repo_name
stringlengths 5
100
| path
stringlengths 4
251
| copies
stringclasses 990
values | size
stringlengths 4
7
| content
stringlengths 499
1.05M
| license
stringclasses 15
values |
---|---|---|---|---|---|
TNT-Samuel/Coding-Projects | DNS Server/Source/Lib/email/_parseaddr.py | 124 | 17199 | # Copyright (C) 2002-2007 Python Software Foundation
# Contact: email-sig@python.org
"""Email address parsing code.
Lifted directly from rfc822.py. This should eventually be rewritten.
"""
__all__ = [
'mktime_tz',
'parsedate',
'parsedate_tz',
'quote',
]
import time, calendar
SPACE = ' '
EMPTYSTRING = ''
COMMASPACE = ', '
# Parse a date field
_monthnames = ['jan', 'feb', 'mar', 'apr', 'may', 'jun', 'jul',
'aug', 'sep', 'oct', 'nov', 'dec',
'january', 'february', 'march', 'april', 'may', 'june', 'july',
'august', 'september', 'october', 'november', 'december']
_daynames = ['mon', 'tue', 'wed', 'thu', 'fri', 'sat', 'sun']
# The timezone table does not include the military time zones defined
# in RFC822, other than Z. According to RFC1123, the description in
# RFC822 gets the signs wrong, so we can't rely on any such time
# zones. RFC1123 recommends that numeric timezone indicators be used
# instead of timezone names.
_timezones = {'UT':0, 'UTC':0, 'GMT':0, 'Z':0,
'AST': -400, 'ADT': -300, # Atlantic (used in Canada)
'EST': -500, 'EDT': -400, # Eastern
'CST': -600, 'CDT': -500, # Central
'MST': -700, 'MDT': -600, # Mountain
'PST': -800, 'PDT': -700 # Pacific
}
def parsedate_tz(data):
"""Convert a date string to a time tuple.
Accounts for military timezones.
"""
res = _parsedate_tz(data)
if not res:
return
if res[9] is None:
res[9] = 0
return tuple(res)
def _parsedate_tz(data):
"""Convert date to extended time tuple.
The last (additional) element is the time zone offset in seconds, except if
the timezone was specified as -0000. In that case the last element is
None. This indicates a UTC timestamp that explicitly declaims knowledge of
the source timezone, as opposed to a +0000 timestamp that indicates the
source timezone really was UTC.
"""
if not data:
return
data = data.split()
# The FWS after the comma after the day-of-week is optional, so search and
# adjust for this.
if data[0].endswith(',') or data[0].lower() in _daynames:
# There's a dayname here. Skip it
del data[0]
else:
i = data[0].rfind(',')
if i >= 0:
data[0] = data[0][i+1:]
if len(data) == 3: # RFC 850 date, deprecated
stuff = data[0].split('-')
if len(stuff) == 3:
data = stuff + data[1:]
if len(data) == 4:
s = data[3]
i = s.find('+')
if i == -1:
i = s.find('-')
if i > 0:
data[3:] = [s[:i], s[i:]]
else:
data.append('') # Dummy tz
if len(data) < 5:
return None
data = data[:5]
[dd, mm, yy, tm, tz] = data
mm = mm.lower()
if mm not in _monthnames:
dd, mm = mm, dd.lower()
if mm not in _monthnames:
return None
mm = _monthnames.index(mm) + 1
if mm > 12:
mm -= 12
if dd[-1] == ',':
dd = dd[:-1]
i = yy.find(':')
if i > 0:
yy, tm = tm, yy
if yy[-1] == ',':
yy = yy[:-1]
if not yy[0].isdigit():
yy, tz = tz, yy
if tm[-1] == ',':
tm = tm[:-1]
tm = tm.split(':')
if len(tm) == 2:
[thh, tmm] = tm
tss = '0'
elif len(tm) == 3:
[thh, tmm, tss] = tm
elif len(tm) == 1 and '.' in tm[0]:
# Some non-compliant MUAs use '.' to separate time elements.
tm = tm[0].split('.')
if len(tm) == 2:
[thh, tmm] = tm
tss = 0
elif len(tm) == 3:
[thh, tmm, tss] = tm
else:
return None
try:
yy = int(yy)
dd = int(dd)
thh = int(thh)
tmm = int(tmm)
tss = int(tss)
except ValueError:
return None
# Check for a yy specified in two-digit format, then convert it to the
# appropriate four-digit format, according to the POSIX standard. RFC 822
# calls for a two-digit yy, but RFC 2822 (which obsoletes RFC 822)
# mandates a 4-digit yy. For more information, see the documentation for
# the time module.
if yy < 100:
# The year is between 1969 and 1999 (inclusive).
if yy > 68:
yy += 1900
# The year is between 2000 and 2068 (inclusive).
else:
yy += 2000
tzoffset = None
tz = tz.upper()
if tz in _timezones:
tzoffset = _timezones[tz]
else:
try:
tzoffset = int(tz)
except ValueError:
pass
if tzoffset==0 and tz.startswith('-'):
tzoffset = None
# Convert a timezone offset into seconds ; -0500 -> -18000
if tzoffset:
if tzoffset < 0:
tzsign = -1
tzoffset = -tzoffset
else:
tzsign = 1
tzoffset = tzsign * ( (tzoffset//100)*3600 + (tzoffset % 100)*60)
# Daylight Saving Time flag is set to -1, since DST is unknown.
return [yy, mm, dd, thh, tmm, tss, 0, 1, -1, tzoffset]
def parsedate(data):
"""Convert a time string to a time tuple."""
t = parsedate_tz(data)
if isinstance(t, tuple):
return t[:9]
else:
return t
def mktime_tz(data):
"""Turn a 10-tuple as returned by parsedate_tz() into a POSIX timestamp."""
if data[9] is None:
# No zone info, so localtime is better assumption than GMT
return time.mktime(data[:8] + (-1,))
else:
t = calendar.timegm(data)
return t - data[9]
def quote(str):
"""Prepare string to be used in a quoted string.
Turns backslash and double quote characters into quoted pairs. These
are the only characters that need to be quoted inside a quoted string.
Does not add the surrounding double quotes.
"""
return str.replace('\\', '\\\\').replace('"', '\\"')
class AddrlistClass:
"""Address parser class by Ben Escoto.
To understand what this class does, it helps to have a copy of RFC 2822 in
front of you.
Note: this class interface is deprecated and may be removed in the future.
Use email.utils.AddressList instead.
"""
def __init__(self, field):
"""Initialize a new instance.
`field' is an unparsed address header field, containing
one or more addresses.
"""
self.specials = '()<>@,:;.\"[]'
self.pos = 0
self.LWS = ' \t'
self.CR = '\r\n'
self.FWS = self.LWS + self.CR
self.atomends = self.specials + self.LWS + self.CR
# Note that RFC 2822 now specifies `.' as obs-phrase, meaning that it
# is obsolete syntax. RFC 2822 requires that we recognize obsolete
# syntax, so allow dots in phrases.
self.phraseends = self.atomends.replace('.', '')
self.field = field
self.commentlist = []
def gotonext(self):
"""Skip white space and extract comments."""
wslist = []
while self.pos < len(self.field):
if self.field[self.pos] in self.LWS + '\n\r':
if self.field[self.pos] not in '\n\r':
wslist.append(self.field[self.pos])
self.pos += 1
elif self.field[self.pos] == '(':
self.commentlist.append(self.getcomment())
else:
break
return EMPTYSTRING.join(wslist)
def getaddrlist(self):
"""Parse all addresses.
Returns a list containing all of the addresses.
"""
result = []
while self.pos < len(self.field):
ad = self.getaddress()
if ad:
result += ad
else:
result.append(('', ''))
return result
def getaddress(self):
"""Parse the next address."""
self.commentlist = []
self.gotonext()
oldpos = self.pos
oldcl = self.commentlist
plist = self.getphraselist()
self.gotonext()
returnlist = []
if self.pos >= len(self.field):
# Bad email address technically, no domain.
if plist:
returnlist = [(SPACE.join(self.commentlist), plist[0])]
elif self.field[self.pos] in '.@':
# email address is just an addrspec
# this isn't very efficient since we start over
self.pos = oldpos
self.commentlist = oldcl
addrspec = self.getaddrspec()
returnlist = [(SPACE.join(self.commentlist), addrspec)]
elif self.field[self.pos] == ':':
# address is a group
returnlist = []
fieldlen = len(self.field)
self.pos += 1
while self.pos < len(self.field):
self.gotonext()
if self.pos < fieldlen and self.field[self.pos] == ';':
self.pos += 1
break
returnlist = returnlist + self.getaddress()
elif self.field[self.pos] == '<':
# Address is a phrase then a route addr
routeaddr = self.getrouteaddr()
if self.commentlist:
returnlist = [(SPACE.join(plist) + ' (' +
' '.join(self.commentlist) + ')', routeaddr)]
else:
returnlist = [(SPACE.join(plist), routeaddr)]
else:
if plist:
returnlist = [(SPACE.join(self.commentlist), plist[0])]
elif self.field[self.pos] in self.specials:
self.pos += 1
self.gotonext()
if self.pos < len(self.field) and self.field[self.pos] == ',':
self.pos += 1
return returnlist
def getrouteaddr(self):
"""Parse a route address (Return-path value).
This method just skips all the route stuff and returns the addrspec.
"""
if self.field[self.pos] != '<':
return
expectroute = False
self.pos += 1
self.gotonext()
adlist = ''
while self.pos < len(self.field):
if expectroute:
self.getdomain()
expectroute = False
elif self.field[self.pos] == '>':
self.pos += 1
break
elif self.field[self.pos] == '@':
self.pos += 1
expectroute = True
elif self.field[self.pos] == ':':
self.pos += 1
else:
adlist = self.getaddrspec()
self.pos += 1
break
self.gotonext()
return adlist
def getaddrspec(self):
"""Parse an RFC 2822 addr-spec."""
aslist = []
self.gotonext()
while self.pos < len(self.field):
preserve_ws = True
if self.field[self.pos] == '.':
if aslist and not aslist[-1].strip():
aslist.pop()
aslist.append('.')
self.pos += 1
preserve_ws = False
elif self.field[self.pos] == '"':
aslist.append('"%s"' % quote(self.getquote()))
elif self.field[self.pos] in self.atomends:
if aslist and not aslist[-1].strip():
aslist.pop()
break
else:
aslist.append(self.getatom())
ws = self.gotonext()
if preserve_ws and ws:
aslist.append(ws)
if self.pos >= len(self.field) or self.field[self.pos] != '@':
return EMPTYSTRING.join(aslist)
aslist.append('@')
self.pos += 1
self.gotonext()
return EMPTYSTRING.join(aslist) + self.getdomain()
def getdomain(self):
"""Get the complete domain name from an address."""
sdlist = []
while self.pos < len(self.field):
if self.field[self.pos] in self.LWS:
self.pos += 1
elif self.field[self.pos] == '(':
self.commentlist.append(self.getcomment())
elif self.field[self.pos] == '[':
sdlist.append(self.getdomainliteral())
elif self.field[self.pos] == '.':
self.pos += 1
sdlist.append('.')
elif self.field[self.pos] in self.atomends:
break
else:
sdlist.append(self.getatom())
return EMPTYSTRING.join(sdlist)
def getdelimited(self, beginchar, endchars, allowcomments=True):
"""Parse a header fragment delimited by special characters.
`beginchar' is the start character for the fragment.
If self is not looking at an instance of `beginchar' then
getdelimited returns the empty string.
`endchars' is a sequence of allowable end-delimiting characters.
Parsing stops when one of these is encountered.
If `allowcomments' is non-zero, embedded RFC 2822 comments are allowed
within the parsed fragment.
"""
if self.field[self.pos] != beginchar:
return ''
slist = ['']
quote = False
self.pos += 1
while self.pos < len(self.field):
if quote:
slist.append(self.field[self.pos])
quote = False
elif self.field[self.pos] in endchars:
self.pos += 1
break
elif allowcomments and self.field[self.pos] == '(':
slist.append(self.getcomment())
continue # have already advanced pos from getcomment
elif self.field[self.pos] == '\\':
quote = True
else:
slist.append(self.field[self.pos])
self.pos += 1
return EMPTYSTRING.join(slist)
def getquote(self):
"""Get a quote-delimited fragment from self's field."""
return self.getdelimited('"', '"\r', False)
def getcomment(self):
"""Get a parenthesis-delimited fragment from self's field."""
return self.getdelimited('(', ')\r', True)
def getdomainliteral(self):
"""Parse an RFC 2822 domain-literal."""
return '[%s]' % self.getdelimited('[', ']\r', False)
def getatom(self, atomends=None):
"""Parse an RFC 2822 atom.
Optional atomends specifies a different set of end token delimiters
(the default is to use self.atomends). This is used e.g. in
getphraselist() since phrase endings must not include the `.' (which
is legal in phrases)."""
atomlist = ['']
if atomends is None:
atomends = self.atomends
while self.pos < len(self.field):
if self.field[self.pos] in atomends:
break
else:
atomlist.append(self.field[self.pos])
self.pos += 1
return EMPTYSTRING.join(atomlist)
def getphraselist(self):
"""Parse a sequence of RFC 2822 phrases.
A phrase is a sequence of words, which are in turn either RFC 2822
atoms or quoted-strings. Phrases are canonicalized by squeezing all
runs of continuous whitespace into one space.
"""
plist = []
while self.pos < len(self.field):
if self.field[self.pos] in self.FWS:
self.pos += 1
elif self.field[self.pos] == '"':
plist.append(self.getquote())
elif self.field[self.pos] == '(':
self.commentlist.append(self.getcomment())
elif self.field[self.pos] in self.phraseends:
break
else:
plist.append(self.getatom(self.phraseends))
return plist
class AddressList(AddrlistClass):
"""An AddressList encapsulates a list of parsed RFC 2822 addresses."""
def __init__(self, field):
AddrlistClass.__init__(self, field)
if field:
self.addresslist = self.getaddrlist()
else:
self.addresslist = []
def __len__(self):
return len(self.addresslist)
def __add__(self, other):
# Set union
newaddr = AddressList(None)
newaddr.addresslist = self.addresslist[:]
for x in other.addresslist:
if not x in self.addresslist:
newaddr.addresslist.append(x)
return newaddr
def __iadd__(self, other):
# Set union, in-place
for x in other.addresslist:
if not x in self.addresslist:
self.addresslist.append(x)
return self
def __sub__(self, other):
# Set difference
newaddr = AddressList(None)
for x in self.addresslist:
if not x in other.addresslist:
newaddr.addresslist.append(x)
return newaddr
def __isub__(self, other):
# Set difference, in-place
for x in other.addresslist:
if x in self.addresslist:
self.addresslist.remove(x)
return self
def __getitem__(self, index):
# Make indexing, slices, and 'in' work
return self.addresslist[index]
| gpl-3.0 |
dmsurti/mayavi | tvtk/tvtk_base.py | 1 | 18638 | """Commonly used code by tvtk objects.
"""
# Author: Prabhu Ramachandran <prabhu_r@users.sf.net>
# Copyright (c) 2004-2015, Enthought, Inc.
# License: BSD Style.
from __future__ import print_function
import sys
import weakref
import os
import logging
import vtk
from traits import api as traits
from traitsui.api import (BooleanEditor, RGBColorEditor, FileEditor)
from . import messenger
# Setup a logger for this module.
logger = logging.getLogger(__name__)
######################################################################
# The TVTK object cache.
######################################################################
class TVTKObjectCache(weakref.WeakValueDictionary):
def __init__(self, *args, **kw):
self._observer_data = {}
weakref.WeakValueDictionary.__init__(self, *args, **kw)
def remove(wr, selfref=weakref.ref(self)):
self = selfref()
if self is not None:
self.teardown_observers(wr.key)
del self.data[wr.key]
self._remove = remove
def setup_observers(self, vtk_obj, event, method):
"""Setup the observer for the VTK object's event.
Parameters
----------
vtk_obj -- The VTK object for which the `event` is
observed.
event -- The VTK event to watch.
method -- The method to be called back when `event` is
fired on the VTK object.
"""
# Setup the observer so the traits are updated even if the
# wrapped VTK object changes.
if hasattr(vtk_obj, 'AddObserver'):
# Some classes like vtkInformation* derive from
# tvtk.ObjectBase which don't support Add/RemoveObserver.
messenger.connect(vtk_obj, event, method)
ob_id = vtk_obj.AddObserver(event, messenger.send)
key = vtk_obj.__this__
od = self._observer_data
if key in od:
od[key].append((vtk_obj, ob_id))
else:
od[key] = [(vtk_obj, ob_id)]
def teardown_observers(self, key):
"""Given the key of the VTK object (vtk_obj.__this__), this
removes the observer for the ModifiedEvent and also disconnects
the messenger.
"""
od = self._observer_data
if key not in od:
return
for vtk_obj, ob_id in od[key]:
try:
# The disconnection sometimes fails at exit.
vtk_obj.RemoveObserver(ob_id)
except AttributeError:
pass
try:
messenger.disconnect(vtk_obj)
except AttributeError:
pass
del od[key]
# The TVTK object cache (`_object_cache`). This caches all the TVTK
# instances using weakrefs. When a VTK object is wrapped via the
# `wrap_vtk` function this cache is checked. The key is the VTK
# object's address. The value of the dict is the TVTK wrapper object.
# If the VTK object address exists in the cache, it is returned by
# `wrap_vtk`. `wrap_vtk` is defined in `tvtk_helper.py` which is
# stored in the ZIP file.
_dummy = None
# This makes the cache work even when the module is reloaded.
for name in ['tvtk_base', 'tvtk.tvtk_base']:
if name in sys.modules:
mod = sys.modules[name]
if hasattr(mod, '_object_cache'):
_dummy = mod._object_cache
del mod
break
if _dummy is not None:
_object_cache = _dummy
else:
_object_cache = TVTKObjectCache()
del _dummy
def get_tvtk_object_from_cache(vtk_obj):
"""Returns the cached TVTK object given a VTK object."""
return _object_cache.get(vtk_obj.__this__)
######################################################################
# Special traits used by the tvtk objects.
######################################################################
true_bool_trait = traits.Trait('true',
{'true': 1, 't': 1, 'yes': 1,
'y': 1, 'on': 1, 1: 1, 'false': 0,
'f': 0, 'no': 0, 'n': 0,
'off': 0, 0: 0, -1:0},
editor=BooleanEditor)
false_bool_trait = traits.Trait('false', true_bool_trait)
class TraitRevPrefixMap(traits.TraitPrefixMap):
"""A reverse mapped TraitPrefixMap. This handler allows for
something like the following::
>>> class A(HasTraits):
... a = Trait('ab', TraitRevPrefixMap({'ab':1, 'cd':2}))
...
>>> a = A()
>>> a.a = 'c'
>>> print a.a
'cd'
>>> a.a = 1
>>> print a.a
'ab'
That is, you can set the trait to the value itself. If multiple
keys map to the same value, one of the valid keys will be used.
"""
def __init__(self, map):
traits.TraitPrefixMap.__init__(self, map)
self._rmap = {}
for key, value in map.items():
self._rmap[value] = key
def validate(self, object, name, value):
try:
if value in self._rmap:
value = self._rmap[value]
if not value in self._map:
match = None
n = len( value )
for key in self.map.keys():
if value == key[:n]:
if match is not None:
match = None
break
match = key
if match is None:
self.error( object, name, value )
self._map[ value ] = match
return self._map[ value ]
except:
self.error( object, name, value )
def info(self):
keys = [repr(x) for x in self._rmap.keys()]
keys.sort()
msg = ' or '.join(keys)
return traits.TraitPrefixMap.info(self) + ' or ' + msg
def vtk_color_trait(default, **metadata):
Range = traits.Range
if default[0] == -1.0:
# Occurs for the vtkTextProperty's color trait. Need to work
# around.
return traits.Trait(default, traits.Tuple(*default),
traits.Tuple(Range(0.0, 1.0),
Range(0.0, 1.0),
Range(0.0, 1.0),
editor=RGBColorEditor),
**metadata)
elif type(default[0]) is float:
return traits.Trait(traits.Tuple(Range(0.0, 1.0, default[0]),
Range(0.0, 1.0, default[1]),
Range(0.0, 1.0, default[2])),
editor=RGBColorEditor, **metadata)
else:
return traits.Trait(
traits.Tuple(
Range(0, 255, default[0]), Range(0, 255, default[1]),
Range(0, 255, default[2]), cols=3
),
**metadata
)
# Special cases for the FileName and FilePrefix
vtk_file_name = traits.Trait(None, None, traits.Str, str,
editor=FileEditor)
vtk_file_prefix = traits.Trait(None, None, traits.Str, str,
editor=(FileEditor, {'truncate_ext': True}))
# The Property class traits are delegated in the Actors.
vtk_property_delegate = traits.Delegate('property', modify=True)
######################################################################
# Utility functions.
######################################################################
def deref_vtk(obj):
"""Dereferences the VTK object from the object if possible."""
if isinstance(obj, TVTKBase):
return obj._vtk_obj
else:
return obj
######################################################################
# 'TVTKBase' class (base class for all tvtk classes):
######################################################################
class TVTKBase(traits.HasStrictTraits):
"""The base class for all TVTK objects. This class encapsulates
key functionality common to all the TVTK classes.
TVTK classes provide a trait wrapped VTK object. They also
primitively picklable. Only the basic state of the object itself
is pickled. References to other VTK objects are NOT pickled.
"""
# This is just a dummy integer (MUST be > 1) that indicates that
# we are updating the traits and there is no need to change the
# underlying VTK object.
DOING_UPDATE = 10
########################################
# Private traits.
# This trait is only used internally and should not activate any
# notifications when set which is why we use `Python`.
_in_set = traits.Python
# The wrapped VTK object.
_vtk_obj = traits.Trait(None, None, vtk.vtkObjectBase())
# Stores the names of the traits that need to be updated.
_updateable_traits_ = traits.Tuple
# List of trait names that are to be included in the full traits view of this object.
_full_traitnames_list_ = traits.List
#################################################################
# `object` interface.
#################################################################
def __init__(self, klass, obj=None, update=True, **traits):
"""Object initialization.
Parameters
----------
- klass: `vtkObjectBase`
A VTK class to wrap. If `obj` is passed, its class must be
the same as `klass` or a subclass of it.
- obj: `vtkObjectBase` (default: None)
An optional VTK object. If passed the passed object is
wrapped. This defaults to `None` where a new VTK instance
of class, `klass` is created.
- update: `bool` (default: True)
If True (default), the traits of the class are automatically
updated based on the state of the wrapped VTK object. If
False, no updation is performed. This is particularly
useful when the object is being unpickled.
- traits: `dict`
A dictionary having the names of the traits as its keys.
This allows a user to set the traits of the object while
creating the object.
"""
# Initialize the Python attribute.
self._in_set = 0
if obj:
assert obj.IsA(klass.__name__)
self._vtk_obj = obj
else:
self._vtk_obj = klass()
# print "INIT", self.__class__.__name__, repr(self._vtk_obj)
# Call the Super class to update the traits.
# Inhibit any updates at this point since we update in the end
# anyway.
self._in_set = 1
super(TVTKBase, self).__init__(**traits)
self._in_set = 0
# Update the traits based on the values of the VTK object.
if update:
self.update_traits()
# Setup observers for the modified event.
self.setup_observers()
_object_cache[self._vtk_obj.__this__] = self
def __getinitargs__(self):
"""This is merely a placeholder so that subclasses can
override this if needed. This is called by `__setstate__`
because `traits.HasTrait` is a newstyle class.
"""
# You usually don't want to call update when calling __init__
# from __setstate__
return (None, 0)
def __getstate__(self):
"""Support for primitive pickling. Only the basic state is
pickled.
"""
self.update_traits()
d = self.__dict__.copy()
for i in ['_vtk_obj', '_in_set', 'reference_count',
'global_warning_display', '__sync_trait__']:
d.pop(i, None)
return d
def __setstate__(self, dict):
"""Support for primitive pickling. Only the basic state is
pickled.
"""
# This is a newstyle class so we need to call init here.
if self._vtk_obj is None:
self.__init__(*self.__getinitargs__())
self._in_set = 1
for i in dict:
# Not enough to update the dict because the vtk object
# needs to be updated.
try:
setattr(self, i, dict[i])
except traits.TraitError as msg:
print("WARNING:", end=' ')
print(msg)
self._in_set = 0
def __str__(self):
"""Return a nice string representation of the object.
This merely returns the result of str on the underlying VTK
object.
"""
return str(self._vtk_obj)
#################################################################
# `HasTraits` interface.
#################################################################
def class_trait_view_elements ( cls ):
""" Returns the ViewElements object associated with the class.
The returned object can be used to access all the view elements
associated with the class.
Overridden here to search through a particular directory for substitute
views to use for this tvtk object. The view should be declared in a
file named <class name>_view. We execute this file and replace any
currently defined view elements with view elements declared in this
file (that have the same name).
"""
# FIXME: This can be enhanced to search for new views also (in addition
# to replacing current views).
view_elements = super(TVTKBase, cls).class_trait_view_elements()
# Get the names of all the currently defined view elements.
names = view_elements.filter_by()
baseDir = os.path.dirname(os.path.abspath(__file__))
viewDir = os.path.join(baseDir, 'view')
try:
module_name = cls.__module__.split('.')[-1]
view_filename = os.path.join(viewDir,
module_name + '_view.py')
result = {}
exec(
compile(
open(view_filename).read(), view_filename, 'exec'
), {}, result
)
for name in names:
if name in result:
view_elements.content[ name ] = result[name]
except Exception:
pass
return view_elements
class_trait_view_elements = classmethod( class_trait_view_elements )
#################################################################
# `TVTKBase` interface.
#################################################################
def setup_observers(self):
"""Add an observer for the ModifiedEvent so the traits are kept
up-to-date with the wrapped VTK object and do it in a way that
avoids reference cycles."""
_object_cache.setup_observers(self._vtk_obj,
'ModifiedEvent',
self.update_traits)
def teardown_observers(self):
"""Remove the observer for the Modified event."""
_object_cache.teardown_observers(self._vtk_obj.__this__)
def update_traits(self, obj=None, event=None):
"""Updates all the 'updateable' traits of the object.
The method works by getting the current value from the wrapped
VTK object. `self._updateable_traits_` stores a tuple of
tuples containing the trait name followed by the name of the
get method to use on the wrapped VTK object.
The `obj` and `event` parameters may be ignored and are not
used in the function. They exist only for compatibility with
the VTK observer callback functions.
"""
if self._in_set:
return
if not hasattr(self, '_updateable_traits_'):
return
self._in_set = self.DOING_UPDATE
vtk_obj = self._vtk_obj
# Save the warning state and turn it off!
warn = vtk.vtkObject.GetGlobalWarningDisplay()
vtk.vtkObject.GlobalWarningDisplayOff()
for name, getter in self._updateable_traits_:
try:
val = getattr(vtk_obj, getter)()
except (AttributeError, TypeError):
pass
else:
if name == 'global_warning_display':
setattr(self, name, warn)
else:
setattr(self, name, val)
# Reset the warning state.
vtk.vtkObject.SetGlobalWarningDisplay(warn)
self._in_set = 0
#################################################################
# Non-public interface.
#################################################################
def _do_change(self, method, val, force_update=False):
"""This is called by the various traits when they change in
order to update the underlying VTK object.
Parameters
----------
- method: `method`
The method to invoke on the VTK object when called.
- val: `Any`
Argument to the method.
- force_update: `bool` (default: False)
If True, `update_traits` is always called at the end.
"""
if self._in_set == self.DOING_UPDATE:
return
vtk_obj = self._vtk_obj
self._in_set += 1
mtime = self._wrapped_mtime(vtk_obj) + 1
try:
method(val)
except TypeError:
if hasattr(val, '__len__'):
method(*val)
else:
raise
self._in_set -= 1
if force_update or self._wrapped_mtime(vtk_obj) > mtime:
self.update_traits()
def _wrap_call(self, vtk_method, *args):
"""This method allows us to safely call a VTK method without
calling `update_traits` during the call. This method is
therefore used to wrap any 'Set' call on a VTK object.
The method returns the output of the vtk_method call.
Parameters
----------
- vtk_method: `method`
The method to invoke safely.
- args: `Any`
Argument to be passed to the method.
"""
vtk_obj = self._vtk_obj
self._in_set += 1
mtime = self._wrapped_mtime(vtk_obj) + 1
ret = vtk_method(*args)
self._in_set -= 1
if self._wrapped_mtime(vtk_obj) > mtime:
self.update_traits()
return ret
def _wrapped_mtime(self, vtk_obj):
"""A simple wrapper for the mtime so tvtk can be used for
`vtk.vtkObjectBase` subclasses that neither have an
`AddObserver` or a `GetMTime` method.
"""
try:
return vtk_obj.GetMTime()
except AttributeError:
return 0
| bsd-3-clause |
TNT-Samuel/Coding-Projects | DNS Server/Source - Copy/Lib/site-packages/pip/_vendor/urllib3/response.py | 23 | 24667 | from __future__ import absolute_import
from contextlib import contextmanager
import zlib
import io
import logging
from socket import timeout as SocketTimeout
from socket import error as SocketError
from ._collections import HTTPHeaderDict
from .exceptions import (
BodyNotHttplibCompatible, ProtocolError, DecodeError, ReadTimeoutError,
ResponseNotChunked, IncompleteRead, InvalidHeader
)
from .packages.six import string_types as basestring, binary_type, PY3
from .packages.six.moves import http_client as httplib
from .connection import HTTPException, BaseSSLError
from .util.response import is_fp_closed, is_response_to_head
log = logging.getLogger(__name__)
class DeflateDecoder(object):
def __init__(self):
self._first_try = True
self._data = binary_type()
self._obj = zlib.decompressobj()
def __getattr__(self, name):
return getattr(self._obj, name)
def decompress(self, data):
if not data:
return data
if not self._first_try:
return self._obj.decompress(data)
self._data += data
try:
decompressed = self._obj.decompress(data)
if decompressed:
self._first_try = False
self._data = None
return decompressed
except zlib.error:
self._first_try = False
self._obj = zlib.decompressobj(-zlib.MAX_WBITS)
try:
return self.decompress(self._data)
finally:
self._data = None
class GzipDecoderState(object):
FIRST_MEMBER = 0
OTHER_MEMBERS = 1
SWALLOW_DATA = 2
class GzipDecoder(object):
def __init__(self):
self._obj = zlib.decompressobj(16 + zlib.MAX_WBITS)
self._state = GzipDecoderState.FIRST_MEMBER
def __getattr__(self, name):
return getattr(self._obj, name)
def decompress(self, data):
ret = binary_type()
if self._state == GzipDecoderState.SWALLOW_DATA or not data:
return ret
while True:
try:
ret += self._obj.decompress(data)
except zlib.error:
previous_state = self._state
# Ignore data after the first error
self._state = GzipDecoderState.SWALLOW_DATA
if previous_state == GzipDecoderState.OTHER_MEMBERS:
# Allow trailing garbage acceptable in other gzip clients
return ret
raise
data = self._obj.unused_data
if not data:
return ret
self._state = GzipDecoderState.OTHER_MEMBERS
self._obj = zlib.decompressobj(16 + zlib.MAX_WBITS)
def _get_decoder(mode):
if mode == 'gzip':
return GzipDecoder()
return DeflateDecoder()
class HTTPResponse(io.IOBase):
"""
HTTP Response container.
Backwards-compatible to httplib's HTTPResponse but the response ``body`` is
loaded and decoded on-demand when the ``data`` property is accessed. This
class is also compatible with the Python standard library's :mod:`io`
module, and can hence be treated as a readable object in the context of that
framework.
Extra parameters for behaviour not present in httplib.HTTPResponse:
:param preload_content:
If True, the response's body will be preloaded during construction.
:param decode_content:
If True, will attempt to decode the body based on the
'content-encoding' header.
:param original_response:
When this HTTPResponse wrapper is generated from an httplib.HTTPResponse
object, it's convenient to include the original for debug purposes. It's
otherwise unused.
:param retries:
The retries contains the last :class:`~urllib3.util.retry.Retry` that
was used during the request.
:param enforce_content_length:
Enforce content length checking. Body returned by server must match
value of Content-Length header, if present. Otherwise, raise error.
"""
CONTENT_DECODERS = ['gzip', 'deflate']
REDIRECT_STATUSES = [301, 302, 303, 307, 308]
def __init__(self, body='', headers=None, status=0, version=0, reason=None,
strict=0, preload_content=True, decode_content=True,
original_response=None, pool=None, connection=None, msg=None,
retries=None, enforce_content_length=False,
request_method=None, request_url=None):
if isinstance(headers, HTTPHeaderDict):
self.headers = headers
else:
self.headers = HTTPHeaderDict(headers)
self.status = status
self.version = version
self.reason = reason
self.strict = strict
self.decode_content = decode_content
self.retries = retries
self.enforce_content_length = enforce_content_length
self._decoder = None
self._body = None
self._fp = None
self._original_response = original_response
self._fp_bytes_read = 0
self.msg = msg
self._request_url = request_url
if body and isinstance(body, (basestring, binary_type)):
self._body = body
self._pool = pool
self._connection = connection
if hasattr(body, 'read'):
self._fp = body
# Are we using the chunked-style of transfer encoding?
self.chunked = False
self.chunk_left = None
tr_enc = self.headers.get('transfer-encoding', '').lower()
# Don't incur the penalty of creating a list and then discarding it
encodings = (enc.strip() for enc in tr_enc.split(","))
if "chunked" in encodings:
self.chunked = True
# Determine length of response
self.length_remaining = self._init_length(request_method)
# If requested, preload the body.
if preload_content and not self._body:
self._body = self.read(decode_content=decode_content)
def get_redirect_location(self):
"""
Should we redirect and where to?
:returns: Truthy redirect location string if we got a redirect status
code and valid location. ``None`` if redirect status and no
location. ``False`` if not a redirect status code.
"""
if self.status in self.REDIRECT_STATUSES:
return self.headers.get('location')
return False
def release_conn(self):
if not self._pool or not self._connection:
return
self._pool._put_conn(self._connection)
self._connection = None
@property
def data(self):
# For backwords-compat with earlier urllib3 0.4 and earlier.
if self._body:
return self._body
if self._fp:
return self.read(cache_content=True)
@property
def connection(self):
return self._connection
def isclosed(self):
return is_fp_closed(self._fp)
def tell(self):
"""
Obtain the number of bytes pulled over the wire so far. May differ from
the amount of content returned by :meth:``HTTPResponse.read`` if bytes
are encoded on the wire (e.g, compressed).
"""
return self._fp_bytes_read
def _init_length(self, request_method):
"""
Set initial length value for Response content if available.
"""
length = self.headers.get('content-length')
if length is not None:
if self.chunked:
# This Response will fail with an IncompleteRead if it can't be
# received as chunked. This method falls back to attempt reading
# the response before raising an exception.
log.warning("Received response with both Content-Length and "
"Transfer-Encoding set. This is expressly forbidden "
"by RFC 7230 sec 3.3.2. Ignoring Content-Length and "
"attempting to process response as Transfer-Encoding: "
"chunked.")
return None
try:
# RFC 7230 section 3.3.2 specifies multiple content lengths can
# be sent in a single Content-Length header
# (e.g. Content-Length: 42, 42). This line ensures the values
# are all valid ints and that as long as the `set` length is 1,
# all values are the same. Otherwise, the header is invalid.
lengths = set([int(val) for val in length.split(',')])
if len(lengths) > 1:
raise InvalidHeader("Content-Length contained multiple "
"unmatching values (%s)" % length)
length = lengths.pop()
except ValueError:
length = None
else:
if length < 0:
length = None
# Convert status to int for comparison
# In some cases, httplib returns a status of "_UNKNOWN"
try:
status = int(self.status)
except ValueError:
status = 0
# Check for responses that shouldn't include a body
if status in (204, 304) or 100 <= status < 200 or request_method == 'HEAD':
length = 0
return length
def _init_decoder(self):
"""
Set-up the _decoder attribute if necessary.
"""
# Note: content-encoding value should be case-insensitive, per RFC 7230
# Section 3.2
content_encoding = self.headers.get('content-encoding', '').lower()
if self._decoder is None and content_encoding in self.CONTENT_DECODERS:
self._decoder = _get_decoder(content_encoding)
def _decode(self, data, decode_content, flush_decoder):
"""
Decode the data passed in and potentially flush the decoder.
"""
try:
if decode_content and self._decoder:
data = self._decoder.decompress(data)
except (IOError, zlib.error) as e:
content_encoding = self.headers.get('content-encoding', '').lower()
raise DecodeError(
"Received response with content-encoding: %s, but "
"failed to decode it." % content_encoding, e)
if flush_decoder and decode_content:
data += self._flush_decoder()
return data
def _flush_decoder(self):
"""
Flushes the decoder. Should only be called if the decoder is actually
being used.
"""
if self._decoder:
buf = self._decoder.decompress(b'')
return buf + self._decoder.flush()
return b''
@contextmanager
def _error_catcher(self):
"""
Catch low-level python exceptions, instead re-raising urllib3
variants, so that low-level exceptions are not leaked in the
high-level api.
On exit, release the connection back to the pool.
"""
clean_exit = False
try:
try:
yield
except SocketTimeout:
# FIXME: Ideally we'd like to include the url in the ReadTimeoutError but
# there is yet no clean way to get at it from this context.
raise ReadTimeoutError(self._pool, None, 'Read timed out.')
except BaseSSLError as e:
# FIXME: Is there a better way to differentiate between SSLErrors?
if 'read operation timed out' not in str(e): # Defensive:
# This shouldn't happen but just in case we're missing an edge
# case, let's avoid swallowing SSL errors.
raise
raise ReadTimeoutError(self._pool, None, 'Read timed out.')
except (HTTPException, SocketError) as e:
# This includes IncompleteRead.
raise ProtocolError('Connection broken: %r' % e, e)
# If no exception is thrown, we should avoid cleaning up
# unnecessarily.
clean_exit = True
finally:
# If we didn't terminate cleanly, we need to throw away our
# connection.
if not clean_exit:
# The response may not be closed but we're not going to use it
# anymore so close it now to ensure that the connection is
# released back to the pool.
if self._original_response:
self._original_response.close()
# Closing the response may not actually be sufficient to close
# everything, so if we have a hold of the connection close that
# too.
if self._connection:
self._connection.close()
# If we hold the original response but it's closed now, we should
# return the connection back to the pool.
if self._original_response and self._original_response.isclosed():
self.release_conn()
def read(self, amt=None, decode_content=None, cache_content=False):
"""
Similar to :meth:`httplib.HTTPResponse.read`, but with two additional
parameters: ``decode_content`` and ``cache_content``.
:param amt:
How much of the content to read. If specified, caching is skipped
because it doesn't make sense to cache partial content as the full
response.
:param decode_content:
If True, will attempt to decode the body based on the
'content-encoding' header.
:param cache_content:
If True, will save the returned data such that the same result is
returned despite of the state of the underlying file object. This
is useful if you want the ``.data`` property to continue working
after having ``.read()`` the file object. (Overridden if ``amt`` is
set.)
"""
self._init_decoder()
if decode_content is None:
decode_content = self.decode_content
if self._fp is None:
return
flush_decoder = False
data = None
with self._error_catcher():
if amt is None:
# cStringIO doesn't like amt=None
data = self._fp.read()
flush_decoder = True
else:
cache_content = False
data = self._fp.read(amt)
if amt != 0 and not data: # Platform-specific: Buggy versions of Python.
# Close the connection when no data is returned
#
# This is redundant to what httplib/http.client _should_
# already do. However, versions of python released before
# December 15, 2012 (http://bugs.python.org/issue16298) do
# not properly close the connection in all cases. There is
# no harm in redundantly calling close.
self._fp.close()
flush_decoder = True
if self.enforce_content_length and self.length_remaining not in (0, None):
# This is an edge case that httplib failed to cover due
# to concerns of backward compatibility. We're
# addressing it here to make sure IncompleteRead is
# raised during streaming, so all calls with incorrect
# Content-Length are caught.
raise IncompleteRead(self._fp_bytes_read, self.length_remaining)
if data:
self._fp_bytes_read += len(data)
if self.length_remaining is not None:
self.length_remaining -= len(data)
data = self._decode(data, decode_content, flush_decoder)
if cache_content:
self._body = data
return data
def stream(self, amt=2**16, decode_content=None):
"""
A generator wrapper for the read() method. A call will block until
``amt`` bytes have been read from the connection or until the
connection is closed.
:param amt:
How much of the content to read. The generator will return up to
much data per iteration, but may return less. This is particularly
likely when using compressed data. However, the empty string will
never be returned.
:param decode_content:
If True, will attempt to decode the body based on the
'content-encoding' header.
"""
if self.chunked and self.supports_chunked_reads():
for line in self.read_chunked(amt, decode_content=decode_content):
yield line
else:
while not is_fp_closed(self._fp):
data = self.read(amt=amt, decode_content=decode_content)
if data:
yield data
@classmethod
def from_httplib(ResponseCls, r, **response_kw):
"""
Given an :class:`httplib.HTTPResponse` instance ``r``, return a
corresponding :class:`urllib3.response.HTTPResponse` object.
Remaining parameters are passed to the HTTPResponse constructor, along
with ``original_response=r``.
"""
headers = r.msg
if not isinstance(headers, HTTPHeaderDict):
if PY3: # Python 3
headers = HTTPHeaderDict(headers.items())
else: # Python 2
headers = HTTPHeaderDict.from_httplib(headers)
# HTTPResponse objects in Python 3 don't have a .strict attribute
strict = getattr(r, 'strict', 0)
resp = ResponseCls(body=r,
headers=headers,
status=r.status,
version=r.version,
reason=r.reason,
strict=strict,
original_response=r,
**response_kw)
return resp
# Backwards-compatibility methods for httplib.HTTPResponse
def getheaders(self):
return self.headers
def getheader(self, name, default=None):
return self.headers.get(name, default)
# Backwards compatibility for http.cookiejar
def info(self):
return self.headers
# Overrides from io.IOBase
def close(self):
if not self.closed:
self._fp.close()
if self._connection:
self._connection.close()
@property
def closed(self):
if self._fp is None:
return True
elif hasattr(self._fp, 'isclosed'):
return self._fp.isclosed()
elif hasattr(self._fp, 'closed'):
return self._fp.closed
else:
return True
def fileno(self):
if self._fp is None:
raise IOError("HTTPResponse has no file to get a fileno from")
elif hasattr(self._fp, "fileno"):
return self._fp.fileno()
else:
raise IOError("The file-like object this HTTPResponse is wrapped "
"around has no file descriptor")
def flush(self):
if self._fp is not None and hasattr(self._fp, 'flush'):
return self._fp.flush()
def readable(self):
# This method is required for `io` module compatibility.
return True
def readinto(self, b):
# This method is required for `io` module compatibility.
temp = self.read(len(b))
if len(temp) == 0:
return 0
else:
b[:len(temp)] = temp
return len(temp)
def supports_chunked_reads(self):
"""
Checks if the underlying file-like object looks like a
httplib.HTTPResponse object. We do this by testing for the fp
attribute. If it is present we assume it returns raw chunks as
processed by read_chunked().
"""
return hasattr(self._fp, 'fp')
def _update_chunk_length(self):
# First, we'll figure out length of a chunk and then
# we'll try to read it from socket.
if self.chunk_left is not None:
return
line = self._fp.fp.readline()
line = line.split(b';', 1)[0]
try:
self.chunk_left = int(line, 16)
except ValueError:
# Invalid chunked protocol response, abort.
self.close()
raise httplib.IncompleteRead(line)
def _handle_chunk(self, amt):
returned_chunk = None
if amt is None:
chunk = self._fp._safe_read(self.chunk_left)
returned_chunk = chunk
self._fp._safe_read(2) # Toss the CRLF at the end of the chunk.
self.chunk_left = None
elif amt < self.chunk_left:
value = self._fp._safe_read(amt)
self.chunk_left = self.chunk_left - amt
returned_chunk = value
elif amt == self.chunk_left:
value = self._fp._safe_read(amt)
self._fp._safe_read(2) # Toss the CRLF at the end of the chunk.
self.chunk_left = None
returned_chunk = value
else: # amt > self.chunk_left
returned_chunk = self._fp._safe_read(self.chunk_left)
self._fp._safe_read(2) # Toss the CRLF at the end of the chunk.
self.chunk_left = None
return returned_chunk
def read_chunked(self, amt=None, decode_content=None):
"""
Similar to :meth:`HTTPResponse.read`, but with an additional
parameter: ``decode_content``.
:param amt:
How much of the content to read. If specified, caching is skipped
because it doesn't make sense to cache partial content as the full
response.
:param decode_content:
If True, will attempt to decode the body based on the
'content-encoding' header.
"""
self._init_decoder()
# FIXME: Rewrite this method and make it a class with a better structured logic.
if not self.chunked:
raise ResponseNotChunked(
"Response is not chunked. "
"Header 'transfer-encoding: chunked' is missing.")
if not self.supports_chunked_reads():
raise BodyNotHttplibCompatible(
"Body should be httplib.HTTPResponse like. "
"It should have have an fp attribute which returns raw chunks.")
with self._error_catcher():
# Don't bother reading the body of a HEAD request.
if self._original_response and is_response_to_head(self._original_response):
self._original_response.close()
return
# If a response is already read and closed
# then return immediately.
if self._fp.fp is None:
return
while True:
self._update_chunk_length()
if self.chunk_left == 0:
break
chunk = self._handle_chunk(amt)
decoded = self._decode(chunk, decode_content=decode_content,
flush_decoder=False)
if decoded:
yield decoded
if decode_content:
# On CPython and PyPy, we should never need to flush the
# decoder. However, on Jython we *might* need to, so
# lets defensively do it anyway.
decoded = self._flush_decoder()
if decoded: # Platform-specific: Jython.
yield decoded
# Chunk content ends with \r\n: discard it.
while True:
line = self._fp.fp.readline()
if not line:
# Some sites may not end with '\r\n'.
break
if line == b'\r\n':
break
# We read everything; close the "file".
if self._original_response:
self._original_response.close()
def geturl(self):
"""
Returns the URL that was the source of this response.
If the request that generated this response redirected, this method
will return the final redirect location.
"""
if self.retries is not None and len(self.retries.history):
return self.retries.history[-1].redirect_location
else:
return self._request_url
| gpl-3.0 |
PaddlePaddle/Paddle | python/paddle/fluid/tests/unittests/dygraph_to_static/test_cast.py | 2 | 5227 | # Copyright (c) 2020 PaddlePaddle Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import unittest
import numpy as np
import paddle.fluid as fluid
from paddle.fluid.dygraph import declarative
SEED = 2020
np.random.seed(SEED)
@declarative
def test_bool_cast(x):
x = fluid.dygraph.to_variable(x)
x = bool(x)
return x
@declarative
def test_int_cast(x):
x = fluid.dygraph.to_variable(x)
x = int(x)
return x
@declarative
def test_float_cast(x):
x = fluid.dygraph.to_variable(x)
x = float(x)
return x
@declarative
def test_not_var_cast(x):
x = int(x)
return x
@declarative
def test_mix_cast(x):
x = fluid.dygraph.to_variable(x)
x = int(x)
x = float(x)
x = bool(x)
x = float(x)
return x
class TestCastBase(unittest.TestCase):
def setUp(self):
self.place = (
fluid.CUDAPlace(0)
if fluid.is_compiled_with_cuda()
else fluid.CPUPlace()
)
self.prepare()
self.set_func()
def prepare(self):
self.input_shape = (16, 32)
self.input_dtype = 'float32'
self.input = (
np.random.binomial(4, 0.3, size=np.product(self.input_shape))
.reshape(self.input_shape)
.astype(self.input_dtype)
)
self.cast_dtype = 'bool'
def set_func(self):
self.func = test_bool_cast
def do_test(self):
with fluid.dygraph.guard():
res = self.func(self.input)
return res
def test_cast_result(self):
res = self.do_test().numpy()
self.assertTrue(
res.dtype == self.cast_dtype,
msg='The target dtype is {}, but the casted dtype is {}.'.format(
self.cast_dtype, res.dtype
),
)
ref_val = self.input.astype(self.cast_dtype)
np.testing.assert_allclose(
res,
ref_val,
rtol=1e-05,
err_msg='The casted value is {}.\nThe correct value is {}.'.format(
res, ref_val
),
)
class TestIntCast(TestCastBase):
def prepare(self):
self.input_shape = (1,)
self.input_dtype = 'float32'
self.input = (
np.random.normal(loc=6, scale=10, size=np.product(self.input_shape))
.reshape(self.input_shape)
.astype(self.input_dtype)
)
self.cast_dtype = 'int32'
def set_func(self):
self.func = test_int_cast
class TestFloatCast(TestCastBase):
def prepare(self):
self.input_shape = (8, 16)
self.input_dtype = 'bool'
self.input = (
np.random.binomial(2, 0.5, size=np.product(self.input_shape))
.reshape(self.input_shape)
.astype(self.input_dtype)
)
self.cast_dtype = 'float32'
def set_func(self):
self.func = test_float_cast
class TestMixCast(TestCastBase):
def prepare(self):
self.input_shape = (8, 32)
self.input_dtype = 'float32'
self.input = (
np.random.normal(loc=6, scale=10, size=np.product(self.input_shape))
.reshape(self.input_shape)
.astype(self.input_dtype)
)
self.cast_int = 'int'
self.cast_float = 'float32'
self.cast_bool = 'bool'
self.cast_dtype = 'float32'
def set_func(self):
self.func = test_mix_cast
def test_cast_result(self):
res = self.do_test().numpy()
self.assertTrue(
res.dtype == self.cast_dtype,
msg='The target dtype is {}, but the casted dtype is {}.'.format(
self.cast_dtype, res.dtype
),
)
ref_val = (
self.input.astype(self.cast_int)
.astype(self.cast_float)
.astype(self.cast_bool)
.astype(self.cast_dtype)
)
np.testing.assert_allclose(
res,
ref_val,
rtol=1e-05,
err_msg='The casted value is {}.\nThe correct value is {}.'.format(
res, ref_val
),
)
class TestNotVarCast(TestCastBase):
def prepare(self):
self.input = 3.14
self.cast_dtype = 'int'
def set_func(self):
self.func = test_not_var_cast
def test_cast_result(self):
res = self.do_test()
self.assertTrue(type(res) == int, msg='The casted dtype is not int.')
ref_val = int(self.input)
self.assertTrue(
res == ref_val,
msg='The casted value is {}.\nThe correct value is {}.'.format(
res, ref_val
),
)
if __name__ == '__main__':
unittest.main()
| apache-2.0 |
astarasikov/iconia-gnu-kernel | tools/perf/scripts/python/failed-syscalls-by-pid.py | 11175 | 2058 | # failed system call counts, by pid
# (c) 2010, Tom Zanussi <tzanussi@gmail.com>
# Licensed under the terms of the GNU GPL License version 2
#
# Displays system-wide failed system call totals, broken down by pid.
# If a [comm] arg is specified, only syscalls called by [comm] are displayed.
import os
import sys
sys.path.append(os.environ['PERF_EXEC_PATH'] + \
'/scripts/python/Perf-Trace-Util/lib/Perf/Trace')
from perf_trace_context import *
from Core import *
from Util import *
usage = "perf script -s syscall-counts-by-pid.py [comm|pid]\n";
for_comm = None
for_pid = None
if len(sys.argv) > 2:
sys.exit(usage)
if len(sys.argv) > 1:
try:
for_pid = int(sys.argv[1])
except:
for_comm = sys.argv[1]
syscalls = autodict()
def trace_begin():
print "Press control+C to stop and show the summary"
def trace_end():
print_error_totals()
def raw_syscalls__sys_exit(event_name, context, common_cpu,
common_secs, common_nsecs, common_pid, common_comm,
id, ret):
if (for_comm and common_comm != for_comm) or \
(for_pid and common_pid != for_pid ):
return
if ret < 0:
try:
syscalls[common_comm][common_pid][id][ret] += 1
except TypeError:
syscalls[common_comm][common_pid][id][ret] = 1
def print_error_totals():
if for_comm is not None:
print "\nsyscall errors for %s:\n\n" % (for_comm),
else:
print "\nsyscall errors:\n\n",
print "%-30s %10s\n" % ("comm [pid]", "count"),
print "%-30s %10s\n" % ("------------------------------", \
"----------"),
comm_keys = syscalls.keys()
for comm in comm_keys:
pid_keys = syscalls[comm].keys()
for pid in pid_keys:
print "\n%s [%d]\n" % (comm, pid),
id_keys = syscalls[comm][pid].keys()
for id in id_keys:
print " syscall: %-16s\n" % syscall_name(id),
ret_keys = syscalls[comm][pid][id].keys()
for ret, val in sorted(syscalls[comm][pid][id].iteritems(), key = lambda(k, v): (v, k), reverse = True):
print " err = %-20s %10d\n" % (strerror(ret), val),
| gpl-2.0 |
dex4er/django | django/contrib/gis/gdal/prototypes/errcheck.py | 220 | 4257 | """
This module houses the error-checking routines used by the GDAL
ctypes prototypes.
"""
from ctypes import c_void_p, string_at
from django.contrib.gis.gdal.error import check_err, OGRException, SRSException
from django.contrib.gis.gdal.libgdal import lgdal
from django.utils import six
# Helper routines for retrieving pointers and/or values from
# arguments passed in by reference.
def arg_byref(args, offset=-1):
"Returns the pointer argument's by-refernece value."
return args[offset]._obj.value
def ptr_byref(args, offset=-1):
"Returns the pointer argument passed in by-reference."
return args[offset]._obj
def check_bool(result, func, cargs):
"Returns the boolean evaluation of the value."
if bool(result): return True
else: return False
### String checking Routines ###
def check_const_string(result, func, cargs, offset=None):
"""
Similar functionality to `check_string`, but does not free the pointer.
"""
if offset:
check_err(result)
ptr = ptr_byref(cargs, offset)
return ptr.value
else:
return result
def check_string(result, func, cargs, offset=-1, str_result=False):
"""
Checks the string output returned from the given function, and frees
the string pointer allocated by OGR. The `str_result` keyword
may be used when the result is the string pointer, otherwise
the OGR error code is assumed. The `offset` keyword may be used
to extract the string pointer passed in by-reference at the given
slice offset in the function arguments.
"""
if str_result:
# For routines that return a string.
ptr = result
if not ptr: s = None
else: s = string_at(result)
else:
# Error-code return specified.
check_err(result)
ptr = ptr_byref(cargs, offset)
# Getting the string value
s = ptr.value
# Correctly freeing the allocated memory beind GDAL pointer
# w/the VSIFree routine.
if ptr: lgdal.VSIFree(ptr)
return s
### DataSource, Layer error-checking ###
### Envelope checking ###
def check_envelope(result, func, cargs, offset=-1):
"Checks a function that returns an OGR Envelope by reference."
env = ptr_byref(cargs, offset)
return env
### Geometry error-checking routines ###
def check_geom(result, func, cargs):
"Checks a function that returns a geometry."
# OGR_G_Clone may return an integer, even though the
# restype is set to c_void_p
if isinstance(result, six.integer_types):
result = c_void_p(result)
if not result:
raise OGRException('Invalid geometry pointer returned from "%s".' % func.__name__)
return result
def check_geom_offset(result, func, cargs, offset=-1):
"Chcks the geometry at the given offset in the C parameter list."
check_err(result)
geom = ptr_byref(cargs, offset=offset)
return check_geom(geom, func, cargs)
### Spatial Reference error-checking routines ###
def check_srs(result, func, cargs):
if isinstance(result, six.integer_types):
result = c_void_p(result)
if not result:
raise SRSException('Invalid spatial reference pointer returned from "%s".' % func.__name__)
return result
### Other error-checking routines ###
def check_arg_errcode(result, func, cargs):
"""
The error code is returned in the last argument, by reference.
Check its value with `check_err` before returning the result.
"""
check_err(arg_byref(cargs))
return result
def check_errcode(result, func, cargs):
"""
Check the error code returned (c_int).
"""
check_err(result)
return
def check_pointer(result, func, cargs):
"Makes sure the result pointer is valid."
if isinstance(result, six.integer_types):
result = c_void_p(result)
if bool(result):
return result
else:
raise OGRException('Invalid pointer returned from "%s"' % func.__name__)
def check_str_arg(result, func, cargs):
"""
This is for the OSRGet[Angular|Linear]Units functions, which
require that the returned string pointer not be freed. This
returns both the double and tring values.
"""
dbl = result
ptr = cargs[-1]._obj
return dbl, ptr.value.decode()
| bsd-3-clause |
taigaio/taiga-back | taiga/projects/migrations/0049_auto_20160629_1443.py | 1 | 6461 | # -*- coding: utf-8 -*-
# Generated by Django 1.9.2 on 2016-06-29 14:43
from __future__ import unicode_literals
import taiga.base.db.models.fields
from django.db import migrations, models
import django.db.models.deletion
import django.contrib.postgres.fields
class Migration(migrations.Migration):
dependencies = [
('projects', '0048_auto_20160615_1508'),
]
operations = [
migrations.CreateModel(
name='EpicStatus',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('name', models.CharField(max_length=255, verbose_name='name')),
('slug', models.SlugField(blank=True, max_length=255, verbose_name='slug')),
('order', models.IntegerField(default=10, verbose_name='order')),
('is_closed', models.BooleanField(default=False, verbose_name='is closed')),
('color', models.CharField(default='#999999', max_length=20, verbose_name='color')),
],
options={
'verbose_name_plural': 'epic statuses',
'ordering': ['project', 'order', 'name'],
'verbose_name': 'epic status',
},
),
migrations.AlterModelOptions(
name='issuestatus',
options={'ordering': ['project', 'order', 'name'], 'verbose_name': 'issue status', 'verbose_name_plural': 'issue statuses'},
),
migrations.AlterModelOptions(
name='issuetype',
options={'ordering': ['project', 'order', 'name'], 'verbose_name': 'issue type', 'verbose_name_plural': 'issue types'},
),
migrations.AlterModelOptions(
name='membership',
options={'ordering': ['project', 'user__full_name', 'user__username', 'user__email', 'email'], 'verbose_name': 'membership', 'verbose_name_plural': 'memberships'},
),
migrations.AlterModelOptions(
name='points',
options={'ordering': ['project', 'order', 'name'], 'verbose_name': 'points', 'verbose_name_plural': 'points'},
),
migrations.AlterModelOptions(
name='priority',
options={'ordering': ['project', 'order', 'name'], 'verbose_name': 'priority', 'verbose_name_plural': 'priorities'},
),
migrations.AlterModelOptions(
name='severity',
options={'ordering': ['project', 'order', 'name'], 'verbose_name': 'severity', 'verbose_name_plural': 'severities'},
),
migrations.AlterModelOptions(
name='taskstatus',
options={'ordering': ['project', 'order', 'name'], 'verbose_name': 'task status', 'verbose_name_plural': 'task statuses'},
),
migrations.AlterModelOptions(
name='userstorystatus',
options={'ordering': ['project', 'order', 'name'], 'verbose_name': 'user story status', 'verbose_name_plural': 'user story statuses'},
),
migrations.AddField(
model_name='project',
name='is_epics_activated',
field=models.BooleanField(default=False, verbose_name='active epics panel'),
),
migrations.AddField(
model_name='projecttemplate',
name='epic_statuses',
field=taiga.base.db.models.fields.JSONField(blank=True, null=True, verbose_name='epic statuses'),
),
migrations.AddField(
model_name='projecttemplate',
name='is_epics_activated',
field=models.BooleanField(default=False, verbose_name='active epics panel'),
),
migrations.AlterField(
model_name='project',
name='anon_permissions',
field=django.contrib.postgres.fields.ArrayField(base_field=models.TextField(choices=[('view_project', 'View project'), ('view_milestones', 'View milestones'), ('view_epics', 'View epic'), ('view_us', 'View user stories'), ('view_tasks', 'View tasks'), ('view_issues', 'View issues'), ('view_wiki_pages', 'View wiki pages'), ('view_wiki_links', 'View wiki links')]), blank=True, default=list, null=True, size=None, verbose_name='anonymous permissions'),
),
migrations.AlterField(
model_name='project',
name='public_permissions',
field=django.contrib.postgres.fields.ArrayField(base_field=models.TextField(choices=[('view_project', 'View project'), ('view_milestones', 'View milestones'), ('add_milestone', 'Add milestone'), ('modify_milestone', 'Modify milestone'), ('delete_milestone', 'Delete milestone'), ('view_epics', 'View epic'), ('add_epic', 'Add epic'), ('modify_epic', 'Modify epic'), ('comment_epic', 'Comment epic'), ('delete_epic', 'Delete epic'), ('view_us', 'View user story'), ('add_us', 'Add user story'), ('modify_us', 'Modify user story'), ('comment_us', 'Comment user story'), ('delete_us', 'Delete user story'), ('view_tasks', 'View tasks'), ('add_task', 'Add task'), ('modify_task', 'Modify task'), ('comment_task', 'Comment task'), ('delete_task', 'Delete task'), ('view_issues', 'View issues'), ('add_issue', 'Add issue'), ('modify_issue', 'Modify issue'), ('comment_issue', 'Comment issue'), ('delete_issue', 'Delete issue'), ('view_wiki_pages', 'View wiki pages'), ('add_wiki_page', 'Add wiki page'), ('modify_wiki_page', 'Modify wiki page'), ('comment_wiki_page', 'Comment wiki page'), ('delete_wiki_page', 'Delete wiki page'), ('view_wiki_links', 'View wiki links'), ('add_wiki_link', 'Add wiki link'), ('modify_wiki_link', 'Modify wiki link'), ('delete_wiki_link', 'Delete wiki link')]), blank=True, default=list, null=True, size=None, verbose_name='user permissions'),
),
migrations.AddField(
model_name='epicstatus',
name='project',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='epic_statuses', to='projects.Project', verbose_name='project'),
),
migrations.AddField(
model_name='project',
name='default_epic_status',
field=models.OneToOneField(blank=True, null=True, on_delete=django.db.models.deletion.SET_NULL, related_name='+', to='projects.EpicStatus', verbose_name='default epic status'),
),
migrations.AlterUniqueTogether(
name='epicstatus',
unique_together=set([('project', 'slug'), ('project', 'name')]),
),
]
| agpl-3.0 |
dex4er/django | django/contrib/gis/gdal/tests/test_geom.py | 109 | 20839 | import json
from binascii import b2a_hex
try:
from django.utils.six.moves import cPickle as pickle
except ImportError:
import pickle
from django.contrib.gis.gdal import HAS_GDAL
from django.contrib.gis.geometry.test_data import TestDataMixin
from django.utils.six.moves import xrange
from django.utils import unittest
from django.utils.unittest import skipUnless
if HAS_GDAL:
from django.contrib.gis.gdal import (OGRGeometry, OGRGeomType,
OGRException, OGRIndexError, SpatialReference, CoordTransform,
GDAL_VERSION)
@skipUnless(HAS_GDAL, "GDAL is required")
class OGRGeomTest(unittest.TestCase, TestDataMixin):
"This tests the OGR Geometry."
def test00a_geomtype(self):
"Testing OGRGeomType object."
# OGRGeomType should initialize on all these inputs.
try:
g = OGRGeomType(1)
g = OGRGeomType(7)
g = OGRGeomType('point')
g = OGRGeomType('GeometrycollectioN')
g = OGRGeomType('LINearrING')
g = OGRGeomType('Unknown')
except:
self.fail('Could not create an OGRGeomType object!')
# Should throw TypeError on this input
self.assertRaises(OGRException, OGRGeomType, 23)
self.assertRaises(OGRException, OGRGeomType, 'fooD')
self.assertRaises(OGRException, OGRGeomType, 9)
# Equivalence can take strings, ints, and other OGRGeomTypes
self.assertEqual(True, OGRGeomType(1) == OGRGeomType(1))
self.assertEqual(True, OGRGeomType(7) == 'GeometryCollection')
self.assertEqual(True, OGRGeomType('point') == 'POINT')
self.assertEqual(False, OGRGeomType('point') == 2)
self.assertEqual(True, OGRGeomType('unknown') == 0)
self.assertEqual(True, OGRGeomType(6) == 'MULtiPolyGON')
self.assertEqual(False, OGRGeomType(1) != OGRGeomType('point'))
self.assertEqual(True, OGRGeomType('POINT') != OGRGeomType(6))
# Testing the Django field name equivalent property.
self.assertEqual('PointField', OGRGeomType('Point').django)
self.assertEqual('GeometryField', OGRGeomType('Unknown').django)
self.assertEqual(None, OGRGeomType('none').django)
# 'Geometry' initialization implies an unknown geometry type.
gt = OGRGeomType('Geometry')
self.assertEqual(0, gt.num)
self.assertEqual('Unknown', gt.name)
def test00b_geomtype_25d(self):
"Testing OGRGeomType object with 25D types."
wkb25bit = OGRGeomType.wkb25bit
self.assertTrue(OGRGeomType(wkb25bit + 1) == 'Point25D')
self.assertTrue(OGRGeomType('MultiLineString25D') == (5 + wkb25bit))
self.assertEqual('GeometryCollectionField', OGRGeomType('GeometryCollection25D').django)
def test01a_wkt(self):
"Testing WKT output."
for g in self.geometries.wkt_out:
geom = OGRGeometry(g.wkt)
self.assertEqual(g.wkt, geom.wkt)
def test01a_ewkt(self):
"Testing EWKT input/output."
for ewkt_val in ('POINT (1 2 3)', 'LINEARRING (0 0,1 1,2 1,0 0)'):
# First with ewkt output when no SRID in EWKT
self.assertEqual(ewkt_val, OGRGeometry(ewkt_val).ewkt)
# No test consumption with an SRID specified.
ewkt_val = 'SRID=4326;%s' % ewkt_val
geom = OGRGeometry(ewkt_val)
self.assertEqual(ewkt_val, geom.ewkt)
self.assertEqual(4326, geom.srs.srid)
def test01b_gml(self):
"Testing GML output."
for g in self.geometries.wkt_out:
geom = OGRGeometry(g.wkt)
exp_gml = g.gml
if GDAL_VERSION >= (1, 8):
# In GDAL 1.8, the non-conformant GML tag <gml:GeometryCollection> was
# replaced with <gml:MultiGeometry>.
exp_gml = exp_gml.replace('GeometryCollection', 'MultiGeometry')
self.assertEqual(exp_gml, geom.gml)
def test01c_hex(self):
"Testing HEX input/output."
for g in self.geometries.hex_wkt:
geom1 = OGRGeometry(g.wkt)
self.assertEqual(g.hex.encode(), geom1.hex)
# Constructing w/HEX
geom2 = OGRGeometry(g.hex)
self.assertEqual(geom1, geom2)
def test01d_wkb(self):
"Testing WKB input/output."
for g in self.geometries.hex_wkt:
geom1 = OGRGeometry(g.wkt)
wkb = geom1.wkb
self.assertEqual(b2a_hex(wkb).upper(), g.hex.encode())
# Constructing w/WKB.
geom2 = OGRGeometry(wkb)
self.assertEqual(geom1, geom2)
def test01e_json(self):
"Testing GeoJSON input/output."
for g in self.geometries.json_geoms:
geom = OGRGeometry(g.wkt)
if not hasattr(g, 'not_equal'):
# Loading jsons to prevent decimal differences
self.assertEqual(json.loads(g.json), json.loads(geom.json))
self.assertEqual(json.loads(g.json), json.loads(geom.geojson))
self.assertEqual(OGRGeometry(g.wkt), OGRGeometry(geom.json))
def test02_points(self):
"Testing Point objects."
prev = OGRGeometry('POINT(0 0)')
for p in self.geometries.points:
if not hasattr(p, 'z'): # No 3D
pnt = OGRGeometry(p.wkt)
self.assertEqual(1, pnt.geom_type)
self.assertEqual('POINT', pnt.geom_name)
self.assertEqual(p.x, pnt.x)
self.assertEqual(p.y, pnt.y)
self.assertEqual((p.x, p.y), pnt.tuple)
def test03_multipoints(self):
"Testing MultiPoint objects."
for mp in self.geometries.multipoints:
mgeom1 = OGRGeometry(mp.wkt) # First one from WKT
self.assertEqual(4, mgeom1.geom_type)
self.assertEqual('MULTIPOINT', mgeom1.geom_name)
mgeom2 = OGRGeometry('MULTIPOINT') # Creating empty multipoint
mgeom3 = OGRGeometry('MULTIPOINT')
for g in mgeom1:
mgeom2.add(g) # adding each point from the multipoints
mgeom3.add(g.wkt) # should take WKT as well
self.assertEqual(mgeom1, mgeom2) # they should equal
self.assertEqual(mgeom1, mgeom3)
self.assertEqual(mp.coords, mgeom2.coords)
self.assertEqual(mp.n_p, mgeom2.point_count)
def test04_linestring(self):
"Testing LineString objects."
prev = OGRGeometry('POINT(0 0)')
for ls in self.geometries.linestrings:
linestr = OGRGeometry(ls.wkt)
self.assertEqual(2, linestr.geom_type)
self.assertEqual('LINESTRING', linestr.geom_name)
self.assertEqual(ls.n_p, linestr.point_count)
self.assertEqual(ls.coords, linestr.tuple)
self.assertEqual(True, linestr == OGRGeometry(ls.wkt))
self.assertEqual(True, linestr != prev)
self.assertRaises(OGRIndexError, linestr.__getitem__, len(linestr))
prev = linestr
# Testing the x, y properties.
x = [tmpx for tmpx, tmpy in ls.coords]
y = [tmpy for tmpx, tmpy in ls.coords]
self.assertEqual(x, linestr.x)
self.assertEqual(y, linestr.y)
def test05_multilinestring(self):
"Testing MultiLineString objects."
prev = OGRGeometry('POINT(0 0)')
for mls in self.geometries.multilinestrings:
mlinestr = OGRGeometry(mls.wkt)
self.assertEqual(5, mlinestr.geom_type)
self.assertEqual('MULTILINESTRING', mlinestr.geom_name)
self.assertEqual(mls.n_p, mlinestr.point_count)
self.assertEqual(mls.coords, mlinestr.tuple)
self.assertEqual(True, mlinestr == OGRGeometry(mls.wkt))
self.assertEqual(True, mlinestr != prev)
prev = mlinestr
for ls in mlinestr:
self.assertEqual(2, ls.geom_type)
self.assertEqual('LINESTRING', ls.geom_name)
self.assertRaises(OGRIndexError, mlinestr.__getitem__, len(mlinestr))
def test06_linearring(self):
"Testing LinearRing objects."
prev = OGRGeometry('POINT(0 0)')
for rr in self.geometries.linearrings:
lr = OGRGeometry(rr.wkt)
#self.assertEqual(101, lr.geom_type.num)
self.assertEqual('LINEARRING', lr.geom_name)
self.assertEqual(rr.n_p, len(lr))
self.assertEqual(True, lr == OGRGeometry(rr.wkt))
self.assertEqual(True, lr != prev)
prev = lr
def test07a_polygons(self):
"Testing Polygon objects."
# Testing `from_bbox` class method
bbox = (-180,-90,180,90)
p = OGRGeometry.from_bbox( bbox )
self.assertEqual(bbox, p.extent)
prev = OGRGeometry('POINT(0 0)')
for p in self.geometries.polygons:
poly = OGRGeometry(p.wkt)
self.assertEqual(3, poly.geom_type)
self.assertEqual('POLYGON', poly.geom_name)
self.assertEqual(p.n_p, poly.point_count)
self.assertEqual(p.n_i + 1, len(poly))
# Testing area & centroid.
self.assertAlmostEqual(p.area, poly.area, 9)
x, y = poly.centroid.tuple
self.assertAlmostEqual(p.centroid[0], x, 9)
self.assertAlmostEqual(p.centroid[1], y, 9)
# Testing equivalence
self.assertEqual(True, poly == OGRGeometry(p.wkt))
self.assertEqual(True, poly != prev)
if p.ext_ring_cs:
ring = poly[0]
self.assertEqual(p.ext_ring_cs, ring.tuple)
self.assertEqual(p.ext_ring_cs, poly[0].tuple)
self.assertEqual(len(p.ext_ring_cs), ring.point_count)
for r in poly:
self.assertEqual('LINEARRING', r.geom_name)
def test07b_closepolygons(self):
"Testing closing Polygon objects."
# Both rings in this geometry are not closed.
poly = OGRGeometry('POLYGON((0 0, 5 0, 5 5, 0 5), (1 1, 2 1, 2 2, 2 1))')
self.assertEqual(8, poly.point_count)
with self.assertRaises(OGRException):
_ = poly.centroid
poly.close_rings()
self.assertEqual(10, poly.point_count) # Two closing points should've been added
self.assertEqual(OGRGeometry('POINT(2.5 2.5)'), poly.centroid)
def test08_multipolygons(self):
"Testing MultiPolygon objects."
prev = OGRGeometry('POINT(0 0)')
for mp in self.geometries.multipolygons:
mpoly = OGRGeometry(mp.wkt)
self.assertEqual(6, mpoly.geom_type)
self.assertEqual('MULTIPOLYGON', mpoly.geom_name)
if mp.valid:
self.assertEqual(mp.n_p, mpoly.point_count)
self.assertEqual(mp.num_geom, len(mpoly))
self.assertRaises(OGRIndexError, mpoly.__getitem__, len(mpoly))
for p in mpoly:
self.assertEqual('POLYGON', p.geom_name)
self.assertEqual(3, p.geom_type)
self.assertEqual(mpoly.wkt, OGRGeometry(mp.wkt).wkt)
def test09a_srs(self):
"Testing OGR Geometries with Spatial Reference objects."
for mp in self.geometries.multipolygons:
# Creating a geometry w/spatial reference
sr = SpatialReference('WGS84')
mpoly = OGRGeometry(mp.wkt, sr)
self.assertEqual(sr.wkt, mpoly.srs.wkt)
# Ensuring that SRS is propagated to clones.
klone = mpoly.clone()
self.assertEqual(sr.wkt, klone.srs.wkt)
# Ensuring all children geometries (polygons and their rings) all
# return the assigned spatial reference as well.
for poly in mpoly:
self.assertEqual(sr.wkt, poly.srs.wkt)
for ring in poly:
self.assertEqual(sr.wkt, ring.srs.wkt)
# Ensuring SRS propagate in topological ops.
a = OGRGeometry(self.geometries.topology_geoms[0].wkt_a, sr)
b = OGRGeometry(self.geometries.topology_geoms[0].wkt_b, sr)
diff = a.difference(b)
union = a.union(b)
self.assertEqual(sr.wkt, diff.srs.wkt)
self.assertEqual(sr.srid, union.srs.srid)
# Instantiating w/an integer SRID
mpoly = OGRGeometry(mp.wkt, 4326)
self.assertEqual(4326, mpoly.srid)
mpoly.srs = SpatialReference(4269)
self.assertEqual(4269, mpoly.srid)
self.assertEqual('NAD83', mpoly.srs.name)
# Incrementing through the multipolyogn after the spatial reference
# has been re-assigned.
for poly in mpoly:
self.assertEqual(mpoly.srs.wkt, poly.srs.wkt)
poly.srs = 32140
for ring in poly:
# Changing each ring in the polygon
self.assertEqual(32140, ring.srs.srid)
self.assertEqual('NAD83 / Texas South Central', ring.srs.name)
ring.srs = str(SpatialReference(4326)) # back to WGS84
self.assertEqual(4326, ring.srs.srid)
# Using the `srid` property.
ring.srid = 4322
self.assertEqual('WGS 72', ring.srs.name)
self.assertEqual(4322, ring.srid)
def test09b_srs_transform(self):
"Testing transform()."
orig = OGRGeometry('POINT (-104.609 38.255)', 4326)
trans = OGRGeometry('POINT (992385.4472045 481455.4944650)', 2774)
# Using an srid, a SpatialReference object, and a CoordTransform object
# or transformations.
t1, t2, t3 = orig.clone(), orig.clone(), orig.clone()
t1.transform(trans.srid)
t2.transform(SpatialReference('EPSG:2774'))
ct = CoordTransform(SpatialReference('WGS84'), SpatialReference(2774))
t3.transform(ct)
# Testing use of the `clone` keyword.
k1 = orig.clone()
k2 = k1.transform(trans.srid, clone=True)
self.assertEqual(k1, orig)
self.assertNotEqual(k1, k2)
prec = 3
for p in (t1, t2, t3, k2):
self.assertAlmostEqual(trans.x, p.x, prec)
self.assertAlmostEqual(trans.y, p.y, prec)
def test09c_transform_dim(self):
"Testing coordinate dimension is the same on transformed geometries."
ls_orig = OGRGeometry('LINESTRING(-104.609 38.255)', 4326)
ls_trans = OGRGeometry('LINESTRING(992385.4472045 481455.4944650)', 2774)
prec = 3
ls_orig.transform(ls_trans.srs)
# Making sure the coordinate dimension is still 2D.
self.assertEqual(2, ls_orig.coord_dim)
self.assertAlmostEqual(ls_trans.x[0], ls_orig.x[0], prec)
self.assertAlmostEqual(ls_trans.y[0], ls_orig.y[0], prec)
def test10_difference(self):
"Testing difference()."
for i in xrange(len(self.geometries.topology_geoms)):
a = OGRGeometry(self.geometries.topology_geoms[i].wkt_a)
b = OGRGeometry(self.geometries.topology_geoms[i].wkt_b)
d1 = OGRGeometry(self.geometries.diff_geoms[i].wkt)
d2 = a.difference(b)
self.assertEqual(d1, d2)
self.assertEqual(d1, a - b) # __sub__ is difference operator
a -= b # testing __isub__
self.assertEqual(d1, a)
def test11_intersection(self):
"Testing intersects() and intersection()."
for i in xrange(len(self.geometries.topology_geoms)):
a = OGRGeometry(self.geometries.topology_geoms[i].wkt_a)
b = OGRGeometry(self.geometries.topology_geoms[i].wkt_b)
i1 = OGRGeometry(self.geometries.intersect_geoms[i].wkt)
self.assertEqual(True, a.intersects(b))
i2 = a.intersection(b)
self.assertEqual(i1, i2)
self.assertEqual(i1, a & b) # __and__ is intersection operator
a &= b # testing __iand__
self.assertEqual(i1, a)
def test12_symdifference(self):
"Testing sym_difference()."
for i in xrange(len(self.geometries.topology_geoms)):
a = OGRGeometry(self.geometries.topology_geoms[i].wkt_a)
b = OGRGeometry(self.geometries.topology_geoms[i].wkt_b)
d1 = OGRGeometry(self.geometries.sdiff_geoms[i].wkt)
d2 = a.sym_difference(b)
self.assertEqual(d1, d2)
self.assertEqual(d1, a ^ b) # __xor__ is symmetric difference operator
a ^= b # testing __ixor__
self.assertEqual(d1, a)
def test13_union(self):
"Testing union()."
for i in xrange(len(self.geometries.topology_geoms)):
a = OGRGeometry(self.geometries.topology_geoms[i].wkt_a)
b = OGRGeometry(self.geometries.topology_geoms[i].wkt_b)
u1 = OGRGeometry(self.geometries.union_geoms[i].wkt)
u2 = a.union(b)
self.assertEqual(u1, u2)
self.assertEqual(u1, a | b) # __or__ is union operator
a |= b # testing __ior__
self.assertEqual(u1, a)
def test14_add(self):
"Testing GeometryCollection.add()."
# Can't insert a Point into a MultiPolygon.
mp = OGRGeometry('MultiPolygon')
pnt = OGRGeometry('POINT(5 23)')
self.assertRaises(OGRException, mp.add, pnt)
# GeometryCollection.add may take an OGRGeometry (if another collection
# of the same type all child geoms will be added individually) or WKT.
for mp in self.geometries.multipolygons:
mpoly = OGRGeometry(mp.wkt)
mp1 = OGRGeometry('MultiPolygon')
mp2 = OGRGeometry('MultiPolygon')
mp3 = OGRGeometry('MultiPolygon')
for poly in mpoly:
mp1.add(poly) # Adding a geometry at a time
mp2.add(poly.wkt) # Adding WKT
mp3.add(mpoly) # Adding a MultiPolygon's entire contents at once.
for tmp in (mp1, mp2, mp3): self.assertEqual(mpoly, tmp)
def test15_extent(self):
"Testing `extent` property."
# The xmin, ymin, xmax, ymax of the MultiPoint should be returned.
mp = OGRGeometry('MULTIPOINT(5 23, 0 0, 10 50)')
self.assertEqual((0.0, 0.0, 10.0, 50.0), mp.extent)
# Testing on the 'real world' Polygon.
poly = OGRGeometry(self.geometries.polygons[3].wkt)
ring = poly.shell
x, y = ring.x, ring.y
xmin, ymin = min(x), min(y)
xmax, ymax = max(x), max(y)
self.assertEqual((xmin, ymin, xmax, ymax), poly.extent)
def test16_25D(self):
"Testing 2.5D geometries."
pnt_25d = OGRGeometry('POINT(1 2 3)')
self.assertEqual('Point25D', pnt_25d.geom_type.name)
self.assertEqual(3.0, pnt_25d.z)
self.assertEqual(3, pnt_25d.coord_dim)
ls_25d = OGRGeometry('LINESTRING(1 1 1,2 2 2,3 3 3)')
self.assertEqual('LineString25D', ls_25d.geom_type.name)
self.assertEqual([1.0, 2.0, 3.0], ls_25d.z)
self.assertEqual(3, ls_25d.coord_dim)
def test17_pickle(self):
"Testing pickle support."
g1 = OGRGeometry('LINESTRING(1 1 1,2 2 2,3 3 3)', 'WGS84')
g2 = pickle.loads(pickle.dumps(g1))
self.assertEqual(g1, g2)
self.assertEqual(4326, g2.srs.srid)
self.assertEqual(g1.srs.wkt, g2.srs.wkt)
def test18_ogrgeometry_transform_workaround(self):
"Testing coordinate dimensions on geometries after transformation."
# A bug in GDAL versions prior to 1.7 changes the coordinate
# dimension of a geometry after it has been transformed.
# This test ensures that the bug workarounds employed within
# `OGRGeometry.transform` indeed work.
wkt_2d = "MULTILINESTRING ((0 0,1 1,2 2))"
wkt_3d = "MULTILINESTRING ((0 0 0,1 1 1,2 2 2))"
srid = 4326
# For both the 2D and 3D MultiLineString, ensure _both_ the dimension
# of the collection and the component LineString have the expected
# coordinate dimension after transform.
geom = OGRGeometry(wkt_2d, srid)
geom.transform(srid)
self.assertEqual(2, geom.coord_dim)
self.assertEqual(2, geom[0].coord_dim)
self.assertEqual(wkt_2d, geom.wkt)
geom = OGRGeometry(wkt_3d, srid)
geom.transform(srid)
self.assertEqual(3, geom.coord_dim)
self.assertEqual(3, geom[0].coord_dim)
self.assertEqual(wkt_3d, geom.wkt)
def test19_equivalence_regression(self):
"Testing equivalence methods with non-OGRGeometry instances."
self.assertNotEqual(None, OGRGeometry('POINT(0 0)'))
self.assertEqual(False, OGRGeometry('LINESTRING(0 0, 1 1)') == 3)
| bsd-3-clause |
AutorestCI/azure-sdk-for-python | azure-cognitiveservices-vision-customvision/azure/cognitiveservices/vision/customvision/training/models/domain.py | 2 | 1248 | # coding=utf-8
# --------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for
# license information.
#
# Code generated by Microsoft (R) AutoRest Code Generator.
# Changes may cause incorrect behavior and will be lost if the code is
# regenerated.
# --------------------------------------------------------------------------
from msrest.serialization import Model
class Domain(Model):
"""Domain.
Variables are only populated by the server, and will be ignored when
sending a request.
:ivar id:
:vartype id: str
:ivar name:
:vartype name: str
:ivar exportable:
:vartype exportable: bool
"""
_validation = {
'id': {'readonly': True},
'name': {'readonly': True},
'exportable': {'readonly': True},
}
_attribute_map = {
'id': {'key': 'Id', 'type': 'str'},
'name': {'key': 'Name', 'type': 'str'},
'exportable': {'key': 'Exportable', 'type': 'bool'},
}
def __init__(self):
super(Domain, self).__init__()
self.id = None
self.name = None
self.exportable = None
| mit |
Azure/azure-sdk-for-python | sdk/keyvault/azure-keyvault-certificates/azure/keyvault/certificates/__init__.py | 1 | 1350 | # ------------------------------------
# Copyright (c) Microsoft Corporation.
# Licensed under the MIT License.
# ------------------------------------
from ._client import CertificateClient
from ._enums import(
CertificatePolicyAction,
KeyCurveName,
KeyType,
CertificateContentType,
KeyUsageType,
WellKnownIssuerNames
)
from ._models import(
AdministratorContact,
CertificateContact,
CertificateIssuer,
CertificateOperation,
CertificateOperationError,
CertificatePolicy,
CertificateProperties,
DeletedCertificate,
IssuerProperties,
LifetimeAction,
KeyVaultCertificate,
KeyVaultCertificateIdentifier
)
from ._shared.client_base import ApiVersion
__all__ = [
"ApiVersion",
"CertificatePolicyAction",
"AdministratorContact",
"CertificateClient",
"CertificateContact",
"CertificateIssuer",
"CertificateOperation",
"CertificateOperationError",
"CertificatePolicy",
"CertificateProperties",
"DeletedCertificate",
"IssuerProperties",
"KeyCurveName",
"KeyType",
"KeyVaultCertificate",
"KeyVaultCertificateIdentifier",
"KeyUsageType",
"LifetimeAction",
"CertificateContentType",
"WellKnownIssuerNames",
"CertificateIssuer",
"IssuerProperties"
]
from ._version import VERSION
__version__ = VERSION
| mit |
aisk/grumpy | third_party/stdlib/unittest_suite.py | 7 | 9938 | """TestSuite"""
import sys
# from . import case
# from . import util
import unittest_case as case
import unittest_util as util
__unittest = True
def _call_if_exists(parent, attr):
func = getattr(parent, attr, lambda: None)
func()
class BaseTestSuite(object):
"""A simple test suite that doesn't provide class or module shared fixtures.
"""
def __init__(self, tests=()):
self._tests = []
self.addTests(tests)
def __repr__(self):
return "<%s tests=%s>" % (util.strclass(self.__class__), list(self))
def __eq__(self, other):
if not isinstance(other, self.__class__):
return NotImplemented
return list(self) == list(other)
def __ne__(self, other):
return not self == other
# Can't guarantee hash invariant, so flag as unhashable
__hash__ = None
def __iter__(self):
return iter(self._tests)
def countTestCases(self):
cases = 0
for test in self:
cases += test.countTestCases()
return cases
def addTest(self, test):
# sanity checks
if not hasattr(test, '__call__'):
# raise TypeError("{} is not callable".format(repr(test)))
raise TypeError("%s is not callable" % (repr(test)))
if isinstance(test, type) and issubclass(test,
(case.TestCase, TestSuite)):
raise TypeError("TestCases and TestSuites must be instantiated "
"before passing them to addTest()")
self._tests.append(test)
def addTests(self, tests):
if isinstance(tests, basestring):
raise TypeError("tests must be an iterable of tests, not a string")
for test in tests:
self.addTest(test)
def run(self, result):
for test in self:
if result.shouldStop:
break
test(result)
return result
def __call__(self, *args, **kwds):
return self.run(*args, **kwds)
def debug(self):
"""Run the tests without collecting errors in a TestResult"""
for test in self:
test.debug()
class TestSuite(BaseTestSuite):
"""A test suite is a composite test consisting of a number of TestCases.
For use, create an instance of TestSuite, then add test case instances.
When all tests have been added, the suite can be passed to a test
runner, such as TextTestRunner. It will run the individual test cases
in the order in which they were added, aggregating the results. When
subclassing, do not forget to call the base class constructor.
"""
def run(self, result, debug=False):
topLevel = False
if getattr(result, '_testRunEntered', False) is False:
result._testRunEntered = topLevel = True
for test in self:
if result.shouldStop:
break
if _isnotsuite(test):
self._tearDownPreviousClass(test, result)
self._handleModuleFixture(test, result)
self._handleClassSetUp(test, result)
result._previousTestClass = test.__class__
if (getattr(test.__class__, '_classSetupFailed', False) or
getattr(result, '_moduleSetUpFailed', False)):
continue
if not debug:
test(result)
else:
test.debug()
if topLevel:
self._tearDownPreviousClass(None, result)
self._handleModuleTearDown(result)
result._testRunEntered = False
return result
def debug(self):
"""Run the tests without collecting errors in a TestResult"""
debug = _DebugResult()
self.run(debug, True)
################################
def _handleClassSetUp(self, test, result):
previousClass = getattr(result, '_previousTestClass', None)
currentClass = test.__class__
if currentClass == previousClass:
return
if result._moduleSetUpFailed:
return
if getattr(currentClass, "__unittest_skip__", False):
return
try:
currentClass._classSetupFailed = False
except TypeError:
# test may actually be a function
# so its class will be a builtin-type
pass
setUpClass = getattr(currentClass, 'setUpClass', None)
if setUpClass is not None:
_call_if_exists(result, '_setupStdout')
try:
setUpClass()
except Exception as e:
if isinstance(result, _DebugResult):
raise
currentClass._classSetupFailed = True
className = util.strclass(currentClass)
errorName = 'setUpClass (%s)' % className
self._addClassOrModuleLevelException(result, e, errorName)
finally:
_call_if_exists(result, '_restoreStdout')
def _get_previous_module(self, result):
previousModule = None
previousClass = getattr(result, '_previousTestClass', None)
if previousClass is not None:
previousModule = previousClass.__module__
return previousModule
def _handleModuleFixture(self, test, result):
previousModule = self._get_previous_module(result)
currentModule = test.__class__.__module__
if currentModule == previousModule:
return
self._handleModuleTearDown(result)
result._moduleSetUpFailed = False
try:
module = sys.modules[currentModule]
except KeyError:
return
setUpModule = getattr(module, 'setUpModule', None)
if setUpModule is not None:
_call_if_exists(result, '_setupStdout')
try:
setUpModule()
except Exception, e:
if isinstance(result, _DebugResult):
raise
result._moduleSetUpFailed = True
errorName = 'setUpModule (%s)' % currentModule
self._addClassOrModuleLevelException(result, e, errorName)
finally:
_call_if_exists(result, '_restoreStdout')
def _addClassOrModuleLevelException(self, result, exception, errorName):
error = _ErrorHolder(errorName)
addSkip = getattr(result, 'addSkip', None)
if addSkip is not None and isinstance(exception, case.SkipTest):
addSkip(error, str(exception))
else:
result.addError(error, sys.exc_info())
def _handleModuleTearDown(self, result):
previousModule = self._get_previous_module(result)
if previousModule is None:
return
if result._moduleSetUpFailed:
return
try:
module = sys.modules[previousModule]
except KeyError:
return
tearDownModule = getattr(module, 'tearDownModule', None)
if tearDownModule is not None:
_call_if_exists(result, '_setupStdout')
try:
tearDownModule()
except Exception as e:
if isinstance(result, _DebugResult):
raise
errorName = 'tearDownModule (%s)' % previousModule
self._addClassOrModuleLevelException(result, e, errorName)
finally:
_call_if_exists(result, '_restoreStdout')
def _tearDownPreviousClass(self, test, result):
previousClass = getattr(result, '_previousTestClass', None)
currentClass = test.__class__
if currentClass == previousClass:
return
if getattr(previousClass, '_classSetupFailed', False):
return
if getattr(result, '_moduleSetUpFailed', False):
return
if getattr(previousClass, "__unittest_skip__", False):
return
tearDownClass = getattr(previousClass, 'tearDownClass', None)
if tearDownClass is not None:
_call_if_exists(result, '_setupStdout')
try:
tearDownClass()
except Exception, e:
if isinstance(result, _DebugResult):
raise
className = util.strclass(previousClass)
errorName = 'tearDownClass (%s)' % className
self._addClassOrModuleLevelException(result, e, errorName)
finally:
_call_if_exists(result, '_restoreStdout')
class _ErrorHolder(object):
"""
Placeholder for a TestCase inside a result. As far as a TestResult
is concerned, this looks exactly like a unit test. Used to insert
arbitrary errors into a test suite run.
"""
# Inspired by the ErrorHolder from Twisted:
# http://twistedmatrix.com/trac/browser/trunk/twisted/trial/runner.py
# attribute used by TestResult._exc_info_to_string
failureException = None
def __init__(self, description):
self.description = description
def id(self):
return self.description
def shortDescription(self):
return None
def __repr__(self):
return "<ErrorHolder description=%r>" % (self.description,)
def __str__(self):
return self.id()
def run(self, result):
# could call result.addError(...) - but this test-like object
# shouldn't be run anyway
pass
def __call__(self, result):
return self.run(result)
def countTestCases(self):
return 0
def _isnotsuite(test):
"A crude way to tell apart testcases and suites with duck-typing"
try:
iter(test)
except TypeError:
return True
return False
class _DebugResult(object):
"Used by the TestSuite to hold previous class when running in debug."
_previousTestClass = None
_moduleSetUpFailed = False
shouldStop = False
| apache-2.0 |
hi-ogawa/hiogawa-blog-ghost | node_modules/grunt-docker/node_modules/docker/node_modules/pygmentize-bundled/vendor/pygments/build-3.3/pygments/styles/emacs.py | 361 | 2486 | # -*- coding: utf-8 -*-
"""
pygments.styles.emacs
~~~~~~~~~~~~~~~~~~~~~
A highlighting style for Pygments, inspired by Emacs.
:copyright: Copyright 2006-2013 by the Pygments team, see AUTHORS.
:license: BSD, see LICENSE for details.
"""
from pygments.style import Style
from pygments.token import Keyword, Name, Comment, String, Error, \
Number, Operator, Generic, Whitespace
class EmacsStyle(Style):
"""
The default style (inspired by Emacs 22).
"""
background_color = "#f8f8f8"
default_style = ""
styles = {
Whitespace: "#bbbbbb",
Comment: "italic #008800",
Comment.Preproc: "noitalic",
Comment.Special: "noitalic bold",
Keyword: "bold #AA22FF",
Keyword.Pseudo: "nobold",
Keyword.Type: "bold #00BB00",
Operator: "#666666",
Operator.Word: "bold #AA22FF",
Name.Builtin: "#AA22FF",
Name.Function: "#00A000",
Name.Class: "#0000FF",
Name.Namespace: "bold #0000FF",
Name.Exception: "bold #D2413A",
Name.Variable: "#B8860B",
Name.Constant: "#880000",
Name.Label: "#A0A000",
Name.Entity: "bold #999999",
Name.Attribute: "#BB4444",
Name.Tag: "bold #008000",
Name.Decorator: "#AA22FF",
String: "#BB4444",
String.Doc: "italic",
String.Interpol: "bold #BB6688",
String.Escape: "bold #BB6622",
String.Regex: "#BB6688",
String.Symbol: "#B8860B",
String.Other: "#008000",
Number: "#666666",
Generic.Heading: "bold #000080",
Generic.Subheading: "bold #800080",
Generic.Deleted: "#A00000",
Generic.Inserted: "#00A000",
Generic.Error: "#FF0000",
Generic.Emph: "italic",
Generic.Strong: "bold",
Generic.Prompt: "bold #000080",
Generic.Output: "#888",
Generic.Traceback: "#04D",
Error: "border:#FF0000"
}
| mit |
benoitsteiner/tensorflow-opencl | tensorflow/contrib/opt/python/training/nadam_optimizer.py | 55 | 4017 | # Copyright 2015 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Nadam for TensorFlow."""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
from tensorflow.python.framework import ops
from tensorflow.python.ops import control_flow_ops
from tensorflow.python.ops import math_ops
from tensorflow.python.ops import state_ops
from tensorflow.python.training import adam
from tensorflow.python.training import training_ops
class NadamOptimizer(adam.AdamOptimizer):
"""Optimizer that implements the Nadam algorithm.
See [Dozat, T., 2015](http://cs229.stanford.edu/proj2015/054_report.pdf).
"""
def _apply_dense(self, grad, var):
m = self.get_slot(var, "m")
v = self.get_slot(var, "v")
return training_ops.apply_adam(
var,
m,
v,
math_ops.cast(self._beta1_power, var.dtype.base_dtype),
math_ops.cast(self._beta2_power, var.dtype.base_dtype),
math_ops.cast(self._lr_t, var.dtype.base_dtype),
math_ops.cast(self._beta1_t, var.dtype.base_dtype),
math_ops.cast(self._beta2_t, var.dtype.base_dtype),
math_ops.cast(self._epsilon_t, var.dtype.base_dtype),
grad,
use_locking=self._use_locking,
use_nesterov=True).op
def _resource_apply_dense(self, grad, var):
m = self.get_slot(var, "m")
v = self.get_slot(var, "v")
return training_ops.resource_apply_adam(
var.handle,
m.handle,
v.handle,
math_ops.cast(self._beta1_power, grad.dtype.base_dtype),
math_ops.cast(self._beta2_power, grad.dtype.base_dtype),
math_ops.cast(self._lr_t, grad.dtype.base_dtype),
math_ops.cast(self._beta1_t, grad.dtype.base_dtype),
math_ops.cast(self._beta2_t, grad.dtype.base_dtype),
math_ops.cast(self._epsilon_t, grad.dtype.base_dtype),
grad,
use_locking=self._use_locking,
use_nesterov=True)
def _apply_sparse_shared(self, grad, var, indices, scatter_add):
beta1_power = math_ops.cast(self._beta1_power, var.dtype.base_dtype)
beta2_power = math_ops.cast(self._beta2_power, var.dtype.base_dtype)
lr_t = math_ops.cast(self._lr_t, var.dtype.base_dtype)
beta1_t = math_ops.cast(self._beta1_t, var.dtype.base_dtype)
beta2_t = math_ops.cast(self._beta2_t, var.dtype.base_dtype)
epsilon_t = math_ops.cast(self._epsilon_t, var.dtype.base_dtype)
lr = (lr_t * math_ops.sqrt(1 - beta2_power) / (1 - beta1_power))
# m_t = beta1 * m + (1 - beta1) * g_t
m = self.get_slot(var, "m")
m_scaled_g_values = grad * (1 - beta1_t)
m_t = state_ops.assign(m, m * beta1_t, use_locking=self._use_locking)
with ops.control_dependencies([m_t]):
m_t = scatter_add(m, indices, m_scaled_g_values)
# m_bar = (1 - beta1) * g_t + beta1 * m_t
m_bar = m_scaled_g_values + beta1_t * m_t
# v_t = beta2 * v + (1 - beta2) * (g_t * g_t)
v = self.get_slot(var, "v")
v_scaled_g_values = (grad * grad) * (1 - beta2_t)
v_t = state_ops.assign(v, v * beta2_t, use_locking=self._use_locking)
with ops.control_dependencies([v_t]):
v_t = scatter_add(v, indices, v_scaled_g_values)
v_sqrt = math_ops.sqrt(v_t)
var_update = state_ops.assign_sub(
var, lr * m_bar / (v_sqrt + epsilon_t), use_locking=self._use_locking)
return control_flow_ops.group(*[var_update, m_bar, v_t])
| apache-2.0 |
vbelakov/h2o | py/testdir_multi_jvm/test_KMeans_libsvm_fvec.py | 8 | 5485 | import unittest
import random, sys, time, os
sys.path.extend(['.','..','../..','py'])
import h2o, h2o_cmd, h2o_browse as h2b, h2o_import as h2i, h2o_kmeans
class Basic(unittest.TestCase):
def tearDown(self):
h2o.check_sandbox_for_errors()
@classmethod
def setUpClass(cls):
global SEED
SEED = h2o.setup_random_seed()
h2o.init(2,java_heap_GB=7)
@classmethod
def tearDownClass(cls):
# wait while I inspect things
# h2o.sleep(1500)
h2o.tear_down_cloud()
def test_KMeans_libsvm_fvec(self):
# hack this into a function so we can call it before and after kmeans
# kmeans is changing the last col to enum?? (and changing the data)
def do_summary_and_inspect():
# SUMMARY******************************************
summaryResult = h2o_cmd.runSummary(key=hex_key)
coltypeList = h2o_cmd.infoFromSummary(summaryResult)
# INSPECT******************************************
inspect = h2o_cmd.runInspect(None, parseResult['destination_key'], timeoutSecs=360)
h2o_cmd.infoFromInspect(inspect, csvFilename)
numRows = inspect['numRows']
numCols = inspect['numCols']
# Now check both inspect and summary
if csvFilename=='covtype.binary.svm':
for k in range(55):
naCnt = inspect['cols'][k]['naCnt']
self.assertEqual(0, naCnt, msg='col %s naCnt %d should be %s' % (k, naCnt, 0))
stype = inspect['cols'][k]['type']
print k, stype
self.assertEqual('Int', stype, msg='col %s type %s should be %s' % (k, stype, 'Int'))
# summary may report type differently than inspect..check it too!
# we could check na here too
for i,c in enumerate(coltypeList):
print "column index: %s column type: %s" % (i, c)
# inspect says 'int?"
assert c=='Numeric', "All cols in covtype.binary.svm should be parsed as Numeric! %s %s" % (i,c)
# just do the import folder once
# make the timeout variable per dataset. it can be 10 secs for covtype 20x (col key creation)
# so probably 10x that for covtype200
csvFilenameList = [
# FIX! fails KMeansScore
("colon-cancer.svm", "cA", 30, 1),
("connect4.svm", "cB", 30, 1),
("covtype.binary.svm", "cC", 30, 1),
# multi-label class
# ("tmc2007_train.svm", "cJ", 30, 1),
("mnist_train.svm", "cM", 30, 1),
("duke.svm", "cD", 30, 1),
# too many features? 150K inspect timeout?
# ("E2006.train.svm", "cE", 30, 1),
("gisette_scale.svm", "cF", 120, 1), #Summary2 is slow with 5001 columns
("mushrooms.svm", "cG", 30, 1),
# ("news20.svm", "cH", 120, 1), #Summary2 is very slow - disable for now
("syn_6_1000_10.svm", "cK", 30, 1),
("syn_0_100_1000.svm", "cL", 30, 1),
]
csvFilenameList = [
("covtype.binary.svm", "cC", 30, 1),
]
### csvFilenameList = random.sample(csvFilenameAll,1)
h2b.browseTheCloud()
lenNodes = len(h2o.nodes)
firstDone = False
importFolderPath = "libsvm"
for (csvFilename, hex_key, timeoutSecs, resultMult) in csvFilenameList:
# have to import each time, because h2o deletes source after parse
csvPathname = importFolderPath + "/" + csvFilename
# PARSE******************************************
# creates csvFilename.hex from file in importFolder dir
parseResult = h2i.import_parse(bucket='home-0xdiag-datasets', path=csvPathname,
hex_key=hex_key, timeoutSecs=2000, doSummary=False)
do_summary_and_inspect()
# KMEANS******************************************
for trial in range(1):
kwargs = {
'k': 3,
'initialization': 'Furthest',
'ignored_cols': None, #range(11, numCols), # THIS BREAKS THE REST API
'max_iter': 10,
# 'normalize': 0,
# reuse the same seed, to get deterministic results (otherwise sometimes fails
'seed': 265211114317615310,
}
# fails if I put this in kwargs..i.e. source = dest
# 'destination_key': parseResult['destination_key'],
timeoutSecs = 600
start = time.time()
kmeans = h2o_cmd.runKMeans(parseResult=parseResult, timeoutSecs=timeoutSecs, **kwargs)
elapsed = time.time() - start
print "kmeans end on ", csvPathname, 'took', elapsed, 'seconds.', \
"%d pct. of timeout" % ((elapsed/timeoutSecs) * 100)
do_summary_and_inspect()
# this does an inspect of the model and prints the clusters
h2o_kmeans.simpleCheckKMeans(self, kmeans, **kwargs)
print "hello"
(centers, tupleResultList) = h2o_kmeans.bigCheckResults(self, kmeans, csvPathname, parseResult, 'd', **kwargs)
do_summary_and_inspect()
if __name__ == '__main__':
h2o.unit_main()
| apache-2.0 |
yaakovs/since-authorAdder | src/DocComment.py | 1 | 1720 | """
author Raviv Rachmiel
author Yaakov Sokolik
since Jan 16, 2017
a father class for getting the edited code file (with since, author and TODO)
will be inherited by any PL doc Comment
"""
class DocComment():
def __init__(self, file_lines):
"""
:param file_lines - list of lines of the code file
"""
self.FileLines = file_lines
def init_doc_comment(self):
"""
:returns the docComment as a string, or None if such doesnt exist
"""
return None
def needs_change(self):
"""
:returns: True if needs change, else false
"""
return False
def get_desc_from_comment(self):
"""
:returns: the description of the class, if there isn't any returns None
"""
return None
def get_author_from_comment(self):
"""
:returns: the author of the class, if there isn't any returns None
"""
return None
def get_since_from_comment(self):
"""
:returns: the since of the class, if there isn't any returns None
"""
return None
def rewrite(self, author, date):
"""
:param author: the author to add to doc comment
:param date: the date to add to doc comment
:returns a string of edited code file
"""
return None
def return_edited_file(self, author, date):
"""
:param author: the author to add to doc comment
:param date: the date to add to doc comment
:returns List of edited code lines or None if no change is needed
"""
if not self.needs_change():
return None
return self.rewrite(author, date)
| mit |
ryfeus/lambda-packs | Lxml_requests/source/wheel/install.py | 93 | 18098 | """
Operations on existing wheel files, including basic installation.
"""
# XXX see patched pip to install
import sys
import warnings
import os.path
import re
import zipfile
import hashlib
import csv
import shutil
try:
_big_number = sys.maxsize
except NameError:
_big_number = sys.maxint
from .decorator import reify
from .util import (urlsafe_b64encode, from_json, urlsafe_b64decode,
native, binary, HashingFile)
from . import signatures
from .pkginfo import read_pkg_info_bytes
from .util import open_for_csv
from .pep425tags import get_supported
from .paths import get_install_paths
# The next major version after this version of the 'wheel' tool:
VERSION_TOO_HIGH = (1, 0)
# Non-greedy matching of an optional build number may be too clever (more
# invalid wheel filenames will match). Separate regex for .dist-info?
WHEEL_INFO_RE = re.compile(
r"""^(?P<namever>(?P<name>.+?)(-(?P<ver>\d.+?))?)
((-(?P<build>\d.*?))?-(?P<pyver>.+?)-(?P<abi>.+?)-(?P<plat>.+?)
\.whl|\.dist-info)$""",
re.VERBOSE).match
def parse_version(version):
"""Use parse_version from pkg_resources or distutils as available."""
global parse_version
try:
from pkg_resources import parse_version
except ImportError:
from distutils.version import LooseVersion as parse_version
return parse_version(version)
class BadWheelFile(ValueError):
pass
class WheelFile(object):
"""Parse wheel-specific attributes from a wheel (.whl) file and offer
basic installation and verification support.
WheelFile can be used to simply parse a wheel filename by avoiding the
methods that require the actual file contents."""
WHEEL_INFO = "WHEEL"
RECORD = "RECORD"
def __init__(self,
filename,
fp=None,
append=False,
context=get_supported):
"""
:param fp: A seekable file-like object or None to open(filename).
:param append: Open archive in append mode.
:param context: Function returning list of supported tags. Wheels
must have the same context to be sortable.
"""
self.filename = filename
self.fp = fp
self.append = append
self.context = context
basename = os.path.basename(filename)
self.parsed_filename = WHEEL_INFO_RE(basename)
if not basename.endswith('.whl') or self.parsed_filename is None:
raise BadWheelFile("Bad filename '%s'" % filename)
def __repr__(self):
return self.filename
@property
def distinfo_name(self):
return "%s.dist-info" % self.parsed_filename.group('namever')
@property
def datadir_name(self):
return "%s.data" % self.parsed_filename.group('namever')
@property
def record_name(self):
return "%s/%s" % (self.distinfo_name, self.RECORD)
@property
def wheelinfo_name(self):
return "%s/%s" % (self.distinfo_name, self.WHEEL_INFO)
@property
def tags(self):
"""A wheel file is compatible with the Cartesian product of the
period-delimited tags in its filename.
To choose a wheel file among several candidates having the same
distribution version 'ver', an installer ranks each triple of
(pyver, abi, plat) that its Python installation can run, sorting
the wheels by the best-ranked tag it supports and then by their
arity which is just len(list(compatibility_tags)).
"""
tags = self.parsed_filename.groupdict()
for pyver in tags['pyver'].split('.'):
for abi in tags['abi'].split('.'):
for plat in tags['plat'].split('.'):
yield (pyver, abi, plat)
compatibility_tags = tags
@property
def arity(self):
"""The number of compatibility tags the wheel declares."""
return len(list(self.compatibility_tags))
@property
def rank(self):
"""
Lowest index of any of this wheel's tags in self.context(), and the
arity e.g. (0, 1)
"""
return self.compatibility_rank(self.context())
@property
def compatible(self):
return self.rank[0] != _big_number # bad API!
# deprecated:
def compatibility_rank(self, supported):
"""Rank the wheel against the supported tags. Smaller ranks are more
compatible!
:param supported: A list of compatibility tags that the current
Python implemenation can run.
"""
preferences = []
for tag in self.compatibility_tags:
try:
preferences.append(supported.index(tag))
# Tag not present
except ValueError:
pass
if len(preferences):
return (min(preferences), self.arity)
return (_big_number, 0)
# deprecated
def supports_current_python(self, x):
assert self.context == x, 'context mismatch'
return self.compatible
# Comparability.
# Wheels are equal if they refer to the same file.
# If two wheels are not equal, compare based on (in this order):
# 1. Name
# 2. Version
# 3. Compatibility rank
# 4. Filename (as a tiebreaker)
@property
def _sort_key(self):
return (self.parsed_filename.group('name'),
parse_version(self.parsed_filename.group('ver')),
tuple(-x for x in self.rank),
self.filename)
def __eq__(self, other):
return self.filename == other.filename
def __ne__(self, other):
return self.filename != other.filename
def __lt__(self, other):
if self.context != other.context:
raise TypeError("{0}.context != {1}.context".format(self, other))
return self._sort_key < other._sort_key
# XXX prune
sn = self.parsed_filename.group('name')
on = other.parsed_filename.group('name')
if sn != on:
return sn < on
sv = parse_version(self.parsed_filename.group('ver'))
ov = parse_version(other.parsed_filename.group('ver'))
if sv != ov:
return sv < ov
# Compatibility
if self.context != other.context:
raise TypeError("{0}.context != {1}.context".format(self, other))
sc = self.rank
oc = other.rank
if sc != None and oc != None and sc != oc:
# Smaller compatibility ranks are "better" than larger ones,
# so we have to reverse the sense of the comparison here!
return sc > oc
elif sc == None and oc != None:
return False
return self.filename < other.filename
def __gt__(self, other):
return other < self
def __le__(self, other):
return self == other or self < other
def __ge__(self, other):
return self == other or other < self
#
# Methods using the file's contents:
#
@reify
def zipfile(self):
mode = "r"
if self.append:
mode = "a"
vzf = VerifyingZipFile(self.fp if self.fp else self.filename, mode)
if not self.append:
self.verify(vzf)
return vzf
@reify
def parsed_wheel_info(self):
"""Parse wheel metadata (the .data/WHEEL file)"""
return read_pkg_info_bytes(self.zipfile.read(self.wheelinfo_name))
def check_version(self):
version = self.parsed_wheel_info['Wheel-Version']
if tuple(map(int, version.split('.'))) >= VERSION_TOO_HIGH:
raise ValueError("Wheel version is too high")
@reify
def install_paths(self):
"""
Consult distutils to get the install paths for our dist. A dict with
('purelib', 'platlib', 'headers', 'scripts', 'data').
We use the name from our filename as the dist name, which means headers
could be installed in the wrong place if the filesystem-escaped name
is different than the Name. Who cares?
"""
name = self.parsed_filename.group('name')
return get_install_paths(name)
def install(self, force=False, overrides={}):
"""
Install the wheel into site-packages.
"""
# Utility to get the target directory for a particular key
def get_path(key):
return overrides.get(key) or self.install_paths[key]
# The base target location is either purelib or platlib
if self.parsed_wheel_info['Root-Is-Purelib'] == 'true':
root = get_path('purelib')
else:
root = get_path('platlib')
# Parse all the names in the archive
name_trans = {}
for info in self.zipfile.infolist():
name = info.filename
# Zip files can contain entries representing directories.
# These end in a '/'.
# We ignore these, as we create directories on demand.
if name.endswith('/'):
continue
# Pathnames in a zipfile namelist are always /-separated.
# In theory, paths could start with ./ or have other oddities
# but this won't happen in practical cases of well-formed wheels.
# We'll cover the simple case of an initial './' as it's both easy
# to do and more common than most other oddities.
if name.startswith('./'):
name = name[2:]
# Split off the base directory to identify files that are to be
# installed in non-root locations
basedir, sep, filename = name.partition('/')
if sep and basedir == self.datadir_name:
# Data file. Target destination is elsewhere
key, sep, filename = filename.partition('/')
if not sep:
raise ValueError("Invalid filename in wheel: {0}".format(name))
target = get_path(key)
else:
# Normal file. Target destination is root
key = ''
target = root
filename = name
# Map the actual filename from the zipfile to its intended target
# directory and the pathname relative to that directory.
dest = os.path.normpath(os.path.join(target, filename))
name_trans[info] = (key, target, filename, dest)
# We're now ready to start processing the actual install. The process
# is as follows:
# 1. Prechecks - is the wheel valid, is its declared architecture
# OK, etc. [[Responsibility of the caller]]
# 2. Overwrite check - do any of the files to be installed already
# exist?
# 3. Actual install - put the files in their target locations.
# 4. Update RECORD - write a suitably modified RECORD file to
# reflect the actual installed paths.
if not force:
for info, v in name_trans.items():
k = info.filename
key, target, filename, dest = v
if os.path.exists(dest):
raise ValueError("Wheel file {0} would overwrite {1}. Use force if this is intended".format(k, dest))
# Get the name of our executable, for use when replacing script
# wrapper hashbang lines.
# We encode it using getfilesystemencoding, as that is "the name of
# the encoding used to convert Unicode filenames into system file
# names".
exename = sys.executable.encode(sys.getfilesystemencoding())
record_data = []
record_name = self.distinfo_name + '/RECORD'
for info, (key, target, filename, dest) in name_trans.items():
name = info.filename
source = self.zipfile.open(info)
# Skip the RECORD file
if name == record_name:
continue
ddir = os.path.dirname(dest)
if not os.path.isdir(ddir):
os.makedirs(ddir)
destination = HashingFile(open(dest, 'wb'))
if key == 'scripts':
hashbang = source.readline()
if hashbang.startswith(b'#!python'):
hashbang = b'#!' + exename + binary(os.linesep)
destination.write(hashbang)
shutil.copyfileobj(source, destination)
reldest = os.path.relpath(dest, root)
reldest.replace(os.sep, '/')
record_data.append((reldest, destination.digest(), destination.length))
destination.close()
source.close()
# preserve attributes (especially +x bit for scripts)
attrs = info.external_attr >> 16
if attrs: # tends to be 0 if Windows.
os.chmod(dest, info.external_attr >> 16)
record_name = os.path.join(root, self.record_name)
with open_for_csv(record_name, 'w+') as record_file:
writer = csv.writer(record_file)
for reldest, digest, length in sorted(record_data):
writer.writerow((reldest, digest, length))
writer.writerow((self.record_name, '', ''))
def verify(self, zipfile=None):
"""Configure the VerifyingZipFile `zipfile` by verifying its signature
and setting expected hashes for every hash in RECORD.
Caller must complete the verification process by completely reading
every file in the archive (e.g. with extractall)."""
sig = None
if zipfile is None:
zipfile = self.zipfile
zipfile.strict = True
record_name = '/'.join((self.distinfo_name, 'RECORD'))
sig_name = '/'.join((self.distinfo_name, 'RECORD.jws'))
# tolerate s/mime signatures:
smime_sig_name = '/'.join((self.distinfo_name, 'RECORD.p7s'))
zipfile.set_expected_hash(record_name, None)
zipfile.set_expected_hash(sig_name, None)
zipfile.set_expected_hash(smime_sig_name, None)
record = zipfile.read(record_name)
record_digest = urlsafe_b64encode(hashlib.sha256(record).digest())
try:
sig = from_json(native(zipfile.read(sig_name)))
except KeyError: # no signature
pass
if sig:
headers, payload = signatures.verify(sig)
if payload['hash'] != "sha256=" + native(record_digest):
msg = "RECORD.sig claimed RECORD hash {0} != computed hash {1}."
raise BadWheelFile(msg.format(payload['hash'],
native(record_digest)))
reader = csv.reader((native(r) for r in record.splitlines()))
for row in reader:
filename = row[0]
hash = row[1]
if not hash:
if filename not in (record_name, sig_name):
sys.stderr.write("%s has no hash!\n" % filename)
continue
algo, data = row[1].split('=', 1)
assert algo == "sha256", "Unsupported hash algorithm"
zipfile.set_expected_hash(filename, urlsafe_b64decode(binary(data)))
class VerifyingZipFile(zipfile.ZipFile):
"""ZipFile that can assert that each of its extracted contents matches
an expected sha256 hash. Note that each file must be completly read in
order for its hash to be checked."""
def __init__(self, file, mode="r",
compression=zipfile.ZIP_STORED,
allowZip64=False):
zipfile.ZipFile.__init__(self, file, mode, compression, allowZip64)
self.strict = False
self._expected_hashes = {}
self._hash_algorithm = hashlib.sha256
def set_expected_hash(self, name, hash):
"""
:param name: name of zip entry
:param hash: bytes of hash (or None for "don't care")
"""
self._expected_hashes[name] = hash
def open(self, name_or_info, mode="r", pwd=None):
"""Return file-like object for 'name'."""
# A non-monkey-patched version would contain most of zipfile.py
ef = zipfile.ZipFile.open(self, name_or_info, mode, pwd)
if isinstance(name_or_info, zipfile.ZipInfo):
name = name_or_info.filename
else:
name = name_or_info
if (name in self._expected_hashes
and self._expected_hashes[name] != None):
expected_hash = self._expected_hashes[name]
try:
_update_crc_orig = ef._update_crc
except AttributeError:
warnings.warn('Need ZipExtFile._update_crc to implement '
'file hash verification (in Python >= 2.7)')
return ef
running_hash = self._hash_algorithm()
if hasattr(ef, '_eof'): # py33
def _update_crc(data):
_update_crc_orig(data)
running_hash.update(data)
if ef._eof and running_hash.digest() != expected_hash:
raise BadWheelFile("Bad hash for file %r" % ef.name)
else:
def _update_crc(data, eof=None):
_update_crc_orig(data, eof=eof)
running_hash.update(data)
if eof and running_hash.digest() != expected_hash:
raise BadWheelFile("Bad hash for file %r" % ef.name)
ef._update_crc = _update_crc
elif self.strict and name not in self._expected_hashes:
raise BadWheelFile("No expected hash for file %r" % ef.name)
return ef
def pop(self):
"""Truncate the last file off this zipfile.
Assumes infolist() is in the same order as the files (true for
ordinary zip files created by Python)"""
if not self.fp:
raise RuntimeError(
"Attempt to pop from ZIP archive that was already closed")
last = self.infolist().pop()
del self.NameToInfo[last.filename]
self.fp.seek(last.header_offset, os.SEEK_SET)
self.fp.truncate()
self._didModify = True
| mit |
nion-software/nionswift | nion/swift/test/DataGroup_test.py | 1 | 26308 | # standard libraries
import copy
import unittest
# third party libraries
import numpy
# local libraries
from nion.swift import Application
from nion.swift import DocumentController
from nion.swift.model import DataGroup
from nion.swift.model import DataItem
from nion.swift.model import Persistence
from nion.swift.test import TestContext
from nion.ui import TestUI
class TestDataGroupClass(unittest.TestCase):
def setUp(self):
TestContext.begin_leaks()
self.app = Application.Application(TestUI.UserInterface(), set_global=False)
def tearDown(self):
TestContext.end_leaks(self)
def test_deep_copy_should_deep_copy_child_data_groups(self):
with TestContext.create_memory_context() as test_context:
document_model = test_context.create_document_model()
data_group = DataGroup.DataGroup()
document_model.append_data_group(data_group)
data_item1 = DataItem.DataItem(numpy.zeros((8, 8), numpy.uint32))
document_model.append_data_item(data_item1)
data_group.append_display_item(document_model.get_display_item_for_data_item(data_item1))
data_group2 = DataGroup.DataGroup()
data_group.append_data_group(data_group2)
# attempt to copy
data_group_copy = copy.deepcopy(data_group)
document_model.append_data_group(data_group_copy)
# make sure data_groups are not shared
self.assertNotEqual(data_group.data_groups[0], data_group_copy.data_groups[0])
def test_deep_copy_should_not_deep_copy_data_items(self):
with TestContext.create_memory_context() as test_context:
document_model = test_context.create_document_model()
data_group = DataGroup.DataGroup()
document_model.append_data_group(data_group)
data_item = DataItem.DataItem(numpy.zeros((8, 8), numpy.uint32))
document_model.append_data_item(data_item)
display_item = document_model.get_display_item_for_data_item(data_item)
data_group.append_display_item(display_item)
data_group_copy = copy.deepcopy(data_group)
document_model.append_data_group(data_group_copy)
display_item_specifier = Persistence.read_persistent_specifier(data_group_copy.display_item_specifiers[0])
self.assertEqual(display_item, document_model.resolve_item_specifier(display_item_specifier))
def test_counted_display_items(self):
# TODO: split test_counted_display_items into separate tests
with TestContext.create_memory_context() as test_context:
document_model = test_context.create_document_model()
data_group = DataGroup.DataGroup()
document_model.append_data_group(data_group)
self.assertEqual(len(data_group.counted_display_items), 0)
self.assertEqual(len(document_model.data_items), 0)
data_item1 = DataItem.DataItem(numpy.zeros((8, 8), numpy.uint32))
document_model.append_data_item(data_item1)
display_item1 = document_model.get_display_item_for_data_item(data_item1)
data_group.append_display_item(display_item1)
# make sure that both top level and data_group see the data item
self.assertEqual(len(document_model.data_items), 1)
self.assertEqual(len(data_group.counted_display_items), 1)
self.assertEqual(list(document_model.display_items), list(data_group.counted_display_items))
self.assertIn(display_item1, list(data_group.counted_display_items.keys()))
# add a child data item and make sure top level and data_group see it
# also check data item.
data_item1a = document_model.get_resample_new(display_item1, display_item1.data_item)
display_item1a = document_model.get_display_item_for_data_item(data_item1a)
data_group.append_display_item(display_item1a)
self.assertEqual(len(document_model.data_items), 2)
self.assertEqual(len(data_group.counted_display_items), 2)
self.assertIn(display_item1, list(data_group.counted_display_items.keys()))
self.assertIn(display_item1a, list(data_group.counted_display_items.keys()))
# add a child data item to the child and make sure top level and data_group match.
# also check data items.
data_item1a1 = document_model.get_resample_new(display_item1a, display_item1a.data_item)
display_item1a1 = document_model.get_display_item_for_data_item(data_item1a1)
data_group.append_display_item(display_item1a1)
self.assertEqual(len(document_model.data_items), 3)
self.assertEqual(len(data_group.counted_display_items), 3)
self.assertIn(display_item1, list(data_group.counted_display_items.keys()))
self.assertIn(display_item1a, list(data_group.counted_display_items.keys()))
self.assertIn(display_item1a1, list(data_group.counted_display_items.keys()))
# now add a data item that already has children
data_item2 = DataItem.DataItem(numpy.zeros((8, 8), numpy.uint32))
document_model.append_data_item(data_item2)
display_item2 = document_model.get_display_item_for_data_item(data_item2)
data_item2a = document_model.get_resample_new(display_item2, display_item2.data_item)
display_item2a = document_model.get_display_item_for_data_item(data_item2a)
data_group.append_display_item(display_item2)
data_group.append_display_item(display_item2a)
self.assertEqual(len(document_model.data_items), 5)
self.assertEqual(len(data_group.counted_display_items), 5)
self.assertIn(data_item2a, document_model.data_items)
self.assertIn(display_item2a, list(data_group.counted_display_items.keys()))
# remove data item without children
document_model.remove_data_item(data_item1a1)
self.assertEqual(len(document_model.data_items), 4)
self.assertEqual(len(data_group.counted_display_items), 4)
# now remove data item with children
document_model.remove_data_item(data_item2)
self.assertEqual(len(document_model.data_items), 2)
self.assertEqual(len(data_group.counted_display_items), 2)
def test_deleting_data_item_removes_it_from_data_group(self):
with TestContext.create_memory_context() as test_context:
document_model = test_context.create_document_model()
data_item = DataItem.DataItem(numpy.random.randn(4, 4))
document_model.append_data_item(data_item)
data_group = DataGroup.DataGroup()
data_group.append_display_item(document_model.get_display_item_for_data_item(data_item))
document_model.append_data_group(data_group)
self.assertEqual(1, len(data_group.display_items))
self.assertEqual(0, len(data_group.data_groups))
document_model.remove_data_item(data_item)
self.assertEqual(0, len(data_group.display_items))
self.assertEqual(0, len(data_group.data_groups))
def test_deleting_data_items_from_data_group_undo_redo(self):
with TestContext.create_memory_context() as test_context:
document_controller = test_context.create_document_controller()
document_model = document_controller.document_model
# setup three data items in a group
data_item1 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item2 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item3 = DataItem.DataItem(numpy.random.randn(4, 4))
document_model.append_data_item(data_item1)
document_model.append_data_item(data_item2)
document_model.append_data_item(data_item3)
data_group = DataGroup.DataGroup()
display_item1 = document_model.get_display_item_for_data_item(data_item1)
display_item2 = document_model.get_display_item_for_data_item(data_item2)
display_item3 = document_model.get_display_item_for_data_item(data_item3)
data_group.append_display_item(display_item1)
data_group.append_display_item(display_item2)
data_group.append_display_item(display_item3)
document_model.append_data_group(data_group)
# remove two of the three
command = DocumentController.DocumentController.RemoveDataGroupDisplayItemsCommand(document_model, data_group, [display_item1, display_item3])
command.perform()
document_controller.push_undo_command(command)
self.assertEqual(1, len(data_group.display_items))
self.assertEqual(0, len(data_group.data_groups))
self.assertEqual(display_item2, data_group.display_items[0])
# undo and check
document_controller.handle_undo()
self.assertEqual(3, len(data_group.display_items))
self.assertEqual(0, len(data_group.data_groups))
self.assertEqual(display_item1, data_group.display_items[0])
self.assertEqual(display_item2, data_group.display_items[1])
self.assertEqual(display_item3, data_group.display_items[2])
# redo and check
document_controller.handle_redo()
self.assertEqual(1, len(data_group.display_items))
self.assertEqual(0, len(data_group.data_groups))
self.assertEqual(display_item2, data_group.display_items[0])
def test_deleting_data_items_out_of_order_from_data_group_undo_redo(self):
with TestContext.create_memory_context() as test_context:
document_controller = test_context.create_document_controller()
document_model = document_controller.document_model
# setup three data items in a group
data_item1 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item2 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item3 = DataItem.DataItem(numpy.random.randn(4, 4))
document_model.append_data_item(data_item1)
document_model.append_data_item(data_item2)
document_model.append_data_item(data_item3)
data_group = DataGroup.DataGroup()
display_item1 = document_model.get_display_item_for_data_item(data_item1)
display_item2 = document_model.get_display_item_for_data_item(data_item2)
display_item3 = document_model.get_display_item_for_data_item(data_item3)
data_group.append_display_item(display_item1)
data_group.append_display_item(display_item2)
data_group.append_display_item(display_item3)
document_model.append_data_group(data_group)
# remove two of the three
command = DocumentController.DocumentController.RemoveDataGroupDisplayItemsCommand(document_model, data_group, [display_item3, display_item1])
command.perform()
document_controller.push_undo_command(command)
self.assertEqual(1, len(data_group.display_items))
self.assertEqual(0, len(data_group.data_groups))
self.assertEqual(display_item2, data_group.display_items[0])
# undo and check
document_controller.handle_undo()
self.assertEqual(3, len(data_group.display_items))
self.assertEqual(0, len(data_group.data_groups))
self.assertEqual(display_item1, data_group.display_items[0])
self.assertEqual(display_item2, data_group.display_items[1])
self.assertEqual(display_item3, data_group.display_items[2])
# redo and check
document_controller.handle_redo()
self.assertEqual(1, len(data_group.display_items))
self.assertEqual(0, len(data_group.data_groups))
self.assertEqual(display_item2, data_group.display_items[0])
def test_insert_data_item_into_data_group_undo_redo(self):
with TestContext.create_memory_context() as test_context:
document_controller = test_context.create_document_controller()
document_model = document_controller.document_model
# setup three data items and put two in a group
data_item1 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item2 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item3 = DataItem.DataItem(numpy.random.randn(4, 4))
document_model.append_data_item(data_item1)
document_model.append_data_item(data_item2)
document_model.append_data_item(data_item3)
data_group = DataGroup.DataGroup()
display_item1 = document_model.get_display_item_for_data_item(data_item1)
display_item2 = document_model.get_display_item_for_data_item(data_item2)
display_item3 = document_model.get_display_item_for_data_item(data_item3)
data_group.append_display_item(display_item1)
data_group.append_display_item(display_item3)
document_model.append_data_group(data_group)
# insert a new one
display_item2 = document_model.get_display_item_for_data_item(data_item2)
command = document_controller.create_insert_data_group_display_items_command(data_group, 1, [display_item2])
command.perform()
document_controller.push_undo_command(command)
self.assertEqual(3, len(data_group.display_items))
self.assertEqual(0, len(data_group.data_groups))
self.assertEqual(display_item1, data_group.display_items[0])
self.assertEqual(display_item2, data_group.display_items[1])
self.assertEqual(display_item3, data_group.display_items[2])
# undo and check
document_controller.handle_undo()
self.assertEqual(2, len(data_group.display_items))
self.assertEqual(0, len(data_group.data_groups))
self.assertEqual(display_item1, data_group.display_items[0])
self.assertEqual(display_item3, data_group.display_items[1])
# redo and check
document_controller.handle_redo()
self.assertEqual(3, len(data_group.display_items))
self.assertEqual(0, len(data_group.data_groups))
self.assertEqual(display_item1, data_group.display_items[0])
self.assertEqual(display_item2, data_group.display_items[1])
self.assertEqual(display_item3, data_group.display_items[2])
def test_insert_data_group_undo_redo(self):
with TestContext.create_memory_context() as test_context:
document_controller = test_context.create_document_controller()
document_model = document_controller.document_model
# setup three data items and put two in a group
data_item1 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item3 = DataItem.DataItem(numpy.random.randn(4, 4))
document_model.append_data_item(data_item1)
document_model.append_data_item(data_item3)
data_group1 = DataGroup.DataGroup()
data_group1.append_display_item(document_model.get_display_item_for_data_item(data_item1))
data_group2 = DataGroup.DataGroup()
data_group3 = DataGroup.DataGroup()
data_group2_uuid = data_group2.uuid
data_group3.append_display_item(document_model.get_display_item_for_data_item(data_item3))
document_model.append_data_group(data_group1)
document_model.append_data_group(data_group3)
# insert the middle data group
command = DocumentController.DocumentController.InsertDataGroupCommand(document_model, document_model._project, 1, data_group2)
command.perform()
document_controller.push_undo_command(command)
self.assertEqual(3, len(document_model.data_groups))
self.assertEqual(data_group1, document_model.data_groups[0])
self.assertEqual(data_group3, document_model.data_groups[2])
self.assertEqual(data_group2_uuid, document_model.data_groups[1].uuid)
# undo and check
document_controller.handle_undo()
self.assertEqual(2, len(document_model.data_groups))
self.assertEqual(data_group1, document_model.data_groups[0])
self.assertEqual(data_group3, document_model.data_groups[1])
# redo and check
document_controller.handle_redo()
self.assertEqual(3, len(document_model.data_groups))
self.assertEqual(data_group1, document_model.data_groups[0])
self.assertEqual(data_group3, document_model.data_groups[2])
self.assertEqual(data_group2_uuid, document_model.data_groups[1].uuid)
def test_remove_data_group_undo_redo(self):
with TestContext.create_memory_context() as test_context:
document_controller = test_context.create_document_controller()
document_model = document_controller.document_model
# setup three data items and put two in a group
data_item1 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item3 = DataItem.DataItem(numpy.random.randn(4, 4))
document_model.append_data_item(data_item1)
document_model.append_data_item(data_item3)
data_group1 = DataGroup.DataGroup()
data_group1.append_display_item(document_model.get_display_item_for_data_item(data_item1))
data_group2 = DataGroup.DataGroup()
data_group3 = DataGroup.DataGroup()
data_group2_uuid = data_group2.uuid
data_group3.append_display_item(document_model.get_display_item_for_data_item(data_item3))
document_model.append_data_group(data_group1)
document_model.append_data_group(data_group2)
document_model.append_data_group(data_group3)
# remove the middle data group
command = DocumentController.DocumentController.RemoveDataGroupCommand(document_model, document_model._project, data_group2)
command.perform()
document_controller.push_undo_command(command)
self.assertEqual(2, len(document_model.data_groups))
self.assertEqual(data_group1, document_model.data_groups[0])
self.assertEqual(data_group3, document_model.data_groups[1])
# undo and check
document_controller.handle_undo()
self.assertEqual(3, len(document_model.data_groups))
self.assertEqual(data_group1, document_model.data_groups[0])
self.assertEqual(data_group3, document_model.data_groups[2])
self.assertEqual(data_group2_uuid, document_model.data_groups[1].uuid)
# redo and check
document_controller.handle_redo()
self.assertEqual(2, len(document_model.data_groups))
self.assertEqual(data_group1, document_model.data_groups[0])
self.assertEqual(data_group3, document_model.data_groups[1])
def test_data_group_rename_undo_redo(self):
with TestContext.create_memory_context() as test_context:
document_controller = test_context.create_document_controller()
document_model = document_controller.document_model
# setup data group
data_group = DataGroup.DataGroup()
data_group.title = "ethel"
document_model.append_data_group(data_group)
# rename
command = document_controller.create_rename_data_group_command(data_group, "fred")
command.perform()
document_controller.push_undo_command(command)
self.assertEqual(data_group.title, "fred")
# undo and check
document_controller.handle_undo()
self.assertEqual(data_group.title, "ethel")
# redo and check
document_controller.handle_redo()
self.assertEqual(data_group.title, "fred")
def test_data_item_removed_implicitly_from_data_group_undo_redo(self):
with TestContext.create_memory_context() as test_context:
document_controller = test_context.create_document_controller()
document_model = document_controller.document_model
# setup three data items and put in a group
data_item1 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item2 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item3 = DataItem.DataItem(numpy.random.randn(4, 4))
document_model.append_data_item(data_item1)
document_model.append_data_item(data_item2)
document_model.append_data_item(data_item3)
data_group = DataGroup.DataGroup()
display_item1 = document_model.get_display_item_for_data_item(data_item1)
display_item2 = document_model.get_display_item_for_data_item(data_item2)
display_item3 = document_model.get_display_item_for_data_item(data_item3)
data_group.append_display_item(display_item1)
data_group.append_display_item(display_item2)
data_group.append_display_item(display_item3)
document_model.append_data_group(data_group)
# remove the 2nd data item
command = document_controller.create_remove_data_items_command([data_item2])
command.perform()
document_controller.push_undo_command(command)
self.assertEqual(2, len(document_model.data_items))
self.assertEqual(2, len(data_group.display_items))
self.assertEqual(document_model.display_items[0], data_group.display_items[0])
self.assertEqual(document_model.display_items[1], data_group.display_items[1])
# undo and check
document_controller.handle_undo()
self.assertEqual(3, len(document_model.data_items))
self.assertEqual(3, len(data_group.display_items))
self.assertEqual(document_model.display_items[0], data_group.display_items[0])
self.assertEqual(document_model.display_items[1], data_group.display_items[1])
self.assertEqual(document_model.display_items[2], data_group.display_items[2])
# redo and check
document_controller.handle_redo()
self.assertEqual(2, len(document_model.data_items))
self.assertEqual(2, len(data_group.display_items))
self.assertEqual(document_model.display_items[0], data_group.display_items[0])
self.assertEqual(document_model.display_items[1], data_group.display_items[1])
def test_inserting_items_data_group_undo_redo(self):
with TestContext.create_memory_context() as test_context:
document_controller = test_context.create_document_controller()
document_model = document_controller.document_model
# setup three data items and put in a group
data_item1 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item3 = DataItem.DataItem(numpy.random.randn(4, 4))
data_item4 = DataItem.DataItem(numpy.random.randn(4, 4))
document_model.append_data_item(data_item1)
document_model.append_data_item(data_item3)
document_model.append_data_item(data_item4)
data_group = DataGroup.DataGroup()
display_item1 = document_model.get_display_item_for_data_item(data_item1)
display_item3 = document_model.get_display_item_for_data_item(data_item3)
display_item4 = document_model.get_display_item_for_data_item(data_item4)
data_group.append_display_item(display_item1)
data_group.append_display_item(display_item3)
document_model.append_data_group(data_group)
self.assertEqual(3, len(document_model.data_items))
self.assertEqual(2, len(data_group.display_items))
self.assertListEqual([data_item1, data_item3, data_item4], list(document_model.data_items))
self.assertListEqual([display_item1, display_item3], list(data_group.display_items))
# insert new items
data_item2 = DataItem.DataItem(numpy.random.randn(4, 4))
document_model.append_data_item(data_item2)
display_item2 = document_model.get_display_item_for_data_item(data_item2)
# hack until InsertDataGroupDisplayItemsCommand handles multiple display items, call it twice
command = DocumentController.DocumentController.InsertDataGroupDisplayItemsCommand(document_controller.document_model, data_group, 1, [display_item2, display_item4])
command.perform()
document_controller.push_undo_command(command)
self.assertEqual(4, len(document_model.data_items))
self.assertEqual(4, len(data_group.display_items))
self.assertListEqual([data_item1, data_item3, data_item4, data_item2], list(document_model.data_items))
self.assertListEqual([display_item1, display_item2, display_item4, display_item3], list(data_group.display_items))
# undo and check
document_controller.handle_undo()
self.assertEqual(4, len(document_model.data_items))
self.assertEqual(2, len(data_group.display_items))
self.assertListEqual([data_item1, data_item3, data_item4, data_item2], list(document_model.data_items))
self.assertListEqual([display_item1, display_item3], list(data_group.display_items))
# redo and check
document_controller.handle_redo()
data_item2 = document_model.data_items[3]
self.assertEqual(4, len(document_model.data_items))
self.assertEqual(4, len(data_group.display_items))
self.assertListEqual([data_item1, data_item3, data_item4, data_item2], list(document_model.data_items))
self.assertListEqual([display_item1, display_item2, display_item4, display_item3], list(data_group.display_items))
if __name__ == '__main__':
unittest.main()
| gpl-3.0 |
ProtractorNinja/qutebrowser | qutebrowser/misc/httpclient.py | 3 | 3706 | # vim: ft=python fileencoding=utf-8 sts=4 sw=4 et:
# Copyright 2014-2015 Florian Bruhin (The Compiler) <mail@qutebrowser.org>
#
# This file is part of qutebrowser.
#
# qutebrowser is free software: you can redistribute it and/or modify
# it under the terms of the GNU General Public License as published by
# the Free Software Foundation, either version 3 of the License, or
# (at your option) any later version.
#
# qutebrowser is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with qutebrowser. If not, see <http://www.gnu.org/licenses/>.
"""A HTTP client based on QNetworkAccessManager."""
import functools
import urllib.request
import urllib.parse
from PyQt5.QtCore import pyqtSignal, QObject, QTimer
from PyQt5.QtNetwork import (QNetworkAccessManager, QNetworkRequest,
QNetworkReply)
class HTTPClient(QObject):
"""A HTTP client based on QNetworkAccessManager.
Intended for APIs, automatically decodes data.
Attributes:
_nam: The QNetworkAccessManager used.
_timers: A {QNetworkReply: QTimer} dict.
Signals:
success: Emitted when the operation succeeded.
arg: The received data.
error: Emitted when the request failed.
arg: The error message, as string.
"""
success = pyqtSignal(str)
error = pyqtSignal(str)
def __init__(self, parent=None):
super().__init__(parent)
self._nam = QNetworkAccessManager(self)
self._timers = {}
def post(self, url, data=None):
"""Create a new POST request.
Args:
url: The URL to post to, as QUrl.
data: A dict of data to send.
"""
if data is None:
data = {}
encoded_data = urllib.parse.urlencode(data).encode('utf-8')
request = QNetworkRequest(url)
request.setHeader(QNetworkRequest.ContentTypeHeader,
'application/x-www-form-urlencoded;charset=utf-8')
reply = self._nam.post(request, encoded_data)
self._handle_reply(reply)
def get(self, url):
"""Create a new GET request.
Emits success/error when done.
Args:
url: The URL to access, as QUrl.
"""
request = QNetworkRequest(url)
reply = self._nam.get(request)
self._handle_reply(reply)
def _handle_reply(self, reply):
"""Handle a new QNetworkReply."""
if reply.isFinished():
self.on_reply_finished(reply)
else:
timer = QTimer(self)
timer.setInterval(10000)
timer.timeout.connect(reply.abort)
timer.start()
self._timers[reply] = timer
reply.finished.connect(functools.partial(
self.on_reply_finished, reply))
def on_reply_finished(self, reply):
"""Read the data and finish when the reply finished.
Args:
reply: The QNetworkReply which finished.
"""
timer = self._timers.pop(reply)
if timer is not None:
timer.stop()
timer.deleteLater()
if reply.error() != QNetworkReply.NoError:
self.error.emit(reply.errorString())
return
try:
data = bytes(reply.readAll()).decode('utf-8')
except UnicodeDecodeError:
self.error.emit("Invalid UTF-8 data received in reply!")
return
self.success.emit(data)
| gpl-3.0 |
ryfeus/lambda-packs | Tensorflow_OpenCV_Nightly/source/tensorflow/contrib/keras/api/keras/models/__init__.py | 56 | 1365 | # Copyright 2016 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Keras models API."""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
from tensorflow.contrib.keras.python.keras.models import load_model
from tensorflow.contrib.keras.python.keras.models import Model
from tensorflow.contrib.keras.python.keras.models import model_from_config
from tensorflow.contrib.keras.python.keras.models import model_from_json
from tensorflow.contrib.keras.python.keras.models import model_from_yaml
from tensorflow.contrib.keras.python.keras.models import save_model
from tensorflow.contrib.keras.python.keras.models import Sequential
del absolute_import
del division
del print_function
| mit |
shakalaca/ASUS_ZenFone_A450CG | linux/kernel/tools/perf/scripts/python/sctop.py | 11175 | 1924 | # system call top
# (c) 2010, Tom Zanussi <tzanussi@gmail.com>
# Licensed under the terms of the GNU GPL License version 2
#
# Periodically displays system-wide system call totals, broken down by
# syscall. If a [comm] arg is specified, only syscalls called by
# [comm] are displayed. If an [interval] arg is specified, the display
# will be refreshed every [interval] seconds. The default interval is
# 3 seconds.
import os, sys, thread, time
sys.path.append(os.environ['PERF_EXEC_PATH'] + \
'/scripts/python/Perf-Trace-Util/lib/Perf/Trace')
from perf_trace_context import *
from Core import *
from Util import *
usage = "perf script -s sctop.py [comm] [interval]\n";
for_comm = None
default_interval = 3
interval = default_interval
if len(sys.argv) > 3:
sys.exit(usage)
if len(sys.argv) > 2:
for_comm = sys.argv[1]
interval = int(sys.argv[2])
elif len(sys.argv) > 1:
try:
interval = int(sys.argv[1])
except ValueError:
for_comm = sys.argv[1]
interval = default_interval
syscalls = autodict()
def trace_begin():
thread.start_new_thread(print_syscall_totals, (interval,))
pass
def raw_syscalls__sys_enter(event_name, context, common_cpu,
common_secs, common_nsecs, common_pid, common_comm,
id, args):
if for_comm is not None:
if common_comm != for_comm:
return
try:
syscalls[id] += 1
except TypeError:
syscalls[id] = 1
def print_syscall_totals(interval):
while 1:
clear_term()
if for_comm is not None:
print "\nsyscall events for %s:\n\n" % (for_comm),
else:
print "\nsyscall events:\n\n",
print "%-40s %10s\n" % ("event", "count"),
print "%-40s %10s\n" % ("----------------------------------------", \
"----------"),
for id, val in sorted(syscalls.iteritems(), key = lambda(k, v): (v, k), \
reverse = True):
try:
print "%-40s %10d\n" % (syscall_name(id), val),
except TypeError:
pass
syscalls.clear()
time.sleep(interval)
| gpl-2.0 |
yongshengwang/hue | desktop/core/ext-py/python-openid-2.2.5/openid/oidutil.py | 143 | 5270 | """This module contains general utility code that is used throughout
the library.
For users of this library, the C{L{log}} function is probably the most
interesting.
"""
__all__ = ['log', 'appendArgs', 'toBase64', 'fromBase64', 'autoSubmitHTML']
import binascii
import sys
import urlparse
from urllib import urlencode
elementtree_modules = [
'lxml.etree',
'xml.etree.cElementTree',
'xml.etree.ElementTree',
'cElementTree',
'elementtree.ElementTree',
]
def autoSubmitHTML(form, title='OpenID transaction in progress'):
return """
<html>
<head>
<title>%s</title>
</head>
<body onload="document.forms[0].submit();">
%s
<script>
var elements = document.forms[0].elements;
for (var i = 0; i < elements.length; i++) {
elements[i].style.display = "none";
}
</script>
</body>
</html>
""" % (title, form)
def importElementTree(module_names=None):
"""Find a working ElementTree implementation, trying the standard
places that such a thing might show up.
>>> ElementTree = importElementTree()
@param module_names: The names of modules to try to use as
ElementTree. Defaults to C{L{elementtree_modules}}
@returns: An ElementTree module
"""
if module_names is None:
module_names = elementtree_modules
for mod_name in module_names:
try:
ElementTree = __import__(mod_name, None, None, ['unused'])
except ImportError:
pass
else:
# Make sure it can actually parse XML
try:
ElementTree.XML('<unused/>')
except (SystemExit, MemoryError, AssertionError):
raise
except:
why = sys.exc_info()[1]
log('Not using ElementTree library %r because it failed to '
'parse a trivial document: %s' % (mod_name, why))
else:
return ElementTree
else:
raise ImportError('No ElementTree library found. '
'You may need to install one. '
'Tried importing %r' % (module_names,)
)
def log(message, level=0):
"""Handle a log message from the OpenID library.
This implementation writes the string it to C{sys.stderr},
followed by a newline.
Currently, the library does not use the second parameter to this
function, but that may change in the future.
To install your own logging hook::
from openid import oidutil
def myLoggingFunction(message, level):
...
oidutil.log = myLoggingFunction
@param message: A string containing a debugging message from the
OpenID library
@type message: str
@param level: The severity of the log message. This parameter is
currently unused, but in the future, the library may indicate
more important information with a higher level value.
@type level: int or None
@returns: Nothing.
"""
sys.stderr.write(message)
sys.stderr.write('\n')
def appendArgs(url, args):
"""Append query arguments to a HTTP(s) URL. If the URL already has
query arguemtns, these arguments will be added, and the existing
arguments will be preserved. Duplicate arguments will not be
detected or collapsed (both will appear in the output).
@param url: The url to which the arguments will be appended
@type url: str
@param args: The query arguments to add to the URL. If a
dictionary is passed, the items will be sorted before
appending them to the URL. If a sequence of pairs is passed,
the order of the sequence will be preserved.
@type args: A dictionary from string to string, or a sequence of
pairs of strings.
@returns: The URL with the parameters added
@rtype: str
"""
if hasattr(args, 'items'):
args = args.items()
args.sort()
else:
args = list(args)
if len(args) == 0:
return url
if '?' in url:
sep = '&'
else:
sep = '?'
# Map unicode to UTF-8 if present. Do not make any assumptions
# about the encodings of plain bytes (str).
i = 0
for k, v in args:
if type(k) is not str:
k = k.encode('UTF-8')
if type(v) is not str:
v = v.encode('UTF-8')
args[i] = (k, v)
i += 1
return '%s%s%s' % (url, sep, urlencode(args))
def toBase64(s):
"""Represent string s as base64, omitting newlines"""
return binascii.b2a_base64(s)[:-1]
def fromBase64(s):
try:
return binascii.a2b_base64(s)
except binascii.Error, why:
# Convert to a common exception type
raise ValueError(why[0])
class Symbol(object):
"""This class implements an object that compares equal to others
of the same type that have the same name. These are distict from
str or unicode objects.
"""
def __init__(self, name):
self.name = name
def __eq__(self, other):
return type(self) is type(other) and self.name == other.name
def __ne__(self, other):
return not (self == other)
def __hash__(self):
return hash((self.__class__, self.name))
def __repr__(self):
return '<Symbol %s>' % (self.name,)
| apache-2.0 |
Sodki/ansible | lib/ansible/modules/network/openswitch/ops_command.py | 69 | 7371 | #!/usr/bin/python
#
# This file is part of Ansible
#
# Ansible is free software: you can redistribute it and/or modify
# it under the terms of the GNU General Public License as published by
# the Free Software Foundation, either version 3 of the License, or
# (at your option) any later version.
#
# Ansible is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with Ansible. If not, see <http://www.gnu.org/licenses/>.
#
ANSIBLE_METADATA = {'metadata_version': '1.0',
'status': ['preview'],
'supported_by': 'community'}
DOCUMENTATION = """
---
module: ops_command
version_added: "2.1"
author: "Peter Sprygada (@privateip)"
short_description: Run arbitrary commands on OpenSwitch devices.
description:
- Sends arbitrary commands to an OpenSwitch node and returns the results
read from the device. This module includes an
argument that will cause the module to wait for a specific condition
before returning or timing out if the condition is not met.
extends_documentation_fragment: openswitch
options:
commands:
description:
- List of commands to send to the remote ops device over the
configured provider. The resulting output from the command
is returned. If the I(wait_for) argument is provided, the
module is not returned until the condition is satisfied or
the number of retires as expired.
required: true
wait_for:
description:
- List of conditions to evaluate against the output of the
command. The task will wait for each condition to be true
before moving forward. If the conditional is not true
within the configured number of retries, the task fails.
See examples.
required: false
default: null
aliases: ['waitfor']
version_added: "2.2"
match:
description:
- The I(match) argument is used in conjunction with the
I(wait_for) argument to specify the match policy. Valid
values are C(all) or C(any). If the value is set to C(all)
then all conditionals in the I(wait_for) must be satisfied. If
the value is set to C(any) then only one of the values must be
satisfied.
required: false
default: all
choices: ['any', 'all']
version_added: "2.2"
retries:
description:
- Specifies the number of retries a command should by tried
before it is considered failed. The command is run on the
target device every retry and evaluated against the
I(wait_for) conditions.
required: false
default: 10
interval:
description:
- Configures the interval in seconds to wait between I(retries)
of the command. If the command does not pass the specified
conditions, the interval indicates how long to wait before
trying the command again.
required: false
default: 1
"""
EXAMPLES = """
# Note: examples below use the following provider dict to handle
# transport and authentication to the node.
---
vars:
cli:
host: "{{ inventory_hostname }}"
username: netop
password: netop
transport: cli
---
- ops_command:
commands:
- show version
provider: "{{ cli }}"
- ops_command:
commands:
- show version
wait_for:
- "result[0] contains OpenSwitch"
provider: "{{ cli }}"
- ops_command:
commands:
- show version
- show interfaces
provider: "{{ cli }}"
"""
RETURN = """
stdout:
description: the set of responses from the commands
returned: always
type: list
sample: ['...', '...']
stdout_lines:
description: The value of stdout split into a list
returned: always
type: list
sample: [['...', '...'], ['...'], ['...']]
failed_conditions:
description: the conditionals that failed
returned: failed
type: list
sample: ['...', '...']
"""
import ansible.module_utils.openswitch
from ansible.module_utils.basic import get_exception
from ansible.module_utils.netcli import CommandRunner
from ansible.module_utils.netcli import AddCommandError, FailedConditionsError
from ansible.module_utils.network import NetworkModule, NetworkError
from ansible.module_utils.six import string_types
VALID_KEYS = ['command', 'prompt', 'response']
def to_lines(stdout):
for item in stdout:
if isinstance(item, string_types):
item = str(item).split('\n')
yield item
def parse_commands(module):
for cmd in module.params['commands']:
if isinstance(cmd, string_types):
cmd = dict(command=cmd, output=None)
elif 'command' not in cmd:
module.fail_json(msg='command keyword argument is required')
elif not set(cmd.keys()).issubset(VALID_KEYS):
module.fail_json(msg='unknown keyword specified')
yield cmd
def main():
spec = dict(
# { command: <str>, prompt: <str>, response: <str> }
commands=dict(type='list', required=True),
wait_for=dict(type='list', aliases=['waitfor']),
match=dict(default='all', choices=['all', 'any']),
retries=dict(default=10, type='int'),
interval=dict(default=1, type='int')
)
module = NetworkModule(argument_spec=spec,
connect_on_load=False,
supports_check_mode=True)
commands = list(parse_commands(module))
conditionals = module.params['wait_for'] or list()
warnings = list()
runner = CommandRunner(module)
for cmd in commands:
if module.check_mode and not cmd['command'].startswith('show'):
warnings.append('only show commands are supported when using '
'check mode, not executing `%s`' % cmd['command'])
else:
if cmd['command'].startswith('conf'):
module.fail_json(msg='ops_command does not support running '
'config mode commands. Please use '
'ops_config instead')
try:
runner.add_command(**cmd)
except AddCommandError:
exc = get_exception()
warnings.append('duplicate command detected: %s' % cmd)
for item in conditionals:
runner.add_conditional(item)
runner.retries = module.params['retries']
runner.interval = module.params['interval']
runner.match = module.params['match']
try:
runner.run()
except FailedConditionsError:
exc = get_exception()
module.fail_json(msg=str(exc), failed_conditions=exc.failed_conditions)
except NetworkError:
exc = get_exception()
module.fail_json(msg=str(exc))
result = dict(changed=False, stdout=list())
for cmd in commands:
try:
output = runner.get_command(cmd['command'])
except ValueError:
output = 'command not executed due to check_mode, see warnings'
result['stdout'].append(output)
result['warnings'] = warnings
result['stdout_lines'] = list(to_lines(result['stdout']))
module.exit_json(**result)
if __name__ == '__main__':
main()
| gpl-3.0 |
andmos/ansible | lib/ansible/modules/cloud/amazon/elb_network_lb.py | 28 | 15357 | #!/usr/bin/python
# Copyright: (c) 2018, Rob White (@wimnat)
# GNU General Public License v3.0+ (see COPYING or https://www.gnu.org/licenses/gpl-3.0.txt)
ANSIBLE_METADATA = {'metadata_version': '1.1',
'status': ['preview'],
'supported_by': 'community'}
DOCUMENTATION = '''
---
module: elb_network_lb
short_description: Manage a Network Load Balancer
description:
- Manage an AWS Network Elastic Load Balancer. See
U(https://aws.amazon.com/blogs/aws/new-network-load-balancer-effortless-scaling-to-millions-of-requests-per-second/) for details.
version_added: "2.6"
requirements: [ boto3 ]
author: "Rob White (@wimnat)"
options:
cross_zone_load_balancing:
description:
- Indicates whether cross-zone load balancing is enabled.
required: false
default: no
type: bool
deletion_protection:
description:
- Indicates whether deletion protection for the ELB is enabled.
required: false
default: no
type: bool
listeners:
description:
- A list of dicts containing listeners to attach to the ELB. See examples for detail of the dict required. Note that listener keys
are CamelCased.
required: false
name:
description:
- The name of the load balancer. This name must be unique within your AWS account, can have a maximum of 32 characters, must contain only alphanumeric
characters or hyphens, and must not begin or end with a hyphen.
required: true
purge_listeners:
description:
- If yes, existing listeners will be purged from the ELB to match exactly what is defined by I(listeners) parameter. If the I(listeners) parameter is
not set then listeners will not be modified
default: yes
type: bool
purge_tags:
description:
- If yes, existing tags will be purged from the resource to match exactly what is defined by I(tags) parameter. If the I(tags) parameter is not set then
tags will not be modified.
required: false
default: yes
type: bool
subnet_mappings:
description:
- A list of dicts containing the IDs of the subnets to attach to the load balancer. You can also specify the allocation ID of an Elastic IP
to attach to the load balancer. You can specify one Elastic IP address per subnet. This parameter is mutually exclusive with I(subnets)
required: false
subnets:
description:
- A list of the IDs of the subnets to attach to the load balancer. You can specify only one subnet per Availability Zone. You must specify subnets from
at least two Availability Zones. Required if state=present. This parameter is mutually exclusive with I(subnet_mappings)
required: false
scheme:
description:
- Internet-facing or internal load balancer. An ELB scheme can not be modified after creation.
required: false
default: internet-facing
choices: [ 'internet-facing', 'internal' ]
state:
description:
- Create or destroy the load balancer.
required: true
choices: [ 'present', 'absent' ]
tags:
description:
- A dictionary of one or more tags to assign to the load balancer.
required: false
wait:
description:
- Whether or not to wait for the network load balancer to reach the desired state.
type: bool
wait_timeout:
description:
- The duration in seconds to wait, used in conjunction with I(wait).
extends_documentation_fragment:
- aws
- ec2
notes:
- Listeners are matched based on port. If a listener's port is changed then a new listener will be created.
- Listener rules are matched based on priority. If a rule's priority is changed then a new rule will be created.
'''
EXAMPLES = '''
# Note: These examples do not set authentication details, see the AWS Guide for details.
# Create an ELB and attach a listener
- elb_network_lb:
name: myelb
subnets:
- subnet-012345678
- subnet-abcdef000
listeners:
- Protocol: TCP # Required. The protocol for connections from clients to the load balancer (Only TCP is available) (case-sensitive).
Port: 80 # Required. The port on which the load balancer is listening.
DefaultActions:
- Type: forward # Required. Only 'forward' is accepted at this time
TargetGroupName: mytargetgroup # Required. The name of the target group
state: present
# Create an ELB with an attached Elastic IP address
- elb_network_lb:
name: myelb
subnet_mappings:
- SubnetId: subnet-012345678
AllocationId: eipalloc-aabbccdd
listeners:
- Protocol: TCP # Required. The protocol for connections from clients to the load balancer (Only TCP is available) (case-sensitive).
Port: 80 # Required. The port on which the load balancer is listening.
DefaultActions:
- Type: forward # Required. Only 'forward' is accepted at this time
TargetGroupName: mytargetgroup # Required. The name of the target group
state: present
# Remove an ELB
- elb_network_lb:
name: myelb
state: absent
'''
RETURN = '''
availability_zones:
description: The Availability Zones for the load balancer.
returned: when state is present
type: list
sample: "[{'subnet_id': 'subnet-aabbccddff', 'zone_name': 'ap-southeast-2a', 'load_balancer_addresses': []}]"
canonical_hosted_zone_id:
description: The ID of the Amazon Route 53 hosted zone associated with the load balancer.
returned: when state is present
type: str
sample: ABCDEF12345678
created_time:
description: The date and time the load balancer was created.
returned: when state is present
type: str
sample: "2015-02-12T02:14:02+00:00"
deletion_protection_enabled:
description: Indicates whether deletion protection is enabled.
returned: when state is present
type: str
sample: true
dns_name:
description: The public DNS name of the load balancer.
returned: when state is present
type: str
sample: internal-my-elb-123456789.ap-southeast-2.elb.amazonaws.com
idle_timeout_timeout_seconds:
description: The idle timeout value, in seconds.
returned: when state is present
type: str
sample: 60
ip_address_type:
description: The type of IP addresses used by the subnets for the load balancer.
returned: when state is present
type: str
sample: ipv4
listeners:
description: Information about the listeners.
returned: when state is present
type: complex
contains:
listener_arn:
description: The Amazon Resource Name (ARN) of the listener.
returned: when state is present
type: str
sample: ""
load_balancer_arn:
description: The Amazon Resource Name (ARN) of the load balancer.
returned: when state is present
type: str
sample: ""
port:
description: The port on which the load balancer is listening.
returned: when state is present
type: int
sample: 80
protocol:
description: The protocol for connections from clients to the load balancer.
returned: when state is present
type: str
sample: HTTPS
certificates:
description: The SSL server certificate.
returned: when state is present
type: complex
contains:
certificate_arn:
description: The Amazon Resource Name (ARN) of the certificate.
returned: when state is present
type: str
sample: ""
ssl_policy:
description: The security policy that defines which ciphers and protocols are supported.
returned: when state is present
type: str
sample: ""
default_actions:
description: The default actions for the listener.
returned: when state is present
type: str
contains:
type:
description: The type of action.
returned: when state is present
type: str
sample: ""
target_group_arn:
description: The Amazon Resource Name (ARN) of the target group.
returned: when state is present
type: str
sample: ""
load_balancer_arn:
description: The Amazon Resource Name (ARN) of the load balancer.
returned: when state is present
type: str
sample: arn:aws:elasticloadbalancing:ap-southeast-2:0123456789:loadbalancer/app/my-elb/001122334455
load_balancer_name:
description: The name of the load balancer.
returned: when state is present
type: str
sample: my-elb
load_balancing_cross_zone_enabled:
description: Indicates whether cross-zone load balancing is enabled.
returned: when state is present
type: str
sample: true
scheme:
description: Internet-facing or internal load balancer.
returned: when state is present
type: str
sample: internal
state:
description: The state of the load balancer.
returned: when state is present
type: dict
sample: "{'code': 'active'}"
tags:
description: The tags attached to the load balancer.
returned: when state is present
type: dict
sample: "{
'Tag': 'Example'
}"
type:
description: The type of load balancer.
returned: when state is present
type: str
sample: network
vpc_id:
description: The ID of the VPC for the load balancer.
returned: when state is present
type: str
sample: vpc-0011223344
'''
from ansible.module_utils.aws.core import AnsibleAWSModule
from ansible.module_utils.ec2 import camel_dict_to_snake_dict, boto3_tag_list_to_ansible_dict, compare_aws_tags
from ansible.module_utils.aws.elbv2 import NetworkLoadBalancer, ELBListeners, ELBListener
def create_or_update_elb(elb_obj):
"""Create ELB or modify main attributes. json_exit here"""
if elb_obj.elb:
# ELB exists so check subnets, security groups and tags match what has been passed
# Subnets
if not elb_obj.compare_subnets():
elb_obj.modify_subnets()
# Tags - only need to play with tags if tags parameter has been set to something
if elb_obj.tags is not None:
# Delete necessary tags
tags_need_modify, tags_to_delete = compare_aws_tags(boto3_tag_list_to_ansible_dict(elb_obj.elb['tags']),
boto3_tag_list_to_ansible_dict(elb_obj.tags), elb_obj.purge_tags)
if tags_to_delete:
elb_obj.delete_tags(tags_to_delete)
# Add/update tags
if tags_need_modify:
elb_obj.modify_tags()
else:
# Create load balancer
elb_obj.create_elb()
# ELB attributes
elb_obj.update_elb_attributes()
elb_obj.modify_elb_attributes()
# Listeners
listeners_obj = ELBListeners(elb_obj.connection, elb_obj.module, elb_obj.elb['LoadBalancerArn'])
listeners_to_add, listeners_to_modify, listeners_to_delete = listeners_obj.compare_listeners()
# Delete listeners
for listener_to_delete in listeners_to_delete:
listener_obj = ELBListener(elb_obj.connection, elb_obj.module, listener_to_delete, elb_obj.elb['LoadBalancerArn'])
listener_obj.delete()
listeners_obj.changed = True
# Add listeners
for listener_to_add in listeners_to_add:
listener_obj = ELBListener(elb_obj.connection, elb_obj.module, listener_to_add, elb_obj.elb['LoadBalancerArn'])
listener_obj.add()
listeners_obj.changed = True
# Modify listeners
for listener_to_modify in listeners_to_modify:
listener_obj = ELBListener(elb_obj.connection, elb_obj.module, listener_to_modify, elb_obj.elb['LoadBalancerArn'])
listener_obj.modify()
listeners_obj.changed = True
# If listeners changed, mark ELB as changed
if listeners_obj.changed:
elb_obj.changed = True
# Get the ELB again
elb_obj.update()
# Get the ELB listeners again
listeners_obj.update()
# Update the ELB attributes
elb_obj.update_elb_attributes()
# Convert to snake_case and merge in everything we want to return to the user
snaked_elb = camel_dict_to_snake_dict(elb_obj.elb)
snaked_elb.update(camel_dict_to_snake_dict(elb_obj.elb_attributes))
snaked_elb['listeners'] = []
for listener in listeners_obj.current_listeners:
snaked_elb['listeners'].append(camel_dict_to_snake_dict(listener))
# Change tags to ansible friendly dict
snaked_elb['tags'] = boto3_tag_list_to_ansible_dict(snaked_elb['tags'])
elb_obj.module.exit_json(changed=elb_obj.changed, **snaked_elb)
def delete_elb(elb_obj):
if elb_obj.elb:
elb_obj.delete()
elb_obj.module.exit_json(changed=elb_obj.changed)
def main():
argument_spec = (
dict(
cross_zone_load_balancing=dict(type='bool'),
deletion_protection=dict(type='bool'),
listeners=dict(type='list',
elements='dict',
options=dict(
Protocol=dict(type='str', required=True),
Port=dict(type='int', required=True),
SslPolicy=dict(type='str'),
Certificates=dict(type='list'),
DefaultActions=dict(type='list', required=True)
)
),
name=dict(required=True, type='str'),
purge_listeners=dict(default=True, type='bool'),
purge_tags=dict(default=True, type='bool'),
subnets=dict(type='list'),
subnet_mappings=dict(type='list'),
scheme=dict(default='internet-facing', choices=['internet-facing', 'internal']),
state=dict(choices=['present', 'absent'], type='str'),
tags=dict(type='dict'),
wait_timeout=dict(type='int'),
wait=dict(type='bool')
)
)
module = AnsibleAWSModule(argument_spec=argument_spec,
mutually_exclusive=[['subnets', 'subnet_mappings']])
# Check for subnets or subnet_mappings if state is present
state = module.params.get("state")
if state == 'present':
if module.params.get("subnets") is None and module.params.get("subnet_mappings") is None:
module.fail_json(msg="'subnets' or 'subnet_mappings' is required when state=present")
# Quick check of listeners parameters
listeners = module.params.get("listeners")
if listeners is not None:
for listener in listeners:
for key in listener.keys():
if key == 'Protocol' and listener[key] != 'TCP':
module.fail_json(msg="'Protocol' must be 'TCP'")
connection = module.client('elbv2')
connection_ec2 = module.client('ec2')
elb = NetworkLoadBalancer(connection, connection_ec2, module)
if state == 'present':
create_or_update_elb(elb)
else:
delete_elb(elb)
if __name__ == '__main__':
main()
| gpl-3.0 |
RO-ny9/python-for-android | python3-alpha/python3-src/Lib/test/test_pep3120.py | 49 | 1280 | # This file is marked as binary in the CVS, to prevent MacCVS from recoding it.
import unittest
from test import support
class PEP3120Test(unittest.TestCase):
def test_pep3120(self):
self.assertEqual(
"Питон".encode("utf-8"),
b'\xd0\x9f\xd0\xb8\xd1\x82\xd0\xbe\xd0\xbd'
)
self.assertEqual(
"\П".encode("utf-8"),
b'\\\xd0\x9f'
)
def test_badsyntax(self):
try:
import test.badsyntax_pep3120
except SyntaxError as msg:
msg = str(msg)
self.assertTrue('UTF-8' in msg or 'utf8' in msg)
else:
self.fail("expected exception didn't occur")
class BuiltinCompileTests(unittest.TestCase):
# Issue 3574.
def test_latin1(self):
# Allow compile() to read Latin-1 source.
source_code = '# coding: Latin-1\nu = "Ç"\n'.encode("Latin-1")
try:
code = compile(source_code, '<dummy>', 'exec')
except SyntaxError:
self.fail("compile() cannot handle Latin-1 source")
ns = {}
exec(code, ns)
self.assertEqual('Ç', ns['u'])
def test_main():
support.run_unittest(PEP3120Test, BuiltinCompileTests)
if __name__=="__main__":
test_main()
| apache-2.0 |
alfa-addon/addon | plugin.video.alfa/channels/kindor.py | 1 | 10042 | # -*- coding: utf-8 -*-
# -*- Channel InkaPelis -*-
# -*- Created for Alfa-addon -*-
# -*- By the Alfa Develop Group -*-
import re
from core import tmdb
from core import httptools
from core import jsontools
from core.item import Item
from core import scrapertools
from core import servertools
from bs4 import BeautifulSoup
from channelselector import get_thumb
from platformcode import config, logger
from channels import filtertools, autoplay
import sys
import base64
PY3 = False
if sys.version_info[0] >= 3: PY3 = True; unicode = str; unichr = chr; long = int
IDIOMAS = {'0': 'LAT', '1': 'CAST', '2': 'VOSE'}
list_language = list(IDIOMAS.values())
list_quality = []
list_servers = [
'fembed',
'streampe',
'gounlimited',
'mystream',
'gvideo'
]
canonical = {
'channel': 'kindor',
'host': config.get_setting("current_host", 'kindor', default=''),
'host_alt': ["https://kindor.pro/"],
'host_black_list': ["https://kindor.vip/", "https://kindor.me/"],
'pattern': '<a\s*href="([^"]+)"\s*class="healog"\s*aria-label="[^"]+">',
'CF': False, 'CF_test': False, 'alfa_s': True
}
host = canonical['host'] or canonical['host_alt'][0]
host_save = host
def mainlist(item):
logger.info()
autoplay.init(item.channel, list_servers, list_quality)
itemlist = list()
itemlist.append(Item(channel=item.channel, title='Peliculas', action='sub_menu',
thumbnail=get_thumb('movies', auto=True)))
itemlist.append(Item(channel=item.channel, title='Series', url=host + 'series', action='sub_menu',
thumbnail=get_thumb('tvshows', auto=True)))
itemlist.append(Item(channel=item.channel, title='Novedades', action='list_all', url=host,
thumbnail=get_thumb('newest', auto=True)))
itemlist.append(Item(channel=item.channel, title='Destacadas', action='list_all', url=host + "destacadas",
thumbnail=get_thumb('hot', auto=True)))
itemlist.append(Item(channel=item.channel, title='Recomendadas', action='list_all', url=host + "recomendadas",
thumbnail=get_thumb('recomended', auto=True)))
itemlist.append(Item(channel=item.channel, title="Buscar...", action="search", url=host + 'buscar/',
thumbnail=get_thumb("search", auto=True)))
autoplay.show_option(item.channel, itemlist)
return itemlist
def sub_menu(item):
logger.info()
itemlist = list()
mode = item.title.lower()
if item.title == "Peliculas":
itemlist.append(Item(channel=item.channel, title='Ultimas', url=host + 'peliculas/estrenos', action='list_all',
thumbnail=get_thumb('all', auto=True)))
itemlist.append(Item(channel=item.channel, title='Todas', url=host + mode, action='list_all',
thumbnail=get_thumb('all', auto=True)))
itemlist.append(Item(channel=item.channel, title='Generos', action='section', mode=mode,
thumbnail=get_thumb('genres', auto=True)))
# itemlist.append(Item(channel=item.channel, title='Por Año', action='section',
# thumbnail=get_thumb('year', auto=True)))
return itemlist
def create_soup(url, referer=None, unescape=False):
logger.info()
if referer:
data = httptools.downloadpage(url, headers={'Referer':referer}, canonical=canonical).data
else:
data = httptools.downloadpage(url, canonical=canonical).data
if unescape:
data = scrapertools.unescape(data)
soup = BeautifulSoup(data, "html5lib", from_encoding="utf-8")
return soup
def section(item):
logger.info()
itemlist = list()
base_url = "%s%s" % (host, item.mode)
soup = create_soup(base_url)
if item.title == "Generos":
matches = soup.find("ul", class_="dropm")
else:
matches = soup.find("ul", class_="releases scrolling")
for elem in matches.find_all("li"):
url = elem.a["href"]
title = elem.a.text
itemlist.append(Item(channel=item.channel, title=title, action="list_all", url=url, first=0))
return itemlist
def list_all(item):
logger.info()
itemlist = list()
soup = create_soup(item.url)
matches = soup.find("section", class_="coys").find_all("div", class_="pogd")
for elem in matches:
url = elem.h3.a["href"]
title = elem.h3.text
try:
thumb = elem.img["data-src"]
except:
thumb = elem.img["src"]
year = "-"
new_item = Item(channel=item.channel, title=title, url=url, thumbnail=thumb,
infoLabels={"year": year})
if "serie/" in url:
new_item.contentSerieName = title
new_item.action = "seasons"
else:
new_item.contentTitle = title
new_item.action = "findvideos"
itemlist.append(new_item)
tmdb.set_infoLabels_itemlist(itemlist, True)
try:
url_next_page = soup.find("div", class_="pazza").find("a", class_="ffas").next_sibling["href"]
except:
return itemlist
if url_next_page and len(matches) > 16:
itemlist.append(Item(channel=item.channel, title="Siguiente >>", url=url_next_page, action='list_all'))
return itemlist
def seasons(item):
logger.info()
itemlist = list()
data = httptools.downloadpage(item.url, canonical=canonical).data
fom, hash = scrapertools.find_single_match(data, "fom:(.*?),hash:'([^']+)'")
json_data = jsontools.load(fom)
infoLabels = item.infoLabels
for elem in json_data:
season = elem
title = "Temporada %s" % season
infoLabels["season"] = season
epi_data = json_data[elem]
itemlist.append(Item(channel=item.channel, title=title, action='episodesxseasons', epi_data=epi_data, hash=hash,
context=filtertools.context(item, list_language, list_quality), infoLabels=infoLabels))
tmdb.set_infoLabels_itemlist(itemlist, True)
itemlist = sorted(itemlist, key=lambda i: i.contentSeason)
if config.get_videolibrary_support() and len(itemlist) > 0:
itemlist.append(
Item(channel=item.channel, title='[COLOR yellow]Añadir esta serie a la videoteca[/COLOR]', url=item.url,
action="add_serie_to_library", extra="episodios", contentSerieName=item.contentSerieName))
return itemlist
def episodios(item):
logger.info()
itemlist = []
templist = seasons(item)
for tempitem in templist:
itemlist += episodesxseasons(tempitem)
return itemlist
def episodesxseasons(item):
logger.info()
itemlist = list()
matches = item.epi_data
infoLabels = item.infoLabels
season = infoLabels["season"]
for epi, info in matches.items():
title = "%sx%s - %s" % (season, epi, info["name"])
json_data = info["all"]
infoLabels["episode"] = epi
itemlist.append(Item(channel=item.channel, title=title, json_data=json_data, action='findvideos',
infoLabels=infoLabels, hash=item.hash))
tmdb.set_infoLabels_itemlist(itemlist, True)
itemlist = sorted(itemlist, key=lambda i: i.contentEpisodeNumber)
return itemlist
def findvideos(item):
logger.info()
itemlist = list()
if item.json_data:
json_data = item.json_data
hash = item.hash
else:
data = httptools.downloadpage(item.url, canonical=canonical).data
json_data = jsontools.load(scrapertools.find_single_match(data, "fom:(\{.*?})"))
hash = scrapertools.find_single_match(data, "hash:'([^']+)'")
for url_info in json_data:
lang = url_info
url = "%s?h=%s" % (base64.b64decode(json_data[url_info]), hash)
itemlist.append(Item(channel=item.channel, title='%s', action='play', url=url,
language=IDIOMAS.get(lang, "VOSE"), infoLabels=item.infoLabels))
itemlist = servertools.get_servers_itemlist(itemlist, lambda i: i.title % i.server)
# Requerido para FilterTools
itemlist = filtertools.get_links(itemlist, item, list_language)
# Requerido para AutoPlay
autoplay.start(itemlist, item)
if config.get_videolibrary_support() and len(itemlist) > 0 and item.extra != 'findvideos':
itemlist.append(Item(channel=item.channel, title='[COLOR yellow]Añadir esta pelicula a la videoteca[/COLOR]',
url=item.url, action="add_pelicula_to_library", extra="findvideos",
contentTitle=item.contentTitle))
return itemlist
def search(item, texto):
logger.info()
try:
texto = texto.replace(" ", "+")
item.url = item.url + texto
if texto != '':
return list_all(item)
else:
return []
# Se captura la excepción, para no interrumpir al buscador global si un canal falla
except:
for line in sys.exc_info():
logger.error("%s" % line)
return []
def newest(categoria):
logger.info()
item = Item()
try:
if categoria in ['peliculas']:
item.url = host + 'peliculas/estrenos'
elif categoria == 'infantiles':
item.url = host + 'peliculas/animacion/'
elif categoria == 'terror':
item.url = host + 'peliculas/terror/'
itemlist = list_all(item)
if itemlist[-1].title == 'Siguiente >>':
itemlist.pop()
except:
import sys
for line in sys.exc_info():
logger.error("{0}".format(line))
return []
return itemlist
| gpl-3.0 |
pnorman/mapnik | scons/scons-local-2.4.1/SCons/Tool/sgicc.py | 6 | 1849 | """SCons.Tool.sgicc
Tool-specific initialization for MIPSPro cc on SGI.
There normally shouldn't be any need to import this module directly.
It will usually be imported through the generic SCons.Tool.Tool()
selection method.
"""
#
# Copyright (c) 2001 - 2015 The SCons Foundation
#
# Permission is hereby granted, free of charge, to any person obtaining
# a copy of this software and associated documentation files (the
# "Software"), to deal in the Software without restriction, including
# without limitation the rights to use, copy, modify, merge, publish,
# distribute, sublicense, and/or sell copies of the Software, and to
# permit persons to whom the Software is furnished to do so, subject to
# the following conditions:
#
# The above copyright notice and this permission notice shall be included
# in all copies or substantial portions of the Software.
#
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY
# KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE
# WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
# NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
# LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
# OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
# WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
#
__revision__ = "src/engine/SCons/Tool/sgicc.py rel_2.4.1:3453:73fefd3ea0b0 2015/11/09 03:25:05 bdbaddog"
import cc
def generate(env):
"""Add Builders and construction variables for gcc to an Environment."""
cc.generate(env)
env['CXX'] = 'CC'
env['SHOBJSUFFIX'] = '.o'
env['STATIC_AND_SHARED_OBJECTS_ARE_THE_SAME'] = 1
def exists(env):
return env.Detect('cc')
# Local Variables:
# tab-width:4
# indent-tabs-mode:nil
# End:
# vim: set expandtab tabstop=4 shiftwidth=4:
| lgpl-2.1 |
RO-ny9/python-for-android | python-modules/twisted/twisted/python/dist.py | 60 | 12329 | """
Distutils convenience functionality.
Don't use this outside of Twisted.
Maintainer: Christopher Armstrong
"""
import sys, os
from distutils.command import build_scripts, install_data, build_ext, build_py
from distutils.errors import CompileError
from distutils import core
from distutils.core import Extension
twisted_subprojects = ["conch", "lore", "mail", "names",
"news", "pair", "runner", "web", "web2",
"words", "vfs"]
class ConditionalExtension(Extension):
"""
An extension module that will only be compiled if certain conditions are
met.
@param condition: A callable of one argument which returns True or False to
indicate whether the extension should be built. The argument is an
instance of L{build_ext_twisted}, which has useful methods for checking
things about the platform.
"""
def __init__(self, *args, **kwargs):
self.condition = kwargs.pop("condition", lambda builder: True)
Extension.__init__(self, *args, **kwargs)
def setup(**kw):
"""
An alternative to distutils' setup() which is specially designed
for Twisted subprojects.
Pass twisted_subproject=projname if you want package and data
files to automatically be found for you.
@param conditionalExtensions: Extensions to optionally build.
@type conditionalExtensions: C{list} of L{ConditionalExtension}
"""
return core.setup(**get_setup_args(**kw))
def get_setup_args(**kw):
if 'twisted_subproject' in kw:
if 'twisted' not in os.listdir('.'):
raise RuntimeError("Sorry, you need to run setup.py from the "
"toplevel source directory.")
projname = kw['twisted_subproject']
projdir = os.path.join('twisted', projname)
kw['packages'] = getPackages(projdir, parent='twisted')
kw['version'] = getVersion(projname)
plugin = "twisted/plugins/twisted_" + projname + ".py"
if os.path.exists(plugin):
kw.setdefault('py_modules', []).append(
plugin.replace("/", ".")[:-3])
kw['data_files'] = getDataFiles(projdir, parent='twisted')
del kw['twisted_subproject']
else:
if 'plugins' in kw:
py_modules = []
for plg in kw['plugins']:
py_modules.append("twisted.plugins." + plg)
kw.setdefault('py_modules', []).extend(py_modules)
del kw['plugins']
if 'cmdclass' not in kw:
kw['cmdclass'] = {
'install_data': install_data_twisted,
'build_scripts': build_scripts_twisted}
if sys.version_info[:3] < (2, 3, 0):
kw['cmdclass']['build_py'] = build_py_twisted
if "conditionalExtensions" in kw:
extensions = kw["conditionalExtensions"]
del kw["conditionalExtensions"]
if 'ext_modules' not in kw:
# This is a workaround for distutils behavior; ext_modules isn't
# actually used by our custom builder. distutils deep-down checks
# to see if there are any ext_modules defined before invoking
# the build_ext command. We need to trigger build_ext regardless
# because it is the thing that does the conditional checks to see
# if it should build any extensions. The reason we have to delay
# the conditional checks until then is that the compiler objects
# are not yet set up when this code is executed.
kw["ext_modules"] = extensions
class my_build_ext(build_ext_twisted):
conditionalExtensions = extensions
kw.setdefault('cmdclass', {})['build_ext'] = my_build_ext
return kw
def getVersion(proj, base="twisted"):
"""
Extract the version number for a given project.
@param proj: the name of the project. Examples are "core",
"conch", "words", "mail".
@rtype: str
@returns: The version number of the project, as a string like
"2.0.0".
"""
if proj == 'core':
vfile = os.path.join(base, '_version.py')
else:
vfile = os.path.join(base, proj, '_version.py')
ns = {'__name__': 'Nothing to see here'}
execfile(vfile, ns)
return ns['version'].base()
# Names that are exluded from globbing results:
EXCLUDE_NAMES = ["{arch}", "CVS", ".cvsignore", "_darcs",
"RCS", "SCCS", ".svn"]
EXCLUDE_PATTERNS = ["*.py[cdo]", "*.s[ol]", ".#*", "*~", "*.py"]
import fnmatch
def _filterNames(names):
"""Given a list of file names, return those names that should be copied.
"""
names = [n for n in names
if n not in EXCLUDE_NAMES]
# This is needed when building a distro from a working
# copy (likely a checkout) rather than a pristine export:
for pattern in EXCLUDE_PATTERNS:
names = [n for n in names
if (not fnmatch.fnmatch(n, pattern))
and (not n.endswith('.py'))]
return names
def relativeTo(base, relativee):
"""
Gets 'relativee' relative to 'basepath'.
i.e.,
>>> relativeTo('/home/', '/home/radix/')
'radix'
>>> relativeTo('.', '/home/radix/Projects/Twisted') # curdir is /home/radix
'Projects/Twisted'
The 'relativee' must be a child of 'basepath'.
"""
basepath = os.path.abspath(base)
relativee = os.path.abspath(relativee)
if relativee.startswith(basepath):
relative = relativee[len(basepath):]
if relative.startswith(os.sep):
relative = relative[1:]
return os.path.join(base, relative)
raise ValueError("%s is not a subpath of %s" % (relativee, basepath))
def getDataFiles(dname, ignore=None, parent=None):
"""
Get all the data files that should be included in this distutils Project.
'dname' should be the path to the package that you're distributing.
'ignore' is a list of sub-packages to ignore. This facilitates
disparate package hierarchies. That's a fancy way of saying that
the 'twisted' package doesn't want to include the 'twisted.conch'
package, so it will pass ['conch'] as the value.
'parent' is necessary if you're distributing a subpackage like
twisted.conch. 'dname' should point to 'twisted/conch' and 'parent'
should point to 'twisted'. This ensures that your data_files are
generated correctly, only using relative paths for the first element
of the tuple ('twisted/conch/*').
The default 'parent' is the current working directory.
"""
parent = parent or "."
ignore = ignore or []
result = []
for directory, subdirectories, filenames in os.walk(dname):
resultfiles = []
for exname in EXCLUDE_NAMES:
if exname in subdirectories:
subdirectories.remove(exname)
for ig in ignore:
if ig in subdirectories:
subdirectories.remove(ig)
for filename in _filterNames(filenames):
resultfiles.append(filename)
if resultfiles:
result.append((relativeTo(parent, directory),
[relativeTo(parent,
os.path.join(directory, filename))
for filename in resultfiles]))
return result
def getPackages(dname, pkgname=None, results=None, ignore=None, parent=None):
"""
Get all packages which are under dname. This is necessary for
Python 2.2's distutils. Pretty similar arguments to getDataFiles,
including 'parent'.
"""
parent = parent or ""
prefix = []
if parent:
prefix = [parent]
bname = os.path.basename(dname)
ignore = ignore or []
if bname in ignore:
return []
if results is None:
results = []
if pkgname is None:
pkgname = []
subfiles = os.listdir(dname)
abssubfiles = [os.path.join(dname, x) for x in subfiles]
if '__init__.py' in subfiles:
results.append(prefix + pkgname + [bname])
for subdir in filter(os.path.isdir, abssubfiles):
getPackages(subdir, pkgname=pkgname + [bname],
results=results, ignore=ignore,
parent=parent)
res = ['.'.join(result) for result in results]
return res
def getScripts(projname, basedir=''):
"""
Returns a list of scripts for a Twisted subproject; this works in
any of an SVN checkout, a project-specific tarball.
"""
scriptdir = os.path.join(basedir, 'bin', projname)
if not os.path.isdir(scriptdir):
# Probably a project-specific tarball, in which case only this
# project's bins are included in 'bin'
scriptdir = os.path.join(basedir, 'bin')
if not os.path.isdir(scriptdir):
return []
thingies = os.listdir(scriptdir)
if '.svn' in thingies:
thingies.remove('.svn')
return filter(os.path.isfile,
[os.path.join(scriptdir, x) for x in thingies])
## Helpers and distutil tweaks
class build_py_twisted(build_py.build_py):
"""
Changes behavior in Python 2.2 to support simultaneous specification of
`packages' and `py_modules'.
"""
def run(self):
if self.py_modules:
self.build_modules()
if self.packages:
self.build_packages()
self.byte_compile(self.get_outputs(include_bytecode=0))
class build_scripts_twisted(build_scripts.build_scripts):
"""Renames scripts so they end with '.py' on Windows."""
def run(self):
build_scripts.build_scripts.run(self)
if not os.name == "nt":
return
for f in os.listdir(self.build_dir):
fpath=os.path.join(self.build_dir, f)
if not fpath.endswith(".py"):
try:
os.unlink(fpath + ".py")
except EnvironmentError, e:
if e.args[1]=='No such file or directory':
pass
os.rename(fpath, fpath + ".py")
class install_data_twisted(install_data.install_data):
"""I make sure data files are installed in the package directory."""
def finalize_options(self):
self.set_undefined_options('install',
('install_lib', 'install_dir')
)
install_data.install_data.finalize_options(self)
class build_ext_twisted(build_ext.build_ext):
"""
Allow subclasses to easily detect and customize Extensions to
build at install-time.
"""
def prepare_extensions(self):
"""
Prepare the C{self.extensions} attribute (used by
L{build_ext.build_ext}) by checking which extensions in
L{conditionalExtensions} should be built. In addition, if we are
building on NT, define the WIN32 macro to 1.
"""
# always define WIN32 under Windows
if os.name == 'nt':
self.define_macros = [("WIN32", 1)]
else:
self.define_macros = []
self.extensions = [x for x in self.conditionalExtensions
if x.condition(self)]
for ext in self.extensions:
ext.define_macros.extend(self.define_macros)
def build_extensions(self):
"""
Check to see which extension modules to build and then build them.
"""
self.prepare_extensions()
build_ext.build_ext.build_extensions(self)
def _remove_conftest(self):
for filename in ("conftest.c", "conftest.o", "conftest.obj"):
try:
os.unlink(filename)
except EnvironmentError:
pass
def _compile_helper(self, content):
conftest = open("conftest.c", "w")
try:
conftest.write(content)
conftest.close()
try:
self.compiler.compile(["conftest.c"], output_dir='')
except CompileError:
return False
return True
finally:
self._remove_conftest()
def _check_header(self, header_name):
"""
Check if the given header can be included by trying to compile a file
that contains only an #include line.
"""
self.compiler.announce("checking for %s ..." % header_name, 0)
return self._compile_helper("#include <%s>\n" % header_name)
| apache-2.0 |
n3wb13/OpenNfrGui-5.0-1 | lib/python/Plugins/Extensions/bmediacenter/src/MC_Settings.py | 1 | 4552 | from Screens.Screen import Screen
from Screens.MessageBox import MessageBox
from Screens.Standby import TryQuitMainloop
#from enigma import getMachineBrand, getMachineName
from Components.ActionMap import ActionMap, NumberActionMap
from Components.Label import Label
from Components.Sources.List import List
from Components.Sources.StaticText import StaticText
from Components.MenuList import MenuList
from Components.ConfigList import ConfigList, ConfigListScreen
from Components.config import *
from Components.Console import Console
from __init__ import _
from os import mkdir
mcpath = "/usr/lib/enigma2/python/Plugins/Extensions/BMediaCenter/skins/defaultHD/images/"
#try:
# from enigma import evfd
#except Exception, e:
# print "Media Center: Import evfd failed"
class MC_Settings(Screen):
def __init__(self, session):
Screen.__init__(self, session)
self.session = session
self.Console = Console()
self.service_name = 'djmount'
self["actions"] = NumberActionMap(["SetupActions","OkCancelActions"],
{
"ok": self.keyOK,
"cancel": self.close,
"left": self.keyLeft,
"right": self.keyRight,
"0": self.keyNumber,
"1": self.keyNumber,
"2": self.keyNumber,
"3": self.keyNumber,
"4": self.keyNumber,
"5": self.keyNumber,
"6": self.keyNumber,
"7": self.keyNumber,
"8": self.keyNumber,
"9": self.keyNumber
}, -1)
self.list = []
self["configlist"] = ConfigList(self.list)
self.list.append(getConfigListEntry(_("Show MC in Main-Menu"), config.plugins.mc_globalsettings.showinmainmenu))
self.list.append(getConfigListEntry(_("Show MC in Extension-Menu"), config.plugins.mc_globalsettings.showinextmenu))
self.list.append(getConfigListEntry(_("UPNP Enabled"), config.plugins.mc_globalsettings.upnp_enable))
def keyLeft(self):
self["configlist"].handleKey(KEY_LEFT)
def keyRight(self):
self["configlist"].handleKey(KEY_RIGHT)
def keyNumber(self, number):
self["configlist"].handleKey(KEY_0 + number)
def keyOK(self):
config.plugins.mc_globalsettings.save()
# if config.plugins.mc_global.vfd.value == "on":
# evfd.getInstance().vfd_write_string(_("Settings"))
if config.plugins.mc_globalsettings.upnp_enable.getValue():
if fileExists("/media/upnp") is False:
mkdir("/media/upnp")
self.Console.ePopen('/usr/bin/opkg list_installed ' + self.service_name, self.checkNetworkState)
else:
self.close()
def checkNetworkState(self, str, retval, extra_args):
if str.find('Collected errors') != -1:
self.session.openWithCallback(self.close, MessageBox, _("A background update check is is progress, please wait a few minutes and try again."), type=MessageBox.TYPE_INFO, timeout=10, close_on_any_key=True)
elif not str:
self.feedscheck = self.session.open(MessageBox,_('Please wait whilst feeds state is checked.'), MessageBox.TYPE_INFO, enable_input = False)
self.feedscheck.setTitle(_('Checking Feeds'))
cmd1 = "opkg update"
self.CheckConsole = Console()
self.CheckConsole.ePopen(cmd1, self.checkNetworkStateFinished)
else:
self.close()
def checkNetworkStateFinished(self, result, retval,extra_args=None):
if result.find('bad address') != -1:
self.session.openWithCallback(self.InstallPackageFailed, MessageBox, _("Your Receiver is not connected to the internet, please check your network settings and try again."), type=MessageBox.TYPE_INFO, timeout=10, close_on_any_key=True)
elif result.find('wget returned 1') != -1 or result.find('wget returned 255') != -1 or result.find('404 Not Found') != -1:
self.session.openWithCallback(self.InstallPackageFailed, MessageBox, _("Sorry feeds are down for maintenance, please try again later."), type=MessageBox.TYPE_INFO, timeout=10, close_on_any_key=True)
else:
self.session.openWithCallback(self.InstallPackage,MessageBox,_('Your Receiver will be restarted after the installation of service\nReady to install %s ?') % (self.service_name), MessageBox.TYPE_YESNO)
def InstallPackage(self, val):
if val:
self.doInstall(self.installComplete, self.service_name)
else:
self.feedscheck.close()
self.close()
def InstallPackageFailed(self, val):
self.feedscheck.close()
self.close()
def doInstall(self, callback, pkgname):
self.message = self.session.open(MessageBox,_("please wait..."), MessageBox.TYPE_INFO, enable_input = False)
self.message.setTitle(_('Installing Service'))
self.Console.ePopen('/usr/bin/opkg install ' + pkgname, callback)
def installComplete(self,result = None, retval = None, extra_args = None):
self.session.open(TryQuitMainloop, 2)
| gpl-2.0 |
themattchan/google-racket-exercises | basic/string1.py | 48 | 3398 | #!/usr/bin/python -tt
# Copyright 2010 Google Inc.
# Licensed under the Apache License, Version 2.0
# http://www.apache.org/licenses/LICENSE-2.0
# Google's Python Class
# http://code.google.com/edu/languages/google-python-class/
# Basic string exercises
# Fill in the code for the functions below. main() is already set up
# to call the functions with a few different inputs,
# printing 'OK' when each function is correct.
# The starter code for each function includes a 'return'
# which is just a placeholder for your code.
# It's ok if you do not complete all the functions, and there
# are some additional functions to try in string2.py.
# A. donuts
# Given an int count of a number of donuts, return a string
# of the form 'Number of donuts: <count>', where <count> is the number
# passed in. However, if the count is 10 or more, then use the word 'many'
# instead of the actual count.
# So donuts(5) returns 'Number of donuts: 5'
# and donuts(23) returns 'Number of donuts: many'
def donuts(count):
# +++your code here+++
return
# B. both_ends
# Given a string s, return a string made of the first 2
# and the last 2 chars of the original string,
# so 'spring' yields 'spng'. However, if the string length
# is less than 2, return instead the empty string.
def both_ends(s):
# +++your code here+++
return
# C. fix_start
# Given a string s, return a string
# where all occurences of its first char have
# been changed to '*', except do not change
# the first char itself.
# e.g. 'babble' yields 'ba**le'
# Assume that the string is length 1 or more.
# Hint: s.replace(stra, strb) returns a version of string s
# where all instances of stra have been replaced by strb.
def fix_start(s):
# +++your code here+++
return
# D. MixUp
# Given strings a and b, return a single string with a and b separated
# by a space '<a> <b>', except swap the first 2 chars of each string.
# e.g.
# 'mix', pod' -> 'pox mid'
# 'dog', 'dinner' -> 'dig donner'
# Assume a and b are length 2 or more.
def mix_up(a, b):
# +++your code here+++
return
# Provided simple test() function used in main() to print
# what each function returns vs. what it's supposed to return.
def test(got, expected):
if got == expected:
prefix = ' OK '
else:
prefix = ' X '
print '%s got: %s expected: %s' % (prefix, repr(got), repr(expected))
# Provided main() calls the above functions with interesting inputs,
# using test() to check if each result is correct or not.
def main():
print 'donuts'
# Each line calls donuts, compares its result to the expected for that call.
test(donuts(4), 'Number of donuts: 4')
test(donuts(9), 'Number of donuts: 9')
test(donuts(10), 'Number of donuts: many')
test(donuts(99), 'Number of donuts: many')
print
print 'both_ends'
test(both_ends('spring'), 'spng')
test(both_ends('Hello'), 'Helo')
test(both_ends('a'), '')
test(both_ends('xyz'), 'xyyz')
print
print 'fix_start'
test(fix_start('babble'), 'ba**le')
test(fix_start('aardvark'), 'a*rdv*rk')
test(fix_start('google'), 'goo*le')
test(fix_start('donut'), 'donut')
print
print 'mix_up'
test(mix_up('mix', 'pod'), 'pox mid')
test(mix_up('dog', 'dinner'), 'dig donner')
test(mix_up('gnash', 'sport'), 'spash gnort')
test(mix_up('pezzy', 'firm'), 'fizzy perm')
# Standard boilerplate to call the main() function.
if __name__ == '__main__':
main()
| apache-2.0 |
drmrd/ansible | lib/ansible/modules/monitoring/zabbix/zabbix_proxy.py | 58 | 12457 | #!/usr/bin/python
# -*- coding: utf-8 -*-
# (c) 2017, Alen Komic
#
# This file is part of Ansible
#
# Ansible is free software: you can redistribute it and/or modify
# it under the terms of the GNU General Public License as published by
# the Free Software Foundation, either version 3 of the License, or
# (at your option) any later version.
#
# Ansible is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with Ansible. If not, see <http://www.gnu.org/licenses/>.
#
from __future__ import (absolute_import, division, print_function)
__metaclass__ = type
ANSIBLE_METADATA = {'metadata_version': '1.1',
'status': ['preview'],
'supported_by': 'community'}
DOCUMENTATION = '''
---
module: zabbix_proxy
short_description: Zabbix proxy creates/deletes/gets/updates
description:
- This module allows you to create, modify, get and delete Zabbix proxy entries.
version_added: "2.5"
author:
- "Alen Komic"
requirements:
- "python >= 2.6"
- "zabbix-api >= 0.5.3"
options:
proxy_name:
description:
- Name of the proxy in Zabbix.
required: true
description:
description:
- Description of the proxy..
required: false
status:
description:
- Type of proxy. (4 - active, 5 - passive)
required: false
choices: ['active', 'passive']
default: "active"
tls_connect:
description:
- Connections to proxy.
required: false
choices: ['no_encryption','PSK','certificate']
default: 'no_encryption'
tls_accept:
description:
- Connections from proxy.
required: false
choices: ['no_encryption','PSK','certificate']
default: 'no_encryption'
tls_issuer:
description:
- Certificate issuer.
required: false
tls_subject:
description:
- Certificate subject.
required: false
tls_psk_identity:
description:
- PSK identity. Required if either I(tls_connect) or I(tls_accept) has PSK enabled.
required: false
tls_psk:
description:
- The preshared key, at least 32 hex digits. Required if either I(tls_connect) or I(tls_accept) has PSK enabled.
required: false
state:
description:
- State of the proxy.
- On C(present), it will create if proxy does not exist or update the proxy if the associated data is different.
- On C(absent) will remove a proxy if it exists.
required: false
choices: ['present', 'absent']
default: "present"
interface:
description:
- Dictionary with params for the interface when proxy is in passive mode
- 'Available values are: dns, ip, main, port, type and useip.'
- Please review the interface documentation for more information on the supported properties
- U(https://www.zabbix.com/documentation/3.2/manual/api/reference/proxy/object#proxy_interface)
required: false
default: {}
extends_documentation_fragment:
- zabbix
'''
EXAMPLES = '''
- name: Create a new proxy or update an existing proxies info
local_action:
module: zabbix_proxy
server_url: http://monitor.example.com
login_user: username
login_password: password
proxy_name: ExampleProxy
description: ExampleProxy
status: active
state: present
interface:
type: 0
main: 1
useip: 1
ip: 10.xx.xx.xx
dns: ""
port: 10050
'''
RETURN = ''' # '''
from ansible.module_utils.basic import AnsibleModule
try:
from zabbix_api import ZabbixAPI
HAS_ZABBIX_API = True
except ImportError:
HAS_ZABBIX_API = False
class Proxy(object):
def __init__(self, module, zbx):
self._module = module
self._zapi = zbx
self.existing_data = None
def proxy_exists(self, proxy_name):
result = self._zapi.proxy.get({
'output': 'extend', 'selectInterface': 'extend',
'filter': {'host': proxy_name}})
if len(result) > 0 and 'proxyid' in result[0]:
self.existing_data = result[0]
return result[0]['proxyid']
else:
return result
def add_proxy(self, data):
try:
if self._module.check_mode:
self._module.exit_json(changed=True)
parameters = {}
for item in data:
if data[item]:
parameters[item] = data[item]
proxy_ids_list = self._zapi.proxy.create(parameters)
self._module.exit_json(changed=True,
result="Successfully added proxy %s (%s)" %
(data['host'], data['status']))
if len(proxy_ids_list) >= 1:
return proxy_ids_list['proxyids'][0]
except Exception as e:
self._module.fail_json(msg="Failed to create proxy %s: %s" %
(data['host'], e))
def delete_proxy(self, proxy_id, proxy_name):
try:
if self._module.check_mode:
self._module.exit_json(changed=True)
self._zapi.proxy.delete([proxy_id])
self._module.exit_json(changed=True,
result="Successfully deleted" +
" proxy %s" % proxy_name)
except Exception as e:
self._module.fail_json(msg="Failed to delete proxy %s: %s" %
(proxy_name, str(e)))
def compile_interface_params(self, new_interface):
old_interface = {}
if 'interface' in self.existing_data and \
len(self.existing_data['interface']) > 0:
old_interface = self.existing_data['interface']
final_interface = old_interface.copy()
final_interface.update(new_interface)
final_interface = dict((k, str(v)) for k, v in final_interface.items())
if final_interface != old_interface:
return final_interface
else:
return {}
def update_proxy(self, proxy_id, data):
try:
if self._module.check_mode:
self._module.exit_json(changed=True)
parameters = {'proxyid': proxy_id}
for item in data:
if data[item] and item in self.existing_data and \
self.existing_data[item] != data[item]:
parameters[item] = data[item]
if 'interface' in parameters:
parameters.pop('interface')
if 'interface' in data and data['status'] == '6':
new_interface = self.compile_interface_params(data['interface'])
if len(new_interface) > 0:
parameters['interface'] = new_interface
if len(parameters) > 1:
self._zapi.proxy.update(parameters)
self._module.exit_json(
changed=True,
result="Successfully updated proxy %s (%s)" %
(data['host'], proxy_id)
)
else:
self._module.exit_json(changed=False)
except Exception as e:
self._module.fail_json(msg="Failed to update proxy %s: %s" %
(data['host'], e))
def main():
module = AnsibleModule(
argument_spec=dict(
server_url=dict(type='str', required=True, aliases=['url']),
login_user=dict(type='str', required=True),
login_password=dict(type='str', required=True, no_log=True),
proxy_name=dict(type='str', required=True),
http_login_user=dict(type='str', required=False, default=None),
http_login_password=dict(type='str', required=False,
default=None, no_log=True),
validate_certs=dict(type='bool', required=False, default=True),
status=dict(default="active", choices=['active', 'passive']),
state=dict(default="present", choices=['present', 'absent']),
description=dict(type='str', required=False),
tls_connect=dict(default='no_encryption',
choices=['no_encryption', 'PSK', 'certificate']),
tls_accept=dict(default='no_encryption',
choices=['no_encryption', 'PSK', 'certificate']),
tls_issuer=dict(type='str', required=False, default=None),
tls_subject=dict(type='str', required=False, default=None),
tls_psk_identity=dict(type='str', required=False, default=None),
tls_psk=dict(type='str', required=False, default=None),
timeout=dict(type='int', default=10),
interface=dict(type='dict', required=False, default={})
),
supports_check_mode=True
)
if not HAS_ZABBIX_API:
module.fail_json(msg="Missing requried zabbix-api module" +
" (check docs or install with:" +
" pip install zabbix-api)")
server_url = module.params['server_url']
login_user = module.params['login_user']
login_password = module.params['login_password']
http_login_user = module.params['http_login_user']
http_login_password = module.params['http_login_password']
validate_certs = module.params['validate_certs']
proxy_name = module.params['proxy_name']
description = module.params['description']
status = module.params['status']
tls_connect = module.params['tls_connect']
tls_accept = module.params['tls_accept']
tls_issuer = module.params['tls_issuer']
tls_subject = module.params['tls_subject']
tls_psk_identity = module.params['tls_psk_identity']
tls_psk = module.params['tls_psk']
state = module.params['state']
timeout = module.params['timeout']
interface = module.params['interface']
# convert enabled to 0; disabled to 1
status = 6 if status == "passive" else 5
if tls_connect == 'certificate':
tls_connect = 4
elif tls_connect == 'PSK':
tls_connect = 2
else:
tls_connect = 1
if tls_accept == 'certificate':
tls_accept = 4
elif tls_accept == 'PSK':
tls_accept = 2
else:
tls_accept = 1
zbx = None
# login to zabbix
try:
zbx = ZabbixAPI(server_url, timeout=timeout,
user=http_login_user,
passwd=http_login_password,
validate_certs=validate_certs)
zbx.login(login_user, login_password)
except Exception as e:
module.fail_json(msg="Failed to connect to Zabbix server: %s" % e)
proxy = Proxy(module, zbx)
# check if proxy already exists
proxy_id = proxy.proxy_exists(proxy_name)
if proxy_id:
if state == "absent":
# remove proxy
proxy.delete_proxy(proxy_id, proxy_name)
else:
proxy.update_proxy(proxy_id, {
'host': proxy_name,
'description': description,
'status': str(status),
'tls_connect': str(tls_connect),
'tls_accept': str(tls_accept),
'tls_issuer': tls_issuer,
'tls_subject': tls_subject,
'tls_psk_identity': tls_psk_identity,
'tls_psk': tls_psk,
'interface': interface
})
else:
if state == "absent":
# the proxy is already deleted.
module.exit_json(changed=False)
proxy_id = proxy.add_proxy(data={
'host': proxy_name,
'description': description,
'status': str(status),
'tls_connect': str(tls_connect),
'tls_accept': str(tls_accept),
'tls_issuer': tls_issuer,
'tls_subject': tls_subject,
'tls_psk_identity': tls_psk_identity,
'tls_psk': tls_psk,
'interface': interface
})
if __name__ == '__main__':
main()
| gpl-3.0 |
manisandro/QGIS | tests/src/python/test_qgsfontbutton.py | 21 | 3420 | # -*- coding: utf-8 -*-
"""QGIS Unit tests for QgsFontButton.
