] && module && !module.nodeType && module,
moduleExports = freeModule && freeModule.exports === freeExports && freeExports,
freeGlobal = objectTypes[typeof global] && global;
if (freeGlobal && (freeGlobal.global === freeGlobal || freeGlobal.window === freeGlobal)) {
root = freeGlobal;
}
var Rx = {
internals: {},
config: {
Promise: root.Promise
},
helpers: { }
};
// Defaults
var noop = Rx.helpers.noop = function () { },
notDefined = Rx.helpers.notDefined = function (x) { return typeof x === 'undefined'; },
isScheduler = Rx.helpers.isScheduler = function (x) { return x instanceof Rx.Scheduler; },
identity = Rx.helpers.identity = function (x) { return x; },
pluck = Rx.helpers.pluck = function (property) { return function (x) { return x[property]; }; },
just = Rx.helpers.just = function (value) { return function () { return value; }; },
defaultNow = Rx.helpers.defaultNow = (function () { return !!Date.now ? Date.now : function () { return +new Date; }; }()),
defaultComparer = Rx.helpers.defaultComparer = function (x, y) { return isEqual(x, y); },
defaultSubComparer = Rx.helpers.defaultSubComparer = function (x, y) { return x > y ? 1 : (x < y ? -1 : 0); },
defaultKeySerializer = Rx.helpers.defaultKeySerializer = function (x) { return x.toString(); },
defaultError = Rx.helpers.defaultError = function (err) { throw err; },
isPromise = Rx.helpers.isPromise = function (p) { return !!p && typeof p.then === 'function'; },
asArray = Rx.helpers.asArray = function () { return Array.prototype.slice.call(arguments); },
not = Rx.helpers.not = function (a) { return !a; },
isFunction = Rx.helpers.isFunction = (function () {
var isFn = function (value) {
return typeof value == 'function' || false;
}
// fallback for older versions of Chrome and Safari
if (isFn(/x/)) {
isFn = function(value) {
return typeof value == 'function' && toString.call(value) == '[object Function]';
};
}
return isFn;
}());
function cloneArray(arr) {
var len = arr.length, a = new Array(len);
for(var i = 0; i < len; i++) { a[i] = arr[i]; }
return a;
}
Rx.config.longStackSupport = false;
var hasStacks = false;
try {
throw new Error();
} catch (e) {
hasStacks = !!e.stack;
}
// All code after this point will be filtered from stack traces reported by RxJS
var rStartingLine = captureLine(), rFileName;
var STACK_JUMP_SEPARATOR = "From previous event:";
function makeStackTraceLong(error, observable) {
// If possible, transform the error stack trace by removing Node and RxJS
// cruft, then concatenating with the stack trace of `observable`.
if (hasStacks &&
observable.stack &&
typeof error === "object" &&
error !== null &&
error.stack &&
error.stack.indexOf(STACK_JUMP_SEPARATOR) === -1
) {
var stacks = [];
for (var o = observable; !!o; o = o.source) {
if (o.stack) {
stacks.unshift(o.stack);
}
}
stacks.unshift(error.stack);
var concatedStacks = stacks.join("\n" + STACK_JUMP_SEPARATOR + "\n");
error.stack = filterStackString(concatedStacks);
}
}
function filterStackString(stackString) {
var lines = stackString.split("\n"),
desiredLines = [];
for (var i = 0, len = lines.length; i < len; i++) {
var line = lines[i];
if (!isInternalFrame(line) && !isNodeFrame(line) && line) {
desiredLines.push(line);
}
}
return desiredLines.join("\n");
}
function isInternalFrame(stackLine) {
var fileNameAndLineNumber = getFileNameAndLineNumber(stackLine);
if (!fileNameAndLineNumber) {
return false;
}
var fileName = fileNameAndLineNumber[0], lineNumber = fileNameAndLineNumber[1];
return fileName === rFileName &&
lineNumber >= rStartingLine &&
lineNumber <= rEndingLine;
}
function isNodeFrame(stackLine) {
return stackLine.indexOf("(module.js:") !== -1 ||
stackLine.indexOf("(node.js:") !== -1;
}
function captureLine() {
if (!hasStacks) { return; }
try {
throw new Error();
} catch (e) {
var lines = e.stack.split("\n");
var firstLine = lines[0].indexOf("@") > 0 ? lines[1] : lines[2];
var fileNameAndLineNumber = getFileNameAndLineNumber(firstLine);
if (!fileNameAndLineNumber) { return; }
rFileName = fileNameAndLineNumber[0];
return fileNameAndLineNumber[1];
}
}
function getFileNameAndLineNumber(stackLine) {
// Named functions: "at functionName (filename:lineNumber:columnNumber)"
var attempt1 = /at .+ \((.+):(\d+):(?:\d+)\)$/.exec(stackLine);
if (attempt1) { return [attempt1[1], Number(attempt1[2])]; }
// Anonymous functions: "at filename:lineNumber:columnNumber"
var attempt2 = /at ([^ ]+):(\d+):(?:\d+)$/.exec(stackLine);
if (attempt2) { return [attempt2[1], Number(attempt2[2])]; }
// Firefox style: "function@filename:lineNumber or @filename:lineNumber"
var attempt3 = /.*@(.+):(\d+)$/.exec(stackLine);
if (attempt3) { return [attempt3[1], Number(attempt3[2])]; }
}
var EmptyError = Rx.EmptyError = function() {
this.message = 'Sequence contains no elements.';
Error.call(this);
};
EmptyError.prototype = Error.prototype;
var ObjectDisposedError = Rx.ObjectDisposedError = function() {
this.message = 'Object has been disposed';
Error.call(this);
};
ObjectDisposedError.prototype = Error.prototype;
var ArgumentOutOfRangeError = Rx.ArgumentOutOfRangeError = function () {
this.message = 'Argument out of range';
Error.call(this);
};
ArgumentOutOfRangeError.prototype = Error.prototype;
var NotSupportedError = Rx.NotSupportedError = function (message) {
this.message = message || 'This operation is not supported';
Error.call(this);
};
NotSupportedError.prototype = Error.prototype;
var NotImplementedError = Rx.NotImplementedError = function (message) {
this.message = message || 'This operation is not implemented';
Error.call(this);
};
NotImplementedError.prototype = Error.prototype;
var notImplemented = Rx.helpers.notImplemented = function () {
throw new NotImplementedError();
};
var notSupported = Rx.helpers.notSupported = function () {
throw new NotSupportedError();
};
// Shim in iterator support
var $iterator$ = (typeof Symbol === 'function' && Symbol.iterator) ||
'_es6shim_iterator_';
// Bug for mozilla version
if (root.Set && typeof new root.Set()['@@iterator'] === 'function') {
$iterator$ = '@@iterator';
}
var doneEnumerator = Rx.doneEnumerator = { done: true, value: undefined };
var isIterable = Rx.helpers.isIterable = function (o) {
return o[$iterator$] !== undefined;
}
var isArrayLike = Rx.helpers.isArrayLike = function (o) {
return o && o.length !== undefined;
}
Rx.helpers.iterator = $iterator$;
var bindCallback = Rx.internals.bindCallback = function (func, thisArg, argCount) {
if (typeof thisArg === 'undefined') { return func; }
switch(argCount) {
case 0:
return function() {
return func.call(thisArg)
};
case 1:
return function(arg) {
return func.call(thisArg, arg);
}
case 2:
return function(value, index) {
return func.call(thisArg, value, index);
};
case 3:
return function(value, index, collection) {
return func.call(thisArg, value, index, collection);
};
}
return function() {
return func.apply(thisArg, arguments);
};
};
/** Used to determine if values are of the language type Object */
var dontEnums = ['toString',
'toLocaleString',
'valueOf',
'hasOwnProperty',
'isPrototypeOf',
'propertyIsEnumerable',
'constructor'],
dontEnumsLength = dontEnums.length;
/** `Object#toString` result shortcuts */
var argsClass = '[object Arguments]',
arrayClass = '[object Array]',
boolClass = '[object Boolean]',
dateClass = '[object Date]',
errorClass = '[object Error]',
funcClass = '[object Function]',
numberClass = '[object Number]',
objectClass = '[object Object]',
regexpClass = '[object RegExp]',
stringClass = '[object String]';
var toString = Object.prototype.toString,
hasOwnProperty = Object.prototype.hasOwnProperty,
supportsArgsClass = toString.call(arguments) == argsClass, // For less <IE9 && FF<4
supportNodeClass,
errorProto = Error.prototype,
objectProto = Object.prototype,
stringProto = String.prototype,
propertyIsEnumerable = objectProto.propertyIsEnumerable;
try {
supportNodeClass = !(toString.call(document) == objectClass && !({ 'toString': 0 } + ''));
} catch (e) {
supportNodeClass = true;
}
var nonEnumProps = {};
nonEnumProps[arrayClass] = nonEnumProps[dateClass] = nonEnumProps[numberClass] = { 'constructor': true, 'toLocaleString': true, 'toString': true, 'valueOf': true };
nonEnumProps[boolClass] = nonEnumProps[stringClass] = { 'constructor': true, 'toString': true, 'valueOf': true };
nonEnumProps[errorClass] = nonEnumProps[funcClass] = nonEnumProps[regexpClass] = { 'constructor': true, 'toString': true };
nonEnumProps[objectClass] = { 'constructor': true };
var support = {};
(function () {
var ctor = function() { this.x = 1; },
props = [];
ctor.prototype = { 'valueOf': 1, 'y': 1 };
for (var key in new ctor) { props.push(key); }
for (key in arguments) { }
// Detect if `name` or `message` properties of `Error.prototype` are enumerable by default.
support.enumErrorProps = propertyIsEnumerable.call(errorProto, 'message') || propertyIsEnumerable.call(errorProto, 'name');
// Detect if `prototype` properties are enumerable by default.
support.enumPrototypes = propertyIsEnumerable.call(ctor, 'prototype');
// Detect if `arguments` object indexes are non-enumerable
support.nonEnumArgs = key != 0;
// Detect if properties shadowing those on `Object.prototype` are non-enumerable.
support.nonEnumShadows = !/valueOf/.test(props);
}(1));
var isObject = Rx.internals.isObject = function(value) {
var type = typeof value;
return value && (type == 'function' || type == 'object') || false;
};
function keysIn(object) {
var result = [];
if (!isObject(object)) {
return result;
}
if (support.nonEnumArgs && object.length && isArguments(object)) {
object = slice.call(object);
}
var skipProto = support.enumPrototypes && typeof object == 'function',
skipErrorProps = support.enumErrorProps && (object === errorProto || object instanceof Error);
for (var key in object) {
if (!(skipProto && key == 'prototype') &&
!(skipErrorProps && (key == 'message' || key == 'name'))) {
result.push(key);
}
}
if (support.nonEnumShadows && object !== objectProto) {
var ctor = object.constructor,
index = -1,
length = dontEnumsLength;
if (object === (ctor && ctor.prototype)) {
var className = object === stringProto ? stringClass : object === errorProto ? errorClass : toString.call(object),
nonEnum = nonEnumProps[className];
}
while (++index < length) {
key = dontEnums[index];
if (!(nonEnum && nonEnum[key]) && hasOwnProperty.call(object, key)) {
result.push(key);
}
}
}
return result;
}
function internalFor(object, callback, keysFunc) {
var index = -1,
props = keysFunc(object),
length = props.length;
while (++index < length) {
var key = props[index];
if (callback(object[key], key, object) === false) {
break;
}
}
return object;
}
function internalForIn(object, callback) {
return internalFor(object, callback, keysIn);
}
function isNode(value) {
// IE < 9 presents DOM nodes as `Object` objects except they have `toString`
// methods that are `typeof` "string" and still can coerce nodes to strings
return typeof value.toString != 'function' && typeof (value + '') == 'string';
}
var isArguments = function(value) {
return (value && typeof value == 'object') ? toString.call(value) == argsClass : false;
}
// fallback for browsers that can't detect `arguments` objects by [[Class]]
if (!supportsArgsClass) {
isArguments = function(value) {
return (value && typeof value == 'object') ? hasOwnProperty.call(value, 'callee') : false;
};
}
var isEqual = Rx.internals.isEqual = function (x, y) {
return deepEquals(x, y, [], []);
};
/** @private
* Used for deep comparison
**/
function deepEquals(a, b, stackA, stackB) {
// exit early for identical values
if (a === b) {
// treat `+0` vs. `-0` as not equal
return a !== 0 || (1 / a == 1 / b);
}
var type = typeof a,
otherType = typeof b;
// exit early for unlike primitive values
if (a === a && (a == null || b == null ||
(type != 'function' && type != 'object' && otherType != 'function' && otherType != 'object'))) {
return false;
}
// compare [[Class]] names
var className = toString.call(a),
otherClass = toString.call(b);
if (className == argsClass) {
className = objectClass;
}
if (otherClass == argsClass) {
otherClass = objectClass;
}
if (className != otherClass) {
return false;
}
switch (className) {
case boolClass:
case dateClass:
// coerce dates and booleans to numbers, dates to milliseconds and booleans
// to `1` or `0` treating invalid dates coerced to `NaN` as not equal
return +a == +b;
case numberClass:
// treat `NaN` vs. `NaN` as equal
return (a != +a) ?
b != +b :
// but treat `-0` vs. `+0` as not equal
(a == 0 ? (1 / a == 1 / b) : a == +b);
case regexpClass:
case stringClass:
// coerce regexes to strings (http://es5.github.io/#x15.10.6.4)
// treat string primitives and their corresponding object instances as equal
return a == String(b);
}
var isArr = className == arrayClass;
if (!isArr) {
// exit for functions and DOM nodes
if (className != objectClass || (!support.nodeClass && (isNode(a) || isNode(b)))) {
return false;
}
// in older versions of Opera, `arguments` objects have `Array` constructors
var ctorA = !support.argsObject && isArguments(a) ? Object : a.constructor,
ctorB = !support.argsObject && isArguments(b) ? Object : b.constructor;
// non `Object` object instances with different constructors are not equal
if (ctorA != ctorB &&
!(hasOwnProperty.call(a, 'constructor') && hasOwnProperty.call(b, 'constructor')) &&
!(isFunction(ctorA) && ctorA instanceof ctorA && isFunction(ctorB) && ctorB instanceof ctorB) &&
('constructor' in a && 'constructor' in b)
) {
return false;
}
}
// assume cyclic structures are equal
// the algorithm for detecting cyclic structures is adapted from ES 5.1
// section 15.12.3, abstract operation `JO` (http://es5.github.io/#x15.12.3)
var initedStack = !stackA;
stackA || (stackA = []);
stackB || (stackB = []);
var length = stackA.length;
while (length--) {
if (stackA[length] == a) {
return stackB[length] == b;
}
}
var size = 0;
var result = true;
// add `a` and `b` to the stack of traversed objects
stackA.push(a);
stackB.push(b);
// recursively compare objects and arrays (susceptible to call stack limits)
if (isArr) {
// compare lengths to determine if a deep comparison is necessary
length = a.length;
size = b.length;
result = size == length;
if (result) {
// deep compare the contents, ignoring non-numeric properties
while (size--) {
var index = length,
value = b[size];
if (!(result = deepEquals(a[size], value, stackA, stackB))) {
break;
}
}
}
}
else {
// deep compare objects using `forIn`, instead of `forOwn`, to avoid `Object.keys`
// which, in this case, is more costly
internalForIn(b, function(value, key, b) {
if (hasOwnProperty.call(b, key)) {
// count the number of properties.
size++;
// deep compare each property value.
return (result = hasOwnProperty.call(a, key) && deepEquals(a[key], value, stackA, stackB));
}
});
if (result) {
// ensure both objects have the same number of properties
internalForIn(a, function(value, key, a) {
if (hasOwnProperty.call(a, key)) {
// `size` will be `-1` if `a` has more properties than `b`
return (result = --size > -1);
}
});
}
}
stackA.pop();
stackB.pop();
return result;
}
var hasProp = {}.hasOwnProperty,
slice = Array.prototype.slice;
var inherits = this.inherits = Rx.internals.inherits = function (child, parent) {
function __() { this.constructor = child; }
__.prototype = parent.prototype;
child.prototype = new __();
};
var addProperties = Rx.internals.addProperties = function (obj) {
for(var sources = [], i = 1, len = arguments.length; i < len; i++) { sources.push(arguments[i]); }
for (var idx = 0, ln = sources.length; idx < ln; idx++) {
var source = sources[idx];
for (var prop in source) {
obj[prop] = source[prop];
}
}
};
// Rx Utils
var addRef = Rx.internals.addRef = function (xs, r) {
return new AnonymousObservable(function (observer) {
return new CompositeDisposable(r.getDisposable(), xs.subscribe(observer));
});
};
function arrayInitialize(count, factory) {
var a = new Array(count);
for (var i = 0; i < count; i++) {
a[i] = factory();
}
return a;
}
var errorObj = {e: {}};
var tryCatchTarget;
function tryCatcher() {
try {
return tryCatchTarget.apply(this, arguments);
} catch (e) {
errorObj.e = e;
return errorObj;
}
}
function tryCatch(fn) {
if (!isFunction(fn)) { throw new TypeError('fn must be a function'); }
tryCatchTarget = fn;
return tryCatcher;
}
function thrower(e) {
throw e;
}
// Utilities
if (!Function.prototype.bind) {
Function.prototype.bind = function (that) {
var target = this,
args = slice.call(arguments, 1);
var bound = function () {
if (this instanceof bound) {
function F() { }
F.prototype = target.prototype;
var self = new F();
var result = target.apply(self, args.concat(slice.call(arguments)));
if (Object(result) === result) {
return result;
}
return self;
} else {
return target.apply(that, args.concat(slice.call(arguments)));
}
};
return bound;
};
}
if (!Array.prototype.forEach) {
Array.prototype.forEach = function (callback, thisArg) {
var T, k;
if (this == null) {
throw new TypeError(" this is null or not defined");
}
var O = Object(this);
var len = O.length >>> 0;
if (typeof callback !== "function") {
throw new TypeError(callback + " is not a function");
}
if (arguments.length > 1) {
T = thisArg;
}
k = 0;
while (k < len) {
var kValue;
if (k in O) {
kValue = O[k];
callback.call(T, kValue, k, O);
}
k++;
}
};
}
var boxedString = Object("a"),
splitString = boxedString[0] != "a" || !(0 in boxedString);
if (!Array.prototype.every) {
Array.prototype.every = function every(fun /*, thisp */) {
var object = Object(this),
self = splitString && {}.toString.call(this) == stringClass ?
this.split("") :
object,
length = self.length >>> 0,
thisp = arguments[1];
if ({}.toString.call(fun) != funcClass) {
throw new TypeError(fun + " is not a function");
}
for (var i = 0; i < length; i++) {
if (i in self && !fun.call(thisp, self[i], i, object)) {
return false;
}
}
return true;
};
}
if (!Array.prototype.map) {
Array.prototype.map = function map(fun /*, thisp*/) {
var object = Object(this),
self = splitString && {}.toString.call(this) == stringClass ?
this.split("") :
object,
length = self.length >>> 0,
result = Array(length),
thisp = arguments[1];
if ({}.toString.call(fun) != funcClass) {
throw new TypeError(fun + " is not a function");
}
for (var i = 0; i < length; i++) {
if (i in self) {
result[i] = fun.call(thisp, self[i], i, object);
}
}
return result;
};
}
if (!Array.prototype.filter) {
Array.prototype.filter = function (predicate) {
var results = [], item, t = new Object(this);
for (var i = 0, len = t.length >>> 0; i < len; i++) {
item = t[i];
if (i in t && predicate.call(arguments[1], item, i, t)) {
results.push(item);
}
}
return results;
};
}
if (!Array.isArray) {
Array.isArray = function (arg) {
return {}.toString.call(arg) == arrayClass;
};
}
if (!Array.prototype.indexOf) {
Array.prototype.indexOf = function indexOf(searchElement) {
var t = Object(this);
var len = t.length >>> 0;
if (len === 0) {
return -1;
}
var n = 0;
if (arguments.length > 1) {
n = Number(arguments[1]);
if (n !== n) {
n = 0;
} else if (n !== 0 && n != Infinity && n !== -Infinity) {
n = (n > 0 || -1) * Math.floor(Math.abs(n));
}
}
if (n >= len) {
return -1;
}
var k = n >= 0 ? n : Math.max(len - Math.abs(n), 0);
for (; k < len; k++) {
if (k in t && t[k] === searchElement) {
return k;
}
}
return -1;
};
}
// Fix for Tessel
if (!Object.prototype.propertyIsEnumerable) {
Object.prototype.propertyIsEnumerable = function (key) {
for (var k in this) { if (k === key) { return true; } }
return false;
};
}
if (!Object.keys) {
Object.keys = (function() {
'use strict';
var hasOwnProperty = Object.prototype.hasOwnProperty,
hasDontEnumBug = !({ toString: null }).propertyIsEnumerable('toString');
return function(obj) {
if (typeof obj !== 'object' && (typeof obj !== 'function' || obj === null)) {
throw new TypeError('Object.keys called on non-object');
}
var result = [], prop, i;
for (prop in obj) {
if (hasOwnProperty.call(obj, prop)) {
result.push(prop);
}
}
if (hasDontEnumBug) {
for (i = 0; i < dontEnumsLength; i++) {
if (hasOwnProperty.call(obj, dontEnums[i])) {
result.push(dontEnums[i]);
}
}
}
return result;
};
}());
}
// Collections
function IndexedItem(id, value) {
this.id = id;
this.value = value;
}
IndexedItem.prototype.compareTo = function (other) {
var c = this.value.compareTo(other.value);
c === 0 && (c = this.id - other.id);
return c;
};
// Priority Queue for Scheduling
var PriorityQueue = Rx.internals.PriorityQueue = function (capacity) {
this.items = new Array(capacity);
this.length = 0;
};
var priorityProto = PriorityQueue.prototype;
priorityProto.isHigherPriority = function (left, right) {
return this.items[left].compareTo(this.items[right]) < 0;
};
priorityProto.percolate = function (index) {
if (index >= this.length || index < 0) { return; }
var parent = index - 1 >> 1;
if (parent < 0 || parent === index) { return; }
if (this.isHigherPriority(index, parent)) {
var temp = this.items[index];
this.items[index] = this.items[parent];
this.items[parent] = temp;
this.percolate(parent);
}
};
priorityProto.heapify = function (index) {
+index || (index = 0);
if (index >= this.length || index < 0) { return; }
var left = 2 * index + 1,
right = 2 * index + 2,
first = index;
if (left < this.length && this.isHigherPriority(left, first)) {
first = left;
}
if (right < this.length && this.isHigherPriority(right, first)) {
first = right;
}
if (first !== index) {
var temp = this.items[index];
this.items[index] = this.items[first];
this.items[first] = temp;
this.heapify(first);
}
};
priorityProto.peek = function () { return this.items[0].value; };
priorityProto.removeAt = function (index) {
this.items[index] = this.items[--this.length];
this.items[this.length] = undefined;
this.heapify();
};
priorityProto.dequeue = function () {
var result = this.peek();
this.removeAt(0);
return result;
};
priorityProto.enqueue = function (item) {
var index = this.length++;
this.items[index] = new IndexedItem(PriorityQueue.count++, item);
this.percolate(index);
};
priorityProto.remove = function (item) {
for (var i = 0; i < this.length; i++) {
if (this.items[i].value === item) {
this.removeAt(i);
return true;
}
}
return false;
};
PriorityQueue.count = 0;
/**
* Represents a group of disposable resources that are disposed together.
* @constructor
*/
var CompositeDisposable = Rx.CompositeDisposable = function () {
var args = [], i, len;
if (Array.isArray(arguments[0])) {
args = arguments[0];
len = args.length;
} else {
len = arguments.length;
args = new Array(len);
for(i = 0; i < len; i++) { args[i] = arguments[i]; }
}
for(i = 0; i < len; i++) {
if (!isDisposable(args[i])) { throw new TypeError('Not a disposable'); }
}
this.disposables = args;
this.isDisposed = false;
this.length = args.length;
};
var CompositeDisposablePrototype = CompositeDisposable.prototype;
/**
* Adds a disposable to the CompositeDisposable or disposes the disposable if the CompositeDisposable is disposed.
* @param {Mixed} item Disposable to add.
*/
CompositeDisposablePrototype.add = function (item) {
if (this.isDisposed) {
item.dispose();
} else {
this.disposables.push(item);
this.length++;
}
};
/**
* Removes and disposes the first occurrence of a disposable from the CompositeDisposable.
* @param {Mixed} item Disposable to remove.
* @returns {Boolean} true if found; false otherwise.
*/
CompositeDisposablePrototype.remove = function (item) {
var shouldDispose = false;
if (!this.isDisposed) {
var idx = this.disposables.indexOf(item);
if (idx !== -1) {
shouldDispose = true;
this.disposables.splice(idx, 1);
this.length--;
item.dispose();
}
}
return shouldDispose;
};
/**
* Disposes all disposables in the group and removes them from the group.
*/
CompositeDisposablePrototype.dispose = function () {
if (!this.isDisposed) {
this.isDisposed = true;
var len = this.disposables.length, currentDisposables = new Array(len);
for(var i = 0; i < len; i++) { currentDisposables[i] = this.disposables[i]; }
this.disposables = [];
this.length = 0;
for (i = 0; i < len; i++) {
currentDisposables[i].dispose();
}
}
};
/**
* Provides a set of static methods for creating Disposables.
* @param {Function} dispose Action to run during the first call to dispose. The action is guaranteed to be run at most once.
*/
var Disposable = Rx.Disposable = function (action) {
this.isDisposed = false;
this.action = action || noop;
};
/** Performs the task of cleaning up resources. */
Disposable.prototype.dispose = function () {
if (!this.isDisposed) {
this.action();
this.isDisposed = true;
}
};
/**
* Creates a disposable object that invokes the specified action when disposed.
* @param {Function} dispose Action to run during the first call to dispose. The action is guaranteed to be run at most once.
* @return {Disposable} The disposable object that runs the given action upon disposal.
*/
var disposableCreate = Disposable.create = function (action) { return new Disposable(action); };
/**
* Gets the disposable that does nothing when disposed.
*/
var disposableEmpty = Disposable.empty = { dispose: noop };
/**
* Validates whether the given object is a disposable
* @param {Object} Object to test whether it has a dispose method
* @returns {Boolean} true if a disposable object, else false.
*/
var isDisposable = Disposable.isDisposable = function (d) {
return d && isFunction(d.dispose);
};
var checkDisposed = Disposable.checkDisposed = function (disposable) {
if (disposable.isDisposed) { throw new ObjectDisposedError(); }
};
var SingleAssignmentDisposable = Rx.SingleAssignmentDisposable = (function () {
function BooleanDisposable () {
this.isDisposed = false;
this.current = null;
}
var booleanDisposablePrototype = BooleanDisposable.prototype;
/**
* Gets the underlying disposable.
* @return The underlying disposable.
*/
booleanDisposablePrototype.getDisposable = function () {
return this.current;
};
/**
* Sets the underlying disposable.
* @param {Disposable} value The new underlying disposable.
*/
booleanDisposablePrototype.setDisposable = function (value) {
var shouldDispose = this.isDisposed;
if (!shouldDispose) {
var old = this.current;
this.current = value;
}
old && old.dispose();
shouldDispose && value && value.dispose();
};
/**
* Disposes the underlying disposable as well as all future replacements.
*/
booleanDisposablePrototype.dispose = function () {
if (!this.isDisposed) {
this.isDisposed = true;
var old = this.current;
this.current = null;
}
old && old.dispose();
};
return BooleanDisposable;
}());
var SerialDisposable = Rx.SerialDisposable = SingleAssignmentDisposable;
/**
* Represents a disposable resource that only disposes its underlying disposable resource when all dependent disposable objects have been disposed.
*/
var RefCountDisposable = Rx.RefCountDisposable = (function () {
function InnerDisposable(disposable) {
this.disposable = disposable;
this.disposable.count++;
this.isInnerDisposed = false;
}
InnerDisposable.prototype.dispose = function () {
if (!this.disposable.isDisposed && !this.isInnerDisposed) {
this.isInnerDisposed = true;
this.disposable.count--;
if (this.disposable.count === 0 && this.disposable.isPrimaryDisposed) {
this.disposable.isDisposed = true;
this.disposable.underlyingDisposable.dispose();
}
}
};
/**
* Initializes a new instance of the RefCountDisposable with the specified disposable.
* @constructor
* @param {Disposable} disposable Underlying disposable.
*/
function RefCountDisposable(disposable) {
this.underlyingDisposable = disposable;
this.isDisposed = false;
this.isPrimaryDisposed = false;
this.count = 0;
}
/**
* Disposes the underlying disposable only when all dependent disposables have been disposed
*/
RefCountDisposable.prototype.dispose = function () {
if (!this.isDisposed && !this.isPrimaryDisposed) {
this.isPrimaryDisposed = true;
if (this.count === 0) {
this.isDisposed = true;
this.underlyingDisposable.dispose();
}
}
};
/**
* Returns a dependent disposable that when disposed decreases the refcount on the underlying disposable.
* @returns {Disposable} A dependent disposable contributing to the reference count that manages the underlying disposable's lifetime.
*/
RefCountDisposable.prototype.getDisposable = function () {
return this.isDisposed ? disposableEmpty : new InnerDisposable(this);
};
return RefCountDisposable;
})();
function ScheduledDisposable(scheduler, disposable) {
this.scheduler = scheduler;
this.disposable = disposable;
this.isDisposed = false;
}
function scheduleItem(s, self) {
if (!self.isDisposed) {
self.isDisposed = true;
self.disposable.dispose();
}
}
ScheduledDisposable.prototype.dispose = function () {
this.scheduler.scheduleWithState(this, scheduleItem);
};
var ScheduledItem = Rx.internals.ScheduledItem = function (scheduler, state, action, dueTime, comparer) {
this.scheduler = scheduler;
this.state = state;
this.action = action;
this.dueTime = dueTime;
this.comparer = comparer || defaultSubComparer;
this.disposable = new SingleAssignmentDisposable();
}
ScheduledItem.prototype.invoke = function () {
this.disposable.setDisposable(this.invokeCore());
};
ScheduledItem.prototype.compareTo = function (other) {
return this.comparer(this.dueTime, other.dueTime);
};
ScheduledItem.prototype.isCancelled = function () {
return this.disposable.isDisposed;
};
ScheduledItem.prototype.invokeCore = function () {
return this.action(this.scheduler, this.state);
};
/** Provides a set of static properties to access commonly used schedulers. */
var Scheduler = Rx.Scheduler = (function () {
function Scheduler(now, schedule, scheduleRelative, scheduleAbsolute) {
this.now = now;
this._schedule = schedule;
this._scheduleRelative = scheduleRelative;
this._scheduleAbsolute = scheduleAbsolute;
}
function invokeAction(scheduler, action) {
action();
return disposableEmpty;
}
var schedulerProto = Scheduler.prototype;
/**
* Schedules an action to be executed.
* @param {Function} action Action to execute.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.schedule = function (action) {
return this._schedule(action, invokeAction);
};
/**
* Schedules an action to be executed.
* @param state State passed to the action to be executed.
* @param {Function} action Action to be executed.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithState = function (state, action) {
return this._schedule(state, action);
};
/**
* Schedules an action to be executed after the specified relative due time.
* @param {Function} action Action to execute.
* @param {Number} dueTime Relative time after which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithRelative = function (dueTime, action) {
return this._scheduleRelative(action, dueTime, invokeAction);
};
/**
* Schedules an action to be executed after dueTime.
* @param state State passed to the action to be executed.
* @param {Function} action Action to be executed.
* @param {Number} dueTime Relative time after which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithRelativeAndState = function (state, dueTime, action) {
return this._scheduleRelative(state, dueTime, action);
};
/**
* Schedules an action to be executed at the specified absolute due time.
* @param {Function} action Action to execute.
* @param {Number} dueTime Absolute time at which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithAbsolute = function (dueTime, action) {
return this._scheduleAbsolute(action, dueTime, invokeAction);
};
/**
* Schedules an action to be executed at dueTime.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to be executed.
* @param {Number}dueTime Absolute time at which to execute the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleWithAbsoluteAndState = function (state, dueTime, action) {
return this._scheduleAbsolute(state, dueTime, action);
};
/** Gets the current time according to the local machine's system clock. */
Scheduler.now = defaultNow;
/**
* Normalizes the specified TimeSpan value to a positive value.
* @param {Number} timeSpan The time span value to normalize.
* @returns {Number} The specified TimeSpan value if it is zero or positive; otherwise, 0
*/
Scheduler.normalize = function (timeSpan) {
timeSpan < 0 && (timeSpan = 0);
return timeSpan;
};
return Scheduler;
}());
var normalizeTime = Scheduler.normalize;
(function (schedulerProto) {
function invokeRecImmediate(scheduler, pair) {
var state = pair.first, action = pair.second, group = new CompositeDisposable(),
recursiveAction = function (state1) {
action(state1, function (state2) {
var isAdded = false, isDone = false,
d = scheduler.scheduleWithState(state2, function (scheduler1, state3) {
if (isAdded) {
group.remove(d);
} else {
isDone = true;
}
recursiveAction(state3);
return disposableEmpty;
});
if (!isDone) {
group.add(d);
isAdded = true;
}
});
};
recursiveAction(state);
return group;
}
function invokeRecDate(scheduler, pair, method) {
var state = pair.first, action = pair.second, group = new CompositeDisposable(),
recursiveAction = function (state1) {
action(state1, function (state2, dueTime1) {
var isAdded = false, isDone = false,
d = scheduler[method](state2, dueTime1, function (scheduler1, state3) {
if (isAdded) {
group.remove(d);
} else {
isDone = true;
}
recursiveAction(state3);
return disposableEmpty;
});
if (!isDone) {
group.add(d);
isAdded = true;
}
});
};
recursiveAction(state);
return group;
}
function scheduleInnerRecursive(action, self) {
action(function(dt) { self(action, dt); });
}
/**
* Schedules an action to be executed recursively.
* @param {Function} action Action to execute recursively. The parameter passed to the action is used to trigger recursive scheduling of the action.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursive = function (action) {
return this.scheduleRecursiveWithState(action, function (_action, self) {
_action(function () { self(_action); }); });
};
/**
* Schedules an action to be executed recursively.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to execute recursively. The last parameter passed to the action is used to trigger recursive scheduling of the action, passing in recursive invocation state.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithState = function (state, action) {
return this.scheduleWithState({ first: state, second: action }, invokeRecImmediate);
};
/**
* Schedules an action to be executed recursively after a specified relative due time.
* @param {Function} action Action to execute recursively. The parameter passed to the action is used to trigger recursive scheduling of the action at the specified relative time.
* @param {Number}dueTime Relative time after which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithRelative = function (dueTime, action) {
return this.scheduleRecursiveWithRelativeAndState(action, dueTime, scheduleInnerRecursive);
};
/**
* Schedules an action to be executed recursively after a specified relative due time.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to execute recursively. The last parameter passed to the action is used to trigger recursive scheduling of the action, passing in the recursive due time and invocation state.
* @param {Number}dueTime Relative time after which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithRelativeAndState = function (state, dueTime, action) {
return this._scheduleRelative({ first: state, second: action }, dueTime, function (s, p) {
return invokeRecDate(s, p, 'scheduleWithRelativeAndState');
});
};
/**
* Schedules an action to be executed recursively at a specified absolute due time.
* @param {Function} action Action to execute recursively. The parameter passed to the action is used to trigger recursive scheduling of the action at the specified absolute time.
* @param {Number}dueTime Absolute time at which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithAbsolute = function (dueTime, action) {
return this.scheduleRecursiveWithAbsoluteAndState(action, dueTime, scheduleInnerRecursive);
};
/**
* Schedules an action to be executed recursively at a specified absolute due time.
* @param {Mixed} state State passed to the action to be executed.
* @param {Function} action Action to execute recursively. The last parameter passed to the action is used to trigger recursive scheduling of the action, passing in the recursive due time and invocation state.
* @param {Number}dueTime Absolute time at which to execute the action for the first time.
* @returns {Disposable} The disposable object used to cancel the scheduled action (best effort).
*/
schedulerProto.scheduleRecursiveWithAbsoluteAndState = function (state, dueTime, action) {
return this._scheduleAbsolute({ first: state, second: action }, dueTime, function (s, p) {
return invokeRecDate(s, p, 'scheduleWithAbsoluteAndState');
});
};
}(Scheduler.prototype));
(function (schedulerProto) {
/**
* Schedules a periodic piece of work by dynamically discovering the scheduler's capabilities. The periodic task will be scheduled using window.setInterval for the base implementation.
* @param {Number} period Period for running the work periodically.
* @param {Function} action Action to be executed.
* @returns {Disposable} The disposable object used to cancel the scheduled recurring action (best effort).
*/
Scheduler.prototype.schedulePeriodic = function (period, action) {
return this.schedulePeriodicWithState(null, period, action);
};
/**
* Schedules a periodic piece of work by dynamically discovering the scheduler's capabilities. The periodic task will be scheduled using window.setInterval for the base implementation.
* @param {Mixed} state Initial state passed to the action upon the first iteration.
* @param {Number} period Period for running the work periodically.
* @param {Function} action Action to be executed, potentially updating the state.
* @returns {Disposable} The disposable object used to cancel the scheduled recurring action (best effort).
*/
Scheduler.prototype.schedulePeriodicWithState = function(state, period, action) {
if (typeof root.setInterval === 'undefined') { throw new NotSupportedError(); }
var s = state;
var id = root.setInterval(function () {
s = action(s);
}, period);
return disposableCreate(function () {
root.clearInterval(id);
});
};
}(Scheduler.prototype));
(function (schedulerProto) {
/**
* Returns a scheduler that wraps the original scheduler, adding exception handling for scheduled actions.
* @param {Function} handler Handler that's run if an exception is caught. The exception will be rethrown if the handler returns false.
* @returns {Scheduler} Wrapper around the original scheduler, enforcing exception handling.
*/
schedulerProto.catchError = schedulerProto['catch'] = function (handler) {
return new CatchScheduler(this, handler);
};
}(Scheduler.prototype));
var SchedulePeriodicRecursive = Rx.internals.SchedulePeriodicRecursive = (function () {
function tick(command, recurse) {
recurse(0, this._period);
try {
this._state = this._action(this._state);
} catch (e) {
this._cancel.dispose();
throw e;
}
}
function SchedulePeriodicRecursive(scheduler, state, period, action) {
this._scheduler = scheduler;
this._state = state;
this._period = period;
this._action = action;
}
SchedulePeriodicRecursive.prototype.start = function () {
var d = new SingleAssignmentDisposable();
this._cancel = d;
d.setDisposable(this._scheduler.scheduleRecursiveWithRelativeAndState(0, this._period, tick.bind(this)));
return d;
};
return SchedulePeriodicRecursive;
}());
/** Gets a scheduler that schedules work immediately on the current thread. */
var immediateScheduler = Scheduler.immediate = (function () {
function scheduleNow(state, action) { return action(this, state); }
return new Scheduler(defaultNow, scheduleNow, notSupported, notSupported);
}());
/**
* Gets a scheduler that schedules work as soon as possible on the current thread.
*/
var currentThreadScheduler = Scheduler.currentThread = (function () {
var queue;
function runTrampoline () {
while (queue.length > 0) {
var item = queue.dequeue();
if (!item.isCancelled()) {
!item.isCancelled() && item.invoke();
}
}
}
function scheduleNow(state, action) {
var si = new ScheduledItem(this, state, action, this.now());
if (!queue) {
queue = new PriorityQueue(4);
queue.enqueue(si);
var result = tryCatch(runTrampoline)();
queue = null;
if (result === errorObj) { return thrower(result.e); }
} else {
queue.enqueue(si);
}
return si.disposable;
}
var currentScheduler = new Scheduler(defaultNow, scheduleNow, notSupported, notSupported);
currentScheduler.scheduleRequired = function () { return !queue; };
currentScheduler.ensureTrampoline = function (action) {
if (!queue) { this.schedule(action); } else { action(); }
};
return currentScheduler;
}());
var scheduleMethod, clearMethod = noop;
var localTimer = (function () {
var localSetTimeout, localClearTimeout = noop;
if ('WScript' in this) {
localSetTimeout = function (fn, time) {
WScript.Sleep(time);
fn();
};
} else if (!!root.setTimeout) {
localSetTimeout = root.setTimeout;
localClearTimeout = root.clearTimeout;
} else {
throw new NotSupportedError();
}
return {
setTimeout: localSetTimeout,
clearTimeout: localClearTimeout
};
}());
var localSetTimeout = localTimer.setTimeout,
localClearTimeout = localTimer.clearTimeout;
(function () {
var reNative = RegExp('^' +
String(toString)
.replace(/[.*+?^${}()|[\]\\]/g, '\\$&')
.replace(/toString| for [^\]]+/g, '.*?') + '$'
);
var setImmediate = typeof (setImmediate = freeGlobal && moduleExports && freeGlobal.setImmediate) == 'function' &&
!reNative.test(setImmediate) && setImmediate,
clearImmediate = typeof (clearImmediate = freeGlobal && moduleExports && freeGlobal.clearImmediate) == 'function' &&
!reNative.test(clearImmediate) && clearImmediate;
function postMessageSupported () {
// Ensure not in a worker
if (!root.postMessage || root.importScripts) { return false; }
var isAsync = false,
oldHandler = root.onmessage;
// Test for async
root.onmessage = function () { isAsync = true; };
root.postMessage('', '*');
root.onmessage = oldHandler;
return isAsync;
}
// Use in order, setImmediate, nextTick, postMessage, MessageChannel, script readystatechanged, setTimeout
if (typeof setImmediate === 'function') {
scheduleMethod = setImmediate;
clearMethod = clearImmediate;
} else if (typeof process !== 'undefined' && {}.toString.call(process) === '[object process]') {
scheduleMethod = process.nextTick;
} else if (postMessageSupported()) {
var MSG_PREFIX = 'ms.rx.schedule' + Math.random(),
tasks = {},
taskId = 0;
var onGlobalPostMessage = function (event) {
// Only if we're a match to avoid any other global events
if (typeof event.data === 'string' && event.data.substring(0, MSG_PREFIX.length) === MSG_PREFIX) {
var handleId = event.data.substring(MSG_PREFIX.length),
action = tasks[handleId];
action();
delete tasks[handleId];
}
}
if (root.addEventListener) {
root.addEventListener('message', onGlobalPostMessage, false);
} else {
root.attachEvent('onmessage', onGlobalPostMessage, false);
}
scheduleMethod = function (action) {
var currentId = taskId++;
tasks[currentId] = action;
root.postMessage(MSG_PREFIX + currentId, '*');
};
} else if (!!root.MessageChannel) {
var channel = new root.MessageChannel(),
channelTasks = {},
channelTaskId = 0;
channel.port1.onmessage = function (event) {
var id = event.data,
action = channelTasks[id];
action();
delete channelTasks[id];
};
scheduleMethod = function (action) {
var id = channelTaskId++;
channelTasks[id] = action;
channel.port2.postMessage(id);
};
} else if ('document' in root && 'onreadystatechange' in root.document.createElement('script')) {
scheduleMethod = function (action) {
var scriptElement = root.document.createElement('script');
scriptElement.onreadystatechange = function () {
action();
scriptElement.onreadystatechange = null;
scriptElement.parentNode.removeChild(scriptElement);
scriptElement = null;
};
root.document.documentElement.appendChild(scriptElement);
};
} else {
scheduleMethod = function (action) { return localSetTimeout(action, 0); };
clearMethod = localClearTimeout;
}
}());
/**
* Gets a scheduler that schedules work via a timed callback based upon platform.
*/
var timeoutScheduler = Scheduler.timeout = (function () {
function scheduleNow(state, action) {
var scheduler = this,
disposable = new SingleAssignmentDisposable();
var id = scheduleMethod(function () {
if (!disposable.isDisposed) {
disposable.setDisposable(action(scheduler, state));
}
});
return new CompositeDisposable(disposable, disposableCreate(function () {
clearMethod(id);
}));
}
function scheduleRelative(state, dueTime, action) {
var scheduler = this,
dt = Scheduler.normalize(dueTime);
if (dt === 0) {
return scheduler.scheduleWithState(state, action);
}
var disposable = new SingleAssignmentDisposable();
var id = localSetTimeout(function () {
if (!disposable.isDisposed) {
disposable.setDisposable(action(scheduler, state));
}
}, dt);
return new CompositeDisposable(disposable, disposableCreate(function () {
localClearTimeout(id);
}));
}
function scheduleAbsolute(state, dueTime, action) {
return this.scheduleWithRelativeAndState(state, dueTime - this.now(), action);
}
return new Scheduler(defaultNow, scheduleNow, scheduleRelative, scheduleAbsolute);
})();
var CatchScheduler = (function (__super__) {
function scheduleNow(state, action) {
return this._scheduler.scheduleWithState(state, this._wrap(action));
}
function scheduleRelative(state, dueTime, action) {
return this._scheduler.scheduleWithRelativeAndState(state, dueTime, this._wrap(action));
}
function scheduleAbsolute(state, dueTime, action) {
return this._scheduler.scheduleWithAbsoluteAndState(state, dueTime, this._wrap(action));
}
inherits(CatchScheduler, __super__);
function CatchScheduler(scheduler, handler) {
this._scheduler = scheduler;
this._handler = handler;
this._recursiveOriginal = null;
this._recursiveWrapper = null;
__super__.call(this, this._scheduler.now.bind(this._scheduler), scheduleNow, scheduleRelative, scheduleAbsolute);
}
CatchScheduler.prototype._clone = function (scheduler) {
return new CatchScheduler(scheduler, this._handler);
};
CatchScheduler.prototype._wrap = function (action) {
var parent = this;
return function (self, state) {
try {
return action(parent._getRecursiveWrapper(self), state);
} catch (e) {
if (!parent._handler(e)) { throw e; }
return disposableEmpty;
}
};
};
CatchScheduler.prototype._getRecursiveWrapper = function (scheduler) {
if (this._recursiveOriginal !== scheduler) {
this._recursiveOriginal = scheduler;
var wrapper = this._clone(scheduler);
wrapper._recursiveOriginal = scheduler;
wrapper._recursiveWrapper = wrapper;
this._recursiveWrapper = wrapper;
}
return this._recursiveWrapper;
};
CatchScheduler.prototype.schedulePeriodicWithState = function (state, period, action) {
var self = this, failed = false, d = new SingleAssignmentDisposable();
d.setDisposable(this._scheduler.schedulePeriodicWithState(state, period, function (state1) {
if (failed) { return null; }
try {
return action(state1);
} catch (e) {
failed = true;
if (!self._handler(e)) { throw e; }
d.dispose();
return null;
}
}));
return d;
};
return CatchScheduler;
}(Scheduler));
/**
* Represents a notification to an observer.
*/
var Notification = Rx.Notification = (function () {
function Notification(kind, value, exception, accept, acceptObservable, toString) {
this.kind = kind;
this.value = value;
this.exception = exception;
this._accept = accept;
this._acceptObservable = acceptObservable;
this.toString = toString;
}
/**
* Invokes the delegate corresponding to the notification or the observer's method corresponding to the notification and returns the produced result.
*
* @memberOf Notification
* @param {Any} observerOrOnNext Delegate to invoke for an OnNext notification or Observer to invoke the notification on..
* @param {Function} onError Delegate to invoke for an OnError notification.
* @param {Function} onCompleted Delegate to invoke for an OnCompleted notification.
* @returns {Any} Result produced by the observation.
*/
Notification.prototype.accept = function (observerOrOnNext, onError, onCompleted) {
return observerOrOnNext && typeof observerOrOnNext === 'object' ?
this._acceptObservable(observerOrOnNext) :
this._accept(observerOrOnNext, onError, onCompleted);
};
/**
* Returns an observable sequence with a single notification.
*
* @memberOf Notifications
* @param {Scheduler} [scheduler] Scheduler to send out the notification calls on.
* @returns {Observable} The observable sequence that surfaces the behavior of the notification upon subscription.
*/
Notification.prototype.toObservable = function (scheduler) {
var self = this;
isScheduler(scheduler) || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.scheduleWithState(self, function (_, notification) {
notification._acceptObservable(observer);
notification.kind === 'N' && observer.onCompleted();
});
});
};
return Notification;
})();
/**
* Creates an object that represents an OnNext notification to an observer.
* @param {Any} value The value contained in the notification.
* @returns {Notification} The OnNext notification containing the value.
*/
var notificationCreateOnNext = Notification.createOnNext = (function () {
function _accept(onNext) { return onNext(this.value); }
function _acceptObservable(observer) { return observer.onNext(this.value); }
function toString() { return 'OnNext(' + this.value + ')'; }
return function (value) {
return new Notification('N', value, null, _accept, _acceptObservable, toString);
};
}());
/**
* Creates an object that represents an OnError notification to an observer.
* @param {Any} error The exception contained in the notification.
* @returns {Notification} The OnError notification containing the exception.
*/
var notificationCreateOnError = Notification.createOnError = (function () {
function _accept (onNext, onError) { return onError(this.exception); }
function _acceptObservable(observer) { return observer.onError(this.exception); }
function toString () { return 'OnError(' + this.exception + ')'; }
return function (e) {
return new Notification('E', null, e, _accept, _acceptObservable, toString);
};
}());
/**
* Creates an object that represents an OnCompleted notification to an observer.
* @returns {Notification} The OnCompleted notification.
*/
var notificationCreateOnCompleted = Notification.createOnCompleted = (function () {
function _accept (onNext, onError, onCompleted) { return onCompleted(); }
function _acceptObservable(observer) { return observer.onCompleted(); }
function toString () { return 'OnCompleted()'; }
return function () {
return new Notification('C', null, null, _accept, _acceptObservable, toString);
};
}());
var Enumerator = Rx.internals.Enumerator = function (next) {
this._next = next;
};
Enumerator.prototype.next = function () {
return this._next();
};
Enumerator.prototype[$iterator$] = function () { return this; }
var Enumerable = Rx.internals.Enumerable = function (iterator) {
this._iterator = iterator;
};
Enumerable.prototype[$iterator$] = function () {
return this._iterator();
};
Enumerable.prototype.concat = function () {
var sources = this;
return new AnonymousObservable(function (o) {
var e = sources[$iterator$]();
var isDisposed, subscription = new SerialDisposable();
var cancelable = immediateScheduler.scheduleRecursive(function (self) {
if (isDisposed) { return; }
try {
var currentItem = e.next();
} catch (ex) {
return o.onError(ex);
}
if (currentItem.done) {
return o.onCompleted();
}
// Check if promise
var currentValue = currentItem.value;
isPromise(currentValue) && (currentValue = observableFromPromise(currentValue));
var d = new SingleAssignmentDisposable();
subscription.setDisposable(d);
d.setDisposable(currentValue.subscribe(
function(x) { o.onNext(x); },
function(err) { o.onError(err); },
self)
);
});
return new CompositeDisposable(subscription, cancelable, disposableCreate(function () {
isDisposed = true;
}));
});
};
Enumerable.prototype.catchError = function () {
var sources = this;
return new AnonymousObservable(function (o) {
var e = sources[$iterator$]();
var isDisposed, subscription = new SerialDisposable();
var cancelable = immediateScheduler.scheduleRecursiveWithState(null, function (lastException, self) {
if (isDisposed) { return; }
try {
var currentItem = e.next();
} catch (ex) {
return observer.onError(ex);
}
if (currentItem.done) {
if (lastException !== null) {
o.onError(lastException);
} else {
o.onCompleted();
}
return;
}
// Check if promise
var currentValue = currentItem.value;
isPromise(currentValue) && (currentValue = observableFromPromise(currentValue));
var d = new SingleAssignmentDisposable();
subscription.setDisposable(d);
d.setDisposable(currentValue.subscribe(
function(x) { o.onNext(x); },
self,
function() { o.onCompleted(); }));
});
return new CompositeDisposable(subscription, cancelable, disposableCreate(function () {
isDisposed = true;
}));
});
};
Enumerable.prototype.catchErrorWhen = function (notificationHandler) {
var sources = this;
return new AnonymousObservable(function (o) {
var exceptions = new Subject(),
notifier = new Subject(),
handled = notificationHandler(exceptions),
notificationDisposable = handled.subscribe(notifier);
var e = sources[$iterator$]();
var isDisposed,
lastException,
subscription = new SerialDisposable();
var cancelable = immediateScheduler.scheduleRecursive(function (self) {
if (isDisposed) { return; }
try {
var currentItem = e.next();
} catch (ex) {
return o.onError(ex);
}
if (currentItem.done) {
if (lastException) {
o.onError(lastException);
} else {
o.onCompleted();
}
return;
}
// Check if promise
var currentValue = currentItem.value;
isPromise(currentValue) && (currentValue = observableFromPromise(currentValue));
var outer = new SingleAssignmentDisposable();
var inner = new SingleAssignmentDisposable();
subscription.setDisposable(new CompositeDisposable(inner, outer));
outer.setDisposable(currentValue.subscribe(
function(x) { o.onNext(x); },
function (exn) {
inner.setDisposable(notifier.subscribe(self, function(ex) {
o.onError(ex);
}, function() {
o.onCompleted();
}));
exceptions.onNext(exn);
},
function() { o.onCompleted(); }));
});
return new CompositeDisposable(notificationDisposable, subscription, cancelable, disposableCreate(function () {
isDisposed = true;
}));
});
};
var enumerableRepeat = Enumerable.repeat = function (value, repeatCount) {
if (repeatCount == null) { repeatCount = -1; }
return new Enumerable(function () {
var left = repeatCount;
return new Enumerator(function () {
if (left === 0) { return doneEnumerator; }
if (left > 0) { left--; }
return { done: false, value: value };
});
});
};
var enumerableOf = Enumerable.of = function (source, selector, thisArg) {
if (selector) {
var selectorFn = bindCallback(selector, thisArg, 3);
}
return new Enumerable(function () {
var index = -1;
return new Enumerator(
function () {
return ++index < source.length ?
{ done: false, value: !selector ? source[index] : selectorFn(source[index], index, source) } :
doneEnumerator;
});
});
};
/**
* Supports push-style iteration over an observable sequence.
*/
var Observer = Rx.Observer = function () { };
/**
* Creates a notification callback from an observer.
* @returns The action that forwards its input notification to the underlying observer.
*/
Observer.prototype.toNotifier = function () {
var observer = this;
return function (n) { return n.accept(observer); };
};
/**
* Hides the identity of an observer.
* @returns An observer that hides the identity of the specified observer.
*/
Observer.prototype.asObserver = function () {
return new AnonymousObserver(this.onNext.bind(this), this.onError.bind(this), this.onCompleted.bind(this));
};
/**
* Checks access to the observer for grammar violations. This includes checking for multiple OnError or OnCompleted calls, as well as reentrancy in any of the observer methods.
* If a violation is detected, an Error is thrown from the offending observer method call.
* @returns An observer that checks callbacks invocations against the observer grammar and, if the checks pass, forwards those to the specified observer.
*/
Observer.prototype.checked = function () { return new CheckedObserver(this); };
/**
* Creates an observer from the specified OnNext, along with optional OnError, and OnCompleted actions.
* @param {Function} [onNext] Observer's OnNext action implementation.
* @param {Function} [onError] Observer's OnError action implementation.
* @param {Function} [onCompleted] Observer's OnCompleted action implementation.
* @returns {Observer} The observer object implemented using the given actions.
*/
var observerCreate = Observer.create = function (onNext, onError, onCompleted) {
onNext || (onNext = noop);
onError || (onError = defaultError);
onCompleted || (onCompleted = noop);
return new AnonymousObserver(onNext, onError, onCompleted);
};
/**
* Creates an observer from a notification callback.
*
* @static
* @memberOf Observer
* @param {Function} handler Action that handles a notification.
* @returns The observer object that invokes the specified handler using a notification corresponding to each message it receives.
*/
Observer.fromNotifier = function (handler, thisArg) {
return new AnonymousObserver(function (x) {
return handler.call(thisArg, notificationCreateOnNext(x));
}, function (e) {
return handler.call(thisArg, notificationCreateOnError(e));
}, function () {
return handler.call(thisArg, notificationCreateOnCompleted());
});
};
/**
* Schedules the invocation of observer methods on the given scheduler.
* @param {Scheduler} scheduler Scheduler to schedule observer messages on.
* @returns {Observer} Observer whose messages are scheduled on the given scheduler.
*/
Observer.prototype.notifyOn = function (scheduler) {
return new ObserveOnObserver(scheduler, this);
};
Observer.prototype.makeSafe = function(disposable) {
return new AnonymousSafeObserver(this._onNext, this._onError, this._onCompleted, disposable);
};
/**
* Abstract base class for implementations of the Observer class.
* This base class enforces the grammar of observers where OnError and OnCompleted are terminal messages.
*/
var AbstractObserver = Rx.internals.AbstractObserver = (function (__super__) {
inherits(AbstractObserver, __super__);
/**
* Creates a new observer in a non-stopped state.
*/
function AbstractObserver() {
this.isStopped = false;
__super__.call(this);
}
// Must be implemented by other observers
AbstractObserver.prototype.next = notImplemented;
AbstractObserver.prototype.error = notImplemented;
AbstractObserver.prototype.completed = notImplemented;
/**
* Notifies the observer of a new element in the sequence.
* @param {Any} value Next element in the sequence.
*/
AbstractObserver.prototype.onNext = function (value) {
if (!this.isStopped) { this.next(value); }
};
/**
* Notifies the observer that an exception has occurred.
* @param {Any} error The error that has occurred.
*/
AbstractObserver.prototype.onError = function (error) {
if (!this.isStopped) {
this.isStopped = true;
this.error(error);
}
};
/**
* Notifies the observer of the end of the sequence.
*/
AbstractObserver.prototype.onCompleted = function () {
if (!this.isStopped) {
this.isStopped = true;
this.completed();
}
};
/**
* Disposes the observer, causing it to transition to the stopped state.
*/
AbstractObserver.prototype.dispose = function () {
this.isStopped = true;
};
AbstractObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.error(e);
return true;
}
return false;
};
return AbstractObserver;
}(Observer));
/**
* Class to create an Observer instance from delegate-based implementations of the on* methods.
*/
var AnonymousObserver = Rx.AnonymousObserver = (function (__super__) {
inherits(AnonymousObserver, __super__);
/**
* Creates an observer from the specified OnNext, OnError, and OnCompleted actions.
* @param {Any} onNext Observer's OnNext action implementation.
* @param {Any} onError Observer's OnError action implementation.
* @param {Any} onCompleted Observer's OnCompleted action implementation.
*/
function AnonymousObserver(onNext, onError, onCompleted) {
__super__.call(this);
this._onNext = onNext;
this._onError = onError;
this._onCompleted = onCompleted;
}
/**
* Calls the onNext action.
* @param {Any} value Next element in the sequence.
*/
AnonymousObserver.prototype.next = function (value) {
this._onNext(value);
};
/**
* Calls the onError action.
* @param {Any} error The error that has occurred.
*/
AnonymousObserver.prototype.error = function (error) {
this._onError(error);
};
/**
* Calls the onCompleted action.
*/
AnonymousObserver.prototype.completed = function () {
this._onCompleted();
};
return AnonymousObserver;
}(AbstractObserver));
var CheckedObserver = (function (__super__) {
inherits(CheckedObserver, __super__);
function CheckedObserver(observer) {
__super__.call(this);
this._observer = observer;
this._state = 0; // 0 - idle, 1 - busy, 2 - done
}
var CheckedObserverPrototype = CheckedObserver.prototype;
CheckedObserverPrototype.onNext = function (value) {
this.checkAccess();
var res = tryCatch(this._observer.onNext).call(this._observer, value);
this._state = 0;
res === errorObj && thrower(res.e);
};
CheckedObserverPrototype.onError = function (err) {
this.checkAccess();
var res = tryCatch(this._observer.onError).call(this._observer, err);
this._state = 2;
res === errorObj && thrower(res.e);
};
CheckedObserverPrototype.onCompleted = function () {
this.checkAccess();
var res = tryCatch(this._observer.onCompleted).call(this._observer);
this._state = 2;
res === errorObj && thrower(res.e);
};
CheckedObserverPrototype.checkAccess = function () {
if (this._state === 1) { throw new Error('Re-entrancy detected'); }
if (this._state === 2) { throw new Error('Observer completed'); }
if (this._state === 0) { this._state = 1; }
};
return CheckedObserver;
}(Observer));
var ScheduledObserver = Rx.internals.ScheduledObserver = (function (__super__) {
inherits(ScheduledObserver, __super__);
function ScheduledObserver(scheduler, observer) {
__super__.call(this);
this.scheduler = scheduler;
this.observer = observer;
this.isAcquired = false;
this.hasFaulted = false;
this.queue = [];
this.disposable = new SerialDisposable();
}
ScheduledObserver.prototype.next = function (value) {
var self = this;
this.queue.push(function () { self.observer.onNext(value); });
};
ScheduledObserver.prototype.error = function (e) {
var self = this;
this.queue.push(function () { self.observer.onError(e); });
};
ScheduledObserver.prototype.completed = function () {
var self = this;
this.queue.push(function () { self.observer.onCompleted(); });
};
ScheduledObserver.prototype.ensureActive = function () {
var isOwner = false, parent = this;
if (!this.hasFaulted && this.queue.length > 0) {
isOwner = !this.isAcquired;
this.isAcquired = true;
}
if (isOwner) {
this.disposable.setDisposable(this.scheduler.scheduleRecursive(function (self) {
var work;
if (parent.queue.length > 0) {
work = parent.queue.shift();
} else {
parent.isAcquired = false;
return;
}
try {
work();
} catch (ex) {
parent.queue = [];
parent.hasFaulted = true;
throw ex;
}
self();
}));
}
};
ScheduledObserver.prototype.dispose = function () {
__super__.prototype.dispose.call(this);
this.disposable.dispose();
};
return ScheduledObserver;
}(AbstractObserver));
var ObserveOnObserver = (function (__super__) {
inherits(ObserveOnObserver, __super__);
function ObserveOnObserver(scheduler, observer, cancel) {
__super__.call(this, scheduler, observer);
this._cancel = cancel;
}
ObserveOnObserver.prototype.next = function (value) {
__super__.prototype.next.call(this, value);
this.ensureActive();
};
ObserveOnObserver.prototype.error = function (e) {
__super__.prototype.error.call(this, e);
this.ensureActive();
};
ObserveOnObserver.prototype.completed = function () {
__super__.prototype.completed.call(this);
this.ensureActive();
};
ObserveOnObserver.prototype.dispose = function () {
__super__.prototype.dispose.call(this);
this._cancel && this._cancel.dispose();
this._cancel = null;
};
return ObserveOnObserver;
})(ScheduledObserver);
var observableProto;
/**
* Represents a push-style collection.
*/
var Observable = Rx.Observable = (function () {
function Observable(subscribe) {
if (Rx.config.longStackSupport && hasStacks) {
try {
throw new Error();
} catch (e) {
this.stack = e.stack.substring(e.stack.indexOf("\n") + 1);
}
var self = this;
this._subscribe = function (observer) {
var oldOnError = observer.onError.bind(observer);
observer.onError = function (err) {
makeStackTraceLong(err, self);
oldOnError(err);
};
return subscribe.call(self, observer);
};
} else {
this._subscribe = subscribe;
}
}
observableProto = Observable.prototype;
/**
* Subscribes an observer to the observable sequence.
* @param {Mixed} [observerOrOnNext] The object that is to receive notifications or an action to invoke for each element in the observable sequence.
* @param {Function} [onError] Action to invoke upon exceptional termination of the observable sequence.
* @param {Function} [onCompleted] Action to invoke upon graceful termination of the observable sequence.
* @returns {Diposable} A disposable handling the subscriptions and unsubscriptions.
*/
observableProto.subscribe = observableProto.forEach = function (observerOrOnNext, onError, onCompleted) {
return this._subscribe(typeof observerOrOnNext === 'object' ?
observerOrOnNext :
observerCreate(observerOrOnNext, onError, onCompleted));
};
/**
* Subscribes to the next value in the sequence with an optional "this" argument.
* @param {Function} onNext The function to invoke on each element in the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Disposable} A disposable handling the subscriptions and unsubscriptions.
*/
observableProto.subscribeOnNext = function (onNext, thisArg) {
return this._subscribe(observerCreate(typeof thisArg !== 'undefined' ? function(x) { onNext.call(thisArg, x); } : onNext));
};
/**
* Subscribes to an exceptional condition in the sequence with an optional "this" argument.
* @param {Function} onError The function to invoke upon exceptional termination of the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Disposable} A disposable handling the subscriptions and unsubscriptions.
*/
observableProto.subscribeOnError = function (onError, thisArg) {
return this._subscribe(observerCreate(null, typeof thisArg !== 'undefined' ? function(e) { onError.call(thisArg, e); } : onError));
};
/**
* Subscribes to the next value in the sequence with an optional "this" argument.
* @param {Function} onCompleted The function to invoke upon graceful termination of the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Disposable} A disposable handling the subscriptions and unsubscriptions.
*/
observableProto.subscribeOnCompleted = function (onCompleted, thisArg) {
return this._subscribe(observerCreate(null, null, typeof thisArg !== 'undefined' ? function() { onCompleted.call(thisArg); } : onCompleted));
};
return Observable;
})();
var ObservableBase = Rx.ObservableBase = (function (__super__) {
inherits(ObservableBase, __super__);
function fixSubscriber(subscriber) {
return subscriber && isFunction(subscriber.dispose) ? subscriber :
isFunction(subscriber) ? disposableCreate(subscriber) : disposableEmpty;
}
function setDisposable(s, state) {
var ado = state[0], self = state[1];
var sub = tryCatch(self.subscribeCore).call(self, ado);
if (sub === errorObj) {
if(!ado.fail(errorObj.e)) { return thrower(errorObj.e); }
}
ado.setDisposable(fixSubscriber(sub));
}
function subscribe(observer) {
var ado = new AutoDetachObserver(observer), state = [ado, this];
if (currentThreadScheduler.scheduleRequired()) {
currentThreadScheduler.scheduleWithState(state, setDisposable);
} else {
setDisposable(null, state);
}
return ado;
}
function ObservableBase() {
__super__.call(this, subscribe);
}
ObservableBase.prototype.subscribeCore = notImplemented;
return ObservableBase;
}(Observable));
/**
* Wraps the source sequence in order to run its observer callbacks on the specified scheduler.
*
* This only invokes observer callbacks on a scheduler. In case the subscription and/or unsubscription actions have side-effects
* that require to be run on a scheduler, use subscribeOn.
*
* @param {Scheduler} scheduler Scheduler to notify observers on.
* @returns {Observable} The source sequence whose observations happen on the specified scheduler.
*/
observableProto.observeOn = function (scheduler) {
var source = this;
return new AnonymousObservable(function (observer) {
return source.subscribe(new ObserveOnObserver(scheduler, observer));
}, source);
};
/**
* Wraps the source sequence in order to run its subscription and unsubscription logic on the specified scheduler. This operation is not commonly used;
* see the remarks section for more information on the distinction between subscribeOn and observeOn.
* This only performs the side-effects of subscription and unsubscription on the specified scheduler. In order to invoke observer
* callbacks on a scheduler, use observeOn.
* @param {Scheduler} scheduler Scheduler to perform subscription and unsubscription actions on.
* @returns {Observable} The source sequence whose subscriptions and unsubscriptions happen on the specified scheduler.
*/
observableProto.subscribeOn = function (scheduler) {
var source = this;
return new AnonymousObservable(function (observer) {
var m = new SingleAssignmentDisposable(), d = new SerialDisposable();
d.setDisposable(m);
m.setDisposable(scheduler.schedule(function () {
d.setDisposable(new ScheduledDisposable(scheduler, source.subscribe(observer)));
}));
return d;
}, source);
};
/**
* Converts a Promise to an Observable sequence
* @param {Promise} An ES6 Compliant promise.
* @returns {Observable} An Observable sequence which wraps the existing promise success and failure.
*/
var observableFromPromise = Observable.fromPromise = function (promise) {
return observableDefer(function () {
var subject = new Rx.AsyncSubject();
promise.then(
function (value) {
subject.onNext(value);
subject.onCompleted();
},
subject.onError.bind(subject));
return subject;
});
};
/*
* Converts an existing observable sequence to an ES6 Compatible Promise
* @example
* var promise = Rx.Observable.return(42).toPromise(RSVP.Promise);
*
* // With config
* Rx.config.Promise = RSVP.Promise;
* var promise = Rx.Observable.return(42).toPromise();
* @param {Function} [promiseCtor] The constructor of the promise. If not provided, it looks for it in Rx.config.Promise.
* @returns {Promise} An ES6 compatible promise with the last value from the observable sequence.
*/
observableProto.toPromise = function (promiseCtor) {
promiseCtor || (promiseCtor = Rx.config.Promise);
if (!promiseCtor) { throw new NotSupportedError('Promise type not provided nor in Rx.config.Promise'); }
var source = this;
return new promiseCtor(function (resolve, reject) {
// No cancellation can be done
var value, hasValue = false;
source.subscribe(function (v) {
value = v;
hasValue = true;
}, reject, function () {
hasValue && resolve(value);
});
});
};
var ToArrayObservable = (function(__super__) {
inherits(ToArrayObservable, __super__);
function ToArrayObservable(source) {
this.source = source;
__super__.call(this);
}
ToArrayObservable.prototype.subscribeCore = function(observer) {
return this.source.subscribe(new ToArrayObserver(observer));
};
return ToArrayObservable;
}(ObservableBase));
function ToArrayObserver(observer) {
this.observer = observer;
this.a = [];
this.isStopped = false;
}
ToArrayObserver.prototype.onNext = function (x) { if(!this.isStopped) { this.a.push(x); } };
ToArrayObserver.prototype.onError = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.observer.onError(e);
}
};
ToArrayObserver.prototype.onCompleted = function () {
if (!this.isStopped) {
this.isStopped = true;
this.observer.onNext(this.a);
this.observer.onCompleted();
}
};
ToArrayObserver.prototype.dispose = function () { this.isStopped = true; }
ToArrayObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.observer.onError(e);
return true;
}
return false;
};
/**
* Creates an array from an observable sequence.
* @returns {Observable} An observable sequence containing a single element with a list containing all the elements of the source sequence.
*/
observableProto.toArray = function () {
return new ToArrayObservable(this);
};
/**
* Creates an observable sequence from a specified subscribe method implementation.
* @example
* var res = Rx.Observable.create(function (observer) { return function () { } );
* var res = Rx.Observable.create(function (observer) { return Rx.Disposable.empty; } );
* var res = Rx.Observable.create(function (observer) { } );
* @param {Function} subscribe Implementation of the resulting observable sequence's subscribe method, returning a function that will be wrapped in a Disposable.
* @returns {Observable} The observable sequence with the specified implementation for the Subscribe method.
*/
Observable.create = Observable.createWithDisposable = function (subscribe, parent) {
return new AnonymousObservable(subscribe, parent);
};
/**
* Returns an observable sequence that invokes the specified factory function whenever a new observer subscribes.
*
* @example
* var res = Rx.Observable.defer(function () { return Rx.Observable.fromArray([1,2,3]); });
* @param {Function} observableFactory Observable factory function to invoke for each observer that subscribes to the resulting sequence or Promise.
* @returns {Observable} An observable sequence whose observers trigger an invocation of the given observable factory function.
*/
var observableDefer = Observable.defer = function (observableFactory) {
return new AnonymousObservable(function (observer) {
var result;
try {
result = observableFactory();
} catch (e) {
return observableThrow(e).subscribe(observer);
}
isPromise(result) && (result = observableFromPromise(result));
return result.subscribe(observer);
});
};
/**
* Returns an empty observable sequence, using the specified scheduler to send out the single OnCompleted message.
*
* @example
* var res = Rx.Observable.empty();
* var res = Rx.Observable.empty(Rx.Scheduler.timeout);
* @param {Scheduler} [scheduler] Scheduler to send the termination call on.
* @returns {Observable} An observable sequence with no elements.
*/
var observableEmpty = Observable.empty = function (scheduler) {
isScheduler(scheduler) || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.schedule(function () {
observer.onCompleted();
});
});
};
var FromObservable = (function(__super__) {
inherits(FromObservable, __super__);
function FromObservable(iterable, mapper, scheduler) {
this.iterable = iterable;
this.mapper = mapper;
this.scheduler = scheduler;
__super__.call(this);
}
FromObservable.prototype.subscribeCore = function (observer) {
var sink = new FromSink(observer, this);
return sink.run();
};
return FromObservable;
}(ObservableBase));
var FromSink = (function () {
function FromSink(observer, parent) {
this.observer = observer;
this.parent = parent;
}
FromSink.prototype.run = function () {
var list = Object(this.parent.iterable),
it = getIterable(list),
observer = this.observer,
mapper = this.parent.mapper;
function loopRecursive(i, recurse) {
try {
var next = it.next();
} catch (e) {
return observer.onError(e);
}
if (next.done) {
return observer.onCompleted();
}
var result = next.value;
if (mapper) {
try {
result = mapper(result, i);
} catch (e) {
return observer.onError(e);
}
}
observer.onNext(result);
recurse(i + 1);
}
return this.parent.scheduler.scheduleRecursiveWithState(0, loopRecursive);
};
return FromSink;
}());
var maxSafeInteger = Math.pow(2, 53) - 1;
function StringIterable(str) {
this._s = s;
}
StringIterable.prototype[$iterator$] = function () {
return new StringIterator(this._s);
};
function StringIterator(str) {
this._s = s;
this._l = s.length;
this._i = 0;
}
StringIterator.prototype[$iterator$] = function () {
return this;
};
StringIterator.prototype.next = function () {
return this._i < this._l ? { done: false, value: this._s.charAt(this._i++) } : doneEnumerator;
};
function ArrayIterable(a) {
this._a = a;
}
ArrayIterable.prototype[$iterator$] = function () {
return new ArrayIterator(this._a);
};
function ArrayIterator(a) {
this._a = a;
this._l = toLength(a);
this._i = 0;
}
ArrayIterator.prototype[$iterator$] = function () {
return this;
};
ArrayIterator.prototype.next = function () {
return this._i < this._l ? { done: false, value: this._a[this._i++] } : doneEnumerator;
};
function numberIsFinite(value) {
return typeof value === 'number' && root.isFinite(value);
}
function isNan(n) {
return n !== n;
}
function getIterable(o) {
var i = o[$iterator$], it;
if (!i && typeof o === 'string') {
it = new StringIterable(o);
return it[$iterator$]();
}
if (!i && o.length !== undefined) {
it = new ArrayIterable(o);
return it[$iterator$]();
}
if (!i) { throw new TypeError('Object is not iterable'); }
return o[$iterator$]();
}
function sign(value) {
var number = +value;
if (number === 0) { return number; }
if (isNaN(number)) { return number; }
return number < 0 ? -1 : 1;
}
function toLength(o) {
var len = +o.length;
if (isNaN(len)) { return 0; }
if (len === 0 || !numberIsFinite(len)) { return len; }
len = sign(len) * Math.floor(Math.abs(len));
if (len <= 0) { return 0; }
if (len > maxSafeInteger) { return maxSafeInteger; }
return len;
}
/**
* This method creates a new Observable sequence from an array-like or iterable object.
* @param {Any} arrayLike An array-like or iterable object to convert to an Observable sequence.
* @param {Function} [mapFn] Map function to call on every element of the array.
* @param {Any} [thisArg] The context to use calling the mapFn if provided.
* @param {Scheduler} [scheduler] Optional scheduler to use for scheduling. If not provided, defaults to Scheduler.currentThread.
*/
var observableFrom = Observable.from = function (iterable, mapFn, thisArg, scheduler) {
if (iterable == null) {
throw new Error('iterable cannot be null.')
}
if (mapFn && !isFunction(mapFn)) {
throw new Error('mapFn when provided must be a function');
}
if (mapFn) {
var mapper = bindCallback(mapFn, thisArg, 2);
}
isScheduler(scheduler) || (scheduler = currentThreadScheduler);
return new FromObservable(iterable, mapper, scheduler);
}
var FromArrayObservable = (function(__super__) {
inherits(FromArrayObservable, __super__);
function FromArrayObservable(args, scheduler) {
this.args = args;
this.scheduler = scheduler;
__super__.call(this);
}
FromArrayObservable.prototype.subscribeCore = function (observer) {
var sink = new FromArraySink(observer, this);
return sink.run();
};
return FromArrayObservable;
}(ObservableBase));
function FromArraySink(observer, parent) {
this.observer = observer;
this.parent = parent;
}
FromArraySink.prototype.run = function () {
var observer = this.observer, args = this.parent.args, len = args.length;
function loopRecursive(i, recurse) {
if (i < len) {
observer.onNext(args[i]);
recurse(i + 1);
} else {
observer.onCompleted();
}
}
return this.parent.scheduler.scheduleRecursiveWithState(0, loopRecursive);
};
/**
* Converts an array to an observable sequence, using an optional scheduler to enumerate the array.
* @deprecated use Observable.from or Observable.of
* @param {Scheduler} [scheduler] Scheduler to run the enumeration of the input sequence on.
* @returns {Observable} The observable sequence whose elements are pulled from the given enumerable sequence.
*/
var observableFromArray = Observable.fromArray = function (array, scheduler) {
isScheduler(scheduler) || (scheduler = currentThreadScheduler);
return new FromArrayObservable(array, scheduler)
};
/**
* Generates an observable sequence by running a state-driven loop producing the sequence's elements, using the specified scheduler to send out observer messages.
*
* @example
* var res = Rx.Observable.generate(0, function (x) { return x < 10; }, function (x) { return x + 1; }, function (x) { return x; });
* var res = Rx.Observable.generate(0, function (x) { return x < 10; }, function (x) { return x + 1; }, function (x) { return x; }, Rx.Scheduler.timeout);
* @param {Mixed} initialState Initial state.
* @param {Function} condition Condition to terminate generation (upon returning false).
* @param {Function} iterate Iteration step function.
* @param {Function} resultSelector Selector function for results produced in the sequence.
* @param {Scheduler} [scheduler] Scheduler on which to run the generator loop. If not provided, defaults to Scheduler.currentThread.
* @returns {Observable} The generated sequence.
*/
Observable.generate = function (initialState, condition, iterate, resultSelector, scheduler) {
isScheduler(scheduler) || (scheduler = currentThreadScheduler);
return new AnonymousObservable(function (observer) {
var first = true, state = initialState;
return scheduler.scheduleRecursive(function (self) {
var hasResult, result;
try {
if (first) {
first = false;
} else {
state = iterate(state);
}
hasResult = condition(state);
if (hasResult) {
result = resultSelector(state);
}
} catch (exception) {
observer.onError(exception);
return;
}
if (hasResult) {
observer.onNext(result);
self();
} else {
observer.onCompleted();
}
});
});
};
/**
* Returns a non-terminating observable sequence, which can be used to denote an infinite duration (e.g. when using reactive joins).
* @returns {Observable} An observable sequence whose observers will never get called.
*/
var observableNever = Observable.never = function () {
return new AnonymousObservable(function () {
return disposableEmpty;
});
};
function observableOf (scheduler, array) {
isScheduler(scheduler) || (scheduler = currentThreadScheduler);
return new FromArrayObservable(array, scheduler);
}
/**
* This method creates a new Observable instance with a variable number of arguments, regardless of number or type of the arguments.
* @returns {Observable} The observable sequence whose elements are pulled from the given arguments.
*/
Observable.of = function () {
var len = arguments.length, args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
return new FromArrayObservable(args, currentThreadScheduler);
};
/**
* This method creates a new Observable instance with a variable number of arguments, regardless of number or type of the arguments.
* @param {Scheduler} scheduler A scheduler to use for scheduling the arguments.
* @returns {Observable} The observable sequence whose elements are pulled from the given arguments.
*/
Observable.ofWithScheduler = function (scheduler) {
var len = arguments.length, args = new Array(len - 1);
for(var i = 1; i < len; i++) { args[i - 1] = arguments[i]; }
return new FromArrayObservable(args, scheduler);
};
/**
* Convert an object into an observable sequence of [key, value] pairs.
* @param {Object} obj The object to inspect.
* @param {Scheduler} [scheduler] Scheduler to run the enumeration of the input sequence on.
* @returns {Observable} An observable sequence of [key, value] pairs from the object.
*/
Observable.pairs = function (obj, scheduler) {
scheduler || (scheduler = Rx.Scheduler.currentThread);
return new AnonymousObservable(function (observer) {
var keys = Object.keys(obj), len = keys.length;
return scheduler.scheduleRecursiveWithState(0, function (idx, self) {
if (idx < len) {
var key = keys[idx];
observer.onNext([key, obj[key]]);
self(idx + 1);
} else {
observer.onCompleted();
}
});
});
};
var RangeObservable = (function(__super__) {
inherits(RangeObservable, __super__);
function RangeObservable(start, count, scheduler) {
this.start = start;
this.count = count;
this.scheduler = scheduler;
__super__.call(this);
}
RangeObservable.prototype.subscribeCore = function (observer) {
var sink = new RangeSink(observer, this);
return sink.run();
};
return RangeObservable;
}(ObservableBase));
var RangeSink = (function () {
function RangeSink(observer, parent) {
this.observer = observer;
this.parent = parent;
}
RangeSink.prototype.run = function () {
var start = this.parent.start, count = this.parent.count, observer = this.observer;
function loopRecursive(i, recurse) {
if (i < count) {
observer.onNext(start + i);
recurse(i + 1);
} else {
observer.onCompleted();
}
}
return this.parent.scheduler.scheduleRecursiveWithState(0, loopRecursive);
};
return RangeSink;
}());
/**
* Generates an observable sequence of integral numbers within a specified range, using the specified scheduler to send out observer messages.
* @param {Number} start The value of the first integer in the sequence.
* @param {Number} count The number of sequential integers to generate.
* @param {Scheduler} [scheduler] Scheduler to run the generator loop on. If not specified, defaults to Scheduler.currentThread.
* @returns {Observable} An observable sequence that contains a range of sequential integral numbers.
*/
Observable.range = function (start, count, scheduler) {
isScheduler(scheduler) || (scheduler = currentThreadScheduler);
return new RangeObservable(start, count, scheduler);
};
/**
* Generates an observable sequence that repeats the given element the specified number of times, using the specified scheduler to send out observer messages.
*
* @example
* var res = Rx.Observable.repeat(42);
* var res = Rx.Observable.repeat(42, 4);
* 3 - res = Rx.Observable.repeat(42, 4, Rx.Scheduler.timeout);
* 4 - res = Rx.Observable.repeat(42, null, Rx.Scheduler.timeout);
* @param {Mixed} value Element to repeat.
* @param {Number} repeatCount [Optiona] Number of times to repeat the element. If not specified, repeats indefinitely.
* @param {Scheduler} scheduler Scheduler to run the producer loop on. If not specified, defaults to Scheduler.immediate.
* @returns {Observable} An observable sequence that repeats the given element the specified number of times.
*/
Observable.repeat = function (value, repeatCount, scheduler) {
isScheduler(scheduler) || (scheduler = currentThreadScheduler);
return observableReturn(value, scheduler).repeat(repeatCount == null ? -1 : repeatCount);
};
/**
* Returns an observable sequence that contains a single element, using the specified scheduler to send out observer messages.
* There is an alias called 'just', and 'returnValue' for browsers <IE9.
* @param {Mixed} value Single element in the resulting observable sequence.
* @param {Scheduler} scheduler Scheduler to send the single element on. If not specified, defaults to Scheduler.immediate.
* @returns {Observable} An observable sequence containing the single specified element.
*/
var observableReturn = Observable['return'] = Observable.just = function (value, scheduler) {
isScheduler(scheduler) || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.schedule(function () {
observer.onNext(value);
observer.onCompleted();
});
});
};
/** @deprecated use return or just */
Observable.returnValue = function () {
//deprecate('returnValue', 'return or just');
return observableReturn.apply(null, arguments);
};
/**
* Returns an observable sequence that terminates with an exception, using the specified scheduler to send out the single onError message.
* There is an alias to this method called 'throwError' for browsers <IE9.
* @param {Mixed} error An object used for the sequence's termination.
* @param {Scheduler} scheduler Scheduler to send the exceptional termination call on. If not specified, defaults to Scheduler.immediate.
* @returns {Observable} The observable sequence that terminates exceptionally with the specified exception object.
*/
var observableThrow = Observable['throw'] = Observable.throwError = function (error, scheduler) {
isScheduler(scheduler) || (scheduler = immediateScheduler);
return new AnonymousObservable(function (observer) {
return scheduler.schedule(function () {
observer.onError(error);
});
});
};
/** @deprecated use #some instead */
Observable.throwException = function () {
//deprecate('throwException', 'throwError');
return Observable.throwError.apply(null, arguments);
};
/**
* Constructs an observable sequence that depends on a resource object, whose lifetime is tied to the resulting observable sequence's lifetime.
* @param {Function} resourceFactory Factory function to obtain a resource object.
* @param {Function} observableFactory Factory function to obtain an observable sequence that depends on the obtained resource.
* @returns {Observable} An observable sequence whose lifetime controls the lifetime of the dependent resource object.
*/
Observable.using = function (resourceFactory, observableFactory) {
return new AnonymousObservable(function (observer) {
var disposable = disposableEmpty, resource, source;
try {
resource = resourceFactory();
resource && (disposable = resource);
source = observableFactory(resource);
} catch (exception) {
return new CompositeDisposable(observableThrow(exception).subscribe(observer), disposable);
}
return new CompositeDisposable(source.subscribe(observer), disposable);
});
};
/**
* Propagates the observable sequence or Promise that reacts first.
* @param {Observable} rightSource Second observable sequence or Promise.
* @returns {Observable} {Observable} An observable sequence that surfaces either of the given sequences, whichever reacted first.
*/
observableProto.amb = function (rightSource) {
var leftSource = this;
return new AnonymousObservable(function (observer) {
var choice,
leftChoice = 'L', rightChoice = 'R',
leftSubscription = new SingleAssignmentDisposable(),
rightSubscription = new SingleAssignmentDisposable();
isPromise(rightSource) && (rightSource = observableFromPromise(rightSource));
function choiceL() {
if (!choice) {
choice = leftChoice;
rightSubscription.dispose();
}
}
function choiceR() {
if (!choice) {
choice = rightChoice;
leftSubscription.dispose();
}
}
leftSubscription.setDisposable(leftSource.subscribe(function (left) {
choiceL();
if (choice === leftChoice) {
observer.onNext(left);
}
}, function (err) {
choiceL();
if (choice === leftChoice) {
observer.onError(err);
}
}, function () {
choiceL();
if (choice === leftChoice) {
observer.onCompleted();
}
}));
rightSubscription.setDisposable(rightSource.subscribe(function (right) {
choiceR();
if (choice === rightChoice) {
observer.onNext(right);
}
}, function (err) {
choiceR();
if (choice === rightChoice) {
observer.onError(err);
}
}, function () {
choiceR();
if (choice === rightChoice) {
observer.onCompleted();
}
}));
return new CompositeDisposable(leftSubscription, rightSubscription);
});
};
/**
* Propagates the observable sequence or Promise that reacts first.
*
* @example
* var = Rx.Observable.amb(xs, ys, zs);
* @returns {Observable} An observable sequence that surfaces any of the given sequences, whichever reacted first.
*/
Observable.amb = function () {
var acc = observableNever(), items = [];
if (Array.isArray(arguments[0])) {
items = arguments[0];
} else {
for(var i = 0, len = arguments.length; i < len; i++) { items.push(arguments[i]); }
}
function func(previous, current) {
return previous.amb(current);
}
for (var i = 0, len = items.length; i < len; i++) {
acc = func(acc, items[i]);
}
return acc;
};
function observableCatchHandler(source, handler) {
return new AnonymousObservable(function (o) {
var d1 = new SingleAssignmentDisposable(), subscription = new SerialDisposable();
subscription.setDisposable(d1);
d1.setDisposable(source.subscribe(function (x) { o.onNext(x); }, function (e) {
try {
var result = handler(e);
} catch (ex) {
return o.onError(ex);
}
isPromise(result) && (result = observableFromPromise(result));
var d = new SingleAssignmentDisposable();
subscription.setDisposable(d);
d.setDisposable(result.subscribe(o));
}, function (x) { o.onCompleted(x); }));
return subscription;
}, source);
}
/**
* Continues an observable sequence that is terminated by an exception with the next observable sequence.
* @example
* 1 - xs.catchException(ys)
* 2 - xs.catchException(function (ex) { return ys(ex); })
* @param {Mixed} handlerOrSecond Exception handler function that returns an observable sequence given the error that occurred in the first sequence, or a second observable sequence used to produce results when an error occurred in the first sequence.
* @returns {Observable} An observable sequence containing the first sequence's elements, followed by the elements of the handler sequence in case an exception occurred.
*/
observableProto['catch'] = observableProto.catchError = observableProto.catchException = function (handlerOrSecond) {
return typeof handlerOrSecond === 'function' ?
observableCatchHandler(this, handlerOrSecond) :
observableCatch([this, handlerOrSecond]);
};
/**
* Continues an observable sequence that is terminated by an exception with the next observable sequence.
* @param {Array | Arguments} args Arguments or an array to use as the next sequence if an error occurs.
* @returns {Observable} An observable sequence containing elements from consecutive source sequences until a source sequence terminates successfully.
*/
var observableCatch = Observable.catchError = Observable['catch'] = Observable.catchException = function () {
var items = [];
if (Array.isArray(arguments[0])) {
items = arguments[0];
} else {
for(var i = 0, len = arguments.length; i < len; i++) { items.push(arguments[i]); }
}
return enumerableOf(items).catchError();
};
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever any of the observable sequences or Promises produces an element.
* This can be in the form of an argument list of observables or an array.
*
* @example
* 1 - obs = observable.combineLatest(obs1, obs2, obs3, function (o1, o2, o3) { return o1 + o2 + o3; });
* 2 - obs = observable.combineLatest([obs1, obs2, obs3], function (o1, o2, o3) { return o1 + o2 + o3; });
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
observableProto.combineLatest = function () {
var len = arguments.length, args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
if (Array.isArray(args[0])) {
args[0].unshift(this);
} else {
args.unshift(this);
}
return combineLatest.apply(this, args);
};
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever any of the observable sequences or Promises produces an element.
*
* @example
* 1 - obs = Rx.Observable.combineLatest(obs1, obs2, obs3, function (o1, o2, o3) { return o1 + o2 + o3; });
* 2 - obs = Rx.Observable.combineLatest([obs1, obs2, obs3], function (o1, o2, o3) { return o1 + o2 + o3; });
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
var combineLatest = Observable.combineLatest = function () {
var len = arguments.length, args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
var resultSelector = args.pop();
Array.isArray(args[0]) && (args = args[0]);
return new AnonymousObservable(function (o) {
var n = args.length,
falseFactory = function () { return false; },
hasValue = arrayInitialize(n, falseFactory),
hasValueAll = false,
isDone = arrayInitialize(n, falseFactory),
values = new Array(n);
function next(i) {
hasValue[i] = true;
if (hasValueAll || (hasValueAll = hasValue.every(identity))) {
try {
var res = resultSelector.apply(null, values);
} catch (e) {
return o.onError(e);
}
o.onNext(res);
} else if (isDone.filter(function (x, j) { return j !== i; }).every(identity)) {
o.onCompleted();
}
}
function done (i) {
isDone[i] = true;
isDone.every(identity) && o.onCompleted();
}
var subscriptions = new Array(n);
for (var idx = 0; idx < n; idx++) {
(function (i) {
var source = args[i], sad = new SingleAssignmentDisposable();
isPromise(source) && (source = observableFromPromise(source));
sad.setDisposable(source.subscribe(function (x) {
values[i] = x;
next(i);
},
function(e) { o.onError(e); },
function () { done(i); }
));
subscriptions[i] = sad;
}(idx));
}
return new CompositeDisposable(subscriptions);
}, this);
};
/**
* Concatenates all the observable sequences. This takes in either an array or variable arguments to concatenate.
* @returns {Observable} An observable sequence that contains the elements of each given sequence, in sequential order.
*/
observableProto.concat = function () {
for(var args = [], i = 0, len = arguments.length; i < len; i++) { args.push(arguments[i]); }
args.unshift(this);
return observableConcat.apply(null, args);
};
/**
* Concatenates all the observable sequences.
* @param {Array | Arguments} args Arguments or an array to concat to the observable sequence.
* @returns {Observable} An observable sequence that contains the elements of each given sequence, in sequential order.
*/
var observableConcat = Observable.concat = function () {
var args;
if (Array.isArray(arguments[0])) {
args = arguments[0];
} else {
args = new Array(arguments.length);
for(var i = 0, len = arguments.length; i < len; i++) { args[i] = arguments[i]; }
}
return enumerableOf(args).concat();
};
/**
* Concatenates an observable sequence of observable sequences.
* @returns {Observable} An observable sequence that contains the elements of each observed inner sequence, in sequential order.
*/
observableProto.concatAll = observableProto.concatObservable = function () {
return this.merge(1);
};
var MergeObservable = (function (__super__) {
inherits(MergeObservable, __super__);
function MergeObservable(source, maxConcurrent) {
this.source = source;
this.maxConcurrent = maxConcurrent;
__super__.call(this);
}
MergeObservable.prototype.subscribeCore = function(observer) {
var g = new CompositeDisposable();
g.add(this.source.subscribe(new MergeObserver(observer, this.maxConcurrent, g)));
return g;
};
return MergeObservable;
}(ObservableBase));
var MergeObserver = (function () {
function MergeObserver(o, max, g) {
this.o = o;
this.max = max;
this.g = g;
this.done = false;
this.q = [];
this.activeCount = 0;
this.isStopped = false;
}
MergeObserver.prototype.handleSubscribe = function (xs) {
var sad = new SingleAssignmentDisposable();
this.g.add(sad);
isPromise(xs) && (xs = observableFromPromise(xs));
sad.setDisposable(xs.subscribe(new InnerObserver(this, sad)));
};
MergeObserver.prototype.onNext = function (innerSource) {
if (this.isStopped) { return; }
if(this.activeCount < this.max) {
this.activeCount++;
this.handleSubscribe(innerSource);
} else {
this.q.push(innerSource);
}
};
MergeObserver.prototype.onError = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.o.onError(e);
}
};
MergeObserver.prototype.onCompleted = function () {
if (!this.isStopped) {
this.isStopped = true;
this.done = true;
this.activeCount === 0 && this.o.onCompleted();
}
};
MergeObserver.prototype.dispose = function() { this.isStopped = true; };
MergeObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.o.onError(e);
return true;
}
return false;
};
function InnerObserver(parent, sad) {
this.parent = parent;
this.sad = sad;
this.isStopped = false;
}
InnerObserver.prototype.onNext = function (x) { if(!this.isStopped) { this.parent.o.onNext(x); } };
InnerObserver.prototype.onError = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.parent.o.onError(e);
}
};
InnerObserver.prototype.onCompleted = function () {
if(!this.isStopped) {
var parent = this.parent;
parent.g.remove(this.sad);
if (parent.q.length > 0) {
parent.handleSubscribe(parent.q.shift());
} else {
parent.activeCount--;
parent.done && parent.activeCount === 0 && parent.o.onCompleted();
}
}
};
InnerObserver.prototype.dispose = function() { this.isStopped = true; };
InnerObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.parent.o.onError(e);
return true;
}
return false;
};
return MergeObserver;
}());
/**
* Merges an observable sequence of observable sequences into an observable sequence, limiting the number of concurrent subscriptions to inner sequences.
* Or merges two observable sequences into a single observable sequence.
*
* @example
* 1 - merged = sources.merge(1);
* 2 - merged = source.merge(otherSource);
* @param {Mixed} [maxConcurrentOrOther] Maximum number of inner observable sequences being subscribed to concurrently or the second observable sequence.
* @returns {Observable} The observable sequence that merges the elements of the inner sequences.
*/
observableProto.merge = function (maxConcurrentOrOther) {
return typeof maxConcurrentOrOther !== 'number' ?
observableMerge(this, maxConcurrentOrOther) :
new MergeObservable(this, maxConcurrentOrOther);
};
/**
* Merges all the observable sequences into a single observable sequence.
* The scheduler is optional and if not specified, the immediate scheduler is used.
* @returns {Observable} The observable sequence that merges the elements of the observable sequences.
*/
var observableMerge = Observable.merge = function () {
var scheduler, sources = [], i, len = arguments.length;
if (!arguments[0]) {
scheduler = immediateScheduler;
for(i = 1; i < len; i++) { sources.push(arguments[i]); }
} else if (isScheduler(arguments[0])) {
scheduler = arguments[0];
for(i = 1; i < len; i++) { sources.push(arguments[i]); }
} else {
scheduler = immediateScheduler;
for(i = 0; i < len; i++) { sources.push(arguments[i]); }
}
if (Array.isArray(sources[0])) {
sources = sources[0];
}
return observableOf(scheduler, sources).mergeAll();
};
var MergeAllObservable = (function (__super__) {
inherits(MergeAllObservable, __super__);
function MergeAllObservable(source) {
this.source = source;
__super__.call(this);
}
MergeAllObservable.prototype.subscribeCore = function (observer) {
var g = new CompositeDisposable(), m = new SingleAssignmentDisposable();
g.add(m);
m.setDisposable(this.source.subscribe(new MergeAllObserver(observer, g)));
return g;
};
return MergeAllObservable;
}(ObservableBase));
var MergeAllObserver = (function() {
function MergeAllObserver(o, g) {
this.o = o;
this.g = g;
this.isStopped = false;
this.done = false;
}
MergeAllObserver.prototype.onNext = function(innerSource) {
if(this.isStopped) { return; }
var sad = new SingleAssignmentDisposable();
this.g.add(sad);
isPromise(innerSource) && (innerSource = observableFromPromise(innerSource));
sad.setDisposable(innerSource.subscribe(new InnerObserver(this, this.g, sad)));
};
MergeAllObserver.prototype.onError = function (e) {
if(!this.isStopped) {
this.isStopped = true;
this.o.onError(e);
}
};
MergeAllObserver.prototype.onCompleted = function () {
if(!this.isStopped) {
this.isStopped = true;
this.done = true;
this.g.length === 1 && this.o.onCompleted();
}
};
MergeAllObserver.prototype.dispose = function() { this.isStopped = true; };
MergeAllObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.o.onError(e);
return true;
}
return false;
};
function InnerObserver(parent, g, sad) {
this.parent = parent;
this.g = g;
this.sad = sad;
this.isStopped = false;
}
InnerObserver.prototype.onNext = function (x) { if (!this.isStopped) { this.parent.o.onNext(x); } };
InnerObserver.prototype.onError = function (e) {
if(!this.isStopped) {
this.isStopped = true;
this.parent.o.onError(e);
}
};
InnerObserver.prototype.onCompleted = function () {
if(!this.isStopped) {
var parent = this.parent;
this.isStopped = true;
parent.g.remove(this.sad);
parent.done && parent.g.length === 1 && parent.o.onCompleted();
}
};
InnerObserver.prototype.dispose = function() { this.isStopped = true; };
InnerObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.parent.o.onError(e);
return true;
}
return false;
};
return MergeAllObserver;
}());
/**
* Merges an observable sequence of observable sequences into an observable sequence.
* @returns {Observable} The observable sequence that merges the elements of the inner sequences.
*/
observableProto.mergeAll = observableProto.mergeObservable = function () {
return new MergeAllObservable(this);
};
var CompositeError = Rx.CompositeError = function(errors) {
this.name = "NotImplementedError";
this.innerErrors = errors;
this.message = 'This contains multiple errors. Check the innerErrors';
Error.call(this);
}
CompositeError.prototype = Error.prototype;
/**
* Flattens an Observable that emits Observables into one Observable, in a way that allows an Observer to
* receive all successfully emitted items from all of the source Observables without being interrupted by
* an error notification from one of them.
*
* This behaves like Observable.prototype.mergeAll except that if any of the merged Observables notify of an
* error via the Observer's onError, mergeDelayError will refrain from propagating that
* error notification until all of the merged Observables have finished emitting items.
* @param {Array | Arguments} args Arguments or an array to merge.
* @returns {Observable} an Observable that emits all of the items emitted by the Observables emitted by the Observable
*/
Observable.mergeDelayError = function() {
var args;
if (Array.isArray(arguments[0])) {
args = arguments[0];
} else {
var len = arguments.length;
args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
}
var source = observableOf(null, args);
return new AnonymousObservable(function (o) {
var group = new CompositeDisposable(),
m = new SingleAssignmentDisposable(),
isStopped = false,
errors = [];
function setCompletion() {
if (errors.length === 0) {
o.onCompleted();
} else if (errors.length === 1) {
o.onError(errors[0]);
} else {
o.onError(new CompositeError(errors));
}
}
group.add(m);
m.setDisposable(source.subscribe(
function (innerSource) {
var innerSubscription = new SingleAssignmentDisposable();
group.add(innerSubscription);
// Check for promises support
isPromise(innerSource) && (innerSource = observableFromPromise(innerSource));
innerSubscription.setDisposable(innerSource.subscribe(
function (x) { o.onNext(x); },
function (e) {
errors.push(e);
group.remove(innerSubscription);
isStopped && group.length === 1 && setCompletion();
},
function () {
group.remove(innerSubscription);
isStopped && group.length === 1 && setCompletion();
}));
},
function (e) {
errors.push(e);
isStopped = true;
group.length === 1 && setCompletion();
},
function () {
isStopped = true;
group.length === 1 && setCompletion();
}));
return group;
});
};
/**
* Continues an observable sequence that is terminated normally or by an exception with the next observable sequence.
* @param {Observable} second Second observable sequence used to produce results after the first sequence terminates.
* @returns {Observable} An observable sequence that concatenates the first and second sequence, even if the first sequence terminates exceptionally.
*/
observableProto.onErrorResumeNext = function (second) {
if (!second) { throw new Error('Second observable is required'); }
return onErrorResumeNext([this, second]);
};
/**
* Continues an observable sequence that is terminated normally or by an exception with the next observable sequence.
*
* @example
* 1 - res = Rx.Observable.onErrorResumeNext(xs, ys, zs);
* 1 - res = Rx.Observable.onErrorResumeNext([xs, ys, zs]);
* @returns {Observable} An observable sequence that concatenates the source sequences, even if a sequence terminates exceptionally.
*/
var onErrorResumeNext = Observable.onErrorResumeNext = function () {
var sources = [];
if (Array.isArray(arguments[0])) {
sources = arguments[0];
} else {
for(var i = 0, len = arguments.length; i < len; i++) { sources.push(arguments[i]); }
}
return new AnonymousObservable(function (observer) {
var pos = 0, subscription = new SerialDisposable(),
cancelable = immediateScheduler.scheduleRecursive(function (self) {
var current, d;
if (pos < sources.length) {
current = sources[pos++];
isPromise(current) && (current = observableFromPromise(current));
d = new SingleAssignmentDisposable();
subscription.setDisposable(d);
d.setDisposable(current.subscribe(observer.onNext.bind(observer), self, self));
} else {
observer.onCompleted();
}
});
return new CompositeDisposable(subscription, cancelable);
});
};
/**
* Returns the values from the source observable sequence only after the other observable sequence produces a value.
* @param {Observable | Promise} other The observable sequence or Promise that triggers propagation of elements of the source sequence.
* @returns {Observable} An observable sequence containing the elements of the source sequence starting from the point the other sequence triggered propagation.
*/
observableProto.skipUntil = function (other) {
var source = this;
return new AnonymousObservable(function (o) {
var isOpen = false;
var disposables = new CompositeDisposable(source.subscribe(function (left) {
isOpen && o.onNext(left);
}, function (e) { o.onError(e); }, function () {
isOpen && o.onCompleted();
}));
isPromise(other) && (other = observableFromPromise(other));
var rightSubscription = new SingleAssignmentDisposable();
disposables.add(rightSubscription);
rightSubscription.setDisposable(other.subscribe(function () {
isOpen = true;
rightSubscription.dispose();
}, function (e) { o.onError(e); }, function () {
rightSubscription.dispose();
}));
return disposables;
}, source);
};
/**
* Transforms an observable sequence of observable sequences into an observable sequence producing values only from the most recent observable sequence.
* @returns {Observable} The observable sequence that at any point in time produces the elements of the most recent inner observable sequence that has been received.
*/
observableProto['switch'] = observableProto.switchLatest = function () {
var sources = this;
return new AnonymousObservable(function (observer) {
var hasLatest = false,
innerSubscription = new SerialDisposable(),
isStopped = false,
latest = 0,
subscription = sources.subscribe(
function (innerSource) {
var d = new SingleAssignmentDisposable(), id = ++latest;
hasLatest = true;
innerSubscription.setDisposable(d);
// Check if Promise or Observable
isPromise(innerSource) && (innerSource = observableFromPromise(innerSource));
d.setDisposable(innerSource.subscribe(
function (x) { latest === id && observer.onNext(x); },
function (e) { latest === id && observer.onError(e); },
function () {
if (latest === id) {
hasLatest = false;
isStopped && observer.onCompleted();
}
}));
},
function (e) { observer.onError(e); },
function () {
isStopped = true;
!hasLatest && observer.onCompleted();
});
return new CompositeDisposable(subscription, innerSubscription);
}, sources);
};
/**
* Returns the values from the source observable sequence until the other observable sequence produces a value.
* @param {Observable | Promise} other Observable sequence or Promise that terminates propagation of elements of the source sequence.
* @returns {Observable} An observable sequence containing the elements of the source sequence up to the point the other sequence interrupted further propagation.
*/
observableProto.takeUntil = function (other) {
var source = this;
return new AnonymousObservable(function (o) {
isPromise(other) && (other = observableFromPromise(other));
return new CompositeDisposable(
source.subscribe(o),
other.subscribe(function () { o.onCompleted(); }, function (e) { o.onError(e); }, noop)
);
}, source);
};
/**
* Merges the specified observable sequences into one observable sequence by using the selector function only when the (first) source observable sequence produces an element.
*
* @example
* 1 - obs = obs1.withLatestFrom(obs2, obs3, function (o1, o2, o3) { return o1 + o2 + o3; });
* 2 - obs = obs1.withLatestFrom([obs2, obs3], function (o1, o2, o3) { return o1 + o2 + o3; });
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
observableProto.withLatestFrom = function () {
var len = arguments.length, args = new Array(len)
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
var resultSelector = args.pop(), source = this;
if (typeof source === 'undefined') {
throw new Error('Source observable not found for withLatestFrom().');
}
if (typeof resultSelector !== 'function') {
throw new Error('withLatestFrom() expects a resultSelector function.');
}
if (Array.isArray(args[0])) {
args = args[0];
}
return new AnonymousObservable(function (observer) {
var falseFactory = function () { return false; },
n = args.length,
hasValue = arrayInitialize(n, falseFactory),
hasValueAll = false,
values = new Array(n);
var subscriptions = new Array(n + 1);
for (var idx = 0; idx < n; idx++) {
(function (i) {
var other = args[i], sad = new SingleAssignmentDisposable();
isPromise(other) && (other = observableFromPromise(other));
sad.setDisposable(other.subscribe(function (x) {
values[i] = x;
hasValue[i] = true;
hasValueAll = hasValue.every(identity);
}, observer.onError.bind(observer), function () {}));
subscriptions[i] = sad;
}(idx));
}
var sad = new SingleAssignmentDisposable();
sad.setDisposable(source.subscribe(function (x) {
var res;
var allValues = [x].concat(values);
if (!hasValueAll) return;
try {
res = resultSelector.apply(null, allValues);
} catch (ex) {
observer.onError(ex);
return;
}
observer.onNext(res);
}, observer.onError.bind(observer), function () {
observer.onCompleted();
}));
subscriptions[n] = sad;
return new CompositeDisposable(subscriptions);
}, this);
};
function zipArray(second, resultSelector) {
var first = this;
return new AnonymousObservable(function (observer) {
var index = 0, len = second.length;
return first.subscribe(function (left) {
if (index < len) {
var right = second[index++], result;
try {
result = resultSelector(left, right);
} catch (e) {
return observer.onError(e);
}
observer.onNext(result);
} else {
observer.onCompleted();
}
}, function (e) { observer.onError(e); }, function () { observer.onCompleted(); });
}, first);
}
function falseFactory() { return false; }
function emptyArrayFactory() { return []; }
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever all of the observable sequences or an array have produced an element at a corresponding index.
* The last element in the arguments must be a function to invoke for each series of elements at corresponding indexes in the args.
*
* @example
* 1 - res = obs1.zip(obs2, fn);
* 1 - res = x1.zip([1,2,3], fn);
* @returns {Observable} An observable sequence containing the result of combining elements of the args using the specified result selector function.
*/
observableProto.zip = function () {
if (Array.isArray(arguments[0])) { return zipArray.apply(this, arguments); }
var len = arguments.length, args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
var parent = this, resultSelector = args.pop();
args.unshift(parent);
return new AnonymousObservable(function (observer) {
var n = args.length,
queues = arrayInitialize(n, emptyArrayFactory),
isDone = arrayInitialize(n, falseFactory);
function next(i) {
var res, queuedValues;
if (queues.every(function (x) { return x.length > 0; })) {
try {
queuedValues = queues.map(function (x) { return x.shift(); });
res = resultSelector.apply(parent, queuedValues);
} catch (ex) {
observer.onError(ex);
return;
}
observer.onNext(res);
} else if (isDone.filter(function (x, j) { return j !== i; }).every(identity)) {
observer.onCompleted();
}
};
function done(i) {
isDone[i] = true;
if (isDone.every(function (x) { return x; })) {
observer.onCompleted();
}
}
var subscriptions = new Array(n);
for (var idx = 0; idx < n; idx++) {
(function (i) {
var source = args[i], sad = new SingleAssignmentDisposable();
isPromise(source) && (source = observableFromPromise(source));
sad.setDisposable(source.subscribe(function (x) {
queues[i].push(x);
next(i);
}, function (e) { observer.onError(e); }, function () {
done(i);
}));
subscriptions[i] = sad;
})(idx);
}
return new CompositeDisposable(subscriptions);
}, parent);
};
/**
* Merges the specified observable sequences into one observable sequence by using the selector function whenever all of the observable sequences have produced an element at a corresponding index.
* @param arguments Observable sources.
* @param {Function} resultSelector Function to invoke for each series of elements at corresponding indexes in the sources.
* @returns {Observable} An observable sequence containing the result of combining elements of the sources using the specified result selector function.
*/
Observable.zip = function () {
var len = arguments.length, args = new Array(len);
for(var i = 0; i < len; i++) { args[i] = arguments[i]; }
var first = args.shift();
return first.zip.apply(first, args);
};
/**
* Merges the specified observable sequences into one observable sequence by emitting a list with the elements of the observable sequences at corresponding indexes.
* @param arguments Observable sources.
* @returns {Observable} An observable sequence containing lists of elements at corresponding indexes.
*/
Observable.zipArray = function () {
var sources;
if (Array.isArray(arguments[0])) {
sources = arguments[0];
} else {
var len = arguments.length;
sources = new Array(len);
for(var i = 0; i < len; i++) { sources[i] = arguments[i]; }
}
return new AnonymousObservable(function (observer) {
var n = sources.length,
queues = arrayInitialize(n, function () { return []; }),
isDone = arrayInitialize(n, function () { return false; });
function next(i) {
if (queues.every(function (x) { return x.length > 0; })) {
var res = queues.map(function (x) { return x.shift(); });
observer.onNext(res);
} else if (isDone.filter(function (x, j) { return j !== i; }).every(identity)) {
observer.onCompleted();
return;
}
};
function done(i) {
isDone[i] = true;
if (isDone.every(identity)) {
observer.onCompleted();
return;
}
}
var subscriptions = new Array(n);
for (var idx = 0; idx < n; idx++) {
(function (i) {
subscriptions[i] = new SingleAssignmentDisposable();
subscriptions[i].setDisposable(sources[i].subscribe(function (x) {
queues[i].push(x);
next(i);
}, function (e) { observer.onError(e); }, function () {
done(i);
}));
})(idx);
}
return new CompositeDisposable(subscriptions);
});
};
/**
* Hides the identity of an observable sequence.
* @returns {Observable} An observable sequence that hides the identity of the source sequence.
*/
observableProto.asObservable = function () {
var source = this;
return new AnonymousObservable(function (o) { return source.subscribe(o); }, this);
};
/**
* Projects each element of an observable sequence into zero or more buffers which are produced based on element count information.
*
* @example
* var res = xs.bufferWithCount(10);
* var res = xs.bufferWithCount(10, 1);
* @param {Number} count Length of each buffer.
* @param {Number} [skip] Number of elements to skip between creation of consecutive buffers. If not provided, defaults to the count.
* @returns {Observable} An observable sequence of buffers.
*/
observableProto.bufferWithCount = function (count, skip) {
if (typeof skip !== 'number') {
skip = count;
}
return this.windowWithCount(count, skip).selectMany(function (x) {
return x.toArray();
}).where(function (x) {
return x.length > 0;
});
};
/**
* Dematerializes the explicit notification values of an observable sequence as implicit notifications.
* @returns {Observable} An observable sequence exhibiting the behavior corresponding to the source sequence's notification values.
*/
observableProto.dematerialize = function () {
var source = this;
return new AnonymousObservable(function (o) {
return source.subscribe(function (x) { return x.accept(o); }, function(e) { o.onError(e); }, function () { o.onCompleted(); });
}, this);
};
/**
* Returns an observable sequence that contains only distinct contiguous elements according to the keySelector and the comparer.
*
* var obs = observable.distinctUntilChanged();
* var obs = observable.distinctUntilChanged(function (x) { return x.id; });
* var obs = observable.distinctUntilChanged(function (x) { return x.id; }, function (x, y) { return x === y; });
*
* @param {Function} [keySelector] A function to compute the comparison key for each element. If not provided, it projects the value.
* @param {Function} [comparer] Equality comparer for computed key values. If not provided, defaults to an equality comparer function.
* @returns {Observable} An observable sequence only containing the distinct contiguous elements, based on a computed key value, from the source sequence.
*/
observableProto.distinctUntilChanged = function (keySelector, comparer) {
var source = this;
comparer || (comparer = defaultComparer);
return new AnonymousObservable(function (o) {
var hasCurrentKey = false, currentKey;
return source.subscribe(function (value) {
var key = value;
if (keySelector) {
try {
key = keySelector(value);
} catch (e) {
o.onError(e);
return;
}
}
if (hasCurrentKey) {
try {
var comparerEquals = comparer(currentKey, key);
} catch (e) {
o.onError(e);
return;
}
}
if (!hasCurrentKey || !comparerEquals) {
hasCurrentKey = true;
currentKey = key;
o.onNext(value);
}
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, this);
};
/**
* Invokes an action for each element in the observable sequence and invokes an action upon graceful or exceptional termination of the observable sequence.
* This method can be used for debugging, logging, etc. of query behavior by intercepting the message stream to run arbitrary actions for messages on the pipeline.
* @param {Function | Observer} observerOrOnNext Action to invoke for each element in the observable sequence or an observer.
* @param {Function} [onError] Action to invoke upon exceptional termination of the observable sequence. Used if only the observerOrOnNext parameter is also a function.
* @param {Function} [onCompleted] Action to invoke upon graceful termination of the observable sequence. Used if only the observerOrOnNext parameter is also a function.
* @returns {Observable} The source sequence with the side-effecting behavior applied.
*/
observableProto['do'] = observableProto.tap = observableProto.doAction = function (observerOrOnNext, onError, onCompleted) {
var source = this, tapObserver = typeof observerOrOnNext === 'function' || typeof observerOrOnNext === 'undefined'?
observerCreate(observerOrOnNext || noop, onError || noop, onCompleted || noop) :
observerOrOnNext;
return new AnonymousObservable(function (observer) {
return source.subscribe(function (x) {
try {
tapObserver.onNext(x);
} catch (e) {
observer.onError(e);
}
observer.onNext(x);
}, function (err) {
try {
tapObserver.onError(err);
} catch (e) {
observer.onError(e);
}
observer.onError(err);
}, function () {
try {
tapObserver.onCompleted();
} catch (e) {
observer.onError(e);
}
observer.onCompleted();
});
}, this);
};
/**
* Invokes an action for each element in the observable sequence.
* This method can be used for debugging, logging, etc. of query behavior by intercepting the message stream to run arbitrary actions for messages on the pipeline.
* @param {Function} onNext Action to invoke for each element in the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} The source sequence with the side-effecting behavior applied.
*/
observableProto.doOnNext = observableProto.tapOnNext = function (onNext, thisArg) {
return this.tap(typeof thisArg !== 'undefined' ? function (x) { onNext.call(thisArg, x); } : onNext);
};
/**
* Invokes an action upon exceptional termination of the observable sequence.
* This method can be used for debugging, logging, etc. of query behavior by intercepting the message stream to run arbitrary actions for messages on the pipeline.
* @param {Function} onError Action to invoke upon exceptional termination of the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} The source sequence with the side-effecting behavior applied.
*/
observableProto.doOnError = observableProto.tapOnError = function (onError, thisArg) {
return this.tap(noop, typeof thisArg !== 'undefined' ? function (e) { onError.call(thisArg, e); } : onError);
};
/**
* Invokes an action upon graceful termination of the observable sequence.
* This method can be used for debugging, logging, etc. of query behavior by intercepting the message stream to run arbitrary actions for messages on the pipeline.
* @param {Function} onCompleted Action to invoke upon graceful termination of the observable sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} The source sequence with the side-effecting behavior applied.
*/
observableProto.doOnCompleted = observableProto.tapOnCompleted = function (onCompleted, thisArg) {
return this.tap(noop, null, typeof thisArg !== 'undefined' ? function () { onCompleted.call(thisArg); } : onCompleted);
};
/**
* Invokes a specified action after the source observable sequence terminates gracefully or exceptionally.
* @param {Function} finallyAction Action to invoke after the source observable sequence terminates.
* @returns {Observable} Source sequence with the action-invoking termination behavior applied.
*/
observableProto['finally'] = observableProto.ensure = function (action) {
var source = this;
return new AnonymousObservable(function (observer) {
var subscription;
try {
subscription = source.subscribe(observer);
} catch (e) {
action();
throw e;
}
return disposableCreate(function () {
try {
subscription.dispose();
} catch (e) {
throw e;
} finally {
action();
}
});
}, this);
};
/**
* @deprecated use #finally or #ensure instead.
*/
observableProto.finallyAction = function (action) {
//deprecate('finallyAction', 'finally or ensure');
return this.ensure(action);
};
/**
* Ignores all elements in an observable sequence leaving only the termination messages.
* @returns {Observable} An empty observable sequence that signals termination, successful or exceptional, of the source sequence.
*/
observableProto.ignoreElements = function () {
var source = this;
return new AnonymousObservable(function (o) {
return source.subscribe(noop, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
/**
* Materializes the implicit notifications of an observable sequence as explicit notification values.
* @returns {Observable} An observable sequence containing the materialized notification values from the source sequence.
*/
observableProto.materialize = function () {
var source = this;
return new AnonymousObservable(function (observer) {
return source.subscribe(function (value) {
observer.onNext(notificationCreateOnNext(value));
}, function (e) {
observer.onNext(notificationCreateOnError(e));
observer.onCompleted();
}, function () {
observer.onNext(notificationCreateOnCompleted());
observer.onCompleted();
});
}, source);
};
/**
* Repeats the observable sequence a specified number of times. If the repeat count is not specified, the sequence repeats indefinitely.
* @param {Number} [repeatCount] Number of times to repeat the sequence. If not provided, repeats the sequence indefinitely.
* @returns {Observable} The observable sequence producing the elements of the given sequence repeatedly.
*/
observableProto.repeat = function (repeatCount) {
return enumerableRepeat(this, repeatCount).concat();
};
/**
* Repeats the source observable sequence the specified number of times or until it successfully terminates. If the retry count is not specified, it retries indefinitely.
* Note if you encounter an error and want it to retry once, then you must use .retry(2);
*
* @example
* var res = retried = retry.repeat();
* var res = retried = retry.repeat(2);
* @param {Number} [retryCount] Number of times to retry the sequence. If not provided, retry the sequence indefinitely.
* @returns {Observable} An observable sequence producing the elements of the given sequence repeatedly until it terminates successfully.
*/
observableProto.retry = function (retryCount) {
return enumerableRepeat(this, retryCount).catchError();
};
/**
* Repeats the source observable sequence upon error each time the notifier emits or until it successfully terminates.
* if the notifier completes, the observable sequence completes.
*
* @example
* var timer = Observable.timer(500);
* var source = observable.retryWhen(timer);
* @param {Observable} [notifier] An observable that triggers the retries or completes the observable with onNext or onCompleted respectively.
* @returns {Observable} An observable sequence producing the elements of the given sequence repeatedly until it terminates successfully.
*/
observableProto.retryWhen = function (notifier) {
return enumerableRepeat(this).catchErrorWhen(notifier);
};
/**
* Applies an accumulator function over an observable sequence and returns each intermediate result. The optional seed value is used as the initial accumulator value.
* For aggregation behavior with no intermediate results, see Observable.aggregate.
* @example
* var res = source.scan(function (acc, x) { return acc + x; });
* var res = source.scan(0, function (acc, x) { return acc + x; });
* @param {Mixed} [seed] The initial accumulator value.
* @param {Function} accumulator An accumulator function to be invoked on each element.
* @returns {Observable} An observable sequence containing the accumulated values.
*/
observableProto.scan = function () {
var hasSeed = false, seed, accumulator, source = this;
if (arguments.length === 2) {
hasSeed = true;
seed = arguments[0];
accumulator = arguments[1];
} else {
accumulator = arguments[0];
}
return new AnonymousObservable(function (o) {
var hasAccumulation, accumulation, hasValue;
return source.subscribe (
function (x) {
!hasValue && (hasValue = true);
try {
if (hasAccumulation) {
accumulation = accumulator(accumulation, x);
} else {
accumulation = hasSeed ? accumulator(seed, x) : x;
hasAccumulation = true;
}
} catch (e) {
o.onError(e);
return;
}
o.onNext(accumulation);
},
function (e) { o.onError(e); },
function () {
!hasValue && hasSeed && o.onNext(seed);
o.onCompleted();
}
);
}, source);
};
/**
* Bypasses a specified number of elements at the end of an observable sequence.
* @description
* This operator accumulates a queue with a length enough to store the first `count` elements. As more elements are
* received, elements are taken from the front of the queue and produced on the result sequence. This causes elements to be delayed.
* @param count Number of elements to bypass at the end of the source sequence.
* @returns {Observable} An observable sequence containing the source sequence elements except for the bypassed ones at the end.
*/
observableProto.skipLast = function (count) {
if (count < 0) { throw new ArgumentOutOfRangeError(); }
var source = this;
return new AnonymousObservable(function (o) {
var q = [];
return source.subscribe(function (x) {
q.push(x);
q.length > count && o.onNext(q.shift());
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
/**
* Prepends a sequence of values to an observable sequence with an optional scheduler and an argument list of values to prepend.
* @example
* var res = source.startWith(1, 2, 3);
* var res = source.startWith(Rx.Scheduler.timeout, 1, 2, 3);
* @param {Arguments} args The specified values to prepend to the observable sequence
* @returns {Observable} The source sequence prepended with the specified values.
*/
observableProto.startWith = function () {
var values, scheduler, start = 0;
if (!!arguments.length && isScheduler(arguments[0])) {
scheduler = arguments[0];
start = 1;
} else {
scheduler = immediateScheduler;
}
for(var args = [], i = start, len = arguments.length; i < len; i++) { args.push(arguments[i]); }
return enumerableOf([observableFromArray(args, scheduler), this]).concat();
};
/**
* Returns a specified number of contiguous elements from the end of an observable sequence.
* @description
* This operator accumulates a buffer with a length enough to store elements count elements. Upon completion of
* the source sequence, this buffer is drained on the result sequence. This causes the elements to be delayed.
* @param {Number} count Number of elements to take from the end of the source sequence.
* @returns {Observable} An observable sequence containing the specified number of elements from the end of the source sequence.
*/
observableProto.takeLast = function (count) {
if (count < 0) { throw new ArgumentOutOfRangeError(); }
var source = this;
return new AnonymousObservable(function (o) {
var q = [];
return source.subscribe(function (x) {
q.push(x);
q.length > count && q.shift();
}, function (e) { o.onError(e); }, function () {
while (q.length > 0) { o.onNext(q.shift()); }
o.onCompleted();
});
}, source);
};
/**
* Returns an array with the specified number of contiguous elements from the end of an observable sequence.
*
* @description
* This operator accumulates a buffer with a length enough to store count elements. Upon completion of the
* source sequence, this buffer is produced on the result sequence.
* @param {Number} count Number of elements to take from the end of the source sequence.
* @returns {Observable} An observable sequence containing a single array with the specified number of elements from the end of the source sequence.
*/
observableProto.takeLastBuffer = function (count) {
var source = this;
return new AnonymousObservable(function (o) {
var q = [];
return source.subscribe(function (x) {
q.push(x);
q.length > count && q.shift();
}, function (e) { o.onError(e); }, function () {
o.onNext(q);
o.onCompleted();
});
}, source);
};
/**
* Projects each element of an observable sequence into zero or more windows which are produced based on element count information.
*
* var res = xs.windowWithCount(10);
* var res = xs.windowWithCount(10, 1);
* @param {Number} count Length of each window.
* @param {Number} [skip] Number of elements to skip between creation of consecutive windows. If not specified, defaults to the count.
* @returns {Observable} An observable sequence of windows.
*/
observableProto.windowWithCount = function (count, skip) {
var source = this;
+count || (count = 0);
Math.abs(count) === Infinity && (count = 0);
if (count <= 0) { throw new ArgumentOutOfRangeError(); }
skip == null && (skip = count);
+skip || (skip = 0);
Math.abs(skip) === Infinity && (skip = 0);
if (skip <= 0) { throw new ArgumentOutOfRangeError(); }
return new AnonymousObservable(function (observer) {
var m = new SingleAssignmentDisposable(),
refCountDisposable = new RefCountDisposable(m),
n = 0,
q = [];
function createWindow () {
var s = new Subject();
q.push(s);
observer.onNext(addRef(s, refCountDisposable));
}
createWindow();
m.setDisposable(source.subscribe(
function (x) {
for (var i = 0, len = q.length; i < len; i++) { q[i].onNext(x); }
var c = n - count + 1;
c >= 0 && c % skip === 0 && q.shift().onCompleted();
++n % skip === 0 && createWindow();
},
function (e) {
while (q.length > 0) { q.shift().onError(e); }
observer.onError(e);
},
function () {
while (q.length > 0) { q.shift().onCompleted(); }
observer.onCompleted();
}
));
return refCountDisposable;
}, source);
};
function concatMap(source, selector, thisArg) {
var selectorFunc = bindCallback(selector, thisArg, 3);
return source.map(function (x, i) {
var result = selectorFunc(x, i, source);
isPromise(result) && (result = observableFromPromise(result));
(isArrayLike(result) || isIterable(result)) && (result = observableFrom(result));
return result;
}).concatAll();
}
/**
* One of the Following:
* Projects each element of an observable sequence to an observable sequence and merges the resulting observable sequences into one observable sequence.
*
* @example
* var res = source.concatMap(function (x) { return Rx.Observable.range(0, x); });
* Or:
* Projects each element of an observable sequence to an observable sequence, invokes the result selector for the source element and each of the corresponding inner sequence's elements, and merges the results into one observable sequence.
*
* var res = source.concatMap(function (x) { return Rx.Observable.range(0, x); }, function (x, y) { return x + y; });
* Or:
* Projects each element of the source observable sequence to the other observable sequence and merges the resulting observable sequences into one observable sequence.
*
* var res = source.concatMap(Rx.Observable.fromArray([1,2,3]));
* @param {Function} selector A transform function to apply to each element or an observable sequence to project each element from the
* source sequence onto which could be either an observable or Promise.
* @param {Function} [resultSelector] A transform function to apply to each element of the intermediate sequence.
* @returns {Observable} An observable sequence whose elements are the result of invoking the one-to-many transform function collectionSelector on each element of the input sequence and then mapping each of those sequence elements and their corresponding source element to a result element.
*/
observableProto.selectConcat = observableProto.concatMap = function (selector, resultSelector, thisArg) {
if (isFunction(selector) && isFunction(resultSelector)) {
return this.concatMap(function (x, i) {
var selectorResult = selector(x, i);
isPromise(selectorResult) && (selectorResult = observableFromPromise(selectorResult));
(isArrayLike(selectorResult) || isIterable(selectorResult)) && (selectorResult = observableFrom(selectorResult));
return selectorResult.map(function (y, i2) {
return resultSelector(x, y, i, i2);
});
});
}
return isFunction(selector) ?
concatMap(this, selector, thisArg) :
concatMap(this, function () { return selector; });
};
/**
* Projects each notification of an observable sequence to an observable sequence and concats the resulting observable sequences into one observable sequence.
* @param {Function} onNext A transform function to apply to each element; the second parameter of the function represents the index of the source element.
* @param {Function} onError A transform function to apply when an error occurs in the source sequence.
* @param {Function} onCompleted A transform function to apply when the end of the source sequence is reached.
* @param {Any} [thisArg] An optional "this" to use to invoke each transform.
* @returns {Observable} An observable sequence whose elements are the result of invoking the one-to-many transform function corresponding to each notification in the input sequence.
*/
observableProto.concatMapObserver = observableProto.selectConcatObserver = function(onNext, onError, onCompleted, thisArg) {
var source = this,
onNextFunc = bindCallback(onNext, thisArg, 2),
onErrorFunc = bindCallback(onError, thisArg, 1),
onCompletedFunc = bindCallback(onCompleted, thisArg, 0);
return new AnonymousObservable(function (observer) {
var index = 0;
return source.subscribe(
function (x) {
var result;
try {
result = onNextFunc(x, index++);
} catch (e) {
observer.onError(e);
return;
}
isPromise(result) && (result = observableFromPromise(result));
observer.onNext(result);
},
function (err) {
var result;
try {
result = onErrorFunc(err);
} catch (e) {
observer.onError(e);
return;
}
isPromise(result) && (result = observableFromPromise(result));
observer.onNext(result);
observer.onCompleted();
},
function () {
var result;
try {
result = onCompletedFunc();
} catch (e) {
observer.onError(e);
return;
}
isPromise(result) && (result = observableFromPromise(result));
observer.onNext(result);
observer.onCompleted();
});
}, this).concatAll();
};
/**
* Returns the elements of the specified sequence or the specified value in a singleton sequence if the sequence is empty.
*
* var res = obs = xs.defaultIfEmpty();
* 2 - obs = xs.defaultIfEmpty(false);
*
* @memberOf Observable#
* @param defaultValue The value to return if the sequence is empty. If not provided, this defaults to null.
* @returns {Observable} An observable sequence that contains the specified default value if the source is empty; otherwise, the elements of the source itself.
*/
observableProto.defaultIfEmpty = function (defaultValue) {
var source = this;
defaultValue === undefined && (defaultValue = null);
return new AnonymousObservable(function (observer) {
var found = false;
return source.subscribe(function (x) {
found = true;
observer.onNext(x);
},
function (e) { observer.onError(e); },
function () {
!found && observer.onNext(defaultValue);
observer.onCompleted();
});
}, source);
};
// Swap out for Array.findIndex
function arrayIndexOfComparer(array, item, comparer) {
for (var i = 0, len = array.length; i < len; i++) {
if (comparer(array[i], item)) { return i; }
}
return -1;
}
function HashSet(comparer) {
this.comparer = comparer;
this.set = [];
}
HashSet.prototype.push = function(value) {
var retValue = arrayIndexOfComparer(this.set, value, this.comparer) === -1;
retValue && this.set.push(value);
return retValue;
};
/**
* Returns an observable sequence that contains only distinct elements according to the keySelector and the comparer.
* Usage of this operator should be considered carefully due to the maintenance of an internal lookup structure which can grow large.
*
* @example
* var res = obs = xs.distinct();
* 2 - obs = xs.distinct(function (x) { return x.id; });
* 2 - obs = xs.distinct(function (x) { return x.id; }, function (a,b) { return a === b; });
* @param {Function} [keySelector] A function to compute the comparison key for each element.
* @param {Function} [comparer] Used to compare items in the collection.
* @returns {Observable} An observable sequence only containing the distinct elements, based on a computed key value, from the source sequence.
*/
observableProto.distinct = function (keySelector, comparer) {
var source = this;
comparer || (comparer = defaultComparer);
return new AnonymousObservable(function (o) {
var hashSet = new HashSet(comparer);
return source.subscribe(function (x) {
var key = x;
if (keySelector) {
try {
key = keySelector(x);
} catch (e) {
o.onError(e);
return;
}
}
hashSet.push(key) && o.onNext(x);
},
function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, this);
};
var MapObservable = (function (__super__) {
inherits(MapObservable, __super__);
function MapObservable(source, selector, thisArg) {
this.source = source;
this.selector = bindCallback(selector, thisArg, 3);
__super__.call(this);
}
MapObservable.prototype.internalMap = function (selector, thisArg) {
var self = this;
return new MapObservable(this.source, function (x, i, o) { return selector(self.selector(x, i, o), i, o); }, thisArg)
};
MapObservable.prototype.subscribeCore = function (observer) {
return this.source.subscribe(new MapObserver(observer, this.selector, this));
};
return MapObservable;
}(ObservableBase));
function MapObserver(observer, selector, source) {
this.observer = observer;
this.selector = selector;
this.source = source;
this.i = 0;
this.isStopped = false;
}
MapObserver.prototype.onNext = function(x) {
if (this.isStopped) { return; }
var result = tryCatch(this.selector).call(this, x, this.i++, this.source);
if (result === errorObj) {
return this.observer.onError(result.e);
}
this.observer.onNext(result);
/*try {
var result = this.selector(x, this.i++, this.source);
} catch (e) {
return this.observer.onError(e);
}
this.observer.onNext(result);*/
};
MapObserver.prototype.onError = function (e) {
if(!this.isStopped) { this.isStopped = true; this.observer.onError(e); }
};
MapObserver.prototype.onCompleted = function () {
if(!this.isStopped) { this.isStopped = true; this.observer.onCompleted(); }
};
MapObserver.prototype.dispose = function() { this.isStopped = true; };
MapObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.observer.onError(e);
return true;
}
return false;
};
/**
* Projects each element of an observable sequence into a new form by incorporating the element's index.
* @param {Function} selector A transform function to apply to each source element; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence whose elements are the result of invoking the transform function on each element of source.
*/
observableProto.map = observableProto.select = function (selector, thisArg) {
var selectorFn = typeof selector === 'function' ? selector : function () { return selector; };
return this instanceof MapObservable ?
this.internalMap(selectorFn, thisArg) :
new MapObservable(this, selectorFn, thisArg);
};
/**
* Retrieves the value of a specified nested property from all elements in
* the Observable sequence.
* @param {Arguments} arguments The nested properties to pluck.
* @returns {Observable} Returns a new Observable sequence of property values.
*/
observableProto.pluck = function () {
var args = arguments, len = arguments.length;
if (len === 0) { throw new Error('List of properties cannot be empty.'); }
return this.map(function (x) {
var currentProp = x;
for (var i = 0; i < len; i++) {
var p = currentProp[args[i]];
if (typeof p !== 'undefined') {
currentProp = p;
} else {
return undefined;
}
}
return currentProp;
});
};
function flatMap(source, selector, thisArg) {
var selectorFunc = bindCallback(selector, thisArg, 3);
return source.map(function (x, i) {
var result = selectorFunc(x, i, source);
isPromise(result) && (result = observableFromPromise(result));
(isArrayLike(result) || isIterable(result)) && (result = observableFrom(result));
return result;
}).mergeAll();
}
/**
* One of the Following:
* Projects each element of an observable sequence to an observable sequence and merges the resulting observable sequences into one observable sequence.
*
* @example
* var res = source.selectMany(function (x) { return Rx.Observable.range(0, x); });
* Or:
* Projects each element of an observable sequence to an observable sequence, invokes the result selector for the source element and each of the corresponding inner sequence's elements, and merges the results into one observable sequence.
*
* var res = source.selectMany(function (x) { return Rx.Observable.range(0, x); }, function (x, y) { return x + y; });
* Or:
* Projects each element of the source observable sequence to the other observable sequence and merges the resulting observable sequences into one observable sequence.
*
* var res = source.selectMany(Rx.Observable.fromArray([1,2,3]));
* @param {Function} selector A transform function to apply to each element or an observable sequence to project each element from the source sequence onto which could be either an observable or Promise.
* @param {Function} [resultSelector] A transform function to apply to each element of the intermediate sequence.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence whose elements are the result of invoking the one-to-many transform function collectionSelector on each element of the input sequence and then mapping each of those sequence elements and their corresponding source element to a result element.
*/
observableProto.selectMany = observableProto.flatMap = function (selector, resultSelector, thisArg) {
if (isFunction(selector) && isFunction(resultSelector)) {
return this.flatMap(function (x, i) {
var selectorResult = selector(x, i);
isPromise(selectorResult) && (selectorResult = observableFromPromise(selectorResult));
(isArrayLike(selectorResult) || isIterable(selectorResult)) && (selectorResult = observableFrom(selectorResult));
return selectorResult.map(function (y, i2) {
return resultSelector(x, y, i, i2);
});
}, thisArg);
}
return isFunction(selector) ?
flatMap(this, selector, thisArg) :
flatMap(this, function () { return selector; });
};
/**
* Projects each notification of an observable sequence to an observable sequence and merges the resulting observable sequences into one observable sequence.
* @param {Function} onNext A transform function to apply to each element; the second parameter of the function represents the index of the source element.
* @param {Function} onError A transform function to apply when an error occurs in the source sequence.
* @param {Function} onCompleted A transform function to apply when the end of the source sequence is reached.
* @param {Any} [thisArg] An optional "this" to use to invoke each transform.
* @returns {Observable} An observable sequence whose elements are the result of invoking the one-to-many transform function corresponding to each notification in the input sequence.
*/
observableProto.flatMapObserver = observableProto.selectManyObserver = function (onNext, onError, onCompleted, thisArg) {
var source = this;
return new AnonymousObservable(function (observer) {
var index = 0;
return source.subscribe(
function (x) {
var result;
try {
result = onNext.call(thisArg, x, index++);
} catch (e) {
observer.onError(e);
return;
}
isPromise(result) && (result = observableFromPromise(result));
observer.onNext(result);
},
function (err) {
var result;
try {
result = onError.call(thisArg, err);
} catch (e) {
observer.onError(e);
return;
}
isPromise(result) && (result = observableFromPromise(result));
observer.onNext(result);
observer.onCompleted();
},
function () {
var result;
try {
result = onCompleted.call(thisArg);
} catch (e) {
observer.onError(e);
return;
}
isPromise(result) && (result = observableFromPromise(result));
observer.onNext(result);
observer.onCompleted();
});
}, source).mergeAll();
};
/**
* Projects each element of an observable sequence into a new sequence of observable sequences by incorporating the element's index and then
* transforms an observable sequence of observable sequences into an observable sequence producing values only from the most recent observable sequence.
* @param {Function} selector A transform function to apply to each source element; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence whose elements are the result of invoking the transform function on each element of source producing an Observable of Observable sequences
* and that at any point in time produces the elements of the most recent inner observable sequence that has been received.
*/
observableProto.selectSwitch = observableProto.flatMapLatest = observableProto.switchMap = function (selector, thisArg) {
return this.select(selector, thisArg).switchLatest();
};
/**
* Bypasses a specified number of elements in an observable sequence and then returns the remaining elements.
* @param {Number} count The number of elements to skip before returning the remaining elements.
* @returns {Observable} An observable sequence that contains the elements that occur after the specified index in the input sequence.
*/
observableProto.skip = function (count) {
if (count < 0) { throw new ArgumentOutOfRangeError(); }
var source = this;
return new AnonymousObservable(function (o) {
var remaining = count;
return source.subscribe(function (x) {
if (remaining <= 0) {
o.onNext(x);
} else {
remaining--;
}
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
/**
* Bypasses elements in an observable sequence as long as a specified condition is true and then returns the remaining elements.
* The element's index is used in the logic of the predicate function.
*
* var res = source.skipWhile(function (value) { return value < 10; });
* var res = source.skipWhile(function (value, index) { return value < 10 || index < 10; });
* @param {Function} predicate A function to test each element for a condition; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence that contains the elements from the input sequence starting at the first element in the linear series that does not pass the test specified by predicate.
*/
observableProto.skipWhile = function (predicate, thisArg) {
var source = this,
callback = bindCallback(predicate, thisArg, 3);
return new AnonymousObservable(function (o) {
var i = 0, running = false;
return source.subscribe(function (x) {
if (!running) {
try {
running = !callback(x, i++, source);
} catch (e) {
o.onError(e);
return;
}
}
running && o.onNext(x);
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
/**
* Returns a specified number of contiguous elements from the start of an observable sequence, using the specified scheduler for the edge case of take(0).
*
* var res = source.take(5);
* var res = source.take(0, Rx.Scheduler.timeout);
* @param {Number} count The number of elements to return.
* @param {Scheduler} [scheduler] Scheduler used to produce an OnCompleted message in case <paramref name="count count</paramref> is set to 0.
* @returns {Observable} An observable sequence that contains the specified number of elements from the start of the input sequence.
*/
observableProto.take = function (count, scheduler) {
if (count < 0) { throw new ArgumentOutOfRangeError(); }
if (count === 0) { return observableEmpty(scheduler); }
var source = this;
return new AnonymousObservable(function (o) {
var remaining = count;
return source.subscribe(function (x) {
if (remaining-- > 0) {
o.onNext(x);
remaining === 0 && o.onCompleted();
}
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
/**
* Returns elements from an observable sequence as long as a specified condition is true.
* The element's index is used in the logic of the predicate function.
* @param {Function} predicate A function to test each element for a condition; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence that contains the elements from the input sequence that occur before the element at which the test no longer passes.
*/
observableProto.takeWhile = function (predicate, thisArg) {
var source = this,
callback = bindCallback(predicate, thisArg, 3);
return new AnonymousObservable(function (o) {
var i = 0, running = true;
return source.subscribe(function (x) {
if (running) {
try {
running = callback(x, i++, source);
} catch (e) {
o.onError(e);
return;
}
if (running) {
o.onNext(x);
} else {
o.onCompleted();
}
}
}, function (e) { o.onError(e); }, function () { o.onCompleted(); });
}, source);
};
var FilterObservable = (function (__super__) {
inherits(FilterObservable, __super__);
function FilterObservable(source, predicate, thisArg) {
this.source = source;
this.predicate = bindCallback(predicate, thisArg, 3);
__super__.call(this);
}
FilterObservable.prototype.subscribeCore = function (observer) {
return this.source.subscribe(new FilterObserver(observer, this.predicate, this));
};
FilterObservable.prototype.internalFilter = function(predicate, thisArg) {
var self = this;
return new FilterObservable(this.source, function(x, i, o) { return self.predicate(x, i, o) && predicate(x, i, o); }, thisArg);
};
return FilterObservable;
}(ObservableBase));
function FilterObserver(observer, predicate, source) {
this.observer = observer;
this.predicate = predicate;
this.source = source;
this.i = 0;
this.isStopped = false;
}
FilterObserver.prototype.onNext = function(x) {
if (this.isStopped) { return; }
var shouldYield = tryCatch(this.predicate).call(this, x, this.i++, this.source);
if (shouldYield === errorObj) {
return this.observer.onError(shouldYield.e);
}
shouldYield && this.observer.onNext(x);
};
FilterObserver.prototype.onError = function (e) {
if(!this.isStopped) { this.isStopped = true; this.observer.onError(e); }
};
FilterObserver.prototype.onCompleted = function () {
if(!this.isStopped) { this.isStopped = true; this.observer.onCompleted(); }
};
FilterObserver.prototype.dispose = function() { this.isStopped = true; };
FilterObserver.prototype.fail = function (e) {
if (!this.isStopped) {
this.isStopped = true;
this.observer.onError(e);
return true;
}
return false;
};
/**
* Filters the elements of an observable sequence based on a predicate by incorporating the element's index.
* @param {Function} predicate A function to test each source element for a condition; the second parameter of the function represents the index of the source element.
* @param {Any} [thisArg] Object to use as this when executing callback.
* @returns {Observable} An observable sequence that contains elements from the input sequence that satisfy the condition.
*/
observableProto.filter = observableProto.where = function (predicate, thisArg) {
return this instanceof FilterObservable ? this.internalFilter(predicate, thisArg) :
new FilterObservable(this, predicate, thisArg);
};
/**
* Executes a transducer to transform the observable sequence
* @param {Transducer} transducer A transducer to execute
* @returns {Observable} An Observable sequence containing the results from the transducer.
*/
observableProto.transduce = function(transducer) {
var source = this;
function transformForObserver(observer) {
return {
init: function() {
return observer;
},
step: function(obs, input) {
return obs.onNext(input);
},
result: function(obs) {
return obs.onCompleted();
}
};
}
return new AnonymousObservable(function(observer) {
var xform = transducer(transformForObserver(observer));
return source.subscribe(
function(v) {
try {
xform.step(observer, v);
} catch (e) {
observer.onError(e);
}
},
observer.onError.bind(observer),
function() { xform.result(observer); }
);
}, source);
};
var AnonymousObservable = Rx.AnonymousObservable = (function (__super__) {
inherits(AnonymousObservable, __super__);
// Fix subscriber to check for undefined or function returned to decorate as Disposable
function fixSubscriber(subscriber) {
return subscriber && isFunction(subscriber.dispose) ? subscriber :
isFunction(subscriber) ? disposableCreate(subscriber) : disposableEmpty;
}
function setDisposable(s, state) {
var ado = state[0], subscribe = state[1];
var sub = tryCatch(subscribe)(ado);
if (sub === errorObj) {
if(!ado.fail(errorObj.e)) { return thrower(errorObj.e); }
}
ado.setDisposable(fixSubscriber(sub));
}
function AnonymousObservable(subscribe, parent) {
this.source = parent;
function s(observer) {
var ado = new AutoDetachObserver(observer), state = [ado, subscribe];
if (currentThreadScheduler.scheduleRequired()) {
currentThreadScheduler.scheduleWithState(state, setDisposable);
} else {
setDisposable(null, state);
}
return ado;
}
__super__.call(this, s);
}
return AnonymousObservable;
}(Observable));
var AutoDetachObserver = (function (__super__) {
inherits(AutoDetachObserver, __super__);
function AutoDetachObserver(observer) {
__super__.call(this);
this.observer = observer;
this.m = new SingleAssignmentDisposable();
}
var AutoDetachObserverPrototype = AutoDetachObserver.prototype;
AutoDetachObserverPrototype.next = function (value) {
var result = tryCatch(this.observer.onNext).call(this.observer, value);
if (result === errorObj) {
this.dispose();
thrower(result.e);
}
};
AutoDetachObserverPrototype.error = function (err) {
var result = tryCatch(this.observer.onError).call(this.observer, err);
this.dispose();
result === errorObj && thrower(result.e);
};
AutoDetachObserverPrototype.completed = function () {
var result = tryCatch(this.observer.onCompleted).call(this.observer);
this.dispose();
result === errorObj && thrower(result.e);
};
AutoDetachObserverPrototype.setDisposable = function (value) { this.m.setDisposable(value); };
AutoDetachObserverPrototype.getDisposable = function () { return this.m.getDisposable(); };
AutoDetachObserverPrototype.dispose = function () {
__super__.prototype.dispose.call(this);
this.m.dispose();
};
return AutoDetachObserver;
}(AbstractObserver));
var InnerSubscription = function (subject, observer) {
this.subject = subject;
this.observer = observer;
};
InnerSubscription.prototype.dispose = function () {
if (!this.subject.isDisposed && this.observer !== null) {
var idx = this.subject.observers.indexOf(this.observer);
this.subject.observers.splice(idx, 1);
this.observer = null;
}
};
/**
* Represents an object that is both an observable sequence as well as an observer.
