text
stringlengths 226
34.5k
|
---|
Does python have one way of doing things?
Question: I have always seen in python articles/books that python is simple and it has
only one way of doing things. I would like someone to **explain to me this
concept** keeping in mind the example below, if I wanted to get the min and
max values of sequence I would do the following;
seq=[1,2,3,4,5,6]
min(seq) #1
max(seq) #6
but I can also do this;
seq[:1] #1
seq[-1] #6
surely this are two ways of doing one simple thing. This confuses me a bit.
Answer: Not that it "has one way of doing things" as more "There should be one-- and
preferably only one --obvious way to do it." (from Zen of Python).
This doesn't exclude possibility of having more than one way of doing things.
We're talking about programming where creativity is one of the most important
skills and finding new ways of solving problems is a must.
In your example you're doing two different things:
1. getting minimum and maximum of the list
2. getting first and the last element of the list
it happens that in this particular case result is exactly the same.
|
Multiple levels of 'collection.defaultdict' in Python
Question: Thanks to some great folks on SO, I discovered the possibilities offered by
`collections.defaultdict`, notably in readability and speed. I have put them
to use with success.
Now I would like to implement three levels of dictionaries, the two top ones
being `defaultdict` and the lowest one being `int`. I don't find the
appropriate way to do this. Here is my attempt:
from collections import defaultdict
d = defaultdict(defaultdict)
a = [("key1", {"a1":22, "a2":33}),
("key2", {"a1":32, "a2":55}),
("key3", {"a1":43, "a2":44})]
for i in a:
d[i[0]] = i[1]
Now this works, but the following, which is the desired behavior, doesn't:
d["key4"]["a1"] + 1
I suspect that I should have declared somewhere that the second level
`defaultdict` is of type `int`, but I didn't find where or how to do so.
The reason I am using `defaultdict` in the first place is to avoid having to
initialize the dictionary for each new key.
Any more elegant suggestion?
Thanks pythoneers!
Answer: Use:
d = defaultdict(lambda: defaultdict(int))
This will create a new `defaultdict(int)` whenever a new key is accessed in
`d`.
|
retrieveing info from python dict with javascript
Question: I have such stuff in my django view:
message = 'sometext'
rating = 7
data = {'message':message, 'rating':rating}
from django.utils import simplejson
return HttpResponse(simplejson.dumps(data))
and this in my site.js:
$.ajax({
'type': "POST",
'url': url,
'data': {'rating':rating},
'success': function(msg){
alert(msg['rating']);
$.modal('<p>'+msg+'</p>');
$(".simplemodal-container").css("height", "auto");
},
'error' : function(){
$.modal('<p>Hinnangu salvestamine ebaõnnestus</p>');
$(".simplemodal-container").css("height", "auto");
}
});
the simplemodel window returns me information like this:
{"rating": "7", "message": "sometext"}
alert on previous line just returns 'undefined'
Why? Whats missing? i read about javascript dictionaries here:
<http://rick.measham.id.au/javascript/hash.htm> and according to that, the
alert should display '7' not 'undefined' or not?
Alan
Answer: Of course it contains that. That's the JSON that has been returned to you. Use
[json2.js](http://www.json.org/js.html) to decode it to JavaScript objects.
|
How to correctly relay TCP traffic between sockets?
Question: I'm trying to write some Python code that will establish an invisible relay
between two TCP sockets. My current technique is to set up two threads, each
one reading and subsequently writing 1kb of data at a time in a particular
direction (i.e. 1 thread for A to B, 1 thread for B to A).
This works for some applications and protocols, but it isn't foolproof -
sometimes particular applications will behave differently when running through
this Python-based relay. Some even crash.
I think that this is because when I finish performing a read on socket A, the
program running there considers its data to have already arrived at B, when in
fact I - the devious man in the middle - have yet to send it to B. In a
situation where B isn't ready to receive the data (whereby `send()` blocks for
a while), we are now in a state where A believes it has successfully sent data
to B, yet I am still holding the data, waiting for the `send()` call to
execute. I think this is the cause of the difference in behaviour that I've
found in some applications, while using my current relaying code. Have I
missed something, or does that sound correct?
If so, my real question is: is there a way around this problem? Is it possible
to only read from socket A when we know that B is ready to receive data? Or is
there another technique that I can use to establish a truly 'invisible' two-
way relay between [already open & established] TCP sockets?
Answer: > Is it possible to only read from socket A when we know that B is ready to
> receive data?
Sure: use
[select.select](http://docs.python.org/library/select.html?highlight=select#select.select)
on both sockets A and B (if it returns saying only one of them is ready, use
it on the other one), and only read from A and write to B when you know
they're both ready. E.g.:
import select
def fromAtoB(A, B):
r, w = select.select([A], [B], [])
if not r: select.select([A], [], [])
elif not w: select.select([], [B], [])
B.sendall(A.recv(4096))
|
Django's post_save signal behaves weirdly with models using multi-table inheritance
Question: Django's post_save signal behaves weirdly with models using multi-table
inheritance
I am noticing an odd behavior in the way Django's post_save signal works when
using a model that has multi-table inheritance.
I have these two models:
class Animal(models.Model):
category = models.CharField(max_length=20)
class Dog(Animal):
color = models.CharField(max_length=10)
I have a post save callback called echo_category:
def echo_category(sender, **kwargs):
print "category: '%s'" % kwargs['instance'].category
post_save.connect(echo_category, sender=Dog)
I have this fixture:
[
{
"pk": 1,
"model": "animal.animal",
"fields": {
"category": "omnivore"
}
},
{
"pk": 1,
"model": "animal.dog",
"fields": {
"color": "brown"
}
}
]
In every part of the program except for in the post_save callback the
following is true:
from animal.models import Dog
Dog.objects.get(pk=1).category == u'omnivore' # True
When I run syncdb and the fixture is installed, the echo_category function is
run. The output from syncdb is:
$ python manage.py syncdb --noinput
Installing json fixture 'initial_data' from '~/my_proj/animal/fixtures'.
category: ''
Installed 2 object(s) from 1 fixture(s)
The weird thing here is that the dog object's category attribute is an empty
string. Why is it not 'omnivore' like it is everywhere else?
As a temporary (hopefully) workaround I reload the object from the database in
the post_save callback:
def echo_category(sender, **kwargs):
instance = kwargs['instance']
instance = sender.objects.get(pk=instance.pk)
print "category: '%s'" % instance.category
post_save.connect(echo_category, sender=Dog)
This works but it is not something I like because I must remember to do it
when the model inherits from another model and it must hit the database again.
The other weird thing is that I must do instance.pk to get the primary key.
The normal 'id' attribute does not work (I cannot use instance.id). I do not
know why this is. Maybe this is related to the reason why the category
attribute is not doing the right thing?
Answer: This is because data loaded from fixture with `loaddata`/`syncdb` command is
saved as _raw_ in the database: only the fields of the resulting model table
get saved, to avoid hitting the database for all the models in the class
hierarchy.
But, when a model is saved _as raw_ , you get an extra `raw` keyword argument
in your signal, so you can handle the case properly. Your signal would end up
like that:
def echo_category(sender, **kwargs):
if kwargs.get('raw', False):
instance = sender.objects.get(pk=kwargs['instance'].pk)
else:
instance = kwargs['instance']
print "category: '%s'" % instance.category
post_save.connect(echo_category, sender=Dog)
This way, you will only get the extra database query when dealing with
fixtures (and I guess that's acceptable in your case).
About your other question:
> The other weird thing is that I must do instance.pk to get the primary key.
> The normal 'id' attribute does not work (I cannot use instance.id). I do not
> know why this is.
`id` and
[`pk`](http://docs.djangoproject.com/en/dev/ref/models/instances/#django.db.models.Model.pk)
have a slightly different semantic. In your example, the `id` of a `Dog`
object is the `AutoField` defined
([automagically](http://docs.djangoproject.com/en/dev/topics/db/models/#id1))
in your `Animal` class. The `pk` however, is an `OneToOneField` (once again,
[automagically
defined](http://docs.djangoproject.com/en/dev/topics/db/models/#id7)) in the
`Dog` class.
In practice, both field have the **same** value all the time. However, as `id`
is a field coming from `Animal`, it won't exists for `Dog` object saved as
_raw_.
Hope that helps.
**EDIT** : this issue has already been reported on django's trac
[here](http://code.djangoproject.com/ticket/13299).
|
Python ctypes and dynamic linking
Question: I'm writing some libraries in C which contain functions that I want to call
from Python via ctypes.
I've done this successfully another library, but that library had only very
vanilla dependencies (namely `fstream`, `math`, `malloc`, `stdio`, `stdlib`).
The other library I'm working on has more complicated dependencies.
For example, I'll try to use `fftw3`. As a test, I'll just try to compile a
simple `.cpp` file containing:
int foo()
{
void *p = fftw_malloc( sizeof(fftw_complex)*64 );
fftw_free(p);
printf("foo called.\n");
return 0;
}
I compile it as:
icpc -Wall -fPIC -c waveprop.cpp -o libwaveprop.o $std_link
icpc -shared -Wl,-soname,libwaveprop.so.1 -o libwaveprop.so.1.0 libwaveprop.o
cp waveprop.so.1.0 /usr/local/lib/
rm waveprop.so.1.0
ln -sf /usr/local/lib/waveprop.so.1.0 /usr/local/lib/waveprop.so
ln -sf /usr/local/lib/waveprop.so.1.0 /usr/local/lib/waveprop.so.1
This all works. Now I test it with another `.cpp` file containing:
int main()
{
foo();
}
Result:
icpc test.cpp -lwaveprop
/lib/../lib64/libwaveprop.so: undefined reference to `fftw_free'
/lib/../lib64/libwaveprop.so: undefined reference to `fftw_malloc'
Which is entirely reasonable. Next I try:
icpc test.cpp -lwaveprop -lfftw3
./a.out
foo called.
Great! But now when I try to load the library with ctypes:
>>> from ctypes import *
>>> print cdll.LoadLibrary('/usr/local/lib/libwaveprop.so.1')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/usr/lib64/python2.6/ctypes/__init__.py", line 431, in LoadLibrary
return self._dlltype(name)
File "/usr/lib64/python2.6/ctypes/__init__.py", line 353, in __init__
self._handle = _dlopen(self._name, mode)
OSError: /usr/local/lib/libwaveprop.so.1: undefined symbol: fftw_free
So it's the same problem, but I have no idea how to resolve it for ctypes.
I've tried various things without any success, and I'm pretty stuck at this
point.
Answer: OK, thanks for your help.
to get this to work I had to include the dependencies when linking (duh). I
had tried this before but got an error, so solve this I had to recompile fftw
with '-fpic' as a CPP flag. all works now.
icpc -Wall -fPIC -c waveprop.cpp -o libwaveprop.o $std_link
icpc -shared -Wl,-soname,libwaveprop.so.1 -o libwaveprop.so.1.0 libwaveprop.o -lfftw3
cp waveprop.so.1.0 /usr/local/lib/
rm waveprop.so.1.0
ln -sf /usr/local/lib/waveprop.so.1.0 /usr/local/lib/waveprop.so
ln -sf /usr/local/lib/waveprop.so.1.0 /usr/local/lib/waveprop.so.1
thanks, -nick
|
"cannot concatenate 'str' and 'list' objects" keeps coming up :(
Question: I'm writing a python program. The program calculates Latin Squares using two
numbers the user enters on a previous page. But but an error keeps coming up,
"cannot concatenate 'str' and 'list' objects" here is the program:
#!/usr/bin/env python
# -*- coding: UTF-8 -*-
# enable debugging
import cgi
import cgitb
cgitb.enable()
def template(file, **vars):
return open(file, 'r').read() % vars
print "Content-type: text/html\n"
print
form = cgi.FieldStorage() # instantiate only once!
num_1 = form.getfirst('num_1')
num_2 = form.getfirst('num_2')
int1r = str(num_1)
int2r = str(num_2)
def calc_range(int2r, int1r):
start = range(int2r, int1r + 1)
end = range(1, int2r)
return start+end
int1 = int(int1r)
int2 = int(int2r)
out_str = ''
for i in range(0, int1):
first_line_num = (int2 + i) % int1
if first_line_num == 0:
first_line_num = int1
line = calc_range(first_line_num, int1)
out_str += line
print template('results.html', output=out_str, title="Latin Squares")
Answer: `range` returns a list object, so when you say
line = calc_range(first_line_num, int1)
You are assigning a list to `line`. This is why `out_str += line` throws the
error.
You can use `str()` to convert a list to a string, or you can build up a
string a different way to get the results you are looking for.
|
Installing Python Script, Maintaining Reference to Python 2.6
Question: I am trying to distribute my Python program. The program relies on version
2.6. I went through the distribution documentation:
<http://docs.python.org/distutils/index.html> and what I have figured out so
far is that I basically need to write a setup.py script. Something like:
setup(name='Distutils',
version='1.0',
description='Python Distribution Utilities',
author='My Name',
author_email='My Email',
url='some URL',
package_dir={'': 'src'},
packages=[''],
)
I would like to ensure that my program uses 2.6 interpreter library when user
install it on their box. What would be the best approach to ensure that my
program uses 2.6 ? Shall I distribute python 2.6 library along with my program
? Is there any alternative approach ?
Answer: One option is to specify the specific version of the Python interpreter using
the hash bang:
#! /usr/bin/env python2.6
Another option is to check
[sys.version_info](http://docs.python.org/dev/library/sys.html#sys.version_info),
for example:
if (sys.version_info[0] != 2) or (sys.version_info[1]<6):
if sys.version_info[0] > 2:
print("Version 2.6+, and not 3.0+ needed.")
sys.exit(1)
else:
print "Version 2.6+, needed. Please upgrade Python."
sys.exit(1)
The hash bang is probably the best option, as it actually ensures that the
script will be interpreted by "python2.6" instead of some other interpreter;
however, there are some disadvantages:
* This will only work on UNIX-like systems that use the hash bang.
* This will won't work if Python2.7 is installed, but not Python 2.6.
As a workaround, what you can do is create a "launcher" Python script that
checks for "python2.6"... "python2.9", which is the last possible version of
the 2.6+ line before Python 3.0+. This launcher script can then invoke your
main program using whichever python interpreter it finds in the search
process. You will have to make your launcher script in a way that uses
elements common to most Python versions.
Borrowing from [test if executable exists in
Python](http://stackoverflow.com/questions/377017/test-if-executable-exists-
in-python):
def which(program):
import os
def is_exe(fpath):
return os.path.exists(fpath) and os.access(fpath, os.X_OK)
fpath, fname = os.path.split(program)
if fpath:
if is_exe(program):
return program
else:
for path in os.environ["PATH"].split(os.pathsep):
exe_file = os.path.join(path, program)
if is_exe(exe_file):
return exe_file
return None
def first_which(progs):
for prog in progs:
progloc = which(prog)
if progloc != None:
return progloc
return None
def main():
interpreter=first_which(["python2.6","python2.7","python2.8","python2.9"])
# Invoke your program using "interpreter"
# You will need to use os.popen or subprocess,
# depending on the version of Python which which
# this launcher script was invoked...
My own opinion is that the method above is more complicated than necessary,
and I would just go with the hash bang... or I would write out the hash bang
to the Python file at deployment time, using a language other than Python (for
which the version wouldn't be an issue... otherwise, it becomes a recursive
problem).
I would also strongly urge you NOT to include a copy of Python in your
software distribution. This will make your download much larger, and it will
annoy users who already have a valid installation of Python available.
Instead, you should simply direct users to download and install the
appropriate version if it isn't available.
|
local variable 'sresult' referenced before assignment
Question: I have had multiple problems trying to use PP. I am running python2.6 and pp
1.6.0 rc3. Using the following test code:
import pp
nodes=('mosura02','mosura03','mosura04','mosura05','mosura06',
'mosura09','mosura10','mosura11','mosura12')
def pptester():
js=pp.Server(ppservers=nodes)
tmp=[]
for i in range(200):
tmp.append(js.submit(ppworktest,(),(),('os',)))
return tmp
def ppworktest():
return os.system("uname -a")
gives me the following result:
In [10]: Exception in thread run_local:
Traceback (most recent call last):
File "/usr/lib64/python2.6/threading.py", line 525, in __bootstrap_inner
self.run()
File "/usr/lib64/python2.6/threading.py", line 477, in run
self.__target(*self.__args, **self.__kwargs)
File "/home/wkerzend/python_coala/lib/python2.6/site-packages/pp.py", line 751, in _run_local
job.finalize(sresult)
UnboundLocalError: local variable 'sresult' referenced before assignment
Exception in thread run_local:
Traceback (most recent call last):
File "/usr/lib64/python2.6/threading.py", line 525, in __bootstrap_inner
self.run()
File "/usr/lib64/python2.6/threading.py", line 477, in run
self.__target(*self.__args, **self.__kwargs)
File "/home/wkerzend/python_coala/lib/python2.6/site-packages/pp.py", line 751, in _run_local
job.finalize(sresult)
UnboundLocalError: local variable 'sresult' referenced before assignment
Exception in thread run_local:
Traceback (most recent call last):
File "/usr/lib64/python2.6/threading.py", line 525, in __bootstrap_inner
self.run()
File "/usr/lib64/python2.6/threading.py", line 477, in run
self.__target(*self.__args, **self.__kwargs)
File "/home/wkerzend/python_coala/lib/python2.6/site-packages/pp.py", line 751, in _run_local
job.finalize(sresult)
UnboundLocalError: local variable 'sresult' referenced before assignment
Exception in thread run_local:
Traceback (most recent call last):
File "/usr/lib64/python2.6/threading.py", line 525, in __bootstrap_inner
self.run()
File "/usr/lib64/python2.6/threading.py", line 477, in run
self.__target(*self.__args, **self.__kwargs)
File "/home/wkerzend/python_coala/lib/python2.6/site-packages/pp.py", line 751, in _run_local
job.finalize(sresult)
UnboundLocalError: local variable 'sresult' referenced before assignment
Any help is greatly appreciated.
Answer: I can't read your code because it's not formatted properly, but I can tell you
your exact problem: you're trying to modify a global variable named "sresult"
from inside a function, but you did not add this line to the beginning of your
function:
`global sresult`
If you don't declare a variable global, Python will assume it's local to the
function if you try to assign it within the function, so when you try to
modify or access it, Python will complain that you haven't yet "bound the
local variable" (that is, assigned it or given it a value).
|
sampling integers uniformly efficiently in python using numpy/scipy
Question: I have a problem where depending on the result of a random coin flip, I have
to sample a random starting position from a string. If the sampling of this
random position is uniform over the string, I thought of two approaches to do
it: one using multinomial from numpy.random, the other using the simple
randint function of Python standard lib. I tested this as follows:
from numpy import *
from numpy.random import multinomial
from random import randint
import time
def use_multinomial(length, num_points):
probs = ones(length)/float(length)
for n in range(num_points):
result = multinomial(1, probs)
def use_rand(length, num_points):
for n in range(num_points):
rand(1, length)
def main():
length = 1700
num_points = 50000
t1 = time.time()
use_multinomial(length, num_points)
t2 = time.time()
print "Multinomial took: %s seconds" %(t2 - t1)
t1 = time.time()
use_rand(length, num_points)
t2 = time.time()
print "Rand took: %s seconds" %(t2 - t1)
if __name__ == '__main__':
main()
The output is:
Multinomial took: 6.58072400093 seconds Rand took: 2.35189199448 seconds
it seems like randint is faster, but it still seems very slow to me. Is there
a vectorized way to get this to be much faster, using numpy or scipy?
thanks.
Answer: I changed your code to actually return values (and used `randint` instead of
`rand` \- isn't that what you meant?) like this...
def use_multinomial(length, num_points):
probs = ones(length)/float(length)
return multinomial(1, probs, num_points)
def use_rand(length, num_points):
return [randint(1,length) for _ in range(num_points)]
Then I tried my own version, using `numpy.random.randint` to generate a numpy
array of random points on the string:
def use_np_randint(length, num_point):
return nprandint(1, length, num_points)
The results:
Multinomial took: 13.6279997826 seconds
Rand took: 0.185000181198 seconds
NP randint took: 0.00100016593933 seconds
Multinomial is obviously really slow comparitively, but is that even what you
want? I thought you said you wanted a uniform distribution? Using numpy's
randint is clearly the fastest of the bunch.
|
Can't import obj in Python on OS X 10.6.3 Snow Leopard - libiconv.2.dylib?
Question: on OS X 10.6.3 Snow Leopard
% python
Python 2.6.1 (r261:67515, Feb 11 2010, 00:51:29)
[GCC 4.2.1 (Apple Inc. build 5646)] on darwin
Type "help", "copyright", "credits" or "license" for more information.
>>> import objc
Traceback (most recent call last):
File "", line 1, in
File "/Library/Python/2.6/site-packages/pyobjc_core-2.2-py2.6-macosx-10.6-universal.egg/objc/__init__.py", line 22, in
_update()
File "/Library/Python/2.6/site-packages/pyobjc_core-2.2-py2.6-macosx-10.6-universal.egg/objc/__init__.py", line 19, in _update
import _objc
ImportError: dlopen(/Library/Python/2.6/site-packages/pyobjc_core-2.2-py2.6-macosx-10.6-universal.egg/objc/_objc.so, 2): Library not loaded: /opt/local/lib/libiconv.2.dylib
Referenced from: /Library/Python/2.6/site-packages/pyobjc_core-2.2-py2.6-macosx-10.6-universal.egg/objc/_objc.so
Reason: Incompatible library version: _objc.so requires version 8.0.0 or later, but libiconv.2.dylib provides version 7.0.0
>>>
\-- what do I need to do?