.. note:: This program is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 2 of the License, or
(at your option) any later version.
"""
__author__ = 'Nyall Dawson'
__date__ = '04/06/2017'
__copyright__ = 'Copyright 2017, The QGIS Project'
# This will get replaced with a git SHA1 when you do a git archive
__revision__ = '$Format:%H$'
import qgis # NOQA
from qgis.core import QgsTextFormat
from qgis.gui import QgsFontButton, QgsMapCanvas
from qgis.testing import start_app, unittest
from qgis.PyQt.QtGui import QColor, QFont
from qgis.PyQt.QtTest import QSignalSpy
from utilities import getTestFont
start_app()
class TestQgsFontButton(unittest.TestCase):
def testGettersSetters(self):
button = QgsFontButton()
canvas = QgsMapCanvas()
button.setDialogTitle('test title')
self.assertEqual(button.dialogTitle(), 'test title')
button.setMapCanvas(canvas)
self.assertEqual(button.mapCanvas(), canvas)
def testSetGetFormat(self):
button = QgsFontButton()
s = QgsTextFormat()
s.setFont(getTestFont())
s.setNamedStyle('Italic')
s.setSize(5)
s.setColor(QColor(255, 0, 0))
s.setOpacity(0.5)
signal_spy = QSignalSpy(button.changed)
button.setTextFormat(s)
self.assertEqual(len(signal_spy), 1)
r = button.textFormat()
self.assertEqual(r.font().family(), 'QGIS Vera Sans')
self.assertEqual(r.namedStyle(), 'Italic')
self.assertEqual(r.size(), 5)
self.assertEqual(r.color(), QColor(255, 0, 0))
self.assertEqual(r.opacity(), 0.5)
def testSetGetFont(self):
button = QgsFontButton()
button.setMode(QgsFontButton.ModeQFont)
self.assertEqual(button.mode(), QgsFontButton.ModeQFont)
s = getTestFont()
s.setPointSize(16)
signal_spy = QSignalSpy(button.changed)
button.setCurrentFont(s)
self.assertEqual(len(signal_spy), 1)
r = button.currentFont()
self.assertEqual(r.family(), 'QGIS Vera Sans')
self.assertEqual(r.styleName(), 'Roman')
self.assertEqual(r.pointSize(), 16)
def testSetColor(self):
button = QgsFontButton()
s = QgsTextFormat()
s.setFont(getTestFont())
s.setNamedStyle('Italic')
s.setSize(5)
s.setColor(QColor(255, 0, 0))
s.setOpacity(0.5)
button.setTextFormat(s)
signal_spy = QSignalSpy(button.changed)
button.setColor(QColor(0, 255, 0))
self.assertEqual(len(signal_spy), 1)
r = button.textFormat()
self.assertEqual(r.font().family(), 'QGIS Vera Sans')
self.assertEqual(r.namedStyle(), 'Italic')
self.assertEqual(r.size(), 5)
self.assertEqual(r.color().name(), QColor(0, 255, 0).name())
self.assertEqual(r.opacity(), 0.5)
# set same color, should not emit signal
button.setColor(QColor(0, 255, 0))
self.assertEqual(len(signal_spy), 1)
# color with transparency - should be stripped
button.setColor(QColor(0, 255, 0, 100))
r = button.textFormat()
self.assertEqual(r.color(), QColor(0, 255, 0))
if __name__ == '__main__':
unittest.main()
| gpl-2.0 |
isnowfy/pydown | pygments/formatters/html.py | 27 | 28066 | # -*- coding: utf-8 -*-
"""
pygments.formatters.html
~~~~~~~~~~~~~~~~~~~~~~~~
Formatter for HTML output.
:copyright: Copyright 2006-2012 by the Pygments team, see AUTHORS.
:license: BSD, see LICENSE for details.
"""
import os
import sys
import StringIO
from pygments.formatter import Formatter
from pygments.token import Token, Text, STANDARD_TYPES
from pygments.util import get_bool_opt, get_int_opt, get_list_opt, bytes
__all__ = ['HtmlFormatter']
_escape_html_table = {
ord('&'): u'&',
ord('<'): u'<',
ord('>'): u'>',
ord('"'): u'"',
ord("'"): u''',
}
def escape_html(text, table=_escape_html_table):
"""Escape &, <, > as well as single and double quotes for HTML."""
return text.translate(table)
def get_random_id():
"""Return a random id for javascript fields."""
from random import random
from time import time
try:
from hashlib import sha1 as sha
except ImportError:
import sha
sha = sha.new
return sha('%s|%s' % (random(), time())).hexdigest()
def _get_ttype_class(ttype):
fname = STANDARD_TYPES.get(ttype)
if fname:
return fname
aname = ''
while fname is None:
aname = '-' + ttype[-1] + aname
ttype = ttype.parent
fname = STANDARD_TYPES.get(ttype)
return fname + aname
CSSFILE_TEMPLATE = '''\
td.linenos { background-color: #f0f0f0; padding-right: 10px; }
span.lineno { background-color: #f0f0f0; padding: 0 5px 0 5px; }
pre { line-height: 125%%; }
%(styledefs)s
'''
DOC_HEADER = '''\
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01//EN"
"http://www.w3.org/TR/html4/strict.dtd">
<html>
<head>
<title>%(title)s</title>
<meta http-equiv="content-type" content="text/html; charset=%(encoding)s">
<style type="text/css">
''' + CSSFILE_TEMPLATE + '''
</style>
</head>
<body>
<h2>%(title)s</h2>
'''
DOC_HEADER_EXTERNALCSS = '''\
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01//EN"
"http://www.w3.org/TR/html4/strict.dtd">
<html>
<head>
<title>%(title)s</title>
<meta http-equiv="content-type" content="text/html; charset=%(encoding)s">
<link rel="stylesheet" href="%(cssfile)s" type="text/css">
</head>
<body>
<h2>%(title)s</h2>
'''
DOC_FOOTER = '''\
</body>
</html>
'''
class HtmlFormatter(Formatter):
r"""
Format tokens as HTML 4 ``<span>`` tags within a ``<pre>`` tag, wrapped
in a ``<div>`` tag. The ``<div>``'s CSS class can be set by the `cssclass`
option.
If the `linenos` option is set to ``"table"``, the ``<pre>`` is
additionally wrapped inside a ``<table>`` which has one row and two
cells: one containing the line numbers and one containing the code.
Example:
.. sourcecode:: html
<div class="highlight" >
<table><tr>
<td class="linenos" title="click to toggle"
onclick="with (this.firstChild.style)
{ display = (display == '') ? 'none' : '' }">
<pre>1
2</pre>
</td>
<td class="code">
<pre><span class="Ke">def </span><span class="NaFu">foo</span>(bar):
<span class="Ke">pass</span>
</pre>
</td>
</tr></table></div>
(whitespace added to improve clarity).
Wrapping can be disabled using the `nowrap` option.
A list of lines can be specified using the `hl_lines` option to make these
lines highlighted (as of Pygments 0.11).
With the `full` option, a complete HTML 4 document is output, including
the style definitions inside a ``<style>`` tag, or in a separate file if
the `cssfile` option is given.
The `get_style_defs(arg='')` method of a `HtmlFormatter` returns a string
containing CSS rules for the CSS classes used by the formatter. The
argument `arg` can be used to specify additional CSS selectors that
are prepended to the classes. A call `fmter.get_style_defs('td .code')`
would result in the following CSS classes:
.. sourcecode:: css
td .code .kw { font-weight: bold; color: #00FF00 }
td .code .cm { color: #999999 }
...
If you have Pygments 0.6 or higher, you can also pass a list or tuple to the
`get_style_defs()` method to request multiple prefixes for the tokens:
.. sourcecode:: python
formatter.get_style_defs(['div.syntax pre', 'pre.syntax'])
The output would then look like this:
.. sourcecode:: css
div.syntax pre .kw,
pre.syntax .kw { font-weight: bold; color: #00FF00 }
div.syntax pre .cm,
pre.syntax .cm { color: #999999 }
...
Additional options accepted:
`nowrap`
If set to ``True``, don't wrap the tokens at all, not even inside a ``<pre>``
tag. This disables most other options (default: ``False``).
`full`
Tells the formatter to output a "full" document, i.e. a complete
self-contained document (default: ``False``).
`title`
If `full` is true, the title that should be used to caption the
document (default: ``''``).
`style`
The style to use, can be a string or a Style subclass (default:
``'default'``). This option has no effect if the `cssfile`
and `noclobber_cssfile` option are given and the file specified in
`cssfile` exists.
`noclasses`
If set to true, token ``<span>`` tags will not use CSS classes, but
inline styles. This is not recommended for larger pieces of code since
it increases output size by quite a bit (default: ``False``).
`classprefix`
Since the token types use relatively short class names, they may clash
with some of your own class names. In this case you can use the
`classprefix` option to give a string to prepend to all Pygments-generated
CSS class names for token types.
Note that this option also affects the output of `get_style_defs()`.
`cssclass`
CSS class for the wrapping ``<div>`` tag (default: ``'highlight'``).
If you set this option, the default selector for `get_style_defs()`
will be this class.
*New in Pygments 0.9:* If you select the ``'table'`` line numbers, the
wrapping table will have a CSS class of this string plus ``'table'``,
the default is accordingly ``'highlighttable'``.
`cssstyles`
Inline CSS styles for the wrapping ``<div>`` tag (default: ``''``).
`prestyles`
Inline CSS styles for the ``<pre>`` tag (default: ``''``). *New in
Pygments 0.11.*
`cssfile`
If the `full` option is true and this option is given, it must be the
name of an external file. If the filename does not include an absolute
path, the file's path will be assumed to be relative to the main output
file's path, if the latter can be found. The stylesheet is then written
to this file instead of the HTML file. *New in Pygments 0.6.*
`noclobber_cssfile`
If `cssfile` is given and the specified file exists, the css file will
not be overwritten. This allows the use of the `full` option in
combination with a user specified css file. Default is ``False``.
*New in Pygments 1.1.*
`linenos`
If set to ``'table'``, output line numbers as a table with two cells,
one containing the line numbers, the other the whole code. This is
copy-and-paste-friendly, but may cause alignment problems with some
browsers or fonts. If set to ``'inline'``, the line numbers will be
integrated in the ``<pre>`` tag that contains the code (that setting
is *new in Pygments 0.8*).
For compatibility with Pygments 0.7 and earlier, every true value
except ``'inline'`` means the same as ``'table'`` (in particular, that
means also ``True``).
The default value is ``False``, which means no line numbers at all.
**Note:** with the default ("table") line number mechanism, the line
numbers and code can have different line heights in Internet Explorer
unless you give the enclosing ``<pre>`` tags an explicit ``line-height``
CSS property (you get the default line spacing with ``line-height:
125%``).
`hl_lines`
Specify a list of lines to be highlighted. *New in Pygments 0.11.*
`linenostart`
The line number for the first line (default: ``1``).
`linenostep`
If set to a number n > 1, only every nth line number is printed.
`linenospecial`
If set to a number n > 0, every nth line number is given the CSS
class ``"special"`` (default: ``0``).
`nobackground`
If set to ``True``, the formatter won't output the background color
for the wrapping element (this automatically defaults to ``False``
when there is no wrapping element [eg: no argument for the
`get_syntax_defs` method given]) (default: ``False``). *New in
Pygments 0.6.*
`lineseparator`
This string is output between lines of code. It defaults to ``"\n"``,
which is enough to break a line inside ``<pre>`` tags, but you can
e.g. set it to ``"<br>"`` to get HTML line breaks. *New in Pygments
0.7.*
`lineanchors`
If set to a nonempty string, e.g. ``foo``, the formatter will wrap each
output line in an anchor tag with a ``name`` of ``foo-linenumber``.
This allows easy linking to certain lines. *New in Pygments 0.9.*
`anchorlinenos`
If set to `True`, will wrap line numbers in <a> tags. Used in
combination with `linenos` and `lineanchors`.
**Subclassing the HTML formatter**
*New in Pygments 0.7.*
The HTML formatter is now built in a way that allows easy subclassing, thus
customizing the output HTML code. The `format()` method calls
`self._format_lines()` which returns a generator that yields tuples of ``(1,
line)``, where the ``1`` indicates that the ``line`` is a line of the
formatted source code.
If the `nowrap` option is set, the generator is the iterated over and the
resulting HTML is output.
Otherwise, `format()` calls `self.wrap()`, which wraps the generator with
other generators. These may add some HTML code to the one generated by
`_format_lines()`, either by modifying the lines generated by the latter,
then yielding them again with ``(1, line)``, and/or by yielding other HTML
code before or after the lines, with ``(0, html)``. The distinction between
source lines and other code makes it possible to wrap the generator multiple
times.
The default `wrap()` implementation adds a ``<div>`` and a ``<pre>`` tag.
A custom `HtmlFormatter` subclass could look like this:
.. sourcecode:: python
class CodeHtmlFormatter(HtmlFormatter):
def wrap(self, source, outfile):
return self._wrap_code(source)
def _wrap_code(self, source):
yield 0, '<code>'
for i, t in source:
if i == 1:
# it's a line of formatted code
t += '<br>'
yield i, t
yield 0, '</code>'
This results in wrapping the formatted lines with a ``<code>`` tag, where the
source lines are broken using ``<br>`` tags.
After calling `wrap()`, the `format()` method also adds the "line numbers"
and/or "full document" wrappers if the respective options are set. Then, all
HTML yielded by the wrapped generator is output.
"""
name = 'HTML'
aliases = ['html']
filenames = ['*.html', '*.htm']
def __init__(self, **options):
Formatter.__init__(self, **options)
self.title = self._decodeifneeded(self.title)
self.nowrap = get_bool_opt(options, 'nowrap', False)
self.noclasses = get_bool_opt(options, 'noclasses', False)
self.classprefix = options.get('classprefix', '')
self.cssclass = self._decodeifneeded(options.get('cssclass', 'highlight'))
self.cssstyles = self._decodeifneeded(options.get('cssstyles', ''))
self.prestyles = self._decodeifneeded(options.get('prestyles', ''))
self.cssfile = self._decodeifneeded(options.get('cssfile', ''))
self.noclobber_cssfile = get_bool_opt(options, 'noclobber_cssfile', False)
linenos = options.get('linenos', False)
if linenos == 'inline':
self.linenos = 2
elif linenos:
# compatibility with <= 0.7
self.linenos = 1
else:
self.linenos = 0
self.linenostart = abs(get_int_opt(options, 'linenostart', 1))
self.linenostep = abs(get_int_opt(options, 'linenostep', 1))
self.linenospecial = abs(get_int_opt(options, 'linenospecial', 0))
self.nobackground = get_bool_opt(options, 'nobackground', False)
self.lineseparator = options.get('lineseparator', '\n')
self.lineanchors = options.get('lineanchors', '')
self.anchorlinenos = options.get('anchorlinenos', False)
self.hl_lines = set()
for lineno in get_list_opt(options, 'hl_lines', []):
try:
self.hl_lines.add(int(lineno))
except ValueError:
pass
self._create_stylesheet()
def _get_css_class(self, ttype):
"""Return the css class of this token type prefixed with
the classprefix option."""
ttypeclass = _get_ttype_class(ttype)
if ttypeclass:
return self.classprefix + ttypeclass
return ''
def _create_stylesheet(self):
t2c = self.ttype2class = {Token: ''}
c2s = self.class2style = {}
for ttype, ndef in self.style:
name = self._get_css_class(ttype)
style = ''
if ndef['color']:
style += 'color: #%s; ' % ndef['color']
if ndef['bold']:
style += 'font-weight: bold; '
if ndef['italic']:
style += 'font-style: italic; '
if ndef['underline']:
style += 'text-decoration: underline; '
if ndef['bgcolor']:
style += 'background-color: #%s; ' % ndef['bgcolor']
if ndef['border']:
style += 'border: 1px solid #%s; ' % ndef['border']
if style:
t2c[ttype] = name
# save len(ttype) to enable ordering the styles by
# hierarchy (necessary for CSS cascading rules!)
c2s[name] = (style[:-2], ttype, len(ttype))
def get_style_defs(self, arg=None):
"""
Return CSS style definitions for the classes produced by the current
highlighting style. ``arg`` can be a string or list of selectors to
insert before the token type classes.
"""
if arg is None:
arg = ('cssclass' in self.options and '.'+self.cssclass or '')
if isinstance(arg, basestring):
args = [arg]
else:
args = list(arg)
def prefix(cls):
if cls:
cls = '.' + cls
tmp = []
for arg in args:
tmp.append((arg and arg + ' ' or '') + cls)
return ', '.join(tmp)
styles = [(level, ttype, cls, style)
for cls, (style, ttype, level) in self.class2style.iteritems()
if cls and style]
styles.sort()
lines = ['%s { %s } /* %s */' % (prefix(cls), style, repr(ttype)[6:])
for (level, ttype, cls, style) in styles]
if arg and not self.nobackground and \
self.style.background_color is not None:
text_style = ''
if Text in self.ttype2class:
text_style = ' ' + self.class2style[self.ttype2class[Text]][0]
lines.insert(0, '%s { background: %s;%s }' %
(prefix(''), self.style.background_color, text_style))
if self.style.highlight_color is not None:
lines.insert(0, '%s.hll { background-color: %s }' %
(prefix(''), self.style.highlight_color))
return '\n'.join(lines)
def _decodeifneeded(self, value):
if isinstance(value, bytes):
if self.encoding:
return value.decode(self.encoding)
return value.decode()
return value
def _wrap_full(self, inner, outfile):
if self.cssfile:
if os.path.isabs(self.cssfile):
# it's an absolute filename
cssfilename = self.cssfile
else:
try:
filename = outfile.name
if not filename or filename[0] == '<':
# pseudo files, e.g. name == '<fdopen>'
raise AttributeError
cssfilename = os.path.join(os.path.dirname(filename),
self.cssfile)
except AttributeError:
print >>sys.stderr, 'Note: Cannot determine output file name, ' \
'using current directory as base for the CSS file name'
cssfilename = self.cssfile
# write CSS file only if noclobber_cssfile isn't given as an option.
try:
if not os.path.exists(cssfilename) or not self.noclobber_cssfile:
cf = open(cssfilename, "w")
cf.write(CSSFILE_TEMPLATE %
{'styledefs': self.get_style_defs('body')})
cf.close()
except IOError, err:
err.strerror = 'Error writing CSS file: ' + err.strerror
raise
yield 0, (DOC_HEADER_EXTERNALCSS %
dict(title = self.title,
cssfile = self.cssfile,
encoding = self.encoding))
else:
yield 0, (DOC_HEADER %
dict(title = self.title,
styledefs = self.get_style_defs('body'),
encoding = self.encoding))
for t, line in inner:
yield t, line
yield 0, DOC_FOOTER
def _wrap_tablelinenos(self, inner):
dummyoutfile = StringIO.StringIO()
lncount = 0
for t, line in inner:
if t:
lncount += 1
dummyoutfile.write(line)
fl = self.linenostart
mw = len(str(lncount + fl - 1))
sp = self.linenospecial
st = self.linenostep
la = self.lineanchors
aln = self.anchorlinenos
nocls = self.noclasses
if sp:
lines = []
for i in range(fl, fl+lncount):
if i % st == 0:
if i % sp == 0:
if aln:
lines.append('<a href="#%s-%d" class="special">%*d</a>' %
(la, i, mw, i))
else:
lines.append('<span class="special">%*d</span>' % (mw, i))
else:
if aln:
lines.append('<a href="#%s-%d">%*d</a>' % (la, i, mw, i))
else:
lines.append('%*d' % (mw, i))
else:
lines.append('')
ls = '\n'.join(lines)
else:
lines = []
for i in range(fl, fl+lncount):
if i % st == 0:
if aln:
lines.append('<a href="#%s-%d">%*d</a>' % (la, i, mw, i))
else:
lines.append('%*d' % (mw, i))
else:
lines.append('')
ls = '\n'.join(lines)
# in case you wonder about the seemingly redundant <div> here: since the
# content in the other cell also is wrapped in a div, some browsers in
# some configurations seem to mess up the formatting...
if nocls:
yield 0, ('<table class="%stable">' % self.cssclass +
'<tr><td><div class="linenodiv" '
'style="background-color: #f0f0f0; padding-right: 10px">'
'<pre style="line-height: 125%">' +
ls + '</pre></div></td><td class="code">')
else:
yield 0, ('<table class="%stable">' % self.cssclass +
'<tr><td class="linenos"><div class="linenodiv"><pre>' +
ls + '</pre></div></td><td class="code">')
yield 0, dummyoutfile.getvalue()
yield 0, '</td></tr></table>'
def _wrap_inlinelinenos(self, inner):
# need a list of lines since we need the width of a single number :(
lines = list(inner)
sp = self.linenospecial
st = self.linenostep
num = self.linenostart
mw = len(str(len(lines) + num - 1))
if self.noclasses:
if sp:
for t, line in lines:
if num%sp == 0:
style = 'background-color: #ffffc0; padding: 0 5px 0 5px'
else:
style = 'background-color: #f0f0f0; padding: 0 5px 0 5px'
yield 1, '<span style="%s">%*s</span> ' % (
style, mw, (num%st and ' ' or num)) + line
num += 1
else:
for t, line in lines:
yield 1, ('<span style="background-color: #f0f0f0; '
'padding: 0 5px 0 5px">%*s</span> ' % (
mw, (num%st and ' ' or num)) + line)
num += 1
elif sp:
for t, line in lines:
yield 1, '<span class="lineno%s">%*s</span> ' % (
num%sp == 0 and ' special' or '', mw,
(num%st and ' ' or num)) + line
num += 1
else:
for t, line in lines:
yield 1, '<span class="lineno">%*s</span> ' % (
mw, (num%st and ' ' or num)) + line
num += 1
def _wrap_lineanchors(self, inner):
s = self.lineanchors
i = self.linenostart - 1 # subtract 1 since we have to increment i *before* yielding
for t, line in inner:
if t:
i += 1
yield 1, '<a name="%s-%d"></a>' % (s, i) + line
else:
yield 0, line
def _wrap_div(self, inner):
style = []
if (self.noclasses and not self.nobackground and
self.style.background_color is not None):
style.append('background: %s' % (self.style.background_color,))
if self.cssstyles:
style.append(self.cssstyles)
style = '; '.join(style)
yield 0, ('<div' + (self.cssclass and ' class="%s"' % self.cssclass)
+ (style and (' style="%s"' % style)) + '>')
for tup in inner:
yield tup
yield 0, '</div>\n'
def _wrap_pre(self, inner):
style = []
if self.prestyles:
style.append(self.prestyles)
if self.noclasses:
style.append('line-height: 125%')
style = '; '.join(style)
yield 0, ('<pre' + (style and ' style="%s"' % style) + '>')
for tup in inner:
yield tup
yield 0, '</pre>'
def _format_lines(self, tokensource):
"""
Just format the tokens, without any wrapping tags.
Yield individual lines.
"""
nocls = self.noclasses
lsep = self.lineseparator
# for <span style=""> lookup only
getcls = self.ttype2class.get
c2s = self.class2style
escape_table = _escape_html_table
lspan = ''
line = ''
for ttype, value in tokensource:
if nocls:
cclass = getcls(ttype)
while cclass is None:
ttype = ttype.parent
cclass = getcls(ttype)
cspan = cclass and '<span style="%s">' % c2s[cclass][0] or ''
else:
cls = self._get_css_class(ttype)
cspan = cls and '<span class="%s">' % cls or ''
parts = value.translate(escape_table).split('\n')
# for all but the last line
for part in parts[:-1]:
if line:
if lspan != cspan:
line += (lspan and '</span>') + cspan + part + \
(cspan and '</span>') + lsep
else: # both are the same
line += part + (lspan and '</span>') + lsep
yield 1, line
line = ''
elif part:
yield 1, cspan + part + (cspan and '</span>') + lsep
else:
yield 1, lsep
# for the last line
if line and parts[-1]:
if lspan != cspan:
line += (lspan and '</span>') + cspan + parts[-1]
lspan = cspan
else:
line += parts[-1]
elif parts[-1]:
line = cspan + parts[-1]
lspan = cspan
# else we neither have to open a new span nor set lspan
if line:
yield 1, line + (lspan and '</span>') + lsep
def _highlight_lines(self, tokensource):
"""
Highlighted the lines specified in the `hl_lines` option by
post-processing the token stream coming from `_format_lines`.
"""
hls = self.hl_lines
for i, (t, value) in enumerate(tokensource):
if t != 1:
yield t, value
if i + 1 in hls: # i + 1 because Python indexes start at 0
if self.noclasses:
style = ''
if self.style.highlight_color is not None:
style = (' style="background-color: %s"' %
(self.style.highlight_color,))
yield 1, '<span%s>%s</span>' % (style, value)
else:
yield 1, '<span class="hll">%s</span>' % value
else:
yield 1, value
def wrap(self, source, outfile):
"""
Wrap the ``source``, which is a generator yielding
individual lines, in custom generators. See docstring
for `format`. Can be overridden.
"""
return self._wrap_div(self._wrap_pre(source))
def format_unencoded(self, tokensource, outfile):
"""
The formatting process uses several nested generators; which of
them are used is determined by the user's options.
Each generator should take at least one argument, ``inner``,
and wrap the pieces of text generated by this.
Always yield 2-tuples: (code, text). If "code" is 1, the text
is part of the original tokensource being highlighted, if it's
0, the text is some piece of wrapping. This makes it possible to
use several different wrappers that process the original source
linewise, e.g. line number generators.
"""
source = self._format_lines(tokensource)
if self.hl_lines:
source = self._highlight_lines(source)
if not self.nowrap:
if self.linenos == 2:
source = self._wrap_inlinelinenos(source)
if self.lineanchors:
source = self._wrap_lineanchors(source)
source = self.wrap(source, outfile)
if self.linenos == 1:
source = self._wrap_tablelinenos(source)
if self.full:
source = self._wrap_full(source, outfile)
for t, piece in source:
outfile.write(piece)
| mit |
wilsonxiao/machinekit | src/hal/user_comps/vismach/pumagui.py | 25 | 4609 | #!/usr/bin/python2.4
# Copyright 2007 John Kasunich and Jeff Epler
#
# This program is free software; you can redistribute it and/or modify
# it under the terms of the GNU General Public License as published by
# the Free Software Foundation; either version 2 of the License, or
# (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with this program; if not, write to the Free Software
# Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
from vismach import *
import hal
c = hal.component("pumagui")
c.newpin("joint1", hal.HAL_FLOAT, hal.HAL_IN)
c.newpin("joint2", hal.HAL_FLOAT, hal.HAL_IN)
c.newpin("joint3", hal.HAL_FLOAT, hal.HAL_IN)
c.newpin("joint4", hal.HAL_FLOAT, hal.HAL_IN)
c.newpin("joint5", hal.HAL_FLOAT, hal.HAL_IN)
c.newpin("joint6", hal.HAL_FLOAT, hal.HAL_IN)
c.newpin("grip", hal.HAL_FLOAT, hal.HAL_IN)
c.ready()
###################
# this stuff is the actual definition of the machine
# ideally it would be in a separate file from the code above
#
# gripper fingers
finger1 = CylinderZ(-0.02, 0.012, 0.1, 0.010)
finger2 = CylinderZ(-0.02, 0.012, 0.1, 0.010)
finger1 = HalRotate([finger1],c,"grip", 40,0,1,0)
finger2 = HalRotate([finger2],c,"grip",-40,0,1,0)
finger1 = Translate([finger1], 0.025,0.0,0.1)
finger2 = Translate([finger2],-0.025,0.0,0.1)
# "hand" - the part the fingers are attached to
# "tooltip" for backplot will be the origin of the hand for now
tooltip = Capture()
link6 = Collection([
tooltip,
Box(-0.060, -0.015, 0.02, 0.060, 0.015, 0.1),
Box(-0.05, -0.05, 0.0, 0.05, 0.05, 0.02)])
# assembly fingers, and make it rotate
link6 = HalRotate([finger1,finger2,link6],c,"joint6",1,0,0,1)
# moving part of wrist joint
link5 = Collection([
CylinderZ( 0.055, 0.060, 0.070, 0.060),
CylinderX(-0.026, 0.050, 0.026, 0.050),
Box(-0.022, -0.050, 0.0, 0.022, 0.050, 0.055)])
# move gripper to end of wrist and attach
link5 = Collection([
link5,
Translate([link6],0,0,0.070)])
# make wrist bend
link5 = HalRotate([link5],c,"joint5",1,1,0,0)
# fixed part of wrist joint (rotates on end of arm)
link4 = Collection([
CylinderX(-0.027, 0.045, -0.055, 0.045),
CylinderX( 0.027, 0.045, 0.055, 0.045),
Box(-0.030, -0.045, -0.060, -0.050, 0.045, 0.0),
Box( 0.030, -0.045, -0.060, 0.050, 0.045, 0.0),
Box(-0.050, -0.050, -0.090, 0.050, 0.050, -0.060)])
# attach wrist, move whole assembly forward so joint 4 is at origin
link4 = Translate([link4,link5], 0, 0, 0.090)
# make joint 4 rotate
link4 = HalRotate([link4],c,"joint4",1,0,0,1)
# next chunk
link3 = Collection([
CylinderX(-0.08, 0.10, 0.08, 0.12),
CylinderZ(0.0, 0.07, 0.7, 0.05)])
# move link4 forward and attach
link3 = Collection([
link3,
Translate([link4],0.0, 0.0, 0.7)])
# move whole assembly over so joint 3 is at origin
link3 = Translate([link3],-0.08, 0.0, 0.0)
# make joint 3 rotate
link3 = HalRotate([link3],c,"joint3",1,1,0,0)
# elbow stuff
link2 = Collection([
CylinderX(-0.1,0.1,-0.09,0.1),
CylinderX(-0.09,0.13,0.09,0.12),
CylinderX(0.09,0.10,0.12,0.08)])
# move elbow to end of upper arm
link2 = Translate([link2],0.0,0.0,1.2)
# rest of upper arm
link2 = Collection([
link2,
CylinderZ(1.2,0.08, 0.0, 0.1),
CylinderX(-0.14,0.17,0.14,0.15)])
# move link 3 into place and attach
link2 = Collection([
link2,
Translate([link3],-0.1,0.0,1.2)])
# move whole assembly over so joint 2 is at origin
link2 = Translate([link2],0.14, 0.0, 0.0)
# make joint 2 rotate
link2 = HalRotate([link2],c,"joint2",1,1,0,0)
# shoulder stuff
link1 = Collection([
CylinderX(0.18,0.14,0.20,0.14),
CylinderX(-0.23,0.18,0.18,0.18),
CylinderX(-0.23,0.17,-0.29,0.13),
Box(-0.15,-0.15,0.0,0.15,0.15,-0.20)])
# move link2 to end and attach
link1 = Collection([
link1,
Translate([link2],0.20,0.0,0.0)])
# move whole assembly up so joint 1 is at origin
link1 = Translate([link1],0.0, 0.0, 0.2)
# make joint 1 rotate
link1 = HalRotate([link1],c,"joint1",1,0,0,1)
# stationary base
link0 = Collection([
CylinderZ(1.9, 0.15, 2.0, 0.15),
CylinderZ(0.05, 0.25, 1.9, 0.13),
CylinderZ(0.00, 0.4, 0.07, 0.4)])
# move link1 to top and attach
link0 = Collection([
link0,
Translate([link1],0.0,0.0,2.0)])
# add a floor
floor = Box(-1.5,-1.5,-0.02,1.5,1.5,0.0)
work = Capture()
model = Collection([link0, floor, work])
main(model, tooltip, work, 5)
| gpl-3.0 |
gvb/odoo | addons/hr_evaluation/report/hr_evaluation_report.py | 307 | 4181 | # -*- coding: utf-8 -*-
##############################################################################
#
# OpenERP, Open Source Management Solution
# Copyright (C) 2004-2010 Tiny SPRL (<http://tiny.be>).
#
# This program is free software: you can redistribute it and/or modify
# it under the terms of the GNU Affero General Public License as
# published by the Free Software Foundation, either version 3 of the
# License, or (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU Affero General Public License for more details.
#
# You should have received a copy of the GNU Affero General Public License
# along with this program. If not, see <http://www.gnu.org/licenses/>.
#
##############################################################################
from openerp import tools
from openerp.osv import fields, osv
class hr_evaluation_report(osv.Model):
_name = "hr.evaluation.report"
_description = "Evaluations Statistics"
_auto = False
_columns = {
'create_date': fields.datetime('Create Date', readonly=True),
'delay_date': fields.float('Delay to Start', digits=(16, 2), readonly=True),
'overpass_delay': fields.float('Overpassed Deadline', digits=(16, 2), readonly=True),
'deadline': fields.date("Deadline", readonly=True),
'request_id': fields.many2one('survey.user_input', 'Request ID', readonly=True),
'closed': fields.date("Close Date", readonly=True), # TDE FIXME master: rename into date_close
'plan_id': fields.many2one('hr_evaluation.plan', 'Plan', readonly=True),
'employee_id': fields.many2one('hr.employee', "Employee", readonly=True),
'rating': fields.selection([
('0', 'Significantly bellow expectations'),
('1', 'Did not meet expectations'),
('2', 'Meet expectations'),
('3', 'Exceeds expectations'),
('4', 'Significantly exceeds expectations'),
], "Overall Rating", readonly=True),
'nbr': fields.integer('# of Requests', readonly=True), # TDE FIXME master: rename into nbr_requests
'state': fields.selection([
('draft', 'Draft'),
('wait', 'Plan In Progress'),
('progress', 'Final Validation'),
('done', 'Done'),
('cancel', 'Cancelled'),
], 'Status', readonly=True),
}
_order = 'create_date desc'
_depends = {
'hr.evaluation.interview': ['evaluation_id', 'id', 'request_id'],
'hr_evaluation.evaluation': [
'create_date', 'date', 'date_close', 'employee_id', 'plan_id',
'rating', 'state',
],
}
def init(self, cr):
tools.drop_view_if_exists(cr, 'hr_evaluation_report')
cr.execute("""
create or replace view hr_evaluation_report as (
select
min(l.id) as id,
s.create_date as create_date,
s.employee_id,
l.request_id,
s.plan_id,
s.rating,
s.date as deadline,
s.date_close as closed,
count(l.*) as nbr,
s.state,
avg(extract('epoch' from age(s.create_date,CURRENT_DATE)))/(3600*24) as delay_date,
avg(extract('epoch' from age(s.date,CURRENT_DATE)))/(3600*24) as overpass_delay
from
hr_evaluation_interview l
LEFT JOIN
hr_evaluation_evaluation s on (s.id=l.evaluation_id)
GROUP BY
s.create_date,
s.state,
s.employee_id,
s.date,
s.date_close,
l.request_id,
s.rating,
s.plan_id
)
""")
# vim:expandtab:smartindent:tabstop=4:softtabstop=4:shiftwidth=4:
| agpl-3.0 |
MalloyPower/parsing-python | front-end/testsuite-python-lib/Python-3.3.0/Lib/test/test_augassign.py | 167 | 7618 | # Augmented assignment test.
from test.support import run_unittest
import unittest
class AugAssignTest(unittest.TestCase):
def testBasic(self):
x = 2
x += 1
x *= 2
x **= 2
x -= 8
x //= 5
x %= 3
x &= 2
x |= 5
x ^= 1
x /= 2
self.assertEqual(x, 3.0)
def test_with_unpacking(self):
self.assertRaises(SyntaxError, compile, "x, b += 3", "<test>", "exec")
def testInList(self):
x = [2]
x[0] += 1
x[0] *= 2
x[0] **= 2
x[0] -= 8
x[0] //= 5
x[0] %= 3
x[0] &= 2
x[0] |= 5
x[0] ^= 1
x[0] /= 2
self.assertEqual(x[0], 3.0)
def testInDict(self):
x = {0: 2}
x[0] += 1
x[0] *= 2
x[0] **= 2
x[0] -= 8
x[0] //= 5
x[0] %= 3
x[0] &= 2
x[0] |= 5
x[0] ^= 1
x[0] /= 2
self.assertEqual(x[0], 3.0)
def testSequences(self):
x = [1,2]
x += [3,4]
x *= 2
self.assertEqual(x, [1, 2, 3, 4, 1, 2, 3, 4])
x = [1, 2, 3]
y = x
x[1:2] *= 2
y[1:2] += [1]
self.assertEqual(x, [1, 2, 1, 2, 3])
self.assertTrue(x is y)
def testCustomMethods1(self):
class aug_test:
def __init__(self, value):
self.val = value
def __radd__(self, val):
return self.val + val
def __add__(self, val):
return aug_test(self.val + val)
class aug_test2(aug_test):
def __iadd__(self, val):
self.val = self.val + val
return self
class aug_test3(aug_test):
def __iadd__(self, val):
return aug_test3(self.val + val)
x = aug_test(1)
y = x
x += 10
self.assertIsInstance(x, aug_test)
self.assertTrue(y is not x)
self.assertEqual(x.val, 11)
x = aug_test2(2)
y = x
x += 10
self.assertTrue(y is x)
self.assertEqual(x.val, 12)
x = aug_test3(3)
y = x
x += 10
self.assertIsInstance(x, aug_test3)
self.assertTrue(y is not x)
self.assertEqual(x.val, 13)
def testCustomMethods2(test_self):
output = []
class testall:
def __add__(self, val):
output.append("__add__ called")
def __radd__(self, val):
output.append("__radd__ called")
def __iadd__(self, val):
output.append("__iadd__ called")
return self
def __sub__(self, val):
output.append("__sub__ called")
def __rsub__(self, val):
output.append("__rsub__ called")
def __isub__(self, val):
output.append("__isub__ called")
return self
def __mul__(self, val):
output.append("__mul__ called")
def __rmul__(self, val):
output.append("__rmul__ called")
def __imul__(self, val):
output.append("__imul__ called")
return self
def __div__(self, val):
output.append("__div__ called")
def __rdiv__(self, val):
output.append("__rdiv__ called")
def __idiv__(self, val):
output.append("__idiv__ called")
return self
def __floordiv__(self, val):
output.append("__floordiv__ called")
return self
def __ifloordiv__(self, val):
output.append("__ifloordiv__ called")
return self
def __rfloordiv__(self, val):
output.append("__rfloordiv__ called")
return self
def __truediv__(self, val):
output.append("__truediv__ called")
return self
def __rtruediv__(self, val):
output.append("__rtruediv__ called")
return self
def __itruediv__(self, val):
output.append("__itruediv__ called")
return self
def __mod__(self, val):
output.append("__mod__ called")
def __rmod__(self, val):
output.append("__rmod__ called")
def __imod__(self, val):
output.append("__imod__ called")
return self
def __pow__(self, val):
output.append("__pow__ called")
def __rpow__(self, val):
output.append("__rpow__ called")
def __ipow__(self, val):
output.append("__ipow__ called")
return self
def __or__(self, val):
output.append("__or__ called")
def __ror__(self, val):
output.append("__ror__ called")
def __ior__(self, val):
output.append("__ior__ called")
return self
def __and__(self, val):
output.append("__and__ called")
def __rand__(self, val):
output.append("__rand__ called")
def __iand__(self, val):
output.append("__iand__ called")
return self
def __xor__(self, val):
output.append("__xor__ called")
def __rxor__(self, val):
output.append("__rxor__ called")
def __ixor__(self, val):
output.append("__ixor__ called")
return self
def __rshift__(self, val):
output.append("__rshift__ called")
def __rrshift__(self, val):
output.append("__rrshift__ called")
def __irshift__(self, val):
output.append("__irshift__ called")
return self
def __lshift__(self, val):
output.append("__lshift__ called")
def __rlshift__(self, val):
output.append("__rlshift__ called")
def __ilshift__(self, val):
output.append("__ilshift__ called")
return self
x = testall()
x + 1
1 + x
x += 1
x - 1
1 - x
x -= 1
x * 1
1 * x
x *= 1
x / 1
1 / x
x /= 1
x // 1
1 // x
x //= 1
x % 1
1 % x
x %= 1
x ** 1
1 ** x
x **= 1
x | 1
1 | x
x |= 1
x & 1
1 & x
x &= 1
x ^ 1
1 ^ x
x ^= 1
x >> 1
1 >> x
x >>= 1
x << 1
1 << x
x <<= 1
test_self.assertEqual(output, '''\
__add__ called
__radd__ called
__iadd__ called
__sub__ called
__rsub__ called
__isub__ called
__mul__ called
__rmul__ called
__imul__ called
__truediv__ called
__rtruediv__ called
__itruediv__ called
__floordiv__ called
__rfloordiv__ called
__ifloordiv__ called
__mod__ called
__rmod__ called
__imod__ called
__pow__ called
__rpow__ called
__ipow__ called
__or__ called
__ror__ called
__ior__ called
__and__ called
__rand__ called
__iand__ called
__xor__ called
__rxor__ called
__ixor__ called
__rshift__ called
__rrshift__ called
__irshift__ called
__lshift__ called
__rlshift__ called
__ilshift__ called
'''.splitlines())
def test_main():
run_unittest(AugAssignTest)
if __name__ == '__main__':
test_main()
| mit |
MalloyPower/parsing-python | front-end/testsuite-python-lib/Python-2.3/Lib/fileinput.py | 10 | 12373 | """Helper class to quickly write a loop over all standard input files.
Typical use is:
import fileinput
for line in fileinput.input():
process(line)
This iterates over the lines of all files listed in sys.argv[1:],
defaulting to sys.stdin if the list is empty. If a filename is '-' it
is also replaced by sys.stdin. To specify an alternative list of
filenames, pass it as the argument to input(). A single file name is
also allowed.
Functions filename(), lineno() return the filename and cumulative line
number of the line that has just been read; filelineno() returns its
line number in the current file; isfirstline() returns true iff the
line just read is the first line of its file; isstdin() returns true
iff the line was read from sys.stdin. Function nextfile() closes the
current file so that the next iteration will read the first line from
the next file (if any); lines not read from the file will not count
towards the cumulative line count; the filename is not changed until
after the first line of the next file has been read. Function close()
closes the sequence.
Before any lines have been read, filename() returns None and both line
numbers are zero; nextfile() has no effect. After all lines have been
read, filename() and the line number functions return the values
pertaining to the last line read; nextfile() has no effect.
All files are opened in text mode. If an I/O error occurs during
opening or reading a file, the IOError exception is raised.
If sys.stdin is used more than once, the second and further use will
return no lines, except perhaps for interactive use, or if it has been
explicitly reset (e.g. using sys.stdin.seek(0)).
Empty files are opened and immediately closed; the only time their
presence in the list of filenames is noticeable at all is when the
last file opened is empty.
It is possible that the last line of a file doesn't end in a newline
character; otherwise lines are returned including the trailing
newline.
Class FileInput is the implementation; its methods filename(),
lineno(), fileline(), isfirstline(), isstdin(), nextfile() and close()
correspond to the functions in the module. In addition it has a
readline() method which returns the next input line, and a
__getitem__() method which implements the sequence behavior. The
sequence must be accessed in strictly sequential order; sequence
access and readline() cannot be mixed.
Optional in-place filtering: if the keyword argument inplace=1 is
passed to input() or to the FileInput constructor, the file is moved
to a backup file and standard output is directed to the input file.
This makes it possible to write a filter that rewrites its input file
in place. If the keyword argument backup=".<some extension>" is also
given, it specifies the extension for the backup file, and the backup
file remains around; by default, the extension is ".bak" and it is
deleted when the output file is closed. In-place filtering is
disabled when standard input is read. XXX The current implementation
does not work for MS-DOS 8+3 filesystems.
Performance: this module is unfortunately one of the slower ways of
processing large numbers of input lines. Nevertheless, a significant
speed-up has been obtained by using readlines(bufsize) instead of
readline(). A new keyword argument, bufsize=N, is present on the
input() function and the FileInput() class to override the default
buffer size.
XXX Possible additions:
- optional getopt argument processing
- specify open mode ('r' or 'rb')
- fileno()
- isatty()
- read(), read(size), even readlines()
"""
import sys, os
__all__ = ["input","close","nextfile","filename","lineno","filelineno",
"isfirstline","isstdin","FileInput"]
_state = None
DEFAULT_BUFSIZE = 8*1024
def input(files=None, inplace=0, backup="", bufsize=0):
"""input([files[, inplace[, backup]]])
Create an instance of the FileInput class. The instance will be used
as global state for the functions of this module, and is also returned
to use during iteration. The parameters to this function will be passed
along to the constructor of the FileInput class.
"""
global _state
if _state and _state._file:
raise RuntimeError, "input() already active"
_state = FileInput(files, inplace, backup, bufsize)
return _state
def close():
"""Close the sequence."""
global _state
state = _state
_state = None
if state:
state.close()
def nextfile():
"""
Close the current file so that the next iteration will read the first
line from the next file (if any); lines not read from the file will
not count towards the cumulative line count. The filename is not
changed until after the first line of the next file has been read.
Before the first line has been read, this function has no effect;
it cannot be used to skip the first file. After the last line of the
last file has been read, this function has no effect.
"""
if not _state:
raise RuntimeError, "no active input()"
return _state.nextfile()
def filename():
"""
Return the name of the file currently being read.
Before the first line has been read, returns None.
"""
if not _state:
raise RuntimeError, "no active input()"
return _state.filename()
def lineno():
"""
Return the cumulative line number of the line that has just been read.
Before the first line has been read, returns 0. After the last line
of the last file has been read, returns the line number of that line.
"""
if not _state:
raise RuntimeError, "no active input()"
return _state.lineno()
def filelineno():
"""
Return the line number in the current file. Before the first line
has been read, returns 0. After the last line of the last file has
been read, returns the line number of that line within the file.
"""
if not _state:
raise RuntimeError, "no active input()"
return _state.filelineno()
def isfirstline():
"""
Returns true the line just read is the first line of its file,
otherwise returns false.
"""
if not _state:
raise RuntimeError, "no active input()"
return _state.isfirstline()
def isstdin():
"""
Returns true if the last line was read from sys.stdin,
otherwise returns false.
"""
if not _state:
raise RuntimeError, "no active input()"
return _state.isstdin()
class FileInput:
"""class FileInput([files[, inplace[, backup]]])
Class FileInput is the implementation of the module; its methods
filename(), lineno(), fileline(), isfirstline(), isstdin(), nextfile()
and close() correspond to the functions of the same name in the module.
In addition it has a readline() method which returns the next
input line, and a __getitem__() method which implements the
sequence behavior. The sequence must be accessed in strictly
sequential order; random access and readline() cannot be mixed.
"""
def __init__(self, files=None, inplace=0, backup="", bufsize=0):
if type(files) == type(''):
files = (files,)
else:
if files is None:
files = sys.argv[1:]
if not files:
files = ('-',)
else:
files = tuple(files)
self._files = files
self._inplace = inplace
self._backup = backup
self._bufsize = bufsize or DEFAULT_BUFSIZE
self._savestdout = None
self._output = None
self._filename = None
self._lineno = 0
self._filelineno = 0
self._file = None
self._isstdin = False
self._backupfilename = None
self._buffer = []
self._bufindex = 0
def __del__(self):
self.close()
def close(self):
self.nextfile()
self._files = ()
def __iter__(self):
return self
def next(self):
try:
line = self._buffer[self._bufindex]
except IndexError:
pass
else:
self._bufindex += 1
self._lineno += 1
self._filelineno += 1
return line
line = self.readline()
if not line:
raise StopIteration
return line
def __getitem__(self, i):
if i != self._lineno:
raise RuntimeError, "accessing lines out of order"
try:
return self.next()
except StopIteration:
raise IndexError, "end of input reached"
def nextfile(self):
savestdout = self._savestdout
self._savestdout = 0
if savestdout:
sys.stdout = savestdout
output = self._output
self._output = 0
if output:
output.close()
file = self._file
self._file = 0
if file and not self._isstdin:
file.close()
backupfilename = self._backupfilename
self._backupfilename = 0
if backupfilename and not self._backup:
try: os.unlink(backupfilename)
except OSError: pass
self._isstdin = False
self._buffer = []
self._bufindex = 0
def readline(self):
try:
line = self._buffer[self._bufindex]
except IndexError:
pass
else:
self._bufindex += 1
self._lineno += 1
self._filelineno += 1
return line
if not self._file:
if not self._files:
return ""
self._filename = self._files[0]
self._files = self._files[1:]
self._filelineno = 0
self._file = None
self._isstdin = False
self._backupfilename = 0
if self._filename == '-':
self._filename = '<stdin>'
self._file = sys.stdin
self._isstdin = True
else:
if self._inplace:
self._backupfilename = (
self._filename + (self._backup or os.extsep+"bak"))
try: os.unlink(self._backupfilename)
except os.error: pass
# The next few lines may raise IOError
os.rename(self._filename, self._backupfilename)
self._file = open(self._backupfilename, "r")
try:
perm = os.fstat(self._file.fileno()).st_mode
except OSError:
self._output = open(self._filename, "w")
else:
fd = os.open(self._filename,
os.O_CREAT | os.O_WRONLY | os.O_TRUNC,
perm)
self._output = os.fdopen(fd, "w")
try:
if hasattr(os, 'chmod'):
os.chmod(self._filename, perm)
except OSError:
pass
self._savestdout = sys.stdout
sys.stdout = self._output
else:
# This may raise IOError
self._file = open(self._filename, "r")
self._buffer = self._file.readlines(self._bufsize)
self._bufindex = 0
if not self._buffer:
self.nextfile()
# Recursive call
return self.readline()
def filename(self):
return self._filename
def lineno(self):
return self._lineno
def filelineno(self):
return self._filelineno
def isfirstline(self):
return self._filelineno == 1
def isstdin(self):
return self._isstdin
def _test():
import getopt
inplace = 0
backup = 0
opts, args = getopt.getopt(sys.argv[1:], "ib:")
for o, a in opts:
if o == '-i': inplace = 1
if o == '-b': backup = a
for line in input(args, inplace=inplace, backup=backup):
if line[-1:] == '\n': line = line[:-1]
if line[-1:] == '\r': line = line[:-1]
print "%d: %s[%d]%s %s" % (lineno(), filename(), filelineno(),
isfirstline() and "*" or "", line)
print "%d: %s[%d]" % (lineno(), filename(), filelineno())
if __name__ == '__main__':
_test()
| mit |
kahopoon/video_autoHandle | upload_video.py | 4 | 6657 | #!/usr/bin/python
import httplib
import httplib2
import os
import random
import sys
import time
from apiclient.discovery import build
from apiclient.errors import HttpError
from apiclient.http import MediaFileUpload
from oauth2client.client import flow_from_clientsecrets
from oauth2client.file import Storage
from oauth2client.tools import argparser, run_flow
# Explicitly tell the underlying HTTP transport library not to retry, since
# we are handling retry logic ourselves.
httplib2.RETRIES = 1
# Maximum number of times to retry before giving up.
MAX_RETRIES = 10
# Always retry when these exceptions are raised.
RETRIABLE_EXCEPTIONS = (httplib2.HttpLib2Error, IOError, httplib.NotConnected,
httplib.IncompleteRead, httplib.ImproperConnectionState,
httplib.CannotSendRequest, httplib.CannotSendHeader,
httplib.ResponseNotReady, httplib.BadStatusLine)
# Always retry when an apiclient.errors.HttpError with one of these status
# codes is raised.
RETRIABLE_STATUS_CODES = [500, 502, 503, 504]
# The CLIENT_SECRETS_FILE variable specifies the name of a file that contains
# the OAuth 2.0 information for this application, including its client_id and
# client_secret. You can acquire an OAuth 2.0 client ID and client secret from
# the Google Developers Console at
# https://console.developers.google.com/.
# Please ensure that you have enabled the YouTube Data API for your project.
# For more information about using OAuth2 to access the YouTube Data API, see:
# https://developers.google.com/youtube/v3/guides/authentication
# For more information about the client_secrets.json file format, see:
# https://developers.google.com/api-client-library/python/guide/aaa_client_secrets
CLIENT_SECRETS_FILE = "client_secrets.json"
# This OAuth 2.0 access scope allows an application to upload files to the
# authenticated user's YouTube channel, but doesn't allow other types of access.
YOUTUBE_UPLOAD_SCOPE = "https://www.googleapis.com/auth/youtube.upload"
YOUTUBE_API_SERVICE_NAME = "youtube"
YOUTUBE_API_VERSION = "v3"
# This variable defines a message to display if the CLIENT_SECRETS_FILE is
# missing.
MISSING_CLIENT_SECRETS_MESSAGE = """
WARNING: Please configure OAuth 2.0
To make this sample run you will need to populate the client_secrets.json file
found at:
%s
with information from the Developers Console
https://console.developers.google.com/
For more information about the client_secrets.json file format, please visit:
https://developers.google.com/api-client-library/python/guide/aaa_client_secrets
""" % os.path.abspath(os.path.join(os.path.dirname(__file__),
CLIENT_SECRETS_FILE))
VALID_PRIVACY_STATUSES = ("public", "private", "unlisted")
def get_authenticated_service(args):
flow = flow_from_clientsecrets(CLIENT_SECRETS_FILE,
scope=YOUTUBE_UPLOAD_SCOPE,
message=MISSING_CLIENT_SECRETS_MESSAGE)
storage = Storage("%s-oauth2.json" % sys.argv[0])
credentials = storage.get()
if credentials is None or credentials.invalid:
credentials = run_flow(flow, storage, args)
return build(YOUTUBE_API_SERVICE_NAME, YOUTUBE_API_VERSION,
http=credentials.authorize(httplib2.Http()))
def initialize_upload(youtube, options):
tags = None
if options.keywords:
tags = options.keywords.split(",")
body=dict(
snippet=dict(
title=options.title,
description=options.description,
tags=tags,
categoryId=options.category
),
status=dict(
privacyStatus=options.privacyStatus
)
)
# Call the API's videos.insert method to create and upload the video.
insert_request = youtube.videos().insert(
part=",".join(body.keys()),
body=body,
# The chunksize parameter specifies the size of each chunk of data, in
# bytes, that will be uploaded at a time. Set a higher value for
# reliable connections as fewer chunks lead to faster uploads. Set a lower
# value for better recovery on less reliable connections.
#
# Setting "chunksize" equal to -1 in the code below means that the entire
# file will be uploaded in a single HTTP request. (If the upload fails,
# it will still be retried where it left off.) This is usually a best
# practice, but if you're using Python older than 2.6 or if you're
# running on App Engine, you should set the chunksize to something like
# 1024 * 1024 (1 megabyte).
media_body=MediaFileUpload(options.file, chunksize=-1, resumable=True)
)
resumable_upload(insert_request)
# This method implements an exponential backoff strategy to resume a
# failed upload.
def resumable_upload(insert_request):
response = None
error = None
retry = 0
while response is None:
try:
print "Uploading file..."
status, response = insert_request.next_chunk()
if 'id' in response:
print "Video id '%s' was successfully uploaded." % response['id']
else:
exit("The upload failed with an unexpected response: %s" % response)
except HttpError, e:
if e.resp.status in RETRIABLE_STATUS_CODES:
error = "A retriable HTTP error %d occurred:\n%s" % (e.resp.status,
e.content)
else:
raise
except RETRIABLE_EXCEPTIONS, e:
error = "A retriable error occurred: %s" % e
if error is not None:
print error
retry += 1
if retry > MAX_RETRIES:
exit("No longer attempting to retry.")
max_sleep = 2 ** retry
sleep_seconds = random.random() * max_sleep
print "Sleeping %f seconds and then retrying..." % sleep_seconds
time.sleep(sleep_seconds)
if __name__ == '__main__':
argparser.add_argument("--file", required=True, help="Video file to upload")
argparser.add_argument("--title", help="Video title", default="Test Title")
argparser.add_argument("--description", help="Video description",
default="Test Description")
argparser.add_argument("--category", default="22",
help="Numeric video category. " +
"See https://developers.google.com/youtube/v3/docs/videoCategories/list")
argparser.add_argument("--keywords", help="Video keywords, comma separated",
default="")
argparser.add_argument("--privacyStatus", choices=VALID_PRIVACY_STATUSES,
default=VALID_PRIVACY_STATUSES[0], help="Video privacy status.")
args = argparser.parse_args()
if not os.path.exists(args.file):
exit("Please specify a valid file using the --file= parameter.")
youtube = get_authenticated_service(args)
try:
initialize_upload(youtube, args)
except HttpError, e:
print "An HTTP error %d occurred:\n%s" % (e.resp.status, e.content)
| mit |
Azure/azure-sdk-for-python | sdk/netapp/azure-mgmt-netapp/azure/mgmt/netapp/operations/_volumes_operations.py | 1 | 182324 | # pylint: disable=too-many-lines
# coding=utf-8
# --------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for license information.
# Code generated by Microsoft (R) AutoRest Code Generator.
# Changes may cause incorrect behavior and will be lost if the code is regenerated.
# --------------------------------------------------------------------------
from typing import Any, Callable, Dict, IO, Iterable, Optional, TypeVar, Union, cast, overload
import urllib.parse
from azure.core.exceptions import (
ClientAuthenticationError,
HttpResponseError,
ResourceExistsError,
ResourceNotFoundError,
ResourceNotModifiedError,
map_error,
)
from azure.core.paging import ItemPaged
from azure.core.pipeline import PipelineResponse
from azure.core.pipeline.transport import HttpResponse
from azure.core.polling import LROPoller, NoPolling, PollingMethod
from azure.core.rest import HttpRequest
from azure.core.tracing.decorator import distributed_trace
from azure.core.utils import case_insensitive_dict
from azure.mgmt.core.exceptions import ARMErrorFormat
from azure.mgmt.core.polling.arm_polling import ARMPolling
from .. import models as _models
from .._serialization import Serializer
from .._vendor import _convert_request, _format_url_section
T = TypeVar("T")
ClsType = Optional[Callable[[PipelineResponse[HttpRequest, HttpResponse], T, Dict[str, Any]], Any]]
_SERIALIZER = Serializer()
_SERIALIZER.client_side_validation = False
def build_list_request(
resource_group_name: str, account_name: str, pool_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
accept = _headers.pop("Accept", "application/json")
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
_headers["Accept"] = _SERIALIZER.header("accept", accept, "str")
return HttpRequest(method="GET", url=_url, params=_params, headers=_headers, **kwargs)
def build_get_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
accept = _headers.pop("Accept", "application/json")
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
_headers["Accept"] = _SERIALIZER.header("accept", accept, "str")
return HttpRequest(method="GET", url=_url, params=_params, headers=_headers, **kwargs)
def build_create_or_update_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
accept = _headers.pop("Accept", "application/json")
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
if content_type is not None:
_headers["Content-Type"] = _SERIALIZER.header("content_type", content_type, "str")
_headers["Accept"] = _SERIALIZER.header("accept", accept, "str")
return HttpRequest(method="PUT", url=_url, params=_params, headers=_headers, **kwargs)
def build_update_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
accept = _headers.pop("Accept", "application/json")
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
if content_type is not None:
_headers["Content-Type"] = _SERIALIZER.header("content_type", content_type, "str")
_headers["Accept"] = _SERIALIZER.header("accept", accept, "str")
return HttpRequest(method="PATCH", url=_url, params=_params, headers=_headers, **kwargs)
def build_delete_request(
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
subscription_id: str,
*,
force_delete: Optional[bool] = None,
**kwargs: Any
) -> HttpRequest:
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
if force_delete is not None:
_params["forceDelete"] = _SERIALIZER.query("force_delete", force_delete, "bool")
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
return HttpRequest(method="DELETE", url=_url, params=_params, **kwargs)
def build_revert_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/revert",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
if content_type is not None:
_headers["Content-Type"] = _SERIALIZER.header("content_type", content_type, "str")
return HttpRequest(method="POST", url=_url, params=_params, headers=_headers, **kwargs)
def build_reset_cifs_password_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/resetCifsPassword",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
return HttpRequest(method="POST", url=_url, params=_params, **kwargs)
def build_break_replication_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/breakReplication",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
if content_type is not None:
_headers["Content-Type"] = _SERIALIZER.header("content_type", content_type, "str")
return HttpRequest(method="POST", url=_url, params=_params, headers=_headers, **kwargs)
def build_reestablish_replication_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/reestablishReplication",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
if content_type is not None:
_headers["Content-Type"] = _SERIALIZER.header("content_type", content_type, "str")
return HttpRequest(method="POST", url=_url, params=_params, headers=_headers, **kwargs)
def build_replication_status_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
accept = _headers.pop("Accept", "application/json")
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/replicationStatus",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
_headers["Accept"] = _SERIALIZER.header("accept", accept, "str")
return HttpRequest(method="GET", url=_url, params=_params, headers=_headers, **kwargs)
def build_list_replications_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
accept = _headers.pop("Accept", "application/json")
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/listReplications",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
_headers["Accept"] = _SERIALIZER.header("accept", accept, "str")
return HttpRequest(method="POST", url=_url, params=_params, headers=_headers, **kwargs)
def build_resync_replication_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/resyncReplication",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
return HttpRequest(method="POST", url=_url, params=_params, **kwargs)
def build_delete_replication_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/deleteReplication",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
return HttpRequest(method="POST", url=_url, params=_params, **kwargs)
def build_authorize_replication_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/authorizeReplication",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
if content_type is not None:
_headers["Content-Type"] = _SERIALIZER.header("content_type", content_type, "str")
return HttpRequest(method="POST", url=_url, params=_params, headers=_headers, **kwargs)
def build_re_initialize_replication_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/reinitializeReplication",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
return HttpRequest(method="POST", url=_url, params=_params, **kwargs)
def build_pool_change_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/poolChange",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
if content_type is not None:
_headers["Content-Type"] = _SERIALIZER.header("content_type", content_type, "str")
return HttpRequest(method="POST", url=_url, params=_params, headers=_headers, **kwargs)
def build_relocate_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/relocate",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
if content_type is not None:
_headers["Content-Type"] = _SERIALIZER.header("content_type", content_type, "str")
return HttpRequest(method="POST", url=_url, params=_params, headers=_headers, **kwargs)
def build_finalize_relocation_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/finalizeRelocation",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
return HttpRequest(method="POST", url=_url, params=_params, **kwargs)
def build_revert_relocation_request(
resource_group_name: str, account_name: str, pool_name: str, volume_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2022-05-01")) # type: str
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/revertRelocation",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url(
"resource_group_name", resource_group_name, "str", max_length=90, min_length=1, pattern=r"^[-\w\._\(\)]+$"
),
"accountName": _SERIALIZER.url("account_name", account_name, "str"),
"poolName": _SERIALIZER.url(
"pool_name", pool_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z0-9][a-zA-Z0-9\-_]{0,63}$"
),
"volumeName": _SERIALIZER.url(
"volume_name", volume_name, "str", max_length=64, min_length=1, pattern=r"^[a-zA-Z][a-zA-Z0-9\-_]{0,63}$"
),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
return HttpRequest(method="POST", url=_url, params=_params, **kwargs)
class VolumesOperations: # pylint: disable=too-many-public-methods
"""
.. warning::
**DO NOT** instantiate this class directly.
Instead, you should access the following operations through
:class:`~azure.mgmt.netapp.NetAppManagementClient`'s
:attr:`volumes` attribute.
"""
models = _models
def __init__(self, *args, **kwargs):
input_args = list(args)
self._client = input_args.pop(0) if input_args else kwargs.pop("client")
self._config = input_args.pop(0) if input_args else kwargs.pop("config")
self._serialize = input_args.pop(0) if input_args else kwargs.pop("serializer")
self._deserialize = input_args.pop(0) if input_args else kwargs.pop("deserializer")
@distributed_trace
def list(
self, resource_group_name: str, account_name: str, pool_name: str, **kwargs: Any
) -> Iterable["_models.Volume"]:
"""Describe all volumes.
List all volumes within the capacity pool.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: An iterator like instance of either Volume or the result of cls(response)
:rtype: ~azure.core.paging.ItemPaged[~azure.mgmt.netapp.models.Volume]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[_models.VolumeList]
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
def prepare_request(next_link=None):
if not next_link:
request = build_list_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self.list.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
else:
# make call to next link with the client's api-version
_parsed_next_link = urllib.parse.urlparse(next_link)
_next_request_params = case_insensitive_dict(
{
key: [urllib.parse.quote(v) for v in value]
for key, value in urllib.parse.parse_qs(_parsed_next_link.query).items()
}
)
_next_request_params["api-version"] = self._config.api_version
request = HttpRequest(
"GET", urllib.parse.urljoin(next_link, _parsed_next_link.path), params=_next_request_params
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
request.method = "GET"
return request
def extract_data(pipeline_response):
deserialized = self._deserialize("VolumeList", pipeline_response)
list_of_elem = deserialized.value
if cls:
list_of_elem = cls(list_of_elem)
return deserialized.next_link or None, iter(list_of_elem)
def get_next(next_link=None):
request = prepare_request(next_link)
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
return pipeline_response
return ItemPaged(get_next, extract_data)
list.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes"} # type: ignore
@distributed_trace
def get(
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> _models.Volume:
"""Describe a volume.
Get the details of the specified volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: Volume or the result of cls(response)
:rtype: ~azure.mgmt.netapp.models.Volume
:raises ~azure.core.exceptions.HttpResponseError:
"""
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[_models.Volume]
request = build_get_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self.get.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
deserialized = self._deserialize("Volume", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
get.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}"} # type: ignore
def _create_or_update_initial(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.Volume, IO],
**kwargs: Any
) -> Optional[_models.Volume]:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[Optional[_models.Volume]]
content_type = content_type or "application/json"
_json = None
_content = None
if isinstance(body, (IO, bytes)):
_content = body
else:
_json = self._serialize.body(body, "Volume")
request = build_create_or_update_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
content_type=content_type,
json=_json,
content=_content,
template_url=self._create_or_update_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 201, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
deserialized = None
if response.status_code == 200:
deserialized = self._deserialize("Volume", pipeline_response)
if response.status_code == 201:
deserialized = self._deserialize("Volume", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
_create_or_update_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}"} # type: ignore
@overload
def begin_create_or_update(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: _models.Volume,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[_models.Volume]:
"""Create or Update a volume.
Create or update the specified volume within the capacity pool.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Volume object supplied in the body of the operation. Required.
:type body: ~azure.mgmt.netapp.models.Volume
:keyword content_type: Body Parameter content-type. Content type parameter for JSON body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either Volume or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.netapp.models.Volume]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@overload
def begin_create_or_update(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: IO,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[_models.Volume]:
"""Create or Update a volume.
Create or update the specified volume within the capacity pool.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Volume object supplied in the body of the operation. Required.
:type body: IO
:keyword content_type: Body Parameter content-type. Content type parameter for binary body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either Volume or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.netapp.models.Volume]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@distributed_trace
def begin_create_or_update(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.Volume, IO],
**kwargs: Any
) -> LROPoller[_models.Volume]:
"""Create or Update a volume.
Create or update the specified volume within the capacity pool.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Volume object supplied in the body of the operation. Is either a model type or a
IO type. Required.
:type body: ~azure.mgmt.netapp.models.Volume or IO
:keyword content_type: Body Parameter content-type. Known values are: 'application/json'.
Default value is None.
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either Volume or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.netapp.models.Volume]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[_models.Volume]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._create_or_update_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
body=body,
api_version=api_version,
content_type=content_type,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response):
deserialized = self._deserialize("Volume", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
if polling is True:
polling_method = cast(
PollingMethod, ARMPolling(lro_delay, lro_options={"final-state-via": "azure-async-operation"}, **kwargs)
) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_create_or_update.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}"} # type: ignore
def _update_initial(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.VolumePatch, IO],
**kwargs: Any
) -> Optional[_models.Volume]:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[Optional[_models.Volume]]
content_type = content_type or "application/json"
_json = None
_content = None
if isinstance(body, (IO, bytes)):
_content = body
else:
_json = self._serialize.body(body, "VolumePatch")
request = build_update_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
content_type=content_type,
json=_json,
content=_content,
template_url=self._update_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
deserialized = None
if response.status_code == 200:
deserialized = self._deserialize("Volume", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
_update_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}"} # type: ignore
@overload
def begin_update(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: _models.VolumePatch,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[_models.Volume]:
"""Update a volume.
Patch the specified volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Volume object supplied in the body of the operation. Required.
:type body: ~azure.mgmt.netapp.models.VolumePatch
:keyword content_type: Body Parameter content-type. Content type parameter for JSON body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either Volume or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.netapp.models.Volume]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@overload
def begin_update(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: IO,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[_models.Volume]:
"""Update a volume.
Patch the specified volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Volume object supplied in the body of the operation. Required.
:type body: IO
:keyword content_type: Body Parameter content-type. Content type parameter for binary body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either Volume or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.netapp.models.Volume]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@distributed_trace
def begin_update(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.VolumePatch, IO],
**kwargs: Any
) -> LROPoller[_models.Volume]:
"""Update a volume.
Patch the specified volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Volume object supplied in the body of the operation. Is either a model type or a
IO type. Required.
:type body: ~azure.mgmt.netapp.models.VolumePatch or IO
:keyword content_type: Body Parameter content-type. Known values are: 'application/json'.
Default value is None.
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either Volume or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.netapp.models.Volume]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[_models.Volume]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._update_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
body=body,
api_version=api_version,
content_type=content_type,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response):
deserialized = self._deserialize("Volume", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
if polling is True:
polling_method = cast(
PollingMethod, ARMPolling(lro_delay, lro_options={"final-state-via": "location"}, **kwargs)
) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_update.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}"} # type: ignore
def _delete_initial( # pylint: disable=inconsistent-return-statements
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
force_delete: Optional[bool] = None,
**kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_delete_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
force_delete=force_delete,
api_version=api_version,
template_url=self._delete_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [202, 204]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_delete_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}"} # type: ignore
@distributed_trace
def begin_delete(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
force_delete: Optional[bool] = None,
**kwargs: Any
) -> LROPoller[None]:
"""Delete a volume.
Delete the specified volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param force_delete: An option to force delete the volume. Will cleanup resources connected to
the particular volume. Default value is None.
:type force_delete: bool
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._delete_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
force_delete=force_delete,
api_version=api_version,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(
PollingMethod, ARMPolling(lro_delay, lro_options={"final-state-via": "location"}, **kwargs)
) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_delete.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}"} # type: ignore
def _revert_initial( # pylint: disable=inconsistent-return-statements
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.VolumeRevert, IO],
**kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
content_type = content_type or "application/json"
_json = None
_content = None
if isinstance(body, (IO, bytes)):
_content = body
else:
_json = self._serialize.body(body, "VolumeRevert")
request = build_revert_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
content_type=content_type,
json=_json,
content=_content,
template_url=self._revert_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_revert_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/revert"} # type: ignore
@overload
def begin_revert(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: _models.VolumeRevert,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Revert a volume to one of its snapshots.
Revert a volume to the snapshot specified in the body.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Object for snapshot to revert supplied in the body of the operation. Required.
:type body: ~azure.mgmt.netapp.models.VolumeRevert
:keyword content_type: Body Parameter content-type. Content type parameter for JSON body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@overload
def begin_revert(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: IO,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Revert a volume to one of its snapshots.
Revert a volume to the snapshot specified in the body.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Object for snapshot to revert supplied in the body of the operation. Required.
:type body: IO
:keyword content_type: Body Parameter content-type. Content type parameter for binary body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@distributed_trace
def begin_revert(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.VolumeRevert, IO],
**kwargs: Any
) -> LROPoller[None]:
"""Revert a volume to one of its snapshots.
Revert a volume to the snapshot specified in the body.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Object for snapshot to revert supplied in the body of the operation. Is either a
model type or a IO type. Required.
:type body: ~azure.mgmt.netapp.models.VolumeRevert or IO
:keyword content_type: Body Parameter content-type. Known values are: 'application/json'.
Default value is None.
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._revert_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
body=body,
api_version=api_version,
content_type=content_type,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(
PollingMethod, ARMPolling(lro_delay, lro_options={"final-state-via": "location"}, **kwargs)
) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_revert.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/revert"} # type: ignore
def _reset_cifs_password_initial( # pylint: disable=inconsistent-return-statements
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_reset_cifs_password_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self._reset_cifs_password_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_reset_cifs_password_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/resetCifsPassword"} # type: ignore
@distributed_trace
def begin_reset_cifs_password(
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> LROPoller[None]:
"""Reset cifs password.
Reset cifs password from volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._reset_cifs_password_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
api_version=api_version,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(PollingMethod, ARMPolling(lro_delay, **kwargs)) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_reset_cifs_password.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/resetCifsPassword"} # type: ignore
def _break_replication_initial( # pylint: disable=inconsistent-return-statements
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Optional[Union[_models.BreakReplicationRequest, IO]] = None,
**kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
content_type = content_type or "application/json"
_json = None
_content = None
if isinstance(body, (IO, bytes)):
_content = body
else:
if body is not None:
_json = self._serialize.body(body, "BreakReplicationRequest")
else:
_json = None
request = build_break_replication_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
content_type=content_type,
json=_json,
content=_content,
template_url=self._break_replication_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_break_replication_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/breakReplication"} # type: ignore
@overload
def begin_break_replication(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Optional[_models.BreakReplicationRequest] = None,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Break volume replication.
Break the replication connection on the destination volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Optional body to force break the replication. Default value is None.
:type body: ~azure.mgmt.netapp.models.BreakReplicationRequest
:keyword content_type: Body Parameter content-type. Content type parameter for JSON body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@overload
def begin_break_replication(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Optional[IO] = None,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Break volume replication.
Break the replication connection on the destination volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Optional body to force break the replication. Default value is None.
:type body: IO
:keyword content_type: Body Parameter content-type. Content type parameter for binary body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@distributed_trace
def begin_break_replication(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Optional[Union[_models.BreakReplicationRequest, IO]] = None,
**kwargs: Any
) -> LROPoller[None]:
"""Break volume replication.
Break the replication connection on the destination volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Optional body to force break the replication. Is either a model type or a IO type.
Default value is None.
:type body: ~azure.mgmt.netapp.models.BreakReplicationRequest or IO
:keyword content_type: Body Parameter content-type. Known values are: 'application/json'.
Default value is None.
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._break_replication_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
body=body,
api_version=api_version,
content_type=content_type,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(
PollingMethod, ARMPolling(lro_delay, lro_options={"final-state-via": "location"}, **kwargs)
) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_break_replication.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/breakReplication"} # type: ignore
def _reestablish_replication_initial( # pylint: disable=inconsistent-return-statements
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.ReestablishReplicationRequest, IO],
**kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
content_type = content_type or "application/json"
_json = None
_content = None
if isinstance(body, (IO, bytes)):
_content = body
else:
_json = self._serialize.body(body, "ReestablishReplicationRequest")
request = build_reestablish_replication_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
content_type=content_type,
json=_json,
content=_content,
template_url=self._reestablish_replication_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_reestablish_replication_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/reestablishReplication"} # type: ignore
@overload
def begin_reestablish_replication(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: _models.ReestablishReplicationRequest,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Re-establish volume replication.
Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or
policy-based snapshots.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: body for the id of the source volume. Required.
:type body: ~azure.mgmt.netapp.models.ReestablishReplicationRequest
:keyword content_type: Body Parameter content-type. Content type parameter for JSON body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@overload
def begin_reestablish_replication(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: IO,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Re-establish volume replication.
Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or
policy-based snapshots.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: body for the id of the source volume. Required.
:type body: IO
:keyword content_type: Body Parameter content-type. Content type parameter for binary body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@distributed_trace
def begin_reestablish_replication(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.ReestablishReplicationRequest, IO],
**kwargs: Any
) -> LROPoller[None]:
"""Re-establish volume replication.
Re-establish a previously deleted replication between 2 volumes that have a common ad-hoc or
policy-based snapshots.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: body for the id of the source volume. Is either a model type or a IO type.
Required.
:type body: ~azure.mgmt.netapp.models.ReestablishReplicationRequest or IO
:keyword content_type: Body Parameter content-type. Known values are: 'application/json'.
Default value is None.
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._reestablish_replication_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
body=body,
api_version=api_version,
content_type=content_type,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(
PollingMethod, ARMPolling(lro_delay, lro_options={"final-state-via": "location"}, **kwargs)
) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_reestablish_replication.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/reestablishReplication"} # type: ignore
@distributed_trace
def replication_status(
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> _models.ReplicationStatus:
"""Get volume replication status.
Get the status of the replication.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: ReplicationStatus or the result of cls(response)
:rtype: ~azure.mgmt.netapp.models.ReplicationStatus
:raises ~azure.core.exceptions.HttpResponseError:
"""
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[_models.ReplicationStatus]
request = build_replication_status_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self.replication_status.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
deserialized = self._deserialize("ReplicationStatus", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
replication_status.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/replicationStatus"} # type: ignore
@distributed_trace
def list_replications(
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> Iterable["_models.Replication"]:
"""List replications for volume.
List all replications for a specified volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: An iterator like instance of either Replication or the result of cls(response)
:rtype: ~azure.core.paging.ItemPaged[~azure.mgmt.netapp.models.Replication]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[_models.ListReplications]
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
def prepare_request(next_link=None):
if not next_link:
request = build_list_replications_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self.list_replications.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
else:
# make call to next link with the client's api-version
_parsed_next_link = urllib.parse.urlparse(next_link)
_next_request_params = case_insensitive_dict(
{
key: [urllib.parse.quote(v) for v in value]
for key, value in urllib.parse.parse_qs(_parsed_next_link.query).items()
}
)
_next_request_params["api-version"] = self._config.api_version
request = HttpRequest(
"GET", urllib.parse.urljoin(next_link, _parsed_next_link.path), params=_next_request_params
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
request.method = "GET"
return request
def extract_data(pipeline_response):
deserialized = self._deserialize("ListReplications", pipeline_response)
list_of_elem = deserialized.value
if cls:
list_of_elem = cls(list_of_elem)
return None, iter(list_of_elem)
def get_next(next_link=None):
request = prepare_request(next_link)
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
return pipeline_response
return ItemPaged(get_next, extract_data)
list_replications.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/listReplications"} # type: ignore
def _resync_replication_initial( # pylint: disable=inconsistent-return-statements
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_resync_replication_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self._resync_replication_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_resync_replication_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/resyncReplication"} # type: ignore
@distributed_trace
def begin_resync_replication(
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> LROPoller[None]:
"""Resync volume replication.
Resync the connection on the destination volume. If the operation is ran on the source volume
it will reverse-resync the connection and sync from destination to source.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._resync_replication_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
api_version=api_version,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(
PollingMethod, ARMPolling(lro_delay, lro_options={"final-state-via": "location"}, **kwargs)
) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_resync_replication.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/resyncReplication"} # type: ignore
def _delete_replication_initial( # pylint: disable=inconsistent-return-statements
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_delete_replication_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self._delete_replication_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_delete_replication_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/deleteReplication"} # type: ignore
@distributed_trace
def begin_delete_replication(
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> LROPoller[None]:
"""Delete volume replication.
Delete the replication connection on the destination volume, and send release to the source
replication.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._delete_replication_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
api_version=api_version,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(
PollingMethod, ARMPolling(lro_delay, lro_options={"final-state-via": "location"}, **kwargs)
) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_delete_replication.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/deleteReplication"} # type: ignore
def _authorize_replication_initial( # pylint: disable=inconsistent-return-statements
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.AuthorizeRequest, IO],
**kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
content_type = content_type or "application/json"
_json = None
_content = None
if isinstance(body, (IO, bytes)):
_content = body
else:
_json = self._serialize.body(body, "AuthorizeRequest")
request = build_authorize_replication_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
content_type=content_type,
json=_json,
content=_content,
template_url=self._authorize_replication_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_authorize_replication_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/authorizeReplication"} # type: ignore
@overload
def begin_authorize_replication(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: _models.AuthorizeRequest,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Authorize source volume replication.
Authorize the replication connection on the source volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Authorize request object supplied in the body of the operation. Required.
:type body: ~azure.mgmt.netapp.models.AuthorizeRequest
:keyword content_type: Body Parameter content-type. Content type parameter for JSON body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@overload
def begin_authorize_replication(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: IO,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Authorize source volume replication.
Authorize the replication connection on the source volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Authorize request object supplied in the body of the operation. Required.
:type body: IO
:keyword content_type: Body Parameter content-type. Content type parameter for binary body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@distributed_trace
def begin_authorize_replication(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.AuthorizeRequest, IO],
**kwargs: Any
) -> LROPoller[None]:
"""Authorize source volume replication.
Authorize the replication connection on the source volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Authorize request object supplied in the body of the operation. Is either a model
type or a IO type. Required.
:type body: ~azure.mgmt.netapp.models.AuthorizeRequest or IO
:keyword content_type: Body Parameter content-type. Known values are: 'application/json'.
Default value is None.
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._authorize_replication_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
body=body,
api_version=api_version,
content_type=content_type,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(
PollingMethod, ARMPolling(lro_delay, lro_options={"final-state-via": "location"}, **kwargs)
) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_authorize_replication.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/authorizeReplication"} # type: ignore
def _re_initialize_replication_initial( # pylint: disable=inconsistent-return-statements
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_re_initialize_replication_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self._re_initialize_replication_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_re_initialize_replication_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/reinitializeReplication"} # type: ignore
@distributed_trace
def begin_re_initialize_replication(
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> LROPoller[None]:
"""ReInitialize volume replication.
Re-Initializes the replication connection on the destination volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._re_initialize_replication_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
api_version=api_version,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(
PollingMethod, ARMPolling(lro_delay, lro_options={"final-state-via": "location"}, **kwargs)
) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_re_initialize_replication.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/reinitializeReplication"} # type: ignore
def _pool_change_initial( # pylint: disable=inconsistent-return-statements
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.PoolChangeRequest, IO],
**kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
content_type = content_type or "application/json"
_json = None
_content = None
if isinstance(body, (IO, bytes)):
_content = body
else:
_json = self._serialize.body(body, "PoolChangeRequest")
request = build_pool_change_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
content_type=content_type,
json=_json,
content=_content,
template_url=self._pool_change_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_pool_change_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/poolChange"} # type: ignore
@overload
def begin_pool_change(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: _models.PoolChangeRequest,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Change pool for volume.
Moves volume to another pool.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Move volume to the pool supplied in the body of the operation. Required.
:type body: ~azure.mgmt.netapp.models.PoolChangeRequest
:keyword content_type: Body Parameter content-type. Content type parameter for JSON body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@overload
def begin_pool_change(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: IO,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Change pool for volume.
Moves volume to another pool.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Move volume to the pool supplied in the body of the operation. Required.
:type body: IO
:keyword content_type: Body Parameter content-type. Content type parameter for binary body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@distributed_trace
def begin_pool_change(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Union[_models.PoolChangeRequest, IO],
**kwargs: Any
) -> LROPoller[None]:
"""Change pool for volume.
Moves volume to another pool.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Move volume to the pool supplied in the body of the operation. Is either a model
type or a IO type. Required.
:type body: ~azure.mgmt.netapp.models.PoolChangeRequest or IO
:keyword content_type: Body Parameter content-type. Known values are: 'application/json'.
Default value is None.
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._pool_change_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
body=body,
api_version=api_version,
content_type=content_type,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(
PollingMethod, ARMPolling(lro_delay, lro_options={"final-state-via": "location"}, **kwargs)
) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_pool_change.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/poolChange"} # type: ignore
def _relocate_initial( # pylint: disable=inconsistent-return-statements
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Optional[Union[_models.RelocateVolumeRequest, IO]] = None,
**kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
content_type = content_type or "application/json"
_json = None
_content = None
if isinstance(body, (IO, bytes)):
_content = body
else:
if body is not None:
_json = self._serialize.body(body, "RelocateVolumeRequest")
else:
_json = None
request = build_relocate_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
content_type=content_type,
json=_json,
content=_content,
template_url=self._relocate_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_relocate_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/relocate"} # type: ignore
@overload
def begin_relocate(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Optional[_models.RelocateVolumeRequest] = None,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Relocate volume.
Relocates volume to a new stamp.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Relocate volume request. Default value is None.
:type body: ~azure.mgmt.netapp.models.RelocateVolumeRequest
:keyword content_type: Body Parameter content-type. Content type parameter for JSON body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@overload
def begin_relocate(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Optional[IO] = None,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[None]:
"""Relocate volume.
Relocates volume to a new stamp.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Relocate volume request. Default value is None.
:type body: IO
:keyword content_type: Body Parameter content-type. Content type parameter for binary body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@distributed_trace
def begin_relocate(
self,
resource_group_name: str,
account_name: str,
pool_name: str,
volume_name: str,
body: Optional[Union[_models.RelocateVolumeRequest, IO]] = None,
**kwargs: Any
) -> LROPoller[None]:
"""Relocate volume.
Relocates volume to a new stamp.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:param body: Relocate volume request. Is either a model type or a IO type. Default value is
None.
:type body: ~azure.mgmt.netapp.models.RelocateVolumeRequest or IO
:keyword content_type: Body Parameter content-type. Known values are: 'application/json'.
Default value is None.
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._relocate_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
body=body,
api_version=api_version,
content_type=content_type,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(PollingMethod, ARMPolling(lro_delay, **kwargs)) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_relocate.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/relocate"} # type: ignore
def _finalize_relocation_initial( # pylint: disable=inconsistent-return-statements
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_finalize_relocation_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self._finalize_relocation_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_finalize_relocation_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/finalizeRelocation"} # type: ignore
@distributed_trace
def begin_finalize_relocation(
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> LROPoller[None]:
"""Finalize volume relocation.
Finalizes the relocation of the volume and cleans up the old volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._finalize_relocation_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
api_version=api_version,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(PollingMethod, ARMPolling(lro_delay, **kwargs)) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_finalize_relocation.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/finalizeRelocation"} # type: ignore
def _revert_relocation_initial( # pylint: disable=inconsistent-return-statements
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_revert_relocation_request(
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self._revert_relocation_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_revert_relocation_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/revertRelocation"} # type: ignore
@distributed_trace
def begin_revert_relocation(
self, resource_group_name: str, account_name: str, pool_name: str, volume_name: str, **kwargs: Any
) -> LROPoller[None]:
"""Revert volume relocation.
Reverts the volume relocation process, cleans up the new volume and starts using the
former-existing volume.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param account_name: The name of the NetApp account. Required.
:type account_name: str
:param pool_name: The name of the capacity pool. Required.
:type pool_name: str
:param volume_name: The name of the volume. Required.
:type volume_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", self._config.api_version)) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._revert_relocation_initial( # type: ignore
resource_group_name=resource_group_name,
account_name=account_name,
pool_name=pool_name,
volume_name=volume_name,
api_version=api_version,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(PollingMethod, ARMPolling(lro_delay, **kwargs)) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_revert_relocation.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.NetApp/netAppAccounts/{accountName}/capacityPools/{poolName}/volumes/{volumeName}/revertRelocation"} # type: ignore
| mit |
Azure/azure-sdk-for-python | sdk/servicefabric/azure-mgmt-servicefabric/azure/mgmt/servicefabric/operations/_services_operations.py | 1 | 47628 | # pylint: disable=too-many-lines
# coding=utf-8
# --------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for license information.
# Code generated by Microsoft (R) AutoRest Code Generator.
# Changes may cause incorrect behavior and will be lost if the code is regenerated.
# --------------------------------------------------------------------------
import sys
from typing import Any, Callable, Dict, IO, Optional, TypeVar, Union, cast, overload
from azure.core.exceptions import (
ClientAuthenticationError,
HttpResponseError,
ResourceExistsError,
ResourceNotFoundError,
ResourceNotModifiedError,
map_error,
)
from azure.core.pipeline import PipelineResponse
from azure.core.pipeline.transport import HttpResponse
from azure.core.polling import LROPoller, NoPolling, PollingMethod
from azure.core.rest import HttpRequest
from azure.core.tracing.decorator import distributed_trace
from azure.core.utils import case_insensitive_dict
from azure.mgmt.core.exceptions import ARMErrorFormat
from azure.mgmt.core.polling.arm_polling import ARMPolling
from .. import models as _models
from .._serialization import Serializer
from .._vendor import _convert_request, _format_url_section
if sys.version_info >= (3, 8):
from typing import Literal # pylint: disable=no-name-in-module, ungrouped-imports
else:
from typing_extensions import Literal # type: ignore # pylint: disable=ungrouped-imports
T = TypeVar("T")
ClsType = Optional[Callable[[PipelineResponse[HttpRequest, HttpResponse], T, Dict[str, Any]], Any]]
_SERIALIZER = Serializer()
_SERIALIZER.client_side_validation = False
def build_get_request(
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
subscription_id: str,
**kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2021-06-01")) # type: Literal["2021-06-01"]
accept = _headers.pop("Accept", "application/json")
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services/{serviceName}",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url("resource_group_name", resource_group_name, "str"),
"clusterName": _SERIALIZER.url("cluster_name", cluster_name, "str"),
"applicationName": _SERIALIZER.url("application_name", application_name, "str"),
"serviceName": _SERIALIZER.url("service_name", service_name, "str"),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
_headers["Accept"] = _SERIALIZER.header("accept", accept, "str")
return HttpRequest(method="GET", url=_url, params=_params, headers=_headers, **kwargs)
def build_create_or_update_request(
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
subscription_id: str,
**kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2021-06-01")) # type: Literal["2021-06-01"]
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
accept = _headers.pop("Accept", "application/json")
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services/{serviceName}",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url("resource_group_name", resource_group_name, "str"),
"clusterName": _SERIALIZER.url("cluster_name", cluster_name, "str"),
"applicationName": _SERIALIZER.url("application_name", application_name, "str"),
"serviceName": _SERIALIZER.url("service_name", service_name, "str"),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
if content_type is not None:
_headers["Content-Type"] = _SERIALIZER.header("content_type", content_type, "str")
_headers["Accept"] = _SERIALIZER.header("accept", accept, "str")
return HttpRequest(method="PUT", url=_url, params=_params, headers=_headers, **kwargs)
def build_update_request(
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
subscription_id: str,
**kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2021-06-01")) # type: Literal["2021-06-01"]
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
accept = _headers.pop("Accept", "application/json")
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services/{serviceName}",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url("resource_group_name", resource_group_name, "str"),
"clusterName": _SERIALIZER.url("cluster_name", cluster_name, "str"),
"applicationName": _SERIALIZER.url("application_name", application_name, "str"),
"serviceName": _SERIALIZER.url("service_name", service_name, "str"),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
if content_type is not None:
_headers["Content-Type"] = _SERIALIZER.header("content_type", content_type, "str")
_headers["Accept"] = _SERIALIZER.header("accept", accept, "str")
return HttpRequest(method="PATCH", url=_url, params=_params, headers=_headers, **kwargs)
def build_delete_request(
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
subscription_id: str,
**kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2021-06-01")) # type: Literal["2021-06-01"]
accept = _headers.pop("Accept", "application/json")
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services/{serviceName}",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url("resource_group_name", resource_group_name, "str"),
"clusterName": _SERIALIZER.url("cluster_name", cluster_name, "str"),
"applicationName": _SERIALIZER.url("application_name", application_name, "str"),
"serviceName": _SERIALIZER.url("service_name", service_name, "str"),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
_headers["Accept"] = _SERIALIZER.header("accept", accept, "str")
return HttpRequest(method="DELETE", url=_url, params=_params, headers=_headers, **kwargs)
def build_list_request(
resource_group_name: str, cluster_name: str, application_name: str, subscription_id: str, **kwargs: Any
) -> HttpRequest:
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2021-06-01")) # type: Literal["2021-06-01"]
accept = _headers.pop("Accept", "application/json")
# Construct URL
_url = kwargs.pop(
"template_url",
"/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services",
) # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, "str"),
"resourceGroupName": _SERIALIZER.url("resource_group_name", resource_group_name, "str"),
"clusterName": _SERIALIZER.url("cluster_name", cluster_name, "str"),
"applicationName": _SERIALIZER.url("application_name", application_name, "str"),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_params["api-version"] = _SERIALIZER.query("api_version", api_version, "str")
# Construct headers
_headers["Accept"] = _SERIALIZER.header("accept", accept, "str")
return HttpRequest(method="GET", url=_url, params=_params, headers=_headers, **kwargs)
class ServicesOperations:
"""
.. warning::
**DO NOT** instantiate this class directly.
Instead, you should access the following operations through
:class:`~azure.mgmt.servicefabric.ServiceFabricManagementClient`'s
:attr:`services` attribute.
"""
models = _models
def __init__(self, *args, **kwargs):
input_args = list(args)
self._client = input_args.pop(0) if input_args else kwargs.pop("client")
self._config = input_args.pop(0) if input_args else kwargs.pop("config")
self._serialize = input_args.pop(0) if input_args else kwargs.pop("serializer")
self._deserialize = input_args.pop(0) if input_args else kwargs.pop("deserializer")
@distributed_trace
def get(
self, resource_group_name: str, cluster_name: str, application_name: str, service_name: str, **kwargs: Any
) -> _models.ServiceResource:
"""Gets a Service Fabric service resource.
Get a Service Fabric service resource created or in the process of being created in the Service
Fabric application resource.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param cluster_name: The name of the cluster resource. Required.
:type cluster_name: str
:param application_name: The name of the application resource. Required.
:type application_name: str
:param service_name: The name of the service resource in the format of
{applicationName}~{serviceName}. Required.
:type service_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: ServiceResource or the result of cls(response)
:rtype: ~azure.mgmt.servicefabric.models.ServiceResource
:raises ~azure.core.exceptions.HttpResponseError:
"""
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop(
"api_version", _params.pop("api-version", self._config.api_version)
) # type: Literal["2021-06-01"]
cls = kwargs.pop("cls", None) # type: ClsType[_models.ServiceResource]
request = build_get_request(
resource_group_name=resource_group_name,
cluster_name=cluster_name,
application_name=application_name,
service_name=service_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self.get.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorModel, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
deserialized = self._deserialize("ServiceResource", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
get.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services/{serviceName}"} # type: ignore
def _create_or_update_initial(
self,
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
parameters: Union[_models.ServiceResource, IO],
**kwargs: Any
) -> _models.ServiceResource:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop(
"api_version", _params.pop("api-version", self._config.api_version)
) # type: Literal["2021-06-01"]
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[_models.ServiceResource]
content_type = content_type or "application/json"
_json = None
_content = None
if isinstance(parameters, (IO, bytes)):
_content = parameters
else:
_json = self._serialize.body(parameters, "ServiceResource")
request = build_create_or_update_request(
resource_group_name=resource_group_name,
cluster_name=cluster_name,
application_name=application_name,
service_name=service_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
content_type=content_type,
json=_json,
content=_content,
template_url=self._create_or_update_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorModel, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
deserialized = self._deserialize("ServiceResource", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
_create_or_update_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services/{serviceName}"} # type: ignore
@overload
def begin_create_or_update(
self,
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
parameters: _models.ServiceResource,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[_models.ServiceResource]:
"""Creates or updates a Service Fabric service resource.
Create or update a Service Fabric service resource with the specified name.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param cluster_name: The name of the cluster resource. Required.
:type cluster_name: str
:param application_name: The name of the application resource. Required.
:type application_name: str
:param service_name: The name of the service resource in the format of
{applicationName}~{serviceName}. Required.
:type service_name: str
:param parameters: The service resource. Required.
:type parameters: ~azure.mgmt.servicefabric.models.ServiceResource
:keyword content_type: Body Parameter content-type. Content type parameter for JSON body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either ServiceResource or the result of
cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.servicefabric.models.ServiceResource]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@overload
def begin_create_or_update(
self,
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
parameters: IO,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[_models.ServiceResource]:
"""Creates or updates a Service Fabric service resource.
Create or update a Service Fabric service resource with the specified name.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param cluster_name: The name of the cluster resource. Required.
:type cluster_name: str
:param application_name: The name of the application resource. Required.
:type application_name: str
:param service_name: The name of the service resource in the format of
{applicationName}~{serviceName}. Required.
:type service_name: str
:param parameters: The service resource. Required.
:type parameters: IO
:keyword content_type: Body Parameter content-type. Content type parameter for binary body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either ServiceResource or the result of
cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.servicefabric.models.ServiceResource]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@distributed_trace
def begin_create_or_update(
self,
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
parameters: Union[_models.ServiceResource, IO],
**kwargs: Any
) -> LROPoller[_models.ServiceResource]:
"""Creates or updates a Service Fabric service resource.
Create or update a Service Fabric service resource with the specified name.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param cluster_name: The name of the cluster resource. Required.
:type cluster_name: str
:param application_name: The name of the application resource. Required.
:type application_name: str
:param service_name: The name of the service resource in the format of
{applicationName}~{serviceName}. Required.
:type service_name: str
:param parameters: The service resource. Is either a model type or a IO type. Required.
:type parameters: ~azure.mgmt.servicefabric.models.ServiceResource or IO
:keyword content_type: Body Parameter content-type. Known values are: 'application/json'.
Default value is None.
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either ServiceResource or the result of
cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.servicefabric.models.ServiceResource]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop(
"api_version", _params.pop("api-version", self._config.api_version)
) # type: Literal["2021-06-01"]
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[_models.ServiceResource]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._create_or_update_initial( # type: ignore
resource_group_name=resource_group_name,
cluster_name=cluster_name,
application_name=application_name,
service_name=service_name,
parameters=parameters,
api_version=api_version,
content_type=content_type,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response):
deserialized = self._deserialize("ServiceResource", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
if polling is True:
polling_method = cast(PollingMethod, ARMPolling(lro_delay, **kwargs)) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_create_or_update.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services/{serviceName}"} # type: ignore
def _update_initial(
self,
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
parameters: Union[_models.ServiceResourceUpdate, IO],
**kwargs: Any
) -> _models.ServiceResource:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop(
"api_version", _params.pop("api-version", self._config.api_version)
) # type: Literal["2021-06-01"]
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[_models.ServiceResource]
content_type = content_type or "application/json"
_json = None
_content = None
if isinstance(parameters, (IO, bytes)):
_content = parameters
else:
_json = self._serialize.body(parameters, "ServiceResourceUpdate")
request = build_update_request(
resource_group_name=resource_group_name,
cluster_name=cluster_name,
application_name=application_name,
service_name=service_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
content_type=content_type,
json=_json,
content=_content,
template_url=self._update_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorModel, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
deserialized = self._deserialize("ServiceResource", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
_update_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services/{serviceName}"} # type: ignore
@overload
def begin_update(
self,
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
parameters: _models.ServiceResourceUpdate,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[_models.ServiceResource]:
"""Updates a Service Fabric service resource.
Update a Service Fabric service resource with the specified name.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param cluster_name: The name of the cluster resource. Required.
:type cluster_name: str
:param application_name: The name of the application resource. Required.
:type application_name: str
:param service_name: The name of the service resource in the format of
{applicationName}~{serviceName}. Required.
:type service_name: str
:param parameters: The service resource for patch operations. Required.
:type parameters: ~azure.mgmt.servicefabric.models.ServiceResourceUpdate
:keyword content_type: Body Parameter content-type. Content type parameter for JSON body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either ServiceResource or the result of
cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.servicefabric.models.ServiceResource]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@overload
def begin_update(
self,
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
parameters: IO,
*,
content_type: str = "application/json",
**kwargs: Any
) -> LROPoller[_models.ServiceResource]:
"""Updates a Service Fabric service resource.
Update a Service Fabric service resource with the specified name.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param cluster_name: The name of the cluster resource. Required.
:type cluster_name: str
:param application_name: The name of the application resource. Required.
:type application_name: str
:param service_name: The name of the service resource in the format of
{applicationName}~{serviceName}. Required.
:type service_name: str
:param parameters: The service resource for patch operations. Required.
:type parameters: IO
:keyword content_type: Body Parameter content-type. Content type parameter for binary body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either ServiceResource or the result of
cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.servicefabric.models.ServiceResource]
:raises ~azure.core.exceptions.HttpResponseError:
"""
@distributed_trace
def begin_update(
self,
resource_group_name: str,
cluster_name: str,
application_name: str,
service_name: str,
parameters: Union[_models.ServiceResourceUpdate, IO],
**kwargs: Any
) -> LROPoller[_models.ServiceResource]:
"""Updates a Service Fabric service resource.
Update a Service Fabric service resource with the specified name.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param cluster_name: The name of the cluster resource. Required.
:type cluster_name: str
:param application_name: The name of the application resource. Required.
:type application_name: str
:param service_name: The name of the service resource in the format of
{applicationName}~{serviceName}. Required.
:type service_name: str
:param parameters: The service resource for patch operations. Is either a model type or a IO
type. Required.
:type parameters: ~azure.mgmt.servicefabric.models.ServiceResourceUpdate or IO
:keyword content_type: Body Parameter content-type. Known values are: 'application/json'.
Default value is None.
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either ServiceResource or the result of
cls(response)
:rtype: ~azure.core.polling.LROPoller[~azure.mgmt.servicefabric.models.ServiceResource]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop(
"api_version", _params.pop("api-version", self._config.api_version)
) # type: Literal["2021-06-01"]
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[_models.ServiceResource]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._update_initial( # type: ignore
resource_group_name=resource_group_name,
cluster_name=cluster_name,
application_name=application_name,
service_name=service_name,
parameters=parameters,
api_version=api_version,
content_type=content_type,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response):
deserialized = self._deserialize("ServiceResource", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
if polling is True:
polling_method = cast(PollingMethod, ARMPolling(lro_delay, **kwargs)) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_update.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services/{serviceName}"} # type: ignore
def _delete_initial( # pylint: disable=inconsistent-return-statements
self, resource_group_name: str, cluster_name: str, application_name: str, service_name: str, **kwargs: Any
) -> None:
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop(
"api_version", _params.pop("api-version", self._config.api_version)
) # type: Literal["2021-06-01"]
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_delete_request(
resource_group_name=resource_group_name,
cluster_name=cluster_name,
application_name=application_name,
service_name=service_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self._delete_initial.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [202, 204]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorModel, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_delete_initial.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services/{serviceName}"} # type: ignore
@distributed_trace
def begin_delete(
self, resource_group_name: str, cluster_name: str, application_name: str, service_name: str, **kwargs: Any
) -> LROPoller[None]:
"""Deletes a Service Fabric service resource.
Delete a Service Fabric service resource with the specified name.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param cluster_name: The name of the cluster resource. Required.
:type cluster_name: str
:param application_name: The name of the application resource. Required.
:type application_name: str
:param service_name: The name of the service resource in the format of
{applicationName}~{serviceName}. Required.
:type service_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises ~azure.core.exceptions.HttpResponseError:
"""
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop(
"api_version", _params.pop("api-version", self._config.api_version)
) # type: Literal["2021-06-01"]
cls = kwargs.pop("cls", None) # type: ClsType[None]
polling = kwargs.pop("polling", True) # type: Union[bool, PollingMethod]
lro_delay = kwargs.pop("polling_interval", self._config.polling_interval)
cont_token = kwargs.pop("continuation_token", None) # type: Optional[str]
if cont_token is None:
raw_result = self._delete_initial( # type: ignore
resource_group_name=resource_group_name,
cluster_name=cluster_name,
application_name=application_name,
service_name=service_name,
api_version=api_version,
cls=lambda x, y, z: x,
headers=_headers,
params=_params,
**kwargs
)
kwargs.pop("error_map", None)
def get_long_running_output(pipeline_response): # pylint: disable=inconsistent-return-statements
if cls:
return cls(pipeline_response, None, {})
if polling is True:
polling_method = cast(PollingMethod, ARMPolling(lro_delay, **kwargs)) # type: PollingMethod
elif polling is False:
polling_method = cast(PollingMethod, NoPolling())
else:
polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output,
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_delete.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services/{serviceName}"} # type: ignore
@distributed_trace
def list(
self, resource_group_name: str, cluster_name: str, application_name: str, **kwargs: Any
) -> _models.ServiceResourceList:
"""Gets the list of service resources created in the specified Service Fabric application
resource.
Gets all service resources created or in the process of being created in the Service Fabric
application resource.
:param resource_group_name: The name of the resource group. Required.
:type resource_group_name: str
:param cluster_name: The name of the cluster resource. Required.
:type cluster_name: str
:param application_name: The name of the application resource. Required.
:type application_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: ServiceResourceList or the result of cls(response)
:rtype: ~azure.mgmt.servicefabric.models.ServiceResourceList
:raises ~azure.core.exceptions.HttpResponseError:
"""
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop(
"api_version", _params.pop("api-version", self._config.api_version)
) # type: Literal["2021-06-01"]
cls = kwargs.pop("cls", None) # type: ClsType[_models.ServiceResourceList]
request = build_list_request(
resource_group_name=resource_group_name,
cluster_name=cluster_name,
application_name=application_name,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self.list.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorModel, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
deserialized = self._deserialize("ServiceResourceList", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
list.metadata = {"url": "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ServiceFabric/clusters/{clusterName}/applications/{applicationName}/services"} # type: ignore
| mit |
Azure/azure-sdk-for-python | sdk/authorization/azure-mgmt-authorization/azure/mgmt/authorization/v2021_03_01_preview/aio/operations/_access_review_instance_operations.py | 1 | 15208 | # pylint: disable=too-many-lines
# coding=utf-8
# --------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for license information.
# Code generated by Microsoft (R) AutoRest Code Generator.
# Changes may cause incorrect behavior and will be lost if the code is regenerated.
# --------------------------------------------------------------------------
from typing import Any, Callable, Dict, Optional, TypeVar
from azure.core.exceptions import (
ClientAuthenticationError,
HttpResponseError,
ResourceExistsError,
ResourceNotFoundError,
ResourceNotModifiedError,
map_error,
)
from azure.core.pipeline import PipelineResponse
from azure.core.pipeline.transport import AsyncHttpResponse
from azure.core.rest import HttpRequest
from azure.core.tracing.decorator_async import distributed_trace_async
from azure.core.utils import case_insensitive_dict
from azure.mgmt.core.exceptions import ARMErrorFormat
from ... import models as _models
from ..._vendor import _convert_request
from ...operations._access_review_instance_operations import (
build_accept_recommendations_request,
build_apply_decisions_request,
build_reset_decisions_request,
build_send_reminders_request,
build_stop_request,
)
T = TypeVar("T")
ClsType = Optional[Callable[[PipelineResponse[HttpRequest, AsyncHttpResponse], T, Dict[str, Any]], Any]]
class AccessReviewInstanceOperations:
"""
.. warning::
**DO NOT** instantiate this class directly.
Instead, you should access the following operations through
:class:`~azure.mgmt.authorization.v2021_03_01_preview.aio.AuthorizationManagementClient`'s
:attr:`access_review_instance` attribute.
"""
models = _models
def __init__(self, *args, **kwargs) -> None:
input_args = list(args)
self._client = input_args.pop(0) if input_args else kwargs.pop("client")
self._config = input_args.pop(0) if input_args else kwargs.pop("config")
self._serialize = input_args.pop(0) if input_args else kwargs.pop("serializer")
self._deserialize = input_args.pop(0) if input_args else kwargs.pop("deserializer")
@distributed_trace_async
async def stop( # pylint: disable=inconsistent-return-statements
self, schedule_definition_id: str, id: str, **kwargs: Any
) -> None:
"""An action to stop an access review instance.
:param schedule_definition_id: The id of the access review schedule definition. Required.
:type schedule_definition_id: str
:param id: The id of the access review instance. Required.
:type id: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: None or the result of cls(response)
:rtype: None
:raises ~azure.core.exceptions.HttpResponseError:
"""
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2021-03-01-preview")) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_stop_request(
schedule_definition_id=schedule_definition_id,
id=id,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self.stop.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = await self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [204]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorDefinition, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
stop.metadata = {"url": "/subscriptions/{subscriptionId}/providers/Microsoft.Authorization/accessReviewScheduleDefinitions/{scheduleDefinitionId}/instances/{id}/stop"} # type: ignore
@distributed_trace_async
async def reset_decisions( # pylint: disable=inconsistent-return-statements
self, schedule_definition_id: str, id: str, **kwargs: Any
) -> None:
"""An action to reset all decisions for an access review instance.
:param schedule_definition_id: The id of the access review schedule definition. Required.
:type schedule_definition_id: str
:param id: The id of the access review instance. Required.
:type id: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: None or the result of cls(response)
:rtype: None
:raises ~azure.core.exceptions.HttpResponseError:
"""
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2021-03-01-preview")) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_reset_decisions_request(
schedule_definition_id=schedule_definition_id,
id=id,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self.reset_decisions.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = await self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [204]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorDefinition, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
reset_decisions.metadata = {"url": "/subscriptions/{subscriptionId}/providers/Microsoft.Authorization/accessReviewScheduleDefinitions/{scheduleDefinitionId}/instances/{id}/resetDecisions"} # type: ignore
@distributed_trace_async
async def apply_decisions( # pylint: disable=inconsistent-return-statements
self, schedule_definition_id: str, id: str, **kwargs: Any
) -> None:
"""An action to apply all decisions for an access review instance.
:param schedule_definition_id: The id of the access review schedule definition. Required.
:type schedule_definition_id: str
:param id: The id of the access review instance. Required.
:type id: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: None or the result of cls(response)
:rtype: None
:raises ~azure.core.exceptions.HttpResponseError:
"""
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2021-03-01-preview")) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_apply_decisions_request(
schedule_definition_id=schedule_definition_id,
id=id,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self.apply_decisions.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = await self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [204]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorDefinition, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
apply_decisions.metadata = {"url": "/subscriptions/{subscriptionId}/providers/Microsoft.Authorization/accessReviewScheduleDefinitions/{scheduleDefinitionId}/instances/{id}/applyDecisions"} # type: ignore
@distributed_trace_async
async def send_reminders( # pylint: disable=inconsistent-return-statements
self, schedule_definition_id: str, id: str, **kwargs: Any
) -> None:
"""An action to send reminders for an access review instance.
:param schedule_definition_id: The id of the access review schedule definition. Required.
:type schedule_definition_id: str
:param id: The id of the access review instance. Required.
:type id: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: None or the result of cls(response)
:rtype: None
:raises ~azure.core.exceptions.HttpResponseError:
"""
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2021-03-01-preview")) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_send_reminders_request(
schedule_definition_id=schedule_definition_id,
id=id,
subscription_id=self._config.subscription_id,
api_version=api_version,
template_url=self.send_reminders.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = await self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [204]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorDefinition, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
send_reminders.metadata = {"url": "/subscriptions/{subscriptionId}/providers/Microsoft.Authorization/accessReviewScheduleDefinitions/{scheduleDefinitionId}/instances/{id}/sendReminders"} # type: ignore
@distributed_trace_async
async def accept_recommendations( # pylint: disable=inconsistent-return-statements
self, schedule_definition_id: str, id: str, **kwargs: Any
) -> None:
"""An action to accept recommendations for decision in an access review instance.
:param schedule_definition_id: The id of the access review schedule definition. Required.
:type schedule_definition_id: str
:param id: The id of the access review instance. Required.
:type id: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: None or the result of cls(response)
:rtype: None
:raises ~azure.core.exceptions.HttpResponseError:
"""
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = kwargs.pop("headers", {}) or {}
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2021-03-01-preview")) # type: str
cls = kwargs.pop("cls", None) # type: ClsType[None]
request = build_accept_recommendations_request(
schedule_definition_id=schedule_definition_id,
id=id,
api_version=api_version,
template_url=self.accept_recommendations.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
request.url = self._client.format_url(request.url) # type: ignore
pipeline_response = await self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [204]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorDefinition, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
accept_recommendations.metadata = {"url": "/providers/Microsoft.Authorization/accessReviewScheduleDefinitions/{scheduleDefinitionId}/instances/{id}/acceptRecommendations"} # type: ignore
| mit |
Azure/azure-sdk-for-python | sdk/healthcareapis/azure-mgmt-healthcareapis/generated_samples/private_link_resources_list_by_service.py | 1 | 1618 | # coding=utf-8
# --------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for license information.
# Code generated by Microsoft (R) AutoRest Code Generator.
# Changes may cause incorrect behavior and will be lost if the code is regenerated.
# --------------------------------------------------------------------------
from azure.identity import DefaultAzureCredential
from azure.mgmt.healthcareapis import HealthcareApisManagementClient
"""
# PREREQUISITES
pip install azure-identity
pip install azure-mgmt-healthcareapis
# USAGE
python private_link_resources_list_by_service.py
Before run the sample, please set the values of the client ID, tenant ID and client secret
of the AAD application as environment variables: AZURE_CLIENT_ID, AZURE_TENANT_ID,
AZURE_CLIENT_SECRET. For more info about how to get the value, please see:
https://docs.microsoft.com/azure/active-directory/develop/howto-create-service-principal-portal
"""
def main():
client = HealthcareApisManagementClient(
credential=DefaultAzureCredential(),
subscription_id="subid",
)
response = client.private_link_resources.list_by_service(
resource_group_name="rgname",
resource_name="service1",
)
print(response)
# x-ms-original-file: specification/healthcareapis/resource-manager/Microsoft.HealthcareApis/stable/2022-06-01/examples/legacy/PrivateLinkResourcesListByService.json
if __name__ == "__main__":
main()
| mit |
anaran/kuma | vendor/packages/pytz/tzfile.py | 477 | 4869 | #!/usr/bin/env python
'''
$Id: tzfile.py,v 1.8 2004/06/03 00:15:24 zenzen Exp $
'''
try:
from cStringIO import StringIO
except ImportError:
from io import StringIO
from datetime import datetime, timedelta
from struct import unpack, calcsize
from pytz.tzinfo import StaticTzInfo, DstTzInfo, memorized_ttinfo
from pytz.tzinfo import memorized_datetime, memorized_timedelta
def _byte_string(s):
"""Cast a string or byte string to an ASCII byte string."""
return s.encode('US-ASCII')
_NULL = _byte_string('\0')
def _std_string(s):
"""Cast a string or byte string to an ASCII string."""
return str(s.decode('US-ASCII'))
def build_tzinfo(zone, fp):
head_fmt = '>4s c 15x 6l'
head_size = calcsize(head_fmt)
(magic, format, ttisgmtcnt, ttisstdcnt,leapcnt, timecnt,
typecnt, charcnt) = unpack(head_fmt, fp.read(head_size))
# Make sure it is a tzfile(5) file
assert magic == _byte_string('TZif'), 'Got magic %s' % repr(magic)
# Read out the transition times, localtime indices and ttinfo structures.
data_fmt = '>%(timecnt)dl %(timecnt)dB %(ttinfo)s %(charcnt)ds' % dict(
timecnt=timecnt, ttinfo='lBB'*typecnt, charcnt=charcnt)
data_size = calcsize(data_fmt)
data = unpack(data_fmt, fp.read(data_size))
# make sure we unpacked the right number of values
assert len(data) == 2 * timecnt + 3 * typecnt + 1
transitions = [memorized_datetime(trans)
for trans in data[:timecnt]]
lindexes = list(data[timecnt:2 * timecnt])
ttinfo_raw = data[2 * timecnt:-1]
tznames_raw = data[-1]
del data
# Process ttinfo into separate structs
ttinfo = []
tznames = {}
i = 0
while i < len(ttinfo_raw):
# have we looked up this timezone name yet?
tzname_offset = ttinfo_raw[i+2]
if tzname_offset not in tznames:
nul = tznames_raw.find(_NULL, tzname_offset)
if nul < 0:
nul = len(tznames_raw)
tznames[tzname_offset] = _std_string(
tznames_raw[tzname_offset:nul])
ttinfo.append((ttinfo_raw[i],
bool(ttinfo_raw[i+1]),
tznames[tzname_offset]))
i += 3
# Now build the timezone object
if len(transitions) == 0:
ttinfo[0][0], ttinfo[0][2]
cls = type(zone, (StaticTzInfo,), dict(
zone=zone,
_utcoffset=memorized_timedelta(ttinfo[0][0]),
_tzname=ttinfo[0][2]))
else:
# Early dates use the first standard time ttinfo
i = 0
while ttinfo[i][1]:
i += 1
if ttinfo[i] == ttinfo[lindexes[0]]:
transitions[0] = datetime.min
else:
transitions.insert(0, datetime.min)
lindexes.insert(0, i)
# calculate transition info
transition_info = []
for i in range(len(transitions)):
inf = ttinfo[lindexes[i]]
utcoffset = inf[0]
if not inf[1]:
dst = 0
else:
for j in range(i-1, -1, -1):
prev_inf = ttinfo[lindexes[j]]
if not prev_inf[1]:
break
dst = inf[0] - prev_inf[0] # dst offset
# Bad dst? Look further. DST > 24 hours happens when
# a timzone has moved across the international dateline.
if dst <= 0 or dst > 3600*3:
for j in range(i+1, len(transitions)):
stdinf = ttinfo[lindexes[j]]
if not stdinf[1]:
dst = inf[0] - stdinf[0]
if dst > 0:
break # Found a useful std time.
tzname = inf[2]
# Round utcoffset and dst to the nearest minute or the
# datetime library will complain. Conversions to these timezones
# might be up to plus or minus 30 seconds out, but it is
# the best we can do.
utcoffset = int((utcoffset + 30) // 60) * 60
dst = int((dst + 30) // 60) * 60
transition_info.append(memorized_ttinfo(utcoffset, dst, tzname))
cls = type(zone, (DstTzInfo,), dict(
zone=zone,
_utc_transition_times=transitions,
_transition_info=transition_info))
return cls()
if __name__ == '__main__':
import os.path
from pprint import pprint
base = os.path.join(os.path.dirname(__file__), 'zoneinfo')
tz = build_tzinfo('Australia/Melbourne',
open(os.path.join(base,'Australia','Melbourne'), 'rb'))
tz = build_tzinfo('US/Eastern',
open(os.path.join(base,'US','Eastern'), 'rb'))
pprint(tz._utc_transition_times)
#print tz.asPython(4)
#print tz.transitions_mapping
| mpl-2.0 |
mccheung/kbengine | kbe/res/scripts/common/Lib/test/test_importlib/util.py | 82 | 7315 | from contextlib import contextmanager
from importlib import util, invalidate_caches
import os.path
from test import support
import unittest
import sys
import types
def import_importlib(module_name):
"""Import a module from importlib both w/ and w/o _frozen_importlib."""
fresh = ('importlib',) if '.' in module_name else ()
frozen = support.import_fresh_module(module_name)
source = support.import_fresh_module(module_name, fresh=fresh,
blocked=('_frozen_importlib',))
return frozen, source
def test_both(test_class, **kwargs):
frozen_tests = types.new_class('Frozen_'+test_class.__name__,
(test_class, unittest.TestCase))
source_tests = types.new_class('Source_'+test_class.__name__,
(test_class, unittest.TestCase))
frozen_tests.__module__ = source_tests.__module__ = test_class.__module__
for attr, (frozen_value, source_value) in kwargs.items():
setattr(frozen_tests, attr, frozen_value)
setattr(source_tests, attr, source_value)
return frozen_tests, source_tests
CASE_INSENSITIVE_FS = True
# Windows is the only OS that is *always* case-insensitive
# (OS X *can* be case-sensitive).
if sys.platform not in ('win32', 'cygwin'):
changed_name = __file__.upper()
if changed_name == __file__:
changed_name = __file__.lower()
if not os.path.exists(changed_name):
CASE_INSENSITIVE_FS = False
def case_insensitive_tests(test):
"""Class decorator that nullifies tests requiring a case-insensitive
file system."""
return unittest.skipIf(not CASE_INSENSITIVE_FS,
"requires a case-insensitive filesystem")(test)
def submodule(parent, name, pkg_dir, content=''):
path = os.path.join(pkg_dir, name + '.py')
with open(path, 'w') as subfile:
subfile.write(content)
return '{}.{}'.format(parent, name), path
@contextmanager
def uncache(*names):
"""Uncache a module from sys.modules.
A basic sanity check is performed to prevent uncaching modules that either
cannot/shouldn't be uncached.
"""
for name in names:
if name in ('sys', 'marshal', 'imp'):
raise ValueError(
"cannot uncache {0}".format(name))
try:
del sys.modules[name]
except KeyError:
pass
try:
yield
finally:
for name in names:
try:
del sys.modules[name]
except KeyError:
pass
@contextmanager
def temp_module(name, content='', *, pkg=False):
conflicts = [n for n in sys.modules if n.partition('.')[0] == name]
with support.temp_cwd(None) as cwd:
with uncache(name, *conflicts):
with support.DirsOnSysPath(cwd):
invalidate_caches()
location = os.path.join(cwd, name)
if pkg:
modpath = os.path.join(location, '__init__.py')
os.mkdir(name)
else:
modpath = location + '.py'
if content is None:
# Make sure the module file gets created.
content = ''
if content is not None:
# not a namespace package
with open(modpath, 'w') as modfile:
modfile.write(content)
yield location
@contextmanager
def import_state(**kwargs):
"""Context manager to manage the various importers and stored state in the
sys module.
The 'modules' attribute is not supported as the interpreter state stores a
pointer to the dict that the interpreter uses internally;
reassigning to sys.modules does not have the desired effect.
"""
originals = {}
try:
for attr, default in (('meta_path', []), ('path', []),
('path_hooks', []),
('path_importer_cache', {})):
originals[attr] = getattr(sys, attr)
if attr in kwargs:
new_value = kwargs[attr]
del kwargs[attr]
else:
new_value = default
setattr(sys, attr, new_value)
if len(kwargs):
raise ValueError(
'unrecognized arguments: {0}'.format(kwargs.keys()))
yield
finally:
for attr, value in originals.items():
setattr(sys, attr, value)
class _ImporterMock:
"""Base class to help with creating importer mocks."""
def __init__(self, *names, module_code={}):
self.modules = {}
self.module_code = {}
for name in names:
if not name.endswith('.__init__'):
import_name = name
else:
import_name = name[:-len('.__init__')]
if '.' not in name:
package = None
elif import_name == name:
package = name.rsplit('.', 1)[0]
else:
package = import_name
module = types.ModuleType(import_name)
module.__loader__ = self
module.__file__ = '<mock __file__>'
module.__package__ = package
module.attr = name
if import_name != name:
module.__path__ = ['<mock __path__>']
self.modules[import_name] = module
if import_name in module_code:
self.module_code[import_name] = module_code[import_name]
def __getitem__(self, name):
return self.modules[name]
def __enter__(self):
self._uncache = uncache(*self.modules.keys())
self._uncache.__enter__()
return self
def __exit__(self, *exc_info):
self._uncache.__exit__(None, None, None)
class mock_modules(_ImporterMock):
"""Importer mock using PEP 302 APIs."""
def find_module(self, fullname, path=None):
if fullname not in self.modules:
return None
else:
return self
def load_module(self, fullname):
if fullname not in self.modules:
raise ImportError
else:
sys.modules[fullname] = self.modules[fullname]
if fullname in self.module_code:
try:
self.module_code[fullname]()
except Exception:
del sys.modules[fullname]
raise
return self.modules[fullname]
class mock_spec(_ImporterMock):
"""Importer mock using PEP 451 APIs."""
def find_spec(self, fullname, path=None, parent=None):
try:
module = self.modules[fullname]
except KeyError:
return None
is_package = hasattr(module, '__path__')
spec = util.spec_from_file_location(
fullname, module.__file__, loader=self,
submodule_search_locations=getattr(module, '__path__', None))
return spec
def create_module(self, spec):
if spec.name not in self.modules:
raise ImportError
return self.modules[spec.name]
def exec_module(self, module):
try:
self.module_code[module.__spec__.name]()
except KeyError:
pass
| lgpl-3.0 |
jguice/iTerm2 | tests/esctest/tests/decaln.py | 31 | 1911 | import esccmd
import escio
from escutil import AssertEQ, AssertScreenCharsInRectEqual, GetCursorPosition, GetScreenSize, Point, Rect, knownBug, optionRequired
class DECALNTests(object):
def test_DECALN_FillsScreen(self):
"""Makes sure DECALN fills the screen with the letter E (could be anything,
but xterm uses E). Testing the whole screen would be slow so we just check
the corners and center."""
esccmd.DECALN()
size = GetScreenSize()
AssertScreenCharsInRectEqual(Rect(1, 1, 1, 1), [ "E" ])
AssertScreenCharsInRectEqual(Rect(size.width(), 1, size.width(), 1), [ "E" ])
AssertScreenCharsInRectEqual(Rect(1, size.height(), 1, size.height()), [ "E" ])
AssertScreenCharsInRectEqual(Rect(size.width(), size.height(), size.width(), size.height()),
[ "E" ])
AssertScreenCharsInRectEqual(Rect(size.width() / 2,
size.height() / 2,
size.width() / 2,
size.height() / 2),
[ "E" ])
def test_DECALN_MovesCursorHome(self):
esccmd.CUP(Point(5, 5))
esccmd.DECALN()
AssertEQ(GetCursorPosition(), Point(1, 1))
def test_DECALN_ClearsMargins(self):
esccmd.DECSET(esccmd.DECLRMM)
esccmd.DECSLRM(2, 3)
esccmd.DECSTBM(4, 5)
esccmd.DECALN()
# Verify we can pass the top margin
esccmd.CUP(Point(2, 4))
esccmd.CUU()
AssertEQ(GetCursorPosition(), Point(2, 3))
# Verify we can pass the bottom margin
esccmd.CUP(Point(2, 5))
esccmd.CUD()
AssertEQ(GetCursorPosition(), Point(2, 6))
# Verify we can pass the left margin
esccmd.CUP(Point(2, 4))
esccmd.CUB()
AssertEQ(GetCursorPosition(), Point(1, 4))
# Verify we can pass the right margin
esccmd.CUP(Point(3, 4))
esccmd.CUF()
AssertEQ(GetCursorPosition(), Point(4, 4))
| gpl-2.0 |
JAOSP/aosp_platform_external_chromium_org | third_party/protobuf/python/google/protobuf/reflection.py | 223 | 6594 | # Protocol Buffers - Google's data interchange format
# Copyright 2008 Google Inc. All rights reserved.
# http://code.google.com/p/protobuf/
#
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions are
# met:
#
# * Redistributions of source code must retain the above copyright
# notice, this list of conditions and the following disclaimer.
# * Redistributions in binary form must reproduce the above
# copyright notice, this list of conditions and the following disclaimer
# in the documentation and/or other materials provided with the
# distribution.
# * Neither the name of Google Inc. nor the names of its
# contributors may be used to endorse or promote products derived from
# this software without specific prior written permission.
#
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
# "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
# LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
# A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
# OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
# SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
# LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
# THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
# (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
# OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
# This code is meant to work on Python 2.4 and above only.
"""Contains a metaclass and helper functions used to create
protocol message classes from Descriptor objects at runtime.
Recall that a metaclass is the "type" of a class.
(A class is to a metaclass what an instance is to a class.)
In this case, we use the GeneratedProtocolMessageType metaclass
to inject all the useful functionality into the classes
output by the protocol compiler at compile-time.
The upshot of all this is that the real implementation
details for ALL pure-Python protocol buffers are *here in
this file*.
"""
__author__ = 'robinson@google.com (Will Robinson)'
from google.protobuf.internal import api_implementation
from google.protobuf import descriptor as descriptor_mod
from google.protobuf import message
_FieldDescriptor = descriptor_mod.FieldDescriptor
if api_implementation.Type() == 'cpp':
if api_implementation.Version() == 2:
from google.protobuf.internal.cpp import cpp_message
_NewMessage = cpp_message.NewMessage
_InitMessage = cpp_message.InitMessage
else:
from google.protobuf.internal import cpp_message
_NewMessage = cpp_message.NewMessage
_InitMessage = cpp_message.InitMessage
else:
from google.protobuf.internal import python_message
_NewMessage = python_message.NewMessage
_InitMessage = python_message.InitMessage
class GeneratedProtocolMessageType(type):
"""Metaclass for protocol message classes created at runtime from Descriptors.
We add implementations for all methods described in the Message class. We
also create properties to allow getting/setting all fields in the protocol
message. Finally, we create slots to prevent users from accidentally
"setting" nonexistent fields in the protocol message, which then wouldn't get
serialized / deserialized properly.
The protocol compiler currently uses this metaclass to create protocol
message classes at runtime. Clients can also manually create their own
classes at runtime, as in this example:
mydescriptor = Descriptor(.....)
class MyProtoClass(Message):
__metaclass__ = GeneratedProtocolMessageType
DESCRIPTOR = mydescriptor
myproto_instance = MyProtoClass()
myproto.foo_field = 23
...
"""
# Must be consistent with the protocol-compiler code in
# proto2/compiler/internal/generator.*.
_DESCRIPTOR_KEY = 'DESCRIPTOR'
def __new__(cls, name, bases, dictionary):
"""Custom allocation for runtime-generated class types.
We override __new__ because this is apparently the only place
where we can meaningfully set __slots__ on the class we're creating(?).
(The interplay between metaclasses and slots is not very well-documented).
Args:
name: Name of the class (ignored, but required by the
metaclass protocol).
bases: Base classes of the class we're constructing.
(Should be message.Message). We ignore this field, but
it's required by the metaclass protocol
dictionary: The class dictionary of the class we're
constructing. dictionary[_DESCRIPTOR_KEY] must contain
a Descriptor object describing this protocol message
type.
Returns:
Newly-allocated class.
"""
descriptor = dictionary[GeneratedProtocolMessageType._DESCRIPTOR_KEY]
bases = _NewMessage(bases, descriptor, dictionary)
superclass = super(GeneratedProtocolMessageType, cls)
new_class = superclass.__new__(cls, name, bases, dictionary)
setattr(descriptor, '_concrete_class', new_class)
return new_class
def __init__(cls, name, bases, dictionary):
"""Here we perform the majority of our work on the class.
We add enum getters, an __init__ method, implementations
of all Message methods, and properties for all fields
in the protocol type.
Args:
name: Name of the class (ignored, but required by the
metaclass protocol).
bases: Base classes of the class we're constructing.
(Should be message.Message). We ignore this field, but
it's required by the metaclass protocol
dictionary: The class dictionary of the class we're
constructing. dictionary[_DESCRIPTOR_KEY] must contain
a Descriptor object describing this protocol message
type.
"""
descriptor = dictionary[GeneratedProtocolMessageType._DESCRIPTOR_KEY]
_InitMessage(descriptor, cls)
superclass = super(GeneratedProtocolMessageType, cls)
superclass.__init__(name, bases, dictionary)
def ParseMessage(descriptor, byte_str):
"""Generate a new Message instance from this Descriptor and a byte string.
Args:
descriptor: Protobuf Descriptor object
byte_str: Serialized protocol buffer byte string
Returns:
Newly created protobuf Message object.
"""
class _ResultClass(message.Message):
__metaclass__ = GeneratedProtocolMessageType
DESCRIPTOR = descriptor
new_msg = _ResultClass()
new_msg.ParseFromString(byte_str)
return new_msg
| bsd-3-clause |
gilt/incubator-airflow | tests/ti_deps/deps/test_dagrun_exists_dep.py | 37 | 1461 | # -*- coding: utf-8 -*-
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import unittest
from airflow.utils.state import State
from mock import Mock, patch
from airflow.models import DAG, DagRun
from airflow.ti_deps.deps.dagrun_exists_dep import DagrunRunningDep
class DagrunRunningDepTest(unittest.TestCase):
@patch('airflow.models.DagRun.find', return_value=())
def test_dagrun_doesnt_exist(self, dagrun_find):
"""
Task instances without dagruns should fail this dep
"""
dag = DAG('test_dag', max_active_runs=2)
ti = Mock(task=Mock(dag=dag), get_dagrun=Mock(return_value=None))
self.assertFalse(DagrunRunningDep().is_met(ti=ti))
def test_dagrun_exists(self):
"""
Task instances with a dagrun should pass this dep
"""
dagrun = DagRun(state=State.RUNNING)
ti = Mock(get_dagrun=Mock(return_value=dagrun))
self.assertTrue(DagrunRunningDep().is_met(ti=ti))
| apache-2.0 |
jguice/iTerm2 | tools/ply/ply-3.4/example/optcalc/calc.py | 164 | 2506 | # -----------------------------------------------------------------------------
# calc.py
#
# A simple calculator with variables. This is from O'Reilly's
# "Lex and Yacc", p. 63.
# -----------------------------------------------------------------------------
import sys
sys.path.insert(0,"../..")
if sys.version_info[0] >= 3:
raw_input = input
tokens = (
'NAME','NUMBER',
'PLUS','MINUS','TIMES','DIVIDE','EQUALS',
'LPAREN','RPAREN',
)
# Tokens
t_PLUS = r'\+'
t_MINUS = r'-'
t_TIMES = r'\*'
t_DIVIDE = r'/'
t_EQUALS = r'='
t_LPAREN = r'\('
t_RPAREN = r'\)'
t_NAME = r'[a-zA-Z_][a-zA-Z0-9_]*'
def t_NUMBER(t):
r'\d+'
try:
t.value = int(t.value)
except ValueError:
print("Integer value too large %s" % t.value)
t.value = 0
return t
t_ignore = " \t"
def t_newline(t):
r'\n+'
t.lexer.lineno += t.value.count("\n")
def t_error(t):
print("Illegal character '%s'" % t.value[0])
t.lexer.skip(1)
# Build the lexer
import ply.lex as lex
lex.lex(optimize=1)
# Parsing rules
precedence = (
('left','PLUS','MINUS'),
('left','TIMES','DIVIDE'),
('right','UMINUS'),
)
# dictionary of names
names = { }
def p_statement_assign(t):
'statement : NAME EQUALS expression'
names[t[1]] = t[3]
def p_statement_expr(t):
'statement : expression'
print(t[1])
def p_expression_binop(t):
'''expression : expression PLUS expression
| expression MINUS expression
| expression TIMES expression
| expression DIVIDE expression'''
if t[2] == '+' : t[0] = t[1] + t[3]
elif t[2] == '-': t[0] = t[1] - t[3]
elif t[2] == '*': t[0] = t[1] * t[3]
elif t[2] == '/': t[0] = t[1] / t[3]
elif t[2] == '<': t[0] = t[1] < t[3]
def p_expression_uminus(t):
'expression : MINUS expression %prec UMINUS'
t[0] = -t[2]
def p_expression_group(t):
'expression : LPAREN expression RPAREN'
t[0] = t[2]
def p_expression_number(t):
'expression : NUMBER'
t[0] = t[1]
def p_expression_name(t):
'expression : NAME'
try:
t[0] = names[t[1]]
except LookupError:
print("Undefined name '%s'" % t[1])
t[0] = 0
def p_error(t):
if t:
print("Syntax error at '%s'" % t.value)
else:
print("Syntax error at EOF")
import ply.yacc as yacc
yacc.yacc(optimize=1)
while 1:
try:
s = raw_input('calc > ')
except EOFError:
break
yacc.parse(s)
| gpl-2.0 |
ryfeus/lambda-packs | Opencv_pil/source/click/_textwrap.py | 277 | 1198 | import textwrap
from contextlib import contextmanager
class TextWrapper(textwrap.TextWrapper):
def _handle_long_word(self, reversed_chunks, cur_line, cur_len, width):
space_left = max(width - cur_len, 1)
if self.break_long_words:
last = reversed_chunks[-1]
cut = last[:space_left]
res = last[space_left:]
cur_line.append(cut)
reversed_chunks[-1] = res
elif not cur_line:
cur_line.append(reversed_chunks.pop())
@contextmanager
def extra_indent(self, indent):
old_initial_indent = self.initial_indent
old_subsequent_indent = self.subsequent_indent
self.initial_indent += indent
self.subsequent_indent += indent
try:
yield
finally:
self.initial_indent = old_initial_indent
self.subsequent_indent = old_subsequent_indent
def indent_only(self, text):
rv = []
for idx, line in enumerate(text.splitlines()):
indent = self.initial_indent
if idx > 0:
indent = self.subsequent_indent
rv.append(indent + line)
return '\n'.join(rv)
| mit |
ryfeus/lambda-packs | Sklearn_x86/source/numpy/typing/tests/data/reveal/fromnumeric.py | 6 | 10131 | """Tests for :mod:`numpy.core.fromnumeric`."""
import numpy as np
A = np.array(True, ndmin=2, dtype=bool)
B = np.array(1.0, ndmin=2, dtype=np.float32)
A.setflags(write=False)
B.setflags(write=False)
a = np.bool_(True)
b = np.float32(1.0)
c = 1.0
d = np.array(1.0, dtype=np.float32) # writeable
reveal_type(np.take(a, 0)) # E: Any
reveal_type(np.take(b, 0)) # E: Any
reveal_type(np.take(c, 0)) # E: Any
reveal_type(np.take(A, 0)) # E: Any
reveal_type(np.take(B, 0)) # E: Any
reveal_type(np.take(A, [0])) # E: Any
reveal_type(np.take(B, [0])) # E: Any
reveal_type(np.reshape(a, 1)) # E: numpy.ndarray[Any, Any]
reveal_type(np.reshape(b, 1)) # E: numpy.ndarray[Any, Any]
reveal_type(np.reshape(c, 1)) # E: numpy.ndarray[Any, Any]
reveal_type(np.reshape(A, 1)) # E: numpy.ndarray[Any, Any]
reveal_type(np.reshape(B, 1)) # E: numpy.ndarray[Any, Any]
reveal_type(np.choose(a, [True, True])) # E: Any
reveal_type(np.choose(A, [True, True])) # E: Any
reveal_type(np.repeat(a, 1)) # E: numpy.ndarray[Any, Any]
reveal_type(np.repeat(b, 1)) # E: numpy.ndarray[Any, Any]
reveal_type(np.repeat(c, 1)) # E: numpy.ndarray[Any, Any]
reveal_type(np.repeat(A, 1)) # E: numpy.ndarray[Any, Any]
reveal_type(np.repeat(B, 1)) # E: numpy.ndarray[Any, Any]
# TODO: Add tests for np.put()
reveal_type(np.swapaxes(A, 0, 0)) # E: numpy.ndarray[Any, Any]
reveal_type(np.swapaxes(B, 0, 0)) # E: numpy.ndarray[Any, Any]
reveal_type(np.transpose(a)) # E: numpy.ndarray[Any, Any]
reveal_type(np.transpose(b)) # E: numpy.ndarray[Any, Any]
reveal_type(np.transpose(c)) # E: numpy.ndarray[Any, Any]
reveal_type(np.transpose(A)) # E: numpy.ndarray[Any, Any]
reveal_type(np.transpose(B)) # E: numpy.ndarray[Any, Any]
reveal_type(np.partition(a, 0, axis=None)) # E: numpy.ndarray[Any, Any]
reveal_type(np.partition(b, 0, axis=None)) # E: numpy.ndarray[Any, Any]
reveal_type(np.partition(c, 0, axis=None)) # E: numpy.ndarray[Any, Any]
reveal_type(np.partition(A, 0)) # E: numpy.ndarray[Any, Any]
reveal_type(np.partition(B, 0)) # E: numpy.ndarray[Any, Any]
reveal_type(np.argpartition(a, 0)) # E: Any
reveal_type(np.argpartition(b, 0)) # E: Any
reveal_type(np.argpartition(c, 0)) # E: Any
reveal_type(np.argpartition(A, 0)) # E: Any
reveal_type(np.argpartition(B, 0)) # E: Any
reveal_type(np.sort(A, 0)) # E: numpy.ndarray[Any, Any]
reveal_type(np.sort(B, 0)) # E: numpy.ndarray[Any, Any]
reveal_type(np.argsort(A, 0)) # E: numpy.ndarray[Any, Any]
reveal_type(np.argsort(B, 0)) # E: numpy.ndarray[Any, Any]
reveal_type(np.argmax(A)) # E: {intp}
reveal_type(np.argmax(B)) # E: {intp}
reveal_type(np.argmax(A, axis=0)) # E: Any
reveal_type(np.argmax(B, axis=0)) # E: Any
reveal_type(np.argmin(A)) # E: {intp}
reveal_type(np.argmin(B)) # E: {intp}
reveal_type(np.argmin(A, axis=0)) # E: Any
reveal_type(np.argmin(B, axis=0)) # E: Any
reveal_type(np.searchsorted(A[0], 0)) # E: {intp}
reveal_type(np.searchsorted(B[0], 0)) # E: {intp}
reveal_type(np.searchsorted(A[0], [0])) # E: numpy.ndarray[Any, Any]
reveal_type(np.searchsorted(B[0], [0])) # E: numpy.ndarray[Any, Any]
reveal_type(np.resize(a, (5, 5))) # E: numpy.ndarray[Any, Any]
reveal_type(np.resize(b, (5, 5))) # E: numpy.ndarray[Any, Any]
reveal_type(np.resize(c, (5, 5))) # E: numpy.ndarray[Any, Any]
reveal_type(np.resize(A, (5, 5))) # E: numpy.ndarray[Any, Any]
reveal_type(np.resize(B, (5, 5))) # E: numpy.ndarray[Any, Any]
reveal_type(np.squeeze(a)) # E: numpy.bool_
reveal_type(np.squeeze(b)) # E: {float32}
reveal_type(np.squeeze(c)) # E: numpy.ndarray[Any, Any]
reveal_type(np.squeeze(A)) # E: numpy.ndarray[Any, Any]
reveal_type(np.squeeze(B)) # E: numpy.ndarray[Any, Any]
reveal_type(np.diagonal(A)) # E: numpy.ndarray[Any, Any]
reveal_type(np.diagonal(B)) # E: numpy.ndarray[Any, Any]
reveal_type(np.trace(A)) # E: Any
reveal_type(np.trace(B)) # E: Any
reveal_type(np.ravel(a)) # E: numpy.ndarray[Any, Any]
reveal_type(np.ravel(b)) # E: numpy.ndarray[Any, Any]
reveal_type(np.ravel(c)) # E: numpy.ndarray[Any, Any]
reveal_type(np.ravel(A)) # E: numpy.ndarray[Any, Any]
reveal_type(np.ravel(B)) # E: numpy.ndarray[Any, Any]
reveal_type(np.nonzero(a)) # E: tuple[numpy.ndarray[Any, Any]]
reveal_type(np.nonzero(b)) # E: tuple[numpy.ndarray[Any, Any]]
reveal_type(np.nonzero(c)) # E: tuple[numpy.ndarray[Any, Any]]
reveal_type(np.nonzero(A)) # E: tuple[numpy.ndarray[Any, Any]]
reveal_type(np.nonzero(B)) # E: tuple[numpy.ndarray[Any, Any]]
reveal_type(np.shape(a)) # E: tuple[builtins.int]
reveal_type(np.shape(b)) # E: tuple[builtins.int]
reveal_type(np.shape(c)) # E: tuple[builtins.int]
reveal_type(np.shape(A)) # E: tuple[builtins.int]
reveal_type(np.shape(B)) # E: tuple[builtins.int]
reveal_type(np.compress([True], a)) # E: numpy.ndarray[Any, Any]
reveal_type(np.compress([True], b)) # E: numpy.ndarray[Any, Any]
reveal_type(np.compress([True], c)) # E: numpy.ndarray[Any, Any]
reveal_type(np.compress([True], A)) # E: numpy.ndarray[Any, Any]
reveal_type(np.compress([True], B)) # E: numpy.ndarray[Any, Any]
reveal_type(np.clip(a, 0, 1.0)) # E: Any
reveal_type(np.clip(b, -1, 1)) # E: Any
reveal_type(np.clip(c, 0, 1)) # E: Any
reveal_type(np.clip(A, 0, 1)) # E: Any
reveal_type(np.clip(B, 0, 1)) # E: Any
reveal_type(np.sum(a)) # E: Any
reveal_type(np.sum(b)) # E: Any
reveal_type(np.sum(c)) # E: Any
reveal_type(np.sum(A)) # E: Any
reveal_type(np.sum(B)) # E: Any
reveal_type(np.sum(A, axis=0)) # E: Any
reveal_type(np.sum(B, axis=0)) # E: Any
reveal_type(np.all(a)) # E: numpy.bool_
reveal_type(np.all(b)) # E: numpy.bool_
reveal_type(np.all(c)) # E: numpy.bool_
reveal_type(np.all(A)) # E: numpy.bool_
reveal_type(np.all(B)) # E: numpy.bool_
reveal_type(np.all(A, axis=0)) # E: Any
reveal_type(np.all(B, axis=0)) # E: Any
reveal_type(np.all(A, keepdims=True)) # E: Any
reveal_type(np.all(B, keepdims=True)) # E: Any
reveal_type(np.any(a)) # E: numpy.bool_
reveal_type(np.any(b)) # E: numpy.bool_
reveal_type(np.any(c)) # E: numpy.bool_
reveal_type(np.any(A)) # E: numpy.bool_
reveal_type(np.any(B)) # E: numpy.bool_
reveal_type(np.any(A, axis=0)) # E: Any
reveal_type(np.any(B, axis=0)) # E: Any
reveal_type(np.any(A, keepdims=True)) # E: Any
reveal_type(np.any(B, keepdims=True)) # E: Any
reveal_type(np.cumsum(a)) # E: numpy.ndarray[Any, Any]
reveal_type(np.cumsum(b)) # E: numpy.ndarray[Any, Any]
reveal_type(np.cumsum(c)) # E: numpy.ndarray[Any, Any]
reveal_type(np.cumsum(A)) # E: numpy.ndarray[Any, Any]
reveal_type(np.cumsum(B)) # E: numpy.ndarray[Any, Any]
reveal_type(np.ptp(a)) # E: Any
reveal_type(np.ptp(b)) # E: Any
reveal_type(np.ptp(c)) # E: Any
reveal_type(np.ptp(A)) # E: Any
reveal_type(np.ptp(B)) # E: Any
reveal_type(np.ptp(A, axis=0)) # E: Any
reveal_type(np.ptp(B, axis=0)) # E: Any
reveal_type(np.ptp(A, keepdims=True)) # E: Any
reveal_type(np.ptp(B, keepdims=True)) # E: Any
reveal_type(np.amax(a)) # E: Any
reveal_type(np.amax(b)) # E: Any
reveal_type(np.amax(c)) # E: Any
reveal_type(np.amax(A)) # E: Any
reveal_type(np.amax(B)) # E: Any
reveal_type(np.amax(A, axis=0)) # E: Any
reveal_type(np.amax(B, axis=0)) # E: Any
reveal_type(np.amax(A, keepdims=True)) # E: Any
reveal_type(np.amax(B, keepdims=True)) # E: Any
reveal_type(np.amin(a)) # E: Any
reveal_type(np.amin(b)) # E: Any
reveal_type(np.amin(c)) # E: Any
reveal_type(np.amin(A)) # E: Any
reveal_type(np.amin(B)) # E: Any
reveal_type(np.amin(A, axis=0)) # E: Any
reveal_type(np.amin(B, axis=0)) # E: Any
reveal_type(np.amin(A, keepdims=True)) # E: Any
reveal_type(np.amin(B, keepdims=True)) # E: Any
reveal_type(np.prod(a)) # E: Any
reveal_type(np.prod(b)) # E: Any
reveal_type(np.prod(c)) # E: Any
reveal_type(np.prod(A)) # E: Any
reveal_type(np.prod(B)) # E: Any
reveal_type(np.prod(A, axis=0)) # E: Any
reveal_type(np.prod(B, axis=0)) # E: Any
reveal_type(np.prod(A, keepdims=True)) # E: Any
reveal_type(np.prod(B, keepdims=True)) # E: Any
reveal_type(np.prod(b, out=d)) # E: Any
reveal_type(np.prod(B, out=d)) # E: Any
reveal_type(np.cumprod(a)) # E: numpy.ndarray[Any, Any]
reveal_type(np.cumprod(b)) # E: numpy.ndarray[Any, Any]
reveal_type(np.cumprod(c)) # E: numpy.ndarray[Any, Any]
reveal_type(np.cumprod(A)) # E: numpy.ndarray[Any, Any]
reveal_type(np.cumprod(B)) # E: numpy.ndarray[Any, Any]
reveal_type(np.ndim(a)) # E: int
reveal_type(np.ndim(b)) # E: int
reveal_type(np.ndim(c)) # E: int
reveal_type(np.ndim(A)) # E: int
reveal_type(np.ndim(B)) # E: int
reveal_type(np.size(a)) # E: int
reveal_type(np.size(b)) # E: int
reveal_type(np.size(c)) # E: int
reveal_type(np.size(A)) # E: int
reveal_type(np.size(B)) # E: int
reveal_type(np.around(a)) # E: Any
reveal_type(np.around(b)) # E: Any
reveal_type(np.around(c)) # E: Any
reveal_type(np.around(A)) # E: Any
reveal_type(np.around(B)) # E: Any
reveal_type(np.mean(a)) # E: Any
reveal_type(np.mean(b)) # E: Any
reveal_type(np.mean(c)) # E: Any
reveal_type(np.mean(A)) # E: Any
reveal_type(np.mean(B)) # E: Any
reveal_type(np.mean(A, axis=0)) # E: Any
reveal_type(np.mean(B, axis=0)) # E: Any
reveal_type(np.mean(A, keepdims=True)) # E: Any
reveal_type(np.mean(B, keepdims=True)) # E: Any
reveal_type(np.mean(b, out=d)) # E: Any
reveal_type(np.mean(B, out=d)) # E: Any
reveal_type(np.std(a)) # E: Any
reveal_type(np.std(b)) # E: Any
reveal_type(np.std(c)) # E: Any
reveal_type(np.std(A)) # E: Any
reveal_type(np.std(B)) # E: Any
reveal_type(np.std(A, axis=0)) # E: Any
reveal_type(np.std(B, axis=0)) # E: Any
reveal_type(np.std(A, keepdims=True)) # E: Any
reveal_type(np.std(B, keepdims=True)) # E: Any
reveal_type(np.std(b, out=d)) # E: Any
reveal_type(np.std(B, out=d)) # E: Any
reveal_type(np.var(a)) # E: Any
reveal_type(np.var(b)) # E: Any
reveal_type(np.var(c)) # E: Any
reveal_type(np.var(A)) # E: Any
reveal_type(np.var(B)) # E: Any
reveal_type(np.var(A, axis=0)) # E: Any
reveal_type(np.var(B, axis=0)) # E: Any
reveal_type(np.var(A, keepdims=True)) # E: Any
reveal_type(np.var(B, keepdims=True)) # E: Any
reveal_type(np.var(b, out=d)) # E: Any
reveal_type(np.var(B, out=d)) # E: Any
| mit |
jnerin/ansible | test/units/modules/network/ironware/test_ironware_config.py | 57 | 6817 | #
# (c) 2016 Red Hat Inc.
#
# This file is part of Ansible
#
# Ansible is free software: you can redistribute it and/or modify
# it under the terms of the GNU General Public License as published by
# the Free Software Foundation, either version 3 of the License, or
# (at your option) any later version.
#
# Ansible is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with Ansible. If not, see <http://www.gnu.org/licenses/>.
# Make coding more python3-ish
from __future__ import (absolute_import, division, print_function)
__metaclass__ = type
import json
from ansible.compat.tests.mock import patch
from ansible.modules.network.ironware import ironware_config
from .ironware_module import TestIronwareModule, load_fixture
from units.modules.utils import set_module_args
class TestIronwareConfigModule(TestIronwareModule):
module = ironware_config
def setUp(self):
super(TestIronwareConfigModule, self).setUp()
self.mock_get_config = patch('ansible.modules.network.ironware.ironware_config.get_config')
self.get_config = self.mock_get_config.start()
self.mock_load_config = patch('ansible.modules.network.ironware.ironware_config.load_config')
self.load_config = self.mock_load_config.start()
self.mock_run_commands = patch('ansible.modules.network.ironware.ironware_config.run_commands')
self.run_commands = self.mock_run_commands.start()
def tearDown(self):
super(TestIronwareConfigModule, self).tearDown()
self.mock_get_config.stop()
self.mock_load_config.stop()
self.mock_run_commands.stop()
def load_fixtures(self, commands=None):
config_file = 'ironware_config_config.cfg'
self.get_config.return_value = load_fixture(config_file)
self.load_config.return_value = None
def execute_module(self, failed=False, changed=False, updates=None, sort=True, defaults=False):
self.load_fixtures(updates)
if failed:
result = self.failed()
self.assertTrue(result['failed'], result)
else:
result = self.changed(changed)
self.assertEqual(result['changed'], changed, result)
if updates is not None:
if sort:
self.assertEqual(sorted(updates), sorted(result['updates']), result['updates'])
else:
self.assertEqual(updates, result['updates'], result['updates'])
return result
def test_ironware_config_unchanged(self):
src = load_fixture('ironware_config_config.cfg')
set_module_args(dict(src=src))
self.execute_module()
def test_ironware_config_src(self):
src = load_fixture('ironware_config_src.cfg')
set_module_args(dict(src=src))
updates = ['hostname foo', 'interface ethernet 1/1',
'no ip address']
self.execute_module(changed=True, updates=updates)
def test_ironware_config_backup(self):
set_module_args(dict(backup=True))
result = self.execute_module()
self.assertIn('__backup__', result)
def test_ironware_config_save_always(self):
self.run_commands.return_value = "hostname foobar"
set_module_args(dict(save_when='always'))
self.execute_module(changed=True)
self.assertEqual(self.run_commands.call_count, 1)
self.assertEqual(self.get_config.call_count, 1)
self.assertEqual(self.load_config.call_count, 0)
def test_ironware_config_lines_wo_parents(self):
set_module_args(dict(lines=['hostname foobar']))
updates = ['hostname foobar']
self.execute_module(changed=True, updates=updates)
def test_ironware_config_lines_w_parents(self):
set_module_args(dict(lines=['disable'], parents=['interface ethernet 1/1']))
updates = ['interface ethernet 1/1', 'disable']
self.execute_module(changed=True, updates=updates)
def test_ironware_config_before(self):
set_module_args(dict(lines=['hostname foo'], before=['test1', 'test2']))
updates = ['test1', 'test2', 'hostname foo']
self.execute_module(changed=True, updates=updates, sort=False)
def test_ironware_config_after(self):
set_module_args(dict(lines=['hostname foo'], after=['test1', 'test2']))
updates = ['hostname foo', 'test1', 'test2']
self.execute_module(changed=True, updates=updates, sort=False)
def test_ironware_config_before_after_no_change(self):
set_module_args(dict(lines=['hostname router'],
before=['test1', 'test2'],
after=['test3', 'test4']))
self.execute_module()
def test_ironware_config_config(self):
config = 'hostname localhost'
set_module_args(dict(lines=['hostname router'], config=config))
updates = ['hostname router']
self.execute_module(changed=True, updates=updates)
def test_ironware_config_replace_block(self):
lines = ['port-name test string', 'test string']
parents = ['interface ethernet 1/1']
set_module_args(dict(lines=lines, replace='block', parents=parents))
updates = parents + lines
self.execute_module(changed=True, updates=updates)
def test_ironware_config_match_none(self):
lines = ['hostname router']
set_module_args(dict(lines=lines, match='none'))
self.execute_module(changed=True, updates=lines)
def test_ironware_config_match_none(self):
lines = ['ip address 1.2.3.4 255.255.255.0', 'port-name test string']
parents = ['interface ethernet 1/1']
set_module_args(dict(lines=lines, parents=parents, match='none'))
updates = parents + lines
self.execute_module(changed=True, updates=updates, sort=False)
def test_ironware_config_match_strict(self):
lines = ['ip address 1.2.3.4 255.255.255.0', 'port-name test string',
'disable']
parents = ['interface ethernet 1/1']
set_module_args(dict(lines=lines, parents=parents, match='strict'))
updates = parents + ['disable']
self.execute_module(changed=True, updates=updates, sort=False)
def test_ironware_config_match_exact(self):
lines = ['ip address 1.2.3.4 255.255.255.0', 'port-name test string',
'disable']
parents = ['interface ethernet 1/1']
set_module_args(dict(lines=lines, parents=parents, match='exact'))
updates = parents + lines
self.execute_module(changed=True, updates=updates, sort=False)
| gpl-3.0 |
ryfeus/lambda-packs | LightGBM_sklearn_scipy_numpy/source/sklearn/preprocessing/__init__.py | 19 | 1447 | """
The :mod:`sklearn.preprocessing` module includes scaling, centering,
normalization, binarization and imputation methods.
"""
from ._function_transformer import FunctionTransformer
from .data import Binarizer
from .data import KernelCenterer
from .data import MinMaxScaler
from .data import MaxAbsScaler
from .data import Normalizer
from .data import RobustScaler
from .data import StandardScaler
from .data import QuantileTransformer
from .data import add_dummy_feature
from .data import binarize
from .data import normalize
from .data import scale
from .data import robust_scale
from .data import maxabs_scale
from .data import minmax_scale
from .data import quantile_transform
from .data import OneHotEncoder
from .data import PolynomialFeatures
from .label import label_binarize
from .label import LabelBinarizer
from .label import LabelEncoder
from .label import MultiLabelBinarizer
from .imputation import Imputer
__all__ = [
'Binarizer',
'FunctionTransformer',
'Imputer',
'KernelCenterer',
'LabelBinarizer',
'LabelEncoder',
'MultiLabelBinarizer',
'MinMaxScaler',
'MaxAbsScaler',
'QuantileTransformer',
'Normalizer',
'OneHotEncoder',
'RobustScaler',
'StandardScaler',
'add_dummy_feature',
'PolynomialFeatures',
'binarize',
'normalize',
'scale',
'robust_scale',
'maxabs_scale',
'minmax_scale',
'label_binarize',
'quantile_transform',
]
| mit |
silky/airflow | airflow/operators/generic_transfer.py | 29 | 2285 | import logging
from airflow.models import BaseOperator
from airflow.utils import apply_defaults
from airflow.hooks.base_hook import BaseHook
class GenericTransfer(BaseOperator):
"""
Moves data from a connection to another, assuming that they both
provide the required methods in their respective hooks. The source hook
needs to expose a `get_records` method, and the destination a
`insert_rows` method.
This is mean to be used on small-ish datasets that fit in memory.
:param sql: SQL query to execute against the source database
:type sql: str
:param destination_table: target table
:type destination_table: str
:param source_conn_id: source connection
:type source_conn_id: str
:param destination_conn_id: source connection
:type destination_conn_id: str
:param preoperator: sql statement or list of statements to be
executed prior to loading the data
:type preoperator: str or list of str
"""
template_fields = ('sql', 'destination_table', 'preoperator')
template_ext = ('.sql', '.hql',)
ui_color = '#b0f07c'
@apply_defaults
def __init__(
self,
sql,
destination_table,
source_conn_id,
destination_conn_id,
preoperator=None,
*args, **kwargs):
super(GenericTransfer, self).__init__(*args, **kwargs)
self.sql = sql
self.destination_table = destination_table
self.source_conn_id = source_conn_id
self.destination_conn_id = destination_conn_id
self.preoperator = preoperator
def execute(self, context):
source_hook = BaseHook.get_hook(self.source_conn_id)
logging.info("Extracting data from {}".format(self.source_conn_id))
logging.info("Executing: \n" + self.sql)
results = source_hook.get_records(self.sql)
destination_hook = BaseHook.get_hook(self.destination_conn_id)
if self.preoperator:
logging.info("Running preoperator")
logging.info(self.preoperator)
destination_hook.run(self.preoperator)
logging.info("Inserting rows into {}".format(self.destination_conn_id))
destination_hook.insert_rows(table=self.destination_table, rows=results)
| apache-2.0 |
Azure/azure-sdk-for-python | sdk/sql/azure-mgmt-sql/generated_samples/list_job_agents_in_a_server.py | 1 | 1572 | # coding=utf-8
# --------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for license information.
# Code generated by Microsoft (R) AutoRest Code Generator.
# Changes may cause incorrect behavior and will be lost if the code is regenerated.
# --------------------------------------------------------------------------
from azure.identity import DefaultAzureCredential
from azure.mgmt.sql import SqlManagementClient
"""
# PREREQUISITES
pip install azure-identity
pip install azure-mgmt-sql
# USAGE
python list_job_agents_in_a_server.py
Before run the sample, please set the values of the client ID, tenant ID and client secret
of the AAD application as environment variables: AZURE_CLIENT_ID, AZURE_TENANT_ID,
AZURE_CLIENT_SECRET. For more info about how to get the value, please see:
https://docs.microsoft.com/azure/active-directory/develop/howto-create-service-principal-portal
"""
def main():
client = SqlManagementClient(
credential=DefaultAzureCredential(),
subscription_id="00000000-1111-2222-3333-444444444444",
)
response = client.job_agents.list_by_server(
resource_group_name="group1",
server_name="server1",
)
for item in response:
print(item)
# x-ms-original-file: specification/sql/resource-manager/Microsoft.Sql/preview/2020-11-01-preview/examples/ListJobAgentsByServer.json
if __name__ == "__main__":
main()
| mit |
AutorestCI/azure-sdk-for-python | azure-keyvault/setup.py | 1 | 2807 | #!/usr/bin/env python
#-------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for
# license information.
#--------------------------------------------------------------------------
import re
import os.path
from io import open
from setuptools import find_packages, setup
try:
from azure_bdist_wheel import cmdclass
except ImportError:
from distutils import log as logger
logger.warn("Wheel is not available, disabling bdist_wheel hook")
cmdclass = {}
# Change the PACKAGE_NAME only to change folder and different name
PACKAGE_NAME = "azure-keyvault"
PACKAGE_PPRINT_NAME = "Key Vault"
# a-b-c => a/b/c
package_folder_path = PACKAGE_NAME.replace('-', '/')
# a-b-c => a.b.c
namespace_name = PACKAGE_NAME.replace('-', '.')
# azure v0.x is not compatible with this package
# azure v0.x used to have a __version__ attribute (newer versions don't)
try:
import azure
try:
ver = azure.__version__
raise Exception(
'This package is incompatible with azure=={}. '.format(ver) +
'Uninstall it with "pip uninstall azure".'
)
except AttributeError:
pass
except ImportError:
pass
# Version extraction inspired from 'requests'
with open(os.path.join(package_folder_path, 'version.py'), 'r') as fd:
version = re.search(r'^VERSION\s*=\s*[\'"]([^\'"]*)[\'"]',
fd.read(), re.MULTILINE).group(1)
if not version:
raise RuntimeError('Cannot find version information')
with open('README.rst', encoding='utf-8') as f:
readme = f.read()
with open('HISTORY.rst', encoding='utf-8') as f:
history = f.read()
setup(
name=PACKAGE_NAME,
version=version,
description='Microsoft Azure {} Client Library for Python'.format(PACKAGE_PPRINT_NAME),
long_description=readme + '\n\n' + history,
license='MIT License',
author='Microsoft Corporation',
author_email='ptvshelp@microsoft.com',
url='https://github.com/Azure/azure-sdk-for-python',
classifiers=[
'Development Status :: 4 - Beta',
'Programming Language :: Python',
'Programming Language :: Python :: 2',
'Programming Language :: Python :: 2.7',
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'License :: OSI Approved :: MIT License',
],
zip_safe=False,
packages=find_packages(),
install_requires=[
'msrestazure>=0.4.15',
'msrest>=0.4.17'
'azure-common~=1.1.5',
],
cmdclass=cmdclass
)
| mit |
cgwalters/hawkey | tests/python/tests/test_reldep.py | 1 | 2961 | #
# Copyright (C) 2012-2013 Red Hat, Inc.
#
# Licensed under the GNU Lesser General Public License Version 2.1
#
# This library is free software; you can redistribute it and/or
# modify it under the terms of the GNU Lesser General Public
# License as published by the Free Software Foundation; either
# version 2.1 of the License, or (at your option) any later version.
#
# This library is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
# Lesser General Public License for more details.
#
# You should have received a copy of the GNU Lesser General Public
# License along with this library; if not, write to the Free Software
# Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
#
from __future__ import absolute_import
from . import base
import hawkey
class Reldep(base.TestCase):
def setUp(self):
self.sack = base.TestSack(repo_dir=self.repo_dir)
self.sack.load_system_repo()
def test_basic(self):
flying = base.by_name(self.sack, "flying")
requires = flying.requires
self.assertLength(requires, 1)
reldep = requires[0]
self.assertEqual(str(reldep), "P-lib >= 3")
def test_custom_creation(self):
reldep_str = "P-lib >= 3"
reldep = hawkey.Reldep(self.sack, reldep_str)
self.assertEqual(reldep_str, str(reldep))
def test_custom_creation_fail(self):
reldep_str = "lane = 4"
self.assertRaises(hawkey.ValueException, hawkey.Reldep, self.sack,
reldep_str)
reldep_str = "P-lib >="
self.assertRaises(hawkey.ValueException, hawkey.Reldep, self.sack,
reldep_str)
def test_custom_querying(self):
reldep = hawkey.Reldep(self.sack, "P-lib = 3-3")
q = hawkey.Query(self.sack).filter(provides=reldep)
self.assertLength(q, 1)
reldep = hawkey.Reldep(self.sack, "P-lib >= 3")
q = hawkey.Query(self.sack).filter(provides=reldep)
self.assertLength(q, 1)
reldep = hawkey.Reldep(self.sack, "P-lib < 3-3")
q = hawkey.Query(self.sack).filter(provides=reldep)
self.assertLength(q, 0)
def test_query_name_only(self):
reldep_str = "P-lib"
reldep = hawkey.Reldep(self.sack, reldep_str)
self.assertEqual(reldep_str, str(reldep))
q = hawkey.Query(self.sack).filter(provides=reldep)
self.assertLength(q, 1)
self.assertEqual(str(q[0]), "penny-lib-4-1.x86_64")
def test_not_crash(self):
self.assertRaises(ValueError, hawkey.Reldep)
def test_non_num_version(self):
reldep_str = 'foo >= 1.0-1.fc20'
with self.assertRaises(hawkey.ValueException) as ctx:
hawkey.Reldep(self.sack, reldep_str)
self.assertEqual(ctx.exception.args[0], "No such reldep: %s" % reldep_str)
| lgpl-2.1 |
anaran/kuma | vendor/packages/diff_match_patch/diff_match_patch_test.py | 319 | 41744 | #!/usr/bin/python2.4
"""Test harness for diff_match_patch.py
Copyright 2006 Google Inc.
http://code.google.com/p/google-diff-match-patch/
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
"""
import sys
import time
import unittest
import diff_match_patch as dmp_module
# Force a module reload. Allows one to edit the DMP module and rerun the tests
# without leaving the Python interpreter.
reload(dmp_module)
class DiffMatchPatchTest(unittest.TestCase):
def setUp(self):
"Test harness for dmp_module."
self.dmp = dmp_module.diff_match_patch()
def diff_rebuildtexts(self, diffs):
# Construct the two texts which made up the diff originally.
text1 = ""
text2 = ""
for x in range(0, len(diffs)):
if diffs[x][0] != dmp_module.diff_match_patch.DIFF_INSERT:
text1 += diffs[x][1]
if diffs[x][0] != dmp_module.diff_match_patch.DIFF_DELETE:
text2 += diffs[x][1]
return (text1, text2)
class DiffTest(DiffMatchPatchTest):
"""DIFF TEST FUNCTIONS"""
def testDiffCommonPrefix(self):
# Detect any common prefix.
# Null case.
self.assertEquals(0, self.dmp.diff_commonPrefix("abc", "xyz"))
# Non-null case.
self.assertEquals(4, self.dmp.diff_commonPrefix("1234abcdef", "1234xyz"))
# Whole case.
self.assertEquals(4, self.dmp.diff_commonPrefix("1234", "1234xyz"))
def testDiffCommonSuffix(self):
# Detect any common suffix.
# Null case.
self.assertEquals(0, self.dmp.diff_commonSuffix("abc", "xyz"))
# Non-null case.
self.assertEquals(4, self.dmp.diff_commonSuffix("abcdef1234", "xyz1234"))
# Whole case.
self.assertEquals(4, self.dmp.diff_commonSuffix("1234", "xyz1234"))
def testDiffCommonOverlap(self):
# Null case.
self.assertEquals(0, self.dmp.diff_commonOverlap("", "abcd"))
# Whole case.
self.assertEquals(3, self.dmp.diff_commonOverlap("abc", "abcd"))
# No overlap.
self.assertEquals(0, self.dmp.diff_commonOverlap("123456", "abcd"))
# Overlap.
self.assertEquals(3, self.dmp.diff_commonOverlap("123456xxx", "xxxabcd"))
# Unicode.
# Some overly clever languages (C#) may treat ligatures as equal to their
# component letters. E.g. U+FB01 == 'fi'
self.assertEquals(0, self.dmp.diff_commonOverlap("fi", u"\ufb01i"))
def testDiffHalfMatch(self):
# Detect a halfmatch.
self.dmp.Diff_Timeout = 1
# No match.
self.assertEquals(None, self.dmp.diff_halfMatch("1234567890", "abcdef"))
self.assertEquals(None, self.dmp.diff_halfMatch("12345", "23"))
# Single Match.
self.assertEquals(("12", "90", "a", "z", "345678"), self.dmp.diff_halfMatch("1234567890", "a345678z"))
self.assertEquals(("a", "z", "12", "90", "345678"), self.dmp.diff_halfMatch("a345678z", "1234567890"))
self.assertEquals(("abc", "z", "1234", "0", "56789"), self.dmp.diff_halfMatch("abc56789z", "1234567890"))
self.assertEquals(("a", "xyz", "1", "7890", "23456"), self.dmp.diff_halfMatch("a23456xyz", "1234567890"))
# Multiple Matches.
self.assertEquals(("12123", "123121", "a", "z", "1234123451234"), self.dmp.diff_halfMatch("121231234123451234123121", "a1234123451234z"))
self.assertEquals(("", "-=-=-=-=-=", "x", "", "x-=-=-=-=-=-=-="), self.dmp.diff_halfMatch("x-=-=-=-=-=-=-=-=-=-=-=-=", "xx-=-=-=-=-=-=-="))
self.assertEquals(("-=-=-=-=-=", "", "", "y", "-=-=-=-=-=-=-=y"), self.dmp.diff_halfMatch("-=-=-=-=-=-=-=-=-=-=-=-=y", "-=-=-=-=-=-=-=yy"))
# Non-optimal halfmatch.
# Optimal diff would be -q+x=H-i+e=lloHe+Hu=llo-Hew+y not -qHillo+x=HelloHe-w+Hulloy
self.assertEquals(("qHillo", "w", "x", "Hulloy", "HelloHe"), self.dmp.diff_halfMatch("qHilloHelloHew", "xHelloHeHulloy"))
# Optimal no halfmatch.
self.dmp.Diff_Timeout = 0
self.assertEquals(None, self.dmp.diff_halfMatch("qHilloHelloHew", "xHelloHeHulloy"))
def testDiffLinesToChars(self):
# Convert lines down to characters.
self.assertEquals(("\x01\x02\x01", "\x02\x01\x02", ["", "alpha\n", "beta\n"]), self.dmp.diff_linesToChars("alpha\nbeta\nalpha\n", "beta\nalpha\nbeta\n"))
self.assertEquals(("", "\x01\x02\x03\x03", ["", "alpha\r\n", "beta\r\n", "\r\n"]), self.dmp.diff_linesToChars("", "alpha\r\nbeta\r\n\r\n\r\n"))
self.assertEquals(("\x01", "\x02", ["", "a", "b"]), self.dmp.diff_linesToChars("a", "b"))
# More than 256 to reveal any 8-bit limitations.
n = 300
lineList = []
charList = []
for x in range(1, n + 1):
lineList.append(str(x) + "\n")
charList.append(unichr(x))
self.assertEquals(n, len(lineList))
lines = "".join(lineList)
chars = "".join(charList)
self.assertEquals(n, len(chars))
lineList.insert(0, "")
self.assertEquals((chars, "", lineList), self.dmp.diff_linesToChars(lines, ""))
def testDiffCharsToLines(self):
# Convert chars up to lines.
diffs = [(self.dmp.DIFF_EQUAL, "\x01\x02\x01"), (self.dmp.DIFF_INSERT, "\x02\x01\x02")]
self.dmp.diff_charsToLines(diffs, ["", "alpha\n", "beta\n"])
self.assertEquals([(self.dmp.DIFF_EQUAL, "alpha\nbeta\nalpha\n"), (self.dmp.DIFF_INSERT, "beta\nalpha\nbeta\n")], diffs)
# More than 256 to reveal any 8-bit limitations.
n = 300
lineList = []
charList = []
for x in range(1, n + 1):
lineList.append(str(x) + "\n")
charList.append(unichr(x))
self.assertEquals(n, len(lineList))
lines = "".join(lineList)
chars = "".join(charList)
self.assertEquals(n, len(chars))
lineList.insert(0, "")
diffs = [(self.dmp.DIFF_DELETE, chars)]
self.dmp.diff_charsToLines(diffs, lineList)
self.assertEquals([(self.dmp.DIFF_DELETE, lines)], diffs)
def testDiffCleanupMerge(self):
# Cleanup a messy diff.
# Null case.
diffs = []
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([], diffs)
# No change case.
diffs = [(self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_DELETE, "b"), (self.dmp.DIFF_INSERT, "c")]
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_DELETE, "b"), (self.dmp.DIFF_INSERT, "c")], diffs)
# Merge equalities.
diffs = [(self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_EQUAL, "b"), (self.dmp.DIFF_EQUAL, "c")]
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "abc")], diffs)
# Merge deletions.
diffs = [(self.dmp.DIFF_DELETE, "a"), (self.dmp.DIFF_DELETE, "b"), (self.dmp.DIFF_DELETE, "c")]
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "abc")], diffs)
# Merge insertions.
diffs = [(self.dmp.DIFF_INSERT, "a"), (self.dmp.DIFF_INSERT, "b"), (self.dmp.DIFF_INSERT, "c")]
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([(self.dmp.DIFF_INSERT, "abc")], diffs)
# Merge interweave.
diffs = [(self.dmp.DIFF_DELETE, "a"), (self.dmp.DIFF_INSERT, "b"), (self.dmp.DIFF_DELETE, "c"), (self.dmp.DIFF_INSERT, "d"), (self.dmp.DIFF_EQUAL, "e"), (self.dmp.DIFF_EQUAL, "f")]
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "ac"), (self.dmp.DIFF_INSERT, "bd"), (self.dmp.DIFF_EQUAL, "ef")], diffs)
# Prefix and suffix detection.
diffs = [(self.dmp.DIFF_DELETE, "a"), (self.dmp.DIFF_INSERT, "abc"), (self.dmp.DIFF_DELETE, "dc")]
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_DELETE, "d"), (self.dmp.DIFF_INSERT, "b"), (self.dmp.DIFF_EQUAL, "c")], diffs)
# Prefix and suffix detection with equalities.
diffs = [(self.dmp.DIFF_EQUAL, "x"), (self.dmp.DIFF_DELETE, "a"), (self.dmp.DIFF_INSERT, "abc"), (self.dmp.DIFF_DELETE, "dc"), (self.dmp.DIFF_EQUAL, "y")]
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "xa"), (self.dmp.DIFF_DELETE, "d"), (self.dmp.DIFF_INSERT, "b"), (self.dmp.DIFF_EQUAL, "cy")], diffs)
# Slide edit left.
diffs = [(self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_INSERT, "ba"), (self.dmp.DIFF_EQUAL, "c")]
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([(self.dmp.DIFF_INSERT, "ab"), (self.dmp.DIFF_EQUAL, "ac")], diffs)
# Slide edit right.
diffs = [(self.dmp.DIFF_EQUAL, "c"), (self.dmp.DIFF_INSERT, "ab"), (self.dmp.DIFF_EQUAL, "a")]
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "ca"), (self.dmp.DIFF_INSERT, "ba")], diffs)
# Slide edit left recursive.
diffs = [(self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_DELETE, "b"), (self.dmp.DIFF_EQUAL, "c"), (self.dmp.DIFF_DELETE, "ac"), (self.dmp.DIFF_EQUAL, "x")]
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "abc"), (self.dmp.DIFF_EQUAL, "acx")], diffs)
# Slide edit right recursive.
diffs = [(self.dmp.DIFF_EQUAL, "x"), (self.dmp.DIFF_DELETE, "ca"), (self.dmp.DIFF_EQUAL, "c"), (self.dmp.DIFF_DELETE, "b"), (self.dmp.DIFF_EQUAL, "a")]
self.dmp.diff_cleanupMerge(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "xca"), (self.dmp.DIFF_DELETE, "cba")], diffs)
def testDiffCleanupSemanticLossless(self):
# Slide diffs to match logical boundaries.
# Null case.
diffs = []
self.dmp.diff_cleanupSemanticLossless(diffs)
self.assertEquals([], diffs)
# Blank lines.
diffs = [(self.dmp.DIFF_EQUAL, "AAA\r\n\r\nBBB"), (self.dmp.DIFF_INSERT, "\r\nDDD\r\n\r\nBBB"), (self.dmp.DIFF_EQUAL, "\r\nEEE")]
self.dmp.diff_cleanupSemanticLossless(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "AAA\r\n\r\n"), (self.dmp.DIFF_INSERT, "BBB\r\nDDD\r\n\r\n"), (self.dmp.DIFF_EQUAL, "BBB\r\nEEE")], diffs)
# Line boundaries.
diffs = [(self.dmp.DIFF_EQUAL, "AAA\r\nBBB"), (self.dmp.DIFF_INSERT, " DDD\r\nBBB"), (self.dmp.DIFF_EQUAL, " EEE")]
self.dmp.diff_cleanupSemanticLossless(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "AAA\r\n"), (self.dmp.DIFF_INSERT, "BBB DDD\r\n"), (self.dmp.DIFF_EQUAL, "BBB EEE")], diffs)
# Word boundaries.
diffs = [(self.dmp.DIFF_EQUAL, "The c"), (self.dmp.DIFF_INSERT, "ow and the c"), (self.dmp.DIFF_EQUAL, "at.")]
self.dmp.diff_cleanupSemanticLossless(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "The "), (self.dmp.DIFF_INSERT, "cow and the "), (self.dmp.DIFF_EQUAL, "cat.")], diffs)
# Alphanumeric boundaries.
diffs = [(self.dmp.DIFF_EQUAL, "The-c"), (self.dmp.DIFF_INSERT, "ow-and-the-c"), (self.dmp.DIFF_EQUAL, "at.")]
self.dmp.diff_cleanupSemanticLossless(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "The-"), (self.dmp.DIFF_INSERT, "cow-and-the-"), (self.dmp.DIFF_EQUAL, "cat.")], diffs)
# Hitting the start.
diffs = [(self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_DELETE, "a"), (self.dmp.DIFF_EQUAL, "ax")]
self.dmp.diff_cleanupSemanticLossless(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "a"), (self.dmp.DIFF_EQUAL, "aax")], diffs)
# Hitting the end.
diffs = [(self.dmp.DIFF_EQUAL, "xa"), (self.dmp.DIFF_DELETE, "a"), (self.dmp.DIFF_EQUAL, "a")]
self.dmp.diff_cleanupSemanticLossless(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "xaa"), (self.dmp.DIFF_DELETE, "a")], diffs)
# Sentence boundaries.
diffs = [(self.dmp.DIFF_EQUAL, "The xxx. The "), (self.dmp.DIFF_INSERT, "zzz. The "), (self.dmp.DIFF_EQUAL, "yyy.")]
self.dmp.diff_cleanupSemanticLossless(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "The xxx."), (self.dmp.DIFF_INSERT, " The zzz."), (self.dmp.DIFF_EQUAL, " The yyy.")], diffs)
def testDiffCleanupSemantic(self):
# Cleanup semantically trivial equalities.
# Null case.
diffs = []
self.dmp.diff_cleanupSemantic(diffs)
self.assertEquals([], diffs)
# No elimination #1.
diffs = [(self.dmp.DIFF_DELETE, "ab"), (self.dmp.DIFF_INSERT, "cd"), (self.dmp.DIFF_EQUAL, "12"), (self.dmp.DIFF_DELETE, "e")]
self.dmp.diff_cleanupSemantic(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "ab"), (self.dmp.DIFF_INSERT, "cd"), (self.dmp.DIFF_EQUAL, "12"), (self.dmp.DIFF_DELETE, "e")], diffs)
# No elimination #2.
diffs = [(self.dmp.DIFF_DELETE, "abc"), (self.dmp.DIFF_INSERT, "ABC"), (self.dmp.DIFF_EQUAL, "1234"), (self.dmp.DIFF_DELETE, "wxyz")]
self.dmp.diff_cleanupSemantic(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "abc"), (self.dmp.DIFF_INSERT, "ABC"), (self.dmp.DIFF_EQUAL, "1234"), (self.dmp.DIFF_DELETE, "wxyz")], diffs)
# Simple elimination.
diffs = [(self.dmp.DIFF_DELETE, "a"), (self.dmp.DIFF_EQUAL, "b"), (self.dmp.DIFF_DELETE, "c")]
self.dmp.diff_cleanupSemantic(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "abc"), (self.dmp.DIFF_INSERT, "b")], diffs)
# Backpass elimination.
diffs = [(self.dmp.DIFF_DELETE, "ab"), (self.dmp.DIFF_EQUAL, "cd"), (self.dmp.DIFF_DELETE, "e"), (self.dmp.DIFF_EQUAL, "f"), (self.dmp.DIFF_INSERT, "g")]
self.dmp.diff_cleanupSemantic(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "abcdef"), (self.dmp.DIFF_INSERT, "cdfg")], diffs)
# Multiple eliminations.
diffs = [(self.dmp.DIFF_INSERT, "1"), (self.dmp.DIFF_EQUAL, "A"), (self.dmp.DIFF_DELETE, "B"), (self.dmp.DIFF_INSERT, "2"), (self.dmp.DIFF_EQUAL, "_"), (self.dmp.DIFF_INSERT, "1"), (self.dmp.DIFF_EQUAL, "A"), (self.dmp.DIFF_DELETE, "B"), (self.dmp.DIFF_INSERT, "2")]
self.dmp.diff_cleanupSemantic(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "AB_AB"), (self.dmp.DIFF_INSERT, "1A2_1A2")], diffs)
# Word boundaries.
diffs = [(self.dmp.DIFF_EQUAL, "The c"), (self.dmp.DIFF_DELETE, "ow and the c"), (self.dmp.DIFF_EQUAL, "at.")]
self.dmp.diff_cleanupSemantic(diffs)
self.assertEquals([(self.dmp.DIFF_EQUAL, "The "), (self.dmp.DIFF_DELETE, "cow and the "), (self.dmp.DIFF_EQUAL, "cat.")], diffs)
# No overlap elimination.
diffs = [(self.dmp.DIFF_DELETE, "abcxx"), (self.dmp.DIFF_INSERT, "xxdef")]
self.dmp.diff_cleanupSemantic(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "abcxx"), (self.dmp.DIFF_INSERT, "xxdef")], diffs)
# Overlap elimination.
diffs = [(self.dmp.DIFF_DELETE, "abcxxx"), (self.dmp.DIFF_INSERT, "xxxdef")]
self.dmp.diff_cleanupSemantic(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "abc"), (self.dmp.DIFF_EQUAL, "xxx"), (self.dmp.DIFF_INSERT, "def")], diffs)
# Reverse overlap elimination.
diffs = [(self.dmp.DIFF_DELETE, "xxxabc"), (self.dmp.DIFF_INSERT, "defxxx")]
self.dmp.diff_cleanupSemantic(diffs)
self.assertEquals([(self.dmp.DIFF_INSERT, "def"), (self.dmp.DIFF_EQUAL, "xxx"), (self.dmp.DIFF_DELETE, "abc")], diffs)
# Two overlap eliminations.
diffs = [(self.dmp.DIFF_DELETE, "abcd1212"), (self.dmp.DIFF_INSERT, "1212efghi"), (self.dmp.DIFF_EQUAL, "----"), (self.dmp.DIFF_DELETE, "A3"), (self.dmp.DIFF_INSERT, "3BC")]
self.dmp.diff_cleanupSemantic(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "abcd"), (self.dmp.DIFF_EQUAL, "1212"), (self.dmp.DIFF_INSERT, "efghi"), (self.dmp.DIFF_EQUAL, "----"), (self.dmp.DIFF_DELETE, "A"), (self.dmp.DIFF_EQUAL, "3"), (self.dmp.DIFF_INSERT, "BC")], diffs)
def testDiffCleanupEfficiency(self):
# Cleanup operationally trivial equalities.
self.dmp.Diff_EditCost = 4
# Null case.
diffs = []
self.dmp.diff_cleanupEfficiency(diffs)
self.assertEquals([], diffs)
# No elimination.
diffs = [(self.dmp.DIFF_DELETE, "ab"), (self.dmp.DIFF_INSERT, "12"), (self.dmp.DIFF_EQUAL, "wxyz"), (self.dmp.DIFF_DELETE, "cd"), (self.dmp.DIFF_INSERT, "34")]
self.dmp.diff_cleanupEfficiency(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "ab"), (self.dmp.DIFF_INSERT, "12"), (self.dmp.DIFF_EQUAL, "wxyz"), (self.dmp.DIFF_DELETE, "cd"), (self.dmp.DIFF_INSERT, "34")], diffs)
# Four-edit elimination.
diffs = [(self.dmp.DIFF_DELETE, "ab"), (self.dmp.DIFF_INSERT, "12"), (self.dmp.DIFF_EQUAL, "xyz"), (self.dmp.DIFF_DELETE, "cd"), (self.dmp.DIFF_INSERT, "34")]
self.dmp.diff_cleanupEfficiency(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "abxyzcd"), (self.dmp.DIFF_INSERT, "12xyz34")], diffs)
# Three-edit elimination.
diffs = [(self.dmp.DIFF_INSERT, "12"), (self.dmp.DIFF_EQUAL, "x"), (self.dmp.DIFF_DELETE, "cd"), (self.dmp.DIFF_INSERT, "34")]
self.dmp.diff_cleanupEfficiency(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "xcd"), (self.dmp.DIFF_INSERT, "12x34")], diffs)
# Backpass elimination.
diffs = [(self.dmp.DIFF_DELETE, "ab"), (self.dmp.DIFF_INSERT, "12"), (self.dmp.DIFF_EQUAL, "xy"), (self.dmp.DIFF_INSERT, "34"), (self.dmp.DIFF_EQUAL, "z"), (self.dmp.DIFF_DELETE, "cd"), (self.dmp.DIFF_INSERT, "56")]
self.dmp.diff_cleanupEfficiency(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "abxyzcd"), (self.dmp.DIFF_INSERT, "12xy34z56")], diffs)
# High cost elimination.
self.dmp.Diff_EditCost = 5
diffs = [(self.dmp.DIFF_DELETE, "ab"), (self.dmp.DIFF_INSERT, "12"), (self.dmp.DIFF_EQUAL, "wxyz"), (self.dmp.DIFF_DELETE, "cd"), (self.dmp.DIFF_INSERT, "34")]
self.dmp.diff_cleanupEfficiency(diffs)
self.assertEquals([(self.dmp.DIFF_DELETE, "abwxyzcd"), (self.dmp.DIFF_INSERT, "12wxyz34")], diffs)
self.dmp.Diff_EditCost = 4
def testDiffPrettyHtml(self):
# Pretty print.
diffs = [(self.dmp.DIFF_EQUAL, "a\n"), (self.dmp.DIFF_DELETE, "<B>b</B>"), (self.dmp.DIFF_INSERT, "c&d")]
self.assertEquals("<span>a¶<br></span><del style=\"background:#ffe6e6;\"><B>b</B></del><ins style=\"background:#e6ffe6;\">c&d</ins>", self.dmp.diff_prettyHtml(diffs))
def testDiffText(self):
# Compute the source and destination texts.
diffs = [(self.dmp.DIFF_EQUAL, "jump"), (self.dmp.DIFF_DELETE, "s"), (self.dmp.DIFF_INSERT, "ed"), (self.dmp.DIFF_EQUAL, " over "), (self.dmp.DIFF_DELETE, "the"), (self.dmp.DIFF_INSERT, "a"), (self.dmp.DIFF_EQUAL, " lazy")]
self.assertEquals("jumps over the lazy", self.dmp.diff_text1(diffs))
self.assertEquals("jumped over a lazy", self.dmp.diff_text2(diffs))
def testDiffDelta(self):
# Convert a diff into delta string.
diffs = [(self.dmp.DIFF_EQUAL, "jump"), (self.dmp.DIFF_DELETE, "s"), (self.dmp.DIFF_INSERT, "ed"), (self.dmp.DIFF_EQUAL, " over "), (self.dmp.DIFF_DELETE, "the"), (self.dmp.DIFF_INSERT, "a"), (self.dmp.DIFF_EQUAL, " lazy"), (self.dmp.DIFF_INSERT, "old dog")]
text1 = self.dmp.diff_text1(diffs)
self.assertEquals("jumps over the lazy", text1)
delta = self.dmp.diff_toDelta(diffs)
self.assertEquals("=4\t-1\t+ed\t=6\t-3\t+a\t=5\t+old dog", delta)
# Convert delta string into a diff.
self.assertEquals(diffs, self.dmp.diff_fromDelta(text1, delta))
# Generates error (19 != 20).
try:
self.dmp.diff_fromDelta(text1 + "x", delta)
self.assertFalse(True)
except ValueError:
# Exception expected.
pass
# Generates error (19 != 18).
try:
self.dmp.diff_fromDelta(text1[1:], delta)
self.assertFalse(True)
except ValueError:
# Exception expected.
pass
# Generates error (%c3%xy invalid Unicode).
try:
self.dmp.diff_fromDelta("", "+%c3xy")
self.assertFalse(True)
except ValueError:
# Exception expected.
pass
# Test deltas with special characters.
diffs = [(self.dmp.DIFF_EQUAL, u"\u0680 \x00 \t %"), (self.dmp.DIFF_DELETE, u"\u0681 \x01 \n ^"), (self.dmp.DIFF_INSERT, u"\u0682 \x02 \\ |")]
text1 = self.dmp.diff_text1(diffs)
self.assertEquals(u"\u0680 \x00 \t %\u0681 \x01 \n ^", text1)
delta = self.dmp.diff_toDelta(diffs)
self.assertEquals("=7\t-7\t+%DA%82 %02 %5C %7C", delta)
# Convert delta string into a diff.
self.assertEquals(diffs, self.dmp.diff_fromDelta(text1, delta))
# Verify pool of unchanged characters.
diffs = [(self.dmp.DIFF_INSERT, "A-Z a-z 0-9 - _ . ! ~ * ' ( ) ; / ? : @ & = + $ , # ")]
text2 = self.dmp.diff_text2(diffs)
self.assertEquals("A-Z a-z 0-9 - _ . ! ~ * \' ( ) ; / ? : @ & = + $ , # ", text2)
delta = self.dmp.diff_toDelta(diffs)
self.assertEquals("+A-Z a-z 0-9 - _ . ! ~ * \' ( ) ; / ? : @ & = + $ , # ", delta)
# Convert delta string into a diff.
self.assertEquals(diffs, self.dmp.diff_fromDelta("", delta))
def testDiffXIndex(self):
# Translate a location in text1 to text2.
self.assertEquals(5, self.dmp.diff_xIndex([(self.dmp.DIFF_DELETE, "a"), (self.dmp.DIFF_INSERT, "1234"), (self.dmp.DIFF_EQUAL, "xyz")], 2))
# Translation on deletion.
self.assertEquals(1, self.dmp.diff_xIndex([(self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_DELETE, "1234"), (self.dmp.DIFF_EQUAL, "xyz")], 3))
def testDiffLevenshtein(self):
# Levenshtein with trailing equality.
self.assertEquals(4, self.dmp.diff_levenshtein([(self.dmp.DIFF_DELETE, "abc"), (self.dmp.DIFF_INSERT, "1234"), (self.dmp.DIFF_EQUAL, "xyz")]))
# Levenshtein with leading equality.
self.assertEquals(4, self.dmp.diff_levenshtein([(self.dmp.DIFF_EQUAL, "xyz"), (self.dmp.DIFF_DELETE, "abc"), (self.dmp.DIFF_INSERT, "1234")]))
# Levenshtein with middle equality.
self.assertEquals(7, self.dmp.diff_levenshtein([(self.dmp.DIFF_DELETE, "abc"), (self.dmp.DIFF_EQUAL, "xyz"), (self.dmp.DIFF_INSERT, "1234")]))
def testDiffBisect(self):
# Normal.
a = "cat"
b = "map"
# Since the resulting diff hasn't been normalized, it would be ok if
# the insertion and deletion pairs are swapped.
# If the order changes, tweak this test as required.
self.assertEquals([(self.dmp.DIFF_DELETE, "c"), (self.dmp.DIFF_INSERT, "m"), (self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_DELETE, "t"), (self.dmp.DIFF_INSERT, "p")], self.dmp.diff_bisect(a, b, sys.maxint))
# Timeout.
self.assertEquals([(self.dmp.DIFF_DELETE, "cat"), (self.dmp.DIFF_INSERT, "map")], self.dmp.diff_bisect(a, b, 0))
def testDiffMain(self):
# Perform a trivial diff.
# Null case.
self.assertEquals([], self.dmp.diff_main("", "", False))
# Equality.
self.assertEquals([(self.dmp.DIFF_EQUAL, "abc")], self.dmp.diff_main("abc", "abc", False))
# Simple insertion.
self.assertEquals([(self.dmp.DIFF_EQUAL, "ab"), (self.dmp.DIFF_INSERT, "123"), (self.dmp.DIFF_EQUAL, "c")], self.dmp.diff_main("abc", "ab123c", False))
# Simple deletion.
self.assertEquals([(self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_DELETE, "123"), (self.dmp.DIFF_EQUAL, "bc")], self.dmp.diff_main("a123bc", "abc", False))
# Two insertions.
self.assertEquals([(self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_INSERT, "123"), (self.dmp.DIFF_EQUAL, "b"), (self.dmp.DIFF_INSERT, "456"), (self.dmp.DIFF_EQUAL, "c")], self.dmp.diff_main("abc", "a123b456c", False))
# Two deletions.
self.assertEquals([(self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_DELETE, "123"), (self.dmp.DIFF_EQUAL, "b"), (self.dmp.DIFF_DELETE, "456"), (self.dmp.DIFF_EQUAL, "c")], self.dmp.diff_main("a123b456c", "abc", False))
# Perform a real diff.
# Switch off the timeout.
self.dmp.Diff_Timeout = 0
# Simple cases.
self.assertEquals([(self.dmp.DIFF_DELETE, "a"), (self.dmp.DIFF_INSERT, "b")], self.dmp.diff_main("a", "b", False))
self.assertEquals([(self.dmp.DIFF_DELETE, "Apple"), (self.dmp.DIFF_INSERT, "Banana"), (self.dmp.DIFF_EQUAL, "s are a"), (self.dmp.DIFF_INSERT, "lso"), (self.dmp.DIFF_EQUAL, " fruit.")], self.dmp.diff_main("Apples are a fruit.", "Bananas are also fruit.", False))
self.assertEquals([(self.dmp.DIFF_DELETE, "a"), (self.dmp.DIFF_INSERT, u"\u0680"), (self.dmp.DIFF_EQUAL, "x"), (self.dmp.DIFF_DELETE, "\t"), (self.dmp.DIFF_INSERT, "\x00")], self.dmp.diff_main("ax\t", u"\u0680x\x00", False))
# Overlaps.
self.assertEquals([(self.dmp.DIFF_DELETE, "1"), (self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_DELETE, "y"), (self.dmp.DIFF_EQUAL, "b"), (self.dmp.DIFF_DELETE, "2"), (self.dmp.DIFF_INSERT, "xab")], self.dmp.diff_main("1ayb2", "abxab", False))
self.assertEquals([(self.dmp.DIFF_INSERT, "xaxcx"), (self.dmp.DIFF_EQUAL, "abc"), (self.dmp.DIFF_DELETE, "y")], self.dmp.diff_main("abcy", "xaxcxabc", False))
self.assertEquals([(self.dmp.DIFF_DELETE, "ABCD"), (self.dmp.DIFF_EQUAL, "a"), (self.dmp.DIFF_DELETE, "="), (self.dmp.DIFF_INSERT, "-"), (self.dmp.DIFF_EQUAL, "bcd"), (self.dmp.DIFF_DELETE, "="), (self.dmp.DIFF_INSERT, "-"), (self.dmp.DIFF_EQUAL, "efghijklmnopqrs"), (self.dmp.DIFF_DELETE, "EFGHIJKLMNOefg")], self.dmp.diff_main("ABCDa=bcd=efghijklmnopqrsEFGHIJKLMNOefg", "a-bcd-efghijklmnopqrs", False))
# Large equality.
self.assertEquals([(self.dmp.DIFF_INSERT, " "), (self.dmp.DIFF_EQUAL,"a"), (self.dmp.DIFF_INSERT,"nd"), (self.dmp.DIFF_EQUAL," [[Pennsylvania]]"), (self.dmp.DIFF_DELETE," and [[New")], self.dmp.diff_main("a [[Pennsylvania]] and [[New", " and [[Pennsylvania]]", False))
# Timeout.
self.dmp.Diff_Timeout = 0.1 # 100ms
a = "`Twas brillig, and the slithy toves\nDid gyre and gimble in the wabe:\nAll mimsy were the borogoves,\nAnd the mome raths outgrabe.\n"
b = "I am the very model of a modern major general,\nI've information vegetable, animal, and mineral,\nI know the kings of England, and I quote the fights historical,\nFrom Marathon to Waterloo, in order categorical.\n"
# Increase the text lengths by 1024 times to ensure a timeout.
for x in range(10):
a = a + a
b = b + b
startTime = time.time()
self.dmp.diff_main(a, b)
endTime = time.time()
# Test that we took at least the timeout period.
self.assertTrue(self.dmp.Diff_Timeout <= endTime - startTime)
# Test that we didn't take forever (be forgiving).
# Theoretically this test could fail very occasionally if the
# OS task swaps or locks up for a second at the wrong moment.
self.assertTrue(self.dmp.Diff_Timeout * 2 > endTime - startTime)
self.dmp.Diff_Timeout = 0
# Test the linemode speedup.
# Must be long to pass the 100 char cutoff.
# Simple line-mode.
a = "1234567890\n" * 13
b = "abcdefghij\n" * 13
self.assertEquals(self.dmp.diff_main(a, b, False), self.dmp.diff_main(a, b, True))
# Single line-mode.
a = "1234567890" * 13
b = "abcdefghij" * 13
self.assertEquals(self.dmp.diff_main(a, b, False), self.dmp.diff_main(a, b, True))
# Overlap line-mode.
a = "1234567890\n" * 13
b = "abcdefghij\n1234567890\n1234567890\n1234567890\nabcdefghij\n1234567890\n1234567890\n1234567890\nabcdefghij\n1234567890\n1234567890\n1234567890\nabcdefghij\n"
texts_linemode = self.diff_rebuildtexts(self.dmp.diff_main(a, b, True))
texts_textmode = self.diff_rebuildtexts(self.dmp.diff_main(a, b, False))
self.assertEquals(texts_textmode, texts_linemode)
# Test null inputs.
try:
self.dmp.diff_main(None, None)
self.assertFalse(True)
except ValueError:
# Exception expected.
pass
class MatchTest(DiffMatchPatchTest):
"""MATCH TEST FUNCTIONS"""
def testMatchAlphabet(self):
# Initialise the bitmasks for Bitap.
self.assertEquals({"a":4, "b":2, "c":1}, self.dmp.match_alphabet("abc"))
self.assertEquals({"a":37, "b":18, "c":8}, self.dmp.match_alphabet("abcaba"))
def testMatchBitap(self):
self.dmp.Match_Distance = 100
self.dmp.Match_Threshold = 0.5
# Exact matches.
self.assertEquals(5, self.dmp.match_bitap("abcdefghijk", "fgh", 5))
self.assertEquals(5, self.dmp.match_bitap("abcdefghijk", "fgh", 0))
# Fuzzy matches.
self.assertEquals(4, self.dmp.match_bitap("abcdefghijk", "efxhi", 0))
self.assertEquals(2, self.dmp.match_bitap("abcdefghijk", "cdefxyhijk", 5))
self.assertEquals(-1, self.dmp.match_bitap("abcdefghijk", "bxy", 1))
# Overflow.
self.assertEquals(2, self.dmp.match_bitap("123456789xx0", "3456789x0", 2))
self.assertEquals(0, self.dmp.match_bitap("abcdef", "xxabc", 4))
self.assertEquals(3, self.dmp.match_bitap("abcdef", "defyy", 4))
self.assertEquals(0, self.dmp.match_bitap("abcdef", "xabcdefy", 0))
# Threshold test.
self.dmp.Match_Threshold = 0.4
self.assertEquals(4, self.dmp.match_bitap("abcdefghijk", "efxyhi", 1))
self.dmp.Match_Threshold = 0.3
self.assertEquals(-1, self.dmp.match_bitap("abcdefghijk", "efxyhi", 1))
self.dmp.Match_Threshold = 0.0
self.assertEquals(1, self.dmp.match_bitap("abcdefghijk", "bcdef", 1))
self.dmp.Match_Threshold = 0.5
# Multiple select.
self.assertEquals(0, self.dmp.match_bitap("abcdexyzabcde", "abccde", 3))
self.assertEquals(8, self.dmp.match_bitap("abcdexyzabcde", "abccde", 5))
# Distance test.
self.dmp.Match_Distance = 10 # Strict location.
self.assertEquals(-1, self.dmp.match_bitap("abcdefghijklmnopqrstuvwxyz", "abcdefg", 24))
self.assertEquals(0, self.dmp.match_bitap("abcdefghijklmnopqrstuvwxyz", "abcdxxefg", 1))
self.dmp.Match_Distance = 1000 # Loose location.
self.assertEquals(0, self.dmp.match_bitap("abcdefghijklmnopqrstuvwxyz", "abcdefg", 24))
def testMatchMain(self):
# Full match.
# Shortcut matches.
self.assertEquals(0, self.dmp.match_main("abcdef", "abcdef", 1000))
self.assertEquals(-1, self.dmp.match_main("", "abcdef", 1))
self.assertEquals(3, self.dmp.match_main("abcdef", "", 3))
self.assertEquals(3, self.dmp.match_main("abcdef", "de", 3))
self.assertEquals(3, self.dmp.match_main("abcdef", "defy", 4))
self.assertEquals(0, self.dmp.match_main("abcdef", "abcdefy", 0))
# Complex match.
self.dmp.Match_Threshold = 0.7
self.assertEquals(4, self.dmp.match_main("I am the very model of a modern major general.", " that berry ", 5))
self.dmp.Match_Threshold = 0.5
# Test null inputs.
try:
self.dmp.match_main(None, None, 0)
self.assertFalse(True)
except ValueError:
# Exception expected.
pass
class PatchTest(DiffMatchPatchTest):
"""PATCH TEST FUNCTIONS"""
def testPatchObj(self):
# Patch Object.
p = dmp_module.patch_obj()
p.start1 = 20
p.start2 = 21
p.length1 = 18
p.length2 = 17
p.diffs = [(self.dmp.DIFF_EQUAL, "jump"), (self.dmp.DIFF_DELETE, "s"), (self.dmp.DIFF_INSERT, "ed"), (self.dmp.DIFF_EQUAL, " over "), (self.dmp.DIFF_DELETE, "the"), (self.dmp.DIFF_INSERT, "a"), (self.dmp.DIFF_EQUAL, "\nlaz")]
strp = str(p)
self.assertEquals("@@ -21,18 +22,17 @@\n jump\n-s\n+ed\n over \n-the\n+a\n %0Alaz\n", strp)
def testPatchFromText(self):
self.assertEquals([], self.dmp.patch_fromText(""))
strp = "@@ -21,18 +22,17 @@\n jump\n-s\n+ed\n over \n-the\n+a\n %0Alaz\n"
self.assertEquals(strp, str(self.dmp.patch_fromText(strp)[0]))
self.assertEquals("@@ -1 +1 @@\n-a\n+b\n", str(self.dmp.patch_fromText("@@ -1 +1 @@\n-a\n+b\n")[0]))
self.assertEquals("@@ -1,3 +0,0 @@\n-abc\n", str(self.dmp.patch_fromText("@@ -1,3 +0,0 @@\n-abc\n")[0]))
self.assertEquals("@@ -0,0 +1,3 @@\n+abc\n", str(self.dmp.patch_fromText("@@ -0,0 +1,3 @@\n+abc\n")[0]))
# Generates error.
try:
self.dmp.patch_fromText("Bad\nPatch\n")
self.assertFalse(True)
except ValueError:
# Exception expected.
pass
def testPatchToText(self):
strp = "@@ -21,18 +22,17 @@\n jump\n-s\n+ed\n over \n-the\n+a\n laz\n"
p = self.dmp.patch_fromText(strp)
self.assertEquals(strp, self.dmp.patch_toText(p))
strp = "@@ -1,9 +1,9 @@\n-f\n+F\n oo+fooba\n@@ -7,9 +7,9 @@\n obar\n-,\n+.\n tes\n"
p = self.dmp.patch_fromText(strp)
self.assertEquals(strp, self.dmp.patch_toText(p))
def testPatchAddContext(self):
self.dmp.Patch_Margin = 4
p = self.dmp.patch_fromText("@@ -21,4 +21,10 @@\n-jump\n+somersault\n")[0]
self.dmp.patch_addContext(p, "The quick brown fox jumps over the lazy dog.")
self.assertEquals("@@ -17,12 +17,18 @@\n fox \n-jump\n+somersault\n s ov\n", str(p))
# Same, but not enough trailing context.
p = self.dmp.patch_fromText("@@ -21,4 +21,10 @@\n-jump\n+somersault\n")[0]
self.dmp.patch_addContext(p, "The quick brown fox jumps.")
self.assertEquals("@@ -17,10 +17,16 @@\n fox \n-jump\n+somersault\n s.\n", str(p))
# Same, but not enough leading context.
p = self.dmp.patch_fromText("@@ -3 +3,2 @@\n-e\n+at\n")[0]
self.dmp.patch_addContext(p, "The quick brown fox jumps.")
self.assertEquals("@@ -1,7 +1,8 @@\n Th\n-e\n+at\n qui\n", str(p))
# Same, but with ambiguity.
p = self.dmp.patch_fromText("@@ -3 +3,2 @@\n-e\n+at\n")[0]
self.dmp.patch_addContext(p, "The quick brown fox jumps. The quick brown fox crashes.")
self.assertEquals("@@ -1,27 +1,28 @@\n Th\n-e\n+at\n quick brown fox jumps. \n", str(p))
def testPatchMake(self):
# Null case.
patches = self.dmp.patch_make("", "")
self.assertEquals("", self.dmp.patch_toText(patches))
text1 = "The quick brown fox jumps over the lazy dog."
text2 = "That quick brown fox jumped over a lazy dog."