* Each notification is broadcasted to all subscribed observers.
*/
var Subject = Rx.Subject = (function (__super__) {
function subscribe(observer) {
checkDisposed(this);
if (!this.isStopped) {
this.observers.push(observer);
return new InnerSubscription(this, observer);
}
if (this.hasError) {
observer.onError(this.error);
return disposableEmpty;
}
observer.onCompleted();
return disposableEmpty;
}
inherits(Subject, __super__);
/**
* Creates a subject.
*/
function Subject() {
__super__.call(this, subscribe);
this.isDisposed = false,
this.isStopped = false,
this.observers = [];
this.hasError = false;
}
addProperties(Subject.prototype, Observer.prototype, {
/**
* Indicates whether the subject has observers subscribed to it.
* @returns {Boolean} Indicates whether the subject has observers subscribed to it.
*/
hasObservers: function () { return this.observers.length > 0; },
/**
* Notifies all subscribed observers about the end of the sequence.
*/
onCompleted: function () {
checkDisposed(this);
if (!this.isStopped) {
this.isStopped = true;
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
os[i].onCompleted();
}
this.observers.length = 0;
}
},
/**
* Notifies all subscribed observers about the exception.
* @param {Mixed} error The exception to send to all observers.
*/
onError: function (error) {
checkDisposed(this);
if (!this.isStopped) {
this.isStopped = true;
this.error = error;
this.hasError = true;
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
os[i].onError(error);
}
this.observers.length = 0;
}
},
/**
* Notifies all subscribed observers about the arrival of the specified element in the sequence.
* @param {Mixed} value The value to send to all observers.
*/
onNext: function (value) {
checkDisposed(this);
if (!this.isStopped) {
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
os[i].onNext(value);
}
}
},
/**
* Unsubscribe all observers and release resources.
*/
dispose: function () {
this.isDisposed = true;
this.observers = null;
}
});
/**
* Creates a subject from the specified observer and observable.
* @param {Observer} observer The observer used to send messages to the subject.
* @param {Observable} observable The observable used to subscribe to messages sent from the subject.
* @returns {Subject} Subject implemented using the given observer and observable.
*/
Subject.create = function (observer, observable) {
return new AnonymousSubject(observer, observable);
};
return Subject;
}(Observable));
/**
* Represents the result of an asynchronous operation.
* The last value before the OnCompleted notification, or the error received through OnError, is sent to all subscribed observers.
*/
var AsyncSubject = Rx.AsyncSubject = (function (__super__) {
function subscribe(observer) {
checkDisposed(this);
if (!this.isStopped) {
this.observers.push(observer);
return new InnerSubscription(this, observer);
}
if (this.hasError) {
observer.onError(this.error);
} else if (this.hasValue) {
observer.onNext(this.value);
observer.onCompleted();
} else {
observer.onCompleted();
}
return disposableEmpty;
}
inherits(AsyncSubject, __super__);
/**
* Creates a subject that can only receive one value and that value is cached for all future observations.
* @constructor
*/
function AsyncSubject() {
__super__.call(this, subscribe);
this.isDisposed = false;
this.isStopped = false;
this.hasValue = false;
this.observers = [];
this.hasError = false;
}
addProperties(AsyncSubject.prototype, Observer, {
/**
* Indicates whether the subject has observers subscribed to it.
* @returns {Boolean} Indicates whether the subject has observers subscribed to it.
*/
hasObservers: function () {
checkDisposed(this);
return this.observers.length > 0;
},
/**
* Notifies all subscribed observers about the end of the sequence, also causing the last received value to be sent out (if any).
*/
onCompleted: function () {
var i, len;
checkDisposed(this);
if (!this.isStopped) {
this.isStopped = true;
var os = cloneArray(this.observers), len = os.length;
if (this.hasValue) {
for (i = 0; i < len; i++) {
var o = os[i];
o.onNext(this.value);
o.onCompleted();
}
} else {
for (i = 0; i < len; i++) {
os[i].onCompleted();
}
}
this.observers.length = 0;
}
},
/**
* Notifies all subscribed observers about the error.
* @param {Mixed} error The Error to send to all observers.
*/
onError: function (error) {
checkDisposed(this);
if (!this.isStopped) {
this.isStopped = true;
this.hasError = true;
this.error = error;
for (var i = 0, os = cloneArray(this.observers), len = os.length; i < len; i++) {
os[i].onError(error);
}
this.observers.length = 0;
}
},
/**
* Sends a value to the subject. The last value received before successful termination will be sent to all subscribed and future observers.
* @param {Mixed} value The value to store in the subject.
*/
onNext: function (value) {
checkDisposed(this);
if (this.isStopped) { return; }
this.value = value;
this.hasValue = true;
},
/**
* Unsubscribe all observers and release resources.
*/
dispose: function () {
this.isDisposed = true;
this.observers = null;
this.exception = null;
this.value = null;
}
});
return AsyncSubject;
}(Observable));
var AnonymousSubject = Rx.AnonymousSubject = (function (__super__) {
inherits(AnonymousSubject, __super__);
function subscribe(observer) {
return this.observable.subscribe(observer);
}
function AnonymousSubject(observer, observable) {
this.observer = observer;
this.observable = observable;
__super__.call(this, subscribe);
}
addProperties(AnonymousSubject.prototype, Observer.prototype, {
onCompleted: function () {
this.observer.onCompleted();
},
onError: function (error) {
this.observer.onError(error);
},
onNext: function (value) {
this.observer.onNext(value);
}
});
return AnonymousSubject;
}(Observable));
if (typeof define == 'function' && typeof define.amd == 'object' && define.amd) {
root.Rx = Rx;
define(function() {
return Rx;
});
} else if (freeExports && freeModule) {
// in Node.js or RingoJS
if (moduleExports) {
(freeModule.exports = Rx).Rx = Rx;
} else {
freeExports.Rx = Rx;
}
} else {
// in a browser or Rhino
root.Rx = Rx;
}
// All code before this point will be filtered from stack traces.
var rEndingLine = captureLine();
}.call(this));
|
src/index.js | ayoisaiah/markdown-previewer | import React from 'react';
import ReactDOM from 'react-dom';
import App from './components/App';
import './stylesheet/css/main.css';
class Index extends React.Component {
constructor(props) {
super(props);
this.toggleMobilePreview = this.toggleMobilePreview.bind(this);
this.handleChange = this.handleChange.bind(this);
this.state = {
markdown: "This is Markdown\n=======\n\nSub-heading\n-----------\n \n### Another sub-heading\n \n### Some code \n\n```javascript\nvar syntaxHighlighting='awesome'; \nalert(syntaxHighlighting); \n```\n\nSimple text formatting: *italic*, **bold**, `inline-code`, ~~strikethrough~~ .\n\nUnordered List:\n\n * apples\n * oranges\n * pears\n\nOrdered List:\n\n 1. apples\n 2. oranges\n 3. pears\n\n## How about Blockquotes?!\n\n > Right, got it \n > Maybe I'm dreaming but I don't think so \n > It doesn't matter....as long as I'm alive. \n > Cheers \n\n ## You forgot images \n\n 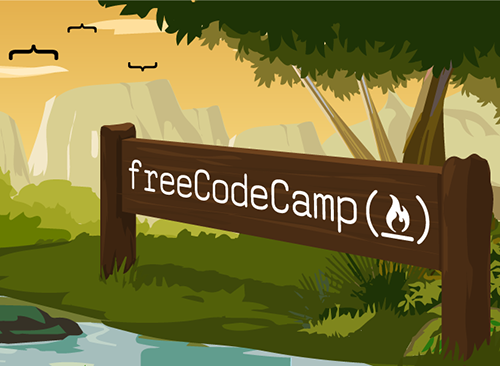\n\n Oops....sorry \n\n [Ayo Isaiah](https://ayoisaiah.com)",
mobilePreview: false
}
}
handleChange(e) {
const content = e.target.value;
this.setState({
markdown: content
});
}
toggleMobilePreview() {
this.setState({ mobilePreview: !this.state.mobilePreview });
}
render() {
return (
<App toggleMobilePreview={this.toggleMobilePreview} mobilePreview={this.state.mobilePreview} markdown={this.state.markdown} handleChange={this.handleChange} />
);
}
}
ReactDOM.render(
<Index />,
document.getElementById('root')
);
|
Examples/UIExplorer/NavigationExperimental/NavigationTabsExample.js | BretJohnson/react-native | /**
* Copyright (c) 2013-present, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* The examples provided by Facebook are for non-commercial testing and
* evaluation purposes only.
*
* Facebook reserves all rights not expressly granted.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS
* OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NON INFRINGEMENT. IN NO EVENT SHALL
* FACEBOOK BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN
* AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
'use strict';
const React = require('react');
const ReactNative = require('react-native');
const {
NavigationExperimental,
ScrollView,
StyleSheet,
View,
} = ReactNative;
const {
Reducer: NavigationReducer,
} = NavigationExperimental;
const NavigationExampleRow = require('./NavigationExampleRow');
const NavigationExampleTabBar = require('./NavigationExampleTabBar');
class ExmpleTabPage extends React.Component {
render() {
const currentTabRoute = this.props.tabs[this.props.index];
return (
<ScrollView style={styles.tabPage}>
<NavigationExampleRow
text={`Current Tab is: ${currentTabRoute.key}`}
/>
{this.props.tabs.map((tab, index) => (
<NavigationExampleRow
key={tab.key}
text={`Go to ${tab.key}`}
onPress={() => {
this.props.onNavigate(NavigationReducer.TabsReducer.JumpToAction(index));
}}
/>
))}
<NavigationExampleRow
text="Exit Tabs Example"
onPress={this.props.onExampleExit}
/>
</ScrollView>
);
}
}
const ExampleTabsReducer = NavigationReducer.TabsReducer({
tabReducers: [
(lastRoute) => lastRoute || {key: 'one'},
(lastRoute) => lastRoute || {key: 'two'},
(lastRoute) => lastRoute || {key: 'three'},
],
});
class NavigationTabsExample extends React.Component {
constructor() {
super();
this.state = ExampleTabsReducer(undefined, {});
}
render() {
return (
<View style={styles.topView}>
<ExmpleTabPage
tabs={this.state.children}
index={this.state.index}
onExampleExit={this.props.onExampleExit}
onNavigate={this.handleAction.bind(this)}
/>
<NavigationExampleTabBar
tabs={this.state.children}
index={this.state.index}
onNavigate={this.handleAction.bind(this)}
/>
</View>
);
}
handleAction(action) {
if (!action) {
return false;
}
const newState = ExampleTabsReducer(this.state, action);
if (newState === this.state) {
return false;
}
this.setState(newState);
return true;
}
handleBackAction() {
return this.handleAction({ type: 'BackAction' });
}
}
const styles = StyleSheet.create({
topView: {
flex: 1,
paddingTop: 30,
},
tabPage: {
backgroundColor: '#E9E9EF',
},
});
module.exports = NavigationTabsExample;
|
ajax/libs/onsen/2.0.0-rc.19/js/onsenui.min.js | kennynaoh/cdnjs | /*! onsenui v2.0.0-rc.19 - 2016-09-15 */
window.CustomEvent||!function(){var CustomEvent;CustomEvent=function(event,params){var evt;return params=params||{bubbles:!1,cancelable:!1,detail:void 0},evt=document.createEvent("CustomEvent"),evt.initCustomEvent(event,params.bubbles,params.cancelable,params.detail),evt},CustomEvent.prototype=window.Event.prototype,window.CustomEvent=CustomEvent}(),"undefined"==typeof WeakMap&&!function(){var defineProperty=Object.defineProperty,counter=Date.now()%1e9,WeakMap=function(){this.name="__st"+(1e9*Math.random()>>>0)+(counter++ +"__")};WeakMap.prototype={set:function(key,value){var entry=key[this.name];return entry&&entry[0]===key?entry[1]=value:defineProperty(key,this.name,{value:[key,value],writable:!0}),this},get:function(key){var entry;return(entry=key[this.name])&&entry[0]===key?entry[1]:void 0},"delete":function(key){var entry=key[this.name];return entry&&entry[0]===key?(entry[0]=entry[1]=void 0,!0):!1},has:function(key){var entry=key[this.name];return entry?entry[0]===key:!1}},window.WeakMap=WeakMap}(),function(global){function scheduleCallback(observer){scheduledObservers.push(observer),isScheduled||(isScheduled=!0,setImmediate(dispatchCallbacks))}function wrapIfNeeded(node){return window.ShadowDOMPolyfill&&window.ShadowDOMPolyfill.wrapIfNeeded(node)||node}function dispatchCallbacks(){isScheduled=!1;var observers=scheduledObservers;scheduledObservers=[],observers.sort(function(o1,o2){return o1.uid_-o2.uid_});var anyNonEmpty=!1;observers.forEach(function(observer){var queue=observer.takeRecords();removeTransientObserversFor(observer),queue.length&&(observer.callback_(queue,observer),anyNonEmpty=!0)}),anyNonEmpty&&dispatchCallbacks()}function removeTransientObserversFor(observer){observer.nodes_.forEach(function(node){var registrations=registrationsTable.get(node);registrations&®istrations.forEach(function(registration){registration.observer===observer&®istration.removeTransientObservers()})})}function forEachAncestorAndObserverEnqueueRecord(target,callback){for(var node=target;node;node=node.parentNode){var registrations=registrationsTable.get(node);if(registrations)for(var j=0;j<registrations.length;j++){var registration=registrations[j],options=registration.options;if(node===target||options.subtree){var record=callback(options);record&®istration.enqueue(record)}}}}function JsMutationObserver(callback){this.callback_=callback,this.nodes_=[],this.records_=[],this.uid_=++uidCounter}function MutationRecord(type,target){this.type=type,this.target=target,this.addedNodes=[],this.removedNodes=[],this.previousSibling=null,this.nextSibling=null,this.attributeName=null,this.attributeNamespace=null,this.oldValue=null}function copyMutationRecord(original){var record=new MutationRecord(original.type,original.target);return record.addedNodes=original.addedNodes.slice(),record.removedNodes=original.removedNodes.slice(),record.previousSibling=original.previousSibling,record.nextSibling=original.nextSibling,record.attributeName=original.attributeName,record.attributeNamespace=original.attributeNamespace,record.oldValue=original.oldValue,record}function getRecord(type,target){return currentRecord=new MutationRecord(type,target)}function getRecordWithOldValue(oldValue){return recordWithOldValue?recordWithOldValue:(recordWithOldValue=copyMutationRecord(currentRecord),recordWithOldValue.oldValue=oldValue,recordWithOldValue)}function clearRecords(){currentRecord=recordWithOldValue=void 0}function recordRepresentsCurrentMutation(record){return record===recordWithOldValue||record===currentRecord}function selectRecord(lastRecord,newRecord){return lastRecord===newRecord?lastRecord:recordWithOldValue&&recordRepresentsCurrentMutation(lastRecord)?recordWithOldValue:null}function Registration(observer,target,options){this.observer=observer,this.target=target,this.options=options,this.transientObservedNodes=[]}if(!global.JsMutationObserver){var setImmediate,registrationsTable=new WeakMap;if(/Trident|Edge/.test(navigator.userAgent))setImmediate=setTimeout;else if(window.setImmediate)setImmediate=window.setImmediate;else{var setImmediateQueue=[],sentinel=String(Math.random());window.addEventListener("message",function(e){if(e.data===sentinel){var queue=setImmediateQueue;setImmediateQueue=[],queue.forEach(function(func){func()})}}),setImmediate=function(func){setImmediateQueue.push(func),window.postMessage(sentinel,"*")}}var isScheduled=!1,scheduledObservers=[],uidCounter=0;JsMutationObserver.prototype={observe:function(target,options){if(target=wrapIfNeeded(target),!options.childList&&!options.attributes&&!options.characterData||options.attributeOldValue&&!options.attributes||options.attributeFilter&&options.attributeFilter.length&&!options.attributes||options.characterDataOldValue&&!options.characterData)throw new SyntaxError;var registrations=registrationsTable.get(target);registrations||registrationsTable.set(target,registrations=[]);for(var registration,i=0;i<registrations.length;i++)if(registrations[i].observer===this){registration=registrations[i],registration.removeListeners(),registration.options=options;break}registration||(registration=new Registration(this,target,options),registrations.push(registration),this.nodes_.push(target)),registration.addListeners()},disconnect:function(){this.nodes_.forEach(function(node){for(var registrations=registrationsTable.get(node),i=0;i<registrations.length;i++){var registration=registrations[i];if(registration.observer===this){registration.removeListeners(),registrations.splice(i,1);break}}},this),this.records_=[]},takeRecords:function(){var copyOfRecords=this.records_;return this.records_=[],copyOfRecords}};var currentRecord,recordWithOldValue;Registration.prototype={enqueue:function(record){var records=this.observer.records_,length=records.length;if(records.length>0){var lastRecord=records[length-1],recordToReplaceLast=selectRecord(lastRecord,record);if(recordToReplaceLast)return void(records[length-1]=recordToReplaceLast)}else scheduleCallback(this.observer);records[length]=record},addListeners:function(){this.addListeners_(this.target)},addListeners_:function(node){var options=this.options;options.attributes&&node.addEventListener("DOMAttrModified",this,!0),options.characterData&&node.addEventListener("DOMCharacterDataModified",this,!0),options.childList&&node.addEventListener("DOMNodeInserted",this,!0),(options.childList||options.subtree)&&node.addEventListener("DOMNodeRemoved",this,!0)},removeListeners:function(){this.removeListeners_(this.target)},removeListeners_:function(node){var options=this.options;options.attributes&&node.removeEventListener("DOMAttrModified",this,!0),options.characterData&&node.removeEventListener("DOMCharacterDataModified",this,!0),options.childList&&node.removeEventListener("DOMNodeInserted",this,!0),(options.childList||options.subtree)&&node.removeEventListener("DOMNodeRemoved",this,!0)},addTransientObserver:function(node){if(node!==this.target){this.addListeners_(node),this.transientObservedNodes.push(node);var registrations=registrationsTable.get(node);registrations||registrationsTable.set(node,registrations=[]),registrations.push(this)}},removeTransientObservers:function(){var transientObservedNodes=this.transientObservedNodes;this.transientObservedNodes=[],transientObservedNodes.forEach(function(node){this.removeListeners_(node);for(var registrations=registrationsTable.get(node),i=0;i<registrations.length;i++)if(registrations[i]===this){registrations.splice(i,1);break}},this)},handleEvent:function(e){switch(e.stopImmediatePropagation(),e.type){case"DOMAttrModified":var name=e.attrName,namespace=e.relatedNode.namespaceURI,target=e.target,record=new getRecord("attributes",target);record.attributeName=name,record.attributeNamespace=namespace;var oldValue=e.attrChange===MutationEvent.ADDITION?null:e.prevValue;forEachAncestorAndObserverEnqueueRecord(target,function(options){return!options.attributes||options.attributeFilter&&options.attributeFilter.length&&-1===options.attributeFilter.indexOf(name)&&-1===options.attributeFilter.indexOf(namespace)?void 0:options.attributeOldValue?getRecordWithOldValue(oldValue):record});break;case"DOMCharacterDataModified":var target=e.target,record=getRecord("characterData",target),oldValue=e.prevValue;forEachAncestorAndObserverEnqueueRecord(target,function(options){return options.characterData?options.characterDataOldValue?getRecordWithOldValue(oldValue):record:void 0});break;case"DOMNodeRemoved":this.addTransientObserver(e.target);case"DOMNodeInserted":var addedNodes,removedNodes,changedNode=e.target;"DOMNodeInserted"===e.type?(addedNodes=[changedNode],removedNodes=[]):(addedNodes=[],removedNodes=[changedNode]);var previousSibling=changedNode.previousSibling,nextSibling=changedNode.nextSibling,record=getRecord("childList",e.target.parentNode);record.addedNodes=addedNodes,record.removedNodes=removedNodes,record.previousSibling=previousSibling,record.nextSibling=nextSibling,forEachAncestorAndObserverEnqueueRecord(e.relatedNode,function(options){return options.childList?record:void 0})}clearRecords()}},global.JsMutationObserver=JsMutationObserver,global.MutationObserver||(global.MutationObserver=JsMutationObserver,JsMutationObserver._isPolyfilled=!0)}}(self),window.animit=function(){"use strict";var TIMEOUT_RATIO=1.4,util={};util.capitalize=function(str){return str.charAt(0).toUpperCase()+str.slice(1)},util.buildTransitionValue=function(params){params.property=params.property||"all",params.duration=params.duration||.4,params.timing=params.timing||"linear";var props=params.property.split(/ +/);return props.map(function(prop){return prop+" "+params.duration+"s "+params.timing}).join(", ")},util.onceOnTransitionEnd=function(element,callback){if(!element)return function(){};var fn=function(event){element==event.target&&(event.stopPropagation(),removeListeners(),callback())},removeListeners=function(){util._transitionEndEvents.forEach(function(eventName){element.removeEventListener(eventName,fn,!1)})};return util._transitionEndEvents.forEach(function(eventName){element.addEventListener(eventName,fn,!1)}),removeListeners},util._transitionEndEvents=function(){return"ontransitionend"in window?["transitionend"]:"onwebkittransitionend"in window?["webkitTransitionEnd"]:"webkit"===util.vendorPrefix||"o"===util.vendorPrefix||"moz"===util.vendorPrefix||"ms"===util.vendorPrefix?[util.vendorPrefix+"TransitionEnd","transitionend"]:[]}(),util._cssPropertyDict=function(){for(var styles=window.getComputedStyle(document.documentElement,""),dict={},a="A".charCodeAt(0),z="z".charCodeAt(0),upper=function(s){return s.substr(1).toUpperCase()},i=0;i<styles.length;i++){var key=styles[i].replace(/^[\-]+/,"").replace(/[\-][a-z]/g,upper).replace(/^moz/,"Moz");a<=key.charCodeAt(0)&&z>=key.charCodeAt(0)&&"cssText"!==key&&"parentText"!==key&&(dict[key]=!0)}return dict}(),util.hasCssProperty=function(name){return name in util._cssPropertyDict},util.vendorPrefix=function(){var styles=window.getComputedStyle(document.documentElement,""),pre=(Array.prototype.slice.call(styles).join("").match(/-(moz|webkit|ms)-/)||""===styles.OLink&&["","o"])[1];return pre}(),util.forceLayoutAtOnce=function(elements,callback){this.batchImmediate(function(){elements.forEach(function(element){element.offsetHeight}),callback()})},util.batchImmediate=function(){var callbacks=[];return function(callback){0===callbacks.length&&setImmediate(function(){var concreateCallbacks=callbacks.slice(0);callbacks=[],concreateCallbacks.forEach(function(callback){callback()})}),callbacks.push(callback)}}(),util.batchAnimationFrame=function(){var callbacks=[],raf=window.requestAnimationFrame||window.webkitRequestAnimationFrame||window.mozRequestAnimationFrame||window.oRequestAnimationFrame||window.msRequestAnimationFrame||function(callback){setTimeout(callback,1e3/60)};return function(callback){0===callbacks.length&&raf(function(){var concreateCallbacks=callbacks.slice(0);callbacks=[],concreateCallbacks.forEach(function(callback){callback()})}),callbacks.push(callback)}}(),util.transitionPropertyName=function(){if(util.hasCssProperty("transitionDuration"))return"transition";if(util.hasCssProperty(util.vendorPrefix+"TransitionDuration"))return util.vendorPrefix+"Transition";throw new Error("Invalid state")}();var Animit=function(element){if(!(this instanceof Animit))return new Animit(element);if(element instanceof HTMLElement)this.elements=[element];else{if("[object Array]"!==Object.prototype.toString.call(element))throw new Error("First argument must be an array or an instance of HTMLElement.");this.elements=element}this.transitionQueue=[],this.lastStyleAttributeDict=[]};return Animit.prototype={transitionQueue:void 0,elements:void 0,play:function(callback){return"function"==typeof callback&&this.transitionQueue.push(function(done){callback(),done()}),this.startAnimation(),this},queue:function(transition,options){var queue=this.transitionQueue;if(transition&&options&&(options.css=transition,transition=new Animit.Transition(options)),transition instanceof Function||transition instanceof Animit.Transition||(transition=transition.css?new Animit.Transition(transition):new Animit.Transition({css:transition})),transition instanceof Function)queue.push(transition);else{if(!(transition instanceof Animit.Transition))throw new Error("Invalid arguments");queue.push(transition.build())}return this},wait:function(seconds){return seconds>0&&this.transitionQueue.push(function(done){setTimeout(done,1e3*seconds)}),this},saveStyle:function(){return this.transitionQueue.push(function(done){this.elements.forEach(function(element,index){for(var css=this.lastStyleAttributeDict[index]={},i=0;i<element.style.length;i++)css[element.style[i]]=element.style[element.style[i]]}.bind(this)),done()}.bind(this)),this},restoreStyle:function(options){function reset(){self.elements.forEach(function(element,index){element.style[transitionName]="none";var css=self.lastStyleAttributeDict[index];if(!css)throw new Error("restoreStyle(): The style is not saved. Invoke saveStyle() before.");self.lastStyleAttributeDict[index]=void 0;for(var i=0,name="";i<element.style.length;i++)name=element.style[i],"undefined"==typeof css[element.style[i]]&&(css[element.style[i]]="");Object.keys(css).forEach(function(key){element.style[key]=css[key]})})}options=options||{};var self=this;if(options.transition&&!options.duration)throw new Error('"options.duration" is required when "options.transition" is enabled.');var transitionName=util.transitionPropertyName;if(options.transition||options.duration&&options.duration>0){var transitionValue=options.transition||"all "+options.duration+"s "+(options.timing||"linear");this.transitionQueue.push(function(done){var timeoutId,elements=this.elements,clearTransition=function(){elements.forEach(function(element){element.style[transitionName]=""})},removeListeners=util.onceOnTransitionEnd(elements[0],function(){clearTimeout(timeoutId),clearTransition(),done()});timeoutId=setTimeout(function(){removeListeners(),clearTransition(),done()},1e3*options.duration*TIMEOUT_RATIO),elements.forEach(function(element,index){var css=self.lastStyleAttributeDict[index];if(!css)throw new Error("restoreStyle(): The style is not saved. Invoke saveStyle() before.");self.lastStyleAttributeDict[index]=void 0;for(var name,i=0,len=element.style.length;len>i;i++)name=element.style[i],void 0===css[name]&&(css[name]="");element.style[transitionName]=transitionValue,Object.keys(css).forEach(function(key){key!==transitionName&&(element.style[key]=css[key])}),element.style[transitionName]=transitionValue})})}else this.transitionQueue.push(function(done){reset(),done()});return this},startAnimation:function(){return this._dequeueTransition(),this},_dequeueTransition:function(){var transition=this.transitionQueue.shift();if(this._currentTransition)throw new Error("Current transition exists.");this._currentTransition=transition;var self=this,called=!1,done=function(){if(called)throw new Error("Invalid state: This callback is called twice.");called=!0,self._currentTransition=void 0,self._dequeueTransition()};transition&&transition.call(this,done)}},Animit.runAll=function(){for(var i=0;i<arguments.length;i++)arguments[i].play()},Animit.Transition=function(options){this.options=options||{},this.options.duration=this.options.duration||0,this.options.timing=this.options.timing||"linear",this.options.css=this.options.css||{},this.options.property=this.options.property||"all"},Animit.Transition.prototype={build:function(){function createActualCssProps(css){var result={};return Object.keys(css).forEach(function(name){var value=css[name];if(util.hasCssProperty(name))return void(result[name]=value);var prefixed=util.vendorPrefix+util.capitalize(name);util.hasCssProperty(prefixed)?result[prefixed]=value:(result[prefixed]=value,result[name]=value)}),result}if(0===Object.keys(this.options.css).length)throw new Error("options.css is required.");var css=createActualCssProps(this.options.css);if(this.options.duration>0){var transitionValue=util.buildTransitionValue(this.options),self=this;return function(callback){var timeoutId,elements=this.elements,timeout=1e3*self.options.duration*TIMEOUT_RATIO,removeListeners=util.onceOnTransitionEnd(elements[0],function(){clearTimeout(timeoutId),callback()});timeoutId=setTimeout(function(){removeListeners(),callback()},timeout),elements.forEach(function(element){element.style[util.transitionPropertyName]=transitionValue,Object.keys(css).forEach(function(name){element.style[name]=css[name]})})}}return this.options.duration<=0?function(callback){var elements=this.elements;elements.forEach(function(element){element.style[util.transitionPropertyName]="",Object.keys(css).forEach(function(name){element.style[name]=css[name]})}),elements.length>0?util.forceLayoutAtOnce(elements,function(){util.batchAnimationFrame(callback)}):util.batchAnimationFrame(callback)}:void 0}},Animit}(),function(){"remove"in Element.prototype||(Element.prototype.remove=function(){this.parentNode&&this.parentNode.removeChild(this)})}(),"document"in self&&("classList"in document.createElement("_")&&(!document.createElementNS||"classList"in document.createElementNS("http://www.w3.org/2000/svg","g"))?!function(){"use strict";var testElement=document.createElement("_");if(testElement.classList.add("c1","c2"),!testElement.classList.contains("c2")){var createMethod=function(method){var original=DOMTokenList.prototype[method];DOMTokenList.prototype[method]=function(token){var i,len=arguments.length;for(i=0;len>i;i++)token=arguments[i],original.call(this,token)}};createMethod("add"),createMethod("remove")}if(testElement.classList.toggle("c3",!1),testElement.classList.contains("c3")){var _toggle=DOMTokenList.prototype.toggle;DOMTokenList.prototype.toggle=function(token,force){return 1 in arguments&&!this.contains(token)==!force?force:_toggle.call(this,token)}}testElement=null}():!function(view){"use strict";if("Element"in view){var classListProp="classList",protoProp="prototype",elemCtrProto=view.Element[protoProp],objCtr=Object,strTrim=String[protoProp].trim||function(){return this.replace(/^\s+|\s+$/g,"")},arrIndexOf=Array[protoProp].indexOf||function(item){for(var i=0,len=this.length;len>i;i++)if(i in this&&this[i]===item)return i;return-1},DOMEx=function(type,message){this.name=type,this.code=DOMException[type],this.message=message},checkTokenAndGetIndex=function(classList,token){if(""===token)throw new DOMEx("SYNTAX_ERR","An invalid or illegal string was specified");if(/\s/.test(token))throw new DOMEx("INVALID_CHARACTER_ERR","String contains an invalid character");return arrIndexOf.call(classList,token)},ClassList=function(elem){for(var trimmedClasses=strTrim.call(elem.getAttribute("class")||""),classes=trimmedClasses?trimmedClasses.split(/\s+/):[],i=0,len=classes.length;len>i;i++)this.push(classes[i]);this._updateClassName=function(){elem.setAttribute("class",this.toString())}},classListProto=ClassList[protoProp]=[],classListGetter=function(){return new ClassList(this)};if(DOMEx[protoProp]=Error[protoProp],classListProto.item=function(i){return this[i]||null},classListProto.contains=function(token){return token+="",-1!==checkTokenAndGetIndex(this,token)},classListProto.add=function(){var token,tokens=arguments,i=0,l=tokens.length,updated=!1;do token=tokens[i]+"",-1===checkTokenAndGetIndex(this,token)&&(this.push(token),updated=!0);while(++i<l);updated&&this._updateClassName()},classListProto.remove=function(){var token,index,tokens=arguments,i=0,l=tokens.length,updated=!1;do for(token=tokens[i]+"",index=checkTokenAndGetIndex(this,token);-1!==index;)this.splice(index,1),updated=!0,index=checkTokenAndGetIndex(this,token);while(++i<l);updated&&this._updateClassName()},classListProto.toggle=function(token,force){token+="";var result=this.contains(token),method=result?force!==!0&&"remove":force!==!1&&"add";return method&&this[method](token),force===!0||force===!1?force:!result},classListProto.toString=function(){return this.join(" ")},objCtr.defineProperty){var classListPropDesc={get:classListGetter,enumerable:!0,configurable:!0};try{objCtr.defineProperty(elemCtrProto,classListProp,classListPropDesc)}catch(ex){-2146823252===ex.number&&(classListPropDesc.enumerable=!1,objCtr.defineProperty(elemCtrProto,classListProp,classListPropDesc))}}else objCtr[protoProp].__defineGetter__&&elemCtrProto.__defineGetter__(classListProp,classListGetter)}}(self)),function(window,document,Object,REGISTER_ELEMENT){"use strict";function ASAP(){var queue=asapQueue.splice(0,asapQueue.length);for(asapTimer=0;queue.length;)queue.shift().call(null,queue.shift())}function loopAndVerify(list,action){for(var i=0,length=list.length;length>i;i++)verifyAndSetupAndAction(list[i],action)}function loopAndSetup(list){for(var node,i=0,length=list.length;length>i;i++)node=list[i],patch(node,protos[getTypeIndex(node)])}function executeAction(action){return function(node){isValidNode(node)&&(verifyAndSetupAndAction(node,action),loopAndVerify(node.querySelectorAll(query),action))}}function getTypeIndex(target){var is=getAttribute.call(target,"is"),nodeName=target.nodeName.toUpperCase(),i=indexOf.call(types,is?PREFIX_IS+is.toUpperCase():PREFIX_TAG+nodeName);return is&&i>-1&&!isInQSA(nodeName,is)?-1:i}function isInQSA(name,type){return-1<query.indexOf(name+'[is="'+type+'"]')}function onDOMAttrModified(e){var node=e.currentTarget,attrChange=e.attrChange,attrName=e.attrName,target=e.target;!notFromInnerHTMLHelper||target&&target!==node||!node[ATTRIBUTE_CHANGED_CALLBACK]||"style"===attrName||e.prevValue===e.newValue||node[ATTRIBUTE_CHANGED_CALLBACK](attrName,attrChange===e[ADDITION]?null:e.prevValue,attrChange===e[REMOVAL]?null:e.newValue)}function onDOMNode(action){var executor=executeAction(action);return function(e){asapQueue.push(executor,e.target),asapTimer&&clearTimeout(asapTimer),asapTimer=setTimeout(ASAP,1)}}function onReadyStateChange(e){dropDomContentLoaded&&(dropDomContentLoaded=!1,e.currentTarget.removeEventListener(DOM_CONTENT_LOADED,onReadyStateChange)),loopAndVerify((e.target||document).querySelectorAll(query),e.detail===DETACHED?DETACHED:ATTACHED),IE8&&purge()}function patchedSetAttribute(name,value){var self=this;setAttribute.call(self,name,value),onSubtreeModified.call(self,{target:self})}function setupNode(node,proto){setPrototype(node,proto),observer?observer.observe(node,attributesObserver):(doesNotSupportDOMAttrModified&&(node.setAttribute=patchedSetAttribute,node[EXPANDO_UID]=getAttributesMirror(node),node[ADD_EVENT_LISTENER](DOM_SUBTREE_MODIFIED,onSubtreeModified)),node[ADD_EVENT_LISTENER](DOM_ATTR_MODIFIED,onDOMAttrModified)),node[CREATED_CALLBACK]&¬FromInnerHTMLHelper&&(node.created=!0,node[CREATED_CALLBACK](),node.created=!1)}function purge(){for(var node,i=0,length=targets.length;length>i;i++)node=targets[i],documentElement.contains(node)||(length--,targets.splice(i--,1),verifyAndSetupAndAction(node,DETACHED))}function throwTypeError(type){throw new Error("A "+type+" type is already registered")}function verifyAndSetupAndAction(node,action){var fn,i=getTypeIndex(node);i>-1&&(patchIfNotAlready(node,protos[i]),i=0,action!==ATTACHED||node[ATTACHED]?action!==DETACHED||node[DETACHED]||(node[ATTACHED]=!1,node[DETACHED]=!0,i=1):(node[DETACHED]=!1,node[ATTACHED]=!0,i=1,IE8&&indexOf.call(targets,node)<0&&targets.push(node)),i&&(fn=node[action+CALLBACK])&&fn.call(node))}function CustomElementRegistry(){}function CERDefine(name,Class,options){var is=options&&options[EXTENDS]||"",CProto=Class.prototype,proto=create(CProto),attributes=Class.observedAttributes||empty,definition={prototype:proto};safeProperty(proto,CREATED_CALLBACK,{value:function(){if(justCreated)justCreated=!1;else if(!this[DRECEV1]){this[DRECEV1]=!0,new Class(this),CProto[CREATED_CALLBACK]&&CProto[CREATED_CALLBACK].call(this);var info=constructors[nodeNames.get(Class)];(!usableCustomElements||info.create.length>1)&¬ifyAttributes(this)}}}),safeProperty(proto,ATTRIBUTE_CHANGED_CALLBACK,{value:function(name){-1<indexOf.call(attributes,name)&&CProto[ATTRIBUTE_CHANGED_CALLBACK].apply(this,arguments)}}),CProto[CONNECTED_CALLBACK]&&safeProperty(proto,ATTACHED_CALLBACK,{value:CProto[CONNECTED_CALLBACK]}),CProto[DISCONNECTED_CALLBACK]&&safeProperty(proto,DETACHED_CALLBACK,{value:CProto[DISCONNECTED_CALLBACK]}),is&&(definition[EXTENDS]=is),document[REGISTER_ELEMENT](name,definition),name=name.toUpperCase(),constructors[name]={constructor:Class,create:is?[is,secondArgument(name)]:[name]},nodeNames.set(Class,name),whenDefined(name),waitingList[name].r()}function get(name){var info=constructors[name.toUpperCase()];return info&&info.constructor}function getIs(options){return"string"==typeof options?options:options&&options.is||""}function notifyAttributes(self){for(var attribute,callback=self[ATTRIBUTE_CHANGED_CALLBACK],attributes=callback?self.attributes:empty,i=attributes.length;i--;)attribute=attributes[i],callback.call(self,attribute.name||attribute.nodeName,null,attribute.value||attribute.nodeValue)}function whenDefined(name){return name=name.toUpperCase(),name in waitingList||(waitingList[name]={},waitingList[name].p=new Promise(function(resolve){waitingList[name].r=resolve})),waitingList[name].p}function polyfillV1(){customElements&&delete window.customElements,defineProperty(window,"customElements",{configurable:!0,value:new CustomElementRegistry}),defineProperty(window,"CustomElementRegistry",{configurable:!0,value:CustomElementRegistry});for(var patchClass=function(name){var Class=window[name];if(Class){window[name]=function(self){var info,isNative;return self||(self=this),self[DRECEV1]||(justCreated=!0,info=constructors[nodeNames.get(self.constructor)],isNative=usableCustomElements&&1===info.create.length,self=isNative?Reflect.construct(Class,empty,info.constructor):document.createElement.apply(document,info.create),self[DRECEV1]=!0,justCreated=!1,isNative||notifyAttributes(self)),self},window[name].prototype=Class.prototype;try{Class.prototype.constructor=window[name]}catch(WebKit){fixGetClass=!0,defineProperty(Class,DRECEV1,{value:window[name]})}}},Classes=htmlClass.get(/^HTML/),i=Classes.length;i--;patchClass(Classes[i]));document.createElement=function(name,options){var is=getIs(options);return is?patchedCreateElement.call(this,name,secondArgument(is)):patchedCreateElement.call(this,name)}}var asapQueue,onSubtreeModified,callDOMAttrModified,getAttributesMirror,observer,patchIfNotAlready,patch,htmlClass=function(info){var i,section,tags,Class,catchClass=/^[A-Z]+[a-z]/,filterBy=function(re){var tag,arr=[];for(tag in register)re.test(tag)&&arr.push(tag);return arr},add=function(Class,tag){tag=tag.toLowerCase(),tag in register||(register[Class]=(register[Class]||[]).concat(tag),register[tag]=register[tag.toUpperCase()]=Class)},register=(Object.create||Object)(null),htmlClass={};for(section in info)for(Class in info[section])for(tags=info[section][Class],register[Class]=tags,i=0;i<tags.length;i++)register[tags[i].toLowerCase()]=register[tags[i].toUpperCase()]=Class;return htmlClass.get=function(tagOrClass){return"string"==typeof tagOrClass?register[tagOrClass]||(catchClass.test(tagOrClass)?[]:""):filterBy(tagOrClass)},htmlClass.set=function(tag,Class){return catchClass.test(tag)?add(tag,Class):add(Class,tag),htmlClass},htmlClass}({collections:{HTMLAllCollection:["all"],HTMLCollection:["forms"],HTMLFormControlsCollection:["elements"],HTMLOptionsCollection:["options"]},elements:{Element:["element"],HTMLAnchorElement:["a"],HTMLAppletElement:["applet"],HTMLAreaElement:["area"],HTMLAttachmentElement:["attachment"],HTMLAudioElement:["audio"],HTMLBRElement:["br"],HTMLBaseElement:["base"],HTMLBodyElement:["body"],HTMLButtonElement:["button"],HTMLCanvasElement:["canvas"],HTMLContentElement:["content"],HTMLDListElement:["dl"],HTMLDataElement:["data"],HTMLDataListElement:["datalist"],HTMLDetailsElement:["details"],HTMLDialogElement:["dialog"],HTMLDirectoryElement:["dir"],HTMLDivElement:["div"],HTMLDocument:["document"],HTMLElement:["element","abbr","address","article","aside","b","bdi","bdo","cite","code","command","dd","dfn","dt","em","figcaption","figure","footer","header","i","kbd","mark","nav","noscript","rp","rt","ruby","s","samp","section","small","strong","sub","summary","sup","u","var","wbr"],HTMLEmbedElement:["embed"],HTMLFieldSetElement:["fieldset"],HTMLFontElement:["font"],HTMLFormElement:["form"],HTMLFrameElement:["frame"],HTMLFrameSetElement:["frameset"],HTMLHRElement:["hr"],HTMLHeadElement:["head"],HTMLHeadingElement:["h1","h2","h3","h4","h5","h6"],HTMLHtmlElement:["html"],HTMLIFrameElement:["iframe"],HTMLImageElement:["img"],HTMLInputElement:["input"],HTMLKeygenElement:["keygen"],HTMLLIElement:["li"],HTMLLabelElement:["label"],HTMLLegendElement:["legend"],HTMLLinkElement:["link"],HTMLMapElement:["map"],HTMLMarqueeElement:["marquee"],HTMLMediaElement:["media"],HTMLMenuElement:["menu"],HTMLMenuItemElement:["menuitem"],HTMLMetaElement:["meta"],HTMLMeterElement:["meter"],HTMLModElement:["del","ins"],HTMLOListElement:["ol"],HTMLObjectElement:["object"],HTMLOptGroupElement:["optgroup"],HTMLOptionElement:["option"],HTMLOutputElement:["output"],HTMLParagraphElement:["p"],HTMLParamElement:["param"],HTMLPictureElement:["picture"],HTMLPreElement:["pre"],HTMLProgressElement:["progress"],HTMLQuoteElement:["blockquote","q","quote"],HTMLScriptElement:["script"],HTMLSelectElement:["select"],HTMLShadowElement:["shadow"],HTMLSlotElement:["slot"],HTMLSourceElement:["source"],HTMLSpanElement:["span"],HTMLStyleElement:["style"],HTMLTableCaptionElement:["caption"],HTMLTableCellElement:["td","th"],HTMLTableColElement:["col","colgroup"],HTMLTableElement:["table"],HTMLTableRowElement:["tr"],HTMLTableSectionElement:["thead","tbody","tfoot"],HTMLTemplateElement:["template"],HTMLTextAreaElement:["textarea"],HTMLTimeElement:["time"],HTMLTitleElement:["title"],HTMLTrackElement:["track"],HTMLUListElement:["ul"],HTMLUnknownElement:["unknown","vhgroupv","vkeygen"],HTMLVideoElement:["video"]},nodes:{Attr:["node"],Audio:["audio"],CDATASection:["node"],CharacterData:["node"],Comment:["#comment"],Document:["#document"],DocumentFragment:["#document-fragment"],DocumentType:["node"],HTMLDocument:["#document"],Image:["img"],Option:["option"],ProcessingInstruction:["node"],ShadowRoot:["#shadow-root"],Text:["#text"],XMLDocument:["xml"]}}),EXPANDO_UID="__"+REGISTER_ELEMENT+(1e5*Math.random()>>0),ADD_EVENT_LISTENER="addEventListener",ATTACHED="attached",CALLBACK="Callback",DETACHED="detached",EXTENDS="extends",ATTRIBUTE_CHANGED_CALLBACK="attributeChanged"+CALLBACK,ATTACHED_CALLBACK=ATTACHED+CALLBACK,CONNECTED_CALLBACK="connected"+CALLBACK,DISCONNECTED_CALLBACK="disconnected"+CALLBACK,CREATED_CALLBACK="created"+CALLBACK,DETACHED_CALLBACK=DETACHED+CALLBACK,ADDITION="ADDITION",MODIFICATION="MODIFICATION",REMOVAL="REMOVAL",DOM_ATTR_MODIFIED="DOMAttrModified",DOM_CONTENT_LOADED="DOMContentLoaded",DOM_SUBTREE_MODIFIED="DOMSubtreeModified",PREFIX_TAG="<",PREFIX_IS="=",validName=/^[A-Z][A-Z0-9]*(?:-[A-Z0-9]+)+$/,invalidNames=["ANNOTATION-XML","COLOR-PROFILE","FONT-FACE","FONT-FACE-SRC","FONT-FACE-URI","FONT-FACE-FORMAT","FONT-FACE-NAME","MISSING-GLYPH"],types=[],protos=[],query="",documentElement=document.documentElement,indexOf=types.indexOf||function(v){
for(var i=this.length;i--&&this[i]!==v;);return i},OP=Object.prototype,hOP=OP.hasOwnProperty,iPO=OP.isPrototypeOf,defineProperty=Object.defineProperty,empty=[],gOPD=Object.getOwnPropertyDescriptor,gOPN=Object.getOwnPropertyNames,gPO=Object.getPrototypeOf,sPO=Object.setPrototypeOf,hasProto=!!Object.__proto__,fixGetClass=!1,DRECEV1="__dreCEv1",customElements=window.customElements,usableCustomElements=!!(customElements&&customElements.define&&customElements.get&&customElements.whenDefined),Dict=Object.create||Object,Map=window.Map||function(){var i,K=[],V=[];return{get:function(k){return V[indexOf.call(K,k)]},set:function(k,v){i=indexOf.call(K,k),0>i?V[K.push(k)-1]=v:V[i]=v}}},Promise=window.Promise||function(fn){function resolve(value){for(done=!0;notify.length;)notify.shift()(value)}var notify=[],done=!1,p={"catch":function(){return p},then:function(cb){return notify.push(cb),done&&setTimeout(resolve,1),p}};return fn(resolve),p},justCreated=!1,constructors=Dict(null),waitingList=Dict(null),nodeNames=new Map,secondArgument=String,create=Object.create||function Bridge(proto){return proto?(Bridge.prototype=proto,new Bridge):this},setPrototype=sPO||(hasProto?function(o,p){return o.__proto__=p,o}:gOPN&&gOPD?function(){function setProperties(o,p){for(var key,names=gOPN(p),i=0,length=names.length;length>i;i++)key=names[i],hOP.call(o,key)||defineProperty(o,key,gOPD(p,key))}return function(o,p){do setProperties(o,p);while((p=gPO(p))&&!iPO.call(p,o));return o}}():function(o,p){for(var key in p)o[key]=p[key];return o}),MutationObserver=window.MutationObserver||window.WebKitMutationObserver,HTMLElementPrototype=(window.HTMLElement||window.Element||window.Node).prototype,IE8=!iPO.call(HTMLElementPrototype,documentElement),safeProperty=IE8?function(o,k,d){return o[k]=d.value,o}:defineProperty,isValidNode=IE8?function(node){return 1===node.nodeType}:function(node){return iPO.call(HTMLElementPrototype,node)},targets=IE8&&[],cloneNode=HTMLElementPrototype.cloneNode,dispatchEvent=HTMLElementPrototype.dispatchEvent,getAttribute=HTMLElementPrototype.getAttribute,hasAttribute=HTMLElementPrototype.hasAttribute,removeAttribute=HTMLElementPrototype.removeAttribute,setAttribute=HTMLElementPrototype.setAttribute,createElement=document.createElement,patchedCreateElement=createElement,attributesObserver=MutationObserver&&{attributes:!0,characterData:!0,attributeOldValue:!0},DOMAttrModified=MutationObserver||function(e){doesNotSupportDOMAttrModified=!1,documentElement.removeEventListener(DOM_ATTR_MODIFIED,DOMAttrModified)},asapTimer=0,setListener=!1,doesNotSupportDOMAttrModified=!0,dropDomContentLoaded=!0,notFromInnerHTMLHelper=!0;REGISTER_ELEMENT in document||(sPO||hasProto?(patchIfNotAlready=function(node,proto){iPO.call(proto,node)||setupNode(node,proto)},patch=setupNode):(patchIfNotAlready=function(node,proto){node[EXPANDO_UID]||(node[EXPANDO_UID]=Object(!0),setupNode(node,proto))},patch=patchIfNotAlready),IE8?(doesNotSupportDOMAttrModified=!1,function(){var descriptor=gOPD(HTMLElementPrototype,ADD_EVENT_LISTENER),addEventListener=descriptor.value,patchedRemoveAttribute=function(name){var e=new CustomEvent(DOM_ATTR_MODIFIED,{bubbles:!0});e.attrName=name,e.prevValue=getAttribute.call(this,name),e.newValue=null,e[REMOVAL]=e.attrChange=2,removeAttribute.call(this,name),dispatchEvent.call(this,e)},patchedSetAttribute=function(name,value){var had=hasAttribute.call(this,name),old=had&&getAttribute.call(this,name),e=new CustomEvent(DOM_ATTR_MODIFIED,{bubbles:!0});setAttribute.call(this,name,value),e.attrName=name,e.prevValue=had?old:null,e.newValue=value,had?e[MODIFICATION]=e.attrChange=1:e[ADDITION]=e.attrChange=0,dispatchEvent.call(this,e)},onPropertyChange=function(e){var event,node=e.currentTarget,superSecret=node[EXPANDO_UID],propertyName=e.propertyName;superSecret.hasOwnProperty(propertyName)&&(superSecret=superSecret[propertyName],event=new CustomEvent(DOM_ATTR_MODIFIED,{bubbles:!0}),event.attrName=superSecret.name,event.prevValue=superSecret.value||null,event.newValue=superSecret.value=node[propertyName]||null,null==event.prevValue?event[ADDITION]=event.attrChange=0:event[MODIFICATION]=event.attrChange=1,dispatchEvent.call(node,event))};descriptor.value=function(type,handler,capture){type===DOM_ATTR_MODIFIED&&this[ATTRIBUTE_CHANGED_CALLBACK]&&this.setAttribute!==patchedSetAttribute&&(this[EXPANDO_UID]={className:{name:"class",value:this.className}},this.setAttribute=patchedSetAttribute,this.removeAttribute=patchedRemoveAttribute,addEventListener.call(this,"propertychange",onPropertyChange)),addEventListener.call(this,type,handler,capture)},defineProperty(HTMLElementPrototype,ADD_EVENT_LISTENER,descriptor)}()):MutationObserver||(documentElement[ADD_EVENT_LISTENER](DOM_ATTR_MODIFIED,DOMAttrModified),documentElement.setAttribute(EXPANDO_UID,1),documentElement.removeAttribute(EXPANDO_UID),doesNotSupportDOMAttrModified&&(onSubtreeModified=function(e){var oldAttributes,newAttributes,key,node=this;if(node===e.target){oldAttributes=node[EXPANDO_UID],node[EXPANDO_UID]=newAttributes=getAttributesMirror(node);for(key in newAttributes){if(!(key in oldAttributes))return callDOMAttrModified(0,node,key,oldAttributes[key],newAttributes[key],ADDITION);if(newAttributes[key]!==oldAttributes[key])return callDOMAttrModified(1,node,key,oldAttributes[key],newAttributes[key],MODIFICATION)}for(key in oldAttributes)if(!(key in newAttributes))return callDOMAttrModified(2,node,key,oldAttributes[key],newAttributes[key],REMOVAL)}},callDOMAttrModified=function(attrChange,currentTarget,attrName,prevValue,newValue,action){var e={attrChange:attrChange,currentTarget:currentTarget,attrName:attrName,prevValue:prevValue,newValue:newValue};e[action]=attrChange,onDOMAttrModified(e)},getAttributesMirror=function(node){for(var attr,name,result={},attributes=node.attributes,i=0,length=attributes.length;length>i;i++)attr=attributes[i],name=attr.name,"setAttribute"!==name&&(result[name]=attr.value);return result})),document[REGISTER_ELEMENT]=function(type,options){if(upperType=type.toUpperCase(),setListener||(setListener=!0,MutationObserver?(observer=function(attached,detached){function checkEmAll(list,callback){for(var i=0,length=list.length;length>i;callback(list[i++]));}return new MutationObserver(function(records){for(var current,node,newValue,i=0,length=records.length;length>i;i++)current=records[i],"childList"===current.type?(checkEmAll(current.addedNodes,attached),checkEmAll(current.removedNodes,detached)):(node=current.target,notFromInnerHTMLHelper&&node[ATTRIBUTE_CHANGED_CALLBACK]&&"style"!==current.attributeName&&(newValue=getAttribute.call(node,current.attributeName),newValue!==current.oldValue&&node[ATTRIBUTE_CHANGED_CALLBACK](current.attributeName,current.oldValue,newValue)))})}(executeAction(ATTACHED),executeAction(DETACHED)),observer.observe(document,{childList:!0,subtree:!0})):(asapQueue=[],document[ADD_EVENT_LISTENER]("DOMNodeInserted",onDOMNode(ATTACHED)),document[ADD_EVENT_LISTENER]("DOMNodeRemoved",onDOMNode(DETACHED))),document[ADD_EVENT_LISTENER](DOM_CONTENT_LOADED,onReadyStateChange),document[ADD_EVENT_LISTENER]("readystatechange",onReadyStateChange),HTMLElementPrototype.cloneNode=function(deep){var node=cloneNode.call(this,!!deep),i=getTypeIndex(node);return i>-1&&patch(node,protos[i]),deep&&loopAndSetup(node.querySelectorAll(query)),node}),-2<indexOf.call(types,PREFIX_IS+upperType)+indexOf.call(types,PREFIX_TAG+upperType)&&throwTypeError(type),!validName.test(upperType)||-1<indexOf.call(invalidNames,upperType))throw new Error("The type "+type+" is invalid");var upperType,i,constructor=function(){return extending?document.createElement(nodeName,upperType):document.createElement(nodeName)},opt=options||OP,extending=hOP.call(opt,EXTENDS),nodeName=extending?options[EXTENDS].toUpperCase():upperType;return extending&&-1<indexOf.call(types,PREFIX_TAG+nodeName)&&throwTypeError(nodeName),i=types.push((extending?PREFIX_IS:PREFIX_TAG)+upperType)-1,query=query.concat(query.length?",":"",extending?nodeName+'[is="'+type.toLowerCase()+'"]':nodeName),constructor.prototype=protos[i]=hOP.call(opt,"prototype")?opt.prototype:create(HTMLElementPrototype),loopAndVerify(document.querySelectorAll(query),ATTACHED),constructor},document.createElement=patchedCreateElement=function(localName,typeExtension){var is=getIs(typeExtension),node=is?createElement.call(document,localName,secondArgument(is)):createElement.call(document,localName),name=""+localName,i=indexOf.call(types,(is?PREFIX_IS:PREFIX_TAG)+(is||name).toUpperCase()),setup=i>-1;return is&&(node.setAttribute("is",is=is.toLowerCase()),setup&&(setup=isInQSA(name.toUpperCase(),is))),notFromInnerHTMLHelper=!document.createElement.innerHTMLHelper,setup&&patch(node,protos[i]),node}),CustomElementRegistry.prototype={constructor:CustomElementRegistry,define:usableCustomElements?function(name,Class,options){options?CERDefine(name,Class,options):(customElements.define(name,Class),name=name.toUpperCase(),constructors[name]={constructor:Class,create:[name]},nodeNames.set(Class,name))}:CERDefine,get:usableCustomElements?function(name){return customElements.get(name)||get(name)}:get,whenDefined:usableCustomElements?function(name){return Promise.race([customElements.whenDefined(name),whenDefined(name)])}:whenDefined},customElements||polyfillV1();try{!function(DRE,options,name){if(options[EXTENDS]="a",DRE.prototype=HTMLAnchorElement.prototype,customElements.define(name,DRE,options),document.createElement(name).getAttribute("is")!==name)throw options}(function DRE(){return Reflect.construct(HTMLAnchorElement,[],DRE)},{},"document-register-element-a")}catch(o_O){polyfillV1()}try{createElement.call(document,"a","a")}catch(FireFox){secondArgument=function(is){return{is:is}}}}(window,document,Object,"registerElement"),function(){"use strict";function FastClick(layer,options){function bind(method,context){return function(){return method.apply(context,arguments)}}var oldOnClick;if(options=options||{},this.trackingClick=!1,this.trackingClickStart=0,this.targetElement=null,this.touchStartX=0,this.touchStartY=0,this.lastTouchIdentifier=0,this.touchBoundary=options.touchBoundary||10,this.layer=layer,this.tapDelay=options.tapDelay||200,this.tapTimeout=options.tapTimeout||700,!FastClick.notNeeded(layer)){for(var methods=["onMouse","onClick","onTouchStart","onTouchMove","onTouchEnd","onTouchCancel"],context=this,i=0,l=methods.length;l>i;i++)context[methods[i]]=bind(context[methods[i]],context);deviceIsAndroid&&(layer.addEventListener("mouseover",this.onMouse,!0),layer.addEventListener("mousedown",this.onMouse,!0),layer.addEventListener("mouseup",this.onMouse,!0)),layer.addEventListener("click",this.onClick,!0),layer.addEventListener("touchstart",this.onTouchStart,!1),layer.addEventListener("touchmove",this.onTouchMove,!1),layer.addEventListener("touchend",this.onTouchEnd,!1),layer.addEventListener("touchcancel",this.onTouchCancel,!1),Event.prototype.stopImmediatePropagation||(layer.removeEventListener=function(type,callback,capture){var rmv=Node.prototype.removeEventListener;"click"===type?rmv.call(layer,type,callback.hijacked||callback,capture):rmv.call(layer,type,callback,capture)},layer.addEventListener=function(type,callback,capture){var adv=Node.prototype.addEventListener;"click"===type?adv.call(layer,type,callback.hijacked||(callback.hijacked=function(event){event.propagationStopped||callback(event)}),capture):adv.call(layer,type,callback,capture)}),"function"==typeof layer.onclick&&(oldOnClick=layer.onclick,layer.addEventListener("click",function(event){oldOnClick(event)},!1),layer.onclick=null)}}var deviceIsWindowsPhone=navigator.userAgent.indexOf("Windows Phone")>=0,deviceIsAndroid=navigator.userAgent.indexOf("Android")>0&&!deviceIsWindowsPhone,deviceIsIOS=/iP(ad|hone|od)/.test(navigator.userAgent)&&!deviceIsWindowsPhone,deviceIsIOS4=deviceIsIOS&&/OS 4_\d(_\d)?/.test(navigator.userAgent),deviceIsIOSWithBadTarget=deviceIsIOS&&/OS [6-7]_\d/.test(navigator.userAgent),deviceIsBlackBerry10=navigator.userAgent.indexOf("BB10")>0;FastClick.prototype.needsClick=function(target){switch(target.nodeName.toLowerCase()){case"button":case"select":case"textarea":if(target.disabled)return!0;break;case"input":if(deviceIsIOS&&"file"===target.type||target.disabled)return!0;break;case"label":case"iframe":case"video":return!0}return/\bneedsclick\b/.test(target.className)},FastClick.prototype.needsFocus=function(target){switch(target.nodeName.toLowerCase()){case"textarea":return!0;case"select":return!deviceIsAndroid;case"input":switch(target.type){case"button":case"checkbox":case"file":case"image":case"radio":case"submit":return!1}return!target.disabled&&!target.readOnly;default:return/\bneedsfocus\b/.test(target.className)}},FastClick.prototype.sendClick=function(targetElement,event){var clickEvent,touch;document.activeElement&&document.activeElement!==targetElement&&document.activeElement.blur(),touch=event.changedTouches[0],clickEvent=document.createEvent("MouseEvents"),clickEvent.initMouseEvent(this.determineEventType(targetElement),!0,!0,window,1,touch.screenX,touch.screenY,touch.clientX,touch.clientY,!1,!1,!1,!1,0,null),clickEvent.forwardedTouchEvent=!0,targetElement.dispatchEvent(clickEvent)},FastClick.prototype.determineEventType=function(targetElement){return deviceIsAndroid&&"select"===targetElement.tagName.toLowerCase()?"mousedown":"click"},FastClick.prototype.focus=function(targetElement){var length;deviceIsIOS&&targetElement.setSelectionRange&&0!==targetElement.type.indexOf("date")&&"time"!==targetElement.type&&"month"!==targetElement.type?(length=targetElement.value.length,targetElement.setSelectionRange(length,length)):targetElement.focus()},FastClick.prototype.updateScrollParent=function(targetElement){var scrollParent,parentElement;if(scrollParent=targetElement.fastClickScrollParent,!scrollParent||!scrollParent.contains(targetElement)){parentElement=targetElement;do{if(parentElement.scrollHeight>parentElement.offsetHeight){scrollParent=parentElement,targetElement.fastClickScrollParent=parentElement;break}parentElement=parentElement.parentElement}while(parentElement)}scrollParent&&(scrollParent.fastClickLastScrollTop=scrollParent.scrollTop)},FastClick.prototype.getTargetElementFromEventTarget=function(eventTarget){return eventTarget.nodeType===Node.TEXT_NODE?eventTarget.parentNode:eventTarget},FastClick.prototype.onTouchStart=function(event){var targetElement,touch,selection;if(event.targetTouches.length>1)return!0;if(targetElement=this.getTargetElementFromEventTarget(event.target),touch=event.targetTouches[0],deviceIsIOS){if(selection=window.getSelection(),selection.rangeCount&&!selection.isCollapsed)return!0;if(!deviceIsIOS4){if(touch.identifier&&touch.identifier===this.lastTouchIdentifier)return event.preventDefault(),!1;this.lastTouchIdentifier=touch.identifier,this.updateScrollParent(targetElement)}}return this.trackingClick=!0,this.trackingClickStart=event.timeStamp,this.targetElement=targetElement,this.touchStartX=touch.pageX,this.touchStartY=touch.pageY,event.timeStamp-this.lastClickTime<this.tapDelay&&event.timeStamp-this.lastClickTime>-1&&event.preventDefault(),!0},FastClick.prototype.touchHasMoved=function(event){var touch=event.changedTouches[0],boundary=this.touchBoundary;return Math.abs(touch.pageX-this.touchStartX)>boundary||Math.abs(touch.pageY-this.touchStartY)>boundary},FastClick.prototype.onTouchMove=function(event){return this.trackingClick?((this.targetElement!==this.getTargetElementFromEventTarget(event.target)||this.touchHasMoved(event))&&(this.trackingClick=!1,this.targetElement=null),!0):!0},FastClick.prototype.findControl=function(labelElement){return void 0!==labelElement.control?labelElement.control:labelElement.htmlFor?document.getElementById(labelElement.htmlFor):labelElement.querySelector("button, input:not([type=hidden]), keygen, meter, output, progress, select, textarea")},FastClick.prototype.onTouchEnd=function(event){var forElement,trackingClickStart,targetTagName,scrollParent,touch,targetElement=this.targetElement;if(!this.trackingClick)return!0;if(event.timeStamp-this.lastClickTime<this.tapDelay&&event.timeStamp-this.lastClickTime>-1)return this.cancelNextClick=!0,!0;if(event.timeStamp-this.trackingClickStart>this.tapTimeout)return!0;if(this.cancelNextClick=!1,this.lastClickTime=event.timeStamp,trackingClickStart=this.trackingClickStart,this.trackingClick=!1,this.trackingClickStart=0,deviceIsIOSWithBadTarget&&(touch=event.changedTouches[0],targetElement=document.elementFromPoint(touch.pageX-window.pageXOffset,touch.pageY-window.pageYOffset)||targetElement,targetElement.fastClickScrollParent=this.targetElement.fastClickScrollParent),targetTagName=targetElement.tagName.toLowerCase(),"label"===targetTagName){if(forElement=this.findControl(targetElement)){if(this.focus(targetElement),deviceIsAndroid)return!1;targetElement=forElement}}else if(this.needsFocus(targetElement))return event.timeStamp-trackingClickStart>100||deviceIsIOS&&window.top!==window&&"input"===targetTagName?(this.targetElement=null,!1):(this.focus(targetElement),this.sendClick(targetElement,event),deviceIsIOS&&"select"===targetTagName||(this.targetElement=null,event.preventDefault()),!1);return deviceIsIOS&&!deviceIsIOS4&&(scrollParent=targetElement.fastClickScrollParent,scrollParent&&scrollParent.fastClickLastScrollTop!==scrollParent.scrollTop)?!0:(this.needsClick(targetElement)||(event.preventDefault(),this.sendClick(targetElement,event)),!1)},FastClick.prototype.onTouchCancel=function(){this.trackingClick=!1,this.targetElement=null},FastClick.prototype.onMouse=function(event){return this.targetElement?event.forwardedTouchEvent?!0:event.cancelable&&(!this.needsClick(this.targetElement)||this.cancelNextClick)?(event.stopImmediatePropagation?event.stopImmediatePropagation():event.propagationStopped=!0,event.stopPropagation(),event.preventDefault(),!1):!0:!0},FastClick.prototype.onClick=function(event){var permitted;return this.trackingClick?(this.targetElement=null,this.trackingClick=!1,!0):"submit"===event.target.type&&0===event.detail?!0:(permitted=this.onMouse(event),permitted||(this.targetElement=null),permitted)},FastClick.prototype.destroy=function(){var layer=this.layer;deviceIsAndroid&&(layer.removeEventListener("mouseover",this.onMouse,!0),layer.removeEventListener("mousedown",this.onMouse,!0),layer.removeEventListener("mouseup",this.onMouse,!0)),layer.removeEventListener("click",this.onClick,!0),layer.removeEventListener("touchstart",this.onTouchStart,!1),layer.removeEventListener("touchmove",this.onTouchMove,!1),layer.removeEventListener("touchend",this.onTouchEnd,!1),layer.removeEventListener("touchcancel",this.onTouchCancel,!1)},FastClick.notNeeded=function(layer){var metaViewport,chromeVersion,blackberryVersion,firefoxVersion;if("undefined"==typeof window.ontouchstart)return!0;if(chromeVersion=+(/Chrome\/([0-9]+)/.exec(navigator.userAgent)||[,0])[1]){if(!deviceIsAndroid)return!0;if(metaViewport=document.querySelector("meta[name=viewport]")){if(-1!==metaViewport.content.indexOf("user-scalable=no"))return!0;if(chromeVersion>31&&document.documentElement.scrollWidth<=window.outerWidth)return!0}}if(deviceIsBlackBerry10&&(blackberryVersion=navigator.userAgent.match(/Version\/([0-9]*)\.([0-9]*)/),blackberryVersion[1]>=10&&blackberryVersion[2]>=3&&(metaViewport=document.querySelector("meta[name=viewport]")))){if(-1!==metaViewport.content.indexOf("user-scalable=no"))return!0;if(document.documentElement.scrollWidth<=window.outerWidth)return!0}return"none"===layer.style.msTouchAction||"manipulation"===layer.style.touchAction?!0:(firefoxVersion=+(/Firefox\/([0-9]+)/.exec(navigator.userAgent)||[,0])[1],firefoxVersion>=27&&(metaViewport=document.querySelector("meta[name=viewport]"),metaViewport&&(-1!==metaViewport.content.indexOf("user-scalable=no")||document.documentElement.scrollWidth<=window.outerWidth))?!0:"none"===layer.style.touchAction||"manipulation"===layer.style.touchAction)},FastClick.attach=function(layer,options){return new FastClick(layer,options)},window.FastClick=FastClick}();var innerHTML=function(document){var initialize,registered,EXTENDS="extends",register=document.registerElement,div=document.createElement("div"),dre="document-register-element",innerHTML=register.innerHTML;if(innerHTML)return innerHTML;try{if(register.call(document,dre,{prototype:Object.create(HTMLElement.prototype,{createdCallback:{value:Object}})}),div.innerHTML="<"+dre+"></"+dre+">","createdCallback"in div.querySelector(dre))return register.innerHTML=function(el,html){return el.innerHTML=html,el}}catch(meh){}return registered=[],initialize=function(el){if(!("createdCallback"in el||"attachedCallback"in el||"detachedCallback"in el||"attributeChangedCallback"in el)){document.createElement.innerHTMLHelper=!0;for(var attr,fc,parentNode=el.parentNode,type=el.getAttribute("is"),name=el.nodeName,node=document.createElement.apply(document,type?[name,type]:[name]),attributes=el.attributes,i=0,length=attributes.length;length>i;i++)attr=attributes[i],node.setAttribute(attr.name,attr.value);for(node.createdCallback&&(node.created=!0,node.createdCallback(),node.created=!1);fc=el.firstChild;)node.appendChild(fc);document.createElement.innerHTMLHelper=!1,parentNode&&parentNode.replaceChild(node,el)}},(document.registerElement=function(type,options){var name=(options[EXTENDS]?options[EXTENDS]+'[is="'+type+'"]':type).toLowerCase();return registered.indexOf(name)<0&®istered.push(name),register.apply(document,arguments)}).innerHTML=function(el,html){el.innerHTML=html;for(var nodes=el.querySelectorAll(registered.join(",")),i=nodes.length;i--;initialize(nodes[i]));return el}}(document),MicroEvent=function(){};MicroEvent.prototype={on:function(event,fct){this._events=this._events||{},this._events[event]=this._events[event]||[],this._events[event].push(fct)},once:function(event,fct){var self=this,wrapper=function(){return self.off(event,wrapper),fct.apply(null,arguments)};this.on(event,wrapper)},off:function(event,fct){this._events=this._events||{},event in this._events!=!1&&this._events[event].splice(this._events[event].indexOf(fct),1)},emit:function(event){if(this._events=this._events||{},event in this._events!=!1)for(var i=0;i<this._events[event].length;i++)this._events[event][i].apply(this,Array.prototype.slice.call(arguments,1))}},MicroEvent.mixin=function(destObject){for(var props=["on","once","off","emit"],i=0;i<props.length;i++)"function"==typeof destObject?destObject.prototype[props[i]]=MicroEvent.prototype[props[i]]:destObject[props[i]]=MicroEvent.prototype[props[i]]},"undefined"!=typeof module&&"exports"in module&&(module.exports=MicroEvent),window.MicroEvent=MicroEvent,function(root){function noop(){}function bind(fn,thisArg){return function(){fn.apply(thisArg,arguments)}}function Promise(fn){if("object"!=typeof this)throw new TypeError("Promises must be constructed via new");if("function"!=typeof fn)throw new TypeError("not a function");this._state=0,this._handled=!1,this._value=void 0,this._deferreds=[],doResolve(fn,this)}function handle(self,deferred){for(;3===self._state;)self=self._value;return 0===self._state?void self._deferreds.push(deferred):(self._handled=!0,void Promise._immediateFn(function(){var cb=1===self._state?deferred.onFulfilled:deferred.onRejected;if(null===cb)return void(1===self._state?resolve:reject)(deferred.promise,self._value);var ret;try{ret=cb(self._value)}catch(e){return void reject(deferred.promise,e)}resolve(deferred.promise,ret)}))}function resolve(self,newValue){try{if(newValue===self)throw new TypeError("A promise cannot be resolved with itself.");if(newValue&&("object"==typeof newValue||"function"==typeof newValue)){var then=newValue.then;if(newValue instanceof Promise)return self._state=3,self._value=newValue,void finale(self);if("function"==typeof then)return void doResolve(bind(then,newValue),self)}self._state=1,self._value=newValue,finale(self)}catch(e){reject(self,e)}}function reject(self,newValue){self._state=2,self._value=newValue,finale(self)}function finale(self){2===self._state&&0===self._deferreds.length&&Promise._immediateFn(function(){self._handled||Promise._unhandledRejectionFn(self._value)});for(var i=0,len=self._deferreds.length;len>i;i++)handle(self,self._deferreds[i]);self._deferreds=null}function Handler(onFulfilled,onRejected,promise){this.onFulfilled="function"==typeof onFulfilled?onFulfilled:null,this.onRejected="function"==typeof onRejected?onRejected:null,this.promise=promise}function doResolve(fn,self){var done=!1;try{fn(function(value){done||(done=!0,resolve(self,value))},function(reason){done||(done=!0,reject(self,reason))})}catch(ex){if(done)return;done=!0,reject(self,ex)}}var setTimeoutFunc=setTimeout;Promise.prototype["catch"]=function(onRejected){return this.then(null,onRejected)},Promise.prototype.then=function(onFulfilled,onRejected){var prom=new this.constructor(noop);return handle(this,new Handler(onFulfilled,onRejected,prom)),prom},Promise.all=function(arr){var args=Array.prototype.slice.call(arr);return new Promise(function(resolve,reject){function res(i,val){try{if(val&&("object"==typeof val||"function"==typeof val)){var then=val.then;if("function"==typeof then)return void then.call(val,function(val){res(i,val)},reject)}args[i]=val,0===--remaining&&resolve(args)}catch(ex){reject(ex)}}if(0===args.length)return resolve([]);for(var remaining=args.length,i=0;i<args.length;i++)res(i,args[i])})},Promise.resolve=function(value){return value&&"object"==typeof value&&value.constructor===Promise?value:new Promise(function(resolve){resolve(value)})},Promise.reject=function(value){return new Promise(function(resolve,reject){reject(value)})},Promise.race=function(values){return new Promise(function(resolve,reject){for(var i=0,len=values.length;len>i;i++)values[i].then(resolve,reject)})},Promise._immediateFn="function"==typeof setImmediate&&function(fn){setImmediate(fn)}||function(fn){setTimeoutFunc(fn,0)},Promise._unhandledRejectionFn=function(err){"undefined"!=typeof console&&console&&console.warn("Possible Unhandled Promise Rejection:",err)},Promise._setImmediateFn=function(fn){Promise._immediateFn=fn},Promise._setUnhandledRejectionFn=function(fn){Promise._unhandledRejectionFn=fn},window.Promise||(window.Promise=Promise)}(this),function(global,undefined){"use strict";function addFromSetImmediateArguments(args){return tasksByHandle[nextHandle]=partiallyApplied.apply(undefined,args),nextHandle++}function partiallyApplied(handler){var args=[].slice.call(arguments,1);return function(){"function"==typeof handler?handler.apply(undefined,args):new Function(""+handler)()}}function runIfPresent(handle){if(currentlyRunningATask)setTimeout(partiallyApplied(runIfPresent,handle),0);else{var task=tasksByHandle[handle];if(task){currentlyRunningATask=!0;try{task()}finally{clearImmediate(handle),currentlyRunningATask=!1}}}}function clearImmediate(handle){delete tasksByHandle[handle]}function installNextTickImplementation(){setImmediate=function(){var handle=addFromSetImmediateArguments(arguments);return process.nextTick(partiallyApplied(runIfPresent,handle)),handle}}function canUsePostMessage(){if(global.postMessage&&!global.importScripts){var postMessageIsAsynchronous=!0,oldOnMessage=global.onmessage;return global.onmessage=function(){postMessageIsAsynchronous=!1},global.postMessage("","*"),global.onmessage=oldOnMessage,postMessageIsAsynchronous}}function installPostMessageImplementation(){var messagePrefix="setImmediate$"+Math.random()+"$",onGlobalMessage=function(event){event.source===global&&"string"==typeof event.data&&0===event.data.indexOf(messagePrefix)&&runIfPresent(+event.data.slice(messagePrefix.length))};global.addEventListener?global.addEventListener("message",onGlobalMessage,!1):global.attachEvent("onmessage",onGlobalMessage),setImmediate=function(){var handle=addFromSetImmediateArguments(arguments);return global.postMessage(messagePrefix+handle,"*"),handle}}function installMessageChannelImplementation(){var channel=new MessageChannel;channel.port1.onmessage=function(event){var handle=event.data;runIfPresent(handle)},setImmediate=function(){var handle=addFromSetImmediateArguments(arguments);return channel.port2.postMessage(handle),handle}}function installReadyStateChangeImplementation(){var html=doc.documentElement;setImmediate=function(){var handle=addFromSetImmediateArguments(arguments),script=doc.createElement("script");return script.onreadystatechange=function(){runIfPresent(handle),script.onreadystatechange=null,html.removeChild(script),script=null},html.appendChild(script),handle}}function installSetTimeoutImplementation(){setImmediate=function(){var handle=addFromSetImmediateArguments(arguments);return setTimeout(partiallyApplied(runIfPresent,handle),0),handle}}if(!global.setImmediate){var setImmediate,nextHandle=1,tasksByHandle={},currentlyRunningATask=!1,doc=global.document,attachTo=Object.getPrototypeOf&&Object.getPrototypeOf(global);attachTo=attachTo&&attachTo.setTimeout?attachTo:global,"[object process]"==={}.toString.call(global.process)?installNextTickImplementation():canUsePostMessage()?installPostMessageImplementation():global.MessageChannel?installMessageChannelImplementation():doc&&"onreadystatechange"in doc.createElement("script")?installReadyStateChangeImplementation():installSetTimeoutImplementation(),attachTo.setImmediate=setImmediate,attachTo.clearImmediate=clearImmediate}}(function(){return this}()),function(){function Viewport(){return this.PRE_IOS7_VIEWPORT="initial-scale=1, maximum-scale=1, user-scalable=no",this.IOS7_VIEWPORT="initial-scale=1, maximum-scale=1, user-scalable=no",this.DEFAULT_VIEWPORT="initial-scale=1, maximum-scale=1, user-scalable=no",this.ensureViewportElement(),this.platform={},this.platform.name=this.getPlatformName(),this.platform.version=this.getPlatformVersion(),this}Viewport.prototype.ensureViewportElement=function(){this.viewportElement=document.querySelector("meta[name=viewport]"),this.viewportElement||(this.viewportElement=document.createElement("meta"),this.viewportElement.name="viewport",document.head.appendChild(this.viewportElement))},Viewport.prototype.setup=function(){function isWebView(){return!!(window.cordova||window.phonegap||window.PhoneGap)}this.viewportElement&&"true"!=this.viewportElement.getAttribute("data-no-adjust")&&(this.viewportElement.getAttribute("content")||("ios"==this.platform.name?this.platform.version>=7&&isWebView()?this.viewportElement.setAttribute("content",this.IOS7_VIEWPORT):this.viewportElement.setAttribute("content",this.PRE_IOS7_VIEWPORT):this.viewportElement.setAttribute("content",this.DEFAULT_VIEWPORT)))},Viewport.prototype.getPlatformName=function(){return navigator.userAgent.match(/Android/i)?"android":navigator.userAgent.match(/iPhone|iPad|iPod/i)?"ios":void 0},Viewport.prototype.getPlatformVersion=function(){var start=window.navigator.userAgent.indexOf("OS ");return window.Number(window.navigator.userAgent.substr(start+3,3).replace("_","."))},window.Viewport=Viewport}(),function(){function getAttributes(element){return Node_get_attributes.call(element)}function setAttribute(element,attribute,value){try{Element_setAttribute.call(element,attribute,value)}catch(e){}}function removeAttribute(element,attribute){Element_removeAttribute.call(element,attribute)}function childNodes(element){return Node_get_childNodes.call(element)}function empty(element){for(;element.childNodes.length;)element.removeChild(element.lastChild)}function insertAdjacentHTML(element,position,html){HTMLElement_insertAdjacentHTMLPropertyDescriptor.value.call(element,position,html)}function inUnsafeMode(){var isUnsafe=!0;try{detectionDiv.innerHTML="<test/>"}catch(ex){isUnsafe=!1}return isUnsafe}function cleanse(html,targetElement){function cleanseAttributes(element){var attributes=getAttributes(element);if(attributes&&attributes.length){for(var events,i=0,len=attributes.length;len>i;i++){var attribute=attributes[i],name=attribute.name;"o"!==name[0]&&"O"!==name[0]||"n"!==name[1]&&"N"!==name[1]||(events=events||[],events.push({
name:attribute.name,value:attribute.value}))}if(events)for(var i=0,len=events.length;len>i;i++){var attribute=events[i];removeAttribute(element,attribute.name),setAttribute(element,"x-"+attribute.name,attribute.value)}}for(var children=childNodes(element),i=0,len=children.length;len>i;i++)cleanseAttributes(children[i])}var cleaner=document.implementation.createHTMLDocument("cleaner");empty(cleaner.documentElement),MSApp.execUnsafeLocalFunction(function(){insertAdjacentHTML(cleaner.documentElement,"afterbegin",html)});var scripts=cleaner.documentElement.querySelectorAll("script");Array.prototype.forEach.call(scripts,function(script){switch(script.type.toLowerCase()){case"":script.type="text/inert";break;case"text/javascript":case"text/ecmascript":case"text/x-javascript":case"text/jscript":case"text/livescript":case"text/javascript1.1":case"text/javascript1.2":case"text/javascript1.3":script.type="text/inert-"+script.type.slice("text/".length);break;case"application/javascript":case"application/ecmascript":case"application/x-javascript":script.type="application/inert-"+script.type.slice("application/".length)}}),cleanseAttributes(cleaner.documentElement);var cleanedNodes=[];return"HTML"===targetElement.tagName?cleanedNodes=Array.prototype.slice.call(document.adoptNode(cleaner.documentElement).childNodes):(cleaner.head&&(cleanedNodes=cleanedNodes.concat(Array.prototype.slice.call(document.adoptNode(cleaner.head).childNodes))),cleaner.body&&(cleanedNodes=cleanedNodes.concat(Array.prototype.slice.call(document.adoptNode(cleaner.body).childNodes)))),cleanedNodes}function cleansePropertySetter(property,setter){var propertyDescriptor=Object.getOwnPropertyDescriptor(HTMLElement.prototype,property),originalSetter=propertyDescriptor.set;Object.defineProperty(HTMLElement.prototype,property,{get:propertyDescriptor.get,set:function(value){if(window.WinJS&&window.WinJS._execUnsafe&&inUnsafeMode())originalSetter.call(this,value);else{var that=this,nodes=cleanse(value,that);MSApp.execUnsafeLocalFunction(function(){setter(propertyDescriptor,that,nodes)})}},enumerable:propertyDescriptor.enumerable,configurable:propertyDescriptor.configurable})}if(window.MSApp&&MSApp.execUnsafeLocalFunction){var Element_setAttribute=Object.getOwnPropertyDescriptor(Element.prototype,"setAttribute").value,Element_removeAttribute=Object.getOwnPropertyDescriptor(Element.prototype,"removeAttribute").value,HTMLElement_insertAdjacentHTMLPropertyDescriptor=Object.getOwnPropertyDescriptor(HTMLElement.prototype,"insertAdjacentHTML"),Node_get_attributes=Object.getOwnPropertyDescriptor(Node.prototype,"attributes").get,Node_get_childNodes=Object.getOwnPropertyDescriptor(Node.prototype,"childNodes").get,detectionDiv=document.createElement("div");cleansePropertySetter("innerHTML",function(propertyDescriptor,target,elements){empty(target);for(var i=0,len=elements.length;len>i;i++)target.appendChild(elements[i])}),cleansePropertySetter("outerHTML",function(propertyDescriptor,target,elements){for(var i=0,len=elements.length;len>i;i++)target.insertAdjacentElement("afterend",elements[i]);target.parentNode.removeChild(target)})}}(),function(global,factory){"object"==typeof exports&&"undefined"!=typeof module?module.exports=factory():"function"==typeof define&&define.amd?define(factory):global.ons=factory()}(this,function(){"use strict";function setup(){GestureDetector.READY||(Event$1.determineEventTypes(),Utils.each(GestureDetector.gestures,function(gesture){Detection.register(gesture)}),Event$1.onTouch(GestureDetector.DOCUMENT,EVENT_MOVE,Detection.detect),Event$1.onTouch(GestureDetector.DOCUMENT,EVENT_END,Detection.detect),GestureDetector.READY=!0)}function isContentReady(element){return element.childNodes.length>0&&setContentReady(element),readyMap.has(element)}function setContentReady(element){readyMap.set(element,!0)}function addCallback(element,fn){queueMap.has(element)||queueMap.set(element,[]),queueMap.get(element).push(fn)}function consumeQueue(element){var callbacks=queueMap.get(element,[])||[];queueMap["delete"](element),callbacks.forEach(function(callback){return callback()})}function contentReady(element,fn){if(addCallback(element,fn),isContentReady(element))return void consumeQueue(element);var observer=new MutationObserver(function(changes){setContentReady(element),consumeQueue(element)});observer.observe(element,{childList:!0,characterData:!0}),setImmediate(function(){setContentReady(element),consumeQueue(element)})}function loadPage(_ref,done){var page=_ref.page,parent=_ref.parent,replace=(_ref.params,_ref.replace);internal.getPageHTMLAsync(page).then(function(html){replace&&(util.propagateAction(parent,"_destroy"),parent.innerHTML="");var element=util.createElement(html.trim());parent.appendChild(element),done({element:element,unload:function(){return element.remove()}})})}function waitDeviceReady(){var unlockDeviceReady=ons._readyLock.lock();window.addEventListener("DOMContentLoaded",function(){ons.isWebView()?window.document.addEventListener("deviceready",unlockDeviceReady,!1):unlockDeviceReady()},!1)}function getElementClass(){if("function"!=typeof HTMLElement){var _BaseElement=function(){};return _BaseElement.prototype=document.createElement("div"),_BaseElement}return HTMLElement}var babelHelpers={};babelHelpers["typeof"]="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(obj){return typeof obj}:function(obj){return obj&&"function"==typeof Symbol&&obj.constructor===Symbol?"symbol":typeof obj},babelHelpers.classCallCheck=function(instance,Constructor){if(!(instance instanceof Constructor))throw new TypeError("Cannot call a class as a function")},babelHelpers.createClass=function(){function defineProperties(target,props){for(var i=0;i<props.length;i++){var descriptor=props[i];descriptor.enumerable=descriptor.enumerable||!1,descriptor.configurable=!0,"value"in descriptor&&(descriptor.writable=!0),Object.defineProperty(target,descriptor.key,descriptor)}}return function(Constructor,protoProps,staticProps){return protoProps&&defineProperties(Constructor.prototype,protoProps),staticProps&&defineProperties(Constructor,staticProps),Constructor}}(),babelHelpers.inherits=function(subClass,superClass){if("function"!=typeof superClass&&null!==superClass)throw new TypeError("Super expression must either be null or a function, not "+typeof superClass);subClass.prototype=Object.create(superClass&&superClass.prototype,{constructor:{value:subClass,enumerable:!1,writable:!0,configurable:!0}}),superClass&&(Object.setPrototypeOf?Object.setPrototypeOf(subClass,superClass):subClass.__proto__=superClass)},babelHelpers.possibleConstructorReturn=function(self,call){if(!self)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return!call||"object"!=typeof call&&"function"!=typeof call?self:call};var unwrap=function(string){return string.slice(1,-1)},isObjectString=function(string){return string.startsWith("{")&&string.endsWith("}")},isArrayString=function(string){return string.startsWith("[")&&string.endsWith("]")},isQuotedString=function(string){return string.startsWith("'")&&string.endsWith("'")||string.startsWith('"')&&string.endsWith('"')},error=function(token,string,originalString){throw new Error("Unexpected token '"+token+"' at position "+(originalString.length-string.length-1)+" in string: '"+originalString+"'")},processToken=function(token,string,originalString){return"true"===token||"false"===token?"true"===token:isQuotedString(token)?unwrap(token):isNaN(token)?isObjectString(token)?parseObject(unwrap(token)):isArrayString(token)?parseArray(unwrap(token)):void error(token,string,originalString):+token},nextToken=function(string){string=string.trimLeft();var limit=string.length;if(":"===string[0]||","===string[0])limit=1;else if("{"===string[0]||"["===string[0]){for(var c=string.charCodeAt(0),nestedObject=1,i=1;i<string.length;i++)if(string.charCodeAt(i)===c)nestedObject++;else if(string.charCodeAt(i)===c+2&&(nestedObject--,0===nestedObject)){limit=i+1;break}}else if("'"===string[0]||'"'===string[0]){for(var _i=1;_i<string.length;_i++)if(string[_i]===string[0]){limit=_i+1;break}}else for(var _i2=1;_i2<string.length;_i2++)if(-1!==[" ",",",":"].indexOf(string[_i2])){limit=_i2;break}return string.slice(0,limit)},parseObject=function(string){var isValidKey=function(key){return/^[A-Z_\$][A-Z0-9_\$]*$/i.test(key)};string=string.trim();for(var originalString=string,object={},readingKey=!0,key=void 0,previousToken=void 0,token=void 0;string.length>0;)if(previousToken=token,token=nextToken(string),string=string.slice(token.length,string.length).trimLeft(),":"===token&&(!readingKey||!previousToken||","===previousToken)||","===token&&readingKey||":"!==token&&","!==token&&previousToken&&","!==previousToken&&":"!==previousToken)error(token,string,originalString);else if(":"===token&&readingKey&&previousToken){if(!isValidKey(previousToken))throw new Error("Invalid key token '"+previousToken+"' at position 0 in string: '"+originalString+"'");key=previousToken,readingKey=!1}else","===token&&!readingKey&&previousToken&&(object[key]=processToken(previousToken,string,originalString),readingKey=!0);return token&&(object[key]=processToken(token,string,originalString)),object},parseArray=function(string){string=string.trim();for(var originalString=string,array=[],previousToken=void 0,token=void 0;string.length>0;)previousToken=token,token=nextToken(string),string=string.slice(token.length,string.length).trimLeft(),","!==token||previousToken&&","!==previousToken?","===token&&array.push(processToken(previousToken,string,originalString)):error(token,string,originalString);return token&&(","!==token?array.push(processToken(token,string,originalString)):error(token,string,originalString)),array},parse=function(string){if(string=string.trim(),isObjectString(string))return parseObject(unwrap(string));if(isArrayString(string))return parseArray(unwrap(string));throw new Error("Provided string must be object or array like: "+string)},util={};util.prepareQuery=function(query){return query instanceof Function?query:function(element){return util.match(element,query)}},util.match=function(element,query){return"."===query[0]?element.classList.contains(query.slice(1)):element.nodeName.toLowerCase()===query},util.findChild=function(element,query){for(var match=util.prepareQuery(query),i=0;i<element.children.length;i++){var node=element.children[i];if(match(node))return node}return null},util.findParent=function(element,query){for(var match=util.prepareQuery(query),parent=element.parentNode;;){if(!parent||parent===document)return null;if(match(parent))return parent;parent=parent.parentNode}},util.isAttached=function(element){for(;document.documentElement!==element;){if(!element)return!1;element=element.parentNode}return!0},util.hasAnyComponentAsParent=function(element){for(;element&&document.documentElement!==element;)if(element=element.parentNode,element&&element.nodeName.toLowerCase().match(/(ons-navigator|ons-tabbar|ons-sliding-menu|ons-split-view)/))return!0;return!1},util.propagateAction=function(element,action){for(var i=0;i<element.childNodes.length;i++){var child=element.childNodes[i];child[action]instanceof Function?child[action]():util.propagateAction(child,action)}},util.create=function(){var selector=arguments.length<=0||void 0===arguments[0]?"":arguments[0],style=arguments.length<=1||void 0===arguments[1]?{}:arguments[1],classList=selector.split("."),element=document.createElement(classList.shift()||"div");return classList.length&&(element.className=classList.join(" ")),util.extend(element.style,style),element},util.createElement=function(html){var wrapper=document.createElement("div");if(innerHTML(wrapper,html),wrapper.children.length>1)throw new Error('"html" must be one wrapper element.');return wrapper.children[0]},util.createFragment=function(html){var wrapper=document.createElement("div");innerHTML(wrapper,html);for(var fragment=document.createDocumentFragment();wrapper.firstChild;)fragment.appendChild(wrapper.firstChild);return fragment},util.extend=function(dst){for(var _len=arguments.length,args=Array(_len>1?_len-1:0),_key=1;_len>_key;_key++)args[_key-1]=arguments[_key];for(var i=0;i<args.length;i++)if(args[i])for(var keys=Object.keys(args[i]),j=0;j<keys.length;j++){var key=keys[j];dst[key]=args[i][key]}return dst},util.arrayFrom=function(arrayLike){return Array.prototype.slice.apply(arrayLike)},util.parseJSONObjectSafely=function(jsonString){var failSafe=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];try{var result=JSON.parse(""+jsonString);if("object"===("undefined"==typeof result?"undefined":babelHelpers["typeof"](result))&&null!==result)return result}catch(e){return failSafe}return failSafe},util.findFromPath=function(path){path=path.split(".");for(var key,el=window;key=path.shift();)el=el[key];return el},util.triggerElementEvent=function(target,eventName){var detail=arguments.length<=2||void 0===arguments[2]?{}:arguments[2],event=new CustomEvent(eventName,{bubbles:!0,cancelable:!0,detail:detail});return Object.keys(detail).forEach(function(key){event[key]=detail[key]}),target.dispatchEvent(event),event},util.hasModifier=function(target,modifierName){return target.hasAttribute("modifier")?target.getAttribute("modifier").split(/\s+/).some(function(e){return e===modifierName}):!1},util.addModifier=function(target,modifierName){if(util.hasModifier(target,modifierName))return!1;modifierName=modifierName.trim();var modifierAttribute=target.getAttribute("modifier")||"";return target.setAttribute("modifier",(modifierAttribute+" "+modifierName).trim()),!0},util.removeModifier=function(target,modifierName){if(!target.getAttribute("modifier"))return!1;var modifiers=target.getAttribute("modifier").split(/\s+/),newModifiers=modifiers.filter(function(item){return item&&item!==modifierName});return target.setAttribute("modifier",newModifiers.join(" ")),modifiers.length!==newModifiers.length},util.updateParentPosition=function(el){!el._parentUpdated&&el.parentElement&&("static"===window.getComputedStyle(el.parentElement).getPropertyValue("position")&&(el.parentElement.style.position="relative"),el._parentUpdated=!0)},util.toggleAttribute=function(element,name,enable){enable?element.setAttribute(name,""):element.removeAttribute(name)},util.bindListeners=function(element,listenerNames){listenerNames.forEach(function(name){var boundName=name.replace(/^_[a-z]/,"_bound"+name[1].toUpperCase());element[boundName]=element[boundName]||element[name].bind(element)})},util.each=function(obj,f){return Object.keys(obj).forEach(function(key){return f(key,obj[key])})},util.updateRipple=function(target){var rippleElement=util.findChild(target,"ons-ripple");target.hasAttribute("ripple")?rippleElement||target.insertBefore(document.createElement("ons-ripple"),target.firstChild):rippleElement&&rippleElement.remove()},util.animationOptionsParse=parse,util.isInteger=function(value){return"number"==typeof value&&isFinite(value)&&Math.floor(value)===value};var Event$1,Utils,Detection,PointerEvent,GestureDetector=function GestureDetector(element,options){return new GestureDetector.Instance(element,options||{})};GestureDetector.defaults={behavior:{touchAction:"pan-y",touchCallout:"none",contentZooming:"none",userDrag:"none",tapHighlightColor:"rgba(0,0,0,0)"}},GestureDetector.DOCUMENT=document,GestureDetector.HAS_POINTEREVENTS=navigator.pointerEnabled||navigator.msPointerEnabled,GestureDetector.HAS_TOUCHEVENTS="ontouchstart"in window,GestureDetector.IS_MOBILE=/mobile|tablet|ip(ad|hone|od)|android|silk/i.test(navigator.userAgent),GestureDetector.NO_MOUSEEVENTS=GestureDetector.HAS_TOUCHEVENTS&&GestureDetector.IS_MOBILE||GestureDetector.HAS_POINTEREVENTS,GestureDetector.CALCULATE_INTERVAL=25;var EVENT_TYPES={},DIRECTION_DOWN=GestureDetector.DIRECTION_DOWN="down",DIRECTION_LEFT=GestureDetector.DIRECTION_LEFT="left",DIRECTION_UP=GestureDetector.DIRECTION_UP="up",DIRECTION_RIGHT=GestureDetector.DIRECTION_RIGHT="right",POINTER_MOUSE=GestureDetector.POINTER_MOUSE="mouse",POINTER_TOUCH=GestureDetector.POINTER_TOUCH="touch",POINTER_PEN=GestureDetector.POINTER_PEN="pen",EVENT_START=GestureDetector.EVENT_START="start",EVENT_MOVE=GestureDetector.EVENT_MOVE="move",EVENT_END=GestureDetector.EVENT_END="end",EVENT_RELEASE=GestureDetector.EVENT_RELEASE="release",EVENT_TOUCH=GestureDetector.EVENT_TOUCH="touch";GestureDetector.READY=!1,GestureDetector.plugins=GestureDetector.plugins||{},GestureDetector.gestures=GestureDetector.gestures||{},Utils=GestureDetector.utils={extend:function(dest,src,merge){for(var key in src)!src.hasOwnProperty(key)||void 0!==dest[key]&&merge||(dest[key]=src[key]);return dest},on:function(element,type,handler){element.addEventListener(type,handler,!1)},off:function(element,type,handler){element.removeEventListener(type,handler,!1)},each:function(obj,iterator,context){var i,len;if("forEach"in obj)obj.forEach(iterator,context);else if(void 0!==obj.length){for(i=0,len=obj.length;len>i;i++)if(iterator.call(context,obj[i],i,obj)===!1)return}else for(i in obj)if(obj.hasOwnProperty(i)&&iterator.call(context,obj[i],i,obj)===!1)return},inStr:function(src,find){return src.indexOf(find)>-1},inArray:function(src,find){if(src.indexOf){var index=src.indexOf(find);return-1===index?!1:index}for(var i=0,len=src.length;len>i;i++)if(src[i]===find)return i;return!1},toArray:function(obj){return Array.prototype.slice.call(obj,0)},hasParent:function(node,parent){for(;node;){if(node==parent)return!0;node=node.parentNode}return!1},getCenter:function(touches){var pageX=[],pageY=[],clientX=[],clientY=[],min=Math.min,max=Math.max;return 1===touches.length?{pageX:touches[0].pageX,pageY:touches[0].pageY,clientX:touches[0].clientX,clientY:touches[0].clientY}:(Utils.each(touches,function(touch){pageX.push(touch.pageX),pageY.push(touch.pageY),clientX.push(touch.clientX),clientY.push(touch.clientY)}),{pageX:(min.apply(Math,pageX)+max.apply(Math,pageX))/2,pageY:(min.apply(Math,pageY)+max.apply(Math,pageY))/2,clientX:(min.apply(Math,clientX)+max.apply(Math,clientX))/2,clientY:(min.apply(Math,clientY)+max.apply(Math,clientY))/2})},getVelocity:function(deltaTime,deltaX,deltaY){return{x:Math.abs(deltaX/deltaTime)||0,y:Math.abs(deltaY/deltaTime)||0}},getAngle:function(touch1,touch2){var x=touch2.clientX-touch1.clientX,y=touch2.clientY-touch1.clientY;return 180*Math.atan2(y,x)/Math.PI},getDirection:function(touch1,touch2){var x=Math.abs(touch1.clientX-touch2.clientX),y=Math.abs(touch1.clientY-touch2.clientY);return x>=y?touch1.clientX-touch2.clientX>0?DIRECTION_LEFT:DIRECTION_RIGHT:touch1.clientY-touch2.clientY>0?DIRECTION_UP:DIRECTION_DOWN},getDistance:function(touch1,touch2){var x=touch2.clientX-touch1.clientX,y=touch2.clientY-touch1.clientY;return Math.sqrt(x*x+y*y)},getScale:function(start,end){return start.length>=2&&end.length>=2?this.getDistance(end[0],end[1])/this.getDistance(start[0],start[1]):1},getRotation:function(start,end){return start.length>=2&&end.length>=2?this.getAngle(end[1],end[0])-this.getAngle(start[1],start[0]):0},isVertical:function(direction){return direction==DIRECTION_UP||direction==DIRECTION_DOWN},setPrefixedCss:function(element,prop,value,toggle){var prefixes=["","Webkit","Moz","O","ms"];prop=Utils.toCamelCase(prop);for(var i=0;i<prefixes.length;i++){var p=prop;if(prefixes[i]&&(p=prefixes[i]+p.slice(0,1).toUpperCase()+p.slice(1)),p in element.style){element.style[p]=(null===toggle||toggle)&&value||"";break}}},toggleBehavior:function(element,props,toggle){if(props&&element&&element.style){Utils.each(props,function(value,prop){Utils.setPrefixedCss(element,prop,value,toggle)});var falseFn=toggle&&function(){return!1};"none"==props.userSelect&&(element.onselectstart=falseFn),"none"==props.userDrag&&(element.ondragstart=falseFn)}},toCamelCase:function(str){return str.replace(/[_-]([a-z])/g,function(s){return s[1].toUpperCase()})}},Event$1=GestureDetector.event={preventMouseEvents:!1,started:!1,shouldDetect:!1,on:function(element,type,handler,hook){var types=type.split(" ");Utils.each(types,function(type){Utils.on(element,type,handler),hook&&hook(type)})},off:function(element,type,handler,hook){var types=type.split(" ");Utils.each(types,function(type){Utils.off(element,type,handler),hook&&hook(type)})},onTouch:function(element,eventType,handler){var self=this,onTouchHandler=function(ev){var triggerType,srcType=ev.type.toLowerCase(),isPointer=GestureDetector.HAS_POINTEREVENTS,isMouse=Utils.inStr(srcType,"mouse");isMouse&&self.preventMouseEvents||(isMouse&&eventType==EVENT_START&&0===ev.button?(self.preventMouseEvents=!1,self.shouldDetect=!0):isPointer&&eventType==EVENT_START?self.shouldDetect=1===ev.buttons||PointerEvent.matchType(POINTER_TOUCH,ev):isMouse||eventType!=EVENT_START||(self.preventMouseEvents=!0,self.shouldDetect=!0),isPointer&&eventType!=EVENT_END&&PointerEvent.updatePointer(eventType,ev),self.shouldDetect&&(triggerType=self.doDetect.call(self,ev,eventType,element,handler)),triggerType==EVENT_END&&(self.preventMouseEvents=!1,self.shouldDetect=!1,PointerEvent.reset()),isPointer&&eventType==EVENT_END&&PointerEvent.updatePointer(eventType,ev))};return this.on(element,EVENT_TYPES[eventType],onTouchHandler),onTouchHandler},doDetect:function(ev,eventType,element,handler){var touchList=this.getTouchList(ev,eventType),touchListLength=touchList.length,triggerType=eventType,triggerChange=touchList.trigger,changedLength=touchListLength;eventType==EVENT_START?triggerChange=EVENT_TOUCH:eventType==EVENT_END&&(triggerChange=EVENT_RELEASE,changedLength=touchList.length-(ev.changedTouches?ev.changedTouches.length:1)),changedLength>0&&this.started&&(triggerType=EVENT_MOVE),this.started=!0;var evData=this.collectEventData(element,triggerType,touchList,ev);return eventType!=EVENT_END&&handler.call(Detection,evData),triggerChange&&(evData.changedLength=changedLength,evData.eventType=triggerChange,handler.call(Detection,evData),evData.eventType=triggerType,delete evData.changedLength),triggerType==EVENT_END&&(handler.call(Detection,evData),this.started=!1),triggerType},determineEventTypes:function(){var types;return types=GestureDetector.HAS_POINTEREVENTS?window.PointerEvent?["pointerdown","pointermove","pointerup pointercancel lostpointercapture"]:["MSPointerDown","MSPointerMove","MSPointerUp MSPointerCancel MSLostPointerCapture"]:GestureDetector.NO_MOUSEEVENTS?["touchstart","touchmove","touchend touchcancel"]:["touchstart mousedown","touchmove mousemove","touchend touchcancel mouseup"],EVENT_TYPES[EVENT_START]=types[0],EVENT_TYPES[EVENT_MOVE]=types[1],EVENT_TYPES[EVENT_END]=types[2],EVENT_TYPES},getTouchList:function(ev,eventType){if(GestureDetector.HAS_POINTEREVENTS)return PointerEvent.getTouchList();if(ev.touches){if(eventType==EVENT_MOVE)return ev.touches;var identifiers=[],concat=[].concat(Utils.toArray(ev.touches),Utils.toArray(ev.changedTouches)),touchList=[];return Utils.each(concat,function(touch){Utils.inArray(identifiers,touch.identifier)===!1&&touchList.push(touch),identifiers.push(touch.identifier)}),touchList}return ev.identifier=1,[ev]},collectEventData:function(element,eventType,touches,ev){var pointerType=POINTER_TOUCH;return Utils.inStr(ev.type,"mouse")||PointerEvent.matchType(POINTER_MOUSE,ev)?pointerType=POINTER_MOUSE:PointerEvent.matchType(POINTER_PEN,ev)&&(pointerType=POINTER_PEN),{center:Utils.getCenter(touches),timeStamp:Date.now(),target:ev.target,touches:touches,eventType:eventType,pointerType:pointerType,srcEvent:ev,preventDefault:function(){var srcEvent=this.srcEvent;srcEvent.preventManipulation&&srcEvent.preventManipulation(),srcEvent.preventDefault&&srcEvent.preventDefault()},stopPropagation:function(){this.srcEvent.stopPropagation()},stopDetect:function(){return Detection.stopDetect()}}}},PointerEvent=GestureDetector.PointerEvent={pointers:{},getTouchList:function(){var touchlist=[];return Utils.each(this.pointers,function(pointer){touchlist.push(pointer)}),touchlist},updatePointer:function(eventType,pointerEvent){eventType==EVENT_END||eventType!=EVENT_END&&1!==pointerEvent.buttons?delete this.pointers[pointerEvent.pointerId]:(pointerEvent.identifier=pointerEvent.pointerId,this.pointers[pointerEvent.pointerId]=pointerEvent)},matchType:function(pointerType,ev){if(!ev.pointerType)return!1;var pt=ev.pointerType,types={};return types[POINTER_MOUSE]=pt===(ev.MSPOINTER_TYPE_MOUSE||POINTER_MOUSE),types[POINTER_TOUCH]=pt===(ev.MSPOINTER_TYPE_TOUCH||POINTER_TOUCH),types[POINTER_PEN]=pt===(ev.MSPOINTER_TYPE_PEN||POINTER_PEN),types[pointerType]},reset:function(){this.pointers={}}},Detection=GestureDetector.detection={gestures:[],current:null,previous:null,stopped:!1,startDetect:function(inst,eventData){this.current||(this.stopped=!1,this.current={inst:inst,startEvent:Utils.extend({},eventData),lastEvent:!1,lastCalcEvent:!1,futureCalcEvent:!1,lastCalcData:{},name:""},this.detect(eventData))},detect:function(eventData){if(this.current&&!this.stopped){eventData=this.extendEventData(eventData);var inst=this.current.inst,instOptions=inst.options;return Utils.each(this.gestures,function(gesture){!this.stopped&&inst.enabled&&instOptions[gesture.name]&&gesture.handler.call(gesture,eventData,inst)},this),this.current&&(this.current.lastEvent=eventData),eventData.eventType==EVENT_END&&this.stopDetect(),eventData}},stopDetect:function(){this.previous=Utils.extend({},this.current),this.current=null,this.stopped=!0},getCalculatedData:function(ev,center,deltaTime,deltaX,deltaY){var cur=this.current,recalc=!1,calcEv=cur.lastCalcEvent,calcData=cur.lastCalcData;calcEv&&ev.timeStamp-calcEv.timeStamp>GestureDetector.CALCULATE_INTERVAL&&(center=calcEv.center,deltaTime=ev.timeStamp-calcEv.timeStamp,deltaX=ev.center.clientX-calcEv.center.clientX,deltaY=ev.center.clientY-calcEv.center.clientY,recalc=!0),ev.eventType!=EVENT_TOUCH&&ev.eventType!=EVENT_RELEASE||(cur.futureCalcEvent=ev),cur.lastCalcEvent&&!recalc||(calcData.velocity=Utils.getVelocity(deltaTime,deltaX,deltaY),calcData.angle=Utils.getAngle(center,ev.center),calcData.direction=Utils.getDirection(center,ev.center),cur.lastCalcEvent=cur.futureCalcEvent||ev,cur.futureCalcEvent=ev),ev.velocityX=calcData.velocity.x,ev.velocityY=calcData.velocity.y,ev.interimAngle=calcData.angle,ev.interimDirection=calcData.direction},extendEventData:function(ev){var cur=this.current,startEv=cur.startEvent,lastEv=cur.lastEvent||startEv;ev.eventType!=EVENT_TOUCH&&ev.eventType!=EVENT_RELEASE||(startEv.touches=[],Utils.each(ev.touches,function(touch){startEv.touches.push({clientX:touch.clientX,clientY:touch.clientY})}));var deltaTime=ev.timeStamp-startEv.timeStamp,deltaX=ev.center.clientX-startEv.center.clientX,deltaY=ev.center.clientY-startEv.center.clientY;return this.getCalculatedData(ev,lastEv.center,deltaTime,deltaX,deltaY),Utils.extend(ev,{startEvent:startEv,deltaTime:deltaTime,deltaX:deltaX,deltaY:deltaY,distance:Utils.getDistance(startEv.center,ev.center),angle:Utils.getAngle(startEv.center,ev.center),direction:Utils.getDirection(startEv.center,ev.center),scale:Utils.getScale(startEv.touches,ev.touches),rotation:Utils.getRotation(startEv.touches,ev.touches)}),ev},register:function(gesture){var options=gesture.defaults||{};return void 0===options[gesture.name]&&(options[gesture.name]=!0),Utils.extend(GestureDetector.defaults,options,!0),gesture.index=gesture.index||1e3,this.gestures.push(gesture),this.gestures.sort(function(a,b){return a.index<b.index?-1:a.index>b.index?1:0}),this.gestures}},GestureDetector.Instance=function(element,options){var self=this;setup(),this.element=element,this.enabled=!0,Utils.each(options,function(value,name){delete options[name],options[Utils.toCamelCase(name)]=value}),this.options=Utils.extend(Utils.extend({},GestureDetector.defaults),options||{}),this.options.behavior&&Utils.toggleBehavior(this.element,this.options.behavior,!0),this.eventStartHandler=Event$1.onTouch(element,EVENT_START,function(ev){self.enabled&&ev.eventType==EVENT_START?Detection.startDetect(self,ev):ev.eventType==EVENT_TOUCH&&Detection.detect(ev)}),this.eventHandlers=[]},GestureDetector.Instance.prototype={on:function(gestures,handler){var self=this;return Event$1.on(self.element,gestures,handler,function(type){self.eventHandlers.push({gesture:type,handler:handler})}),self},off:function(gestures,handler){var self=this;return Event$1.off(self.element,gestures,handler,function(type){var index=Utils.inArray({gesture:type,handler:handler});index!==!1&&self.eventHandlers.splice(index,1)}),self},trigger:function(gesture,eventData){eventData||(eventData={});var event=GestureDetector.DOCUMENT.createEvent("Event");event.initEvent(gesture,!0,!0),event.gesture=eventData;var element=this.element;return Utils.hasParent(eventData.target,element)&&(element=eventData.target),element.dispatchEvent(event),this},enable:function(state){return this.enabled=state,this},dispose:function(){var i,eh;for(Utils.toggleBehavior(this.element,this.options.behavior,!1),i=-1;eh=this.eventHandlers[++i];)Utils.off(this.element,eh.gesture,eh.handler);return this.eventHandlers=[],Event$1.off(this.element,EVENT_TYPES[EVENT_START],this.eventStartHandler),null}},function(name){function dragGesture(ev,inst){var cur=Detection.current;if(!(inst.options.dragMaxTouches>0&&ev.touches.length>inst.options.dragMaxTouches))switch(ev.eventType){case EVENT_START:triggered=!1;break;case EVENT_MOVE:if(ev.distance<inst.options.dragMinDistance&&cur.name!=name)return;var startCenter=cur.startEvent.center;if(cur.name!=name&&(cur.name=name,inst.options.dragDistanceCorrection&&ev.distance>0)){var factor=Math.abs(inst.options.dragMinDistance/ev.distance);startCenter.pageX+=ev.deltaX*factor,startCenter.pageY+=ev.deltaY*factor,startCenter.clientX+=ev.deltaX*factor,startCenter.clientY+=ev.deltaY*factor,ev=Detection.extendEventData(ev)}(cur.lastEvent.dragLockToAxis||inst.options.dragLockToAxis&&inst.options.dragLockMinDistance<=ev.distance)&&(ev.dragLockToAxis=!0);var lastDirection=cur.lastEvent.direction;ev.dragLockToAxis&&lastDirection!==ev.direction&&(Utils.isVertical(lastDirection)?ev.direction=ev.deltaY<0?DIRECTION_UP:DIRECTION_DOWN:ev.direction=ev.deltaX<0?DIRECTION_LEFT:DIRECTION_RIGHT),triggered||(inst.trigger(name+"start",ev),triggered=!0),inst.trigger(name,ev),inst.trigger(name+ev.direction,ev);var isVertical=Utils.isVertical(ev.direction);(inst.options.dragBlockVertical&&isVertical||inst.options.dragBlockHorizontal&&!isVertical)&&ev.preventDefault();break;case EVENT_RELEASE:triggered&&ev.changedLength<=inst.options.dragMaxTouches&&(inst.trigger(name+"end",ev),triggered=!1);break;case EVENT_END:triggered=!1}}var triggered=!1;GestureDetector.gestures.Drag={name:name,index:50,handler:dragGesture,defaults:{dragMinDistance:10,dragDistanceCorrection:!0,dragMaxTouches:1,dragBlockHorizontal:!1,dragBlockVertical:!1,dragLockToAxis:!1,dragLockMinDistance:25}}}("drag"),GestureDetector.gestures.Gesture={name:"gesture",index:1337,handler:function(ev,inst){inst.trigger(this.name,ev)}},function(name){function holdGesture(ev,inst){var options=inst.options,current=Detection.current;switch(ev.eventType){case EVENT_START:clearTimeout(timer),current.name=name,timer=setTimeout(function(){current&¤t.name==name&&inst.trigger(name,ev)},options.holdTimeout);break;case EVENT_MOVE:ev.distance>options.holdThreshold&&clearTimeout(timer);break;case EVENT_RELEASE:clearTimeout(timer)}}var timer;GestureDetector.gestures.Hold={name:name,index:10,defaults:{holdTimeout:500,holdThreshold:2},handler:holdGesture}}("hold"),GestureDetector.gestures.Release={name:"release",index:1/0,handler:function(ev,inst){ev.eventType==EVENT_RELEASE&&inst.trigger(this.name,ev)}},GestureDetector.gestures.Swipe={name:"swipe",index:40,defaults:{swipeMinTouches:1,swipeMaxTouches:1,swipeVelocityX:.6,swipeVelocityY:.6},handler:function(ev,inst){if(ev.eventType==EVENT_RELEASE){var touches=ev.touches.length,options=inst.options;if(touches<options.swipeMinTouches||touches>options.swipeMaxTouches)return;(ev.velocityX>options.swipeVelocityX||ev.velocityY>options.swipeVelocityY)&&(inst.trigger(this.name,ev),inst.trigger(this.name+ev.direction,ev));
}}},function(name){function tapGesture(ev,inst){var sincePrev,didDoubleTap,options=inst.options,current=Detection.current,prev=Detection.previous;switch(ev.eventType){case EVENT_START:hasMoved=!1;break;case EVENT_MOVE:hasMoved=hasMoved||ev.distance>options.tapMaxDistance;break;case EVENT_END:!Utils.inStr(ev.srcEvent.type,"cancel")&&ev.deltaTime<options.tapMaxTime&&!hasMoved&&(sincePrev=prev&&prev.lastEvent&&ev.timeStamp-prev.lastEvent.timeStamp,didDoubleTap=!1,prev&&prev.name==name&&sincePrev&&sincePrev<options.doubleTapInterval&&ev.distance<options.doubleTapDistance&&(inst.trigger("doubletap",ev),didDoubleTap=!0),didDoubleTap&&!options.tapAlways||(current.name=name,inst.trigger(current.name,ev)))}}var hasMoved=!1;GestureDetector.gestures.Tap={name:name,index:100,handler:tapGesture,defaults:{tapMaxTime:250,tapMaxDistance:10,tapAlways:!0,doubleTapDistance:20,doubleTapInterval:300}}}("tap"),GestureDetector.gestures.Touch={name:"touch",index:-(1/0),defaults:{preventDefault:!1,preventMouse:!1},handler:function(ev,inst){return inst.options.preventMouse&&ev.pointerType==POINTER_MOUSE?void ev.stopDetect():(inst.options.preventDefault&&ev.preventDefault(),void(ev.eventType==EVENT_TOUCH&&inst.trigger("touch",ev)))}},function(name){function transformGesture(ev,inst){switch(ev.eventType){case EVENT_START:triggered=!1;break;case EVENT_MOVE:if(ev.touches.length<2)return;var scaleThreshold=Math.abs(1-ev.scale),rotationThreshold=Math.abs(ev.rotation);if(scaleThreshold<inst.options.transformMinScale&&rotationThreshold<inst.options.transformMinRotation)return;Detection.current.name=name,triggered||(inst.trigger(name+"start",ev),triggered=!0),inst.trigger(name,ev),rotationThreshold>inst.options.transformMinRotation&&inst.trigger("rotate",ev),scaleThreshold>inst.options.transformMinScale&&(inst.trigger("pinch",ev),inst.trigger("pinch"+(ev.scale<1?"in":"out"),ev));break;case EVENT_RELEASE:triggered&&ev.changedLength<2&&(inst.trigger(name+"end",ev),triggered=!1)}}var triggered=!1;GestureDetector.gestures.Transform={name:name,index:45,defaults:{transformMinScale:.01,transformMinRotation:1},handler:transformGesture}}("transform");var Platform=function(){function Platform(){babelHelpers.classCallCheck(this,Platform),this._renderPlatform=null}return babelHelpers.createClass(Platform,[{key:"select",value:function(platform){"string"==typeof platform&&(this._renderPlatform=platform.trim().toLowerCase())}},{key:"isWebView",value:function(){if("loading"===document.readyState||"uninitialized"==document.readyState)throw new Error("isWebView() method is available after dom contents loaded.");return!!(window.cordova||window.phonegap||window.PhoneGap)}},{key:"isIOS",value:function(){return this._renderPlatform?"ios"===this._renderPlatform:"object"!==("undefined"==typeof device?"undefined":babelHelpers["typeof"](device))||/browser/i.test(device.platform)?/iPhone|iPad|iPod/i.test(navigator.userAgent):/iOS/i.test(device.platform)}},{key:"isAndroid",value:function(){return this._renderPlatform?"android"===this._renderPlatform:"object"!==("undefined"==typeof device?"undefined":babelHelpers["typeof"](device))||/browser/i.test(device.platform)?/Android/i.test(navigator.userAgent):/Android/i.test(device.platform)}},{key:"isAndroidPhone",value:function(){return/Android/i.test(navigator.userAgent)&&/Mobile/i.test(navigator.userAgent)}},{key:"isAndroidTablet",value:function(){return/Android/i.test(navigator.userAgent)&&!/Mobile/i.test(navigator.userAgent)}},{key:"isWP",value:function(){return this._renderPlatform?"wp"===this._renderPlatform:"object"!==("undefined"==typeof device?"undefined":babelHelpers["typeof"](device))||/browser/i.test(device.platform)?/Windows Phone|IEMobile|WPDesktop/i.test(navigator.userAgent):/Win32NT|WinCE/i.test(device.platform)}},{key:"isIPhone",value:function(){return/iPhone/i.test(navigator.userAgent)}},{key:"isIPad",value:function(){return/iPad/i.test(navigator.userAgent)}},{key:"isIPod",value:function(){return/iPod/i.test(navigator.userAgent)}},{key:"isBlackBerry",value:function(){return this._renderPlatform?"blackberry"===this._renderPlatform:"object"!==("undefined"==typeof device?"undefined":babelHelpers["typeof"](device))||/browser/i.test(device.platform)?/BlackBerry|RIM Tablet OS|BB10/i.test(navigator.userAgent):/BlackBerry/i.test(device.platform)}},{key:"isOpera",value:function(){return this._renderPlatform?"opera"===this._renderPlatform:!!window.opera||navigator.userAgent.indexOf(" OPR/")>=0}},{key:"isFirefox",value:function(){return this._renderPlatform?"firefox"===this._renderPlatform:"undefined"!=typeof InstallTrigger}},{key:"isSafari",value:function(){return this._renderPlatform?"safari"===this._renderPlatform:Object.prototype.toString.call(window.HTMLElement).indexOf("Constructor")>0}},{key:"isChrome",value:function(){return this._renderPlatform?"chrome"===this._renderPlatform:!(!window.chrome||window.opera||navigator.userAgent.indexOf(" OPR/")>=0||navigator.userAgent.indexOf(" Edge/")>=0)}},{key:"isIE",value:function(){return this._renderPlatform?"ie"===this._renderPlatform:!!document.documentMode}},{key:"isEdge",value:function(){return this._renderPlatform?"edge"===this._renderPlatform:navigator.userAgent.indexOf(" Edge/")>=0}},{key:"isIOS7above",value:function(){if("object"===("undefined"==typeof device?"undefined":babelHelpers["typeof"](device))&&!/browser/i.test(device.platform))return/iOS/i.test(device.platform)&&parseInt(device.version.split(".")[0])>=7;if(/iPhone|iPad|iPod/i.test(navigator.userAgent)){var ver=(navigator.userAgent.match(/\b[0-9]+_[0-9]+(?:_[0-9]+)?\b/)||[""])[0].replace(/_/g,".");return parseInt(ver.split(".")[0])>=7}return!1}},{key:"getMobileOS",value:function(){return this.isAndroid()?"android":this.isIOS()?"ios":this.isWP()?"wp":"other"}},{key:"getIOSDevice",value:function(){return this.isIPhone()?"iphone":this.isIPad()?"ipad":this.isIPod()?"ipod":"na"}}]),Platform}(),platform=new Platform,notification={};notification._createAlertDialog=function(title,message,buttonLabels,primaryButtonIndex,modifier,animation,id,_callback,messageIsHTML,cancelable,promptDialog,autofocus,placeholder,defaultValue,submitOnEnter,compile){compile=compile||function(object){return object};var titleElementHTML="string"==typeof title?'<div class="alert-dialog-title"></div>':"",dialogElement=document.createElement("ons-alert-dialog");innerHTML(dialogElement,"\n "+titleElementHTML+'\n <div class="alert-dialog-content"></div>\n <div class="alert-dialog-footer"></div>\n '),id&&dialogElement.setAttribute("id",id);var titleElement=dialogElement.querySelector(".alert-dialog-title"),messageElement=dialogElement.querySelector(".alert-dialog-content"),footerElement=dialogElement.querySelector(".alert-dialog-footer"),inputElement=void 0,result={};result.promise=new Promise(function(resolve,reject){result.resolve=resolve,result.reject=reject}),modifier=modifier||dialogElement.getAttribute("modifier"),"string"==typeof title&&(titleElement.textContent=title),titleElement=null,dialogElement.setAttribute("animation",animation),messageIsHTML?innerHTML(messageElement,message):messageElement.textContent=message,promptDialog&&(inputElement=util.createElement('<input class="text-input text-input--underbar" type="text"></input>'),modifier&&inputElement.classList.add("text-input--"+modifier),inputElement.setAttribute("placeholder",placeholder),inputElement.value=defaultValue,inputElement.style.width="100%",inputElement.style.marginTop="10px",messageElement.appendChild(inputElement),submitOnEnter&&inputElement.addEventListener("keypress",function(event){13===event.keyCode&&dialogElement.hide({callback:function(){_callback(inputElement.value),result.resolve(inputElement.value),dialogElement.remove(),dialogElement=null}})},!1)),document.body.appendChild(dialogElement),compile(dialogElement),buttonLabels.length<=2&&footerElement.classList.add("alert-dialog-footer--one");for(var createButton=function(i){var buttonElement=util.createElement('<button class="alert-dialog-button"></button>');buttonElement.appendChild(document.createTextNode(buttonLabels[i])),i==primaryButtonIndex&&buttonElement.classList.add("alert-dialog-button--primal"),buttonLabels.length<=2&&buttonElement.classList.add("alert-dialog-button--one");var onClick=function onClick(){buttonElement.removeEventListener("click",onClick,!1),dialogElement.hide({callback:function(){promptDialog?(_callback(inputElement.value),result.resolve(inputElement.value)):(_callback(i),result.resolve(i)),dialogElement.remove(),dialogElement=inputElement=buttonElement=null}})};buttonElement.addEventListener("click",onClick,!1),footerElement.appendChild(buttonElement)},i=0;i<buttonLabels.length;i++)createButton(i);return cancelable&&(dialogElement.cancelable=!0,dialogElement.addEventListener("dialog-cancel",function(){promptDialog?(_callback(null),result.reject(null)):(_callback(-1),result.reject(-1)),setTimeout(function(){dialogElement.remove(),dialogElement=null,inputElement=null})},!1)),setImmediate(function(){dialogElement.show({callback:function(){inputElement&&promptDialog&&autofocus&&inputElement.focus()}})}),messageElement=footerElement=null,modifier&&(dialogElement.setAttribute("modifier",""),dialogElement.setAttribute("modifier",modifier)),result.promise},notification._alertOriginal=function(message){var options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];"string"==typeof message?options.message=message:options=message;var defaults={buttonLabel:"OK",animation:"default",title:"Alert",callback:function(){}};if(options=util.extend({},defaults,options),!options.message&&!options.messageHTML)throw new Error("Alert dialog must contain a message.");return notification._createAlertDialog(options.title,options.message||options.messageHTML,[options.buttonLabel],0,options.modifier,options.animation,options.id,options.callback,!options.message,!1,!1,!1,"","",!1,options.compile)},notification.alert=notification._alertOriginal,notification._confirmOriginal=function(message){var options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];"string"==typeof message?options.message=message:options=message;var defaults={buttonLabels:["Cancel","OK"],primaryButtonIndex:1,animation:"default",title:"Confirm",callback:function(){},cancelable:!1};if(options=util.extend({},defaults,options),!options.message&&!options.messageHTML)throw new Error("Confirm dialog must contain a message.");return notification._createAlertDialog(options.title,options.message||options.messageHTML,options.buttonLabels,options.primaryButtonIndex,options.modifier,options.animation,options.id,options.callback,!options.message,options.cancelable,!1,!1,"","",!1,options.compile)},notification.confirm=notification._confirmOriginal,notification._promptOriginal=function(message){var options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];"string"==typeof message?options.message=message:options=message;var defaults={buttonLabel:"OK",animation:"default",title:"Alert",defaultValue:"",placeholder:"",callback:function(){},cancelable:!1,autofocus:!0,submitOnEnter:!0};if(options=util.extend({},defaults,options),!options.message&&!options.messageHTML)throw new Error("Prompt dialog must contain a message.");return notification._createAlertDialog(options.title,options.message||options.messageHTML,[options.buttonLabel],0,options.modifier,options.animation,options.id,options.callback,!options.message,options.cancelable,!0,options.autofocus,options.placeholder,options.defaultValue,options.submitOnEnter,options.compile)},notification.prompt=notification._promptOriginal;var pageAttributeExpression={_variables:{},defineVariable:function(name,value){var overwrite=arguments.length<=2||void 0===arguments[2]?!1:arguments[2];if("string"!=typeof name)throw new Error("Variable name must be a string.");if("string"!=typeof value&&"function"!=typeof value)throw new Error("Variable value must be a string or a function.");if(this._variables.hasOwnProperty(name)&&!overwrite)throw new Error('"'+name+'" is already defined.');this._variables[name]=value},getVariable:function(name){return this._variables.hasOwnProperty(name)?this._variables[name]:null},removeVariable:function(name){delete this._variables[name]},getAllVariables:function(){return this._variables},_parsePart:function(part){var c=void 0,inInterpolation=!1,currentIndex=0,tokens=[];if(0===part.length)throw new Error("Unable to parse empty string.");for(var i=0;i<part.length;i++)if(c=part.charAt(i),"$"===c&&"{"===part.charAt(i+1)){if(inInterpolation)throw new Error("Nested interpolation not supported.");var token=part.substring(currentIndex,i);token.length>0&&tokens.push(part.substring(currentIndex,i)),currentIndex=i,inInterpolation=!0}else if("}"===c){if(!inInterpolation)throw new Error("} must be preceeded by ${");var _token=part.substring(currentIndex,i+1);_token.length>0&&tokens.push(part.substring(currentIndex,i+1)),currentIndex=i+1,inInterpolation=!1}if(inInterpolation)throw new Error("Unterminated interpolation.");return tokens.push(part.substring(currentIndex,part.length)),tokens},_replaceToken:function(token){var re=/^\${(.*?)}$/,match=token.match(re);if(match){var name=match[1].trim(),variable=this.getVariable(name);if(null===variable)throw new Error('Variable "'+name+'" does not exist.');if("string"==typeof variable)return variable;var rv=variable();if("string"!=typeof rv)throw new Error("Must return a string.");return rv}return token},_replaceTokens:function(tokens){return tokens.map(this._replaceToken.bind(this))},_parseExpression:function(expression){return expression.split(",").map(function(part){return part.trim()}).map(this._parsePart.bind(this)).map(this._replaceTokens.bind(this)).map(function(part){return part.join("")})},evaluate:function(expression){return expression?this._parseExpression(expression):[]}};pageAttributeExpression.defineVariable("mobileOS",platform.getMobileOS()),pageAttributeExpression.defineVariable("iOSDevice",platform.getIOSDevice()),pageAttributeExpression.defineVariable("runtime",function(){return platform.isWebView()?"cordova":"browser"});var internal={};internal.config={autoStatusBarFill:!0,animationsDisabled:!1},internal.nullElement=window.document.createElement("div"),internal.isEnabledAutoStatusBarFill=function(){return!!internal.config.autoStatusBarFill},internal.normalizePageHTML=function(html){return html=(""+html).trim(),html.match(/^<ons-page/)||(html="<ons-page _muted>"+html+"</ons-page>"),html},internal.waitDOMContentLoaded=function(callback){"loading"===window.document.readyState||"uninitialized"==window.document.readyState?window.document.addEventListener("DOMContentLoaded",callback):setImmediate(callback)},internal.autoStatusBarFill=function(action){var onReady=function onReady(){internal.shouldFillStatusBar()&&action(),document.removeEventListener("deviceready",onReady),document.removeEventListener("DOMContentLoaded",onReady)};"object"===("undefined"==typeof device?"undefined":babelHelpers["typeof"](device))?document.addEventListener("deviceready",onReady):-1===["complete","interactive"].indexOf(document.readyState)?document.addEventListener("DOMContentLoaded",function(){onReady()}):onReady()},internal.shouldFillStatusBar=function(){return internal.isEnabledAutoStatusBarFill()&&platform.isWebView()&&platform.isIOS7above()},internal.templateStore={_storage:{},get:function(key){return internal.templateStore._storage[key]||null},set:function(key,template){internal.templateStore._storage[key]=template}},window.document.addEventListener("_templateloaded",function(e){"ons-template"===e.target.nodeName.toLowerCase()&&internal.templateStore.set(e.templateId,e.template)},!1),window.document.addEventListener("DOMContentLoaded",function(){function register(query){for(var templates=window.document.querySelectorAll(query),i=0;i<templates.length;i++)internal.templateStore.set(templates[i].getAttribute("id"),templates[i].textContent)}register('script[type="text/ons-template"]'),register('script[type="text/template"]'),register('script[type="text/ng-template"]')},!1),internal.getTemplateHTMLAsync=function(page){return new Promise(function(resolve,reject){setImmediate(function(){var cache=internal.templateStore.get(page);if(cache){var html="string"==typeof cache?cache:cache[1];resolve(html)}else!function(){var xhr=new XMLHttpRequest;xhr.open("GET",page,!0),xhr.onload=function(response){var html=xhr.responseText;xhr.status>=400&&xhr.status<600?reject(html):resolve(html)},xhr.onerror=function(){throw new Error("The page is not found: "+page)},xhr.send(null)}()})})},internal.getPageHTMLAsync=function(page){var pages=pageAttributeExpression.evaluate(page),getPage=function getPage(page){return"string"!=typeof page?Promise.reject("Must specify a page."):internal.getTemplateHTMLAsync(page).then(function(html){return internal.normalizePageHTML(html)},function(error){return 0===pages.length?Promise.reject(error):getPage(pages.shift())}).then(function(html){return internal.normalizePageHTML(html)})};return getPage(pages.shift())};var AnimatorFactory=function(){function AnimatorFactory(opts){if(babelHelpers.classCallCheck(this,AnimatorFactory),this._animators=opts.animators,this._baseClass=opts.baseClass,this._baseClassName=opts.baseClassName||opts.baseClass.name,this._animation=opts.defaultAnimation||"default",this._animationOptions=opts.defaultAnimationOptions||{},!this._animators[this._animation])throw new Error("No such animation: "+this._animation)}return babelHelpers.createClass(AnimatorFactory,[{key:"setAnimationOptions",value:function(options){this._animationOptions=options}},{key:"newAnimator",value:function(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],defaultAnimator=arguments[1],animator=null;if(options.animation instanceof this._baseClass)return options.animation;var Animator=null;if("string"==typeof options.animation&&(Animator=this._animators[options.animation]),!Animator&&defaultAnimator)animator=defaultAnimator;else{Animator=Animator||this._animators[this._animation];var animationOpts=util.extend({},this._animationOptions,options.animationOptions||{},internal.config.animationsDisabled?{duration:0,delay:0}:{});animator=new Animator(animationOpts),"function"==typeof animator&&(animator=new animator(animationOpts))}if(!(animator instanceof this._baseClass))throw new Error('"animator" is not an instance of '+this._baseClassName+".");return animator}}],[{key:"parseAnimationOptionsString",value:function(jsonString){try{if("string"==typeof jsonString){var result=util.animationOptionsParse(jsonString);if("object"===("undefined"==typeof result?"undefined":babelHelpers["typeof"](result))&&null!==result)return result;console.error('"animation-options" attribute must be a JSON object string: '+jsonString)}return{}}catch(e){return console.error('"animation-options" attribute must be a JSON object string: '+jsonString),{}}}}]),AnimatorFactory}(),ModifierUtil=function(){function ModifierUtil(){babelHelpers.classCallCheck(this,ModifierUtil)}return babelHelpers.createClass(ModifierUtil,null,[{key:"diff",value:function(last,current){function makeDict(modifier){var dict={};return ModifierUtil.split(modifier).forEach(function(token){return dict[token]=token}),dict}last=makeDict((""+last).trim()),current=makeDict((""+current).trim());var removed=Object.keys(last).reduce(function(result,token){return current[token]||result.push(token),result},[]),added=Object.keys(current).reduce(function(result,token){return last[token]||result.push(token),result},[]);return{added:added,removed:removed}}},{key:"applyDiffToClassList",value:function(diff,classList,template){diff.added.map(function(modifier){return template.replace(/\*/g,modifier)}).forEach(function(klass){return classList.add(klass)}),diff.removed.map(function(modifier){return template.replace(/\*/g,modifier)}).forEach(function(klass){return classList.remove(klass)})}},{key:"applyDiffToElement",value:function(diff,element,scheme){var matches=function(e,s){return(e.matches||e.webkitMatchesSelector||e.mozMatchesSelector||e.msMatchesSelector).call(e,s)};for(var selector in scheme)if(scheme.hasOwnProperty(selector))for(var targetElements=!selector||matches(element,selector)?[element]:element.querySelectorAll(selector),i=0;i<targetElements.length;i++)ModifierUtil.applyDiffToClassList(diff,targetElements[i].classList,scheme[selector])}},{key:"onModifierChanged",value:function(last,current,element,scheme){return ModifierUtil.applyDiffToElement(ModifierUtil.diff(last,current),element,scheme)}},{key:"initModifier",value:function(element,scheme){var modifier=element.getAttribute("modifier");"string"==typeof modifier&&ModifierUtil.applyDiffToElement({removed:[],added:ModifierUtil.split(modifier)},element,scheme)}},{key:"split",value:function(modifier){return"string"!=typeof modifier?[]:modifier.trim().split(/ +/).filter(function(token){return""!==token})}}]),ModifierUtil}(),LazyRepeatDelegate=function(){function LazyRepeatDelegate(userDelegate){var templateElement=arguments.length<=1||void 0===arguments[1]?null:arguments[1];if(babelHelpers.classCallCheck(this,LazyRepeatDelegate),"object"!==("undefined"==typeof userDelegate?"undefined":babelHelpers["typeof"](userDelegate))||null===userDelegate)throw Error('"delegate" parameter must be an object.');if(this._userDelegate=userDelegate,!(templateElement instanceof Element)&&null!==templateElement)throw Error('"templateElement" parameter must be an instance of Element or null.');this._templateElement=templateElement}return babelHelpers.createClass(LazyRepeatDelegate,[{key:"hasRenderFunction",value:function(){return this._userDelegate._render instanceof Function}},{key:"_render",value:function(items,height){this._userDelegate._render(items,height)}},{key:"loadItemElement",value:function(index,parent,done){if(this._userDelegate.loadItemElement instanceof Function)this._userDelegate.loadItemElement(index,parent,function(element){return done({element:element})});else{var element=this._userDelegate.createItemContent(index,this._templateElement);if(!(element instanceof Element))throw Error("createItemContent() must return an instance of Element.");parent.appendChild(element),done({element:element})}}},{key:"countItems",value:function(){var count=this._userDelegate.countItems();if("number"!=typeof count)throw Error("countItems() must return a number.");return count}},{key:"updateItem",value:function(index,item){this._userDelegate.updateItemContent instanceof Function&&this._userDelegate.updateItemContent(index,item)}},{key:"calculateItemHeight",value:function(index){if(this._userDelegate.calculateItemHeight instanceof Function){var height=this._userDelegate.calculateItemHeight(index);if("number"!=typeof height)throw Error("calculateItemHeight() must return a number.");return height}return 0}},{key:"destroyItem",value:function(index,item){this._userDelegate.destroyItem instanceof Function&&this._userDelegate.destroyItem(index,item)}},{key:"destroy",value:function(){this._userDelegate.destroy instanceof Function&&this._userDelegate.destroy(),this._userDelegate=this._templateElement=null}},{key:"itemHeight",get:function(){return this._userDelegate.itemHeight}}]),LazyRepeatDelegate}(),LazyRepeatProvider=function(){function LazyRepeatProvider(wrapperElement,delegate){if(babelHelpers.classCallCheck(this,LazyRepeatProvider),!(delegate instanceof LazyRepeatDelegate))throw Error('"delegate" parameter must be an instance of LazyRepeatDelegate.');if(this._wrapperElement=wrapperElement,this._delegate=delegate,"ons-list"===wrapperElement.tagName.toLowerCase()&&wrapperElement.classList.add("lazy-list"),this._pageContent=util.findParent(wrapperElement,".page__content"),!this._pageContent)throw new Error("ons-lazy-repeat must be a descendant of an <ons-page> or an element.");this._topPositions=[],this._renderedItems={},this._delegate.itemHeight||this._delegate.calculateItemHeight(0)||(this._unknownItemHeight=!0),this._addEventListeners(),this._onChange()}return babelHelpers.createClass(LazyRepeatProvider,[{key:"_checkItemHeight",value:function(callback){var _this=this;this._delegate.loadItemElement(0,this._wrapperElement,function(item){if(!_this._unknownItemHeight)throw Error("Invalid state");var done=function(){_this._wrapperElement.removeChild(item.element),delete _this._unknownItemHeight,callback()};if(_this._itemHeight=item.element.offsetHeight,_this._itemHeight>0)return void done();var lastVisibility=_this._wrapperElement.style.visibility;_this._wrapperElement.style.visibility="hidden",item.element.style.visibility="hidden",setImmediate(function(){if(_this._itemHeight=item.element.offsetHeight,0==_this._itemHeight)throw Error("Invalid state: this._itemHeight must be greater than zero.");_this._wrapperElement.style.visibility=lastVisibility,done()})})}},{key:"_countItems",value:function(){return this._delegate.countItems()}},{key:"_getItemHeight",value:function(i){return this.staticItemHeight||this._delegate.calculateItemHeight(i)}},{key:"_onChange",value:function(){this._render()}},{key:"refresh",value:function(){this._removeAllElements(),this._onChange()}},{key:"_render",value:function(){var _this2=this;if(this._unknownItemHeight)return this._checkItemHeight(this._render.bind(this));var items=this._getItemsInView();if(this._delegate.hasRenderFunction&&this._delegate.hasRenderFunction())return this._delegate._render(items,this._listHeight),null;var keep={};items.forEach(function(item){_this2._renderElement(item),keep[item.index]=!0}),Object.keys(this._renderedItems).forEach(function(key){return keep[key]||_this2._removeElement(key)}),this._wrapperElement.style.height=this._listHeight+"px"}},{key:"_renderElement",value:function(_ref){var _this3=this,index=_ref.index,top=_ref.top,item=this._renderedItems[index];return item?(this._delegate.updateItem(index,item),void(item.element.style.top=top+"px")):void this._delegate.loadItemElement(index,this._wrapperElement,function(item){util.extend(item.element.style,{position:"absolute",top:top+"px",left:0,right:0}),_this3._renderedItems[index]=item})}},{key:"_removeElement",value:function(index){var item=this._renderedItems[index];this._delegate.destroyItem(index,item),item.element.parentElement&&item.element.parentElement.removeChild(item.element),delete this._renderedItems[index]}},{key:"_removeAllElements",value:function(){var _this4=this;Object.keys(this._renderedItems).forEach(function(key){return _this4._removeElement(key)})}},{key:"_calculateStartIndex",value:function(current){var start=0,end=this._itemCount-1;if(this.staticItemHeight)return parseInt(-current/this.staticItemHeight);for(;;){var middle=Math.floor((start+end)/2),value=current+this._topPositions[middle];if(start>end)return 0;if(0>=value&&value+this._getItemHeight(middle)>0)return middle;isNaN(value)||value>=0?end=middle-1:start=middle+1}}},{key:"_recalculateTopPositions",value:function(){Math.min(this._topPositions.length,this._itemCount);this._topPositions[0]=0;for(var _l,i=1;_l>i;i++)this._topPositions[i]=this._topPositions[i-1]+this._getItemHeight(i)}},{key:"_getItemsInView",value:function(){var offset=this._wrapperElement.getBoundingClientRect().top,limit=4*window.innerHeight-offset,count=this._countItems();count!==this._itemCount&&(this._itemCount=count,this._recalculateTopPositions());for(var i=Math.max(0,this._calculateStartIndex(offset)-30),items=[],top=this._topPositions[i];count>i&&limit>top;i++)i>=this._topPositions.length&&(this._topPositions.length+=100),this._topPositions[i]=top,items.push({top:top,index:i}),top+=this._getItemHeight(i);return this._listHeight=top,items}},{key:"_debounce",value:function(func,wait,immediate){var timeout=void 0;return function(){var _this5=this,_arguments=arguments,callNow=immediate&&!timeout;clearTimeout(timeout),callNow?func.apply(this,arguments):timeout=setTimeout(function(){timeout=null,func.apply(_this5,_arguments)},wait)}}},{key:"_doubleFireOnTouchend",value:function(){this._render(),this._debounce(this._render.bind(this),100)}},{key:"_addEventListeners",value:function(){util.bindListeners(this,["_onChange","_doubleFireOnTouchend"]),platform.isIOS()&&(this._boundOnChange=this._debounce(this._boundOnChange,30)),this._pageContent.addEventListener("scroll",this._boundOnChange,!0),platform.isIOS()&&(this._pageContent.addEventListener("touchmove",this._boundOnChange,!0),this._pageContent.addEventListener("touchend",this._boundDoubleFireOnTouchend,!0)),window.document.addEventListener("resize",this._boundOnChange,!0)}},{key:"_removeEventListeners",value:function(){this._pageContent.removeEventListener("scroll",this._boundOnChange,!0),platform.isIOS()&&(this._pageContent.removeEventListener("touchmove",this._boundOnChange,!0),this._pageContent.removeEventListener("touchend",this._boundDoubleFireOnTouchend,!0)),window.document.removeEventListener("resize",this._boundOnChange,!0)}},{key:"destroy",value:function(){this._removeAllElements(),this._delegate.destroy(),this._parentElement=this._delegate=this._renderedItems=null,this._removeEventListeners()}},{key:"staticItemHeight",get:function(){return this._delegate.itemHeight||this._itemHeight}}]),LazyRepeatProvider}();internal.AnimatorFactory=AnimatorFactory,internal.ModifierUtil=ModifierUtil,internal.LazyRepeatProvider=LazyRepeatProvider,internal.LazyRepeatDelegate=LazyRepeatDelegate;var create=function(){var obj={_isPortrait:!1,isPortrait:function(){return this._isPortrait()},isLandscape:function(){return!this.isPortrait()},_init:function(){return document.addEventListener("DOMContentLoaded",this._onDOMContentLoaded.bind(this),!1),"orientation"in window?window.addEventListener("orientationchange",this._onOrientationChange.bind(this),!1):window.addEventListener("resize",this._onResize.bind(this),!1),this._isPortrait=function(){return window.innerHeight>window.innerWidth},this},_onDOMContentLoaded:function(){this._installIsPortraitImplementation(),this.emit("change",{isPortrait:this.isPortrait()})},_installIsPortraitImplementation:function(){var isPortrait=window.innerWidth<window.innerHeight;"orientation"in window?window.orientation%180===0?this._isPortrait=function(){return 0===Math.abs(window.orientation%180)?isPortrait:!isPortrait}:this._isPortrait=function(){return 90===Math.abs(window.orientation%180)?isPortrait:!isPortrait}:this._isPortrait=function(){return window.innerHeight>window.innerWidth}},_onOrientationChange:function(){var _this=this,isPortrait=this._isPortrait(),nIter=0,interval=setInterval(function(){nIter++;var w=window.innerWidth,h=window.innerHeight;isPortrait&&h>=w||!isPortrait&&w>=h?(_this.emit("change",{isPortrait:isPortrait}),clearInterval(interval)):50===nIter&&(_this.emit("change",{isPortrait:isPortrait}),clearInterval(interval))},20)},_onResize:function(){this.emit("change",{isPortrait:this.isPortrait()})}};return MicroEvent.mixin(obj),obj},orientation=create()._init(),softwareKeyboard=new MicroEvent;softwareKeyboard._visible=!1;var onShow=function(){softwareKeyboard._visible=!0,softwareKeyboard.emit("show")},onHide=function(){softwareKeyboard._visible=!1,softwareKeyboard.emit("hide")},bindEvents=function(){return"undefined"!=typeof Keyboard?(Keyboard.onshow=onShow,Keyboard.onhide=onHide,softwareKeyboard.emit("init",{visible:Keyboard.isVisible}),!0):"undefined"!=typeof cordova.plugins&&"undefined"!=typeof cordova.plugins.Keyboard?(window.addEventListener("native.keyboardshow",onShow),window.addEventListener("native.keyboardhide",onHide),softwareKeyboard.emit("init",{visible:cordova.plugins.Keyboard.isVisible}),!0):!1},noPluginError=function(){console.warn("ons-keyboard: Cordova Keyboard plugin is not present.")};document.addEventListener("deviceready",function(){bindEvents()||((document.querySelector("[ons-keyboard-active]")||document.querySelector("[ons-keyboard-inactive]"))&&noPluginError(),softwareKeyboard.on=noPluginError)});var util$1={_ready:!1,_domContentLoaded:!1,_onDOMContentLoaded:function(){util$1._domContentLoaded=!0,platform.isWebView()?window.document.addEventListener("deviceready",function(){util$1._ready=!0;