Answer: First I'd try to temporary move `/Library/Python/2.6/site-
packages/pyobjc_core-2.2-py2.6-macosx-10.6-universal.egg/` to somewhere else
and try the import statement again. This will enable the OS to import the
version of `objc` that came with the OS by default (this is in
`/System/Library`). By using the version in `/System/Library`, it works for
me:
>>> import objc
>>> print objc.__file__
/System/Library/Frameworks/Python.framework/Versions/2.6/Extras/lib/python/PyObjC/objc/__init__.pyc
You might also consider moving `/opt/local/lib/libiconv.2.dylib` to somewhere
else. It looks outdated and OS X Snow Leopard has its own copy of
`libiconv.2.dylib` in `/usr/lib` IIRC.
|
python file manipulation
Question: I have a directory /tmp/dir with two types of file names
> /tmp/dir/abc-something-server.log
>
> /tmp/dir/xyz-something-server.log
>
> ..
>
> ..
and
> /tmp/dir/something-client.log
I need append a few lines (these lines are constant) to files end with
"client.log"
> line 1
>
> line 2
>
> line 3
>
> line 4
append these four lines to files end with "client.log"
Yes I found open () "a" option will provide the desired result. but how to
select the correct file that is, exclude server.log and choose client.log ?
and For files end with "server.log"
I needed to append after a keyword say "After-this". "server.log " file has
multiple entries of "After-this" I need to find the first entry of "After-
this" and append above said four lines keep the remaining file as it is.
Any help will be great appreciated :) Thanks in advance.
Answer: not tested
import os,glob,fileinput
root="/tmp"
path=os.path.join(root,"dir")
alines=["line 1\n","line 2\n","line 3\n","line 4\n"]
os.chdir(path)
# for clients
for clientfile in glob.glob("*.client.log"):
data=open(clientfile).readlines()
data.append(alines)
open("temp","w").write(''.join(data))
os.rename("temp",clientfile)
for svrfile in glob.glob("*.server.log"):
f=0
for line in fileinput.FileInput(svrfile,inplace=1):
ind=line.find("After-this")
if ind!=-1 and not f:
line=line[:ind+10] + ''.join(alines) + line[ind+10:]
f=1
print line
|
deciding among subprocess, multiprocessing, and thread in Python?
Question: I'd like to parallelize my Python program so that it can make use of multiple
processors on the machine that it runs on. My parallelization is very simple,
in that all the parallel "threads" of the program are independent and write
their output to separate files. I don't need the threads to exchange
information but it is imperative that I know when the threads finish since
some steps of my pipeline depend on their output.
Portability is important, in that I'd like this to run on any Python version
on Mac, Linux and Windows. Given these constraints, which is the most
appropriate Python module for implementing this? I am trying to decide between
thread, subprocess and multiprocessing, which all seem to provide related
functionality.
Any thoughts on this? I'd like the simplest solution that's portable.
Thanks.
Answer: For me this is actually pretty simple:
# The _subprocess_ option:
`subprocess` is **for running other executables** \--- it's basically a
wrapper around `os.fork()` and `os.execve()` with some support for optional
plumbing (setting up PIPEs to and from the subprocesses. (Obviously other OS
inter-process communications (IPC) mechanisms, such as sockets, SysV shared
memory and message queues can be used --- but usually you're using subprocess
to run third party binary executables and are going to be stuck with whatever
interfaces and IPC channels those support).
Commonly one uses `subprocess` synchronously --- simply calling some external
utility and reading back its output or awaiting its completion (perhaps
reading its results from a temporary file, or after its posted them to some
database). However one can spawn hundreds of subprocesses and poll them. My
own personal favorite utility does exactly that. **The biggest disadvantage**
of the `subprocess` module is that its I/O support is generally blocking.
There is a draft [PEP-3145](https://www.python.org/dev/peps/pep-3145/) to fix
that in some future version of Python 3.x and an alternative
[asyncproc](http://www.lysator.liu.se/~bellman/download/asyncproc.py) (Warning
that leads right to the download, not to any sort of documentation nor
README). I've also found that it's relatively easy to just import `fcntl` and
manipulate your `Popen` PIPE file descriptors directly --- though I don't know
if this is portable to non-UNIX platforms.
`subprocess` **has almost no event handling support** ... **though** you can
use the `signal` module and plain old-school UNIX/Linux signals --- killing
your processes softly, as it were.
# The _multiprocessing_ option:
`multiprocessing` is **for running functions within your existing (Python)
code** with support for more flexible communications among the family of
processes. In particular it's best to build your `multiprocessing` IPC around
the module's `Queue` objects where possible, but you can also use `Event`
objects and various other features (some of which are, presumably, built
around `mmap` support on platforms where that support is sufficient).
Python's `multiprocessing` module is intended to provide interfaces and
features which are very **similar to** `threading` while allowing CPython to
scale your processing among multiple CPUs/cores despite the GIL. It leverages
all the fine-grained SMP locking and coherency effort that was done by
developers of your OS kernel.
# The _threading_ option:
`threading` is **for a fairly narrow range of applications which are I/O
bound** (don't need to scale across multiple CPU cores) and which benefit from
the extremely low latency and switching overhead of thread switching (with
shared core memory) vs. process/context switching. On Linux this is almost the
empty set (Linux process switch times are extremely close to its thread-
switches).
`threading` suffers from **two major disadvantages in Python**. One, of
course, is implementation specific --- mostly affecting CPython. That's the
GIL (Global Interpreter Lock). For the most part, most CPython programs will
not benefit from the availability of more than two CPUs (cores) and often
performance will _suffer_ from the GIL locking contention. The larger issue
which is not implementation specific, is that threads share the same memory,
signal handlers, file descriptors and certain other OS resources. Thus the
programmer must be extremely careful about object locking, exception handling
and other aspects of their code which are both subtle and which can kill,
stall, or deadlock the entire process (suite of threads).
By comparison the `multiprocessing` model gives each process its own memory,
file descriptors, etc. A crash or unhandled exception in any one of them will
only kill that resource and robustly handling the disappearance of a child or
sibling process can be considerably easier than debugging, isolating and
fixing or working around similar issues in threads.
* (Note: use of `threading` with major Python systems, such as Numpy, may suffer considerably less from GIL contention then most of your own Python code would. That's because they've been specifically engineered to do so).
# The _twisted_ option:
It's also worth noting that [Twisted](http://twistedmatrix.com/) offers yet
another alternative which is both **elegant and very challenging to
understand**. Basically, at the risk of over simplifying to the point where
fans of Twisted may storm my home with pitchforks and torches, Twisted
provides and event-driven co-operative multi-tasking within any (single)
process.
To understand how this is possible one should read about the features of
`select()` (which can be built around the _select()_ or _poll()_ or similar OS
system calls). Basically it's all driven by the ability to make a request of
the OS to sleep pending any activity on a list of file descriptors or some
timeout. The awakening from each of these calls to `select()` is an event ---
either one involving input available (readable) on some number of sockets or
file descriptors, or buffering space becoming available on some other
descriptors or sockets (writable), or some exceptional conditions (TCP out-of-
band PUSH'd packets, for example), or a TIMEOUT.
Thus the Twisted programming model is built around handling these events then
looping on the resulting "main" handler, allowing it to dispatch the events to
your handlers.
I personally think of the name, **_Twisted_** as evocative of the programming
model ... since your approach to the problem must be, in some sense, "twisted"
inside out. Rather than conceiving of your program as a series of operations
on input data and outputs or results, you're writing your program as a service
or daemon and defining how it reacts to various events. (In fact the core
"main loop" of a Twisted program is (usually? always?) a `reactor()`.
The **major challenges to using Twisted** involve twisting your mind around
the event driven model and also eschewing the use of any class libraries or
toolkits which are not written to co-operate within the Twisted framework.
This is why Twisted supplies its own modules for SSH protocol handling, for
curses, and its own subprocess/popen functions, and many other modules and
protocol handlers which, at first blush, would seem to duplicate things in the
Python standard libraries.
I think it's useful to understand Twisted on a conceptual level even if you
never intend to use it. It may give insights into performance, contention, and
event handling in your threading, multiprocessing and even subprocess handling
as well as any distributed processing you undertake.
# The _distributed_ option:
Yet another realm of processing you haven't asked about, but which is worth
considering, is that of **_distributed_** processing. There are many Python
tools and frameworks for distributed processing and parallel computation.
Personally I think the easiest to use is one which is least often considered
to be in that space.
It is almost trivial to build distributed processing around
[Redis](http://redis.io/). The entire key store can be used to store work
units and results, Redis LISTs can be used as `Queue()` like object, and the
PUB/SUB support can be used for `Event`-like handling. You can hash your keys
and use values, replicated across a loose cluster of Redis instances, to store
the topology and hash-token mappings to provide consistent hashing and fail-
over for scaling beyond the capacity of any single instance for co-ordinating
your workers and marshaling data (pickled, JSON, BSON, or YAML) among them.
Of course as you start to build a larger scale and more sophisticated solution
around Redis you are re-implementing many of the features that have already
been solved using Hadoop, Zookeeper, Cassandra and so on. Those also have
modules for Python access to their services.
# Conclusion
There you have the gamut of processing alternatives for Python, from single
threaded, with simple synchronous calls to sub-processes, pools of polled
subprocesses, threaded and multiprocessing, event-driven co-operative multi-
tasking, and out to distributed processing.
|
How to pass an IronPython instance method to a (C#) function parameter of type `Func<Foo>`
Question: I am trying to assign an IronPython instance method to a C# `Func<Foo>`
parameter.
In C# I would have a method like:
public class CSharpClass
{
public void DoSomething(Func<Foo> something)
{
var foo = something()
}
}
And call it from IronPython like this:
class IronPythonClass:
def foobar(self):
return Foo()
CSharpClass().DoSomething(foobar)
But I'm getting the following error:
**TypeError: expected Func[Foo], got instancemethod**
Answer: OK. I think I might have found a solution:
import clr
clr.AddReference('System')
clr.AddReference('System.Core')
from System import Func
class IronPythonClass:
def foobar(self):
return Foo()
CSharpClass().DoSomething(Func[Foo](foobar))
The interesting bit is the `Func[Foo]`constructor :)
|
What is the fastest way to send 100,000 HTTP requests in Python?
Question: I am opening a file which has 100,000 url's. I need to send an http request to
each url and print the status code. I am using Python 2.6, and so far looked
at the many confusing ways Python implements threading/concurrency. I have
even looked at the python
[concurrence](http://opensource.hyves.org/concurrence) library, but cannot
figure out how to write this program correctly. Has anyone come across a
similar problem? I guess generally I need to know how to perform thousands of
tasks in Python as fast as possible - I suppose that means 'concurrently'.
Thank you, Igor
Answer: Twistedless solution:
from urlparse import urlparse
from threading import Thread
import httplib, sys
from Queue import Queue
concurrent = 200
def doWork():
while True:
url = q.get()
status, url = getStatus(url)
doSomethingWithResult(status, url)
q.task_done()
def getStatus(ourl):
try:
url = urlparse(ourl)
conn = httplib.HTTPConnection(url.netloc)
conn.request("HEAD", url.path)
res = conn.getresponse()
return res.status, ourl
except:
return "error", ourl
def doSomethingWithResult(status, url):
print status, url
q = Queue(concurrent * 2)
for i in range(concurrent):
t = Thread(target=doWork)
t.daemon = True
t.start()
try:
for url in open('urllist.txt'):
q.put(url.strip())
q.join()
except KeyboardInterrupt:
sys.exit(1)
This one is slighty faster than the twisted solution and uses less CPU.
|
Python - multiple copies of output when using multiprocessing
Question: > **Possible Duplicate:**
> [Multiprocessing launching too many instances of Python
> VM](http://stackoverflow.com/questions/1923706/multiprocessing-launching-
> too-many-instances-of-python-vm)
Module run via `python myscript.py` (not shell input)
import uuid
import time
import multiprocessing
def sleep_then_write(content):
time.sleep(5)
print(content)
if __name__ == '__main__':
for i in range(15):
p = multiprocessing.Process(target=sleep_then_write,
args=('Hello World',))
p.start()
print('Ah, what a hard day of threading...')
This script output the following:
Ah, what a hard day of threading...
Ah, what a hard day of threading...
Ah, what a hard day of threading...
Ah, what a hard day of threading...
Ah, what a hard day of threading...
Ah, what a hard day of threading...
Ah, what a hard day of threading...
Ah, what a hard day of threading...
Ah, what a hard day of threading...
AAh, what a hard day of threading..
h, what a hard day of threading...
Ah, what a hard day of threading...
Ah, what a hard day of threading...
Ah, what a hard day of threading...
Ah, what a hard day of threading...
Ah, what a hard day of threading...
Hello World
Hello World
Hello World
Hello World
Hello World
Hello World
Hello World
Hello World
Hello World
Hello World
Hello World
Hello World
Hello World
Hello World
Hello World
Firstly, why the heck did it print the bottom statement sixteen times (one for
each process) instead of just the one time?
Second, notice the **`AAh,`** and **`h,`** about half way down; that was the
real output. This makes me wary of using threads ever, now.
(Windows XP, Python 2.6.4, Core 2 Duo)
Answer: multiprocessing works by starting several processes. Each process loads a copy
of your script (that way it has access to the "target" function), and then
runs the target function.
You get the bottom print statement 16 times because the statement is sitting
out there by itself and gets printed when you load the module. Put it inside a
**main** block and it wont:
if __name__ == "__main__":
print('Ah, what a hard day of threading...')
Regarding the "AAh" - you have multiple processes going and they'll produce
output as they run, so you simply have the "A" from one process next to the
"Ah" from another.
When dealing with multi process or multi threaded environments you have to
think through locking and communication. This is not unique to
multiprocessing; any concurrent library will have the same issues.
|
How to evaluate a custom math expression in Python
Question: I'm writing a custom dice rolling parser (snicker if you must) in python.
Basically, I want to use standard math evaluation but add the 'd' operator:
#xdy
sum = 0
for each in range(x):
sum += randInt(1, y)
return sum
So that, for example, 1d6+2d6+2d6-72+4d100 = (5)+(1+1)+(6+2)-72+(5+39+38+59) =
84
I was using regex to replace all 'd's with the sum and then using eval, but my
regex fell apart when dealing with parentheses on either side. Is there a
faster way to go about this than implementing my own recursive parsing?
Perhaps adding an operator to eval?
Edit: I seem to have given a bad example, as the above example works with my
current version. What I'm looking for is some way to evaluate, say,
(5+(6d6))d(7-2*(1d4)).
By "fell apart", I just meant that my current regex expression failed. I have
been too vague about my failure, sorry for the confusion. Here's my current
code:
def evalDice(roll_matchgroup):
roll_split = roll_matchgroup.group('roll').split('d')
print roll_split
roll_list = []
for die in range(int(roll_split[0])):
roll = random.randint(1,int(roll_split[1]))
roll_list.append(roll)
def EvalRoll(roll):
if not roll: return 0
rollPattern = re.compile('(?P<roll>\d*d\d+)')
roll_string = rollPattern.sub(evalDice, roll.lower())
for this, "1d6+4d100" works just fine, but "(1d6+4)d100" or even "1d6+4d(100)"
fails.
Answer: You could use a [callback
function](http://docs.python.org/library/re.html#re.sub) with `re.sub`. When
you follow the link, search down to the paragraph beginning with "If repl is a
function..."
import re
import random
def xdy(matchobj):
x,y=map(int,matchobj.groups())
s = 0
for each in range(x):
s += random.randint(1, y)
return str(s)
s='1d6+2d6+2d6-72+4d100'
t=re.sub('(\d+)d(\d+)',xdy,s)
print(t)
# 5+10+8-72+197
print(eval(t))
# 148
|
How would I merged nested dictionaries in a list in python?
Question: for example if i had the result
[{'Germany': {"Luge - Men's Singles": 'Gold'}},
{'Germany': {"Luge - Men's Singles": 'Silver'}},
{'Italy': {"Luge - Men's Singles": 'Bronze'}}]
[{'Germany': {"Luge - Women's Singles": 'Gold'}},
{'Austria': {"Luge - Women's Singles": 'Silver'}},
{'Germany': {"Luge - Women's Singles": 'Bronze'}}]
[{'Austria': {'Luge - Doubles': 'Gold'}},
{'Latvia': {'Luge - Doubles': 'Silver'}},
{'Germany': {'Luge - Doubles': 'Bronze'}}]
how would I sort this so that all of the events germany and so on had won
could be under one single title. i.e germany would be germany:Luge - Men's
Singles: Gold, Silver, Luge - Women's Singles: Gold, Bronze, Luge - Doubles:
Bronze.
thanks for any help
EDIT: this is a straight copy and paste from the python shell now to help
confusion:
[{'Germany': {"Luge - Men's Singles": 'Gold'}}, {'Germany': {"Luge - Men's
Singles": 'Silver'}}, {'Italy': {"Luge - Men's Singles": 'Bronze'}}]
[{'Germany': {"Luge - Women's Singles": 'Gold'}}, {'Austria': {"Luge - Women's
Singles": 'Silver'}}, {'Germany': {"Luge - Women's Singles": 'Bronze'}}]
[{'Austria': {'Luge - Doubles': 'Gold'}}, {'Latvia': {'Luge - Doubles':
'Silver'}}, {'Germany': {'Luge - Doubles': 'Bronze'}}]
sorry about that im new to this site. It loops round 3 time one for each
different event and i was wondering if i could get the desired from merging it
after the final loop?
Answer:
import collections
merged_result = collections.defaultdict(list)
for L in listoflistsofdicts:
for d in L:
for k in d:
merged_result[k].append(d[k])
or if you just have a list of dicts instead of a list of lists of dicts (hard
to say from your Q!-), then just the
for d in listofdicts:
for k in d:
merged_result[k].append(d[k])
part of the loop.
If you want strings rather than lists as the values of `merged_result` then
after the above code add
for k in merged_result:
merged_result[k] = ', '.join(merged_result[k])
or, equivalently (but building a new plain dict instead of the defaultdict):
merged_result = dict((k, ', '.join(v)) for k, v in merged_result.iteritems())
(this assumes Python 2.* -- in Python 3, use `.items` instead of
`.iteritems`).
**Edit** : looking at the sample code it looks like it's invalid syntax for a
list of list of dicts (missing commas) so I showed how to deal with that, too.
|
Why urllib.urlopen doesn't (seem to) work with Stack Overflow?
Question: I need to retrieve my info from Stack Overflow. The web page that I want to
retrieve is something like this.
http://stackoverflow.com/users/260127/prosseek
When I run the script, it doesn't seem return any results.
import urllib
sock = urllib.urlopen("http://stackoverflow.com/users/260127/prosseek")
htmlSource = sock.read()
sock.close()
print htmlSource
whereas I get almost instant result with this.
import urllib
sock = urllib.urlopen("http://diveintopython.net/")
htmlSource = sock.read()
sock.close()
print htmlSource
What might be wrong?
PS. I don't know wether this should be asked at MetaStackOverflow or not.
Answer: It "works" for me - the page returned is a 404 error. Try putting the URL
<http://stackoverflow.com/users/XXXXX/prosseek> into your browser and see for
yourself.
I think you want to use <http://stackoverflow.com/users/260127/prosseek>
instead.
|
Unique user ID in a Pylons web application
Question: What is the best way to create a unique user ID in Python, using
[UUID](http://docs.python.org/library/uuid.html)?
Answer: I'd go with uuid
from uuid import uuid4
def new_user_id():
return uuid4().hex
|
Python Pre-testing for exceptions when coverage fails
Question: I recently came across a simple but nasty bug. I had a list and I wanted to
find the smallest member in it. I used Python's built-in min(). Everything
worked great until in some strange scenario the list was empty (due to strange
user input I could not have anticipated). My application crashed with a
ValueError (BTW - not documented in the official docs).
I have very extensive unit tests and I regularly check coverage to avoid
surprises like this. I also use Pylint (everything is integrated in PyDev) and
I never ignore warnings, yet I failed to catch this bug before my users did.
Is there anything I can change **in my methodology** to avoid these kind of
runtime errors? (which would have been caught at compile time in Java / C#?).
I'm looking for something more than wrapping my code with a big try-except.
What else can I do? How many other build in Python functions are hiding nasty
surprises like this???
Answer: The problem here is that malformed external input crashed your program. The
solution is to exhaustively unit test possible input scenarios at the
boundaries of your code. You say your unit tests are 'extensive', but you
clearly hadn't tested for this possibility. Code coverage is a useful tool,
but it's important to remember that covering code is **not** the same as
thoroughly testing it. Thorough testing is a combination of covering usage
scenarios as well as lines of code.
The methodology I use is to trust internal callers, but never to trust
external callers or input. So I explicitly **don't** unit test for the empty
list case in any code beyond the first function that receives the external
input. But that input function should be **exhaustively** covered.
In this case I think the library's exception is reasonable behaviour - it
makes no sense to ask for the `min` of an empty list. The library can't
legitimately set a value such as 0 for you, since you may be dealing with
negative numbers, for example.
I think the empty list should never have reached the code that asks for the
`min` \- it should have been identified at input, and either raised an
exception there, or set it to 0 if that works for you, or whatever else it is
that does work for you.
|
error in a pygame code
Question:
# INTIALISATION
import pygame, math, sys
from pygame.locals import *
screen = pygame.display.set_mode((1024, 768))
car = pygame.image.load('car.png')
clock = pygame.time.Clock()
k_up = k_down = k_left = k_right = 0
speed = direction = 0
position = (100, 100)
TURN_SPEED = 5
ACCELERATION = 2
MAX_FORWARD_SPEED = 10
MAX_REVERSE_SPEED = 5
BLACK = (0,0,0)
while 1:
# USER INPUT
clock.tick(30)
for event in pygame.event.get():
if not hasattr(event, 'key'): continue
down = event.type == KEYDOWN # key down or up?
if event.key == K_RIGHT: k_right = down * 5
elif event.key == K_LEFT: k_left = down * 5
elif event.key == K_UP: k_up = down * 2
elif event.key == K_DOWN: k_down = down * 2
elif event.key == K_ESCAPE: sys.exit(0) # quit the game
screen.fill(BLACK)
# SIMULATION
# .. new speed and direction based on acceleration and turn
speed += (k_up + k_down)
if speed > MAX_FORWARD_SPEED: speed = MAX_FORWARD_SPEED
if speed < MAX_REVERSE_SPEED: speed = MAX_REVERSE_SPEED
direction += (k_right + k_left)
# .. new position based on current position, speed and direction
x, y = position
rad = direction * math.pi / 180
x += speed*math.sin(rad)
y += speed*math.cos(rad)
position = (x, y)
# RENDERING
# .. rotate the car image for direction
rotated = pygame.transform.rotate(car, direction)
# .. position the car on screen
rect = rotated.get_rect()
rect.center = position
# .. render the car to screen
screen.blit(rotated, rect)
pygame.display.flip()
enter code here
the error i get is this Non-ASCII character '\xc2' in file race1.py on line
13, but no encoding declared; see <http://www.python.org/peps/pep-0263.html>
for details
Not able to understand what the error is and how to get rid of it?