# Text2+Text1 inputs.
expectedPatch = "@@ -1,8 +1,7 @@\n Th\n-at\n+e\n qui\n@@ -21,17 +21,18 @@\n jump\n-ed\n+s\n over \n-a\n+the\n laz\n"
# The second patch must be "-21,17 +21,18", not "-22,17 +21,18" due to rolling context.
patches = self.dmp.patch_make(text2, text1)
self.assertEquals(expectedPatch, self.dmp.patch_toText(patches))
# Text1+Text2 inputs.
expectedPatch = "@@ -1,11 +1,12 @@\n Th\n-e\n+at\n quick b\n@@ -22,18 +22,17 @@\n jump\n-s\n+ed\n over \n-the\n+a\n laz\n"
patches = self.dmp.patch_make(text1, text2)
self.assertEquals(expectedPatch, self.dmp.patch_toText(patches))
# Diff input.
diffs = self.dmp.diff_main(text1, text2, False)
patches = self.dmp.patch_make(diffs)
self.assertEquals(expectedPatch, self.dmp.patch_toText(patches))
# Text1+Diff inputs.
patches = self.dmp.patch_make(text1, diffs)
self.assertEquals(expectedPatch, self.dmp.patch_toText(patches))
# Text1+Text2+Diff inputs (deprecated).
patches = self.dmp.patch_make(text1, text2, diffs)
self.assertEquals(expectedPatch, self.dmp.patch_toText(patches))
# Character encoding.
patches = self.dmp.patch_make("`1234567890-=[]\\;',./", "~!@#$%^&*()_+{}|:\"<>?")
self.assertEquals("@@ -1,21 +1,21 @@\n-%601234567890-=%5B%5D%5C;',./\n+~!@#$%25%5E&*()_+%7B%7D%7C:%22%3C%3E?\n", self.dmp.patch_toText(patches))
# Character decoding.
diffs = [(self.dmp.DIFF_DELETE, "`1234567890-=[]\\;',./"), (self.dmp.DIFF_INSERT, "~!@#$%^&*()_+{}|:\"<>?")]
self.assertEquals(diffs, self.dmp.patch_fromText("@@ -1,21 +1,21 @@\n-%601234567890-=%5B%5D%5C;',./\n+~!@#$%25%5E&*()_+%7B%7D%7C:%22%3C%3E?\n")[0].diffs)
# Long string with repeats.
text1 = ""
for x in range(100):
text1 += "abcdef"
text2 = text1 + "123"
expectedPatch = "@@ -573,28 +573,31 @@\n cdefabcdefabcdefabcdefabcdef\n+123\n"
patches = self.dmp.patch_make(text1, text2)
self.assertEquals(expectedPatch, self.dmp.patch_toText(patches))
# Test null inputs.
try:
self.dmp.patch_make(None, None)
self.assertFalse(True)
except ValueError:
# Exception expected.
pass
def testPatchSplitMax(self):
# Assumes that Match_MaxBits is 32.
patches = self.dmp.patch_make("abcdefghijklmnopqrstuvwxyz01234567890", "XabXcdXefXghXijXklXmnXopXqrXstXuvXwxXyzX01X23X45X67X89X0")
self.dmp.patch_splitMax(patches)
self.assertEquals("@@ -1,32 +1,46 @@\n+X\n ab\n+X\n cd\n+X\n ef\n+X\n gh\n+X\n ij\n+X\n kl\n+X\n mn\n+X\n op\n+X\n qr\n+X\n st\n+X\n uv\n+X\n wx\n+X\n yz\n+X\n 012345\n@@ -25,13 +39,18 @@\n zX01\n+X\n 23\n+X\n 45\n+X\n 67\n+X\n 89\n+X\n 0\n", self.dmp.patch_toText(patches))
patches = self.dmp.patch_make("abcdef1234567890123456789012345678901234567890123456789012345678901234567890uvwxyz", "abcdefuvwxyz")
oldToText = self.dmp.patch_toText(patches)
self.dmp.patch_splitMax(patches)
self.assertEquals(oldToText, self.dmp.patch_toText(patches))
patches = self.dmp.patch_make("1234567890123456789012345678901234567890123456789012345678901234567890", "abc")
self.dmp.patch_splitMax(patches)
self.assertEquals("@@ -1,32 +1,4 @@\n-1234567890123456789012345678\n 9012\n@@ -29,32 +1,4 @@\n-9012345678901234567890123456\n 7890\n@@ -57,14 +1,3 @@\n-78901234567890\n+abc\n", self.dmp.patch_toText(patches))
patches = self.dmp.patch_make("abcdefghij , h : 0 , t : 1 abcdefghij , h : 0 , t : 1 abcdefghij , h : 0 , t : 1", "abcdefghij , h : 1 , t : 1 abcdefghij , h : 1 , t : 1 abcdefghij , h : 0 , t : 1")
self.dmp.patch_splitMax(patches)
self.assertEquals("@@ -2,32 +2,32 @@\n bcdefghij , h : \n-0\n+1\n , t : 1 abcdef\n@@ -29,32 +29,32 @@\n bcdefghij , h : \n-0\n+1\n , t : 1 abcdef\n", self.dmp.patch_toText(patches))
def testPatchAddPadding(self):
# Both edges full.
patches = self.dmp.patch_make("", "test")
self.assertEquals("@@ -0,0 +1,4 @@\n+test\n", self.dmp.patch_toText(patches))
self.dmp.patch_addPadding(patches)
self.assertEquals("@@ -1,8 +1,12 @@\n %01%02%03%04\n+test\n %01%02%03%04\n", self.dmp.patch_toText(patches))
# Both edges partial.
patches = self.dmp.patch_make("XY", "XtestY")
self.assertEquals("@@ -1,2 +1,6 @@\n X\n+test\n Y\n", self.dmp.patch_toText(patches))
self.dmp.patch_addPadding(patches)
self.assertEquals("@@ -2,8 +2,12 @@\n %02%03%04X\n+test\n Y%01%02%03\n", self.dmp.patch_toText(patches))
# Both edges none.
patches = self.dmp.patch_make("XXXXYYYY", "XXXXtestYYYY")
self.assertEquals("@@ -1,8 +1,12 @@\n XXXX\n+test\n YYYY\n", self.dmp.patch_toText(patches))
self.dmp.patch_addPadding(patches)
self.assertEquals("@@ -5,8 +5,12 @@\n XXXX\n+test\n YYYY\n", self.dmp.patch_toText(patches))
def testPatchApply(self):
self.dmp.Match_Distance = 1000
self.dmp.Match_Threshold = 0.5
self.dmp.Patch_DeleteThreshold = 0.5
# Null case.
patches = self.dmp.patch_make("", "")
results = self.dmp.patch_apply(patches, "Hello world.")
self.assertEquals(("Hello world.", []), results)
# Exact match.
patches = self.dmp.patch_make("The quick brown fox jumps over the lazy dog.", "That quick brown fox jumped over a lazy dog.")
results = self.dmp.patch_apply(patches, "The quick brown fox jumps over the lazy dog.")
self.assertEquals(("That quick brown fox jumped over a lazy dog.", [True, True]), results)
# Partial match.
results = self.dmp.patch_apply(patches, "The quick red rabbit jumps over the tired tiger.")
self.assertEquals(("That quick red rabbit jumped over a tired tiger.", [True, True]), results)
# Failed match.
results = self.dmp.patch_apply(patches, "I am the very model of a modern major general.")
self.assertEquals(("I am the very model of a modern major general.", [False, False]), results)
# Big delete, small change.
patches = self.dmp.patch_make("x1234567890123456789012345678901234567890123456789012345678901234567890y", "xabcy")
results = self.dmp.patch_apply(patches, "x123456789012345678901234567890-----++++++++++-----123456789012345678901234567890y")
self.assertEquals(("xabcy", [True, True]), results)
# Big delete, big change 1.
patches = self.dmp.patch_make("x1234567890123456789012345678901234567890123456789012345678901234567890y", "xabcy")
results = self.dmp.patch_apply(patches, "x12345678901234567890---------------++++++++++---------------12345678901234567890y")
self.assertEquals(("xabc12345678901234567890---------------++++++++++---------------12345678901234567890y", [False, True]), results)
# Big delete, big change 2.
self.dmp.Patch_DeleteThreshold = 0.6
patches = self.dmp.patch_make("x1234567890123456789012345678901234567890123456789012345678901234567890y", "xabcy")
results = self.dmp.patch_apply(patches, "x12345678901234567890---------------++++++++++---------------12345678901234567890y")
self.assertEquals(("xabcy", [True, True]), results)
self.dmp.Patch_DeleteThreshold = 0.5
# Compensate for failed patch.
self.dmp.Match_Threshold = 0.0
self.dmp.Match_Distance = 0
patches = self.dmp.patch_make("abcdefghijklmnopqrstuvwxyz--------------------1234567890", "abcXXXXXXXXXXdefghijklmnopqrstuvwxyz--------------------1234567YYYYYYYYYY890")
results = self.dmp.patch_apply(patches, "ABCDEFGHIJKLMNOPQRSTUVWXYZ--------------------1234567890")
self.assertEquals(("ABCDEFGHIJKLMNOPQRSTUVWXYZ--------------------1234567YYYYYYYYYY890", [False, True]), results)
self.dmp.Match_Threshold = 0.5
self.dmp.Match_Distance = 1000
# No side effects.
patches = self.dmp.patch_make("", "test")
patchstr = self.dmp.patch_toText(patches)
results = self.dmp.patch_apply(patches, "")
self.assertEquals(patchstr, self.dmp.patch_toText(patches))
# No side effects with major delete.
patches = self.dmp.patch_make("The quick brown fox jumps over the lazy dog.", "Woof")
patchstr = self.dmp.patch_toText(patches)
self.dmp.patch_apply(patches, "The quick brown fox jumps over the lazy dog.")
self.assertEquals(patchstr, self.dmp.patch_toText(patches))
# Edge exact match.
patches = self.dmp.patch_make("", "test")
self.dmp.patch_apply(patches, "")
self.assertEquals(("test", [True]), results)
# Near edge exact match.
patches = self.dmp.patch_make("XY", "XtestY")
results = self.dmp.patch_apply(patches, "XY")
self.assertEquals(("XtestY", [True]), results)
# Edge partial match.
patches = self.dmp.patch_make("y", "y123")
results = self.dmp.patch_apply(patches, "x")
self.assertEquals(("x123", [True]), results)
if __name__ == "__main__":
unittest.main()
| mpl-2.0 |
krafczyk/spack | var/spack/repos/builtin/packages/vardictjava/package.py | 5 | 1996 | ##############################################################################
# Copyright (c) 2013-2018, Lawrence Livermore National Security, LLC.
# Produced at the Lawrence Livermore National Laboratory.
#
# This file is part of Spack.
# Created by Todd Gamblin, tgamblin@llnl.gov, All rights reserved.
# LLNL-CODE-647188
#
# For details, see https://github.com/spack/spack
# Please also see the NOTICE and LICENSE files for our notice and the LGPL.
#
# This program is free software; you can redistribute it and/or modify
# it under the terms of the GNU Lesser General Public License (as
# published by the Free Software Foundation) version 2.1, February 1999.
#
# This program is distributed in the hope that it will be useful, but
# WITHOUT ANY WARRANTY; without even the IMPLIED WARRANTY OF
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the terms and
# conditions of the GNU Lesser General Public License for more details.
#
# You should have received a copy of the GNU Lesser General Public
# License along with this program; if not, write to the Free Software
# Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
##############################################################################
from spack import *
import glob
class Vardictjava(Package):
"""VarDictJava is a variant discovery program written in Java.
It is a partial Java port of VarDict variant caller."""
homepage = "https://github.com/AstraZeneca-NGS/VarDictJava"
url = "https://github.com/AstraZeneca-NGS/VarDictJava/releases/download/v1.5.1/VarDict-1.5.1.tar"
version('1.5.1', '8c0387bcc1f7dc696b04e926c48b27e6')
version('1.4.4', '6b2d7e1e5502b875760fc9938a0fe5e0')
depends_on('java@8:', type='run')
def install(self, spec, prefix):
mkdirp(prefix.bin)
install('bin/VarDict', prefix.bin)
mkdirp(prefix.lib)
files = [x for x in glob.glob("lib/*jar")]
for f in files:
install(f, prefix.lib)
| lgpl-2.1 |
strogo/djpcms | djpcms/contrib/flowrepo/cms/plugins.py | 1 | 7819 | from django.contrib.auth.models import User
from django.contrib.contenttypes.models import ContentType
from djpcms import get_site
from djpcms.template import loader
from djpcms.plugins import DJPplugin
from djpcms import forms
from djpcms.utils.html import htmlwrap, Paginator
from djpcms.views import appsite
from djpcms.contrib.flowrepo.models import FlowItem
from djpcms.contrib.flowrepo.forms import FlowItemSelector, ChangeImage, ChangeCategory
class LinkedItemForm(forms.Form):
content_types = forms.ModelMultipleChoiceField(ContentType.objects.all(),
required = False,
label = 'model')
display = forms.ChoiceField(choices = (('list','list'),
('detail','detail')), initial = 'list')
inverse = forms.BooleanField(initial = False, required = False)
class FlowItemSelection(DJPplugin):
name = 'flowitem-selection'
description = 'Items Selection'
form = FlowItemSelector
def render(self, djp, wrapper, prefix,
visibility = None, item_per_page = 10,
content_type = None, tags = '',
**kwargs):
request = djp.request
qs = FlowItem.objects.selection(request.user,
types = content_type,
visibility = visibility,
tags = tags)
if not qs:
return None
pa = Paginator(djp.request, qs, item_per_page)
return loader.render_to_string(['flowitem_list.html',
'djpcms/components/object_list.html'],
{'items': self.paginator(djp,pa)})
def paginator(self, djp, pa):
site = get_site()
appmodel = site.for_model(FlowItem)
qs = pa.qs
for obj in qs:
object = obj.object
model = object.__class__
objmodel = site.for_model(model) or appmodel
if objmodel:
content = objmodel.object_content(djp, obj)
tname = '%s_list_item.html' % model.__name__.lower()
yield loader.render_to_string(['components/%s' % tname,
'flowrepo/%s' % tname,
'flowrepo/flowitem_list_item.html'],
content)
class ImagePlugin(DJPplugin):
'''
Plugin for displaying a single image on a content block
'''
name = 'image'
description = 'Display Image'
form = ChangeImage
def render(self, djp, wrapper, prefix, image = None, class_name = None, **kwargs):
try:
img = Image.objects.get(id = int(image))
if class_name:
class_name = '%s image-plugin'
else:
class_name = 'image-plugin'
cn = ' class="%s"' % class_name
return '<img src="%s" title="%s" alt="%s"%s/>' % (img.url,img.name,img.name,cn)
except:
return u''
class CategoryLinks(DJPplugin):
name = 'category-links'
description = "Display links for a category"
form = ChangeCategory
def render(self, djp, wrapper, prefix, category_name = None, **kwargs):
if category_name:
qs = Category.objects.filter(type__id = int(category_name))
else:
return u''
if not qs:
return u''
return loader.render_to_string(['category_list.html',
'components/category_list.html',
'flowrepo/category_list.html'],
{'items': self.paginator(djp,qs)})
def paginator(self, djp, qs):
from djpcms.views import appsite
appmodel = appsite.site.for_model(Category)
for obj in qs:
if appmodel:
content = appmodel.object_content(djp, obj)
yield loader.render_to_string(['category_list_item.html',
'components/category_list_item.html',
'flowrepo/category_list_item.html'],
content)
def edit_form(self, djp, category_name = None, **kwargs):
if category_name:
return EditContentForm(**form_kwargs(request = djp.request,
initial = {'category_name': category_name},
withrequest = True))
class LinkedItems(DJPplugin):
'''
Very very useful plugin for displaying a list of linked item
to the flowitem object
'''
name = 'flowrepo-linked'
description = "Related Items"
form = LinkedItemForm
def render(self, djp, wrapper, prefix, content_types = None, display = 'list', inverse = False, **kwargs):
from djpcms.views import appsite
instance = djp.instance
if not isinstance(instance,FlowItem):
return
qs = instance.items.all()
lqs = list(qs)
if content_types:
qs = qs.filter(content_type__id__in = content_types)
if not qs:
return ''
request = djp.request
return loader.render_to_string(['report_draft_list.html',
'flowrepo/related_list.html'],
{'items': self.paginator(djp,wrapper,qs,display)})
def paginator(self, djp, wrapper, qs, display):
for obj in qs:
object = obj.object
opts = object._meta
appmodel = appsite.site.for_model(object.__class__)
if display == 'detail':
if appmodel:
yield appmodel.render_object(djp, wrapper)
else:
yield loader.render_to_string(['%s/%s.html' % (opts.app_label,opts.module_name),
'djpcms/components/object.html'],
{'item':object})
url = None
r = ''
try:
url = object.url
except:
appmodel = appsite.site.for_model(object.__class__)
if appmodel:
url = appmodel.viewurl(djp.request,object)
if url:
try:
name = object.name
if not name:
name = str(object)
except:
name = str(object)
link = htmlwrap('a', name)
link._attrs['title'] = name
link._attrs['href'] = url
if not url.startswith('/'):
link._attrs['target'] = "_blank"
r = link.render()
yield r
class AddLinkedItems(DJPplugin):
name = 'flowrepo-add-linked'
description = "Add Linked item urls"
def render(self, djp, wrapper, prefix, **kwargs):
from djpcms.views import appsite
instance = djp.instance
if not isinstance(instance,FlowItem):
return
qs = FlowRelated.objects.filter(item = instance)
if not qs:
return
request = djp.request
return loader.render_to_string(['report_draft_list.html',
'flowrepo/report_draft_list.html'],
{'items': self.paginator(djp,qs)})
| bsd-3-clause |
xyzz/vcmi-build | project/jni/python/src/Lib/test/test_StringIO.py | 54 | 4284 | # Tests StringIO and cStringIO
import unittest
import StringIO
import cStringIO
import types
from test import test_support
class TestGenericStringIO(unittest.TestCase):
# use a class variable MODULE to define which module is being tested
# Line of data to test as string
_line = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ!'
# Constructor to use for the test data (._line is passed to this
# constructor)
constructor = str
def setUp(self):
self._line = self.constructor(self._line)
self._lines = self.constructor((self._line + '\n') * 5)
self._fp = self.MODULE.StringIO(self._lines)
def test_reads(self):
eq = self.assertEqual
self.assertRaises(TypeError, self._fp.seek)
eq(self._fp.read(10), self._line[:10])
eq(self._fp.readline(), self._line[10:] + '\n')
eq(len(self._fp.readlines(60)), 2)
def test_writes(self):
f = self.MODULE.StringIO()
self.assertRaises(TypeError, f.seek)
f.write(self._line[:6])
f.seek(3)
f.write(self._line[20:26])
f.write(self._line[52])
self.assertEqual(f.getvalue(), 'abcuvwxyz!')
def test_writelines(self):
f = self.MODULE.StringIO()
f.writelines([self._line[0], self._line[1], self._line[2]])
f.seek(0)
self.assertEqual(f.getvalue(), 'abc')
def test_writelines_error(self):
def errorGen():
yield 'a'
raise KeyboardInterrupt()
f = self.MODULE.StringIO()
self.assertRaises(KeyboardInterrupt, f.writelines, errorGen())
def test_truncate(self):
eq = self.assertEqual
f = self.MODULE.StringIO()
f.write(self._lines)
f.seek(10)
f.truncate()
eq(f.getvalue(), 'abcdefghij')
f.truncate(5)
eq(f.getvalue(), 'abcde')
f.write('xyz')
eq(f.getvalue(), 'abcdexyz')
self.assertRaises(IOError, f.truncate, -1)
f.close()
self.assertRaises(ValueError, f.write, 'frobnitz')
def test_closed_flag(self):
f = self.MODULE.StringIO()
self.assertEqual(f.closed, False)
f.close()
self.assertEqual(f.closed, True)
f = self.MODULE.StringIO("abc")
self.assertEqual(f.closed, False)
f.close()
self.assertEqual(f.closed, True)
def test_isatty(self):
f = self.MODULE.StringIO()
self.assertRaises(TypeError, f.isatty, None)
self.assertEqual(f.isatty(), False)
f.close()
self.assertRaises(ValueError, f.isatty)
def test_iterator(self):
eq = self.assertEqual
unless = self.failUnless
eq(iter(self._fp), self._fp)
# Does this object support the iteration protocol?
unless(hasattr(self._fp, '__iter__'))
unless(hasattr(self._fp, 'next'))
i = 0
for line in self._fp:
eq(line, self._line + '\n')
i += 1
eq(i, 5)
self._fp.close()
self.assertRaises(ValueError, self._fp.next)
class TestStringIO(TestGenericStringIO):
MODULE = StringIO
def test_unicode(self):
if not test_support.have_unicode: return
# The StringIO module also supports concatenating Unicode
# snippets to larger Unicode strings. This is tested by this
# method. Note that cStringIO does not support this extension.
f = self.MODULE.StringIO()
f.write(self._line[:6])
f.seek(3)
f.write(unicode(self._line[20:26]))
f.write(unicode(self._line[52]))
s = f.getvalue()
self.assertEqual(s, unicode('abcuvwxyz!'))
self.assertEqual(type(s), types.UnicodeType)
class TestcStringIO(TestGenericStringIO):
MODULE = cStringIO
import sys
if sys.platform.startswith('java'):
# Jython doesn't have a buffer object, so we just do a useless
# fake of the buffer tests.
buffer = str
class TestBufferStringIO(TestStringIO):
constructor = buffer
class TestBuffercStringIO(TestcStringIO):
constructor = buffer
def test_main():
test_support.run_unittest(
TestStringIO,
TestcStringIO,
TestBufferStringIO,
TestBuffercStringIO
)
if __name__ == '__main__':
test_main()
| lgpl-2.1 |
gvb/odoo | addons/hw_posbox_homepage/__openerp__.py | 307 | 1691 | # -*- coding: utf-8 -*-
##############################################################################
#
# OpenERP, Open Source Management Solution
# Copyright (C) 2004-2010 Tiny SPRL (<http://tiny.be>).
#
# This program is free software: you can redistribute it and/or modify
# it under the terms of the GNU Affero General Public License as
# published by the Free Software Foundation, either version 3 of the
# License, or (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU Affero General Public License for more details.
#
# You should have received a copy of the GNU Affero General Public License
# along with this program. If not, see <http://www.gnu.org/licenses/>.
#
##############################################################################
{
'name': 'PosBox Homepage',
'version': '1.0',
'category': 'Hardware Drivers',
'sequence': 6,
'website': 'https://www.odoo.com/page/point-of-sale',
'summary': 'A homepage for the PosBox',
'description': """
PosBox Homepage
===============
This module overrides openerp web interface to display a simple
Homepage that explains what's the posbox and show the status,
and where to find documentation.
If you activate this module, you won't be able to access the
regular openerp interface anymore.
""",
'author': 'OpenERP SA',
'depends': ['hw_proxy'],
'installable': False,
'auto_install': False,
}
# vim:expandtab:smartindent:tabstop=4:softtabstop=4:shiftwidth=4:
| agpl-3.0 |
kitanata/resume | env/lib/python2.7/site-packages/PyPDF2/filters.py | 3 | 11911 | # vim: sw=4:expandtab:foldmethod=marker
#
# Copyright (c) 2006, Mathieu Fenniak
# All rights reserved.
#
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions are
# met:
#
# * Redistributions of source code must retain the above copyright notice,
# this list of conditions and the following disclaimer.
# * Redistributions in binary form must reproduce the above copyright notice,
# this list of conditions and the following disclaimer in the documentation
# and/or other materials provided with the distribution.
# * The name of the author may not be used to endorse or promote products
# derived from this software without specific prior written permission.
#
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
# AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
# IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
# ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
# LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
# CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
# SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
# INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
# CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
# ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
# POSSIBILITY OF SUCH DAMAGE.
"""
Implementation of stream filters for PDF.
"""
__author__ = "Mathieu Fenniak"
__author_email__ = "biziqe@mathieu.fenniak.net"
from .utils import PdfReadError, ord_, chr_
from sys import version_info
if version_info < ( 3, 0 ):
from cStringIO import StringIO
else:
from io import StringIO
try:
import zlib
def decompress(data):
return zlib.decompress(data)
def compress(data):
return zlib.compress(data)
except ImportError:
# Unable to import zlib. Attempt to use the System.IO.Compression
# library from the .NET framework. (IronPython only)
import System
from System import IO, Collections, Array
def _string_to_bytearr(buf):
retval = Array.CreateInstance(System.Byte, len(buf))
for i in range(len(buf)):
retval[i] = ord(buf[i])
return retval
def _bytearr_to_string(bytes):
retval = ""
for i in range(bytes.Length):
retval += chr(bytes[i])
return retval
def _read_bytes(stream):
ms = IO.MemoryStream()
buf = Array.CreateInstance(System.Byte, 2048)
while True:
bytes = stream.Read(buf, 0, buf.Length)
if bytes == 0:
break
else:
ms.Write(buf, 0, bytes)
retval = ms.ToArray()
ms.Close()
return retval
def decompress(data):
bytes = _string_to_bytearr(data)
ms = IO.MemoryStream()
ms.Write(bytes, 0, bytes.Length)
ms.Position = 0 # fseek 0
gz = IO.Compression.DeflateStream(ms, IO.Compression.CompressionMode.Decompress)
bytes = _read_bytes(gz)
retval = _bytearr_to_string(bytes)
gz.Close()
return retval
def compress(data):
bytes = _string_to_bytearr(data)
ms = IO.MemoryStream()
gz = IO.Compression.DeflateStream(ms, IO.Compression.CompressionMode.Compress, True)
gz.Write(bytes, 0, bytes.Length)
gz.Close()
ms.Position = 0 # fseek 0
bytes = ms.ToArray()
retval = _bytearr_to_string(bytes)
ms.Close()
return retval
class FlateDecode(object):
def decode(data, decodeParms):
data = decompress(data)
predictor = 1
if decodeParms:
try:
predictor = decodeParms.get("/Predictor", 1)
except AttributeError:
pass # usually an array with a null object was read
# predictor 1 == no predictor
if predictor != 1:
columns = decodeParms["/Columns"]
# PNG prediction:
if predictor >= 10 and predictor <= 15:
output = StringIO()
# PNG prediction can vary from row to row
rowlength = columns + 1
assert len(data) % rowlength == 0
prev_rowdata = (0,) * rowlength
for row in range(len(data) // rowlength):
rowdata = [ord_(x) for x in data[(row*rowlength):((row+1)*rowlength)]]
filterByte = rowdata[0]
if filterByte == 0:
pass
elif filterByte == 1:
for i in range(2, rowlength):
rowdata[i] = (rowdata[i] + rowdata[i-1]) % 256
elif filterByte == 2:
for i in range(1, rowlength):
rowdata[i] = (rowdata[i] + prev_rowdata[i]) % 256
else:
# unsupported PNG filter
raise PdfReadError("Unsupported PNG filter %r" % filterByte)
prev_rowdata = rowdata
output.write(''.join([chr(x) for x in rowdata[1:]]))
data = output.getvalue()
else:
# unsupported predictor
raise PdfReadError("Unsupported flatedecode predictor %r" % predictor)
return data
decode = staticmethod(decode)
def encode(data):
return compress(data)
encode = staticmethod(encode)
class ASCIIHexDecode(object):
def decode(data, decodeParms=None):
retval = ""
char = ""
x = 0
while True:
c = data[x]
if c == ">":
break
elif c.isspace():
x += 1
continue
char += c
if len(char) == 2:
retval += chr(int(char, base=16))
char = ""
x += 1
assert char == ""
return retval
decode = staticmethod(decode)
class LZWDecode(object):
"""Taken from:
http://www.java2s.com/Open-Source/Java-Document/PDF/PDF-Renderer/com/sun/pdfview/decode/LZWDecode.java.htm
"""
class decoder(object):
def __init__(self, data):
self.STOP=257
self.CLEARDICT=256
self.data=data
self.bytepos=0
self.bitpos=0
self.dict=[""]*4096
for i in range(256):
self.dict[i]=chr(i)
self.resetDict()
def resetDict(self):
self.dictlen=258
self.bitspercode=9
def nextCode(self):
fillbits=self.bitspercode
value=0
while fillbits>0 :
if self.bytepos >= len(self.data):
return -1
nextbits=ord(self.data[self.bytepos])
bitsfromhere=8-self.bitpos
if bitsfromhere>fillbits:
bitsfromhere=fillbits
value |= (((nextbits >> (8-self.bitpos-bitsfromhere)) &
(0xff >> (8-bitsfromhere))) <<
(fillbits-bitsfromhere))
fillbits -= bitsfromhere
self.bitpos += bitsfromhere
if self.bitpos >=8:
self.bitpos=0
self.bytepos = self.bytepos+1
return value
def decode(self):
""" algorithm derived from:
http://www.rasip.fer.hr/research/compress/algorithms/fund/lz/lzw.html
and the PDFReference
"""
cW = self.CLEARDICT;
baos=""
while True:
pW = cW;
cW = self.nextCode();
if cW == -1:
raise PdfReadError("Missed the stop code in LZWDecode!")
if cW == self.STOP:
break;
elif cW == self.CLEARDICT:
self.resetDict();
elif pW == self.CLEARDICT:
baos+=self.dict[cW]
else:
if cW < self.dictlen:
baos += self.dict[cW]
p=self.dict[pW]+self.dict[cW][0]
self.dict[self.dictlen]=p
self.dictlen+=1
else:
p=self.dict[pW]+self.dict[pW][0]
baos+=p
self.dict[self.dictlen] = p;
self.dictlen+=1
if (self.dictlen >= (1 << self.bitspercode) - 1 and
self.bitspercode < 12):
self.bitspercode+=1
return baos
@staticmethod
def decode(data,decodeParams=None):
return LZWDecode.decoder(data).decode()
class ASCII85Decode(object):
def decode(data, decodeParms=None):
retval = ""
group = []
x = 0
hitEod = False
# remove all whitespace from data
data = [y for y in data if not (y in ' \n\r\t')]
while not hitEod:
c = data[x]
if len(retval) == 0 and c == "<" and data[x+1] == "~":
x += 2
continue
#elif c.isspace():
# x += 1
# continue
elif c == 'z':
assert len(group) == 0
retval += '\x00\x00\x00\x00'
x += 1
continue
elif c == "~" and data[x+1] == ">":
if len(group) != 0:
# cannot have a final group of just 1 char
assert len(group) > 1
cnt = len(group) - 1
group += [ 85, 85, 85 ]
hitEod = cnt
else:
break
else:
c = ord(c) - 33
assert c >= 0 and c < 85
group += [ c ]
if len(group) >= 5:
b = group[0] * (85**4) + \
group[1] * (85**3) + \
group[2] * (85**2) + \
group[3] * 85 + \
group[4]
assert b < (2**32 - 1)
c4 = chr((b >> 0) % 256)
c3 = chr((b >> 8) % 256)
c2 = chr((b >> 16) % 256)
c1 = chr(b >> 24)
retval += (c1 + c2 + c3 + c4)
if hitEod:
retval = retval[:-4+hitEod]
group = []
x += 1
return retval
decode = staticmethod(decode)
def decodeStreamData(stream):
from .generic import NameObject
filters = stream.get("/Filter", ())
if len(filters) and not isinstance(filters[0], NameObject):
# we have a single filter instance
filters = (filters,)
data = stream._data
for filterType in filters:
if filterType == "/FlateDecode":
data = FlateDecode.decode(data, stream.get("/DecodeParms"))
elif filterType == "/ASCIIHexDecode":
data = ASCIIHexDecode.decode(data)
elif filterType == "/LZWDecode":
data = LZWDecode.decode(data, stream.get("/DecodeParms"))
elif filterType == "/ASCII85Decode":
data = ASCII85Decode.decode(data)
elif filterType == "/Crypt":
decodeParams = stream.get("/DecodeParams", {})
if "/Name" not in decodeParams and "/Type" not in decodeParams:
pass
else:
raise NotImplementedError("/Crypt filter with /Name or /Type not supported yet")
else:
# unsupported filter
raise NotImplementedError("unsupported filter %s" % filterType)
return data
| mit |
agentr13/python-phonenumbers | python/phonenumbers/geodata/data4.py | 2 | 694125 | """Per-prefix data, mapping each prefix to a dict of locale:name.
Auto-generated file, do not edit by hand.
"""
from ..util import u
# Copyright (C) 2011-2015 The Libphonenumber Authors
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
data = {
'35236':{'de': 'Hesperingen/Kockelscheuer/Roeser', 'en': 'Hesperange/Kockelscheuer/Roeser', 'fr': 'Hesperange/Kockelscheuer/Roeser'},
'35237':{'de': 'Leudelingen/Ehlingen/Monnerich', 'en': 'Leudelange/Ehlange/Mondercange', 'fr': 'Leudelange/Ehlange/Mondercange'},
'35234':{'de': 'Rammeldingen/Senningerberg', 'en': 'Rameldange/Senningerberg', 'fr': 'Rameldange/Senningerberg'},
'35235':{'de': 'Sandweiler/Mutfort/Roodt-sur-Syre', 'en': 'Sandweiler/Moutfort/Roodt-sur-Syre', 'fr': 'Sandweiler/Moutfort/Roodt-sur-Syre'},
'35232':{'de': 'Kanton Mersch', 'en': 'Mersch', 'fr': 'Mersch'},
'35233':{'de': 'Walferdingen', 'en': 'Walferdange', 'fr': 'Walferdange'},
'35230':{'de': 'Kanton Capellen/Kehlen', 'en': 'Capellen/Kehlen', 'fr': 'Capellen/Kehlen'},
'35547':{'en': u('Kam\u00ebz/Vor\u00eb/Paskuqan/Zall-Herr/Burxull\u00eb/Prez\u00eb, Tiran\u00eb')},
'35548':{'en': u('Kashar/Vaqar/Ndroq/Pez\u00eb/Fark\u00eb/Dajt, Tiran\u00eb')},
'35549':{'en': u('Petrel\u00eb/Baldushk/B\u00ebrzhit\u00eb/Krrab\u00eb/Shengjergj/Zall-Bastar, Tiran\u00eb')},
'35239':{'de': 'Windhof/Steinfort', 'en': 'Windhof/Steinfort', 'fr': 'Windhof/Steinfort'},
'3332660':{'en': 'Sainte-Menehould', 'fr': 'Sainte-Menehould'},
'35231':{'de': 'Bartringen', 'en': 'Bertrange/Mamer/Munsbach/Strassen', 'fr': 'Bertrange/Mamer/Munsbach/Strassen'},
'3593120':{'bg': u('\u0425\u0440\u0430\u0431\u0440\u0438\u043d\u043e'), 'en': 'Hrabrino'},
'3332669':{'en': u('Ch\u00e2lons-en-Champagne'), 'fr': u('Ch\u00e2lons-en-Champagne')},
'435550':{'de': u('Th\u00fcringen'), 'en': u('Th\u00fcringen')},
'441779':{'en': 'Peterhead'},
'441778':{'en': 'Bourne'},
'441777':{'en': 'Retford'},
'441776':{'en': 'Stranraer'},
'441775':{'en': 'Spalding'},
'441773':{'en': 'Ripley'},
'441772':{'en': 'Preston'},
'441771':{'en': 'Maud'},
'441770':{'en': 'Isle of Arran'},
'3596026':{'bg': u('\u041c\u0430\u043a\u0430\u0440\u0438\u043e\u043f\u043e\u043b\u0441\u043a\u043e'), 'en': 'Makariopolsko'},
'3596027':{'bg': u('\u0414\u0440\u0430\u043b\u0444\u0430'), 'en': 'Dralfa'},
'3596024':{'bg': u('\u0420\u0443\u0435\u0446'), 'en': 'Ruets'},
'3596025':{'bg': u('\u0410\u043b\u0432\u0430\u043d\u043e\u0432\u043e'), 'en': 'Alvanovo'},
'3316468':{'en': 'Champs-sur-Marne', 'fr': 'Champs-sur-Marne'},
'3316469':{'en': 'Fontainebleau', 'fr': 'Fontainebleau'},
'3596020':{'bg': u('\u041b\u0438\u043b\u044f\u043a'), 'en': 'Lilyak'},
'3346758':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3316464':{'en': 'Melun', 'fr': 'Melun'},
'3316465':{'en': 'Coulommiers', 'fr': 'Coulommiers'},
'3316466':{'en': 'Bussy-Saint-Georges', 'fr': 'Bussy-Saint-Georges'},
'3316467':{'en': 'Villeparisis', 'fr': 'Villeparisis'},
'3316460':{'en': 'Provins', 'fr': 'Provins'},
'3316461':{'en': 'Champs-sur-Marne', 'fr': 'Champs-sur-Marne'},
'3316462':{'en': 'Lognes', 'fr': 'Lognes'},
'3316463':{'en': u('Cr\u00e9cy-la-Chapelle'), 'fr': u('Cr\u00e9cy-la-Chapelle')},
'3349008':{'en': 'Lauris', 'fr': 'Lauris'},
'3349009':{'en': 'Pertuis', 'fr': 'Pertuis'},
'435556':{'de': 'Schruns', 'en': 'Schruns'},
'3349002':{'en': u('Mori\u00e8res-l\u00e8s-Avignon'), 'fr': u('Mori\u00e8res-l\u00e8s-Avignon')},
'3349003':{'en': 'Le Pontet', 'fr': 'Le Pontet'},
'3349001':{'en': 'Caumont-sur-Durance', 'fr': 'Caumont-sur-Durance'},
'3349006':{'en': 'Cavaillon', 'fr': 'Cavaillon'},
'3349007':{'en': 'La Tour d\'Aigues', 'fr': 'La Tour d\'Aigues'},
'3349004':{'en': 'Apt', 'fr': 'Apt'},
'3349005':{'en': 'Roussillon', 'fr': 'Roussillon'},
'435558':{'de': 'Gaschurn', 'en': 'Gaschurn'},
'37426699':{'am': u('\u053f\u0578\u0569\u056b'), 'en': 'Koti', 'ru': u('\u041a\u043e\u0447\u0438')},
'37426696':{'am': u('\u0548\u057d\u056f\u0565\u057a\u0561\u0580'), 'en': 'Voskepar', 'ru': u('\u0412\u043e\u0441\u043a\u0435\u043f\u0430\u0440')},
'37426695':{'am': u('\u0536\u0578\u0580\u0561\u056f\u0561\u0576'), 'en': 'Zorakan', 'ru': u('\u0417\u043e\u0440\u0430\u043a\u0430\u043d')},
'37426693':{'am': u('\u0532\u0561\u0572\u0561\u0576\u056b\u057d'), 'en': 'Baghanis', 'ru': u('\u0411\u0430\u0433\u0430\u043d\u0438\u0441')},
'37426692':{'am': u('\u0531\u0580\u0573\u056b\u057d'), 'en': 'Archis', 'ru': u('\u0410\u0440\u0447\u0438\u0441')},
'35931397':{'bg': u('\u0425\u0440\u0438\u0441\u0442\u043e \u0414\u0430\u043d\u043e\u0432\u043e'), 'en': 'Hristo Danovo'},
'35931396':{'bg': u('\u0414\u043e\u043c\u043b\u044f\u043d'), 'en': 'Domlyan'},
'35931395':{'bg': u('\u0418\u0433\u0430\u043d\u043e\u0432\u043e'), 'en': 'Iganovo'},
'35931394':{'bg': u('\u0412\u0430\u0441\u0438\u043b \u041b\u0435\u0432\u0441\u043a\u0438, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Vasil Levski, Plovdiv'},
'35931393':{'bg': u('\u0412\u043e\u0439\u043d\u044f\u0433\u043e\u0432\u043e'), 'en': 'Voynyagovo'},
'35931392':{'bg': u('\u0414\u044a\u0431\u0435\u043d\u0435'), 'en': 'Dabene'},
'3323560':{'en': 'Bois-Guillaume', 'fr': 'Bois-Guillaume'},
'35931390':{'bg': u('\u041c\u043e\u0441\u043a\u043e\u0432\u0435\u0446'), 'en': 'Moskovets'},
'35931398':{'bg': u('\u0421\u043b\u0430\u0442\u0438\u043d\u0430, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Slatina, Plovdiv'},
'3522735':{'de': 'Sandweiler/Mutfort/Roodt-sur-Syre', 'en': 'Sandweiler/Moutfort/Roodt-sur-Syre', 'fr': 'Sandweiler/Moutfort/Roodt-sur-Syre'},
'3522734':{'de': 'Rammeldingen/Senningerberg', 'en': 'Rameldange/Senningerberg', 'fr': 'Rameldange/Senningerberg'},
'3522737':{'de': 'Leudelingen/Ehlingen/Monnerich', 'en': 'Leudelange/Ehlange/Mondercange', 'fr': 'Leudelange/Ehlange/Mondercange'},
'3522736':{'de': 'Hesperingen/Kockelscheuer/Roeser', 'en': 'Hesperange/Kockelscheuer/Roeser', 'fr': 'Hesperange/Kockelscheuer/Roeser'},
'3522731':{'de': 'Bartringen', 'en': 'Bertrange/Mamer/Munsbach/Strassen', 'fr': 'Bertrange/Mamer/Munsbach/Strassen'},
'3522730':{'de': 'Kanton Capellen/Kehlen', 'en': 'Capellen/Kehlen', 'fr': 'Capellen/Kehlen'},
'3522733':{'de': 'Walferdingen', 'en': 'Walferdange', 'fr': 'Walferdange'},
'3522732':{'de': 'Lintgen/Kanton Mersch/Steinfort', 'en': 'Lintgen/Mersch/Steinfort', 'fr': 'Lintgen/Mersch/Steinfort'},
'3349913':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3522739':{'de': 'Windhof/Steinfort', 'en': 'Windhof/Steinfort', 'fr': 'Windhof/Steinfort'},
'35937422':{'bg': u('\u0414\u043e\u043b\u043d\u0438 \u0413\u043b\u0430\u0432\u0430\u043d\u0430\u043a'), 'en': 'Dolni Glavanak'},
'35937421':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u043f\u043e\u043b\u0435'), 'en': 'Dolno pole'},
'35937420':{'bg': u('\u041f\u043e\u0434\u043a\u0440\u0435\u043f\u0430'), 'en': 'Podkrepa'},
'441368':{'en': 'Dunbar'},
'441369':{'en': 'Dunoon'},
'441364':{'en': 'Ashburton'},
'441366':{'en': 'Downham Market'},
'441367':{'en': 'Faringdon'},
'441360':{'en': 'Killearn'},
'441361':{'en': 'Duns'},
'441362':{'en': 'Dereham'},
'441363':{'en': 'Crediton'},
'3338792':{'en': 'Saint-Avold', 'fr': 'Saint-Avold'},
'3338793':{'en': 'Creutzwald', 'fr': 'Creutzwald'},
'3338822':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338791':{'en': 'Saint-Avold', 'fr': 'Saint-Avold'},
'3338796':{'en': 'Bitche', 'fr': 'Bitche'},
'3338797':{'en': 'Sarralbe', 'fr': 'Sarralbe'},
'3338794':{'en': 'Faulquemont', 'fr': 'Faulquemont'},
'3338795':{'en': 'Sarreguemines', 'fr': 'Sarreguemines'},
'3338828':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338829':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338798':{'en': 'Sarreguemines', 'fr': 'Sarreguemines'},
'3323138':{'en': 'Caen', 'fr': 'Caen'},
'437619':{'de': 'Kirchham', 'en': 'Kirchham'},
'3323130':{'en': 'Caen', 'fr': 'Caen'},
'3323131':{'en': 'Lisieux', 'fr': 'Lisieux'},
'3323137':{'en': 'Courseulles-sur-Mer', 'fr': 'Courseulles-sur-Mer'},
'3323134':{'en': 'Caen', 'fr': 'Caen'},
'3323135':{'en': 'Mondeville', 'fr': 'Mondeville'},
'432289':{'de': 'Matzen', 'en': 'Matzen'},
'432288':{'de': 'Auersthal', 'en': 'Auersthal'},
'432283':{'de': 'Angern an der March', 'en': 'Angern an der March'},
'432282':{'de': u('G\u00e4nserndorf'), 'en': u('G\u00e4nserndorf')},
'432285':{'de': 'Marchegg', 'en': 'Marchegg'},
'432284':{'de': 'Oberweiden', 'en': 'Oberweiden'},
'432287':{'de': 'Strasshof an der Nordbahn', 'en': 'Strasshof an der Nordbahn'},
'432286':{'de': 'Obersiebenbrunn', 'en': 'Obersiebenbrunn'},
'437614':{'de': 'Vorchdorf', 'en': 'Vorchdorf'},
'3323394':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'3323395':{'en': 'Valognes', 'fr': 'Valognes'},
'3323393':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'3323390':{'en': 'Granville', 'fr': 'Granville'},
'3323391':{'en': 'Granville', 'fr': 'Granville'},
'3355308':{'en': u('P\u00e9rigueux'), 'fr': u('P\u00e9rigueux')},
'3355309':{'en': u('P\u00e9rigueux'), 'fr': u('P\u00e9rigueux')},
'3346970':{'en': 'Lyon', 'fr': 'Lyon'},
'437612':{'de': 'Gmunden', 'en': 'Gmunden'},
'433857':{'de': u('Neuberg an der M\u00fcrz'), 'en': u('Neuberg an der M\u00fcrz')},
'35930517':{'bg': u('\u0417\u0430\u0431\u044a\u0440\u0434\u043e'), 'en': 'Zabardo'},
'3332530':{'en': 'Chaumont', 'fr': 'Chaumont'},
'432756':{'de': 'Sankt Leonhard am Forst', 'en': 'St. Leonhard am Forst'},
'3332532':{'en': 'Chaumont', 'fr': 'Chaumont'},
'37428494':{'am': u('\u053d\u0576\u0571\u0578\u0580\u0565\u057d\u056f'), 'en': 'Khndzoresk', 'ru': u('\u0425\u043d\u0434\u0437\u043e\u0440\u0435\u0441\u043a')},
'432753':{'de': 'Gansbach', 'en': 'Gansbach'},
'3332535':{'en': 'Chaumont', 'fr': 'Chaumont'},
'37428491':{'am': u('\u0540\u0561\u0580\u056a\u056b\u057d'), 'en': 'Harzhis', 'ru': u('\u0410\u0440\u0436\u0438\u0441')},
'3332539':{'en': 'Nogent-sur-Seine', 'fr': 'Nogent-sur-Seine'},
'37428499':{'am': u('\u053f\u0578\u057c\u0576\u056b\u0571\u0578\u0580'), 'en': 'Kornidzor', 'ru': u('\u041a\u043e\u0440\u043d\u0438\u0434\u0437\u043e\u0440')},
'390586':{'en': 'Livorno', 'it': 'Livorno'},
'4412291':{'en': 'Barrow-in-Furness/Millom'},
'4412290':{'en': 'Barrow-in-Furness/Millom'},
'4412293':{'en': 'Millom'},
'4412292':{'en': 'Barrow-in-Furness'},
'4412295':{'en': 'Barrow-in-Furness'},
'4412294':{'en': 'Barrow-in-Furness'},
'4412297':{'en': 'Millom'},
'4412296':{'en': 'Barrow-in-Furness'},
'4412299':{'en': 'Millom'},
'4412298':{'en': 'Barrow-in-Furness'},
'3593762':{'bg': u('\u0418\u0437\u0432\u043e\u0440\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Izvorovo, Hask.'},
'3593763':{'bg': u('\u0411\u044a\u043b\u0433\u0430\u0440\u0438\u043d'), 'en': 'Balgarin'},
'3593766':{'bg': u('\u0411\u0438\u0441\u0435\u0440'), 'en': 'Biser'},
'3593767':{'bg': u('\u0411\u0440\u0430\u043d\u0438\u0446\u0430'), 'en': 'Branitsa'},
'3593764':{'bg': u('\u041f\u043e\u043b\u044f\u043d\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Polyanovo, Hask.'},
'3593765':{'bg': u('\u0418\u0432\u0430\u043d\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Ivanovo, Hask.'},
'3593768':{'bg': u('\u0414\u043e\u0441\u0438\u0442\u0435\u0435\u0432\u043e'), 'en': 'Dositeevo'},
'3593769':{'bg': u('\u041e\u0440\u0435\u0448\u0435\u0446, \u0425\u0430\u0441\u043a.'), 'en': 'Oreshets, Hask.'},
'359722':{'bg': u('\u0421\u0430\u043c\u043e\u043a\u043e\u0432'), 'en': 'Samokov'},
'434842':{'de': 'Sillian', 'en': 'Sillian'},
'433884':{'de': 'Wegscheid', 'en': 'Wegscheid'},
'433885':{'de': 'Greith', 'en': 'Greith'},
'433886':{'de': 'Weichselboden', 'en': 'Weichselboden'},
'433882':{'de': 'Mariazell', 'en': 'Mariazell'},
'3353305':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3332151':{'en': 'Arras', 'fr': 'Arras'},
'433619':{'de': 'Oppenberg', 'en': 'Oppenberg'},
'441562':{'en': 'Kidderminster'},
'441563':{'en': 'Kilmarnock'},
'441560':{'en': 'Moscow'},
'441561':{'en': 'Laurencekirk'},
'441566':{'en': 'Launceston'},
'441567':{'en': 'Killin'},
'441564':{'en': 'Lapworth'},
'441565':{'en': 'Knutsford'},
'441568':{'en': 'Leominster'},
'441569':{'en': 'Stonehaven'},
'355597':{'en': u('Kodovjat/Poro\u00e7an/Kukur/Lenie, Gramsh')},
'355596':{'en': u('Pishaj/Sult/Tunj\u00eb/Kushov\u00eb/Sk\u00ebnderbegas, Gramsh')},
'355595':{'en': u('Quk\u00ebs/Rajc\u00eb, Librazhd')},
'355594':{'en': 'Hotolisht/Polis/Stravaj, Librazhd'},
'355593':{'en': u('Lunik/Orenj\u00eb/Stebleve, Librazhd')},
'355592':{'en': u('Qend\u00ebr, Librazhd')},
'355591':{'en': u('P\u00ebrrenjas, Librazhd')},
'437945':{'de': 'Sankt Oswald bei Freistadt', 'en': 'St. Oswald bei Freistadt'},
'437944':{'de': 'Sandl', 'en': 'Sandl'},
'437947':{'de': 'Kefermarkt', 'en': 'Kefermarkt'},
'437946':{'de': 'Gutau', 'en': 'Gutau'},
'3596909':{'bg': u('\u041c\u0430\u043b\u043a\u0430 \u0416\u0435\u043b\u044f\u0437\u043d\u0430'), 'en': 'Malka Zhelyazna'},
'3596908':{'bg': u('\u0413\u043b\u043e\u0433\u043e\u0432\u043e'), 'en': 'Glogovo'},
'437943':{'de': 'Windhaag bei Freistadt', 'en': 'Windhaag bei Freistadt'},
'437942':{'de': 'Freistadt', 'en': 'Freistadt'},
'3596905':{'bg': u('\u0414\u0438\u0432\u0447\u043e\u0432\u043e\u0442\u043e'), 'en': 'Divchovoto'},
'3596907':{'bg': u('\u0413\u0440\u0430\u0434\u0435\u0436\u043d\u0438\u0446\u0430'), 'en': 'Gradezhnitsa'},
'3596906':{'bg': u('\u0427\u0435\u0440\u043d\u0438 \u0412\u0438\u0442'), 'en': 'Cherni Vit'},
'3596901':{'bg': u('\u0413\u043b\u043e\u0436\u0435\u043d\u0435, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Glozhene, Lovech'},
'3596900':{'bg': u('\u0412\u0430\u0441\u0438\u043b\u044c\u043e\u0432\u043e'), 'en': 'Vasilyovo'},
'3596902':{'bg': u('\u0420\u0438\u0431\u0430\u0440\u0438\u0446\u0430, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Ribaritsa, Lovech'},
'35938':{'bg': u('\u0425\u0430\u0441\u043a\u043e\u0432\u043e'), 'en': 'Haskovo'},
'35932':{'bg': u('\u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Plovdiv'},
'35934':{'bg': u('\u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Pazardzhik'},
'3324072':{'en': 'Nort-sur-Erdre', 'fr': 'Nort-sur-Erdre'},
'3324073':{'en': 'Nantes', 'fr': 'Nantes'},
'3324070':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'3324071':{'en': 'Nantes', 'fr': 'Nantes'},
'3324076':{'en': 'Nantes', 'fr': 'Nantes'},
'3324077':{'en': u('Suc\u00e9-sur-Erdre'), 'fr': u('Suc\u00e9-sur-Erdre')},
'3324074':{'en': 'Nantes', 'fr': 'Nantes'},
'3324075':{'en': u('Rez\u00e9'), 'fr': u('Rez\u00e9')},
'3324078':{'en': 'Saint-Philbert-de-Grand-Lieu', 'fr': 'Saint-Philbert-de-Grand-Lieu'},
'3324079':{'en': 'Blain', 'fr': 'Blain'},
'3346674':{'en': 'Milhaud', 'fr': 'Milhaud'},
'354462':{'en': 'Akureyri'},
'43463':{'de': 'Klagenfurt', 'en': 'Klagenfurt'},
'3329919':{'en': 'Saint-Malo', 'fr': 'Saint-Malo'},
'3347615':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347342':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347617':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347340':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347611':{'en': 'Le Bourg d\'Oisans', 'fr': 'Le Bourg d\'Oisans'},
'3347344':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3596022':{'bg': u('\u041f\u0440\u043e\u0431\u0443\u0434\u0430, \u0422\u044a\u0440\u0433.'), 'en': 'Probuda, Targ.'},
'3347618':{'en': 'Meylan', 'fr': 'Meylan'},
'3596023':{'bg': u('\u041f\u043e\u0434\u0433\u043e\u0440\u0438\u0446\u0430'), 'en': 'Podgoritsa'},
'3329951':{'en': 'Rennes', 'fr': 'Rennes'},
'3596021':{'bg': u('\u0411\u0443\u0445\u043e\u0432\u0446\u0438'), 'en': 'Buhovtsi'},
'3329957':{'en': 'Guichen', 'fr': 'Guichen'},
'3346757':{'en': 'Gignac', 'fr': 'Gignac'},
'3323898':{'en': 'Montargis', 'fr': 'Montargis'},
'3346756':{'en': 'La Grande Motte', 'fr': 'La Grande Motte'},
'3323895':{'en': u('Ch\u00e2teau-Renard'), 'fr': u('Ch\u00e2teau-Renard')},
'3323897':{'en': 'Courtenay', 'fr': 'Courtenay'},
'3323896':{'en': u('Ferri\u00e8res-en-G\u00e2tinais'), 'fr': u('Ferri\u00e8res-en-G\u00e2tinais')},
'3323891':{'en': 'Neuville-aux-Bois', 'fr': 'Neuville-aux-Bois'},
'3323890':{'en': 'Bellegarde', 'fr': 'Bellegarde'},
'3323893':{'en': 'Montargis', 'fr': 'Montargis'},
'3323892':{'en': 'Dordives', 'fr': 'Dordives'},
'3346754':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346753':{'en': u('S\u00e8te'), 'fr': u('S\u00e8te')},
'3346752':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346751':{'en': u('S\u00e8te'), 'fr': u('S\u00e8te')},
'3346750':{'en': u('P\u00e9rols'), 'fr': u('P\u00e9rols')},
'3323096':{'en': 'Rennes', 'fr': 'Rennes'},
'390766':{'it': 'Civitavecchia'},
'3347964':{'en': 'Saint-Jean-de-Maurienne', 'fr': 'Saint-Jean-de-Maurienne'},
'3347962':{'en': u('Chamb\u00e9ry'), 'fr': u('Chamb\u00e9ry')},
'3347961':{'en': 'Aix-les-Bains', 'fr': 'Aix-les-Bains'},
'3347960':{'en': u('Chamb\u00e9ry'), 'fr': u('Chamb\u00e9ry')},
'3347969':{'en': u('Chamb\u00e9ry'), 'fr': u('Chamb\u00e9ry')},
'3347968':{'en': u('Chamb\u00e9ry'), 'fr': u('Chamb\u00e9ry')},
'3329640':{'en': 'Guingamp', 'fr': 'Guingamp'},
'3329641':{'en': 'Saint-Cast-le-Guildo', 'fr': 'Saint-Cast-le-Guildo'},
'3329646':{'en': 'Lannion', 'fr': 'Lannion'},
'3329647':{'en': 'Ploubezre', 'fr': 'Ploubezre'},
'3329644':{'en': 'Guingamp', 'fr': 'Guingamp'},
'3329645':{'en': u('B\u00e9gard'), 'fr': u('B\u00e9gard')},
'3329648':{'en': 'Lannion', 'fr': 'Lannion'},
'3329649':{'en': 'Perros-Guirec', 'fr': 'Perros-Guirec'},
'3323271':{'en': 'Vernon', 'fr': 'Vernon'},
'3323270':{'en': 'Yvetot', 'fr': 'Yvetot'},
'3323274':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323277':{'en': 'Gaillon', 'fr': 'Gaillon'},
'3323276':{'en': 'Rouen', 'fr': 'Rouen'},
'3323279':{'en': 'Montivilliers', 'fr': 'Montivilliers'},
'3351685':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'390985':{'it': 'Scalea'},
'390984':{'it': 'Cosenza'},
'390983':{'it': 'Rossano'},
'390982':{'it': 'Paola'},
'390981':{'it': 'Castrovillari'},
'3358274':{'en': 'Toulouse', 'fr': 'Toulouse'},
'42158':{'en': u('Ro\u017e\u0148ava')},
'42151':{'en': u('Pre\u0161ov')},
'42153':{'en': u('Spi\u0161sk\u00e1 Nov\u00e1 Ves')},
'42152':{'en': 'Poprad'},
'42155':{'en': u('Ko\u0161ice')},
'42154':{'en': 'Bardejov'},
'42157':{'en': u('Humenn\u00e9')},
'42156':{'en': 'Michalovce'},
'390187':{'en': 'La Spezia', 'it': 'La Spezia'},
'390185':{'en': 'Genoa', 'it': 'Rapallo'},
'390184':{'it': 'Sanremo'},
'390183':{'en': 'Imperia', 'it': 'Imperia'},
'390182':{'it': 'Albenga'},
'390174':{'it': u('Mondov\u00ec')},
'3345069':{'en': 'Seynod', 'fr': 'Seynod'},
'3345062':{'en': u('Valli\u00e8res'), 'fr': u('Valli\u00e8res')},
'3345064':{'en': 'Rumilly', 'fr': 'Rumilly'},
'3345065':{'en': 'Annecy', 'fr': 'Annecy'},
'3345066':{'en': 'Annecy', 'fr': 'Annecy'},
'3345067':{'en': 'Annecy', 'fr': 'Annecy'},
'3317430':{'en': 'Paris', 'fr': 'Paris'},
'3338587':{'en': 'Chagny', 'fr': 'Chagny'},
'3338586':{'en': 'Autun', 'fr': 'Autun'},
'3338585':{'en': 'Gueugnon', 'fr': 'Gueugnon'},
'3338581':{'en': 'Paray-le-Monial', 'fr': 'Paray-le-Monial'},
'3338580':{'en': 'Le Creusot', 'fr': 'Le Creusot'},
'3338589':{'en': 'Bourbon-Lancy', 'fr': 'Bourbon-Lancy'},
'3338588':{'en': 'Digoin', 'fr': 'Digoin'},
'3332277':{'en': 'Doullens', 'fr': 'Doullens'},
'3332275':{'en': 'Albert', 'fr': 'Albert'},
'3332274':{'en': 'Albert', 'fr': 'Albert'},
'3332272':{'en': 'Amiens', 'fr': 'Amiens'},
'3332271':{'en': 'Amiens', 'fr': 'Amiens'},
'3332270':{'en': 'Rivery', 'fr': 'Rivery'},
'3347145':{'en': 'Aurillac', 'fr': 'Aurillac'},
'3332278':{'en': 'Montdidier', 'fr': 'Montdidier'},
'437718':{'de': 'Waldkirchen am Wesen', 'en': 'Waldkirchen am Wesen'},
'437719':{'de': 'Taufkirchen an der Pram', 'en': 'Taufkirchen an der Pram'},
'437711':{'de': 'Suben', 'en': 'Suben'},
'437712':{'de': u('Sch\u00e4rding'), 'en': u('Sch\u00e4rding')},
'437713':{'de': 'Schardenberg', 'en': 'Schardenberg'},
'437714':{'de': 'Esternberg', 'en': 'Esternberg'},
'437716':{'de': u('M\u00fcnzkirchen'), 'en': u('M\u00fcnzkirchen')},
'437717':{'de': 'Sankt Aegidi', 'en': 'St. Aegidi'},
'37166':{'en': 'Riga'},
'37167':{'en': 'Riga'},
'3352460':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3352461':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'37424297':{'am': u('\u0531\u0576\u056b \u053f\u0561\u0575\u0561\u0580\u0561\u0576'), 'en': 'Ani Kayaran', 'ru': u('\u0410\u043d\u0438 \u041a\u0430\u044f\u0440\u0430\u043d')},
'3356363':{'en': 'Montauban', 'fr': 'Montauban'},
'3356362':{'en': 'Castres', 'fr': 'Castres'},
'3356361':{'en': 'Mazamet', 'fr': 'Mazamet'},
'3356360':{'en': 'Albi', 'fr': 'Albi'},
'3356366':{'en': 'Montauban', 'fr': 'Montauban'},
'3347428':{'en': 'Bourgoin-Jallieu', 'fr': 'Bourgoin-Jallieu'},
'3347429':{'en': 'Roussillon', 'fr': 'Roussillon'},
'3347422':{'en': 'Bourg-en-Bresse', 'fr': 'Bourg-en-Bresse'},
'3347423':{'en': 'Bourg-en-Bresse', 'fr': 'Bourg-en-Bresse'},
'3347420':{'en': u('La C\u00f4te Saint Andr\u00e9'), 'fr': u('La C\u00f4te Saint Andr\u00e9')},
'3347421':{'en': 'Bourg-en-Bresse', 'fr': 'Bourg-en-Bresse'},
'3347427':{'en': 'L\'Isle d\'Abeau', 'fr': 'L\'Isle d\'Abeau'},
'3347424':{'en': 'Bourg-en-Bresse', 'fr': 'Bourg-en-Bresse'},
'3347425':{'en': 'Viriat', 'fr': 'Viriat'},
'3598192':{'bg': u('\u0425\u043e\u0442\u0430\u043d\u0446\u0430'), 'en': 'Hotantsa'},
'3598194':{'bg': u('\u0421\u0430\u043d\u0434\u0440\u043e\u0432\u043e'), 'en': 'Sandrovo'},
'333839':{'en': 'Nancy', 'fr': 'Nancy'},
'3598196':{'bg': u('\u041d\u0438\u0441\u043e\u0432\u043e'), 'en': 'Nisovo'},
'333835':{'en': u('Vand\u0153uvre-l\u00e8s-Nancy'), 'fr': u('Vand\u0153uvre-l\u00e8s-Nancy')},
'432949':{'de': 'Niederfladnitz', 'en': 'Niederfladnitz'},
'3329888':{'en': 'Morlaix', 'fr': 'Morlaix'},
'35937424':{'bg': u('\u041a\u043e\u0437\u043b\u0435\u0446'), 'en': 'Kozlets'},
'35937423':{'bg': u('\u0413\u043e\u043b\u0435\u043c\u0430\u043d\u0446\u0438'), 'en': 'Golemantsi'},
'3342210':{'en': 'Cannes', 'fr': 'Cannes'},
'3347288':{'en': 'Beynost', 'fr': 'Beynost'},
'3347289':{'en': u('V\u00e9nissieux'), 'fr': u('V\u00e9nissieux')},
'3347284':{'en': 'Lyon', 'fr': 'Lyon'},
'3347285':{'en': 'Lyon', 'fr': 'Lyon'},
'3347282':{'en': 'Villeurbanne', 'fr': 'Villeurbanne'},
'3347283':{'en': 'Lyon', 'fr': 'Lyon'},
'3347280':{'en': 'Lyon', 'fr': 'Lyon'},
'3347281':{'en': 'Bron', 'fr': 'Bron'},
'3316446':{'en': 'Orsay', 'fr': 'Orsay'},
'3316447':{'en': 'Massy', 'fr': 'Massy'},
'3316445':{'en': 'Montigny-sur-Loing', 'fr': 'Montigny-sur-Loing'},
'3316443':{'en': 'Pontault-Combault', 'fr': 'Pontault-Combault'},
'3316440':{'en': u('Ozoir-la-Ferri\u00e8re'), 'fr': u('Ozoir-la-Ferri\u00e8re')},
'3316441':{'en': 'Savigny-le-Temple', 'fr': 'Savigny-le-Temple'},
'3316448':{'en': 'Longjumeau', 'fr': 'Longjumeau'},
'3316449':{'en': 'Marcoussis', 'fr': 'Marcoussis'},
'3346779':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3329880':{'en': 'Brest', 'fr': 'Brest'},
'3596042':{'bg': u('\u0418\u043b\u0438\u0439\u043d\u043e'), 'en': 'Iliyno'},
'3596043':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u041d\u043e\u0432\u043a\u043e\u0432\u043e'), 'en': 'Dolno Novkovo'},
'3596044':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u041a\u043e\u0437\u0430\u0440\u0435\u0432\u043e'), 'en': 'Dolno Kozarevo'},
'3596046':{'bg': u('\u0412\u0440\u0430\u043d\u0438 \u043a\u043e\u043d'), 'en': 'Vrani kon'},
'3329881':{'en': u('Ch\u00e2teauneuf-du-Faou'), 'fr': u('Ch\u00e2teauneuf-du-Faou')},
'3346771':{'en': 'Lunel', 'fr': 'Lunel'},
'3596049':{'bg': u('\u041a\u0430\u043c\u0431\u0443\u0440\u043e\u0432\u043e'), 'en': 'Kamburovo'},
'3346773':{'en': 'Ganges', 'fr': 'Ganges'},
'3346772':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346775':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346774':{'en': u('S\u00e8te'), 'fr': u('S\u00e8te')},
'3346777':{'en': 'Marseillan', 'fr': 'Marseillan'},
'3346776':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3329883':{'en': 'Lesneven', 'fr': 'Lesneven'},
'3593029':{'bg': u('\u041f\u0435\u0442\u043a\u043e\u0432\u043e, \u0421\u043c\u043e\u043b.'), 'en': 'Petkovo, Smol.'},
'34920':{'en': u('\u00c1vila'), 'es': u('\u00c1vila')},
'3329886':{'en': 'Chateaulin', 'fr': 'Chateaulin'},
'436548':{'de': 'Niedernsill', 'en': 'Niedernsill'},
'436549':{'de': 'Piesendorf', 'en': 'Piesendorf'},
'436546':{'de': u('Fusch an der Gro\u00dfglocknerstra\u00dfe'), 'en': 'Fusch an der Grossglocknerstrasse'},
'436547':{'de': 'Kaprun', 'en': 'Kaprun'},
'436544':{'de': 'Rauris', 'en': 'Rauris'},
'3329887':{'en': u('Pont-l\'Abb\u00e9'), 'fr': u('Pont-l\'Abb\u00e9')},
'436542':{'de': 'Zell am See', 'en': 'Zell am See'},
'436543':{'de': 'Taxenbach', 'en': 'Taxenbach'},
'436541':{'de': 'Saalbach', 'en': 'Saalbach'},
'3338618':{'en': 'Auxerre', 'fr': 'Auxerre'},
'3338820':{'en': 'Souffelweyersheim', 'fr': 'Souffelweyersheim'},
'3338821':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338823':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'333225':{'en': 'Amiens', 'fr': 'Amiens'},
'3338824':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338825':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338808':{'en': 'Barr', 'fr': 'Barr'},
'3338809':{'en': 'Niederbronn-les-Bains', 'fr': 'Niederbronn-les-Bains'},
'3338778':{'en': 'Bouzonville', 'fr': 'Bouzonville'},
'3338779':{'en': 'Boulay-Moselle', 'fr': 'Boulay-Moselle'},
'3338802':{'en': 'Saverne', 'fr': 'Saverne'},
'3338803':{'en': 'Saverne', 'fr': 'Saverne'},
'3338776':{'en': 'Metz', 'fr': 'Metz'},
'3338777':{'en': 'Vigy', 'fr': 'Vigy'},
'3338806':{'en': 'Haguenau', 'fr': 'Haguenau'},
'3338771':{'en': 'Hagondange', 'fr': 'Hagondange'},
'3338772':{'en': 'Hagondange', 'fr': 'Hagondange'},
'3338805':{'en': 'Haguenau', 'fr': 'Haguenau'},
'35947201':{'bg': u('\u0418\u0437\u0433\u0440\u0435\u0432, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Izgrev, Yambol'},
'35947202':{'bg': u('\u0416\u0440\u0435\u0431\u0438\u043d\u043e'), 'en': 'Zhrebino'},
'35947203':{'bg': u('\u0422\u0440\u044a\u043d\u043a\u043e\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Trankovo, Yambol'},
'35947204':{'bg': u('\u041f\u0447\u0435\u043b\u0430'), 'en': 'Pchela'},
'3354639':{'en': 'Royan', 'fr': 'Royan'},
'3354638':{'en': 'Royan', 'fr': 'Royan'},
'3354637':{'en': 'Nieul-sur-Mer', 'fr': 'Nieul-sur-Mer'},
'3354636':{'en': 'La Tremblade', 'fr': 'La Tremblade'},
'331835':{'en': 'Paris', 'fr': 'Paris'},
'3354632':{'en': u('Saint-Jean-d\'Ang\u00e9ly'), 'fr': u('Saint-Jean-d\'Ang\u00e9ly')},
'3354631':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3354630':{'en': u('Sainte-Marie-de-R\u00e9'), 'fr': u('Sainte-Marie-de-R\u00e9')},
'3531':{'en': 'Dublin'},
'331838':{'en': 'Paris', 'fr': 'Paris'},
'331839':{'en': 'Paris', 'fr': 'Paris'},
'3355323':{'en': 'Eymet', 'fr': 'Eymet'},
'3355320':{'en': 'Marmande', 'fr': 'Marmande'},
'3355327':{'en': 'Bergerac', 'fr': 'Bergerac'},
'3346712':{'en': 'Mauguio', 'fr': 'Mauguio'},
'3349281':{'en': 'Barcelonnette', 'fr': 'Barcelonnette'},
'3349283':{'en': 'Castellane', 'fr': 'Castellane'},
'3349284':{'en': 'Pra-Loup', 'fr': 'Pra-Loup'},
'3349285':{'en': u('La Br\u00e9ole'), 'fr': u('La Br\u00e9ole')},
'3349287':{'en': 'Manosque', 'fr': 'Manosque'},
'390864':{'it': 'Sulmona'},
'3349289':{'en': u('Saint-Andr\u00e9-les-Alpes'), 'fr': u('Saint-Andr\u00e9-les-Alpes')},
'390861':{'it': 'Teramo'},
'390862':{'en': 'L\'Aquila', 'it': 'L\'Aquila'},
'390863':{'it': 'Avezzano'},
'3354989':{'en': 'Vivonne', 'fr': 'Vivonne'},
'3354988':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354981':{'en': u('Maul\u00e9on'), 'fr': u('Maul\u00e9on')},
'3354980':{'en': 'Cerizay', 'fr': 'Cerizay'},
'3354983':{'en': 'Montmorillon', 'fr': 'Montmorillon'},
'3354982':{'en': 'Bressuire', 'fr': 'Bressuire'},
'3354985':{'en': u('Ch\u00e2tellerault'), 'fr': u('Ch\u00e2tellerault')},
'3346711':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'371645':{'en': 'Balvi'},
'371644':{'en': 'Gulbene'},
'371647':{'en': 'Valka'},
'371646':{'en': u('R\u0113zekne')},
'371641':{'en': u('C\u0113sis')},
'371640':{'en': u('Limba\u017ei')},
'371643':{'en': u('Al\u016bksne')},
'371642':{'en': 'Valmiera'},
'371649':{'en': 'Aizkraukle'},
'371648':{'en': 'Madona'},
'3593561':{'bg': u('\u0421\u0435\u043f\u0442\u0435\u043c\u0432\u0440\u0438'), 'en': 'Septemvri'},
'3593562':{'bg': u('\u0421\u043b\u0430\u0432\u043e\u0432\u0438\u0446\u0430, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Slavovitsa, Pazardzhik'},
'3593563':{'bg': u('\u0412\u0430\u0440\u0432\u0430\u0440\u0430, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Varvara, Pazardzhik'},
'3593564':{'bg': u('\u0421\u0435\u043c\u0447\u0438\u043d\u043e\u0432\u043e'), 'en': 'Semchinovo'},
'3593566':{'bg': u('\u0411\u043e\u0448\u0443\u043b\u044f'), 'en': 'Boshulya'},
'3593567':{'bg': u('\u041a\u043e\u0432\u0430\u0447\u0435\u0432\u043e, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Kovachevo, Pazardzhik'},
'3593568':{'bg': u('\u0412\u0438\u043d\u043e\u0433\u0440\u0430\u0434\u0435\u0446'), 'en': 'Vinogradets'},
'3593569':{'bg': u('\u041a\u0430\u0440\u0430\u0431\u0443\u043d\u0430\u0440'), 'en': 'Karabunar'},
'3346710':{'en': 'Montpellier', 'fr': 'Montpellier'},
'432731':{'de': 'Idolsberg', 'en': 'Idolsberg'},
'432733':{'de': u('Sch\u00f6nberg am Kamp'), 'en': u('Sch\u00f6nberg am Kamp')},
'432732':{'de': 'Krems an der Donau', 'en': 'Krems an der Donau'},
'3332048':{'en': 'Nieppe', 'fr': 'Nieppe'},
'3332049':{'en': 'Lille', 'fr': 'Lille'},
'432736':{'de': 'Paudorf', 'en': 'Paudorf'},
'432739':{'de': 'Tiefenfucha', 'en': 'Tiefenfucha'},
'3332045':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332047':{'en': 'Villeneuve-d\'Ascq', 'fr': 'Villeneuve-d\'Ascq'},
'3332040':{'en': 'Lille', 'fr': 'Lille'},
'3332041':{'en': 'Villeneuve-d\'Ascq', 'fr': 'Villeneuve-d\'Ascq'},
'3332042':{'en': 'Lille', 'fr': 'Lille'},
'3332043':{'en': 'Villeneuve-d\'Ascq', 'fr': 'Villeneuve-d\'Ascq'},
'3323514':{'en': 'Rouen', 'fr': 'Rouen'},
'3323515':{'en': 'Rouen', 'fr': 'Rouen'},
'3323510':{'en': u('F\u00e9camp'), 'fr': u('F\u00e9camp')},
'3323512':{'en': 'Mont-Saint-Aignan', 'fr': 'Mont-Saint-Aignan'},
'3355589':{'en': 'Dun-le-Palestel', 'fr': 'Dun-le-Palestel'},
'3355586':{'en': 'Brive-la-Gaillarde', 'fr': 'Brive-la-Gaillarde'},
'3355587':{'en': 'Brive-la-Gaillarde', 'fr': 'Brive-la-Gaillarde'},
'3355584':{'en': 'Allassac', 'fr': 'Allassac'},
'3329923':{'en': u('Saint-Gr\u00e9goire'), 'fr': u('Saint-Gr\u00e9goire')},
'3323519':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3329921':{'en': 'Saint-Malo', 'fr': 'Saint-Malo'},
'3329920':{'en': 'Saint-Malo', 'fr': 'Saint-Malo'},
'3349424':{'en': 'Toulon', 'fr': 'Toulon'},
'3349425':{'en': 'Six-Fours-les-Plages', 'fr': 'Six-Fours-les-Plages'},
'3349426':{'en': 'Saint-Cyr-sur-Mer', 'fr': 'Saint-Cyr-sur-Mer'},
'3349427':{'en': 'Toulon', 'fr': 'Toulon'},
'3349420':{'en': 'Toulon', 'fr': 'Toulon'},
'3349421':{'en': 'La Garde', 'fr': 'La Garde'},
'3349422':{'en': 'Toulon', 'fr': 'Toulon'},
'3349423':{'en': 'Toulon', 'fr': 'Toulon'},
'3593784':{'bg': u('\u041a\u043e\u043d\u0441\u0442\u0430\u043d\u0442\u0438\u043d\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Konstantinovo, Hask.'},
'3593785':{'bg': u('\u0414\u0440\u044f\u043d\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Dryanovo, Hask.'},
'3593786':{'bg': u('\u041d\u0430\u0432\u044a\u0441\u0435\u043d'), 'en': 'Navasen'},
'3593787':{'bg': u('\u0422\u044f\u043d\u0435\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Tyanevo, Hask.'},
'3349428':{'en': 'Cuers', 'fr': 'Cuers'},
'3349429':{'en': 'Bandol', 'fr': 'Bandol'},
'3593782':{'bg': u('\u041a\u0430\u043b\u0443\u0433\u0435\u0440\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Kalugerovo, Hask.'},
'3593783':{'bg': u('\u0421\u0432\u0438\u0440\u043a\u043e\u0432\u043e'), 'en': 'Svirkovo'},
'441548':{'en': 'Kingsbridge'},
'441549':{'en': 'Lairg'},
'441544':{'en': 'Kington'},
'441545':{'en': 'Llanarth'},
'441546':{'en': 'Lochgilphead'},
'441547':{'en': 'Knighton'},
'441540':{'en': 'Kingussie'},
'441542':{'en': 'Keith'},
'441543':{'en': 'Cannock'},
'3324896':{'en': 'Saint-Amand-Montrond', 'fr': 'Saint-Amand-Montrond'},
'3599110':{'bg': u('\u0412\u0438\u0440\u043e\u0432\u0441\u043a\u043e'), 'en': 'Virovsko'},
'373263':{'en': 'Leova', 'ro': 'Leova', 'ru': u('\u041b\u0435\u043e\u0432\u0430')},
'373262':{'en': 'Singerei', 'ro': u('S\u00eengerei'), 'ru': u('\u0421\u044b\u043d\u0436\u0435\u0440\u0435\u0439')},
'373265':{'en': 'Anenii Noi', 'ro': 'Anenii Noi', 'ru': u('\u0410\u043d\u0435\u043d\u0438\u0439 \u041d\u043e\u0439')},
'373264':{'en': 'Nisporeni', 'ro': 'Nisporeni', 'ru': u('\u041d\u0438\u0441\u043f\u043e\u0440\u0435\u043d\u044c')},
'373269':{'en': 'Hincesti', 'ro': u('H\u00eence\u015fti'), 'ru': u('\u0425\u044b\u043d\u0447\u0435\u0448\u0442\u044c')},
'373268':{'en': 'Ialoveni', 'ro': 'Ialoveni', 'ru': u('\u042f\u043b\u043e\u0432\u0435\u043d\u044c')},
'3355302':{'en': u('P\u00e9rigueux'), 'fr': u('P\u00e9rigueux')},
'3324094':{'en': u('Treilli\u00e8res'), 'fr': u('Treilli\u00e8res')},
'3324095':{'en': 'Nantes', 'fr': 'Nantes'},
'3324096':{'en': 'Ancenis', 'fr': 'Ancenis'},
'435522':{'de': 'Feldkirch', 'en': 'Feldkirch'},
'3324090':{'en': 'Trignac', 'fr': 'Trignac'},
'3324091':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'3324092':{'en': 'Saint-Herblain', 'fr': 'Saint-Herblain'},
'3324093':{'en': 'Nantes', 'fr': 'Nantes'},
'3355306':{'en': u('P\u00e9rigueux'), 'fr': u('P\u00e9rigueux')},
'441467':{'en': 'Inverurie'},
'355311':{'en': u('Ku\u00e7ov\u00eb')},
'355313':{'en': u('Ballsh, Mallakast\u00ebr')},
'355312':{'en': u('\u00c7orovod\u00eb, Skrapar')},
'441465':{'en': 'Girvan'},
'3356272':{'en': 'Toulouse', 'fr': 'Toulouse'},
'441463':{'en': 'Inverness'},
'441461':{'en': 'Gretna'},
'441460':{'en': 'Chard'},
'3595784':{'bg': u('\u0412\u0440\u0430\u0447\u0430\u043d\u0446\u0438'), 'en': 'Vrachantsi'},
'3347369':{'en': 'Cournon-d\'Auvergne', 'fr': 'Cournon-d\'Auvergne'},
'3595783':{'bg': u('\u041a\u043e\u0442\u043b\u0435\u043d\u0446\u0438'), 'en': 'Kotlentsi'},
'3595781':{'bg': u('\u0421\u0432\u043e\u0431\u043e\u0434\u0430, \u0414\u043e\u0431\u0440.'), 'en': 'Svoboda, Dobr.'},
'3347365':{'en': 'Le Mont Dore', 'fr': 'Le Mont Dore'},
'3347364':{'en': 'Riom', 'fr': 'Riom'},
'3347367':{'en': 'Riom', 'fr': 'Riom'},
'3347361':{'en': 'Lempdes', 'fr': 'Lempdes'},
'3347360':{'en': 'Nohanent', 'fr': 'Nohanent'},
'3347362':{'en': 'Orcines', 'fr': 'Orcines'},
'3596527':{'bg': u('\u041e\u0434\u044a\u0440\u043d\u0435'), 'en': 'Odarne'},
'3596526':{'bg': u('\u0412\u044a\u0440\u0431\u0438\u0446\u0430, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Varbitsa, Pleven'},
'3596525':{'bg': u('\u0411\u044a\u0440\u043a\u0430\u0447'), 'en': 'Barkach'},
'3596524':{'bg': u('\u041f\u0435\u0442\u044a\u0440\u043d\u0438\u0446\u0430'), 'en': 'Petarnitsa'},
'3596523':{'bg': u('\u041a\u0440\u0443\u0448\u043e\u0432\u0438\u0446\u0430, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Krushovitsa, Pleven'},
'3596522':{'bg': u('\u0417\u0433\u0430\u043b\u0435\u0432\u043e'), 'en': 'Zgalevo'},
'3596521':{'bg': u('\u0421\u0430\u0434\u043e\u0432\u0435\u0446'), 'en': 'Sadovets'},
'3596520':{'bg': u('\u041d\u0438\u043a\u043e\u043b\u0430\u0435\u0432\u043e, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Nikolaevo, Pleven'},
'3596529':{'bg': u('\u041a\u043e\u0438\u043b\u043e\u0432\u0446\u0438'), 'en': 'Koilovtsi'},
'3596528':{'bg': u('\u0412\u044a\u043b\u0447\u0438\u0442\u0440\u044a\u043d'), 'en': 'Valchitran'},
'436229':{'de': 'Hof bei Salzburg', 'en': 'Hof bei Salzburg'},
'4414349':{'en': 'Bellingham'},
'4414348':{'en': 'Hexham'},
'4414345':{'en': 'Haltwhistle'},
'3315842':{'en': 'Choisy-le-Roi', 'fr': 'Choisy-le-Roi'},
'4414347':{'en': 'Hexham'},
'4414346':{'en': 'Hexham'},
'4414341':{'en': 'Bellingham/Haltwhistle/Hexham'},
'4414340':{'en': 'Bellingham/Haltwhistle/Hexham'},
'4414343':{'en': 'Haltwhistle'},
'4414342':{'en': 'Bellingham'},
'359570':{'bg': u('\u041a\u0430\u0432\u0430\u0440\u043d\u0430'), 'en': 'Kavarna'},
'359579':{'bg': u('\u0410\u043b\u0431\u0435\u043d\u0430'), 'en': 'Albena'},
'35961203':{'bg': u('\u0415\u043c\u0435\u043d'), 'en': 'Emen'},
'3347941':{'en': u('Val-d\'Is\u00e8re'), 'fr': u('Val-d\'Is\u00e8re')},
'3343440':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3347942':{'en': 'Belley', 'fr': 'Belley'},
'3347944':{'en': u('Chamb\u00e9ry'), 'fr': u('Chamb\u00e9ry')},
'3597438':{'bg': u('\u041a\u0430\u0442\u0443\u043d\u0446\u0438'), 'en': 'Katuntsi'},
'3343443':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3597928':{'bg': u('\u0413\u044a\u0440\u043b\u044f\u043d\u043e'), 'en': 'Garlyano'},
'3597929':{'bg': u('\u0421\u043e\u0432\u043e\u043b\u044f\u043d\u043e'), 'en': 'Sovolyano'},
'3323218':{'en': 'Rouen', 'fr': 'Rouen'},
'390473':{'it': 'Merano'},
'390472':{'it': 'Bressanone'},
'390471':{'en': 'Bolzano/Bozen', 'it': 'Bolzano'},
'3323212':{'en': 'Rouen', 'fr': 'Rouen'},
'3323211':{'en': 'Le Grand Quevilly', 'fr': 'Le Grand Quevilly'},
'3323210':{'en': 'Rouen', 'fr': 'Rouen'},
'3323214':{'en': 'Dieppe', 'fr': 'Dieppe'},
'3597124':{'bg': u('\u0411\u0435\u043b\u0447\u0438\u043d\u0441\u043a\u0438 \u0431\u0430\u043d\u0438'), 'en': 'Belchinski bani'},
'3597125':{'bg': u('\u0413\u043e\u0432\u0435\u0434\u0430\u0440\u0446\u0438'), 'en': 'Govedartsi'},
'3597126':{'bg': u('\u0413\u043e\u0440\u043d\u0438 \u041e\u043a\u043e\u043b'), 'en': 'Gorni Okol'},
'3597127':{'bg': u('\u0428\u0438\u0440\u043e\u043a\u0438 \u0434\u043e\u043b'), 'en': 'Shiroki dol'},
'3597120':{'bg': u('\u0414\u043e\u043b\u043d\u0430 \u0431\u0430\u043d\u044f'), 'en': 'Dolna banya'},
'3597123':{'bg': u('\u041a\u043e\u0432\u0430\u0447\u0435\u0432\u0446\u0438, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Kovachevtsi, Sofia'},
'3597129':{'bg': u('\u0420\u0430\u0434\u0443\u0438\u043b'), 'en': 'Raduil'},
'3597927':{'bg': u('\u0422\u0440\u0435\u043a\u043b\u044f\u043d\u043e'), 'en': 'Treklyano'},
'3597436':{'bg': u('\u041b\u0435\u0432\u0443\u043d\u043e\u0432\u043e'), 'en': 'Levunovo'},
'3597437':{'bg': u('\u041c\u0435\u043b\u043d\u0438\u043a'), 'en': 'Melnik'},
'3342244':{'en': 'Toulon', 'fr': 'Toulon'},
'3597434':{'bg': u('\u0421\u0442\u0440\u0443\u043c\u044f\u043d\u0438'), 'en': 'Strumyani'},
'3597923':{'bg': u('\u0412\u0440\u0430\u0442\u0446\u0430'), 'en': 'Vrattsa'},
'3345042':{'en': 'Saint-Genis-Pouilly', 'fr': 'Saint-Genis-Pouilly'},
'3597744':{'bg': u('\u0415\u043b\u043e\u0432\u0434\u043e\u043b, \u041f\u0435\u0440\u043d\u0438\u043a'), 'en': 'Elovdol, Pernik'},
'3345040':{'en': 'Ferney-Voltaire', 'fr': 'Ferney-Voltaire'},
'3345041':{'en': 'Gex', 'fr': 'Gex'},
'3345045':{'en': 'Annecy', 'fr': 'Annecy'},
'3345048':{'en': 'Bellegarde-sur-Valserine', 'fr': 'Bellegarde-sur-Valserine'},
'3345049':{'en': 'Saint-Julien-en-Genevois', 'fr': 'Saint-Julien-en-Genevois'},
'3345739':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3326249':{'en': 'Saint-Pierre', 'fr': 'Saint-Pierre'},
'3326248':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326247':{'en': 'Salazie', 'fr': 'Salazie'},
'3326246':{'en': u('Saint-Andr\u00e9'), 'fr': u('Saint-Andr\u00e9')},
'3326245':{'en': 'Saint-Paul', 'fr': 'Saint-Paul'},
'3326244':{'en': 'Saint-Paul', 'fr': 'Saint-Paul'},
'3326243':{'en': 'Le Port', 'fr': 'Le Port'},
'3326242':{'en': 'Le Port', 'fr': 'Le Port'},
'3326241':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326240':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3317413':{'en': 'Saint-Germain-en-Laye', 'fr': 'Saint-Germain-en-Laye'},
'3597743':{'bg': u('\u0414\u0438\u0432\u043b\u044f'), 'en': 'Divlya'},
'3751562':{'be': u('\u0421\u043b\u043e\u043d\u0456\u043c'), 'en': 'Slonim', 'ru': u('\u0421\u043b\u043e\u043d\u0438\u043c')},
'3751563':{'be': u('\u0414\u0437\u044f\u0442\u043b\u0430\u0432\u0430, \u0413\u0440\u043e\u0434\u0437\u0435\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Dyatlovo, Grodno Region', 'ru': u('\u0414\u044f\u0442\u043b\u043e\u0432\u043e, \u0413\u0440\u043e\u0434\u043d\u0435\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751564':{'be': u('\u0417\u044d\u043b\u044c\u0432\u0430, \u0413\u0440\u043e\u0434\u0437\u0435\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Zelva, Grodno Region', 'ru': u('\u0417\u0435\u043b\u044c\u0432\u0430, \u0413\u0440\u043e\u0434\u043d\u0435\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3332709':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3332708':{'en': 'Douai', 'fr': 'Douai'},
'433473':{'de': 'Straden', 'en': 'Straden'},
'433472':{'de': 'Mureck', 'en': 'Mureck'},
'433475':{'de': u('H\u00fcrth'), 'en': u('H\u00fcrth')},
'433474':{'de': 'Deutsch Goritz', 'en': 'Deutsch Goritz'},
'433477':{'de': 'Sankt Peter am Ottersbach', 'en': 'St. Peter am Ottersbach'},
'433476':{'de': 'Bad Radkersburg', 'en': 'Bad Radkersburg'},
'375222':{'be': u('\u041c\u0430\u0433\u0456\u043b\u0451\u045e'), 'en': 'Mogilev', 'ru': u('\u041c\u043e\u0433\u0438\u043b\u0435\u0432')},
'375225':{'be': u('\u0411\u0430\u0431\u0440\u0443\u0439\u0441\u043a'), 'en': 'Babruysk', 'ru': u('\u0411\u043e\u0431\u0440\u0443\u0439\u0441\u043a')},
'35963202':{'bg': u('\u0414\u0440\u0430\u0433\u043e\u043c\u0438\u0440\u043e\u0432\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Dragomirovo, V. Tarnovo'},
'35963203':{'bg': u('\u0425\u0430\u0434\u0436\u0438\u0434\u0438\u043c\u0438\u0442\u0440\u043e\u0432\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Hadzhidimitrovo, V. Tarnovo'},
'35963204':{'bg': u('\u0414\u0435\u043b\u044f\u043d\u043e\u0432\u0446\u0438'), 'en': 'Delyanovtsi'},
'35963205':{'bg': u('\u0427\u0435\u0440\u0432\u0435\u043d\u0430'), 'en': 'Chervena'},
'35346':{'en': 'Navan/Kells/Trim/Edenderry/Enfield'},
'35347':{'en': 'Monaghan/Clones'},
'3356161':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356160':{'en': 'Pamiers', 'fr': 'Pamiers'},
'3356163':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356162':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356165':{'en': 'Foix', 'fr': 'Foix'},
'35344':{'en': 'Mullingar/Castlepollard/Tyrrellspass'},
'3356167':{'en': 'Pamiers', 'fr': 'Pamiers'},
'3356166':{'en': 'Saint-Girons', 'fr': 'Saint-Girons'},
'3344262':{'en': 'Aubagne', 'fr': 'Aubagne'},
'35342':{'en': 'Dundalk/Carrickmacross/Castleblaney'},
'3344264':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'432757':{'de': u('P\u00f6chlarn'), 'en': u('P\u00f6chlarn')},
'35341':{'en': 'Drogheda/Ardee'},
'37428495':{'am': u('\u0547\u056b\u0576\u0578\u0582\u0570\u0561\u0575\u0580'), 'en': 'Shinuhayr', 'ru': u('\u0428\u0438\u043d\u0443\u0430\u0439\u0440')},
'34983':{'en': 'Valladolid', 'es': 'Valladolid'},
'34982':{'en': 'Lugo', 'es': 'Lugo'},
'34981':{'en': u('Coru\u00f1a'), 'es': u('Coru\u00f1a')},
'34980':{'en': 'Zamora', 'es': 'Zamora'},
'3593043':{'bg': u('\u0417\u043c\u0435\u0438\u0446\u0430'), 'en': 'Zmeitsa'},
'34986':{'en': 'Pontevedra', 'es': 'Pontevedra'},
'3593041':{'bg': u('\u0414\u0435\u0432\u0438\u043d'), 'en': 'Devin'},
'34984':{'en': 'Asturias', 'es': 'Asturias'},
'34988':{'en': 'Ourense', 'es': 'Orense'},
'3593049':{'bg': u('\u0411\u0435\u0434\u0435\u043d'), 'en': 'Beden'},
'3751514':{'be': u('\u0428\u0447\u0443\u0447\u044b\u043d, \u0413\u0440\u043e\u0434\u0437\u0435\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Shchuchin, Grodno Region', 'ru': u('\u0429\u0443\u0447\u0438\u043d, \u0413\u0440\u043e\u0434\u043d\u0435\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3599559':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u041a\u043e\u0432\u0430\u0447\u0438\u0446\u0430'), 'en': 'Gorna Kovachitsa'},
'3599558':{'bg': u('\u0413\u0430\u0432\u0440\u0438\u043b \u0413\u0435\u043d\u043e\u0432\u043e'), 'en': 'Gavril Genovo'},
'3599557':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u0426\u0435\u0440\u043e\u0432\u0435\u043d\u0435'), 'en': 'Gorno Tserovene'},
'3599556':{'bg': u('\u0413\u043e\u0432\u0435\u0436\u0434\u0430'), 'en': 'Govezhda'},
'3599555':{'bg': u('\u041a\u043e\u043f\u0438\u043b\u043e\u0432\u0446\u0438, \u041c\u043e\u043d\u0442.'), 'en': 'Kopilovtsi, Mont.'},
'3599554':{'bg': u('\u0427\u0438\u043f\u0440\u043e\u0432\u0446\u0438'), 'en': 'Chiprovtsi'},
'3599553':{'bg': u('\u041f\u0440\u0435\u0432\u0430\u043b\u0430'), 'en': 'Prevala'},
'3599552':{'bg': u('\u0411\u0435\u043b\u0438\u043c\u0435\u043b'), 'en': 'Belimel'},
'3347638':{'en': 'Saint-Marcellin', 'fr': 'Saint-Marcellin'},
'3599550':{'bg': u('\u041c\u0438\u0442\u0440\u043e\u0432\u0446\u0438'), 'en': 'Mitrovtsi'},
'3324793':{'en': 'Chinon', 'fr': 'Chinon'},
'3324791':{'en': 'Loches', 'fr': 'Loches'},
'3324797':{'en': 'Bourgueil', 'fr': 'Bourgueil'},
'3324796':{'en': 'Langeais', 'fr': 'Langeais'},
'3324798':{'en': 'Chinon', 'fr': 'Chinon'},
'35278':{'de': 'Junglinster', 'en': 'Junglinster', 'fr': 'Junglinster'},
'35279':{'de': 'Berdorf/Consdorf', 'en': 'Berdorf/Consdorf', 'fr': 'Berdorf/Consdorf'},
'3598628':{'bg': u('\u0426\u0430\u0440 \u0410\u0441\u0435\u043d, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Tsar Asen, Silistra'},
'35272':{'de': 'Echternach', 'en': 'Echternach', 'fr': 'Echternach'},
'35273':{'de': 'Rosport', 'en': 'Rosport', 'fr': 'Rosport'},
'35271':{'de': 'Betzdorf', 'en': 'Betzdorf', 'fr': 'Betzdorf'},
'35276':{'de': 'Wormeldingen', 'en': 'Wormeldange', 'fr': 'Wormeldange'},
'35274':{'de': 'Wasserbillig', 'en': 'Wasserbillig', 'fr': 'Wasserbillig'},
'35275':{'de': 'Distrikt Grevenmacher', 'en': 'Grevenmacher', 'fr': 'Grevenmacher'},
'3353531':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'359361':{'bg': u('\u041a\u044a\u0440\u0434\u0436\u0430\u043b\u0438'), 'en': 'Kardzhali'},
'441489':{'en': 'Bishops Waltham'},
'441488':{'en': 'Hungerford'},
'441481':{'en': 'Guernsey'},
'3598622':{'bg': u('\u0410\u043b\u0435\u043a\u043e\u0432\u043e, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Alekovo, Silistra'},
'441483':{'en': 'Guildford'},
'441482':{'en': 'Kingston-upon-Hull'},
'441485':{'en': 'Hunstanton'},
'441484':{'en': 'Huddersfield'},
'441487':{'en': 'Warboys'},
'3598623':{'bg': u('\u0413\u043e\u043b\u0435\u0448, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Golesh, Silistra'},
'3356345':{'en': u('Saint-Ju\u00e9ry'), 'fr': u('Saint-Ju\u00e9ry')},
'3356347':{'en': 'Albi', 'fr': 'Albi'},
'3356346':{'en': 'Albi', 'fr': 'Albi'},
'3356343':{'en': 'Albi', 'fr': 'Albi'},
'3356342':{'en': 'Graulhet', 'fr': 'Graulhet'},
'3356349':{'en': 'Albi', 'fr': 'Albi'},
'3356348':{'en': 'Albi', 'fr': 'Albi'},
'351274':{'en': u('Proen\u00e7a-a-Nova'), 'pt': u('Proen\u00e7a-a-Nova')},
'441733':{'en': 'Peterborough'},
'441732':{'en': 'Sevenoaks'},
'351275':{'en': u('Covilh\u00e3'), 'pt': u('Covilh\u00e3')},
'441737':{'en': 'Redhill'},
'441736':{'en': 'Penzance'},
'441738':{'en': 'Perth'},
'3346713':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3316421':{'en': 'Chelles', 'fr': 'Chelles'},
'3324135':{'en': 'Angers', 'fr': 'Angers'},
'3324134':{'en': 'Angers', 'fr': 'Angers'},
'3346716':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346715':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3316427':{'en': 'Villeparisis', 'fr': 'Villeparisis'},
'3316428':{'en': 'Nemours', 'fr': 'Nemours'},
'3346718':{'en': u('S\u00e8te'), 'fr': u('S\u00e8te')},
'35584':{'en': u('Gjirokast\u00ebr')},
'35585':{'en': u('Sarand\u00eb')},
'3596068':{'bg': u('\u0411\u043e\u0436\u0443\u0440\u043a\u0430'), 'en': 'Bozhurka'},
'3596069':{'bg': u('\u0412\u0430\u0441\u0438\u043b \u041b\u0435\u0432\u0441\u043a\u0438, \u0422\u044a\u0440\u0433.'), 'en': 'Vasil Levski, Targ.'},
'39039':{'en': 'Monza', 'it': 'Monza'},
'35582':{'en': u('Kor\u00e7\u00eb')},
'35583':{'en': 'Pogradec'},
'3596062':{'bg': u('\u0421\u0442\u0440\u0430\u0436\u0430, \u0422\u044a\u0440\u0433.'), 'en': 'Strazha, Targ.'},
'3596063':{'bg': u('\u0411\u0430\u044f\u0447\u0435\u0432\u043e'), 'en': 'Bayachevo'},
'3596060':{'bg': u('\u041e\u0432\u0447\u0430\u0440\u043e\u0432\u043e, \u0422\u044a\u0440\u0433.'), 'en': 'Ovcharovo, Targ.'},
'3596061':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u043e \u0421\u043e\u043a\u043e\u043b\u043e\u0432\u043e'), 'en': 'Golyamo Sokolovo'},
'3596066':{'bg': u('\u0411\u0443\u0439\u043d\u043e\u0432\u043e, \u0422\u044a\u0440\u0433.'), 'en': 'Buynovo, Targ.'},
'3596067':{'bg': u('\u041a\u0440\u0430\u043b\u0435\u0432\u043e, \u0422\u044a\u0440\u0433.'), 'en': 'Kralevo, Targ.'},
'3596064':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u043e \u041d\u043e\u0432\u043e'), 'en': 'Golyamo Novo'},
'3596065':{'bg': u('\u0411\u0438\u0441\u0442\u0440\u0430, \u0422\u044a\u0440\u0433.'), 'en': 'Bistra, Targ.'},
'3336707':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3336708':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'351278':{'en': 'Mirandela', 'pt': 'Mirandela'},
'3324038':{'en': u('Cou\u00ebron'), 'fr': u('Cou\u00ebron')},
'436562':{'de': 'Mittersill', 'en': 'Mittersill'},
'3347685':{'en': 'Grenoble', 'fr': 'Grenoble'},
'436564':{'de': 'Krimml', 'en': 'Krimml'},
'436565':{'de': u('Neukirchen am Gro\u00dfvenediger'), 'en': 'Neukirchen am Grossvenediger'},
'436566':{'de': 'Bramberg am Wildkogel', 'en': 'Bramberg am Wildkogel'},
'3338631':{'en': 'Avallon', 'fr': 'Avallon'},
'3338630':{'en': 'Luzy', 'fr': 'Luzy'},
'3338635':{'en': 'Saint-Florentin', 'fr': 'Saint-Florentin'},
'3338634':{'en': 'Avallon', 'fr': 'Avallon'},
'3338636':{'en': 'Nevers', 'fr': 'Nevers'},
'3338639':{'en': 'Pouilly-sur-Loire', 'fr': 'Pouilly-sur-Loire'},
'3338756':{'en': 'Metz', 'fr': 'Metz'},
'441874':{'en': 'Brecon'},
'3338755':{'en': 'Metz', 'fr': 'Metz'},
'3338753':{'en': 'Amanvillers', 'fr': 'Amanvillers'},
'3338750':{'en': 'Metz', 'fr': 'Metz'},
'3338751':{'en': u('Maizi\u00e8res-l\u00e8s-Metz'), 'fr': u('Maizi\u00e8res-l\u00e8s-Metz')},
'3751775':{'be': u('\u0416\u043e\u0434\u0437\u0456\u043d\u0430'), 'en': 'Zhodino', 'ru': u('\u0416\u043e\u0434\u0438\u043d\u043e')},
'3751774':{'be': u('\u041b\u0430\u0433\u043e\u0439\u0441\u043a'), 'en': 'Lahoysk', 'ru': u('\u041b\u043e\u0433\u043e\u0439\u0441\u043a')},
'3751776':{'be': u('\u0421\u043c\u0430\u043b\u044f\u0432\u0456\u0447\u044b'), 'en': 'Smalyavichy', 'ru': u('\u0421\u043c\u043e\u043b\u0435\u0432\u0438\u0447\u0438')},
'3751771':{'be': u('\u0412\u0456\u043b\u0435\u0439\u043a\u0430'), 'en': 'Vileyka', 'ru': u('\u0412\u0438\u043b\u0435\u0439\u043a\u0430')},
'3751770':{'be': u('\u041d\u044f\u0441\u0432\u0456\u0436'), 'en': 'Nesvizh', 'ru': u('\u041d\u0435\u0441\u0432\u0438\u0436')},
'3338758':{'en': 'Moyeuvre-Grande', 'fr': 'Moyeuvre-Grande'},
'3751772':{'be': u('\u0412\u0430\u043b\u043e\u0436\u044b\u043d'), 'en': 'Volozhin', 'ru': u('\u0412\u043e\u043b\u043e\u0436\u0438\u043d')},
'35955504':{'bg': u('\u0411\u043e\u0433\u0434\u0430\u043d\u043e\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Bogdanovo, Burgas'},
'35955505':{'bg': u('\u0414\u0440\u0430\u0447\u0435\u0432\u043e'), 'en': 'Drachevo'},
'35955502':{'bg': u('\u0421\u0443\u0445\u043e\u0434\u043e\u043b, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Suhodol, Burgas'},
'3338258':{'en': 'Florange', 'fr': 'Florange'},
'3332066':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332067':{'en': 'Villeneuve-d\'Ascq', 'fr': 'Villeneuve-d\'Ascq'},
'3593548':{'bg': u('\u041f\u0430\u0448\u043e\u0432\u043e'), 'en': 'Pashovo'},
'3332065':{'en': u('Marcq-en-Bar\u0153ul'), 'fr': u('Marcq-en-Bar\u0153ul')},
'3332062':{'en': 'Seclin', 'fr': 'Seclin'},
'3332063':{'en': 'Lille', 'fr': 'Lille'},
'3332060':{'en': 'Wattignies', 'fr': 'Wattignies'},
'3593542':{'bg': u('\u0420\u0430\u043a\u0438\u0442\u043e\u0432\u043e'), 'en': 'Rakitovo'},
'3593543':{'bg': u('\u0414\u043e\u0440\u043a\u043e\u0432\u043e'), 'en': 'Dorkovo'},
'3593547':{'bg': u('\u0421\u044a\u0440\u043d\u0438\u0446\u0430, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Sarnitsa, Pazardzhik'},
'3332068':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3332069':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'432719':{'de': u('Dro\u00df'), 'en': 'Dross'},
'432718':{'de': 'Lichtenau im Waldviertel', 'en': 'Lichtenau im Waldviertel'},
'432249':{'de': u('Gro\u00df-Enzersdorf'), 'en': 'Gross-Enzersdorf'},
'34875':{'en': 'Soria', 'es': 'Soria'},
'3338436':{'en': 'Delle', 'fr': 'Delle'},
'432245':{'de': 'Wolkersdorf im Weinviertel', 'en': 'Wolkersdorf im Weinviertel'},
'432244':{'de': 'Langenzersdorf', 'en': 'Langenzersdorf'},
'432247':{'de': 'Deutsch-Wagram', 'en': 'Deutsch-Wagram'},
'432246':{'de': 'Gerasdorf bei Wien', 'en': 'Gerasdorf bei Wien'},
'432717':{'de': 'Unter-Meisling', 'en': 'Unter-Meisling'},
'432716':{'de': u('Gf\u00f6hl'), 'en': u('Gf\u00f6hl')},
'432243':{'de': 'Klosterneuburg', 'en': 'Klosterneuburg'},
'432242':{'de': u('Sankt Andr\u00e4-W\u00f6rdern'), 'en': u('St. Andr\u00e4-W\u00f6rdern')},
'3355876':{'en': 'Saint-Sever', 'fr': 'Saint-Sever'},
'3355877':{'en': 'Saint-Vincent-de-Tyrosse', 'fr': 'Saint-Vincent-de-Tyrosse'},
'3355874':{'en': 'Dax', 'fr': 'Dax'},
'3355875':{'en': 'Mont-de-Marsan', 'fr': 'Mont-de-Marsan'},
'3355872':{'en': 'Capbreton', 'fr': 'Capbreton'},
'3355873':{'en': 'Peyrehorade', 'fr': 'Peyrehorade'},
'3355871':{'en': 'Aire-sur-l\'Adour', 'fr': 'Aire-sur-l\'Adour'},
'3338921':{'en': 'Colmar', 'fr': 'Colmar'},
'3355878':{'en': 'Biscarrosse', 'fr': 'Biscarrosse'},
'3355879':{'en': 'Hagetmau', 'fr': 'Hagetmau'},
'3329909':{'en': 'Montfort-sur-Meu', 'fr': 'Montfort-sur-Meu'},
'3341359':{'en': 'Marseille', 'fr': 'Marseille'},
'3323538':{'en': 'Lillebonne', 'fr': 'Lillebonne'},
'3323536':{'en': 'Canteleu', 'fr': 'Canteleu'},
'3323537':{'en': 'Duclair', 'fr': 'Duclair'},
'3338430':{'en': 'Lure', 'fr': 'Lure'},
'3329905':{'en': 'Bruz', 'fr': 'Bruz'},
'3323530':{'en': 'Montivilliers', 'fr': 'Montivilliers'},
'3323531':{'en': 'Bolbec', 'fr': 'Bolbec'},
'3332393':{'en': 'Soissons', 'fr': 'Soissons'},
'3349408':{'en': 'La Garde', 'fr': 'La Garde'},
'3349409':{'en': 'Toulon', 'fr': 'Toulon'},
'3349406':{'en': 'La Seyne sur Mer', 'fr': 'La Seyne sur Mer'},
'3349407':{'en': 'Six-Fours-les-Plages', 'fr': 'Six-Fours-les-Plages'},
'3349404':{'en': u('Carc\u00e8s'), 'fr': u('Carc\u00e8s')},
'3349403':{'en': 'Toulon', 'fr': 'Toulon'},
'3349400':{'en': u('Hy\u00e8res'), 'fr': u('Hy\u00e8res')},
'3349401':{'en': u('Hy\u00e8res'), 'fr': u('Hy\u00e8res')},
'441904':{'en': 'York'},
'441905':{'en': 'Worcester'},
'441900':{'en': 'Workington'},
'441902':{'en': 'Wolverhampton'},
'441903':{'en': 'Worthing'},
'441908':{'en': 'Milton Keynes'},
'432611':{'de': 'Mannersdorf an der Rabnitz', 'en': 'Mannersdorf an der Rabnitz'},
'3355701':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'433883':{'de': 'Terz', 'en': 'Terz'},
'3323301':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'437232':{'de': u('Sankt Martin im M\u00fchlkreis'), 'en': u('St. Martin im M\u00fchlkreis')},
'3323303':{'en': u('\u00c9queurdreville-Hainneville'), 'fr': u('\u00c9queurdreville-Hainneville')},
'3332934':{'en': u('\u00c9pinal'), 'fr': u('\u00c9pinal')},
'3332935':{'en': u('\u00c9pinal'), 'fr': u('\u00c9pinal')},
'3332937':{'en': 'Mirecourt', 'fr': 'Mirecourt'},
'3332930':{'en': 'Le Val-d\'Ajol', 'fr': 'Le Val-d\'Ajol'},
'3332931':{'en': u('\u00c9pinal'), 'fr': u('\u00c9pinal')},
'3332932':{'en': u('\u00c9loyes'), 'fr': u('\u00c9loyes')},
'3332933':{'en': u('\u00c9pinal'), 'fr': u('\u00c9pinal')},
'3324304':{'en': 'Mayenne', 'fr': 'Mayenne'},
'3324305':{'en': u('Ern\u00e9e'), 'fr': u('Ern\u00e9e')},
'3324307':{'en': u('Ch\u00e2teau-Gontier'), 'fr': u('Ch\u00e2teau-Gontier')},
'3332938':{'en': 'Charmes', 'fr': 'Charmes'},
'3324301':{'en': u('\u00c9vron'), 'fr': u('\u00c9vron')},
'437231':{'de': 'Herzogsdorf', 'en': 'Herzogsdorf'},
'433624':{'de': 'Pichl-Kainisch', 'en': 'Pichl-Kainisch'},
'3355393':{'en': 'Casteljaloux', 'fr': 'Casteljaloux'},
'433622':{'de': 'Bad Aussee', 'en': 'Bad Aussee'},
'3323308':{'en': u('\u00c9queurdreville-Hainneville'), 'fr': u('\u00c9queurdreville-Hainneville')},
'373249':{'en': 'Glodeni', 'ro': 'Glodeni', 'ru': u('\u0413\u043b\u043e\u0434\u0435\u043d\u044c')},
'3329821':{'en': 'Landerneau', 'fr': 'Landerneau'},
'3596770':{'bg': u('\u041f\u043b\u0430\u0447\u043a\u043e\u0432\u0446\u0438'), 'en': 'Plachkovtsi'},
'373243':{'en': 'Causeni', 'ro': u('C\u0103u\u015feni'), 'ru': u('\u041a\u044d\u0443\u0448\u0435\u043d\u044c')},
'3329826':{'en': 'Pleyben', 'fr': 'Pleyben'},
'373241':{'en': 'Cimislia', 'ro': u('Cimi\u015flia'), 'ru': u('\u0427\u0438\u043c\u0438\u0448\u043b\u0438\u044f')},
'373247':{'en': 'Briceni', 'ro': 'Briceni', 'ru': u('\u0411\u0440\u0438\u0447\u0435\u043d\u044c')},
'373246':{'en': u('Edine\u0163'), 'ro': u('Edine\u0163'), 'ru': u('\u0415\u0434\u0438\u043d\u0435\u0446')},
'373533':{'en': 'Tiraspol', 'ro': 'Tiraspol', 'ru': u('\u0422\u0438\u0440\u0430\u0441\u043f\u043e\u043b')},
'3329827':{'en': 'Crozon', 'fr': 'Crozon'},
'3329824':{'en': 'Landivisiau', 'fr': 'Landivisiau'},
'35953223':{'bg': u('\u0426\u044a\u0440\u043a\u0432\u0438\u0446\u0430'), 'en': 'Tsarkvitsa'},
'35953222':{'bg': u('\u041c\u0430\u0440\u043a\u043e\u0432\u043e, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Markovo, Shumen'},
'35953221':{'bg': u('\u0421\u0442\u043e\u044f\u043d \u041c\u0438\u0445\u0430\u0439\u043b\u043e\u0432\u0441\u043a\u0438'), 'en': 'Stoyan Mihaylovski'},
'35953220':{'bg': u('\u041f\u0430\u043c\u0443\u043a\u0447\u0438\u0438, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Pamukchii, Shumen'},
'354421':{'en': u('Keflav\u00edk')},
'437236':{'de': 'Pregarten', 'en': 'Pregarten'},
'3349279':{'en': 'Oraison', 'fr': 'Oraison'},
'43316':{'de': 'Graz', 'en': 'Graz'},
'441877':{'en': 'Callander'},
'3522455':{'de': 'Esch-sur-Alzette/Monnerich', 'en': 'Esch-sur-Alzette/Mondercange', 'fr': 'Esch-sur-Alzette/Mondercange'},
'3347535':{'en': 'Aubenas', 'fr': 'Aubenas'},
'437234':{'de': 'Ottensheim', 'en': 'Ottensheim'},
'3315864':{'en': 'Vincennes', 'fr': 'Vincennes'},
'3315860':{'en': 'Paris', 'fr': 'Paris'},
'3315862':{'en': 'Paris', 'fr': 'Paris'},
'3315869':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'441824':{'en': 'Ruthin'},
'359559':{'bg': u('\u041a\u0430\u0440\u043d\u043e\u0431\u0430\u0442'), 'en': 'Karnobat'},
'359558':{'bg': u('\u0410\u0439\u0442\u043e\u0441'), 'en': 'Aytos'},
'359556':{'bg': u('\u041e\u0431\u0437\u043e\u0440'), 'en': 'Obzor'},
'359554':{'bg': u('\u0421\u043b\u044a\u043d\u0447\u0435\u0432 \u0431\u0440\u044f\u0433'), 'en': 'Sunny Beach'},
'359550':{'bg': u('\u0421\u043e\u0437\u043e\u043f\u043e\u043b'), 'en': 'Sozopol'},
'434826':{'de': u('M\u00f6rtschach'), 'en': u('M\u00f6rtschach')},
'434825':{'de': u('Gro\u00dfkirchheim'), 'en': 'Grosskirchheim'},
'434824':{'de': 'Heiligenblut', 'en': 'Heiligenblut'},
'434823':{'de': 'Tresdorf, Rangersdorf', 'en': 'Tresdorf, Rangersdorf'},
'434822':{'de': 'Winklern', 'en': 'Winklern'},
'441828':{'en': 'Coupar Angus'},
'3329687':{'en': 'Dinan', 'fr': 'Dinan'},
'3329684':{'en': u('Planco\u00ebt'), 'fr': u('Planco\u00ebt')},
'3329685':{'en': 'Dinan', 'fr': 'Dinan'},
'3329680':{'en': 'Broons', 'fr': 'Broons'},
'37422298':{'am': u('\u0531\u057c\u056b\u0576\u057b'), 'en': 'Arinj', 'ru': u('\u0410\u0440\u0438\u043d\u0434\u0436')},
'37422297':{'am': u('\u0533\u0565\u0572\u0561\u0577\u0565\u0576'), 'en': 'Geghashen', 'ru': u('\u0413\u0435\u0445\u0430\u0448\u0435\u043d')},
'37422296':{'am': u('\u054a\u057f\u0572\u0576\u056b'), 'en': 'Ptghni', 'ru': u('\u041f\u0442\u0445\u043d\u0438')},
'3347920':{'en': 'Aussois', 'fr': 'Aussois'},
'37422293':{'am': u('\u0531\u0580\u0561\u0574\u0578\u0582\u057d'), 'en': 'Aramus', 'ru': u('\u0410\u0440\u0430\u043c\u0443\u0441')},
'3347925':{'en': 'La Motte Servolex', 'fr': 'La Motte Servolex'},
'3347924':{'en': 'Moutiers Tarentaise', 'fr': 'Moutiers Tarentaise'},
'3316986':{'en': 'Gif-sur-Yvette', 'fr': 'Gif-sur-Yvette'},
'3316983':{'en': 'Yerres', 'fr': 'Yerres'},
'3316981':{'en': 'Massy', 'fr': 'Massy'},
'3342004':{'en': 'Ajaccio', 'fr': 'Ajaccio'},
'435526':{'de': 'Laterns', 'en': 'Laterns'},
'3316989':{'en': 'Soisy-sur-Seine', 'fr': 'Soisy-sur-Seine'},
'3316988':{'en': u('Br\u00e9tigny-sur-Orge'), 'fr': u('Br\u00e9tigny-sur-Orge')},
'3597106':{'bg': u('\u042f\u043c\u043d\u0430'), 'en': 'Yamna'},
'3597104':{'bg': u('\u041b\u044a\u0433\u0430'), 'en': 'Laga'},
'3597105':{'bg': u('\u041c\u0430\u043b\u043a\u0438 \u0418\u0441\u043a\u044a\u0440'), 'en': 'Malki Iskar'},
'3597102':{'bg': u('\u041b\u043e\u043f\u044f\u043d'), 'en': 'Lopyan'},
'3597103':{'bg': u('\u0411\u0440\u0443\u0441\u0435\u043d, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Brusen, Sofia'},
'3336138':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'441228':{'en': 'Carlisle'},
'441225':{'en': 'Bath'},
'441224':{'en': 'Aberdeen'},
'441227':{'en': 'Canterbury'},
'441226':{'en': 'Barnsley'},
'441223':{'en': 'Cambridge'},
'3338010':{'en': 'Dijon', 'fr': 'Dijon'},
'3345713':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3323231':{'en': u('\u00c9vreux'), 'fr': u('\u00c9vreux')},
'3323230':{'en': 'Conches-en-Ouche', 'fr': 'Conches-en-Ouche'},
'3323233':{'en': u('\u00c9vreux'), 'fr': u('\u00c9vreux')},
'3323232':{'en': 'Verneuil-sur-Avre', 'fr': 'Verneuil-sur-Avre'},
'3323239':{'en': u('\u00c9vreux'), 'fr': u('\u00c9vreux')},
'3323238':{'en': u('\u00c9vreux'), 'fr': u('\u00c9vreux')},
'3355398':{'en': 'Agen', 'fr': 'Agen'},
'437759':{'de': 'Antiesenhofen', 'en': 'Antiesenhofen'},
'3332233':{'en': 'Amiens', 'fr': 'Amiens'},
'3332232':{'en': 'Doullens', 'fr': 'Doullens'},
'3332231':{'en': 'Abbeville', 'fr': 'Abbeville'},
'437757':{'de': 'Gurten', 'en': 'Gurten'},
'3355512':{'en': 'Limoges', 'fr': 'Limoges'},
'437751':{'de': 'Sankt Martin im Innkreis', 'en': 'St. Martin im Innkreis'},
'3332235':{'en': 'Moreuil', 'fr': 'Moreuil'},
'437753':{'de': 'Eberschwang', 'en': 'Eberschwang'},
'437941':{'de': u('Neumarkt im M\u00fchlkreis'), 'en': u('Neumarkt im M\u00fchlkreis')},
'433453':{'de': 'Ehrenhausen', 'en': 'Ehrenhausen'},
'433452':{'de': 'Leibnitz', 'en': 'Leibnitz'},
'433457':{'de': u('Gleinst\u00e4tten'), 'en': u('Gleinst\u00e4tten')},
'433456':{'de': 'Fresing', 'en': 'Fresing'},
'433455':{'de': 'Arnfels', 'en': 'Arnfels'},
'433454':{'de': 'Leutschach', 'en': 'Leutschach'},
'3593933':{'bg': u('\u0421\u0442\u0440\u0430\u043d\u0441\u043a\u043e'), 'en': 'Stransko'},
'3593932':{'bg': u('\u0411\u043e\u0434\u0440\u043e\u0432\u043e'), 'en': 'Bodrovo'},
'3593931':{'bg': u('\u041a\u0430\u0441\u043d\u0430\u043a\u043e\u0432\u043e'), 'en': 'Kasnakovo'},
'3593937':{'bg': u('\u042f\u0431\u044a\u043b\u043a\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Yabalkovo, Hask.'},
'3593936':{'bg': u('\u0413\u043e\u0440\u0441\u043a\u0438 \u0438\u0437\u0432\u043e\u0440, \u0425\u0430\u0441\u043a.'), 'en': 'Gorski izvor, Hask.'},
'3593935':{'bg': u('\u0412\u044a\u0440\u0431\u0438\u0446\u0430, \u0425\u0430\u0441\u043a.'), 'en': 'Varbitsa, Hask.'},
'3593934':{'bg': u('\u0421\u043a\u043e\u0431\u0435\u043b\u0435\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Skobelevo, Hask.'},
'40269':{'en': 'Sibiu', 'ro': 'Sibiu'},
'40268':{'en': u('Bra\u0219ov'), 'ro': u('Bra\u0219ov')},
'40261':{'en': 'Satu Mare', 'ro': 'Satu Mare'},
'40260':{'en': u('S\u0103laj'), 'ro': u('S\u0103laj')},
'40263':{'en': u('Bistri\u021ba-N\u0103s\u0103ud'), 'ro': u('Bistri\u021ba-N\u0103s\u0103ud')},
'40262':{'en': u('Maramure\u0219'), 'ro': u('Maramure\u0219')},
'40265':{'en': u('Mure\u0219'), 'ro': u('Mure\u0219')},
'40264':{'en': 'Cluj', 'ro': 'Cluj'},
'40267':{'en': 'Covasna', 'ro': 'Covasna'},
'40266':{'en': 'Harghita', 'ro': 'Harghita'},
'35963579':{'bg': u('\u0420\u0430\u043b\u0435\u0432\u043e'), 'en': 'Ralevo'},
'3598185':{'bg': u('\u0421\u0435\u043d\u043e\u0432\u043e'), 'en': 'Senovo'},
'370451':{'en': 'Pasvalys'},
'370450':{'en': u('Bir\u017eai')},
'370459':{'en': u('Kupi\u0161kis')},
'370458':{'en': u('Roki\u0161kis')},
'434229':{'de': u('Krumpendorf am W\u00f6rther See'), 'en': u('Krumpendorf am W\u00f6rther See')},
'434228':{'de': 'Feistritz im Rosental', 'en': 'Feistritz im Rosental'},
'441872':{'en': 'Truro'},
'434225':{'de': 'Grafenstein', 'en': 'Grafenstein'},
'3593111':{'bg': u('\u041f\u044a\u0440\u0432\u0435\u043d\u0435\u0446, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Parvenets, Plovdiv'},
'3349378':{'en': 'Beausoleil', 'fr': 'Beausoleil'},
'434226':{'de': 'Sankt Margareten im Rosental', 'en': 'St. Margareten im Rosental'},
'434221':{'de': 'Gallizien', 'en': 'Gallizien'},
'434220':{'de': u('K\u00f6ttmannsdorf'), 'en': u('K\u00f6ttmannsdorf')},
'434223':{'de': 'Maria Saal', 'en': 'Maria Saal'},
'3593110':{'bg': u('\u041f\u043e\u043f\u043e\u0432\u0438\u0446\u0430'), 'en': 'Popovitsa'},
'3593112':{'bg': u('\u041c\u0430\u0440\u043a\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Markovo, Plovdiv'},
'3356102':{'en': 'Foix', 'fr': 'Foix'},
'3356101':{'en': 'Lavelanet', 'fr': 'Lavelanet'},
'3356100':{'en': u('Lab\u00e8ge'), 'fr': u('Lab\u00e8ge')},
'3356107':{'en': 'Tournefeuille', 'fr': 'Tournefeuille'},
'3356105':{'en': u('Tarascon-sur-Ari\u00e8ge'), 'fr': u('Tarascon-sur-Ari\u00e8ge')},
'3356104':{'en': 'Saint-Girons', 'fr': 'Saint-Girons'},
'359301':{'bg': u('\u0421\u043c\u043e\u043b\u044f\u043d'), 'en': 'Smolyan'},
'359306':{'bg': u('\u0420\u0443\u0434\u043e\u0437\u0435\u043c'), 'en': 'Rudozem'},
'34851':{'en': u('M\u00e1laga'), 'es': u('M\u00e1laga')},
'359308':{'bg': u('\u041c\u0430\u0434\u0430\u043d, \u0421\u043c\u043e\u043b.'), 'en': 'Madan, Smol.'},
'359309':{'bg': u('\u041f\u0430\u043c\u043f\u043e\u0440\u043e\u0432\u043e'), 'en': 'Pamporovo'},
'34850':{'en': u('Almer\u00eda'), 'es': u('\u00c1lmer\u00eda')},
'441255':{'en': 'Clacton-on-Sea'},
'3316402':{'en': 'Lagny-sur-Marne', 'fr': 'Lagny-sur-Marne'},
'3355390':{'en': u('Rib\u00e9rac'), 'fr': u('Rib\u00e9rac')},
'35951537':{'bg': u('\u0427\u0435\u0440\u043d\u0435\u0432\u043e'), 'en': 'Chernevo'},
'35951536':{'bg': u('\u041d\u0438\u043a\u043e\u043b\u0430\u0435\u0432\u043a\u0430'), 'en': 'Nikolaevka'},
'35951539':{'bg': u('\u041b\u0435\u0432\u0441\u043a\u0438, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Levski, Varna'},
'35951538':{'bg': u('\u0418\u0437\u0433\u0440\u0435\u0432, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Izgrev, Varna'},
'441253':{'en': 'Blackpool'},
'3316403':{'en': 'Coulommiers', 'fr': 'Coulommiers'},
'3343276':{'en': 'Avignon', 'fr': 'Avignon'},
'3343274':{'en': 'Avignon', 'fr': 'Avignon'},
'3343270':{'en': 'Avignon', 'fr': 'Avignon'},
'3338381':{'en': u('Pont-\u00e0-Mousson'), 'fr': u('Pont-\u00e0-Mousson')},
'3338380':{'en': u('Pont-\u00e0-Mousson'), 'fr': u('Pont-\u00e0-Mousson')},
'3316408':{'en': 'Nangis', 'fr': 'Nangis'},
'3316409':{'en': 'Melun', 'fr': 'Melun'},
'3338385':{'en': 'Nancy', 'fr': 'Nancy'},
'3338384':{'en': u('Pont-\u00e0-Mousson'), 'fr': u('Pont-\u00e0-Mousson')},
'3346735':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3346734':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3316400':{'en': 'Provins', 'fr': 'Provins'},
'3346736':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3346731':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3316407':{'en': 'Tournan-en-Brie', 'fr': 'Tournan-en-Brie'},
'3346732':{'en': u('S\u00e9rignan'), 'fr': u('S\u00e9rignan')},
'35250':{'de': 'Bascharage/Petingen/Rodingen', 'en': 'Bascharage/Petange/Rodange', 'fr': 'Bascharage/Petange/Rodange'},
'35251':{'de': u('D\u00fcdelingen/Bettemburg/Livingen'), 'en': 'Dudelange/Bettembourg/Livange', 'fr': 'Dudelange/Bettembourg/Livange'},
'35252':{'de': u('D\u00fcdelingen'), 'en': 'Dudelange', 'fr': 'Dudelange'},
'35253':{'de': 'Esch-sur-Alzette', 'en': 'Esch-sur-Alzette', 'fr': 'Esch-sur-Alzette'},
'35254':{'de': 'Esch-sur-Alzette', 'en': 'Esch-sur-Alzette', 'fr': 'Esch-sur-Alzette'},
'35255':{'de': 'Esch-sur-Alzette/Monnerich', 'en': 'Esch-sur-Alzette/Mondercange', 'fr': 'Esch-sur-Alzette/Mondercange'},
'35256':{'de': u('R\u00fcmelingen'), 'en': 'Rumelange', 'fr': 'Rumelange'},
'35257':{'de': 'Esch-sur-Alzette/Schifflingen', 'en': 'Esch-sur-Alzette/Schifflange', 'fr': 'Esch-sur-Alzette/Schifflange'},
'35258':{'de': 'Differdingen', 'en': 'Differdange', 'fr': 'Differdange'},
'35259':{'de': 'Soleuvre', 'en': 'Soleuvre', 'fr': 'Soleuvre'},
'39050':{'en': 'Pisa', 'it': 'Pisa'},
'3332652':{'en': 'Vertus', 'fr': 'Vertus'},
'39055':{'en': 'Florence', 'it': 'Firenze'},
'3347028':{'en': u('Montlu\u00e7on'), 'fr': u('Montlu\u00e7on')},
'3347029':{'en': u('Montlu\u00e7on'), 'fr': u('Montlu\u00e7on')},
'3343010':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3343017':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3347020':{'en': 'Moulins', 'fr': 'Moulins'},
'3338659':{'en': 'Nevers', 'fr': 'Nevers'},
'3338653':{'en': 'Appoigny', 'fr': 'Appoigny'},
'3338652':{'en': 'Auxerre', 'fr': 'Auxerre'},
'3338651':{'en': 'Auxerre', 'fr': 'Auxerre'},
'3338657':{'en': 'Nevers', 'fr': 'Nevers'},
'3338656':{'en': u('Brienon-sur-Arman\u00e7on'), 'fr': u('Brienon-sur-Arman\u00e7on')},
'3338655':{'en': 'Tonnerre', 'fr': 'Tonnerre'},
'3338654':{'en': 'Tonnerre', 'fr': 'Tonnerre'},
'3338730':{'en': 'Metz', 'fr': 'Metz'},
'3338731':{'en': 'Metz', 'fr': 'Metz'},
'3338732':{'en': 'Metz', 'fr': 'Metz'},
'3338733':{'en': 'Metz', 'fr': 'Metz'},
'3338734':{'en': 'Metz', 'fr': 'Metz'},
'3338735':{'en': 'Metz', 'fr': 'Metz'},
'3338736':{'en': 'Metz', 'fr': 'Metz'},
'3338737':{'en': 'Metz', 'fr': 'Metz'},
'3338738':{'en': 'Augny', 'fr': 'Augny'},
'3338739':{'en': 'Metz', 'fr': 'Metz'},
'3645':{'en': 'Kisvarda', 'hu': u('Kisv\u00e1rda')},
'3644':{'en': u('M\u00e1t\u00e9szalka'), 'hu': u('M\u00e1t\u00e9szalka')},
'3642':{'en': 'Nyiregyhaza', 'hu': u('Ny\u00edregyh\u00e1za')},
'3335580':{'en': 'Metz', 'fr': 'Metz'},
'35961503':{'bg': u('\u0427\u0430\u043a\u0430\u043b\u0438'), 'en': 'Chakali'},
'35961502':{'bg': u('\u0413\u043e\u0440\u0441\u043a\u043e \u041d\u043e\u0432\u043e \u0421\u0435\u043b\u043e'), 'en': 'Gorsko Novo Selo'},
'3522792':{'de': 'Kanton Clerf/Fischbach/Hosingen', 'en': 'Clervaux/Fischbach/Hosingen', 'fr': 'Clervaux/Fischbach/Hosingen'},
'3522797':{'de': 'Huldingen', 'en': 'Huldange', 'fr': 'Huldange'},
'3522795':{'de': 'Wiltz', 'en': 'Wiltz', 'fr': 'Wiltz'},
'3522799':{'de': 'Ulflingen', 'en': 'Troisvierges', 'fr': 'Troisvierges'},
'35930205':{'bg': u('\u0421\u0442\u044a\u0440\u043d\u0438\u0446\u0430'), 'en': 'Starnitsa'},
'35930200':{'bg': u('\u0417\u0430\u0433\u0440\u0430\u0436\u0434\u0435\u043d, \u0421\u043c\u043e\u043b.'), 'en': 'Zagrazhden, Smol.'},
'432267':{'de': 'Sierndorf', 'en': 'Sierndorf'},
'3332001':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3332002':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332003':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3332004':{'en': u('Mons-en-Bar\u0153ul'), 'fr': u('Mons-en-Bar\u0153ul')},
'3332005':{'en': 'Villeneuve-d\'Ascq', 'fr': 'Villeneuve-d\'Ascq'},
'3332006':{'en': 'Lille', 'fr': 'Lille'},
'3332007':{'en': 'Loos', 'fr': 'Loos'},
'3332008':{'en': u('P\u00e9renchies'), 'fr': u('P\u00e9renchies')},
'3332009':{'en': 'Lomme', 'fr': 'Lomme'},
'432269':{'de': 'Niederfellabrunn', 'en': 'Niederfellabrunn'},
'432268':{'de': u('Gro\u00dfmugl'), 'en': 'Grossmugl'},
'3354676':{'en': u('Saint-Georges-d\'Ol\u00e9ron'), 'fr': u('Saint-Georges-d\'Ol\u00e9ron')},
'3354675':{'en': u('Dolus-d\'Ol\u00e9ron'), 'fr': u('Dolus-d\'Ol\u00e9ron')},
'3354674':{'en': 'Saintes', 'fr': 'Saintes'},
'3324048':{'en': 'Nantes', 'fr': 'Nantes'},
'3329963':{'en': 'Rennes', 'fr': 'Rennes'},
'3329962':{'en': 'Vern-sur-Seiche', 'fr': 'Vern-sur-Seiche'},
'3329961':{'en': u('Pl\u00e9lan-le-Grand'), 'fr': u('Pl\u00e9lan-le-Grand')},
'3329960':{'en': u('Pac\u00e9'), 'fr': u('Pac\u00e9')},
'3329967':{'en': 'Rennes', 'fr': 'Rennes'},
'3329966':{'en': 'Melesse', 'fr': 'Melesse'},
'3329965':{'en': 'Rennes', 'fr': 'Rennes'},
'3329964':{'en': 'Saint-Gilles', 'fr': 'Saint-Gilles'},
'3329969':{'en': u('G\u00e9vez\u00e9'), 'fr': u('G\u00e9vez\u00e9')},
'3329968':{'en': u('Liffr\u00e9'), 'fr': u('Liffr\u00e9')},
'3323559':{'en': 'Bois-Guillaume', 'fr': 'Bois-Guillaume'},
'3323550':{'en': 'Eu', 'fr': 'Eu'},
'3323551':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323552':{'en': 'Rouen', 'fr': 'Rouen'},
'3323553':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323554':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323556':{'en': 'Yvetot', 'fr': 'Yvetot'},
'3323557':{'en': 'Saint-Valery-en-Caux', 'fr': 'Saint-Valery-en-Caux'},
'3349468':{'en': 'Draguignan', 'fr': 'Draguignan'},
'3349469':{'en': 'Brignoles', 'fr': 'Brignoles'},
'3349460':{'en': 'Le Luc', 'fr': 'Le Luc'},
'3349461':{'en': 'Toulon', 'fr': 'Toulon'},
'3349462':{'en': 'Toulon', 'fr': 'Toulon'},
'3349463':{'en': 'Ollioules', 'fr': 'Ollioules'},
'3349464':{'en': 'Cavalaire-sur-Mer', 'fr': 'Cavalaire-sur-Mer'},
'3349465':{'en': u('Hy\u00e8res'), 'fr': u('Hy\u00e8res')},
'3349466':{'en': 'La Crau', 'fr': 'La Crau'},
'3349467':{'en': 'Draguignan', 'fr': 'Draguignan'},
'35941489':{'bg': u('\u0411\u043e\u0437\u0434\u0443\u0433\u0430\u043d\u043e\u0432\u043e'), 'en': 'Bozduganovo'},
'3355729':{'en': 'Merignac', 'fr': u('M\u00e9rignac')},
'3355726':{'en': 'Pessac', 'fr': 'Pessac'},
'3355724':{'en': u('Saint-\u00c9milion'), 'fr': u('Saint-\u00c9milion')},
'3355725':{'en': 'Libourne', 'fr': 'Libourne'},
'3355722':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'433535':{'de': 'Krakaudorf', 'en': 'Krakaudorf'},
'3324326':{'en': 'Laval', 'fr': 'Laval'},
'3324324':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324323':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324321':{'en': 'Arnage', 'fr': 'Arnage'},
'3324328':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324329':{'en': u('Bonn\u00e9table'), 'fr': u('Bonn\u00e9table')},
'437255':{'de': 'Losenstein', 'en': 'Losenstein'},
'437254':{'de': u('Gro\u00dframing'), 'en': 'Grossraming'},
'437257':{'de': u('Gr\u00fcnburg'), 'en': u('Gr\u00fcnburg')},
'437256':{'de': 'Ternberg', 'en': 'Ternberg'},
'437251':{'de': 'Schiedlberg', 'en': 'Schiedlberg'},
'437250':{'de': 'Maria Neustift', 'en': 'Maria Neustift'},
'437253':{'de': 'Wolfern', 'en': 'Wolfern'},
'437252':{'de': 'Steyr', 'en': 'Steyr'},
'437259':{'de': 'Sierning', 'en': 'Sierning'},
'437258':{'de': 'Bad Hall', 'en': 'Bad Hall'},
'381290':{'en': 'Urosevac', 'sr': u('Uro\u0161evac')},
'4418518':{'en': 'Stornoway'},
'4418519':{'en': 'Great Bernera'},
'437282':{'de': 'Neufelden', 'en': 'Neufelden'},
'441709':{'en': 'Rotherham'},
'4418510':{'en': 'Great Bernera/Stornoway'},
'4418511':{'en': 'Great Bernera/Stornoway'},
'4418512':{'en': 'Stornoway'},
'3356733':{'en': 'Toulouse', 'fr': 'Toulouse'},
'4418514':{'en': 'Great Bernera'},
'4418515':{'en': 'Stornoway'},
'435633':{'de': u('H\u00e4gerau'), 'en': u('H\u00e4gerau')},
'4418517':{'en': 'Stornoway'},
'432813':{'de': 'Arbesbach', 'en': 'Arbesbach'},
'3599748':{'bg': u('\u0414\u044a\u043b\u0433\u043e\u0434\u0435\u043b\u0446\u0438'), 'en': 'Dalgodeltsi'},
'3599749':{'bg': u('\u0427\u0435\u0440\u043d\u0438 \u0432\u0440\u044a\u0445, \u041c\u043e\u043d\u0442.'), 'en': 'Cherni vrah, Mont.'},
'3599746':{'bg': u('\u0420\u0430\u0437\u0433\u0440\u0430\u0434, \u041c\u043e\u043d\u0442.'), 'en': 'Razgrad, Mont.'},
'3599747':{'bg': u('\u041c\u043e\u043a\u0440\u0435\u0448, \u041c\u043e\u043d\u0442.'), 'en': 'Mokresh, Mont.'},
'3599744':{'bg': u('\u0412\u044a\u043b\u0447\u0435\u0434\u0440\u044a\u043c'), 'en': 'Valchedram'},
'3599745':{'bg': u('\u0417\u043b\u0430\u0442\u0438\u044f, \u041c\u043e\u043d\u0442.'), 'en': 'Zlatia, Mont.'},
'3599742':{'bg': u('\u042f\u043a\u0438\u043c\u043e\u0432\u043e'), 'en': 'Yakimovo'},
'3599740':{'bg': u('\u0421\u0435\u043f\u0442\u0435\u043c\u0432\u0440\u0438\u0439\u0446\u0438, \u041c\u043e\u043d\u0442.'), 'en': 'Septemvriytsi, Mont.'},
'3599741':{'bg': u('\u0414\u043e\u043b\u043d\u0438 \u0426\u0438\u0431\u044a\u0440'), 'en': 'Dolni Tsibar'},
'374237':{'am': u('\u0531\u0580\u0561\u0584\u057d/\u0531\u0580\u0574\u0561\u057e\u056b\u0580/\u0540\u0578\u056f\u057f\u0565\u0574\u0562\u0565\u0580/\u053c\u0565\u0576\u0578\u0582\u0572\u056b/\u0544\u0565\u056e\u0561\u0574\u0578\u0580/\u0536\u0561\u0580\u0569\u0578\u0576\u0584'), 'en': 'Araks/Armavir/Hoktember/Lenughi/Metsamor/Zartonk', 'ru': u('\u0410\u0440\u0430\u043a\u0441/\u0410\u0440\u043c\u0430\u0432\u0438\u0440/\u041e\u043a\u0442\u0435\u043c\u0431\u0435\u0440/\u041b\u0435\u043d\u0443\u0445\u0438/\u041c\u0435\u0446\u0430\u043c\u043e\u0440/\u0417\u0430\u0440\u0442\u043e\u043d\u043a')},
'374236':{'am': u('\u0531\u0575\u0576\u0569\u0561\u057a/\u0544\u0561\u057d\u056b\u057d/\u0546\u0578\u0580 \u053d\u0561\u0580\u0562\u0565\u0580\u0564/\u0546\u0578\u0580\u0561\u0562\u0561\u0581'), 'en': 'Ayntap/Masis/Nor Kharberd/Norabats', 'ru': u('\u041c\u0430\u0441\u0438\u0441/\u041d\u043e\u0440 \u0425\u0430\u0440\u0431\u0435\u0440\u0434/\u041d\u043e\u0440\u0430\u0431\u0430\u0446')},
'374235':{'am': u('\u0531\u0580\u057f\u0561\u0577\u0561\u057f/\u0531\u0575\u0563\u0565\u0566\u0561\u0580\u0564/\u0534\u0561\u056c\u0561\u0580/\u0554\u0561\u0572\u0581\u0580\u0561\u0577\u0565\u0576/\u0544\u056d\u0579\u0575\u0561\u0576/\u0547\u0561\u0570\u0578\u0582\u0574\u0575\u0561\u0576'), 'en': 'Artashat/Aygezard/Dalar/Kaghtsrashen/Mkhchyan/Shahumyan', 'ru': u('\u0410\u0440\u0442\u0430\u0448\u0430\u0442/\u0410\u0439\u0433\u0435\u0437\u0430\u0440\u0434/\u0414\u0430\u043b\u0430\u0440/\u041a\u0430\u0445\u0446\u0440\u0430\u0448\u0435\u043d/\u041c\u0445\u0447\u044f\u043d/\u0428\u0430\u0443\u043c\u044f\u043d')},
'374234':{'am': u('\u054e\u0565\u0564\u056b/\u0548\u057d\u056f\u0565\u057f\u0561\u0583/\u0531\u0580\u0561\u0580\u0561\u057f'), 'en': 'Vedi/Vosketap/Ararat', 'ru': u('\u0412\u0435\u0434\u0438/\u0412\u043e\u0441\u043a\u0435\u0442\u0430\u043f/\u0410\u0440\u0430\u0440\u0430\u0442')},
'374233':{'am': u('\u0532\u0561\u0572\u0580\u0561\u0574\u0575\u0561\u0576/\u053c\u0565\u057c\u0576\u0561\u0563\u0578\u0563'), 'en': 'Baghramyan/Lernagog', 'ru': u('\u0411\u0430\u0433\u0440\u0430\u043c\u044f\u043d/\u041b\u0435\u0440\u043d\u0430\u0433\u043e\u0433')},
'374232':{'am': u('\u0531\u0577\u057f\u0561\u0580\u0561\u056f/\u0531\u0572\u0571\u0584/\u053f\u0561\u0580\u0562\u056b/\u0555\u0577\u0561\u056f\u0561\u0576'), 'en': 'Aghdzq/Ashtarak/Karbi/Oshakan', 'ru': u('\u0410\u0448\u0442\u0430\u0440\u0430\u043a/\u0410\u0445\u0446\u043a/\u041a\u0430\u0440\u0431\u0438/\u041e\u0448\u0430\u043a\u0430\u043d')},
'374231':{'am': u('\u054e\u0561\u0572\u0561\u0580\u0577\u0561\u057a\u0561\u057f/\u0544\u0578\u0582\u057d\u0561\u056c\u0565\u057c/\u0553\u0561\u0580\u0561\u0584\u0561\u0580/\u0536\u057e\u0561\u0580\u0569\u0576\u0578\u0581'), 'en': 'Echmiadzin/Musaler/Parakar/Zvartnots', 'ru': u('\u042d\u0447\u043c\u0438\u0430\u0434\u0437\u0438\u043d/\u041c\u0443\u0441\u0430\u043b\u0435\u0440/\u041f\u0430\u0440\u0430\u043a\u044f\u0440/\u0417\u0432\u0430\u0440\u0442\u043d\u043e\u0446')},
'374238':{'am': u('\u0531\u0580\u0561\u0580\u0561\u057f/\u0531\u057e\u0577\u0561\u0580/\u054d\u0578\u0582\u0580\u0565\u0576\u0561\u057e\u0561\u0576/\u0535\u0580\u0561\u057d\u056d'), 'en': 'Ararat/Avshar/Surenavan/Yeraskh', 'ru': u('\u0410\u0440\u0430\u0440\u0430\u0442/\u0410\u0432\u0448\u0430\u0440/\u0421\u0443\u0440\u0435\u043d\u0430\u0432\u0430\u043d/\u0415\u0440\u0430\u0441\u0445')},
'3596569':{'bg': u('\u041b\u0435\u043d\u043a\u043e\u0432\u043e'), 'en': 'Lenkovo'},
'3596568':{'bg': u('\u0414\u044a\u0431\u043e\u0432\u0430\u043d'), 'en': 'Dabovan'},
'3315888':{'en': 'Issy-les-Moulineaux', 'fr': 'Issy-les-Moulineaux'},
'3596563':{'bg': u('\u0411\u0440\u0435\u0441\u0442, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Brest, Pleven'},
'3596562':{'bg': u('\u0413\u0438\u0433\u0435\u043d'), 'en': 'Gigen'},
'3596561':{'bg': u('\u0413\u0443\u043b\u044f\u043d\u0446\u0438'), 'en': 'Gulyantsi'},
'3347612':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3596567':{'bg': u('\u0421\u043e\u043c\u043e\u0432\u0438\u0442'), 'en': 'Somovit'},
'3596566':{'bg': u('\u0414\u043e\u043b\u043d\u0438 \u0412\u0438\u0442'), 'en': 'Dolni Vit'},
'3596565':{'bg': u('\u041c\u0438\u043b\u043a\u043e\u0432\u0438\u0446\u0430'), 'en': 'Milkovitsa'},
'3596564':{'bg': u('\u0417\u0430\u0433\u0440\u0430\u0436\u0434\u0435\u043d, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Zagrazhden, Pleven'},
'441676':{'en': 'Meriden'},
'359538':{'bg': u('\u0412\u0435\u043b\u0438\u043a\u0438 \u041f\u0440\u0435\u0441\u043b\u0430\u0432'), 'en': 'Veliki Preslav'},
'441674':{'en': 'Montrose'},
'3346747':{'en': 'Montpellier', 'fr': 'Montpellier'},
'441672':{'en': 'Marlborough'},
'441673':{'en': 'Market Rasen'},
'441670':{'en': 'Morpeth'},
'441671':{'en': 'Newton Stewart'},
'359537':{'bg': u('\u041d\u043e\u0432\u0438 \u043f\u0430\u0437\u0430\u0440, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Novi pazar, Shumen'},
'3346741':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346742':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346743':{'en': u('M\u00e8ze'), 'fr': u('M\u00e8ze')},
'3347905':{'en': 'Modane', 'fr': 'Modane'},
'3347904':{'en': 'Bourg-Saint-Maurice', 'fr': 'Bourg-Saint-Maurice'},
'3347907':{'en': 'Bourg-Saint-Maurice', 'fr': 'Bourg-Saint-Maurice'},
'3347906':{'en': u('Val-d\'Is\u00e8re'), 'fr': u('Val-d\'Is\u00e8re')},
'434847':{'de': 'Obertilliach', 'en': 'Obertilliach'},
'434846':{'de': 'Abfaltersbach', 'en': 'Abfaltersbach'},
'434848':{'de': 'Kartitsch', 'en': 'Kartitsch'},
'3347908':{'en': 'Courchevel Saint Bon', 'fr': 'Courchevel Saint Bon'},
'390439':{'it': 'Feltre'},
'390438':{'it': 'Conegliano'},
'432216':{'de': 'Leopoldsdorf im Marchfelde', 'en': 'Leopoldsdorf im Marchfelde'},
'390433':{'it': 'Tolmezzo'},
'390432':{'en': 'Udine', 'it': 'Udine'},
'390431':{'it': 'Cervignano del Friuli'},
'390437':{'it': 'Belluno'},
'390436':{'it': 'Cortina d\'Ampezzo'},
'390435':{'it': 'Pieve di Cadore'},
'390434':{'it': 'Pordenone'},
'3597168':{'bg': u('\u0411\u043e\u0432'), 'en': 'Bov'},
'3597169':{'bg': u('\u0422\u043e\u043c\u043f\u0441\u044a\u043d'), 'en': 'Tompsan'},
'441205':{'en': 'Boston'},
'441204':{'en': 'Bolton'},
'441202':{'en': 'Bournemouth'},
'441200':{'en': 'Clitheroe'},
'374322':{'am': u('\u054e\u0561\u0576\u0561\u0571\u0578\u0580/\u0533\u0578\u0582\u0563\u0561\u0580\u0584'), 'en': 'Vanadzor/Gugark region', 'ru': u('\u0412\u0430\u043d\u0430\u0434\u0437\u043e\u0440/\u0413\u0443\u0433\u0430\u0440\u043a')},
'3343737':{'en': 'Lyon', 'fr': 'Lyon'},
'3597162':{'bg': u('\u041b\u0430\u043a\u0430\u0442\u043d\u0438\u043a'), 'en': 'Lakatnik'},
'3597163':{'bg': u('\u0418\u0441\u043a\u0440\u0435\u0446'), 'en': 'Iskrets'},
'3597164':{'bg': u('\u0420\u0435\u0431\u0440\u043e\u0432\u043e'), 'en': 'Rebrovo'},
'3597165':{'bg': u('\u041c\u0438\u043b\u0430\u043d\u043e\u0432\u043e, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Milanovo, Sofia'},
'3597166':{'bg': u('\u0412\u043b\u0430\u0434\u043e \u0422\u0440\u0438\u0447\u043a\u043e\u0432'), 'en': 'Vlado Trichkov'},
'3597167':{'bg': u('\u0426\u0435\u0440\u043e\u0432\u043e, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Tserovo, Sofia'},
'3742549':{'am': u('\u0544\u0565\u056e\u0561\u057e\u0561\u0576'), 'en': 'Metsavan', 'ru': u('\u041c\u0435\u0446\u0430\u0432\u0430\u043d')},
'3752239':{'be': u('\u0428\u043a\u043b\u043e\u045e'), 'en': 'Shklov', 'ru': u('\u0428\u043a\u043b\u043e\u0432')},
'3752238':{'be': u('\u041a\u0440\u0430\u0441\u043d\u0430\u043f\u043e\u043b\u043b\u0435, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Krasnopolye, Mogilev Region', 'ru': u('\u041a\u0440\u0430\u0441\u043d\u043e\u043f\u043e\u043b\u044c\u0435, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752235':{'be': u('\u0410\u0441\u0456\u043f\u043e\u0432\u0456\u0447\u044b'), 'en': 'Osipovichi', 'ru': u('\u041e\u0441\u0438\u043f\u043e\u0432\u0438\u0447\u0438')},
'3752234':{'be': u('\u041a\u0440\u0443\u0433\u043b\u0430\u0435, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Krugloye, Mogilev Region', 'ru': u('\u041a\u0440\u0443\u0433\u043b\u043e\u0435, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752237':{'be': u('\u041a\u0456\u0440\u0430\u045e\u0441\u043a, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Kirovsk, Mogilev Region', 'ru': u('\u041a\u0438\u0440\u043e\u0432\u0441\u043a, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752236':{'be': u('\u041a\u043b\u0456\u0447\u0430\u045e, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Klichev, Mogilev Region', 'ru': u('\u041a\u043b\u0438\u0447\u0435\u0432, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752231':{'be': u('\u0411\u044b\u0445\u0430\u045e, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Byhov, Mogilev Region', 'ru': u('\u0411\u044b\u0445\u043e\u0432, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752230':{'be': u('\u0413\u043b\u0443\u0441\u043a, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Glusk, Mogilev Region', 'ru': u('\u0413\u043b\u0443\u0441\u043a, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752233':{'be': u('\u0413\u043e\u0440\u043a\u0456, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Gorki, Mogilev Region', 'ru': u('\u0413\u043e\u0440\u043a\u0438, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752232':{'be': u('\u0411\u044f\u043b\u044b\u043d\u0456\u0447\u044b, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Belynichi, Mogilev Region', 'ru': u('\u0411\u0435\u043b\u044b\u043d\u0438\u0447\u0438, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'37410':{'am': u('\u0535\u0580\u0587\u0561\u0576/\u054b\u0580\u057e\u0565\u056a'), 'en': 'Yerevan/Jrvezh', 'ru': u('\u0415\u0440\u0435\u0432\u0430\u043d/\u0414\u0436\u0440\u0432\u0435\u0436')},
'37411':{'am': u('\u0535\u0580\u0587\u0561\u0576'), 'en': 'Yerevan', 'ru': u('\u0415\u0440\u0435\u0432\u0430\u043d')},
'35963570':{'bg': u('\u0421\u0442\u0430\u0440\u043e\u0441\u0435\u043b\u0446\u0438'), 'en': 'Staroseltsi'},
'3595517':{'bg': u('\u0420\u0430\u0432\u043d\u0435\u0446, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Ravnets, Burgas'},
'35963573':{'bg': u('\u0414\u0438\u0441\u0435\u0432\u0438\u0446\u0430'), 'en': 'Disevitsa'},
'35963574':{'bg': u('\u0422\u043e\u0434\u043e\u0440\u043e\u0432\u043e, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Todorovo, Pleven'},
'3323788':{'en': 'Chartres', 'fr': 'Chartres'},
'35963576':{'bg': u('\u0422\u0443\u0447\u0435\u043d\u0438\u0446\u0430'), 'en': 'Tuchenitsa'},
'3595516':{'bg': u('\u0418\u043d\u0434\u0436\u0435 \u0432\u043e\u0439\u0432\u043e\u0434\u0430'), 'en': 'Indzhe voyvoda'},
'35963578':{'bg': u('\u041e\u043f\u0430\u043d\u0435\u0446, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Opanets, Pleven'},
'3323784':{'en': 'Chartres', 'fr': 'Chartres'},
'3323781':{'en': 'La Loupe', 'fr': 'La Loupe'},
'3595515':{'bg': u('\u041a\u0430\u043c\u0435\u043d\u043e'), 'en': 'Kameno'},
'3323783':{'en': u('\u00c9pernon'), 'fr': u('\u00c9pernon')},
'432262':{'de': 'Korneuburg', 'en': 'Korneuburg'},
'35971227':{'bg': u('\u0411\u0435\u043b\u0438 \u0418\u0441\u043a\u044a\u0440'), 'en': 'Beli Iskar'},
'432522':{'de': 'Laa an der Thaya', 'en': 'Laa an der Thaya'},
'432523':{'de': 'Kirchstetten, Neudorf bei Staatz', 'en': 'Kirchstetten, Neudorf bei Staatz'},
'432524':{'de': 'Kautendorf', 'en': 'Kautendorf'},
'432525':{'de': 'Gnadendorf', 'en': 'Gnadendorf'},
'432526':{'de': 'Stronsdorf', 'en': 'Stronsdorf'},
'432527':{'de': 'Wulzeshofen', 'en': 'Wulzeshofen'},
'3595511':{'bg': u('\u041b\u0443\u043a\u043e\u0439\u043b \u041d\u0435\u0444\u0442\u043e\u0445\u0438\u043c'), 'en': 'Lukoil Neftochim'},
'35971224':{'bg': u('\u0428\u0438\u043f\u043e\u0447\u0430\u043d\u0435'), 'en': 'Shipochane'},
'3345087':{'en': 'Annemasse', 'fr': 'Annemasse'},
'3345084':{'en': 'Annemasse', 'fr': 'Annemasse'},
'3345083':{'en': 'Thonon-les-Bains', 'fr': 'Thonon-les-Bains'},
'3345081':{'en': 'Thonon-les-Bains', 'fr': 'Thonon-les-Bains'},
'3593667':{'bg': u('\u041f\u043e\u043a\u0440\u043e\u0432\u0430\u043d'), 'en': 'Pokrovan'},
'3593666':{'bg': u('\u041f\u043e\u043f\u0441\u043a\u043e'), 'en': 'Popsko'},
'3593665':{'bg': u('\u0421\u0432\u0438\u0440\u0430\u0447\u0438'), 'en': 'Svirachi'},
'3593664':{'bg': u('\u041f\u043b\u0435\u0432\u0443\u043d'), 'en': 'Plevun'},
'3593662':{'bg': u('\u0416\u0435\u043b\u0435\u0437\u0438\u043d\u043e'), 'en': 'Zhelezino'},
'3345088':{'en': 'Annecy', 'fr': 'Annecy'},
'3345089':{'en': 'Cluses', 'fr': 'Cluses'},
'3332743':{'en': 'Denain', 'fr': 'Denain'},
'3332742':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3332741':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3332740':{'en': u('Vieux-Cond\u00e9'), 'fr': u('Vieux-Cond\u00e9')},
'3332747':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3332746':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3332745':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3332744':{'en': 'Denain', 'fr': 'Denain'},
'3332748':{'en': 'Saint-Amand-les-Eaux', 'fr': 'Saint-Amand-les-Eaux'},
'3332219':{'en': 'Abbeville', 'fr': 'Abbeville'},
'40247':{'en': 'Teleorman', 'ro': 'Teleorman'},
'40246':{'en': 'Giurgiu', 'ro': 'Giurgiu'},
'40245':{'en': u('D\u00e2mbovi\u021ba'), 'ro': u('D\u00e2mbovi\u021ba')},
'40244':{'en': 'Prahova', 'ro': 'Prahova'},
'3356129':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3352407':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'40241':{'en': u('Constan\u021ba'), 'ro': u('Constan\u021ba')},
'3347553':{'en': u('Mont\u00e9limar'), 'fr': u('Mont\u00e9limar')},
'3356125':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356124':{'en': 'Balma', 'fr': 'Balma'},
'3356126':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356121':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356120':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356123':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356122':{'en': 'Toulouse', 'fr': 'Toulouse'},
'37423191':{'am': u('\u054e\u0561\u0579\u0565'), 'en': 'Vache', 'ru': u('\u0412\u0430\u0447\u0435')},
'37423190':{'am': u('\u0532\u0561\u0572\u0580\u0561\u0574\u0575\u0561\u0576'), 'en': 'Baghramyan', 'ru': u('\u0411\u0430\u0433\u0440\u0430\u043c\u044f\u043d')},
'37423195':{'am': u('\u0546\u0578\u0580\u0561\u056f\u0565\u0580\u057f'), 'en': 'Norakert', 'ru': u('\u041d\u043e\u0440\u0430\u043a\u0435\u0440\u0442')},
'37423199':{'am': u('\u053d\u0578\u0580\u0578\u0576\u0584'), 'en': 'Khoronk', 'ru': u('\u0425\u043e\u0440\u043e\u043d\u043a')},
'37423198':{'am': u('\u054b\u0580\u0561\u057c\u0561\u057f'), 'en': 'Jrarat', 'ru': u('\u0414\u0436\u0440\u0430\u0440\u0430\u0442')},
'433834':{'de': u('Wald am Schoberpa\u00df'), 'en': 'Wald am Schoberpass'},
'437475':{'de': 'Hausmening, Neuhofen an der Ybbs', 'en': 'Hausmening, Neuhofen an der Ybbs'},
'441449':{'en': 'Stowmarket'},
'437474':{'de': 'Euratsfeld', 'en': 'Euratsfeld'},
'441445':{'en': 'Gairloch'},
'441444':{'en': 'Haywards Heath'},
'441446':{'en': 'Barry'},
'437477':{'de': 'Sankt Peter in der Au', 'en': 'St. Peter in der Au'},
'441443':{'en': 'Pontypridd'},
'441442':{'en': 'Hemel Hempstead'},
'331585':{'en': 'Paris', 'fr': 'Paris'},
'437471':{'de': 'Neustadtl an der Donau', 'en': 'Neustadtl an der Donau'},
'3599513':{'bg': u('\u0411\u043e\u0439\u0447\u0438\u043d\u043e\u0432\u0446\u0438'), 'en': 'Boychinovtsi'},
'3599512':{'bg': u('\u0411\u0435\u043b\u0438 \u0431\u0440\u0435\u0433'), 'en': 'Beli breg'},
'3599517':{'bg': u('\u041a\u043e\u0431\u0438\u043b\u044f\u043a'), 'en': 'Kobilyak'},
'3599516':{'bg': u('\u041b\u0435\u0445\u0447\u0435\u0432\u043e'), 'en': 'Lehchevo'},
'3599515':{'bg': u('\u041c\u0430\u0434\u0430\u043d, \u041c\u043e\u043d\u0442.'), 'en': 'Madan, Mont.'},
'3599514':{'bg': u('\u0412\u043b\u0430\u0434\u0438\u043c\u0438\u0440\u043e\u0432\u043e, \u041c\u043e\u043d\u0442.'), 'en': 'Vladimirovo, Mont.'},
'3356381':{'en': 'Gaillac', 'fr': 'Gaillac'},
'3356380':{'en': 'Albi', 'fr': 'Albi'},
'3356383':{'en': 'Lavaur', 'fr': 'Lavaur'},
'3599518':{'bg': u('\u041c\u044a\u0440\u0447\u0435\u0432\u043e'), 'en': 'Marchevo'},
'3347228':{'en': 'Saint-Priest', 'fr': 'Saint-Priest'},
'3347596':{'en': 'Pierrelatte', 'fr': 'Pierrelatte'},
'3343250':{'en': 'Cavaillon', 'fr': 'Cavaillon'},
'3347226':{'en': u('Saint-Andr\u00e9-de-Corcy'), 'fr': u('Saint-Andr\u00e9-de-Corcy')},
'3347227':{'en': 'Caluire-et-Cuire', 'fr': 'Caluire-et-Cuire'},
'3347592':{'en': u('Mont\u00e9limar'), 'fr': u('Mont\u00e9limar')},
'3347221':{'en': u('V\u00e9nissieux'), 'fr': u('V\u00e9nissieux')},
'3347222':{'en': u('Lyon Saint Exup\u00e9ry A\u00e9roport'), 'fr': u('Lyon Saint Exup\u00e9ry A\u00e9roport')},
'3347591':{'en': u('Mont\u00e9limar'), 'fr': u('Mont\u00e9limar')},
'3593036':{'bg': u('\u041c\u043e\u0433\u0438\u043b\u0438\u0446\u0430'), 'en': 'Mogilitsa'},
'3593037':{'bg': u('\u0421\u0438\u0432\u0438\u043d\u043e'), 'en': 'Sivino'},
'39070':{'en': 'Cagliari', 'it': 'Cagliari'},
'39071':{'en': 'Ancona', 'it': 'Ancona'},
'3324179':{'en': 'Angers', 'fr': 'Angers'},
'3324178':{'en': 'Chalonnes-sur-Loire', 'fr': 'Chalonnes-sur-Loire'},
'39075':{'en': 'Perugia', 'it': 'Perugia'},
'3324173':{'en': 'Angers', 'fr': 'Angers'},
'3324172':{'en': 'Angers', 'fr': 'Angers'},
'3324171':{'en': 'Cholet', 'fr': 'Cholet'},
'3593035':{'bg': u('\u0412\u044a\u0440\u0431\u0438\u043d\u0430'), 'en': 'Varbina'},
'35935418':{'bg': u('\u041a\u0440\u044a\u0441\u0442\u0430\u0432\u0430'), 'en': 'Krastava'},
'35935419':{'bg': u('\u0421\u0432\u0435\u0442\u0430 \u041f\u0435\u0442\u043a\u0430'), 'en': 'Sveta Petka'},
'42144':{'en': u('Liptovsk\u00fd Mikul\u00e1\u0161')},
'3347048':{'en': 'Moulins', 'fr': 'Moulins'},
'3593039':{'bg': u('\u041f\u043e\u043b\u043a\u043e\u0432\u043d\u0438\u043a \u0421\u0435\u0440\u0430\u0444\u0438\u043c\u043e\u0432\u043e'), 'en': 'Polkovnik Serafimovo'},
'3347044':{'en': 'Moulins', 'fr': 'Moulins'},
'3347045':{'en': u('Saint-Pour\u00e7ain-sur-Sioule'), 'fr': u('Saint-Pour\u00e7ain-sur-Sioule')},
'3347046':{'en': 'Moulins', 'fr': 'Moulins'},
'435331':{'de': 'Brandenberg', 'en': 'Brandenberg'},
'3338677':{'en': 'Decize', 'fr': 'Decize'},
'3338676':{'en': 'Moux-en-Morvan', 'fr': 'Moux-en-Morvan'},
'3338671':{'en': 'Nevers', 'fr': 'Nevers'},
'3338670':{'en': u('La Charit\u00e9 sur Loire'), 'fr': u('La Charit\u00e9 sur Loire')},
'435336':{'de': 'Alpbach', 'en': 'Alpbach'},
'3338672':{'en': 'Auxerre', 'fr': 'Auxerre'},
'435338':{'de': 'Kundl', 'en': 'Kundl'},
'435339':{'de': u('Wildsch\u00f6nau'), 'en': u('Wildsch\u00f6nau')},
'433578':{'de': 'Obdach', 'en': 'Obdach'},
'35984269':{'bg': u('\u041d\u0435\u0434\u043e\u043a\u043b\u0430\u043d'), 'en': 'Nedoklan'},
'35984266':{'bg': u('\u041f\u0440\u043e\u0441\u0442\u043e\u0440\u043d\u043e'), 'en': 'Prostorno'},
'3666':{'en': 'Bekescsaba', 'hu': u('B\u00e9k\u00e9scsaba')},
'3663':{'en': 'Szentes', 'hu': 'Szentes'},
'3662':{'en': 'Szeged', 'hu': 'Szeged'},
'432556':{'de': u('Gro\u00dfkrut'), 'en': 'Grosskrut'},
'3669':{'en': 'Mohacs', 'hu': u('Moh\u00e1cs')},
'3668':{'en': 'Oroshaza', 'hu': u('Orosh\u00e1za')},
'3596174':{'bg': u('\u0414\u0440\u0430\u0433\u0430\u043d\u043e\u0432\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Draganovo, V. Tarnovo'},
'3596175':{'bg': u('\u041f\u044a\u0440\u0432\u043e\u043c\u0430\u0439\u0446\u0438'), 'en': 'Parvomaytsi'},
'3338718':{'en': 'Metz', 'fr': 'Metz'},
'3596177':{'bg': u('\u042f\u043d\u0442\u0440\u0430, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Yantra, V. Tarnovo'},
'3596173':{'bg': u('\u0414\u043e\u043b\u043d\u0430 \u041e\u0440\u044f\u0445\u043e\u0432\u0438\u0446\u0430'), 'en': 'Dolna Oryahovitsa'},
'3338713':{'en': 'Forbach', 'fr': 'Forbach'},
'3338716':{'en': 'Metz', 'fr': 'Metz'},
'3338717':{'en': 'Metz', 'fr': 'Metz'},
'3338715':{'en': 'Metz', 'fr': 'Metz'},
'3338815':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'433576':{'de': 'Bretstein', 'en': 'Bretstein'},
'3338814':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3343038':{'en': u('Al\u00e8s'), 'fr': u('Al\u00e8s')},
'3316024':{'en': 'Meaux', 'fr': 'Meaux'},
'3316025':{'en': 'Meaux', 'fr': 'Meaux'},
'3316026':{'en': 'Claye-Souilly', 'fr': 'Claye-Souilly'},
'3316027':{'en': 'Claye-Souilly', 'fr': 'Claye-Souilly'},
'3316020':{'en': 'Chelles', 'fr': 'Chelles'},
'3316021':{'en': 'Mitry-Mory', 'fr': 'Mitry-Mory'},
'3316022':{'en': u('La Fert\u00e9 sous Jouarre'), 'fr': u('La Fert\u00e9 sous Jouarre')},
'3316023':{'en': 'Meaux', 'fr': 'Meaux'},
'334938':{'en': 'Nice', 'fr': 'Nice'},
'3316028':{'en': 'Pontault-Combault', 'fr': 'Pontault-Combault'},
'3316029':{'en': 'Pontault-Combault', 'fr': 'Pontault-Combault'},
'3338810':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338813':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338812':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'4415074':{'en': 'Alford (Lincs)'},
'3349991':{'en': u('Clermont-l\'H\u00e9rault'), 'fr': u('Clermont-l\'H\u00e9rault')},
'434255':{'de': 'Arnoldstein', 'en': 'Arnoldstein'},
'437284':{'de': 'Oberkappel', 'en': 'Oberkappel'},
'434253':{'de': 'Sankt Jakob im Rosental', 'en': 'St. Jakob im Rosental'},
'3332028':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3332029':{'en': u('La Bass\u00e9e'), 'fr': u('La Bass\u00e9e')},
'35951314':{'bg': u('\u0412\u043e\u0439\u0432\u043e\u0434\u0438\u043d\u043e'), 'en': 'Voyvodino'},
'437218':{'de': u('Gro\u00dftraberg'), 'en': 'Grosstraberg'},
'3332022':{'en': 'Lomme', 'fr': 'Lomme'},
'3332023':{'en': 'Bondues', 'fr': 'Bondues'},
'3332020':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332021':{'en': 'Lille', 'fr': 'Lille'},
'3332026':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3332027':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3332024':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3332025':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3354659':{'en': u('Saint-Jean-d\'Ang\u00e9ly'), 'fr': u('Saint-Jean-d\'Ang\u00e9ly')},
'3354658':{'en': 'Matha', 'fr': 'Matha'},
'3354656':{'en': u('Ch\u00e2telaillon-Plage'), 'fr': u('Ch\u00e2telaillon-Plage')},
'3354650':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3354652':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3323572':{'en': 'Rouen', 'fr': 'Rouen'},
'3323573':{'en': 'Rouen', 'fr': 'Rouen'},
'3323570':{'en': 'Rouen', 'fr': 'Rouen'},
'3323571':{'en': 'Rouen', 'fr': 'Rouen'},
'3329949':{'en': u('Val-d\'Iz\u00e9'), 'fr': u('Val-d\'Iz\u00e9')},
'3329948':{'en': 'Dol-de-Bretagne', 'fr': 'Dol-de-Bretagne'},
'3323574':{'en': u('D\u00e9ville-l\u00e8s-Rouen'), 'fr': u('D\u00e9ville-l\u00e8s-Rouen')},
'37425691':{'am': u('\u053f\u0578\u0582\u0580\u0569\u0561\u0576'), 'en': 'Kurtan', 'ru': u('\u041a\u0443\u0440\u0442\u0430\u043d')},
'3355524':{'en': 'Brive-la-Gaillarde', 'fr': 'Brive-la-Gaillarde'},
'3323578':{'en': 'Elbeuf', 'fr': 'Elbeuf'},
'3329946':{'en': 'Dinard', 'fr': 'Dinard'},
'3329941':{'en': 'Chantepie', 'fr': 'Chantepie'},
'3329940':{'en': 'Saint-Malo', 'fr': 'Saint-Malo'},
'3355522':{'en': 'Brive-la-Gaillarde', 'fr': 'Brive-la-Gaillarde'},
'3355835':{'en': u('Saint-Paul-l\u00e8s-Dax'), 'fr': u('Saint-Paul-l\u00e8s-Dax')},
'3349334':{'en': 'Antibes Juan les Pins', 'fr': 'Antibes Juan les Pins'},
'3349335':{'en': 'Menton', 'fr': 'Menton'},
'3349336':{'en': 'Grasse', 'fr': 'Grasse'},
'3349337':{'en': 'Nice', 'fr': 'Nice'},
'3349446':{'en': 'Toulon', 'fr': 'Toulon'},
'3349331':{'en': 'Saint-Laurent-du-Var', 'fr': 'Saint-Laurent-du-Var'},
'3349332':{'en': 'La Colle sur Loup', 'fr': 'La Colle sur Loup'},
'3349445':{'en': 'Puget-sur-Argens', 'fr': 'Puget-sur-Argens'},
'3349449':{'en': 'Sainte-Maxime', 'fr': 'Sainte-Maxime'},
'3349338':{'en': 'Cannes', 'fr': 'Cannes'},
'3349339':{'en': 'Cannes', 'fr': 'Cannes'},
'437212':{'de': 'Zwettl an der Rodl', 'en': 'Zwettl an der Rodl'},
'3327202':{'en': 'Nantes', 'fr': 'Nantes'},
'437214':{'de': 'Reichenthal', 'en': 'Reichenthal'},
'3324023':{'en': 'Batz-sur-Mer', 'fr': 'Batz-sur-Mer'},
'3355748':{'en': 'Libourne', 'fr': 'Libourne'},
'3355749':{'en': 'Coutras', 'fr': 'Coutras'},
'3355740':{'en': 'Castillon-la-Bataille', 'fr': 'Castillon-la-Bataille'},
'3355742':{'en': 'Blaye', 'fr': 'Blaye'},
'3355743':{'en': u('Saint-Andr\u00e9-de-Cubzac'), 'fr': u('Saint-Andr\u00e9-de-Cubzac')},
'3355746':{'en': 'Sainte-Foy-la-Grande', 'fr': 'Sainte-Foy-la-Grande'},
'3332688':{'en': 'Reims', 'fr': 'Reims'},
'3332689':{'en': 'Reims', 'fr': 'Reims'},
'3332686':{'en': 'Reims', 'fr': 'Reims'},
'3332687':{'en': 'Reims', 'fr': 'Reims'},
'3332684':{'en': 'Reims', 'fr': 'Reims'},
'3332685':{'en': 'Reims', 'fr': 'Reims'},
'3332682':{'en': 'Reims', 'fr': 'Reims'},
'3332683':{'en': 'Reims', 'fr': 'Reims'},
'3332680':{'en': u('S\u00e9zanne'), 'fr': u('S\u00e9zanne')},
'3594729':{'bg': u('\u041a\u0438\u0440\u0438\u043b\u043e\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Kirilovo, Yambol'},
'3594728':{'bg': u('\u041c\u0435\u043b\u043d\u0438\u0446\u0430'), 'en': 'Melnitsa'},
'3594727':{'bg': u('\u041c\u0430\u043b\u044a\u043a \u043c\u0430\u043d\u0430\u0441\u0442\u0438\u0440'), 'en': 'Malak manastir'},
'3594726':{'bg': u('\u041c\u0430\u043b\u043e\u043c\u0438\u0440\u043e\u0432\u043e'), 'en': 'Malomirovo'},
'3594725':{'bg': u('\u041b\u0435\u0441\u043e\u0432\u043e'), 'en': 'Lesovo'},
'3594724':{'bg': u('\u0420\u0430\u0437\u0434\u0435\u043b, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Razdel, Yambol'},
'3594723':{'bg': u('\u0411\u043e\u044f\u043d\u043e\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Boyanovo, Yambol'},
'3524':{'de': 'Luxemburg', 'en': 'Luxembourg City', 'fr': 'Luxembourg-Ville'},
'3324340':{'en': u('Chang\u00e9'), 'fr': u('Chang\u00e9')},
'3324341':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324342':{'en': u('\u00c9commoy'), 'fr': u('\u00c9commoy')},
'3324343':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324344':{'en': u('Ch\u00e2teau-du-Loir'), 'fr': u('Ch\u00e2teau-du-Loir')},
'3324348':{'en': u('La Fl\u00e8che'), 'fr': u('La Fl\u00e8che')},
'3324349':{'en': 'Laval', 'fr': 'Laval'},
'3332978':{'en': 'Ligny-en-Barrois', 'fr': 'Ligny-en-Barrois'},
'3332979':{'en': 'Bar-le-Duc', 'fr': 'Bar-le-Duc'},
'437279':{'de': 'Haibach ob der Donau', 'en': 'Haibach ob der Donau'},
'437278':{'de': 'Neukirchen am Walde', 'en': 'Neukirchen am Walde'},
'437277':{'de': 'Waizenkirchen', 'en': 'Waizenkirchen'},
'437276':{'de': 'Peuerbach', 'en': 'Peuerbach'},
'437274':{'de': 'Alkoven', 'en': 'Alkoven'},
'437273':{'de': 'Aschach an der Donau', 'en': 'Aschach an der Donau'},
'437272':{'de': 'Eferding', 'en': 'Eferding'},
'3332976':{'en': 'Bar-le-Duc', 'fr': 'Bar-le-Duc'},
'3332977':{'en': 'Bar-le-Duc', 'fr': 'Bar-le-Duc'},
'3348311':{'en': 'Draguignan', 'fr': 'Draguignan'},
'3348312':{'en': u('Fr\u00e9jus'), 'fr': u('Fr\u00e9jus')},
'3348315':{'en': 'Antibes', 'fr': 'Antibes'},
'3348314':{'en': 'Cannes', 'fr': 'Cannes'},
'3348316':{'en': 'Toulon', 'fr': 'Toulon'},
'3726':{'en': 'Tallinn/Harju County'},
'3356244':{'en': 'Tarbes', 'fr': 'Tarbes'},
'3356246':{'en': 'Lourdes', 'fr': 'Lourdes'},
'3356711':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356241':{'en': u('Saint-P\u00e9-de-Bigorre'), 'fr': u('Saint-P\u00e9-de-Bigorre')},
'3356242':{'en': 'Lourdes', 'fr': 'Lourdes'},
'3356248':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3325167':{'en': 'Les Herbiers', 'fr': 'Les Herbiers'},
'3325166':{'en': 'Les Herbiers', 'fr': 'Les Herbiers'},
'3325165':{'en': u('Mortagne-sur-S\u00e8vre'), 'fr': u('Mortagne-sur-S\u00e8vre')},
'3325164':{'en': 'Les Herbiers', 'fr': 'Les Herbiers'},
'3325163':{'en': u('Mortagne-sur-S\u00e8vre'), 'fr': u('Mortagne-sur-S\u00e8vre')},
'3325162':{'en': 'La Roche sur Yon', 'fr': 'La Roche sur Yon'},
'3325160':{'en': 'Saint-Gilles-Croix-de-Vie', 'fr': 'Saint-Gilles-Croix-de-Vie'},
'3325169':{'en': 'Fontenay-le-Comte', 'fr': 'Fontenay-le-Comte'},
'3325168':{'en': 'Challans', 'fr': 'Challans'},
'3346960':{'en': 'Lyon', 'fr': 'Lyon'},
'3595733':{'bg': u('\u041a\u0430\u0440\u0434\u0430\u043c, \u0414\u043e\u0431\u0440.'), 'en': 'Kardam, Dobr.'},
'331830':{'en': 'Paris', 'fr': 'Paris'},
'3595732':{'bg': u('\u041f\u0435\u0442\u043b\u0435\u0448\u043a\u043e\u0432\u043e'), 'en': 'Petleshkovo'},
'3344254':{'en': 'Venelles', 'fr': 'Venelles'},
'3344255':{'en': 'Istres', 'fr': 'Istres'},
'3344256':{'en': 'Istres', 'fr': 'Istres'},
'3344257':{'en': 'Lambesc', 'fr': 'Lambesc'},
'35351':{'en': 'Waterford/Carrick-on-Suir/New Ross/Kilmacthomas'},
'3344251':{'en': 'Gardanne', 'fr': 'Gardanne'},
'3344252':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'35352':{'en': 'Clonmel/Cahir/Killenaule'},
'35359':{'en': 'Carlow/Muine Bheag/Athy/Baltinglass'},
'35358':{'en': 'Dungarvan'},
'3599728':{'bg': u('\u0421\u043b\u0438\u0432\u0430\u0442\u0430'), 'en': 'Slivata'},
'3599729':{'bg': u('\u0420\u0430\u0441\u043e\u0432\u043e'), 'en': 'Rasovo'},
'3599720':{'bg': u('\u041a\u043e\u0432\u0430\u0447\u0438\u0446\u0430'), 'en': 'Kovachitsa'},
'3599721':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u0426\u0435\u0440\u043e\u0432\u0435\u043d\u0435'), 'en': 'Dolno Tserovene'},
'3599722':{'bg': u('\u0421\u0442\u0430\u043b\u0438\u0439\u0441\u043a\u0430 \u043c\u0430\u0445\u0430\u043b\u0430'), 'en': 'Staliyska mahala'},
'3599723':{'bg': u('\u0422\u0440\u0430\u0439\u043a\u043e\u0432\u043e'), 'en': 'Traykovo'},
'3599724':{'bg': u('\u0421\u0442\u0430\u043d\u0435\u0432\u043e'), 'en': 'Stanevo'},
'3599725':{'bg': u('\u041a\u043e\u043c\u043e\u0449\u0438\u0446\u0430'), 'en': 'Komoshtitsa'},
'3349957':{'en': u('S\u00e8te'), 'fr': u('S\u00e8te')},
'3599727':{'bg': u('\u041c\u0435\u0434\u043a\u043e\u0432\u0435\u0446'), 'en': 'Medkovets'},
'3347699':{'en': 'Claix', 'fr': 'Claix'},
'3347698':{'en': 'Claix', 'fr': 'Claix'},
'3347695':{'en': 'Villard-de-Lans', 'fr': 'Villard-de-Lans'},
'3347694':{'en': 'Villard-de-Lans', 'fr': 'Villard-de-Lans'},
'3347697':{'en': 'Pontcharra', 'fr': 'Pontcharra'},
'3347696':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347691':{'en': 'Rives', 'fr': 'Rives'},
'3347690':{'en': 'Meylan', 'fr': 'Meylan'},
'3347692':{'en': 'Crolles', 'fr': 'Crolles'},
'374253':{'am': u('\u0531\u056c\u0561\u057e\u0565\u0580\u0564\u056b/\u0555\u0571\u0578\u0582\u0576/\u053e\u0561\u0572\u056f\u0561\u0577\u0561\u057f/\u0539\u0578\u0582\u0574\u0561\u0576\u0575\u0561\u0576'), 'en': 'Alaverdi/Odzun/Tsaghkashat/Tumanyan', 'ru': u('\u0410\u043b\u0430\u0432\u0435\u0440\u0434\u0438/\u041e\u0434\u0437\u0443\u043d/\u0426\u0430\u0445\u043a\u0430\u0448\u0430\u0442/\u0422\u0443\u043c\u0430\u043d\u044f\u043d')},
'374252':{'am': u('\u0531\u057a\u0561\u0580\u0561\u0576'), 'en': 'Aparan', 'ru': u('\u0410\u043f\u0430\u0440\u0430\u043d')},
'374255':{'am': u('\u054d\u057a\u056b\u057f\u0561\u056f'), 'en': 'Spitak region', 'ru': u('\u0421\u043f\u0438\u0442\u0430\u043a')},
'3595739':{'bg': u('\u041f\u0447\u0435\u043b\u0430\u0440\u043e\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Pchelarovo, Dobr.'},
'374257':{'am': u('\u0531\u0580\u0561\u0563\u0561\u056e'), 'en': 'Aragats', 'ru': u('\u0410\u0440\u0430\u0433\u0430\u0446')},
'374256':{'am': u('\u054d\u057f\u0565\u0583\u0561\u0576\u0561\u057e\u0561\u0576/\u0532\u0578\u057e\u0561\u0571\u0578\u0580'), 'en': 'Bovadzor/Stepanavan', 'ru': u('\u0421\u0442\u0435\u043f\u0430\u043d\u0430\u0432\u0430\u043d/\u0411\u043e\u0432\u0430\u0434\u0437\u043e\u0440')},
'3595738':{'bg': u('\u0421\u043f\u0430\u0441\u043e\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Spasovo, Dobr.'},
'3349923':{'en': 'Montpellier', 'fr': 'Montpellier'},
'435510':{'de': u('Dam\u00fcls'), 'en': u('Dam\u00fcls')},
'373293':{'en': 'Vulcanesti', 'ro': u('Vulc\u0103ne\u015fti'), 'ru': u('\u0412\u0443\u043b\u043a\u044d\u043d\u0435\u0448\u0442\u044c')},
'359517':{'bg': u('\u0414\u044a\u043b\u0433\u043e\u043f\u043e\u043b'), 'en': 'Dalgopol'},
'359519':{'bg': u('\u0414\u0435\u0432\u043d\u044f'), 'en': 'Devnya'},
'359518':{'bg': u('\u041f\u0440\u043e\u0432\u0430\u0434\u0438\u044f'), 'en': 'Provadia'},
'441650':{'en': 'Cemmaes Road'},
'441651':{'en': 'Oldmeldrum'},
'441652':{'en': 'Brigg'},
'441653':{'en': 'Malton'},
'441654':{'en': 'Machynlleth'},
'441655':{'en': 'Maybole'},
'441656':{'en': 'Bridgend'},
'441659':{'en': 'Sanquhar'},
'355373':{'en': u('Krutje/Bubullim\u00eb/Allkaj, Lushnj\u00eb')},
'355372':{'en': u('Karbunar\u00eb/Fier-Shegan/Hysgjokaj/Ballagat, Lushnj\u00eb')},
'355371':{'en': u('Divjak\u00eb, Lushnj\u00eb')},
'355377':{'en': u('Qend\u00ebr/Greshic\u00eb/Hekal, Mallakast\u00ebr')},
'355376':{'en': u('Dushk/T\u00ebrbuf, Lushnj\u00eb')},
'355375':{'en': u('Golem/Grabian/Remas, Lushnj\u00eb')},
'355374':{'en': u('Gradisht\u00eb/Kolonj\u00eb, Lushnj\u00eb')},
'355378':{'en': u('Aranitas/Ngracan/Selit\u00eb/Fratar/Kut\u00eb, Mallakast\u00ebr')},
'3597918':{'bg': u('\u042f\u0431\u044a\u043b\u043a\u043e\u0432\u043e, \u041a\u044e\u0441\u0442.'), 'en': 'Yabalkovo, Kyust.'},
'3596545':{'bg': u('\u041b\u044e\u0431\u0435\u043d\u043e\u0432\u043e, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Lyubenovo, Pleven'},
'3596544':{'bg': u('\u041d\u043e\u0432\u0430\u0447\u0435\u043d\u0435, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Novachene, Pleven'},
'3596547':{'bg': u('\u0414\u0440\u0430\u0433\u0430\u0448 \u0432\u043e\u0439\u0432\u043e\u0434\u0430'), 'en': 'Dragash voyvoda'},
'3596546':{'bg': u('\u041b\u043e\u0437\u0438\u0446\u0430, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Lozitsa, Pleven'},
'3596541':{'bg': u('\u041d\u0438\u043a\u043e\u043f\u043e\u043b'), 'en': 'Nikopol'},
'3596540':{'bg': u('\u0410\u0441\u0435\u043d\u043e\u0432\u043e, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Asenovo, Pleven'},
'3596543':{'bg': u('\u041c\u0443\u0441\u0435\u043b\u0438\u0435\u0432\u043e'), 'en': 'Muselievo'},
'3596542':{'bg': u('\u0412\u044a\u0431\u0435\u043b, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Vabel, Pleven'},
'3596549':{'bg': u('\u0421\u0430\u043d\u0430\u0434\u0438\u043d\u043e\u0432\u043e'), 'en': 'Sanadinovo'},
'3596548':{'bg': u('\u0414\u0435\u0431\u043e\u0432\u043e'), 'en': 'Debovo'},
'3597142':{'bg': u('\u041a\u043e\u0441\u0442\u0435\u043d\u0435\u0446'), 'en': 'Kostenets'},
'3597143':{'bg': u('\u0412\u0430\u043a\u0430\u0440\u0435\u043b'), 'en': 'Vakarel'},
'3597146':{'bg': u('\u0427\u0435\u0440\u043d\u044c\u043e\u0432\u043e'), 'en': 'Chernyovo'},
'3597147':{'bg': u('\u041f\u0447\u0435\u043b\u0438\u043d, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Pchelin, Sofia'},
'3597144':{'bg': u('\u041a\u043e\u0441\u0442\u0435\u043d\u0435\u0446'), 'en': 'Kostenets'},
'3597145':{'bg': u('\u041c\u0438\u0440\u043e\u0432\u043e, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Mirovo, Sofia'},
'3597148':{'bg': u('\u041c\u0443\u0445\u043e\u0432\u043e'), 'en': 'Muhovo'},
'3597149':{'bg': u('\u0416\u0438\u0432\u043a\u043e\u0432\u043e, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Zhivkovo, Sofia'},
'441261':{'en': 'Banff'},
'441260':{'en': 'Congleton'},
'441263':{'en': 'Cromer'},
'441262':{'en': 'Bridlington'},
'441264':{'en': 'Andover'},
'441267':{'en': 'Carmarthen'},
'441269':{'en': 'Ammanford'},
'441268':{'en': 'Basildon'},
'3332357':{'en': 'Tergnier', 'fr': 'Tergnier'},
'3597913':{'bg': u('\u0420\u0430\u0448\u043a\u0430 \u0413\u0440\u0430\u0449\u0438\u0446\u0430'), 'en': 'Rashka Grashtitsa'},
'3343757':{'en': 'Lyon', 'fr': 'Lyon'},
'35974401':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u0414\u0440\u0430\u0433\u043b\u0438\u0449\u0435'), 'en': 'Gorno Draglishte'},
'35974402':{'bg': u('\u0413\u043e\u0434\u043b\u0435\u0432\u043e'), 'en': 'Godlevo'},
'35974403':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u0414\u0440\u0430\u0433\u043b\u0438\u0449\u0435'), 'en': 'Dolno Draglishte'},
'35974404':{'bg': u('\u0411\u0430\u0431\u044f\u043a'), 'en': 'Babyak'},
'35974405':{'bg': u('\u041a\u0440\u0430\u0438\u0449\u0435, \u0411\u043b\u0430\u0433.'), 'en': 'Kraishte, Blag.'},
'35974406':{'bg': u('\u0414\u043e\u0431\u044a\u0440\u0441\u043a\u043e'), 'en': 'Dobarsko'},
'35974407':{'bg': u('\u041a\u0440\u0435\u043c\u0435\u043d, \u0411\u043b\u0430\u0433.'), 'en': 'Kremen, Blag.'},
'35974408':{'bg': u('\u041e\u0431\u0438\u0434\u0438\u043c'), 'en': 'Obidim'},
'35974409':{'bg': u('\u041c\u0435\u0441\u0442\u0430'), 'en': 'Mesta'},
'35984749':{'bg': u('\u0421\u0435\u0432\u0430\u0440'), 'en': 'Sevar'},
'35984740':{'bg': u('\u0411\u0438\u0441\u0435\u0440\u0446\u0438'), 'en': 'Bisertsi'},
'35984743':{'bg': u('\u0411\u0440\u0435\u0441\u0442\u043e\u0432\u0435\u043d\u0435'), 'en': 'Brestovene'},
'35984744':{'bg': u('\u0411\u0435\u043b\u043e\u0432\u0435\u0446'), 'en': 'Belovets'},
'35984745':{'bg': u('\u0412\u043b\u0430\u0434\u0438\u043c\u0438\u0440\u043e\u0432\u0446\u0438'), 'en': 'Vladimirovtsi'},
'3326229':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326228':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326225':{'en': 'Saint-Pierre', 'fr': 'Saint-Pierre'},
'3326224':{'en': 'Saint-Paul', 'fr': 'Saint-Paul'},
'3326227':{'en': 'Le Tampon', 'fr': 'Le Tampon'},
'3326226':{'en': 'Saint-Louis', 'fr': 'Saint-Louis'},
'3326221':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326220':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326223':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326222':{'en': 'Saint-Paul', 'fr': 'Saint-Paul'},
'35967394':{'bg': u('\u0411\u043e\u0433\u0430\u0442\u043e\u0432\u043e'), 'en': 'Bogatovo'},
'35967395':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u0420\u043e\u0441\u0438\u0446\u0430'), 'en': 'Gorna Rositsa'},
'35967396':{'bg': u('\u0411\u0435\u0440\u0438\u0435\u0432\u043e'), 'en': 'Berievo'},
'35967397':{'bg': u('\u0420\u044f\u0445\u043e\u0432\u0446\u0438\u0442\u0435'), 'en': 'Ryahovtsite'},
'35967390':{'bg': u('\u0428\u0443\u043c\u0430\u0442\u0430'), 'en': 'Shumata'},
'35967391':{'bg': u('\u0421\u0442\u043e\u043b\u044a\u0442'), 'en': 'Stolat'},
'35967392':{'bg': u('\u042f\u0432\u043e\u0440\u0435\u0446'), 'en': 'Yavorets'},
'35967393':{'bg': u('\u0414\u0443\u0448\u0435\u0432\u043e'), 'en': 'Dushevo'},
'35967398':{'bg': u('\u0414\u0430\u043c\u044f\u043d\u043e\u0432\u043e'), 'en': 'Damyanovo'},
'35967399':{'bg': u('\u041c\u0430\u043b\u043a\u0438 \u0412\u044a\u0440\u0448\u0435\u0446'), 'en': 'Malki Varshets'},
'3599529':{'bg': u('\u0411\u043e\u0440\u043e\u0432\u0446\u0438'), 'en': 'Borovtsi'},
'441885':{'en': 'Pencombe'},
'441884':{'en': 'Tiverton'},
'441887':{'en': 'Aberfeldy'},
'441886':{'en': 'Bromyard (Knightwick/Leigh Sinton)'},
'441880':{'en': 'Tarbert'},
'441883':{'en': 'Caterham'},
'441882':{'en': 'Kinloch Rannoch'},
'4415399':{'en': 'Kendal'},
'441889':{'en': 'Rugeley'},
'441888':{'en': 'Turriff'},
'433843':{'de': 'Sankt Michael in Obersteiermark', 'en': 'St. Michael in Obersteiermark'},
'3359638':{'en': 'Le Robert', 'fr': 'Le Robert'},
'3359639':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3593648':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u043e \u041a\u0430\u043c\u0435\u043d\u044f\u043d\u0435'), 'en': 'Golyamo Kamenyane'},
'3593641':{'bg': u('\u041a\u0440\u0443\u043c\u043e\u0432\u0433\u0440\u0430\u0434'), 'en': 'Krumovgrad'},
'3593643':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u0430 \u0427\u0438\u043d\u043a\u0430'), 'en': 'Golyama Chinka'},
'3593642':{'bg': u('\u041f\u043e\u0442\u043e\u0447\u043d\u0438\u0446\u0430'), 'en': 'Potochnitsa'},
'3593645':{'bg': u('\u0410\u0432\u0440\u0435\u043d, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Avren, Kardzh.'},
'3593644':{'bg': u('\u0415\u0433\u0440\u0435\u043a'), 'en': 'Egrek'},
'3593647':{'bg': u('\u0427\u0435\u0440\u043d\u0438\u0447\u0435\u0432\u043e, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Chernichevo, Kardzh.'},
'3593646':{'bg': u('\u0422\u043e\u043a\u0430\u0447\u043a\u0430'), 'en': 'Tokachka'},
'3355990':{'en': 'Pau', 'fr': 'Pau'},
'3355993':{'en': 'Ustaritz', 'fr': 'Ustaritz'},
'3355992':{'en': 'Pau', 'fr': 'Pau'},
'3355998':{'en': 'Pau', 'fr': 'Pau'},
'3593025':{'bg': u('\u0411\u0430\u043d\u0438\u0442\u0435'), 'en': 'Banite'},
'3593024':{'bg': u('\u0422\u044a\u0440\u044a\u043d'), 'en': 'Taran'},
'3593027':{'bg': u('\u0421\u043b\u0430\u0432\u0435\u0439\u043d\u043e'), 'en': 'Slaveyno'},
'3593026':{'bg': u('\u0421\u043c\u0438\u043b\u044f\u043d'), 'en': 'Smilyan'},
'3593020':{'bg': u('\u0414\u0430\u0432\u0438\u0434\u043a\u043e\u0432\u043e'), 'en': 'Davidkovo'},
'3593023':{'bg': u('\u041c\u043e\u043c\u0447\u0438\u043b\u043e\u0432\u0446\u0438'), 'en': 'Momchilovtsi'},
'3593022':{'bg': u('\u0412\u0438\u0435\u0432\u043e'), 'en': 'Vievo'},
'34925':{'en': 'Toledo', 'es': 'Toledo'},
'34924':{'en': 'Badajoz', 'es': 'Badajoz'},
'3329882':{'en': u('Pont-l\'Abb\u00e9'), 'fr': u('Pont-l\'Abb\u00e9')},
'34926':{'en': 'Ciudad Real', 'es': 'Ciudad Real'},
'34921':{'en': 'Segovia', 'es': 'Segovia'},
'3593028':{'bg': u('\u0410\u0440\u0434\u0430'), 'en': 'Arda'},
'34923':{'en': 'Salamanca', 'es': 'Salamanca'},
'34922':{'en': 'Tenerife', 'es': 'Tenerife'},
'3332765':{'en': 'Maubeuge', 'fr': 'Maubeuge'},
'3332764':{'en': 'Maubeuge', 'fr': 'Maubeuge'},
'3332761':{'en': 'Avesnes-sur-Helpe', 'fr': 'Avesnes-sur-Helpe'},
'3332760':{'en': 'Fourmies', 'fr': 'Fourmies'},
'3332763':{'en': 'Hautmont', 'fr': 'Hautmont'},
'3332762':{'en': 'Maubeuge', 'fr': 'Maubeuge'},
'437744':{'de': 'Munderfing', 'en': 'Munderfing'},
'434263':{'de': u('H\u00fcttenberg'), 'en': u('H\u00fcttenberg')},
'434262':{'de': 'Treibach', 'en': 'Treibach'},
'434265':{'de': 'Weitensfeld im Gurktal', 'en': 'Weitensfeld im Gurktal'},
'434264':{'de': 'Klein Sankt Paul', 'en': 'Klein St. Paul'},
'434267':{'de': 'Metnitz', 'en': 'Metnitz'},
'434266':{'de': u('Stra\u00dfburg'), 'en': 'Strassburg'},
'434269':{'de': 'Flattnitz', 'en': 'Flattnitz'},
'434268':{'de': 'Friesach', 'en': 'Friesach'},