},!1):util$1._ready=!0},addBackButtonListener:function(fn){if(!this._domContentLoaded)throw new Error("This method is available after DOMContentLoaded");this._ready?window.document.addEventListener("backbutton",fn,!1):window.document.addEventListener("deviceready",function(){window.document.addEventListener("backbutton",fn,!1)})},removeBackButtonListener:function(fn){if(!this._domContentLoaded)throw new Error("This method is available after DOMContentLoaded");this._ready?window.document.removeEventListener("backbutton",fn,!1):window.document.addEventListener("deviceready",function(){window.document.removeEventListener("backbutton",fn,!1)})}};window.addEventListener("DOMContentLoaded",function(){return util$1._onDOMContentLoaded()},!1);var HandlerRepository={_store:{},_genId:function(){var i=0;return function(){return i++}}(),set:function(element,handler){element.dataset.deviceBackButtonHandlerId&&this.remove(element);var id=element.dataset.deviceBackButtonHandlerId=HandlerRepository._genId();this._store[id]=handler},remove:function(element){element.dataset.deviceBackButtonHandlerId&&(delete this._store[element.dataset.deviceBackButtonHandlerId],delete element.dataset.deviceBackButtonHandlerId)},get:function(element){if(element.dataset.deviceBackButtonHandlerId){var id=element.dataset.deviceBackButtonHandlerId;if(!this._store[id])throw new Error;return this._store[id]}},has:function(element){if(!element.dataset)return!1;var id=element.dataset.deviceBackButtonHandlerId;return!!this._store[id]}},DeviceBackButtonDispatcher=function(){function DeviceBackButtonDispatcher(){babelHelpers.classCallCheck(this,DeviceBackButtonDispatcher),this._isEnabled=!1,this._boundCallback=this._callback.bind(this)}return babelHelpers.createClass(DeviceBackButtonDispatcher,[{key:"enable",value:function(){this._isEnabled||(util$1.addBackButtonListener(this._boundCallback),this._isEnabled=!0)}},{key:"disable",value:function(){this._isEnabled&&(util$1.removeBackButtonListener(this._boundCallback),this._isEnabled=!1)}},{key:"fireDeviceBackButtonEvent",value:function(){var event=document.createEvent("Event");event.initEvent("backbutton",!0,!0),document.dispatchEvent(event)}},{key:"_callback",value:function(){this._dispatchDeviceBackButtonEvent()}},{key:"createHandler",value:function(element,callback){if(!(element instanceof HTMLElement))throw new Error("element must be an instance of HTMLElement");if(!(callback instanceof Function))throw new Error("callback must be an instance of Function");var handler={_callback:callback,_element:element,disable:function(){HandlerRepository.remove(element)},setListener:function(callback){this._callback=callback},enable:function(){HandlerRepository.set(element,this)},isEnabled:function(){return HandlerRepository.get(element)===this},destroy:function(){HandlerRepository.remove(element),this._callback=this._element=null}};return handler.enable(),handler}},{key:"_dispatchDeviceBackButtonEvent",value:function(){function createEvent(element){return{_element:element,callParentHandler:function(){for(var parent=this._element.parentNode;parent;){if(handler=HandlerRepository.get(parent))return handler._callback(createEvent(parent));parent=parent.parentNode}}}}var tree=this._captureTree(),element=this._findHandlerLeafElement(tree),handler=HandlerRepository.get(element);handler._callback(createEvent(element))}},{key:"_captureTree",value:function(){function createTree(element){return{element:element,children:Array.prototype.concat.apply([],arrayOf(element.children).map(function(childElement){if("none"===childElement.style.display)return[];if(0===childElement.children.length&&!HandlerRepository.has(childElement))return[];var result=createTree(childElement);return 0!==result.children.length||HandlerRepository.has(result.element)?[result]:[]}))}}function arrayOf(target){for(var result=[],i=0;i<target.length;i++)result.push(target[i]);return result}return createTree(document.body)}},{key:"_findHandlerLeafElement",value:function(tree){function find(node){return 0===node.children.length?node.element:1===node.children.length?find(node.children[0]):node.children.map(function(childNode){return childNode.element}).reduce(function(left,right){if(!left)return right;var leftZ=parseInt(window.getComputedStyle(left,"").zIndex,10),rightZ=parseInt(window.getComputedStyle(right,"").zIndex,10);if(!isNaN(leftZ)&&!isNaN(rightZ))return leftZ>rightZ?left:right;throw new Error("Capturing backbutton-handler is failure.")},null)}return find(tree)}}]),DeviceBackButtonDispatcher}(),deviceBackButtonDispatcher=new DeviceBackButtonDispatcher,autoStyleEnabled=!0,modifiersMap={quiet:"material--flat",light:"material--flat",outline:"material--flat",cta:"","large--quiet":"material--flat large","large--cta":"large",noborder:"",chevron:"",tappable:""},platforms={};platforms.android=function(element){if(!/ons-fab|ons-speed-dial|ons-progress/.test(element.tagName.toLowerCase())&&!/material/.test(element.getAttribute("modifier"))){var oldModifier=element.getAttribute("modifier")||"",newModifier=oldModifier.trim().split(/\s+/).map(function(e){return modifiersMap.hasOwnProperty(e)?modifiersMap[e]:e});newModifier.unshift("material"),element.setAttribute("modifier",newModifier.join(" ").trim())}!/ons-button|ons-list-item|ons-fab|ons-speed-dial|ons-tab$/.test(element.tagName.toLowerCase())||element.hasAttribute("ripple")||util.findChild(element,"ons-ripple")||("ons-list-item"===element.tagName.toLowerCase()?element.hasAttribute("tappable")&&(element.setAttribute("ripple",""),element.removeAttribute("tappable")):element.setAttribute("ripple",""))},platforms.ios=function(element){/material/.test(element.getAttribute("modifier"))&&(util.removeModifier(element,"material"),util.removeModifier(element,"material--flat")&&util.addModifier(element,util.removeModifier(element,"large")?"large--quiet":"quiet"),element.getAttribute("modifier")||element.removeAttribute("modifier")),element.hasAttribute("ripple")&&("ons-list-item"===element.tagName.toLowerCase()&&element.setAttribute("tappable",""),element.removeAttribute("ripple"))};var unlocked={android:!0},prepareAutoStyle=function(element,force){if(autoStyleEnabled&&!element.hasAttribute("disable-auto-styling")){var mobileOS=platform.getMobileOS();platforms.hasOwnProperty(mobileOS)&&(unlocked.hasOwnProperty(mobileOS)||force)&&platforms[mobileOS](element)}},autoStyle={isEnabled:function(){return autoStyleEnabled},enable:function(){return autoStyleEnabled=!0},disable:function(){return autoStyleEnabled=!1},prepare:prepareAutoStyle},generateId=function(){var i=0;return function(){return i++}}(),DoorLock=function(){function DoorLock(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];babelHelpers.classCallCheck(this,DoorLock),this._lockList=[],this._waitList=[],this._log=options.log||function(){}}return babelHelpers.createClass(DoorLock,[{key:"lock",value:function(){var _this=this,unlock=function unlock(){_this._unlock(unlock)};return unlock.id=generateId(),this._lockList.push(unlock),this._log("lock: "+unlock.id),unlock}},{key:"_unlock",value:function(fn){var index=this._lockList.indexOf(fn);if(-1===index)throw new Error("This function is not registered in the lock list.");this._lockList.splice(index,1),this._log("unlock: "+fn.id),this._tryToFreeWaitList()}},{key:"_tryToFreeWaitList",value:function(){for(;!this.isLocked()&&this._waitList.length>0;)this._waitList.shift()()}},{key:"waitUnlock",value:function(callback){if(!(callback instanceof Function))throw new Error("The callback param must be a function.");this.isLocked()?this._waitList.push(callback):callback()}},{key:"isLocked",value:function(){return this._lockList.length>0}}]),DoorLock}(),readyMap=new WeakMap,queueMap=new WeakMap,PageLoader=function(){function PageLoader(fn){babelHelpers.classCallCheck(this,PageLoader),this._loader=fn instanceof Function?fn:loadPage}return babelHelpers.createClass(PageLoader,[{key:"load",value:function(_ref2,done){var page=_ref2.page,parent=_ref2.parent,_ref2$params=_ref2.params,params=void 0===_ref2$params?{}:_ref2$params,replace=_ref2.replace;this._loader({page:page,parent:parent,params:params,replace:replace},function(result){if(!(result.element instanceof Element))throw Error("target.element must be an instance of Element.");if(!(result.unload instanceof Function))throw Error("target.unload must be an instance of Function.");done(result)},params)}},{key:"internalLoader",set:function(fn){if(!(fn instanceof Function))throw Error("First parameter must be an instance of Function");this._loader=fn},get:function(){return this._loader}}]),PageLoader}(),defaultPageLoader=new PageLoader,instantPageLoader=new PageLoader(function(_ref3,done){var page=_ref3.page,parent=_ref3.parent,replace=(_ref3.params,_ref3.replace);replace&&(util.propagateAction(parent,"_destroy"),parent.innerHTML="");var element=util.createElement(page.trim());parent.appendChild(element),done({element:element,unload:function(){return element.remove()}})}),ons={};ons._util=util,ons._deviceBackButtonDispatcher=deviceBackButtonDispatcher,ons._internal=internal,ons.GestureDetector=GestureDetector,ons.platform=platform,ons.softwareKeyboard=softwareKeyboard,ons.pageAttributeExpression=pageAttributeExpression,ons.orientation=orientation,ons.notification=notification,ons._animationOptionsParser=parse,ons._autoStyle=autoStyle,ons._DoorLock=DoorLock,ons._contentReady=contentReady,ons.defaultPageLoader=defaultPageLoader,ons.PageLoader=PageLoader,ons._readyLock=new DoorLock,ons.platform.select((window.location.search.match(/platform=([\w-]+)/)||[])[1]),waitDeviceReady(),ons.isReady=function(){return!ons._readyLock.isLocked()},ons.isWebView=ons.platform.isWebView,ons.ready=function(callback){ons.isReady()?callback():ons._readyLock.waitUnlock(callback)},ons.setDefaultDeviceBackButtonListener=function(listener){ons._defaultDeviceBackButtonHandler.setListener(listener)},ons.disableDeviceBackButtonHandler=function(){ons._deviceBackButtonDispatcher.disable()},ons.enableDeviceBackButtonHandler=function(){ons._deviceBackButtonDispatcher.enable()},ons.enableAutoStatusBarFill=function(){if(ons.isReady())throw new Error("This method must be called before ons.isReady() is true.");ons._internal.config.autoStatusBarFill=!0},ons.disableAutoStatusBarFill=function(){if(ons.isReady())throw new Error("This method must be called before ons.isReady() is true.");ons._internal.config.autoStatusBarFill=!1},ons.disableAnimations=function(){ons._internal.config.animationsDisabled=!0},ons.enableAnimations=function(){ons._internal.config.animationsDisabled=!1},ons.disableAutoStyling=ons._autoStyle.disable,ons.enableAutoStyling=ons._autoStyle.enable,ons.forcePlatformStyling=function(newPlatform){ons.enableAutoStyling(),ons.platform.select(newPlatform||"ios"),ons._util.arrayFrom(document.querySelectorAll("*")).forEach(function(element){"ons-if"===element.tagName.toLowerCase()?element._platformUpdate():element.tagName.match(/^ons-/i)&&(ons._autoStyle.prepare(element,!0),"ons-tabbar"===element.tagName.toLowerCase()&&element._updatePosition())})},ons._createPopoverOriginal=function(page){var options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];if(!page)throw new Error("Page url must be defined.");return ons._internal.getPageHTMLAsync(page).then(function(html){html=html.match(/<ons-popover/gi)?"<div>"+html+"</div>":"<ons-popover>"+html+"</ons-popover>";var div=ons._util.createElement("<div>"+html+"</div>"),popover=div.querySelector("ons-popover");return document.body.appendChild(popover),options.link instanceof Function&&options.link(popover),popover})},ons.createPopover=ons._createPopoverOriginal,ons._createDialogOriginal=function(page){var options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];if(!page)throw new Error("Page url must be defined.");return ons._internal.getPageHTMLAsync(page).then(function(html){html=html.match(/<ons-dialog/gi)?"<div>"+html+"</div>":"<ons-dialog>"+html+"</ons-dialog>";var div=ons._util.createElement("<div>"+html+"</div>"),dialog=div.querySelector("ons-dialog");return document.body.appendChild(dialog),options.link instanceof Function&&options.link(dialog),dialog})},ons.createDialog=ons._createDialogOriginal,ons._createAlertDialogOriginal=function(page){var options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];if(!page)throw new Error("Page url must be defined.");return ons._internal.getPageHTMLAsync(page).then(function(html){html=html.match(/<ons-alert-dialog/gi)?"<div>"+html+"</div>":"<ons-alert-dialog>"+html+"</ons-alert-dialog>";var div=ons._util.createElement("<div>"+html+"</div>"),alertDialog=div.querySelector("ons-alert-dialog");return document.body.appendChild(alertDialog),options.link instanceof Function&&options.link(alertDialog),alertDialog})},ons.createAlertDialog=ons._createAlertDialogOriginal,ons._resolveLoadingPlaceholderOriginal=function(page,link){var elements=ons._util.arrayFrom(window.document.querySelectorAll("[ons-loading-placeholder]"));if(!(elements.length>0))throw new Error("No ons-loading-placeholder exists.");elements.filter(function(element){return!element.getAttribute("page")}).forEach(function(element){element.setAttribute("ons-loading-placeholder",page),ons._resolveLoadingPlaceholder(element,page,link)})},ons.resolveLoadingPlaceholder=ons._resolveLoadingPlaceholderOriginal,ons._setupLoadingPlaceHolders=function(){ons.ready(function(){var elements=ons._util.arrayFrom(window.document.querySelectorAll("[ons-loading-placeholder]"));elements.forEach(function(element){var page=element.getAttribute("ons-loading-placeholder");"string"==typeof page&&ons._resolveLoadingPlaceholder(element,page)})})},ons._resolveLoadingPlaceholder=function(element,page,link){link=link||function(element,done){done()},ons._internal.getPageHTMLAsync(page).then(function(html){for(;element.firstChild;)element.removeChild(element.firstChild);var contentElement=ons._util.createElement("<div>"+html+"</div>");contentElement.style.display="none",element.appendChild(contentElement),link(contentElement,function(){contentElement.style.display=""})})["catch"](function(error){throw new Error("Unabled to resolve placeholder: "+error)})},window._superSecretOns=ons;var BaseElement=function(_getElementClass){function BaseElement(self){var _this,_ret;return babelHelpers.classCallCheck(this,BaseElement),self=_this=babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(BaseElement).call(this,self)),self.init(),_ret=self,babelHelpers.possibleConstructorReturn(_this,_ret)}return babelHelpers.inherits(BaseElement,_getElementClass),babelHelpers.createClass(BaseElement,[{key:"init",value:function(){}}]),BaseElement}(getElementClass()),TemplateElement=function(_BaseElement){function TemplateElement(){return babelHelpers.classCallCheck(this,TemplateElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(TemplateElement).apply(this,arguments))}return babelHelpers.inherits(TemplateElement,_BaseElement),babelHelpers.createClass(TemplateElement,[{key:"init",value:function(){for(this.template=this.innerHTML;this.firstChild;)this.removeChild(this.firstChild)}},{key:"connectedCallback",value:function(){var event=new CustomEvent("_templateloaded",{bubbles:!0,cancelable:!0});event.template=this.template,event.templateId=this.getAttribute("id"),this.dispatchEvent(event)}}]),TemplateElement}(BaseElement);customElements.define("ons-template",TemplateElement);var IfElement=function(_BaseElement){function IfElement(){return babelHelpers.classCallCheck(this,IfElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(IfElement).apply(this,arguments))}return babelHelpers.inherits(IfElement,_BaseElement),babelHelpers.createClass(IfElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){if(null!==platform._renderPlatform)_this2._platformUpdate();else if(!_this2._isAllowedPlatform()){for(;_this2.childNodes[0];)_this2.childNodes[0].remove();_this2._platformUpdate()}}),this._onOrientationChange()}},{key:"connectedCallback",value:function(){orientation.on("change",this._onOrientationChange.bind(this))}},{key:"attributeChangedCallback",value:function(name){"orientation"===name&&this._onOrientationChange()}},{key:"disconnectedCallback",value:function(){orientation.off("change",this._onOrientationChange)}},{key:"_platformUpdate",value:function(){this.style.display=this._isAllowedPlatform()?"":"none"}},{key:"_isAllowedPlatform",value:function(){return!this.getAttribute("platform")||this.getAttribute("platform").split(/\s+/).indexOf(platform.getMobileOS())>=0}},{key:"_onOrientationChange",value:function(){if(this.hasAttribute("orientation")&&this._isAllowedPlatform()){var conditionalOrientation=this.getAttribute("orientation").toLowerCase(),currentOrientation=orientation.isPortrait()?"portrait":"landscape";this.style.display=conditionalOrientation===currentOrientation?"":"none"}}}],[{key:"observedAttributes",get:function(){return["orientation"]}}]),IfElement}(BaseElement);customElements.define("ons-if",IfElement);var AlertDialogAnimator=function(){function AlertDialogAnimator(){var _ref=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_ref$timing=_ref.timing,timing=void 0===_ref$timing?"linear":_ref$timing,_ref$delay=_ref.delay,delay=void 0===_ref$delay?0:_ref$delay,_ref$duration=_ref.duration,duration=void 0===_ref$duration?.2:_ref$duration;babelHelpers.classCallCheck(this,AlertDialogAnimator),this.timing=timing,this.delay=delay,this.duration=duration}return babelHelpers.createClass(AlertDialogAnimator,[{key:"show",value:function(dialog,done){done()}},{key:"hide",value:function(dialog,done){done()}}]),AlertDialogAnimator}(),AndroidAlertDialogAnimator=function(_AlertDialogAnimator){function AndroidAlertDialogAnimator(){var _ref2=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_ref2$timing=_ref2.timing,timing=void 0===_ref2$timing?"cubic-bezier(.1, .7, .4, 1)":_ref2$timing,_ref2$duration=_ref2.duration,duration=void 0===_ref2$duration?.2:_ref2$duration,_ref2$delay=_ref2.delay,delay=void 0===_ref2$delay?0:_ref2$delay;return babelHelpers.classCallCheck(this,AndroidAlertDialogAnimator),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(AndroidAlertDialogAnimator).call(this,{duration:duration,timing:timing,delay:delay}))}return babelHelpers.inherits(AndroidAlertDialogAnimator,_AlertDialogAnimator),babelHelpers.createClass(AndroidAlertDialogAnimator,[{key:"show",value:function(dialog,callback){callback=callback?callback:function(){},animit.runAll(animit(dialog._mask).queue({opacity:0}).wait(this.delay).queue({opacity:1},{duration:this.duration,timing:this.timing}),animit(dialog._dialog).saveStyle().queue({css:{transform:"translate3d(-50%, -50%, 0) scale3d(0.9, 0.9, 1.0)",opacity:0},duration:0}).wait(this.delay).queue({css:{transform:"translate3d(-50%, -50%, 0) scale3d(1.0, 1.0, 1.0)",opacity:1},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}))}},{key:"hide",value:function(dialog,callback){callback=callback?callback:function(){},animit.runAll(animit(dialog._mask).queue({opacity:1}).wait(this.delay).queue({opacity:0},{duration:this.duration,timing:this.timing}),animit(dialog._dialog).saveStyle().queue({css:{transform:"translate3d(-50%, -50%, 0) scale3d(1.0, 1.0, 1.0)",opacity:1},duration:0}).wait(this.delay).queue({css:{transform:"translate3d(-50%, -50%, 0) scale3d(0.9, 0.9, 1.0)",opacity:0},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}))}}]),AndroidAlertDialogAnimator}(AlertDialogAnimator),IOSAlertDialogAnimator=function(_AlertDialogAnimator2){function IOSAlertDialogAnimator(){var _ref3=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_ref3$timing=_ref3.timing,timing=void 0===_ref3$timing?"cubic-bezier(.1, .7, .4, 1)":_ref3$timing,_ref3$duration=_ref3.duration,duration=void 0===_ref3$duration?.2:_ref3$duration,_ref3$delay=_ref3.delay,delay=void 0===_ref3$delay?0:_ref3$delay;return babelHelpers.classCallCheck(this,IOSAlertDialogAnimator),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(IOSAlertDialogAnimator).call(this,{duration:duration,timing:timing,delay:delay}))}return babelHelpers.inherits(IOSAlertDialogAnimator,_AlertDialogAnimator2),babelHelpers.createClass(IOSAlertDialogAnimator,[{key:"show",value:function(dialog,callback){callback=callback?callback:function(){},animit.runAll(animit(dialog._mask).queue({opacity:0}).wait(this.delay).queue({opacity:1},{duration:this.duration,timing:this.timing}),animit(dialog._dialog).saveStyle().queue({css:{transform:"translate3d(-50%, -50%, 0) scale3d(1.3, 1.3, 1.0)",opacity:0},duration:0}).wait(this.delay).queue({css:{transform:"translate3d(-50%, -50%, 0) scale3d(1.0, 1.0, 1.0)",opacity:1},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}))}},{key:"hide",value:function(dialog,callback){callback=callback?callback:function(){},animit.runAll(animit(dialog._mask).queue({opacity:1}).wait(this.delay).queue({opacity:0},{duration:this.duration,timing:this.timing}),animit(dialog._dialog).saveStyle().queue({css:{opacity:1},duration:0}).wait(this.delay).queue({css:{opacity:0},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}))}}]),IOSAlertDialogAnimator}(AlertDialogAnimator),scheme={".alert-dialog":"alert-dialog--*",".alert-dialog-container":"alert-dialog-container--*",".alert-dialog-title":"alert-dialog-title--*",".alert-dialog-content":"alert-dialog-content--*",".alert-dialog-footer":"alert-dialog-footer--*",".alert-dialog-button":"alert-dialog-button--*",".alert-dialog-footer--one":"alert-dialog-footer--one--*",".alert-dialog-button--one":"alert-dialog-button--one--*",".alert-dialog-button--primal":"alert-dialog-button--primal--*",".alert-dialog-mask":"alert-dialog-mask--*"},_animatorDict={none:AlertDialogAnimator,"default":function(){return platform.isAndroid()?AndroidAlertDialogAnimator:IOSAlertDialogAnimator},fade:function(){return platform.isAndroid()?AndroidAlertDialogAnimator:IOSAlertDialogAnimator}},AlertDialogElement=function(_BaseElement){function AlertDialogElement(){return babelHelpers.classCallCheck(this,AlertDialogElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(AlertDialogElement).apply(this,arguments))}return babelHelpers.inherits(AlertDialogElement,_BaseElement),babelHelpers.createClass(AlertDialogElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){return _this2._compile()}),this._visible=!1,this._doorLock=new DoorLock,this._boundCancel=this._cancel.bind(this),this._updateAnimatorFactory()}},{key:"_updateAnimatorFactory",value:function(){this._animatorFactory=new AnimatorFactory({animators:_animatorDict,baseClass:AlertDialogAnimator,baseClassName:"AlertDialogAnimator",defaultAnimation:this.getAttribute("animation")})}},{key:"_compile",value:function(){autoStyle.prepare(this),this.style.display="none";var content=document.createDocumentFragment();if(!this._mask&&!this._dialog)for(;this.firstChild;)content.appendChild(this.firstChild);if(!this._mask){var mask=document.createElement("div");mask.classList.add("alert-dialog-mask"),this.insertBefore(mask,this.children[0])}if(!this._dialog){var dialog=document.createElement("div");dialog.classList.add("alert-dialog"),this.insertBefore(dialog,null)}if(!util.findChild(this._dialog,".alert-dialog-container")){var container=document.createElement("div");container.classList.add("alert-dialog-container"),this._dialog.appendChild(container)}this._dialog.children[0].appendChild(content),this._dialog.style.zIndex=20001,this._mask.style.zIndex=2e4,this.getAttribute("mask-color")&&(this._mask.style.backgroundColor=this.getAttribute("mask-color")),ModifierUtil.initModifier(this,scheme)}},{key:"show",value:function(){var _this3=this,options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_cancel2=!1,callback=options.callback||function(){};if(options.animationOptions=util.extend(options.animationOptions||{},AnimatorFactory.parseAnimationOptionsString(this.getAttribute("animation-options"))),util.triggerElementEvent(this,"preshow",{alertDialog:this,cancel:function(){_cancel2=!0}}),_cancel2)return Promise.reject("Canceled in preshow event.");var _ret=function(){var tryShow=function(){var unlock=_this3._doorLock.lock(),animator=_this3._animatorFactory.newAnimator(options);return _this3.style.display="block",_this3._mask.style.opacity="1",new Promise(function(resolve){contentReady(_this3,function(){animator.show(_this3,function(){_this3._visible=!0,unlock(),util.triggerElementEvent(_this3,"postshow",{alertDialog:_this3}),callback(),resolve(_this3)})})})};return{v:new Promise(function(resolve){_this3._doorLock.waitUnlock(function(){return resolve(tryShow())})})}}();return"object"===("undefined"==typeof _ret?"undefined":babelHelpers["typeof"](_ret))?_ret.v:void 0}},{key:"hide",value:function(){var _this4=this,options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_cancel3=!1,callback=options.callback||function(){};if(options.animationOptions=util.extend(options.animationOptions||{},AnimatorFactory.parseAnimationOptionsString(this.getAttribute("animation-options"))),util.triggerElementEvent(this,"prehide",{alertDialog:this,cancel:function(){_cancel3=!0}}),_cancel3)return Promise.reject("Canceled in prehide event.");var _ret2=function(){var tryHide=function(){var unlock=_this4._doorLock.lock(),animator=_this4._animatorFactory.newAnimator(options);return new Promise(function(resolve){contentReady(_this4,function(){animator.hide(_this4,function(){_this4.style.display="none",_this4._visible=!1,unlock(),util.triggerElementEvent(_this4,"posthide",{alertDialog:_this4}),callback(),resolve(_this4)})})})};return{v:new Promise(function(resolve){_this4._doorLock.waitUnlock(function(){return resolve(tryHide())})})}}();return"object"===("undefined"==typeof _ret2?"undefined":babelHelpers["typeof"](_ret2))?_ret2.v:void 0}},{key:"_cancel",value:function(){var _this5=this;this.cancelable&&!this._running&&(this._running=!0,this.hide({callback:function(){_this5._running=!1,util.triggerElementEvent(_this5,"dialog-cancel")}}))}},{key:"connectedCallback",value:function(){var _this6=this;this.onDeviceBackButton=function(e){return _this6.cancelable?_this6._cancel():e.callParentHandler()},contentReady(this,function(){_this6._mask.addEventListener("click",_this6._boundCancel,!1)})}},{key:"disconnectedCallback",value:function(){this._backButtonHandler.destroy(),this._backButtonHandler=null,this._mask.removeEventListener("click",this._boundCancel.bind(this),!1)}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme):void("animation"===name&&this._updateAnimatorFactory())}},{key:"_mask",get:function(){return util.findChild(this,".alert-dialog-mask")}},{key:"_dialog",get:function(){return util.findChild(this,".alert-dialog")}},{key:"_titleElement",get:function(){return util.findChild(this._dialog.children[0],".alert-dialog-title")}},{key:"_contentElement",get:function(){return util.findChild(this._dialog.children[0],".alert-dialog-content")}},{key:"disabled",set:function(value){return util.toggleAttribute(this,"disabled",value)},get:function(){return this.hasAttribute("disabled")}},{key:"cancelable",set:function(value){return util.toggleAttribute(this,"cancelable",value)},get:function(){return this.hasAttribute("cancelable")}},{key:"visible",get:function(){return this._visible}},{key:"onDeviceBackButton",get:function(){return this._backButtonHandler},set:function(callback){this._backButtonHandler&&this._backButtonHandler.destroy(),this._backButtonHandler=deviceBackButtonDispatcher.createHandler(this,callback)}}],[{key:"registerAnimator",value:function(name,Animator){if(!(Animator.prototype instanceof AlertDialogAnimator))throw new Error('"Animator" param must inherit OnsAlertDialogElement.AlertDialogAnimator');_animatorDict[name]=Animator}},{key:"observedAttributes",get:function(){return["modifier","animation"]}},{key:"AlertDialogAnimator",get:function(){return AlertDialogAnimator}}]),AlertDialogElement}(BaseElement);customElements.define("ons-alert-dialog",AlertDialogElement);var scheme$1={"":"back-button--*",".back-button__icon":"back-button--*__icon",".back-button__label":"back-button--*__label"},BackButtonElement=function(_BaseElement){function BackButtonElement(){return babelHelpers.classCallCheck(this,BackButtonElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(BackButtonElement).apply(this,arguments))}return babelHelpers.inherits(BackButtonElement,_BaseElement),babelHelpers.createClass(BackButtonElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){_this2._compile()}),this._options={},this._boundOnClick=this._onClick.bind(this)}},{key:"_compile",value:function(){if(autoStyle.prepare(this),this.classList.add("back-button"),!util.findChild(this,".back-button__label")){for(var label=util.create("span.back-button__label");this.childNodes[0];)label.appendChild(this.childNodes[0]);this.appendChild(label)}if(!util.findChild(this,".back-button__icon")){var icon=util.create("span.back-button__icon");this.insertBefore(icon,this.children[0])}ModifierUtil.initModifier(this,scheme$1)}},{key:"_onClick",value:function(){if(this.onClick)this.onClick.apply(this);else{var navigator=util.findParent(this,"ons-navigator");navigator&&navigator.popPage(this.options)}}},{key:"connectedCallback",value:function(){this.addEventListener("click",this._boundOnClick,!1)}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$1):void 0}},{key:"disconnectedCallback",value:function(){this.removeEventListener("click",this._boundOnClick,!1)}},{key:"show",value:function(){this.style.display="inline-block"}},{key:"hide",value:function(){this.style.display="none"}},{key:"options",get:function(){return this._options},set:function(object){this._options=object}}],[{key:"observedAttributes",get:function(){return["modifier"]}}]),BackButtonElement}(BaseElement);customElements.define("ons-back-button",BackButtonElement);var scheme$2={"":"bottom-bar--*"},BottomToolbarElement=function(_BaseElement){function BottomToolbarElement(){return babelHelpers.classCallCheck(this,BottomToolbarElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(BottomToolbarElement).apply(this,arguments))}return babelHelpers.inherits(BottomToolbarElement,_BaseElement),babelHelpers.createClass(BottomToolbarElement,[{key:"init",value:function(){this.classList.add("bottom-bar"),ModifierUtil.initModifier(this,scheme$2)}},{key:"connectedCallback",value:function(){util.match(this.parentNode,"ons-page")&&this.parentNode.classList.add("page-with-bottom-toolbar")}},{key:"attributeChangedCallback",value:function(name,last,current){"modifier"===name&&ModifierUtil.onModifierChanged(last,current,this,scheme$2)}}],[{key:"observedAttributes",get:function(){return["modifier"]}}]),BottomToolbarElement}(BaseElement);customElements.define("ons-bottom-toolbar",BottomToolbarElement);var scheme$3={"":"button--*"},ButtonElement=function(_BaseElement){function ButtonElement(){return babelHelpers.classCallCheck(this,ButtonElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(ButtonElement).apply(this,arguments))}return babelHelpers.inherits(ButtonElement,_BaseElement),babelHelpers.createClass(ButtonElement,[{key:"init",value:function(){this.hasAttribute("_compiled")||this._compile()}},{key:"attributeChangedCallback",value:function(name,last,current){switch(name){case"modifier":ModifierUtil.onModifierChanged(last,current,this,scheme$3);break;case"ripple":this._updateRipple()}}},{key:"_compile",value:function(){autoStyle.prepare(this),this.classList.add("button"),this._updateRipple(),ModifierUtil.initModifier(this,scheme$3),this.setAttribute("_compiled","");
}},{key:"_updateRipple",value:function(){util.updateRipple(this)}},{key:"disabled",set:function(value){return util.toggleAttribute(this,"disabled",value)},get:function(){return this.hasAttribute("disabled")}}],[{key:"observedAttributes",get:function(){return["modifier","ripple"]}}]),ButtonElement}(BaseElement);customElements.define("ons-button",ButtonElement);var scheme$4={"":"carousel-item--*"},CarouselItemElement=function(_BaseElement){function CarouselItemElement(){return babelHelpers.classCallCheck(this,CarouselItemElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(CarouselItemElement).apply(this,arguments))}return babelHelpers.inherits(CarouselItemElement,_BaseElement),babelHelpers.createClass(CarouselItemElement,[{key:"init",value:function(){this.style.width="100%",ModifierUtil.initModifier(this,scheme$4)}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$4):void 0}}],[{key:"observedAttributes",get:function(){return["modifier"]}}]),CarouselItemElement}(BaseElement);customElements.define("ons-carousel-item",CarouselItemElement);var VerticalModeTrait={_getScrollDelta:function(event){return event.gesture.deltaY},_getScrollVelocity:function(event){return event.gesture.velocityY},_getElementSize:function(){return this._currentElementSize||(this._currentElementSize=this.getBoundingClientRect().height),this._currentElementSize},_generateScrollTransform:function(scroll){return"translate3d(0px, "+-scroll+"px, 0px)"},_updateDimensionData:function(){this._style=window.getComputedStyle(this),this._dimensions=this.getBoundingClientRect()},_updateOffset:function(){if(this.centered){var height=(this._dimensions.height||0)-parseInt(this._style.paddingTop,10)-parseInt(this._style.paddingBottom,10);this._offset=-(height-this._getCarouselItemSize())/2}},_layoutCarouselItems:function(){for(var children=this._getCarouselItemElements(),sizeAttr=this._getCarouselItemSizeAttr(),sizeInfo=this._decomposeSizeString(sizeAttr),i=0;i<children.length;i++)children[i].style.position="absolute",children[i].style.height=sizeAttr,children[i].style.visibility="visible",children[i].style.top=i*sizeInfo.number+sizeInfo.unit},_setup:function(){this._updateDimensionData(),this._updateOffset(),this._layoutCarouselItems()}},HorizontalModeTrait={_getScrollDelta:function(event){return event.gesture.deltaX},_getScrollVelocity:function(event){return event.gesture.velocityX},_getElementSize:function(){return this._currentElementSize||(this._currentElementSize=this.getBoundingClientRect().width),this._currentElementSize},_generateScrollTransform:function(scroll){return"translate3d("+-scroll+"px, 0px, 0px)"},_updateDimensionData:function(){this._style=window.getComputedStyle(this),this._dimensions=this.getBoundingClientRect()},_updateOffset:function(){if(this.centered){var width=(this._dimensions.width||0)-parseInt(this._style.paddingLeft,10)-parseInt(this._style.paddingRight,10);this._offset=-(width-this._getCarouselItemSize())/2}},_layoutCarouselItems:function(){for(var children=this._getCarouselItemElements(),sizeAttr=this._getCarouselItemSizeAttr(),sizeInfo=this._decomposeSizeString(sizeAttr),i=0;i<children.length;i++)children[i].style.position="absolute",children[i].style.width=sizeAttr,children[i].style.visibility="visible",children[i].style.left=i*sizeInfo.number+sizeInfo.unit},_setup:function(){this._updateDimensionData(),this._updateOffset(),this._layoutCarouselItems()}},CarouselElement=function(_BaseElement){function CarouselElement(){return babelHelpers.classCallCheck(this,CarouselElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(CarouselElement).apply(this,arguments))}return babelHelpers.inherits(CarouselElement,_BaseElement),babelHelpers.createClass(CarouselElement,[{key:"init",value:function(){this._doorLock=new DoorLock,this._scroll=0,this._offset=0,this._lastActiveIndex=0,this._boundOnDrag=this._onDrag.bind(this),this._boundOnDragEnd=this._onDragEnd.bind(this),this._boundOnResize=this._onResize.bind(this),this._mixin(this._isVertical()?VerticalModeTrait:HorizontalModeTrait)}},{key:"_onResize",value:function(){var i=this._scroll/this._currentElementSize;delete this._currentElementSize,this.setActiveIndex(i)}},{key:"_onDirectionChange",value:function(){this._isVertical()?(this.style.overflowX="auto",this.style.overflowY=""):(this.style.overflowX="",this.style.overflowY="auto"),this.refresh()}},{key:"_saveLastState",value:function(){this._lastState={elementSize:this._getCarouselItemSize(),carouselElementCount:this.itemCount,width:this._getCarouselItemSize()*this.itemCount}}},{key:"_getCarouselItemSize",value:function(){var sizeAttr=this._getCarouselItemSizeAttr(),sizeInfo=this._decomposeSizeString(sizeAttr),elementSize=this._getElementSize();if("%"===sizeInfo.unit)return Math.round(sizeInfo.number/100*elementSize);if("px"===sizeInfo.unit)return sizeInfo.number;throw new Error("Invalid state")}},{key:"_getInitialIndex",value:function(){var index=parseInt(this.getAttribute("initial-index"),10);return"number"!=typeof index||isNaN(index)?0:Math.max(Math.min(index,this.itemCount-1),0)}},{key:"_getCarouselItemSizeAttr",value:function(){var attrName="item-"+(this._isVertical()?"height":"width"),itemSizeAttr=(""+this.getAttribute(attrName)).trim();return itemSizeAttr.match(/^\d+(px|%)$/)?itemSizeAttr:"100%"}},{key:"_decomposeSizeString",value:function(size){var matches=size.match(/^(\d+)(px|%)/);return{number:parseInt(matches[1],10),unit:matches[2]}}},{key:"_setupInitialIndex",value:function(){this._scroll=(this._offset||0)+this._getCarouselItemSize()*this._getInitialIndex(),this._lastActiveIndex=this._getInitialIndex(),this._scrollTo(this._scroll)}},{key:"setActiveIndex",value:function(index){var _this2=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];if(options&&"object"!=("undefined"==typeof options?"undefined":babelHelpers["typeof"](options)))throw new Error("options must be an object. You supplied "+options);options.animationOptions=util.extend({duration:.3,timing:"cubic-bezier(.1, .7, .1, 1)"},options.animationOptions||{},this.hasAttribute("animation-options")?util.animationOptionsParse(this.getAttribute("animation-options")):{}),index=Math.max(0,Math.min(index,this.itemCount-1));var scroll=(this._offset||0)+this._getCarouselItemSize()*index,max=this._calculateMaxScroll();return this._scroll=Math.max(0,Math.min(max,scroll)),this._scrollTo(this._scroll,options).then(function(){return _this2._tryFirePostChangeEvent(),_this2})}},{key:"getActiveIndex",value:function(){var scroll=this._scroll-(this._offset||0),count=this.itemCount,size=this._getCarouselItemSize();if(0>scroll)return 0;var i=void 0;for(i=0;count>i;i++)if(scroll>=size*i&&size*(i+1)>scroll)return i;return i}},{key:"next",value:function(options){return this.setActiveIndex(this.getActiveIndex()+1,options)}},{key:"prev",value:function(options){return this.setActiveIndex(this.getActiveIndex()-1,options)}},{key:"_isEnabledChangeEvent",value:function(){var elementSize=this._getElementSize(),carouselItemSize=this._getCarouselItemSize();return this.autoScroll&&elementSize===carouselItemSize}},{key:"_isVertical",value:function(){return"vertical"===this.getAttribute("direction")}},{key:"_prepareEventListeners",value:function(){var _this3=this;this._gestureDetector=new GestureDetector(this,{dragMinDistance:1,dragLockToAxis:!0}),this._mutationObserver=new MutationObserver(function(){return _this3.refresh()}),this._updateSwipeable(),this._updateAutoRefresh(),window.addEventListener("resize",this._boundOnResize,!0)}},{key:"_removeEventListeners",value:function(){this._gestureDetector.dispose(),this._gestureDetector=null,this._mutationObserver.disconnect(),this._mutationObserver=null,window.removeEventListener("resize",this._boundOnResize,!0)}},{key:"_updateSwipeable",value:function(){this._gestureDetector&&(this.swipeable?(this._gestureDetector.on("drag dragleft dragright dragup dragdown swipe swipeleft swiperight swipeup swipedown",this._boundOnDrag),this._gestureDetector.on("dragend",this._boundOnDragEnd)):(this._gestureDetector.off("drag dragleft dragright dragup dragdown swipe swipeleft swiperight swipeup swipedown",this._boundOnDrag),this._gestureDetector.off("dragend",this._boundOnDragEnd)))}},{key:"_updateAutoRefresh",value:function(){this._mutationObserver&&(this.hasAttribute("auto-refresh")?this._mutationObserver.observe(this,{childList:!0}):this._mutationObserver.disconnect())}},{key:"_tryFirePostChangeEvent",value:function(){var currentIndex=this.getActiveIndex();if(this._lastActiveIndex!==currentIndex){var lastActiveIndex=this._lastActiveIndex;this._lastActiveIndex=currentIndex,util.triggerElementEvent(this,"postchange",{carousel:this,activeIndex:currentIndex,lastActiveIndex:lastActiveIndex})}}},{key:"_isWrongDirection",value:function(d){return this._isVertical()?"left"===d||"right"===d:"up"===d||"down"===d}},{key:"_onDrag",value:function(event){if(!this._isWrongDirection(event.gesture.direction)){event.stopPropagation(),this._lastDragEvent=event;var scroll=this._scroll-this._getScrollDelta(event);this._scrollTo(scroll),event.gesture.preventDefault(),this._tryFirePostChangeEvent()}}},{key:"_onDragEnd",value:function(event){var _this4=this;if(this._lastDragEvent){if(this._currentElementSize=void 0,this._scroll=this._scroll-this._getScrollDelta(event),this._isOverScroll(this._scroll)){var waitForAction=!1;util.triggerElementEvent(this,"overscroll",{carousel:this,activeIndex:this.getActiveIndex(),direction:this._getOverScrollDirection(),waitToReturn:function(promise){waitForAction=!0,promise.then(function(){return _this4._scrollToKillOverScroll()})}}),waitForAction||this._scrollToKillOverScroll()}else this._startMomentumScroll();this._lastDragEvent=null,event.gesture.preventDefault()}}},{key:"_mixin",value:function(trait){Object.keys(trait).forEach(function(key){this[key]=trait[key]}.bind(this))}},{key:"_startMomentumScroll",value:function(){if(this._lastDragEvent){var velocity=this._getScrollVelocity(this._lastDragEvent),duration=.3,scrollDelta=100*duration*velocity,scroll=this._normalizeScrollPosition(this._scroll+(this._getScrollDelta(this._lastDragEvent)>0?-scrollDelta:scrollDelta));this._scroll=scroll,animit(this._getCarouselItemElements()).queue({transform:this._generateScrollTransform(this._scroll)},{duration:duration,timing:"cubic-bezier(.1, .7, .1, 1)"}).queue(function(done){done(),this._tryFirePostChangeEvent()}.bind(this)).play()}}},{key:"_normalizeScrollPosition",value:function(scroll){var max=this._calculateMaxScroll();if(!this.autoScroll)return Math.max(0,Math.min(max,scroll));for(var arr=[],size=this._getCarouselItemSize(),nbrOfItems=this.itemCount,i=0;nbrOfItems>i;i++)i*size+this._offset<max&&arr.push(i*size+this._offset);arr.push(max),arr.sort(function(left,right){return left=Math.abs(left-scroll),right=Math.abs(right-scroll),left-right}),arr=arr.filter(function(item,pos){return!pos||item!=arr[pos-1]});var lastScroll=this._lastActiveIndex*size+this._offset,scrollRatio=Math.abs(scroll-lastScroll)/size,result=arr[0];return scrollRatio<=this.autoScrollRatio?result=lastScroll:1>scrollRatio&&arr[0]===lastScroll&&arr.length>1&&(result=arr[1]),Math.max(0,Math.min(max,result))}},{key:"_getCarouselItemElements",value:function(){return util.arrayFrom(this.children).filter(function(child){return"ons-carousel-item"===child.nodeName.toLowerCase()})}},{key:"_scrollTo",value:function(scroll){var _this5=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1],isOverscrollable=this.overscrollable,normalizeScroll=function(scroll){var ratio=.35;if(0>scroll)return isOverscrollable?Math.round(scroll*ratio):0;var maxScroll=_this5._calculateMaxScroll();return scroll>maxScroll?isOverscrollable?maxScroll+Math.round((scroll-maxScroll)*ratio):maxScroll:scroll};return new Promise(function(resolve){animit(_this5._getCarouselItemElements()).queue({transform:_this5._generateScrollTransform(normalizeScroll(scroll))},"none"!==options.animation?options.animationOptions:{}).play(function(){options.callback instanceof Function&&options.callback(),resolve()})})}},{key:"_calculateMaxScroll",value:function(){var max=this.itemCount*this._getCarouselItemSize()-this._getElementSize();return Math.ceil(0>max?0:max)}},{key:"_isOverScroll",value:function(scroll){return 0>scroll||scroll>this._calculateMaxScroll()}},{key:"_getOverScrollDirection",value:function(){return this._isVertical()?this._scroll<=0?"up":"down":this._scroll<=0?"left":"right"}},{key:"_scrollToKillOverScroll",value:function(){var duration=.4;if(this._scroll<0)return animit(this._getCarouselItemElements()).queue({transform:this._generateScrollTransform(0)},{duration:duration,timing:"cubic-bezier(.1, .4, .1, 1)"}).queue(function(done){done(),this._tryFirePostChangeEvent()}.bind(this)).play(),void(this._scroll=0);var maxScroll=this._calculateMaxScroll();return maxScroll<this._scroll?(animit(this._getCarouselItemElements()).queue({transform:this._generateScrollTransform(maxScroll)},{duration:duration,timing:"cubic-bezier(.1, .4, .1, 1)"}).queue(function(done){done(),this._tryFirePostChangeEvent()}.bind(this)).play(),void(this._scroll=maxScroll)):void 0}},{key:"refresh",value:function(){if(0!==this._getCarouselItemSize()){if(this._mixin(this._isVertical()?VerticalModeTrait:HorizontalModeTrait),this._setup(),this._lastState&&this._lastState.width>0){var scroll=this._scroll;this._isOverScroll(scroll)?this._scrollToKillOverScroll():(this.autoScroll&&(scroll=this._normalizeScrollPosition(scroll)),this._scrollTo(scroll))}this._saveLastState(),util.triggerElementEvent(this,"refresh",{carousel:this})}}},{key:"first",value:function(options){return this.setActiveIndex(0,options)}},{key:"last",value:function(options){this.setActiveIndex(Math.max(this.itemCount-1,0),options)}},{key:"connectedCallback",value:function(){var _this6=this;this._prepareEventListeners(),this._setup(),this._setupInitialIndex(),this._saveLastState(),0===this.offsetHeight&&setImmediate(function(){return _this6.refresh()})}},{key:"attributeChangedCallback",value:function(name,last,current){switch(name){case"swipeable":this._updateSwipeable();break;case"auto-refresh":this._updateAutoRefresh();break;case"direction":this._onDirectionChange()}}},{key:"disconnectedCallback",value:function(){this._removeEventListeners()}},{key:"itemCount",get:function(){return this._getCarouselItemElements().length}},{key:"autoScrollRatio",get:function(){var attr=this.getAttribute("auto-scroll-ratio");if(!attr)return.5;var scrollRatio=parseFloat(attr);if(0>scrollRatio||scrollRatio>1)throw new Error("Invalid ratio.");return isNaN(scrollRatio)?.5:scrollRatio},set:function(ratio){if(0>ratio||ratio>1)throw new Error("Invalid ratio.");this.setAttribute("auto-scroll-ratio",ratio)}},{key:"swipeable",get:function(){return this.hasAttribute("swipeable")},set:function(value){return util.toggleAttribute(this,"swipeable",value)}},{key:"autoScroll",get:function(){return this.hasAttribute("auto-scroll")},set:function(value){return util.toggleAttribute(this,"auto-scroll",value)}},{key:"disabled",get:function(){return this.hasAttribute("disabled")},set:function(value){return util.toggleAttribute(this,"disabled",value)}},{key:"overscrollable",get:function(){return this.hasAttribute("overscrollable")},set:function(value){return util.toggleAttribute(this,"overscrollable",value)}},{key:"centered",get:function(){return this.hasAttribute("centered")},set:function(value){return util.toggleAttribute(this,"centered",value)}}],[{key:"observedAttributes",get:function(){return["swipeable","auto-refresh","direction"]}}]),CarouselElement}(BaseElement);customElements.define("ons-carousel",CarouselElement);var ColElement=function(_BaseElement){function ColElement(){return babelHelpers.classCallCheck(this,ColElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(ColElement).apply(this,arguments))}return babelHelpers.inherits(ColElement,_BaseElement),babelHelpers.createClass(ColElement,[{key:"init",value:function(){this.getAttribute("width")&&this._updateWidth()}},{key:"attributeChangedCallback",value:function(name,last,current){"width"===name&&this._updateWidth()}},{key:"_updateWidth",value:function(){var width=this.getAttribute("width");"string"==typeof width&&(width=(""+width).trim(),width=width.match(/^\d+$/)?width+"%":width,this.style.webkitBoxFlex="0",this.style.webkitFlex="0 0 "+width,this.style.mozBoxFlex="0",this.style.mozFlex="0 0 "+width,this.style.msFlex="0 0 "+width,this.style.flex="0 0 "+width,this.style.maxWidth=width)}}],[{key:"observedAttributes",get:function(){return["width"]}}]),ColElement}(BaseElement);customElements.define("ons-col",ColElement);var DialogAnimator=function(){function DialogAnimator(){var _ref=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_ref$timing=_ref.timing,timing=void 0===_ref$timing?"linear":_ref$timing,_ref$delay=_ref.delay,delay=void 0===_ref$delay?0:_ref$delay,_ref$duration=_ref.duration,duration=void 0===_ref$duration?.2:_ref$duration;babelHelpers.classCallCheck(this,DialogAnimator),this.timing=timing,this.delay=delay,this.duration=duration}return babelHelpers.createClass(DialogAnimator,[{key:"show",value:function(dialog,done){done()}},{key:"hide",value:function(dialog,done){done()}}]),DialogAnimator}(),AndroidDialogAnimator=function(_DialogAnimator){function AndroidDialogAnimator(){var _ref2=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_ref2$timing=_ref2.timing,timing=void 0===_ref2$timing?"ease-in-out":_ref2$timing,_ref2$delay=_ref2.delay,delay=void 0===_ref2$delay?0:_ref2$delay,_ref2$duration=_ref2.duration,duration=void 0===_ref2$duration?.3:_ref2$duration;return babelHelpers.classCallCheck(this,AndroidDialogAnimator),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(AndroidDialogAnimator).call(this,{timing:timing,delay:delay,duration:duration}))}return babelHelpers.inherits(AndroidDialogAnimator,_DialogAnimator),babelHelpers.createClass(AndroidDialogAnimator,[{key:"show",value:function(dialog,callback){callback=callback?callback:function(){},animit.runAll(animit(dialog._mask).queue({opacity:0}).wait(this.delay).queue({opacity:1},{duration:this.duration,timing:this.timing}),animit(dialog._dialog).saveStyle().queue({css:{transform:"translate3d(-50%, -60%, 0)",opacity:0},duration:0}).wait(this.delay).queue({css:{transform:"translate3d(-50%, -50%, 0)",opacity:1},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}))}},{key:"hide",value:function(dialog,callback){callback=callback?callback:function(){},animit.runAll(animit(dialog._mask).queue({opacity:1}).wait(this.delay).queue({opacity:0},{duration:this.duration,timing:this.timing}),animit(dialog._dialog).saveStyle().queue({css:{transform:"translate3d(-50%, -50%, 0)",opacity:1},duration:0}).wait(this.delay).queue({css:{transform:"translate3d(-50%, -60%, 0)",opacity:0},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}))}}]),AndroidDialogAnimator}(DialogAnimator),IOSDialogAnimator=function(_DialogAnimator2){function IOSDialogAnimator(){var _ref3=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_ref3$timing=_ref3.timing,timing=void 0===_ref3$timing?"ease-in-out":_ref3$timing,_ref3$delay=_ref3.delay,delay=void 0===_ref3$delay?0:_ref3$delay,_ref3$duration=_ref3.duration,duration=void 0===_ref3$duration?.3:_ref3$duration;return babelHelpers.classCallCheck(this,IOSDialogAnimator),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(IOSDialogAnimator).call(this,{timing:timing,delay:delay,duration:duration}))}return babelHelpers.inherits(IOSDialogAnimator,_DialogAnimator2),babelHelpers.createClass(IOSDialogAnimator,[{key:"show",value:function(dialog,callback){callback=callback?callback:function(){},animit.runAll(animit(dialog._mask).queue({opacity:0}).wait(this.delay).queue({opacity:1},{duration:this.duration,timing:this.timing}),animit(dialog._dialog).saveStyle().queue({css:{transform:"translate3d(-50%, 300%, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3d(-50%, -50%, 0)"},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}))}},{key:"hide",value:function(dialog,callback){callback=callback?callback:function(){},animit.runAll(animit(dialog._mask).queue({opacity:1}).wait(this.delay).queue({opacity:0},{duration:this.duration,timing:this.timing}),animit(dialog._dialog).saveStyle().queue({css:{transform:"translate3d(-50%, -50%, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3d(-50%, 300%, 0)"},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}))}}]),IOSDialogAnimator}(DialogAnimator),SlideDialogAnimator=function(_DialogAnimator3){function SlideDialogAnimator(){var _ref4=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_ref4$timing=_ref4.timing,timing=void 0===_ref4$timing?"cubic-bezier(.1, .7, .4, 1)":_ref4$timing,_ref4$delay=_ref4.delay,delay=void 0===_ref4$delay?0:_ref4$delay,_ref4$duration=_ref4.duration,duration=void 0===_ref4$duration?.2:_ref4$duration;return babelHelpers.classCallCheck(this,SlideDialogAnimator),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(SlideDialogAnimator).call(this,{timing:timing,delay:delay,duration:duration}))}return babelHelpers.inherits(SlideDialogAnimator,_DialogAnimator3),babelHelpers.createClass(SlideDialogAnimator,[{key:"show",value:function(dialog,callback){callback=callback?callback:function(){},animit.runAll(animit(dialog._mask).queue({opacity:0}).wait(this.delay).queue({opacity:1},{duration:this.duration,timing:this.timing}),animit(dialog._dialog).saveStyle().queue({css:{transform:"translate3D(-50%, -350%, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(-50%, -50%, 0)"},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}))}},{key:"hide",value:function(dialog,callback){callback=callback?callback:function(){},animit.runAll(animit(dialog._mask).queue({opacity:1}).wait(this.delay).queue({opacity:0},{duration:this.duration,timing:this.timing}),animit(dialog._dialog).saveStyle().queue({css:{transform:"translate3D(-50%, -50%, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(-50%, -350%, 0)"},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}))}}]),SlideDialogAnimator}(DialogAnimator),scheme$5={".dialog":"dialog--*",".dialog-container":"dialog-container--*",".dialog-mask":"dialog-mask--*"},_animatorDict$1={"default":function(){return platform.isAndroid()?AndroidDialogAnimator:IOSDialogAnimator},slide:SlideDialogAnimator,none:DialogAnimator},DialogElement=function(_BaseElement){function DialogElement(){return babelHelpers.classCallCheck(this,DialogElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(DialogElement).apply(this,arguments))}return babelHelpers.inherits(DialogElement,_BaseElement),babelHelpers.createClass(DialogElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){return _this2._compile()}),this._visible=!1,this._doorLock=new DoorLock,this._boundCancel=this._cancel.bind(this),this._updateAnimatorFactory()}},{key:"_updateAnimatorFactory",value:function(){this._animatorFactory=new AnimatorFactory({animators:_animatorDict$1,baseClass:DialogAnimator,baseClassName:"DialogAnimator",defaultAnimation:this.getAttribute("animation")})}},{key:"_compile",value:function(){if(autoStyle.prepare(this),this.style.display="none",!this._dialog){var dialog=document.createElement("div");dialog.classList.add("dialog");var container=document.createElement("div");for(dialog.classList.add("dialog-container"),dialog.appendChild(container);this.firstChild;)container.appendChild(this.firstChild);this.appendChild(dialog)}if(!this._mask){var mask=document.createElement("div");mask.classList.add("dialog-mask"),this.insertBefore(mask,this.firstChild)}this._dialog.style.zIndex=20001,this._mask.style.zIndex=2e4,this.setAttribute("status-bar-fill",""),ModifierUtil.initModifier(this,scheme$5)}},{key:"_cancel",value:function(){var _this3=this;this.cancelable&&!this._running&&(this._running=!0,this.hide({callback:function(){_this3._running=!1,util.triggerElementEvent(_this3,"dialog-cancel")}}))}},{key:"show",value:function(){var _this4=this,options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_cancel2=!1,callback=options.callback||function(){};if(options.animationOptions=util.extend(options.animationOptions||{},AnimatorFactory.parseAnimationOptionsString(this.getAttribute("animation-options"))),util.triggerElementEvent(this,"preshow",{dialog:this,cancel:function(){_cancel2=!0}}),_cancel2)return Promise.reject("Canceled in preshow event.");var _ret=function(){var tryShow=function(){var unlock=_this4._doorLock.lock(),animator=_this4._animatorFactory.newAnimator(options);return _this4.style.display="block",_this4._mask.style.opacity="1",new Promise(function(resolve){contentReady(_this4,function(){animator.show(_this4,function(){_this4._visible=!0,unlock(),util.triggerElementEvent(_this4,"postshow",{dialog:_this4}),callback(),resolve(_this4)})})})};return{v:new Promise(function(resolve){_this4._doorLock.waitUnlock(function(){return resolve(tryShow())})})}}();return"object"===("undefined"==typeof _ret?"undefined":babelHelpers["typeof"](_ret))?_ret.v:void 0}},{key:"hide",value:function(){var _this5=this,options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_cancel3=!1,callback=options.callback||function(){};if(options.animationOptions=util.extend(options.animationOptions||{},AnimatorFactory.parseAnimationOptionsString(this.getAttribute("animation-options"))),util.triggerElementEvent(this,"prehide",{dialog:this,cancel:function(){_cancel3=!0}}),_cancel3)return Promise.reject("Canceled in prehide event.");var _ret2=function(){var tryHide=function(){var unlock=_this5._doorLock.lock(),animator=_this5._animatorFactory.newAnimator(options);return new Promise(function(resolve){contentReady(_this5,function(){animator.hide(_this5,function(){_this5.style.display="none",_this5._visible=!1,unlock(),util.triggerElementEvent(_this5,"posthide",{dialog:_this5}),callback(),resolve(_this5)})})})};return{v:new Promise(function(resolve){_this5._doorLock.waitUnlock(function(){return resolve(tryHide())})})}}();return"object"===("undefined"==typeof _ret2?"undefined":babelHelpers["typeof"](_ret2))?_ret2.v:void 0}},{key:"connectedCallback",value:function(){var _this6=this;this.onDeviceBackButton=function(e){return _this6.cancelable?_this6._cancel():e.callParentHandler()},contentReady(this,function(){_this6._mask.addEventListener("click",_this6._boundCancel,!1)})}},{key:"disconnectedCallback",value:function(){this._backButtonHandler.destroy(),this._backButtonHandler=null,this._mask.removeEventListener("click",this._boundCancel.bind(this),!1)}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$5):void("animation"===name&&this._updateAnimatorFactory())}},{key:"_mask",get:function(){return util.findChild(this,".dialog-mask")}},{key:"_dialog",get:function(){return util.findChild(this,".dialog")}},{key:"onDeviceBackButton",get:function(){return this._backButtonHandler},set:function(callback){this._backButtonHandler&&this._backButtonHandler.destroy(),this._backButtonHandler=deviceBackButtonDispatcher.createHandler(this,callback)}},{key:"visible",get:function(){return this._visible}},{key:"disabled",set:function(value){return util.toggleAttribute(this,"disabled",value)},get:function(){return this.hasAttribute("disabled")}},{key:"cancelable",set:function(value){return util.toggleAttribute(this,"cancelable",value)},get:function(){return this.hasAttribute("cancelable")}}],[{key:"registerAnimator",value:function(name,Animator){if(!(Animator.prototype instanceof DialogAnimator))throw new Error('"Animator" param must inherit OnsDialogElement.DialogAnimator');_animatorDict$1[name]=Animator}},{key:"observedAttributes",get:function(){return["modifier","animation"]}},{key:"DialogAnimator",get:function(){return DialogAnimator}}]),DialogElement}(BaseElement);customElements.define("ons-dialog",DialogElement);var scheme$6={"":"fab--*"},FabElement=function(_BaseElement){function FabElement(){return babelHelpers.classCallCheck(this,FabElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(FabElement).apply(this,arguments))}return babelHelpers.inherits(FabElement,_BaseElement),babelHelpers.createClass(FabElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){_this2._compile()})}},{key:"_compile",value:function(){var _this3=this;autoStyle.prepare(this),this.classList.add("fab"),util.findChild(this,".fab__icon")||!function(){var content=document.createElement("span");content.classList.add("fab__icon"),util.arrayFrom(_this3.childNodes).forEach(function(element){element.tagName&&"ons-ripple"===element.tagName.toLowerCase()||content.appendChild(element)}),_this3.appendChild(content)}(),this._updateRipple(),ModifierUtil.initModifier(this,scheme$6),this._updatePosition(),this.show()}},{key:"attributeChangedCallback",value:function(name,last,current){switch(name){case"modifier":ModifierUtil.onModifierChanged(last,current,this,scheme$6);break;case"ripple":this._updateRipple();break;case"position":this._updatePosition()}}},{key:"_show",value:function(){this.show()}},{key:"_hide",value:function(){this.hide()}},{key:"_updateRipple",value:function(){util.updateRipple(this)}},{key:"_updatePosition",value:function(){var position=this.getAttribute("position");switch(this.classList.remove("fab--top__left","fab--bottom__right","fab--bottom__left","fab--top__right","fab--top__center","fab--bottom__center"),position){case"top right":case"right top":this.classList.add("fab--top__right");break;case"top left":case"left top":this.classList.add("fab--top__left");break;case"bottom right":case"right bottom":this.classList.add("fab--bottom__right");break;case"bottom left":case"left bottom":this.classList.add("fab--bottom__left");break;case"center top":case"top center":this.classList.add("fab--top__center");break;case"center bottom":case"bottom center":this.classList.add("fab--bottom__center")}}},{key:"show",value:function(){arguments.length<=0||void 0===arguments[0]?{}:arguments[0];this.style.transform="scale(1)",this.style.webkitTransform="scale(1)"}},{key:"hide",value:function(){arguments.length<=0||void 0===arguments[0]?{}:arguments[0];this.style.transform="scale(0)",this.style.webkitTransform="scale(0)"}},{key:"toggle",value:function(){this.visible?this.hide():this.show()}},{key:"disabled",set:function(value){return util.toggleAttribute(this,"disabled",value)},get:function(){return this.hasAttribute("disabled")}},{key:"visible",get:function(){return"scale(1)"===this.style.transform&&"none"!==this.style.display}}],[{key:"observedAttributes",get:function(){return["modifier","ripple","position"]}}]),FabElement}(BaseElement);customElements.define("ons-fab",FabElement);var GestureDetectorElement=function(_BaseElement){function GestureDetectorElement(){return babelHelpers.classCallCheck(this,GestureDetectorElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(GestureDetectorElement).apply(this,arguments))}return babelHelpers.inherits(GestureDetectorElement,_BaseElement),babelHelpers.createClass(GestureDetectorElement,[{key:"init",value:function(){this._gestureDetector=new GestureDetector(this)}}]),GestureDetectorElement}(BaseElement);customElements.define("ons-gesture-detector",GestureDetectorElement);var IconElement=function(_BaseElement){function IconElement(){return babelHelpers.classCallCheck(this,IconElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(IconElement).apply(this,arguments))}return babelHelpers.inherits(IconElement,_BaseElement),
babelHelpers.createClass(IconElement,[{key:"init",value:function(){this._compile()}},{key:"attributeChangedCallback",value:function(name,last,current){-1!==["icon","size","modifier"].indexOf(name)&&this._update()}},{key:"_compile",value:function(){autoStyle.prepare(this),this._update()}},{key:"_update",value:function(){var _this2=this;this._cleanClassAttribute();var _buildClassAndStyle2=this._buildClassAndStyle(this._getAttribute("icon"),this._getAttribute("size")),classList=_buildClassAndStyle2.classList,style=_buildClassAndStyle2.style;util.extend(this.style,style),classList.forEach(function(className){return _this2.classList.add(className)})}},{key:"_getAttribute",value:function(attr){var parts=(this.getAttribute(attr)||"").split(/\s*,\s*/),def=parts[0],md=parts[1];return md=(md||"").split(/\s*:\s*/),(util.hasModifier(this,md[0])?md[1]:def)||""}},{key:"_cleanClassAttribute",value:function(){var _this3=this;util.arrayFrom(this.classList).filter(function(className){return/^(fa$|fa-|ion-|zmdi-)/.test(className)}).forEach(function(className){return _this3.classList.remove(className)}),this.classList.remove("zmdi"),this.classList.remove("ons-icon--ion")}},{key:"_buildClassAndStyle",value:function(iconName,size){var classList=["ons-icon"],style={};return 0===iconName.indexOf("ion-")?(classList.push(iconName),classList.push("ons-icon--ion")):0===iconName.indexOf("fa-")?(classList.push(iconName),classList.push("fa")):0===iconName.indexOf("md-")?(classList.push("zmdi"),classList.push("zmdi-"+iconName.split(/\-(.+)?/)[1])):(classList.push("fa"),classList.push("fa-"+iconName)),size.match(/^[1-5]x|lg$/)?(classList.push("fa-"+size),this.style.removeProperty("font-size")):style.fontSize=size,{classList:classList,style:style}}}],[{key:"observedAttributes",get:function(){return["icon","size","modifier"]}}]),IconElement}(BaseElement);customElements.define("ons-icon",IconElement);var LazyRepeatElement=function(_BaseElement){function LazyRepeatElement(){return babelHelpers.classCallCheck(this,LazyRepeatElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(LazyRepeatElement).apply(this,arguments))}return babelHelpers.inherits(LazyRepeatElement,_BaseElement),babelHelpers.createClass(LazyRepeatElement,[{key:"connectedCallback",value:function(){util.updateParentPosition(this),this.hasAttribute("delegate")&&(this.delegate=window[this.getAttribute("delegate")])}},{key:"refresh",value:function(){this._lazyRepeatProvider&&this._lazyRepeatProvider.refresh()}},{key:"attributeChangedCallback",value:function(name,last,current){}},{key:"disconnectedCallback",value:function(){this._lazyRepeatProvider&&(this._lazyRepeatProvider.destroy(),this._lazyRepeatProvider=null)}},{key:"delegate",set:function(userDelegate){this._lazyRepeatProvider&&this._lazyRepeatProvider.destroy(),!this._templateElement&&this.children[0]&&(this._templateElement=this.removeChild(this.children[0]));var delegate=new LazyRepeatDelegate(userDelegate,this._templateElement||null);this._lazyRepeatProvider=new LazyRepeatProvider(this.parentElement,delegate)},get:function(){throw new Error("This property can only be used to set the delegate object.")}}]),LazyRepeatElement}(BaseElement);customElements.define("ons-lazy-repeat",LazyRepeatElement);var scheme$7={"":"list__header--*"},ListHeaderElement=function(_BaseElement){function ListHeaderElement(){return babelHelpers.classCallCheck(this,ListHeaderElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(ListHeaderElement).apply(this,arguments))}return babelHelpers.inherits(ListHeaderElement,_BaseElement),babelHelpers.createClass(ListHeaderElement,[{key:"init",value:function(){this.hasAttribute("_compiled")||this._compile()}},{key:"_compile",value:function(){autoStyle.prepare(this),this.classList.add("list__header"),ModifierUtil.initModifier(this,scheme$7),this.setAttribute("_compiled","")}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$7):void 0}}],[{key:"observedAttributes",get:function(){return["modifier"]}}]),ListHeaderElement}(BaseElement);customElements.define("ons-list-header",ListHeaderElement);var scheme$8={".list__item":"list__item--*",".list__item__left":"list__item--*__left",".list__item__center":"list__item--*__center",".list__item__right":"list__item--*__right",".list__item__label":"list__item--*__label",".list__item__title":"list__item--*__title",".list__item__subtitle":"list__item--*__subtitle",".list__item__thumbnail":"list__item--*__thumbnail",".list__item__icon":"list__item--*__icon"},ListItemElement=function(_BaseElement){function ListItemElement(){return babelHelpers.classCallCheck(this,ListItemElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(ListItemElement).apply(this,arguments))}return babelHelpers.inherits(ListItemElement,_BaseElement),babelHelpers.createClass(ListItemElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){_this2._compile()})}},{key:"_compile",value:function(){this.classList.add("list__item");for(var left=void 0,center=void 0,right=void 0,i=0;i<this.children.length;i++){var el=this.children[i];el.classList.contains("left")?(el.classList.add("list__item__left"),left=el):el.classList.contains("center")?center=el:el.classList.contains("right")&&(el.classList.add("list__item__right"),right=el)}if(!center){if(center=document.createElement("div"),left||right)for(var _i=this.childNodes.length-1;_i>=0;_i--){var _el=this.childNodes[_i];_el!==left&&_el!==right&¢er.insertBefore(_el,center.firstChild)}else for(;this.childNodes[0];)center.appendChild(this.childNodes[0]);this.insertBefore(center,right||null)}center.classList.add("center"),center.classList.add("list__item__center"),this._updateRipple(),ModifierUtil.initModifier(this,scheme$8),autoStyle.prepare(this)}},{key:"attributeChangedCallback",value:function(name,last,current){switch(name){case"modifier":ModifierUtil.onModifierChanged(last,current,this,scheme$8);break;case"ripple":this._updateRipple()}}},{key:"connectedCallback",value:function(){this.addEventListener("drag",this._onDrag),this.addEventListener("touchstart",this._onTouch),this.addEventListener("mousedown",this._onTouch),this.addEventListener("touchend",this._onRelease),this.addEventListener("touchmove",this._onRelease),this.addEventListener("touchcancel",this._onRelease),this.addEventListener("mouseup",this._onRelease),this.addEventListener("mouseout",this._onRelease),this.addEventListener("touchleave",this._onRelease),this._originalBackgroundColor=this.style.backgroundColor,this.tapped=!1}},{key:"disconnectedCallback",value:function(){this.removeEventListener("drag",this._onDrag),this.removeEventListener("touchstart",this._onTouch),this.removeEventListener("mousedown",this._onTouch),this.removeEventListener("touchend",this._onRelease),this.removeEventListener("touchmove",this._onRelease),this.removeEventListener("touchcancel",this._onRelease),this.removeEventListener("mouseup",this._onRelease),this.removeEventListener("mouseout",this._onRelease),this.removeEventListener("touchleave",this._onRelease)}},{key:"_updateRipple",value:function(){util.updateRipple(this)}},{key:"_onDrag",value:function(event){var gesture=event.gesture;this._shouldLockOnDrag()&&["left","right"].indexOf(gesture.direction)>-1&&gesture.preventDefault()}},{key:"_onTouch",value:function(){this.tapped||(this.tapped=!0,this.style.transition=this._transition,this.style.webkitTransition=this._transition,this.style.MozTransition=this._transition,this._tappable&&(this.style.backgroundColor&&(this._originalBackgroundColor=this.style.backgroundColor),this.style.backgroundColor=this._tapBackgroundColor,this.style.boxShadow="0px -1px 0px 0px "+this._tapBackgroundColor))}},{key:"_onRelease",value:function(){this.tapped=!1,this.style.transition="",this.style.webkitTransition="",this.style.MozTransition="",this.style.backgroundColor=this._originalBackgroundColor||"",this.style.boxShadow=""}},{key:"_shouldLockOnDrag",value:function(){return this.hasAttribute("lock-on-drag")}},{key:"_transition",get:function(){return"background-color 0.0s linear 0.02s, box-shadow 0.0s linear 0.02s"}},{key:"_tappable",get:function(){return this.hasAttribute("tappable")}},{key:"_tapBackgroundColor",get:function(){return this.getAttribute("tap-background-color")||"#d9d9d9"}}],[{key:"observedAttributes",get:function(){return["modifier","ripple"]}}]),ListItemElement}(BaseElement);customElements.define("ons-list-item",ListItemElement);var scheme$9={"":"list--*"},ListElement=function(_BaseElement){function ListElement(){return babelHelpers.classCallCheck(this,ListElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(ListElement).apply(this,arguments))}return babelHelpers.inherits(ListElement,_BaseElement),babelHelpers.createClass(ListElement,[{key:"init",value:function(){this.hasAttribute("_compiled")||this._compile()}},{key:"_compile",value:function(){autoStyle.prepare(this),this.classList.add("list"),ModifierUtil.initModifier(this,scheme$9),this.setAttribute("_compiled","")}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$9):void 0}}],[{key:"observedAttributes",get:function(){return["modifier"]}}]),ListElement}(BaseElement);customElements.define("ons-list",ListElement);var scheme$10={".text-input":"text-input--*",".text-input__label":"text-input--*__label",".radio-button":"radio-button--*",".radio-button__input":"radio-button--*__input",".radio-button__checkmark":"radio-button--*__checkmark",".checkbox":"checkbox--*",".checkbox__input":"checkbox--*__input",".checkbox__checkmark":"checkbox--*__checkmark"},INPUT_ATTRIBUTES=["autocapitalize","autocomplete","autocorrect","autofocus","disabled","inputmode","max","maxlength","min","minlength","name","pattern","placeholder","readonly","size","step","type","validator","value"],InputElement=function(_BaseElement){function InputElement(){return babelHelpers.classCallCheck(this,InputElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(InputElement).apply(this,arguments))}return babelHelpers.inherits(InputElement,_BaseElement),babelHelpers.createClass(InputElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){_this2._compile(),_this2.attributeChangedCallback("checked",null,_this2.getAttribute("checked"))}),this._boundOnInput=this._onInput.bind(this),this._boundOnFocusin=this._onFocusin.bind(this),this._boundDelegateEvent=this._delegateEvent.bind(this)}},{key:"_compile",value:function(){if(autoStyle.prepare(this),0===this.children.length){var helper=document.createElement("span");helper.classList.add("_helper");var container=document.createElement("label");container.appendChild(document.createElement("input")),container.appendChild(helper);var label=document.createElement("span");switch(label.classList.add("input-label"),util.arrayFrom(this.childNodes).forEach(function(element){return label.appendChild(element)}),this.hasAttribute("content-left")?container.insertBefore(label,container.firstChild):container.appendChild(label),this.appendChild(container),this.getAttribute("type")){case"checkbox":this.classList.add("checkbox"),this._input.classList.add("checkbox__input"),this._helper.classList.add("checkbox__checkmark"),this._updateBoundAttributes();break;case"radio":this.classList.add("radio-button"),this._input.classList.add("radio-button__input"),this._helper.classList.add("radio-button__checkmark"),this._updateBoundAttributes();break;default:this._input.classList.add("text-input"),this._helper.classList.add("text-input__label"),this._input.parentElement.classList.add("text-input__container"),this._updateLabel(),this._updateBoundAttributes(),this._updateLabelClass()}this.hasAttribute("input-id")&&(this._input.id=this.getAttribute("input-id")),ModifierUtil.initModifier(this,scheme$10)}}},{key:"attributeChangedCallback",value:function(name,last,current){var _this3=this;if("modifier"===name)return contentReady(this,function(){return ModifierUtil.onModifierChanged(last,current,_this3,scheme$10)});if("placeholder"===name)return contentReady(this,function(){return _this3._updateLabel()});if("input-id"===name&&contentReady(this,function(){return _this3._input.id=current}),"checked"===name)this.checked=null!==current;else if(INPUT_ATTRIBUTES.indexOf(name)>=0)return contentReady(this,function(){return _this3._updateBoundAttributes()})}},{key:"connectedCallback",value:function(){var _this4=this;contentReady(this,function(){"checkbox"!==_this4._input.type&&"radio"!==_this4._input.type&&(_this4._input.addEventListener("input",_this4._boundOnInput),_this4._input.addEventListener("focusin",_this4._boundOnFocusin),_this4._input.addEventListener("focusout",_this4._boundOnFocusout)),_this4._input.addEventListener("focus",_this4._boundDelegateEvent),_this4._input.addEventListener("blur",_this4._boundDelegateEvent)})}},{key:"disconnectedCallback",value:function(){var _this5=this;contentReady(this,function(){_this5._input.removeEventListener("input",_this5._boundOnInput),_this5._input.removeEventListener("focusin",_this5._boundOnFocusin),_this5._input.removeEventListener("focus",_this5._boundDelegateEvent),_this5._input.removeEventListener("blur",_this5._boundDelegateEvent)})}},{key:"_setLabel",value:function(value){"undefined"!=typeof this._helper.textContent?this._helper.textContent=value:this._helper.innerText=value}},{key:"_updateLabel",value:function(){this._setLabel(this.hasAttribute("placeholder")?this.getAttribute("placeholder"):"")}},{key:"_updateBoundAttributes",value:function(){var _this6=this;INPUT_ATTRIBUTES.forEach(function(attr){_this6.hasAttribute(attr)?_this6._input.setAttribute(attr,_this6.getAttribute(attr)):_this6._input.removeAttribute(attr)})}},{key:"_updateLabelClass",value:function(){""===this.value?this._helper.classList.remove("text-input--material__label--active"):-1===["checkbox","radio"].indexOf(this.getAttribute("type"))&&this._helper.classList.add("text-input--material__label--active")}},{key:"_delegateEvent",value:function(event){var e=new CustomEvent(event.type,{bubbles:!1,cancelable:!0});return this.dispatchEvent(e)}},{key:"_onInput",value:function(event){this._updateLabelClass()}},{key:"_onFocusin",value:function(event){this._updateLabelClass()}},{key:"_input",get:function(){return this.querySelector("input")}},{key:"_helper",get:function(){return this.querySelector("._helper")}},{key:"value",get:function(){return null===this._input?this.getAttribute("value"):this._input.value},set:function(val){var _this7=this;return this.setAttribute("value",val),contentReady(this,function(){_this7._input.value=val,_this7._onInput()}),val}},{key:"checked",get:function(){return this._input.checked},set:function(val){var _this8=this;contentReady(this,function(){_this8._input.checked=val})}},{key:"disabled",set:function(value){return util.toggleAttribute(this,"disabled",value)},get:function(){return this.hasAttribute("disabled")}},{key:"_isTextInput",get:function(){return"radio"!==this.type&&"checkbox"!==this.type}},{key:"type",get:function(){return this.getAttribute("type")}}],[{key:"observedAttributes",get:function(){return["modifier","placeholder","input-id","checked"].concat(INPUT_ATTRIBUTES)}}]),InputElement}(BaseElement);customElements.define("ons-input",InputElement);var ModalAnimator=function(){function ModalAnimator(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];babelHelpers.classCallCheck(this,ModalAnimator),this.delay=0,this.duration=.2,this.timing=options.timing||this.timing,this.duration=void 0!==options.duration?options.duration:this.duration,this.delay=void 0!==options.delay?options.delay:this.delay}return babelHelpers.createClass(ModalAnimator,[{key:"show",value:function(modal,callback){callback()}},{key:"hide",value:function(modal,callback){callback()}}]),ModalAnimator}(),FadeModalAnimator=function(_ModalAnimator){function FadeModalAnimator(options){return babelHelpers.classCallCheck(this,FadeModalAnimator),options.timing=options.timing||"linear",options.duration=options.duration||"0.3",options.delay=options.delay||0,babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(FadeModalAnimator).call(this,options))}return babelHelpers.inherits(FadeModalAnimator,_ModalAnimator),babelHelpers.createClass(FadeModalAnimator,[{key:"show",value:function(modal,callback){callback=callback?callback:function(){},animit(modal).queue({opacity:0}).wait(this.delay).queue({opacity:1},{duration:this.duration,timing:this.timing}).queue(function(done){callback(),done()}).play()}},{key:"hide",value:function(modal,callback){callback=callback?callback:function(){},animit(modal).queue({opacity:1}).wait(this.delay).queue({opacity:0},{duration:this.duration,timing:this.timing}).queue(function(done){callback(),done()}).play()}}]),FadeModalAnimator}(ModalAnimator),scheme$11={"":"modal--*",modal__content:"modal--*__content"},_animatorDict$2={"default":ModalAnimator,fade:FadeModalAnimator,none:ModalAnimator},ModalElement=function(_BaseElement){function ModalElement(){return babelHelpers.classCallCheck(this,ModalElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(ModalElement).apply(this,arguments))}return babelHelpers.inherits(ModalElement,_BaseElement),babelHelpers.createClass(ModalElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){_this2._compile()}),this._doorLock=new DoorLock,this._animatorFactory=new AnimatorFactory({animators:_animatorDict$2,baseClass:ModalAnimator,baseClassName:"ModalAnimator",defaultAnimation:this.getAttribute("animation")})}},{key:"_compile",value:function(){if(this.style.display="none",this.style.zIndex=10001,this.classList.add("modal"),!util.findChild(this,".modal__content")){var content=document.createElement("div");for(content.classList.add("modal__content");this.childNodes[0];){var node=this.childNodes[0];this.removeChild(node),content.insertBefore(node,null)}this.appendChild(content)}ModifierUtil.initModifier(this,scheme$11)}},{key:"disconnectedCallback",value:function(){this._backButtonHandler&&this._backButtonHandler.destroy()}},{key:"connectedCallback",value:function(){this.onDeviceBackButton=function(){}}},{key:"show",value:function(){var _this3=this,options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];options.animationOptions=util.extend(options.animationOptions||{},AnimatorFactory.parseAnimationOptionsString(this.getAttribute("animation-options")));var callback=options.callback||function(){},tryShow=function(){var unlock=_this3._doorLock.lock(),animator=_this3._animatorFactory.newAnimator(options);return new Promise(function(resolve){contentReady(_this3,function(){_this3.style.display="table",animator.show(_this3,function(){unlock(),callback(),resolve(_this3)})})})};return new Promise(function(resolve){_this3._doorLock.waitUnlock(function(){return resolve(tryShow())})})}},{key:"toggle",value:function(){return this.visible?this.hide.apply(this,arguments):this.show.apply(this,arguments)}},{key:"hide",value:function(){var _this4=this,options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];options.animationOptions=util.extend(options.animationOptions||{},AnimatorFactory.parseAnimationOptionsString(this.getAttribute("animation-options")));var callback=options.callback||function(){},tryHide=function(){var unlock=_this4._doorLock.lock(),animator=_this4._animatorFactory.newAnimator(options);return new Promise(function(resolve){contentReady(_this4,function(){animator.hide(_this4,function(){_this4.style.display="none",unlock(),callback(),resolve(_this4)})})})};return new Promise(function(resolve){_this4._doorLock.waitUnlock(function(){return resolve(tryHide())})})}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$11):void 0}},{key:"onDeviceBackButton",get:function(){return this._backButtonHandler},set:function(handler){this._backButtonHandler&&this._backButtonHandler.destroy(),this._backButtonHandler=deviceBackButtonDispatcher.createHandler(this,handler)}},{key:"visible",get:function(){return"none"!==this.style.display}}],[{key:"registerAnimator",value:function(name,Animator){if(!(Animator.prototype instanceof ModalAnimator))throw new Error('"Animator" param must inherit OnsModalElement.ModalAnimator');_animatorDict$2[name]=Animator}},{key:"observedAttributes",get:function(){return["modifier"]}},{key:"ModalAnimator",get:function(){return ModalAnimator}}]),ModalElement}(BaseElement);customElements.define("ons-modal",ModalElement);var NavigatorTransitionAnimator=function(){function NavigatorTransitionAnimator(options){babelHelpers.classCallCheck(this,NavigatorTransitionAnimator),options=util.extend({timing:"linear",duration:"0.4",delay:"0"},options||{}),this.timing=options.timing,this.duration=options.duration,this.delay=options.delay}return babelHelpers.createClass(NavigatorTransitionAnimator,[{key:"push",value:function(enterPage,leavePage,callback){callback()}},{key:"pop",value:function(enterPage,leavePage,callback){callback()}}],[{key:"extend",value:function(){var properties=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],extendedAnimator=this,newAnimator=function(){extendedAnimator.apply(this,arguments),util.extend(this,properties)};return newAnimator.prototype=this.prototype,newAnimator}}]),NavigatorTransitionAnimator}(),IOSSlideNavigatorTransitionAnimator=function(_NavigatorTransitionA){function IOSSlideNavigatorTransitionAnimator(options){babelHelpers.classCallCheck(this,IOSSlideNavigatorTransitionAnimator),options=util.extend({duration:.4,timing:"ease",delay:0},options||{});var _this=babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(IOSSlideNavigatorTransitionAnimator).call(this,options));return _this.backgroundMask=util.createElement('\n <div style="position: absolute; width: 100%; height: 100%;\n background-color: black; opacity: 0; z-index: 2"></div>\n '),_this}return babelHelpers.inherits(IOSSlideNavigatorTransitionAnimator,_NavigatorTransitionA),babelHelpers.createClass(IOSSlideNavigatorTransitionAnimator,[{key:"_decompose",value:function(page){var toolbar=page._getToolbarElement(),left=toolbar._getToolbarLeftItemsElement(),right=toolbar._getToolbarRightItemsElement(),excludeBackButton=function(elements){for(var result=[],i=0;i<elements.length;i++)"ons-back-button"!==elements[i].nodeName.toLowerCase()&&result.push(elements[i]);return result},other=[].concat(0===left.children.length?left:excludeBackButton(left.children)).concat(0===right.children.length?right:excludeBackButton(right.children));return{toolbarCenter:toolbar._getToolbarCenterItemsElement(),backButtonIcon:toolbar._getToolbarBackButtonIconElement(),backButtonLabel:toolbar._getToolbarBackButtonLabelElement(),other:other,content:page._getContentElement(),background:page._getBackgroundElement(),toolbar:toolbar,bottomToolbar:page._getBottomToolbarElement()}}},{key:"_shouldAnimateToolbar",value:function(enterPage,leavePage){var bothPageHasToolbar=enterPage._canAnimateToolbar()&&leavePage._canAnimateToolbar(),noMaterialToolbar=!enterPage._getToolbarElement().classList.contains("navigation-bar--material")&&!leavePage._getToolbarElement().classList.contains("navigation-bar--material");return bothPageHasToolbar&&noMaterialToolbar}},{key:"_calculateDelta",value:function(element,decomposition){var title=void 0,label=void 0,pageRect=element.getBoundingClientRect();if(decomposition.backButtonLabel.classList.contains("back-button__label")){var labelRect=decomposition.backButtonLabel.getBoundingClientRect();title=Math.round(pageRect.width/2-labelRect.width/2-labelRect.left)}else title=Math.round(pageRect.width/2*.6);return decomposition.backButtonIcon.classList.contains("back-button__icon")&&(label=decomposition.backButtonIcon.getBoundingClientRect().right-2),{title:title,label:label}}},{key:"push",value:function(enterPage,leavePage,callback){var _this2=this;this.backgroundMask.remove(),leavePage.parentNode.insertBefore(this.backgroundMask,leavePage.nextSibling),contentReady(enterPage,function(){var enterPageDecomposition=_this2._decompose(enterPage),leavePageDecomposition=_this2._decompose(leavePage),delta=_this2._calculateDelta(leavePage,enterPageDecomposition),maskClear=animit(_this2.backgroundMask).saveStyle().queue({opacity:0,transform:"translate3d(0, 0, 0)"}).wait(_this2.delay).queue({opacity:.05},{duration:_this2.duration,timing:_this2.timing}).restoreStyle().queue(function(done){_this2.backgroundMask.remove(),done()}),shouldAnimateToolbar=_this2._shouldAnimateToolbar(enterPage,leavePage);if(shouldAnimateToolbar){var enterPageToolbarHeight=enterPageDecomposition.toolbar.getBoundingClientRect().height+"px";_this2.backgroundMask.style.top=enterPageToolbarHeight,animit.runAll(maskClear,animit([enterPageDecomposition.content,enterPageDecomposition.bottomToolbar,enterPageDecomposition.background]).saveStyle().queue({css:{transform:"translate3D(100%, 0px, 0px)"},duration:0}).wait(_this2.delay).queue({css:{transform:"translate3D(0px, 0px, 0px)"},duration:_this2.duration,timing:_this2.timing}).restoreStyle(),animit(enterPageDecomposition.toolbar).saveStyle().queue({css:{opacity:0},duration:0}).queue({css:{opacity:1},duration:_this2.duration,timing:_this2.timing}).restoreStyle(),animit(enterPageDecomposition.background).queue({css:{top:enterPageToolbarHeight},duration:0}),animit(enterPageDecomposition.toolbarCenter).saveStyle().queue({css:{transform:"translate3d(125%, 0, 0)",opacity:1},duration:0}).wait(_this2.delay).queue({css:{transform:"translate3d(0, 0, 0)",opacity:1},duration:_this2.duration,timing:_this2.timing}).restoreStyle(),animit(enterPageDecomposition.backButtonLabel).saveStyle().queue({css:{transform:"translate3d("+delta.title+"px, 0, 0)",opacity:0},duration:0}).wait(_this2.delay).queue({css:{transform:"translate3d(0, 0, 0)",opacity:1},duration:_this2.duration,timing:_this2.timing}).restoreStyle(),animit(enterPageDecomposition.other).saveStyle().queue({css:{opacity:0},duration:0}).wait(_this2.delay).queue({css:{opacity:1},duration:_this2.duration,timing:_this2.timing}).restoreStyle(),animit([leavePageDecomposition.content,leavePageDecomposition.bottomToolbar,leavePageDecomposition.background]).saveStyle().queue({css:{transform:"translate3D(0, 0, 0)"},duration:0}).wait(_this2.delay).queue({css:{transform:"translate3D(-25%, 0px, 0px)"},duration:_this2.duration,timing:_this2.timing}).restoreStyle().queue(function(done){callback(),done()}),animit(leavePageDecomposition.toolbarCenter).saveStyle().queue({css:{transform:"translate3d(0, 0, 0)",opacity:1},duration:0}).wait(_this2.delay).queue({css:{transform:"translate3d(-"+delta.title+"px, 0, 0)",opacity:0},duration:_this2.duration,timing:_this2.timing}).restoreStyle(),animit(leavePageDecomposition.backButtonLabel).saveStyle().queue({css:{transform:"translate3d(0, 0, 0)",opacity:1},duration:0}).wait(_this2.delay).queue({css:{transform:"translate3d(-"+delta.label+"px, 0, 0)",opacity:0},duration:_this2.duration,timing:_this2.timing}).restoreStyle(),animit(leavePageDecomposition.other).saveStyle().queue({css:{opacity:1},duration:0}).wait(_this2.delay).queue({css:{opacity:0},duration:_this2.duration,timing:_this2.timing}).restoreStyle())}else animit.runAll(maskClear,animit(enterPage).saveStyle().queue({css:{transform:"translate3D(100%, 0px, 0px)"},duration:0}).wait(_this2.delay).queue({css:{transform:"translate3D(0px, 0px, 0px)"},duration:_this2.duration,timing:_this2.timing}).restoreStyle(),animit(leavePage).saveStyle().queue({css:{transform:"translate3D(0, 0, 0)"},duration:0}).wait(_this2.delay).queue({css:{transform:"translate3D(-25%, 0px, 0px)"},duration:_this2.duration,timing:_this2.timing}).restoreStyle().queue(function(done){callback(),done()}))})}},{key:"pop",value:function(enterPage,leavePage,done){this.backgroundMask.remove(),enterPage.parentNode.insertBefore(this.backgroundMask,enterPage.nextSibling);var enterPageDecomposition=this._decompose(enterPage),leavePageDecomposition=this._decompose(leavePage),delta=this._calculateDelta(leavePage,leavePageDecomposition),maskClear=animit(this.backgroundMask).saveStyle().queue({opacity:.1,transform:"translate3d(0, 0, 0)"}).wait(this.delay).queue({opacity:0},{duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){done()}),shouldAnimateToolbar=this._shouldAnimateToolbar(enterPage,leavePage);if(shouldAnimateToolbar){var enterPageToolbarHeight=enterPageDecomposition.toolbar.getBoundingClientRect().height+"px";this.backgroundMask.style.top=enterPageToolbarHeight,animit.runAll(maskClear,animit([enterPageDecomposition.content,enterPageDecomposition.bottomToolbar,enterPageDecomposition.background]).saveStyle().queue({css:{transform:"translate3D(-25%, 0px, 0px)",opacity:.9},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0px, 0px, 0px)",opacity:1},duration:this.duration,timing:this.timing}).restoreStyle(),animit(enterPageDecomposition.toolbarCenter).saveStyle().queue({css:{transform:"translate3d(-"+delta.title+"px, 0, 0)",opacity:0},duration:0}).wait(this.delay).queue({css:{transform:"translate3d(0, 0, 0)",opacity:1},duration:this.duration,timing:this.timing}).restoreStyle(),animit(enterPageDecomposition.backButtonLabel).saveStyle().queue({css:{transform:"translate3d(-"+delta.label+"px, 0, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3d(0, 0, 0)"},duration:this.duration,timing:this.timing}).restoreStyle(),animit(enterPageDecomposition.other).saveStyle().queue({css:{opacity:0},duration:0}).wait(this.delay).queue({css:{opacity:1},duration:this.duration,timing:this.timing}).restoreStyle(),animit(leavePageDecomposition.background).queue({css:{top:enterPageToolbarHeight},duration:0}),animit([leavePageDecomposition.content,leavePageDecomposition.bottomToolbar,leavePageDecomposition.background]).queue({css:{transform:"translate3D(0px, 0px, 0px)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(100%, 0px, 0px)"},duration:this.duration,timing:this.timing}).wait(0).queue(function(finish){this.backgroundMask.remove(),done(),finish()}.bind(this)),animit(leavePageDecomposition.toolbar).queue({css:{opacity:1},duration:0}).queue({css:{opacity:0},duration:this.duration,timing:this.timing}),animit(leavePageDecomposition.toolbarCenter).queue({css:{transform:"translate3d(0, 0, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3d(125%, 0, 0)"},duration:this.duration,timing:this.timing}),animit(leavePageDecomposition.backButtonLabel).queue({css:{transform:"translate3d(0, 0, 0)",opacity:1},duration:0}).wait(this.delay).queue({css:{transform:"translate3d("+delta.title+"px, 0, 0)",opacity:0},duration:this.duration,timing:this.timing}))}else animit.runAll(maskClear,animit(enterPage).saveStyle().queue({css:{transform:"translate3D(-25%, 0px, 0px)",opacity:.9},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0px, 0px, 0px)",opacity:1},duration:this.duration,timing:this.timing}).restoreStyle(),animit(leavePage).queue({css:{transform:"translate3D(0px, 0px, 0px)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(100%, 0px, 0px)"},duration:this.duration,timing:this.timing}).queue(function(finish){this.backgroundMask.remove(),done(),finish()}.bind(this)))}}]),IOSSlideNavigatorTransitionAnimator}(NavigatorTransitionAnimator),IOSLiftNavigatorTransitionAnimator=function(_NavigatorTransitionA){function IOSLiftNavigatorTransitionAnimator(options){babelHelpers.classCallCheck(this,IOSLiftNavigatorTransitionAnimator),options=util.extend({duration:.4,timing:"cubic-bezier(.1, .7, .1, 1)",delay:0},options||{});var _this=babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(IOSLiftNavigatorTransitionAnimator).call(this,options));return _this.backgroundMask=util.createElement('\n <div style="position: absolute; width: 100%; height: 100%;\n background: linear-gradient(black, white);"></div>\n '),