Answer: You have a non-ASCII character on line 13. Python doesn't accept UTF-8 in
source files unless you put a special comment at the top of your file:
# encoding: UTF-8
|
SQLAlchemy custom sorting algorithms when using SQL indexes
Question: Is it possible to write custom collation functions with indexes in SQLAlchemy?
SQLite for example allows specifying the sorting function at a C level as
[`sqlite3_create_collation()`](http://www.sqlite.org/datatype3.html).
An implementation of some of the Unicode collation algorithm has been provided
by James Tauber
[here](http://jtauber.com/blog/2006/01/27/python_unicode_collation_algorithm/),
which for example sorts all the "a"'s close together whether they have accents
on them or not.
Other examples of why this might be useful is for different alphabet orders
(languages other than English) and sorting numeric values (sorting `10` after
`9` rather than codepoint order.)
Is this possible in SQLAlchemy? If not, is it supported by the `pysqlite3` or
`MySQLdb` modules, or for any other SQL database modules supported by python
for that matter?
Any information would be greatly appreciated.
Answer: Below is an example demonstrating unicode collation algorithm for sqlite:
from sqlalchemy import *
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
from pyuca import Collator
metadata = MetaData()
Base = declarative_base(metadata=metadata)
class Item(Base):
__tablename__ = 'Item'
id = Column(Integer, primary_key=True)
value = Column(String, nullable=False)
collator = Collator('allkeys.txt')
def collate_unicode(value1, value2):
return cmp(collator.sort_key(value1), collator.sort_key(value2))
engine = create_engine('sqlite://')
engine.raw_connection().create_collation('unicode', collate_unicode)
metadata.create_all(engine)
session = sessionmaker(engine)()
for word in [u"ĉambr", u"ĉar", u"car'", u"carin'", u"ĉe", u"ĉef'",
u"centjar'", u"centr'", u"cerb'", u"cert'", u"ĉes'", u"ceter'"]:
item = Item(value=word)
session.add(item)
session.commit()
for item in session.query(Item).order_by(collate(Item.value, 'unicode')):
print item.value
|
how to measure running time of algorithms in python
Question: > **Possible Duplicates:**
> [Accurate timing of functions in
> python](http://stackoverflow.com/questions/889900/accurate-timing-of-
> functions-in-python)
> [accurately measure time python function
> takes](http://stackoverflow.com/questions/1685221/accurately-measure-time-
> python-function-takes)
How can i mesure and compare the running times of my algorithms written in
python .Also point me to a nice algorithms site/forum like stackoverflow if
you can.
Answer: The module [`timeit`](http://docs.python.org/library/timeit.html) is useful
for this and is included in the standard Python distribution.
Example:
import timeit
timeit.Timer('for i in xrange(10): oct(i)').timeit()
|
Counting longest occurence of repeated sequence in Python
Question: What's the easiest way to count the longest consecutive repeat of a certain
character in a string? For example, the longest consecutive repeat of "b" in
the following string:
my_str = "abcdefgfaabbbffbbbbbbfgbb"
would be 6, since other consecutive repeats are shorter (3 and 2,
respectively.) How can I do this in Python?
thanks.
Answer: How about a regex example:
import re
my_str = "abcdefgfaabbbffbbbbbbfgbb"
len(max(re.compile("(b+b)*").findall(my_str))) #changed the regex from (b+b) to (b+b)*
# max([len(i) for i in re.compile("(b+b)").findall(my_str)]) also works
**Edit, Mine vs. interjays**
x=timeit.Timer(stmt='import itertools;my_str = "abcdefgfaabbbffbbbbbbfgbb";max(len(list(y)) for (c,y) in itertools.groupby(my_str) if c=="b")')
x.timeit()
22.759046077728271
x=timeit.Timer(stmt='import re;my_str = "abcdefgfaabbbffbbbbbbfgbb";len(max(re.compile("(b+b)").findall(my_str)))')
x.timeit()
8.4770550727844238
|
Problems with Routing URLs using CGI and Bottle.py
Question: I've been having difficulty getting anything more than a simple index / to
return correctly using bottle.py in a CGI environment. When I try to return
/hello I get a 404 response. However, if I request /index.py/hello
import bottle
from bottle import route
@route('/')
def index():
return 'Index'
@route('/hello')
def hello():
return 'Hello'
if __name__ == '__main__':
from wsgiref.handlers import CGIHandler
CGIHandler().run(bottle.default_app())
And here is my .htaccess file
DirectoryIndex index.py
<ifmodule mod_rewrite.c="">
RewriteEngine on
RewriteBase /
RewriteCond %{REQUEST_FILENAME} !-f
RewriteRule ^(.*)$ /index.py/$1 [L]
</ifmodule>
I copied much of the code from here as I'm using DH and it seemed relevant:
<http://blog.coderonfire.com/2010/02/running-bottle-python-micro-
framework.html>
Thanks for helping.
Answer: The problem is that the `<ifmodule>` block is not relevant to your Apache
server and the directives to mod_rewrite are not working. Start with the
following `.htaccess` and then if you have a need, add the block according to
your current apache version.
DirectoryIndex index.py
RewriteEngine on
RewriteCond %{REQUEST_FILENAME} !-f
RewriteRule ^(.*)$ /index.py/$1 [L]
|
Twisted Python getPage
Question: I tried to get support on this but I am TOTALLY confused.
Here's my code:
from twisted.internet import reactor
from twisted.web.client import getPage
from twisted.web.error import Error
from twisted.internet.defer import DeferredList
from sys import argv
class GrabPage:
def __init__(self, page):
self.page = page
def start(self, *args):
if args == ():
# We apparently don't need authentication for this
d1 = getPage(self.page)
else:
if len(args) == 2:
# We have our login information
d1 = getPage(self.page, headers={"Authorization": " ".join(args)})
else:
raise Exception('Missing parameters')
d1.addCallback(self.pageCallback)
dl = DeferredList([d1])
d1.addErrback(self.errorHandler)
dl.addCallback(self.listCallback)
def errorHandler(self,result):
# Bad thingy!
pass
def pageCallback(self, result):
return result
def listCallback(self, result):
print result
a = GrabPage('http://www.google.com')
data = a.start() # Not the HTML
I wish to get the HTML out which is given to pageCallback when start() is
called. This has been a pita for me. Ty! And sorry for my sucky coding.
Answer: You're missing the basics of how Twisted operates. It all revolves around the
`reactor`, which you're never even running. Think of the reactor like this:

Until you start the reactor, by setting up deferreds all you're doing is
chaining them with no events from which to fire.
I recommend you give the [Twisted Intro](http://krondo.com/blog/?page_id=1327)
by [Dave Peticolas](http://krondo.com/) a read. It's quick and it really gives
you all the missing information that the Twisted documentation doesn't.
Anyways, here is the most basic usage example of `getPage` as possible:
from twisted.web.client import getPage
from twisted.internet import reactor
url = 'http://aol.com'
def print_and_stop(output):
print output
if reactor.running:
reactor.stop()
if __name__ == '__main__':
print 'fetching', url
d = getPage(url)
d.addCallback(print_and_stop)
reactor.run()
Since `getPage` returns a deferred, I'm adding the callback `print_and_stop`
to the deferred chain. After that, I start the `reactor`. The reactor fires
`getPage`, which then fires `print_and_stop` which prints the data from
aol.com and then stops the reactor.
**Edit to show a working example of OP's code:**
class GrabPage:
def __init__(self, page):
self.page = page
########### I added this:
self.data = None
def start(self, *args):
if args == ():
# We apparently don't need authentication for this
d1 = getPage(self.page)
else:
if len(args) == 2:
# We have our login information
d1 = getPage(self.page, headers={"Authorization": " ".join(args)})
else:
raise Exception('Missing parameters')
d1.addCallback(self.pageCallback)
dl = DeferredList([d1])
d1.addErrback(self.errorHandler)
dl.addCallback(self.listCallback)
def errorHandler(self,result):
# Bad thingy!
pass
def pageCallback(self, result):
########### I added this, to hold the data:
self.data = result
return result
def listCallback(self, result):
print result
# Added for effect:
if reactor.running:
reactor.stop()
a = GrabPage('http://google.com')
########### Just call it without assigning to data
#data = a.start() # Not the HTML
a.start()
########### I added this:
if not reactor.running:
reactor.run()
########### Reference the data attribute from the class
data = a.data
print '------REACTOR STOPPED------'
print
########### First 100 characters of a.data:
print '------a.data[:100]------'
print data[:100]
|
Why do I get a TypeError: 'module' object is not callable when trying to import the random module?
Question: I am using Python 2.6 and am trying to run a simple random number generator
program (random.py):
import random
for i in range(5):
# random float: 0.0 <= number < 1.0
print random.random(),
# random float: 10 <= number < 20
print random.uniform(10, 20),
# random integer: 100 <= number <= 1000
print random.randint(100, 1000),
# random integer: even numbers in 100 <= number < 1000
print random.randrange(100, 1000, 2)
I'm now receiving the following error:
C:\Users\Developer\Documents\PythonDemo>python random.py
Traceback (most recent call last):
File "random.py", line 3, in <module>
import random
File "C:\Users\Developer\Documents\PythonDemo\random.py", line 8, in <module>
print random.random(),
TypeError: 'module' object is not callable
C:\Users\Developer\Documents\PythonDemo>
I've looked at the Python docs and this version of Python supports random. Is
there something else I'm missing?
Answer: Name your file something else. In Python a script _is_ a module, whose name is
determined by the filename. So when you start out your file `random.py` with
`import random` you are creating a loop in the module structure.
|
Resizing image with Python with locked aspect ratio
Question: How should I resize an image with Python script so that it would automatically
adjust the Height ratio to the Width used? I'm using the following code:
def Do(Environment):
# Resize
App.Do( Environment, 'Resize', {
'AspectRatio': 1.33333,
'CurrentDimensionUnits': App.Constants.UnitsOfMeasure.Pixels,
'CurrentResolutionUnits': App.Constants.ResolutionUnits.PixelsPerIn,
'Height': 1440,
'MaintainAspectRatio': True,
'Resample': True,
'ResampleType': App.Constants.ResampleType.SmartSize,
'ResizeAllLayers': True,
'Resolution': 72,
'Width': 1920,
})
Using this code works perfectly if the aspect ratio of an image is the same as
the one defined in the code - i.e. 1.33333. But how should I make it work with
images that do not have this ratio? For me, what is important is that the new
Width is 1920; Height has to be able to adjust automatically. Any ideas which
part of my code should be altered and how?
Answer: According to [this forum post](http://www.webmaster-talk.com/jasc-paint-shop-
pro/28772-resize-batch-script.html),
> the magic word is None
- i.e. change
'Height': 1440,
to
'Height': None,
As we found out in the comments below, you also have to set `AspectRatio` to
`None`.
|
Extend django.core.paginator Paginator to work with Google App Engine
Question: How would one extend the
[`Paginator`](http://docs.djangoproject.com/en/dev/topics/pagination/#django.core.paginator.Paginator)
class in
[`django.core.paginator`](http://code.djangoproject.com/browser/django/trunk/django/core/paginator.py)
to work with Google App Engine
[`queries`](http://code.google.com/appengine/docs/python/datastore/queryclass.html)?
Answer: The following changes are necessary:
from django.core.paginator import Paginator, Page
class GAEPaginator(Paginator):
def page(self, number):
"Returns a Page object for the given 1-based page number."
number = self.validate_number(number)
offset = (number - 1) * self.per_page
if offset+self.per_page + self.orphans >= self.count:
top = self.count
return Page(self.object_list.fetch(self.per_page, offset), number, self)
It would be nice of Paginator was aware of GAE cursors.
Please feel free to edit this answer with improvements.
|
exceptions with python unicode encode/decode functions (why doesn't errors=ignore actually ignore them??)
Question: Does anyone know why the string conversion functions throw exceptions when
errors="ignore" is passed? How can I convert from regular Python string
objects to unicode without errors being thrown? Thanks very much!
python -c "import codecs; codecs.open('tmp', 'wb', encoding='utf8', errors='ignore').write('кошка')"
returns
Traceback (most recent call last):
File "", line 1, in
File "/usr/lib/python2.6/codecs.py", line 686, in write
return self.writer.write(data)
File "/usr/lib/python2.6/codecs.py", line 351, in write
data, consumed = self.encode(object, self.errors)
UnicodeDecodeError: 'ascii' codec can't decode byte 0xd0 in position 0:
ordinal not in range(128)
**EDIT** \-- thanks for the responses, but does anyone know how to convert the
literal above, not using the "u" prefix? The reason being is that you could,
of course, be dealing with something that wasn't a constant :)
Answer: The `write` method (in Python 2) takes a unicode object, and you're passing it
a str -- so the `encode` call in `codecs.py` line 351 is first trying to build
a unicode object (with the default codec, 'ascii'). Fix is easy: change the
`write` call to
write(u'кошка')
The `u` prefix tells Python you're using a Unicode object, and it should be
fine.
|
Case insensitive string columns in SQLAlchemy?
Question: can i create a case insensitive string column in sqlalchemy? im using sqlite,
and theres probaby a way to do it through DB by changing collation, but i want
to keep it in sqlalchemy/python.
Answer: SQLAlchemy doesn't seem to allow COLLATE clauses at the table creation (DDL)
stage by default, but I finally figured out a way to get this working on
SQLAlchemy 0.6+. Unfortunately, it involves a bit of subclassing and
decorating, but it's reasonably compact.
from sqlalchemy import *
from sqlalchemy.ext.compiler import compiles
from sqlalchemy.types import TypeDecorator
class CI_String(TypeDecorator):
""" Case-insensitive String subclass definition"""
impl = String
def __init__(self, length, **kwargs):
if kwargs.get('collate'):
if kwargs['collate'].upper() not in ['BINARY','NOCASE','RTRIM']:
raise TypeError("%s is not a valid SQLite collation" % kwargs['collate'])
self.collation = kwargs.pop('collate').upper()
super(CI_String, self).__init__(length=length, **kwargs)
@compiles(CI_String, 'sqlite')
def compile_ci_string(element, compiler, **kwargs):
base_visit = compiler.visit_string(element, **kwargs)
if element.collation:
return "%s COLLATE %s" % (base_visit, element.collation)
else:
return base_visit
The new string type can then be used normally for creating tables:
just_a_table = Table('table_name', metadata,
Column('case_insensitive', CI_String(8, collate='NOCASE'), nullable=False))
Hope someone finds this useful!
|
Extract images from PDF without resampling, in python?
Question: How might one extract all images from a pdf document, at native resolution and
format? (Meaning extract tiff as tiff, jpeg as jpeg, etc. and without
resampling). Layout is unimportant, I don't care were the source image is
located on the page.
I'm using python 2.7 but can use 3.x if required.
Answer: Often in a PDF, the image is simply stored as-is. For example, a PDF with a
jpg inserted will have a range of bytes somewhere in the middle that when
extracted is a valid jpg file. You can use this to very simply extract byte
ranges from the PDF. I wrote about this some time ago, with sample code:
[Extracting JPGs from
PDFs](http://nedbatchelder.com/blog/200712/extracting_jpgs_from_pdfs.html).
|
matplotlib: working with range in x-axis
Question: I'm trying to do a basic line graph here, but I can't seem to figure out how
to adjust my x axis.
And here is the error I get when I try adjusting my range.
from pylab import *
plot ( range(0,11),[9,4,5,2,3,5,7,12,2,3],'.-',label='sample1' )
plot ( range(0,11),[12,5,33,2,4,5,3,3,22,10],'o-',label='sample2' )
xlabel('x axis')
ylabel('y axis')
title('my sample graphs')
legend(('sample1','sample2'))
savefig("sampleg.png",dpi=(640/8))
show()
File "C:\Python26\lib\site-packages\matplotlib\axes.py", line 228, in _xy_from_xy
raise ValueError("x and y must have same first dimension")
ValueError: x and y must have same first dimension
I want my range to be a list of strings: ["12/1/2007","12/1/2008",
"12/1/2009","12/1/2010"]
Any suggestions?
* * *
Honestly, I found the code online and was trying to rewrite it to properly
understand it. I think I'm going to start from scratch so that I know what I'm
doing but I need help on where to start.
I posted another question which explains what I want to do here:
[Using PyLab to create a 2D graph from two separate
lists](http://stackoverflow.com/questions/2722216/using-pylab-to-
create-a-2d-graph-from-two-separate-lists)
Answer: `range(0,11)` should be `range(0,10)`.
|
PyFacebook with Pylons
Question: I'd like to implement PyFacebook in my Python + Pylons application. Where
should I include the package? What's the cleanest way to import it? What
directory should I put the files in? Thanks!
Answer: Most of your libraries are on your pythonpath, which mostly is lib/site-
packages. You should just install those and most installers will make sure
they're on your python-path. Then you should be able to import them normally.
|
Process list on Linux via Python
Question: How can I get running process list using Python on Linux?
Answer: IMO looking at the `/proc` filesystem is less nasty than hacking the text
output of `ps`.
import os
pids = [pid for pid in os.listdir('/proc') if pid.isdigit()]
for pid in pids:
try:
print open(os.path.join('/proc', pid, 'cmdline'), 'rb').read()
except IOError: # proc has already terminated
continue
|
WxPython multiple grid instances
Question: Does anybody know how I can get multiple instances of the same grid to display
on one frame? Whenever I create more than 1 instance of the same object, the
display of the original grid widget completely collapses and I'm left unable
to do anything with it.
For reference, here's the code:
import wx
import wx.grid as gridlib
class levelGrid(gridlib.Grid):
def __init__(self, parent, rows, columns):
gridlib.Grid.__init__(self, parent, -1)
self.moveTo = None
self.CreateGrid(rows, columns)
self.SetDefaultColSize(32)
self.SetDefaultRowSize(32)
self.SetColLabelSize(0)
self.SetRowLabelSize(0)
self.SetDefaultCellBackgroundColour(wx.BLACK)
self.EnableDragGridSize(False)
class mainFrame(wx.Frame):
def __init__(self, parent, id, title):
wx.Frame.__init__(self, parent, id, title, size=(768, 576))
editor = levelGrid(self, 25, 25)
panel1 = wx.Panel(editor, -1)
#vbox = wx.BoxSizer(wx.VERTICAL)
#vbox.Add(editor, 1, wx.EXPAND | wx.ALL, 5)
#selector = levelGrid(self, 1, 25)
#vbox.Add(selector, 1, wx.EXPAND |wx.BOTTOM, 5)
self.Centre()
self.Show(True)
app = wx.App()
mainFrame(None, -1, "SLAE")
app.MainLoop()
Answer: You will need to add sizer(vbox) to a panel, so you should be doing this
1. Create a panel inside frame
2. Create 2 level Grids as child of panel
3. Add levelGrids to vbox sizer
4. Add sizer to panel
e.g.
class mainFrame(wx.Frame):
def __init__(self, parent, id, title):
wx.Frame.__init__(self, parent, id, title, size=(768, 576))
panel = wx.Panel(self, -1)
editor = levelGrid(panel, 15, 25)
selector = levelGrid(panel, 1, 25)
selector.SetDefaultCellBackgroundColour(wx.BLUE)
vbox = wx.BoxSizer(wx.VERTICAL)
vbox.Add(editor, 0, wx.EXPAND | wx.ALL, 5)
vbox.Add(selector, 1, wx.EXPAND |wx.BOTTOM, 5)
panel.SetSizerAndFit(vbox)
self.Centre()
self.Show(True)
|
Problems inserting file data into sqlite database using python
Question: I'm trying to open an image file in python and add that data to an sqlite
table. I created the table using: "CREATE TABLE "images" ("id" INTEGER PRIMARY
KEY AUTOINCREMENT NOT NULL , "description" VARCHAR, "image" BLOB );"
I am trying to add the image to the db using:
imageFile = open(imageName, 'rb')
b = sqlite3.Binary(imageFile.read())
targetCursor.execute("INSERT INTO images (image) values(?)", (b,))
targetCursor.execute("SELECT id from images")
for id in targetCursor:
imageid= id[0]
targetCursor.execute("INSERT INTO %s (questionID,imageID) values(?,?)" % table, (questionId, imageid))
When I print the value of 'b' it looks like binary data but when I call:
'select image from images where id = 1' I get '????' printed to the console.
Anyone know what I'm doing wrong?
Answer: It works for me with Python 2.6.4, pysqlite (sqlite3.version) 2.4.1, and a png
test image. You have to unpack the tuple.
>>> import sqlite3
>>> conn = sqlite3.connect(":memory:")
>>> targetCursor = conn.cursor()
>>> imageName = "blue.png"
>>> imageFile = open(imageName, 'rb')
>>> b = sqlite3.Binary(imageFile.read())
>>> print b
�PNG
▒
IHDR@%
��sRGB��� pHYs
��▒tIME�
0�\"▒'S�A�:hVO\��8�}^c��"]IEND�B`�
>>> targetCursor.execute("create table images (id integer primary key, image BLOB)")
<sqlite3.Cursor object at 0xb7688e00>
>>> targetCursor.execute("insert into images (image) values(?)", (b,))
<sqlite3.Cursor object at 0xb7688e00>
>>> targetCursor.execute("SELECT image from images where id = 1")
<sqlite3.Cursor object at 0xb7688e00>
>>> for image, in targetCursor:
... print image
...