'432725':{'de': 'Frankenfels', 'en': 'Frankenfels'},
'3332505':{'en': 'Saint-Dizier', 'fr': 'Saint-Dizier'},
'3344271':{'en': 'La Ciotat', 'fr': 'La Ciotat'},
'3332507':{'en': 'Saint-Dizier', 'fr': 'Saint-Dizier'},
'3332506':{'en': 'Saint-Dizier', 'fr': 'Saint-Dizier'},
'3332501':{'en': 'Chaumont', 'fr': 'Chaumont'},
'3332503':{'en': 'Chaumont', 'fr': 'Chaumont'},
'3595395':{'bg': u('\u0427\u0435\u0440\u043d\u043e\u043e\u043a\u043e\u0432\u043e, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Chernookovo, Shumen'},
'3595394':{'bg': u('\u0411\u044f\u043b\u0430 \u0440\u0435\u043a\u0430, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Byala reka, Shumen'},
'3595397':{'bg': u('\u041c\u0435\u0442\u043e\u0434\u0438\u0435\u0432\u043e, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Metodievo, Shumen'},
'3595396':{'bg': u('\u041b\u043e\u0432\u0435\u0446, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Lovets, Shumen'},
'3595391':{'bg': u('\u0412\u044a\u0440\u0431\u0438\u0446\u0430, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Varbitsa, Shumen'},
'441462':{'en': 'Hitchin'},
'3595393':{'bg': u('\u0418\u0432\u0430\u043d\u043e\u0432\u043e, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Ivanovo, Shumen'},
'3595392':{'bg': u('\u041c\u0435\u043d\u0433\u0438\u0448\u0435\u0432\u043e'), 'en': 'Mengishevo'},
'441469':{'en': 'Killingholme'},
'437684':{'de': 'Frankenmarkt', 'en': 'Frankenmarkt'},
'437682':{'de': u('V\u00f6cklamarkt'), 'en': u('V\u00f6cklamarkt')},
'437683':{'de': 'Frankenburg am Hausruck', 'en': 'Frankenburg am Hausruck'},
'437664':{'de': 'Weyregg am Attersee', 'en': 'Weyregg am Attersee'},
'3594554':{'bg': u('\u0422\u0440\u0430\u043f\u043e\u043a\u043b\u043e\u0432\u043e'), 'en': 'Trapoklovo'},
'3594556':{'bg': u('\u0421\u043e\u0442\u0438\u0440\u044f'), 'en': 'Sotirya'},
'3594557':{'bg': u('\u0411\u0438\u043a\u043e\u0432\u043e'), 'en': 'Bikovo'},
'3594551':{'bg': u('\u0411\u044f\u043b\u0430, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Byala, Sliven'},
'3594552':{'bg': u('\u0421\u0442\u0430\u0440\u0430 \u0440\u0435\u043a\u0430, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Stara reka, Sliven'},
'3594553':{'bg': u('\u0420\u0430\u043a\u043e\u0432\u043e, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Rakovo, Sliven'},
'441790':{'en': 'Spilsby'},
'441793':{'en': 'Swindon'},
'441792':{'en': 'Swansea'},
'441795':{'en': 'Sittingbourne'},
'441794':{'en': 'Romsey'},
'441797':{'en': 'Rye'},
'441796':{'en': 'Pitlochry'},
'441799':{'en': 'Saffron Walden'},
'441798':{'en': 'Pulborough'},
'3324151':{'en': 'Saumur', 'fr': 'Saumur'},
'3324150':{'en': 'Saumur', 'fr': 'Saumur'},
'3324153':{'en': 'Saumur', 'fr': 'Saumur'},
'3598629':{'bg': u('\u0421\u043c\u0438\u043b\u0435\u0446, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Smilets, Silistra'},
'3598626':{'bg': u('\u0421\u0440\u0435\u0434\u0438\u0449\u0435, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Sredishte, Silistra'},
'3598627':{'bg': u('\u0411\u0430\u0431\u0443\u043a'), 'en': 'Babuk'},
'3598624':{'bg': u('\u041a\u0430\u043b\u0438\u043f\u0435\u0442\u0440\u043e\u0432\u043e'), 'en': 'Kalipetrovo'},
'3598625':{'bg': u('\u041e\u0432\u0435\u043d'), 'en': 'Oven'},
'3324159':{'en': u('Dou\u00e9-la-Fontaine'), 'fr': u('Dou\u00e9-la-Fontaine')},
'3324158':{'en': 'Cholet', 'fr': 'Cholet'},
'35295':{'de': 'Wiltz', 'en': 'Wiltz', 'fr': 'Wiltz'},
'35297':{'de': 'Huldingen', 'en': 'Huldange', 'fr': 'Huldange'},
'35292':{'de': 'Kanton Clerf/Fischbach/Hosingen', 'en': 'Clervaux/Fischbach/Hosingen', 'fr': 'Clervaux/Fischbach/Hosingen'},
'39099':{'en': 'Taranto'},
'39095':{'en': 'Catania', 'it': 'Catania'},
'35299':{'de': 'Ulflingen', 'en': 'Troisvierges', 'fr': 'Troisvierges'},
'39090':{'en': 'Messina', 'it': 'Messina'},
'39091':{'en': 'Palermo', 'it': 'Palermo'},
'3355595':{'en': 'Meymac', 'fr': 'Meymac'},
'354561':{'en': u('Reykjav\u00edk/Vesturb\u00e6r/Mi\u00f0b\u00e6rinn')},
'354562':{'en': u('Reykjav\u00edk/Vesturb\u00e6r/Mi\u00f0b\u00e6rinn')},
'35931993':{'bg': u('\u0421\u044a\u0440\u043d\u0435\u0433\u043e\u0440'), 'en': 'Sarnegor'},
'35931992':{'bg': u('\u041f\u044a\u0434\u0430\u0440\u0441\u043a\u043e'), 'en': 'Padarsko'},
'35931995':{'bg': u('\u0421\u0442\u0440\u0435\u043b\u0446\u0438, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Streltsi, Plovdiv'},
'35931997':{'bg': u('\u0417\u043b\u0430\u0442\u043e\u0441\u0435\u043b'), 'en': 'Zlatosel'},
'35931996':{'bg': u('\u0421\u0432\u0435\u0436\u0435\u043d'), 'en': 'Svezhen'},
'35931998':{'bg': u('\u0427\u043e\u0431\u0430'), 'en': 'Choba'},
'3355848':{'en': 'Vieux-Boucau-les-Bains', 'fr': 'Vieux-Boucau-les-Bains'},
'435358':{'de': 'Ellmau', 'en': 'Ellmau'},
'435359':{'de': 'Hochfilzen', 'en': 'Hochfilzen'},
'435352':{'de': 'Sankt Johann in Tirol', 'en': 'St. Johann in Tirol'},
'435353':{'de': 'Waidring', 'en': 'Waidring'},
'3338695':{'en': 'Sens', 'fr': 'Sens'},
'3338694':{'en': 'Auxerre', 'fr': 'Auxerre'},
'3338693':{'en': 'Nevers', 'fr': 'Nevers'},
'3338692':{'en': 'Migennes', 'fr': 'Migennes'},
'435354':{'de': 'Fieberbrunn', 'en': 'Fieberbrunn'},
'435355':{'de': 'Jochberg', 'en': 'Jochberg'},
'35953436':{'bg': u('\u0427\u0435\u0440\u043d\u043e\u0433\u043b\u0430\u0432\u0446\u0438'), 'en': 'Chernoglavtsi'},
'35953437':{'bg': u('\u0413\u0430\u0431\u0440\u0438\u0446\u0430, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Gabritsa, Shumen'},
'35953434':{'bg': u('\u042f\u0441\u0435\u043d\u043a\u043e\u0432\u043e'), 'en': 'Yasenkovo'},
'35953435':{'bg': u('\u0418\u0437\u0433\u0440\u0435\u0432, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Izgrev, Shumen'},
'3347207':{'en': 'Lyon', 'fr': 'Lyon'},
'3347204':{'en': 'Vaulx-en-Velin', 'fr': 'Vaulx-en-Velin'},
'3347205':{'en': u('D\u00e9cines-Charpieu'), 'fr': u('D\u00e9cines-Charpieu')},
'3355841':{'en': 'Soustons', 'fr': 'Soustons'},
'3347200':{'en': 'Lyon', 'fr': 'Lyon'},
'3347201':{'en': 'Rillieux-la-Pape', 'fr': 'Rillieux-la-Pape'},
'3347208':{'en': u('Neuville-sur-Sa\u00f4ne'), 'fr': u('Neuville-sur-Sa\u00f4ne')},
'3347209':{'en': 'Saint-Priest', 'fr': 'Saint-Priest'},
'3596158':{'bg': u('\u0421\u0440\u0435\u0434\u043d\u0438 \u043a\u043e\u043b\u0438\u0431\u0438'), 'en': 'Sredni kolibi'},
'354551':{'en': u('Reykjav\u00edk/Vesturb\u00e6r/Mi\u00f0b\u00e6rinn')},
'3596156':{'bg': u('\u0420\u043e\u0434\u0438\u043d\u0430'), 'en': 'Rodina'},
'3596157':{'bg': u('\u0421\u043b\u0438\u0432\u043e\u0432\u0438\u0446\u0430'), 'en': 'Slivovitsa'},
'3596154':{'bg': u('\u0411\u0443\u0439\u043d\u043e\u0432\u0446\u0438'), 'en': 'Buynovtsi'},
'3596155':{'bg': u('\u041a\u043e\u043d\u0441\u0442\u0430\u043d\u0442\u0438\u043d'), 'en': 'Konstantin'},
'3596152':{'bg': u('\u0411\u0435\u0431\u0440\u043e\u0432\u043e'), 'en': 'Bebrovo'},
'3596151':{'bg': u('\u0415\u043b\u0435\u043d\u0430, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Elena, V. Tarnovo'},
'3593328':{'bg': u('\u0414\u043e\u043b\u043d\u043e\u0441\u043b\u0430\u0432'), 'en': 'Dolnoslav'},
'3347067':{'en': 'Bourbon-l\'Archambault', 'fr': 'Bourbon-l\'Archambault'},
'3347064':{'en': 'Commentry', 'fr': 'Commentry'},
'3351607':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3316008':{'en': 'Chelles', 'fr': 'Chelles'},
'3316009':{'en': 'Meaux', 'fr': 'Meaux'},
'3316006':{'en': 'Torcy', 'fr': 'Torcy'},
'3316007':{'en': 'Lagny-sur-Marne', 'fr': 'Lagny-sur-Marne'},
'3316005':{'en': 'Torcy', 'fr': 'Torcy'},
'3316002':{'en': u('Ozoir-la-Ferri\u00e8re'), 'fr': u('Ozoir-la-Ferri\u00e8re')},
'3316003':{'en': u('Dammartin-en-Go\u00eble'), 'fr': u('Dammartin-en-Go\u00eble')},
'3593320':{'bg': u('\u041e\u0440\u0435\u0448\u0435\u0446, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Oreshets, Plovdiv'},
'359707':{'bg': u('\u0421\u0430\u043f\u0430\u0440\u0435\u0432\u0430 \u0431\u0430\u043d\u044f'), 'en': 'Sapareva banya'},
'3593321':{'bg': u('\u0422\u043e\u043f\u043e\u043b\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Topolovo, Plovdiv'},
'359702':{'bg': u('\u0411\u043e\u0431\u043e\u0432 \u0434\u043e\u043b'), 'en': 'Bobov dol'},
'359701':{'bg': u('\u0414\u0443\u043f\u043d\u0438\u0446\u0430'), 'en': 'Dupnitsa'},
'3329056':{'en': 'Rennes', 'fr': 'Rennes'},
'3593324':{'bg': u('\u0418\u0437\u0431\u0435\u0433\u043b\u0438\u0438'), 'en': 'Izbeglii'},
'3339023':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3339022':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3339020':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'35943616':{'bg': u('\u0422\u0443\u0440\u0438\u044f'), 'en': 'Turia'},
'390578':{'it': 'Chianciano Terme'},
'390577':{'en': 'Siena', 'it': 'Siena'},
'390574':{'en': 'Prato', 'it': 'Prato'},
'390575':{'en': 'Arezzo', 'it': 'Arezzo'},
'390572':{'it': 'Montecatini Terme'},
'390573':{'it': 'Pistoia'},
'390571':{'it': 'Empoli'},
'3338932':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3348805':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3338426':{'en': 'Belfort', 'fr': 'Belfort'},
'3323598':{'en': 'Rouen', 'fr': 'Rouen'},
'3323595':{'en': 'Yvetot', 'fr': 'Yvetot'},
'3323597':{'en': 'Saint-Valery-en-Caux', 'fr': 'Saint-Valery-en-Caux'},
'3323590':{'en': 'Gournay-en-Bray', 'fr': 'Gournay-en-Bray'},
'3323591':{'en': 'Barentin', 'fr': 'Barentin'},
'3323592':{'en': 'Barentin', 'fr': 'Barentin'},
'3347246':{'en': u('Pont-de-Ch\u00e9ruy'), 'fr': u('Pont-de-Ch\u00e9ruy')},
'3349318':{'en': 'Nice', 'fr': 'Nice'},
'3349319':{'en': 'Saint-Laurent-du-Var', 'fr': 'Saint-Laurent-du-Var'},
'3349316':{'en': 'Nice', 'fr': 'Nice'},
'3349317':{'en': 'Nice', 'fr': 'Nice'},
'3349314':{'en': 'Saint-Laurent-du-Var', 'fr': 'Saint-Laurent-du-Var'},
'3349312':{'en': 'Valbonne', 'fr': 'Valbonne'},
'3349313':{'en': 'Nice', 'fr': 'Nice'},
'3347247':{'en': 'Chassieu', 'fr': 'Chassieu'},
'3751641':{'be': u('\u0416\u0430\u0431\u0456\u043d\u043a\u0430, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Zhabinka, Brest Region', 'ru': u('\u0416\u0430\u0431\u0438\u043d\u043a\u0430, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751643':{'be': u('\u0411\u044f\u0440\u043e\u0437\u0430, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Bereza, Brest Region', 'ru': u('\u0411\u0435\u0440\u0435\u0437\u0430, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751642':{'be': u('\u041a\u043e\u0431\u0440\u044b\u043d'), 'en': 'Kobryn', 'ru': u('\u041a\u043e\u0431\u0440\u0438\u043d')},
'3751645':{'be': u('\u0406\u0432\u0430\u0446\u044d\u0432\u0456\u0447\u044b, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Ivatsevichi, Brest Region', 'ru': u('\u0418\u0432\u0430\u0446\u0435\u0432\u0438\u0447\u0438, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751644':{'be': u('\u0414\u0440\u0430\u0433\u0456\u0447\u044b\u043d, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Drogichin, Brest Region', 'ru': u('\u0414\u0440\u043e\u0433\u0438\u0447\u0438\u043d, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751647':{'be': u('\u041b\u0443\u043d\u0456\u043d\u0435\u0446, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Luninets, Brest Region', 'ru': u('\u041b\u0443\u043d\u0438\u043d\u0435\u0446, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751646':{'be': u('\u0413\u0430\u043d\u0446\u0430\u0432\u0456\u0447\u044b, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Gantsevichi, Brest Region', 'ru': u('\u0413\u0430\u043d\u0446\u0435\u0432\u0438\u0447\u0438, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'37041':{'en': u('\u0160iauliai')},
'37046':{'en': u('Klaip\u0117da')},
'37045':{'en': u('Panev\u0117\u017eys')},
'331755':{'en': 'Paris', 'fr': 'Paris'},
'3329787':{'en': 'Lorient', 'fr': 'Lorient'},
'432959':{'de': 'Sitzendorf an der Schmida', 'en': 'Sitzendorf an der Schmida'},
'432958':{'de': 'Maissau', 'en': 'Maissau'},
'432955':{'de': u('Gro\u00dfweikersdorf'), 'en': 'Grossweikersdorf'},
'432954':{'de': u('G\u00f6llersdorf'), 'en': u('G\u00f6llersdorf')},
'432957':{'de': 'Hohenwarth', 'en': 'Hohenwarth'},
'432956':{'de': 'Ziersdorf', 'en': 'Ziersdorf'},
'432951':{'de': 'Guntersdorf', 'en': 'Guntersdorf'},
'432953':{'de': 'Nappersdorf', 'en': 'Nappersdorf'},
'432952':{'de': 'Hollabrunn', 'en': 'Hollabrunn'},
'3355506':{'en': 'Limoges', 'fr': 'Limoges'},
'433579':{'de': u('P\u00f6ls'), 'en': u('P\u00f6ls')},
'3355504':{'en': 'Limoges', 'fr': 'Limoges'},
'3355505':{'en': 'Limoges', 'fr': 'Limoges'},
'3355502':{'en': 'Saint-Junien', 'fr': 'Saint-Junien'},
'3355503':{'en': 'Rochechouart', 'fr': 'Rochechouart'},
'3355501':{'en': 'Limoges', 'fr': 'Limoges'},
'433571':{'de': u('M\u00f6derbrugg'), 'en': u('M\u00f6derbrugg')},
'433572':{'de': 'Judenburg', 'en': 'Judenburg'},
'433573':{'de': 'Fohnsdorf', 'en': 'Fohnsdorf'},
'433574':{'de': 'Pusterwald', 'en': 'Pusterwald'},
'433575':{'de': 'Sankt Johann am Tauern', 'en': 'St. Johann am Tauern'},
'3355508':{'en': 'Saint-Yrieix-la-Perche', 'fr': 'Saint-Yrieix-la-Perche'},
'433577':{'de': 'Zeltweg', 'en': 'Zeltweg'},
'3332952':{'en': u('Saint-Di\u00e9-des-Vosges'), 'fr': u('Saint-Di\u00e9-des-Vosges')},
'3324369':{'en': 'Laval', 'fr': 'Laval'},
'3332956':{'en': u('Saint-Di\u00e9-des-Vosges'), 'fr': u('Saint-Di\u00e9-des-Vosges')},
'3332957':{'en': 'Senones', 'fr': 'Senones'},
'3332955':{'en': u('Saint-Di\u00e9-des-Vosges'), 'fr': u('Saint-Di\u00e9-des-Vosges')},
'3324362':{'en': u('Sabl\u00e9-sur-Sarthe'), 'fr': u('Sabl\u00e9-sur-Sarthe')},
'3324363':{'en': 'Saint-Calais', 'fr': 'Saint-Calais'},
'3324360':{'en': u('La Fert\u00e9 Bernard'), 'fr': u('La Fert\u00e9 Bernard')},
'3324366':{'en': 'Laval', 'fr': 'Laval'},
'3324367':{'en': 'Laval', 'fr': 'Laval'},
'437219':{'de': u('Vorderwei\u00dfenbach'), 'en': 'Vorderweissenbach'},
'3594122':{'bg': u('\u0415\u043b\u0435\u043d\u0438\u043d\u043e'), 'en': 'Elenino'},
'437211':{'de': u('Reichenau im M\u00fchlkreis'), 'en': u('Reichenau im M\u00fchlkreis')},
'35996':{'bg': u('\u041c\u043e\u043d\u0442\u0430\u043d\u0430'), 'en': 'Montana'},
'437213':{'de': 'Bad Leonfelden', 'en': 'Bad Leonfelden'},
'35994':{'bg': u('\u0412\u0438\u0434\u0438\u043d'), 'en': 'Vidin'},
'437215':{'de': u('Hellmons\u00f6dt'), 'en': u('Hellmons\u00f6dt')},
'35992':{'bg': u('\u0412\u0440\u0430\u0446\u0430'), 'en': 'Vratsa'},
'437217':{'de': u('Sankt Veit im M\u00fchlkreis'), 'en': u('St. Veit im M\u00fchlkreis')},
'437216':{'de': 'Helfenberg', 'en': 'Helfenberg'},
'433682':{'de': 'Stainach', 'en': 'Stainach'},
'433683':{'de': 'Donnersbach', 'en': 'Donnersbach'},
'433680':{'de': 'Donnersbachwald', 'en': 'Donnersbachwald'},
'433686':{'de': 'Haus', 'en': 'Haus'},
'433687':{'de': 'Schladming', 'en': 'Schladming'},
'433684':{'de': 'Sankt Martin am Grimming', 'en': 'St. Martin am Grimming'},
'433685':{'de': u('Gr\u00f6bming'), 'en': u('Gr\u00f6bming')},
'3324129':{'en': 'Cholet', 'fr': 'Cholet'},
'433688':{'de': 'Tauplitz', 'en': 'Tauplitz'},
'433689':{'de': u('Sankt Nikolai im S\u00f6lktal'), 'en': u('St. Nikolai im S\u00f6lktal')},
'432632':{'de': 'Pernitz', 'en': 'Pernitz'},
'3356268':{'en': 'Lectoure', 'fr': 'Lectoure'},
'4212':{'en': 'Bratislava'},
'3356267':{'en': 'Gimont', 'fr': 'Gimont'},
'3356262':{'en': 'Samatan', 'fr': 'Samatan'},
'3356263':{'en': 'Auch', 'fr': 'Auch'},
'3356260':{'en': 'Auch', 'fr': 'Auch'},
'3356261':{'en': 'Auch', 'fr': 'Auch'},
'4416866':{'en': 'Newtown'},
'3325144':{'en': 'La Roche sur Yon', 'fr': 'La Roche sur Yon'},
'3325147':{'en': 'La Roche sur Yon', 'fr': 'La Roche sur Yon'},
'4416867':{'en': 'Llanidloes'},
'3325149':{'en': 'Challans', 'fr': 'Challans'},
'4416864':{'en': 'Llanidloes'},
'4416865':{'en': 'Newtown'},
'4416862':{'en': 'Llanidloes'},
'35371':{'en': 'Sligo/Manorhamilton/Carrick-on-Shannon'},
'4416860':{'en': 'Newtown/Llanidloes'},
'3344278':{'en': 'Rognac', 'fr': 'Rognac'},
'3344279':{'en': 'Vitrolles', 'fr': 'Vitrolles'},
'3344276':{'en': u('Ch\u00e2teauneuf-les-Martigues'), 'fr': u('Ch\u00e2teauneuf-les-Martigues')},
'3344277':{'en': 'Marignane', 'fr': 'Marignane'},
'3344274':{'en': u('Berre-l\'\u00c9tang'), 'fr': u('Berre-l\'\u00c9tang')},
'3344275':{'en': 'Vitrolles', 'fr': 'Vitrolles'},
'3344272':{'en': 'Aubagne', 'fr': 'Aubagne'},
'3344273':{'en': 'Carnoux-en-Provence', 'fr': 'Carnoux-en-Provence'},
'3344270':{'en': 'Aubagne', 'fr': 'Aubagne'},
'3324001':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'390331':{'en': 'Varese', 'it': 'Busto Arsizio'},
'390332':{'en': 'Varese', 'it': 'Varese'},
'351265':{'en': u('Set\u00fabal'), 'pt': u('Set\u00fabal')},
'351266':{'en': u('\u00c9vora'), 'pt': u('\u00c9vora')},
'351261':{'en': 'Torres Vedras', 'pt': 'Torres Vedras'},
'351263':{'en': 'Vila Franca de Xira', 'pt': 'Vila Franca de Xira'},
'351262':{'en': 'Caldas da Rainha', 'pt': 'Caldas da Rainha'},
'351269':{'en': u('Santiago do Cac\u00e9m'), 'pt': u('Santiago do Cac\u00e9m')},
'351268':{'en': 'Estremoz', 'pt': 'Estremoz'},
'373552':{'en': 'Bender', 'ro': 'Bender', 'ru': u('\u0411\u0435\u043d\u0434\u0435\u0440')},
'441685':{'en': 'Merthyr Tydfil'},
'373557':{'en': 'Slobozia', 'ro': 'Slobozia', 'ru': u('\u0421\u043b\u043e\u0431\u043e\u0437\u0438\u044f')},
'373555':{'en': 'Ribnita', 'ro': u('R\u00eebni\u0163a'), 'ru': u('\u0420\u044b\u0431\u043d\u0438\u0446\u0430')},
'371684':{'en': u('Liep\u0101ja')},
'441638':{'en': 'Newmarket'},
'441639':{'en': 'Neath'},
'441633':{'en': 'Newport'},
'441630':{'en': 'Market Drayton'},
'441631':{'en': 'Oban'},
'441636':{'en': 'Newark-on-Trent'},
'441637':{'en': 'Newquay'},
'441634':{'en': 'Medway'},
'441635':{'en': 'Newbury'},
'432638':{'de': 'Winzendorf-Muthmannsdorf', 'en': 'Winzendorf-Muthmannsdorf'},
'355398':{'en': u('Sevaster/Brataj/Hore-Vranisht, Vlor\u00eb')},
'355395':{'en': u('Novosel\u00eb, Vlor\u00eb')},
'355394':{'en': u('Qend\u00ebr, Vlor\u00eb')},
'355397':{'en': u('Vllahin\u00eb/Kote, Vlor\u00eb')},
'355396':{'en': u('Shushic\u00eb/Armen, Vlor\u00eb')},
'355391':{'en': u('Orikum, Vlor\u00eb')},
'355393':{'en': u('Himar\u00eb, Vlor\u00eb')},
'355392':{'en': u('Selenic\u00eb, Vlor\u00eb')},
'35941179':{'bg': u('\u0425\u0430\u043d \u0410\u0441\u043f\u0430\u0440\u0443\u0445\u043e\u0432\u043e'), 'en': 'Han Asparuhovo'},
'436483':{'de': u('G\u00f6riach'), 'en': u('G\u00f6riach')},
'37426791':{'am': u('\u0546\u0561\u057e\u0578\u0582\u0580'), 'en': 'Navur', 'ru': u('\u041d\u0430\u0432\u0443\u0440')},
'35977229':{'bg': u('\u0413\u044a\u043b\u044a\u0431\u043d\u0438\u043a'), 'en': 'Galabnik'},
'37426797':{'am': u('\u0546\u0578\u0580\u0561\u0577\u0565\u0576'), 'en': 'Norashen', 'ru': u('\u041d\u043e\u0440\u0430\u0448\u0435\u043d')},
'37426794':{'am': u('\u0539\u0578\u057e\u0578\u0582\u0566'), 'en': 'Tovuz', 'ru': u('\u0422\u043e\u0432\u0443\u0437')},
'436484':{'de': 'Lessach', 'en': 'Lessach'},
'3336664':{'en': 'Lille', 'fr': 'Lille'},
'35977226':{'bg': u('\u0414\u0435\u0431\u0435\u043b\u0438 \u043b\u0430\u0433'), 'en': 'Debeli lag'},
'35977221':{'bg': u('\u041a\u043e\u043d\u0434\u043e\u0444\u0440\u0435\u0439'), 'en': 'Kondofrey'},
'35977222':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u0414\u0438\u043a\u0430\u043d\u044f'), 'en': 'Gorna Dikanya'},
'3343770':{'en': 'Lyon', 'fr': 'Lyon'},
'3355645':{'en': 'Pessac', 'fr': 'Pessac'},
'441248':{'en': 'Bangor (Gwynedd)'},
'432672':{'de': 'Berndorf', 'en': 'Berndorf'},
'441243':{'en': 'Chichester'},
'441242':{'en': 'Cheltenham'},
'441241':{'en': 'Arbroath'},
'441246':{'en': 'Chesterfield'},
'441245':{'en': 'Chelmsford'},
'441244':{'en': 'Chester'},
'3355644':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'35984768':{'bg': u('\u0421\u0438\u043d\u044f \u0432\u043e\u0434\u0430'), 'en': 'Sinya voda'},
'35984769':{'bg': u('\u0413\u043e\u0440\u043e\u0446\u0432\u0435\u0442'), 'en': 'Gorotsvet'},
'35984766':{'bg': u('\u041a\u0430\u043c\u0435\u043d\u0430\u0440, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Kamenar, Razgrad'},
'35984764':{'bg': u('\u0421\u0435\u0439\u0434\u043e\u043b'), 'en': 'Seydol'},
'35984765':{'bg': u('\u0412\u0435\u0441\u0435\u043b\u0438\u043d\u0430'), 'en': 'Veselina'},
'35984763':{'bg': u('\u0411\u0435\u043b\u0438 \u041b\u043e\u043c'), 'en': 'Beli Lom'},
'35984760':{'bg': u('\u0422\u0440\u0430\u043f\u0438\u0449\u0435'), 'en': 'Trapishte'},
'35984761':{'bg': u('\u0413\u0440\u0430\u0434\u0438\u043d\u0430, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Gradina, Razgrad'},
'3354592':{'en': u('Angoul\u00eame'), 'fr': u('Angoul\u00eame')},
'3354593':{'en': u('Angoul\u00eame'), 'fr': u('Angoul\u00eame')},
'3354590':{'en': u('Angoul\u00eame'), 'fr': u('Angoul\u00eame')},
'3354591':{'en': u('Angoul\u00eame'), 'fr': u('Angoul\u00eame')},
'3354594':{'en': u('Angoul\u00eame'), 'fr': u('Angoul\u00eame')},
'3354595':{'en': u('Angoul\u00eame'), 'fr': u('Angoul\u00eame')},
'3354598':{'en': 'Chalais', 'fr': 'Chalais'},
'37246':{'en': u('K\u00e4rdla')},
'3338529':{'en': u('M\u00e2con'), 'fr': u('M\u00e2con')},
'3338528':{'en': 'La Clayette', 'fr': 'La Clayette'},
'3323291':{'en': u('Saint-\u00c9tienne-du-Rouvray'), 'fr': u('Saint-\u00c9tienne-du-Rouvray')},
'3323290':{'en': 'Dieppe', 'fr': 'Dieppe'},
'3323297':{'en': u('Neufch\u00e2tel-en-Bray'), 'fr': u('Neufch\u00e2tel-en-Bray')},
'3323296':{'en': 'Elbeuf', 'fr': 'Elbeuf'},
'3323294':{'en': 'Barentin', 'fr': 'Barentin'},
'3338521':{'en': u('M\u00e2con'), 'fr': u('M\u00e2con')},
'3338520':{'en': u('M\u00e2con'), 'fr': u('M\u00e2con')},
'3338522':{'en': u('M\u00e2con'), 'fr': u('M\u00e2con')},
'3338525':{'en': 'Marcigny', 'fr': 'Marcigny'},
'3338526':{'en': 'Chauffailles', 'fr': 'Chauffailles'},
'390961':{'en': 'Catanzaro', 'it': 'Catanzaro'},
'390963':{'en': 'Vibo Valentia', 'it': 'Vibo Valentia'},
'390962':{'en': 'Crotone', 'it': 'Crotone'},
'3349811':{'en': u('Saint-Rapha\u00ebl'), 'fr': u('Saint-Rapha\u00ebl')},
'3349810':{'en': 'Draguignan', 'fr': 'Draguignan'},
'390967':{'it': 'Soverato'},
'3349812':{'en': u('Fr\u00e9jus'), 'fr': u('Fr\u00e9jus')},
'390968':{'it': 'Lamezia Terme'},
'3593623':{'bg': u('\u0411\u043e\u0439\u043d\u043e'), 'en': 'Boyno'},
'3593622':{'bg': u('\u0421\u0442\u0440\u0435\u043c\u0446\u0438'), 'en': 'Stremtsi'},
'3593626':{'bg': u('\u041f\u0435\u0440\u043f\u0435\u0440\u0435\u043a'), 'en': 'Perperek'},
'3593625':{'bg': u('\u0428\u0438\u0440\u043e\u043a\u043e \u043f\u043e\u043b\u0435'), 'en': 'Shiroko pole'},
'3593624':{'bg': u('\u0427\u0438\u0444\u043b\u0438\u043a, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Chiflik, Kardzh.'},
'3593629':{'bg': u('\u041c\u043e\u0441\u0442'), 'en': 'Most'},
'3593628':{'bg': u('\u041c\u0438\u043b\u0430\u0434\u0438\u043d\u043e\u0432\u043e'), 'en': 'Miladinovo'},
'3355977':{'en': 'Lescar', 'fr': 'Lescar'},
'3355974':{'en': 'Anglet', 'fr': 'Anglet'},
'3355972':{'en': 'Lons', 'fr': 'Lons'},
'3355970':{'en': 'Hasparren', 'fr': 'Hasparren'},
'390385':{'it': 'Stradella'},
'390384':{'it': 'Mortara'},
'390386':{'it': 'Ostiglia'},
'390381':{'it': 'Vigevano'},
'390383':{'it': 'Voghera'},
'390382':{'en': 'Pavia', 'it': 'Pavia'},
'35941173':{'bg': u('\u0411\u0440\u0430\u0442\u044f \u041a\u0443\u043d\u0447\u0435\u0432\u0438'), 'en': 'Bratya Kunchevi'},
'3752159':{'be': u('\u0420\u0430\u0441\u043e\u043d\u044b, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Rossony, Vitebsk Region', 'ru': u('\u0420\u043e\u0441\u0441\u043e\u043d\u044b, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'434248':{'de': 'Treffen', 'en': 'Treffen'},
'434243':{'de': 'Bodensdorf', 'en': 'Bodensdorf'},
'3346322':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'434240':{'de': 'Bad Kleinkirchheim', 'en': 'Bad Kleinkirchheim'},
'434247':{'de': 'Afritz', 'en': 'Afritz'},
'434246':{'de': 'Radenthein', 'en': 'Radenthein'},
'3355526':{'en': 'Tulle', 'fr': 'Tulle'},
'434244':{'de': 'Bad Bleiberg', 'en': 'Bad Bleiberg'},
'3593104':{'bg': u('\u042f\u0433\u043e\u0434\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Yagodovo, Plovdiv'},
'34849':{'en': 'Guadalajara', 'es': 'Guadalajara'},
'441249':{'en': 'Chippenham'},
'441400':{'en': 'Honington'},
'441403':{'en': 'Horsham'},
'3593109':{'bg': u('\u041b\u0438\u043b\u043a\u043e\u0432\u043e'), 'en': 'Lilkovo'},
'441405':{'en': 'Goole'},
'441404':{'en': 'Honiton'},
'441407':{'en': 'Holyhead'},
'441406':{'en': 'Holbeach'},
'441409':{'en': 'Holsworthy'},
'441408':{'en': 'Golspie'},
'3598424':{'bg': u('\u0426\u0430\u0440 \u041a\u0430\u043b\u043e\u044f\u043d, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Tsar Kaloyan, Razgrad'},
'3317852':{'en': 'Versailles', 'fr': 'Versailles'},
'3317853':{'en': u('Asni\u00e8res-sur-Seine'), 'fr': u('Asni\u00e8res-sur-Seine')},
'3317854':{'en': u('Cr\u00e9teil'), 'fr': u('Cr\u00e9teil')},
'3334476':{'en': 'Thourotte', 'fr': 'Thourotte'},
'3595119':{'bg': u('\u0418\u0433\u043d\u0430\u0442\u0438\u0435\u0432\u043e'), 'en': 'Ignatievo'},
'3334474':{'en': 'Nogent-sur-Oise', 'fr': 'Nogent-sur-Oise'},
'3334473':{'en': 'Liancourt', 'fr': 'Liancourt'},
'3334472':{'en': 'Pont-Sainte-Maxence', 'fr': 'Pont-Sainte-Maxence'},
'3334471':{'en': 'Nogent-sur-Oise', 'fr': 'Nogent-sur-Oise'},
'3334470':{'en': 'Pont-Sainte-Maxence', 'fr': 'Pont-Sainte-Maxence'},
'3595112':{'bg': u('\u0411\u0435\u043b\u043e\u0441\u043b\u0430\u0432'), 'en': 'Beloslav'},
'3595117':{'bg': u('\u0411\u043e\u0442\u0435\u0432\u043e, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Botevo, Varna'},
'3595116':{'bg': u('\u041a\u0440\u0443\u043c\u043e\u0432\u043e, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Krumovo, Varna'},
'3334479':{'en': 'Troissereux', 'fr': 'Troissereux'},
'3334478':{'en': u('Saint-Just-en-Chauss\u00e9e'), 'fr': u('Saint-Just-en-Chauss\u00e9e')},
'3598648':{'bg': u('\u0421\u0435\u043a\u0443\u043b\u043e\u0432\u043e'), 'en': 'Sekulovo'},
'3598649':{'bg': u('\u042f\u0440\u0435\u0431\u0438\u0446\u0430'), 'en': 'Yarebitsa'},
'4418475':{'en': 'Thurso'},
'4418474':{'en': 'Thurso'},
'4418473':{'en': 'Thurso'},
'4418472':{'en': 'Thurso'},
'4418471':{'en': 'Thurso/Tongue'},
'3338328':{'en': 'Nancy', 'fr': 'Nancy'},
'3338327':{'en': 'Nancy', 'fr': 'Nancy'},
'3598641':{'bg': u('\u041e\u043a\u043e\u0440\u0448'), 'en': 'Okorsh'},
'3338325':{'en': 'Ludres', 'fr': 'Ludres'},
'3338324':{'en': 'Liverdun', 'fr': 'Liverdun'},
'3338323':{'en': 'Velaine-en-Haye', 'fr': 'Velaine-en-Haye'},
'3338322':{'en': u('Bouxi\u00e8res-aux-Dames'), 'fr': u('Bouxi\u00e8res-aux-Dames')},
'3598646':{'bg': u('\u0412\u043e\u043a\u0438\u043b'), 'en': 'Vokil'},
'3598647':{'bg': u('\u041f\u0430\u0438\u0441\u0438\u0435\u0432\u043e'), 'en': 'Paisievo'},
'3332641':{'en': u('Vitry-le-Fran\u00e7ois'), 'fr': u('Vitry-le-Fran\u00e7ois')},
'3332646':{'en': 'Reims', 'fr': 'Reims'},
'3332647':{'en': 'Reims', 'fr': 'Reims'},
'435374':{'de': 'Walchsee', 'en': 'Walchsee'},
'3332354':{'en': 'Vailly-sur-Aisne', 'fr': 'Vailly-sur-Aisne'},
'435376':{'de': 'Thiersee', 'en': 'Thiersee'},
'42037':{'en': u('Plze\u0148 Region')},
'435372':{'de': 'Kufstein', 'en': 'Kufstein'},
'435373':{'de': 'Ebbs', 'en': 'Ebbs'},
'3332356':{'en': u('La F\u00e8re'), 'fr': u('La F\u00e8re')},
'3356520':{'en': 'Cahors', 'fr': 'Cahors'},
'3356523':{'en': 'Cahors', 'fr': 'Cahors'},
'3356522':{'en': 'Cahors', 'fr': 'Cahors'},
'3356527':{'en': 'Souillac', 'fr': 'Souillac'},
'3332824':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'3332825':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'3347260':{'en': 'Lyon', 'fr': 'Lyon'},
'3347261':{'en': 'Lyon', 'fr': 'Lyon'},
'3347265':{'en': 'Villeurbanne', 'fr': 'Villeurbanne'},
'3347266':{'en': 'Oullins', 'fr': 'Oullins'},
'3347267':{'en': 'Saint-Genis-Laval', 'fr': 'Saint-Genis-Laval'},
'3347268':{'en': 'Lyon', 'fr': 'Lyon'},
'3347269':{'en': 'Lyon', 'fr': 'Lyon'},
'3356768':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3629':{'en': 'Monor', 'hu': 'Monor'},
'3346680':{'en': u('Sommi\u00e8res'), 'fr': u('Sommi\u00e8res')},
'3346685':{'en': 'Saint-Jean-du-Gard', 'fr': 'Saint-Jean-du-Gard'},
'3346684':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346687':{'en': 'Saint-Gilles', 'fr': 'Saint-Gilles'},
'3346686':{'en': u('Al\u00e8s'), 'fr': u('Al\u00e8s')},
'3346689':{'en': u('Bagnols-sur-C\u00e8ze'), 'fr': u('Bagnols-sur-C\u00e8ze')},
'3346688':{'en': 'Vauvert', 'fr': 'Vauvert'},
'3623':{'en': u('Biatorb\u00e1gy'), 'hu': u('Biatorb\u00e1gy')},
'3622':{'en': u('Sz\u00e9kesfeh\u00e9rv\u00e1r'), 'hu': u('Sz\u00e9kesfeh\u00e9rv\u00e1r')},
'3625':{'en': 'Dunaujvaros', 'hu': u('Duna\u00fajv\u00e1ros')},
'3624':{'en': u('Szigetszentmikl\u00f3s'), 'hu': u('Szigetszentmikl\u00f3s')},
'3627':{'en': 'Vac', 'hu': u('V\u00e1c')},
'3626':{'en': 'Szentendre', 'hu': 'Szentendre'},
'3596138':{'bg': u('\u041d\u0435\u0434\u0430\u043d'), 'en': 'Nedan'},
'3599116':{'bg': u('\u041a\u043e\u0441\u0442\u0435\u043b\u0435\u0432\u043e'), 'en': 'Kostelevo'},
'3599115':{'bg': u('\u0427\u0438\u0440\u0435\u043d'), 'en': 'Chiren'},
'3599113':{'bg': u('\u041c\u0440\u0430\u043c\u043e\u0440\u0435\u043d'), 'en': 'Mramoren'},
'3599112':{'bg': u('\u0411\u0430\u043d\u0438\u0446\u0430'), 'en': 'Banitsa'},
'3599111':{'bg': u('\u0427\u0435\u043b\u043e\u043f\u0435\u043a'), 'en': 'Chelopek'},
'3329885':{'en': 'Landerneau', 'fr': 'Landerneau'},
'3596132':{'bg': u('\u041a\u0430\u0440\u0430\u0438\u0441\u0435\u043d'), 'en': 'Karaisen'},
'3596133':{'bg': u('\u041c\u0438\u0445\u0430\u043b\u0446\u0438'), 'en': 'Mihaltsi'},
'3596134':{'bg': u('\u0411\u044f\u043b\u0430 \u0447\u0435\u0440\u043a\u0432\u0430, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Byala cherkva, V. Tarnovo'},
'3596135':{'bg': u('\u0412\u044a\u0440\u0431\u043e\u0432\u043a\u0430'), 'en': 'Varbovka'},
'3596136':{'bg': u('\u0421\u0443\u0445\u0438\u043d\u0434\u043e\u043b'), 'en': 'Suhindol'},
'3596137':{'bg': u('\u0411\u0443\u0442\u043e\u0432\u043e'), 'en': 'Butovo'},
'3594583':{'bg': u('\u041a\u0438\u043f\u0438\u043b\u043e\u0432\u043e'), 'en': 'Kipilovo'},
'3594582':{'bg': u('\u0413\u0440\u0430\u0434\u0435\u0446, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Gradets, Sliven'},
'432674':{'de': u('Wei\u00dfenbach an der Triesting'), 'en': 'Weissenbach an der Triesting'},
'3594580':{'bg': u('\u0411\u043e\u0440\u0438\u043d\u0446\u0438'), 'en': 'Borintsi'},
'3594587':{'bg': u('\u042f\u0431\u043b\u0430\u043d\u043e\u0432\u043e'), 'en': 'Yablanovo'},
'3316068':{'en': 'Melun', 'fr': 'Melun'},
'3316069':{'en': 'Bois-le-Roi', 'fr': 'Bois-le-Roi'},
'3594585':{'bg': u('\u0416\u0435\u0440\u0430\u0432\u043d\u0430'), 'en': 'Zheravna'},
'3316060':{'en': 'Combs-la-Ville', 'fr': 'Combs-la-Ville'},
'3316062':{'en': 'Brie-Comte-Robert', 'fr': 'Brie-Comte-Robert'},
'3316063':{'en': 'Savigny-le-Temple', 'fr': 'Savigny-le-Temple'},
'3316065':{'en': 'Saint-Fargeau-Ponthierry', 'fr': 'Saint-Fargeau-Ponthierry'},
'42038':{'en': 'South Bohemian Region'},
'3347884':{'en': 'Villeurbanne', 'fr': 'Villeurbanne'},
'3347885':{'en': 'Villeurbanne', 'fr': 'Villeurbanne'},
'3347886':{'en': 'Oullins', 'fr': 'Oullins'},
'3347887':{'en': u('Charbonni\u00e8res-les-Bains'), 'fr': u('Charbonni\u00e8res-les-Bains')},
'3347880':{'en': 'Vaulx-en-Velin', 'fr': 'Vaulx-en-Velin'},
'359725':{'bg': u('\u0415\u043b\u0438\u043d \u041f\u0435\u043b\u0438\u043d'), 'en': 'Elin Pelin'},
'359726':{'bg': u('\u0421\u0432\u043e\u0433\u0435'), 'en': 'Svoge'},
'3347883':{'en': 'Lyon', 'fr': 'Lyon'},
'359728':{'bg': u('\u0417\u043b\u0430\u0442\u0438\u0446\u0430'), 'en': 'Zlatitsa'},
'359729':{'bg': u('\u0413\u043e\u0434\u0435\u0447'), 'en': 'Godech'},
'3347888':{'en': 'Rillieux-la-Pape', 'fr': 'Rillieux-la-Pape'},
'3347889':{'en': 'Lyon', 'fr': 'Lyon'},
'433137':{'de': u('S\u00f6ding'), 'en': u('S\u00f6ding')},
'3329078':{'en': 'Rennes', 'fr': 'Rennes'},
'3522488':{'de': 'Mertzig/Wahl', 'en': 'Mertzig/Wahl', 'fr': 'Mertzig/Wahl'},
'3358134':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3522483':{'de': 'Vianden', 'en': 'Vianden', 'fr': 'Vianden'},
'3522481':{'de': u('Ettelbr\u00fcck/Reckange-sur-Mess'), 'en': 'Ettelbruck/Reckange-sur-Mess', 'fr': 'Ettelbruck/Reckange-sur-Mess'},
'3522480':{'de': 'Diekirch', 'en': 'Diekirch', 'fr': 'Diekirch'},
'3522487':{'de': 'Fels', 'en': 'Larochette', 'fr': 'Larochette'},
'3522485':{'de': 'Bissen/Roost', 'en': 'Bissen/Roost', 'fr': 'Bissen/Roost'},
'3522484':{'de': 'Han/Lesse', 'en': 'Han/Lesse', 'fr': 'Han/Lesse'},
'37424293':{'am': u('\u0547\u056b\u0580\u0561\u056f\u0561\u057e\u0561\u0576'), 'en': 'Shirakavan', 'ru': u('\u0428\u0438\u0440\u0430\u043a\u0430\u0432\u0430\u043d')},
'390734':{'en': 'Fermo', 'it': 'Fermo'},
'3349487':{'en': 'La Seyne sur Mer', 'fr': 'La Seyne sur Mer'},
'390736':{'it': 'Ascoli Piceno'},
'3349485':{'en': 'Draguignan', 'fr': 'Draguignan'},
'3349482':{'en': u('Saint-Rapha\u00ebl'), 'fr': u('Saint-Rapha\u00ebl')},
'3349483':{'en': u('Saint-Rapha\u00ebl'), 'fr': u('Saint-Rapha\u00ebl')},
'390732':{'en': 'Ancona', 'it': 'Fabriano'},
'3349481':{'en': u('Fr\u00e9jus'), 'fr': u('Fr\u00e9jus')},
'3349488':{'en': 'Sanary-sur-Mer', 'fr': 'Sanary-sur-Mer'},
'3349489':{'en': 'Toulon', 'fr': 'Toulon'},
'3522627':{'de': 'Belair, Luxemburg', 'en': 'Belair, Luxembourg', 'fr': 'Belair, Luxembourg'},
'3349379':{'en': 'Contes', 'fr': 'Contes'},
'3522625':{'de': 'Luxemburg', 'en': 'Luxembourg', 'fr': 'Luxembourg'},
'3522623':{'de': 'Bad Mondorf', 'en': 'Mondorf-les-Bains/Bascharage/Noerdange/Remich', 'fr': 'Mondorf-les-Bains/Bascharage/Noerdange/Remich'},
'3522622':{'de': 'Luxemburg', 'en': 'Luxembourg City', 'fr': 'Luxembourg-Ville'},
'3522621':{'de': 'Weicherdingen', 'en': 'Weicherdange', 'fr': 'Weicherdange'},
'3349370':{'en': 'Grasse', 'fr': 'Grasse'},
'3349371':{'en': 'Nice', 'fr': 'Nice'},
'3349372':{'en': 'Nice', 'fr': 'Nice'},
'3349373':{'en': 'Cagnes-sur-Mer', 'fr': 'Cagnes-sur-Mer'},
'3349374':{'en': 'Antibes Juan les Pins', 'fr': 'Antibes Juan les Pins'},
'3349375':{'en': 'Mougins', 'fr': 'Mougins'},
'3522629':{'de': 'Luxemburg/Kockelscheuer', 'en': 'Luxembourg/Kockelscheuer', 'fr': 'Luxembourg/Kockelscheuer'},
'3349377':{'en': 'Roquefort-les-Pins', 'fr': 'Roquefort-les-Pins'},
'390341':{'en': 'Lecco', 'it': 'Lecco'},
'432673':{'de': 'Altenmarkt an der Triesting', 'en': 'Altenmarkt an der Triesting'},
'3355605':{'en': u('Saint-M\u00e9dard-en-Jalles'), 'fr': u('Saint-M\u00e9dard-en-Jalles')},
'3594763':{'bg': u('\u0418\u0440\u0435\u0447\u0435\u043a\u043e\u0432\u043e'), 'en': 'Irechekovo'},
'3594762':{'bg': u('\u0412\u043e\u0434\u0435\u043d\u0438\u0447\u0430\u043d\u0435'), 'en': 'Vodenichane'},
'3594761':{'bg': u('\u0421\u0442\u0440\u0430\u043b\u0434\u0436\u0430'), 'en': 'Straldzha'},
'3594764':{'bg': u('\u041c\u0430\u043b\u0435\u043d\u043e\u0432\u043e'), 'en': 'Malenovo'},
'3594768':{'bg': u('\u0417\u0438\u043c\u043d\u0438\u0446\u0430, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Zimnitsa, Yambol'},
'433512':{'de': 'Knittelfeld', 'en': 'Knittelfeld'},
'433513':{'de': 'Bischoffeld', 'en': 'Bischoffeld'},
'3355604':{'en': 'Talence', 'fr': 'Talence'},
'433516':{'de': 'Kleinlobming', 'en': 'Kleinlobming'},
'433514':{'de': 'Seckau', 'en': 'Seckau'},
'433515':{'de': 'Sankt Lorenzen bei Knittelfeld', 'en': 'St. Lorenzen bei Knittelfeld'},
'3348681':{'en': 'Avignon', 'fr': 'Avignon'},
'3355561':{'en': u('Gu\u00e9ret'), 'fr': u('Gu\u00e9ret')},
'3355563':{'en': 'La Souterraine', 'fr': 'La Souterraine'},
'3355564':{'en': 'Bourganeuf', 'fr': 'Bourganeuf'},
'3355566':{'en': 'Aubusson', 'fr': 'Aubusson'},
'3355568':{'en': 'Bellac', 'fr': 'Bellac'},
'3355569':{'en': 'Eymoutiers', 'fr': 'Eymoutiers'},
'40346':{'en': 'Giurgiu', 'ro': 'Giurgiu'},
'3355607':{'en': 'Pessac', 'fr': 'Pessac'},
'40344':{'en': 'Prahova', 'ro': 'Prahova'},
'40345':{'en': u('D\u00e2mbovi\u021ba'), 'ro': u('D\u00e2mbovi\u021ba')},
'40342':{'en': u('C\u0103l\u0103ra\u0219i'), 'ro': u('C\u0103l\u0103ra\u0219i')},
'40343':{'en': u('Ialomi\u021ba'), 'ro': u('Ialomi\u021ba')},
'40340':{'en': 'Tulcea', 'ro': 'Tulcea'},
'3332429':{'en': 'Sedan', 'fr': 'Sedan'},
'3332427':{'en': 'Sedan', 'fr': 'Sedan'},
'437239':{'de': 'Lichtenberg', 'en': 'Lichtenberg'},
'437238':{'de': 'Mauthausen', 'en': 'Mauthausen'},
'40348':{'en': u('Arge\u0219'), 'ro': u('Arge\u0219')},
'40349':{'en': 'Olt', 'ro': 'Olt'},
'3348426':{'en': 'Marseille', 'fr': 'Marseille'},
'3348357':{'en': 'Toulon', 'fr': 'Toulon'},
'3348350':{'en': 'Nice', 'fr': 'Nice'},
'3355788':{'en': u('Ludon-M\u00e9doc'), 'fr': u('Ludon-M\u00e9doc')},
'3355789':{'en': 'Pessac', 'fr': 'Pessac'},
'3355785':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355787':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355780':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355781':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355783':{'en': 'Cestas', 'fr': 'Cestas'},
'3356200':{'en': 'Saint-Gaudens', 'fr': 'Saint-Gaudens'},
'390966':{'it': 'Palmi'},
'3356205':{'en': 'Auch', 'fr': 'Auch'},
'3356206':{'en': 'Fleurance', 'fr': 'Fleurance'},
'3356207':{'en': 'L\'Isle Jourdain', 'fr': 'L\'Isle Jourdain'},
'3325129':{'en': u('Lu\u00e7on'), 'fr': u('Lu\u00e7on')},
'3325123':{'en': 'Les Sables d\'Olonne', 'fr': 'Les Sables d\'Olonne'},
'3325121':{'en': 'Les Sables d\'Olonne', 'fr': 'Les Sables d\'Olonne'},
'3325126':{'en': 'Saint-Gilles-Croix-de-Vie', 'fr': 'Saint-Gilles-Croix-de-Vie'},
'435475':{'de': 'Feichten', 'en': 'Feichten'},
'3325124':{'en': 'La Roche sur Yon', 'fr': 'La Roche sur Yon'},
'3324830':{'en': 'Saint-Germain-du-Puy', 'fr': 'Saint-Germain-du-Puy'},
'3344218':{'en': 'Aubagne', 'fr': 'Aubagne'},
'3344210':{'en': 'Vitrolles', 'fr': 'Vitrolles'},
'3344211':{'en': 'Istres', 'fr': 'Istres'},
'3344212':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'435474':{'de': 'Pfunds', 'en': 'Pfunds'},
'3344215':{'en': 'Vitrolles', 'fr': 'Vitrolles'},
'3344216':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344217':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'434761':{'de': 'Stockenboi', 'en': 'Stockenboi'},
'434762':{'de': 'Spittal an der Drau', 'en': 'Spittal an der Drau'},
'434766':{'de': 'Millstatt', 'en': 'Millstatt'},
'434767':{'de': 'Rothenthurn', 'en': 'Rothenthurn'},
'432614':{'de': 'Kleinwarasdorf', 'en': 'Kleinwarasdorf'},
'434769':{'de': u('M\u00f6llbr\u00fccke'), 'en': u('M\u00f6llbr\u00fccke')},
'351249':{'en': 'Torres Novas', 'pt': 'Torres Novas'},
'3347603':{'en': 'Grenoble', 'fr': 'Grenoble'},
'351245':{'en': 'Portalegre', 'pt': 'Portalegre'},
'351244':{'en': 'Leiria', 'pt': 'Leiria'},
'351243':{'en': u('Santar\u00e9m'), 'pt': u('Santar\u00e9m')},
'351242':{'en': u('Ponte de S\u00f4r'), 'pt': u('Ponte de S\u00f4r')},
'351241':{'en': 'Abrantes', 'pt': 'Abrantes'},
'3347601':{'en': 'Grenoble', 'fr': 'Grenoble'},
'435332':{'de': u('W\u00f6rgl'), 'en': u('W\u00f6rgl')},
'435583':{'de': 'Lech', 'en': 'Lech'},
'435582':{'de': u('Kl\u00f6sterle'), 'en': u('Kl\u00f6sterle')},
'435585':{'de': 'Dalaas', 'en': 'Dalaas'},
'3347607':{'en': 'Tullins', 'fr': 'Tullins'},
'39010':{'en': 'Genoa', 'it': 'Genova'},
'3596047':{'bg': u('\u0417\u0435\u043b\u0435\u043d\u0430 \u043c\u043e\u0440\u0430\u0432\u0430'), 'en': 'Zelena morava'},
'3596048':{'bg': u('\u0418\u0437\u0432\u043e\u0440\u043e\u0432\u043e, \u0422\u044a\u0440\u0433.'), 'en': 'Izvorovo, Targ.'},
'3595580':{'bg': u('\u0422\u0440\u043e\u044f\u043d\u043e\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Troyanovo, Burgas'},
'3595589':{'bg': u('\u0412\u0438\u043d\u0430\u0440\u0441\u043a\u043e'), 'en': 'Vinarsko'},
'441780':{'en': 'Stamford'},
'441875':{'en': 'Tranent'},
'3596728':{'bg': u('\u0411\u0443\u0440\u044f'), 'en': 'Burya'},
'3596725':{'bg': u('\u0413\u043e\u0441\u0442\u0438\u043b\u0438\u0446\u0430'), 'en': 'Gostilitsa'},
'3596724':{'bg': u('\u042f\u043d\u0442\u0440\u0430, \u0413\u0430\u0431\u0440.'), 'en': 'Yantra, Gabr.'},
'3596727':{'bg': u('\u0413\u0430\u043d\u0447\u043e\u0432\u0435\u0446'), 'en': 'Ganchovets'},
'3596726':{'bg': u('\u0421\u043a\u0430\u043b\u0441\u043a\u043e'), 'en': 'Skalsko'},
'3596720':{'bg': u('\u041a\u0435\u0440\u0435\u043a\u0430'), 'en': 'Kereka'},
'3596723':{'bg': u('\u0426\u0430\u0440\u0435\u0432\u0430 \u043b\u0438\u0432\u0430\u0434\u0430'), 'en': 'Tsareva livada'},
'3596722':{'bg': u('\u0421\u043e\u043a\u043e\u043b\u043e\u0432\u043e, \u0413\u0430\u0431\u0440.'), 'en': 'Sokolovo, Gabr.'},
'3347747':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347746':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347744':{'en': 'Roanne', 'fr': 'Roanne'},
'3347743':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347742':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347741':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347740':{'en': 'Firminy', 'fr': 'Firminy'},
'441876':{'en': 'Lochmaddy'},
'3347749':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'432610':{'de': 'Horitschon', 'en': 'Horitschon'},
'3349363':{'en': 'Vallauris', 'fr': 'Vallauris'},
'436468':{'de': 'Werfen', 'en': 'Werfen'},
'35965617':{'bg': u('\u0418\u0441\u043a\u044a\u0440, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Iskar, Pleven'},
'436467':{'de': u('M\u00fchlbach am Hochk\u00f6nig'), 'en': u('M\u00fchlbach am Hochk\u00f6nig')},
'436466':{'de': 'Werfenweng', 'en': 'Werfenweng'},
'436461':{'de': u('Dienten am Hochk\u00f6nig'), 'en': u('Dienten am Hochk\u00f6nig')},
'3349361':{'en': 'Antibes Juan les Pins', 'fr': 'Antibes Juan les Pins'},
'436463':{'de': u('Annaberg-Lung\u00f6tz'), 'en': u('Annaberg-Lung\u00f6tz')},
'436462':{'de': 'Bischofshofen', 'en': 'Bischofshofen'},
'3338156':{'en': 'Valdahon', 'fr': 'Valdahon'},
'3338150':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338151':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338152':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338153':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3316907':{'en': 'Gif-sur-Yvette', 'fr': 'Gif-sur-Yvette'},
'3349367':{'en': 'Antibes Juan les Pins', 'fr': 'Antibes Juan les Pins'},
'3316905':{'en': 'Savigny-sur-Orge', 'fr': 'Savigny-sur-Orge'},
'3316904':{'en': 'Morsang-sur-Orge', 'fr': 'Morsang-sur-Orge'},
'3316903':{'en': 'Montgeron', 'fr': 'Montgeron'},
'3316902':{'en': 'Ris-Orangis', 'fr': 'Ris-Orangis'},
'3316901':{'en': u('Montlh\u00e9ry'), 'fr': u('Montlh\u00e9ry')},
'3349366':{'en': 'Peymeinade', 'fr': 'Peymeinade'},
'3596589':{'bg': u('\u041a\u0443\u043b\u0438\u043d\u0430 \u0432\u043e\u0434\u0430'), 'en': 'Kulina voda'},
'3596588':{'bg': u('\u0414\u0435\u043a\u043e\u0432'), 'en': 'Dekov'},
'3349497':{'en': 'Saint-Tropez', 'fr': 'Saint-Tropez'},
'3596581':{'bg': u('\u0411\u044f\u043b\u0430 \u0432\u043e\u0434\u0430, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Byala voda, Pleven'},
'3596580':{'bg': u('\u0422\u0430\u0442\u0430\u0440\u0438'), 'en': 'Tatari'},
'3349364':{'en': 'Vallauris', 'fr': 'Vallauris'},
'3596587':{'bg': u('\u041f\u0435\u0442\u043e\u043a\u043b\u0430\u0434\u0435\u043d\u0446\u0438'), 'en': 'Petokladentsi'},
'441870':{'en': 'Isle of Benbecula'},
'3332640':{'en': 'Reims', 'fr': 'Reims'},
'3343790':{'en': 'Lyon', 'fr': 'Lyon'},
'3343791':{'en': 'Lyon', 'fr': 'Lyon'},
'441784':{'en': 'Staines'},
'3683':{'en': 'Keszthely', 'hu': 'Keszthely'},
'4414372':{'en': 'Clynderwen (Clunderwen)'},
'4414373':{'en': 'Clynderwen (Clunderwen)'},
'441785':{'en': 'Stafford'},
'3682':{'en': 'Kaposvar', 'hu': u('Kaposv\u00e1r')},
'3354578':{'en': 'Barbezieux-Saint-Hilaire', 'fr': 'Barbezieux-Saint-Hilaire'},
'3329711':{'en': 'Plouay', 'fr': 'Plouay'},
'3354570':{'en': 'Montbron', 'fr': 'Montbron'},
'3354571':{'en': u('Roumazi\u00e8res-Loubert'), 'fr': u('Roumazi\u00e8res-Loubert')},
'4414378':{'en': 'Haverfordwest'},
'4414379':{'en': 'Haverfordwest'},
'436545':{'de': u('Bruck an der Gro\u00dfglocknerstra\u00dfe'), 'en': 'Bruck an der Grossglocknerstrasse'},
'390934':{'en': 'Caltanissetta and Enna', 'it': 'Caltanissetta'},
'3597186':{'bg': u('\u0410\u043d\u0442\u043e\u043d'), 'en': 'Anton'},
'35952':{'bg': u('\u0412\u0430\u0440\u043d\u0430'), 'en': 'Varna'},
'3597184':{'bg': u('\u041a\u043e\u043f\u0440\u0438\u0432\u0449\u0438\u0446\u0430'), 'en': 'Koprivshtitsa'},
'3597185':{'bg': u('\u0427\u0435\u043b\u043e\u043f\u0435\u0447'), 'en': 'Chelopech'},
'3597182':{'bg': u('\u041c\u0438\u0440\u043a\u043e\u0432\u043e'), 'en': 'Mirkovo'},
'3315830':{'en': 'Paris', 'fr': 'Paris'},
'3597181':{'bg': u('\u041f\u0438\u0440\u0434\u043e\u043f'), 'en': 'Pirdop'},
'390942':{'en': 'Catania', 'it': 'Taormina'},
'390941':{'it': 'Patti'},
'3597188':{'bg': u('\u041f\u0435\u0442\u0440\u0438\u0447, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Petrich, Sofia'},
'3597189':{'bg': u('\u0427\u0430\u0432\u0434\u0430\u0440, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Chavdar, Sofia'},
'3359670':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3359671':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3359672':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3359673':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3359674':{'en': 'Le Vauclin', 'fr': 'Le Vauclin'},
'3359675':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3359676':{'en': 'Sainte-Anne', 'fr': 'Sainte-Anne'},
'3359677':{'en': 'Ducos', 'fr': 'Ducos'},
'3359678':{'en': 'Basse-Pointe', 'fr': 'Basse-Pointe'},
'3359679':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3322728':{'en': 'Eu', 'fr': 'Eu'},
'435278':{'de': 'Navis', 'en': 'Navis'},
'3355660':{'en': u('L\u00e8ge-Cap-Ferret'), 'fr': u('L\u00e8ge-Cap-Ferret')},
'34961':{'en': 'Valencia', 'es': 'Valencia'},
'34960':{'en': 'Valencia', 'es': 'Valencia'},
'34963':{'en': 'Valencia', 'es': 'Valencia'},
'34962':{'en': 'Valencia', 'es': 'Valencia'},
'34965':{'en': 'Alicante', 'es': 'Alicante'},
'34964':{'en': u('Castell\u00f3n'), 'es': u('Castell\u00f3n')},
'34967':{'en': 'Albacete', 'es': 'Albacete'},
'34966':{'en': 'Alicante', 'es': 'Alicante'},
'3355951':{'en': 'Saint-Jean-de-Luz', 'fr': 'Saint-Jean-de-Luz'},
'34968':{'en': 'Murcia', 'es': 'Murcia'},
'3355953':{'en': 'Pontacq', 'fr': 'Pontacq'},
'3355952':{'en': 'Anglet', 'fr': 'Anglet'},
'3355955':{'en': 'Bayonne', 'fr': 'Bayonne'},
'3329841':{'en': 'Brest', 'fr': 'Brest'},
'3355957':{'en': 'Anglet', 'fr': 'Anglet'},
'3355956':{'en': 'Saint-Martin-de-Seignanx', 'fr': 'Saint-Martin-de-Seignanx'},
'333884':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3684':{'en': 'Siofok', 'hu': u('Si\u00f3fok')},
'35993212':{'bg': u('\u041a\u0430\u0440\u0431\u0438\u043d\u0446\u0438'), 'en': 'Karbintsi'},
'435272':{'de': 'Steinach am Brenner', 'en': 'Steinach am Brenner'},
'3359094':{'en': 'Petit Bourg', 'fr': 'Petit Bourg'},
'35827':{'en': 'Turku/Pori', 'fi': 'Turku/Pori', 'se': u('\u00c5bo/Bj\u00f6rneborg')},
'3359097':{'en': 'Grand-Bourg', 'fr': 'Grand-Bourg'},
'3359090':{'en': u('Pointe-\u00e0-Pitre'), 'fr': u('Pointe-\u00e0-Pitre')},
'3359091':{'en': 'Les Abymes', 'fr': 'Les Abymes'},
'3359092':{'en': 'Trois Rivieres', 'fr': 'Trois Rivieres'},
'35826':{'en': 'Turku/Pori', 'fi': 'Turku/Pori', 'se': u('\u00c5bo/Bj\u00f6rneborg')},
'3359098':{'en': 'Vieux Habitants', 'fr': 'Vieux Habitants'},
'3359099':{'en': 'Basse Terre', 'fr': 'Basse Terre'},
'35824':{'en': 'Turku/Pori', 'fi': 'Turku/Pori', 'se': u('\u00c5bo/Bj\u00f6rneborg')},
'35823':{'en': 'Turku/Pori', 'fi': 'Turku/Pori', 'se': u('\u00c5bo/Bj\u00f6rneborg')},
'3344295':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'437448':{'de': 'Kematen an der Ybbs', 'en': 'Kematen an der Ybbs'},
'3344296':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'35396':{'en': 'Ballina'},
'35399':{'en': 'Kilronan'},
'35398':{'en': 'Westport'},
'3332624':{'en': 'Reims', 'fr': 'Reims'},
'441429':{'en': 'Hartlepool'},
'441428':{'en': 'Haslemere'},
'3595351':{'bg': u('\u0421\u043c\u044f\u0434\u043e\u0432\u043e'), 'en': 'Smyadovo'},
'441422':{'en': 'Halifax'},
'3595353':{'bg': u('\u0412\u0435\u0441\u0435\u043b\u0438\u043d\u043e\u0432\u043e, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Veselinovo, Shumen'},
'3595352':{'bg': u('\u042f\u043d\u043a\u043e\u0432\u043e'), 'en': 'Yankovo'},
'441427':{'en': 'Gainsborough'},
'3595354':{'bg': u('\u0420\u0438\u0448'), 'en': 'Rish'},
'441425':{'en': 'Ringwood'},
'441424':{'en': 'Hastings'},
'35974207':{'bg': u('\u0413\u0435\u043d\u0435\u0440\u0430\u043b \u0422\u043e\u0434\u043e\u0440\u043e\u0432'), 'en': 'General Todorov'},
'3332855':{'en': 'Lille', 'fr': 'Lille'},
'3332853':{'en': 'Lille', 'fr': 'Lille'},
'3332852':{'en': 'Lille', 'fr': 'Lille'},
'3332851':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'3332850':{'en': 'Bailleul', 'fr': 'Bailleul'},
'437442':{'de': 'Waidhofen an der Ybbs', 'en': 'Waidhofen an der Ybbs'},
'3332859':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'3332858':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'35937705':{'bg': u('\u041f\u044a\u0441\u0442\u0440\u043e\u0433\u043e\u0440'), 'en': 'Pastrogor'},
'437444':{'de': 'Opponitz', 'en': 'Opponitz'},
'3315742':{'en': 'Pantin', 'fr': 'Pantin'},
'437445':{'de': 'Hollenstein an der Ybbs', 'en': 'Hollenstein an der Ybbs'},
'3594518':{'bg': u('\u0422\u043e\u043f\u043e\u043b\u0447\u0430\u043d\u0435'), 'en': 'Topolchane'},
'3594519':{'bg': u('\u0421\u0430\u043c\u0443\u0438\u043b\u043e\u0432\u043e, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Samuilovo, Sliven'},
'3594510':{'bg': u('\u0416\u0435\u043b\u044e \u0432\u043e\u0439\u0432\u043e\u0434\u0430'), 'en': 'Zhelyu voyvoda'},
'3594511':{'bg': u('\u0421\u043b\u0438\u0432\u0435\u043d\u0441\u043a\u0438 \u043c\u0438\u043d\u0435\u0440\u0430\u043b\u043d\u0438 \u0431\u0430\u043d\u0438'), 'en': 'Slivenski mineralni bani'},
'3594512':{'bg': u('\u0411\u043b\u0430\u0442\u0435\u0446, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Blatets, Sliven'},
'3594513':{'bg': u('\u0413\u0430\u0432\u0440\u0430\u0438\u043b\u043e\u0432\u043e'), 'en': 'Gavrailovoc'},
'3594514':{'bg': u('\u041a\u0440\u0443\u0448\u0430\u0440\u0435'), 'en': 'Krushare'},
'3594515':{'bg': u('\u041c\u043e\u043a\u0440\u0435\u043d'), 'en': 'Mokren'},
'3594516':{'bg': u('\u041a\u0435\u0440\u043c\u0435\u043d'), 'en': 'Kermen'},
'3594517':{'bg': u('\u0418\u0447\u0435\u0440\u0430'), 'en': 'Ichera'},
'3598662':{'bg': u('\u0414\u043e\u0431\u0440\u043e\u0442\u0438\u0446\u0430, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Dobrotitsa, Silistra'},
'3334450':{'en': 'Clermont', 'fr': 'Clermont'},
'3334453':{'en': 'Senlis', 'fr': 'Senlis'},
'3334452':{'en': u('M\u00e9ru'), 'fr': u('M\u00e9ru')},
'3334455':{'en': 'Creil', 'fr': 'Creil'},
'3598667':{'bg': u('\u0411\u0435\u043b\u0438\u0446\u0430, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Belitsa, Silistra'},
'3334457':{'en': 'Chantilly', 'fr': 'Chantilly'},
'3334456':{'en': 'Saint-Leu-d\'Esserent', 'fr': 'Saint-Leu-d\'Esserent'},
'3334459':{'en': u('Cr\u00e9py-en-Valois'), 'fr': u('Cr\u00e9py-en-Valois')},
'3334458':{'en': 'Chantilly', 'fr': 'Chantilly'},
'3598668':{'bg': u('\u041f\u043e\u043f\u0438\u043d\u0430'), 'en': 'Popina'},
'35936702':{'bg': u('\u0413\u043e\u0440\u0441\u043a\u0438 \u0438\u0437\u0432\u043e\u0440, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Gorski izvor, Kardzh.'},
'432248':{'de': 'Markgrafneusiedl', 'en': 'Markgrafneusiedl'},
'3324191':{'en': u('Brissac-Quinc\u00e9'), 'fr': u('Brissac-Quinc\u00e9')},
'3324190':{'en': u('Chevir\u00e9-le-Rouge'), 'fr': u('Chevir\u00e9-le-Rouge')},
'3324193':{'en': u('Saint-Barth\u00e9lemy-d\'Anjou'), 'fr': u('Saint-Barth\u00e9lemy-d\'Anjou')},
'3324192':{'en': u('Segr\u00e9'), 'fr': u('Segr\u00e9')},
'355211':{'en': 'Koplik'},
'355212':{'en': u('Puk\u00eb')},
'355213':{'en': 'Bajram Curri'},
'355214':{'en': u('Krum\u00eb')},
'355215':{'en': u('Lezh\u00eb')},
'355216':{'en': u('Rr\u00ebshen')},
'355217':{'en': 'Burrel'},
'355218':{'en': 'Peshkopi'},
'355219':{'en': u('Bulqiz\u00eb')},
'3349456':{'en': 'Grimaud', 'fr': 'Grimaud'},
'3356549':{'en': 'Saint-Affrique', 'fr': 'Saint-Affrique'},
'4021':{'en': 'Bucharest and Ilfov County', 'ro': u('Bucure\u0219ti \u0219i jude\u021bul Ilfov')},
'3356543':{'en': 'Decazeville', 'fr': 'Decazeville'},
'3356542':{'en': 'Rodez', 'fr': 'Rodez'},
'3356541':{'en': 'Gourdon', 'fr': 'Gourdon'},
'3356545':{'en': 'Villefranche-de-Rouergue', 'fr': 'Villefranche-de-Rouergue'},
'42057':{'en': u('Zl\u00edn Region')},
'4418906':{'en': 'Ayton'},
'3595135':{'bg': u('\u0421\u0442\u0435\u0444\u0430\u043d \u041a\u0430\u0440\u0430\u0434\u0436\u0430, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Stefan Karadzha, Varna'},
'3595134':{'bg': u('\u0427\u0435\u0440\u0432\u0435\u043d\u0446\u0438'), 'en': 'Cherventsi'},
'3347248':{'en': 'Saint-Pierre-de-Chandieu', 'fr': 'Saint-Pierre-de-Chandieu'},
'3347249':{'en': 'Givors', 'fr': 'Givors'},
'3595131':{'bg': u('\u0412\u044a\u043b\u0447\u0438 \u0434\u043e\u043b'), 'en': 'Valchi dol'},
'3595130':{'bg': u('\u0413\u0435\u043d\u0435\u0440\u0430\u043b \u041a\u0438\u0441\u0435\u043b\u043e\u0432\u043e'), 'en': 'General Kiselovo'},
'3595133':{'bg': u('\u0413\u0435\u043d\u0435\u0440\u0430\u043b \u041a\u043e\u043b\u0435\u0432\u043e, \u0412\u0430\u0440\u043d\u0430'), 'en': 'General Kolevo, Varna'},
'3595132':{'bg': u('\u041c\u0438\u0445\u0430\u043b\u0438\u0447, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Mihalich, Varna'},
'3347242':{'en': u('Fontaines-sur-Sa\u00f4ne'), 'fr': u('Fontaines-sur-Sa\u00f4ne')},
'3343892':{'en': 'Crolles', 'fr': 'Crolles'},
'3347240':{'en': 'Lyon', 'fr': 'Lyon'},
'3347241':{'en': 'Lyon', 'fr': 'Lyon'},
'3347530':{'en': u('Saint-Agr\u00e8ve'), 'fr': u('Saint-Agr\u00e8ve')},
'3347531':{'en': 'Saint-Rambert-d\'Albon', 'fr': 'Saint-Rambert-d\'Albon'},
'3347244':{'en': 'Villeurbanne', 'fr': 'Villeurbanne'},
'3347245':{'en': 'Meyzieu', 'fr': 'Meyzieu'},
'3596112':{'bg': u('\u0421\u0430\u043c\u043e\u0432\u043e\u0434\u0435\u043d\u0435'), 'en': 'Samovodene'},
'3596113':{'bg': u('\u0411\u0430\u043b\u0432\u0430\u043d'), 'en': 'Balvan'},
'3596111':{'bg': u('\u041a\u044a\u043f\u0438\u043d\u043e\u0432\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Kapinovo, V. Tarnovo'},
'3596116':{'bg': u('\u0413\u043e\u043b\u0435\u043c\u0430\u043d\u0438\u0442\u0435'), 'en': 'Golemanite'},
'3596117':{'bg': u('\u0414\u0435\u0431\u0435\u043b\u0435\u0446, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Debelets, V. Tarnovo'},
'3596114':{'bg': u('\u041a\u0438\u043b\u0438\u0444\u0430\u0440\u0435\u0432\u043e'), 'en': 'Kilifarevo'},
'3596115':{'bg': u('\u0420\u0435\u0441\u0435\u043d'), 'en': 'Resen'},
'3596118':{'bg': u('\u0412\u043e\u043d\u0435\u0449\u0430 \u0432\u043e\u0434\u0430'), 'en': 'Voneshta voda'},
'3596119':{'bg': u('\u0414\u0438\u0447\u0438\u043d'), 'en': 'Dichin'},
'3689':{'en': 'Papa', 'hu': u('P\u00e1pa')},
'3599131':{'bg': u('\u0414\u043e\u0431\u0440\u043e\u043b\u0435\u0432\u043e'), 'en': 'Dobrolevo'},
'3599130':{'bg': u('\u0422\u043b\u0430\u0447\u0435\u043d\u0435'), 'en': 'Tlachene'},
'3599133':{'bg': u('\u041a\u043e\u043c\u0430\u0440\u0435\u0432\u043e, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Komarevo, Vratsa'},
'3599132':{'bg': u('\u041a\u043d\u0435\u0436\u0430'), 'en': 'Knezha'},
'3599135':{'bg': u('\u0411\u044a\u0440\u0434\u0430\u0440\u0441\u043a\u0438 \u0433\u0435\u0440\u0430\u043d'), 'en': 'Bardarski geran'},
'3599134':{'bg': u('\u0422\u044a\u0440\u043d\u0430\u043a'), 'en': 'Tarnak'},
'3599137':{'bg': u('\u041f\u043e\u043f\u0438\u0446\u0430'), 'en': 'Popitsa'},
'3599136':{'bg': u('\u0413\u0430\u043b\u0438\u0447\u0435'), 'en': 'Galiche'},
'3599139':{'bg': u('\u0422\u044a\u0440\u043d\u0430\u0432\u0430, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Tarnava, Vratsa'},
'3599138':{'bg': u('\u0410\u043b\u0442\u0438\u043c\u0438\u0440'), 'en': 'Altimir'},
'435554':{'de': 'Sonntag', 'en': 'Sonntag'},
'3353441':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3353440':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3353443':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3353442':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3353445':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3353444':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3353446':{'en': 'Muret', 'fr': 'Muret'},
'3338774':{'en': 'Metz', 'fr': 'Metz'},
'3338775':{'en': 'Metz', 'fr': 'Metz'},
'432616':{'de': 'Lockenhaus', 'en': 'Lockenhaus'},
'432617':{'de': u('Dra\u00dfmarkt'), 'en': 'Drassmarkt'},
'35984393':{'bg': u('\u0412\u0430\u0437\u043e\u0432\u043e'), 'en': 'Vazovo'},
'3338770':{'en': 'Hagondange', 'fr': 'Hagondange'},
'40357':{'en': 'Arad', 'ro': 'Arad'},
'35984394':{'bg': u('\u0414\u0443\u0445\u043e\u0432\u0435\u0446'), 'en': 'Duhovets'},
'432615':{'de': 'Lutzmannsburg', 'en': 'Lutzmannsburg'},
'3685':{'en': 'Marcali', 'hu': 'Marcali'},
'432612':{'de': 'Oberpullendorf', 'en': 'Oberpullendorf'},
'3338773':{'en': 'Ennery', 'fr': 'Ennery'},
'4413878':{'en': 'Dumfries'},
'432613':{'de': 'Deutschkreutz', 'en': 'Deutschkreutz'},
'42054':{'en': 'South Moravian Region'},
'42055':{'en': 'Moravian-Silesian Region'},
'359748':{'bg': u('\u0421\u0438\u043c\u0438\u0442\u043b\u0438'), 'en': 'Simitli'},
'359749':{'bg': u('\u0411\u0430\u043d\u0441\u043a\u043e'), 'en': 'Bansko'},
'40353':{'en': 'Gorj', 'ro': 'Gorj'},
'42053':{'en': 'South Moravian Region'},
'40352':{'en': u('Mehedin\u021bi'), 'ro': u('Mehedin\u021bi')},
'359746':{'bg': u('\u0421\u0430\u043d\u0434\u0430\u043d\u0441\u043a\u0438'), 'en': 'Sandanski'},
'359747':{'bg': u('\u0420\u0430\u0437\u043b\u043e\u0433'), 'en': 'Razlog'},
'359745':{'bg': u('\u041f\u0435\u0442\u0440\u0438\u0447, \u0411\u043b\u0430\u0433.'), 'en': 'Petrich, Blag.'},
'435417':{'de': 'Roppen', 'en': 'Roppen'},
'434242':{'de': 'Villach', 'en': 'Villach'},
'435557':{'de': 'Sankt Gallenkirch', 'en': 'St. Gallenkirch'},
'390532':{'en': 'Ferrara', 'it': 'Ferrara'},
'390533':{'it': 'Comacchio'},
'390536':{'it': 'Sassuolo'},
'390534':{'it': 'Porretta Terme'},
'390535':{'it': 'Mirandola'},
'437228':{'de': 'Kematen an der Krems', 'en': 'Kematen an der Krems'},
'437229':{'de': 'Traun', 'en': 'Traun'},
'35969248':{'bg': u('\u0421\u043a\u043e\u0431\u0435\u043b\u0435\u0432\u043e, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Skobelevo, Lovech'},
'35969249':{'bg': u('\u0414\u043e\u0439\u0440\u0435\u043d\u0446\u0438'), 'en': 'Doyrentsi'},
'434245':{'de': 'Feistritz an der Drau', 'en': 'Feistritz an der Drau'},
'35969240':{'bg': u('\u0425\u043b\u0435\u0432\u0435\u043d\u0435'), 'en': 'Hlevene'},
'35969241':{'bg': u('\u0419\u043e\u0433\u043b\u0430\u0432'), 'en': 'Yoglav'},
'35969242':{'bg': u('\u041f\u0440\u0435\u0441\u044f\u043a\u0430'), 'en': 'Presyaka'},
'35969243':{'bg': u('\u041a\u0430\u0437\u0430\u0447\u0435\u0432\u043e'), 'en': 'Kazachevo'},
'35969244':{'bg': u('\u0422\u0435\u043f\u0430\u0432\u0430'), 'en': 'Tepava'},
'35969245':{'bg': u('\u0414\u0435\u0432\u0435\u0442\u0430\u043a\u0438'), 'en': 'Devetaki'},
'35969247':{'bg': u('\u0413\u043e\u0441\u0442\u0438\u043d\u044f'), 'en': 'Gostinya'},
'3316042':{'en': 'Serris', 'fr': 'Serris'},
'3316043':{'en': 'Serris', 'fr': 'Serris'},
'3316046':{'en': 'Brunoy', 'fr': 'Brunoy'},
'3316047':{'en': 'Brunoy', 'fr': 'Brunoy'},
'3316044':{'en': 'Meaux', 'fr': 'Meaux'},
'3316048':{'en': 'Athis-Mons', 'fr': 'Athis-Mons'},
'3349352':{'en': 'Nice', 'fr': 'Nice'},
'3349353':{'en': 'Nice', 'fr': 'Nice'},
'3349351':{'en': 'Nice', 'fr': 'Nice'},
'3349356':{'en': 'Nice', 'fr': 'Nice'},
'3349357':{'en': 'Menton', 'fr': 'Menton'},
'3349354':{'en': 'Nice', 'fr': 'Nice'},
'3349355':{'en': 'Nice', 'fr': 'Nice'},
'3349358':{'en': 'Vence', 'fr': 'Vence'},
'3349359':{'en': 'Tourrettes-sur-Loup', 'fr': 'Tourrettes-sur-Loup'},
'435356':{'de': u('Kitzb\u00fchel'), 'en': u('Kitzb\u00fchel')},
'371683':{'en': u('J\u0113kabpils')},
'371682':{'en': 'Valmiera'},
'3338958':{'en': 'Sainte-Marie-aux-Mines', 'fr': 'Sainte-Marie-aux-Mines'},
'3338959':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'371686':{'en': 'Jelgava'},
'3338486':{'en': 'Lons-le-Saunier', 'fr': 'Lons-le-Saunier'},
'3338487':{'en': 'Lons-le-Saunier', 'fr': 'Lons-le-Saunier'},
'3338956':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3338482':{'en': 'Dole', 'fr': 'Dole'},
'3338951':{'en': 'Wittenheim', 'fr': 'Wittenheim'},
'3338952':{'en': 'Wittenheim', 'fr': 'Wittenheim'},
'3338953':{'en': 'Wittenheim', 'fr': 'Wittenheim'},
'331719':{'en': 'Paris', 'fr': 'Paris'},
'3354634':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'432915':{'de': 'Drosendorf-Zissersdorf', 'en': 'Drosendorf-Zissersdorf'},
'432914':{'de': 'Japons', 'en': 'Japons'},
'3332198':{'en': 'Saint-Omer', 'fr': 'Saint-Omer'},
'3332199':{'en': 'Boulogne-sur-Mer', 'fr': 'Boulogne-sur-Mer'},
'331713':{'en': 'Paris', 'fr': 'Paris'},
'331712':{'en': 'Paris', 'fr': 'Paris'},
'3332194':{'en': u('\u00c9taples'), 'fr': u('\u00c9taples')},
'3596982':{'bg': u('\u042a\u0433\u043b\u0435\u043d'), 'en': 'aglen'},
'331717':{'en': 'Paris', 'fr': 'Paris'},
'331716':{'en': 'Paris', 'fr': 'Paris'},
'331715':{'en': 'Paris', 'fr': 'Paris'},
'3594749':{'bg': u('\u0420\u0443\u0436\u0438\u0446\u0430, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Ruzhitsa, Yambol'},
'3594748':{'bg': u('\u0412\u043e\u0434\u0435\u043d, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Voden, Yambol'},
'3594745':{'bg': u('\u0428\u0430\u0440\u043a\u043e\u0432\u043e'), 'en': 'Sharkovo'},
'3594744':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u043e \u041a\u0440\u0443\u0448\u0435\u0432\u043e'), 'en': 'Golyamo Krushevo'},
'3594747':{'bg': u('\u0414\u0435\u043d\u043d\u0438\u0446\u0430, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Dennitsa, Yambol'},
'3594746':{'bg': u('\u041f\u043e\u043f\u043e\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Popovo, Yambol'},
'3594741':{'bg': u('\u0411\u043e\u043b\u044f\u0440\u043e\u0432\u043e'), 'en': 'Bolyarovo'},
'3594743':{'bg': u('\u041c\u0430\u043c\u0430\u0440\u0447\u0435\u0432\u043e'), 'en': 'Mamarchevo'},
'3594742':{'bg': u('\u0421\u0442\u0435\u0444\u0430\u043d \u041a\u0430\u0440\u0430\u0434\u0436\u043e\u0432\u043e'), 'en': 'Stefan Karadzhovo'},
'3346805':{'en': 'Prades', 'fr': 'Prades'},
'433534':{'de': 'Stadl an der Mur', 'en': 'Stadl an der Mur'},
'3596989':{'bg': u('\u0422\u043e\u0440\u043e\u0441'), 'en': 'Toros'},
'433536':{'de': 'Sankt Peter am Kammersberg', 'en': 'St. Peter am Kammersberg'},
'433537':{'de': 'Sankt Georgen ob Murau', 'en': 'St. Georgen ob Murau'},
'433532':{'de': 'Murau', 'en': 'Murau'},
'3346808':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3355549':{'en': 'Limoges', 'fr': 'Limoges'},
'3355542':{'en': 'Limoges', 'fr': 'Limoges'},
'3355543':{'en': 'Limoges', 'fr': 'Limoges'},
'3355541':{'en': u('Gu\u00e9ret'), 'fr': u('Gu\u00e9ret')},
'3355546':{'en': 'Ussel', 'fr': 'Ussel'},
'3355545':{'en': 'Limoges', 'fr': 'Limoges'},
'40368':{'en': u('Bra\u0219ov'), 'ro': u('Bra\u0219ov')},
'40369':{'en': 'Sibiu', 'ro': 'Sibiu'},
'432629':{'de': u('Warth, Nieder\u00f6sterreich'), 'en': 'Warth, Lower Austria'},
'432628':{'de': 'Felixdorf', 'en': 'Felixdorf'},
'3332440':{'en': 'Revin', 'fr': 'Revin'},
'3332441':{'en': 'Fumay', 'fr': 'Fumay'},
'3332442':{'en': 'Givet', 'fr': 'Givet'},
'40367':{'en': 'Covasna', 'ro': 'Covasna'},
'40360':{'en': u('S\u0103laj'), 'ro': u('S\u0103laj')},
'40361':{'en': 'Satu Mare', 'ro': 'Satu Mare'},
'40362':{'en': u('Maramure\u0219'), 'ro': u('Maramure\u0219')},
'40363':{'en': u('Bistri\u021ba-N\u0103s\u0103ud'), 'ro': u('Bistri\u021ba-N\u0103s\u0103ud')},
'3359425':{'en': 'Cayenne', 'fr': 'Cayenne'},
'3359427':{'en': 'Saint-Laurent-du-Maroni', 'fr': 'Saint-Laurent-du-Maroni'},
'3359422':{'en': 'Kourou', 'fr': 'Kourou'},
'390964':{'it': 'Locri'},
'3359429':{'en': 'Cayenne', 'fr': 'Cayenne'},
'3359428':{'en': 'Cayenne', 'fr': 'Cayenne'},
'3593164':{'bg': u('\u0414\u044a\u043b\u0431\u043e\u043a \u0438\u0437\u0432\u043e\u0440'), 'en': 'Dalbok izvor'},
'3593165':{'bg': u('\u041a\u0430\u0440\u0430\u0434\u0436\u0430\u043b\u043e\u0432\u043e'), 'en': 'Karadzhalovo'},
'3593166':{'bg': u('\u0411\u044f\u043b\u0430 \u0440\u0435\u043a\u0430, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Byala reka, Plovdiv'},
'3593167':{'bg': u('\u0411\u0440\u044f\u0433\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Bryagovo, Plovdiv'},
'3597139':{'bg': u('\u0414\u0436\u0443\u0440\u043e\u0432\u043e'), 'en': 'Dzhurovo'},
'3593162':{'bg': u('\u0413\u0440\u0430\u0434\u0438\u043d\u0430, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Gradina, Plovdiv'},
'3593163':{'bg': u('\u0418\u0441\u043a\u0440\u0430, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Iskra, Plovdiv'},
'3593168':{'bg': u('\u0415\u0437\u0435\u0440\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Ezerovo, Plovdiv'},
'3317956':{'en': u('Cr\u00e9teil'), 'fr': u('Cr\u00e9teil')},
'3317951':{'en': 'Argenteuil', 'fr': 'Argenteuil'},
'3595145':{'bg': u('\u0413\u0440\u043e\u0437\u0434\u044c\u043e\u0432\u043e'), 'en': 'Grozdyovo'},
'3325105':{'en': 'La Roche sur Yon', 'fr': 'La Roche sur Yon'},
'3325456':{'en': 'Blois', 'fr': 'Blois'},
'3325455':{'en': 'Blois', 'fr': 'Blois'},
'3325453':{'en': u('Ch\u00e2teauroux'), 'fr': u('Ch\u00e2teauroux')},
'3325451':{'en': 'Blois', 'fr': 'Blois'},
'3325450':{'en': 'Vineuil', 'fr': 'Vineuil'},
'3325108':{'en': 'La Roche sur Yon', 'fr': 'La Roche sur Yon'},
'37425694':{'am': u('\u0531\u0563\u0561\u0580\u0561\u056f'), 'en': 'Agarak', 'ru': u('\u0410\u0433\u0430\u0440\u0430\u043a')},
'3325458':{'en': 'Blois', 'fr': 'Blois'},
'3595724':{'bg': u('\u0414\u0440\u043e\u043f\u043b\u0430, \u0414\u043e\u0431\u0440.'), 'en': 'Dropla, Dobr.'},
'3324850':{'en': 'Bourges', 'fr': 'Bourges'},
'3324852':{'en': 'Vierzon', 'fr': 'Vierzon'},
'3324854':{'en': 'Sancerre', 'fr': 'Sancerre'},
'3324855':{'en': 'Saint-Florent-sur-Cher', 'fr': 'Saint-Florent-sur-Cher'},
'3324857':{'en': u('Mehun-sur-Y\u00e8vre'), 'fr': u('Mehun-sur-Y\u00e8vre')},
'3324858':{'en': u('Aubigny-sur-N\u00e8re'), 'fr': u('Aubigny-sur-N\u00e8re')},
'3324859':{'en': 'Dun-sur-Auron', 'fr': 'Dun-sur-Auron'},
'37425695':{'am': u('\u053c\u0565\u057b\u0561\u0576'), 'en': 'Lejan', 'ru': u('\u041b\u0435\u0436\u0430\u043d')},
'370315':{'en': 'Alytus'},
'370310':{'en': u('Var\u0117na')},
'370313':{'en': 'Druskininkai'},
'370318':{'en': 'Lazdijai'},
'370319':{'en': u('Bir\u0161tonas/Prienai')},
'333254':{'en': 'Troyes', 'fr': 'Troyes'},
'4413885':{'en': 'Stanhope (Eastgate)'},
'3356220':{'en': 'Portet-sur-Garonne', 'fr': 'Portet-sur-Garonne'},
'3356221':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356226':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356227':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356224':{'en': u('Lab\u00e8ge'), 'fr': u('Lab\u00e8ge')},
'4417685':{'en': 'Penrith'},
'4417684':{'en': 'Pooley Bridge'},
'3356228':{'en': 'Condom', 'fr': 'Condom'},
'3356229':{'en': u('Montr\u00e9al'), 'fr': u('Montr\u00e9al')},
'4417681':{'en': 'Penrith'},
'4417680':{'en': 'Penrith'},
'4417683':{'en': 'Appleby'},
'4417682':{'en': 'Penrith'},
'4413884':{'en': 'Bishop Auckland'},
'359697':{'bg': u('\u041b\u0443\u043a\u043e\u0432\u0438\u0442'), 'en': 'Lukovit'},
'390865':{'en': 'Isernia', 'it': 'Isernia'},
'4413883':{'en': 'Bishop Auckland'},
'42147':{'en': u('Lu\u010denec')},
'3344232':{'en': u('G\u00e9menos'), 'fr': u('G\u00e9menos')},
'3344230':{'en': 'Gignac-la-Nerthe', 'fr': 'Gignac-la-Nerthe'},
'3344231':{'en': 'Marignane', 'fr': 'Marignane'},
'3344236':{'en': 'Aubagne', 'fr': 'Aubagne'},
'3344237':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344234':{'en': 'Vitrolles', 'fr': 'Vitrolles'},
'3344238':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344239':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3347761':{'en': 'Firminy', 'fr': 'Firminy'},
'3347760':{'en': 'Charlieu', 'fr': 'Charlieu'},
'35969031':{'bg': u('\u0413\u0430\u043b\u0430\u0442\u0430'), 'en': 'Galata'},
'3347989':{'en': 'Ugine', 'fr': 'Ugine'},
'3347988':{'en': 'Aix-les-Bains', 'fr': 'Aix-les-Bains'},
'3347767':{'en': 'Roanne', 'fr': 'Roanne'},
'3343404':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3347984':{'en': u('Montm\u00e9lian'), 'fr': u('Montm\u00e9lian')},
'3343400':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3347983':{'en': 'Saint-Jean-de-Maurienne', 'fr': 'Saint-Jean-de-Maurienne'},
'4413881':{'en': 'Bishop Auckland/Stanhope (Eastgate)'},
'35931258':{'bg': u('\u0413\u043b\u0430\u0432\u0430\u0442\u0430\u0440'), 'en': 'Glavatar'},
'3316929':{'en': 'Orsay', 'fr': 'Orsay'},
'3316928':{'en': 'Orsay', 'fr': 'Orsay'},
'3316921':{'en': 'Juvisy-sur-Orge', 'fr': 'Juvisy-sur-Orge'},
'3316920':{'en': 'Massy', 'fr': 'Massy'},
'3316923':{'en': 'Cerny', 'fr': 'Cerny'},
'3316925':{'en': u('Sainte-Genevi\u00e8ve-des-Bois'), 'fr': u('Sainte-Genevi\u00e8ve-des-Bois')},
'3316924':{'en': u('Viry-Ch\u00e2tillon'), 'fr': u('Viry-Ch\u00e2tillon')},
'3316927':{'en': 'Lardy', 'fr': 'Lardy'},
'3316926':{'en': 'Arpajon', 'fr': 'Arpajon'},
'3355680':{'en': 'Talence', 'fr': 'Talence'},
'4413880':{'en': 'Bishop Auckland/Stanhope (Eastgate)'},
'441920':{'en': 'Ware'},
'35984728':{'bg': u('\u0420\u0430\u043a\u043e\u0432\u0441\u043a\u0438, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Rakovski, Razgrad'},
'35984729':{'bg': u('\u0415\u0437\u0435\u0440\u0447\u0435'), 'en': 'Ezerche'},
'3327776':{'en': 'Rouen', 'fr': 'Rouen'},
'35984722':{'bg': u('\u042f\u0441\u0435\u043d\u043e\u0432\u0435\u0446'), 'en': 'Yasenovets'},
'35984723':{'bg': u('\u0414\u044f\u043d\u043a\u043e\u0432\u043e'), 'en': 'Dyankovo'},
'35984720':{'bg': u('\u0422\u043e\u043f\u0447\u0438\u0438'), 'en': 'Topchii'},
'35984721':{'bg': u('\u041b\u0438\u043f\u043d\u0438\u043a'), 'en': 'Lipnik'},
'35984726':{'bg': u('\u0423\u0448\u0438\u043d\u0446\u0438'), 'en': 'Ushintsi'},
'35984727':{'bg': u('\u041a\u0430\u043c\u0435\u043d\u043e\u0432\u043e, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Kamenovo, Razgrad'},
'35984725':{'bg': u('\u041a\u0438\u0447\u0435\u043d\u0438\u0446\u0430'), 'en': 'Kichenitsa'},
'43512':{'de': 'Innsbruck', 'en': 'Innsbruck'},
'3329736':{'en': 'Hennebont', 'fr': 'Hennebont'},
'3329737':{'en': 'Lorient', 'fr': 'Lorient'},
'3329734':{'en': 'Guiscriff', 'fr': 'Guiscriff'},
'3329735':{'en': 'Lorient', 'fr': 'Lorient'},
'3329732':{'en': 'Pont-Scorff', 'fr': 'Pont-Scorff'},
'3329733':{'en': 'Plouay', 'fr': 'Plouay'},
'3329730':{'en': 'Quiberon', 'fr': 'Quiberon'},
'3329731':{'en': 'Le Palais', 'fr': 'Le Palais'},
'3595148':{'bg': u('\u0420\u0443\u0434\u043d\u0438\u043a, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Rudnik, Varna'},
'3338569':{'en': 'Montceau-les-Mines', 'fr': 'Montceau-les-Mines'},
'3338568':{'en': 'Blanzy', 'fr': 'Blanzy'},
'3338567':{'en': 'Montceau-les-Mines', 'fr': 'Montceau-les-Mines'},
'3595149':{'bg': u('\u0413\u043e\u043b\u0438\u0446\u0430'), 'en': 'Golitsa'},
'4417688':{'en': 'Penrith'},
'3359658':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3359652':{'en': 'Le Morne Rouge', 'fr': 'Le Morne Rouge'},
'3359653':{'en': 'Le Lorrain', 'fr': 'Le Lorrain'},
'3359650':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3359651':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3359656':{'en': 'Ducos', 'fr': 'Ducos'},
'3359657':{'en': 'Saint Joseph', 'fr': 'Saint Joseph'},
'3359654':{'en': u('Le Fran\u00e7ois'), 'fr': u('Le Fran\u00e7ois')},
'3359655':{'en': 'Le Morne Vert', 'fr': 'Le Morne Vert'},
'435224':{'de': 'Wattens', 'en': 'Wattens'},
'3329866':{'en': u('Pont-l\'Abb\u00e9'), 'fr': u('Pont-l\'Abb\u00e9')},
'3355684':{'en': 'Talence', 'fr': 'Talence'},
'3355687':{'en': 'Villenave-d\'Ornon', 'fr': 'Villenave-d\'Ornon'},
'3355686':{'en': 'Cenon', 'fr': 'Cenon'},
'3355681':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3329863':{'en': 'Morlaix', 'fr': 'Morlaix'},
'3355683':{'en': 'Arcachon', 'fr': 'Arcachon'},
'3329861':{'en': u('Ploun\u00e9vez-Lochrist'), 'fr': u('Ploun\u00e9vez-Lochrist')},
'3355939':{'en': 'Oloron-Sainte-Marie', 'fr': 'Oloron-Sainte-Marie'},
'3355938':{'en': u('Salies-de-B\u00e9arn'), 'fr': u('Salies-de-B\u00e9arn')},
'3355689':{'en': 'Gradignan', 'fr': 'Gradignan'},
'3355688':{'en': 'Salles', 'fr': 'Salles'},
'3329868':{'en': 'Landivisiau', 'fr': 'Landivisiau'},
'3329869':{'en': u('Saint-Pol-de-L\u00e9on'), 'fr': u('Saint-Pol-de-L\u00e9on')},
'3595118':{'bg': u('\u0412\u043e\u0434\u0438\u0446\u0430, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Voditsa, Varna'},
'34949':{'en': 'Guadalajara', 'es': 'Guadalajara'},
'34948':{'en': 'Navarre', 'es': 'Navarra'},
'34947':{'en': 'Burgos', 'es': 'Burgos'},
'34946':{'en': 'Vizcaya', 'es': 'Vizcaya'},
'34945':{'en': 'Araba', 'es': u('\u00c1lava')},
'34944':{'en': 'Vizcaya', 'es': 'Vizcaya'},
'34943':{'en': u('Guip\u00fazcoa'), 'es': u('Guip\u00fazcoa')},
'34942':{'en': 'Cantabria', 'es': 'Cantabria'},
'34941':{'en': 'La Rioja', 'es': 'La Rioja'},
'35971337':{'bg': u('\u041a\u0430\u043b\u0443\u0433\u0435\u0440\u043e\u0432\u043e, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Kalugerovo, Sofia'},
'3332295':{'en': 'Amiens', 'fr': 'Amiens'},
'3332294':{'en': 'Hangest-en-Santerre', 'fr': 'Hangest-en-Santerre'},
'3332297':{'en': 'Amiens', 'fr': 'Amiens'},
'3332296':{'en': 'Corbie', 'fr': 'Corbie'},
'3332291':{'en': 'Amiens', 'fr': 'Amiens'},
'3332292':{'en': 'Amiens', 'fr': 'Amiens'},
'441395':{'en': 'Budleigh Salterton'},
'3344203':{'en': 'Aubagne', 'fr': 'Aubagne'},
'441397':{'en': 'Fort William'},
'3344202':{'en': 'Les Pennes Mirabeau', 'fr': 'Les Pennes Mirabeau'},
'432735':{'de': 'Hadersdorf am Kamp', 'en': 'Hadersdorf am Kamp'},
'3344201':{'en': 'Cassis', 'fr': 'Cassis'},
'432734':{'de': 'Langenlois', 'en': 'Langenlois'},
'3344206':{'en': 'Port-de-Bouc', 'fr': 'Port-de-Bouc'},
'35362':{'en': 'Tipperary/Cashel'},
'432738':{'de': 'Fels am Wagram', 'en': 'Fels am Wagram'},
'441466':{'en': 'Huntly'},
'35363':{'en': 'Charleville'},
'3594356':{'bg': u('\u0425\u0430\u0434\u0436\u0438\u0434\u0438\u043c\u0438\u0442\u0440\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Hadzhidimitrovo, St. Zagora'},
'3594357':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u0421\u0430\u0445\u0440\u0430\u043d\u0435'), 'en': 'Gorno Sahrane'},
'3594354':{'bg': u('\u0417\u0438\u043c\u043d\u0438\u0446\u0430, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Zimnitsa, St. Zagora'},
'3594355':{'bg': u('\u0411\u0443\u0437\u043e\u0432\u0433\u0440\u0430\u0434'), 'en': 'Buzovgrad'},
'3594352':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u0427\u0435\u0440\u043a\u043e\u0432\u0438\u0449\u0435'), 'en': 'Gorno Cherkovishte'},
'3594353':{'bg': u('\u0421\u0440\u0435\u0434\u043d\u043e\u0433\u043e\u0440\u043e\u0432\u043e'), 'en': 'Srednogorovo'},
'3594350':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u0418\u0437\u0432\u043e\u0440\u043e\u0432\u043e'), 'en': 'Gorno Izvorovo'},
'3594351':{'bg': u('\u041a\u043e\u043f\u0440\u0438\u043d\u043a\u0430'), 'en': 'Koprinka'},
'3594358':{'bg': u('\u0421\u043a\u043e\u0431\u0435\u043b\u0435\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Skobelevo, St. Zagora'},
'3594359':{'bg': u('\u0410\u0441\u0435\u043d, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Asen, St. Zagora'},
'4418470':{'en': 'Thurso/Tongue'},
'3598640':{'bg': u('\u041f\u0440\u0430\u0432\u0434\u0430, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Pravda, Silistra'},
'441464':{'en': 'Insch'},
'3315763':{'en': 'Montrouge', 'fr': 'Montrouge'},
'435357':{'de': 'Kirchberg in Tirol', 'en': 'Kirchberg in Tirol'},
'3315769':{'en': 'Rueil-Malmaison', 'fr': 'Rueil-Malmaison'},
'3598643':{'bg': u('\u0417\u043b\u0430\u0442\u043e\u043a\u043b\u0430\u0441'), 'en': 'Zlatoklas'},
'3598644':{'bg': u('\u0427\u0435\u0440\u043d\u043e\u043b\u0438\u043a'), 'en': 'Chernolik'},
'3598645':{'bg': u('\u041c\u0435\u0436\u0434\u0435\u043d'), 'en': 'Mezhden'},
'3317549':{'en': 'Boulogne-Billancourt', 'fr': 'Boulogne-Billancourt'},
'4418479':{'en': 'Tongue'},
'3317540':{'en': 'Argenteuil', 'fr': 'Argenteuil'},
'3317542':{'en': 'Paris', 'fr': 'Paris'},
'4418478':{'en': 'Thurso'},
'3338363':{'en': 'Toul', 'fr': 'Toul'},
'3334439':{'en': u('Cr\u00e9py-en-Valois'), 'fr': u('Cr\u00e9py-en-Valois')},
'3334438':{'en': u('Compi\u00e8gne'), 'fr': u('Compi\u00e8gne')},
'3338367':{'en': 'Nancy', 'fr': 'Nancy'},
'3338364':{'en': 'Toul', 'fr': 'Toul'},
'3334432':{'en': 'Senlis', 'fr': 'Senlis'},
'3334431':{'en': 'Pont-Sainte-Maxence', 'fr': 'Pont-Sainte-Maxence'},
'3334430':{'en': u('Compi\u00e8gne'), 'fr': u('Compi\u00e8gne')},
'3334437':{'en': 'Jaux', 'fr': 'Jaux'},
'3334436':{'en': u('Compi\u00e8gne'), 'fr': u('Compi\u00e8gne')},
'441440':{'en': 'Haverhill'},
'3355608':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3595333':{'bg': u('\u0425\u0430\u043d \u041a\u0440\u0443\u043c'), 'en': 'Han Krum'},
'3595332':{'bg': u('\u0414\u0440\u0430\u0433\u043e\u0435\u0432\u043e'), 'en': 'Dragoevo'},
'3595330':{'bg': u('\u0417\u043b\u0430\u0442\u0430\u0440'), 'en': 'Zlatar'},
'3595337':{'bg': u('\u041a\u043e\u0447\u043e\u0432\u043e'), 'en': 'Kochovo'},
'3595336':{'bg': u('\u0418\u043c\u0440\u0435\u043d\u0447\u0435\u0432\u043e'), 'en': 'Imrenchevo'},
'3595335':{'bg': u('\u041c\u0438\u043b\u0430\u043d\u043e\u0432\u043e, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Milanovo, Shumen'},
'3595334':{'bg': u('\u041e\u0441\u043c\u0430\u0440'), 'en': 'Osmar'},
'35941279':{'bg': u('\u0421\u0442\u0440\u0435\u043b\u0435\u0446, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Strelets, St. Zagora'},
'3355858':{'en': 'Dax', 'fr': 'Dax'},
'3595338':{'bg': u('\u0422\u0440\u043e\u0438\u0446\u0430'), 'en': 'Troitsa'},
'3356565':{'en': 'Rieupeyroux', 'fr': 'Rieupeyroux'},
'3356567':{'en': 'Rodez', 'fr': 'Rodez'},
'355279':{'en': u('Gryk-\u00c7aj\u00eb/Ujmisht/Bushtrice/Kalis, Kuk\u00ebs')},
'3356561':{'en': 'Millau', 'fr': 'Millau'},
'3329929':{'en': 'Rennes', 'fr': 'Rennes'},
'355272':{'en': u('Qerret/Qel\u00ebz/Gjegjan, Puk\u00eb')},
'355273':{'en': u('Iball\u00eb/Fierz\u00eb/Blerim/Qaf\u00eb-Mali, Puk\u00eb')},
'355270':{'en': u('Kolsh/Surroj/Arren/Malzi, Kuk\u00ebs')},
'355271':{'en': u('Fush\u00eb-Arr\u00ebz/Rrap\u00eb, Puk\u00eb')},
'355276':{'en': 'Fajza/Golaj/Gjinaj, Has'},
'3356568':{'en': 'Rodez', 'fr': 'Rodez'},
'355274':{'en': u('Tropoj\u00eb/Llugaj/Margegaj, Tropoj\u00eb')},
'355275':{'en': u('Bujan/Fierz\u00eb/Bytyc/Lekbiba, Tropoj\u00eb')},
'3329927':{'en': 'Rennes', 'fr': 'Rennes'},
'3597925':{'bg': u('\u0413\u044e\u0435\u0448\u0435\u0432\u043e'), 'en': 'Gyueshevo'},
'3599339':{'bg': u('\u041c\u0430\u043a\u0440\u0435\u0448'), 'en': 'Makresh'},
'3329926':{'en': 'Rennes', 'fr': 'Rennes'},
'3599337':{'bg': u('\u0413\u0440\u0430\u043c\u0430\u0434\u0430'), 'en': 'Gramada'},
'3599336':{'bg': u('\u0421\u0442\u0430\u0440\u043e\u043f\u0430\u0442\u0438\u0446\u0430'), 'en': 'Staropatitsa'},
'3599335':{'bg': u('\u0426\u0430\u0440 \u041f\u0435\u0442\u0440\u043e\u0432\u043e'), 'en': 'Tsar Petrovo'},
'3599333':{'bg': u('\u0411\u043e\u0439\u043d\u0438\u0446\u0430'), 'en': 'Boynitsa'},
'3355856':{'en': 'Dax', 'fr': 'Dax'},
'3599330':{'bg': u('\u0420\u0430\u0431\u0440\u043e\u0432\u043e'), 'en': 'Rabrovo'},
'3329922':{'en': 'Rennes', 'fr': 'Rennes'},
'3597926':{'bg': u('\u041a\u043e\u043d\u044f\u0432\u043e'), 'en': 'Konyavo'},
'3355852':{'en': 'Saint-Martin-d\'Oney', 'fr': 'Saint-Martin-d\'Oney'},
'433463':{'de': 'Stainz', 'en': 'Stainz'},
'3353428':{'en': 'Auterive', 'fr': 'Auterive'},
'3597920':{'bg': u('\u0421\u043a\u0440\u0438\u043d\u044f\u043d\u043e'), 'en': 'Skrinyano'},
'3353425':{'en': 'Toulouse', 'fr': 'Toulouse'},
'433113':{'de': 'Pischelsdorf in der Steiermark', 'en': 'Pischelsdorf in der Steiermark'},
'433468':{'de': 'Sankt Oswald ob Eibiswald', 'en': 'St. Oswald ob Eibiswald'},
'437443':{'de': 'Ybbsitz', 'en': 'Ybbsitz'},
'435375':{'de': u('K\u00f6ssen'), 'en': u('K\u00f6ssen')},
'3597921':{'bg': u('\u0416\u0438\u043b\u0435\u043d\u0446\u0438'), 'en': 'Zhilentsi'},
'3593781':{'bg': u('\u0421\u0438\u043c\u0435\u043e\u043d\u043e\u0432\u0433\u0440\u0430\u0434'), 'en': 'Simeonovgrad'},
'3339180':{'en': u('Bruay-la-Buissi\u00e8re'), 'fr': u('Bruay-la-Buissi\u00e8re')},
'3339183':{'en': 'Carvin', 'fr': 'Carvin'},
'42032':{'en': 'Central Bohemian Region'},
'37422393':{'am': u('\u0544\u0565\u0572\u0580\u0561\u0571\u0578\u0580'), 'en': 'Meghradzor', 'ru': u('\u041c\u0435\u0445\u0440\u0430\u0434\u0437\u043e\u0440')},
'37422391':{'am': u('\u053c\u0565\u057c\u0576\u0561\u0576\u056b\u057d\u057f'), 'en': 'Lernanist', 'ru': u('\u041b\u0435\u0440\u043d\u0430\u043d\u0438\u0441\u0442')},
'37422397':{'am': u('\u054d\u0578\u056c\u0561\u056f'), 'en': 'Solak', 'ru': u('\u0421\u043e\u043b\u0430\u043a')},
'37422394':{'am': u('\u0553\u0575\u0578\u0582\u0576\u056b\u0584'), 'en': 'Pyunik', 'ru': u('\u041f\u044e\u043d\u0438\u043a')},
'42035':{'en': 'Karlovy Vary Region'},
'3597922':{'bg': u('\u0414\u0440\u0430\u0433\u043e\u0432\u0438\u0449\u0438\u0446\u0430, \u041a\u044e\u0441\u0442.'), 'en': 'Dragovishtitsa, Kyust.'},
'37422398':{'am': u('\u0532\u057b\u0576\u056b'), 'en': 'Bjni', 'ru': u('\u0411\u0436\u043d\u0438')},
'42039':{'en': 'South Bohemian Region'},
'3347772':{'en': 'Roanne', 'fr': 'Roanne'},
'3522449':{'de': 'Howald', 'en': 'Howald', 'fr': 'Howald'},
'3522448':{'de': 'Contern/Foetz', 'en': 'Contern/Foetz', 'fr': 'Contern/Foetz'},
'3522447':{'de': 'Lintgen', 'en': 'Lintgen', 'fr': 'Lintgen'},
'3596966':{'bg': u('\u0428\u0438\u043f\u043a\u043e\u0432\u043e'), 'en': 'Shipkovo'},
'3522445':{'de': 'Diedrich', 'en': 'Diedrich', 'fr': 'Diedrich'},
'3522443':{'de': 'Findel/Kirchberg', 'en': 'Findel/Kirchberg', 'fr': 'Findel/Kirchberg'},
'3522442':{'de': 'Plateau de Kirchberg', 'en': 'Plateau de Kirchberg', 'fr': 'Plateau de Kirchberg'},
'3522440':{'de': 'Howald', 'en': 'Howald', 'fr': 'Howald'},
'3742680':{'am': u('\u0534\u056b\u056c\u056b\u057b\u0561\u0576'), 'en': 'Dilijan', 'ru': u('\u0414\u0438\u043b\u0438\u0436\u0430\u043d')},
'3596965':{'bg': u('\u0411\u0435\u043b\u0438 \u041e\u0441\u044a\u043c'), 'en': 'Beli Osam'},
'437563':{'de': 'Spital am Pyhrn', 'en': 'Spital am Pyhrn'},
'40237':{'en': 'Vrancea', 'ro': 'Vrancea'},
'3345617':{'en': 'Grenoble', 'fr': 'Grenoble'},
'40232':{'en': u('Ia\u0219i'), 'ro': u('Ia\u0219i')},
'3522667':{'de': u('D\u00fcdelingen'), 'en': 'Dudelange', 'fr': 'Dudelange'},
'37424496':{'am': u('\u0544\u0565\u056e \u0544\u0561\u0576\u0569\u0561\u0577'), 'en': 'Mets Mantash', 'ru': u('\u041c\u0435\u0446 \u041c\u0430\u043d\u0442\u0430\u0448')},
'3338976':{'en': 'Guebwiller', 'fr': 'Guebwiller'},
'3338977':{'en': 'Munster', 'fr': 'Munster'},
'3338974':{'en': 'Guebwiller', 'fr': 'Guebwiller'},
'3338975':{'en': 'Cernay', 'fr': 'Cernay'},
'3338973':{'en': 'Ribeauville', 'fr': 'Ribeauville'},
'3338970':{'en': 'Saint-Louis', 'fr': 'Saint-Louis'},
'3338971':{'en': 'Orbey', 'fr': 'Orbey'},
'437564':{'de': 'Hinterstoder', 'en': 'Hinterstoder'},
'3338978':{'en': 'Kaysersberg', 'fr': 'Kaysersberg'},
'3338979':{'en': 'Colmar', 'fr': 'Colmar'},
'3323864':{'en': 'Saint-Denis-en-Val', 'fr': 'Saint-Denis-en-Val'},
'3323865':{'en': 'Loury', 'fr': 'Loury'},
'3323866':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323867':{'en': 'Gien', 'fr': 'Gien'},
'3323861':{'en': 'Saint-Jean-de-Braye', 'fr': 'Saint-Jean-de-Braye'},
'3323862':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323863':{'en': 'Olivet', 'fr': 'Olivet'},
'3323868':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323869':{'en': 'Olivet', 'fr': 'Olivet'},
'437413':{'de': 'Marbach an der Donau', 'en': 'Marbach an der Donau'},
'437412':{'de': 'Ybbs an der Donau', 'en': 'Ybbs an der Donau'},
'44131':{'en': 'Edinburgh'},
'437416':{'de': 'Wieselburg', 'en': 'Wieselburg'},
'437415':{'de': 'Altenmarkt, Yspertal', 'en': 'Altenmarkt, Yspertal'},
'437414':{'de': 'Weins-Isperdorf', 'en': 'Weins-Isperdorf'},
'40238':{'en': u('Buz\u0103u'), 'ro': u('Buz\u0103u')},
'40239':{'en': u('Br\u0103ila'), 'ro': u('Br\u0103ila')},
'3346821':{'en': 'Saint-Cyprien', 'fr': 'Saint-Cyprien'},
'3346820':{'en': 'Quillan', 'fr': 'Quillan'},
'3346823':{'en': 'Castelnaudary', 'fr': 'Castelnaudary'},
'3346822':{'en': 'Elne', 'fr': 'Elne'},
'3346825':{'en': 'Carcassonne', 'fr': 'Carcassonne'},
'3346827':{'en': u('L\u00e9zignan-Corbi\u00e8res'), 'fr': u('L\u00e9zignan-Corbi\u00e8res')},
'3346826':{'en': 'Carcassonne', 'fr': 'Carcassonne'},
'3346829':{'en': 'Estagel', 'fr': 'Estagel'},
'3346828':{'en': 'Saint-Laurent-de-la-Salanque', 'fr': 'Saint-Laurent-de-la-Salanque'},
'432649':{'de': u('M\u00f6nichkirchen'), 'en': u('M\u00f6nichkirchen')},
'432648':{'de': 'Hochneukirchen', 'en': 'Hochneukirchen'},
'432641':{'de': 'Kirchberg am Wechsel', 'en': 'Kirchberg am Wechsel'},
'432643':{'de': 'Lichtenegg', 'en': 'Lichtenegg'},
'381230':{'en': 'Kikinda', 'sr': 'Kikinda'},
'432645':{'de': 'Wiesmath', 'en': 'Wiesmath'},
'3596960':{'bg': u('\u0411\u0435\u043b\u0438\u0448'), 'en': 'Belish'},
'432647':{'de': u('Krumbach, Nieder\u00f6sterreich'), 'en': 'Krumbach, Lower Austria'},
'432646':{'de': 'Kirchschlag in der Buckligen Welt', 'en': 'Kirchschlag in der Buckligen Welt'},
'390324':{'en': 'Verbano-Cusio-Ossola', 'it': 'Domodossola'},
'441626':{'en': 'Newton Abbot'},
'34888':{'en': 'Ourense', 'es': 'Orense'},
'3593148':{'bg': u('\u0419\u043e\u0430\u043a\u0438\u043c \u0413\u0440\u0443\u0435\u0432\u043e'), 'en': 'Yoakim Gruevo'},
'3593149':{'bg': u('\u0426\u0430\u043b\u0430\u043f\u0438\u0446\u0430'), 'en': 'Tsalapitsa'},
'3593146':{'bg': u('\u041a\u0443\u0440\u0442\u043e\u0432\u043e \u041a\u043e\u043d\u0430\u0440\u0435'), 'en': 'Kurtovo Konare'},
'34883':{'en': 'Valladolid', 'es': 'Valladolid'},
'34880':{'en': 'Zamora', 'es': 'Zamora'},
'34881':{'en': u('La Coru\u00f1a'), 'es': u('La Coru\u00f1a')},
'34886':{'en': 'Pontevedra', 'es': 'Pontevedra'},
'3593143':{'bg': u('\u041f\u0435\u0440\u0443\u0449\u0438\u0446\u0430'), 'en': 'Perushtitsa'},
'34884':{'en': 'Asturias', 'es': 'Asturias'},
'3325470':{'en': u('Mont-pr\u00e9s-Chambord'), 'fr': u('Mont-pr\u00e9s-Chambord')},
'3325473':{'en': u('Vend\u00f4me'), 'fr': u('Vend\u00f4me')},
'3325475':{'en': 'Saint-Aignan', 'fr': 'Saint-Aignan'},
'3325474':{'en': 'Blois', 'fr': 'Blois'},
'3325477':{'en': u('Vend\u00f4me'), 'fr': u('Vend\u00f4me')},
'3325476':{'en': 'Romorantin-Lanthenay', 'fr': 'Romorantin-Lanthenay'},
'3325479':{'en': 'Contres', 'fr': 'Contres'},
'3325478':{'en': 'Blois', 'fr': 'Blois'},
'3324878':{'en': 'Sancerre', 'fr': 'Sancerre'},
'42031':{'en': 'Central Bohemian Region'},
'3324873':{'en': 'Argent-sur-Sauldre', 'fr': 'Argent-sur-Sauldre'},
'3324870':{'en': 'Bourges', 'fr': 'Bourges'},
'3324871':{'en': 'Vierzon', 'fr': 'Vierzon'},
'3324874':{'en': 'Sancoins', 'fr': 'Sancoins'},
'3324875':{'en': 'Vierzon', 'fr': 'Vierzon'},
'433116':{'de': 'Kirchbach in Steiermark', 'en': 'Kirchbach in Steiermark'},
'433117':{'de': 'Eggersdorf bei Graz', 'en': 'Eggersdorf bei Graz'},
'375162':{'be': u('\u0411\u0440\u044d\u0441\u0442'), 'en': 'Brest', 'ru': u('\u0411\u0440\u0435\u0441\u0442')},
'375163':{'be': u('\u0411\u0430\u0440\u0430\u043d\u0430\u0432\u0456\u0447\u044b'), 'en': 'Baranovichi', 'ru': u('\u0411\u0430\u0440\u0430\u043d\u043e\u0432\u0438\u0447\u0438')},
'433112':{'de': 'Gleisdorf', 'en': 'Gleisdorf'},
'375165':{'be': u('\u041f\u0456\u043d\u0441\u043a'), 'en': 'Pinsk', 'ru': u('\u041f\u0438\u043d\u0441\u043a')},
'3628':{'en': 'Godollo', 'hu': u('G\u00f6d\u00f6ll\u0151')},
'433118':{'de': 'Sinabelkirchen', 'en': 'Sinabelkirchen'},
'433119':{'de': 'Sankt Marein bei Graz', 'en': 'St. Marein bei Graz'},
'3597447':{'bg': u('\u0414\u043e\u0431\u0440\u0438\u043d\u0438\u0449\u0435'), 'en': 'Dobrinishte'},
'3347168':{'en': 'Mauriac', 'fr': 'Mauriac'},
'3347167':{'en': 'Mauriac', 'fr': 'Mauriac'},
'3347166':{'en': 'Monistrol-sur-Loire', 'fr': 'Monistrol-sur-Loire'},
'3347165':{'en': 'Yssingeaux', 'fr': 'Yssingeaux'},
'3347164':{'en': 'Aurillac', 'fr': 'Aurillac'},
'3347163':{'en': 'Aurillac', 'fr': 'Aurillac'},
'359596':{'bg': u('\u041f\u043e\u043c\u043e\u0440\u0438\u0435'), 'en': 'Pomorie'},
'3347160':{'en': 'Saint-Flour', 'fr': 'Saint-Flour'},
'3598147':{'bg': u('\u0411\u0430\u043d\u0438\u0441\u043a\u0430'), 'en': 'Baniska'},
'3598145':{'bg': u('\u0422\u0440\u044a\u0441\u0442\u0435\u043d\u0438\u043a, \u0420\u0443\u0441\u0435'), 'en': 'Trastenik, Ruse'},
'3598144':{'bg': u('\u0411\u0430\u0442\u0438\u0448\u043d\u0438\u0446\u0430'), 'en': 'Batishnitsa'},
'3598143':{'bg': u('\u041e\u0431\u0440\u0435\u0442\u0435\u043d\u0438\u043a'), 'en': 'Obretenik'},
'3598142':{'bg': u('\u0411\u044a\u0437\u043e\u0432\u0435\u0446, \u0420\u0443\u0441\u0435'), 'en': 'Bazovets, Ruse'},
'3598141':{'bg': u('\u0414\u0432\u0435 \u043c\u043e\u0433\u0438\u043b\u0438'), 'en': 'Dve mogili'},
'3598140':{'bg': u('\u0411\u043e\u0440\u043e\u0432\u043e, \u0420\u0443\u0441\u0435'), 'en': 'Borovo, Ruse'},
'3598149':{'bg': u('\u041a\u0430\u0446\u0435\u043b\u043e\u0432\u043e'), 'en': 'Katselovo'},
'3598148':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u0410\u0431\u043b\u0430\u043d\u043e\u0432\u043e'), 'en': 'Gorno Ablanovo'},
'3599117':{'bg': u('\u041a\u0440\u0438\u0432\u043e\u0434\u043e\u043b'), 'en': 'Krivodol'},
'35863':{'en': 'Vaasa', 'fi': 'Vaasa', 'se': 'Vasa'},
'35862':{'en': 'Vaasa', 'fi': 'Vaasa', 'se': 'Vasa'},
'35861':{'en': 'Vaasa', 'fi': 'Vaasa', 'se': 'Vasa'},
'35867':{'en': 'Vaasa', 'fi': 'Vaasa', 'se': 'Vasa'},
'35866':{'en': 'Vaasa', 'fi': 'Vaasa', 'se': 'Vasa'},
'35865':{'en': 'Vaasa', 'fi': 'Vaasa', 'se': 'Vasa'},
'35864':{'en': 'Vaasa', 'fi': 'Vaasa', 'se': 'Vasa'},
'35868':{'en': 'Vaasa', 'fi': 'Vaasa', 'se': 'Vasa'},
'3336978':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3347702':{'en': u('Andr\u00e9zieux-Bouth\u00e9on'), 'fr': u('Andr\u00e9zieux-Bouth\u00e9on')},
'3347701':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3336977':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3347706':{'en': 'Veauche', 'fr': 'Veauche'},
'3316943':{'en': 'Ris-Orangis', 'fr': 'Ris-Orangis'},
'3316942':{'en': 'Draveil', 'fr': 'Draveil'},
'3316941':{'en': 'Igny', 'fr': 'Igny'},
'3316940':{'en': 'Draveil', 'fr': 'Draveil'},
'3316947':{'en': u('\u00c9vry'), 'fr': u('\u00c9vry')},
'3316946':{'en': u('Sainte-Genevi\u00e8ve-des-Bois'), 'fr': u('Sainte-Genevi\u00e8ve-des-Bois')},
'3316945':{'en': 'Juvisy-sur-Orge', 'fr': 'Juvisy-sur-Orge'},
'3316944':{'en': 'Savigny-sur-Orge', 'fr': 'Savigny-sur-Orge'},
'3316949':{'en': 'Yerres', 'fr': 'Yerres'},
'3316948':{'en': 'Yerres', 'fr': 'Yerres'},
'3599119':{'bg': u('\u0422\u0438\u0448\u0435\u0432\u0438\u0446\u0430'), 'en': 'Tishevitsa'},
'3343426':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3343422':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3597415':{'bg': u('\u0421\u0435\u043b\u0438\u0449\u0435, \u0411\u043b\u0430\u0433.'), 'en': 'Selishte, Blag.'},
'3343428':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3343429':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3329758':{'en': 'Baden', 'fr': 'Baden'},
'3329750':{'en': 'Quiberon', 'fr': 'Quiberon'},
'3329752':{'en': 'Carnac', 'fr': 'Carnac'},
'3329753':{'en': 'Elven', 'fr': 'Elven'},
'3329754':{'en': 'Vannes', 'fr': 'Vannes'},
'3329755':{'en': 'Belz', 'fr': 'Belz'},
'3329756':{'en': 'Auray', 'fr': 'Auray'},
'3329757':{'en': 'Baden', 'fr': 'Baden'},
'3596321':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u0421\u0442\u0443\u0434\u0435\u043d\u0430'), 'en': 'Gorna Studena'},
'3596323':{'bg': u('\u0411\u044a\u043b\u0433\u0430\u0440\u0441\u043a\u043e \u0441\u043b\u0438\u0432\u043e\u0432\u043e'), 'en': 'Balgarsko slivovo'},
'3596322':{'bg': u('\u0410\u043b\u0435\u043a\u043e\u0432\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Alekovo, V. Tarnovo'},
'3596325':{'bg': u('\u041a\u043e\u0437\u043b\u043e\u0432\u0435\u0446'), 'en': 'Kozlovets'},
'3596324':{'bg': u('\u0412\u0430\u0440\u0434\u0438\u043c'), 'en': 'Vardim'},
'3596327':{'bg': u('\u041e\u0432\u0447\u0430 \u043c\u043e\u0433\u0438\u043b\u0430'), 'en': 'Ovcha mogila'},
'3596326':{'bg': u('\u041c\u043e\u0440\u0430\u0432\u0430'), 'en': 'Morava'},
'3596329':{'bg': u('\u0426\u0430\u0440\u0435\u0432\u0435\u0446, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Tsarevets, V. Tarnovo'},
'3596328':{'bg': u('\u041e\u0440\u0435\u0448'), 'en': 'Oresh'},
'3323189':{'en': 'Honfleur', 'fr': 'Honfleur'},
'3323188':{'en': 'Deauville', 'fr': 'Deauville'},
'3323183':{'en': 'Caen', 'fr': 'Caen'},
'3323182':{'en': 'Caen', 'fr': 'Caen'},
'3323181':{'en': 'Deauville', 'fr': 'Deauville'},
'3323187':{'en': 'Villers-sur-Mer', 'fr': 'Villers-sur-Mer'},
'3323186':{'en': 'Caen', 'fr': 'Caen'},
'3323185':{'en': 'Caen', 'fr': 'Caen'},
'3323184':{'en': 'Caen', 'fr': 'Caen'},
'3322854':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'3322853':{'en': 'Saint-Brevin-les-Pins', 'fr': 'Saint-Brevin-les-Pins'},
'432859':{'de': 'Brand-Nagelberg', 'en': 'Brand-Nagelberg'},
'3354531':{'en': 'Ruffec', 'fr': 'Ruffec'},
'3354532':{'en': 'Cognac', 'fr': 'Cognac'},
'3354535':{'en': 'Cognac', 'fr': 'Cognac'},
'3354536':{'en': 'Cognac', 'fr': 'Cognac'},
'3354537':{'en': u('Angoul\u00eame'), 'fr': u('Angoul\u00eame')},
'3354538':{'en': u('Angoul\u00eame'), 'fr': u('Angoul\u00eame')},
'3354539':{'en': 'Chasseneuil-sur-Bonnieure', 'fr': 'Chasseneuil-sur-Bonnieure'},
'3597741':{'bg': u('\u0417\u0435\u043c\u0435\u043d'), 'en': 'Zemen'},
'3329800':{'en': 'Brest', 'fr': 'Brest'},
'3323326':{'en': u('Alen\u00e7on'), 'fr': u('Alen\u00e7on')},
'3329802':{'en': 'Brest', 'fr': 'Brest'},
'3323324':{'en': 'L\'Aigle', 'fr': 'L\'Aigle'},
'3323323':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'3323322':{'en': 'Tourlaville', 'fr': 'Tourlaville'},
'3355669':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3323320':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'3355915':{'en': 'Anglet', 'fr': 'Anglet'},
'3329809':{'en': 'Pont-Aven', 'fr': 'Pont-Aven'},
'3355664':{'en': u('L\u00e9ognan'), 'fr': u('L\u00e9ognan')},
'3355663':{'en': 'Langon', 'fr': 'Langon'},
'3323329':{'en': u('Alen\u00e7on'), 'fr': u('Alen\u00e7on')},
'3323328':{'en': u('Alen\u00e7on'), 'fr': u('Alen\u00e7on')},
'3349257':{'en': 'Veynes', 'fr': 'Veynes'},
'3349256':{'en': 'Gap', 'fr': 'Gap'},
'355861':{'en': u('Maliq, Kor\u00e7\u00eb')},
'355860':{'en': u('Trebinj\u00eb/Proptisht/Vel\u00e7an, Pogradec')},
'3349253':{'en': 'Gap', 'fr': 'Gap'},
'3349252':{'en': 'Gap', 'fr': 'Gap'},
'3349251':{'en': 'Gap', 'fr': 'Gap'},
'3349702':{'en': 'Cagnes-sur-Mer', 'fr': 'Cagnes-sur-Mer'},
'355869':{'en': u('\u00c7\u00ebrav\u00eb/Dardhas, Pogradec')},
'355868':{'en': u('Bu\u00e7imas/Udenisht, Pogradec')},
'3349708':{'en': 'Nice', 'fr': 'Nice'},
'3349259':{'en': 'Cannes', 'fr': 'Cannes'},
'3349258':{'en': 'Veynes', 'fr': 'Veynes'},
'432855':{'de': 'Waldenstein', 'en': 'Waldenstein'},
'441809':{'en': 'Tomdoun'},
'441808':{'en': 'Tomatin'},
'441805':{'en': 'Torrington'},
'441807':{'en': 'Ballindalloch'},
'441806':{'en': 'Shetland'},
'3597193':{'bg': u('\u0413\u043e\u043b\u0435\u0448, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Golesh, Sofia'},
'441803':{'en': 'Torquay'},
'3594330':{'bg': u('\u041d\u0438\u043a\u043e\u043b\u0430\u0435\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Nikolaevo, St. Zagora'},
'3594331':{'bg': u('\u0413\u0443\u0440\u043a\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Gurkovo, St. Zagora'},
'3594332':{'bg': u('\u0412\u0435\u0442\u0440\u0435\u043d, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Vetren, St. Zagora'},
'3594333':{'bg': u('\u0414\u044a\u0431\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Dabovo, St. Zagora'},
'3317702':{'en': 'Argenteuil', 'fr': 'Argenteuil'},
'3594335':{'bg': u('\u0420\u044a\u0436\u0435\u043d\u0430'), 'en': 'Razhena'},
'3594336':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u0418\u0437\u0432\u043e\u0440\u043e\u0432\u043e'), 'en': 'Dolno Izvorovo'},
'3594337':{'bg': u('\u042f\u0441\u0435\u043d\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Yasenovo, St. Zagora'},
'3594338':{'bg': u('\u041a\u0440\u044a\u043d'), 'en': 'Kran'},
'3594339':{'bg': u('\u042e\u043b\u0438\u0435\u0432\u043e'), 'en': 'Yulievo'},
'3598448':{'bg': u('\u0422\u0435\u0440\u0442\u0435\u0440'), 'en': 'Terter'},
'3598442':{'bg': u('\u0417\u0430\u0432\u0435\u0442, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Zavet, Razgrad'},
'35981262':{'bg': u('\u0411\u0438\u0441\u0442\u0440\u0435\u043d\u0446\u0438'), 'en': 'Bistrentsi'},
'3598445':{'bg': u('\u042e\u043f\u0435\u0440'), 'en': 'Yuper'},
'3322325':{'en': u('Saint-Gr\u00e9goire'), 'fr': u('Saint-Gr\u00e9goire')},
'437353':{'de': 'Gaflenz', 'en': 'Gaflenz'},
'3322320':{'en': 'Rennes', 'fr': 'Rennes'},
'3322321':{'en': 'Rennes', 'fr': 'Rennes'},
'3322322':{'en': 'Montreuil-sur-Ille', 'fr': 'Montreuil-sur-Ille'},
'437357':{'de': 'Kleinreifling', 'en': 'Kleinreifling'},
'35930458':{'bg': u('\u041b\u044e\u0431\u0447\u0430'), 'en': 'Lyubcha'},
'3332816':{'en': 'Seclin', 'fr': 'Seclin'},
'3332814':{'en': 'Lille', 'fr': 'Lille'},
'359720':{'bg': u('\u0415\u0442\u0440\u043e\u043f\u043e\u043b\u0435'), 'en': 'Etropole'},
'3338349':{'en': 'Pompey', 'fr': 'Pompey'},
'3338348':{'en': 'Dombasle-sur-Meurthe', 'fr': 'Dombasle-sur-Meurthe'},
'359721':{'bg': u('\u041a\u043e\u0441\u0442\u0438\u043d\u0431\u0440\u043e\u0434'), 'en': 'Kostinbrod'},
'3338345':{'en': 'Dombasle-sur-Meurthe', 'fr': 'Dombasle-sur-Meurthe'},
'3338344':{'en': u('Vand\u0153uvre-l\u00e8s-Nancy'), 'fr': u('Vand\u0153uvre-l\u00e8s-Nancy')},
'3338347':{'en': 'Neuves-Maisons', 'fr': 'Neuves-Maisons'},
'3338341':{'en': 'Nancy', 'fr': 'Nancy'},
'3338340':{'en': 'Nancy', 'fr': 'Nancy'},
'3338343':{'en': 'Toul', 'fr': 'Toul'},
'359723':{'bg': u('\u0411\u043e\u0442\u0435\u0432\u0433\u0440\u0430\u0434'), 'en': 'Botevgrad'},
'359724':{'bg': u('\u0418\u0445\u0442\u0438\u043c\u0430\u043d'), 'en': 'Ihtiman'},
'434224':{'de': 'Pischeldorf', 'en': 'Pischeldorf'},
'359727':{'bg': u('\u0421\u043b\u0438\u0432\u043d\u0438\u0446\u0430, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Slivnitsa, Sofia'},
'3595319':{'bg': u('\u0414\u0440\u0443\u043c\u0435\u0432\u043e'), 'en': 'Drumevo'},
'3595318':{'bg': u('\u0421\u0440\u0435\u0434\u043d\u044f'), 'en': 'Srednya'},
'3595315':{'bg': u('\u0426\u0430\u0440\u0435\u0432 \u0431\u0440\u043e\u0434'), 'en': 'Tsarev brod'},
'3595314':{'bg': u('\u0411\u0435\u043b\u043e\u043a\u043e\u043f\u0438\u0442\u043e\u0432\u043e'), 'en': 'Belokopitovo'},
'3595317':{'bg': u('\u0418\u0432\u0430\u043d\u0441\u043a\u0438'), 'en': 'Ivanski'},
'3595316':{'bg': u('\u0421\u0430\u043b\u043c\u0430\u043d\u043e\u0432\u043e'), 'en': 'Salmanovo'},
'3595311':{'bg': u('\u0413\u0440\u0430\u0434\u0438\u0449\u0435, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Gradishte, Shumen'},
'3595310':{'bg': u('\u0420\u0430\u0434\u043a\u043e \u0414\u0438\u043c\u0438\u0442\u0440\u0438\u0435\u0432\u043e'), 'en': 'Radko Dimitrievo'},
'3595313':{'bg': u('\u041c\u0430\u0434\u0430\u0440\u0430'), 'en': 'Madara'},
'3595312':{'bg': u('\u0414\u0438\u0431\u0438\u0447'), 'en': 'Dibich'},
'432146':{'de': 'Nickelsdorf', 'en': 'Nickelsdorf'},
'433329':{'de': 'Jennersdorf', 'en': 'Jennersdorf'},
'3356580':{'en': 'Montbazens', 'fr': 'Montbazens'},
'3599319':{'bg': u('\u0413\u044a\u043c\u0437\u043e\u0432\u043e'), 'en': 'Gamzovo'},
'3599318':{'bg': u('\u0411\u0443\u043a\u043e\u0432\u0435\u0446, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Bukovets, Vidin'},
'432145':{'de': 'Prellenkirchen', 'en': 'Prellenkirchen'},
'3599311':{'bg': u('\u041a\u0443\u0442\u043e\u0432\u043e'), 'en': 'Kutovo'},
'432142':{'de': 'Gattendorf', 'en': 'Gattendorf'},
'3599313':{'bg': u('\u041a\u0430\u043f\u0438\u0442\u0430\u043d\u043e\u0432\u0446\u0438'), 'en': 'Kapitanovtsi'},
'3599312':{'bg': u('\u0411\u0440\u0435\u0433\u043e\u0432\u043e, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Bregovo, Vidin'},
'3599315':{'bg': u('\u0413\u0440\u0430\u0434\u0435\u0446, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Gradets, Vidin'},
'3599314':{'bg': u('\u0414\u0443\u043d\u0430\u0432\u0446\u0438, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Dunavtsi, Vidin'},
'3599317':{'bg': u('\u0410\u0440\u0447\u0430\u0440'), 'en': 'Archar'},
'3332364':{'en': 'Saint-Quentin', 'fr': 'Saint-Quentin'},
'3347570':{'en': u('Romans-sur-Is\u00e8re'), 'fr': u('Romans-sur-Is\u00e8re')},
'3347571':{'en': u('Romans-sur-Is\u00e8re'), 'fr': u('Romans-sur-Is\u00e8re')},
'3347572':{'en': u('Romans-sur-Is\u00e8re'), 'fr': u('Romans-sur-Is\u00e8re')},
'3347575':{'en': 'Valence', 'fr': 'Valence'},
'3347576':{'en': 'Crest', 'fr': 'Crest'},
'433858':{'de': u('Mitterdorf im M\u00fcrztal'), 'en': u('Mitterdorf im M\u00fcrztal')},
'3347578':{'en': 'Valence', 'fr': 'Valence'},
'3347579':{'en': 'Valence', 'fr': 'Valence'},
'433855':{'de': 'Krieglach', 'en': 'Krieglach'},
'433854':{'de': 'Langenwang', 'en': 'Langenwang'},
'433853':{'de': 'Spital am Semmering', 'en': 'Spital am Semmering'},
'433852':{'de': u('M\u00fcrzzuschlag'), 'en': u('M\u00fcrzzuschlag')},
'435524':{'de': 'Satteins', 'en': 'Satteins'},
'3334415':{'en': 'Beauvais', 'fr': 'Beauvais'},
'3334414':{'en': 'Beauvais', 'fr': 'Beauvais'},
'3334411':{'en': 'Beauvais', 'fr': 'Beauvais'},
'3334410':{'en': 'Beauvais', 'fr': 'Beauvais'},
'331565':{'en': 'Paris', 'fr': 'Paris'},
'35971398':{'bg': u('\u041e\u0441\u0438\u043a\u043e\u0432\u0438\u0446\u0430'), 'en': 'Osikovitsa'},
'35981268':{'bg': u('\u041a\u0440\u0438\u0432\u0438\u043d\u0430, \u0420\u0443\u0441\u0435'), 'en': 'Krivina, Ruse'},
'3599175':{'bg': u('\u041e\u0441\u0442\u0440\u043e\u0432'), 'en': 'Ostrov'},
'3599174':{'bg': u('\u0413\u043e\u0440\u043d\u0438 \u0412\u0430\u0434\u0438\u043d'), 'en': 'Gorni Vadin'},
'3599176':{'bg': u('\u041b\u0435\u0441\u043a\u043e\u0432\u0435\u0446, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Leskovets, Vratsa'},
'3599171':{'bg': u('\u041e\u0440\u044f\u0445\u043e\u0432\u043e, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Oryahovo, Vratsa'},
'3599173':{'bg': u('\u0413\u0430\u043b\u043e\u0432\u043e'), 'en': 'Galovo'},
'3599172':{'bg': u('\u0421\u0435\u043b\u0430\u043d\u043e\u0432\u0446\u0438'), 'en': 'Selanovtsi'},
'433583':{'de': 'Unzmarkt', 'en': 'Unzmarkt'},
'3353154':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3353401':{'en': 'Pamiers', 'fr': 'Pamiers'},
'35984778':{'bg': u('\u0411\u043e\u0433\u0434\u0430\u043d\u0446\u0438, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Bogdantsi, Razgrad'},
'3353409':{'en': 'Foix', 'fr': 'Foix'},
'3594593':{'bg': u('\u0428\u0438\u0432\u0430\u0447\u0435\u0432\u043e'), 'en': 'Shivachevo'},
'435248':{'de': 'Steinberg am Rofan', 'en': 'Steinberg am Rofan'},
'3594597':{'bg': u('\u0411\u043e\u0440\u043e\u0432 \u0434\u043e\u043b'), 'en': 'Borov dol'},
'3347394':{'en': 'Puy-Guillaume', 'fr': 'Puy-Guillaume'},
'3347390':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347391':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347392':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347393':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347398':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3329701':{'en': 'Vannes', 'fr': 'Vannes'},
'3346629':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346628':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346627':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346626':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346625':{'en': u('Bess\u00e8ges'), 'fr': u('Bess\u00e8ges')},
'3346624':{'en': 'Saint-Ambroix', 'fr': 'Saint-Ambroix'},
'3346623':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346622':{'en': u('Uz\u00e8s'), 'fr': u('Uz\u00e8s')},
'3346621':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346620':{'en': 'Bouillargues', 'fr': 'Bouillargues'},
'3597755':{'bg': u('\u0412\u0435\u043b\u043a\u043e\u0432\u0446\u0438, \u041f\u0435\u0440\u043d\u0438\u043a'), 'en': 'Velkovtsi, Pernik'},
'3597754':{'bg': u('\u041a\u043e\u0448\u0430\u0440\u0435\u0432\u043e'), 'en': 'Kosharevo'},
'3597753':{'bg': u('\u041d\u043e\u0435\u0432\u0446\u0438'), 'en': 'Noevtsi'},
'3597752':{'bg': u('\u0420\u0435\u0436\u0430\u043d\u0446\u0438'), 'en': 'Rezhantsi'},
'3597751':{'bg': u('\u0411\u0440\u0435\u0437\u043d\u0438\u043a'), 'en': 'Breznik'},
'433326':{'de': 'Stegersbach', 'en': 'Stegersbach'},
'3522467':{'de': u('D\u00fcdelingen'), 'en': 'Dudelange', 'fr': 'Dudelange'},
'3316086':{'en': 'Bondoufle', 'fr': 'Bondoufle'},
'3316087':{'en': u('\u00c9vry'), 'fr': u('\u00c9vry')},
'3316084':{'en': u('Br\u00e9tigny-sur-Orge'), 'fr': u('Br\u00e9tigny-sur-Orge')},
'3316085':{'en': u('Br\u00e9tigny-sur-Orge'), 'fr': u('Br\u00e9tigny-sur-Orge')},
'3316082':{'en': 'Lardy', 'fr': 'Lardy'},
'3316083':{'en': 'Arpajon', 'fr': 'Arpajon'},
'3316080':{'en': u('\u00c9tr\u00e9chy'), 'fr': u('\u00c9tr\u00e9chy')},
'3316081':{'en': 'Dourdan', 'fr': 'Dourdan'},
'3316088':{'en': 'Corbeil-Essonnes', 'fr': 'Corbeil-Essonnes'},
'3316089':{'en': 'Corbeil-Essonnes', 'fr': 'Corbeil-Essonnes'},
'3522645':{'de': 'Diedrich', 'en': 'Diedrich', 'fr': 'Diedrich'},
'3522647':{'de': 'Lintgen', 'en': 'Lintgen', 'fr': 'Lintgen'},
'3522640':{'de': 'Howald', 'en': 'Howald', 'fr': 'Howald'},
'3522643':{'de': 'Findel/Kirchberg', 'en': 'Findel/Kirchberg', 'fr': 'Findel/Kirchberg'},
'3522642':{'de': 'Plateau de Kirchberg', 'en': 'Plateau de Kirchberg', 'fr': 'Plateau de Kirchberg'},
'3522649':{'de': 'Howald', 'en': 'Howald', 'fr': 'Howald'},
'3522648':{'de': 'Contern/Foetz', 'en': 'Contern/Foetz', 'fr': 'Contern/Foetz'},
'3742377':{'am': u('\u0544\u0580\u0563\u0561\u0577\u0561\u057f'), 'en': 'Mrgashat', 'ru': u('\u041c\u0440\u0433\u0430\u0448\u0430\u0442')},
'37517':{'be': u('\u041c\u0456\u043d\u0441\u043a'), 'en': 'Minsk', 'ru': u('\u041c\u0438\u043d\u0441\u043a')},
'3742379':{'am': u('\u0546\u0561\u056c\u0562\u0561\u0576\u0564\u0575\u0561\u0576'), 'en': 'Nalbandian', 'ru': u('\u041d\u0430\u043b\u0431\u0430\u043d\u0434\u044f\u043d')},
'3323849':{'en': 'Olivet', 'fr': 'Olivet'},
'3323844':{'en': 'Beaugency', 'fr': 'Beaugency'},
'3323845':{'en': u('Cl\u00e9ry-Saint-Andr\u00e9'), 'fr': u('Cl\u00e9ry-Saint-Andr\u00e9')},
'3323842':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323843':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323841':{'en': 'Sandillon', 'fr': 'Sandillon'},
'4415391':{'en': 'Kendal'},
'4415390':{'en': 'Kendal'},
'4415393':{'en': 'Kendal'},
'44118':{'en': 'Reading'},
'4415395':{'en': 'Grange-over-Sands'},
'4415394':{'en': 'Hawkshead'},
'4415397':{'en': 'Kendal'},
'4415396':{'en': 'Sedbergh'},
'44113':{'en': 'Leeds'},
'4415398':{'en': 'Kendal'},
'44117':{'en': 'Bristol'},
'44116':{'en': 'Leicester'},
'44115':{'en': 'Nottingham'},
'44114':{'en': 'Sheffield'},
'3355914':{'en': 'Pau', 'fr': 'Pau'},
'3348665':{'en': 'Avignon', 'fr': 'Avignon'},
'3346849':{'en': 'Gruissan', 'fr': 'Gruissan'},
'3346848':{'en': 'Sigean', 'fr': 'Sigean'},
'3346842':{'en': 'Narbonne', 'fr': 'Narbonne'},
'3346841':{'en': 'Narbonne', 'fr': 'Narbonne'},
'3346840':{'en': 'Leucate', 'fr': 'Leucate'},
'3346847':{'en': 'Carcassonne', 'fr': 'Carcassonne'},
'441726':{'en': 'St Austell'},
'3346844':{'en': 'Saint-Laurent-de-la-Cabrerisse', 'fr': 'Saint-Laurent-de-la-Cabrerisse'},
'3349396':{'en': 'Nice', 'fr': 'Nice'},
'3349397':{'en': 'Nice', 'fr': 'Nice'},
'3349394':{'en': 'Cannes', 'fr': 'Cannes'},
'3349395':{'en': 'Antibes Juan les Pins', 'fr': 'Antibes Juan les Pins'},
'3349392':{'en': 'Nice', 'fr': 'Nice'},
'3349393':{'en': 'Mandelieu-la-Napoule', 'fr': 'Mandelieu-la-Napoule'},
'3349390':{'en': 'Cannes', 'fr': 'Cannes'},
'3349398':{'en': 'Nice', 'fr': 'Nice'},
'3349399':{'en': 'Cannes', 'fr': 'Cannes'},
'3348914':{'en': 'Nice', 'fr': 'Nice'},
'433148':{'de': 'Kainach bei Voitsberg', 'en': 'Kainach bei Voitsberg'},
'3596988':{'bg': u('\u041f\u0435\u0449\u0435\u0440\u043d\u0430'), 'en': 'Peshterna'},
'3593128':{'bg': u('\u0417\u043b\u0430\u0442\u0438\u0442\u0440\u0430\u043f'), 'en': 'Zlatitrap'},
'3593129':{'bg': u('\u0421\u043a\u0443\u0442\u0430\u0440\u0435'), 'en': 'Skutare'},
'3332662':{'en': u('Vitry-le-Fran\u00e7ois'), 'fr': u('Vitry-le-Fran\u00e7ois')},
'3332664':{'en': u('Ch\u00e2lons-en-Champagne'), 'fr': u('Ch\u00e2lons-en-Champagne')},
'3332665':{'en': u('Ch\u00e2lons-en-Champagne'), 'fr': u('Ch\u00e2lons-en-Champagne')},
'3332668':{'en': u('Ch\u00e2lons-en-Champagne'), 'fr': u('Ch\u00e2lons-en-Champagne')},
'3593121':{'bg': u('\u0420\u043e\u0433\u043e\u0448'), 'en': 'Rogosh'},
'3593122':{'bg': u('\u041c\u0430\u043d\u043e\u043b\u0435'), 'en': 'Manole'},
'3593123':{'bg': u('\u041a\u0430\u043b\u043e\u044f\u043d\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Kaloyanovo, Plovdiv'},
'3593124':{'bg': u('\u041a\u0430\u043b\u0435\u043a\u043e\u0432\u0435\u0446'), 'en': 'Kalekovets'},
'3593125':{'bg': u('\u0420\u044a\u0436\u0435\u0432\u043e \u041a\u043e\u043d\u0430\u0440\u0435'), 'en': 'Razhevo Konare'},
'3593126':{'bg': u('\u0422\u0440\u0443\u0434'), 'en': 'Trud'},
'3593127':{'bg': u('\u0426\u0430\u0440\u0430\u0446\u043e\u0432\u043e'), 'en': 'Tsaratsovo'},
'435243':{'de': 'Maurach', 'en': 'Maurach'},
'3346766':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3316432':{'en': 'Montereau-Fault-Yonne', 'fr': 'Montereau-Fault-Yonne'},
'3355588':{'en': 'Brive-la-Gaillarde', 'fr': 'Brive-la-Gaillarde'},
'3316430':{'en': 'Lagny-sur-Marne', 'fr': 'Lagny-sur-Marne'},
'3316437':{'en': 'Melun', 'fr': 'Melun'},
'435244':{'de': 'Jenbach', 'en': 'Jenbach'},
'3346760':{'en': 'Montpellier', 'fr': 'Montpellier'},
'390131':{'en': 'Alessandria', 'it': 'Alessandria'},
'3346761':{'en': 'Montpellier', 'fr': 'Montpellier'},
'35959409':{'bg': u('\u0427\u0435\u0440\u0435\u0448\u0430'), 'en': 'Cheresha'},
'35959408':{'bg': u('\u0420\u044a\u0436\u0438\u0446\u0430'), 'en': 'Razhitsa'},
'432667':{'de': 'Schwarzau im Gebirge', 'en': 'Schwarzau im Gebirge'},
'432666':{'de': 'Reichenau', 'en': 'Reichenau'},
'432665':{'de': 'Prein an der Rax', 'en': 'Prein an der Rax'},
'432664':{'de': 'Semmering', 'en': 'Semmering'},
'35959400':{'bg': u('\u0414\u044a\u0441\u043a\u043e\u0442\u043d\u0430'), 'en': 'Daskotna'},
'35959403':{'bg': u('\u0420\u0435\u0447\u0438\u0446\u0430'), 'en': 'Rechitsa'},
'35959405':{'bg': u('\u0417\u0430\u0439\u0447\u0430\u0440'), 'en': 'Zaychar'},
'35959404':{'bg': u('\u042f\u0441\u0435\u043d\u043e\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Yasenovo, Burgas'},
'35959407':{'bg': u('\u0421\u0438\u043d\u0438 \u0440\u0438\u0434'), 'en': 'Sini rid'},
'35959406':{'bg': u('\u0420\u0430\u0437\u0431\u043e\u0439\u043d\u0430, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Razboyna, Burgas'},
'433132':{'de': 'Kumberg', 'en': 'Kumberg'},
'433133':{'de': 'Nestelbach', 'en': 'Nestelbach'},
'433134':{'de': 'Heiligenkreuz am Waasen', 'en': 'Heiligenkreuz am Waasen'},
'433135':{'de': 'Kalsdorf bei Graz', 'en': 'Kalsdorf bei Graz'},
'433136':{'de': 'Dobl', 'en': 'Dobl'},
'390735':{'en': 'Ascoli Piceno', 'it': 'San Benedetto del Tronto'},
'390737':{'en': 'Macerata', 'it': 'Camerino'},
'435246':{'de': 'Achenkirch', 'en': 'Achenkirch'},
'390731':{'en': 'Ancona', 'it': 'Jesi'},
'35974386':{'bg': u('\u041f\u0438\u0440\u0438\u043d'), 'en': 'Pirin'},
'390345':{'it': 'San Pellegrino Terme'},
'390733':{'en': 'Macerata', 'it': 'Macerata'},
'35974388':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u0421\u043f\u0430\u043d\u0447\u0435\u0432\u043e'), 'en': 'Gorno Spanchevo'},
'3595782':{'bg': u('\u0411\u0435\u043d\u043a\u043e\u0432\u0441\u043a\u0438, \u0414\u043e\u0431\u0440.'), 'en': 'Benkovski, Dobr.'},
'3347148':{'en': 'Aurillac', 'fr': 'Aurillac'},
'3347498':{'en': 'Villars-les-Dombes', 'fr': 'Villars-les-Dombes'},
'3347493':{'en': 'Bourgoin-Jallieu', 'fr': 'Bourgoin-Jallieu'},
'3347143':{'en': 'Aurillac', 'fr': 'Aurillac'},
'3347490':{'en': u('Cr\u00e9mieu'), 'fr': u('Cr\u00e9mieu')},
'3347497':{'en': 'La Tour du Pin', 'fr': 'La Tour du Pin'},
'3347496':{'en': 'Villefontaine', 'fr': 'Villefontaine'},
'3347495':{'en': 'Saint-Quentin-Fallavier', 'fr': 'Saint-Quentin-Fallavier'},
'3347494':{'en': 'Saint-Quentin-Fallavier', 'fr': 'Saint-Quentin-Fallavier'},
'3598161':{'bg': u('\u0412\u0435\u0442\u043e\u0432\u043e'), 'en': 'Vetovo'},
'3598163':{'bg': u('\u0411\u044a\u0437\u044a\u043d'), 'en': 'Bazan'},
'3598165':{'bg': u('\u0421\u043c\u0438\u0440\u043d\u0435\u043d\u0441\u043a\u0438, \u0420\u0443\u0441\u0435'), 'en': 'Smirnenski, Ruse'},
'3598164':{'bg': u('\u041f\u0438\u0441\u0430\u043d\u0435\u0446'), 'en': 'Pisanets'},
'3598167':{'bg': u('\u0426\u0435\u0440\u043e\u0432\u0435\u0446'), 'en': 'Tserovets'},
'3598166':{'bg': u('\u0421\u0432\u0430\u043b\u0435\u043d\u0438\u043a'), 'en': 'Svalenik'},
'3338288':{'en': 'Thionville', 'fr': 'Thionville'},
'3338289':{'en': 'Villerupt', 'fr': 'Villerupt'},
'4416971':{'en': 'Brampton'},
'3338282':{'en': 'Thionville', 'fr': 'Thionville'},
'3338284':{'en': 'Hayange', 'fr': 'Hayange'},
'3338285':{'en': 'Hayange', 'fr': 'Hayange'},
'3338286':{'en': 'Uckange', 'fr': 'Uckange'},
'4416973':{'en': 'Wigton'},
'3599782':{'bg': u('\u0411\u0443\u043a\u043e\u0432\u0435\u0446, \u041c\u043e\u043d\u0442.'), 'en': 'Bukovets, Mont.'},
'3599783':{'bg': u('\u0411\u0440\u0443\u0441\u0430\u0440\u0446\u0438'), 'en': 'Brusartsi'},
'3599787':{'bg': u('\u0421\u043c\u0438\u0440\u043d\u0435\u043d\u0441\u043a\u0438, \u041c\u043e\u043d\u0442.'), 'en': 'Smirnenski, Mont.'},
'3599784':{'bg': u('\u041a\u0438\u0441\u0435\u043b\u0435\u0432\u043e'), 'en': 'Kiselevo'},
'3599785':{'bg': u('\u0412\u0430\u0441\u0438\u043b\u043e\u0432\u0446\u0438, \u041c\u043e\u043d\u0442.'), 'en': 'Vasilovtsi, Mont.'},
'4416976':{'en': 'Brampton'},
'4416977':{'en': 'Brampton'},
'3338132':{'en': u('Montb\u00e9liard'), 'fr': u('Montb\u00e9liard')},
'3338130':{'en': 'Audincourt', 'fr': 'Audincourt'},
'3338131':{'en': u('Montb\u00e9liard'), 'fr': u('Montb\u00e9liard')},
'3338136':{'en': 'Audincourt', 'fr': 'Audincourt'},
'3338137':{'en': 'Valentigney', 'fr': 'Valentigney'},
'3338134':{'en': 'Audincourt', 'fr': 'Audincourt'},
'3338135':{'en': 'Audincourt', 'fr': 'Audincourt'},
'435477':{'de': u('T\u00f6sens'), 'en': u('T\u00f6sens')},
'435476':{'de': 'Serfaus', 'en': 'Serfaus'},
'3338138':{'en': 'Pontarlier', 'fr': 'Pontarlier'},
'3338139':{'en': 'Pontarlier', 'fr': 'Pontarlier'},
'435473':{'de': 'Nauders', 'en': 'Nauders'},
'435472':{'de': 'Prutz', 'en': 'Prutz'},
'390771':{'it': 'Formia'},
'433587':{'de': u('Sch\u00f6nberg-Lachtal'), 'en': u('Sch\u00f6nberg-Lachtal')},
'4162':{'de': 'Olten', 'en': 'Olten', 'fr': 'Olten', 'it': 'Olten'},
'4161':{'de': 'Basel', 'en': 'Basel', 'fr': u('B\u00e2le'), 'it': 'Basilea'},
'3349376':{'en': 'Saint-Jean-Cap-Ferrat', 'fr': 'Saint-Jean-Cap-Ferrat'},
'3522628':{'de': 'Luxemburg', 'en': 'Luxembourg City', 'fr': 'Luxembourg-Ville'},
'4416970':{'en': 'Brampton'},
'35951125':{'bg': u('\u041a\u043e\u043d\u0441\u0442\u0430\u043d\u0442\u0438\u043d\u043e\u0432\u043e, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Konstantinovo, Varna'},
'390773':{'it': 'Latina'},
'3594111':{'bg': u('\u0421\u0442\u0430\u0440\u043e\u0437\u0430\u0433\u043e\u0440\u0441\u043a\u0438 \u0431\u0430\u043d\u0438'), 'en': 'Starozagorski bani'},
'3347725':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347727':{'en': 'Feurs', 'fr': 'Feurs'},
'3347726':{'en': 'Feurs', 'fr': 'Feurs'},
'3347721':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3343445':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3347723':{'en': 'Roanne', 'fr': 'Roanne'},
'3347722':{'en': 'Saint-Chamond', 'fr': 'Saint-Chamond'},
'3597924':{'bg': u('\u0428\u0438\u0448\u043a\u043e\u0432\u0446\u0438'), 'en': 'Shishkovtsi'},
'3597433':{'bg': u('\u041a\u0440\u0435\u0441\u043d\u0430'), 'en': 'Kresna'},
'3597430':{'bg': u('\u0414\u0430\u043c\u044f\u043d\u0438\u0446\u0430'), 'en': 'Damyanitsa'},
'3593718':{'bg': u('\u0412\u043e\u0439\u0432\u043e\u0434\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Voyvodovo, Hask.'},
'3347729':{'en': 'Saint-Chamond', 'fr': 'Saint-Chamond'},
'3347728':{'en': u('Panissi\u00e8res'), 'fr': u('Panissi\u00e8res')},
'3336958':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3597435':{'bg': u('\u0421\u043a\u043b\u0430\u0432\u0435'), 'en': 'Sklave'},
'4416972':{'en': 'Brampton'},
'3493':{'en': 'Barcelona', 'es': 'Barcelona'},
'3491':{'en': 'Madrid', 'es': 'Madrid'},
'441688':{'en': 'Isle of Mull - Tobermory'},
'4414344':{'en': 'Bellingham'},
'390775':{'it': 'Frosinone'},
'390776':{'en': 'Frosinone', 'it': 'Cassino'},
'441681':{'en': 'Isle of Mull - Fionnphort'},
'4416974':{'en': 'Raughton Head'},
'441680':{'en': 'Isle of Mull - Craignure'},
'441687':{'en': 'Mallaig'},
'3349094':{'en': u('Ch\u00e2teaurenard'), 'fr': u('Ch\u00e2teaurenard')},
'3349097':{'en': 'Saintes-Maries-de-la-Mer', 'fr': 'Saintes-Maries-de-la-Mer'},
'3349096':{'en': 'Arles', 'fr': 'Arles'},
'3349091':{'en': 'Tarascon', 'fr': 'Tarascon'},
'3349090':{'en': u('Ch\u00e2teaurenard'), 'fr': u('Ch\u00e2teaurenard')},
'3349093':{'en': 'Arles', 'fr': 'Arles'},
'3349092':{'en': u('Saint-R\u00e9my-de-Provence'), 'fr': u('Saint-R\u00e9my-de-Provence')},
'441684':{'en': 'Malvern'},
'3349098':{'en': 'Arles', 'fr': 'Arles'},
'3598118':{'bg': u('\u041d\u0438\u043a\u043e\u043b\u043e\u0432\u043e, \u0420\u0443\u0441\u0435'), 'en': 'Nikolovo, Ruse'},
'3329772':{'en': u('Plo\u00ebrmel'), 'fr': u('Plo\u00ebrmel')},
'3329773':{'en': u('Plo\u00ebrmel'), 'fr': u('Plo\u00ebrmel')},
'3329770':{'en': 'Guer', 'fr': 'Guer'},
'3329776':{'en': 'Lanester', 'fr': 'Lanester'},
'3329774':{'en': u('Plo\u00ebrmel'), 'fr': u('Plo\u00ebrmel')},
'3355649':{'en': u('B\u00e8gles'), 'fr': u('B\u00e8gles')},
'3355648':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3329828':{'en': 'Le Relecq Kerhuon', 'fr': 'Le Relecq Kerhuon'},
'3329829':{'en': u('Saint-Pol-de-L\u00e9on'), 'fr': u('Saint-Pol-de-L\u00e9on')},
'3323305':{'en': u('Saint-L\u00f4'), 'fr': u('Saint-L\u00f4')},
'3323306':{'en': u('Saint-L\u00f4'), 'fr': u('Saint-L\u00f4')},
'3355641':{'en': u('Lesparre-M\u00e9doc'), 'fr': u('Lesparre-M\u00e9doc')},
'3355640':{'en': 'Cenon', 'fr': 'Cenon'},
'3355643':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355642':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355397':{'en': u('N\u00e9rac'), 'fr': u('N\u00e9rac')},
'3355396':{'en': 'Agen', 'fr': 'Agen'},
'3355647':{'en': 'Merignac', 'fr': u('M\u00e9rignac')},
'3355646':{'en': 'Pessac', 'fr': 'Pessac'},
'3349271':{'en': 'Manosque', 'fr': 'Manosque'},
'3349270':{'en': 'Manosque', 'fr': 'Manosque'},
'3349273':{'en': 'Banon', 'fr': 'Banon'},
'3349272':{'en': 'Manosque', 'fr': 'Manosque'},
'3349275':{'en': 'Forcalquier', 'fr': 'Forcalquier'},
'3349276':{'en': 'Reillanne', 'fr': 'Reillanne'},
'355885':{'en': u('Memaliaj, Tepelen\u00eb')},
'355884':{'en': u('Dropull i Posht\u00ebm/Dropull i Sip\u00ebrm, Gjirokast\u00ebr')},
'355887':{'en': u('Qesarat/Krah\u00ebs/Luftinje/Buz, Tepelen\u00eb')},
'355886':{'en': u('Qend\u00ebr/Kurvelesh/Lop\u00ebz, Tepelen\u00eb')},
'355881':{'en': u('Libohov\u00eb/Qend\u00ebr, Gjirokast\u00ebr')},
'435242':{'de': 'Schwaz', 'en': 'Schwaz'},
'355883':{'en': u('Lunxheri/Odrie/Zagorie/Pogon, Gjirokast\u00ebr')},
'355882':{'en': u('Cepo/Picar/Lazarat/Atigon, Gjirokast\u00ebr')},
'441827':{'en': 'Tamworth'},
'40253':{'en': 'Gorj', 'ro': 'Gorj'},
'441825':{'en': 'Uckfield'},
'3598113':{'bg': u('\u041d\u043e\u0432\u043e \u0441\u0435\u043b\u043e, \u0420\u0443\u0441\u0435'), 'en': 'Novo selo, Ruse'},
'441823':{'en': 'Taunton'},
'441822':{'en': 'Tavistock'},
'441821':{'en': 'Kinrossie'},
'3598114':{'bg': u('\u041f\u0438\u0440\u0433\u043e\u0432\u043e'), 'en': 'Pirgovo'},
'441829':{'en': 'Tarporley'},
'3598115':{'bg': u('\u0427\u0435\u0440\u0432\u0435\u043d\u0430 \u0432\u043e\u0434\u0430'), 'en': 'Chervena voda'},
'3598116':{'bg': u('\u0418\u0432\u0430\u043d\u043e\u0432\u043e, \u0420\u0443\u0441\u0435'), 'en': 'Ivanovo, Ruse'},
'432878':{'de': 'Traunstein', 'en': 'Traunstein'},
'432876':{'de': 'Els', 'en': 'Els'},
'432877':{'de': 'Grainbrunn', 'en': 'Grainbrunn'},
'432874':{'de': 'Martinsberg', 'en': 'Martinsberg'},
'432875':{'de': 'Grafenschlag', 'en': 'Grafenschlag'},
'432872':{'de': 'Ottenschlag', 'en': 'Ottenschlag'},
'432873':{'de': 'Kottes', 'en': 'Kottes'},
'37235':{'en': u('Narva/Sillam\u00e4e')},
'3596738':{'bg': u('\u0414\u043e\u0431\u0440\u043e\u043c\u0438\u0440\u043a\u0430'), 'en': 'Dobromirka'},
'37232':{'en': 'Rakvere'},
'37233':{'en': u('Kohtla-J\u00e4rve')},
'37238':{'en': 'Paide'},
'35941276':{'bg': u('\u041c\u043e\u0433\u0438\u043b\u0430, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Mogila, St. Zagora'},
'3332836':{'en': 'Lille', 'fr': 'Lille'},
'35941275':{'bg': u('\u041b\u044f\u0441\u043a\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Lyaskovo, St. Zagora'},
'3332833':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332340':{'en': 'Chauny', 'fr': 'Chauny'},
'35941274':{'bg': u('\u0421\u0430\u043c\u0443\u0438\u043b\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Samuilovo, St. Zagora'},
'3332838':{'en': 'Lille', 'fr': 'Lille'},
'3329857':{'en': 'Briec', 'fr': 'Briec'},
'3329856':{'en': 'Fouesnant', 'fr': 'Fouesnant'},
'3315721':{'en': 'Montrouge', 'fr': 'Montrouge'},
'3329855':{'en': 'Quimper', 'fr': 'Quimper'},
'390521':{'en': 'Parma', 'it': 'Parma'},
'3329854':{'en': 'Plogastel-Saint-Germain', 'fr': 'Plogastel-Saint-Germain'},
'3329853':{'en': 'Quimper', 'fr': 'Quimper'},
'3317581':{'en': 'Cergy', 'fr': 'Cergy'},
'3329852':{'en': 'Quimper', 'fr': 'Quimper'},
'3329851':{'en': 'Fouesnant', 'fr': 'Fouesnant'},
'40258':{'en': 'Alba', 'ro': 'Alba'},
'35941484':{'bg': u('\u0417\u0435\u043c\u043b\u0435\u043d'), 'en': 'Zemlen'},
'3329850':{'en': 'Concarneau', 'fr': 'Concarneau'},
'35941480':{'bg': u('\u041a\u043e\u043b\u0430\u0440\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Kolarovo, St. Zagora'},
'390523':{'en': 'Piacenza', 'it': 'Piacenza'},
'3332869':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'38129':{'en': 'Prizren', 'sr': 'Prizren'},
'38128':{'en': 'Kosovska Mitrovica', 'sr': 'Kosovska Mitrovica'},
'38121':{'en': 'Novi Sad', 'sr': 'Novi Sad'},
'38120':{'en': 'Novi Pazar', 'sr': 'Novi Pazar'},
'38123':{'en': 'Zrenjanin', 'sr': 'Zrenjanin'},
'38122':{'en': 'Sremska Mitrovica', 'sr': 'Sremska Mitrovica'},
'38125':{'en': 'Sombor', 'sr': 'Sombor'},
'38124':{'en': 'Subotica', 'sr': 'Subotica'},
'38127':{'en': 'Prokuplje', 'sr': 'Prokuplje'},
'38126':{'en': 'Smederevo', 'sr': 'Smederevo'},
'37447732':{'am': u('\u0532\u0565\u0580\u0571\u0578\u0580/\u0554\u0561\u0577\u0561\u0569\u0561\u0572\u056b \u0577\u0580\u057b\u0561\u0576'), 'en': 'Berdzor/Kashatagh', 'ru': u('\u0411\u0435\u0440\u0434\u0437\u043e\u0440/\u041a\u0430\u0448\u0430\u0442\u0430\u0445')},
'37423376':{'am': u('\u0534\u0561\u056c\u0561\u0580\u056b\u056f'), 'en': 'Dalarik', 'ru': u('\u0414\u0430\u043b\u0430\u0440\u0438\u043a')},
'37423375':{'am': u('\u0554\u0561\u0580\u0561\u056f\u0565\u0580\u057f'), 'en': 'Karakert', 'ru': u('\u041a\u0430\u0440\u0430\u043a\u0435\u0440\u0442')},
'37423374':{'am': u('\u0544\u0575\u0561\u057d\u0576\u056b\u056f\u0575\u0561\u0576'), 'en': 'Myasnikyan', 'ru': u('\u041c\u044f\u0441\u043d\u0438\u043a\u044f\u043d')},
'3347559':{'en': 'Chabeuil', 'fr': 'Chabeuil'},
'370380':{'en': u('\u0160al\u010dininkai')},
'3343870':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347550':{'en': u('Mont\u00e9limar'), 'fr': u('Mont\u00e9limar')},
'3347551':{'en': u('Mont\u00e9limar'), 'fr': u('Mont\u00e9limar')},
'3347556':{'en': 'Valence', 'fr': 'Valence'},
'3347557':{'en': u('Portes-l\u00e8s-Valence'), 'fr': u('Portes-l\u00e8s-Valence')},
'3347554':{'en': u('Bourg-Saint-And\u00e9ol'), 'fr': u('Bourg-Saint-And\u00e9ol')},
'3347555':{'en': 'Valence', 'fr': 'Valence'},
'3347940':{'en': 'Tignes', 'fr': 'Tignes'},
'359418':{'bg': u('\u0413\u044a\u043b\u044a\u0431\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Galabovo, St. Zagora'},
'370528':{'en': u('Elektr\u0117nai/Trakai')},
'359416':{'bg': u('\u0427\u0438\u0440\u043f\u0430\u043d'), 'en': 'Chirpan'},
'359417':{'bg': u('\u0420\u0430\u0434\u043d\u0435\u0432\u043e'), 'en': 'Radnevo'},
'3693':{'en': 'Nagykanizsa', 'hu': 'Nagykanizsa'},
'441599':{'en': 'Kyle'},
'441598':{'en': 'Lynton'},
'441597':{'en': 'Llandrindod Wells'},
'441595':{'en': 'Lerwick, Foula & Fair Isle'},
'35991888':{'bg': u('\u0412\u0435\u0441\u043b\u0435\u0446, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Veslets, Vratsa'},
'441593':{'en': 'Lybster'},
'441592':{'en': 'Kirkcaldy'},
'441591':{'en': 'Llanwrtyd Wells'},
'432728':{'de': 'Wienerbruck', 'en': 'Wienerbruck'},
'35957308':{'bg': u('\u0427\u0435\u0440\u043d\u043e\u043e\u043a\u043e\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Chernookovo, Dobr.'},
'437233':{'de': 'Feldkirchen an der Donau', 'en': 'Feldkirchen an der Donau'},
'40347':{'en': 'Teleorman', 'ro': 'Teleorman'},
'35957304':{'bg': u('\u0414\u044a\u0431\u043e\u0432\u0438\u043a'), 'en': 'Dabovik'},
'35957305':{'bg': u('\u0420\u043e\u0441\u0438\u0446\u0430, \u0414\u043e\u0431\u0440.'), 'en': 'Rositsa, Dobr.'},
'35957306':{'bg': u('\u0418\u0437\u0432\u043e\u0440\u043e\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Izvorovo, Dobr.'},
'35957307':{'bg': u('\u0416\u0438\u0442\u0435\u043d, \u0414\u043e\u0431\u0440.'), 'en': 'Zhiten, Dobr.'},
'3595737':{'bg': u('\u041b\u044e\u043b\u044f\u043a\u043e\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Lyulyakovo, Dobr.'},
'3595736':{'bg': u('\u0412\u0430\u0441\u0438\u043b\u0435\u0432\u043e'), 'en': 'Vasilevo'},
'3595735':{'bg': u('\u041a\u0440\u0430\u0441\u0435\u043d, \u0414\u043e\u0431\u0440.'), 'en': 'Krasen, Dobr.'},
'3595734':{'bg': u('\u041f\u0440\u0435\u0441\u0435\u043b\u0435\u043d\u0446\u0438'), 'en': 'Preselentsi'},
'3347808':{'en': 'Caluire-et-Cuire', 'fr': 'Caluire-et-Cuire'},
'3347809':{'en': 'Lyon', 'fr': 'Lyon'},
'3595731':{'bg': u('\u0413\u0435\u043d\u0435\u0440\u0430\u043b \u0422\u043e\u0448\u0435\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'General Toshevo, Dobr.'},
'4413886':{'en': 'Bishop Auckland'},
'3347804':{'en': 'Meyzieu', 'fr': 'Meyzieu'},
'3347805':{'en': 'Brignais', 'fr': 'Brignais'},
'3347806':{'en': 'Montluel', 'fr': 'Montluel'},
'3347807':{'en': 'Givors', 'fr': 'Givors'},
'3347800':{'en': 'Lyon', 'fr': 'Lyon'},
'3347801':{'en': 'Lyon', 'fr': 'Lyon'},
'3347802':{'en': 'Saint-Symphorien-d\'Ozon', 'fr': 'Saint-Symphorien-d\'Ozon'},
'3347803':{'en': 'Villeurbanne', 'fr': 'Villeurbanne'},
'373291':{'en': 'Ceadir Lunga', 'ro': u('Cead\u00eer Lunga'), 'ru': u('\u0427\u0430\u0434\u044b\u0440-\u041b\u0443\u043d\u0433\u0430')},
'3355933':{'en': 'Serres-Castet', 'fr': 'Serres-Castet'},
'35984462':{'bg': u('\u041e\u0441\u0442\u0440\u043e\u0432\u043e'), 'en': 'Ostrovo'},
'373294':{'en': 'Taraclia', 'ro': 'Taraclia', 'ru': u('\u0422\u0430\u0440\u0430\u043a\u043b\u0438\u044f')},
'437235':{'de': 'Gallneukirchen', 'en': 'Gallneukirchen'},
'373297':{'en': 'Basarabeasca', 'ro': 'Basarabeasca', 'ru': u('\u0411\u0430\u0441\u0430\u0440\u0430\u0431\u044f\u0441\u043a\u0430')},
'373298':{'en': 'Comrat', 'ro': 'Comrat', 'ru': u('\u041a\u043e\u043c\u0440\u0430\u0442')},
'373299':{'en': 'Cahul', 'ro': 'Cahul', 'ru': u('\u041a\u0430\u0433\u0443\u043b')},
'40341':{'en': u('Constan\u021ba'), 'ro': u('Constan\u021ba')},
'3596737':{'bg': u('\u041a\u0440\u0443\u0448\u0435\u0432\u043e, \u0413\u0430\u0431\u0440.'), 'en': 'Krushevo, Gabr.'},
'4413882':{'en': 'Stanhope (Eastgate)'},
'35984467':{'bg': u('\u0421\u0435\u0441\u043b\u0430\u0432'), 'en': 'Seslav'},
'3346601':{'en': 'Bellegarde', 'fr': 'Bellegarde'},
'3346603':{'en': u('Uz\u00e8s'), 'fr': u('Uz\u00e8s')},
'3346602':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346605':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346604':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'40233':{'en': u('Neam\u021b'), 'ro': u('Neam\u021b')},
'436219':{'de': 'Obertrum am See', 'en': 'Obertrum am See'},
'436216':{'de': 'Neumarkt am Wallersee', 'en': 'Neumarkt am Wallersee'},
'436217':{'de': 'Mattsee', 'en': 'Mattsee'},
'436214':{'de': 'Henndorf am Wallersee', 'en': 'Henndorf am Wallersee'},
'436215':{'de': u('Stra\u00dfwalchen'), 'en': 'Strasswalchen'},
'436212':{'de': 'Seekirchen am Wallersee', 'en': 'Seekirchen am Wallersee'},
'436213':{'de': 'Oberhofen am Irrsee', 'en': 'Oberhofen am Irrsee'},
'3355931':{'en': 'Anglet', 'fr': 'Anglet'},
'40230':{'en': 'Suceava', 'ro': 'Suceava'},
'3694':{'en': 'Szombathely', 'hu': 'Szombathely'},
'37426299':{'am': u('\u0535\u0580\u0561\u0576\u0578\u057d'), 'en': 'Eranos', 'ru': u('\u0415\u0440\u0430\u043d\u043e\u0441')},
'35931792':{'bg': u('\u0427\u0435\u0440\u043d\u0438\u0447\u0435\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Chernichevo, Plovdiv'},
'35931791':{'bg': u('\u0411\u0435\u0433\u043e\u0432\u043e'), 'en': 'Begovo'},
'390474':{'it': 'Brunico'},
'3338939':{'en': 'Cernay', 'fr': 'Cernay'},
'3338428':{'en': 'Belfort', 'fr': 'Belfort'},
'3338424':{'en': 'Lons-le-Saunier', 'fr': 'Lons-le-Saunier'},
'3338933':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3338930':{'en': 'Colmar', 'fr': 'Colmar'},
'3338931':{'en': 'Sausheim', 'fr': 'Sausheim'},
'3338936':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3338421':{'en': 'Belfort', 'fr': 'Belfort'},
'3338422':{'en': 'Belfort', 'fr': 'Belfort'},
'3338935':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3323828':{'en': 'Montargis', 'fr': 'Montargis'},
'3323821':{'en': 'Saint-Jean-de-Braye', 'fr': 'Saint-Jean-de-Braye'},
'3323822':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323824':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323825':{'en': 'Olivet', 'fr': 'Olivet'},
'3346865':{'en': 'Narbonne', 'fr': 'Narbonne'},
'3346864':{'en': 'Rivesaltes', 'fr': 'Rivesaltes'},
'3346867':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3346866':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3346861':{'en': 'Perpignan', 'fr': 'Perpignan'},
'4416863':{'en': 'Llanidloes'},
'3346863':{'en': 'Pia', 'fr': 'Pia'},
'3346862':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3354623':{'en': 'Saint-Palais-sur-Mer', 'fr': 'Saint-Palais-sur-Mer'},
'3346868':{'en': 'Perpignan', 'fr': 'Perpignan'},
'4416868':{'en': 'Newtown'},
'4416869':{'en': 'Newtown'},
'355293':{'en': u('Kastriot/Muhur/Selisht\u00eb, Dib\u00ebr')},
'355296':{'en': u('Fush\u00eb-Bulqiz\u00eb/Shupenz\u00eb/Zerqan, Bulqiz\u00eb')},
'355297':{'en': u('Gjorice/Ostren/Trebisht/Martanesh, Bulqiz\u00eb')},
'3752154':{'be': u('\u0428\u0430\u0440\u043a\u043e\u045e\u0448\u0447\u044b\u043d\u0430, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Sharkovshchina, Vitebsk Region', 'ru': u('\u0428\u0430\u0440\u043a\u043e\u0432\u0449\u0438\u043d\u0430, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752155':{'be': u('\u041f\u0430\u0441\u0442\u0430\u0432\u044b'), 'en': 'Postavy', 'ru': u('\u041f\u043e\u0441\u0442\u0430\u0432\u044b')},
'3752156':{'be': u('\u0413\u043b\u044b\u0431\u043e\u043a\u0430\u0435'), 'en': 'Glubokoye', 'ru': u('\u0413\u043b\u0443\u0431\u043e\u043a\u043e\u0435')},
'3752157':{'be': u('\u0414\u043e\u043a\u0448\u044b\u0446\u044b, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Dokshitsy, Vitebsk Region', 'ru': u('\u0414\u043e\u043a\u0448\u0438\u0446\u044b, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752151':{'be': u('\u0412\u0435\u0440\u0445\u043d\u044f\u0434\u0437\u0432\u0456\u043d\u0441\u043a, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Verhnedvinsk, Vitebsk Region', 'ru': u('\u0412\u0435\u0440\u0445\u043d\u0435\u0434\u0432\u0438\u043d\u0441\u043a, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752152':{'be': u('\u041c\u0456\u0451\u0440\u044b, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Miory, Vitebsk Region', 'ru': u('\u041c\u0438\u043e\u0440\u044b, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752153':{'be': u('\u0411\u0440\u0430\u0441\u043b\u0430\u045e'), 'en': 'Braslav', 'ru': u('\u0411\u0440\u0430\u0441\u043b\u0430\u0432')},
'3325651':{'en': 'Rennes', 'fr': 'Rennes'},
'3752158':{'be': u('\u0423\u0448\u0430\u0447\u044b, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Ushachi, Vitebsk Region', 'ru': u('\u0423\u0448\u0430\u0447\u0438, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3325654':{'en': 'Lorient', 'fr': 'Lorient'},
'3593102':{'bg': u('\u041a\u0430\u0440\u0430\u0434\u0436\u043e\u0432\u043e'), 'en': 'Karadzhovo'},
'3593103':{'bg': u('\u041c\u0438\u043b\u0435\u0432\u043e'), 'en': 'Milevo'},
'3593100':{'bg': u('\u0411\u0435\u043b\u0430\u0449\u0438\u0446\u0430'), 'en': 'Belashtitsa'},
'3593101':{'bg': u('\u0412\u043e\u0439\u0432\u043e\u0434\u0438\u043d\u043e\u0432\u043e'), 'en': 'Voyvodinovo'},
'3593106':{'bg': u('\u0421\u0442\u0440\u043e\u0435\u0432\u043e'), 'en': 'Stroevo'},
'3593107':{'bg': u('\u0413\u0440\u0430\u0444 \u0418\u0433\u043d\u0430\u0442\u0438\u0435\u0432\u043e'), 'en': 'Graf Ignatievo'},
'34848':{'en': 'Navarre', 'es': 'Navarra'},
'3593105':{'bg': u('\u041c\u0430\u043d\u043e\u043b\u0441\u043a\u043e \u041a\u043e\u043d\u0430\u0440\u0435'), 'en': 'Manolsko Konare'},
'34847':{'en': 'Burgos', 'es': 'Burgos'},
'3593108':{'bg': u('\u0411\u043e\u0439\u043a\u043e\u0432\u043e'), 'en': 'Boykovo'},
'34845':{'en': 'Araba', 'es': u('\u00c1lava')},
'34842':{'en': 'Cantabria', 'es': 'Cantabria'},
'34843':{'en': u('Guip\u00fazcoa'), 'es': u('Guip\u00fazcoa')},
'34841':{'en': 'La Rioja', 'es': 'La Rioja'},
'3332648':{'en': 'Fismes', 'fr': 'Fismes'},
'3332138':{'en': 'Saint-Omer', 'fr': 'Saint-Omer'},
'3332134':{'en': 'Calais', 'fr': 'Calais'},
'3332136':{'en': 'Calais', 'fr': 'Calais'},
'3332137':{'en': 'Carvin', 'fr': 'Carvin'},
'3332130':{'en': 'Boulogne-sur-Mer', 'fr': 'Boulogne-sur-Mer'},
'3332131':{'en': 'Boulogne-sur-Mer', 'fr': 'Boulogne-sur-Mer'},
'3325435':{'en': 'Levroux', 'fr': 'Levroux'},
'3325434':{'en': u('Ch\u00e2teauroux'), 'fr': u('Ch\u00e2teauroux')},
'3325437':{'en': 'Le Blanc', 'fr': 'Le Blanc'},
'3325436':{'en': 'Ardentes', 'fr': 'Ardentes'},
'3325431':{'en': 'Cluis', 'fr': 'Cluis'},
'433338':{'de': 'Lafnitz', 'en': 'Lafnitz'},
'3325432':{'en': 'Montrichard', 'fr': 'Montrichard'},
'433336':{'de': 'Waldbach', 'en': 'Waldbach'},
'433337':{'de': 'Vorau', 'en': 'Vorau'},
'433334':{'de': 'Kaindorf', 'en': 'Kaindorf'},
'433335':{'de': u('P\u00f6llau'), 'en': u('P\u00f6llau')},
'433332':{'de': 'Hartberg', 'en': 'Hartberg'},
'3325438':{'en': u('Ch\u00e2tillon-sur-Indre'), 'fr': u('Ch\u00e2tillon-sur-Indre')},
'433331':{'de': 'Sankt Lorenzen am Wechsel', 'en': 'St. Lorenzen am Wechsel'},
'35941019':{'bg': u('\u0412\u0435\u043d\u0435\u0446, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Venets, St. Zagora'},
'35941018':{'bg': u('\u041a\u043d\u044f\u0436\u0435\u0432\u0441\u043a\u043e'), 'en': 'Knyazhevsko'},
'3349510':{'en': 'Ajaccio', 'fr': 'Ajaccio'},
'35960458':{'bg': u('\u0412\u0435\u0440\u0435\u043d\u0446\u0438'), 'en': 'Verentsi'},
'35960454':{'bg': u('\u0417\u043c\u0435\u0439\u043d\u043e'), 'en': 'Zmeyno'},
'35960453':{'bg': u('\u041c\u043e\u0440\u0430\u0432\u043a\u0430'), 'en': 'Moravka'},
'35960450':{'bg': u('\u0414\u043e\u043b\u043d\u0430 \u0425\u0443\u0431\u0430\u0432\u043a\u0430'), 'en': 'Dolna Hubavka'},
'35960451':{'bg': u('\u041e\u0431\u0438\u0442\u0435\u043b'), 'en': 'Obitel'},
'432689':{'de': 'Hornstein', 'en': 'Hornstein'},
'432688':{'de': 'Steinbrunn', 'en': 'Steinbrunn'},
'432685':{'de': 'Rust', 'en': 'Rust'},
'432684':{'de': u('Sch\u00fctzen am Gebirge'), 'en': u('Sch\u00fctzen am Gebirge')},
'432687':{'de': 'Siegendorf', 'en': 'Siegendorf'},
'432686':{'de': u('Dra\u00dfburg'), 'en': 'Drassburg'},
'432680':{'de': 'Sankt Margarethen im Burgenland', 'en': 'St. Margarethen im Burgenland'},
'432683':{'de': 'Purbach am Neusiedler See', 'en': 'Purbach am Neusiedler See'},
'432682':{'de': 'Eisenstadt', 'en': 'Eisenstadt'},
'433158':{'de': 'Sankt Anna am Aigen', 'en': 'St. Anna am Aigen'},
'433159':{'de': 'Bad Gleichenberg', 'en': 'Bad Gleichenberg'},
'441436':{'en': 'Helensburgh'},
'433152':{'de': 'Feldbach', 'en': 'Feldbach'},
'433153':{'de': 'Riegersburg', 'en': 'Riegersburg'},
'433150':{'de': 'Paldau', 'en': 'Paldau'},
'433151':{'de': 'Gnas', 'en': 'Gnas'},
'433157':{'de': 'Kapfenstein', 'en': 'Kapfenstein'},
'433155':{'de': 'Fehring', 'en': 'Fehring'},
'441438':{'en': 'Stevenage'},
'3356287':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356288':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356289':{'en': 'Saint-Jean', 'fr': 'Saint-Jean'},
'3317930':{'en': 'Rueil-Malmaison', 'fr': 'Rueil-Malmaison'},
'3594109':{'bg': u('\u0422\u0440\u0430\u043a\u0438\u044f'), 'en': 'Trakia'},
'3594108':{'bg': u('\u0412\u0430\u0441\u0438\u043b \u041b\u0435\u0432\u0441\u043a\u0438, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Vasil Levski, St. Zagora'},
'3594107':{'bg': u('\u0421\u0440\u0435\u0434\u0435\u0446, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Sredets, St. Zagora'},
'3594106':{'bg': u('\u041f\u044a\u0441\u0442\u0440\u0435\u043d'), 'en': 'Pastren'},
'3594105':{'bg': u('\u0411\u044f\u043b\u043e \u043f\u043e\u043b\u0435'), 'en': 'Byalo pole'},
'3594104':{'bg': u('\u041a\u0440\u0430\u0432\u0438\u043d\u043e'), 'en': 'Kravino'},
'3594103':{'bg': u('\u0411\u044f\u043b \u0438\u0437\u0432\u043e\u0440, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Byal izvor, St. Zagora'},
'3594102':{'bg': u('\u042f\u0441\u0442\u0440\u0435\u0431\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Yastrebovo, St. Zagora'},
'3594101':{'bg': u('\u041e\u043f\u0430\u043d'), 'en': 'Opan'},
'3594100':{'bg': u('\u0421\u0442\u043e\u043b\u0435\u0442\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Stoletovo, St. Zagora'},
'441698':{'en': 'Motherwell'},
'355291':{'en': u('Tomin/Luzni, Dib\u00ebr')},
'441694':{'en': 'Church Stretton'},
'441695':{'en': 'Skelmersdale'},
'441690':{'en': 'Betws-y-Coed'},
'441691':{'en': 'Oswestry'},
'441692':{'en': 'North Walsham'},
'441431':{'en': 'Helmsdale'},
'3324744':{'en': 'Saint-Pierre-des-Corps', 'fr': 'Saint-Pierre-des-Corps'},
'3324745':{'en': 'Azay-le-Rideau', 'fr': 'Azay-le-Rideau'},
'3324746':{'en': 'Tours', 'fr': 'Tours'},
'3324747':{'en': 'Tours', 'fr': 'Tours'},
'435279':{'de': 'Sankt Jodok am Brenner', 'en': 'St. Jodok am Brenner'},
'3324741':{'en': 'Tours', 'fr': 'Tours'},
'3324742':{'en': 'Fondettes', 'fr': 'Fondettes'},
'3324743':{'en': 'Truyes', 'fr': 'Truyes'},
'435275':{'de': 'Trins', 'en': 'Trins'},
'435274':{'de': 'Gries am Brenner', 'en': 'Gries am Brenner'},
'435276':{'de': 'Gschnitz', 'en': 'Gschnitz'},
'3324748':{'en': u('Chambray-l\u00e8s-Tours'), 'fr': u('Chambray-l\u00e8s-Tours')},
'3324749':{'en': 'Fondettes', 'fr': 'Fondettes'},
'435273':{'de': 'Matrei am Brenner', 'en': 'Matrei am Brenner'},
'3597439':{'bg': u('\u041f\u043b\u043e\u0441\u043a\u0438'), 'en': 'Ploski'},
'3344290':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344291':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'35825':{'en': 'Turku/Pori', 'fi': 'Turku/Pori', 'se': u('\u00c5bo/Bj\u00f6rneborg')},
'3344293':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344294':{'en': 'Bouc-Bel-air', 'fr': 'Bouc-Bel-air'},
'35822':{'en': 'Turku/Pori', 'fi': 'Turku/Pori', 'se': u('\u00c5bo/Bj\u00f6rneborg')},
'35821':{'en': 'Turku/Pori', 'fi': 'Turku/Pori', 'se': u('\u00c5bo/Bj\u00f6rneborg')},
'3344297':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344298':{'en': 'La Ciotat', 'fr': 'La Ciotat'},
'3344299':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3324000':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'35828':{'en': 'Turku/Pori', 'fi': 'Turku/Pori', 'se': u('\u00c5bo/Bj\u00f6rneborg')},
'4413872':{'en': 'Dumfries'},
'435418':{'de': u('Sch\u00f6nwies'), 'en': u('Sch\u00f6nwies')},
'4413870':{'en': 'Dumfries'},
'4413871':{'en': 'Dumfries'},
'4413876':{'en': 'Dumfries'},
'4413877':{'en': 'Dumfries'},
'4413874':{'en': 'Dumfries'},
'4413875':{'en': 'Dumfries'},
'435413':{'de': 'Sankt Leonhard im Pitztal', 'en': 'St. Leonhard im Pitztal'},
'435412':{'de': 'Imst', 'en': 'Imst'},
'435414':{'de': 'Wenns', 'en': 'Wenns'},
'331812':{'en': 'Paris', 'fr': 'Paris'},
'4144':{'de': u('Z\u00fcrich'), 'en': 'Zurich', 'fr': 'Zurich', 'it': 'Zurigo'},
'4141':{'de': 'Luzern', 'en': 'Lucerne', 'fr': 'Lucerne', 'it': 'Lucerna'},
'4143':{'de': u('Z\u00fcrich'), 'en': 'Zurich', 'fr': 'Zurich', 'it': 'Zurigo'},
'3347120':{'en': 'Murat', 'fr': 'Murat'},
'3595759':{'bg': u('\u041a\u043e\u0447\u043c\u0430\u0440'), 'en': 'Kochmar'},
'35535':{'en': 'Lushnje'},
'35534':{'en': 'Fier'},
'35533':{'en': u('Vlor\u00eb')},
'35532':{'en': 'Berat'},
'355278':{'en': u('Bicaj/Topojan/Shishtavec, Kuk\u00ebs')},
'3595758':{'bg': u('\u041a\u043b\u0430\u0434\u0435\u043d\u0446\u0438'), 'en': 'Kladentsi'},
'3356247':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3597713':{'bg': u('\u0420\u0443\u0434\u0430\u0440\u0446\u0438'), 'en': 'Rudartsi'},
'37426596':{'am': u('\u054e\u0561\u0570\u0561\u0576'), 'en': 'Vahan', 'ru': u('\u0412\u0430\u0430\u043d')},
'3597711':{'bg': u('\u041a\u043b\u0430\u0434\u043d\u0438\u0446\u0430'), 'en': 'Kladnitsa'},
'3323148':{'en': 'Lisieux', 'fr': 'Lisieux'},
'3323147':{'en': 'Caen', 'fr': 'Caen'},
'3323146':{'en': 'Caen', 'fr': 'Caen'},
'3323144':{'en': 'Caen', 'fr': 'Caen'},
'3323143':{'en': 'Caen', 'fr': 'Caen'},
'3323140':{'en': 'Falaise', 'fr': 'Falaise'},
'3597715':{'bg': u('\u0421\u0442\u0443\u0434\u0435\u043d\u0430, \u041f\u0435\u0440\u043d\u0438\u043a'), 'en': 'Studena, Pernik'},
'3349079':{'en': 'Pertuis', 'fr': 'Pertuis'},
'3349078':{'en': 'Cavaillon', 'fr': 'Cavaillon'},
'3349077':{'en': 'Cucuron', 'fr': 'Cucuron'},
'3349076':{'en': 'Cavaillon', 'fr': 'Cavaillon'},
'3349074':{'en': 'Apt', 'fr': 'Apt'},
'3349073':{'en': 'Plan-d\'Orgon', 'fr': 'Plan-d\'Orgon'},
'3349072':{'en': 'Gordes', 'fr': 'Gordes'},
'3349071':{'en': 'Cavaillon', 'fr': 'Cavaillon'},
'3349070':{'en': u('Courth\u00e9zon'), 'fr': u('Courth\u00e9zon')},
'3322812':{'en': 'Challans', 'fr': 'Challans'},
'3322813':{'en': 'Fontenay-le-Comte', 'fr': 'Fontenay-le-Comte'},
'3322811':{'en': 'Saint-Jean-de-Monts', 'fr': 'Saint-Jean-de-Monts'},
'3595108':{'bg': u('\u0421\u0430\u0434\u043e\u0432\u043e, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Sadovo, Varna'},
'3323368':{'en': 'Avranches', 'fr': 'Avranches'},
'390323':{'it': 'Baveno'},
'390322':{'en': 'Novara', 'it': 'Arona'},
'390321':{'en': 'Novara', 'it': 'Novara'},
'3323362':{'en': 'Flers', 'fr': 'Flers'},
'3323367':{'en': 'Argentan', 'fr': 'Argentan'},
'3598677':{'bg': u('\u0421\u0440\u0435\u0431\u044a\u0440\u043d\u0430'), 'en': 'Srebarna'},
'3323365':{'en': 'Flers', 'fr': 'Flers'},
'3323364':{'en': 'Flers', 'fr': 'Flers'},
'3349219':{'en': 'La Roquette sur Siagne', 'fr': 'La Roquette sur Siagne'},
'3349218':{'en': 'Le Cannet', 'fr': 'Le Cannet'},
'37424996':{'am': u('\u0546\u0565\u0580\u0584\u056b\u0576 \u0532\u0561\u0566\u0574\u0561\u0562\u0565\u0580\u0564'), 'en': 'Nerkin Bazmaberd', 'ru': u('\u041d\u0435\u0440\u043a\u0438\u043d \u0411\u0430\u0437\u043c\u0430\u0431\u0435\u0440\u0434')},
'37424997':{'am': u('\u0544\u0561\u057d\u057f\u0561\u0580\u0561'), 'en': 'Mastara', 'ru': u('\u041c\u0430\u0441\u0442\u0430\u0440\u0430')},
'3349213':{'en': 'Cagnes-sur-Mer', 'fr': 'Cagnes-sur-Mer'},
'3349212':{'en': 'Saint-Laurent-du-Var', 'fr': 'Saint-Laurent-du-Var'},
'3349210':{'en': 'Menton', 'fr': 'Menton'},
'3349217':{'en': 'Nice', 'fr': 'Nice'},
'436232':{'de': 'Mondsee', 'en': 'Mondsee'},
'3349215':{'en': 'Nice', 'fr': 'Nice'},
'3349214':{'en': 'Nice', 'fr': 'Nice'},
'441841':{'en': 'Newquay (Padstow)'},
'441840':{'en': 'Camelford'},
'441843':{'en': 'Thanet'},
'441842':{'en': 'Thetford'},
'441845':{'en': 'Thirsk'},
'3598673':{'bg': u('\u0410\u043b\u0444\u0430\u0442\u0430\u0440'), 'en': 'Alfatar'},
'441848':{'en': 'Thornhill'},
'3598672':{'bg': u('\u0411\u0440\u0430\u0434\u0432\u0430\u0440\u0438'), 'en': 'Bradvari'},
'3338895':{'en': 'Obernai', 'fr': 'Obernai'},
'3338894':{'en': 'Wissembourg', 'fr': 'Wissembourg'},
'3338891':{'en': 'Saverne', 'fr': 'Saverne'},
'3338893':{'en': 'Haguenau', 'fr': 'Haguenau'},
'3338892':{'en': u('S\u00e9lestat'), 'fr': u('S\u00e9lestat')},
'3338898':{'en': 'Erstein', 'fr': 'Erstein'},
'3332587':{'en': 'Langres', 'fr': 'Langres'},
'3332581':{'en': 'Troyes', 'fr': 'Troyes'},
'3332580':{'en': 'Troyes', 'fr': 'Troyes'},
'3332583':{'en': 'Troyes', 'fr': 'Troyes'},
'3332582':{'en': 'Troyes', 'fr': 'Troyes'},
'3598678':{'bg': u('\u0421\u0440\u0430\u0446\u0438\u043c\u0438\u0440, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Sratsimir, Silistra'},
'432858':{'de': 'Moorbad Harbach', 'en': 'Moorbad Harbach'},
'432256':{'de': 'Leobersdorf', 'en': 'Leobersdorf'},
'432257':{'de': 'Klausen-Leopoldsdorf', 'en': 'Klausen-Leopoldsdorf'},
'432852':{'de': u('Gm\u00fcnd'), 'en': u('Gm\u00fcnd')},
'432853':{'de': 'Schrems', 'en': 'Schrems'},
'432854':{'de': 'Kirchberg am Walde', 'en': 'Kirchberg am Walde'},
'432254':{'de': 'Ebreichsdorf', 'en': 'Ebreichsdorf'},
'432856':{'de': 'Weitra', 'en': 'Weitra'},
'432857':{'de': u('Bad Gro\u00dfpertholz'), 'en': 'Bad Grosspertholz'},
'432255':{'de': 'Deutsch Brodersdorf', 'en': 'Deutsch Brodersdorf'},
'432252':{'de': 'Baden', 'en': 'Baden'},
'432253':{'de': 'Oberwaltersdorf', 'en': 'Oberwaltersdorf'},
'3355622':{'en': 'Arcachon', 'fr': 'Arcachon'},
'3355627':{'en': 'Podensac', 'fr': 'Podensac'},
'3355626':{'en': 'Mios', 'fr': 'Mios'},
'3355625':{'en': 'Bazas', 'fr': 'Bazas'},
'3355624':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355629':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355628':{'en': 'Eysines', 'fr': 'Eysines'},
'3332361':{'en': 'Guise', 'fr': 'Guise'},
'432147':{'de': 'Zurndorf', 'en': 'Zurndorf'},
'432144':{'de': 'Deutsch Jahrndorf', 'en': 'Deutsch Jahrndorf'},
'3332362':{'en': 'Saint-Quentin', 'fr': 'Saint-Quentin'},
'3332365':{'en': 'Saint-Quentin', 'fr': 'Saint-Quentin'},
'331569':{'en': 'Paris', 'fr': 'Paris'},
'3332367':{'en': 'Saint-Quentin', 'fr': 'Saint-Quentin'},
'3332369':{'en': u('Ch\u00e2teau-Thierry'), 'fr': u('Ch\u00e2teau-Thierry')},
'3332368':{'en': 'Saint-Quentin', 'fr': 'Saint-Quentin'},
'331566':{'en': 'Paris', 'fr': 'Paris'},
'434768':{'de': 'Kleblach-Lind', 'en': 'Kleblach-Lind'},
'3594592':{'bg': u('\u0411\u044f\u043b\u0430 \u043f\u0430\u043b\u0430\u043d\u043a\u0430'), 'en': 'Byala palanka'},
'3354937':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354930':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3594595':{'bg': u('\u0421\u0431\u043e\u0440\u0438\u0449\u0435'), 'en': 'Sborishte'},
'3354932':{'en': 'Aiffres', 'fr': 'Aiffres'},
'3354933':{'en': 'Niort', 'fr': 'Niort'},
'3594599':{'bg': u('\u0427\u0435\u0440\u0432\u0435\u043d\u0430\u043a\u043e\u0432\u043e'), 'en': 'Chervenakovo'},