_this}return babelHelpers.inherits(IOSLiftNavigatorTransitionAnimator,_NavigatorTransitionA),babelHelpers.createClass(IOSLiftNavigatorTransitionAnimator,[{key:"push",value:function(enterPage,leavePage,callback){var _this2=this;this.backgroundMask.remove(),leavePage.parentNode.insertBefore(this.backgroundMask,leavePage);var maskClear=animit(this.backgroundMask).wait(this.delay+this.duration).queue(function(done){_this2.backgroundMask.remove(),done()});animit.runAll(maskClear,animit(enterPage).saveStyle().queue({css:{transform:"translate3D(0, 100%, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 0, 0)"},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}),animit(leavePage).queue({css:{transform:"translate3D(0, 0, 0)",opacity:1},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, -10%, 0)",opacity:.9},duration:this.duration,timing:this.timing}))}},{key:"pop",value:function(enterPage,leavePage,callback){var _this3=this;this.backgroundMask.remove(),enterPage.parentNode.insertBefore(this.backgroundMask,enterPage),animit.runAll(animit(this.backgroundMask).wait(this.delay+this.duration).queue(function(done){_this3.backgroundMask.remove(),done()}),animit(enterPage).queue({css:{transform:"translate3D(0, -10%, 0)",opacity:.9},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 0, 0)",opacity:1},duration:this.duration,timing:this.timing}).queue(function(done){callback(),done()}),animit(leavePage).queue({css:{transform:"translate3D(0, 0, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 100%, 0)"},duration:this.duration,timing:this.timing}))}}]),IOSLiftNavigatorTransitionAnimator}(NavigatorTransitionAnimator),IOSFadeNavigatorTransitionAnimator=function(_NavigatorTransitionA){function IOSFadeNavigatorTransitionAnimator(options){return babelHelpers.classCallCheck(this,IOSFadeNavigatorTransitionAnimator),options=util.extend({timing:"linear",duration:"0.4",delay:"0"},options||{}),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(IOSFadeNavigatorTransitionAnimator).call(this,options))}return babelHelpers.inherits(IOSFadeNavigatorTransitionAnimator,_NavigatorTransitionA),babelHelpers.createClass(IOSFadeNavigatorTransitionAnimator,[{key:"push",value:function(enterPage,leavePage,callback){animit.runAll(animit([enterPage._getContentElement(),enterPage._getBackgroundElement()]).saveStyle().queue({css:{transform:"translate3D(0, 0, 0)",opacity:0},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 0, 0)",opacity:1},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}),animit(enterPage._getToolbarElement()).saveStyle().queue({css:{transform:"translate3D(0, 0, 0)",opacity:0},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 0, 0)",opacity:1},duration:this.duration,timing:this.timing}).restoreStyle())}},{key:"pop",value:function(enterPage,leavePage,callback){animit.runAll(animit([leavePage._getContentElement(),leavePage._getBackgroundElement()]).queue({css:{transform:"translate3D(0, 0, 0)",opacity:1},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 0, 0)",opacity:0},duration:this.duration,timing:this.timing}).queue(function(done){callback(),done()}),animit(leavePage._getToolbarElement()).queue({css:{transform:"translate3D(0, 0, 0)",opacity:1},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 0, 0)",opacity:0},duration:this.duration,timing:this.timing}))}}]),IOSFadeNavigatorTransitionAnimator}(NavigatorTransitionAnimator),MDSlideNavigatorTransitionAnimator=function(_NavigatorTransitionA){function MDSlideNavigatorTransitionAnimator(options){babelHelpers.classCallCheck(this,MDSlideNavigatorTransitionAnimator),options=util.extend({duration:.3,timing:"cubic-bezier(.1, .7, .4, 1)",delay:0},options||{});var _this=babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(MDSlideNavigatorTransitionAnimator).call(this,options));return _this.backgroundMask=util.createElement('\n <div style="position: absolute; width: 100%; height: 100%; z-index: 2;\n background-color: black; opacity: 0;"></div>\n '),_this.blackMaskOpacity=.4,_this}return babelHelpers.inherits(MDSlideNavigatorTransitionAnimator,_NavigatorTransitionA),babelHelpers.createClass(MDSlideNavigatorTransitionAnimator,[{key:"push",value:function(enterPage,leavePage,callback){var _this2=this;this.backgroundMask.remove(),leavePage.parentElement.insertBefore(this.backgroundMask,leavePage.nextSibling),animit.runAll(animit(this.backgroundMask).saveStyle().queue({opacity:0,transform:"translate3d(0, 0, 0)"}).wait(this.delay).queue({opacity:this.blackMaskOpacity},{duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){_this2.backgroundMask.remove(),done()}),animit(enterPage).saveStyle().queue({css:{transform:"translate3D(100%, 0, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 0, 0)"},duration:this.duration,timing:this.timing}).restoreStyle(),animit(leavePage).saveStyle().queue({css:{transform:"translate3D(0, 0, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(-45%, 0px, 0px)"},duration:this.duration,timing:this.timing}).restoreStyle().wait(.2).queue(function(done){callback(),done()}))}},{key:"pop",value:function(enterPage,leavePage,done){var _this3=this;this.backgroundMask.remove(),enterPage.parentNode.insertBefore(this.backgroundMask,enterPage.nextSibling),animit.runAll(animit(this.backgroundMask).saveStyle().queue({opacity:this.blackMaskOpacity,transform:"translate3d(0, 0, 0)"}).wait(this.delay).queue({opacity:0},{duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){_this3.backgroundMask.remove(),done()}),animit(enterPage).saveStyle().queue({css:{transform:"translate3D(-45%, 0px, 0px)",opacity:.9},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0px, 0px, 0px)",opacity:1},duration:this.duration,timing:this.timing}).restoreStyle(),animit(leavePage).queue({css:{transform:"translate3D(0px, 0px, 0px)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(100%, 0px, 0px)"},duration:this.duration,timing:this.timing}).wait(.2).queue(function(finish){done(),finish()}))}}]),MDSlideNavigatorTransitionAnimator}(NavigatorTransitionAnimator),MDLiftNavigatorTransitionAnimator=function(_NavigatorTransitionA){function MDLiftNavigatorTransitionAnimator(options){babelHelpers.classCallCheck(this,MDLiftNavigatorTransitionAnimator),options=util.extend({duration:.4,timing:"cubic-bezier(.1, .7, .1, 1)",delay:.05},options||{});var _this=babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(MDLiftNavigatorTransitionAnimator).call(this,options));return _this.backgroundMask=util.createElement('\n <div style="position: absolute; width: 100%; height: 100%;\n background-color: black;"></div>\n '),_this}return babelHelpers.inherits(MDLiftNavigatorTransitionAnimator,_NavigatorTransitionA),babelHelpers.createClass(MDLiftNavigatorTransitionAnimator,[{key:"push",value:function(enterPage,leavePage,callback){var _this2=this;this.backgroundMask.remove(),leavePage.parentNode.insertBefore(this.backgroundMask,leavePage);var maskClear=animit(this.backgroundMask).wait(this.delay+this.duration).queue(function(done){_this2.backgroundMask.remove(),done()});animit.runAll(maskClear,animit(enterPage).saveStyle().queue({css:{transform:"translate3D(0, 100%, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 0, 0)"},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}),animit(leavePage).queue({css:{opacity:1},duration:0}).queue({css:{opacity:.4},duration:this.duration,timing:this.timing}))}},{key:"pop",value:function(enterPage,leavePage,callback){var _this3=this;this.backgroundMask.remove(),enterPage.parentNode.insertBefore(this.backgroundMask,enterPage),animit.runAll(animit(this.backgroundMask).wait(this.delay+this.duration).queue(function(done){_this3.backgroundMask.remove(),done()}),animit(enterPage).queue({css:{transform:"translate3D(0, 0, 0)",opacity:.4},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 0, 0)",opacity:1},duration:this.duration,timing:this.timing}).queue(function(done){callback(),done()}),animit(leavePage).queue({css:{transform:"translate3D(0, 0, 0)"},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 100%, 0)"},duration:this.duration,timing:this.timing}))}}]),MDLiftNavigatorTransitionAnimator}(NavigatorTransitionAnimator),MDFadeNavigatorTransitionAnimator=function(_NavigatorTransitionA){function MDFadeNavigatorTransitionAnimator(options){return babelHelpers.classCallCheck(this,MDFadeNavigatorTransitionAnimator),options=util.extend({timing:"ease-out",duration:"0.25",delay:"0"},options||{}),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(MDFadeNavigatorTransitionAnimator).call(this,options))}return babelHelpers.inherits(MDFadeNavigatorTransitionAnimator,_NavigatorTransitionA),babelHelpers.createClass(MDFadeNavigatorTransitionAnimator,[{key:"push",value:function(enterPage,leavePage,callback){animit.runAll(animit(enterPage).saveStyle().queue({css:{transform:"translate3D(0, 42px, 0)",opacity:0},duration:0}).wait(this.delay).queue({css:{transform:"translate3D(0, 0, 0)",opacity:1},duration:this.duration,timing:this.timing}).restoreStyle().queue(function(done){callback(),done()}))}},{key:"pop",value:function(enterPage,leavePage,callback){animit.runAll(animit(leavePage).queue({css:{transform:"translate3D(0, 0, 0)"},duration:0}).wait(.15).queue({css:{transform:"translate3D(0, 38px, 0)"},duration:this.duration,timing:this.timing}).queue(function(done){callback(),done()}),animit(leavePage).queue({css:{opacity:1},duration:0}).wait(.04).queue({css:{opacity:0},duration:this.duration,timing:this.timing}))}}]),MDFadeNavigatorTransitionAnimator}(NavigatorTransitionAnimator),NoneNavigatorTransitionAnimator=function(_NavigatorTransitionA){function NoneNavigatorTransitionAnimator(options){return babelHelpers.classCallCheck(this,NoneNavigatorTransitionAnimator),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(NoneNavigatorTransitionAnimator).call(this,options))}return babelHelpers.inherits(NoneNavigatorTransitionAnimator,_NavigatorTransitionA),babelHelpers.createClass(NoneNavigatorTransitionAnimator,[{key:"push",value:function(enterPage,leavePage,callback){callback()}},{key:"pop",value:function(enterPage,leavePage,callback){callback()}}]),NoneNavigatorTransitionAnimator}(NavigatorTransitionAnimator),_animatorDict$3={"default":function(){return platform.isAndroid()?MDFadeNavigatorTransitionAnimator:IOSSlideNavigatorTransitionAnimator},slide:function(){return platform.isAndroid()?MDSlideNavigatorTransitionAnimator:IOSSlideNavigatorTransitionAnimator},lift:function(){return platform.isAndroid()?MDLiftNavigatorTransitionAnimator:IOSLiftNavigatorTransitionAnimator},fade:function(){return platform.isAndroid()?MDFadeNavigatorTransitionAnimator:IOSFadeNavigatorTransitionAnimator},"slide-ios":IOSSlideNavigatorTransitionAnimator,"slide-md":MDSlideNavigatorTransitionAnimator,"lift-ios":IOSLiftNavigatorTransitionAnimator,"lift-md":MDLiftNavigatorTransitionAnimator,"fade-ios":IOSFadeNavigatorTransitionAnimator,"fade-md":MDFadeNavigatorTransitionAnimator,none:NoneNavigatorTransitionAnimator},rewritables={ready:function(navigatorElement,callback){callback()},link:function(navigatorElement,target,options,callback){callback(target)}},NavigatorElement=function(_BaseElement){function NavigatorElement(){return babelHelpers.classCallCheck(this,NavigatorElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(NavigatorElement).apply(this,arguments))}return babelHelpers.inherits(NavigatorElement,_BaseElement),babelHelpers.createClass(NavigatorElement,[{key:"init",value:function(){this._isRunning=!1,this._pageLoader=defaultPageLoader,this._updateAnimatorFactory()}},{key:"_getPageTarget",value:function(){return this._page||this.getAttribute("page")}},{key:"connectedCallback",value:function(){var _this2=this;this.onDeviceBackButton=this._onDeviceBackButton.bind(this),rewritables.ready(this,function(){if(0===_this2.pages.length&&_this2._getPageTarget()&&_this2.pushPage(_this2._getPageTarget(),{animation:"none"}),_this2.pages.length>0){for(var i=0;i<_this2.pages.length;i++)if("ONS-PAGE"!==_this2.pages[i].nodeName)throw new Error("The children of <ons-navigator> need to be of type <ons-page>");_this2.topPage&&setImmediate(function(){_this2.topPage._show(),_this2._updateLastPageBackButton()})}else contentReady(_this2,function(){0===_this2.pages.length&&_this2._getPageTarget()&&_this2.pushPage(_this2._getPageTarget(),{animation:"none"})})})}},{key:"_updateAnimatorFactory",value:function(){this._animatorFactory=new AnimatorFactory({animators:_animatorDict$3,baseClass:NavigatorTransitionAnimator,baseClassName:"NavigatorTransitionAnimator",defaultAnimation:this.getAttribute("animation")})}},{key:"disconnectedCallback",value:function(){this._backButtonHandler.destroy(),this._backButtonHandler=null}},{key:"attributeChangedCallback",value:function(name,last,current){"animation"===name&&this._updateAnimatorFactory()}},{key:"popPage",value:function(){var _this3=this,options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],_preparePageAndOption=this._preparePageAndOptions(null,options);options=_preparePageAndOption.options;var popUpdate=function(){return new Promise(function(resolve){_this3.pages[_this3.pages.length-1]._destroy(),resolve()})};if(!options.refresh)return this._popPage(options,popUpdate);var index=this.pages.length-2,oldPage=this.pages[index];if(!oldPage.name)throw new Error("Refresh option cannot be used with pages directly inside the Navigator. Use ons-template instead.");return new Promise(function(resolve){var options={page:oldPage.name,parent:_this3,params:oldPage.pushedOptions.data};_this3._pageLoader.load(options,function(_ref){var element=_ref.element,unload=_ref.unload;element=util.extend(element,{name:oldPage.name,data:oldPage.data,pushedOptions:oldPage.pushedOptions,unload:unload}),rewritables.link(_this3,element,oldPage.options,function(element){_this3.insertBefore(element,oldPage?oldPage:null),oldPage._destroy(),resolve()})})}).then(function(){return _this3._popPage(options,popUpdate)})}},{key:"_popPage",value:function(options){var _this4=this,update=arguments.length<=1||void 0===arguments[1]?function(){return Promise.resolve()}:arguments[1];if(this._isRunning)return Promise.reject("popPage is already running.");if(this.pages.length<=1)return Promise.reject("ons-navigator's page stack is empty.");if(this._emitPrePopEvent())return Promise.reject("Canceled in prepop event.");var length=this.pages.length;return this._isRunning=!0,this.pages[length-2].updateBackButton(length-2>0),new Promise(function(resolve){var leavePage=_this4.pages[length-1],enterPage=_this4.pages[length-2];enterPage.style.display="block",options.animation=options.animation||leavePage.pushedOptions.animation,options.animationOptions=util.extend({},leavePage.pushedOptions.animationOptions,options.animationOptions||{}),options.data&&(enterPage.data=util.extend({},enterPage.data||{},options.data||{}));var callback=function(){update().then(function(){_this4._isRunning=!1,enterPage._show(),util.triggerElementEvent(_this4,"postpop",{leavePage:leavePage,enterPage:enterPage,navigator:_this4}),"function"==typeof options.callback&&options.callback(),resolve(enterPage)})};leavePage._hide();var animator=_this4._animatorFactory.newAnimator(options);animator.pop(_this4.pages[length-2],_this4.pages[length-1],callback)})["catch"](function(){return _this4._isRunning=!1})}},{key:"pushPage",value:function(page){var _this5=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1],_preparePageAndOption2=this._preparePageAndOptions(page,options);page=_preparePageAndOption2.page,options=_preparePageAndOption2.options;var prepare=function(element,unload){_this5._verifyPageElement(element),element=util.extend(element,{name:options.page,data:options.data,unload:unload}),element.style.display="none"};return options.pageHTML?this._pushPage(options,function(){return new Promise(function(resolve){instantPageLoader.load({page:options.pageHTML,parent:_this5,params:options.data},function(_ref2){var element=_ref2.element,unload=_ref2.unload;prepare(element,unload),resolve()})})}):this._pushPage(options,function(){return new Promise(function(resolve){_this5._pageLoader.load({page:page,parent:_this5,params:options.data},function(_ref3){var element=_ref3.element,unload=_ref3.unload;prepare(element,unload),resolve()})})})}},{key:"_pushPage",value:function(){var _this6=this,options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0],update=arguments.length<=1||void 0===arguments[1]?function(){return Promise.resolve()}:arguments[1];if(this._isRunning)return Promise.reject("pushPage is already running.");if(this._emitPrePushEvent())return Promise.reject("Canceled in prepush event.");this._isRunning=!0;var animationOptions=AnimatorFactory.parseAnimationOptionsString(this.getAttribute("animation-options"));options=util.extend({},this.options||{},{animationOptions:animationOptions},options);var animator=this._animatorFactory.newAnimator(options);return update().then(function(){var pageLength=_this6.pages.length,enterPage=_this6.pages[pageLength-1],leavePage=_this6.pages[pageLength-2];if("ONS-PAGE"!==enterPage.nodeName)throw new Error("Only elements of type <ons-page> can be pushed to the navigator");return enterPage.updateBackButton(pageLength-1),enterPage.pushedOptions=util.extend({},enterPage.pushedOptions||{},options||{}),enterPage.data=util.extend({},enterPage.data||{},options.data||{}),enterPage.name=enterPage.name||options.page,enterPage.unload=enterPage.unload||options.unload,new Promise(function(resolve){var done=function(){_this6._isRunning=!1,leavePage&&(leavePage.style.display="none"),setImmediate(function(){return enterPage._show()}),util.triggerElementEvent(_this6,"postpush",{leavePage:leavePage,enterPage:enterPage,navigator:_this6}),"function"==typeof options.callback&&options.callback(),resolve(enterPage)};enterPage.style.display="none";var push=function(){enterPage.style.display="block",leavePage?(leavePage._hide(),animator.push(enterPage,leavePage,done)):done()};options._linked?push():rewritables.link(_this6,enterPage,options,push)})})["catch"](function(error){throw _this6._isRunning=!1,error})}},{key:"replacePage",value:function(page){var _this7=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];return this.pushPage(page,options).then(function(resolvedValue){return _this7.pages.length>1&&_this7.pages[_this7.pages.length-2]._destroy(),_this7._updateLastPageBackButton(),Promise.resolve(resolvedValue)})}},{key:"insertPage",value:function(index,page){var _this8=this,options=arguments.length<=2||void 0===arguments[2]?{}:arguments[2],_preparePageAndOption3=this._preparePageAndOptions(page,options);if(page=_preparePageAndOption3.page,options=_preparePageAndOption3.options,index=this._normalizeIndex(index),index>=this.pages.length)return this.pushPage(page,options);page="string"==typeof options.pageHTML?options.pageHTML:page;var loader="string"==typeof options.pageHTML?instantPageLoader:this._pageLoader;return new Promise(function(resolve){loader.load({page:page,parent:_this8},function(_ref4){var element=_ref4.element,unload=_ref4.unload;_this8._verifyPageElement(element),element=util.extend(element,{name:options.page,data:options.data,pushedOptions:options,unload:unload}),options.animationOptions=util.extend({},AnimatorFactory.parseAnimationOptionsString(_this8.getAttribute("animation-options")),options.animationOptions||{}),element.style.display="none",_this8.insertBefore(element,_this8.pages[index]),_this8.topPage.updateBackButton(!0),rewritables.link(_this8,element,options,function(element){setTimeout(function(){element=null,resolve(_this8.pages[index])},1e3/60)})})})}},{key:"resetToPage",value:function(page){var _this9=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1],_preparePageAndOption4=this._preparePageAndOptions(page,options);page=_preparePageAndOption4.page,options=_preparePageAndOption4.options,options.animator||options.animation||(options.animation="none");var callback=options.callback;return options.callback=function(){for(;_this9.pages.length>1;)_this9.pages[0]._destroy();_this9.pages[0].updateBackButton(!1),callback&&callback()},options.page||options.pageHTML||!this._getPageTarget()||(page=options.page=this._getPageTarget()),this.pushPage(page,options)}},{key:"bringPageTop",value:function(item){var options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];if(-1===["number","string"].indexOf("undefined"==typeof item?"undefined":babelHelpers["typeof"](item)))throw new Error("First argument must be a page name or the index of an existing page. You supplied "+item);var index="number"==typeof item?this._normalizeIndex(item):this._lastIndexOfPage(item),page=this.pages[index];if(0>index)return this.pushPage(item,options);var _preparePageAndOption5=this._preparePageAndOptions(page,options);if(options=_preparePageAndOption5.options,index===this.pages.length-1)return Promise.resolve(page);if(!page)throw new Error("Failed to find item "+item);return this._isRunning?Promise.reject("pushPage is already running."):this._emitPrePushEvent()?Promise.reject("Canceled in prepush event."):(util.extend(options,{page:page.name,_linked:!0}),page.style.display="none",page.setAttribute("_skipinit",""),page.parentNode.appendChild(page),this._pushPage(options))}},{key:"_preparePageAndOptions",value:function(page){var options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];if("object"!=("undefined"==typeof options?"undefined":babelHelpers["typeof"](options)))throw new Error("options must be an object. You supplied "+options);return null!==page&&void 0!==page||!options.page||(page=options.page),options=util.extend({},this.options||{},options,{page:page}),{page:page,options:options}}},{key:"_updateLastPageBackButton",value:function(){var index=this.pages.length-1;index>=0&&this.pages[index].updateBackButton(index>0)}},{key:"_normalizeIndex",value:function(index){return index>=0?index:Math.abs(this.pages.length+index)%this.pages.length}},{key:"_onDeviceBackButton",value:function(event){this.pages.length>1?this.popPage():event.callParentHandler()}},{key:"_lastIndexOfPage",value:function(pageName){var index=void 0;for(index=this.pages.length-1;index>=0&&this.pages[index].name!==pageName;index--);return index}},{key:"_emitPreEvent",value:function(name){var data=arguments.length<=1||void 0===arguments[1]?{}:arguments[1],isCanceled=!1;return util.triggerElementEvent(this,"pre"+name,util.extend({navigator:this,currentPage:this.pages[this.pages.length-1],cancel:function(){return isCanceled=!0}},data)),isCanceled}},{key:"_emitPrePushEvent",value:function(){return this._emitPreEvent("push")}},{key:"_emitPrePopEvent",value:function(){var l=this.pages.length;return this._emitPreEvent("pop",{leavePage:this.pages[l-1],enterPage:this.pages[l-2]})}},{key:"_createPageElement",value:function(templateHTML){var pageElement=util.createElement(internal.normalizePageHTML(templateHTML));return this._verifyPageElement(pageElement),pageElement}},{key:"_verifyPageElement",value:function(element){if("ons-page"!==element.nodeName.toLowerCase())throw new Error('You must supply an "ons-page" element to "ons-navigator".')}},{key:"_show",value:function(){this.topPage&&this.topPage._show()}},{key:"_hide",value:function(){this.topPage&&this.topPage._hide()}},{key:"_destroy",value:function(){for(var i=this.pages.length-1;i>=0;i--)this.pages[i]._destroy();this.remove()}},{key:"animatorFactory",get:function(){return this._animatorFactory}},{key:"pageLoader",get:function(){return this._pageLoader},set:function(pageLoader){if(!(pageLoader instanceof PageLoader))throw Error("First parameter must be an instance of PageLoader.");this._pageLoader=pageLoader}},{key:"page",get:function(){return this._page},set:function(page){this._page=page}},{key:"onDeviceBackButton",get:function(){return this._backButtonHandler},set:function(callback){this._backButtonHandler&&this._backButtonHandler.destroy(),this._backButtonHandler=deviceBackButtonDispatcher.createHandler(this,callback)}},{key:"topPage",get:function(){return this.pages[this.pages.length-1]||null}},{key:"pages",get:function(){return util.arrayFrom(this.children).filter(function(n){return"ONS-PAGE"===n.tagName})}},{key:"options",get:function(){return this._options},set:function(object){this._options=object}},{key:"_isRunning",set:function(value){this.setAttribute("_is-running",value?"true":"false")},get:function(){return JSON.parse(this.getAttribute("_is-running"))}}],[{key:"registerAnimator",value:function(name,Animator){if(!(Animator.prototype instanceof NavigatorTransitionAnimator))throw new Error('"Animator" param must inherit NavigatorElement.NavigatorTransitionAnimator');_animatorDict$3[name]=Animator}},{key:"observedAttributes",get:function(){return["animation"]}},{key:"animators",get:function(){return _animatorDict$3}},{key:"NavigatorTransitionAnimator",get:function(){return NavigatorTransitionAnimator}},{key:"rewritables",get:function(){return rewritables}}]),NavigatorElement}(BaseElement);customElements.define("ons-navigator",NavigatorElement);var scheme$13={"":"navigation-bar--*",".navigation-bar__left":"navigation-bar--*__left",".navigation-bar__center":"navigation-bar--*__center",".navigation-bar__right":"navigation-bar--*__right"},ToolbarElement=function(_BaseElement){function ToolbarElement(){return babelHelpers.classCallCheck(this,ToolbarElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(ToolbarElement).apply(this,arguments))}return babelHelpers.inherits(ToolbarElement,_BaseElement),babelHelpers.createClass(ToolbarElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){_this2.hasAttribute("_compiled")||_this2._compile()})}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$13):void 0}},{key:"_getToolbarLeftItemsElement",value:function(){return this.querySelector(".left")||internal.nullElement}},{key:"_getToolbarCenterItemsElement",value:function(){return this.querySelector(".center")||internal.nullElement}},{key:"_getToolbarRightItemsElement",value:function(){return this.querySelector(".right")||internal.nullElement}},{key:"_getToolbarBackButtonLabelElement",value:function(){return this.querySelector("ons-back-button .back-button__label")||internal.nullElement}},{key:"_getToolbarBackButtonIconElement",value:function(){return this.querySelector("ons-back-button .back-button__icon")||internal.nullElement}},{key:"_compile",value:function(){autoStyle.prepare(this),this.classList.add("navigation-bar"),this._ensureToolbarItemElements(),ModifierUtil.initModifier(this,scheme$13),this.setAttribute("_compiled","")}},{key:"_ensureToolbarItemElements",value:function(){for(var i=this.childNodes.length-1;i>=0;i--)1!=this.childNodes[i].nodeType&&this.removeChild(this.childNodes[i]);var center=this._ensureToolbarElement("center");if(center.classList.add("navigation-bar__title"),1!==this.children.length||!this.children[0].classList.contains("center")){var left=this._ensureToolbarElement("left"),right=this._ensureToolbarElement("right");this.children[0]===left&&this.children[1]===center&&this.children[2]===right||(this.appendChild(left),this.appendChild(center),this.appendChild(right))}}},{key:"_ensureToolbarElement",value:function(name){var element=util.findChild(this,"."+name)||util.create("."+name);return element.classList.add("navigation-bar__"+name),element}}],[{key:"observedAttributes",get:function(){return["modifier"]}}]),ToolbarElement}(BaseElement);customElements.define("ons-toolbar",ToolbarElement);var scheme$12={"":"page--*",".page__content":"page--*__content",".page__background":"page--*__background"},nullToolbarElement=document.createElement("ons-toolbar"),PageElement=function(_BaseElement){function PageElement(){return babelHelpers.classCallCheck(this,PageElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(PageElement).apply(this,arguments))}return babelHelpers.inherits(PageElement,_BaseElement),babelHelpers.createClass(PageElement,[{key:"init",value:function(){var _this2=this;this.classList.add("page"),contentReady(this,function(){_this2.hasAttribute("_compiled")||_this2._compile(),_this2._isShown=!1,_this2._contentElement=_this2._getContentElement(),_this2._isMuted=_this2.hasAttribute("_muted"),_this2._skipInit=_this2.hasAttribute("_skipinit"),_this2.pushedOptions={}})}},{key:"connectedCallback",value:function(){var _this3=this;contentReady(this,function(){_this3._isMuted||(_this3._skipInit?_this3.removeAttribute("_skipinit"):setImmediate(function(){return util.triggerElementEvent(_this3,"init")})),util.hasAnyComponentAsParent(_this3)||setImmediate(function(){return _this3._show()}),_this3._tryToFillStatusBar(),_this3.hasAttribute("on-infinite-scroll")&&_this3.attributeChangedCallback("on-infinite-scroll",null,_this3.getAttribute("on-infinite-scroll"))})}},{key:"updateBackButton",value:function(show){this.backButton&&(show?this.backButton.show():this.backButton.hide())}},{key:"_tryToFillStatusBar",value:function(){var _this4=this;internal.autoStatusBarFill(function(){var filled=util.findParent(_this4,function(e){return e.hasAttribute("status-bar-fill")});util.toggleAttribute(_this4,"status-bar-fill",!filled&&(_this4._canAnimateToolbar()||!_this4._hasAPageControlChild()))})}},{key:"_hasAPageControlChild",value:function(){return util.findChild(this._contentElement,function(e){return e.nodeName.match(/ons-(splitter|sliding-menu|navigator|tabbar)/i)})}},{key:"_onScroll",value:function(){var _this5=this,c=this._contentElement,overLimit=(c.scrollTop+c.clientHeight)/c.scrollHeight>=this._infiniteScrollLimit;this._onInfiniteScroll&&!this._loadingContent&&overLimit&&(this._loadingContent=!0,this._onInfiniteScroll(function(){return _this5._loadingContent=!1}))}},{key:"_getContentElement",value:function(){var result=util.findChild(this,".page__content");if(result)return result;throw Error('fail to get ".page__content" element.')}},{key:"_canAnimateToolbar",value:function(){return util.findChild(this,"ons-toolbar")?!0:!!util.findChild(this._contentElement,function(el){return util.match(el,"ons-toolbar")&&!el.hasAttribute("inline")})}},{key:"_getBackgroundElement",value:function(){var result=util.findChild(this,".page__background");if(result)return result;throw Error('fail to get ".page__background" element.')}},{key:"_getBottomToolbarElement",value:function(){return util.findChild(this,"ons-bottom-toolbar")||internal.nullElement}},{key:"_getToolbarElement",value:function(){return util.findChild(this,"ons-toolbar")||nullToolbarElement}},{key:"attributeChangedCallback",value:function(name,last,current){var _this6=this;return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$12):void("_muted"===name?this._isMuted=this.hasAttribute("_muted"):"_skipinit"===name?this._skipInit=this.hasAttribute("_skipinit"):"on-infinite-scroll"===name&&(null===current?this.onInfiniteScroll=null:this.onInfiniteScroll=function(done){var f=util.findFromPath(current);_this6.onInfiniteScroll=f,f(done)}))}},{key:"_compile",value:function(){var _this7=this;if(autoStyle.prepare(this),util.findChild(this,".content")&&util.findChild(this,".content").classList.add("page__content"),util.findChild(this,".background")&&util.findChild(this,".background").classList.add("page__background"),util.findChild(this,".page__content")||!function(){var content=util.create(".page__content");util.arrayFrom(_this7.childNodes).forEach(function(node){(1!==node.nodeType||_this7._elementShouldBeMoved(node))&&content.appendChild(node);
});var prevNode=util.findChild(_this7,".page__background")||util.findChild(_this7,"ons-toolbar");_this7.insertBefore(content,prevNode&&prevNode.nextSibling)}(),!util.findChild(this,".page__background")){var background=util.create(".page__background");this.insertBefore(background,util.findChild(this,".page__content"))}ModifierUtil.initModifier(this,scheme$12),this.setAttribute("_compiled","")}},{key:"_elementShouldBeMoved",value:function(el){if(el.classList.contains("page__background"))return!1;var tagName=el.tagName.toLowerCase();if("ons-fab"===tagName)return!el.hasAttribute("position");var fixedElements=["ons-toolbar","ons-bottom-toolbar","ons-modal","ons-speed-dial"];return el.hasAttribute("inline")||-1===fixedElements.indexOf(tagName)}},{key:"_show",value:function(){!this._isShown&&util.isAttached(this)&&(this._isShown=!0,this._isMuted||util.triggerElementEvent(this,"show"),util.propagateAction(this._contentElement,"_show"))}},{key:"_hide",value:function(){this._isShown&&(this._isShown=!1,this._isMuted||util.triggerElementEvent(this,"hide"),util.propagateAction(this._contentElement,"_hide"))}},{key:"_destroy",value:function(){this._hide(),this._isMuted||util.triggerElementEvent(this,"destroy"),this.onDeviceBackButton&&this.onDeviceBackButton.destroy(),util.propagateAction(this._contentElement,"_destroy"),this.unload instanceof Function&&this.unload(),this.remove()}},{key:"name",set:function(str){this.setAttribute("name",str)},get:function(){return this.getAttribute("name")}},{key:"backButton",get:function(){return this.querySelector("ons-back-button")}},{key:"onInfiniteScroll",set:function(value){if(null===value)return this._onInfiniteScroll=null,void this._contentElement.removeEventListener("scroll",this._boundOnScroll);if(!(value instanceof Function))throw new Error("onInfiniteScroll must be a function or null");this._onInfiniteScroll||(this._infiniteScrollLimit=.9,this._boundOnScroll=this._onScroll.bind(this),this._contentElement.addEventListener("scroll",this._boundOnScroll)),this._onInfiniteScroll=value},get:function(){return this._onInfiniteScroll}},{key:"onDeviceBackButton",get:function(){return this._backButtonHandler},set:function(callback){this._backButtonHandler&&this._backButtonHandler.destroy(),this._backButtonHandler=deviceBackButtonDispatcher.createHandler(this,callback)}}],[{key:"observedAttributes",get:function(){return["modifier","_muted","_skipinit","on-infinite-scroll"]}}]),PageElement}(BaseElement);customElements.define("ons-page",PageElement);var PopoverAnimator=function(){function PopoverAnimator(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];babelHelpers.classCallCheck(this,PopoverAnimator),this.options=util.extend({timing:"cubic-bezier(.1, .7, .4, 1)",duration:.2,delay:0},options)}return babelHelpers.createClass(PopoverAnimator,[{key:"show",value:function(popover,callback){callback()}},{key:"hide",value:function(popover,callback){callback()}},{key:"_animate",value:function(element,_ref){var from=_ref.from,to=_ref.to,options=_ref.options,callback=_ref.callback,_ref$restore=_ref.restore,restore=void 0===_ref$restore?!1:_ref$restore,animation=_ref.animation;return options=util.extend({},this.options,options),animation&&(from=animation.from,to=animation.to),animation=animit(element),restore&&(animation=animation.saveStyle()),animation=animation.queue(from).wait(options.delay).queue({css:to,duration:options.duration,timing:options.timing}),restore&&(animation=animation.restoreStyle()),callback&&(animation=animation.queue(function(done){callback(),done()})),animation}},{key:"_animateAll",value:function(element,animations){var _this=this;Object.keys(animations).forEach(function(key){return _this._animate(element[key],animations[key]).play()})}}]),PopoverAnimator}(),fade={out:{from:{opacity:1},to:{opacity:0}},"in":{from:{opacity:0},to:{opacity:1}}},MDFadePopoverAnimator=function(_PopoverAnimator){function MDFadePopoverAnimator(){return babelHelpers.classCallCheck(this,MDFadePopoverAnimator),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(MDFadePopoverAnimator).apply(this,arguments))}return babelHelpers.inherits(MDFadePopoverAnimator,_PopoverAnimator),babelHelpers.createClass(MDFadePopoverAnimator,[{key:"show",value:function(popover,callback){this._animateAll(popover,{_mask:fade["in"],_popover:{animation:fade["in"],restore:!0,callback:callback}})}},{key:"hide",value:function(popover,callback){this._animateAll(popover,{_mask:fade.out,_popover:{animation:fade.out,restore:!0,callback:callback}})}}]),MDFadePopoverAnimator}(PopoverAnimator),IOSFadePopoverAnimator=function(_MDFadePopoverAnimato){function IOSFadePopoverAnimator(){return babelHelpers.classCallCheck(this,IOSFadePopoverAnimator),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(IOSFadePopoverAnimator).apply(this,arguments))}return babelHelpers.inherits(IOSFadePopoverAnimator,_MDFadePopoverAnimato),babelHelpers.createClass(IOSFadePopoverAnimator,[{key:"show",value:function(popover,callback){this._animateAll(popover,{_mask:fade["in"],_popover:{from:{transform:"scale3d(1.3, 1.3, 1.0)",opacity:0},to:{transform:"scale3d(1.0, 1.0, 1.0)",opacity:1},restore:!0,callback:callback}})}}]),IOSFadePopoverAnimator}(MDFadePopoverAnimator),scheme$14={".popover":"popover--*",".popover-mask":"popover-mask--*",".popover__container":"popover__container--*",".popover__content":"popover__content--*",".popover__arrow":"popover__arrow--*"},_animatorDict$4={"default":function(){return platform.isAndroid()?MDFadePopoverAnimator:IOSFadePopoverAnimator},none:PopoverAnimator,"fade-ios":IOSFadePopoverAnimator,"fade-md":MDFadePopoverAnimator},templateSource=util.createFragment('\n <div class="popover-mask"></div>\n <div class="popover__container">\n <div class="popover__content"></div>\n <div class="popover__arrow"></div>\n </div>\n'),positions={up:"bottom",left:"right",down:"top",right:"left"},PopoverElement=(Object.keys(positions),function(_BaseElement){function PopoverElement(){return babelHelpers.classCallCheck(this,PopoverElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(PopoverElement).apply(this,arguments))}return babelHelpers.inherits(PopoverElement,_BaseElement),babelHelpers.createClass(PopoverElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){_this2._compile(),_this2._initAnimatorFactory()}),this._doorLock=new DoorLock,this._boundOnChange=this._onChange.bind(this),this._boundCancel=this._cancel.bind(this)}},{key:"_initAnimatorFactory",value:function(){var factory=new AnimatorFactory({animators:_animatorDict$4,baseClass:PopoverAnimator,baseClassName:"PopoverAnimator",defaultAnimation:this.getAttribute("animation")||"default"});this._animator=function(options){return factory.newAnimator(options)}}},{key:"_positionPopover",value:function(target){var radius=this._radius,el=this._content,margin=this._margin,pos=target.getBoundingClientRect(),isMD=util.hasModifier(this,"material"),cover=isMD&&this.hasAttribute("cover-target"),distance={top:pos.top-margin,left:pos.left-margin,right:window.innerWidth-pos.right-margin,bottom:window.innerHeight-pos.bottom-margin},_calculateDirections2=this._calculateDirections(distance),vertical=_calculateDirections2.vertical,primary=_calculateDirections2.primary,secondary=_calculateDirections2.secondary;this._popover.classList.add("popover--"+primary);var offset=cover?0:(vertical?pos.height:pos.width)+(isMD?0:14);this.style[primary]=Math.max(0,distance[primary]+offset)+margin+"px",el.style[primary]=0;var l=vertical?"width":"height",sizes=function(style){return{width:parseInt(style.getPropertyValue("width")),height:parseInt(style.getPropertyValue("height"))}}(window.getComputedStyle(el));el.style[secondary]=Math.max(0,distance[secondary]-(sizes[l]-pos[l])/2)+"px",this._arrow.style[secondary]=Math.max(radius,distance[secondary]+pos[l]/2)+"px",this._setTransformOrigin(distance,sizes,pos,primary),el.removeAttribute("data-animit-orig-style")}},{key:"_setTransformOrigin",value:function(distance,sizes,pos,primary){var calc=function(a,o,l){return primary===a?sizes[l]/2:distance[a]+(primary===o?-sizes[l]:sizes[l]-pos[l])/2},x=calc("left","right","width")+"px",y=calc("top","bottom","height")+"px";util.extend(this._popover.style,{transformOrigin:x+" "+y,webkitTransformOriginX:x,webkitTransformOriginY:y})}},{key:"_calculateDirections",value:function(distance){var options=(this.getAttribute("direction")||"up down left right").split(/\s+/).map(function(e){return positions[e]}),primary=options.sort(function(a,b){return distance[a]-distance[b]})[0],vertical=-1!==["top","bottom"].indexOf(primary),secondary=void 0;return secondary=vertical?distance.left<distance.right?"left":"right":distance.top<distance.bottom?"top":"bottom",{vertical:vertical,primary:primary,secondary:secondary}}},{key:"_clearStyles",value:function(){var _this3=this;["top","bottom","left","right"].forEach(function(e){_this3._arrow.style[e]=_this3._content.style[e]=_this3.style[e]="",_this3._popover.classList.remove("popover--"+e)})}},{key:"_onChange",value:function(){var _this4=this;setImmediate(function(){_this4._currentTarget&&_this4._positionPopover(_this4._currentTarget)})}},{key:"_compile",value:function(){if(autoStyle.prepare(this),!this.classList.contains("popover")){this.classList.add("popover");var hasDefaultContainer=this._popover&&this._content;if(hasDefaultContainer){if(!this._mask){var mask=document.createElement("div");mask.classList.add("popover-mask"),this.insertBefore(mask,this.firstChild)}if(!this._arrow){var arrow=document.createElement("div");arrow.classList.add("popover__arrow"),this._popover.appendChild(arrow)}}else{for(var template=templateSource.cloneNode(!0),content=template.querySelector(".popover__content");this.childNodes[0];)content.appendChild(this.childNodes[0]);this.appendChild(template)}this.hasAttribute("style")&&(this._popover.setAttribute("style",this.getAttribute("style")),this.removeAttribute("style")),this.hasAttribute("mask-color")&&(this._mask.style.backgroundColor=this.getAttribute("mask-color")),ModifierUtil.initModifier(this,scheme$14)}}},{key:"_prepareAnimationOptions",value:function(options){if(options.animation&&!(options.animation in _animatorDict$4))throw new Error("Animator "+options.animation+" is not registered.");options.animationOptions=util.extend(AnimatorFactory.parseAnimationOptionsString(this.getAttribute("animation-options")),options.animationOptions||{})}},{key:"_executeAction",value:function(actions){var _this5=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1],callback=options.callback,action=actions.action,before=actions.before,after=actions.after;this._prepareAnimationOptions(options);var canceled=!1;return util.triggerElementEvent(this,"pre"+action,{popover:this,cancel:function(){return canceled=!0}}),canceled?Promise.reject("Canceled in pre"+action+" event."):new Promise(function(resolve){_this5._doorLock.waitUnlock(function(){var unlock=_this5._doorLock.lock();before&&before(),contentReady(_this5,function(){_this5._animator(options)[action](_this5,function(){after&&after(),unlock(),util.triggerElementEvent(_this5,"post"+action,{popover:_this5}),callback&&callback(),resolve(_this5)})})})})}},{key:"show",value:function(target){var _this6=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];if("string"==typeof target?target=document.querySelector(target):target instanceof Event&&(target=target.target),!(target instanceof HTMLElement))throw new Error("Invalid target");return this._executeAction({action:"show",before:function(){_this6.style.display="block",_this6._currentTarget=target,_this6._positionPopover(target)}},options)}},{key:"hide",value:function(){var _this7=this,options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];return this._executeAction({action:"hide",after:function(){_this7.style.display="none",_this7._clearStyles()}},options)}},{key:"_resetBackButtonHandler",value:function(){var _this8=this;this.onDeviceBackButton=function(e){return _this8.cancelable?_this8._cancel():e.callParentHandler()}}},{key:"connectedCallback",value:function(){var _this9=this;this._resetBackButtonHandler(),contentReady(this,function(){_this9._margin=_this9._margin||parseInt(window.getComputedStyle(_this9).getPropertyValue("top")),_this9._radius=parseInt(window.getComputedStyle(_this9._content).getPropertyValue("border-top-left-radius")),_this9._mask.addEventListener("click",_this9._boundCancel,!1),_this9._resetBackButtonHandler(),window.addEventListener("resize",_this9._boundOnChange,!1)})}},{key:"disconnectedCallback",value:function(){var _this10=this;contentReady(this,function(){_this10._mask.removeEventListener("click",_this10._boundCancel,!1),_this10._backButtonHandler.destroy(),_this10._backButtonHandler=null,window.removeEventListener("resize",_this10._boundOnChange,!1)})}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$14):"direction"===name?this._boundOnChange():void("animation"===name&&this._initAnimatorFactory())}},{key:"_cancel",value:function(){var _this11=this;this.cancelable&&this.hide({callback:function(){util.triggerElementEvent(_this11,"dialog-cancel")}})}},{key:"_mask",get:function(){return util.findChild(this,".popover-mask")}},{key:"_popover",get:function(){return util.findChild(this,".popover__container")}},{key:"_content",get:function(){return util.findChild(this._popover,".popover__content")}},{key:"_arrow",get:function(){return util.findChild(this._popover,".popover__arrow")}},{key:"visible",get:function(){return"none"!==window.getComputedStyle(this).getPropertyValue("display")}},{key:"cancelable",set:function(value){return util.toggleAttribute(this,"cancelable",value)},get:function(){return this.hasAttribute("cancelable")}},{key:"onDeviceBackButton",get:function(){return this._backButtonHandler},set:function(callback){this._backButtonHandler&&this._backButtonHandler.destroy(),this._backButtonHandler=deviceBackButtonDispatcher.createHandler(this,callback)}}],[{key:"registerAnimator",value:function(name,Animator){if(!(Animator.prototype instanceof PopoverAnimator))throw new Error('"Animator" param must inherit PopoverAnimator');_animatorDict$4[name]=Animator}},{key:"observedAttributes",get:function(){return["modifier","direction","animation"]}},{key:"PopoverAnimator",get:function(){return PopoverAnimator}}]),PopoverElement}(BaseElement));customElements.define("ons-popover",PopoverElement);var scheme$15={".progress-bar":"progress-bar--*",".progress-bar__primary":"progress-bar__primary--*",".progress-bar__secondary":"progress-bar__secondary--*"},template=util.createElement('\n <div class="progress-bar">\n <div class="progress-bar__secondary"></div>\n <div class="progress-bar__primary"></div>\n </div>\n'),ProgressBarElement=function(_BaseElement){function ProgressBarElement(){return babelHelpers.classCallCheck(this,ProgressBarElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(ProgressBarElement).apply(this,arguments))}return babelHelpers.inherits(ProgressBarElement,_BaseElement),babelHelpers.createClass(ProgressBarElement,[{key:"init",value:function(){this.hasAttribute("_compiled")||this._compile()}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$15):void("value"===name||"secondary-value"===name?this._updateValue():"indeterminate"===name&&this._updateDeterminate())}},{key:"_updateDeterminate",value:function(){this.hasAttribute("indeterminate")?(this._template.classList.add("progress-bar--indeterminate"),this._template.classList.remove("progress-bar--determinate")):(this._template.classList.add("progress-bar--determinate"),this._template.classList.remove("progress-bar--indeterminate"))}},{key:"_updateValue",value:function(){this._primary.style.width=this.hasAttribute("value")?this.getAttribute("value")+"%":"0%",this._secondary.style.width=this.hasAttribute("secondary-value")?this.getAttribute("secondary-value")+"%":"0%"}},{key:"_compile",value:function(){this._template=template.cloneNode(!0),this._primary=this._template.childNodes[3],this._secondary=this._template.childNodes[1],this._updateDeterminate(),this._updateValue(),this.appendChild(this._template),ModifierUtil.initModifier(this,scheme$15),this.setAttribute("_compiled","")}},{key:"value",set:function(value){if("number"!=typeof value||0>value||value>100)throw new Error("Invalid value");this.setAttribute("value",Math.floor(value))},get:function(){return parseInt(this.getAttribute("value")||"0")}},{key:"secondaryValue",set:function(value){if("number"!=typeof value||0>value||value>100)throw new Error("Invalid value");this.setAttribute("secondary-value",Math.floor(value))},get:function(){return parseInt(this.getAttribute("secondary-value")||"0")}},{key:"indeterminate",set:function(value){value?this.setAttribute("indeterminate",""):this.removeAttribute("indeterminate")},get:function(){return this.hasAttribute("indeterminate")}}],[{key:"observedAttributes",get:function(){return["modifier","value","secondary-value","indeterminate"]}}]),ProgressBarElement}(BaseElement);customElements.define("ons-progress-bar",ProgressBarElement);var scheme$16={".progress-circular":"progress-circular--*",".progress-circular__primary":"progress-circular__primary--*",".progress-circular__secondary":"progress-circular__secondary--*"},template$1=util.createElement('\n <svg class="progress-circular">\n <circle class="progress-circular__secondary" cx="50%" cy="50%" r="40%" fill="none" stroke-width="10%" stroke-miterlimit="10"/>\n <circle class="progress-circular__primary" cx="50%" cy="50%" r="40%" fill="none" stroke-width="10%" stroke-miterlimit="10"/>\n </svg>\n'),ProgressCircularElement=function(_BaseElement){function ProgressCircularElement(){return babelHelpers.classCallCheck(this,ProgressCircularElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(ProgressCircularElement).apply(this,arguments))}return babelHelpers.inherits(ProgressCircularElement,_BaseElement),babelHelpers.createClass(ProgressCircularElement,[{key:"init",value:function(){this.hasAttribute("_compiled")||this._compile()}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$16):void("value"===name||"secondary-value"===name?this._updateValue():"indeterminate"===name&&this._updateDeterminate())}},{key:"_updateDeterminate",value:function(){this.hasAttribute("indeterminate")?(this._template.classList.add("progress-circular--indeterminate"),this._template.classList.remove("progress-circular--determinate")):(this._template.classList.add("progress-circular--determinate"),this._template.classList.remove("progress-circular--indeterminate"))}},{key:"_updateValue",value:function(){if(this.hasAttribute("value")){var per=Math.ceil(251.32*this.getAttribute("value")*.01);this._primary.style["stroke-dasharray"]=per+"%, 251.32%"}if(this.hasAttribute("secondary-value")){var _per=Math.ceil(251.32*this.getAttribute("secondary-value")*.01);this._secondary.style["stroke-dasharray"]=_per+"%, 251.32%"}}},{key:"_compile",value:function(){this._template=template$1.cloneNode(!0),this._primary=this._template.childNodes[3],this._secondary=this._template.childNodes[1],this._updateDeterminate(),this._updateValue(),this.appendChild(this._template),ModifierUtil.initModifier(this,scheme$16),this.setAttribute("_compiled","")}},{key:"value",set:function(value){if("number"!=typeof value||0>value||value>100)throw new Error("Invalid value");this.setAttribute("value",Math.floor(value))},get:function(){return parseInt(this.getAttribute("value")||"0")}},{key:"secondaryValue",set:function(value){if("number"!=typeof value||0>value||value>100)throw new Error("Invalid value");this.setAttribute("secondary-value",Math.floor(value))},get:function(){return parseInt(this.getAttribute("secondary-value")||"0")}},{key:"indeterminate",set:function(value){value?this.setAttribute("indeterminate",""):this.removeAttribute("indeterminate")},get:function(){return this.hasAttribute("indeterminate")}}],[{key:"observedAttributes",get:function(){return["modifier","value","secondary-value","indeterminate"]}}]),ProgressCircularElement}(BaseElement);customElements.define("ons-progress-circular",ProgressCircularElement);var STATE_INITIAL="initial",STATE_PREACTION="preaction",STATE_ACTION="action",removeTransform=function(el){el.style.transform="",el.style.WebkitTransform="",el.style.transition="",el.style.WebkitTransition=""},PullHookElement=function(_BaseElement){function PullHookElement(){return babelHelpers.classCallCheck(this,PullHookElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(PullHookElement).apply(this,arguments))}return babelHelpers.inherits(PullHookElement,_BaseElement),babelHelpers.createClass(PullHookElement,[{key:"init",value:function(){this._boundOnDrag=this._onDrag.bind(this),this._boundOnDragStart=this._onDragStart.bind(this),this._boundOnDragEnd=this._onDragEnd.bind(this),this._boundOnScroll=this._onScroll.bind(this),this._setState(STATE_INITIAL,!0)}},{key:"_setStyle",value:function(){var height=this.height;this.style.height=height+"px",this.style.lineHeight=height+"px",this.style.marginTop="-1px",this._pageElement.style.marginTop="-"+height+"px"}},{key:"_onScroll",value:function(event){var element=this._pageElement;element.scrollTop<0&&(element.scrollTop=0)}},{key:"_generateTranslationTransform",value:function(scroll){return"translate3d(0px, "+scroll+"px, 0px)"}},{key:"_onDrag",value:function(event){var _this2=this;if(!this.disabled){if(platform.isAndroid()){var element=this._pageElement;element.scrollTop=this._startScroll-event.gesture.deltaY,element.scrollTop<window.innerHeight&&"up"!==event.gesture.direction&&event.gesture.preventDefault()}if(0===this._currentTranslation&&0===this._getCurrentScroll()){this._transitionDragLength=event.gesture.deltaY;var direction=event.gesture.interimDirection;"down"===direction?this._transitionDragLength-=1:this._transitionDragLength+=1}var scroll=Math.max(event.gesture.deltaY-this._startScroll,0);this._thresholdHeightEnabled()&&scroll>=this.thresholdHeight?(event.gesture.stopDetect(),setImmediate(function(){return _this2._finish()})):scroll>=this.height?this._setState(STATE_PREACTION):this._setState(STATE_INITIAL),event.stopPropagation(),this._translateTo(scroll)}}},{key:"_onDragStart",value:function(event){this.disabled||(this._startScroll=this._getCurrentScroll())}},{key:"_onDragEnd",value:function(event){if(!this.disabled&&this._currentTranslation>0){var scroll=this._currentTranslation;scroll>this.height?this._finish():this._translateTo(0,{animate:!0})}}},{key:"_finish",value:function(){var _this3=this;this._setState(STATE_ACTION),this._translateTo(this.height,{animate:!0});var action=this.onAction||function(done){return done()};action(function(){_this3._translateTo(0,{animate:!0}),_this3._setState(STATE_INITIAL)})}},{key:"_thresholdHeightEnabled",value:function(){var th=this.thresholdHeight;return th>0&&th>=this.height}},{key:"_setState",value:function(state,noEvent){var lastState=this._getState();this.setAttribute("state",state),noEvent||lastState===this._getState()||util.triggerElementEvent(this,"changestate",{pullHook:this,state:state,lastState:lastState})}},{key:"_getState",value:function(){return this.getAttribute("state")}},{key:"_getCurrentScroll",value:function(){return this._pageElement.scrollTop}},{key:"_isContentFixed",value:function(){return this.hasAttribute("fixed-content")}},{key:"_getScrollableElement",value:function(){return this._isContentFixed()?this:this._pageElement}},{key:"_translateTo",value:function(scroll){var _this4=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];if(0!=this._currentTranslation||0!=scroll){var done=function(){if(0===scroll){var el=_this4._getScrollableElement();removeTransform(el)}options.callback&&options.callback()};this._currentTranslation=scroll,options.animate?animit(this._getScrollableElement()).queue({transform:this._generateTranslationTransform(scroll)},{duration:.3,timing:"cubic-bezier(.1, .7, .1, 1)"}).play(done):animit(this._getScrollableElement()).queue({transform:this._generateTranslationTransform(scroll)}).play(done)}}},{key:"_disableDragLock",value:function(){this._dragLockDisabled=!0,this._destroyEventListeners(),this._createEventListeners()}},{key:"_createEventListeners",value:function(){this._gestureDetector=new GestureDetector(this._pageElement,{dragMinDistance:1,dragDistanceCorrection:!1,dragLockToAxis:!this._dragLockDisabled}),this._gestureDetector.on("dragup dragdown",this._boundOnDrag),this._gestureDetector.on("dragstart",this._boundOnDragStart),this._gestureDetector.on("dragend",this._boundOnDragEnd),this._pageElement.addEventListener("scroll",this._boundOnScroll,!1)}},{key:"_destroyEventListeners",value:function(){this._gestureDetector&&(this._gestureDetector.off("dragup dragdown",this._boundOnDrag),this._gestureDetector.off("dragstart",this._boundOnDragStart),this._gestureDetector.off("dragend",this._boundOnDragEnd),this._gestureDetector.dispose(),this._gestureDetector=null),this._pageElement.removeEventListener("scroll",this._boundOnScroll,!1)}},{key:"connectedCallback",value:function(){this._currentTranslation=0,this._pageElement=this.parentNode,this._createEventListeners(),this._setStyle()}},{key:"disconnectedCallback",value:function(){this._pageElement.style.marginTop="",this._destroyEventListeners()}},{key:"attributeChangedCallback",value:function(name,last,current){"height"===name&&this._setStyle()}},{key:"height",set:function(value){if(!util.isInteger(value))throw new Error("The height must be an integer");this.setAttribute("height",value+"px")},get:function(){return parseInt(this.getAttribute("height")||"64",10)}},{key:"thresholdHeight",set:function(value){if(!util.isInteger(value))throw new Error("The threshold height must be an integer");this.setAttribute("threshold-height",value+"px")},get:function(){return parseInt(this.getAttribute("threshold-height")||"96",10)}},{key:"state",get:function(){return this._getState()}},{key:"pullDistance",get:function(){return this._currentTranslation}},{key:"disabled",set:function(value){return util.toggleAttribute(this,"disabled",value)},get:function(){return this.hasAttribute("disabled")}}],[{key:"observedAttributes",get:function(){return["height"]}},{key:"STATE_INITIAL",get:function(){return STATE_INITIAL}},{key:"STATE_PREACTION",get:function(){return STATE_PREACTION}},{key:"STATE_ACTION",get:function(){return STATE_ACTION}}]),PullHookElement}(BaseElement);customElements.define("ons-pull-hook",PullHookElement);var AnimatorCSS=function(){function AnimatorCSS(){babelHelpers.classCallCheck(this,AnimatorCSS),this._queue=[],this._index=0}return babelHelpers.createClass(AnimatorCSS,[{key:"animate",value:function(el,final){var duration=arguments.length<=2||void 0===arguments[2]?200:arguments[2],start=(new Date).getTime(),initial={},stopped=!1,next=!1,timeout=!1,properties=Object.keys(final),updateStyles=function(){var s=window.getComputedStyle(el);properties.forEach(s.getPropertyValue.bind(s)),s=el.offsetHeight},result={stop:function(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];timeout&&clearTimeout(timeout);var k=Math.min(1,((new Date).getTime()-start)/duration);return properties.forEach(function(i){el.style[i]=(1-k)*initial[i]+k*final[i]+("opacity"==i?"":"px")}),el.style.transitionDuration="0s",options.stopNext?next=!1:stopped||(stopped=!0,next&&next()),result},then:function(cb){return next=cb,stopped&&next&&next(),result},speed:function(newDuration){return internal.config.animationsDisabled&&(newDuration=0),stopped||!function(){timeout&&clearTimeout(timeout);var passed=(new Date).getTime()-start,k=passed/duration,remaining=newDuration*(1-k);properties.forEach(function(i){el.style[i]=(1-k)*initial[i]+k*final[i]+("opacity"==i?"":"px")}),updateStyles(),start=el.speedUpTime,duration=remaining,el.style.transitionDuration=duration/1e3+"s",properties.forEach(function(i){el.style[i]=final[i]+("opacity"==i?"":"px")}),timeout=setTimeout(result.stop,remaining)}(),result},finish:function(){var milliseconds=arguments.length<=0||void 0===arguments[0]?50:arguments[0],k=((new Date).getTime()-start)/duration;return result.speed(milliseconds/(1-k)),result}};if(el.hasAttribute("disabled")||stopped||internal.config.animationsDisabled)return result;var style=window.getComputedStyle(el);return properties.forEach(function(e){var v=parseFloat(style.getPropertyValue(e));initial[e]=isNaN(v)?0:v}),stopped||(el.style.transitionProperty=properties.join(","),el.style.transitionDuration=duration/1e3+"s",properties.forEach(function(e){el.style[e]=final[e]+("opacity"==e?"":"px")})),timeout=setTimeout(result.stop,duration),this._onStopAnimations(el,result.stop),result}}]),babelHelpers.createClass(AnimatorCSS,[{key:"_onStopAnimations",value:function(el,listener){var queue=this._queue,i=this._index++;queue[el]=queue[el]||[],queue[el][i]=function(options){return delete queue[el][i],queue[el]&&0==queue[el].length&&delete queue[el],listener(options)}}},{key:"stopAnimations",value:function(el){var _this=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];return Array.isArray(el)?el.forEach(function(el){_this.stopAnimations(el,options)}):void(this._queue[el]||[]).forEach(function(e){e(options||{})})}},{key:"stopAll",value:function(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];this.stopAnimations(Object.keys(this._queue),options)}},{key:"fade",value:function(el){var duration=arguments.length<=1||void 0===arguments[1]?200:arguments[1];return this.animate(el,{opacity:0},duration)}}]),AnimatorCSS}(),RippleElement=function(_BaseElement){function RippleElement(){return babelHelpers.classCallCheck(this,RippleElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(RippleElement).apply(this,arguments))}return babelHelpers.inherits(RippleElement,_BaseElement),babelHelpers.createClass(RippleElement,[{key:"init",value:function(){var _this2=this;this.classList.add("ripple"),this.hasAttribute("_compiled")?(this._background=this.getElementsByClassName("ripple__background")[0],this._wave=this.getElementsByClassName("ripple__wave")[0]):this._compile(),this._animator=new AnimatorCSS,["color","center","start-radius","background"].forEach(function(e){_this2.attributeChangedCallback(e,null,_this2.getAttribute(e))})}},{key:"_compile",value:function(){var _this3=this;["_wave","_background"].forEach(function(e){_this3[e]=document.createElement("div"),_this3[e].classList.add("ripple_"+e),_this3.appendChild(_this3[e])}),this.setAttribute("_compiled","")}},{key:"_calculateCoords",value:function(e){var x,y,h,w,r,b=this.getBoundingClientRect();return this._center?(x=b.width/2,y=b.height/2,r=Math.sqrt(x*x+y*y)):(x=(e.clientX||e.changedTouches[0].clientX)-b.left,y=(e.clientY||e.changedTouches[0].clientY)-b.top,h=Math.max(y,b.height-y),w=Math.max(x,b.width-x),r=Math.sqrt(h*h+w*w)),{x:x,y:y,r:r}}},{key:"_rippleAnimation",value:function(e){var duration=arguments.length<=1||void 0===arguments[1]?300:arguments[1],_animator=this._animator,_wave=this._wave,_background=this._background,_minR=this._minR,_calculateCoords2=this._calculateCoords(e),x=_calculateCoords2.x,y=_calculateCoords2.y,r=_calculateCoords2.r;return _animator.stopAll({stopNext:1}),_animator.animate(_background,{opacity:1},duration),util.extend(_wave.style,{opacity:1,top:y-_minR+"px",left:x-_minR+"px",width:2*_minR+"px",height:2*_minR+"px"}),_animator.animate(_wave,{top:y-r,left:x-r,height:2*r,width:2*r},duration)}},{key:"_updateParent",value:function(){if(!this._parentUpdated&&this.parentNode){var computedStyle=window.getComputedStyle(this.parentNode);"static"===computedStyle.getPropertyValue("position")&&(this.parentNode.style.position="relative"),
this._parentUpdated=!0}}},{key:"_onTap",value:function(e){var _this4=this;this.disabled||(this._updateParent(),this._rippleAnimation(e.gesture.srcEvent).then(function(){_this4._animator.fade(_this4._wave),_this4._animator.fade(_this4._background)}))}},{key:"_onHold",value:function(e){this.disabled||(this._updateParent(),this._holding=this._rippleAnimation(e.gesture.srcEvent,2e3),document.addEventListener("release",this._boundOnRelease))}},{key:"_onRelease",value:function(e){var _this5=this;this._holding&&(this._holding.speed(300).then(function(){_this5._animator.stopAll({stopNext:!0}),_this5._animator.fade(_this5._wave),_this5._animator.fade(_this5._background)}),this._holding=!1),document.removeEventListener("release",this._boundOnRelease)}},{key:"_onDragStart",value:function(e){return this._holding?this._onRelease(e):void(-1!=["left","right"].indexOf(e.gesture.direction)&&this._onTap(e))}},{key:"connectedCallback",value:function(){this._parentNode=this.parentNode,this._boundOnTap=this._onTap.bind(this),this._boundOnHold=this._onHold.bind(this),this._boundOnDragStart=this._onDragStart.bind(this),this._boundOnRelease=this._onRelease.bind(this),internal.config.animationsDisabled?this.disabled=!0:(this._parentNode.addEventListener("tap",this._boundOnTap),this._parentNode.addEventListener("hold",this._boundOnHold),this._parentNode.addEventListener("dragstart",this._boundOnDragStart))}},{key:"disconnectedCallback",value:function(){var pn=this._parentNode||this.parentNode;pn.removeEventListener("tap",this._boundOnTap),pn.removeEventListener("hold",this._boundOnHold),pn.removeEventListener("dragstart",this._boundOnDragStart)}},{key:"attributeChangedCallback",value:function(name,last,current){"start-radius"===name&&(this._minR=Math.max(0,parseFloat(current)||0)),"color"===name&¤t&&(this._wave.style.background=current,this.hasAttribute("background")||(this._background.style.background=current)),"background"===name&&(current||last)&&("none"===current?(this._background.setAttribute("disabled","disabled"),this._background.style.background="transparent"):(this._background.hasAttribute("disabled")&&this._background.removeAttribute("disabled"),this._background.style.background=current)),"center"===name&&(this._center=null!=current&&"false"!=current)}},{key:"disabled",set:function(value){return util.toggleAttribute(this,"disabled",value)},get:function(){return this.hasAttribute("disabled")}}],[{key:"observedAttributes",get:function(){return["start-radius","color","background","center"]}}]),RippleElement}(BaseElement);customElements.define("ons-ripple",RippleElement);var RowElement=function(_BaseElement){function RowElement(){return babelHelpers.classCallCheck(this,RowElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(RowElement).apply(this,arguments))}return babelHelpers.inherits(RowElement,_BaseElement),RowElement}(BaseElement);customElements.define("ons-row",RowElement);var scheme$17={"":"speed-dial__item--*"},SpeedDialItemElement=function(_BaseElement){function SpeedDialItemElement(){return babelHelpers.classCallCheck(this,SpeedDialItemElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(SpeedDialItemElement).apply(this,arguments))}return babelHelpers.inherits(SpeedDialItemElement,_BaseElement),babelHelpers.createClass(SpeedDialItemElement,[{key:"init",value:function(){this._compile(),this._boundOnClick=this._onClick.bind(this)}},{key:"attributeChangedCallback",value:function(name,last,current){switch(name){case"modifier":ModifierUtil.onModifierChanged(last,current,this,scheme$17);break;case"ripple":this._updateRipple()}}},{key:"connectedCallback",value:function(){this.addEventListener("click",this._boundOnClick,!1)}},{key:"disconnectedCallback",value:function(){this.removeEventListener("click",this._boundOnClick,!1)}},{key:"_updateRipple",value:function(){util.updateRipple(this)}},{key:"_onClick",value:function(e){e.stopPropagation()}},{key:"_compile",value:function(){autoStyle.prepare(this),this.classList.add("fab"),this.classList.add("fab--mini"),this.classList.add("speed-dial__item"),this._updateRipple(),ModifierUtil.initModifier(this,scheme$17)}}],[{key:"observedAttributes",get:function(){return["modifier","ripple"]}}]),SpeedDialItemElement}(BaseElement);customElements.define("ons-speed-dial-item",SpeedDialItemElement);var styler=function styler(element,style){return styler.css.apply(styler,arguments)};styler.css=function(element,styles){var keys=Object.keys(styles);return keys.forEach(function(key){key in element.style?element.style[key]=styles[key]:styler._prefix(key)in element.style?element.style[styler._prefix(key)]=styles[key]:console.warn("No such style property: "+key)}),element},styler._prefix=function(){var styles=window.getComputedStyle(document.documentElement,""),prefix=(Array.prototype.slice.call(styles).join("").match(/-(moz|webkit|ms)-/)||""===styles.OLink&&["","o"])[1];return function(name){return prefix+name.substr(0,1).toUpperCase()+name.substr(1)}}(),styler.clear=function(element){styler._clear(element)},styler._clear=function(element){for(var len=element.style.length,style=element.style,keys=[],i=0;len>i;i++)keys.push(style[i]);keys.forEach(function(key){style[key]=""})};var scheme$18={"":"speed-dial--*"},SpeedDialElement=function(_BaseElement){function SpeedDialElement(){return babelHelpers.classCallCheck(this,SpeedDialElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(SpeedDialElement).apply(this,arguments))}return babelHelpers.inherits(SpeedDialElement,_BaseElement),babelHelpers.createClass(SpeedDialElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){_this2._compile()}),this._shown=!0,this._itemShown=!1,this._boundOnClick=this._onClick.bind(this)}},{key:"_compile",value:function(){this.classList.contains("speed__dial")||(this.classList.add("speed__dial"),autoStyle.prepare(this),this._updateRipple(),ModifierUtil.initModifier(this,scheme$18),this.hasAttribute("direction")?this._updateDirection(this.getAttribute("direction")):this._updateDirection("up")),this._updatePosition()}},{key:"attributeChangedCallback",value:function(name,last,current){var _this3=this;switch(name){case"modifier":ModifierUtil.onModifierChanged(last,current,this,scheme$18);break;case"ripple":contentReady(this,function(){return _this3._updateRipple()});break;case"direction":contentReady(this,function(){return _this3._updateDirection(current)});break;case"position":contentReady(this,function(){return _this3._updatePosition()})}}},{key:"connectedCallback",value:function(){this.addEventListener("click",this._boundOnClick,!1)}},{key:"disconnectedCallback",value:function(){this.removeEventListener("click",this._boundOnClick,!1)}},{key:"_onClick",value:function(e){!this.disabled&&this._shown&&this.toggleItems()}},{key:"_show",value:function(){this.inline||this.show()}},{key:"_hide",value:function(){this.inline||this.hide()}},{key:"_updateRipple",value:function(){var fab=util.findChild(this,"ons-fab");fab&&(this.hasAttribute("ripple")?fab.setAttribute("ripple",""):fab.removeAttribute("ripple"))}},{key:"_updateDirection",value:function(direction){for(var children=this.items,i=0;i<children.length;i++)styler(children[i],{transitionDelay:25*i+"ms",bottom:"auto",right:"auto",top:"auto",left:"auto"});switch(direction){case"up":for(var _i=0;_i<children.length;_i++)children[_i].style.bottom=72+56*_i+"px",children[_i].style.right="8px";break;case"down":for(var _i2=0;_i2<children.length;_i2++)children[_i2].style.top=72+56*_i2+"px",children[_i2].style.left="8px";break;case"left":for(var _i3=0;_i3<children.length;_i3++)children[_i3].style.top="8px",children[_i3].style.right=72+56*_i3+"px";break;case"right":for(var _i4=0;_i4<children.length;_i4++)children[_i4].style.top="8px",children[_i4].style.left=72+56*_i4+"px";break;default:throw new Error("Argument must be one of up, down, left or right.")}}},{key:"_updatePosition",value:function(){var position=this.getAttribute("position");switch(this.classList.remove("fab--top__left","fab--bottom__right","fab--bottom__left","fab--top__right","fab--top__center","fab--bottom__center"),position){case"top right":case"right top":this.classList.add("fab--top__right");break;case"top left":case"left top":this.classList.add("fab--top__left");break;case"bottom right":case"right bottom":this.classList.add("fab--bottom__right");break;case"bottom left":case"left bottom":this.classList.add("fab--bottom__left");break;case"center top":case"top center":this.classList.add("fab--top__center");break;case"center bottom":case"bottom center":this.classList.add("fab--bottom__center")}}},{key:"show",value:function(){arguments.length<=0||void 0===arguments[0]?{}:arguments[0];this.querySelector("ons-fab").show(),this._shown=!0}},{key:"hide",value:function(){var _this4=this;arguments.length<=0||void 0===arguments[0]?{}:arguments[0];this.hideItems(),setTimeout(function(){_this4.querySelector("ons-fab").hide()},200),this._shown=!1}},{key:"showItems",value:function(){if(this.hasAttribute("direction")?this._updateDirection(this.getAttribute("direction")):this._updateDirection("up"),!this._itemShown)for(var children=this.items,i=0;i<children.length;i++)styler(children[i],{transform:"scale(1)",transitionDelay:25*i+"ms"});this._itemShown=!0,util.triggerElementEvent(this,"open")}},{key:"hideItems",value:function(){if(this._itemShown)for(var children=this.items,i=0;i<children.length;i++)styler(children[i],{transform:"scale(0)",transitionDelay:25*(children.length-i)+"ms"});this._itemShown=!1,util.triggerElementEvent(this,"close")}},{key:"isOpen",value:function(){return this._itemShown}},{key:"toggle",value:function(){this.visible?this.hide():this.show()}},{key:"toggleItems",value:function(){this.isOpen()?this.hideItems():this.showItems()}},{key:"items",get:function(){return util.arrayFrom(this.querySelectorAll("ons-speed-dial-item"))}},{key:"disabled",set:function(value){return value&&this.hideItems(),util.arrayFrom(this.children).forEach(function(e){util.match(e,".fab")&&util.toggleAttribute(e,"disabled",value)}),util.toggleAttribute(this,"disabled",value)},get:function(){return this.hasAttribute("disabled")}},{key:"inline",get:function(){return this.hasAttribute("inline")}},{key:"visible",get:function(){return this._shown&&"none"!==this.style.display}}],[{key:"observedAttributes",get:function(){return["modifier","ripple","direction","position"]}}]),SpeedDialElement}(BaseElement);customElements.define("ons-speed-dial",SpeedDialElement);var rewritables$1={ready:function(element,callback){setImmediate(callback)},link:function(element,target,options,callback){callback(target)}},SplitterContentElement=function(_BaseElement){function SplitterContentElement(){return babelHelpers.classCallCheck(this,SplitterContentElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(SplitterContentElement).apply(this,arguments))}return babelHelpers.inherits(SplitterContentElement,_BaseElement),babelHelpers.createClass(SplitterContentElement,[{key:"init",value:function(){var _this2=this;this._page=null,this._pageLoader=defaultPageLoader,contentReady(this,function(){var page=_this2._getPageTarget();page&&_this2.load(page)})}},{key:"connectedCallback",value:function(){if(!util.match(this.parentNode,"ons-splitter"))throw new Error('"ons-splitter-content" must have "ons-splitter" as parentNode.')}},{key:"_getPageTarget",value:function(){return this._page||this.getAttribute("page")}},{key:"disconnectedCallback",value:function(){}},{key:"attributeChangedCallback",value:function(name,last,current){}},{key:"load",value:function(page){var _this3=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];this._page=page;var callback=options.callback||function(){};return new Promise(function(resolve){_this3._pageLoader.load({page:page,parent:_this3,replace:!0},function(_ref){var element=_ref.element;_ref.unload;rewritables$1.link(_this3,element,options,function(fragment){setImmediate(function(){return _this3._show()}),callback(),resolve(_this3.firstChild)})})})}},{key:"_show",value:function(){util.propagateAction(this,"_show")}},{key:"_hide",value:function(){util.propagateAction(this,"_hide")}},{key:"_destroy",value:function(){util.propagateAction(this,"_destroy"),this.remove()}},{key:"page",get:function(){return this._page},set:function(page){this._page=page}},{key:"pageLoader",get:function(){return this._pageLoader},set:function(loader){if(!(loader instanceof PageLoader))throw Error("First parameter must be an instance of PageLoader");this._pageLoader=loader}}],[{key:"observedAttributes",get:function(){return[]}},{key:"rewritables",get:function(){return rewritables$1}}]),SplitterContentElement}(BaseElement);customElements.define("ons-splitter-content",SplitterContentElement);var SplitterMaskElement=function(_BaseElement){function SplitterMaskElement(){return babelHelpers.classCallCheck(this,SplitterMaskElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(SplitterMaskElement).apply(this,arguments))}return babelHelpers.inherits(SplitterMaskElement,_BaseElement),babelHelpers.createClass(SplitterMaskElement,[{key:"init",value:function(){this._boundOnClick=this._onClick.bind(this)}},{key:"_onClick",value:function(event){util.match(this.parentNode,"ons-splitter")&&this.parentNode._sides.forEach(function(side){return side.close("left")["catch"](function(){})}),event.stopPropagation()}},{key:"attributeChangedCallback",value:function(name,last,current){}},{key:"connectedCallback",value:function(){this.addEventListener("click",this._boundOnClick)}},{key:"disconnectedCallback",value:function(){this.removeEventListener("click",this._boundOnClick)}}],[{key:"observedAttributes",get:function(){return[]}}]),SplitterMaskElement}(BaseElement);customElements.define("ons-splitter-mask",SplitterMaskElement);var SplitterAnimator=function(){function SplitterAnimator(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];babelHelpers.classCallCheck(this,SplitterAnimator),this._options={timing:"cubic-bezier(.1, .7, .1, 1)",duration:"0.3",delay:"0"},this.updateOptions(options)}return babelHelpers.createClass(SplitterAnimator,[{key:"updateOptions",value:function(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];util.extend(this._options,options),this._timing=this._options.timing,this._duration=this._options.duration,this._delay=this._options.delay}},{key:"activate",value:function(sideElement){var _this=this,splitter=sideElement.parentNode;contentReady(splitter,function(){_this._side=sideElement,_this._content=splitter.content,_this._mask=splitter.mask})}},{key:"inactivate",value:function(){this._content=this._side=this._mask=null}},{key:"translate",value:function(distance){animit(this._side).queue({transform:"translate3d("+(this.minus+distance)+"px, 0px, 0px)"}).play()}},{key:"open",value:function(done){animit.runAll(animit(this._side).wait(this._delay).queue({transform:"translate3d("+this.minus+"100%, 0px, 0px)"},{duration:this._duration,timing:this._timing}).queue(function(callback){callback(),done&&done()}),animit(this._mask).wait(this._delay).queue({display:"block"}).queue({opacity:"1"},{duration:this._duration,timing:"linear"}))}},{key:"close",value:function(done){var _this2=this;animit.runAll(animit(this._side).wait(this._delay).queue({transform:"translate3d(0px, 0px, 0px)"},{duration:this._duration,timing:this._timing}).queue(function(callback){_this2._side.style.webkitTransition="",done&&done(),callback()}),animit(this._mask).wait(this._delay).queue({opacity:"0"},{duration:this._duration,timing:"linear"}).queue({display:"none"}))}},{key:"minus",get:function(){return"right"===this._side._side?"-":""}}]),SplitterAnimator}(),_animatorDict$5={"default":SplitterAnimator,overlay:SplitterAnimator},SplitterElement=function(_BaseElement){function SplitterElement(){return babelHelpers.classCallCheck(this,SplitterElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(SplitterElement).apply(this,arguments))}return babelHelpers.inherits(SplitterElement,_BaseElement),babelHelpers.createClass(SplitterElement,[{key:"_getSide",value:function(side){var element=util.findChild(this,function(e){return util.match(e,"ons-splitter-side")&&e.getAttribute("side")===side});return element}},{key:"_onDeviceBackButton",value:function(event){this._sides.some(function(s){return s.isOpen?s.close():!1})||event.callParentHandler()}},{key:"_onModeChange",value:function(e){var _this2=this;e.target.parentNode&&contentReady(this,function(){_this2._layout()})}},{key:"_layout",value:function(){var _this3=this;this._sides.forEach(function(side){_this3.content.style[side._side]="split"===side.mode?side._width:0})}},{key:"init",value:function(){var _this4=this;this._boundOnModeChange=this._onModeChange.bind(this),contentReady(this,function(){_this4._compile(),_this4._layout()})}},{key:"_compile",value:function(){this.mask||this.appendChild(document.createElement("ons-splitter-mask"))}},{key:"connectedCallback",value:function(){this.onDeviceBackButton=this._onDeviceBackButton.bind(this),this.addEventListener("modechange",this._boundOnModeChange,!1)}},{key:"disconnectedCallback",value:function(){this._backButtonHandler.destroy(),this._backButtonHandler=null,this.removeEventListener("modechange",this._boundOnModeChange,!1)}},{key:"attributeChangedCallback",value:function(name,last,current){}},{key:"_show",value:function(){util.propagateAction(this,"_show")}},{key:"_hide",value:function(){util.propagateAction(this,"_hide")}},{key:"_destroy",value:function(){util.propagateAction(this,"_destroy"),this.remove()}},{key:"left",get:function(){return this._getSide("left")}},{key:"right",get:function(){return this._getSide("right")}},{key:"_sides",get:function(){return[this.left,this.right].filter(function(e){return e})}},{key:"content",get:function(){return util.findChild(this,"ons-splitter-content")}},{key:"mask",get:function(){return util.findChild(this,"ons-splitter-mask")}},{key:"onDeviceBackButton",get:function(){return this._backButtonHandler},set:function(callback){this._backButtonHandler&&this._backButtonHandler.destroy(),this._backButtonHandler=deviceBackButtonDispatcher.createHandler(this,callback)}}],[{key:"registerAnimator",value:function(name,Animator){if(!(Animator instanceof SplitterAnimator))throw new Error("Animator parameter must be an instance of SplitterAnimator.");_animatorDict$5[name]=Animator}},{key:"SplitterAnimator",get:function(){return SplitterAnimator}},{key:"animators",get:function(){return _animatorDict$5}}]),SplitterElement}(BaseElement);customElements.define("ons-splitter",SplitterElement);var SPLIT_MODE="split",COLLAPSE_MODE="collapse",CLOSED_STATE="closed",OPEN_STATE="open",CHANGING_STATE="changing",WATCHED_ATTRIBUTES=["animation","width","side","collapse","swipeable","swipe-target-width","animation-options","open-threshold"],rewritables$2={ready:function(splitterSideElement,callback){setImmediate(callback)},link:function(splitterSideElement,target,options,callback){callback(target)}},CollapseDetection=function(){function CollapseDetection(element,target){babelHelpers.classCallCheck(this,CollapseDetection),this._element=element,this._boundOnChange=this._onChange.bind(this),target&&this.changeTarget(target)}return babelHelpers.createClass(CollapseDetection,[{key:"changeTarget",value:function(target){this.disable(),this._target=target,target&&(this._orientation=-1!==["portrait","landscape"].indexOf(target),this.activate())}},{key:"_match",value:function(value){return this._orientation?this._target===(value.isPortrait?"portrait":"landscape"):value.matches}},{key:"_onChange",value:function(value){this._element._updateMode(this._match(value)?COLLAPSE_MODE:SPLIT_MODE)}},{key:"activate",value:function(){this._orientation?(orientation.on("change",this._boundOnChange),this._onChange({isPortrait:orientation.isPortrait()})):(this._queryResult=window.matchMedia(this._target),this._queryResult.addListener(this._boundOnChange),this._onChange(this._queryResult))}},{key:"disable",value:function(){this._orientation?orientation.off("change",this._boundOnChange):this._queryResult&&(this._queryResult.removeListener(this._boundOnChange),this._queryResult=null)}}]),CollapseDetection}(),widthToPx=function(width,parent){var value=parseInt(width,10),px=/px/.test(width);return px?value:Math.round(parent.offsetWidth*value/100)},CollapseMode=function(){function CollapseMode(element){babelHelpers.classCallCheck(this,CollapseMode),this._active=!1,this._state=CLOSED_STATE,this._element=element,this._lock=new DoorLock}return babelHelpers.createClass(CollapseMode,[{key:"_animator",get:function(){return this._element._animator}}]),babelHelpers.createClass(CollapseMode,[{key:"isOpen",value:function(){return this._active&&this._state!==CLOSED_STATE}},{key:"handleGesture",value:function(e){!this._active||this._lock.isLocked()||this._isOpenOtherSideMenu()||("dragstart"===e.type?this._onDragStart(e):this._ignoreDrag||("dragend"===e.type?this._onDragEnd(e):this._onDrag(e)))}},{key:"_onDragStart",value:function(event){var scrolling=!/left|right/.test(event.gesture.direction),distance="left"===this._element._side?event.gesture.center.clientX:window.innerWidth-event.gesture.center.clientX,area=this._element._swipeTargetWidth,isOpen=this.isOpen();this._ignoreDrag=scrolling||area&&distance>area&&!isOpen,this._width=widthToPx(this._element._width,this._element.parentNode),this._startDistance=this._distance=isOpen?this._width:0}},{key:"_onDrag",value:function(event){event.gesture.preventDefault();var delta="left"===this._element._side?event.gesture.deltaX:-event.gesture.deltaX,distance=Math.max(0,Math.min(this._width,this._startDistance+delta));distance!==this._distance&&(this._animator.translate(distance),this._distance=distance,this._state=CHANGING_STATE)}},{key:"_onDragEnd",value:function(event){var distance=this._distance,width=this._width,el=this._element,direction=event.gesture.interimDirection,shouldOpen=el._side!==direction&&distance>width*el._threshold;this.executeAction(shouldOpen?"open":"close"),this._ignoreDrag=!0}},{key:"layout",value:function(){this._active&&this._state===OPEN_STATE&&this._animator.open()}},{key:"enterMode",value:function(){this._active||(this._active=!0,this.layout())}},{key:"exitMode",value:function(){this._active=!1}},{key:"_isOpenOtherSideMenu",value:function(){var _this=this;return util.arrayFrom(this._element.parentElement.children).some(function(e){return util.match(e,"ons-splitter-side")&&e!==_this._element&&e.isOpen})}},{key:"executeAction",value:function(name){var _this2=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1],FINAL_STATE="open"===name?OPEN_STATE:CLOSED_STATE;if(!this._active)return Promise.resolve(!1);if(this._state===FINAL_STATE)return Promise.resolve(this._element);if(this._lock.isLocked())return Promise.reject("Splitter side is locked.");if("open"===name&&this._isOpenOtherSideMenu())return Promise.reject("Another menu is already open.");if(this._element._emitEvent("pre"+name))return Promise.reject("Canceled in pre"+name+" event.");var callback=options.callback,unlock=this._lock.lock(),done=function(){_this2._state=FINAL_STATE,_this2.layout(),unlock(),_this2._element._emitEvent("post"+name),callback&&callback()};return options.withoutAnimation?(done(),Promise.resolve(this._element)):(this._state=CHANGING_STATE,new Promise(function(resolve){_this2._animator[name](function(){done(),resolve(_this2._element)})}))}}]),CollapseMode}(),SplitterSideElement=function(_BaseElement){function SplitterSideElement(){return babelHelpers.classCallCheck(this,SplitterSideElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(SplitterSideElement).apply(this,arguments))}return babelHelpers.inherits(SplitterSideElement,_BaseElement),babelHelpers.createClass(SplitterSideElement,[{key:"init",value:function(){var _this4=this;this._page=null,this._pageLoader=defaultPageLoader,this._collapseMode=new CollapseMode(this),this._collapseDetection=new CollapseDetection(this),this._animatorFactory=new AnimatorFactory({animators:SplitterElement.animators,baseClass:SplitterAnimator,baseClassName:"SplitterAnimator",defaultAnimation:this.getAttribute("animation")}),this._boundHandleGesture=function(e){return _this4._collapseMode.handleGesture(e)},this._watchedAttributes=WATCHED_ATTRIBUTES,contentReady(this,function(){rewritables$2.ready(_this4,function(){var page=_this4._getPageTarget();page&&_this4.load(page)})})}},{key:"connectedCallback",value:function(){var _this5=this;if(!util.match(this.parentNode,"ons-splitter"))throw new Error("Parent must be an ons-splitter element.");this._gestureDetector=new GestureDetector(this.parentElement,{dragMinDistance:1}),contentReady(this,function(){_this5._watchedAttributes.forEach(function(e){return _this5._update(e)})}),this.hasAttribute("side")||this.setAttribute("side","left")}},{key:"_getPageTarget",value:function(){return this._page||this.getAttribute("page")}},{key:"disconnectedCallback",value:function(){this._collapseDetection.disable(),this._gestureDetector.dispose(),this._gestureDetector=null}},{key:"attributeChangedCallback",value:function(name,last,current){this._update(name,current)}},{key:"_update",value:function(name,value){return name="_update"+name.split("-").map(function(e){return e[0].toUpperCase()+e.slice(1)}).join(""),this[name](value)}},{key:"_emitEvent",value:function(name){if("pre"!==name.slice(0,3))return util.triggerElementEvent(this,name,{side:this});var isCanceled=!1;return util.triggerElementEvent(this,name,{side:this,cancel:function(){return isCanceled=!0}}),isCanceled}},{key:"_updateCollapse",value:function(){var value=arguments.length<=0||void 0===arguments[0]?this.getAttribute("collapse"):arguments[0];return null===value||"split"===value?(this._collapseDetection.disable(),this._updateMode(SPLIT_MODE)):""===value||"collapse"===value?(this._collapseDetection.disable(),this._updateMode(COLLAPSE_MODE)):void this._collapseDetection.changeTarget(value)}},{key:"_updateMode",value:function(mode){mode!==this._mode&&(this._mode=mode,this._collapseMode[mode===COLLAPSE_MODE?"enterMode":"exitMode"](),this.setAttribute("mode",mode),util.triggerElementEvent(this,"modechange",{side:this,mode:mode}))}},{key:"_updateOpenThreshold",value:function(){var threshold=arguments.length<=0||void 0===arguments[0]?this.getAttribute("open-threshold"):arguments[0];this._threshold=Math.max(0,Math.min(1,parseFloat(threshold)||.3))}},{key:"_updateSwipeable",value:function(){var swipeable=arguments.length<=0||void 0===arguments[0]?this.getAttribute("swipeable"):arguments[0],action=null===swipeable?"off":"on";this._gestureDetector&&this._gestureDetector[action]("dragstart dragleft dragright dragend",this._boundHandleGesture)}},{key:"_updateSwipeTargetWidth",value:function(){var value=arguments.length<=0||void 0===arguments[0]?this.getAttribute("swipe-target-width"):arguments[0];this._swipeTargetWidth=Math.max(0,parseInt(value)||0)}},{key:"_updateWidth",value:function(){this.style.width=this._width}},{key:"_updateSide",value:function(){var side=arguments.length<=0||void 0===arguments[0]?this.getAttribute("side"):arguments[0];this._side="right"===side?side:"left"}},{key:"_updateAnimation",value:function(){var animation=arguments.length<=0||void 0===arguments[0]?this.getAttribute("animation"):arguments[0];this._animator=this._animatorFactory.newAnimator({animation:animation}),this._animator.activate(this)}},{key:"_updateAnimationOptions",value:function(){var value=arguments.length<=0||void 0===arguments[0]?this.getAttribute("animation-options"):arguments[0];this._animator.updateOptions(AnimatorFactory.parseAnimationOptionsString(value))}},{key:"open",value:function(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];return this._collapseMode.executeAction("open",options)}},{key:"close",value:function(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];return this._collapseMode.executeAction("close",options)}},{key:"toggle",value:function(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];return this.isOpen?this.close(options):this.open(options)}},{key:"load",value:function(page){var _this6=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];this._page=page;var callback=options.callback||function(){};return new Promise(function(resolve){_this6._pageLoader.load({page:page,parent:_this6,replace:!0},function(_ref){var element=_ref.element;_ref.unload;rewritables$2.link(_this6,element,options,function(fragment){setImmediate(function(){return _this6._show()}),callback(),resolve(_this6.firstChild)})})})}},{key:"_show",value:function(){util.propagateAction(this,"_show")}},{key:"_hide",value:function(){util.propagateAction(this,"_hide")}},{key:"_destroy",value:function(){util.propagateAction(this,"_destroy"),this.remove()}},{key:"_width",get:function(){var width=this.getAttribute("width");return/^\d+(px|%)$/.test(width)?width:"80%"},set:function(value){this.setAttribute("width",value)}},{key:"page",get:function(){return this._page},set:function(page){this._page=page}},{key:"pageLoader",get:function(){return this._pageLoader},set:function(loader){if(!(loader instanceof PageLoader))throw Error("First parameter must be an instance of PageLoader.");this._pageLoader=loader}},{key:"mode",get:function(){return this._mode}},{key:"isOpen",get:function(){return this._collapseMode.isOpen()}}],[{key:"observedAttributes",get:function(){return WATCHED_ATTRIBUTES}},{key:"rewritables",get:function(){return rewritables$2}}]),SplitterSideElement}(BaseElement);customElements.define("ons-splitter-side",SplitterSideElement);var scheme$19={"":"switch--*",".switch__input":"switch--*__input",".switch__handle":"switch--*__handle",".switch__toggle":"switch--*__toggle"},template$2=util.createFragment('\n <input type="checkbox" class="switch__input">\n <div class="switch__toggle">\n <div class="switch__handle">\n <div class="switch__touch"></div>\n </div>\n </div>\n'),locations={ios:[1,21],material:[0,16]},SwitchElement=function(_BaseElement){function SwitchElement(){return babelHelpers.classCallCheck(this,SwitchElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(SwitchElement).apply(this,arguments))}return babelHelpers.inherits(SwitchElement,_BaseElement),babelHelpers.createClass(SwitchElement,[{key:"init",value:function(){var _this2=this;this.hasAttribute("_compiled")||this._compile(),this._checkbox=this.querySelector(".switch__input"),this._handle=this.querySelector(".switch__handle"),["checked","disabled","modifier","name","input-id"].forEach(function(e){_this2.attributeChangedCallback(e,null,_this2.getAttribute(e))})}},{key:"_compile",value:function(){autoStyle.prepare(this),this.classList.add("switch"),this.appendChild(template$2.cloneNode(!0)),this.setAttribute("_compiled","")}},{key:"disconnectedCallback",value:function(){this._checkbox.removeEventListener("change",this._onChange),this.removeEventListener("dragstart",this._onDragStart),this.removeEventListener("hold",this._onHold),this.removeEventListener("tap",this.click),this.removeEventListener("click",this._onClick),this._gestureDetector.dispose()}},{key:"connectedCallback",value:function(){this._checkbox.addEventListener("change",this._onChange),this._gestureDetector=new GestureDetector(this,{dragMinDistance:1,holdTimeout:251}),this.addEventListener("dragstart",this._onDragStart),this.addEventListener("hold",this._onHold),this.addEventListener("tap",this.click),this._boundOnRelease=this._onRelease.bind(this),this.addEventListener("click",this._onClick)}},{key:"_onChange",value:function(){this.checked?this.parentNode.setAttribute("checked",""):this.parentNode.removeAttribute("checked")}},{key:"_onClick",value:function(ev){ev.target.classList.contains("switch__touch")&&ev.preventDefault()}},{key:"click",value:function(){this.disabled||(this.checked=!this.checked)}},{key:"_getPosition",
value:function(e){var l=this._locations;return Math.min(l[1],Math.max(l[0],this._startX+e.gesture.deltaX))}},{key:"_onHold",value:function(e){this.disabled||(this.classList.add("switch--active"),document.addEventListener("release",this._boundOnRelease))}},{key:"_onDragStart",value:function(e){return this.disabled||-1===["left","right"].indexOf(e.gesture.direction)?void this.classList.remove("switch--active"):(e.stopPropagation(),this.classList.add("switch--active"),this._startX=this._locations[this.checked?1:0],this.addEventListener("drag",this._onDrag),void document.addEventListener("release",this._boundOnRelease))}},{key:"_onDrag",value:function(e){e.gesture.srcEvent.preventDefault(),this._handle.style.left=this._getPosition(e)+"px"}},{key:"_onRelease",value:function(e){var l=this._locations,position=this._getPosition(e);this.checked=position>=(l[0]+l[1])/2,this.removeEventListener("drag",this._onDrag),document.removeEventListener("release",this._boundOnRelease),this._handle.style.left="",this.classList.remove("switch--active")}},{key:"attributeChangedCallback",value:function(name,last,current){switch(name){case"modifier":this._isMaterial=-1!==(current||"").indexOf("material"),this._locations=locations[this._isMaterial?"material":"ios"],ModifierUtil.onModifierChanged(last,current,this,scheme$19);break;case"input-id":this._checkbox.id=current;break;case"checked":this._checkbox.checked=null!==current,util.toggleAttribute(this._checkbox,name,null!==current);break;case"disabled":util.toggleAttribute(this._checkbox,name,null!==current)}}},{key:"checked",get:function(){return this._checkbox.checked},set:function(value){return!!value!==this._checkbox.checked?(this._checkbox.click(),this._checkbox.checked=!!value,util.toggleAttribute(this,"checked",this.checked)):void 0}},{key:"disabled",get:function(){return this._checkbox.disabled},set:function(value){return this._checkbox.disabled=value,util.toggleAttribute(this,"disabled",this.disabled)}},{key:"checkbox",get:function(){return this._checkbox}}],[{key:"observedAttributes",get:function(){return["modifier","input-id","checked","disabled"]}}]),SwitchElement}(BaseElement);customElements.define("ons-switch",SwitchElement);var TabbarAnimator=function(){function TabbarAnimator(){var options=arguments.length<=0||void 0===arguments[0]?{}:arguments[0];babelHelpers.classCallCheck(this,TabbarAnimator),this.timing=options.timing||"linear",this.duration=void 0!==options.duration?options.duration:"0.4",this.delay=void 0!==options.delay?options.delay:"0"}return babelHelpers.createClass(TabbarAnimator,[{key:"apply",value:function(enterPage,leavePage,enterPageIndex,leavePageIndex,done){throw new Error("This method must be implemented.")}}]),TabbarAnimator}(),TabbarNoneAnimator=function(_TabbarAnimator){function TabbarNoneAnimator(){return babelHelpers.classCallCheck(this,TabbarNoneAnimator),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(TabbarNoneAnimator).apply(this,arguments))}return babelHelpers.inherits(TabbarNoneAnimator,_TabbarAnimator),babelHelpers.createClass(TabbarNoneAnimator,[{key:"apply",value:function(enterPage,leavePage,enterIndex,leaveIndex,done){setTimeout(done,1e3/60)}}]),TabbarNoneAnimator}(TabbarAnimator),TabbarFadeAnimator=function(_TabbarAnimator2){function TabbarFadeAnimator(options){return babelHelpers.classCallCheck(this,TabbarFadeAnimator),options.timing=void 0!==options.timing?options.timing:"linear",options.duration=void 0!==options.duration?options.duration:"0.4",options.delay=void 0!==options.delay?options.delay:"0",babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(TabbarFadeAnimator).call(this,options))}return babelHelpers.inherits(TabbarFadeAnimator,_TabbarAnimator2),babelHelpers.createClass(TabbarFadeAnimator,[{key:"apply",value:function(enterPage,leavePage,enterPageIndex,leavePageIndex,done){animit.runAll(animit(enterPage).saveStyle().queue({transform:"translate3D(0, 0, 0)",opacity:0}).wait(this.delay).queue({transform:"translate3D(0, 0, 0)",opacity:1},{duration:this.duration,timing:this.timing}).restoreStyle().queue(function(callback){done(),callback()}),animit(leavePage).queue({transform:"translate3D(0, 0, 0)",opacity:1}).wait(this.delay).queue({transform:"translate3D(0, 0, 0)",opacity:0},{duration:this.duration,timing:this.timing}))}}]),TabbarFadeAnimator}(TabbarAnimator),TabbarSlideAnimator=function(_TabbarAnimator3){function TabbarSlideAnimator(options){return babelHelpers.classCallCheck(this,TabbarSlideAnimator),options.timing=void 0!==options.timing?options.timing:"ease-in",options.duration=void 0!==options.duration?options.duration:"0.15",options.delay=void 0!==options.delay?options.delay:"0",babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(TabbarSlideAnimator).call(this,options))}return babelHelpers.inherits(TabbarSlideAnimator,_TabbarAnimator3),babelHelpers.createClass(TabbarSlideAnimator,[{key:"apply",value:function(enterPage,leavePage,enterIndex,leaveIndex,done){var sgn=enterIndex>leaveIndex;animit.runAll(animit(enterPage).saveStyle().queue({transform:"translate3D("+(sgn?"":"-")+"100%, 0, 0)"}).wait(this.delay).queue({transform:"translate3D(0, 0, 0)"},{duration:this.duration,timing:this.timing}).restoreStyle().queue(function(callback){done(),callback()}),animit(leavePage).queue({transform:"translate3D(0, 0, 0)"}).wait(this.delay).queue({transform:"translate3D("+(sgn?"-":"")+"100%, 0, 0)"},{duration:this.duration,timing:this.timing}))}}]),TabbarSlideAnimator}(TabbarAnimator),scheme$21={".tab-bar__content":"tab-bar--*__content",".tab-bar":"tab-bar--*"},_animatorDict$6={"default":TabbarNoneAnimator,fade:TabbarFadeAnimator,slide:TabbarSlideAnimator,none:TabbarNoneAnimator},rewritables$3={ready:function(tabbarElement,callback){callback()},link:function(tabbarElement,target,options,callback){callback(target)},unlink:function(tabbarElement,target,callback){callback(target)}},generateId$1=function(){var i=0;return function(){return"ons-tabbar-gen-"+i++}}(),TabbarElement=function(_BaseElement){function TabbarElement(){return babelHelpers.classCallCheck(this,TabbarElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(TabbarElement).apply(this,arguments))}return babelHelpers.inherits(TabbarElement,_BaseElement),babelHelpers.createClass(TabbarElement,[{key:"init",value:function(){var _this2=this;this._tabbarId=generateId$1(),contentReady(this,function(){_this2._compile();for(var content=_this2._contentElement,i=0;i<content.children.length;i++)content.children[i].style.display="none";var activeIndex=_this2.getAttribute("activeIndex"),tabbar=_this2._tabbarElement;activeIndex&&tabbar.children.length>activeIndex&&tabbar.children[activeIndex].setAttribute("active","true"),autoStyle.prepare(_this2),ModifierUtil.initModifier(_this2,scheme$21),_this2._animatorFactory=new AnimatorFactory({animators:_animatorDict$6,baseClass:TabbarAnimator,baseClassName:"TabbarAnimator",defaultAnimation:_this2.getAttribute("animation")})})}},{key:"connectedCallback",value:function(){var _this3=this;contentReady(this,function(){return _this3._updatePosition()})}},{key:"_compile",value:function(){if(this._contentElement&&this._tabbarElement){var content=util.findChild(this,".tab-bar__content"),bar=util.findChild(this,".tab-bar");content.classList.add("ons-tab-bar__content"),bar.classList.add("ons-tab-bar__footer"),bar.classList.add("ons-tabbar-inner")}else{for(var _content=util.create(".ons-tab-bar__content.tab-bar__content"),tabbar=util.create(".tab-bar.ons-tab-bar__footer.ons-tabbar-inner");this.firstChild;)tabbar.appendChild(this.firstChild);this.appendChild(_content),this.appendChild(tabbar)}}},{key:"_updatePosition",value:function(){var _this4=this,position=arguments.length<=0||void 0===arguments[0]?this.getAttribute("position"):arguments[0],top=this._top="top"===position||"auto"===position&&platform.isAndroid(),action=top?util.addModifier:util.removeModifier;action(this,"top");var page=util.findParent(this,"ons-page");page&&(this.style.top=top?window.getComputedStyle(page._getContentElement(),null).getPropertyValue("padding-top"):"",util.match(page.firstChild,"ons-toolbar")&&action(page.firstChild,"noshadow")),internal.autoStatusBarFill(function(){var filled=util.findParent(_this4,function(e){return e.hasAttribute("status-bar-fill")});util.toggleAttribute(_this4,"status-bar-fill",top&&!filled)})}},{key:"_getTabbarElement",value:function(){return util.findChild(this,".tab-bar")}},{key:"loadPage",value:function(page){var _this5=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];return new Promise(function(resolve){var tab=_this5._tabbarElement.children[0]||new TabElement;tab._loadPage(page,_this5._contentElement,function(pageElement){resolve(_this5._loadPageDOMAsync(pageElement,options))})})}},{key:"_loadPageDOMAsync",value:function(pageElement){var _this6=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];return new Promise(function(resolve){rewritables$3.link(_this6,pageElement,options,function(pageElement){_this6._contentElement.appendChild(pageElement),-1!==_this6.getActiveTabIndex()?resolve(_this6._switchPage(pageElement,options)):(options.callback instanceof Function&&options.callback(),_this6._oldPageElement=pageElement,resolve(pageElement))})})}},{key:"getTabbarId",value:function(){return this._tabbarId}},{key:"_getCurrentPageElement",value:function(){for(var pages=this._contentElement.children,page=null,i=0;i<pages.length;i++)if("none"!==pages[i].style.display){page=pages[i];break}if(page&&"ons-page"!==page.nodeName.toLowerCase())throw new Error('Invalid state: page element must be a "ons-page" element.');return page}},{key:"_switchPage",value:function(element,options){var oldPageElement=this._oldPageElement||internal.nullElement;this._oldPageElement=element;var animator=this._animatorFactory.newAnimator(options);return new Promise(function(resolve){oldPageElement!==internal.nullElement&&oldPageElement._hide(),animator.apply(element,oldPageElement,options.selectedTabIndex,options.previousTabIndex,function(){oldPageElement!==internal.nullElement&&(oldPageElement.style.display="none"),element.style.display="block",element._show(),options.callback instanceof Function&&options.callback(),resolve(element)})})}},{key:"setActiveTab",value:function(index){var _this7=this,options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];if(options&&"object"!=("undefined"==typeof options?"undefined":babelHelpers["typeof"](options)))throw new Error("options must be an object. You supplied "+options);options.animationOptions=util.extend(options.animationOptions||{},AnimatorFactory.parseAnimationOptionsString(this.getAttribute("animation-options"))),!options.animation&&this.hasAttribute("animation")&&(options.animation=this.getAttribute("animation"));var previousTab=this._getActiveTabElement(),selectedTab=this._getTabElement(index),previousTabIndex=this.getActiveTabIndex(),selectedTabIndex=index,previousPageElement=this._getCurrentPageElement();if(!selectedTab)return Promise.reject("Specified index does not match any tab.");if(selectedTabIndex===previousTabIndex)return util.triggerElementEvent(this,"reactive",{index:selectedTabIndex,tabItem:selectedTab}),Promise.resolve(previousPageElement);var canceled=!1;if(util.triggerElementEvent(this,"prechange",{index:selectedTabIndex,tabItem:selectedTab,cancel:function(){return canceled=!0}}),canceled)return selectedTab.setInactive(),previousTab&&previousTab.setActive(),Promise.reject("Canceled in prechange event.");selectedTab.setActive();var needLoad=!options.keepPage;if(util.arrayFrom(this._getTabbarElement().children).forEach(function(tab){tab!=selectedTab?tab.setInactive():needLoad||util.triggerElementEvent(_this7,"postchange",{index:selectedTabIndex,tabItem:selectedTab})}),!needLoad)return new Promise(function(resolve){_this7._contentElement.appendChild(selectedTab.pageElement),selectedTab.pageElement.style.display="block",resolve(_this7._loadPersistentPageDOM(selectedTab.pageElement,params))});var _ret=function(){var removeElement=!1;(!previousTab&&previousPageElement||previousTab&&previousTab._pageElement!==previousPageElement)&&(removeElement=!0);var params={callback:function(){util.triggerElementEvent(_this7,"postchange",{index:selectedTabIndex,tabItem:selectedTab}),options.callback instanceof Function&&options.callback()},previousTabIndex:previousTabIndex,selectedTabIndex:selectedTabIndex};options.animation&&(params.animation=options.animation),params.animationOptions=options.animationOptions||{};var link=function(element,callback){rewritables$3.link(_this7,element,options,callback)};return{v:new Promise(function(resolve){selectedTab._loadPageElement(_this7._contentElement,function(pageElement){pageElement.style.display="block",resolve(_this7._loadPersistentPageDOM(pageElement,params))},link)})}}();return"object"===("undefined"==typeof _ret?"undefined":babelHelpers["typeof"](_ret))?_ret.v:void 0}},{key:"_loadPersistentPageDOM",value:function(element){var options=arguments.length<=1||void 0===arguments[1]?{}:arguments[1];return util.isAttached(element)||this._contentElement.appendChild(element),element.removeAttribute("style"),this._switchPage(element,options)}},{key:"setTabbarVisibility",value:function(visible){this._contentElement.style[this._top?"top":"bottom"]=visible?"":"0px",this._getTabbarElement().style.display=visible?"":"none"}},{key:"getActiveTabIndex",value:function(){for(var tabs=this._getTabbarElement().children,i=0;i<tabs.length;i++)if(tabs[i]instanceof TabElement&&tabs[i].isActive&&tabs[i].isActive())return i;return-1}},{key:"_getActiveTabElement",value:function(){return this._getTabElement(this.getActiveTabIndex())}},{key:"_getTabElement",value:function(index){return this._getTabbarElement().children[index]}},{key:"disconnectedCallback",value:function(){}},{key:"_show",value:function(){var currentPageElement=this._getCurrentPageElement();currentPageElement&¤tPageElement._show()}},{key:"_hide",value:function(){var currentPageElement=this._getCurrentPageElement();currentPageElement&¤tPageElement._hide()}},{key:"_destroy",value:function(){for(var pages=this._contentElement.children,i=pages.length-1;i>=0;i--)pages[i]._destroy();this.remove()}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$21):void 0}},{key:"_contentElement",get:function(){return util.findChild(this,".tab-bar__content")}},{key:"_tabbarElement",get:function(){return util.findChild(this,".tab-bar")}},{key:"pages",get:function(){return util.arrayFrom(this._contentElement.children)}}],[{key:"registerAnimator",value:function(name,Animator){if(!(Animator.prototype instanceof TabbarAnimator))throw new Error('"Animator" param must inherit TabbarElement.TabbarAnimator');_animatorDict$6[name]=Animator}},{key:"observedAttributes",get:function(){return["modifier"]}},{key:"rewritables",get:function(){return rewritables$3}},{key:"TabbarAnimator",get:function(){return TabbarAnimator}}]),TabbarElement}(BaseElement);customElements.define("ons-tabbar",TabbarElement);var scheme$20={"":"tab-bar--*__item",".tab-bar__button":"tab-bar--*__button"},templateSource$1=util.createElement('\n <div>\n <input type="radio" style="display: none">\n <button class="tab-bar__button tab-bar-inner"></button>\n </div>\n'),defaultInnerTemplateSource=util.createElement('\n <div>\n <div class="tab-bar__icon">\n <ons-icon icon="ion-cloud"></ons-icon>\n </div>\n <div class="tab-bar__label">label</div>\n </div>\n'),TabElement=function(_BaseElement){function TabElement(){return babelHelpers.classCallCheck(this,TabElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(TabElement).apply(this,arguments))}return babelHelpers.inherits(TabElement,_BaseElement),babelHelpers.createClass(TabElement,[{key:"init",value:function(){var _this2=this;this._pageLoader=defaultPageLoader,this._page=null,this.hasAttribute("label")||this.hasAttribute("icon")?this.hasAttribute("_compiled")||this._compile():contentReady(this,function(){_this2.hasAttribute("_compiled")||_this2._compile()}),this._boundOnClick=this._onClick.bind(this)}},{key:"_getPageTarget",value:function(){return this.page||this.getAttribute("page")}},{key:"_compile",value:function(){autoStyle.prepare(this);for(var fragment=document.createDocumentFragment(),hasChildren=!1;this.childNodes[0];){var node=this.childNodes[0];this.removeChild(node),fragment.appendChild(node),node.nodeType==Node.ELEMENT_NODE&&(hasChildren=!0)}for(var template=templateSource$1.cloneNode(!0);template.children[0];)this.appendChild(template.children[0]);this.classList.add("tab-bar__item");var button=util.findChild(this,".tab-bar__button");hasChildren?(button.appendChild(fragment),this._hasDefaultTemplate=!1):(this._hasDefaultTemplate=!0,this._updateDefaultTemplate()),ModifierUtil.initModifier(this,scheme$20),this._updateRipple(),this.setAttribute("_compiled","")}},{key:"_updateRipple",value:function(){}},{key:"_updateDefaultTemplate",value:function(){function getLabelElement(){return self.querySelector(".tab-bar__label")}function getIconElement(){return self.querySelector("ons-icon")}if(this._hasDefaultTemplate){var button=util.findChild(this,".tab-bar__button");if(0==button.children.length){for(var template=defaultInnerTemplateSource.cloneNode(!0);template.children[0];)button.appendChild(template.children[0]);button.querySelector(".tab-bar__icon")||button.insertBefore(template.querySelector(".tab-bar__icon"),button.firstChild),button.querySelector(".tab-bar__label")||button.appendChild(template.querySelector(".tab-bar__label"))}var self=this,icon=this.getAttribute("icon"),label=this.getAttribute("label");if("string"==typeof icon)getIconElement().setAttribute("icon",icon);else{var wrapper=button.querySelector(".tab-bar__icon");wrapper&&wrapper.remove()}if("string"==typeof label)getLabelElement().textContent=label;else{var _label=getLabelElement();_label&&_label.remove()}}}},{key:"_onClick",value:function(){var tabbar=this._findTabbarElement();tabbar&&tabbar.setActiveTab(this._findTabIndex())}},{key:"setActive",value:function(){var radio=util.findChild(this,"input");radio.checked=!0,this.classList.add("active"),util.arrayFrom(this.querySelectorAll("[ons-tab-inactive], ons-tab-inactive")).forEach(function(element){return element.style.display="none"}),util.arrayFrom(this.querySelectorAll("[ons-tab-active], ons-tab-active")).forEach(function(element){return element.style.display="inherit"})}},{key:"setInactive",value:function(){var radio=util.findChild(this,"input");radio.checked=!1,this.classList.remove("active"),util.arrayFrom(this.querySelectorAll("[ons-tab-inactive], ons-tab-inactive")).forEach(function(element){return element.style.display="inherit"}),util.arrayFrom(this.querySelectorAll("[ons-tab-active], ons-tab-active")).forEach(function(element){return element.style.display="none"})}},{key:"_loadPageElement",value:function(parent,callback,link){var _this3=this;if(this._loadedPage||this._getPageTarget())this._loadedPage?callback(this._loadedPage.element):this._pageLoader.load({page:this._getPageTarget(),parent:parent},function(page){_this3._loadedPage=page,link(page.element,function(element){page.element=element,callback(page.element)})});else{var pages=this._findTabbarElement().pages,index=this._findTabIndex();callback(pages[index])}}},{key:"_loadPage",value:function(page,parent,callback){this._pageLoader.load({page:page,parent:parent},function(page){callback(page.element)})}},{key:"isActive",value:function(){return this.classList.contains("active")}},{key:"disconnectedCallback",value:function(){this.removeEventListener("click",this._boundOnClick,!1),this._loadedPage&&(this._loadedPage.unload(),this._loadedPage=null)}},{key:"connectedCallback",value:function(){var _this4=this;contentReady(this,function(){_this4._ensureElementPosition();var tabbar=_this4._findTabbarElement();if(tabbar.hasAttribute("modifier")){var prefix=_this4.hasAttribute("modifier")?_this4.getAttribute("modifier")+" ":"";_this4.setAttribute("modifier",prefix+tabbar.getAttribute("modifier"))}if(_this4.hasAttribute("active"))!function(){var tabIndex=_this4._findTabIndex();TabbarElement.rewritables.ready(tabbar,function(){setImmediate(function(){return tabbar.setActiveTab(tabIndex,{animation:"none"})})})}();else{var onReady=function(){_this4._getPageTarget()&&_this4._loadPageElement(tabbar._contentElement,function(pageElement){pageElement.style.display="none",tabbar._contentElement.appendChild(pageElement)},function(pageElement,done){TabbarElement.rewritables.link(tabbar,pageElement,{},function(element){return done(element)})})};TabbarElement.rewritables.ready(tabbar,onReady)}_this4.addEventListener("click",_this4._boundOnClick,!1)})}},{key:"_findTabbarElement",value:function(){return this.parentNode&&"ons-tabbar"===this.parentNode.nodeName.toLowerCase()?this.parentNode:this.parentNode.parentNode&&"ons-tabbar"===this.parentNode.parentNode.nodeName.toLowerCase()?this.parentNode.parentNode:null}},{key:"_findTabIndex",value:function(){for(var elements=this.parentNode.children,i=0;i<elements.length;i++)if(this===elements[i])return i}},{key:"_ensureElementPosition",value:function(){if(!this._findTabbarElement())throw new Error("This ons-tab element is must be child of ons-tabbar element.")}},{key:"attributeChangedCallback",value:function(name,last,current){var _this5=this;switch(name){case"modifier":contentReady(this,function(){return ModifierUtil.onModifierChanged(last,current,_this5,scheme$20)});break;case"ripple":contentReady(this,function(){return _this5._updateRipple()});break;case"icon":case"label":contentReady(this,function(){return _this5._updateDefaultTemplate()});break;case"page":"string"==typeof current&&(this._page=current)}}},{key:"page",set:function(page){this._page=page},get:function(){return this._page}},{key:"pageLoader",set:function(loader){if(!(loader instanceof PageLoader))throw Error("First parameter must be an instance of PageLoader.");this._pageLoader=loader},get:function(){return this._pageLoader}},{key:"pageElement",get:function(){if(this._loadedPage)return this._loadedPage.element;var tabbar=this._findTabbarElement(),index=this._findTabIndex();return tabbar._contentElement.children[index]}}],[{key:"observedAttributes",get:function(){return["modifier","ripple","icon","label","page"]}}]),TabElement}(BaseElement);customElements.define("ons-tab",TabElement);var scheme$22={"":"toolbar-button--*"},ToolbarButtonElement=function(_BaseElement){function ToolbarButtonElement(){return babelHelpers.classCallCheck(this,ToolbarButtonElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(ToolbarButtonElement).apply(this,arguments))}return babelHelpers.inherits(ToolbarButtonElement,_BaseElement),babelHelpers.createClass(ToolbarButtonElement,[{key:"init",value:function(){this.hasAttribute("_compiled")||this._compile()}},{key:"_compile",value:function(){autoStyle.prepare(this),this.classList.add("toolbar-button"),ModifierUtil.initModifier(this,scheme$22),this.setAttribute("_compiled","")}},{key:"attributeChangedCallback",value:function(name,last,current){return"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$22):void 0}},{key:"disabled",set:function(value){return util.toggleAttribute(this,"disabled",value)},get:function(){return this.hasAttribute("disabled")}}],[{key:"observedAttributes",get:function(){return["modifier"]}}]),ToolbarButtonElement}(BaseElement);customElements.define("ons-toolbar-button",ToolbarButtonElement);var scheme$23={".range":"range--*",".range__left":"range--*__left"},templateSource$2=util.createElement('<div>\n <div class="range__left"></div>\n <input type="range" class="range">\n</div>'),INPUT_ATTRIBUTES$1=["autofocus","disabled","inputmode","max","min","name","placeholder","readonly","size","step","validator","value"],RangeElement=function(_BaseElement){function RangeElement(){return babelHelpers.classCallCheck(this,RangeElement),babelHelpers.possibleConstructorReturn(this,Object.getPrototypeOf(RangeElement).apply(this,arguments))}return babelHelpers.inherits(RangeElement,_BaseElement),babelHelpers.createClass(RangeElement,[{key:"init",value:function(){var _this2=this;contentReady(this,function(){_this2.hasAttribute("_compiled")||_this2._compile(),_this2._updateBoundAttributes(),_this2._onChange()})}},{key:"_compile",value:function(){if(autoStyle.prepare(this),!util.findChild(this,".range__left")||!util.findChild(this,"input"))for(var template=templateSource$2.cloneNode(!0);template.children[0];)this.appendChild(template.children[0]);ModifierUtil.initModifier(this,scheme$23),this.setAttribute("_compiled","")}},{key:"_onChange",value:function(){this._left.style.width=100*this._ratio+"%"}},{key:"_onDragstart",value:function(e){e.stopPropagation(),e.gesture.stopPropagation()}},{key:"attributeChangedCallback",value:function(name,last,current){var _this3=this;"modifier"===name?ModifierUtil.onModifierChanged(last,current,this,scheme$23):INPUT_ATTRIBUTES$1.indexOf(name)>=0&&contentReady(this,function(){_this3._updateBoundAttributes(),"min"!==name&&"max"!==name||_this3._onChange()})}},{key:"connectedCallback",value:function(){this.addEventListener("dragstart",this._onDragstart),this.addEventListener("input",this._onChange)}},{key:"disconnectedCallback",value:function(){this.removeEventListener("dragstart",this._onDragstart),this.removeEventListener("input",this._onChange)}},{key:"_updateBoundAttributes",value:function(){var _this4=this;INPUT_ATTRIBUTES$1.forEach(function(attr){_this4.hasAttribute(attr)?_this4._input.setAttribute(attr,_this4.getAttribute(attr)):_this4._input.removeAttribute(attr)})}},{key:"_ratio",get:function(){var min=""===this._input.min?0:parseInt(this._input.min),max=""===this._input.max?100:parseInt(this._input.max);return(this.value-min)/(max-min)}},{key:"_input",get:function(){return this.querySelector("input")}},{key:"_left",get:function(){return this.querySelector(".range__left")}},{key:"disabled",set:function(value){return util.toggleAttribute(this,"disabled",value)},get:function(){return this.hasAttribute("disabled")}},{key:"value",get:function(){return this._input.value},set:function(val){var _this5=this;contentReady(this,function(){_this5._input.value=val,_this5._onChange()})}}],[{key:"observedAttributes",get:function(){return["modifier"].concat(INPUT_ATTRIBUTES$1)}}]),RangeElement}(BaseElement);return customElements.define("ons-range",RangeElement),ons.TemplateElement=TemplateElement,ons.IfElement=IfElement,ons.AlertDialogElement=AlertDialogElement,ons.BackButtonElement=BackButtonElement,ons.BottomToolbarElement=BottomToolbarElement,ons.ButtonElement=ButtonElement,ons.CarouselItemElement=CarouselItemElement,ons.CarouselElement=CarouselElement,ons.ColElement=ColElement,ons.DialogElement=DialogElement,ons.FabElement=FabElement,ons.GestureDetectorElement=GestureDetectorElement,ons.IconElement=IconElement,ons.LazyRepeatElement=LazyRepeatElement,ons.ListHeaderElement=ListHeaderElement,ons.ListItemElement=ListItemElement,ons.ListElement=ListElement,ons.InputElement=InputElement,ons.ModalElement=ModalElement,ons.NavigatorElement=NavigatorElement,ons.PageElement=PageElement,ons.PopoverElement=PopoverElement,ons.ProgressBarElement=ProgressBarElement,ons.ProgressCircularElement=ProgressCircularElement,ons.PullHookElement=PullHookElement,ons.RippleElement=RippleElement,ons.RowElement=RowElement,ons.SpeedDialItemElement=SpeedDialItemElement,ons.SpeedDialElement=SpeedDialElement,ons.SplitterContentElement=SplitterContentElement,ons.SplitterMaskElement=SplitterMaskElement,ons.SplitterSideElement=SplitterSideElement,ons.SplitterElement=SplitterElement,ons.SwitchElement=SwitchElement,ons.TabElement=TabElement,ons.TabbarElement=TabbarElement,ons.ToolbarButtonElement=ToolbarButtonElement,ons.ToolbarElement=ToolbarElement,ons.RangeElement=RangeElement,window.addEventListener("load",function(){ons.fastClick=FastClick.attach(document.body)},!1),window.addEventListener("DOMContentLoaded",function(){ons._deviceBackButtonDispatcher.enable(),ons._defaultDeviceBackButtonHandler=ons._deviceBackButtonDispatcher.createHandler(window.document.body,function(){navigator.app.exitApp()}),document.body._gestureDetector=new ons.GestureDetector(document.body)},!1),ons.ready(function(){ons._setupLoadingPlaceHolders()}),(new Viewport).setup(),ons}); |
node_modules/react-icons/md/highlight-off.js | bengimbel/Solstice-React-Contacts-Project |
import React from 'react'
import Icon from 'react-icon-base'
const MdHighlightOff = props => (
<Icon viewBox="0 0 40 40" {...props}>
<g><path d="m20 33.4c7.3 0 13.4-6.1 13.4-13.4s-6.1-13.4-13.4-13.4-13.4 6.1-13.4 13.4 6.1 13.4 13.4 13.4z m0-30c9.2 0 16.6 7.4 16.6 16.6s-7.4 16.6-16.6 16.6-16.6-7.4-16.6-16.6 7.4-16.6 16.6-16.6z m4.3 10l2.3 2.3-4.3 4.3 4.3 4.3-2.3 2.3-4.3-4.3-4.3 4.3-2.3-2.3 4.3-4.3-4.3-4.3 2.3-2.3 4.3 4.3z"/></g>
</Icon>
)
export default MdHighlightOff
|
src/components/NotFoundPage/NotFoundPage.js | tarkanlar/eskimo | /*
* React.js Starter Kit
* Copyright (c) 2014 Konstantin Tarkus (@koistya), KriaSoft LLC.
*
* This source code is licensed under the MIT license found in the
* LICENSE.txt file in the root directory of this source tree.
*/
//import './NotFoundPage.less';
import React from 'react'; // eslint-disable-line no-unused-vars
class NotFoundPage {
render() {
return (
<div>
<h1>Page Not Found</h1>
<p>Sorry, but the page you were trying to view does not exist.</p>
</div>
);
}
}
export default NotFoundPage;
|
app/javascript/mastodon/features/standalone/compose/index.js | PlantsNetwork/mastodon | import React from 'react';
import ComposeFormContainer from '../../compose/containers/compose_form_container';
import NotificationsContainer from '../../ui/containers/notifications_container';
import LoadingBarContainer from '../../ui/containers/loading_bar_container';
import ModalContainer from '../../ui/containers/modal_container';
export default class Compose extends React.PureComponent {
render () {
return (
<div>
<ComposeFormContainer />
<NotificationsContainer />
<ModalContainer />
<LoadingBarContainer className='loading-bar' />
</div>
);
}
}
|
vendor/bundle/ruby/2.1.0/gems/rails-4.2.3/guides/assets/javascripts/jquery.min.js | ralzate/Aerosanidad-Correciones | /*! jQuery v1.7.2 jquery.com | jquery.org/license */