�PNG
▒
IHDR@%
��sRGB��� pHYs
��▒tIME�
0�\"▒'S�A�:hVO\��8�}^c��"]IEND�B`�
|
Python `if x is not None` or `if not x is None`?
Question: I've always thought of the `if not x is None` version to be more clear, but
Google's [style guide](http://google-
styleguide.googlecode.com/svn/trunk/pyguide.html?showone=True/False_evaluations#True/False_evaluations)
implies (based on this excerpt) that they use `if x is not None`. Is there any
minor performance difference (I'm assuming not), and is there any case where
one really doesn't fit (making the other a clear winner for my convention)?*
*I'm referring to any singleton, rather than just `None`.
> ...to compare singletons like None. Use is or is not.
Answer: There's no performance difference, as they compile to the same bytecode:
Python 2.6.2 (r262:71600, Apr 15 2009, 07:20:39)
>>> import dis
>>> def f(x):
... return x is not None
...
>>> dis.dis(f)
2 0 LOAD_FAST 0 (x)
3 LOAD_CONST 0 (None)
6 COMPARE_OP 9 (is not)
9 RETURN_VALUE
>>> def g(x):
... return not x is None
...
>>> dis.dis(g)
2 0 LOAD_FAST 0 (x)
3 LOAD_CONST 0 (None)
6 COMPARE_OP 9 (is not)
9 RETURN_VALUE
Stylistically, I try to avoid `not x is y`. Although the compiler will always
treat it as `not (x is y)`, a human reader might misunderstand the construct
as `(not x) is y`. If I write `x is not y` then there is no ambiguity.
|
Hashing a python function to regenerate output when the function is modified
Question: I have a python function that has a deterministic result. It takes a long time
to run and generates a large output:
def time_consuming_function():
# lots_of_computing_time to come up with the_result
return the_result
I modify `time_consuming_function` from time to time, but I would like to
avoid having it run again while it's unchanged. [`time_consuming_function`
only depends on functions that are immutable for the purposes considered here;
i.e. it might have functions from Python libraries but not from other pieces
of my code that I'd change.] The solution that suggests itself to me is to
cache the output and also cache some "hash" of the function. If the hash
changes, the function will have been modified, and we have to re-generate the
output.
Is this possible or ridiculous?
* * *
**Updated** : based on the answers, it looks like what I want to do is to
"memoize" `time_consuming_function`, except instead of (or in addition to)
arguments passed into an invariant function, I want to account for a function
that itself will change.
Answer: If I understand your problem, I think I'd tackle it like this. It's a touch
evil, but I think it's more reliable and on-point than the other solutions I
see here.
import inspect
import functools
import json
def memoize_zeroadic_function_to_disk(memo_filename):
def decorator(f):
try:
with open(memo_filename, 'r') as fp:
cache = json.load(fp)
except IOError:
# file doesn't exist yet
cache = {}
source = inspect.getsource(f)
@functools.wraps(f)
def wrapper():
if source not in cache:
cache[source] = f()
with open(memo_filename, 'w') as fp:
json.dump(cache, fp)
return cache[source]
return wrapper
return decorator
@memoize_zeroadic_function_to_disk(...SOME PATH HERE...)
def time_consuming_function():
# lots_of_computing_time to come up with the_result
return the_result
|
How to display a page in my browser with python code that is run locally on my computer with "GAE" SDK?
Question: When I run this code on my computer with the help of "Google App Engine SDK",
it displays (in my browser) the HTML code of the Google home page:
from google.appengine.api import urlfetch
url = "http://www.google.com/"
result = urlfetch.fetch(url)
print result.content
How can I make it display the page itself? I mean I want to see that page in
my browser the way it would normally be seen by any user of the internet.
* * *
Update 1:
I see I have received a few questions that look a bit complicated to me,
although I definitely remember I was able to do it, and it was very simple,
except i don't remember what exactly i changed then in this code.
Perhaps, I didn't give You all enough details on how I run this code and where
I found it. So, let me tell You what I did. I only installed Python 2.5 on my
computer and then downloaded "Google App Engine SDK" and installed it, too.
Following the instructions on "GAE" page
(<http://code.google.com/appengine/docs/python/gettingstarted/helloworld.html>)
I created a directory and named it “My_test”, then I created a “my_test.py” in
it containing that small piece of the code that I mentioned in my question.
Then, continuing to follow on the said instructions, I created an “app.yaml”
file in it, in which my “my_test.py” file was mentioned. After that in “Google
App Engine Launcher” I found “My_test” directory and clicked on Run button,
and then on Browse. Then, having visited this URL <http://localhost:8080/> in
my web browser, I saw the results.
I definitely remember I was able to display any page in my browser in this
way, and it was very simple, except I don’t remember what exactly I changed in
the code (it was a slight change). Now, all I can see is a raw HTML code of a
page, but not a page itself.
* * *
Update 2:
(this update is my response to wescpy)
Hello, wescpy!!! I've tried Your updated code and something didn't work well
there. Perhaps, it's because I am not using a certain framework that I am
supposed to use for this code. Please, take a look at this screen shot (I
guess You'll need to right-click this image to see it in better resolution):
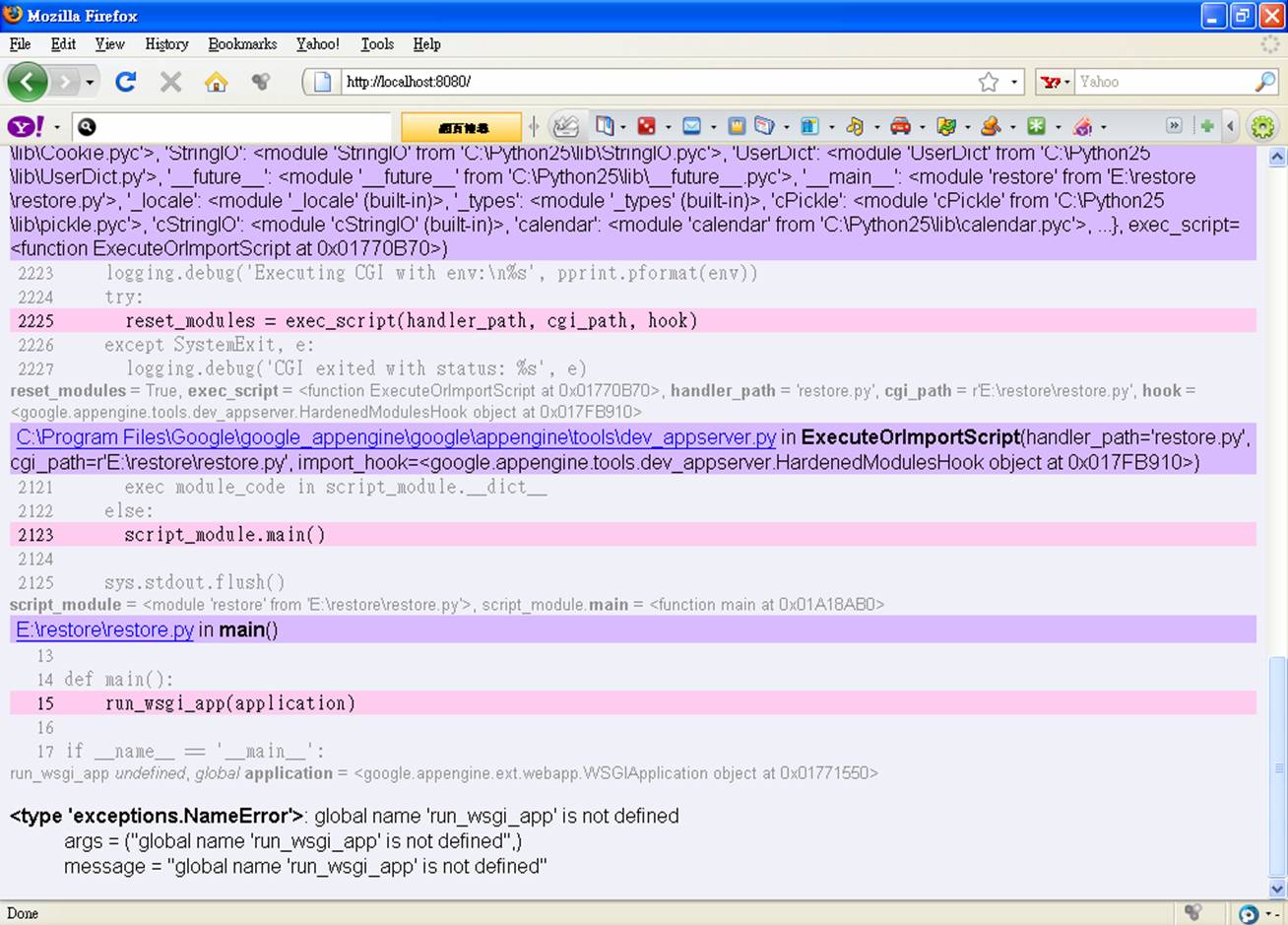
Answer: Is not that easy, you have to parse content and adjust relative to absolute
paths for images and javascripts.
Anyway, give it a try adding the correct Content-Type:
from google.appengine.api import urlfetch
url = "http://www.google.com/"
result = urlfetch.fetch(url)
print 'Content-Type: text/html'
print ''
print result.content
|
How to install pyobjc on SnowLeopard's non-default python installation
Question: I'm having problems installing pyobjc on SnowLeopard.
It came with python 2.6 but I need 2.5 so I have installed 2.5 successfully.
After that I have installed xcode. After that I have installed pyobjc with
"easy_install-2.5 pyobjc"
But when I start my python 2.5 and from cmd line try to import Foundation, it
says "no module named Foundation"
I tried to do `export PYTHONPATH="/Library/Python/2.5/site-
packages/pyobjc_core-2.2-py2.5-macosx-10.6-i386.egg/objc"` before starting
python interpreter but still no luck (this .egg directory is the only
directory pyobjc installation made, and there are several more egg files there
in site-packages... in objc subdir there is **init**.py file)
Of course, from 2.6 everything works fine. How do I find out what's wrong and
what should i do?
When I print sys.modules from python 2.6 I find that objc that gets imported
is basically from the same install location "/Library/Python/2.6/site-
packages/pyobjc_core-2.2-py2.6-macosx-10.6-universal.egg/objc/", so why it
won't work for 2.5?
Answer: Ok, found what's wrong.
My SnowLeopard came with BOTH python 2.6 (default) and 2.5 installed
XCode installed objc for both.
So basically I have broken my pythonpath etc with additional python 2.5 and
objc manual installations, somehow libraries weren't compatible (mine and
original python are both 2.5.4 but slightly different release and what's more
important probably built with different build options)
What I did is: making sure I start everything with original python2.5 (on my
system it's in /usr/bin/python2.5), removing wrong entries from
easy_install.pth in site-packages, and adding the path to PyObjc to
easy_install.pth.
Sorry for not finding out sooner, but I hope this will be helpful to someone
in the future!
|
Why does Python's __import__ require fromlist?
Question: In Python, if you want to programmatically import a module, you can do:
module = __import__('module_name')
If you want to import a submodule, you would think it would be a simple matter
of:
module = __import__('module_name.submodule')
Of course, this doesn't work; you just get `module_name` again. You have to
do:
module = __import__('module_name.submodule', fromlist=['blah'])
**Why?** The actual value of `fromlist` don't seem to matter at all, as long
as it's non-empty. What is the point of requiring an argument, then ignoring
its values?
Most stuff in Python seems to be done for good reason, but for the life of me,
I can't come up with any reasonable explanation for this behavior to exist.
Answer: In fact, the behaviour of `__import__()` is entirely because of the
implementation of the `import` statement, which calls `__import__()`. There's
basically five slightly different ways `__import__()` can be called by
`import` (with two main categories):
import pkg
import pkg.mod
from pkg import mod, mod2
from pkg.mod import func, func2
from pkg.mod import submod
In the first _and_ the second case, the `import` statement should assign the
"left-most" module object to the "left-most" name: `pkg`. After `import
pkg.mod` you can do `pkg.mod.func()` because the `import` statement introduced
the local name `pkg`, which is a module object that has a `mod` attribute. So,
the `__import__()` function has to return the "left-most" module object so it
can be assigned to `pkg`. Those two import statements thus translate into:
pkg = __import__('pkg')
pkg = __import__('pkg.mod')
In the third, fourth and fifth case, the `import` statement has to do more
work: it has to assign to (potentially) multiple names, which it has to get
from the module object. The `__import__()` function can only return one
object, and there's no real reason to make it retrieve each of those names
from the module object (and it would make the implementation a lot more
complicated.) So the simple approach would be something like (for the third
case):
tmp = __import__('pkg')
mod = tmp.mod
mod2 = tmp.mod2
However, that won't work if `pkg` is a package and `mod` or `mod2` are modules
in that package _that are not already imported_ , as they are in the third and
fifth case. The `__import__()` function needs to know that `mod` and `mod2`
are names that the `import` statement will want to have accessible, so that it
can see if they are modules and try to import them too. So the call is closer
to:
tmp = __import__('pkg', fromlist=['mod', 'mod2'])
mod = tmp.mod
mod2 = tmp.mod2
which causes `__import__()` to try and load `pkg.mod` and `pkg.mod2` as well
as `pkg` (but if `mod` or `mod2` don't exist, it's not an error in the
`__import__()` call; producing an error is left to the `import` statement.)
But that still isn't the right thing for the fourth and fifth example, because
if the call were so:
tmp = __import__('pkg.mod', fromlist=['submod'])
submod = tmp.submod
then `tmp` would end up being `pkg`, as before, and not the `pkg.mod` module
you want to get the `submod` attribute from. The implementation could have
decided to make it so the `import` statement does extra work, splitting the
package name on `.` like the `__import__()` function already does and
traversing the names, but this would have meant duplicating some of the
effort. So, instead, the implementation made `__import__()` return the _right-
most_ module instead of the _left-most_ one **if and only if** fromlist is
passed and not empty.
(The `import pkg as p` and `from pkg import mod as m` syntax doesn't change
anything about this story except which local names get assigned to -- the
`__import__()` function sees nothing different when `as` is used, it all
remains in the `import` statement implementation.)
|
Common elements between two lists not using sets in Python
Question: I want count the same elements of two lists. Lists can have duplicate
elements, so I can't convert this to sets and use & operator.
a=[2,2,1,1]
b=[1,1,3,3]
set(a) & set(b) work
a & b don't work
It is possible to do it withoud set and dictonary?
Answer: In Python 3.x (and Python 2.7, when it's released), you can use
[collections.Counter](http://docs.python.org/dev/library/collections.html#collections.Counter)
for this:
>>> from collections import Counter
>>> list((Counter([2,2,1,1]) & Counter([1,3,3,1])).elements())
[1, 1]
Here's an alternative using
[collections.defaultdict](http://docs.python.org/library/collections.html#collections.defaultdict)
(available in Python 2.5 and later). It has the nice property that the order
of the result is deterministic (it essentially corresponds to the order of the
second list).
from collections import defaultdict
def list_intersection(list1, list2):
bag = defaultdict(int)
for elt in list1:
bag[elt] += 1
result = []
for elt in list2:
if elt in bag:
# remove elt from bag, making sure
# that bag counts are kept positive
if bag[elt] == 1:
del bag[elt]
else:
bag[elt] -= 1
result.append(elt)
return result
For both these solutions, the number of occurrences of any given element `x`
in the output list is the minimum of the numbers of occurrences of `x` in the
two input lists. It's not clear from your question whether this is the
behavior that you want.
|
Django: text fixture fails to load
Question: Did a dumpdata of my project, then in my new test I added it to fixtures.
from django.test import TestCase
class TestGoal(TestCase):
fixtures = ['test_data.json']
def test_goal(self):
"""
Tests that 1 + 1 always equals 2.
"""
self.failUnlessEqual(1 + 1, 2)
When running the test I get:
> Problem installing fixture 'XXX/fixtures/test_data.json':
>
> DoesNotExist: XXX matching query does not exist.
But manually doing loaddata ~~works fine~~ does not when the db is empty. I do
a dropdb, createdb a simple syncdb the try loaddata and it fails, same error.
Any clue?
Python version 2.6.5, Django 1.1.1
Answer: Perhaps you have some foreign key troubles. If you have a model that contains
a foreign key referring to another model but the other model doesn't exist,
you'll get this error.
This can happen for a couple of reasons: if you are pointing to a model in
another app that you didn't include in the test_data.json dump, you'll have
trouble.
Also, if foreign keys change, this can break serialization -- this is
especially problematic with automatically created fields like
[permissions](http://docs.djangoproject.com/en/dev/topics/auth/#id1) or
[generic
relations](http://docs.djangoproject.com/en/dev/ref/contrib/contenttypes/#id1).
Django 1.2 supports [natural
keys](http://docs.djangoproject.com/en/dev/topics/serialization/#natural-
keys), which are a way to serialize using the "natural" representation of a
model as a foreign key rather than an ID which might change.
|
python script problem once build and package it
Question: I've written python script to scan wifi and send data to the server, I set
interval value, so it keep on scanning and send the data, it read from
config.txt file where i set the interval value to scan, I also add yes/no in
my config file, so is 'no' it will scan only once and if 'yes' it will scan
according to the interval level,
my code as below
import time,.....
from threading import Event, Thread
class RepeatTimer(Thread):
def __init__(self, interval, function, iterations=0, args=[], kwargs={}):
Thread.__init__(self)
self.interval = interval
self.function = function
self.iterations = iterations
self.args = args
self.kwargs = kwargs
self.finished = Event()
def run(self):
count = 0
while not self.finished.is_set() and (self.iterations <= 0 or count < self.iterations):
self.finished.wait(self.interval)
if not self.finished.is_set():
self.function(*self.args, **self.kwargs)
count += 1
def cancel(self):
self.finished.set()
def scanWifi(self):
#scanning process and sending data done here
obj = JW()
if status == "yes":
t = RepeatTimer(int(intervalTime),obj.scanWifi)
t.start()
else:
obj.scanWifi()
once I package my code, its only run when I set my config file set to 'no'
where it scan only once, but when I set my config file to 'yes', there is no
progress at all, so I found that there is problem with my class
RepeatTimer(Timer) once build, but don't know how to solve
can anyone help me
thanks
Answer: I think the problem is in the loop condition. Supposing that `is_set()`
returns `False`, the second part is always `False`. While is `intervalTime` is
not known, i think that it is positive (does has sense a negative interval
time?) and `count` is never lesser than `self.iterations`: they are both `0`.
But the code you posted is too few, it is not given to know how exactly works.
while not self.finished.is_set() and (self.iterations <= 0 or count < self.iterations):
|
Can ElementTree be told to preserve the order of attributes?
Question: I've written a fairly simple filter in python using ElementTree to munge the
contexts of some xml files. And it works, more or less.
But it reorders the attributes of various tags, and I'd like it to not do
that.
Does anyone know a switch I can throw to make it keep them in specified order?
## Context for this
I'm working with and on a particle physics tool that has a complex, but oddly
limited configuration system based on xml files. Among the many things setup
that way are the paths to various static data files. These paths are hardcoded
into the existing xml and there are no facilities for setting or varying them
based on environment variables, and in our local installation they are
necessarily in a different place.
This isn't a disaster because the combined source- and build-control tool
we're using allows us to shadow certain files with local copies. But even
thought the data fields are static the xml isn't, so I've written a script for
fixing the paths, but with the attribute rearrangement diffs between the local
and master versions are harder to read than necessary.
* * *
This is my first time taking ElementTree for a spin (and only my fifth or
sixth python project) so maybe I'm just doing it wrong.
Abstracted for simplicity the code looks like this:
tree = elementtree.ElementTree.parse(inputfile)
i = tree.getiterator()
for e in i:
e.text = filter(e.text)
tree.write(outputfile)
Reasonable or dumb?
* * *
Related links:
* [How can I get the order of an element attribute list using Python xml.sax?](http://stackoverflow.com/questions/998514/how-can-i-get-the-order-of-an-element-attribute-list-using-python-xml-sax)
* [Preserve order of attributes when modifying with minidom](http://stackoverflow.com/questions/662624/preserve-order-of-attributes-when-modifying-with-minidom)
Answer: Nope. ElementTree uses a dictionary to store attribute values, so it's
inherently unordered.
Even DOM doesn't guarantee you attribute ordering, and DOM exposes a lot more
detail of the XML infoset than ElementTree does. (There are some DOMs that do
offer it as a feature, but it's not standard.)
Can it be fixed? Maybe. Here's a stab at it that replaces the dictionary when
parsing with an ordered one
([`collections.OrderedDict()`](https://docs.python.org/2/library/collections.html#ordereddict-
objects)).
from xml.etree import ElementTree
from collections import OrderedDict
import StringIO
class OrderedXMLTreeBuilder(ElementTree.XMLTreeBuilder):
def _start_list(self, tag, attrib_in):
fixname = self._fixname
tag = fixname(tag)
attrib = OrderedDict()
if attrib_in:
for i in range(0, len(attrib_in), 2):
attrib[fixname(attrib_in[i])] = self._fixtext(attrib_in[i+1])
return self._target.start(tag, attrib)
>>> xmlf = StringIO.StringIO('<a b="c" d="e" f="g" j="k" h="i"/>')
>>> tree = ElementTree.ElementTree()
>>> root = tree.parse(xmlf, OrderedXMLTreeBuilder())
>>> root.attrib
OrderedDict([('b', 'c'), ('d', 'e'), ('f', 'g'), ('j', 'k'), ('h', 'i')])
Looks potentially promising.
>>> s = StringIO.StringIO()
>>> tree.write(s)
>>> s.getvalue()
'<a b="c" d="e" f="g" h="i" j="k" />'
Bah, the serialiser outputs them in canonical order.
This looks like the line to blame, in `ElementTree._write`:
items.sort() # lexical order
Subclassing or monkey-patching that is going to be annoying as it's right in
the middle of a big method.