'3354938':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354939':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3348038':{'en': 'Grenoble', 'fr': 'Grenoble'},
'437433':{'de': 'Wallsee', 'en': 'Wallsee'},
'355277':{'en': u('Shtiqen/T\u00ebrthore/Zapod, Kuk\u00ebs')},
'441432':{'en': 'Hereford'},
'35941116':{'bg': u('\u0421\u043b\u0430\u0434\u044a\u043a \u041a\u043b\u0430\u0434\u0435\u043d\u0435\u0446'), 'en': 'Sladak Kladenets'},
'3593717':{'bg': u('\u041a\u043e\u043d\u0443\u0448, \u0425\u0430\u0441\u043a.'), 'en': 'Konush, Hask.'},
'35941115':{'bg': u('\u0411\u043e\u0440\u0438\u043b\u043e\u0432\u043e'), 'en': 'Borilovo'},
'3593713':{'bg': u('\u0414\u0438\u043d\u0435\u0432\u043e'), 'en': 'Dinevo'},
'3593712':{'bg': u('\u041c\u0430\u043b\u0435\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Malevo, Hask.'},
'3593711':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u0411\u043e\u0442\u0435\u0432\u043e'), 'en': 'Dolno Botevo'},
'3593710':{'bg': u('\u0423\u0437\u0443\u043d\u0434\u0436\u043e\u0432\u043e'), 'en': 'Uzundzhovo'},
'3329938':{'en': 'Rennes', 'fr': 'Rennes'},
'3593719':{'bg': u('\u041a\u043d\u0438\u0436\u043e\u0432\u043d\u0438\u043a'), 'en': 'Knizhovnik'},
'3329939':{'en': 'Saint-Aubin-du-Cormier', 'fr': 'Saint-Aubin-du-Cormier'},
'3522688':{'de': 'Mertzig/Wahl', 'en': 'Mertzig/Wahl', 'fr': 'Mertzig/Wahl'},
'3599355':{'bg': u('\u041a\u043e\u0441\u043e\u0432\u043e, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Kosovo, Vidin'},
'3599354':{'bg': u('\u0410\u043d\u0442\u0438\u043c\u043e\u0432\u043e, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Antimovo, Vidin'},
'3599356':{'bg': u('\u041a\u0430\u043b\u0435\u043d\u0438\u043a, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Kalenik, Vidin'},
'3599351':{'bg': u('\u0418\u0437\u0432\u043e\u0440, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Izvor, Vidin'},
'3599353':{'bg': u('\u041a\u043e\u0448\u0430\u0432\u0430'), 'en': 'Koshava'},
'3599352':{'bg': u('\u0414\u0440\u0443\u0436\u0431\u0430'), 'en': 'Druzhba'},
'3343812':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3329935':{'en': 'Rennes', 'fr': 'Rennes'},
'3325355':{'en': 'Nantes', 'fr': 'Nantes'},
'35941118':{'bg': u('\u041e\u0441\u0442\u0440\u0430 \u043c\u043e\u0433\u0438\u043b\u0430, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Ostra mogila, St. Zagora'},
'3598664':{'bg': u('\u041f\u043e\u043b\u044f\u043d\u0430, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Polyana, Silistra'},
'35941119':{'bg': u('\u0415\u043b\u0445\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Elhovo, St. Zagora'},
'35935252':{'bg': u('\u0422\u043e\u043f\u043e\u043b\u0438 \u0434\u043e\u043b'), 'en': 'Topoli dol'},
'3349411':{'en': 'La Seyne-sur-Mer', 'fr': 'La Seyne-sur-Mer'},
'35935251':{'bg': u('\u0411\u0440\u0430\u0442\u0430\u043d\u0438\u0446\u0430'), 'en': 'Bratanitsa'},
'35935256':{'bg': u('\u0421\u0431\u043e\u0440, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Sbor, Pazardzhik'},
'35935257':{'bg': u('\u0421\u0430\u0440\u0430\u044f'), 'en': 'Saraya'},
'35935254':{'bg': u('\u0414\u043e\u0431\u0440\u043e\u0432\u043d\u0438\u0446\u0430'), 'en': 'Dobrovnitsa'},
'35935255':{'bg': u('\u0420\u043e\u0441\u0435\u043d, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Rosen, Pazardzhik'},
'35935258':{'bg': u('\u0426\u0430\u0440 \u0410\u0441\u0435\u043d, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Tsar Asen, Pazardzhik'},
'3349413':{'en': u('Solli\u00e8s-Pont'), 'fr': u('Solli\u00e8s-Pont')},
'37428195':{'am': u('\u0544\u0561\u056c\u056b\u0577\u056f\u0561'), 'en': 'Malishka', 'ru': u('\u041c\u0430\u043b\u0438\u0448\u043a\u0430')},
'3329639':{'en': 'Dinan', 'fr': 'Dinan'},
'3598665':{'bg': u('\u0418\u0441\u043a\u0440\u0430, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Iskra, Silistra'},
'359431':{'bg': u('\u041a\u0430\u0437\u0430\u043d\u043b\u044a\u043a'), 'en': 'Kazanlak'},
'37428191':{'am': u('\u0531\u0580\u0583\u056b'), 'en': 'Arpi', 'ru': u('\u0410\u0440\u043f\u0438')},
'3596992':{'bg': u('\u0417\u043b\u0430\u0442\u043d\u0430 \u041f\u0430\u043d\u0435\u0433\u0430'), 'en': 'Zlatna Panega'},
'3596990':{'bg': u('\u041c\u0430\u043b\u044a\u043a \u0438\u0437\u0432\u043e\u0440, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Malak izvor, Lovech'},
'3596991':{'bg': u('\u042f\u0431\u043b\u0430\u043d\u0438\u0446\u0430'), 'en': 'Yablanitsa'},
'3596997':{'bg': u('\u0414\u043e\u0431\u0440\u0435\u0432\u0446\u0438, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Dobrevtsi, Lovech'},
'3596994':{'bg': u('\u0411\u0440\u0435\u0441\u0442\u043d\u0438\u0446\u0430, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Brestnitsa, Lovech'},
'355562':{'en': 'Milot/Fushe-Kuqe, Kurbin'},
'355563':{'en': u('Fush\u00eb-Kruj\u00eb')},
'355292':{'en': u('Maqellar\u00eb/Melan, Dib\u00ebr')},
'355561':{'en': 'Mamurras, Kurbin'},
'355294':{'en': u('Arras/Fush\u00eb-\u00c7idh\u00ebn/Lur\u00eb, Dib\u00ebr')},
'355295':{'en': u('Sllov\u00eb/Zall-Dardh\u00eb/Zall-Re\u00e7/Kala e Dodes, Dib\u00ebr')},
'355564':{'en': u('Nik\u00ebl/Bubq, Kruje')},
'355565':{'en': 'Koder-Thumane/Cudhi, Kruje'},
'3347826':{'en': 'Bron', 'fr': 'Bron'},
'3347827':{'en': 'Lyon', 'fr': 'Lyon'},
'3347824':{'en': 'Lyon', 'fr': 'Lyon'},
'3347825':{'en': 'Lyon', 'fr': 'Lyon'},
'3347822':{'en': u('Fontaines-sur-Sa\u00f4ne'), 'fr': u('Fontaines-sur-Sa\u00f4ne')},
'3347823':{'en': 'Caluire-et-Cuire', 'fr': 'Caluire-et-Cuire'},
'3347820':{'en': 'Saint-Priest', 'fr': 'Saint-Priest'},
'3347821':{'en': 'Saint-Priest', 'fr': 'Saint-Priest'},
'3347828':{'en': 'Lyon', 'fr': 'Lyon'},
'3347829':{'en': 'Lyon', 'fr': 'Lyon'},
'3595711':{'bg': u('\u041e\u0432\u0447\u0430\u0440\u043e\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Ovcharovo, Dobr.'},
'3595710':{'bg': u('\u041f\u043e\u0431\u0435\u0434\u0430, \u0414\u043e\u0431\u0440.'), 'en': 'Pobeda, Dobr.'},
'3595713':{'bg': u('\u0421\u0442\u0435\u0444\u0430\u043d\u043e\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Stefanovo, Dobr.'},
'3595712':{'bg': u('\u0421\u0442\u043e\u0436\u0435\u0440'), 'en': 'Stozher'},
'3595715':{'bg': u('\u041f\u043e\u043f\u0433\u0440\u0438\u0433\u043e\u0440\u043e\u0432\u043e'), 'en': 'Popgrigorovo'},
'3595714':{'bg': u('\u041a\u0430\u0440\u0430\u043f\u0435\u043b\u0438\u0442'), 'en': 'Karapelit'},
'3595717':{'bg': u('\u0412\u0435\u0434\u0440\u0438\u043d\u0430'), 'en': 'Vedrina'},
'3595716':{'bg': u('\u041f\u0430\u0441\u043a\u0430\u043b\u0435\u0432\u043e'), 'en': 'Paskalevo'},
'3595719':{'bg': u('\u0414\u043e\u043d\u0447\u0435\u0432\u043e'), 'en': 'Donchevo'},
'3595718':{'bg': u('\u0421\u043c\u043e\u043b\u043d\u0438\u0446\u0430'), 'en': 'Smolnitsa'},
'3324007':{'en': 'Derval', 'fr': 'Derval'},
'3346662':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346661':{'en': 'Anduze', 'fr': 'Anduze'},
'3346660':{'en': u('Saint-Christol-l\u00e8s-Al\u00e8s'), 'fr': u('Saint-Christol-l\u00e8s-Al\u00e8s')},
'3346667':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3324002':{'en': 'Sainte-Pazanne', 'fr': 'Sainte-Pazanne'},
'3346665':{'en': 'Mende', 'fr': 'Mende'},
'3346664':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346669':{'en': 'Langogne', 'fr': 'Langogne'},
'3346668':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3324009':{'en': u('Lir\u00e9'), 'fr': u('Lir\u00e9')},
'3324008':{'en': 'Nantes', 'fr': 'Nantes'},
'3597719':{'bg': u('\u042f\u0440\u0434\u0436\u0438\u043b\u043e\u0432\u0446\u0438'), 'en': 'Yardzhilovtsi'},
'3597718':{'bg': u('\u0414\u0440\u0430\u0433\u0438\u0447\u0435\u0432\u043e'), 'en': 'Dragichevo'},
'3522429':{'de': 'Luxemburg/Kockelscheuer', 'en': 'Luxembourg/Kockelscheuer', 'fr': 'Luxembourg/Kockelscheuer'},
'3522428':{'de': 'Luxemburg', 'en': 'Luxembourg City', 'fr': 'Luxembourg-Ville'},
'3522425':{'de': 'Luxemburg', 'en': 'Luxembourg', 'fr': 'Luxembourg'},
'3597712':{'bg': u('\u0411\u0430\u0442\u0430\u043d\u043e\u0432\u0446\u0438'), 'en': 'Batanovtsi'},
'3522427':{'de': 'Belair, Luxemburg', 'en': 'Belair, Luxembourg', 'fr': 'Belair, Luxembourg'},
'3522421':{'de': 'Weicherdingen', 'en': 'Weicherdange', 'fr': 'Weicherdange'},
'3522423':{'de': 'Bad Mondorf', 'en': 'Mondorf-les-Bains/Bascharage/Noerdange/Remich', 'fr': 'Mondorf-les-Bains/Bascharage/Noerdange/Remich'},
'3522422':{'de': 'Luxemburg', 'en': 'Luxembourg City', 'fr': 'Luxembourg-Ville'},
'35931403':{'bg': u('\u0422\u0440\u0438\u0432\u043e\u0434\u0438\u0446\u0438'), 'en': 'Trivoditsi'},
'35931402':{'bg': u('\u0421\u043a\u043e\u0431\u0435\u043b\u0435\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Skobelevo, Plovdiv'},
'35931401':{'bg': u('\u041a\u0430\u0434\u0438\u0435\u0432\u043e'), 'en': 'Kadievo'},
'3742625':{'am': u('\u054e\u0561\u0580\u0564\u0565\u0576\u056b\u056f'), 'en': 'Vardenik', 'ru': u('\u0412\u0430\u0440\u0434\u0435\u043d\u0438\u043a')},
'436233':{'de': 'Oberwang', 'en': 'Oberwang'},
'436234':{'de': 'Zell am Moos', 'en': 'Zell am Moos'},
'436235':{'de': 'Thalgau', 'en': 'Thalgau'},
'3329637':{'en': 'Lannion', 'fr': 'Lannion'},
'3329636':{'en': u('Plouguern\u00e9vel'), 'fr': u('Plouguern\u00e9vel')},
'3329635':{'en': u('Plestin-les-Gr\u00e8ves'), 'fr': u('Plestin-les-Gr\u00e8ves')},
'3329633':{'en': 'Saint-Brieuc', 'fr': 'Saint-Brieuc'},
'3329631':{'en': 'Lamballe', 'fr': 'Lamballe'},
'3329630':{'en': 'Lamballe', 'fr': 'Lamballe'},
'3522681':{'de': u('Ettelbr\u00fcck/Reckange-sur-Mess'), 'en': 'Ettelbruck/Reckange-sur-Mess', 'fr': 'Ettelbruck/Reckange-sur-Mess'},
'3522680':{'de': 'Diekirch', 'en': 'Diekirch', 'fr': 'Diekirch'},
'3522683':{'de': 'Vianden', 'en': 'Vianden', 'fr': 'Vianden'},
'3522685':{'de': 'Bissen/Roost', 'en': 'Bissen/Roost', 'fr': 'Bissen/Roost'},
'3522684':{'de': 'Han/Lesse', 'en': 'Han/Lesse', 'fr': 'Han/Lesse'},
'3522687':{'de': 'Fels', 'en': 'Larochette', 'fr': 'Larochette'},
'3329638':{'en': 'Plouaret', 'fr': 'Plouaret'},
'3323807':{'en': 'Montargis', 'fr': 'Montargis'},
'3354729':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'433615':{'de': 'Trieben', 'en': 'Trieben'},
'433617':{'de': 'Gaishorn am See', 'en': 'Gaishorn am See'},
'3346888':{'en': 'Banyuls-sur-Mer', 'fr': 'Banyuls-sur-Mer'},
'3346887':{'en': u('C\u00e9ret'), 'fr': u('C\u00e9ret')},
'3346886':{'en': u('Le Barcar\u00e8s'), 'fr': u('Le Barcar\u00e8s')},
'3346885':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3346884':{'en': u('Ille-sur-T\u00eat'), 'fr': u('Ille-sur-T\u00eat')},
'3346883':{'en': 'Le Boulou', 'fr': 'Le Boulou'},
'3346882':{'en': 'Collioure', 'fr': 'Collioure'},
'3346881':{'en': u('Argel\u00e8s-sur-Mer'), 'fr': u('Argel\u00e8s-sur-Mer')},
'3346880':{'en': 'Canet-en-Roussillon', 'fr': 'Canet-en-Roussillon'},
'34864':{'en': u('Castell\u00f3n'), 'es': u('Castell\u00f3n')},
'34865':{'en': 'Alicante', 'es': 'Alicante'},
'34867':{'en': 'Albacete', 'es': 'Albacete'},
'34860':{'en': 'Valencia', 'es': 'Valencia'},
'34868':{'en': 'Murcia', 'es': 'Murcia'},
'34869':{'en': 'Cuenca', 'es': 'Cuenca'},
'3332116':{'en': 'Arras', 'fr': 'Arras'},
'3332117':{'en': 'Calais', 'fr': 'Calais'},
'3332114':{'en': 'Lens', 'fr': 'Lens'},
'3332115':{'en': 'Arras', 'fr': 'Arras'},
'3332113':{'en': 'Lens', 'fr': 'Lens'},
'3332110':{'en': 'Boulogne-sur-Mer', 'fr': 'Boulogne-sur-Mer'},
'3332119':{'en': 'Calais', 'fr': 'Calais'},
'3594584':{'bg': u('\u0422\u0438\u0447\u0430'), 'en': 'Ticha'},
'355866':{'en': u('Libonik/Vreshtaz, Kor\u00e7\u00eb')},
'44151':{'en': 'Liverpool'},
'3325189':{'en': 'Nantes', 'fr': 'Nantes'},
'3325185':{'en': 'Sainte-Luce-sur-Loire', 'fr': 'Sainte-Luce-sur-Loire'},
'3325184':{'en': 'Nantes', 'fr': 'Nantes'},
'3325186':{'en': 'Nantes', 'fr': 'Nantes'},
'3325181':{'en': 'Nantes', 'fr': 'Nantes'},
'3325180':{'en': 'Nantes', 'fr': 'Nantes'},
'3325183':{'en': 'Nantes', 'fr': 'Nantes'},
'3325182':{'en': 'Nantes', 'fr': 'Nantes'},
'3347257':{'en': 'Lyon', 'fr': 'Lyon'},
'3522757':{'de': 'Esch-sur-Alzette/Schifflingen', 'en': 'Esch-sur-Alzette/Schifflange', 'fr': 'Esch-sur-Alzette/Schifflange'},
'435282':{'de': 'Zell am Ziller', 'en': 'Zell am Ziller'},
'3522756':{'de': u('R\u00fcmelingen'), 'en': 'Rumelange', 'fr': 'Rumelange'},
'390172':{'it': 'Savigliano'},
'3349537':{'en': 'Saint-Florent', 'fr': 'Saint-Florent'},
'3349534':{'en': 'Bastia', 'fr': 'Bastia'},
'390171':{'en': 'Cuneo', 'it': 'Cuneo'},
'3349532':{'en': 'Bastia', 'fr': 'Bastia'},
'3349533':{'en': 'Bastia', 'fr': 'Bastia'},
'3349530':{'en': 'Bastia', 'fr': 'Bastia'},
'3349531':{'en': 'Bastia', 'fr': 'Bastia'},
'35974347':{'bg': u('\u0420\u0430\u0437\u0434\u043e\u043b'), 'en': 'Razdol'},
'35974346':{'bg': u('\u0426\u0430\u043f\u0430\u0440\u0435\u0432\u043e'), 'en': 'Tsaparevo'},
'35974348':{'bg': u('\u0418\u0433\u0440\u0430\u043b\u0438\u0449\u0435'), 'en': 'Igralishte'},
'3593046':{'bg': u('\u0411\u0430\u0440\u0443\u0442\u0438\u043d'), 'en': 'Barutin'},
'3593045':{'bg': u('\u0414\u043e\u0441\u043f\u0430\u0442'), 'en': 'Dospat'},
'3594129':{'bg': u('\u041c\u0430\u0434\u0436\u0435\u0440\u0438\u0442\u043e'), 'en': 'Madzherito'},
'3593044':{'bg': u('\u041b\u044f\u0441\u043a\u043e\u0432\u043e, \u0421\u043c\u043e\u043b.'), 'en': 'Lyaskovo, Smol.'},
'3594121':{'bg': u('\u041b\u044e\u043b\u044f\u043a'), 'en': 'Lyulyak'},
'34987':{'en': u('Le\u00f3n'), 'es': u('Le\u00f3n')},
'3594123':{'bg': u('\u0411\u043e\u0433\u043e\u043c\u0438\u043b\u043e\u0432\u043e'), 'en': 'Bogomilovo'},
'3522758':{'de': 'Soleuvre/Differdingen', 'en': 'Soleuvre/Differdange', 'fr': 'Soleuvre/Differdange'},
'3594125':{'bg': u('\u041c\u0438\u0445\u0430\u0439\u043b\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Mihaylovo, St. Zagora'},
'3594124':{'bg': u('\u0417\u043c\u0435\u0439\u043e\u0432\u043e'), 'en': 'Zmeyovo'},
'3593042':{'bg': u('\u0411\u043e\u0440\u0438\u043d\u043e'), 'en': 'Borino'},
'3598125':{'bg': u('\u041a\u043e\u043f\u0440\u0438\u0432\u0435\u0446'), 'en': 'Koprivets'},
'3598124':{'bg': u('\u041d\u043e\u0432\u0433\u0440\u0430\u0434'), 'en': 'Novgrad'},
'3598127':{'bg': u('\u041a\u0430\u0440\u0430\u043c\u0430\u043d\u043e\u0432\u043e'), 'en': 'Karamanovo'},
'435254':{'de': u('S\u00f6lden'), 'en': u('S\u00f6lden')},
'435253':{'de': u('L\u00e4ngenfeld'), 'en': u('L\u00e4ngenfeld')},
'34985':{'en': 'Asturias', 'es': 'Asturias'},
'3598123':{'bg': u('\u0411\u043e\u0441\u0438\u043b\u043a\u043e\u0432\u0446\u0438'), 'en': 'Bosilkovtsi'},
'3598122':{'bg': u('\u0426\u0435\u043d\u043e\u0432\u043e, \u0420\u0443\u0441\u0435'), 'en': 'Tsenovo, Ruse'},
'3593040':{'bg': u('\u0422\u0440\u0438\u0433\u0440\u0430\u0434'), 'en': 'Trigrad'},
'3598129':{'bg': u('\u041b\u043e\u043c \u0427\u0435\u0440\u043a\u043e\u0432\u043d\u0430'), 'en': 'Lom Cherkovna'},
'3598128':{'bg': u('\u041f\u043e\u043b\u0441\u043a\u043e \u041a\u043e\u0441\u043e\u0432\u043e'), 'en': 'Polsko Kosovo'},
'3324768':{'en': u('Jou\u00e9-l\u00e8s-Tours'), 'fr': u('Jou\u00e9-l\u00e8s-Tours')},
'3324766':{'en': 'Tours', 'fr': 'Tours'},
'3324767':{'en': u('Jou\u00e9-l\u00e8s-Tours'), 'fr': u('Jou\u00e9-l\u00e8s-Tours')},
'3324764':{'en': 'Tours', 'fr': 'Tours'},
'3324765':{'en': 'Sainte-Maure-de-Touraine', 'fr': 'Sainte-Maure-de-Touraine'},
'3324763':{'en': 'Saint-Pierre-des-Corps', 'fr': 'Saint-Pierre-des-Corps'},
'3324760':{'en': 'Tours', 'fr': 'Tours'},
'3324761':{'en': 'Tours', 'fr': 'Tours'},
'433174':{'de': 'Birkfeld', 'en': 'Birkfeld'},
'433175':{'de': 'Anger', 'en': 'Anger'},
'433176':{'de': 'Stubenberg', 'en': 'Stubenberg'},
'433177':{'de': 'Puch bei Weiz', 'en': 'Puch bei Weiz'},
'433170':{'de': 'Fischbach', 'en': 'Fischbach'},
'433171':{'de': 'Gasen', 'en': 'Gasen'},
'433172':{'de': 'Weiz', 'en': 'Weiz'},
'433173':{'de': 'Ratten', 'en': 'Ratten'},
'433178':{'de': 'Sankt Ruprecht an der Raab', 'en': 'St. Ruprecht an der Raab'},
'433179':{'de': 'Passail', 'en': 'Passail'},
'441305':{'en': 'Dorchester'},
'4418516':{'en': 'Great Bernera'},
'441302':{'en': 'Doncaster'},
'441871':{'en': 'Castlebay'},
'441300':{'en': 'Cerne Abbas'},
'441873':{'en': 'Abergavenny'},
'4122':{'de': 'Genf', 'en': 'Geneva', 'fr': u('Gen\u00e8ve'), 'it': 'Ginevra'},
'4121':{'de': 'Lausanne', 'en': 'Lausanne', 'fr': 'Lausanne', 'it': 'Losanna'},
'4127':{'de': 'Sitten', 'en': 'Sion', 'fr': 'Sion', 'it': 'Sion'},
'4126':{'de': 'Freiburg', 'en': 'Fribourg', 'fr': 'Fribourg', 'it': 'Friburgo'},
'4124':{'de': 'Yverdon/Aigle', 'en': 'Yverdon/Aigle', 'fr': 'Yverdon/Aigle', 'it': 'Yverdon/Aigle'},
'3595526':{'bg': u('\u041a\u043b\u0438\u043a\u0430\u0447'), 'en': 'Klikach'},
'3595527':{'bg': u('\u0421\u043e\u043a\u043e\u043b\u043e\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Sokolovo, Burgas'},
'3595524':{'bg': u('\u0415\u043a\u0437\u0430\u0440\u0445 \u0410\u043d\u0442\u0438\u043c\u043e\u0432\u043e'), 'en': 'Ekzarh Antimovo'},
'3595525':{'bg': u('\u0414\u0435\u0432\u0435\u0442\u0430\u043a'), 'en': 'Devetak'},
'3347109':{'en': 'Le Puy en Velay', 'fr': 'Le Puy en Velay'},
'3595523':{'bg': u('\u041a\u0440\u0443\u043c\u043e\u0432\u043e \u0433\u0440\u0430\u0434\u0438\u0449\u0435'), 'en': 'Krumovo gradishte'},
'3595520':{'bg': u('\u0427\u0435\u0440\u043a\u043e\u0432\u043e'), 'en': 'Cherkovo'},
'3595521':{'bg': u('\u0412\u0435\u043d\u0435\u0446, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Venets, Burgas'},
'3347105':{'en': 'Le Puy en Velay', 'fr': 'Le Puy en Velay'},
'3347456':{'en': 'Ampuis', 'fr': 'Ampuis'},
'3347455':{'en': u('Ch\u00e2tillon-sur-Chalaronne'), 'fr': u('Ch\u00e2tillon-sur-Chalaronne')},
'3347106':{'en': 'Le Puy en Velay', 'fr': 'Le Puy en Velay'},
'3347453':{'en': 'Vienne', 'fr': 'Vienne'},
'3595528':{'bg': u('\u041d\u0435\u0432\u0435\u0441\u0442\u0438\u043d\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Nevestino, Burgas'},
'3347102':{'en': 'Le Puy en Velay', 'fr': 'Le Puy en Velay'},
'38932':{'en': 'Stip/Probistip/Sveti Nikole/Radovis'},
'38933':{'en': 'Kocani/Berovo/Delcevo/Vinica'},
'38931':{'en': 'Kumanovo/Kriva Palanka/Kratovo'},
'38934':{'en': 'Gevgelija/Valandovo/Strumica/Dojran'},
'3599551':{'bg': u('\u0413\u0435\u043e\u0440\u0433\u0438 \u0414\u0430\u043c\u044f\u043d\u043e\u0432\u043e'), 'en': 'Georgi Damyanovo'},
'3338784':{'en': 'Forbach', 'fr': 'Forbach'},
'3597157':{'bg': u('\u0421\u0430\u0440\u0430\u043d\u0446\u0438'), 'en': 'Sarantsi'},
'4191':{'de': 'Bellinzona', 'en': 'Bellinzona', 'fr': 'Bellinzona', 'it': 'Bellinzona'},
'3316909':{'en': 'Chilly-Mazarin', 'fr': 'Chilly-Mazarin'},
'3336919':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3316906':{'en': 'Ris-Orangis', 'fr': 'Ris-Orangis'},
'3336914':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3316900':{'en': u('Quincy-sous-S\u00e9nart'), 'fr': u('Quincy-sous-S\u00e9nart')},
'432841':{'de': 'Vitis', 'en': 'Vitis'},
'359608':{'bg': u('\u041f\u043e\u043f\u043e\u0432\u043e, \u0422\u044a\u0440\u0433.'), 'en': 'Popovo, Targ.'},
'359605':{'bg': u('\u041e\u043c\u0443\u0440\u0442\u0430\u0433'), 'en': 'Omurtag'},
'359601':{'bg': u('\u0422\u044a\u0440\u0433\u043e\u0432\u0438\u0449\u0435'), 'en': 'Targovishte'},
'3338781':{'en': 'Freyming-Merlebach', 'fr': 'Freyming-Merlebach'},
'3323169':{'en': u('Cond\u00e9-sur-Noireau'), 'fr': u('Cond\u00e9-sur-Noireau')},
'3323168':{'en': 'Vire', 'fr': 'Vire'},
'3323161':{'en': 'Lisieux', 'fr': 'Lisieux'},
'3323162':{'en': 'Lisieux', 'fr': 'Lisieux'},
'3323165':{'en': u('Pont-l\'\u00c9v\u00eaque'), 'fr': u('Pont-l\'\u00c9v\u00eaque')},
'3323164':{'en': u('Pont-l\'\u00c9v\u00eaque'), 'fr': u('Pont-l\'\u00c9v\u00eaque')},
'3323167':{'en': 'Vire', 'fr': 'Vire'},
'3323166':{'en': 'Vire', 'fr': 'Vire'},
'3349059':{'en': 'Mallemort', 'fr': 'Mallemort'},
'3349058':{'en': 'Miramas', 'fr': 'Miramas'},
'3349051':{'en': 'Orange', 'fr': 'Orange'},
'3349050':{'en': 'Miramas', 'fr': 'Miramas'},
'3349053':{'en': 'Salon-de-Provence', 'fr': 'Salon-de-Provence'},
'3349052':{'en': 'Arles', 'fr': 'Arles'},
'3349055':{'en': u('P\u00e9lissanne'), 'fr': u('P\u00e9lissanne')},
'3349054':{'en': 'Fontvieille', 'fr': 'Fontvieille'},
'3349057':{'en': u('Eygui\u00e8res'), 'fr': u('Eygui\u00e8res')},
'3349056':{'en': 'Salon-de-Provence', 'fr': 'Salon-de-Provence'},
'441899':{'en': 'Biggar'},
'3354005':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3354007':{'en': 'Bayonne', 'fr': 'Bayonne'},
'3349235':{'en': 'Seyne-les-Alpes', 'fr': 'Seyne-les-Alpes'},
'390343':{'en': 'Sondrio', 'it': 'Chiavenna'},
'3349236':{'en': 'Digne-les-Bains', 'fr': 'Digne-les-Bains'},
'3349231':{'en': 'Digne-les-Bains', 'fr': 'Digne-les-Bains'},
'3349230':{'en': 'Digne-les-Bains', 'fr': 'Digne-les-Bains'},
'3349941':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3349232':{'en': 'Digne-les-Bains', 'fr': 'Digne-les-Bains'},
'35961602':{'bg': u('\u0426\u0430\u0440\u0441\u043a\u0438 \u0438\u0437\u0432\u043e\u0440'), 'en': 'Tsarski izvor'},
'35961603':{'bg': u('\u041b\u043e\u0437\u0435\u043d, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Lozen, V. Tarnovo'},
'35961604':{'bg': u('\u041c\u0438\u0440\u043e\u0432\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Mirovo, V. Tarnovo'},
'3349238':{'en': 'Vallauris', 'fr': 'Vallauris'},
'35961606':{'bg': u('\u0412\u043b\u0430\u0434\u0438\u0441\u043b\u0430\u0432'), 'en': 'Vladislav'},
'35961607':{'bg': u('\u0411\u0430\u043b\u043a\u0430\u043d\u0446\u0438, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Balkantsi, V. Tarnovo'},
'3522745':{'de': 'Diedrich', 'en': 'Diedrich', 'fr': 'Diedrich'},
'3522747':{'de': 'Lintgen', 'en': 'Lintgen', 'fr': 'Lintgen'},
'3522740':{'de': 'Howald', 'en': 'Howald', 'fr': 'Howald'},
'3522742':{'de': 'Plateau de Kirchberg', 'en': 'Plateau de Kirchberg', 'fr': 'Plateau de Kirchberg'},
'3522743':{'de': 'Findel/Kirchberg', 'en': 'Findel/Kirchberg', 'fr': 'Findel/Kirchberg'},
'3597036':{'bg': u('\u0411\u0430\u043b\u0430\u043d\u043e\u0432\u043e'), 'en': 'Balanovo'},
'3597034':{'bg': u('\u0414\u0436\u0435\u0440\u043c\u0430\u043d'), 'en': 'Dzherman'},
'3597035':{'bg': u('\u0427\u0435\u0440\u0432\u0435\u043d \u0431\u0440\u0435\u0433'), 'en': 'Cherven breg'},
'3522748':{'de': 'Contern/Foetz', 'en': 'Contern/Foetz', 'fr': 'Contern/Foetz'},
'3522749':{'de': 'Howald', 'en': 'Howald', 'fr': 'Howald'},
'3597030':{'bg': u('\u0420\u0435\u0441\u0438\u043b\u043e\u0432\u043e'), 'en': 'Resilovo'},
'3597031':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u041a\u043e\u0437\u043d\u0438\u0446\u0430'), 'en': 'Gorna Koznitsa'},
'441869':{'en': 'Bicester'},
'37428197':{'am': u('\u0535\u056c\u0583\u056b\u0576'), 'en': 'Yelpin', 'ru': u('\u0415\u043b\u043f\u0438\u043d')},
'441863':{'en': 'Ardgay'},
'441862':{'en': 'Tain'},
'3336265':{'en': 'Lille', 'fr': 'Lille'},
'441865':{'en': 'Oxford'},
'441864':{'en': 'Abington (Crawford)'},
'3338879':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338878':{'en': 'Lingolsheim', 'fr': 'Lingolsheim'},
'3338877':{'en': 'Lingolsheim', 'fr': 'Lingolsheim'},
'3338876':{'en': 'Lingolsheim', 'fr': 'Lingolsheim'},
'3338875':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338874':{'en': 'Benfeld', 'fr': 'Benfeld'},
'3338873':{'en': 'Haguenau', 'fr': 'Haguenau'},
'3338872':{'en': 'Schweighouse-sur-Moder', 'fr': 'Schweighouse-sur-Moder'},
'3338871':{'en': 'Saverne', 'fr': 'Saverne'},
'432784':{'de': 'Perschling', 'en': 'Perschling'},
'432786':{'de': u('Oberw\u00f6lbling'), 'en': u('Oberw\u00f6lbling')},
'37428194':{'am': u('\u0531\u0580\u0565\u0576\u056b'), 'en': 'Areni', 'ru': u('\u0410\u0440\u0435\u043d\u0438')},
'432782':{'de': 'Herzogenburg', 'en': 'Herzogenburg'},
'432783':{'de': 'Traismauer', 'en': 'Traismauer'},
'37278':{'en': u('V\u00f5ru')},
'37279':{'en': u('P\u00f5lva')},
'37273':{'en': 'Tartu'},
'37274':{'en': 'Tartu'},
'37275':{'en': 'Tartu'},
'37276':{'en': 'Valga'},
'37277':{'en': u('J\u00f5geva')},
'3323345':{'en': 'Coutances', 'fr': 'Coutances'},
'3323344':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'35981266':{'bg': u('\u0421\u0442\u044a\u0440\u043c\u0435\u043d, \u0420\u0443\u0441\u0435'), 'en': 'Starmen, Ruse'},
'3355609':{'en': 'Soulac-sur-Mer', 'fr': 'Soulac-sur-Mer'},
'3323340':{'en': 'Valognes', 'fr': 'Valognes'},
'3355359':{'en': u('Sarlat-la-Can\u00e9da'), 'fr': u('Sarlat-la-Can\u00e9da')},
'3323342':{'en': 'Carentan', 'fr': 'Carentan'},
'3355357':{'en': 'Bergerac', 'fr': 'Bergerac'},
'3355356':{'en': 'Nontron', 'fr': 'Nontron'},
'3355355':{'en': 'Thiviers', 'fr': 'Thiviers'},
'3355606':{'en': 'Lormont', 'fr': 'Lormont'},
'3355353':{'en': u('P\u00e9rigueux'), 'fr': u('P\u00e9rigueux')},
'3355600':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355603':{'en': 'Lacanau', 'fr': 'Lacanau'},
'3355602':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3322342':{'en': 'Rennes', 'fr': 'Rennes'},
'3322343':{'en': 'Montfort-sur-Meu', 'fr': 'Montfort-sur-Meu'},
'3322340':{'en': 'Rennes', 'fr': 'Rennes'},
'3332308':{'en': 'Saint-Quentin', 'fr': 'Saint-Quentin'},
'3322346':{'en': 'Rennes', 'fr': 'Rennes'},
'432165':{'de': 'Hainburg a.d. Donau', 'en': 'Hainburg a.d. Donau'},
'3322344':{'en': 'Rennes', 'fr': 'Rennes'},
'3322345':{'en': u('Cesson-S\u00e9vign\u00e9'), 'fr': u('Cesson-S\u00e9vign\u00e9')},
'432168':{'de': 'Mannersdorf am Leithagebirge', 'en': 'Mannersdorf am Leithagebirge'},
'432169':{'de': 'Trautmannsdorf an der Leitha', 'en': 'Trautmannsdorf an der Leitha'},
'3322348':{'en': 'Rennes', 'fr': 'Rennes'},
'437667':{'de': 'Sankt Georgen im Attergau', 'en': 'St. Georgen im Attergau'},
'3332307':{'en': 'Bohain-en-Vermandois', 'fr': 'Bohain-en-Vermandois'},
'3332306':{'en': 'Saint-Quentin', 'fr': 'Saint-Quentin'},
'3332305':{'en': 'Saint-Quentin', 'fr': 'Saint-Quentin'},
'3332304':{'en': 'Saint-Quentin', 'fr': 'Saint-Quentin'},
'435517':{'de': 'Riezlern', 'en': 'Riezlern'},
'3354918':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354919':{'en': u('Ch\u00e2tellerault'), 'fr': u('Ch\u00e2tellerault')},
'3354917':{'en': 'Niort', 'fr': 'Niort'},
'3354911':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3359032':{'en': 'Baie Mahault', 'fr': 'Baie Mahault'},
'3359038':{'en': 'Baie Mahault', 'fr': 'Baie Mahault'},
'432814':{'de': 'Langschlag', 'en': 'Langschlag'},
'435518':{'de': 'Mellau', 'en': 'Mellau'},
'435519':{'de': u('Schr\u00f6cken'), 'en': u('Schr\u00f6cken')},
'3595959':{'bg': u('\u0417\u0432\u0435\u0437\u0434\u0435\u0446'), 'en': 'Zvezdets'},
'3595958':{'bg': u('\u0413\u0440\u0430\u043c\u0430\u0442\u0438\u043a\u043e\u0432\u043e'), 'en': 'Gramatikovo'},
'3595952':{'bg': u('\u041c\u0430\u043b\u043a\u043e \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Malko Tarnovo'},
'432816':{'de': 'Karlstift', 'en': 'Karlstift'},
'3353351':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3343837':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3332379':{'en': 'Laon', 'fr': 'Laon'},
'359453':{'bg': u('\u041a\u043e\u0442\u0435\u043b'), 'en': 'Kotel'},
'35935502':{'bg': u('\u0424\u043e\u0442\u0438\u043d\u043e\u0432\u043e, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Fotinovo, Pazardzhik'},
'359457':{'bg': u('\u041d\u043e\u0432\u0430 \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Nova Zagora'},
'359454':{'bg': u('\u0422\u0432\u044a\u0440\u0434\u0438\u0446\u0430, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Tvarditsa, Sliven'},
'35935501':{'bg': u('\u0420\u0430\u0432\u043d\u043e\u0433\u043e\u0440'), 'en': 'Ravnogor'},
'3324395':{'en': u('Sabl\u00e9-sur-Sarthe'), 'fr': u('Sabl\u00e9-sur-Sarthe')},
'3324394':{'en': u('La Fl\u00e8che'), 'fr': u('La Fl\u00e8che')},
'3324392':{'en': u('Sabl\u00e9-sur-Sarthe'), 'fr': u('Sabl\u00e9-sur-Sarthe')},
'3324391':{'en': 'Laval', 'fr': 'Laval'},
'35960382':{'bg': u('\u041a\u043e\u0432\u0430\u0447\u0435\u0432\u0435\u0446'), 'en': 'Kovachevets'},
'35960383':{'bg': u('\u0411\u0435\u0440\u043a\u043e\u0432\u0441\u043a\u0438'), 'en': 'Berkovski'},
'35960380':{'bg': u('\u0414\u0440\u0438\u043d\u043e\u0432\u043e'), 'en': 'Drinovo'},
'35960386':{'bg': u('\u0412\u043e\u0434\u0438\u0446\u0430, \u0422\u044a\u0440\u0433.'), 'en': 'Voditsa, Targ.'},
'35960387':{'bg': u('\u0413\u043b\u043e\u0433\u0438\u043d\u043a\u0430'), 'en': 'Gloginka'},
'35960384':{'bg': u('\u0413\u0430\u0433\u043e\u0432\u043e'), 'en': 'Gagovo'},
'35960385':{'bg': u('\u041b\u043e\u043c\u0446\u0438'), 'en': 'Lomtsi'},
'441480':{'en': 'Huntingdon'},
'3347840':{'en': 'Heyrieux', 'fr': 'Heyrieux'},
'3347841':{'en': 'Bron', 'fr': 'Bron'},
'3347842':{'en': 'Lyon', 'fr': 'Lyon'},
'3347667':{'en': 'Voiron', 'fr': 'Voiron'},
'3347844':{'en': 'Mornant', 'fr': 'Mornant'},
'3347661':{'en': 'Meylan', 'fr': 'Meylan'},
'3347846':{'en': 'Irigny', 'fr': 'Irigny'},
'3347847':{'en': 'Lyon', 'fr': 'Lyon'},
'3347848':{'en': 'Saint-Martin-en-Haut', 'fr': 'Saint-Martin-en-Haut'},
'3347849':{'en': u('D\u00e9cines-Charpieu'), 'fr': u('D\u00e9cines-Charpieu')},
'3347668':{'en': 'Vizille', 'fr': 'Vizille'},
'374286':{'am': u('\u0544\u0565\u0572\u0580\u056b/\u0531\u0563\u0561\u0580\u0561\u056f'), 'en': 'Meghri/Agarak', 'ru': u('\u041c\u0435\u0433\u0440\u0438/\u0410\u0433\u0430\u0440\u0430\u043a')},
'374287':{'am': u('\u054b\u0565\u0580\u0574\u0578\u0582\u056f'), 'en': 'Jermuk', 'ru': u('\u0414\u0436\u0435\u0440\u043c\u0443\u043a')},
'374284':{'am': u('\u0533\u0578\u0580\u056b\u057d/\u054e\u0565\u0580\u056b\u0577\u0565\u0576'), 'en': 'Goris/Verishen', 'ru': u('\u0413\u043e\u0440\u0438\u0441/\u0412\u0435\u0440\u0438\u0448\u0435\u043d')},
'374285':{'am': u('\u0534\u0561\u057e\u056b\u0569 \u0532\u0565\u056f/\u0554\u0561\u057b\u0561\u0580\u0561\u0576/\u053f\u0561\u057a\u0561\u0576'), 'en': 'Davit Bek/Kajaran/Kapan', 'ru': u('\u0414\u0430\u0432\u0438\u0442 \u0411\u0435\u043a/\u041a\u0430\u0434\u0436\u0430\u0440\u0430\u043d/\u041a\u0430\u043f\u0430\u043d')},
'374282':{'am': u('\u054e\u0561\u0575\u0584'), 'en': 'Vayk region', 'ru': u('\u0412\u0430\u0439\u043a')},
'374281':{'am': u('\u0533\u0565\u057f\u0561\u0583/\u054d\u0561\u056c\u056c\u056b/\u0535\u0572\u0565\u0563\u0576\u0561\u0571\u0578\u0580'), 'en': 'Getap/Salli/Yeghegnadzor', 'ru': u('\u0413\u0435\u0442\u0430\u043f/\u0421\u0430\u043b\u043b\u0438/\u0415\u0445\u0435\u0433\u043d\u0430\u0434\u0437\u043e\u0440')},
'3595773':{'bg': u('\u041b\u043e\u0437\u0435\u043d\u0435\u0446, \u0414\u043e\u0431\u0440.'), 'en': 'Lozenets, Dobr.'},
'3595772':{'bg': u('\u0422\u0435\u043b\u0435\u0440\u0438\u0433'), 'en': 'Telerig'},
'3595771':{'bg': u('\u041a\u0440\u0443\u0448\u0430\u0440\u0438'), 'en': 'Krushari'},
'3595776':{'bg': u('\u0427\u0435\u0440\u043d\u0430, \u0414\u043e\u0431\u0440.'), 'en': 'Cherna, Dobr.'},
'3595775':{'bg': u('\u041f\u043e\u043b\u043a\u043e\u0432\u043d\u0438\u043a \u0414\u044f\u043a\u043e\u0432\u043e'), 'en': 'Polkovnik Dyakovo'},
'3595774':{'bg': u('\u041a\u043e\u0440\u0438\u0442\u0435\u043d'), 'en': 'Koriten'},
'3338051':{'en': u('Chen\u00f4ve'), 'fr': u('Chen\u00f4ve')},
'3338050':{'en': 'Dijon', 'fr': 'Dijon'},
'3338053':{'en': 'Dijon', 'fr': 'Dijon'},
'3338052':{'en': u('Chen\u00f4ve'), 'fr': u('Chen\u00f4ve')},
'3324025':{'en': 'Sainte-Luce-sur-Loire', 'fr': 'Sainte-Luce-sur-Loire'},
'3324024':{'en': u('Gu\u00e9rande'), 'fr': u('Gu\u00e9rande')},
'3338057':{'en': 'Talant', 'fr': 'Talant'},
'3338056':{'en': u('Fontaine-l\u00e8s-Dijon'), 'fr': u('Fontaine-l\u00e8s-Dijon')},
'3338059':{'en': 'Dijon', 'fr': 'Dijon'},
'3338058':{'en': 'Dijon', 'fr': 'Dijon'},
'3346640':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346643':{'en': u('Al\u00e8s'), 'fr': u('Al\u00e8s')},
'3346642':{'en': 'Aumont-Aubrac', 'fr': 'Aumont-Aubrac'},
'390173':{'it': 'Alba'},
'3329611':{'en': 'Ploumagoar', 'fr': 'Ploumagoar'},
'3329615':{'en': u('Tr\u00e9beurden'), 'fr': u('Tr\u00e9beurden')},
'3329614':{'en': 'Lannion', 'fr': 'Lannion'},
'3329616':{'en': u('Plou\u00e9zec'), 'fr': u('Plou\u00e9zec')},
'3338460':{'en': 'Les Rousses', 'fr': 'Les Rousses'},
'3338462':{'en': 'Lure', 'fr': 'Lure'},
'3338464':{'en': 'Gray', 'fr': 'Gray'},
'3338465':{'en': 'Gray', 'fr': 'Gray'},
'3338466':{'en': 'Arbois', 'fr': 'Arbois'},
'3338469':{'en': 'Dole', 'fr': 'Dole'},
'390175':{'it': 'Saluzzo'},
'3597735':{'bg': u('\u041b\u0435\u0432\u0430 \u0440\u0435\u043a\u0430'), 'en': 'Leva reka'},
'3597734':{'bg': u('\u0413\u043b\u0430\u0432\u0430\u043d\u043e\u0432\u0446\u0438, \u041f\u0435\u0440\u043d\u0438\u043a'), 'en': 'Glavanovtsi, Pernik'},
'3597731':{'bg': u('\u0422\u0440\u044a\u043d'), 'en': 'Tran'},
'3597733':{'bg': u('\u0424\u0438\u043b\u0438\u043f\u043e\u0432\u0446\u0438'), 'en': 'Filipovtsi'},
'3597732':{'bg': u('\u0412\u0443\u043a\u0430\u043d'), 'en': 'Vukan'},
'441730':{'en': 'Petersfield'},
'3323752':{'en': 'Nogent-le-Rotrou', 'fr': 'Nogent-le-Rotrou'},
'3323753':{'en': 'Nogent-le-Rotrou', 'fr': 'Nogent-le-Rotrou'},
'3323750':{'en': 'Dreux', 'fr': 'Dreux'},
'3323751':{'en': 'Nogent-le-Roi', 'fr': 'Nogent-le-Roi'},
'3332170':{'en': 'Lens', 'fr': 'Lens'},
'3332171':{'en': 'Arras', 'fr': 'Arras'},
'3332172':{'en': u('Li\u00e9vin'), 'fr': u('Li\u00e9vin')},
'3332174':{'en': 'Carvin', 'fr': 'Carvin'},
'3332175':{'en': u('H\u00e9nin-Beaumont'), 'fr': u('H\u00e9nin-Beaumont')},
'3332176':{'en': u('H\u00e9nin-Beaumont'), 'fr': u('H\u00e9nin-Beaumont')},
'3332177':{'en': 'Leforest', 'fr': 'Leforest'},
'3332178':{'en': 'Lens', 'fr': 'Lens'},
'390481':{'en': 'Gorizia', 'it': 'Gorizia'},
'3354747':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3597183':{'bg': u('\u0414\u0443\u0448\u0430\u043d\u0446\u0438'), 'en': 'Dushantsi'},
'3316420':{'en': 'Coulommiers', 'fr': 'Coulommiers'},
'3324136':{'en': 'Angers', 'fr': 'Angers'},
'3316422':{'en': 'Fontainebleau', 'fr': 'Fontainebleau'},
'42051':{'en': 'South Moravian Region'},
'3345037':{'en': 'Annemasse', 'fr': 'Annemasse'},
'3345035':{'en': 'Saint-Julien-en-Genevois', 'fr': 'Saint-Julien-en-Genevois'},
'3345034':{'en': u('Samo\u00ebns'), 'fr': u('Samo\u00ebns')},
'3345033':{'en': 'Annecy', 'fr': 'Annecy'},
'3316425':{'en': u('Fontenay-Tr\u00e9signy'), 'fr': u('Fontenay-Tr\u00e9signy')},
'3349558':{'en': 'Bastia', 'fr': 'Bastia'},
'3349559':{'en': 'Penta-di-Casinca', 'fr': 'Penta-di-Casinca'},
'3316426':{'en': 'Chelles', 'fr': 'Chelles'},
'3349550':{'en': 'Ajaccio', 'fr': 'Ajaccio'},
'3349551':{'en': 'Ajaccio', 'fr': 'Ajaccio'},
'3349552':{'en': 'Ajaccio', 'fr': 'Ajaccio'},
'3324130':{'en': u('Chemill\u00e9'), 'fr': u('Chemill\u00e9')},
'3349555':{'en': 'Bastia', 'fr': 'Bastia'},
'3349556':{'en': 'Ghisonaccia', 'fr': 'Ghisonaccia'},
'3349557':{'en': u('Al\u00e9ria'), 'fr': u('Al\u00e9ria')},
'3348974':{'en': 'Nice', 'fr': 'Nice'},
'3317464':{'en': 'Paris', 'fr': 'Paris'},
'3348979':{'en': 'Toulon', 'fr': 'Toulon'},
'3317462':{'en': 'Boulogne-Billancourt', 'fr': 'Boulogne-Billancourt'},
'351289':{'en': 'Faro', 'pt': 'Faro'},
'390722':{'it': 'Urbino'},
'351283':{'en': 'Odemira', 'pt': 'Odemira'},
'351282':{'en': u('Portim\u00e3o'), 'pt': u('Portim\u00e3o')},
'351281':{'en': 'Tavira', 'pt': 'Tavira'},
'351286':{'en': 'Castro Verde', 'pt': 'Castro Verde'},
'351285':{'en': 'Moura', 'pt': 'Moura'},
'351284':{'en': 'Beja', 'pt': 'Beja'},
'3332794':{'en': 'Douai', 'fr': 'Douai'},
'3332795':{'en': 'Douai', 'fr': 'Douai'},
'3332796':{'en': 'Douai', 'fr': 'Douai'},
'3332797':{'en': 'Douai', 'fr': 'Douai'},
'3332792':{'en': 'Aniche', 'fr': 'Aniche'},
'3332793':{'en': 'Douai', 'fr': 'Douai'},
'3332798':{'en': 'Douai', 'fr': 'Douai'},
'3332799':{'en': 'Douai', 'fr': 'Douai'},
'3594143':{'bg': u('\u0421\u044a\u0440\u043d\u0435\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Sarnevo, St. Zagora'},
'3594142':{'bg': u('\u0422\u0440\u043e\u044f\u043d\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Troyanovo, St. Zagora'},
'3594140':{'bg': u('\u041f\u043e\u043b\u0441\u043a\u0438 \u0413\u0440\u0430\u0434\u0435\u0446'), 'en': 'Polski Gradets'},
'3594147':{'bg': u('\u041b\u044e\u0431\u0435\u043d\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Lyubenovo, St. Zagora'},
'3594146':{'bg': u('\u0414\u0438\u043d\u044f'), 'en': 'Dinya'},
'3594145':{'bg': u('\u0417\u043d\u0430\u043c\u0435\u043d\u043e\u0441\u0435\u0446'), 'en': 'Znamenosets'},
'3594144':{'bg': u('\u041a\u043e\u0432\u0430\u0447\u0435\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Kovachevo, St. Zagora'},
'3594149':{'bg': u('\u0422\u0440\u044a\u043d\u043a\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Trankovo, St. Zagora'},
'390143':{'it': 'Novi Ligure'},
'435230':{'de': 'Sellrain', 'en': 'Sellrain'},
'435232':{'de': 'Kematen in Tirol', 'en': 'Kematen in Tirol'},
'435234':{'de': 'Axams', 'en': 'Axams'},
'435236':{'de': 'Gries im Sellrain', 'en': 'Gries im Sellrain'},
'435239':{'de': u('K\u00fchtai'), 'en': u('K\u00fchtai')},
'435238':{'de': 'Zirl', 'en': 'Zirl'},
'39035':{'en': 'Bergamo', 'it': 'Bergamo'},
'390142':{'it': 'Casale Monferrato'},
'3324705':{'en': 'Tours', 'fr': 'Tours'},
'39030':{'en': 'Brescia', 'it': 'Brescia'},
'39031':{'en': 'Como', 'it': 'Como'},
'432715':{'de': u('Wei\u00dfenkirchen in der Wachau'), 'en': 'Weissenkirchen in der Wachau'},
'359848':{'bg': u('\u041a\u0443\u0431\u0440\u0430\u0442'), 'en': 'Kubrat'},
'3338190':{'en': u('Montb\u00e9liard'), 'fr': u('Montb\u00e9liard')},
'3338191':{'en': u('Montb\u00e9liard'), 'fr': u('Montb\u00e9liard')},
'3338192':{'en': 'Pont-de-Roide', 'fr': 'Pont-de-Roide'},
'3338194':{'en': u('Montb\u00e9liard'), 'fr': u('Montb\u00e9liard')},
'3338195':{'en': u('Montb\u00e9liard'), 'fr': u('Montb\u00e9liard')},
'3338197':{'en': u('Montb\u00e9liard'), 'fr': u('Montb\u00e9liard')},
'3338198':{'en': u('Montb\u00e9liard'), 'fr': u('Montb\u00e9liard')},
'3338199':{'en': u('Montb\u00e9liard'), 'fr': u('Montb\u00e9liard')},
'3347479':{'en': 'Beaurepaire', 'fr': 'Beaurepaire'},
'3347478':{'en': 'Vienne', 'fr': 'Vienne'},
'3892':{'en': 'Skopje'},
'3347473':{'en': 'Oyonnax', 'fr': 'Oyonnax'},
'3347475':{'en': 'Nantua', 'fr': 'Nantua'},
'3347477':{'en': 'Oyonnax', 'fr': 'Oyonnax'},
'3347476':{'en': u('Montr\u00e9al-la-Cluse'), 'fr': u('Montr\u00e9al-la-Cluse')},
'3346788':{'en': u('Clermont-l\'H\u00e9rault'), 'fr': u('Clermont-l\'H\u00e9rault')},
'3346789':{'en': 'Cessenon-sur-Orb', 'fr': 'Cessenon-sur-Orb'},
'3346780':{'en': 'Frontignan', 'fr': 'Frontignan'},
'3346781':{'en': 'Le Vigan', 'fr': 'Le Vigan'},
'3346783':{'en': 'Lunel', 'fr': 'Lunel'},
'3346784':{'en': u('Saint-G\u00e9ly-du-Fesc'), 'fr': u('Saint-G\u00e9ly-du-Fesc')},
'3346785':{'en': u('Fabr\u00e8gues'), 'fr': u('Fabr\u00e8gues')},
'434286':{'de': u('Wei\u00dfbriach'), 'en': 'Weissbriach'},
'437948':{'de': u('Hirschbach im M\u00fchlkreis'), 'en': u('Hirschbach im M\u00fchlkreis')},
'3347783':{'en': 'Rive-de-Gier', 'fr': 'Rive-de-Gier'},
'3347781':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347780':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347789':{'en': 'Firminy', 'fr': 'Firminy'},
'3594126':{'bg': u('\u0425\u0440\u0438\u0449\u0435\u043d\u0438'), 'en': 'Hrishteni'},
'435256':{'de': 'Untergurgl', 'en': 'Untergurgl'},
'37428695':{'am': u('\u0547\u057e\u0561\u0576\u056b\u0571\u0578\u0580'), 'en': 'Shvanidzor', 'ru': u('\u0428\u0432\u0430\u043d\u0438\u0434\u0437\u043e\u0440')},
'435255':{'de': 'Umhausen', 'en': 'Umhausen'},
'437230':{'de': 'Altenberg bei Linz', 'en': 'Altenberg bei Linz'},
'435252':{'de': 'Oetz', 'en': 'Oetz'},
'441746':{'en': 'Bridgnorth'},
'441747':{'en': 'Shaftesbury'},
'441744':{'en': 'St Helens'},
'436563':{'de': 'Uttendorf', 'en': 'Uttendorf'},
'441743':{'en': 'Shrewsbury'},
'441740':{'en': 'Sedgefield'},
'441748':{'en': 'Richmond'},
'441749':{'en': 'Shepton Mallet'},
'3316494':{'en': u('\u00c9tampes'), 'fr': u('\u00c9tampes')},
'3316497':{'en': u('\u00c9vry'), 'fr': u('\u00c9vry')},
'3316496':{'en': 'Corbeil-Essonnes', 'fr': 'Corbeil-Essonnes'},
'3316491':{'en': 'Limours en Hurepoix', 'fr': 'Limours en Hurepoix'},
'3316490':{'en': 'Arpajon', 'fr': 'Arpajon'},
'3316493':{'en': 'Ballancourt-sur-Essonne', 'fr': 'Ballancourt-sur-Essonne'},
'3316492':{'en': 'Arpajon', 'fr': 'Arpajon'},
'3316499':{'en': 'Mennecy', 'fr': 'Mennecy'},
'3316498':{'en': u('Milly-la-For\u00eat'), 'fr': u('Milly-la-For\u00eat')},
'3349033':{'en': u('B\u00e9darrides'), 'fr': u('B\u00e9darrides')},
'3338937':{'en': 'Thann', 'fr': 'Thann'},
'3349031':{'en': 'Le Pontet', 'fr': 'Le Pontet'},
'3349030':{'en': u('Boll\u00e8ne'), 'fr': u('Boll\u00e8ne')},
'3349037':{'en': 'Camaret-sur-Aigues', 'fr': 'Camaret-sur-Aigues'},
'3349036':{'en': 'Vaison-la-Romaine', 'fr': 'Vaison-la-Romaine'},
'3349035':{'en': u('Valr\u00e9as'), 'fr': u('Valr\u00e9as')},
'3349034':{'en': 'Orange', 'fr': 'Orange'},
'3349039':{'en': 'Sorgues', 'fr': 'Sorgues'},
'3349038':{'en': 'L\'Isle sur la Sorgue', 'fr': 'L\'Isle sur la Sorgue'},
'3751631':{'be': u('\u041a\u0430\u043c\u044f\u043d\u0435\u0446, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Kamenets, Brest Region', 'ru': u('\u041a\u0430\u043c\u0435\u043d\u0435\u0446, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751632':{'be': u('\u041f\u0440\u0443\u0436\u0430\u043d\u044b, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Pruzhany, Brest Region', 'ru': u('\u041f\u0440\u0443\u0436\u0430\u043d\u044b, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751633':{'be': u('\u041b\u044f\u0445\u0430\u0432\u0456\u0447\u044b, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Lyakhovichi, Brest Region', 'ru': u('\u041b\u044f\u0445\u043e\u0432\u0438\u0447\u0438, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3342777':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3349961':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3349963':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3349962':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3349964':{'en': 'Montpellier', 'fr': 'Montpellier'},
'390363':{'en': 'Bergamo', 'it': 'Treviglio'},
'390362':{'en': 'Cremona/Monza', 'it': 'Seregno'},
'390365':{'en': 'Brescia', 'it': u('Sal\u00f2')},
'390364':{'en': 'Brescia', 'it': 'Breno'},
'3597058':{'bg': u('\u0421\u0442\u043e\u0431'), 'en': 'Stob'},
'441335':{'en': 'Ashbourne'},
'441334':{'en': 'St Andrews'},
'441333':{'en': 'Peat Inn (Leven (Fife))'},
'441332':{'en': 'Derby'},
'441330':{'en': 'Banchory'},
'3522767':{'de': u('D\u00fcdelingen'), 'en': 'Dudelange', 'fr': 'Dudelange'},
'3597052':{'bg': u('\u041f\u0430\u0441\u0442\u0440\u0430'), 'en': 'Pastra'},
'3597053':{'bg': u('\u041a\u043e\u0447\u0435\u0440\u0438\u043d\u043e\u0432\u043e'), 'en': 'Kocherinovo'},
'3597054':{'bg': u('\u0420\u0438\u043b\u0430'), 'en': 'Rila'},
'3338246':{'en': 'Briey', 'fr': 'Briey'},
'3597056':{'bg': u('\u041c\u0443\u0440\u0441\u0430\u043b\u0435\u0432\u043e'), 'en': 'Mursalevo'},
'3597057':{'bg': u('\u041c\u0430\u043b\u043e \u0441\u0435\u043b\u043e'), 'en': 'Malo selo'},
'437237':{'de': 'Sankt Georgen an der Gusen', 'en': 'St. Georgen an der Gusen'},
'333272':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3338858':{'en': u('S\u00e9lestat'), 'fr': u('S\u00e9lestat')},
'3323106':{'en': 'Caen', 'fr': 'Caen'},
'3332188':{'en': 'Saint-Omer', 'fr': 'Saint-Omer'},
'3338851':{'en': 'Brumath', 'fr': 'Brumath'},
'3752344':{'be': u('\u0411\u0440\u0430\u0433\u0456\u043d, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Bragin, Gomel Region', 'ru': u('\u0411\u0440\u0430\u0433\u0438\u043d, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3323109':{'en': 'Vire', 'fr': 'Vire'},
'3338852':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338855':{'en': 'Geispolsheim', 'fr': 'Geispolsheim'},
'3338854':{'en': 'Betschdorf', 'fr': 'Betschdorf'},
'3338856':{'en': 'Oberhausbergen', 'fr': 'Oberhausbergen'},
'3332099':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332098':{'en': u('Marcq-en-Bar\u0153ul'), 'fr': u('Marcq-en-Bar\u0153ul')},
'432768':{'de': 'Sankt Aegyd am Neuwalde', 'en': 'St. Aegyd am Neuwalde'},
'432769':{'de': u('T\u00fcrnitz'), 'en': u('T\u00fcrnitz')},
'3332093':{'en': 'Lille', 'fr': 'Lille'},
'3332092':{'en': 'Lomme', 'fr': 'Lomme'},
'3332091':{'en': 'Villeneuve-d\'Ascq', 'fr': 'Villeneuve-d\'Ascq'},
'3332090':{'en': 'Seclin', 'fr': 'Seclin'},
'432762':{'de': 'Lilienfeld', 'en': 'Lilienfeld'},
'432763':{'de': u('Sankt Veit an der G\u00f6lsen'), 'en': u('St. Veit an der G\u00f6lsen')},
'3332095':{'en': 'Wattignies', 'fr': 'Wattignies'},
'3332094':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3354688':{'en': 'Rochefort', 'fr': 'Rochefort'},
'3354687':{'en': 'Rochefort', 'fr': 'Rochefort'},
'3354684':{'en': 'Fouras', 'fr': 'Fouras'},
'3354685':{'en': 'Marennes', 'fr': 'Marennes'},
'3354682':{'en': 'Rochefort', 'fr': 'Rochefort'},
'3355959':{'en': 'Bayonne', 'fr': 'Bayonne'},
'3355958':{'en': 'Anglet', 'fr': 'Anglet'},
'3355379':{'en': 'Tonneins', 'fr': 'Tonneins'},
'3355371':{'en': 'Fumel', 'fr': 'Fumel'},
'3355370':{'en': 'Villeneuve-sur-Lot', 'fr': 'Villeneuve-sur-Lot'},
'3355373':{'en': 'Bergerac', 'fr': 'Bergerac'},
'3355375':{'en': 'Montayral', 'fr': 'Montayral'},
'3355374':{'en': 'Bergerac', 'fr': 'Bergerac'},
'3355377':{'en': 'Agen', 'fr': 'Agen'},
'3329849':{'en': 'Brest', 'fr': 'Brest'},
'34969':{'en': 'Cuenca', 'es': 'Cuenca'},
'3355950':{'en': 'Bayonne', 'fr': 'Bayonne'},
'3354971':{'en': 'Parthenay', 'fr': 'Parthenay'},
'3354973':{'en': 'Niort', 'fr': 'Niort'},
'3354974':{'en': 'Bressuire', 'fr': 'Bressuire'},
'3329846':{'en': 'Brest', 'fr': 'Brest'},
'3354977':{'en': 'Niort', 'fr': 'Niort'},
'434782':{'de': 'Obervellach', 'en': 'Obervellach'},
'3359052':{'en': u('Saint Barth\u00e9l\u00e9my'), 'fr': u('Saint Barth\u00e9l\u00e9my')},
'3329847':{'en': 'Brest', 'fr': 'Brest'},
'434783':{'de': u('Rei\u00dfeck'), 'en': 'Reisseck'},
'3329840':{'en': 'Plougastel-Daoulas', 'fr': 'Plougastel-Daoulas'},
'3329842':{'en': 'Brest', 'fr': 'Brest'},
'3593538':{'bg': u('\u0415\u043b\u0448\u0438\u0446\u0430'), 'en': 'Elshitsa'},
'3593537':{'bg': u('\u041f\u0430\u043d\u0430\u0433\u044e\u0440\u0441\u043a\u0438 \u043a\u043e\u043b\u043e\u043d\u0438\u0438'), 'en': 'Panagyurski kolonii'},
'3593536':{'bg': u('\u0411\u0430\u043d\u044f, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Banya, Pazardzhik'},
'3593535':{'bg': u('\u041b\u0435\u0432\u0441\u043a\u0438, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Levski, Pazardzhik'},
'3329843':{'en': 'Brest', 'fr': 'Brest'},
'3593533':{'bg': u('\u0411\u044a\u0442\u0430'), 'en': 'Bata'},
'3593532':{'bg': u('\u0421\u0442\u0440\u0435\u043b\u0447\u0430'), 'en': 'Strelcha'},
'3593530':{'bg': u('\u041f\u043e\u0438\u0431\u0440\u0435\u043d\u0435'), 'en': 'Poibrene'},
'434784':{'de': 'Mallnitz', 'en': 'Mallnitz'},
'434785':{'de': u('Au\u00dferfragant'), 'en': 'Ausserfragant'},
'3329998':{'en': u('Louvign\u00e9-du-D\u00e9sert'), 'fr': u('Louvign\u00e9-du-D\u00e9sert')},
'3329999':{'en': u('Foug\u00e8res'), 'fr': u('Foug\u00e8res')},
'3355883':{'en': 'Biscarrosse', 'fr': 'Biscarrosse'},
'3355882':{'en': 'Biscarrosse', 'fr': 'Biscarrosse'},
'3329994':{'en': u('Foug\u00e8res'), 'fr': u('Foug\u00e8res')},
'3329992':{'en': 'Guignen', 'fr': 'Guignen'},
'3329993':{'en': 'Carentoir', 'fr': 'Carentoir'},
'3355885':{'en': 'Mont-de-Marsan', 'fr': 'Mont-de-Marsan'},
'3341320':{'en': 'Marseille', 'fr': 'Marseille'},
'3593759':{'bg': u('\u0411\u043e\u0440\u0438\u0441\u043b\u0430\u0432\u0446\u0438'), 'en': 'Borislavtsi'},
'3593758':{'bg': u('\u0419\u0435\u0440\u0443\u0441\u0430\u043b\u0438\u043c\u043e\u0432\u043e'), 'en': 'Yerusalimovo'},
'3593753':{'bg': u('\u041e\u0440\u044f\u0445\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Oryahovo, Hask.'},
'3593752':{'bg': u('\u041c\u0430\u043b\u043a\u043e \u0433\u0440\u0430\u0434\u0438\u0449\u0435'), 'en': 'Malko gradishte'},
'3593751':{'bg': u('\u041b\u044e\u0431\u0438\u043c\u0435\u0446'), 'en': 'Lyubimets'},
'3593757':{'bg': u('\u0413\u0435\u043e\u0440\u0433\u0438 \u0414\u043e\u0431\u0440\u0435\u0432\u043e'), 'en': 'Georgi Dobrevo'},
'3593756':{'bg': u('\u0412\u044a\u043b\u0447\u0435 \u043f\u043e\u043b\u0435'), 'en': 'Valche pole'},
'3593755':{'bg': u('\u0411\u0435\u043b\u0438\u0446\u0430, \u0425\u0430\u0441\u043a.'), 'en': 'Belitsa, Hask.'},
'3593754':{'bg': u('\u041b\u043e\u0437\u0435\u043d, \u0425\u0430\u0441\u043a.'), 'en': 'Lozen, Hask.'},
'3325397':{'en': 'Nantes', 'fr': 'Nantes'},
'3334494':{'en': u('Cr\u00e9py-en-Valois'), 'fr': u('Cr\u00e9py-en-Valois')},
'3334497':{'en': u('Compi\u00e8gne'), 'fr': u('Compi\u00e8gne')},
'3334496':{'en': 'Thourotte', 'fr': 'Thourotte'},
'3334491':{'en': 'La Croix-Saint-Ouen', 'fr': 'La Croix-Saint-Ouen'},
'3334490':{'en': u('Compi\u00e8gne'), 'fr': u('Compi\u00e8gne')},
'3334493':{'en': 'Noyon', 'fr': 'Noyon'},
'359478':{'bg': u('\u0415\u043b\u0445\u043e\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Elhovo, Yambol'},
'359470':{'bg': u('\u0422\u043e\u043f\u043e\u043b\u043e\u0432\u0433\u0440\u0430\u0434'), 'en': 'Topolovgrad'},
'441538':{'en': 'Ipstones'},
'441535':{'en': 'Keighley'},
'441534':{'en': 'Jersey'},
'441536':{'en': 'Kettering'},
'441531':{'en': 'Ledbury'},
'441530':{'en': 'Coalville'},
'3596956':{'bg': u('\u041b\u043e\u043c\u0435\u0446, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Lomets, Lovech'},
'3596957':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u0430 \u0416\u0435\u043b\u044f\u0437\u043d\u0430'), 'en': 'Golyama Zhelyazna'},
'3596954':{'bg': u('\u0412\u0440\u0430\u0431\u0435\u0432\u043e'), 'en': 'Vrabevo'},
'3596955':{'bg': u('\u0414\u044a\u043b\u0431\u043e\u043a \u0434\u043e\u043b'), 'en': 'Dalbok dol'},
'3329942':{'en': u('Laill\u00e9'), 'fr': u('Laill\u00e9')},
'3596953':{'bg': u('\u0411\u043e\u0440\u0438\u043c\u0430'), 'en': 'Borima'},
'3596950':{'bg': u('\u0413\u0443\u043c\u043e\u0449\u043d\u0438\u043a'), 'en': 'Gumoshtnik'},
'3596958':{'bg': u('\u0410\u043f\u0440\u0438\u043b\u0446\u0438, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Apriltsi, Lovech'},
'3596959':{'bg': u('\u0414\u0435\u0431\u043d\u0435\u0432\u043e'), 'en': 'Debnevo'},
'35966':{'bg': u('\u0413\u0430\u0431\u0440\u043e\u0432\u043e'), 'en': 'Gabrovo'},
'35964':{'bg': u('\u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Pleven'},
'35962':{'bg': u('\u0412\u0435\u043b\u0438\u043a\u043e \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Veliko Tarnovo'},
'35968':{'bg': u('\u041b\u043e\u0432\u0435\u0447'), 'en': 'Lovech'},
'3595755':{'bg': u('\u041a\u043e\u043b\u0430\u0440\u0446\u0438'), 'en': 'Kolartsi'},
'3595754':{'bg': u('\u0417\u044a\u0440\u043d\u0435\u0432\u043e'), 'en': 'Zarnevo'},
'3347868':{'en': 'Villeurbanne', 'fr': 'Villeurbanne'},
'3347869':{'en': 'Lyon', 'fr': 'Lyon'},
'3595751':{'bg': u('\u0422\u0435\u0440\u0432\u0435\u043b, \u0414\u043e\u0431\u0440.'), 'en': 'Tervel, Dobr.'},
'3595750':{'bg': u('\u041a\u0430\u0431\u043b\u0435\u0448\u043a\u043e\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Kableshkovo, Dobr.'},
'3347648':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347649':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347862':{'en': 'Lyon', 'fr': 'Lyon'},
'3347647':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347860':{'en': 'Lyon', 'fr': 'Lyon'},
'3347861':{'en': 'Lyon', 'fr': 'Lyon'},
'3347866':{'en': 'Dardilly', 'fr': 'Dardilly'},
'3347643':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347864':{'en': 'Lyon', 'fr': 'Lyon'},
'3347865':{'en': 'Lyon', 'fr': 'Lyon'},
'435576':{'de': 'Hohenems', 'en': 'Hohenems'},
'435577':{'de': 'Lustenau', 'en': 'Lustenau'},
'435574':{'de': 'Bregenz', 'en': 'Bregenz'},
'432633':{'de': 'Markt Piesting', 'en': 'Markt Piesting'},
'435572':{'de': 'Dornbirn', 'en': 'Dornbirn'},
'435573':{'de': u('H\u00f6rbranz'), 'en': u('H\u00f6rbranz')},
'373236':{'en': 'Ungheni', 'ro': 'Ungheni', 'ru': u('\u0423\u043d\u0433\u0435\u043d\u044c')},
'373237':{'en': 'Straseni', 'ro': u('Str\u0103\u015feni'), 'ru': u('\u0421\u0442\u0440\u044d\u0448\u0435\u043d\u044c')},
'373235':{'en': 'Orhei', 'ro': 'Orhei', 'ru': u('\u041e\u0440\u0445\u0435\u0439')},
'373230':{'en': 'Soroca', 'ro': 'Soroca', 'ru': u('\u0421\u043e\u0440\u043e\u043a\u0430')},
'373231':{'en': u('Bal\u0163i'), 'ro': u('B\u0103l\u0163i'), 'ru': u('\u0411\u044d\u043b\u0446\u044c')},
'3324049':{'en': 'Nantes', 'fr': 'Nantes'},
'3338038':{'en': 'Dijon', 'fr': 'Dijon'},
'3324043':{'en': 'Nantes', 'fr': 'Nantes'},
'3338032':{'en': 'Brazey-en-Plaine', 'fr': 'Brazey-en-Plaine'},
'3338031':{'en': 'Auxonne', 'fr': 'Auxonne'},
'3338030':{'en': 'Dijon', 'fr': 'Dijon'},
'3338037':{'en': 'Auxonne', 'fr': 'Auxonne'},
'3324046':{'en': 'Nantes', 'fr': 'Nantes'},
'3324045':{'en': 'Donges', 'fr': 'Donges'},
'3338034':{'en': 'Gevrey-Chambertin', 'fr': 'Gevrey-Chambertin'},
'3332453':{'en': 'Nouzonville', 'fr': 'Nouzonville'},
'3597178':{'bg': u('\u041f\u0440\u043e\u043b\u0435\u0448\u0430'), 'en': 'Prolesha'},
'3742667':{'am': u('\u0532\u0565\u0580\u0564\u0561\u057e\u0561\u0576'), 'en': 'Berdavan', 'ru': u('\u0411\u0435\u0440\u0434\u0430\u0432\u0430\u043d')},
'3742665':{'am': u('\u053f\u0578\u0572\u0562'), 'en': 'Koghb', 'ru': u('\u041a\u043e\u0445\u0431')},
'436274':{'de': 'Lamprechtshausen', 'en': 'Lamprechtshausen'},
'436276':{'de': u('Nu\u00dfdorf am Haunsberg'), 'en': 'Nussdorf am Haunsberg'},
'436277':{'de': 'Sankt Pantaleon', 'en': 'St. Pantaleon'},
'436272':{'de': 'Oberndorf bei Salzburg', 'en': 'Oberndorf bei Salzburg'},
'3332456':{'en': u('Charleville-M\u00e9zi\u00e8res'), 'fr': u('Charleville-M\u00e9zi\u00e8res')},
'334700':{'en': u('Montlu\u00e7on'), 'fr': u('Montlu\u00e7on')},
'3597533':{'bg': u('\u041e\u0441\u0438\u043a\u043e\u0432\u043e, \u0411\u043b\u0430\u0433.'), 'en': 'Osikovo, Blag.'},
'3597532':{'bg': u('\u0411\u0443\u043a\u043e\u0432\u043e, \u0411\u043b\u0430\u0433.'), 'en': 'Bukovo, Blag.'},
'3597531':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u0414\u0440\u044f\u043d\u043e\u0432\u043e'), 'en': 'Dolno Dryanovo'},
'3329679':{'en': 'Pordic', 'fr': 'Pordic'},
'3329678':{'en': 'Saint-Brieuc', 'fr': 'Saint-Brieuc'},
'3329673':{'en': 'Binic', 'fr': 'Binic'},
'3329672':{'en': 'Erquy', 'fr': 'Erquy'},
'3329671':{'en': u('Tr\u00e9gueux'), 'fr': u('Tr\u00e9gueux')},
'3329670':{'en': 'Saint-Quay-Portrieux', 'fr': 'Saint-Quay-Portrieux'},
'3329677':{'en': 'Saint-Brieuc', 'fr': 'Saint-Brieuc'},
'3329676':{'en': 'Ploufragan', 'fr': 'Ploufragan'},
'3329675':{'en': 'Saint-Brieuc', 'fr': 'Saint-Brieuc'},
'3329674':{'en': u('Pl\u00e9rin'), 'fr': u('Pl\u00e9rin')},
'3338449':{'en': 'Fougerolles', 'fr': 'Fougerolles'},
'3338442':{'en': 'Moirans-en-Montagne', 'fr': 'Moirans-en-Montagne'},
'3338443':{'en': 'Lons-le-Saunier', 'fr': 'Lons-le-Saunier'},
'3338440':{'en': 'Luxeuil-les-Bains', 'fr': 'Luxeuil-les-Bains'},
'3338446':{'en': u('H\u00e9ricourt'), 'fr': u('H\u00e9ricourt')},
'3338447':{'en': 'Lons-le-Saunier', 'fr': 'Lons-le-Saunier'},
'3338445':{'en': 'Saint-Claude', 'fr': 'Saint-Claude'},
'441580':{'en': 'Cranbrook'},
'35961107':{'bg': u('\u041c\u043e\u043c\u0438\u043d \u0441\u0431\u043e\u0440'), 'en': 'Momin sbor'},
'35961106':{'bg': u('\u041d\u043e\u0432\u043e \u0441\u0435\u043b\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Novo selo, V. Tarnovo'},
'35961105':{'bg': u('\u041f\u0440\u0438\u0441\u043e\u0432\u043e'), 'en': 'Prisovo'},
'35961104':{'bg': u('\u0412\u043e\u0434\u043e\u043b\u0435\u0439'), 'en': 'Vodoley'},
'35961103':{'bg': u('\u0420\u0443\u0441\u0430\u043b\u044f'), 'en': 'Rusalya'},
'35961102':{'bg': u('\u041f\u0447\u0435\u043b\u0438\u0449\u0435'), 'en': 'Pchelishte'},
'35961101':{'bg': u('\u0412\u0435\u043b\u0447\u0435\u0432\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Velchevo, V. Tarnovo'},
'3595522':{'bg': u('\u0418\u0441\u043a\u0440\u0430, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Iskra, Burgas'},
'35961109':{'bg': u('\u0412\u044a\u0433\u043b\u0435\u0432\u0446\u0438'), 'en': 'Vaglevtsi'},
'3323562':{'en': 'Rouen', 'fr': 'Rouen'},
'3596967':{'bg': u('\u041a\u0430\u043b\u0435\u0439\u0446\u0430'), 'en': 'Kaleytsa'},
'3347104':{'en': 'Le Puy en Velay', 'fr': 'Le Puy en Velay'},
'3347107':{'en': 'Le Puy-en-Velay', 'fr': 'Le Puy-en-Velay'},
'3596964':{'bg': u('\u0412\u0435\u043b\u0447\u0435\u0432\u043e, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Velchevo, Lovech'},
'3596963':{'bg': u('\u0411\u0430\u043b\u043a\u0430\u043d\u0435\u0446'), 'en': 'Balkanets'},
'3596962':{'bg': u('\u0427\u0435\u0440\u043d\u0438 \u041e\u0441\u044a\u043c'), 'en': 'Cherni Osam'},
'3347450':{'en': 'Vonnas', 'fr': 'Vonnas'},
'441583':{'en': 'Carradale'},
'3752132':{'be': u('\u041b\u0435\u043f\u0435\u043b\u044c'), 'en': 'Lepel', 'ru': u('\u041b\u0435\u043f\u0435\u043b\u044c')},
'3347677':{'en': u('Dom\u00e8ne'), 'fr': u('Dom\u00e8ne')},
'3752130':{'be': u('\u0428\u0443\u043c\u0456\u043b\u0456\u043d\u0430, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Shumilino, Vitebsk Region', 'ru': u('\u0428\u0443\u043c\u0438\u043b\u0438\u043d\u043e, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752131':{'be': u('\u0411\u0435\u0448\u0430\u043d\u043a\u043e\u0432\u0456\u0447\u044b, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Beshenkovichi, Vitebsk Region', 'ru': u('\u0411\u0435\u0448\u0435\u043d\u043a\u043e\u0432\u0438\u0447\u0438, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3341363':{'en': 'Marseille', 'fr': 'Marseille'},
'3752137':{'be': u('\u0414\u0443\u0431\u0440\u043e\u045e\u043d\u0430, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Dubrovno, Vitebsk Region', 'ru': u('\u0414\u0443\u0431\u0440\u043e\u0432\u043d\u043e, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752135':{'be': u('\u0421\u044f\u043d\u043d\u043e, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Senno, Vitebsk Region', 'ru': u('\u0421\u0435\u043d\u043d\u043e, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752138':{'be': u('\u041b\u0451\u0437\u043d\u0430, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Liozno, Vitebsk Region', 'ru': u('\u041b\u0438\u043e\u0437\u043d\u043e, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752139':{'be': u('\u0413\u0430\u0440\u0430\u0434\u043e\u043a, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Gorodok, Vitebsk Region', 'ru': u('\u0413\u043e\u0440\u043e\u0434\u043e\u043a, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3347675':{'en': u('Saint-Egr\u00e8ve'), 'fr': u('Saint-Egr\u00e8ve')},
'34828':{'en': 'Las Palmas', 'es': 'Las Palmas'},
'34820':{'en': u('\u00c1vila'), 'es': u('\u00c1vila')},
'34821':{'en': 'Segovia', 'es': 'Segovia'},
'34822':{'en': 'Tenerife', 'es': 'Tenerife'},
'34823':{'en': 'Salamanca', 'es': 'Salamanca'},
'34824':{'en': 'Badajoz', 'es': 'Badajoz'},
'34825':{'en': 'Toledo', 'es': 'Toledo'},
'34826':{'en': 'Ciudad Real', 'es': 'Ciudad Real'},
'3347672':{'en': 'Vif', 'fr': 'Vif'},
'3332153':{'en': u('Bruay-la-Buissi\u00e8re'), 'fr': u('Bruay-la-Buissi\u00e8re')},
'3347670':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3332156':{'en': u('B\u00e9thune'), 'fr': u('B\u00e9thune')},
'3332157':{'en': u('B\u00e9thune'), 'fr': u('B\u00e9thune')},
'3332154':{'en': 'Lillers', 'fr': 'Lillers'},
'441290':{'en': 'Cumnock'},
'441291':{'en': 'Chepstow'},
'441292':{'en': 'Ayr'},
'441293':{'en': 'Crawley'},
'441294':{'en': 'Ardrossan'},
'441295':{'en': 'Banbury'},
'441296':{'en': 'Aylesbury'},
'441297':{'en': 'Axminster'},
'441298':{'en': 'Buxton'},
'3354764':{'en': 'Anglet', 'fr': 'Anglet'},
'3349572':{'en': 'Porto-Vecchio', 'fr': 'Porto-Vecchio'},
'3349573':{'en': 'Bonifacio', 'fr': 'Bonifacio'},
'3349570':{'en': 'Porto-Vecchio', 'fr': 'Porto-Vecchio'},
'3349576':{'en': 'Propriano', 'fr': 'Propriano'},
'3349577':{'en': u('Sart\u00e8ne'), 'fr': u('Sart\u00e8ne')},
'3345017':{'en': 'Thonon-les-Bains', 'fr': 'Thonon-les-Bains'},
'3345019':{'en': u('S\u00e9vrier'), 'fr': u('S\u00e9vrier')},
'3349578':{'en': 'Levie', 'fr': 'Levie'},
'35951103':{'bg': u('\u041b\u044e\u0431\u0435\u043d \u041a\u0430\u0440\u0430\u0432\u0435\u043b\u043e\u0432\u043e'), 'en': 'Lyuben Karavelovo'},
'441239':{'en': 'Cardigan'},
'3348999':{'en': u('Fr\u00e9jus'), 'fr': u('Fr\u00e9jus')},
'3348996':{'en': 'Toulon', 'fr': 'Toulon'},
'3348992':{'en': 'Nice', 'fr': 'Nice'},
'441235':{'en': 'Abingdon'},
'3325490':{'en': 'Blois', 'fr': 'Blois'},
'3325497':{'en': 'Salbris', 'fr': 'Salbris'},
'433359':{'de': 'Loipersdorf-Kitzladen', 'en': 'Loipersdorf-Kitzladen'},
'3325495':{'en': 'Romorantin-Lanthenay', 'fr': 'Romorantin-Lanthenay'},
'433354':{'de': 'Bernstein', 'en': 'Bernstein'},
'433355':{'de': 'Stadtschlaining', 'en': 'Stadtschlaining'},
'433356':{'de': 'Markt Allhau', 'en': 'Markt Allhau'},
'433357':{'de': 'Pinkafeld', 'en': 'Pinkafeld'},
'433352':{'de': 'Oberwart', 'en': 'Oberwart'},
'433353':{'de': u('Obersch\u00fctzen'), 'en': u('Obersch\u00fctzen')},
'35936402':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u043a\u0443\u043b\u0430'), 'en': 'Gorna kula'},
'3324014':{'en': 'Nantes', 'fr': 'Nantes'},
'3324720':{'en': 'Tours', 'fr': 'Tours'},
'3324721':{'en': 'Tours', 'fr': 'Tours'},
'3324727':{'en': 'Saint-Avertin', 'fr': 'Saint-Avertin'},
'3338065':{'en': 'Dijon', 'fr': 'Dijon'},
'435213':{'de': 'Scharnitz', 'en': 'Scharnitz'},
'435212':{'de': 'Seefeld in Tirol', 'en': 'Seefeld in Tirol'},
'3324728':{'en': u('Chambray-l\u00e8s-Tours'), 'fr': u('Chambray-l\u00e8s-Tours')},
'3324016':{'en': 'Nantes', 'fr': 'Nantes'},
'435214':{'de': 'Leutasch', 'en': 'Leutasch'},
'35881':{'en': 'Oulu', 'fi': 'Oulu', 'se': u('Ule\u00e5borg')},
'3356199':{'en': 'Toulouse', 'fr': 'Toulouse'},
'35883':{'en': 'Oulu', 'fi': 'Oulu', 'se': u('Ule\u00e5borg')},
'35882':{'en': 'Oulu', 'fi': 'Oulu', 'se': u('Ule\u00e5borg')},
'35885':{'en': 'Oulu', 'fi': 'Oulu', 'se': u('Ule\u00e5borg')},
'35884':{'en': 'Oulu', 'fi': 'Oulu', 'se': u('Ule\u00e5borg')},
'35887':{'en': 'Oulu', 'fi': 'Oulu', 'se': u('Ule\u00e5borg')},
'35886':{'en': 'Oulu', 'fi': 'Oulu', 'se': u('Ule\u00e5borg')},
'35888':{'en': 'Oulu', 'fi': 'Oulu', 'se': u('Ule\u00e5borg')},
'3356192':{'en': 'Cugnaux', 'fr': 'Cugnaux'},
'3356195':{'en': 'Saint-Gaudens', 'fr': 'Saint-Gaudens'},
'3356197':{'en': u('Caz\u00e8res'), 'fr': u('Caz\u00e8res')},
'359866':{'bg': u('\u0422\u0443\u0442\u0440\u0430\u043a\u0430\u043d'), 'en': 'Tutrakan'},
'359864':{'bg': u('\u0414\u0443\u043b\u043e\u0432\u043e'), 'en': 'Dulovo'},
'3346676':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3338063':{'en': 'Dijon', 'fr': 'Dijon'},
'35974204':{'bg': u('\u0413\u0435\u0433\u0430'), 'en': 'Gega'},
'35974202':{'bg': u('\u041a\u043b\u044e\u0447'), 'en': 'Klyuch'},
'35974203':{'bg': u('\u0420\u0443\u043f\u0438\u0442\u0435'), 'en': 'Rupite'},
'3356334':{'en': 'Graulhet', 'fr': 'Graulhet'},
'3356335':{'en': 'Castres', 'fr': 'Castres'},
'3356336':{'en': 'Carmaux', 'fr': 'Carmaux'},
'3356337':{'en': 'Lacaune', 'fr': 'Lacaune'},
'3356332':{'en': 'Castelsarrasin', 'fr': 'Castelsarrasin'},
'3338068':{'en': 'Dijon', 'fr': 'Dijon'},
'35974201':{'bg': u('\u041a\u0430\u043f\u0430\u0442\u043e\u0432\u043e'), 'en': 'Kapatovo'},
'3356338':{'en': 'Albi', 'fr': 'Albi'},
'3356339':{'en': 'Valence', 'fr': 'Valence'},
'3347412':{'en': 'Oyonnax', 'fr': 'Oyonnax'},
'3347411':{'en': 'Salaise-sur-Sanne', 'fr': 'Salaise-sur-Sanne'},
'3347416':{'en': 'Vienne', 'fr': 'Vienne'},
'3347414':{'en': 'Bourg-en-Bresse', 'fr': 'Bourg-en-Bresse'},
'3347419':{'en': 'Bourgoin-Jallieu', 'fr': 'Bourgoin-Jallieu'},
'35971506':{'bg': u('\u0413\u0430\u0431\u0440\u0430'), 'en': 'Gabra'},
'35971504':{'bg': u('\u0411\u0435\u043b\u043e\u043f\u043e\u043f\u0446\u0438'), 'en': 'Belopoptsi'},
'35971505':{'bg': u('\u0427\u0443\u0440\u0435\u043a'), 'en': 'Churek'},
'35971502':{'bg': u('\u0415\u043b\u0435\u0448\u043d\u0438\u0446\u0430, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Eleshnitsa, Sofia'},
'35971503':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u041a\u0430\u043c\u0430\u0440\u0446\u0438'), 'en': 'Dolno Kamartsi'},
'35225':{'de': 'Luxemburg', 'en': 'Luxembourg', 'fr': 'Luxembourg'},
'35224':{'de': 'Luxemburg', 'en': 'Luxembourg', 'fr': 'Luxembourg'},
'35555':{'en': u('Kavaj\u00eb')},
'35554':{'en': 'Elbasan'},
'35553':{'en': u('La\u00e7, Kurbin')},
'35552':{'en': u('Durr\u00ebs')},
'35223':{'de': 'Bad Mondorf', 'en': 'Mondorf-les-Bains/Bascharage/Noerdange/Remich', 'fr': 'Mondorf-les-Bains/Bascharage/Noerdange/Remich'},
'35222':{'de': 'Luxemburg', 'en': 'Luxembourg City', 'fr': 'Luxembourg-Ville'},
'3596030':{'bg': u('\u0421\u0432\u0435\u0442\u043b\u0435\u043d, \u0422\u044a\u0440\u0433.'), 'en': 'Svetlen, Targ.'},
'3596033':{'bg': u('\u041c\u0435\u0434\u043e\u0432\u0438\u043d\u0430'), 'en': 'Medovina'},
'3596032':{'bg': u('\u0417\u0430\u0440\u0430\u0435\u0432\u043e'), 'en': 'Zaraevo'},
'35229':{'de': 'Luxemburg', 'en': 'Luxembourg/Kockelscheuer', 'fr': 'Luxembourg/Kockelscheuer'},
'35228':{'de': 'Luxemburg', 'en': 'Luxembourg City', 'fr': 'Luxembourg-Ville'},
'3596036':{'bg': u('\u0421\u0430\u0434\u0438\u043d\u0430'), 'en': 'Sadina'},
'359391':{'bg': u('\u0414\u0438\u043c\u0438\u0442\u0440\u043e\u0432\u0433\u0440\u0430\u0434'), 'en': 'Dimitrovgrad'},
'3329806':{'en': u('Riec-sur-B\u00e9lon'), 'fr': u('Riec-sur-B\u00e9lon')},
'437949':{'de': u('Rainbach im M\u00fchlkreis'), 'en': u('Rainbach im M\u00fchlkreis')},
'436226':{'de': 'Fuschl am See', 'en': 'Fuschl am See'},
'436224':{'de': 'Hintersee', 'en': 'Hintersee'},
'441760':{'en': 'Swaffham'},
'441761':{'en': 'Temple Cloud'},
'441763':{'en': 'Royston'},
'441764':{'en': 'Crieff'},
'441765':{'en': 'Ripon'},
'441766':{'en': 'Porthmadog'},
'441767':{'en': 'Sandy'},
'441769':{'en': 'South Molton'},
'3316479':{'en': 'Dammarie-les-Lys', 'fr': 'Dammarie-les-Lys'},
'3316478':{'en': 'Nemours', 'fr': 'Nemours'},
'3316477':{'en': 'Bussy-Saint-Georges', 'fr': 'Bussy-Saint-Georges'},
'3316476':{'en': 'Bussy-Saint-Georges', 'fr': 'Bussy-Saint-Georges'},
'3316475':{'en': 'Coulommiers', 'fr': 'Coulommiers'},
'3316472':{'en': 'Chelles', 'fr': 'Chelles'},
'3316471':{'en': 'Melun', 'fr': 'Melun'},
'3316470':{'en': 'Montereau-Fault-Yonne', 'fr': 'Montereau-Fault-Yonne'},
'435245':{'de': u('Hinterri\u00df'), 'en': 'Hinterriss'},
'3349018':{'en': 'Arles', 'fr': 'Arles'},
'3349014':{'en': 'Avignon', 'fr': 'Avignon'},
'3349017':{'en': 'Miramas', 'fr': 'Miramas'},
'3349016':{'en': 'Avignon', 'fr': 'Avignon'},
'3349011':{'en': 'Orange', 'fr': 'Orange'},
'3349013':{'en': 'Avignon', 'fr': 'Avignon'},
'35969613':{'bg': u('\u0427\u0438\u0444\u043b\u0438\u043a, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Chiflik, Lovech'},
'35969612':{'bg': u('\u0422\u0435\u0440\u0437\u0438\u0439\u0441\u043a\u043e, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Terziysko, Lovech'},
'3593549':{'bg': u('\u0413\u0440\u0430\u0448\u0435\u0432\u043e'), 'en': 'Grashevo'},
'35969616':{'bg': u('\u0421\u0442\u0430\u0440\u043e \u0441\u0435\u043b\u043e, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Staro selo, Lovech'},
'35969615':{'bg': u('\u0411\u0430\u043b\u0430\u0431\u0430\u043d\u0441\u043a\u043e'), 'en': 'Balabansko'},
'35969614':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u0442\u0440\u0430\u043f\u0435'), 'en': 'Gorno trape'},
'436228':{'de': 'Faistenau', 'en': 'Faistenau'},
'35931387':{'bg': u('\u041f\u0440\u043e\u043b\u043e\u043c'), 'en': 'Prolom'},
'3349451':{'en': u('Fr\u00e9jus'), 'fr': u('Fr\u00e9jus')},
'35931388':{'bg': u('\u0411\u0435\u0433\u0443\u043d\u0446\u0438'), 'en': 'Beguntsi'},
'3598663':{'bg': u('\u0421\u0438\u0442\u043e\u0432\u043e, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Sitovo, Silistra'},
'3349902':{'en': u('S\u00e8te'), 'fr': u('S\u00e8te')},
'3349906':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3349904':{'en': u('S\u00e8te'), 'fr': u('S\u00e8te')},
'441350':{'en': 'Dunkeld'},
'441353':{'en': 'Ely'},
'441352':{'en': 'Mold'},
'441355':{'en': 'East Kilbride'},
'3593544':{'bg': u('\u041a\u043e\u0441\u0442\u0430\u043d\u0434\u043e\u0432\u043e'), 'en': 'Kostandovo'},
'441357':{'en': 'Strathaven'},
'441356':{'en': 'Brechin'},
'441359':{'en': 'Pakenham'},
'441358':{'en': 'Ellon'},
'3593545':{'bg': u('\u0414\u0440\u0430\u0433\u0438\u043d\u043e\u0432\u043e'), 'en': 'Draginovo'},
'3338833':{'en': 'Schiltigheim', 'fr': 'Schiltigheim'},
'3338832':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338831':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338830':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338837':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338836':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338835':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338834':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338839':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338838':{'en': 'Molsheim', 'fr': 'Molsheim'},
'3338789':{'en': u('Far\u00e9bersviller'), 'fr': u('Far\u00e9bersviller')},
'3338788':{'en': 'Forbach', 'fr': 'Forbach'},
'334918':{'en': 'Marseille', 'fr': 'Marseille'},
'334919':{'en': 'Marseille', 'fr': 'Marseille'},
'3323129':{'en': 'Caen', 'fr': 'Caen'},
'3323128':{'en': 'Houlgate', 'fr': 'Houlgate'},
'35936700':{'bg': u('\u0428\u043e\u043f\u0446\u0438'), 'en': 'Shoptsi'},
'334912':{'en': 'Marseille', 'fr': 'Marseille'},
'334913':{'en': 'Marseille', 'fr': 'Marseille'},
'3323127':{'en': 'Caen', 'fr': 'Caen'},
'334911':{'en': 'Marseille', 'fr': 'Marseille'},
'334917':{'en': 'Marseille', 'fr': 'Marseille'},
'334914':{'en': 'Marseille', 'fr': 'Marseille'},
'334915':{'en': 'Marseille', 'fr': 'Marseille'},
'432748':{'de': 'Kilb', 'en': 'Kilb'},
'432749':{'de': 'Prinzersdorf', 'en': 'Prinzersdorf'},
'3598669':{'bg': u('\u0413\u0430\u0440\u0432\u0430\u043d, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Garvan, Silistra'},
'35951108':{'bg': u('\u0418\u0437\u0432\u043e\u0440\u0441\u043a\u043e'), 'en': 'Izvorsko'},
'432741':{'de': 'Flinsbach', 'en': 'Flinsbach'},
'432742':{'de': u('Sankt P\u00f6lten'), 'en': u('St. P\u00f6lten')},
'432743':{'de': u('B\u00f6heimkirchen'), 'en': u('B\u00f6heimkirchen')},
'432744':{'de': u('Kasten bei B\u00f6heimkirchen'), 'en': u('Kasten bei B\u00f6heimkirchen')},
'432745':{'de': 'Pyhra', 'en': 'Pyhra'},
'432746':{'de': 'Wilhelmsburg', 'en': 'Wilhelmsburg'},
'432747':{'de': 'Ober-Grafendorf', 'en': 'Ober-Grafendorf'},
'432713':{'de': 'Spitz', 'en': 'Spitz'},
'432712':{'de': 'Aggsbach', 'en': 'Aggsbach'},
'432711':{'de': u('D\u00fcrnstein'), 'en': u('D\u00fcrnstein')},
'3355313':{'en': u('P\u00e9rigueux'), 'fr': u('P\u00e9rigueux')},
'3323389':{'en': 'Avranches', 'fr': 'Avranches'},
'3323388':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'390835':{'it': 'Matera'},
'390833':{'it': 'Gallipoli'},
'390832':{'en': 'Lecce', 'it': 'Lecce'},
'390831':{'it': 'Brindisi'},
'3323381':{'en': u('Alen\u00e7on'), 'fr': u('Alen\u00e7on')},
'3323380':{'en': u('Alen\u00e7on'), 'fr': u('Alen\u00e7on')},
'3323382':{'en': u('Alen\u00e7on'), 'fr': u('Alen\u00e7on')},
'3323385':{'en': 'Mortagne-au-Perche', 'fr': 'Mortagne-au-Perche'},
'3323384':{'en': 'L\'Aigle', 'fr': 'L\'Aigle'},
'3323387':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'35967774':{'bg': u('\u0411\u0435\u043b\u0438\u0446\u0430, \u0413\u0430\u0431\u0440.'), 'en': 'Belitsa, Gabr.'},
'437764':{'de': 'Riedau', 'en': 'Riedau'},
'35930528':{'bg': u('\u041c\u0430\u043d\u0430\u0441\u0442\u0438\u0440, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Manastir, Plovdiv'},
'437767':{'de': 'Eggerding', 'en': 'Eggerding'},
'437766':{'de': 'Andorf', 'en': 'Andorf'},
'3593519':{'bg': u('\u0412\u0435\u043b\u0438\u0447\u043a\u043e\u0432\u043e, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Velichkovo, Pazardzhik'},
'3332714':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3332527':{'en': 'Bar-sur-Aube', 'fr': 'Bar-sur-Aube'},
'3332525':{'en': 'Romilly-sur-Seine', 'fr': 'Romilly-sur-Seine'},
'3332524':{'en': 'Romilly-sur-Seine', 'fr': 'Romilly-sur-Seine'},
'3593511':{'bg': u('\u041e\u0433\u043d\u044f\u043d\u043e\u0432\u043e, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Ognyanovo, Pazardzhik'},
'3593510':{'bg': u('\u041e\u0432\u0447\u0435\u043f\u043e\u043b\u0446\u0438'), 'en': 'Ovchepoltsi'},
'3332529':{'en': 'Bar-sur-Seine', 'fr': 'Bar-sur-Seine'},
'3593512':{'bg': u('\u0425\u0430\u0434\u0436\u0438\u0435\u0432\u043e'), 'en': 'Hadzhievo'},
'3593515':{'bg': u('\u041a\u0430\u043b\u0443\u0433\u0435\u0440\u043e\u0432\u043e, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Kalugerovo, Pazardzhik'},
'3593514':{'bg': u('\u0427\u0435\u0440\u043d\u043e\u0433\u043e\u0440\u043e\u0432\u043e, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Chernogorovo, Pazardzhik'},
'3593517':{'bg': u('\u041b\u0435\u0441\u0438\u0447\u043e\u0432\u043e'), 'en': 'Lesichovo'},
'3593516':{'bg': u('\u0426\u0440\u044a\u043d\u0447\u0430, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Tsrancha, Pazardzhik'},
'37423486':{'am': u('\u0548\u0582\u0580\u0581\u0561\u0571\u0578\u0580'), 'en': 'Urtsadzor', 'ru': u('\u0423\u0440\u0446\u0430\u0434\u0437\u043e\u0440')},
'37423481':{'am': u('\u0531\u0575\u0563\u0561\u057e\u0561\u0576'), 'en': 'Aygavan', 'ru': u('\u0410\u0439\u0433\u0430\u0432\u0430\u043d')},
'3595913':{'bg': u('\u041a\u0440\u0443\u0448\u0435\u0432\u0435\u0446'), 'en': 'Krushevets'},
'3595912':{'bg': u('\u041f\u043e\u043b\u0441\u043a\u0438 \u0438\u0437\u0432\u043e\u0440'), 'en': 'Polski izvor'},
'3595910':{'bg': u('\u0427\u0435\u0440\u043d\u0438 \u0432\u0440\u044a\u0445, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Cherni vrah, Burgas'},
'3595917':{'bg': u('\u0418\u0437\u0432\u043e\u0440, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Izvor, Burgas'},
'3595916':{'bg': u('\u0420\u043e\u0441\u0435\u043d, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Rosen, Burgas'},
'3595915':{'bg': u('\u0411\u044a\u043b\u0433\u0430\u0440\u043e\u0432\u043e'), 'en': 'Balgarovo'},
'3595914':{'bg': u('\u0410\u0442\u0438\u044f'), 'en': 'Atia'},
'3595919':{'bg': u('\u041c\u0430\u0440\u0438\u043d\u043a\u0430'), 'en': 'Marinka'},
'3595918':{'bg': u('\u0420\u0443\u0441\u043e\u043a\u0430\u0441\u0442\u0440\u043e'), 'en': 'Rusokastro'},
'3593775':{'bg': u('\u0413\u0435\u043d\u0435\u0440\u0430\u043b\u043e\u0432\u043e'), 'en': 'Generalovo'},
'3593774':{'bg': u('\u041b\u0435\u0432\u043a\u0430'), 'en': 'Levka'},
'3593777':{'bg': u('\u041c\u0435\u0437\u0435\u043a'), 'en': 'Mezek'},
'3593776':{'bg': u('\u0420\u0430\u0439\u043a\u043e\u0432\u0430 \u043c\u043e\u0433\u0438\u043b\u0430'), 'en': 'Raykova mogila'},
'3593773':{'bg': u('\u041a\u0430\u043f\u0438\u0442\u0430\u043d \u0410\u043d\u0434\u0440\u0435\u0435\u0432\u043e'), 'en': 'Kapitan Andreevo'},
'3593772':{'bg': u('\u041c\u043e\u043c\u043a\u043e\u0432\u043e'), 'en': 'Momkovo'},
'37428151':{'am': u('\u053d\u0561\u0579\u056b\u056f'), 'en': 'Khachik', 'ru': u('\u0425\u0430\u0447\u0438\u043a')},
'3593779':{'bg': u('\u0421\u0438\u0432\u0430 \u0440\u0435\u043a\u0430'), 'en': 'Siva reka'},
'3593778':{'bg': u('\u0421\u0442\u0443\u0434\u0435\u043d\u0430, \u0425\u0430\u0441\u043a.'), 'en': 'Studena, Hask.'},
'3341307':{'en': 'Carpentras', 'fr': 'Carpentras'},
'35961397':{'bg': u('\u041c\u0443\u0441\u0438\u043d\u0430'), 'en': 'Musina'},
'434350':{'de': 'Bad Sankt Leonhard im Lavanttal', 'en': 'Bad St. Leonhard im Lavanttal'},
'434353':{'de': 'Prebl', 'en': 'Prebl'},
'434352':{'de': 'Wolfsberg', 'en': 'Wolfsberg'},
'434355':{'de': 'Gemmersdorf', 'en': 'Gemmersdorf'},
'434354':{'de': 'Preitenegg', 'en': 'Preitenegg'},
'434357':{'de': 'Sankt Paul im Lavanttal', 'en': 'St. Paul im Lavanttal'},
'434356':{'de': u('Lavam\u00fcnd'), 'en': u('Lavam\u00fcnd')},
'434359':{'de': 'Reichenfels', 'en': 'Reichenfels'},
'434358':{'de': u('Sankt Andr\u00e4'), 'en': u('St. Andr\u00e4')},
'35941338':{'bg': u('\u0426\u0435\u043b\u0438\u043d\u0430'), 'en': 'Tselina'},
'3329900':{'en': u('Ch\u00e2teaubourg'), 'fr': u('Ch\u00e2teaubourg')},
'35941336':{'bg': u('\u042f\u0437\u0434\u0430\u0447'), 'en': 'Yazdach'},
'35941337':{'bg': u('\u0421\u044a\u0440\u043d\u0435\u0432\u0435\u0446'), 'en': 'Sarnevets'},
'35941334':{'bg': u('\u0421\u044a\u0435\u0434\u0438\u043d\u0435\u043d\u0438\u0435, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Saedinenie, St. Zagora'},
'35941335':{'bg': u('\u041c\u043e\u0433\u0438\u043b\u043e\u0432\u043e'), 'en': 'Mogilovo'},
'35941332':{'bg': u('\u041d\u0430\u0439\u0434\u0435\u043d\u043e\u0432\u043e'), 'en': 'Naydenovo'},
'35941333':{'bg': u('\u0421\u0440\u0435\u0434\u043d\u043e \u0433\u0440\u0430\u0434\u0438\u0449\u0435'), 'en': 'Sredno gradishte'},
'35941330':{'bg': u('\u0426\u0435\u043d\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Tsenovo, St. Zagora'},
'35941331':{'bg': u('\u0413\u0440\u0430\u043d\u0438\u0442'), 'en': 'Granit'},
'35951429':{'bg': u('\u0421\u043e\u043b\u043d\u0438\u043a'), 'en': 'Solnik'},
'35951428':{'bg': u('\u0413\u043e\u0441\u043f\u043e\u0434\u0438\u043d\u043e\u0432\u043e, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Gospodinovo, Varna'},
'3329906':{'en': 'Montauban-de-Bretagne', 'fr': 'Montauban-de-Bretagne'},
'4418904':{'en': 'Coldstream'},
'355588':{'en': u('Rras\u00eb/Fierz\u00eb/Kajan/Grekan, Elbasan')},
'355589':{'en': u('Karin\u00eb/Gjocaj/Shez\u00eb, Peqin')},
'3596932':{'bg': u('\u041c\u0438\u043a\u0440\u0435'), 'en': 'Mikre'},
'3596933':{'bg': u('\u0413\u043e\u043b\u0435\u0446'), 'en': 'Golets'},
'3596934':{'bg': u('\u041a\u0430\u0442\u0443\u043d\u0435\u0446'), 'en': 'Katunets'},
'3332396':{'en': u('Villers-Cotter\u00eats'), 'fr': u('Villers-Cotter\u00eats')},
'3596937':{'bg': u('\u0421\u043e\u043a\u043e\u043b\u043e\u0432\u043e, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Sokolovo, Lovech'},
'355580':{'en': u('P\u00ebrparim/Pajov\u00eb, Peqin')},
'355581':{'en': u('C\u00ebrrik, Elbasan')},
'355582':{'en': 'Belsh, Elbasan'},
'355583':{'en': 'Bradashesh/Shirgjan, Elbasan'},
'355584':{'en': u('Labinot-Fush\u00eb/Labinot-Mal/Funar\u00eb/Gracen, Elbasan')},
'355585':{'en': u('Shushic\u00eb/Tregan/Gjinar/Zavalin\u00eb, Elbasan')},
'355586':{'en': u('Gjergjan/Pap\u00ebr/Shal\u00ebs, Elbasan')},
'355587':{'en': 'Gostime/Klos/Mollas, Elbasan'},
'4418903':{'en': 'Coldstream'},
'335345':{'en': 'Toulouse', 'fr': 'Toulouse'},
'335346':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3346649':{'en': 'Mende', 'fr': 'Mende'},
'35944':{'bg': u('\u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Sliven'},
'35946':{'bg': u('\u042f\u043c\u0431\u043e\u043b'), 'en': 'Yambol'},
'35942':{'bg': u('\u0421\u0442\u0430\u0440\u0430 \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Stara Zagora'},
'3338476':{'en': 'Vesoul', 'fr': 'Vesoul'},
'3347629':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347621':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347622':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347623':{'en': u('\u00c9chirolles'), 'fr': u('\u00c9chirolles')},
'3347624':{'en': 'Eybens', 'fr': 'Eybens'},
'3347625':{'en': u('Saint-Martin-d\'H\u00e8res'), 'fr': u('Saint-Martin-d\'H\u00e8res')},
'3347626':{'en': 'Fontaine', 'fr': 'Fontaine'},
'3347627':{'en': 'Fontaine', 'fr': 'Fontaine'},
'3324065':{'en': 'Bouguenais', 'fr': 'Bouguenais'},
'373219':{'en': 'Dnestrovsk', 'ro': 'Dnestrovsk', 'ru': u('\u0414\u043d\u0435\u0441\u0442\u0440\u043e\u0432\u0441\u043a')},
'435552':{'de': 'Bludenz', 'en': 'Bludenz'},
'3324066':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'3324061':{'en': 'Pornichet', 'fr': 'Pornichet'},
'3324060':{'en': 'La Baule Escoublac', 'fr': 'La Baule Escoublac'},
'3324063':{'en': 'Orvault', 'fr': 'Orvault'},
'3324062':{'en': u('Gu\u00e9rande'), 'fr': u('Gu\u00e9rande')},
'373210':{'en': 'Grigoriopol', 'ro': 'Grigoriopol', 'ru': u('\u0413\u0440\u0438\u0433\u043e\u0440\u0438\u043e\u043f\u043e\u043b\u044c')},
'435559':{'de': 'Brand', 'en': 'Brand'},
'3324069':{'en': 'Nantes', 'fr': 'Nantes'},
'3324068':{'en': 'Carquefou', 'fr': 'Carquefou'},
'373216':{'en': 'Camenca', 'ro': 'Camenca', 'ru': u('\u041a\u0430\u043c\u0435\u043d\u043a\u0430')},
'4418901':{'en': 'Coldstream/Ayton'},
'35991203':{'bg': u('\u041b\u0438\u043a'), 'en': 'Lik'},
'35991202':{'bg': u('\u041a\u0443\u043d\u0438\u043d\u043e'), 'en': 'Kunino'},
'35991201':{'bg': u('\u041b\u044e\u0442\u0438 \u0431\u0440\u043e\u0434'), 'en': 'Lyuti brod'},
'4418477':{'en': 'Tongue'},
'4418900':{'en': 'Coldstream/Ayton'},
'4418476':{'en': 'Tongue'},
'3355514':{'en': 'Limoges', 'fr': 'Limoges'},
'441909':{'en': 'Worksop'},
'3347336':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347337':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347334':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347335':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347333':{'en': 'Volvic', 'fr': 'Volvic'},
'3347330':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347331':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3347338':{'en': 'Riom', 'fr': 'Riom'},
'3315818':{'en': 'Paris', 'fr': 'Paris'},
'3316138':{'en': 'Montigny-le-Bretonneux', 'fr': 'Montigny-le-Bretonneux'},
'3342216':{'en': 'Nice', 'fr': 'Nice'},
'3316137':{'en': 'Montigny-le-Bretonneux', 'fr': 'Montigny-le-Bretonneux'},
'3316130':{'en': 'Sartrouville', 'fr': 'Sartrouville'},
'3329655':{'en': 'Paimpol', 'fr': 'Paimpol'},
'3329654':{'en': u('Plestin-les-Gr\u00e8ves'), 'fr': u('Plestin-les-Gr\u00e8ves')},
'3329656':{'en': 'Illifaut', 'fr': 'Illifaut'},
'3329650':{'en': 'Lamballe', 'fr': 'Lamballe'},
'3329652':{'en': 'Langueux', 'fr': 'Langueux'},
'3329658':{'en': u('Pl\u00e9rin'), 'fr': u('Pl\u00e9rin')},
'35961608':{'bg': u('\u0413\u043e\u0440\u0441\u043a\u0438 \u0421\u0435\u043d\u043e\u0432\u0435\u0446'), 'en': 'Gorski Senovets'},
'3323246':{'en': 'Bernay', 'fr': 'Bernay'},
'3323247':{'en': 'Bernay', 'fr': 'Bernay'},
'3323240':{'en': 'Louviers', 'fr': 'Louviers'},
'3323241':{'en': 'Pont-Audemer', 'fr': 'Pont-Audemer'},
'3323243':{'en': 'Bernay', 'fr': 'Bernay'},
'3323718':{'en': 'Chartres', 'fr': 'Chartres'},
'390342':{'en': 'Sondrio', 'it': 'Sondrio'},
'3349943':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'390444':{'en': 'Vicenza', 'it': 'Vicenza'},
'390445':{'en': 'Vicenza', 'it': 'Schio'},
'390442':{'it': 'Legnago'},
'433832':{'de': 'Kraubath an der Mur', 'en': 'Kraubath an der Mur'},
'390344':{'en': 'Como', 'it': 'Menaggio'},
'390346':{'en': 'Bergamo', 'it': 'Clusone'},
'3595137':{'bg': u('\u041a\u0430\u043b\u043e\u044f\u043d'), 'en': 'Kaloyan'},
'3595136':{'bg': u('\u0411\u0440\u0435\u0441\u0442\u0430\u043a'), 'en': 'Brestak'},
'35961605':{'bg': u('\u041d\u043e\u0432\u043e \u0433\u0440\u0430\u0434\u0438\u0449\u0435'), 'en': 'Novo gradishte'},
'3345079':{'en': 'Morzine', 'fr': 'Morzine'},
'3345078':{'en': 'Passy', 'fr': 'Passy'},
'35951127':{'bg': u('\u0420\u0430\u0437\u0434\u0435\u043b\u043d\u0430, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Razdelna, Varna'},
'3347243':{'en': 'Villeurbanne', 'fr': 'Villeurbanne'},
'3345073':{'en': u('Ch\u00e2tel'), 'fr': u('Ch\u00e2tel')},
'3345072':{'en': 'Sciez', 'fr': 'Sciez'},
'3345071':{'en': 'Thonon-les-Bains', 'fr': 'Thonon-les-Bains'},
'3345070':{'en': 'Thonon-les-Bains', 'fr': 'Thonon-les-Bains'},
'3345076':{'en': 'Lugrin', 'fr': 'Lugrin'},
'3345075':{'en': u('\u00c9vian-les-Bains'), 'fr': u('\u00c9vian-les-Bains')},
'3345074':{'en': 'Morzine', 'fr': 'Morzine'},
'3326298':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326290':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326291':{'en': 'Saint-Louis', 'fr': 'Saint-Louis'},
'3326292':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326293':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326294':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326296':{'en': 'Saint-Pierre', 'fr': 'Saint-Pierre'},
'3326297':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3751511':{'be': u('\u0412\u044f\u043b\u0456\u043a\u0430\u044f \u0411\u0435\u0440\u0430\u0441\u0442\u0430\u0432\u0456\u0446\u0430, \u0413\u0440\u043e\u0434\u0437\u0435\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Vyalikaya Byerastavitsa, Grodno Region', 'ru': u('\u0411\u0435\u0440\u0435\u0441\u0442\u043e\u0432\u0438\u0446\u0430, \u0413\u0440\u043e\u0434\u043d\u0435\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751513':{'be': u('\u0421\u0432\u0456\u0441\u043b\u0430\u0447, \u0413\u0440\u043e\u0434\u0437\u0435\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Svisloch, Grodno Region', 'ru': u('\u0421\u0432\u0438\u0441\u043b\u043e\u0447\u044c, \u0413\u0440\u043e\u0434\u043d\u0435\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751512':{'be': u('\u0412\u0430\u045e\u043a\u0430\u0432\u044b\u0441\u043a'), 'en': 'Volkovysk', 'ru': u('\u0412\u043e\u043b\u043a\u043e\u0432\u044b\u0441\u043a')},
'3751515':{'be': u('\u041c\u0430\u0441\u0442\u044b, \u0413\u0440\u043e\u0434\u0437\u0435\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Mosty, Grodno Region', 'ru': u('\u041c\u043e\u0441\u0442\u044b, \u0413\u0440\u043e\u0434\u043d\u0435\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3347532':{'en': 'Annonay', 'fr': 'Annonay'},
'3347533':{'en': 'Annonay', 'fr': 'Annonay'},
'35974325':{'bg': u('\u041b\u0438\u043b\u044f\u043d\u043e\u0432\u043e'), 'en': 'Lilyanovo'},
'35974324':{'bg': u('\u0421\u0442\u0440\u0443\u043c\u0430'), 'en': 'Struma'},
'35974327':{'bg': u('\u041d\u043e\u0432\u043e \u0414\u0435\u043b\u0447\u0435\u0432\u043e'), 'en': 'Novo Delchevo'},
'3596736':{'bg': u('\u0413\u0440\u0430\u0434\u043d\u0438\u0446\u0430, \u0413\u0430\u0431\u0440.'), 'en': 'Gradnitsa, Gabr.'},
'35974323':{'bg': u('\u041b\u043e\u0437\u0435\u043d\u0438\u0446\u0430'), 'en': 'Lozenitsa'},
'35974322':{'bg': u('\u041f\u0435\u0442\u0440\u043e\u0432\u043e, \u0411\u043b\u0430\u0433.'), 'en': 'Petrovo, Blag.'},
'3597039':{'bg': u('\u0421\u0430\u043c\u043e\u0440\u0430\u043d\u043e\u0432\u043e'), 'en': 'Samoranovo'},
'437729':{'de': 'Neukirchen an der Enknach', 'en': 'Neukirchen an der Enknach'},
'437728':{'de': 'Schwand im Innkreis', 'en': 'Schwand im Innkreis'},
'437724':{'de': 'Mauerkirchen', 'en': 'Mauerkirchen'},
'437727':{'de': 'Ach', 'en': 'Ach'},
'437723':{'de': 'Altheim', 'en': 'Altheim'},
'437722':{'de': 'Braunau am Inn', 'en': 'Braunau am Inn'},
'375212':{'be': u('\u0412\u0456\u0446\u0435\u0431\u0441\u043a'), 'en': 'Vitebsk', 'ru': u('\u0412\u0438\u0442\u0435\u0431\u0441\u043a')},
'375216':{'be': u('\u041e\u0440\u0448\u0430'), 'en': 'Orsha', 'ru': u('\u041e\u0440\u0448\u0430')},
'375214':{'be': u('\u041f\u043e\u043b\u0430\u0446\u043a/\u041d\u0430\u0432\u0430\u043f\u043e\u043b\u0430\u0446\u043a'), 'en': 'Polotsk/Navapolatsk', 'ru': u('\u041f\u043e\u043b\u043e\u0446\u043a/\u041d\u043e\u0432\u043e\u043f\u043e\u043b\u043e\u0446\u043a')},
'3597032':{'bg': u('\u042f\u0445\u0438\u043d\u043e\u0432\u043e'), 'en': 'Yahinovo'},
'3597033':{'bg': u('\u041a\u0440\u0430\u0439\u043d\u0438\u0446\u0438'), 'en': 'Kraynitsi'},
'3338222':{'en': u('J\u0153uf'), 'fr': u('J\u0153uf')},
'3338223':{'en': 'Longwy', 'fr': 'Longwy'},
'3338226':{'en': 'Longuyon', 'fr': 'Longuyon'},
'3338224':{'en': 'Longwy', 'fr': 'Longwy'},
'3338225':{'en': 'Longwy', 'fr': 'Longwy'},
'441866':{'en': 'Kilchrenan'},
'37423290':{'am': u('\u0555\u0570\u0561\u0576\u0561\u057e\u0561\u0576'), 'en': 'Ohanavan', 'ru': u('\u041e\u0433\u0430\u043d\u0430\u0432\u0430\u043d')},
'37423294':{'am': u('\u0532\u0575\u0578\u0582\u0580\u0561\u056f\u0561\u0576'), 'en': 'Byurakan', 'ru': u('\u0411\u044e\u0440\u0430\u043a\u0430\u043d')},
'3347439':{'en': 'Pont-d\'Ain', 'fr': 'Pont-d\'Ain'},
'3347438':{'en': u('Amb\u00e9rieu-en-Bugey'), 'fr': u('Amb\u00e9rieu-en-Bugey')},
'3347435':{'en': 'Hauteville-Lompnes', 'fr': 'Hauteville-Lompnes'},
'3347434':{'en': u('Amb\u00e9rieu-en-Bugey'), 'fr': u('Amb\u00e9rieu-en-Bugey')},
'3347437':{'en': 'Poncin', 'fr': 'Poncin'},
'3347431':{'en': 'Vienne', 'fr': 'Vienne'},
'3347433':{'en': u('Les Aveni\u00e8res'), 'fr': u('Les Aveni\u00e8res')},
'3347432':{'en': 'Bourg-en-Bresse', 'fr': 'Bourg-en-Bresse'},
'4181':{'de': 'Chur', 'en': 'Chur', 'fr': 'Coire', 'it': 'Coira'},
'3598187':{'bg': u('\u0422\u0435\u0442\u043e\u0432\u043e'), 'en': 'Tetovo'},
'333807':{'en': 'Dijon', 'fr': 'Dijon'},
'3598184':{'bg': u('\u0413\u043b\u043e\u0434\u0436\u0435\u0432\u043e'), 'en': 'Glodzhevo'},
'3341175':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3599547':{'bg': u('\u0412\u0438\u043d\u0438\u0449\u0435'), 'en': 'Vinishte'},
'3338165':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3596590':{'bg': u('\u0420\u0430\u043a\u0438\u0442\u0430, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Rakita, Pleven'},
'3594722':{'bg': u('\u0413\u0440\u0430\u043d\u0438\u0442\u043e\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Granitovo, Yambol'},
'3324308':{'en': 'Gorron', 'fr': 'Gorron'},
'3324309':{'en': u('Ch\u00e2teau-Gontier'), 'fr': u('Ch\u00e2teau-Gontier')},
'3347291':{'en': 'Lyon', 'fr': 'Lyon'},
'3347290':{'en': u('V\u00e9nissieux'), 'fr': u('V\u00e9nissieux')},
'3347293':{'en': u('D\u00e9cines-Charpieu'), 'fr': u('D\u00e9cines-Charpieu')},
'434237':{'de': 'Miklauzhof', 'en': 'Miklauzhof'},
'3347298':{'en': 'Lyon', 'fr': 'Lyon'},
'441708':{'en': 'Romford'},
'434235':{'de': 'Bleiburg', 'en': 'Bleiburg'},
'441702':{'en': 'Southend-on-Sea'},
'434232':{'de': u('V\u00f6lkermarkt'), 'en': u('V\u00f6lkermarkt')},
'441700':{'en': 'Rothesay'},
'441706':{'en': 'Rochdale'},
'441707':{'en': 'Welwyn Garden City'},
'441704':{'en': 'Southport'},
'3332939':{'en': 'Thaon-les-Vosges', 'fr': 'Thaon-les-Vosges'},
'3316459':{'en': 'Dourdan', 'fr': 'Dourdan'},
'3316458':{'en': 'Breuillet', 'fr': 'Breuillet'},
'3346748':{'en': 'Frontignan', 'fr': 'Frontignan'},
'3346749':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3346744':{'en': u('Lod\u00e8ve'), 'fr': u('Lod\u00e8ve')},
'3346745':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346746':{'en': u('S\u00e8te'), 'fr': u('S\u00e8te')},
'3316452':{'en': 'Melun', 'fr': 'Melun'},
'3346740':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3316454':{'en': 'Longjumeau', 'fr': 'Longjumeau'},
'3316457':{'en': 'Mennecy', 'fr': 'Mennecy'},
'3316456':{'en': u('Saint-Ch\u00e9ron'), 'fr': u('Saint-Ch\u00e9ron')},
'3522722':{'de': 'Luxemburg', 'en': 'Luxembourg City', 'fr': 'Luxembourg-Ville'},
'3522723':{'de': 'Bad Mondorf', 'en': 'Mondorf-les-Bains/Bascharage/Noerdange/Remich', 'fr': 'Mondorf-les-Bains/Bascharage/Noerdange/Remich'},
'3522721':{'de': 'Weicherdingen', 'en': 'Weicherdange', 'fr': 'Weicherdange'},
'3522727':{'de': 'Belair, Luxemburg', 'en': 'Belair, Luxembourg', 'fr': 'Belair, Luxembourg'},
'3522725':{'de': 'Luxemburg', 'en': 'Luxembourg', 'fr': 'Luxembourg'},
'3522728':{'de': 'Luxemburg', 'en': 'Luxembourg City', 'fr': 'Luxembourg-Ville'},
'3522729':{'de': 'Luxemburg/Kockelscheuer', 'en': 'Luxembourg/Kockelscheuer', 'fr': 'Luxembourg/Kockelscheuer'},
'333232':{'en': 'Laon', 'fr': 'Laon'},
'441379':{'en': 'Diss'},
'35931108':{'bg': u('\u0411\u043e\u0433\u0434\u0430\u043d\u0438\u0446\u0430'), 'en': 'Bogdanitsa'},
'441373':{'en': 'Frome'},
'441372':{'en': 'Esher'},
'441371':{'en': 'Great Dunmow'},
'441377':{'en': 'Driffield'},
'441376':{'en': 'Braintree'},
'441375':{'en': 'Grays Thurrock'},
'3699':{'en': 'Sopron', 'hu': 'Sopron'},
'3338819':{'en': 'Schiltigheim', 'fr': 'Schiltigheim'},
'3338818':{'en': 'Schiltigheim', 'fr': 'Schiltigheim'},
'3338769':{'en': 'Metz', 'fr': 'Metz'},
'3338768':{'en': 'Metz', 'fr': 'Metz'},
'3338767':{'en': 'Rombas', 'fr': 'Rombas'},
'3338766':{'en': 'Metz', 'fr': 'Metz'},
'3338765':{'en': 'Metz', 'fr': 'Metz'},
'3338816':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338763':{'en': 'Metz', 'fr': 'Metz'},
'3338762':{'en': 'Metz', 'fr': 'Metz'},
'3338761':{'en': u('Sainte-Marie-aux-Ch\u00eanes'), 'fr': u('Sainte-Marie-aux-Ch\u00eanes')},
'3338760':{'en': 'Ars-sur-Moselle', 'fr': 'Ars-sur-Moselle'},
'35984392':{'bg': u('\u0411\u0435\u043b\u0438\u043d\u0446\u0438'), 'en': 'Belintsi'},
'3324785':{'en': 'Tours', 'fr': 'Tours'},
'3346701':{'en': 'Agde', 'fr': 'Agde'},
'3324786':{'en': 'Tours', 'fr': 'Tours'},
'433623':{'de': 'Bad Mitterndorf', 'en': 'Bad Mitterndorf'},
'3342665':{'en': 'Lyon', 'fr': 'Lyon'},
'3342663':{'en': 'Lyon', 'fr': 'Lyon'},
'3596998':{'bg': u('\u0413\u043e\u043b\u044f\u043c \u0438\u0437\u0432\u043e\u0440, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Golyam izvor, Lovech'},
'3355335':{'en': u('P\u00e9rigueux'), 'fr': u('P\u00e9rigueux')},
'3355331':{'en': u('Sarlat-la-Can\u00e9da'), 'fr': u('Sarlat-la-Can\u00e9da')},
'3355330':{'en': u('Sarlat-la-Can\u00e9da'), 'fr': u('Sarlat-la-Can\u00e9da')},
'3349293':{'en': 'Antibes Juan les Pins', 'fr': 'Antibes Juan les Pins'},
'3349292':{'en': 'Mougins', 'fr': 'Mougins'},
'3349291':{'en': 'Antibes Juan les Pins', 'fr': 'Antibes Juan les Pins'},
'3349290':{'en': 'Antibes Juan les Pins', 'fr': 'Antibes Juan les Pins'},
'3349297':{'en': 'Mandelieu-la-Napoule', 'fr': 'Mandelieu-la-Napoule'},
'3349296':{'en': 'Valbonne', 'fr': 'Valbonne'},
'3349295':{'en': 'Vallauris', 'fr': 'Vallauris'},
'3349294':{'en': 'Valbonne', 'fr': 'Valbonne'},
'3349299':{'en': 'Cannes', 'fr': 'Cannes'},
'3349298':{'en': 'Cannes', 'fr': 'Cannes'},
'371656':{'en': u('Kr\u0101slava')},
'371657':{'en': 'Ludza'},
'371654':{'en': 'Daugavpils'},
'371655':{'en': 'Ogre'},
'371652':{'en': u('J\u0113kabpils')},
'371653':{'en': u('Prei\u013ci')},
'371650':{'en': 'Ogre'},
'371651':{'en': 'Aizkraukle'},
'371658':{'en': 'Daugavpils'},
'371659':{'en': u('C\u0113sis')},
'35981264':{'bg': u('\u041f\u0438\u043f\u0435\u0440\u043a\u043e\u0432\u043e'), 'en': 'Piperkovo'},
'432722':{'de': 'Kirchberg an der Pielach', 'en': 'Kirchberg an der Pielach'},
'432723':{'de': 'Rabenstein an der Pielach', 'en': 'Rabenstein an der Pielach'},
'432726':{'de': 'Puchenstuben', 'en': 'Puchenstuben'},
'432724':{'de': 'Schwarzenbach an der Pielach', 'en': 'Schwarzenbach an der Pielach'},
'3332058':{'en': 'Wavrin', 'fr': 'Wavrin'},
'3332057':{'en': 'Lille', 'fr': 'Lille'},
'3332056':{'en': 'Lille', 'fr': 'Lille'},
'3332055':{'en': 'Lille', 'fr': 'Lille'},
'3332054':{'en': 'Lille', 'fr': 'Lille'},
'3332053':{'en': 'Lille', 'fr': 'Lille'},
'3332052':{'en': 'Lille', 'fr': 'Lille'},
'3332051':{'en': 'Lille', 'fr': 'Lille'},
'3332502':{'en': 'Chaumont', 'fr': 'Chaumont'},
'3323343':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'42056':{'en': u('Vyso\u010dina Region')},
'3323507':{'en': 'Rouen', 'fr': 'Rouen'},
'3323506':{'en': 'Dieppe', 'fr': 'Dieppe'},
'3355593':{'en': u('\u00c9gletons'), 'fr': u('\u00c9gletons')},
'3355592':{'en': u('Malemort-sur-Corr\u00e8ze'), 'fr': u('Malemort-sur-Corr\u00e8ze')},
'3323503':{'en': 'Rouen', 'fr': 'Rouen'},
'3355849':{'en': u('L\u00e9on'), 'fr': u('L\u00e9on')},
'3355596':{'en': 'Bort-les-Orgues', 'fr': 'Bort-les-Orgues'},
'3355847':{'en': 'Magescq', 'fr': 'Magescq'},
'3355846':{'en': 'Mont-de-Marsan', 'fr': 'Mont-de-Marsan'},
'373244':{'en': 'Calarasi', 'ro': u('C\u0103l\u0103ra\u015fi'), 'ru': u('\u041a\u044d\u043b\u044d\u0440\u0430\u0448\u044c')},
'3355843':{'en': 'Soorts-Hossegor', 'fr': 'Soorts-Hossegor'},
'3355842':{'en': 'Lit-et-Mixe', 'fr': 'Lit-et-Mixe'},
'3323509':{'en': 'Gournay-en-Bray', 'fr': 'Gournay-en-Bray'},
'3323508':{'en': u('Darn\u00e9tal'), 'fr': u('Darn\u00e9tal')},
'3349437':{'en': 'Brignoles', 'fr': 'Brignoles'},
'3349436':{'en': 'Toulon', 'fr': 'Toulon'},
'3349435':{'en': u('Hy\u00e8res'), 'fr': u('Hy\u00e8res')},
'3349434':{'en': 'Six-Fours-les-Plages', 'fr': 'Six-Fours-les-Plages'},
'3349433':{'en': u('Solli\u00e8s-Pont'), 'fr': u('Solli\u00e8s-Pont')},
'3349432':{'en': 'Bandol', 'fr': 'Bandol'},
'3349431':{'en': 'Toulon', 'fr': 'Toulon'},
'3349430':{'en': 'La Seyne sur Mer', 'fr': 'La Seyne sur Mer'},
'3355601':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3349439':{'en': 'Montauroux', 'fr': 'Montauroux'},
'3349438':{'en': u('Hy\u00e8res'), 'fr': u('Hy\u00e8res')},
'3355350':{'en': 'Terrasson-Lavilledieu', 'fr': 'Terrasson-Lavilledieu'},
'432160':{'de': 'Jois', 'en': 'Jois'},
'42058':{'en': 'Olomouc Region'},
'432162':{'de': 'Bruck an der Leitha', 'en': 'Bruck an der Leitha'},
'42059':{'en': 'Moravian-Silesian Region'},
'35941354':{'bg': u('\u041c\u0430\u043b\u043a\u043e \u0422\u0440\u044a\u043d\u043e\u0432\u043e'), 'en': 'Malko Tranovo'},
'35941355':{'bg': u('\u042f\u0432\u043e\u0440\u043e\u0432\u043e'), 'en': 'Yavorovo'},
'35941356':{'bg': u('\u0420\u0443\u043f\u043a\u0438\u0442\u0435'), 'en': 'Rupkite'},
'3322341':{'en': u('Br\u00e9al-sous-Montfort'), 'fr': u('Br\u00e9al-sous-Montfort')},
'35941350':{'bg': u('\u041c\u0438\u0440\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Mirovo, St. Zagora'},
'35941351':{'bg': u('\u041f\u0430\u0440\u0442\u0438\u0437\u0430\u043d\u0438\u043d'), 'en': 'Partizanin'},
'35941352':{'bg': u('\u0412\u0438\u043d\u0430\u0440\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Vinarovo, St. Zagora'},
'35941353':{'bg': u('\u041f\u043b\u043e\u0434\u043e\u0432\u0438\u0442\u043e\u0432\u043e'), 'en': 'Plodovitovo'},
'432164':{'de': 'Rohrau', 'en': 'Rohrau'},
'35941358':{'bg': u('\u041e\u043f\u044a\u043b\u0447\u0435\u043d\u0435\u0446'), 'en': 'Opalchenets'},
'35941359':{'bg': u('\u0418\u0437\u0432\u043e\u0440\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Izvorovo, St. Zagora'},
'432166':{'de': 'Parndorf', 'en': 'Parndorf'},
'432167':{'de': 'Neusiedl am See', 'en': 'Neusiedl am See'},
'3346709':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'437665':{'de': 'Unterach am Attersee', 'en': 'Unterach am Attersee'},
'437666':{'de': 'Attersee', 'en': 'Attersee'},
'441571':{'en': 'Lochinver'},
'441570':{'en': 'Lampeter'},
'441573':{'en': 'Kelso'},
'441572':{'en': 'Oakham'},
'441575':{'en': 'Kirriemuir'},
'441577':{'en': 'Kinross'},
'441576':{'en': 'Lockerbie'},
'441579':{'en': 'Liskeard'},
'441578':{'en': 'Lauder'},
'437662':{'de': 'Seewalchen am Attersee', 'en': 'Seewalchen am Attersee'},
'3324881':{'en': u('Aubigny-sur-N\u00e8re'), 'fr': u('Aubigny-sur-N\u00e8re')},
'3324880':{'en': 'Cuffy', 'fr': 'Cuffy'},
'437663':{'de': 'Steinbach am Attersee', 'en': 'Steinbach am Attersee'},
'3596918':{'bg': u('\u0414\u0440\u0435\u043d\u043e\u0432'), 'en': 'Drenov'},
'3596919':{'bg': u('\u0421\u043b\u0430\u0442\u0438\u043d\u0430, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Slatina, Lovech'},
'3596912':{'bg': u('\u0411\u0430\u0445\u043e\u0432\u0438\u0446\u0430'), 'en': 'Bahovitsa'},
'3596913':{'bg': u('\u0421\u043b\u0430\u0432\u044f\u043d\u0438'), 'en': 'Slavyani'},
'3596910':{'bg': u('\u041c\u0430\u043b\u0438\u043d\u043e\u0432\u043e'), 'en': 'Malinovo'},
'3596911':{'bg': u('\u041b\u0438\u0441\u0435\u0446, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Lisets, Lovech'},
'3596916':{'bg': u('\u0411\u0440\u0435\u0441\u0442\u043e\u0432\u043e, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Brestovo, Lovech'},
'3596917':{'bg': u('\u0411\u044a\u043b\u0433\u0430\u0440\u0435\u043d\u0435, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Balgarene, Lovech'},
'3596914':{'bg': u('\u0421\u043b\u0438\u0432\u0435\u043a'), 'en': 'Slivek'},
'3596915':{'bg': u('\u0421\u043c\u043e\u0447\u0430\u043d'), 'en': 'Smochan'},
'373272':{'en': 'Soldanesti', 'ro': u('\u015eold\u0103ne\u015fti'), 'ru': u('\u0428\u043e\u043b\u0434\u044d\u043d\u0435\u0448\u0442\u044c')},
'373273':{'en': 'Cantemir', 'ro': 'Cantemir', 'ru': u('\u041a\u0430\u043d\u0442\u0435\u043c\u0438\u0440')},
'373271':{'en': u('Ocni\u0163a'), 'ro': u('Ocni\u0163a'), 'ru': u('\u041e\u043a\u043d\u0438\u0446\u0430')},
'3324089':{'en': 'Nantes', 'fr': 'Nantes'},
'3324086':{'en': u('Cou\u00ebron'), 'fr': u('Cou\u00ebron')},
'3324085':{'en': 'Saint-Herblain', 'fr': 'Saint-Herblain'},
'3324084':{'en': 'Nantes', 'fr': 'Nantes'},
'3324083':{'en': 'Ancenis', 'fr': 'Ancenis'},
'3324082':{'en': 'Pornic', 'fr': 'Pornic'},
'3324081':{'en': u('Ch\u00e2teaubriant'), 'fr': u('Ch\u00e2teaubriant')},
'3324080':{'en': u('Saint-S\u00e9bastien-sur-Loire'), 'fr': u('Saint-S\u00e9bastien-sur-Loire')},
'433328':{'de': 'Kukmirn', 'en': 'Kukmirn'},
'3353198':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3347351':{'en': 'Thiers', 'fr': 'Thiers'},
'3347600':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347353':{'en': u('Courpi\u00e8re'), 'fr': u('Courpi\u00e8re')},
'3347354':{'en': 'Brassac-les-Mines', 'fr': 'Brassac-les-Mines'},
'3347355':{'en': 'Issoire', 'fr': 'Issoire'},
'3347604':{'en': 'Meylan', 'fr': 'Meylan'},
'3347605':{'en': 'Voiron', 'fr': 'Voiron'},
'3347608':{'en': 'Crolles', 'fr': 'Crolles'},
'3347609':{'en': u('\u00c9chirolles'), 'fr': u('\u00c9chirolles')},
'3906':{'en': 'Rome', 'it': 'Roma'},
'3335412':{'en': 'Nancy', 'fr': 'Nancy'},
'4414371':{'en': 'Haverfordwest/Clynderwen (Clunderwen)'},
'3323888':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323889':{'en': 'Montargis', 'fr': 'Montargis'},
'4414374':{'en': 'Clynderwen (Clunderwen)'},
'4414375':{'en': 'Clynderwen (Clunderwen)'},
'4414376':{'en': 'Haverfordwest'},
'4414377':{'en': 'Haverfordwest'},
'3315836':{'en': 'Paris', 'fr': 'Paris'},
'3323883':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3315834':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3323881':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323886':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323884':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323885':{'en': 'Montargis', 'fr': 'Montargis'},
'3325113':{'en': 'Nantes', 'fr': 'Nantes'},
'3752248':{'be': u('\u0414\u0440\u044b\u0431\u0456\u043d, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Dribin, Mogilev Region', 'ru': u('\u0414\u0440\u0438\u0431\u0438\u043d, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752244':{'be': u('\u041a\u043b\u0456\u043c\u0430\u0432\u0456\u0447\u044b, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Klimovichi, Mogilev Region', 'ru': u('\u041a\u043b\u0438\u043c\u043e\u0432\u0438\u0447\u0438, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752245':{'be': u('\u041a\u0430\u0441\u0446\u044e\u043a\u043e\u0432\u0456\u0447\u044b, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Kostyukovichi, Mogilev Region', 'ru': u('\u041a\u043e\u0441\u0442\u044e\u043a\u043e\u0432\u0438\u0447\u0438, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752246':{'be': u('\u0421\u043b\u0430\u045e\u0433\u0430\u0440\u0430\u0434, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Slavgorod, Mogilev Region', 'ru': u('\u0421\u043b\u0430\u0432\u0433\u043e\u0440\u043e\u0434, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752247':{'be': u('\u0425\u043e\u0446\u0456\u043c\u0441\u043a, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Khotimsk, Mogilev Region', 'ru': u('\u0425\u043e\u0442\u0438\u043c\u0441\u043a, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752240':{'be': u('\u041c\u0441\u0446\u0456\u0441\u043b\u0430\u045e'), 'en': 'Mstislavl', 'ru': u('\u041c\u0441\u0442\u0438\u0441\u043b\u0430\u0432\u043b\u044c')},
'3752241':{'be': u('\u041a\u0440\u044b\u0447\u0430\u045e, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Krichev, Mogilev Region', 'ru': u('\u041a\u0440\u0438\u0447\u0435\u0432, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752242':{'be': u('\u0427\u0430\u0432\u0443\u0441\u044b, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Chausy, Mogilev Region', 'ru': u('\u0427\u0430\u0443\u0441\u044b, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752243':{'be': u('\u0427\u044d\u0440\u044b\u043a\u0430\u045e, \u041c\u0430\u0433\u0456\u043b\u0451\u045e\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Cherikov, Mogilev Region', 'ru': u('\u0427\u0435\u0440\u0438\u043a\u043e\u0432, \u041c\u043e\u0433\u0438\u043b\u0435\u0432\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3347975':{'en': u('Chamb\u00e9ry'), 'fr': u('Chamb\u00e9ry')},
'3347970':{'en': u('Chamb\u00e9ry'), 'fr': u('Chamb\u00e9ry')},
'3347971':{'en': u('Chamb\u00e9ry'), 'fr': u('Chamb\u00e9ry')},
'3347972':{'en': 'Challes-les-Eaux', 'fr': 'Challes-les-Eaux'},
'3323269':{'en': u('\u00c9couis'), 'fr': u('\u00c9couis')},
'3323264':{'en': 'Vernon', 'fr': 'Vernon'},
'3323262':{'en': u('\u00c9vreux'), 'fr': u('\u00c9vreux')},
'3323261':{'en': 'Val-de-Reuil', 'fr': 'Val-de-Reuil'},
'3597137':{'bg': u('\u0421\u043a\u0440\u0430\u0432\u0435\u043d\u0430'), 'en': 'Skravena'},
'3597136':{'bg': u('\u041d\u043e\u0432\u0430\u0447\u0435\u043d\u0435, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Novachene, Sofia'},
'3597135':{'bg': u('\u0422\u0440\u0443\u0434\u043e\u0432\u0435\u0446'), 'en': 'Trudovets'},
'3597134':{'bg': u('\u0412\u0440\u0430\u0447\u0435\u0448'), 'en': 'Vrachesh'},
'3597133':{'bg': u('\u041f\u0440\u0430\u0432\u0435\u0446'), 'en': 'Pravets'},
'3597132':{'bg': u('\u0420\u0430\u0434\u043e\u0442\u0438\u043d\u0430'), 'en': 'Radotina'},
'390461':{'en': 'Trento', 'it': 'Trento'},
'390462':{'it': 'Cavalese'},
'390463':{'it': 'Cles'},
'390464':{'it': 'Rovereto'},
'390465':{'it': 'Tione di Trento'},
'35971221':{'bg': u('\u042f\u0440\u043b\u043e\u0432\u043e'), 'en': 'Yarlovo'},
'3597138':{'bg': u('\u041b\u0438\u0442\u0430\u043a\u043e\u0432\u043e'), 'en': 'Litakovo'},
'42148':{'en': u('Bansk\u00e1 Bystrica')},
'42142':{'en': u('Pova\u017esk\u00e1 Bystrica')},
'42143':{'en': 'Martin'},
'42141':{'en': u('\u017dilina')},
'42146':{'en': 'Prievidza'},
'35974496':{'bg': u('\u0424\u0438\u043b\u0438\u043f\u043e\u0432\u043e, \u0411\u043b\u0430\u0433.'), 'en': 'Filipovo, Blag.'},
'35974495':{'bg': u('\u042e\u0440\u0443\u043a\u043e\u0432\u043e'), 'en': 'Yurukovo'},
'42145':{'en': 'Zvolen'},
'3345055':{'en': 'Chamonix-Mont-Blanc', 'fr': 'Chamonix-Mont-Blanc'},
'3324124':{'en': 'Angers', 'fr': 'Angers'},
'3345057':{'en': 'Annecy', 'fr': 'Annecy'},
'3345056':{'en': 'Bellegarde-sur-Valserine', 'fr': 'Bellegarde-sur-Valserine'},
'3345051':{'en': 'Annecy', 'fr': 'Annecy'},
'3345053':{'en': 'Chamonix-Mont-Blanc', 'fr': 'Chamonix-Mont-Blanc'},
'3345052':{'en': 'Annecy', 'fr': 'Annecy'},
'3345058':{'en': 'Sallanches', 'fr': 'Sallanches'},
'3346703':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3324120':{'en': 'Angers', 'fr': 'Angers'},
'3324122':{'en': 'Angers', 'fr': 'Angers'},
'3324123':{'en': 'Angers', 'fr': 'Angers'},
'3338594':{'en': u('Chalon-sur-Sa\u00f4ne'), 'fr': u('Chalon-sur-Sa\u00f4ne')},
'3338597':{'en': u('Chalon-sur-Sa\u00f4ne'), 'fr': u('Chalon-sur-Sa\u00f4ne')},
'3338590':{'en': u('Chalon-sur-Sa\u00f4ne'), 'fr': u('Chalon-sur-Sa\u00f4ne')},
'3338592':{'en': 'Buxy', 'fr': 'Buxy'},
'3338593':{'en': u('Chalon-sur-Sa\u00f4ne'), 'fr': u('Chalon-sur-Sa\u00f4ne')},
'3338598':{'en': u('Ch\u00e2tenoy-le-Royal'), 'fr': u('Ch\u00e2tenoy-le-Royal')},
'3332266':{'en': 'Amiens', 'fr': 'Amiens'},
'3332260':{'en': 'Saint-Valery-sur-Somme', 'fr': 'Saint-Valery-sur-Somme'},
'3332261':{'en': 'Friville-Escarbotin', 'fr': 'Friville-Escarbotin'},
'3316410':{'en': 'Melun', 'fr': 'Melun'},
'35971225':{'bg': u('\u041d\u043e\u0432\u043e \u0441\u0435\u043b\u043e, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Novo selo, Sofia'},
'433462':{'de': 'Deutschlandsberg', 'en': 'Deutschlandsberg'},
'39049':{'en': 'Padova', 'it': 'Padova'},
'433460':{'de': 'Soboth', 'en': 'Soboth'},
'433461':{'de': u('Trah\u00fctten'), 'en': u('Trah\u00fctten')},
'433466':{'de': 'Eibiswald', 'en': 'Eibiswald'},
'433467':{'de': 'Schwanberg', 'en': 'Schwanberg'},
'433464':{'de': u('Gro\u00df Sankt Florian'), 'en': 'Gross St. Florian'},
'433465':{'de': u('P\u00f6lfing-Brunn'), 'en': u('P\u00f6lfing-Brunn')},
'375236':{'be': u('\u041c\u0430\u0437\u044b\u0440'), 'en': 'Mozyr', 'ru': u('\u041c\u043e\u0437\u044b\u0440\u044c')},
'433469':{'de': 'Sankt Oswald im Freiland', 'en': 'St. Oswald im Freiland'},
'375232':{'be': u('\u0413\u043e\u043c\u0435\u043b\u044c'), 'en': 'Gomel', 'ru': u('\u0413\u043e\u043c\u0435\u043b\u044c')},
'40236':{'en': u('Gala\u021bi'), 'ro': u('Gala\u021bi')},
'37424492':{'am': u('\u0553\u0561\u0576\u056b\u056f'), 'en': 'Panik', 'ru': u('\u041f\u0430\u043d\u0438\u043a')},
'40234':{'en': u('Bac\u0103u'), 'ro': u('Bac\u0103u')},
'40235':{'en': 'Vaslui', 'ro': 'Vaslui'},
'3356158':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356159':{'en': 'Toulouse', 'fr': 'Toulouse'},
'37424495':{'am': u('\u0531\u0580\u0587\u0577\u0561\u057f'), 'en': 'Arevshat', 'ru': u('\u0410\u0440\u0435\u0432\u0448\u0430\u0442')},
'40231':{'en': u('Boto\u0219ani'), 'ro': u('Boto\u0219ani')},
'3356154':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356155':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356156':{'en': 'Muret', 'fr': 'Muret'},
'3356157':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356150':{'en': 'Auterive', 'fr': 'Auterive'},
'3356151':{'en': 'Muret', 'fr': 'Muret'},
'3356152':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356153':{'en': 'Toulouse', 'fr': 'Toulouse'},
'39040':{'en': 'Trieste', 'it': 'Trieste'},
'3356378':{'en': 'Albi', 'fr': 'Albi'},
'3336710':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3347030':{'en': 'Vichy', 'fr': 'Vichy'},
'3356371':{'en': 'Castres', 'fr': 'Castres'},
'3356372':{'en': 'Castres', 'fr': 'Castres'},
'3356373':{'en': u('Labrugui\u00e8re'), 'fr': u('Labrugui\u00e8re')},
'3356376':{'en': 'Carmaux', 'fr': 'Carmaux'},
'3356377':{'en': 'Albi', 'fr': 'Albi'},
'370469':{'en': 'Neringa'},
'370460':{'en': 'Palanga'},
'35267':{'de': u('D\u00fcdelingen'), 'en': 'Dudelange', 'fr': 'Dudelange'},
'359359':{'bg': u('\u0412\u0435\u043b\u0438\u043d\u0433\u0440\u0430\u0434'), 'en': 'Velingrad'},
'35971302':{'bg': u('\u0411\u043e\u0436\u0435\u043d\u0438\u0446\u0430'), 'en': 'Bozhenitsa'},
'359357':{'bg': u('\u041f\u0430\u043d\u0430\u0433\u044e\u0440\u0438\u0449\u0435'), 'en': 'Panagyurishte'},
'359350':{'bg': u('\u041f\u0435\u0449\u0435\u0440\u0430, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Peshtera, Pazardzhik'},
'441499':{'en': 'Inveraray'},
'441492':{'en': 'Colwyn Bay'},
'441493':{'en': 'Great Yarmouth'},
'441490':{'en': 'Corwen'},
'441491':{'en': 'Henley-on-Thames'},
'441496':{'en': 'Port Ellen'},
'441497':{'en': 'Hay-on-Wye'},
'441494':{'en': 'High Wycombe'},
'441495':{'en': 'Pontypool'},
'435223':{'de': 'Hall in Tirol', 'en': 'Hall in Tirol'},
'441724':{'en': 'Scunthorpe'},
'441725':{'en': 'Rockbourne'},
'35971304':{'bg': u('\u041b\u0438\u043f\u043d\u0438\u0446\u0430, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Lipnitsa, Sofia'},
'441727':{'en': 'St Albans'},
'441720':{'en': 'Isles of Scilly'},
'441721':{'en': 'Peebles'},
'441722':{'en': 'Salisbury'},
'441723':{'en': 'Scarborough'},
'435226':{'de': 'Neustift im Stubaital', 'en': 'Neustift im Stubaital'},
'441728':{'en': 'Saxmundham'},
'441729':{'en': 'Settle'},
'3316433':{'en': 'Meaux', 'fr': 'Meaux'},
'3346767':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346764':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346765':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346762':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3346763':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3316435':{'en': 'Meaux', 'fr': 'Meaux'},
'3316434':{'en': 'Meaux', 'fr': 'Meaux'},
'3316439':{'en': 'Melun', 'fr': 'Melun'},
'435225':{'de': 'Fulpmes', 'en': 'Fulpmes'},
'3346768':{'en': 'Palavas-les-Flots', 'fr': 'Palavas-les-Flots'},
'3346769':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3596074':{'bg': u('\u041b\u044e\u0431\u0438\u0447\u0435\u0432\u043e'), 'en': 'Lyubichevo'},
'3596077':{'bg': u('\u0421\u0442\u0435\u0432\u0440\u0435\u043a'), 'en': 'Stevrek'},
'3596076':{'bg': u('\u0422\u0430\u0439\u043c\u0438\u0449\u0435'), 'en': 'Taymishte'},
'3596071':{'bg': u('\u0410\u043d\u0442\u043e\u043d\u043e\u0432\u043e'), 'en': 'Antonovo'},
'3598138':{'bg': u('\u0411\u0440\u044a\u0448\u043b\u0435\u043d'), 'en': 'Brashlen'},
'3596072':{'bg': u('\u0414\u043e\u0431\u0440\u043e\u0442\u0438\u0446\u0430, \u0422\u044a\u0440\u0433.'), 'en': 'Dobrotitsa, Targ.'},
'3343044':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3338954':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3338955':{'en': 'Wittelsheim', 'fr': 'Wittelsheim'},
'3336807':{'en': 'Colmar', 'fr': 'Colmar'},
'37426397':{'am': u('\u0531\u0566\u0561\u057f\u0561\u0574\u0578\u0582\u057f'), 'en': 'Azatamut', 'ru': u('\u0410\u0437\u0430\u0442\u0430\u043c\u0443\u0442')},
'37426392':{'am': u('\u0531\u0579\u0561\u057b\u0578\u0582\u0580'), 'en': 'Achajur', 'ru': u('\u0410\u0447\u0430\u0434\u0436\u0443\u0440')},
'432913':{'de': u('H\u00f6tzelsdorf'), 'en': u('H\u00f6tzelsdorf')},
'432912':{'de': 'Geras', 'en': 'Geras'},
'437435':{'de': 'Sankt Valentin', 'en': 'St. Valentin'},
'3325378':{'en': 'Nantes', 'fr': 'Nantes'},
'437434':{'de': 'Haag', 'en': 'Haag'},
'432916':{'de': 'Riegersburg, Hardegg', 'en': 'Riegersburg, Hardegg'},
'3332196':{'en': 'Calais', 'fr': 'Calais'},
'441337':{'en': 'Ladybank'},
'3332197':{'en': 'Calais', 'fr': 'Calais'},
'3332626':{'en': u('Ch\u00e2lons-en-Champagne'), 'fr': u('Ch\u00e2lons-en-Champagne')},
'3354628':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3354629':{'en': u('Ars-en-R\u00e9'), 'fr': u('Ars-en-R\u00e9')},
'3354625':{'en': u('Authon-Eb\u00e9on'), 'fr': u('Authon-Eb\u00e9on')},
'3354627':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3332621':{'en': u('Ch\u00e2lons-en-Champagne'), 'fr': u('Ch\u00e2lons-en-Champagne')},
'3332622':{'en': u('Ch\u00e2lons-en-Champagne'), 'fr': u('Ch\u00e2lons-en-Champagne')},
'34973':{'en': 'Lleida', 'es': u('L\u00e9rida')},
'390873':{'it': 'Vasto'},
'390872':{'it': 'Lanciano'},
'390871':{'it': 'Chieti'},
'390875':{'it': 'Termoli'},
'390874':{'en': 'Campobasso', 'it': 'Campobasso'},
'34979':{'en': 'Palencia', 'es': 'Palencia'},
'3593559':{'bg': u('\u041a\u0430\u043f\u0438\u0442\u0430\u043d \u0414\u0438\u043c\u0438\u0442\u0440\u0438\u0435\u0432\u043e'), 'en': 'Kapitan Dimitrievo'},
'3593558':{'bg': u('\u0418\u0441\u043f\u0435\u0440\u0438\u0445\u043e\u0432\u043e'), 'en': 'Isperihovo'},
'3593555':{'bg': u('\u041d\u043e\u0432\u0430 \u043c\u0430\u0445\u0430\u043b\u0430, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Nova mahala, Pazardzhik'},
'3593554':{'bg': u('\u041a\u043e\u0437\u0430\u0440\u0441\u043a\u043e'), 'en': 'Kozarsko'},
'3593557':{'bg': u('\u0411\u044f\u0433\u0430'), 'en': 'Byaga'},
'3593556':{'bg': u('\u0420\u0430\u0434\u0438\u043b\u043e\u0432\u043e'), 'en': 'Radilovo'},
'3593553':{'bg': u('\u0411\u0430\u0442\u0430\u043a, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Batak, Pazardzhik'},
'3593552':{'bg': u('\u0411\u0440\u0430\u0446\u0438\u0433\u043e\u0432\u043e'), 'en': 'Bratsigovo'},
'3332078':{'en': 'Lille', 'fr': 'Lille'},
'432258':{'de': 'Alland', 'en': 'Alland'},
'432259':{'de': u('M\u00fcnchendorf'), 'en': u('M\u00fcnchendorf')},
'3332071':{'en': 'Orchies', 'fr': 'Orchies'},
'3332070':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332073':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332072':{'en': u('Marcq-en-Bar\u0153ul'), 'fr': u('Marcq-en-Bar\u0153ul')},
'3332075':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332074':{'en': 'Lille', 'fr': 'Lille'},
'3332077':{'en': u('Armenti\u00e8res'), 'fr': u('Armenti\u00e8res')},
'3332076':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3323521':{'en': 'Le Havre', 'fr': 'Le Havre'},
'35941117':{'bg': u('\u041a\u0430\u0437\u0430\u043d\u043a\u0430'), 'en': 'Kazanka'},
'35941114':{'bg': u('\u041b\u043e\u0437\u0435\u043d, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Lozen, St. Zagora'},
'3323522':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323525':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323524':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323526':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323529':{'en': u('F\u00e9camp'), 'fr': u('F\u00e9camp')},
'3323528':{'en': u('F\u00e9camp'), 'fr': u('F\u00e9camp')},
'3329936':{'en': 'Rennes', 'fr': 'Rennes'},
'3329937':{'en': u('Ch\u00e2teaugiron'), 'fr': u('Ch\u00e2teaugiron')},
'3329930':{'en': 'Rennes', 'fr': 'Rennes'},
'3329931':{'en': 'Rennes', 'fr': 'Rennes'},
'3329932':{'en': 'Rennes', 'fr': 'Rennes'},
'3329933':{'en': 'Rennes', 'fr': 'Rennes'},
'3332383':{'en': u('Ch\u00e2teau-Thierry'), 'fr': u('Ch\u00e2teau-Thierry')},
'3349410':{'en': 'Six-Fours-les-Plages', 'fr': 'Six-Fours-les-Plages'},
'3332381':{'en': 'Ham', 'fr': 'Ham'},
'3349412':{'en': u('Hy\u00e8res'), 'fr': u('Hy\u00e8res')},
'3349415':{'en': 'Bormes-les-Mimosas', 'fr': 'Bormes-les-Mimosas'},
'37428193':{'am': u('\u0531\u0572\u0561\u057e\u0576\u0561\u0571\u0578\u0580'), 'en': 'Aghavnadzor', 'ru': u('\u0410\u0433\u0430\u0432\u043d\u0430\u0434\u0437\u043e\u0440')},
'3349417':{'en': u('Fr\u00e9jus'), 'fr': u('Fr\u00e9jus')},
'3332384':{'en': u('Ch\u00e2teau-Thierry'), 'fr': u('Ch\u00e2teau-Thierry')},
'3349419':{'en': u('Saint-Rapha\u00ebl'), 'fr': u('Saint-Rapha\u00ebl')},
'3349418':{'en': 'Toulon', 'fr': 'Toulon'},
'37428198':{'am': u('\u054c\u056b\u0576\u0564'), 'en': 'Rind', 'ru': u('\u0420\u0438\u043d\u0434')},
'37428199':{'am': u('\u0547\u0561\u057f\u056b\u0576'), 'en': 'Shatin', 'ru': u('\u0428\u0430\u0442\u0438\u043d')},
'3354996':{'en': 'Thouars', 'fr': 'Thouars'},
'3354997':{'en': 'Civray', 'fr': 'Civray'},
'3354994':{'en': 'Parthenay', 'fr': 'Parthenay'},
'3354993':{'en': u('Ch\u00e2tellerault'), 'fr': u('Ch\u00e2tellerault')},
'3354990':{'en': u('Naintr\u00e9'), 'fr': u('Naintr\u00e9')},
'3354991':{'en': 'Montmorillon', 'fr': 'Montmorillon'},
'3354998':{'en': 'Loudun', 'fr': 'Loudun'},
'3355717':{'en': 'Biganos', 'fr': 'Biganos'},
'433618':{'de': 'Hohentauern', 'en': 'Hohentauern'},
'3355715':{'en': 'La Teste de Buch', 'fr': 'La Teste de Buch'},
'3355714':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355712':{'en': 'Gradignan', 'fr': 'Gradignan'},
'3355710':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'433611':{'de': 'Johnsbach', 'en': 'Johnsbach'},
'433613':{'de': 'Admont', 'en': 'Admont'},
'433612':{'de': 'Liezen', 'en': 'Liezen'},
'3323737':{'en': 'Senonches', 'fr': 'Senonches'},
'433614':{'de': 'Rottenmann', 'en': 'Rottenmann'},
'3355719':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'433616':{'de': 'Selzthal', 'en': 'Selzthal'},
'433533':{'de': 'Turrach', 'en': 'Turrach'},
'3656':{'en': 'Szolnok', 'hu': 'Szolnok'},
'3657':{'en': 'Jaszbereny', 'hu': u('J\u00e1szber\u00e9ny')},
'3594798':{'bg': u('\u0421\u0430\u0432\u0438\u043d\u043e'), 'en': 'Savino'},
'3594799':{'bg': u('\u0413\u043e\u043b\u044f\u043c \u043c\u0430\u043d\u0430\u0441\u0442\u0438\u0440'), 'en': 'Golyam manastir'},
'3594796':{'bg': u('\u0413\u0435\u043d\u0435\u0440\u0430\u043b \u0422\u043e\u0448\u0435\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'General Toshevo, Yambol'},
'3594797':{'bg': u('\u0413\u044a\u043b\u044a\u0431\u0438\u043d\u0446\u0438'), 'en': 'Galabintsi'},
'3594794':{'bg': u('\u041e\u0432\u0447\u0438 \u043a\u043b\u0430\u0434\u0435\u043d\u0435\u0446'), 'en': 'Ovchi kladenets'},
'3594795':{'bg': u('\u0421\u043a\u0430\u043b\u0438\u0446\u0430'), 'en': 'Skalitsa'},
'3594792':{'bg': u('\u0411\u043e\u0442\u0435\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Botevo, Yambol'},
'3594793':{'bg': u('\u0411\u043e\u044f\u0434\u0436\u0438\u043a'), 'en': 'Boyadzhik'},
'441559':{'en': 'Llandysul'},
'441558':{'en': 'Llandeilo'},
'441553':{'en': 'Kings Lynn'},
'441550':{'en': 'Llandovery'},
'441557':{'en': 'Kirkcudbright'},
'441556':{'en': 'Castle Douglas'},
'441555':{'en': 'Lanark'},
'441554':{'en': 'Llanelli'},
'3332925':{'en': 'La Bresse', 'fr': 'La Bresse'},
'3332923':{'en': 'Remiremont', 'fr': 'Remiremont'},
'3332922':{'en': 'Remiremont', 'fr': 'Remiremont'},
'3324316':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324314':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3332929':{'en': u('\u00c9pinal'), 'fr': u('\u00c9pinal')},
'37422299':{'am': u('\u053f\u0578\u057f\u0561\u0575\u0584'), 'en': 'Kotayk', 'ru': u('\u041a\u043e\u0442\u0430\u0439\u043a')},
'435514':{'de': 'Bezau', 'en': 'Bezau'},
'435515':{'de': 'Au', 'en': 'Au'},
'435516':{'de': 'Doren', 'en': 'Doren'},
'37422294':{'am': u('\u0531\u0580\u0566\u0576\u056b'), 'en': 'Arzni', 'ru': u('\u0410\u0440\u0437\u043d\u0438')},
'373258':{'en': 'Telenesti', 'ro': u('Telene\u015fti'), 'ru': u('\u0422\u0435\u043b\u0435\u043d\u0435\u0448\u0442\u044c')},
'373259':{'en': 'Falesti', 'ro': u('F\u0103le\u015fti'), 'ru': u('\u0424\u044d\u043b\u0435\u0448\u0442\u044c')},
'435512':{'de': 'Egg', 'en': 'Egg'},
'435513':{'de': 'Hittisau', 'en': 'Hittisau'},
'373254':{'en': 'Rezina', 'ro': 'Rezina', 'ru': u('\u0420\u0435\u0437\u0438\u043d\u0430')},
'373256':{'en': 'Riscani', 'ro': u('R\u00ee\u015fcani'), 'ru': u('\u0420\u044b\u0448\u043a\u0430\u043d\u044c')},
'373250':{'en': 'Floresti', 'ro': u('Flore\u015fti'), 'ru': u('\u0424\u043b\u043e\u0440\u0435\u0448\u0442\u044c')},
'373251':{'en': 'Donduseni', 'ro': u('Dondu\u015feni'), 'ru': u('\u0414\u043e\u043d\u0434\u0443\u0448\u0435\u043d\u044c')},
'373252':{'en': 'Drochia', 'ro': 'Drochia', 'ru': u('\u0414\u0440\u043e\u043a\u0438\u044f')},
'37422291':{'am': u('\u0532\u0561\u056c\u0561\u0570\u0578\u057e\u056b\u057f/\u053f\u0561\u0574\u0561\u0580\u056b\u057d'), 'en': 'Balahovit/Kamaris', 'ru': u('\u0411\u0430\u043b\u0430\u043e\u0432\u0438\u0442/\u041a\u0430\u043c\u0430\u0440\u0438\u0441')},
'37422290':{'am': u('\u0544\u0561\u0575\u0561\u056f\u0578\u057e\u057d\u056f\u056b'), 'en': 'Mayakovsky', 'ru': u('\u041c\u0430\u044f\u043a\u043e\u0432\u0441\u043a\u0438\u0439')},
'35953234':{'bg': u('\u0417\u043b\u0430\u0442\u043d\u0430 \u043d\u0438\u0432\u0430'), 'en': 'Zlatna niva'},
'40364':{'en': 'Cluj', 'ro': 'Cluj'},
'40365':{'en': u('Mure\u0219'), 'ro': u('Mure\u0219')},
'40366':{'en': 'Harghita', 'ro': 'Harghita'},
'432624':{'de': 'Ebenfurth', 'en': 'Ebenfurth'},
'432623':{'de': 'Pottendorf', 'en': 'Pottendorf'},
'3316980':{'en': u('Montlh\u00e9ry'), 'fr': u('Montlh\u00e9ry')},
'35960388':{'bg': u('\u0413\u043e\u0440\u0438\u0446\u0430, \u0422\u044a\u0440\u0433.'), 'en': 'Goritsa, Targ.'},
'432622':{'de': 'Wiener Neustadt', 'en': 'Wiener Neustadt'},
'35960389':{'bg': u('\u041a\u0430\u0440\u0434\u0430\u043c, \u0422\u044a\u0440\u0433.'), 'en': 'Kardam, Targ.'},
'432621':{'de': 'Sieggraben', 'en': 'Sieggraben'},
'3329848':{'en': u('Ploudalm\u00e9zeau'), 'fr': u('Ploudalm\u00e9zeau')},
'432620':{'de': 'Willendorf', 'en': 'Willendorf'},
'35961305':{'bg': u('\u041b\u0435\u0441\u0438\u0447\u0435\u0440\u0438'), 'en': 'Lesicheri'},
'35961304':{'bg': u('\u0414\u0438\u043c\u0447\u0430'), 'en': 'Dimcha'},
'35961307':{'bg': u('\u0421\u0442\u0430\u043c\u0431\u043e\u043b\u043e\u0432\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Stambolovo, V. Tarnovo'},
'35961306':{'bg': u('\u041f\u0430\u0442\u0440\u0435\u0448'), 'en': 'Patresh'},
'35961301':{'bg': u('\u0411\u044f\u043b\u0430 \u0440\u0435\u043a\u0430, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Byala reka, V. Tarnovo'},
'35961303':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u041b\u0438\u043f\u043d\u0438\u0446\u0430'), 'en': 'Gorna Lipnitsa'},
'35961302':{'bg': u('\u0411\u0430\u0442\u0430\u043a, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Batak, V. Tarnovo'},
'35961309':{'bg': u('\u0413\u043e\u0440\u0441\u043a\u043e \u041a\u0430\u043b\u0443\u0433\u0435\u0440\u043e\u0432\u043e'), 'en': 'Gorsko Kalugerovo'},
'35961308':{'bg': u('\u0412\u0438\u0448\u043e\u0432\u0433\u0440\u0430\u0434'), 'en': 'Vishovgrad'},
'3347373':{'en': 'Lezoux', 'fr': 'Lezoux'},
'3347377':{'en': 'Cournon-d\'Auvergne', 'fr': 'Cournon-d\'Auvergne'},
'3347374':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'3596534':{'bg': u('\u041c\u0430\u043b\u0447\u0438\u043a\u0430'), 'en': 'Malchika'},
'3596535':{'bg': u('\u041a\u043e\u0437\u0430\u0440 \u0411\u0435\u043b\u0435\u043d\u0435'), 'en': 'Kozar Belene'},
'3596536':{'bg': u('\u0410\u0441\u043f\u0430\u0440\u0443\u0445\u043e\u0432\u043e, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Asparuhovo, Pleven'},
'3596537':{'bg': u('\u0410\u0441\u0435\u043d\u043e\u0432\u0446\u0438'), 'en': 'Asenovtsi'},
'3596530':{'bg': u('\u0422\u0440\u044a\u043d\u0447\u043e\u0432\u0438\u0446\u0430'), 'en': 'Tranchovitsa'},
'3596531':{'bg': u('\u0418\u0437\u0433\u0440\u0435\u0432, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Izgrev, Pleven'},
'3596532':{'bg': u('\u0411\u044a\u043b\u0433\u0430\u0440\u0435\u043d\u0435, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Balgarene, Pleven'},
'3596533':{'bg': u('\u0421\u0442\u0435\u0436\u0435\u0440\u043e\u0432\u043e'), 'en': 'Stezherovo'},
'37428794':{'am': u('\u0533\u0576\u0564\u0565\u057e\u0561\u0566'), 'en': 'Gndevaz', 'ru': u('\u0413\u043d\u0434\u0435\u0432\u0430\u0437')},
'3596538':{'bg': u('\u041e\u0431\u043d\u043e\u0432\u0430'), 'en': 'Obnova'},
'3596539':{'bg': u('\u0413\u0440\u0430\u0434\u0438\u0449\u0435, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Gradishte, Pleven'},
'3329844':{'en': 'Brest', 'fr': 'Brest'},
'437562':{'de': 'Windischgarsten', 'en': 'Windischgarsten'},
'3595559':{'bg': u('\u041a\u0443\u0431\u0430\u0434\u0438\u043d'), 'en': 'Kubadin'},
'3595558':{'bg': u('\u0414\u0435\u0431\u0435\u043b\u0442'), 'en': 'Debelt'},
'3347952':{'en': 'Aix-les-Bains', 'fr': 'Aix-les-Bains'},
'3329691':{'en': 'Perros-Guirec', 'fr': 'Perros-Guirec'},
'3329692':{'en': u('Tr\u00e9guier'), 'fr': u('Tr\u00e9guier')},
'3329695':{'en': 'Pontrieux', 'fr': 'Pontrieux'},
'3329694':{'en': 'Saint-Brieuc', 'fr': 'Saint-Brieuc'},
'3323208':{'en': 'Rouen', 'fr': 'Rouen'},
'3323209':{'en': 'Louviers', 'fr': 'Louviers'},
'3349620':{'en': 'Marseille', 'fr': 'Marseille'},
'3347665':{'en': 'Voiron', 'fr': 'Voiron'},
'441782':{'en': 'Stoke-on-Trent'},
'3347666':{'en': 'Entre-Deux-Guiers', 'fr': 'Entre-Deux-Guiers'},
'3597110':{'bg': u('\u041e\u043f\u0438\u0446\u0432\u0435\u0442'), 'en': 'Opitsvet'},
'3343703':{'en': 'Bourgoin-Jallieu', 'fr': 'Bourgoin-Jallieu'},
'3343702':{'en': 'Vienne', 'fr': 'Vienne'},
'3597117':{'bg': u('\u0413\u0440\u0430\u0434\u0435\u0446, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Gradets, Sofia'},
'3597116':{'bg': u('\u041f\u0435\u0442\u044a\u0440\u0447'), 'en': 'Petarch'},
'3597119':{'bg': u('\u0414\u0440\u044a\u043c\u0448\u0430'), 'en': 'Dramsha'},
'3597118':{'bg': u('\u0414\u0440\u0430\u0433\u043e\u0432\u0438\u0449\u0438\u0446\u0430, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Dragovishtitsa, Sofia'},
'3347845':{'en': 'Vaugneray', 'fr': 'Vaugneray'},
'3347662':{'en': u('Saint-Martin-d\'H\u00e8res'), 'fr': u('Saint-Martin-d\'H\u00e8res')},
'3742570':{'am': u('\u053e\u0561\u0572\u056f\u0561\u0570\u0578\u057e\u056b\u057f'), 'en': 'Tsakhkahovit region', 'ru': u('\u0426\u0430\u0445\u043a\u0430\u0445\u043e\u0432\u0438\u0442')},
'441236':{'en': 'Coatbridge'},
'441237':{'en': 'Bideford'},
'441234':{'en': 'Bedford'},
'3347663':{'en': 'Grenoble', 'fr': 'Grenoble'},
'441233':{'en': 'Ashford (Kent)'},
'437566':{'de': u('Rosenau am Hengstpa\u00df'), 'en': 'Rosenau am Hengstpass'},
'3326258':{'en': u('Saint-Andr\u00e9'), 'fr': u('Saint-Andr\u00e9')},
'3326259':{'en': 'Le Tampon', 'fr': 'Le Tampon'},
'3326254':{'en': 'Saint-Leu', 'fr': 'Saint-Leu'},
'3326255':{'en': 'Saint-Paul', 'fr': 'Saint-Paul'},
'3326256':{'en': 'Saint-Joseph', 'fr': 'Saint-Joseph'},
'3326257':{'en': 'Le Tampon', 'fr': 'Le Tampon'},
'3326250':{'en': u('Saint-Beno\u00eet'), 'fr': u('Saint-Beno\u00eet')},
'3326251':{'en': u('Saint-Beno\u00eet'), 'fr': u('Saint-Beno\u00eet')},
'3326252':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326253':{'en': 'Sainte-Marie', 'fr': 'Sainte-Marie'},
'437565':{'de': 'Sankt Pankraz', 'en': 'St. Pankraz'},
'437763':{'de': 'Kopfing im Innkreis', 'en': 'Kopfing im Innkreis'},
'437762':{'de': 'Raab', 'en': 'Raab'},
'437765':{'de': 'Lambrechten', 'en': 'Lambrechten'},
'3332719':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3332248':{'en': 'Corbie', 'fr': 'Corbie'},
'3332249':{'en': 'Camon', 'fr': 'Camon'},
'3332246':{'en': 'Amiens', 'fr': 'Amiens'},
'3332247':{'en': 'Amiens', 'fr': 'Amiens'},
'3332244':{'en': 'Amiens', 'fr': 'Amiens'},
'3332245':{'en': 'Amiens', 'fr': 'Amiens'},
'3332243':{'en': 'Amiens', 'fr': 'Amiens'},
'3332241':{'en': 'Ailly-sur-Noye', 'fr': 'Ailly-sur-Noye'},
'35963564':{'bg': u('\u0411\u0438\u0432\u043e\u043b\u0430\u0440\u0435'), 'en': 'Bivolare'},
'437588':{'de': 'Ried im Traunkreis', 'en': 'Ried im Traunkreis'},
'3324020':{'en': 'Nantes', 'fr': 'Nantes'},
'437585':{'de': 'Klaus an der Pyhrnbahn', 'en': 'Klaus an der Pyhrnbahn'},
'437584':{'de': 'Molln', 'en': 'Molln'},
'437587':{'de': 'Wartberg an der Krems', 'en': 'Wartberg an der Krems'},
'437586':{'de': 'Pettenbach', 'en': 'Pettenbach'},
'3352436':{'en': 'Pau', 'fr': 'Pau'},
'437583':{'de': u('Kremsm\u00fcnster'), 'en': u('Kremsm\u00fcnster')},
'437582':{'de': 'Kirchdorf an der Krems', 'en': 'Kirchdorf an der Krems'},
'3324022':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'3338055':{'en': 'Dijon', 'fr': 'Dijon'},
'3338054':{'en': 'Dijon', 'fr': 'Dijon'},
'3324027':{'en': 'Saint-Brevin-les-Pins', 'fr': 'Saint-Brevin-les-Pins'},
'3593054':{'bg': u('\u041f\u043e\u0434\u0432\u0438\u0441, \u0421\u043c\u043e\u043b.'), 'en': 'Podvis, Smol.'},
'3593055':{'bg': u('\u0415\u043b\u0445\u043e\u0432\u0435\u0446'), 'en': 'Elhovets'},
'3593056':{'bg': u('\u0427\u0435\u043f\u0438\u043d\u0446\u0438, \u0421\u043c\u043e\u043b.'), 'en': 'Chepintsi, Smol.'},
'3593057':{'bg': u('\u041f\u043b\u043e\u0432\u0434\u0438\u0432\u0446\u0438'), 'en': 'Plovdivtsi'},
'3593050':{'bg': u('\u0411\u0443\u043a\u043e\u0432\u0430 \u043f\u043e\u043b\u044f\u043d\u0430'), 'en': 'Bukova polyana'},
'3593051':{'bg': u('\u0427\u0435\u043f\u0435\u043b\u0430\u0440\u0435'), 'en': 'Chepelare'},
'3593052':{'bg': u('\u041b\u044a\u043a\u0438, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Laki, Plovdiv'},
'3593053':{'bg': u('\u0425\u0432\u043e\u0439\u043d\u0430'), 'en': 'Hvoyna'},
'3593058':{'bg': u('\u041c\u0443\u0433\u043b\u0430'), 'en': 'Mugla'},
'3593059':{'bg': u('\u041a\u0443\u0442\u0435\u043b\u0430'), 'en': 'Kutela'},
'3599548':{'bg': u('\u041a\u0440\u0430\u043f\u0447\u0435\u043d\u0435'), 'en': 'Krapchene'},
'3599549':{'bg': u('\u0414\u043e\u043b\u043d\u0430 \u0420\u0438\u043a\u0441\u0430'), 'en': 'Dolna Riksa'},
'3599544':{'bg': u('\u0421\u0442\u0443\u0434\u0435\u043d\u043e \u0431\u0443\u0447\u0435'), 'en': 'Studeno buche'},
'3599545':{'bg': u('\u0413\u0430\u0431\u0440\u043e\u0432\u043d\u0438\u0446\u0430'), 'en': 'Gabrovnitsa'},
'3599546':{'bg': u('\u0421\u043b\u0430\u0432\u043e\u0442\u0438\u043d'), 'en': 'Slavotin'},
'35963568':{'bg': u('\u0411\u0443\u043a\u043e\u0432\u043b\u044a\u043a'), 'en': 'Bukovlak'},
'3599540':{'bg': u('\u0411\u0435\u043b\u043e\u0442\u0438\u043d\u0446\u0438'), 'en': 'Belotintsi'},
'3599541':{'bg': u('\u0414\u043e\u043a\u0442\u043e\u0440 \u0419\u043e\u0441\u0438\u0444\u043e\u0432\u043e'), 'en': 'Doktor Yosifovo'},
'3599542':{'bg': u('\u0421\u043c\u043e\u043b\u044f\u043d\u043e\u0432\u0446\u0438'), 'en': 'Smolyanovtsi'},
'370448':{'en': u('Plung\u0117')},
'370449':{'en': u('\u0160ilal\u0117')},
'434238':{'de': 'Eisenkappel-Vellach', 'en': 'Eisenkappel-Vellach'},
'434239':{'de': 'Sankt Kanzian am Klopeiner See', 'en': 'St. Kanzian am Klopeiner See'},
'370440':{'en': 'Skuodas'},
'370441':{'en': u('\u0160ilut\u0117')},
'434234':{'de': 'Ruden', 'en': 'Ruden'},
'370443':{'en': u('Ma\u017eeikiai')},
'370444':{'en': u('Tel\u0161iai')},
'370445':{'en': 'Kretinga'},
'370446':{'en': u('Taurag\u0117')},
'370447':{'en': 'Jurbarkas'},
'3324780':{'en': u('Jou\u00e9-l\u00e8s-Tours'), 'fr': u('Jou\u00e9-l\u00e8s-Tours')},
'3356175':{'en': 'Ramonville-Saint-Agne', 'fr': 'Ramonville-Saint-Agne'},
'3356172':{'en': 'Portet-sur-Garonne', 'fr': 'Portet-sur-Garonne'},
'3356173':{'en': 'Ramonville-Saint-Agne', 'fr': 'Ramonville-Saint-Agne'},
'3356170':{'en': 'Aucamville', 'fr': 'Aucamville'},
'3356171':{'en': 'Blagnac', 'fr': 'Blagnac'},
'3324788':{'en': 'Tours', 'fr': 'Tours'},
'3356178':{'en': 'Colomiers', 'fr': 'Colomiers'},
'3356179':{'en': u('Bagn\u00e8res-de-Luchon'), 'fr': u('Bagn\u00e8res-de-Luchon')},
'359373':{'bg': u('\u0425\u0430\u0440\u043c\u0430\u043d\u043b\u0438'), 'en': 'Harmanli'},
'359379':{'bg': u('\u0421\u0432\u0438\u043b\u0435\u043d\u0433\u0440\u0430\u0434'), 'en': 'Svilengrad'},
'3356351':{'en': 'Castres', 'fr': 'Castres'},
'3356357':{'en': 'Gaillac', 'fr': 'Gaillac'},
'3356354':{'en': 'Albi', 'fr': 'Albi'},
'3356358':{'en': 'Lavaur', 'fr': 'Lavaur'},
'3356359':{'en': 'Castres', 'fr': 'Castres'},
'3343280':{'en': u('Boll\u00e8ne'), 'fr': u('Boll\u00e8ne')},
'3343281':{'en': 'Orange', 'fr': 'Orange'},
'3902':{'en': 'Milan', 'it': 'Milano'},
'3343285':{'en': 'Carpentras', 'fr': 'Carpentras'},
'37432298':{'am': u('\u053c\u0565\u0580\u0574\u0578\u0576\u057f\u0578\u057e\u0578'), 'en': 'Lermontovo', 'ru': u('\u041b\u0435\u0440\u043c\u043e\u043d\u0442\u043e\u0432\u043e')},
'37432299':{'am': u('\u054e\u0561\u0570\u0561\u0563\u0576\u056b'), 'en': 'Vahagni', 'ru': u('\u0412\u0430\u0430\u0433\u043d\u0438')},
'37432296':{'am': u('\u0544\u0561\u0580\u0563\u0561\u0570\u0578\u057e\u056b\u057f'), 'en': 'Margahovit', 'ru': u('\u041c\u0430\u0440\u0433\u0430\u043e\u0432\u0438\u0442')},
'37432297':{'am': u('\u0541\u0578\u0580\u0561\u0563\u0565\u057f'), 'en': 'Dzoraget', 'ru': u('\u0414\u0437\u043e\u0440\u0430\u0433\u0435\u0442')},
'37432294':{'am': u('\u053c\u0565\u057c\u0576\u0561\u057a\u0561\u057f'), 'en': 'Lernapat', 'ru': u('\u041b\u0435\u0440\u043d\u0430\u043f\u0430\u0442')},
'37432295':{'am': u('\u0535\u0572\u0565\u0563\u0576\u0578\u0582\u057f'), 'en': 'Yeghegnut', 'ru': u('\u0415\u0445\u0435\u0433\u043d\u0443\u0442')},
'37432293':{'am': u('\u0553\u0561\u0574\u0562\u0561\u056f'), 'en': 'Pambak', 'ru': u('\u041f\u0430\u043c\u0431\u0430\u043a')},
'3346700':{'en': 'Agde', 'fr': 'Agde'},
'3324125':{'en': 'Angers', 'fr': 'Angers'},
'3346702':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3324127':{'en': 'Angers', 'fr': 'Angers'},
'3346704':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346706':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346707':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3316414':{'en': 'Melun', 'fr': 'Melun'},
'3316417':{'en': 'Serris', 'fr': 'Serris'},
'3316411':{'en': 'Torcy', 'fr': 'Torcy'},
'3332994':{'en': u('Neufch\u00e2teau'), 'fr': u('Neufch\u00e2teau')},
'3316413':{'en': 'Moissy-Cramayel', 'fr': 'Moissy-Cramayel'},
'3316412':{'en': 'Lagny-sur-Marne', 'fr': 'Lagny-sur-Marne'},
'35247':{'de': 'Lintgen', 'en': 'Lintgen', 'fr': 'Lintgen'},
'35245':{'de': 'Diedrich', 'en': 'Diedrich', 'fr': 'Diedrich'},
'35243':{'de': 'Findel/Kirchberg', 'en': 'Findel/Kirchberg', 'fr': 'Findel/Kirchberg'},
'35242':{'de': 'Plateau de Kirchberg', 'en': 'Plateau de Kirchberg', 'fr': 'Plateau de Kirchberg'},
'35240':{'de': 'Howald', 'en': 'Howald', 'fr': 'Howald'},
'39041':{'en': 'Venice', 'it': 'Venezia'},
'3332992':{'en': 'Commercy', 'fr': 'Commercy'},
'39045':{'en': 'Verona', 'it': 'Verona'},
'35249':{'de': 'Howald', 'en': 'Howald', 'fr': 'Howald'},
'35248':{'de': 'Contern/Foetz', 'en': 'Contern/Foetz', 'fr': 'Contern/Foetz'},
'3347031':{'en': 'Vichy', 'fr': 'Vichy'},
'3336711':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3347032':{'en': 'Bellerive-sur-Allier', 'fr': 'Bellerive-sur-Allier'},
'3347035':{'en': 'Moulins', 'fr': 'Moulins'},
'3347034':{'en': 'Dompierre-sur-Besbre', 'fr': 'Dompierre-sur-Besbre'},
'3343067':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3332991':{'en': 'Commercy', 'fr': 'Commercy'},
'35965165':{'bg': u('\u041f\u0438\u0441\u0430\u0440\u043e\u0432\u043e, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Pisarovo, Pleven'},
'3338628':{'en': 'Cosne-Cours-sur-Loire', 'fr': 'Cosne-Cours-sur-Loire'},
'3338626':{'en': 'Cosne-Cours-sur-Loire', 'fr': 'Cosne-Cours-sur-Loire'},
'3338627':{'en': 'Clamecy', 'fr': 'Clamecy'},
'3338624':{'en': 'Clamecy', 'fr': 'Clamecy'},
'3338625':{'en': 'Decize', 'fr': 'Decize'},
'3338622':{'en': 'Lormes', 'fr': 'Lormes'},
'3338623':{'en': 'Nevers', 'fr': 'Nevers'},
'3338620':{'en': 'Corbigny', 'fr': 'Corbigny'},
'3338621':{'en': 'Nevers', 'fr': 'Nevers'},
'3338317':{'en': 'Nancy', 'fr': 'Nancy'},
'437283':{'de': 'Sarleinsbach', 'en': 'Sarleinsbach'},
'3338723':{'en': 'Sarrebourg', 'fr': 'Sarrebourg'},
'3338721':{'en': 'Metz', 'fr': 'Metz'},
'3338720':{'en': 'Metz', 'fr': 'Metz'},
'3338727':{'en': 'Sarreguemines', 'fr': 'Sarreguemines'},
'3659':{'en': 'Karcag', 'hu': 'Karcag'},
'3338724':{'en': 'Phalsbourg', 'fr': 'Phalsbourg'},
'3654':{'en': 'Berettyoujfalu', 'hu': u('Beretty\u00f3\u00fajfalu')},
'3338729':{'en': 'Saint-Avold', 'fr': 'Saint-Avold'},
'3338728':{'en': 'Sarreguemines', 'fr': 'Sarreguemines'},
'3652':{'en': 'Debrecen', 'hu': 'Debrecen'},
'3653':{'en': 'Cegled', 'hu': u('Cegl\u00e9d')},
'432278':{'de': 'Absdorf', 'en': 'Absdorf'},
'3334448':{'en': 'Beauvais', 'fr': 'Beauvais'},
'432279':{'de': 'Kirchberg am Wagram', 'en': 'Kirchberg am Wagram'},
'3334449':{'en': 'Chaumont-en-Vexin', 'fr': 'Chaumont-en-Vexin'},
'3324058':{'en': 'Nantes', 'fr': 'Nantes'},
'3598696':{'bg': u('\u0421\u0443\u0445\u043e\u0434\u043e\u043b, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Suhodol, Silistra'},
'3598695':{'bg': u('\u041d\u043e\u0436\u0430\u0440\u0435\u0432\u043e'), 'en': 'Nozharevo'},
'3598694':{'bg': u('\u0417\u0435\u0431\u0438\u043b'), 'en': 'Zebil'},
'437281':{'de': u('Aigen im M\u00fchlkreis'), 'en': u('Aigen im M\u00fchlkreis')},
'3347642':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3328552':{'en': 'Nantes', 'fr': 'Nantes'},
'3324188':{'en': 'Angers', 'fr': 'Angers'},
'3338319':{'en': 'Nancy', 'fr': 'Nancy'},
'441786':{'en': 'Stirling'},
'432276':{'de': 'Reidling', 'en': 'Reidling'},
'432277':{'de': 'Zwentendorf', 'en': 'Zwentendorf'},
'433588':{'de': 'Katsch an der Mur', 'en': 'Katsch an der Mur'},
'437758':{'de': 'Obernberg am Inn', 'en': 'Obernberg am Inn'},
'3329913':{'en': 'Melesse', 'fr': 'Melesse'},
'3332013':{'en': 'Lille', 'fr': 'Lille'},
'3332012':{'en': 'Lille', 'fr': 'Lille'},
'3332011':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3332017':{'en': 'Lille', 'fr': 'Lille'},
'3332015':{'en': 'Lille', 'fr': 'Lille'},
'3332014':{'en': 'Lille', 'fr': 'Lille'},
'3332019':{'en': 'Villeneuve-d\'Ascq', 'fr': 'Villeneuve-d\'Ascq'},
'3332018':{'en': 'Lille', 'fr': 'Lille'},
'3354606':{'en': 'Royan', 'fr': 'Royan'},
'3354607':{'en': u('Surg\u00e8res'), 'fr': u('Surg\u00e8res')},
'3354605':{'en': 'Royan', 'fr': 'Royan'},
'3354602':{'en': 'Saujon', 'fr': 'Saujon'},
'3354600':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'437755':{'de': 'Mettmach', 'en': 'Mettmach'},
'432271':{'de': 'Ried am Riederberg', 'en': 'Ried am Riederberg'},
'432272':{'de': 'Tulln an der Donau', 'en': 'Tulln an der Donau'},
'432273':{'de': 'Tulbing', 'en': 'Tulbing'},
'432274':{'de': 'Sieghartskirchen', 'en': 'Sieghartskirchen'},
'432275':{'de': 'Atzenbrugg', 'en': 'Atzenbrugg'},
'3354608':{'en': 'Royan', 'fr': 'Royan'},
'3354609':{'en': u('Saint-Martin-de-R\u00e9'), 'fr': u('Saint-Martin-de-R\u00e9')},
'3329916':{'en': 'Dinard', 'fr': 'Dinard'},
'3329917':{'en': u('Foug\u00e8res'), 'fr': u('Foug\u00e8res')},
'3329914':{'en': 'Rennes', 'fr': 'Rennes'},
'433582':{'de': 'Scheifling', 'en': 'Scheifling'},
'35941178':{'bg': u('\u041e\u0440\u044f\u0445\u043e\u0432\u0438\u0446\u0430, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Oryahovitsa, St. Zagora'},
'3355806':{'en': 'Mont-de-Marsan', 'fr': 'Mont-de-Marsan'},
'3355805':{'en': 'Mont-de-Marsan', 'fr': 'Mont-de-Marsan'},
'35941174':{'bg': u('\u041f\u043e\u0434\u0441\u043b\u043e\u043d, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Podslon, St. Zagora'},
'35941175':{'bg': u('\u041a\u043e\u043b\u0435\u043d\u0430'), 'en': 'Kolena'},
'3355809':{'en': 'Mimizan', 'fr': 'Mimizan'},
'3355808':{'en': 'Pissos', 'fr': 'Pissos'},
'433585':{'de': 'Sankt Lambrecht', 'en': 'St. Lambrecht'},
'35941171':{'bg': u('\u0414\u044a\u043b\u0431\u043e\u043a\u0438'), 'en': 'Dalboki'},
'3329918':{'en': u('Saint-Brice-en-Cogl\u00e8s'), 'fr': u('Saint-Brice-en-Cogl\u00e8s')},
'3325065':{'en': 'Caen', 'fr': 'Caen'},
'3323549':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323548':{'en': 'Le Havre', 'fr': 'Le Havre'},
'35941172':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u0411\u043e\u0442\u0435\u0432\u043e'), 'en': 'Gorno Botevo'},
'3323543':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323542':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323541':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323540':{'en': 'Dieppe', 'fr': 'Dieppe'},
'3323547':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323546':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323545':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3323544':{'en': 'Le Havre', 'fr': 'Le Havre'},
'3349479':{'en': 'Ramatuelle', 'fr': 'Ramatuelle'},
'3349478':{'en': 'Saint-Maximin-la-Sainte-Baume', 'fr': 'Saint-Maximin-la-Sainte-Baume'},
'3349473':{'en': 'Vidauban', 'fr': 'Vidauban'},
'3349471':{'en': 'Bormes-les-Mimosas', 'fr': 'Bormes-les-Mimosas'},
'3349477':{'en': 'Barjols', 'fr': 'Barjols'},
'3349476':{'en': 'Fayence', 'fr': 'Fayence'},
'3349475':{'en': 'La Garde', 'fr': 'La Garde'},
'3349474':{'en': 'Sanary-sur-Mer', 'fr': 'Sanary-sur-Mer'},
'441913':{'en': 'Durham'},
'441912':{'en': 'Tyneside'},
'441911':{'en': 'Tyneside/Durham/Sunderland'},
'434231':{'de': 'Mittertrixen', 'en': 'Mittertrixen'},
'441917':{'en': 'Sunderland'},
'441916':{'en': 'Tyneside'},
'441915':{'en': 'Sunderland'},
'441914':{'en': 'Tyneside'},
'3322940':{'en': 'Briec', 'fr': 'Briec'},
'441919':{'en': 'Durham'},
'441918':{'en': 'Tyneside'},
'3355730':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355732':{'en': 'Saint-Ciers-sur-Gironde', 'fr': 'Saint-Ciers-sur-Gironde'},
'3355735':{'en': u('B\u00e8gles'), 'fr': u('B\u00e8gles')},
'3355734':{'en': 'Tresses', 'fr': 'Tresses'},
'3347769':{'en': 'Charlieu', 'fr': 'Charlieu'},
'34928':{'en': 'Las Palmas', 'es': 'Las Palmas'},
'335495':{'en': 'Poitiers', 'fr': 'Poitiers'},
'432988':{'de': u('Neup\u00f6lla'), 'en': u('Neup\u00f6lla')},
'432989':{'de': 'Brunn an der Wild', 'en': 'Brunn an der Wild'},
'432982':{'de': 'Horn', 'en': 'Horn'},
'432983':{'de': 'Sigmundsherberg', 'en': 'Sigmundsherberg'},
'432986':{'de': 'Irnfritz', 'en': 'Irnfritz'},
'432987':{'de': 'Sankt Leonhard am Hornerwald', 'en': 'St. Leonhard am Hornerwald'},
'432984':{'de': 'Eggenburg', 'en': 'Eggenburg'},
'432985':{'de': 'Gars am Kamp', 'en': 'Gars am Kamp'},
'3317348':{'en': 'Boulogne-Billancourt', 'fr': 'Boulogne-Billancourt'},
'4417689':{'en': 'Penrith'},
'359938':{'bg': u('\u041a\u0443\u043b\u0430'), 'en': 'Kula'},
'3318372':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3324339':{'en': 'Le Mans', 'fr': 'Le Mans'},
'437247':{'de': 'Kematen am Innbach', 'en': 'Kematen am Innbach'},
'437244':{'de': 'Sattledt', 'en': 'Sattledt'},
'437245':{'de': 'Lambach', 'en': 'Lambach'},
'437242':{'de': 'Wels', 'en': 'Wels'},
'437243':{'de': 'Marchtrenk', 'en': 'Marchtrenk'},
'437240':{'de': 'Sipbachzell', 'en': 'Sipbachzell'},
'437241':{'de': 'Steinerkirchen an der Traun', 'en': 'Steinerkirchen an der Traun'},
'3324330':{'en': 'Mayenne', 'fr': 'Mayenne'},
'3324332':{'en': 'Mayenne', 'fr': 'Mayenne'},
'437224':{'de': 'Sankt Florian', 'en': 'St. Florian'},
'4417687':{'en': 'Keswick'},
'437248':{'de': 'Grieskirchen', 'en': 'Grieskirchen'},
'437249':{'de': 'Bad Schallerbach', 'en': 'Bad Schallerbach'},
'4417686':{'en': 'Penrith'},
'381280':{'en': 'Gnjilane', 'sr': 'Gnjilane'},
'373215':{'en': 'Dubasari', 'ro': u('Dub\u0103sari'), 'ru': u('\u0414\u0443\u0431\u044d\u0441\u0430\u0440\u044c')},
'433633':{'de': 'Landl', 'en': 'Landl'},
'433632':{'de': 'Sankt Gallen', 'en': 'St. Gallen'},
'433631':{'de': 'Unterlaussa', 'en': 'Unterlaussa'},
'433637':{'de': 'Gams bei Hieflau', 'en': 'Gams bei Hieflau'},
'433636':{'de': 'Wildalpen', 'en': 'Wildalpen'},
'433635':{'de': 'Radmer', 'en': 'Radmer'},
'433634':{'de': 'Hieflau', 'en': 'Hieflau'},
'437225':{'de': 'Hargelsberg', 'en': 'Hargelsberg'},
'433638':{'de': 'Palfau', 'en': 'Palfau'},
'35981886':{'bg': u('\u0427\u0435\u0440\u0435\u0448\u043e\u0432\u043e, \u0420\u0443\u0441\u0435'), 'en': 'Chereshovo, Ruse'},
'374223':{'am': u('\u0540\u0561\u0576\u0584\u0561\u057e\u0561\u0576/\u0540\u0580\u0561\u0566\u0564\u0561\u0576/\u053e\u0561\u0572\u056f\u0561\u0571\u0578\u0580'), 'en': 'Hankavan/Hrazdan/Tsakhkadzor', 'ru': u('\u0410\u043d\u043a\u0430\u0432\u0430\u043d/\u0420\u0430\u0437\u0434\u0430\u043d/\u0426\u0430\u0445\u043a\u0430\u0434\u0437\u043e\u0440')},
'374224':{'am': u('\u0554\u0561\u0576\u0561\u0584\u0565\u057c\u0561\u057e\u0561\u0576/\u0546\u0578\u0580 \u0533\u0565\u0572\u056b/\u0546\u0578\u0580 \u0540\u0561\u0573\u0568\u0576/\u0535\u0572\u057e\u0561\u0580\u0564'), 'en': 'Kanakeravan/Nor Geghi/Nor Hajn/Yeghvard', 'ru': u('\u041a\u0430\u043d\u0430\u043a\u0435\u0440\u0430\u0432\u0430\u043d/\u041d\u043e\u0440 \u0413\u0435\u0445\u0438/\u041d\u043e\u0440 \u0410\u0447\u043d/\u0415\u0433\u0432\u0430\u0440\u0434')},
'374226':{'am': u('\u0549\u0561\u0580\u0565\u0576\u0581\u0561\u057e\u0561\u0576'), 'en': 'Charentsavan', 'ru': u('\u0427\u0430\u0440\u0435\u043d\u0446\u0430\u0432\u0430\u043d')},
'35993342':{'bg': u('\u041a\u0438\u0440\u0435\u0435\u0432\u043e'), 'en': 'Kireevo'},
'437226':{'de': 'Wilhering', 'en': 'Wilhering'},
'3356722':{'en': 'Toulouse', 'fr': 'Toulouse'},
'34927':{'en': u('C\u00e1ceres'), 'es': u('C\u00e1ceres')},
'441910':{'en': 'Tyneside/Durham/Sunderland'},
'437227':{'de': 'Neuhofen an der Krems', 'en': 'Neuhofen an der Krems'},
'437221':{'de': u('H\u00f6rsching'), 'en': u('H\u00f6rsching')},
'3596518':{'bg': u('\u0420\u0438\u0431\u0435\u043d'), 'en': 'Riben'},
'3596519':{'bg': u('\u0411\u0435\u0433\u043b\u0435\u0436'), 'en': 'Beglezh'},
'3596516':{'bg': u('\u0418\u0441\u043a\u044a\u0440, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Iskar, Pleven'},
'3596517':{'bg': u('\u041f\u043e\u0434\u0435\u043c'), 'en': 'Podem'},
'3596514':{'bg': u('\u0414\u043e\u043b\u043d\u0438 \u0414\u044a\u0431\u043d\u0438\u043a'), 'en': 'Dolni Dabnik'},
'3596515':{'bg': u('\u0421\u043b\u0430\u0432\u044f\u043d\u043e\u0432\u043e, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Slavyanovo, Pleven'},
'3596512':{'bg': u('\u0413\u043e\u0440\u043d\u0438 \u0414\u044a\u0431\u043d\u0438\u043a'), 'en': 'Gorni Dabnik'},
'3596513':{'bg': u('\u041f\u043e\u0440\u0434\u0438\u043c'), 'en': 'Pordim'},
'3596510':{'bg': u('\u0422\u043e\u0442\u043b\u0435\u0431\u0435\u043d'), 'en': 'Totleben'},
'3596511':{'bg': u('\u041f\u043e\u0431\u0435\u0434\u0430, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Pobeda, Pleven'},
'437223':{'de': 'Enns', 'en': 'Enns'},
'433867':{'de': 'Pernegg an der Mur', 'en': 'Pernegg an der Mur'},
'434852':{'de': 'Lienz', 'en': 'Lienz'},
'434853':{'de': 'Ainet', 'en': 'Ainet'},
'434855':{'de': 'Assling', 'en': 'Assling'},
'434858':{'de': 'Nikolsdorf', 'en': 'Nikolsdorf'},