(function(a,b){function cy(a){return f.isWindow(a)?a:a.nodeType===9?a.defaultView||a.parentWindow:!1}function cu(a){if(!cj[a]){var b=c.body,d=f("<"+a+">").appendTo(b),e=d.css("display");d.remove();if(e==="none"||e===""){ck||(ck=c.createElement("iframe"),ck.frameBorder=ck.width=ck.height=0),b.appendChild(ck);if(!cl||!ck.createElement)cl=(ck.contentWindow||ck.contentDocument).document,cl.write((f.support.boxModel?"<!doctype html>":"")+"<html><body>"),cl.close();d=cl.createElement(a),cl.body.appendChild(d),e=f.css(d,"display"),b.removeChild(ck)}cj[a]=e}return cj[a]}function ct(a,b){var c={};f.each(cp.concat.apply([],cp.slice(0,b)),function(){c[this]=a});return c}function cs(){cq=b}function cr(){setTimeout(cs,0);return cq=f.now()}function ci(){try{return new a.ActiveXObject("Microsoft.XMLHTTP")}catch(b){}}function ch(){try{return new a.XMLHttpRequest}catch(b){}}function cb(a,c){a.dataFilter&&(c=a.dataFilter(c,a.dataType));var d=a.dataTypes,e={},g,h,i=d.length,j,k=d[0],l,m,n,o,p;for(g=1;g<i;g++){if(g===1)for(h in a.converters)typeof h=="string"&&(e[h.toLowerCase()]=a.converters[h]);l=k,k=d[g];if(k==="*")k=l;else if(l!=="*"&&l!==k){m=l+" "+k,n=e[m]||e["* "+k];if(!n){p=b;for(o in e){j=o.split(" ");if(j[0]===l||j[0]==="*"){p=e[j[1]+" "+k];if(p){o=e[o],o===!0?n=p:p===!0&&(n=o);break}}}}!n&&!p&&f.error("No conversion from "+m.replace(" "," to ")),n!==!0&&(c=n?n(c):p(o(c)))}}return c}function ca(a,c,d){var e=a.contents,f=a.dataTypes,g=a.responseFields,h,i,j,k;for(i in g)i in d&&(c[g[i]]=d[i]);while(f[0]==="*")f.shift(),h===b&&(h=a.mimeType||c.getResponseHeader("content-type"));if(h)for(i in e)if(e[i]&&e[i].test(h)){f.unshift(i);break}if(f[0]in d)j=f[0];else{for(i in d){if(!f[0]||a.converters[i+" "+f[0]]){j=i;break}k||(k=i)}j=j||k}if(j){j!==f[0]&&f.unshift(j);return d[j]}}function b_(a,b,c,d){if(f.isArray(b))f.each(b,function(b,e){c||bD.test(a)?d(a,e):b_(a+"["+(typeof e=="object"?b:"")+"]",e,c,d)});else if(!c&&f.type(b)==="object")for(var e in b)b_(a+"["+e+"]",b[e],c,d);else d(a,b)}function b$(a,c){var d,e,g=f.ajaxSettings.flatOptions||{};for(d in c)c[d]!==b&&((g[d]?a:e||(e={}))[d]=c[d]);e&&f.extend(!0,a,e)}function bZ(a,c,d,e,f,g){f=f||c.dataTypes[0],g=g||{},g[f]=!0;var h=a[f],i=0,j=h?h.length:0,k=a===bS,l;for(;i<j&&(k||!l);i++)l=h[i](c,d,e),typeof l=="string"&&(!k||g[l]?l=b:(c.dataTypes.unshift(l),l=bZ(a,c,d,e,l,g)));(k||!l)&&!g["*"]&&(l=bZ(a,c,d,e,"*",g));return l}function bY(a){return function(b,c){typeof b!="string"&&(c=b,b="*");if(f.isFunction(c)){var d=b.toLowerCase().split(bO),e=0,g=d.length,h,i,j;for(;e<g;e++)h=d[e],j=/^\+/.test(h),j&&(h=h.substr(1)||"*"),i=a[h]=a[h]||[],i[j?"unshift":"push"](c)}}}function bB(a,b,c){var d=b==="width"?a.offsetWidth:a.offsetHeight,e=b==="width"?1:0,g=4;if(d>0){if(c!=="border")for(;e<g;e+=2)c||(d-=parseFloat(f.css(a,"padding"+bx[e]))||0),c==="margin"?d+=parseFloat(f.css(a,c+bx[e]))||0:d-=parseFloat(f.css(a,"border"+bx[e]+"Width"))||0;return d+"px"}d=by(a,b);if(d<0||d==null)d=a.style[b];if(bt.test(d))return d;d=parseFloat(d)||0;if(c)for(;e<g;e+=2)d+=parseFloat(f.css(a,"padding"+bx[e]))||0,c!=="padding"&&(d+=parseFloat(f.css(a,"border"+bx[e]+"Width"))||0),c==="margin"&&(d+=parseFloat(f.css(a,c+bx[e]))||0);return d+"px"}function bo(a){var b=c.createElement("div");bh.appendChild(b),b.innerHTML=a.outerHTML;return b.firstChild}function bn(a){var b=(a.nodeName||"").toLowerCase();b==="input"?bm(a):b!=="script"&&typeof a.getElementsByTagName!="undefined"&&f.grep(a.getElementsByTagName("input"),bm)}function bm(a){if(a.type==="checkbox"||a.type==="radio")a.defaultChecked=a.checked}function bl(a){return typeof a.getElementsByTagName!="undefined"?a.getElementsByTagName("*"):typeof a.querySelectorAll!="undefined"?a.querySelectorAll("*"):[]}function bk(a,b){var c;b.nodeType===1&&(b.clearAttributes&&b.clearAttributes(),b.mergeAttributes&&b.mergeAttributes(a),c=b.nodeName.toLowerCase(),c==="object"?b.outerHTML=a.outerHTML:c!=="input"||a.type!=="checkbox"&&a.type!=="radio"?c==="option"?b.selected=a.defaultSelected:c==="input"||c==="textarea"?b.defaultValue=a.defaultValue:c==="script"&&b.text!==a.text&&(b.text=a.text):(a.checked&&(b.defaultChecked=b.checked=a.checked),b.value!==a.value&&(b.value=a.value)),b.removeAttribute(f.expando),b.removeAttribute("_submit_attached"),b.removeAttribute("_change_attached"))}function bj(a,b){if(b.nodeType===1&&!!f.hasData(a)){var c,d,e,g=f._data(a),h=f._data(b,g),i=g.events;if(i){delete h.handle,h.events={};for(c in i)for(d=0,e=i[c].length;d<e;d++)f.event.add(b,c,i[c][d])}h.data&&(h.data=f.extend({},h.data))}}function bi(a,b){return f.nodeName(a,"table")?a.getElementsByTagName("tbody")[0]||a.appendChild(a.ownerDocument.createElement("tbody")):a}function U(a){var b=V.split("|"),c=a.createDocumentFragment();if(c.createElement)while(b.length)c.createElement(b.pop());return c}function T(a,b,c){b=b||0;if(f.isFunction(b))return f.grep(a,function(a,d){var e=!!b.call(a,d,a);return e===c});if(b.nodeType)return f.grep(a,function(a,d){return a===b===c});if(typeof b=="string"){var d=f.grep(a,function(a){return a.nodeType===1});if(O.test(b))return f.filter(b,d,!c);b=f.filter(b,d)}return f.grep(a,function(a,d){return f.inArray(a,b)>=0===c})}function S(a){return!a||!a.parentNode||a.parentNode.nodeType===11}function K(){return!0}function J(){return!1}function n(a,b,c){var d=b+"defer",e=b+"queue",g=b+"mark",h=f._data(a,d);h&&(c==="queue"||!f._data(a,e))&&(c==="mark"||!f._data(a,g))&&setTimeout(function(){!f._data(a,e)&&!f._data(a,g)&&(f.removeData(a,d,!0),h.fire())},0)}function m(a){for(var b in a){if(b==="data"&&f.isEmptyObject(a[b]))continue;if(b!=="toJSON")return!1}return!0}function l(a,c,d){if(d===b&&a.nodeType===1){var e="data-"+c.replace(k,"-$1").toLowerCase();d=a.getAttribute(e);if(typeof d=="string"){try{d=d==="true"?!0:d==="false"?!1:d==="null"?null:f.isNumeric(d)?+d:j.test(d)?f.parseJSON(d):d}catch(g){}f.data(a,c,d)}else d=b}return d}function h(a){var b=g[a]={},c,d;a=a.split(/\s+/);for(c=0,d=a.length;c<d;c++)b[a[c]]=!0;return b}var c=a.document,d=a.navigator,e=a.location,f=function(){function J(){if(!e.isReady){try{c.documentElement.doScroll("left")}catch(a){setTimeout(J,1);return}e.ready()}}var e=function(a,b){return new e.fn.init(a,b,h)},f=a.jQuery,g=a.$,h,i=/^(?:[^#<]*(<[\w\W]+>)[^>]*$|#([\w\-]*)$)/,j=/\S/,k=/^\s+/,l=/\s+$/,m=/^<(\w+)\s*\/?>(?:<\/\1>)?$/,n=/^[\],:{}\s]*$/,o=/\\(?:["\\\/bfnrt]|u[0-9a-fA-F]{4})/g,p=/"[^"\\\n\r]*"|true|false|null|-?\d+(?:\.\d*)?(?:[eE][+\-]?\d+)?/g,q=/(?:^|:|,)(?:\s*\[)+/g,r=/(webkit)[ \/]([\w.]+)/,s=/(opera)(?:.*version)?[ \/]([\w.]+)/,t=/(msie) ([\w.]+)/,u=/(mozilla)(?:.*? rv:([\w.]+))?/,v=/-([a-z]|[0-9])/ig,w=/^-ms-/,x=function(a,b){return(b+"").toUpperCase()},y=d.userAgent,z,A,B,C=Object.prototype.toString,D=Object.prototype.hasOwnProperty,E=Array.prototype.push,F=Array.prototype.slice,G=String.prototype.trim,H=Array.prototype.indexOf,I={};e.fn=e.prototype={constructor:e,init:function(a,d,f){var g,h,j,k;if(!a)return this;if(a.nodeType){this.context=this[0]=a,this.length=1;return this}if(a==="body"&&!d&&c.body){this.context=c,this[0]=c.body,this.selector=a,this.length=1;return this}if(typeof a=="string"){a.charAt(0)!=="<"||a.charAt(a.length-1)!==">"||a.length<3?g=i.exec(a):g=[null,a,null];if(g&&(g[1]||!d)){if(g[1]){d=d instanceof e?d[0]:d,k=d?d.ownerDocument||d:c,j=m.exec(a),j?e.isPlainObject(d)?(a=[c.createElement(j[1])],e.fn.attr.call(a,d,!0)):a=[k.createElement(j[1])]:(j=e.buildFragment([g[1]],[k]),a=(j.cacheable?e.clone(j.fragment):j.fragment).childNodes);return e.merge(this,a)}h=c.getElementById(g[2]);if(h&&h.parentNode){if(h.id!==g[2])return f.find(a);this.length=1,this[0]=h}this.context=c,this.selector=a;return this}return!d||d.jquery?(d||f).find(a):this.constructor(d).find(a)}if(e.isFunction(a))return f.ready(a);a.selector!==b&&(this.selector=a.selector,this.context=a.context);return e.makeArray(a,this)},selector:"",jquery:"1.7.2",length:0,size:function(){return this.length},toArray:function(){return F.call(this,0)},get:function(a){return a==null?this.toArray():a<0?this[this.length+a]:this[a]},pushStack:function(a,b,c){var d=this.constructor();e.isArray(a)?E.apply(d,a):e.merge(d,a),d.prevObject=this,d.context=this.context,b==="find"?d.selector=this.selector+(this.selector?" ":"")+c:b&&(d.selector=this.selector+"."+b+"("+c+")");return d},each:function(a,b){return e.each(this,a,b)},ready:function(a){e.bindReady(),A.add(a);return this},eq:function(a){a=+a;return a===-1?this.slice(a):this.slice(a,a+1)},first:function(){return this.eq(0)},last:function(){return this.eq(-1)},slice:function(){return this.pushStack(F.apply(this,arguments),"slice",F.call(arguments).join(","))},map:function(a){return this.pushStack(e.map(this,function(b,c){return a.call(b,c,b)}))},end:function(){return this.prevObject||this.constructor(null)},push:E,sort:[].sort,splice:[].splice},e.fn.init.prototype=e.fn,e.extend=e.fn.extend=function(){var a,c,d,f,g,h,i=arguments[0]||{},j=1,k=arguments.length,l=!1;typeof i=="boolean"&&(l=i,i=arguments[1]||{},j=2),typeof i!="object"&&!e.isFunction(i)&&(i={}),k===j&&(i=this,--j);for(;j<k;j++)if((a=arguments[j])!=null)for(c in a){d=i[c],f=a[c];if(i===f)continue;l&&f&&(e.isPlainObject(f)||(g=e.isArray(f)))?(g?(g=!1,h=d&&e.isArray(d)?d:[]):h=d&&e.isPlainObject(d)?d:{},i[c]=e.extend(l,h,f)):f!==b&&(i[c]=f)}return i},e.extend({noConflict:function(b){a.$===e&&(a.$=g),b&&a.jQuery===e&&(a.jQuery=f);return e},isReady:!1,readyWait:1,holdReady:function(a){a?e.readyWait++:e.ready(!0)},ready:function(a){if(a===!0&&!--e.readyWait||a!==!0&&!e.isReady){if(!c.body)return setTimeout(e.ready,1);e.isReady=!0;if(a!==!0&&--e.readyWait>0)return;A.fireWith(c,[e]),e.fn.trigger&&e(c).trigger("ready").off("ready")}},bindReady:function(){if(!A){A=e.Callbacks("once memory");if(c.readyState==="complete")return setTimeout(e.ready,1);if(c.addEventListener)c.addEventListener("DOMContentLoaded",B,!1),a.addEventListener("load",e.ready,!1);else if(c.attachEvent){c.attachEvent("onreadystatechange",B),a.attachEvent("onload",e.ready);var b=!1;try{b=a.frameElement==null}catch(d){}c.documentElement.doScroll&&b&&J()}}},isFunction:function(a){return e.type(a)==="function"},isArray:Array.isArray||function(a){return e.type(a)==="array"},isWindow:function(a){return a!=null&&a==a.window},isNumeric:function(a){return!isNaN(parseFloat(a))&&isFinite(a)},type:function(a){return a==null?String(a):I[C.call(a)]||"object"},isPlainObject:function(a){if(!a||e.type(a)!=="object"||a.nodeType||e.isWindow(a))return!1;try{if(a.constructor&&!D.call(a,"constructor")&&!D.call(a.constructor.prototype,"isPrototypeOf"))return!1}catch(c){return!1}var d;for(d in a);return d===b||D.call(a,d)},isEmptyObject:function(a){for(var b in a)return!1;return!0},error:function(a){throw new Error(a)},parseJSON:function(b){if(typeof b!="string"||!b)return null;b=e.trim(b);if(a.JSON&&a.JSON.parse)return a.JSON.parse(b);if(n.test(b.replace(o,"@").replace(p,"]").replace(q,"")))return(new Function("return "+b))();e.error("Invalid JSON: "+b)},parseXML:function(c){if(typeof c!="string"||!c)return null;var d,f;try{a.DOMParser?(f=new DOMParser,d=f.parseFromString(c,"text/xml")):(d=new ActiveXObject("Microsoft.XMLDOM"),d.async="false",d.loadXML(c))}catch(g){d=b}(!d||!d.documentElement||d.getElementsByTagName("parsererror").length)&&e.error("Invalid XML: "+c);return d},noop:function(){},globalEval:function(b){b&&j.test(b)&&(a.execScript||function(b){a.eval.call(a,b)})(b)},camelCase:function(a){return a.replace(w,"ms-").replace(v,x)},nodeName:function(a,b){return a.nodeName&&a.nodeName.toUpperCase()===b.toUpperCase()},each:function(a,c,d){var f,g=0,h=a.length,i=h===b||e.isFunction(a);if(d){if(i){for(f in a)if(c.apply(a[f],d)===!1)break}else for(;g<h;)if(c.apply(a[g++],d)===!1)break}else if(i){for(f in a)if(c.call(a[f],f,a[f])===!1)break}else for(;g<h;)if(c.call(a[g],g,a[g++])===!1)break;return a},trim:G?function(a){return a==null?"":G.call(a)}:function(a){return a==null?"":(a+"").replace(k,"").replace(l,"")},makeArray:function(a,b){var c=b||[];if(a!=null){var d=e.type(a);a.length==null||d==="string"||d==="function"||d==="regexp"||e.isWindow(a)?E.call(c,a):e.merge(c,a)}return c},inArray:function(a,b,c){var d;if(b){if(H)return H.call(b,a,c);d=b.length,c=c?c<0?Math.max(0,d+c):c:0;for(;c<d;c++)if(c in b&&b[c]===a)return c}return-1},merge:function(a,c){var d=a.length,e=0;if(typeof c.length=="number")for(var f=c.length;e<f;e++)a[d++]=c[e];else while(c[e]!==b)a[d++]=c[e++];a.length=d;return a},grep:function(a,b,c){var d=[],e;c=!!c;for(var f=0,g=a.length;f<g;f++)e=!!b(a[f],f),c!==e&&d.push(a[f]);return d},map:function(a,c,d){var f,g,h=[],i=0,j=a.length,k=a instanceof e||j!==b&&typeof j=="number"&&(j>0&&a[0]&&a[j-1]||j===0||e.isArray(a));if(k)for(;i<j;i++)f=c(a[i],i,d),f!=null&&(h[h.length]=f);else for(g in a)f=c(a[g],g,d),f!=null&&(h[h.length]=f);return h.concat.apply([],h)},guid:1,proxy:function(a,c){if(typeof c=="string"){var d=a[c];c=a,a=d}if(!e.isFunction(a))return b;var f=F.call(arguments,2),g=function(){return a.apply(c,f.concat(F.call(arguments)))};g.guid=a.guid=a.guid||g.guid||e.guid++;return g},access:function(a,c,d,f,g,h,i){var j,k=d==null,l=0,m=a.length;if(d&&typeof d=="object"){for(l in d)e.access(a,c,l,d[l],1,h,f);g=1}else if(f!==b){j=i===b&&e.isFunction(f),k&&(j?(j=c,c=function(a,b,c){return j.call(e(a),c)}):(c.call(a,f),c=null));if(c)for(;l<m;l++)c(a[l],d,j?f.call(a[l],l,c(a[l],d)):f,i);g=1}return g?a:k?c.call(a):m?c(a[0],d):h},now:function(){return(new Date).getTime()},uaMatch:function(a){a=a.toLowerCase();var b=r.exec(a)||s.exec(a)||t.exec(a)||a.indexOf("compatible")<0&&u.exec(a)||[];return{browser:b[1]||"",version:b[2]||"0"}},sub:function(){function a(b,c){return new a.fn.init(b,c)}e.extend(!0,a,this),a.superclass=this,a.fn=a.prototype=this(),a.fn.constructor=a,a.sub=this.sub,a.fn.init=function(d,f){f&&f instanceof e&&!(f instanceof a)&&(f=a(f));return e.fn.init.call(this,d,f,b)},a.fn.init.prototype=a.fn;var b=a(c);return a},browser:{}}),e.each("Boolean Number String Function Array Date RegExp Object".split(" "),function(a,b){I["[object "+b+"]"]=b.toLowerCase()}),z=e.uaMatch(y),z.browser&&(e.browser[z.browser]=!0,e.browser.version=z.version),e.browser.webkit&&(e.browser.safari=!0),j.test(" ")&&(k=/^[\s\xA0]+/,l=/[\s\xA0]+$/),h=e(c),c.addEventListener?B=function(){c.removeEventListener("DOMContentLoaded",B,!1),e.ready()}:c.attachEvent&&(B=function(){c.readyState==="complete"&&(c.detachEvent("onreadystatechange",B),e.ready())});return e}(),g={};f.Callbacks=function(a){a=a?g[a]||h(a):{};var c=[],d=[],e,i,j,k,l,m,n=function(b){var d,e,g,h,i;for(d=0,e=b.length;d<e;d++)g=b[d],h=f.type(g),h==="array"?n(g):h==="function"&&(!a.unique||!p.has(g))&&c.push(g)},o=function(b,f){f=f||[],e=!a.memory||[b,f],i=!0,j=!0,m=k||0,k=0,l=c.length;for(;c&&m<l;m++)if(c[m].apply(b,f)===!1&&a.stopOnFalse){e=!0;break}j=!1,c&&(a.once?e===!0?p.disable():c=[]:d&&d.length&&(e=d.shift(),p.fireWith(e[0],e[1])))},p={add:function(){if(c){var a=c.length;n(arguments),j?l=c.length:e&&e!==!0&&(k=a,o(e[0],e[1]))}return this},remove:function(){if(c){var b=arguments,d=0,e=b.length;for(;d<e;d++)for(var f=0;f<c.length;f++)if(b[d]===c[f]){j&&f<=l&&(l--,f<=m&&m--),c.splice(f--,1);if(a.unique)break}}return this},has:function(a){if(c){var b=0,d=c.length;for(;b<d;b++)if(a===c[b])return!0}return!1},empty:function(){c=[];return this},disable:function(){c=d=e=b;return this},disabled:function(){return!c},lock:function(){d=b,(!e||e===!0)&&p.disable();return this},locked:function(){return!d},fireWith:function(b,c){d&&(j?a.once||d.push([b,c]):(!a.once||!e)&&o(b,c));return this},fire:function(){p.fireWith(this,arguments);return this},fired:function(){return!!i}};return p};var i=[].slice;f.extend({Deferred:function(a){var b=f.Callbacks("once memory"),c=f.Callbacks("once memory"),d=f.Callbacks("memory"),e="pending",g={resolve:b,reject:c,notify:d},h={done:b.add,fail:c.add,progress:d.add,state:function(){return e},isResolved:b.fired,isRejected:c.fired,then:function(a,b,c){i.done(a).fail(b).progress(c);return this},always:function(){i.done.apply(i,arguments).fail.apply(i,arguments);return this},pipe:function(a,b,c){return f.Deferred(function(d){f.each({done:[a,"resolve"],fail:[b,"reject"],progress:[c,"notify"]},function(a,b){var c=b[0],e=b[1],g;f.isFunction(c)?i[a](function(){g=c.apply(this,arguments),g&&f.isFunction(g.promise)?g.promise().then(d.resolve,d.reject,d.notify):d[e+"With"](this===i?d:this,[g])}):i[a](d[e])})}).promise()},promise:function(a){if(a==null)a=h;else for(var b in h)a[b]=h[b];return a}},i=h.promise({}),j;for(j in g)i[j]=g[j].fire,i[j+"With"]=g[j].fireWith;i.done(function(){e="resolved"},c.disable,d.lock).fail(function(){e="rejected"},b.disable,d.lock),a&&a.call(i,i);return i},when:function(a){function m(a){return function(b){e[a]=arguments.length>1?i.call(arguments,0):b,j.notifyWith(k,e)}}function l(a){return function(c){b[a]=arguments.length>1?i.call(arguments,0):c,--g||j.resolveWith(j,b)}}var b=i.call(arguments,0),c=0,d=b.length,e=Array(d),g=d,h=d,j=d<=1&&a&&f.isFunction(a.promise)?a:f.Deferred(),k=j.promise();if(d>1){for(;c<d;c++)b[c]&&b[c].promise&&f.isFunction(b[c].promise)?b[c].promise().then(l(c),j.reject,m(c)):--g;g||j.resolveWith(j,b)}else j!==a&&j.resolveWith(j,d?[a]:[]);return k}}),f.support=function(){var b,d,e,g,h,i,j,k,l,m,n,o,p=c.createElement("div"),q=c.documentElement;p.setAttribute("className","t"),p.innerHTML=" <link/><table></table><a href='/a' style='top:1px;float:left;opacity:.55;'>a</a><input type='checkbox'/>",d=p.getElementsByTagName("*"),e=p.getElementsByTagName("a")[0];if(!d||!d.length||!e)return{};g=c.createElement("select"),h=g.appendChild(c.createElement("option")),i=p.getElementsByTagName("input")[0],b={leadingWhitespace:p.firstChild.nodeType===3,tbody:!p.getElementsByTagName("tbody").length,htmlSerialize:!!p.getElementsByTagName("link").length,style:/top/.test(e.getAttribute("style")),hrefNormalized:e.getAttribute("href")==="/a",opacity:/^0.55/.test(e.style.opacity),cssFloat:!!e.style.cssFloat,checkOn:i.value==="on",optSelected:h.selected,getSetAttribute:p.className!=="t",enctype:!!c.createElement("form").enctype,html5Clone:c.createElement("nav").cloneNode(!0).outerHTML!=="<:nav></:nav>",submitBubbles:!0,changeBubbles:!0,focusinBubbles:!1,deleteExpando:!0,noCloneEvent:!0,inlineBlockNeedsLayout:!1,shrinkWrapBlocks:!1,reliableMarginRight:!0,pixelMargin:!0},f.boxModel=b.boxModel=c.compatMode==="CSS1Compat",i.checked=!0,b.noCloneChecked=i.cloneNode(!0).checked,g.disabled=!0,b.optDisabled=!h.disabled;try{delete p.test}catch(r){b.deleteExpando=!1}!p.addEventListener&&p.attachEvent&&p.fireEvent&&(p.attachEvent("onclick",function(){b.noCloneEvent=!1}),p.cloneNode(!0).fireEvent("onclick")),i=c.createElement("input"),i.value="t",i.setAttribute("type","radio"),b.radioValue=i.value==="t",i.setAttribute("checked","checked"),i.setAttribute("name","t"),p.appendChild(i),j=c.createDocumentFragment(),j.appendChild(p.lastChild),b.checkClone=j.cloneNode(!0).cloneNode(!0).lastChild.checked,b.appendChecked=i.checked,j.removeChild(i),j.appendChild(p);if(p.attachEvent)for(n in{submit:1,change:1,focusin:1})m="on"+n,o=m in p,o||(p.setAttribute(m,"return;"),o=typeof p[m]=="function"),b[n+"Bubbles"]=o;j.removeChild(p),j=g=h=p=i=null,f(function(){var d,e,g,h,i,j,l,m,n,q,r,s,t,u=c.getElementsByTagName("body")[0];!u||(m=1,t="padding:0;margin:0;border:",r="position:absolute;top:0;left:0;width:1px;height:1px;",s=t+"0;visibility:hidden;",n="style='"+r+t+"5px solid #000;",q="<div "+n+"display:block;'><div style='"+t+"0;display:block;overflow:hidden;'></div></div>"+"<table "+n+"' cellpadding='0' cellspacing='0'>"+"<tr><td></td></tr></table>",d=c.createElement("div"),d.style.cssText=s+"width:0;height:0;position:static;top:0;margin-top:"+m+"px",u.insertBefore(d,u.firstChild),p=c.createElement("div"),d.appendChild(p),p.innerHTML="<table><tr><td style='"+t+"0;display:none'></td><td>t</td></tr></table>",k=p.getElementsByTagName("td"),o=k[0].offsetHeight===0,k[0].style.display="",k[1].style.display="none",b.reliableHiddenOffsets=o&&k[0].offsetHeight===0,a.getComputedStyle&&(p.innerHTML="",l=c.createElement("div"),l.style.width="0",l.style.marginRight="0",p.style.width="2px",p.appendChild(l),b.reliableMarginRight=(parseInt((a.getComputedStyle(l,null)||{marginRight:0}).marginRight,10)||0)===0),typeof p.style.zoom!="undefined"&&(p.innerHTML="",p.style.width=p.style.padding="1px",p.style.border=0,p.style.overflow="hidden",p.style.display="inline",p.style.zoom=1,b.inlineBlockNeedsLayout=p.offsetWidth===3,p.style.display="block",p.style.overflow="visible",p.innerHTML="<div style='width:5px;'></div>",b.shrinkWrapBlocks=p.offsetWidth!==3),p.style.cssText=r+s,p.innerHTML=q,e=p.firstChild,g=e.firstChild,i=e.nextSibling.firstChild.firstChild,j={doesNotAddBorder:g.offsetTop!==5,doesAddBorderForTableAndCells:i.offsetTop===5},g.style.position="fixed",g.style.top="20px",j.fixedPosition=g.offsetTop===20||g.offsetTop===15,g.style.position=g.style.top="",e.style.overflow="hidden",e.style.position="relative",j.subtractsBorderForOverflowNotVisible=g.offsetTop===-5,j.doesNotIncludeMarginInBodyOffset=u.offsetTop!==m,a.getComputedStyle&&(p.style.marginTop="1%",b.pixelMargin=(a.getComputedStyle(p,null)||{marginTop:0}).marginTop!=="1%"),typeof d.style.zoom!="undefined"&&(d.style.zoom=1),u.removeChild(d),l=p=d=null,f.extend(b,j))});return b}();var j=/^(?:\{.*\}|\[.*\])$/,k=/([A-Z])/g;f.extend({cache:{},uuid:0,expando:"jQuery"+(f.fn.jquery+Math.random()).replace(/\D/g,""),noData:{embed:!0,object:"clsid:D27CDB6E-AE6D-11cf-96B8-444553540000",applet:!0},hasData:function(a){a=a.nodeType?f.cache[a[f.expando]]:a[f.expando];return!!a&&!m(a)},data:function(a,c,d,e){if(!!f.acceptData(a)){var g,h,i,j=f.expando,k=typeof c=="string",l=a.nodeType,m=l?f.cache:a,n=l?a[j]:a[j]&&j,o=c==="events";if((!n||!m[n]||!o&&!e&&!m[n].data)&&k&&d===b)return;n||(l?a[j]=n=++f.uuid:n=j),m[n]||(m[n]={},l||(m[n].toJSON=f.noop));if(typeof c=="object"||typeof c=="function")e?m[n]=f.extend(m[n],c):m[n].data=f.extend(m[n].data,c);g=h=m[n],e||(h.data||(h.data={}),h=h.data),d!==b&&(h[f.camelCase(c)]=d);if(o&&!h[c])return g.events;k?(i=h[c],i==null&&(i=h[f.camelCase(c)])):i=h;return i}},removeData:function(a,b,c){if(!!f.acceptData(a)){var d,e,g,h=f.expando,i=a.nodeType,j=i?f.cache:a,k=i?a[h]:h;if(!j[k])return;if(b){d=c?j[k]:j[k].data;if(d){f.isArray(b)||(b in d?b=[b]:(b=f.camelCase(b),b in d?b=[b]:b=b.split(" ")));for(e=0,g=b.length;e<g;e++)delete d[b[e]];if(!(c?m:f.isEmptyObject)(d))return}}if(!c){delete j[k].data;if(!m(j[k]))return}f.support.deleteExpando||!j.setInterval?delete j[k]:j[k]=null,i&&(f.support.deleteExpando?delete a[h]:a.removeAttribute?a.removeAttribute(h):a[h]=null)}},_data:function(a,b,c){return f.data(a,b,c,!0)},acceptData:function(a){if(a.nodeName){var b=f.noData[a.nodeName.toLowerCase()];if(b)return b!==!0&&a.getAttribute("classid")===b}return!0}}),f.fn.extend({data:function(a,c){var d,e,g,h,i,j=this[0],k=0,m=null;if(a===b){if(this.length){m=f.data(j);if(j.nodeType===1&&!f._data(j,"parsedAttrs")){g=j.attributes;for(i=g.length;k<i;k++)h=g[k].name,h.indexOf("data-")===0&&(h=f.camelCase(h.substring(5)),l(j,h,m[h]));f._data(j,"parsedAttrs",!0)}}return m}if(typeof a=="object")return this.each(function(){f.data(this,a)});d=a.split(".",2),d[1]=d[1]?"."+d[1]:"",e=d[1]+"!";return f.access(this,function(c){if(c===b){m=this.triggerHandler("getData"+e,[d[0]]),m===b&&j&&(m=f.data(j,a),m=l(j,a,m));return m===b&&d[1]?this.data(d[0]):m}d[1]=c,this.each(function(){var b=f(this);b.triggerHandler("setData"+e,d),f.data(this,a,c),b.triggerHandler("changeData"+e,d)})},null,c,arguments.length>1,null,!1)},removeData:function(a){return this.each(function(){f.removeData(this,a)})}}),f.extend({_mark:function(a,b){a&&(b=(b||"fx")+"mark",f._data(a,b,(f._data(a,b)||0)+1))},_unmark:function(a,b,c){a!==!0&&(c=b,b=a,a=!1);if(b){c=c||"fx";var d=c+"mark",e=a?0:(f._data(b,d)||1)-1;e?f._data(b,d,e):(f.removeData(b,d,!0),n(b,c,"mark"))}},queue:function(a,b,c){var d;if(a){b=(b||"fx")+"queue",d=f._data(a,b),c&&(!d||f.isArray(c)?d=f._data(a,b,f.makeArray(c)):d.push(c));return d||[]}},dequeue:function(a,b){b=b||"fx";var c=f.queue(a,b),d=c.shift(),e={};d==="inprogress"&&(d=c.shift()),d&&(b==="fx"&&c.unshift("inprogress"),f._data(a,b+".run",e),d.call(a,function(){f.dequeue(a,b)},e)),c.length||(f.removeData(a,b+"queue "+b+".run",!0),n(a,b,"queue"))}}),f.fn.extend({queue:function(a,c){var d=2;typeof a!="string"&&(c=a,a="fx",d--);if(arguments.length<d)return f.queue(this[0],a);return c===b?this:this.each(function(){var b=f.queue(this,a,c);a==="fx"&&b[0]!=="inprogress"&&f.dequeue(this,a)})},dequeue:function(a){return this.each(function(){f.dequeue(this,a)})},delay:function(a,b){a=f.fx?f.fx.speeds[a]||a:a,b=b||"fx";return this.queue(b,function(b,c){var d=setTimeout(b,a);c.stop=function(){clearTimeout(d)}})},clearQueue:function(a){return this.queue(a||"fx",[])},promise:function(a,c){function m(){--h||d.resolveWith(e,[e])}typeof a!="string"&&(c=a,a=b),a=a||"fx";var d=f.Deferred(),e=this,g=e.length,h=1,i=a+"defer",j=a+"queue",k=a+"mark",l;while(g--)if(l=f.data(e[g],i,b,!0)||(f.data(e[g],j,b,!0)||f.data(e[g],k,b,!0))&&f.data(e[g],i,f.Callbacks("once memory"),!0))h++,l.add(m);m();return d.promise(c)}});var o=/[\n\t\r]/g,p=/\s+/,q=/\r/g,r=/^(?:button|input)$/i,s=/^(?:button|input|object|select|textarea)$/i,t=/^a(?:rea)?$/i,u=/^(?:autofocus|autoplay|async|checked|controls|defer|disabled|hidden|loop|multiple|open|readonly|required|scoped|selected)$/i,v=f.support.getSetAttribute,w,x,y;f.fn.extend({attr:function(a,b){return f.access(this,f.attr,a,b,arguments.length>1)},removeAttr:function(a){return this.each(function(){f.removeAttr(this,a)})},prop:function(a,b){return f.access(this,f.prop,a,b,arguments.length>1)},removeProp:function(a){a=f.propFix[a]||a;return this.each(function(){try{this[a]=b,delete this[a]}catch(c){}})},addClass:function(a){var b,c,d,e,g,h,i;if(f.isFunction(a))return this.each(function(b){f(this).addClass(a.call(this,b,this.className))});if(a&&typeof a=="string"){b=a.split(p);for(c=0,d=this.length;c<d;c++){e=this[c];if(e.nodeType===1)if(!e.className&&b.length===1)e.className=a;else{g=" "+e.className+" ";for(h=0,i=b.length;h<i;h++)~g.indexOf(" "+b[h]+" ")||(g+=b[h]+" ");e.className=f.trim(g)}}}return this},removeClass:function(a){var c,d,e,g,h,i,j;if(f.isFunction(a))return this.each(function(b){f(this).removeClass(a.call(this,b,this.className))});if(a&&typeof a=="string"||a===b){c=(a||"").split(p);for(d=0,e=this.length;d<e;d++){g=this[d];if(g.nodeType===1&&g.className)if(a){h=(" "+g.className+" ").replace(o," ");for(i=0,j=c.length;i<j;i++)h=h.replace(" "+c[i]+" "," ");g.className=f.trim(h)}else g.className=""}}return this},toggleClass:function(a,b){var c=typeof a,d=typeof b=="boolean";if(f.isFunction(a))return this.each(function(c){f(this).toggleClass(a.call(this,c,this.className,b),b)});return this.each(function(){if(c==="string"){var e,g=0,h=f(this),i=b,j=a.split(p);while(e=j[g++])i=d?i:!h.hasClass(e),h[i?"addClass":"removeClass"](e)}else if(c==="undefined"||c==="boolean")this.className&&f._data(this,"__className__",this.className),this.className=this.className||a===!1?"":f._data(this,"__className__")||""})},hasClass:function(a){var b=" "+a+" ",c=0,d=this.length;for(;c<d;c++)if(this[c].nodeType===1&&(" "+this[c].className+" ").replace(o," ").indexOf(b)>-1)return!0;return!1},val:function(a){var c,d,e,g=this[0];{if(!!arguments.length){e=f.isFunction(a);return this.each(function(d){var g=f(this),h;if(this.nodeType===1){e?h=a.call(this,d,g.val()):h=a,h==null?h="":typeof h=="number"?h+="":f.isArray(h)&&(h=f.map(h,function(a){return a==null?"":a+""})),c=f.valHooks[this.type]||f.valHooks[this.nodeName.toLowerCase()];if(!c||!("set"in c)||c.set(this,h,"value")===b)this.value=h}})}if(g){c=f.valHooks[g.type]||f.valHooks[g.nodeName.toLowerCase()];if(c&&"get"in c&&(d=c.get(g,"value"))!==b)return d;d=g.value;return typeof d=="string"?d.replace(q,""):d==null?"":d}}}}),f.extend({valHooks:{option:{get:function(a){var b=a.attributes.value;return!b||b.specified?a.value:a.text}},select:{get:function(a){var b,c,d,e,g=a.selectedIndex,h=[],i=a.options,j=a.type==="select-one";if(g<0)return null;c=j?g:0,d=j?g+1:i.length;for(;c<d;c++){e=i[c];if(e.selected&&(f.support.optDisabled?!e.disabled:e.getAttribute("disabled")===null)&&(!e.parentNode.disabled||!f.nodeName(e.parentNode,"optgroup"))){b=f(e).val();if(j)return b;h.push(b)}}if(j&&!h.length&&i.length)return f(i[g]).val();return h},set:function(a,b){var c=f.makeArray(b);f(a).find("option").each(function(){this.selected=f.inArray(f(this).val(),c)>=0}),c.length||(a.selectedIndex=-1);return c}}},attrFn:{val:!0,css:!0,html:!0,text:!0,data:!0,width:!0,height:!0,offset:!0},attr:function(a,c,d,e){var g,h,i,j=a.nodeType;if(!!a&&j!==3&&j!==8&&j!==2){if(e&&c in f.attrFn)return f(a)[c](d);if(typeof a.getAttribute=="undefined")return f.prop(a,c,d);i=j!==1||!f.isXMLDoc(a),i&&(c=c.toLowerCase(),h=f.attrHooks[c]||(u.test(c)?x:w));if(d!==b){if(d===null){f.removeAttr(a,c);return}if(h&&"set"in h&&i&&(g=h.set(a,d,c))!==b)return g;a.setAttribute(c,""+d);return d}if(h&&"get"in h&&i&&(g=h.get(a,c))!==null)return g;g=a.getAttribute(c);return g===null?b:g}},removeAttr:function(a,b){var c,d,e,g,h,i=0;if(b&&a.nodeType===1){d=b.toLowerCase().split(p),g=d.length;for(;i<g;i++)e=d[i],e&&(c=f.propFix[e]||e,h=u.test(e),h||f.attr(a,e,""),a.removeAttribute(v?e:c),h&&c in a&&(a[c]=!1))}},attrHooks:{type:{set:function(a,b){if(r.test(a.nodeName)&&a.parentNode)f.error("type property can't be changed");else if(!f.support.radioValue&&b==="radio"&&f.nodeName(a,"input")){var c=a.value;a.setAttribute("type",b),c&&(a.value=c);return b}}},value:{get:function(a,b){if(w&&f.nodeName(a,"button"))return w.get(a,b);return b in a?a.value:null},set:function(a,b,c){if(w&&f.nodeName(a,"button"))return w.set(a,b,c);a.value=b}}},propFix:{tabindex:"tabIndex",readonly:"readOnly","for":"htmlFor","class":"className",maxlength:"maxLength",cellspacing:"cellSpacing",cellpadding:"cellPadding",rowspan:"rowSpan",colspan:"colSpan",usemap:"useMap",frameborder:"frameBorder",contenteditable:"contentEditable"},prop:function(a,c,d){var e,g,h,i=a.nodeType;if(!!a&&i!==3&&i!==8&&i!==2){h=i!==1||!f.isXMLDoc(a),h&&(c=f.propFix[c]||c,g=f.propHooks[c]);return d!==b?g&&"set"in g&&(e=g.set(a,d,c))!==b?e:a[c]=d:g&&"get"in g&&(e=g.get(a,c))!==null?e:a[c]}},propHooks:{tabIndex:{get:function(a){var c=a.getAttributeNode("tabindex");return c&&c.specified?parseInt(c.value,10):s.test(a.nodeName)||t.test(a.nodeName)&&a.href?0:b}}}}),f.attrHooks.tabindex=f.propHooks.tabIndex,x={get:function(a,c){var d,e=f.prop(a,c);return e===!0||typeof e!="boolean"&&(d=a.getAttributeNode(c))&&d.nodeValue!==!1?c.toLowerCase():b},set:function(a,b,c){var d;b===!1?f.removeAttr(a,c):(d=f.propFix[c]||c,d in a&&(a[d]=!0),a.setAttribute(c,c.toLowerCase()));return c}},v||(y={name:!0,id:!0,coords:!0},w=f.valHooks.button={get:function(a,c){var d;d=a.getAttributeNode(c);return d&&(y[c]?d.nodeValue!=="":d.specified)?d.nodeValue:b},set:function(a,b,d){var e=a.getAttributeNode(d);e||(e=c.createAttribute(d),a.setAttributeNode(e));return e.nodeValue=b+""}},f.attrHooks.tabindex.set=w.set,f.each(["width","height"],function(a,b){f.attrHooks[b]=f.extend(f.attrHooks[b],{set:function(a,c){if(c===""){a.setAttribute(b,"auto");return c}}})}),f.attrHooks.contenteditable={get:w.get,set:function(a,b,c){b===""&&(b="false"),w.set(a,b,c)}}),f.support.hrefNormalized||f.each(["href","src","width","height"],function(a,c){f.attrHooks[c]=f.extend(f.attrHooks[c],{get:function(a){var d=a.getAttribute(c,2);return d===null?b:d}})}),f.support.style||(f.attrHooks.style={get:function(a){return a.style.cssText.toLowerCase()||b},set:function(a,b){return a.style.cssText=""+b}}),f.support.optSelected||(f.propHooks.selected=f.extend(f.propHooks.selected,{get:function(a){var b=a.parentNode;b&&(b.selectedIndex,b.parentNode&&b.parentNode.selectedIndex);return null}})),f.support.enctype||(f.propFix.enctype="encoding"),f.support.checkOn||f.each(["radio","checkbox"],function(){f.valHooks[this]={get:function(a){return a.getAttribute("value")===null?"on":a.value}}}),f.each(["radio","checkbox"],function(){f.valHooks[this]=f.extend(f.valHooks[this],{set:function(a,b){if(f.isArray(b))return a.checked=f.inArray(f(a).val(),b)>=0}})});var z=/^(?:textarea|input|select)$/i,A=/^([^\.]*)?(?:\.(.+))?$/,B=/(?:^|\s)hover(\.\S+)?\b/,C=/^key/,D=/^(?:mouse|contextmenu)|click/,E=/^(?:focusinfocus|focusoutblur)$/,F=/^(\w*)(?:#([\w\-]+))?(?:\.([\w\-]+))?$/,G=function(
a){var b=F.exec(a);b&&(b[1]=(b[1]||"").toLowerCase(),b[3]=b[3]&&new RegExp("(?:^|\\s)"+b[3]+"(?:\\s|$)"));return b},H=function(a,b){var c=a.attributes||{};return(!b[1]||a.nodeName.toLowerCase()===b[1])&&(!b[2]||(c.id||{}).value===b[2])&&(!b[3]||b[3].test((c["class"]||{}).value))},I=function(a){return f.event.special.hover?a:a.replace(B,"mouseenter$1 mouseleave$1")};f.event={add:function(a,c,d,e,g){var h,i,j,k,l,m,n,o,p,q,r,s;if(!(a.nodeType===3||a.nodeType===8||!c||!d||!(h=f._data(a)))){d.handler&&(p=d,d=p.handler,g=p.selector),d.guid||(d.guid=f.guid++),j=h.events,j||(h.events=j={}),i=h.handle,i||(h.handle=i=function(a){return typeof f!="undefined"&&(!a||f.event.triggered!==a.type)?f.event.dispatch.apply(i.elem,arguments):b},i.elem=a),c=f.trim(I(c)).split(" ");for(k=0;k<c.length;k++){l=A.exec(c[k])||[],m=l[1],n=(l[2]||"").split(".").sort(),s=f.event.special[m]||{},m=(g?s.delegateType:s.bindType)||m,s=f.event.special[m]||{},o=f.extend({type:m,origType:l[1],data:e,handler:d,guid:d.guid,selector:g,quick:g&&G(g),namespace:n.join(".")},p),r=j[m];if(!r){r=j[m]=[],r.delegateCount=0;if(!s.setup||s.setup.call(a,e,n,i)===!1)a.addEventListener?a.addEventListener(m,i,!1):a.attachEvent&&a.attachEvent("on"+m,i)}s.add&&(s.add.call(a,o),o.handler.guid||(o.handler.guid=d.guid)),g?r.splice(r.delegateCount++,0,o):r.push(o),f.event.global[m]=!0}a=null}},global:{},remove:function(a,b,c,d,e){var g=f.hasData(a)&&f._data(a),h,i,j,k,l,m,n,o,p,q,r,s;if(!!g&&!!(o=g.events)){b=f.trim(I(b||"")).split(" ");for(h=0;h<b.length;h++){i=A.exec(b[h])||[],j=k=i[1],l=i[2];if(!j){for(j in o)f.event.remove(a,j+b[h],c,d,!0);continue}p=f.event.special[j]||{},j=(d?p.delegateType:p.bindType)||j,r=o[j]||[],m=r.length,l=l?new RegExp("(^|\\.)"+l.split(".").sort().join("\\.(?:.*\\.)?")+"(\\.|$)"):null;for(n=0;n<r.length;n++)s=r[n],(e||k===s.origType)&&(!c||c.guid===s.guid)&&(!l||l.test(s.namespace))&&(!d||d===s.selector||d==="**"&&s.selector)&&(r.splice(n--,1),s.selector&&r.delegateCount--,p.remove&&p.remove.call(a,s));r.length===0&&m!==r.length&&((!p.teardown||p.teardown.call(a,l)===!1)&&f.removeEvent(a,j,g.handle),delete o[j])}f.isEmptyObject(o)&&(q=g.handle,q&&(q.elem=null),f.removeData(a,["events","handle"],!0))}},customEvent:{getData:!0,setData:!0,changeData:!0},trigger:function(c,d,e,g){if(!e||e.nodeType!==3&&e.nodeType!==8){var h=c.type||c,i=[],j,k,l,m,n,o,p,q,r,s;if(E.test(h+f.event.triggered))return;h.indexOf("!")>=0&&(h=h.slice(0,-1),k=!0),h.indexOf(".")>=0&&(i=h.split("."),h=i.shift(),i.sort());if((!e||f.event.customEvent[h])&&!f.event.global[h])return;c=typeof c=="object"?c[f.expando]?c:new f.Event(h,c):new f.Event(h),c.type=h,c.isTrigger=!0,c.exclusive=k,c.namespace=i.join("."),c.namespace_re=c.namespace?new RegExp("(^|\\.)"+i.join("\\.(?:.*\\.)?")+"(\\.|$)"):null,o=h.indexOf(":")<0?"on"+h:"";if(!e){j=f.cache;for(l in j)j[l].events&&j[l].events[h]&&f.event.trigger(c,d,j[l].handle.elem,!0);return}c.result=b,c.target||(c.target=e),d=d!=null?f.makeArray(d):[],d.unshift(c),p=f.event.special[h]||{};if(p.trigger&&p.trigger.apply(e,d)===!1)return;r=[[e,p.bindType||h]];if(!g&&!p.noBubble&&!f.isWindow(e)){s=p.delegateType||h,m=E.test(s+h)?e:e.parentNode,n=null;for(;m;m=m.parentNode)r.push([m,s]),n=m;n&&n===e.ownerDocument&&r.push([n.defaultView||n.parentWindow||a,s])}for(l=0;l<r.length&&!c.isPropagationStopped();l++)m=r[l][0],c.type=r[l][1],q=(f._data(m,"events")||{})[c.type]&&f._data(m,"handle"),q&&q.apply(m,d),q=o&&m[o],q&&f.acceptData(m)&&q.apply(m,d)===!1&&c.preventDefault();c.type=h,!g&&!c.isDefaultPrevented()&&(!p._default||p._default.apply(e.ownerDocument,d)===!1)&&(h!=="click"||!f.nodeName(e,"a"))&&f.acceptData(e)&&o&&e[h]&&(h!=="focus"&&h!=="blur"||c.target.offsetWidth!==0)&&!f.isWindow(e)&&(n=e[o],n&&(e[o]=null),f.event.triggered=h,e[h](),f.event.triggered=b,n&&(e[o]=n));return c.result}},dispatch:function(c){c=f.event.fix(c||a.event);var d=(f._data(this,"events")||{})[c.type]||[],e=d.delegateCount,g=[].slice.call(arguments,0),h=!c.exclusive&&!c.namespace,i=f.event.special[c.type]||{},j=[],k,l,m,n,o,p,q,r,s,t,u;g[0]=c,c.delegateTarget=this;if(!i.preDispatch||i.preDispatch.call(this,c)!==!1){if(e&&(!c.button||c.type!=="click")){n=f(this),n.context=this.ownerDocument||this;for(m=c.target;m!=this;m=m.parentNode||this)if(m.disabled!==!0){p={},r=[],n[0]=m;for(k=0;k<e;k++)s=d[k],t=s.selector,p[t]===b&&(p[t]=s.quick?H(m,s.quick):n.is(t)),p[t]&&r.push(s);r.length&&j.push({elem:m,matches:r})}}d.length>e&&j.push({elem:this,matches:d.slice(e)});for(k=0;k<j.length&&!c.isPropagationStopped();k++){q=j[k],c.currentTarget=q.elem;for(l=0;l<q.matches.length&&!c.isImmediatePropagationStopped();l++){s=q.matches[l];if(h||!c.namespace&&!s.namespace||c.namespace_re&&c.namespace_re.test(s.namespace))c.data=s.data,c.handleObj=s,o=((f.event.special[s.origType]||{}).handle||s.handler).apply(q.elem,g),o!==b&&(c.result=o,o===!1&&(c.preventDefault(),c.stopPropagation()))}}i.postDispatch&&i.postDispatch.call(this,c);return c.result}},props:"attrChange attrName relatedNode srcElement altKey bubbles cancelable ctrlKey currentTarget eventPhase metaKey relatedTarget shiftKey target timeStamp view which".split(" "),fixHooks:{},keyHooks:{props:"char charCode key keyCode".split(" "),filter:function(a,b){a.which==null&&(a.which=b.charCode!=null?b.charCode:b.keyCode);return a}},mouseHooks:{props:"button buttons clientX clientY fromElement offsetX offsetY pageX pageY screenX screenY toElement".split(" "),filter:function(a,d){var e,f,g,h=d.button,i=d.fromElement;a.pageX==null&&d.clientX!=null&&(e=a.target.ownerDocument||c,f=e.documentElement,g=e.body,a.pageX=d.clientX+(f&&f.scrollLeft||g&&g.scrollLeft||0)-(f&&f.clientLeft||g&&g.clientLeft||0),a.pageY=d.clientY+(f&&f.scrollTop||g&&g.scrollTop||0)-(f&&f.clientTop||g&&g.clientTop||0)),!a.relatedTarget&&i&&(a.relatedTarget=i===a.target?d.toElement:i),!a.which&&h!==b&&(a.which=h&1?1:h&2?3:h&4?2:0);return a}},fix:function(a){if(a[f.expando])return a;var d,e,g=a,h=f.event.fixHooks[a.type]||{},i=h.props?this.props.concat(h.props):this.props;a=f.Event(g);for(d=i.length;d;)e=i[--d],a[e]=g[e];a.target||(a.target=g.srcElement||c),a.target.nodeType===3&&(a.target=a.target.parentNode),a.metaKey===b&&(a.metaKey=a.ctrlKey);return h.filter?h.filter(a,g):a},special:{ready:{setup:f.bindReady},load:{noBubble:!0},focus:{delegateType:"focusin"},blur:{delegateType:"focusout"},beforeunload:{setup:function(a,b,c){f.isWindow(this)&&(this.onbeforeunload=c)},teardown:function(a,b){this.onbeforeunload===b&&(this.onbeforeunload=null)}}},simulate:function(a,b,c,d){var e=f.extend(new f.Event,c,{type:a,isSimulated:!0,originalEvent:{}});d?f.event.trigger(e,null,b):f.event.dispatch.call(b,e),e.isDefaultPrevented()&&c.preventDefault()}},f.event.handle=f.event.dispatch,f.removeEvent=c.removeEventListener?function(a,b,c){a.removeEventListener&&a.removeEventListener(b,c,!1)}:function(a,b,c){a.detachEvent&&a.detachEvent("on"+b,c)},f.Event=function(a,b){if(!(this instanceof f.Event))return new f.Event(a,b);a&&a.type?(this.originalEvent=a,this.type=a.type,this.isDefaultPrevented=a.defaultPrevented||a.returnValue===!1||a.getPreventDefault&&a.getPreventDefault()?K:J):this.type=a,b&&f.extend(this,b),this.timeStamp=a&&a.timeStamp||f.now(),this[f.expando]=!0},f.Event.prototype={preventDefault:function(){this.isDefaultPrevented=K;var a=this.originalEvent;!a||(a.preventDefault?a.preventDefault():a.returnValue=!1)},stopPropagation:function(){this.isPropagationStopped=K;var a=this.originalEvent;!a||(a.stopPropagation&&a.stopPropagation(),a.cancelBubble=!0)},stopImmediatePropagation:function(){this.isImmediatePropagationStopped=K,this.stopPropagation()},isDefaultPrevented:J,isPropagationStopped:J,isImmediatePropagationStopped:J},f.each({mouseenter:"mouseover",mouseleave:"mouseout"},function(a,b){f.event.special[a]={delegateType:b,bindType:b,handle:function(a){var c=this,d=a.relatedTarget,e=a.handleObj,g=e.selector,h;if(!d||d!==c&&!f.contains(c,d))a.type=e.origType,h=e.handler.apply(this,arguments),a.type=b;return h}}}),f.support.submitBubbles||(f.event.special.submit={setup:function(){if(f.nodeName(this,"form"))return!1;f.event.add(this,"click._submit keypress._submit",function(a){var c=a.target,d=f.nodeName(c,"input")||f.nodeName(c,"button")?c.form:b;d&&!d._submit_attached&&(f.event.add(d,"submit._submit",function(a){a._submit_bubble=!0}),d._submit_attached=!0)})},postDispatch:function(a){a._submit_bubble&&(delete a._submit_bubble,this.parentNode&&!a.isTrigger&&f.event.simulate("submit",this.parentNode,a,!0))},teardown:function(){if(f.nodeName(this,"form"))return!1;f.event.remove(this,"._submit")}}),f.support.changeBubbles||(f.event.special.change={setup:function(){if(z.test(this.nodeName)){if(this.type==="checkbox"||this.type==="radio")f.event.add(this,"propertychange._change",function(a){a.originalEvent.propertyName==="checked"&&(this._just_changed=!0)}),f.event.add(this,"click._change",function(a){this._just_changed&&!a.isTrigger&&(this._just_changed=!1,f.event.simulate("change",this,a,!0))});return!1}f.event.add(this,"beforeactivate._change",function(a){var b=a.target;z.test(b.nodeName)&&!b._change_attached&&(f.event.add(b,"change._change",function(a){this.parentNode&&!a.isSimulated&&!a.isTrigger&&f.event.simulate("change",this.parentNode,a,!0)}),b._change_attached=!0)})},handle:function(a){var b=a.target;if(this!==b||a.isSimulated||a.isTrigger||b.type!=="radio"&&b.type!=="checkbox")return a.handleObj.handler.apply(this,arguments)},teardown:function(){f.event.remove(this,"._change");return z.test(this.nodeName)}}),f.support.focusinBubbles||f.each({focus:"focusin",blur:"focusout"},function(a,b){var d=0,e=function(a){f.event.simulate(b,a.target,f.event.fix(a),!0)};f.event.special[b]={setup:function(){d++===0&&c.addEventListener(a,e,!0)},teardown:function(){--d===0&&c.removeEventListener(a,e,!0)}}}),f.fn.extend({on:function(a,c,d,e,g){var h,i;if(typeof a=="object"){typeof c!="string"&&(d=d||c,c=b);for(i in a)this.on(i,c,d,a[i],g);return this}d==null&&e==null?(e=c,d=c=b):e==null&&(typeof c=="string"?(e=d,d=b):(e=d,d=c,c=b));if(e===!1)e=J;else if(!e)return this;g===1&&(h=e,e=function(a){f().off(a);return h.apply(this,arguments)},e.guid=h.guid||(h.guid=f.guid++));return this.each(function(){f.event.add(this,a,e,d,c)})},one:function(a,b,c,d){return this.on(a,b,c,d,1)},off:function(a,c,d){if(a&&a.preventDefault&&a.handleObj){var e=a.handleObj;f(a.delegateTarget).off(e.namespace?e.origType+"."+e.namespace:e.origType,e.selector,e.handler);return this}if(typeof a=="object"){for(var g in a)this.off(g,c,a[g]);return this}if(c===!1||typeof c=="function")d=c,c=b;d===!1&&(d=J);return this.each(function(){f.event.remove(this,a,d,c)})},bind:function(a,b,c){return this.on(a,null,b,c)},unbind:function(a,b){return this.off(a,null,b)},live:function(a,b,c){f(this.context).on(a,this.selector,b,c);return this},die:function(a,b){f(this.context).off(a,this.selector||"**",b);return this},delegate:function(a,b,c,d){return this.on(b,a,c,d)},undelegate:function(a,b,c){return arguments.length==1?this.off(a,"**"):this.off(b,a,c)},trigger:function(a,b){return this.each(function(){f.event.trigger(a,b,this)})},triggerHandler:function(a,b){if(this[0])return f.event.trigger(a,b,this[0],!0)},toggle:function(a){var b=arguments,c=a.guid||f.guid++,d=0,e=function(c){var e=(f._data(this,"lastToggle"+a.guid)||0)%d;f._data(this,"lastToggle"+a.guid,e+1),c.preventDefault();return b[e].apply(this,arguments)||!1};e.guid=c;while(d<b.length)b[d++].guid=c;return this.click(e)},hover:function(a,b){return this.mouseenter(a).mouseleave(b||a)}}),f.each("blur focus focusin focusout load resize scroll unload click dblclick mousedown mouseup mousemove mouseover mouseout mouseenter mouseleave change select submit keydown keypress keyup error contextmenu".split(" "),function(a,b){f.fn[b]=function(a,c){c==null&&(c=a,a=null);return arguments.length>0?this.on(b,null,a,c):this.trigger(b)},f.attrFn&&(f.attrFn[b]=!0),C.test(b)&&(f.event.fixHooks[b]=f.event.keyHooks),D.test(b)&&(f.event.fixHooks[b]=f.event.mouseHooks)}),function(){function x(a,b,c,e,f,g){for(var h=0,i=e.length;h<i;h++){var j=e[h];if(j){var k=!1;j=j[a];while(j){if(j[d]===c){k=e[j.sizset];break}if(j.nodeType===1){g||(j[d]=c,j.sizset=h);if(typeof b!="string"){if(j===b){k=!0;break}}else if(m.filter(b,[j]).length>0){k=j;break}}j=j[a]}e[h]=k}}}function w(a,b,c,e,f,g){for(var h=0,i=e.length;h<i;h++){var j=e[h];if(j){var k=!1;j=j[a];while(j){if(j[d]===c){k=e[j.sizset];break}j.nodeType===1&&!g&&(j[d]=c,j.sizset=h);if(j.nodeName.toLowerCase()===b){k=j;break}j=j[a]}e[h]=k}}}var a=/((?:\((?:\([^()]+\)|[^()]+)+\)|\[(?:\[[^\[\]]*\]|['"][^'"]*['"]|[^\[\]'"]+)+\]|\\.|[^ >+~,(\[\\]+)+|[>+~])(\s*,\s*)?((?:.|\r|\n)*)/g,d="sizcache"+(Math.random()+"").replace(".",""),e=0,g=Object.prototype.toString,h=!1,i=!0,j=/\\/g,k=/\r\n/g,l=/\W/;[0,0].sort(function(){i=!1;return 0});var m=function(b,d,e,f){e=e||[],d=d||c;var h=d;if(d.nodeType!==1&&d.nodeType!==9)return[];if(!b||typeof b!="string")return e;var i,j,k,l,n,q,r,t,u=!0,v=m.isXML(d),w=[],x=b;do{a.exec(""),i=a.exec(x);if(i){x=i[3],w.push(i[1]);if(i[2]){l=i[3];break}}}while(i);if(w.length>1&&p.exec(b))if(w.length===2&&o.relative[w[0]])j=y(w[0]+w[1],d,f);else{j=o.relative[w[0]]?[d]:m(w.shift(),d);while(w.length)b=w.shift(),o.relative[b]&&(b+=w.shift()),j=y(b,j,f)}else{!f&&w.length>1&&d.nodeType===9&&!v&&o.match.ID.test(w[0])&&!o.match.ID.test(w[w.length-1])&&(n=m.find(w.shift(),d,v),d=n.expr?m.filter(n.expr,n.set)[0]:n.set[0]);if(d){n=f?{expr:w.pop(),set:s(f)}:m.find(w.pop(),w.length===1&&(w[0]==="~"||w[0]==="+")&&d.parentNode?d.parentNode:d,v),j=n.expr?m.filter(n.expr,n.set):n.set,w.length>0?k=s(j):u=!1;while(w.length)q=w.pop(),r=q,o.relative[q]?r=w.pop():q="",r==null&&(r=d),o.relative[q](k,r,v)}else k=w=[]}k||(k=j),k||m.error(q||b);if(g.call(k)==="[object Array]")if(!u)e.push.apply(e,k);else if(d&&d.nodeType===1)for(t=0;k[t]!=null;t++)k[t]&&(k[t]===!0||k[t].nodeType===1&&m.contains(d,k[t]))&&e.push(j[t]);else for(t=0;k[t]!=null;t++)k[t]&&k[t].nodeType===1&&e.push(j[t]);else s(k,e);l&&(m(l,h,e,f),m.uniqueSort(e));return e};m.uniqueSort=function(a){if(u){h=i,a.sort(u);if(h)for(var b=1;b<a.length;b++)a[b]===a[b-1]&&a.splice(b--,1)}return a},m.matches=function(a,b){return m(a,null,null,b)},m.matchesSelector=function(a,b){return m(b,null,null,[a]).length>0},m.find=function(a,b,c){var d,e,f,g,h,i;if(!a)return[];for(e=0,f=o.order.length;e<f;e++){h=o.order[e];if(g=o.leftMatch[h].exec(a)){i=g[1],g.splice(1,1);if(i.substr(i.length-1)!=="\\"){g[1]=(g[1]||"").replace(j,""),d=o.find[h](g,b,c);if(d!=null){a=a.replace(o.match[h],"");break}}}}d||(d=typeof b.getElementsByTagName!="undefined"?b.getElementsByTagName("*"):[]);return{set:d,expr:a}},m.filter=function(a,c,d,e){var f,g,h,i,j,k,l,n,p,q=a,r=[],s=c,t=c&&c[0]&&m.isXML(c[0]);while(a&&c.length){for(h in o.filter)if((f=o.leftMatch[h].exec(a))!=null&&f[2]){k=o.filter[h],l=f[1],g=!1,f.splice(1,1);if(l.substr(l.length-1)==="\\")continue;s===r&&(r=[]);if(o.preFilter[h]){f=o.preFilter[h](f,s,d,r,e,t);if(!f)g=i=!0;else if(f===!0)continue}if(f)for(n=0;(j=s[n])!=null;n++)j&&(i=k(j,f,n,s),p=e^i,d&&i!=null?p?g=!0:s[n]=!1:p&&(r.push(j),g=!0));if(i!==b){d||(s=r),a=a.replace(o.match[h],"");if(!g)return[];break}}if(a===q)if(g==null)m.error(a);else break;q=a}return s},m.error=function(a){throw new Error("Syntax error, unrecognized expression: "+a)};var n=m.getText=function(a){var b,c,d=a.nodeType,e="";if(d){if(d===1||d===9||d===11){if(typeof a.textContent=="string")return a.textContent;if(typeof a.innerText=="string")return a.innerText.replace(k,"");for(a=a.firstChild;a;a=a.nextSibling)e+=n(a)}else if(d===3||d===4)return a.nodeValue}else for(b=0;c=a[b];b++)c.nodeType!==8&&(e+=n(c));return e},o=m.selectors={order:["ID","NAME","TAG"],match:{ID:/#((?:[\w\u00c0-\uFFFF\-]|\\.)+)/,CLASS:/\.((?:[\w\u00c0-\uFFFF\-]|\\.)+)/,NAME:/\[name=['"]*((?:[\w\u00c0-\uFFFF\-]|\\.)+)['"]*\]/,ATTR:/\[\s*((?:[\w\u00c0-\uFFFF\-]|\\.)+)\s*(?:(\S?=)\s*(?:(['"])(.*?)\3|(#?(?:[\w\u00c0-\uFFFF\-]|\\.)*)|)|)\s*\]/,TAG:/^((?:[\w\u00c0-\uFFFF\*\-]|\\.)+)/,CHILD:/:(only|nth|last|first)-child(?:\(\s*(even|odd|(?:[+\-]?\d+|(?:[+\-]?\d*)?n\s*(?:[+\-]\s*\d+)?))\s*\))?/,POS:/:(nth|eq|gt|lt|first|last|even|odd)(?:\((\d*)\))?(?=[^\-]|$)/,PSEUDO:/:((?:[\w\u00c0-\uFFFF\-]|\\.)+)(?:\((['"]?)((?:\([^\)]+\)|[^\(\)]*)+)\2\))?/},leftMatch:{},attrMap:{"class":"className","for":"htmlFor"},attrHandle:{href:function(a){return a.getAttribute("href")},type:function(a){return a.getAttribute("type")}},relative:{"+":function(a,b){var c=typeof b=="string",d=c&&!l.test(b),e=c&&!d;d&&(b=b.toLowerCase());for(var f=0,g=a.length,h;f<g;f++)if(h=a[f]){while((h=h.previousSibling)&&h.nodeType!==1);a[f]=e||h&&h.nodeName.toLowerCase()===b?h||!1:h===b}e&&m.filter(b,a,!0)},">":function(a,b){var c,d=typeof b=="string",e=0,f=a.length;if(d&&!l.test(b)){b=b.toLowerCase();for(;e<f;e++){c=a[e];if(c){var g=c.parentNode;a[e]=g.nodeName.toLowerCase()===b?g:!1}}}else{for(;e<f;e++)c=a[e],c&&(a[e]=d?c.parentNode:c.parentNode===b);d&&m.filter(b,a,!0)}},"":function(a,b,c){var d,f=e++,g=x;typeof b=="string"&&!l.test(b)&&(b=b.toLowerCase(),d=b,g=w),g("parentNode",b,f,a,d,c)},"~":function(a,b,c){var d,f=e++,g=x;typeof b=="string"&&!l.test(b)&&(b=b.toLowerCase(),d=b,g=w),g("previousSibling",b,f,a,d,c)}},find:{ID:function(a,b,c){if(typeof b.getElementById!="undefined"&&!c){var d=b.getElementById(a[1]);return d&&d.parentNode?[d]:[]}},NAME:function(a,b){if(typeof b.getElementsByName!="undefined"){var c=[],d=b.getElementsByName(a[1]);for(var e=0,f=d.length;e<f;e++)d[e].getAttribute("name")===a[1]&&c.push(d[e]);return c.length===0?null:c}},TAG:function(a,b){if(typeof b.getElementsByTagName!="undefined")return b.getElementsByTagName(a[1])}},preFilter:{CLASS:function(a,b,c,d,e,f){a=" "+a[1].replace(j,"")+" ";if(f)return a;for(var g=0,h;(h=b[g])!=null;g++)h&&(e^(h.className&&(" "+h.className+" ").replace(/[\t\n\r]/g," ").indexOf(a)>=0)?c||d.push(h):c&&(b[g]=!1));return!1},ID:function(a){return a[1].replace(j,"")},TAG:function(a,b){return a[1].replace(j,"").toLowerCase()},CHILD:function(a){if(a[1]==="nth"){a[2]||m.error(a[0]),a[2]=a[2].replace(/^\+|\s*/g,"");var b=/(-?)(\d*)(?:n([+\-]?\d*))?/.exec(a[2]==="even"&&"2n"||a[2]==="odd"&&"2n+1"||!/\D/.test(a[2])&&"0n+"+a[2]||a[2]);a[2]=b[1]+(b[2]||1)-0,a[3]=b[3]-0}else a[2]&&m.error(a[0]);a[0]=e++;return a},ATTR:function(a,b,c,d,e,f){var g=a[1]=a[1].replace(j,"");!f&&o.attrMap[g]&&(a[1]=o.attrMap[g]),a[4]=(a[4]||a[5]||"").replace(j,""),a[2]==="~="&&(a[4]=" "+a[4]+" ");return a},PSEUDO:function(b,c,d,e,f){if(b[1]==="not")if((a.exec(b[3])||"").length>1||/^\w/.test(b[3]))b[3]=m(b[3],null,null,c);else{var g=m.filter(b[3],c,d,!0^f);d||e.push.apply(e,g);return!1}else if(o.match.POS.test(b[0])||o.match.CHILD.test(b[0]))return!0;return b},POS:function(a){a.unshift(!0);return a}},filters:{enabled:function(a){return a.disabled===!1&&a.type!=="hidden"},disabled:function(a){return a.disabled===!0},checked:function(a){return a.checked===!0},selected:function(a){a.parentNode&&a.parentNode.selectedIndex;return a.selected===!0},parent:function(a){return!!a.firstChild},empty:function(a){return!a.firstChild},has:function(a,b,c){return!!m(c[3],a).length},header:function(a){return/h\d/i.test(a.nodeName)},text:function(a){var b=a.getAttribute("type"),c=a.type;return a.nodeName.toLowerCase()==="input"&&"text"===c&&(b===c||b===null)},radio:function(a){return a.nodeName.toLowerCase()==="input"&&"radio"===a.type},checkbox:function(a){return a.nodeName.toLowerCase()==="input"&&"checkbox"===a.type},file:function(a){return a.nodeName.toLowerCase()==="input"&&"file"===a.type},password:function(a){return a.nodeName.toLowerCase()==="input"&&"password"===a.type},submit:function(a){var b=a.nodeName.toLowerCase();return(b==="input"||b==="button")&&"submit"===a.type},image:function(a){return a.nodeName.toLowerCase()==="input"&&"image"===a.type},reset:function(a){var b=a.nodeName.toLowerCase();return(b==="input"||b==="button")&&"reset"===a.type},button:function(a){var b=a.nodeName.toLowerCase();return b==="input"&&"button"===a.type||b==="button"},input:function(a){return/input|select|textarea|button/i.test(a.nodeName)},focus:function(a){return a===a.ownerDocument.activeElement}},setFilters:{first:function(a,b){return b===0},last:function(a,b,c,d){return b===d.length-1},even:function(a,b){return b%2===0},odd:function(a,b){return b%2===1},lt:function(a,b,c){return b<c[3]-0},gt:function(a,b,c){return b>c[3]-0},nth:function(a,b,c){return c[3]-0===b},eq:function(a,b,c){return c[3]-0===b}},filter:{PSEUDO:function(a,b,c,d){var e=b[1],f=o.filters[e];if(f)return f(a,c,b,d);if(e==="contains")return(a.textContent||a.innerText||n([a])||"").indexOf(b[3])>=0;if(e==="not"){var g=b[3];for(var h=0,i=g.length;h<i;h++)if(g[h]===a)return!1;return!0}m.error(e)},CHILD:function(a,b){var c,e,f,g,h,i,j,k=b[1],l=a;switch(k){case"only":case"first":while(l=l.previousSibling)if(l.nodeType===1)return!1;if(k==="first")return!0;l=a;case"last":while(l=l.nextSibling)if(l.nodeType===1)return!1;return!0;case"nth":c=b[2],e=b[3];if(c===1&&e===0)return!0;f=b[0],g=a.parentNode;if(g&&(g[d]!==f||!a.nodeIndex)){i=0;for(l=g.firstChild;l;l=l.nextSibling)l.nodeType===1&&(l.nodeIndex=++i);g[d]=f}j=a.nodeIndex-e;return c===0?j===0:j%c===0&&j/c>=0}},ID:function(a,b){return a.nodeType===1&&a.getAttribute("id")===b},TAG:function(a,b){return b==="*"&&a.nodeType===1||!!a.nodeName&&a.nodeName.toLowerCase()===b},CLASS:function(a,b){return(" "+(a.className||a.getAttribute("class"))+" ").indexOf(b)>-1},ATTR:function(a,b){var c=b[1],d=m.attr?m.attr(a,c):o.attrHandle[c]?o.attrHandle[c](a):a[c]!=null?a[c]:a.getAttribute(c),e=d+"",f=b[2],g=b[4];return d==null?f==="!=":!f&&m.attr?d!=null:f==="="?e===g:f==="*="?e.indexOf(g)>=0:f==="~="?(" "+e+" ").indexOf(g)>=0:g?f==="!="?e!==g:f==="^="?e.indexOf(g)===0:f==="$="?e.substr(e.length-g.length)===g:f==="|="?e===g||e.substr(0,g.length+1)===g+"-":!1:e&&d!==!1},POS:function(a,b,c,d){var e=b[2],f=o.setFilters[e];if(f)return f(a,c,b,d)}}},p=o.match.POS,q=function(a,b){return"\\"+(b-0+1)};for(var r in o.match)o.match[r]=new RegExp(o.match[r].source+/(?![^\[]*\])(?![^\(]*\))/.source),o.leftMatch[r]=new RegExp(/(^(?:.|\r|\n)*?)/.source+o.match[r].source.replace(/\\(\d+)/g,q));o.match.globalPOS=p;var s=function(a,b){a=Array.prototype.slice.call(a,0);if(b){b.push.apply(b,a);return b}return a};try{Array.prototype.slice.call(c.documentElement.childNodes,0)[0].nodeType}catch(t){s=function(a,b){var c=0,d=b||[];if(g.call(a)==="[object Array]")Array.prototype.push.apply(d,a);else if(typeof a.length=="number")for(var e=a.length;c<e;c++)d.push(a[c]);else for(;a[c];c++)d.push(a[c]);return d}}var u,v;c.documentElement.compareDocumentPosition?u=function(a,b){if(a===b){h=!0;return 0}if(!a.compareDocumentPosition||!b.compareDocumentPosition)return a.compareDocumentPosition?-1:1;return a.compareDocumentPosition(b)&4?-1:1}:(u=function(a,b){if(a===b){h=!0;return 0}if(a.sourceIndex&&b.sourceIndex)return a.sourceIndex-b.sourceIndex;var c,d,e=[],f=[],g=a.parentNode,i=b.parentNode,j=g;if(g===i)return v(a,b);if(!g)return-1;if(!i)return 1;while(j)e.unshift(j),j=j.parentNode;j=i;while(j)f.unshift(j),j=j.parentNode;c=e.length,d=f.length;for(var k=0;k<c&&k<d;k++)if(e[k]!==f[k])return v(e[k],f[k]);return k===c?v(a,f[k],-1):v(e[k],b,1)},v=function(a,b,c){if(a===b)return c;var d=a.nextSibling;while(d){if(d===b)return-1;d=d.nextSibling}return 1}),function(){var a=c.createElement("div"),d="script"+(new Date).getTime(),e=c.documentElement;a.innerHTML="<a name='"+d+"'/>",e.insertBefore(a,e.firstChild),c.getElementById(d)&&(o.find.ID=function(a,c,d){if(typeof c.getElementById!="undefined"&&!d){var e=c.getElementById(a[1]);return e?e.id===a[1]||typeof e.getAttributeNode!="undefined"&&e.getAttributeNode("id").nodeValue===a[1]?[e]:b:[]}},o.filter.ID=function(a,b){var c=typeof a.getAttributeNode!="undefined"&&a.getAttributeNode("id");return a.nodeType===1&&c&&c.nodeValue===b}),e.removeChild(a),e=a=null}(),function(){var a=c.createElement("div");a.appendChild(c.createComment("")),a.getElementsByTagName("*").length>0&&(o.find.TAG=function(a,b){var c=b.getElementsByTagName(a[1]);if(a[1]==="*"){var d=[];for(var e=0;c[e];e++)c[e].nodeType===1&&d.push(c[e]);c=d}return c}),a.innerHTML="<a href='#'></a>",a.firstChild&&typeof a.firstChild.getAttribute!="undefined"&&a.firstChild.getAttribute("href")!=="#"&&(o.attrHandle.href=function(a){return a.getAttribute("href",2)}),a=null}(),c.querySelectorAll&&function(){var a=m,b=c.createElement("div"),d="__sizzle__";b.innerHTML="<p class='TEST'></p>";if(!b.querySelectorAll||b.querySelectorAll(".TEST").length!==0){m=function(b,e,f,g){e=e||c;if(!g&&!m.isXML(e)){var h=/^(\w+$)|^\.([\w\-]+$)|^#([\w\-]+$)/.exec(b);if(h&&(e.nodeType===1||e.nodeType===9)){if(h[1])return s(e.getElementsByTagName(b),f);if(h[2]&&o.find.CLASS&&e.getElementsByClassName)return s(e.getElementsByClassName(h[2]),f)}if(e.nodeType===9){if(b==="body"&&e.body)return s([e.body],f);if(h&&h[3]){var i=e.getElementById(h[3]);if(!i||!i.parentNode)return s([],f);if(i.id===h[3])return s([i],f)}try{return s(e.querySelectorAll(b),f)}catch(j){}}else if(e.nodeType===1&&e.nodeName.toLowerCase()!=="object"){var k=e,l=e.getAttribute("id"),n=l||d,p=e.parentNode,q=/^\s*[+~]/.test(b);l?n=n.replace(/'/g,"\\$&"):e.setAttribute("id",n),q&&p&&(e=e.parentNode);try{if(!q||p)return s(e.querySelectorAll("[id='"+n+"'] "+b),f)}catch(r){}finally{l||k.removeAttribute("id")}}}return a(b,e,f,g)};for(var e in a)m[e]=a[e];b=null}}(),function(){var a=c.documentElement,b=a.matchesSelector||a.mozMatchesSelector||a.webkitMatchesSelector||a.msMatchesSelector;if(b){var d=!b.call(c.createElement("div"),"div"),e=!1;try{b.call(c.documentElement,"[test!='']:sizzle")}catch(f){e=!0}m.matchesSelector=function(a,c){c=c.replace(/\=\s*([^'"\]]*)\s*\]/g,"='$1']");if(!m.isXML(a))try{if(e||!o.match.PSEUDO.test(c)&&!/!=/.test(c)){var f=b.call(a,c);if(f||!d||a.document&&a.document.nodeType!==11)return f}}catch(g){}return m(c,null,null,[a]).length>0}}}(),function(){var a=c.createElement("div");a.innerHTML="<div class='test e'></div><div class='test'></div>";if(!!a.getElementsByClassName&&a.getElementsByClassName("e").length!==0){a.lastChild.className="e";if(a.getElementsByClassName("e").length===1)return;o.order.splice(1,0,"CLASS"),o.find.CLASS=function(a,b,c){if(typeof b.getElementsByClassName!="undefined"&&!c)return b.getElementsByClassName(a[1])},a=null}}(),c.documentElement.contains?m.contains=function(a,b){return a!==b&&(a.contains?a.contains(b):!0)}:c.documentElement.compareDocumentPosition?m.contains=function(a,b){return!!(a.compareDocumentPosition(b)&16)}:m.contains=function(){return!1},m.isXML=function(a){var b=(a?a.ownerDocument||a:0).documentElement;return b?b.nodeName!=="HTML":!1};var y=function(a,b,c){var d,e=[],f="",g=b.nodeType?[b]:b;while(d=o.match.PSEUDO.exec(a))f+=d[0],a=a.replace(o.match.PSEUDO,"");a=o.relative[a]?a+"*":a;for(var h=0,i=g.length;h<i;h++)m(a,g[h],e,c);return m.filter(f,e)};m.attr=f.attr,m.selectors.attrMap={},f.find=m,f.expr=m.selectors,f.expr[":"]=f.expr.filters,f.unique=m.uniqueSort,f.text=m.getText,f.isXMLDoc=m.isXML,f.contains=m.contains}();var L=/Until$/,M=/^(?:parents|prevUntil|prevAll)/,N=/,/,O=/^.[^:#\[\.,]*$/,P=Array.prototype.slice,Q=f.expr.match.globalPOS,R={children:!0,contents:!0,next:!0,prev:!0};f.fn.extend({find:function(a){var b=this,c,d;if(typeof a!="string")return f(a).filter(function(){for(c=0,d=b.length;c<d;c++)if(f.contains(b[c],this))return!0});var e=this.pushStack("","find",a),g,h,i;for(c=0,d=this.length;c<d;c++){g=e.length,f.find(a,this[c],e);if(c>0)for(h=g;h<e.length;h++)for(i=0;i<g;i++)if(e[i]===e[h]){e.splice(h--,1);break}}return e},has:function(a){var b=f(a);return this.filter(function(){for(var a=0,c=b.length;a<c;a++)if(f.contains(this,b[a]))return!0})},not:function(a){return this.pushStack(T(this,a,!1),"not",a)},filter:function(a){return this.pushStack(T(this,a,!0),"filter",a)},is:function(a){return!!a&&(typeof a=="string"?Q.test(a)?f(a,this.context).index(this[0])>=0:f.filter(a,this).length>0:this.filter(a).length>0)},closest:function(a,b){var c=[],d,e,g=this[0];if(f.isArray(a)){var h=1;while(g&&g.ownerDocument&&g!==b){for(d=0;d<a.length;d++)f(g).is(a[d])&&c.push({selector:a[d],elem:g,level:h});g=g.parentNode,h++}return c}var i=Q.test(a)||typeof a!="string"?f(a,b||this.context):0;for(d=0,e=this.length;d<e;d++){g=this[d];while(g){if(i?i.index(g)>-1:f.find.matchesSelector(g,a)){c.push(g);break}g=g.parentNode;if(!g||!g.ownerDocument||g===b||g.nodeType===11)break}}c=c.length>1?f.unique(c):c;return this.pushStack(c,"closest",a)},index:function(a){if(!a)return this[0]&&this[0].parentNode?this.prevAll().length:-1;if(typeof a=="string")return f.inArray(this[0],f(a));return f.inArray(a.jquery?a[0]:a,this)},add:function(a,b){var c=typeof a=="string"?f(a,b):f.makeArray(a&&a.nodeType?[a]:a),d=f.merge(this.get(),c);return this.pushStack(S(c[0])||S(d[0])?d:f.unique(d))},andSelf:function(){return this.add(this.prevObject)}}),f.each({parent:function(a){var b=a.parentNode;return b&&b.nodeType!==11?b:null},parents:function(a){return f.dir(a,"parentNode")},parentsUntil:function(a,b,c){return f.dir(a,"parentNode",c)},next:function(a){return f.nth(a,2,"nextSibling")},prev:function(a){return f.nth(a,2,"previousSibling")},nextAll:function(a){return f.dir(a,"nextSibling")},prevAll:function(a){return f.dir(a,"previousSibling")},nextUntil:function(a,b,c){return f.dir(a,"nextSibling",c)},prevUntil:function(a,b,c){return f.dir(a,"previousSibling",c)},siblings:function(a){return f.sibling((a.parentNode||{}).firstChild,a)},children:function(a){return f.sibling(a.firstChild)},contents:function(a){return f.nodeName(a,"iframe")?a.contentDocument||a.contentWindow.document:f.makeArray(a.childNodes)}},function(a,b){f.fn[a]=function(c,d){var e=f.map(this,b,c);L.test(a)||(d=c),d&&typeof d=="string"&&(e=f.filter(d,e)),e=this.length>1&&!R[a]?f.unique(e):e,(this.length>1||N.test(d))&&M.test(a)&&(e=e.reverse());return this.pushStack(e,a,P.call(arguments).join(","))}}),f.extend({filter:function(a,b,c){c&&(a=":not("+a+")");return b.length===1?f.find.matchesSelector(b[0],a)?[b[0]]:[]:f.find.matches(a,b)},dir:function(a,c,d){var e=[],g=a[c];while(g&&g.nodeType!==9&&(d===b||g.nodeType!==1||!f(g).is(d)))g.nodeType===1&&e.push(g),g=g[c];return e},nth:function(a,b,c,d){b=b||1;var e=0;for(;a;a=a[c])if(a.nodeType===1&&++e===b)break;return a},sibling:function(a,b){var c=[];for(;a;a=a.nextSibling)a.nodeType===1&&a!==b&&c.push(a);return c}});var V="abbr|article|aside|audio|bdi|canvas|data|datalist|details|figcaption|figure|footer|header|hgroup|mark|meter|nav|output|progress|section|summary|time|video",W=/ jQuery\d+="(?:\d+|null)"/g,X=/^\s+/,Y=/<(?!area|br|col|embed|hr|img|input|link|meta|param)(([\w:]+)[^>]*)\/>/ig,Z=/<([\w:]+)/,$=/<tbody/i,_=/<|&#?\w+;/,ba=/<(?:script|style)/i,bb=/<(?:script|object|embed|option|style)/i,bc=new RegExp("<(?:"+V+")[\\s/>]","i"),bd=/checked\s*(?:[^=]|=\s*.checked.)/i,be=/\/(java|ecma)script/i,bf=/^\s*<!(?:\[CDATA\[|\-\-)/,bg={option:[1,"<select multiple='multiple'>","</select>"],legend:[1,"<fieldset>","</fieldset>"],thead:[1,"<table>","</table>"],tr:[2,"<table><tbody>","</tbody></table>"],td:[3,"<table><tbody><tr>","</tr></tbody></table>"],col:[2,"<table><tbody></tbody><colgroup>","</colgroup></table>"],area:[1,"<map>","</map>"],_default:[0,"",""]},bh=U(c);bg.optgroup=bg.option,bg.tbody=bg.tfoot=bg.colgroup=bg.caption=bg.thead,bg.th=bg.td,f.support.htmlSerialize||(bg._default=[1,"div<div>","</div>"]),f.fn.extend({text:function(a){return f.access(this,function(a){return a===b?f.text(this):this.empty().append((this[0]&&this[0].ownerDocument||c).createTextNode(a))},null,a,arguments.length)},wrapAll:function(a){if(f.isFunction(a))return this.each(function(b){f(this).wrapAll(a.call(this,b))});if(this[0]){var b=f(a,this[0].ownerDocument).eq(0).clone(!0);this[0].parentNode&&b.insertBefore(this[0]),b.map(function(){var a=this;while(a.firstChild&&a.firstChild.nodeType===1)a=a.firstChild;return a}).append(this)}return this},wrapInner:function(a){if(f.isFunction(a))return this.each(function(b){f(this).wrapInner(a.call(this,b))});return this.each(function(){var b=f(this),c=b.contents();c.length?c.wrapAll(a):b.append(a)})},wrap:function(a){var b=f.isFunction(a);return this.each(function(c){f(this).wrapAll(b?a.call(this,c):a)})},unwrap:function(){return this.parent().each(function(){f.nodeName(this,"body")||f(this).replaceWith(this.childNodes)}).end()},append:function(){return this.domManip(arguments,!0,function(a){this.nodeType===1&&this.appendChild(a)})},prepend:function(){return this.domManip(arguments,!0,function(a){this.nodeType===1&&this.insertBefore(a,this.firstChild)})},before:function(){if(this[0]&&this[0].parentNode)return this.domManip(arguments,!1,function(a){this.parentNode.insertBefore(a,this)});if(arguments.length){var a=f
.clean(arguments);a.push.apply(a,this.toArray());return this.pushStack(a,"before",arguments)}},after:function(){if(this[0]&&this[0].parentNode)return this.domManip(arguments,!1,function(a){this.parentNode.insertBefore(a,this.nextSibling)});if(arguments.length){var a=this.pushStack(this,"after",arguments);a.push.apply(a,f.clean(arguments));return a}},remove:function(a,b){for(var c=0,d;(d=this[c])!=null;c++)if(!a||f.filter(a,[d]).length)!b&&d.nodeType===1&&(f.cleanData(d.getElementsByTagName("*")),f.cleanData([d])),d.parentNode&&d.parentNode.removeChild(d);return this},empty:function(){for(var a=0,b;(b=this[a])!=null;a++){b.nodeType===1&&f.cleanData(b.getElementsByTagName("*"));while(b.firstChild)b.removeChild(b.firstChild)}return this},clone:function(a,b){a=a==null?!1:a,b=b==null?a:b;return this.map(function(){return f.clone(this,a,b)})},html:function(a){return f.access(this,function(a){var c=this[0]||{},d=0,e=this.length;if(a===b)return c.nodeType===1?c.innerHTML.replace(W,""):null;if(typeof a=="string"&&!ba.test(a)&&(f.support.leadingWhitespace||!X.test(a))&&!bg[(Z.exec(a)||["",""])[1].toLowerCase()]){a=a.replace(Y,"<$1></$2>");try{for(;d<e;d++)c=this[d]||{},c.nodeType===1&&(f.cleanData(c.getElementsByTagName("*")),c.innerHTML=a);c=0}catch(g){}}c&&this.empty().append(a)},null,a,arguments.length)},replaceWith:function(a){if(this[0]&&this[0].parentNode){if(f.isFunction(a))return this.each(function(b){var c=f(this),d=c.html();c.replaceWith(a.call(this,b,d))});typeof a!="string"&&(a=f(a).detach());return this.each(function(){var b=this.nextSibling,c=this.parentNode;f(this).remove(),b?f(b).before(a):f(c).append(a)})}return this.length?this.pushStack(f(f.isFunction(a)?a():a),"replaceWith",a):this},detach:function(a){return this.remove(a,!0)},domManip:function(a,c,d){var e,g,h,i,j=a[0],k=[];if(!f.support.checkClone&&arguments.length===3&&typeof j=="string"&&bd.test(j))return this.each(function(){f(this).domManip(a,c,d,!0)});if(f.isFunction(j))return this.each(function(e){var g=f(this);a[0]=j.call(this,e,c?g.html():b),g.domManip(a,c,d)});if(this[0]){i=j&&j.parentNode,f.support.parentNode&&i&&i.nodeType===11&&i.childNodes.length===this.length?e={fragment:i}:e=f.buildFragment(a,this,k),h=e.fragment,h.childNodes.length===1?g=h=h.firstChild:g=h.firstChild;if(g){c=c&&f.nodeName(g,"tr");for(var l=0,m=this.length,n=m-1;l<m;l++)d.call(c?bi(this[l],g):this[l],e.cacheable||m>1&&l<n?f.clone(h,!0,!0):h)}k.length&&f.each(k,function(a,b){b.src?f.ajax({type:"GET",global:!1,url:b.src,async:!1,dataType:"script"}):f.globalEval((b.text||b.textContent||b.innerHTML||"").replace(bf,"/*$0*/")),b.parentNode&&b.parentNode.removeChild(b)})}return this}}),f.buildFragment=function(a,b,d){var e,g,h,i,j=a[0];b&&b[0]&&(i=b[0].ownerDocument||b[0]),i.createDocumentFragment||(i=c),a.length===1&&typeof j=="string"&&j.length<512&&i===c&&j.charAt(0)==="<"&&!bb.test(j)&&(f.support.checkClone||!bd.test(j))&&(f.support.html5Clone||!bc.test(j))&&(g=!0,h=f.fragments[j],h&&h!==1&&(e=h)),e||(e=i.createDocumentFragment(),f.clean(a,i,e,d)),g&&(f.fragments[j]=h?e:1);return{fragment:e,cacheable:g}},f.fragments={},f.each({appendTo:"append",prependTo:"prepend",insertBefore:"before",insertAfter:"after",replaceAll:"replaceWith"},function(a,b){f.fn[a]=function(c){var d=[],e=f(c),g=this.length===1&&this[0].parentNode;if(g&&g.nodeType===11&&g.childNodes.length===1&&e.length===1){e[b](this[0]);return this}for(var h=0,i=e.length;h<i;h++){var j=(h>0?this.clone(!0):this).get();f(e[h])[b](j),d=d.concat(j)}return this.pushStack(d,a,e.selector)}}),f.extend({clone:function(a,b,c){var d,e,g,h=f.support.html5Clone||f.isXMLDoc(a)||!bc.test("<"+a.nodeName+">")?a.cloneNode(!0):bo(a);if((!f.support.noCloneEvent||!f.support.noCloneChecked)&&(a.nodeType===1||a.nodeType===11)&&!f.isXMLDoc(a)){bk(a,h),d=bl(a),e=bl(h);for(g=0;d[g];++g)e[g]&&bk(d[g],e[g])}if(b){bj(a,h);if(c){d=bl(a),e=bl(h);for(g=0;d[g];++g)bj(d[g],e[g])}}d=e=null;return h},clean:function(a,b,d,e){var g,h,i,j=[];b=b||c,typeof b.createElement=="undefined"&&(b=b.ownerDocument||b[0]&&b[0].ownerDocument||c);for(var k=0,l;(l=a[k])!=null;k++){typeof l=="number"&&(l+="");if(!l)continue;if(typeof l=="string")if(!_.test(l))l=b.createTextNode(l);else{l=l.replace(Y,"<$1></$2>");var m=(Z.exec(l)||["",""])[1].toLowerCase(),n=bg[m]||bg._default,o=n[0],p=b.createElement("div"),q=bh.childNodes,r;b===c?bh.appendChild(p):U(b).appendChild(p),p.innerHTML=n[1]+l+n[2];while(o--)p=p.lastChild;if(!f.support.tbody){var s=$.test(l),t=m==="table"&&!s?p.firstChild&&p.firstChild.childNodes:n[1]==="<table>"&&!s?p.childNodes:[];for(i=t.length-1;i>=0;--i)f.nodeName(t[i],"tbody")&&!t[i].childNodes.length&&t[i].parentNode.removeChild(t[i])}!f.support.leadingWhitespace&&X.test(l)&&p.insertBefore(b.createTextNode(X.exec(l)[0]),p.firstChild),l=p.childNodes,p&&(p.parentNode.removeChild(p),q.length>0&&(r=q[q.length-1],r&&r.parentNode&&r.parentNode.removeChild(r)))}var u;if(!f.support.appendChecked)if(l[0]&&typeof (u=l.length)=="number")for(i=0;i<u;i++)bn(l[i]);else bn(l);l.nodeType?j.push(l):j=f.merge(j,l)}if(d){g=function(a){return!a.type||be.test(a.type)};for(k=0;j[k];k++){h=j[k];if(e&&f.nodeName(h,"script")&&(!h.type||be.test(h.type)))e.push(h.parentNode?h.parentNode.removeChild(h):h);else{if(h.nodeType===1){var v=f.grep(h.getElementsByTagName("script"),g);j.splice.apply(j,[k+1,0].concat(v))}d.appendChild(h)}}}return j},cleanData:function(a){var b,c,d=f.cache,e=f.event.special,g=f.support.deleteExpando;for(var h=0,i;(i=a[h])!=null;h++){if(i.nodeName&&f.noData[i.nodeName.toLowerCase()])continue;c=i[f.expando];if(c){b=d[c];if(b&&b.events){for(var j in b.events)e[j]?f.event.remove(i,j):f.removeEvent(i,j,b.handle);b.handle&&(b.handle.elem=null)}g?delete i[f.expando]:i.removeAttribute&&i.removeAttribute(f.expando),delete d[c]}}}});var bp=/alpha\([^)]*\)/i,bq=/opacity=([^)]*)/,br=/([A-Z]|^ms)/g,bs=/^[\-+]?(?:\d*\.)?\d+$/i,bt=/^-?(?:\d*\.)?\d+(?!px)[^\d\s]+$/i,bu=/^([\-+])=([\-+.\de]+)/,bv=/^margin/,bw={position:"absolute",visibility:"hidden",display:"block"},bx=["Top","Right","Bottom","Left"],by,bz,bA;f.fn.css=function(a,c){return f.access(this,function(a,c,d){return d!==b?f.style(a,c,d):f.css(a,c)},a,c,arguments.length>1)},f.extend({cssHooks:{opacity:{get:function(a,b){if(b){var c=by(a,"opacity");return c===""?"1":c}return a.style.opacity}}},cssNumber:{fillOpacity:!0,fontWeight:!0,lineHeight:!0,opacity:!0,orphans:!0,widows:!0,zIndex:!0,zoom:!0},cssProps:{"float":f.support.cssFloat?"cssFloat":"styleFloat"},style:function(a,c,d,e){if(!!a&&a.nodeType!==3&&a.nodeType!==8&&!!a.style){var g,h,i=f.camelCase(c),j=a.style,k=f.cssHooks[i];c=f.cssProps[i]||i;if(d===b){if(k&&"get"in k&&(g=k.get(a,!1,e))!==b)return g;return j[c]}h=typeof d,h==="string"&&(g=bu.exec(d))&&(d=+(g[1]+1)*+g[2]+parseFloat(f.css(a,c)),h="number");if(d==null||h==="number"&&isNaN(d))return;h==="number"&&!f.cssNumber[i]&&(d+="px");if(!k||!("set"in k)||(d=k.set(a,d))!==b)try{j[c]=d}catch(l){}}},css:function(a,c,d){var e,g;c=f.camelCase(c),g=f.cssHooks[c],c=f.cssProps[c]||c,c==="cssFloat"&&(c="float");if(g&&"get"in g&&(e=g.get(a,!0,d))!==b)return e;if(by)return by(a,c)},swap:function(a,b,c){var d={},e,f;for(f in b)d[f]=a.style[f],a.style[f]=b[f];e=c.call(a);for(f in b)a.style[f]=d[f];return e}}),f.curCSS=f.css,c.defaultView&&c.defaultView.getComputedStyle&&(bz=function(a,b){var c,d,e,g,h=a.style;b=b.replace(br,"-$1").toLowerCase(),(d=a.ownerDocument.defaultView)&&(e=d.getComputedStyle(a,null))&&(c=e.getPropertyValue(b),c===""&&!f.contains(a.ownerDocument.documentElement,a)&&(c=f.style(a,b))),!f.support.pixelMargin&&e&&bv.test(b)&&bt.test(c)&&(g=h.width,h.width=c,c=e.width,h.width=g);return c}),c.documentElement.currentStyle&&(bA=function(a,b){var c,d,e,f=a.currentStyle&&a.currentStyle[b],g=a.style;f==null&&g&&(e=g[b])&&(f=e),bt.test(f)&&(c=g.left,d=a.runtimeStyle&&a.runtimeStyle.left,d&&(a.runtimeStyle.left=a.currentStyle.left),g.left=b==="fontSize"?"1em":f,f=g.pixelLeft+"px",g.left=c,d&&(a.runtimeStyle.left=d));return f===""?"auto":f}),by=bz||bA,f.each(["height","width"],function(a,b){f.cssHooks[b]={get:function(a,c,d){if(c)return a.offsetWidth!==0?bB(a,b,d):f.swap(a,bw,function(){return bB(a,b,d)})},set:function(a,b){return bs.test(b)?b+"px":b}}}),f.support.opacity||(f.cssHooks.opacity={get:function(a,b){return bq.test((b&&a.currentStyle?a.currentStyle.filter:a.style.filter)||"")?parseFloat(RegExp.$1)/100+"":b?"1":""},set:function(a,b){var c=a.style,d=a.currentStyle,e=f.isNumeric(b)?"alpha(opacity="+b*100+")":"",g=d&&d.filter||c.filter||"";c.zoom=1;if(b>=1&&f.trim(g.replace(bp,""))===""){c.removeAttribute("filter");if(d&&!d.filter)return}c.filter=bp.test(g)?g.replace(bp,e):g+" "+e}}),f(function(){f.support.reliableMarginRight||(f.cssHooks.marginRight={get:function(a,b){return f.swap(a,{display:"inline-block"},function(){return b?by(a,"margin-right"):a.style.marginRight})}})}),f.expr&&f.expr.filters&&(f.expr.filters.hidden=function(a){var b=a.offsetWidth,c=a.offsetHeight;return b===0&&c===0||!f.support.reliableHiddenOffsets&&(a.style&&a.style.display||f.css(a,"display"))==="none"},f.expr.filters.visible=function(a){return!f.expr.filters.hidden(a)}),f.each({margin:"",padding:"",border:"Width"},function(a,b){f.cssHooks[a+b]={expand:function(c){var d,e=typeof c=="string"?c.split(" "):[c],f={};for(d=0;d<4;d++)f[a+bx[d]+b]=e[d]||e[d-2]||e[0];return f}}});var bC=/%20/g,bD=/\[\]$/,bE=/\r?\n/g,bF=/#.*$/,bG=/^(.*?):[ \t]*([^\r\n]*)\r?$/mg,bH=/^(?:color|date|datetime|datetime-local|email|hidden|month|number|password|range|search|tel|text|time|url|week)$/i,bI=/^(?:about|app|app\-storage|.+\-extension|file|res|widget):$/,bJ=/^(?:GET|HEAD)$/,bK=/^\/\//,bL=/\?/,bM=/<script\b[^<]*(?:(?!<\/script>)<[^<]*)*<\/script>/gi,bN=/^(?:select|textarea)/i,bO=/\s+/,bP=/([?&])_=[^&]*/,bQ=/^([\w\+\.\-]+:)(?:\/\/([^\/?#:]*)(?::(\d+))?)?/,bR=f.fn.load,bS={},bT={},bU,bV,bW=["*/"]+["*"];try{bU=e.href}catch(bX){bU=c.createElement("a"),bU.href="",bU=bU.href}bV=bQ.exec(bU.toLowerCase())||[],f.fn.extend({load:function(a,c,d){if(typeof a!="string"&&bR)return bR.apply(this,arguments);if(!this.length)return this;var e=a.indexOf(" ");if(e>=0){var g=a.slice(e,a.length);a=a.slice(0,e)}var h="GET";c&&(f.isFunction(c)?(d=c,c=b):typeof c=="object"&&(c=f.param(c,f.ajaxSettings.traditional),h="POST"));var i=this;f.ajax({url:a,type:h,dataType:"html",data:c,complete:function(a,b,c){c=a.responseText,a.isResolved()&&(a.done(function(a){c=a}),i.html(g?f("<div>").append(c.replace(bM,"")).find(g):c)),d&&i.each(d,[c,b,a])}});return this},serialize:function(){return f.param(this.serializeArray())},serializeArray:function(){return this.map(function(){return this.elements?f.makeArray(this.elements):this}).filter(function(){return this.name&&!this.disabled&&(this.checked||bN.test(this.nodeName)||bH.test(this.type))}).map(function(a,b){var c=f(this).val();return c==null?null:f.isArray(c)?f.map(c,function(a,c){return{name:b.name,value:a.replace(bE,"\r\n")}}):{name:b.name,value:c.replace(bE,"\r\n")}}).get()}}),f.each("ajaxStart ajaxStop ajaxComplete ajaxError ajaxSuccess ajaxSend".split(" "),function(a,b){f.fn[b]=function(a){return this.on(b,a)}}),f.each(["get","post"],function(a,c){f[c]=function(a,d,e,g){f.isFunction(d)&&(g=g||e,e=d,d=b);return f.ajax({type:c,url:a,data:d,success:e,dataType:g})}}),f.extend({getScript:function(a,c){return f.get(a,b,c,"script")},getJSON:function(a,b,c){return f.get(a,b,c,"json")},ajaxSetup:function(a,b){b?b$(a,f.ajaxSettings):(b=a,a=f.ajaxSettings),b$(a,b);return a},ajaxSettings:{url:bU,isLocal:bI.test(bV[1]),global:!0,type:"GET",contentType:"application/x-www-form-urlencoded; charset=UTF-8",processData:!0,async:!0,accepts:{xml:"application/xml, text/xml",html:"text/html",text:"text/plain",json:"application/json, text/javascript","*":bW},contents:{xml:/xml/,html:/html/,json:/json/},responseFields:{xml:"responseXML",text:"responseText"},converters:{"* text":a.String,"text html":!0,"text json":f.parseJSON,"text xml":f.parseXML},flatOptions:{context:!0,url:!0}},ajaxPrefilter:bY(bS),ajaxTransport:bY(bT),ajax:function(a,c){function w(a,c,l,m){if(s!==2){s=2,q&&clearTimeout(q),p=b,n=m||"",v.readyState=a>0?4:0;var o,r,u,w=c,x=l?ca(d,v,l):b,y,z;if(a>=200&&a<300||a===304){if(d.ifModified){if(y=v.getResponseHeader("Last-Modified"))f.lastModified[k]=y;if(z=v.getResponseHeader("Etag"))f.etag[k]=z}if(a===304)w="notmodified",o=!0;else try{r=cb(d,x),w="success",o=!0}catch(A){w="parsererror",u=A}}else{u=w;if(!w||a)w="error",a<0&&(a=0)}v.status=a,v.statusText=""+(c||w),o?h.resolveWith(e,[r,w,v]):h.rejectWith(e,[v,w,u]),v.statusCode(j),j=b,t&&g.trigger("ajax"+(o?"Success":"Error"),[v,d,o?r:u]),i.fireWith(e,[v,w]),t&&(g.trigger("ajaxComplete",[v,d]),--f.active||f.event.trigger("ajaxStop"))}}typeof a=="object"&&(c=a,a=b),c=c||{};var d=f.ajaxSetup({},c),e=d.context||d,g=e!==d&&(e.nodeType||e instanceof f)?f(e):f.event,h=f.Deferred(),i=f.Callbacks("once memory"),j=d.statusCode||{},k,l={},m={},n,o,p,q,r,s=0,t,u,v={readyState:0,setRequestHeader:function(a,b){if(!s){var c=a.toLowerCase();a=m[c]=m[c]||a,l[a]=b}return this},getAllResponseHeaders:function(){return s===2?n:null},getResponseHeader:function(a){var c;if(s===2){if(!o){o={};while(c=bG.exec(n))o[c[1].toLowerCase()]=c[2]}c=o[a.toLowerCase()]}return c===b?null:c},overrideMimeType:function(a){s||(d.mimeType=a);return this},abort:function(a){a=a||"abort",p&&p.abort(a),w(0,a);return this}};h.promise(v),v.success=v.done,v.error=v.fail,v.complete=i.add,v.statusCode=function(a){if(a){var b;if(s<2)for(b in a)j[b]=[j[b],a[b]];else b=a[v.status],v.then(b,b)}return this},d.url=((a||d.url)+"").replace(bF,"").replace(bK,bV[1]+"//"),d.dataTypes=f.trim(d.dataType||"*").toLowerCase().split(bO),d.crossDomain==null&&(r=bQ.exec(d.url.toLowerCase()),d.crossDomain=!(!r||r[1]==bV[1]&&r[2]==bV[2]&&(r[3]||(r[1]==="http:"?80:443))==(bV[3]||(bV[1]==="http:"?80:443)))),d.data&&d.processData&&typeof d.data!="string"&&(d.data=f.param(d.data,d.traditional)),bZ(bS,d,c,v);if(s===2)return!1;t=d.global,d.type=d.type.toUpperCase(),d.hasContent=!bJ.test(d.type),t&&f.active++===0&&f.event.trigger("ajaxStart");if(!d.hasContent){d.data&&(d.url+=(bL.test(d.url)?"&":"?")+d.data,delete d.data),k=d.url;if(d.cache===!1){var x=f.now(),y=d.url.replace(bP,"$1_="+x);d.url=y+(y===d.url?(bL.test(d.url)?"&":"?")+"_="+x:"")}}(d.data&&d.hasContent&&d.contentType!==!1||c.contentType)&&v.setRequestHeader("Content-Type",d.contentType),d.ifModified&&(k=k||d.url,f.lastModified[k]&&v.setRequestHeader("If-Modified-Since",f.lastModified[k]),f.etag[k]&&v.setRequestHeader("If-None-Match",f.etag[k])),v.setRequestHeader("Accept",d.dataTypes[0]&&d.accepts[d.dataTypes[0]]?d.accepts[d.dataTypes[0]]+(d.dataTypes[0]!=="*"?", "+bW+"; q=0.01":""):d.accepts["*"]);for(u in d.headers)v.setRequestHeader(u,d.headers[u]);if(d.beforeSend&&(d.beforeSend.call(e,v,d)===!1||s===2)){v.abort();return!1}for(u in{success:1,error:1,complete:1})v[u](d[u]);p=bZ(bT,d,c,v);if(!p)w(-1,"No Transport");else{v.readyState=1,t&&g.trigger("ajaxSend",[v,d]),d.async&&d.timeout>0&&(q=setTimeout(function(){v.abort("timeout")},d.timeout));try{s=1,p.send(l,w)}catch(z){if(s<2)w(-1,z);else throw z}}return v},param:function(a,c){var d=[],e=function(a,b){b=f.isFunction(b)?b():b,d[d.length]=encodeURIComponent(a)+"="+encodeURIComponent(b)};c===b&&(c=f.ajaxSettings.traditional);if(f.isArray(a)||a.jquery&&!f.isPlainObject(a))f.each(a,function(){e(this.name,this.value)});else for(var g in a)b_(g,a[g],c,e);return d.join("&").replace(bC,"+")}}),f.extend({active:0,lastModified:{},etag:{}});var cc=f.now(),cd=/(\=)\?(&|$)|\?\?/i;f.ajaxSetup({jsonp:"callback",jsonpCallback:function(){return f.expando+"_"+cc++}}),f.ajaxPrefilter("json jsonp",function(b,c,d){var e=typeof b.data=="string"&&/^application\/x\-www\-form\-urlencoded/.test(b.contentType);if(b.dataTypes[0]==="jsonp"||b.jsonp!==!1&&(cd.test(b.url)||e&&cd.test(b.data))){var g,h=b.jsonpCallback=f.isFunction(b.jsonpCallback)?b.jsonpCallback():b.jsonpCallback,i=a[h],j=b.url,k=b.data,l="$1"+h+"$2";b.jsonp!==!1&&(j=j.replace(cd,l),b.url===j&&(e&&(k=k.replace(cd,l)),b.data===k&&(j+=(/\?/.test(j)?"&":"?")+b.jsonp+"="+h))),b.url=j,b.data=k,a[h]=function(a){g=[a]},d.always(function(){a[h]=i,g&&f.isFunction(i)&&a[h](g[0])}),b.converters["script json"]=function(){g||f.error(h+" was not called");return g[0]},b.dataTypes[0]="json";return"script"}}),f.ajaxSetup({accepts:{script:"text/javascript, application/javascript, application/ecmascript, application/x-ecmascript"},contents:{script:/javascript|ecmascript/},converters:{"text script":function(a){f.globalEval(a);return a}}}),f.ajaxPrefilter("script",function(a){a.cache===b&&(a.cache=!1),a.crossDomain&&(a.type="GET",a.global=!1)}),f.ajaxTransport("script",function(a){if(a.crossDomain){var d,e=c.head||c.getElementsByTagName("head")[0]||c.documentElement;return{send:function(f,g){d=c.createElement("script"),d.async="async",a.scriptCharset&&(d.charset=a.scriptCharset),d.src=a.url,d.onload=d.onreadystatechange=function(a,c){if(c||!d.readyState||/loaded|complete/.test(d.readyState))d.onload=d.onreadystatechange=null,e&&d.parentNode&&e.removeChild(d),d=b,c||g(200,"success")},e.insertBefore(d,e.firstChild)},abort:function(){d&&d.onload(0,1)}}}});var ce=a.ActiveXObject?function(){for(var a in cg)cg[a](0,1)}:!1,cf=0,cg;f.ajaxSettings.xhr=a.ActiveXObject?function(){return!this.isLocal&&ch()||ci()}:ch,function(a){f.extend(f.support,{ajax:!!a,cors:!!a&&"withCredentials"in a})}(f.ajaxSettings.xhr()),f.support.ajax&&f.ajaxTransport(function(c){if(!c.crossDomain||f.support.cors){var d;return{send:function(e,g){var h=c.xhr(),i,j;c.username?h.open(c.type,c.url,c.async,c.username,c.password):h.open(c.type,c.url,c.async);if(c.xhrFields)for(j in c.xhrFields)h[j]=c.xhrFields[j];c.mimeType&&h.overrideMimeType&&h.overrideMimeType(c.mimeType),!c.crossDomain&&!e["X-Requested-With"]&&(e["X-Requested-With"]="XMLHttpRequest");try{for(j in e)h.setRequestHeader(j,e[j])}catch(k){}h.send(c.hasContent&&c.data||null),d=function(a,e){var j,k,l,m,n;try{if(d&&(e||h.readyState===4)){d=b,i&&(h.onreadystatechange=f.noop,ce&&delete cg[i]);if(e)h.readyState!==4&&h.abort();else{j=h.status,l=h.getAllResponseHeaders(),m={},n=h.responseXML,n&&n.documentElement&&(m.xml=n);try{m.text=h.responseText}catch(a){}try{k=h.statusText}catch(o){k=""}!j&&c.isLocal&&!c.crossDomain?j=m.text?200:404:j===1223&&(j=204)}}}catch(p){e||g(-1,p)}m&&g(j,k,m,l)},!c.async||h.readyState===4?d():(i=++cf,ce&&(cg||(cg={},f(a).unload(ce)),cg[i]=d),h.onreadystatechange=d)},abort:function(){d&&d(0,1)}}}});var cj={},ck,cl,cm=/^(?:toggle|show|hide)$/,cn=/^([+\-]=)?([\d+.\-]+)([a-z%]*)$/i,co,cp=[["height","marginTop","marginBottom","paddingTop","paddingBottom"],["width","marginLeft","marginRight","paddingLeft","paddingRight"],["opacity"]],cq;f.fn.extend({show:function(a,b,c){var d,e;if(a||a===0)return this.animate(ct("show",3),a,b,c);for(var g=0,h=this.length;g<h;g++)d=this[g],d.style&&(e=d.style.display,!f._data(d,"olddisplay")&&e==="none"&&(e=d.style.display=""),(e===""&&f.css(d,"display")==="none"||!f.contains(d.ownerDocument.documentElement,d))&&f._data(d,"olddisplay",cu(d.nodeName)));for(g=0;g<h;g++){d=this[g];if(d.style){e=d.style.display;if(e===""||e==="none")d.style.display=f._data(d,"olddisplay")||""}}return this},hide:function(a,b,c){if(a||a===0)return this.animate(ct("hide",3),a,b,c);var d,e,g=0,h=this.length;for(;g<h;g++)d=this[g],d.style&&(e=f.css(d,"display"),e!=="none"&&!f._data(d,"olddisplay")&&f._data(d,"olddisplay",e));for(g=0;g<h;g++)this[g].style&&(this[g].style.display="none");return this},_toggle:f.fn.toggle,toggle:function(a,b,c){var d=typeof a=="boolean";f.isFunction(a)&&f.isFunction(b)?this._toggle.apply(this,arguments):a==null||d?this.each(function(){var b=d?a:f(this).is(":hidden");f(this)[b?"show":"hide"]()}):this.animate(ct("toggle",3),a,b,c);return this},fadeTo:function(a,b,c,d){return this.filter(":hidden").css("opacity",0).show().end().animate({opacity:b},a,c,d)},animate:function(a,b,c,d){function g(){e.queue===!1&&f._mark(this);var b=f.extend({},e),c=this.nodeType===1,d=c&&f(this).is(":hidden"),g,h,i,j,k,l,m,n,o,p,q;b.animatedProperties={};for(i in a){g=f.camelCase(i),i!==g&&(a[g]=a[i],delete a[i]);if((k=f.cssHooks[g])&&"expand"in k){l=k.expand(a[g]),delete a[g];for(i in l)i in a||(a[i]=l[i])}}for(g in a){h=a[g],f.isArray(h)?(b.animatedProperties[g]=h[1],h=a[g]=h[0]):b.animatedProperties[g]=b.specialEasing&&b.specialEasing[g]||b.easing||"swing";if(h==="hide"&&d||h==="show"&&!d)return b.complete.call(this);c&&(g==="height"||g==="width")&&(b.overflow=[this.style.overflow,this.style.overflowX,this.style.overflowY],f.css(this,"display")==="inline"&&f.css(this,"float")==="none"&&(!f.support.inlineBlockNeedsLayout||cu(this.nodeName)==="inline"?this.style.display="inline-block":this.style.zoom=1))}b.overflow!=null&&(this.style.overflow="hidden");for(i in a)j=new f.fx(this,b,i),h=a[i],cm.test(h)?(q=f._data(this,"toggle"+i)||(h==="toggle"?d?"show":"hide":0),q?(f._data(this,"toggle"+i,q==="show"?"hide":"show"),j[q]()):j[h]()):(m=cn.exec(h),n=j.cur(),m?(o=parseFloat(m[2]),p=m[3]||(f.cssNumber[i]?"":"px"),p!=="px"&&(f.style(this,i,(o||1)+p),n=(o||1)/j.cur()*n,f.style(this,i,n+p)),m[1]&&(o=(m[1]==="-="?-1:1)*o+n),j.custom(n,o,p)):j.custom(n,h,""));return!0}var e=f.speed(b,c,d);if(f.isEmptyObject(a))return this.each(e.complete,[!1]);a=f.extend({},a);return e.queue===!1?this.each(g):this.queue(e.queue,g)},stop:function(a,c,d){typeof a!="string"&&(d=c,c=a,a=b),c&&a!==!1&&this.queue(a||"fx",[]);return this.each(function(){function h(a,b,c){var e=b[c];f.removeData(a,c,!0),e.stop(d)}var b,c=!1,e=f.timers,g=f._data(this);d||f._unmark(!0,this);if(a==null)for(b in g)g[b]&&g[b].stop&&b.indexOf(".run")===b.length-4&&h(this,g,b);else g[b=a+".run"]&&g[b].stop&&h(this,g,b);for(b=e.length;b--;)e[b].elem===this&&(a==null||e[b].queue===a)&&(d?e[b](!0):e[b].saveState(),c=!0,e.splice(b,1));(!d||!c)&&f.dequeue(this,a)})}}),f.each({slideDown:ct("show",1),slideUp:ct("hide",1),slideToggle:ct("toggle",1),fadeIn:{opacity:"show"},fadeOut:{opacity:"hide"},fadeToggle:{opacity:"toggle"}},function(a,b){f.fn[a]=function(a,c,d){return this.animate(b,a,c,d)}}),f.extend({speed:function(a,b,c){var d=a&&typeof a=="object"?f.extend({},a):{complete:c||!c&&b||f.isFunction(a)&&a,duration:a,easing:c&&b||b&&!f.isFunction(b)&&b};d.duration=f.fx.off?0:typeof d.duration=="number"?d.duration:d.duration in f.fx.speeds?f.fx.speeds[d.duration]:f.fx.speeds._default;if(d.queue==null||d.queue===!0)d.queue="fx";d.old=d.complete,d.complete=function(a){f.isFunction(d.old)&&d.old.call(this),d.queue?f.dequeue(this,d.queue):a!==!1&&f._unmark(this)};return d},easing:{linear:function(a){return a},swing:function(a){return-Math.cos(a*Math.PI)/2+.5}},timers:[],fx:function(a,b,c){this.options=b,this.elem=a,this.prop=c,b.orig=b.orig||{}}}),f.fx.prototype={update:function(){this.options.step&&this.options.step.call(this.elem,this.now,this),(f.fx.step[this.prop]||f.fx.step._default)(this)},cur:function(){if(this.elem[this.prop]!=null&&(!this.elem.style||this.elem.style[this.prop]==null))return this.elem[this.prop];var a,b=f.css(this.elem,this.prop);return isNaN(a=parseFloat(b))?!b||b==="auto"?0:b:a},custom:function(a,c,d){function h(a){return e.step(a)}var e=this,g=f.fx;this.startTime=cq||cr(),this.end=c,this.now=this.start=a,this.pos=this.state=0,this.unit=d||this.unit||(f.cssNumber[this.prop]?"":"px"),h.queue=this.options.queue,h.elem=this.elem,h.saveState=function(){f._data(e.elem,"fxshow"+e.prop)===b&&(e.options.hide?f._data(e.elem,"fxshow"+e.prop,e.start):e.options.show&&f._data(e.elem,"fxshow"+e.prop,e.end))},h()&&f.timers.push(h)&&!co&&(co=setInterval(g.tick,g.interval))},show:function(){var a=f._data(this.elem,"fxshow"+this.prop);this.options.orig[this.prop]=a||f.style(this.elem,this.prop),this.options.show=!0,a!==b?this.custom(this.cur(),a):this.custom(this.prop==="width"||this.prop==="height"?1:0,this.cur()),f(this.elem).show()},hide:function(){this.options.orig[this.prop]=f._data(this.elem,"fxshow"+this.prop)||f.style(this.elem,this.prop),this.options.hide=!0,this.custom(this.cur(),0)},step:function(a){var b,c,d,e=cq||cr(),g=!0,h=this.elem,i=this.options;if(a||e>=i.duration+this.startTime){this.now=this.end,this.pos=this.state=1,this.update(),i.animatedProperties[this.prop]=!0;for(b in i.animatedProperties)i.animatedProperties[b]!==!0&&(g=!1);if(g){i.overflow!=null&&!f.support.shrinkWrapBlocks&&f.each(["","X","Y"],function(a,b){h.style["overflow"+b]=i.overflow[a]}),i.hide&&f(h).hide();if(i.hide||i.show)for(b in i.animatedProperties)f.style(h,b,i.orig[b]),f.removeData(h,"fxshow"+b,!0),f.removeData(h,"toggle"+b,!0);d=i.complete,d&&(i.complete=!1,d.call(h))}return!1}i.duration==Infinity?this.now=e:(c=e-this.startTime,this.state=c/i.duration,this.pos=f.easing[i.animatedProperties[this.prop]](this.state,c,0,1,i.duration),this.now=this.start+(this.end-this.start)*this.pos),this.update();return!0}},f.extend(f.fx,{tick:function(){var a,b=f.timers,c=0;for(;c<b.length;c++)a=b[c],!a()&&b[c]===a&&b.splice(c--,1);b.length||f.fx.stop()},interval:13,stop:function(){clearInterval(co),co=null},speeds:{slow:600,fast:200,_default:400},step:{opacity:function(a){f.style(a.elem,"opacity",a.now)},_default:function(a){a.elem.style&&a.elem.style[a.prop]!=null?a.elem.style[a.prop]=a.now+a.unit:a.elem[a.prop]=a.now}}}),f.each(cp.concat.apply([],cp),function(a,b){b.indexOf("margin")&&(f.fx.step[b]=function(a){f.style(a.elem,b,Math.max(0,a.now)+a.unit)})}),f.expr&&f.expr.filters&&(f.expr.filters.animated=function(a){return f.grep(f.timers,function(b){return a===b.elem}).length});var cv,cw=/^t(?:able|d|h)$/i,cx=/^(?:body|html)$/i;"getBoundingClientRect"in c.documentElement?cv=function(a,b,c,d){try{d=a.getBoundingClientRect()}catch(e){}if(!d||!f.contains(c,a))return d?{top:d.top,left:d.left}:{top:0,left:0};var g=b.body,h=cy(b),i=c.clientTop||g.clientTop||0,j=c.clientLeft||g.clientLeft||0,k=h.pageYOffset||f.support.boxModel&&c.scrollTop||g.scrollTop,l=h.pageXOffset||f.support.boxModel&&c.scrollLeft||g.scrollLeft,m=d.top+k-i,n=d.left+l-j;return{top:m,left:n}}:cv=function(a,b,c){var d,e=a.offsetParent,g=a,h=b.body,i=b.defaultView,j=i?i.getComputedStyle(a,null):a.currentStyle,k=a.offsetTop,l=a.offsetLeft;while((a=a.parentNode)&&a!==h&&a!==c){if(f.support.fixedPosition&&j.position==="fixed")break;d=i?i.getComputedStyle(a,null):a.currentStyle,k-=a.scrollTop,l-=a.scrollLeft,a===e&&(k+=a.offsetTop,l+=a.offsetLeft,f.support.doesNotAddBorder&&(!f.support.doesAddBorderForTableAndCells||!cw.test(a.nodeName))&&(k+=parseFloat(d.borderTopWidth)||0,l+=parseFloat(d.borderLeftWidth)||0),g=e,e=a.offsetParent),f.support.subtractsBorderForOverflowNotVisible&&d.overflow!=="visible"&&(k+=parseFloat(d.borderTopWidth)||0,l+=parseFloat(d.borderLeftWidth)||0),j=d}if(j.position==="relative"||j.position==="static")k+=h.offsetTop,l+=h.offsetLeft;f.support.fixedPosition&&j.position==="fixed"&&(k+=Math.max(c.scrollTop,h.scrollTop),l+=Math.max(c.scrollLeft,h.scrollLeft));return{top:k,left:l}},f.fn.offset=function(a){if(arguments.length)return a===b?this:this.each(function(b){f.offset.setOffset(this,a,b)});var c=this[0],d=c&&c.ownerDocument;if(!d)return null;if(c===d.body)return f.offset.bodyOffset(c);return cv(c,d,d.documentElement)},f.offset={bodyOffset:function(a){var b=a.offsetTop,c=a.offsetLeft;f.support.doesNotIncludeMarginInBodyOffset&&(b+=parseFloat(f.css(a,"marginTop"))||0,c+=parseFloat(f.css(a,"marginLeft"))||0);return{top:b,left:c}},setOffset:function(a,b,c){var d=f.css(a,"position");d==="static"&&(a.style.position="relative");var e=f(a),g=e.offset(),h=f.css(a,"top"),i=f.css(a,"left"),j=(d==="absolute"||d==="fixed")&&f.inArray("auto",[h,i])>-1,k={},l={},m,n;j?(l=e.position(),m=l.top,n=l.left):(m=parseFloat(h)||0,n=parseFloat(i)||0),f.isFunction(b)&&(b=b.call(a,c,g)),b.top!=null&&(k.top=b.top-g.top+m),b.left!=null&&(k.left=b.left-g.left+n),"using"in b?b.using.call(a,k):e.css(k)}},f.fn.extend({position:function(){if(!this[0])return null;var a=this[0],b=this.offsetParent(),c=this.offset(),d=cx.test(b[0].nodeName)?{top:0,left:0}:b.offset();c.top-=parseFloat(f.css(a,"marginTop"))||0,c.left-=parseFloat(f.css(a,"marginLeft"))||0,d.top+=parseFloat(f.css(b[0],"borderTopWidth"))||0,d.left+=parseFloat(f.css(b[0],"borderLeftWidth"))||0;return{top:c.top-d.top,left:c.left-d.left}},offsetParent:function(){return this.map(function(){var a=this.offsetParent||c.body;while(a&&!cx.test(a.nodeName)&&f.css(a,"position")==="static")a=a.offsetParent;return a})}}),f.each({scrollLeft:"pageXOffset",scrollTop:"pageYOffset"},function(a,c){var d=/Y/.test(c);f.fn[a]=function(e){return f.access(this,function(a,e,g){var h=cy(a);if(g===b)return h?c in h?h[c]:f.support.boxModel&&h.document.documentElement[e]||h.document.body[e]:a[e];h?h.scrollTo(d?f(h).scrollLeft():g,d?g:f(h).scrollTop()):a[e]=g},a,e,arguments.length,null)}}),f.each({Height:"height",Width:"width"},function(a,c){var d="client"+a,e="scroll"+a,g="offset"+a;f.fn["inner"+a]=function(){var a=this[0];return a?a.style?parseFloat(f.css(a,c,"padding")):this[c]():null},f.fn["outer"+a]=function(a){var b=this[0];return b?b.style?parseFloat(f.css(b,c,a?"margin":"border")):this[c]():null},f.fn[c]=function(a){return f.access(this,function(a,c,h){var i,j,k,l;if(f.isWindow(a)){i=a.document,j=i.documentElement[d];return f.support.boxModel&&j||i.body&&i.body[d]||j}if(a.nodeType===9){i=a.documentElement;if(i[d]>=i[e])return i[d];return Math.max(a.body[e],i[e],a.body[g],i[g])}if(h===b){k=f.css(a,c),l=parseFloat(k);return f.isNumeric(l)?l:k}f(a).css(c,h)},c,a,arguments.length,null)}}),a.jQuery=a.$=f,typeof define=="function"&&define.amd&&define.amd.jQuery&&define("jquery",[],function(){return f})})(window); |
src/renderers/dom/shared/__tests__/ReactDOMTextComponent-test.js | stevemao/react | /**
* Copyright 2013-2015, Facebook, Inc.
* All rights reserved.
*
* This source code is licensed under the BSD-style license found in the
* LICENSE file in the root directory of this source tree. An additional grant
* of patent rights can be found in the PATENTS file in the same directory.
*
* @emails react-core
*/
'use strict';
var React;
var ReactDOM;
var ReactDOMServer;
describe('ReactDOMTextComponent', function() {
beforeEach(function() {
React = require('React');
ReactDOM = require('ReactDOM');
ReactDOMServer = require('ReactDOMServer');
});
it('should escape the rootID', function() {
var ThisThingShouldBeEscaped = '">>> LULZ <<<"';
var ThisThingWasBeEscaped = '">>> LULZ <<<"';
var thing = <div><span key={ThisThingShouldBeEscaped}>LULZ</span></div>;
var html = ReactDOMServer.renderToString(thing);
expect(html).not.toContain(ThisThingShouldBeEscaped);
expect(html).toContain(ThisThingWasBeEscaped);
});
it('updates a mounted text component in place', function() {
var el = document.createElement('div');
var inst = ReactDOM.render(<div>{'foo'}{'bar'}</div>, el);
var foo = ReactDOM.findDOMNode(inst).children[0];
var bar = ReactDOM.findDOMNode(inst).children[1];
expect(foo.tagName).toBe('SPAN');
expect(bar.tagName).toBe('SPAN');
inst = ReactDOM.render(<div>{'baz'}{'qux'}</div>, el);
// After the update, the spans should have stayed in place (as opposed to
// getting unmounted and remounted)
expect(ReactDOM.findDOMNode(inst).children[0]).toBe(foo);
expect(ReactDOM.findDOMNode(inst).children[1]).toBe(bar);
});
});
|
src/components/Registration.js | apasashkov/treasy-client | import React, { Component } from 'react';
import Modal from './Modal';
import SignUpForm from './SignUpForm';
class Registration extends Component {
render() {
return (
<Modal id="RegistrationForm--Modal">
<h2> Sign Up! </h2>
<SignUpForm />
</Modal>
);
}
}
export default Registration; |
src/js/components/share/linkedin-share-link.js | navidonskis/silverstripe-react-theme | import React from 'react';
import ShareLink from './share-link';
class LinkedInShareLink extends ShareLink {
shareUrl() {
let title = decodeURIComponent(this.getWindowTitle());
let currentLink = this.state.currentLink;
return `https://twitter.com/intent/tweet?text=${title}&url=${currentLink}`;
}
render() {
return (
<a className={'twitter ' + this.props.className} href={this.state.currentLink}
onClick={event => this.onClick(event)}>
<svg className="icon fill small default">
<use xlinkHref="#twitter"></use>
</svg>
</a>
);
}
}
export default LinkedInShareLink; |
src/components/routes/user/login.js | fredmarques/petshop | import './User.css';
import React, { Component } from 'react';
import { Field, reduxForm } from 'redux-form';
import { Link } from 'react-router-dom';
import { connect } from 'react-redux';
import { loginAdmin, loginUser } from '../../../actions/login';
import { loginStatus, loginMode } from '../../../reducers/login';
class Login extends Component {
renderField(field) {
const { meta: { touched, error } } = field;
const className = '';
return (
<div className={className}>
<input className="form-control"
type={field.type}
placeholder={field.placeholder}
{...field.input} />
<div className="text-help">
{touched ? error : ''}
</div>
</div>
);
}
onSubmit(values) {
if (values.email === 'admin' && values.password === 'admin') {
this.props.loginAdmin(values.email, values.password);
} else {
this.props.loginUser(values.email, values.password);
}
values.email = '';
values.password = '';
}
render() {
const { handleSubmit } = this.props;
return (
<div className={'loginForm'}>
<h3>Acesse sua conta</h3>
<form onSubmit={handleSubmit(this.onSubmit.bind(this))} className={'form-inline'}>
<Field
name="email"
label="Email"
placeholder="E-mail"
type="text"
component={this.renderField}
/>
<Field
name="password"
label="Password"
placeholder="Senha"
type="password"
component={this.renderField}
/>
<button type="submit" className="btn btn-primary">Entrar</button>
<Link to="/" className="btn btn-danger">Cancelar</Link>
<br/>
<p> Não possui cadastro ainda? </p>
<Link to="/logon" className="btn btn-primary">Cadastrar</Link>
</form>
</div>
);
}
}
const mapStateToProps = (state) => {
return {
status: loginStatus(state),
mode: loginMode(state)
}
};
export default reduxForm({
form: 'Login'
})(
connect(mapStateToProps, { loginAdmin, loginUser })(Login)
); |
src/index.js | taylordevereaux/ppu-compare | import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
// Css
import '../node_modules/bootstrap/dist/css/bootstrap.css';
import './index.css';
import './theme/dist/toolkit-inverse.css';
// Utils
import registerServiceWorker from './utilities/registerServiceWorker';
// Javascript
import jquery from "jquery";
import popper from 'popper.js';
import tether from 'tether';
// import theme from './theme/dist/toolkit.js';
/* eslint-disable */
var jQuery = window.$ = window.jQuery = jquery;
var Popper = window.Popper = popper;
var Tether = window.Tether = tether;
var theme = require('./theme/dist/toolkit.js');
/* eslint-enable */
ReactDOM.render(<App />, document.getElementById('root'));
registerServiceWorker(); |
lib/sparks/generators/template/vendor/assets/javascripts/polymer-all/platform/tools/doc/themes/footstrap/assets/vendor/jquery/jquery-1.8.2.min.js | revans/sparks | /*! jQuery v1.8.2 jquery.com | jquery.org/license */
(function(a,b){function G(a){var b=F[a]={};return p.each(a.split(s),function(a,c){b[c]=!0}),b}function J(a,c,d){if(d===b&&a.nodeType===1){var e="data-"+c.replace(I,"-$1").toLowerCase();d=a.getAttribute(e);if(typeof d=="string"){try{d=d==="true"?!0:d==="false"?!1:d==="null"?null:+d+""===d?+d:H.test(d)?p.parseJSON(d):d}catch(f){}p.data(a,c,d)}else d=b}return d}function K(a){var b;for(b in a){if(b==="data"&&p.isEmptyObject(a[b]))continue;if(b!=="toJSON")return!1}return!0}function ba(){return!1}function bb(){return!0}function bh(a){return!a||!a.parentNode||a.parentNode.nodeType===11}function bi(a,b){do a=a[b];while(a&&a.nodeType!==1);return a}function bj(a,b,c){b=b||0;if(p.isFunction(b))return p.grep(a,function(a,d){var e=!!b.call(a,d,a);return e===c});if(b.nodeType)return p.grep(a,function(a,d){return a===b===c});if(typeof b=="string"){var d=p.grep(a,function(a){return a.nodeType===1});if(be.test(b))return p.filter(b,d,!c);b=p.filter(b,d)}return p.grep(a,function(a,d){return p.inArray(a,b)>=0===c})}function bk(a){var b=bl.split("|"),c=a.createDocumentFragment();if(c.createElement)while(b.length)c.createElement(b.pop());return c}function bC(a,b){return a.getElementsByTagName(b)[0]||a.appendChild(a.ownerDocument.createElement(b))}function bD(a,b){if(b.nodeType!==1||!p.hasData(a))return;var c,d,e,f=p._data(a),g=p._data(b,f),h=f.events;if(h){delete g.handle,g.events={};for(c in h)for(d=0,e=h[c].length;d<e;d++)p.event.add(b,c,h[c][d])}g.data&&(g.data=p.extend({},g.data))}function bE(a,b){var c;if(b.nodeType!==1)return;b.clearAttributes&&b.clearAttributes(),b.mergeAttributes&&b.mergeAttributes(a),c=b.nodeName.toLowerCase(),c==="object"?(b.parentNode&&(b.outerHTML=a.outerHTML),p.support.html5Clone&&a.innerHTML&&!p.trim(b.innerHTML)&&(b.innerHTML=a.innerHTML)):c==="input"&&bv.test(a.type)?(b.defaultChecked=b.checked=a.checked,b.value!==a.value&&(b.value=a.value)):c==="option"?b.selected=a.defaultSelected:c==="input"||c==="textarea"?b.defaultValue=a.defaultValue:c==="script"&&b.text!==a.text&&(b.text=a.text),b.removeAttribute(p.expando)}function bF(a){return typeof a.getElementsByTagName!="undefined"?a.getElementsByTagName("*"):typeof a.querySelectorAll!="undefined"?a.querySelectorAll("*"):[]}function bG(a){bv.test(a.type)&&(a.defaultChecked=a.checked)}function bY(a,b){if(b in a)return b;var c=b.charAt(0).toUpperCase()+b.slice(1),d=b,e=bW.length;while(e--){b=bW[e]+c;if(b in a)return b}return d}function bZ(a,b){return a=b||a,p.css(a,"display")==="none"||!p.contains(a.ownerDocument,a)}function b$(a,b){var c,d,e=[],f=0,g=a.length;for(;f<g;f++){c=a[f];if(!c.style)continue;e[f]=p._data(c,"olddisplay"),b?(!e[f]&&c.style.display==="none"&&(c.style.display=""),c.style.display===""&&bZ(c)&&(e[f]=p._data(c,"olddisplay",cc(c.nodeName)))):(d=bH(c,"display"),!e[f]&&d!=="none"&&p._data(c,"olddisplay",d))}for(f=0;f<g;f++){c=a[f];if(!c.style)continue;if(!b||c.style.display==="none"||c.style.display==="")c.style.display=b?e[f]||"":"none"}return a}function b_(a,b,c){var d=bP.exec(b);return d?Math.max(0,d[1]-(c||0))+(d[2]||"px"):b}function ca(a,b,c,d){var e=c===(d?"border":"content")?4:b==="width"?1:0,f=0;for(;e<4;e+=2)c==="margin"&&(f+=p.css(a,c+bV[e],!0)),d?(c==="content"&&(f-=parseFloat(bH(a,"padding"+bV[e]))||0),c!=="margin"&&(f-=parseFloat(bH(a,"border"+bV[e]+"Width"))||0)):(f+=parseFloat(bH(a,"padding"+bV[e]))||0,c!=="padding"&&(f+=parseFloat(bH(a,"border"+bV[e]+"Width"))||0));return f}function cb(a,b,c){var d=b==="width"?a.offsetWidth:a.offsetHeight,e=!0,f=p.support.boxSizing&&p.css(a,"boxSizing")==="border-box";if(d<=0||d==null){d=bH(a,b);if(d<0||d==null)d=a.style[b];if(bQ.test(d))return d;e=f&&(p.support.boxSizingReliable||d===a.style[b]),d=parseFloat(d)||0}return d+ca(a,b,c||(f?"border":"content"),e)+"px"}function cc(a){if(bS[a])return bS[a];var b=p("<"+a+">").appendTo(e.body),c=b.css("display");b.remove();if(c==="none"||c===""){bI=e.body.appendChild(bI||p.extend(e.createElement("iframe"),{frameBorder:0,width:0,height:0}));if(!bJ||!bI.createElement)bJ=(bI.contentWindow||bI.contentDocument).document,bJ.write("<!doctype html><html><body>"),bJ.close();b=bJ.body.appendChild(bJ.createElement(a)),c=bH(b,"display"),e.body.removeChild(bI)}return bS[a]=c,c}function ci(a,b,c,d){var e;if(p.isArray(b))p.each(b,function(b,e){c||ce.test(a)?d(a,e):ci(a+"["+(typeof e=="object"?b:"")+"]",e,c,d)});else if(!c&&p.type(b)==="object")for(e in b)ci(a+"["+e+"]",b[e],c,d);else d(a,b)}function cz(a){return function(b,c){typeof b!="string"&&(c=b,b="*");var d,e,f,g=b.toLowerCase().split(s),h=0,i=g.length;if(p.isFunction(c))for(;h<i;h++)d=g[h],f=/^\+/.test(d),f&&(d=d.substr(1)||"*"),e=a[d]=a[d]||[],e[f?"unshift":"push"](c)}}function cA(a,c,d,e,f,g){f=f||c.dataTypes[0],g=g||{},g[f]=!0;var h,i=a[f],j=0,k=i?i.length:0,l=a===cv;for(;j<k&&(l||!h);j++)h=i[j](c,d,e),typeof h=="string"&&(!l||g[h]?h=b:(c.dataTypes.unshift(h),h=cA(a,c,d,e,h,g)));return(l||!h)&&!g["*"]&&(h=cA(a,c,d,e,"*",g)),h}function cB(a,c){var d,e,f=p.ajaxSettings.flatOptions||{};for(d in c)c[d]!==b&&((f[d]?a:e||(e={}))[d]=c[d]);e&&p.extend(!0,a,e)}function cC(a,c,d){var e,f,g,h,i=a.contents,j=a.dataTypes,k=a.responseFields;for(f in k)f in d&&(c[k[f]]=d[f]);while(j[0]==="*")j.shift(),e===b&&(e=a.mimeType||c.getResponseHeader("content-type"));if(e)for(f in i)if(i[f]&&i[f].test(e)){j.unshift(f);break}if(j[0]in d)g=j[0];else{for(f in d){if(!j[0]||a.converters[f+" "+j[0]]){g=f;break}h||(h=f)}g=g||h}if(g)return g!==j[0]&&j.unshift(g),d[g]}function cD(a,b){var c,d,e,f,g=a.dataTypes.slice(),h=g[0],i={},j=0;a.dataFilter&&(b=a.dataFilter(b,a.dataType));if(g[1])for(c in a.converters)i[c.toLowerCase()]=a.converters[c];for(;e=g[++j];)if(e!=="*"){if(h!=="*"&&h!==e){c=i[h+" "+e]||i["* "+e];if(!c)for(d in i){f=d.split(" ");if(f[1]===e){c=i[h+" "+f[0]]||i["* "+f[0]];if(c){c===!0?c=i[d]:i[d]!==!0&&(e=f[0],g.splice(j--,0,e));break}}}if(c!==!0)if(c&&a["throws"])b=c(b);else try{b=c(b)}catch(k){return{state:"parsererror",error:c?k:"No conversion from "+h+" to "+e}}}h=e}return{state:"success",data:b}}function cL(){try{return new a.XMLHttpRequest}catch(b){}}function cM(){try{return new a.ActiveXObject("Microsoft.XMLHTTP")}catch(b){}}function cU(){return setTimeout(function(){cN=b},0),cN=p.now()}function cV(a,b){p.each(b,function(b,c){var d=(cT[b]||[]).concat(cT["*"]),e=0,f=d.length;for(;e<f;e++)if(d[e].call(a,b,c))return})}function cW(a,b,c){var d,e=0,f=0,g=cS.length,h=p.Deferred().always(function(){delete i.elem}),i=function(){var b=cN||cU(),c=Math.max(0,j.startTime+j.duration-b),d=1-(c/j.duration||0),e=0,f=j.tweens.length;for(;e<f;e++)j.tweens[e].run(d);return h.notifyWith(a,[j,d,c]),d<1&&f?c:(h.resolveWith(a,[j]),!1)},j=h.promise({elem:a,props:p.extend({},b),opts:p.extend(!0,{specialEasing:{}},c),originalProperties:b,originalOptions:c,startTime:cN||cU(),duration:c.duration,tweens:[],createTween:function(b,c,d){var e=p.Tween(a,j.opts,b,c,j.opts.specialEasing[b]||j.opts.easing);return j.tweens.push(e),e},stop:function(b){var c=0,d=b?j.tweens.length:0;for(;c<d;c++)j.tweens[c].run(1);return b?h.resolveWith(a,[j,b]):h.rejectWith(a,[j,b]),this}}),k=j.props;cX(k,j.opts.specialEasing);for(;e<g;e++){d=cS[e].call(j,a,k,j.opts);if(d)return d}return cV(j,k),p.isFunction(j.opts.start)&&j.opts.start.call(a,j),p.fx.timer(p.extend(i,{anim:j,queue:j.opts.queue,elem:a})),j.progress(j.opts.progress).done(j.opts.done,j.opts.complete).fail(j.opts.fail).always(j.opts.always)}function cX(a,b){var c,d,e,f,g;for(c in a){d=p.camelCase(c),e=b[d],f=a[c],p.isArray(f)&&(e=f[1],f=a[c]=f[0]),c!==d&&(a[d]=f,delete a[c]),g=p.cssHooks[d];if(g&&"expand"in g){f=g.expand(f),delete a[d];for(c in f)c in a||(a[c]=f[c],b[c]=e)}else b[d]=e}}function cY(a,b,c){var d,e,f,g,h,i,j,k,l=this,m=a.style,n={},o=[],q=a.nodeType&&bZ(a);c.queue||(j=p._queueHooks(a,"fx"),j.unqueued==null&&(j.unqueued=0,k=j.empty.fire,j.empty.fire=function(){j.unqueued||k()}),j.unqueued++,l.always(function(){l.always(function(){j.unqueued--,p.queue(a,"fx").length||j.empty.fire()})})),a.nodeType===1&&("height"in b||"width"in b)&&(c.overflow=[m.overflow,m.overflowX,m.overflowY],p.css(a,"display")==="inline"&&p.css(a,"float")==="none"&&(!p.support.inlineBlockNeedsLayout||cc(a.nodeName)==="inline"?m.display="inline-block":m.zoom=1)),c.overflow&&(m.overflow="hidden",p.support.shrinkWrapBlocks||l.done(function(){m.overflow=c.overflow[0],m.overflowX=c.overflow[1],m.overflowY=c.overflow[2]}));for(d in b){f=b[d];if(cP.exec(f)){delete b[d];if(f===(q?"hide":"show"))continue;o.push(d)}}g=o.length;if(g){h=p._data(a,"fxshow")||p._data(a,"fxshow",{}),q?p(a).show():l.done(function(){p(a).hide()}),l.done(function(){var b;p.removeData(a,"fxshow",!0);for(b in n)p.style(a,b,n[b])});for(d=0;d<g;d++)e=o[d],i=l.createTween(e,q?h[e]:0),n[e]=h[e]||p.style(a,e),e in h||(h[e]=i.start,q&&(i.end=i.start,i.start=e==="width"||e==="height"?1:0))}}function cZ(a,b,c,d,e){return new cZ.prototype.init(a,b,c,d,e)}function c$(a,b){var c,d={height:a},e=0;b=b?1:0;for(;e<4;e+=2-b)c=bV[e],d["margin"+c]=d["padding"+c]=a;return b&&(d.opacity=d.width=a),d}function da(a){return p.isWindow(a)?a:a.nodeType===9?a.defaultView||a.parentWindow:!1}var c,d,e=a.document,f=a.location,g=a.navigator,h=a.jQuery,i=a.$,j=Array.prototype.push,k=Array.prototype.slice,l=Array.prototype.indexOf,m=Object.prototype.toString,n=Object.prototype.hasOwnProperty,o=String.prototype.trim,p=function(a,b){return new p.fn.init(a,b,c)},q=/[\-+]?(?:\d*\.|)\d+(?:[eE][\-+]?\d+|)/.source,r=/\S/,s=/\s+/,t=/^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g,u=/^(?:[^#<]*(<[\w\W]+>)[^>]*$|#([\w\-]*)$)/,v=/^<(\w+)\s*\/?>(?:<\/\1>|)$/,w=/^[\],:{}\s]*$/,x=/(?:^|:|,)(?:\s*\[)+/g,y=/\\(?:["\\\/bfnrt]|u[\da-fA-F]{4})/g,z=/"[^"\\\r\n]*"|true|false|null|-?(?:\d\d*\.|)\d+(?:[eE][\-+]?\d+|)/g,A=/^-ms-/,B=/-([\da-z])/gi,C=function(a,b){return(b+"").toUpperCase()},D=function(){e.addEventListener?(e.removeEventListener("DOMContentLoaded",D,!1),p.ready()):e.readyState==="complete"&&(e.detachEvent("onreadystatechange",D),p.ready())},E={};p.fn=p.prototype={constructor:p,init:function(a,c,d){var f,g,h,i;if(!a)return this;if(a.nodeType)return this.context=this[0]=a,this.length=1,this;if(typeof a=="string"){a.charAt(0)==="<"&&a.charAt(a.length-1)===">"&&a.length>=3?f=[null,a,null]:f=u.exec(a);if(f&&(f[1]||!c)){if(f[1])return c=c instanceof p?c[0]:c,i=c&&c.nodeType?c.ownerDocument||c:e,a=p.parseHTML(f[1],i,!0),v.test(f[1])&&p.isPlainObject(c)&&this.attr.call(a,c,!0),p.merge(this,a);g=e.getElementById(f[2]);if(g&&g.parentNode){if(g.id!==f[2])return d.find(a);this.length=1,this[0]=g}return this.context=e,this.selector=a,this}return!c||c.jquery?(c||d).find(a):this.constructor(c).find(a)}return p.isFunction(a)?d.ready(a):(a.selector!==b&&(this.selector=a.selector,this.context=a.context),p.makeArray(a,this))},selector:"",jquery:"1.8.2",length:0,size:function(){return this.length},toArray:function(){return k.call(this)},get:function(a){return a==null?this.toArray():a<0?this[this.length+a]:this[a]},pushStack:function(a,b,c){var d=p.merge(this.constructor(),a);return d.prevObject=this,d.context=this.context,b==="find"?d.selector=this.selector+(this.selector?" ":"")+c:b&&(d.selector=this.selector+"."+b+"("+c+")"),d},each:function(a,b){return p.each(this,a,b)},ready:function(a){return p.ready.promise().done(a),this},eq:function(a){return a=+a,a===-1?this.slice(a):this.slice(a,a+1)},first:function(){return this.eq(0)},last:function(){return this.eq(-1)},slice:function(){return this.pushStack(k.apply(this,arguments),"slice",k.call(arguments).join(","))},map:function(a){return this.pushStack(p.map(this,function(b,c){return a.call(b,c,b)}))},end:function(){return this.prevObject||this.constructor(null)},push:j,sort:[].sort,splice:[].splice},p.fn.init.prototype=p.fn,p.extend=p.fn.extend=function(){var a,c,d,e,f,g,h=arguments[0]||{},i=1,j=arguments.length,k=!1;typeof h=="boolean"&&(k=h,h=arguments[1]||{},i=2),typeof h!="object"&&!p.isFunction(h)&&(h={}),j===i&&(h=this,--i);for(;i<j;i++)if((a=arguments[i])!=null)for(c in a){d=h[c],e=a[c];if(h===e)continue;k&&e&&(p.isPlainObject(e)||(f=p.isArray(e)))?(f?(f=!1,g=d&&p.isArray(d)?d:[]):g=d&&p.isPlainObject(d)?d:{},h[c]=p.extend(k,g,e)):e!==b&&(h[c]=e)}return h},p.extend({noConflict:function(b){return a.$===p&&(a.$=i),b&&a.jQuery===p&&(a.jQuery=h),p},isReady:!1,readyWait:1,holdReady:function(a){a?p.readyWait++:p.ready(!0)},ready:function(a){if(a===!0?--p.readyWait:p.isReady)return;if(!e.body)return setTimeout(p.ready,1);p.isReady=!0;if(a!==!0&&--p.readyWait>0)return;d.resolveWith(e,[p]),p.fn.trigger&&p(e).trigger("ready").off("ready")},isFunction:function(a){return p.type(a)==="function"},isArray:Array.isArray||function(a){return p.type(a)==="array"},isWindow:function(a){return a!=null&&a==a.window},isNumeric:function(a){return!isNaN(parseFloat(a))&&isFinite(a)},type:function(a){return a==null?String(a):E[m.call(a)]||"object"},isPlainObject:function(a){if(!a||p.type(a)!=="object"||a.nodeType||p.isWindow(a))return!1;try{if(a.constructor&&!n.call(a,"constructor")&&!n.call(a.constructor.prototype,"isPrototypeOf"))return!1}catch(c){return!1}var d;for(d in a);return d===b||n.call(a,d)},isEmptyObject:function(a){var b;for(b in a)return!1;return!0},error:function(a){throw new Error(a)},parseHTML:function(a,b,c){var d;return!a||typeof a!="string"?null:(typeof b=="boolean"&&(c=b,b=0),b=b||e,(d=v.exec(a))?[b.createElement(d[1])]:(d=p.buildFragment([a],b,c?null:[]),p.merge([],(d.cacheable?p.clone(d.fragment):d.fragment).childNodes)))},parseJSON:function(b){if(!b||typeof b!="string")return null;b=p.trim(b);if(a.JSON&&a.JSON.parse)return a.JSON.parse(b);if(w.test(b.replace(y,"@").replace(z,"]").replace(x,"")))return(new Function("return "+b))();p.error("Invalid JSON: "+b)},parseXML:function(c){var d,e;if(!c||typeof c!="string")return null;try{a.DOMParser?(e=new DOMParser,d=e.parseFromString(c,"text/xml")):(d=new ActiveXObject("Microsoft.XMLDOM"),d.async="false",d.loadXML(c))}catch(f){d=b}return(!d||!d.documentElement||d.getElementsByTagName("parsererror").length)&&p.error("Invalid XML: "+c),d},noop:function(){},globalEval:function(b){b&&r.test(b)&&(a.execScript||function(b){a.eval.call(a,b)})(b)},camelCase:function(a){return a.replace(A,"ms-").replace(B,C)},nodeName:function(a,b){return a.nodeName&&a.nodeName.toLowerCase()===b.toLowerCase()},each:function(a,c,d){var e,f=0,g=a.length,h=g===b||p.isFunction(a);if(d){if(h){for(e in a)if(c.apply(a[e],d)===!1)break}else for(;f<g;)if(c.apply(a[f++],d)===!1)break}else if(h){for(e in a)if(c.call(a[e],e,a[e])===!1)break}else for(;f<g;)if(c.call(a[f],f,a[f++])===!1)break;return a},trim:o&&!o.call(" ")?function(a){return a==null?"":o.call(a)}:function(a){return a==null?"":(a+"").replace(t,"")},makeArray:function(a,b){var c,d=b||[];return a!=null&&(c=p.type(a),a.length==null||c==="string"||c==="function"||c==="regexp"||p.isWindow(a)?j.call(d,a):p.merge(d,a)),d},inArray:function(a,b,c){var d;if(b){if(l)return l.call(b,a,c);d=b.length,c=c?c<0?Math.max(0,d+c):c:0;for(;c<d;c++)if(c in b&&b[c]===a)return c}return-1},merge:function(a,c){var d=c.length,e=a.length,f=0;if(typeof d=="number")for(;f<d;f++)a[e++]=c[f];else while(c[f]!==b)a[e++]=c[f++];return a.length=e,a},grep:function(a,b,c){var d,e=[],f=0,g=a.length;c=!!c;for(;f<g;f++)d=!!b(a[f],f),c!==d&&e.push(a[f]);return e},map:function(a,c,d){var e,f,g=[],h=0,i=a.length,j=a instanceof p||i!==b&&typeof i=="number"&&(i>0&&a[0]&&a[i-1]||i===0||p.isArray(a));if(j)for(;h<i;h++)e=c(a[h],h,d),e!=null&&(g[g.length]=e);else for(f in a)e=c(a[f],f,d),e!=null&&(g[g.length]=e);return g.concat.apply([],g)},guid:1,proxy:function(a,c){var d,e,f;return typeof c=="string"&&(d=a[c],c=a,a=d),p.isFunction(a)?(e=k.call(arguments,2),f=function(){return a.apply(c,e.concat(k.call(arguments)))},f.guid=a.guid=a.guid||p.guid++,f):b},access:function(a,c,d,e,f,g,h){var i,j=d==null,k=0,l=a.length;if(d&&typeof d=="object"){for(k in d)p.access(a,c,k,d[k],1,g,e);f=1}else if(e!==b){i=h===b&&p.isFunction(e),j&&(i?(i=c,c=function(a,b,c){return i.call(p(a),c)}):(c.call(a,e),c=null));if(c)for(;k<l;k++)c(a[k],d,i?e.call(a[k],k,c(a[k],d)):e,h);f=1}return f?a:j?c.call(a):l?c(a[0],d):g},now:function(){return(new Date).getTime()}}),p.ready.promise=function(b){if(!d){d=p.Deferred();if(e.readyState==="complete")setTimeout(p.ready,1);else if(e.addEventListener)e.addEventListener("DOMContentLoaded",D,!1),a.addEventListener("load",p.ready,!1);else{e.attachEvent("onreadystatechange",D),a.attachEvent("onload",p.ready);var c=!1;try{c=a.frameElement==null&&e.documentElement}catch(f){}c&&c.doScroll&&function g(){if(!p.isReady){try{c.doScroll("left")}catch(a){return setTimeout(g,50)}p.ready()}}()}}return d.promise(b)},p.each("Boolean Number String Function Array Date RegExp Object".split(" "),function(a,b){E["[object "+b+"]"]=b.toLowerCase()}),c=p(e);var F={};p.Callbacks=function(a){a=typeof a=="string"?F[a]||G(a):p.extend({},a);var c,d,e,f,g,h,i=[],j=!a.once&&[],k=function(b){c=a.memory&&b,d=!0,h=f||0,f=0,g=i.length,e=!0;for(;i&&h<g;h++)if(i[h].apply(b[0],b[1])===!1&&a.stopOnFalse){c=!1;break}e=!1,i&&(j?j.length&&k(j.shift()):c?i=[]:l.disable())},l={add:function(){if(i){var b=i.length;(function d(b){p.each(b,function(b,c){var e=p.type(c);e==="function"&&(!a.unique||!l.has(c))?i.push(c):c&&c.length&&e!=="string"&&d(c)})})(arguments),e?g=i.length:c&&(f=b,k(c))}return this},remove:function(){return i&&p.each(arguments,function(a,b){var c;while((c=p.inArray(b,i,c))>-1)i.splice(c,1),e&&(c<=g&&g--,c<=h&&h--)}),this},has:function(a){return p.inArray(a,i)>-1},empty:function(){return i=[],this},disable:function(){return i=j=c=b,this},disabled:function(){return!i},lock:function(){return j=b,c||l.disable(),this},locked:function(){return!j},fireWith:function(a,b){return b=b||[],b=[a,b.slice?b.slice():b],i&&(!d||j)&&(e?j.push(b):k(b)),this},fire:function(){return l.fireWith(this,arguments),this},fired:function(){return!!d}};return l},p.extend({Deferred:function(a){var b=[["resolve","done",p.Callbacks("once memory"),"resolved"],["reject","fail",p.Callbacks("once memory"),"rejected"],["notify","progress",p.Callbacks("memory")]],c="pending",d={state:function(){return c},always:function(){return e.done(arguments).fail(arguments),this},then:function(){var a=arguments;return p.Deferred(function(c){p.each(b,function(b,d){var f=d[0],g=a[b];e[d[1]](p.isFunction(g)?function(){var a=g.apply(this,arguments);a&&p.isFunction(a.promise)?a.promise().done(c.resolve).fail(c.reject).progress(c.notify):c[f+"With"](this===e?c:this,[a])}:c[f])}),a=null}).promise()},promise:function(a){return a!=null?p.extend(a,d):d}},e={};return d.pipe=d.then,p.each(b,function(a,f){var g=f[2],h=f[3];d[f[1]]=g.add,h&&g.add(function(){c=h},b[a^1][2].disable,b[2][2].lock),e[f[0]]=g.fire,e[f[0]+"With"]=g.fireWith}),d.promise(e),a&&a.call(e,e),e},when:function(a){var b=0,c=k.call(arguments),d=c.length,e=d!==1||a&&p.isFunction(a.promise)?d:0,f=e===1?a:p.Deferred(),g=function(a,b,c){return function(d){b[a]=this,c[a]=arguments.length>1?k.call(arguments):d,c===h?f.notifyWith(b,c):--e||f.resolveWith(b,c)}},h,i,j;if(d>1){h=new Array(d),i=new Array(d),j=new Array(d);for(;b<d;b++)c[b]&&p.isFunction(c[b].promise)?c[b].promise().done(g(b,j,c)).fail(f.reject).progress(g(b,i,h)):--e}return e||f.resolveWith(j,c),f.promise()}}),p.support=function(){var b,c,d,f,g,h,i,j,k,l,m,n=e.createElement("div");n.setAttribute("className","t"),n.innerHTML=" <link/><table></table><a href='/a'>a</a><input type='checkbox'/>",c=n.getElementsByTagName("*"),d=n.getElementsByTagName("a")[0],d.style.cssText="top:1px;float:left;opacity:.5";if(!c||!c.length)return{};f=e.createElement("select"),g=f.appendChild(e.createElement("option")),h=n.getElementsByTagName("input")[0],b={leadingWhitespace:n.firstChild.nodeType===3,tbody:!n.getElementsByTagName("tbody").length,htmlSerialize:!!n.getElementsByTagName("link").length,style:/top/.test(d.getAttribute("style")),hrefNormalized:d.getAttribute("href")==="/a",opacity:/^0.5/.test(d.style.opacity),cssFloat:!!d.style.cssFloat,checkOn:h.value==="on",optSelected:g.selected,getSetAttribute:n.className!=="t",enctype:!!e.createElement("form").enctype,html5Clone:e.createElement("nav").cloneNode(!0).outerHTML!=="<:nav></:nav>",boxModel:e.compatMode==="CSS1Compat",submitBubbles:!0,changeBubbles:!0,focusinBubbles:!1,deleteExpando:!0,noCloneEvent:!0,inlineBlockNeedsLayout:!1,shrinkWrapBlocks:!1,reliableMarginRight:!0,boxSizingReliable:!0,pixelPosition:!1},h.checked=!0,b.noCloneChecked=h.cloneNode(!0).checked,f.disabled=!0,b.optDisabled=!g.disabled;try{delete n.test}catch(o){b.deleteExpando=!1}!n.addEventListener&&n.attachEvent&&n.fireEvent&&(n.attachEvent("onclick",m=function(){b.noCloneEvent=!1}),n.cloneNode(!0).fireEvent("onclick"),n.detachEvent("onclick",m)),h=e.createElement("input"),h.value="t",h.setAttribute("type","radio"),b.radioValue=h.value==="t",h.setAttribute("checked","checked"),h.setAttribute("name","t"),n.appendChild(h),i=e.createDocumentFragment(),i.appendChild(n.lastChild),b.checkClone=i.cloneNode(!0).cloneNode(!0).lastChild.checked,b.appendChecked=h.checked,i.removeChild(h),i.appendChild(n);if(n.attachEvent)for(k in{submit:!0,change:!0,focusin:!0})j="on"+k,l=j in n,l||(n.setAttribute(j,"return;"),l=typeof n[j]=="function"),b[k+"Bubbles"]=l;return p(function(){var c,d,f,g,h="padding:0;margin:0;border:0;display:block;overflow:hidden;",i=e.getElementsByTagName("body")[0];if(!i)return;c=e.createElement("div"),c.style.cssText="visibility:hidden;border:0;width:0;height:0;position:static;top:0;margin-top:1px",i.insertBefore(c,i.firstChild),d=e.createElement("div"),c.appendChild(d),d.innerHTML="<table><tr><td></td><td>t</td></tr></table>",f=d.getElementsByTagName("td"),f[0].style.cssText="padding:0;margin:0;border:0;display:none",l=f[0].offsetHeight===0,f[0].style.display="",f[1].style.display="none",b.reliableHiddenOffsets=l&&f[0].offsetHeight===0,d.innerHTML="",d.style.cssText="box-sizing:border-box;-moz-box-sizing:border-box;-webkit-box-sizing:border-box;padding:1px;border:1px;display:block;width:4px;margin-top:1%;position:absolute;top:1%;",b.boxSizing=d.offsetWidth===4,b.doesNotIncludeMarginInBodyOffset=i.offsetTop!==1,a.getComputedStyle&&(b.pixelPosition=(a.getComputedStyle(d,null)||{}).top!=="1%",b.boxSizingReliable=(a.getComputedStyle(d,null)||{width:"4px"}).width==="4px",g=e.createElement("div"),g.style.cssText=d.style.cssText=h,g.style.marginRight=g.style.width="0",d.style.width="1px",d.appendChild(g),b.reliableMarginRight=!parseFloat((a.getComputedStyle(g,null)||{}).marginRight)),typeof d.style.zoom!="undefined"&&(d.innerHTML="",d.style.cssText=h+"width:1px;padding:1px;display:inline;zoom:1",b.inlineBlockNeedsLayout=d.offsetWidth===3,d.style.display="block",d.style.overflow="visible",d.innerHTML="<div></div>",d.firstChild.style.width="5px",b.shrinkWrapBlocks=d.offsetWidth!==3,c.style.zoom=1),i.removeChild(c),c=d=f=g=null}),i.removeChild(n),c=d=f=g=h=i=n=null,b}();var H=/(?:\{[\s\S]*\}|\[[\s\S]*\])$/,I=/([A-Z])/g;p.extend({cache:{},deletedIds:[],uuid:0,expando:"jQuery"+(p.fn.jquery+Math.random()).replace(/\D/g,""),noData:{embed:!0,object:"clsid:D27CDB6E-AE6D-11cf-96B8-444553540000",applet:!0},hasData:function(a){return a=a.nodeType?p.cache[a[p.expando]]:a[p.expando],!!a&&!K(a)},data:function(a,c,d,e){if(!p.acceptData(a))return;var f,g,h=p.expando,i=typeof c=="string",j=a.nodeType,k=j?p.cache:a,l=j?a[h]:a[h]&&h;if((!l||!k[l]||!e&&!k[l].data)&&i&&d===b)return;l||(j?a[h]=l=p.deletedIds.pop()||p.guid++:l=h),k[l]||(k[l]={},j||(k[l].toJSON=p.noop));if(typeof c=="object"||typeof c=="function")e?k[l]=p.extend(k[l],c):k[l].data=p.extend(k[l].data,c);return f=k[l],e||(f.data||(f.data={}),f=f.data),d!==b&&(f[p.camelCase(c)]=d),i?(g=f[c],g==null&&(g=f[p.camelCase(c)])):g=f,g},removeData:function(a,b,c){if(!p.acceptData(a))return;var d,e,f,g=a.nodeType,h=g?p.cache:a,i=g?a[p.expando]:p.expando;if(!h[i])return;if(b){d=c?h[i]:h[i].data;if(d){p.isArray(b)||(b in d?b=[b]:(b=p.camelCase(b),b in d?b=[b]:b=b.split(" ")));for(e=0,f=b.length;e<f;e++)delete d[b[e]];if(!(c?K:p.isEmptyObject)(d))return}}if(!c){delete h[i].data;if(!K(h[i]))return}g?p.cleanData([a],!0):p.support.deleteExpando||h!=h.window?delete h[i]:h[i]=null},_data:function(a,b,c){return p.data(a,b,c,!0)},acceptData:function(a){var b=a.nodeName&&p.noData[a.nodeName.toLowerCase()];return!b||b!==!0&&a.getAttribute("classid")===b}}),p.fn.extend({data:function(a,c){var d,e,f,g,h,i=this[0],j=0,k=null;if(a===b){if(this.length){k=p.data(i);if(i.nodeType===1&&!p._data(i,"parsedAttrs")){f=i.attributes;for(h=f.length;j<h;j++)g=f[j].name,g.indexOf("data-")||(g=p.camelCase(g.substring(5)),J(i,g,k[g]));p._data(i,"parsedAttrs",!0)}}return k}return typeof a=="object"?this.each(function(){p.data(this,a)}):(d=a.split(".",2),d[1]=d[1]?"."+d[1]:"",e=d[1]+"!",p.access(this,function(c){if(c===b)return k=this.triggerHandler("getData"+e,[d[0]]),k===b&&i&&(k=p.data(i,a),k=J(i,a,k)),k===b&&d[1]?this.data(d[0]):k;d[1]=c,this.each(function(){var b=p(this);b.triggerHandler("setData"+e,d),p.data(this,a,c),b.triggerHandler("changeData"+e,d)})},null,c,arguments.length>1,null,!1))},removeData:function(a){return this.each(function(){p.removeData(this,a)})}}),p.extend({queue:function(a,b,c){var d;if(a)return b=(b||"fx")+"queue",d=p._data(a,b),c&&(!d||p.isArray(c)?d=p._data(a,b,p.makeArray(c)):d.push(c)),d||[]},dequeue:function(a,b){b=b||"fx";var c=p.queue(a,b),d=c.length,e=c.shift(),f=p._queueHooks(a,b),g=function(){p.dequeue(a,b)};e==="inprogress"&&(e=c.shift(),d--),e&&(b==="fx"&&c.unshift("inprogress"),delete f.stop,e.call(a,g,f)),!d&&f&&f.empty.fire()},_queueHooks:function(a,b){var c=b+"queueHooks";return p._data(a,c)||p._data(a,c,{empty:p.Callbacks("once memory").add(function(){p.removeData(a,b+"queue",!0),p.removeData(a,c,!0)})})}}),p.fn.extend({queue:function(a,c){var d=2;return typeof a!="string"&&(c=a,a="fx",d--),arguments.length<d?p.queue(this[0],a):c===b?this:this.each(function(){var b=p.queue(this,a,c);p._queueHooks(this,a),a==="fx"&&b[0]!=="inprogress"&&p.dequeue(this,a)})},dequeue:function(a){return this.each(function(){p.dequeue(this,a)})},delay:function(a,b){return a=p.fx?p.fx.speeds[a]||a:a,b=b||"fx",this.queue(b,function(b,c){var d=setTimeout(b,a);c.stop=function(){clearTimeout(d)}})},clearQueue:function(a){return this.queue(a||"fx",[])},promise:function(a,c){var d,e=1,f=p.Deferred(),g=this,h=this.length,i=function(){--e||f.resolveWith(g,[g])};typeof a!="string"&&(c=a,a=b),a=a||"fx";while(h--)d=p._data(g[h],a+"queueHooks"),d&&d.empty&&(e++,d.empty.add(i));return i(),f.promise(c)}});var L,M,N,O=/[\t\r\n]/g,P=/\r/g,Q=/^(?:button|input)$/i,R=/^(?:button|input|object|select|textarea)$/i,S=/^a(?:rea|)$/i,T=/^(?:autofocus|autoplay|async|checked|controls|defer|disabled|hidden|loop|multiple|open|readonly|required|scoped|selected)$/i,U=p.support.getSetAttribute;p.fn.extend({attr:function(a,b){return p.access(this,p.attr,a,b,arguments.length>1)},removeAttr:function(a){return this.each(function(){p.removeAttr(this,a)})},prop:function(a,b){return p.access(this,p.prop,a,b,arguments.length>1)},removeProp:function(a){return a=p.propFix[a]||a,this.each(function(){try{this[a]=b,delete this[a]}catch(c){}})},addClass:function(a){var b,c,d,e,f,g,h;if(p.isFunction(a))return this.each(function(b){p(this).addClass(a.call(this,b,this.className))});if(a&&typeof a=="string"){b=a.split(s);for(c=0,d=this.length;c<d;c++){e=this[c];if(e.nodeType===1)if(!e.className&&b.length===1)e.className=a;else{f=" "+e.className+" ";for(g=0,h=b.length;g<h;g++)f.indexOf(" "+b[g]+" ")<0&&(f+=b[g]+" ");e.className=p.trim(f)}}}return this},removeClass:function(a){var c,d,e,f,g,h,i;if(p.isFunction(a))return this.each(function(b){p(this).removeClass(a.call(this,b,this.className))});if(a&&typeof a=="string"||a===b){c=(a||"").split(s);for(h=0,i=this.length;h<i;h++){e=this[h];if(e.nodeType===1&&e.className){d=(" "+e.className+" ").replace(O," ");for(f=0,g=c.length;f<g;f++)while(d.indexOf(" "+c[f]+" ")>=0)d=d.replace(" "+c[f]+" "," ");e.className=a?p.trim(d):""}}}return this},toggleClass:function(a,b){var c=typeof a,d=typeof b=="boolean";return p.isFunction(a)?this.each(function(c){p(this).toggleClass(a.call(this,c,this.className,b),b)}):this.each(function(){if(c==="string"){var e,f=0,g=p(this),h=b,i=a.split(s);while(e=i[f++])h=d?h:!g.hasClass(e),g[h?"addClass":"removeClass"](e)}else if(c==="undefined"||c==="boolean")this.className&&p._data(this,"__className__",this.className),this.className=this.className||a===!1?"":p._data(this,"__className__")||""})},hasClass:function(a){var b=" "+a+" ",c=0,d=this.length;for(;c<d;c++)if(this[c].nodeType===1&&(" "+this[c].className+" ").replace(O," ").indexOf(b)>=0)return!0;return!1},val:function(a){var c,d,e,f=this[0];if(!arguments.length){if(f)return c=p.valHooks[f.type]||p.valHooks[f.nodeName.toLowerCase()],c&&"get"in c&&(d=c.get(f,"value"))!==b?d:(d=f.value,typeof d=="string"?d.replace(P,""):d==null?"":d);return}return e=p.isFunction(a),this.each(function(d){var f,g=p(this);if(this.nodeType!==1)return;e?f=a.call(this,d,g.val()):f=a,f==null?f="":typeof f=="number"?f+="":p.isArray(f)&&(f=p.map(f,function(a){return a==null?"":a+""})),c=p.valHooks[this.type]||p.valHooks[this.nodeName.toLowerCase()];if(!c||!("set"in c)||c.set(this,f,"value")===b)this.value=f})}}),p.extend({valHooks:{option:{get:function(a){var b=a.attributes.value;return!b||b.specified?a.value:a.text}},select:{get:function(a){var b,c,d,e,f=a.selectedIndex,g=[],h=a.options,i=a.type==="select-one";if(f<0)return null;c=i?f:0,d=i?f+1:h.length;for(;c<d;c++){e=h[c];if(e.selected&&(p.support.optDisabled?!e.disabled:e.getAttribute("disabled")===null)&&(!e.parentNode.disabled||!p.nodeName(e.parentNode,"optgroup"))){b=p(e).val();if(i)return b;g.push(b)}}return i&&!g.length&&h.length?p(h[f]).val():g},set:function(a,b){var c=p.makeArray(b);return p(a).find("option").each(function(){this.selected=p.inArray(p(this).val(),c)>=0}),c.length||(a.selectedIndex=-1),c}}},attrFn:{},attr:function(a,c,d,e){var f,g,h,i=a.nodeType;if(!a||i===3||i===8||i===2)return;if(e&&p.isFunction(p.fn[c]))return p(a)[c](d);if(typeof a.getAttribute=="undefined")return p.prop(a,c,d);h=i!==1||!p.isXMLDoc(a),h&&(c=c.toLowerCase(),g=p.attrHooks[c]||(T.test(c)?M:L));if(d!==b){if(d===null){p.removeAttr(a,c);return}return g&&"set"in g&&h&&(f=g.set(a,d,c))!==b?f:(a.setAttribute(c,d+""),d)}return g&&"get"in g&&h&&(f=g.get(a,c))!==null?f:(f=a.getAttribute(c),f===null?b:f)},removeAttr:function(a,b){var c,d,e,f,g=0;if(b&&a.nodeType===1){d=b.split(s);for(;g<d.length;g++)e=d[g],e&&(c=p.propFix[e]||e,f=T.test(e),f||p.attr(a,e,""),a.removeAttribute(U?e:c),f&&c in a&&(a[c]=!1))}},attrHooks:{type:{set:function(a,b){if(Q.test(a.nodeName)&&a.parentNode)p.error("type property can't be changed");else if(!p.support.radioValue&&b==="radio"&&p.nodeName(a,"input")){var c=a.value;return a.setAttribute("type",b),c&&(a.value=c),b}}},value:{get:function(a,b){return L&&p.nodeName(a,"button")?L.get(a,b):b in a?a.value:null},set:function(a,b,c){if(L&&p.nodeName(a,"button"))return L.set(a,b,c);a.value=b}}},propFix:{tabindex:"tabIndex",readonly:"readOnly","for":"htmlFor","class":"className",maxlength:"maxLength",cellspacing:"cellSpacing",cellpadding:"cellPadding",rowspan:"rowSpan",colspan:"colSpan",usemap:"useMap",frameborder:"frameBorder",contenteditable:"contentEditable"},prop:function(a,c,d){var e,f,g,h=a.nodeType;if(!a||h===3||h===8||h===2)return;return g=h!==1||!p.isXMLDoc(a),g&&(c=p.propFix[c]||c,f=p.propHooks[c]),d!==b?f&&"set"in f&&(e=f.set(a,d,c))!==b?e:a[c]=d:f&&"get"in f&&(e=f.get(a,c))!==null?e:a[c]},propHooks:{tabIndex:{get:function(a){var c=a.getAttributeNode("tabindex");return c&&c.specified?parseInt(c.value,10):R.test(a.nodeName)||S.test(a.nodeName)&&a.href?0:b}}}}),M={get:function(a,c){var d,e=p.prop(a,c);return e===!0||typeof e!="boolean"&&(d=a.getAttributeNode(c))&&d.nodeValue!==!1?c.toLowerCase():b},set:function(a,b,c){var d;return b===!1?p.removeAttr(a,c):(d=p.propFix[c]||c,d in a&&(a[d]=!0),a.setAttribute(c,c.toLowerCase())),c}},U||(N={name:!0,id:!0,coords:!0},L=p.valHooks.button={get:function(a,c){var d;return d=a.getAttributeNode(c),d&&(N[c]?d.value!=="":d.specified)?d.value:b},set:function(a,b,c){var d=a.getAttributeNode(c);return d||(d=e.createAttribute(c),a.setAttributeNode(d)),d.value=b+""}},p.each(["width","height"],function(a,b){p.attrHooks[b]=p.extend(p.attrHooks[b],{set:function(a,c){if(c==="")return a.setAttribute(b,"auto"),c}})}),p.attrHooks.contenteditable={get:L.get,set:function(a,b,c){b===""&&(b="false"),L.set(a,b,c)}}),p.support.hrefNormalized||p.each(["href","src","width","height"],function(a,c){p.attrHooks[c]=p.extend(p.attrHooks[c],{get:function(a){var d=a.getAttribute(c,2);return d===null?b:d}})}),p.support.style||(p.attrHooks.style={get:function(a){return a.style.cssText.toLowerCase()||b},set:function(a,b){return a.style.cssText=b+""}}),p.support.optSelected||(p.propHooks.selected=p.extend(p.propHooks.selected,{get:function(a){var b=a.parentNode;return b&&(b.selectedIndex,b.parentNode&&b.parentNode.selectedIndex),null}})),p.support.enctype||(p.propFix.enctype="encoding"),p.support.checkOn||p.each(["radio","checkbox"],function(){p.valHooks[this]={get:function(a){return a.getAttribute("value")===null?"on":a.value}}}),p.each(["radio","checkbox"],function(){p.valHooks[this]=p.extend(p.valHooks[this],{set:function(a,b){if(p.isArray(b))return a.checked=p.inArray(p(a).val(),b)>=0}})});var V=/^(?:textarea|input|select)$/i,W=/^([^\.]