Unless you did something nasty like subclass `OrderedDict` and hack `items` to
return a special subclass of `list` that ignores calls to `sort()`. Nah,
probably that's even worse and I should go to bed before I come up with
anything more horrible than that.
|
Python: Plot some data (matplotlib) without GIL
Question: my problem is the GIL of course. While I'm analysing data it would be nice to
present some plots in between (so it's not too boring waiting for results)
But the GIL prevents this (and this is bringing me to the point of asking
myself if Python was such a good idea in the first place).
I can only display the plot, wait till the user closes it and commence
calculations after that. A waste of time obviously.
I already tried the subprocess and multiprocessing modules but can't seem to
get them to work.
Any thoughts on this one? Thanks
Edit: Ok so it's not the GIL but show().
Answer: This is not a problem from matplotlib or the GIL.
In matplotlib You can open as many figures as you want and have them in the
screen while your application continues doing other things.
You must use matplotlib in interactive mode. This probably is your problem.
from matplotlib import interactive
interactive(True)
this should be at the top of your imports
|
how to exit recursive math formula and still get an answer
Question: i wrote this python code, which from wolfram alpha says that its supposed to
return the factorial of any positive value (i probably messed up somewhere),
integer or not:
from math import *
def double_factorial(n):
if int(n) == n:
n = int(n)
if [0,1].__contains__(n):
return 1
a = (n&1) + 2
b = 1
while a<=n:
b*=a
a+= 2
return float(b)
else:
return factorials(n/2) * 2**(n/2) *(pi/2)**(.25 *(-1+cos(n * pi)))
def factorials(n):
return pi**(.5 * sin(n*pi)**2) * 2**(-n + .25 * (-1 + cos(2*n*pi))) * double_factorial(2*n)
the problem is , say i input pi to 6 decimal places. 2*n will not become a
float with 0 as its decimals any time soon, so the equation turns out to be
pi**(.5 * sin(n*pi)**2) * 2**(-n + .25 * (-1 + cos(2*n*pi))) * double_factorial(loop(loop(loop(...)))))
how would i stop the recursion and still get the answer?
ive had suggestions to add an index to the definitions or something, but the
problem is, if the code stops when it reaches an index, there is still no
answer to put back into the previous "nests" or whatever you call them
Answer: You defined `f` in terms of `g` and `g` in terms of `f`. But you don't just
have a circular definition with no base point to start the recursion. You have
something worse. The definition of `f` is actually the definition of `g`
inverted. `f` is precisely undoing what `g` did and vice versa. If you're
trying to implement gamma yourself (ie. not using the one that's already there
in the libraries) then you need to use a formula that expresses gamma in terms
of something else that you know how to evaluate. Just using one formula and
its inversion like that is a method that will fail for almost any problem you
apply it to.
|
Is this a good approach to execute a list of operations on a data structure in Python?
Question: I have a dictionary of data, the key is the file name and the value is another
dictionary of its attribute values. Now I'd like to pass this data structure
to various functions, each of which runs some test on the attribute and
returns True/False.
One approach would be to call each function one by one explicitly from the
main code. However I can do something like this:
#MYmodule.py
class Mymodule:
def MYfunc1(self):
...
def MYfunc2(self):
...
#main.py
import Mymodule
...
#fill the data structure
...
#Now call all the functions in Mymodule one by one
for funcs in dir(Mymodule):
if funcs[:2]=='MY':
result=Mymodule.__dict__.get(funcs)(dataStructure)
The advantage of this approach is that implementation of main class needn't
change when I add more logic/tests to MYmodule.
Is this a good way to solve the problem at hand? Are there better alternatives
to this solution?
Answer: I'd say a better and much more Pythonic approach would be to define a
[decorator](http://www.artima.com/weblogs/viewpost.jsp?thread=240808) to
indicate which functions you want to use:
class MyFunc(object):
funcs = []
def __init__(self, func):
self.funcs.append(func)
@MyFunc
def foo():
return 5
@MyFunc
def bar():
return 10
def quux():
# Not decorated, so will not be in MyFunc
return 20
for func in MyFunc.funcs:
print func()
Output:
5
10
Essentially you're performing the same logic: taking only functions who were
defined in a particular manner and applying them to a specific set of data.
|
How to compile OpenGL with a python C++ extension using distutils on Mac OSX?
Question: When I try it I get:
> ImportError:
> dlopen(/Library/Frameworks/Python.framework/Versions/2.5/lib/python2.5/site-
> packages/cscalelib.so, 2): Symbol not found: _glBindFramebufferEXT
> Referenced from:
> /Library/Frameworks/Python.framework/Versions/2.5/lib/python2.5/site-
> packages/cscalelib.so Expected in: dynamic lookup
I've tried all sort of things in the setup.py file. What do I actually need to
put in it to link to OpenGL properly? My code compiles fine so there's no
point putting that on there. Here is setup.py
from distutils.core import setup, Extension
module1 = Extension('cscalelib',
extra_compile_args = ["-framework OpenGL", "-lm", "-lGL", "-lGLU"],
sources = ['cscalelib.cpp'])
setup (name = 'cscalelib',
version = '0.1',
description = 'Test for setup_framebuffer',
ext_modules = [module1])
Answer: I didn't realise I had to remove the build directory. Now it imports
correctly.
For anyone that needs to know you need: `extra_link_args=['-framework',
'OpenGL']` Delete the build directory and try it again. It will work.
|
Access to module denied from within GAE dev server
Question: I am developing an app for GAE.
Having installed the "feedparser" module with setuptools, I tried importing it
(with "import feedparser") statement. However, the module does not load and
when I look at the dev_appserver.py debug log on screen, I see the following:
Access to module file denied: /usr/local/lib/python2.6/dist-packages/feedparser-4.1-py2.6.egg/feedparser.py
So GAE dev server cannot access the module but I can't figure out why. The
path is correct and the file is accessible.
I am fairly new to Python/Django/GAE - what am I missing?
Answer: App Engine runs Python code in a sandbox, and only authorized standard library
modules & packages can be imported from your application.
as @mg has mentioned, if you want to allow for 3rd-party modules & packages,
you need to bundle them with your application. to do that specifically for
feedparser, just drop the `feedparser.py` file into your top-level App Engine
directory (where your `app.yaml`, `index.yaml`, and `main.py` are located).
(UPDATED Oct 2011) also keep in mind the hard limits:
* max total number of files (app files and static files): 3,000 (upped to 10k in 1.5.5, Oct 2011)
* max size of an application or static file: 10MB (upped to 32MB in 1.5.5)
* max total size of all application and static files: 150MB
if you want to save on the total number of files, you can put a wad of `.py`
files in a ZIP so you only pay for one file. although this article is slightly
out-of-date -- recommending bundling of Django 1.0 which is now included --
the technique of bundling modules & packages into ZIP files still apply:
<http://code.google.com/appengine/articles/django10_zipimport.html>
Official page in the docs which discusses the file limitations:
<http://code.google.com/appengine/docs/python/runtime.html#Pure_Python>
(UPDATED Nov 2011): The link below features a list of whitelisted Python
modules/packages with C code for 2.5. The Python 2.7 runtime frees up many
restrictions so much so that the whitelist has become a blacklist. Here are
the allowed/whitelisted 2.5 C modules as well as the disallowed/blacklisted
2.7 C modules:
<http://code.google.com/appengine/kb/libraries.html>
|
Import module stored in a cStringIO data structure vs. physical disk file
Question: Is there a way to import a Python module stored in a cStringIO data structure
vs. physical disk file?
It looks like "imp.load_compiled(name, pathname[, file])" is what I need, but
the description of this method (and similar methods) has the following
disclaimer:
Quote: "The file argument is the byte-compiled code file, open for reading in
binary mode, from the beginning. It must currently be a real file object, not
a user-defined class emulating a file." [1]
I tried using a cStringIO object vs. a real file object, but the help
documentation is correct - only a real file object can be used.
Any ideas on why these modules would impose such a restriction or is this just
an historical artifact?
Are there any techniques I can use to avoid this physical file requirement?
Thanks, Malcolm
[1] <http://docs.python.org/library/imp.html#imp.load_module>
Answer: Something like this perhaps?
import types
import sys
src = """
def hello(who):
print 'hello', who
"""
def module_from_text(modulename, src):
if modulename in sys.modules:
module = sys.modules[modulename]
else:
module = sys.modules[modulename] = types.ModuleType(modulename)
exec compile(src, '<no-file>', 'exec') in module.__dict__
return module
module_from_text('flup', src)
import flup
flup.hello('world')
Which prints:
hello world
**EDIT** :
Evaluating code in this way treads nearish the realm of writing custom
importers. It may be useful to look at [PEP
302](http://www.python.org/dev/peps/pep-0302/), and Doug Hellmann's [PyMOTW:
Modules and Imports](http://www.doughellmann.com/PyMOTW/sys/imports.html).
|
Selenium Webdriver example in Python
Question: I had written a scipt in Java with Webdriver and it worked fine and below is
the code for the sample
import org.junit.After;
import org.junit.AfterClass;
import org.junit.Before;
import org.junit.BeforeClass;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebDriverBackedSelenium;
import org.openqa.selenium.firefox.FirefoxDriver;
import com.thoughtworks.selenium.Selenium;
import java.util.*;
import java.lang.Thread.*;
public class Login {
@BeforeClass
public static void setUpBeforeClass() throws Exception {
}
@AfterClass
public static void tearDownAfterClass() throws Exception {
}
@Before
public void setUp() throws Exception {
}
@After
public void tearDown() throws Exception {
}
public static void main(String[] args) {
WebDriver driver = new FirefoxDriver();
Selenium selenium = new WebDriverBackedSelenium(driver, "http://192.168.10.10:8080/");
selenium.open("/");
selenium.keyPress("name=user_id", "admin");
}
}
}
But my requirement is to implement the same in python with webdriver, can you
please let me know how this can be done with the above example and webdriver
binaries and how to do setup for the same
Answer: Did you read the instructions at [_python bindings for
WebDriver_](http://code.google.com/p/selenium/wiki/PythonBindings) ?
[`example2.py`](http://code.google.com/p/selenium/source/browse/trunk/py/test/selenium/webdriver/common/example2.py)
is clear, although not a direct translation of your code:
import unittest
from google_one_box import GoogleOneBox
from selenium.firefox.webdriver import WebDriver
class ExampleTest2(unittest.TestCase):
"""This example shows how to use the page object pattern.
For more information about this pattern, see:
http://code.google.com/p/webdriver/wiki/PageObjects
"""
def setUp(self):
self._driver = WebDriver()
def tearDown(self):
self._driver.quit()
def testSearch(self):
google = GoogleOneBox(self._driver, "http://www.google.com")
res = google.search_for("cheese")
self.assertTrue(res.link_contains_match_for("Wikipedia"))
if __name__ == "__main__":
unittest.main()
A test module,
[GoogleOneBox](http://code.google.com/p/selenium/source/browse/trunk/py/test/selenium/webdriver/common/google_one_box.py),
models a page that has a google search bar (the url moved a little).
|
Setting timeouts to parse webpages using python lxml
Question: I am using python lxml library to parse html pages:
import lxml.html
# this might run indefinitely
page = lxml.html.parse('http://stackoverflow.com/')
Is there any way to set timeout for parsing?
Answer: It looks to be using `urllib.urlopen` as the opener, but the easiest way to do
this would just to modify the default timeout for the socket handler.
import socket
timeout = 10
socket.setdefaulttimeout(timeout)
Of course this is a quick-and-dirty solution.
|
Ruby LESS gem equivalent in Python
Question: The Ruby [LESS gem](http://lesscss.org/) looks awesome - and I am working on a
Python/Pylons web project where it would be highly useful. CSS is, as someone
we're all familiar with [recently wrote
about](http://www.codinghorror.com/blog/2010/04/whats-wrong-with-css.html),
clunky in some important ways. So I'd like to make it easier on myself.
Is there an existing Python module or library that provides parallel
functionality?
Answer: I have need for a Python lesscss compiler too, so have started work on one
here: <http://code.google.com/p/lesscss-python/>
Version 0.0.1 has been released, with no support for namespaces/accessors. It
is probably riddled with bugs too.
Please feel free to chip in with bug reports/coding or have a look at less-js
<http://fadeyev.net/2010/06/19/lessjs-will-obsolete-css/>.
|
Import Error when use templatetags in Django
Question: Well, when I'm trying to use 'inclusion' in Django, I met some confused
problems that I can't solve it by myself.
There is the structures for my project.
MyProject---
App1---
__init__.py
models.py
test.py
urls.py
views.py
App2---
...
template---
App1---
some htmls
App2---
...
templatetags---
__init__.py
inclusion_cld_tags.py
manage.py
urls.py
__init__.py
settings.py
I have registered templatetags folder in the settings.py (Both in Installed
APPS & TEMPLATE_DIRS). But when I want to use {% load inclusion_test %} in my
html, it raise an exception like this:
'inclusion_cld_tags' is not a valid tag library: Could not load template library from django.templatetags.inclusion_cld_tags, No module named inclusion_cld_tags
I think there is nothing wrong with my import work, how can I do with that?
Thanks for help!
My django version: 1.0+ My Python version: 2.6.4
Answer: 1. The templatetags folder [should live in the app folder](http://docs.djangoproject.com/en/dev/howto/custom-template-tags/#code-layout):
App1---
__init__.py
models.py
test.py
urls.py
views.py
templatetags---
__init__.py
inclusion_test.py
...
2. Did you registered the tag?
Example:
register = template.Library()
@register.inclusion_tag('platform/templatetags/pagination_links.html')
def pagination_links(page, per_page, link):
|
Howto ignore specific undefined variables in Pydev Eclipse
Question: I'm writing a crossplatform python script on windows using Eclipse with the
Pydev plugin. The script makes use of the `os.symlink()` and `os.readlink()`
methods if the current platform isn't NT.
Since the `os.symlink()` and `os.readlink()` methods aren't available on the
Windows platform Pydev flags them as undefined variables--like so:
[](http://www.flickr.com/photos/chris_nullptr/4582418253/)
### Question:
Is there a way to ignore specific undefined variable name errors _without_
modifying my source file?
**edit** : I found a way to ignore undefined variable errors from [this
answer](http://stackoverflow.com/questions/2112715/how-do-i-fix-pydev-
undefined-variable-from-import-errors/2248987#2248987) on stackoverflow.
I'll leave the question open in case there is a way to solve this using
project file or Pydev setting.
Answer: I use pydev + pylint.
With pylint you can add which messages to ignore in the
Preferences>Pydev>Pylint>"Aggruments to pass to pylint" section.
--disable-msg=W0232,F0401
You can ignore messages in-line as well with comments:
os.symlink(target, symlink) # IGNORE:<MessageID>
Mouse-over the "x" where the line numbers are to see the message id.
|
Difference between URLLIB2 call in IDLE and from Django?
Question: The following piece of code works as expected when running in a local install
of django apache 2.2
fx = urllib2.Request(f);
fx.add_header('User-Agent','Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US) AppleWebKit/525.19 (KHTML, like Gecko) Chrome/1.0.154.36 Safari/525.19');
url_opened = urllib2.urlopen(fx);
However when I enter that code into IDLE on the same machine I get the
following error:
url_opened = urllib2.urlopen(fx);
File "C:\Python25\lib\urllib2.py", line 124, in urlopen
return _opener.open(url, data)
File "C:\Python25\lib\urllib2.py", line 387, in open
response = meth(req, response)
File "C:\Python25\lib\urllib2.py", line 498, in http_response
'http', request, response, code, msg, hdrs)
File "C:\Python25\lib\urllib2.py", line 425, in error
return self._call_chain(*args)
File "C:\Python25\lib\urllib2.py", line 360, in _call_chain
result = func(*args)
File "C:\Python25\lib\urllib2.py", line 506, in http_error_default
raise HTTPError(req.get_full_url(), code, msg, hdrs, fp)
HTTPError: HTTP Error 407: Proxy Authentication Required
Any ideas?
Answer: `urllib` and `urllib2` I think look at environment variables for proxies if
one isn't set programatically. Maybe the proxy environment variables haven't
been set properly in IDLE?
Compare the output of the following from IDLE to the Django program:
import os, pprint
for k in os.environ:
if 'proxy' in k.lower(): # look for proxy environment variables
print k, os.environ[k]
**EDIT:** Quoting
<http://docs.python.org/library/urllib2.html#urllib2.ProxyHandler>:
Cause requests to go through a proxy. If proxies is given, it must be a
dictionary mapping protocol names to URLs of proxies. The default is to read the
list of proxies from the environment variables. If no proxy environment
variables are set, in a Windows environment, proxy settings are obtained from
the Internet Settings section and in a Mac OS X environment, proxy
information is retrieved from the OS X System Configuration Framework.
To disable autodetected proxy pass an empty dictionary.
Maybe Django creates a `ProxyHandler`? Try calling `urllib2.ProxyHandler()` in
IDLE.
|
socket.error: [Errno 10013] An attempt was made to access a socket in a way forbidden by its access permissions
Question: I'm trying to create a custom TCP stack using Python 2.6.5 on Windows 7 to
serve valid http page requests on port 80 locally. But, I've run into a snag
with what seems like Windows 7 tightened up security. This code worked on
Vista.
Here's my sample code:
import SocketServer
import struct
class MyTCPHandler(SocketServer.BaseRequestHandler):
def handle(self):
headerText = """HTTP/1.0 200 OK
Date: Fri, 31 Dec 1999 23:59:59 GMT
Content-Type: text/html
Content-Length: 1354"""
bodyText = "<html><body>some page</body></html>"
self.request.send(headerText + "\n" + bodyText)
if __name__ == "__main__":
HOST, PORT = "localhost", 80
server = SocketServer.TCPServer((HOST, PORT), MyTCPHandler)
server.serve_forever()
> **C:\python>python TestServer.py** Traceback (most recent call last):
> File "TestServer.py", line 19, in server = SocketServer.TCPServer((HOST,
> PORT), MyTCPHandler) File "C:\Python26\lib\SocketServer.py", line 400, in
> **init** self.server_bind() File "C:\Python26\lib\SocketServer.py", line
> 411, in server_bind self.socket.bind(self.server_address) File "", line 1,
> in bind
>
> socket.error: [Errno 10013] An attempt was made to access a socket in a way
> forbidden by its access permissions
How exactly do I get this to work on Windows 7?
[Edit on 5/5/2010 @ 2344 PDT] This
[answer](http://stackoverflow.com/questions/550032/what-causes-python-socket-
error) explains that the error is caused by the need for elevated / superuser
privileges when accessing ports lower than 1024. I'm going to try using a
higher port number to see if that works. However, I still would like to know
why my local admin account can't access port 80.
Answer: I just encountered the same issue, my system is Win7. just use the command on
terminal like: netstat -na|findstr port, you will see the port has been used.
So if you want to start the server without this message, you can change other
port that not been used.
|
show() doesn't redraw anymore
Question: I am working in linux and I don't know why using python and matplotlib
commands draws me only once the chart I want. The first time I call show() the
plot is drawn, wihtout any problem, but not the second time and the following.
I close the window showing the chart between the two calls. Do you know why
and hot to fix it?
Thanks AFG
from numpy import *
from pylab import *
data = array( [ 1,2,3,4,5] )
plot(data)
[<matplotlib.lines.Line2D object at 0x90c98ac>]
show() # this call shows me a plot
#..now I close the window...
data = array( [ 1,2,3,4,5,6] )
plot(data)
[<matplotlib.lines.Line2D object at 0x92dafec>]
show() # this one doesn't shows me anything
Answer: in windows this works perfect:
from pylab import *
plot([1,2,3,4])
[<matplotlib.lines.Line2D object at 0x03442C10>]
#close window here
plot([1,2,3,4])
[<matplotlib.lines.Line2D object at 0x035BC570>]
did you try with:
from matplotlib import interactive
interactive(True)
sometimes matplotlib produces some headaches because we have to remember that
some options are set in matplotlibrc (such as the backend or the interactive
parameters). If you use matplotlib from different editors (IDLE-tk, pycrust-
wxpython) or alternating interactive with scripting, then you have to take
into account that the configuration that works in one mode could give you
problems in the other mode and must be modified programmatically or using a
dedicated configuration file.
The example I give, works directly (and without show()) because in
matplotlibrc I have interactive set to True as default
|
How can I turn a single element in a list into multiple elements using Python?
Question: I have a list of elements, and each element consists of four separate values
that are separated by tabs:
['A\tB\tC\tD', 'Q\tW\tE\tR', etc.]
What I want is to create a larger list without the tabs, so that each value is
a separate element:
['A', 'B', 'C', 'D', 'Q', 'W', 'E', 'R', etc.]
How can I do that in Python? I need it for my coursework, due tonight
(midnight GMT) and I'm completely stumped.
Answer: All at once:
'\t'.join(['A\tB\tC\tD', 'Q\tW\tE\tR']).split('\t')
One at a time:
[c for s in ['A\tB\tC\tD', 'Q\tW\tE\tR'] for c in s.split('\t')]
Or if all elements are single letters:
[c for s in ['A\tB\tC\tD', 'Q\tW\tE\tR'] for c in s[::2]]
If there could be quoted tabs then:
import csv
rows = csv.reader(['A\t"\t"\tB\tC\tD', 'Q\tW\tE\tR'], delimiter='\t')
[c for s in rows for c in s] # or list(itertools.chain.from_iterable(rows))
|
Python3 error: "Import error: No module name urllib2"
Question: Here's my code:
import urllib2.request
response = urllib2.urlopen("http://www.google.com")
html = response.read()
print(html)
Any help?
Answer: As stated in the urllib2 documentation at
<http://docs.python.org/library/urllib2.html>:
> The urllib2 module has been split across several modules in Python 3.0 named
> urllib.request and urllib.error. The 2to3 tool will automatically adapt
> imports when converting your sources to 3
So you should instead be saying
from urllib.request import urlopen
html = urlopen("http://www.google.com/")
print(html)
Your current, now-edited code sample is incorrect because you are saying
`urllib.urlopen("http://www.google.com/")` instead of just
`urlopen("http://www.google.com/")`.
|
How can i do this using a Python Regex?