'3347939':{'en': 'Albertville', 'fr': 'Albertville'},
'37426272':{'am': u('\u053c\u056b\u0573\u0584'), 'en': 'Lichk', 'ru': u('\u041b\u0438\u0447\u043a')},
'3347932':{'en': 'Albertville', 'fr': 'Albertville'},
'3347933':{'en': u('Chamb\u00e9ry'), 'fr': u('Chamb\u00e9ry')},
'3347934':{'en': 'Aix-les-Bains', 'fr': 'Aix-les-Bains'},
'3347935':{'en': 'Aix-les-Bains', 'fr': 'Aix-les-Bains'},
'3347937':{'en': 'Albertville', 'fr': 'Albertville'},
'3316995':{'en': 'Angerville', 'fr': 'Angerville'},
'3316996':{'en': 'Savigny-sur-Orge', 'fr': 'Savigny-sur-Orge'},
'3316990':{'en': 'Mennecy', 'fr': 'Mennecy'},
'3316991':{'en': u('\u00c9vry'), 'fr': u('\u00c9vry')},
'3316992':{'en': u('\u00c9tampes'), 'fr': u('\u00c9tampes')},
'3349222':{'en': u('Brian\u00e7on'), 'fr': u('Brian\u00e7on')},
'390428':{'it': 'Tarvisio'},
'390429':{'it': 'Este'},
'390424':{'en': 'Vicenza', 'it': 'Bassano del Grappa'},
'390425':{'en': 'Rovigo', 'it': 'Rovigo'},
'390426':{'en': 'Rovigo', 'it': 'Adria'},
'390427':{'it': 'Spilimbergo'},
'390932':{'it': 'Ragusa'},
'390421':{'en': 'Venice', 'it': u('San Don\u00e0 di Piave')},
'390422':{'en': 'Treviso', 'it': 'Treviso'},
'390423':{'en': 'Treviso', 'it': 'Montebelluna'},
'3343720':{'en': 'Givors', 'fr': 'Givors'},
'3343723':{'en': 'Lyon', 'fr': 'Lyon'},
'3343722':{'en': 'Craponne', 'fr': 'Craponne'},
'3343725':{'en': 'Saint-Priest', 'fr': 'Saint-Priest'},
'3343724':{'en': 'Lyon', 'fr': 'Lyon'},
'3338785':{'en': 'Forbach', 'fr': 'Forbach'},
'3343728':{'en': 'Lyon', 'fr': 'Lyon'},
'3597177':{'bg': u('\u0410\u043b\u0434\u043e\u043c\u0438\u0440\u043e\u0432\u0446\u0438'), 'en': 'Aldomirovtsi'},
'3597176':{'bg': u('\u0425\u0440\u0430\u0431\u044a\u0440\u0441\u043a\u043e'), 'en': 'Hrabarsko'},
'3597175':{'bg': u('\u0413\u0430\u0431\u0435\u0440'), 'en': 'Gaber'},
'3349227':{'en': 'Saint-Laurent-du-Var', 'fr': 'Saint-Laurent-du-Var'},
'3349224':{'en': 'Saint-Chaffrey', 'fr': 'Saint-Chaffrey'},
'3595123':{'bg': u('\u0420\u0430\u0432\u043d\u0430, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Ravna, Varna'},
'3355675':{'en': 'Gradignan', 'fr': 'Gradignan'},
'390376':{'en': 'Mantua', 'it': 'Mantova'},
'3345099':{'en': 'Divonne-les-Bains', 'fr': 'Divonne-les-Bains'},
'3345098':{'en': 'Cluses', 'fr': 'Cluses'},
'390377':{'it': 'Codogno'},
'3326272':{'en': 'Sainte-Marie', 'fr': 'Sainte-Marie'},
'3326270':{'en': 'Saint-Paul', 'fr': 'Saint-Paul'},
'3326271':{'en': 'Le Port', 'fr': 'Le Port'},
'3345091':{'en': 'Sallanches', 'fr': 'Sallanches'},
'3345090':{'en': u('Ar\u00e2ches-la-Frasse'), 'fr': u('Ar\u00e2ches-la-Frasse')},
'3345093':{'en': 'Saint-Gervais-les-Bains', 'fr': 'Saint-Gervais-les-Bains'},
'3345092':{'en': 'Annemasse', 'fr': 'Annemasse'},
'3345095':{'en': 'Annemasse', 'fr': 'Annemasse'},
'3345094':{'en': 'Douvaine', 'fr': 'Douvaine'},
'3345097':{'en': 'Bonneville', 'fr': 'Bonneville'},
'3345096':{'en': 'Cluses', 'fr': 'Cluses'},
'3317117':{'en': 'Boulogne-Billancourt', 'fr': 'Boulogne-Billancourt'},
'3317119':{'en': 'Paris', 'fr': 'Paris'},
'3317118':{'en': 'Paris', 'fr': 'Paris'},
'3595129':{'bg': u('\u0411\u043b\u044a\u0441\u043a\u043e\u0432\u043e'), 'en': 'Blaskovo'},
'3323223':{'en': u('\u00c9vreux'), 'fr': u('\u00c9vreux')},
'3323220':{'en': 'Pont-Audemer', 'fr': 'Pont-Audemer'},
'3323221':{'en': 'Vernon', 'fr': 'Vernon'},
'3323227':{'en': 'Gisors', 'fr': 'Gisors'},
'3323225':{'en': 'Louviers', 'fr': 'Louviers'},
'3323228':{'en': u('\u00c9vreux'), 'fr': u('\u00c9vreux')},
'3323229':{'en': 'Breteuil sur Iton', 'fr': 'Breteuil sur Iton'},
'437748':{'de': 'Eggelsberg', 'en': 'Eggelsberg'},
'35969032':{'bg': u('\u0411\u044a\u043b\u0433\u0430\u0440\u0441\u043a\u0438 \u0438\u0437\u0432\u043e\u0440'), 'en': 'Balgarski izvor'},
'3332220':{'en': 'Abbeville', 'fr': 'Abbeville'},
'437742':{'de': 'Mattighofen', 'en': 'Mattighofen'},
'3332222':{'en': 'Amiens', 'fr': 'Amiens'},
'3332224':{'en': 'Abbeville', 'fr': 'Abbeville'},
'3332225':{'en': 'Rue', 'fr': 'Rue'},
'3332226':{'en': 'Cayeux-sur-Mer', 'fr': 'Cayeux-sur-Mer'},
'3332227':{'en': 'Le Crotoy', 'fr': 'Le Crotoy'},
'3597043':{'bg': u('\u0413\u043e\u043b\u0435\u043c\u043e \u0441\u0435\u043b\u043e'), 'en': 'Golemo selo'},
'3597042':{'bg': u('\u041a\u043e\u0440\u043a\u0438\u043d\u0430'), 'en': 'Korkina'},
'3593920':{'bg': u('\u0417\u043b\u0430\u0442\u043e\u043f\u043e\u043b\u0435'), 'en': 'Zlatopole'},
'3347985':{'en': u('Chamb\u00e9ry'), 'fr': u('Chamb\u00e9ry')},
'3593922':{'bg': u('\u0411\u0440\u043e\u0434'), 'en': 'Brod'},
'3593691':{'bg': u('\u0427\u0435\u0440\u043d\u043e\u043e\u0447e\u043d\u0435'), 'en': 'Chernoochene'},
'3593696':{'bg': u('\u041f\u0447\u0435\u043b\u0430\u0440\u043e\u0432\u043e, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Pchelarovo, Kardzh.'},
'3593925':{'bg': u('\u041a\u0440\u0443\u043c'), 'en': 'Krum'},
'3593926':{'bg': u('\u0414\u043e\u0431\u0440\u0438\u0447, \u0425\u0430\u0441\u043a.'), 'en': 'Dobrich, Hask.'},
'3347768':{'en': 'Roanne', 'fr': 'Roanne'},
'3593928':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u0411\u0435\u043b\u0435\u0432\u043e'), 'en': 'Dolno Belevo'},
'3593929':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u043e \u0410\u0441\u0435\u043d\u043e\u0432\u043e'), 'en': 'Golyamo Asenovo'},
'3593699':{'bg': u('\u0413\u0430\u0431\u0440\u043e\u0432\u043e, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Gabrovo, Kardzh.'},
'3347981':{'en': 'Belley', 'fr': 'Belley'},
'433833':{'de': 'Traboch', 'en': 'Traboch'},
'3332732':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3332733':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3332730':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'3332731':{'en': 'Denain', 'fr': 'Denain'},
'3593076':{'bg': u('\u0426\u0430\u0446\u0430\u0440\u043e\u0432\u0446\u0438'), 'en': 'Tsatsarovtsi'},
'3593077':{'bg': u('\u0421\u0440\u0435\u0434\u0435\u0446, \u0421\u043c\u043e\u043b.'), 'en': 'Sredets, Smol.'},
'3593074':{'bg': u('\u0415\u0440\u043c\u0430 \u0440\u0435\u043a\u0430'), 'en': 'Erma reka'},
'3593075':{'bg': u('\u0414\u043e\u043b\u0435\u043d, \u0421\u043c\u043e\u043b.'), 'en': 'Dolen, Smol.'},
'3593072':{'bg': u('\u041d\u0435\u0434\u0435\u043b\u0438\u043d\u043e'), 'en': 'Nedelino'},
'3593073':{'bg': u('\u0421\u0442\u0430\u0440\u0446\u0435\u0432\u043e'), 'en': 'Startsevo'},
'3332739':{'en': 'Jeumont', 'fr': 'Jeumont'},
'370422':{'en': u('Radvili\u0161kis')},
'370421':{'en': 'Pakruojis'},
'370426':{'en': u('Joni\u0161kis')},
'370427':{'en': u('Kelm\u0117')},
'370425':{'en': u('Akmen\u0117')},
'370428':{'en': 'Raseiniai'},
'3599129':{'bg': u('\u0421\u0438\u043d\u044c\u043e \u0431\u044a\u0440\u0434\u043e'), 'en': 'Sinyo bardo'},
'3599127':{'bg': u('\u041b\u044e\u0442\u0438\u0434\u043e\u043b'), 'en': 'Lyutidol'},
'434212':{'de': 'Sankt Veit an der Glan', 'en': 'St. Veit an der Glan'},
'3316419':{'en': 'Savigny-le-Temple', 'fr': 'Savigny-le-Temple'},
'434214':{'de': u('Br\u00fcckl'), 'en': u('Br\u00fcckl')},
'434215':{'de': 'Liebenfels', 'en': 'Liebenfels'},
'35971587':{'bg': u('\u0413\u043e\u043b\u0435\u043c\u0430 \u0420\u0430\u043a\u043e\u0432\u0438\u0446\u0430'), 'en': 'Golema Rakovitsa'},
'3356111':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356112':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356113':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356114':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356115':{'en': 'Colomiers', 'fr': 'Colomiers'},
'3356116':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356117':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3599121':{'bg': u('\u0426\u0430\u0440\u0435\u0432\u0435\u0446, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Tsarevets, Vratsa'},
'3353540':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3679':{'en': 'Baja', 'hu': 'Baja'},
'359318':{'bg': u('\u0421\u044a\u0435\u0434\u0438\u043d\u0435\u043d\u0438\u0435, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Saedinenie, Plovdiv'},
'3742830':{'am': u('\u054d\u056b\u057d\u056b\u0561\u0576'), 'en': 'Sisian', 'ru': u('\u0421\u0438\u0441\u0438\u0430\u043d')},
'441458':{'en': 'Glastonbury'},
'441456':{'en': 'Glenurquhart'},
'441457':{'en': 'Glossop'},
'441454':{'en': 'Chipping Sodbury'},
'441455':{'en': 'Hinckley'},
'441452':{'en': 'Gloucester'},
'441453':{'en': 'Dursley'},
'441450':{'en': 'Hawick'},
'39051':{'en': 'Bologna', 'it': 'Bologna'},
'437246':{'de': 'Gunskirchen', 'en': 'Gunskirchen'},
'441788':{'en': 'Rugby'},
'3599567':{'bg': u('\u0414\u043e\u043b\u043d\u0430 \u0412\u0435\u0440\u0435\u043d\u0438\u0446\u0430'), 'en': 'Dolna Verenitsa'},
'3599564':{'bg': u('\u0421\u0442\u0443\u0431\u0435\u043b'), 'en': 'Stubel'},
'3599560':{'bg': u('\u0411\u0435\u0437\u0434\u0435\u043d\u0438\u0446\u0430'), 'en': 'Bezdenitsa'},
'3599561':{'bg': u('\u0421\u0443\u043c\u0435\u0440'), 'en': 'Sumer'},
'3599568':{'bg': u('\u0411\u043b\u0430\u0433\u043e\u0432\u043e, \u041c\u043e\u043d\u0442.'), 'en': 'Blagovo, Mont.'},
'3599569':{'bg': u('\u041b\u0438\u043f\u0435\u043d'), 'en': 'Lipen'},
'3347581':{'en': 'Valence', 'fr': 'Valence'},
'3347580':{'en': u('Saint-P\u00e9ray'), 'fr': u('Saint-P\u00e9ray')},
'3347583':{'en': u('Bourg-l\u00e8s-Valence'), 'fr': u('Bourg-l\u00e8s-Valence')},
'3347582':{'en': 'Valence', 'fr': 'Valence'},
'3347584':{'en': u('Pont-de-l\'Is\u00e8re'), 'fr': u('Pont-de-l\'Is\u00e8re')},
'3347239':{'en': 'Saint-Genis-Laval', 'fr': 'Saint-Genis-Laval'},
'3347238':{'en': 'Tassin-la-Demi-Lune', 'fr': 'Tassin-la-Demi-Lune'},
'3347589':{'en': 'Aubenas', 'fr': 'Aubenas'},
'3347588':{'en': 'Vallon-Pont-d\'Arc', 'fr': 'Vallon-Pont-d\'Arc'},
'3347235':{'en': 'Lyon', 'fr': 'Lyon'},
'3347234':{'en': 'Lyon', 'fr': 'Lyon'},
'3347233':{'en': 'Lyon', 'fr': 'Lyon'},
'3347232':{'en': 'Lyon', 'fr': 'Lyon'},
'3347231':{'en': 'Brignais', 'fr': 'Brignais'},
'3332908':{'en': 'Vittel', 'fr': 'Vittel'},
'432825':{'de': u('G\u00f6pfritz an der Wild'), 'en': u('G\u00f6pfritz an der Wild')},
'434227':{'de': 'Ferlach', 'en': 'Ferlach'},
'432824':{'de': 'Allentsteig', 'en': 'Allentsteig'},
'3346728':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3346729':{'en': 'Mauguio', 'fr': 'Mauguio'},
'3346722':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346723':{'en': u('B\u00e9darieux'), 'fr': u('B\u00e9darieux')},
'3346720':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3324105':{'en': 'Angers', 'fr': 'Angers'},
'3346726':{'en': 'Le Cap d\'Agde', 'fr': 'Le Cap d\'Agde'},
'3346727':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346724':{'en': 'Montagnac', 'fr': 'Montagnac'},
'3346725':{'en': 'Paulhan', 'fr': 'Paulhan'},
'432829':{'de': 'Schweiggers', 'en': 'Schweiggers'},
'432828':{'de': 'Rappottenstein', 'en': 'Rappottenstein'},
'35931308':{'bg': u('\u0411\u043e\u0433\u0434\u0430\u043d, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Bogdan, Plovdiv'},
'35931309':{'bg': u('\u041a\u043b\u0438\u043c\u0435\u043d\u0442, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Kliment, Plovdiv'},
'3338648':{'en': 'Auxerre', 'fr': 'Auxerre'},
'3338649':{'en': 'Auxerre', 'fr': 'Auxerre'},
'3338640':{'en': u('Mon\u00e9teau'), 'fr': u('Mon\u00e9teau')},
'3338642':{'en': 'Chablis', 'fr': 'Chablis'},
'3338643':{'en': 'Saint-Florentin', 'fr': 'Saint-Florentin'},
'3338644':{'en': 'Toucy', 'fr': 'Toucy'},
'3338646':{'en': 'Auxerre', 'fr': 'Auxerre'},
'35937604':{'bg': u('\u0412\u044a\u0440\u0431\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Varbovo, Hask.'},
'3742499':{'am': u('\u0531\u0580\u0561\u0563\u0561\u056e\u0561\u057e\u0561\u0576'), 'en': 'Aragatsavan', 'ru': u('\u0410\u0440\u0430\u0433\u0430\u0446\u0430\u0432\u0430\u043d')},
'3336847':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3596167':{'bg': u('\u041a\u0435\u0441\u0430\u0440\u0435\u0432\u043e'), 'en': 'Kesarevo'},
'3596166':{'bg': u('\u0412\u0438\u043d\u043e\u0433\u0440\u0430\u0434'), 'en': 'Vinograd'},
'3596165':{'bg': u('\u0410\u0441\u0435\u043d\u043e\u0432\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Asenovo, V. Tarnovo'},
'3596164':{'bg': u('\u0411\u0440\u044f\u0433\u043e\u0432\u0438\u0446\u0430'), 'en': 'Bryagovitsa'},
'3596163':{'bg': u('\u041a\u0430\u043c\u0435\u043d, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Kamen, V. Tarnovo'},
'3596161':{'bg': u('\u0421\u0442\u0440\u0430\u0436\u0438\u0446\u0430, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Strazhitsa, V. Tarnovo'},
'3338705':{'en': u('Ch\u00e2teau-Salins'), 'fr': u('Ch\u00e2teau-Salins')},
'3338704':{'en': 'Freyming-Merlebach', 'fr': 'Freyming-Merlebach'},
'3338706':{'en': 'Bitche', 'fr': 'Bitche'},
'3676':{'en': 'Kecskemet', 'hu': u('Kecskem\u00e9t')},
'3677':{'en': 'Kiskunhalas', 'hu': 'Kiskunhalas'},
'3338703':{'en': 'Sarrebourg', 'fr': 'Sarrebourg'},
'3596168':{'bg': u('\u0421\u0443\u0448\u0438\u0446\u0430, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Sushitsa, V. Tarnovo'},
'3316037':{'en': 'Torcy', 'fr': 'Torcy'},
'3316036':{'en': u('Mont\u00e9vrain'), 'fr': u('Mont\u00e9vrain')},
'3316035':{'en': 'Saint-Thibault-des-Vignes', 'fr': 'Saint-Thibault-des-Vignes'},
'3316034':{'en': 'Pontault-Combault', 'fr': 'Pontault-Combault'},
'3316032':{'en': 'Meaux', 'fr': 'Meaux'},
'3316031':{'en': 'Lagny-sur-Marne', 'fr': 'Lagny-sur-Marne'},
'3342602':{'en': 'Lyon', 'fr': 'Lyon'},
'3316039':{'en': 'Fontainebleau', 'fr': 'Fontainebleau'},
'3522780':{'de': 'Diekirch', 'en': 'Diekirch', 'fr': 'Diekirch'},
'3522781':{'de': u('Ettelbr\u00fcck/Reckange-sur-Mess'), 'en': 'Ettelbruck/Reckange-sur-Mess', 'fr': 'Ettelbruck/Reckange-sur-Mess'},
'3522783':{'de': 'Vianden', 'en': 'Vianden', 'fr': 'Vianden'},
'3522784':{'de': 'Han/Lesse', 'en': 'Han/Lesse', 'fr': 'Han/Lesse'},
'3522785':{'de': 'Bissen/Roost', 'en': 'Bissen/Roost', 'fr': 'Bissen/Roost'},
'3522787':{'de': 'Fels', 'en': 'Larochette', 'fr': 'Larochette'},
'3522788':{'de': 'Mertzig/Wahl', 'en': 'Mertzig/Wahl', 'fr': 'Mertzig/Wahl'},
'371634':{'en': 'Liepaja'},
'371635':{'en': 'Ventspils'},
'371636':{'en': 'Ventspils'},
'371637':{'en': 'Dobele'},
'371630':{'en': 'Jelgava'},
'371631':{'en': 'Tukums'},
'371632':{'en': 'Talsi'},
'371633':{'en': 'Kuldiga'},
'371638':{'en': 'Saldus'},
'371639':{'en': 'Bauska'},
'3674':{'en': 'Szekszard', 'hu': u('Szeksz\u00e1rd')},
'432212':{'de': 'Orth an der Donau', 'en': 'Orth an der Donau'},
'432213':{'de': 'Lassee', 'en': 'Lassee'},
'3332039':{'en': 'Comines', 'fr': 'Comines'},
'432214':{'de': 'Kopfstetten', 'en': 'Kopfstetten'},
'432215':{'de': 'Probstdorf', 'en': 'Probstdorf'},
'3332035':{'en': u('Armenti\u00e8res'), 'fr': u('Armenti\u00e8res')},
'3332034':{'en': 'Villeneuve-d\'Ascq', 'fr': 'Villeneuve-d\'Ascq'},
'3332036':{'en': 'Tourcoing', 'fr': 'Tourcoing'},
'3332031':{'en': 'Lille', 'fr': 'Lille'},
'3332030':{'en': 'Lille', 'fr': 'Lille'},
'3332033':{'en': 'Villeneuve-d\'Ascq', 'fr': 'Villeneuve-d\'Ascq'},
'3332032':{'en': 'Seclin', 'fr': 'Seclin'},
'4415078':{'en': 'Alford (Lincs)'},
'4415079':{'en': 'Alford (Lincs)'},
'3354667':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3354668':{'en': 'Puilboreau', 'fr': 'Puilboreau'},
'4415075':{'en': 'Spilsby (Horncastle)'},
'4415076':{'en': 'Louth'},
'4415077':{'en': 'Louth'},
'4415070':{'en': 'Louth/Alford (Lincs)/Spilsby (Horncastle)'},
'374222':{'am': u('\u0531\u0562\u0578\u057e\u0575\u0561\u0576/\u0531\u056f\u0578\u0582\u0576\u0584/\u0532\u0575\u0578\u0582\u0580\u0565\u0572\u0561\u057e\u0561\u0576/\u0546\u0578\u0580 \u0533\u0575\u0578\u0582\u0572/\u054e\u0565\u0580\u056b\u0576 \u054a\u057f\u0572\u0576\u056b'), 'en': 'Abovyan/Akunk/Byureghavan/Nor Gyugh/Verin Ptghni', 'ru': u('\u0410\u0431\u043e\u0432\u044f\u043d/\u0410\u043a\u0443\u043d\u043a/\u0411\u044e\u0440\u0435\u0433\u0430\u0432\u0430\u043d/\u041d\u043e\u0440 \u0413\u044e\u0445/\u0412\u0435\u0440\u0438\u043d \u041f\u0442\u0445\u043d\u0438')},
'4415072':{'en': 'Spilsby (Horncastle)'},
'3358160':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3329970':{'en': 'Sixt-sur-Aff', 'fr': 'Sixt-sur-Aff'},
'3329971':{'en': 'Redon', 'fr': 'Redon'},
'3323567':{'en': 'Grand-Couronne', 'fr': 'Grand-Couronne'},
'3323566':{'en': u('Saint-\u00c9tienne-du-Rouvray'), 'fr': u('Saint-\u00c9tienne-du-Rouvray')},
'3329974':{'en': u('Vitr\u00e9'), 'fr': u('Vitr\u00e9')},
'3329975':{'en': u('Vitr\u00e9'), 'fr': u('Vitr\u00e9')},
'3323563':{'en': 'Rouen', 'fr': 'Rouen'},
'3355538':{'en': 'Limoges', 'fr': 'Limoges'},
'3329978':{'en': 'Rennes', 'fr': 'Rennes'},
'3329979':{'en': 'Rennes', 'fr': 'Rennes'},
'3355535':{'en': 'Limoges', 'fr': 'Limoges'},
'3355534':{'en': 'Limoges', 'fr': 'Limoges'},
'3323569':{'en': 'Le Grand Quevilly', 'fr': 'Le Grand Quevilly'},
'3323568':{'en': 'Petit-Couronne', 'fr': 'Petit-Couronne'},
'3355531':{'en': 'Limoges', 'fr': 'Limoges'},
'3355530':{'en': 'Limoges', 'fr': 'Limoges'},
'3349327':{'en': 'Nice', 'fr': 'Nice'},
'3349326':{'en': 'Nice', 'fr': 'Nice'},
'3349457':{'en': u('Hy\u00e8res'), 'fr': u('Hy\u00e8res')},
'3349324':{'en': 'Vence', 'fr': 'Vence'},
'3349323':{'en': 'Isola', 'fr': 'Isola'},
'3349450':{'en': 'Draguignan', 'fr': 'Draguignan'},
'3349453':{'en': u('Fr\u00e9jus'), 'fr': u('Fr\u00e9jus')},
'3349452':{'en': u('Fr\u00e9jus'), 'fr': u('Fr\u00e9jus')},
'3349459':{'en': 'Saint-Maximin-la-Sainte-Baume', 'fr': 'Saint-Maximin-la-Sainte-Baume'},
'3349458':{'en': 'Carqueiranne', 'fr': 'Carqueiranne'},
'3349329':{'en': 'Carros', 'fr': 'Carros'},
'3349328':{'en': 'Menton', 'fr': 'Menton'},
'3322962':{'en': 'Landerneau', 'fr': 'Landerneau'},
'3355347':{'en': 'Agen', 'fr': 'Agen'},
'3355340':{'en': 'Villeneuve-sur-Lot', 'fr': 'Villeneuve-sur-Lot'},
'4413879':{'en': 'Dumfries'},
'3355759':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355758':{'en': 'Saint-Savin', 'fr': 'Saint-Savin'},
'3355752':{'en': 'La Teste de Buch', 'fr': 'La Teste de Buch'},
'3355751':{'en': 'Libourne', 'fr': 'Libourne'},
'3355750':{'en': 'Libourne', 'fr': 'Libourne'},
'3355755':{'en': 'Libourne', 'fr': 'Libourne'},
'3355754':{'en': 'Cenon', 'fr': 'Cenon'},
'3332691':{'en': 'Reims', 'fr': 'Reims'},
'437485':{'de': 'Gaming', 'en': 'Gaming'},
'437486':{'de': 'Lunz am See', 'en': 'Lunz am See'},
'437487':{'de': 'Gresten', 'en': 'Gresten'},
'437480':{'de': 'Langau, Gaming', 'en': 'Langau, Gaming'},
'437482':{'de': 'Scheibbs', 'en': 'Scheibbs'},
'437483':{'de': 'Oberndorf an der Melk', 'en': 'Oberndorf an der Melk'},
'437488':{'de': 'Steinakirchen am Forst', 'en': 'Steinakirchen am Forst'},
'437489':{'de': 'Purgstall an der Erlauf', 'en': 'Purgstall an der Erlauf'},
'437673':{'de': 'Schwanenstadt', 'en': 'Schwanenstadt'},
'437672':{'de': u('V\u00f6cklabruck'), 'en': u('V\u00f6cklabruck')},
'3324353':{'en': 'Laval', 'fr': 'Laval'},
'3324352':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324350':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324356':{'en': 'Laval', 'fr': 'Laval'},
'3324354':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324359':{'en': 'Laval', 'fr': 'Laval'},
'3324358':{'en': 'Laval', 'fr': 'Laval'},
'437676':{'de': 'Ottnang am Hausruck', 'en': 'Ottnang am Hausruck'},
'3315670':{'en': 'Rungis Complexe', 'fr': 'Rungis Complexe'},
'3315671':{'en': u('Cr\u00e9teil'), 'fr': u('Cr\u00e9teil')},
'437262':{'de': 'Perg', 'en': 'Perg'},
'3332968':{'en': u('\u00c9pinal'), 'fr': u('\u00c9pinal')},
'3315674':{'en': 'Sucy-en-Brie', 'fr': 'Sucy-en-Brie'},
'437265':{'de': 'Pabneukirchen', 'en': 'Pabneukirchen'},
'437266':{'de': 'Bad Kreuzen', 'en': 'Bad Kreuzen'},
'437267':{'de': u('M\u00f6nchdorf'), 'en': u('M\u00f6nchdorf')},
'3332963':{'en': u('G\u00e9rardmer'), 'fr': u('G\u00e9rardmer')},
'3332962':{'en': 'Remiremont', 'fr': 'Remiremont'},
'3332960':{'en': u('G\u00e9rardmer'), 'fr': u('G\u00e9rardmer')},
'3332966':{'en': u('Plombi\u00e8res-les-Bains'), 'fr': u('Plombi\u00e8res-les-Bains')},
'3332965':{'en': 'Rambervillers', 'fr': 'Rambervillers'},
'3332964':{'en': u('\u00c9pinal'), 'fr': u('\u00c9pinal')},
'3356257':{'en': 'Balma', 'fr': 'Balma'},
'3356256':{'en': 'Tarbes', 'fr': 'Tarbes'},
'3356706':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356253':{'en': 'Tarbes', 'fr': 'Tarbes'},
'3356251':{'en': 'Tarbes', 'fr': 'Tarbes'},
'3338095':{'en': 'Is-sur-Tille', 'fr': 'Is-sur-Tille'},
'3338097':{'en': 'Semur-en-Auxois', 'fr': 'Semur-en-Auxois'},
'3338091':{'en': u('Ch\u00e2tillon-sur-Seine'), 'fr': u('Ch\u00e2tillon-sur-Seine')},
'3338090':{'en': 'Pouilly-en-Auxois', 'fr': 'Pouilly-en-Auxois'},
'3338092':{'en': 'Montbard', 'fr': 'Montbard'},
'390525':{'it': 'Fornovo di Taro'},
'390524':{'it': 'Fidenza'},
'3345038':{'en': 'Annemasse', 'fr': 'Annemasse'},
'3344247':{'en': 'Fos-sur-Mer', 'fr': 'Fos-sur-Mer'},
'3344246':{'en': 'Vitrolles', 'fr': 'Vitrolles'},
'3344245':{'en': 'Carry-le-Rouet', 'fr': 'Carry-le-Rouet'},
'3344244':{'en': 'Carry-le-Rouet', 'fr': 'Carry-le-Rouet'},
'3344243':{'en': 'Martigues', 'fr': 'Martigues'},
'3344242':{'en': 'Martigues', 'fr': 'Martigues'},
'3344241':{'en': 'Vitrolles', 'fr': 'Vitrolles'},
'3344240':{'en': 'Martigues', 'fr': 'Martigues'},
'3344249':{'en': 'Martigues', 'fr': 'Martigues'},
'3344248':{'en': u('Port-Saint-Louis-du-Rh\u00f4ne'), 'fr': u('Port-Saint-Louis-du-Rh\u00f4ne')},
'3355591':{'en': 'Beaulieu-sur-Dordogne', 'fr': 'Beaulieu-sur-Dordogne'},
'3595944':{'bg': u('\u0420\u0443\u0435\u043d, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Ruen, Burgas'},
'374242':{'am': u('\u0544\u0561\u0580\u0561\u056c\u056b\u056f/\u054d\u0561\u057c\u0576\u0561\u0572\u0562\u0575\u0578\u0582\u0580'), 'en': 'Maralik/Sarnaghbyur', 'ru': u('\u041c\u0430\u0440\u0430\u043b\u0438\u043a/\u0421\u0430\u0440\u043d\u0430\u0445\u0431\u044e\u0440')},
'374246':{'am': u('\u0531\u0574\u0561\u057d\u056b\u0561'), 'en': 'Amasia region', 'ru': u('\u0410\u043c\u0430\u0441\u0438\u044f')},
'374244':{'am': u('\u0531\u0580\u0569\u056b\u056f/\u054a\u0565\u0574\u0566\u0561\u0577\u0565\u0576'), 'en': 'Artik/Pemzashen', 'ru': u('\u0410\u0440\u0442\u0438\u043a/\u041f\u0435\u043c\u0437\u0430\u0448\u0435\u043d')},
'374245':{'am': u('\u0531\u0577\u0578\u0581\u0584'), 'en': 'Ashotsk region', 'ru': u('\u0410\u0448\u043e\u0446\u043a')},
'374249':{'am': u('\u0539\u0561\u056c\u056b\u0576'), 'en': 'Talin', 'ru': u('\u0422\u0430\u043b\u0438\u043d')},
'3596578':{'bg': u('\u0420\u0435\u0441\u0435\u043b\u0435\u0446'), 'en': 'Reselets'},
'3596579':{'bg': u('\u0420\u0443\u043f\u0446\u0438, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Ruptsi, Pleven'},
'3596570':{'bg': u('\u0414\u0435\u0432\u0435\u043d\u0446\u0438'), 'en': 'Deventsi'},
'3596571':{'bg': u('\u041b\u0435\u043f\u0438\u0446\u0430'), 'en': 'Lepitsa'},
'3596572':{'bg': u('\u0421\u0443\u0445\u0430\u0447\u0435'), 'en': 'Suhache'},
'3596573':{'bg': u('\u041a\u043e\u0439\u043d\u0430\u0440\u0435'), 'en': 'Koynare'},
'3596574':{'bg': u('\u0427\u043e\u043c\u0430\u043a\u043e\u0432\u0446\u0438'), 'en': 'Chomakovtsi'},
'3596575':{'bg': u('\u0422\u0435\u043b\u0438\u0448'), 'en': 'Telish'},
'3596576':{'bg': u('\u0420\u0430\u0434\u043e\u043c\u0438\u0440\u0446\u0438'), 'en': 'Radomirtsi'},
'3596577':{'bg': u('\u0411\u0440\u0435\u0441\u0442\u0435'), 'en': 'Breste'},
'441665':{'en': 'Alnwick'},
'441664':{'en': 'Melton Mowbray'},
'441667':{'en': 'Nairn'},
'441666':{'en': 'Malmesbury'},
'441661':{'en': 'Prudhoe'},
'441663':{'en': 'New Mills'},
'441669':{'en': 'Rothbury'},
'441668':{'en': 'Bamburgh'},
'355364':{'en': u('V\u00ebrtop/Terpan, Berat')},
'355365':{'en': u('Sinj\u00eb/Cukalat, Berat')},
'355366':{'en': u('Poshnj\u00eb/Kutalli, Berat')},
'355367':{'en': u('Perondi/Kozar\u00eb, Ku\u00e7ov\u00eb')},
'355360':{'en': u('Leshnje/Potom/\u00c7epan/Gjerb\u00ebs/Zhep\u00eb, Skrapar')},
'355361':{'en': 'Ura Vajgurore, Berat'},
'355362':{'en': 'Velabisht/Roshnik, Berat'},
'355363':{'en': 'Otllak/Lumas, Berat'},
'4414370':{'en': 'Haverfordwest/Clynderwen (Clunderwen)'},
'355368':{'en': u('Poli\u00e7an/Bogov\u00eb, Skrapar')},
'355369':{'en': u('Qend\u00ebr/Vendresh\u00eb, Skrapar')},
'3347910':{'en': 'Albertville', 'fr': 'Albertville'},
'434879':{'de': 'Sankt Veit in Defereggen', 'en': 'St. Veit in Defereggen'},
'434874':{'de': 'Virgen', 'en': 'Virgen'},
'434875':{'de': 'Matrei in Osttirol', 'en': 'Matrei in Osttirol'},
'434876':{'de': u('Kals am Gro\u00dfglockner'), 'en': 'Kals am Grossglockner'},
'434877':{'de': u('Pr\u00e4graten am Gro\u00dfvenediger'), 'en': u('Pr\u00e4graten am Grossvenediger')},
'434872':{'de': 'Huben', 'en': 'Huben'},
'434873':{'de': 'Sankt Jakob in Defereggen', 'en': 'St. Jakob in Defereggen'},
'3317463':{'en': 'Levallois-Perret', 'fr': 'Levallois-Perret'},
'3597155':{'bg': u('\u041b\u0435\u0441\u043d\u043e\u0432\u043e'), 'en': 'Lesnovo'},
'3597154':{'bg': u('\u0421\u0442\u043e\u043b\u043d\u0438\u043a'), 'en': 'Stolnik'},
'35961703':{'bg': u('\u0412\u044a\u0440\u0431\u0438\u0446\u0430, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Varbitsa, V. Tarnovo'},
'3597156':{'bg': u('\u0420\u0430\u0432\u043d\u043e \u043f\u043e\u043b\u0435'), 'en': 'Ravno pole'},
'35961705':{'bg': u('\u0413\u043e\u0440\u0441\u043a\u0438 \u0434\u043e\u043b\u0435\u043d \u0422\u0440\u044a\u043c\u0431\u0435\u0448'), 'en': 'Gorski dolen Trambesh'},
'35961704':{'bg': u('\u041f\u0440\u0430\u0432\u0434\u0430, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Pravda, V. Tarnovo'},
'35961706':{'bg': u('\u041f\u0438\u0441\u0430\u0440\u0435\u0432\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Pisarevo, V. Tarnovo'},
'35941149':{'bg': u('\u041b\u043e\u0432\u0435\u0446, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Lovets, St. Zagora'},
'3597159':{'bg': u('\u0410\u043f\u0440\u0438\u043b\u043e\u0432\u043e, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Aprilovo, Sofia'},
'3597158':{'bg': u('\u0414\u043e\u0433\u0430\u043d\u043e\u0432\u043e'), 'en': 'Doganovo'},
'441273':{'en': 'Brighton'},
'441270':{'en': 'Crewe'},
'3343748':{'en': 'Lyon', 'fr': 'Lyon'},
'441276':{'en': 'Camberley'},
'441277':{'en': 'Brentwood'},
'441274':{'en': 'Bradford'},
'441275':{'en': 'Clevedon'},
'3343743':{'en': 'Villeurbanne', 'fr': 'Villeurbanne'},
'3343742':{'en': 'Lyon', 'fr': 'Lyon'},
'441278':{'en': 'Bridgwater'},
'3343740':{'en': 'Caluire-et-Cuire', 'fr': 'Caluire-et-Cuire'},
'3343747':{'en': 'Villeurbanne', 'fr': 'Villeurbanne'},
'3343746':{'en': 'Lyon', 'fr': 'Lyon'},
'3343745':{'en': 'Vaulx-en-Velin', 'fr': 'Vaulx-en-Velin'},
'3343744':{'en': 'Meyzieu', 'fr': 'Meyzieu'},
'432263':{'de': u('Gro\u00dfru\u00dfbach'), 'en': 'Grossrussbach'},
'3338383':{'en': u('Pont-\u00e0-Mousson'), 'fr': u('Pont-\u00e0-Mousson')},
'3338382':{'en': u('Pont-\u00e0-Mousson'), 'fr': u('Pont-\u00e0-Mousson')},
'3346739':{'en': 'Servian', 'fr': 'Servian'},
'3346738':{'en': 'Saint-Chinian', 'fr': 'Saint-Chinian'},
'3751591':{'be': u('\u0410\u0441\u0442\u0440\u0430\u0432\u0435\u0446, \u0413\u0440\u043e\u0434\u0437\u0435\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Ostrovets, Grodno Region', 'ru': u('\u041e\u0441\u0442\u0440\u043e\u0432\u0435\u0446, \u0413\u0440\u043e\u0434\u043d\u0435\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751593':{'be': u('\u0410\u0448\u043c\u044f\u043d\u044b'), 'en': 'Oshmyany', 'ru': u('\u041e\u0448\u043c\u044f\u043d\u044b')},
'3751592':{'be': u('\u0421\u043c\u0430\u0440\u0433\u043e\u043d\u044c'), 'en': 'Smorgon', 'ru': u('\u0421\u043c\u043e\u0440\u0433\u043e\u043d\u044c')},
'3751595':{'be': u('\u0406\u045e\u0435, \u0413\u0440\u043e\u0434\u0437\u0435\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Ivye, Grodno Region', 'ru': u('\u0418\u0432\u044c\u0435, \u0413\u0440\u043e\u0434\u043d\u0435\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751594':{'be': u('\u0412\u043e\u0440\u0430\u043d\u0430\u0432\u0430, \u0413\u0440\u043e\u0434\u0437\u0435\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Voronovo, Grodno Region', 'ru': u('\u0412\u043e\u0440\u043e\u043d\u043e\u0432\u043e, \u0413\u0440\u043e\u0434\u043d\u0435\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751597':{'be': u('\u041d\u0430\u0432\u0430\u0433\u0440\u0443\u0434\u0430\u043a'), 'en': 'Novogrudok', 'ru': u('\u041d\u043e\u0432\u043e\u0433\u0440\u0443\u0434\u043e\u043a')},
'3751596':{'be': u('\u041a\u0430\u0440\u044d\u043b\u0456\u0447\u044b, \u0413\u0440\u043e\u0434\u0437\u0435\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Korelichi, Grodno Region', 'ru': u('\u041a\u043e\u0440\u0435\u043b\u0438\u0447\u0438, \u0413\u0440\u043e\u0434\u043d\u0435\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'35963563':{'bg': u('\u0411\u043e\u0440\u0438\u0441\u043b\u0430\u0432'), 'en': 'Borislav'},
'3324119':{'en': 'Angers', 'fr': 'Angers'},
'3323798':{'en': 'Cloyes-sur-le-Loir', 'fr': 'Cloyes-sur-le-Loir'},
'3323799':{'en': 'Voves', 'fr': 'Voves'},
'35963567':{'bg': u('\u0413\u0440\u0430\u0434\u0438\u043d\u0430, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Gradina, Pleven'},
'35963566':{'bg': u('\u0411\u0440\u044a\u0448\u043b\u044f\u043d\u0438\u0446\u0430'), 'en': 'Brashlyanitsa'},
'35963565':{'bg': u('\u041c\u0435\u0447\u043a\u0430, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Mechka, Pleven'},
'3324118':{'en': 'Angers', 'fr': 'Angers'},
'35963569':{'bg': u('\u041a\u0430\u043c\u0435\u043d\u0435\u0446, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Kamenets, Pleven'},
'3323791':{'en': 'Chartres', 'fr': 'Chartres'},
'3323797':{'en': 'Arrou', 'fr': 'Arrou'},
'441896':{'en': 'Galashiels'},
'441895':{'en': 'Uxbridge'},
'441892':{'en': 'Tunbridge Wells'},
'3346730':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3316405':{'en': 'Brie-Comte-Robert', 'fr': 'Brie-Comte-Robert'},
'3324028':{'en': u('Ch\u00e2teaubriant'), 'fr': u('Ch\u00e2teaubriant')},
'3345744':{'en': 'Chamonix-Mont-Blanc', 'fr': 'Chamonix-Mont-Blanc'},
'39059':{'en': 'Modena', 'it': 'Modena'},
'3593678':{'bg': u('\u0427\u0430\u043a\u0430\u043b\u0430\u0440\u043e\u0432\u043e'), 'en': 'Chakalarovo'},
'3593679':{'bg': u('\u041a\u0438\u0440\u043a\u043e\u0432\u043e'), 'en': 'Kirkovo'},
'3593674':{'bg': u('\u0421\u0430\u043c\u043e\u0434\u0438\u0432\u0430'), 'en': 'Samodiva'},
'3593675':{'bg': u('\u0424\u043e\u0442\u0438\u043d\u043e\u0432\u043e, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Fotinovo, Kardzh.'},
'3593676':{'bg': u('\u0411\u0435\u043d\u043a\u043e\u0432\u0441\u043a\u0438, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Benkovski, Kardzh.'},
'3593677':{'bg': u('\u0414\u0440\u0430\u043d\u0433\u043e\u0432\u043e, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Drangovo, Kardzh.'},
'3593671':{'bg': u('\u041f\u043e\u0434\u043a\u043e\u0432\u0430'), 'en': 'Podkova'},
'3593672':{'bg': u('\u0427\u043e\u0440\u0431\u0430\u0434\u0436\u0438\u0439\u0441\u043a\u043e'), 'en': 'Chorbadzhiysko'},
'3593673':{'bg': u('\u0422\u0438\u0445\u043e\u043c\u0438\u0440'), 'en': 'Tihomir'},
'3595948':{'bg': u('\u0421\u043d\u044f\u0433\u043e\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Snyagovo, Burgas'},
'3595949':{'bg': u('\u041f\u043b\u0430\u043d\u0438\u043d\u0438\u0446\u0430, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Planinitsa, Burgas'},
'3329899':{'en': 'Carhaix-Plouguer', 'fr': 'Carhaix-Plouguer'},
'3329898':{'en': 'Quimper', 'fr': 'Quimper'},
'3329893':{'en': 'Carhaix-Plouguer', 'fr': 'Carhaix-Plouguer'},
'3329892':{'en': 'Douarnenez', 'fr': 'Douarnenez'},
'3329891':{'en': u('Ploz\u00e9vet'), 'fr': u('Ploz\u00e9vet')},
'3329890':{'en': 'Quimper', 'fr': 'Quimper'},
'3329897':{'en': 'Concarneau', 'fr': 'Concarneau'},
'3329896':{'en': u('Quimperl\u00e9'), 'fr': u('Quimperl\u00e9')},
'3329895':{'en': 'Quimper', 'fr': 'Quimper'},
'3593019':{'bg': u('\u041f\u0438\u0441\u0430\u043d\u0438\u0446\u0430'), 'en': 'Pisanitsa'},
'432535':{'de': 'Hohenau an der March', 'en': 'Hohenau an der March'},
'3332753':{'en': 'Maubeuge', 'fr': 'Maubeuge'},
'432533':{'de': 'Neusiedl an der Zaya', 'en': 'Neusiedl an der Zaya'},
'432532':{'de': 'Zistersdorf', 'en': 'Zistersdorf'},
'3332756':{'en': 'Avesnes-sur-Helpe', 'fr': 'Avesnes-sur-Helpe'},
'3595945':{'bg': u('\u0414\u043e\u0431\u0440\u043e\u043c\u0438\u0440'), 'en': 'Dobromir'},
'3332758':{'en': 'Maubeuge', 'fr': 'Maubeuge'},
'3332209':{'en': 'Moreuil', 'fr': 'Moreuil'},
'432538':{'de': u('Velm-G\u00f6tzendorf'), 'en': u('Velm-G\u00f6tzendorf')},
'434272':{'de': u('P\u00f6rtschach am W\u00f6rther See'), 'en': u('P\u00f6rtschach am W\u00f6rther See')},
'434273':{'de': 'Reifnitz', 'en': 'Reifnitz'},
'434271':{'de': 'Steuerberg', 'en': 'Steuerberg'},
'434276':{'de': u('Feldkirchen in K\u00e4rnten'), 'en': u('Feldkirchen in K\u00e4rnten')},
'434277':{'de': 'Glanegg', 'en': 'Glanegg'},
'434274':{'de': u('Velden am W\u00f6rther See'), 'en': u('Velden am W\u00f6rther See')},
'434275':{'de': 'Ebene Reichenau', 'en': 'Ebene Reichenau'},
'434278':{'de': 'Gnesau', 'en': 'Gnesau'},
'434279':{'de': 'Sirnitz', 'en': 'Sirnitz'},
'433856':{'de': 'Veitsch', 'en': 'Veitsch'},
'332482':{'en': 'Bourges', 'fr': 'Bourges'},
'40250':{'en': u('V\u00e2lcea'), 'ro': u('V\u00e2lcea')},
'40251':{'en': 'Dolj', 'ro': 'Dolj'},
'40252':{'en': u('Mehedin\u021bi'), 'ro': u('Mehedin\u021bi')},
'3356139':{'en': 'Saint-Orens-de-Gameville', 'fr': 'Saint-Orens-de-Gameville'},
'40254':{'en': 'Hunedoara', 'ro': 'Hunedoara'},
'40255':{'en': u('Cara\u0219-Severin'), 'ro': u('Cara\u0219-Severin')},
'40256':{'en': u('Timi\u0219'), 'ro': u('Timi\u0219')},
'40257':{'en': 'Arad', 'ro': 'Arad'},
'3356132':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356133':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356130':{'en': 'Colomiers', 'fr': 'Colomiers'},
'3356131':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356136':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356134':{'en': 'Toulouse', 'fr': 'Toulouse'},
'359339':{'bg': u('\u0421\u0442\u0430\u043c\u0431\u043e\u043b\u0438\u0439\u0441\u043a\u0438, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Stamboliyski, Plovdiv'},
'359331':{'bg': u('\u0410\u0441\u0435\u043d\u043e\u0432\u0433\u0440\u0430\u0434'), 'en': 'Asenovgrad'},
'359337':{'bg': u('\u0425\u0438\u0441\u0430\u0440\u044f'), 'en': 'Hisarya'},
'359336':{'bg': u('\u041f\u044a\u0440\u0432\u043e\u043c\u0430\u0439, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Parvomay, Plovdiv'},
'359335':{'bg': u('\u041a\u0430\u0440\u043b\u043e\u0432\u043e'), 'en': 'Karlovo'},
'441478':{'en': 'Isle of Skye - Portree'},
'441479':{'en': 'Grantown-on-Spey'},
'441470':{'en': 'Isle of Skye - Edinbane'},
'441471':{'en': 'Isle of Skye - Broadford'},
'441472':{'en': 'Grimsby'},
'441473':{'en': 'Ipswich'},
'441474':{'en': 'Gravesend'},
'441475':{'en': 'Greenock'},
'441476':{'en': 'Grantham'},
'441477':{'en': 'Holmes Chapel'},
'3356397':{'en': 'Mazamet', 'fr': 'Mazamet'},
'3356392':{'en': 'Montauban', 'fr': 'Montauban'},
'3356393':{'en': 'Caussade', 'fr': 'Caussade'},
'3356391':{'en': 'Montauban', 'fr': 'Montauban'},
'3356398':{'en': 'Mazamet', 'fr': 'Mazamet'},
'3347219':{'en': 'Lyon', 'fr': 'Lyon'},
'3347218':{'en': u('\u00c9cully'), 'fr': u('\u00c9cully')},
'3595146':{'bg': u('\u0413\u043e\u0440\u0435\u043d \u0447\u0438\u0444\u043b\u0438\u043a'), 'en': 'Goren chiflik'},
'3595147':{'bg': u('\u041f\u0447\u0435\u043b\u043d\u0438\u043a, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Pchelnik, Varna'},
'3595140':{'bg': u('\u0428\u043a\u043e\u0440\u043f\u0438\u043b\u043e\u0432\u0446\u0438'), 'en': 'Shkorpilovtsi'},
'3595141':{'bg': u('\u0421\u0442\u0430\u0440\u043e \u041e\u0440\u044f\u0445\u043e\u0432\u043e'), 'en': 'Staro Oryahovo'},
'3595142':{'bg': u('\u0414\u043e\u043b\u043d\u0438 \u0447\u0438\u0444\u043b\u0438\u043a'), 'en': 'Dolni chiflik'},
'3595143':{'bg': u('\u0411\u044f\u043b\u0430, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Byala, Varna'},
'3347210':{'en': 'Lyon', 'fr': 'Lyon'},
'3347213':{'en': 'Lyon', 'fr': 'Lyon'},
'3347212':{'en': 'Lyon', 'fr': 'Lyon'},
'3347215':{'en': 'Bron', 'fr': 'Bron'},
'3347214':{'en': 'Bron', 'fr': 'Bron'},
'3347217':{'en': 'Dardilly', 'fr': 'Dardilly'},
'3347216':{'en': u('Sainte-Foy-l\u00e8s-Lyon'), 'fr': u('Sainte-Foy-l\u00e8s-Lyon')},
'3598633':{'bg': u('\u0421\u0442\u0430\u0440\u043e \u0441\u0435\u043b\u043e, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Staro selo, Silistra'},
'3598632':{'bg': u('\u0417\u0430\u0444\u0438\u0440\u043e\u0432\u043e'), 'en': 'Zafirovo'},
'3598635':{'bg': u('\u0426\u0430\u0440 \u0421\u0430\u043c\u0443\u0438\u043b'), 'en': 'Tsar Samuil'},
'3598634':{'bg': u('\u041d\u043e\u0432\u0430 \u0427\u0435\u0440\u043d\u0430'), 'en': 'Nova Cherna'},
'3598637':{'bg': u('\u041c\u0430\u043b\u044a\u043a \u041f\u0440\u0435\u0441\u043b\u0430\u0432\u0435\u0446'), 'en': 'Malak Preslavets'},
'3598636':{'bg': u('\u0413\u043b\u0430\u0432\u0438\u043d\u0438\u0446\u0430, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Glavinitsa, Silistra'},
'3598639':{'bg': u('\u041a\u043e\u043b\u0430\u0440\u043e\u0432\u043e, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Kolarovo, Silistra'},
'3598638':{'bg': u('\u0411\u043e\u0433\u0434\u0430\u043d\u0446\u0438, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Bogdantsi, Silistra'},
'3324168':{'en': 'Angers', 'fr': 'Angers'},
'3338969':{'en': 'Saint-Louis', 'fr': 'Saint-Louis'},
'39089':{'en': 'Salerno', 'it': 'Salerno'},
'3324160':{'en': 'Angers', 'fr': 'Angers'},
'3324162':{'en': 'Cholet', 'fr': 'Cholet'},
'3324163':{'en': 'Beaupreau', 'fr': 'Beaupreau'},
'39081':{'en': 'Naples', 'it': 'Napoli'},
'3324165':{'en': 'Cholet', 'fr': 'Cholet'},
'3324166':{'en': 'Angers', 'fr': 'Angers'},
'3324167':{'en': 'Saumur', 'fr': 'Saumur'},
'35288':{'de': 'Mertzig/Wahl', 'en': 'Mertzig/Wahl', 'fr': 'Mertzig/Wahl'},
'35283':{'de': 'Vianden', 'en': 'Vianden', 'fr': 'Vianden'},
'35281':{'de': u('Ettelbr\u00fcck'), 'en': 'Ettelbruck', 'fr': 'Ettelbruck'},
'35280':{'de': 'Diekirch', 'en': 'Diekirch', 'fr': 'Diekirch'},
'35287':{'de': 'Fels', 'en': 'Larochette', 'fr': 'Larochette'},
'35285':{'de': 'Bissen/Roost', 'en': 'Bissen/Roost', 'fr': 'Bissen/Roost'},
'35284':{'de': 'Han/Lesse', 'en': 'Han/Lesse', 'fr': 'Han/Lesse'},
'35931324':{'bg': u('\u041c\u0440\u0430\u0447\u0435\u043d\u0438\u043a'), 'en': 'Mrachenik'},
'3338662':{'en': 'Joigny', 'fr': 'Joigny'},
'3338661':{'en': 'Nevers', 'fr': 'Nevers'},
'3338666':{'en': 'Villeneuve-la-Guyard', 'fr': 'Villeneuve-la-Guyard'},
'3338667':{'en': 'Pont-sur-Yonne', 'fr': 'Pont-sur-Yonne'},
'3338664':{'en': 'Sens', 'fr': 'Sens'},
'3338665':{'en': 'Sens', 'fr': 'Sens'},
'3338668':{'en': u('Pr\u00e9mery'), 'fr': u('Pr\u00e9mery')},
'3338967':{'en': 'Saint-Louis', 'fr': 'Saint-Louis'},
'3355685':{'en': u('B\u00e8gles'), 'fr': u('B\u00e8gles')},
'3355932':{'en': 'Pau', 'fr': 'Pau'},
'3329864':{'en': 'Quimper', 'fr': 'Quimper'},
'3596141':{'bg': u('\u041f\u043e\u043b\u0441\u043a\u0438 \u0422\u0440\u044a\u043c\u0431\u0435\u0448'), 'en': 'Polski Trambesh'},
'3596143':{'bg': u('\u041c\u0430\u0441\u043b\u0430\u0440\u0435\u0432\u043e'), 'en': 'Maslarevo'},
'3355930':{'en': 'Pau', 'fr': 'Pau'},
'3596145':{'bg': u('\u0421\u0442\u0440\u0430\u0445\u0438\u043b\u043e\u0432\u043e'), 'en': 'Strahilovo'},
'3596144':{'bg': u('\u0414\u043e\u043b\u043d\u0430 \u041b\u0438\u043f\u043d\u0438\u0446\u0430'), 'en': 'Dolna Lipnitsa'},
'3596147':{'bg': u('\u0418\u0432\u0430\u043d\u0447\u0430, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Ivancha, V. Tarnovo'},
'3596146':{'bg': u('\u041f\u043e\u043b\u0441\u043a\u0438 \u0421\u0435\u043d\u043e\u0432\u0435\u0446'), 'en': 'Polski Senovets'},
'3596149':{'bg': u('\u041a\u0443\u0446\u0438\u043d\u0430'), 'en': 'Kutsina'},
'3329862':{'en': 'Morlaix', 'fr': 'Morlaix'},
'3355936':{'en': 'Oloron-Sainte-Marie', 'fr': 'Oloron-Sainte-Marie'},
'3329860':{'en': 'Concarneau', 'fr': 'Concarneau'},
'3355682':{'en': 'Andernos-les-Bains', 'fr': 'Andernos-les-Bains'},
'3316011':{'en': 'Massy', 'fr': 'Massy'},
'3316010':{'en': 'Palaiseau', 'fr': 'Palaiseau'},
'3316013':{'en': 'Massy', 'fr': 'Massy'},
'3316012':{'en': 'Gif-sur-Yvette', 'fr': 'Gif-sur-Yvette'},
'3316015':{'en': u('Sainte-Genevi\u00e8ve-des-Bois'), 'fr': u('Sainte-Genevi\u00e8ve-des-Bois')},
'3316014':{'en': 'Palaiseau', 'fr': 'Palaiseau'},
'3316017':{'en': 'Torcy', 'fr': 'Torcy'},
'3316016':{'en': u('Sainte-Genevi\u00e8ve-des-Bois'), 'fr': u('Sainte-Genevi\u00e8ve-des-Bois')},
'3316019':{'en': 'Igny', 'fr': 'Igny'},
'3316018':{'en': 'Lieusaint', 'fr': 'Lieusaint'},
'4418902':{'en': 'Coldstream'},
'37425353':{'am': u('\u0547\u0576\u0578\u0572'), 'en': 'Shnogh', 'ru': u('\u0428\u043d\u043e\u0445')},
'37425352':{'am': u('\u0531\u056d\u0569\u0561\u056c\u0561'), 'en': 'Akhtala', 'ru': u('\u0410\u0445\u0442\u0430\u043b\u0430')},
'3338989':{'en': 'Saint-Louis', 'fr': 'Saint-Louis'},
'37425357':{'am': u('\u0539\u0578\u0582\u0574\u0561\u0576\u0575\u0561\u0576'), 'en': 'Tumanyan', 'ru': u('\u0422\u0443\u043c\u0430\u043d\u044f\u043d')},
'37425356':{'am': u('\u0543\u0578\u0573\u056f\u0561\u0576'), 'en': 'Chochkan', 'ru': u('\u0427\u043e\u0447\u043a\u0430\u043d')},
'3338980':{'en': 'Colmar', 'fr': 'Colmar'},
'390583':{'en': 'Lucca', 'it': 'Lucca'},
'3339057':{'en': u('S\u00e9lestat'), 'fr': u('S\u00e9lestat')},
'390587':{'it': 'Pontedera'},
'37428427':{'am': u('\u054e\u0565\u0580\u056b\u0577\u0565\u0576'), 'en': 'Verishen', 'ru': u('\u0412\u0435\u0440\u0438\u0448\u0435\u043d')},
'390585':{'en': 'Massa-Carrara', 'it': 'Massa'},
'390584':{'it': 'Viareggio'},
'390588':{'it': 'Volterra'},
'432238':{'de': 'Kaltenleutgeben', 'en': 'Kaltenleutgeben'},
'432239':{'de': 'Breitenfurt bei Wien', 'en': 'Breitenfurt bei Wien'},
'432234':{'de': 'Gramatneusiedl', 'en': 'Gramatneusiedl'},
'432235':{'de': 'Maria-Lanzendorf', 'en': 'Maria-Lanzendorf'},
'432236':{'de': u('M\u00f6dling'), 'en': u('M\u00f6dling')},
'432237':{'de': 'Gaaden', 'en': 'Gaaden'},
'432230':{'de': 'Schwadorf', 'en': 'Schwadorf'},
'432231':{'de': 'Purkersdorf', 'en': 'Purkersdorf'},
'432232':{'de': 'Fischamend', 'en': 'Fischamend'},
'432233':{'de': u('Pre\u00dfbaum'), 'en': 'Pressbaum'},
'3354648':{'en': 'Jonzac', 'fr': 'Jonzac'},
'3354642':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3354643':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3354641':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3354647':{'en': u('Saint-Pierre-d\'Ol\u00e9ron'), 'fr': u('Saint-Pierre-d\'Ol\u00e9ron')},
'3354644':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3354645':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3355518':{'en': 'Brive-la-Gaillarde', 'fr': 'Brive-la-Gaillarde'},
'3329959':{'en': 'Rennes', 'fr': 'Rennes'},
'3323589':{'en': 'Rouen', 'fr': 'Rouen'},
'3323588':{'en': 'Rouen', 'fr': 'Rouen'},
'3355511':{'en': 'Limoges', 'fr': 'Limoges'},
'3355510':{'en': 'Limoges', 'fr': 'Limoges'},
'3329950':{'en': 'Rennes', 'fr': 'Rennes'},
'3323584':{'en': 'Dieppe', 'fr': 'Dieppe'},
'3329956':{'en': 'Saint-Malo', 'fr': 'Saint-Malo'},
'3323582':{'en': 'Dieppe', 'fr': 'Dieppe'},
'3323581':{'en': 'Elbeuf', 'fr': 'Elbeuf'},
'3329955':{'en': 'Betton', 'fr': 'Betton'},
'3349301':{'en': 'Beaulieu-sur-Mer', 'fr': 'Beaulieu-sur-Mer'},
'3349300':{'en': 'Valbonne', 'fr': 'Valbonne'},
'3349303':{'en': u('Roquebilli\u00e8re'), 'fr': u('Roquebilli\u00e8re')},
'3349305':{'en': u('Puget-Th\u00e9niers'), 'fr': u('Puget-Th\u00e9niers')},
'3349304':{'en': 'Nice', 'fr': 'Nice'},
'3349307':{'en': 'Saint-Laurent-du-Var', 'fr': 'Saint-Laurent-du-Var'},
'3349306':{'en': 'Cannes', 'fr': 'Cannes'},
'3349309':{'en': 'Grasse', 'fr': 'Grasse'},
'3349308':{'en': 'Carros', 'fr': 'Carros'},
'390765':{'it': 'Poggio Mirteto'},
'390763':{'it': 'Orvieto'},
'390761':{'it': 'Viterbo'},
'3329884':{'en': 'Guipavas', 'fr': 'Guipavas'},
'3349723':{'en': 'Antibes Juan les Pins', 'fr': 'Antibes Juan les Pins'},
'3349721':{'en': 'Antibes Juan les Pins', 'fr': 'Antibes Juan les Pins'},
'3649':{'en': 'Mezokovesd', 'hu': u('Mez\u0151k\u00f6vesd')},
'3648':{'en': 'Ozd', 'hu': u('\u00d3zd')},
'3647':{'en': 'Szerencs', 'hu': 'Szerencs'},
'432948':{'de': 'Weitersfeld', 'en': 'Weitersfeld'},
'3646':{'en': 'Miskolc', 'hu': 'Miskolc'},
'432946':{'de': 'Pulkau', 'en': 'Pulkau'},
'432947':{'de': 'Theras', 'en': 'Theras'},
'432944':{'de': 'Haugsdorf', 'en': 'Haugsdorf'},
'432945':{'de': 'Zellerndorf', 'en': 'Zellerndorf'},
'432942':{'de': 'Retz', 'en': 'Retz'},
'432943':{'de': 'Obritz', 'en': 'Obritz'},
'3594738':{'bg': u('\u0425\u043b\u044f\u0431\u043e\u0432\u043e'), 'en': 'Hlyabovo'},
'3594739':{'bg': u('\u0420\u0430\u0434\u043e\u0432\u0435\u0446'), 'en': 'Radovets'},
'441394':{'en': 'Felixstowe'},
'3594734':{'bg': u('\u0421\u0440\u0435\u043c'), 'en': 'Srem'},
'3594736':{'bg': u('\u0421\u0432\u0435\u0442\u043b\u0438\u043d\u0430'), 'en': 'Svetlina'},
'3594737':{'bg': u('\u0421\u0438\u043d\u0430\u043f\u043e\u0432\u043e'), 'en': 'Sinapovo'},
'3594730':{'bg': u('\u041a\u043d\u044f\u0436\u0435\u0432\u043e'), 'en': 'Knyazhevo'},
'3594732':{'bg': u('\u0423\u0441\u0442\u0440\u0435\u043c'), 'en': 'Ustrem'},
'3594733':{'bg': u('\u041e\u0440\u043b\u043e\u0432 \u0434\u043e\u043b'), 'en': 'Orlov dol'},
'35981462':{'bg': u('\u0412\u043e\u043b\u043e\u0432\u043e'), 'en': 'Volovo'},
'35981463':{'bg': u('\u041c\u043e\u0433\u0438\u043b\u0438\u043d\u043e'), 'en': 'Mogilino'},
'35981461':{'bg': u('\u041a\u0430\u0440\u0430\u043d \u0412\u044a\u0440\u0431\u043e\u0432\u043a\u0430'), 'en': 'Karan Varbovka'},
'35981466':{'bg': u('\u041f\u043e\u043c\u0435\u043d'), 'en': 'Pomen'},
'35981464':{'bg': u('\u041e\u0441\u0442\u0440\u0438\u0446\u0430, \u0420\u0443\u0441\u0435'), 'en': 'Ostritsa, Ruse'},
'35981465':{'bg': u('\u0411\u0430\u0442\u0438\u043d'), 'en': 'Batin'},
'3332945':{'en': 'Bar-le-Duc', 'fr': 'Bar-le-Duc'},
'3332941':{'en': u('Raon-l\'\u00c9tape'), 'fr': u('Raon-l\'\u00c9tape')},
'3324378':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3332942':{'en': u('Saint-Di\u00e9-des-Vosges'), 'fr': u('Saint-Di\u00e9-des-Vosges')},
'3324375':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324374':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324377':{'en': 'Le Mans', 'fr': 'Le Mans'},
'441392':{'en': 'Exeter'},
'3324371':{'en': u('La Fert\u00e9 Bernard'), 'fr': u('La Fert\u00e9 Bernard')},
'3324802':{'en': 'Bourges', 'fr': 'Bourges'},
'3324372':{'en': 'Le Mans', 'fr': 'Le Mans'},
'441398':{'en': 'Dulverton'},
'3355776':{'en': 'Andernos-les-Bains', 'fr': 'Andernos-les-Bains'},
'3355771':{'en': 'Marcheprime', 'fr': 'Marcheprime'},
'3355773':{'en': 'Gujan-Mestras', 'fr': 'Gujan-Mestras'},
'3355772':{'en': 'Arcachon', 'fr': 'Arcachon'},
'3355778':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3356271':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356270':{'en': 'Montesquiou', 'fr': 'Montesquiou'},
'3356273':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3324011':{'en': 'La Baule Escoublac', 'fr': 'La Baule Escoublac'},
'3356275':{'en': 'Aucamville', 'fr': 'Aucamville'},
'3356274':{'en': 'Blagnac', 'fr': 'Blagnac'},
'4202':{'en': 'Prague'},
'3325174':{'en': 'Pornic', 'fr': 'Pornic'},
'3325175':{'en': 'La Baule Escoublac', 'fr': 'La Baule Escoublac'},
'3325176':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'3325177':{'en': 'Nantes', 'fr': 'Nantes'},
'3325170':{'en': u('Rez\u00e9'), 'fr': u('Rez\u00e9')},
'3325172':{'en': 'Nantes', 'fr': 'Nantes'},
'3325173':{'en': u('Gu\u00e9rande'), 'fr': u('Gu\u00e9rande')},
'3325178':{'en': 'Orvault', 'fr': 'Orvault'},
'3325179':{'en': 'Vertou', 'fr': 'Vertou'},
'3344261':{'en': 'Trets', 'fr': 'Trets'},
'3344260':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344263':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'35345':{'en': 'Naas/Kildare/Curragh'},
'3344265':{'en': 'Gardanne', 'fr': 'Gardanne'},
'35343':{'en': 'Longford/Granard'},
'3344267':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344266':{'en': 'Puyloubier', 'fr': 'Puyloubier'},
'3344269':{'en': u('Cabri\u00e8s'), 'fr': u('Cabri\u00e8s')},
'3344268':{'en': 'Fuveau', 'fr': 'Fuveau'},
'3599719':{'bg': u('\u0410\u0441\u043f\u0430\u0440\u0443\u0445\u043e\u0432\u043e, \u041c\u043e\u043d\u0442.'), 'en': 'Asparuhovo, Mont.'},
'35349':{'en': 'Cavan/Cootehill/Oldcastle/Belturbet'},
'351276':{'en': 'Chaves', 'pt': 'Chaves'},
'351277':{'en': 'Idanha-a-Nova', 'pt': 'Idanha-a-Nova'},
'3347688':{'en': 'Saint-Pierre-de-Chartreuse', 'fr': 'Saint-Pierre-de-Chartreuse'},
'3347689':{'en': 'Saint-Martin-d\'Uriage', 'fr': 'Saint-Martin-d\'Uriage'},
'351272':{'en': 'Castelo Branco', 'pt': 'Castelo Branco'},
'351273':{'en': u('Bragan\u00e7a'), 'pt': u('Bragan\u00e7a')},
'351271':{'en': 'Guarda', 'pt': 'Guarda'},
'3347681':{'en': u('La Mure d\'Is\u00e8re'), 'fr': u('La Mure d\'Is\u00e8re')},
'3347686':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347687':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347684':{'en': 'Grenoble', 'fr': 'Grenoble'},
'351279':{'en': 'Moncorvo', 'pt': 'Moncorvo'},
'374269':{'am': u('\u054e\u0561\u0580\u0564\u0565\u0576\u056b\u057d'), 'en': 'Vardenis', 'ru': u('\u0412\u0430\u0440\u0434\u0435\u043d\u0438\u0441')},
'374264':{'am': u('\u0533\u0561\u057e\u0561\u057c/\u054d\u0561\u0580\u0578\u0582\u056d\u0561\u0576'), 'en': 'Gavar/Sarukhan', 'ru': u('\u0413\u0430\u0432\u0430\u0440/\u0421\u0430\u0440\u0443\u0445\u0430\u043d')},
'374265':{'am': u('\u0543\u0561\u0574\u0562\u0561\u0580\u0561\u056f'), 'en': 'Tchambarak', 'ru': u('\u0427\u0430\u043c\u0431\u0430\u0440\u0430\u043a')},
'374266':{'am': u('\u0532\u0565\u0580\u0564\u0561\u057e\u0561\u0576/\u053f\u0578\u0572\u0562/\u0546\u0578\u0575\u0565\u0574\u0562\u0565\u0580\u0575\u0561\u0576'), 'en': 'Berdavan/Koghb/Noyemberyan', 'ru': u('\u0411\u0435\u0440\u0434\u0430\u0432\u0430\u043d/\u041a\u043e\u0445\u0431/\u041d\u043e\u0435\u043c\u0431\u0435\u0440\u044f\u043d')},
'374267':{'am': u('\u0531\u0575\u0563\u0565\u057a\u0561\u0580/\u0532\u0565\u0580\u0564'), 'en': 'Aygepar/Berd', 'ru': u('\u0410\u0439\u0433\u0435\u043f\u0430\u0440/\u0411\u0435\u0440\u0434')},
'374261':{'am': u('\u054d\u0587\u0561\u0576'), 'en': 'Sevan', 'ru': u('\u0421\u0435\u0432\u0430\u043d')},
'374262':{'am': u('\u0544\u0561\u0580\u057f\u0578\u0582\u0576\u056b'), 'en': 'Martuni', 'ru': u('\u041c\u0430\u0440\u0442\u0443\u043d\u0438')},
'374263':{'am': u('\u053b\u057b\u0587\u0561\u0576/\u0531\u0566\u0561\u057f\u0561\u0574\u0578\u0582\u057f/\u0533\u0565\u057f\u0561\u0570\u0578\u057e\u056b\u057f/\u0535\u0576\u0578\u0584\u0561\u057e\u0561\u0576'), 'en': 'Azatamut/Getahovit/Ijevan/Yenokavan', 'ru': u('\u0410\u0437\u0430\u0442\u0430\u043c\u0443\u0442/\u0413\u0435\u0442\u0430\u0445\u043e\u0432\u0438\u0442/\u0418\u0434\u0436\u0435\u0432\u0430\u043d/\u0415\u043d\u043e\u043a\u0430\u0432\u0430\u043d')},
'432627':{'de': 'Pitten', 'en': 'Pitten'},
'432626':{'de': 'Mattersburg', 'en': 'Mattersburg'},
'441647':{'en': 'Moretonhampstead'},
'441646':{'en': 'Milford Haven'},
'441644':{'en': 'New Galloway'},
'441643':{'en': 'Minehead'},
'441642':{'en': 'Middlesbrough'},
'441641':{'en': 'Strathy'},
'3596938':{'bg': u('\u041a\u0430\u043b\u0435\u043d\u0438\u043a, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Kalenik, Lovech'},
'355388':{'en': 'Levan, Fier'},
'355389':{'en': u('Dermenas/Topoj\u00eb, Fier')},
'355386':{'en': 'Kuman/Kurjan/Strum/Ruzhdie, Fier'},
'355387':{'en': 'Cakran/Frakull, Fier'},
'355384':{'en': u('Mbrostar Ura/LIibofsh\u00eb, Fier')},
'355385':{'en': u('Port\u00ebz/Zhar\u00ebz, Fier')},
'355382':{'en': 'Roskovec, Fier'},
'355383':{'en': u('Qend\u00ebr, Fier')},
'432625':{'de': 'Bad Sauerbrunn', 'en': 'Bad Sauerbrunn'},
'355381':{'en': 'Patos, Fier'},
'390935':{'it': 'Enna'},
'390933':{'en': 'Caltanissetta', 'it': 'Caltagirone'},
'3596939':{'bg': u('\u0414\u0440\u0430\u0433\u0430\u043d\u0430'), 'en': 'Dragana'},
'390931':{'it': 'Siracusa'},
'3336673':{'en': 'Lille', 'fr': 'Lille'},
'3336672':{'en': 'Lille', 'fr': 'Lille'},
'3597179':{'bg': u('\u0413\u043e\u043b\u0435\u043c\u043e \u041c\u0430\u043b\u043e\u0432\u043e'), 'en': 'Golemo Malovo'},
'35978':{'bg': u('\u041a\u044e\u0441\u0442\u0435\u043d\u0434\u0438\u043b'), 'en': 'Kyustendil'},
'3596552':{'bg': u('\u0414\u043e\u043b\u043d\u0430 \u041c\u0438\u0442\u0440\u043e\u043f\u043e\u043b\u0438\u044f'), 'en': 'Dolna Mitropolia'},
'3596553':{'bg': u('\u041e\u0440\u044f\u0445\u043e\u0432\u0438\u0446\u0430, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Oryahovitsa, Pleven'},
'3596550':{'bg': u('\u0421\u0442\u0430\u0432\u0435\u0440\u0446\u0438'), 'en': 'Stavertsi'},
'3596551':{'bg': u('\u0422\u0440\u044a\u0441\u0442\u0435\u043d\u0438\u043a, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Trastenik, Pleven'},
'3596556':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u041c\u0438\u0442\u0440\u043e\u043f\u043e\u043b\u0438\u044f'), 'en': 'Gorna Mitropolia'},
'3596557':{'bg': u('\u0411\u0440\u0435\u0433\u0430\u0440\u0435'), 'en': 'Bregare'},
'3596554':{'bg': u('\u041a\u0440\u0443\u0448\u043e\u0432\u0435\u043d\u0435'), 'en': 'Krushovene'},
'3596555':{'bg': u('\u0411\u0430\u0439\u043a\u0430\u043b'), 'en': 'Baykal'},
'3596558':{'bg': u('\u0421\u043b\u0430\u0432\u043e\u0432\u0438\u0446\u0430, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Slavovitsa, Pleven'},
'3596559':{'bg': u('\u0413\u043e\u0441\u0442\u0438\u043b\u044f'), 'en': 'Gostilya'},
'3343765':{'en': 'Lyon', 'fr': 'Lyon'},
'3343764':{'en': 'Lyon', 'fr': 'Lyon'},
'3343766':{'en': 'Lyon', 'fr': 'Lyon'},
'441258':{'en': 'Blandford'},
'441259':{'en': 'Alloa'},
'3597172':{'bg': u('\u0414\u0440\u0430\u0433\u043e\u043c\u0430\u043d'), 'en': 'Dragoman'},
'441254':{'en': 'Blackburn'},
'3347655':{'en': 'Saint-Laurent-du-Pont', 'fr': 'Saint-Laurent-du-Pont'},
'441256':{'en': 'Basingstoke'},
'441257':{'en': 'Coppull'},
'441250':{'en': 'Blairgowrie'},
'441252':{'en': 'Aldershot'},
'3347654':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347659':{'en': 'Saint-Martin-d\'Uriage', 'fr': 'Saint-Martin-d\'Uriage'},
'3323002':{'en': 'Rennes', 'fr': 'Rennes'},
'3597174':{'bg': u('\u041a\u0430\u043b\u043e\u0442\u0438\u043d\u0430'), 'en': 'Kalotina'},
'3354584':{'en': 'Confolens', 'fr': 'Confolens'},
'3326238':{'en': 'Les Avirons', 'fr': 'Les Avirons'},
'3326239':{'en': 'Saint-Louis', 'fr': 'Saint-Louis'},
'3354581':{'en': 'Jarnac', 'fr': 'Jarnac'},
'3354583':{'en': 'Segonzac', 'fr': 'Segonzac'},
'3354582':{'en': 'Cognac', 'fr': 'Cognac'},
'3326232':{'en': 'Saint-Paul', 'fr': 'Saint-Paul'},
'3326233':{'en': 'Saint-Paul', 'fr': 'Saint-Paul'},
'3326230':{'en': 'Saint-Denis', 'fr': 'Saint-Denis'},
'3326231':{'en': 'Saint-Pierre', 'fr': 'Saint-Pierre'},
'3354589':{'en': 'Chabanais', 'fr': 'Chabanais'},
'3326237':{'en': 'Saint-Joseph', 'fr': 'Saint-Joseph'},
'3326234':{'en': 'Saint-Leu', 'fr': 'Saint-Leu'},
'3326235':{'en': 'Saint-Pierre', 'fr': 'Saint-Pierre'},
'37447':{'am': u('\u053c\u0565\u057c\u0576\u0561\u0575\u056b\u0576-\u0542\u0561\u0580\u0561\u0562\u0561\u0572'), 'en': 'Nagorno-Karabakh', 'ru': u('\u041d\u0430\u0433\u043e\u0440\u043d\u044b\u0439 \u041a\u0430\u0440\u0430\u0431\u0430\u0445')},
'437754':{'de': 'Waldzell', 'en': 'Waldzell'},
'390972':{'it': 'Melfi'},
'390973':{'it': 'Lagonegro'},
'390971':{'it': 'Potenza'},
'390976':{'it': 'Muro Lucano'},
'390974':{'en': 'Salerno', 'it': 'Vallo della Lucania'},
'390975':{'en': 'Potenza', 'it': 'Sala Consilina'},
'3593657':{'bg': u('\u0416\u044a\u043b\u0442\u0443\u0448\u0430'), 'en': 'Zhaltusha'},
'3593652':{'bg': u('\u0411\u044f\u043b \u0438\u0437\u0432\u043e\u0440, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Byal izvor, Kardzh.'},
'3593653':{'bg': u('\u041c\u043b\u0435\u0447\u0438\u043d\u043e'), 'en': 'Mlechino'},
'3593651':{'bg': u('\u0410\u0440\u0434\u0438\u043d\u043e'), 'en': 'Ardino'},
'3593658':{'bg': u('\u041f\u0430\u0434\u0438\u043d\u0430, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Padina, Kardzh.'},
'3355982':{'en': 'Pau', 'fr': 'Pau'},
'3355983':{'en': 'Pau', 'fr': 'Pau'},
'3355980':{'en': 'Pau', 'fr': 'Pau'},
'3355981':{'en': 'Lescar', 'fr': 'Lescar'},
'437750':{'de': 'Andrichsfurt', 'en': 'Andrichsfurt'},
'3355984':{'en': 'Pau', 'fr': 'Pau'},
'3355985':{'en': 'Saint-Jean-de-Luz', 'fr': 'Saint-Jean-de-Luz'},
'3355988':{'en': 'Arette', 'fr': 'Arette'},
'3593032':{'bg': u('\u0421\u0440\u0435\u0434\u043d\u043e\u0433\u043e\u0440\u0446\u0438'), 'en': 'Srednogortsi'},
'355814':{'en': u('Tepelen\u00eb')},
'355815':{'en': u('Delvin\u00eb')},
'355812':{'en': u('Ersek\u00eb, Kolonj\u00eb')},
'355813':{'en': u('P\u00ebrmet')},
'3593034':{'bg': u('\u041b\u0435\u0432\u043e\u0447\u0435\u0432\u043e'), 'en': 'Levochevo'},
'355811':{'en': 'Bilisht, Devoll'},
'3593038':{'bg': u('\u0427\u043e\u043a\u043c\u0430\u043d\u043e\u0432\u043e'), 'en': 'Chokmanovo'},
'3324039':{'en': 'Saint-Brevin-les-Pins', 'fr': 'Saint-Brevin-les-Pins'},
'3324036':{'en': 'Vallet', 'fr': 'Vallet'},
'3332778':{'en': 'Cambrai', 'fr': 'Cambrai'},
'432552':{'de': 'Poysdorf', 'en': 'Poysdorf'},
'432555':{'de': 'Herrnbaumgarten', 'en': 'Herrnbaumgarten'},
'432554':{'de': u('St\u00fctzenhofen'), 'en': u('St\u00fctzenhofen')},
'432557':{'de': 'Bernhardsthal', 'en': 'Bernhardsthal'},
'3324037':{'en': 'Nantes', 'fr': 'Nantes'},
'3332772':{'en': 'Cambrai', 'fr': 'Cambrai'},
'3332773':{'en': 'Cambrai', 'fr': 'Cambrai'},
'3332770':{'en': 'Cambrai', 'fr': 'Cambrai'},
'3332771':{'en': 'Douai', 'fr': 'Douai'},
'3332776':{'en': 'Caudry', 'fr': 'Caudry'},
'3324034':{'en': 'Vertou', 'fr': 'Vertou'},
'3332774':{'en': 'Cambrai', 'fr': 'Cambrai'},
'3332775':{'en': 'Caudry', 'fr': 'Caudry'},
'3324035':{'en': 'Nantes', 'fr': 'Nantes'},
'434258':{'de': 'Gummern', 'en': 'Gummern'},
'434254':{'de': 'Faak am See', 'en': 'Faak am See'},
'3346656':{'en': u('Al\u00e8s'), 'fr': u('Al\u00e8s')},
'434256':{'de': u('N\u00f6tsch im Gailtal'), 'en': u('N\u00f6tsch im Gailtal')},
'434257':{'de': u('F\u00fcrnitz'), 'en': u('F\u00fcrnitz')},
'434252':{'de': 'Wernberg', 'en': 'Wernberg'},
'3338043':{'en': 'Dijon', 'fr': 'Dijon'},
'437752':{'de': 'Ried im Innkreis', 'en': 'Ried im Innkreis'},
'3324030':{'en': 'Nantes', 'fr': 'Nantes'},
'3338041':{'en': 'Dijon', 'fr': 'Dijon'},
'39015':{'en': 'Biella', 'it': 'Biella'},
'433383':{'de': 'Burgau', 'en': 'Burgau'},
'3334440':{'en': u('Compi\u00e8gne'), 'fr': u('Compi\u00e8gne')},
'3355517':{'en': 'Brive-la-Gaillarde', 'fr': 'Brive-la-Gaillarde'},
'3598431':{'bg': u('\u0418\u0441\u043f\u0435\u0440\u0438\u0445'), 'en': 'Isperih'},
'3599528':{'bg': u('\u0413\u0430\u0433\u0430\u043d\u0438\u0446\u0430'), 'en': 'Gaganitsa'},
'3594562':{'bg': u('\u041a\u0440\u0438\u0432\u0430 \u043a\u0440\u0443\u0448\u0430'), 'en': 'Kriva krusha'},
'3594564':{'bg': u('\u041d\u043e\u0432\u043e\u0441\u0435\u043b\u0435\u0446'), 'en': 'Novoselets'},
'3594567':{'bg': u('\u0411\u0430\u043d\u044f, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Banya, Sliven'},
'3594566':{'bg': u('\u041f\u0438\u0442\u043e\u0432\u043e'), 'en': 'Pitovo'},
'3599522':{'bg': u('\u041a\u043e\u0442\u0435\u043d\u043e\u0432\u0446\u0438'), 'en': 'Kotenovtsi'},
'3599523':{'bg': u('\u0411\u044a\u0440\u0437\u0438\u044f'), 'en': 'Barzia'},
'3599520':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u041e\u0437\u0438\u0440\u043e\u0432\u043e'), 'en': 'Gorno Ozirovo'},
'3599521':{'bg': u('\u0417\u0430\u043c\u0444\u0438\u0440\u043e\u0432\u043e'), 'en': 'Zamfirovo'},
'3599526':{'bg': u('\u0421\u043b\u0430\u0442\u0438\u043d\u0430, \u041c\u043e\u043d\u0442.'), 'en': 'Slatina, Mont.'},
'3599527':{'bg': u('\u0412\u044a\u0440\u0448\u0435\u0446'), 'en': 'Varshets'},
'3599524':{'bg': u('\u042f\u0433\u043e\u0434\u043e\u0432\u043e, \u041c\u043e\u043d\u0442.'), 'en': 'Yagodovo, Mont.'},
'3599525':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u041e\u0437\u0438\u0440\u043e\u0432\u043e'), 'en': 'Dolno Ozirovo'},
'3595168':{'bg': u('\u041f\u0435\u0442\u0440\u043e\u0432 \u0434\u043e\u043b, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Petrov dol, Varna'},
'3595169':{'bg': u('\u041c\u043e\u043c\u0447\u0438\u043b\u043e\u0432\u043e'), 'en': 'Momchilovo'},
'3595166':{'bg': u('\u0414\u043e\u0431\u0440\u043e\u043f\u043b\u043e\u0434\u043d\u043e'), 'en': 'Dobroplodno'},
'3595167':{'bg': u('\u0412\u0435\u043d\u0447\u0430\u043d'), 'en': 'Venchan'},
'3595164':{'bg': u('\u041d\u0435\u043e\u0444\u0438\u0442 \u0420\u0438\u043b\u0441\u043a\u0438'), 'en': 'Neofit Rilski'},
'3595165':{'bg': u('\u041d\u0435\u0432\u0448\u0430'), 'en': 'Nevsha'},
'3595162':{'bg': u('\u0411\u0435\u043b\u043e\u0433\u0440\u0430\u0434\u0435\u0446'), 'en': 'Belogradets'},
'3595163':{'bg': u('\u041c\u043b\u0430\u0434\u0430 \u0433\u0432\u0430\u0440\u0434\u0438\u044f'), 'en': 'Mlada gvardia'},
'3595161':{'bg': u('\u0412\u0435\u0442\u0440\u0438\u043d\u043e'), 'en': 'Vetrino'},
'3324143':{'en': 'Angers', 'fr': 'Angers'},
'3324140':{'en': 'Saumur', 'fr': 'Saumur'},
'3324146':{'en': 'Cholet', 'fr': 'Cholet'},
'3324147':{'en': 'Angers', 'fr': 'Angers'},
'3324144':{'en': 'Angers', 'fr': 'Angers'},
'3324148':{'en': 'Angers', 'fr': 'Angers'},
'3324149':{'en': 'Cholet', 'fr': 'Cholet'},
'39011':{'en': 'Turin', 'it': 'Torino'},
'3332471':{'en': 'Vouziers', 'fr': 'Vouziers'},
'354552':{'en': u('Reykjav\u00edk/Vesturb\u00e6r/Mi\u00f0b\u00e6rinn')},
'432266':{'de': 'Stockerau', 'en': 'Stockerau'},
'432265':{'de': 'Hausleiten', 'en': 'Hausleiten'},
'437263':{'de': 'Bad Zell', 'en': 'Bad Zell'},
'3356514':{'en': 'Figeac', 'fr': 'Figeac'},
'432264':{'de': u('R\u00fcckersdorf, Harmannsdorf'), 'en': u('R\u00fcckersdorf, Harmannsdorf')},
'3338685':{'en': u('Ch\u00e2teau-Chinon(Ville)'), 'fr': u('Ch\u00e2teau-Chinon(Ville)')},
'3338687':{'en': 'Villeneuve-sur-Yonne', 'fr': 'Villeneuve-sur-Yonne'},
'3338680':{'en': 'Migennes', 'fr': 'Migennes'},
'3338681':{'en': 'Vermenton', 'fr': 'Vermenton'},
'3338682':{'en': 'Noyers sur Serein', 'fr': 'Noyers sur Serein'},
'3338683':{'en': 'Sens', 'fr': 'Sens'},
'43732':{'de': 'Linz', 'en': 'Linz'},
'3347273':{'en': 'Lyon', 'fr': 'Lyon'},
'3347272':{'en': 'Lyon', 'fr': 'Lyon'},
'3347271':{'en': 'Lyon', 'fr': 'Lyon'},
'3347270':{'en': 'Lyon', 'fr': 'Lyon'},
'3347277':{'en': 'Lyon', 'fr': 'Lyon'},
'3347276':{'en': 'Lyon', 'fr': 'Lyon'},
'3347275':{'en': 'Lyon', 'fr': 'Lyon'},
'3347274':{'en': 'Lyon', 'fr': 'Lyon'},
'3347279':{'en': 'Saint-Priest', 'fr': 'Saint-Priest'},
'3347278':{'en': 'Lyon', 'fr': 'Lyon'},
'432663':{'de': 'Schottwien', 'en': 'Schottwien'},
'3636':{'en': 'Eger', 'hu': 'Eger'},
'3637':{'en': 'Gyongyos', 'hu': u('Gy\u00f6ngy\u00f6s')},
'3634':{'en': 'Tatabanya', 'hu': u('Tatab\u00e1nya')},
'3635':{'en': 'Balassagyarmat', 'hu': 'Balassagyarmat'},
'3632':{'en': 'Salgotarjan', 'hu': u('Salg\u00f3tarj\u00e1n')},
'3633':{'en': 'Esztergom', 'hu': 'Esztergom'},
'3324137':{'en': 'Angers', 'fr': 'Angers'},
'3596129':{'bg': u('\u0413\u0430\u0431\u0440\u043e\u0432\u0446\u0438'), 'en': 'Gabrovtsi'},
'3596128':{'bg': u('\u0425\u043e\u0442\u043d\u0438\u0446\u0430'), 'en': 'Hotnitsa'},
'3596123':{'bg': u('\u0411\u0443\u043a\u043e\u0432\u0435\u0446, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Bukovets, V. Tarnovo'},
'3596122':{'bg': u('\u0411\u0435\u043b\u044f\u043a\u043e\u0432\u0435\u0446'), 'en': 'Belyakovets'},
'3596121':{'bg': u('\u041d\u0438\u043a\u044e\u043f'), 'en': 'Nikyup'},
'432662':{'de': 'Gloggnitz', 'en': 'Gloggnitz'},
'3596126':{'bg': u('\u0426\u0435\u0440\u043e\u0432\u0430 \u043a\u043e\u0440\u0438\u044f'), 'en': 'Tserova koria'},
'3596125':{'bg': u('\u041f\u0443\u0448\u0435\u0432\u043e'), 'en': 'Pushevo'},
'3596124':{'bg': u('\u041b\u0435\u0434\u0435\u043d\u0438\u043a'), 'en': 'Ledenik'},
'3355618':{'en': 'Merignac', 'fr': u('M\u00e9rignac')},
'3338389':{'en': u('Lun\u00e9ville'), 'fr': u('Lun\u00e9ville')},
'3347099':{'en': 'Lapalisse', 'fr': 'Lapalisse'},
'3347098':{'en': 'Vichy', 'fr': 'Vichy'},
'3347097':{'en': 'Vichy', 'fr': 'Vichy'},
'3347096':{'en': 'Vichy', 'fr': 'Vichy'},
'374312':{'am': u('\u0533\u0575\u0578\u0582\u0574\u0580\u056b/\u0531\u056d\u0578\u0582\u0580\u0575\u0561\u0576'), 'en': 'Gyumri/Akhuryan region', 'ru': u('\u0413\u044e\u043c\u0440\u0438/\u0410\u0445\u0443\u0440\u044f\u043d')},
'3347090':{'en': 'Gannat', 'fr': 'Gannat'},
'3316079':{'en': u('\u00c9vry'), 'fr': u('\u00c9vry')},
'3316078':{'en': u('\u00c9vry'), 'fr': u('\u00c9vry')},
'435523':{'de': u('G\u00f6tzis'), 'en': u('G\u00f6tzis')},
'3316072':{'en': 'Fontainebleau', 'fr': 'Fontainebleau'},
'3316071':{'en': 'Fontainebleau', 'fr': 'Fontainebleau'},
'3316070':{'en': 'Moret-sur-Loing', 'fr': 'Moret-sur-Loing'},
'3316077':{'en': u('\u00c9vry'), 'fr': u('\u00c9vry')},
'3316075':{'en': 'Soisy-sur-Seine', 'fr': 'Soisy-sur-Seine'},
'3316074':{'en': 'Fontainebleau', 'fr': 'Fontainebleau'},
'441745':{'en': 'Rhyl'},
'433581':{'de': u('Oberw\u00f6lz'), 'en': u('Oberw\u00f6lz')},
'3347897':{'en': 'Rillieux-la-Pape', 'fr': 'Rillieux-la-Pape'},
'3347896':{'en': 'Chaponnay', 'fr': 'Chaponnay'},
'3347895':{'en': 'Lyon', 'fr': 'Lyon'},
'3347894':{'en': 'Villeurbanne', 'fr': 'Villeurbanne'},
'3347893':{'en': 'Lyon', 'fr': 'Lyon'},
'3347892':{'en': 'Lyon', 'fr': 'Lyon'},
'3347891':{'en': u('Neuville-sur-Sa\u00f4ne'), 'fr': u('Neuville-sur-Sa\u00f4ne')},
'3347890':{'en': 'Genas', 'fr': 'Genas'},
'3338064':{'en': 'Saulieu', 'fr': 'Saulieu'},
'37422675':{'am': u('\u0531\u056c\u0561\u057a\u0561\u0580\u057d/\u054e\u0561\u0580\u0564\u0561\u0576\u0561\u057e\u0561\u0576\u0584'), 'en': 'Alapars/Vardanavank', 'ru': u('\u0410\u043b\u0430\u043f\u0430\u0440\u0441/\u0412\u0430\u0440\u0434\u0430\u043d\u0430\u0432\u0430\u043d\u043a')},
'37422672':{'am': u('\u0531\u0580\u0566\u0561\u056f\u0561\u0576'), 'en': 'Arzakan', 'ru': u('\u0410\u0440\u0437\u0430\u043a\u0430\u043d')},
'437269':{'de': 'Baumgartenberg', 'en': 'Baumgartenberg'},
'435525':{'de': 'Nenzing', 'en': 'Nenzing'},
'35930257':{'bg': u('\u0412\u0438\u0448\u043d\u0435\u0432\u043e'), 'en': 'Vishnevo'},
'35930256':{'bg': u('\u0413\u044a\u043b\u044a\u0431\u043e\u0432\u043e, \u0421\u043c\u043e\u043b.'), 'en': 'Galabovo, Smol.'},
'39019':{'it': 'Savona'},
'432163':{'de': 'Petronell-Carnuntum', 'en': 'Petronell-Carnuntum'},
'434710':{'de': 'Oberdrauburg', 'en': 'Oberdrauburg'},
'390565':{'en': 'Livorno', 'it': 'Piombino'},
'390564':{'it': 'Grosseto'},
'390566':{'it': 'Follonica'},
'35991180':{'bg': u('\u041b\u0435\u0441\u0443\u0440\u0430'), 'en': 'Lesura'},
'3338066':{'en': 'Dijon', 'fr': 'Dijon'},
'35991182':{'bg': u('\u041e\u0441\u0435\u043d, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Osen, Vratsa'},
'437743':{'de': 'Maria Schmolln', 'en': 'Maria Schmolln'},
'35991183':{'bg': u('\u0424\u0443\u0440\u0435\u043d'), 'en': 'Furen'},
'433327':{'de': 'Sankt Michael im Burgenland', 'en': 'St. Michael im Burgenland'},
'3324131':{'en': 'Angers', 'fr': 'Angers'},
'3338067':{'en': 'Dijon', 'fr': 'Dijon'},
'437747':{'de': 'Kirchberg bei Mattighofen', 'en': 'Kirchberg bei Mattighofen'},
'437746':{'de': 'Friedburg', 'en': 'Friedburg'},
'3349499':{'en': 'Vidauban', 'fr': 'Vidauban'},
'3349498':{'en': 'Le Beausset', 'fr': 'Le Beausset'},
'3349369':{'en': 'Le Cannet', 'fr': 'Le Cannet'},
'3349368':{'en': 'Cannes', 'fr': 'Cannes'},
'437745':{'de': 'Lochen', 'en': 'Lochen'},
'3349491':{'en': 'Toulon', 'fr': 'Toulon'},
'3349362':{'en': 'Nice', 'fr': 'Nice'},
'3349493':{'en': 'Toulon', 'fr': 'Toulon'},
'3349492':{'en': 'Toulon', 'fr': 'Toulon'},
'3349495':{'en': u('Saint-Rapha\u00ebl'), 'fr': u('Saint-Rapha\u00ebl')},
'3349494':{'en': 'La Seyne sur Mer', 'fr': 'La Seyne sur Mer'},
'3349365':{'en': 'Biot', 'fr': 'Biot'},
'3349496':{'en': 'Sainte-Maxime', 'fr': 'Sainte-Maxime'},
'35931708':{'bg': u('\u041f\u0435\u0441\u043d\u043e\u043f\u043e\u0439, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Pesnopoy, Plovdiv'},
'35931709':{'bg': u('\u041c\u0438\u0445\u0438\u043b\u0446\u0438'), 'en': 'Mihiltsi'},
'35931700':{'bg': u('\u0411\u0435\u043b\u043e\u0432\u0438\u0446\u0430'), 'en': 'Belovitsa'},
'35931701':{'bg': u('\u041a\u0440\u044a\u0441\u0442\u0435\u0432\u0438\u0447'), 'en': 'Krastevich'},
'35931702':{'bg': u('\u0414\u043e\u043b\u043d\u0430 \u043c\u0430\u0445\u0430\u043b\u0430, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Dolna mahala, Plovdiv'},
'35931703':{'bg': u('\u0416\u0438\u0442\u043d\u0438\u0446\u0430, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Zhitnitsa, Plovdiv'},
'35931704':{'bg': u('\u0418\u0432\u0430\u043d \u0412\u0430\u0437\u043e\u0432\u043e'), 'en': 'Ivan Vazovo'},
'35931705':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u043c\u0430\u0445\u0430\u043b\u0430'), 'en': 'Gorna mahala'},
'35931706':{'bg': u('\u0421\u0443\u0445\u043e\u0437\u0435\u043c'), 'en': 'Suhozem'},
'35931707':{'bg': u('\u0427\u0435\u0440\u043d\u043e\u0437\u0435\u043c\u0435\u043d'), 'en': 'Chernozemen'},
'433584':{'de': 'Neumarkt in Steiermark', 'en': 'Neumarkt in Steiermark'},
'3355613':{'en': 'Merignac', 'fr': u('M\u00e9rignac')},
'3751655':{'be': u('\u0421\u0442\u043e\u043b\u0456\u043d, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Stolin, Brest Region', 'ru': u('\u0421\u0442\u043e\u043b\u0438\u043d, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751652':{'be': u('\u0406\u0432\u0430\u043d\u0430\u0432\u0430, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Ivanovo, Brest Region', 'ru': u('\u0418\u0432\u0430\u043d\u043e\u0432\u043e, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3347251':{'en': u('V\u00e9nissieux'), 'fr': u('V\u00e9nissieux')},
'3751651':{'be': u('\u041c\u0430\u043b\u0430\u0440\u044b\u0442\u0430, \u0411\u0440\u044d\u0441\u0446\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Malorita, Brest Region', 'ru': u('\u041c\u0430\u043b\u043e\u0440\u0438\u0442\u0430, \u0411\u0440\u0435\u0441\u0442\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3338061':{'en': 'Nuits-Saint-Georges', 'fr': 'Nuits-Saint-Georges'},
'3349032':{'en': 'Le Pontet', 'fr': 'Le Pontet'},
'3594716':{'bg': u('\u0412\u0435\u0441\u0435\u043b\u0438\u043d\u043e\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Veselinovo, Yambol'},
'3594717':{'bg': u('\u0427\u0430\u0440\u0433\u0430\u043d'), 'en': 'Chargan'},
'3594714':{'bg': u('\u0414\u0440\u0430\u0436\u0435\u0432\u043e'), 'en': 'Drazhevo'},
'3594715':{'bg': u('\u041a\u0430\u043b\u0447\u0435\u0432\u043e'), 'en': 'Kalchevo'},
'3594712':{'bg': u('\u041a\u0430\u0431\u0438\u043b\u0435'), 'en': 'Kabile'},
'3594713':{'bg': u('\u0421\u0442\u0430\u0440\u0430 \u0440\u0435\u043a\u0430, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Stara reka, Yambol'},
'3594710':{'bg': u('\u0411\u043e\u043b\u044f\u0440\u0441\u043a\u043e'), 'en': 'Bolyarsko'},
'3594711':{'bg': u('\u0411\u0435\u0437\u043c\u0435\u0440, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Bezmer, Yambol'},
'35937706':{'bg': u('\u0427\u0435\u0440\u043d\u043e\u0434\u044a\u0431'), 'en': 'Chernodab'},
'35937707':{'bg': u('\u0429\u0438\u0442'), 'en': 'Shtit'},
'35937704':{'bg': u('\u041c\u043b\u0430\u0434\u0438\u043d\u043e\u0432\u043e'), 'en': 'Mladinovo'},
'3347250':{'en': u('V\u00e9nissieux'), 'fr': u('V\u00e9nissieux')},
'35937702':{'bg': u('\u041c\u0443\u0441\u0442\u0440\u0430\u043a'), 'en': 'Mustrak'},
'35937703':{'bg': u('\u0414\u0438\u043c\u0438\u0442\u0440\u043e\u0432\u0447\u0435'), 'en': 'Dimitrovche'},
'3594718':{'bg': u('\u0420\u043e\u0437\u0430'), 'en': 'Roza'},
'35937701':{'bg': u('\u0421\u043b\u0430\u0434\u0443\u043d'), 'en': 'Sladun'},
'3338062':{'en': 'Nuits-Saint-Georges', 'fr': 'Nuits-Saint-Georges'},
'433586':{'de': u('M\u00fchlen'), 'en': u('M\u00fchlen')},
'4418907':{'en': 'Ayton'},
'3355572':{'en': 'Ussel', 'fr': 'Ussel'},
'4418905':{'en': 'Ayton'},
'3355570':{'en': 'Aixe-sur-Vienne', 'fr': 'Aixe-sur-Vienne'},
'3355577':{'en': 'Limoges', 'fr': 'Limoges'},
'3355576':{'en': 'Bessines-sur-Gartempe', 'fr': 'Bessines-sur-Gartempe'},
'3355575':{'en': 'Saint-Yrieix-la-Perche', 'fr': 'Saint-Yrieix-la-Perche'},
'3355574':{'en': 'Brive-la-Gaillarde', 'fr': 'Brive-la-Gaillarde'},
'3355579':{'en': 'Limoges', 'fr': 'Limoges'},
'4418909':{'en': 'Ayton'},
'4418908':{'en': 'Coldstream'},
'40355':{'en': u('Cara\u0219-Severin'), 'ro': u('Cara\u0219-Severin')},
'40354':{'en': 'Hunedoara', 'ro': 'Hunedoara'},
'3332433':{'en': u('Charleville-M\u00e9zi\u00e8res'), 'fr': u('Charleville-M\u00e9zi\u00e8res')},
'40356':{'en': u('Timi\u0219'), 'ro': u('Timi\u0219')},
'40351':{'en': 'Dolj', 'ro': 'Dolj'},
'40350':{'en': u('V\u00e2lcea'), 'ro': u('V\u00e2lcea')},
'3332437':{'en': u('Charleville-M\u00e9zi\u00e8res'), 'fr': u('Charleville-M\u00e9zi\u00e8res')},
'3332436':{'en': u('Charleville-M\u00e9zi\u00e8res'), 'fr': u('Charleville-M\u00e9zi\u00e8res')},
'3332439':{'en': 'Rethel', 'fr': 'Rethel'},
'3332438':{'en': 'Rethel', 'fr': 'Rethel'},
'40359':{'en': 'Bihor', 'ro': 'Bihor'},
'40358':{'en': 'Alba', 'ro': 'Alba'},
'432618':{'de': 'Markt Sankt Martin', 'en': 'Markt St. Martin'},
'432619':{'de': 'Lackendorf', 'en': 'Lackendorf'},
'35982':{'bg': u('\u0420\u0443\u0441\u0435'), 'en': 'Ruse'},
'35984':{'bg': u('\u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Razgrad'},
'35986':{'bg': u('\u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Silistra'},
'437432':{'de': 'Strengberg', 'en': 'Strengberg'},
'3355799':{'en': 'Villenave-d\'Ornon', 'fr': 'Villenave-d\'Ornon'},
'3355798':{'en': 'Langon', 'fr': 'Langon'},
'3355796':{'en': 'Gradignan', 'fr': 'Gradignan'},
'3355795':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355794':{'en': u('Saint-Andr\u00e9-de-Cubzac'), 'fr': u('Saint-Andr\u00e9-de-Cubzac')},
'3355793':{'en': 'Eysines', 'fr': 'Eysines'},
'3355792':{'en': 'Merignac', 'fr': u('M\u00e9rignac')},
'3356219':{'en': 'Ramonville-Saint-Agne', 'fr': 'Ramonville-Saint-Agne'},
'3356212':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356211':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356217':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356216':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356214':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356560':{'en': 'Millau', 'fr': 'Millau'},
'3325158':{'en': 'Saint-Jean-de-Monts', 'fr': 'Saint-Jean-de-Monts'},
'3325159':{'en': 'Saint-Jean-de-Monts', 'fr': 'Saint-Jean-de-Monts'},
'3325156':{'en': u('Lu\u00e7on'), 'fr': u('Lu\u00e7on')},
'3325157':{'en': 'Pouzauges', 'fr': 'Pouzauges'},
'3325154':{'en': 'Saint-Hilaire-de-Riez', 'fr': 'Saint-Hilaire-de-Riez'},
'3325155':{'en': 'Saint-Gilles-Croix-de-Vie', 'fr': 'Saint-Gilles-Croix-de-Vie'},
'3325153':{'en': 'Saint-Martin-de-Fraigneau', 'fr': 'Saint-Martin-de-Fraigneau'},
'3325150':{'en': 'Fontenay-le-Comte', 'fr': 'Fontenay-le-Comte'},
'3593923':{'bg': u('\u0420\u0430\u0434\u0438\u0435\u0432\u043e'), 'en': 'Radievo'},
'3593924':{'bg': u('\u041a\u0440\u0435\u043f\u043e\u0441\u0442'), 'en': 'Krepost'},
'3705':{'en': 'Vilnius'},
'3344209':{'en': 'Marignane', 'fr': 'Marignane'},
'3344208':{'en': 'La Ciotat', 'fr': 'La Ciotat'},
'35368':{'en': 'Listowel'},
'35369':{'en': 'Newcastle West'},
'35364':{'en': 'Killarney/Rathmore'},
'35365':{'en': 'Ennis/Ennistymon/Kilrush'},
'35366':{'en': 'Tralee/Dingle/Killorglin/Cahersiveen'},
'35367':{'en': 'Nenagh'},
'3344207':{'en': 'Martigues', 'fr': 'Martigues'},
'35361':{'en': 'Limerick/Scariff'},
'3344205':{'en': 'Fos-sur-Mer', 'fr': 'Fos-sur-Mer'},
'3344204':{'en': 'Roquevaire', 'fr': 'Roquevaire'},
'370349':{'en': 'Jonava'},
'370347':{'en': u('K\u0117dainiai')},
'370346':{'en': u('Kai\u0161iadorys')},
'370345':{'en': u('\u0160akiai')},
'370343':{'en': u('Marijampol\u0117')},
'370342':{'en': u('Vilkavi\u0161kis')},
'370340':{'en': u('Ukmerg\u0117')},
'351251':{'en': u('Valen\u00e7a'), 'pt': u('Valen\u00e7a')},
'351252':{'en': u('V. N. de Famalic\u00e3o'), 'pt': u('V. N. de Famalic\u00e3o')},
'351253':{'en': 'Braga', 'pt': 'Braga'},
'351254':{'en': u('Peso da R\u00e9gua'), 'pt': u('Peso da R\u00e9gua')},
'351255':{'en': 'Penafiel', 'pt': 'Penafiel'},
'351256':{'en': u('S. Jo\u00e3o da Madeira'), 'pt': u('S. Jo\u00e3o da Madeira')},
'351258':{'en': 'Viana do Castelo', 'pt': 'Viana do Castelo'},
'351259':{'en': 'Vila Real', 'pt': 'Vila Real'},
'3347237':{'en': 'Bron', 'fr': 'Bron'},
'3599338':{'bg': u('\u0428\u0438\u0448\u0435\u043d\u0446\u0438'), 'en': 'Shishentsi'},
'3347236':{'en': 'Lyon', 'fr': 'Lyon'},
'3595590':{'bg': u('\u0416\u0438\u0442\u043e\u0441\u0432\u044f\u0442'), 'en': 'Zhitosvyat'},
'3595599':{'bg': u('\u0425\u0430\u0434\u0436\u0438\u0438\u0442\u0435'), 'en': 'Hadzhiite'},
'441629':{'en': 'Matlock'},
'441628':{'en': 'Maidenhead'},
'441621':{'en': 'Maldon'},
'441620':{'en': 'North Berwick'},
'441623':{'en': 'Mansfield'},
'441622':{'en': 'Maidstone'},
'441625':{'en': 'Macclesfield'},
'441624':{'en': 'Isle of Man'},
'3599332':{'bg': u('\u0420\u0430\u043a\u043e\u0432\u0438\u0446\u0430'), 'en': 'Rakovitsa'},
'3596710':{'bg': u('\u0414\u043e\u043d\u0438\u043d\u043e'), 'en': 'Donino'},
'3596711':{'bg': u('\u041a\u043e\u0437\u0438 \u0440\u043e\u0433'), 'en': 'Kozi rog'},
'3596712':{'bg': u('\u0413\u044a\u0431\u0435\u043d\u0435'), 'en': 'Gabene'},
'3596713':{'bg': u('\u0412\u0440\u0430\u043d\u0438\u043b\u043e\u0432\u0446\u0438'), 'en': 'Vranilovtsi'},
'3596714':{'bg': u('\u041f\u043e\u043f\u043e\u0432\u0446\u0438'), 'en': 'Popovtsi'},
'3596716':{'bg': u('\u0416\u044a\u043b\u0442\u0435\u0448'), 'en': 'Zhaltesh'},
'3596717':{'bg': u('\u041b\u0435\u0441\u0438\u0447\u0430\u0440\u043a\u0430'), 'en': 'Lesicharka'},
'3596718':{'bg': u('\u0414\u0440\u0430\u0433\u0430\u043d\u043e\u0432\u0446\u0438'), 'en': 'Draganovtsi'},
'3593534':{'bg': u('\u041f\u043e\u043f\u0438\u043d\u0446\u0438'), 'en': 'Popintsi'},
'3597448':{'bg': u('\u0411\u0430\u0447\u0435\u0432\u043e'), 'en': 'Bachevo'},
'3355674':{'en': 'Lormont', 'fr': 'Lormont'},
'3597446':{'bg': u('\u0415\u043b\u0435\u0448\u043d\u0438\u0446\u0430, \u0411\u043b\u0430\u0433.'), 'en': 'Eleshnitsa, Blag.'},
'3597445':{'bg': u('\u0411\u0430\u043d\u044f, \u0411\u043b\u0430\u0433.'), 'en': 'Banya, Blag.'},
'3597444':{'bg': u('\u0411\u0435\u043b\u0438\u0446\u0430, \u0411\u043b\u0430\u0433.'), 'en': 'Belitsa, Blag.'},
'3597442':{'bg': u('\u042f\u043a\u043e\u0440\u0443\u0434\u0430'), 'en': 'Yakoruda'},
'436478':{'de': 'Zederhaus', 'en': 'Zederhaus'},
'436479':{'de': 'Muhr', 'en': 'Muhr'},
'436476':{'de': 'Sankt Margarethen im Lungau', 'en': 'St. Margarethen im Lungau'},
'436477':{'de': 'Sankt Michael im Lungau', 'en': 'St. Michael im Lungau'},
'436474':{'de': 'Tamsweg', 'en': 'Tamsweg'},
'436475':{'de': 'Ramingstein', 'en': 'Ramingstein'},
'436472':{'de': 'Mauterndorf', 'en': 'Mauterndorf'},
'436473':{'de': 'Mariapfarr', 'en': 'Mariapfarr'},
'436470':{'de': 'Atzmannsdorf', 'en': 'Atzmannsdorf'},
'436471':{'de': 'Tweng', 'en': 'Tweng'},
'3338147':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338146':{'en': 'Pontarlier', 'fr': 'Pontarlier'},
'3338141':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338140':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338148':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'390371':{'en': 'Lodi', 'it': 'Lodi'},
'390925':{'en': 'Agrigento', 'it': 'Sciacca'},
'3595115':{'bg': u('\u0413\u0435\u043d\u0435\u0440\u0430\u043b \u041a\u0430\u043d\u0442\u0430\u0440\u0434\u0436\u0438\u0435\u0432\u043e'), 'en': 'General Kantardzhievo'},
'3343785':{'en': 'Rillieux-la-Pape', 'fr': 'Rillieux-la-Pape'},
'3597192':{'bg': u('\u0413\u0438\u043d\u0446\u0438'), 'en': 'Gintsi'},
'390924':{'en': 'Trapani', 'it': 'Alcamo'},
'3595114':{'bg': u('\u0415\u0437\u0435\u0440\u043e\u0432\u043e, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Ezerovo, Varna'},
'35857':{'en': 'Kymi', 'fi': 'Kymi', 'se': 'Kymmene'},
'35854':{'en': 'Kymi', 'fi': 'Kymi', 'se': 'Kymmene'},
'35855':{'en': 'Kymi', 'fi': 'Kymi', 'se': 'Kymmene'},
'35984774':{'bg': u('\u0413\u043e\u043b\u044f\u043c \u0438\u0437\u0432\u043e\u0440, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Golyam izvor, Razgrad'},
'35984776':{'bg': u('\u0425\u044a\u0440\u0441\u043e\u0432\u043e, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Harsovo, Razgrad'},
'35984779':{'bg': u('\u0417\u0434\u0440\u0430\u0432\u0435\u0446, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Zdravets, Razgrad'},
'35852':{'en': 'Kymi', 'fi': 'Kymi', 'se': 'Kymmene'},
'35853':{'en': 'Kymi', 'fi': 'Kymi', 'se': 'Kymmene'},
'3329705':{'en': u('Qu\u00e9ven'), 'fr': u('Qu\u00e9ven')},
'3329702':{'en': 'Guidel', 'fr': 'Guidel'},
'3354569':{'en': 'Champniers', 'fr': 'Champniers'},
'3354568':{'en': 'Gond-Pontouvre', 'fr': 'Gond-Pontouvre'},
'3354567':{'en': 'La Couronne', 'fr': 'La Couronne'},
'3354566':{'en': u('Roullet-Saint-Est\u00e8phe'), 'fr': u('Roullet-Saint-Est\u00e8phe')},
'35327':{'en': 'Bantry'},
'3354563':{'en': 'La Rochefoucauld', 'fr': 'La Rochefoucauld'},
'3354561':{'en': u('Angoul\u00eame'), 'fr': u('Angoul\u00eame')},
'3329708':{'en': 'Baud', 'fr': 'Baud'},
'3338534':{'en': u('M\u00e2con'), 'fr': u('M\u00e2con')},
'3338532':{'en': 'Tournus', 'fr': 'Tournus'},
'3338531':{'en': 'Replonges', 'fr': 'Replonges'},
'3342789':{'en': 'Lyon', 'fr': 'Lyon'},
'3338538':{'en': u('M\u00e2con'), 'fr': u('M\u00e2con')},
'3338539':{'en': u('M\u00e2con'), 'fr': u('M\u00e2con')},
'3323281':{'en': 'Rouen', 'fr': 'Rouen'},
'3323282':{'en': 'Maromme', 'fr': 'Maromme'},
'3323283':{'en': 'Rouen', 'fr': 'Rouen'},
'3323284':{'en': 'Notre-Dame-de-Gravenchon', 'fr': 'Notre-Dame-de-Gravenchon'},
'3323289':{'en': 'Gournay-en-Bray', 'fr': 'Gournay-en-Bray'},
'3349803':{'en': 'Toulon', 'fr': 'Toulon'},
'3349800':{'en': 'Toulon', 'fr': 'Toulon'},
'3349805':{'en': 'Saint-Maximin-la-Sainte-Baume', 'fr': 'Saint-Maximin-la-Sainte-Baume'},
'3359663':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3359662':{'en': u('Rivi\u00e8re-Pilote'), 'fr': u('Rivi\u00e8re-Pilote')},
'3359661':{'en': 'Schoelcher', 'fr': 'Schoelcher'},
'3359660':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3359667':{'en': 'Gros-Morne', 'fr': 'Gros-Morne'},
'4416975':{'en': 'Brampton'},
'3359665':{'en': 'Le Robert', 'fr': 'Le Robert'},
'3359664':{'en': 'Fort de France', 'fr': 'Fort de France'},
'4416978':{'en': 'Brampton'},
'4416979':{'en': 'Brampton'},
'3359669':{'en': 'Sainte Marie', 'fr': 'Sainte Marie'},
'3359668':{'en': u('Rivi\u00e8re-Sal\u00e9e'), 'fr': u('Rivi\u00e8re-Sal\u00e9e')},
'3593631':{'bg': u('\u041c\u043e\u043c\u0447\u0438\u043b\u0433\u0440\u0430\u0434'), 'en': 'Momchilgrad'},
'3593632':{'bg': u('\u0414\u0436\u0435\u0431\u0435\u043b'), 'en': 'Dzhebel'},
'3593633':{'bg': u('\u0420\u043e\u0433\u043e\u0437\u0447\u0435'), 'en': 'Rogozche'},
'3593634':{'bg': u('\u041f\u0440\u0438\u043f\u0435\u043a, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Pripek, Kardzh.'},
'436434':{'de': 'Bad Gastein', 'en': 'Bad Gastein'},
'3593636':{'bg': u('\u0420\u0430\u0432\u0435\u043d'), 'en': 'Raven'},
'3593637':{'bg': u('\u0413\u0440\u0443\u0435\u0432\u043e'), 'en': 'Gruevo'},
'3593638':{'bg': u('\u0417\u0432\u0435\u0437\u0434\u0435\u043b'), 'en': 'Zvezdel'},
'3593639':{'bg': u('\u041d\u0430\u043d\u043e\u0432\u0438\u0446\u0430, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Nanovitsa, Kardzh.'},
'3347774':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347775':{'en': 'Rive-de-Gier', 'fr': 'Rive-de-Gier'},
'3347996':{'en': u('Chamb\u00e9ry'), 'fr': u('Chamb\u00e9ry')},
'3593079':{'bg': u('\u041a\u043e\u0437\u0430\u0440\u043a\u0430'), 'en': 'Kozarka'},
'35941270':{'bg': u('\u041c\u0430\u043b\u043a\u0430 \u0412\u0435\u0440\u0435\u044f'), 'en': 'Malka Vereya'},
'35941277':{'bg': u('\u0417\u0430\u0433\u043e\u0440\u0435'), 'en': 'Zagore'},
'3355969':{'en': 'Orthez', 'fr': 'Orthez'},
'3329859':{'en': 'Rosporden', 'fr': 'Rosporden'},
'3329858':{'en': 'Penmarch', 'fr': 'Penmarch'},
'3355964':{'en': 'Tarnos', 'fr': 'Tarnos'},
'3355965':{'en': 'Saint-Palais', 'fr': 'Saint-Palais'},
'3355966':{'en': 'Navarrenx', 'fr': 'Navarrenx'},
'3355967':{'en': 'Orthez', 'fr': 'Orthez'},
'3355960':{'en': 'Mourenx', 'fr': 'Mourenx'},
'3355961':{'en': 'Nay', 'fr': 'Nay'},
'3355962':{'en': 'Lons', 'fr': 'Lons'},
'3355963':{'en': 'Anglet', 'fr': 'Anglet'},
'435446':{'de': 'Sankt Anton am Arlberg', 'en': 'St. Anton am Arlberg'},
'435444':{'de': 'Ischgl', 'en': 'Ischgl'},
'435445':{'de': 'Kappl', 'en': 'Kappl'},
'432573':{'de': 'Wilfersdorf', 'en': 'Wilfersdorf'},
'432572':{'de': 'Mistelbach', 'en': 'Mistelbach'},
'3593071':{'bg': u('\u0417\u043b\u0430\u0442\u043e\u0433\u0440\u0430\u0434'), 'en': 'Zlatograd'},
'432577':{'de': 'Asparn an der Zaya', 'en': 'Asparn an der Zaya'},
'432576':{'de': 'Ernstbrunn', 'en': 'Ernstbrunn'},
'432575':{'de': 'Ladendorf', 'en': 'Ladendorf'},
'432574':{'de': 'Gaweinstal', 'en': 'Gaweinstal'},
'3359087':{'en': u('Saint Barth\u00e9l\u00e9my'), 'fr': u('Saint Barth\u00e9l\u00e9my')},
'3359086':{'en': 'Capesterre Belle Eau', 'fr': 'Capesterre Belle Eau'},
'3359085':{'en': 'Sainte-Anne', 'fr': 'Sainte-Anne'},
'3359084':{'en': 'Le Gosier', 'fr': 'Le Gosier'},
'3359083':{'en': u('Pointe-\u00e0-Pitre'), 'fr': u('Pointe-\u00e0-Pitre')},
'3359082':{'en': u('Pointe-\u00e0-Pitre'), 'fr': u('Pointe-\u00e0-Pitre')},
'3359081':{'en': 'Basse Terre', 'fr': 'Basse Terre'},
'3359080':{'en': 'Saint-Claude', 'fr': 'Saint-Claude'},
'435441':{'de': 'See', 'en': 'See'},
'3359089':{'en': 'Les Abymes', 'fr': 'Les Abymes'},
'3359088':{'en': 'Sainte-Anne', 'fr': 'Sainte-Anne'},
'432536':{'de': u('Dr\u00f6sing'), 'en': u('Dr\u00f6sing')},
'435448':{'de': 'Pettneu am Arlberg', 'en': 'Pettneu am Arlberg'},
'3594369':{'bg': u('\u0410\u043b\u0435\u043a\u0441\u0430\u043d\u0434\u0440\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Aleksandrovo, St. Zagora'},
'3594368':{'bg': u('\u0422\u044a\u0440\u043d\u0438\u0447\u0435\u043d\u0438'), 'en': 'Tarnicheni'},
'3594367':{'bg': u('\u0422\u044a\u0436\u0430'), 'en': 'Tazha'},
'3594364':{'bg': u('\u041e\u0441\u0435\u0442\u0435\u043d\u043e\u0432\u043e'), 'en': 'Osetenovo'},
'3594363':{'bg': u('\u0413\u0430\u0431\u0430\u0440\u0435\u0432\u043e'), 'en': 'Gabarevo'},
'3594362':{'bg': u('\u041c\u0430\u043d\u043e\u043b\u043e\u0432\u043e'), 'en': 'Manolovo'},
'3594361':{'bg': u('\u041f\u0430\u0432\u0435\u043b \u0431\u0430\u043d\u044f'), 'en': 'Pavel banya'},
'3595368':{'bg': u('\u0422\u044a\u043a\u0430\u0447'), 'en': 'Takach'},
'441439':{'en': 'Helmsley'},
'3595365':{'bg': u('\u041b\u044f\u0442\u043d\u043e'), 'en': 'Lyatno'},
'3595366':{'bg': u('\u0411\u0440\u0430\u043d\u0438\u0447\u0435\u0432\u043e'), 'en': 'Branichevo'},
'3595367':{'bg': u('\u0422\u043e\u0434\u043e\u0440 \u0418\u043a\u043e\u043d\u043e\u043c\u043e\u0432\u043e'), 'en': 'Todor Ikonomovo'},
'3595361':{'bg': u('\u041a\u0430\u043e\u043b\u0438\u043d\u043e\u0432\u043e'), 'en': 'Kaolinovo'},
'3595362':{'bg': u('\u041a\u043b\u0438\u043c\u0435\u043d\u0442, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Kliment, Shumen'},
'3595363':{'bg': u('\u0413\u0443\u0441\u043b\u0430'), 'en': 'Gusla'},
'3752345':{'be': u('\u041a\u0430\u043b\u0456\u043d\u043a\u0430\u0432\u0456\u0447\u044b'), 'en': 'Kalinkovichi', 'ru': u('\u041a\u0430\u043b\u0438\u043d\u043a\u043e\u0432\u0438\u0447\u0438')},
'3338853':{'en': 'Drusenheim', 'fr': 'Drusenheim'},
'3752346':{'be': u('\u0425\u043e\u0439\u043d\u0456\u043a\u0456, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Khoiniki, Gomel Region', 'ru': u('\u0425\u043e\u0439\u043d\u0438\u043a\u0438, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752340':{'be': u('\u0420\u044d\u0447\u044b\u0446\u0430'), 'en': 'Rechitsa', 'ru': u('\u0420\u0435\u0447\u0438\u0446\u0430')},
'3752342':{'be': u('\u0421\u0432\u0435\u0442\u043b\u0430\u0433\u043e\u0440\u0441\u043a'), 'en': 'Svetlogorsk', 'ru': u('\u0421\u0432\u0435\u0442\u043b\u043e\u0433\u043e\u0440\u0441\u043a')},
'441435':{'en': 'Heathfield'},
'3334464':{'en': 'Creil', 'fr': 'Creil'},
'3598674':{'bg': u('\u041f\u0440\u043e\u0444\u0435\u0441\u043e\u0440 \u0418\u0448\u0438\u0440\u043a\u043e\u0432\u043e'), 'en': 'Profesor Ishirkovo'},
'3334466':{'en': 'Nogent-sur-Oise', 'fr': 'Nogent-sur-Oise'},
'3598676':{'bg': u('\u0412\u0435\u0442\u0440\u0435\u043d, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Vetren, Silistra'},
'3334460':{'en': 'Senlis', 'fr': 'Senlis'},
'3334462':{'en': 'Chantilly', 'fr': 'Chantilly'},
'3334463':{'en': 'Senlis', 'fr': 'Senlis'},
'3595100':{'bg': u('\u0421\u0438\u043d\u0434\u0435\u043b'), 'en': 'Sindel'},
'3595101':{'bg': u('\u0414\u044a\u0431\u0440\u0430\u0432\u0438\u043d\u043e'), 'en': 'Dabravino'},
'3595102':{'bg': u('\u041f\u0430\u0434\u0438\u043d\u0430, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Padina, Varna'},
'3598679':{'bg': u('\u041a\u0430\u0439\u043d\u0430\u0440\u0434\u0436\u0430'), 'en': 'Kaynardzha'},
'3595105':{'bg': u('\u041f\u0440\u0438\u0441\u0435\u043b\u0446\u0438, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Priseltsi, Varna'},
'3595106':{'bg': u('\u0410\u0432\u0440\u0435\u043d, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Avren, Varna'},
'3338338':{'en': 'Champigneulles', 'fr': 'Champigneulles'},
'3338339':{'en': 'Nancy', 'fr': 'Nancy'},
'3338335':{'en': 'Nancy', 'fr': 'Nancy'},
'3338336':{'en': 'Nancy', 'fr': 'Nancy'},
'3338337':{'en': 'Nancy', 'fr': 'Nancy'},
'3338330':{'en': 'Nancy', 'fr': 'Nancy'},
'3338332':{'en': 'Nancy', 'fr': 'Nancy'},
'3338333':{'en': 'Nancy', 'fr': 'Nancy'},
'3355694':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'434213':{'de': 'Launsdorf', 'en': 'Launsdorf'},
'432766':{'de': 'Kleinzell', 'en': 'Kleinzell'},
'353504':{'en': 'Thurles'},
'353505':{'en': 'Roscrea'},
'432767':{'de': 'Hohenberg', 'en': 'Hohenberg'},
'390721':{'it': 'Pesaro'},
'432764':{'de': 'Hainfeld', 'en': 'Hainfeld'},
'432765':{'de': 'Kaumberg', 'en': 'Kaumberg'},
'35391':{'en': 'Galway/Gort/Loughrea'},
'432812':{'de': u('Gro\u00df Gerungs'), 'en': 'Gross Gerungs'},
'3356538':{'en': u('Saint-C\u00e9r\u00e9'), 'fr': u('Saint-C\u00e9r\u00e9')},
'3356539':{'en': 'Bretenoux', 'fr': 'Bretenoux'},
'3356534':{'en': 'Figeac', 'fr': 'Figeac'},
'3356535':{'en': 'Cahors', 'fr': 'Cahors'},
'3356530':{'en': 'Cahors', 'fr': 'Cahors'},
'35390':{'en': 'Athlone/Ballinasloe/Portumna/Roscommon'},
'3347259':{'en': 'Tassin-la-Demi-Lune', 'fr': 'Tassin-la-Demi-Lune'},
'3347529':{'en': 'Le Cheylard', 'fr': 'Le Cheylard'},
'3347528':{'en': 'Buis-les-Baronnies', 'fr': 'Buis-les-Baronnies'},
'3347526':{'en': 'Nyons', 'fr': 'Nyons'},
'3347525':{'en': 'Crest', 'fr': 'Crest'},
'3347256':{'en': 'Lyon', 'fr': 'Lyon'},
'3347523':{'en': 'Saint-Vallier', 'fr': 'Saint-Vallier'},
'3347522':{'en': 'Die', 'fr': 'Die'},
'3347253':{'en': 'Lyon', 'fr': 'Lyon'},
'3347252':{'en': 'Dardilly', 'fr': 'Dardilly'},
'35393':{'en': 'Tuam'},
'4414239':{'en': 'Boroughbridge'},
'4414238':{'en': 'Harrogate'},
'4414235':{'en': 'Harrogate'},
'4414234':{'en': 'Boroughbridge'},
'4414237':{'en': 'Harrogate'},
'4414236':{'en': 'Harrogate'},
'4414231':{'en': 'Harrogate/Boroughbridge'},
'4414230':{'en': 'Harrogate/Boroughbridge'},
'4414233':{'en': 'Boroughbridge'},
'4414232':{'en': 'Harrogate'},
'435553':{'de': 'Raggal', 'en': 'Raggal'},
'441433':{'en': 'Hathersage'},
'3323724':{'en': 'Illiers-Combray', 'fr': 'Illiers-Combray'},
'35395':{'en': 'Clifden'},
'332282':{'en': 'Nantes', 'fr': 'Nantes'},
'35394':{'en': 'Castlebar/Claremorris/Castlerea/Ballinrobe'},
'37426374':{'am': u('\u0531\u0575\u0563\u0565\u0570\u0578\u057e\u056b\u057f'), 'en': 'Aygehovit', 'ru': u('\u0410\u0439\u0433\u0435\u0445\u043e\u0432\u0438\u0442')},
'42049':{'en': u('Hradec Kr\u00e1lov\u00e9 Region')},
'42048':{'en': 'Liberec Region'},
'35397':{'en': 'Belmullet'},
'42041':{'en': u('\u00dast\u00ed nad Labem Region')},
'42047':{'en': u('\u00dast\u00ed nad Labem Region')},
'42046':{'en': 'Pardubice Region'},
'390547':{'it': 'Cesena'},
'390546':{'it': 'Faenza'},
'390545':{'en': 'Ravenna', 'it': 'Lugo'},
'390544':{'it': 'Ravenna'},
'390543':{'en': u('Forl\u00ec-Cesena'), 'it': u('Forl\u00ec')},
'390542':{'it': 'Imola'},
'390541':{'en': 'Rimini', 'it': 'Rimini'},
'390549':{'en': 'San Marino', 'it': 'Repubblica di San Marino'},
'3349169':{'en': 'Marseille', 'fr': 'Marseille'},
'3349168':{'en': 'Allauch', 'fr': 'Allauch'},
'3349161':{'en': 'Marseille', 'fr': 'Marseille'},
'3349160':{'en': 'Marseille', 'fr': 'Marseille'},
'3349163':{'en': 'Marseille', 'fr': 'Marseille'},
'3349162':{'en': 'Marseille', 'fr': 'Marseille'},
'3349165':{'en': 'Marseille', 'fr': 'Marseille'},
'3349164':{'en': 'Marseille', 'fr': 'Marseille'},
'3349167':{'en': 'Marseille', 'fr': 'Marseille'},
'3349166':{'en': 'Marseille', 'fr': 'Marseille'},
'3522499':{'de': 'Ulflingen', 'en': 'Troisvierges', 'fr': 'Troisvierges'},
'3522492':{'de': 'Kanton Clerf/Fischbach/Hosingen', 'en': 'Clervaux/Fischbach/Hosingen', 'fr': 'Clervaux/Fischbach/Hosingen'},
'3522495':{'de': 'Wiltz', 'en': 'Wiltz', 'fr': 'Wiltz'},
'3522497':{'de': 'Huldingen', 'en': 'Huldange', 'fr': 'Huldange'},
'3316055':{'en': 'Nemours', 'fr': 'Nemours'},
'3316054':{'en': u('Dammartin-en-Go\u00eble'), 'fr': u('Dammartin-en-Go\u00eble')},
'3316057':{'en': 'Montereau-Fault-Yonne', 'fr': 'Montereau-Fault-Yonne'},
'3316056':{'en': 'Melun', 'fr': 'Melun'},
'3316059':{'en': 'Melun', 'fr': 'Melun'},
'3316058':{'en': 'Provins', 'fr': 'Provins'},
'3349345':{'en': 'Le Cannet', 'fr': 'Le Cannet'},
'3349344':{'en': 'Nice', 'fr': 'Nice'},
'3349347':{'en': 'Cannes', 'fr': 'Cannes'},
'3349346':{'en': 'Le Cannet', 'fr': 'Le Cannet'},
'3349341':{'en': 'Menton', 'fr': 'Menton'},
'3349340':{'en': 'Grasse', 'fr': 'Grasse'},
'3349343':{'en': 'Cannes', 'fr': 'Cannes'},
'3349349':{'en': 'Mandelieu-la-Napoule', 'fr': 'Mandelieu-la-Napoule'},
'3349348':{'en': 'Cannes', 'fr': 'Cannes'},
'3522634':{'de': 'Rammeldingen/Senningerberg', 'en': 'Rameldange/Senningerberg', 'fr': 'Rameldange/Senningerberg'},
'3522635':{'de': 'Sandweiler/Mutfort/Roodt-sur-Syre', 'en': 'Sandweiler/Moutfort/Roodt-sur-Syre', 'fr': 'Sandweiler/Moutfort/Roodt-sur-Syre'},
'3522636':{'de': 'Hesperingen/Kockelscheuer/Roeser', 'en': 'Hesperange/Kockelscheuer/Roeser', 'fr': 'Hesperange/Kockelscheuer/Roeser'},
'3522637':{'de': 'Leudelingen/Ehlingen/Monnerich', 'en': 'Leudelange/Ehlange/Mondercange', 'fr': 'Leudelange/Ehlange/Mondercange'},
'3522630':{'de': 'Kanton Capellen/Kehlen', 'en': 'Capellen/Kehlen', 'fr': 'Capellen/Kehlen'},
'3522631':{'de': 'Bartringen', 'en': 'Bertrange/Mamer/Munsbach/Strassen', 'fr': 'Bertrange/Mamer/Munsbach/Strassen'},
'3522632':{'de': 'Lintgen/Kanton Mersch/Steinfort', 'en': 'Lintgen/Mersch/Steinfort', 'fr': 'Lintgen/Mersch/Steinfort'},
'3522633':{'de': 'Walferdingen', 'en': 'Walferdange', 'fr': 'Walferdange'},
'3522639':{'de': 'Windhof/Steinfort', 'en': 'Windhof/Steinfort', 'fr': 'Windhof/Steinfort'},
'35984738':{'bg': u('\u041a\u0438\u0442\u0430\u043d\u0447\u0435\u0432\u043e'), 'en': 'Kitanchevo'},
'37037':{'en': 'Kaunas'},
'436138':{'de': 'Sankt Wolfgang im Salzkammergut', 'en': 'St. Wolfgang im Salzkammergut'},
'436137':{'de': 'Strobl', 'en': 'Strobl'},
'436136':{'de': 'Gosau', 'en': 'Gosau'},
'436135':{'de': 'Bad Goisern', 'en': 'Bad Goisern'},
'436134':{'de': 'Hallstatt', 'en': 'Hallstatt'},
'436133':{'de': 'Ebensee', 'en': 'Ebensee'},
'436132':{'de': 'Bad Ischl', 'en': 'Bad Ischl'},
'436131':{'de': 'Obertraun', 'en': 'Obertraun'},
'390965':{'en': 'Reggio Calabria', 'it': 'Reggio di Calabria'},
'3338490':{'en': 'Belfort', 'fr': 'Belfort'},
'3338493':{'en': 'Luxeuil-les-Bains', 'fr': 'Luxeuil-les-Bains'},
'3338495':{'en': 'Saulx', 'fr': 'Saulx'},
'3338497':{'en': 'Vesoul', 'fr': 'Vesoul'},
'3338496':{'en': 'Vesoul', 'fr': 'Vesoul'},
'3338946':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3338945':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3338944':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3338943':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3338942':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3338941':{'en': 'Colmar', 'fr': 'Colmar'},
'3338940':{'en': 'Altkirch', 'fr': 'Altkirch'},
'3332180':{'en': 'Boulogne-sur-Mer', 'fr': 'Boulogne-sur-Mer'},
'35984736':{'bg': u('\u041f\u043e\u0434\u0430\u0439\u0432\u0430'), 'en': 'Podayva'},
'3332185':{'en': 'Calais', 'fr': 'Calais'},
'3332187':{'en': 'Boulogne-sur-Mer', 'fr': 'Boulogne-sur-Mer'},
'3332636':{'en': 'Reims', 'fr': 'Reims'},
'3332635':{'en': 'Reims', 'fr': 'Reims'},
'3332632':{'en': u('\u00c9pernay'), 'fr': u('\u00c9pernay')},
'3594770':{'bg': u('\u0413\u0435\u043d\u0435\u0440\u0430\u043b \u0418\u043d\u0437\u043e\u0432\u043e'), 'en': 'General Inzovo'},
'3594771':{'bg': u('\u041c\u0430\u043b\u043e\u043c\u0438\u0440, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Malomir, Yambol'},
'3594772':{'bg': u('\u0421\u0438\u043c\u0435\u043e\u043d\u043e\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Simeonovo, Yambol'},
'3594773':{'bg': u('\u041e\u043a\u043e\u043f'), 'en': 'Okop'},
'3594774':{'bg': u('\u041a\u0440\u0443\u043c\u043e\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Krumovo, Yambol'},
'3594775':{'bg': u('\u041a\u0430\u0440\u0430\u0432\u0435\u043b\u043e\u0432\u043e, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Karavelovo, Yambol'},
'3354792':{'en': 'Pau', 'fr': 'Pau'},
'3594777':{'bg': u('\u0422\u0435\u043d\u0435\u0432\u043e'), 'en': 'Tenevo'},
'3594778':{'bg': u('\u041f\u043e\u0431\u0435\u0434\u0430, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Pobeda, Yambol'},
'3594779':{'bg': u('\u0425\u0430\u043d\u043e\u0432\u043e'), 'en': 'Hanovo'},
'4418513':{'en': 'Stornoway'},
'432143':{'de': 'Kittsee', 'en': 'Kittsee'},
'3348695':{'en': 'Marseille', 'fr': 'Marseille'},
'3355558':{'en': 'Nexon', 'fr': 'Nexon'},
'3355556':{'en': u('Saint-L\u00e9onard-de-Noblat'), 'fr': u('Saint-L\u00e9onard-de-Noblat')},
'3355551':{'en': u('Gu\u00e9ret'), 'fr': u('Gu\u00e9ret')},
'3355550':{'en': 'Limoges', 'fr': 'Limoges'},
'3355553':{'en': 'Nantiat', 'fr': 'Nantiat'},
'3355552':{'en': u('Gu\u00e9ret'), 'fr': u('Gu\u00e9ret')},
'432630':{'de': 'Ternitz', 'en': 'Ternitz'},
'432631':{'de': u('P\u00f6ttsching'), 'en': u('P\u00f6ttsching')},
'3332459':{'en': u('Charleville-M\u00e9zi\u00e8res'), 'fr': u('Charleville-M\u00e9zi\u00e8res')},
'3332458':{'en': u('Charleville-M\u00e9zi\u00e8res'), 'fr': u('Charleville-M\u00e9zi\u00e8res')},
'432634':{'de': 'Gutenstein', 'en': 'Gutenstein'},
'432635':{'de': 'Neunkirchen', 'en': 'Neunkirchen'},
'432636':{'de': 'Puchberg am Schneeberg', 'en': 'Puchberg am Schneeberg'},
'432637':{'de': u('Gr\u00fcnbach am Schneeberg'), 'en': u('Gr\u00fcnbach am Schneeberg')},
'3332981':{'en': u('\u00c9pinal'), 'fr': u('\u00c9pinal')},
'432639':{'de': 'Bad Fischau', 'en': 'Bad Fischau'},
'3332983':{'en': 'Verdun', 'fr': 'Verdun'},
'3332982':{'en': u('\u00c9pinal'), 'fr': u('\u00c9pinal')},
'3332457':{'en': u('Charleville-M\u00e9zi\u00e8res'), 'fr': u('Charleville-M\u00e9zi\u00e8res')},
'3332984':{'en': 'Verdun', 'fr': 'Verdun'},
'3332455':{'en': u('Charleville-M\u00e9zi\u00e8res'), 'fr': u('Charleville-M\u00e9zi\u00e8res')},
'3332986':{'en': 'Verdun', 'fr': 'Verdun'},
'3348345':{'en': 'Nice', 'fr': 'Nice'},
'3349565':{'en': 'Calvi', 'fr': 'Calvi'},
'3354979':{'en': 'Niort', 'fr': 'Niort'},
'390141':{'en': 'Asti', 'it': 'Asti'},
'3593177':{'bg': u('\u041d\u043e\u0432\u043e \u0416\u0435\u043b\u0435\u0437\u0430\u0440\u0435'), 'en': 'Novo Zhelezare'},
'3593176':{'bg': u('\u0421\u0442\u0430\u0440\u043e\u0441\u0435\u043b'), 'en': 'Starosel'},
'3593175':{'bg': u('\u0414\u044a\u043b\u0433\u043e \u043f\u043e\u043b\u0435, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Dalgo pole, Plovdiv'},
'3593174':{'bg': u('\u0421\u0442\u0430\u0440\u043e \u0416\u0435\u043b\u0435\u0437\u0430\u0440\u0435'), 'en': 'Staro Zhelezare'},
'3593173':{'bg': u('\u041f\u0430\u043d\u0438\u0447\u0435\u0440\u0438'), 'en': 'Panicheri'},
'3349561':{'en': 'Corte', 'fr': 'Corte'},
'37428396':{'am': u('\u0531\u0576\u0563\u0565\u0572\u0561\u056f\u0578\u0569'), 'en': 'Angehakot', 'ru': u('\u0410\u043d\u0433\u0435\u0445\u0430\u043a\u043e\u0442')},
'3593178':{'bg': u('\u041a\u0440\u0430\u0441\u043d\u043e\u0432\u043e'), 'en': 'Krasnovo'},
'3345023':{'en': 'Annecy-le-Vieux', 'fr': 'Annecy-le-Vieux'},
'3325138':{'en': 'Mouilleron-le-Captif', 'fr': 'Mouilleron-le-Captif'},
'3325139':{'en': u('Noirmoutier-en-l\'\u00cele'), 'fr': u('Noirmoutier-en-l\'\u00cele')},
'3325130':{'en': 'La Tranche sur Mer', 'fr': 'La Tranche sur Mer'},
'3325132':{'en': 'Les Sables d\'Olonne', 'fr': 'Les Sables d\'Olonne'},
'3325133':{'en': 'Jard-sur-Mer', 'fr': 'Jard-sur-Mer'},
'3325135':{'en': 'Challans', 'fr': 'Challans'},
'3325136':{'en': 'La Roche sur Yon', 'fr': 'La Roche sur Yon'},
'3325137':{'en': 'La Roche sur Yon', 'fr': 'La Roche sur Yon'},
'351238':{'en': 'Seia', 'pt': 'Seia'},
'351239':{'en': 'Coimbra', 'pt': 'Coimbra'},
'441844':{'en': 'Thame'},
'351232':{'en': 'Viseu', 'pt': 'Viseu'},
'351233':{'en': 'Figueira da Foz', 'pt': 'Figueira da Foz'},
'351231':{'en': 'Mealhada', 'pt': 'Mealhada'},
'351236':{'en': 'Pombal', 'pt': 'Pombal'},
'351234':{'en': 'Aveiro', 'pt': 'Aveiro'},
'351235':{'en': 'Arganil', 'pt': 'Arganil'},
'3355690':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3356234':{'en': 'Tarbes', 'fr': 'Tarbes'},
'3356237':{'en': 'Tarbes', 'fr': 'Tarbes'},
'3356236':{'en': 'Tarbes', 'fr': 'Tarbes'},
'3356230':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356232':{'en': 'Juillan', 'fr': 'Juillan'},
'3356238':{'en': 'Tarbes', 'fr': 'Tarbes'},
'3355691':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'441603':{'en': 'Norwich'},
'441600':{'en': 'Monmouth'},
'441606':{'en': 'Northwich'},
'441604':{'en': 'Northampton'},
'441609':{'en': 'Northallerton'},
'441608':{'en': 'Chipping Norton'},
'3347586':{'en': 'Valence', 'fr': 'Valence'},
'3344224':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344227':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344226':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344221':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344220':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344223':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3344222':{'en': 'Bouc-Bel-air', 'fr': 'Bouc-Bel-air'},
'3596732':{'bg': u('\u0421\u0435\u043d\u043d\u0438\u043a'), 'en': 'Sennik'},
'3596733':{'bg': u('\u041a\u043e\u0440\u043c\u044f\u043d\u0441\u043a\u043e'), 'en': 'Kormyansko'},
'3344229':{'en': 'Trets', 'fr': 'Trets'},
'3344228':{'en': 'Ventabren', 'fr': 'Ventabren'},
'3596734':{'bg': u('\u041f\u0435\u0442\u043a\u043e \u0421\u043b\u0430\u0432\u0435\u0439\u043a\u043e\u0432'), 'en': 'Petko Slaveykov'},
'3347754':{'en': 'Chazelles-sur-Lyon', 'fr': 'Chazelles-sur-Lyon'},
'3347755':{'en': u('Andr\u00e9zieux-Bouth\u00e9on'), 'fr': u('Andr\u00e9zieux-Bouth\u00e9on')},
'3347756':{'en': 'Firminy', 'fr': 'Firminy'},
'3347757':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3336981':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3347751':{'en': 'Saint-Genest-Malifaux', 'fr': 'Saint-Genest-Malifaux'},
'3347752':{'en': 'Saint-Just-Saint-Rambert', 'fr': 'Saint-Just-Saint-Rambert'},
'3347753':{'en': 'Sorbiers', 'fr': 'Sorbiers'},
'3343260':{'en': u('Saint-R\u00e9my-de-Provence'), 'fr': u('Saint-R\u00e9my-de-Provence')},
'3347758':{'en': 'Montbrison', 'fr': 'Montbrison'},
'3347759':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3343411':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3343410':{'en': 'Perpignan', 'fr': 'Perpignan'},
'436458':{'de': u('H\u00fcttau'), 'en': u('H\u00fcttau')},
'3343262':{'en': u('Ch\u00e2teaurenard'), 'fr': u('Ch\u00e2teaurenard')},
'436452':{'de': 'Radstadt', 'en': 'Radstadt'},
'436453':{'de': 'Filzmoos', 'en': 'Filzmoos'},
'436454':{'de': 'Mandling', 'en': 'Mandling'},
'436455':{'de': 'Untertauern', 'en': 'Untertauern'},
'436456':{'de': 'Obertauern', 'en': 'Obertauern'},
'436457':{'de': 'Flachau', 'en': 'Flachau'},
'3338161':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338162':{'en': 'Ornans', 'fr': 'Ornans'},
'3316918':{'en': 'Les Ulis', 'fr': 'Les Ulis'},
'3338164':{'en': u('Ma\u00eeche'), 'fr': u('Ma\u00eeche')},
'3338167':{'en': 'Morteau', 'fr': 'Morteau'},
'3596591':{'bg': u('\u0413\u043e\u0440\u043d\u0438\u043a'), 'en': 'Gornik'},
'3316914':{'en': 'Marolles-en-Hurepoix', 'fr': 'Marolles-en-Hurepoix'},
'3338168':{'en': 'Villers-le-Lac', 'fr': 'Villers-le-Lac'},
'3316910':{'en': 'Chilly-Mazarin', 'fr': 'Chilly-Mazarin'},
'3316911':{'en': 'Lisses', 'fr': 'Lisses'},
'3316912':{'en': u('Viry-Ch\u00e2tillon'), 'fr': u('Viry-Ch\u00e2tillon')},
'35984717':{'bg': u('\u041f\u043e\u0431\u0438\u0442 \u043a\u0430\u043c\u044a\u043a, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Pobit kamak, Razgrad'},
'35984713':{'bg': u('\u0411\u043b\u0430\u0433\u043e\u0435\u0432\u043e, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Blagoevo, Razgrad'},
'35984712':{'bg': u('\u041a\u043e\u0441\u0442\u0430\u043d\u0434\u0435\u043d\u0435\u0446'), 'en': 'Kostandenets'},
'35984711':{'bg': u('\u041c\u043e\u0440\u0442\u0430\u0433\u043e\u043d\u043e\u0432\u043e'), 'en': 'Mortagonovo'},
'35984710':{'bg': u('\u041e\u0441\u0435\u043d\u0435\u0446'), 'en': 'Osenets'},
'35984719':{'bg': u('\u0411\u0430\u043b\u043a\u0430\u043d\u0441\u043a\u0438'), 'en': 'Balkanski'},
'35984718':{'bg': u('\u0414\u0440\u044f\u043d\u043e\u0432\u0435\u0446, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Dryanovets, Razgrad'},
'3329721':{'en': 'Lorient', 'fr': 'Lorient'},
'3329723':{'en': 'Gourin', 'fr': 'Gourin'},
'3329722':{'en': 'Guer', 'fr': 'Guer'},
'3329725':{'en': 'Pontivy', 'fr': 'Pontivy'},
'3329724':{'en': 'Auray', 'fr': 'Auray'},
'3329727':{'en': 'Pontivy', 'fr': 'Pontivy'},
'3329726':{'en': 'Questembert', 'fr': 'Questembert'},
'3329729':{'en': 'Auray', 'fr': 'Auray'},
'3329728':{'en': u('S\u00e9glien'), 'fr': u('S\u00e9glien')},
'3338551':{'en': 'Tournus', 'fr': 'Tournus'},
'3338552':{'en': 'Autun', 'fr': 'Autun'},
'3338553':{'en': 'Digoin', 'fr': 'Digoin'},
'3338555':{'en': 'Le Creusot', 'fr': 'Le Creusot'},
'3338556':{'en': 'Le Creusot', 'fr': 'Le Creusot'},
'3338557':{'en': 'Montceau-les-Mines', 'fr': 'Montceau-les-Mines'},
'3338558':{'en': 'Montceau-les-Mines', 'fr': 'Montceau-les-Mines'},
'3338559':{'en': 'Cluny', 'fr': 'Cluny'},
'3335728':{'en': 'Metz', 'fr': 'Metz'},
'3332651':{'en': u('\u00c9pernay'), 'fr': u('\u00c9pernay')},
'3346721':{'en': 'Agde', 'fr': 'Agde'},
'3692':{'en': 'Zalaegerszeg', 'hu': 'Zalaegerszeg'},
'3359648':{'en': u('Rivi\u00e8re-Sal\u00e9e'), 'fr': u('Rivi\u00e8re-Sal\u00e9e')},
'3359642':{'en': 'Fort de France', 'fr': 'Fort de France'},
'3593348':{'bg': u('\u0414\u043e\u0431\u0440\u0430\u043b\u044a\u043a'), 'en': 'Dobralak'},
'3593349':{'bg': u('\u0411\u043e\u044f\u043d\u0446\u0438'), 'en': 'Boyantsi'},
'3593344':{'bg': u('\u041d\u043e\u0432\u0430\u043a\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Novakovo, Plovdiv'},
'3593345':{'bg': u('\u041b\u044f\u0441\u043a\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Lyaskovo, Plovdiv'},
'3593346':{'bg': u('\u041c\u0443\u043b\u0434\u0430\u0432\u0430'), 'en': 'Muldava'},
'3593347':{'bg': u('\u041b\u0435\u043d\u043e\u0432\u043e'), 'en': 'Lenovo'},
'3593340':{'bg': u('\u041d\u043e\u0432\u0438 \u0438\u0437\u0432\u043e\u0440'), 'en': 'Novi izvor'},
'3593341':{'bg': u('\u041a\u043e\u043d\u0443\u0448, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Konush, Plovdiv'},
'3593342':{'bg': u('\u041d\u0430\u0440\u0435\u0447\u0435\u043d\u0441\u043a\u0438 \u0431\u0430\u043d\u0438'), 'en': 'Narechenski bani'},
'3593343':{'bg': u('\u041a\u043e\u0437\u0430\u043d\u043e\u0432\u043e'), 'en': 'Kozanovo'},
'3355946':{'en': 'Bayonne', 'fr': 'Bayonne'},
'3355947':{'en': 'Ciboure', 'fr': 'Ciboure'},
'3355944':{'en': 'Saint-Pierre-d\'Irube', 'fr': 'Saint-Pierre-d\'Irube'},
'3355945':{'en': 'Labenne', 'fr': 'Labenne'},
'3355942':{'en': 'Anglet', 'fr': 'Anglet'},
'3355943':{'en': 'Biarritz', 'fr': 'Biarritz'},
'3355940':{'en': 'Pau', 'fr': 'Pau'},
'3355941':{'en': 'Biarritz', 'fr': 'Biarritz'},
'3355948':{'en': 'Hendaye', 'fr': 'Hendaye'},
'34972':{'en': 'Gerona', 'es': 'Gerona'},
'3329878':{'en': 'Pleyber-Christ', 'fr': 'Pleyber-Christ'},
'34971':{'en': 'Balearic Islands', 'es': 'Baleares'},
'34976':{'en': 'Zaragoza', 'es': 'Zaragoza'},
'34977':{'en': 'Tarragona', 'es': 'Tarragona'},
'34974':{'en': 'Huesca', 'es': 'Huesca'},
'34975':{'en': 'Soria', 'es': 'Soria'},
'3329871':{'en': u('Clohars-Carno\u00ebt'), 'fr': u('Clohars-Carno\u00ebt')},
'3329870':{'en': 'Audierne', 'fr': 'Audierne'},
'34978':{'en': 'Teruel', 'es': 'Teruel'},
'3329872':{'en': 'Guerlesquin', 'fr': 'Guerlesquin'},
'3329875':{'en': 'Douarnenez', 'fr': 'Douarnenez'},
'3329874':{'en': 'Douarnenez', 'fr': 'Douarnenez'},
'3695':{'en': 'Sarvar', 'hu': u('S\u00e1rv\u00e1r')},
'441386':{'en': 'Evesham'},
'441384':{'en': 'Dudley'},
'441382':{'en': 'Dundee'},
'441383':{'en': 'Dunfermline'},
'441380':{'en': 'Devizes'},
'441381':{'en': 'Fortrose'},
'441389':{'en': 'Dumbarton'},
'3696':{'en': 'Gyor', 'hu': u('Gy\u0151r')},
'3597717':{'bg': u('\u0414\u0438\u0432\u043e\u0442\u0438\u043d\u043e'), 'en': 'Divotino'},
'3329990':{'en': 'Nivillac', 'fr': 'Nivillac'},
'3352498':{'en': 'Pau', 'fr': 'Pau'},
'3594348':{'bg': u('\u0414\u0443\u043d\u0430\u0432\u0446\u0438, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Dunavtsi, St. Zagora'},
'3594341':{'bg': u('\u0427\u0435\u0440\u0433\u0430\u043d\u043e\u0432\u043e'), 'en': 'Cherganovo'},
'3594340':{'bg': u('\u041f\u0430\u043d\u0438\u0447\u0435\u0440\u0435\u0432\u043e'), 'en': 'Panicherevo'},
'3594343':{'bg': u('\u041a\u043e\u043d\u0430\u0440\u0435, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Konare, St. Zagora'},
'3594342':{'bg': u('\u041e\u0432\u043e\u0449\u043d\u0438\u043a'), 'en': 'Ovoshtnik'},
'3594345':{'bg': u('\u0420\u0430\u0434\u0443\u043d\u0446\u0438'), 'en': 'Raduntsi'},
'3594344':{'bg': u('\u0428\u0430\u043d\u043e\u0432\u043e'), 'en': 'Shanovo'},
'3594347':{'bg': u('\u0420\u043e\u0437\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Rozovo, St. Zagora'},
'3594346':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u043e \u0414\u0440\u044f\u043d\u043e\u0432\u043e'), 'en': 'Golyamo Dryanovo'},
'3596560':{'bg': u('\u041a\u0440\u0435\u0442\u0430, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Kreta, Pleven'},
'3598477':{'bg': u('\u0421\u0430\u043c\u0443\u0438\u043b'), 'en': 'Samuil'},
'3598475':{'bg': u('\u041b\u043e\u0437\u043d\u0438\u0446\u0430, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Loznitsa, Razgrad'},
'3742359':{'am': u('\u0546\u0578\u0580\u0561\u0577\u0565\u0576'), 'en': 'Norashen', 'ru': u('\u041d\u043e\u0440\u0430\u0448\u0435\u043d')},
'3332844':{'en': 'Hazebrouck', 'fr': 'Hazebrouck'},
'3332841':{'en': 'Hazebrouck', 'fr': 'Hazebrouck'},
'3332849':{'en': 'Bailleul', 'fr': 'Bailleul'},
'3597714':{'bg': u('\u041c\u0435\u0449\u0438\u0446\u0430'), 'en': 'Meshtitsa'},
'3594525':{'bg': u('\u041e\u043c\u0430\u0440\u0447\u0435\u0432\u043e, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Omarchevo, Sliven'},
'3594524':{'bg': u('\u041a\u0430\u043c\u0435\u043d\u043e\u0432\u043e, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Kamenovo, Sliven'},
'3594527':{'bg': u('\u0417\u0430\u0433\u043e\u0440\u0446\u0438, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Zagortsi, Sliven'},
'3594526':{'bg': u('\u041c\u043b\u0435\u043a\u0430\u0440\u0435\u0432\u043e'), 'en': 'Mlekarevo'},
'3594520':{'bg': u('\u041a\u043e\u043d\u044c\u043e\u0432\u043e'), 'en': 'Konyovo'},
'3594523':{'bg': u('\u0421\u0442\u043e\u0438\u043b \u0432\u043e\u0439\u0432\u043e\u0434\u0430'), 'en': 'Stoil voyvoda'},
'3594522':{'bg': u('\u041a\u043e\u0440\u0442\u0435\u043d'), 'en': 'Korten'},
'3594529':{'bg': u('\u0421\u044a\u0434\u0438\u0435\u0432\u043e, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Sadievo, Sliven'},
'3594528':{'bg': u('\u041b\u044e\u0431\u0435\u043d\u043e\u0432\u0430 \u043c\u0430\u0445\u0430\u043b\u0430'), 'en': 'Lyubenova mahala'},
'3324186':{'en': 'Angers', 'fr': 'Angers'},
'3324187':{'en': 'Angers', 'fr': 'Angers'},
'3338315':{'en': 'Ludres', 'fr': 'Ludres'},
'3324183':{'en': 'Saumur', 'fr': 'Saumur'},
'3324180':{'en': 'Maze', 'fr': 'Maze'},
'3324181':{'en': 'Angers', 'fr': 'Angers'},
'3598697':{'bg': u('\u0421\u043e\u043a\u043e\u043b, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Sokol, Silistra'},
'3334447':{'en': 'Auneuil', 'fr': 'Auneuil'},
'3334444':{'en': 'Noyon', 'fr': 'Noyon'},
'3334445':{'en': 'Beauvais', 'fr': 'Beauvais'},
'3598693':{'bg': u('\u0417\u0432\u0435\u043d\u0438\u043c\u0438\u0440'), 'en': 'Zvenimir'},
'3598692':{'bg': u('\u0421\u0442\u0435\u0444\u0430\u043d \u041a\u0430\u0440\u0430\u0434\u0436\u0430, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Stefan Karadzha, Silistra'},
'3338318':{'en': 'Nancy', 'fr': 'Nancy'},
'3324189':{'en': u('Baug\u00e9'), 'fr': u('Baug\u00e9')},
'3595346':{'bg': u('\u041a\u0430\u043c\u0435\u043d\u044f\u043a, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Kamenyak, Shumen'},
'3595347':{'bg': u('\u0416\u0438\u0432\u043a\u043e\u0432\u043e, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Zhivkovo, Shumen'},
'3595344':{'bg': u('\u0412\u0435\u043b\u0438\u043d\u043e'), 'en': 'Velino'},
'3595345':{'bg': u('\u0420\u0430\u0437\u0432\u0438\u0433\u043e\u0440\u043e\u0432\u043e'), 'en': 'Razvigorovo'},
'3595342':{'bg': u('\u041a\u0430\u043f\u0438\u0442\u0430\u043d \u041f\u0435\u0442\u043a\u043e'), 'en': 'Kapitan Petko'},
'3595343':{'bg': u('\u0412\u0435\u043d\u0435\u0446, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Venets, Shumen'},
'3595340':{'bg': u('\u0412\u0438\u0441\u043e\u043a\u0430 \u043f\u043e\u043b\u044f\u043d\u0430, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Visoka polyana, Shumen'},
'3595341':{'bg': u('\u0425\u0438\u0442\u0440\u0438\u043d\u043e'), 'en': 'Hitrino'},
'359936':{'bg': u('\u0411\u0435\u043b\u043e\u0433\u0440\u0430\u0434\u0447\u0438\u043a'), 'en': 'Belogradchik'},
'3595348':{'bg': u('\u0422\u0440\u0435\u043c'), 'en': 'Trem'},
'3595349':{'bg': u('\u0421\u0442\u0443\u0434\u0435\u043d\u0438\u0446\u0430'), 'en': 'Studenitsa'},
'441677':{'en': 'Bedale'},
'3324738':{'en': 'Tours', 'fr': 'Tours'},
'4031':{'en': 'Bucharest and Ilfov County', 'ro': u('Bucure\u0219ti \u0219i jude\u021bul Ilfov')},
'3356559':{'en': 'Millau', 'fr': 'Millau'},
'441675':{'en': 'Coleshill'},
'3356550':{'en': 'Figeac', 'fr': 'Figeac'},
'3356551':{'en': 'Espalion', 'fr': 'Espalion'},
'3356553':{'en': 'Cahors', 'fr': 'Cahors'},
'433866':{'de': 'Breitenau am Hochlantsch', 'en': 'Breitenau am Hochlantsch'},
'3597912':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u0413\u0440\u0430\u0449\u0438\u0446\u0430'), 'en': 'Gorna Grashtitsa'},
'433864':{'de': u('Sankt Marein im M\u00fcrztal'), 'en': u('St. Marein im M\u00fcrztal')},
'433865':{'de': 'Kindberg', 'en': 'Kindberg'},
'433862':{'de': 'Bruck an der Mur', 'en': 'Bruck an der Mur'},
'433863':{'de': 'Turnau', 'en': 'Turnau'},
'433861':{'de': 'Aflenz', 'en': 'Aflenz'},
'35941113':{'bg': u('\u041f\u0440\u044f\u043f\u043e\u0440\u0435\u0446, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Pryaporets, St. Zagora'},
'433868':{'de': u('Trag\u00f6\u00df'), 'en': u('Trag\u00f6ss')},
'433869':{'de': 'Sankt Katharein an der Laming', 'en': 'St. Katharein an der Laming'},
'3595122':{'bg': u('\u0421\u043b\u0430\u0432\u0435\u0439\u043a\u043e\u0432\u043e, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Slaveykovo, Varna'},
'3347508':{'en': u('Tournon-sur-Rh\u00f4ne'), 'fr': u('Tournon-sur-Rh\u00f4ne')},
'3595120':{'bg': u('\u0411\u043e\u0437\u0432\u0435\u043b\u0438\u0439\u0441\u043a\u043e'), 'en': 'Bozveliysko'},
'3595121':{'bg': u('\u0422\u0443\u0442\u0440\u0430\u043a\u0430\u043d\u0446\u0438'), 'en': 'Tutrakantsi'},
'3595126':{'bg': u('\u0427\u0435\u0440\u043a\u043e\u0432\u043d\u0430, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Cherkovna, Varna'},
'3595127':{'bg': u('\u041c\u0430\u043d\u0430\u0441\u0442\u0438\u0440, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Manastir, Varna'},
'3595124':{'bg': u('\u041a\u043e\u043c\u0430\u0440\u0435\u0432\u043e, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Komarevo, Varna'},
'3595125':{'bg': u('\u0413\u0440\u0430\u0434\u0438\u043d\u0430\u0440\u043e\u0432\u043e'), 'en': 'Gradinarovo'},
'3347501':{'en': u('Mont\u00e9limar'), 'fr': u('Mont\u00e9limar')},
'3347500':{'en': u('Mont\u00e9limar'), 'fr': u('Mont\u00e9limar')},
'3595128':{'bg': u('\u0416\u0438\u0442\u043d\u0438\u0446\u0430, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Zhitnitsa, Varna'},
'3347502':{'en': u('Romans-sur-Is\u00e8re'), 'fr': u('Romans-sur-Is\u00e8re')},
'3347505':{'en': u('Romans-sur-Is\u00e8re'), 'fr': u('Romans-sur-Is\u00e8re')},
'3347504':{'en': 'Pierrelatte', 'fr': 'Pierrelatte'},
'3347507':{'en': u('Tournon-sur-Rh\u00f4ne'), 'fr': u('Tournon-sur-Rh\u00f4ne')},
'3355692':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355693':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355922':{'en': 'Biarritz', 'fr': 'Biarritz'},
'3355923':{'en': 'Biarritz', 'fr': 'Biarritz'},
'441678':{'en': 'Bala'},
'3599128':{'bg': u('\u0415\u043b\u0438\u0441\u0435\u0439\u043d\u0430'), 'en': 'Eliseyna'},
'3356182':{'en': 'Grenade', 'fr': 'Grenade'},
'3599126':{'bg': u('\u041a\u0430\u043c\u0435\u043d\u043e \u043f\u043e\u043b\u0435'), 'en': 'Kameno pole'},
'3329817':{'en': 'Crozon', 'fr': 'Crozon'},
'3599124':{'bg': u('\u0422\u0438\u043f\u0447\u0435\u043d\u0438\u0446\u0430'), 'en': 'Tipchenitsa'},
'3599125':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u0411\u0435\u0448\u043e\u0432\u0438\u0446\u0430'), 'en': 'Gorna Beshovitsa'},
'3599122':{'bg': u('\u0417\u0432\u0435\u0440\u0438\u043d\u043e'), 'en': 'Zverino'},
'3599123':{'bg': u('\u0420\u043e\u043c\u0430\u043d'), 'en': 'Roman'},
'3329816':{'en': 'Crozon', 'fr': 'Crozon'},
'3355926':{'en': 'Saint-Jean-de-Luz', 'fr': 'Saint-Jean-de-Luz'},
'3355927':{'en': 'Pau', 'fr': 'Pau'},
'3355698':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3339190':{'en': 'Boulogne-sur-Mer', 'fr': 'Boulogne-sur-Mer'},
'355874':{'en': u('Ho\u00e7isht/Miras, Devoll')},
'355875':{'en': u('K\u00eblcyr\u00eb, P\u00ebrmet')},
'359751':{'bg': u('\u0413\u043e\u0446\u0435 \u0414\u0435\u043b\u0447\u0435\u0432'), 'en': 'Gotse Delchev'},
'359750':{'bg': u('\u0411\u043e\u0440\u043e\u0432\u0435\u0446, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Borovets, Sofia'},
'390884':{'en': 'Foggia', 'it': 'Manfredonia'},
'390885':{'it': 'Cerignola'},
'390883':{'en': 'Andria Barletta Trani', 'it': 'Andria'},
'3349103':{'en': 'Marseille', 'fr': 'Marseille'},
'3349102':{'en': 'Marseille', 'fr': 'Marseille'},
'3349101':{'en': 'Marseille', 'fr': 'Marseille'},
'390522':{'en': 'Reggio Emilia', 'it': 'Reggio nell\'Emilia'},
'3349107':{'en': 'Allauch', 'fr': 'Allauch'},
'3349106':{'en': 'Marseille', 'fr': 'Marseille'},
'3349105':{'en': 'Marseille', 'fr': 'Marseille'},
'3349104':{'en': 'Marseille', 'fr': 'Marseille'},
'3349109':{'en': 'Marseille', 'fr': 'Marseille'},
'3349108':{'en': 'Marseille', 'fr': 'Marseille'},
'3678':{'en': 'Kiskoros', 'hu': u('Kisk\u0151r\u00f6s')},
'3323877':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323874':{'en': u('Ingr\u00e9'), 'fr': u('Ingr\u00e9')},
'3323873':{'en': 'Saran', 'fr': 'Saran'},
'3338961':{'en': 'Illzach', 'fr': 'Illzach'},
'3338960':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3338965':{'en': 'Rixheim', 'fr': 'Rixheim'},
'3338964':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3323879':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3338966':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'4413873':{'en': 'Langholm'},
'3672':{'en': 'Pecs', 'hu': u('P\u00e9cs')},
'3742227':{'am': u('\u0533\u0561\u057c\u0576\u056b'), 'en': 'Garni', 'ru': u('\u0413\u0430\u0440\u043d\u0438')},
'3673':{'en': 'Szigetvar', 'hu': u('Szigetv\u00e1r')},
'3594752':{'bg': u('\u041f\u044a\u0440\u0432\u0435\u043d\u0435\u0446, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Parvenets, Yambol'},
'3594753':{'bg': u('\u0417\u043e\u0440\u043d\u0438\u0446\u0430, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Zornitsa, Yambol'},
'3742229':{'am': u('\u0536\u0578\u057e\u0584'), 'en': 'Zovk', 'ru': u('\u0417\u043e\u0432\u043a')},
'3594751':{'bg': u('\u0412\u043e\u0439\u043d\u0438\u043a\u0430'), 'en': 'Voynika'},
'3594756':{'bg': u('\u041f\u043e\u043b\u044f\u043d\u0430, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Polyana, Yambol'},
'3594757':{'bg': u('\u041d\u0435\u0434\u044f\u043b\u0441\u043a\u043e'), 'en': 'Nedyalsko'},
'3594754':{'bg': u('\u041a\u0430\u043c\u0435\u043d\u0435\u0446, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Kamenets, Yambol'},
'3594755':{'bg': u('\u0422\u0430\u043c\u0430\u0440\u0438\u043d\u043e'), 'en': 'Tamarino'},
'3346810':{'en': 'Carcassonne', 'fr': 'Carcassonne'},
'3346811':{'en': 'Carcassonne', 'fr': 'Carcassonne'},
'3596169':{'bg': u('\u0411\u043b\u0430\u0433\u043e\u0435\u0432\u043e, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Blagoevo, V. Tarnovo'},
'3675':{'en': 'Paks', 'hu': 'Paks'},
'3348418':{'en': 'Marseille', 'fr': 'Marseille'},
'3359432':{'en': 'Kourou', 'fr': 'Kourou'},
'3359430':{'en': 'Cayenne', 'fr': 'Cayenne'},
'3359431':{'en': 'Cayenne', 'fr': 'Cayenne'},
'3359437':{'en': 'Cayenne', 'fr': 'Cayenne'},
'3359434':{'en': 'Saint-Laurent-du-Maroni', 'fr': 'Saint-Laurent-du-Maroni'},
'3359435':{'en': 'Matoury', 'fr': 'Matoury'},
'3359438':{'en': 'Cayenne', 'fr': 'Cayenne'},
'3359439':{'en': 'Cayenne', 'fr': 'Cayenne'},
'3593151':{'bg': u('\u0420\u0430\u043a\u043e\u0432\u0441\u043a\u0438, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Rakovski, Plovdiv'},
'3593153':{'bg': u('\u0421\u0442\u0440\u044f\u043c\u0430'), 'en': 'Stryama'},
'3593155':{'bg': u('\u041c\u043e\u043c\u0438\u043d\u043e \u0441\u0435\u043b\u043e'), 'en': 'Momino selo'},
'3593154':{'bg': u('\u0427\u0430\u043b\u044a\u043a\u043e\u0432\u0438'), 'en': 'Chalakovi'},
'3593157':{'bg': u('\u0411\u043e\u043b\u044f\u0440\u0438\u043d\u043e'), 'en': 'Bolyarino'},
'3593156':{'bg': u('\u0428\u0438\u0448\u043c\u0430\u043d\u0446\u0438'), 'en': 'Shishmantsi'},
'3593159':{'bg': u('\u0411\u0435\u043b\u043e\u0437\u0435\u043c'), 'en': 'Belozem'},
'435442':{'de': 'Landeck', 'en': 'Landeck'},
'3595139':{'bg': u('\u041e\u0431\u043e\u0440\u0438\u0449\u0435, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Oborishte, Varna'},
'3325112':{'en': 'La Chapelle sur Erdre', 'fr': 'La Chapelle sur Erdre'},
'3325445':{'en': 'Blois', 'fr': 'Blois'},
'3325110':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'3325111':{'en': u('Rez\u00e9'), 'fr': u('Rez\u00e9')},
'3325116':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'3325441':{'en': 'Lye', 'fr': 'Lye'},
'3325442':{'en': 'Blois', 'fr': 'Blois'},
'3325443':{'en': 'Blois', 'fr': 'Blois'},
'433387':{'de': u('S\u00f6chau'), 'en': u('S\u00f6chau')},
'433386':{'de': u('Gro\u00dfsteinbach'), 'en': 'Grosssteinbach'},
'433385':{'de': 'Ilz', 'en': 'Ilz'},
'3325448':{'en': u('La Ch\u00e2tre'), 'fr': u('La Ch\u00e2tre')},
'433382':{'de': u('F\u00fcrstenfeld'), 'en': u('F\u00fcrstenfeld')},
'435443':{'de': u('Galt\u00fcr'), 'en': u('Galt\u00fcr')},
'3595138':{'bg': u('\u0414\u043e\u0431\u0440\u043e\u0442\u0438\u0447'), 'en': 'Dobrotich'},
'3324869':{'en': 'Bourges', 'fr': 'Bourges'},
'3324868':{'en': 'Bourges', 'fr': 'Bourges'},
'3324865':{'en': 'Bourges', 'fr': 'Bourges'},
'3324867':{'en': 'Bourges', 'fr': 'Bourges'},
'3324866':{'en': 'Bourges', 'fr': 'Bourges'},
'3324861':{'en': u('Ch\u00e2teaumeillant'), 'fr': u('Ch\u00e2teaumeillant')},
'375154':{'be': u('\u041b\u0456\u0434\u0430'), 'en': 'Lida', 'ru': u('\u041b\u0438\u0434\u0430')},
'432642':{'de': 'Aspangberg-Sankt Peter', 'en': 'Aspangberg-St. Peter'},
'375152':{'be': u('\u0413\u0440\u043e\u0434\u043d\u0430'), 'en': 'Grodno', 'ru': u('\u0413\u0440\u043e\u0434\u043d\u043e')},
'432644':{'de': 'Grimmenstein', 'en': 'Grimmenstein'},
'434718':{'de': 'Dellach', 'en': 'Dellach'},
'3596952':{'bg': u('\u041e\u0440\u0435\u0448\u0430\u043a, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Oreshak, Lovech'},
'434713':{'de': 'Techendorf', 'en': 'Techendorf'},
'434712':{'de': 'Greifenburg', 'en': 'Greifenburg'},
'434715':{'de': u('K\u00f6tschach-Mauthen'), 'en': u('K\u00f6tschach-Mauthen')},
'434714':{'de': 'Dellach im Drautal', 'en': 'Dellach im Drautal'},
'434717':{'de': 'Steinfeld', 'en': 'Steinfeld'},
'434716':{'de': 'Lesachtal', 'en': 'Lesachtal'},
'3347867':{'en': 'Saint-Fons', 'fr': 'Saint-Fons'},
'4413399':{'en': 'Ballater'},
'4413398':{'en': 'Aboyne'},
'4413397':{'en': 'Ballater'},
'4413396':{'en': 'Ballater'},
'4413395':{'en': 'Aboyne'},
'4413394':{'en': 'Ballater'},
'4413393':{'en': 'Aboyne'},
'4413392':{'en': 'Aboyne'},
'4413391':{'en': 'Aboyne/Ballater'},
'4413390':{'en': 'Aboyne/Ballater'},
'35856':{'en': 'Kymi', 'fi': 'Kymi', 'se': 'Kymmene'},
'35321':{'en': 'Cork/Kinsale/Coachford'},
'35322':{'en': 'Mallow'},
'35323':{'en': 'Bandon'},
'35324':{'en': 'Youghal'},
'35325':{'en': 'Fermoy'},
'35326':{'en': 'Macroom'},
'35851':{'en': 'Kymi', 'fi': 'Kymi', 'se': 'Kymmene'},
'35328':{'en': 'Skibbereen'},
'35329':{'en': 'Kanturk'},
'35858':{'en': 'Kymi', 'fi': 'Kymi', 'se': 'Kymmene'},
'361':{'en': 'Budapest', 'hu': 'Budapest'},
'436432':{'de': 'Bad Hofgastein', 'en': 'Bad Hofgastein'},
'436433':{'de': 'Dorfgastein', 'en': 'Dorfgastein'},
'434843':{'de': u('Au\u00dfervillgraten'), 'en': 'Ausservillgraten'},
'3347778':{'en': 'Roanne', 'fr': 'Roanne'},
'3347779':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'390921':{'en': 'Palermo', 'it': u('Cefal\u00f9')},
'3343433':{'en': u('B\u00e9ziers'), 'fr': u('B\u00e9ziers')},
'3343432':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3343435':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3347773':{'en': 'Saint-Paul-en-Jarez', 'fr': 'Saint-Paul-en-Jarez'},
'3347770':{'en': 'Roanne', 'fr': 'Roanne'},
'3347771':{'en': 'Roanne', 'fr': 'Roanne'},
'3316936':{'en': u('\u00c9vry'), 'fr': u('\u00c9vry')},
'435447':{'de': 'Flirsch', 'en': 'Flirsch'},
'3316934':{'en': 'Longjumeau', 'fr': 'Longjumeau'},
'3316935':{'en': u('Bi\u00e8vres'), 'fr': u('Bi\u00e8vres')},
'3316932':{'en': 'Massy', 'fr': 'Massy'},
'390923':{'it': 'Trapani'},
'3316930':{'en': 'Massy', 'fr': 'Massy'},
'3316931':{'en': 'Palaiseau', 'fr': 'Palaiseau'},
'390922':{'en': 'Agrigento', 'it': 'Agrigento'},
'3316938':{'en': 'Athis-Mons', 'fr': 'Athis-Mons'},
'3316939':{'en': 'Brunoy', 'fr': 'Brunoy'},
'441207':{'en': 'Consett'},
'441206':{'en': 'Colchester'},
'3595757':{'bg': u('\u0411\u0435\u0437\u043c\u0435\u0440, \u0414\u043e\u0431\u0440.'), 'en': 'Bezmer, Dobr.'},
'3595756':{'bg': u('\u0411\u043e\u0436\u0430\u043d'), 'en': 'Bozhan'},
'3595753':{'bg': u('\u041e\u0440\u043b\u044f\u043a'), 'en': 'Orlyak'},
'35984735':{'bg': u('\u0421\u0432\u0435\u0449\u0430\u0440\u0438'), 'en': 'Sveshtari'},
'35984734':{'bg': u('\u0422\u043e\u0434\u043e\u0440\u043e\u0432\u043e, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Todorovo, Razgrad'},
'35984737':{'bg': u('\u0420\u0430\u0439\u043d\u0438\u043d\u043e'), 'en': 'Raynino'},
'3595752':{'bg': u('\u041d\u043e\u0432\u0430 \u041a\u0430\u043c\u0435\u043d\u0430'), 'en': 'Nova Kamena'},
'35984730':{'bg': u('\u0413\u043e\u043b\u044f\u043c \u041f\u043e\u0440\u043e\u0432\u0435\u0446'), 'en': 'Golyam Porovets'},
'35984733':{'bg': u('\u041b\u0443\u0434\u043e\u0433\u043e\u0440\u0446\u0438'), 'en': 'Ludogortsi'},
'35984732':{'bg': u('\u0419\u043e\u043d\u043a\u043e\u0432\u043e'), 'en': 'Yonkovo'},
'3347646':{'en': 'Grenoble', 'fr': 'Grenoble'},
'441209':{'en': 'Redruth'},
'441208':{'en': 'Bodmin'},
'3347644':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3329749':{'en': 'Questembert', 'fr': 'Questembert'},
'3329748':{'en': 'Sarzeau', 'fr': 'Sarzeau'},
'3329743':{'en': 'Theix', 'fr': 'Theix'},
'3329742':{'en': 'Vannes', 'fr': 'Vannes'},
'3329741':{'en': 'Sarzeau', 'fr': 'Sarzeau'},
'3329740':{'en': 'Vannes', 'fr': 'Vannes'},
'3329747':{'en': 'Vannes', 'fr': 'Vannes'},
'3329746':{'en': 'Vannes', 'fr': 'Vannes'},
'435449':{'de': u('Flie\u00df'), 'en': 'Fliess'},
'3329744':{'en': 'Arradon', 'fr': 'Arradon'},
'34882':{'en': 'Lugo', 'es': 'Lugo'},
'3338578':{'en': 'Montchanin', 'fr': 'Montchanin'},
'3347640':{'en': u('\u00c9chirolles'), 'fr': u('\u00c9chirolles')},
'3593147':{'bg': u('\u041d\u043e\u0432\u043e \u0441\u0435\u043b\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Novo selo, Plovdiv'},
'3338573':{'en': 'Le Creusot', 'fr': 'Le Creusot'},
'3347641':{'en': 'Meylan', 'fr': 'Meylan'},
'3338577':{'en': 'Le Creusot', 'fr': 'Le Creusot'},
'3338575':{'en': 'Louhans', 'fr': 'Louhans'},
'3593145':{'bg': u('\u041a\u0440\u0438\u0447\u0438\u043c'), 'en': 'Krichim'},
'3593142':{'bg': u('\u0411\u0440\u0435\u0441\u0442\u043e\u0432\u0438\u0446\u0430, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Brestovitsa, Plovdiv'},
'3355925':{'en': 'Bayonne', 'fr': 'Bayonne'},
'34887':{'en': u('Le\u00f3n'), 'es': u('Le\u00f3n')},
'435575':{'de': 'Langen bei Bregenz', 'en': 'Langen bei Bregenz'},
'37425295':{'am': u('\u0531\u0580\u057f\u0561\u057e\u0561\u0576'), 'en': 'Artavan', 'ru': u('\u0410\u0440\u0442\u0430\u0432\u0430\u043d')},
'435578':{'de': u('H\u00f6chst'), 'en': u('H\u00f6chst')},
'3354525':{'en': u('Angoul\u00eame'), 'fr': u('Angoul\u00eame')},
'3354529':{'en': 'Ruffec', 'fr': 'Ruffec'},
'435579':{'de': 'Alberschwende', 'en': 'Alberschwende'},
'3322844':{'en': 'Nantes', 'fr': 'Nantes'},
'3355920':{'en': 'Hendaye', 'fr': 'Hendaye'},
'3355921':{'en': 'Monein', 'fr': 'Monein'},
'3329811':{'en': 'Douarnenez', 'fr': 'Douarnenez'},
'3329810':{'en': 'Quimper', 'fr': 'Quimper'},
'3355696':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355697':{'en': 'Merignac', 'fr': u('M\u00e9rignac')},
'3329815':{'en': 'Morlaix', 'fr': 'Morlaix'},
'3355695':{'en': 'Blanquefort', 'fr': 'Blanquefort'},
'3355928':{'en': u('Maul\u00e9on-Licharre'), 'fr': u('Maul\u00e9on-Licharre')},
'3355929':{'en': 'Hasparren', 'fr': 'Hasparren'},
'3329819':{'en': u('Saint-Pol-de-L\u00e9on'), 'fr': u('Saint-Pol-de-L\u00e9on')},
'3355699':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'34958':{'en': 'Granada', 'es': 'Granada'},
'34959':{'en': 'Huelva', 'es': 'Huelva'},
'355876':{'en': u('Qend\u00ebr/Frash\u00ebr/Petran/\u00c7arshov\u00eb, P\u00ebrmet')},
'355877':{'en': u('Dishnic\u00eb/Suk\u00eb/Ballaban, P\u00ebrmet')},
'390882':{'en': 'Foggia', 'it': 'San Severo'},
'355871':{'en': u('Leskovik/Barmash/Novosel\u00eb, Kolonj\u00eb')},
'355872':{'en': u('Qend\u00ebr Ersek\u00eb/Mollas/\u00c7lirim, Kolonj\u00eb')},
'355873':{'en': u('Qend\u00ebr Bilisht/Prog\u00ebr, Devoll')},
'34950':{'en': u('Almer\u00eda'), 'es': u('Almer\u00eda')},
'34951':{'en': u('M\u00e1laga'), 'es': u('M\u00e1laga')},
'34952':{'en': u('M\u00e1laga'), 'es': u('M\u00e1laga')},
'34953':{'en': u('Ja\u00e9n'), 'es': u('Ja\u00e9n')},
'34954':{'en': 'Seville', 'es': 'Sevilla'},
'34955':{'en': 'Seville', 'es': 'Sevilla'},
'34956':{'en': u('C\u00e1diz'), 'es': u('C\u00e1diz')},
'34957':{'en': 'Cordova', 'es': u('C\u00f3rdoba')},
'3332288':{'en': u('Rosi\u00e8res-en-Santerre'), 'fr': u('Rosi\u00e8res-en-Santerre')},
'3332289':{'en': 'Amiens', 'fr': 'Amiens'},
'3332283':{'en': 'Chaulnes', 'fr': 'Chaulnes'},
'3332280':{'en': 'Amiens', 'fr': 'Amiens'},
'3332286':{'en': 'Roisel', 'fr': 'Roisel'},
'3332287':{'en': 'Roye', 'fr': 'Roye'},
'3332284':{'en': u('P\u00e9ronne'), 'fr': u('P\u00e9ronne')},
'35947192':{'bg': u('\u0417\u0430\u0432\u043e\u0439'), 'en': 'Zavoy'},
'35947193':{'bg': u('\u041c\u043e\u0433\u0438\u043b\u0430, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Mogila, Yambol'},
'3324042':{'en': 'Le Pouliguen', 'fr': 'Le Pouliguen'},
'3324041':{'en': 'Nantes', 'fr': 'Nantes'},
'3324040':{'en': 'Nantes', 'fr': 'Nantes'},
'3332868':{'en': 'Bergues', 'fr': 'Bergues'},
'3324047':{'en': 'Nantes', 'fr': 'Nantes'},
'3322330':{'en': 'Rennes', 'fr': 'Rennes'},
'3332864':{'en': 'Coudekerque-Branche', 'fr': 'Coudekerque-Branche'},
'3687':{'en': 'Tapolca', 'hu': 'Tapolca'},
'3594323':{'bg': u('\u0422\u0443\u043b\u043e\u0432\u043e'), 'en': 'Tulovo'},
'3594322':{'bg': u('\u042f\u0433\u043e\u0434\u0430'), 'en': 'Yagoda'},
'3594321':{'bg': u('\u041c\u044a\u0433\u043b\u0438\u0436'), 'en': 'Maglizh'},
'3594327':{'bg': u('\u0428\u0435\u0439\u043d\u043e\u0432\u043e'), 'en': 'Sheynovo'},
'3594326':{'bg': u('\u0415\u043d\u0438\u043d\u0430'), 'en': 'Enina'},
'3594325':{'bg': u('\u041a\u044a\u043d\u0447\u0435\u0432\u043e'), 'en': 'Kanchevo'},
'3594324':{'bg': u('\u0428\u0438\u043f\u043a\u0430'), 'en': 'Shipka'},
'3594329':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u0421\u0430\u0445\u0440\u0430\u043d\u0435'), 'en': 'Dolno Sahrane'},
'3322335':{'en': 'Rennes', 'fr': 'Rennes'},
'3322331':{'en': 'Bain-de-Bretagne', 'fr': 'Bain-de-Bretagne'},
'3315714':{'en': 'Pantin', 'fr': 'Pantin'},
'3332866':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'3315719':{'en': 'Antony', 'fr': 'Antony'},
'3332865':{'en': 'Wormhout', 'fr': 'Wormhout'},
'3332863':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'3332860':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'3332861':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'3334428':{'en': 'Creil', 'fr': 'Creil'},
'3334429':{'en': 'Saint-Martin-Longueau', 'fr': 'Saint-Martin-Longueau'},
'3338373':{'en': u('Lun\u00e9ville'), 'fr': u('Lun\u00e9ville')},
'3338374':{'en': u('Lun\u00e9ville'), 'fr': u('Lun\u00e9ville')},
'3338375':{'en': 'Baccarat', 'fr': 'Baccarat'},
'3338376':{'en': u('Lun\u00e9ville'), 'fr': u('Lun\u00e9ville')},
'3334420':{'en': u('Compi\u00e8gne'), 'fr': u('Compi\u00e8gne')},
'3334421':{'en': 'Lamorlaye', 'fr': 'Lamorlaye'},
'3334422':{'en': u('M\u00e9ru'), 'fr': u('M\u00e9ru')},
'3334423':{'en': u('Compi\u00e8gne'), 'fr': u('Compi\u00e8gne')},
'3334424':{'en': 'Creil', 'fr': 'Creil'},
'3334425':{'en': 'Creil', 'fr': 'Creil'},
'3334426':{'en': 'Neuilly-en-Thelle', 'fr': 'Neuilly-en-Thelle'},
'3334427':{'en': u('Pr\u00e9cy-sur-Oise'), 'fr': u('Pr\u00e9cy-sur-Oise')},
'436278':{'de': 'Ostermiething', 'en': 'Ostermiething'},
'390144':{'it': 'Acqui Terme'},
'437479':{'de': 'Ardagger', 'en': 'Ardagger'},
'359915':{'bg': u('\u0411\u044f\u043b\u0430 \u0421\u043b\u0430\u0442\u0438\u043d\u0430'), 'en': 'Byala Slatina'},
'359910':{'bg': u('\u041c\u0435\u0437\u0434\u0440\u0430'), 'en': 'Mezdra'},
'3595320':{'bg': u('\u041f\u0435\u0442 \u043c\u043e\u0433\u0438\u043b\u0438, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Pet mogili, Shumen'},
'3595321':{'bg': u('\u041f\u0440\u0430\u0432\u0435\u043d\u0446\u0438'), 'en': 'Praventsi'},
'3595323':{'bg': u('\u041f\u043b\u0438\u0441\u043a\u0430'), 'en': 'Pliska'},
'3595324':{'bg': u('\u0425\u044a\u0440\u0441\u043e\u0432\u043e, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Harsovo, Shumen'},
'3595325':{'bg': u('\u0412\u043e\u0439\u0432\u043e\u0434\u0430'), 'en': 'Voyvoda'},
'3595326':{'bg': u('\u0412\u044a\u0440\u0431\u044f\u043d\u0435'), 'en': 'Varbyane'},
'3595327':{'bg': u('\u041a\u0430\u0441\u043f\u0438\u0447\u0430\u043d, \u0428\u0443\u043c\u0435\u043d'), 'en': 'Kaspichan, Shumen'},
'3595328':{'bg': u('\u041d\u0438\u043a\u043e\u043b\u0430 \u041a\u043e\u0437\u043b\u0435\u0432\u043e'), 'en': 'Nikola Kozlevo'},
'3595329':{'bg': u('\u041c\u0438\u0440\u043e\u0432\u0446\u0438'), 'en': 'Mirovtsi'},
'437478':{'de': 'Oed-Oehling', 'en': 'Oed-Oehling'},
'355269':{'en': u('Kastrat/Shkrel/Kelmend, Mal\u00ebsi e Madhe')},
'3356573':{'en': 'Rodez', 'fr': 'Rodez'},
'3356577':{'en': 'Rodez', 'fr': 'Rodez'},
'3356575':{'en': 'Rodez', 'fr': 'Rodez'},
'355261':{'en': u('Vau-Dej\u00ebs')},
'355263':{'en': u('Pult/Shal\u00eb/Shosh/Temal/Shllak, Shkod\u00ebr')},
'355262':{'en': u('Rrethinat/Ana-Malit, Shkod\u00ebr')},
'355265':{'en': u('Vig-Mnel\u00eb/Hajmel, Shkod\u00ebr')},
'355264':{'en': u('Postrib\u00eb/Gur i Zi')},
'355267':{'en': u('Daj\u00e7/Velipoj\u00eb, Shkod\u00ebr')},
'355266':{'en': u('Bushat/B\u00ebrdic\u00eb, Shkod\u00ebr')},
'3599328':{'bg': u('\u0414\u043e\u043b\u043d\u0438 \u041b\u043e\u043c'), 'en': 'Dolni Lom'},
'3599329':{'bg': u('\u0420\u0430\u0431\u0438\u0448\u0430'), 'en': 'Rabisha'},
'3599324':{'bg': u('\u0420\u0443\u0436\u0438\u043d\u0446\u0438'), 'en': 'Ruzhintsi'},
'3599325':{'bg': u('\u0411\u0435\u043b\u043e \u043f\u043e\u043b\u0435, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Belo pole, Vidin'},
'3599326':{'bg': u('\u0413\u043e\u0440\u043d\u0438 \u041b\u043e\u043c'), 'en': 'Gorni Lom'},
'3599327':{'bg': u('\u0427\u0443\u043f\u0440\u0435\u043d\u0435'), 'en': 'Chuprene'},
'3599320':{'bg': u('\u0421\u0442\u0430\u043a\u0435\u0432\u0446\u0438'), 'en': 'Stakevtsi'},
'3599322':{'bg': u('\u041e\u0440\u0435\u0448\u0435\u0446, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Oreshets, Vidin'},
'3599323':{'bg': u('\u0414\u0440\u0435\u043d\u043e\u0432\u0435\u0446'), 'en': 'Drenovets'},
'3347563':{'en': u('Saulce-sur-Rh\u00f4ne'), 'fr': u('Saulce-sur-Rh\u00f4ne')},
'3347562':{'en': u('La Voulte sur Rh\u00f4ne'), 'fr': u('La Voulte sur Rh\u00f4ne')},
'3347561':{'en': u('Livron-sur-Dr\u00f4me'), 'fr': u('Livron-sur-Dr\u00f4me')},
'3347560':{'en': u('\u00c9toile-sur-Rh\u00f4ne'), 'fr': u('\u00c9toile-sur-Rh\u00f4ne')},
'3347567':{'en': 'Annonay', 'fr': 'Annonay'},
'433845':{'de': 'Mautern in Steiermark', 'en': 'Mautern in Steiermark'},
'433846':{'de': 'Kalwang', 'en': 'Kalwang'},
'3347564':{'en': 'Privas', 'fr': 'Privas'},
'433848':{'de': 'Eisenerz', 'en': 'Eisenerz'},
'433849':{'de': 'Vordernberg', 'en': 'Vordernberg'},
'3347569':{'en': 'Annonay', 'fr': 'Annonay'},
'3347568':{'en': 'Hauterives', 'fr': 'Hauterives'},
'433114':{'de': 'Markt Hartmannsdorf', 'en': 'Markt Hartmannsdorf'},
'3599148':{'bg': u('\u0411\u044a\u0440\u043a\u0430\u0447\u0435\u0432\u043e'), 'en': 'Barkachevo'},
'3599149':{'bg': u('\u0411\u0443\u043a\u043e\u0432\u0435\u0446, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Bukovets, Vratsa'},
'433115':{'de': 'Studenzen', 'en': 'Studenzen'},
'3599140':{'bg': u('\u0413\u0430\u0431\u0430\u0440\u0435'), 'en': 'Gabare'},
'3599141':{'bg': u('\u041c\u0430\u043b\u043e\u0440\u0430\u0434'), 'en': 'Malorad'},
'3599142':{'bg': u('\u041b\u0430\u0437\u0430\u0440\u043e\u0432\u043e'), 'en': 'Lazarovo'},
'3599143':{'bg': u('\u0415\u043d\u0438\u0446\u0430'), 'en': 'Enitsa'},
'3599144':{'bg': u('\u041d\u0438\u0432\u044f\u043d\u0438\u043d'), 'en': 'Nivyanin'},
'3599145':{'bg': u('\u0411\u0440\u0435\u043d\u0438\u0446\u0430, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Brenitsa, Vratsa'},
'3599146':{'bg': u('\u0421\u043e\u043a\u043e\u043b\u0430\u0440\u0435'), 'en': 'Sokolare'},
'3599147':{'bg': u('\u0411\u043e\u0440\u043e\u0432\u0430\u043d'), 'en': 'Borovan'},
'3353439':{'en': 'Colomiers', 'fr': 'Colomiers'},
'35963571':{'bg': u('\u0411\u0440\u0435\u0441\u0442\u043e\u0432\u0435\u0446'), 'en': 'Brestovets'},
'3353430':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3353431':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3353433':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3353436':{'en': 'Blagnac', 'fr': 'Blagnac'},
'435635':{'de': 'Elmen', 'en': 'Elmen'},
'435634':{'de': 'Elbigenalp', 'en': 'Elbigenalp'},
'432714':{'de': 'Rossatz', 'en': 'Rossatz'},
'35963575':{'bg': u('\u0411\u043e\u0445\u043e\u0442'), 'en': 'Bohot'},
'35963577':{'bg': u('\u041f\u0435\u043b\u0438\u0448\u0430\u0442'), 'en': 'Pelishat'},
'3347386':{'en': u('Ch\u00e2tel-Guyon'), 'fr': u('Ch\u00e2tel-Guyon')},
'3347385':{'en': u('Saint-\u00c9loy-les-Mines'), 'fr': u('Saint-\u00c9loy-les-Mines')},
'3347384':{'en': 'Cournon-d\'Auvergne', 'fr': 'Cournon-d\'Auvergne'},
'3347383':{'en': u('Pont-du-Ch\u00e2teau'), 'fr': u('Pont-du-Ch\u00e2teau')},
'3347382':{'en': 'Ambert', 'fr': 'Ambert'},
'3347381':{'en': 'La Bourboule', 'fr': 'La Bourboule'},
'3347380':{'en': 'Thiers', 'fr': 'Thiers'},
'435632':{'de': 'Stanzach', 'en': 'Stanzach'},
'3347389':{'en': 'Issoire', 'fr': 'Issoire'},
'441753':{'en': 'Slough'},
'4415071':{'en': 'Louth/Alford (Lincs)/Spilsby (Horncastle)'},
'437476':{'de': 'Aschbach-Markt', 'en': 'Aschbach-Markt'},
'4415073':{'en': 'Louth'},
'3323565':{'en': u('Saint-\u00c9tienne-du-Rouvray'), 'fr': u('Saint-\u00c9tienne-du-Rouvray')},
'3323564':{'en': 'Oissel', 'fr': 'Oissel'},
'35974321':{'bg': u('\u0425\u044a\u0440\u0441\u043e\u0432\u043e, \u0411\u043b\u0430\u0433.'), 'en': 'Harsovo, Blag.'},
'3329972':{'en': 'Redon', 'fr': 'Redon'},
'3329973':{'en': 'Combourg', 'fr': 'Combourg'},
'3522458':{'de': 'Soleuvre/Differdingen', 'en': 'Soleuvre/Differdange', 'fr': 'Soleuvre/Differdange'},
'3522459':{'de': 'Soleuvre', 'en': 'Soleuvre', 'fr': 'Soleuvre'},
'3522454':{'de': 'Esch-sur-Alzette', 'en': 'Esch-sur-Alzette', 'fr': 'Esch-sur-Alzette'},
'3323561':{'en': 'Bois-Guillaume', 'fr': 'Bois-Guillaume'},
'3522456':{'de': u('R\u00fcmelingen'), 'en': 'Rumelange', 'fr': 'Rumelange'},
'3522457':{'de': 'Esch-sur-Alzette/Schifflingen', 'en': 'Esch-sur-Alzette/Schifflange', 'fr': 'Esch-sur-Alzette/Schifflange'},
'3522450':{'de': 'Bascharage/Petingen/Rodingen', 'en': 'Bascharage/Petange/Rodange', 'fr': 'Bascharage/Petange/Rodange'},
'3522451':{'de': u('D\u00fcdelingen/Bettemburg/Livingen'), 'en': 'Dudelange/Bettembourg/Livange', 'fr': 'Dudelange/Bettembourg/Livange'},
'3522452':{'de': u('D\u00fcdelingen'), 'en': 'Dudelange', 'fr': 'Dudelange'},
'3522453':{'de': 'Esch-sur-Alzette', 'en': 'Esch-sur-Alzette', 'fr': 'Esch-sur-Alzette'},
'3355539':{'en': 'Couzeix', 'fr': 'Couzeix'},
'3329977':{'en': 'Chartres-de-Bretagne', 'fr': 'Chartres-de-Bretagne'},
'3355537':{'en': 'Limoges', 'fr': 'Limoges'},
'3316091':{'en': u('\u00c9vry'), 'fr': u('\u00c9vry')},
'3316092':{'en': 'Villebon-sur-Yvette', 'fr': 'Villebon-sur-Yvette'},
'3316096':{'en': 'Montereau-Fault-Yonne', 'fr': 'Montereau-Fault-Yonne'},
'441420':{'en': 'Alton'},
'3355533':{'en': 'Limoges', 'fr': 'Limoges'},
'437473':{'de': 'Blindenmarkt', 'en': 'Blindenmarkt'},
'3355532':{'en': 'Limoges', 'fr': 'Limoges'},
'3522678':{'de': 'Junglinster', 'en': 'Junglinster', 'fr': 'Junglinster'},
'3522679':{'de': 'Berdorf/Consdorf', 'en': 'Berdorf/Consdorf', 'fr': 'Berdorf/Consdorf'},
'3522671':{'de': 'Betzdorf', 'en': 'Betzdorf', 'fr': 'Betzdorf'},
'3522672':{'de': 'Echternach', 'en': 'Echternach', 'fr': 'Echternach'},
'3522673':{'de': 'Rosport', 'en': 'Rosport', 'fr': 'Rosport'},
'3522674':{'de': 'Wasserbillig', 'en': 'Wasserbillig', 'fr': 'Wasserbillig'},
'3522675':{'de': 'Distrikt Grevenmacher-sur-Moselle', 'en': 'Grevenmacher-sur-Moselle', 'fr': 'Grevenmacher-sur-Moselle'},
'3522676':{'de': 'Wormeldingen', 'en': 'Wormeldange', 'fr': 'Wormeldange'},
'3338906':{'en': 'Brunstatt', 'fr': 'Brunstatt'},
'3349454':{'en': 'Cogolin', 'fr': 'Cogolin'},
'437472':{'de': 'Amstetten', 'en': 'Amstetten'},
'3338908':{'en': 'Altkirch', 'fr': 'Altkirch'},
'3323851':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323853':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323852':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323855':{'en': 'Saint-Jean-de-Braye', 'fr': 'Saint-Jean-de-Braye'},
'3323854':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323856':{'en': u('Orl\u00e9ans'), 'fr': u('Orl\u00e9ans')},
'3323859':{'en': 'Jargeau', 'fr': 'Jargeau'},
'3323858':{'en': u('Ch\u00e2teauneuf-sur-Loire'), 'fr': u('Ch\u00e2teauneuf-sur-Loire')},
'3349322':{'en': 'Cagnes-sur-Mer', 'fr': 'Cagnes-sur-Mer'},
'3349321':{'en': 'Nice', 'fr': 'Nice'},
'3349320':{'en': 'Cagnes-sur-Mer', 'fr': 'Cagnes-sur-Mer'},
'44121':{'en': 'Birmingham'},
'3346839':{'en': u('Am\u00e9lie-les-Bains-Palalda'), 'fr': u('Am\u00e9lie-les-Bains-Palalda')},
'3346837':{'en': 'Saint-Cyprien', 'fr': 'Saint-Cyprien'},
'3346834':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3346835':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3346832':{'en': 'Narbonne', 'fr': 'Narbonne'},
'3346833':{'en': 'Coursan', 'fr': 'Coursan'},
'3346830':{'en': 'Font-Romeu-Odeillo-Via', 'fr': 'Font-Romeu-Odeillo-Via'},
'3346831':{'en': 'Limoux', 'fr': 'Limoux'},
'35930419':{'bg': u('\u042f\u0433\u043e\u0434\u0438\u043d\u0430'), 'en': 'Yagodina'},
'35930418':{'bg': u('\u0411\u0443\u0439\u043d\u043e\u0432\u043e, \u0421\u043c\u043e\u043b.'), 'en': 'Buynovo, Smol.'},
'35930410':{'bg': u('\u0411\u0440\u0435\u0437\u0435, \u0421\u043c\u043e\u043b.'), 'en': 'Breze, Smol.'},
'35930417':{'bg': u('\u0413\u0440\u043e\u0445\u043e\u0442\u043d\u043e'), 'en': 'Grohotno'},
'35930416':{'bg': u('\u0413\u044c\u043e\u0432\u0440\u0435\u043d'), 'en': 'Gyovren'},
'3593138':{'bg': u('\u0412\u0435\u0434\u0440\u0430\u0440\u0435'), 'en': 'Vedrare'},
'3332677':{'en': 'Reims', 'fr': 'Reims'},
'3332674':{'en': u('Vitry-le-Fran\u00e7ois'), 'fr': u('Vitry-le-Fran\u00e7ois')},
'3593133':{'bg': u('\u041a\u0430\u043b\u043e\u0444\u0435\u0440'), 'en': 'Kalofer'},
'3593132':{'bg': u('\u0411\u0430\u043d\u044f, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Banya, Plovdiv'},
'3332679':{'en': 'Reims', 'fr': 'Reims'},
'3593130':{'bg': u('\u041a\u0430\u0440\u0430\u0432\u0435\u043b\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Karavelovo, Plovdiv'},
'3593137':{'bg': u('\u041a\u043b\u0438\u0441\u0443\u0440\u0430, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Klisura, Plovdiv'},
'3593136':{'bg': u('\u0420\u043e\u0437\u0438\u043d\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Rozino, Plovdiv'},
'3593135':{'bg': u('\u041a\u044a\u0440\u043d\u0430\u0440\u0435'), 'en': 'Karnare'},
'3593134':{'bg': u('\u0421\u043e\u043f\u043e\u0442, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Sopot, Plovdiv'},
'355268':{'en': u('Qend\u00ebr/Gruemir\u00eb, Mal\u00ebsi e Madhe')},
'35941357':{'bg': u('\u0417\u0435\u0442\u044c\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Zetyovo, St. Zagora'},
'3325467':{'en': u('Vend\u00f4me'), 'fr': u('Vend\u00f4me')},
'3325460':{'en': u('Ch\u00e2teauroux'), 'fr': u('Ch\u00e2teauroux')},
'3325461':{'en': u('Ch\u00e2teauroux'), 'fr': u('Ch\u00e2teauroux')},
'40337':{'en': 'Vrancea', 'ro': 'Vrancea'},
'40336':{'en': u('Gala\u021bi'), 'ro': u('Gala\u021bi')},
'40335':{'en': 'Vaslui', 'ro': 'Vaslui'},
'40334':{'en': u('Bac\u0103u'), 'ro': u('Bac\u0103u')},
'40333':{'en': u('Neam\u021b'), 'ro': u('Neam\u021b')},
'40332':{'en': u('Ia\u0219i'), 'ro': u('Ia\u0219i')},
'40331':{'en': u('Boto\u0219ani'), 'ro': u('Boto\u0219ani')},
'40330':{'en': 'Suceava', 'ro': 'Suceava'},
'437288':{'de': 'Ulrichsberg', 'en': 'Ulrichsberg'},
'437289':{'de': u('Rohrbach in Ober\u00f6sterreich'), 'en': u('Rohrbach in Ober\u00f6sterreich')},
'3356578':{'en': 'Rodez', 'fr': 'Rodez'},
'40339':{'en': u('Br\u0103ila'), 'ro': u('Br\u0103ila')},
'40338':{'en': u('Buz\u0103u'), 'ro': u('Buz\u0103u')},
'434733':{'de': 'Malta', 'en': 'Malta'},
'434732':{'de': u('Gm\u00fcnd in K\u00e4rnten'), 'en': u('Gm\u00fcnd in K\u00e4rnten')},