*|)(?:\.(.+)|)$/,X=/(?:^|\s)hover(\.\S+|)\b/,Y=/^key/,Z=/^(?:mouse|contextmenu)|click/,$=/^(?:focusinfocus|focusoutblur)$/,_=function(a){return p.event.special.hover?a:a.replace(X,"mouseenter$1 mouseleave$1")};p.event={add:function(a,c,d,e,f){var g,h,i,j,k,l,m,n,o,q,r;if(a.nodeType===3||a.nodeType===8||!c||!d||!(g=p._data(a)))return;d.handler&&(o=d,d=o.handler,f=o.selector),d.guid||(d.guid=p.guid++),i=g.events,i||(g.events=i={}),h=g.handle,h||(g.handle=h=function(a){return typeof p!="undefined"&&(!a||p.event.triggered!==a.type)?p.event.dispatch.apply(h.elem,arguments):b},h.elem=a),c=p.trim(_(c)).split(" ");for(j=0;j<c.length;j++){k=W.exec(c[j])||[],l=k[1],m=(k[2]||"").split(".").sort(),r=p.event.special[l]||{},l=(f?r.delegateType:r.bindType)||l,r=p.event.special[l]||{},n=p.extend({type:l,origType:k[1],data:e,handler:d,guid:d.guid,selector:f,needsContext:f&&p.expr.match.needsContext.test(f),namespace:m.join(".")},o),q=i[l];if(!q){q=i[l]=[],q.delegateCount=0;if(!r.setup||r.setup.call(a,e,m,h)===!1)a.addEventListener?a.addEventListener(l,h,!1):a.attachEvent&&a.attachEvent("on"+l,h)}r.add&&(r.add.call(a,n),n.handler.guid||(n.handler.guid=d.guid)),f?q.splice(q.delegateCount++,0,n):q.push(n),p.event.global[l]=!0}a=null},global:{},remove:function(a,b,c,d,e){var f,g,h,i,j,k,l,m,n,o,q,r=p.hasData(a)&&p._data(a);if(!r||!(m=r.events))return;b=p.trim(_(b||"")).split(" ");for(f=0;f<b.length;f++){g=W.exec(b[f])||[],h=i=g[1],j=g[2];if(!h){for(h in m)p.event.remove(a,h+b[f],c,d,!0);continue}n=p.event.special[h]||{},h=(d?n.delegateType:n.bindType)||h,o=m[h]||[],k=o.length,j=j?new RegExp("(^|\\.)"+j.split(".").sort().join("\\.(?:.*\\.|)")+"(\\.|$)"):null;for(l=0;l<o.length;l++)q=o[l],(e||i===q.origType)&&(!c||c.guid===q.guid)&&(!j||j.test(q.namespace))&&(!d||d===q.selector||d==="**"&&q.selector)&&(o.splice(l--,1),q.selector&&o.delegateCount--,n.remove&&n.remove.call(a,q));o.length===0&&k!==o.length&&((!n.teardown||n.teardown.call(a,j,r.handle)===!1)&&p.removeEvent(a,h,r.handle),delete m[h])}p.isEmptyObject(m)&&(delete r.handle,p.removeData(a,"events",!0))},customEvent:{getData:!0,setData:!0,changeData:!0},trigger:function(c,d,f,g){if(!f||f.nodeType!==3&&f.nodeType!==8){var h,i,j,k,l,m,n,o,q,r,s=c.type||c,t=[];if($.test(s+p.event.triggered))return;s.indexOf("!")>=0&&(s=s.slice(0,-1),i=!0),s.indexOf(".")>=0&&(t=s.split("."),s=t.shift(),t.sort());if((!f||p.event.customEvent[s])&&!p.event.global[s])return;c=typeof c=="object"?c[p.expando]?c:new p.Event(s,c):new p.Event(s),c.type=s,c.isTrigger=!0,c.exclusive=i,c.namespace=t.join("."),c.namespace_re=c.namespace?new RegExp("(^|\\.)"+t.join("\\.(?:.*\\.|)")+"(\\.|$)"):null,m=s.indexOf(":")<0?"on"+s:"";if(!f){h=p.cache;for(j in h)h[j].events&&h[j].events[s]&&p.event.trigger(c,d,h[j].handle.elem,!0);return}c.result=b,c.target||(c.target=f),d=d!=null?p.makeArray(d):[],d.unshift(c),n=p.event.special[s]||{};if(n.trigger&&n.trigger.apply(f,d)===!1)return;q=[[f,n.bindType||s]];if(!g&&!n.noBubble&&!p.isWindow(f)){r=n.delegateType||s,k=$.test(r+s)?f:f.parentNode;for(l=f;k;k=k.parentNode)q.push([k,r]),l=k;l===(f.ownerDocument||e)&&q.push([l.defaultView||l.parentWindow||a,r])}for(j=0;j<q.length&&!c.isPropagationStopped();j++)k=q[j][0],c.type=q[j][1],o=(p._data(k,"events")||{})[c.type]&&p._data(k,"handle"),o&&o.apply(k,d),o=m&&k[m],o&&p.acceptData(k)&&o.apply&&o.apply(k,d)===!1&&c.preventDefault();return c.type=s,!g&&!c.isDefaultPrevented()&&(!n._default||n._default.apply(f.ownerDocument,d)===!1)&&(s!=="click"||!p.nodeName(f,"a"))&&p.acceptData(f)&&m&&f[s]&&(s!=="focus"&&s!=="blur"||c.target.offsetWidth!==0)&&!p.isWindow(f)&&(l=f[m],l&&(f[m]=null),p.event.triggered=s,f[s](),p.event.triggered=b,l&&(f[m]=l)),c.result}return},dispatch:function(c){c=p.event.fix(c||a.event);var d,e,f,g,h,i,j,l,m,n,o=(p._data(this,"events")||{})[c.type]||[],q=o.delegateCount,r=k.call(arguments),s=!c.exclusive&&!c.namespace,t=p.event.special[c.type]||{},u=[];r[0]=c,c.delegateTarget=this;if(t.preDispatch&&t.preDispatch.call(this,c)===!1)return;if(q&&(!c.button||c.type!=="click"))for(f=c.target;f!=this;f=f.parentNode||this)if(f.disabled!==!0||c.type!=="click"){h={},j=[];for(d=0;d<q;d++)l=o[d],m=l.selector,h[m]===b&&(h[m]=l.needsContext?p(m,this).index(f)>=0:p.find(m,this,null,[f]).length),h[m]&&j.push(l);j.length&&u.push({elem:f,matches:j})}o.length>q&&u.push({elem:this,matches:o.slice(q)});for(d=0;d<u.length&&!c.isPropagationStopped();d++){i=u[d],c.currentTarget=i.elem;for(e=0;e<i.matches.length&&!c.isImmediatePropagationStopped();e++){l=i.matches[e];if(s||!c.namespace&&!l.namespace||c.namespace_re&&c.namespace_re.test(l.namespace))c.data=l.data,c.handleObj=l,g=((p.event.special[l.origType]||{}).handle||l.handler).apply(i.elem,r),g!==b&&(c.result=g,g===!1&&(c.preventDefault(),c.stopPropagation()))}}return t.postDispatch&&t.postDispatch.call(this,c),c.result},props:"attrChange attrName relatedNode srcElement altKey bubbles cancelable ctrlKey currentTarget eventPhase metaKey relatedTarget shiftKey target timeStamp view which".split(" "),fixHooks:{},keyHooks:{props:"char charCode key keyCode".split(" "),filter:function(a,b){return a.which==null&&(a.which=b.charCode!=null?b.charCode:b.keyCode),a}},mouseHooks:{props:"button buttons clientX clientY fromElement offsetX offsetY pageX pageY screenX screenY toElement".split(" "),filter:function(a,c){var d,f,g,h=c.button,i=c.fromElement;return a.pageX==null&&c.clientX!=null&&(d=a.target.ownerDocument||e,f=d.documentElement,g=d.body,a.pageX=c.clientX+(f&&f.scrollLeft||g&&g.scrollLeft||0)-(f&&f.clientLeft||g&&g.clientLeft||0),a.pageY=c.clientY+(f&&f.scrollTop||g&&g.scrollTop||0)-(f&&f.clientTop||g&&g.clientTop||0)),!a.relatedTarget&&i&&(a.relatedTarget=i===a.target?c.toElement:i),!a.which&&h!==b&&(a.which=h&1?1:h&2?3:h&4?2:0),a}},fix:function(a){if(a[p.expando])return a;var b,c,d=a,f=p.event.fixHooks[a.type]||{},g=f.props?this.props.concat(f.props):this.props;a=p.Event(d);for(b=g.length;b;)c=g[--b],a[c]=d[c];return a.target||(a.target=d.srcElement||e),a.target.nodeType===3&&(a.target=a.target.parentNode),a.metaKey=!!a.metaKey,f.filter?f.filter(a,d):a},special:{load:{noBubble:!0},focus:{delegateType:"focusin"},blur:{delegateType:"focusout"},beforeunload:{setup:function(a,b,c){p.isWindow(this)&&(this.onbeforeunload=c)},teardown:function(a,b){this.onbeforeunload===b&&(this.onbeforeunload=null)}}},simulate:function(a,b,c,d){var e=p.extend(new p.Event,c,{type:a,isSimulated:!0,originalEvent:{}});d?p.event.trigger(e,null,b):p.event.dispatch.call(b,e),e.isDefaultPrevented()&&c.preventDefault()}},p.event.handle=p.event.dispatch,p.removeEvent=e.removeEventListener?function(a,b,c){a.removeEventListener&&a.removeEventListener(b,c,!1)}:function(a,b,c){var d="on"+b;a.detachEvent&&(typeof a[d]=="undefined"&&(a[d]=null),a.detachEvent(d,c))},p.Event=function(a,b){if(this instanceof p.Event)a&&a.type?(this.originalEvent=a,this.type=a.type,this.isDefaultPrevented=a.defaultPrevented||a.returnValue===!1||a.getPreventDefault&&a.getPreventDefault()?bb:ba):this.type=a,b&&p.extend(this,b),this.timeStamp=a&&a.timeStamp||p.now(),this[p.expando]=!0;else return new p.Event(a,b)},p.Event.prototype={preventDefault:function(){this.isDefaultPrevented=bb;var a=this.originalEvent;if(!a)return;a.preventDefault?a.preventDefault():a.returnValue=!1},stopPropagation:function(){this.isPropagationStopped=bb;var a=this.originalEvent;if(!a)return;a.stopPropagation&&a.stopPropagation(),a.cancelBubble=!0},stopImmediatePropagation:function(){this.isImmediatePropagationStopped=bb,this.stopPropagation()},isDefaultPrevented:ba,isPropagationStopped:ba,isImmediatePropagationStopped:ba},p.each({mouseenter:"mouseover",mouseleave:"mouseout"},function(a,b){p.event.special[a]={delegateType:b,bindType:b,handle:function(a){var c,d=this,e=a.relatedTarget,f=a.handleObj,g=f.selector;if(!e||e!==d&&!p.contains(d,e))a.type=f.origType,c=f.handler.apply(this,arguments),a.type=b;return c}}}),p.support.submitBubbles||(p.event.special.submit={setup:function(){if(p.nodeName(this,"form"))return!1;p.event.add(this,"click._submit keypress._submit",function(a){var c=a.target,d=p.nodeName(c,"input")||p.nodeName(c,"button")?c.form:b;d&&!p._data(d,"_submit_attached")&&(p.event.add(d,"submit._submit",function(a){a._submit_bubble=!0}),p._data(d,"_submit_attached",!0))})},postDispatch:function(a){a._submit_bubble&&(delete a._submit_bubble,this.parentNode&&!a.isTrigger&&p.event.simulate("submit",this.parentNode,a,!0))},teardown:function(){if(p.nodeName(this,"form"))return!1;p.event.remove(this,"._submit")}}),p.support.changeBubbles||(p.event.special.change={setup:function(){if(V.test(this.nodeName)){if(this.type==="checkbox"||this.type==="radio")p.event.add(this,"propertychange._change",function(a){a.originalEvent.propertyName==="checked"&&(this._just_changed=!0)}),p.event.add(this,"click._change",function(a){this._just_changed&&!a.isTrigger&&(this._just_changed=!1),p.event.simulate("change",this,a,!0)});return!1}p.event.add(this,"beforeactivate._change",function(a){var b=a.target;V.test(b.nodeName)&&!p._data(b,"_change_attached")&&(p.event.add(b,"change._change",function(a){this.parentNode&&!a.isSimulated&&!a.isTrigger&&p.event.simulate("change",this.parentNode,a,!0)}),p._data(b,"_change_attached",!0))})},handle:function(a){var b=a.target;if(this!==b||a.isSimulated||a.isTrigger||b.type!=="radio"&&b.type!=="checkbox")return a.handleObj.handler.apply(this,arguments)},teardown:function(){return p.event.remove(this,"._change"),!V.test(this.nodeName)}}),p.support.focusinBubbles||p.each({focus:"focusin",blur:"focusout"},function(a,b){var c=0,d=function(a){p.event.simulate(b,a.target,p.event.fix(a),!0)};p.event.special[b]={setup:function(){c++===0&&e.addEventListener(a,d,!0)},teardown:function(){--c===0&&e.removeEventListener(a,d,!0)}}}),p.fn.extend({on:function(a,c,d,e,f){var g,h;if(typeof a=="object"){typeof c!="string"&&(d=d||c,c=b);for(h in a)this.on(h,c,d,a[h],f);return this}d==null&&e==null?(e=c,d=c=b):e==null&&(typeof c=="string"?(e=d,d=b):(e=d,d=c,c=b));if(e===!1)e=ba;else if(!e)return this;return f===1&&(g=e,e=function(a){return p().off(a),g.apply(this,arguments)},e.guid=g.guid||(g.guid=p.guid++)),this.each(function(){p.event.add(this,a,e,d,c)})},one:function(a,b,c,d){return this.on(a,b,c,d,1)},off:function(a,c,d){var e,f;if(a&&a.preventDefault&&a.handleObj)return e=a.handleObj,p(a.delegateTarget).off(e.namespace?e.origType+"."+e.namespace:e.origType,e.selector,e.handler),this;if(typeof a=="object"){for(f in a)this.off(f,c,a[f]);return this}if(c===!1||typeof c=="function")d=c,c=b;return d===!1&&(d=ba),this.each(function(){p.event.remove(this,a,d,c)})},bind:function(a,b,c){return this.on(a,null,b,c)},unbind:function(a,b){return this.off(a,null,b)},live:function(a,b,c){return p(this.context).on(a,this.selector,b,c),this},die:function(a,b){return p(this.context).off(a,this.selector||"**",b),this},delegate:function(a,b,c,d){return this.on(b,a,c,d)},undelegate:function(a,b,c){return arguments.length===1?this.off(a,"**"):this.off(b,a||"**",c)},trigger:function(a,b){return this.each(function(){p.event.trigger(a,b,this)})},triggerHandler:function(a,b){if(this[0])return p.event.trigger(a,b,this[0],!0)},toggle:function(a){var b=arguments,c=a.guid||p.guid++,d=0,e=function(c){var e=(p._data(this,"lastToggle"+a.guid)||0)%d;return p._data(this,"lastToggle"+a.guid,e+1),c.preventDefault(),b[e].apply(this,arguments)||!1};e.guid=c;while(d<b.length)b[d++].guid=c;return this.click(e)},hover:function(a,b){return this.mouseenter(a).mouseleave(b||a)}}),p.each("blur focus focusin focusout load resize scroll unload click dblclick mousedown mouseup mousemove mouseover mouseout mouseenter mouseleave change select submit keydown keypress keyup error contextmenu".split(" "),function(a,b){p.fn[b]=function(a,c){return c==null&&(c=a,a=null),arguments.length>0?this.on(b,null,a,c):this.trigger(b)},Y.test(b)&&(p.event.fixHooks[b]=p.event.keyHooks),Z.test(b)&&(p.event.fixHooks[b]=p.event.mouseHooks)}),function(a,b){function bc(a,b,c,d){c=c||[],b=b||r;var e,f,i,j,k=b.nodeType;if(!a||typeof a!="string")return c;if(k!==1&&k!==9)return[];i=g(b);if(!i&&!d)if(e=P.exec(a))if(j=e[1]){if(k===9){f=b.getElementById(j);if(!f||!f.parentNode)return c;if(f.id===j)return c.push(f),c}else if(b.ownerDocument&&(f=b.ownerDocument.getElementById(j))&&h(b,f)&&f.id===j)return c.push(f),c}else{if(e[2])return w.apply(c,x.call(b.getElementsByTagName(a),0)),c;if((j=e[3])&&_&&b.getElementsByClassName)return w.apply(c,x.call(b.getElementsByClassName(j),0)),c}return bp(a.replace(L,"$1"),b,c,d,i)}function bd(a){return function(b){var c=b.nodeName.toLowerCase();return c==="input"&&b.type===a}}function be(a){return function(b){var c=b.nodeName.toLowerCase();return(c==="input"||c==="button")&&b.type===a}}function bf(a){return z(function(b){return b=+b,z(function(c,d){var e,f=a([],c.length,b),g=f.length;while(g--)c[e=f[g]]&&(c[e]=!(d[e]=c[e]))})})}function bg(a,b,c){if(a===b)return c;var d=a.nextSibling;while(d){if(d===b)return-1;d=d.nextSibling}return 1}function bh(a,b){var c,d,f,g,h,i,j,k=C[o][a];if(k)return b?0:k.slice(0);h=a,i=[],j=e.preFilter;while(h){if(!c||(d=M.exec(h)))d&&(h=h.slice(d[0].length)),i.push(f=[]);c=!1;if(d=N.exec(h))f.push(c=new q(d.shift())),h=h.slice(c.length),c.type=d[0].replace(L," ");for(g in e.filter)(d=W[g].exec(h))&&(!j[g]||(d=j[g](d,r,!0)))&&(f.push(c=new q(d.shift())),h=h.slice(c.length),c.type=g,c.matches=d);if(!c)break}return b?h.length:h?bc.error(a):C(a,i).slice(0)}function bi(a,b,d){var e=b.dir,f=d&&b.dir==="parentNode",g=u++;return b.first?function(b,c,d){while(b=b[e])if(f||b.nodeType===1)return a(b,c,d)}:function(b,d,h){if(!h){var i,j=t+" "+g+" ",k=j+c;while(b=b[e])if(f||b.nodeType===1){if((i=b[o])===k)return b.sizset;if(typeof i=="string"&&i.indexOf(j)===0){if(b.sizset)return b}else{b[o]=k;if(a(b,d,h))return b.sizset=!0,b;b.sizset=!1}}}else while(b=b[e])if(f||b.nodeType===1)if(a(b,d,h))return b}}function bj(a){return a.length>1?function(b,c,d){var e=a.length;while(e--)if(!a[e](b,c,d))return!1;return!0}:a[0]}function bk(a,b,c,d,e){var f,g=[],h=0,i=a.length,j=b!=null;for(;h<i;h++)if(f=a[h])if(!c||c(f,d,e))g.push(f),j&&b.push(h);return g}function bl(a,b,c,d,e,f){return d&&!d[o]&&(d=bl(d)),e&&!e[o]&&(e=bl(e,f)),z(function(f,g,h,i){if(f&&e)return;var j,k,l,m=[],n=[],o=g.length,p=f||bo(b||"*",h.nodeType?[h]:h,[],f),q=a&&(f||!b)?bk(p,m,a,h,i):p,r=c?e||(f?a:o||d)?[]:g:q;c&&c(q,r,h,i);if(d){l=bk(r,n),d(l,[],h,i),j=l.length;while(j--)if(k=l[j])r[n[j]]=!(q[n[j]]=k)}if(f){j=a&&r.length;while(j--)if(k=r[j])f[m[j]]=!(g[m[j]]=k)}else r=bk(r===g?r.splice(o,r.length):r),e?e(null,g,r,i):w.apply(g,r)})}function bm(a){var b,c,d,f=a.length,g=e.relative[a[0].type],h=g||e.relative[" "],i=g?1:0,j=bi(function(a){return a===b},h,!0),k=bi(function(a){return y.call(b,a)>-1},h,!0),m=[function(a,c,d){return!g&&(d||c!==l)||((b=c).nodeType?j(a,c,d):k(a,c,d))}];for(;i<f;i++)if(c=e.relative[a[i].type])m=[bi(bj(m),c)];else{c=e.filter[a[i].type].apply(null,a[i].matches);if(c[o]){d=++i;for(;d<f;d++)if(e.relative[a[d].type])break;return bl(i>1&&bj(m),i>1&&a.slice(0,i-1).join("").replace(L,"$1"),c,i<d&&bm(a.slice(i,d)),d<f&&bm(a=a.slice(d)),d<f&&a.join(""))}m.push(c)}return bj(m)}function bn(a,b){var d=b.length>0,f=a.length>0,g=function(h,i,j,k,m){var n,o,p,q=[],s=0,u="0",x=h&&[],y=m!=null,z=l,A=h||f&&e.find.TAG("*",m&&i.parentNode||i),B=t+=z==null?1:Math.E;y&&(l=i!==r&&i,c=g.el);for(;(n=A[u])!=null;u++){if(f&&n){for(o=0;p=a[o];o++)if(p(n,i,j)){k.push(n);break}y&&(t=B,c=++g.el)}d&&((n=!p&&n)&&s--,h&&x.push(n))}s+=u;if(d&&u!==s){for(o=0;p=b[o];o++)p(x,q,i,j);if(h){if(s>0)while(u--)!x[u]&&!q[u]&&(q[u]=v.call(k));q=bk(q)}w.apply(k,q),y&&!h&&q.length>0&&s+b.length>1&&bc.uniqueSort(k)}return y&&(t=B,l=z),x};return g.el=0,d?z(g):g}function bo(a,b,c,d){var e=0,f=b.length;for(;e<f;e++)bc(a,b[e],c,d);return c}function bp(a,b,c,d,f){var g,h,j,k,l,m=bh(a),n=m.length;if(!d&&m.length===1){h=m[0]=m[0].slice(0);if(h.length>2&&(j=h[0]).type==="ID"&&b.nodeType===9&&!f&&e.relative[h[1].type]){b=e.find.ID(j.matches[0].replace(V,""),b,f)[0];if(!b)return c;a=a.slice(h.shift().length)}for(g=W.POS.test(a)?-1:h.length-1;g>=0;g--){j=h[g];if(e.relative[k=j.type])break;if(l=e.find[k])if(d=l(j.matches[0].replace(V,""),R.test(h[0].type)&&b.parentNode||b,f)){h.splice(g,1),a=d.length&&h.join("");if(!a)return w.apply(c,x.call(d,0)),c;break}}}return i(a,m)(d,b,f,c,R.test(a)),c}function bq(){}var c,d,e,f,g,h,i,j,k,l,m=!0,n="undefined",o=("sizcache"+Math.random()).replace(".",""),q=String,r=a.document,s=r.documentElement,t=0,u=0,v=[].pop,w=[].push,x=[].slice,y=[].indexOf||function(a){var b=0,c=this.length;for(;b<c;b++)if(this[b]===a)return b;return-1},z=function(a,b){return a[o]=b==null||b,a},A=function(){var a={},b=[];return z(function(c,d){return b.push(c)>e.cacheLength&&delete a[b.shift()],a[c]=d},a)},B=A(),C=A(),D=A(),E="[\\x20\\t\\r\\n\\f]",F="(?:\\\\.|[-\\w]|[^\\x00-\\xa0])+",G=F.replace("w","w#"),H="([*^$|!~]?=)",I="\\["+E+"*("+F+")"+E+"*(?:"+H+E+"*(?:(['\"])((?:\\\\.|[^\\\\])*?)\\3|("+G+")|)|)"+E+"*\\]",J=":("+F+")(?:\\((?:(['\"])((?:\\\\.|[^\\\\])*?)\\2|([^()[\\]]*|(?:(?:"+I+")|[^:]|\\\\.)*|.*))\\)|)",K=":(even|odd|eq|gt|lt|nth|first|last)(?:\\("+E+"*((?:-\\d)?\\d*)"+E+"*\\)|)(?=[^-]|$)",L=new RegExp("^"+E+"+|((?:^|[^\\\\])(?:\\\\.)*)"+E+"+$","g"),M=new RegExp("^"+E+"*,"+E+"*"),N=new RegExp("^"+E+"*([\\x20\\t\\r\\n\\f>+~])"+E+"*"),O=new RegExp(J),P=/^(?:#([\w\-]+)|(\w+)|\.([\w\-]+))$/,Q=/^:not/,R=/[\x20\t\r\n\f]*[+~]/,S=/:not\($/,T=/h\d/i,U=/input|select|textarea|button/i,V=/\\(?!\\)/g,W={ID:new RegExp("^#("+F+")"),CLASS:new RegExp("^\\.("+F+")"),NAME:new RegExp("^\\[name=['\"]?("+F+")['\"]?\\]"),TAG:new RegExp("^("+F.replace("w","w*")+")"),ATTR:new RegExp("^"+I),PSEUDO:new RegExp("^"+J),POS:new RegExp(K,"i"),CHILD:new RegExp("^:(only|nth|first|last)-child(?:\\("+E+"*(even|odd|(([+-]|)(\\d*)n|)"+E+"*(?:([+-]|)"+E+"*(\\d+)|))"+E+"*\\)|)","i"),needsContext:new RegExp("^"+E+"*[>+~]|"+K,"i")},X=function(a){var b=r.createElement("div");try{return a(b)}catch(c){return!1}finally{b=null}},Y=X(function(a){return a.appendChild(r.createComment("")),!a.getElementsByTagName("*").length}),Z=X(function(a){return a.innerHTML="<a href='#'></a>",a.firstChild&&typeof a.firstChild.getAttribute!==n&&a.firstChild.getAttribute("href")==="#"}),$=X(function(a){a.innerHTML="<select></select>";var b=typeof a.lastChild.getAttribute("multiple");return b!=="boolean"&&b!=="string"}),_=X(function(a){return a.innerHTML="<div class='hidden e'></div><div class='hidden'></div>",!a.getElementsByClassName||!a.getElementsByClassName("e").length?!1:(a.lastChild.className="e",a.getElementsByClassName("e").length===2)}),ba=X(function(a){a.id=o+0,a.innerHTML="<a name='"+o+"'></a><div name='"+o+"'></div>",s.insertBefore(a,s.firstChild);var b=r.getElementsByName&&r.getElementsByName(o).length===2+r.getElementsByName(o+0).length;return d=!r.getElementById(o),s.removeChild(a),b});try{x.call(s.childNodes,0)[0].nodeType}catch(bb){x=function(a){var b,c=[];for(;b=this[a];a++)c.push(b);return c}}bc.matches=function(a,b){return bc(a,null,null,b)},bc.matchesSelector=function(a,b){return bc(b,null,null,[a]).length>0},f=bc.getText=function(a){var b,c="",d=0,e=a.nodeType;if(e){if(e===1||e===9||e===11){if(typeof a.textContent=="string")return a.textContent;for(a=a.firstChild;a;a=a.nextSibling)c+=f(a)}else if(e===3||e===4)return a.nodeValue}else for(;b=a[d];d++)c+=f(b);return c},g=bc.isXML=function(a){var b=a&&(a.ownerDocument||a).documentElement;return b?b.nodeName!=="HTML":!1},h=bc.contains=s.contains?function(a,b){var c=a.nodeType===9?a.documentElement:a,d=b&&b.parentNode;return a===d||!!(d&&d.nodeType===1&&c.contains&&c.contains(d))}:s.compareDocumentPosition?function(a,b){return b&&!!(a.compareDocumentPosition(b)&16)}:function(a,b){while(b=b.parentNode)if(b===a)return!0;return!1},bc.attr=function(a,b){var c,d=g(a);return d||(b=b.toLowerCase()),(c=e.attrHandle[b])?c(a):d||$?a.getAttribute(b):(c=a.getAttributeNode(b),c?typeof a[b]=="boolean"?a[b]?b:null:c.specified?c.value:null:null)},e=bc.selectors={cacheLength:50,createPseudo:z,match:W,attrHandle:Z?{}:{href:function(a){return a.getAttribute("href",2)},type:function(a){return a.getAttribute("type")}},find:{ID:d?function(a,b,c){if(typeof b.getElementById!==n&&!c){var d=b.getElementById(a);return d&&d.parentNode?[d]:[]}}:function(a,c,d){if(typeof c.getElementById!==n&&!d){var e=c.getElementById(a);return e?e.id===a||typeof e.getAttributeNode!==n&&e.getAttributeNode("id").value===a?[e]:b:[]}},TAG:Y?function(a,b){if(typeof b.getElementsByTagName!==n)return b.getElementsByTagName(a)}:function(a,b){var c=b.getElementsByTagName(a);if(a==="*"){var d,e=[],f=0;for(;d=c[f];f++)d.nodeType===1&&e.push(d);return e}return c},NAME:ba&&function(a,b){if(typeof b.getElementsByName!==n)return b.getElementsByName(name)},CLASS:_&&function(a,b,c){if(typeof b.getElementsByClassName!==n&&!c)return b.getElementsByClassName(a)}},relative:{">":{dir:"parentNode",first:!0}," ":{dir:"parentNode"},"+":{dir:"previousSibling",first:!0},"~":{dir:"previousSibling"}},preFilter:{ATTR:function(a){return a[1]=a[1].replace(V,""),a[3]=(a[4]||a[5]||"").replace(V,""),a[2]==="~="&&(a[3]=" "+a[3]+" "),a.slice(0,4)},CHILD:function(a){return a[1]=a[1].toLowerCase(),a[1]==="nth"?(a[2]||bc.error(a[0]),a[3]=+(a[3]?a[4]+(a[5]||1):2*(a[2]==="even"||a[2]==="odd")),a[4]=+(a[6]+a[7]||a[2]==="odd")):a[2]&&bc.error(a[0]),a},PSEUDO:function(a){var b,c;if(W.CHILD.test(a[0]))return null;if(a[3])a[2]=a[3];else if(b=a[4])O.test(b)&&(c=bh(b,!0))&&(c=b.indexOf(")",b.length-c)-b.length)&&(b=b.slice(0,c),a[0]=a[0].slice(0,c)),a[2]=b;return a.slice(0,3)}},filter:{ID:d?function(a){return a=a.replace(V,""),function(b){return b.getAttribute("id")===a}}:function(a){return a=a.replace(V,""),function(b){var c=typeof b.getAttributeNode!==n&&b.getAttributeNode("id");return c&&c.value===a}},TAG:function(a){return a==="*"?function(){return!0}:(a=a.replace(V,"").toLowerCase(),function(b){return b.nodeName&&b.nodeName.toLowerCase()===a})},CLASS:function(a){var b=B[o][a];return b||(b=B(a,new RegExp("(^|"+E+")"+a+"("+E+"|$)"))),function(a){return b.test(a.className||typeof a.getAttribute!==n&&a.getAttribute("class")||"")}},ATTR:function(a,b,c){return function(d,e){var f=bc.attr(d,a);return f==null?b==="!=":b?(f+="",b==="="?f===c:b==="!="?f!==c:b==="^="?c&&f.indexOf(c)===0:b==="*="?c&&f.indexOf(c)>-1:b==="$="?c&&f.substr(f.length-c.length)===c:b==="~="?(" "+f+" ").indexOf(c)>-1:b==="|="?f===c||f.substr(0,c.length+1)===c+"-":!1):!0}},CHILD:function(a,b,c,d){return a==="nth"?function(a){var b,e,f=a.parentNode;if(c===1&&d===0)return!0;if(f){e=0;for(b=f.firstChild;b;b=b.nextSibling)if(b.nodeType===1){e++;if(a===b)break}}return e-=d,e===c||e%c===0&&e/c>=0}:function(b){var c=b;switch(a){case"only":case"first":while(c=c.previousSibling)if(c.nodeType===1)return!1;if(a==="first")return!0;c=b;case"last":while(c=c.nextSibling)if(c.nodeType===1)return!1;return!0}}},PSEUDO:function(a,b){var c,d=e.pseudos[a]||e.setFilters[a.toLowerCase()]||bc.error("unsupported pseudo: "+a);return d[o]?d(b):d.length>1?(c=[a,a,"",b],e.setFilters.hasOwnProperty(a.toLowerCase())?z(function(a,c){var e,f=d(a,b),g=f.length;while(g--)e=y.call(a,f[g]),a[e]=!(c[e]=f[g])}):function(a){return d(a,0,c)}):d}},pseudos:{not:z(function(a){var b=[],c=[],d=i(a.replace(L,"$1"));return d[o]?z(function(a,b,c,e){var f,g=d(a,null,e,[]),h=a.length;while(h--)if(f=g[h])a[h]=!(b[h]=f)}):function(a,e,f){return b[0]=a,d(b,null,f,c),!c.pop()}}),has:z(function(a){return function(b){return bc(a,b).length>0}}),contains:z(function(a){return function(b){return(b.textContent||b.innerText||f(b)).indexOf(a)>-1}}),enabled:function(a){return a.disabled===!1},disabled:function(a){return a.disabled===!0},checked:function(a){var b=a.nodeName.toLowerCase();return b==="input"&&!!a.checked||b==="option"&&!!a.selected},selected:function(a){return a.parentNode&&a.parentNode.selectedIndex,a.selected===!0},parent:function(a){return!e.pseudos.empty(a)},empty:function(a){var b;a=a.firstChild;while(a){if(a.nodeName>"@"||(b=a.nodeType)===3||b===4)return!1;a=a.nextSibling}return!0},header:function(a){return T.test(a.nodeName)},text:function(a){var b,c;return a.nodeName.toLowerCase()==="input"&&(b=a.type)==="text"&&((c=a.getAttribute("type"))==null||c.toLowerCase()===b)},radio:bd("radio"),checkbox:bd("checkbox"),file:bd("file"),password:bd("password"),image:bd("image"),submit:be("submit"),reset:be("reset"),button:function(a){var b=a.nodeName.toLowerCase();return b==="input"&&a.type==="button"||b==="button"},input:function(a){return U.test(a.nodeName)},focus:function(a){var b=a.ownerDocument;return a===b.activeElement&&(!b.hasFocus||b.hasFocus())&&(!!a.type||!!a.href)},active:function(a){return a===a.ownerDocument.activeElement},first:bf(function(a,b,c){return[0]}),last:bf(function(a,b,c){return[b-1]}),eq:bf(function(a,b,c){return[c<0?c+b:c]}),even:bf(function(a,b,c){for(var d=0;d<b;d+=2)a.push(d);return a}),odd:bf(function(a,b,c){for(var d=1;d<b;d+=2)a.push(d);return a}),lt:bf(function(a,b,c){for(var d=c<0?c+b:c;--d>=0;)a.push(d);return a}),gt:bf(function(a,b,c){for(var d=c<0?c+b:c;++d<b;)a.push(d);return a})}},j=s.compareDocumentPosition?function(a,b){return a===b?(k=!0,0):(!a.compareDocumentPosition||!b.compareDocumentPosition?a.compareDocumentPosition:a.compareDocumentPosition(b)&4)?-1:1}:function(a,b){if(a===b)return k=!0,0;if(a.sourceIndex&&b.sourceIndex)return a.sourceIndex-b.sourceIndex;var c,d,e=[],f=[],g=a.parentNode,h=b.parentNode,i=g;if(g===h)return bg(a,b);if(!g)return-1;if(!h)return 1;while(i)e.unshift(i),i=i.parentNode;i=h;while(i)f.unshift(i),i=i.parentNode;c=e.length,d=f.length;for(var j=0;j<c&&j<d;j++)if(e[j]!==f[j])return bg(e[j],f[j]);return j===c?bg(a,f[j],-1):bg(e[j],b,1)},[0,0].sort(j),m=!k,bc.uniqueSort=function(a){var b,c=1;k=m,a.sort(j);if(k)for(;b=a[c];c++)b===a[c-1]&&a.splice(c--,1);return a},bc.error=function(a){throw new Error("Syntax error, unrecognized expression: "+a)},i=bc.compile=function(a,b){var c,d=[],e=[],f=D[o][a];if(!f){b||(b=bh(a)),c=b.length;while(c--)f=bm(b[c]),f[o]?d.push(f):e.push(f);f=D(a,bn(e,d))}return f},r.querySelectorAll&&function(){var a,b=bp,c=/'|\\/g,d=/\=[\x20\t\r\n\f]*([^'"\]]*)[\x20\t\r\n\f]*\]/g,e=[":focus"],f=[":active",":focus"],h=s.matchesSelector||s.mozMatchesSelector||s.webkitMatchesSelector||s.oMatchesSelector||s.msMatchesSelector;X(function(a){a.innerHTML="<select><option selected=''></option></select>",a.querySelectorAll("[selected]").length||e.push("\\["+E+"*(?:checked|disabled|ismap|multiple|readonly|selected|value)"),a.querySelectorAll(":checked").length||e.push(":checked")}),X(function(a){a.innerHTML="<p test=''></p>",a.querySelectorAll("[test^='']").length&&e.push("[*^$]="+E+"*(?:\"\"|'')"),a.innerHTML="<input type='hidden'/>",a.querySelectorAll(":enabled").length||e.push(":enabled",":disabled")}),e=new RegExp(e.join("|")),bp=function(a,d,f,g,h){if(!g&&!h&&(!e||!e.test(a))){var i,j,k=!0,l=o,m=d,n=d.nodeType===9&&a;if(d.nodeType===1&&d.nodeName.toLowerCase()!=="object"){i=bh(a),(k=d.getAttribute("id"))?l=k.replace(c,"\\$&"):d.setAttribute("id",l),l="[id='"+l+"'] ",j=i.length;while(j--)i[j]=l+i[j].join("");m=R.test(a)&&d.parentNode||d,n=i.join(",")}if(n)try{return w.apply(f,x.call(m.querySelectorAll(n),0)),f}catch(p){}finally{k||d.removeAttribute("id")}}return b(a,d,f,g,h)},h&&(X(function(b){a=h.call(b,"div");try{h.call(b,"[test!='']:sizzle"),f.push("!=",J)}catch(c){}}),f=new RegExp(f.join("|")),bc.matchesSelector=function(b,c){c=c.replace(d,"='$1']");if(!g(b)&&!f.test(c)&&(!e||!e.test(c)))try{var i=h.call(b,c);if(i||a||b.document&&b.document.nodeType!==11)return i}catch(j){}return bc(c,null,null,[b]).length>0})}(),e.pseudos.nth=e.pseudos.eq,e.filters=bq.prototype=e.pseudos,e.setFilters=new bq,bc.attr=p.attr,p.find=bc,p.expr=bc.selectors,p.expr[":"]=p.expr.pseudos,p.unique=bc.uniqueSort,p.text=bc.getText,p.isXMLDoc=bc.isXML,p.contains=bc.contains}(a);var bc=/Until$/,bd=/^(?:parents|prev(?:Until|All))/,be=/^.[^:#\[\.,]*$/,bf=p.expr.match.needsContext,bg={children:!0,contents:!0,next:!0,prev:!0};p.fn.extend({find:function(a){var b,c,d,e,f,g,h=this;if(typeof a!="string")return p(a).filter(function(){for(b=0,c=h.length;b<c;b++)if(p.contains(h[b],this))return!0});g=this.pushStack("","find",a);for(b=0,c=this.length;b<c;b++){d=g.length,p.find(a,this[b],g);if(b>0)for(e=d;e<g.length;e++)for(f=0;f<d;f++)if(g[f]===g[e]){g.splice(e--,1);break}}return g},has:function(a){var b,c=p(a,this),d=c.length;return this.filter(function(){for(b=0;b<d;b++)if(p.contains(this,c[b]))return!0})},not:function(a){return this.pushStack(bj(this,a,!1),"not",a)},filter:function(a){return this.pushStack(bj(this,a,!0),"filter",a)},is:function(a){return!!a&&(typeof a=="string"?bf.test(a)?p(a,this.context).index(this[0])>=0:p.filter(a,this).length>0:this.filter(a).length>0)},closest:function(a,b){var c,d=0,e=this.length,f=[],g=bf.test(a)||typeof a!="string"?p(a,b||this.context):0;for(;d<e;d++){c=this[d];while(c&&c.ownerDocument&&c!==b&&c.nodeType!==11){if(g?g.index(c)>-1:p.find.matchesSelector(c,a)){f.push(c);break}c=c.parentNode}}return f=f.length>1?p.unique(f):f,this.pushStack(f,"closest",a)},index:function(a){return a?typeof a=="string"?p.inArray(this[0],p(a)):p.inArray(a.jquery?a[0]:a,this):this[0]&&this[0].parentNode?this.prevAll().length:-1},add:function(a,b){var c=typeof a=="string"?p(a,b):p.makeArray(a&&a.nodeType?[a]:a),d=p.merge(this.get(),c);return this.pushStack(bh(c[0])||bh(d[0])?d:p.unique(d))},addBack:function(a){return this.add(a==null?this.prevObject:this.prevObject.filter(a))}}),p.fn.andSelf=p.fn.addBack,p.each({parent:function(a){var b=a.parentNode;return b&&b.nodeType!==11?b:null},parents:function(a){return p.dir(a,"parentNode")},parentsUntil:function(a,b,c){return p.dir(a,"parentNode",c)},next:function(a){return bi(a,"nextSibling")},prev:function(a){return bi(a,"previousSibling")},nextAll:function(a){return p.dir(a,"nextSibling")},prevAll:function(a){return p.dir(a,"previousSibling")},nextUntil:function(a,b,c){return p.dir(a,"nextSibling",c)},prevUntil:function(a,b,c){return p.dir(a,"previousSibling",c)},siblings:function(a){return p.sibling((a.parentNode||{}).firstChild,a)},children:function(a){return p.sibling(a.firstChild)},contents:function(a){return p.nodeName(a,"iframe")?a.contentDocument||a.contentWindow.document:p.merge([],a.childNodes)}},function(a,b){p.fn[a]=function(c,d){var e=p.map(this,b,c);return bc.test(a)||(d=c),d&&typeof d=="string"&&(e=p.filter(d,e)),e=this.length>1&&!bg[a]?p.unique(e):e,this.length>1&&bd.test(a)&&(e=e.reverse()),this.pushStack(e,a,k.call(arguments).join(","))}}),p.extend({filter:function(a,b,c){return c&&(a=":not("+a+")"),b.length===1?p.find.matchesSelector(b[0],a)?[b[0]]:[]:p.find.matches(a,b)},dir:function(a,c,d){var e=[],f=a[c];while(f&&f.nodeType!==9&&(d===b||f.nodeType!==1||!p(f).is(d)))f.nodeType===1&&e.push(f),f=f[c];return e},sibling:function(a,b){var c=[];for(;a;a=a.nextSibling)a.nodeType===1&&a!==b&&c.push(a);return c}});var bl="abbr|article|aside|audio|bdi|canvas|data|datalist|details|figcaption|figure|footer|header|hgroup|mark|meter|nav|output|progress|section|summary|time|video",bm=/ jQuery\d+="(?:null|\d+)"/g,bn=/^\s+/,bo=/<(?!area|br|col|embed|hr|img|input|link|meta|param)(([\w:]+)[^>]*)\/>/gi,bp=/<([\w:]+)/,bq=/<tbody/i,br=/<|&#?\w+;/,bs=/<(?:script|style|link)/i,bt=/<(?:script|object|embed|option|style)/i,bu=new RegExp("<(?:"+bl+")[\\s/>]","i"),bv=/^(?:checkbox|radio)$/,bw=/checked\s*(?:[^=]|=\s*.checked.)/i,bx=/\/(java|ecma)script/i,by=/^\s*<!(?:\[CDATA\[|\-\-)|[\]\-]{2}>\s*$/g,bz={option:[1,"<select multiple='multiple'>","</select>"],legend:[1,"<fieldset>","</fieldset>"],thead:[1,"<table>","</table>"],tr:[2,"<table><tbody>","</tbody></table>"],td:[3,"<table><tbody><tr>","</tr></tbody></table>"],col:[2,"<table><tbody></tbody><colgroup>","</colgroup></table>"],area:[1,"<map>","</map>"],_default:[0,"",""]},bA=bk(e),bB=bA.appendChild(e.createElement("div"));bz.optgroup=bz.option,bz.tbody=bz.tfoot=bz.colgroup=bz.caption=bz.thead,bz.th=bz.td,p.support.htmlSerialize||(bz._default=[1,"X<div>","</div>"]),p.fn.extend({text:function(a){return p.access(this,function(a){return a===b?p.text(this):this.empty().append((this[0]&&this[0].ownerDocument||e).createTextNode(a))},null,a,arguments.length)},wrapAll:function(a){if(p.isFunction(a))return this.each(function(b){p(this).wrapAll(a.call(this,b))});if(this[0]){var b=p(a,this[0].ownerDocument).eq(0).clone(!0);this[0].parentNode&&b.insertBefore(this[0]),b.map(function(){var a=this;while(a.firstChild&&a.firstChild.nodeType===1)a=a.firstChild;return a}).append(this)}return this},wrapInner:function(a){return p.isFunction(a)?this.each(function(b){p(this).wrapInner(a.call(this,b))}):this.each(function(){var b=p(this),c=b.contents();c.length?c.wrapAll(a):b.append(a)})},wrap:function(a){var b=p.isFunction(a);return this.each(function(c){p(this).wrapAll(b?a.call(this,c):a)})},unwrap:function(){return this.parent().each(function(){p.nodeName(this,"body")||p(this).replaceWith(this.childNodes)}).end()},append:function(){return this.domManip(arguments,!0,function(a){(this.nodeType===1||this.nodeType===11)&&this.appendChild(a)})},prepend:function(){return this.domManip(arguments,!0,function(a){(this.nodeType===1||this.nodeType===11)&&this.insertBefore(a,this.firstChild)})},before:function(){if(!bh(this[0]))return this.domManip(arguments,!1,function(a){this.parentNode.insertBefore(a,this)});if(arguments.length){var a=p.clean(arguments);return this.pushStack(p.merge(a,this),"before",this.selector)}},after:function(){if(!bh(this[0]))return this.domManip(arguments,!1,function(a){this.parentNode.insertBefore(a,this.nextSibling)});if(arguments.length){var a=p.clean(arguments);return this.pushStack(p.merge(this,a),"after",this.selector)}},remove:function(a,b){var c,d=0;for(;(c=this[d])!=null;d++)if(!a||p.filter(a,[c]).length)!b&&c.nodeType===1&&(p.cleanData(c.getElementsByTagName("*")),p.cleanData([c])),c.parentNode&&c.parentNode.removeChild(c);return this},empty:function(){var a,b=0;for(;(a=this[b])!=null;b++){a.nodeType===1&&p.cleanData(a.getElementsByTagName("*"));while(a.firstChild)a.removeChild(a.firstChild)}return this},clone:function(a,b){return a=a==null?!1:a,b=b==null?a:b,this.map(function(){return p.clone(this,a,b)})},html:function(a){return p.access(this,function(a){var c=this[0]||{},d=0,e=this.length;if(a===b)return c.nodeType===1?c.innerHTML.replace(bm,""):b;if(typeof a=="string"&&!bs.test(a)&&(p.support.htmlSerialize||!bu.test(a))&&(p.support.leadingWhitespace||!bn.test(a))&&!bz[(bp.exec(a)||["",""])[1].toLowerCase()]){a=a.replace(bo,"<$1></$2>");try{for(;d<e;d++)c=this[d]||{},c.nodeType===1&&(p.cleanData(c.getElementsByTagName("*")),c.innerHTML=a);c=0}catch(f){}}c&&this.empty().append(a)},null,a,arguments.length)},replaceWith:function(a){return bh(this[0])?this.length?this.pushStack(p(p.isFunction(a)?a():a),"replaceWith",a):this:p.isFunction(a)?this.each(function(b){var c=p(this),d=c.html();c.replaceWith(a.call(this,b,d))}):(typeof a!="string"&&(a=p(a).detach()),this.each(function(){var b=this.nextSibling,c=this.parentNode;p(this).remove(),b?p(b).before(a):p(c).append(a)}))},detach:function(a){return this.remove(a,!0)},domManip:function(a,c,d){a=[].concat.apply([],a);var e,f,g,h,i=0,j=a[0],k=[],l=this.length;if(!p.support.checkClone&&l>1&&typeof j=="string"&&bw.test(j))return this.each(function(){p(this).domManip(a,c,d)});if(p.isFunction(j))return this.each(function(e){var f=p(this);a[0]=j.call(this,e,c?f.html():b),f.domManip(a,c,d)});if(this[0]){e=p.buildFragment(a,this,k),g=e.fragment,f=g.firstChild,g.childNodes.length===1&&(g=f);if(f){c=c&&p.nodeName(f,"tr");for(h=e.cacheable||l-1;i<l;i++)d.call(c&&p.nodeName(this[i],"table")?bC(this[i],"tbody"):this[i],i===h?g:p.clone(g,!0,!0))}g=f=null,k.length&&p.each(k,function(a,b){b.src?p.ajax?p.ajax({url:b.src,type:"GET",dataType:"script",async:!1,global:!1,"throws":!0}):p.error("no ajax"):p.globalEval((b.text||b.textContent||b.innerHTML||"").replace(by,"")),b.parentNode&&b.parentNode.removeChild(b)})}return this}}),p.buildFragment=function(a,c,d){var f,g,h,i=a[0];return c=c||e,c=!c.nodeType&&c[0]||c,c=c.ownerDocument||c,a.length===1&&typeof i=="string"&&i.length<512&&c===e&&i.charAt(0)==="<"&&!bt.test(i)&&(p.support.checkClone||!bw.test(i))&&(p.support.html5Clone||!bu.test(i))&&(g=!0,f=p.fragments[i],h=f!==b),f||(f=c.createDocumentFragment(),p.clean(a,c,f,d),g&&(p.fragments[i]=h&&f)),{fragment:f,cacheable:g}},p.fragments={},p.each({appendTo:"append",prependTo:"prepend",insertBefore:"before",insertAfter:"after",replaceAll:"replaceWith"},function(a,b){p.fn[a]=function(c){var d,e=0,f=[],g=p(c),h=g.length,i=this.length===1&&this[0].parentNode;if((i==null||i&&i.nodeType===11&&i.childNodes.length===1)&&h===1)return g[b](this[0]),this;for(;e<h;e++)d=(e>0?this.clone(!0):this).get(),p(g[e])[b](d),f=f.concat(d);return this.pushStack(f,a,g.selector)}}),p.extend({clone:function(a,b,c){var d,e,f,g;p.support.html5Clone||p.isXMLDoc(a)||!bu.test("<"+a.nodeName+">")?g=a.cloneNode(!0):(bB.innerHTML=a.outerHTML,bB.removeChild(g=bB.firstChild));if((!p.support.noCloneEvent||!p.support.noCloneChecked)&&(a.nodeType===1||a.nodeType===11)&&!p.isXMLDoc(a)){bE(a,g),d=bF(a),e=bF(g);for(f=0;d[f];++f)e[f]&&bE(d[f],e[f])}if(b){bD(a,g);if(c){d=bF(a),e=bF(g);for(f=0;d[f];++f)bD(d[f],e[f])}}return d=e=null,g},clean:function(a,b,c,d){var f,g,h,i,j,k,l,m,n,o,q,r,s=b===e&&bA,t=[];if(!b||typeof b.createDocumentFragment=="undefined")b=e;for(f=0;(h=a[f])!=null;f++){typeof h=="number"&&(h+="");if(!h)continue;if(typeof h=="string")if(!br.test(h))h=b.createTextNode(h);else{s=s||bk(b),l=b.createElement("div"),s.appendChild(l),h=h.replace(bo,"<$1></$2>"),i=(bp.exec(h)||["",""])[1].toLowerCase(),j=bz[i]||bz._default,k=j[0],l.innerHTML=j[1]+h+j[2];while(k--)l=l.lastChild;if(!p.support.tbody){m=bq.test(h),n=i==="table"&&!m?l.firstChild&&l.firstChild.childNodes:j[1]==="<table>"&&!m?l.childNodes:[];for(g=n.length-1;g>=0;--g)p.nodeName(n[g],"tbody")&&!n[g].childNodes.length&&n[g].parentNode.removeChild(n[g])}!p.support.leadingWhitespace&&bn.test(h)&&l.insertBefore(b.createTextNode(bn.exec(h)[0]),l.firstChild),h=l.childNodes,l.parentNode.removeChild(l)}h.nodeType?t.push(h):p.merge(t,h)}l&&(h=l=s=null);if(!p.support.appendChecked)for(f=0;(h=t[f])!=null;f++)p.nodeName(h,"input")?bG(h):typeof h.getElementsByTagName!="undefined"&&p.grep(h.getElementsByTagName("input"),bG);if(c){q=function(a){if(!a.type||bx.test(a.type))return d?d.push(a.parentNode?a.parentNode.removeChild(a):a):c.appendChild(a)};for(f=0;(h=t[f])!=null;f++)if(!p.nodeName(h,"script")||!q(h))c.appendChild(h),typeof h.getElementsByTagName!="undefined"&&(r=p.grep(p.merge([],h.getElementsByTagName("script")),q),t.splice.apply(t,[f+1,0].concat(r)),f+=r.length)}return t},cleanData:function(a,b){var c,d,e,f,g=0,h=p.expando,i=p.cache,j=p.support.deleteExpando,k=p.event.special;for(;(e=a[g])!=null;g++)if(b||p.acceptData(e)){d=e[h],c=d&&i[d];if(c){if(c.events)for(f in c.events)k[f]?p.event.remove(e,f):p.removeEvent(e,f,c.handle);i[d]&&(delete i[d],j?delete e[h]:e.removeAttribute?e.removeAttribute(h):e[h]=null,p.deletedIds.push(d))}}}}),function(){var a,b;p.uaMatch=function(a){a=a.toLowerCase();var b=/(chrome)[ \/]([\w.]+)/.exec(a)||/(webkit)[ \/]([\w.]+)/.exec(a)||/(opera)(?:.*version|)[ \/]([\w.]+)/.exec(a)||/(msie) ([\w.]+)/.exec(a)||a.indexOf("compatible")<0&&/(mozilla)(?:.*? rv:([\w.]+)|)/.exec(a)||[];return{browser:b[1]||"",version:b[2]||"0"}},a=p.uaMatch(g.userAgent),b={},a.browser&&(b[a.browser]=!0,b.version=a.version),b.chrome?b.webkit=!0:b.webkit&&(b.safari=!0),p.browser=b,p.sub=function(){function a(b,c){return new a.fn.init(b,c)}p.extend(!0,a,this),a.superclass=this,a.fn=a.prototype=this(),a.fn.constructor=a,a.sub=this.sub,a.fn.init=function c(c,d){return d&&d instanceof p&&!(d instanceof a)&&(d=a(d)),p.fn.init.call(this,c,d,b)},a.fn.init.prototype=a.fn;var b=a(e);return a}}();var bH,bI,bJ,bK=/alpha\([^)]*\)/i,bL=/opacity=([^)]*)/,bM=/^(top|right|bottom|left)$/,bN=/^(none|table(?!-c[ea]).+)/,bO=/^margin/,bP=new RegExp("^("+q+")(.*)$","i"),bQ=new RegExp("^("+q+")(?!px)[a-z%]+$","i"),bR=new RegExp("^([-+])=("+q+")","i"),bS={},bT={position:"absolute",visibility:"hidden",display:"block"},bU={letterSpacing:0,fontWeight:400},bV=["Top","Right","Bottom","Left"],bW=["Webkit","O","Moz","ms"],bX=p.fn.toggle;p.fn.extend({css:function(a,c){return p.access(this,function(a,c,d){return d!==b?p.style(a,c,d):p.css(a,c)},a,c,arguments.length>1)},show:function(){return b$(this,!0)},hide:function(){return b$(this)},toggle:function(a,b){var c=typeof a=="boolean";return p.isFunction(a)&&p.isFunction(b)?bX.apply(this,arguments):this.each(function(){(c?a:bZ(this))?p(this).show():p(this).hide()})}}),p.extend({cssHooks:{opacity:{get:function(a,b){if(b){var c=bH(a,"opacity");return c===""?"1":c}}}},cssNumber:{fillOpacity:!0,fontWeight:!0,lineHeight:!0,opacity:!0,orphans:!0,widows:!0,zIndex:!0,zoom:!0},cssProps:{"float":p.support.cssFloat?"cssFloat":"styleFloat"},style:function(a,c,d,e){if(!a||a.nodeType===3||a.nodeType===8||!a.style)return;var f,g,h,i=p.camelCase(c),j=a.style;c=p.cssProps[i]||(p.cssProps[i]=bY(j,i)),h=p.cssHooks[c]||p.cssHooks[i];if(d===b)return h&&"get"in h&&(f=h.get(a,!1,e))!==b?f:j[c];g=typeof d,g==="string"&&(f=bR.exec(d))&&(d=(f[1]+1)*f[2]+parseFloat(p.css(a,c)),g="number");if(d==null||g==="number"&&isNaN(d))return;g==="number"&&!p.cssNumber[i]&&(d+="px");if(!h||!("set"in h)||(d=h.set(a,d,e))!==b)try{j[c]=d}catch(k){}},css:function(a,c,d,e){var f,g,h,i=p.camelCase(c);return c=p.cssProps[i]||(p.cssProps[i]=bY(a.style,i)),h=p.cssHooks[c]||p.cssHooks[i],h&&"get"in h&&(f=h.get(a,!0,e)),f===b&&(f=bH(a,c)),f==="normal"&&c in bU&&(f=bU[c]),d||e!==b?(g=parseFloat(f),d||p.isNumeric(g)?g||0:f):f},swap:function(a,b,c){var d,e,f={};for(e in b)f[e]=a.style[e],a.style[e]=b[e];d=c.call(a);for(e in b)a.style[e]=f[e];return d}}),a.getComputedStyle?bH=function(b,c){var d,e,f,g,h=a.getComputedStyle(b,null),i=b.style;return h&&(d=h[c],d===""&&!p.contains(b.ownerDocument,b)&&(d=p.style(b,c)),bQ.test(d)&&bO.test(c)&&(e=i.width,f=i.minWidth,g=i.maxWidth,i.minWidth=i.maxWidth=i.width=d,d=h.width,i.width=e,i.minWidth=f,i.maxWidth=g)),d}:e.documentElement.currentStyle&&(bH=function(a,b){var c,d,e=a.currentStyle&&a.currentStyle[b],f=a.style;return e==null&&f&&f[b]&&(e=f[b]),bQ.test(e)&&!bM.test(b)&&(c=f.left,d=a.runtimeStyle&&a.runtimeStyle.left,d&&(a.runtimeStyle.left=a.currentStyle.left),f.left=b==="fontSize"?"1em":e,e=f.pixelLeft+"px",f.left=c,d&&(a.runtimeStyle.left=d)),e===""?"auto":e}),p.each(["height","width"],function(a,b){p.cssHooks[b]={get:function(a,c,d){if(c)return a.offsetWidth===0&&bN.test(bH(a,"display"))?p.swap(a,bT,function(){return cb(a,b,d)}):cb(a,b,d)},set:function(a,c,d){return b_(a,c,d?ca(a,b,d,p.support.boxSizing&&p.css(a,"boxSizing")==="border-box"):0)}}}),p.support.opacity||(p.cssHooks.opacity={get:function(a,b){return bL.test((b&&a.currentStyle?a.currentStyle.filter:a.style.filter)||"")?.01*parseFloat(RegExp.$1)+"":b?"1":""},set:function(a,b){var c=a.style,d=a.currentStyle,e=p.isNumeric(b)?"alpha(opacity="+b*100+")":"",f=d&&d.filter||c.filter||"";c.zoom=1;if(b>=1&&p.trim(f.replace(bK,""))===""&&c.removeAttribute){c.removeAttribute("filter");if(d&&!d.filter)return}c.filter=bK.test(f)?f.replace(bK,e):f+" "+e}}),p(function(){p.support.reliableMarginRight||(p.cssHooks.marginRight={get:function(a,b){return p.swap(a,{display:"inline-block"},function(){if(b)return bH(a,"marginRight")})}}),!p.support.pixelPosition&&p.fn.position&&p.each(["top","left"],function(a,b){p.cssHooks[b]={get:function(a,c){if(c){var d=bH(a,b);return bQ.test(d)?p(a).position()[b]+"px":d}}}})}),p.expr&&p.expr.filters&&(p.expr.filters.hidden=function(a){return a.offsetWidth===0&&a.offsetHeight===0||!p.support.reliableHiddenOffsets&&(a.style&&a.style.display||bH(a,"display"))==="none"},p.expr.filters.visible=function(a){return!p.expr.filters.hidden(a)}),p.each({margin:"",padding:"",border:"Width"},function(a,b){p.cssHooks[a+b]={expand:function(c){var d,e=typeof c=="string"?c.split(" "):[c],f={};for(d=0;d<4;d++)f[a+bV[d]+b]=e[d]||e[d-2]||e[0];return f}},bO.test(a)||(p.cssHooks[a+b].set=b_)});var cd=/%20/g,ce=/\[\]$/,cf=/\r?\n/g,cg=/^(?:color|date|datetime|datetime-local|email|hidden|month|number|password|range|search|tel|text|time|url|week)$/i,ch=/^(?:select|textarea)/i;p.fn.extend({serialize:function(){return p.param(this.serializeArray())},serializeArray:function(){return this.map(function(){return this.elements?p.makeArray(this.elements):this}).filter(function(){return this.name&&!this.disabled&&(this.checked||ch.test(this.nodeName)||cg.test(this.type))}).map(function(a,b){var c=p(this).val();return c==null?null:p.isArray(c)?p.map(c,function(a,c){return{name:b.name,value:a.replace(cf,"\r\n")}}):{name:b.name,value:c.replace(cf,"\r\n")}}).get()}}),p.param=function(a,c){var d,e=[],f=function(a,b){b=p.isFunction(b)?b():b==null?"":b,e[e.length]=encodeURIComponent(a)+"="+encodeURIComponent(b)};c===b&&(c=p.ajaxSettings&&p.ajaxSettings.traditional);if(p.isArray(a)||a.jquery&&!p.isPlainObject(a))p.each(a,function(){f(this.name,this.value)});else for(d in a)ci(d,a[d],c,f);return e.join("&").replace(cd,"+")};var cj,ck,cl=/#.*$/,cm=/^(.*?):[ \t]*([^\r\n]*)\r?$/mg,cn=/^(?:about|app|app\-storage|.+\-extension|file|res|widget):$/,co=/^(?:GET|HEAD)$/,cp=/^\/\//,cq=/\?/,cr=/<script\b[^<]*(?:(?!<\/script>)<[^<]*)*<\/script>/gi,cs=/([?&])_=[^&]*/,ct=/^([\w\+\.\-]+:)(?:\/\/([^\/?#:]*)(?::(\d+)|)|)/,cu=p.fn.load,cv={},cw={},cx=["*/"]+["*"];try{ck=f.href}catch(cy){ck=e.createElement("a"),ck.href="",ck=ck.href}cj=ct.exec(ck.toLowerCase())||[],p.fn.load=function(a,c,d){if(typeof a!="string"&&cu)return cu.apply(this,arguments);if(!this.length)return this;var e,f,g,h=this,i=a.indexOf(" ");return i>=0&&(e=a.slice(i,a.length),a=a.slice(0,i)),p.isFunction(c)?(d=c,c=b):c&&typeof c=="object"&&(f="POST"),p.ajax({url:a,type:f,dataType:"html",data:c,complete:function(a,b){d&&h.each(d,g||[a.responseText,b,a])}}).done(function(a){g=arguments,h.html(e?p("<div>").append(a.replace(cr,"")).find(e):a)}),this},p.each("ajaxStart ajaxStop ajaxComplete ajaxError ajaxSuccess ajaxSend".split(" "),function(a,b){p.fn[b]=function(a){return this.on(b,a)}}),p.each(["get","post"],function(a,c){p[c]=function(a,d,e,f){return p.isFunction(d)&&(f=f||e,e=d,d=b),p.ajax({type:c,url:a,data:d,success:e,dataType:f})}}),p.extend({getScript:function(a,c){return p.get(a,b,c,"script")},getJSON:function(a,b,c){return p.get(a,b,c,"json")},ajaxSetup:function(a,b){return b?cB(a,p.ajaxSettings):(b=a,a=p.ajaxSettings),cB(a,b),a},ajaxSettings:{url:ck,isLocal:cn.test(cj[1]),global:!0,type:"GET",contentType:"application/x-www-form-urlencoded; charset=UTF-8",processData:!0,async:!0,accepts:{xml:"application/xml, text/xml",html:"text/html",text:"text/plain",json:"application/json, text/javascript","*":cx},contents:{xml:/xml/,html:/html/,json:/json/},responseFields:{xml:"responseXML",text:"responseText"},converters:{"* text":a.String,"text html":!0,"text json":p.parseJSON,"text xml":p.parseXML},flatOptions:{context:!0,url:!0}},ajaxPrefilter:cz(cv),ajaxTransport:cz(cw),ajax:function(a,c){function y(a,c,f,i){var k,s,t,u,w,y=c;if(v===2)return;v=2,h&&clearTimeout(h),g=b,e=i||"",x.readyState=a>0?4:0,f&&(u=cC(l,x,f));if(a>=200&&a<300||a===304)l.ifModified&&(w=x.getResponseHeader("Last-Modified"),w&&(p.lastModified[d]=w),w=x.getResponseHeader("Etag"),w&&(p.etag[d]=w)),a===304?(y="notmodified",k=!0):(k=cD(l,u),y=k.state,s=k.data,t=k.error,k=!t);else{t=y;if(!y||a)y="error",a<0&&(a=0)}x.status=a,x.statusText=(c||y)+"",k?o.resolveWith(m,[s,y,x]):o.rejectWith(m,[x,y,t]),x.statusCode(r),r=b,j&&n.trigger("ajax"+(k?"Success":"Error"),[x,l,k?s:t]),q.fireWith(m,[x,y]),j&&(n.trigger("ajaxComplete",[x,l]),--p.active||p.event.trigger("ajaxStop"))}typeof a=="object"&&(c=a,a=b),c=c||{};var d,e,f,g,h,i,j,k,l=p.ajaxSetup({},c),m=l.context||l,n=m!==l&&(m.nodeType||m instanceof p)?p(m):p.event,o=p.Deferred(),q=p.Callbacks("once memory"),r=l.statusCode||{},t={},u={},v=0,w="canceled",x={readyState:0,setRequestHeader:function(a,b){if(!v){var c=a.toLowerCase();a=u[c]=u[c]||a,t[a]=b}return this},getAllResponseHeaders:function(){return v===2?e:null},getResponseHeader:function(a){var c;if(v===2){if(!f){f={};while(c=cm.exec(e))f[c[1].toLowerCase()]=c[2]}c=f[a.toLowerCase()]}return c===b?null:c},overrideMimeType:function(a){return v||(l.mimeType=a),this},abort:function(a){return a=a||w,g&&g.abort(a),y(0,a),this}};o.promise(x),x.success=x.done,x.error=x.fail,x.complete=q.add,x.statusCode=function(a){if(a){var b;if(v<2)for(b in a)r[b]=[r[b],a[b]];else b=a[x.status],x.always(b)}return this},l.url=((a||l.url)+"").replace(cl,"").replace(cp,cj[1]+"//"),l.dataTypes=p.trim(l.dataType||"*").toLowerCase().split(s),l.crossDomain==null&&(i=ct.exec(l.url.toLowerCase())||!1,l.crossDomain=i&&i.join(":")+(i[3]?"":i[1]==="http:"?80:443)!==cj.join(":")+(cj[3]?"":cj[1]==="http:"?80:443)),l.data&&l.processData&&typeof l.data!="string"&&(l.data=p.param(l.data,l.traditional)),cA(cv,l,c,x);if(v===2)return x;j=l.global,l.type=l.type.toUpperCase(),l.hasContent=!co.test(l.type),j&&p.active++===0&&p.event.trigger("ajaxStart");if(!l.hasContent){l.data&&(l.url+=(cq.test(l.url)?"&":"?")+l.data,delete l.data),d=l.url;if(l.cache===!1){var z=p.now(),A=l.url.replace(cs,"$1_="+z);l.url=A+(A===l.url?(cq.test(l.url)?"&":"?")+"_="+z:"")}}(l.data&&l.hasContent&&l.contentType!==!1||c.contentType)&&x.setRequestHeader("Content-Type",l.contentType),l.ifModified&&(d=d||l.url,p.lastModified[d]&&x.setRequestHeader("If-Modified-Since",p.lastModified[d]),p.etag[d]&&x.setRequestHeader("If-None-Match",p.etag[d])),x.setRequestHeader("Accept",l.dataTypes[0]&&l.accepts[l.dataTypes[0]]?l.accepts[l.dataTypes[0]]+(l.dataTypes[0]!=="*"?", "+cx+"; q=0.01":""):l.accepts["*"]);for(k in l.headers)x.setRequestHeader(k,l.headers[k]);if(!l.beforeSend||l.beforeSend.call(m,x,l)!==!1&&v!==2){w="abort";for(k in{success:1,error:1,complete:1})x[k](l[k]);g=cA(cw,l,c,x);if(!g)y(-1,"No Transport");else{x.readyState=1,j&&n.trigger("ajaxSend",[x,l]),l.async&&l.timeout>0&&(h=setTimeout(function(){x.abort("timeout")},l.timeout));try{v=1,g.send(t,y)}catch(B){if(v<2)y(-1,B);else throw B}}return x}return x.abort()},active:0,lastModified:{},etag:{}});var cE=[],cF=/\?/,cG=/(=)\?(?=&|$)|\?\?/,cH=p.now();p.ajaxSetup({jsonp:"callback",jsonpCallback:function(){var a=cE.pop()||p.expando+"_"+cH++;return this[a]=!0,a}}),p.ajaxPrefilter("json jsonp",function(c,d,e){var f,g,h,i=c.data,j=c.url,k=c.jsonp!==!1,l=k&&cG.test(j),m=k&&!l&&typeof i=="string"&&!(c.contentType||"").indexOf("application/x-www-form-urlencoded")&&cG.test(i);if(c.dataTypes[0]==="jsonp"||l||m)return f=c.jsonpCallback=p.isFunction(c.jsonpCallback)?c.jsonpCallback():c.jsonpCallback,g=a[f],l?c.url=j.replace(cG,"$1"+f):m?c.data=i.replace(cG,"$1"+f):k&&(c.url+=(cF.test(j)?"&":"?")+c.jsonp+"="+f),c.converters["script json"]=function(){return h||p.error(f+" was not called"),h[0]},c.dataTypes[0]="json",a[f]=function(){h=arguments},e.always(function(){a[f]=g,c[f]&&(c.jsonpCallback=d.jsonpCallback,cE.push(f)),h&&p.isFunction(g)&&g(h[0]),h=g=b}),"script"}),p.ajaxSetup({accepts:{script:"text/javascript, application/javascript, application/ecmascript, application/x-ecmascript"},contents:{script:/javascript|ecmascript/},converters:{"text script":function(a){return p.globalEval(a),a}}}),p.ajaxPrefilter("script",function(a){a.cache===b&&(a.cache=!1),a.crossDomain&&(a.type="GET",a.global=!1)}),p.ajaxTransport("script",function(a){if(a.crossDomain){var c,d=e.head||e.getElementsByTagName("head")[0]||e.documentElement;return{send:function(f,g){c=e.createElement("script"),c.async="async",a.scriptCharset&&(c.charset=a.scriptCharset),c.src=a.url,c.onload=c.onreadystatechange=function(a,e){if(e||!c.readyState||/loaded|complete/.test(c.readyState))c.onload=c.onreadystatechange=null,d&&c.parentNode&&d.removeChild(c),c=b,e||g(200,"success")},d.insertBefore(c,d.firstChild)},abort:function(){c&&c.onload(0,1)}}}});var cI,cJ=a.ActiveXObject?function(){for(var a in cI)cI[a](0,1)}:!1,cK=0;p.ajaxSettings.xhr=a.ActiveXObject?function(){return!this.isLocal&&cL()||cM()}:cL,function(a){p.extend(p.support,{ajax:!!a,cors:!!a&&"withCredentials"in a})}(p.ajaxSettings.xhr()),p.support.ajax&&p.ajaxTransport(function(c){if(!c.crossDomain||p.support.cors){var d;return{send:function(e,f){var g,h,i=c.xhr();c.username?i.open(c.type,c.url,c.async,c.username,c.password):i.open(c.type,c.url,c.async);if(c.xhrFields)for(h in c.xhrFields)i[h]=c.xhrFields[h];c.mimeType&&i.overrideMimeType&&i.overrideMimeType(c.mimeType),!c.crossDomain&&!e["X-Requested-With"]&&(e["X-Requested-With"]="XMLHttpRequest");try{for(h in e)i.setRequestHeader(h,e[h])}catch(j){}i.send(c.hasContent&&c.data||null),d=function(a,e){var h,j,k,l,m;try{if(d&&(e||i.readyState===4)){d=b,g&&(i.onreadystatechange=p.noop,cJ&&delete cI[g]);if(e)i.readyState!==4&&i.abort();else{h=i.status,k=i.getAllResponseHeaders(),l={},m=i.responseXML,m&&m.documentElement&&(l.xml=m);try{l.text=i.responseText}catch(a){}try{j=i.statusText}catch(n){j=""}!h&&c.isLocal&&!c.crossDomain?h=l.text?200:404:h===1223&&(h=204)}}}catch(o){e||f(-1,o)}l&&f(h,j,l,k)},c.async?i.readyState===4?setTimeout(d,0):(g=++cK,cJ&&(cI||(cI={},p(a).unload(cJ)),cI[g]=d),i.onreadystatechange=d):d()},abort:function(){d&&d(0,1)}}}});var cN,cO,cP=/^(?:toggle|show|hide)$/,cQ=new RegExp("^(?:([-+])=|)("+q+")([a-z%]*)$","i"),cR=/queueHooks$/,cS=[cY],cT={"*":[function(a,b){var c,d,e=this.createTween(a,b),f=cQ.exec(b),g=e.cur(),h=+g||0,i=1,j=20;if(f){c=+f[2],d=f[3]||(p.cssNumber[a]?"":"px");if(d!=="px"&&h){h=p.css(e.elem,a,!0)||c||1;do i=i||".5",h=h/i,p.style(e.elem,a,h+d);while(i!==(i=e.cur()/g)&&i!==1&&--j)}e.unit=d,e.start=h,e.end=f[1]?h+(f[1]+1)*c:c}return e}]};p.Animation=p.extend(cW,{tweener:function(a,b){p.isFunction(a)?(b=a,a=["*"]):a=a.split(" ");var c,d=0,e=a.length;for(;d<e;d++)c=a[d],cT[c]=cT[c]||[],cT[c].unshift(b)},prefilter:function(a,b){b?cS.unshift(a):cS.push(a)}}),p.Tween=cZ,cZ.prototype={constructor:cZ,init:function(a,b,c,d,e,f){this.elem=a,this.prop=c,this.easing=e||"swing",this.options=b,this.start=this.now=this.cur(),this.end=d,this.unit=f||(p.cssNumber[c]?"":"px")},cur:function(){var a=cZ.propHooks[this.prop];return a&&a.get?a.get(this):cZ.propHooks._default.get(this)},run:function(a){var b,c=cZ.propHooks[this.prop];return this.options.duration?this.pos=b=p.easing[this.easing](a,this.options.duration*a,0,1,this.options.duration):this.pos=b=a,this.now=(this.end-this.start)*b+this.start,this.options.step&&this.options.step.call(this.elem,this.now,this),c&&c.set?c.set(this):cZ.propHooks._default.set(this),this}},cZ.prototype.init.prototype=cZ.prototype,cZ.propHooks={_default:{get:function(a){var b;return a.elem[a.prop]==null||!!a.elem.style&&a.elem.style[a.prop]!=null?(b=p.css(a.elem,a.prop,!1,""),!b||b==="auto"?0:b):a.elem[a.prop]},set:function(a){p.fx.step[a.prop]?p.fx.step[a.prop](a):a.elem.style&&(a.elem.style[p.cssProps[a.prop]]!=null||p.cssHooks[a.prop])?p.style(a.elem,a.prop,a.now+a.unit):a.elem[a.prop]=a.now}}},cZ.propHooks.scrollTop=cZ.propHooks.scrollLeft={set:function(a){a.elem.nodeType&&a.elem.parentNode&&(a.elem[a.prop]=a.now)}},p.each(["toggle","show","hide"],function(a,b){var c=p.fn[b];p.fn[b]=function(d,e,f){return d==null||typeof d=="boolean"||!a&&p.isFunction(d)&&p.isFunction(e)?c.apply(this,arguments):this.animate(c$(b,!0),d,e,f)}}),p.fn.extend({fadeTo:function(a,b,c,d){return this.filter(bZ).css("opacity",0).show().end().animate({opacity:b},a,c,d)},animate:function(a,b,c,d){var e=p.isEmptyObject(a),f=p.speed(b,c,d),g=function(){var b=cW(this,p.extend({},a),f);e&&b.stop(!0)};return e||f.queue===!1?this.each(g):this.queue(f.queue,g)},stop:function(a,c,d){var e=function(a){var b=a.stop;delete a.stop,b(d)};return typeof a!="string"&&(d=c,c=a,a=b),c&&a!==!1&&this.queue(a||"fx",[]),this.each(function(){var b=!0,c=a!=null&&a+"queueHooks",f=p.timers,g=p._data(this);if(c)g[c]&&g[c].stop&&e(g[c]);else for(c in g)g[c]&&g[c].stop&&cR.test(c)&&e(g[c]);for(c=f.length;c--;)f[c].elem===this&&(a==null||f[c].queue===a)&&(f[c].anim.stop(d),b=!1,f.splice(c,1));(b||!d)&&p.dequeue(this,a)})}}),p.each({slideDown:c$("show"),slideUp:c$("hide"),slideToggle:c$("toggle"),fadeIn:{opacity:"show"},fadeOut:{opacity:"hide"},fadeToggle:{opacity:"toggle"}},function(a,b){p.fn[a]=function(a,c,d){return this.animate(b,a,c,d)}}),p.speed=function(a,b,c){var d=a&&typeof a=="object"?p.extend({},a):{complete:c||!c&&b||p.isFunction(a)&&a,duration:a,easing:c&&b||b&&!p.isFunction(b)&&b};d.duration=p.fx.off?0:typeof d.duration=="number"?d.duration:d.duration in p.fx.speeds?p.fx.speeds[d.duration]:p.fx.speeds._default;if(d.queue==null||d.queue===!0)d.queue="fx";return d.old=d.complete,d.complete=function(){p.isFunction(d.old)&&d.old.call(this),d.queue&&p.dequeue(this,d.queue)},d},p.easing={linear:function(a){return a},swing:function(a){return.5-Math.cos(a*Math.PI)/2}},p.timers=[],p.fx=cZ.prototype.init,p.fx.tick=function(){var a,b=p.timers,c=0;for(;c<b.length;c++)a=b[c],!a()&&b[c]===a&&b.splice(c--,1);b.length||p.fx.stop()},p.fx.timer=function(a){a()&&p.timers.push(a)&&!cO&&(cO=setInterval(p.fx.tick,p.fx.interval))},p.fx.interval=13,p.fx.stop=function(){clearInterval(cO),cO=null},p.fx.speeds={slow:600,fast:200,_default:400},p.fx.step={},p.expr&&p.expr.filters&&(p.expr.filters.animated=function(a){return p.grep(p.timers,function(b){return a===b.elem}).length});var c_=/^(?:body|html)$/i;p.fn.offset=function(a){if(arguments.length)return a===b?this:this.each(function(b){p.offset.setOffset(this,a,b)});var c,d,e,f,g,h,i,j={top:0,left:0},k=this[0],l=k&&k.ownerDocument;if(!l)return;return(d=l.body)===k?p.offset.bodyOffset(k):(c=l.documentElement,p.contains(c,k)?(typeof k.getBoundingClientRect!="undefined"&&(j=k.getBoundingClientRect()),e=da(l),f=c.clientTop||d.clientTop||0,g=c.clientLeft||d.clientLeft||0,h=e.pageYOffset||c.scrollTop,i=e.pageXOffset||c.scrollLeft,{top:j.top+h-f,left:j.left+i-g}):j)},p.offset={bodyOffset:function(a){var b=a.offsetTop,c=a.offsetLeft;return p.support.doesNotIncludeMarginInBodyOffset&&(b+=parseFloat(p.css(a,"marginTop"))||0,c+=parseFloat(p.css(a,"marginLeft"))||0),{top:b,left:c}},setOffset:function(a,b,c){var d=p.css(a,"position");d==="static"&&(a.style.position="relative");var e=p(a),f=e.offset(),g=p.css(a,"top"),h=p.css(a,"left"),i=(d==="absolute"||d==="fixed")&&p.inArray("auto",[g,h])>-1,j={},k={},l,m;i?(k=e.position(),l=k.top,m=k.left):(l=parseFloat(g)||0,m=parseFloat(h)||0),p.isFunction(b)&&(b=b.call(a,c,f)),b.top!=null&&(j.top=b.top-f.top+l),b.left!=null&&(j.left=b.left-f.left+m),"using"in b?b.using.call(a,j):e.css(j)}},p.fn.extend({position:function(){if(!this[0])return;var a=this[0],b=this.offsetParent(),c=this.offset(),d=c_.test(b[0].nodeName)?{top:0,left:0}:b.offset();return c.top-=parseFloat(p.css(a,"marginTop"))||0,c.left-=parseFloat(p.css(a,"marginLeft"))||0,d.top+=parseFloat(p.css(b[0],"borderTopWidth"))||0,d.left+=parseFloat(p.css(b[0],"borderLeftWidth"))||0,{top:c.top-d.top,left:c.left-d.left}},offsetParent:function(){return this.map(function(){var a=this.offsetParent||e.body;while(a&&!c_.test(a.nodeName)&&p.css(a,"position")==="static")a=a.offsetParent;return a||e.body})}}),p.each({scrollLeft:"pageXOffset",scrollTop:"pageYOffset"},function(a,c){var d=/Y/.test(c);p.fn[a]=function(e){return p.access(this,function(a,e,f){var g=da(a);if(f===b)return g?c in g?g[c]:g.document.documentElement[e]:a[e];g?g.scrollTo(d?p(g).scrollLeft():f,d?f:p(g).scrollTop()):a[e]=f},a,e,arguments.length,null)}}),p.each({Height:"height",Width:"width"},function(a,c){p.each({padding:"inner"+a,content:c,"":"outer"+a},function(d,e){p.fn[e]=function(e,f){var g=arguments.length&&(d||typeof e!="boolean"),h=d||(e===!0||f===!0?"margin":"border");return p.access(this,function(c,d,e){var f;return p.isWindow(c)?c.document.documentElement["client"+a]:c.nodeType===9?(f=c.documentElement,Math.max(c.body["scroll"+a],f["scroll"+a],c.body["offset"+a],f["offset"+a],f["client"+a])):e===b?p.css(c,d,e,h):p.style(c,d,e,h)},c,g?e:b,g,null)}})}),a.jQuery=a.$=p,typeof define=="function"&&define.amd&&define.amd.jQuery&&define("jquery",[],function(){return p})})(window); |
src/components/pages/grocerylist/grocerylistDetails.js | emilmannfeldt/ettkilomjol | import React, { Component } from 'react';
import List from '@material-ui/core/List';
import Divider from '@material-ui/core/Divider';
import ListItem from '@material-ui/core/ListItem';
import ChevronLeftIcon from '@material-ui/icons/ChevronLeft';
import PrintIcon from '@material-ui/icons/PrintOutlined';
import IconButton from '@material-ui/core/IconButton';
import PropTypes from 'prop-types';
import Grid from '@material-ui/core/Grid';
import NewGroceryItem from './newGroceryItem';
import GroceryItem from './groceryItem';
import { fire } from '../../../base';
function printPage() {
window.print();
}
class GrocerylistDetails extends Component {
constructor(props) {
super(props);
this.state = {
};
this.updateItem = this.updateItem.bind(this);
this.createItem = this.createItem.bind(this);
this.deleteItem = this.deleteItem.bind(this);
}
createItem(item) {
const { grocerylist } = this.props;
fire.database().ref(`users/${fire.auth().currentUser.uid}/grocerylists/${grocerylist.name}/items`).push(item);
}
updateItem(item, key) {
const { grocerylist } = this.props;
fire.database().ref(`users/${fire.auth().currentUser.uid}/grocerylists/${grocerylist.name}/items/${key}`).update(item);
}
deleteItem(key) {
const { grocerylist } = this.props;
const items = {};
items[key] = null;
fire.database().ref(`users/${fire.auth().currentUser.uid}/grocerylists/${grocerylist.name}/items`).update(items);
}
render() {
const {
returnFunc, grocerylist, foods, units,
} = this.props;
return (
<Grid item container xs={12} className="grocerylist-details--container">
<List className="grocerylist-itemlist">
<ListItem className="grocerylist-header text-big">
<IconButton onClick={returnFunc}>
<ChevronLeftIcon />
</IconButton>
{grocerylist.name}
<IconButton onClick={printPage} className="print-btn">
<PrintIcon />
</IconButton>
</ListItem>
<NewGroceryItem foods={foods} units={units} createItem={this.createItem} grocerylistItems={grocerylist.items} />
{grocerylist.items
&& grocerylist.items.map((grocerytItem, index) => (
<div key={grocerytItem.key}>
<GroceryItem
itemId={index}
foods={foods}
units={units}
updateItem={this.updateItem}
groceryItem={grocerytItem}
deleteItem={this.deleteItem}
itemList={grocerylist.items}
/>
{index === grocerylist.items.length - 1 ? (null) : (<Divider />)}
</div>
))
}
</List>
</Grid>
);
}
}
GrocerylistDetails.propTypes = {
grocerylist: PropTypes.object.isRequired,
foods: PropTypes.array.isRequired,
units: PropTypes.any.isRequired,
returnFunc: PropTypes.func.isRequired,
};
export default GrocerylistDetails;
|
src/index.js | yuri/notality | import './styles/styles.css';
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import { ReduxRouter } from 'redux-router';
import configureStore from './store/configureStore';
const store = configureStore({});
import {fetchNotes} from './actions/notes';
store.dispatch(fetchNotes());
ReactDOM.render(
<div>
<Provider store={ store }>
<ReduxRouter />
</Provider>
</div>,
document.getElementById('root')
);
|
packages/react-router-website/modules/components/Guide.js | goblortikus/react-router | import React from 'react'
import PropTypes from 'prop-types'
import { Redirect, Route } from 'react-router-dom'
import { Block } from 'jsxstyle'
import ScrollToDoc from './ScrollToDoc'
import MarkdownViewer from './MarkdownViewer'
// almost identical to `API`, but I'm lazy rn
const Guide = ({ match, data }) => {
const { params: { mod, header: headerParam, environment } } = match
const doc = data.guides.find(doc => mod === doc.title.slug)
const header = doc && headerParam ? doc.headers.find(h => h.slug === headerParam) : null
return !doc ? (
<Redirect to={`/${environment}`}/>
) : (
<Block
className="api-doc-wrapper"
fontSize="80%"
>
<Block className="api-doc">
<ScrollToDoc doc={doc} header={header}/>
<MarkdownViewer html={doc.markup}/>
</Block>
<Route
path={`${match.path}/:header`}
render={({ match: { params: { header: slug }}}) => {
const header = doc.headers.find(h => h.slug === slug )
return header ? (
<ScrollToDoc doc={doc} header={header}/>
) : (
<Redirect to={`/${environment}/guides/${mod}`}/>
)
}}
/>
</Block>
)
}
Guide.propTypes = {
match: PropTypes.object,
data: PropTypes.object
}
export default Guide
|
node_modules/react-router/es6/Route.js | ddigioia/react_router_practice_1 | import React from 'react';
import invariant from 'invariant';
import { createRouteFromReactElement } from './RouteUtils';
import { component, components } from './InternalPropTypes';
var _React$PropTypes = React.PropTypes;
var string = _React$PropTypes.string;
var func = _React$PropTypes.func;
/**
* A <Route> is used to declare which components are rendered to the
* page when the URL matches a given pattern.
*
* Routes are arranged in a nested tree structure. When a new URL is
* requested, the tree is searched depth-first to find a route whose
* path matches the URL. When one is found, all routes in the tree
* that lead to it are considered "active" and their components are
* rendered into the DOM, nested in the same order as in the tree.
*/
var Route = React.createClass({
displayName: 'Route',
statics: {
createRouteFromReactElement: createRouteFromReactElement
},
propTypes: {
path: string,
component: component,
components: components,
getComponent: func,
getComponents: func
},
/* istanbul ignore next: sanity check */
render: function render() {
!false ? process.env.NODE_ENV !== 'production' ? invariant(false, '<Route> elements are for router configuration only and should not be rendered') : invariant(false) : void 0;
}
});
export default Route; |
tests/containers/GuessFeedback.spec.js | LaunchAcademy/name-game | import GuessFeedback from '../../src/containers/GuessFeedback'
import { mount } from 'enzyme'
import React from 'react'
describe('Guess Feedback', () => {
let guess
let component
describe('correct guesses', () => {
beforeEach(() => {
guess = {
correct: true,
identity: {
name: 'Foo'
}
}
component = mount(
<GuessFeedback guess={guess} />
)
})
it('affirms the guess was right', () => {
expect(component.text()).to.contain('Yes')
})
it('sets the "correct" class name', () => {
expect(component.find("p.correct")).to.be.present()
})
})
describe('incorrect guesses', () => {
beforeEach(() => {
guess = {
correct: false,
identity: {
name: 'Foo'
}
}
component = mount(
<GuessFeedback guess={guess} />
)
})
it('affirms the guess was right', () => {
expect(component.text()).to.contain('Nope')
})
it('sets the "correct" class name', () => {
expect(component.find("p.incorrect")).to.be.present()
})
})
})
|
node_modules/material-ui/svg-icons/image/filter-2.js | Jacob-Elder/react-socket.io-messaging-v2 | 'use strict';
Object.defineProperty(exports, "__esModule", {
value: true
});
var _react = require('react');
var _react2 = _interopRequireDefault(_react);
var _pure = require('recompose/pure');
var _pure2 = _interopRequireDefault(_pure);
var _SvgIcon = require('../../SvgIcon');
var _SvgIcon2 = _interopRequireDefault(_SvgIcon);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
var ImageFilter2 = function ImageFilter2(props) {
return _react2.default.createElement(
_SvgIcon2.default,
props,
_react2.default.createElement('path', { d: 'M3 5H1v16c0 1.1.9 2 2 2h16v-2H3V5zm18-4H7c-1.1 0-2 .9-2 2v14c0 1.1.9 2 2 2h14c1.1 0 2-.9 2-2V3c0-1.1-.9-2-2-2zm0 16H7V3h14v14zm-4-4h-4v-2h2c1.1 0 2-.89 2-2V7c0-1.11-.9-2-2-2h-4v2h4v2h-2c-1.1 0-2 .89-2 2v4h6v-2z' })
);
};
ImageFilter2 = (0, _pure2.default)(ImageFilter2);
ImageFilter2.displayName = 'ImageFilter2';
ImageFilter2.muiName = 'SvgIcon';
exports.default = ImageFilter2; |
src/components/CompanyAndJobTitle/TimeAndSalary/Helmet.js | goodjoblife/GoodJobShare | import React from 'react';
import Helmet from 'react-helmet';
import { formatTitle, formatCanonicalPath } from 'utils/helmetHelper';
import { IMG_HOST, SITE_NAME } from '../../../constants/helmetData';
import { pageType as PAGE_TYPE } from '../../../constants/companyJobTitle';
const CompanySalaryWorkTimeHelmet = ({
companyName,
salaryWorkTimes,
salaryWorkTimeStatistics,
page,
}) => {
// title
const title = `${companyName} 薪水&加班狀況 - 第${page}頁`;
// description
let description = `目前還沒有${companyName}的薪水、加班狀況資料。分享你的薪水、加班狀況,一起讓職場更透明。`;
if (salaryWorkTimes && salaryWorkTimes.length > 0) {
description = `查看${salaryWorkTimes.length}筆由${companyName}內部員工提供的薪水、加班狀況資料。`;
}
// canonical url
let url = formatCanonicalPath(`/companies/${companyName}/salary-work-times`);
if (page > 1) {
url = formatCanonicalPath(
`/companies/${companyName}/salary-work-times?p=${page}`,
);
}
return (
<Helmet>
<title itemProp="name" lang="zh-TW">
{title}
</title>
<meta name="description" content={description} />
<meta property="og:title" content={formatTitle(title, SITE_NAME)} />
<meta property="og:description" content={description} />
<meta
name="keywords"
content={`${companyName}薪水, ${companyName}薪資, ${companyName}加班狀況, ${companyName}工時`}
/>
<meta property="og:url" content={url} />
<meta
property="og:image"
content={`${IMG_HOST}/og/time-and-salary.jpg`}
/>
<link rel="canonical" href={url} />
</Helmet>
);
};
const JobTitleSalaryWorkTimeHelmet = ({
jobTitle,
salaryWorkTimes,
salaryWorkTimeStatistics,
page,
}) => {
// title
const title = `${jobTitle} 薪水&加班狀況 - 第${page}頁`;
// description
let description = `目前還沒有${jobTitle}的薪水、加班狀況資料。分享你的薪水、加班狀況,一起讓職場更透明。`;
if (salaryWorkTimes && salaryWorkTimes.length > 0) {
description = `查看${salaryWorkTimes.length}筆由${jobTitle}提供的薪水、加班狀況資料。`;
}
// canonical url
let url = formatCanonicalPath(`/job-titles/${jobTitle}/salary-work-times`);
if (page > 1) {
url = formatCanonicalPath(
`/job-titles/${jobTitle}/salary-work-times?p=${page}`,
);
}
return (
<Helmet>
<title itemProp="name" lang="zh-TW">
{title}
</title>
<meta name="description" content={description} />
<meta property="og:title" content={formatTitle(title, SITE_NAME)} />
<meta property="og:description" content={description} />
<meta
name="keywords"
content={`${jobTitle}薪水, ${jobTitle}薪資, ${jobTitle}加班狀況, ${jobTitle}工時`}
/>
<meta property="og:url" content={url} />
<meta
property="og:image"
content={`${IMG_HOST}/og/time-and-salary.jpg`}
/>
<link rel="canonical" href={url} />
</Helmet>
);
};
export default props => {
if (props.pageType === PAGE_TYPE.JOB_TITLE) {
return (
<JobTitleSalaryWorkTimeHelmet {...props} jobTitle={props.pageName} />
);
} else if (props.pageType === PAGE_TYPE.COMPANY) {
return (
<CompanySalaryWorkTimeHelmet {...props} companyName={props.pageName} />
);
} else {
return null;
}
};
|
src/PageHeader.js | Cellule/react-bootstrap | import React from 'react';
import classNames from 'classnames';
const PageHeader = React.createClass({
render() {
return (
<div {...this.props} className={classNames(this.props.className, 'page-header')}>
<h1>{this.props.children}</h1>
</div>
);
}
});
export default PageHeader;
|
tests/components/TextField/TextField.spec.js | Venryx/ThesisMap | import React from 'react'
import TextField from 'components/TextField'
import { shallow } from 'enzyme'
describe('(Component) TextField', () => {
let _component
beforeEach(() => {
_component = shallow(
<TextField
type='text'
label='some label'
touched={false}
input={{}}
/>
)
})
it.skip('throws for no type', () => {
expect(shallow(<TextField />)).to.throw(Error)
})
it.skip('Renders div', () => {
const firstDiv = _component.find('div')
expect(firstDiv).to.exist
})
})
|
ajax/libs/core-js/0.4.3/core.js | vfonic/cdnjs | /**
* Core.js 0.4.3
* https://github.com/zloirock/core-js
* License: http://rock.mit-license.org
* © 2015 Denis Pushkarev
*/
!function(returnThis, framework, undefined){
'use strict';
/******************************************************************************
* Module : common *
******************************************************************************/
var global = returnThis()
// Shortcuts for [[Class]] & property names
, OBJECT = 'Object'
, FUNCTION = 'Function'
, ARRAY = 'Array'
, STRING = 'String'
, NUMBER = 'Number'
, REGEXP = 'RegExp'
, DATE = 'Date'
, MAP = 'Map'
, SET = 'Set'
, WEAKMAP = 'WeakMap'
, WEAKSET = 'WeakSet'
, SYMBOL = 'Symbol'
, PROMISE = 'Promise'
, MATH = 'Math'
, ARGUMENTS = 'Arguments'
, PROTOTYPE = 'prototype'
, CONSTRUCTOR = 'constructor'
, TO_STRING = 'toString'
, TO_STRING_TAG = TO_STRING + 'Tag'
, TO_LOCALE = 'toLocaleString'
, HAS_OWN = 'hasOwnProperty'
, FOR_EACH = 'forEach'
, ITERATOR = 'iterator'
, FF_ITERATOR = '@@' + ITERATOR
, PROCESS = 'process'
, CREATE_ELEMENT = 'createElement'
// Aliases global objects and prototypes
, Function = global[FUNCTION]
, Object = global[OBJECT]
, Array = global[ARRAY]
, String = global[STRING]
, Number = global[NUMBER]
, RegExp = global[REGEXP]
, Date = global[DATE]
, Map = global[MAP]
, Set = global[SET]
, WeakMap = global[WEAKMAP]
, WeakSet = global[WEAKSET]
, Symbol = global[SYMBOL]
, Math = global[MATH]
, TypeError = global.TypeError
, setTimeout = global.setTimeout
, setImmediate = global.setImmediate
, clearImmediate = global.clearImmediate
, process = global[PROCESS]
, nextTick = process && process.nextTick
, document = global.document
, html = document && document.documentElement
, navigator = global.navigator
, define = global.define
, ArrayProto = Array[PROTOTYPE]
, ObjectProto = Object[PROTOTYPE]
, FunctionProto = Function[PROTOTYPE]
, Infinity = 1 / 0
, DOT = '.';
// http://jsperf.com/core-js-isobject
function isObject(it){
return it != null && (typeof it == 'object' || typeof it == 'function');
}
function isFunction(it){
return typeof it == 'function';
}
// Native function?
var isNative = ctx(/./.test, /\[native code\]\s*\}\s*$/, 1);
// Object internal [[Class]] or toStringTag
// http://people.mozilla.org/~jorendorff/es6-draft.html#sec-object.prototype.tostring
var buildIn = {
Undefined: 1, Null: 1, Array: 1, String: 1, Arguments: 1,
Function: 1, Error: 1, Boolean: 1, Number: 1, Date: 1, RegExp:1
} , toString = ObjectProto[TO_STRING];
function setToStringTag(it, tag, stat){
if(it && !has(it = stat ? it : it[PROTOTYPE], SYMBOL_TAG))hidden(it, SYMBOL_TAG, tag);
}
function cof(it){
return it == undefined ? it === undefined
? 'Undefined' : 'Null' : toString.call(it).slice(8, -1);
}
function classof(it){
var klass = cof(it), tag;
return klass == OBJECT && (tag = it[SYMBOL_TAG]) ? has(buildIn, tag) ? '~' + tag : tag : klass;
}
// Function
var call = FunctionProto.call
, apply = FunctionProto.apply
, REFERENCE_GET;
// Partial apply
function part(/* ...args */){
var length = arguments.length
, args = Array(length)
, i = 0
, _ = path._
, holder = false;
while(length > i)if((args[i] = arguments[i++]) === _)holder = true;
return partial(this, args, length, holder, _, false);
}
// Internal partial application & context binding
function partial(fn, argsPart, lengthPart, holder, _, bind, context){
assertFunction(fn);
return function(/* ...args */){
var that = bind ? context : this
, length = arguments.length
, i = 0, j = 0, args;
if(!holder && !length)return invoke(fn, argsPart, that);
args = argsPart.slice();
if(holder)for(;lengthPart > i; i++)if(args[i] === _)args[i] = arguments[j++];
while(length > j)args.push(arguments[j++]);
return invoke(fn, args, that);
}
}
// Optional / simple context binding
function ctx(fn, that, length){
assertFunction(fn);
if(~length && that === undefined)return fn;
switch(length){
case 1: return function(a){
return fn.call(that, a);
}
case 2: return function(a, b){
return fn.call(that, a, b);
}
case 3: return function(a, b, c){
return fn.call(that, a, b, c);
}
} return function(/* ...args */){
return fn.apply(that, arguments);
}
}
// Fast apply
// http://jsperf.lnkit.com/fast-apply/5
function invoke(fn, args, that){
var un = that === undefined;
switch(args.length | 0){
case 0: return un ? fn()
: fn.call(that);
case 1: return un ? fn(args[0])
: fn.call(that, args[0]);
case 2: return un ? fn(args[0], args[1])
: fn.call(that, args[0], args[1]);
case 3: return un ? fn(args[0], args[1], args[2])
: fn.call(that, args[0], args[1], args[2]);
case 4: return un ? fn(args[0], args[1], args[2], args[3])
: fn.call(that, args[0], args[1], args[2], args[3]);
case 5: return un ? fn(args[0], args[1], args[2], args[3], args[4])
: fn.call(that, args[0], args[1], args[2], args[3], args[4]);
} return fn.apply(that, args);
}
function construct(target, argumentsList){
var instance = create(target[PROTOTYPE])
, result = apply.call(target, instance, argumentsList);
return isObject(result) ? result : instance;
}
// Object:
var create = Object.create
, getPrototypeOf = Object.getPrototypeOf
, setPrototypeOf = Object.setPrototypeOf
, defineProperty = Object.defineProperty
, defineProperties = Object.defineProperties
, getOwnDescriptor = Object.getOwnPropertyDescriptor
, getKeys = Object.keys
, getNames = Object.getOwnPropertyNames
, getSymbols = Object.getOwnPropertySymbols
, has = ctx(call, ObjectProto[HAS_OWN], 2)
// Dummy, fix for not array-like ES3 string in es5 module
, ES5Object = Object;
function returnIt(it){
return it;
}
function get(object, key){
if(has(object, key))return object[key];
}
function ownKeys(it){
return getSymbols ? getNames(it).concat(getSymbols(it)) : getNames(it);
}
// 19.1.2.1 Object.assign(target, source, ...)
var assign = Object.assign || function(target, source){
var T = Object(assertDefined(target))
, l = arguments.length
, i = 1;
while(l > i){
var S = ES5Object(arguments[i++])
, keys = getKeys(S)
, length = keys.length
, j = 0
, key;
while(length > j)T[key = keys[j++]] = S[key];
}
return T;
}
function keyOf(object, el){
var O = ES5Object(object)
, keys = getKeys(O)
, length = keys.length
, index = 0
, key;
while(length > index)if(O[key = keys[index++]] === el)return key;
}
// Array
// array('str1,str2,str3') => ['str1', 'str2', 'str3']
function array(it){
return String(it).split(',');
}
var push = ArrayProto.push
, unshift = ArrayProto.unshift
, slice = ArrayProto.slice
, splice = ArrayProto.splice
, indexOf = ArrayProto.indexOf
, forEach = ArrayProto[FOR_EACH];
/*
* 0 -> forEach
* 1 -> map
* 2 -> filter
* 3 -> some
* 4 -> every
* 5 -> find
* 6 -> findIndex
*/
function createArrayMethod(type){
var isMap = type == 1
, isFilter = type == 2
, isSome = type == 3
, isEvery = type == 4
, isFindIndex = type == 6
, noholes = type == 5 || isFindIndex;
return function(callbackfn/*, that = undefined */){
var O = Object(assertDefined(this))
, that = arguments[1]
, self = ES5Object(O)
, f = ctx(callbackfn, that, 3)
, length = toLength(self.length)
, index = 0
, result = isMap ? Array(length) : isFilter ? [] : undefined
, val, res;
for(;length > index; index++)if(noholes || index in self){
val = self[index];
res = f(val, index, O);
if(type){
if(isMap)result[index] = res; // map
else if(res)switch(type){
case 3: return true; // some
case 5: return val; // find
case 6: return index; // findIndex
case 2: result.push(val); // filter
} else if(isEvery)return false; // every
}
}
return isFindIndex ? -1 : isSome || isEvery ? isEvery : result;
}
}
function createArrayContains(isContains){
return function(el /*, fromIndex = 0 */){
var O = ES5Object(assertDefined(this))
, length = toLength(O.length)
, index = toIndex(arguments[1], length);
if(isContains && el != el){
for(;length > index; index++)if(sameNaN(O[index]))return isContains || index;
} else for(;length > index; index++)if(isContains || index in O){
if(O[index] === el)return isContains || index;
} return !isContains && -1;
}
}
function generic(A, B){
// strange IE quirks mode bug -> use typeof vs isFunction
return typeof A == 'function' ? A : B;
}
// Math
var MAX_SAFE_INTEGER = 0x1fffffffffffff // pow(2, 53) - 1 == 9007199254740991
, ceil = Math.ceil
, floor = Math.floor
, max = Math.max
, min = Math.min
, random = Math.random
, trunc = Math.trunc || function(it){
return (it > 0 ? floor : ceil)(it);
}
// 20.1.2.4 Number.isNaN(number)
function sameNaN(number){
return number != number;
}
// 7.1.4 ToInteger
function toInteger(it){
return isNaN(it) ? 0 : trunc(it);
}
// 7.1.15 ToLength
function toLength(it){
return it > 0 ? min(toInteger(it), MAX_SAFE_INTEGER) : 0;
}
function toIndex(index, length){
var index = toInteger(index);
return index < 0 ? max(index + length, 0) : min(index, length);
}
function createReplacer(regExp, replace, isStatic){
var replacer = isObject(replace) ? function(part){
return replace[part];
} : replace;
return function(it){
return String(isStatic ? it : this).replace(regExp, replacer);
}
}
function createPointAt(toString){
return function(pos){
var s = String(assertDefined(this))
, i = toInteger(pos)
, l = s.length
, a, b;
if(i < 0 || i >= l)return toString ? '' : undefined;
a = s.charCodeAt(i);
return a < 0xd800 || a > 0xdbff || i + 1 === l || (b = s.charCodeAt(i + 1)) < 0xdc00 || b > 0xdfff
? toString ? s.charAt(i) : a
: toString ? s.slice(i, i + 2) : (a - 0xd800 << 10) + (b - 0xdc00) + 0x10000;
}
}
// Assertion & errors
var REDUCE_ERROR = 'Reduce of empty object with no initial value';
function assert(condition, msg1, msg2){
if(!condition)throw TypeError(msg2 ? msg1 + msg2 : msg1);
}
function assertDefined(it){
if(it == undefined)throw TypeError('Function called on null or undefined');
return it;
}
function assertFunction(it){
assert(isFunction(it), it, ' is not a function!');
return it;
}
function assertObject(it){
assert(isObject(it), it, ' is not an object!');
return it;
}
function assertInstance(it, Constructor, name){
assert(it instanceof Constructor, name, ": use the 'new' operator!");
}
// Property descriptors & Symbol
function descriptor(bitmap, value){
return {
enumerable : !(bitmap & 1),
configurable: !(bitmap & 2),
writable : !(bitmap & 4),
value : value
}
}
function simpleSet(object, key, value){
object[key] = value;
return object;
}
function createDefiner(bitmap){
return DESC ? function(object, key, value){
return defineProperty(object, key, descriptor(bitmap, value));
} : simpleSet;
}
function uid(key){
return SYMBOL + '(' + key + ')_' + (++sid + random())[TO_STRING](36);
}
function getWellKnownSymbol(name, setter){
return (Symbol && Symbol[name]) || (setter ? Symbol : safeSymbol)(SYMBOL + DOT + name);
}
// The engine works fine with descriptors? Thank's IE8 for his funny defineProperty.
var DESC = !!function(){try{return defineProperty({}, DOT, ObjectProto)}catch(e){}}()
, sid = 0
, hidden = createDefiner(1)
, set = Symbol ? simpleSet : hidden
, safeSymbol = Symbol || uid;
function assignHidden(target, src){
for(var key in src)hidden(target, key, src[key]);
return target;
}
var SYMBOL_UNSCOPABLES = getWellKnownSymbol('unscopables')
, ArrayUnscopables = ArrayProto[SYMBOL_UNSCOPABLES] || {};
// Iterators
var SYMBOL_ITERATOR = getWellKnownSymbol(ITERATOR)
, SYMBOL_TAG = getWellKnownSymbol(TO_STRING_TAG)
, SUPPORT_FF_ITER = FF_ITERATOR in ArrayProto
, ITER = safeSymbol('iter')
, KEY = 1
, VALUE = 2
, Iterators = {}
, IteratorPrototype = {}
, NATIVE_ITERATORS = SYMBOL_ITERATOR in ArrayProto
// Safari define byggy iterators w/o `next`
, BUGGY_ITERATORS = 'keys' in ArrayProto && !('next' in [].keys());
// 25.1.2.1.1 %IteratorPrototype%[@@iterator]()
setIterator(IteratorPrototype, returnThis);
function setIterator(O, value){
hidden(O, SYMBOL_ITERATOR, value);
// Add iterator for FF iterator protocol
SUPPORT_FF_ITER && hidden(O, FF_ITERATOR, value);
}
function createIterator(Constructor, NAME, next, proto){
Constructor[PROTOTYPE] = create(proto || IteratorPrototype, {next: descriptor(1, next)});
setToStringTag(Constructor, NAME + ' Iterator');
}
function defineIterator(Constructor, NAME, value, DEFAULT){
var proto = Constructor[PROTOTYPE]
, iter = get(proto, SYMBOL_ITERATOR) || get(proto, FF_ITERATOR) || (DEFAULT && get(proto, DEFAULT)) || value;
if(framework){
// Define iterator
setIterator(proto, iter);
if(iter !== value){
var iterProto = getPrototypeOf(iter.call(new Constructor));
// Set @@toStringTag to native iterators
setToStringTag(iterProto, NAME + ' Iterator', true);
// FF fix
has(proto, FF_ITERATOR) && setIterator(iterProto, returnThis);
}
}
// Plug for library
Iterators[NAME] = iter;
// FF & v8 fix
Iterators[NAME + ' Iterator'] = returnThis;
return iter;
}
function defineStdIterators(Base, NAME, Constructor, next, DEFAULT, IS_SET){
function createIter(kind){
return function(){
return new Constructor(this, kind);
}
}
createIterator(Constructor, NAME, next);
var entries = createIter(KEY+VALUE)
, values = createIter(VALUE);
if(DEFAULT == VALUE)values = defineIterator(Base, NAME, values, 'values');
else entries = defineIterator(Base, NAME, entries, 'entries');
if(DEFAULT){
$define(PROTO + FORCED * BUGGY_ITERATORS, NAME, {
entries: entries,
keys: IS_SET ? values : createIter(KEY),
values: values
});
}
}
function iterResult(done, value){
return {value: value, done: !!done};
}
function isIterable(it){
var O = Object(it)
, Symbol = global[SYMBOL]
, hasExt = !!(Symbol && Symbol[ITERATOR] && Symbol[ITERATOR] in O);
return hasExt || SYMBOL_ITERATOR in O || has(Iterators, classof(O));
}
function getIterator(it){
var Symbol = global[SYMBOL]
, ext = Symbol && Symbol[ITERATOR] && it[Symbol[ITERATOR]]
, getIter = ext || it[SYMBOL_ITERATOR] || Iterators[classof(it)];
return assertObject(getIter.call(it));
}
function stepCall(fn, value, entries){
return entries ? invoke(fn, value) : fn(value);
}
function forOf(iterable, entries, fn, that){
var iterator = getIterator(iterable)
, f = ctx(fn, that, entries ? 2 : 1)
, step;
while(!(step = iterator.next()).done)if(stepCall(f, step.value, entries) === false)return;
}
// core
var NODE = cof(process) == PROCESS
, core = {}
, path = framework ? global : core
, old = global.core
// type bitmap
, FORCED = 1
, GLOBAL = 2
, STATIC = 4
, PROTO = 8
, BIND = 16
, WRAP = 32;
function $define(type, name, source){
var key, own, out, exp
, isGlobal = type & GLOBAL
, target = isGlobal ? global : (type & STATIC)
? global[name] : (global[name] || ObjectProto)[PROTOTYPE]
, exports = isGlobal ? core : core[name] || (core[name] = {});
if(isGlobal)source = name;
for(key in source){
// there is a similar native
own = !(type & FORCED) && target && key in target
&& (!isFunction(target[key]) || isNative(target[key]));
// export native or passed
out = (own ? target : source)[key];
// bind timers to global for call from export context
if(type & BIND && own)exp = ctx(out, global);
// wrap global constructors for prevent change them in library
else if(type & WRAP && !framework && target[key] == out){
exp = function(param){
return this instanceof out ? new out(param) : out(param);
}
exp[PROTOTYPE] = out[PROTOTYPE];
} else exp = type & PROTO && isFunction(out) ? ctx(call, out) : out;
// export
if(exports[key] != out)hidden(exports, key, exp);
// extend global
if(framework && target && !own){
if(isGlobal)target[key] = out;
else delete target[key] && hidden(target, key, out);
}
}
}
// CommonJS export
if(typeof module != 'undefined' && module.exports)module.exports = core;
// RequireJS export
if(isFunction(define) && define.amd)define(function(){return core});
// Export to global object
if(!NODE || framework){
core.noConflict = function(){
global.core = old;
return core;
}
global.core = core;
}
/******************************************************************************
* Module : es5 *
******************************************************************************/
// ECMAScript 5 shim
!function(IS_ENUMERABLE, Empty, _classof, $PROTO){
if(!DESC){
getOwnDescriptor = function(O, P){
if(has(O, P))return descriptor(!ObjectProto[IS_ENUMERABLE].call(O, P), O[P]);
};
defineProperty = function(O, P, Attributes){
if('value' in Attributes)assertObject(O)[P] = Attributes.value;
return O;
};
defineProperties = function(O, Properties){
assertObject(O);
var keys = getKeys(Properties)
, length = keys.length
, i = 0
, P, Attributes;
while(length > i){
P = keys[i++];
Attributes = Properties[P];
if('value' in Attributes)O[P] = Attributes.value;
}
return O;
};
}
$define(STATIC + FORCED * !DESC, OBJECT, {
// 19.1.2.6 / 15.2.3.3 Object.getOwnPropertyDescriptor(O, P)
getOwnPropertyDescriptor: getOwnDescriptor,
// 19.1.2.4 / 15.2.3.6 Object.defineProperty(O, P, Attributes)
defineProperty: defineProperty,
// 19.1.2.3 / 15.2.3.7 Object.defineProperties(O, Properties)
defineProperties: defineProperties
});
// IE 8- don't enum bug keys
var keys1 = [CONSTRUCTOR, HAS_OWN, 'isPrototypeOf', IS_ENUMERABLE, TO_LOCALE, TO_STRING, 'valueOf']
// Additional keys for getOwnPropertyNames
, keys2 = keys1.concat('length', PROTOTYPE)
, keysLen1 = keys1.length;
// Create object with `null` prototype: use iframe Object with cleared prototype
function createDict(){
// Thrash, waste and sodomy: IE GC bug
var iframe = document[CREATE_ELEMENT]('iframe')
, i = keysLen1
, iframeDocument;
iframe.style.display = 'none';
html.appendChild(iframe);
iframe.src = 'javascript:';
// createDict = iframe.contentWindow.Object;
// html.removeChild(iframe);
iframeDocument = iframe.contentWindow.document;
iframeDocument.open();
iframeDocument.write('<script>document.F=Object</script>');
iframeDocument.close();
createDict = iframeDocument.F;
while(i--)delete createDict[PROTOTYPE][keys1[i]];
return createDict();
}
function createGetKeys(names, length, isNames){
return function(object){
var O = ES5Object(assertDefined(object))
, i = 0
, result = []
, key;
for(key in O)if(key != $PROTO)has(O, key) && result.push(key);
// Don't enum bug & hidden keys
while(length > i)if(has(O, key = names[i++])){
~indexOf.call(result, key) || result.push(key);
}
return result;
}
}
function isPrimitive(it){ return !isObject(it) }
$define(STATIC, OBJECT, {
// 19.1.2.9 / 15.2.3.2 Object.getPrototypeOf(O)
getPrototypeOf: getPrototypeOf = getPrototypeOf || function(O){
if(has(assertObject(O), $PROTO))return O[$PROTO];
if(isFunction(O[CONSTRUCTOR]) && O instanceof O[CONSTRUCTOR]){
return O[CONSTRUCTOR][PROTOTYPE];
} return O instanceof Object ? ObjectProto : null;
},
// 19.1.2.7 / 15.2.3.4 Object.getOwnPropertyNames(O)
getOwnPropertyNames: getNames = getNames || createGetKeys(keys2, keys2.length, true),
// 19.1.2.2 / 15.2.3.5 Object.create(O [, Properties])
create: create = create || function(O, /*?*/Properties){
var result
if(O !== null){
Empty[PROTOTYPE] = assertObject(O);
result = new Empty();
Empty[PROTOTYPE] = null;
// add "__proto__" for Object.getPrototypeOf shim
if(result[CONSTRUCTOR][PROTOTYPE] !== O)result[$PROTO] = O;
} else result = createDict();
return Properties === undefined ? result : defineProperties(result, Properties);
},
// 19.1.2.14 / 15.2.3.14 Object.keys(O)
keys: getKeys = getKeys || createGetKeys(keys1, keysLen1, false),
// 19.1.2.17 / 15.2.3.8 Object.seal(O)
seal: returnIt, // <- cap
// 19.1.2.5 / 15.2.3.9 Object.freeze(O)
freeze: returnIt, // <- cap
// 19.1.2.15 / 15.2.3.10 Object.preventExtensions(O)
preventExtensions: returnIt, // <- cap
// 19.1.2.13 / 15.2.3.11 Object.isSealed(O)
isSealed: isPrimitive, // <- cap
// 19.1.2.12 / 15.2.3.12 Object.isFrozen(O)
isFrozen: isPrimitive, // <- cap
// 19.1.2.11 / 15.2.3.13 Object.isExtensible(O)
isExtensible: isObject // <- cap
});
// 19.2.3.2 / 15.3.4.5 Function.prototype.bind(thisArg, args...)
$define(PROTO, FUNCTION, {
bind: function(that /*, args... */){
var fn = assertFunction(this)
, partArgs = slice.call(arguments, 1);
function bound(/* args... */){
var args = partArgs.concat(slice.call(arguments));
return (this instanceof bound ? construct : invoke)(fn, args, that);
}
return bound;
}
});
// Fix for not array-like ES3 string
function arrayMethodFix(fn){
return function(){
return fn.apply(ES5Object(this), arguments);
}
}
if(!(0 in Object(DOT) && DOT[0] == DOT)){
ES5Object = function(it){
return cof(it) == STRING ? it.split('') : Object(it);
}
slice = arrayMethodFix(slice);
}
$define(PROTO + FORCED * (ES5Object != Object), ARRAY, {
slice: slice,
join: arrayMethodFix(ArrayProto.join)
});
// 22.1.2.2 / 15.4.3.2 Array.isArray(arg)
$define(STATIC, ARRAY, {
isArray: function(arg){
return cof(arg) == ARRAY
}
});
function createArrayReduce(isRight){
return function(callbackfn, memo){
assertFunction(callbackfn);
var O = ES5Object(this)
, length = toLength(O.length)
, index = isRight ? length - 1 : 0
, i = isRight ? -1 : 1;
if(2 > arguments.length)for(;;){
if(index in O){
memo = O[index];
index += i;
break;
}
index += i;
assert(isRight ? index >= 0 : length > index, REDUCE_ERROR);
}
for(;isRight ? index >= 0 : length > index; index += i)if(index in O){
memo = callbackfn(memo, O[index], index, this);
}
return memo;
}
}
$define(PROTO, ARRAY, {
// 22.1.3.10 / 15.4.4.18 Array.prototype.forEach(callbackfn [, thisArg])
forEach: forEach = forEach || createArrayMethod(0),
// 22.1.3.15 / 15.4.4.19 Array.prototype.map(callbackfn [, thisArg])
map: createArrayMethod(1),
// 22.1.3.7 / 15.4.4.20 Array.prototype.filter(callbackfn [, thisArg])
filter: createArrayMethod(2),
// 22.1.3.23 / 15.4.4.17 Array.prototype.some(callbackfn [, thisArg])
some: createArrayMethod(3),
// 22.1.3.5 / 15.4.4.16 Array.prototype.every(callbackfn [, thisArg])
every: createArrayMethod(4),
// 22.1.3.18 / 15.4.4.21 Array.prototype.reduce(callbackfn [, initialValue])
reduce: createArrayReduce(false),
// 22.1.3.19 / 15.4.4.22 Array.prototype.reduceRight(callbackfn [, initialValue])
reduceRight: createArrayReduce(true),
// 22.1.3.11 / 15.4.4.14 Array.prototype.indexOf(searchElement [, fromIndex])
indexOf: indexOf = indexOf || createArrayContains(false),
// 22.1.3.14 / 15.4.4.15 Array.prototype.lastIndexOf(searchElement [, fromIndex])
lastIndexOf: function(el, fromIndex /* = @[*-1] */){
var O = ES5Object(this)
, length = toLength(O.length)
, index = length - 1;
if(arguments.length > 1)index = min(index, toInteger(fromIndex));
if(index < 0)index = toLength(length + index);
for(;index >= 0; index--)if(index in O)if(O[index] === el)return index;
return -1;
}
});
// 21.1.3.25 / 15.5.4.20 String.prototype.trim()
$define(PROTO, STRING, {trim: createReplacer(/^\s*([\s\S]*\S)?\s*$/, '$1')});
// 20.3.3.1 / 15.9.4.4 Date.now()
$define(STATIC, DATE, {now: function(){
return +new Date;
}});
if(_classof(function(){return arguments}()) == OBJECT)classof = function(it){
var cof = _classof(it);
return cof == OBJECT && isFunction(it.callee) ? ARGUMENTS : cof;
}
}('propertyIsEnumerable', Function(), classof, safeSymbol(PROTOTYPE));
/******************************************************************************
* Module : global *
******************************************************************************/
$define(GLOBAL + FORCED, {global: global});
/******************************************************************************
* Module : es6_symbol *
******************************************************************************/
// ECMAScript 6 symbols shim
!function(TAG, SymbolRegistry, setter){
// 19.4.1.1 Symbol([description])
if(!isNative(Symbol)){
Symbol = function(description){
assert(!(this instanceof Symbol), SYMBOL + ' is not a ' + CONSTRUCTOR);
var tag = uid(description);
DESC && setter && defineProperty(ObjectProto, tag, {
configurable: true,
set: function(value){
hidden(this, tag, value);
}
});
return set(create(Symbol[PROTOTYPE]), TAG, tag);
}
hidden(Symbol[PROTOTYPE], TO_STRING, function(){
return this[TAG];
});
}
$define(GLOBAL + WRAP, {Symbol: Symbol});
var symbolStatics = {
// 19.4.2.1 Symbol.for(key)
'for': function(key){
return has(SymbolRegistry, key += '')
? SymbolRegistry[key]
: SymbolRegistry[key] = Symbol(key);
},
// 19.4.2.4 Symbol.iterator
iterator: SYMBOL_ITERATOR,
// 19.4.2.5 Symbol.keyFor(sym)
keyFor: part.call(keyOf, SymbolRegistry),
// 19.4.2.13 Symbol.toStringTag
toStringTag: SYMBOL_TAG = getWellKnownSymbol(TO_STRING_TAG, true),
// 19.4.2.14 Symbol.unscopables
unscopables: SYMBOL_UNSCOPABLES,
pure: safeSymbol,
set: set,
useSetter: function(){setter = true},
useSimple: function(){setter = false}
};
// 19.4.2.2 Symbol.hasInstance
// 19.4.2.3 Symbol.isConcatSpreadable
// 19.4.2.6 Symbol.match
// 19.4.2.8 Symbol.replace
// 19.4.2.9 Symbol.search
// 19.4.2.10 Symbol.species
// 19.4.2.11 Symbol.split
// 19.4.2.12 Symbol.toPrimitive
forEach.call(array('hasInstance,isConcatSpreadable,match,replace,search,' +
'species,split,toPrimitive'), function(it){
symbolStatics[it] = getWellKnownSymbol(it);
}
);
$define(STATIC, SYMBOL, symbolStatics);
setToStringTag(Symbol, SYMBOL);
// 26.1.11 Reflect.ownKeys(target)
$define(GLOBAL, {Reflect: {ownKeys: ownKeys}});
}(safeSymbol('tag'), {}, true);
/******************************************************************************
* Module : es6 *
******************************************************************************/
// ECMAScript 6 shim
!function(RegExpProto, isFinite, tmp, NAME){
var RangeError = global.RangeError
// 20.1.2.3 Number.isInteger(number)
, isInteger = Number.isInteger || function(it){
return !isObject(it) && isFinite(it) && floor(it) === it;
}
// 20.2.2.28 Math.sign(x)
, sign = Math.sign || function sign(it){
return (it = +it) == 0 || it != it ? it : it < 0 ? -1 : 1;
}
, pow = Math.pow
, abs = Math.abs
, exp = Math.exp
, log = Math.log
, sqrt = Math.sqrt
, fcc = String.fromCharCode
, at = createPointAt(true);
var objectStatic = {
// 19.1.3.1 Object.assign(target, source)
assign: assign,
// 19.1.3.10 Object.is(value1, value2)
is: function(x, y){
return x === y ? x !== 0 || 1 / x === 1 / y : x != x && y != y;
}
};
// 19.1.3.19 Object.setPrototypeOf(O, proto)
// Works with __proto__ only. Old v8 can't works with null proto objects.
'__proto__' in ObjectProto && function(buggy, set){
try {
set = ctx(call, getOwnDescriptor(ObjectProto, '__proto__').set, 2);
set({}, ArrayProto);
} catch(e){ buggy = true }
objectStatic.setPrototypeOf = setPrototypeOf = setPrototypeOf || function(O, proto){
assertObject(O);
assert(proto === null || isObject(proto), proto, ": can't set as prototype!");
if(buggy)O.__proto__ = proto;
else set(O, proto);
return O;
}
}();
$define(STATIC, OBJECT, objectStatic);
// 20.2.2.5 Math.asinh(x)
function asinh(x){
return !isFinite(x = +x) || x == 0 ? x : x < 0 ? -asinh(-x) : log(x + sqrt(x * x + 1));
}
$define(STATIC, NUMBER, {
// 20.1.2.1 Number.EPSILON
EPSILON: pow(2, -52),
// 20.1.2.2 Number.isFinite(number)
isFinite: function(it){
return typeof it == 'number' && isFinite(it);
},
// 20.1.2.3 Number.isInteger(number)
isInteger: isInteger,
// 20.1.2.4 Number.isNaN(number)
isNaN: sameNaN,
// 20.1.2.5 Number.isSafeInteger(number)
isSafeInteger: function(number){
return isInteger(number) && abs(number) <= MAX_SAFE_INTEGER;
},
// 20.1.2.6 Number.MAX_SAFE_INTEGER
MAX_SAFE_INTEGER: MAX_SAFE_INTEGER,
// 20.1.2.10 Number.MIN_SAFE_INTEGER
MIN_SAFE_INTEGER: -MAX_SAFE_INTEGER,
// 20.1.2.12 Number.parseFloat(string)
parseFloat: parseFloat,
// 20.1.2.13 Number.parseInt(string, radix)
parseInt: parseInt
});
$define(STATIC, MATH, {
// 20.2.2.3 Math.acosh(x)
acosh: function(x){
return x < 1 ? NaN : log(x + sqrt(x * x - 1));
},
// 20.2.2.5 Math.asinh(x)
asinh: asinh,
// 20.2.2.7 Math.atanh(x)
atanh: function(x){
return x == 0 ? +x : log((1 + +x) / (1 - x)) / 2;
},
// 20.2.2.9 Math.cbrt(x)
cbrt: function(x){
return sign(x) * pow(abs(x), 1 / 3);
},
// 20.2.2.11 Math.clz32(x)
clz32: function(x){
return (x >>>= 0) ? 32 - x[TO_STRING](2).length : 32;
},
// 20.2.2.12 Math.cosh(x)
cosh: function(x){
return (exp(x) + exp(-x)) / 2;
},
// 20.2.2.14 Math.expm1(x)
expm1: function(x){
return x == 0 ? +x : x > -1e-6 && x < 1e-6 ? +x + x * x / 2 : exp(x) - 1;
},
// 20.2.2.16 Math.fround(x)
// TODO: fallback for IE9-
fround: function(x){
return new Float32Array([x])[0];
},
// 20.2.2.17 Math.hypot([value1[, value2[, … ]]])
// TODO: work with very large & small numbers
hypot: function(value1, value2){
var sum = 0
, length = arguments.length
, value;
while(length--){
value = +arguments[length];
if(value == Infinity || value == -Infinity)return Infinity;
sum += value * value;
}
return sqrt(sum);
},
// 20.2.2.18 Math.imul(x, y)
imul: function(x, y){
var UInt16 = 0xffff
, xn = +x
, yn = +y
, xl = UInt16 & xn
, yl = UInt16 & yn;
return 0 | xl * yl + ((UInt16 & xn >>> 16) * yl + xl * (UInt16 & yn >>> 16) << 16 >>> 0);
},
// 20.2.2.20 Math.log1p(x)
log1p: function(x){
return x > -1e-8 && x < 1e-8 ? x - x * x / 2 : log(1 + +x);
},
// 20.2.2.21 Math.log10(x)
log10: function(x){
return log(x) / Math.LN10;
},
// 20.2.2.22 Math.log2(x)
log2: function(x){
return log(x) / Math.LN2;
},
// 20.2.2.28 Math.sign(x)
sign: sign,
// 20.2.2.30 Math.sinh(x)
sinh: function(x){
return x == 0 ? +x : (exp(x) - exp(-x)) / 2;
},
// 20.2.2.33 Math.tanh(x)
tanh: function(x){
return isFinite(x) ? x == 0 ? +x : (exp(x) - exp(-x)) / (exp(x) + exp(-x)) : sign(x);
},
// 20.2.2.34 Math.trunc(x)
trunc: trunc
});
// 20.2.1.9 Math[@@toStringTag]
setToStringTag(Math, MATH, true);
function assertNotRegExp(it){
if(cof(it) == REGEXP)throw TypeError();
}
$define(STATIC, STRING, {
// 21.1.2.2 String.fromCodePoint(...codePoints)
fromCodePoint: function(x){
var res = []
, len = arguments.length
, i = 0
, code
while(len > i){
code = +arguments[i++];
if(toIndex(code, 0x10ffff) !== code)throw RangeError(code + ' is not a valid code point');
res.push(code < 0x10000
? fcc(code)
: fcc(((code -= 0x10000) >> 10) + 0xd800, code % 0x400 + 0xdc00)
);
} return res.join('');
},
// 21.1.2.4 String.raw(callSite, ...substitutions)
raw: function(callSite){
var raw = ES5Object(assertDefined(callSite.raw))
, len = toLength(raw.length)
, sln = arguments.length
, res = []
, i = 0;
while(len > i){
res.push(String(raw[i++]));
if(i < sln)res.push(String(arguments[i]));
} return res.join('');
}
});
$define(PROTO, STRING, {
// 21.1.3.3 String.prototype.codePointAt(pos)
codePointAt: createPointAt(false),
// 21.1.3.6 String.prototype.endsWith(searchString [, endPosition])
endsWith: function(searchString /*, endPosition = @length */){
assertNotRegExp(searchString);
var that = String(assertDefined(this))
, endPosition = arguments[1]
, len = toLength(that.length)
, end = endPosition === undefined ? len : min(toLength(endPosition), len);
searchString += '';
return that.slice(end - searchString.length, end) === searchString;
},
// 21.1.3.7 String.prototype.includes(searchString, position = 0)
includes: function(searchString /*, position = 0 */){
assertNotRegExp(searchString);
return !!~String(assertDefined(this)).indexOf(searchString, arguments[1]);
},
// 21.1.3.13 String.prototype.repeat(count)
repeat: function(count){
var str = String(assertDefined(this))
, res = ''
, n = toInteger(count);
if(0 > n || n == Infinity)throw RangeError("Count can't be negative");
for(;n > 0; (n >>>= 1) && (str += str))if(n & 1)res += str;
return res;
},
// 21.1.3.18 String.prototype.startsWith(searchString [, position ])
startsWith: function(searchString /*, position = 0 */){
assertNotRegExp(searchString);
var that = String(assertDefined(this))
, index = toLength(min(arguments[1], that.length));
searchString += '';
return that.slice(index, index + searchString.length) === searchString;
}
});
// 21.1.3.27 String.prototype[@@iterator]()
defineStdIterators(String, STRING, function(iterated){
set(this, ITER, {o: String(iterated), i: 0});
// 21.1.5.2.1 %StringIteratorPrototype%.next()
}, function(){
var iter = this[ITER]
, O = iter.o
, index = iter.i
, point;
if(index >= O.length)return iterResult(1);
point = at.call(O, index);
iter.i += point.length;
return iterResult(0, point);
});
$define(STATIC, ARRAY, {
// 22.1.2.1 Array.from(arrayLike, mapfn = undefined, thisArg = undefined)
from: function(arrayLike/*, mapfn = undefined, thisArg = undefined*/){
var O = Object(assertDefined(arrayLike))
, result = new (generic(this, Array))
, mapfn = arguments[1]
, that = arguments[2]
, mapping = mapfn !== undefined
, f = mapping ? ctx(mapfn, that, 2) : undefined
, index = 0
, length;
if(isIterable(O))for(var iter = getIterator(O), step; !(step = iter.next()).done; index++){
result[index] = mapping ? f(step.value, index) : step.value;
} else for(length = toLength(O.length); length > index; index++){
result[index] = mapping ? f(O[index], index) : O[index];
}
result.length = index;
return result;
},
// 22.1.2.3 Array.of( ...items)
of: function(/* ...args */){
var index = 0
, length = arguments.length
, result = new (generic(this, Array))(length);
while(length > index)result[index] = arguments[index++];
result.length = length;
return result;
}
});
$define(PROTO, ARRAY, {
// 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
copyWithin: function(target /* = 0 */, start /* = 0, end = @length */){
var O = Object(assertDefined(this))
, len = toLength(O.length)
, to = toIndex(target, len)
, from = toIndex(start, len)
, end = arguments[2]
, fin = end === undefined ? len : toIndex(end, len)
, count = min(fin - from, len - to)
, inc = 1;
if(from < to && to < from + count){
inc = -1;
from = from + count - 1;
to = to + count - 1;
}
while(count-- > 0){
if(from in O)O[to] = O[from];
else delete O[to];
to += inc;
from += inc;
} return O;
},
// 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
fill: function(value /*, start = 0, end = @length */){
var O = Object(assertDefined(this))
, length = toLength(O.length)
, index = toIndex(arguments[1], length)
, end = arguments[2]
, endPos = end === undefined ? length : toIndex(end, length);
while(endPos > index)O[index++] = value;
return O;
},
// 22.1.3.8 Array.prototype.find(predicate, thisArg = undefined)
find: createArrayMethod(5),
// 22.1.3.9 Array.prototype.findIndex(predicate, thisArg = undefined)
findIndex: createArrayMethod(6)
});
// 22.1.3.4 Array.prototype.entries()
// 22.1.3.13 Array.prototype.keys()
// 22.1.3.29 Array.prototype.values()
// 22.1.3.30 Array.prototype[@@iterator]()
defineStdIterators(Array, ARRAY, function(iterated, kind){
set(this, ITER, {o: ES5Object(iterated), i: 0, k: kind});
// 22.1.5.2.1 %ArrayIteratorPrototype%.next()
}, function(){
var iter = this[ITER]
, O = iter.o
, kind = iter.k
, index = iter.i++;
if(!O || index >= O.length)return iter.o = undefined, iterResult(1);
if(kind == KEY) return iterResult(0, index);
if(kind == VALUE)return iterResult(0, O[index]);
return iterResult(0, [index, O[index]]);
}, VALUE);
// argumentsList[@@iterator] is %ArrayProto_values% (9.4.4.6, 9.4.4.7)
Iterators[ARGUMENTS] = Iterators[ARRAY];
// 24.3.3 JSON[@@toStringTag]
setToStringTag(global.JSON, 'JSON', true);
function WrappedRegExp(pattern, flags){
return new RegExp(cof(pattern) == REGEXP && flags !== undefined
? pattern.source : pattern, flags);
}
function wrapObjectMethod(key, MODE){
var fn = Object[key];
try { fn(DOT) }
catch(e){
Object[key] =
MODE == 1 ? function(it){ return isObject(it) ? fn(it) : it } :
MODE == 2 ? function(it){ return isObject(it) ? fn(it) : true } :
MODE == 3 ? function(it){ return isObject(it) ? fn(it) : false } :
MODE == 4 ? function(it, key){ return fn(Object(assertDefined(it)), key) } :
function(it){ return fn(Object(assertDefined(it))) }
}
}
if(framework){
// Object static methods accept primitives
wrapObjectMethod('freeze', 1);
wrapObjectMethod('seal', 1);
wrapObjectMethod('preventExtensions', 1);
wrapObjectMethod('isFrozen', 2);
wrapObjectMethod('isSealed', 2);
wrapObjectMethod('isExtensible', 3);
wrapObjectMethod('getOwnPropertyDescriptor', 4);
wrapObjectMethod('getPrototypeOf');
wrapObjectMethod('keys');
wrapObjectMethod('getOwnPropertyNames');
// 19.1.3.6 Object.prototype.toString()
tmp[SYMBOL_TAG] = DOT;
if(cof(tmp) != DOT)hidden(ObjectProto, TO_STRING, function(){
return '[object ' + classof(this) + ']';
});
// 19.2.4.2 name
NAME in FunctionProto || defineProperty(FunctionProto, NAME, {
configurable: true,
get: function(){
var match = String(this).match(/^\s*function ([^ (]*)/)
, name = match ? match[1] : '';
has(this, NAME) || defineProperty(this, NAME, descriptor(5, name));
return name;
}
});
// RegExp allows a regex with flags as the pattern
if(DESC && !function(){try{return RegExp(/a/g, 'i') == '/a/i'}catch(e){}}()){
forEach.call(getNames(RegExp), function(key){
key in WrappedRegExp || defineProperty(WrappedRegExp, key, {
configurable: true,
get: function(){ return RegExp[key] },
set: function(it){ RegExp[key] = it }
});
});
RegExpProto[CONSTRUCTOR] = WrappedRegExp;
WrappedRegExp[PROTOTYPE] = RegExpProto;
hidden(global, REGEXP, WrappedRegExp);
}
// 21.2.5.3 get RegExp.prototype.flags()
if(/./g.flags != 'g')defineProperty(RegExpProto, 'flags', {
configurable: true,
get: createReplacer(/^.*\/(\w*)$/, '$1')
});
// 22.1.3.31 Array.prototype[@@unscopables]
forEach.call(array('find,findIndex,fill,copyWithin,entries,keys,values'), function(it){
ArrayUnscopables[it] = true;
});
SYMBOL_UNSCOPABLES in ArrayProto || hidden(ArrayProto, SYMBOL_UNSCOPABLES, ArrayUnscopables);
}
}(RegExp[PROTOTYPE], isFinite, {}, 'name');
/******************************************************************************
* Module : immediate *
******************************************************************************/
// setImmediate shim
// Node.js 0.9+ & IE10+ has setImmediate, else:
isFunction(setImmediate) && isFunction(clearImmediate) || function(ONREADYSTATECHANGE){
var postMessage = global.postMessage
, addEventListener = global.addEventListener
, MessageChannel = global.MessageChannel
, counter = 0
, queue = {}
, defer, channel, port;
setImmediate = function(fn){
var args = [], i = 1;
while(arguments.length > i)args.push(arguments[i++]);
queue[++counter] = function(){
invoke(isFunction(fn) ? fn : Function(fn), args);
}
defer(counter);
return counter;
}
clearImmediate = function(id){
delete queue[id];
}
function run(id){
if(has(queue, id)){
var fn = queue[id];
delete queue[id];
fn();
}
}
function listner(event){
run(event.data);
}
// Node.js 0.8-
if(NODE){
defer = function(id){
nextTick(part.call(run, id));
}
// Modern browsers, skip implementation for WebWorkers
// IE8 has postMessage, but it's sync & typeof its postMessage is object
} else if(addEventListener && isFunction(postMessage) && !global.importScripts){
defer = function(id){
postMessage(id, '*');
}
addEventListener('message', listner, false);
// WebWorkers
} else if(isFunction(MessageChannel)){
channel = new MessageChannel;
port = channel.port2;
channel.port1.onmessage = listner;
defer = ctx(port.postMessage, port, 1);
// IE8-
} else if(document && ONREADYSTATECHANGE in document[CREATE_ELEMENT]('script')){
defer = function(id){
html.appendChild(document[CREATE_ELEMENT]('script'))[ONREADYSTATECHANGE] = function(){
html.removeChild(this);
run(id);
}
}
// Rest old browsers
} else {
defer = function(id){
setTimeout(part.call(run, id), 0);
}
}
}('onreadystatechange');
$define(GLOBAL + BIND, {
setImmediate: setImmediate,
clearImmediate: clearImmediate
});
/******************************************************************************
* Module : es6_promise *
******************************************************************************/
// ES6 promises shim
// Based on https://github.com/getify/native-promise-only/
!function(Promise, test){
isFunction(Promise) && isFunction(Promise.resolve)
&& Promise.resolve(test = new Promise(Function())) == test
|| function(asap, DEF){
function isThenable(o){
var then;
if(isObject(o))then = o.then;
return isFunction(then) ? then : false;
}
function notify(def){
var chain = def.chain;
chain.length && asap(function(){
var msg = def.msg
, ok = def.state == 1
, i = 0;
while(chain.length > i)!function(react){
var cb = ok ? react.ok : react.fail
, ret, then;
try {
if(cb){
ret = cb === true ? msg : cb(msg);
if(ret === react.P){
react.rej(TypeError(PROMISE + '-chain cycle'));
} else if(then = isThenable(ret)){
then.call(ret, react.res, react.rej);
} else react.res(ret);
} else react.rej(msg);
} catch(err){
react.rej(err);
}
}(chain[i++]);
chain.length = 0;
});
}
function resolve(msg){
var def = this
, then, wrapper;
if(def.done)return;
def.done = true;
def = def.def || def; // unwrap
try {
if(then = isThenable(msg)){
wrapper = {def: def, done: false}; // wrap
then.call(msg, ctx(resolve, wrapper, 1), ctx(reject, wrapper, 1));
} else {
def.msg = msg;
def.state = 1;
notify(def);
}
} catch(err){
reject.call(wrapper || {def: def, done: false}, err); // wrap
}
}
function reject(msg){
var def = this;
if(def.done)return;
def.done = true;
def = def.def || def; // unwrap
def.msg = msg;
def.state = 2;
notify(def);
}
// 25.4.3.1 Promise(executor)
Promise = function(executor){
assertFunction(executor);
assertInstance(this, Promise, PROMISE);
var def = {chain: [], state: 0, done: false, msg: undefined};
hidden(this, DEF, def);
try {
executor(ctx(resolve, def, 1), ctx(reject, def, 1));
} catch(err){
reject.call(def, err);
}
}
assignHidden(Promise[PROTOTYPE], {
// 25.4.5.3 Promise.prototype.then(onFulfilled, onRejected)
then: function(onFulfilled, onRejected){
var react = {
ok: isFunction(onFulfilled) ? onFulfilled : true,
fail: isFunction(onRejected) ? onRejected : false
} , P = react.P = new this[CONSTRUCTOR](function(resolve, reject){
react.res = assertFunction(resolve);
react.rej = assertFunction(reject);
}), def = this[DEF];
def.chain.push(react);
def.state && notify(def);
return P;
},
// 25.4.5.1 Promise.prototype.catch(onRejected)
'catch': function(onRejected){
return this.then(undefined, onRejected);
}
});
assignHidden(Promise, {
// 25.4.4.1 Promise.all(iterable)
all: function(iterable){
var Promise = this
, values = [];
return new Promise(function(resolve, reject){
forOf(iterable, false, push, values);
var remaining = values.length
, results = Array(remaining);
if(remaining)forEach.call(values, function(promise, index){
Promise.resolve(promise).then(function(value){
results[index] = value;
--remaining || resolve(results);
}, reject);
});
else resolve(results);
});
},
// 25.4.4.4 Promise.race(iterable)
race: function(iterable){
var Promise = this;
return new Promise(function(resolve, reject){
forOf(iterable, false, function(promise){
Promise.resolve(promise).then(resolve, reject);
});
});
},
// 25.4.4.5 Promise.reject(r)
reject: function(r){
return new this(function(resolve, reject){
reject(r);
});
},
// 25.4.4.6 Promise.resolve(x)
resolve: function(x){
return isObject(x) && getPrototypeOf(x) === this[PROTOTYPE]
? x : new this(function(resolve, reject){
resolve(x);
});
}
});
}(nextTick || setImmediate, safeSymbol('def'));
setToStringTag(Promise, PROMISE);
$define(GLOBAL + FORCED * !isNative(Promise), {Promise: Promise});
}(global[PROMISE]);
/******************************************************************************
* Module : es6_collections *
******************************************************************************/
// ECMAScript 6 collections shim
!function(){
var UID = safeSymbol('uid')
, DATA = safeSymbol('data')
, WEAK = safeSymbol('weak')
, LAST = safeSymbol('last')
, FIRST = safeSymbol('first')
, SIZE = DESC ? safeSymbol('size') : 'size'
, uid = 0;
function getCollection(C, NAME, methods, commonMethods, isMap, isWeak){
var ADDER = isMap ? 'set' : 'add'
, proto = C && C[PROTOTYPE]
, O = {};
function initFromIterable(that, iterable){
if(iterable != undefined)forOf(iterable, isMap, that[ADDER], that);
return that;
}
function fixSVZ(key, chain){
var method = proto[key];
framework && hidden(proto, key, function(a, b){
var result = method.call(this, a === 0 ? 0 : a, b);
return chain ? this : result;
});
}
if(!isNative(C) || !(isWeak || (!BUGGY_ITERATORS && has(proto, 'entries')))){
// create collection constructor
C = isWeak
? function(iterable){
assertInstance(this, C, NAME);
set(this, UID, uid++);
initFromIterable(this, iterable);
}
: function(iterable){
var that = this;
assertInstance(that, C, NAME);
set(that, DATA, create(null));
set(that, SIZE, 0);
set(that, LAST, undefined);
set(that, FIRST, undefined);
initFromIterable(that, iterable);
};
assignHidden(assignHidden(C[PROTOTYPE], methods), commonMethods);
isWeak || defineProperty(C[PROTOTYPE], 'size', {get: function(){
return assertDefined(this[SIZE]);
}});
} else {
var Native = C
, inst = new C
, chain = inst[ADDER](isWeak ? {} : -0, 1)
, buggyZero;
// wrap to init collections from iterable
if(!NATIVE_ITERATORS || !C.length){
C = function(iterable){
assertInstance(this, C, NAME);
return initFromIterable(new Native, iterable);
}
C[PROTOTYPE] = proto;
}
isWeak || inst[FOR_EACH](function(val, key){
buggyZero = 1 / key === -Infinity;
});
// fix converting -0 key to +0
if(buggyZero){
fixSVZ('delete');
fixSVZ('has');
isMap && fixSVZ('get');
}
// + fix .add & .set for chaining
if(buggyZero || chain !== inst)fixSVZ(ADDER, true);
}
setToStringTag(C, NAME);
O[NAME] = C;
$define(GLOBAL + WRAP + FORCED * !isNative(C), O);
// add .keys, .values, .entries, [@@iterator]
// 23.1.3.4, 23.1.3.8, 23.1.3.11, 23.1.3.12, 23.2.3.5, 23.2.3.8, 23.2.3.10, 23.2.3.11
isWeak || defineStdIterators(C, NAME, function(iterated, kind){
set(this, ITER, {o: iterated, k: kind});
}, function(){
var iter = this[ITER]
, O = iter.o
, kind = iter.k
, entry = iter.l;
while(entry && entry.r)entry = entry.p;
if(!O || !(iter.l = entry = entry ? entry.n : O[FIRST]))return iter.o = undefined, iterResult(1);
if(kind == KEY) return iterResult(0, entry.k);
if(kind == VALUE)return iterResult(0, entry.v);
return iterResult(0, [entry.k, entry.v]);
}, isMap ? KEY+VALUE : VALUE, !isMap);
return C;
}
function fastKey(it, create){
// return it with 'S' prefix if it's string or with 'P' prefix for over primitives
if(!isObject(it))return (typeof it == 'string' ? 'S' : 'P') + it;
// if it hasn't object id - add next
if(!has(it, UID)){
if(create)hidden(it, UID, ++uid);
else return '';
}
// return object id with 'O' prefix
return 'O' + it[UID];
}
function def(that, key, value){
var index = fastKey(key, true)
, data = that[DATA]
, last = that[LAST]
, entry;
if(index in data)data[index].v = value;
else {
entry = data[index] = {k: key, v: value, p: last};
if(!that[FIRST])that[FIRST] = entry;
if(last)last.n = entry;
that[LAST] = entry;
that[SIZE]++;
} return that;
}
function del(that, index){
var data = that[DATA]
, entry = data[index]
, next = entry.n
, prev = entry.p;
delete data[index];
entry.r = true;
if(prev)prev.n = next;
if(next)next.p = prev;
if(that[FIRST] == entry)that[FIRST] = next;
if(that[LAST] == entry)that[LAST] = prev;
that[SIZE]--;
}
var collectionMethods = {
// 23.1.3.1 Map.prototype.clear()
// 23.2.3.2 Set.prototype.clear()
clear: function(){
for(var index in this[DATA])del(this, index);
},
// 23.1.3.3 Map.prototype.delete(key)
// 23.2.3.4 Set.prototype.delete(value)
'delete': function(key){
var index = fastKey(key)
, contains = index in this[DATA];
if(contains)del(this, index);
return contains;
},
// 23.2.3.6 Set.prototype.forEach(callbackfn, thisArg = undefined)
// 23.1.3.5 Map.prototype.forEach(callbackfn, thisArg = undefined)
forEach: function(callbackfn /*, that = undefined */){
var f = ctx(callbackfn, arguments[1], 3)
, entry;
while(entry = entry ? entry.n : this[FIRST]){
f(entry.v, entry.k, this);
while(entry && entry.r)entry = entry.p;
}
},
// 23.1.3.7 Map.prototype.has(key)
// 23.2.3.7 Set.prototype.has(value)
has: function(key){
return fastKey(key) in this[DATA];
}
}
// 23.1 Map Objects
Map = getCollection(Map, MAP, {
// 23.1.3.6 Map.prototype.get(key)
get: function(key){
var entry = this[DATA][fastKey(key)];
return entry && entry.v;
},
// 23.1.3.9 Map.prototype.set(key, value)
set: function(key, value){
return def(this, key === 0 ? 0 : key, value);
}
}, collectionMethods, true);
// 23.2 Set Objects
Set = getCollection(Set, SET, {
// 23.2.3.1 Set.prototype.add(value)
add: function(value){
return def(this, value = value === 0 ? 0 : value, value);
}
}, collectionMethods);
function setWeak(that, key, value){
has(assertObject(key), WEAK) || hidden(key, WEAK, {});
key[WEAK][that[UID]] = value;
return that;
}
function hasWeak(key){
return isObject(key) && has(key, WEAK) && has(key[WEAK], this[UID]);
}
var weakMethods = {
// 23.3.3.2 WeakMap.prototype.delete(key)
// 23.4.3.3 WeakSet.prototype.delete(value)
'delete': function(key){
return hasWeak.call(this, key) && delete key[WEAK][this[UID]];
},
// 23.3.3.4 WeakMap.prototype.has(key)
// 23.4.3.4 WeakSet.prototype.has(value)
has: hasWeak
};
// 23.3 WeakMap Objects
WeakMap = getCollection(WeakMap, WEAKMAP, {
// 23.3.3.3 WeakMap.prototype.get(key)
get: function(key){
if(isObject(key) && has(key, WEAK))return key[WEAK][this[UID]];
},
// 23.3.3.5 WeakMap.prototype.set(key, value)
set: function(key, value){
return setWeak(this, key, value);
}
}, weakMethods, true, true);
// 23.4 WeakSet Objects
WeakSet = getCollection(WeakSet, WEAKSET, {
// 23.4.3.1 WeakSet.prototype.add(value)
add: function(value){
return setWeak(this, value, true);
}
}, weakMethods, false, true);
}();
/******************************************************************************
* Module : es6_reflect *
******************************************************************************/
!function(){
function Enumerate(iterated){
var keys = [], key;
for(key in iterated)keys.push(key);
set(this, ITER, {o: iterated, a: keys, i: 0});
}
createIterator(Enumerate, OBJECT, function(){
var iter = this[ITER]
, keys = iter.a
, key;
do {
if(iter.i >= keys.length)return iterResult(1);
} while(!((key = keys[iter.i++]) in iter.o));
return iterResult(0, key);
});
function wrap(fn){
return function(it){
assertObject(it);
try {
return fn.apply(undefined, arguments), true;
} catch(e){
return false;
}
}
}
function reflectGet(target, propertyKey/*, receiver*/){
var receiver = arguments.length < 3 ? target : arguments[2]
, desc = getOwnDescriptor(assertObject(target), propertyKey), proto;
if(desc)return desc.get ? desc.get.call(receiver) : desc.value;
return isObject(proto = getPrototypeOf(target)) ? reflectGet(proto, propertyKey, receiver) : undefined;
}
function reflectSet(target, propertyKey, V/*, receiver*/){
var receiver = arguments.length < 4 ? target : arguments[3]
, desc = getOwnDescriptor(assertObject(target), propertyKey), proto;
if(desc){
if(desc.writable === false)return false;
if(desc.set)return desc.set.call(receiver, V), true;
}
if(isObject(proto = getPrototypeOf(target)))return reflectSet(proto, propertyKey, V, receiver);
desc = getOwnDescriptor(receiver, propertyKey) || descriptor(0);
desc.value = V;
return defineProperty(receiver, propertyKey, desc), true;
}
var reflect = {
// 26.1.1 Reflect.apply(target, thisArgument, argumentsList)
apply: ctx(call, apply, 3),
// 26.1.2 Reflect.construct(target, argumentsList)
construct: construct,
// 26.1.3 Reflect.defineProperty(target, propertyKey, attributes)
defineProperty: wrap(defineProperty),
// 26.1.4 Reflect.deleteProperty(target, propertyKey)
deleteProperty: function(target, propertyKey){
var desc = getOwnDescriptor(assertObject(target), propertyKey);
return desc && !desc.configurable ? false : delete target[propertyKey];
},