Question: I am trying to properly extract _methods_ definitions that are generated by
comtypes for Com Interfaces using a regex. Furthermore some of them are blank
which causes even more problems for me.
Basically i have this:
IXMLSerializerAlt._methods_ = [
COMMETHOD([helpstring(u'Loads an object from an XML string.')], HRESULT, 'LoadFromString',
( ['in'], BSTR, 'XML' ),
( ['in'], BSTR, 'TypeName' ),
( ['in'], BSTR, 'TypeNamespaceURI' ),
( ['retval', 'out'], POINTER(POINTER(IUnknown)), 'obj' )),
]
class EnvironmentManager(CoClass):
u'Singleton object that manages different environments (collections of configuration information).'
_reg_clsid_ = GUID('{8A626D49-5F5E-47D9-9463-0B802E9C4167}')
_idlflags_ = []
_typelib_path_ = typelib_path
_reg_typelib_ = ('{5E1F7BC3-67C5-4AEE-8EC6-C4B73AAC42ED}', 1, 0)
INumberFormat._methods_ = [
]
I want to extract both the IXMLSerializerAlt and INumberFormat methods
definitions however i cant figure out a proper regex. E.g. for IXMLSerializer
i want to extract this:
IXMLSerializerAlt._methods_ = [
COMMETHOD([helpstring(u'Loads an object from an XML string.')], HRESULT, 'LoadFromString',
( ['in'], BSTR, 'XML' ),
( ['in'], BSTR, 'TypeName' ),
( ['in'], BSTR, 'TypeNamespaceURI' ),
( ['retval', 'out'], POINTER(POINTER(IUnknown)), 'obj' )),
]
This regex in my mind this should work:
^\w+\._methods_\s=\s\[$
(^.+$)*
^]$
Im checking my regex's using kodos however i cannot figure out a way to make
this work.
Answer: You're missing the newline characters between `$` and `^`, and may not be
using the `re.MULTILINE` flag which allows those to anchor at the start and
end of lines. The following (compiled with `re.MULTILINE`) would match:
\w+\._methods_\s=\s\[$(?:\n^.+$)*\n^\]$
However, here's a slightly simpliifed regex that will also match your
examples:
>>> s = '''...\nIXMLSerializerAlt._methods_ = [\n COMMETHOD([helpstring(u'Loads an object from an XML string.')], HRESULT, 'LoadFromString',\n ( ['in'], BSTR, 'XML' ),\n ( ['in'], BSTR, 'TypeName' ),\n ( ['in'], BSTR, 'TypeNamespaceURI' ),\n ( ['retval', 'out'], POINTER(POINTER(IUnknown)), 'obj' )),\n]\n...'''
>>> import re
>>> re.findall(r'^\w+\._methods_\s=\s\[$.*?^\]$', s, re.DOTALL | re.MULTILINE)
["IXMLSerializerAlt._methods_ = [\n COMMETHOD([helpstring(u'Loads an object from an XML string.')], HRESULT, 'LoadFromString',\n ( ['in'], BSTR, 'XML' ),\n ( ['in'], BSTR, 'TypeName' ),\n ( ['in'], BSTR, 'TypeNamespaceURI' ),\n ( ['retval', 'out'], POINTER(POINTER(IUnknown)), 'obj' )),\n]"]
|
Python Finding all packages inside a package, even when in an egg
Question: Given a Python package, how can I automatically find all its sub-packages?
I used to have a function that would just browse the file system, looking for
folders that have an `__init__.py*` file in them, but now I need a method that
would work even if the whole package is in an egg.
Answer: [pkgutil](http://docs.python.org/library/pkgutil.html) could be helpfull.
Also see [this SO question.](http://stackoverflow.com/questions/1707709/list-
all-the-modules-that-are-part-of-a-python-package), this is a code example
form [that question](http://stackoverflow.com/questions/1707709/list-all-the-
modules-that-are-part-of-a-python-package).
[kaizer.se](http://stackoverflow.com/questions/1707709/list-all-the-modules-
that-are-part-of-a-python-package/1707786#1707786)
import pkgutil
# this is the package we are inspecting -- for example 'email' from stdlib
import email
package = email
for importer, modname, ispkg in pkgutil.iter_modules(package.__path__):
print "Found submodule %s (is a package: %s)" % (modname, ispkg)
[~unutbu](http://stackoverflow.com/questions/1707709/list-all-the-modules-
that-are-part-of-a-python-package/1708706#1708706)
import pkgutil
for importer, modname, ispkg in pkgutil.walk_packages(path=None, onerror=lambda x: None):
print(modname)
|
Python 3.1 twitter post with installed library,
Question: I'd like to be able to post twitter messages from python 3.0. None of the
twitter API I have looked at support python 3.1. Since the post proceedure
only requires this :
JSON: curl -u username:password -d status="your message here" http://api.twitter.com/1/statuses/update.json
I was wondering if it is possible with the standard libraries to format this
so a message could be sent. My head says it should be possible.
Answer: Try this:
import urllib.request
import urllib.parse
import base64
def encode_credentials(username, password):
byte_creds = '{}:{}'.format(username, password).encode('utf-8')
return base64.b64encode(byte_creds).decode('utf-8')
def tweet(username, password, message):
encoded_msg = urllib.parse.urlencode({'status': message})
credentials = encode_credentials(username, password)
request = urllib.request.Request(
'http://api.twitter.com/1/statuses/update.json')
request.add_header('Authorization', 'Basic ' + credentials)
urllib.request.urlopen(request, encoded_msg)
Then call `tweet('username', 'password', 'Hello twitter from Python3!')`.
The
[`urlencode`](http://docs.python.org/py3k/library/urllib.parse.html#urllib.parse.urlencode)
function prepares the message for the `HTTP POST` request.
The
[`Request`](http://docs.python.org/py3k/library/urllib.request.html#urllib.request.Request)
object uses HTTP authentication as explained here:
<http://en.wikipedia.org/wiki/Basic_access_authentication>
The
[`urlopen`](http://docs.python.org/py3k/library/urllib.request.html#urllib.request.urlopen)
methods sends the request to the twitter. When you pass it some data, it uses
`POST`, otherwise `GET`.
This uses only parts of the Python3 standard library. However, if you want to
work with HTTP, you should reconsider using third-party libraries. This [Dive
Into Python 3 chapter](http://diveintopython3.org/http-web-services.html)
explains how and why.
|
converting a treebank of vertical trees to s-expressions
Question: I have a collection of parse trees, and they are in this ascii representation
where indentation determines the structure (and closing brackets are
implicit). I need to convert them to s-expressions so that parentheses
determine the structure. It's a little bit like python's significant
whitespace vs. braces. The input format is a vertical representation of trees,
like so:
STA:fcl
=S:np
==DN:pron-dem("tia" <*> <Dem> <Du> <dem> DET P NOM) Tiaj
==H:n("akuzo" <act> <sd> P NOM) akuzoj
=fA:adv("certe") certe
=P:v-fin("dauxri" <va+TEMP> <mv> FUT VFIN) dauxros
.
Should become:
(STA:fcl (S:np (DN:pron-dem Tiaj) (H:n akuzoj)) (fA:adv certe) (P:v-fin dauxros) .)
I have code that _almost_ does it, but not quite. There's always a missing
paren somewhere; it's getting very frustrating. Should I use a proper parser,
maybe a CFG? The current (messy) code is at
<http://github.com/andreasvc/eodop/blob/master/arbobanko.py>
Answer: Focusing only on the example you're giving in this Q, and the Q's title about
converting vertical trees to S-expressions, something like...:
import re
import sys
samp='''S
=NP
==(DT +def) the
== (N +ani) man
=VP
==V walks'''.splitlines()
relinelev = re.compile(r'(=*)(.*)')
reclean = re.compile(r'\s*\((\S+)[^)]*\)')
def clean(line):
return reclean.sub(r'\1', line)
def reparse(tree=samp):
stack = [-1]
for line in tree:
equals, rest = relinelev.match(line).groups()
linelev = len(equals)
while linelev < stack[-1]:
sys.stdout.softspace = False
print ')',
curlev = stack.pop()
if linelev == stack[-1]:
sys.stdout.softspace = False
print ')',
else:
stack.append(linelev)
print '(%s' % clean(rest),
while stack[-1] >= 0:
sys.stdout.softspace = False
print ')',
stack.pop()
print
reparse()
seems to work, and outputs
(S (NP (DT the) (N man)) (VP (V walks)))
I realize you're trying to do much more "cleaning" than I'm doing here, but
that can be concentrated in the `clean` function, leaving `reparse` to deal
with the Q's title. If you don't want to print as you go, but rather return
the result as a string, the changes are of course quite minor:
def reparse(tree=samp):
stack = [-1]
result = []
for line in tree:
equals, rest = relinelev.match(line).groups()
linelev = len(equals)
while linelev < stack[-1]:
result[-1] += ')'
curlev = stack.pop()
if linelev == stack[-1]:
result[-1] += ')'
else:
stack.append(linelev)
result.append('(%s' % clean(rest))
while stack[-1] >= 0:
result[-1] += ')'
stack.pop()
return ' '.join(result)
|
Windows 7 Task Scheduler
Question: Very new to this, and I have no idea where to start.
I want to schedule a python script using Task Scheduler in Windows 7. When I
add a "New Action", I place the following command as the script/program :
`c:\python25\python.exe`
As the argument, I add the full path to the location of my python script
`path\script.py`
Here is my script:
import datetime
import csv
import os
now = datetime.datetime.now()
print str(now)
os.chdir('C:/Users/Brock/Desktop/')
print os.getcwd()
writer = csv.writer(open("test task.csv", "wb"))
row = ('This is a test', str(now))
writer.writerow(row)
I got an error saying the script could not run. Any help you can provide to
get me up and running will be very much appreciated!
Thanks,
Brock
Answer: Not sure how to do it properly through the GUI, but [see here for a nice
solution](http://stackoverflow.com/questions/2725754/schedule-python-script-
windows-7) involving the `schtasks` command.
|
Bit of python help
Question: I've tried to get this to work, but it just freezes. It should display a
pyramid, but all it does is.. halts.
from graphics import *
valid_colours = ['red', 'blue', 'yellow', 'green']
colour = ['', '', '']
while True:
colour[0] = raw_input("Enter your first colour: ")
colour[1] = raw_input("Enter your second colour: ")
colour[2] = raw_input("Enter your third colour: ")
if ((colour[0] and colour[1] and colour[2]) in valid_colours):
break
while True:
width = raw_input("Enter a width between 2-7: ")
if width.isdigit():
if (int(width) <= 7 and int(width) >= 2):
break
width = int(width)
win = GraphWin("My Mini Project ", 1000, 1000) # 1000 \ 20 = 50
win.setCoords(0 , 0 , 20, 20)
p1 = [0, 2]
while width > 0:
p = [1, 3]
loopWidth = 0
while loopWidth < width:
loopWidth = loopWidth + 1
c = 0
while c <= 10:
c = c + 1
if c % 2:
colour = "white"
else:
colour = "red"
rectangle = Rectangle(Point(p[0],p1[0]), Point(p[1], p1[1]))
rectangle.setFill(colour)
rectangle.setOutline("black")
rectangle.draw(win)
p[0] = p[0] + 0.2
p1[0] = p1[0] + 0.2
p[0] = p[0] - 2
p1[0] = p1[0] - 2
p[0] = p[0] + 2
p[1] = p[1] + 2
width = width - 1
p1[0] = p1[0] + 2
p1[1] = p1[1] + 2
Answer: * Well first the color-input loop does not do what you want it to do.
The 'and' in `if ((colour[0] and colour[1] and colour[2]) in valid_colours):`
compares their _string_ values to each other, where any non-empty string
evaluates as True. The expression evaluates to colour[2], assuming it is the
last non-empty string, you can prove this with: `print (colour[0] and
colour[1] and colour[2])`
Change to: `if (colour[0] in valid_colours and colour[1] in valid_colours and
colour[2] in valid_colours):`
* Your main rectangle-drawing loop: The intent of the following code is to iterate from 1..width (inclusively)
:
barloopWidth = 0
while loopWidth < width:
loopWidth = loopWidth + 1
do stuff using (loopWidth + 1)
so replace it with: for loopWidth in range(1,width+1):
* The loop iterating c and colour through 6 loops of white,red can be rewritten using '*' (the sequence replication operator):
for colour in ['white','red']*6:
So your main loop becomes:
while width > 0:
p = [1, 3]
for loopWidth in range(1,width+1):
for colour in ['white','red']*6:
#Draw your rectangles
p[0] += 2
p1[0] += 2
p[0] -= 2
p1[0] -= 2
p[0] = p[0] + 2
p[1] = p[1] + 2
width = width - 1
p1[0] += 2
p1[1] += 2
* As to why it hangs on your pyramid coordinates loop, debug it yourself using print: `print 'Drawing rectangle from (%d,%d) to (%d,%d)'% (p[0],p1[0],p[1],p1[1])`
You might find it helpful for testing to isolate that code info a function
draw_pyramid(), away from get_colors().
|
deploying a war to tomcat using python
Question: I'm trying to deploy a war to a Apache Tomcat server (Build 6.0.24) using
python (2.4.2) as part of a build process.
I'm using the following code
import urllib2
import base64
war_file_contents = open('war_file.war','rb').read()
username='some_user'
password='some_pwd'
base64string = base64.encodestring('%s:%s' % (username, password))[:-1]
authheader = "Basic %s" % base64string
opener = urllib2.build_opener(urllib2.HTTPHandler)
request = urllib2.Request('http://localhost:8080/manager/deploy?path=war_file', data=war_file_contents)
request.add_header('Content-Type', 'application/octet-stream')
request.add_header("Authorization", authheader)
request.get_method = lambda: 'PUT'
url = opener.open(request)
the url.code is 200, and the url.msg is "OK". However the web archive doesn't
appear on the manager list applications page.
Thanks.
Answer: Okay, figured it out.
The urllib2.Request line needs to have a slash in front of the path so:-
request = urllib2.Request('http://localhost:8080/manager/deploy?path=/war_file', data=war_file_contents)
All then works fine.
|
Best data-structure to use for two ended sorted list
Question: I need a collection data-structure that can do the following:
* Be sorted
* Allow me to quickly pop values off the front _and_ back of the list O(log n)
* Remain sorted after I insert a new value
* Allow a user-specified comparison function, as I will be storing tuples and want to sort on a particular value
* Thread-safety is not required
* Optionally allow efficient haskey() lookups (I'm happy to maintain a separate hash-table for this though)
My thoughts at this stage are that I need a priority queue _and_ a hash table,
although I don't know if I can quickly pop values off _both_ ends of a
priority queue. Another possibility is simply maintaining an OrderedDictionary
and doing an insertion sort it every-time I add more data to it.
Because I'm interested in performance for a moderate number of items (I would
estimate less than 200,000), I am unsure about what asymptotic performance I
require for these operations. n will not grow infinitely, so a low constant
performance `k` in `k * O(n)` may be as important `O(n)`. That said, I would
prefer that both the insert and pop operations take `O(log n)` time.
Furthermore, are there any particular implementations in Python? I would
really like to avoid writing this code myself.
Answer: You might get good performance for these kinds of operations using
[`blist`](http://pypi.python.org/pypi/blist/) or a database (such as the
[sqlite](http://docs.python.org/library/sqlite3.html) which is in the stdlib).
|
Converting a bash script to python (small script)
Question: I’ve a bash script I’ve been using for a Linux environment but now I have to
use it on a Windows platform and want to convert the bash script to a python
script which I can run.
The bash script is rather simple (I think) and I’ve tried to convert it by
google by way around but can’t convert it successfully.
The bash script looks like this:
runs=5
queries=50
outfile=outputfile.txt
date >> $outfile
echo -e "\n---------------------------------"
echo -e "\n----------- Normal --------------"
echo -e "\n---------------------------------"
echo -e "\n----------- Normal --------------" >> $outfile
for ((r = 1; r < ($runs + 1); r++))
do
echo -e "Run $r of $runs\n"
db2 FLUSH PACKAGE CACHE DYNAMIC
python reads.py -r1 -pquery1.sql -q$queries -shotelspec -k6 -a5 >> $outfile
done
The main command, the python read.py … etc. is another python file I’ve been
given and have the arguments as you see.
I know it is a lot to ask for, but it would really help me out if someone
could convert this to a python script I can use or at least give me some hints
and directions.
Sincerely
Mestika
# Added per request:
This is what I've written but without success:
runs=5
queries=50
outfile=ReadsAgain.txt
file = open("results.txt", "ab")
print "\n---------------------------------"
print "\n----------- Normal --------------"
print "\n---------------------------------"
file.write("\n----------- Normal --------------\n")
print "\n------------- Query without Index --------------"
file.write("\n------------- Query without Index --------------\n")
for r = 1; r < (%s + 1); r++ % runs
print "Run %s of %s \n" % r % runs
db2 FLUSH PACKAGE CACHE DYNAMIC
output = python reads.py -r1 -pquery1.sql -q$queries -shotelspec -k6 -a5
file.write(output)
file.close()
Answer: ## Answer
Let's break it down into pieces. Especially the pieces you got wrong. :)
* * *
### Assignment
>
> outfile=ReadsAgain.txt
>
It should come to little surprise that you need to put quotes around strings.
On the other hand, you have the luxury of putting spaces around the `=` for
readability.
outfilename = "ReadsAgain.txt"
* * *
### Variable expansion →
[`str.format`](http://docs.python.org/library/stdtypes.html#str.format) (or,
the [`%`](http://docs.python.org/library/stdtypes.html#string-formatting-
operations) operation)
>
> python reads.py <snip/> -q$queries <snip/>
>
So you know how to do the redirection already, but how do you do the variable
expansion? You can use [the `format`
method](http://docs.python.org/library/stdtypes.html#str.format) (v2.6+):
command = "python reads.py -r1 -pquery1.sql -q{0} -shotelspec -k6 -a5".format(queries)
You can alternatively use [the `%`
operator](http://docs.python.org/library/stdtypes.html#string-formatting-
operations):
#since queries is a number, use %d as a placeholder
command = "python reads.py -r1 -pquery1.sql -q%d -shotelspec -k6 -a5" % queries
* * *
### C-style loop → [Object-oriented-style
loop](http://docs.python.org/tutorial/controlflow.html#for-statements)
>
> for ((r = 1; r < ($runs + 1); r++)) do done
>
Looping in Python is different from C-style iteration. What happens in Python
is you iterate over an iterable object, like for example a list. Here, you are
trying to do something `runs` times, so you would do this:
for r in range(runs):
#loop body here
`range(runs)` is equivalent to `[0,1,...,runs-1]`, a list of `runs = 5`
integer elements. So you'll be repeating the body `runs` times. At every
cicle, `r` is assigned the next item of the list. This is thus completely
equivalent to what you are doing in Bash.
If you're feeling daring, use
[`xrange`](http://docs.python.org/library/functions.html#xrange) instead. It's
completely equivalent but uses more advanced language features (so it is
harder to explain in layman's terms) but consumes less resources.
* * *
### Output redirection → [the `subprocess`
module](http://docs.python.org/library/subprocess.html?highlight=subprocess#using-
the-subprocess-module)
The "tougher" part, if you will: executing a program and getting its output.
[Google to the
rescue!](http://www.google.com/search?q=how+to+get+the+output+of+a+program+python)
Obviously, the top hit is a stackoverflow question: [this
one](http://stackoverflow.com/questions/748028/how-to-get-output-of-exe-in-
python-script). You can hide all the complexity behind it with a simple
function:
import subprocess, shlex
def get_output_of(command):
args = shlex.split(command)
return subprocess.Popen(args,
stdout=subprocess.PIPE).communicate()[0]
# this only returns stdout
So:
>
> python reads.py -r1 -pquery1.sql -q$queries -shotelspec -k6 -a5 >>
> $outfile
>
becomes:
command = "python reads.py -r1 -pquery1.sql -q%s -shotelspec -k6 -a5" % queries
read_result = get_output_of(command)
* * *
### Don't over-`subprocess`, batteries are included
Optionally, consider that you can get pretty much the same output of `date`
with the following:
import time
time_now = time.strftime("%c", time.localtime()) # Sat May 15 15:42:47 2010
(Note the absence of the time zone information. This should be the subject of
[another question](http://stackoverflow.com/questions/ask), if it is important
to you.)
* * *
### How your program should look like
The final result should then look like this:
import subprocess, shlex, time
def get_output_of(command):
#... body of get_output_of
#... more functions ...
if __name__ = "__main__":
#only execute the following if you are calling this .py file directly,
#and not, say, importing it
#... initialization ...
with file("outputfile.txt", "a") as output_file: #alternative way to open files, v2.5+
#... write date and other stuff ...
for r in range(runs):
#... loop body here ...
* * *
## Post scriptum
That must look pretty horrible when compared to the relatively simple and
short Bash script, right? Python is not a specialized language: it aims to do
everything reasonably well, but isn't built directly for running programs and
getting the output of those.
Still, you wouldn't _normally_ write a database engine in Bash, right? It's
different tools for different jobs. Here, unless you're planning to make some
changes that would be non-trivial to write with that language, [Ba]sh was
definitely the right choice.
|
How to encrypt a file using a Public Key in C#?
Question: I have a 2048 bits public key (asymmetric, RSA) of which I know the Modulus
and Exponent and need to encrypt a string.
Ideally, I want to encrypt a small string in c# and later on decrypt it with
python.
Answer: You will have to carefully check all standards involved, these encryptions
should be cross-platform but it's vulnerable. It helps if you have a setup
where you can inspect all the various byte-arrays, you don't want to be
chasing an error that comes from an ASCII/UTF8 encoding difference.