'359590':{'bg': u('\u0426\u0430\u0440\u0435\u0432\u043e'), 'en': 'Tsarevo'},
'434736':{'de': 'Innerkrems', 'en': 'Innerkrems'},
'434735':{'de': u('Kremsbr\u00fccke'), 'en': u('Kremsbr\u00fccke')},
'434734':{'de': 'Rennweg', 'en': 'Rennweg'},
'375177':{'be': u('\u0411\u0430\u0440\u044b\u0441\u0430\u045e'), 'en': 'Borisov', 'ru': u('\u0411\u043e\u0440\u0438\u0441\u043e\u0432')},
'375176':{'be': u('\u041c\u0430\u043b\u0430\u0434\u0437\u0435\u0447\u043d\u0430'), 'en': 'Molodechno', 'ru': u('\u041c\u043e\u043b\u043e\u0434\u0435\u0447\u043d\u043e')},
'375174':{'be': u('\u0421\u0430\u043b\u0456\u0433\u043e\u0440\u0441\u043a'), 'en': 'Soligorsk', 'ru': u('\u0421\u043e\u043b\u0438\u0433\u043e\u0440\u0441\u043a')},
'35122':{'en': 'Porto', 'pt': 'Porto'},
'35121':{'en': 'Lisbon', 'pt': 'Lisboa'},
'3593661':{'bg': u('\u0418\u0432\u0430\u0439\u043b\u043e\u0432\u0433\u0440\u0430\u0434'), 'en': 'Ivaylovgrad'},
'3347178':{'en': u('Riom-\u00e9s-Montagnes'), 'fr': u('Riom-\u00e9s-Montagnes')},
'3347174':{'en': 'Brioude', 'fr': 'Brioude'},
'3347175':{'en': 'Monistrol-sur-Loire', 'fr': 'Monistrol-sur-Loire'},
'3347177':{'en': 'Langeac', 'fr': 'Langeac'},
'435284':{'de': 'Gerlos', 'en': 'Gerlos'},
'435285':{'de': 'Mayrhofen', 'en': 'Mayrhofen'},
'3598156':{'bg': u('\u0427\u0435\u0440\u0432\u0435\u043d, \u0420\u0443\u0441\u0435'), 'en': 'Cherven, Ruse'},
'435287':{'de': 'Tux', 'en': 'Tux'},
'3598150':{'bg': u('\u0421\u0435\u043c\u0435\u0440\u0434\u0436\u0438\u0435\u0432\u043e'), 'en': 'Semerdzhievo'},
'3598151':{'bg': u('\u041f\u0440\u043e\u0441\u0435\u043d\u0430'), 'en': 'Prosena'},
'3598152':{'bg': u('\u041a\u0440\u0430\u0441\u0435\u043d, \u0420\u0443\u0441\u0435'), 'en': 'Krasen, Ruse'},
'435283':{'de': 'Kaltenbach', 'en': 'Kaltenbach'},
'3598158':{'bg': u('\u041c\u0435\u0447\u043a\u0430, \u0420\u0443\u0441\u0435'), 'en': 'Mechka, Ruse'},
'3598159':{'bg': u('\u041a\u043e\u0448\u043e\u0432'), 'en': 'Koshov'},
'3338291':{'en': 'Aumetz', 'fr': 'Aumetz'},
'3347719':{'en': 'Saint-Chamond', 'fr': 'Saint-Chamond'},
'436418':{'de': 'Kleinarl', 'en': 'Kleinarl'},
'3347710':{'en': 'Firminy', 'fr': 'Firminy'},
'436415':{'de': 'Schwarzach im Pongau', 'en': 'Schwarzach im Pongau'},
'436416':{'de': 'Lend', 'en': 'Lend'},
'436417':{'de': u('H\u00fcttschlag'), 'en': u('H\u00fcttschlag')},
'433842':{'de': 'Leoben', 'en': 'Leoben'},
'436412':{'de': 'Sankt Johann im Pongau', 'en': 'St. Johann im Pongau'},
'436413':{'de': 'Wagrain', 'en': 'Wagrain'},
'3338125':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3316951':{'en': u('Sainte-Genevi\u00e8ve-des-Bois'), 'fr': u('Sainte-Genevi\u00e8ve-des-Bois')},
'3316952':{'en': 'Montgeron', 'fr': 'Montgeron'},
'3316953':{'en': 'Massy', 'fr': 'Massy'},
'3338121':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3316956':{'en': 'Juvisy-sur-Orge', 'fr': 'Juvisy-sur-Orge'},
'3316957':{'en': 'Athis-Mons', 'fr': 'Athis-Mons'},
'433844':{'de': 'Kammern im Liesingtal', 'en': 'Kammern im Liesingtal'},
'437484':{'de': u('G\u00f6stling an der Ybbs'), 'en': u('G\u00f6stling an der Ybbs')},
'4171':{'de': 'St. Gallen', 'en': 'St. Gallen', 'fr': 'St. Gall', 'it': 'San Gallo'},
'433847':{'de': 'Trofaiach', 'en': 'Trofaiach'},
'35991668':{'bg': u('\u041c\u0430\u043d\u0430\u0441\u0442\u0438\u0440\u0438\u0449\u0435'), 'en': 'Manastirishte'},
'35961108':{'bg': u('\u041f\u043b\u0430\u043a\u043e\u0432\u043e'), 'en': 'Plakovo'},
'3597428':{'bg': u('\u0413\u0430\u0431\u0440\u0435\u043d\u0435'), 'en': 'Gabrene'},
'3597939':{'bg': u('\u0426\u044a\u0440\u0432\u0430\u0440\u0438\u0446\u0430'), 'en': 'Tsarvaritsa'},
'3597938':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u0423\u0439\u043d\u043e'), 'en': 'Dolno Uyno'},
'3597425':{'bg': u('\u041a\u0443\u043b\u0430\u0442\u0430'), 'en': 'Kulata'},
'3597424':{'bg': u('\u041a\u044a\u0440\u043d\u0430\u043b\u043e\u0432\u043e'), 'en': 'Karnalovo'},
'3597427':{'bg': u('\u041f\u044a\u0440\u0432\u043e\u043c\u0430\u0439, \u0411\u043b\u0430\u0433.'), 'en': 'Parvomay, Blag.'},
'3597426':{'bg': u('\u041c\u0430\u0440\u0438\u043a\u043e\u0441\u0442\u0438\u043d\u043e\u0432\u043e'), 'en': 'Marikostinovo'},
'3597933':{'bg': u('\u0413\u0440\u0430\u043c\u0430\u0436\u0434\u0430\u043d\u043e'), 'en': 'Gramazhdano'},
'3597932':{'bg': u('\u0428\u0438\u043f\u043e\u0447\u0430\u043d\u043e'), 'en': 'Shipochano'},
'3597423':{'bg': u('\u041a\u043e\u043b\u0430\u0440\u043e\u0432\u043e, \u0411\u043b\u0430\u0433.'), 'en': 'Kolarovo, Blag.'},
'3597422':{'bg': u('\u0422\u043e\u043f\u043e\u043b\u043d\u0438\u0446\u0430, \u0411\u043b\u0430\u0433.'), 'en': 'Topolnitsa, Blag.'},
'35967309':{'bg': u('\u0410\u0433\u0430\u0442\u043e\u0432\u043e'), 'en': 'Agatovo'},
'35967308':{'bg': u('\u041b\u043e\u0432\u043d\u0438\u0434\u043e\u043b'), 'en': 'Lovnidol'},
'35967307':{'bg': u('\u041c\u043b\u0435\u0447\u0435\u0432\u043e'), 'en': 'Mlechevo'},
'35967306':{'bg': u('\u0413\u0440\u0430\u0434\u0438\u0449\u0435, \u0413\u0430\u0431\u0440.'), 'en': 'Gradishte, Gabr.'},
'35967305':{'bg': u('\u0421\u0442\u043e\u043a\u0438\u0442\u0435'), 'en': 'Stokite'},
'35967304':{'bg': u('\u041a\u0440\u0430\u043c\u043e\u043b\u0438\u043d'), 'en': 'Kramolin'},
'35967303':{'bg': u('\u0411\u0430\u0442\u043e\u0448\u0435\u0432\u043e'), 'en': 'Batoshevo'},
'35967302':{'bg': u('\u041a\u0440\u044a\u0432\u0435\u043d\u0438\u043a'), 'en': 'Kravenik'},
'35967301':{'bg': u('\u0418\u0434\u0438\u043b\u0435\u0432\u043e'), 'en': 'Idilevo'},
'3323198':{'en': 'Deauville', 'fr': 'Deauville'},
'3323190':{'en': 'Falaise', 'fr': 'Falaise'},
'3323191':{'en': 'Cabourg', 'fr': 'Cabourg'},
'3323192':{'en': 'Bayeux', 'fr': 'Bayeux'},
'3323193':{'en': 'Caen', 'fr': 'Caen'},
'3323194':{'en': 'Caen', 'fr': 'Caen'},
'3323195':{'en': u('H\u00e9rouville-Saint-Clair'), 'fr': u('H\u00e9rouville-Saint-Clair')},
'3323196':{'en': 'Ouistreham', 'fr': 'Ouistreham'},
'3323197':{'en': 'Ouistreham', 'fr': 'Ouistreham'},
'3329765':{'en': 'Languidic', 'fr': 'Languidic'},
'3329764':{'en': 'Lorient', 'fr': 'Lorient'},
'3329766':{'en': 'Grand-Champ', 'fr': 'Grand-Champ'},
'35947356':{'bg': u('\u041c\u0440\u0430\u043c\u043e\u0440, \u042f\u043c\u0431\u043e\u043b'), 'en': 'Mramor, Yambol'},
'3329763':{'en': 'Vannes', 'fr': 'Vannes'},
'3329762':{'en': 'Vannes', 'fr': 'Vannes'},
'3329769':{'en': 'Vannes', 'fr': 'Vannes'},
'3329768':{'en': 'Vannes', 'fr': 'Vannes'},
'3323336':{'en': 'Argentan', 'fr': 'Argentan'},
'3329839':{'en': 'Bannalec', 'fr': 'Bannalec'},
'3355679':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3323332':{'en': u('Alen\u00e7on'), 'fr': u('Alen\u00e7on')},
'3355902':{'en': 'Pau', 'fr': 'Pau'},
'3355903':{'en': 'Anglet', 'fr': 'Anglet'},
'3329837':{'en': 'Plabennec', 'fr': 'Plabennec'},
'3355901':{'en': 'Biarritz', 'fr': 'Biarritz'},
'3355670':{'en': u('Saint-M\u00e9dard-en-Jalles'), 'fr': u('Saint-M\u00e9dard-en-Jalles')},
'3329830':{'en': 'Landerneau', 'fr': 'Landerneau'},
'3329833':{'en': 'Brest', 'fr': 'Brest'},
'3329832':{'en': 'Saint-Renan', 'fr': 'Saint-Renan'},
'3349244':{'en': 'Savines-le-Lac', 'fr': 'Savines-le-Lac'},
'3349245':{'en': 'Guillestre', 'fr': 'Guillestre'},
'3349247':{'en': 'Nice', 'fr': 'Nice'},
'3349241':{'en': 'Menton', 'fr': 'Menton'},
'3349242':{'en': 'Grasse', 'fr': 'Grasse'},
'3349243':{'en': 'Embrun', 'fr': 'Embrun'},
'355894':{'en': u('Livadhja/Dhiv\u00ebr, Sarand\u00eb')},
'355895':{'en': u('Finiq/Mesopotam/Vergo, Delvin\u00eb')},
'355892':{'en': u('Aliko/Lukov\u00eb, Sarand\u00eb')},
'3349249':{'en': 'Gap', 'fr': 'Gap'},
'355891':{'en': u('Konispol/Xare/Markat, Sarand\u00eb')},
'4415243':{'en': 'Lancaster'},
'4415242':{'en': 'Hornby'},
'4415241':{'en': 'Lancaster'},
'37424973':{'am': u('\u053f\u0561\u0569\u0576\u0561\u0572\u0562\u0575\u0578\u0582\u0580'), 'en': 'Katnaghbyur', 'ru': u('\u041a\u0430\u0442\u043d\u0430\u0445\u0431\u044e\u0440')},
'4415247':{'en': 'Lancaster'},
'4415246':{'en': 'Lancaster'},
'4415245':{'en': 'Lancaster'},
'4415244':{'en': 'Lancaster'},
'4415249':{'en': 'Lancaster'},
'4415248':{'en': 'Lancaster'},
'3751797':{'be': u('\u041c\u044f\u0434\u0437\u0435\u043b'), 'en': 'Myadel', 'ru': u('\u041c\u044f\u0434\u0435\u043b\u044c')},
'3751796':{'be': u('\u041a\u0440\u0443\u043f\u043a\u0456, \u041c\u0456\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Krupki, Minsk Region', 'ru': u('\u041a\u0440\u0443\u043f\u043a\u0438, \u041c\u0438\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751795':{'be': u('\u0421\u043b\u0443\u0446\u043a'), 'en': 'Slutsk', 'ru': u('\u0421\u043b\u0443\u0446\u043a')},
'3751794':{'be': u('\u041b\u044e\u0431\u0430\u043d\u044c, \u041c\u0456\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Lyuban, Minsk Region', 'ru': u('\u041b\u044e\u0431\u0430\u043d\u044c, \u041c\u0438\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751793':{'be': u('\u041a\u043b\u0435\u0446\u043a, \u041c\u0456\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Kletsk, Minsk Region', 'ru': u('\u041a\u043b\u0435\u0446\u043a, \u041c\u0438\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751792':{'be': u('\u0421\u0442\u0430\u0440\u044b\u044f \u0414\u0430\u0440\u043e\u0433\u0456, \u041c\u0456\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Starye Dorogi, Minsk Region', 'ru': u('\u0421\u0442\u0430\u0440\u044b\u0435 \u0414\u043e\u0440\u043e\u0433\u0438, \u041c\u0438\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'441830':{'en': 'Kirkwhelpington'},
'441832':{'en': 'Clopton'},
'441833':{'en': 'Barnard Castle'},
'441834':{'en': 'Narberth'},
'441835':{'en': 'St Boswells'},
'441837':{'en': 'Okehampton'},
'3322310':{'en': u('P\u00e9nestin'), 'fr': u('P\u00e9nestin')},
'3322315':{'en': 'Cancale', 'fr': 'Cancale'},
'437618':{'de': u('Neukirchen, Altm\u00fcnster'), 'en': u('Neukirchen, Altm\u00fcnster')},
'3322316':{'en': 'Combourg', 'fr': 'Combourg'},
'437615':{'de': 'Scharnstein', 'en': 'Scharnstein'},
'3322318':{'en': 'Saint-Malo', 'fr': 'Saint-Malo'},
'437617':{'de': 'Traunkirchen', 'en': 'Traunkirchen'},
'437616':{'de': u('Gr\u00fcnau im Almtal'), 'en': u('Gr\u00fcnau im Almtal')},
'3332804':{'en': 'Lille', 'fr': 'Lille'},
'437613':{'de': 'Laakirchen', 'en': 'Laakirchen'},
'3332807':{'en': 'Lille', 'fr': 'Lille'},
'437260':{'de': 'Waldhausen', 'en': 'Waldhausen'},
'3324029':{'en': 'Nantes', 'fr': 'Nantes'},
'437261':{'de': u('Sch\u00f6nau im M\u00fchlkreis'), 'en': u('Sch\u00f6nau im M\u00fchlkreis')},
'3593927':{'bg': u('\u0427\u0435\u0440\u043d\u043e\u0433\u043e\u0440\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Chernogorovo, Hask.'},
'3315673':{'en': 'Sucy-en-Brie', 'fr': 'Sucy-en-Brie'},
'3752354':{'be': u('\u0415\u043b\u044c\u0441\u043a, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Yelsk, Gomel Region', 'ru': u('\u0415\u043b\u044c\u0441\u043a, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'437264':{'de': 'Windhaag bei Perg', 'en': 'Windhaag bei Perg'},
'435337':{'de': 'Brixlegg', 'en': 'Brixlegg'},
'3752133':{'be': u('\u0427\u0430\u0448\u043d\u0456\u043a\u0456, \u0412\u0456\u0446\u0435\u0431\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Chashniki, Vitebsk Region', 'ru': u('\u0427\u0430\u0448\u043d\u0438\u043a\u0438, \u0412\u0438\u0442\u0435\u0431\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'437268':{'de': 'Grein', 'en': 'Grein'},
'3315679':{'en': 'Paris', 'fr': 'Paris'},
'3752136':{'be': u('\u0422\u0430\u043b\u0430\u0447\u044b\u043d'), 'en': 'Tolochin', 'ru': u('\u0422\u043e\u043b\u043e\u0447\u0438\u043d')},
'359971':{'bg': u('\u041b\u043e\u043c'), 'en': 'Lom'},
'359973':{'bg': u('\u041a\u043e\u0437\u043b\u043e\u0434\u0443\u0439'), 'en': 'Kozloduy'},
'434236':{'de': 'Eberndorf', 'en': 'Eberndorf'},
'432774':{'de': 'Innermanzing', 'en': 'Innermanzing'},
'3356598':{'en': 'Saint-Affrique', 'fr': 'Saint-Affrique'},
'3356597':{'en': 'Saint-Affrique', 'fr': 'Saint-Affrique'},
'432772':{'de': 'Neulengbach', 'en': 'Neulengbach'},
'3325320':{'en': 'Angers', 'fr': 'Angers'},
'3347549':{'en': 'Le Teil', 'fr': 'Le Teil'},
'3343849':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347545':{'en': 'Saint-Donat-sur-l\'Herbasse', 'fr': 'Saint-Donat-sur-l\'Herbasse'},
'3347544':{'en': 'Valence', 'fr': 'Valence'},
'3347541':{'en': 'Valence', 'fr': 'Valence'},
'3347540':{'en': 'Valence', 'fr': 'Valence'},
'3347543':{'en': 'Valence', 'fr': 'Valence'},
'3347542':{'en': 'Valence', 'fr': 'Valence'},
'3334402':{'en': 'Beauvais', 'fr': 'Beauvais'},
'3334403':{'en': 'Noailles', 'fr': 'Noailles'},
'3334406':{'en': 'Beauvais', 'fr': 'Beauvais'},
'3334407':{'en': 'Bresles', 'fr': 'Bresles'},
'3334405':{'en': 'Beauvais', 'fr': 'Beauvais'},
'3334409':{'en': 'Noyon', 'fr': 'Noyon'},
'3599162':{'bg': u('\u041c\u0438\u0445\u0430\u0439\u043b\u043e\u0432\u043e, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Mihaylovo, Vratsa'},
'3599163':{'bg': u('\u0425\u044a\u0440\u043b\u0435\u0446'), 'en': 'Harlets'},
'3599160':{'bg': u('\u0413\u043b\u043e\u0436\u0435\u043d\u0435, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Glozhene, Vratsa'},
'3599161':{'bg': u('\u041c\u0438\u0437\u0438\u044f'), 'en': 'Mizia'},
'3599166':{'bg': u('\u0425\u0430\u0439\u0440\u0435\u0434\u0438\u043d'), 'en': 'Hayredin'},
'3599167':{'bg': u('\u041b\u0438\u043f\u043d\u0438\u0446\u0430, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Lipnitsa, Vratsa'},
'3599164':{'bg': u('\u041a\u0440\u0443\u0448\u043e\u0432\u0438\u0446\u0430, \u0412\u0440\u0430\u0446\u0430'), 'en': 'Krushovitsa, Vratsa'},
'3599165':{'bg': u('\u0421\u043e\u0444\u0440\u043e\u043d\u0438\u0435\u0432\u043e'), 'en': 'Sofronievo'},
'3599168':{'bg': u('\u0411\u0443\u0442\u0430\u043d'), 'en': 'Butan'},
'3599169':{'bg': u('\u0420\u043e\u0433\u043e\u0437\u0435\u043d'), 'en': 'Rogozen'},
'3323730':{'en': 'Chartres', 'fr': 'Chartres'},
'3353414':{'en': 'Saint-Girons', 'fr': 'Saint-Girons'},
'3323731':{'en': 'Auneau', 'fr': 'Auneau'},
'35374':{'en': 'Letterkenny/Donegal/Dungloe/Buncrana'},
'441581':{'en': 'New Luce'},
'441582':{'en': 'Luton'},
'3323733':{'en': 'Chartres', 'fr': 'Chartres'},
'441584':{'en': 'Ludlow'},
'441586':{'en': 'Campbeltown'},
'441588':{'en': 'Bishops Castle'},
'3323734':{'en': 'Chartres', 'fr': 'Chartres'},
'3323735':{'en': 'Chartres', 'fr': 'Chartres'},
'3323736':{'en': 'Chartres', 'fr': 'Chartres'},
'40243':{'en': u('Ialomi\u021ba'), 'ro': u('Ialomi\u021ba')},
'34827':{'en': u('C\u00e1ceres'), 'es': u('C\u00e1ceres')},
'40242':{'en': u('C\u0103l\u0103ra\u0219i'), 'ro': u('C\u0103l\u0103ra\u0219i')},
'434233':{'de': 'Griffen', 'en': 'Griffen'},
'40240':{'en': 'Tulcea', 'ro': 'Tulcea'},
'433339':{'de': 'Friedberg', 'en': 'Friedberg'},
'3347818':{'en': 'Lyon', 'fr': 'Lyon'},
'3347817':{'en': 'Lyon', 'fr': 'Lyon'},
'3347816':{'en': 'Brindas', 'fr': 'Brindas'},
'3347814':{'en': 'Lyon', 'fr': 'Lyon'},
'434230':{'de': 'Globasnitz', 'en': 'Globasnitz'},
'3593693':{'bg': u('\u041b\u044f\u0441\u043a\u043e\u0432\u043e, \u041a\u044a\u0440\u0434\u0436.'), 'en': 'Lyaskovo, Kardzh.'},
'3346638':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346639':{'en': 'Pont-Saint-Esprit', 'fr': 'Pont-Saint-Esprit'},
'3346634':{'en': 'La Grand Combe', 'fr': 'La Grand Combe'},
'359777':{'bg': u('\u0420\u0430\u0434\u043e\u043c\u0438\u0440'), 'en': 'Radomir'},
'3346636':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3346637':{'en': 'Remoulins', 'fr': 'Remoulins'},
'3346630':{'en': u('Al\u00e8s'), 'fr': u('Al\u00e8s')},
'3346631':{'en': u('Saint-Ch\u00e9ly-d\'Apcher'), 'fr': u('Saint-Ch\u00e9ly-d\'Apcher')},
'3346632':{'en': 'Marvejols', 'fr': 'Marvejols'},
'3346633':{'en': u('Bagnols-sur-C\u00e8ze'), 'fr': u('Bagnols-sur-C\u00e8ze')},
'3522476':{'de': 'Wormeldingen', 'en': 'Wormeldange', 'fr': 'Wormeldange'},
'3597745':{'bg': u('\u0415\u0433\u044a\u043b\u043d\u0438\u0446\u0430'), 'en': 'Egalnitsa'},
'3522474':{'de': 'Wasserbillig', 'en': 'Wasserbillig', 'fr': 'Wasserbillig'},
'3522475':{'de': 'Distrikt Grevenmacher-sur-Moselle', 'en': 'Grevenmacher-sur-Moselle', 'fr': 'Grevenmacher-sur-Moselle'},
'3522472':{'de': 'Echternach', 'en': 'Echternach', 'fr': 'Echternach'},
'3522473':{'de': 'Rosport', 'en': 'Rosport', 'fr': 'Rosport'},
'3597742':{'bg': u('\u041a\u0430\u043b\u0438\u0449\u0435'), 'en': 'Kalishte'},
'3522471':{'de': 'Betzdorf', 'en': 'Betzdorf', 'fr': 'Betzdorf'},
'3522478':{'de': 'Junglinster', 'en': 'Junglinster', 'fr': 'Junglinster'},
'3522479':{'de': 'Berdorf/Consdorf', 'en': 'Berdorf/Consdorf', 'fr': 'Berdorf/Consdorf'},
'37426796':{'am': u('\u0544\u0578\u057d\u0565\u057d\u0563\u0565\u0572'), 'en': 'Mosesgegh', 'ru': u('\u041c\u043e\u0441\u0435\u0441\u0433\u0435\u0445')},
'3355924':{'en': 'Biarritz', 'fr': 'Biarritz'},
'437285':{'de': u('Hofkirchen im M\u00fchlkreis'), 'en': u('Hofkirchen im M\u00fchlkreis')},
'3522652':{'de': u('D\u00fcdelingen'), 'en': 'Dudelange', 'fr': 'Dudelange'},
'3522653':{'de': 'Esch-sur-Alzette', 'en': 'Esch-sur-Alzette', 'fr': 'Esch-sur-Alzette'},
'3522650':{'de': 'Bascharage/Petingen/Rodingen', 'en': 'Bascharage/Petange/Rodange', 'fr': 'Bascharage/Petange/Rodange'},
'3522651':{'de': u('D\u00fcdelingen/Bettemburg/Livingen'), 'en': 'Dudelange/Bettembourg/Livange', 'fr': 'Dudelange/Bettembourg/Livange'},
'3522656':{'de': u('R\u00fcmelingen'), 'en': 'Rumelange', 'fr': 'Rumelange'},
'3522657':{'de': 'Esch-sur-Alzette/Schifflingen', 'en': 'Esch-sur-Alzette/Schifflange', 'fr': 'Esch-sur-Alzette/Schifflange'},
'3522654':{'de': 'Esch-sur-Alzette', 'en': 'Esch-sur-Alzette', 'fr': 'Esch-sur-Alzette'},
'3522655':{'de': 'Esch-sur-Alzette/Monnerich', 'en': 'Esch-sur-Alzette/Mondercange', 'fr': 'Esch-sur-Alzette/Mondercange'},
'3522658':{'de': 'Soleuvre/Differdingen', 'en': 'Soleuvre/Differdange', 'fr': 'Soleuvre/Differdange'},
'3522659':{'de': 'Soleuvre', 'en': 'Soleuvre', 'fr': 'Soleuvre'},
'3742363':{'am': u('\u0531\u0575\u0576\u0569\u0561\u057a'), 'en': 'Aintab', 'ru': u('\u0410\u0439\u043d\u0442\u0430\u043f')},
'441299':{'en': 'Bewdley'},
'3338929':{'en': 'Colmar', 'fr': 'Colmar'},
'3338437':{'en': 'Poligny', 'fr': 'Poligny'},
'3338924':{'en': 'Colmar', 'fr': 'Colmar'},
'3338927':{'en': 'Wintzenheim', 'fr': 'Wintzenheim'},
'3338433':{'en': 'Morez', 'fr': 'Morez'},
'3338920':{'en': 'Colmar', 'fr': 'Colmar'},
'3338923':{'en': 'Colmar', 'fr': 'Colmar'},
'3338922':{'en': 'Sainte-Croix-en-Plaine', 'fr': 'Sainte-Croix-en-Plaine'},
'3323838':{'en': 'Gien', 'fr': 'Gien'},
'3323833':{'en': 'Puiseaux', 'fr': 'Puiseaux'},
'3323831':{'en': 'Briare', 'fr': 'Briare'},
'3323830':{'en': 'Pithiviers', 'fr': 'Pithiviers'},
'3323837':{'en': 'Briare', 'fr': 'Briare'},
'3323836':{'en': 'Sully-sur-Loire', 'fr': 'Sully-sur-Loire'},
'3323835':{'en': u('Saint-Beno\u00eet-sur-Loire'), 'fr': u('Saint-Beno\u00eet-sur-Loire')},
'3323834':{'en': 'Malesherbes', 'fr': 'Malesherbes'},
'3358295':{'en': 'Toulouse', 'fr': 'Toulouse'},
'433333':{'de': 'Sebersdorf', 'en': 'Sebersdorf'},
'3348671':{'en': 'Carpentras', 'fr': 'Carpentras'},
'3346858':{'en': 'Narbonne', 'fr': 'Narbonne'},
'3346859':{'en': 'Saint-Paul-de-Fenouillet', 'fr': 'Saint-Paul-de-Fenouillet'},
'3346850':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3346851':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3346852':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3346853':{'en': 'Thuir', 'fr': 'Thuir'},
'3346854':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3346855':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3346856':{'en': 'Perpignan', 'fr': 'Perpignan'},
'3346857':{'en': 'Millas', 'fr': 'Millas'},
'390781':{'it': 'Iglesias'},
'390783':{'en': 'Oristano', 'it': 'Oristano'},
'390782':{'it': 'Lanusei'},
'390785':{'it': 'Macomer'},
'390784':{'it': 'Nuoro'},
'390789':{'en': 'Sassari', 'it': 'Olbia'},
'3348905':{'en': 'Nice', 'fr': 'Nice'},
'3348900':{'en': 'Nice', 'fr': 'Nice'},
'3348902':{'en': 'Antibes', 'fr': 'Antibes'},
'3348903':{'en': 'Nice', 'fr': 'Nice'},
'3593115':{'bg': u('\u041a\u0443\u043a\u043b\u0435\u043d'), 'en': 'Kuklen'},
'3593114':{'bg': u('\u0411\u0440\u0435\u0441\u0442\u043d\u0438\u043a'), 'en': 'Brestnik'},
'3593117':{'bg': u('\u041a\u0430\u0442\u0443\u043d\u0438\u0446\u0430'), 'en': 'Katunitsa'},
'3593116':{'bg': u('\u041a\u0440\u0443\u043c\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Krumovo, Plovdiv'},
'34859':{'en': 'Huelva', 'es': 'Huelva'},
'34858':{'en': 'Granada', 'es': 'Granada'},
'3593113':{'bg': u('\u0411\u0440\u0430\u043d\u0438\u043f\u043e\u043b\u0435'), 'en': 'Branipole'},
'3345010':{'en': 'Annecy', 'fr': 'Annecy'},
'34854':{'en': 'Seville', 'es': 'Sevilla'},
'34857':{'en': 'Cordova', 'es': u('C\u00f3rdoba')},
'34856':{'en': u('C\u00e1diz'), 'es': u('C\u00e1diz')},
'3593119':{'bg': u('\u0413\u044a\u043b\u044a\u0431\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Galabovo, Plovdiv'},
'3593118':{'bg': u('\u0421\u0430\u0434\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Sadovo, Plovdiv'},
'34853':{'en': u('Ja\u00e9n'), 'es': u('Ja\u00e9n')},
'3332129':{'en': 'Bully-les-Mines', 'fr': 'Bully-les-Mines'},
'3332128':{'en': 'Lens', 'fr': 'Lens'},
'3332655':{'en': u('\u00c9pernay'), 'fr': u('\u00c9pernay')},
'3332654':{'en': u('\u00c9pernay'), 'fr': u('\u00c9pernay')},
'3332656':{'en': u('\u00c9pernay'), 'fr': u('\u00c9pernay')},
'3332123':{'en': 'Arras', 'fr': 'Arras'},
'3332650':{'en': 'Reims', 'fr': 'Reims'},
'3332121':{'en': 'Arras', 'fr': 'Arras'},
'3332120':{'en': u('H\u00e9nin-Beaumont'), 'fr': u('H\u00e9nin-Beaumont')},
'3325408':{'en': u('Ch\u00e2teauroux'), 'fr': u('Ch\u00e2teauroux')},
'35951104':{'bg': u('\u0414\u043e\u043b\u0438\u0449\u0435, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Dolishte, Varna'},
'3325400':{'en': u('Valen\u00e7ay'), 'fr': u('Valen\u00e7ay')},
'3325401':{'en': 'Argenton-sur-Creuse', 'fr': 'Argenton-sur-Creuse'},
'3325402':{'en': u('Buzan\u00e7ais'), 'fr': u('Buzan\u00e7ais')},
'3325403':{'en': 'Issoudun', 'fr': 'Issoudun'},
'35951106':{'bg': u('\u041e\u0441\u0435\u043d\u043e\u0432\u043e, \u0412\u0430\u0440\u043d\u0430'), 'en': 'Osenovo, Varna'},
'3325406':{'en': 'Aigurande', 'fr': 'Aigurande'},
'3325407':{'en': u('Ch\u00e2teauroux'), 'fr': u('Ch\u00e2teauroux')},
'390125':{'en': 'Turin', 'it': 'Ivrea'},
'390124':{'it': 'Rivarolo Canavese'},
'3349505':{'en': 'Marseille', 'fr': 'Marseille'},
'390121':{'it': 'Pinerolo'},
'390123':{'it': 'Lanzo Torinese'},
'390122':{'en': 'Turin', 'it': 'Susa'},
'3349508':{'en': 'Marseille', 'fr': 'Marseille'},
'3358197':{'en': 'Toulouse', 'fr': 'Toulouse'},
'433127':{'de': 'Peggau', 'en': 'Peggau'},
'433126':{'de': 'Frohnleiten', 'en': 'Frohnleiten'},
'433125':{'de': u('\u00dcbelbach'), 'en': u('\u00dcbelbach')},
'433124':{'de': 'Gratkorn', 'en': 'Gratkorn'},
'433123':{'de': 'Sankt Oswald bei Plankenwarth', 'en': 'St. Oswald bei Plankenwarth'},
'37423779':{'am': u('\u0532\u0561\u0574\u0562\u0561\u056f\u0561\u0577\u0561\u057f'), 'en': 'Bambakashat', 'ru': u('\u0411\u0430\u043c\u0431\u0430\u043a\u0430\u0448\u0430\u0442')},
'3356293':{'en': 'Tarbes', 'fr': 'Tarbes'},
'3356292':{'en': 'Cauterets', 'fr': 'Cauterets'},
'3356291':{'en': u('Bagn\u00e8res-de-Bigorre'), 'fr': u('Bagn\u00e8res-de-Bigorre')},
'3356290':{'en': 'Ibos', 'fr': 'Ibos'},
'3356297':{'en': u('Argel\u00e8s-Gazost'), 'fr': u('Argel\u00e8s-Gazost')},
'3356295':{'en': u('Bagn\u00e8res-de-Bigorre'), 'fr': u('Bagn\u00e8res-de-Bigorre')},
'3356294':{'en': 'Lourdes', 'fr': 'Lourdes'},
'3356298':{'en': 'Lannemezan', 'fr': 'Lannemezan'},
'35959694':{'bg': u('\u0413\u0430\u0431\u0435\u0440\u043e\u0432\u043e'), 'en': 'Gaberovo'},
'3347489':{'en': 'Amplepuis', 'fr': 'Amplepuis'},
'35971228':{'bg': u('\u041c\u0430\u0440\u0438\u0446\u0430'), 'en': 'Maritsa'},
'3595519':{'bg': u('\u0417\u0438\u0434\u0430\u0440\u043e\u0432\u043e'), 'en': 'Zidarovo'},
'3595518':{'bg': u('\u0420\u0443\u0434\u043d\u0438\u043a, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Rudnik, Burgas'},
'3347480':{'en': 'Morestel', 'fr': 'Morestel'},
'3347481':{'en': 'Oyonnax', 'fr': 'Oyonnax'},
'3347482':{'en': 'Saint-Quentin-Fallavier', 'fr': 'Saint-Quentin-Fallavier'},
'35971220':{'bg': u('\u0413\u0443\u0446\u0430\u043b'), 'en': 'Gutsal'},
'3595513':{'bg': u('\u0413\u0430\u0431\u044a\u0440'), 'en': 'Gabar'},
'3347485':{'en': 'Vienne', 'fr': 'Vienne'},
'3347486':{'en': 'Roussillon', 'fr': 'Roussillon'},
'3347487':{'en': u('P\u00e9lussin'), 'fr': u('P\u00e9lussin')},
'3324757':{'en': 'Amboise', 'fr': 'Amboise'},
'3324755':{'en': 'Luynes', 'fr': 'Luynes'},
'3324754':{'en': 'Tours', 'fr': 'Tours'},
'3324753':{'en': u('Jou\u00e9-l\u00e8s-Tours'), 'fr': u('Jou\u00e9-l\u00e8s-Tours')},
'3324752':{'en': 'Vouvray', 'fr': 'Vouvray'},
'3324751':{'en': 'Tours', 'fr': 'Tours'},
'3324750':{'en': 'Montlouis-sur-Loire', 'fr': 'Montlouis-sur-Loire'},
'435266':{'de': u('\u00d6tztal-Bahnhof'), 'en': u('\u00d6tztal-Bahnhof')},
'3595153':{'bg': u('\u0421\u0443\u0432\u043e\u0440\u043e\u0432\u043e'), 'en': 'Suvorovo'},
'435264':{'de': 'Mieming', 'en': 'Mieming'},
'435265':{'de': 'Nassereith', 'en': 'Nassereith'},
'435262':{'de': 'Telfs', 'en': 'Telfs'},
'435263':{'de': 'Silz', 'en': 'Silz'},
'3324759':{'en': 'Loches', 'fr': 'Loches'},
'3344283':{'en': 'La Ciotat', 'fr': 'La Ciotat'},
'3344282':{'en': 'Aubagne', 'fr': 'Aubagne'},
'3344281':{'en': 'Martigues', 'fr': 'Martigues'},
'3344280':{'en': 'Martigues', 'fr': 'Martigues'},
'3344287':{'en': 'Rognac', 'fr': 'Rognac'},
'3344286':{'en': u('Port-Saint-Louis-du-Rh\u00f4ne'), 'fr': u('Port-Saint-Louis-du-Rh\u00f4ne')},
'3344285':{'en': u('Berre-l\'\u00c9tang'), 'fr': u('Berre-l\'\u00c9tang')},
'3344284':{'en': 'Aubagne', 'fr': 'Aubagne'},
'3344289':{'en': 'Vitrolles', 'fr': 'Vitrolles'},
'3344288':{'en': 'Marignane', 'fr': 'Marignane'},
'3347224':{'en': 'Givors', 'fr': 'Givors'},
'35818':{'en': u('\u00c5land Islands'), 'fi': 'Ahvenanmaa', 'se': u('\u00c5land')},
'35819':{'en': 'Nylandia', 'fi': 'Uusimaa', 'se': 'Nyland'},
'35813':{'en': 'North Karelia', 'fi': 'Pohjois-Karjala', 'se': 'Norra Karelen'},
'35816':{'en': 'Lapland', 'fi': 'Lappi', 'se': 'Lappland'},
'35817':{'en': 'Kuopio', 'fi': 'Kuopio', 'se': 'Kuopio'},
'35814':{'en': 'Central Finland', 'fi': 'Keski-Suomi', 'se': 'Mellersta Finland'},
'35815':{'en': 'Mikkeli', 'fi': 'Mikkeli', 'se': 'St Michel'},
'3347220':{'en': 'Lyon', 'fr': 'Lyon'},
'3347593':{'en': 'Aubenas', 'fr': 'Aubenas'},
'331820':{'en': 'Paris', 'fr': 'Paris'},
'3316978':{'en': u('\u00c9tampes'), 'fr': u('\u00c9tampes')},
'3316979':{'en': 'Chilly-Mazarin', 'fr': 'Chilly-Mazarin'},
'3347223':{'en': 'Saint-Priest', 'fr': 'Saint-Priest'},
'3316975':{'en': 'Massy', 'fr': 'Massy'},
'4152':{'de': 'Winterthur', 'en': 'Winterthur', 'fr': 'Winterthour', 'it': 'Winterthur'},
'4156':{'de': 'Baden', 'en': 'Baden', 'fr': 'Baden', 'it': 'Baden'},
'4155':{'de': 'Rapperswil', 'en': 'Rapperswil', 'fr': 'Rapperswil', 'it': 'Rapperswil'},
'3347156':{'en': 'Yssingeaux', 'fr': 'Yssingeaux'},
'3347150':{'en': 'Brioude', 'fr': 'Brioude'},
'3347159':{'en': 'Yssingeaux', 'fr': 'Yssingeaux'},
'390744':{'it': 'Terni'},
'38948':{'en': 'Prilep/Krusevo'},
'38943':{'en': 'Veles/Kavadarci/Negotino'},
'38942':{'en': 'Gostivar'},
'38947':{'en': 'Bitola/Demir Hisar/Resen'},
'38946':{'en': 'Ohrid/Struga/Debar'},
'38945':{'en': 'Kicevo/Makedonski Brod'},
'38944':{'en': 'Tetovo'},
'3347732':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347733':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347730':{'en': 'Sury-le-Comtal', 'fr': 'Sury-le-Comtal'},
'3347731':{'en': 'Saint-Chamond', 'fr': 'Saint-Chamond'},
'3347736':{'en': u('Andr\u00e9zieux-Bouth\u00e9on'), 'fr': u('Andr\u00e9zieux-Bouth\u00e9on')},
'3347737':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347734':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347735':{'en': 'Aurec-sur-Loire', 'fr': 'Aurec-sur-Loire'},
'3597911':{'bg': u('\u0413\u0440\u0430\u043d\u0438\u0446\u0430'), 'en': 'Granitsa'},
'3597910':{'bg': u('\u0411\u0435\u0440\u0441\u0438\u043d'), 'en': 'Bersin'},
'3347738':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347739':{'en': 'Bourg-Argental', 'fr': 'Bourg-Argental'},
'3597915':{'bg': u('\u041d\u0435\u0432\u0435\u0441\u0442\u0438\u043d\u043e, \u041a\u044e\u0441\u0442.'), 'en': 'Nevestino, Kyust.'},
'3597914':{'bg': u('\u0412\u0430\u043a\u0441\u0435\u0432\u043e'), 'en': 'Vaksevo'},
'3597917':{'bg': u('\u0422\u0430\u0432\u0430\u043b\u0438\u0447\u0435\u0432\u043e'), 'en': 'Tavalichevo'},
'3597916':{'bg': u('\u0411\u0430\u0433\u0440\u0435\u043d\u0446\u0438'), 'en': 'Bagrentsi'},
'390746':{'it': 'Rieti'},
'3596359':{'bg': u('\u0413\u043b\u0430\u0432\u0430'), 'en': 'Glava'},
'3596352':{'bg': u('\u0414\u043e\u043b\u043d\u0438 \u041b\u0443\u043a\u043e\u0432\u0438\u0442'), 'en': 'Dolni Lukovit'},
'3596356':{'bg': u('\u041a\u043e\u043c\u0430\u0440\u0435\u0432\u043e, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Komarevo, Pleven'},
'3596357':{'bg': u('\u042f\u0441\u0435\u043d, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Yasen, Pleven'},
'359631':{'bg': u('\u0421\u0432\u0438\u0449\u043e\u0432'), 'en': 'Svishtov'},
'39079':{'en': 'Sassari', 'it': 'Sassari'},
'3752339':{'be': u('\u0420\u0430\u0433\u0430\u0447\u043e\u045e'), 'en': 'Rogachev', 'ru': u('\u0420\u043e\u0433\u0430\u0447\u0435\u0432')},
'37425251':{'am': u('\u0554\u0578\u0582\u0579\u0561\u056f'), 'en': 'Kuchak', 'ru': u('\u041a\u0443\u0447\u0430\u043a')},
'3752334':{'be': u('\u0416\u043b\u043e\u0431\u0456\u043d'), 'en': 'Zhlobin', 'ru': u('\u0416\u043b\u043e\u0431\u0438\u043d')},
'3752336':{'be': u('\u0411\u0443\u0434\u0430-\u041a\u0430\u0448\u0430\u043b\u0451\u0432\u0430, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Budo-Koshelevo, Gomel Region', 'ru': u('\u0411\u0443\u0434\u0430-\u041a\u043e\u0448\u0435\u043b\u0435\u0432\u043e, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752337':{'be': u('\u041a\u0430\u0440\u043c\u0430, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Korma, Gomel Region', 'ru': u('\u041a\u043e\u0440\u043c\u0430, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752330':{'be': u('\u0412\u0435\u0442\u043a\u0430, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Vetka, Gomel Region', 'ru': u('\u0412\u0435\u0442\u043a\u0430, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752332':{'be': u('\u0427\u0430\u0447\u044d\u0440\u0441\u043a, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Chechersk, Gomel Region', 'ru': u('\u0427\u0435\u0447\u0435\u0440\u0441\u043a, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752333':{'be': u('\u0414\u043e\u0431\u0440\u0443\u0448, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Dobrush, Gomel Region', 'ru': u('\u0414\u043e\u0431\u0440\u0443\u0448, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3349082':{'en': 'Avignon', 'fr': 'Avignon'},
'3349083':{'en': 'Sorgues', 'fr': 'Sorgues'},
'3349080':{'en': 'Avignon', 'fr': 'Avignon'},
'3349081':{'en': 'Avignon', 'fr': 'Avignon'},
'3349086':{'en': 'Avignon', 'fr': 'Avignon'},
'3349087':{'en': 'Avignon', 'fr': 'Avignon'},
'3349084':{'en': 'Avignon', 'fr': 'Avignon'},
'3349085':{'en': 'Avignon', 'fr': 'Avignon'},
'3593322':{'bg': u('\u0417\u043b\u0430\u0442\u043e\u0432\u0440\u044a\u0445'), 'en': 'Zlatovrah'},
'3593323':{'bg': u('\u0411\u043e\u043b\u044f\u0440\u0446\u0438, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Bolyartsi, Plovdiv'},
'3349088':{'en': 'Avignon', 'fr': 'Avignon'},
'3349089':{'en': 'Avignon', 'fr': 'Avignon'},
'3593326':{'bg': u('\u041f\u0430\u0442\u0440\u0438\u0430\u0440\u0445 \u0415\u0432\u0442\u0438\u043c\u043e\u0432\u043e'), 'en': 'Patriarh Evtimovo'},
'3593327':{'bg': u('\u0411\u0430\u0447\u043a\u043e\u0432\u043e'), 'en': 'Bachkovo'},
'3359095':{'en': 'Petit Bourg', 'fr': 'Petit Bourg'},
'3593325':{'bg': u('\u0427\u0435\u0440\u0432\u0435\u043d, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Cherven, Plovdiv'},
'390743':{'it': 'Spoleto'},
'3322808':{'en': 'Nantes', 'fr': 'Nantes'},
'441451':{'en': 'Stow-on-the-Wold'},
'3322803':{'en': 'Saint-Herblain', 'fr': 'Saint-Herblain'},
'3322802':{'en': 'Vigneux-de-Bretagne', 'fr': 'Vigneux-de-Bretagne'},
'3329789':{'en': 'Lanester', 'fr': 'Lanester'},
'3329788':{'en': 'Lorient', 'fr': 'Lorient'},
'3354071':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3329786':{'en': 'Ploemeur', 'fr': 'Ploemeur'},
'3329785':{'en': 'Hennebont', 'fr': 'Hennebont'},
'3329784':{'en': 'Lorient', 'fr': 'Lorient'},
'3329783':{'en': 'Lorient', 'fr': 'Lorient'},
'3329782':{'en': 'Ploemeur', 'fr': 'Ploemeur'},
'3329781':{'en': 'Lanester', 'fr': 'Lanester'},
'3329780':{'en': u('Qu\u00e9ven'), 'fr': u('Qu\u00e9ven')},
'390742':{'it': 'Foligno'},
'3329845':{'en': 'Brest', 'fr': 'Brest'},
'3323319':{'en': 'Coutances', 'fr': 'Coutances'},
'3355380':{'en': u('Montpon-M\u00e9nest\u00e9rol'), 'fr': u('Montpon-M\u00e9nest\u00e9rol')},
'3323317':{'en': 'Coutances', 'fr': 'Coutances'},
'3323312':{'en': 'Argentan', 'fr': 'Argentan'},
'3323310':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'3349268':{'en': 'Peyruis', 'fr': 'Peyruis'},
'3349267':{'en': 'Serres', 'fr': 'Serres'},
'3349264':{'en': u('Ch\u00e2teau-Arnoux-Saint-Auban'), 'fr': u('Ch\u00e2teau-Arnoux-Saint-Auban')},
'3349265':{'en': u('Laragne-Mont\u00e9glin'), 'fr': u('Laragne-Mont\u00e9glin')},
'3349260':{'en': 'Grasse', 'fr': 'Grasse'},
'3349261':{'en': 'Sisteron', 'fr': 'Sisteron'},
'441852':{'en': 'Kilmelford'},
'441856':{'en': 'Orkney'},
'441857':{'en': 'Sanday'},
'441854':{'en': 'Ullapool'},
'441855':{'en': 'Ballachulish'},
'441858':{'en': 'Market Harborough'},
'441859':{'en': 'Harris'},
'3593588':{'bg': u('\u041c\u0435\u043d\u0435\u043d\u043a\u044c\u043e\u0432\u043e'), 'en': 'Menenkyovo'},
'3593589':{'bg': u('\u0426\u0435\u0440\u043e\u0432\u043e, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Tserovo, Pazardzhik'},
'3593586':{'bg': u('\u0411\u043e\u0440\u0438\u043c\u0435\u0447\u043a\u043e\u0432\u043e'), 'en': 'Borimechkovo'},
'3593587':{'bg': u('\u0421\u0435\u0441\u0442\u0440\u0438\u043c\u043e'), 'en': 'Sestrimo'},
'3593584':{'bg': u('\u0412\u0435\u0442\u0440\u0435\u043d, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Vetren, Pazardzhik'},
'3593585':{'bg': u('\u0410\u043a\u0430\u043d\u0434\u0436\u0438\u0435\u0432\u043e'), 'en': 'Akandzhievo'},
'3593582':{'bg': u('\u041c\u043e\u043c\u0438\u043d\u0430 \u043a\u043b\u0438\u0441\u0443\u0440\u0430'), 'en': 'Momina klisura'},
'3593583':{'bg': u('\u0413\u0430\u0431\u0440\u043e\u0432\u0438\u0446\u0430'), 'en': 'Gabrovitsa'},
'3593581':{'bg': u('\u0411\u0435\u043b\u043e\u0432\u043e'), 'en': 'Belovo'},
'432865':{'de': 'Litschau', 'en': 'Litschau'},
'432864':{'de': 'Kautzen', 'en': 'Kautzen'},
'432863':{'de': 'Eggern', 'en': 'Eggern'},
'432862':{'de': 'Heidenreichstein', 'en': 'Heidenreichstein'},
'37243':{'en': 'Viljandi'},
'37245':{'en': 'Kuressaare'},
'37244':{'en': u('P\u00e4rnu')},
'37247':{'en': 'Haapsalu'},
'3317028':{'en': 'Boulogne-Billancourt', 'fr': 'Boulogne-Billancourt'},
'37248':{'en': 'Rapla'},
'3355656':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355657':{'en': 'Eysines', 'fr': 'Eysines'},
'3355654':{'en': 'La Teste de Buch', 'fr': 'La Teste de Buch'},
'3355655':{'en': 'Merignac', 'fr': u('M\u00e9rignac')},
'3355652':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355650':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355651':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'433358':{'de': 'Litzelsdorf', 'en': 'Litzelsdorf'},
'3355659':{'en': 'Pauillac', 'fr': 'Pauillac'},
'3332822':{'en': 'Bourbourg', 'fr': 'Bourbourg'},
'3332823':{'en': 'Gravelines', 'fr': 'Gravelines'},
'3332820':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'3332821':{'en': 'Grande-Synthe', 'fr': 'Grande-Synthe'},
'3332826':{'en': u('T\u00e9teghem'), 'fr': u('T\u00e9teghem')},
'3332352':{'en': 'Chauny', 'fr': 'Chauny'},
'3332353':{'en': 'Soissons', 'fr': 'Soissons'},
'3332828':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'3332829':{'en': 'Dunkirk', 'fr': 'Dunkerque'},
'3332358':{'en': 'Hirson', 'fr': 'Hirson'},
'3332359':{'en': 'Soissons', 'fr': 'Soissons'},
'3354927':{'en': 'Melle', 'fr': 'Melle'},
'3354926':{'en': u('Mauz\u00e9-sur-le-Mignon'), 'fr': u('Mauz\u00e9-sur-le-Mignon')},
'3359093':{'en': u('Pointe-\u00e0-Pitre'), 'fr': u('Pointe-\u00e0-Pitre')},
'3354924':{'en': 'Niort', 'fr': 'Niort'},
'3354923':{'en': u('Ch\u00e2tellerault'), 'fr': u('Ch\u00e2tellerault')},
'3594586':{'bg': u('\u041d\u0435\u0439\u043a\u043e\u0432\u043e, \u0421\u043b\u0438\u0432\u0435\u043d'), 'en': 'Neykovo, Sliven'},
'3354921':{'en': u('Ch\u00e2tellerault'), 'fr': u('Ch\u00e2tellerault')},
'3354920':{'en': u('Ch\u00e2tellerault'), 'fr': u('Ch\u00e2tellerault')},
'3594588':{'bg': u('\u0424\u0438\u043b\u0430\u0440\u0435\u0442\u043e\u0432\u043e'), 'en': 'Filaretovo'},
'3354928':{'en': 'Niort', 'fr': 'Niort'},
'3593743':{'bg': u('\u0421\u0438\u043b\u0435\u043d'), 'en': 'Silen'},
'3593695':{'bg': u('\u041a\u043e\u043c\u0443\u043d\u0438\u0433\u0430'), 'en': 'Komuniga'},
'432755':{'de': 'Mank', 'en': 'Mank'},
'435333':{'de': u('S\u00f6ll'), 'en': u('S\u00f6ll')},
'3593746':{'bg': u('\u0421\u0438\u0440\u0430\u043a\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Sirakovo, Hask.'},
'435334':{'de': 'Westendorf', 'en': 'Westendorf'},
'435335':{'de': 'Hopfgarten im Brixental', 'en': 'Hopfgarten im Brixental'},
'3324723':{'en': 'Amboise', 'fr': 'Amboise'},
'432754':{'de': 'Loosdorf', 'en': 'Loosdorf'},
'35936401':{'bg': u('\u0421\u0442\u0440\u0430\u043d\u0434\u0436\u0435\u0432\u043e'), 'en': 'Strandzhevo'},
'359953':{'bg': u('\u0411\u0435\u0440\u043a\u043e\u0432\u0438\u0446\u0430'), 'en': 'Berkovitsa'},
'3324725':{'en': u('Chambray-l\u00e8s-Tours'), 'fr': u('Chambray-l\u00e8s-Tours')},
'38138':{'en': 'Pristina', 'sr': u('Pri\u0161tina')},
'38139':{'en': 'Pec', 'sr': u('Pe\u0107')},
'38132':{'en': 'Cacak', 'sr': u('\u010ca\u010dak')},
'38133':{'en': 'Prijepolje', 'sr': 'Prijepolje'},
'38130':{'en': 'Bor', 'sr': 'Bor'},
'38131':{'en': 'Uzice', 'sr': u('U\u017eice')},
'38136':{'en': 'Kraljevo', 'sr': 'Kraljevo'},
'38137':{'en': 'Krusevac', 'sr': u('Kru\u0161evac')},
'38134':{'en': 'Kragujevac', 'sr': 'Kragujevac'},
'38135':{'en': 'Jagodina', 'sr': 'Jagodina'},
'432752':{'de': 'Melk', 'en': 'Melk'},
'3598699':{'bg': u('\u0422\u044a\u0440\u043d\u043e\u0432\u0446\u0438, \u0421\u0438\u043b\u0438\u0441\u0442\u0440\u0430'), 'en': 'Tarnovtsi, Silistra'},
'35995276':{'bg': u('\u0414\u0440\u0430\u0433\u0430\u043d\u0438\u0446\u0430'), 'en': 'Draganitsa'},
'35995277':{'bg': u('\u0427\u0435\u0440\u043a\u0430\u0441\u043a\u0438'), 'en': 'Cherkaski'},
'3598698':{'bg': u('\u0428\u0443\u043c\u0435\u043d\u0446\u0438'), 'en': 'Shumentsi'},
'3325345':{'en': 'Nantes', 'fr': 'Nantes'},
'435673':{'de': 'Ehrwald', 'en': 'Ehrwald'},
'435672':{'de': 'Reutte', 'en': 'Reutte'},
'435675':{'de': 'Tannheim', 'en': 'Tannheim'},
'435674':{'de': 'Bichlbach', 'en': 'Bichlbach'},
'435677':{'de': 'Vils', 'en': 'Vils'},
'435676':{'de': 'Jungholz', 'en': 'Jungholz'},
'435678':{'de': u('Wei\u00dfenbach am Lech'), 'en': 'Weissenbach am Lech'},
'3599188':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u041f\u0435\u0449\u0435\u043d\u0435'), 'en': 'Gorno Peshtene'},
'3599189':{'bg': u('\u041f\u0430\u0432\u043e\u043b\u0447\u0435'), 'en': 'Pavolche'},
'3329801':{'en': 'Brest', 'fr': 'Brest'},
'3599184':{'bg': u('\u041e\u0445\u043e\u0434\u0435\u043d'), 'en': 'Ohoden'},
'3599185':{'bg': u('\u0411\u0435\u043b\u0438 \u0418\u0437\u0432\u043e\u0440'), 'en': 'Beli Izvor'},
'3599186':{'bg': u('\u0417\u0433\u043e\u0440\u0438\u0433\u0440\u0430\u0434'), 'en': 'Zgorigrad'},
'3599187':{'bg': u('\u041b\u044e\u0442\u0430\u0434\u0436\u0438\u043a'), 'en': 'Lyutadzhik'},
'3599180':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u043e \u041f\u0435\u0449\u0435\u043d\u0435'), 'en': 'Golyamo Peshtene'},
'3599181':{'bg': u('\u041a\u0440\u0430\u0432\u043e\u0434\u0435\u0440'), 'en': 'Kravoder'},
'3599182':{'bg': u('\u0414\u0435\u0432\u0435\u043d\u0435'), 'en': 'Devene'},
'3599183':{'bg': u('\u041b\u0438\u043b\u044f\u0447\u0435'), 'en': 'Lilyache'},
'3329803':{'en': 'Brest', 'fr': 'Brest'},
'3329804':{'en': 'Lannilis', 'fr': 'Lannilis'},
'3329805':{'en': 'Brest', 'fr': 'Brest'},
'3323453':{'en': 'Tours', 'fr': 'Tours'},
'3329807':{'en': 'Guilers', 'fr': 'Guilers'},
'3596985':{'bg': u('\u0420\u0443\u043c\u044f\u043d\u0446\u0435\u0432\u043e'), 'en': 'Rumyantsevo'},
'3596984':{'bg': u('\u0411\u0435\u0436\u0430\u043d\u043e\u0432\u043e, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Bezhanovo, Lovech'},
'3596987':{'bg': u('\u041a\u0430\u0440\u043b\u0443\u043a\u043e\u0432\u043e'), 'en': 'Karlukovo'},
'3596986':{'bg': u('\u0414\u044a\u0431\u0435\u043d'), 'en': 'Daben'},
'3596981':{'bg': u('\u041f\u0435\u0442\u0440\u0435\u0432\u0435\u043d\u0435'), 'en': 'Petrevene'},
'3596980':{'bg': u('\u0411\u0435\u043b\u0435\u043d\u0446\u0438'), 'en': 'Belentsi'},
'3596983':{'bg': u('\u0414\u0435\u0440\u043c\u0430\u043d\u0446\u0438'), 'en': 'Dermantsi'},
'3355666':{'en': 'Gujan-Mestras', 'fr': 'Gujan-Mestras'},
'355514':{'en': 'Librazhd'},
'355513':{'en': 'Gramsh'},
'355512':{'en': 'Peqin'},
'355511':{'en': 'Kruje'},
'3355911':{'en': 'Pau', 'fr': 'Pau'},
'3347839':{'en': 'Lyon', 'fr': 'Lyon'},
'3347838':{'en': 'Lyon', 'fr': 'Lyon'},
'3595726':{'bg': u('\u0426\u0430\u0440\u0438\u0447\u0438\u043d\u043e'), 'en': 'Tsarichino'},
'3595727':{'bg': u('\u0421\u0435\u043d\u043e\u043a\u043e\u0441, \u0414\u043e\u0431\u0440.'), 'en': 'Senokos, Dobr.'},
'3349333':{'en': 'Antibes Juan les Pins', 'fr': 'Antibes Juan les Pins'},
'3595723':{'bg': u('\u0413\u0443\u0440\u043a\u043e\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Gurkovo, Dobr.'},
'3347831':{'en': 'Meyzieu', 'fr': 'Meyzieu'},
'3347830':{'en': 'Lyon', 'fr': 'Lyon'},
'3347833':{'en': u('\u00c9cully'), 'fr': u('\u00c9cully')},
'3355912':{'en': 'Serres-Castet', 'fr': 'Serres-Castet'},
'3347835':{'en': 'Champagne-au-Mont-d\'Or', 'fr': 'Champagne-au-Mont-d\'Or'},
'3347834':{'en': 'Tassin-la-Demi-Lune', 'fr': 'Tassin-la-Demi-Lune'},
'3347837':{'en': 'Lyon', 'fr': 'Lyon'},
'3347836':{'en': 'Lyon', 'fr': 'Lyon'},
'355863':{'en': u('Drenov\u00eb/Mollaj, Kor\u00e7\u00eb')},
'355862':{'en': u('Qend\u00ebr, Kor\u00e7\u00eb')},
'3349255':{'en': u('Orci\u00e8res'), 'fr': u('Orci\u00e8res')},
'3349706':{'en': 'Cannes', 'fr': 'Cannes'},
'40248':{'en': u('Arge\u0219'), 'ro': u('Arge\u0219')},
'355867':{'en': u('Pojan/Liqenas, Kor\u00e7\u00eb')},
'3349700':{'en': 'Nice', 'fr': 'Nice'},
'355865':{'en': u('Gor\u00eb/Pirg/Moglic\u00eb, Kor\u00e7\u00eb')},
'355864':{'en': u('Voskop/Voskopoj\u00eb/Vithkuq/Lekas, Kor\u00e7\u00eb')},
'3596176':{'bg': u('\u041f\u043e\u043b\u0438\u043a\u0440\u0430\u0438\u0449\u0435'), 'en': 'Polikraishte'},
'432758':{'de': u('P\u00f6ggstall'), 'en': u('P\u00f6ggstall')},
'35961402':{'bg': u('\u0421\u0442\u0435\u0444\u0430\u043d \u0421\u0442\u0430\u043c\u0431\u043e\u043b\u043e\u0432\u043e'), 'en': 'Stefan Stambolovo'},
'35961403':{'bg': u('\u041e\u0440\u043b\u043e\u0432\u0435\u0446'), 'en': 'Orlovets'},
'35961406':{'bg': u('\u041a\u0430\u0440\u0430\u043d\u0446\u0438'), 'en': 'Karantsi'},
'35961405':{'bg': u('\u041f\u0435\u0442\u043a\u043e \u041a\u0430\u0440\u0430\u0432\u0435\u043b\u043e\u0432\u043e'), 'en': 'Petko Karavelovo'},
'3348612':{'en': 'Marseille', 'fr': 'Marseille'},
'3346872':{'en': 'Carcassonne', 'fr': 'Carcassonne'},
'3346873':{'en': 'Canet-en-Roussillon', 'fr': 'Canet-en-Roussillon'},
'3346871':{'en': 'Carcassonne', 'fr': 'Carcassonne'},
'3346876':{'en': 'Bram', 'fr': 'Bram'},
'3346877':{'en': 'Carcassonne', 'fr': 'Carcassonne'},
'3346875':{'en': 'Narbonne', 'fr': 'Narbonne'},
'3346878':{'en': u('Tr\u00e8bes'), 'fr': u('Tr\u00e8bes')},
'3596178':{'bg': u('\u0421\u0442\u0440\u0435\u043b\u0435\u0446, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Strelets, V. Tarnovo'},
'3596179':{'bg': u('\u041a\u0440\u0443\u0448\u0435\u0442\u043e'), 'en': 'Krusheto'},
'3348929':{'en': 'Toulon', 'fr': 'Toulon'},
'3348924':{'en': 'Nice', 'fr': 'Nice'},
'3348922':{'en': 'Nice', 'fr': 'Nice'},
'3323766':{'en': u('Ch\u00e2teaudun'), 'fr': u('Ch\u00e2teaudun')},
'3323765':{'en': 'Tremblay-les-Villages', 'fr': 'Tremblay-les-Villages'},
'3323764':{'en': u('\u00c9zy-sur-Eure'), 'fr': u('\u00c9zy-sur-Eure')},
'35930457':{'bg': u('\u041a\u044a\u0441\u0430\u043a'), 'en': 'Kasak'},
'35930456':{'bg': u('\u0427\u0430\u0432\u0434\u0430\u0440, \u0421\u043c\u043e\u043b.'), 'en': 'Chavdar, Smol.'},
'34879':{'en': 'Palencia', 'es': 'Palencia'},
'34878':{'en': 'Teruel', 'es': 'Teruel'},
'34873':{'en': 'Lleida', 'es': u('L\u00e9rida')},
'34872':{'en': 'Gerona', 'es': 'Gerona'},
'34871':{'en': 'Balearic Islands', 'es': 'Baleares'},
'34877':{'en': 'Tarragona', 'es': 'Tarragona'},
'34876':{'en': 'Zaragoza', 'es': 'Zaragoza'},
'35930459':{'bg': u('\u0411\u0440\u044a\u0449\u0435\u043d'), 'en': 'Brashten'},
'34874':{'en': 'Huesca', 'es': 'Huesca'},
'3332109':{'en': 'Berck sur Mer', 'fr': 'Berck sur Mer'},
'44161':{'en': 'Manchester'},
'3332101':{'en': u('B\u00e9thune'), 'fr': u('B\u00e9thune')},
'3332100':{'en': 'Calais', 'fr': 'Calais'},
'3332103':{'en': 'Saint-Pol-sur-Ternoise', 'fr': 'Saint-Pol-sur-Ternoise'},
'3332105':{'en': 'Le Touquet Paris Plage', 'fr': 'Le Touquet Paris Plage'},
'3332106':{'en': 'Montreuil', 'fr': 'Montreuil'},
'3325422':{'en': u('Ch\u00e2teauroux'), 'fr': u('Ch\u00e2teauroux')},
'3325421':{'en': 'Issoudun', 'fr': 'Issoudun'},
'3325426':{'en': 'Villedieu-sur-Indre', 'fr': 'Villedieu-sur-Indre'},
'3325427':{'en': u('Ch\u00e2teauroux'), 'fr': u('Ch\u00e2teauroux')},
'3325424':{'en': 'Argenton-sur-Creuse', 'fr': 'Argenton-sur-Creuse'},
'433325':{'de': 'Heiligenkreuz im Lafnitztal', 'en': 'Heiligenkreuz im Lafnitztal'},
'433324':{'de': 'Strem', 'en': 'Strem'},
'3325428':{'en': 'Le Blanc', 'fr': 'Le Blanc'},
'3325429':{'en': 'Niherne', 'fr': 'Niherne'},
'433323':{'de': 'Eberau', 'en': 'Eberau'},
'433322':{'de': u('G\u00fcssing'), 'en': u('G\u00fcssing')},
'441501':{'en': 'Harthill'},
'3349521':{'en': 'Ajaccio', 'fr': 'Ajaccio'},
'3349520':{'en': 'Ajaccio', 'fr': 'Ajaccio'},
'3349523':{'en': 'Ajaccio', 'fr': 'Ajaccio'},
'3349522':{'en': 'Ajaccio', 'fr': 'Ajaccio'},
'3349525':{'en': 'Grosseto-Prugna', 'fr': 'Grosseto-Prugna'},
'3349527':{'en': 'Piana', 'fr': 'Piana'},
'3349526':{'en': u('Carg\u00e8se'), 'fr': u('Carg\u00e8se')},
'3349528':{'en': 'Sagone', 'fr': 'Sagone'},
'433149':{'de': 'Geistthal', 'en': 'Geistthal'},
'3596923':{'bg': u('\u0413\u043e\u0440\u0430\u043d'), 'en': 'Goran'},
'3596922':{'bg': u('\u041b\u0435\u0448\u043d\u0438\u0446\u0430, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Leshnitsa, Lovech'},
'433141':{'de': 'Hirschegg', 'en': 'Hirschegg'},
'433140':{'de': u('Sankt Martin am W\u00f6llmi\u00dfberg'), 'en': u('St. Martin am W\u00f6llmissberg')},
'433143':{'de': 'Krottendorf', 'en': 'Krottendorf'},
'433142':{'de': 'Voitsberg', 'en': 'Voitsberg'},
'433145':{'de': 'Edelschrott', 'en': 'Edelschrott'},
'433144':{'de': u('K\u00f6flach'), 'en': u('K\u00f6flach')},
'433147':{'de': 'Salla', 'en': 'Salla'},
'433146':{'de': 'Modriach', 'en': 'Modriach'},
'3596920':{'bg': u('\u041b\u0435\u0441\u0438\u0434\u0440\u0435\u043d'), 'en': 'Lesidren'},
'3596927':{'bg': u('\u0423\u043c\u0430\u0440\u0435\u0432\u0446\u0438'), 'en': 'Umarevtsi'},
'3596926':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u041f\u0430\u0432\u043b\u0438\u043a\u0435\u043d\u0435'), 'en': 'Gorno Pavlikene'},
'3596925':{'bg': u('\u0412\u043b\u0430\u0434\u0438\u043d\u044f'), 'en': 'Vladinya'},
'3594118':{'bg': u('\u041f\u0430\u043c\u0443\u043a\u0447\u0438\u0438, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Pamukchii, St. Zagora'},
'3594115':{'bg': u('\u041a\u0438\u0440\u0438\u043b\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Kirilovo, St. Zagora'},
'3594116':{'bg': u('\u0420\u0430\u043a\u0438\u0442\u043d\u0438\u0446\u0430, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Rakitnitsa, St. Zagora'},
'37423572':{'am': u('\u0531\u0580\u0587\u0577\u0561\u057f'), 'en': 'Arevshat', 'ru': u('\u0410\u0440\u0435\u0432\u0448\u0430\u0442')},
'3323357':{'en': u('Saint-L\u00f4'), 'fr': u('Saint-L\u00f4')},
'3594112':{'bg': u('\u0411\u044a\u0434\u0435\u0449\u0435'), 'en': 'Badeshte'},
'3594113':{'bg': u('\u041f\u0440\u0435\u0441\u043b\u0430\u0432\u0435\u043d'), 'en': 'Preslaven'},
'3595539':{'bg': u('\u0427\u0435\u0440\u043d\u043e\u0433\u0440\u0430\u0434'), 'en': 'Chernograd'},
'3595538':{'bg': u('\u041a\u0430\u0440\u0430\u043d\u043e\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Karanovo, Burgas'},
'441689':{'en': 'Orpington'},
'3596928':{'bg': u('\u041a\u044a\u043a\u0440\u0438\u043d\u0430'), 'en': 'Kakrina'},
'3592':{'bg': u('\u0421\u043e\u0444\u0438\u044f'), 'en': 'Sofia'},
'441683':{'en': 'Moffat'},
'3595530':{'bg': u('\u041f\u0435\u0449\u0435\u0440\u0441\u043a\u043e'), 'en': 'Peshtersko'},
'3595533':{'bg': u('\u041f\u0438\u0440\u043d\u0435'), 'en': 'Pirne'},
'3595532':{'bg': u('\u0422\u043e\u043f\u043e\u043b\u0438\u0446\u0430'), 'en': 'Topolitsa'},
'3595535':{'bg': u('\u041b\u044f\u0441\u043a\u043e\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Lyaskovo, Burgas'},
'3595534':{'bg': u('\u041a\u0430\u0440\u0430\u0433\u0435\u043e\u0440\u0433\u0438\u0435\u0432\u043e'), 'en': 'Karageorgievo'},
'3595537':{'bg': u('\u0421\u044a\u0434\u0438\u0435\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Sadievo, Burgas'},
'3595536':{'bg': u('\u041c\u044a\u0433\u043b\u0435\u043d'), 'en': 'Maglen'},
'3324771':{'en': u('Chambray-l\u00e8s-Tours'), 'fr': u('Chambray-l\u00e8s-Tours')},
'3324770':{'en': 'Tours', 'fr': 'Tours'},
'3324773':{'en': u('Jou\u00e9-l\u00e8s-Tours'), 'fr': u('Jou\u00e9-l\u00e8s-Tours')},
'3324775':{'en': 'Tours', 'fr': 'Tours'},
'3324774':{'en': 'Saint-Avertin', 'fr': 'Saint-Avertin'},
'3338259':{'en': 'Thionville', 'fr': 'Thionville'},
'3324776':{'en': 'Tours', 'fr': 'Tours'},
'3338257':{'en': 'Florange', 'fr': 'Florange'},
'3338256':{'en': 'Yutz', 'fr': 'Yutz'},
'3338255':{'en': 'Cattenom', 'fr': 'Cattenom'},
'3338254':{'en': 'Thionville', 'fr': 'Thionville'},
'3338253':{'en': 'Thionville', 'fr': 'Thionville'},
'3338252':{'en': 'Audun-le-Tiche', 'fr': 'Audun-le-Tiche'},
'3338251':{'en': 'Thionville', 'fr': 'Thionville'},
'3598117':{'bg': u('\u041c\u0430\u0440\u0442\u0435\u043d'), 'en': 'Marten'},
'35831':{'en': 'Tavastia', 'fi': u('H\u00e4me'), 'se': 'Tavastland'},
'35832':{'en': 'Tavastia', 'fi': u('H\u00e4me'), 'se': 'Tavastland'},
'35833':{'en': 'Tavastia', 'fi': u('H\u00e4me'), 'se': 'Tavastland'},
'35834':{'en': 'Tavastia', 'fi': u('H\u00e4me'), 'se': 'Tavastland'},
'35835':{'en': 'Tavastia', 'fi': u('H\u00e4me'), 'se': 'Tavastland'},
'35836':{'en': 'Tavastia', 'fi': u('H\u00e4me'), 'se': 'Tavastland'},
'35837':{'en': 'Tavastia', 'fi': u('H\u00e4me'), 'se': 'Tavastland'},
'35838':{'en': 'Tavastia', 'fi': u('H\u00e4me'), 'se': 'Tavastland'},
'3597152':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u041c\u0430\u043b\u0438\u043d\u0430'), 'en': 'Gorna Malina'},
'35954':{'bg': u('\u0428\u0443\u043c\u0435\u043d'), 'en': 'Shumen'},
'35984469':{'bg': u('\u0411\u043e\u0436\u0443\u0440\u043e\u0432\u043e, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Bozhurovo, Razgrad'},
'4413889':{'en': 'Bishop Auckland'},
'4413888':{'en': 'Bishop Auckland'},
'4413887':{'en': 'Bishop Auckland'},
'3594334':{'bg': u('\u0415\u043b\u0445\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430, \u043e\u0431\u0449. \u041d\u0438\u043a\u043e\u043b\u0430\u0435\u0432\u043e'), 'en': 'Elhovo, St. Zagora, mun. Nikolaevo'},
'35984463':{'bg': u('\u041f\u0440\u0435\u043b\u0435\u0437'), 'en': 'Prelez'},
'331800':{'en': 'Paris', 'fr': 'Paris'},
'35984465':{'bg': u('\u0421\u0430\u0432\u0438\u043d'), 'en': 'Savin'},
'35984464':{'bg': u('\u0412\u0435\u0441\u0435\u043b\u0435\u0446, \u0420\u0430\u0437\u0433\u0440\u0430\u0434'), 'en': 'Veselets, Razgrad'},
'331805':{'en': 'Paris', 'fr': 'Paris'},
'35984466':{'bg': u('\u0417\u0432\u044a\u043d\u0430\u0440\u0446\u0438'), 'en': 'Zvanartsi'},
'390881':{'en': 'Foggia', 'it': 'Foggia'},
'4131':{'de': 'Bern', 'en': 'Berne', 'fr': 'Berne', 'it': 'Berna'},
'4132':{'de': 'Biel/Neuenburg/Solothurn/Jura', 'en': u('Bienne/Neuch\u00e2tel/Soleure/Jura'), 'fr': u('Bienne/Neuch\u00e2tel/Soleure/Jura'), 'it': u('Bienne/Neuch\u00e2tel/Soletta/Giura')},
'4133':{'de': 'Thun', 'en': 'Thun', 'fr': 'Thoune', 'it': 'Thun'},
'4134':{'de': 'Burgdorf/Langnau i.E.', 'en': 'Burgdorf/Langnau i.E.', 'fr': 'Burgdorf/Langnau i.E.', 'it': 'Burgdorf/Langnau i.E.'},
'441271':{'en': 'Barnstaple'},
'3596039':{'bg': u('\u041e\u043f\u0430\u043a\u0430'), 'en': 'Opaka'},
'441323':{'en': 'Eastbourne'},
'35524':{'en': u('Kuk\u00ebs')},
'3323358':{'en': 'Avranches', 'fr': 'Avranches'},
'35522':{'en': u('Shkod\u00ebr')},
'35221':{'de': 'Weicherdingen', 'en': 'Weicherdange', 'fr': 'Weicherdange'},
'441279':{'en': 'Bishops Stortford'},
'4416861':{'en': 'Newtown/Llanidloes'},
'3332670':{'en': u('Ch\u00e2lons-en-Champagne'), 'fr': u('Ch\u00e2lons-en-Champagne')},
'3336907':{'en': 'Mulhouse', 'fr': 'Mulhouse'},
'3596035':{'bg': u('\u041f\u0430\u043b\u0430\u043c\u0430\u0440\u0446\u0430'), 'en': 'Palamartsa'},
'3596034':{'bg': u('\u0421\u043b\u0430\u0432\u044f\u043d\u043e\u0432\u043e, \u0422\u044a\u0440\u0433.'), 'en': 'Slavyanovo, Targ.'},
'359610':{'bg': u('\u041f\u0430\u0432\u043b\u0438\u043a\u0435\u043d\u0438, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Pavlikeni, V. Tarnovo'},
'359615':{'bg': u('\u0417\u043b\u0430\u0442\u0430\u0440\u0438\u0446\u0430'), 'en': 'Zlataritsa'},
'359618':{'bg': u('\u0413\u043e\u0440\u043d\u0430 \u041e\u0440\u044f\u0445\u043e\u0432\u0438\u0446\u0430'), 'en': 'Gorna Oryahovitsa'},
'359619':{'bg': u('\u041b\u044f\u0441\u043a\u043e\u0432\u0435\u0446, \u0412. \u0422\u044a\u0440\u043d\u043e\u0432\u043e'), 'en': 'Lyaskovets, V. Tarnovo'},
'3323154':{'en': u('H\u00e9rouville-Saint-Clair'), 'fr': u('H\u00e9rouville-Saint-Clair')},
'3323150':{'en': 'Caen', 'fr': 'Caen'},
'3323151':{'en': 'Bayeux', 'fr': 'Bayeux'},
'3323152':{'en': 'Caen', 'fr': 'Caen'},
'3323153':{'en': 'Caen', 'fr': 'Caen'},
'3349068':{'en': 'Cadenet', 'fr': 'Cadenet'},
'3349069':{'en': 'Mazan', 'fr': 'Mazan'},
'3349064':{'en': 'Sault', 'fr': 'Sault'},
'3349065':{'en': 'Sarrians', 'fr': 'Sarrians'},
'3349066':{'en': 'Monteux', 'fr': 'Monteux'},
'3349067':{'en': 'Carpentras', 'fr': 'Carpentras'},
'3349060':{'en': 'Carpentras', 'fr': 'Carpentras'},
'3349061':{'en': 'Pernes-les-Fontaines', 'fr': 'Pernes-les-Fontaines'},
'3349062':{'en': 'Caromb', 'fr': 'Caromb'},
'3349063':{'en': 'Carpentras', 'fr': 'Carpentras'},
'3323378':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'3323379':{'en': 'Avranches', 'fr': 'Avranches'},
'3324059':{'en': 'Nantes', 'fr': 'Nantes'},
'3323371':{'en': 'Carentan', 'fr': 'Carentan'},
'3323372':{'en': u('Saint-L\u00f4'), 'fr': u('Saint-L\u00f4')},
'3323376':{'en': 'Coutances', 'fr': 'Coutances'},
'3323377':{'en': u('Saint-L\u00f4'), 'fr': u('Saint-L\u00f4')},
'3349208':{'en': 'Carros', 'fr': 'Carros'},
'3349209':{'en': 'Nice', 'fr': 'Nice'},
'3522755':{'de': 'Esch-sur-Alzette/Monnerich', 'en': 'Esch-sur-Alzette/Mondercange', 'fr': 'Esch-sur-Alzette/Mondercange'},
'3522754':{'de': 'Esch-sur-Alzette', 'en': 'Esch-sur-Alzette', 'fr': 'Esch-sur-Alzette'},
'3522753':{'de': 'Esch-sur-Alzette', 'en': 'Esch-sur-Alzette', 'fr': 'Esch-sur-Alzette'},
'3522752':{'de': u('D\u00fcdelingen'), 'en': 'Dudelange', 'fr': 'Dudelange'},
'3522751':{'de': u('D\u00fcdelingen/Bettemburg/Livingen'), 'en': 'Dudelange/Bettembourg/Livange', 'fr': 'Dudelange/Bettembourg/Livange'},
'3522750':{'de': 'Bascharage/Petingen/Rodingen', 'en': 'Bascharage/Petange/Rodange', 'fr': 'Bascharage/Petange/Rodange'},
'3349200':{'en': 'Nice', 'fr': 'Nice'},
'3349202':{'en': 'Cagnes-sur-Mer', 'fr': 'Cagnes-sur-Mer'},
'3349204':{'en': 'Nice', 'fr': 'Nice'},
'3522759':{'de': 'Soleuvre', 'en': 'Soleuvre', 'fr': 'Soleuvre'},
'3349207':{'en': 'Nice', 'fr': 'Nice'},
'441878':{'en': 'Lochboisdale'},
'441879':{'en': 'Scarinish'},
'441308':{'en': 'Bridport'},
'441309':{'en': 'Forres'},
'441306':{'en': 'Dorking'},
'441307':{'en': 'Forfar'},
'441304':{'en': 'Dover'},
'35931620':{'bg': u('\u0414\u043e\u0431\u0440\u0438 \u0434\u043e\u043b, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Dobri dol, Plovdiv'},
'35931627':{'bg': u('\u041a\u0440\u0443\u0448\u0435\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Krushevo, Plovdiv'},
'441303':{'en': 'Folkestone'},
'335614':{'en': 'Toulouse', 'fr': 'Toulouse'},
'441301':{'en': 'Arrochar'},
'3338882':{'en': u('S\u00e9lestat'), 'fr': u('S\u00e9lestat')},
'3338883':{'en': 'Schiltigheim', 'fr': 'Schiltigheim'},
'3338881':{'en': 'Schiltigheim', 'fr': 'Schiltigheim'},
'3338887':{'en': 'Wasselonne', 'fr': 'Wasselonne'},
'3338884':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'381390':{'en': 'Dakovica', 'sr': 'Dakovica'},
'3338889':{'en': 'Ingwiller', 'fr': 'Ingwiller'},
'3324052':{'en': 'Nantes', 'fr': 'Nantes'},
'437355':{'de': 'Weyer', 'en': 'Weyer'},
'432847':{'de': u('Gro\u00df-Siegharts'), 'en': 'Gross-Siegharts'},
'432846':{'de': 'Raabs an der Thaya', 'en': 'Raabs an der Thaya'},
'3332590':{'en': 'Bourbonne-les-Bains', 'fr': 'Bourbonne-les-Bains'},
'432843':{'de': 'Dobersberg', 'en': 'Dobersberg'},
'432842':{'de': 'Waidhofen an der Thaya', 'en': 'Waidhofen an der Thaya'},
'3332594':{'en': 'Joinville', 'fr': 'Joinville'},
'3324054':{'en': 'Clisson', 'fr': 'Clisson'},
'432849':{'de': 'Schwarzenau', 'en': 'Schwarzenau'},
'432848':{'de': 'Pfaffenschlag bei Waidhofen', 'en': 'Pfaffenschlag bei Waidhofen'},
'3324057':{'en': u('H\u00e9ric'), 'fr': u('H\u00e9ric')},
'3317000':{'en': 'Chelles', 'fr': 'Chelles'},
'3355630':{'en': 'Cadaujac', 'fr': 'Cadaujac'},
'3355631':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355632':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355633':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355634':{'en': 'Merignac', 'fr': u('M\u00e9rignac')},
'3355635':{'en': 'Blanquefort', 'fr': 'Blanquefort'},
'3355636':{'en': 'Pessac', 'fr': 'Pessac'},
'3355637':{'en': 'Talence', 'fr': 'Talence'},
'3355638':{'en': u('Ambar\u00e8s-et-Lagrave'), 'fr': u('Ambar\u00e8s-et-Lagrave')},
'3355639':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3322355':{'en': u('Vitr\u00e9'), 'fr': u('Vitr\u00e9')},
'3322351':{'en': u('Foug\u00e8res'), 'fr': u('Foug\u00e8res')},
'3322350':{'en': 'Bruz', 'fr': 'Bruz'},
'3322352':{'en': 'Saint-Malo', 'fr': 'Saint-Malo'},
'3332376':{'en': 'Soissons', 'fr': 'Soissons'},
'3332375':{'en': 'Soissons', 'fr': 'Soissons'},
'3332373':{'en': 'Soissons', 'fr': 'Soissons'},
'3354901':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354900':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354903':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354902':{'en': u('Ch\u00e2tellerault'), 'fr': u('Ch\u00e2tellerault')},
'3354906':{'en': 'Niort', 'fr': 'Niort'},
'3354909':{'en': 'Niort', 'fr': 'Niort'},
'3354908':{'en': 'Chauray', 'fr': 'Chauray'},
'3359029':{'en': u('Saint Barth\u00e9l\u00e9my'), 'fr': u('Saint Barth\u00e9l\u00e9my')},
'3359028':{'en': 'Sainte Rose', 'fr': 'Sainte Rose'},
'3598675':{'bg': u('\u0410\u0439\u0434\u0435\u043c\u0438\u0440'), 'en': 'Aydemir'},
'3359021':{'en': u('Pointe-\u00e0-Pitre'), 'fr': u('Pointe-\u00e0-Pitre')},
'3359020':{'en': 'Les Abymes', 'fr': 'Les Abymes'},
'3359023':{'en': 'Le Moule', 'fr': 'Le Moule'},
'3359022':{'en': 'Petit-Canal', 'fr': 'Petit-Canal'},
'3359025':{'en': 'Baie Mahault', 'fr': 'Baie Mahault'},
'3359024':{'en': u('Morne-\u00c0-l\'Eau'), 'fr': u('Morne-\u00c0-l\'Eau')},
'3359027':{'en': u('Saint Barth\u00e9l\u00e9my'), 'fr': u('Saint Barth\u00e9l\u00e9my')},
'3359026':{'en': 'Baie Mahault', 'fr': 'Baie Mahault'},
'3595529':{'bg': u('\u041a\u0440\u0443\u0448\u043e\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Krushovo, Burgas'},
'35975214':{'bg': u('\u0413\u0430\u0439\u0442\u0430\u043d\u0438\u043d\u043e\u0432\u043e'), 'en': 'Gaytaninovo'},
'35975215':{'bg': u('\u0422\u0435\u0448\u043e\u0432\u043e'), 'en': 'Teshovo'},
'38110':{'en': 'Pirot', 'sr': 'Pirot'},
'38111':{'en': 'Belgrade', 'sr': 'Beograd'},
'38112':{'en': 'Pozarevac', 'sr': u('Po\u017earevac')},
'38113':{'en': 'Pancevo', 'sr': u('Pan\u010devo')},
'38114':{'en': 'Valjevo', 'sr': 'Valjevo'},
'38115':{'en': 'Sabac', 'sr': u('\u0160abac')},
'38116':{'en': 'Leskovac', 'sr': 'Leskovac'},
'38117':{'en': 'Vranje', 'sr': 'Vranje'},
'38118':{'en': 'Nis', 'sr': u('Ni\u0161')},
'38119':{'en': 'Zajecar', 'sr': u('Zaje\u010dar')},
'437280':{'de': u('Schwarzenberg am B\u00f6hmerwald'), 'en': u('Schwarzenberg am B\u00f6hmerwald')},
'3593704':{'bg': u('\u0411\u0440\u044f\u0433\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Bryagovo, Hask.'},
'3593705':{'bg': u('\u0412\u044a\u0433\u043b\u0430\u0440\u043e\u0432\u043e'), 'en': 'Vaglarovo'},
'3593706':{'bg': u('\u0422\u044a\u043d\u043a\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Tankovo, Hask.'},
'3593707':{'bg': u('\u041d\u0438\u043a\u043e\u043b\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Nikolovo, Hask.'},
'3593700':{'bg': u('\u0422\u0440\u0430\u043a\u0438\u0435\u0446'), 'en': 'Trakiets'},
'3593701':{'bg': u('\u0415\u043b\u0435\u043d\u0430, \u0425\u0430\u0441\u043a.'), 'en': 'Elena, Hask.'},
'3593702':{'bg': u('\u0426\u0430\u0440\u0435\u0432\u0430 \u043f\u043e\u043b\u044f\u043d\u0430'), 'en': 'Tsareva polyana'},
'3593703':{'bg': u('\u0416\u044a\u043b\u0442\u0438 \u0431\u0440\u044f\u0433'), 'en': 'Zhalti bryag'},
'437286':{'de': u('Lembach im M\u00fchlkreis'), 'en': u('Lembach im M\u00fchlkreis')},
'3593708':{'bg': u('\u041e\u0440\u043b\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Orlovo, Hask.'},
'3593709':{'bg': u('\u041a\u0430\u0440\u0430\u043c\u0430\u043d\u0446\u0438'), 'en': 'Karamantsi'},
'437287':{'de': u('Peilstein im M\u00fchlviertel'), 'en': u('Peilstein im M\u00fchlviertel')},
'3599348':{'bg': u('\u041d\u0435\u0433\u043e\u0432\u0430\u043d\u043e\u0432\u0446\u0438'), 'en': 'Negovanovtsi'},
'3599349':{'bg': u('\u0421\u043b\u0430\u043d\u043e\u0442\u0440\u044a\u043d'), 'en': 'Slanotran'},
'3593921':{'bg': u('\u041c\u0435\u0440\u0438\u0447\u043b\u0435\u0440\u0438'), 'en': 'Merichleri'},
'3599342':{'bg': u('\u0418\u043d\u043e\u0432\u043e'), 'en': 'Inovo'},
'3599343':{'bg': u('\u0413\u043e\u043c\u043e\u0442\u0430\u0440\u0446\u0438'), 'en': 'Gomotartsi'},
'3599340':{'bg': u('\u0421\u0435\u043f\u0442\u0435\u043c\u0432\u0440\u0438\u0439\u0446\u0438, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Septemvriytsi, Vidin'},
'3599341':{'bg': u('\u0414\u0438\u043c\u043e\u0432\u043e, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Dimovo, Vidin'},
'3599346':{'bg': u('\u0421\u0438\u043d\u0430\u0433\u043e\u0432\u0446\u0438'), 'en': 'Sinagovtsi'},
'3599347':{'bg': u('\u0411\u0435\u043b\u0430 \u0420\u0430\u0434\u0430'), 'en': 'Bela Rada'},
'3599344':{'bg': u('\u0412\u0440\u044a\u0432'), 'en': 'Vrav'},
'3599345':{'bg': u('\u0412\u0438\u043d\u0430\u0440\u043e\u0432\u043e, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Vinarovo, Vidin'},
'3343802':{'en': u('Saint-Egr\u00e8ve'), 'fr': u('Saint-Egr\u00e8ve')},
'3339041':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3339040':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'355287':{'en': u('Klos/Su\u00e7/Lis, Mat')},
'355286':{'en': u('Ka\u00e7inar/Orosh/Fan, Mirdit\u00eb')},
'355285':{'en': u('Kthjell\u00eb/Selit\u00eb, Mirdit\u00eb')},
'355284':{'en': u('Rubik, Mirdit\u00eb')},
'355283':{'en': u('Kolsh/Zejmen/Sh\u00ebnkoll, Lezh\u00eb')},
'355282':{'en': u('Kallmet/Blinisht/Daj\u00e7/Ungrej, Lezh\u00eb')},
'355281':{'en': u('Sh\u00ebngjin/Balldre, Lezh\u00eb')},
'35963561':{'bg': u('\u0413\u0440\u0438\u0432\u0438\u0446\u0430'), 'en': 'Grivitsa'},
'355289':{'en': u('Ul\u00ebz/Rukaj/Derjan/Macukull, Mat')},
'355288':{'en': u('Baz/Komsi/Gurr\u00eb/Xib\u00ebr, Mat')},
'355579':{'en': u('Luz i Vog\u00ebl/Kryevidh/Helm\u00ebs, Kavaj\u00eb')},
'355578':{'en': u('Synej/Golem, Kavaj\u00eb')},
'35963560':{'bg': u('\u0420\u0430\u0434\u0438\u0448\u0435\u0432\u043e'), 'en': 'Radishevo'},
'3596969':{'bg': u('\u0411\u0435\u043a\u043b\u0435\u043c\u0435\u0442\u043e'), 'en': 'Beklemeto'},
'3596968':{'bg': u('\u0414\u043e\u0431\u0440\u043e\u0434\u0430\u043d'), 'en': 'Dobrodan'},
'355571':{'en': u('Shijak, Durr\u00ebs')},
'355570':{'en': u('Gos\u00eb/Lekaj/Sinaballaj, Kavaj\u00eb')},
'355573':{'en': u('Sukth, Durr\u00ebs')},
'355572':{'en': u('Man\u00ebz, Durr\u00ebs')},
'355575':{'en': u('Xhafzotaj/Maminas, Durr\u00ebs')},
'355574':{'en': u('Rashbull/Gjepalaj, Durr\u00ebs')},
'355577':{'en': u('Rrogozhin\u00eb, Kavaj\u00eb')},
'355576':{'en': u('Katund i Ri/Ishem, Durr\u00ebs')},
'3347853':{'en': 'Lyon', 'fr': 'Lyon'},
'3347852':{'en': 'Lyon', 'fr': 'Lyon'},
'3347851':{'en': 'Oullins', 'fr': 'Oullins'},
'3347850':{'en': 'Oullins', 'fr': 'Oullins'},
'3347857':{'en': 'Craponne', 'fr': 'Craponne'},
'3347856':{'en': 'Saint-Genis-Laval', 'fr': 'Saint-Genis-Laval'},
'3347855':{'en': 'Miribel', 'fr': 'Miribel'},
'3347854':{'en': 'Lyon', 'fr': 'Lyon'},
'3347859':{'en': u('Sainte-Foy-l\u00e8s-Lyon'), 'fr': u('Sainte-Foy-l\u00e8s-Lyon')},
'3347858':{'en': 'Lyon', 'fr': 'Lyon'},
'3347679':{'en': 'Les Deux Alpes', 'fr': 'Les Deux Alpes'},
'3347678':{'en': 'Vizille', 'fr': 'Vizille'},
'3346670':{'en': u('N\u00eemes'), 'fr': u('N\u00eemes')},
'3324015':{'en': u('Gu\u00e9rande'), 'fr': u('Gu\u00e9rande')},
'3346672':{'en': 'Lussan', 'fr': 'Lussan'},
'3324017':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'3338060':{'en': 'Dijon', 'fr': 'Dijon'},
'3346675':{'en': 'Marguerittes', 'fr': 'Marguerittes'},
'3324012':{'en': 'Nantes', 'fr': 'Nantes'},
'3324013':{'en': u('Rez\u00e9'), 'fr': u('Rez\u00e9')},
'3346678':{'en': u('Al\u00e8s'), 'fr': u('Al\u00e8s')},
'3346679':{'en': 'Laudun-l\'Ardoise', 'fr': 'Laudun-l\'Ardoise'},
'3324018':{'en': 'Nantes', 'fr': 'Nantes'},
'3324019':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'436227':{'de': 'Sankt Gilgen', 'en': 'St. Gilgen'},
'35941145':{'bg': u('\u0410\u0440\u043d\u0430\u0443\u0442\u0438\u0442\u043e'), 'en': 'Arnautito'},
'436225':{'de': 'Eugendorf', 'en': 'Eugendorf'},
'3522439':{'de': 'Windhof/Steinfort', 'en': 'Windhof/Steinfort', 'fr': 'Windhof/Steinfort'},
'436223':{'de': 'Anthering', 'en': 'Anthering'},
'436221':{'de': 'Koppl', 'en': 'Koppl'},
'35941144':{'bg': u('\u041a\u0430\u043b\u043e\u044f\u043d\u043e\u0432\u0435\u0446'), 'en': 'Kaloyanovets'},
'3522432':{'de': 'Lintgen/Kanton Mersch/Steinfort', 'en': 'Lintgen/Mersch/Steinfort', 'fr': 'Lintgen/Mersch/Steinfort'},
'3522433':{'de': 'Walferdingen', 'en': 'Walferdange', 'fr': 'Walferdange'},
'3522430':{'de': 'Kanton Capellen/Kehlen', 'en': 'Capellen/Kehlen', 'fr': 'Capellen/Kehlen'},
'3522431':{'de': 'Bartringen', 'en': 'Bertrange/Mamer/Munsbach/Strassen', 'fr': 'Bertrange/Mamer/Munsbach/Strassen'},
'3522436':{'de': 'Hesperingen/Kockelscheuer/Roeser', 'en': 'Hesperange/Kockelscheuer/Roeser', 'fr': 'Hesperange/Kockelscheuer/Roeser'},
'3522437':{'de': 'Leudelingen/Ehlingen/Monnerich', 'en': 'Leudelange/Ehlange/Mondercange', 'fr': 'Leudelange/Ehlange/Mondercange'},
'3522434':{'de': 'Rammeldingen/Senningerberg', 'en': 'Rameldange/Senningerberg', 'fr': 'Rameldange/Senningerberg'},
'3522435':{'de': 'Sandweiler/Mutfort/Roodt-sur-Syre', 'en': 'Sandweiler/Moutfort/Roodt-sur-Syre', 'fr': 'Sandweiler/Moutfort/Roodt-sur-Syre'},
'35941146':{'bg': u('\u0425\u0440\u0438\u0441\u0442\u0438\u044f\u043d\u043e\u0432\u043e'), 'en': 'Hristianovo'},
'3355528':{'en': 'Argentat', 'fr': 'Argentat'},
'3323577':{'en': 'Elbeuf', 'fr': 'Elbeuf'},
'334732':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'334731':{'en': 'Clermont-Ferrand', 'fr': 'Clermont-Ferrand'},
'35961393':{'bg': u('\u0413\u043e\u0440\u0441\u043a\u043e \u041a\u043e\u0441\u043e\u0432\u043e'), 'en': 'Gorsko Kosovo'},
'35961391':{'bg': u('\u0421\u043b\u043e\u043c\u0435\u0440'), 'en': 'Slomer'},
'3329947':{'en': u('Janz\u00e9'), 'fr': u('Janz\u00e9')},
'35961394':{'bg': u('\u0414\u044a\u0441\u043a\u043e\u0442'), 'en': 'Daskot'},
'35961395':{'bg': u('\u041f\u0430\u0441\u043a\u0430\u043b\u0435\u0432\u0435\u0446'), 'en': 'Paskalevets'},
'3329620':{'en': 'Paimpol', 'fr': 'Paimpol'},
'3329623':{'en': 'Perros-Guirec', 'fr': 'Perros-Guirec'},
'3355520':{'en': 'Tulle', 'fr': 'Tulle'},
'3329628':{'en': u('Loud\u00e9ac'), 'fr': u('Loud\u00e9ac')},
'3329629':{'en': 'Rostrenen', 'fr': 'Rostrenen'},
'3338473':{'en': 'Salins-les-Bains', 'fr': 'Salins-les-Bains'},
'3338472':{'en': 'Dole', 'fr': 'Dole'},
'3329943':{'en': 'Bain-de-Bretagne', 'fr': 'Bain-de-Bretagne'},
'3338475':{'en': 'Vesoul', 'fr': 'Vesoul'},
'3338474':{'en': 'Mollans', 'fr': 'Mollans'},
'3338479':{'en': 'Dole', 'fr': 'Dole'},
'3355523':{'en': 'Brive-la-Gaillarde', 'fr': 'Brive-la-Gaillarde'},
'3349442':{'en': 'Toulon', 'fr': 'Toulon'},
'3335462':{'en': 'Metz', 'fr': 'Metz'},
'3349443':{'en': 'Grimaud', 'fr': 'Grimaud'},
'3349440':{'en': u('Fr\u00e9jus'), 'fr': u('Fr\u00e9jus')},
'3349441':{'en': 'Toulon', 'fr': 'Toulon'},
'390774':{'en': 'Rome', 'it': 'Tivoli'},
'3349447':{'en': 'Draguignan', 'fr': 'Draguignan'},
'3349444':{'en': u('Fr\u00e9jus'), 'fr': u('Fr\u00e9jus')},
'3348633':{'en': 'La Ciotat', 'fr': 'La Ciotat'},
'3348631':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3348634':{'en': 'Avignon', 'fr': 'Avignon'},
'3346894':{'en': 'Castelnaudary', 'fr': 'Castelnaudary'},
'3346895':{'en': u('Argel\u00e8s-sur-Mer'), 'fr': u('Argel\u00e8s-sur-Mer')},
'3346896':{'en': 'Prades', 'fr': 'Prades'},
'3346890':{'en': 'Narbonne', 'fr': 'Narbonne'},
'3346892':{'en': u('Saint-Est\u00e8ve'), 'fr': u('Saint-Est\u00e8ve')},
'3346893':{'en': 'Moussan', 'fr': 'Moussan'},
'3522697':{'de': 'Huldingen', 'en': 'Huldange', 'fr': 'Huldange'},
'3522695':{'de': 'Wiltz', 'en': 'Wiltz', 'fr': 'Wiltz'},
'3522692':{'de': 'Kanton Clerf/Fischbach/Hosingen', 'en': 'Clervaux/Fischbach/Hosingen', 'fr': 'Clervaux/Fischbach/Hosingen'},
'3597187':{'bg': u('\u0411\u0443\u043d\u043e\u0432\u043e, \u0421\u043e\u0444\u0438\u044f'), 'en': 'Bunovo, Sofia'},
'3522699':{'de': 'Ulflingen', 'en': 'Troisvierges', 'fr': 'Troisvierges'},
'3325629':{'en': 'Brest', 'fr': 'Brest'},
'3323741':{'en': 'Anet', 'fr': 'Anet'},
'34810':{'en': 'Madrid', 'es': 'Madrid'},
'3323742':{'en': 'Dreux', 'fr': 'Dreux'},
'3323745':{'en': u('Ch\u00e2teaudun'), 'fr': u('Ch\u00e2teaudun')},
'3323744':{'en': u('Ch\u00e2teaudun'), 'fr': u('Ch\u00e2teaudun')},
'3323747':{'en': 'Bonneval', 'fr': 'Bonneval'},
'3323746':{'en': 'Dreux', 'fr': 'Dreux'},
'35930475':{'bg': u('\u0421\u0442\u043e\u043c\u0430\u043d\u0435\u0432\u043e'), 'en': 'Stomanevo'},
'35930476':{'bg': u('\u0421\u0435\u043b\u0447\u0430'), 'en': 'Selcha'},
'35930472':{'bg': u('\u041c\u0438\u0445\u0430\u043b\u043a\u043e\u0432\u043e'), 'en': 'Mihalkovo'},
'3332163':{'en': u('B\u00e9thune'), 'fr': u('B\u00e9thune')},
'3332162':{'en': u('Bruay-la-Buissi\u00e8re'), 'fr': u('Bruay-la-Buissi\u00e8re')},
'3332160':{'en': 'Arras', 'fr': 'Arras'},
'3332167':{'en': 'Lens', 'fr': 'Lens'},
'3332169':{'en': 'Wingles', 'fr': 'Wingles'},
'3332168':{'en': u('B\u00e9thune'), 'fr': u('B\u00e9thune')},
'3354733':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'44141':{'en': 'Glasgow'},
'431':{'de': 'Wien', 'en': 'Vienna'},
'3325193':{'en': 'Challans', 'fr': 'Challans'},
'3325190':{'en': 'Bretignolles-sur-Mer', 'fr': 'Bretignolles-sur-Mer'},
'3325194':{'en': 'Chantonnay', 'fr': 'Chantonnay'},
'3325195':{'en': 'Les Sables d\'Olonne', 'fr': 'Les Sables d\'Olonne'},
'390161':{'en': 'Vercelli', 'it': 'Vercelli'},
'390163':{'it': 'Borgosesia'},
'390165':{'en': 'Aosta Valley', 'it': 'Aosta'},
'3349546':{'en': 'Corte', 'fr': 'Corte'},
'390166':{'en': 'Aosta Valley', 'it': 'Saint-Vincent'},
'351295':{'en': u('Angra do Hero\u00edsmo'), 'pt': u('Angra do Hero\u00edsmo')},
'351296':{'en': 'Ponta Delgada', 'pt': 'Ponta Delgada'},
'351291':{'en': 'Funchal', 'pt': 'Funchal'},
'351292':{'en': 'Horta', 'pt': 'Horta'},
'3332787':{'en': 'Douai', 'fr': 'Douai'},
'3332786':{'en': 'Somain', 'fr': 'Somain'},
'3332785':{'en': 'Caudry', 'fr': 'Caudry'},
'3332784':{'en': u('Le Cateau Cambr\u00e9sis'), 'fr': u('Le Cateau Cambr\u00e9sis')},
'3332783':{'en': 'Cambrai', 'fr': 'Cambrai'},
'3332782':{'en': 'Cambrai', 'fr': 'Cambrai'},
'3332781':{'en': 'Cambrai', 'fr': 'Cambrai'},
'3332788':{'en': 'Douai', 'fr': 'Douai'},
'3594136':{'bg': u('\u0427\u0435\u0440\u043d\u0430 \u0433\u043e\u0440\u0430, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Cherna gora, St. Zagora'},
'3594137':{'bg': u('\u0412\u0435\u0440\u0435\u043d'), 'en': 'Veren'},
'3594134':{'bg': u('\u0411\u0440\u0430\u0442\u044f \u0414\u0430\u0441\u043a\u0430\u043b\u043e\u0432\u0438'), 'en': 'Bratya Daskalovi'},
'3594132':{'bg': u('\u041e\u0440\u0438\u0437\u043e\u0432\u043e'), 'en': 'Orizovo'},
'3594130':{'bg': u('\u0421\u043f\u0430\u0441\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Spasovo, St. Zagora'},
'435286':{'de': 'Ginzling', 'en': 'Ginzling'},
'3594138':{'bg': u('\u0413\u0438\u0442\u0430'), 'en': 'Gita'},
'3594139':{'bg': u('\u0421\u0432\u043e\u0431\u043e\u0434\u0430, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Svoboda, St. Zagora'},
'4414301':{'en': 'North Cave/Market Weighton'},
'4414300':{'en': 'North Cave/Market Weighton'},
'435280':{'de': u('Hochf\u00fcgen'), 'en': u('Hochf\u00fcgen')},
'4414303':{'en': 'North Cave'},
'3598132':{'bg': u('\u042e\u0434\u0435\u043b\u043d\u0438\u043a'), 'en': 'Yudelnik'},
'3598133':{'bg': u('\u0420\u044f\u0445\u043e\u0432\u043e'), 'en': 'Ryahovo'},
'3598131':{'bg': u('\u0411\u043e\u0440\u0438\u0441\u043e\u0432\u043e, \u0420\u0443\u0441\u0435'), 'en': 'Borisovo, Ruse'},
'3598136':{'bg': u('\u0421\u0442\u0430\u043c\u0431\u043e\u043b\u043e\u0432\u043e, \u0420\u0443\u0441\u0435'), 'en': 'Stambolovo, Ruse'},
'3598137':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u043e \u0412\u0440\u0430\u043d\u043e\u0432\u043e'), 'en': 'Golyamo Vranovo'},
'3598134':{'bg': u('\u041c\u0430\u043b\u043a\u043e \u0412\u0440\u0430\u043d\u043e\u0432\u043e'), 'en': 'Malko Vranovo'},
'3598135':{'bg': u('\u0411\u0430\u0431\u043e\u0432\u043e'), 'en': 'Babovo'},
'333854':{'en': u('Chalon-sur-Sa\u00f4ne'), 'fr': u('Chalon-sur-Sa\u00f4ne')},
'4414306':{'en': 'Market Weighton'},
'3688':{'en': 'Veszprem', 'hu': u('Veszpr\u00e9m')},
'435288':{'de': u('F\u00fcgen'), 'en': u('F\u00fcgen')},
'435289':{'de': u('H\u00e4usling'), 'en': u('H\u00e4usling')},
'370389':{'en': 'Utena'},
'370383':{'en': u('Mol\u0117tai')},
'370382':{'en': u('\u0160irvintos')},
'370381':{'en': u('Anyk\u0161\u010diai')},
'3599316':{'bg': u('\u041d\u043e\u0432\u043e \u0441\u0435\u043b\u043e, \u0412\u0438\u0434\u0438\u043d'), 'en': 'Novo selo, Vidin'},
'370387':{'en': u('\u0160ven\u010dionys')},
'370386':{'en': 'Ignalina/Visaginas'},
'370385':{'en': 'Zarasai'},
'3338183':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338182':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338181':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338180':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338187':{'en': 'Saint-Vit', 'fr': 'Saint-Vit'},
'3338185':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3338184':{'en': 'Baume-les-Dames', 'fr': 'Baume-les-Dames'},
'3338188':{'en': u('Besan\u00e7on'), 'fr': u('Besan\u00e7on')},
'3596028':{'bg': u('\u0412\u0430\u0440\u0434\u0443\u043d'), 'en': 'Vardun'},
'433859':{'de': u('M\u00fcrzsteg'), 'en': u('M\u00fcrzsteg')},
'3596029':{'bg': u('\u041d\u0430\u0434\u0430\u0440\u0435\u0432\u043e'), 'en': 'Nadarevo'},
'3595553':{'bg': u('\u041e\u0440\u043b\u0438\u043d\u0446\u0438'), 'en': 'Orlintsi'},
'3595552':{'bg': u('\u0414\u044e\u043b\u0435\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Dyulevo, Burgas'},
'3595551':{'bg': u('\u0421\u0440\u0435\u0434\u0435\u0446, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Sredets, Burgas'},
'3595557':{'bg': u('\u0411\u0438\u0441\u0442\u0440\u0435\u0446, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Bistrets, Burgas'},
'3595556':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u043e \u0411\u0443\u043a\u043e\u0432\u043e'), 'en': 'Golyamo Bukovo'},
'3595555':{'bg': u('\u0424\u0430\u043a\u0438\u044f'), 'en': 'Fakia'},
'3595554':{'bg': u('\u041c\u043e\u043c\u0438\u043d\u0430 \u0446\u044a\u0440\u043a\u0432\u0430'), 'en': 'Momina tsarkva'},
'3347445':{'en': 'Bourg-en-Bresse', 'fr': 'Bourg-en-Bresse'},
'3347446':{'en': u('Amb\u00e9rieu-en-Bugey'), 'fr': u('Amb\u00e9rieu-en-Bugey')},
'3347447':{'en': 'Bourg-en-Bresse', 'fr': 'Bourg-en-Bresse'},
'3347440':{'en': 'Lagnieu', 'fr': 'Lagnieu'},
'3347443':{'en': 'Bourgoin-Jallieu', 'fr': 'Bourgoin-Jallieu'},
'3346799':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346798':{'en': u('P\u00e9zenas'), 'fr': u('P\u00e9zenas')},
'3346793':{'en': 'Capestang', 'fr': 'Capestang'},
'3346792':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3346791':{'en': 'Lunel', 'fr': 'Lunel'},
'3346797':{'en': u('Saint-Pons-de-Thomi\u00e8res'), 'fr': u('Saint-Pons-de-Thomi\u00e8res')},
'3346796':{'en': u('Clermont-l\'H\u00e9rault'), 'fr': u('Clermont-l\'H\u00e9rault')},
'3346795':{'en': u('B\u00e9darieux'), 'fr': u('B\u00e9darieux')},
'3346794':{'en': 'Agde', 'fr': 'Agde'},
'331771':{'en': 'Paris', 'fr': 'Paris'},
'3334412':{'en': 'Beauvais', 'fr': 'Beauvais'},
'359678':{'bg': u('\u0422\u0435\u0442\u0435\u0432\u0435\u043d'), 'en': 'Teteven'},
'359670':{'bg': u('\u0422\u0440\u043e\u044f\u043d, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Troyan, Lovech'},
'359676':{'bg': u('\u0414\u0440\u044f\u043d\u043e\u0432\u043e, \u0413\u0430\u0431\u0440.'), 'en': 'Dryanovo, Gabr.'},
'359677':{'bg': u('\u0422\u0440\u044f\u0432\u043d\u0430'), 'en': 'Tryavna'},
'359675':{'bg': u('\u0421\u0435\u0432\u043b\u0438\u0435\u0432\u043e'), 'en': 'Sevlievo'},
'441354':{'en': 'Chatteris'},
'3349047':{'en': 'Saint-Martin-de-Crau', 'fr': 'Saint-Martin-de-Crau'},
'3349044':{'en': 'Salon-de-Provence', 'fr': 'Salon-de-Provence'},
'3349045':{'en': 'Salon-de-Provence', 'fr': 'Salon-de-Provence'},
'3349042':{'en': 'Salon-de-Provence', 'fr': 'Salon-de-Provence'},
'3349043':{'en': 'Tarascon', 'fr': 'Tarascon'},
'3349040':{'en': u('Boll\u00e8ne'), 'fr': u('Boll\u00e8ne')},
'3349041':{'en': 'Visan', 'fr': 'Visan'},
'3351649':{'en': 'La Rochelle', 'fr': 'La Rochelle'},
'3349048':{'en': 'Entraigues-sur-la-Sorgue', 'fr': 'Entraigues-sur-la-Sorgue'},
'3349049':{'en': 'Arles', 'fr': 'Arles'},
'3354039':{'en': 'Bayonne', 'fr': 'Bayonne'},
'3332751':{'en': 'Valenciennes', 'fr': 'Valenciennes'},
'436414':{'de': u('Gro\u00dfarl'), 'en': 'Grossarl'},
'432534':{'de': 'Niedersulz', 'en': 'Niedersulz'},
'3349954':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3349223':{'en': u('L\'Argenti\u00e8re la Bess\u00e9e'), 'fr': u('L\'Argenti\u00e8re la Bess\u00e9e')},
'3349220':{'en': u('Brian\u00e7on'), 'fr': u('Brian\u00e7on')},
'3349221':{'en': u('Brian\u00e7on'), 'fr': u('Brian\u00e7on')},
'3349951':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3349952':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3349953':{'en': 'Montpellier', 'fr': 'Montpellier'},
'390374':{'it': 'Soresina'},
'390375':{'it': 'Casalmaggiore'},
'3349228':{'en': 'Mougins', 'fr': 'Mougins'},
'3349229':{'en': 'Nice', 'fr': 'Nice'},
'3349958':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3338787':{'en': 'Forbach', 'fr': 'Forbach'},
'390372':{'en': 'Cremona', 'it': 'Cremona'},
'390373':{'en': 'Cremona', 'it': 'Crema'},
'3522771':{'de': 'Betzdorf', 'en': 'Betzdorf', 'fr': 'Betzdorf'},
'3522773':{'de': 'Rosport', 'en': 'Rosport', 'fr': 'Rosport'},
'3522772':{'de': 'Echternach', 'en': 'Echternach', 'fr': 'Echternach'},
'3522775':{'de': 'Distrikt Grevenmacher-sur-Moselle', 'en': 'Grevenmacher-sur-Moselle', 'fr': 'Grevenmacher-sur-Moselle'},
'3522774':{'de': 'Wasserbillig', 'en': 'Wasserbillig', 'fr': 'Wasserbillig'},
'3522776':{'de': 'Wormeldingen', 'en': 'Wormeldange', 'fr': 'Wormeldange'},
'3522779':{'de': 'Berdorf/Consdorf', 'en': 'Berdorf/Consdorf', 'fr': 'Berdorf/Consdorf'},
'3522778':{'de': 'Junglinster', 'en': 'Junglinster', 'fr': 'Junglinster'},
'3597041':{'bg': u('\u0428\u0430\u0442\u0440\u043e\u0432\u043e'), 'en': 'Shatrovo'},
'3597047':{'bg': u('\u0423\u0441\u043e\u0439\u043a\u0430'), 'en': 'Usoyka'},
'3597046':{'bg': u('\u0411\u043e\u0431\u043e\u0448\u0435\u0432\u043e'), 'en': 'Boboshevo'},
'3597045':{'bg': u('\u0413\u043e\u043b\u0435\u043c \u0412\u044a\u0440\u0431\u043e\u0432\u043d\u0438\u043a'), 'en': 'Golem Varbovnik'},
'3338780':{'en': u('Maizi\u00e8res-l\u00e8s-Metz'), 'fr': u('Maizi\u00e8res-l\u00e8s-Metz')},
'441328':{'en': 'Fakenham'},
'441329':{'en': 'Fareham'},
'35931603':{'bg': u('\u0412\u0438\u043d\u0438\u0446\u0430'), 'en': 'Vinitsa'},
'35931602':{'bg': u('\u0422\u0430\u0442\u0430\u0440\u0435\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Tatarevo, Plovdiv'},
'35931605':{'bg': u('\u0411\u0443\u043a\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Bukovo, Plovdiv'},
'35931604':{'bg': u('\u0414\u0440\u0430\u0433\u043e\u0439\u043d\u043e\u0432\u043e'), 'en': 'Dragoynovo'},
'35931606':{'bg': u('\u0412\u043e\u0434\u0435\u043d, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Voden, Plovdiv'},
'441320':{'en': 'Fort Augustus'},
'441322':{'en': 'Dartford'},
'3338782':{'en': 'Creutzwald', 'fr': 'Creutzwald'},
'441324':{'en': 'Falkirk'},
'441325':{'en': 'Darlington'},
'441326':{'en': 'Falmouth'},
'441327':{'en': 'Daventry'},
'3323174':{'en': 'Caen', 'fr': 'Caen'},
'3323175':{'en': 'Caen', 'fr': 'Caen'},
'3323172':{'en': 'Colombelles', 'fr': 'Colombelles'},
'3323173':{'en': 'Caen', 'fr': 'Caen'},
'3323170':{'en': 'Caen', 'fr': 'Caen'},
'3323171':{'en': 'Carpiquet', 'fr': 'Carpiquet'},
'3338864':{'en': 'Fegersheim', 'fr': 'Fegersheim'},
'3338865':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338866':{'en': 'Illkirch-Graffenstaden', 'fr': 'Illkirch-Graffenstaden'},
'3338867':{'en': 'Illkirch-Graffenstaden', 'fr': 'Illkirch-Graffenstaden'},
'3338860':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338861':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3338862':{'en': 'Schiltigheim', 'fr': 'Schiltigheim'},
'3338863':{'en': 'Bischwiller', 'fr': 'Bischwiller'},
'432823':{'de': u('Gro\u00dfglobnitz'), 'en': 'Grossglobnitz'},
'432822':{'de': u('Zwettl-Nieder\u00f6sterreich'), 'en': 'Zwettl, Lower Austria'},
'3332578':{'en': 'Troyes', 'fr': 'Troyes'},
'3332579':{'en': 'Sainte-Savine', 'fr': 'Sainte-Savine'},
'432827':{'de': u('Sch\u00f6nbach'), 'en': u('Sch\u00f6nbach')},
'432826':{'de': 'Rastenfeld', 'en': 'Rastenfeld'},
'3332574':{'en': 'Troyes', 'fr': 'Troyes'},
'3332575':{'en': 'Troyes', 'fr': 'Troyes'},
'3332576':{'en': 'Troyes', 'fr': 'Troyes'},
'3332571':{'en': 'Troyes', 'fr': 'Troyes'},
'3332572':{'en': 'Troyes', 'fr': 'Troyes'},
'3332573':{'en': 'Troyes', 'fr': 'Troyes'},
'35937606':{'bg': u('\u0428\u0438\u0448\u043c\u0430\u043d\u043e\u0432\u043e'), 'en': 'Shishmanovo'},
'3354693':{'en': 'Saintes', 'fr': 'Saintes'},
'3354692':{'en': 'Saintes', 'fr': 'Saintes'},
'35937603':{'bg': u('\u0420\u043e\u0433\u043e\u0437\u0438\u043d\u043e\u0432\u043e'), 'en': 'Rogozinovo'},
'35937602':{'bg': u('\u0427\u0435\u0440\u043d\u0430 \u043c\u043e\u0433\u0438\u043b\u0430, \u0425\u0430\u0441\u043a.'), 'en': 'Cherna mogila, Hask.'},
'3354697':{'en': 'Saintes', 'fr': 'Saintes'},
'3354699':{'en': 'Rochefort', 'fr': 'Rochefort'},
'3354698':{'en': 'Saintes', 'fr': 'Saintes'},
'3554':{'en': 'Tirana'},
'3329952':{'en': 'Bruz', 'fr': 'Bruz'},
'3346931':{'en': 'Bourgoin-Jallieu', 'fr': 'Bourgoin-Jallieu'},
'3323353':{'en': 'Cherbourg-Octeville', 'fr': 'Cherbourg-Octeville'},
'3323350':{'en': 'Granville', 'fr': 'Granville'},
'3355348':{'en': 'Agen', 'fr': 'Agen'},
'3355349':{'en': 'Villeneuve-sur-Lot', 'fr': 'Villeneuve-sur-Lot'},
'3355612':{'en': 'Merignac', 'fr': u('M\u00e9rignac')},
'3355345':{'en': u('P\u00e9rigueux'), 'fr': u('P\u00e9rigueux')},
'3355346':{'en': u('P\u00e9rigueux'), 'fr': u('P\u00e9rigueux')},
'3355611':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355616':{'en': 'Eysines', 'fr': 'Eysines'},
'3355617':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3355615':{'en': 'Pessac', 'fr': 'Pessac'},
'3329953':{'en': 'Rennes', 'fr': 'Rennes'},
'37424300':{'am': u('\u0531\u056d\u0578\u0582\u0580\u0575\u0561\u0576/\u0531\u057c\u0561\u0583\u056b/\u053f\u0561\u0574\u0578/\u0544\u0578\u0582\u057d\u0561\u0575\u0565\u056c\u0575\u0561\u0576'), 'en': 'Akhuryan/Arapi/Kamo/Musayelyan', 'ru': u('\u0410\u0445\u0443\u0440\u044f\u043d/\u0410\u0440\u0430\u043f\u0438/\u041a\u0430\u043c\u043e/\u041c\u0443\u0441\u0430\u0435\u043b\u044f\u043d')},
'432177':{'de': 'Podersdorf am See', 'en': 'Podersdorf am See'},
'432176':{'de': 'Tadten', 'en': 'Tadten'},
'432175':{'de': 'Apetlon', 'en': 'Apetlon'},
'432174':{'de': 'Wallern im Burgenland', 'en': 'Wallern im Burgenland'},
'432173':{'de': 'Gols', 'en': 'Gols'},
'432172':{'de': 'Frauenkirchen', 'en': 'Frauenkirchen'},
'437675':{'de': 'Ampflwang im Hausruckwald', 'en': 'Ampflwang im Hausruckwald'},
'437674':{'de': 'Attnang-Puchheim', 'en': 'Attnang-Puchheim'},
'3354968':{'en': 'Thouars', 'fr': 'Thouars'},
'3354962':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354961':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354960':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354966':{'en': 'Thouars', 'fr': 'Thouars'},
'3354965':{'en': 'Bressuire', 'fr': 'Bressuire'},
'3354964':{'en': 'Parthenay', 'fr': 'Parthenay'},
'3359041':{'en': 'Baie Mahault', 'fr': 'Baie Mahault'},
'3359048':{'en': 'Les Abymes', 'fr': 'Les Abymes'},
'3323120':{'en': 'Saint-Pierre-sur-Dives', 'fr': 'Saint-Pierre-sur-Dives'},
'3329981':{'en': 'Saint-Malo', 'fr': 'Saint-Malo'},
'3329983':{'en': u('Cesson-S\u00e9vign\u00e9'), 'fr': u('Cesson-S\u00e9vign\u00e9')},
'3329982':{'en': 'Saint-Malo', 'fr': 'Saint-Malo'},
'3329985':{'en': u('Pac\u00e9'), 'fr': u('Pac\u00e9')},
'3329984':{'en': 'Rennes', 'fr': 'Rennes'},
'3329987':{'en': 'Rennes', 'fr': 'Rennes'},
'3329986':{'en': 'Rennes', 'fr': 'Rennes'},
'3329989':{'en': 'Cancale', 'fr': 'Cancale'},
'3329988':{'en': 'Pleurtuit', 'fr': 'Pleurtuit'},
'3595946':{'bg': u('\u0422\u0440\u044a\u043d\u0430\u043a'), 'en': 'Tranak'},
'3595947':{'bg': u('\u041f\u0440\u043e\u0441\u0435\u043d\u0438\u043a'), 'en': 'Prosenik'},
'3595941':{'bg': u('\u0421\u043a\u0430\u043b\u0430\u043a, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Skalak, Burgas'},
'3595942':{'bg': u('\u041b\u044e\u043b\u044f\u043a\u043e\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Lyulyakovo, Burgas'},
'3595943':{'bg': u('\u0412\u0440\u0435\u0441\u043e\u0432\u043e'), 'en': 'Vresovo'},
'3593728':{'bg': u('\u0421\u043b\u0430\u0432\u044f\u043d\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Slavyanovo, Hask.'},
'3593729':{'bg': u('\u041a\u0440\u0438\u0432\u043e \u043f\u043e\u043b\u0435'), 'en': 'Krivo pole'},
'3593726':{'bg': u('\u041a\u043b\u043e\u043a\u043e\u0442\u043d\u0438\u0446\u0430'), 'en': 'Klokotnitsa'},
'3593727':{'bg': u('\u041d\u043e\u0432\u0430 \u041d\u0430\u0434\u0435\u0436\u0434\u0430'), 'en': 'Nova Nadezhda'},
'3593724':{'bg': u('\u0421\u0443\u0441\u0430\u043c'), 'en': 'Susam'},
'3593725':{'bg': u('\u0421\u0442\u0430\u043c\u0431\u043e\u043b\u0438\u0439\u0441\u043a\u0438, \u0425\u0430\u0441\u043a.'), 'en': 'Stamboliyski, Hask.'},
'3593722':{'bg': u('\u041c\u0438\u043d\u0435\u0440\u0430\u043b\u043d\u0438 \u0431\u0430\u043d\u0438, \u0425\u0430\u0441\u043a.'), 'en': 'Mineralni bani, Hask.'},
'3593720':{'bg': u('\u041c\u0430\u0434\u0436\u0430\u0440\u043e\u0432\u043e'), 'en': 'Madzharovo'},
'3593721':{'bg': u('\u0421\u0442\u0430\u043c\u0431\u043e\u043b\u043e\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Stambolovo, Hask.'},
'3353348':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'3329954':{'en': 'Rennes', 'fr': 'Rennes'},
'37423699':{'am': u('\u0534\u0561\u0577\u057f\u0561\u057e\u0561\u0576'), 'en': 'Dashtavan', 'ru': u('\u0414\u0430\u0448\u0442\u0430\u0432\u0430\u043d')},
'374471':{'am': u('\u054d\u057f\u0565\u0583\u0561\u0576\u0561\u056f\u0565\u0580\u057f'), 'en': 'Stepanakert', 'ru': u('\u0421\u0442\u0435\u043f\u0430\u043d\u0430\u043a\u0435\u0440\u0442')},
'374475':{'am': u('\u0540\u0561\u0580\u0564\u0580\u0578\u0582\u0569'), 'en': 'Hadrut', 'ru': u('\u0413\u0430\u0434\u0440\u0443\u0442')},
'374474':{'am': u('\u0544\u0561\u0580\u057f\u0561\u056f\u0565\u0580\u057f'), 'en': 'Martakert', 'ru': u('\u041c\u0430\u0440\u0442\u0430\u043a\u0435\u0440\u0442')},
'374477':{'am': u('\u0547\u0578\u0582\u0577\u056b'), 'en': 'Shushi', 'ru': u('\u0428\u0443\u0448\u0438')},
'374476':{'am': u('\u0531\u057d\u056f\u0565\u0580\u0561\u0576'), 'en': 'Askeran', 'ru': u('\u0410\u0441\u043a\u0435\u0440\u0430\u043d')},
'374479':{'am': u('\u054d\u057f\u0565\u0583\u0561\u0576\u0561\u056f\u0565\u0580\u057f'), 'en': 'Stepanakert', 'ru': u('\u0421\u0442\u0435\u043f\u0430\u043d\u0430\u043a\u0435\u0440\u0442')},
'374478':{'am': u('\u0544\u0561\u0580\u057f\u0578\u0582\u0576\u056b'), 'en': 'Martuni', 'ru': u('\u041c\u0430\u0440\u0442\u0443\u043d\u0438')},
'441528':{'en': 'Laggan'},
'441529':{'en': 'Sleaford'},
'441526':{'en': 'Martin'},
'441527':{'en': 'Redditch'},
'441525':{'en': 'Leighton Buzzard'},
'441522':{'en': 'Lincoln'},
'441520':{'en': 'Lochcarron'},
'3324384':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324385':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324386':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324380':{'en': 'Allonnes', 'fr': 'Allonnes'},
'3324381':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324382':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324383':{'en': 'Le Mans', 'fr': 'Le Mans'},
'3324389':{'en': u('Connerr\u00e9'), 'fr': u('Connerr\u00e9')},
'3315681':{'en': 'Paris', 'fr': 'Paris'},
'3596948':{'bg': u('\u041a\u044a\u0440\u043f\u0430\u0447\u0435\u0432\u043e'), 'en': 'Karpachevo'},
'3315683':{'en': 'Colombes', 'fr': 'Colombes'},
'35976':{'bg': u('\u041f\u0435\u0440\u043d\u0438\u043a'), 'en': 'Pernik'},
'35973':{'bg': u('\u0411\u043b\u0430\u0433\u043e\u0435\u0432\u0433\u0440\u0430\u0434'), 'en': 'Blagoevgrad'},
'3315686':{'en': u('Chennevi\u00e8res-sur-Marne'), 'fr': u('Chennevi\u00e8res-sur-Marne')},
'3596941':{'bg': u('\u041b\u0435\u0442\u043d\u0438\u0446\u0430'), 'en': 'Letnitsa'},
'3315688':{'en': 'Paris', 'fr': 'Paris'},
'3596943':{'bg': u('\u0413\u043e\u0440\u0441\u043a\u043e \u0421\u043b\u0438\u0432\u043e\u0432\u043e'), 'en': 'Gorsko Slivovo'},
'3596942':{'bg': u('\u0410\u043b\u0435\u043a\u0441\u0430\u043d\u0434\u0440\u043e\u0432\u043e, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Aleksandrovo, Lovech'},
'3596944':{'bg': u('\u041a\u0440\u0443\u0448\u0443\u043d\u0430'), 'en': 'Krushuna'},
'3596946':{'bg': u('\u0427\u0430\u0432\u0434\u0430\u0440\u0446\u0438'), 'en': 'Chavdartsi'},
'3347651':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347650':{'en': 'Voreppe', 'fr': 'Voreppe'},
'3347653':{'en': 'Fontaine', 'fr': 'Fontaine'},
'3347652':{'en': 'Saint-Ismier', 'fr': 'Saint-Ismier'},
'3347879':{'en': 'Vaulx-en-Velin', 'fr': 'Vaulx-en-Velin'},
'3347878':{'en': 'Lyon', 'fr': 'Lyon'},
'3347656':{'en': 'Grenoble', 'fr': 'Grenoble'},
'3347875':{'en': 'Lyon', 'fr': 'Lyon'},
'3347874':{'en': 'Lyon', 'fr': 'Lyon'},
'3347877':{'en': 'Lyon', 'fr': 'Lyon'},
'3347876':{'en': 'Lyon', 'fr': 'Lyon'},
'3347871':{'en': 'Lyon', 'fr': 'Lyon'},
'3347870':{'en': 'Saint-Fons', 'fr': 'Saint-Fons'},
'3347873':{'en': 'Givors', 'fr': 'Givors'},
'3347872':{'en': 'Lyon', 'fr': 'Lyon'},
'3595768':{'bg': u('\u041e\u0434\u0440\u0438\u043d\u0446\u0438, \u0414\u043e\u0431\u0440.'), 'en': 'Odrintsi, Dobr.'},
'3595769':{'bg': u('\u0425\u0438\u0442\u043e\u0432\u043e'), 'en': 'Hitovo'},
'40259':{'en': 'Bihor', 'ro': 'Bihor'},
'3595760':{'bg': u('\u0411\u043e\u0436\u0443\u0440\u043e\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Bozhurovo, Dobr.'},
'3595761':{'bg': u('\u0411\u0430\u0442\u043e\u0432\u043e'), 'en': 'Batovo'},
'3595762':{'bg': u('\u0421\u0442\u0435\u0444\u0430\u043d \u041a\u0430\u0440\u0430\u0434\u0436\u0430, \u0414\u043e\u0431\u0440.'), 'en': 'Stefan Karadzha, Dobr.'},
'3595763':{'bg': u('\u041f\u043b\u0430\u0447\u0438\u0434\u043e\u043b'), 'en': 'Plachidol'},
'3595764':{'bg': u('\u0412\u043b\u0430\u0434\u0438\u043c\u0438\u0440\u043e\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Vladimirovo, Dobr.'},
'3595765':{'bg': u('\u041b\u043e\u0432\u0447\u0430\u043d\u0446\u0438'), 'en': 'Lovchantsi'},
'3595766':{'bg': u('\u041c\u0435\u0442\u043e\u0434\u0438\u0435\u0432\u043e, \u0414\u043e\u0431\u0440.'), 'en': 'Metodievo, Dobr.'},
'3595767':{'bg': u('\u0416\u0438\u0442\u043d\u0438\u0446\u0430, \u0414\u043e\u0431\u0440.'), 'en': 'Zhitnitsa, Dobr.'},
'3329838':{'en': u('Ploudalm\u00e9zeau'), 'fr': u('Ploudalm\u00e9zeau')},
'3346659':{'en': 'Beaucaire', 'fr': 'Beaucaire'},
'3338048':{'en': 'Quetigny', 'fr': 'Quetigny'},
'3338049':{'en': 'Dijon', 'fr': 'Dijon'},
'3338046':{'en': 'Quetigny', 'fr': 'Quetigny'},
'3346653':{'en': 'Aigues-Mortes', 'fr': 'Aigues-Mortes'},
'3338044':{'en': 'Dijon', 'fr': 'Dijon'},
'3338045':{'en': 'Dijon', 'fr': 'Dijon'},
'3338042':{'en': 'Dijon', 'fr': 'Dijon'},
'3346657':{'en': 'Montfrin', 'fr': 'Montfrin'},
'3346654':{'en': u('Al\u00e8s'), 'fr': u('Al\u00e8s')},
'3346655':{'en': u('Al\u00e8s'), 'fr': u('Al\u00e8s')},
'436241':{'de': 'Sankt Koloman', 'en': 'St. Koloman'},
'436240':{'de': 'Krispl', 'en': 'Krispl'},
'436243':{'de': 'Abtenau', 'en': 'Abtenau'},
'436242':{'de': u('Ru\u00dfbach am Pa\u00df Gsch\u00fctt'), 'en': u('Russbach am Pass Gsch\u00fctt')},
'436245':{'de': 'Hallein', 'en': 'Hallein'},
'436244':{'de': 'Golling an der Salzach', 'en': 'Golling an der Salzach'},
'436247':{'de': u('Gro\u00dfgmain'), 'en': 'Grossgmain'},
'436246':{'de': u('Gr\u00f6dig'), 'en': u('Gr\u00f6dig')},
'3338089':{'en': 'Montbard', 'fr': 'Montbard'},
'390836':{'it': 'Maglie'},
'3597546':{'bg': u('\u0421\u043b\u0430\u0449\u0435\u043d'), 'en': 'Slashten'},
'3597547':{'bg': u('\u0412\u044a\u043b\u043a\u043e\u0441\u0435\u043b'), 'en': 'Valkosel'},
'3597544':{'bg': u('\u041e\u0441\u0438\u043d\u0430'), 'en': 'Osina'},
'3597545':{'bg': u('\u041a\u043e\u0447\u0430\u043d'), 'en': 'Kochan'},
'35991184':{'bg': u('\u0420\u0430\u043a\u0435\u0432\u043e'), 'en': 'Rakevo'},
'35991185':{'bg': u('\u041f\u0443\u0434\u0440\u0438\u044f'), 'en': 'Pudria'},
'35991186':{'bg': u('\u0411\u0430\u0443\u0440\u0435\u043d\u0435'), 'en': 'Baurene'},
'3597541':{'bg': u('\u0421\u0430\u0442\u043e\u0432\u0447\u0430'), 'en': 'Satovcha'},
'35991188':{'bg': u('\u0413\u0430\u043b\u0430\u0442\u0438\u043d'), 'en': 'Galatin'},
'35991189':{'bg': u('\u0422\u0440\u0438 \u043a\u043b\u0430\u0434\u0435\u043d\u0446\u0438'), 'en': 'Tri kladentsi'},
'432815':{'de': u('Gro\u00dfsch\u00f6nau'), 'en': u('Grosssch\u00f6nau')},
'3597548':{'bg': u('\u0413\u043e\u0434\u0435\u0448\u0435\u0432\u043e'), 'en': 'Godeshevo'},
'3597549':{'bg': u('\u0414\u043e\u043b\u0435\u043d, \u0411\u043b\u0430\u0433.'), 'en': 'Dolen, Blag.'},
'441289':{'en': 'Berwick-upon-Tweed'},
'441288':{'en': 'Bude'},
'3329601':{'en': 'Saint-Brieuc', 'fr': 'Saint-Brieuc'},
'3338458':{'en': 'Belfort', 'fr': 'Belfort'},
'3338454':{'en': 'Belfort', 'fr': 'Belfort'},
'3338457':{'en': 'Belfort', 'fr': 'Belfort'},
'3338456':{'en': 'Beaucourt', 'fr': 'Beaucourt'},
'3338452':{'en': 'Champagnole', 'fr': 'Champagnole'},
'441284':{'en': 'Bury St Edmunds'},
'3597723':{'bg': u('\u0414\u043e\u043b\u043d\u0438 \u0420\u0430\u043a\u043e\u0432\u0435\u0446'), 'en': 'Dolni Rakovets'},
'3597720':{'bg': u('\u041f\u0440\u0438\u0431\u043e\u0439'), 'en': 'Priboy'},
'3597726':{'bg': u('\u0414\u0440\u0435\u043d'), 'en': 'Dren'},
'3597727':{'bg': u('\u041a\u043e\u0432\u0430\u0447\u0435\u0432\u0446\u0438, \u041f\u0435\u0440\u043d\u0438\u043a'), 'en': 'Kovachevtsi, Pernik'},
'3597724':{'bg': u('\u0418\u0437\u0432\u043e\u0440, \u041f\u0435\u0440\u043d\u0438\u043a'), 'en': 'Izvor, Pernik'},
'3597725':{'bg': u('\u041a\u043b\u0435\u043d\u043e\u0432\u0438\u043a'), 'en': 'Klenovik'},
'3597728':{'bg': u('\u0414\u0440\u0443\u0433\u0430\u043d'), 'en': 'Drugan'},
'3597729':{'bg': u('\u0414\u043e\u043b\u043d\u0430 \u0414\u0438\u043a\u0430\u043d\u044f'), 'en': 'Dolna Dikanya'},
'3344292':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'3316104':{'en': 'Sartrouville', 'fr': 'Sartrouville'},
'3345681':{'en': 'Thonon-les-Bains', 'fr': 'Thonon-les-Bains'},
'437956':{'de': u('Unterwei\u00dfenbach'), 'en': 'Unterweissenbach'},
'3597937':{'bg': u('\u0420\u044a\u0436\u0434\u0430\u0432\u0438\u0446\u0430'), 'en': 'Razhdavitsa'},
'3323728':{'en': 'Chartres', 'fr': 'Chartres'},
'3597936':{'bg': u('\u0421\u043b\u043e\u043a\u043e\u0449\u0438\u0446\u0430'), 'en': 'Slokoshtitsa'},
'3323723':{'en': 'Courville-sur-Eure', 'fr': 'Courville-sur-Eure'},
'3323721':{'en': 'Chartres', 'fr': 'Chartres'},
'3323720':{'en': 'Chartres', 'fr': 'Chartres'},
'3323727':{'en': 'Maintenon', 'fr': 'Maintenon'},
'3597935':{'bg': u('\u0414\u043e\u043b\u043d\u043e \u0441\u0435\u043b\u043e'), 'en': 'Dolno selo'},
'34830':{'en': 'Barcelona', 'es': 'Barcelona'},
'3597934':{'bg': u('\u0411\u0443\u043d\u043e\u0432\u043e, \u041a\u044e\u0441\u0442.'), 'en': 'Bunovo, Kyust.'},
'3332149':{'en': u('H\u00e9nin-Beaumont'), 'fr': u('H\u00e9nin-Beaumont')},
'3332145':{'en': u('Li\u00e9vin'), 'fr': u('Li\u00e9vin')},
'3332144':{'en': u('Li\u00e9vin'), 'fr': u('Li\u00e9vin')},
'3332146':{'en': 'Calais', 'fr': 'Calais'},
'3332143':{'en': 'Lens', 'fr': 'Lens'},
'3332142':{'en': 'Lens', 'fr': 'Lens'},
'3597930':{'bg': u('\u0415\u0440\u0435\u043c\u0438\u044f'), 'en': 'Eremia'},
'3597048':{'bg': u('\u0411\u043b\u0430\u0436\u0438\u0435\u0432\u043e'), 'en': 'Blazhievo'},
'3598111':{'bg': u('\u0429\u0440\u044a\u043a\u043b\u0435\u0432\u043e'), 'en': 'Shtraklevo'},
'3345024':{'en': 'Meythet', 'fr': 'Meythet'},
'3345025':{'en': 'Bonneville', 'fr': 'Bonneville'},
'3345026':{'en': 'Thonon-les-Bains', 'fr': 'Thonon-les-Bains'},
'3345020':{'en': 'Divonne-les-Bains', 'fr': 'Divonne-les-Bains'},
'3345021':{'en': u('Meg\u00e8ve'), 'fr': u('Meg\u00e8ve')},
'3345022':{'en': 'Meythet', 'fr': 'Meythet'},
'3349562':{'en': 'Calenzana', 'fr': 'Calenzana'},
'3345028':{'en': 'Ferney-Voltaire', 'fr': 'Ferney-Voltaire'},
'3348966':{'en': 'Toulon', 'fr': 'Toulon'},
'3348968':{'en': 'Cannes', 'fr': 'Cannes'},
'3345001':{'en': 'Rumilly', 'fr': 'Rumilly'},
'35971798':{'bg': u('\u0412\u0430\u0441\u0438\u043b\u043e\u0432\u0446\u0438,\u0421\u043e\u0444.'), 'en': 'Vasilovtsi,Sof.'},
'437953':{'de': 'Liebenau', 'en': 'Liebenau'},
'3594152':{'bg': u('\u041e\u0431\u0440\u0443\u0447\u0438\u0449\u0435'), 'en': 'Obruchishte'},
'3594153':{'bg': u('\u041c\u044a\u0434\u0440\u0435\u0446, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Madrets, St. Zagora'},
'3594154':{'bg': u('\u041c\u0435\u0434\u043d\u0438\u043a\u0430\u0440\u043e\u0432\u043e'), 'en': 'Mednikarovo'},
'3594155':{'bg': u('\u0413\u043b\u0430\u0432\u0430\u043d, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Glavan, St. Zagora'},
'3594156':{'bg': u('\u0410\u043f\u0440\u0438\u043b\u043e\u0432\u043e, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Aprilovo, St. Zagora'},
'3594157':{'bg': u('\u0420\u0430\u0437\u0434\u0435\u043b\u043d\u0430, \u0421\u0442. \u0417\u0430\u0433\u043e\u0440\u0430'), 'en': 'Razdelna, St. Zagora'},
'3594158':{'bg': u('\u0418\u0441\u043a\u0440\u0438\u0446\u0430'), 'en': 'Iskritsa'},
'433363':{'de': 'Rechnitz', 'en': 'Rechnitz'},
'433362':{'de': u('Gro\u00dfpetersdorf'), 'en': 'Grosspetersdorf'},
'433365':{'de': u('Deutsch Sch\u00fctzen-Eisenberg'), 'en': u('Deutsch Sch\u00fctzen-Eisenberg')},
'433364':{'de': 'Hannersdorf', 'en': 'Hannersdorf'},
'433366':{'de': 'Kohfidisch', 'en': 'Kohfidisch'},
'35971471':{'bg': u('\u041e\u0447\u0443\u0448\u0430'), 'en': 'Ochusha'},
'441594':{'en': 'Lydney'},
'3595144':{'bg': u('\u041a\u0430\u043c\u0447\u0438\u044f'), 'en': 'Kamchia'},
'3324737':{'en': 'Tours', 'fr': 'Tours'},
'3324736':{'en': 'Tours', 'fr': 'Tours'},
'3324731':{'en': 'Tours', 'fr': 'Tours'},
'3324730':{'en': 'Amboise', 'fr': 'Amboise'},
'3324732':{'en': 'Saint-Pierre-des-Corps', 'fr': 'Saint-Pierre-des-Corps'},
'3324739':{'en': 'Tours', 'fr': 'Tours'},
'35357':{'en': 'Portlaoise/Abbeyleix/Tullamore/Birr'},
'3356189':{'en': 'Saint-Gaudens', 'fr': 'Saint-Gaudens'},
'35356':{'en': 'Kilkenny/Castlecomer/Freshford'},
'3344250':{'en': 'Rognes', 'fr': 'Rognes'},
'3356180':{'en': 'Toulouse', 'fr': 'Toulouse'},
'3356187':{'en': 'Carbonne', 'fr': 'Carbonne'},
'3356186':{'en': 'Tournefeuille', 'fr': 'Tournefeuille'},
'441787':{'en': 'Sudbury'},
'37423798':{'am': u('\u0547\u0565\u0576\u0561\u057e\u0561\u0576'), 'en': 'Shenavan', 'ru': u('\u0428\u0435\u043d\u0430\u0432\u0430\u043d')},
'35991401':{'bg': u('\u0412\u0440\u0430\u043d\u044f\u043a'), 'en': 'Vranyak'},
'35353':{'en': 'Wexford/Enniscorthy/Ferns/Gorey'},
'3593518':{'bg': u('\u0414\u0440\u0430\u0433\u043e\u0440'), 'en': 'Dragor'},
'3344253':{'en': 'Rousset', 'fr': 'Rousset'},
'37423794':{'am': u('\u0544\u0561\u0580\u0563\u0561\u0580\u0561'), 'en': 'Margara', 'ru': u('\u041c\u0430\u0440\u0433\u0430\u0440\u0430')},
'37423796':{'am': u('\u054f\u0561\u0576\u0571\u0578\u0582\u057f'), 'en': 'Tandzut', 'ru': u('\u0422\u0430\u043d\u0434\u0437\u0443\u0442')},
'433185':{'de': 'Preding', 'en': 'Preding'},
'433184':{'de': 'Wolfsberg im Schwarzautal', 'en': 'Wolfsberg im Schwarzautal'},
'353404':{'en': 'Wicklow'},
'353402':{'en': 'Arklow'},
'433183':{'de': 'Sankt Georgen an der Stiefing', 'en': 'St. Georgen an der Stiefing'},
'433182':{'de': 'Wildon', 'en': 'Wildon'},
'441789':{'en': 'Stratford-upon-Avon'},
'3356323':{'en': 'Montauban', 'fr': 'Montauban'},
'3356321':{'en': 'Montauban', 'fr': 'Montauban'},
'3356320':{'en': 'Montauban', 'fr': 'Montauban'},
'3344258':{'en': 'Gardanne', 'fr': 'Gardanne'},
'3356329':{'en': 'Valence', 'fr': 'Valence'},
'3344259':{'en': 'Aix-en-Provence', 'fr': 'Aix-en-Provence'},
'35971306':{'bg': u('\u0420\u0430\u0448\u043a\u043e\u0432\u043e'), 'en': 'Rashkovo'},
'3593513':{'bg': u('\u041c\u0430\u043b\u043e \u041a\u043e\u043d\u0430\u0440\u0435'), 'en': 'Malo Konare'},
'3347466':{'en': 'Belleville', 'fr': 'Belleville'},
'3347464':{'en': 'Thizy', 'fr': 'Thizy'},
'3347465':{'en': u('Villefranche-sur-Sa\u00f4ne'), 'fr': u('Villefranche-sur-Sa\u00f4ne')},
'3347462':{'en': u('Villefranche-sur-Sa\u00f4ne'), 'fr': u('Villefranche-sur-Sa\u00f4ne')},
'3347463':{'en': 'Tarare', 'fr': 'Tarare'},
'3347460':{'en': u('Villefranche-sur-Sa\u00f4ne'), 'fr': u('Villefranche-sur-Sa\u00f4ne')},
'3347461':{'en': 'Meximieux', 'fr': 'Meximieux'},
'3347468':{'en': u('Villefranche-sur-Sa\u00f4ne'), 'fr': u('Villefranche-sur-Sa\u00f4ne')},
'3595579':{'bg': u('\u0412\u0435\u0434\u0440\u043e\u0432\u043e'), 'en': 'Vedrovo'},
'3595578':{'bg': u('\u0422\u0435\u0440\u0437\u0438\u0439\u0441\u043a\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Terziysko, Burgas'},
'441590':{'en': 'Lymington'},
'3595575':{'bg': u('\u041f\u0440\u0438\u043b\u0435\u043f, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Prilep, Burgas'},
'3595574':{'bg': u('\u0421\u044a\u0435\u0434\u0438\u043d\u0435\u043d\u0438\u0435, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Saedinenie, Burgas'},
'3595577':{'bg': u('\u041f\u043e\u0434\u0432\u0438\u0441, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Podvis, Burgas'},
'3595576':{'bg': u('\u041b\u043e\u0437\u0430\u0440\u0435\u0432\u043e'), 'en': 'Lozarevo'},
'3595571':{'bg': u('\u0421\u0443\u043d\u0433\u0443\u0440\u043b\u0430\u0440\u0435'), 'en': 'Sungurlare'},
'3595570':{'bg': u('\u041c\u0430\u043d\u043e\u043b\u0438\u0447'), 'en': 'Manolich'},
'3595573':{'bg': u('\u0412\u0435\u0437\u0435\u043d\u043a\u043e\u0432\u043e'), 'en': 'Vezenkovo'},
'3595572':{'bg': u('\u0411\u0435\u0440\u043e\u043d\u043e\u0432\u043e'), 'en': 'Beronovo'},
'3596004':{'bg': u('\u041c\u0430\u043a\u043e\u0432\u043e'), 'en': 'Makovo'},
'3596006':{'bg': u('\u041f\u0440\u0435\u0441\u0438\u044f\u043d'), 'en': 'Presian'},
'3596007':{'bg': u('\u0420\u0430\u043b\u0438\u0446\u0430'), 'en': 'Ralitsa'},
'3596001':{'bg': u('\u0427\u0435\u0440\u043a\u043e\u0432\u043d\u0430, \u0422\u044a\u0440\u0433.'), 'en': 'Cherkovna, Targ.'},
'3596002':{'bg': u('\u0421\u044a\u0435\u0434\u0438\u043d\u0435\u043d\u0438\u0435, \u0422\u044a\u0440\u0433.'), 'en': 'Saedinenie, Targ.'},
'3596003':{'bg': u('\u041f\u0440\u0435\u0441\u0435\u043b\u0435\u0446'), 'en': 'Preselets'},
'3347790':{'en': u('Roche-la-Moli\u00e8re'), 'fr': u('Roche-la-Moli\u00e8re')},
'3347791':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347792':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347793':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347795':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'3347796':{'en': 'Montbrison', 'fr': 'Montbrison'},
'3599726':{'bg': u('\u0417\u0430\u043c\u0444\u0438\u0440'), 'en': 'Zamfir'},
'3324169':{'en': u('Avrill\u00e9'), 'fr': u('Avrill\u00e9')},
'359650':{'bg': u('\u041b\u0435\u0432\u0441\u043a\u0438, \u041f\u043b\u0435\u0432\u0435\u043d'), 'en': 'Levski, Pleven'},
'359658':{'bg': u('\u0411\u0435\u043b\u0435\u043d\u0435'), 'en': 'Belene'},
'359659':{'bg': u('\u0427\u0435\u0440\u0432\u0435\u043d \u0431\u0440\u044f\u0433'), 'en': 'Cherven bryag'},
'441754':{'en': 'Skegness'},
'441757':{'en': 'Selby'},
'441756':{'en': 'Skipton'},
'441751':{'en': 'Pickering'},
'441750':{'en': 'Selkirk'},
'3597044':{'bg': u('\u0411\u0430\u0431\u0438\u043d\u043e'), 'en': 'Babino'},
'441752':{'en': 'Plymouth'},
'441759':{'en': 'Pocklington'},
'441758':{'en': 'Pwllheli'},
'39085':{'en': 'Pescara', 'it': 'Pescara'},
'3316480':{'en': 'Lognes', 'fr': 'Lognes'},
'3316486':{'en': 'Les Ulis', 'fr': 'Les Ulis'},
'3316487':{'en': 'Melun', 'fr': 'Melun'},
'3316488':{'en': 'Moissy-Cramayel', 'fr': 'Moissy-Cramayel'},
'3349020':{'en': 'L\'Isle sur la Sorgue', 'fr': 'L\'Isle sur la Sorgue'},
'3349021':{'en': 'L\'Isle sur la Sorgue', 'fr': 'L\'Isle sur la Sorgue'},
'3349022':{'en': u('Saint-Saturnin-l\u00e8s-Avignon'), 'fr': u('Saint-Saturnin-l\u00e8s-Avignon')},
'3349023':{'en': 'Caumont-sur-Durance', 'fr': 'Caumont-sur-Durance'},
'3349024':{'en': u('Ch\u00e2teaurenard'), 'fr': u('Ch\u00e2teaurenard')},
'3349025':{'en': u('Villeneuve-l\u00e8s-Avignon'), 'fr': u('Villeneuve-l\u00e8s-Avignon')},
'3349026':{'en': 'Pujaut', 'fr': 'Pujaut'},
'3349027':{'en': 'Avignon', 'fr': 'Avignon'},
'3349028':{'en': 'Vaison-la-Romaine', 'fr': 'Vaison-la-Romaine'},
'3349029':{'en': 'Piolenc', 'fr': 'Piolenc'},
'39080':{'en': 'Bari', 'it': 'Bari'},
'436588':{'de': 'Lofer', 'en': 'Lofer'},
'436589':{'de': 'Unken', 'en': 'Unken'},
'436582':{'de': 'Saalfelden am Steinernen Meer', 'en': 'Saalfelden am Steinernen Meer'},
'436583':{'de': 'Leogang', 'en': 'Leogang'},
'436584':{'de': 'Maria Alm am Steinernen Meer', 'en': 'Maria Alm am Steinernen Meer'},
'3342764':{'en': u('Saint-\u00c9tienne'), 'fr': u('Saint-\u00c9tienne')},
'374254':{'am': u('\u054f\u0561\u0577\u056b\u0580'), 'en': 'Tashir', 'ru': u('\u0422\u0430\u0448\u0438\u0440')},
'390828':{'it': 'Battipaglia'},
'3323586':{'en': 'Eu', 'fr': 'Eu'},
'3349977':{'en': 'Montpellier', 'fr': 'Montpellier'},
'3349974':{'en': 'Montpellier', 'fr': 'Montpellier'},
'390823':{'en': 'Caserta', 'it': 'Caserta'},
'390824':{'en': 'Benevento', 'it': 'Benevento'},
'390825':{'en': 'Avellino', 'it': 'Avellino'},
'390827':{'it': 'Sant\'Angelo dei Lombardi'},
'441342':{'en': 'East Grinstead'},
'441343':{'en': 'Elgin'},
'441340':{'en': 'Craigellachie (Aberlour)'},
'441341':{'en': 'Barmouth'},
'441346':{'en': 'Fraserburgh'},
'441347':{'en': 'Easingwold'},
'441344':{'en': 'Bracknell'},
'441348':{'en': 'Fishguard'},
'441349':{'en': 'Dingwall'},
'40249':{'en': 'Olt', 'ro': 'Olt'},
'3751719':{'be': u('\u041a\u0430\u043f\u044b\u043b\u044c, \u041c\u0456\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Kopyl, Minsk Region', 'ru': u('\u041a\u043e\u043f\u044b\u043b\u044c, \u041c\u0438\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751718':{'be': u('\u0423\u0437\u0434\u0430, \u041c\u0456\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Uzda, Minsk Region', 'ru': u('\u0423\u0437\u0434\u0430, \u041c\u0438\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751717':{'be': u('\u0421\u0442\u0430\u045e\u0431\u0446\u044b'), 'en': 'Stolbtsy', 'ru': u('\u0421\u0442\u043e\u043b\u0431\u0446\u044b')},
'3751716':{'be': u('\u0414\u0437\u044f\u0440\u0436\u044b\u043d\u0441\u043a'), 'en': 'Dzerzhinsk', 'ru': u('\u0414\u0437\u0435\u0440\u0436\u0438\u043d\u0441\u043a')},
'3751715':{'be': u('\u0411\u0435\u0440\u0430\u0437\u0456\u043d\u043e, \u041c\u0456\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Berezino, Minsk Region', 'ru': u('\u0411\u0435\u0440\u0435\u0437\u0438\u043d\u043e, \u041c\u0438\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3751714':{'be': u('\u0427\u044d\u0440\u0432\u0435\u043d\u044c'), 'en': 'Cherven', 'ru': u('\u0427\u0435\u0440\u0432\u0435\u043d\u044c')},
'3751713':{'be': u('\u041c\u0430\u0440\u2019\u0456\u043d\u0430 \u0413\u043e\u0440\u043a\u0430, \u041c\u0456\u043d\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Maryina Gorka, Minsk Region', 'ru': u('\u041c\u0430\u0440\u044c\u0438\u043d\u0430 \u0413\u043e\u0440\u043a\u0430, \u041c\u0438\u043d\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'333260':{'en': 'Reims', 'fr': 'Reims'},
'3323110':{'en': 'Bayeux', 'fr': 'Bayeux'},
'3323114':{'en': 'Deauville', 'fr': 'Deauville'},
'3323115':{'en': 'Caen', 'fr': 'Caen'},
'3752353':{'be': u('\u0416\u044b\u0442\u043a\u0430\u0432\u0456\u0447\u044b, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Zhitkovichi, Gomel Region', 'ru': u('\u0416\u0438\u0442\u043a\u043e\u0432\u0438\u0447\u0438, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752350':{'be': u('\u041f\u0435\u0442\u0440\u044b\u043a\u0430\u045e, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Petrikov, Gomel Region', 'ru': u('\u041f\u0435\u0442\u0440\u0438\u043a\u043e\u0432, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752356':{'be': u('\u041b\u0435\u043b\u044c\u0447\u044b\u0446\u044b, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Lelchitsy, Gomel Region', 'ru': u('\u041b\u0435\u043b\u044c\u0447\u0438\u0446\u044b, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3752357':{'be': u('\u0410\u043a\u0446\u044f\u0431\u0440\u0441\u043a\u0456, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Oktyabrskiy, Gomel Region', 'ru': u('\u041e\u043a\u0442\u044f\u0431\u0440\u044c\u0441\u043a\u0438\u0439, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'334961':{'en': 'Marseille', 'fr': 'Marseille'},
'3752355':{'be': u('\u041d\u0430\u0440\u043e\u045e\u043b\u044f, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Narovlya, Gomel Region', 'ru': u('\u041d\u0430\u0440\u043e\u0432\u043b\u044f, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3332088':{'en': 'Lille', 'fr': 'Lille'},
'3332089':{'en': u('Marcq-en-Bar\u0153ul'), 'fr': u('Marcq-en-Bar\u0153ul')},
'3332080':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332081':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332082':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332083':{'en': 'Roubaix', 'fr': 'Roubaix'},
'3332085':{'en': u('Ann\u0153ullin'), 'fr': u('Ann\u0153ullin')},
'432773':{'de': 'Eichgraben', 'en': 'Eichgraben'},
'3332087':{'en': 'Lesquin', 'fr': 'Lesquin'},
'3355366':{'en': 'Agen', 'fr': 'Agen'},
'3355364':{'en': 'Marmande', 'fr': 'Marmande'},
'3355365':{'en': u('N\u00e9rac'), 'fr': u('N\u00e9rac')},
'3355363':{'en': 'Bergerac', 'fr': 'Bergerac'},
'3355361':{'en': 'Bergerac', 'fr': 'Bergerac'},
'3355369':{'en': 'Agen', 'fr': 'Agen'},
'3354945':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354944':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354947':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354946':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354941':{'en': 'Poitiers', 'fr': 'Poitiers'},
'3354943':{'en': 'Vivonne', 'fr': 'Vivonne'},
'3354949':{'en': 'Chasseneuil-du-Poitou', 'fr': 'Chasseneuil-du-Poitou'},
'3359068':{'en': 'Les Abymes', 'fr': 'Les Abymes'},
'3359060':{'en': 'Baie Mahault', 'fr': 'Baie Mahault'},
'3332556':{'en': 'Saint-Dizier', 'fr': 'Saint-Dizier'},
'3593528':{'bg': u('\u0413\u043e\u0432\u0435\u0434\u0430\u0440\u0435'), 'en': 'Govedare'},
'3593529':{'bg': u('\u041c\u043e\u043a\u0440\u0438\u0449\u0435'), 'en': 'Mokrishte'},
'3593524':{'bg': u('\u0410\u043f\u0440\u0438\u043b\u0446\u0438, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Apriltsi, Pazardzhik'},
'3593526':{'bg': u('\u0414\u0438\u043d\u043a\u0430\u0442\u0430'), 'en': 'Dinkata'},
'3593527':{'bg': u('\u0410\u043b\u0435\u043a\u043e \u041a\u043e\u043d\u0441\u0442\u0430\u043d\u0442\u0438\u043d\u043e\u0432\u043e'), 'en': 'Aleko Konstantinovo'},
'3593520':{'bg': u('\u041c\u0438\u0440\u044f\u043d\u0446\u0438'), 'en': 'Miryantsi'},
'3593521':{'bg': u('\u0417\u0432\u044a\u043d\u0438\u0447\u0435\u0432\u043e'), 'en': 'Zvanichevo'},
'3593522':{'bg': u('\u0413\u0435\u043b\u0435\u043c\u0435\u043d\u043e\u0432\u043e'), 'en': 'Gelemenovo'},
'3593523':{'bg': u('\u0421\u0438\u043d\u0438\u0442\u0435\u0432\u043e'), 'en': 'Sinitevo'},
'37423498':{'am': u('\u054f\u0561\u0583\u0565\u0580\u0561\u056f\u0561\u0576'), 'en': 'Taperakan', 'ru': u('\u0422\u0430\u043f\u0435\u0440\u0430\u043a\u0430\u043d')},
'37423492':{'am': u('\u0544\u0561\u0580\u057f\u056b\u0580\u0578\u057d\u0575\u0561\u0576'), 'en': 'Martirosyan', 'ru': u('\u041c\u0430\u0440\u0442\u0438\u0440\u043e\u0441\u044f\u043d')},
'37423497':{'am': u('\u0553\u0578\u0584\u0580 \u054e\u0565\u0564\u056b'), 'en': 'Pokr Vedi', 'ru': u('\u041f\u043e\u043a\u0440 \u0412\u0435\u0434\u0438')},
'3595967':{'bg': u('\u0411\u0430\u0442\u0430'), 'en': 'Bata'},
'3595968':{'bg': u('\u041a\u0430\u0431\u043b\u0435\u0448\u043a\u043e\u0432\u043e, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Kableshkovo, Burgas'},
'3595969':{'bg': u('\u0413\u044a\u043b\u044a\u0431\u0435\u0446, \u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Galabets, Burgas'},
'3341339':{'en': 'Avignon', 'fr': 'Avignon'},
'35947353':{'bg': u('\u0411\u044a\u043b\u0433\u0430\u0440\u0441\u043a\u0430 \u043f\u043e\u043b\u044f\u043d\u0430'), 'en': 'Balgarska polyana'},
'3355890':{'en': 'Dax', 'fr': 'Dax'},
'3355891':{'en': u('Saint-Paul-l\u00e8s-Dax'), 'fr': u('Saint-Paul-l\u00e8s-Dax')},
'3355897':{'en': 'Mugron', 'fr': 'Mugron'},
'3593748':{'bg': u('\u041b\u044f\u0441\u043a\u043e\u0432\u0435\u0446, \u0425\u0430\u0441\u043a.'), 'en': 'Lyaskovets, Hask.'},
'3593749':{'bg': u('\u0413\u0430\u0440\u0432\u0430\u043d\u043e\u0432\u043e'), 'en': 'Garvanovo'},
'3332336':{'en': 'Ham', 'fr': 'Ham'},
'3593740':{'bg': u('\u041f\u0447\u0435\u043b\u0430\u0440\u0438'), 'en': 'Pchelari'},
'3593741':{'bg': u('\u041c\u0430\u043d\u0434\u0440\u0430'), 'en': 'Mandra'},
'3332338':{'en': 'Chauny', 'fr': 'Chauny'},
'3332339':{'en': 'Chauny', 'fr': 'Chauny'},
'3593744':{'bg': u('\u0421\u044a\u0440\u043d\u0438\u0446\u0430, \u0425\u0430\u0441\u043a.'), 'en': 'Sarnitsa, Hask.'},
'3593745':{'bg': u('\u041c\u0430\u043b\u044a\u043a \u0438\u0437\u0432\u043e\u0440, \u0425\u0430\u0441\u043a.'), 'en': 'Malak izvor, Hask.'},
'3324944':{'en': 'Nantes', 'fr': 'Nantes'},
'3593747':{'bg': u('\u0422\u0430\u0442\u0430\u0440\u0435\u0432\u043e, \u0425\u0430\u0441\u043a.'), 'en': 'Tatarevo, Hask.'},
'35947354':{'bg': u('\u041a\u0430\u043c\u0435\u043d\u043d\u0430 \u0440\u0435\u043a\u0430'), 'en': 'Kamenna reka'},
'3349560':{'en': u('L\'\u00cele-Rousse'), 'fr': u('L\'\u00cele-Rousse')},
'3334488':{'en': 'Nanteuil-le-Haudouin', 'fr': 'Nanteuil-le-Haudouin'},
'3334483':{'en': u('Margny-l\u00e8s-Compi\u00e8gne'), 'fr': u('Margny-l\u00e8s-Compi\u00e8gne')},
'3334486':{'en': u('Compi\u00e8gne'), 'fr': u('Compi\u00e8gne')},
'3334487':{'en': u('Cr\u00e9py-en-Valois'), 'fr': u('Cr\u00e9py-en-Valois')},
'35941339':{'bg': u('\u0414\u0438\u043c\u0438\u0442\u0440\u0438\u0435\u0432\u043e'), 'en': 'Dimitrievo'},
'3355908':{'en': 'Saint-Jean-de-Luz', 'fr': 'Saint-Jean-de-Luz'},
'441508':{'en': 'Brooke'},
'441509':{'en': 'Loughborough'},
'4415392':{'en': 'Kendal'},
'3355678':{'en': 'Cestas', 'fr': 'Cestas'},
'441502':{'en': 'Lowestoft'},
'441503':{'en': 'Looe'},
'441505':{'en': 'Johnstone'},
'441506':{'en': 'Bathgate'},
'3323331':{'en': u('Alen\u00e7on'), 'fr': u('Alen\u00e7on')},
'35960373':{'bg': u('\u041f\u043e\u0441\u0430\u0431\u0438\u043d\u0430'), 'en': 'Posabina'},
'35960372':{'bg': u('\u041a\u0440\u0435\u043f\u0447\u0430'), 'en': 'Krepcha'},
'3596921':{'bg': u('\u0410\u0431\u043b\u0430\u043d\u0438\u0446\u0430, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Ablanitsa, Lovech'},
'35960370':{'bg': u('\u0413\u043e\u043b\u044f\u043c\u043e \u0433\u0440\u0430\u0434\u0438\u0449\u0435'), 'en': 'Golyamo gradishte'},
'35960377':{'bg': u('\u0410\u043f\u0440\u0438\u043b\u043e\u0432\u043e, \u0422\u044a\u0440\u0433.'), 'en': 'Aprilovo, Targ.'},
'35960376':{'bg': u('\u041b\u044e\u0431\u043b\u0435\u043d'), 'en': 'Lyublen'},
'35960375':{'bg': u('\u0413\u044a\u0440\u0447\u0438\u043d\u043e\u0432\u043e'), 'en': 'Garchinovo'},
'35960374':{'bg': u('\u0413\u043e\u0440\u0441\u043a\u043e \u0410\u0431\u043b\u0430\u043d\u043e\u0432\u043e'), 'en': 'Gorsko Ablanovo'},
'3596929':{'bg': u('\u0420\u0430\u0434\u044e\u0432\u0435\u043d\u0435'), 'en': 'Radyuvene'},
'35960378':{'bg': u('\u0426\u0430\u0440 \u0410\u0441\u0435\u043d, \u0422\u044a\u0440\u0433.'), 'en': 'Tsar Asen, Targ.'},
'3329835':{'en': u('Quimperl\u00e9'), 'fr': u('Quimperl\u00e9')},
'35956':{'bg': u('\u0411\u0443\u0440\u0433\u0430\u0441'), 'en': 'Burgas'},
'3329834':{'en': 'Brest', 'fr': 'Brest'},
'35958':{'bg': u('\u0414\u043e\u0431\u0440\u0438\u0447'), 'en': 'Dobrich'},
'3355676':{'en': 'Langon', 'fr': 'Langon'},
'3595742':{'bg': u('\u0420\u0430\u043a\u043e\u0432\u0441\u043a\u0438, \u0414\u043e\u0431\u0440.'), 'en': 'Rakovski, Dobr.'},
'3595743':{'bg': u('\u0428\u0430\u0431\u043b\u0430'), 'en': 'Shabla'},
'3595740':{'bg': u('\u0413\u043e\u0440\u0438\u0447\u0430\u043d\u0435'), 'en': 'Gorichane'},
'3355677':{'en': u('Ambar\u00e8s-et-Lagrave'), 'fr': u('Ambar\u00e8s-et-Lagrave')},
'3595746':{'bg': u('\u0411\u0435\u043b\u0433\u0443\u043d'), 'en': 'Belgun'},
'3595747':{'bg': u('\u0412\u0430\u043a\u043b\u0438\u043d\u043e'), 'en': 'Vaklino'},
'3595745':{'bg': u('\u0412\u0440\u0430\u043d\u0438\u043d\u043e'), 'en': 'Vranino'},
'3347633':{'en': u('\u00c9chirolles'), 'fr': u('\u00c9chirolles')},
'3355906':{'en': u('Juran\u00e7on'), 'fr': u('Juran\u00e7on')},
'3595748':{'bg': u('\u0414\u0443\u0440\u0430\u043d\u043a\u0443\u043b\u0430\u043a'), 'en': 'Durankulak'},
'3595749':{'bg': u('\u041a\u0440\u0430\u043f\u0435\u0446, \u0414\u043e\u0431\u0440.'), 'en': 'Krapets, Dobr.'},
'3347637':{'en': 'Le Pont de Beauvoisin', 'fr': 'Le Pont de Beauvoisin'},
'3347636':{'en': 'Vinay', 'fr': 'Vinay'},
'3347635':{'en': 'Moirans', 'fr': 'Moirans'},
'3355905':{'en': 'Laruns', 'fr': 'Laruns'},
'3338028':{'en': 'Dijon', 'fr': 'Dijon'},
'3338029':{'en': 'Brazey-en-Plaine', 'fr': 'Brazey-en-Plaine'},
'3324050':{'en': 'Nantes', 'fr': 'Nantes'},
'3338022':{'en': 'Beaune', 'fr': 'Beaune'},
'3324053':{'en': 'Saint-Nazaire', 'fr': 'Saint-Nazaire'},
'3338024':{'en': 'Beaune', 'fr': 'Beaune'},
'3338025':{'en': 'Beaune', 'fr': 'Beaune'},
'3338027':{'en': 'Auxonne', 'fr': 'Auxonne'},
'3346652':{'en': u('Al\u00e8s'), 'fr': u('Al\u00e8s')},
'3742675':{'am': u('\u0531\u0580\u056e\u057e\u0561\u0562\u0565\u0580\u0564'), 'en': 'Artsvaberd', 'ru': u('\u0410\u0440\u0446\u0432\u0430\u0431\u0435\u0440\u0434')},
'355893':{'en': u('Ksamil, Sarand\u00eb')},
'3597520':{'bg': u('\u041a\u043e\u0440\u043d\u0438\u0446\u0430'), 'en': 'Kornitsa'},
'3597521':{'bg': u('\u041a\u043e\u043f\u0440\u0438\u0432\u043b\u0435\u043d'), 'en': 'Koprivlen'},
'3597522':{'bg': u('\u0414\u044a\u0431\u043d\u0438\u0446\u0430'), 'en': 'Dabnitsa'},
'3597523':{'bg': u('\u0413\u044a\u0440\u043c\u0435\u043d'), 'en': 'Garmen'},
'3597524':{'bg': u('\u0410\u0431\u043b\u0430\u043d\u0438\u0446\u0430, \u0411\u043b\u0430\u0433.'), 'en': 'Ablanitsa, Blag.'},
'3597525':{'bg': u('\u0411\u0430\u043d\u0438\u0447\u0430\u043d'), 'en': 'Banichan'},
'3597526':{'bg': u('\u0420\u0438\u0431\u043d\u043e\u0432\u043e'), 'en': 'Ribnovo'},
'3597527':{'bg': u('\u0413\u043e\u0440\u043d\u043e \u0414\u0440\u044f\u043d\u043e\u0432\u043e'), 'en': 'Gorno Dryanovo'},
'3597528':{'bg': u('\u0425\u0430\u0434\u0436\u0438\u0434\u0438\u043c\u043e\u0432\u043e'), 'en': 'Hadzhidimovo'},
'3597529':{'bg': u('\u0411\u0440\u0435\u0437\u043d\u0438\u0446\u0430'), 'en': 'Breznitsa'},
'373248':{'en': 'Criuleni', 'ro': 'Criuleni', 'ru': u('\u041a\u0440\u0438\u0443\u043b\u0435\u043d\u044c')},
'3329668':{'en': 'Saint-Brieuc', 'fr': 'Saint-Brieuc'},
'3329669':{'en': 'Binic', 'fr': 'Binic'},
'3593030':{'bg': u('\u0428\u0438\u0440\u043e\u043a\u0430 \u043b\u044a\u043a\u0430'), 'en': 'Shiroka laka'},
'3329660':{'en': 'Saint-Brieuc', 'fr': 'Saint-Brieuc'},
'3329661':{'en': 'Saint-Brieuc', 'fr': 'Saint-Brieuc'},
'3329662':{'en': 'Saint-Brieuc', 'fr': 'Saint-Brieuc'},
'3329663':{'en': u('Pl\u00e9neuf-Val-Andr\u00e9'), 'fr': u('Pl\u00e9neuf-Val-Andr\u00e9')},
'3329664':{'en': u('Pl\u00e9dran'), 'fr': u('Pl\u00e9dran')},
'3329665':{'en': 'Lanvollon', 'fr': 'Lanvollon'},
'3329666':{'en': u('Loud\u00e9ac'), 'fr': u('Loud\u00e9ac')},
'3315805':{'en': 'Paris', 'fr': 'Paris'},
'4415240':{'en': 'Lancaster'},
'3346651':{'en': 'Le Grau du Roi', 'fr': 'Le Grau du Roi'},
'4414309':{'en': 'Market Weighton'},
'4414308':{'en': 'Market Weighton'},
'43662':{'de': 'Salzburg', 'en': 'Salzburg'},
'35935393':{'bg': u('\u0421\u043c\u0438\u043b\u0435\u0446, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Smilets, Pazardzhik'},
'35935392':{'bg': u('\u0414\u044e\u043b\u0435\u0432\u043e, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Dyulevo, Pazardzhik'},
'35935391':{'bg': u('\u0411\u043b\u0430\u0442\u043d\u0438\u0446\u0430'), 'en': 'Blatnitsa'},
'4414302':{'en': 'North Cave'},
'4414305':{'en': 'North Cave'},
'4414304':{'en': 'North Cave'},
'4414307':{'en': 'Market Weighton'},
'35935394':{'bg': u('\u041e\u0431\u043e\u0440\u0438\u0449\u0435, \u041f\u0430\u0437\u0430\u0440\u0434\u0436\u0438\u043a'), 'en': 'Oborishte, Pazardzhik'},
'3596142':{'bg': u('\u041e\u0431\u0435\u0434\u0438\u043d\u0435\u043d\u0438\u0435'), 'en': 'Obedinenie'},
'37422453':{'am': u('\u054a\u057c\u0578\u0577\u0575\u0561\u0576'), 'en': 'Proshyan', 'ru': u('\u041f\u0440\u043e\u0448\u044f\u043d')},
'37422452':{'am': u('\u0536\u0578\u057e\u0578\u0582\u0576\u056b'), 'en': 'Zovuni', 'ru': u('\u0417\u043e\u0432\u0443\u043d\u0438')},
'37422454':{'am': u('\u0531\u0580\u0563\u0565\u056c'), 'en': 'Argel', 'ru': u('\u0410\u0440\u0433\u0435\u043b')},
'35967193':{'bg': u('\u041a\u043c\u0435\u0442\u043e\u0432\u0446\u0438'), 'en': 'Kmetovtsi'},
'35967194':{'bg': u('\u0413\u0440\u044a\u0431\u043b\u0435\u0432\u0446\u0438'), 'en': 'Grablevtsi'},
'3596148':{'bg': u('\u041f\u0430\u0432\u0435\u043b'), 'en': 'Pavel'},
'441838':{'en': 'Dalmally'},
'3328537':{'en': 'Nantes', 'fr': 'Nantes'},
'3323255':{'en': 'Gisors', 'fr': 'Gisors'},
'3323254':{'en': 'Les Andelys', 'fr': 'Les Andelys'},
'3323253':{'en': 'Gaillon', 'fr': 'Gaillon'},
'3323251':{'en': 'Vernon', 'fr': 'Vernon'},
'3323250':{'en': 'Louviers', 'fr': 'Louviers'},
'3323259':{'en': 'Val-de-Reuil', 'fr': 'Val-de-Reuil'},
'3323258':{'en': 'Nonancourt', 'fr': 'Nonancourt'},
'3593198':{'bg': u('\u0427\u0435\u0445\u043b\u0430\u0440\u0435'), 'en': 'Chehlare'},
'37428375':{'am': u('\u0539\u0561\u057d\u056b\u056f'), 'en': 'Tasik', 'ru': u('\u0422\u0430\u0441\u0438\u043a')},
'3593195':{'bg': u('\u0420\u043e\u0437\u043e\u0432\u0435\u0446'), 'en': 'Rozovets'},
'3593194':{'bg': u('\u0417\u0435\u043b\u0435\u043d\u0438\u043a\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Zelenikovo, Plovdiv'},
'3593197':{'bg': u('\u0422\u044e\u0440\u043a\u043c\u0435\u043d'), 'en': 'Tyurkmen'},
'3593196':{'bg': u('\u0414\u0440\u0430\u043d\u0433\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Drangovo, Plovdiv'},
'3593191':{'bg': u('\u0411\u0440\u0435\u0437\u043e\u0432\u043e, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Brezovo, Plovdiv'},
'3593190':{'bg': u('\u0412\u044a\u0440\u0431\u0435\u043d, \u041f\u043b\u043e\u0432\u0434\u0438\u0432'), 'en': 'Varben, Plovdiv'},
'3593193':{'bg': u('\u0411\u043e\u0440\u0435\u0446'), 'en': 'Borets'},
'3593192':{'bg': u('\u0411\u0430\u0431\u0435\u043a'), 'en': 'Babek'},
'373242':{'en': 'Stefan Voda', 'ro': u('\u015etefan Vod\u0103'), 'ru': u('\u0428\u0442\u0435\u0444\u0430\u043d \u0412\u043e\u0434\u044d')},
'42133':{'en': 'Trnava'},
'42132':{'en': u('Tren\u010d\u00edn')},
'42131':{'en': u('Dunajsk\u00e1 Streda')},
'3354779':{'en': 'Bordeaux', 'fr': 'Bordeaux'},
'42137':{'en': 'Nitra'},
'42136':{'en': 'Levice'},
'42135':{'en': u('Nov\u00e9 Z\u00e1mky')},
'42134':{'en': 'Senica'},
'441287':{'en': 'Guisborough'},
'441286':{'en': 'Caernarfon'},
'441285':{'en': 'Cirencester'},
'42138':{'en': u('Topo\u013e\u010dany')},
'441283':{'en': 'Burton-on-Trent'},
'441282':{'en': 'Burnley'},
'441280':{'en': 'Buckingham'},
'3338826':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'3345008':{'en': 'Annecy', 'fr': 'Annecy'},
'3345009':{'en': 'Annecy-le-Vieux', 'fr': 'Annecy-le-Vieux'},
'3345007':{'en': 'Bonneville', 'fr': 'Bonneville'},
'3345005':{'en': 'Annecy', 'fr': 'Annecy'},
'3345002':{'en': u('Th\u00f4nes'), 'fr': u('Th\u00f4nes')},
'3345003':{'en': 'La Roche sur Foron', 'fr': 'La Roche sur Foron'},
'3338827':{'en': 'Strasbourg', 'fr': 'Strasbourg'},
'37322':{'en': 'Chisinau', 'ro': u('Chi\u015fin\u0103u'), 'ru': u('\u041a\u0438\u0448\u0438\u043d\u044d\u0443')},
'3348982':{'en': 'Cannes', 'fr': 'Cannes'},
'3596930':{'bg': u('\u0421\u043b\u0430\u0432\u0449\u0438\u0446\u0430'), 'en': 'Slavshtitsa'},
'3596931':{'bg': u('\u0423\u0433\u044a\u0440\u0447\u0438\u043d'), 'en': 'Ugarchin'},
'437954':{'de': 'Sankt Georgen am Walde', 'en': 'St. Georgen am Walde'},
'437955':{'de': u('K\u00f6nigswiesen'), 'en': u('K\u00f6nigswiesen')},
'437736':{'de': 'Pram', 'en': 'Pram'},
'437952':{'de': 'Weitersfelden', 'en': 'Weitersfelden'},
'437734':{'de': 'Hofkirchen an der Trattnach', 'en': 'Hofkirchen an der Trattnach'},
'437735':{'de': 'Gaspoltshofen', 'en': 'Gaspoltshofen'},
'437732':{'de': 'Haag am Hausruck', 'en': 'Haag am Hausruck'},
'437733':{'de': 'Neumarkt im Hausruckkreis', 'en': 'Neumarkt im Hausruckkreis'},
'3596935':{'bg': u('\u0421\u043e\u043f\u043e\u0442, \u041b\u043e\u0432\u0435\u0447'), 'en': 'Sopot, Lovech'},
'3349707':{'en': 'Nice', 'fr': 'Nice'},
'3317234':{'en': 'Paris', 'fr': 'Paris'},
'35971338':{'bg': u('\u041e\u0441\u0438\u043a\u043e\u0432\u0441\u043a\u0430 \u041b\u0430\u043a\u0430\u0432\u0438\u0446\u0430'), 'en': 'Osikovska Lakavitsa'},
'3325481':{'en': 'Mer', 'fr': 'Mer'},
'3325484':{'en': u('Buzan\u00e7ais'), 'fr': u('Buzan\u00e7ais')},
'3325485':{'en': 'Montoire-sur-le-Loir', 'fr': 'Montoire-sur-le-Loir'},
'3325487':{'en': 'Saint-Laurent-Nouan', 'fr': 'Saint-Laurent-Nouan'},
'3325488':{'en': 'Lamotte-Beuvron', 'fr': 'Lamotte-Beuvron'},
'3325489':{'en': u('Vend\u00f4me'), 'fr': u('Vend\u00f4me')},
'3752347':{'be': u('\u041b\u043e\u0435\u045e, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u0432\u043e\u0431\u043b\u0430\u0441\u0446\u044c'), 'en': 'Loyev, Gomel Region', 'ru': u('\u041b\u043e\u0435\u0432, \u0413\u043e\u043c\u0435\u043b\u044c\u0441\u043a\u0430\u044f \u043e\u0431\u043b\u0430\u0441\u0442\u044c')},
'3338239':{'en': 'Longuyon', 'fr': 'Longuyon'},
'3338234':{'en': 'Thionville', 'fr': 'Thionville'},
'3338233':{'en': 'Jarny', 'fr': 'Jarny'},
'35892':{'en': 'Helsinki', 'fi': 'Helsinki', 'se': 'Helsingfors'},
'35893':{'en': 'Helsinki', 'fi': 'Helsinki', 'se': 'Helsingfors'},
'35891':{'en': 'Helsinki', 'fi': 'Helsinki', 'se': 'Helsingfors'},
'35896':{'en': 'Helsinki', 'fi': 'Helsinki', 'se': 'Helsingfors'},
'35897':{'en': 'Helsinki', 'fi': 'Helsinki', 'se': 'Helsingfors'},
'35894':{'en': 'Helsinki', 'fi': 'Helsinki', 'se': 'Helsingfors'},
'35895':{'en': 'Helsinki', 'fi': 'Helsinki', 'se': 'Helsingfors'},
'35898':{'en': 'Helsinki', 'fi': 'Helsinki', 'se': 'Helsingfors'},
'359817':{'bg': u('\u0411\u044f\u043b\u0430, \u0420\u0443\u0441\u0435'), 'en': 'Byala, Ruse'},
'3356303':{'en': 'Montauban', 'fr': 'Montauban'},
'3356305':{'en': 'Moissac', 'fr': 'Moissac'},
'3356304':{'en': 'Moissac', 'fr': 'Moissac'},
'3349703':{'en': 'Nice', 'fr': 'Nice'},
'3347400':{'en': u('Tr\u00e9voux'), 'fr': u('Tr\u00e9voux')},
'3347402':{'en': u('Villefranche-sur-Sa\u00f4ne'), 'fr': u('Villefranche-sur-Sa\u00f4ne')},
'3347405':{'en': 'Tarare', 'fr': 'Tarare'},
'3347406':{'en': 'Belleville', 'fr': 'Belleville'},
'3347407':{'en': u('Villefranche-sur-Sa\u00f4ne'), 'fr': u('Villefranche-sur-Sa\u00f4ne')},
'3347408':{'en': u('Tr\u00e9voux'), 'fr': u('Tr\u00e9voux')},
'3347409':{'en': u('Villefranche-sur-Sa\u00f4ne'), 'fr': u('Villefranche-sur-Sa\u00f4ne')},
'434285':{'de': u('Tr\u00f6polach'), 'en': u('Tr\u00f6polach')},
'434284':{'de': 'Kirchbach', 'en': 'Kirchbach'},
'434283':{'de': 'Sankt Stefan im Gailtal', 'en': 'St. Stefan im Gailtal'},
'434282':{'de': 'Hermagor', 'en': 'Hermagor'},
'3341164':{'en': 'Perpignan', 'fr': 'Perpignan'},
}
| apache-2.0 |
FedoraScientific/salome-smesh | src/Tools/MacMesh/MacMesh/SharpAngle.py | 1 | 15412 | # Copyright (C) 2007-2014 CEA/DEN, EDF R&D, OPEN CASCADE
# Copyright (C) 2003-2007 OPEN CASCADE, EADS/CCR, LIP6, CEA/DEN,
# CEDRAT, EDF R&D, LEG, PRINCIPIA R&D, BUREAU VERITAS
# This library is free software; you can redistribute it and/or
# modify it under the terms of the GNU Lesser General Public
# License as published by the Free Software Foundation; either
# version 2.1 of the License, or (at your option) any later version.
# This library is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
# Lesser General Public License for more details.
# You should have received a copy of the GNU Lesser General Public
# License along with this library; if not, write to the Free Software
# Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
# See http://www.salome-platform.org/ or email : webmaster.salome@opencascade.com
# This is an automation of the sharp angle object, with a corner at (X0,Y0), side length : Extension and a fine local meshing : LocalMeshing
# The corner orientation is defined as NE (North-East) , NW (North-West), SE, or SW. The object's "arm" is 8/14 of Extension
# | | 8 6
# ------- ---------
# ----> | | <----
# | NW NE | oo
# _____| |_____
import sys, math, commands
CWD = commands.getoutput('pwd')
sys.path.append(CWD)
from MacObject import *
from CompositeBox import *
import Config, GenFunctions
def SharpAngleOut (X0 , Y0 , DX , DY , DLocal, LocalMeshing , CornerOrientation , NLevels, **args) :
if DLocal == 'auto' : DLocal = float(min(DX,DY))
BoxSide = DLocal/(2.**(NLevels+1))
InternalMeshing = int(math.ceil(BoxSide/(3*LocalMeshing)))
InternalMeshing = InternalMeshing+InternalMeshing%2 # An even number is needed, otherwise the objects would not be compatible once created
if InternalMeshing == 0 : InternalMeshing = 2 # This sets a minimum meshing condition in order to avoid an error. The user is notified of the value considered for the local meshing
print "Possible Local meshing is :", BoxSide/(3*InternalMeshing), "\nThis value is returned by this function for your convenience"
DirPar = {'NE' : lambda : ['NE', 'NW', 'SE', 'EW', 'NW', 'SN', 'SN', 'NE', 'WE', 'WE', 'SE', 'NS'],
'NW' : lambda : ['NW', 'NE', 'SW', 'WE', 'NE', 'SN', 'SN', 'NW', 'EW', 'EW', 'SW', 'NS'],
'SE' : lambda : ['SE', 'SW', 'NE', 'EW', 'SW', 'NS', 'NS', 'SE', 'WE', 'WE', 'NE', 'SN'],
'SW' : lambda : ['SW', 'SE', 'NW', 'WE', 'SE', 'NS', 'NS', 'SW', 'EW', 'EW', 'NW', 'SN'], }[CornerOrientation]()
CoefVer = {'NE' : lambda : 1,
'NW' : lambda : 1,
'SE' : lambda : -1,
'SW' : lambda : -1, }[CornerOrientation]()
CoefHor = {'NE' : lambda : 1,
'NW' : lambda : -1,
'SE' : lambda : 1,
'SW' : lambda : -1, }[CornerOrientation]()
ToLook = {'NE' : lambda : [0,2,1,3],
'NW' : lambda : [0,3,1,2],
'SE' : lambda : [1,2,0,3],
'SW' : lambda : [1,3,0,2], }[CornerOrientation]()
if args.__contains__('groups') :
GroupNames = args['groups']
else : GroupNames = [None, None, None, None, None, None]
GN00 = GroupArray(ToLook[0],GroupNames[0])
GN01 = GroupArray(ToLook[1],GroupNames[1])
GN1 = GroupArray([ToLook[0],ToLook[1]],[GroupNames[0],GroupNames[5]])
GN7 = GroupArray([ToLook[0],ToLook[1]],[GroupNames[4],GroupNames[1]])
if DY == DLocal :
GN2 = GroupArray([ToLook[1],ToLook[2]],[GroupNames[5],GroupNames[2]])
GN3 = GroupArray(ToLook[2],GroupNames[2])
if DX == DLocal:
GN4 = GroupArray([ToLook[2],ToLook[3]],[GroupNames[2],GroupNames[3]])
GN5 = GroupArray(ToLook[3],GroupNames[3])
GN6 = GroupArray([ToLook[3],ToLook[0]],[GroupNames[3],GroupNames[4]])
else :
GN4 = GroupArray(ToLook[2],GroupNames[2])
GN5 = [None,None,None,None]
GN6 = GroupArray(ToLook[0],GroupNames[4])
GN21 = GroupArray([ToLook[3],ToLook[0],ToLook[2]],[GroupNames[3],GroupNames[4],GroupNames[2]])
else :
GN2 = GroupArray(ToLook[1],GroupNames[5])
GN3 = [None,None,None,None]
if DX == DLocal:
GN4 = GroupArray(ToLook[3],GroupNames[3])
GN5 = GroupArray(ToLook[3],GroupNames[3])
GN6 = GroupArray([ToLook[3],ToLook[0]],[GroupNames[3],GroupNames[4]])
GN22 = GroupArray([ToLook[1],ToLook[2],ToLook[3]],[GroupNames[5],GroupNames[2],GroupNames[3]])
else :
GN4 = [None,None,None,None]
GN5 = [None,None,None,None]
GN6 = GroupArray(ToLook[0],GroupNames[4])
GN21 = GroupArray([ToLook[3],ToLook[0]],[GroupNames[3],GroupNames[4]])
GN22 = GroupArray([ToLook[1],ToLook[2]],[GroupNames[5],GroupNames[2]])
GN23 = GroupArray([ToLook[2],ToLook[3]],[GroupNames[2],GroupNames[3]])
Obj = []
Obj.append(MacObject('BoxAng32',[(X0+CoefHor*BoxSide/2,Y0+CoefVer*BoxSide/2),(BoxSide,BoxSide)],[InternalMeshing,DirPar[0]]))
Obj.append(MacObject('BoxAng32',[(X0-CoefHor*BoxSide/2,Y0+CoefVer*BoxSide/2),(BoxSide,BoxSide)],['auto',DirPar[1]], groups = GroupArray(ToLook[0],GroupNames[0])))
Obj.append(MacObject('BoxAng32',[(X0+CoefHor*BoxSide/2,Y0-CoefVer*BoxSide/2),(BoxSide,BoxSide)],['auto',DirPar[2]], groups = GroupArray(ToLook[1],GroupNames[1])))
for N in range (1,NLevels+1):
n = N-1
if N < NLevels :
Obj.append(MacObject('Box42',[(X0-CoefHor*BoxSide*(2**n)*3/2,Y0+CoefVer*(2**n)*BoxSide/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[3]] , groups = GN00))
Obj.append(MacObject('BoxAng32',[(X0-CoefHor*(2**n)*BoxSide*3/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[4]] ))
Obj.append(MacObject('Box42',[(X0-CoefHor*(2**n)*BoxSide/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[5]] ))
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[6]] ))
Obj.append(MacObject('BoxAng32',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[7]] ))
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0+CoefVer*(2**n)*BoxSide/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[8]] ))
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0-CoefVer*(2**n)*BoxSide/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[9]] ))
Obj.append(MacObject('BoxAng32',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0-CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[10]] ))
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide/2,Y0-CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[11]] , groups = GN01))
else :
Obj.append(MacObject('Box42',[(X0-CoefHor*BoxSide*(2**n)*3/2,Y0+CoefVer*(2**n)*BoxSide/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[3]] , groups = GN1))
Obj.append(MacObject('BoxAng32',[(X0-CoefHor*(2**n)*BoxSide*3/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[4]] , groups = GN2))
Obj.append(MacObject('Box42',[(X0-CoefHor*(2**n)*BoxSide/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[5]] , groups = GN3))
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[6]] , groups = GN3))
Obj.append(MacObject('BoxAng32',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[7]] , groups = GN4))
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0+CoefVer*(2**n)*BoxSide/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[8]] , groups = GN5))
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0-CoefVer*(2**n)*BoxSide/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[9]] , groups = GN5))
Obj.append(MacObject('BoxAng32',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0-CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[10]], groups = GN6))
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide/2,Y0-CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[11]] , groups = GN7))
OuterMeshing = (3/2)*InternalMeshing*2**(NLevels-1)
OuterSegLength = (DLocal/OuterMeshing)
if DX > DLocal :
dX = DX - DLocal
Obj.append(MacObject('CompBoxF',[(X0+CoefHor*(DX)/2.,Y0),(dX,DLocal)],['auto'], groups = GN21))
if DY > DLocal :
dY = DY - DLocal
if DX > DLocal :
Obj.append(MacObject('CompBoxF',[(X0+CoefHor*DX/2.,Y0+CoefVer*(DY)/2.),(DX-DLocal,dY)],['auto'], groups = GN23))
Obj.append(MacObject('CompBoxF',[(X0,Y0+CoefVer*(DY)/2.),(DLocal,dY)],['auto'], groups = GN22))
return Obj
def SharpAngleIn (X0 , Y0 , DX , DY , DLocal, LocalMeshing , CornerOrientation , NLevels, **args) :
if DLocal == 'auto' : DLocal = float(min(DX,DY))
BoxSide = DLocal/(2.**(NLevels))
InternalMeshing = int(math.ceil(BoxSide/(3*LocalMeshing)))
InternalMeshing = InternalMeshing+InternalMeshing%2 # An even number is needed, otherwise the objects would not be compatible once created
if InternalMeshing == 0 : InternalMeshing = 2 # This sets a minimum meshing condition in order to avoid an error. The user is notified of the value considered for the local meshing
print "Possible Local meshing is :", BoxSide/(3*InternalMeshing), "\nThis value is returned by this function for your convenience..."
DirPar = {'NE' : lambda : ['NE', 'SN', 'NE', 'WE'],
'NW' : lambda : ['NW', 'SN', 'NW', 'EW'],
'SE' : lambda : ['SE', 'NS', 'SE', 'WE'],
'SW' : lambda : ['SW', 'NS', 'SW', 'EW'], }[CornerOrientation]()
CoefVer = {'NE' : lambda : 1,
'NW' : lambda : 1,
'SE' : lambda : -1,
'SW' : lambda : -1, }[CornerOrientation]()
CoefHor = {'NE' : lambda : 1,
'NW' : lambda : -1,
'SE' : lambda : 1,
'SW' : lambda : -1, }[CornerOrientation]()
ToLook = {'NE' : lambda : [0,2,1,3],
'NW' : lambda : [0,3,1,2],
'SE' : lambda : [1,2,0,3],
'SW' : lambda : [1,3,0,2], }[CornerOrientation]()
if args.__contains__('groups') :
GroupNames = args['groups']
else : GroupNames = [None, None, None, None]
GN01 = GroupArray([ToLook[0],ToLook[1]],[GroupNames[ToLook[0]],GroupNames[ToLook[1]]])
GN02 = GroupArray(ToLook[1],GroupNames[ToLook[1]])
GN03 = [None, None, None, None]
GN04 = GroupArray(ToLook[0],GroupNames[ToLook[0]])
if DY == DLocal :
GN05 = GroupArray([ToLook[1],ToLook[2]],[GroupNames[ToLook[1]],GroupNames[ToLook[2]]])
GN08 = GroupArray([ToLook[0],ToLook[2],ToLook[3]],[GroupNames[ToLook[0]],GroupNames[ToLook[2]],GroupNames[ToLook[3]]])
if DX == DLocal:
GN06 = GroupArray([ToLook[2],ToLook[3]],[GroupNames[ToLook[2]],GroupNames[ToLook[3]]])
GN07 = GroupArray([ToLook[0],ToLook[3]],[GroupNames[ToLook[0]],GroupNames[ToLook[3]]])
else :
GN06 = GroupArray(ToLook[2],GroupNames[ToLook[2]])
GN07 = GroupArray(ToLook[0],GroupNames[ToLook[0]])
else :
GN05 = GroupArray(ToLook[1],GroupNames[ToLook[1]])
if DX == DLocal :
GN06 = GroupArray(ToLook[3],GroupNames[ToLook[3]])
GN07 = GroupArray([ToLook[0],ToLook[3]],[GroupNames[ToLook[0]],GroupNames[ToLook[3]]])
GN10 = GroupArray([ToLook[1],ToLook[2],ToLook[3]],[GroupNames[ToLook[1]],GroupNames[ToLook[2]],GroupNames[ToLook[3]]])
else :
GN06 = [None, None, None, None]
GN07 = GroupArray(ToLook[0],GroupNames[ToLook[0]])
GN08 = GroupArray([ToLook[0],ToLook[3]],[GroupNames[ToLook[0]],GroupNames[ToLook[3]]])
GN09 = GroupArray([ToLook[2],ToLook[3]],[GroupNames[ToLook[2]],GroupNames[ToLook[3]]])
GN10 = GroupArray([ToLook[1],ToLook[2]],[GroupNames[ToLook[1]],GroupNames[ToLook[2]]])
Obj = []
Obj.append(MacObject('BoxAng32',[(X0+CoefHor*BoxSide/2,Y0+CoefVer*BoxSide/2),(BoxSide,BoxSide)],[InternalMeshing,DirPar[0]],groups = GN01))
for N in range (1,NLevels+1):
n = N-1
if N < NLevels :
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[1]],groups = GN02))
Obj.append(MacObject('BoxAng32',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[2]],groups = GN03))
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0+CoefVer*(2**n)*BoxSide/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[3]],groups = GN04))
else :
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[1]],groups = GN05))
Obj.append(MacObject('BoxAng32',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0+CoefVer*(2**n)*BoxSide*3/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[2]],groups = GN06))
Obj.append(MacObject('Box42',[(X0+CoefHor*(2**n)*BoxSide*3/2,Y0+CoefVer*(2**n)*BoxSide/2),((2**n)*BoxSide,(2**n)*BoxSide)],['auto',DirPar[3]],groups = GN07))
OuterMeshing = (3/2)*InternalMeshing*2**(NLevels-1)
OuterSegLength = (DLocal/OuterMeshing)
if DX > DLocal :
dX = DX - DLocal
Obj = Obj + CompositeBox(X0+CoefHor*(DLocal+dX/2.),Y0+CoefVer*(DLocal)/2.,dX,DLocal, groups = GN08)
if DY > DLocal :
dY = DY - DLocal
if DX > DLocal :
Obj = Obj + CompositeBox(X0+CoefHor*(DLocal+(DX-DLocal)/2.),Y0+CoefVer*(DLocal+dY/2.),DX-DLocal,dY, groups = GN09)
Obj = Obj + CompositeBox(X0+CoefHor*DLocal/2,Y0+CoefVer*(DLocal+dY/2.),DLocal,dY,groups = GN10)
return Obj
def GroupArray(indices, GroupNames) :
if type(indices) is int :
indices = [indices]
GroupNames = [GroupNames]
Output = [None,None,None,None]
for i, ind in enumerate(indices) :
Output[ind] = GroupNames[i]
return Output
| lgpl-2.1 |
JioCloud/nova | nova/tests/unit/fake_notifier.py | 59 | 2755 | # Copyright 2013 Red Hat, Inc.
#
# Licensed under the Apache License, Version 2.0 (the "License"); you may
# not use this file except in compliance with the License. You may obtain
# a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
# License for the specific language governing permissions and limitations
# under the License.
import collections
import functools
import oslo_messaging as messaging
from oslo_serialization import jsonutils
from nova import rpc
NOTIFICATIONS = []
def reset():
del NOTIFICATIONS[:]
FakeMessage = collections.namedtuple('Message',
['publisher_id', 'priority',
'event_type', 'payload', 'context'])
class FakeNotifier(object):
def __init__(self, transport, publisher_id, serializer=None):
self.transport = transport
self.publisher_id = publisher_id
self._serializer = serializer or messaging.serializer.NoOpSerializer()
for priority in ['debug', 'info', 'warn', 'error', 'critical']:
setattr(self, priority,
functools.partial(self._notify, priority.upper()))
def prepare(self, publisher_id=None):
if publisher_id is None:
publisher_id = self.publisher_id
return self.__class__(self.transport, publisher_id,
serializer=self._serializer)
def _notify(self, priority, ctxt, event_type, payload):
payload = self._serializer.serialize_entity(ctxt, payload)
# NOTE(sileht): simulate the kombu serializer
# this permit to raise an exception if something have not
# been serialized correctly
jsonutils.to_primitive(payload)
# NOTE(melwitt): Try to serialize the context, as the rpc would.
# An exception will be raised if something is wrong
# with the context.
self._serializer.serialize_context(ctxt)
msg = FakeMessage(self.publisher_id, priority, event_type,
payload, ctxt)
NOTIFICATIONS.append(msg)
def stub_notifier(stubs):
stubs.Set(messaging, 'Notifier', FakeNotifier)
if rpc.NOTIFIER:
stubs.Set(rpc, 'NOTIFIER',
FakeNotifier(rpc.NOTIFIER.transport,
rpc.NOTIFIER.publisher_id,
serializer=getattr(rpc.NOTIFIER, '_serializer',
None)))
| apache-2.0 |
ryfeus/lambda-packs | Sklearn_x86/source/numpy/core/_ufunc_config.py | 6 | 13387 | """
Functions for changing global ufunc configuration
This provides helpers which wrap `umath.geterrobj` and `umath.seterrobj`
"""
import collections.abc
import contextlib
from .overrides import set_module
from .umath import (
UFUNC_BUFSIZE_DEFAULT,
ERR_IGNORE, ERR_WARN, ERR_RAISE, ERR_CALL, ERR_PRINT, ERR_LOG, ERR_DEFAULT,
SHIFT_DIVIDEBYZERO, SHIFT_OVERFLOW, SHIFT_UNDERFLOW, SHIFT_INVALID,
)
from . import umath
__all__ = [
"seterr", "geterr", "setbufsize", "getbufsize", "seterrcall", "geterrcall",
"errstate",
]
_errdict = {"ignore": ERR_IGNORE,
"warn": ERR_WARN,
"raise": ERR_RAISE,
"call": ERR_CALL,
"print": ERR_PRINT,
"log": ERR_LOG}
_errdict_rev = {value: key for key, value in _errdict.items()}
@set_module('numpy')
def seterr(all=None, divide=None, over=None, under=None, invalid=None):
"""
Set how floating-point errors are handled.
Note that operations on integer scalar types (such as `int16`) are
handled like floating point, and are affected by these settings.
Parameters
----------
all : {'ignore', 'warn', 'raise', 'call', 'print', 'log'}, optional
Set treatment for all types of floating-point errors at once:
- ignore: Take no action when the exception occurs.
- warn: Print a `RuntimeWarning` (via the Python `warnings` module).
- raise: Raise a `FloatingPointError`.
- call: Call a function specified using the `seterrcall` function.
- print: Print a warning directly to ``stdout``.
- log: Record error in a Log object specified by `seterrcall`.
The default is not to change the current behavior.
divide : {'ignore', 'warn', 'raise', 'call', 'print', 'log'}, optional
Treatment for division by zero.
over : {'ignore', 'warn', 'raise', 'call', 'print', 'log'}, optional
Treatment for floating-point overflow.
under : {'ignore', 'warn', 'raise', 'call', 'print', 'log'}, optional
Treatment for floating-point underflow.
invalid : {'ignore', 'warn', 'raise', 'call', 'print', 'log'}, optional
Treatment for invalid floating-point operation.
Returns
-------
old_settings : dict
Dictionary containing the old settings.
See also
--------
seterrcall : Set a callback function for the 'call' mode.
geterr, geterrcall, errstate
Notes
-----
The floating-point exceptions are defined in the IEEE 754 standard [1]_:
- Division by zero: infinite result obtained from finite numbers.
- Overflow: result too large to be expressed.
- Underflow: result so close to zero that some precision
was lost.
- Invalid operation: result is not an expressible number, typically
indicates that a NaN was produced.