// 26.1.5 Reflect.enumerate(target)
enumerate: function(target){
return new Enumerate(assertObject(target));
},
// 26.1.6 Reflect.get(target, propertyKey [, receiver])
get: reflectGet,
// 26.1.7 Reflect.getOwnPropertyDescriptor(target, propertyKey)
getOwnPropertyDescriptor: getOwnDescriptor,
// 26.1.8 Reflect.getPrototypeOf(target)
getPrototypeOf: getPrototypeOf,
// 26.1.9 Reflect.has(target, propertyKey)
has: function(target, propertyKey){
return propertyKey in target;
},
// 26.1.10 Reflect.isExtensible(target)
isExtensible: Object.isExtensible || function(target){
return !!assertObject(target);
},
// 26.1.11 Reflect.ownKeys(target)
ownKeys: ownKeys,
// 26.1.12 Reflect.preventExtensions(target)
preventExtensions: wrap(Object.preventExtensions || returnIt),
// 26.1.13 Reflect.set(target, propertyKey, V [, receiver])
set: reflectSet
}
// 26.1.14 Reflect.setPrototypeOf(target, proto)
if(setPrototypeOf)reflect.setPrototypeOf = function(target, proto){
return setPrototypeOf(assertObject(target), proto), true;
};
$define(GLOBAL, {Reflect: {}});
$define(STATIC, 'Reflect', reflect);
}();
/******************************************************************************
* Module : es7 *
******************************************************************************/
!function(){
$define(PROTO, ARRAY, {
// https://github.com/domenic/Array.prototype.includes
includes: createArrayContains(true)
});
$define(PROTO, STRING, {
// https://github.com/mathiasbynens/String.prototype.at
at: createPointAt(true)
});
function createObjectToArray(isEntries){
return function(object){
var O = ES5Object(object)
, keys = getKeys(object)
, length = keys.length
, i = 0
, result = Array(length)
, key;
if(isEntries)while(length > i)result[i] = [key = keys[i++], O[key]];
else while(length > i)result[i] = O[keys[i++]];
return result;
}
}
$define(STATIC, OBJECT, {
// https://github.com/rwaldron/tc39-notes/blob/master/es6/2014-04/apr-9.md#51-objectentries-objectvalues
values: createObjectToArray(false),
entries: createObjectToArray(true)
});
$define(STATIC, REGEXP, {
// https://gist.github.com/kangax/9698100
escape: createReplacer(/([\\\-[\]{}()*+?.,^$|])/g, '\\$1', true)
});
}();
/******************************************************************************
* Module : es7_refs *
******************************************************************************/
// https://github.com/zenparsing/es-abstract-refs
!function(REFERENCE){
REFERENCE_GET = getWellKnownSymbol(REFERENCE+'Get', true);
var REFERENCE_SET = getWellKnownSymbol(REFERENCE+SET, true)
, REFERENCE_DELETE = getWellKnownSymbol(REFERENCE+'Delete', true);
$define(STATIC, SYMBOL, {
referenceGet: REFERENCE_GET,
referenceSet: REFERENCE_SET,
referenceDelete: REFERENCE_DELETE
});
hidden(FunctionProto, REFERENCE_GET, returnThis);
function setMapMethods(Constructor){
if(Constructor){
var MapProto = Constructor[PROTOTYPE];
hidden(MapProto, REFERENCE_GET, MapProto.get);
hidden(MapProto, REFERENCE_SET, MapProto.set);
hidden(MapProto, REFERENCE_DELETE, MapProto['delete']);
}
}
setMapMethods(Map);
setMapMethods(WeakMap);
}('reference');
/******************************************************************************
* Module : dict *
******************************************************************************/
!function(DICT){
function Dict(iterable){
var dict = create(null);
if(iterable != undefined){
if(isIterable(iterable)){
for(var iter = getIterator(iterable), step, value; !(step = iter.next()).done;){
value = step.value;
dict[value[0]] = value[1];
}
} else assign(dict, iterable);
}
return dict;
}
Dict[PROTOTYPE] = null;
function DictIterator(iterated, kind){
set(this, ITER, {o: ES5Object(iterated), a: getKeys(iterated), i: 0, k: kind});
}
createIterator(DictIterator, DICT, function(){
var iter = this[ITER]
, O = iter.o
, keys = iter.a
, kind = iter.k
, key;
do {
if(iter.i >= keys.length)return iterResult(1);
} while(!has(O, key = keys[iter.i++]));
if(kind == KEY) return iterResult(0, key);
if(kind == VALUE)return iterResult(0, O[key]);
return iterResult(0, [key, O[key]]);
});
function createDictIter(kind){
return function(it){
return new DictIterator(it, kind);
}
}
/*
* 0 -> forEach
* 1 -> map
* 2 -> filter
* 3 -> some
* 4 -> every
* 5 -> find
* 6 -> findKey
* 7 -> mapPairs
*/
function createDictMethod(type){
var isMap = type == 1
, isEvery = type == 4;
return function(object, callbackfn, that /* = undefined */){
var f = ctx(callbackfn, that, 3)
, O = ES5Object(object)
, result = isMap || type == 7 || type == 2 ? new (generic(this, Dict)) : undefined
, key, val, res;
for(key in O)if(has(O, key)){
val = O[key];
res = f(val, key, object);
if(type){
if(isMap)result[key] = res; // map
else if(res)switch(type){
case 2: result[key] = val; break // filter
case 3: return true; // some
case 5: return val; // find
case 6: return key; // findKey
case 7: result[res[0]] = res[1]; // mapPairs
} else if(isEvery)return false; // every
}
}
return type == 3 || isEvery ? isEvery : result;
}
}
function createDictReduce(isTurn){
return function(object, mapfn, init){
assertFunction(mapfn);
var O = ES5Object(object)
, keys = getKeys(O)
, length = keys.length
, i = 0
, memo, key, result;
if(isTurn)memo = init == undefined ? new (generic(this, Dict)) : Object(init);
else if(arguments.length < 3){
assert(length, REDUCE_ERROR);
memo = O[keys[i++]];
} else memo = Object(init);
while(length > i)if(has(O, key = keys[i++])){
result = mapfn(memo, O[key], key, object);
if(isTurn){
if(result === false)break;
} else memo = result;
}
return memo;
}
}
var findKey = createDictMethod(6);
function includes(object, el){
return (el == el ? keyOf(object, el) : findKey(object, sameNaN)) !== undefined;
}
var dictMethods = {
keys: createDictIter(KEY),
values: createDictIter(VALUE),
entries: createDictIter(KEY+VALUE),
forEach: createDictMethod(0),
map: createDictMethod(1),
filter: createDictMethod(2),
some: createDictMethod(3),
every: createDictMethod(4),
find: createDictMethod(5),
findKey: findKey,
mapPairs:createDictMethod(7),
reduce: createDictReduce(false),
turn: createDictReduce(true),
keyOf: keyOf,
includes:includes,
// Has / get / set own property
has: has,
get: get,
set: createDefiner(0),
isDict: function(it){
return isObject(it) && getPrototypeOf(it) === Dict[PROTOTYPE];
}
};
if(REFERENCE_GET)for(var key in dictMethods)!function(fn){
function method(){
for(var args = [this], i = 0; i < arguments.length;)args.push(arguments[i++]);
return invoke(fn, args);
}
fn[REFERENCE_GET] = function(){
return method;
}
}(dictMethods[key]);
$define(GLOBAL + FORCED, {Dict: assignHidden(Dict, dictMethods)});
}('Dict');
/******************************************************************************
* Module : $for *
******************************************************************************/
!function(ENTRIES, FN){
function $for(iterable, entries){
if(!(this instanceof $for))return new $for(iterable, entries);
this[ITER] = getIterator(iterable);
this[ENTRIES] = !!entries;
}
createIterator($for, 'Wrapper', function(){
return this[ITER].next();
});
var $forProto = $for[PROTOTYPE];
setIterator($forProto, function(){
return this[ITER]; // unwrap
});
function createChainIterator(next){
function Iter(I, fn, that){
this[ITER] = getIterator(I);
this[ENTRIES] = I[ENTRIES];
this[FN] = ctx(fn, that, I[ENTRIES] ? 2 : 1);
}
createIterator(Iter, 'Chain', next, $forProto);
setIterator(Iter[PROTOTYPE], returnThis); // override $forProto iterator
return Iter;
}
var MapIter = createChainIterator(function(){
var step = this[ITER].next();
return step.done ? step : iterResult(0, stepCall(this[FN], step.value, this[ENTRIES]));
});
var FilterIter = createChainIterator(function(){
for(;;){
var step = this[ITER].next();
if(step.done || stepCall(this[FN], step.value, this[ENTRIES]))return step;
}
});
assignHidden($forProto, {
of: function(fn, that){
forOf(this, this[ENTRIES], fn, that);
},
array: function(fn, that){
var result = [];
forOf(fn != undefined ? this.map(fn, that) : this, false, push, result);
return result;
},
filter: function(fn, that){
return new FilterIter(this, fn, that);
},
map: function(fn, that){
return new MapIter(this, fn, that);
}
});
$for.isIterable = isIterable;
$for.getIterator = getIterator;
$define(GLOBAL + FORCED, {$for: $for});
}('entries', safeSymbol('fn'));
/******************************************************************************
* Module : timers *
******************************************************************************/
// ie9- setTimeout & setInterval additional parameters fix
!function(MSIE){
function wrap(set){
return MSIE ? function(fn, time /*, ...args */){
return set(invoke(part, slice.call(arguments, 2), isFunction(fn) ? fn : Function(fn)), time);
} : set;
}
$define(GLOBAL + BIND + FORCED * MSIE, {
setTimeout: setTimeout = wrap(setTimeout),
setInterval: wrap(setInterval)
});
// ie9- dirty check
}(!!navigator && /MSIE .\./.test(navigator.userAgent));
/******************************************************************************
* Module : binding *
******************************************************************************/
!function(_, toLocaleString){
// Placeholder
core._ = path._ = path._ || {};
$define(PROTO + FORCED, FUNCTION, {
part: part,
by: function(that){
var fn = this
, _ = path._
, holder = false
, length = arguments.length
, isThat = that === _
, i = +!isThat
, indent = i
, it, args;
if(isThat){
it = fn;
fn = call;
} else it = that;
if(length < 2)return ctx(fn, it, -1);
args = Array(length - indent);
while(length > i)if((args[i - indent] = arguments[i++]) === _)holder = true;
return partial(fn, args, length, holder, _, true, it);
},
only: function(numberArguments, that /* = @ */){
var fn = assertFunction(this)
, n = toLength(numberArguments)
, isThat = arguments.length > 1;
return function(/* ...args */){
var length = min(n, arguments.length)
, args = Array(length)
, i = 0;
while(length > i)args[i] = arguments[i++];
return invoke(fn, args, isThat ? that : this);
}
}
});
function tie(key){
var that = this
, bound = {};
return hidden(that, _, function(key){
if(key === undefined || !(key in that))return toLocaleString.call(that);
return has(bound, key) ? bound[key] : (bound[key] = ctx(that[key], that, -1));
})[_](key);
}
hidden(path._, TO_STRING, function(){
return _;
});
hidden(ObjectProto, _, tie);
DESC || hidden(ArrayProto, _, tie);
// IE8- dirty hack - redefined toLocaleString is not enumerable
}(DESC ? uid('tie') : TO_LOCALE, ObjectProto[TO_LOCALE]);
/******************************************************************************
* Module : object *
******************************************************************************/
!function(){
function define(target, mixin){
var keys = ownKeys(ES5Object(mixin))
, length = keys.length
, i = 0, key;
while(length > i)defineProperty(target, key = keys[i++], getOwnDescriptor(mixin, key));
return target;
};
$define(STATIC + FORCED, OBJECT, {
isObject: isObject,
classof: classof,
define: define,
make: function(proto, mixin){
return define(create(proto), mixin);
}
});
}();
/******************************************************************************
* Module : array *
******************************************************************************/
$define(PROTO + FORCED, ARRAY, {
turn: function(fn, target /* = [] */){
assertFunction(fn);
var memo = target == undefined ? [] : Object(target)
, O = ES5Object(this)
, length = toLength(O.length)
, index = 0;
while(length > index)if(fn(memo, O[index], index++, this) === false)break;
return memo;
}
});
if(framework)ArrayUnscopables.turn = true;
/******************************************************************************
* Module : array_statics *
******************************************************************************/
// JavaScript 1.6 / Strawman array statics shim
!function(arrayStatics){
function setArrayStatics(keys, length){
forEach.call(array(keys), function(key){
if(key in ArrayProto)arrayStatics[key] = ctx(call, ArrayProto[key], length);
});
}
setArrayStatics('pop,reverse,shift,keys,values,entries', 1);
setArrayStatics('indexOf,every,some,forEach,map,filter,find,findIndex,includes', 3);
setArrayStatics('join,slice,concat,push,splice,unshift,sort,lastIndexOf,' +
'reduce,reduceRight,copyWithin,fill,turn');
$define(STATIC, ARRAY, arrayStatics);
}({});
/******************************************************************************
* Module : number *
******************************************************************************/
!function(numberMethods){
function NumberIterator(iterated){
set(this, ITER, {l: toLength(iterated), i: 0});
}
createIterator(NumberIterator, NUMBER, function(){
var iter = this[ITER]
, i = iter.i++;
return i < iter.l ? iterResult(0, i) : iterResult(1);
});
defineIterator(Number, NUMBER, function(){
return new NumberIterator(this);
});
numberMethods.random = function(lim /* = 0 */){
var a = +this
, b = lim == undefined ? 0 : +lim
, m = min(a, b);
return random() * (max(a, b) - m) + m;
};
forEach.call(array(
// ES3:
'round,floor,ceil,abs,sin,asin,cos,acos,tan,atan,exp,sqrt,max,min,pow,atan2,' +
// ES6:
'acosh,asinh,atanh,cbrt,clz32,cosh,expm1,hypot,imul,log1p,log10,log2,sign,sinh,tanh,trunc'
), function(key){
var fn = Math[key];
if(fn)numberMethods[key] = function(/* ...args */){
// ie9- dont support strict mode & convert `this` to object -> convert it to number
var args = [+this]
, i = 0;
while(arguments.length > i)args.push(arguments[i++]);
return invoke(fn, args);
}
}
);
$define(PROTO + FORCED, NUMBER, numberMethods);
}({});
/******************************************************************************
* Module : string *
******************************************************************************/
!function(){
var escapeHTMLDict = {
'&': '&',
'<': '<',
'>': '>',
'"': '"',
"'": '''
}, unescapeHTMLDict = {}, key;
for(key in escapeHTMLDict)unescapeHTMLDict[escapeHTMLDict[key]] = key;
$define(PROTO + FORCED, STRING, {
escapeHTML: createReplacer(/[&<>"']/g, escapeHTMLDict),
unescapeHTML: createReplacer(/&(?:amp|lt|gt|quot|apos);/g, unescapeHTMLDict)
});
}();
/******************************************************************************
* Module : date *
******************************************************************************/
!function(formatRegExp, flexioRegExp, locales, current, SECONDS, MINUTES, HOURS, MONTH, YEAR){
function createFormat(prefix){
return function(template, locale /* = current */){
var that = this
, dict = locales[has(locales, locale) ? locale : current];
function get(unit){
return that[prefix + unit]();
}
return String(template).replace(formatRegExp, function(part){
switch(part){
case 's' : return get(SECONDS); // Seconds : 0-59
case 'ss' : return lz(get(SECONDS)); // Seconds : 00-59
case 'm' : return get(MINUTES); // Minutes : 0-59
case 'mm' : return lz(get(MINUTES)); // Minutes : 00-59
case 'h' : return get(HOURS); // Hours : 0-23
case 'hh' : return lz(get(HOURS)); // Hours : 00-23
case 'D' : return get(DATE); // Date : 1-31
case 'DD' : return lz(get(DATE)); // Date : 01-31
case 'W' : return dict[0][get('Day')]; // Day : Понедельник
case 'N' : return get(MONTH) + 1; // Month : 1-12
case 'NN' : return lz(get(MONTH) + 1); // Month : 01-12
case 'M' : return dict[2][get(MONTH)]; // Month : Январь
case 'MM' : return dict[1][get(MONTH)]; // Month : Января
case 'Y' : return get(YEAR); // Year : 2014
case 'YY' : return lz(get(YEAR) % 100); // Year : 14
} return part;
});
}
}
function lz(num){
return num > 9 ? num : '0' + num;
}
function addLocale(lang, locale){
function split(index){
var result = [];
forEach.call(array(locale.months), function(it){
result.push(it.replace(flexioRegExp, '$' + index));
});
return result;
}
locales[lang] = [array(locale.weekdays), split(1), split(2)];
return core;
}
$define(PROTO + FORCED, DATE, {
format: createFormat('get'),
formatUTC: createFormat('getUTC')
});
addLocale(current, {
weekdays: 'Sunday,Monday,Tuesday,Wednesday,Thursday,Friday,Saturday',
months: 'January,February,March,April,May,June,July,August,September,October,November,December'
});
addLocale('ru', {
weekdays: 'Воскресенье,Понедельник,Вторник,Среда,Четверг,Пятница,Суббота',
months: 'Январ:я|ь,Феврал:я|ь,Март:а|,Апрел:я|ь,Ма:я|й,Июн:я|ь,' +
'Июл:я|ь,Август:а|,Сентябр:я|ь,Октябр:я|ь,Ноябр:я|ь,Декабр:я|ь'
});
core.locale = function(locale){
return has(locales, locale) ? current = locale : current;
};
core.addLocale = addLocale;
}(/\b\w\w?\b/g, /:(.*)\|(.*)$/, {}, 'en', 'Seconds', 'Minutes', 'Hours', 'Month', 'FullYear');
/******************************************************************************
* Module : console *
******************************************************************************/
!function(console, enabled){
var _console = {
enable: function(){ enabled = true },
disable: function(){ enabled = false }
};
// Methods from:
// https://github.com/DeveloperToolsWG/console-object/blob/master/api.md
// https://developer.mozilla.org/en-US/docs/Web/API/console
forEach.call(array('assert,clear,count,debug,dir,dirxml,error,exception,group,' +
'groupCollapsed,groupEnd,info,isIndependentlyComposed,log,markTimeline,profile,' +
'profileEnd,table,time,timeEnd,timeline,timelineEnd,timeStamp,trace,warn'),
function(key){
var fn = console[key];
_console[key] = function(){
if(enabled && fn)return apply.call(fn, console, arguments);
};
}
);
// console methods in some browsers are not configurable
try {
framework && delete global.console;
} catch(e){}
$define(GLOBAL + FORCED, {console: _console});
}(global.console || {}, true);
}(Function('return this'), true); |
ajax/libs/unitegallery/1.7.28/js/jquery-11.0.min.js | emmy41124/cdnjs | /*! jQuery v1.11.0 | (c) 2005, 2014 jQuery Foundation, Inc. | jquery.org/license */
!function(a,b){"object"==typeof module&&"object"==typeof module.exports?module.exports=a.document?b(a,!0):function(a){if(!a.document)throw new Error("jQuery requires a window with a document");return b(a)}:b(a)}("undefined"!=typeof window?window:this,function(a,b){var c=[],d=c.slice,e=c.concat,f=c.push,g=c.indexOf,h={},i=h.toString,j=h.hasOwnProperty,k="".trim,l={},m="1.11.0",n=function(a,b){return new n.fn.init(a,b)},o=/^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g,p=/^-ms-/,q=/-([\da-z])/gi,r=function(a,b){return b.toUpperCase()};n.fn=n.prototype={jquery:m,constructor:n,selector:"",length:0,toArray:function(){return d.call(this)},get:function(a){return null!=a?0>a?this[a+this.length]:this[a]:d.call(this)},pushStack:function(a){var b=n.merge(this.constructor(),a);return b.prevObject=this,b.context=this.context,b},each:function(a,b){return n.each(this,a,b)},map:function(a){return this.pushStack(n.map(this,function(b,c){return a.call(b,c,b)}))},slice:function(){return this.pushStack(d.apply(this,arguments))},first:function(){return this.eq(0)},last:function(){return this.eq(-1)},eq:function(a){var b=this.length,c=+a+(0>a?b:0);return this.pushStack(c>=0&&b>c?[this[c]]:[])},end:function(){return this.prevObject||this.constructor(null)},push:f,sort:c.sort,splice:c.splice},n.extend=n.fn.extend=function(){var a,b,c,d,e,f,g=arguments[0]||{},h=1,i=arguments.length,j=!1;for("boolean"==typeof g&&(j=g,g=arguments[h]||{},h++),"object"==typeof g||n.isFunction(g)||(g={}),h===i&&(g=this,h--);i>h;h++)if(null!=(e=arguments[h]))for(d in e)a=g[d],c=e[d],g!==c&&(j&&c&&(n.isPlainObject(c)||(b=n.isArray(c)))?(b?(b=!1,f=a&&n.isArray(a)?a:[]):f=a&&n.isPlainObject(a)?a:{},g[d]=n.extend(j,f,c)):void 0!==c&&(g[d]=c));return g},n.extend({expando:"jQuery"+(m+Math.random()).replace(/\D/g,""),isReady:!0,error:function(a){throw new Error(a)},noop:function(){},isFunction:function(a){return"function"===n.type(a)},isArray:Array.isArray||function(a){return"array"===n.type(a)},isWindow:function(a){return null!=a&&a==a.window},isNumeric:function(a){return a-parseFloat(a)>=0},isEmptyObject:function(a){var b;for(b in a)return!1;return!0},isPlainObject:function(a){var b;if(!a||"object"!==n.type(a)||a.nodeType||n.isWindow(a))return!1;try{if(a.constructor&&!j.call(a,"constructor")&&!j.call(a.constructor.prototype,"isPrototypeOf"))return!1}catch(c){return!1}if(l.ownLast)for(b in a)return j.call(a,b);for(b in a);return void 0===b||j.call(a,b)},type:function(a){return null==a?a+"":"object"==typeof a||"function"==typeof a?h[i.call(a)]||"object":typeof a},globalEval:function(b){b&&n.trim(b)&&(a.execScript||function(b){a.eval.call(a,b)})(b)},camelCase:function(a){return a.replace(p,"ms-").replace(q,r)},nodeName:function(a,b){return a.nodeName&&a.nodeName.toLowerCase()===b.toLowerCase()},each:function(a,b,c){var d,e=0,f=a.length,g=s(a);if(c){if(g){for(;f>e;e++)if(d=b.apply(a[e],c),d===!1)break}else for(e in a)if(d=b.apply(a[e],c),d===!1)break}else if(g){for(;f>e;e++)if(d=b.call(a[e],e,a[e]),d===!1)break}else for(e in a)if(d=b.call(a[e],e,a[e]),d===!1)break;return a},trim:k&&!k.call("\ufeff\xa0")?function(a){return null==a?"":k.call(a)}:function(a){return null==a?"":(a+"").replace(o,"")},makeArray:function(a,b){var c=b||[];return null!=a&&(s(Object(a))?n.merge(c,"string"==typeof a?[a]:a):f.call(c,a)),c},inArray:function(a,b,c){var d;if(b){if(g)return g.call(b,a,c);for(d=b.length,c=c?0>c?Math.max(0,d+c):c:0;d>c;c++)if(c in b&&b[c]===a)return c}return-1},merge:function(a,b){var c=+b.length,d=0,e=a.length;while(c>d)a[e++]=b[d++];if(c!==c)while(void 0!==b[d])a[e++]=b[d++];return a.length=e,a},grep:function(a,b,c){for(var d,e=[],f=0,g=a.length,h=!c;g>f;f++)d=!b(a[f],f),d!==h&&e.push(a[f]);return e},map:function(a,b,c){var d,f=0,g=a.length,h=s(a),i=[];if(h)for(;g>f;f++)d=b(a[f],f,c),null!=d&&i.push(d);else for(f in a)d=b(a[f],f,c),null!=d&&i.push(d);return e.apply([],i)},guid:1,proxy:function(a,b){var c,e,f;return"string"==typeof b&&(f=a[b],b=a,a=f),n.isFunction(a)?(c=d.call(arguments,2),e=function(){return a.apply(b||this,c.concat(d.call(arguments)))},e.guid=a.guid=a.guid||n.guid++,e):void 0},now:function(){return+new Date},support:l}),n.each("Boolean Number String Function Array Date RegExp Object Error".split(" "),function(a,b){h["[object "+b+"]"]=b.toLowerCase()});function s(a){var b=a.length,c=n.type(a);return"function"===c||n.isWindow(a)?!1:1===a.nodeType&&b?!0:"array"===c||0===b||"number"==typeof b&&b>0&&b-1 in a}var t=function(a){var b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s="sizzle"+-new Date,t=a.document,u=0,v=0,w=eb(),x=eb(),y=eb(),z=function(a,b){return a===b&&(j=!0),0},A="undefined",B=1<<31,C={}.hasOwnProperty,D=[],E=D.pop,F=D.push,G=D.push,H=D.slice,I=D.indexOf||function(a){for(var b=0,c=this.length;c>b;b++)if(this[b]===a)return b;return-1},J="checked|selected|async|autofocus|autoplay|controls|defer|disabled|hidden|ismap|loop|multiple|open|readonly|required|scoped",K="[\\x20\\t\\r\\n\\f]",L="(?:\\\\.|[\\w-]|[^\\x00-\\xa0])+",M=L.replace("w","w#"),N="\\["+K+"*("+L+")"+K+"*(?:([*^$|!~]?=)"+K+"*(?:(['\"])((?:\\\\.|[^\\\\])*?)\\3|("+M+")|)|)"+K+"*\\]",O=":("+L+")(?:\\(((['\"])((?:\\\\.|[^\\\\])*?)\\3|((?:\\\\.|[^\\\\()[\\]]|"+N.replace(3,8)+")*)|.*)\\)|)",P=new RegExp("^"+K+"+|((?:^|[^\\\\])(?:\\\\.)*)"+K+"+$","g"),Q=new RegExp("^"+K+"*,"+K+"*"),R=new RegExp("^"+K+"*([>+~]|"+K+")"+K+"*"),S=new RegExp("="+K+"*([^\\]'\"]*?)"+K+"*\\]","g"),T=new RegExp(O),U=new RegExp("^"+M+"$"),V={ID:new RegExp("^#("+L+")"),CLASS:new RegExp("^\\.("+L+")"),TAG:new RegExp("^("+L.replace("w","w*")+")"),ATTR:new RegExp("^"+N),PSEUDO:new RegExp("^"+O),CHILD:new RegExp("^:(only|first|last|nth|nth-last)-(child|of-type)(?:\\("+K+"*(even|odd|(([+-]|)(\\d*)n|)"+K+"*(?:([+-]|)"+K+"*(\\d+)|))"+K+"*\\)|)","i"),bool:new RegExp("^(?:"+J+")$","i"),needsContext:new RegExp("^"+K+"*[>+~]|:(even|odd|eq|gt|lt|nth|first|last)(?:\\("+K+"*((?:-\\d)?\\d*)"+K+"*\\)|)(?=[^-]|$)","i")},W=/^(?:input|select|textarea|button)$/i,X=/^h\d$/i,Y=/^[^{]+\{\s*\[native \w/,Z=/^(?:#([\w-]+)|(\w+)|\.([\w-]+))$/,$=/[+~]/,_=/'|\\/g,ab=new RegExp("\\\\([\\da-f]{1,6}"+K+"?|("+K+")|.)","ig"),bb=function(a,b,c){var d="0x"+b-65536;return d!==d||c?b:0>d?String.fromCharCode(d+65536):String.fromCharCode(d>>10|55296,1023&d|56320)};try{G.apply(D=H.call(t.childNodes),t.childNodes),D[t.childNodes.length].nodeType}catch(cb){G={apply:D.length?function(a,b){F.apply(a,H.call(b))}:function(a,b){var c=a.length,d=0;while(a[c++]=b[d++]);a.length=c-1}}}function db(a,b,d,e){var f,g,h,i,j,m,p,q,u,v;if((b?b.ownerDocument||b:t)!==l&&k(b),b=b||l,d=d||[],!a||"string"!=typeof a)return d;if(1!==(i=b.nodeType)&&9!==i)return[];if(n&&!e){if(f=Z.exec(a))if(h=f[1]){if(9===i){if(g=b.getElementById(h),!g||!g.parentNode)return d;if(g.id===h)return d.push(g),d}else if(b.ownerDocument&&(g=b.ownerDocument.getElementById(h))&&r(b,g)&&g.id===h)return d.push(g),d}else{if(f[2])return G.apply(d,b.getElementsByTagName(a)),d;if((h=f[3])&&c.getElementsByClassName&&b.getElementsByClassName)return G.apply(d,b.getElementsByClassName(h)),d}if(c.qsa&&(!o||!o.test(a))){if(q=p=s,u=b,v=9===i&&a,1===i&&"object"!==b.nodeName.toLowerCase()){m=ob(a),(p=b.getAttribute("id"))?q=p.replace(_,"\\$&"):b.setAttribute("id",q),q="[id='"+q+"'] ",j=m.length;while(j--)m[j]=q+pb(m[j]);u=$.test(a)&&mb(b.parentNode)||b,v=m.join(",")}if(v)try{return G.apply(d,u.querySelectorAll(v)),d}catch(w){}finally{p||b.removeAttribute("id")}}}return xb(a.replace(P,"$1"),b,d,e)}function eb(){var a=[];function b(c,e){return a.push(c+" ")>d.cacheLength&&delete b[a.shift()],b[c+" "]=e}return b}function fb(a){return a[s]=!0,a}function gb(a){var b=l.createElement("div");try{return!!a(b)}catch(c){return!1}finally{b.parentNode&&b.parentNode.removeChild(b),b=null}}function hb(a,b){var c=a.split("|"),e=a.length;while(e--)d.attrHandle[c[e]]=b}function ib(a,b){var c=b&&a,d=c&&1===a.nodeType&&1===b.nodeType&&(~b.sourceIndex||B)-(~a.sourceIndex||B);if(d)return d;if(c)while(c=c.nextSibling)if(c===b)return-1;return a?1:-1}function jb(a){return function(b){var c=b.nodeName.toLowerCase();return"input"===c&&b.type===a}}function kb(a){return function(b){var c=b.nodeName.toLowerCase();return("input"===c||"button"===c)&&b.type===a}}function lb(a){return fb(function(b){return b=+b,fb(function(c,d){var e,f=a([],c.length,b),g=f.length;while(g--)c[e=f[g]]&&(c[e]=!(d[e]=c[e]))})})}function mb(a){return a&&typeof a.getElementsByTagName!==A&&a}c=db.support={},f=db.isXML=function(a){var b=a&&(a.ownerDocument||a).documentElement;return b?"HTML"!==b.nodeName:!1},k=db.setDocument=function(a){var b,e=a?a.ownerDocument||a:t,g=e.defaultView;return e!==l&&9===e.nodeType&&e.documentElement?(l=e,m=e.documentElement,n=!f(e),g&&g!==g.top&&(g.addEventListener?g.addEventListener("unload",function(){k()},!1):g.attachEvent&&g.attachEvent("onunload",function(){k()})),c.attributes=gb(function(a){return a.className="i",!a.getAttribute("className")}),c.getElementsByTagName=gb(function(a){return a.appendChild(e.createComment("")),!a.getElementsByTagName("*").length}),c.getElementsByClassName=Y.test(e.getElementsByClassName)&&gb(function(a){return a.innerHTML="<div class='a'></div><div class='a i'></div>",a.firstChild.className="i",2===a.getElementsByClassName("i").length}),c.getById=gb(function(a){return m.appendChild(a).id=s,!e.getElementsByName||!e.getElementsByName(s).length}),c.getById?(d.find.ID=function(a,b){if(typeof b.getElementById!==A&&n){var c=b.getElementById(a);return c&&c.parentNode?[c]:[]}},d.filter.ID=function(a){var b=a.replace(ab,bb);return function(a){return a.getAttribute("id")===b}}):(delete d.find.ID,d.filter.ID=function(a){var b=a.replace(ab,bb);return function(a){var c=typeof a.getAttributeNode!==A&&a.getAttributeNode("id");return c&&c.value===b}}),d.find.TAG=c.getElementsByTagName?function(a,b){return typeof b.getElementsByTagName!==A?b.getElementsByTagName(a):void 0}:function(a,b){var c,d=[],e=0,f=b.getElementsByTagName(a);if("*"===a){while(c=f[e++])1===c.nodeType&&d.push(c);return d}return f},d.find.CLASS=c.getElementsByClassName&&function(a,b){return typeof b.getElementsByClassName!==A&&n?b.getElementsByClassName(a):void 0},p=[],o=[],(c.qsa=Y.test(e.querySelectorAll))&&(gb(function(a){a.innerHTML="<select t=''><option selected=''></option></select>",a.querySelectorAll("[t^='']").length&&o.push("[*^$]="+K+"*(?:''|\"\")"),a.querySelectorAll("[selected]").length||o.push("\\["+K+"*(?:value|"+J+")"),a.querySelectorAll(":checked").length||o.push(":checked")}),gb(function(a){var b=e.createElement("input");b.setAttribute("type","hidden"),a.appendChild(b).setAttribute("name","D"),a.querySelectorAll("[name=d]").length&&o.push("name"+K+"*[*^$|!~]?="),a.querySelectorAll(":enabled").length||o.push(":enabled",":disabled"),a.querySelectorAll("*,:x"),o.push(",.*:")})),(c.matchesSelector=Y.test(q=m.webkitMatchesSelector||m.mozMatchesSelector||m.oMatchesSelector||m.msMatchesSelector))&&gb(function(a){c.disconnectedMatch=q.call(a,"div"),q.call(a,"[s!='']:x"),p.push("!=",O)}),o=o.length&&new RegExp(o.join("|")),p=p.length&&new RegExp(p.join("|")),b=Y.test(m.compareDocumentPosition),r=b||Y.test(m.contains)?function(a,b){var c=9===a.nodeType?a.documentElement:a,d=b&&b.parentNode;return a===d||!(!d||1!==d.nodeType||!(c.contains?c.contains(d):a.compareDocumentPosition&&16&a.compareDocumentPosition(d)))}:function(a,b){if(b)while(b=b.parentNode)if(b===a)return!0;return!1},z=b?function(a,b){if(a===b)return j=!0,0;var d=!a.compareDocumentPosition-!b.compareDocumentPosition;return d?d:(d=(a.ownerDocument||a)===(b.ownerDocument||b)?a.compareDocumentPosition(b):1,1&d||!c.sortDetached&&b.compareDocumentPosition(a)===d?a===e||a.ownerDocument===t&&r(t,a)?-1:b===e||b.ownerDocument===t&&r(t,b)?1:i?I.call(i,a)-I.call(i,b):0:4&d?-1:1)}:function(a,b){if(a===b)return j=!0,0;var c,d=0,f=a.parentNode,g=b.parentNode,h=[a],k=[b];if(!f||!g)return a===e?-1:b===e?1:f?-1:g?1:i?I.call(i,a)-I.call(i,b):0;if(f===g)return ib(a,b);c=a;while(c=c.parentNode)h.unshift(c);c=b;while(c=c.parentNode)k.unshift(c);while(h[d]===k[d])d++;return d?ib(h[d],k[d]):h[d]===t?-1:k[d]===t?1:0},e):l},db.matches=function(a,b){return db(a,null,null,b)},db.matchesSelector=function(a,b){if((a.ownerDocument||a)!==l&&k(a),b=b.replace(S,"='$1']"),!(!c.matchesSelector||!n||p&&p.test(b)||o&&o.test(b)))try{var d=q.call(a,b);if(d||c.disconnectedMatch||a.document&&11!==a.document.nodeType)return d}catch(e){}return db(b,l,null,[a]).length>0},db.contains=function(a,b){return(a.ownerDocument||a)!==l&&k(a),r(a,b)},db.attr=function(a,b){(a.ownerDocument||a)!==l&&k(a);var e=d.attrHandle[b.toLowerCase()],f=e&&C.call(d.attrHandle,b.toLowerCase())?e(a,b,!n):void 0;return void 0!==f?f:c.attributes||!n?a.getAttribute(b):(f=a.getAttributeNode(b))&&f.specified?f.value:null},db.error=function(a){throw new Error("Syntax error, unrecognized expression: "+a)},db.uniqueSort=function(a){var b,d=[],e=0,f=0;if(j=!c.detectDuplicates,i=!c.sortStable&&a.slice(0),a.sort(z),j){while(b=a[f++])b===a[f]&&(e=d.push(f));while(e--)a.splice(d[e],1)}return i=null,a},e=db.getText=function(a){var b,c="",d=0,f=a.nodeType;if(f){if(1===f||9===f||11===f){if("string"==typeof a.textContent)return a.textContent;for(a=a.firstChild;a;a=a.nextSibling)c+=e(a)}else if(3===f||4===f)return a.nodeValue}else while(b=a[d++])c+=e(b);return c},d=db.selectors={cacheLength:50,createPseudo:fb,match:V,attrHandle:{},find:{},relative:{">":{dir:"parentNode",first:!0}," ":{dir:"parentNode"},"+":{dir:"previousSibling",first:!0},"~":{dir:"previousSibling"}},preFilter:{ATTR:function(a){return a[1]=a[1].replace(ab,bb),a[3]=(a[4]||a[5]||"").replace(ab,bb),"~="===a[2]&&(a[3]=" "+a[3]+" "),a.slice(0,4)},CHILD:function(a){return a[1]=a[1].toLowerCase(),"nth"===a[1].slice(0,3)?(a[3]||db.error(a[0]),a[4]=+(a[4]?a[5]+(a[6]||1):2*("even"===a[3]||"odd"===a[3])),a[5]=+(a[7]+a[8]||"odd"===a[3])):a[3]&&db.error(a[0]),a},PSEUDO:function(a){var b,c=!a[5]&&a[2];return V.CHILD.test(a[0])?null:(a[3]&&void 0!==a[4]?a[2]=a[4]:c&&T.test(c)&&(b=ob(c,!0))&&(b=c.indexOf(")",c.length-b)-c.length)&&(a[0]=a[0].slice(0,b),a[2]=c.slice(0,b)),a.slice(0,3))}},filter:{TAG:function(a){var b=a.replace(ab,bb).toLowerCase();return"*"===a?function(){return!0}:function(a){return a.nodeName&&a.nodeName.toLowerCase()===b}},CLASS:function(a){var b=w[a+" "];return b||(b=new RegExp("(^|"+K+")"+a+"("+K+"|$)"))&&w(a,function(a){return b.test("string"==typeof a.className&&a.className||typeof a.getAttribute!==A&&a.getAttribute("class")||"")})},ATTR:function(a,b,c){return function(d){var e=db.attr(d,a);return null==e?"!="===b:b?(e+="","="===b?e===c:"!="===b?e!==c:"^="===b?c&&0===e.indexOf(c):"*="===b?c&&e.indexOf(c)>-1:"$="===b?c&&e.slice(-c.length)===c:"~="===b?(" "+e+" ").indexOf(c)>-1:"|="===b?e===c||e.slice(0,c.length+1)===c+"-":!1):!0}},CHILD:function(a,b,c,d,e){var f="nth"!==a.slice(0,3),g="last"!==a.slice(-4),h="of-type"===b;return 1===d&&0===e?function(a){return!!a.parentNode}:function(b,c,i){var j,k,l,m,n,o,p=f!==g?"nextSibling":"previousSibling",q=b.parentNode,r=h&&b.nodeName.toLowerCase(),t=!i&&!h;if(q){if(f){while(p){l=b;while(l=l[p])if(h?l.nodeName.toLowerCase()===r:1===l.nodeType)return!1;o=p="only"===a&&!o&&"nextSibling"}return!0}if(o=[g?q.firstChild:q.lastChild],g&&t){k=q[s]||(q[s]={}),j=k[a]||[],n=j[0]===u&&j[1],m=j[0]===u&&j[2],l=n&&q.childNodes[n];while(l=++n&&l&&l[p]||(m=n=0)||o.pop())if(1===l.nodeType&&++m&&l===b){k[a]=[u,n,m];break}}else if(t&&(j=(b[s]||(b[s]={}))[a])&&j[0]===u)m=j[1];else while(l=++n&&l&&l[p]||(m=n=0)||o.pop())if((h?l.nodeName.toLowerCase()===r:1===l.nodeType)&&++m&&(t&&((l[s]||(l[s]={}))[a]=[u,m]),l===b))break;return m-=e,m===d||m%d===0&&m/d>=0}}},PSEUDO:function(a,b){var c,e=d.pseudos[a]||d.setFilters[a.toLowerCase()]||db.error("unsupported pseudo: "+a);return e[s]?e(b):e.length>1?(c=[a,a,"",b],d.setFilters.hasOwnProperty(a.toLowerCase())?fb(function(a,c){var d,f=e(a,b),g=f.length;while(g--)d=I.call(a,f[g]),a[d]=!(c[d]=f[g])}):function(a){return e(a,0,c)}):e}},pseudos:{not:fb(function(a){var b=[],c=[],d=g(a.replace(P,"$1"));return d[s]?fb(function(a,b,c,e){var f,g=d(a,null,e,[]),h=a.length;while(h--)(f=g[h])&&(a[h]=!(b[h]=f))}):function(a,e,f){return b[0]=a,d(b,null,f,c),!c.pop()}}),has:fb(function(a){return function(b){return db(a,b).length>0}}),contains:fb(function(a){return function(b){return(b.textContent||b.innerText||e(b)).indexOf(a)>-1}}),lang:fb(function(a){return U.test(a||"")||db.error("unsupported lang: "+a),a=a.replace(ab,bb).toLowerCase(),function(b){var c;do if(c=n?b.lang:b.getAttribute("xml:lang")||b.getAttribute("lang"))return c=c.toLowerCase(),c===a||0===c.indexOf(a+"-");while((b=b.parentNode)&&1===b.nodeType);return!1}}),target:function(b){var c=a.location&&a.location.hash;return c&&c.slice(1)===b.id},root:function(a){return a===m},focus:function(a){return a===l.activeElement&&(!l.hasFocus||l.hasFocus())&&!!(a.type||a.href||~a.tabIndex)},enabled:function(a){return a.disabled===!1},disabled:function(a){return a.disabled===!0},checked:function(a){var b=a.nodeName.toLowerCase();return"input"===b&&!!a.checked||"option"===b&&!!a.selected},selected:function(a){return a.parentNode&&a.parentNode.selectedIndex,a.selected===!0},empty:function(a){for(a=a.firstChild;a;a=a.nextSibling)if(a.nodeType<6)return!1;return!0},parent:function(a){return!d.pseudos.empty(a)},header:function(a){return X.test(a.nodeName)},input:function(a){return W.test(a.nodeName)},button:function(a){var b=a.nodeName.toLowerCase();return"input"===b&&"button"===a.type||"button"===b},text:function(a){var b;return"input"===a.nodeName.toLowerCase()&&"text"===a.type&&(null==(b=a.getAttribute("type"))||"text"===b.toLowerCase())},first:lb(function(){return[0]}),last:lb(function(a,b){return[b-1]}),eq:lb(function(a,b,c){return[0>c?c+b:c]}),even:lb(function(a,b){for(var c=0;b>c;c+=2)a.push(c);return a}),odd:lb(function(a,b){for(var c=1;b>c;c+=2)a.push(c);return a}),lt:lb(function(a,b,c){for(var d=0>c?c+b:c;--d>=0;)a.push(d);return a}),gt:lb(function(a,b,c){for(var d=0>c?c+b:c;++d<b;)a.push(d);return a})}},d.pseudos.nth=d.pseudos.eq;for(b in{radio:!0,checkbox:!0,file:!0,password:!0,image:!0})d.pseudos[b]=jb(b);for(b in{submit:!0,reset:!0})d.pseudos[b]=kb(b);function nb(){}nb.prototype=d.filters=d.pseudos,d.setFilters=new nb;function ob(a,b){var c,e,f,g,h,i,j,k=x[a+" "];if(k)return b?0:k.slice(0);h=a,i=[],j=d.preFilter;while(h){(!c||(e=Q.exec(h)))&&(e&&(h=h.slice(e[0].length)||h),i.push(f=[])),c=!1,(e=R.exec(h))&&(c=e.shift(),f.push({value:c,type:e[0].replace(P," ")}),h=h.slice(c.length));for(g in d.filter)!(e=V[g].exec(h))||j[g]&&!(e=j[g](e))||(c=e.shift(),f.push({value:c,type:g,matches:e}),h=h.slice(c.length));if(!c)break}return b?h.length:h?db.error(a):x(a,i).slice(0)}function pb(a){for(var b=0,c=a.length,d="";c>b;b++)d+=a[b].value;return d}function qb(a,b,c){var d=b.dir,e=c&&"parentNode"===d,f=v++;return b.first?function(b,c,f){while(b=b[d])if(1===b.nodeType||e)return a(b,c,f)}:function(b,c,g){var h,i,j=[u,f];if(g){while(b=b[d])if((1===b.nodeType||e)&&a(b,c,g))return!0}else while(b=b[d])if(1===b.nodeType||e){if(i=b[s]||(b[s]={}),(h=i[d])&&h[0]===u&&h[1]===f)return j[2]=h[2];if(i[d]=j,j[2]=a(b,c,g))return!0}}}function rb(a){return a.length>1?function(b,c,d){var e=a.length;while(e--)if(!a[e](b,c,d))return!1;return!0}:a[0]}function sb(a,b,c,d,e){for(var f,g=[],h=0,i=a.length,j=null!=b;i>h;h++)(f=a[h])&&(!c||c(f,d,e))&&(g.push(f),j&&b.push(h));return g}function tb(a,b,c,d,e,f){return d&&!d[s]&&(d=tb(d)),e&&!e[s]&&(e=tb(e,f)),fb(function(f,g,h,i){var j,k,l,m=[],n=[],o=g.length,p=f||wb(b||"*",h.nodeType?[h]:h,[]),q=!a||!f&&b?p:sb(p,m,a,h,i),r=c?e||(f?a:o||d)?[]:g:q;if(c&&c(q,r,h,i),d){j=sb(r,n),d(j,[],h,i),k=j.length;while(k--)(l=j[k])&&(r[n[k]]=!(q[n[k]]=l))}if(f){if(e||a){if(e){j=[],k=r.length;while(k--)(l=r[k])&&j.push(q[k]=l);e(null,r=[],j,i)}k=r.length;while(k--)(l=r[k])&&(j=e?I.call(f,l):m[k])>-1&&(f[j]=!(g[j]=l))}}else r=sb(r===g?r.splice(o,r.length):r),e?e(null,g,r,i):G.apply(g,r)})}function ub(a){for(var b,c,e,f=a.length,g=d.relative[a[0].type],i=g||d.relative[" "],j=g?1:0,k=qb(function(a){return a===b},i,!0),l=qb(function(a){return I.call(b,a)>-1},i,!0),m=[function(a,c,d){return!g&&(d||c!==h)||((b=c).nodeType?k(a,c,d):l(a,c,d))}];f>j;j++)if(c=d.relative[a[j].type])m=[qb(rb(m),c)];else{if(c=d.filter[a[j].type].apply(null,a[j].matches),c[s]){for(e=++j;f>e;e++)if(d.relative[a[e].type])break;return tb(j>1&&rb(m),j>1&&pb(a.slice(0,j-1).concat({value:" "===a[j-2].type?"*":""})).replace(P,"$1"),c,e>j&&ub(a.slice(j,e)),f>e&&ub(a=a.slice(e)),f>e&&pb(a))}m.push(c)}return rb(m)}function vb(a,b){var c=b.length>0,e=a.length>0,f=function(f,g,i,j,k){var m,n,o,p=0,q="0",r=f&&[],s=[],t=h,v=f||e&&d.find.TAG("*",k),w=u+=null==t?1:Math.random()||.1,x=v.length;for(k&&(h=g!==l&&g);q!==x&&null!=(m=v[q]);q++){if(e&&m){n=0;while(o=a[n++])if(o(m,g,i)){j.push(m);break}k&&(u=w)}c&&((m=!o&&m)&&p--,f&&r.push(m))}if(p+=q,c&&q!==p){n=0;while(o=b[n++])o(r,s,g,i);if(f){if(p>0)while(q--)r[q]||s[q]||(s[q]=E.call(j));s=sb(s)}G.apply(j,s),k&&!f&&s.length>0&&p+b.length>1&&db.uniqueSort(j)}return k&&(u=w,h=t),r};return c?fb(f):f}g=db.compile=function(a,b){var c,d=[],e=[],f=y[a+" "];if(!f){b||(b=ob(a)),c=b.length;while(c--)f=ub(b[c]),f[s]?d.push(f):e.push(f);f=y(a,vb(e,d))}return f};function wb(a,b,c){for(var d=0,e=b.length;e>d;d++)db(a,b[d],c);return c}function xb(a,b,e,f){var h,i,j,k,l,m=ob(a);if(!f&&1===m.length){if(i=m[0]=m[0].slice(0),i.length>2&&"ID"===(j=i[0]).type&&c.getById&&9===b.nodeType&&n&&d.relative[i[1].type]){if(b=(d.find.ID(j.matches[0].replace(ab,bb),b)||[])[0],!b)return e;a=a.slice(i.shift().value.length)}h=V.needsContext.test(a)?0:i.length;while(h--){if(j=i[h],d.relative[k=j.type])break;if((l=d.find[k])&&(f=l(j.matches[0].replace(ab,bb),$.test(i[0].type)&&mb(b.parentNode)||b))){if(i.splice(h,1),a=f.length&&pb(i),!a)return G.apply(e,f),e;break}}}return g(a,m)(f,b,!n,e,$.test(a)&&mb(b.parentNode)||b),e}return c.sortStable=s.split("").sort(z).join("")===s,c.detectDuplicates=!!j,k(),c.sortDetached=gb(function(a){return 1&a.compareDocumentPosition(l.createElement("div"))}),gb(function(a){return a.innerHTML="<a href='#'></a>","#"===a.firstChild.getAttribute("href")})||hb("type|href|height|width",function(a,b,c){return c?void 0:a.getAttribute(b,"type"===b.toLowerCase()?1:2)}),c.attributes&&gb(function(a){return a.innerHTML="<input/>",a.firstChild.setAttribute("value",""),""===a.firstChild.getAttribute("value")})||hb("value",function(a,b,c){return c||"input"!==a.nodeName.toLowerCase()?void 0:a.defaultValue}),gb(function(a){return null==a.getAttribute("disabled")})||hb(J,function(a,b,c){var d;return c?void 0:a[b]===!0?b.toLowerCase():(d=a.getAttributeNode(b))&&d.specified?d.value:null}),db}(a);n.find=t,n.expr=t.selectors,n.expr[":"]=n.expr.pseudos,n.unique=t.uniqueSort,n.text=t.getText,n.isXMLDoc=t.isXML,n.contains=t.contains;var u=n.expr.match.needsContext,v=/^<(\w+)\s*\/?>(?:<\/\1>|)$/,w=/^.[^:#\[\.,]*$/;function x(a,b,c){if(n.isFunction(b))return n.grep(a,function(a,d){return!!b.call(a,d,a)!==c});if(b.nodeType)return n.grep(a,function(a){return a===b!==c});if("string"==typeof b){if(w.test(b))return n.filter(b,a,c);b=n.filter(b,a)}return n.grep(a,function(a){return n.inArray(a,b)>=0!==c})}n.filter=function(a,b,c){var d=b[0];return c&&(a=":not("+a+")"),1===b.length&&1===d.nodeType?n.find.matchesSelector(d,a)?[d]:[]:n.find.matches(a,n.grep(b,function(a){return 1===a.nodeType}))},n.fn.extend({find:function(a){var b,c=[],d=this,e=d.length;if("string"!=typeof a)return this.pushStack(n(a).filter(function(){for(b=0;e>b;b++)if(n.contains(d[b],this))return!0}));for(b=0;e>b;b++)n.find(a,d[b],c);return c=this.pushStack(e>1?n.unique(c):c),c.selector=this.selector?this.selector+" "+a:a,c},filter:function(a){return this.pushStack(x(this,a||[],!1))},not:function(a){return this.pushStack(x(this,a||[],!0))},is:function(a){return!!x(this,"string"==typeof a&&u.test(a)?n(a):a||[],!1).length}});var y,z=a.document,A=/^(?:\s*(<[\w\W]+>)[^>]*|#([\w-]*))$/,B=n.fn.init=function(a,b){var c,d;if(!a)return this;if("string"==typeof a){if(c="<"===a.charAt(0)&&">"===a.charAt(a.length-1)&&a.length>=3?[null,a,null]:A.exec(a),!c||!c[1]&&b)return!b||b.jquery?(b||y).find(a):this.constructor(b).find(a);if(c[1]){if(b=b instanceof n?b[0]:b,n.merge(this,n.parseHTML(c[1],b&&b.nodeType?b.ownerDocument||b:z,!0)),v.test(c[1])&&n.isPlainObject(b))for(c in b)n.isFunction(this[c])?this[c](b[c]):this.attr(c,b[c]);return this}if(d=z.getElementById(c[2]),d&&d.parentNode){if(d.id!==c[2])return y.find(a);this.length=1,this[0]=d}return this.context=z,this.selector=a,this}return a.nodeType?(this.context=this[0]=a,this.length=1,this):n.isFunction(a)?"undefined"!=typeof y.ready?y.ready(a):a(n):(void 0!==a.selector&&(this.selector=a.selector,this.context=a.context),n.makeArray(a,this))};B.prototype=n.fn,y=n(z);var C=/^(?:parents|prev(?:Until|All))/,D={children:!0,contents:!0,next:!0,prev:!0};n.extend({dir:function(a,b,c){var d=[],e=a[b];while(e&&9!==e.nodeType&&(void 0===c||1!==e.nodeType||!n(e).is(c)))1===e.nodeType&&d.push(e),e=e[b];return d},sibling:function(a,b){for(var c=[];a;a=a.nextSibling)1===a.nodeType&&a!==b&&c.push(a);return c}}),n.fn.extend({has:function(a){var b,c=n(a,this),d=c.length;return this.filter(function(){for(b=0;d>b;b++)if(n.contains(this,c[b]))return!0})},closest:function(a,b){for(var c,d=0,e=this.length,f=[],g=u.test(a)||"string"!=typeof a?n(a,b||this.context):0;e>d;d++)for(c=this[d];c&&c!==b;c=c.parentNode)if(c.nodeType<11&&(g?g.index(c)>-1:1===c.nodeType&&n.find.matchesSelector(c,a))){f.push(c);break}return this.pushStack(f.length>1?n.unique(f):f)},index:function(a){return a?"string"==typeof a?n.inArray(this[0],n(a)):n.inArray(a.jquery?a[0]:a,this):this[0]&&this[0].parentNode?this.first().prevAll().length:-1},add:function(a,b){return this.pushStack(n.unique(n.merge(this.get(),n(a,b))))},addBack:function(a){return this.add(null==a?this.prevObject:this.prevObject.filter(a))}});function E(a,b){do a=a[b];while(a&&1!==a.nodeType);return a}n.each({parent:function(a){var b=a.parentNode;return b&&11!==b.nodeType?b:null},parents:function(a){return n.dir(a,"parentNode")},parentsUntil:function(a,b,c){return n.dir(a,"parentNode",c)},next:function(a){return E(a,"nextSibling")},prev:function(a){return E(a,"previousSibling")},nextAll:function(a){return n.dir(a,"nextSibling")},prevAll:function(a){return n.dir(a,"previousSibling")},nextUntil:function(a,b,c){return n.dir(a,"nextSibling",c)},prevUntil:function(a,b,c){return n.dir(a,"previousSibling",c)},siblings:function(a){return n.sibling((a.parentNode||{}).firstChild,a)},children:function(a){return n.sibling(a.firstChild)},contents:function(a){return n.nodeName(a,"iframe")?a.contentDocument||a.contentWindow.document:n.merge([],a.childNodes)}},function(a,b){n.fn[a]=function(c,d){var e=n.map(this,b,c);return"Until"!==a.slice(-5)&&(d=c),d&&"string"==typeof d&&(e=n.filter(d,e)),this.length>1&&(D[a]||(e=n.unique(e)),C.test(a)&&(e=e.reverse())),this.pushStack(e)}});var F=/\S+/g,G={};function H(a){var b=G[a]={};return n.each(a.match(F)||[],function(a,c){b[c]=!0}),b}n.Callbacks=function(a){a="string"==typeof a?G[a]||H(a):n.extend({},a);var b,c,d,e,f,g,h=[],i=!a.once&&[],j=function(l){for(c=a.memory&&l,d=!0,f=g||0,g=0,e=h.length,b=!0;h&&e>f;f++)if(h[f].apply(l[0],l[1])===!1&&a.stopOnFalse){c=!1;break}b=!1,h&&(i?i.length&&j(i.shift()):c?h=[]:k.disable())},k={add:function(){if(h){var d=h.length;!function f(b){n.each(b,function(b,c){var d=n.type(c);"function"===d?a.unique&&k.has(c)||h.push(c):c&&c.length&&"string"!==d&&f(c)})}(arguments),b?e=h.length:c&&(g=d,j(c))}return this},remove:function(){return h&&n.each(arguments,function(a,c){var d;while((d=n.inArray(c,h,d))>-1)h.splice(d,1),b&&(e>=d&&e--,f>=d&&f--)}),this},has:function(a){return a?n.inArray(a,h)>-1:!(!h||!h.length)},empty:function(){return h=[],e=0,this},disable:function(){return h=i=c=void 0,this},disabled:function(){return!h},lock:function(){return i=void 0,c||k.disable(),this},locked:function(){return!i},fireWith:function(a,c){return!h||d&&!i||(c=c||[],c=[a,c.slice?c.slice():c],b?i.push(c):j(c)),this},fire:function(){return k.fireWith(this,arguments),this},fired:function(){return!!d}};return k},n.extend({Deferred:function(a){var b=[["resolve","done",n.Callbacks("once memory"),"resolved"],["reject","fail",n.Callbacks("once memory"),"rejected"],["notify","progress",n.Callbacks("memory")]],c="pending",d={state:function(){return c},always:function(){return e.done(arguments).fail(arguments),this},then:function(){var a=arguments;return n.Deferred(function(c){n.each(b,function(b,f){var g=n.isFunction(a[b])&&a[b];e[f[1]](function(){var a=g&&g.apply(this,arguments);a&&n.isFunction(a.promise)?a.promise().done(c.resolve).fail(c.reject).progress(c.notify):c[f[0]+"With"](this===d?c.promise():this,g?[a]:arguments)})}),a=null}).promise()},promise:function(a){return null!=a?n.extend(a,d):d}},e={};return d.pipe=d.then,n.each(b,function(a,f){var g=f[2],h=f[3];d[f[1]]=g.add,h&&g.add(function(){c=h},b[1^a][2].disable,b[2][2].lock),e[f[0]]=function(){return e[f[0]+"With"](this===e?d:this,arguments),this},e[f[0]+"With"]=g.fireWith}),d.promise(e),a&&a.call(e,e),e},when:function(a){var b=0,c=d.call(arguments),e=c.length,f=1!==e||a&&n.isFunction(a.promise)?e:0,g=1===f?a:n.Deferred(),h=function(a,b,c){return function(e){b[a]=this,c[a]=arguments.length>1?d.call(arguments):e,c===i?g.notifyWith(b,c):--f||g.resolveWith(b,c)}},i,j,k;if(e>1)for(i=new Array(e),j=new Array(e),k=new Array(e);e>b;b++)c[b]&&n.isFunction(c[b].promise)?c[b].promise().done(h(b,k,c)).fail(g.reject).progress(h(b,j,i)):--f;return f||g.resolveWith(k,c),g.promise()}});var I;n.fn.ready=function(a){return n.ready.promise().done(a),this},n.extend({isReady:!1,readyWait:1,holdReady:function(a){a?n.readyWait++:n.ready(!0)},ready:function(a){if(a===!0?!--n.readyWait:!n.isReady){if(!z.body)return setTimeout(n.ready);n.isReady=!0,a!==!0&&--n.readyWait>0||(I.resolveWith(z,[n]),n.fn.trigger&&n(z).trigger("ready").off("ready"))}}});function J(){z.addEventListener?(z.removeEventListener("DOMContentLoaded",K,!1),a.removeEventListener("load",K,!1)):(z.detachEvent("onreadystatechange",K),a.detachEvent("onload",K))}function K(){(z.addEventListener||"load"===event.type||"complete"===z.readyState)&&(J(),n.ready())}n.ready.promise=function(b){if(!I)if(I=n.Deferred(),"complete"===z.readyState)setTimeout(n.ready);else if(z.addEventListener)z.addEventListener("DOMContentLoaded",K,!1),a.addEventListener("load",K,!1);else{z.attachEvent("onreadystatechange",K),a.attachEvent("onload",K);var c=!1;try{c=null==a.frameElement&&z.documentElement}catch(d){}c&&c.doScroll&&!function e(){if(!n.isReady){try{c.doScroll("left")}catch(a){return setTimeout(e,50)}J(),n.ready()}}()}return I.promise(b)};var L="undefined",M;for(M in n(l))break;l.ownLast="0"!==M,l.inlineBlockNeedsLayout=!1,n(function(){var a,b,c=z.getElementsByTagName("body")[0];c&&(a=z.createElement("div"),a.style.cssText="border:0;width:0;height:0;position:absolute;top:0;left:-9999px;margin-top:1px",b=z.createElement("div"),c.appendChild(a).appendChild(b),typeof b.style.zoom!==L&&(b.style.cssText="border:0;margin:0;width:1px;padding:1px;display:inline;zoom:1",(l.inlineBlockNeedsLayout=3===b.offsetWidth)&&(c.style.zoom=1)),c.removeChild(a),a=b=null)}),function(){var a=z.createElement("div");if(null==l.deleteExpando){l.deleteExpando=!0;try{delete a.test}catch(b){l.deleteExpando=!1}}a=null}(),n.acceptData=function(a){var b=n.noData[(a.nodeName+" ").toLowerCase()],c=+a.nodeType||1;return 1!==c&&9!==c?!1:!b||b!==!0&&a.getAttribute("classid")===b};var N=/^(?:\{[\w\W]*\}|\[[\w\W]*\])$/,O=/([A-Z])/g;function P(a,b,c){if(void 0===c&&1===a.nodeType){var d="data-"+b.replace(O,"-$1").toLowerCase();if(c=a.getAttribute(d),"string"==typeof c){try{c="true"===c?!0:"false"===c?!1:"null"===c?null:+c+""===c?+c:N.test(c)?n.parseJSON(c):c}catch(e){}n.data(a,b,c)}else c=void 0}return c}function Q(a){var b;for(b in a)if(("data"!==b||!n.isEmptyObject(a[b]))&&"toJSON"!==b)return!1;return!0}function R(a,b,d,e){if(n.acceptData(a)){var f,g,h=n.expando,i=a.nodeType,j=i?n.cache:a,k=i?a[h]:a[h]&&h;if(k&&j[k]&&(e||j[k].data)||void 0!==d||"string"!=typeof b)return k||(k=i?a[h]=c.pop()||n.guid++:h),j[k]||(j[k]=i?{}:{toJSON:n.noop}),("object"==typeof b||"function"==typeof b)&&(e?j[k]=n.extend(j[k],b):j[k].data=n.extend(j[k].data,b)),g=j[k],e||(g.data||(g.data={}),g=g.data),void 0!==d&&(g[n.camelCase(b)]=d),"string"==typeof b?(f=g[b],null==f&&(f=g[n.camelCase(b)])):f=g,f
}}function S(a,b,c){if(n.acceptData(a)){var d,e,f=a.nodeType,g=f?n.cache:a,h=f?a[n.expando]:n.expando;if(g[h]){if(b&&(d=c?g[h]:g[h].data)){n.isArray(b)?b=b.concat(n.map(b,n.camelCase)):b in d?b=[b]:(b=n.camelCase(b),b=b in d?[b]:b.split(" ")),e=b.length;while(e--)delete d[b[e]];if(c?!Q(d):!n.isEmptyObject(d))return}(c||(delete g[h].data,Q(g[h])))&&(f?n.cleanData([a],!0):l.deleteExpando||g!=g.window?delete g[h]:g[h]=null)}}}n.extend({cache:{},noData:{"applet ":!0,"embed ":!0,"object ":"clsid:D27CDB6E-AE6D-11cf-96B8-444553540000"},hasData:function(a){return a=a.nodeType?n.cache[a[n.expando]]:a[n.expando],!!a&&!Q(a)},data:function(a,b,c){return R(a,b,c)},removeData:function(a,b){return S(a,b)},_data:function(a,b,c){return R(a,b,c,!0)},_removeData:function(a,b){return S(a,b,!0)}}),n.fn.extend({data:function(a,b){var c,d,e,f=this[0],g=f&&f.attributes;if(void 0===a){if(this.length&&(e=n.data(f),1===f.nodeType&&!n._data(f,"parsedAttrs"))){c=g.length;while(c--)d=g[c].name,0===d.indexOf("data-")&&(d=n.camelCase(d.slice(5)),P(f,d,e[d]));n._data(f,"parsedAttrs",!0)}return e}return"object"==typeof a?this.each(function(){n.data(this,a)}):arguments.length>1?this.each(function(){n.data(this,a,b)}):f?P(f,a,n.data(f,a)):void 0},removeData:function(a){return this.each(function(){n.removeData(this,a)})}}),n.extend({queue:function(a,b,c){var d;return a?(b=(b||"fx")+"queue",d=n._data(a,b),c&&(!d||n.isArray(c)?d=n._data(a,b,n.makeArray(c)):d.push(c)),d||[]):void 0},dequeue:function(a,b){b=b||"fx";var c=n.queue(a,b),d=c.length,e=c.shift(),f=n._queueHooks(a,b),g=function(){n.dequeue(a,b)};"inprogress"===e&&(e=c.shift(),d--),e&&("fx"===b&&c.unshift("inprogress"),delete f.stop,e.call(a,g,f)),!d&&f&&f.empty.fire()},_queueHooks:function(a,b){var c=b+"queueHooks";return n._data(a,c)||n._data(a,c,{empty:n.Callbacks("once memory").add(function(){n._removeData(a,b+"queue"),n._removeData(a,c)})})}}),n.fn.extend({queue:function(a,b){var c=2;return"string"!=typeof a&&(b=a,a="fx",c--),arguments.length<c?n.queue(this[0],a):void 0===b?this:this.each(function(){var c=n.queue(this,a,b);n._queueHooks(this,a),"fx"===a&&"inprogress"!==c[0]&&n.dequeue(this,a)})},dequeue:function(a){return this.each(function(){n.dequeue(this,a)})},clearQueue:function(a){return this.queue(a||"fx",[])},promise:function(a,b){var c,d=1,e=n.Deferred(),f=this,g=this.length,h=function(){--d||e.resolveWith(f,[f])};"string"!=typeof a&&(b=a,a=void 0),a=a||"fx";while(g--)c=n._data(f[g],a+"queueHooks"),c&&c.empty&&(d++,c.empty.add(h));return h(),e.promise(b)}});var T=/[+-]?(?:\d*\.|)\d+(?:[eE][+-]?\d+|)/.source,U=["Top","Right","Bottom","Left"],V=function(a,b){return a=b||a,"none"===n.css(a,"display")||!n.contains(a.ownerDocument,a)},W=n.access=function(a,b,c,d,e,f,g){var h=0,i=a.length,j=null==c;if("object"===n.type(c)){e=!0;for(h in c)n.access(a,b,h,c[h],!0,f,g)}else if(void 0!==d&&(e=!0,n.isFunction(d)||(g=!0),j&&(g?(b.call(a,d),b=null):(j=b,b=function(a,b,c){return j.call(n(a),c)})),b))for(;i>h;h++)b(a[h],c,g?d:d.call(a[h],h,b(a[h],c)));return e?a:j?b.call(a):i?b(a[0],c):f},X=/^(?:checkbox|radio)$/i;!function(){var a=z.createDocumentFragment(),b=z.createElement("div"),c=z.createElement("input");if(b.setAttribute("className","t"),b.innerHTML=" <link/><table></table><a href='/a'>a</a>",l.leadingWhitespace=3===b.firstChild.nodeType,l.tbody=!b.getElementsByTagName("tbody").length,l.htmlSerialize=!!b.getElementsByTagName("link").length,l.html5Clone="<:nav></:nav>"!==z.createElement("nav").cloneNode(!0).outerHTML,c.type="checkbox",c.checked=!0,a.appendChild(c),l.appendChecked=c.checked,b.innerHTML="<textarea>x</textarea>",l.noCloneChecked=!!b.cloneNode(!0).lastChild.defaultValue,a.appendChild(b),b.innerHTML="<input type='radio' checked='checked' name='t'/>",l.checkClone=b.cloneNode(!0).cloneNode(!0).lastChild.checked,l.noCloneEvent=!0,b.attachEvent&&(b.attachEvent("onclick",function(){l.noCloneEvent=!1}),b.cloneNode(!0).click()),null==l.deleteExpando){l.deleteExpando=!0;try{delete b.test}catch(d){l.deleteExpando=!1}}a=b=c=null}(),function(){var b,c,d=z.createElement("div");for(b in{submit:!0,change:!0,focusin:!0})c="on"+b,(l[b+"Bubbles"]=c in a)||(d.setAttribute(c,"t"),l[b+"Bubbles"]=d.attributes[c].expando===!1);d=null}();var Y=/^(?:input|select|textarea)$/i,Z=/^key/,$=/^(?:mouse|contextmenu)|click/,_=/^(?:focusinfocus|focusoutblur)$/,ab=/^([^.]*)(?:\.(.+)|)$/;function bb(){return!0}function cb(){return!1}function db(){try{return z.activeElement}catch(a){}}n.event={global:{},add:function(a,b,c,d,e){var f,g,h,i,j,k,l,m,o,p,q,r=n._data(a);if(r){c.handler&&(i=c,c=i.handler,e=i.selector),c.guid||(c.guid=n.guid++),(g=r.events)||(g=r.events={}),(k=r.handle)||(k=r.handle=function(a){return typeof n===L||a&&n.event.triggered===a.type?void 0:n.event.dispatch.apply(k.elem,arguments)},k.elem=a),b=(b||"").match(F)||[""],h=b.length;while(h--)f=ab.exec(b[h])||[],o=q=f[1],p=(f[2]||"").split(".").sort(),o&&(j=n.event.special[o]||{},o=(e?j.delegateType:j.bindType)||o,j=n.event.special[o]||{},l=n.extend({type:o,origType:q,data:d,handler:c,guid:c.guid,selector:e,needsContext:e&&n.expr.match.needsContext.test(e),namespace:p.join(".")},i),(m=g[o])||(m=g[o]=[],m.delegateCount=0,j.setup&&j.setup.call(a,d,p,k)!==!1||(a.addEventListener?a.addEventListener(o,k,!1):a.attachEvent&&a.attachEvent("on"+o,k))),j.add&&(j.add.call(a,l),l.handler.guid||(l.handler.guid=c.guid)),e?m.splice(m.delegateCount++,0,l):m.push(l),n.event.global[o]=!0);a=null}},remove:function(a,b,c,d,e){var f,g,h,i,j,k,l,m,o,p,q,r=n.hasData(a)&&n._data(a);if(r&&(k=r.events)){b=(b||"").match(F)||[""],j=b.length;while(j--)if(h=ab.exec(b[j])||[],o=q=h[1],p=(h[2]||"").split(".").sort(),o){l=n.event.special[o]||{},o=(d?l.delegateType:l.bindType)||o,m=k[o]||[],h=h[2]&&new RegExp("(^|\\.)"+p.join("\\.(?:.*\\.|)")+"(\\.|$)"),i=f=m.length;while(f--)g=m[f],!e&&q!==g.origType||c&&c.guid!==g.guid||h&&!h.test(g.namespace)||d&&d!==g.selector&&("**"!==d||!g.selector)||(m.splice(f,1),g.selector&&m.delegateCount--,l.remove&&l.remove.call(a,g));i&&!m.length&&(l.teardown&&l.teardown.call(a,p,r.handle)!==!1||n.removeEvent(a,o,r.handle),delete k[o])}else for(o in k)n.event.remove(a,o+b[j],c,d,!0);n.isEmptyObject(k)&&(delete r.handle,n._removeData(a,"events"))}},trigger:function(b,c,d,e){var f,g,h,i,k,l,m,o=[d||z],p=j.call(b,"type")?b.type:b,q=j.call(b,"namespace")?b.namespace.split("."):[];if(h=l=d=d||z,3!==d.nodeType&&8!==d.nodeType&&!_.test(p+n.event.triggered)&&(p.indexOf(".")>=0&&(q=p.split("."),p=q.shift(),q.sort()),g=p.indexOf(":")<0&&"on"+p,b=b[n.expando]?b:new n.Event(p,"object"==typeof b&&b),b.isTrigger=e?2:3,b.namespace=q.join("."),b.namespace_re=b.namespace?new RegExp("(^|\\.)"+q.join("\\.(?:.*\\.|)")+"(\\.|$)"):null,b.result=void 0,b.target||(b.target=d),c=null==c?[b]:n.makeArray(c,[b]),k=n.event.special[p]||{},e||!k.trigger||k.trigger.apply(d,c)!==!1)){if(!e&&!k.noBubble&&!n.isWindow(d)){for(i=k.delegateType||p,_.test(i+p)||(h=h.parentNode);h;h=h.parentNode)o.push(h),l=h;l===(d.ownerDocument||z)&&o.push(l.defaultView||l.parentWindow||a)}m=0;while((h=o[m++])&&!b.isPropagationStopped())b.type=m>1?i:k.bindType||p,f=(n._data(h,"events")||{})[b.type]&&n._data(h,"handle"),f&&f.apply(h,c),f=g&&h[g],f&&f.apply&&n.acceptData(h)&&(b.result=f.apply(h,c),b.result===!1&&b.preventDefault());if(b.type=p,!e&&!b.isDefaultPrevented()&&(!k._default||k._default.apply(o.pop(),c)===!1)&&n.acceptData(d)&&g&&d[p]&&!n.isWindow(d)){l=d[g],l&&(d[g]=null),n.event.triggered=p;try{d[p]()}catch(r){}n.event.triggered=void 0,l&&(d[g]=l)}return b.result}},dispatch:function(a){a=n.event.fix(a);var b,c,e,f,g,h=[],i=d.call(arguments),j=(n._data(this,"events")||{})[a.type]||[],k=n.event.special[a.type]||{};if(i[0]=a,a.delegateTarget=this,!k.preDispatch||k.preDispatch.call(this,a)!==!1){h=n.event.handlers.call(this,a,j),b=0;while((f=h[b++])&&!a.isPropagationStopped()){a.currentTarget=f.elem,g=0;while((e=f.handlers[g++])&&!a.isImmediatePropagationStopped())(!a.namespace_re||a.namespace_re.test(e.namespace))&&(a.handleObj=e,a.data=e.data,c=((n.event.special[e.origType]||{}).handle||e.handler).apply(f.elem,i),void 0!==c&&(a.result=c)===!1&&(a.preventDefault(),a.stopPropagation()))}return k.postDispatch&&k.postDispatch.call(this,a),a.result}},handlers:function(a,b){var c,d,e,f,g=[],h=b.delegateCount,i=a.target;if(h&&i.nodeType&&(!a.button||"click"!==a.type))for(;i!=this;i=i.parentNode||this)if(1===i.nodeType&&(i.disabled!==!0||"click"!==a.type)){for(e=[],f=0;h>f;f++)d=b[f],c=d.selector+" ",void 0===e[c]&&(e[c]=d.needsContext?n(c,this).index(i)>=0:n.find(c,this,null,[i]).length),e[c]&&e.push(d);e.length&&g.push({elem:i,handlers:e})}return h<b.length&&g.push({elem:this,handlers:b.slice(h)}),g},fix:function(a){if(a[n.expando])return a;var b,c,d,e=a.type,f=a,g=this.fixHooks[e];g||(this.fixHooks[e]=g=$.test(e)?this.mouseHooks:Z.test(e)?this.keyHooks:{}),d=g.props?this.props.concat(g.props):this.props,a=new n.Event(f),b=d.length;while(b--)c=d[b],a[c]=f[c];return a.target||(a.target=f.srcElement||z),3===a.target.nodeType&&(a.target=a.target.parentNode),a.metaKey=!!a.metaKey,g.filter?g.filter(a,f):a},props:"altKey bubbles cancelable ctrlKey currentTarget eventPhase metaKey relatedTarget shiftKey target timeStamp view which".split(" "),fixHooks:{},keyHooks:{props:"char charCode key keyCode".split(" "),filter:function(a,b){return null==a.which&&(a.which=null!=b.charCode?b.charCode:b.keyCode),a}},mouseHooks:{props:"button buttons clientX clientY fromElement offsetX offsetY pageX pageY screenX screenY toElement".split(" "),filter:function(a,b){var c,d,e,f=b.button,g=b.fromElement;return null==a.pageX&&null!=b.clientX&&(d=a.target.ownerDocument||z,e=d.documentElement,c=d.body,a.pageX=b.clientX+(e&&e.scrollLeft||c&&c.scrollLeft||0)-(e&&e.clientLeft||c&&c.clientLeft||0),a.pageY=b.clientY+(e&&e.scrollTop||c&&c.scrollTop||0)-(e&&e.clientTop||c&&c.clientTop||0)),!a.relatedTarget&&g&&(a.relatedTarget=g===a.target?b.toElement:g),a.which||void 0===f||(a.which=1&f?1:2&f?3:4&f?2:0),a}},special:{load:{noBubble:!0},focus:{trigger:function(){if(this!==db()&&this.focus)try{return this.focus(),!1}catch(a){}},delegateType:"focusin"},blur:{trigger:function(){return this===db()&&this.blur?(this.blur(),!1):void 0},delegateType:"focusout"},click:{trigger:function(){return n.nodeName(this,"input")&&"checkbox"===this.type&&this.click?(this.click(),!1):void 0},_default:function(a){return n.nodeName(a.target,"a")}},beforeunload:{postDispatch:function(a){void 0!==a.result&&(a.originalEvent.returnValue=a.result)}}},simulate:function(a,b,c,d){var e=n.extend(new n.Event,c,{type:a,isSimulated:!0,originalEvent:{}});d?n.event.trigger(e,null,b):n.event.dispatch.call(b,e),e.isDefaultPrevented()&&c.preventDefault()}},n.removeEvent=z.removeEventListener?function(a,b,c){a.removeEventListener&&a.removeEventListener(b,c,!1)}:function(a,b,c){var d="on"+b;a.detachEvent&&(typeof a[d]===L&&(a[d]=null),a.detachEvent(d,c))},n.Event=function(a,b){return this instanceof n.Event?(a&&a.type?(this.originalEvent=a,this.type=a.type,this.isDefaultPrevented=a.defaultPrevented||void 0===a.defaultPrevented&&(a.returnValue===!1||a.getPreventDefault&&a.getPreventDefault())?bb:cb):this.type=a,b&&n.extend(this,b),this.timeStamp=a&&a.timeStamp||n.now(),void(this[n.expando]=!0)):new n.Event(a,b)},n.Event.prototype={isDefaultPrevented:cb,isPropagationStopped:cb,isImmediatePropagationStopped:cb,preventDefault:function(){var a=this.originalEvent;this.isDefaultPrevented=bb,a&&(a.preventDefault?a.preventDefault():a.returnValue=!1)},stopPropagation:function(){var a=this.originalEvent;this.isPropagationStopped=bb,a&&(a.stopPropagation&&a.stopPropagation(),a.cancelBubble=!0)},stopImmediatePropagation:function(){this.isImmediatePropagationStopped=bb,this.stopPropagation()}},n.each({mouseenter:"mouseover",mouseleave:"mouseout"},function(a,b){n.event.special[a]={delegateType:b,bindType:b,handle:function(a){var c,d=this,e=a.relatedTarget,f=a.handleObj;return(!e||e!==d&&!n.contains(d,e))&&(a.type=f.origType,c=f.handler.apply(this,arguments),a.type=b),c}}}),l.submitBubbles||(n.event.special.submit={setup:function(){return n.nodeName(this,"form")?!1:void n.event.add(this,"click._submit keypress._submit",function(a){var b=a.target,c=n.nodeName(b,"input")||n.nodeName(b,"button")?b.form:void 0;c&&!n._data(c,"submitBubbles")&&(n.event.add(c,"submit._submit",function(a){a._submit_bubble=!0}),n._data(c,"submitBubbles",!0))})},postDispatch:function(a){a._submit_bubble&&(delete a._submit_bubble,this.parentNode&&!a.isTrigger&&n.event.simulate("submit",this.parentNode,a,!0))},teardown:function(){return n.nodeName(this,"form")?!1:void n.event.remove(this,"._submit")}}),l.changeBubbles||(n.event.special.change={setup:function(){return Y.test(this.nodeName)?(("checkbox"===this.type||"radio"===this.type)&&(n.event.add(this,"propertychange._change",function(a){"checked"===a.originalEvent.propertyName&&(this._just_changed=!0)}),n.event.add(this,"click._change",function(a){this._just_changed&&!a.isTrigger&&(this._just_changed=!1),n.event.simulate("change",this,a,!0)})),!1):void n.event.add(this,"beforeactivate._change",function(a){var b=a.target;Y.test(b.nodeName)&&!n._data(b,"changeBubbles")&&(n.event.add(b,"change._change",function(a){!this.parentNode||a.isSimulated||a.isTrigger||n.event.simulate("change",this.parentNode,a,!0)}),n._data(b,"changeBubbles",!0))})},handle:function(a){var b=a.target;return this!==b||a.isSimulated||a.isTrigger||"radio"!==b.type&&"checkbox"!==b.type?a.handleObj.handler.apply(this,arguments):void 0},teardown:function(){return n.event.remove(this,"._change"),!Y.test(this.nodeName)}}),l.focusinBubbles||n.each({focus:"focusin",blur:"focusout"},function(a,b){var c=function(a){n.event.simulate(b,a.target,n.event.fix(a),!0)};n.event.special[b]={setup:function(){var d=this.ownerDocument||this,e=n._data(d,b);e||d.addEventListener(a,c,!0),n._data(d,b,(e||0)+1)},teardown:function(){var d=this.ownerDocument||this,e=n._data(d,b)-1;e?n._data(d,b,e):(d.removeEventListener(a,c,!0),n._removeData(d,b))}}}),n.fn.extend({on:function(a,b,c,d,e){var f,g;if("object"==typeof a){"string"!=typeof b&&(c=c||b,b=void 0);for(f in a)this.on(f,b,c,a[f],e);return this}if(null==c&&null==d?(d=b,c=b=void 0):null==d&&("string"==typeof b?(d=c,c=void 0):(d=c,c=b,b=void 0)),d===!1)d=cb;else if(!d)return this;return 1===e&&(g=d,d=function(a){return n().off(a),g.apply(this,arguments)},d.guid=g.guid||(g.guid=n.guid++)),this.each(function(){n.event.add(this,a,d,c,b)})},one:function(a,b,c,d){return this.on(a,b,c,d,1)},off:function(a,b,c){var d,e;if(a&&a.preventDefault&&a.handleObj)return d=a.handleObj,n(a.delegateTarget).off(d.namespace?d.origType+"."+d.namespace:d.origType,d.selector,d.handler),this;if("object"==typeof a){for(e in a)this.off(e,b,a[e]);return this}return(b===!1||"function"==typeof b)&&(c=b,b=void 0),c===!1&&(c=cb),this.each(function(){n.event.remove(this,a,c,b)})},trigger:function(a,b){return this.each(function(){n.event.trigger(a,b,this)})},triggerHandler:function(a,b){var c=this[0];return c?n.event.trigger(a,b,c,!0):void 0}});function eb(a){var b=fb.split("|"),c=a.createDocumentFragment();if(c.createElement)while(b.length)c.createElement(b.pop());return c}var fb="abbr|article|aside|audio|bdi|canvas|data|datalist|details|figcaption|figure|footer|header|hgroup|mark|meter|nav|output|progress|section|summary|time|video",gb=/ jQuery\d+="(?:null|\d+)"/g,hb=new RegExp("<(?:"+fb+")[\\s/>]","i"),ib=/^\s+/,jb=/<(?!area|br|col|embed|hr|img|input|link|meta|param)(([\w:]+)[^>]*)\/>/gi,kb=/<([\w:]+)/,lb=/<tbody/i,mb=/<|&#?\w+;/,nb=/<(?:script|style|link)/i,ob=/checked\s*(?:[^=]|=\s*.checked.)/i,pb=/^$|\/(?:java|ecma)script/i,qb=/^true\/(.*)/,rb=/^\s*<!(?:\[CDATA\[|--)|(?:\]\]|--)>\s*$/g,sb={option:[1,"<select multiple='multiple'>","</select>"],legend:[1,"<fieldset>","</fieldset>"],area:[1,"<map>","</map>"],param:[1,"<object>","</object>"],thead:[1,"<table>","</table>"],tr:[2,"<table><tbody>","</tbody></table>"],col:[2,"<table><tbody></tbody><colgroup>","</colgroup></table>"],td:[3,"<table><tbody><tr>","</tr></tbody></table>"],_default:l.htmlSerialize?[0,"",""]:[1,"X<div>","</div>"]},tb=eb(z),ub=tb.appendChild(z.createElement("div"));sb.optgroup=sb.option,sb.tbody=sb.tfoot=sb.colgroup=sb.caption=sb.thead,sb.th=sb.td;function vb(a,b){var c,d,e=0,f=typeof a.getElementsByTagName!==L?a.getElementsByTagName(b||"*"):typeof a.querySelectorAll!==L?a.querySelectorAll(b||"*"):void 0;if(!f)for(f=[],c=a.childNodes||a;null!=(d=c[e]);e++)!b||n.nodeName(d,b)?f.push(d):n.merge(f,vb(d,b));return void 0===b||b&&n.nodeName(a,b)?n.merge([a],f):f}function wb(a){X.test(a.type)&&(a.defaultChecked=a.checked)}function xb(a,b){return n.nodeName(a,"table")&&n.nodeName(11!==b.nodeType?b:b.firstChild,"tr")?a.getElementsByTagName("tbody")[0]||a.appendChild(a.ownerDocument.createElement("tbody")):a}function yb(a){return a.type=(null!==n.find.attr(a,"type"))+"/"+a.type,a}function zb(a){var b=qb.exec(a.type);return b?a.type=b[1]:a.removeAttribute("type"),a}function Ab(a,b){for(var c,d=0;null!=(c=a[d]);d++)n._data(c,"globalEval",!b||n._data(b[d],"globalEval"))}function Bb(a,b){if(1===b.nodeType&&n.hasData(a)){var c,d,e,f=n._data(a),g=n._data(b,f),h=f.events;if(h){delete g.handle,g.events={};for(c in h)for(d=0,e=h[c].length;e>d;d++)n.event.add(b,c,h[c][d])}g.data&&(g.data=n.extend({},g.data))}}function Cb(a,b){var c,d,e;if(1===b.nodeType){if(c=b.nodeName.toLowerCase(),!l.noCloneEvent&&b[n.expando]){e=n._data(b);for(d in e.events)n.removeEvent(b,d,e.handle);b.removeAttribute(n.expando)}"script"===c&&b.text!==a.text?(yb(b).text=a.text,zb(b)):"object"===c?(b.parentNode&&(b.outerHTML=a.outerHTML),l.html5Clone&&a.innerHTML&&!n.trim(b.innerHTML)&&(b.innerHTML=a.innerHTML)):"input"===c&&X.test(a.type)?(b.defaultChecked=b.checked=a.checked,b.value!==a.value&&(b.value=a.value)):"option"===c?b.defaultSelected=b.selected=a.defaultSelected:("input"===c||"textarea"===c)&&(b.defaultValue=a.defaultValue)}}n.extend({clone:function(a,b,c){var d,e,f,g,h,i=n.contains(a.ownerDocument,a);if(l.html5Clone||n.isXMLDoc(a)||!hb.test("<"+a.nodeName+">")?f=a.cloneNode(!0):(ub.innerHTML=a.outerHTML,ub.removeChild(f=ub.firstChild)),!(l.noCloneEvent&&l.noCloneChecked||1!==a.nodeType&&11!==a.nodeType||n.isXMLDoc(a)))for(d=vb(f),h=vb(a),g=0;null!=(e=h[g]);++g)d[g]&&Cb(e,d[g]);if(b)if(c)for(h=h||vb(a),d=d||vb(f),g=0;null!=(e=h[g]);g++)Bb(e,d[g]);else Bb(a,f);return d=vb(f,"script"),d.length>0&&Ab(d,!i&&vb(a,"script")),d=h=e=null,f},buildFragment:function(a,b,c,d){for(var e,f,g,h,i,j,k,m=a.length,o=eb(b),p=[],q=0;m>q;q++)if(f=a[q],f||0===f)if("object"===n.type(f))n.merge(p,f.nodeType?[f]:f);else if(mb.test(f)){h=h||o.appendChild(b.createElement("div")),i=(kb.exec(f)||["",""])[1].toLowerCase(),k=sb[i]||sb._default,h.innerHTML=k[1]+f.replace(jb,"<$1></$2>")+k[2],e=k[0];while(e--)h=h.lastChild;if(!l.leadingWhitespace&&ib.test(f)&&p.push(b.createTextNode(ib.exec(f)[0])),!l.tbody){f="table"!==i||lb.test(f)?"<table>"!==k[1]||lb.test(f)?0:h:h.firstChild,e=f&&f.childNodes.length;while(e--)n.nodeName(j=f.childNodes[e],"tbody")&&!j.childNodes.length&&f.removeChild(j)}n.merge(p,h.childNodes),h.textContent="";while(h.firstChild)h.removeChild(h.firstChild);h=o.lastChild}else p.push(b.createTextNode(f));h&&o.removeChild(h),l.appendChecked||n.grep(vb(p,"input"),wb),q=0;while(f=p[q++])if((!d||-1===n.inArray(f,d))&&(g=n.contains(f.ownerDocument,f),h=vb(o.appendChild(f),"script"),g&&Ab(h),c)){e=0;while(f=h[e++])pb.test(f.type||"")&&c.push(f)}return h=null,o},cleanData:function(a,b){for(var d,e,f,g,h=0,i=n.expando,j=n.cache,k=l.deleteExpando,m=n.event.special;null!=(d=a[h]);h++)if((b||n.acceptData(d))&&(f=d[i],g=f&&j[f])){if(g.events)for(e in g.events)m[e]?n.event.remove(d,e):n.removeEvent(d,e,g.handle);j[f]&&(delete j[f],k?delete d[i]:typeof d.removeAttribute!==L?d.removeAttribute(i):d[i]=null,c.push(f))}}}),n.fn.extend({text:function(a){return W(this,function(a){return void 0===a?n.text(this):this.empty().append((this[0]&&this[0].ownerDocument||z).createTextNode(a))},null,a,arguments.length)},append:function(){return this.domManip(arguments,function(a){if(1===this.nodeType||11===this.nodeType||9===this.nodeType){var b=xb(this,a);b.appendChild(a)}})},prepend:function(){return this.domManip(arguments,function(a){if(1===this.nodeType||11===this.nodeType||9===this.nodeType){var b=xb(this,a);b.insertBefore(a,b.firstChild)}})},before:function(){return this.domManip(arguments,function(a){this.parentNode&&this.parentNode.insertBefore(a,this)})},after:function(){return this.domManip(arguments,function(a){this.parentNode&&this.parentNode.insertBefore(a,this.nextSibling)})},remove:function(a,b){for(var c,d=a?n.filter(a,this):this,e=0;null!=(c=d[e]);e++)b||1!==c.nodeType||n.cleanData(vb(c)),c.parentNode&&(b&&n.contains(c.ownerDocument,c)&&Ab(vb(c,"script")),c.parentNode.removeChild(c));return this},empty:function(){for(var a,b=0;null!=(a=this[b]);b++){1===a.nodeType&&n.cleanData(vb(a,!1));while(a.firstChild)a.removeChild(a.firstChild);a.options&&n.nodeName(a,"select")&&(a.options.length=0)}return this},clone:function(a,b){return a=null==a?!1:a,b=null==b?a:b,this.map(function(){return n.clone(this,a,b)})},html:function(a){return W(this,function(a){var b=this[0]||{},c=0,d=this.length;if(void 0===a)return 1===b.nodeType?b.innerHTML.replace(gb,""):void 0;if(!("string"!=typeof a||nb.test(a)||!l.htmlSerialize&&hb.test(a)||!l.leadingWhitespace&&ib.test(a)||sb[(kb.exec(a)||["",""])[1].toLowerCase()])){a=a.replace(jb,"<$1></$2>");try{for(;d>c;c++)b=this[c]||{},1===b.nodeType&&(n.cleanData(vb(b,!1)),b.innerHTML=a);b=0}catch(e){}}b&&this.empty().append(a)},null,a,arguments.length)},replaceWith:function(){var a=arguments[0];return this.domManip(arguments,function(b){a=this.parentNode,n.cleanData(vb(this)),a&&a.replaceChild(b,this)}),a&&(a.length||a.nodeType)?this:this.remove()},detach:function(a){return this.remove(a,!0)},domManip:function(a,b){a=e.apply([],a);var c,d,f,g,h,i,j=0,k=this.length,m=this,o=k-1,p=a[0],q=n.isFunction(p);if(q||k>1&&"string"==typeof p&&!l.checkClone&&ob.test(p))return this.each(function(c){var d=m.eq(c);q&&(a[0]=p.call(this,c,d.html())),d.domManip(a,b)});if(k&&(i=n.buildFragment(a,this[0].ownerDocument,!1,this),c=i.firstChild,1===i.childNodes.length&&(i=c),c)){for(g=n.map(vb(i,"script"),yb),f=g.length;k>j;j++)d=i,j!==o&&(d=n.clone(d,!0,!0),f&&n.merge(g,vb(d,"script"))),b.call(this[j],d,j);if(f)for(h=g[g.length-1].ownerDocument,n.map(g,zb),j=0;f>j;j++)d=g[j],pb.test(d.type||"")&&!n._data(d,"globalEval")&&n.contains(h,d)&&(d.src?n._evalUrl&&n._evalUrl(d.src):n.globalEval((d.text||d.textContent||d.innerHTML||"").replace(rb,"")));i=c=null}return this}}),n.each({appendTo:"append",prependTo:"prepend",insertBefore:"before",insertAfter:"after",replaceAll:"replaceWith"},function(a,b){n.fn[a]=function(a){for(var c,d=0,e=[],g=n(a),h=g.length-1;h>=d;d++)c=d===h?this:this.clone(!0),n(g[d])[b](c),f.apply(e,c.get());return this.pushStack(e)}});var Db,Eb={};function Fb(b,c){var d=n(c.createElement(b)).appendTo(c.body),e=a.getDefaultComputedStyle?a.getDefaultComputedStyle(d[0]).display:n.css(d[0],"display");return d.detach(),e}function Gb(a){var b=z,c=Eb[a];return c||(c=Fb(a,b),"none"!==c&&c||(Db=(Db||n("<iframe frameborder='0' width='0' height='0'/>")).appendTo(b.documentElement),b=(Db[0].contentWindow||Db[0].contentDocument).document,b.write(),b.close(),c=Fb(a,b),Db.detach()),Eb[a]=c),c}!function(){var a,b,c=z.createElement("div"),d="-webkit-box-sizing:content-box;-moz-box-sizing:content-box;box-sizing:content-box;display:block;padding:0;margin:0;border:0";c.innerHTML=" <link/><table></table><a href='/a'>a</a><input type='checkbox'/>",a=c.getElementsByTagName("a")[0],a.style.cssText="float:left;opacity:.5",l.opacity=/^0.5/.test(a.style.opacity),l.cssFloat=!!a.style.cssFloat,c.style.backgroundClip="content-box",c.cloneNode(!0).style.backgroundClip="",l.clearCloneStyle="content-box"===c.style.backgroundClip,a=c=null,l.shrinkWrapBlocks=function(){var a,c,e,f;if(null==b){if(a=z.getElementsByTagName("body")[0],!a)return;f="border:0;width:0;height:0;position:absolute;top:0;left:-9999px",c=z.createElement("div"),e=z.createElement("div"),a.appendChild(c).appendChild(e),b=!1,typeof e.style.zoom!==L&&(e.style.cssText=d+";width:1px;padding:1px;zoom:1",e.innerHTML="<div></div>",e.firstChild.style.width="5px",b=3!==e.offsetWidth),a.removeChild(c),a=c=e=null}return b}}();var Hb=/^margin/,Ib=new RegExp("^("+T+")(?!px)[a-z%]+$","i"),Jb,Kb,Lb=/^(top|right|bottom|left)$/;a.getComputedStyle?(Jb=function(a){return a.ownerDocument.defaultView.getComputedStyle(a,null)},Kb=function(a,b,c){var d,e,f,g,h=a.style;return c=c||Jb(a),g=c?c.getPropertyValue(b)||c[b]:void 0,c&&(""!==g||n.contains(a.ownerDocument,a)||(g=n.style(a,b)),Ib.test(g)&&Hb.test(b)&&(d=h.width,e=h.minWidth,f=h.maxWidth,h.minWidth=h.maxWidth=h.width=g,g=c.width,h.width=d,h.minWidth=e,h.maxWidth=f)),void 0===g?g:g+""}):z.documentElement.currentStyle&&(Jb=function(a){return a.currentStyle},Kb=function(a,b,c){var d,e,f,g,h=a.style;return c=c||Jb(a),g=c?c[b]:void 0,null==g&&h&&h[b]&&(g=h[b]),Ib.test(g)&&!Lb.test(b)&&(d=h.left,e=a.runtimeStyle,f=e&&e.left,f&&(e.left=a.currentStyle.left),h.left="fontSize"===b?"1em":g,g=h.pixelLeft+"px",h.left=d,f&&(e.left=f)),void 0===g?g:g+""||"auto"});function Mb(a,b){return{get:function(){var c=a();if(null!=c)return c?void delete this.get:(this.get=b).apply(this,arguments)}}}!function(){var b,c,d,e,f,g,h=z.createElement("div"),i="border:0;width:0;height:0;position:absolute;top:0;left:-9999px",j="-webkit-box-sizing:content-box;-moz-box-sizing:content-box;box-sizing:content-box;display:block;padding:0;margin:0;border:0";h.innerHTML=" <link/><table></table><a href='/a'>a</a><input type='checkbox'/>",b=h.getElementsByTagName("a")[0],b.style.cssText="float:left;opacity:.5",l.opacity=/^0.5/.test(b.style.opacity),l.cssFloat=!!b.style.cssFloat,h.style.backgroundClip="content-box",h.cloneNode(!0).style.backgroundClip="",l.clearCloneStyle="content-box"===h.style.backgroundClip,b=h=null,n.extend(l,{reliableHiddenOffsets:function(){if(null!=c)return c;var a,b,d,e=z.createElement("div"),f=z.getElementsByTagName("body")[0];if(f)return e.setAttribute("className","t"),e.innerHTML=" <link/><table></table><a href='/a'>a</a><input type='checkbox'/>",a=z.createElement("div"),a.style.cssText=i,f.appendChild(a).appendChild(e),e.innerHTML="<table><tr><td></td><td>t</td></tr></table>",b=e.getElementsByTagName("td"),b[0].style.cssText="padding:0;margin:0;border:0;display:none",d=0===b[0].offsetHeight,b[0].style.display="",b[1].style.display="none",c=d&&0===b[0].offsetHeight,f.removeChild(a),e=f=null,c},boxSizing:function(){return null==d&&k(),d},boxSizingReliable:function(){return null==e&&k(),e},pixelPosition:function(){return null==f&&k(),f},reliableMarginRight:function(){var b,c,d,e;if(null==g&&a.getComputedStyle){if(b=z.getElementsByTagName("body")[0],!b)return;c=z.createElement("div"),d=z.createElement("div"),c.style.cssText=i,b.appendChild(c).appendChild(d),e=d.appendChild(z.createElement("div")),e.style.cssText=d.style.cssText=j,e.style.marginRight=e.style.width="0",d.style.width="1px",g=!parseFloat((a.getComputedStyle(e,null)||{}).marginRight),b.removeChild(c)}return g}});function k(){var b,c,h=z.getElementsByTagName("body")[0];h&&(b=z.createElement("div"),c=z.createElement("div"),b.style.cssText=i,h.appendChild(b).appendChild(c),c.style.cssText="-webkit-box-sizing:border-box;-moz-box-sizing:border-box;box-sizing:border-box;position:absolute;display:block;padding:1px;border:1px;width:4px;margin-top:1%;top:1%",n.swap(h,null!=h.style.zoom?{zoom:1}:{},function(){d=4===c.offsetWidth}),e=!0,f=!1,g=!0,a.getComputedStyle&&(f="1%"!==(a.getComputedStyle(c,null)||{}).top,e="4px"===(a.getComputedStyle(c,null)||{width:"4px"}).width),h.removeChild(b),c=h=null)}}(),n.swap=function(a,b,c,d){var e,f,g={};for(f in b)g[f]=a.style[f],a.style[f]=b[f];e=c.apply(a,d||[]);for(f in b)a.style[f]=g[f];return e};var Nb=/alpha\([^)]*\)/i,Ob=/opacity\s*=\s*([^)]*)/,Pb=/^(none|table(?!-c[ea]).+)/,Qb=new RegExp("^("+T+")(.*)$","i"),Rb=new RegExp("^([+-])=("+T+")","i"),Sb={position:"absolute",visibility:"hidden",display:"block"},Tb={letterSpacing:0,fontWeight:400},Ub=["Webkit","O","Moz","ms"];function Vb(a,b){if(b in a)return b;var c=b.charAt(0).toUpperCase()+b.slice(1),d=b,e=Ub.length;while(e--)if(b=Ub[e]+c,b in a)return b;return d}function Wb(a,b){for(var c,d,e,f=[],g=0,h=a.length;h>g;g++)d=a[g],d.style&&(f[g]=n._data(d,"olddisplay"),c=d.style.display,b?(f[g]||"none"!==c||(d.style.display=""),""===d.style.display&&V(d)&&(f[g]=n._data(d,"olddisplay",Gb(d.nodeName)))):f[g]||(e=V(d),(c&&"none"!==c||!e)&&n._data(d,"olddisplay",e?c:n.css(d,"display"))));for(g=0;h>g;g++)d=a[g],d.style&&(b&&"none"!==d.style.display&&""!==d.style.display||(d.style.display=b?f[g]||"":"none"));return a}function Xb(a,b,c){var d=Qb.exec(b);return d?Math.max(0,d[1]-(c||0))+(d[2]||"px"):b}function Yb(a,b,c,d,e){for(var f=c===(d?"border":"content")?4:"width"===b?1:0,g=0;4>f;f+=2)"margin"===c&&(g+=n.css(a,c+U[f],!0,e)),d?("content"===c&&(g-=n.css(a,"padding"+U[f],!0,e)),"margin"!==c&&(g-=n.css(a,"border"+U[f]+"Width",!0,e))):(g+=n.css(a,"padding"+U[f],!0,e),"padding"!==c&&(g+=n.css(a,"border"+U[f]+"Width",!0,e)));return g}function Zb(a,b,c){var d=!0,e="width"===b?a.offsetWidth:a.offsetHeight,f=Jb(a),g=l.boxSizing()&&"border-box"===n.css(a,"boxSizing",!1,f);if(0>=e||null==e){if(e=Kb(a,b,f),(0>e||null==e)&&(e=a.style[b]),Ib.test(e))return e;d=g&&(l.boxSizingReliable()||e===a.style[b]),e=parseFloat(e)||0}return e+Yb(a,b,c||(g?"border":"content"),d,f)+"px"}n.extend({cssHooks:{opacity:{get:function(a,b){if(b){var c=Kb(a,"opacity");return""===c?"1":c}}}},cssNumber:{columnCount:!0,fillOpacity:!0,fontWeight:!0,lineHeight:!0,opacity:!0,order:!0,orphans:!0,widows:!0,zIndex:!0,zoom:!0},cssProps:{"float":l.cssFloat?"cssFloat":"styleFloat"},style:function(a,b,c,d){if(a&&3!==a.nodeType&&8!==a.nodeType&&a.style){var e,f,g,h=n.camelCase(b),i=a.style;if(b=n.cssProps[h]||(n.cssProps[h]=Vb(i,h)),g=n.cssHooks[b]||n.cssHooks[h],void 0===c)return g&&"get"in g&&void 0!==(e=g.get(a,!1,d))?e:i[b];if(f=typeof c,"string"===f&&(e=Rb.exec(c))&&(c=(e[1]+1)*e[2]+parseFloat(n.css(a,b)),f="number"),null!=c&&c===c&&("number"!==f||n.cssNumber[h]||(c+="px"),l.clearCloneStyle||""!==c||0!==b.indexOf("background")||(i[b]="inherit"),!(g&&"set"in g&&void 0===(c=g.set(a,c,d)))))try{i[b]="",i[b]=c}catch(j){}}},css:function(a,b,c,d){var e,f,g,h=n.camelCase(b);return b=n.cssProps[h]||(n.cssProps[h]=Vb(a.style,h)),g=n.cssHooks[b]||n.cssHooks[h],g&&"get"in g&&(f=g.get(a,!0,c)),void 0===f&&(f=Kb(a,b,d)),"normal"===f&&b in Tb&&(f=Tb[b]),""===c||c?(e=parseFloat(f),c===!0||n.isNumeric(e)?e||0:f):f}}),n.each(["height","width"],function(a,b){n.cssHooks[b]={get:function(a,c,d){return c?0===a.offsetWidth&&Pb.test(n.css(a,"display"))?n.swap(a,Sb,function(){return Zb(a,b,d)}):Zb(a,b,d):void 0},set:function(a,c,d){var e=d&&Jb(a);return Xb(a,c,d?Yb(a,b,d,l.boxSizing()&&"border-box"===n.css(a,"boxSizing",!1,e),e):0)}}}),l.opacity||(n.cssHooks.opacity={get:function(a,b){return Ob.test((b&&a.currentStyle?a.currentStyle.filter:a.style.filter)||"")?.01*parseFloat(RegExp.$1)+"":b?"1":""},set:function(a,b){var c=a.style,d=a.currentStyle,e=n.isNumeric(b)?"alpha(opacity="+100*b+")":"",f=d&&d.filter||c.filter||"";c.zoom=1,(b>=1||""===b)&&""===n.trim(f.replace(Nb,""))&&c.removeAttribute&&(c.removeAttribute("filter"),""===b||d&&!d.filter)||(c.filter=Nb.test(f)?f.replace(Nb,e):f+" "+e)}}),n.cssHooks.marginRight=Mb(l.reliableMarginRight,function(a,b){return b?n.swap(a,{display:"inline-block"},Kb,[a,"marginRight"]):void 0}),n.each({margin:"",padding:"",border:"Width"},function(a,b){n.cssHooks[a+b]={expand:function(c){for(var d=0,e={},f="string"==typeof c?c.split(" "):[c];4>d;d++)e[a+U[d]+b]=f[d]||f[d-2]||f[0];return e}},Hb.test(a)||(n.cssHooks[a+b].set=Xb)}),n.fn.extend({css:function(a,b){return W(this,function(a,b,c){var d,e,f={},g=0;if(n.isArray(b)){for(d=Jb(a),e=b.length;e>g;g++)f[b[g]]=n.css(a,b[g],!1,d);return f}return void 0!==c?n.style(a,b,c):n.css(a,b)
},a,b,arguments.length>1)},show:function(){return Wb(this,!0)},hide:function(){return Wb(this)},toggle:function(a){return"boolean"==typeof a?a?this.show():this.hide():this.each(function(){V(this)?n(this).show():n(this).hide()})}});function $b(a,b,c,d,e){return new $b.prototype.init(a,b,c,d,e)}n.Tween=$b,$b.prototype={constructor:$b,init:function(a,b,c,d,e,f){this.elem=a,this.prop=c,this.easing=e||"swing",this.options=b,this.start=this.now=this.cur(),this.end=d,this.unit=f||(n.cssNumber[c]?"":"px")},cur:function(){var a=$b.propHooks[this.prop];return a&&a.get?a.get(this):$b.propHooks._default.get(this)},run:function(a){var b,c=$b.propHooks[this.prop];return this.pos=b=this.options.duration?n.easing[this.easing](a,this.options.duration*a,0,1,this.options.duration):a,this.now=(this.end-this.start)*b+this.start,this.options.step&&this.options.step.call(this.elem,this.now,this),c&&c.set?c.set(this):$b.propHooks._default.set(this),this}},$b.prototype.init.prototype=$b.prototype,$b.propHooks={_default:{get:function(a){var b;return null==a.elem[a.prop]||a.elem.style&&null!=a.elem.style[a.prop]?(b=n.css(a.elem,a.prop,""),b&&"auto"!==b?b:0):a.elem[a.prop]},set:function(a){n.fx.step[a.prop]?n.fx.step[a.prop](a):a.elem.style&&(null!=a.elem.style[n.cssProps[a.prop]]||n.cssHooks[a.prop])?n.style(a.elem,a.prop,a.now+a.unit):a.elem[a.prop]=a.now}}},$b.propHooks.scrollTop=$b.propHooks.scrollLeft={set:function(a){a.elem.nodeType&&a.elem.parentNode&&(a.elem[a.prop]=a.now)}},n.easing={linear:function(a){return a},swing:function(a){return.5-Math.cos(a*Math.PI)/2}},n.fx=$b.prototype.init,n.fx.step={};var _b,ac,bc=/^(?:toggle|show|hide)$/,cc=new RegExp("^(?:([+-])=|)("+T+")([a-z%]*)$","i"),dc=/queueHooks$/,ec=[jc],fc={"*":[function(a,b){var c=this.createTween(a,b),d=c.cur(),e=cc.exec(b),f=e&&e[3]||(n.cssNumber[a]?"":"px"),g=(n.cssNumber[a]||"px"!==f&&+d)&&cc.exec(n.css(c.elem,a)),h=1,i=20;if(g&&g[3]!==f){f=f||g[3],e=e||[],g=+d||1;do h=h||".5",g/=h,n.style(c.elem,a,g+f);while(h!==(h=c.cur()/d)&&1!==h&&--i)}return e&&(g=c.start=+g||+d||0,c.unit=f,c.end=e[1]?g+(e[1]+1)*e[2]:+e[2]),c}]};function gc(){return setTimeout(function(){_b=void 0}),_b=n.now()}function hc(a,b){var c,d={height:a},e=0;for(b=b?1:0;4>e;e+=2-b)c=U[e],d["margin"+c]=d["padding"+c]=a;return b&&(d.opacity=d.width=a),d}function ic(a,b,c){for(var d,e=(fc[b]||[]).concat(fc["*"]),f=0,g=e.length;g>f;f++)if(d=e[f].call(c,b,a))return d}function jc(a,b,c){var d,e,f,g,h,i,j,k,m=this,o={},p=a.style,q=a.nodeType&&V(a),r=n._data(a,"fxshow");c.queue||(h=n._queueHooks(a,"fx"),null==h.unqueued&&(h.unqueued=0,i=h.empty.fire,h.empty.fire=function(){h.unqueued||i()}),h.unqueued++,m.always(function(){m.always(function(){h.unqueued--,n.queue(a,"fx").length||h.empty.fire()})})),1===a.nodeType&&("height"in b||"width"in b)&&(c.overflow=[p.overflow,p.overflowX,p.overflowY],j=n.css(a,"display"),k=Gb(a.nodeName),"none"===j&&(j=k),"inline"===j&&"none"===n.css(a,"float")&&(l.inlineBlockNeedsLayout&&"inline"!==k?p.zoom=1:p.display="inline-block")),c.overflow&&(p.overflow="hidden",l.shrinkWrapBlocks()||m.always(function(){p.overflow=c.overflow[0],p.overflowX=c.overflow[1],p.overflowY=c.overflow[2]}));for(d in b)if(e=b[d],bc.exec(e)){if(delete b[d],f=f||"toggle"===e,e===(q?"hide":"show")){if("show"!==e||!r||void 0===r[d])continue;q=!0}o[d]=r&&r[d]||n.style(a,d)}if(!n.isEmptyObject(o)){r?"hidden"in r&&(q=r.hidden):r=n._data(a,"fxshow",{}),f&&(r.hidden=!q),q?n(a).show():m.done(function(){n(a).hide()}),m.done(function(){var b;n._removeData(a,"fxshow");for(b in o)n.style(a,b,o[b])});for(d in o)g=ic(q?r[d]:0,d,m),d in r||(r[d]=g.start,q&&(g.end=g.start,g.start="width"===d||"height"===d?1:0))}}function kc(a,b){var c,d,e,f,g;for(c in a)if(d=n.camelCase(c),e=b[d],f=a[c],n.isArray(f)&&(e=f[1],f=a[c]=f[0]),c!==d&&(a[d]=f,delete a[c]),g=n.cssHooks[d],g&&"expand"in g){f=g.expand(f),delete a[d];for(c in f)c in a||(a[c]=f[c],b[c]=e)}else b[d]=e}function lc(a,b,c){var d,e,f=0,g=ec.length,h=n.Deferred().always(function(){delete i.elem}),i=function(){if(e)return!1;for(var b=_b||gc(),c=Math.max(0,j.startTime+j.duration-b),d=c/j.duration||0,f=1-d,g=0,i=j.tweens.length;i>g;g++)j.tweens[g].run(f);return h.notifyWith(a,[j,f,c]),1>f&&i?c:(h.resolveWith(a,[j]),!1)},j=h.promise({elem:a,props:n.extend({},b),opts:n.extend(!0,{specialEasing:{}},c),originalProperties:b,originalOptions:c,startTime:_b||gc(),duration:c.duration,tweens:[],createTween:function(b,c){var d=n.Tween(a,j.opts,b,c,j.opts.specialEasing[b]||j.opts.easing);return j.tweens.push(d),d},stop:function(b){var c=0,d=b?j.tweens.length:0;if(e)return this;for(e=!0;d>c;c++)j.tweens[c].run(1);return b?h.resolveWith(a,[j,b]):h.rejectWith(a,[j,b]),this}}),k=j.props;for(kc(k,j.opts.specialEasing);g>f;f++)if(d=ec[f].call(j,a,k,j.opts))return d;return n.map(k,ic,j),n.isFunction(j.opts.start)&&j.opts.start.call(a,j),n.fx.timer(n.extend(i,{elem:a,anim:j,queue:j.opts.queue})),j.progress(j.opts.progress).done(j.opts.done,j.opts.complete).fail(j.opts.fail).always(j.opts.always)}n.Animation=n.extend(lc,{tweener:function(a,b){n.isFunction(a)?(b=a,a=["*"]):a=a.split(" ");for(var c,d=0,e=a.length;e>d;d++)c=a[d],fc[c]=fc[c]||[],fc[c].unshift(b)},prefilter:function(a,b){b?ec.unshift(a):ec.push(a)}}),n.speed=function(a,b,c){var d=a&&"object"==typeof a?n.extend({},a):{complete:c||!c&&b||n.isFunction(a)&&a,duration:a,easing:c&&b||b&&!n.isFunction(b)&&b};return d.duration=n.fx.off?0:"number"==typeof d.duration?d.duration:d.duration in n.fx.speeds?n.fx.speeds[d.duration]:n.fx.speeds._default,(null==d.queue||d.queue===!0)&&(d.queue="fx"),d.old=d.complete,d.complete=function(){n.isFunction(d.old)&&d.old.call(this),d.queue&&n.dequeue(this,d.queue)},d},n.fn.extend({fadeTo:function(a,b,c,d){return this.filter(V).css("opacity",0).show().end().animate({opacity:b},a,c,d)},animate:function(a,b,c,d){var e=n.isEmptyObject(a),f=n.speed(b,c,d),g=function(){var b=lc(this,n.extend({},a),f);(e||n._data(this,"finish"))&&b.stop(!0)};return g.finish=g,e||f.queue===!1?this.each(g):this.queue(f.queue,g)},stop:function(a,b,c){var d=function(a){var b=a.stop;delete a.stop,b(c)};return"string"!=typeof a&&(c=b,b=a,a=void 0),b&&a!==!1&&this.queue(a||"fx",[]),this.each(function(){var b=!0,e=null!=a&&a+"queueHooks",f=n.timers,g=n._data(this);if(e)g[e]&&g[e].stop&&d(g[e]);else for(e in g)g[e]&&g[e].stop&&dc.test(e)&&d(g[e]);for(e=f.length;e--;)f[e].elem!==this||null!=a&&f[e].queue!==a||(f[e].anim.stop(c),b=!1,f.splice(e,1));(b||!c)&&n.dequeue(this,a)})},finish:function(a){return a!==!1&&(a=a||"fx"),this.each(function(){var b,c=n._data(this),d=c[a+"queue"],e=c[a+"queueHooks"],f=n.timers,g=d?d.length:0;for(c.finish=!0,n.queue(this,a,[]),e&&e.stop&&e.stop.call(this,!0),b=f.length;b--;)f[b].elem===this&&f[b].queue===a&&(f[b].anim.stop(!0),f.splice(b,1));for(b=0;g>b;b++)d[b]&&d[b].finish&&d[b].finish.call(this);delete c.finish})}}),n.each(["toggle","show","hide"],function(a,b){var c=n.fn[b];n.fn[b]=function(a,d,e){return null==a||"boolean"==typeof a?c.apply(this,arguments):this.animate(hc(b,!0),a,d,e)}}),n.each({slideDown:hc("show"),slideUp:hc("hide"),slideToggle:hc("toggle"),fadeIn:{opacity:"show"},fadeOut:{opacity:"hide"},fadeToggle:{opacity:"toggle"}},function(a,b){n.fn[a]=function(a,c,d){return this.animate(b,a,c,d)}}),n.timers=[],n.fx.tick=function(){var a,b=n.timers,c=0;for(_b=n.now();c<b.length;c++)a=b[c],a()||b[c]!==a||b.splice(c--,1);b.length||n.fx.stop(),_b=void 0},n.fx.timer=function(a){n.timers.push(a),a()?n.fx.start():n.timers.pop()},n.fx.interval=13,n.fx.start=function(){ac||(ac=setInterval(n.fx.tick,n.fx.interval))},n.fx.stop=function(){clearInterval(ac),ac=null},n.fx.speeds={slow:600,fast:200,_default:400},n.fn.delay=function(a,b){return a=n.fx?n.fx.speeds[a]||a:a,b=b||"fx",this.queue(b,function(b,c){var d=setTimeout(b,a);c.stop=function(){clearTimeout(d)}})},function(){var a,b,c,d,e=z.createElement("div");e.setAttribute("className","t"),e.innerHTML=" <link/><table></table><a href='/a'>a</a><input type='checkbox'/>",a=e.getElementsByTagName("a")[0],c=z.createElement("select"),d=c.appendChild(z.createElement("option")),b=e.getElementsByTagName("input")[0],a.style.cssText="top:1px",l.getSetAttribute="t"!==e.className,l.style=/top/.test(a.getAttribute("style")),l.hrefNormalized="/a"===a.getAttribute("href"),l.checkOn=!!b.value,l.optSelected=d.selected,l.enctype=!!z.createElement("form").enctype,c.disabled=!0,l.optDisabled=!d.disabled,b=z.createElement("input"),b.setAttribute("value",""),l.input=""===b.getAttribute("value"),b.value="t",b.setAttribute("type","radio"),l.radioValue="t"===b.value,a=b=c=d=e=null}();var mc=/\r/g;n.fn.extend({val:function(a){var b,c,d,e=this[0];{if(arguments.length)return d=n.isFunction(a),this.each(function(c){var e;1===this.nodeType&&(e=d?a.call(this,c,n(this).val()):a,null==e?e="":"number"==typeof e?e+="":n.isArray(e)&&(e=n.map(e,function(a){return null==a?"":a+""})),b=n.valHooks[this.type]||n.valHooks[this.nodeName.toLowerCase()],b&&"set"in b&&void 0!==b.set(this,e,"value")||(this.value=e))});if(e)return b=n.valHooks[e.type]||n.valHooks[e.nodeName.toLowerCase()],b&&"get"in b&&void 0!==(c=b.get(e,"value"))?c:(c=e.value,"string"==typeof c?c.replace(mc,""):null==c?"":c)}}}),n.extend({valHooks:{option:{get:function(a){var b=n.find.attr(a,"value");return null!=b?b:n.text(a)}},select:{get:function(a){for(var b,c,d=a.options,e=a.selectedIndex,f="select-one"===a.type||0>e,g=f?null:[],h=f?e+1:d.length,i=0>e?h:f?e:0;h>i;i++)if(c=d[i],!(!c.selected&&i!==e||(l.optDisabled?c.disabled:null!==c.getAttribute("disabled"))||c.parentNode.disabled&&n.nodeName(c.parentNode,"optgroup"))){if(b=n(c).val(),f)return b;g.push(b)}return g},set:function(a,b){var c,d,e=a.options,f=n.makeArray(b),g=e.length;while(g--)if(d=e[g],n.inArray(n.valHooks.option.get(d),f)>=0)try{d.selected=c=!0}catch(h){d.scrollHeight}else d.selected=!1;return c||(a.selectedIndex=-1),e}}}}),n.each(["radio","checkbox"],function(){n.valHooks[this]={set:function(a,b){return n.isArray(b)?a.checked=n.inArray(n(a).val(),b)>=0:void 0}},l.checkOn||(n.valHooks[this].get=function(a){return null===a.getAttribute("value")?"on":a.value})});var nc,oc,pc=n.expr.attrHandle,qc=/^(?:checked|selected)$/i,rc=l.getSetAttribute,sc=l.input;n.fn.extend({attr:function(a,b){return W(this,n.attr,a,b,arguments.length>1)},removeAttr:function(a){return this.each(function(){n.removeAttr(this,a)})}}),n.extend({attr:function(a,b,c){var d,e,f=a.nodeType;if(a&&3!==f&&8!==f&&2!==f)return typeof a.getAttribute===L?n.prop(a,b,c):(1===f&&n.isXMLDoc(a)||(b=b.toLowerCase(),d=n.attrHooks[b]||(n.expr.match.bool.test(b)?oc:nc)),void 0===c?d&&"get"in d&&null!==(e=d.get(a,b))?e:(e=n.find.attr(a,b),null==e?void 0:e):null!==c?d&&"set"in d&&void 0!==(e=d.set(a,c,b))?e:(a.setAttribute(b,c+""),c):void n.removeAttr(a,b))},removeAttr:function(a,b){var c,d,e=0,f=b&&b.match(F);if(f&&1===a.nodeType)while(c=f[e++])d=n.propFix[c]||c,n.expr.match.bool.test(c)?sc&&rc||!qc.test(c)?a[d]=!1:a[n.camelCase("default-"+c)]=a[d]=!1:n.attr(a,c,""),a.removeAttribute(rc?c:d)},attrHooks:{type:{set:function(a,b){if(!l.radioValue&&"radio"===b&&n.nodeName(a,"input")){var c=a.value;return a.setAttribute("type",b),c&&(a.value=c),b}}}}}),oc={set:function(a,b,c){return b===!1?n.removeAttr(a,c):sc&&rc||!qc.test(c)?a.setAttribute(!rc&&n.propFix[c]||c,c):a[n.camelCase("default-"+c)]=a[c]=!0,c}},n.each(n.expr.match.bool.source.match(/\w+/g),function(a,b){var c=pc[b]||n.find.attr;pc[b]=sc&&rc||!qc.test(b)?function(a,b,d){var e,f;return d||(f=pc[b],pc[b]=e,e=null!=c(a,b,d)?b.toLowerCase():null,pc[b]=f),e}:function(a,b,c){return c?void 0:a[n.camelCase("default-"+b)]?b.toLowerCase():null}}),sc&&rc||(n.attrHooks.value={set:function(a,b,c){return n.nodeName(a,"input")?void(a.defaultValue=b):nc&&nc.set(a,b,c)}}),rc||(nc={set:function(a,b,c){var d=a.getAttributeNode(c);return d||a.setAttributeNode(d=a.ownerDocument.createAttribute(c)),d.value=b+="","value"===c||b===a.getAttribute(c)?b:void 0}},pc.id=pc.name=pc.coords=function(a,b,c){var d;return c?void 0:(d=a.getAttributeNode(b))&&""!==d.value?d.value:null},n.valHooks.button={get:function(a,b){var c=a.getAttributeNode(b);return c&&c.specified?c.value:void 0},set:nc.set},n.attrHooks.contenteditable={set:function(a,b,c){nc.set(a,""===b?!1:b,c)}},n.each(["width","height"],function(a,b){n.attrHooks[b]={set:function(a,c){return""===c?(a.setAttribute(b,"auto"),c):void 0}}})),l.style||(n.attrHooks.style={get:function(a){return a.style.cssText||void 0},set:function(a,b){return a.style.cssText=b+""}});var tc=/^(?:input|select|textarea|button|object)$/i,uc=/^(?:a|area)$/i;n.fn.extend({prop:function(a,b){return W(this,n.prop,a,b,arguments.length>1)},removeProp:function(a){return a=n.propFix[a]||a,this.each(function(){try{this[a]=void 0,delete this[a]}catch(b){}})}}),n.extend({propFix:{"for":"htmlFor","class":"className"},prop:function(a,b,c){var d,e,f,g=a.nodeType;if(a&&3!==g&&8!==g&&2!==g)return f=1!==g||!n.isXMLDoc(a),f&&(b=n.propFix[b]||b,e=n.propHooks[b]),void 0!==c?e&&"set"in e&&void 0!==(d=e.set(a,c,b))?d:a[b]=c:e&&"get"in e&&null!==(d=e.get(a,b))?d:a[b]},propHooks:{tabIndex:{get:function(a){var b=n.find.attr(a,"tabindex");return b?parseInt(b,10):tc.test(a.nodeName)||uc.test(a.nodeName)&&a.href?0:-1}}}}),l.hrefNormalized||n.each(["href","src"],function(a,b){n.propHooks[b]={get:function(a){return a.getAttribute(b,4)}}}),l.optSelected||(n.propHooks.selected={get:function(a){var b=a.parentNode;return b&&(b.selectedIndex,b.parentNode&&b.parentNode.selectedIndex),null}}),n.each(["tabIndex","readOnly","maxLength","cellSpacing","cellPadding","rowSpan","colSpan","useMap","frameBorder","contentEditable"],function(){n.propFix[this.toLowerCase()]=this}),l.enctype||(n.propFix.enctype="encoding");var vc=/[\t\r\n\f]/g;n.fn.extend({addClass:function(a){var b,c,d,e,f,g,h=0,i=this.length,j="string"==typeof a&&a;if(n.isFunction(a))return this.each(function(b){n(this).addClass(a.call(this,b,this.className))});if(j)for(b=(a||"").match(F)||[];i>h;h++)if(c=this[h],d=1===c.nodeType&&(c.className?(" "+c.className+" ").replace(vc," "):" ")){f=0;while(e=b[f++])d.indexOf(" "+e+" ")<0&&(d+=e+" ");g=n.trim(d),c.className!==g&&(c.className=g)}return this},removeClass:function(a){var b,c,d,e,f,g,h=0,i=this.length,j=0===arguments.length||"string"==typeof a&&a;if(n.isFunction(a))return this.each(function(b){n(this).removeClass(a.call(this,b,this.className))});if(j)for(b=(a||"").match(F)||[];i>h;h++)if(c=this[h],d=1===c.nodeType&&(c.className?(" "+c.className+" ").replace(vc," "):"")){f=0;while(e=b[f++])while(d.indexOf(" "+e+" ")>=0)d=d.replace(" "+e+" "," ");g=a?n.trim(d):"",c.className!==g&&(c.className=g)}return this},toggleClass:function(a,b){var c=typeof a;return"boolean"==typeof b&&"string"===c?b?this.addClass(a):this.removeClass(a):this.each(n.isFunction(a)?function(c){n(this).toggleClass(a.call(this,c,this.className,b),b)}:function(){if("string"===c){var b,d=0,e=n(this),f=a.match(F)||[];while(b=f[d++])e.hasClass(b)?e.removeClass(b):e.addClass(b)}else(c===L||"boolean"===c)&&(this.className&&n._data(this,"__className__",this.className),this.className=this.className||a===!1?"":n._data(this,"__className__")||"")})},hasClass:function(a){for(var b=" "+a+" ",c=0,d=this.length;d>c;c++)if(1===this[c].nodeType&&(" "+this[c].className+" ").replace(vc," ").indexOf(b)>=0)return!0;return!1}}),n.each("blur focus focusin focusout load resize scroll unload click dblclick mousedown mouseup mousemove mouseover mouseout mouseenter mouseleave change select submit keydown keypress keyup error contextmenu".split(" "),function(a,b){n.fn[b]=function(a,c){return arguments.length>0?this.on(b,null,a,c):this.trigger(b)}}),n.fn.extend({hover:function(a,b){return this.mouseenter(a).mouseleave(b||a)},bind:function(a,b,c){return this.on(a,null,b,c)},unbind:function(a,b){return this.off(a,null,b)},delegate:function(a,b,c,d){return this.on(b,a,c,d)},undelegate:function(a,b,c){return 1===arguments.length?this.off(a,"**"):this.off(b,a||"**",c)}});var wc=n.now(),xc=/\?/,yc=/(,)|(\[|{)|(}|])|"(?:[^"\\\r\n]|\\["\\\/bfnrt]|\\u[\da-fA-F]{4})*"\s*:?|true|false|null|-?(?!0\d)\d+(?:\.\d+|)(?:[eE][+-]?\d+|)/g;n.parseJSON=function(b){if(a.JSON&&a.JSON.parse)return a.JSON.parse(b+"");var c,d=null,e=n.trim(b+"");return e&&!n.trim(e.replace(yc,function(a,b,e,f){return c&&b&&(d=0),0===d?a:(c=e||b,d+=!f-!e,"")}))?Function("return "+e)():n.error("Invalid JSON: "+b)},n.parseXML=function(b){var c,d;if(!b||"string"!=typeof b)return null;try{a.DOMParser?(d=new DOMParser,c=d.parseFromString(b,"text/xml")):(c=new ActiveXObject("Microsoft.XMLDOM"),c.async="false",c.loadXML(b))}catch(e){c=void 0}return c&&c.documentElement&&!c.getElementsByTagName("parsererror").length||n.error("Invalid XML: "+b),c};var zc,Ac,Bc=/#.*$/,Cc=/([?&])_=[^&]*/,Dc=/^(.*?):[ \t]*([^\r\n]*)\r?$/gm,Ec=/^(?:about|app|app-storage|.+-extension|file|res|widget):$/,Fc=/^(?:GET|HEAD)$/,Gc=/^\/\//,Hc=/^([\w.+-]+:)(?:\/\/(?:[^\/?#]*@|)([^\/?#:]*)(?::(\d+)|)|)/,Ic={},Jc={},Kc="*/".concat("*");try{Ac=location.href}catch(Lc){Ac=z.createElement("a"),Ac.href="",Ac=Ac.href}zc=Hc.exec(Ac.toLowerCase())||[];function Mc(a){return function(b,c){"string"!=typeof b&&(c=b,b="*");var d,e=0,f=b.toLowerCase().match(F)||[];if(n.isFunction(c))while(d=f[e++])"+"===d.charAt(0)?(d=d.slice(1)||"*",(a[d]=a[d]||[]).unshift(c)):(a[d]=a[d]||[]).push(c)}}function Nc(a,b,c,d){var e={},f=a===Jc;function g(h){var i;return e[h]=!0,n.each(a[h]||[],function(a,h){var j=h(b,c,d);return"string"!=typeof j||f||e[j]?f?!(i=j):void 0:(b.dataTypes.unshift(j),g(j),!1)}),i}return g(b.dataTypes[0])||!e["*"]&&g("*")}function Oc(a,b){var c,d,e=n.ajaxSettings.flatOptions||{};for(d in b)void 0!==b[d]&&((e[d]?a:c||(c={}))[d]=b[d]);return c&&n.extend(!0,a,c),a}function Pc(a,b,c){var d,e,f,g,h=a.contents,i=a.dataTypes;while("*"===i[0])i.shift(),void 0===e&&(e=a.mimeType||b.getResponseHeader("Content-Type"));if(e)for(g in h)if(h[g]&&h[g].test(e)){i.unshift(g);break}if(i[0]in c)f=i[0];else{for(g in c){if(!i[0]||a.converters[g+" "+i[0]]){f=g;break}d||(d=g)}f=f||d}return f?(f!==i[0]&&i.unshift(f),c[f]):void 0}function Qc(a,b,c,d){var e,f,g,h,i,j={},k=a.dataTypes.slice();if(k[1])for(g in a.converters)j[g.toLowerCase()]=a.converters[g];f=k.shift();while(f)if(a.responseFields[f]&&(c[a.responseFields[f]]=b),!i&&d&&a.dataFilter&&(b=a.dataFilter(b,a.dataType)),i=f,f=k.shift())if("*"===f)f=i;else if("*"!==i&&i!==f){if(g=j[i+" "+f]||j["* "+f],!g)for(e in j)if(h=e.split(" "),h[1]===f&&(g=j[i+" "+h[0]]||j["* "+h[0]])){g===!0?g=j[e]:j[e]!==!0&&(f=h[0],k.unshift(h[1]));break}if(g!==!0)if(g&&a["throws"])b=g(b);else try{b=g(b)}catch(l){return{state:"parsererror",error:g?l:"No conversion from "+i+" to "+f}}}return{state:"success",data:b}}n.extend({active:0,lastModified:{},etag:{},ajaxSettings:{url:Ac,type:"GET",isLocal:Ec.test(zc[1]),global:!0,processData:!0,async:!0,contentType:"application/x-www-form-urlencoded; charset=UTF-8",accepts:{"*":Kc,text:"text/plain",html:"text/html",xml:"application/xml, text/xml",json:"application/json, text/javascript"},contents:{xml:/xml/,html:/html/,json:/json/},responseFields:{xml:"responseXML",text:"responseText",json:"responseJSON"},converters:{"* text":String,"text html":!0,"text json":n.parseJSON,"text xml":n.parseXML},flatOptions:{url:!0,context:!0}},ajaxSetup:function(a,b){return b?Oc(Oc(a,n.ajaxSettings),b):Oc(n.ajaxSettings,a)},ajaxPrefilter:Mc(Ic),ajaxTransport:Mc(Jc),ajax:function(a,b){"object"==typeof a&&(b=a,a=void 0),b=b||{};var c,d,e,f,g,h,i,j,k=n.ajaxSetup({},b),l=k.context||k,m=k.context&&(l.nodeType||l.jquery)?n(l):n.event,o=n.Deferred(),p=n.Callbacks("once memory"),q=k.statusCode||{},r={},s={},t=0,u="canceled",v={readyState:0,getResponseHeader:function(a){var b;if(2===t){if(!j){j={};while(b=Dc.exec(f))j[b[1].toLowerCase()]=b[2]}b=j[a.toLowerCase()]}return null==b?null:b},getAllResponseHeaders:function(){return 2===t?f:null},setRequestHeader:function(a,b){var c=a.toLowerCase();return t||(a=s[c]=s[c]||a,r[a]=b),this},overrideMimeType:function(a){return t||(k.mimeType=a),this},statusCode:function(a){var b;if(a)if(2>t)for(b in a)q[b]=[q[b],a[b]];else v.always(a[v.status]);return this},abort:function(a){var b=a||u;return i&&i.abort(b),x(0,b),this}};if(o.promise(v).complete=p.add,v.success=v.done,v.error=v.fail,k.url=((a||k.url||Ac)+"").replace(Bc,"").replace(Gc,zc[1]+"//"),k.type=b.method||b.type||k.method||k.type,k.dataTypes=n.trim(k.dataType||"*").toLowerCase().match(F)||[""],null==k.crossDomain&&(c=Hc.exec(k.url.toLowerCase()),k.crossDomain=!(!c||c[1]===zc[1]&&c[2]===zc[2]&&(c[3]||("http:"===c[1]?"80":"443"))===(zc[3]||("http:"===zc[1]?"80":"443")))),k.data&&k.processData&&"string"!=typeof k.data&&(k.data=n.param(k.data,k.traditional)),Nc(Ic,k,b,v),2===t)return v;h=k.global,h&&0===n.active++&&n.event.trigger("ajaxStart"),k.type=k.type.toUpperCase(),k.hasContent=!Fc.test(k.type),e=k.url,k.hasContent||(k.data&&(e=k.url+=(xc.test(e)?"&":"?")+k.data,delete k.data),k.cache===!1&&(k.url=Cc.test(e)?e.replace(Cc,"$1_="+wc++):e+(xc.test(e)?"&":"?")+"_="+wc++)),k.ifModified&&(n.lastModified[e]&&v.setRequestHeader("If-Modified-Since",n.lastModified[e]),n.etag[e]&&v.setRequestHeader("If-None-Match",n.etag[e])),(k.data&&k.hasContent&&k.contentType!==!1||b.contentType)&&v.setRequestHeader("Content-Type",k.contentType),v.setRequestHeader("Accept",k.dataTypes[0]&&k.accepts[k.dataTypes[0]]?k.accepts[k.dataTypes[0]]+("*"!==k.dataTypes[0]?", "+Kc+"; q=0.01":""):k.accepts["*"]);for(d in k.headers)v.setRequestHeader(d,k.headers[d]);if(k.beforeSend&&(k.beforeSend.call(l,v,k)===!1||2===t))return v.abort();u="abort";for(d in{success:1,error:1,complete:1})v[d](k[d]);if(i=Nc(Jc,k,b,v)){v.readyState=1,h&&m.trigger("ajaxSend",[v,k]),k.async&&k.timeout>0&&(g=setTimeout(function(){v.abort("timeout")},k.timeout));try{t=1,i.send(r,x)}catch(w){if(!(2>t))throw w;x(-1,w)}}else x(-1,"No Transport");function x(a,b,c,d){var j,r,s,u,w,x=b;2!==t&&(t=2,g&&clearTimeout(g),i=void 0,f=d||"",v.readyState=a>0?4:0,j=a>=200&&300>a||304===a,c&&(u=Pc(k,v,c)),u=Qc(k,u,v,j),j?(k.ifModified&&(w=v.getResponseHeader("Last-Modified"),w&&(n.lastModified[e]=w),w=v.getResponseHeader("etag"),w&&(n.etag[e]=w)),204===a||"HEAD"===k.type?x="nocontent":304===a?x="notmodified":(x=u.state,r=u.data,s=u.error,j=!s)):(s=x,(a||!x)&&(x="error",0>a&&(a=0))),v.status=a,v.statusText=(b||x)+"",j?o.resolveWith(l,[r,x,v]):o.rejectWith(l,[v,x,s]),v.statusCode(q),q=void 0,h&&m.trigger(j?"ajaxSuccess":"ajaxError",[v,k,j?r:s]),p.fireWith(l,[v,x]),h&&(m.trigger("ajaxComplete",[v,k]),--n.active||n.event.trigger("ajaxStop")))}return v},getJSON:function(a,b,c){return n.get(a,b,c,"json")},getScript:function(a,b){return n.get(a,void 0,b,"script")}}),n.each(["get","post"],function(a,b){n[b]=function(a,c,d,e){return n.isFunction(c)&&(e=e||d,d=c,c=void 0),n.ajax({url:a,type:b,dataType:e,data:c,success:d})}}),n.each(["ajaxStart","ajaxStop","ajaxComplete","ajaxError","ajaxSuccess","ajaxSend"],function(a,b){n.fn[b]=function(a){return this.on(b,a)}}),n._evalUrl=function(a){return n.ajax({url:a,type:"GET",dataType:"script",async:!1,global:!1,"throws":!0})},n.fn.extend({wrapAll:function(a){if(n.isFunction(a))return this.each(function(b){n(this).wrapAll(a.call(this,b))});if(this[0]){var b=n(a,this[0].ownerDocument).eq(0).clone(!0);this[0].parentNode&&b.insertBefore(this[0]),b.map(function(){var a=this;while(a.firstChild&&1===a.firstChild.nodeType)a=a.firstChild;return a}).append(this)}return this},wrapInner:function(a){return this.each(n.isFunction(a)?function(b){n(this).wrapInner(a.call(this,b))}:function(){var b=n(this),c=b.contents();c.length?c.wrapAll(a):b.append(a)})},wrap:function(a){var b=n.isFunction(a);return this.each(function(c){n(this).wrapAll(b?a.call(this,c):a)})},unwrap:function(){return this.parent().each(function(){n.nodeName(this,"body")||n(this).replaceWith(this.childNodes)}).end()}}),n.expr.filters.hidden=function(a){return a.offsetWidth<=0&&a.offsetHeight<=0||!l.reliableHiddenOffsets()&&"none"===(a.style&&a.style.display||n.css(a,"display"))},n.expr.filters.visible=function(a){return!n.expr.filters.hidden(a)};var Rc=/%20/g,Sc=/\[\]$/,Tc=/\r?\n/g,Uc=/^(?:submit|button|image|reset|file)$/i,Vc=/^(?:input|select|textarea|keygen)/i;function Wc(a,b,c,d){var e;if(n.isArray(b))n.each(b,function(b,e){c||Sc.test(a)?d(a,e):Wc(a+"["+("object"==typeof e?b:"")+"]",e,c,d)});else if(c||"object"!==n.type(b))d(a,b);else for(e in b)Wc(a+"["+e+"]",b[e],c,d)}n.param=function(a,b){var c,d=[],e=function(a,b){b=n.isFunction(b)?b():null==b?"":b,d[d.length]=encodeURIComponent(a)+"="+encodeURIComponent(b)};if(void 0===b&&(b=n.ajaxSettings&&n.ajaxSettings.traditional),n.isArray(a)||a.jquery&&!n.isPlainObject(a))n.each(a,function(){e(this.name,this.value)});else for(c in a)Wc(c,a[c],b,e);return d.join("&").replace(Rc,"+")},n.fn.extend({serialize:function(){return n.param(this.serializeArray())},serializeArray:function(){return this.map(function(){var a=n.prop(this,"elements");return a?n.makeArray(a):this}).filter(function(){var a=this.type;return this.name&&!n(this).is(":disabled")&&Vc.test(this.nodeName)&&!Uc.test(a)&&(this.checked||!X.test(a))}).map(function(a,b){var c=n(this).val();return null==c?null:n.isArray(c)?n.map(c,function(a){return{name:b.name,value:a.replace(Tc,"\r\n")}}):{name:b.name,value:c.replace(Tc,"\r\n")}}).get()}}),n.ajaxSettings.xhr=void 0!==a.ActiveXObject?function(){return!this.isLocal&&/^(get|post|head|put|delete|options)$/i.test(this.type)&&$c()||_c()}:$c;var Xc=0,Yc={},Zc=n.ajaxSettings.xhr();a.ActiveXObject&&n(a).on("unload",function(){for(var a in Yc)Yc[a](void 0,!0)}),l.cors=!!Zc&&"withCredentials"in Zc,Zc=l.ajax=!!Zc,Zc&&n.ajaxTransport(function(a){if(!a.crossDomain||l.cors){var b;return{send:function(c,d){var e,f=a.xhr(),g=++Xc;if(f.open(a.type,a.url,a.async,a.username,a.password),a.xhrFields)for(e in a.xhrFields)f[e]=a.xhrFields[e];a.mimeType&&f.overrideMimeType&&f.overrideMimeType(a.mimeType),a.crossDomain||c["X-Requested-With"]||(c["X-Requested-With"]="XMLHttpRequest");for(e in c)void 0!==c[e]&&f.setRequestHeader(e,c[e]+"");f.send(a.hasContent&&a.data||null),b=function(c,e){var h,i,j;if(b&&(e||4===f.readyState))if(delete Yc[g],b=void 0,f.onreadystatechange=n.noop,e)4!==f.readyState&&f.abort();else{j={},h=f.status,"string"==typeof f.responseText&&(j.text=f.responseText);try{i=f.statusText}catch(k){i=""}h||!a.isLocal||a.crossDomain?1223===h&&(h=204):h=j.text?200:404}j&&d(h,i,j,f.getAllResponseHeaders())},a.async?4===f.readyState?setTimeout(b):f.onreadystatechange=Yc[g]=b:b()},abort:function(){b&&b(void 0,!0)}}}});function $c(){try{return new a.XMLHttpRequest}catch(b){}}function _c(){try{return new a.ActiveXObject("Microsoft.XMLHTTP")}catch(b){}}n.ajaxSetup({accepts:{script:"text/javascript, application/javascript, application/ecmascript, application/x-ecmascript"},contents:{script:/(?:java|ecma)script/},converters:{"text script":function(a){return n.globalEval(a),a}}}),n.ajaxPrefilter("script",function(a){void 0===a.cache&&(a.cache=!1),a.crossDomain&&(a.type="GET",a.global=!1)}),n.ajaxTransport("script",function(a){if(a.crossDomain){var b,c=z.head||n("head")[0]||z.documentElement;return{send:function(d,e){b=z.createElement("script"),b.async=!0,a.scriptCharset&&(b.charset=a.scriptCharset),b.src=a.url,b.onload=b.onreadystatechange=function(a,c){(c||!b.readyState||/loaded|complete/.test(b.readyState))&&(b.onload=b.onreadystatechange=null,b.parentNode&&b.parentNode.removeChild(b),b=null,c||e(200,"success"))},c.insertBefore(b,c.firstChild)},abort:function(){b&&b.onload(void 0,!0)}}}});var ad=[],bd=/(=)\?(?=&|$)|\?\?/;n.ajaxSetup({jsonp:"callback",jsonpCallback:function(){var a=ad.pop()||n.expando+"_"+wc++;return this[a]=!0,a}}),n.ajaxPrefilter("json jsonp",function(b,c,d){var e,f,g,h=b.jsonp!==!1&&(bd.test(b.url)?"url":"string"==typeof b.data&&!(b.contentType||"").indexOf("application/x-www-form-urlencoded")&&bd.test(b.data)&&"data");return h||"jsonp"===b.dataTypes[0]?(e=b.jsonpCallback=n.isFunction(b.jsonpCallback)?b.jsonpCallback():b.jsonpCallback,h?b[h]=b[h].replace(bd,"$1"+e):b.jsonp!==!1&&(b.url+=(xc.test(b.url)?"&":"?")+b.jsonp+"="+e),b.converters["script json"]=function(){return g||n.error(e+" was not called"),g[0]},b.dataTypes[0]="json",f=a[e],a[e]=function(){g=arguments},d.always(function(){a[e]=f,b[e]&&(b.jsonpCallback=c.jsonpCallback,ad.push(e)),g&&n.isFunction(f)&&f(g[0]),g=f=void 0}),"script"):void 0}),n.parseHTML=function(a,b,c){if(!a||"string"!=typeof a)return null;"boolean"==typeof b&&(c=b,b=!1),b=b||z;var d=v.exec(a),e=!c&&[];return d?[b.createElement(d[1])]:(d=n.buildFragment([a],b,e),e&&e.length&&n(e).remove(),n.merge([],d.childNodes))};var cd=n.fn.load;n.fn.load=function(a,b,c){if("string"!=typeof a&&cd)return cd.apply(this,arguments);var d,e,f,g=this,h=a.indexOf(" ");return h>=0&&(d=a.slice(h,a.length),a=a.slice(0,h)),n.isFunction(b)?(c=b,b=void 0):b&&"object"==typeof b&&(f="POST"),g.length>0&&n.ajax({url:a,type:f,dataType:"html",data:b}).done(function(a){e=arguments,g.html(d?n("<div>").append(n.parseHTML(a)).find(d):a)}).complete(c&&function(a,b){g.each(c,e||[a.responseText,b,a])}),this},n.expr.filters.animated=function(a){return n.grep(n.timers,function(b){return a===b.elem}).length};var dd=a.document.documentElement;function ed(a){return n.isWindow(a)?a:9===a.nodeType?a.defaultView||a.parentWindow:!1}n.offset={setOffset:function(a,b,c){var d,e,f,g,h,i,j,k=n.css(a,"position"),l=n(a),m={};"static"===k&&(a.style.position="relative"),h=l.offset(),f=n.css(a,"top"),i=n.css(a,"left"),j=("absolute"===k||"fixed"===k)&&n.inArray("auto",[f,i])>-1,j?(d=l.position(),g=d.top,e=d.left):(g=parseFloat(f)||0,e=parseFloat(i)||0),n.isFunction(b)&&(b=b.call(a,c,h)),null!=b.top&&(m.top=b.top-h.top+g),null!=b.left&&(m.left=b.left-h.left+e),"using"in b?b.using.call(a,m):l.css(m)}},n.fn.extend({offset:function(a){if(arguments.length)return void 0===a?this:this.each(function(b){n.offset.setOffset(this,a,b)});var b,c,d={top:0,left:0},e=this[0],f=e&&e.ownerDocument;if(f)return b=f.documentElement,n.contains(b,e)?(typeof e.getBoundingClientRect!==L&&(d=e.getBoundingClientRect()),c=ed(f),{top:d.top+(c.pageYOffset||b.scrollTop)-(b.clientTop||0),left:d.left+(c.pageXOffset||b.scrollLeft)-(b.clientLeft||0)}):d},position:function(){if(this[0]){var a,b,c={top:0,left:0},d=this[0];return"fixed"===n.css(d,"position")?b=d.getBoundingClientRect():(a=this.offsetParent(),b=this.offset(),n.nodeName(a[0],"html")||(c=a.offset()),c.top+=n.css(a[0],"borderTopWidth",!0),c.left+=n.css(a[0],"borderLeftWidth",!0)),{top:b.top-c.top-n.css(d,"marginTop",!0),left:b.left-c.left-n.css(d,"marginLeft",!0)}}},offsetParent:function(){return this.map(function(){var a=this.offsetParent||dd;while(a&&!n.nodeName(a,"html")&&"static"===n.css(a,"position"))a=a.offsetParent;return a||dd})}}),n.each({scrollLeft:"pageXOffset",scrollTop:"pageYOffset"},function(a,b){var c=/Y/.test(b);n.fn[a]=function(d){return W(this,function(a,d,e){var f=ed(a);return void 0===e?f?b in f?f[b]:f.document.documentElement[d]:a[d]:void(f?f.scrollTo(c?n(f).scrollLeft():e,c?e:n(f).scrollTop()):a[d]=e)},a,d,arguments.length,null)}}),n.each(["top","left"],function(a,b){n.cssHooks[b]=Mb(l.pixelPosition,function(a,c){return c?(c=Kb(a,b),Ib.test(c)?n(a).position()[b]+"px":c):void 0})}),n.each({Height:"height",Width:"width"},function(a,b){n.each({padding:"inner"+a,content:b,"":"outer"+a},function(c,d){n.fn[d]=function(d,e){var f=arguments.length&&(c||"boolean"!=typeof d),g=c||(d===!0||e===!0?"margin":"border");return W(this,function(b,c,d){var e;return n.isWindow(b)?b.document.documentElement["client"+a]:9===b.nodeType?(e=b.documentElement,Math.max(b.body["scroll"+a],e["scroll"+a],b.body["offset"+a],e["offset"+a],e["client"+a])):void 0===d?n.css(b,c,g):n.style(b,c,d,g)},b,f?d:void 0,f,null)}})}),n.fn.size=function(){return this.length},n.fn.andSelf=n.fn.addBack,"function"==typeof define&&define.amd&&define("jquery",[],function(){return n});var fd=a.jQuery,gd=a.$;return n.noConflict=function(b){return a.$===n&&(a.$=gd),b&&a.jQuery===n&&(a.jQuery=fd),n},typeof b===L&&(a.jQuery=a.$=n),n});
|
actor-apps/app-web/src/app/components/common/MessageItem.react.js | TimurTarasenko/actor-platform | import _ from 'lodash';
import React from 'react';
import { PureRenderMixin } from 'react/addons';
import memoize from 'memoizee';
import classNames from 'classnames';
import emojify from 'emojify.js';
import hljs from 'highlight.js';
import marked from 'marked';
import emojiCharacters from 'emoji-named-characters';
import Lightbox from 'jsonlylightbox';
import VisibilitySensor from 'react-visibility-sensor';
import AvatarItem from 'components/common/AvatarItem.react';
import DialogActionCreators from 'actions/DialogActionCreators';
import { MessageContentTypes } from 'constants/ActorAppConstants';
let lastMessageSenderId,
lastMessageContentType;
var MessageItem = React.createClass({
displayName: 'MessageItem',
propTypes: {
message: React.PropTypes.object.isRequired,
newDay: React.PropTypes.bool,
onVisibilityChange: React.PropTypes.func
},
mixins: [PureRenderMixin],
onClick() {
DialogActionCreators.selectDialogPeerUser(this.props.message.sender.peer.id);
},
onVisibilityChange(isVisible) {
this.props.onVisibilityChange(this.props.message, isVisible);
},
render() {
const message = this.props.message;
const newDay = this.props.newDay;
let header,
visibilitySensor,
leftBlock;
let isSameSender = message.sender.peer.id === lastMessageSenderId &&
lastMessageContentType !== MessageContentTypes.SERVICE &&
!newDay;
let messageClassName = classNames({
'message': true,
'row': true,
'message--same-sender': isSameSender
});
if (isSameSender) {
leftBlock = (
<div className="message__info text-right">
<time className="message__timestamp">{message.date}</time>
<MessageItem.State message={message}/>
</div>
);
} else {
leftBlock = (
<div className="message__info message__info--avatar">
<a onClick={this.onClick}>
<AvatarItem image={message.sender.avatar}
placeholder={message.sender.placeholder}
title={message.sender.title}/>
</a>
</div>
);
header = (
<header className="message__header">
<h3 className="message__sender">
<a onClick={this.onClick}>{message.sender.title}</a>
</h3>
<time className="message__timestamp">{message.date}</time>
<MessageItem.State message={message}/>
</header>
);
}
if (message.content.content === MessageContentTypes.SERVICE) {
leftBlock = null;
header = null;
}
if (this.props.onVisibilityChange) {
visibilitySensor = <VisibilitySensor onChange={this.onVisibilityChange}/>;
}
lastMessageSenderId = message.sender.peer.id;
lastMessageContentType = message.content.content;
return (
<li className={messageClassName}>
{leftBlock}
<div className="message__body col-xs">
{header}
<MessageItem.Content content={message.content}/>
{visibilitySensor}
</div>
</li>
);
}
});
emojify.setConfig({
mode: 'img',
img_dir: 'assets/img/emoji' // eslint-disable-line
});
const mdRenderer = new marked.Renderer();
// target _blank for links
mdRenderer.link = function(href, title, text) {
let external, newWindow, out;
external = /^https?:\/\/.+$/.test(href);
newWindow = external || title === 'newWindow';
out = '<a href=\"' + href + '\"';
if (newWindow) {
out += ' target="_blank"';
}
if (title && title !== 'newWindow') {
out += ' title=\"' + title + '\"';
}
return (out + '>' + text + '</a>');
};
const markedOptions = {
sanitize: true,
breaks: true,
highlight: function (code) {
return hljs.highlightAuto(code).value;
},
renderer: mdRenderer
};
const inversedEmojiCharacters = _.invert(_.mapValues(emojiCharacters, (e) => e.character));
const emojiVariants = _.map(Object.keys(inversedEmojiCharacters), function(name) {
return name.replace(/\+/g, '\\+');
});
const emojiRegexp = new RegExp('(' + emojiVariants.join('|') + ')', 'gi');
const processText = function(text) {
let markedText = marked(text, markedOptions);
// need hack with replace because of https://github.com/Ranks/emojify.js/issues/127
const noPTag = markedText.replace(/<p>/g, '<p> ');
let emojifiedText = emojify
.replace(noPTag.replace(emojiRegexp, (match) => ':' + inversedEmojiCharacters[match] + ':'));
return emojifiedText;
};
const memoizedProcessText = memoize(processText, {
length: 1000,
maxAge: 60 * 60 * 1000,
max: 10000
});
// lightbox init
const lightbox = new Lightbox();
const lightboxOptions = {
animation: false,
controlClose: '<i class="material-icons">close</i>'
};
lightbox.load(lightboxOptions);
MessageItem.Content = React.createClass({
propTypes: {
content: React.PropTypes.object.isRequired
},
mixins: [PureRenderMixin],
getInitialState() {
return {
isImageLoaded: false
};
},
render() {
const content = this.props.content;
const isImageLoaded = this.state.isImageLoaded;
let contentClassName = classNames('message__content', {
'message__content--service': content.content === MessageContentTypes.SERVICE,
'message__content--text': content.content === MessageContentTypes.TEXT,
'message__content--photo': content.content === MessageContentTypes.PHOTO,
'message__content--photo--loaded': isImageLoaded,
'message__content--document': content.content === MessageContentTypes.DOCUMENT,
'message__content--unsupported': content.content === MessageContentTypes.UNSUPPORTED
});
switch (content.content) {
case 'service':
return (
<div className={contentClassName}>
{content.text}
</div>
);
case 'text':
return (
<div className={contentClassName}
dangerouslySetInnerHTML={{__html: memoizedProcessText(content.text)}}>
</div>
);
case 'photo':
const preview = <img className="photo photo--preview" src={content.preview}/>;
const k = content.w / 300;
const photoMessageStyes = {
width: Math.round(content.w / k),
height: Math.round(content.h / k)
};
let original,
preloader;
if (content.fileUrl) {
original = (
<img className="photo photo--original"
height={content.h}
onClick={this.openLightBox}
onLoad={this.imageLoaded}
src={content.fileUrl}
width={content.w}/>
);
}
if (content.isUploading === true || isImageLoaded === false) {
preloader =
<div className="preloader"><div/><div/><div/><div/><div/></div>;
}
return (
<div className={contentClassName} style={photoMessageStyes}>
{preview}
{original}
{preloader}
<svg dangerouslySetInnerHTML={{__html: '<filter id="blur-effect"><feGaussianBlur stdDeviation="3"/></filter>'}}></svg>
</div>
);
case 'document':
contentClassName = classNames(contentClassName, 'row');
let availableActions;
if (content.isUploading === true) {
availableActions = <span>Loading...</span>;
} else {
availableActions = <a href={content.fileUrl}>Open</a>;
}
return (
<div className={contentClassName}>
<div className="document row">
<div className="document__icon">
<i className="material-icons">attach_file</i>
</div>
<div className="col-xs">
<span className="document__filename">{content.fileName}</span>
<div className="document__meta">
<span className="document__meta__size">{content.fileSize}</span>
<span className="document__meta__ext">{content.fileExtension}</span>
</div>
<div className="document__actions">
{availableActions}
</div>
</div>
</div>
<div className="col-xs"></div>
</div>
);
default:
}
},
imageLoaded() {
this.setState({isImageLoaded: true});
},
openLightBox() {
lightbox.open(this.props.content.fileUrl);
}
});
MessageItem.State = React.createClass({
propTypes: {
message: React.PropTypes.object.isRequired
},
render() {
const message = this.props.message;
if (message.content.content === MessageContentTypes.SERVICE) {
return null;
} else {
let icon = null;
switch(message.state) {
case 'pending':
icon = <i className="status status--penging material-icons">access_time</i>;
break;
case 'sent':
icon = <i className="status status--sent material-icons">done</i>;
break;
case 'received':
icon = <i className="status status--received material-icons">done_all</i>;
break;
case 'read':
icon = <i className="status status--read material-icons">done_all</i>;
break;
case 'error':
icon = <i className="status status--error material-icons">report_problem</i>;
break;
default:
}
return (
<div className="message__status">{icon}</div>
);
}
}
});
export default MessageItem;
|
src/svg-icons/file/cloud-download.js | pradel/material-ui | import React from 'react';
import pure from 'recompose/pure';
import SvgIcon from '../../SvgIcon';
let FileCloudDownload = (props) => (
<SvgIcon {...props}>
<path d="M19.35 10.04C18.67 6.59 15.64 4 12 4 9.11 4 6.6 5.64 5.35 8.04 2.34 8.36 0 10.91 0 14c0 3.31 2.69 6 6 6h13c2.76 0 5-2.24 5-5 0-2.64-2.05-4.78-4.65-4.96zM17 13l-5 5-5-5h3V9h4v4h3z"/>
</SvgIcon>
);
FileCloudDownload = pure(FileCloudDownload);
FileCloudDownload.displayName = 'FileCloudDownload';
export default FileCloudDownload;
|
jiuzhou/common/WebScene.js | Cowboy1995/JZWXZ | /**
* Copyright (c) 2017-present, Liu Jinyong
* All rights reserved.