Your title mentions a 'file', note that RSA only encrypts data up to the key
size. It is usually used to encrypt (MD5/SHA1) hashes or keys for other
encryptions. The RSA classes have extended support for signing and verifying
signatures.
You will find the most relevant steps [here
(MSDN)](http://msdn.microsoft.com/en-
us/library/system.security.cryptography.rsacryptoserviceprovider.aspx). Note
that you will have to import the keys whereas the example exports them.
|
regular expression with special chars
Question: I need a regular expression to validate string with one or more of these
characters:
* a-z
* A-Z
* '
* àòèéùì
* simple white space
FOR EXAMPLE these string are valide:
D' argon calabrò
maryòn l' Ancol
these string are NOT valide:
hello38239
my_house
work [tab] with me
I tryed this:
re.match(r"^[a-zA-Z 'òàèéìù]+$", string )
It seems to work in my python shell but in Django I get this error:
SyntaxError at /home/
("Non-ASCII character '\\xc3' ...
Why ?
* * *
Edit:
I have added # -_\- coding: utf-8 -_ \- at the top of my forms.py but the
strings with à,è,ò,ù,é or ì doesn't match never.
This is my forms.py clean method:
def clean_title(self):
if(re.match(r"^[a-zA-Z 'òàèéìù]+$", self.cleaned_data['title'].strip())):
return self.cleaned_data['title'].strip()
raise forms.ValidationError(_("This title is not valid."))
Answer: If you user Non-ASCII characters in your python source files you should add
proper encoding to the top of your source file like this:
# -*- coding: utf-8 -*-
utf_string='čćžđšp'
[Defining Python Source Code
Encodings](http://www.python.org/dev/peps/pep-0263/)
This seems to work fine for me:
>>> import re
>>> mystring = "D' argon calabrò"
>>> matched = re.match(r"^([a-zA-Z 'òàèéìù]+)$", mystring)
>>> print matched.groups()
("D' argon calabr\xc3\xb2",)
|
How do you connect remotely using Python + Webdriver
Question: I am trying to figure out how to connect to my remote webdriver instance.
This is the code I am currently using:
from selenium.remote.webdriver import WebDriver
driver = WebDriver("http://172.16.205.129:4444", "firefox", "ANY")
driver.get('http://google.com')
driver.quit()
I find that I can't connect and will get a connection refused, or if i play
around with the code, occasionally a time out.
Any help is appreciated.
Answer: You need to ensure `selenium` server hub and node are set up before the test.
Please [refer to this](http://code.google.com/p/selenium/wiki/Grid2).
Example on hub run
java -jar selenium-server-standalone-2.5.0.jar -role hub
Example on node to connect to hub ,run
java -jar selenium-server-standalone-2.5.0.jar -role webdriver -hub
http://localhost:4444/grid/register -port 5555
You can have as many nodes as you want.
|
Scheduling a task on python
Question: I want to run a program that runs a function every 4 hours. What is the least
consuming way to do so?
Answer: Simlest way I can think of (in python since the post is tagged with python):
import time
while True:
do_task()
time.sleep(4 * 60 * 60) # 4 hours * 60 minutes * 60 seconds
|
What's the fastest way to strip and replace a document of high unicode characters using Python?
Question: I am looking to replace from a large document all high unicode characters,
such as accented Es, left and right quotes, etc., with "normal" counterparts
in the low range, such as a regular 'E', and straight quotes. I need to
perform this on a very large document rather often. I see an example of this
in what I think might be perl here:
<http://www.designmeme.com/mtplugins/lowdown.txt>
Is there a fast way of doing this in Python without using
s.replace(...).replace(...).replace(...)...? I've tried this on just a few
characters to replace and the document stripping became really slow.
EDIT, my version of unutbu's code that doesn't seem to work:
# -*- coding: iso-8859-15 -*-
import unidecode
def ascii_map():
data={}
for num in range(256):
h=num
filename='x{num:02x}'.format(num=num)
try:
mod = __import__('unidecode.'+filename,
fromlist=True)
except ImportError:
pass
else:
for l,val in enumerate(mod.data):
i=h<<8
i+=l
if i >= 0x80:
data[i]=unicode(val)
return data
if __name__=='__main__':
s = u'“fancy“fancy2'
print(s.translate(ascii_map()))
Answer:
# -*- encoding: utf-8 -*-
import unicodedata
def shoehorn_unicode_into_ascii(s):
return unicodedata.normalize('NFKD', s).encode('ascii','ignore')
if __name__=='__main__':
s = u"éèêàùçÇ"
print(shoehorn_unicode_into_ascii(s))
# eeeaucC
Note, as @Mark Tolonen kindly points out, the method above removes some
characters like ß‘’“”. If the above code truncates characters that you wish
translated, then you may have to use the string's `translate` method to
manually fix these problems. Another option is to use
[unidecode](http://code.zemanta.com/tsolc/unidecode/) (see [J.F. Sebastian's
answer](http://stackoverflow.com/questions/2854230/whats-the-fastest-way-to-
strip-and-replace-a-document-of-high-unicode-characters/2876950#2876950)).
When you have a large unicode string, using its `translate` method will be
much much faster than using the `replace` method.
**Edit:** `unidecode` has a more complete mapping of unicode codepoints to
ascii. However, `unidecode.unidecode` loops through the string character-by-
character (in a Python loop), which is slower than using the `translate`
method.
The following helper function uses `unidecode`'s data files, and the
`translate` method to attain better speed, especially for long strings.
In my tests on 1-6 MB text files, using `ascii_map` is about 4-6 times faster
than `unidecode.unidecode`.
# -*- coding: utf-8 -*-
import unidecode
def ascii_map():
data={}
for num in range(256):
h=num
filename='x{num:02x}'.format(num=num)
try:
mod = __import__('unidecode.'+filename,
fromlist=True)
except ImportError:
pass
else:
for l,val in enumerate(mod.data):
i=h<<8
i+=l
if i >= 0x80:
data[i]=unicode(val)
return data
if __name__=='__main__':
s = u"éèêàùçÇ"
print(s.translate(ascii_map()))
# eeeaucC
Edit2: Rhubarb, if `# -*- encoding: utf-8 -*-` is causing a SyntaxError, try
`# -*- encoding: cp1252 -*-`. What encoding to declare depends on what
encoding your text editor uses to save the file. Linux tends to use utf-8, and
(it seems perhaps) Windows tends to cp1252.
|
Any other way to import data files(like .csv) in python sqlite3 module ? [not insert one by one]
Question: In sqlite3's client CLI, there is " .import file TABLE_name " to do it.
But, I do not want to install sqlite3 to my server at present.
In python sqlite3 module, we can creat and edit a DB.
But, I have not found a way to import data-file to a TABLE, except inserting
rows one by one.
Any other way?
Answer: You could insert at one shot using
[executemany](http://docs.python.org/library/sqlite3.html#using-shortcut-
methods) command instead of inserting one by one
Lets say I have users.csv with following contents
"Hugo","Boss"
"Calvin","Klein"
and basically open with csv module and pass it to .executemany function
import csv,sqlite3
persons= csv.reader(open("users.csv"))
con = sqlite3.connect(":memory:")
con.execute("create table person(firstname, lastname)")
con.executemany("insert into person(firstname, lastname) values (?, ?)", persons)
for row in con.execute("select firstname, lastname from person"):
print row
#(u'Hugo', u'Boss')
#(u'Calvin', u'Klein')
|
Why doesn't appending binary pickles work?
Question: I know this isn't exactly how the pickle module was intended to be used, but I
would have thought this would work. I'm using Python 3.1.2
Here's the background code:
import pickle
FILEPATH='/tmp/tempfile'
class HistoryFile():
"""
Persistent store of a history file
Each line should be a separate Python object
Usually, pickle is used to make a file for each object,
but here, I'm trying to use the append mode of writing a file to store a sequence
"""
def validate(self, obj):
"""
Returns whether or not obj is the right Pythonic object
"""
return True
def add(self, obj):
if self.validate(obj):
with open(FILEPATH, mode='ba') as f: # appending, not writing
f.write(pickle.dumps(obj))
else:
raise "Did not validate"
def unpack(self):
"""
Go through each line in the file and put each python object
into a list, which is returned
"""
lst = []
with open(FILEPATH, mode='br') as f:
# problem must be here, does it not step through the file?
for l in f:
lst.append(pickle.loads(l))
return lst
Now, when I run it, it only prints out the first object that is passed to the
class.
if __name__ == '__main__':
L = HistoryFile()
L.add('a')
L.add('dfsdfs')
L.add(['dfdkfjdf', 'errree', 'cvcvcxvx'])
print(L.unpack()) # only prints the first item, 'a'!
Is this because it's seeing an early EOF? Maybe appending is intended only for
ascii? (in which case, why is it letting me do mode='ba'?) Is there a much
simpler duh way to do this?
Answer: Why would you think appending binary pickles would produce a single pickle?!
Pickling lets you put (and get back) several items one after the other, so
obviously it must be a "self-terminating" serialization format. Forget lines
and just get them back! For example:
>>> import pickle
>>> import cStringIO
>>> s = cStringIO.StringIO()
>>> pickle.dump(23, s)
>>> pickle.dump(45, s)
>>> s.seek(0)
>>> pickle.load(s)
23
>>> pickle.load(s)
45
>>> pickle.load(s)
Traceback (most recent call last):
...
EOFError
>>>
just catch the `EOFError` to tell you when you're done unpickling.
|
using sqlite3 with lua
Question: I'm trying to use sqlite3 with lua (am already using c++, but I'm a n00b with
lua- I read [this](http://stackoverflow.com/questions/356160/which-game-
scripting-language-is-better-to-use-lua-or-python/358583#358583)) but I'm
getting the following when trying to build the library or whatever:
C:\lib\lsqlite3-7>mingw32-make
process_begin: CreateProcess(NULL, pkg-config --version, ...) failed.
makefile:53: *** windows32. Stop.
I'm not at all surprised at a makefile failing but I can't do them (is it
spaces or tabs? where is it they have to go?), I would have thought there was
a binary for windows?
Any simple answers appreciated. I haven't got the time to learn make or
install cygwin or whatever.
Answer: You might consider using LuaSQL as your wrapper instead, since it is already
included in the [Lua for Windows](http://code.google.com/p/luaforwindows/)
package and so it is likely already on your system. It includes support for
SQLite3 among other databases.
It does look like you are trying to build the latest release of lsqlite3, but
that this release was not packaged to be easily built on or for Windows. I
didn't attempt to track down a binary since LuaForge only has a source
package. It looks like building it successfully will require manually editing
the Makefile, and then running the Makefile under either MSYS or Cygwin so
that the *nix utilities it assumes exist are available to it.
**Update: June, 2012**
Since writing this answer, I've had occasion to consider using `lsqlite3`
myself for a project that will not require the flexibility of binding to many
distinct database providers, so building in a tight dependency on SQLite makes
sense. Given that, the `lsqlite3` wrapper is a good fit due to its small size
and clean implementation of a lot of the important bits of the SQLite C API.
To build it, I used [MSYS](http://www.mingw.org/wiki/msys) which provides a
minimal unix-like command prompt and supporting utilities for use with the
MinGW port of GCC. In that environment, I had to make a small modification to
the Makefile to force it to use manual configuration, and to provide the
configuration details identifying where `lua.h`, `lua51.dll`, `sqlite3.h`, and
`sqlite3.dll` were hiding on my system.
The only real trick to that is to correctly map Windows path names to MSYS
path names by changing `C:` to `/c/`, and to avoid using any folder names with
spaces in them. So with Lua for Windows on `C:\Program Files (x86)\Lua\5.1`,
that maps to `/c/PROGRA~2/Lua/5.1`, using the Windows 8.3 short name to avoid
the spaces.
A key point to recommend MSYS over other unix-like build environments on
Windows is that it is specifically intended for this purpose. The programs and
DLLs build from it are free of any unexpected dependencies. Specifically, this
is unlike Cygwin which is intended to provide a complete unix-like experience
layered on top of Windows.
With `lsqlite3.dll` freshly built, all I had to do was install it somewhere on
my Lua `package.cpath` along with `sqlite3.dll` and the examples and test
cases all appear to work.
|
Execute a BASH command in Python-- in the same process
Question: I need to execute the command `. /home/db2v95/sqllib/db2profile` before I can
`import ibm_db_dbi` in Python 2.6.
Executing it before I enter Python works:
baldurb@gigur:~$ . /home/db2v95/sqllib/db2profile
baldurb@gigur:~$ python
Python 2.6.4 (r264:75706, Dec 7 2009, 18:45:15)
[GCC 4.4.1] on linux2
Type "help", "copyright", "credits" or "license" for more information.
>>> import ibm_db_dbi
>>>
but executing it in Python using `os.system(".
/home/db2v95/sqllib/db2profile")` or `subprocess.Popen([".
/home/db2v95/sqllib/db2profile"])` results in an error. What am I doing wrong?
**Edit:** this is the error I receive:
> Traceback (most recent call last):
> File "<file>.py", line 8, in
> <module>
> subprocess.Popen([". /home/db2v95/sqllib/db2profile"])
> File
> "/usr/lib/python2.6/subprocess.py",
> line 621, in __init__
> errread, errwrite) File "/usr/lib/python2.6/subprocess.py",
> line 1126, in _execute_child
> raise child_exception OSError: [Errno 2] No such file or directory
Answer: You are calling a '.' shell command. This command means 'execute this shell
file in current process'. You cannot execute shell file in Python process as
Python is not a shell script interpreter.
The `/home/b2v95/sqllib/db2profile` probably sets some shell environment
variables. If you read it using `system()` function, then the variables will
be only changed in the shell executed and will not be visible in the process
calling that shell (your script).
You can only load this file before starting your python script – you could
make a shell wrapper script which would do `. /home/b2v95/sqllib/db2profile`
and execute your python script.
Other way would be to see what the `db2profile` contains. If that are only
`NAME=value` lines, you could parse it in your python script and update
`os.environ` with the data obtained. If script does something more (like
calling something else to obtain the values) you can reimplement whole script
in Python.
**Update** An idea: read the script into python, pipe it (using Popen) to the
shell, after the script write `env` command to the same shell and read the
output. This way you will get all the variables defined in the shell. Now you
can read the variables.
Something like this:
shell = subprocess.Popen(["sh"], stdin=subprocess.PIPE, stdout=subprocess.PIPE)
script = open("/home/db2v95/sqllib/db2profile", "r").read()
shell.stdin.write(script + "\n")
shell.stdin.write("env\n")
shell.stdin.close()
for line in shell.stdout:
name, value = line.strip().split("=", 1)
os.environ[name] = value
|
Problems with Threading in Python 2.5, KeyError: 51, Help debugging?
Question: I have a python script which runs a particular script large number of times
(for monte carlo purpose) and the way I have scripted it is that, I queue up
the script the desired number of times it should be run then I spawn threads
and each thread runs the script once and again when its done.
Once the script in a particular thread is finished, the output is written to a
file by accessing a lock (so my guess was that only one thread accesses the
lock at a given time). Once the lock is released by one thread, the next
thread accesses it and adds its output to the previously written file and
rewrites it.
I am not facing a problem when the number of iterations is small like 10 or 20
but when its large like 50 or 150, python returns a KeyError: 51 telling me
element doesn't exist and the error it points out to is within the lock which
puzzles me since only one thread should access the lock at once and I do not
expect an error.
This is the class I use:
class errorclass(threading.Thread):
def __init__(self, queue):
self.__queue=queue
threading.Thread.__init__(self)
def run(self):
while 1:
item = self.__queue.get()
if item is None: break
result = myfunction()
lock = threading.RLock()
lock.acquire()
ADD entries from current thread to entries in file and
REWRITE FILE
lock.release()
queue = Queue.Queue()
for i in range(threads):
errorclass(queue).start()
for i in range(desired iterations):
queue.put(i)
for i in range(threads):
queue.put(None)
Python returns with KeyError: 51 for large number of desired iterations during
the adding/write file operation after lock access, I am wondering if this is
the correct way to use the lock since every thread has a lock operation rather
than every thread accessing a shared lock? What would be the way to rectify
this?
Answer: What you have right now is a _new lock_ for every _iteration_ in each thread's
`run` method. In effect, there is no locking going on at all. If you want to
protect writes to a file, you need to make sure that _all_ threads that access
the same file use the same lock object. The simplest way to do that is to
create it at the global level:
lock = threading.RLock()
class errorclass(...):
...
def run(self)
... # get items and process
with lock:
ADD entries from current thread to entries in file and REWRITE FILE
You'll need to use `from __future__ import with_statement` to use the `with`
statement in Python 2.5, but it makes sure you never forget to unlock the
lock, not even when an error occurs. (If you need Python 2.4-and-earlier
compatibility, you'll have to use a try/finally instead.)
|
POSTing a form using Python and Curl
Question: I am relatively new (as in a few days) to Python - I am looking for an example
that would show me how to post a form to a website (say www.example.com).
I already know how to use Curl. Infact, I have written C+++ code that does
exactly the same thing (i.e. POST a form using Curl), but I would like some
starting point (a few lines from which I can build on), which will show me how
to do this using Python.
Answer: Here is an example using urllib and urllib2 for both POST and GET:
**POST** \- If `urlopen()` has a second parameter then it is a POST request.
import urllib
import urllib2
url = 'http://www.example.com'
values = {'var' : 500}
data = urllib.urlencode(values)
response = urllib2.urlopen(url, data)
page = response.read()
**GET** \- If `urlopen()` has a single parameter then it is a GET request.
import urllib
import urllib2
url = 'http://www.example.com'
values = {'var' : 500}
data = urllib.urlencode(values)
fullurl = url + '?' + data
response = urllib2.urlopen(fullurl)
page = response.read()
You could also use `curl` if you call it using `os.system()`.
Here are some helpful links:
<http://docs.python.org/library/urllib2.html#urllib2.urlopen>
<http://docs.python.org/library/os.html#os.system>
|
Python: avoiding fraction simplification
Question: I'm working on a music app' in Python and would like to use the fractions
module to handle time signatures amongst other things. My problem is that
fractions get simplified, i.e.:
>>> from fractions import Fraction
>>> x = Fraction(4, 4)
>>> x
Fraction(1, 1)
However, it is important from a musical point of view that 4/4 stays 4/4 even
though it equals 1. Is there any built-in way to avoid that behaviour?
Thanks!
Answer: Yes: make a custom class for it.
Musical time signatures are not fractions, so it doesn't make sense to
represent them with a math class.
|
Autoproperty failing in IronPython works in Python?
Question: I have this following python code, it works fine in python but fails with the
following error in IronPython 2.6 any ideas as to why?
======================================================================
ERROR: testAutoProp (__main__.testProperty)
----------------------------------------------------------------------
Traceback (most recent call last):
File "oproperty.py", line 66, in testAutoProp
a.x = 200
File "oproperty.py", line 31, in __set__
getattr(obj, self.fset.__name__)(value)
AttributeError: 'A' object has no attribute '<lambda$48>'
----------------------------------------------------------------------
Ran 1 test in 0.234s
FAILED (errors=1)
Here is the code
#provides an overridable version of the property keyword
import unittest
#provides an overridable version of the property keyword
class OProperty(object):
"""Based on the emulation of PyProperty_Type() in Objects/descrobject.c"""
def __init__(self, fget=None, fset=None, fdel=None, doc=None):
self.fget = fget
self.fset = fset
self.fdel = fdel
self.__doc__ = doc
def __get__(self, obj, objtype=None):
if obj is None:
return self
if self.fget is None:
raise AttributeError, "unreadable attribute"
if self.fget.__name__ == '<lambda>' or not self.fget.__name__:
return self.fget(obj)
else:
return getattr(obj, self.fget.__name__)()
def __set__(self, obj, value):
if self.fset is None:
raise AttributeError, "can't set attribute"
if self.fset.__name__ == '<lambda>' or not self.fset.__name__:
self.fset(obj, value)
else:
getattr(obj, self.fset.__name__)(value)
def __delete__(self, obj):
if self.fdel is None:
raise AttributeError, "can't delete attribute"
if self.fdel.__name__ == '<lambda>' or not self.fdel.__name__:
self.fdel(obj)
else:
getattr(obj, self.fdel.__name__)()
def autoProperty( attrname, desc ):
"Try to auto gen getters and setting for any property type"
getFn = lambda self: getattr( self, attrname )
setFn = lambda self, v: setattr( self, attrname, v )
#set the corresponding function in the descriptor
desc.fget = getFn
desc.fset = setFn
#if there is a blank colname let X property sort it out
#if hasattr( desc, "colname") and desc.colname == "":
# i = 0
# while attrname[ i ] == "_":
# i = i + 1
# desc.colname = attrname[i:]
return desc
class testProperty(unittest.TestCase):
def testAutoProp(self):
class A(object):
def __init__(self):
self._x = 50
x = autoProperty( "_x", OProperty() )
a = A()
a.x = 200
if __name__ == "__main__":
unittest.main()
Answer: Just looking at your code and traceback, it looks to me as though lambdas on
IronPython have a name such as `<lambda$48>` instead of just `<lambda>`. That
means your test `if self.fset.__name__ == '<lambda>' or not
self.fset.__name__:` will take the wrong branch.
Try:
if self.fset.__name__.startswith('<lambda') or not self.fset.__name__:
and so on.
|
python: importing modules with incorrect import statements => unexhaustive info from resulting ImportError
Question: I have a funny problem I'd like to ask you guys ('n gals) about.
I'm importing some module A that is importing some non-existent module B. Of
course this will result in an ImportError.
This is what A.py looks like
import B
Now let's import A
>>> import A
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/tmp/importtest/A.py", line 1, in <module>
import B
ImportError: No module named B
Alright, on to the problem. How can I know if this ImportError results from
importing A or from some corrupt import inside A _without_ looking at the
error's string representation.
The difference is that either A is not there or does have incorrect import
statements.
Hope you can help me out...
Cheers bb
Answer: There is the [`imp`](http://docs.python.org/library/imp.html) module in the
standard lib, so you could do:
>>> import imp
>>> imp.find_module('collections')
(<_io.TextIOWrapper name=4 encoding='utf-8'>, 'C:\\Program Files\\Python31\\lib\\collections.py', ('.py', 'U', 1))
>>> imp.find_module('col')
Traceback (most recent call last):
File "<pyshell#2>", line 1, in <module>
imp.find_module('col')
ImportError: No module named col
which raises `ImportError` when module is not found. As it's not trying to
import that module it's completely independent on whether `ImportError` will
be raised by that particular module.
And of course there's a `imp.load_module` to actually load that module.
|
Python3 function annotations for type hinting versus Boo
Question: I've started on a medium-sized project in python, and I decided to use python
3 because I'm not using any large external libraries and py3k has some nice
new syntactic sugar and more importantly function annotations. However, it
seems like none of WingIDE, Pydev, or pycharm actually have any support for
type hinting using function annotations. If I want something resembling static
typing in python, is switching to boo a reasonable option?
Answer: Boo is a **great** Python-like statically-typed language, but keep in mind
that there [more differences than just static
typing](http://boo.codehaus.org/Gotchas+for+Python+Users). Actually you can
also do [duck typing on Boo](http://boo.codehaus.org/Duck+Typing).
Technically, I'd say the biggest difference is that Boo runs on Mono/.Net so
the libraries and framework are _totally_ different.
[SharpDevelop](http://boo.codehaus.org/Boo+AddIn+For+SharpDevelop) and
[MonoDevelop](http://monodevelop.com) both have good support for Boo. There's
also a [Visual Studio 2010 plugin that adds Boo
support](http://visualstudiogallery.msdn.microsoft.com/en-
us/8d15c62f-de0d-42f0-863a-daa8330d977a). It's still alpha, yet already
usable.
|
Python - Removing duplicates from a string
Question:
def remove_duplicates(strng):
"""
Returns a string which is the same as the argument except only the
first occurrence of each letter is present. Upper and lower case
letters are treated as different. Only duplicate letters are removed,
other characters such as spaces or numbers are not changed.
>>> remove_duplicates('apple')
'aple'
>>> remove_duplicates('Mississippi')
'Misp'
>>> remove_duplicates('The quick brown fox jumps over the lazy dog')
'The quick brown fx jmps v t lazy dg'
>>> remove_duplicates('121 balloons 2 u')
'121 balons 2 u'
"""
s = strng.split()
return strng.replace(s[0],"")
Writing a function to get rid of duplicate letters but so far have been
playing around for an hour and can't get anything. Help would be appreciated,
thanks.
Answer: Not the most efficient, but the most straightforward way is:
>>> s = 'The quick brown fox jumps over the lazy dog'
>>> import string
>>> n = ''
>>> for i in s:
if i not in string.ascii_letters:
n += i
elif i not in n:
n += i
>>> n
'The quick brown fx jmps v t lazy dg'
|
Python Textwrap - forcing 'hard' breaks
Question: I am trying to use textwrap to format an import file that is quite particular
in how it is formatted. Basically, it is as follows (line length shortened for
simplicity):
abcdef <- Ok line
abcdef
ghijk <- Note leading space to indicate wrapped line
lm
Now, I have got code to work as follows:
wrapper = TextWrapper(width=80, subsequent_indent=' ', break_long_words=True, break_on_hyphens=False)
for l in lines:
wrapline=wrapper.wrap(l)
This works nearly perfectly, however, the text wrapping code doesn't do a hard
break at the 80 character mark, it tries to be smart and break on a space (at
approx 20 chars in).
I have got round this by replacing all spaces in the string list with a unique
character (#), wrapping them and then removing the character, but surely there
must be a cleaner way?
N.B Any possible answers need to work on Python 2.4 - sorry!
Answer: It sounds like you are disabling most of the functionality of TextWrapper, and
then trying to add a little of your own. I think you'd be better off writing
your own function or class. If I understand you right, you're simply looking
for lines longer than 80 chars, and breaking them at the 80-char mark, and
indenting the remainder by one space.
For example, this:
s = """\
This line is fine.
This line is very long and should wrap, It'll end up on a few lines.
A short line.
"""
def hard_wrap(s, n, indent):
wrapped = ""
n_next = n - len(indent)
for l in s.split('\n'):
first, rest = l[:n], l[n:]
wrapped += first + "\n"
while rest:
next, rest = rest[:n_next], rest[n_next:]
wrapped += indent + next + "\n"
return wrapped
print hard_wrap(s, 20, " ")
produces:
This line is fine.
This line is very lo
ng and should wrap,
It'll end up on a
few lines.
A short line.
|
How can I time a code segment for testing performance with Pythons timeit?
Question: I've a python script which works just as it should but I need to write the
time for the execution. I've googled that I should use
[`timeit`](https://docs.python.org/2/library/timeit.html) but I can't seem to
get it to work.
My Python script looks like this:
import sys
import getopt
import timeit
import random
import os
import re
import ibm_db
import time
from string import maketrans
myfile = open("results_update.txt", "a")
for r in range(100):
rannumber = random.randint(0, 100)
update = "update TABLE set val = %i where MyCount >= '2010' and MyCount < '2012' and number = '250'" % rannumber
#print rannumber
conn = ibm_db.pconnect("dsn=myDB","usrname","secretPWD")
for r in range(5):
print "Run %s\n" % r
ibm_db.execute(query_stmt)
query_stmt = ibm_db.prepare(conn, update)
myfile.close()
ibm_db.close(conn)
What I need it the time it takes the execution of the query and written to the
file "results_update.txt". The purpose is to test an update statement for my
database with different indexes and tuning mechanisms.
Answer: you can use time.time ot time.clock before and after the block you want to
time
import time
t0 = time.time()
code_block
t1 = time.time()
total = t1-t0
this method is not as exact as timeit (it does not average several runs) but
it is straighforward.
time.time() (in windows and linux) and time.clock(in linux) have not enough
resolution for fast functions (you get total = 0). In this case or if you want
to average the time elapsed by several runs, you have to manually call the
function multiple times (As I think you already do in you example code and
timeit does automatically when you set its _number_ argument)
import time
def myfast():
code
n = 10000
t0 = time.time()
for i in range(n): myfast()
t1 = time.time()
total_n = t1-t0
in windows, as Corey stated in the comment, time.clock() has much higher
precision (microsecond instead of second) and must be prefered to time.time().
|
How to write this snippet in Python?
Question: I am learning Python (I have a C/C++ background).
I need to write something practical in Python though, whilst learning. I have
the following pseudocode (my first attempt at writing a Python script, since
reading about Python yesterday). Hopefully, the snippet details the logic of
what I want to do. BTW I am using python 2.6 on Ubuntu Karmic.
Assume the script is invoked as: script_name.py directory_path
import csv, sys, os, glob
# Can I declare that the function accepts a dictionary as first arg?
def getItemValue(item, key, defval)
return !item.haskey(key) ? defval : item[key]
dirname = sys.argv[1]
# declare some default values here
weight, is_male, default_city_id = 100, true, 1
# fetch some data from a database table into a nested dictionary, indexed by a string
curr_dict = load_dict_from_db('foo')
#iterate through all the files matching *.csv in the specified folder
for infile in glob.glob( os.path.join(dirname, '*.csv') ):
#get the file name (without the '.csv' extension)
code = infile[0:-4]
# open file, and iterate through the rows of the current file (a CSV file)
f = open(infile, 'rt')
try:
reader = csv.reader(f)
for row in reader:
#lookup the id for the code in the dictionary
id = curr_dict[code]['id']
name = row['name']
address1 = row['address1']
address2 = row['address2']
city_id = getItemValue(row, 'city_id', default_city_id)
# insert row to database table
finally:
f.close()
I have the following questions:
1. Is the code written in a Pythonic enough way (is there a better way of implementing it)?
2. Given a table with a schema like shown below, how may I write a Python function that fetches data from the table and returns is in a dictionary indexed by string (name).
3. How can I insert the row data into the table (actually I would like to use a transaction if possible, and commit just before the file is closed)
Table schema:
create table demo (id int, name varchar(32), weight float, city_id int);
BTW, my backend database is postgreSQL
**[Edit]**
Wayne et al:
To clarify, what I want is a set of rows. Each row can be indexed by a key (so
that means the rows container is a dictionary (right)?. Ok, now once we have
retrieved a row by using the key, I also want to be able to access the
'columns' in the row - meaning that the row data itself is a dictionary. I
dont know if Python supports multidimensional array syntax when dealing with
dictionaries - but the following statement will help explain how I intend to
conceptually use the data returned from the db. A statement like
dataset['joe']['weight'] will first fetch the row data indexed by the key
'joe' (which is a dictionary) and then index that dictionary for the key
'weight'. I want to know how to build such a dictionary of dictionaries from
the retrieved data in a Pythonic way like you did before.
A simplistic way would be to write something like:
import pyodbc
mydict = {}
cnxn = pyodbc.connect(params)
cursor = cnxn.cursor()
cursor.execute("select user_id, user_name from users"):
for row in cursor:
mydict[row.id] = row
Is this correct/can it be written in a more pythonic way?
Answer: to get the value from the dictionary you need to use
[`.get`](http://docs.python.org/library/stdtypes.html#dict.get) method of the
`dict`:
>>> d = {1: 2}
>>> d.get(1, 3)
2
>>> d.get(5, 3)
3
This will remove the need for `getItemValue` function. I wont' comment on the
existing syntax since it's clearly alien to Python. Correct syntax for the
ternary in Python is:
true_val if true_false_check else false_val
>>> 'a' if False else 'b'
'b'
But as I'm saying below, you don't need it at all.
If you're using Python > 2.6, you should use `with` statement over the `try-
finally`:
with open(infile) as f:
reader = csv.reader(f)
... etc
Seeing that you want to have `row` as dictionary, you should be using
[`csv.DictReader`](http://docs.python.org/library/csv.html#csv.DictReader) and
not a simple `csv. reader`. However, it is unnecessary in your case. Your sql
query could just be constructed to access the fields of the `row` dict. In
this case you wouldn't need to create separate items `city_id`, `name`, etc.
To add default `city_id` to `row` if it doesn't exist, you could use
[`.setdefault`](http://docs.python.org/library/stdtypes.html#dict.setdefault)
method:
>>> d
{1: 2}
>>> d.setdefault(1, 3)
2
>>> d
{1: 2}
>>> d.setdefault(3, 3)
3
>>> d
{1: 2, 3: 3}
and for `id`, simply `row[id] = curr_dict[code]['id']`
When slicing, you could skip `0`:
>>> 'abc.txt'[:-4]
'abc'
Generally, Python's library provide a `fetchone`, `fetchmany`, `fetchall`
methods on cursor, which return `Row` object, that might support dict-like
access or return a simple tuple. It will depend on the particular module
you're using.
|
Python, store a dict in a database
Question: What's the best way to store and retrieve a python dict in a database?
Answer: If you are not specifically interested into using a traditionally SQL
database, such as MySQL, you could look into unstructured document databases
where documents naturally map to python dictionaries, for example
[MongoDB](http://www.mongodb.org/). The MongoDB [python
bindings](http://api.mongodb.org/python/1.6%2B/index.html) allow you to just
insert dicts in the DB, and query them based on the values in the dict. See
for example the code below from the
[tutorial](http://api.mongodb.org/python/1.6%2B/tutorial.html):
>>> from pymongo import Connection
>>> connection = Connection()
>>> db = connection['test-database']
>>> import datetime
>>> post = {"author": "Mike",
... "text": "My first blog post!",
... "tags": ["mongodb", "python", "pymongo"],
... "date": datetime.datetime.utcnow()}
>>> posts = db.posts
>>> posts.insert(post)
ObjectId('...')
>>> posts.find_one({"author": "Mike"})
{u'date': datetime.datetime(...), u'text': u'My first blog post!', u'_id': ObjectId('...'), u'author': u'Mike', u'tags': [u'mongodb', u'python', u'pymongo']}
|
Python timezone issue?
Question: im having troubles with parsing a feed and getting the time. i am using
dateutil.parser
from dateutil.parser import parse
print updated, parse(updated ), parse( updated ).utcoffset()
this should be a time in cali, output
2010-05-20T11:00:00.000-07:00 2010-05-20 11:00:00.000000-07:00 -1 day, 17:00:00
why is the offset -1 day 17 hours?
this is causing me issues when i try to do things with it
Answer: "Minus 7 hours" is equal to "minus 1 day, plus 17 hours". So probably the time
zone offset is correct and just displayed in a weird way.
|
how to import a 'zip' file to my .py
Question: when i use <http://github.com/joshthecoder/tweepy-examples> ,
i find :
import tweepy
in the appengine\oauth_example\handlers.py
but i can't find a tweepy file or tweepy's 'py' file, except a tweepy.zip
file,
i don't think this is right,cauz i never import a zip file,
i find this in app.py:
import sys
sys.path.insert(0, 'tweepy.zip')
why ?
how to import a zip file..
thanks
**updated**
a.py :
import sys
sys.path.insert(0, 'b.zip')
import b
print b
b.zip:
b file
|-----__init__.py
|-----c.py
c.py:
cc='ccccc'
the error is :
> "D:\Python25\pythonw.exe" "D:\zjm_code\a.py"
Traceback (most recent call last):
File "D:\zjm_code\a.py", line 9, in <module>
import b
ImportError: No module named b
**updated2**
it is ok now ,
the error's reason is : i rename b.rar to b.zip
Answer: The name of the zip file is irrelevent when searching for modules - this
allows you to include version numbers in the file name, such as
`my_b_package.1.2.3.zip`.
To import from a zip file, you need to replicate the full package structure
within it. In this case, you need a package `b`, with the `__init__.py` and
`c.py` modules.
I.e:
b.zip
|
| -- b <dir>
| -- __init__.py
| -- c.py
|
Printing to STDOUT and log file while removing ANSI color codes
Question: I have the following functions for colorizing my screen messages:
def error(string):
return '\033[31;1m' + string + '\033[0m'
def standout(string):
return '\033[34;1m' + string + '\033[0m'
I use them as follows:
print error('There was a problem with the program')
print "This is normal " + standout("and this stands out")
I want to log the output to a file (in addition to STDOUT) WITHOUT the ANSI
color codes, hopefully without having to add a second "logging" line to each
`print` statement.
The reason is that if you simply `python program.py > out` then the file `out`
will have the ANSI color codes, which look terrible if you open in a plain
text editor.
Any advice?
Answer: The `sys.stdout.isatty` function might be able to help:
from sys import stdout
def error(string, is_tty=stdout.isatty()):
return ('\033[31;1m' + string + '\033[0m') if is_tty else string
def standout(string, is_tty=stdout.isatty()):
return ('\033[34;1m' + string + '\033[0m') if is_tty else string
That's actually one of the few uses I can think of to use a default argument
that isn't set to `None` because default arguments are evaluated at compile
time in Python rather than at runtime like in C++...
Also the behaviour can be explicitly overridden if you really need to, though
that doesn't let you manipulate stdout itself when it's redirected. Is there
any reason why you're not using the `logging` module (perhaps you didn't know
about it)?
|
Compiling ODE on windows without Visual Studio (for PyODE)
Question: I'm new to compiling programs written by someone else, so I hope I'm not
missing anything obvious.
What I am really trying to do is install PyODE, and I think I managed that
just fine, but when running the PyODE examples I get an error:
Traceback (most recent call last):
File "C:\Python26\pyode-examples\tutorial3.py", line 12, in <module>
import ode
ImportError: DLL load failed: The specified module could not be found.
which assume means that it can't find ODE installed. I thought PyODE came
bundled with ODE but I guess not.. so now I'm trying to compile ODE according
to these instructions:
<http://opende.sourceforge.net/wiki/index.php/Manual_%28Install_and_Use%29>
but they all seem to revolve around creating configuration files for visual
studio which I don't have.
Could someone please clue me in on the proper procedure here? Thanks! :) -Leav
Answer: My Problem was completley unrelated to whether or not you could compile ODE
without visual studio.
Simply copied ODE.dll which was in the python directory into the example's
directory.
Thanks Thomas! :)
|
How can I use COM and USB ports within Cygwin?
Question: I want to send/receive data from my Arduino board with a Python script. I
would like to do it using Python and its pySerial module which seems to fit my
needs. So I installed Python and pySerial within cygwin (windows XP behind).
The Python script is rather straightforward:
$ cat example.py
#print "testing my COM26 port using python"
import serial
ser = serial.Serial()
ser.baudrate = 9600
ser.port = 26
ser
ser.open()
ser.isOpen()
However at runtime I get the following error.
$ python example.py
Traceback (most recent call last):
File "example.py", line 9, in <module>
ser.open()
File "/usr/lib/python2.5/site-packages/serial/serialposix.py", line 276, in open
raise SerialException("could not open port %s: %s" % (self._port, msg))
serial.serialutil.SerialException: could not open port 26: [Errno 2] No such file or directory: '/dev/com27'
> Could not open port 26: [Errno 2] No such file or directory: '/dev/com27'
How do I know my Arduino is connected to port COM27?
Well, it's simple. The Arduino IDE says so, I can send and receive data from
the Serial Port Monitor tool for the IDE using that port. Besides, I managed
to get the hyperterminal working using that port too.
However, it seems Cygwin is not aware of such USB and COM ports:
$ ls -lah /dev
total 4,0K
.
..
fd -> /proc/self/fd
mqueue
shm
stderr -> /proc/self/fd/2
stdin -> /proc/self/fd/0
stdout -> /proc/self/fd/1
It should be mentioned that I am running this on a Dell laptop that has no
classic serial COM port, just USB ports. (So I guess it's plain normal for
instance that `/dev/com1` does not exist.)
I don't know if I'm asking correctly, but my question is: how can I configure
Cygwin so that it becomes _aware_ of this COM27 port?
Answer: If Hyperterminal can access it, then it's installed as a "virtual COM port".
Cygwin will let you access it as **/dev/ttyS26** (called COM27 by Windows).
You may still have an issue with the input blocking until a CR is received--I
do. (Trying to solve _that_ , is how I found _this_.)
|
Correct way to do timer function in Python
Question: I have a GUI application that needs to do something simple in the background
(update a wx python progress bar, but that doesn't really matter). I see that
there is a threading.timer class.. but there seems to be no way to make it
repeat. So if I use the timer, I end up having to make a new thread on every
single execution... like :
import threading
import time
def DoTheDew():
print "I did it"
t = threading.Timer(1, function=DoTheDew)
t.daemon = True
t.start()
if __name__ == '__main__':
t = threading.Timer(1, function=DoTheDew)
t.daemon = True
t.start()
time.sleep(10)
This seems like I am making a bunch of threads that do 1 silly thing and die..
why not write it as :
import threading
import time
def DoTheDew():
while True:
print "I did it"
time.sleep(1)
if __name__ == '__main__':
t = threading.Thread(target=DoTheDew)
t.daemon = True
t.start()
time.sleep(10)
Am I missing some way to make a timer keep doing something? Either of these
options seems silly... I am looking for a timer more like a java.util.Timer
that can schedule the thread to happen every second... If there isn't a way in
Python, which of my above methods is better and why?
Answer: A pattern more like this is probably what you should be doing, but it's hard
to say because you didn't provide many details.
def do_background_work(self):
# do work on a background thread, posting updates to the
# GUI thread with CallAfter
while True:
# do stuff
wx.CallAfter(self.update_progress, percent_complete)
def update_progress(self, percent_complete):
# update the progress bar from the GUI thread
self.gauge.SetValue(percent_complete)
def on_start_button(self, event):
# start doing background work when the user hits a button
thread = threading.Thread(target=self.do_background_work)
thread.setDaemon(True)
thread.start()
|
Trouble importing a Python module
Question: I have a Python project with 2 files: epic.py site.py
in the epic.py I have the lines
from site import *
bark()
in site.py I have the lines
def bark():
print('arf!')
when I try to run epic.py, it returns "bark is not defined"
this is weird.
Answer: Try renaming site.py to mysite.py or something like that because there is a
standard Python `site` module.
|
Sending file over socket
Question: I'm have a problem sending data as a file from one end of a socket to the
other. What's happening is that both the server and client are trying to read
the file so the file never gets sent. I was wondering how to have the client
block until the server's completed reading the file sent from the client.
I have this working with raw packets using send and recv, but figured this was
a cleaner solution...
**Client:**
1. connects to server creating socket connection
2. creates a file on socket and sends data
3. waits for file from server
**Server:**
1. waits for file from client
**Complete interraction:**
1. client sends data to server
2. server sends data to client
Edit
The code I'm actually looking at was posted in this question, but I think the
Eventlet stuff scared people...
<http://stackoverflow.com/questions/2909358/eventlet-client-server>
Underneath its just using Python sockets so I figured I'd simplify the
question and ask about just the core socket issue...
Answer: It sounds like you have the client sending the file and then waiting for the
server response, but if you don't give the server an indication that it has
completely read the file, recv() on the server side will hang waiting for more
data. You can call shutdown(SHUT_WR) on the client-side once the client is
done sending. This informs the server that once it has read all the data sent
that there is no more.
A very basic example (sending one data blob to server and receiving one data
blob in response):
**server**
>>> from socket import *
>>> s=socket()
>>> s.bind(('',8000))
>>> s.listen(1)
>>> c,a = s.accept() # will hang here until a client connects
>>> recvd=''
>>> while True:
... data = c.recv(1024)
... if not data: break # recv() will return '' only if the client calls shutdown(SHUT_WR) or close()
... recvd += data
...
>>> recvd
'a message'
>>> c.sendall('a response')
>>> c.close() # done with client, could loop back to accept here
>>> s.close() # done with server
**client**
>>> from socket import *
>>> s=socket()
>>> s.connect(('localhost',8000))
>>> s.sendall('a message')
>>> s.shutdown(SHUT_WR) # tells server you're done sending, so recv won't wait for more
>>> recvd=''
>>> while True:
... data = s.recv(1024)
... if not data: break
... recvd += data
...
>>> recvd
'a response'
>>> s.close()
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.