*
* https://github.com/huanxsd/MeiTuan
* @flow
*/
//import liraries
import React, { Component } from 'react';
import { View, Text, StyleSheet, WebView, InteractionManager } from 'react-native';
// create a component
class WebScene extends Component {
static navigationOptions = ({ navigation }) => ({
headerStyle: { backgroundColor: 'white' },
title: navigation.state.params.title,
});
state: {
source: Object
}
constructor(props: Object) {
super(props)
this.state = {
source: {}
}
this.props.navigation.setParams({ title: '加载中'})
}
componentDidMount() {
InteractionManager.runAfterInteractions(() => {
this.setState({ source: { uri: this.props.navigation.state.params.url } })
})
}
render() {
return (
<View style={styles.container}>
<WebView
ref='webView'
automaticallyAdjustContentInsets={false}
style={styles.webView}
source={this.state.source}
onLoadEnd={(e) => this.onLoadEnd(e)}
scalesPageToFit={true}
/>
</View>
);
}
onLoadEnd(e: any) {
if (e.nativeEvent.title.length > 0) {
this.props.navigation.setParams({ title: e.nativeEvent.title })
}
}
}
// define your styles
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#2c3e50',
},
webView: {
flex: 1,
backgroundColor: 'white',
}
});
//make this component available to the app
export default WebScene;
|
ajax/libs/yui/3.10.0/datatable-body/datatable-body-coverage.js | CarlosOhh/cdnjs | if (typeof __coverage__ === 'undefined') { __coverage__ = {}; }
if (!__coverage__['build/datatable-body/datatable-body.js']) {
__coverage__['build/datatable-body/datatable-body.js'] = {"path":"build/datatable-body/datatable-body.js","s":{"1":0,"2":0,"3":0,"4":0,"5":0,"6":0,"7":0,"8":0,"9":0,"10":0,"11":0,"12":0,"13":0,"14":0,"15":0,"16":0,"17":0,"18":0,"19":0,"20":0,"21":0,"22":0,"23":0,"24":0,"25":0,"26":0,"27":0,"28":0,"29":0,"30":0,"31":0,"32":0,"33":0,"34":0,"35":0,"36":0,"37":0,"38":0,"39":0,"40":0,"41":0,"42":0,"43":0,"44":0,"45":0,"46":0,"47":0,"48":0,"49":0,"50":0,"51":0,"52":0,"53":0,"54":0,"55":0,"56":0,"57":0,"58":0,"59":0,"60":0,"61":0,"62":0,"63":0,"64":0,"65":0,"66":0,"67":0,"68":0,"69":0,"70":0,"71":0,"72":0,"73":0,"74":0,"75":0,"76":0,"77":0,"78":0,"79":0,"80":0,"81":0,"82":0,"83":0,"84":0,"85":0,"86":0,"87":0,"88":0,"89":0,"90":0,"91":0,"92":0,"93":0,"94":0,"95":0,"96":0,"97":0,"98":0,"99":0,"100":0,"101":0,"102":0,"103":0,"104":0,"105":0,"106":0,"107":0,"108":0,"109":0,"110":0,"111":0,"112":0,"113":0,"114":0,"115":0,"116":0,"117":0,"118":0,"119":0,"120":0,"121":0,"122":0,"123":0,"124":0,"125":0,"126":0,"127":0,"128":0,"129":0,"130":0,"131":0,"132":0,"133":0,"134":0,"135":0,"136":0,"137":0,"138":0,"139":0,"140":0,"141":0,"142":0,"143":0,"144":0,"145":0,"146":0},"b":{"1":[0,0],"2":[0,0],"3":[0,0],"4":[0,0],"5":[0,0],"6":[0,0],"7":[0,0],"8":[0,0],"9":[0,0,0,0],"10":[0,0],"11":[0,0],"12":[0,0],"13":[0,0],"14":[0,0],"15":[0,0],"16":[0,0],"17":[0,0],"18":[0,0],"19":[0,0],"20":[0,0],"21":[0,0],"22":[0,0],"23":[0,0],"24":[0,0],"25":[0,0],"26":[0,0],"27":[0,0],"28":[0,0],"29":[0,0],"30":[0,0],"31":[0,0],"32":[0,0],"33":[0,0],"34":[0,0],"35":[0,0],"36":[0,0],"37":[0,0],"38":[0,0],"39":[0,0],"40":[0,0],"41":[0,0],"42":[0,0],"43":[0,0],"44":[0,0],"45":[0,0],"46":[0,0],"47":[0,0],"48":[0,0,0],"49":[0,0],"50":[0,0],"51":[0,0],"52":[0,0],"53":[0,0],"54":[0,0],"55":[0,0],"56":[0,0],"57":[0,0],"58":[0,0],"59":[0,0],"60":[0,0],"61":[0,0]},"f":{"1":0,"2":0,"3":0,"4":0,"5":0,"6":0,"7":0,"8":0,"9":0,"10":0,"11":0,"12":0,"13":0,"14":0,"15":0,"16":0,"17":0,"18":0,"19":0,"20":0,"21":0,"22":0},"fnMap":{"1":{"name":"(anonymous_1)","line":1,"loc":{"start":{"line":1,"column":26},"end":{"line":1,"column":45}}},"2":{"name":"(anonymous_2)","line":213,"loc":{"start":{"line":213,"column":13},"end":{"line":213,"column":36}}},"3":{"name":"(anonymous_3)","line":266,"loc":{"start":{"line":266,"column":18},"end":{"line":266,"column":30}}},"4":{"name":"(anonymous_4)","line":291,"loc":{"start":{"line":291,"column":15},"end":{"line":291,"column":31}}},"5":{"name":"(anonymous_5)","line":303,"loc":{"start":{"line":303,"column":36},"end":{"line":303,"column":52}}},"6":{"name":"(anonymous_6)","line":325,"loc":{"start":{"line":325,"column":12},"end":{"line":325,"column":26}}},"7":{"name":"(anonymous_7)","line":432,"loc":{"start":{"line":432,"column":12},"end":{"line":432,"column":24}}},"8":{"name":"(anonymous_8)","line":475,"loc":{"start":{"line":475,"column":25},"end":{"line":475,"column":37}}},"9":{"name":"(anonymous_9)","line":489,"loc":{"start":{"line":489,"column":22},"end":{"line":489,"column":34}}},"10":{"name":"(anonymous_10)","line":506,"loc":{"start":{"line":506,"column":27},"end":{"line":506,"column":39}}},"11":{"name":"(anonymous_11)","line":530,"loc":{"start":{"line":530,"column":26},"end":{"line":530,"column":52}}},"12":{"name":"(anonymous_12)","line":547,"loc":{"start":{"line":547,"column":22},"end":{"line":547,"column":47}}},"13":{"name":"(anonymous_13)","line":594,"loc":{"start":{"line":594,"column":12},"end":{"line":594,"column":24}}},"14":{"name":"(anonymous_14)","line":625,"loc":{"start":{"line":625,"column":21},"end":{"line":625,"column":40}}},"15":{"name":"(anonymous_15)","line":630,"loc":{"start":{"line":630,"column":22},"end":{"line":630,"column":46}}},"16":{"name":"(anonymous_16)","line":673,"loc":{"start":{"line":673,"column":20},"end":{"line":673,"column":53}}},"17":{"name":"(anonymous_17)","line":738,"loc":{"start":{"line":738,"column":24},"end":{"line":738,"column":43}}},"18":{"name":"(anonymous_18)","line":788,"loc":{"start":{"line":788,"column":25},"end":{"line":788,"column":37}}},"19":{"name":"(anonymous_19)","line":806,"loc":{"start":{"line":806,"column":22},"end":{"line":806,"column":34}}},"20":{"name":"(anonymous_20)","line":819,"loc":{"start":{"line":819,"column":16},"end":{"line":819,"column":28}}},"21":{"name":"(anonymous_21)","line":843,"loc":{"start":{"line":843,"column":15},"end":{"line":843,"column":35}}},"22":{"name":"(anonymous_22)","line":869,"loc":{"start":{"line":869,"column":17},"end":{"line":869,"column":35}}}},"statementMap":{"1":{"start":{"line":1,"column":0},"end":{"line":912,"column":78}},"2":{"start":{"line":11,"column":0},"end":{"line":20,"column":32}},"3":{"start":{"line":115,"column":0},"end":{"line":909,"column":3}},"4":{"start":{"line":214,"column":8},"end":{"line":215,"column":45}},"5":{"start":{"line":217,"column":8},"end":{"line":246,"column":9}},"6":{"start":{"line":218,"column":12},"end":{"line":223,"column":13}},"7":{"start":{"line":219,"column":16},"end":{"line":219,"column":58}},"8":{"start":{"line":220,"column":16},"end":{"line":220,"column":64}},"9":{"start":{"line":221,"column":19},"end":{"line":223,"column":13}},"10":{"start":{"line":222,"column":16},"end":{"line":222,"column":76}},"11":{"start":{"line":225,"column":12},"end":{"line":245,"column":13}},"12":{"start":{"line":226,"column":16},"end":{"line":226,"column":66}},"13":{"start":{"line":227,"column":16},"end":{"line":235,"column":17}},"14":{"start":{"line":229,"column":20},"end":{"line":234,"column":21}},"15":{"start":{"line":230,"column":41},"end":{"line":230,"column":57}},"16":{"start":{"line":230,"column":58},"end":{"line":230,"column":64}},"17":{"start":{"line":231,"column":41},"end":{"line":231,"column":56}},"18":{"start":{"line":231,"column":57},"end":{"line":231,"column":63}},"19":{"start":{"line":232,"column":41},"end":{"line":232,"column":56}},"20":{"start":{"line":232,"column":57},"end":{"line":232,"column":63}},"21":{"start":{"line":233,"column":41},"end":{"line":233,"column":57}},"22":{"start":{"line":233,"column":58},"end":{"line":233,"column":64}},"23":{"start":{"line":237,"column":16},"end":{"line":244,"column":17}},"24":{"start":{"line":238,"column":20},"end":{"line":239,"column":58}},"25":{"start":{"line":240,"column":20},"end":{"line":240,"column":62}},"26":{"start":{"line":242,"column":20},"end":{"line":242,"column":61}},"27":{"start":{"line":243,"column":20},"end":{"line":243,"column":67}},"28":{"start":{"line":248,"column":8},"end":{"line":248,"column":28}},"29":{"start":{"line":267,"column":8},"end":{"line":268,"column":17}},"30":{"start":{"line":270,"column":8},"end":{"line":277,"column":9}},"31":{"start":{"line":271,"column":12},"end":{"line":271,"column":60}},"32":{"start":{"line":273,"column":12},"end":{"line":273,"column":38}},"33":{"start":{"line":274,"column":12},"end":{"line":274,"column":48}},"34":{"start":{"line":275,"column":12},"end":{"line":276,"column":49}},"35":{"start":{"line":292,"column":8},"end":{"line":295,"column":19}},"36":{"start":{"line":297,"column":8},"end":{"line":310,"column":9}},"37":{"start":{"line":298,"column":12},"end":{"line":300,"column":13}},"38":{"start":{"line":299,"column":16},"end":{"line":299,"column":45}},"39":{"start":{"line":302,"column":12},"end":{"line":309,"column":13}},"40":{"start":{"line":303,"column":16},"end":{"line":305,"column":25}},"41":{"start":{"line":304,"column":20},"end":{"line":304,"column":67}},"42":{"start":{"line":307,"column":16},"end":{"line":308,"column":72}},"43":{"start":{"line":312,"column":8},"end":{"line":312,"column":30}},"44":{"start":{"line":326,"column":8},"end":{"line":327,"column":23}},"45":{"start":{"line":329,"column":8},"end":{"line":337,"column":9}},"46":{"start":{"line":330,"column":12},"end":{"line":332,"column":13}},"47":{"start":{"line":331,"column":16},"end":{"line":331,"column":73}},"48":{"start":{"line":334,"column":12},"end":{"line":336,"column":36}},"49":{"start":{"line":339,"column":8},"end":{"line":339,"column":19}},"50":{"start":{"line":433,"column":8},"end":{"line":437,"column":65}},"51":{"start":{"line":440,"column":8},"end":{"line":440,"column":41}},"52":{"start":{"line":442,"column":8},"end":{"line":446,"column":9}},"53":{"start":{"line":443,"column":12},"end":{"line":443,"column":57}},"54":{"start":{"line":445,"column":12},"end":{"line":445,"column":54}},"55":{"start":{"line":448,"column":8},"end":{"line":450,"column":9}},"56":{"start":{"line":449,"column":12},"end":{"line":449,"column":37}},"57":{"start":{"line":452,"column":8},"end":{"line":452,"column":35}},"58":{"start":{"line":454,"column":8},"end":{"line":454,"column":22}},"59":{"start":{"line":456,"column":8},"end":{"line":456,"column":20}},"60":{"start":{"line":476,"column":8},"end":{"line":476,"column":22}},"61":{"start":{"line":493,"column":8},"end":{"line":493,"column":22}},"62":{"start":{"line":507,"column":8},"end":{"line":507,"column":41}},"63":{"start":{"line":509,"column":8},"end":{"line":513,"column":9}},"64":{"start":{"line":510,"column":12},"end":{"line":510,"column":40}},"65":{"start":{"line":511,"column":12},"end":{"line":511,"column":38}},"66":{"start":{"line":512,"column":12},"end":{"line":512,"column":26}},"67":{"start":{"line":515,"column":8},"end":{"line":517,"column":9}},"68":{"start":{"line":516,"column":12},"end":{"line":516,"column":26}},"69":{"start":{"line":531,"column":8},"end":{"line":535,"column":25}},"70":{"start":{"line":538,"column":8},"end":{"line":542,"column":9}},"71":{"start":{"line":539,"column":12},"end":{"line":541,"column":13}},"72":{"start":{"line":540,"column":16},"end":{"line":540,"column":35}},"73":{"start":{"line":544,"column":8},"end":{"line":584,"column":9}},"74":{"start":{"line":545,"column":12},"end":{"line":545,"column":43}},"75":{"start":{"line":547,"column":12},"end":{"line":583,"column":15}},"76":{"start":{"line":548,"column":16},"end":{"line":554,"column":56}},"77":{"start":{"line":557,"column":16},"end":{"line":582,"column":17}},"78":{"start":{"line":558,"column":20},"end":{"line":558,"column":50}},"79":{"start":{"line":559,"column":20},"end":{"line":581,"column":21}},"80":{"start":{"line":560,"column":24},"end":{"line":560,"column":57}},"81":{"start":{"line":562,"column":24},"end":{"line":580,"column":25}},"82":{"start":{"line":563,"column":28},"end":{"line":563,"column":80}},"83":{"start":{"line":564,"column":28},"end":{"line":564,"column":52}},"84":{"start":{"line":566,"column":28},"end":{"line":566,"column":66}},"85":{"start":{"line":567,"column":28},"end":{"line":567,"column":55}},"86":{"start":{"line":568,"column":28},"end":{"line":568,"column":79}},"87":{"start":{"line":570,"column":28},"end":{"line":570,"column":78}},"88":{"start":{"line":572,"column":28},"end":{"line":579,"column":29}},"89":{"start":{"line":578,"column":32},"end":{"line":578,"column":51}},"90":{"start":{"line":595,"column":8},"end":{"line":597,"column":59}},"91":{"start":{"line":599,"column":8},"end":{"line":602,"column":9}},"92":{"start":{"line":600,"column":12},"end":{"line":601,"column":51}},"93":{"start":{"line":604,"column":8},"end":{"line":608,"column":9}},"94":{"start":{"line":605,"column":12},"end":{"line":607,"column":48}},"95":{"start":{"line":626,"column":8},"end":{"line":627,"column":22}},"96":{"start":{"line":629,"column":8},"end":{"line":633,"column":9}},"97":{"start":{"line":630,"column":12},"end":{"line":632,"column":21}},"98":{"start":{"line":631,"column":16},"end":{"line":631,"column":67}},"99":{"start":{"line":635,"column":8},"end":{"line":635,"column":20}},"100":{"start":{"line":674,"column":8},"end":{"line":682,"column":53}},"101":{"start":{"line":684,"column":8},"end":{"line":721,"column":9}},"102":{"start":{"line":685,"column":12},"end":{"line":685,"column":31}},"103":{"start":{"line":686,"column":12},"end":{"line":686,"column":34}},"104":{"start":{"line":687,"column":12},"end":{"line":687,"column":39}},"105":{"start":{"line":689,"column":12},"end":{"line":689,"column":46}},"106":{"start":{"line":691,"column":12},"end":{"line":712,"column":13}},"107":{"start":{"line":692,"column":16},"end":{"line":700,"column":18}},"108":{"start":{"line":703,"column":16},"end":{"line":703,"column":67}},"109":{"start":{"line":706,"column":16},"end":{"line":708,"column":17}},"110":{"start":{"line":707,"column":20},"end":{"line":707,"column":48}},"111":{"start":{"line":710,"column":16},"end":{"line":710,"column":71}},"112":{"start":{"line":711,"column":16},"end":{"line":711,"column":64}},"113":{"start":{"line":714,"column":12},"end":{"line":716,"column":13}},"114":{"start":{"line":715,"column":16},"end":{"line":715,"column":49}},"115":{"start":{"line":718,"column":12},"end":{"line":718,"column":70}},"116":{"start":{"line":720,"column":12},"end":{"line":720,"column":67}},"117":{"start":{"line":723,"column":8},"end":{"line":723,"column":55}},"118":{"start":{"line":739,"column":8},"end":{"line":742,"column":69}},"119":{"start":{"line":744,"column":8},"end":{"line":777,"column":9}},"120":{"start":{"line":745,"column":12},"end":{"line":745,"column":33}},"121":{"start":{"line":746,"column":12},"end":{"line":746,"column":30}},"122":{"start":{"line":747,"column":12},"end":{"line":747,"column":37}},"123":{"start":{"line":748,"column":12},"end":{"line":748,"column":38}},"124":{"start":{"line":750,"column":12},"end":{"line":751,"column":72}},"125":{"start":{"line":753,"column":12},"end":{"line":760,"column":14}},"126":{"start":{"line":761,"column":12},"end":{"line":769,"column":13}},"127":{"start":{"line":762,"column":16},"end":{"line":768,"column":17}},"128":{"start":{"line":763,"column":20},"end":{"line":763,"column":49}},"129":{"start":{"line":764,"column":23},"end":{"line":768,"column":17}},"130":{"start":{"line":765,"column":20},"end":{"line":765,"column":81}},"131":{"start":{"line":767,"column":20},"end":{"line":767,"column":94}},"132":{"start":{"line":771,"column":12},"end":{"line":774,"column":13}},"133":{"start":{"line":773,"column":16},"end":{"line":773,"column":41}},"134":{"start":{"line":776,"column":12},"end":{"line":776,"column":80}},"135":{"start":{"line":779,"column":8},"end":{"line":781,"column":11}},"136":{"start":{"line":789,"column":8},"end":{"line":790,"column":36}},"137":{"start":{"line":792,"column":8},"end":{"line":794,"column":9}},"138":{"start":{"line":793,"column":12},"end":{"line":793,"column":43}},"139":{"start":{"line":807,"column":8},"end":{"line":809,"column":12}},"140":{"start":{"line":820,"column":8},"end":{"line":820,"column":73}},"141":{"start":{"line":844,"column":8},"end":{"line":844,"column":75}},"142":{"start":{"line":870,"column":8},"end":{"line":870,"column":32}},"143":{"start":{"line":872,"column":8},"end":{"line":875,"column":10}},"144":{"start":{"line":876,"column":8},"end":{"line":876,"column":25}},"145":{"start":{"line":878,"column":8},"end":{"line":878,"column":51}},"146":{"start":{"line":879,"column":8},"end":{"line":879,"column":52}}},"branchMap":{"1":{"line":217,"type":"if","locations":[{"start":{"line":217,"column":8},"end":{"line":217,"column":8}},{"start":{"line":217,"column":8},"end":{"line":217,"column":8}}]},"2":{"line":217,"type":"binary-expr","locations":[{"start":{"line":217,"column":12},"end":{"line":217,"column":16}},{"start":{"line":217,"column":20},"end":{"line":217,"column":25}}]},"3":{"line":218,"type":"if","locations":[{"start":{"line":218,"column":12},"end":{"line":218,"column":12}},{"start":{"line":218,"column":12},"end":{"line":218,"column":12}}]},"4":{"line":220,"type":"binary-expr","locations":[{"start":{"line":220,"column":23},"end":{"line":220,"column":26}},{"start":{"line":220,"column":30},"end":{"line":220,"column":63}}]},"5":{"line":221,"type":"if","locations":[{"start":{"line":221,"column":19},"end":{"line":221,"column":19}},{"start":{"line":221,"column":19},"end":{"line":221,"column":19}}]},"6":{"line":225,"type":"if","locations":[{"start":{"line":225,"column":12},"end":{"line":225,"column":12}},{"start":{"line":225,"column":12},"end":{"line":225,"column":12}}]},"7":{"line":225,"type":"binary-expr","locations":[{"start":{"line":225,"column":16},"end":{"line":225,"column":20}},{"start":{"line":225,"column":24},"end":{"line":225,"column":29}}]},"8":{"line":227,"type":"if","locations":[{"start":{"line":227,"column":16},"end":{"line":227,"column":16}},{"start":{"line":227,"column":16},"end":{"line":227,"column":16}}]},"9":{"line":229,"type":"switch","locations":[{"start":{"line":230,"column":24},"end":{"line":230,"column":64}},{"start":{"line":231,"column":24},"end":{"line":231,"column":63}},{"start":{"line":232,"column":24},"end":{"line":232,"column":63}},{"start":{"line":233,"column":24},"end":{"line":233,"column":64}}]},"10":{"line":237,"type":"if","locations":[{"start":{"line":237,"column":16},"end":{"line":237,"column":16}},{"start":{"line":237,"column":16},"end":{"line":237,"column":16}}]},"11":{"line":243,"type":"binary-expr","locations":[{"start":{"line":243,"column":28},"end":{"line":243,"column":31}},{"start":{"line":243,"column":35},"end":{"line":243,"column":66}}]},"12":{"line":248,"type":"binary-expr","locations":[{"start":{"line":248,"column":15},"end":{"line":248,"column":19}},{"start":{"line":248,"column":23},"end":{"line":248,"column":27}}]},"13":{"line":270,"type":"if","locations":[{"start":{"line":270,"column":8},"end":{"line":270,"column":8}},{"start":{"line":270,"column":8},"end":{"line":270,"column":8}}]},"14":{"line":270,"type":"binary-expr","locations":[{"start":{"line":270,"column":12},"end":{"line":270,"column":16}},{"start":{"line":270,"column":20},"end":{"line":270,"column":37}}]},"15":{"line":297,"type":"if","locations":[{"start":{"line":297,"column":8},"end":{"line":297,"column":8}},{"start":{"line":297,"column":8},"end":{"line":297,"column":8}}]},"16":{"line":298,"type":"if","locations":[{"start":{"line":298,"column":12},"end":{"line":298,"column":12}},{"start":{"line":298,"column":12},"end":{"line":298,"column":12}}]},"17":{"line":302,"type":"if","locations":[{"start":{"line":302,"column":12},"end":{"line":302,"column":12}},{"start":{"line":302,"column":12},"end":{"line":302,"column":12}}]},"18":{"line":307,"type":"binary-expr","locations":[{"start":{"line":307,"column":25},"end":{"line":307,"column":28}},{"start":{"line":308,"column":20},"end":{"line":308,"column":71}}]},"19":{"line":312,"type":"binary-expr","locations":[{"start":{"line":312,"column":15},"end":{"line":312,"column":21}},{"start":{"line":312,"column":25},"end":{"line":312,"column":29}}]},"20":{"line":329,"type":"if","locations":[{"start":{"line":329,"column":8},"end":{"line":329,"column":8}},{"start":{"line":329,"column":8},"end":{"line":329,"column":8}}]},"21":{"line":330,"type":"if","locations":[{"start":{"line":330,"column":12},"end":{"line":330,"column":12}},{"start":{"line":330,"column":12},"end":{"line":330,"column":12}}]},"22":{"line":331,"type":"binary-expr","locations":[{"start":{"line":331,"column":21},"end":{"line":331,"column":66}},{"start":{"line":331,"column":70},"end":{"line":331,"column":72}}]},"23":{"line":331,"type":"cond-expr","locations":[{"start":{"line":331,"column":42},"end":{"line":331,"column":60}},{"start":{"line":331,"column":63},"end":{"line":331,"column":65}}]},"24":{"line":334,"type":"cond-expr","locations":[{"start":{"line":335,"column":16},"end":{"line":335,"column":46}},{"start":{"line":336,"column":16},"end":{"line":336,"column":35}}]},"25":{"line":436,"type":"binary-expr","locations":[{"start":{"line":436,"column":22},"end":{"line":436,"column":36}},{"start":{"line":437,"column":23},"end":{"line":437,"column":63}}]},"26":{"line":442,"type":"if","locations":[{"start":{"line":442,"column":8},"end":{"line":442,"column":8}},{"start":{"line":442,"column":8},"end":{"line":442,"column":8}}]},"27":{"line":448,"type":"if","locations":[{"start":{"line":448,"column":8},"end":{"line":448,"column":8}},{"start":{"line":448,"column":8},"end":{"line":448,"column":8}}]},"28":{"line":509,"type":"if","locations":[{"start":{"line":509,"column":8},"end":{"line":509,"column":8}},{"start":{"line":509,"column":8},"end":{"line":509,"column":8}}]},"29":{"line":515,"type":"if","locations":[{"start":{"line":515,"column":8},"end":{"line":515,"column":8}},{"start":{"line":515,"column":8},"end":{"line":515,"column":8}}]},"30":{"line":539,"type":"if","locations":[{"start":{"line":539,"column":12},"end":{"line":539,"column":12}},{"start":{"line":539,"column":12},"end":{"line":539,"column":12}}]},"31":{"line":544,"type":"if","locations":[{"start":{"line":544,"column":8},"end":{"line":544,"column":8}},{"start":{"line":544,"column":8},"end":{"line":544,"column":8}}]},"32":{"line":544,"type":"binary-expr","locations":[{"start":{"line":544,"column":12},"end":{"line":544,"column":16}},{"start":{"line":544,"column":20},"end":{"line":544,"column":37}}]},"33":{"line":557,"type":"if","locations":[{"start":{"line":557,"column":16},"end":{"line":557,"column":16}},{"start":{"line":557,"column":16},"end":{"line":557,"column":16}}]},"34":{"line":562,"type":"if","locations":[{"start":{"line":562,"column":24},"end":{"line":562,"column":24}},{"start":{"line":562,"column":24},"end":{"line":562,"column":24}}]},"35":{"line":564,"type":"binary-expr","locations":[{"start":{"line":564,"column":34},"end":{"line":564,"column":41}},{"start":{"line":564,"column":45},"end":{"line":564,"column":51}}]},"36":{"line":568,"type":"binary-expr","locations":[{"start":{"line":568,"column":50},"end":{"line":568,"column":70}},{"start":{"line":568,"column":74},"end":{"line":568,"column":78}}]},"37":{"line":572,"type":"if","locations":[{"start":{"line":572,"column":28},"end":{"line":572,"column":28}},{"start":{"line":572,"column":28},"end":{"line":572,"column":28}}]},"38":{"line":599,"type":"if","locations":[{"start":{"line":599,"column":8},"end":{"line":599,"column":8}},{"start":{"line":599,"column":8},"end":{"line":599,"column":8}}]},"39":{"line":604,"type":"if","locations":[{"start":{"line":604,"column":8},"end":{"line":604,"column":8}},{"start":{"line":604,"column":8},"end":{"line":604,"column":8}}]},"40":{"line":604,"type":"binary-expr","locations":[{"start":{"line":604,"column":12},"end":{"line":604,"column":21}},{"start":{"line":604,"column":25},"end":{"line":604,"column":44}}]},"41":{"line":629,"type":"if","locations":[{"start":{"line":629,"column":8},"end":{"line":629,"column":8}},{"start":{"line":629,"column":8},"end":{"line":629,"column":8}}]},"42":{"line":679,"type":"cond-expr","locations":[{"start":{"line":679,"column":40},"end":{"line":679,"column":54}},{"start":{"line":679,"column":57},"end":{"line":679,"column":72}}]},"43":{"line":681,"type":"binary-expr","locations":[{"start":{"line":681,"column":19},"end":{"line":681,"column":28}},{"start":{"line":681,"column":32},"end":{"line":681,"column":36}}]},"44":{"line":687,"type":"binary-expr","locations":[{"start":{"line":687,"column":20},"end":{"line":687,"column":27}},{"start":{"line":687,"column":31},"end":{"line":687,"column":38}}]},"45":{"line":691,"type":"if","locations":[{"start":{"line":691,"column":12},"end":{"line":691,"column":12}},{"start":{"line":691,"column":12},"end":{"line":691,"column":12}}]},"46":{"line":706,"type":"if","locations":[{"start":{"line":706,"column":16},"end":{"line":706,"column":16}},{"start":{"line":706,"column":16},"end":{"line":706,"column":16}}]},"47":{"line":714,"type":"if","locations":[{"start":{"line":714,"column":12},"end":{"line":714,"column":12}},{"start":{"line":714,"column":12},"end":{"line":714,"column":12}}]},"48":{"line":714,"type":"binary-expr","locations":[{"start":{"line":714,"column":16},"end":{"line":714,"column":35}},{"start":{"line":714,"column":39},"end":{"line":714,"column":53}},{"start":{"line":714,"column":57},"end":{"line":714,"column":69}}]},"49":{"line":715,"type":"binary-expr","locations":[{"start":{"line":715,"column":24},"end":{"line":715,"column":42}},{"start":{"line":715,"column":46},"end":{"line":715,"column":48}}]},"50":{"line":718,"type":"cond-expr","locations":[{"start":{"line":718,"column":44},"end":{"line":718,"column":49}},{"start":{"line":718,"column":52},"end":{"line":718,"column":69}}]},"51":{"line":747,"type":"binary-expr","locations":[{"start":{"line":747,"column":22},"end":{"line":747,"column":29}},{"start":{"line":747,"column":33},"end":{"line":747,"column":36}}]},"52":{"line":750,"type":"cond-expr","locations":[{"start":{"line":751,"column":24},"end":{"line":751,"column":66}},{"start":{"line":751,"column":69},"end":{"line":751,"column":71}}]},"53":{"line":750,"type":"binary-expr","locations":[{"start":{"line":750,"column":23},"end":{"line":750,"column":35}},{"start":{"line":750,"column":39},"end":{"line":750,"column":41}}]},"54":{"line":757,"type":"binary-expr","locations":[{"start":{"line":757,"column":28},"end":{"line":757,"column":41}},{"start":{"line":757,"column":45},"end":{"line":757,"column":47}}]},"55":{"line":761,"type":"if","locations":[{"start":{"line":761,"column":12},"end":{"line":761,"column":12}},{"start":{"line":761,"column":12},"end":{"line":761,"column":12}}]},"56":{"line":762,"type":"if","locations":[{"start":{"line":762,"column":16},"end":{"line":762,"column":16}},{"start":{"line":762,"column":16},"end":{"line":762,"column":16}}]},"57":{"line":764,"type":"if","locations":[{"start":{"line":764,"column":23},"end":{"line":764,"column":23}},{"start":{"line":764,"column":23},"end":{"line":764,"column":23}}]},"58":{"line":765,"type":"binary-expr","locations":[{"start":{"line":765,"column":57},"end":{"line":765,"column":66}},{"start":{"line":765,"column":70},"end":{"line":765,"column":74}}]},"59":{"line":771,"type":"if","locations":[{"start":{"line":771,"column":12},"end":{"line":771,"column":12}},{"start":{"line":771,"column":12},"end":{"line":771,"column":12}}]},"60":{"line":776,"type":"binary-expr","locations":[{"start":{"line":776,"column":33},"end":{"line":776,"column":49}},{"start":{"line":776,"column":53},"end":{"line":776,"column":65}}]},"61":{"line":844,"type":"binary-expr","locations":[{"start":{"line":844,"column":15},"end":{"line":844,"column":36}},{"start":{"line":844,"column":41},"end":{"line":844,"column":73}}]}},"code":["(function () { YUI.add('datatable-body', function (Y, NAME) {","","/**","View class responsible for rendering the `<tbody>` section of a table. Used as","the default `bodyView` for `Y.DataTable.Base` and `Y.DataTable` classes.","","@module datatable","@submodule datatable-body","@since 3.5.0","**/","var Lang = Y.Lang,"," isArray = Lang.isArray,"," isNumber = Lang.isNumber,"," isString = Lang.isString,"," fromTemplate = Lang.sub,"," htmlEscape = Y.Escape.html,"," toArray = Y.Array,"," bind = Y.bind,"," YObject = Y.Object,"," valueRegExp = /\\{value\\}/g;","","/**","View class responsible for rendering the `<tbody>` section of a table. Used as","the default `bodyView` for `Y.DataTable.Base` and `Y.DataTable` classes.","","Translates the provided `modelList` into a rendered `<tbody>` based on the data","in the constituent Models, altered or ammended by any special column","configurations.","","The `columns` configuration, passed to the constructor, determines which","columns will be rendered.","","The rendering process involves constructing an HTML template for a complete row","of data, built by concatenating a customized copy of the instance's","`CELL_TEMPLATE` into the `ROW_TEMPLATE` once for each column. This template is","then populated with values from each Model in the `modelList`, aggregating a","complete HTML string of all row and column data. A `<tbody>` Node is then created from the markup and any column `nodeFormatter`s are applied.","","Supported properties of the column objects include:",""," * `key` - Used to link a column to an attribute in a Model."," * `name` - Used for columns that don't relate to an attribute in the Model"," (`formatter` or `nodeFormatter` only) if the implementer wants a"," predictable name to refer to in their CSS."," * `cellTemplate` - Overrides the instance's `CELL_TEMPLATE` for cells in this"," column only."," * `formatter` - Used to customize or override the content value from the"," Model. These do not have access to the cell or row Nodes and should"," return string (HTML) content."," * `nodeFormatter` - Used to provide content for a cell as well as perform any"," custom modifications on the cell or row Node that could not be performed by"," `formatter`s. Should be used sparingly for better performance."," * `emptyCellValue` - String (HTML) value to use if the Model data for a"," column, or the content generated by a `formatter`, is the empty string,"," `null`, or `undefined`."," * `allowHTML` - Set to `true` if a column value, `formatter`, or"," `emptyCellValue` can contain HTML. This defaults to `false` to protect"," against XSS."," * `className` - Space delimited CSS classes to add to all `<td>`s in a column.","","A column `formatter` can be:",""," * a function, as described below."," * a string which can be:"," * the name of a pre-defined formatter function"," which can be located in the `Y.DataTable.BodyView.Formatters` hash using the"," value of the `formatter` property as the index."," * A template that can use the `{value}` placeholder to include the value"," for the current cell or the name of any field in the underlaying model"," also enclosed in curly braces. Any number and type of these placeholders"," can be used.","","Column `formatter`s are passed an object (`o`) with the following properties:",""," * `value` - The current value of the column's associated attribute, if any."," * `data` - An object map of Model keys to their current values."," * `record` - The Model instance."," * `column` - The column configuration object for the current column."," * `className` - Initially empty string to allow `formatter`s to add CSS"," classes to the cell's `<td>`."," * `rowIndex` - The zero-based row number."," * `rowClass` - Initially empty string to allow `formatter`s to add CSS"," classes to the cell's containing row `<tr>`.","","They may return a value or update `o.value` to assign specific HTML content. A","returned value has higher precedence.","","Column `nodeFormatter`s are passed an object (`o`) with the following","properties:",""," * `value` - The current value of the column's associated attribute, if any."," * `td` - The `<td>` Node instance."," * `cell` - The `<div>` liner Node instance if present, otherwise, the `<td>`."," When adding content to the cell, prefer appending into this property."," * `data` - An object map of Model keys to their current values."," * `record` - The Model instance."," * `column` - The column configuration object for the current column."," * `rowIndex` - The zero-based row number.","","They are expected to inject content into the cell's Node directly, including","any \"empty\" cell content. Each `nodeFormatter` will have access through the","Node API to all cells and rows in the `<tbody>`, but not to the `<table>`, as","it will not be attached yet.","","If a `nodeFormatter` returns `false`, the `o.td` and `o.cell` Nodes will be","`destroy()`ed to remove them from the Node cache and free up memory. The DOM","elements will remain as will any content added to them. _It is highly","advisable to always return `false` from your `nodeFormatter`s_.","","@class BodyView","@namespace DataTable","@extends View","@since 3.5.0","**/","Y.namespace('DataTable').BodyView = Y.Base.create('tableBody', Y.View, [], {"," // -- Instance properties -------------------------------------------------",""," /**"," HTML template used to create table cells.",""," @property CELL_TEMPLATE"," @type {HTML}"," @default '<td {headers} class=\"{className}\">{content}</td>'"," @since 3.5.0"," **/"," CELL_TEMPLATE: '<td {headers} class=\"{className}\">{content}</td>',",""," /**"," CSS class applied to even rows. This is assigned at instantiation.",""," For DataTable, this will be `yui3-datatable-even`.",""," @property CLASS_EVEN"," @type {String}"," @default 'yui3-table-even'"," @since 3.5.0"," **/"," //CLASS_EVEN: null",""," /**"," CSS class applied to odd rows. This is assigned at instantiation.",""," When used by DataTable instances, this will be `yui3-datatable-odd`.",""," @property CLASS_ODD"," @type {String}"," @default 'yui3-table-odd'"," @since 3.5.0"," **/"," //CLASS_ODD: null",""," /**"," HTML template used to create table rows.",""," @property ROW_TEMPLATE"," @type {HTML}"," @default '<tr id=\"{rowId}\" data-yui3-record=\"{clientId}\" class=\"{rowClass}\">{content}</tr>'"," @since 3.5.0"," **/"," ROW_TEMPLATE : '<tr id=\"{rowId}\" data-yui3-record=\"{clientId}\" class=\"{rowClass}\">{content}</tr>',",""," /**"," The object that serves as the source of truth for column and row data."," This property is assigned at instantiation from the `host` property of"," the configuration object passed to the constructor.",""," @property host"," @type {Object}"," @default (initially unset)"," @since 3.5.0"," **/"," //TODO: should this be protected?"," //host: null,",""," /**"," HTML templates used to create the `<tbody>` containing the table rows.",""," @property TBODY_TEMPLATE"," @type {HTML}"," @default '<tbody class=\"{className}\">{content}</tbody>'"," @since 3.6.0"," **/"," TBODY_TEMPLATE: '<tbody class=\"{className}\"></tbody>',",""," // -- Public methods ------------------------------------------------------",""," /**"," Returns the `<td>` Node from the given row and column index. Alternately,"," the `seed` can be a Node. If so, the nearest ancestor cell is returned."," If the `seed` is a cell, it is returned. If there is no cell at the given"," coordinates, `null` is returned.",""," Optionally, include an offset array or string to return a cell near the"," cell identified by the `seed`. The offset can be an array containing the"," number of rows to shift followed by the number of columns to shift, or one"," of \"above\", \"below\", \"next\", or \"previous\".",""," <pre><code>// Previous cell in the previous row"," var cell = table.getCell(e.target, [-1, -1]);",""," // Next cell"," var cell = table.getCell(e.target, 'next');"," var cell = table.getCell(e.taregt, [0, 1];</pre></code>",""," @method getCell"," @param {Number[]|Node} seed Array of row and column indexes, or a Node that"," is either the cell itself or a descendant of one."," @param {Number[]|String} [shift] Offset by which to identify the returned"," cell Node"," @return {Node}"," @since 3.5.0"," **/"," getCell: function (seed, shift) {"," var tbody = this.tbodyNode,"," row, cell, index, rowIndexOffset;",""," if (seed && tbody) {"," if (isArray(seed)) {"," row = tbody.get('children').item(seed[0]);"," cell = row && row.get('children').item(seed[1]);"," } else if (Y.instanceOf(seed, Y.Node)) {"," cell = seed.ancestor('.' + this.getClassName('cell'), true);"," }",""," if (cell && shift) {"," rowIndexOffset = tbody.get('firstChild.rowIndex');"," if (isString(shift)) {"," // TODO this should be a static object map"," switch (shift) {"," case 'above' : shift = [-1, 0]; break;"," case 'below' : shift = [1, 0]; break;"," case 'next' : shift = [0, 1]; break;"," case 'previous': shift = [0, -1]; break;"," }"," }",""," if (isArray(shift)) {"," index = cell.get('parentNode.rowIndex') +"," shift[0] - rowIndexOffset;"," row = tbody.get('children').item(index);",""," index = cell.get('cellIndex') + shift[1];"," cell = row && row.get('children').item(index);"," }"," }"," }",""," return cell || null;"," },",""," /**"," Returns the generated CSS classname based on the input. If the `host`"," attribute is configured, it will attempt to relay to its `getClassName`"," or use its static `NAME` property as a string base.",""," If `host` is absent or has neither method nor `NAME`, a CSS classname"," will be generated using this class's `NAME`.",""," @method getClassName"," @param {String} token* Any number of token strings to assemble the"," classname from."," @return {String}"," @protected"," @since 3.5.0"," **/"," getClassName: function () {"," var host = this.host,"," args;",""," if (host && host.getClassName) {"," return host.getClassName.apply(host, arguments);"," } else {"," args = toArray(arguments);"," args.unshift(this.constructor.NAME);"," return Y.ClassNameManager.getClassName"," .apply(Y.ClassNameManager, args);"," }"," },",""," /**"," Returns the Model associated to the row Node or id provided. Passing the"," Node or id for a descendant of the row also works.",""," If no Model can be found, `null` is returned.",""," @method getRecord"," @param {String|Node} seed Row Node or `id`, or one for a descendant of a row"," @return {Model}"," @since 3.5.0"," **/"," getRecord: function (seed) {"," var modelList = this.get('modelList'),"," tbody = this.tbodyNode,"," row = null,"," record;",""," if (tbody) {"," if (isString(seed)) {"," seed = tbody.one('#' + seed);"," }",""," if (Y.instanceOf(seed, Y.Node)) {"," row = seed.ancestor(function (node) {"," return node.get('parentNode').compareTo(tbody);"," }, true);",""," record = row &&"," modelList.getByClientId(row.getData('yui3-record'));"," }"," }",""," return record || null;"," },",""," /**"," Returns the `<tr>` Node from the given row index, Model, or Model's"," `clientId`. If the rows haven't been rendered yet, or if the row can't be"," found by the input, `null` is returned.",""," @method getRow"," @param {Number|String|Model} id Row index, Model instance, or clientId"," @return {Node}"," @since 3.5.0"," **/"," getRow: function (id) {"," var tbody = this.tbodyNode,"," row = null;",""," if (tbody) {"," if (id) {"," id = this._idMap[id.get ? id.get('clientId') : id] || id;"," }",""," row = isNumber(id) ?"," tbody.get('children').item(id) :"," tbody.one('#' + id);"," }",""," return row;"," },",""," /**"," Creates the table's `<tbody>` content by assembling markup generated by"," populating the `ROW\\_TEMPLATE`, and `CELL\\_TEMPLATE` templates with content"," from the `columns` and `modelList` attributes.",""," The rendering process happens in three stages:",""," 1. A row template is assembled from the `columns` attribute (see"," `_createRowTemplate`)",""," 2. An HTML string is built up by concatening the application of the data in"," each Model in the `modelList` to the row template. For cells with"," `formatter`s, the function is called to generate cell content. Cells"," with `nodeFormatter`s are ignored. For all other cells, the data value"," from the Model attribute for the given column key is used. The"," accumulated row markup is then inserted into the container.",""," 3. If any column is configured with a `nodeFormatter`, the `modelList` is"," iterated again to apply the `nodeFormatter`s.",""," Supported properties of the column objects include:",""," * `key` - Used to link a column to an attribute in a Model."," * `name` - Used for columns that don't relate to an attribute in the Model"," (`formatter` or `nodeFormatter` only) if the implementer wants a"," predictable name to refer to in their CSS."," * `cellTemplate` - Overrides the instance's `CELL_TEMPLATE` for cells in"," this column only."," * `formatter` - Used to customize or override the content value from the"," Model. These do not have access to the cell or row Nodes and should"," return string (HTML) content."," * `nodeFormatter` - Used to provide content for a cell as well as perform"," any custom modifications on the cell or row Node that could not be"," performed by `formatter`s. Should be used sparingly for better"," performance."," * `emptyCellValue` - String (HTML) value to use if the Model data for a"," column, or the content generated by a `formatter`, is the empty string,"," `null`, or `undefined`."," * `allowHTML` - Set to `true` if a column value, `formatter`, or"," `emptyCellValue` can contain HTML. This defaults to `false` to protect"," against XSS."," * `className` - Space delimited CSS classes to add to all `<td>`s in a"," column.",""," Column `formatter`s are passed an object (`o`) with the following"," properties:",""," * `value` - The current value of the column's associated attribute, if"," any."," * `data` - An object map of Model keys to their current values."," * `record` - The Model instance."," * `column` - The column configuration object for the current column."," * `className` - Initially empty string to allow `formatter`s to add CSS"," classes to the cell's `<td>`."," * `rowIndex` - The zero-based row number."," * `rowClass` - Initially empty string to allow `formatter`s to add CSS"," classes to the cell's containing row `<tr>`.",""," They may return a value or update `o.value` to assign specific HTML"," content. A returned value has higher precedence.",""," Column `nodeFormatter`s are passed an object (`o`) with the following"," properties:",""," * `value` - The current value of the column's associated attribute, if"," any."," * `td` - The `<td>` Node instance."," * `cell` - The `<div>` liner Node instance if present, otherwise, the"," `<td>`. When adding content to the cell, prefer appending into this"," property."," * `data` - An object map of Model keys to their current values."," * `record` - The Model instance."," * `column` - The column configuration object for the current column."," * `rowIndex` - The zero-based row number.",""," They are expected to inject content into the cell's Node directly, including"," any \"empty\" cell content. Each `nodeFormatter` will have access through the"," Node API to all cells and rows in the `<tbody>`, but not to the `<table>`,"," as it will not be attached yet.",""," If a `nodeFormatter` returns `false`, the `o.td` and `o.cell` Nodes will be"," `destroy()`ed to remove them from the Node cache and free up memory. The"," DOM elements will remain as will any content added to them. _It is highly"," advisable to always return `false` from your `nodeFormatter`s_.",""," @method render"," @return {BodyView} The instance"," @chainable"," @since 3.5.0"," **/"," render: function () {"," var table = this.get('container'),"," data = this.get('modelList'),"," columns = this.get('columns'),"," tbody = this.tbodyNode ||"," (this.tbodyNode = this._createTBodyNode());",""," // Needed for mutation"," this._createRowTemplate(columns);",""," if (data) {"," tbody.setHTML(this._createDataHTML(columns));",""," this._applyNodeFormatters(tbody, columns);"," }",""," if (tbody.get('parentNode') !== table) {"," table.appendChild(tbody);"," }",""," this._afterRenderCleanup();",""," this.bindUI();",""," return this;"," },",""," // -- Protected and private methods ---------------------------------------"," /**"," Handles changes in the source's columns attribute. Redraws the table data.",""," @method _afterColumnsChange"," @param {EventFacade} e The `columnsChange` event object"," @protected"," @since 3.5.0"," **/"," // TODO: Preserve existing DOM"," // This will involve parsing and comparing the old and new column configs"," // and reacting to four types of changes:"," // 1. formatter, nodeFormatter, emptyCellValue changes"," // 2. column deletions"," // 3. column additions"," // 4. column moves (preserve cells)"," _afterColumnsChange: function () {"," this.render();"," },",""," /**"," Handles modelList changes, including additions, deletions, and updates.",""," Modifies the existing table DOM accordingly.",""," @method _afterDataChange"," @param {EventFacade} e The `change` event from the ModelList"," @protected"," @since 3.5.0"," **/"," _afterDataChange: function () {"," //var type = e.type.slice(e.type.lastIndexOf(':') + 1);",""," // TODO: Isolate changes"," this.render();"," },",""," /**"," Handles replacement of the modelList.",""," Rerenders the `<tbody>` contents.",""," @method _afterModelListChange"," @param {EventFacade} e The `modelListChange` event"," @protected"," @since 3.6.0"," **/"," _afterModelListChange: function () {"," var handles = this._eventHandles;",""," if (handles.dataChange) {"," handles.dataChange.detach();"," delete handles.dataChange;"," this.bindUI();"," }",""," if (this.tbodyNode) {"," this.render();"," }"," },",""," /**"," Iterates the `modelList`, and calls any `nodeFormatter`s found in the"," `columns` param on the appropriate cell Nodes in the `tbody`.",""," @method _applyNodeFormatters"," @param {Node} tbody The `<tbody>` Node whose columns to update"," @param {Object[]} columns The column configurations"," @protected"," @since 3.5.0"," **/"," _applyNodeFormatters: function (tbody, columns) {"," var host = this.host,"," data = this.get('modelList'),"," formatters = [],"," linerQuery = '.' + this.getClassName('liner'),"," rows, i, len;",""," // Only iterate the ModelList again if there are nodeFormatters"," for (i = 0, len = columns.length; i < len; ++i) {"," if (columns[i].nodeFormatter) {"," formatters.push(i);"," }"," }",""," if (data && formatters.length) {"," rows = tbody.get('childNodes');",""," data.each(function (record, index) {"," var formatterData = {"," data : record.toJSON(),"," record : record,"," rowIndex : index"," },"," row = rows.item(index),"," i, len, col, key, cells, cell, keep;","",""," if (row) {"," cells = row.get('childNodes');"," for (i = 0, len = formatters.length; i < len; ++i) {"," cell = cells.item(formatters[i]);",""," if (cell) {"," col = formatterData.column = columns[formatters[i]];"," key = col.key || col.id;",""," formatterData.value = record.get(key);"," formatterData.td = cell;"," formatterData.cell = cell.one(linerQuery) || cell;",""," keep = col.nodeFormatter.call(host,formatterData);",""," if (keep === false) {"," // Remove from the Node cache to reduce"," // memory footprint. This also purges events,"," // which you shouldn't be scoping to a cell"," // anyway. You've been warned. Incidentally,"," // you should always return false. Just sayin."," cell.destroy(true);"," }"," }"," }"," }"," });"," }"," },",""," /**"," Binds event subscriptions from the UI and the host (if assigned).",""," @method bindUI"," @protected"," @since 3.5.0"," **/"," bindUI: function () {"," var handles = this._eventHandles,"," modelList = this.get('modelList'),"," changeEvent = modelList.model.NAME + ':change';",""," if (!handles.columnsChange) {"," handles.columnsChange = this.after('columnsChange',"," bind('_afterColumnsChange', this));"," }",""," if (modelList && !handles.dataChange) {"," handles.dataChange = modelList.after("," ['add', 'remove', 'reset', changeEvent],"," bind('_afterDataChange', this));"," }"," },",""," /**"," Iterates the `modelList` and applies each Model to the `_rowTemplate`,"," allowing any column `formatter` or `emptyCellValue` to override cell"," content for the appropriate column. The aggregated HTML string is"," returned.",""," @method _createDataHTML"," @param {Object[]} columns The column configurations to customize the"," generated cell content or class names"," @return {HTML} The markup for all Models in the `modelList`, each applied"," to the `_rowTemplate`"," @protected"," @since 3.5.0"," **/"," _createDataHTML: function (columns) {"," var data = this.get('modelList'),"," html = '';",""," if (data) {"," data.each(function (model, index) {"," html += this._createRowHTML(model, index, columns);"," }, this);"," }",""," return html;"," },",""," /**"," Applies the data of a given Model, modified by any column formatters and"," supplemented by other template values to the instance's `_rowTemplate` (see"," `_createRowTemplate`). The generated string is then returned.",""," The data from Model's attributes is fetched by `toJSON` and this data"," object is appended with other properties to supply values to {placeholders}"," in the template. For a template generated from a Model with 'foo' and 'bar'"," attributes, the data object would end up with the following properties"," before being used to populate the `_rowTemplate`:",""," * `clientID` - From Model, used the assign the `<tr>`'s 'id' attribute."," * `foo` - The value to populate the 'foo' column cell content. This"," value will be the value stored in the Model's `foo` attribute, or the"," result of the column's `formatter` if assigned. If the value is '',"," `null`, or `undefined`, and the column's `emptyCellValue` is assigned,"," that value will be used."," * `bar` - Same for the 'bar' column cell content."," * `foo-className` - String of CSS classes to apply to the `<td>`."," * `bar-className` - Same."," * `rowClass` - String of CSS classes to apply to the `<tr>`. This"," will be the odd/even class per the specified index plus any additional"," classes assigned by column formatters (via `o.rowClass`).",""," Because this object is available to formatters, any additional properties"," can be added to fill in custom {placeholders} in the `_rowTemplate`.",""," @method _createRowHTML"," @param {Model} model The Model instance to apply to the row template"," @param {Number} index The index the row will be appearing"," @param {Object[]} columns The column configurations"," @return {HTML} The markup for the provided Model, less any `nodeFormatter`s"," @protected"," @since 3.5.0"," **/"," _createRowHTML: function (model, index, columns) {"," var data = model.toJSON(),"," clientId = model.get('clientId'),"," values = {"," rowId : this._getRowId(clientId),"," clientId: clientId,"," rowClass: (index % 2) ? this.CLASS_ODD : this.CLASS_EVEN"," },"," host = this.host || this,"," i, len, col, token, value, formatterData;",""," for (i = 0, len = columns.length; i < len; ++i) {"," col = columns[i];"," value = data[col.key];"," token = col._id || col.key;",""," values[token + '-className'] = '';",""," if (col._formatterFn) {"," formatterData = {"," value : value,"," data : data,"," column : col,"," record : model,"," className: '',"," rowClass : '',"," rowIndex : index"," };",""," // Formatters can either return a value"," value = col._formatterFn.call(host, formatterData);",""," // or update the value property of the data obj passed"," if (value === undefined) {"," value = formatterData.value;"," }",""," values[token + '-className'] = formatterData.className;"," values.rowClass += ' ' + formatterData.rowClass;"," }",""," if (value === undefined || value === null || value === '') {"," value = col.emptyCellValue || '';"," }",""," values[token] = col.allowHTML ? value : htmlEscape(value);",""," values.rowClass = values.rowClass.replace(/\\s+/g, ' ');"," }",""," return fromTemplate(this._rowTemplate, values);"," },",""," /**"," Creates a custom HTML template string for use in generating the markup for"," individual table rows with {placeholder}s to capture data from the Models"," in the `modelList` attribute or from column `formatter`s.",""," Assigns the `_rowTemplate` property.",""," @method _createRowTemplate"," @param {Object[]} columns Array of column configuration objects"," @protected"," @since 3.5.0"," **/"," _createRowTemplate: function (columns) {"," var html = '',"," cellTemplate = this.CELL_TEMPLATE,"," F = Y.DataTable.BodyView.Formatters,"," i, len, col, key, token, headers, tokenValues, formatter;",""," for (i = 0, len = columns.length; i < len; ++i) {"," col = columns[i];"," key = col.key;"," token = col._id || key;"," formatter = col.formatter;"," // Only include headers if there are more than one"," headers = (col._headers || []).length > 1 ?"," 'headers=\"' + col._headers.join(' ') + '\"' : '';",""," tokenValues = {"," content : '{' + token + '}',"," headers : headers,"," className: this.getClassName('col', token) + ' ' +"," (col.className || '') + ' ' +"," this.getClassName('cell') +"," ' {' + token + '-className}'"," };"," if (formatter) {"," if (Lang.isFunction(formatter)) {"," col._formatterFn = formatter;"," } else if (formatter in F) {"," col._formatterFn = F[formatter].call(this.host || this, col);"," } else {"," tokenValues.content = formatter.replace(valueRegExp, tokenValues.content);"," }"," }",""," if (col.nodeFormatter) {"," // Defer all node decoration to the formatter"," tokenValues.content = '';"," }",""," html += fromTemplate(col.cellTemplate || cellTemplate, tokenValues);"," }",""," this._rowTemplate = fromTemplate(this.ROW_TEMPLATE, {"," content: html"," });"," },"," /**"," Cleans up temporary values created during rendering."," @method _afterRenderCleanup"," @private"," */"," _afterRenderCleanup: function () {"," var columns = this.get('columns'),"," i, len = columns.length;",""," for (i = 0;i < len; i+=1) {"," delete columns[i]._formatterFn;"," }",""," },",""," /**"," Creates the `<tbody>` node that will store the data rows.",""," @method _createTBodyNode"," @return {Node}"," @protected"," @since 3.6.0"," **/"," _createTBodyNode: function () {"," return Y.Node.create(fromTemplate(this.TBODY_TEMPLATE, {"," className: this.getClassName('data')"," }));"," },",""," /**"," Destroys the instance.",""," @method destructor"," @protected"," @since 3.5.0"," **/"," destructor: function () {"," (new Y.EventHandle(YObject.values(this._eventHandles))).detach();"," },",""," /**"," Holds the event subscriptions needing to be detached when the instance is"," `destroy()`ed.",""," @property _eventHandles"," @type {Object}"," @default undefined (initially unset)"," @protected"," @since 3.5.0"," **/"," //_eventHandles: null,",""," /**"," Returns the row ID associated with a Model's clientId.",""," @method _getRowId"," @param {String} clientId The Model clientId"," @return {String}"," @protected"," **/"," _getRowId: function (clientId) {"," return this._idMap[clientId] || (this._idMap[clientId] = Y.guid());"," },",""," /**"," Map of Model clientIds to row ids.",""," @property _idMap"," @type {Object}"," @protected"," **/"," //_idMap,",""," /**"," Initializes the instance. Reads the following configuration properties in"," addition to the instance attributes:",""," * `columns` - (REQUIRED) The initial column information"," * `host` - The object to serve as source of truth for column info and"," for generating class names",""," @method initializer"," @param {Object} config Configuration data"," @protected"," @since 3.5.0"," **/"," initializer: function (config) {"," this.host = config.host;",""," this._eventHandles = {"," modelListChange: this.after('modelListChange',"," bind('_afterModelListChange', this))"," };"," this._idMap = {};",""," this.CLASS_ODD = this.getClassName('odd');"," this.CLASS_EVEN = this.getClassName('even');",""," }",""," /**"," The HTML template used to create a full row of markup for a single Model in"," the `modelList` plus any customizations defined in the column"," configurations.",""," @property _rowTemplate"," @type {HTML}"," @default (initially unset)"," @protected"," @since 3.5.0"," **/"," //_rowTemplate: null","},{"," /**"," Hash of formatting functions for cell contents.",""," This property can be populated with a hash of formatting functions by the developer"," or a set of pre-defined functions can be loaded via the `datatable-formatters` module.",""," See: [DataTable.BodyView.Formatters](./DataTable.BodyView.Formatters.html)"," @property Formatters"," @type Object"," @since 3.8.0"," @static"," **/"," Formatters: {}","});","","","}, '@VERSION@', {\"requires\": [\"datatable-core\", \"view\", \"classnamemanager\"]});","","}());"]};
}
var __cov_lwdQmRpEAfazeg1nWBy89g = __coverage__['build/datatable-body/datatable-body.js'];
__cov_lwdQmRpEAfazeg1nWBy89g.s['1']++;YUI.add('datatable-body',function(Y,NAME){__cov_lwdQmRpEAfazeg1nWBy89g.f['1']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['2']++;var Lang=Y.Lang,isArray=Lang.isArray,isNumber=Lang.isNumber,isString=Lang.isString,fromTemplate=Lang.sub,htmlEscape=Y.Escape.html,toArray=Y.Array,bind=Y.bind,YObject=Y.Object,valueRegExp=/\{value\}/g;__cov_lwdQmRpEAfazeg1nWBy89g.s['3']++;Y.namespace('DataTable').BodyView=Y.Base.create('tableBody',Y.View,[],{CELL_TEMPLATE:'<td {headers} class="{className}">{content}</td>',ROW_TEMPLATE:'<tr id="{rowId}" data-yui3-record="{clientId}" class="{rowClass}">{content}</tr>',TBODY_TEMPLATE:'<tbody class="{className}"></tbody>',getCell:function(seed,shift){__cov_lwdQmRpEAfazeg1nWBy89g.f['2']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['4']++;var tbody=this.tbodyNode,row,cell,index,rowIndexOffset;__cov_lwdQmRpEAfazeg1nWBy89g.s['5']++;if((__cov_lwdQmRpEAfazeg1nWBy89g.b['2'][0]++,seed)&&(__cov_lwdQmRpEAfazeg1nWBy89g.b['2'][1]++,tbody)){__cov_lwdQmRpEAfazeg1nWBy89g.b['1'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['6']++;if(isArray(seed)){__cov_lwdQmRpEAfazeg1nWBy89g.b['3'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['7']++;row=tbody.get('children').item(seed[0]);__cov_lwdQmRpEAfazeg1nWBy89g.s['8']++;cell=(__cov_lwdQmRpEAfazeg1nWBy89g.b['4'][0]++,row)&&(__cov_lwdQmRpEAfazeg1nWBy89g.b['4'][1]++,row.get('children').item(seed[1]));}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['3'][1]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['9']++;if(Y.instanceOf(seed,Y.Node)){__cov_lwdQmRpEAfazeg1nWBy89g.b['5'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['10']++;cell=seed.ancestor('.'+this.getClassName('cell'),true);}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['5'][1]++;}}__cov_lwdQmRpEAfazeg1nWBy89g.s['11']++;if((__cov_lwdQmRpEAfazeg1nWBy89g.b['7'][0]++,cell)&&(__cov_lwdQmRpEAfazeg1nWBy89g.b['7'][1]++,shift)){__cov_lwdQmRpEAfazeg1nWBy89g.b['6'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['12']++;rowIndexOffset=tbody.get('firstChild.rowIndex');__cov_lwdQmRpEAfazeg1nWBy89g.s['13']++;if(isString(shift)){__cov_lwdQmRpEAfazeg1nWBy89g.b['8'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['14']++;switch(shift){case'above':__cov_lwdQmRpEAfazeg1nWBy89g.b['9'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['15']++;shift=[-1,0];__cov_lwdQmRpEAfazeg1nWBy89g.s['16']++;break;case'below':__cov_lwdQmRpEAfazeg1nWBy89g.b['9'][1]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['17']++;shift=[1,0];__cov_lwdQmRpEAfazeg1nWBy89g.s['18']++;break;case'next':__cov_lwdQmRpEAfazeg1nWBy89g.b['9'][2]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['19']++;shift=[0,1];__cov_lwdQmRpEAfazeg1nWBy89g.s['20']++;break;case'previous':__cov_lwdQmRpEAfazeg1nWBy89g.b['9'][3]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['21']++;shift=[0,-1];__cov_lwdQmRpEAfazeg1nWBy89g.s['22']++;break;}}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['8'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['23']++;if(isArray(shift)){__cov_lwdQmRpEAfazeg1nWBy89g.b['10'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['24']++;index=cell.get('parentNode.rowIndex')+shift[0]-rowIndexOffset;__cov_lwdQmRpEAfazeg1nWBy89g.s['25']++;row=tbody.get('children').item(index);__cov_lwdQmRpEAfazeg1nWBy89g.s['26']++;index=cell.get('cellIndex')+shift[1];__cov_lwdQmRpEAfazeg1nWBy89g.s['27']++;cell=(__cov_lwdQmRpEAfazeg1nWBy89g.b['11'][0]++,row)&&(__cov_lwdQmRpEAfazeg1nWBy89g.b['11'][1]++,row.get('children').item(index));}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['10'][1]++;}}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['6'][1]++;}}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['1'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['28']++;return(__cov_lwdQmRpEAfazeg1nWBy89g.b['12'][0]++,cell)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['12'][1]++,null);},getClassName:function(){__cov_lwdQmRpEAfazeg1nWBy89g.f['3']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['29']++;var host=this.host,args;__cov_lwdQmRpEAfazeg1nWBy89g.s['30']++;if((__cov_lwdQmRpEAfazeg1nWBy89g.b['14'][0]++,host)&&(__cov_lwdQmRpEAfazeg1nWBy89g.b['14'][1]++,host.getClassName)){__cov_lwdQmRpEAfazeg1nWBy89g.b['13'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['31']++;return host.getClassName.apply(host,arguments);}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['13'][1]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['32']++;args=toArray(arguments);__cov_lwdQmRpEAfazeg1nWBy89g.s['33']++;args.unshift(this.constructor.NAME);__cov_lwdQmRpEAfazeg1nWBy89g.s['34']++;return Y.ClassNameManager.getClassName.apply(Y.ClassNameManager,args);}},getRecord:function(seed){__cov_lwdQmRpEAfazeg1nWBy89g.f['4']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['35']++;var modelList=this.get('modelList'),tbody=this.tbodyNode,row=null,record;__cov_lwdQmRpEAfazeg1nWBy89g.s['36']++;if(tbody){__cov_lwdQmRpEAfazeg1nWBy89g.b['15'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['37']++;if(isString(seed)){__cov_lwdQmRpEAfazeg1nWBy89g.b['16'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['38']++;seed=tbody.one('#'+seed);}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['16'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['39']++;if(Y.instanceOf(seed,Y.Node)){__cov_lwdQmRpEAfazeg1nWBy89g.b['17'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['40']++;row=seed.ancestor(function(node){__cov_lwdQmRpEAfazeg1nWBy89g.f['5']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['41']++;return node.get('parentNode').compareTo(tbody);},true);__cov_lwdQmRpEAfazeg1nWBy89g.s['42']++;record=(__cov_lwdQmRpEAfazeg1nWBy89g.b['18'][0]++,row)&&(__cov_lwdQmRpEAfazeg1nWBy89g.b['18'][1]++,modelList.getByClientId(row.getData('yui3-record')));}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['17'][1]++;}}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['15'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['43']++;return(__cov_lwdQmRpEAfazeg1nWBy89g.b['19'][0]++,record)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['19'][1]++,null);},getRow:function(id){__cov_lwdQmRpEAfazeg1nWBy89g.f['6']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['44']++;var tbody=this.tbodyNode,row=null;__cov_lwdQmRpEAfazeg1nWBy89g.s['45']++;if(tbody){__cov_lwdQmRpEAfazeg1nWBy89g.b['20'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['46']++;if(id){__cov_lwdQmRpEAfazeg1nWBy89g.b['21'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['47']++;id=(__cov_lwdQmRpEAfazeg1nWBy89g.b['22'][0]++,this._idMap[id.get?(__cov_lwdQmRpEAfazeg1nWBy89g.b['23'][0]++,id.get('clientId')):(__cov_lwdQmRpEAfazeg1nWBy89g.b['23'][1]++,id)])||(__cov_lwdQmRpEAfazeg1nWBy89g.b['22'][1]++,id);}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['21'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['48']++;row=isNumber(id)?(__cov_lwdQmRpEAfazeg1nWBy89g.b['24'][0]++,tbody.get('children').item(id)):(__cov_lwdQmRpEAfazeg1nWBy89g.b['24'][1]++,tbody.one('#'+id));}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['20'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['49']++;return row;},render:function(){__cov_lwdQmRpEAfazeg1nWBy89g.f['7']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['50']++;var table=this.get('container'),data=this.get('modelList'),columns=this.get('columns'),tbody=(__cov_lwdQmRpEAfazeg1nWBy89g.b['25'][0]++,this.tbodyNode)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['25'][1]++,this.tbodyNode=this._createTBodyNode());__cov_lwdQmRpEAfazeg1nWBy89g.s['51']++;this._createRowTemplate(columns);__cov_lwdQmRpEAfazeg1nWBy89g.s['52']++;if(data){__cov_lwdQmRpEAfazeg1nWBy89g.b['26'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['53']++;tbody.setHTML(this._createDataHTML(columns));__cov_lwdQmRpEAfazeg1nWBy89g.s['54']++;this._applyNodeFormatters(tbody,columns);}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['26'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['55']++;if(tbody.get('parentNode')!==table){__cov_lwdQmRpEAfazeg1nWBy89g.b['27'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['56']++;table.appendChild(tbody);}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['27'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['57']++;this._afterRenderCleanup();__cov_lwdQmRpEAfazeg1nWBy89g.s['58']++;this.bindUI();__cov_lwdQmRpEAfazeg1nWBy89g.s['59']++;return this;},_afterColumnsChange:function(){__cov_lwdQmRpEAfazeg1nWBy89g.f['8']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['60']++;this.render();},_afterDataChange:function(){__cov_lwdQmRpEAfazeg1nWBy89g.f['9']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['61']++;this.render();},_afterModelListChange:function(){__cov_lwdQmRpEAfazeg1nWBy89g.f['10']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['62']++;var handles=this._eventHandles;__cov_lwdQmRpEAfazeg1nWBy89g.s['63']++;if(handles.dataChange){__cov_lwdQmRpEAfazeg1nWBy89g.b['28'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['64']++;handles.dataChange.detach();__cov_lwdQmRpEAfazeg1nWBy89g.s['65']++;delete handles.dataChange;__cov_lwdQmRpEAfazeg1nWBy89g.s['66']++;this.bindUI();}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['28'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['67']++;if(this.tbodyNode){__cov_lwdQmRpEAfazeg1nWBy89g.b['29'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['68']++;this.render();}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['29'][1]++;}},_applyNodeFormatters:function(tbody,columns){__cov_lwdQmRpEAfazeg1nWBy89g.f['11']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['69']++;var host=this.host,data=this.get('modelList'),formatters=[],linerQuery='.'+this.getClassName('liner'),rows,i,len;__cov_lwdQmRpEAfazeg1nWBy89g.s['70']++;for(i=0,len=columns.length;i<len;++i){__cov_lwdQmRpEAfazeg1nWBy89g.s['71']++;if(columns[i].nodeFormatter){__cov_lwdQmRpEAfazeg1nWBy89g.b['30'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['72']++;formatters.push(i);}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['30'][1]++;}}__cov_lwdQmRpEAfazeg1nWBy89g.s['73']++;if((__cov_lwdQmRpEAfazeg1nWBy89g.b['32'][0]++,data)&&(__cov_lwdQmRpEAfazeg1nWBy89g.b['32'][1]++,formatters.length)){__cov_lwdQmRpEAfazeg1nWBy89g.b['31'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['74']++;rows=tbody.get('childNodes');__cov_lwdQmRpEAfazeg1nWBy89g.s['75']++;data.each(function(record,index){__cov_lwdQmRpEAfazeg1nWBy89g.f['12']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['76']++;var formatterData={data:record.toJSON(),record:record,rowIndex:index},row=rows.item(index),i,len,col,key,cells,cell,keep;__cov_lwdQmRpEAfazeg1nWBy89g.s['77']++;if(row){__cov_lwdQmRpEAfazeg1nWBy89g.b['33'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['78']++;cells=row.get('childNodes');__cov_lwdQmRpEAfazeg1nWBy89g.s['79']++;for(i=0,len=formatters.length;i<len;++i){__cov_lwdQmRpEAfazeg1nWBy89g.s['80']++;cell=cells.item(formatters[i]);__cov_lwdQmRpEAfazeg1nWBy89g.s['81']++;if(cell){__cov_lwdQmRpEAfazeg1nWBy89g.b['34'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['82']++;col=formatterData.column=columns[formatters[i]];__cov_lwdQmRpEAfazeg1nWBy89g.s['83']++;key=(__cov_lwdQmRpEAfazeg1nWBy89g.b['35'][0]++,col.key)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['35'][1]++,col.id);__cov_lwdQmRpEAfazeg1nWBy89g.s['84']++;formatterData.value=record.get(key);__cov_lwdQmRpEAfazeg1nWBy89g.s['85']++;formatterData.td=cell;__cov_lwdQmRpEAfazeg1nWBy89g.s['86']++;formatterData.cell=(__cov_lwdQmRpEAfazeg1nWBy89g.b['36'][0]++,cell.one(linerQuery))||(__cov_lwdQmRpEAfazeg1nWBy89g.b['36'][1]++,cell);__cov_lwdQmRpEAfazeg1nWBy89g.s['87']++;keep=col.nodeFormatter.call(host,formatterData);__cov_lwdQmRpEAfazeg1nWBy89g.s['88']++;if(keep===false){__cov_lwdQmRpEAfazeg1nWBy89g.b['37'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['89']++;cell.destroy(true);}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['37'][1]++;}}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['34'][1]++;}}}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['33'][1]++;}});}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['31'][1]++;}},bindUI:function(){__cov_lwdQmRpEAfazeg1nWBy89g.f['13']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['90']++;var handles=this._eventHandles,modelList=this.get('modelList'),changeEvent=modelList.model.NAME+':change';__cov_lwdQmRpEAfazeg1nWBy89g.s['91']++;if(!handles.columnsChange){__cov_lwdQmRpEAfazeg1nWBy89g.b['38'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['92']++;handles.columnsChange=this.after('columnsChange',bind('_afterColumnsChange',this));}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['38'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['93']++;if((__cov_lwdQmRpEAfazeg1nWBy89g.b['40'][0]++,modelList)&&(__cov_lwdQmRpEAfazeg1nWBy89g.b['40'][1]++,!handles.dataChange)){__cov_lwdQmRpEAfazeg1nWBy89g.b['39'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['94']++;handles.dataChange=modelList.after(['add','remove','reset',changeEvent],bind('_afterDataChange',this));}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['39'][1]++;}},_createDataHTML:function(columns){__cov_lwdQmRpEAfazeg1nWBy89g.f['14']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['95']++;var data=this.get('modelList'),html='';__cov_lwdQmRpEAfazeg1nWBy89g.s['96']++;if(data){__cov_lwdQmRpEAfazeg1nWBy89g.b['41'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['97']++;data.each(function(model,index){__cov_lwdQmRpEAfazeg1nWBy89g.f['15']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['98']++;html+=this._createRowHTML(model,index,columns);},this);}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['41'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['99']++;return html;},_createRowHTML:function(model,index,columns){__cov_lwdQmRpEAfazeg1nWBy89g.f['16']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['100']++;var data=model.toJSON(),clientId=model.get('clientId'),values={rowId:this._getRowId(clientId),clientId:clientId,rowClass:index%2?(__cov_lwdQmRpEAfazeg1nWBy89g.b['42'][0]++,this.CLASS_ODD):(__cov_lwdQmRpEAfazeg1nWBy89g.b['42'][1]++,this.CLASS_EVEN)},host=(__cov_lwdQmRpEAfazeg1nWBy89g.b['43'][0]++,this.host)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['43'][1]++,this),i,len,col,token,value,formatterData;__cov_lwdQmRpEAfazeg1nWBy89g.s['101']++;for(i=0,len=columns.length;i<len;++i){__cov_lwdQmRpEAfazeg1nWBy89g.s['102']++;col=columns[i];__cov_lwdQmRpEAfazeg1nWBy89g.s['103']++;value=data[col.key];__cov_lwdQmRpEAfazeg1nWBy89g.s['104']++;token=(__cov_lwdQmRpEAfazeg1nWBy89g.b['44'][0]++,col._id)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['44'][1]++,col.key);__cov_lwdQmRpEAfazeg1nWBy89g.s['105']++;values[token+'-className']='';__cov_lwdQmRpEAfazeg1nWBy89g.s['106']++;if(col._formatterFn){__cov_lwdQmRpEAfazeg1nWBy89g.b['45'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['107']++;formatterData={value:value,data:data,column:col,record:model,className:'',rowClass:'',rowIndex:index};__cov_lwdQmRpEAfazeg1nWBy89g.s['108']++;value=col._formatterFn.call(host,formatterData);__cov_lwdQmRpEAfazeg1nWBy89g.s['109']++;if(value===undefined){__cov_lwdQmRpEAfazeg1nWBy89g.b['46'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['110']++;value=formatterData.value;}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['46'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['111']++;values[token+'-className']=formatterData.className;__cov_lwdQmRpEAfazeg1nWBy89g.s['112']++;values.rowClass+=' '+formatterData.rowClass;}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['45'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['113']++;if((__cov_lwdQmRpEAfazeg1nWBy89g.b['48'][0]++,value===undefined)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['48'][1]++,value===null)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['48'][2]++,value==='')){__cov_lwdQmRpEAfazeg1nWBy89g.b['47'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['114']++;value=(__cov_lwdQmRpEAfazeg1nWBy89g.b['49'][0]++,col.emptyCellValue)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['49'][1]++,'');}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['47'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['115']++;values[token]=col.allowHTML?(__cov_lwdQmRpEAfazeg1nWBy89g.b['50'][0]++,value):(__cov_lwdQmRpEAfazeg1nWBy89g.b['50'][1]++,htmlEscape(value));__cov_lwdQmRpEAfazeg1nWBy89g.s['116']++;values.rowClass=values.rowClass.replace(/\s+/g,' ');}__cov_lwdQmRpEAfazeg1nWBy89g.s['117']++;return fromTemplate(this._rowTemplate,values);},_createRowTemplate:function(columns){__cov_lwdQmRpEAfazeg1nWBy89g.f['17']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['118']++;var html='',cellTemplate=this.CELL_TEMPLATE,F=Y.DataTable.BodyView.Formatters,i,len,col,key,token,headers,tokenValues,formatter;__cov_lwdQmRpEAfazeg1nWBy89g.s['119']++;for(i=0,len=columns.length;i<len;++i){__cov_lwdQmRpEAfazeg1nWBy89g.s['120']++;col=columns[i];__cov_lwdQmRpEAfazeg1nWBy89g.s['121']++;key=col.key;__cov_lwdQmRpEAfazeg1nWBy89g.s['122']++;token=(__cov_lwdQmRpEAfazeg1nWBy89g.b['51'][0]++,col._id)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['51'][1]++,key);__cov_lwdQmRpEAfazeg1nWBy89g.s['123']++;formatter=col.formatter;__cov_lwdQmRpEAfazeg1nWBy89g.s['124']++;headers=((__cov_lwdQmRpEAfazeg1nWBy89g.b['53'][0]++,col._headers)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['53'][1]++,[])).length>1?(__cov_lwdQmRpEAfazeg1nWBy89g.b['52'][0]++,'headers="'+col._headers.join(' ')+'"'):(__cov_lwdQmRpEAfazeg1nWBy89g.b['52'][1]++,'');__cov_lwdQmRpEAfazeg1nWBy89g.s['125']++;tokenValues={content:'{'+token+'}',headers:headers,className:this.getClassName('col',token)+' '+((__cov_lwdQmRpEAfazeg1nWBy89g.b['54'][0]++,col.className)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['54'][1]++,''))+' '+this.getClassName('cell')+' {'+token+'-className}'};__cov_lwdQmRpEAfazeg1nWBy89g.s['126']++;if(formatter){__cov_lwdQmRpEAfazeg1nWBy89g.b['55'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['127']++;if(Lang.isFunction(formatter)){__cov_lwdQmRpEAfazeg1nWBy89g.b['56'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['128']++;col._formatterFn=formatter;}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['56'][1]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['129']++;if(formatter in F){__cov_lwdQmRpEAfazeg1nWBy89g.b['57'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['130']++;col._formatterFn=F[formatter].call((__cov_lwdQmRpEAfazeg1nWBy89g.b['58'][0]++,this.host)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['58'][1]++,this),col);}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['57'][1]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['131']++;tokenValues.content=formatter.replace(valueRegExp,tokenValues.content);}}}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['55'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['132']++;if(col.nodeFormatter){__cov_lwdQmRpEAfazeg1nWBy89g.b['59'][0]++;__cov_lwdQmRpEAfazeg1nWBy89g.s['133']++;tokenValues.content='';}else{__cov_lwdQmRpEAfazeg1nWBy89g.b['59'][1]++;}__cov_lwdQmRpEAfazeg1nWBy89g.s['134']++;html+=fromTemplate((__cov_lwdQmRpEAfazeg1nWBy89g.b['60'][0]++,col.cellTemplate)||(__cov_lwdQmRpEAfazeg1nWBy89g.b['60'][1]++,cellTemplate),tokenValues);}__cov_lwdQmRpEAfazeg1nWBy89g.s['135']++;this._rowTemplate=fromTemplate(this.ROW_TEMPLATE,{content:html});},_afterRenderCleanup:function(){__cov_lwdQmRpEAfazeg1nWBy89g.f['18']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['136']++;var columns=this.get('columns'),i,len=columns.length;__cov_lwdQmRpEAfazeg1nWBy89g.s['137']++;for(i=0;i<len;i+=1){__cov_lwdQmRpEAfazeg1nWBy89g.s['138']++;delete columns[i]._formatterFn;}},_createTBodyNode:function(){__cov_lwdQmRpEAfazeg1nWBy89g.f['19']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['139']++;return Y.Node.create(fromTemplate(this.TBODY_TEMPLATE,{className:this.getClassName('data')}));},destructor:function(){__cov_lwdQmRpEAfazeg1nWBy89g.f['20']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['140']++;new Y.EventHandle(YObject.values(this._eventHandles)).detach();},_getRowId:function(clientId){__cov_lwdQmRpEAfazeg1nWBy89g.f['21']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['141']++;return(__cov_lwdQmRpEAfazeg1nWBy89g.b['61'][0]++,this._idMap[clientId])||(__cov_lwdQmRpEAfazeg1nWBy89g.b['61'][1]++,this._idMap[clientId]=Y.guid());},initializer:function(config){__cov_lwdQmRpEAfazeg1nWBy89g.f['22']++;__cov_lwdQmRpEAfazeg1nWBy89g.s['142']++;this.host=config.host;__cov_lwdQmRpEAfazeg1nWBy89g.s['143']++;this._eventHandles={modelListChange:this.after('modelListChange',bind('_afterModelListChange',this))};__cov_lwdQmRpEAfazeg1nWBy89g.s['144']++;this._idMap={};__cov_lwdQmRpEAfazeg1nWBy89g.s['145']++;this.CLASS_ODD=this.getClassName('odd');__cov_lwdQmRpEAfazeg1nWBy89g.s['146']++;this.CLASS_EVEN=this.getClassName('even');}},{Formatters:{}});},'@VERSION@',{'requires':['datatable-core','view','classnamemanager']});
|
src/controls/ColorPicker/Component/index.js | michalko/draft-wyswig | /* @flow */
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import classNames from 'classnames';
import { stopPropagation } from '../../../utils/common';
import Option from '../../../components/Option';
import './styles.css';
class LayoutComponent extends Component {
static propTypes = {
expanded: PropTypes.bool,
onExpandEvent: PropTypes.func,
onChange: PropTypes.func,
config: PropTypes.object,
currentState: PropTypes.object,
translations: PropTypes.object,
};
state: Object = {
currentStyle: 'color',
};
componentWillReceiveProps(props) {
if (!this.props.expanded && props.expanded) {
this.setState({
currentStyle: 'color',
});
}
}
onChange: Function = (color: string): void => {
const { onChange } = this.props;
const { currentStyle } = this.state;
onChange(currentStyle, color);
}
setCurrentStyleColor: Function = (): void => {
this.setState({
currentStyle: 'color',
});
};
setCurrentStyleBgcolor: Function = (): void => {
this.setState({
currentStyle: 'bgcolor',
});
};
renderModal: Function = (): Object => {
const {
config: { popupClassName, colors },
currentState: { color, bgColor },
translations,
} = this.props;
const { currentStyle } = this.state;
const currentSelectedColor = (currentStyle === 'color') ? color : bgColor;
return (
<div
className={classNames('rdw-colorpicker-modal', popupClassName)}
onClick={stopPropagation}
>
<span className="rdw-colorpicker-modal-header">
<span
className={classNames(
'rdw-colorpicker-modal-style-label',
{ 'rdw-colorpicker-modal-style-label-active': currentStyle === 'color' },
)}
onClick={this.setCurrentStyleColor}
>
{translations['components.controls.colorpicker.text']}
</span>
<span
className={classNames(
'rdw-colorpicker-modal-style-label',
{ 'rdw-colorpicker-modal-style-label-active': currentStyle === 'bgcolor' },
)}
onClick={this.setCurrentStyleBgcolor}
>
{translations['components.controls.colorpicker.background']}
</span>
</span>
<span className="rdw-colorpicker-modal-options">
{
colors.map((c, index) =>
(<Option
value={c}
key={index}
className="rdw-colorpicker-option"
activeClassName="rdw-colorpicker-option-active"
active={currentSelectedColor === c}
onClick={this.onChange}
>
<span
style={{ backgroundColor: c }}
className="rdw-colorpicker-cube"
/>
</Option>))
}
</span>
</div>
);
};
render(): Object {
const {
config: { icon, className, title },
expanded,
onExpandEvent,
translations,
} = this.props;
return (
<div
className="rdw-colorpicker-wrapper"
aria-haspopup="true"
aria-expanded={expanded}
aria-label="rdw-color-picker"
title={title || translations['components.controls.colorpicker.colorpicker']}
>
<Option
onClick={onExpandEvent}
className={classNames(className)}
>
<img
src={icon}
alt=""
/>
</Option>
{expanded ? this.renderModal() : undefined}
</div>
);
}
}
export default LayoutComponent;
|
__test__/ReduxBlockUi.spec.js | Availity/react-block-ui | import React from 'react';
import { shallow } from 'enzyme';
import ReduxBlockUi from 'react-block-ui/redux';
import * as BlockUiMiddleware from '../src/reduxMiddleware';
import BlockUi from 'react-block-ui';
describe('ReduxBlockUi', function() {
it('should render a "blockUi"', () => {
const wrapper = shallow(<ReduxBlockUi>Yo!</ReduxBlockUi>);
expect(wrapper.type()).to.equal(BlockUi);
});
it('should allow "manual" blocking', () => {
const wrapper = shallow(<ReduxBlockUi blocking>Yo!</ReduxBlockUi>);
expect(wrapper.prop('blocking')).to.be.true;
});
it('should trigger on change when the blocking amount changes', () => {
const spy = sinon.spy();
const wrapper = shallow(<ReduxBlockUi block="fetch" onChange={spy}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.middleware({type: 'fetch'});
expect(spy).to.have.been.calledWith(1, 0);
});
it('should not have a negative blocking value', () => {
const wrapper = shallow(<ReduxBlockUi unblock="success">Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.blocking = 0;
wrapper.setState({blocking: 0});
instance.middleware({type: 'success'});
expect(wrapper.state('blocking')).to.equal(0);
});
describe('mounting', () => {
beforeEach(() => {
this.register = sinon.spy(BlockUiMiddleware, 'register');
});
afterEach(() => {
this.register.restore();
});
it('should register the instance in the middleware', () => {
const wrapper = shallow(<ReduxBlockUi>Yo!</ReduxBlockUi>);
const fn = wrapper.instance().middleware;
expect(this.register).to.have.been.calledWith(fn);
});
});
describe('unmounting', () => {
beforeEach(() => {
this.unregister = sinon.spy(BlockUiMiddleware, 'unregister');
});
afterEach(() => {
this.unregister.restore();
});
it('should unregister the instance in the middleware', () => {
const wrapper = shallow(<ReduxBlockUi>Yo!</ReduxBlockUi>);
const fn = wrapper.instance().middleware;
wrapper.unmount();
expect(this.unregister).to.have.been.calledWith(fn);
});
});
describe('block prop', () => {
describe('with a string value', () => {
it('should block when the value matches the action type', () => {
const wrapper = shallow(<ReduxBlockUi block="fetch">Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.middleware({type: 'fetch'});
expect(wrapper.state('blocking')).to.equal(1);
});
it('should not block when the value does not match the action type', () => {
const wrapper = shallow(<ReduxBlockUi block="fetch">Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.middleware({type: 'no-fetch'});
expect(wrapper.state('blocking')).to.equal(0);
});
});
describe('with a RegExp value', () => {
it('should block when the value matches the action type', () => {
const wrapper = shallow(<ReduxBlockUi block={/fetch/}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.middleware({type: 'fetching'});
expect(wrapper.state('blocking')).to.equal(1);
});
it('should not block when the value does not match the action type', () => {
const wrapper = shallow(<ReduxBlockUi block={/fetch/}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.middleware({type: 'something-else'});
expect(wrapper.state('blocking')).to.equal(0);
});
});
describe('with a function value', () => {
it('should block when the function returns true', () => {
const wrapper = shallow(<ReduxBlockUi block={() => true}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.middleware({type: 'fetching'});
expect(wrapper.state('blocking')).to.equal(1);
});
it('should not block when the function does not return true', () => {
const wrapper = shallow(<ReduxBlockUi block={() => undefined}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.middleware({type: 'something-else'});
expect(wrapper.state('blocking')).to.equal(0);
});
});
describe('with an array value', () => {
it('should block when any of the cases are match', () => {
const wrapper = shallow(<ReduxBlockUi block={['something', /idk/, () => true]}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.middleware({type: 'fetching'});
expect(wrapper.state('blocking')).to.equal(1);
});
it('should not block when none of the cases match', () => {
const wrapper = shallow(<ReduxBlockUi block={['nothing', /fetch/, () => undefined]}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.middleware({type: 'something-else'});
expect(wrapper.state('blocking')).to.equal(0);
});
});
});
describe('unblock prop', () => {
describe('with a string value', () => {
it('should unblock when the value matches the action type', () => {
const wrapper = shallow(<ReduxBlockUi unblock="success">Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.blocking = 1;
wrapper.setState({blocking: 1});
instance.middleware({type: 'success'});
expect(wrapper.state('blocking')).to.equal(0);
});
it('should not unblock when the value does not match the action type', () => {
const wrapper = shallow(<ReduxBlockUi unblock="success">Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.blocking = 1;
wrapper.setState({blocking: 1});
instance.middleware({type: 'no-success'});
expect(wrapper.state('blocking')).to.equal(1);
});
});
describe('with a RegExp value', () => {
it('should unblock when the value matches the action type', () => {
const wrapper = shallow(<ReduxBlockUi unblock={/success/}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.blocking = 1;
wrapper.setState({blocking: 1});
instance.middleware({type: 'successful'});
expect(wrapper.state('blocking')).to.equal(0);
});
it('should not unblock when the value does not match the action type', () => {
const wrapper = shallow(<ReduxBlockUi unblock={/success/}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.blocking = 1;
wrapper.setState({blocking: 1});
instance.middleware({type: 'something-else'});
expect(wrapper.state('blocking')).to.equal(1);
});
});
describe('with a function value', () => {
it('should unblock when the function returns true', () => {
const wrapper = shallow(<ReduxBlockUi unblock={() => true}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.blocking = 1;
wrapper.setState({blocking: 1});
instance.middleware({type: 'successful'});
expect(wrapper.state('blocking')).to.equal(0);
});
it('should not unblock when the function does not return true', () => {
const wrapper = shallow(<ReduxBlockUi unblock={() => undefined}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.blocking = 1;
wrapper.setState({blocking: 1});
instance.middleware({type: 'something-else'});
expect(wrapper.state('blocking')).to.equal(1);
});
});
describe('with an array value', () => {
it('should unblock when any of the cases are match', () => {
const wrapper = shallow(<ReduxBlockUi unblock={['something', /idk/, () => true]}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.blocking = 1;
wrapper.setState({blocking: 1});
instance.middleware({type: 'successful'});
expect(wrapper.state('blocking')).to.equal(0);
});
it('should not unblock when none of the cases match', () => {
const wrapper = shallow(<ReduxBlockUi unblock={['nothing', /success/, () => undefined]}>Yo!</ReduxBlockUi>);
const instance = wrapper.instance();
instance.blocking = 1;
wrapper.setState({blocking: 1});
instance.middleware({type: 'something-else'});
expect(wrapper.state('blocking')).to.equal(1);
});
});
});
});
|
packages/benchmarks/src/implementations/react-native-web/Tweet/IconDirectMessage.js | css-components/styled-components | /* eslint-disable react/prop-types */
import { createElement } from 'react-native';
import React from 'react';
import styles from './styles';
const IconDirectMessage = props =>
createElement('svg', {
children: (
<g>
<path d="M43.34 14H12.66L28 27.946z" />
<path d="M51.392 14.789L30.018 34.22c-.009.008-.028.006-.039.012-.563.5-1.266.768-1.98.768-.72 0-1.442-.258-2.017-.78L4.609 14.79A3.957 3.957 0 0 0 3 18v37a1.998 1.998 0 0 0 2 2c.464 0 .924-.162 1.292-.473L19 46h30c2.243 0 4-1.757 4-4V18a3.96 3.96 0 0 0-1.608-3.211z" />
</g>
),
style: [styles.icon, props.style],
viewBox: '0 0 56 72'
});
IconDirectMessage.metadata = { height: 72, width: 56 };
export default IconDirectMessage;
|
src/App.js | SpencerCDixon/drag-and-drop-example | import React, { Component } from 'react';
export default class App extends Component {
render() {
return (
<h1>Hello, world.</h1>
);
}
}
|
src/js/main.js | betaflight/betaflight-configurator | import { i18n } from './localization';
$(document).ready(function () {
useGlobalNodeFunctions();
if (typeof cordovaApp === 'undefined') {
appReady();
}
});
function useGlobalNodeFunctions() {
// The global functions of Node continue working on background. This is good to continue flashing,
// for example, when the window is minimized
if (GUI.isNWJS()) {
console.log("Replacing timeout/interval functions with Node versions");
window.setTimeout = global.setTimeout;
window.clearTimeout = global.clearTimeout;
window.setInterval = global.setInterval;
window.clearInterval = global.clearInterval;
}
}
function readConfiguratorVersionMetadata() {
const manifest = chrome.runtime.getManifest();
CONFIGURATOR.productName = manifest.productName;
CONFIGURATOR.version = manifest.version;
CONFIGURATOR.gitRevision = manifest.gitRevision;
}
function appReady() {
readConfiguratorVersionMetadata();
i18n.init(function() {
startProcess();
checkSetupAnalytics(function (analyticsService) {
analyticsService.sendEvent(analyticsService.EVENT_CATEGORIES.APPLICATION, 'SelectedLanguage', i18n.selectedLanguage);
});
initializeSerialBackend();
});
}
function checkSetupAnalytics(callback) {
if (!analytics) {
setTimeout(function () {
ConfigStorage.get(['userId', 'analyticsOptOut', 'checkForConfiguratorUnstableVersions' ], function (result) {
if (!analytics) {
setupAnalytics(result);
}
callback(analytics);
});
});
} else if (callback) {
callback(analytics);
}
}
function getBuildType() {
return GUI.Mode;
}
function setupAnalytics(result) {
let userId;
if (result.userId) {
userId = result.userId;
} else {
const uid = new ShortUniqueId();
userId = uid.randomUUID(13);
ConfigStorage.set({ 'userId': userId });
}
const optOut = !!result.analyticsOptOut;
const checkForDebugVersions = !!result.checkForConfiguratorUnstableVersions;
const debugMode = typeof process === "object" && process.versions['nw-flavor'] === 'sdk';
window.analytics = new Analytics('UA-123002063-1', userId, CONFIGURATOR.productName, CONFIGURATOR.version, CONFIGURATOR.gitRevision, GUI.operating_system,
checkForDebugVersions, optOut, debugMode, getBuildType());
function logException(exception) {
analytics.sendException(exception.stack);
}
if (typeof process === "object") {
process.on('uncaughtException', logException);
}
analytics.sendEvent(analytics.EVENT_CATEGORIES.APPLICATION, 'AppStart', { sessionControl: 'start' });
$('.connect_b a.connect').removeClass('disabled');
$('.firmware_b a.flash').removeClass('disabled');
}
function closeSerial() {
// automatically close the port when application closes
const connectionId = serial.connectionId;
if (connectionId && CONFIGURATOR.connectionValid && !CONFIGURATOR.virtualMode) {
// code below is handmade MSP message (without pretty JS wrapper), it behaves exactly like MSP.send_message
// sending exit command just in case the cli tab was open.
// reset motors to default (mincommand)
let bufferOut = new ArrayBuffer(5),
bufView = new Uint8Array(bufferOut);
bufView[0] = 0x65; // e
bufView[1] = 0x78; // x
bufView[2] = 0x69; // i
bufView[3] = 0x74; // t
bufView[4] = 0x0D; // enter
const sendFn = (serial.connectionType === 'serial' ? chrome.serial.send : chrome.sockets.tcp.send);
sendFn(connectionId, bufferOut, function () {
console.log('Send exit');
});
setTimeout(function() {
bufferOut = new ArrayBuffer(22);
bufView = new Uint8Array(bufferOut);
let checksum = 0;
bufView[0] = 36; // $
bufView[1] = 77; // M
bufView[2] = 60; // <
bufView[3] = 16; // data length
bufView[4] = 214; // MSP_SET_MOTOR
checksum = bufView[3] ^ bufView[4];
for (let i = 0; i < 16; i += 2) {
bufView[i + 5] = FC.MOTOR_CONFIG.mincommand & 0x00FF;
bufView[i + 6] = FC.MOTOR_CONFIG.mincommand >> 8;
checksum ^= bufView[i + 5];
checksum ^= bufView[i + 6];
}
bufView[5 + 16] = checksum;
sendFn(connectionId, bufferOut, function () {
serial.disconnect();
});
}, 100);
} else if (connectionId) {
serial.disconnect();
}
}
function closeHandler() {
if (!GUI.isCordova()) {
this.hide();
}
analytics.sendEvent(analytics.EVENT_CATEGORIES.APPLICATION, 'AppClose', { sessionControl: 'end' });
closeSerial();
if (!GUI.isCordova()) {
this.close(true);
}
}
//Process to execute to real start the app
function startProcess() {
// translate to user-selected language
i18n.localizePage();
GUI.log(i18n.getMessage('infoVersionOs', { operatingSystem: GUI.operating_system }));
GUI.log(i18n.getMessage('infoVersionConfigurator', { configuratorVersion: CONFIGURATOR.getDisplayVersion() }));
if (GUI.isNWJS()) {
let nwWindow = GUI.nwGui.Window.get();
nwWindow.on('new-win-policy', function(frame, url, policy) {
// do not open the window
policy.ignore();
// and open it in external browser
GUI.nwGui.Shell.openExternal(url);
});
nwWindow.on('close', closeHandler);
// TODO: Remove visibilitychange Listener when upgrading to NW2
// capture Command H on MacOS and change it to minimize
document.addEventListener("visibilitychange", function() {
if (GUI.operating_system === "MacOS" && document.visibilityState === "hidden") {
nwWindow.minimize();
}
}, false);
} else if (GUI.isCordova()) {
window.addEventListener('beforeunload', closeHandler);
document.addEventListener('backbutton', function(e) {
e.preventDefault();
navigator.notification.confirm(
i18n.getMessage('cordovaExitAppMessage'),
function(stat) {
if (stat === 1) {
navigator.app.exitApp();
}
},
i18n.getMessage('cordovaExitAppTitle'),
[i18n.getMessage('yes'),i18n.getMessage('no')],
);
});
}
$('.connect_b a.connect').removeClass('disabled');
// with Vue reactive system we don't need to call these,
// our view is reactive to model changes
// updateTopBarVersion();
if (!GUI.isOther()) {
checkForConfiguratorUpdates();
}
// log webgl capability
// it would seem the webgl "enabling" through advanced settings will be ignored in the future
// and webgl will be supported if gpu supports it by default (canary 40.0.2175.0), keep an eye on this one
document.createElement('canvas');
// log library versions in console to make version tracking easier
console.log(`Libraries: jQuery - ${$.fn.jquery}, d3 - ${d3.version}, three.js - ${THREE.REVISION}`);
// Tabs
$("#tabs ul.mode-connected li").click(function() {
// store the first class of the current tab (omit things like ".active")
const tabName = $(this).attr("class").split(' ')[0];
const tabNameWithoutPrefix = tabName.substring(4);
if (tabNameWithoutPrefix !== "cli") {
// Don't store 'cli' otherwise you can never connect to another tab.
ConfigStorage.set(
{lastTab: tabName},
);
}
});
if (GUI.isCordova()) {
UI_PHONES.init();
}
const ui_tabs = $('#tabs > ul');
$('a', ui_tabs).click(function () {
if ($(this).parent().hasClass('active') === false && !GUI.tab_switch_in_progress) { // only initialize when the tab isn't already active
const self = this;
const tabClass = $(self).parent().prop('class');
const tabRequiresConnection = $(self).parent().hasClass('mode-connected');
const tab = tabClass.substring(4);
const tabName = $(self).text();
if (tabRequiresConnection && !CONFIGURATOR.connectionValid) {
GUI.log(i18n.getMessage('tabSwitchConnectionRequired'));
return;
}
if (GUI.connect_lock) { // tab switching disabled while operation is in progress
GUI.log(i18n.getMessage('tabSwitchWaitForOperation'));
return;
}
if (GUI.allowedTabs.indexOf(tab) < 0 && tab === "firmware_flasher") {
if (GUI.connected_to || GUI.connecting_to) {
$('a.connect').click();
} else {
serial.disconnect();
}
$('div.open_firmware_flasher a.flash').click();
} else if (GUI.allowedTabs.indexOf(tab) < 0) {
GUI.log(i18n.getMessage('tabSwitchUpgradeRequired', [tabName]));
return;
}
GUI.tab_switch_in_progress = true;
GUI.tab_switch_cleanup(function () {
// disable active firmware flasher if it was active
if ($('div#flashbutton a.flash_state').hasClass('active') && $('div#flashbutton a.flash').hasClass('active')) {
$('div#flashbutton a.flash_state').removeClass('active');
$('div#flashbutton a.flash').removeClass('active');
}
// disable previously active tab highlight
$('li', ui_tabs).removeClass('active');
// Highlight selected tab
$(self).parent().addClass('active');
// detach listeners and remove element data
const content = $('#content');
content.empty();
// display loading screen
$('#cache .data-loading').clone().appendTo(content);
function content_ready() {
GUI.tab_switch_in_progress = false;
}
checkSetupAnalytics(function (analyticsService) {
analyticsService.sendAppView(tab);
});
switch (tab) {
case 'landing':
import("./tabs/landing").then(({ landing }) =>
landing.initialize(content_ready),
);
break;
case 'changelog':
import("./tabs/static_tab").then(({ staticTab }) =>
staticTab.initialize("changelog", content_ready),
);
break;
case 'privacy_policy':
import("./tabs/static_tab").then(({ staticTab }) =>
staticTab.initialize("privacy_policy", content_ready),
);
break;
case 'options':
import("./tabs/options").then(({ options }) =>
options.initialize(content_ready),
);
break;
case 'firmware_flasher':
import("./tabs/firmware_flasher").then(({ firmware_flasher }) =>
firmware_flasher.initialize(content_ready),
);
break;
case 'help':
import('./tabs/help').then(({ help }) => help.initialize(content_ready));
break;
case 'auxiliary':
TABS.auxiliary.initialize(content_ready);
break;
case 'adjustments':
TABS.adjustments.initialize(content_ready);
break;
case 'ports':
TABS.ports.initialize(content_ready);
break;
case 'led_strip':
TABS.led_strip.initialize(content_ready);
break;
case 'failsafe':
TABS.failsafe.initialize(content_ready);
break;
case 'transponder':
TABS.transponder.initialize(content_ready);
break;
case 'osd':
TABS.osd.initialize(content_ready);
break;
case 'vtx':
TABS.vtx.initialize(content_ready);
break;
case 'power':
TABS.power.initialize(content_ready);
break;
case 'setup':
TABS.setup.initialize(content_ready);
break;
case 'setup_osd':
TABS.setup_osd.initialize(content_ready);
break;
case 'configuration':
TABS.configuration.initialize(content_ready);
break;
case 'pid_tuning':
TABS.pid_tuning.initialize(content_ready);
break;
case 'receiver':
TABS.receiver.initialize(content_ready);
break;
case 'servos':
TABS.servos.initialize(content_ready);
break;
case 'gps':
TABS.gps.initialize(content_ready);
break;
case 'motors':
TABS.motors.initialize(content_ready);
break;
case 'sensors':
TABS.sensors.initialize(content_ready);
break;
case 'logging':
TABS.logging.initialize(content_ready);
break;
case 'onboard_logging':
TABS.onboard_logging.initialize(content_ready);
break;
case 'cli':
TABS.cli.initialize(content_ready, GUI.nwGui);
break;
case 'presets':
TABS.presets.initialize(content_ready, GUI.nwGui);
break;
default:
console.log(`Tab not found: ${tab}`);
}
});
}
});
$('#tabs ul.mode-disconnected li a:first').click();
// listen to all input change events and adjust the value within limits if necessary
$("#content").on('focus', 'input[type="number"]', function () {
const element = $(this);
const val = element.val();
if (!isNaN(val)) {
element.data('previousValue', parseFloat(val));
}
});
$("#content").on('keydown', 'input[type="number"]', function (e) {
// whitelist all that we need for numeric control
const whitelist = [
96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, // numpad and standard number keypad
109, 189, // minus on numpad and in standard keyboard
8, 46, 9, // backspace, delete, tab
190, 110, // decimal point
37, 38, 39, 40, 13, // arrows and enter
];
if (whitelist.indexOf(e.keyCode) === -1) {
e.preventDefault();
}
});
$("#content").on('change', 'input[type="number"]', function () {
const element = $(this);
const min = parseFloat(element.prop('min'));
const max = parseFloat(element.prop('max'));
const step = parseFloat(element.prop('step'));
let val = parseFloat(element.val());
// only adjust minimal end if bound is set
if (element.prop('min') && val < min) {
element.val(min);
val = min;
}
// only adjust maximal end if bound is set
if (element.prop('max') && val > max) {
element.val(max);
val = max;
}
// if entered value is illegal use previous value instead
if (isNaN(val)) {
element.val(element.data('previousValue'));
val = element.data('previousValue');
}
// if step is not set or step is int and value is float use previous value instead
if ((isNaN(step) || step % 1 === 0) && val % 1 !== 0) {
element.val(element.data('previousValue'));
val = element.data('previousValue');
}
// if step is set and is float and value is int, convert to float, keep decimal places in float according to step *experimental*
if (!isNaN(step) && step % 1 !== 0) {
const decimal_places = String(step).split('.')[1].length;
if (val % 1 === 0 || String(val).split('.')[1].length !== decimal_places) {
element.val(val.toFixed(decimal_places));
}
}
});
$("#showlog").on('click', function () {
let state = $(this).data('state');
if (state) {
setTimeout(function() {
const command_log = $('div#log');
command_log.scrollTop($('div.wrapper', command_log).height());
}, 200);
$("#log").removeClass('active');
$("#tab-content-container").removeClass('logopen');
$("#scrollicon").removeClass('active');
ConfigStorage.set({'logopen': false});
state = false;
} else {
$("#log").addClass('active');
$("#tab-content-container").addClass('logopen');
$("#scrollicon").addClass('active');
ConfigStorage.set({'logopen': true});
state = true;
}
$(this).text(state ? i18n.getMessage('logActionHide') : i18n.getMessage('logActionShow'));
$(this).data('state', state);
});
ConfigStorage.get('logopen', function (result) {
if (result.logopen) {
$("#showlog").trigger('click');
}
});
ConfigStorage.get('permanentExpertMode', function (result) {
const expertModeCheckbox = 'input[name="expertModeCheckbox"]';
if (result.permanentExpertMode) {
$(expertModeCheckbox).prop('checked', true);
}
$(expertModeCheckbox).on("change", () => {
const checked = $(expertModeCheckbox).is(':checked');
checkSetupAnalytics(function (analyticsService) {
analyticsService.setDimension(analyticsService.DIMENSIONS.CONFIGURATOR_EXPERT_MODE, checked ? 'On' : 'Off');
});
if (FC.FEATURE_CONFIG && FC.FEATURE_CONFIG.features !== 0) {
updateTabList(FC.FEATURE_CONFIG.features);
}
if (GUI.active_tab) {
TABS[GUI.active_tab]?.expertModeChanged?.(checked);
}
});
$(expertModeCheckbox).trigger("change");
});
ConfigStorage.get('cliAutoComplete', function (result) {
CliAutoComplete.setEnabled(typeof result.cliAutoComplete == 'undefined' || result.cliAutoComplete); // On by default
});
ConfigStorage.get('darkTheme', function (result) {
if (result.darkTheme === undefined || typeof result.darkTheme !== "number") {
// sets dark theme to auto if not manually changed
setDarkTheme(2);
} else {
setDarkTheme(result.darkTheme);
}
});
if (GUI.isCordova()) {
let darkMode = false;
const checkDarkMode = function() {
cordova.plugins.ThemeDetection.isDarkModeEnabled(function(success) {
if (success.value !== darkMode) {
darkMode = success.value;
DarkTheme.autoSet();
}
});
};
setInterval(checkDarkMode, 500);
} else {
window.matchMedia("(prefers-color-scheme: dark)").addEventListener("change", function() {
DarkTheme.autoSet();
});
}
}
function setDarkTheme(enabled) {
DarkTheme.setConfig(enabled);
checkSetupAnalytics(function (analyticsService) {
analyticsService.sendEvent(analyticsService.EVENT_CATEGORIES.APPLICATION, 'DarkTheme', enabled);
});
}
function checkForConfiguratorUpdates() {
const releaseChecker = new ReleaseChecker('configurator', 'https://api.github.com/repos/betaflight/betaflight-configurator/releases');
releaseChecker.loadReleaseData(notifyOutdatedVersion);
}
function notifyOutdatedVersion(releaseData) {
ConfigStorage.get('checkForConfiguratorUnstableVersions', function (result) {
let showUnstableReleases = false;
if (result.checkForConfiguratorUnstableVersions) {
showUnstableReleases = true;
}
const versions = releaseData.filter(function (version) {
const semVerVersion = semver.parse(version.tag_name);
if (semVerVersion && (showUnstableReleases || semVerVersion.prerelease.length === 0)) {
return version;
} else {
return null;
}
}).sort(function (v1, v2) {
try {
return semver.compare(v2.tag_name, v1.tag_name);
} catch (e) {
return false;
}
});
if (versions.length > 0) {
CONFIGURATOR.latestVersion = versions[0].tag_name;
CONFIGURATOR.latestVersionReleaseUrl = versions[0].html_url;
}
if (semver.lt(CONFIGURATOR.version, CONFIGURATOR.latestVersion)) {
const message = i18n.getMessage('configuratorUpdateNotice', [CONFIGURATOR.latestVersion, CONFIGURATOR.latestVersionReleaseUrl]);
GUI.log(message);
const dialog = $('.dialogConfiguratorUpdate')[0];
$('.dialogConfiguratorUpdate-content').html(message);
$('.dialogConfiguratorUpdate-closebtn').click(function() {
dialog.close();
});
$('.dialogConfiguratorUpdate-websitebtn').click(function() {
dialog.close();
window.open(CONFIGURATOR.latestVersionReleaseUrl, '_blank');
});
dialog.showModal();
}
});
}
function isExpertModeEnabled() {
return $('input[name="expertModeCheckbox"]').is(':checked');
}
function updateTabList(features) {
if (isExpertModeEnabled()) {
$('#tabs ul.mode-connected li.tab_failsafe').show();
$('#tabs ul.mode-connected li.tab_adjustments').show();
$('#tabs ul.mode-connected li.tab_servos').show();
$('#tabs ul.mode-connected li.tab_sensors').show();
$('#tabs ul.mode-connected li.tab_logging').show();
} else {
$('#tabs ul.mode-connected li.tab_failsafe').hide();
$('#tabs ul.mode-connected li.tab_adjustments').hide();
$('#tabs ul.mode-connected li.tab_servos').hide();
$('#tabs ul.mode-connected li.tab_sensors').hide();
$('#tabs ul.mode-connected li.tab_logging').hide();
}
if (features.isEnabled('GPS') && isExpertModeEnabled()) {
$('#tabs ul.mode-connected li.tab_gps').show();
} else {
$('#tabs ul.mode-connected li.tab_gps').hide();
}
if (features.isEnabled('LED_STRIP')) {
$('#tabs ul.mode-connected li.tab_led_strip').show();
} else {
$('#tabs ul.mode-connected li.tab_led_strip').hide();
}
if (features.isEnabled('TRANSPONDER')) {
$('#tabs ul.mode-connected li.tab_transponder').show();
} else {
$('#tabs ul.mode-connected li.tab_transponder').hide();
}
if (features.isEnabled('OSD')) {
$('#tabs ul.mode-connected li.tab_osd').show();
} else {
$('#tabs ul.mode-connected li.tab_osd').hide();
}
if (semver.gte(FC.CONFIG.apiVersion, API_VERSION_1_36)) {
$('#tabs ul.mode-connected li.tab_power').show();
} else {
$('#tabs ul.mode-connected li.tab_power').hide();
}
if (semver.gte(FC.CONFIG.apiVersion, API_VERSION_1_42)) {
$('#tabs ul.mode-connected li.tab_vtx').show();
} else {
$('#tabs ul.mode-connected li.tab_vtx').hide();
}
}
function zeroPad(value, width) {
let valuePadded = String(value);
while (valuePadded.length < width) {
valuePadded = `0${value}`;
}
return valuePadded;
}
function generateFilename(prefix, suffix) {
const date = new Date();
let filename = prefix;
if (FC.CONFIG) {
if (FC.CONFIG.flightControllerIdentifier) {
filename = `${FC.CONFIG.flightControllerIdentifier}_${filename}`;
}
if(FC.CONFIG.name && FC.CONFIG.name.trim() !== '') {
filename = `${filename}_${FC.CONFIG.name.trim().replace(' ', '_')}`;
}
}
const yyyymmdd = `${date.getFullYear()}${zeroPad(date.getMonth() + 1, 2)}${zeroPad(date.getDate(), 2)}`;
const hhmmss = `${zeroPad(date.getHours(), 2)}${zeroPad(date.getMinutes(), 2)}${zeroPad(date.getSeconds(), 2)}`;
filename = `${filename}_${yyyymmdd}_${hhmmss}`;
return `${filename}.${suffix}`;
}
function showErrorDialog(message) {
const dialog = $('.dialogError')[0];
$('.dialogError-content').html(message);
$('.dialogError-closebtn').click(function() {
dialog.close();
});
dialog.showModal();
}
// TODO: all of these are used as globals in other parts.
// once moved to modules extract to own module.
window.googleAnalytics = analytics;
window.analytics = null;
window.showErrorDialog = showErrorDialog;
window.generateFilename = generateFilename;
window.updateTabList = updateTabList;
window.isExpertModeEnabled = isExpertModeEnabled;
window.checkForConfiguratorUpdates = checkForConfiguratorUpdates;
window.setDarkTheme = setDarkTheme;
window.appReady = appReady;
window.checkSetupAnalytics = checkSetupAnalytics;
|
ajax/libs/react-slick/0.13.0/react-slick.min.js | kennynaoh/cdnjs | !function(t,e){"object"==typeof exports&&"object"==typeof module?module.exports=e(require("react"),require("react-dom")):"function"==typeof define&&define.amd?define(["react","react-dom"],e):"object"==typeof exports?exports.Slider=e(require("react"),require("react-dom")):t.Slider=e(t.React,t.ReactDOM)}(this,function(t,e){return function(t){function e(s){if(i[s])return i[s].exports;var r=i[s]={exports:{},id:s,loaded:!1};return t[s].call(r.exports,r,r.exports,e),r.loaded=!0,r.exports}var i={};return e.m=t,e.c=i,e.p="",e(0)}([function(t,e,i){"use strict";t.exports=i(1)},function(t,e,i){"use strict";function s(t){return t&&t.__esModule?t:{"default":t}}var r=i(2),n=s(r),o=i(3),a=i(8),l=s(a),d=i(15),u=s(d),c=i(17),h=s(c),p=i(10),f=s(p),S=n["default"].createClass({displayName:"Slider",mixins:[h["default"]],getInitialState:function(){return{breakpoint:null}},componentDidMount:function(){var t=this;if(this.props.responsive){var e=this.props.responsive.map(function(t){return t.breakpoint});e.sort(function(t,e){return t-e}),e.forEach(function(i,s){var r;r=0===s?(0,u["default"])({minWidth:0,maxWidth:i}):(0,u["default"])({minWidth:e[s-1],maxWidth:i}),t.media(r,function(){t.setState({breakpoint:i})})});var i=(0,u["default"])({minWidth:e.slice(-1)[0]});this.media(i,function(){t.setState({breakpoint:null})})}},render:function(){var t,e,i=this;this.state.breakpoint?(e=this.props.responsive.filter(function(t){return t.breakpoint===i.state.breakpoint}),t="unslick"===e[0].settings?"unslick":(0,l["default"])({},this.props,e[0].settings)):t=(0,l["default"])({},f["default"],this.props);var s=this.props.children;return Array.isArray(s)||(s=[s]),s=s.filter(function(t){return!!t}),"unslick"===t?n["default"].createElement("div",null,s):n["default"].createElement(o.InnerSlider,t,s)}});t.exports=S},function(e,i){e.exports=t},function(t,e,i){"use strict";function s(t){return t&&t.__esModule?t:{"default":t}}e.__esModule=!0,e.InnerSlider=void 0;var r=Object.assign||function(t){for(var e=1;e<arguments.length;e++){var i=arguments[e];for(var s in i)Object.prototype.hasOwnProperty.call(i,s)&&(t[s]=i[s])}return t},n=i(2),o=s(n),a=i(4),l=s(a),d=i(7),u=s(d),c=i(9),h=s(c),p=i(10),f=s(p),S=i(11),v=s(S),g=i(12),w=i(13),m=i(14);e.InnerSlider=o["default"].createClass({displayName:"InnerSlider",mixins:[u["default"],l["default"]],getInitialState:function(){return r({},h["default"],{currentSlide:this.props.initialSlide})},getDefaultProps:function(){return f["default"]},componentWillMount:function(){this.props.init&&this.props.init(),this.setState({mounted:!0});for(var t=[],e=0;e<o["default"].Children.count(this.props.children);e++)e>=this.state.currentSlide&&e<this.state.currentSlide+this.props.slidesToShow&&t.push(e);this.props.lazyLoad&&0===this.state.lazyLoadedList.length&&this.setState({lazyLoadedList:t})},componentDidMount:function(){this.initialize(this.props),this.adaptHeight(),window.addEventListener?window.addEventListener("resize",this.onWindowResized):window.attachEvent("onresize",this.onWindowResized)},componentWillUnmount:function(){this.animationEndCallback&&clearTimeout(this.animationEndCallback),window.addEventListener?window.removeEventListener("resize",this.onWindowResized):window.detachEvent("onresize",this.onWindowResized),this.state.autoPlayTimer&&window.clearInterval(this.state.autoPlayTimer)},componentWillReceiveProps:function(t){this.props.slickGoTo!=t.slickGoTo?this.changeSlide({message:"index",index:t.slickGoTo,currentSlide:this.state.currentSlide}):this.update(t)},componentDidUpdate:function(){this.adaptHeight()},onWindowResized:function(){this.update(this.props),this.setState({animating:!1})},render:function(){var t,e=(0,v["default"])("slick-initialized","slick-slider",this.props.className),i={fade:this.props.fade,cssEase:this.props.cssEase,speed:this.props.speed,infinite:this.props.infinite,centerMode:this.props.centerMode,currentSlide:this.state.currentSlide,lazyLoad:this.props.lazyLoad,lazyLoadedList:this.state.lazyLoadedList,rtl:this.props.rtl,slideWidth:this.state.slideWidth,slidesToShow:this.props.slidesToShow,slideCount:this.state.slideCount,trackStyle:this.state.trackStyle,variableWidth:this.props.variableWidth};if(this.props.dots===!0&&this.state.slideCount>=this.props.slidesToShow){var s={dotsClass:this.props.dotsClass,slideCount:this.state.slideCount,slidesToShow:this.props.slidesToShow,currentSlide:this.state.currentSlide,slidesToScroll:this.props.slidesToScroll,clickHandler:this.changeSlide};t=o["default"].createElement(w.Dots,s)}var n,a,l={infinite:this.props.infinite,centerMode:this.props.centerMode,currentSlide:this.state.currentSlide,slideCount:this.state.slideCount,slidesToShow:this.props.slidesToShow,prevArrow:this.props.prevArrow,nextArrow:this.props.nextArrow,clickHandler:this.changeSlide};this.props.arrows&&(n=o["default"].createElement(m.PrevArrow,l),a=o["default"].createElement(m.NextArrow,l));var d=null;return this.props.vertical===!1?this.props.centerMode===!0&&(d={padding:"0px "+this.props.centerPadding}):this.props.centerMode===!0&&(d={padding:this.props.centerPadding+" 0px"}),o["default"].createElement("div",{className:e,onMouseEnter:this.onInnerSliderEnter,onMouseLeave:this.onInnerSliderLeave},o["default"].createElement("div",{ref:"list",className:"slick-list",style:d,onMouseDown:this.swipeStart,onMouseMove:this.state.dragging?this.swipeMove:null,onMouseUp:this.swipeEnd,onMouseLeave:this.state.dragging?this.swipeEnd:null,onTouchStart:this.swipeStart,onTouchMove:this.state.dragging?this.swipeMove:null,onTouchEnd:this.swipeEnd,onTouchCancel:this.state.dragging?this.swipeEnd:null},o["default"].createElement(g.Track,r({ref:"track"},i),this.props.children)),n,a,t)}})},function(t,e,i){"use strict";function s(t){return t&&t.__esModule?t:{"default":t}}e.__esModule=!0;var r=i(5),n=i(7),o=(s(n),i(8)),a=s(o),l={changeSlide:function(t){var e,i,s,r,n,o=this.props,a=o.slidesToScroll,l=o.slidesToShow,d=this.state,u=d.slideCount,c=d.currentSlide;if(r=u%a!==0,e=r?0:(u-c)%a,"previous"===t.message)s=0===e?a:l-e,n=c-s,this.props.lazyLoad&&(i=c-s,n=-1===i?u-1:i);else if("next"===t.message)s=0===e?a:e,n=c+s,this.props.lazyLoad&&(n=(c+a)%u+e);else if("dots"===t.message){if(n=t.index*t.slidesToScroll,n===t.currentSlide)return}else if("index"===t.message&&(n=t.index,n===t.currentSlide))return;this.slideHandler(n)},keyHandler:function(t){},selectHandler:function(t){},swipeStart:function(t){var e,i;this.props.swipe===!1||"ontouchend"in document&&this.props.swipe===!1||this.props.draggable===!1&&-1!==t.type.indexOf("mouse")||(e=void 0!==t.touches?t.touches[0].pageX:t.clientX,i=void 0!==t.touches?t.touches[0].pageY:t.clientY,this.setState({dragging:!0,touchObject:{startX:e,startY:i,curX:e,curY:i}}))},swipeMove:function(t){if(!this.state.dragging)return void t.preventDefault();if(!this.state.animating){var e,i,s,n=this.state.touchObject;i=(0,r.getTrackLeft)((0,a["default"])({slideIndex:this.state.currentSlide,trackRef:this.refs.track},this.props,this.state)),n.curX=t.touches?t.touches[0].pageX:t.clientX,n.curY=t.touches?t.touches[0].pageY:t.clientY,n.swipeLength=Math.round(Math.sqrt(Math.pow(n.curX-n.startX,2))),s=(this.props.rtl===!1?1:-1)*(n.curX>n.startX?1:-1);var o=this.state.currentSlide,l=Math.ceil(this.state.slideCount/this.props.slidesToScroll),d=this.swipeDirection(this.state.touchObject),u=n.swipeLength;this.props.infinite===!1&&(0===o&&"right"===d||o+1>=l&&"left"===d)&&(u=n.swipeLength*this.props.edgeFriction,this.state.edgeDragged===!1&&this.props.edgeEvent&&(this.props.edgeEvent(d),this.setState({edgeDragged:!0}))),this.state.swiped===!1&&this.props.swipeEvent&&(this.props.swipeEvent(d),this.setState({swiped:!0})),e=i+u*s,this.setState({touchObject:n,swipeLeft:e,trackStyle:(0,r.getTrackCSS)((0,a["default"])({left:e},this.props,this.state))}),Math.abs(n.curX-n.startX)<.8*Math.abs(n.curY-n.startY)||n.swipeLength>4&&t.preventDefault()}},swipeEnd:function(t){if(!this.state.dragging)return void t.preventDefault();var e=this.state.touchObject,i=this.state.listWidth/this.props.touchThreshold,s=this.swipeDirection(e);if(this.setState({dragging:!1,edgeDragged:!1,swiped:!1,swipeLeft:null,touchObject:{}}),e.swipeLength)if(e.swipeLength>i)t.preventDefault(),"left"===s?this.slideHandler(this.state.currentSlide+this.props.slidesToScroll):"right"===s?this.slideHandler(this.state.currentSlide-this.props.slidesToScroll):this.slideHandler(this.state.currentSlide);else{var n=(0,r.getTrackLeft)((0,a["default"])({slideIndex:this.state.currentSlide,trackRef:this.refs.track},this.props,this.state));this.setState({trackStyle:(0,r.getTrackAnimateCSS)((0,a["default"])({left:n},this.props,this.state))})}},onInnerSliderEnter:function(t){this.props.autoplay&&this.props.pauseOnHover&&this.pause()},onInnerSliderLeave:function(t){this.props.autoplay&&this.props.pauseOnHover&&this.autoPlay()}};e["default"]=l},function(t,e,i){"use strict";function s(t){return t&&t.__esModule?t:{"default":t}}e.__esModule=!0,e.getTrackLeft=e.getTrackAnimateCSS=e.getTrackCSS=void 0;var r=i(6),n=s(r),o=function(t,e){return e.reduce(function(e,i){return e&&t.hasOwnProperty(i)},!0)?null:console.error("Keys Missing",t)},a=e.getTrackCSS=function(t){o(t,["left","variableWidth","slideCount","slidesToShow","slideWidth"]);var e;e=t.variableWidth?(t.slideCount+2*t.slidesToShow)*t.slideWidth:t.centerMode?(t.slideCount+2*(t.slidesToShow+1))*t.slideWidth:(t.slideCount+2*t.slidesToShow)*t.slideWidth;var i={opacity:1,width:e,WebkitTransform:"translate3d("+t.left+"px, 0px, 0px)",transform:"translate3d("+t.left+"px, 0px, 0px)",transition:"",WebkitTransition:"",msTransform:"translateX("+t.left+"px)"};return!window.addEventListener&&window.attachEvent&&(i.marginLeft=t.left+"px"),i};e.getTrackAnimateCSS=function(t){o(t,["left","variableWidth","slideCount","slidesToShow","slideWidth","speed","cssEase"]);var e=a(t);return e.WebkitTransition="-webkit-transform "+t.speed+"ms "+t.cssEase,e.transition="transform "+t.speed+"ms "+t.cssEase,e},e.getTrackLeft=function(t){o(t,["slideIndex","trackRef","infinite","centerMode","slideCount","slidesToShow","slidesToScroll","slideWidth","listWidth","variableWidth"]);var e,i,s=0;if(t.fade)return 0;if(t.infinite&&(t.slideCount>t.slidesToShow&&(s=t.slideWidth*t.slidesToShow*-1),t.slideCount%t.slidesToScroll!==0&&t.slideIndex+t.slidesToScroll>t.slideCount&&t.slideCount>t.slidesToShow&&(s=t.slideIndex>t.slideCount?(t.slidesToShow-(t.slideIndex-t.slideCount))*t.slideWidth*-1:t.slideCount%t.slidesToScroll*t.slideWidth*-1)),t.centerMode&&(t.infinite?s+=t.slideWidth*Math.floor(t.slidesToShow/2):s=t.slideWidth*Math.floor(t.slidesToShow/2)),e=t.slideIndex*t.slideWidth*-1+s,t.variableWidth===!0){var r;t.slideCount<=t.slidesToShow||t.infinite===!1?i=n["default"].findDOMNode(t.trackRef).childNodes[t.slideIndex]:(r=t.slideIndex+t.slidesToShow,i=n["default"].findDOMNode(t.trackRef).childNodes[r]),e=i?-1*i.offsetLeft:0,t.centerMode===!0&&(i=t.infinite===!1?n["default"].findDOMNode(t.trackRef).children[t.slideIndex]:n["default"].findDOMNode(t.trackRef).children[t.slideIndex+t.slidesToShow+1],e=i?-1*i.offsetLeft:0,e+=(t.listWidth-i.offsetWidth)/2)}return e}},function(t,i){t.exports=e},function(t,e,i){"use strict";function s(t){return t&&t.__esModule?t:{"default":t}}e.__esModule=!0;var r=i(2),n=s(r),o=i(6),a=s(o),l=i(5),d=i(8),u=s(d),c={initialize:function(t){var e=n["default"].Children.count(t.children),i=this.getWidth(a["default"].findDOMNode(this.refs.list)),s=this.getWidth(a["default"].findDOMNode(this.refs.track)),r=s/t.slidesToShow,o=t.rtl?e-1-t.initialSlide:t.initialSlide;this.setState({slideCount:e,slideWidth:r,listWidth:i,trackWidth:s,currentSlide:o},function(){var e=(0,l.getTrackLeft)((0,u["default"])({slideIndex:this.state.currentSlide,trackRef:this.refs.track},t,this.state)),i=(0,l.getTrackCSS)((0,u["default"])({left:e},t,this.state));this.setState({trackStyle:i}),this.autoPlay()})},update:function(t){var e=n["default"].Children.count(t.children),i=this.getWidth(a["default"].findDOMNode(this.refs.list)),s=this.getWidth(a["default"].findDOMNode(this.refs.track)),r=this.getWidth(a["default"].findDOMNode(this))/t.slidesToShow;t.autoplay||this.pause(),this.setState({slideCount:e,slideWidth:r,listWidth:i,trackWidth:s},function(){var e=(0,l.getTrackLeft)((0,u["default"])({slideIndex:this.state.currentSlide,trackRef:this.refs.track},t,this.state)),i=(0,l.getTrackCSS)((0,u["default"])({left:e},t,this.state));this.setState({trackStyle:i})})},getWidth:function(t){return t.getBoundingClientRect().width||t.offsetWidth},adaptHeight:function(){if(this.props.adaptiveHeight){var t='[data-index="'+this.state.currentSlide+'"]';if(this.refs.list){var e=a["default"].findDOMNode(this.refs.list);e.style.height=e.querySelector(t).offsetHeight+"px"}}},slideHandler:function(t){var e,i,s,r,n,o=this;if(!this.props.waitForAnimate||!this.state.animating){if(this.props.fade)return i=this.state.currentSlide,e=0>t?t+this.state.slideCount:t>=this.state.slideCount?t-this.state.slideCount:t,this.props.lazyLoad&&this.state.lazyLoadedList.indexOf(e)<0&&this.setState({lazyLoadedList:this.state.lazyLoadedList.concat(e)}),n=function(){o.setState({animating:!1}),o.props.afterChange&&o.props.afterChange(e),delete o.animationEndCallback},this.setState({animating:!0,currentSlide:e},function(){this.animationEndCallback=setTimeout(n,this.props.speed)}),this.props.beforeChange&&this.props.beforeChange(this.state.currentSlide,e),void this.autoPlay();if(e=t,i=0>e?this.props.infinite===!1?0:this.state.slideCount%this.props.slidesToScroll!==0?this.state.slideCount-this.state.slideCount%this.props.slidesToScroll:this.state.slideCount+e:e>=this.state.slideCount?this.props.infinite===!1?this.state.slideCount-this.props.slidesToShow:this.state.slideCount%this.props.slidesToScroll!==0?0:e-this.state.slideCount:e,s=(0,l.getTrackLeft)((0,u["default"])({slideIndex:e,trackRef:this.refs.track},this.props,this.state)),r=(0,l.getTrackLeft)((0,u["default"])({slideIndex:i,trackRef:this.refs.track},this.props,this.state)),this.props.infinite===!1&&(s=r),this.props.beforeChange&&this.props.beforeChange(this.state.currentSlide,i),this.props.lazyLoad){for(var a=!0,d=[],c=e;c<e+this.props.slidesToShow;c++)a=a&&this.state.lazyLoadedList.indexOf(c)>=0,a||d.push(c);a||this.setState({lazyLoadedList:this.state.lazyLoadedList.concat(d)})}if(this.props.useCSS===!1)this.setState({currentSlide:i,trackStyle:(0,l.getTrackCSS)((0,u["default"])({left:r},this.props,this.state))},function(){this.props.afterChange&&this.props.afterChange(i)});else{var h={animating:!1,currentSlide:i,trackStyle:(0,l.getTrackCSS)((0,u["default"])({left:r},this.props,this.state)),swipeLeft:null};n=function(){o.setState(h),o.props.afterChange&&o.props.afterChange(i),delete o.animationEndCallback},this.setState({animating:!0,currentSlide:i,trackStyle:(0,l.getTrackAnimateCSS)((0,u["default"])({left:s},this.props,this.state))},function(){this.animationEndCallback=setTimeout(n,this.props.speed)})}this.autoPlay()}},swipeDirection:function(t){var e,i,s,r;return e=t.startX-t.curX,i=t.startY-t.curY,s=Math.atan2(i,e),r=Math.round(180*s/Math.PI),0>r&&(r=360-Math.abs(r)),45>=r&&r>=0||360>=r&&r>=315?this.props.rtl===!1?"left":"right":r>=135&&225>=r?this.props.rtl===!1?"right":"left":"vertical"},autoPlay:function(){var t=this;if(!this.state.autoPlayTimer){var e=function(){if(t.state.mounted){var e=t.props.rtl?t.state.currentSlide-t.props.slidesToScroll:t.state.currentSlide+t.props.slidesToScroll;t.slideHandler(e)}};this.props.autoplay&&this.setState({autoPlayTimer:window.setInterval(e,this.props.autoplaySpeed)})}},pause:function(){this.state.autoPlayTimer&&(window.clearInterval(this.state.autoPlayTimer),this.setState({autoPlayTimer:null}))}};e["default"]=c},function(t,e){"use strict";function i(t){if(null===t||void 0===t)throw new TypeError("Object.assign cannot be called with null or undefined");return Object(t)}function s(){try{if(!Object.assign)return!1;var t=new String("abc");if(t[5]="de","5"===Object.getOwnPropertyNames(t)[0])return!1;for(var e={},i=0;10>i;i++)e["_"+String.fromCharCode(i)]=i;var s=Object.getOwnPropertyNames(e).map(function(t){return e[t]});if("0123456789"!==s.join(""))return!1;var r={};return"abcdefghijklmnopqrst".split("").forEach(function(t){r[t]=t}),"abcdefghijklmnopqrst"===Object.keys(Object.assign({},r)).join("")}catch(n){return!1}}var r=Object.prototype.hasOwnProperty,n=Object.prototype.propertyIsEnumerable;t.exports=s()?Object.assign:function(t,e){for(var s,o,a=i(t),l=1;l<arguments.length;l++){s=Object(arguments[l]);for(var d in s)r.call(s,d)&&(a[d]=s[d]);if(Object.getOwnPropertySymbols){o=Object.getOwnPropertySymbols(s);for(var u=0;u<o.length;u++)n.call(s,o[u])&&(a[o[u]]=s[o[u]])}}return a}},function(t,e){"use strict";var i={animating:!1,dragging:!1,autoPlayTimer:null,currentDirection:0,currentLeft:null,currentSlide:0,direction:1,slideCount:null,slideWidth:null,swipeLeft:null,touchObject:{startX:0,startY:0,curX:0,curY:0},lazyLoadedList:[],initialized:!1,edgeDragged:!1,swiped:!1,trackStyle:{},trackWidth:0};t.exports=i},function(t,e){"use strict";var i={className:"",adaptiveHeight:!1,arrows:!0,autoplay:!1,autoplaySpeed:3e3,centerMode:!1,centerPadding:"50px",cssEase:"ease",dots:!1,dotsClass:"slick-dots",draggable:!0,easing:"linear",edgeFriction:.35,fade:!1,focusOnSelect:!1,infinite:!0,initialSlide:0,lazyLoad:!1,pauseOnHover:!1,responsive:null,rtl:!1,slide:"div",slidesToShow:1,slidesToScroll:1,speed:500,swipe:!0,swipeToSlide:!1,touchMove:!0,touchThreshold:5,useCSS:!0,variableWidth:!1,vertical:!1,waitForAnimate:!0,afterChange:null,beforeChange:null,edgeEvent:null,init:null,swipeEvent:null,nextArrow:null,prevArrow:null};t.exports=i},function(t,e,i){var s,r;/*!
Copyright (c) 2016 Jed Watson.
Licensed under the MIT License (MIT), see
http://jedwatson.github.io/classnames
*/
!function(){"use strict";function i(){for(var t=[],e=0;e<arguments.length;e++){var s=arguments[e];if(s){var r=typeof s;if("string"===r||"number"===r)t.push(s);else if(Array.isArray(s))t.push(i.apply(null,s));else if("object"===r)for(var o in s)n.call(s,o)&&s[o]&&t.push(o)}}return t.join(" ")}var n={}.hasOwnProperty;"undefined"!=typeof t&&t.exports?t.exports=i:(s=[],r=function(){return i}.apply(e,s),!(void 0!==r&&(t.exports=r)))}()},function(t,e,i){"use strict";function s(t){return t&&t.__esModule?t:{"default":t}}e.__esModule=!0,e.Track=void 0;var r=i(2),n=s(r),o=i(8),a=s(o),l=i(11),d=s(l),u=function(t){var e,i,s,r,n;return n=t.rtl?t.slideCount-1-t.index:t.index,s=0>n||n>=t.slideCount,t.centerMode?(r=Math.floor(t.slidesToShow/2),i=(n-t.currentSlide)%t.slideCount===0,n>t.currentSlide-r-1&&n<=t.currentSlide+r&&(e=!0)):e=t.currentSlide<=n&&n<t.currentSlide+t.slidesToShow,(0,d["default"])({"slick-slide":!0,"slick-active":e,"slick-center":i,"slick-cloned":s})},c=function(t){var e={};return void 0!==t.variableWidth&&t.variableWidth!==!1||(e.width=t.slideWidth),t.fade&&(e.position="relative",e.left=-t.index*t.slideWidth,e.opacity=t.currentSlide===t.index?1:0,e.transition="opacity "+t.speed+"ms "+t.cssEase,e.WebkitTransition="opacity "+t.speed+"ms "+t.cssEase),e},h=function(t,e){return null===t.key||void 0===t.key?e:t.key},p=function(t){var e,i,s=[],r=[],o=[],l=n["default"].Children.count(t.children);return n["default"].Children.forEach(t.children,function(p,f){i=!t.lazyLoad|(t.lazyLoad&&t.lazyLoadedList.indexOf(f)>=0)?p:n["default"].createElement("div",null);var S,v=c((0,a["default"])({},t,{index:f})),g=u((0,a["default"])({index:f},t));if(S=i.props.className?(0,d["default"])(g,i.props.className):g,s.push(n["default"].cloneElement(i,{key:"original"+h(i,f),"data-index":f,className:S,style:(0,a["default"])({},i.props.style||{},v)})),t.infinite&&t.fade===!1){var w=t.variableWidth?t.slidesToShow+1:t.slidesToShow;f>=l-w&&(e=-(l-f),r.push(n["default"].cloneElement(i,{key:"precloned"+h(i,e),"data-index":e,className:S,style:(0,a["default"])({},i.props.style||{},v)}))),w>f&&(e=l+f,o.push(n["default"].cloneElement(i,{key:"postcloned"+h(i,e),"data-index":e,className:S,style:(0,a["default"])({},i.props.style||{},v)})))}}),t.rtl?r.concat(s,o).reverse():r.concat(s,o)};e.Track=n["default"].createClass({displayName:"Track",render:function(){var t=p(this.props);return n["default"].createElement("div",{className:"slick-track",style:this.props.trackStyle},t)}})},function(t,e,i){"use strict";function s(t){return t&&t.__esModule?t:{"default":t}}e.__esModule=!0,e.Dots=void 0;var r=i(2),n=s(r),o=i(11),a=s(o),l=function(t){var e;return e=Math.ceil(t.slideCount/t.slidesToScroll)};e.Dots=n["default"].createClass({displayName:"Dots",clickHandler:function(t,e){e.preventDefault(),this.props.clickHandler(t)},render:function(){var t=this,e=l({slideCount:this.props.slideCount,slidesToScroll:this.props.slidesToScroll}),i=Array.apply(null,Array(e+1).join("0").split("")).map(function(e,i){var s=i*t.props.slidesToScroll,r=i*t.props.slidesToScroll+(t.props.slidesToScroll-1),o=(0,a["default"])({"slick-active":t.props.currentSlide>=s&&t.props.currentSlide<=r}),l={message:"dots",index:i,slidesToScroll:t.props.slidesToScroll,currentSlide:t.props.currentSlide};return n["default"].createElement("li",{key:i,className:o},n["default"].createElement("button",{onClick:t.clickHandler.bind(t,l)},i+1))});return n["default"].createElement("ul",{className:this.props.dotsClass,style:{display:"block"}},i)}})},function(t,e,i){"use strict";function s(t){return t&&t.__esModule?t:{"default":t}}e.__esModule=!0,e.NextArrow=e.PrevArrow=void 0;var r=Object.assign||function(t){for(var e=1;e<arguments.length;e++){var i=arguments[e];for(var s in i)Object.prototype.hasOwnProperty.call(i,s)&&(t[s]=i[s])}return t},n=i(2),o=s(n),a=i(11),l=s(a);e.PrevArrow=o["default"].createClass({displayName:"PrevArrow",clickHandler:function(t,e){e&&e.preventDefault(),this.props.clickHandler(t,e)},render:function(){var t={"slick-arrow":!0,"slick-prev":!0},e=this.clickHandler.bind(this,{message:"previous"});!this.props.infinite&&(0===this.props.currentSlide||this.props.slideCount<=this.props.slidesToShow)&&(t["slick-disabled"]=!0,e=null);var i,s={key:"0","data-role":"none",className:(0,l["default"])(t),style:{display:"block"},onClick:e};return i=this.props.prevArrow?o["default"].cloneElement(this.props.prevArrow,s):o["default"].createElement("button",r({key:"0",type:"button"},s)," Previous")}}),e.NextArrow=o["default"].createClass({displayName:"NextArrow",clickHandler:function(t,e){e&&e.preventDefault(),this.props.clickHandler(t,e)},render:function(){var t={"slick-arrow":!0,"slick-next":!0},e=this.clickHandler.bind(this,{message:"next"});this.props.infinite||(this.props.centerMode&&this.props.currentSlide>=this.props.slideCount-1?(t["slick-disabled"]=!0,e=null):this.props.currentSlide>=this.props.slideCount-this.props.slidesToShow&&(t["slick-disabled"]=!0,e=null),this.props.slideCount<=this.props.slidesToShow&&(t["slick-disabled"]=!0,e=null));var i,s={key:"1","data-role":"none",className:(0,l["default"])(t),style:{display:"block"},onClick:e};return i=this.props.nextArrow?o["default"].cloneElement(this.props.nextArrow,s):o["default"].createElement("button",r({key:"1",type:"button"},s)," Next")}})},function(t,e,i){var s=i(16),r=function(t){var e=/[height|width]$/;return e.test(t)},n=function(t){var e="",i=Object.keys(t);return i.forEach(function(n,o){var a=t[n];n=s(n),r(n)&&"number"==typeof a&&(a+="px"),e+=a===!0?n:a===!1?"not "+n:"("+n+": "+a+")",o<i.length-1&&(e+=" and ")}),e},o=function(t){var e="";return"string"==typeof t?t:t instanceof Array?(t.forEach(function(i,s){e+=n(i),s<t.length-1&&(e+=", ")}),e):n(t)};t.exports=o},function(t,e){var i=function(t){return t.replace(/[A-Z]/g,function(t){return"-"+t.toLowerCase()}).toLowerCase()};t.exports=i},function(t,e,i){var s=i(18),r=s&&i(19),n=i(15),o={media:function(t,e){t=n(t),"function"==typeof e&&(e={match:e}),r.register(t,e),this._responsiveMediaHandlers||(this._responsiveMediaHandlers=[]),this._responsiveMediaHandlers.push({query:t,handler:e})},componentWillUnmount:function(){this._responsiveMediaHandlers&&this._responsiveMediaHandlers.forEach(function(t){r.unregister(t.query,t.handler)})}};t.exports=o},function(t,e){var i=!("undefined"==typeof window||!window.document||!window.document.createElement);t.exports=i},function(t,e,i){var s;!function(r,n,o){var a=window.matchMedia;"undefined"!=typeof t&&t.exports?t.exports=o(a):(s=function(){return n[r]=o(a)}.call(e,i,e,t),!(void 0!==s&&(t.exports=s)))}("enquire",this,function(t){"use strict";function e(t,e){var i,s=0,r=t.length;for(s;r>s&&(i=e(t[s],s),i!==!1);s++);}function i(t){return"[object Array]"===Object.prototype.toString.apply(t)}function s(t){return"function"==typeof t}function r(t){this.options=t,!t.deferSetup&&this.setup()}function n(e,i){this.query=e,this.isUnconditional=i,this.handlers=[],this.mql=t(e);var s=this;this.listener=function(t){s.mql=t,s.assess()},this.mql.addListener(this.listener)}function o(){if(!t)throw new Error("matchMedia not present, legacy browsers require a polyfill");this.queries={},this.browserIsIncapable=!t("only all").matches}return r.prototype={setup:function(){this.options.setup&&this.options.setup(),this.initialised=!0},on:function(){!this.initialised&&this.setup(),this.options.match&&this.options.match()},off:function(){this.options.unmatch&&this.options.unmatch()},destroy:function(){this.options.destroy?this.options.destroy():this.off()},equals:function(t){return this.options===t||this.options.match===t}},n.prototype={addHandler:function(t){var e=new r(t);this.handlers.push(e),this.matches()&&e.on()},removeHandler:function(t){var i=this.handlers;e(i,function(e,s){return e.equals(t)?(e.destroy(),!i.splice(s,1)):void 0})},matches:function(){return this.mql.matches||this.isUnconditional},clear:function(){e(this.handlers,function(t){t.destroy()}),this.mql.removeListener(this.listener),this.handlers.length=0},assess:function(){var t=this.matches()?"on":"off";e(this.handlers,function(e){e[t]()})}},o.prototype={register:function(t,r,o){var a=this.queries,l=o&&this.browserIsIncapable;return a[t]||(a[t]=new n(t,l)),s(r)&&(r={match:r}),i(r)||(r=[r]),e(r,function(e){a[t].addHandler(e)}),this},unregister:function(t,e){var i=this.queries[t];return i&&(e?i.removeHandler(e):(i.clear(),delete this.queries[t])),this}},new o})}])}); |
src/__tests__/Button.spec.js | reactstrap/reactstrap | import React from 'react';
import { shallow, mount } from 'enzyme';
import { Button } from '../';
describe('Button', () => {
it('should render children', () => {
const wrapper = shallow(<Button>Ello world</Button>);
expect(wrapper.text()).toBe('Ello world');
});
it('should render custom element', () => {
const Link = props => <a href="/home" {...props}>{props.children}</a>;
const wrapper = mount(<Button tag={Link}>Home</Button>);
expect(wrapper.find('a').hostNodes().length).toBe(1);
expect(wrapper.text()).toBe('Home');
});
it('should render an anchor element if href exists', () => {
const wrapper = mount(<Button href="/home">Home</Button>);
expect(wrapper.find('a').hostNodes().length).toBe(1);
expect(wrapper.text()).toBe('Home');
});
it('should render type as undefined by default when tag is "button"', () => {
const wrapper = mount(<Button>Home</Button>);
expect(wrapper.find('button').hostNodes().prop('type')).toBe(undefined);
expect(wrapper.text()).toBe('Home');
});
it('should render type as "button" by default when tag is "button" and onClick is provided', () => {
const wrapper = mount(<Button onClick={() => {}}>Home</Button>);
expect(wrapper.find('button').hostNodes().prop('type')).toBe('button');
expect(wrapper.text()).toBe('Home');
});
it('should render type as user defined when defined by the user', () => {
const wrapper = mount(<Button type="submit">Home</Button>);
expect(wrapper.find('button').hostNodes().prop('type')).toBe('submit');
expect(wrapper.text()).toBe('Home');
});
it('should not render type by default when the type is not defined and the tag is not "button"', () => {
const wrapper = mount(<Button tag="a">Home</Button>);
expect(wrapper.find('a').hostNodes().prop('type')).toBe(undefined);
expect(wrapper.text()).toBe('Home');
});
it('should not render type by default when the type is not defined and the href is defined', () => {
const wrapper = mount(<Button href="#">Home</Button>);
expect(wrapper.find('a').hostNodes().prop('type')).toBe(undefined);
expect(wrapper.text()).toBe('Home');
});
it('should render buttons with default color', () => {
const wrapper = shallow(<Button>Default Button</Button>);
expect(wrapper.hasClass('btn-secondary')).toBe(true);
});
it('should render buttons with other colors', () => {
const wrapper = shallow(<Button color="danger">Default Button</Button>);
expect(wrapper.hasClass('btn-danger')).toBe(true);
});
it('should render buttons with outline variant', () => {
const wrapper = shallow(<Button outline>Default Button</Button>);
expect(wrapper.hasClass('btn-outline-secondary')).toBe(true);
});
it('should render buttons with outline variant with different colors', () => {
const wrapper = shallow(<Button outline color="info">Default Button</Button>);
expect(wrapper.hasClass('btn-outline-info')).toBe(true);
});
it('should render buttons at different sizes', () => {
const small = shallow(<Button size="sm">Small Button</Button>);
const large = shallow(<Button size="lg">Large Button</Button>);
expect(small.hasClass('btn-sm')).toBe(true);
expect(large.hasClass('btn-lg')).toBe(true);
});
it('should render block level buttons', () => {
const block = shallow(<Button block>Block Level Button</Button>);
expect(block.hasClass('d-block w-100')).toBe(true);
});
it('should render close icon with custom child and props', () => {
const testChild = 'close this thing';
const wrapper = shallow(<Button close>{testChild}</Button>);
expect(wrapper.contains(testChild));
});
describe('onClick', () => {
it('calls props.onClick if it exists', () => {
const onClick = jest.fn();
const wrapper = mount(<Button onClick={onClick}>Testing Click</Button>);
wrapper.find('button').hostNodes().simulate('click');
expect(onClick).toHaveBeenCalled();
});
it('returns the value returned by props.onClick', () => {
const onClick = jest.fn(() => 1234);
const wrapper = mount(<Button onClick={onClick}>Testing Click</Button>);
const result = wrapper.find('button').props().onClick();
expect(onClick).toHaveBeenCalled();
expect(result).toEqual(1234);
});
it('is not called when disabled', () => {
const e = createSpyObj('e', ['preventDefault']);
const wrapper = mount(<Button>Testing Click</Button>);
wrapper.instance().onClick(e);
expect(e.preventDefault).not.toHaveBeenCalled();
wrapper.setProps({ disabled: true });
wrapper.instance().onClick(e);
expect(e.preventDefault).toHaveBeenCalled();
});
});
});
|
index.ios.js | buildreactnative/assemblies | import React, { Component } from 'react';
import {
AppRegistry,
ActivityIndicator,
View,
Navigator,
AsyncStorage
} from 'react-native';
import Landing from './application/components/Landing';
import Register from './application/components/accounts/Register';
import RegisterConfirmation from './application/components/accounts/RegisterConfirmation';
import Login from './application/components/accounts/Login';
import Dashboard from './application/components/Dashboard';
import Loading from './application/components/shared/Loading';
import { Headers } from './application/fixtures';
import { extend } from 'underscore';
import { API, DEV } from './application/config';
import { globals } from './application/styles';
class assembliesTutorial extends Component {
constructor(){
super();
this.logout = this.logout.bind(this);
this.updateUser = this.updateUser.bind(this);
this.state = {
user : null,
ready : false,
initialRoute : 'Landing',
}
}
componentDidMount(){
this._loadLoginCredentials()
}
async _loadLoginCredentials(){
try {
let sid = await AsyncStorage.getItem('sid');
console.log('SID', sid);
if (sid){
this.fetchUser(sid);
} else {
this.ready();
}
} catch (err) {
this.ready(err);
}
}
ready(err){
this.setState({ ready: true });
}
fetchUser(sid){
fetch(`${API}/users/me`, { headers: extend(Headers, { 'Set-Cookie': `sid=${sid}`})})
.then(response => response.json())
.then(user => this.setState({ user, ready: true, initialRoute: 'Dashboard' }))
.catch(err => this.ready(err))
.done();
}
logout(){
this.nav.push({ name: 'Landing' })
}
updateUser(user){
this.setState({ user });
}
render() {
if ( ! this.state.ready ) { return <Loading /> }
return (
<Navigator
style={globals.flex}
ref={(el) => this.nav = el }
initialRoute={{ name: this.state.initialRoute }}
renderScene={(route, navigator) => {
switch(route.name){
case 'Landing':
return (
<Landing navigator={navigator}/>
);
case 'Dashboard':
return (
<Dashboard
updateUser={this.updateUser}
navigator={navigator}
logout={this.logout}
user={this.state.user}
/>
);
case 'Register':
return (
<Register navigator={navigator}/>
);
case 'RegisterConfirmation':
return (
<RegisterConfirmation
{...route}
updateUser={this.updateUser}
navigator={navigator}
/>
);
case 'Login':
return (
<Login
navigator={navigator}
updateUser={this.updateUser}
/>
);
}
}}
/>
);
}
}
AppRegistry.registerComponent('assembliesTutorial', () => assembliesTutorial);
|
frontend/app/components/Nav/NavLink.js | briancappello/flask-react-spa | import React from 'react'
import PropTypes from 'prop-types'
import { NavLink } from 'react-router-dom'
import isFunction from 'lodash/isFunction'
import { ROUTE_MAP } from 'routes'
export default class LoadableNavLink extends React.Component {
static propTypes = {
children: PropTypes.node,
to: PropTypes.string,
params: PropTypes.object,
}
constructor(props) {
super(props)
this.route = ROUTE_MAP[props.to]
}
componentWillReceiveProps(nextProps) {
this.route = ROUTE_MAP[nextProps.to]
}
maybePreloadComponent = () => {
if (!this.route) {
return
}
const { component } = this.route
if (isFunction(component.preload)) {
component.preload()
}
}
render() {
const { children, to, params, ...props } = this.props
return (
<NavLink {...props}
activeClassName="active"
onMouseOver={this.maybePreloadComponent}
to={this.route ? this.route.toPath(params) : to}
>
{children || this.route.label}
</NavLink>
)
}
}
|
accounts-ui/app/components/reset.js | CloudBoost/cloudboost | import React from 'react';
import {Link} from 'react-router'
import axios from 'axios'
import CircularProgress from 'material-ui/CircularProgress'
class Reset extends React.Component {
constructor() {
super()
this.state = {
errorMessage: '',
email: '',
success: false,
progress: false
}
}
componentDidMount() {
if(__isBrowser) document.title = "CloudBoost | Forgot Password"
if (!__isDevelopment) {
/****Tracking*********/
mixpanel.track('Portal:Visited ForgotPassword Page', {"Visited": "Visited ForgotPassword page in portal!"});
/****End of Tracking*****/
}
}
reset(e) {
e.preventDefault()
this.setProgress(true)
let postData = {
email: this.state.email
}
axios.post(USER_SERVICE_URL + "/user/ResetPassword", postData).then(function(data) {
this.setProgress(false)
this.state.email = ''
this.state.success = true;
this.state['errorMessage'] = ''
this.setState(this.state)
}.bind(this), function(err) {
this.setProgress(false)
this.state['errorMessage'] = 'We dont have an account with this email. Please try again.'
if (err.response == undefined) {
this.state['errorMessage'] = "Sorry, we currently cannot process your request, please try again later."
}
this.state.email = ''
this.setState(this.state)
}.bind(this))
if (!__isDevelopment) {
/****Tracking*********/
mixpanel.track('Portal:Clicked ResetPassword Button', {"Clicked": "ResetPassword Button in portal!"});
/****End of Tracking*****/
}
}
changeHandler(which, e) {
this.state[which] = e.target.value
this.setState(this.state)
}
setProgress(which) {
this.state.progress = which
this.setState(this.state)
}
render() {
return (
<div>
<div className={this.state.progress
? 'loader'
: 'hide'}>
<CircularProgress color="#4E8EF7" size={50} thickness={6}/>
</div>
<div id="login" className={!this.state.progress
? ''
: 'hide'}>
<div id="image">
<img className="logo" src="public/assets/images/CbLogoIcon.png"/>
</div>
<div id="headLine" className={!this.state.success
? ''
: 'hide'}>
<h3 className="tacenter hfont">Reset your password.</h3>
</div>
<div id="box" className={!this.state.success
? ''
: 'hide'}>
<h5 className="tacenter bfont">Enter your email and we'll reset the password for you.</h5>
</div>
<div id="headLine" className={this.state.success
? ''
: 'hide'}>
<h3 className="tacenter hfont">Reset password email sent.</h3>
</div>
<div id="box" className={this.state.success
? ''
: 'hide'}>
<h5 className="tacenter bfont">We've sent you reset password email. Please make sure you check spam.</h5>
</div>
<div className={!this.state.success
? 'loginbox'
: 'hide'}>
<h5 className="tacenter red">{this.state.errorMessage}</h5>
<form onSubmit={this.reset.bind(this)}>
<input type="email" value={this.state.email} onChange={this.changeHandler.bind(this, 'email')} className="loginInput from-control" placeholder="Your Email." disabled={this.state.successReset} required/>
<button className="loginbtn" type="submit">
Reset Password
</button>
</form>
<Link to={ LOGIN_URL }>
<span className="forgotpw fl">Login.</span>
</Link>
<Link to={ SIGNUP_URL }>
<span className="forgotpw fr">
<span className="greydonthaveaccnt">Dont have an account? </span>
Sign Up.</span>
</Link>
</div>
<div className={this.state.success
? 'loginbox'
: 'hide'}>
<h5 className="tacenter">Want to login?
<Link to={ LOGIN_URL }>
<span className="forgotpw">Log in.
</span>
</Link>
</h5>
</div>
</div>
</div>
);
}
}
export default Reset;
|
test/ListGroupSpec.js | andrew-d/react-bootstrap | import React from 'react';
import ReactTestUtils from 'react/lib/ReactTestUtils';
import ListGroup from '../src/ListGroup';
import ListGroupItem from '../src/ListGroupItem';
describe('ListGroup', function () {
it('Should output a "div" with the class "list-group"', function () {
let instance = ReactTestUtils.renderIntoDocument(
<ListGroup/>
);
assert.equal(React.findDOMNode(instance).nodeName, 'DIV');
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(instance, 'list-group'));
});
it('Should support a single "ListGroupItem" child', function () {
let instance = ReactTestUtils.renderIntoDocument(
<ListGroup>
<ListGroupItem>Only Child</ListGroupItem>
</ListGroup>
);
let items = ReactTestUtils.scryRenderedComponentsWithType(instance, ListGroupItem);
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(items[0], 'list-group-item'));
});
it('Should support a single "ListGroupItem" child contained in an array', function () {
let child = [<ListGroupItem key={42}>Only Child in array</ListGroupItem>];
let instance = ReactTestUtils.renderIntoDocument(
<ListGroup>
{child}
</ListGroup>
);
let items = ReactTestUtils.scryRenderedComponentsWithType(instance, ListGroupItem);
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(items[0], 'list-group-item'));
});
it('Should output a "ul" when single "ListGroupItem" child is a list item', function () {
let instance = ReactTestUtils.renderIntoDocument(
<ListGroup>
<ListGroupItem>Only Child</ListGroupItem>
</ListGroup>
);
assert.equal(React.findDOMNode(instance).nodeName, 'UL');
assert.equal(React.findDOMNode(instance).firstChild.nodeName, 'LI');
});
it('Should output a "div" when single "ListGroupItem" child is an anchor', function () {
let instance = ReactTestUtils.renderIntoDocument(
<ListGroup>
<ListGroupItem href="#test">Only Child</ListGroupItem>
</ListGroup>
);
assert.equal(React.findDOMNode(instance).nodeName, 'DIV');
assert.equal(React.findDOMNode(instance).firstChild.nodeName, 'A');
});
it('Should support multiple "ListGroupItem" children', function () {
let instance = ReactTestUtils.renderIntoDocument(
<ListGroup>
<ListGroupItem>1st Child</ListGroupItem>
<ListGroupItem>2nd Child</ListGroupItem>
</ListGroup>
);
let items = ReactTestUtils.scryRenderedComponentsWithType(instance, ListGroupItem);
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(items[0], 'list-group-item'));
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(items[1], 'list-group-item'));
});
it('Should support multiple "ListGroupItem" children including a subset contained in an array', function () {
let itemArray = [
<ListGroupItem key={0}>2nd Child nested</ListGroupItem>,
<ListGroupItem key={1}>3rd Child nested</ListGroupItem>
];
let instance = ReactTestUtils.renderIntoDocument(
<ListGroup>
<ListGroupItem>1st Child</ListGroupItem>
{itemArray}
<ListGroupItem>4th Child</ListGroupItem>
</ListGroup>
);
let items = ReactTestUtils.scryRenderedComponentsWithType(instance, ListGroupItem);
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(items[0], 'list-group-item'));
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(items[1], 'list-group-item'));
});
it('Should output a "ul" when children are list items', function () {
let instance = ReactTestUtils.renderIntoDocument(
<ListGroup>
<ListGroupItem>1st Child</ListGroupItem>
<ListGroupItem>2nd Child</ListGroupItem>
</ListGroup>
);
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(instance, 'list-group'));
assert.equal(React.findDOMNode(instance).nodeName, 'UL');
assert.equal(React.findDOMNode(instance).firstChild.nodeName, 'LI');
assert.equal(React.findDOMNode(instance).lastChild.nodeName, 'LI');
});
it('Should output a "div" when "ListGroupItem" children are anchors and spans', function () {
let instance = ReactTestUtils.renderIntoDocument(
<ListGroup>
<ListGroupItem href="#test">1st Child</ListGroupItem>
<ListGroupItem>2nd Child</ListGroupItem>
</ListGroup>
);
assert.equal(React.findDOMNode(instance).nodeName, 'DIV');
assert.equal(React.findDOMNode(instance).firstChild.nodeName, 'A');
assert.equal(React.findDOMNode(instance).lastChild.nodeName, 'SPAN');
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(instance, 'list-group'));
});
it('Should output a "div" when "ListGroupItem" children have an onClick handler', function () {
let instance = ReactTestUtils.renderIntoDocument(
<ListGroup>
<ListGroupItem onClick={() => null}>1st Child</ListGroupItem>
<ListGroupItem>2nd Child</ListGroupItem>
</ListGroup>
);
assert.equal(React.findDOMNode(instance).nodeName, 'DIV');
assert.equal(React.findDOMNode(instance).firstChild.nodeName, 'A');
assert.equal(React.findDOMNode(instance).lastChild.nodeName, 'SPAN');
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(instance, 'list-group'));
});
it('Should support an element id through "id" prop', function () {
let instance = ReactTestUtils.renderIntoDocument(
<ListGroup id="testItem">
<ListGroupItem>Child</ListGroupItem>
</ListGroup>
);
assert.ok(ReactTestUtils.findRenderedDOMComponentWithClass(instance, 'list-group'));
assert.equal(React.findDOMNode(instance).nodeName, 'UL');
assert.equal(React.findDOMNode(instance).id, 'testItem');
});
});
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.