.. [1] https://en.wikipedia.org/wiki/IEEE_754
Examples
--------
>>> old_settings = np.seterr(all='ignore') #seterr to known value
>>> np.seterr(over='raise')
{'divide': 'ignore', 'over': 'ignore', 'under': 'ignore', 'invalid': 'ignore'}
>>> np.seterr(**old_settings) # reset to default
{'divide': 'ignore', 'over': 'raise', 'under': 'ignore', 'invalid': 'ignore'}
>>> np.int16(32000) * np.int16(3)
30464
>>> old_settings = np.seterr(all='warn', over='raise')
>>> np.int16(32000) * np.int16(3)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
FloatingPointError: overflow encountered in short_scalars
>>> old_settings = np.seterr(all='print')
>>> np.geterr()
{'divide': 'print', 'over': 'print', 'under': 'print', 'invalid': 'print'}
>>> np.int16(32000) * np.int16(3)
30464
"""
pyvals = umath.geterrobj()
old = geterr()
if divide is None:
divide = all or old['divide']
if over is None:
over = all or old['over']
if under is None:
under = all or old['under']
if invalid is None:
invalid = all or old['invalid']
maskvalue = ((_errdict[divide] << SHIFT_DIVIDEBYZERO) +
(_errdict[over] << SHIFT_OVERFLOW) +
(_errdict[under] << SHIFT_UNDERFLOW) +
(_errdict[invalid] << SHIFT_INVALID))
pyvals[1] = maskvalue
umath.seterrobj(pyvals)
return old
@set_module('numpy')
def geterr():
"""
Get the current way of handling floating-point errors.
Returns
-------
res : dict
A dictionary with keys "divide", "over", "under", and "invalid",
whose values are from the strings "ignore", "print", "log", "warn",
"raise", and "call". The keys represent possible floating-point
exceptions, and the values define how these exceptions are handled.
See Also
--------
geterrcall, seterr, seterrcall
Notes
-----
For complete documentation of the types of floating-point exceptions and
treatment options, see `seterr`.
Examples
--------
>>> np.geterr()
{'divide': 'warn', 'over': 'warn', 'under': 'ignore', 'invalid': 'warn'}
>>> np.arange(3.) / np.arange(3.)
array([nan, 1., 1.])
>>> oldsettings = np.seterr(all='warn', over='raise')
>>> np.geterr()
{'divide': 'warn', 'over': 'raise', 'under': 'warn', 'invalid': 'warn'}
>>> np.arange(3.) / np.arange(3.)
array([nan, 1., 1.])
"""
maskvalue = umath.geterrobj()[1]
mask = 7
res = {}
val = (maskvalue >> SHIFT_DIVIDEBYZERO) & mask
res['divide'] = _errdict_rev[val]
val = (maskvalue >> SHIFT_OVERFLOW) & mask
res['over'] = _errdict_rev[val]
val = (maskvalue >> SHIFT_UNDERFLOW) & mask
res['under'] = _errdict_rev[val]
val = (maskvalue >> SHIFT_INVALID) & mask
res['invalid'] = _errdict_rev[val]
return res
@set_module('numpy')
def setbufsize(size):
"""
Set the size of the buffer used in ufuncs.
Parameters
----------
size : int
Size of buffer.
"""
if size > 10e6:
raise ValueError("Buffer size, %s, is too big." % size)
if size < 5:
raise ValueError("Buffer size, %s, is too small." % size)
if size % 16 != 0:
raise ValueError("Buffer size, %s, is not a multiple of 16." % size)
pyvals = umath.geterrobj()
old = getbufsize()
pyvals[0] = size
umath.seterrobj(pyvals)
return old
@set_module('numpy')
def getbufsize():
"""
Return the size of the buffer used in ufuncs.
Returns
-------
getbufsize : int
Size of ufunc buffer in bytes.
"""
return umath.geterrobj()[0]
@set_module('numpy')
def seterrcall(func):
"""
Set the floating-point error callback function or log object.
There are two ways to capture floating-point error messages. The first
is to set the error-handler to 'call', using `seterr`. Then, set
the function to call using this function.
The second is to set the error-handler to 'log', using `seterr`.
Floating-point errors then trigger a call to the 'write' method of
the provided object.
Parameters
----------
func : callable f(err, flag) or object with write method
Function to call upon floating-point errors ('call'-mode) or
object whose 'write' method is used to log such message ('log'-mode).
The call function takes two arguments. The first is a string describing
the type of error (such as "divide by zero", "overflow", "underflow",
or "invalid value"), and the second is the status flag. The flag is a
byte, whose four least-significant bits indicate the type of error, one
of "divide", "over", "under", "invalid"::
[0 0 0 0 divide over under invalid]
In other words, ``flags = divide + 2*over + 4*under + 8*invalid``.
If an object is provided, its write method should take one argument,
a string.
Returns
-------
h : callable, log instance or None
The old error handler.
See Also
--------
seterr, geterr, geterrcall
Examples
--------
Callback upon error:
>>> def err_handler(type, flag):
... print("Floating point error (%s), with flag %s" % (type, flag))
...
>>> saved_handler = np.seterrcall(err_handler)
>>> save_err = np.seterr(all='call')
>>> np.array([1, 2, 3]) / 0.0
Floating point error (divide by zero), with flag 1
array([inf, inf, inf])
>>> np.seterrcall(saved_handler)
<function err_handler at 0x...>
>>> np.seterr(**save_err)
{'divide': 'call', 'over': 'call', 'under': 'call', 'invalid': 'call'}
Log error message:
>>> class Log:
... def write(self, msg):
... print("LOG: %s" % msg)
...
>>> log = Log()
>>> saved_handler = np.seterrcall(log)
>>> save_err = np.seterr(all='log')
>>> np.array([1, 2, 3]) / 0.0
LOG: Warning: divide by zero encountered in true_divide
array([inf, inf, inf])
>>> np.seterrcall(saved_handler)
<numpy.core.numeric.Log object at 0x...>
>>> np.seterr(**save_err)
{'divide': 'log', 'over': 'log', 'under': 'log', 'invalid': 'log'}
"""
if func is not None and not isinstance(func, collections.abc.Callable):
if (not hasattr(func, 'write') or
not isinstance(func.write, collections.abc.Callable)):
raise ValueError("Only callable can be used as callback")
pyvals = umath.geterrobj()
old = geterrcall()
pyvals[2] = func
umath.seterrobj(pyvals)
return old
@set_module('numpy')
def geterrcall():
"""
Return the current callback function used on floating-point errors.
When the error handling for a floating-point error (one of "divide",
"over", "under", or "invalid") is set to 'call' or 'log', the function
that is called or the log instance that is written to is returned by
`geterrcall`. This function or log instance has been set with
`seterrcall`.
Returns
-------
errobj : callable, log instance or None
The current error handler. If no handler was set through `seterrcall`,
``None`` is returned.
See Also
--------
seterrcall, seterr, geterr
Notes
-----
For complete documentation of the types of floating-point exceptions and
treatment options, see `seterr`.
Examples
--------
>>> np.geterrcall() # we did not yet set a handler, returns None
>>> oldsettings = np.seterr(all='call')
>>> def err_handler(type, flag):
... print("Floating point error (%s), with flag %s" % (type, flag))
>>> oldhandler = np.seterrcall(err_handler)
>>> np.array([1, 2, 3]) / 0.0
Floating point error (divide by zero), with flag 1
array([inf, inf, inf])
>>> cur_handler = np.geterrcall()
>>> cur_handler is err_handler
True
"""
return umath.geterrobj()[2]
class _unspecified:
pass
_Unspecified = _unspecified()
@set_module('numpy')
class errstate(contextlib.ContextDecorator):
"""
errstate(**kwargs)
Context manager for floating-point error handling.
Using an instance of `errstate` as a context manager allows statements in
that context to execute with a known error handling behavior. Upon entering
the context the error handling is set with `seterr` and `seterrcall`, and
upon exiting it is reset to what it was before.
.. versionchanged:: 1.17.0
`errstate` is also usable as a function decorator, saving
a level of indentation if an entire function is wrapped.
See :py:class:`contextlib.ContextDecorator` for more information.
Parameters
----------
kwargs : {divide, over, under, invalid}
Keyword arguments. The valid keywords are the possible floating-point
exceptions. Each keyword should have a string value that defines the
treatment for the particular error. Possible values are
{'ignore', 'warn', 'raise', 'call', 'print', 'log'}.
See Also
--------
seterr, geterr, seterrcall, geterrcall
Notes
-----
For complete documentation of the types of floating-point exceptions and
treatment options, see `seterr`.
Examples
--------
>>> olderr = np.seterr(all='ignore') # Set error handling to known state.
>>> np.arange(3) / 0.
array([nan, inf, inf])
>>> with np.errstate(divide='warn'):
... np.arange(3) / 0.
array([nan, inf, inf])
>>> np.sqrt(-1)
nan
>>> with np.errstate(invalid='raise'):
... np.sqrt(-1)
Traceback (most recent call last):
File "<stdin>", line 2, in <module>
FloatingPointError: invalid value encountered in sqrt
Outside the context the error handling behavior has not changed:
>>> np.geterr()
{'divide': 'ignore', 'over': 'ignore', 'under': 'ignore', 'invalid': 'ignore'}
"""
def __init__(self, *, call=_Unspecified, **kwargs):
self.call = call
self.kwargs = kwargs
def __enter__(self):
self.oldstate = seterr(**self.kwargs)
if self.call is not _Unspecified:
self.oldcall = seterrcall(self.call)
def __exit__(self, *exc_info):
seterr(**self.oldstate)
if self.call is not _Unspecified:
seterrcall(self.oldcall)
def _setdef():
defval = [UFUNC_BUFSIZE_DEFAULT, ERR_DEFAULT, None]
umath.seterrobj(defval)
# set the default values
_setdef()
| mit |
tragiclifestories/django | tests/handlers/tests.py | 254 | 8672 | # -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.core.handlers.wsgi import WSGIHandler, WSGIRequest, get_script_name
from django.core.signals import request_finished, request_started
from django.db import close_old_connections, connection
from django.test import (
RequestFactory, SimpleTestCase, TransactionTestCase, override_settings,
)
from django.utils import six
from django.utils.encoding import force_str
class HandlerTests(SimpleTestCase):
def setUp(self):
request_started.disconnect(close_old_connections)
def tearDown(self):
request_started.connect(close_old_connections)
# Mangle settings so the handler will fail
@override_settings(MIDDLEWARE_CLASSES=42)
def test_lock_safety(self):
"""
Tests for bug #11193 (errors inside middleware shouldn't leave
the initLock locked).
"""
# Try running the handler, it will fail in load_middleware
handler = WSGIHandler()
self.assertEqual(handler.initLock.locked(), False)
with self.assertRaises(Exception):
handler(None, None)
self.assertEqual(handler.initLock.locked(), False)
def test_bad_path_info(self):
"""Tests for bug #15672 ('request' referenced before assignment)"""
environ = RequestFactory().get('/').environ
environ['PATH_INFO'] = b'\xed' if six.PY2 else '\xed'
handler = WSGIHandler()
response = handler(environ, lambda *a, **k: None)
self.assertEqual(response.status_code, 400)
def test_non_ascii_query_string(self):
"""
Test that non-ASCII query strings are properly decoded (#20530, #22996).
"""
environ = RequestFactory().get('/').environ
raw_query_strings = [
b'want=caf%C3%A9', # This is the proper way to encode 'café'
b'want=caf\xc3\xa9', # UA forgot to quote bytes
b'want=caf%E9', # UA quoted, but not in UTF-8
b'want=caf\xe9', # UA forgot to convert Latin-1 to UTF-8 and to quote (typical of MSIE)
]
got = []
for raw_query_string in raw_query_strings:
if six.PY3:
# Simulate http.server.BaseHTTPRequestHandler.parse_request handling of raw request
environ['QUERY_STRING'] = str(raw_query_string, 'iso-8859-1')
else:
environ['QUERY_STRING'] = raw_query_string
request = WSGIRequest(environ)
got.append(request.GET['want'])
if six.PY2:
self.assertListEqual(got, ['café', 'café', 'café', 'café'])
else:
# On Python 3, %E9 is converted to the unicode replacement character by parse_qsl
self.assertListEqual(got, ['café', 'café', 'caf\ufffd', 'café'])
def test_non_ascii_cookie(self):
"""Test that non-ASCII cookies set in JavaScript are properly decoded (#20557)."""
environ = RequestFactory().get('/').environ
raw_cookie = 'want="café"'
if six.PY3:
raw_cookie = raw_cookie.encode('utf-8').decode('iso-8859-1')
environ['HTTP_COOKIE'] = raw_cookie
request = WSGIRequest(environ)
# If would be nicer if request.COOKIES returned unicode values.
# However the current cookie parser doesn't do this and fixing it is
# much more work than fixing #20557. Feel free to remove force_str()!
self.assertEqual(request.COOKIES['want'], force_str("café"))
def test_invalid_unicode_cookie(self):
"""
Invalid cookie content should result in an absent cookie, but not in a
crash while trying to decode it (#23638).
"""
environ = RequestFactory().get('/').environ
environ['HTTP_COOKIE'] = 'x=W\x03c(h]\x8e'
request = WSGIRequest(environ)
# We don't test COOKIES content, as the result might differ between
# Python version because parsing invalid content became stricter in
# latest versions.
self.assertIsInstance(request.COOKIES, dict)
@override_settings(ROOT_URLCONF='handlers.urls')
def test_invalid_multipart_boundary(self):
"""
Invalid boundary string should produce a "Bad Request" response, not a
server error (#23887).
"""
environ = RequestFactory().post('/malformed_post/').environ
environ['CONTENT_TYPE'] = 'multipart/form-data; boundary=WRONG\x07'
handler = WSGIHandler()
response = handler(environ, lambda *a, **k: None)
# Expect "bad request" response
self.assertEqual(response.status_code, 400)
@override_settings(ROOT_URLCONF='handlers.urls')
class TransactionsPerRequestTests(TransactionTestCase):
available_apps = []
def test_no_transaction(self):
response = self.client.get('/in_transaction/')
self.assertContains(response, 'False')
def test_auto_transaction(self):
old_atomic_requests = connection.settings_dict['ATOMIC_REQUESTS']
try:
connection.settings_dict['ATOMIC_REQUESTS'] = True
response = self.client.get('/in_transaction/')
finally:
connection.settings_dict['ATOMIC_REQUESTS'] = old_atomic_requests
self.assertContains(response, 'True')
def test_no_auto_transaction(self):
old_atomic_requests = connection.settings_dict['ATOMIC_REQUESTS']
try:
connection.settings_dict['ATOMIC_REQUESTS'] = True
response = self.client.get('/not_in_transaction/')
finally:
connection.settings_dict['ATOMIC_REQUESTS'] = old_atomic_requests
self.assertContains(response, 'False')
@override_settings(ROOT_URLCONF='handlers.urls')
class SignalsTests(SimpleTestCase):
def setUp(self):
self.signals = []
self.signaled_environ = None
request_started.connect(self.register_started)
request_finished.connect(self.register_finished)
def tearDown(self):
request_started.disconnect(self.register_started)
request_finished.disconnect(self.register_finished)
def register_started(self, **kwargs):
self.signals.append('started')
self.signaled_environ = kwargs.get('environ')
def register_finished(self, **kwargs):
self.signals.append('finished')
def test_request_signals(self):
response = self.client.get('/regular/')
self.assertEqual(self.signals, ['started', 'finished'])
self.assertEqual(response.content, b"regular content")
self.assertEqual(self.signaled_environ, response.wsgi_request.environ)
def test_request_signals_streaming_response(self):
response = self.client.get('/streaming/')
self.assertEqual(self.signals, ['started'])
self.assertEqual(b''.join(response.streaming_content), b"streaming content")
self.assertEqual(self.signals, ['started', 'finished'])
@override_settings(ROOT_URLCONF='handlers.urls')
class HandlerSuspiciousOpsTest(SimpleTestCase):
def test_suspiciousop_in_view_returns_400(self):
response = self.client.get('/suspicious/')
self.assertEqual(response.status_code, 400)
@override_settings(ROOT_URLCONF='handlers.urls')
class HandlerNotFoundTest(SimpleTestCase):
def test_invalid_urls(self):
response = self.client.get('~%A9helloworld')
self.assertEqual(response.status_code, 404)
self.assertContains(response, '~%A9helloworld', status_code=404)
response = self.client.get('d%aao%aaw%aan%aal%aao%aaa%aad%aa/')
self.assertEqual(response.status_code, 404)
self.assertContains(response, 'd%AAo%AAw%AAn%AAl%AAo%AAa%AAd%AA', status_code=404)
response = self.client.get('/%E2%99%E2%99%A5/')
self.assertEqual(response.status_code, 404)
self.assertContains(response, '%E2%99\u2665', status_code=404)
response = self.client.get('/%E2%98%8E%E2%A9%E2%99%A5/')
self.assertEqual(response.status_code, 404)
self.assertContains(response, '\u260e%E2%A9\u2665', status_code=404)
def test_environ_path_info_type(self):
environ = RequestFactory().get('/%E2%A8%87%87%A5%E2%A8%A0').environ
self.assertIsInstance(environ['PATH_INFO'], six.text_type)
class ScriptNameTests(SimpleTestCase):
def test_get_script_name(self):
# Regression test for #23173
# Test first without PATH_INFO
script_name = get_script_name({'SCRIPT_URL': '/foobar/'})
self.assertEqual(script_name, '/foobar/')
script_name = get_script_name({'SCRIPT_URL': '/foobar/', 'PATH_INFO': '/'})
self.assertEqual(script_name, '/foobar')
| bsd-3-clause |
vbelakov/h2o | py/testdir_single_jvm/test_insert_na.py | 9 | 2513 | import unittest, time, sys, random
sys.path.extend(['.','..','../..','py'])
import h2o, h2o_cmd, h2o_glm, h2o_import as h2i, h2o_jobs, h2o_exec as h2e, h2o_util
DO_POLL = False
class Basic(unittest.TestCase):
def tearDown(self):
h2o.check_sandbox_for_errors()
@classmethod
def setUpClass(cls):
global SEED
SEED = h2o.setup_random_seed()
h2o.init(java_heap_GB=4)
@classmethod
def tearDownClass(cls):
h2o.tear_down_cloud()
def test_insert_na(self):
csvFilename = 'covtype.data'
csvPathname = 'standard/' + csvFilename
hex_key = "covtype.hex"
parseResult = h2i.import_parse(bucket='home-0xdiag-datasets', path=csvPathname, hex_key=hex_key, schema='local', timeoutSecs=20)
print "Just insert some NAs and see what happens"
inspect = h2o_cmd.runInspect(key=hex_key)
origNumRows = inspect['numRows']
origNumCols = inspect['numCols']
missing_fraction = 0.1
# every iteration, we add 0.1 more from the unmarked to the marked (missing)
expectedMissing = missing_fraction * origNumRows # per col
for trial in range(2):
fs = h2o.nodes[0].insert_missing_values(key=hex_key, missing_fraction=missing_fraction, seed=SEED)
print "fs", h2o.dump_json(fs)
inspect = h2o_cmd.runInspect(key=hex_key)
numRows = inspect['numRows']
numCols = inspect['numCols']
expected = .1 * numRows
# Each column should get .10 random NAs per iteration. Within 10%?
missingValuesList = h2o_cmd.infoFromInspect(inspect)
print "missingValuesList", missingValuesList
for mv in missingValuesList:
# h2o_util.assertApproxEqual(mv, expectedMissing, tol=0.01, msg='mv %s is not approx. expected %s' % (mv, expectedMissing))
self.assertAlmostEqual(mv, expectedMissing, delta=0.1 * mv, msg='mv %s is not approx. expected %s' % (mv, expectedMissing))
self.assertEqual(origNumRows, numRows)
self.assertEqual(origNumCols, numCols)
summaryResult = h2o_cmd.runSummary(key=hex_key)
# h2o_cmd.infoFromSummary(summaryResult)
print "trial", trial
print "expectedMissing:", expectedMissing
print "I don't understand why the values don't increase every iteration. It seems to stay stuck with the first effect"
if __name__ == '__main__':
h2o.unit_main()
| apache-2.0 |
ryfeus/lambda-packs | Skimage_numpy/source/scipy/signal/lti_conversion.py | 41 | 13971 | """
ltisys -- a collection of functions to convert linear time invariant systems
from one representation to another.
"""
from __future__ import division, print_function, absolute_import
import numpy
import numpy as np
from numpy import (r_, eye, atleast_2d, poly, dot,
asarray, product, zeros, array, outer)
from scipy import linalg
from .filter_design import tf2zpk, zpk2tf, normalize
__all__ = ['tf2ss', 'abcd_normalize', 'ss2tf', 'zpk2ss', 'ss2zpk',
'cont2discrete']
def tf2ss(num, den):
r"""Transfer function to state-space representation.
Parameters
----------
num, den : array_like
Sequences representing the coefficients of the numerator and
denominator polynomials, in order of descending degree. The
denominator needs to be at least as long as the numerator.
Returns
-------
A, B, C, D : ndarray
State space representation of the system, in controller canonical
form.
Examples
--------
Convert the transfer function:
.. math:: H(s) = \frac{s^2 + 3s + 3}{s^2 + 2s + 1}
>>> num = [1, 3, 3]
>>> den = [1, 2, 1]
to the state-space representation:
.. math::
\dot{\textbf{x}}(t) =
\begin{bmatrix} -2 & -1 \\ 1 & 0 \end{bmatrix} \textbf{x}(t) +
\begin{bmatrix} 1 \\ 0 \end{bmatrix} \textbf{u}(t) \\
\textbf{y}(t) = \begin{bmatrix} 1 & 2 \end{bmatrix} \textbf{x}(t) +
\begin{bmatrix} 1 \end{bmatrix} \textbf{u}(t)
>>> from scipy.signal import tf2ss
>>> A, B, C, D = tf2ss(num, den)
>>> A
array([[-2., -1.],
[ 1., 0.]])
>>> B
array([[ 1.],
[ 0.]])
>>> C
array([[ 1., 2.]])
>>> D
array([[ 1.]])
"""
# Controller canonical state-space representation.
# if M+1 = len(num) and K+1 = len(den) then we must have M <= K
# states are found by asserting that X(s) = U(s) / D(s)
# then Y(s) = N(s) * X(s)
#
# A, B, C, and D follow quite naturally.
#
num, den = normalize(num, den) # Strips zeros, checks arrays
nn = len(num.shape)
if nn == 1:
num = asarray([num], num.dtype)
M = num.shape[1]
K = len(den)
if M > K:
msg = "Improper transfer function. `num` is longer than `den`."
raise ValueError(msg)
if M == 0 or K == 0: # Null system
return (array([], float), array([], float), array([], float),
array([], float))
# pad numerator to have same number of columns has denominator
num = r_['-1', zeros((num.shape[0], K - M), num.dtype), num]
if num.shape[-1] > 0:
D = atleast_2d(num[:, 0])
else:
# We don't assign it an empty array because this system
# is not 'null'. It just doesn't have a non-zero D
# matrix. Thus, it should have a non-zero shape so that
# it can be operated on by functions like 'ss2tf'
D = array([[0]], float)
if K == 1:
D = D.reshape(num.shape)
return (zeros((1, 1)), zeros((1, D.shape[1])),
zeros((D.shape[0], 1)), D)
frow = -array([den[1:]])
A = r_[frow, eye(K - 2, K - 1)]
B = eye(K - 1, 1)
C = num[:, 1:] - outer(num[:, 0], den[1:])
D = D.reshape((C.shape[0], B.shape[1]))
return A, B, C, D
def _none_to_empty_2d(arg):
if arg is None:
return zeros((0, 0))
else:
return arg
def _atleast_2d_or_none(arg):
if arg is not None:
return atleast_2d(arg)
def _shape_or_none(M):
if M is not None:
return M.shape
else:
return (None,) * 2
def _choice_not_none(*args):
for arg in args:
if arg is not None:
return arg
def _restore(M, shape):
if M.shape == (0, 0):
return zeros(shape)
else:
if M.shape != shape:
raise ValueError("The input arrays have incompatible shapes.")
return M
def abcd_normalize(A=None, B=None, C=None, D=None):
"""Check state-space matrices and ensure they are two-dimensional.
If enough information on the system is provided, that is, enough
properly-shaped arrays are passed to the function, the missing ones
are built from this information, ensuring the correct number of
rows and columns. Otherwise a ValueError is raised.
Parameters
----------
A, B, C, D : array_like, optional
State-space matrices. All of them are None (missing) by default.
See `ss2tf` for format.
Returns
-------
A, B, C, D : array
Properly shaped state-space matrices.
Raises
------
ValueError
If not enough information on the system was provided.
"""
A, B, C, D = map(_atleast_2d_or_none, (A, B, C, D))
MA, NA = _shape_or_none(A)
MB, NB = _shape_or_none(B)
MC, NC = _shape_or_none(C)
MD, ND = _shape_or_none(D)
p = _choice_not_none(MA, MB, NC)
q = _choice_not_none(NB, ND)
r = _choice_not_none(MC, MD)
if p is None or q is None or r is None:
raise ValueError("Not enough information on the system.")
A, B, C, D = map(_none_to_empty_2d, (A, B, C, D))
A = _restore(A, (p, p))
B = _restore(B, (p, q))
C = _restore(C, (r, p))
D = _restore(D, (r, q))
return A, B, C, D
def ss2tf(A, B, C, D, input=0):
r"""State-space to transfer function.
A, B, C, D defines a linear state-space system with `p` inputs,
`q` outputs, and `n` state variables.
Parameters
----------
A : array_like
State (or system) matrix of shape ``(n, n)``
B : array_like
Input matrix of shape ``(n, p)``
C : array_like
Output matrix of shape ``(q, n)``
D : array_like
Feedthrough (or feedforward) matrix of shape ``(q, p)``
input : int, optional
For multiple-input systems, the index of the input to use.
Returns
-------
num : 2-D ndarray
Numerator(s) of the resulting transfer function(s). `num` has one row
for each of the system's outputs. Each row is a sequence representation
of the numerator polynomial.
den : 1-D ndarray
Denominator of the resulting transfer function(s). `den` is a sequence
representation of the denominator polynomial.
Examples
--------
Convert the state-space representation:
.. math::
\dot{\textbf{x}}(t) =
\begin{bmatrix} -2 & -1 \\ 1 & 0 \end{bmatrix} \textbf{x}(t) +
\begin{bmatrix} 1 \\ 0 \end{bmatrix} \textbf{u}(t) \\
\textbf{y}(t) = \begin{bmatrix} 1 & 2 \end{bmatrix} \textbf{x}(t) +
\begin{bmatrix} 1 \end{bmatrix} \textbf{u}(t)
>>> A = [[-2, -1], [1, 0]]
>>> B = [[1], [0]] # 2-dimensional column vector
>>> C = [[1, 2]] # 2-dimensional row vector
>>> D = 1
to the transfer function:
.. math:: H(s) = \frac{s^2 + 3s + 3}{s^2 + 2s + 1}
>>> from scipy.signal import ss2tf
>>> ss2tf(A, B, C, D)
(array([[1, 3, 3]]), array([ 1., 2., 1.]))
"""
# transfer function is C (sI - A)**(-1) B + D
# Check consistency and make them all rank-2 arrays
A, B, C, D = abcd_normalize(A, B, C, D)
nout, nin = D.shape
if input >= nin:
raise ValueError("System does not have the input specified.")
# make SIMO from possibly MIMO system.
B = B[:, input:input + 1]
D = D[:, input:input + 1]
try:
den = poly(A)
except ValueError:
den = 1
if (product(B.shape, axis=0) == 0) and (product(C.shape, axis=0) == 0):
num = numpy.ravel(D)
if (product(D.shape, axis=0) == 0) and (product(A.shape, axis=0) == 0):
den = []
return num, den
num_states = A.shape[0]
type_test = A[:, 0] + B[:, 0] + C[0, :] + D
num = numpy.zeros((nout, num_states + 1), type_test.dtype)
for k in range(nout):
Ck = atleast_2d(C[k, :])
num[k] = poly(A - dot(B, Ck)) + (D[k] - 1) * den
return num, den
def zpk2ss(z, p, k):
"""Zero-pole-gain representation to state-space representation
Parameters
----------
z, p : sequence
Zeros and poles.
k : float
System gain.
Returns
-------
A, B, C, D : ndarray
State space representation of the system, in controller canonical
form.
"""
return tf2ss(*zpk2tf(z, p, k))
def ss2zpk(A, B, C, D, input=0):
"""State-space representation to zero-pole-gain representation.
A, B, C, D defines a linear state-space system with `p` inputs,
`q` outputs, and `n` state variables.
Parameters
----------
A : array_like
State (or system) matrix of shape ``(n, n)``
B : array_like
Input matrix of shape ``(n, p)``
C : array_like
Output matrix of shape ``(q, n)``
D : array_like
Feedthrough (or feedforward) matrix of shape ``(q, p)``
input : int, optional
For multiple-input systems, the index of the input to use.
Returns
-------
z, p : sequence
Zeros and poles.
k : float
System gain.
"""
return tf2zpk(*ss2tf(A, B, C, D, input=input))
def cont2discrete(system, dt, method="zoh", alpha=None):
"""
Transform a continuous to a discrete state-space system.
Parameters
----------
system : a tuple describing the system or an instance of `lti`
The following gives the number of elements in the tuple and
the interpretation:
* 1: (instance of `lti`)
* 2: (num, den)
* 3: (zeros, poles, gain)
* 4: (A, B, C, D)
dt : float
The discretization time step.
method : {"gbt", "bilinear", "euler", "backward_diff", "zoh"}, optional
Which method to use:
* gbt: generalized bilinear transformation
* bilinear: Tustin's approximation ("gbt" with alpha=0.5)
* euler: Euler (or forward differencing) method ("gbt" with alpha=0)
* backward_diff: Backwards differencing ("gbt" with alpha=1.0)
* zoh: zero-order hold (default)
alpha : float within [0, 1], optional
The generalized bilinear transformation weighting parameter, which
should only be specified with method="gbt", and is ignored otherwise
Returns
-------
sysd : tuple containing the discrete system
Based on the input type, the output will be of the form
* (num, den, dt) for transfer function input
* (zeros, poles, gain, dt) for zeros-poles-gain input
* (A, B, C, D, dt) for state-space system input
Notes
-----
By default, the routine uses a Zero-Order Hold (zoh) method to perform
the transformation. Alternatively, a generalized bilinear transformation
may be used, which includes the common Tustin's bilinear approximation,
an Euler's method technique, or a backwards differencing technique.
The Zero-Order Hold (zoh) method is based on [1]_, the generalized bilinear
approximation is based on [2]_ and [3]_.
References
----------
.. [1] http://en.wikipedia.org/wiki/Discretization#Discretization_of_linear_state_space_models
.. [2] http://techteach.no/publications/discretetime_signals_systems/discrete.pdf
.. [3] G. Zhang, X. Chen, and T. Chen, Digital redesign via the generalized
bilinear transformation, Int. J. Control, vol. 82, no. 4, pp. 741-754,
2009.
(http://www.ece.ualberta.ca/~gfzhang/research/ZCC07_preprint.pdf)
"""
if len(system) == 1:
return system.to_discrete()
if len(system) == 2:
sysd = cont2discrete(tf2ss(system[0], system[1]), dt, method=method,
alpha=alpha)
return ss2tf(sysd[0], sysd[1], sysd[2], sysd[3]) + (dt,)
elif len(system) == 3:
sysd = cont2discrete(zpk2ss(system[0], system[1], system[2]), dt,
method=method, alpha=alpha)
return ss2zpk(sysd[0], sysd[1], sysd[2], sysd[3]) + (dt,)
elif len(system) == 4:
a, b, c, d = system
else:
raise ValueError("First argument must either be a tuple of 2 (tf), "
"3 (zpk), or 4 (ss) arrays.")
if method == 'gbt':
if alpha is None:
raise ValueError("Alpha parameter must be specified for the "
"generalized bilinear transform (gbt) method")
elif alpha < 0 or alpha > 1:
raise ValueError("Alpha parameter must be within the interval "
"[0,1] for the gbt method")
if method == 'gbt':
# This parameter is used repeatedly - compute once here
ima = np.eye(a.shape[0]) - alpha*dt*a
ad = linalg.solve(ima, np.eye(a.shape[0]) + (1.0-alpha)*dt*a)
bd = linalg.solve(ima, dt*b)
# Similarly solve for the output equation matrices
cd = linalg.solve(ima.transpose(), c.transpose())
cd = cd.transpose()
dd = d + alpha*np.dot(c, bd)
elif method == 'bilinear' or method == 'tustin':
return cont2discrete(system, dt, method="gbt", alpha=0.5)
elif method == 'euler' or method == 'forward_diff':
return cont2discrete(system, dt, method="gbt", alpha=0.0)
elif method == 'backward_diff':
return cont2discrete(system, dt, method="gbt", alpha=1.0)
elif method == 'zoh':
# Build an exponential matrix
em_upper = np.hstack((a, b))
# Need to stack zeros under the a and b matrices
em_lower = np.hstack((np.zeros((b.shape[1], a.shape[0])),
np.zeros((b.shape[1], b.shape[1]))))
em = np.vstack((em_upper, em_lower))
ms = linalg.expm(dt * em)
# Dispose of the lower rows
ms = ms[:a.shape[0], :]
ad = ms[:, 0:a.shape[1]]
bd = ms[:, a.shape[1]:]
cd = c
dd = d
else:
raise ValueError("Unknown transformation method '%s'" % method)
return ad, bd, cd, dd, dt
| mit |
cglatot/PokeManager | pogo/POGOProtos/Networking/Requests/Messages/DownloadSettingsMessage_pb2.py | 16 | 2402 | # Generated by the protocol buffer compiler. DO NOT EDIT!
# source: POGOProtos/Networking/Requests/Messages/DownloadSettingsMessage.proto
import sys
_b=sys.version_info[0]<3 and (lambda x:x) or (lambda x:x.encode('latin1'))
from google.protobuf import descriptor as _descriptor
from google.protobuf import message as _message
from google.protobuf import reflection as _reflection
from google.protobuf import symbol_database as _symbol_database
from google.protobuf import descriptor_pb2
# @@protoc_insertion_point(imports)
_sym_db = _symbol_database.Default()
DESCRIPTOR = _descriptor.FileDescriptor(
name='POGOProtos/Networking/Requests/Messages/DownloadSettingsMessage.proto',
package='POGOProtos.Networking.Requests.Messages',
syntax='proto3',
serialized_pb=_b('\nEPOGOProtos/Networking/Requests/Messages/DownloadSettingsMessage.proto\x12\'POGOProtos.Networking.Requests.Messages\"\'\n\x17\x44ownloadSettingsMessage\x12\x0c\n\x04hash\x18\x01 \x01(\tb\x06proto3')
)
_sym_db.RegisterFileDescriptor(DESCRIPTOR)
_DOWNLOADSETTINGSMESSAGE = _descriptor.Descriptor(
name='DownloadSettingsMessage',
full_name='POGOProtos.Networking.Requests.Messages.DownloadSettingsMessage',
filename=None,
file=DESCRIPTOR,
containing_type=None,
fields=[
_descriptor.FieldDescriptor(
name='hash', full_name='POGOProtos.Networking.Requests.Messages.DownloadSettingsMessage.hash', index=0,
number=1, type=9, cpp_type=9, label=1,
has_default_value=False, default_value=_b("").decode('utf-8'),
message_type=None, enum_type=None, containing_type=None,
is_extension=False, extension_scope=None,
options=None),
],
extensions=[
],
nested_types=[],
enum_types=[
],
options=None,
is_extendable=False,
syntax='proto3',
extension_ranges=[],
oneofs=[
],
serialized_start=114,
serialized_end=153,
)
DESCRIPTOR.message_types_by_name['DownloadSettingsMessage'] = _DOWNLOADSETTINGSMESSAGE
DownloadSettingsMessage = _reflection.GeneratedProtocolMessageType('DownloadSettingsMessage', (_message.Message,), dict(
DESCRIPTOR = _DOWNLOADSETTINGSMESSAGE,
__module__ = 'POGOProtos.Networking.Requests.Messages.DownloadSettingsMessage_pb2'
# @@protoc_insertion_point(class_scope:POGOProtos.Networking.Requests.Messages.DownloadSettingsMessage)
))
_sym_db.RegisterMessage(DownloadSettingsMessage)
# @@protoc_insertion_point(module_scope)
| mit |
android-ia/platform_external_chromium_org | third_party/protobuf/python/google/protobuf/service.py | 590 | 9131 | # Protocol Buffers - Google's data interchange format
# Copyright 2008 Google Inc. All rights reserved.
# http://code.google.com/p/protobuf/
#
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions are
# met:
#
# * Redistributions of source code must retain the above copyright
# notice, this list of conditions and the following disclaimer.
# * Redistributions in binary form must reproduce the above
# copyright notice, this list of conditions and the following disclaimer
# in the documentation and/or other materials provided with the
# distribution.
# * Neither the name of Google Inc. nor the names of its
# contributors may be used to endorse or promote products derived from
# this software without specific prior written permission.
#
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
# "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
# LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
# A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
# OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
# SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
# LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
# DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
# THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
# (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
# OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
"""DEPRECATED: Declares the RPC service interfaces.
This module declares the abstract interfaces underlying proto2 RPC
services. These are intended to be independent of any particular RPC
implementation, so that proto2 services can be used on top of a variety
of implementations. Starting with version 2.3.0, RPC implementations should
not try to build on these, but should instead provide code generator plugins
which generate code specific to the particular RPC implementation. This way
the generated code can be more appropriate for the implementation in use
and can avoid unnecessary layers of indirection.
"""
__author__ = 'petar@google.com (Petar Petrov)'
class RpcException(Exception):
"""Exception raised on failed blocking RPC method call."""
pass
class Service(object):
"""Abstract base interface for protocol-buffer-based RPC services.
Services themselves are abstract classes (implemented either by servers or as
stubs), but they subclass this base interface. The methods of this
interface can be used to call the methods of the service without knowing
its exact type at compile time (analogous to the Message interface).
"""
def GetDescriptor():
"""Retrieves this service's descriptor."""
raise NotImplementedError
def CallMethod(self, method_descriptor, rpc_controller,
request, done):
"""Calls a method of the service specified by method_descriptor.
If "done" is None then the call is blocking and the response
message will be returned directly. Otherwise the call is asynchronous
and "done" will later be called with the response value.
In the blocking case, RpcException will be raised on error.
Preconditions:
* method_descriptor.service == GetDescriptor
* request is of the exact same classes as returned by
GetRequestClass(method).
* After the call has started, the request must not be modified.
* "rpc_controller" is of the correct type for the RPC implementation being
used by this Service. For stubs, the "correct type" depends on the
RpcChannel which the stub is using.
Postconditions:
* "done" will be called when the method is complete. This may be
before CallMethod() returns or it may be at some point in the future.
* If the RPC failed, the response value passed to "done" will be None.
Further details about the failure can be found by querying the
RpcController.
"""
raise NotImplementedError
def GetRequestClass(self, method_descriptor):
"""Returns the class of the request message for the specified method.
CallMethod() requires that the request is of a particular subclass of
Message. GetRequestClass() gets the default instance of this required
type.
Example:
method = service.GetDescriptor().FindMethodByName("Foo")
request = stub.GetRequestClass(method)()
request.ParseFromString(input)
service.CallMethod(method, request, callback)
"""
raise NotImplementedError
def GetResponseClass(self, method_descriptor):
"""Returns the class of the response message for the specified method.
This method isn't really needed, as the RpcChannel's CallMethod constructs
the response protocol message. It's provided anyway in case it is useful
for the caller to know the response type in advance.
"""
raise NotImplementedError
class RpcController(object):
"""An RpcController mediates a single method call.
The primary purpose of the controller is to provide a way to manipulate
settings specific to the RPC implementation and to find out about RPC-level
errors. The methods provided by the RpcController interface are intended
to be a "least common denominator" set of features which we expect all
implementations to support. Specific implementations may provide more
advanced features (e.g. deadline propagation).
"""
# Client-side methods below
def Reset(self):
"""Resets the RpcController to its initial state.
After the RpcController has been reset, it may be reused in
a new call. Must not be called while an RPC is in progress.
"""
raise NotImplementedError
def Failed(self):
"""Returns true if the call failed.
After a call has finished, returns true if the call failed. The possible
reasons for failure depend on the RPC implementation. Failed() must not
be called before a call has finished. If Failed() returns true, the
contents of the response message are undefined.
"""
raise NotImplementedError
def ErrorText(self):
"""If Failed is true, returns a human-readable description of the error."""
raise NotImplementedError
def StartCancel(self):
"""Initiate cancellation.
Advises the RPC system that the caller desires that the RPC call be
canceled. The RPC system may cancel it immediately, may wait awhile and
then cancel it, or may not even cancel the call at all. If the call is
canceled, the "done" callback will still be called and the RpcController
will indicate that the call failed at that time.
"""
raise NotImplementedError
# Server-side methods below
def SetFailed(self, reason):
"""Sets a failure reason.
Causes Failed() to return true on the client side. "reason" will be
incorporated into the message returned by ErrorText(). If you find
you need to return machine-readable information about failures, you
should incorporate it into your response protocol buffer and should
NOT call SetFailed().
"""
raise NotImplementedError
def IsCanceled(self):
"""Checks if the client cancelled the RPC.
If true, indicates that the client canceled the RPC, so the server may
as well give up on replying to it. The server should still call the
final "done" callback.
"""
raise NotImplementedError
def NotifyOnCancel(self, callback):
"""Sets a callback to invoke on cancel.
Asks that the given callback be called when the RPC is canceled. The
callback will always be called exactly once. If the RPC completes without
being canceled, the callback will be called after completion. If the RPC
has already been canceled when NotifyOnCancel() is called, the callback
will be called immediately.
NotifyOnCancel() must be called no more than once per request.
"""
raise NotImplementedError
class RpcChannel(object):
"""Abstract interface for an RPC channel.
An RpcChannel represents a communication line to a service which can be used
to call that service's methods. The service may be running on another
machine. Normally, you should not use an RpcChannel directly, but instead
construct a stub {@link Service} wrapping it. Example:
Example:
RpcChannel channel = rpcImpl.Channel("remotehost.example.com:1234")
RpcController controller = rpcImpl.Controller()
MyService service = MyService_Stub(channel)
service.MyMethod(controller, request, callback)
"""
def CallMethod(self, method_descriptor, rpc_controller,
request, response_class, done):
"""Calls the method identified by the descriptor.
Call the given method of the remote service. The signature of this
procedure looks the same as Service.CallMethod(), but the requirements
are less strict in one important way: the request object doesn't have to
be of any specific class as long as its descriptor is method.input_type.
"""
raise NotImplementedError
| bsd-3-clause |
DarkArtek/FFXIVITAFC | allauth/socialaccount/providers/linkedin_oauth2/provider.py | 6 | 2294 | from allauth.socialaccount import app_settings
from allauth.socialaccount.providers.base import ProviderAccount
from allauth.socialaccount.providers.oauth2.provider import OAuth2Provider
class LinkedInOAuth2Account(ProviderAccount):
def get_profile_url(self):
return self.account.extra_data.get('publicProfileUrl')
def get_avatar_url(self):
# try to return the higher res picture-urls::(original) first
try:
return self.account.extra_data['pictureUrls']['values'][0]
except:
# if we can't get higher res for any reason, we'll just return the
# low res
pass
return self.account.extra_data.get('pictureUrl')
def to_str(self):
dflt = super(LinkedInOAuth2Account, self).to_str()
name = self.account.extra_data.get('name', dflt)
first_name = self.account.extra_data.get('firstName', None)
last_name = self.account.extra_data.get('lastName', None)
if first_name and last_name:
name = first_name + ' ' + last_name
return name
class LinkedInOAuth2Provider(OAuth2Provider):
id = 'linkedin_oauth2'
# Name is displayed to ordinary users -- don't include protocol
name = 'LinkedIn'
account_class = LinkedInOAuth2Account
def extract_uid(self, data):
return str(data['id'])
def get_profile_fields(self):
default_fields = ['id',
'first-name',
'last-name',
'email-address',
'picture-url',
# picture-urls::(original) is higher res
'picture-urls::(original)',
'public-profile-url']
fields = self.get_settings().get('PROFILE_FIELDS',
default_fields)
return fields
def get_default_scope(self):
scope = []
if app_settings.QUERY_EMAIL:
scope.append('r_emailaddress')
return scope
def extract_common_fields(self, data):
return dict(email=data.get('emailAddress'),
first_name=data.get('firstName'),
last_name=data.get('lastName'))
provider_classes = [LinkedInOAuth2Provider]
| unlicense |
mccheung/kbengine | kbe/res/scripts/common/Lib/site-packages/pip/_vendor/colorama/ansi.py | 523 | 1039 | # Copyright Jonathan Hartley 2013. BSD 3-Clause license, see LICENSE file.
'''
This module generates ANSI character codes to printing colors to terminals.
See: http://en.wikipedia.org/wiki/ANSI_escape_code
'''
CSI = '\033['
def code_to_chars(code):
return CSI + str(code) + 'm'
class AnsiCodes(object):
def __init__(self, codes):
for name in dir(codes):
if not name.startswith('_'):
value = getattr(codes, name)
setattr(self, name, code_to_chars(value))
class AnsiFore:
BLACK = 30
RED = 31
GREEN = 32
YELLOW = 33
BLUE = 34
MAGENTA = 35
CYAN = 36
WHITE = 37
RESET = 39
class AnsiBack:
BLACK = 40
RED = 41
GREEN = 42
YELLOW = 43
BLUE = 44
MAGENTA = 45
CYAN = 46
WHITE = 47
RESET = 49
class AnsiStyle:
BRIGHT = 1
DIM = 2
NORMAL = 22
RESET_ALL = 0
Fore = AnsiCodes( AnsiFore )
Back = AnsiCodes( AnsiBack )
Style = AnsiCodes( AnsiStyle )
| lgpl-3.0 |
dec5e/twemproxy | tests/lib/utils.py | 41 | 2990 | import os
import re
import sys
import time
import copy
import thread
import socket
import threading
import logging
import inspect
import argparse
import telnetlib
import redis
import random
import redis
import json
import glob
import commands
from collections import defaultdict
from argparse import RawTextHelpFormatter
from string import Template
PWD = os.path.dirname(os.path.realpath(__file__))
WORKDIR = os.path.join(PWD, '../')
def getenv(key, default):
if key in os.environ:
return os.environ[key]
return default
logfile = getenv('T_LOGFILE', 'log/t.log')
if logfile == '-':
logging.basicConfig(level=logging.DEBUG,
format="%(asctime)-15s [%(threadName)s] [%(levelname)s] %(message)s")
else:
logging.basicConfig(filename=logfile, level=logging.DEBUG,
format="%(asctime)-15s [%(threadName)s] [%(levelname)s] %(message)s")
logging.info("test running")
def strstr(s1, s2):
return s1.find(s2) != -1
def lets_sleep(SLEEP_TIME = 0.1):
time.sleep(SLEEP_TIME)
def TT(template, args): #todo: modify all
return Template(template).substitute(args)
def TTCMD(template, args): #todo: modify all
'''
Template for cmd (we will replace all spaces)
'''
ret = TT(template, args)
return re.sub(' +', ' ', ret)
def nothrow(ExceptionToCheck=Exception, logger=None):
def deco_retry(f):
def f_retry(*args, **kwargs):
try:
return f(*args, **kwargs)
except ExceptionToCheck, e:
if logger:
logger.info(e)
else:
print str(e)
return f_retry # true decorator
return deco_retry
@nothrow(Exception)
def test_nothrow():
raise Exception('exception: xx')
def json_encode(j):
return json.dumps(j, indent=4, cls=MyEncoder)
def json_decode(j):
return json.loads(j)
#commands dose not work on windows..
def system(cmd, log_fun=logging.info):
if log_fun: log_fun(cmd)
r = commands.getoutput(cmd)
return r
def shorten(s, l=80):
if len(s)<=l:
return s
return s[:l-3] + '...'
def assert_true(a):
assert a, 'assert fail: except true, got %s' % a
def assert_equal(a, b):
assert a == b, 'assert fail: %s vs %s' % (shorten(str(a)), shorten(str(b)))
def assert_raises(exception_cls, callable, *args, **kwargs):
try:
callable(*args, **kwargs)
except exception_cls as e:
return e
except Exception as e:
assert False, 'assert_raises %s but raised: %s' % (exception_cls, e)
assert False, 'assert_raises %s but nothing raise' % (exception_cls)
def assert_fail(err_response, callable, *args, **kwargs):
try:
callable(*args, **kwargs)
except Exception as e:
assert re.search(err_response, str(e)), \
'assert "%s" but got "%s"' % (err_response, e)
return
assert False, 'assert_fail %s but nothing raise' % (err_response)
if __name__ == "__main__":
test_nothrow()
| apache-2.0 |
Azure/azure-sdk-for-python | sdk/synapse/azure-synapse-artifacts/azure/synapse/artifacts/aio/operations/_workspace_git_repo_management_operations.py | 1 | 8070 | # pylint: disable=too-many-lines
# coding=utf-8
# --------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for license information.
# Code generated by Microsoft (R) AutoRest Code Generator.
# Changes may cause incorrect behavior and will be lost if the code is regenerated.
# --------------------------------------------------------------------------
from typing import Any, Callable, Dict, IO, Optional, TypeVar, Union, overload
from azure.core.exceptions import (
ClientAuthenticationError,
HttpResponseError,
ResourceExistsError,
ResourceNotFoundError,
ResourceNotModifiedError,
map_error,
)
from azure.core.pipeline import PipelineResponse
from azure.core.pipeline.transport import AsyncHttpResponse
from azure.core.rest import HttpRequest
from azure.core.tracing.decorator_async import distributed_trace_async
from azure.core.utils import case_insensitive_dict
from ... import models as _models
from ..._vendor import _convert_request
from ...operations._workspace_git_repo_management_operations import build_get_git_hub_access_token_request
T = TypeVar("T")
ClsType = Optional[Callable[[PipelineResponse[HttpRequest, AsyncHttpResponse], T, Dict[str, Any]], Any]]
class WorkspaceGitRepoManagementOperations:
"""
.. warning::
**DO NOT** instantiate this class directly.
Instead, you should access the following operations through
:class:`~azure.synapse.artifacts.aio.ArtifactsClient`'s
:attr:`workspace_git_repo_management` attribute.
"""
models = _models
def __init__(self, *args, **kwargs) -> None:
input_args = list(args)
self._client = input_args.pop(0) if input_args else kwargs.pop("client")
self._config = input_args.pop(0) if input_args else kwargs.pop("config")
self._serialize = input_args.pop(0) if input_args else kwargs.pop("serializer")
self._deserialize = input_args.pop(0) if input_args else kwargs.pop("deserializer")
@overload
async def get_git_hub_access_token(
self,
git_hub_access_token_request: _models.GitHubAccessTokenRequest,
client_request_id: Optional[str] = None,
*,
content_type: str = "application/json",
**kwargs: Any
) -> _models.GitHubAccessTokenResponse:
"""Get the GitHub access token.
:param git_hub_access_token_request: Required.
:type git_hub_access_token_request: ~azure.synapse.artifacts.models.GitHubAccessTokenRequest
:param client_request_id: Can provide a guid, which is helpful for debugging and to provide
better customer support. Default value is None.
:type client_request_id: str
:keyword content_type: Body Parameter content-type. Content type parameter for JSON body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: GitHubAccessTokenResponse or the result of cls(response)
:rtype: ~azure.synapse.artifacts.models.GitHubAccessTokenResponse
:raises ~azure.core.exceptions.HttpResponseError:
"""
@overload
async def get_git_hub_access_token(
self,
git_hub_access_token_request: IO,
client_request_id: Optional[str] = None,
*,
content_type: str = "application/json",
**kwargs: Any
) -> _models.GitHubAccessTokenResponse:
"""Get the GitHub access token.
:param git_hub_access_token_request: Required.
:type git_hub_access_token_request: IO
:param client_request_id: Can provide a guid, which is helpful for debugging and to provide
better customer support. Default value is None.
:type client_request_id: str
:keyword content_type: Body Parameter content-type. Content type parameter for binary body.
Default value is "application/json".
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: GitHubAccessTokenResponse or the result of cls(response)
:rtype: ~azure.synapse.artifacts.models.GitHubAccessTokenResponse
:raises ~azure.core.exceptions.HttpResponseError:
"""
@distributed_trace_async
async def get_git_hub_access_token(
self,
git_hub_access_token_request: Union[_models.GitHubAccessTokenRequest, IO],
client_request_id: Optional[str] = None,
**kwargs: Any
) -> _models.GitHubAccessTokenResponse:
"""Get the GitHub access token.
:param git_hub_access_token_request: Is either a model type or a IO type. Required.
:type git_hub_access_token_request: ~azure.synapse.artifacts.models.GitHubAccessTokenRequest or
IO
:param client_request_id: Can provide a guid, which is helpful for debugging and to provide
better customer support. Default value is None.
:type client_request_id: str
:keyword content_type: Body Parameter content-type. Known values are: 'application/json'.
Default value is None.
:paramtype content_type: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: GitHubAccessTokenResponse or the result of cls(response)
:rtype: ~azure.synapse.artifacts.models.GitHubAccessTokenResponse
:raises ~azure.core.exceptions.HttpResponseError:
"""
error_map = {
401: ClientAuthenticationError,
404: ResourceNotFoundError,
409: ResourceExistsError,
304: ResourceNotModifiedError,
}
error_map.update(kwargs.pop("error_map", {}) or {})
_headers = case_insensitive_dict(kwargs.pop("headers", {}) or {})
_params = case_insensitive_dict(kwargs.pop("params", {}) or {})
api_version = kwargs.pop("api_version", _params.pop("api-version", "2020-12-01")) # type: str
content_type = kwargs.pop("content_type", _headers.pop("Content-Type", None)) # type: Optional[str]
cls = kwargs.pop("cls", None) # type: ClsType[_models.GitHubAccessTokenResponse]
content_type = content_type or "application/json"
_json = None
_content = None
if isinstance(git_hub_access_token_request, (IO, bytes)):
_content = git_hub_access_token_request
else:
_json = self._serialize.body(git_hub_access_token_request, "GitHubAccessTokenRequest")
request = build_get_git_hub_access_token_request(
client_request_id=client_request_id,
api_version=api_version,
content_type=content_type,
json=_json,
content=_content,
template_url=self.get_git_hub_access_token.metadata["url"],
headers=_headers,
params=_params,
)
request = _convert_request(request)
path_format_arguments = {
"endpoint": self._serialize.url("self._config.endpoint", self._config.endpoint, "str", skip_quote=True),
}
request.url = self._client.format_url(request.url, **path_format_arguments) # type: ignore
pipeline_response = await self._client._pipeline.run( # type: ignore # pylint: disable=protected-access
request, stream=False, **kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response)
deserialized = self._deserialize("GitHubAccessTokenResponse", pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
get_git_hub_access_token.metadata = {"url": "/getGitHubAccessToken"} # type: ignore
| mit |
chromium/chromium | net/data/ov_name_constraints/generate-certs.py | 7 | 8427 | #!/usr/bin/env python
# Copyright 2018 The Chromium Authors
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
import os
import sys
sys.path += ['..']
import gencerts
# Generate the keys -- the same key is used between all intermediate certs and
# between all leaf certs.
root_key = gencerts.get_or_generate_rsa_key(2048,
gencerts.create_key_path('root'))
i_key = gencerts.get_or_generate_rsa_key(2048, gencerts.create_key_path('i'))
leaf_key = gencerts.get_or_generate_rsa_key(2048,
gencerts.create_key_path('leaf'))
# Self-signed root certificate.
root = gencerts.create_self_signed_root_certificate('Root')
root.set_key(root_key)
# Preserve the ordering of the distinguished name in CSRs when issuing
# certificates. This must be in the BASE ('ca') section.
root.config.get_section('ca').set_property('preserve', 'yes')
gencerts.write_string_to_file(root.get_cert_pem(), 'root.pem')
## Create intermediate certs
# Intermediate with two organizations as two distinct SETs, ordered O1 and O2
i_o1_o2 = gencerts.create_intermediate_certificate('I1', root)
i_o1_o2.set_key(i_key)
dn = i_o1_o2.get_subject()
dn.clear_properties()
dn.add_property('0.organizationName', 'O1')
dn.add_property('1.organizationName', 'O2')
gencerts.write_string_to_file(i_o1_o2.get_cert_pem(), 'int-o1-o2.pem')
# Intermediate with two organizations as two distinct SETs, ordered O2 and O1
i_o2_o1 = gencerts.create_intermediate_certificate('I2', root)
i_o2_o1.set_key(i_key)
dn = i_o2_o1.get_subject()
dn.clear_properties()
dn.add_property('0.organizationName', 'O2')
dn.add_property('1.organizationName', 'O1')
gencerts.write_string_to_file(i_o2_o1.get_cert_pem(), 'int-o2-o1.pem')
# Intermediate with a single organization name, O3
i_o3 = gencerts.create_intermediate_certificate('I3', root)
i_o3.set_key(i_key)
dn = i_o3.get_subject()
dn.clear_properties()
dn.add_property('organizationName', 'O3')
gencerts.write_string_to_file(i_o3.get_cert_pem(), 'int-o3.pem')
# Intermediate with a single organization name, O1, encoded as BMPString
i_bmp_o1 = gencerts.create_intermediate_certificate('I4', root)
i_bmp_o1.set_key(i_key)
# 2048 = 0x0800, B_ASN1_BMPSTRING
i_bmp_o1.config.get_section('req').set_property('string_mask', 'MASK:2048')
i_bmp_o1.config.get_section('req').set_property('utf8', 'no')
dn = i_bmp_o1.get_subject()
dn.clear_properties()
dn.add_property('organizationName', 'O1')
gencerts.write_string_to_file(i_bmp_o1.get_cert_pem(), 'int-bmp-o1.pem')
# Intermediate with two organizations as a single SET, ordered O1 and O2
i_o1_plus_o2 = gencerts.create_intermediate_certificate('I5', root)
i_o1_plus_o2.set_key(i_key)
dn = i_o1_plus_o2.get_subject()
dn.clear_properties()
dn.add_property('organizationName', 'O1')
dn.add_property('+organizationName', 'O2')
gencerts.write_string_to_file(i_o1_plus_o2.get_cert_pem(), 'int-o1-plus-o2.pem')
# Intermediate with no organization name (not BR compliant)
i_cn = gencerts.create_intermediate_certificate('I6', root)
i_cn.set_key(i_key)
dn = i_cn.get_subject()
dn.clear_properties()
dn.add_property('commonName', 'O1')
gencerts.write_string_to_file(i_cn.get_cert_pem(), 'int-cn.pem')
## Create name-constrained intermediate certs
# Create a name-constrained intermediate that has O1 as a permitted
# organizationName in a directoryName nameConstraint
nc_permit_o1 = gencerts.create_intermediate_certificate('NC1', root)
nc_permit_o1.set_key(i_key)
nc_permit_o1.get_extensions().set_property('nameConstraints', 'critical,@nc')
nc = nc_permit_o1.config.get_section('nc')
nc.add_property('permitted;dirName.1', 'nc_1')
nc_1 = nc_permit_o1.config.get_section('nc_1')
nc_1.add_property('organizationName', 'O1')
gencerts.write_string_to_file(nc_permit_o1.get_cert_pem(),
'nc-int-permit-o1.pem')
# Create a name-constrained intermediate that has O1 as a permitted
# organizationName, but encoded as a BMPString within a directoryName
# nameConstraint
nc_permit_bmp_o1 = gencerts.create_intermediate_certificate('NC2', root)
nc_permit_bmp_o1.set_key(i_key)
# 2048 = 0x0800, B_ASN1_BMPSTRING
nc_permit_bmp_o1.config.get_section('req').set_property('string_mask',
'MASK:2048')
nc_permit_bmp_o1.config.get_section('req').set_property('utf8', 'no')
nc = nc_permit_bmp_o1.config.get_section('nc')
nc.add_property('permitted;dirName.1', 'nc_1')
nc_1 = nc_permit_bmp_o1.config.get_section('nc_1')
nc_1.add_property('organizationName', 'O1')
gencerts.write_string_to_file(nc_permit_bmp_o1.get_cert_pem(),
'nc-int-permit-bmp-o1.pem')
# Create a name-constrained intermediate that has O1 as a permitted
# commonName in a directoryName nameConstraint
nc_permit_cn = gencerts.create_intermediate_certificate('NC3', root)
nc_permit_cn.set_key(i_key)
nc_permit_cn.get_extensions().set_property('nameConstraints', 'critical,@nc')
nc = nc_permit_cn.config.get_section('nc')
nc.add_property('permitted;dirName.1', 'nc_1')
nc_1 = nc_permit_cn.config.get_section('nc_1')
nc_1.add_property('commonName', 'O1')
gencerts.write_string_to_file(nc_permit_cn.get_cert_pem(),
'nc-int-permit-cn.pem')
# Create a name-constrainted intermediate that has O1 as an excluded
# commonName in a directoryName nameConstraint
nc_exclude_o1 = gencerts.create_intermediate_certificate('NC4', root)
nc_exclude_o1.set_key(i_key)
nc_exclude_o1.get_extensions().set_property('nameConstraints', 'critical,@nc')
nc = nc_exclude_o1.config.get_section('nc')
nc.add_property('excluded;dirName.1', 'nc_1')
nc_1 = nc_exclude_o1.config.get_section('nc_1')
nc_1.add_property('organizationName', 'O1')
gencerts.write_string_to_file(nc_exclude_o1.get_cert_pem(),
'nc-int-exclude-o1.pem')
# Create a name-constrained intermediate that does not have a directoryName
# nameConstraint
nc_permit_dns = gencerts.create_intermediate_certificate('NC5', root)
nc_permit_dns.set_key(i_key)
nc_permit_dns.get_extensions().set_property('nameConstraints', 'critical,@nc')
nc = nc_permit_dns.config.get_section('nc')
nc.add_property('permitted;DNS.1', 'test.invalid')
gencerts.write_string_to_file(nc_permit_dns.get_cert_pem(),
'nc-int-permit-dns.pem')
# Create a name-constrained intermediate with multiple directoryName
# nameConstraints
nc_permit_o2_o1_o3 = gencerts.create_intermediate_certificate('NC6', root)
nc_permit_o2_o1_o3.set_key(i_key)
nc_permit_o2_o1_o3.get_extensions().set_property('nameConstraints',
'critical,@nc')
nc = nc_permit_o2_o1_o3.config.get_section('nc')
nc.add_property('permitted;dirName.1', 'nc_1')
nc_1 = nc_permit_o2_o1_o3.config.get_section('nc_1')
nc_1.add_property('organizationName', 'O2')
nc.add_property('permitted;dirName.2', 'nc_2')
nc_2 = nc_permit_o2_o1_o3.config.get_section('nc_2')
nc_2.add_property('organizationName', 'O1')
nc.add_property('permitted;dirName.3', 'nc_3')
nc_3 = nc_permit_o2_o1_o3.config.get_section('nc_3')
nc_3.add_property('organizationName', 'O3')
gencerts.write_string_to_file(nc_permit_o2_o1_o3.get_cert_pem(),
'nc-int-permit-o2-o1-o3.pem')
## Create leaf certs (note: The issuer name does not matter for these tests)
# Leaf missing an organization name
leaf_no_o = gencerts.create_end_entity_certificate('L1', root)
leaf_no_o.set_key(leaf_key)
dn = leaf_no_o.get_subject()
dn.clear_properties()
dn.add_property('commonName', 'O1')
gencerts.write_string_to_file(leaf_no_o.get_cert_pem(), 'leaf-no-o.pem')
# Leaf with two organizations as two distinct SETs, ordered O1 and O2
leaf_o1_o2 = gencerts.create_end_entity_certificate('L2', root)
leaf_o1_o2.set_key(leaf_key)
dn = leaf_o1_o2.get_subject()
dn.clear_properties()
dn.add_property('0.organizationName', 'O1')
dn.add_property('1.organizationName', 'O2')
dn.add_property('commonName', 'Leaf')
gencerts.write_string_to_file(leaf_o1_o2.get_cert_pem(), 'leaf-o1-o2.pem')
# Leaf with a single organization name, O1
leaf_o1 = gencerts.create_end_entity_certificate('L3', root)
leaf_o1.set_key(leaf_key)
dn = leaf_o1.get_subject()
dn.clear_properties()
dn.add_property('0.organizationName', 'O1')
dn.add_property('commonName', 'Leaf')
gencerts.write_string_to_file(leaf_o1.get_cert_pem(), 'leaf-o1.pem')
| bsd-3-clause |
ghl3/spark-testing-base | python/sparktestingbase/test/reuse_spark_context_test.py | 10 | 1317 | #
# Licensed to the Apache Software Foundation (ASF) under one or more
# contributor license agreements. See the NOTICE file distributed with
# this work for additional information regarding copyright ownership.
# The ASF licenses this file to You under the Apache License, Version 2.0
# (the "License"); you may not use this file except in compliance with
# the License. You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#
"""Simple test example"""
from sparktestingbase.testcase import SparkTestingBaseReuse
import unittest2
class ReuseSparkContextTest(SparkTestingBaseReuse):
"""Test that we re-use the spark context when asked to."""
def test_samecontext_1(self):
"""Set a system property"""
self.sc.setLocalProperty("pandas", "123")
def test_samecontext_2(self):
"""Test that we have the same context."""
assert self.sc.getLocalProperty("pandas") == "123"
if __name__ == "__main__":
unittest2.main()
| apache-2.0 |
luwei0917/awsemmd_script | experiment_functions.py | 1 | 2597 | #!/usr/bin/env python3
import os
import argparse
import sys
from time import sleep
import subprocess
def clean():
os.system("rm results/ga.dat")
os.system("rm results/gb.dat")
os.system("rm results/gaa.dat")
os.system("rm results/ga_highest.dat")
os.system("rm results/gb_highest.dat")
def gagb():
os.system("cp ~/opt/gagb/*.pdb .")
os.system("awk '{if ((!((($1>0 && $1<25) || ($1>159 && $1<200) ) && $3>-10) ) ) print }' dump.lammpstrj > data_test")
os.system("python2 ~/opt/script/CalcQValue.py 2lhc_part.pdb data_test test")
os.system("tail -n 50 test | awk '{s+=$1} END {print s/50}' > gaa")
os.system("tail -n 50 test | sort | tail -n 1 > ga_highest")
os.system("python2 ~/opt/script/CalcQValue.py 2lhc.pdb dump.lammpstrj test2")
os.system("tail -n 50 test2 | sort | tail -n 1 > gb_highest")
os.system("tail -n +40 final.pdb | ghead -n -15 > final_chosen.pdb")
os.system("python2 ~/opt/small_script/CalcQValueFrom2Pdb.py 2lhc_part.pdb final_chosen.pdb > ga")
os.system("python2 ~/opt/small_script/CalcQValueFrom2Pdb.py 2lhd.pdb final.pdb > gb")
os.system("cat ga_highest >> ../../results/ga_highest.dat")
os.system("cat gb_highest >> ../../results/gb_highest.dat")
os.system("cat gaa >> ../../results/gaa.dat")
os.system("cat ga >> ../../results/ga.dat")
os.system("cat gb >> ../../results/gb.dat")
# os.system("> results/cross_q")
# for i in range(n):
# for j in range(0, i):
# os.system("echo '"+str(i)+" "+str(j)+" \c' >> results/cross_q")
# os.system("python2 ~/opt/CalcQValue.py analysis/"+str(i)+"/final \
# analysis/"+str(j)+"/final.txt >> results/cross_q ")
# exec(open("config.py").read())
# n = number_of_run
# steps = simulation_steps
# os.system("mkdir -p results")
# for i in range(n):
# # analysis
# os.system("mkdir -p analysis/"+str(i))
# os.chdir("analysis/"+str(i))
#
# sys.stdout = open("final.txt", "w")
# print('ITEM: TIMESTEP')
# time_step = simulation_steps
# with open('dump.lammpstrj') as input_data:
# # Skips text before the beginning of the interesting block:
# for line in input_data:
# if line.strip() == str(time_step):
# print(line.strip()) # Or whatever test is needed
# break
# # Reads text until the end of the block:
# for line in input_data: # This keeps reading the file
# if line.strip() == 'ITEM: TIMESTEP':
# break
# print(line.strip())
# sys.stdout.close()
#
# os.chdir("../..")
| mit |
Azure/azure-sdk-for-python | sdk/communication/azure-communication-rooms/azure/communication/rooms/_shared/user_credential.py | 8 | 6391 | # -------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for
# license information.
# --------------------------------------------------------------------------
from threading import Lock, Condition, Timer, TIMEOUT_MAX, Event
from datetime import timedelta
from typing import Any
import six
from .utils import get_current_utc_as_int
from .utils import create_access_token
class CommunicationTokenCredential(object):
"""Credential type used for authenticating to an Azure Communication service.
:param str token: The token used to authenticate to an Azure Communication service.
:keyword token_refresher: The sync token refresher to provide capacity to fetch a fresh token.
The returned token must be valid (expiration date must be in the future).
:paramtype token_refresher: Callable[[], AccessToken]
:keyword bool proactive_refresh: Whether to refresh the token proactively or not.
If the proactive refreshing is enabled ('proactive_refresh' is true), the credential will use
a background thread to attempt to refresh the token within 10 minutes before the cached token expires,
the proactive refresh will request a new token by calling the 'token_refresher' callback.
When 'proactive_refresh' is enabled, the Credential object must be either run within a context manager
or the 'close' method must be called once the object usage has been finished.
:raises: TypeError if paramater 'token' is not a string
:raises: ValueError if the 'proactive_refresh' is enabled without providing the 'token_refresher' callable.
"""
_ON_DEMAND_REFRESHING_INTERVAL_MINUTES = 2
_DEFAULT_AUTOREFRESH_INTERVAL_MINUTES = 10
def __init__(self, token: str, **kwargs: Any):
if not isinstance(token, six.string_types):
raise TypeError("Token must be a string.")
self._token = create_access_token(token)
self._token_refresher = kwargs.pop('token_refresher', None)
self._proactive_refresh = kwargs.pop('proactive_refresh', False)
if(self._proactive_refresh and self._token_refresher is None):
raise ValueError("When 'proactive_refresh' is True, 'token_refresher' must not be None.")
self._timer = None
self._lock = Condition(Lock())
self._some_thread_refreshing = False
self._is_closed = Event()
def get_token(self, *scopes, **kwargs): # pylint: disable=unused-argument
# type (*str, **Any) -> AccessToken
"""The value of the configured token.
:rtype: ~azure.core.credentials.AccessToken
"""
if self._proactive_refresh and self._is_closed.is_set():
raise RuntimeError("An instance of CommunicationTokenCredential cannot be reused once it has been closed.")
if not self._token_refresher or not self._is_token_expiring_soon(self._token):
return self._token
self._update_token_and_reschedule()
return self._token
def _update_token_and_reschedule(self):
should_this_thread_refresh = False
with self._lock:
while self._is_token_expiring_soon(self._token):
if self._some_thread_refreshing:
if self._is_token_valid(self._token):
return self._token
self._wait_till_lock_owner_finishes_refreshing()
else:
should_this_thread_refresh = True
self._some_thread_refreshing = True
break
if should_this_thread_refresh:
try:
new_token = self._token_refresher()
if not self._is_token_valid(new_token):
raise ValueError(
"The token returned from the token_refresher is expired.")
with self._lock:
self._token = new_token
self._some_thread_refreshing = False
self._lock.notify_all()
except:
with self._lock:
self._some_thread_refreshing = False
self._lock.notify_all()
raise
if self._proactive_refresh:
self._schedule_refresh()
return self._token
def _schedule_refresh(self):
if self._is_closed.is_set():
return
if self._timer is not None:
self._timer.cancel()
token_ttl = self._token.expires_on - get_current_utc_as_int()
if self._is_token_expiring_soon(self._token):
# Schedule the next refresh for when it reaches a certain percentage of the remaining lifetime.
timespan = token_ttl // 2
else:
# Schedule the next refresh for when it gets in to the soon-to-expire window.
timespan = token_ttl - timedelta(
minutes=self._DEFAULT_AUTOREFRESH_INTERVAL_MINUTES).total_seconds()
if timespan <= TIMEOUT_MAX:
self._timer = Timer(timespan, self._update_token_and_reschedule)
self._timer.daemon = True
self._timer.start()
def _wait_till_lock_owner_finishes_refreshing(self):
self._lock.release()
self._lock.acquire()
def _is_token_expiring_soon(self, token):
if self._proactive_refresh:
interval = timedelta(
minutes=self._DEFAULT_AUTOREFRESH_INTERVAL_MINUTES)
else:
interval = timedelta(
minutes=self._ON_DEMAND_REFRESHING_INTERVAL_MINUTES)
return ((token.expires_on - get_current_utc_as_int())
< interval.total_seconds())
@classmethod
def _is_token_valid(cls, token):
return get_current_utc_as_int() < token.expires_on
def __enter__(self):
if self._proactive_refresh:
if self._is_closed.is_set():
raise RuntimeError(
"An instance of CommunicationTokenCredential cannot be reused once it has been closed.")
self._schedule_refresh()
return self
def __exit__(self, *args):
self.close()
def close(self) -> None:
if self._timer is not None:
self._timer.cancel()
self._timer = None
self._is_closed.set()
| mit |
Azure/azure-sdk-for-python | sdk/datamigration/azure-mgmt-datamigration/setup.py | 1 | 2835 | #!/usr/bin/env python
#-------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for
# license information.
#--------------------------------------------------------------------------
import re
import os.path
from io import open
from setuptools import find_packages, setup
# Change the PACKAGE_NAME only to change folder and different name
PACKAGE_NAME = "azure-mgmt-datamigration"
PACKAGE_PPRINT_NAME = "Data Migration"
# a-b-c => a/b/c
package_folder_path = PACKAGE_NAME.replace('-', '/')
# a-b-c => a.b.c
namespace_name = PACKAGE_NAME.replace('-', '.')
# Version extraction inspired from 'requests'
with open(os.path.join(package_folder_path, 'version.py')
if os.path.exists(os.path.join(package_folder_path, 'version.py'))
else os.path.join(package_folder_path, '_version.py'), 'r') as fd:
version = re.search(r'^VERSION\s*=\s*[\'"]([^\'"]*)[\'"]',
fd.read(), re.MULTILINE).group(1)
if not version:
raise RuntimeError('Cannot find version information')
with open('README.md', encoding='utf-8') as f:
readme = f.read()
with open('CHANGELOG.md', encoding='utf-8') as f:
changelog = f.read()
setup(
name=PACKAGE_NAME,
version=version,
description='Microsoft Azure {} Client Library for Python'.format(PACKAGE_PPRINT_NAME),
long_description=readme + '\n\n' + changelog,
long_description_content_type='text/markdown',
license='MIT License',
author='Microsoft Corporation',
author_email='azpysdkhelp@microsoft.com',
url='https://github.com/Azure/azure-sdk-for-python',
keywords="azure, azure sdk", # update with search keywords relevant to the azure service / product
classifiers=[
'Development Status :: 5 - Production/Stable',
'Programming Language :: Python',
'Programming Language :: Python :: 3 :: Only',
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: 3.9',
'Programming Language :: Python :: 3.10',
'Programming Language :: Python :: 3.11',
'License :: OSI Approved :: MIT License',
],
zip_safe=False,
packages=find_packages(exclude=[
'tests',
# Exclude packages that will be covered by PEP420 or nspkg
'azure',
'azure.mgmt',
]),
include_package_data=True,
package_data={
'pytyped': ['py.typed'],
},
install_requires=[
"msrest>=0.7.1",
"azure-common~=1.1",
"azure-mgmt-core>=1.3.2,<2.0.0",
"typing-extensions>=4.3.0; python_version<'3.8.0'",
],
python_requires=">=3.7"
)
| mit |
Azure/azure-sdk-for-python | sdk/digitaltwins/azure-mgmt-digitaltwins/azure/mgmt/digitaltwins/v2020_12_01/operations/_private_endpoint_connections_operations.py | 1 | 26644 | # pylint: disable=too-many-lines
# coding=utf-8
# --------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for license information.
# Code generated by Microsoft (R) AutoRest Code Generator.
# Changes may cause incorrect behavior and will be lost if the code is regenerated.
# --------------------------------------------------------------------------
from typing import Any, Callable, Dict, Optional, TypeVar, Union
from msrest import Serializer
from azure.core.exceptions import ClientAuthenticationError, HttpResponseError, ResourceExistsError, ResourceNotFoundError, map_error
from azure.core.pipeline import PipelineResponse
from azure.core.pipeline.transport import HttpResponse
from azure.core.polling import LROPoller, NoPolling, PollingMethod
from azure.core.rest import HttpRequest
from azure.core.tracing.decorator import distributed_trace
from azure.mgmt.core.exceptions import ARMErrorFormat
from azure.mgmt.core.polling.arm_polling import ARMPolling
from .. import models as _models
from .._vendor import _convert_request, _format_url_section
T = TypeVar('T')
JSONType = Any
ClsType = Optional[Callable[[PipelineResponse[HttpRequest, HttpResponse], T, Dict[str, Any]], Any]]
_SERIALIZER = Serializer()
_SERIALIZER.client_side_validation = False
def build_list_request(
subscription_id: str,
resource_group_name: str,
resource_name: str,
**kwargs: Any
) -> HttpRequest:
api_version = kwargs.pop('api_version', "2020-12-01") # type: str
accept = "application/json"
# Construct URL
_url = kwargs.pop("template_url", "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DigitalTwins/digitalTwinsInstances/{resourceName}/privateEndpointConnections") # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, 'str'),
"resourceGroupName": _SERIALIZER.url("resource_group_name", resource_group_name, 'str', max_length=90, min_length=1),
"resourceName": _SERIALIZER.url("resource_name", resource_name, 'str', max_length=63, min_length=3, pattern=r'^(?!-)[A-Za-z0-9-]{3,63}(?<!-)$'),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_query_parameters = kwargs.pop("params", {}) # type: Dict[str, Any]
_query_parameters['api-version'] = _SERIALIZER.query("api_version", api_version, 'str')
# Construct headers
_header_parameters = kwargs.pop("headers", {}) # type: Dict[str, Any]
_header_parameters['Accept'] = _SERIALIZER.header("accept", accept, 'str')
return HttpRequest(
method="GET",
url=_url,
params=_query_parameters,
headers=_header_parameters,
**kwargs
)
def build_get_request(
subscription_id: str,
resource_group_name: str,
resource_name: str,
private_endpoint_connection_name: str,
**kwargs: Any
) -> HttpRequest:
api_version = kwargs.pop('api_version', "2020-12-01") # type: str
accept = "application/json"
# Construct URL
_url = kwargs.pop("template_url", "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DigitalTwins/digitalTwinsInstances/{resourceName}/privateEndpointConnections/{privateEndpointConnectionName}") # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, 'str'),
"resourceGroupName": _SERIALIZER.url("resource_group_name", resource_group_name, 'str', max_length=90, min_length=1),
"resourceName": _SERIALIZER.url("resource_name", resource_name, 'str', max_length=63, min_length=3, pattern=r'^(?!-)[A-Za-z0-9-]{3,63}(?<!-)$'),
"privateEndpointConnectionName": _SERIALIZER.url("private_endpoint_connection_name", private_endpoint_connection_name, 'str'),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_query_parameters = kwargs.pop("params", {}) # type: Dict[str, Any]
_query_parameters['api-version'] = _SERIALIZER.query("api_version", api_version, 'str')
# Construct headers
_header_parameters = kwargs.pop("headers", {}) # type: Dict[str, Any]
_header_parameters['Accept'] = _SERIALIZER.header("accept", accept, 'str')
return HttpRequest(
method="GET",
url=_url,
params=_query_parameters,
headers=_header_parameters,
**kwargs
)
def build_delete_request_initial(
subscription_id: str,
resource_group_name: str,
resource_name: str,
private_endpoint_connection_name: str,
**kwargs: Any
) -> HttpRequest:
api_version = kwargs.pop('api_version', "2020-12-01") # type: str
accept = "application/json"
# Construct URL
_url = kwargs.pop("template_url", "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DigitalTwins/digitalTwinsInstances/{resourceName}/privateEndpointConnections/{privateEndpointConnectionName}") # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, 'str'),
"resourceGroupName": _SERIALIZER.url("resource_group_name", resource_group_name, 'str', max_length=90, min_length=1),
"resourceName": _SERIALIZER.url("resource_name", resource_name, 'str', max_length=63, min_length=3, pattern=r'^(?!-)[A-Za-z0-9-]{3,63}(?<!-)$'),
"privateEndpointConnectionName": _SERIALIZER.url("private_endpoint_connection_name", private_endpoint_connection_name, 'str'),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_query_parameters = kwargs.pop("params", {}) # type: Dict[str, Any]
_query_parameters['api-version'] = _SERIALIZER.query("api_version", api_version, 'str')
# Construct headers
_header_parameters = kwargs.pop("headers", {}) # type: Dict[str, Any]
_header_parameters['Accept'] = _SERIALIZER.header("accept", accept, 'str')
return HttpRequest(
method="DELETE",
url=_url,
params=_query_parameters,
headers=_header_parameters,
**kwargs
)
def build_create_or_update_request_initial(
subscription_id: str,
resource_group_name: str,
resource_name: str,
private_endpoint_connection_name: str,
*,
json: JSONType = None,
content: Any = None,
**kwargs: Any
) -> HttpRequest:
api_version = kwargs.pop('api_version', "2020-12-01") # type: str
content_type = kwargs.pop('content_type', None) # type: Optional[str]
accept = "application/json"
# Construct URL
_url = kwargs.pop("template_url", "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DigitalTwins/digitalTwinsInstances/{resourceName}/privateEndpointConnections/{privateEndpointConnectionName}") # pylint: disable=line-too-long
path_format_arguments = {
"subscriptionId": _SERIALIZER.url("subscription_id", subscription_id, 'str'),
"resourceGroupName": _SERIALIZER.url("resource_group_name", resource_group_name, 'str', max_length=90, min_length=1),
"resourceName": _SERIALIZER.url("resource_name", resource_name, 'str', max_length=63, min_length=3, pattern=r'^(?!-)[A-Za-z0-9-]{3,63}(?<!-)$'),
"privateEndpointConnectionName": _SERIALIZER.url("private_endpoint_connection_name", private_endpoint_connection_name, 'str'),
}
_url = _format_url_section(_url, **path_format_arguments)
# Construct parameters
_query_parameters = kwargs.pop("params", {}) # type: Dict[str, Any]
_query_parameters['api-version'] = _SERIALIZER.query("api_version", api_version, 'str')
# Construct headers
_header_parameters = kwargs.pop("headers", {}) # type: Dict[str, Any]
if content_type is not None:
_header_parameters['Content-Type'] = _SERIALIZER.header("content_type", content_type, 'str')
_header_parameters['Accept'] = _SERIALIZER.header("accept", accept, 'str')
return HttpRequest(
method="PUT",
url=_url,
params=_query_parameters,
headers=_header_parameters,
json=json,
content=content,
**kwargs
)
class PrivateEndpointConnectionsOperations(object):
"""PrivateEndpointConnectionsOperations operations.
You should not instantiate this class directly. Instead, you should create a Client instance that
instantiates it for you and attaches it as an attribute.
:ivar models: Alias to model classes used in this operation group.
:type models: ~azure.mgmt.digitaltwins.v2020_12_01.models
:param client: Client for service requests.
:param config: Configuration of service client.
:param serializer: An object model serializer.
:param deserializer: An object model deserializer.
"""
models = _models
def __init__(self, client, config, serializer, deserializer):
self._client = client
self._serialize = serializer
self._deserialize = deserializer
self._config = config
@distributed_trace
def list(
self,
resource_group_name: str,
resource_name: str,
**kwargs: Any
) -> "_models.PrivateEndpointConnectionsResponse":
"""List private endpoint connection properties.
:param resource_group_name: The name of the resource group that contains the
DigitalTwinsInstance.
:type resource_group_name: str
:param resource_name: The name of the DigitalTwinsInstance.
:type resource_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: PrivateEndpointConnectionsResponse, or the result of cls(response)
:rtype: ~azure.mgmt.digitaltwins.v2020_12_01.models.PrivateEndpointConnectionsResponse
:raises: ~azure.core.exceptions.HttpResponseError
"""
cls = kwargs.pop('cls', None) # type: ClsType["_models.PrivateEndpointConnectionsResponse"]
error_map = {
401: ClientAuthenticationError, 404: ResourceNotFoundError, 409: ResourceExistsError
}
error_map.update(kwargs.pop('error_map', {}))
api_version = kwargs.pop('api_version', "2020-12-01") # type: str
request = build_list_request(
subscription_id=self._config.subscription_id,
resource_group_name=resource_group_name,
resource_name=resource_name,
api_version=api_version,
template_url=self.list.metadata['url'],
)
request = _convert_request(request)
request.url = self._client.format_url(request.url)
pipeline_response = self._client._pipeline.run( # pylint: disable=protected-access
request,
stream=False,
**kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorResponse, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
deserialized = self._deserialize('PrivateEndpointConnectionsResponse', pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
list.metadata = {'url': "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DigitalTwins/digitalTwinsInstances/{resourceName}/privateEndpointConnections"} # type: ignore
@distributed_trace
def get(
self,
resource_group_name: str,
resource_name: str,
private_endpoint_connection_name: str,
**kwargs: Any
) -> "_models.PrivateEndpointConnection":
"""Get private endpoint connection properties for the given private endpoint.
:param resource_group_name: The name of the resource group that contains the
DigitalTwinsInstance.
:type resource_group_name: str
:param resource_name: The name of the DigitalTwinsInstance.
:type resource_name: str
:param private_endpoint_connection_name: The name of the private endpoint connection.
:type private_endpoint_connection_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:return: PrivateEndpointConnection, or the result of cls(response)
:rtype: ~azure.mgmt.digitaltwins.v2020_12_01.models.PrivateEndpointConnection
:raises: ~azure.core.exceptions.HttpResponseError
"""
cls = kwargs.pop('cls', None) # type: ClsType["_models.PrivateEndpointConnection"]
error_map = {
401: ClientAuthenticationError, 404: ResourceNotFoundError, 409: ResourceExistsError
}
error_map.update(kwargs.pop('error_map', {}))
api_version = kwargs.pop('api_version', "2020-12-01") # type: str
request = build_get_request(
subscription_id=self._config.subscription_id,
resource_group_name=resource_group_name,
resource_name=resource_name,
private_endpoint_connection_name=private_endpoint_connection_name,
api_version=api_version,
template_url=self.get.metadata['url'],
)
request = _convert_request(request)
request.url = self._client.format_url(request.url)
pipeline_response = self._client._pipeline.run( # pylint: disable=protected-access
request,
stream=False,
**kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
error = self._deserialize.failsafe_deserialize(_models.ErrorResponse, pipeline_response)
raise HttpResponseError(response=response, model=error, error_format=ARMErrorFormat)
deserialized = self._deserialize('PrivateEndpointConnection', pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
get.metadata = {'url': "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DigitalTwins/digitalTwinsInstances/{resourceName}/privateEndpointConnections/{privateEndpointConnectionName}"} # type: ignore
def _delete_initial( # pylint: disable=inconsistent-return-statements
self,
resource_group_name: str,
resource_name: str,
private_endpoint_connection_name: str,
**kwargs: Any
) -> None:
cls = kwargs.pop('cls', None) # type: ClsType[None]
error_map = {
401: ClientAuthenticationError, 404: ResourceNotFoundError, 409: ResourceExistsError
}
error_map.update(kwargs.pop('error_map', {}))
api_version = kwargs.pop('api_version', "2020-12-01") # type: str
request = build_delete_request_initial(
subscription_id=self._config.subscription_id,
resource_group_name=resource_group_name,
resource_name=resource_name,
private_endpoint_connection_name=private_endpoint_connection_name,
api_version=api_version,
template_url=self._delete_initial.metadata['url'],
)
request = _convert_request(request)
request.url = self._client.format_url(request.url)
pipeline_response = self._client._pipeline.run( # pylint: disable=protected-access
request,
stream=False,
**kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202, 204]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if cls:
return cls(pipeline_response, None, {})
_delete_initial.metadata = {'url': "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DigitalTwins/digitalTwinsInstances/{resourceName}/privateEndpointConnections/{privateEndpointConnectionName}"} # type: ignore
@distributed_trace
def begin_delete( # pylint: disable=inconsistent-return-statements
self,
resource_group_name: str,
resource_name: str,
private_endpoint_connection_name: str,
**kwargs: Any
) -> LROPoller[None]:
"""Delete private endpoint connection with the specified name.
:param resource_group_name: The name of the resource group that contains the
DigitalTwinsInstance.
:type resource_group_name: str
:param resource_name: The name of the DigitalTwinsInstance.
:type resource_name: str
:param private_endpoint_connection_name: The name of the private endpoint connection.
:type private_endpoint_connection_name: str
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either None or the result of cls(response)
:rtype: ~azure.core.polling.LROPoller[None]
:raises: ~azure.core.exceptions.HttpResponseError
"""
api_version = kwargs.pop('api_version', "2020-12-01") # type: str
polling = kwargs.pop('polling', True) # type: Union[bool, PollingMethod]
cls = kwargs.pop('cls', None) # type: ClsType[None]
lro_delay = kwargs.pop(
'polling_interval',
self._config.polling_interval
)
cont_token = kwargs.pop('continuation_token', None) # type: Optional[str]
if cont_token is None:
raw_result = self._delete_initial(
resource_group_name=resource_group_name,
resource_name=resource_name,
private_endpoint_connection_name=private_endpoint_connection_name,
api_version=api_version,
cls=lambda x,y,z: x,
**kwargs
)
kwargs.pop('error_map', None)
def get_long_running_output(pipeline_response):
if cls:
return cls(pipeline_response, None, {})
if polling is True: polling_method = ARMPolling(lro_delay, **kwargs)
elif polling is False: polling_method = NoPolling()
else: polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_delete.metadata = {'url': "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DigitalTwins/digitalTwinsInstances/{resourceName}/privateEndpointConnections/{privateEndpointConnectionName}"} # type: ignore
def _create_or_update_initial(
self,
resource_group_name: str,
resource_name: str,
private_endpoint_connection_name: str,
private_endpoint_connection: "_models.PrivateEndpointConnection",
**kwargs: Any
) -> "_models.PrivateEndpointConnection":
cls = kwargs.pop('cls', None) # type: ClsType["_models.PrivateEndpointConnection"]
error_map = {
401: ClientAuthenticationError, 404: ResourceNotFoundError, 409: ResourceExistsError
}
error_map.update(kwargs.pop('error_map', {}))
api_version = kwargs.pop('api_version', "2020-12-01") # type: str
content_type = kwargs.pop('content_type', "application/json") # type: Optional[str]
_json = self._serialize.body(private_endpoint_connection, 'PrivateEndpointConnection')
request = build_create_or_update_request_initial(
subscription_id=self._config.subscription_id,
resource_group_name=resource_group_name,
resource_name=resource_name,
private_endpoint_connection_name=private_endpoint_connection_name,
api_version=api_version,
content_type=content_type,
json=_json,
template_url=self._create_or_update_initial.metadata['url'],
)
request = _convert_request(request)
request.url = self._client.format_url(request.url)
pipeline_response = self._client._pipeline.run( # pylint: disable=protected-access
request,
stream=False,
**kwargs
)
response = pipeline_response.http_response
if response.status_code not in [200, 202]:
map_error(status_code=response.status_code, response=response, error_map=error_map)
raise HttpResponseError(response=response, error_format=ARMErrorFormat)
if response.status_code == 200:
deserialized = self._deserialize('PrivateEndpointConnection', pipeline_response)
if response.status_code == 202:
deserialized = self._deserialize('PrivateEndpointConnection', pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
_create_or_update_initial.metadata = {'url': "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DigitalTwins/digitalTwinsInstances/{resourceName}/privateEndpointConnections/{privateEndpointConnectionName}"} # type: ignore
@distributed_trace
def begin_create_or_update(
self,
resource_group_name: str,
resource_name: str,
private_endpoint_connection_name: str,
private_endpoint_connection: "_models.PrivateEndpointConnection",
**kwargs: Any
) -> LROPoller["_models.PrivateEndpointConnection"]:
"""Update the status of a private endpoint connection with the given name.
:param resource_group_name: The name of the resource group that contains the
DigitalTwinsInstance.
:type resource_group_name: str
:param resource_name: The name of the DigitalTwinsInstance.
:type resource_name: str
:param private_endpoint_connection_name: The name of the private endpoint connection.
:type private_endpoint_connection_name: str
:param private_endpoint_connection: The private endpoint connection with updated properties.
:type private_endpoint_connection:
~azure.mgmt.digitaltwins.v2020_12_01.models.PrivateEndpointConnection
:keyword callable cls: A custom type or function that will be passed the direct response
:keyword str continuation_token: A continuation token to restart a poller from a saved state.
:keyword polling: By default, your polling method will be ARMPolling. Pass in False for this
operation to not poll, or pass in your own initialized polling object for a personal polling
strategy.
:paramtype polling: bool or ~azure.core.polling.PollingMethod
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no
Retry-After header is present.
:return: An instance of LROPoller that returns either PrivateEndpointConnection or the result
of cls(response)
:rtype:
~azure.core.polling.LROPoller[~azure.mgmt.digitaltwins.v2020_12_01.models.PrivateEndpointConnection]
:raises: ~azure.core.exceptions.HttpResponseError
"""
api_version = kwargs.pop('api_version', "2020-12-01") # type: str
content_type = kwargs.pop('content_type', "application/json") # type: Optional[str]
polling = kwargs.pop('polling', True) # type: Union[bool, PollingMethod]
cls = kwargs.pop('cls', None) # type: ClsType["_models.PrivateEndpointConnection"]
lro_delay = kwargs.pop(
'polling_interval',
self._config.polling_interval
)
cont_token = kwargs.pop('continuation_token', None) # type: Optional[str]
if cont_token is None:
raw_result = self._create_or_update_initial(
resource_group_name=resource_group_name,
resource_name=resource_name,
private_endpoint_connection_name=private_endpoint_connection_name,
private_endpoint_connection=private_endpoint_connection,
api_version=api_version,
content_type=content_type,
cls=lambda x,y,z: x,
**kwargs
)
kwargs.pop('error_map', None)
def get_long_running_output(pipeline_response):
response = pipeline_response.http_response
deserialized = self._deserialize('PrivateEndpointConnection', pipeline_response)
if cls:
return cls(pipeline_response, deserialized, {})
return deserialized
if polling is True: polling_method = ARMPolling(lro_delay, **kwargs)
elif polling is False: polling_method = NoPolling()
else: polling_method = polling
if cont_token:
return LROPoller.from_continuation_token(
polling_method=polling_method,
continuation_token=cont_token,
client=self._client,
deserialization_callback=get_long_running_output
)
return LROPoller(self._client, raw_result, get_long_running_output, polling_method)
begin_create_or_update.metadata = {'url': "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DigitalTwins/digitalTwinsInstances/{resourceName}/privateEndpointConnections/{privateEndpointConnectionName}"} # type: ignore
| mit |
phenoxim/nova | nova/tests/unit/api/openstack/compute/test_extended_volumes.py | 1 | 9589 | # Copyright 2013 OpenStack Foundation
# All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License"); you may
# not use this file except in compliance with the License. You may obtain
# a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
# License for the specific language governing permissions and limitations
# under the License.
import mock
from oslo_serialization import jsonutils
from nova.api.openstack.compute import (extended_volumes
as extended_volumes_v21)
from nova.api.openstack import wsgi as os_wsgi
from nova import context as nova_context
from nova import objects
from nova.objects import instance as instance_obj
from nova import test
from nova.tests.unit.api.openstack import fakes
from nova.tests.unit import fake_block_device
from nova.tests.unit import fake_instance
from nova.tests import uuidsentinel as uuids
UUID1 = '00000000-0000-0000-0000-000000000001'
UUID2 = '00000000-0000-0000-0000-000000000002'
UUID3 = '00000000-0000-0000-0000-000000000003'
def fake_compute_get(*args, **kwargs):
inst = fakes.stub_instance(1, uuid=UUID1)
return fake_instance.fake_instance_obj(args[1], **inst)
def fake_compute_get_all(*args, **kwargs):
db_list = [
fakes.stub_instance(1, uuid=UUID1),
fakes.stub_instance(2, uuid=UUID2),
]
fields = instance_obj.INSTANCE_DEFAULT_FIELDS
return instance_obj._make_instance_list(args[1],
objects.InstanceList(),
db_list, fields)
def fake_bdms_get_all_by_instance_uuids(*args, **kwargs):
return [
fake_block_device.FakeDbBlockDeviceDict({
'id': 1,
'volume_id': 'some_volume_1',
'instance_uuid': UUID1,
'source_type': 'volume',
'destination_type': 'volume',
'delete_on_termination': True,
}),
fake_block_device.FakeDbBlockDeviceDict({
'id': 2,
'volume_id': 'some_volume_2',
'instance_uuid': UUID2,
'source_type': 'volume',
'destination_type': 'volume',
'delete_on_termination': False,
}),
fake_block_device.FakeDbBlockDeviceDict({
'id': 3,
'volume_id': 'some_volume_3',
'instance_uuid': UUID2,
'source_type': 'volume',
'destination_type': 'volume',
'delete_on_termination': False,
}),
]
class ExtendedVolumesTestV21(test.TestCase):
content_type = 'application/json'
prefix = 'os-extended-volumes:'
exp_volumes_show = [{'id': 'some_volume_1'}]
exp_volumes_detail = [
[{'id': 'some_volume_1'}],
[{'id': 'some_volume_2'}, {'id': 'some_volume_3'}],
]
wsgi_api_version = os_wsgi.DEFAULT_API_VERSION
def setUp(self):
super(ExtendedVolumesTestV21, self).setUp()
fakes.stub_out_nw_api(self)
fakes.stub_out_secgroup_api(self)
self.stub_out('nova.compute.api.API.get', fake_compute_get)
self.stub_out('nova.compute.api.API.get_all', fake_compute_get_all)
self.stub_out('nova.db.block_device_mapping_get_all_by_instance_uuids',
fake_bdms_get_all_by_instance_uuids)
self._setUp()
self.app = self._setup_app()
return_server = fakes.fake_instance_get()
self.stub_out('nova.db.instance_get_by_uuid', return_server)
def _setup_app(self):
return fakes.wsgi_app_v21()
def _setUp(self):
self.Controller = extended_volumes_v21.ExtendedVolumesController()
self.stub_out('nova.volume.cinder.API.get', lambda *a, **k: None)
def _make_request(self, url, body=None):
req = fakes.HTTPRequest.blank('/v2/fake/servers' + url)
req.headers['Accept'] = self.content_type
req.headers = {os_wsgi.API_VERSION_REQUEST_HEADER:
'compute %s' % self.wsgi_api_version}
if body:
req.body = jsonutils.dump_as_bytes(body)
req.method = 'POST'
req.content_type = self.content_type
res = req.get_response(self.app)
return res
def _get_server(self, body):
return jsonutils.loads(body).get('server')
def _get_servers(self, body):
return jsonutils.loads(body).get('servers')
def test_show(self):
res = self._make_request('/%s' % UUID1)
self.assertEqual(200, res.status_int)
server = self._get_server(res.body)
actual = server.get('%svolumes_attached' % self.prefix)
self.assertEqual(self.exp_volumes_show, actual)
@mock.patch.object(objects.InstanceMappingList, 'get_by_instance_uuids')
def test_detail(self, mock_get_by_instance_uuids):
mock_get_by_instance_uuids.return_value = [
objects.InstanceMapping(
instance_uuid=UUID1,
cell_mapping=objects.CellMapping(
uuid=uuids.cell1,
transport_url='fake://nowhere/',
database_connection=uuids.cell1)),
objects.InstanceMapping(
instance_uuid=UUID2,
cell_mapping=objects.CellMapping(
uuid=uuids.cell1,
transport_url='fake://nowhere/',
database_connection=uuids.cell1))]
res = self._make_request('/detail')
mock_get_by_instance_uuids.assert_called_once_with(
test.MatchType(nova_context.RequestContext), [UUID1, UUID2])
self.assertEqual(200, res.status_int)
for i, server in enumerate(self._get_servers(res.body)):
actual = server.get('%svolumes_attached' % self.prefix)
self.assertEqual(self.exp_volumes_detail[i], actual)
@mock.patch.object(objects.InstanceMappingList, 'get_by_instance_uuids')
@mock.patch('nova.context.scatter_gather_cells')
def test_detail_with_cell_failures(self, mock_sg,
mock_get_by_instance_uuids):
mock_get_by_instance_uuids.return_value = [
objects.InstanceMapping(
instance_uuid=UUID1,
cell_mapping=objects.CellMapping(
uuid=uuids.cell1,
transport_url='fake://nowhere/',
database_connection=uuids.cell1)),
objects.InstanceMapping(
instance_uuid=UUID2,
cell_mapping=objects.CellMapping(
uuid=uuids.cell2,
transport_url='fake://nowhere/',
database_connection=uuids.cell2))
]
bdm = fake_bdms_get_all_by_instance_uuids()
fake_bdm = fake_block_device.fake_bdm_object(
nova_context.RequestContext, bdm[0])
mock_sg.return_value = {
uuids.cell1: {UUID1: [fake_bdm]},
uuids.cell2: nova_context.raised_exception_sentinel
}
res = self._make_request('/detail')
mock_get_by_instance_uuids.assert_called_once_with(
test.MatchType(nova_context.RequestContext), [UUID1, UUID2])
self.assertEqual(200, res.status_int)
# we would get an empty list for the second instance
# which is in the down cell, however this would printed
# in the logs.
for i, server in enumerate(self._get_servers(res.body)):
actual = server.get('%svolumes_attached' % self.prefix)
if i == 0:
self.assertEqual(self.exp_volumes_detail[i], actual)
else:
self.assertEqual([], actual)
class ExtendedVolumesTestV23(ExtendedVolumesTestV21):
exp_volumes_show = [
{'id': 'some_volume_1', 'delete_on_termination': True},
]
exp_volumes_detail = [
[
{'id': 'some_volume_1', 'delete_on_termination': True},
],
[
{'id': 'some_volume_2', 'delete_on_termination': False},
{'id': 'some_volume_3', 'delete_on_termination': False},
],
]
wsgi_api_version = '2.3'
class ExtendedVolumesEnforcementV21(test.NoDBTestCase):
def setUp(self):
super(ExtendedVolumesEnforcementV21, self).setUp()
self.controller = extended_volumes_v21.ExtendedVolumesController()
self.req = fakes.HTTPRequest.blank('')
@mock.patch.object(extended_volumes_v21.ExtendedVolumesController,
'_extend_server')
def test_extend_show_policy_failed(self, mock_extend):
rule_name = 'os_compute_api:os-extended-volumes'
self.policy.set_rules({rule_name: "project:non_fake"})
# Pass ResponseObj as None, the code shouldn't touch the None.
self.controller.show(self.req, None, fakes.FAKE_UUID)
self.assertFalse(mock_extend.called)
@mock.patch.object(extended_volumes_v21.ExtendedVolumesController,
'_extend_server')
def test_extend_detail_policy_failed(self, mock_extend):
rule_name = 'os_compute_api:os-extended-volumes'
self.policy.set_rules({rule_name: "project:non_fake"})
# Pass ResponseObj as None, the code shouldn't touch the None.
self.controller.detail(self.req, None)
self.assertFalse(mock_extend.called)
| apache-2.0 |
Azure/azure-sdk-for-python | sdk/redisenterprise/azure-mgmt-redisenterprise/setup.py | 1 | 2837 | #!/usr/bin/env python
#-------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for
# license information.
#--------------------------------------------------------------------------
import re
import os.path
from io import open
from setuptools import find_packages, setup
# Change the PACKAGE_NAME only to change folder and different name
PACKAGE_NAME = "azure-mgmt-redisenterprise"
PACKAGE_PPRINT_NAME = "Redis Enterprise Management"
# a-b-c => a/b/c
package_folder_path = PACKAGE_NAME.replace('-', '/')
# a-b-c => a.b.c
namespace_name = PACKAGE_NAME.replace('-', '.')
# Version extraction inspired from 'requests'
with open(os.path.join(package_folder_path, 'version.py')
if os.path.exists(os.path.join(package_folder_path, 'version.py'))
else os.path.join(package_folder_path, '_version.py'), 'r') as fd:
version = re.search(r'^VERSION\s*=\s*[\'"]([^\'"]*)[\'"]',
fd.read(), re.MULTILINE).group(1)
if not version:
raise RuntimeError('Cannot find version information')
with open('README.md', encoding='utf-8') as f:
readme = f.read()
with open('CHANGELOG.md', encoding='utf-8') as f:
changelog = f.read()
setup(
name=PACKAGE_NAME,
version=version,
description='Microsoft Azure {} Client Library for Python'.format(PACKAGE_PPRINT_NAME),
long_description=readme + '\n\n' + changelog,
long_description_content_type='text/markdown',
license='MIT License',
author='Microsoft Corporation',
author_email='azpysdkhelp@microsoft.com',
url='https://github.com/Azure/azure-sdk-for-python',
keywords="azure, azure sdk", # update with search keywords relevant to the azure service / product
classifiers=[
'Development Status :: 4 - Beta',
'Programming Language :: Python',
'Programming Language :: Python :: 3 :: Only',
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: 3.9',
'Programming Language :: Python :: 3.10',
'Programming Language :: Python :: 3.11',
'License :: OSI Approved :: MIT License',
],
zip_safe=False,
packages=find_packages(exclude=[
'tests',
# Exclude packages that will be covered by PEP420 or nspkg
'azure',
'azure.mgmt',
]),
include_package_data=True,
package_data={
'pytyped': ['py.typed'],
},
install_requires=[
"msrest>=0.7.1",
"azure-common~=1.1",
"azure-mgmt-core>=1.3.2,<2.0.0",
"typing-extensions>=4.3.0; python_version<'3.8.0'",
],
python_requires=">=3.7"
)
| mit |
Azure/azure-sdk-for-python | sdk/oep/azure-mgmt-oep/azure/mgmt/oep/aio/_configuration.py | 1 | 3868 | # coding=utf-8
# --------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for license information.
# Code generated by Microsoft (R) AutoRest Code Generator.
# Changes may cause incorrect behavior and will be lost if the code is regenerated.
# --------------------------------------------------------------------------
import sys
from typing import Any, TYPE_CHECKING
from azure.core.configuration import Configuration
from azure.core.pipeline import policies
from azure.mgmt.core.policies import ARMHttpLoggingPolicy, AsyncARMChallengeAuthenticationPolicy
from .._version import VERSION
if sys.version_info >= (3, 8):
from typing import Literal # pylint: disable=no-name-in-module, ungrouped-imports
else:
from typing_extensions import Literal # type: ignore # pylint: disable=ungrouped-imports
if TYPE_CHECKING:
# pylint: disable=unused-import,ungrouped-imports
from azure.core.credentials_async import AsyncTokenCredential
class OpenEnergyPlatformManagementServiceAPIsConfiguration(
Configuration
): # pylint: disable=too-many-instance-attributes
"""Configuration for OpenEnergyPlatformManagementServiceAPIs.
Note that all parameters used to create this instance are saved as instance
attributes.
:param credential: Credential needed for the client to connect to Azure. Required.
:type credential: ~azure.core.credentials_async.AsyncTokenCredential
:param subscription_id: The ID of the target subscription. Required.
:type subscription_id: str
:keyword api_version: Api Version. Default value is "2022-04-04-preview". Note that overriding
this default value may result in unsupported behavior.
:paramtype api_version: str
"""
def __init__(self, credential: "AsyncTokenCredential", subscription_id: str, **kwargs: Any) -> None:
super(OpenEnergyPlatformManagementServiceAPIsConfiguration, self).__init__(**kwargs)
api_version = kwargs.pop("api_version", "2022-04-04-preview") # type: Literal["2022-04-04-preview"]
if credential is None:
raise ValueError("Parameter 'credential' must not be None.")
if subscription_id is None:
raise ValueError("Parameter 'subscription_id' must not be None.")
self.credential = credential
self.subscription_id = subscription_id
self.api_version = api_version
self.credential_scopes = kwargs.pop("credential_scopes", ["https://management.azure.com/.default"])
kwargs.setdefault("sdk_moniker", "mgmt-oep/{}".format(VERSION))
self._configure(**kwargs)
def _configure(self, **kwargs: Any) -> None:
self.user_agent_policy = kwargs.get("user_agent_policy") or policies.UserAgentPolicy(**kwargs)
self.headers_policy = kwargs.get("headers_policy") or policies.HeadersPolicy(**kwargs)
self.proxy_policy = kwargs.get("proxy_policy") or policies.ProxyPolicy(**kwargs)
self.logging_policy = kwargs.get("logging_policy") or policies.NetworkTraceLoggingPolicy(**kwargs)
self.http_logging_policy = kwargs.get("http_logging_policy") or ARMHttpLoggingPolicy(**kwargs)
self.retry_policy = kwargs.get("retry_policy") or policies.AsyncRetryPolicy(**kwargs)
self.custom_hook_policy = kwargs.get("custom_hook_policy") or policies.CustomHookPolicy(**kwargs)
self.redirect_policy = kwargs.get("redirect_policy") or policies.AsyncRedirectPolicy(**kwargs)
self.authentication_policy = kwargs.get("authentication_policy")
if self.credential and not self.authentication_policy:
self.authentication_policy = AsyncARMChallengeAuthenticationPolicy(
self.credential, *self.credential_scopes, **kwargs
)
| mit |
jpajuelo/wirecloud | src/urls.py | 2 | 1105 | # -*- coding: utf-8 -*-
# urls.py used as base for developing wirecloud.
from django.conf.urls import include, url
from django.contrib import admin
from django.contrib.auth import views as django_auth
from django.contrib.staticfiles.urls import staticfiles_urlpatterns
from wirecloud.commons import authentication as wc_auth
import wirecloud.platform.urls
admin.autodiscover()
urlpatterns = (
# Catalogue
url(r'^catalogue/', include('wirecloud.catalogue.urls')),
# Proxy
url(r'^cdp/', include('wirecloud.proxy.urls')),
# Login/logout
url(r'^login/?$', django_auth.login, name="login"),
url(r'^logout/?$', wc_auth.logout, name="logout"),
url(r'^admin/logout/?$', wc_auth.logout),
# Admin interface
url(r'^admin/', include(admin.site.urls)),
)
urlpatterns += wirecloud.platform.urls.urlpatterns
urlpatterns += tuple(staticfiles_urlpatterns())
handler400 = "wirecloud.commons.views.bad_request"
handler403 = "wirecloud.commons.views.permission_denied"
handler404 = "wirecloud.commons.views.page_not_found"
handler500 = "wirecloud.commons.views.server_error"
| agpl-3.0 |
tkas/osmose-backend | analysers/analyser_osmosis_orphan_nodes_cluster.py | 4 | 2872 | #!/usr/bin/env python
#-*- coding: utf-8 -*-
###########################################################################
## ##
## Copyrights Frédéric Rodrigo 2011 ##
## ##
## This program is free software: you can redistribute it and/or modify ##
## it under the terms of the GNU General Public License as published by ##
## the Free Software Foundation, either version 3 of the License, or ##
## (at your option) any later version. ##
## ##
## This program is distributed in the hope that it will be useful, ##
## but WITHOUT ANY WARRANTY; without even the implied warranty of ##
## MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the ##
## GNU General Public License for more details. ##
## ##
## You should have received a copy of the GNU General Public License ##
## along with this program. If not, see <http://www.gnu.org/licenses/>. ##
## ##
###########################################################################
from modules.Stablehash import stablehash64
from modules.OsmoseTranslation import T_
from .Analyser_Osmosis import Analyser_Osmosis
sql10 = """
SELECT
ST_AsText(ST_Centroid(geom))
FROM
(
SELECT
(ST_Dump(ST_MemUnion(ST_Buffer(geom, 0.001, 'quad_segs=2')))).geom AS geom
FROM
(
SELECT geom
FROM nodes
LEFT JOIN way_nodes ON
nodes.id = way_nodes.node_id
WHERE
way_nodes.node_id IS NULL AND
tags = ''::hstore AND
version = 1
LIMIT 3000
) AS n
) AS t
WHERE
ST_Area(geom) > 1e-5
"""
class Analyser_Osmosis_Orphan_Nodes_Cluster(Analyser_Osmosis):
def __init__(self, config, logger = None):
Analyser_Osmosis.__init__(self, config, logger)
self.classs[1] = self.def_class(item = 1080, level = 1, tags = ['geom', 'building', 'fix:chair'],
title = T_('Orphan nodes cluster'),
detail = T_(
'''Nodes in the vicinity without tag and not part of a way.'''),
fix = T_(
'''Find the origin of these nodes. Probably resulting from bad import.
Contact the contributor submiting the nodes or remove those.'''),
example = T_(
'''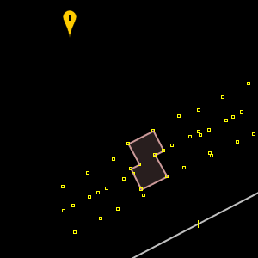
Group of orphan nodes.'''))
def analyser_osmosis_common(self):
self.run(sql10, lambda res: {"class":1, "subclass":stablehash64(res[0]), "data":[self.positionAsText]} )
| gpl-3.0 |
ryfeus/lambda-packs | ONNX/lambda-onnx/numpy/core/tests/test_getlimits.py | 11 | 4297 | """ Test functions for limits module.
"""
import numpy as np
from numpy.core import finfo, iinfo
from numpy import half, single, double, longdouble
from numpy.testing import assert_equal, assert_, assert_raises
from numpy.core.getlimits import _discovered_machar, _float_ma
##################################################
class TestPythonFloat:
def test_singleton(self):
ftype = finfo(float)
ftype2 = finfo(float)
assert_equal(id(ftype), id(ftype2))
class TestHalf:
def test_singleton(self):
ftype = finfo(half)
ftype2 = finfo(half)
assert_equal(id(ftype), id(ftype2))
class TestSingle:
def test_singleton(self):
ftype = finfo(single)
ftype2 = finfo(single)
assert_equal(id(ftype), id(ftype2))
class TestDouble:
def test_singleton(self):
ftype = finfo(double)
ftype2 = finfo(double)
assert_equal(id(ftype), id(ftype2))
class TestLongdouble:
def test_singleton(self):
ftype = finfo(longdouble)
ftype2 = finfo(longdouble)
assert_equal(id(ftype), id(ftype2))
class TestFinfo:
def test_basic(self):
dts = list(zip(['f2', 'f4', 'f8', 'c8', 'c16'],
[np.float16, np.float32, np.float64, np.complex64,
np.complex128]))
for dt1, dt2 in dts:
for attr in ('bits', 'eps', 'epsneg', 'iexp', 'machar', 'machep',
'max', 'maxexp', 'min', 'minexp', 'negep', 'nexp',
'nmant', 'precision', 'resolution', 'tiny'):
assert_equal(getattr(finfo(dt1), attr),
getattr(finfo(dt2), attr), attr)
assert_raises(ValueError, finfo, 'i4')
class TestIinfo:
def test_basic(self):
dts = list(zip(['i1', 'i2', 'i4', 'i8',
'u1', 'u2', 'u4', 'u8'],
[np.int8, np.int16, np.int32, np.int64,
np.uint8, np.uint16, np.uint32, np.uint64]))
for dt1, dt2 in dts:
for attr in ('bits', 'min', 'max'):
assert_equal(getattr(iinfo(dt1), attr),
getattr(iinfo(dt2), attr), attr)
assert_raises(ValueError, iinfo, 'f4')
def test_unsigned_max(self):
types = np.sctypes['uint']
for T in types:
assert_equal(iinfo(T).max, T(-1))
class TestRepr:
def test_iinfo_repr(self):
expected = "iinfo(min=-32768, max=32767, dtype=int16)"
assert_equal(repr(np.iinfo(np.int16)), expected)
def test_finfo_repr(self):
expected = "finfo(resolution=1e-06, min=-3.4028235e+38," + \
" max=3.4028235e+38, dtype=float32)"
assert_equal(repr(np.finfo(np.float32)), expected)
def test_instances():
iinfo(10)
finfo(3.0)
def assert_ma_equal(discovered, ma_like):
# Check MachAr-like objects same as calculated MachAr instances
for key, value in discovered.__dict__.items():
assert_equal(value, getattr(ma_like, key))
if hasattr(value, 'shape'):
assert_equal(value.shape, getattr(ma_like, key).shape)
assert_equal(value.dtype, getattr(ma_like, key).dtype)
def test_known_types():
# Test we are correctly compiling parameters for known types
for ftype, ma_like in ((np.float16, _float_ma[16]),
(np.float32, _float_ma[32]),
(np.float64, _float_ma[64])):
assert_ma_equal(_discovered_machar(ftype), ma_like)
# Suppress warning for broken discovery of double double on PPC
with np.errstate(all='ignore'):
ld_ma = _discovered_machar(np.longdouble)
bytes = np.dtype(np.longdouble).itemsize
if (ld_ma.it, ld_ma.maxexp) == (63, 16384) and bytes in (12, 16):
# 80-bit extended precision
assert_ma_equal(ld_ma, _float_ma[80])
elif (ld_ma.it, ld_ma.maxexp) == (112, 16384) and bytes == 16:
# IEE 754 128-bit
assert_ma_equal(ld_ma, _float_ma[128])
def test_plausible_finfo():
# Assert that finfo returns reasonable results for all types
for ftype in np.sctypes['float'] + np.sctypes['complex']:
info = np.finfo(ftype)
assert_(info.nmant > 1)
assert_(info.minexp < -1)
assert_(info.maxexp > 1)
| mit |
ryfeus/lambda-packs | Keras_tensorflow_nightly/source2.7/tensorflow/contrib/image/python/ops/image_ops.py | 6 | 21743 | # Copyright 2016 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Python layer for image_ops."""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
from tensorflow.contrib.image.ops import gen_image_ops
from tensorflow.contrib.util import loader
from tensorflow.python.framework import common_shapes
from tensorflow.python.framework import constant_op
from tensorflow.python.framework import dtypes
from tensorflow.python.framework import ops
from tensorflow.python.ops import array_ops
from tensorflow.python.ops import control_flow_ops
from tensorflow.python.ops import linalg_ops
from tensorflow.python.ops import math_ops
from tensorflow.python.platform import resource_loader
_image_ops_so = loader.load_op_library(
resource_loader.get_path_to_datafile("_image_ops.so"))
_IMAGE_DTYPES = set(
[dtypes.uint8, dtypes.int32, dtypes.int64, dtypes.float32, dtypes.float64])
ops.RegisterShape("ImageConnectedComponents")(common_shapes.call_cpp_shape_fn)
ops.RegisterShape("ImageProjectiveTransform")(common_shapes.call_cpp_shape_fn)
def rotate(images, angles, interpolation="NEAREST", name=None):
"""Rotate image(s) counterclockwise by the passed angle(s) in radians.
Args:
images: A tensor of shape (num_images, num_rows, num_columns, num_channels)
(NHWC), (num_rows, num_columns, num_channels) (HWC), or
(num_rows, num_columns) (HW). The rank must be statically known (the
shape is not `TensorShape(None)`.
angles: A scalar angle to rotate all images by, or (if images has rank 4)
a vector of length num_images, with an angle for each image in the batch.
interpolation: Interpolation mode. Supported values: "NEAREST", "BILINEAR".
name: The name of the op.
Returns:
Image(s) with the same type and shape as `images`, rotated by the given
angle(s). Empty space due to the rotation will be filled with zeros.
Raises:
TypeError: If `image` is an invalid type.
"""
with ops.name_scope(name, "rotate"):
image_or_images = ops.convert_to_tensor(images)
if image_or_images.dtype.base_dtype not in _IMAGE_DTYPES:
raise TypeError("Invalid dtype %s." % image_or_images.dtype)
elif image_or_images.get_shape().ndims is None:
raise TypeError("image_or_images rank must be statically known")
elif len(image_or_images.get_shape()) == 2:
images = image_or_images[None, :, :, None]
elif len(image_or_images.get_shape()) == 3:
images = image_or_images[None, :, :, :]
elif len(image_or_images.get_shape()) == 4:
images = image_or_images
else:
raise TypeError("Images should have rank between 2 and 4.")
image_height = math_ops.cast(array_ops.shape(images)[1],
dtypes.float32)[None]
image_width = math_ops.cast(array_ops.shape(images)[2],
dtypes.float32)[None]
output = transform(
images,
angles_to_projective_transforms(angles, image_height, image_width),
interpolation=interpolation)
if image_or_images.get_shape().ndims is None:
raise TypeError("image_or_images rank must be statically known")
elif len(image_or_images.get_shape()) == 2:
return output[0, :, :, 0]
elif len(image_or_images.get_shape()) == 3:
return output[0, :, :, :]
else:
return output
def translate(images, translations, interpolation="NEAREST", name=None):
"""Translate image(s) by the passed vectors(s).
Args:
images: A tensor of shape (num_images, num_rows, num_columns, num_channels)
(NHWC), (num_rows, num_columns, num_channels) (HWC), or
(num_rows, num_columns) (HW). The rank must be statically known (the
shape is not `TensorShape(None)`.
translations: A vector representing [dx, dy] or (if images has rank 4)
a matrix of length num_images, with a [dx, dy] vector for each image in
the batch.
interpolation: Interpolation mode. Supported values: "NEAREST", "BILINEAR".
name: The name of the op.
Returns:
Image(s) with the same type and shape as `images`, translated by the given
vector(s). Empty space due to the translation will be filled with zeros.
Raises:
TypeError: If `image` is an invalid type.
"""
with ops.name_scope(name, "translate"):
return transform(
images,
translations_to_projective_transforms(translations),
interpolation=interpolation)
def angles_to_projective_transforms(angles,
image_height,
image_width,
name=None):
"""Returns projective transform(s) for the given angle(s).
Args:
angles: A scalar angle to rotate all images by, or (for batches of images)
a vector with an angle to rotate each image in the batch. The rank must
be statically known (the shape is not `TensorShape(None)`.
image_height: Height of the image(s) to be transformed.
image_width: Width of the image(s) to be transformed.
Returns:
A tensor of shape (num_images, 8). Projective transforms which can be given
to `tf.contrib.image.transform`.
"""
with ops.name_scope(name, "angles_to_projective_transforms"):
angle_or_angles = ops.convert_to_tensor(
angles, name="angles", dtype=dtypes.float32)
if len(angle_or_angles.get_shape()) == 0: # pylint: disable=g-explicit-length-test
angles = angle_or_angles[None]
elif len(angle_or_angles.get_shape()) == 1:
angles = angle_or_angles
else:
raise TypeError("Angles should have rank 0 or 1.")
x_offset = ((image_width - 1) - (math_ops.cos(angles) *
(image_width - 1) - math_ops.sin(angles) *
(image_height - 1))) / 2.0
y_offset = ((image_height - 1) - (math_ops.sin(angles) *
(image_width - 1) + math_ops.cos(angles) *
(image_height - 1))) / 2.0
num_angles = array_ops.shape(angles)[0]
return array_ops.concat(
values=[
math_ops.cos(angles)[:, None],
-math_ops.sin(angles)[:, None],
x_offset[:, None],
math_ops.sin(angles)[:, None],
math_ops.cos(angles)[:, None],
y_offset[:, None],
array_ops.zeros((num_angles, 2), dtypes.float32),
],
axis=1)
def translations_to_projective_transforms(translations, name=None):
"""Returns projective transform(s) for the given translation(s).
Args:
translations: A 2-element list representing [dx, dy] or a matrix of
2-element lists representing [dx, dy] to translate for each image
(for a batch of images). The rank must be statically known (the shape
is not `TensorShape(None)`.
name: The name of the op.
Returns:
A tensor of shape (num_images, 8) projective transforms which can be given
to `tf.contrib.image.transform`.
"""
with ops.name_scope(name, "translations_to_projective_transforms"):
translation_or_translations = ops.convert_to_tensor(
translations, name="translations", dtype=dtypes.float32)
if translation_or_translations.get_shape().ndims is None:
raise TypeError(
"translation_or_translations rank must be statically known")
elif len(translation_or_translations.get_shape()) == 1:
translations = translation_or_translations[None]
elif len(translation_or_translations.get_shape()) == 2:
translations = translation_or_translations
else:
raise TypeError("Translations should have rank 1 or 2.")
num_translations = array_ops.shape(translations)[0]
# The translation matrix looks like:
# [[1 0 -dx]
# [0 1 -dy]
# [0 0 1]]
# where the last entry is implicit.
# Translation matrices are always float32.
return array_ops.concat(
values=[
array_ops.ones((num_translations, 1), dtypes.float32),
array_ops.zeros((num_translations, 1), dtypes.float32),
-translations[:, 0, None],
array_ops.zeros((num_translations, 1), dtypes.float32),
array_ops.ones((num_translations, 1), dtypes.float32),
-translations[:, 1, None],
array_ops.zeros((num_translations, 2), dtypes.float32),
],
axis=1)
def transform(images, transforms, interpolation="NEAREST", name=None):
"""Applies the given transform(s) to the image(s).
Args:
images: A tensor of shape (num_images, num_rows, num_columns, num_channels)
(NHWC), (num_rows, num_columns, num_channels) (HWC), or
(num_rows, num_columns) (HW). The rank must be statically known (the
shape is not `TensorShape(None)`.
transforms: Projective transform matrix/matrices. A vector of length 8 or
tensor of size N x 8. If one row of transforms is
[a0, a1, a2, b0, b1, b2, c0, c1], then it maps the *output* point
`(x, y)` to a transformed *input* point
`(x', y') = ((a0 x + a1 y + a2) / k, (b0 x + b1 y + b2) / k)`,
where `k = c0 x + c1 y + 1`. The transforms are *inverted* compared to
the transform mapping input points to output points. Note that gradients
are not backpropagated into transformation parameters.
interpolation: Interpolation mode. Supported values: "NEAREST", "BILINEAR".
Returns:
Image(s) with the same type and shape as `images`, with the given
transform(s) applied. Transformed coordinates outside of the input image
will be filled with zeros.
Raises:
TypeError: If `image` is an invalid type.
"""
with ops.name_scope(name, "transform"):
image_or_images = ops.convert_to_tensor(images, name="images")
transform_or_transforms = ops.convert_to_tensor(
transforms, name="transforms", dtype=dtypes.float32)
if image_or_images.dtype.base_dtype not in _IMAGE_DTYPES:
raise TypeError("Invalid dtype %s." % image_or_images.dtype)
elif image_or_images.get_shape().ndims is None:
raise TypeError("image_or_images rank must be statically known")
elif len(image_or_images.get_shape()) == 2:
images = image_or_images[None, :, :, None]
elif len(image_or_images.get_shape()) == 3:
images = image_or_images[None, :, :, :]
elif len(image_or_images.get_shape()) == 4:
images = image_or_images
else:
raise TypeError("Images should have rank between 2 and 4.")
if len(transform_or_transforms.get_shape()) == 1:
transforms = transform_or_transforms[None]
elif transform_or_transforms.get_shape().ndims is None:
raise TypeError(
"transform_or_transforms rank must be statically known")
elif len(transform_or_transforms.get_shape()) == 2:
transforms = transform_or_transforms
else:
raise TypeError("Transforms should have rank 1 or 2.")
output = gen_image_ops.image_projective_transform(
images, transforms, interpolation=interpolation.upper())
if len(image_or_images.get_shape()) == 2:
return output[0, :, :, 0]
elif len(image_or_images.get_shape()) == 3:
return output[0, :, :, :]
else:
return output
def compose_transforms(*transforms):
"""Composes the transforms tensors.
Args:
*transforms: List of image projective transforms to be composed. Each
transform is length 8 (single transform) or shape (N, 8) (batched
transforms). The shapes of all inputs must be equal, and at least one
input must be given.
Returns:
A composed transform tensor. When passed to `tf.contrib.image.transform`,
equivalent to applying each of the given transforms to the image in
order.
"""
assert transforms, "transforms cannot be empty"
with ops.name_scope("compose_transforms"):
composed = flat_transforms_to_matrices(transforms[0])
for tr in transforms[1:]:
# Multiply batches of matrices.
composed = math_ops.matmul(composed, flat_transforms_to_matrices(tr))
return matrices_to_flat_transforms(composed)
def flat_transforms_to_matrices(transforms):
"""Converts `tf.contrib.image` projective transforms to affine matrices.
Note that the output matrices map output coordinates to input coordinates. For
the forward transformation matrix, call `tf.linalg.inv` on the result.
Args:
transforms: Vector of length 8, or batches of transforms with shape
`(N, 8)`.
Returns:
3D tensor of matrices with shape `(N, 3, 3)`. The output matrices map the
*output coordinates* (in homogeneous coordinates) of each transform to the
corresponding *input coordinates*.
Raises:
ValueError: If `transforms` have an invalid shape.
"""
with ops.name_scope("flat_transforms_to_matrices"):
transforms = ops.convert_to_tensor(transforms, name="transforms")
if transforms.shape.ndims not in (1, 2):
raise ValueError("Transforms should be 1D or 2D, got: %s" % transforms)
# Make the transform(s) 2D in case the input is a single transform.
transforms = array_ops.reshape(transforms, constant_op.constant([-1, 8]))
num_transforms = array_ops.shape(transforms)[0]
# Add a column of ones for the implicit last entry in the matrix.
return array_ops.reshape(
array_ops.concat(
[transforms, array_ops.ones([num_transforms, 1])], axis=1),
constant_op.constant([-1, 3, 3]))
def matrices_to_flat_transforms(transform_matrices):
"""Converts affine matrices to `tf.contrib.image` projective transforms.
Note that we expect matrices that map output coordinates to input coordinates.
To convert forward transformation matrices, call `tf.linalg.inv` on the
matrices and use the result here.
Args:
transform_matrices: One or more affine transformation matrices, for the
reverse transformation in homogeneous coordinates. Shape `(3, 3)` or
`(N, 3, 3)`.
Returns:
2D tensor of flat transforms with shape `(N, 8)`, which may be passed into
`tf.contrib.image.transform`.
Raises:
ValueError: If `transform_matrices` have an invalid shape.
"""
with ops.name_scope("matrices_to_flat_transforms"):
transform_matrices = ops.convert_to_tensor(
transform_matrices, name="transform_matrices")
if transform_matrices.shape.ndims not in (2, 3):
raise ValueError(
"Matrices should be 2D or 3D, got: %s" % transform_matrices)
# Flatten each matrix.
transforms = array_ops.reshape(transform_matrices,
constant_op.constant([-1, 9]))
# Divide each matrix by the last entry (normally 1).
transforms /= transforms[:, 8:9]
return transforms[:, :8]
@ops.RegisterGradient("ImageProjectiveTransform")
def _image_projective_transform_grad(op, grad):
"""Computes the gradient for ImageProjectiveTransform."""
images = op.inputs[0]
transforms = op.inputs[1]
interpolation = op.get_attr("interpolation")
image_or_images = ops.convert_to_tensor(images, name="images")
transform_or_transforms = ops.convert_to_tensor(
transforms, name="transforms", dtype=dtypes.float32)
if image_or_images.dtype.base_dtype not in _IMAGE_DTYPES:
raise TypeError("Invalid dtype %s." % image_or_images.dtype)
if len(image_or_images.get_shape()) == 2:
images = image_or_images[None, :, :, None]
elif len(image_or_images.get_shape()) == 3:
images = image_or_images[None, :, :, :]
elif len(image_or_images.get_shape()) == 4:
images = image_or_images
else:
raise TypeError("Images should have rank between 2 and 4")
if len(transform_or_transforms.get_shape()) == 1:
transforms = transform_or_transforms[None]
elif len(transform_or_transforms.get_shape()) == 2:
transforms = transform_or_transforms
else:
raise TypeError("Transforms should have rank 1 or 2.")
# Invert transformations
transforms = flat_transforms_to_matrices(transforms=transforms)
inverse = linalg_ops.matrix_inverse(transforms)
transforms = matrices_to_flat_transforms(inverse)
output = gen_image_ops.image_projective_transform(
grad, transforms, interpolation=interpolation)
if len(image_or_images.get_shape()) == 2:
return [output[0, :, :, 0], None]
elif len(image_or_images.get_shape()) == 3:
return [output[0, :, :, :], None]
else:
return [output, None]
def bipartite_match(distance_mat,
num_valid_rows,
top_k=-1,
name="bipartite_match"):
"""Find bipartite matching based on a given distance matrix.
A greedy bi-partite matching algorithm is used to obtain the matching with
the (greedy) minimum distance.
Args:
distance_mat: A 2-D float tensor of shape `[num_rows, num_columns]`. It is a
pair-wise distance matrix between the entities represented by each row and
each column. It is an asymmetric matrix. The smaller the distance is, the
more similar the pairs are. The bipartite matching is to minimize the
distances.
num_valid_rows: A scalar or a 1-D tensor with one element describing the
number of valid rows of distance_mat to consider for the bipartite
matching. If set to be negative, then all rows from `distance_mat` are
used.
top_k: A scalar that specifies the number of top-k matches to retrieve.
If set to be negative, then is set according to the maximum number of
matches from `distance_mat`.
name: The name of the op.
Returns:
row_to_col_match_indices: A vector of length num_rows, which is the number
of rows of the input `distance_matrix`. If `row_to_col_match_indices[i]`
is not -1, row i is matched to column `row_to_col_match_indices[i]`.
col_to_row_match_indices: A vector of length num_columns, which is the
number of columns of the input ditance matrix.
If `col_to_row_match_indices[j]` is not -1, column j is matched to row
`col_to_row_match_indices[j]`.
"""
result = gen_image_ops.bipartite_match(
distance_mat, num_valid_rows, top_k, name=name)
return result
def connected_components(images):
"""Labels the connected components in a batch of images.
A component is a set of pixels in a single input image, which are all adjacent
and all have the same non-zero value. The components using a squared
connectivity of one (all True entries are joined with their neighbors above,
below, left, and right). Components across all images have consecutive ids 1
through n. Components are labeled according to the first pixel of the
component appearing in row-major order (lexicographic order by
image_index_in_batch, row, col). Zero entries all have an output id of 0.
This op is equivalent with `scipy.ndimage.measurements.label` on a 2D array
with the default structuring element (which is the connectivity used here).
Args:
images: A 2D (H, W) or 3D (N, H, W) Tensor of boolean image(s).
Returns:
Components with the same shape as `images`. False entries in `images` have
value 0, and all True entries map to a component id > 0.
Raises:
TypeError: if `images` is not 2D or 3D.
"""
with ops.name_scope("connected_components"):
image_or_images = ops.convert_to_tensor(images, name="images")
if len(image_or_images.get_shape()) == 2:
images = image_or_images[None, :, :]
elif len(image_or_images.get_shape()) == 3:
images = image_or_images
else:
raise TypeError(
"images should have rank 2 (HW) or 3 (NHW). Static shape is %s" %
image_or_images.get_shape())
components = gen_image_ops.image_connected_components(images)
# TODO(ringwalt): Component id renaming should be done in the op, to avoid
# constructing multiple additional large tensors.
components_flat = array_ops.reshape(components, [-1])
unique_ids, id_index = array_ops.unique(components_flat)
id_is_zero = array_ops.where(math_ops.equal(unique_ids, 0))[:, 0]
# Map each nonzero id to consecutive values.
nonzero_consecutive_ids = math_ops.range(
array_ops.shape(unique_ids)[0] - array_ops.shape(id_is_zero)[0]) + 1
def no_zero():
# No need to insert a zero into the ids.
return nonzero_consecutive_ids
def has_zero():
# Insert a zero in the consecutive ids where zero appears in unique_ids.
# id_is_zero has length 1.
zero_id_ind = math_ops.to_int32(id_is_zero[0])
ids_before = nonzero_consecutive_ids[:zero_id_ind]
ids_after = nonzero_consecutive_ids[zero_id_ind:]
return array_ops.concat([ids_before, [0], ids_after], axis=0)
new_ids = control_flow_ops.cond(
math_ops.equal(array_ops.shape(id_is_zero)[0], 0), no_zero, has_zero)
components = array_ops.reshape(
array_ops.gather(new_ids, id_index), array_ops.shape(components))
if len(image_or_images.get_shape()) == 2:
return components[0, :, :]
else:
return components
ops.NotDifferentiable("BipartiteMatch")
ops.NotDifferentiable("ImageConnectedComponents")
| mit |
ryfeus/lambda-packs | Rasterio_osgeo_shapely_PIL_pyproj_numpy/source/numpy/lib/_iotools.py | 72 | 32062 | """A collection of functions designed to help I/O with ascii files.
"""
from __future__ import division, absolute_import, print_function
__docformat__ = "restructuredtext en"
import sys
import numpy as np
import numpy.core.numeric as nx
from numpy.compat import asbytes, bytes, asbytes_nested, basestring
if sys.version_info[0] >= 3:
from builtins import bool, int, float, complex, object, str
unicode = str
else:
from __builtin__ import bool, int, float, complex, object, unicode, str
if sys.version_info[0] >= 3:
def _bytes_to_complex(s):
return complex(s.decode('ascii'))
def _bytes_to_name(s):
return s.decode('ascii')
else:
_bytes_to_complex = complex
_bytes_to_name = str
def _is_string_like(obj):
"""
Check whether obj behaves like a string.
"""
try:
obj + ''
except (TypeError, ValueError):
return False
return True
def _is_bytes_like(obj):
"""
Check whether obj behaves like a bytes object.
"""
try:
obj + asbytes('')
except (TypeError, ValueError):
return False
return True
def _to_filehandle(fname, flag='r', return_opened=False):
"""
Returns the filehandle corresponding to a string or a file.
If the string ends in '.gz', the file is automatically unzipped.
Parameters
----------
fname : string, filehandle
Name of the file whose filehandle must be returned.
flag : string, optional
Flag indicating the status of the file ('r' for read, 'w' for write).
return_opened : boolean, optional
Whether to return the opening status of the file.
"""
if _is_string_like(fname):
if fname.endswith('.gz'):
import gzip
fhd = gzip.open(fname, flag)
elif fname.endswith('.bz2'):
import bz2
fhd = bz2.BZ2File(fname)
else:
fhd = file(fname, flag)
opened = True
elif hasattr(fname, 'seek'):
fhd = fname
opened = False
else:
raise ValueError('fname must be a string or file handle')
if return_opened:
return fhd, opened
return fhd
def has_nested_fields(ndtype):
"""
Returns whether one or several fields of a dtype are nested.
Parameters
----------
ndtype : dtype
Data-type of a structured array.
Raises
------
AttributeError
If `ndtype` does not have a `names` attribute.
Examples
--------
>>> dt = np.dtype([('name', 'S4'), ('x', float), ('y', float)])
>>> np.lib._iotools.has_nested_fields(dt)
False
"""
for name in ndtype.names or ():
if ndtype[name].names:
return True
return False
def flatten_dtype(ndtype, flatten_base=False):
"""
Unpack a structured data-type by collapsing nested fields and/or fields
with a shape.
Note that the field names are lost.
Parameters
----------
ndtype : dtype
The datatype to collapse
flatten_base : {False, True}, optional
Whether to transform a field with a shape into several fields or not.
Examples
--------
>>> dt = np.dtype([('name', 'S4'), ('x', float), ('y', float),
... ('block', int, (2, 3))])
>>> np.lib._iotools.flatten_dtype(dt)
[dtype('|S4'), dtype('float64'), dtype('float64'), dtype('int32')]
>>> np.lib._iotools.flatten_dtype(dt, flatten_base=True)
[dtype('|S4'), dtype('float64'), dtype('float64'), dtype('int32'),
dtype('int32'), dtype('int32'), dtype('int32'), dtype('int32'),
dtype('int32')]
"""
names = ndtype.names
if names is None:
if flatten_base:
return [ndtype.base] * int(np.prod(ndtype.shape))
return [ndtype.base]
else:
types = []
for field in names:
info = ndtype.fields[field]
flat_dt = flatten_dtype(info[0], flatten_base)
types.extend(flat_dt)
return types
class LineSplitter(object):
"""
Object to split a string at a given delimiter or at given places.
Parameters
----------
delimiter : str, int, or sequence of ints, optional
If a string, character used to delimit consecutive fields.
If an integer or a sequence of integers, width(s) of each field.
comments : str, optional
Character used to mark the beginning of a comment. Default is '#'.
autostrip : bool, optional
Whether to strip each individual field. Default is True.
"""
def autostrip(self, method):
"""
Wrapper to strip each member of the output of `method`.
Parameters
----------
method : function
Function that takes a single argument and returns a sequence of
strings.
Returns
-------
wrapped : function
The result of wrapping `method`. `wrapped` takes a single input
argument and returns a list of strings that are stripped of
white-space.
"""
return lambda input: [_.strip() for _ in method(input)]
#
def __init__(self, delimiter=None, comments=asbytes('#'), autostrip=True):
self.comments = comments
# Delimiter is a character
if isinstance(delimiter, unicode):
delimiter = delimiter.encode('ascii')
if (delimiter is None) or _is_bytes_like(delimiter):
delimiter = delimiter or None
_handyman = self._delimited_splitter
# Delimiter is a list of field widths
elif hasattr(delimiter, '__iter__'):
_handyman = self._variablewidth_splitter
idx = np.cumsum([0] + list(delimiter))
delimiter = [slice(i, j) for (i, j) in zip(idx[:-1], idx[1:])]
# Delimiter is a single integer
elif int(delimiter):
(_handyman, delimiter) = (
self._fixedwidth_splitter, int(delimiter))
else:
(_handyman, delimiter) = (self._delimited_splitter, None)
self.delimiter = delimiter
if autostrip:
self._handyman = self.autostrip(_handyman)
else:
self._handyman = _handyman
#
def _delimited_splitter(self, line):
if self.comments is not None:
line = line.split(self.comments)[0]
line = line.strip(asbytes(" \r\n"))
if not line:
return []
return line.split(self.delimiter)
#
def _fixedwidth_splitter(self, line):
if self.comments is not None:
line = line.split(self.comments)[0]
line = line.strip(asbytes("\r\n"))
if not line:
return []
fixed = self.delimiter
slices = [slice(i, i + fixed) for i in range(0, len(line), fixed)]
return [line[s] for s in slices]
#
def _variablewidth_splitter(self, line):
if self.comments is not None:
line = line.split(self.comments)[0]
if not line:
return []
slices = self.delimiter
return [line[s] for s in slices]
#
def __call__(self, line):
return self._handyman(line)
class NameValidator(object):
"""
Object to validate a list of strings to use as field names.
The strings are stripped of any non alphanumeric character, and spaces
are replaced by '_'. During instantiation, the user can define a list
of names to exclude, as well as a list of invalid characters. Names in
the exclusion list are appended a '_' character.
Once an instance has been created, it can be called with a list of
names, and a list of valid names will be created. The `__call__`
method accepts an optional keyword "default" that sets the default name
in case of ambiguity. By default this is 'f', so that names will
default to `f0`, `f1`, etc.
Parameters
----------
excludelist : sequence, optional
A list of names to exclude. This list is appended to the default
list ['return', 'file', 'print']. Excluded names are appended an
underscore: for example, `file` becomes `file_` if supplied.
deletechars : str, optional
A string combining invalid characters that must be deleted from the
names.
case_sensitive : {True, False, 'upper', 'lower'}, optional
* If True, field names are case-sensitive.
* If False or 'upper', field names are converted to upper case.
* If 'lower', field names are converted to lower case.
The default value is True.
replace_space : '_', optional
Character(s) used in replacement of white spaces.
Notes
-----
Calling an instance of `NameValidator` is the same as calling its
method `validate`.
Examples
--------
>>> validator = np.lib._iotools.NameValidator()
>>> validator(['file', 'field2', 'with space', 'CaSe'])
['file_', 'field2', 'with_space', 'CaSe']
>>> validator = np.lib._iotools.NameValidator(excludelist=['excl'],
deletechars='q',
case_sensitive='False')
>>> validator(['excl', 'field2', 'no_q', 'with space', 'CaSe'])
['excl_', 'field2', 'no_', 'with_space', 'case']
"""
#
defaultexcludelist = ['return', 'file', 'print']
defaultdeletechars = set("""~!@#$%^&*()-=+~\|]}[{';: /?.>,<""")
#
def __init__(self, excludelist=None, deletechars=None,
case_sensitive=None, replace_space='_'):
# Process the exclusion list ..
if excludelist is None:
excludelist = []
excludelist.extend(self.defaultexcludelist)
self.excludelist = excludelist
# Process the list of characters to delete
if deletechars is None:
delete = self.defaultdeletechars
else:
delete = set(deletechars)
delete.add('"')
self.deletechars = delete
# Process the case option .....
if (case_sensitive is None) or (case_sensitive is True):
self.case_converter = lambda x: x
elif (case_sensitive is False) or case_sensitive.startswith('u'):
self.case_converter = lambda x: x.upper()
elif case_sensitive.startswith('l'):
self.case_converter = lambda x: x.lower()
else:
msg = 'unrecognized case_sensitive value %s.' % case_sensitive
raise ValueError(msg)
#
self.replace_space = replace_space
def validate(self, names, defaultfmt="f%i", nbfields=None):
"""
Validate a list of strings as field names for a structured array.
Parameters
----------
names : sequence of str
Strings to be validated.
defaultfmt : str, optional
Default format string, used if validating a given string
reduces its length to zero.
nbfields : integer, optional
Final number of validated names, used to expand or shrink the
initial list of names.
Returns
-------
validatednames : list of str
The list of validated field names.
Notes
-----
A `NameValidator` instance can be called directly, which is the
same as calling `validate`. For examples, see `NameValidator`.
"""
# Initial checks ..............
if (names is None):
if (nbfields is None):
return None
names = []
if isinstance(names, basestring):
names = [names, ]
if nbfields is not None:
nbnames = len(names)
if (nbnames < nbfields):
names = list(names) + [''] * (nbfields - nbnames)
elif (nbnames > nbfields):
names = names[:nbfields]
# Set some shortcuts ...........
deletechars = self.deletechars
excludelist = self.excludelist
case_converter = self.case_converter
replace_space = self.replace_space
# Initializes some variables ...
validatednames = []
seen = dict()
nbempty = 0
#
for item in names:
item = case_converter(item).strip()
if replace_space:
item = item.replace(' ', replace_space)
item = ''.join([c for c in item if c not in deletechars])
if item == '':
item = defaultfmt % nbempty
while item in names:
nbempty += 1
item = defaultfmt % nbempty
nbempty += 1
elif item in excludelist:
item += '_'
cnt = seen.get(item, 0)
if cnt > 0:
validatednames.append(item + '_%d' % cnt)
else:
validatednames.append(item)
seen[item] = cnt + 1
return tuple(validatednames)
#
def __call__(self, names, defaultfmt="f%i", nbfields=None):
return self.validate(names, defaultfmt=defaultfmt, nbfields=nbfields)
def str2bool(value):
"""
Tries to transform a string supposed to represent a boolean to a boolean.
Parameters
----------
value : str
The string that is transformed to a boolean.
Returns
-------
boolval : bool
The boolean representation of `value`.
Raises
------
ValueError
If the string is not 'True' or 'False' (case independent)
Examples
--------
>>> np.lib._iotools.str2bool('TRUE')
True
>>> np.lib._iotools.str2bool('false')
False
"""
value = value.upper()
if value == asbytes('TRUE'):
return True
elif value == asbytes('FALSE'):
return False
else:
raise ValueError("Invalid boolean")
class ConverterError(Exception):
"""
Exception raised when an error occurs in a converter for string values.
"""
pass
class ConverterLockError(ConverterError):
"""
Exception raised when an attempt is made to upgrade a locked converter.
"""
pass
class ConversionWarning(UserWarning):
"""
Warning issued when a string converter has a problem.
Notes
-----
In `genfromtxt` a `ConversionWarning` is issued if raising exceptions
is explicitly suppressed with the "invalid_raise" keyword.
"""
pass
class StringConverter(object):
"""
Factory class for function transforming a string into another object
(int, float).
After initialization, an instance can be called to transform a string
into another object. If the string is recognized as representing a
missing value, a default value is returned.
Attributes
----------
func : function
Function used for the conversion.
default : any
Default value to return when the input corresponds to a missing
value.
type : type
Type of the output.
_status : int
Integer representing the order of the conversion.
_mapper : sequence of tuples
Sequence of tuples (dtype, function, default value) to evaluate in
order.
_locked : bool
Holds `locked` parameter.
Parameters
----------
dtype_or_func : {None, dtype, function}, optional
If a `dtype`, specifies the input data type, used to define a basic
function and a default value for missing data. For example, when
`dtype` is float, the `func` attribute is set to `float` and the
default value to `np.nan`. If a function, this function is used to
convert a string to another object. In this case, it is recommended
to give an associated default value as input.
default : any, optional
Value to return by default, that is, when the string to be
converted is flagged as missing. If not given, `StringConverter`
tries to supply a reasonable default value.
missing_values : sequence of str, optional
Sequence of strings indicating a missing value.
locked : bool, optional
Whether the StringConverter should be locked to prevent automatic
upgrade or not. Default is False.
"""
#
_mapper = [(nx.bool_, str2bool, False),
(nx.integer, int, -1)]
# On 32-bit systems, we need to make sure that we explicitly include
# nx.int64 since ns.integer is nx.int32.
if nx.dtype(nx.integer).itemsize < nx.dtype(nx.int64).itemsize:
_mapper.append((nx.int64, int, -1))
_mapper.extend([(nx.floating, float, nx.nan),
(complex, _bytes_to_complex, nx.nan + 0j),
(nx.longdouble, nx.longdouble, nx.nan),
(nx.string_, bytes, asbytes('???'))])
(_defaulttype, _defaultfunc, _defaultfill) = zip(*_mapper)
@classmethod
def _getdtype(cls, val):
"""Returns the dtype of the input variable."""
return np.array(val).dtype
#
@classmethod
def _getsubdtype(cls, val):
"""Returns the type of the dtype of the input variable."""
return np.array(val).dtype.type
#
# This is a bit annoying. We want to return the "general" type in most
# cases (ie. "string" rather than "S10"), but we want to return the
# specific type for datetime64 (ie. "datetime64[us]" rather than
# "datetime64").
@classmethod
def _dtypeortype(cls, dtype):
"""Returns dtype for datetime64 and type of dtype otherwise."""
if dtype.type == np.datetime64:
return dtype
return dtype.type
#
@classmethod
def upgrade_mapper(cls, func, default=None):
"""
Upgrade the mapper of a StringConverter by adding a new function and
its corresponding default.
The input function (or sequence of functions) and its associated
default value (if any) is inserted in penultimate position of the
mapper. The corresponding type is estimated from the dtype of the
default value.
Parameters
----------
func : var
Function, or sequence of functions
Examples
--------
>>> import dateutil.parser
>>> import datetime
>>> dateparser = datetustil.parser.parse
>>> defaultdate = datetime.date(2000, 1, 1)
>>> StringConverter.upgrade_mapper(dateparser, default=defaultdate)
"""
# Func is a single functions
if hasattr(func, '__call__'):
cls._mapper.insert(-1, (cls._getsubdtype(default), func, default))
return
elif hasattr(func, '__iter__'):
if isinstance(func[0], (tuple, list)):
for _ in func:
cls._mapper.insert(-1, _)
return
if default is None:
default = [None] * len(func)
else:
default = list(default)
default.append([None] * (len(func) - len(default)))
for (fct, dft) in zip(func, default):
cls._mapper.insert(-1, (cls._getsubdtype(dft), fct, dft))
#
def __init__(self, dtype_or_func=None, default=None, missing_values=None,
locked=False):
# Convert unicode (for Py3)
if isinstance(missing_values, unicode):
missing_values = asbytes(missing_values)
elif isinstance(missing_values, (list, tuple)):
missing_values = asbytes_nested(missing_values)
# Defines a lock for upgrade
self._locked = bool(locked)
# No input dtype: minimal initialization
if dtype_or_func is None:
self.func = str2bool
self._status = 0
self.default = default or False
dtype = np.dtype('bool')
else:
# Is the input a np.dtype ?
try:
self.func = None
dtype = np.dtype(dtype_or_func)
except TypeError:
# dtype_or_func must be a function, then
if not hasattr(dtype_or_func, '__call__'):
errmsg = ("The input argument `dtype` is neither a"
" function nor a dtype (got '%s' instead)")
raise TypeError(errmsg % type(dtype_or_func))
# Set the function
self.func = dtype_or_func
# If we don't have a default, try to guess it or set it to
# None
if default is None:
try:
default = self.func(asbytes('0'))
except ValueError:
default = None
dtype = self._getdtype(default)
# Set the status according to the dtype
_status = -1
for (i, (deftype, func, default_def)) in enumerate(self._mapper):
if np.issubdtype(dtype.type, deftype):
_status = i
if default is None:
self.default = default_def
else:
self.default = default
break
# if a converter for the specific dtype is available use that
last_func = func
for (i, (deftype, func, default_def)) in enumerate(self._mapper):
if dtype.type == deftype:
_status = i
last_func = func
if default is None:
self.default = default_def
else:
self.default = default
break
func = last_func
if _status == -1:
# We never found a match in the _mapper...
_status = 0
self.default = default
self._status = _status
# If the input was a dtype, set the function to the last we saw
if self.func is None:
self.func = func
# If the status is 1 (int), change the function to
# something more robust.
if self.func == self._mapper[1][1]:
if issubclass(dtype.type, np.uint64):
self.func = np.uint64
elif issubclass(dtype.type, np.int64):
self.func = np.int64
else:
self.func = lambda x: int(float(x))
# Store the list of strings corresponding to missing values.
if missing_values is None:
self.missing_values = set([asbytes('')])
else:
if isinstance(missing_values, bytes):
missing_values = missing_values.split(asbytes(","))
self.missing_values = set(list(missing_values) + [asbytes('')])
#
self._callingfunction = self._strict_call
self.type = self._dtypeortype(dtype)
self._checked = False
self._initial_default = default
#
def _loose_call(self, value):
try:
return self.func(value)
except ValueError:
return self.default
#
def _strict_call(self, value):
try:
# We check if we can convert the value using the current function
new_value = self.func(value)
# In addition to having to check whether func can convert the
# value, we also have to make sure that we don't get overflow
# errors for integers.
if self.func is int:
try:
np.array(value, dtype=self.type)
except OverflowError:
raise ValueError
# We're still here so we can now return the new value
return new_value
except ValueError:
if value.strip() in self.missing_values:
if not self._status:
self._checked = False
return self.default
raise ValueError("Cannot convert string '%s'" % value)
#
def __call__(self, value):
return self._callingfunction(value)
#
def upgrade(self, value):
"""
Find the best converter for a given string, and return the result.
The supplied string `value` is converted by testing different
converters in order. First the `func` method of the
`StringConverter` instance is tried, if this fails other available
converters are tried. The order in which these other converters
are tried is determined by the `_status` attribute of the instance.
Parameters
----------
value : str
The string to convert.
Returns
-------
out : any
The result of converting `value` with the appropriate converter.
"""
self._checked = True
try:
return self._strict_call(value)
except ValueError:
# Raise an exception if we locked the converter...
if self._locked:
errmsg = "Converter is locked and cannot be upgraded"
raise ConverterLockError(errmsg)
_statusmax = len(self._mapper)
# Complains if we try to upgrade by the maximum
_status = self._status
if _status == _statusmax:
errmsg = "Could not find a valid conversion function"
raise ConverterError(errmsg)
elif _status < _statusmax - 1:
_status += 1
(self.type, self.func, default) = self._mapper[_status]
self._status = _status
if self._initial_default is not None:
self.default = self._initial_default
else:
self.default = default
return self.upgrade(value)
def iterupgrade(self, value):
self._checked = True
if not hasattr(value, '__iter__'):
value = (value,)
_strict_call = self._strict_call
try:
for _m in value:
_strict_call(_m)
except ValueError:
# Raise an exception if we locked the converter...
if self._locked:
errmsg = "Converter is locked and cannot be upgraded"
raise ConverterLockError(errmsg)
_statusmax = len(self._mapper)
# Complains if we try to upgrade by the maximum
_status = self._status
if _status == _statusmax:
raise ConverterError(
"Could not find a valid conversion function"
)
elif _status < _statusmax - 1:
_status += 1
(self.type, self.func, default) = self._mapper[_status]
if self._initial_default is not None:
self.default = self._initial_default
else:
self.default = default
self._status = _status
self.iterupgrade(value)
def update(self, func, default=None, testing_value=None,
missing_values=asbytes(''), locked=False):
"""
Set StringConverter attributes directly.
Parameters
----------
func : function
Conversion function.
default : any, optional
Value to return by default, that is, when the string to be
converted is flagged as missing. If not given,
`StringConverter` tries to supply a reasonable default value.
testing_value : str, optional
A string representing a standard input value of the converter.
This string is used to help defining a reasonable default
value.
missing_values : sequence of str, optional
Sequence of strings indicating a missing value.
locked : bool, optional
Whether the StringConverter should be locked to prevent
automatic upgrade or not. Default is False.
Notes
-----
`update` takes the same parameters as the constructor of
`StringConverter`, except that `func` does not accept a `dtype`
whereas `dtype_or_func` in the constructor does.
"""
self.func = func
self._locked = locked
# Don't reset the default to None if we can avoid it
if default is not None:
self.default = default
self.type = self._dtypeortype(self._getdtype(default))
else:
try:
tester = func(testing_value or asbytes('1'))
except (TypeError, ValueError):
tester = None
self.type = self._dtypeortype(self._getdtype(tester))
# Add the missing values to the existing set
if missing_values is not None:
if _is_bytes_like(missing_values):
self.missing_values.add(missing_values)
elif hasattr(missing_values, '__iter__'):
for val in missing_values:
self.missing_values.add(val)
else:
self.missing_values = []
def easy_dtype(ndtype, names=None, defaultfmt="f%i", **validationargs):
"""
Convenience function to create a `np.dtype` object.
The function processes the input `dtype` and matches it with the given
names.
Parameters
----------
ndtype : var
Definition of the dtype. Can be any string or dictionary recognized
by the `np.dtype` function, or a sequence of types.
names : str or sequence, optional
Sequence of strings to use as field names for a structured dtype.
For convenience, `names` can be a string of a comma-separated list
of names.
defaultfmt : str, optional
Format string used to define missing names, such as ``"f%i"``
(default) or ``"fields_%02i"``.
validationargs : optional
A series of optional arguments used to initialize a
`NameValidator`.
Examples
--------
>>> np.lib._iotools.easy_dtype(float)
dtype('float64')
>>> np.lib._iotools.easy_dtype("i4, f8")
dtype([('f0', '<i4'), ('f1', '<f8')])
>>> np.lib._iotools.easy_dtype("i4, f8", defaultfmt="field_%03i")
dtype([('field_000', '<i4'), ('field_001', '<f8')])
>>> np.lib._iotools.easy_dtype((int, float, float), names="a,b,c")
dtype([('a', '<i8'), ('b', '<f8'), ('c', '<f8')])
>>> np.lib._iotools.easy_dtype(float, names="a,b,c")
dtype([('a', '<f8'), ('b', '<f8'), ('c', '<f8')])
"""
try:
ndtype = np.dtype(ndtype)
except TypeError:
validate = NameValidator(**validationargs)
nbfields = len(ndtype)
if names is None:
names = [''] * len(ndtype)
elif isinstance(names, basestring):
names = names.split(",")
names = validate(names, nbfields=nbfields, defaultfmt=defaultfmt)
ndtype = np.dtype(dict(formats=ndtype, names=names))
else:
nbtypes = len(ndtype)
# Explicit names
if names is not None:
validate = NameValidator(**validationargs)
if isinstance(names, basestring):
names = names.split(",")
# Simple dtype: repeat to match the nb of names
if nbtypes == 0:
formats = tuple([ndtype.type] * len(names))
names = validate(names, defaultfmt=defaultfmt)
ndtype = np.dtype(list(zip(names, formats)))
# Structured dtype: just validate the names as needed
else:
ndtype.names = validate(names, nbfields=nbtypes,
defaultfmt=defaultfmt)
# No implicit names
elif (nbtypes > 0):
validate = NameValidator(**validationargs)
# Default initial names : should we change the format ?
if ((ndtype.names == tuple("f%i" % i for i in range(nbtypes))) and
(defaultfmt != "f%i")):
ndtype.names = validate([''] * nbtypes, defaultfmt=defaultfmt)
# Explicit initial names : just validate
else:
ndtype.names = validate(ndtype.names, defaultfmt=defaultfmt)
return ndtype
| mit |
ryfeus/lambda-packs | Sklearn_scipy_numpy/source/numpy/core/records.py | 12 | 29431 | """
Record Arrays
=============
Record arrays expose the fields of structured arrays as properties.
Most commonly, ndarrays contain elements of a single type, e.g. floats,
integers, bools etc. However, it is possible for elements to be combinations
of these using structured types, such as::
>>> a = np.array([(1, 2.0), (1, 2.0)], dtype=[('x', int), ('y', float)])
>>> a
array([(1, 2.0), (1, 2.0)],
dtype=[('x', '<i4'), ('y', '<f8')])
Here, each element consists of two fields: x (and int), and y (a float).
This is known as a structured array. The different fields are analogous
to columns in a spread-sheet. The different fields can be accessed as
one would a dictionary::
>>> a['x']
array([1, 1])
>>> a['y']
array([ 2., 2.])
Record arrays allow us to access fields as properties::
>>> ar = np.rec.array(a)
>>> ar.x
array([1, 1])
>>> ar.y
array([ 2., 2.])
"""
from __future__ import division, absolute_import, print_function
import sys
import os
from . import numeric as sb
from . import numerictypes as nt
from numpy.compat import isfileobj, bytes, long
# All of the functions allow formats to be a dtype
__all__ = ['record', 'recarray', 'format_parser']
ndarray = sb.ndarray
_byteorderconv = {'b':'>',
'l':'<',
'n':'=',
'B':'>',
'L':'<',
'N':'=',
'S':'s',
's':'s',
'>':'>',
'<':'<',
'=':'=',
'|':'|',
'I':'|',
'i':'|'}
# formats regular expression
# allows multidimension spec with a tuple syntax in front
# of the letter code '(2,3)f4' and ' ( 2 , 3 ) f4 '
# are equally allowed
numfmt = nt.typeDict
def find_duplicate(list):
"""Find duplication in a list, return a list of duplicated elements"""
dup = []
for i in range(len(list)):
if (list[i] in list[i + 1:]):
if (list[i] not in dup):
dup.append(list[i])
return dup
class format_parser:
"""
Class to convert formats, names, titles description to a dtype.
After constructing the format_parser object, the dtype attribute is
the converted data-type:
``dtype = format_parser(formats, names, titles).dtype``
Attributes
----------
dtype : dtype
The converted data-type.
Parameters
----------
formats : str or list of str
The format description, either specified as a string with
comma-separated format descriptions in the form ``'f8, i4, a5'``, or
a list of format description strings in the form
``['f8', 'i4', 'a5']``.
names : str or list/tuple of str
The field names, either specified as a comma-separated string in the
form ``'col1, col2, col3'``, or as a list or tuple of strings in the
form ``['col1', 'col2', 'col3']``.
An empty list can be used, in that case default field names
('f0', 'f1', ...) are used.
titles : sequence
Sequence of title strings. An empty list can be used to leave titles
out.
aligned : bool, optional
If True, align the fields by padding as the C-compiler would.
Default is False.
byteorder : str, optional
If specified, all the fields will be changed to the
provided byte-order. Otherwise, the default byte-order is
used. For all available string specifiers, see `dtype.newbyteorder`.
See Also
--------
dtype, typename, sctype2char
Examples
--------
>>> np.format_parser(['f8', 'i4', 'a5'], ['col1', 'col2', 'col3'],
... ['T1', 'T2', 'T3']).dtype
dtype([(('T1', 'col1'), '<f8'), (('T2', 'col2'), '<i4'),
(('T3', 'col3'), '|S5')])
`names` and/or `titles` can be empty lists. If `titles` is an empty list,
titles will simply not appear. If `names` is empty, default field names
will be used.
>>> np.format_parser(['f8', 'i4', 'a5'], ['col1', 'col2', 'col3'],
... []).dtype
dtype([('col1', '<f8'), ('col2', '<i4'), ('col3', '|S5')])
>>> np.format_parser(['f8', 'i4', 'a5'], [], []).dtype
dtype([('f0', '<f8'), ('f1', '<i4'), ('f2', '|S5')])
"""
def __init__(self, formats, names, titles, aligned=False, byteorder=None):
self._parseFormats(formats, aligned)
self._setfieldnames(names, titles)
self._createdescr(byteorder)
self.dtype = self._descr
def _parseFormats(self, formats, aligned=0):
""" Parse the field formats """
if formats is None:
raise ValueError("Need formats argument")
if isinstance(formats, list):
if len(formats) < 2:
formats.append('')
formats = ','.join(formats)
dtype = sb.dtype(formats, aligned)
fields = dtype.fields
if fields is None:
dtype = sb.dtype([('f1', dtype)], aligned)
fields = dtype.fields
keys = dtype.names
self._f_formats = [fields[key][0] for key in keys]
self._offsets = [fields[key][1] for key in keys]
self._nfields = len(keys)
def _setfieldnames(self, names, titles):
"""convert input field names into a list and assign to the _names
attribute """
if (names):
if (type(names) in [list, tuple]):
pass
elif isinstance(names, str):
names = names.split(',')
else:
raise NameError("illegal input names %s" % repr(names))
self._names = [n.strip() for n in names[:self._nfields]]
else:
self._names = []
# if the names are not specified, they will be assigned as
# "f0, f1, f2,..."
# if not enough names are specified, they will be assigned as "f[n],
# f[n+1],..." etc. where n is the number of specified names..."
self._names += ['f%d' % i for i in range(len(self._names),
self._nfields)]
# check for redundant names
_dup = find_duplicate(self._names)
if _dup:
raise ValueError("Duplicate field names: %s" % _dup)
if (titles):
self._titles = [n.strip() for n in titles[:self._nfields]]
else:
self._titles = []
titles = []
if (self._nfields > len(titles)):
self._titles += [None] * (self._nfields - len(titles))
def _createdescr(self, byteorder):
descr = sb.dtype({'names':self._names,
'formats':self._f_formats,
'offsets':self._offsets,
'titles':self._titles})
if (byteorder is not None):
byteorder = _byteorderconv[byteorder[0]]
descr = descr.newbyteorder(byteorder)
self._descr = descr
class record(nt.void):
"""A data-type scalar that allows field access as attribute lookup.
"""
# manually set name and module so that this class's type shows up
# as numpy.record when printed
__name__ = 'record'
__module__ = 'numpy'
def __repr__(self):
return self.__str__()
def __str__(self):
return str(self.item())
def __getattribute__(self, attr):
if attr in ['setfield', 'getfield', 'dtype']:
return nt.void.__getattribute__(self, attr)
try:
return nt.void.__getattribute__(self, attr)
except AttributeError:
pass
fielddict = nt.void.__getattribute__(self, 'dtype').fields
res = fielddict.get(attr, None)
if res:
obj = self.getfield(*res[:2])
# if it has fields return a record,
# otherwise return the object
try:
dt = obj.dtype
except AttributeError:
#happens if field is Object type
return obj
if dt.fields:
return obj.view((self.__class__, obj.dtype.fields))
return obj
else:
raise AttributeError("'record' object has no "
"attribute '%s'" % attr)
def __setattr__(self, attr, val):
if attr in ['setfield', 'getfield', 'dtype']:
raise AttributeError("Cannot set '%s' attribute" % attr)
fielddict = nt.void.__getattribute__(self, 'dtype').fields
res = fielddict.get(attr, None)
if res:
return self.setfield(val, *res[:2])
else:
if getattr(self, attr, None):
return nt.void.__setattr__(self, attr, val)
else:
raise AttributeError("'record' object has no "
"attribute '%s'" % attr)
def __getitem__(self, indx):
obj = nt.void.__getitem__(self, indx)
# copy behavior of record.__getattribute__,
if isinstance(obj, nt.void) and obj.dtype.fields:
return obj.view((self.__class__, obj.dtype.fields))
else:
# return a single element
return obj
def pprint(self):
"""Pretty-print all fields."""
# pretty-print all fields
names = self.dtype.names
maxlen = max(len(name) for name in names)
rows = []
fmt = '%% %ds: %%s' % maxlen
for name in names:
rows.append(fmt % (name, getattr(self, name)))
return "\n".join(rows)
# The recarray is almost identical to a standard array (which supports
# named fields already) The biggest difference is that it can use
# attribute-lookup to find the fields and it is constructed using
# a record.
# If byteorder is given it forces a particular byteorder on all
# the fields (and any subfields)
class recarray(ndarray):
"""Construct an ndarray that allows field access using attributes.
Arrays may have a data-types containing fields, analogous
to columns in a spread sheet. An example is ``[(x, int), (y, float)]``,
where each entry in the array is a pair of ``(int, float)``. Normally,
these attributes are accessed using dictionary lookups such as ``arr['x']``
and ``arr['y']``. Record arrays allow the fields to be accessed as members
of the array, using ``arr.x`` and ``arr.y``.
Parameters
----------
shape : tuple
Shape of output array.
dtype : data-type, optional
The desired data-type. By default, the data-type is determined
from `formats`, `names`, `titles`, `aligned` and `byteorder`.
formats : list of data-types, optional
A list containing the data-types for the different columns, e.g.
``['i4', 'f8', 'i4']``. `formats` does *not* support the new
convention of using types directly, i.e. ``(int, float, int)``.
Note that `formats` must be a list, not a tuple.
Given that `formats` is somewhat limited, we recommend specifying
`dtype` instead.
names : tuple of str, optional
The name of each column, e.g. ``('x', 'y', 'z')``.
buf : buffer, optional
By default, a new array is created of the given shape and data-type.
If `buf` is specified and is an object exposing the buffer interface,
the array will use the memory from the existing buffer. In this case,
the `offset` and `strides` keywords are available.
Other Parameters
----------------
titles : tuple of str, optional
Aliases for column names. For example, if `names` were
``('x', 'y', 'z')`` and `titles` is
``('x_coordinate', 'y_coordinate', 'z_coordinate')``, then
``arr['x']`` is equivalent to both ``arr.x`` and ``arr.x_coordinate``.
byteorder : {'<', '>', '='}, optional
Byte-order for all fields.
aligned : bool, optional
Align the fields in memory as the C-compiler would.
strides : tuple of ints, optional
Buffer (`buf`) is interpreted according to these strides (strides
define how many bytes each array element, row, column, etc.
occupy in memory).
offset : int, optional
Start reading buffer (`buf`) from this offset onwards.
order : {'C', 'F'}, optional
Row-major (C-style) or column-major (Fortran-style) order.
Returns
-------
rec : recarray
Empty array of the given shape and type.
See Also
--------
rec.fromrecords : Construct a record array from data.
record : fundamental data-type for `recarray`.
format_parser : determine a data-type from formats, names, titles.
Notes
-----
This constructor can be compared to ``empty``: it creates a new record
array but does not fill it with data. To create a record array from data,
use one of the following methods:
1. Create a standard ndarray and convert it to a record array,
using ``arr.view(np.recarray)``
2. Use the `buf` keyword.
3. Use `np.rec.fromrecords`.
Examples
--------
Create an array with two fields, ``x`` and ``y``:
>>> x = np.array([(1.0, 2), (3.0, 4)], dtype=[('x', float), ('y', int)])
>>> x
array([(1.0, 2), (3.0, 4)],
dtype=[('x', '<f8'), ('y', '<i4')])
>>> x['x']
array([ 1., 3.])
View the array as a record array:
>>> x = x.view(np.recarray)
>>> x.x
array([ 1., 3.])
>>> x.y
array([2, 4])
Create a new, empty record array:
>>> np.recarray((2,),
... dtype=[('x', int), ('y', float), ('z', int)]) #doctest: +SKIP
rec.array([(-1073741821, 1.2249118382103472e-301, 24547520),
(3471280, 1.2134086255804012e-316, 0)],
dtype=[('x', '<i4'), ('y', '<f8'), ('z', '<i4')])
"""
# manually set name and module so that this class's type shows
# up as "numpy.recarray" when printed
__name__ = 'recarray'
__module__ = 'numpy'
def __new__(subtype, shape, dtype=None, buf=None, offset=0, strides=None,
formats=None, names=None, titles=None,
byteorder=None, aligned=False, order='C'):
if dtype is not None:
descr = sb.dtype(dtype)
else:
descr = format_parser(formats, names, titles, aligned, byteorder)._descr
if buf is None:
self = ndarray.__new__(subtype, shape, (record, descr), order=order)
else:
self = ndarray.__new__(subtype, shape, (record, descr),
buffer=buf, offset=offset,
strides=strides, order=order)
return self
def __array_finalize__(self, obj):
if self.dtype.type is not record:
# if self.dtype is not np.record, invoke __setattr__ which will
# convert it to a record if it is a void dtype.
self.dtype = self.dtype
def __getattribute__(self, attr):
# See if ndarray has this attr, and return it if so. (note that this
# means a field with the same name as an ndarray attr cannot be
# accessed by attribute).
try:
return object.__getattribute__(self, attr)
except AttributeError: # attr must be a fieldname
pass
# look for a field with this name
fielddict = ndarray.__getattribute__(self, 'dtype').fields
try:
res = fielddict[attr][:2]
except (TypeError, KeyError):
raise AttributeError("recarray has no attribute %s" % attr)
obj = self.getfield(*res)
# At this point obj will always be a recarray, since (see
# PyArray_GetField) the type of obj is inherited. Next, if obj.dtype is
# non-structured, convert it to an ndarray. Then if obj is structured
# with void type convert it to the same dtype.type (eg to preserve
# numpy.record type if present), since nested structured fields do not
# inherit type. Don't do this for non-void structures though.
if obj.dtype.fields:
if issubclass(obj.dtype.type, nt.void):
return obj.view(dtype=(self.dtype.type, obj.dtype))
return obj
else:
return obj.view(ndarray)
# Save the dictionary.
# If the attr is a field name and not in the saved dictionary
# Undo any "setting" of the attribute and do a setfield
# Thus, you can't create attributes on-the-fly that are field names.
def __setattr__(self, attr, val):
# Automatically convert (void) structured types to records
# (but not non-void structures, subarrays, or non-structured voids)
if attr == 'dtype' and issubclass(val.type, nt.void) and val.fields:
val = sb.dtype((record, val))
newattr = attr not in self.__dict__
try:
ret = object.__setattr__(self, attr, val)
except:
fielddict = ndarray.__getattribute__(self, 'dtype').fields or {}
if attr not in fielddict:
exctype, value = sys.exc_info()[:2]
raise exctype(value)
else:
fielddict = ndarray.__getattribute__(self, 'dtype').fields or {}
if attr not in fielddict:
return ret
if newattr:
# We just added this one or this setattr worked on an
# internal attribute.
try:
object.__delattr__(self, attr)
except:
return ret
try:
res = fielddict[attr][:2]
except (TypeError, KeyError):
raise AttributeError("record array has no attribute %s" % attr)
return self.setfield(val, *res)
def __getitem__(self, indx):
obj = super(recarray, self).__getitem__(indx)
# copy behavior of getattr, except that here
# we might also be returning a single element
if isinstance(obj, ndarray):
if obj.dtype.fields:
obj = obj.view(type(self))
if issubclass(obj.dtype.type, nt.void):
return obj.view(dtype=(self.dtype.type, obj.dtype))
return obj
else:
return obj.view(type=ndarray)
else:
# return a single element
return obj
def __repr__(self):
# get data/shape string. logic taken from numeric.array_repr
if self.size > 0 or self.shape == (0,):
lst = sb.array2string(self, separator=', ')
else:
# show zero-length shape unless it is (0,)
lst = "[], shape=%s" % (repr(self.shape),)
if (self.dtype.type is record
or (not issubclass(self.dtype.type, nt.void))):
# If this is a full record array (has numpy.record dtype),
# or if it has a scalar (non-void) dtype with no records,
# represent it using the rec.array function. Since rec.array
# converts dtype to a numpy.record for us, convert back
# to non-record before printing
plain_dtype = self.dtype
if plain_dtype.type is record:
plain_dtype = sb.dtype((nt.void, plain_dtype))
lf = '\n'+' '*len("rec.array(")
return ('rec.array(%s, %sdtype=%s)' %
(lst, lf, plain_dtype))
else:
# otherwise represent it using np.array plus a view
# This should only happen if the user is playing
# strange games with dtypes.
lf = '\n'+' '*len("array(")
return ('array(%s, %sdtype=%s).view(numpy.recarray)' %
(lst, lf, str(self.dtype)))
def field(self, attr, val=None):
if isinstance(attr, int):
names = ndarray.__getattribute__(self, 'dtype').names
attr = names[attr]
fielddict = ndarray.__getattribute__(self, 'dtype').fields
res = fielddict[attr][:2]
if val is None:
obj = self.getfield(*res)
if obj.dtype.fields:
return obj
return obj.view(ndarray)
else:
return self.setfield(val, *res)
def fromarrays(arrayList, dtype=None, shape=None, formats=None,
names=None, titles=None, aligned=False, byteorder=None):
""" create a record array from a (flat) list of arrays
>>> x1=np.array([1,2,3,4])
>>> x2=np.array(['a','dd','xyz','12'])
>>> x3=np.array([1.1,2,3,4])
>>> r = np.core.records.fromarrays([x1,x2,x3],names='a,b,c')
>>> print r[1]
(2, 'dd', 2.0)
>>> x1[1]=34
>>> r.a
array([1, 2, 3, 4])
"""
arrayList = [sb.asarray(x) for x in arrayList]
if shape is None or shape == 0:
shape = arrayList[0].shape
if isinstance(shape, int):
shape = (shape,)
if formats is None and dtype is None:
# go through each object in the list to see if it is an ndarray
# and determine the formats.
formats = []
for obj in arrayList:
if not isinstance(obj, ndarray):
raise ValueError("item in the array list must be an ndarray.")
formats.append(obj.dtype.str)
formats = ','.join(formats)
if dtype is not None:
descr = sb.dtype(dtype)
_names = descr.names
else:
parsed = format_parser(formats, names, titles, aligned, byteorder)
_names = parsed._names
descr = parsed._descr
# Determine shape from data-type.
if len(descr) != len(arrayList):
raise ValueError("mismatch between the number of fields "
"and the number of arrays")
d0 = descr[0].shape
nn = len(d0)
if nn > 0:
shape = shape[:-nn]
for k, obj in enumerate(arrayList):
nn = len(descr[k].shape)
testshape = obj.shape[:len(obj.shape) - nn]
if testshape != shape:
raise ValueError("array-shape mismatch in array %d" % k)
_array = recarray(shape, descr)
# populate the record array (makes a copy)
for i in range(len(arrayList)):
_array[_names[i]] = arrayList[i]
return _array
# shape must be 1-d if you use list of lists...
def fromrecords(recList, dtype=None, shape=None, formats=None, names=None,
titles=None, aligned=False, byteorder=None):
""" create a recarray from a list of records in text form
The data in the same field can be heterogeneous, they will be promoted
to the highest data type. This method is intended for creating
smaller record arrays. If used to create large array without formats
defined
r=fromrecords([(2,3.,'abc')]*100000)
it can be slow.
If formats is None, then this will auto-detect formats. Use list of
tuples rather than list of lists for faster processing.
>>> r=np.core.records.fromrecords([(456,'dbe',1.2),(2,'de',1.3)],
... names='col1,col2,col3')
>>> print r[0]
(456, 'dbe', 1.2)
>>> r.col1
array([456, 2])
>>> r.col2
array(['dbe', 'de'],
dtype='|S3')
>>> import pickle
>>> print pickle.loads(pickle.dumps(r))
[(456, 'dbe', 1.2) (2, 'de', 1.3)]
"""
nfields = len(recList[0])
if formats is None and dtype is None: # slower
obj = sb.array(recList, dtype=object)
arrlist = [sb.array(obj[..., i].tolist()) for i in range(nfields)]
return fromarrays(arrlist, formats=formats, shape=shape, names=names,
titles=titles, aligned=aligned, byteorder=byteorder)
if dtype is not None:
descr = sb.dtype((record, dtype))
else:
descr = format_parser(formats, names, titles, aligned, byteorder)._descr
try:
retval = sb.array(recList, dtype=descr)
except TypeError: # list of lists instead of list of tuples
if (shape is None or shape == 0):
shape = len(recList)
if isinstance(shape, (int, long)):
shape = (shape,)
if len(shape) > 1:
raise ValueError("Can only deal with 1-d array.")
_array = recarray(shape, descr)
for k in range(_array.size):
_array[k] = tuple(recList[k])
return _array
else:
if shape is not None and retval.shape != shape:
retval.shape = shape
res = retval.view(recarray)
return res
def fromstring(datastring, dtype=None, shape=None, offset=0, formats=None,
names=None, titles=None, aligned=False, byteorder=None):
""" create a (read-only) record array from binary data contained in
a string"""
if dtype is None and formats is None:
raise ValueError("Must have dtype= or formats=")
if dtype is not None:
descr = sb.dtype(dtype)
else:
descr = format_parser(formats, names, titles, aligned, byteorder)._descr
itemsize = descr.itemsize
if (shape is None or shape == 0 or shape == -1):
shape = (len(datastring) - offset) / itemsize
_array = recarray(shape, descr, buf=datastring, offset=offset)
return _array
def get_remaining_size(fd):
try:
fn = fd.fileno()
except AttributeError:
return os.path.getsize(fd.name) - fd.tell()
st = os.fstat(fn)
size = st.st_size - fd.tell()
return size
def fromfile(fd, dtype=None, shape=None, offset=0, formats=None,
names=None, titles=None, aligned=False, byteorder=None):
"""Create an array from binary file data
If file is a string then that file is opened, else it is assumed
to be a file object.
>>> from tempfile import TemporaryFile
>>> a = np.empty(10,dtype='f8,i4,a5')
>>> a[5] = (0.5,10,'abcde')
>>>
>>> fd=TemporaryFile()
>>> a = a.newbyteorder('<')
>>> a.tofile(fd)
>>>
>>> fd.seek(0)
>>> r=np.core.records.fromfile(fd, formats='f8,i4,a5', shape=10,
... byteorder='<')
>>> print r[5]
(0.5, 10, 'abcde')
>>> r.shape
(10,)
"""
if (shape is None or shape == 0):
shape = (-1,)
elif isinstance(shape, (int, long)):
shape = (shape,)
name = 0
if isinstance(fd, str):
name = 1
fd = open(fd, 'rb')
if (offset > 0):
fd.seek(offset, 1)
size = get_remaining_size(fd)
if dtype is not None:
descr = sb.dtype(dtype)
else:
descr = format_parser(formats, names, titles, aligned, byteorder)._descr
itemsize = descr.itemsize
shapeprod = sb.array(shape).prod()
shapesize = shapeprod * itemsize
if shapesize < 0:
shape = list(shape)
shape[shape.index(-1)] = size / -shapesize
shape = tuple(shape)
shapeprod = sb.array(shape).prod()
nbytes = shapeprod * itemsize
if nbytes > size:
raise ValueError(
"Not enough bytes left in file for specified shape and type")
# create the array
_array = recarray(shape, descr)
nbytesread = fd.readinto(_array.data)
if nbytesread != nbytes:
raise IOError("Didn't read as many bytes as expected")
if name:
fd.close()
return _array
def array(obj, dtype=None, shape=None, offset=0, strides=None, formats=None,
names=None, titles=None, aligned=False, byteorder=None, copy=True):
"""Construct a record array from a wide-variety of objects.
"""
if ((isinstance(obj, (type(None), str)) or isfileobj(obj)) and
(formats is None) and (dtype is None)):
raise ValueError("Must define formats (or dtype) if object is "
"None, string, or an open file")
kwds = {}
if dtype is not None:
dtype = sb.dtype(dtype)
elif formats is not None:
dtype = format_parser(formats, names, titles,
aligned, byteorder)._descr
else:
kwds = {'formats': formats,
'names': names,
'titles': titles,
'aligned': aligned,
'byteorder': byteorder
}
if obj is None:
if shape is None:
raise ValueError("Must define a shape if obj is None")
return recarray(shape, dtype, buf=obj, offset=offset, strides=strides)
elif isinstance(obj, bytes):
return fromstring(obj, dtype, shape=shape, offset=offset, **kwds)
elif isinstance(obj, (list, tuple)):
if isinstance(obj[0], (tuple, list)):
return fromrecords(obj, dtype=dtype, shape=shape, **kwds)
else:
return fromarrays(obj, dtype=dtype, shape=shape, **kwds)
elif isinstance(obj, recarray):
if dtype is not None and (obj.dtype != dtype):
new = obj.view(dtype)
else:
new = obj
if copy:
new = new.copy()
return new
elif isfileobj(obj):
return fromfile(obj, dtype=dtype, shape=shape, offset=offset)
elif isinstance(obj, ndarray):
if dtype is not None and (obj.dtype != dtype):
new = obj.view(dtype)
else:
new = obj
if copy:
new = new.copy()
return new.view(recarray)
else:
interface = getattr(obj, "__array_interface__", None)
if interface is None or not isinstance(interface, dict):
raise ValueError("Unknown input type")
obj = sb.array(obj)
if dtype is not None and (obj.dtype != dtype):
obj = obj.view(dtype)
return obj.view(recarray)
| mit |
ryfeus/lambda-packs | Keras_tensorflow/source/tensorflow/contrib/specs/python/specs_lib.py | 162 | 6145 | # Copyright 2016 The TensorFlow Authors. All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
# ==============================================================================
"""Implement the "specs" DSL for describing deep networks."""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import importlib
import operator
import re
from six import exec_
QUOTED = re.compile(r"""
"([^"\\]|\\.)*" |
'([^'\\]|\\.)*'
""", re.VERBOSE)
KEYWORDS = re.compile(r"""\b(import|while|def|exec)\b""")
debug_ = False
def check_keywords(spec):
"""Check for common Python keywords in spec.
This function discourages the use of complex constructs
in TensorFlow specs; it doesn't completely prohibit them
(if necessary, we could check the AST).
Args:
spec: spec string
Raises:
ValueError: raised if spec contains a prohibited keyword.
"""
spec = re.sub(QUOTED, "", spec)
match = re.search(KEYWORDS, spec)
if match:
raise ValueError("keyword '%s' found in spec" % match.group(1))
def get_positional(args, kw, kw_overrides=False):
"""Interpolates keyword arguments into argument lists.
If `kw` contains keywords of the form "_0", "_1", etc., these
are positionally interpolated into the argument list.
Args:
args: argument list
kw: keyword dictionary
kw_overrides: key/value pairs that override kw
Returns:
(new_args, new_kw), new argument lists and keyword dictionaries
with values interpolated.
"""
new_kw = {k: v for k, v in kw.items() if k[0] != "_"}
if len(new_kw) == len(kw):
return args, kw
new_args = list(args)
for key, value in kw.items():
if key[0] != "_": continue
index = int(key[1:])
while len(new_args) <= index:
new_args += [None]
if kw_overrides or new_args[index] is None:
new_args[index] = value
return new_args, new_kw
class Composable(object):
"""A composable function.
This defines the operators common to all composable objects.
Currently defines copmosition (via "|") and repeated application
(via "**"), and maps addition ("+") and multiplication ("*")
as "(f + g)(x) = f(x) + g(x)".
"""
def __or__(self, f):
return Composition(self, f)
def __add__(self, g):
return Operator(operator.add, self, g)
def __mul__(self, g):
return Operator(operator.mul, self, g)
def __pow__(self, n):
assert n >= 0
if n == 0:
return Function(lambda x, *args, **kw: x)
result = self
for _ in range(n-1):
result = Composition(result, self)
return result
class Callable(Composable):
"""A composable function that simply defers to a callable function.
"""
def __init__(self, f):
self.f = f
def funcall(self, x):
return self.f(x)
class Operator(Composable):
"""A wrapper for an operator.
This takes an operator and an argument list and returns
the result of applying the operator to the results of applying
the functions in the argument list.
"""
def __init__(self, op, *args):
self.op = op
self.funs = args
def funcall(self, x):
outputs = [f.funcall(x) for f in self.funs]
return self.op(*outputs)
class Function(Composable):
"""A composable function wrapper for a regular Python function.
This overloads the regular __call__ operator for currying, i.e.,
arguments passed to __call__ are remembered for the eventual
function application.
The final function application happens via the `of` method.
"""
def __init__(self, f, *args, **kw):
if not callable(f):
raise ValueError("%s: is not callable" % f)
self.f = f
self.args = list(args)
self.kw = kw
def __call__(self, *args, **kw):
new_args = list(args) + self.args
new_kw = self.kw.copy()
new_kw.update(kw)
return Function(self.f, *new_args, **new_kw)
# TODO(tmb) The `of` method may be renamed to `function`.
def funcall(self, x):
args, kw = get_positional(self.args, self.kw)
if debug_:
print("DEBUG:", self.f, x, args, kw)
return self.f(x, *args, **kw)
class Composition(Composable):
"""A function composition.
This simply composes its two argument functions when
applied to a final argument via `of`.
"""
def __init__(self, f, g):
self.f = f
self.g = g
def funcall(self, x):
return self.g.funcall(self.f.funcall(x))
# These are DSL names, not Python names
# pylint: disable=invalid-name, exec-used
def External(module_name, function_name):
"""Import a function from an external module.
Note that the `module_name` must be a module name
that works with the usual import mechanisms. Shorthands
like "tf.nn" will not work.
Args:
module_name: name of the module
function_name: name of the function within the module
Returns:
Function-wrapped value of symbol.
"""
module = importlib.import_module(module_name)
return Function(vars(module)[function_name])
def Import(statements):
"""Import a function by exec.
Args:
statements: Python statements
Returns:
Function-wrapped value of `f`.
Raises:
ValueError: the statements didn't define a value for "f"
"""
environ = {}
exec_(statements, environ)
if "f" not in environ:
raise ValueError("failed to define \"f\": %s", statements)
f = environ["f"]
return Function(f)
# pylint: enable=invalid-name, exec-used
def debug(mode=True):
"""Turn on/off debugging mode.
Debugging mode prints more information about the construction
of a network.
Args:
mode: True if turned on, False otherwise
"""
global debug_
debug_ = mode
| mit |
Azure/azure-sdk-for-python | sdk/rdbms/azure-mgmt-rdbms/azure/mgmt/rdbms/mariadb/aio/_maria_db_management_client.py | 1 | 12385 | # coding=utf-8
# --------------------------------------------------------------------------
# Copyright (c) Microsoft Corporation. All rights reserved.
# Licensed under the MIT License. See License.txt in the project root for license information.
# Code generated by Microsoft (R) AutoRest Code Generator.
# Changes may cause incorrect behavior and will be lost if the code is regenerated.
# --------------------------------------------------------------------------
from typing import Any, Optional, TYPE_CHECKING
from azure.core.pipeline.transport import AsyncHttpResponse, HttpRequest
from azure.mgmt.core import AsyncARMPipelineClient
from msrest import Deserializer, Serializer
if TYPE_CHECKING:
# pylint: disable=unused-import,ungrouped-imports
from azure.core.credentials_async import AsyncTokenCredential
from ._configuration import MariaDBManagementClientConfiguration
from .operations import ServersOperations
from .operations import ReplicasOperations
from .operations import FirewallRulesOperations
from .operations import VirtualNetworkRulesOperations
from .operations import DatabasesOperations
from .operations import ConfigurationsOperations
from .operations import ServerParametersOperations
from .operations import LogFilesOperations
from .operations import RecoverableServersOperations
from .operations import ServerBasedPerformanceTierOperations
from .operations import LocationBasedPerformanceTierOperations
from .operations import CheckNameAvailabilityOperations
from .operations import Operations
from .operations import QueryTextsOperations
from .operations import TopQueryStatisticsOperations
from .operations import WaitStatisticsOperations
from .operations import MariaDBManagementClientOperationsMixin
from .operations import AdvisorsOperations
from .operations import RecommendedActionsOperations
from .operations import LocationBasedRecommendedActionSessionsOperationStatusOperations
from .operations import LocationBasedRecommendedActionSessionsResultOperations
from .operations import PrivateEndpointConnectionsOperations
from .operations import PrivateLinkResourcesOperations
from .operations import ServerSecurityAlertPoliciesOperations
from .. import models
class MariaDBManagementClient(MariaDBManagementClientOperationsMixin):
"""The Microsoft Azure management API provides create, read, update, and delete functionality for Azure MariaDB resources including servers, databases, firewall rules, VNET rules, log files and configurations with new business model.
:ivar servers: ServersOperations operations
:vartype servers: azure.mgmt.rdbms.mariadb.aio.operations.ServersOperations
:ivar replicas: ReplicasOperations operations
:vartype replicas: azure.mgmt.rdbms.mariadb.aio.operations.ReplicasOperations
:ivar firewall_rules: FirewallRulesOperations operations
:vartype firewall_rules: azure.mgmt.rdbms.mariadb.aio.operations.FirewallRulesOperations
:ivar virtual_network_rules: VirtualNetworkRulesOperations operations
:vartype virtual_network_rules: azure.mgmt.rdbms.mariadb.aio.operations.VirtualNetworkRulesOperations
:ivar databases: DatabasesOperations operations
:vartype databases: azure.mgmt.rdbms.mariadb.aio.operations.DatabasesOperations
:ivar configurations: ConfigurationsOperations operations
:vartype configurations: azure.mgmt.rdbms.mariadb.aio.operations.ConfigurationsOperations
:ivar server_parameters: ServerParametersOperations operations
:vartype server_parameters: azure.mgmt.rdbms.mariadb.aio.operations.ServerParametersOperations
:ivar log_files: LogFilesOperations operations
:vartype log_files: azure.mgmt.rdbms.mariadb.aio.operations.LogFilesOperations
:ivar recoverable_servers: RecoverableServersOperations operations
:vartype recoverable_servers: azure.mgmt.rdbms.mariadb.aio.operations.RecoverableServersOperations
:ivar server_based_performance_tier: ServerBasedPerformanceTierOperations operations
:vartype server_based_performance_tier: azure.mgmt.rdbms.mariadb.aio.operations.ServerBasedPerformanceTierOperations
:ivar location_based_performance_tier: LocationBasedPerformanceTierOperations operations
:vartype location_based_performance_tier: azure.mgmt.rdbms.mariadb.aio.operations.LocationBasedPerformanceTierOperations
:ivar check_name_availability: CheckNameAvailabilityOperations operations
:vartype check_name_availability: azure.mgmt.rdbms.mariadb.aio.operations.CheckNameAvailabilityOperations
:ivar operations: Operations operations
:vartype operations: azure.mgmt.rdbms.mariadb.aio.operations.Operations
:ivar query_texts: QueryTextsOperations operations
:vartype query_texts: azure.mgmt.rdbms.mariadb.aio.operations.QueryTextsOperations
:ivar top_query_statistics: TopQueryStatisticsOperations operations
:vartype top_query_statistics: azure.mgmt.rdbms.mariadb.aio.operations.TopQueryStatisticsOperations
:ivar wait_statistics: WaitStatisticsOperations operations
:vartype wait_statistics: azure.mgmt.rdbms.mariadb.aio.operations.WaitStatisticsOperations
:ivar advisors: AdvisorsOperations operations
:vartype advisors: azure.mgmt.rdbms.mariadb.aio.operations.AdvisorsOperations
:ivar recommended_actions: RecommendedActionsOperations operations
:vartype recommended_actions: azure.mgmt.rdbms.mariadb.aio.operations.RecommendedActionsOperations
:ivar location_based_recommended_action_sessions_operation_status: LocationBasedRecommendedActionSessionsOperationStatusOperations operations
:vartype location_based_recommended_action_sessions_operation_status: azure.mgmt.rdbms.mariadb.aio.operations.LocationBasedRecommendedActionSessionsOperationStatusOperations
:ivar location_based_recommended_action_sessions_result: LocationBasedRecommendedActionSessionsResultOperations operations
:vartype location_based_recommended_action_sessions_result: azure.mgmt.rdbms.mariadb.aio.operations.LocationBasedRecommendedActionSessionsResultOperations
:ivar private_endpoint_connections: PrivateEndpointConnectionsOperations operations
:vartype private_endpoint_connections: azure.mgmt.rdbms.mariadb.aio.operations.PrivateEndpointConnectionsOperations
:ivar private_link_resources: PrivateLinkResourcesOperations operations
:vartype private_link_resources: azure.mgmt.rdbms.mariadb.aio.operations.PrivateLinkResourcesOperations
:ivar server_security_alert_policies: ServerSecurityAlertPoliciesOperations operations
:vartype server_security_alert_policies: azure.mgmt.rdbms.mariadb.aio.operations.ServerSecurityAlertPoliciesOperations
:param credential: Credential needed for the client to connect to Azure.
:type credential: ~azure.core.credentials_async.AsyncTokenCredential
:param subscription_id: The ID of the target subscription.
:type subscription_id: str
:param str base_url: Service URL
:keyword int polling_interval: Default waiting time between two polls for LRO operations if no Retry-After header is present.
"""
def __init__(
self,
credential: "AsyncTokenCredential",
subscription_id: str,
base_url: Optional[str] = None,
**kwargs: Any
) -> None:
if not base_url:
base_url = 'https://management.azure.com'
self._config = MariaDBManagementClientConfiguration(credential, subscription_id, **kwargs)
self._client = AsyncARMPipelineClient(base_url=base_url, config=self._config, **kwargs)
client_models = {k: v for k, v in models.__dict__.items() if isinstance(v, type)}
self._serialize = Serializer(client_models)
self._serialize.client_side_validation = False
self._deserialize = Deserializer(client_models)
self.servers = ServersOperations(
self._client, self._config, self._serialize, self._deserialize)
self.replicas = ReplicasOperations(
self._client, self._config, self._serialize, self._deserialize)
self.firewall_rules = FirewallRulesOperations(
self._client, self._config, self._serialize, self._deserialize)
self.virtual_network_rules = VirtualNetworkRulesOperations(
self._client, self._config, self._serialize, self._deserialize)
self.databases = DatabasesOperations(
self._client, self._config, self._serialize, self._deserialize)
self.configurations = ConfigurationsOperations(
self._client, self._config, self._serialize, self._deserialize)
self.server_parameters = ServerParametersOperations(
self._client, self._config, self._serialize, self._deserialize)
self.log_files = LogFilesOperations(
self._client, self._config, self._serialize, self._deserialize)
self.recoverable_servers = RecoverableServersOperations(
self._client, self._config, self._serialize, self._deserialize)
self.server_based_performance_tier = ServerBasedPerformanceTierOperations(
self._client, self._config, self._serialize, self._deserialize)
self.location_based_performance_tier = LocationBasedPerformanceTierOperations(
self._client, self._config, self._serialize, self._deserialize)
self.check_name_availability = CheckNameAvailabilityOperations(
self._client, self._config, self._serialize, self._deserialize)
self.operations = Operations(
self._client, self._config, self._serialize, self._deserialize)
self.query_texts = QueryTextsOperations(
self._client, self._config, self._serialize, self._deserialize)
self.top_query_statistics = TopQueryStatisticsOperations(
self._client, self._config, self._serialize, self._deserialize)
self.wait_statistics = WaitStatisticsOperations(
self._client, self._config, self._serialize, self._deserialize)
self.advisors = AdvisorsOperations(
self._client, self._config, self._serialize, self._deserialize)
self.recommended_actions = RecommendedActionsOperations(
self._client, self._config, self._serialize, self._deserialize)
self.location_based_recommended_action_sessions_operation_status = LocationBasedRecommendedActionSessionsOperationStatusOperations(
self._client, self._config, self._serialize, self._deserialize)
self.location_based_recommended_action_sessions_result = LocationBasedRecommendedActionSessionsResultOperations(
self._client, self._config, self._serialize, self._deserialize)
self.private_endpoint_connections = PrivateEndpointConnectionsOperations(
self._client, self._config, self._serialize, self._deserialize)
self.private_link_resources = PrivateLinkResourcesOperations(
self._client, self._config, self._serialize, self._deserialize)
self.server_security_alert_policies = ServerSecurityAlertPoliciesOperations(
self._client, self._config, self._serialize, self._deserialize)
async def _send_request(self, http_request: HttpRequest, **kwargs: Any) -> AsyncHttpResponse:
"""Runs the network request through the client's chained policies.
:param http_request: The network request you want to make. Required.
:type http_request: ~azure.core.pipeline.transport.HttpRequest
:keyword bool stream: Whether the response payload will be streamed. Defaults to True.
:return: The response of your network call. Does not do error handling on your response.
:rtype: ~azure.core.pipeline.transport.AsyncHttpResponse
"""
path_format_arguments = {
'subscriptionId': self._serialize.url("self._config.subscription_id", self._config.subscription_id, 'str', min_length=1),
}
http_request.url = self._client.format_url(http_request.url, **path_format_arguments)
stream = kwargs.pop("stream", True)
pipeline_response = await self._client._pipeline.run(http_request, stream=stream, **kwargs)
return pipeline_response.http_response
async def close(self) -> None:
await self._client.close()
async def __aenter__(self) -> "MariaDBManagementClient":
await self._client.__aenter__()
return self
async def __aexit__(self, *exc_details) -> None:
await self._client.__aexit__(*exc_details)
| mit |
rkhmelichek/zookeeper | src/contrib/monitoring/test.py | 114 | 8195 | #! /usr/bin/env python
# Licensed to the Apache Software Foundation (ASF) under one
# or more contributor license agreements. See the NOTICE file
# distributed with this work for additional information
# regarding copyright ownership. The ASF licenses this file
# to you under the Apache License, Version 2.0 (the
# "License"); you may not use this file except in compliance
# with the License. You may obtain a copy of the License at
# http://www.apache.org/licenses/LICENSE-2.0
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
import unittest
import socket
import sys
from StringIO import StringIO
from check_zookeeper import ZooKeeperServer, NagiosHandler, CactiHandler, GangliaHandler
ZK_MNTR_OUTPUT = """zk_version\t3.4.0--1, built on 06/19/2010 15:07 GMT
zk_avg_latency\t1
zk_max_latency\t132
zk_min_latency\t0
zk_packets_received\t640
zk_packets_sent\t639
zk_outstanding_requests\t0
zk_server_state\tfollower
zk_znode_count\t4
zk_watch_count\t0
zk_ephemerals_count\t0
zk_approximate_data_size\t27
zk_open_file_descriptor_count\t22
zk_max_file_descriptor_count\t1024
"""
ZK_MNTR_OUTPUT_WITH_BROKEN_LINES = """zk_version\t3.4.0
zk_avg_latency\t23
broken-line
"""
ZK_STAT_OUTPUT = """Zookeeper version: 3.3.0-943314, built on 05/11/2010 22:20 GMT
Clients:
/0:0:0:0:0:0:0:1:34564[0](queued=0,recved=1,sent=0)
Latency min/avg/max: 0/40/121
Received: 11
Sent: 10
Outstanding: 0
Zxid: 0x700000003
Mode: follower
Node count: 4
"""
class SocketMock(object):
def __init__(self):
self.sent = []
def settimeout(self, timeout):
self.timeout = timeout
def connect(self, address):
self.address = address
def send(self, data):
self.sent.append(data)
return len(data)
def recv(self, size):
return ZK_MNTR_OUTPUT[:size]
def close(self): pass
class ZK33xSocketMock(SocketMock):
def __init__(self):
SocketMock.__init__(self)
self.got_stat_cmd = False
def recv(self, size):
if 'stat' in self.sent:
return ZK_STAT_OUTPUT[:size]
else:
return ''
class UnableToConnectSocketMock(SocketMock):
def connect(self, _):
raise socket.error('[Errno 111] Connection refused')
def create_server_mock(socket_class):
class ZooKeeperServerMock(ZooKeeperServer):
def _create_socket(self):
return socket_class()
return ZooKeeperServerMock()
class TestCheckZookeeper(unittest.TestCase):
def setUp(self):
self.zk = ZooKeeperServer()
def test_parse_valid_line(self):
key, value = self.zk._parse_line('something\t5')
self.assertEqual(key, 'something')
self.assertEqual(value, 5)
def test_parse_line_raises_exception_on_invalid_output(self):
invalid_lines = ['something', '', 'a\tb\tc', '\t1']
for line in invalid_lines:
self.assertRaises(ValueError, self.zk._parse_line, line)
def test_parser_on_valid_output(self):
data = self.zk._parse(ZK_MNTR_OUTPUT)
self.assertEqual(len(data), 14)
self.assertEqual(data['zk_znode_count'], 4)
def test_parse_should_ignore_invalid_lines(self):
data = self.zk._parse(ZK_MNTR_OUTPUT_WITH_BROKEN_LINES)
self.assertEqual(len(data), 2)
def test_parse_stat_valid_output(self):
data = self.zk._parse_stat(ZK_STAT_OUTPUT)
result = {
'zk_version' : '3.3.0-943314, built on 05/11/2010 22:20 GMT',
'zk_min_latency' : 0,
'zk_avg_latency' : 40,
'zk_max_latency' : 121,
'zk_packets_received': 11,
'zk_packets_sent': 10,
'zk_server_state': 'follower',
'zk_znode_count': 4
}
for k, v in result.iteritems():
self.assertEqual(v, data[k])
def test_recv_valid_output(self):
zk = create_server_mock(SocketMock)
data = zk.get_stats()
self.assertEqual(len(data), 14)
self.assertEqual(data['zk_znode_count'], 4)
def test_socket_unable_to_connect(self):
zk = create_server_mock(UnableToConnectSocketMock)
self.assertRaises(socket.error, zk.get_stats)
def test_use_stat_cmd_if_mntr_is_not_available(self):
zk = create_server_mock(ZK33xSocketMock)
data = zk.get_stats()
self.assertEqual(data['zk_version'], '3.3.0-943314, built on 05/11/2010 22:20 GMT')
class HandlerTestCase(unittest.TestCase):
def setUp(self):
try:
sys._stdout
except:
sys._stdout = sys.stdout
sys.stdout = StringIO()
def tearDown(self):
sys.stdout = sys._stdout
def output(self):
sys.stdout.seek(0)
return sys.stdout.read()
class TestNagiosHandler(HandlerTestCase):
def _analyze(self, w, c, k, stats):
class Opts(object):
warning = w
critical = c
key = k
return NagiosHandler().analyze(Opts(), {'localhost:2181':stats})
def test_ok_status(self):
r = self._analyze(10, 20, 'a', {'a': 5})
self.assertEqual(r, 0)
self.assertEqual(self.output(), 'Ok "a"!|localhost:2181=5;10;20\n')
r = self._analyze(20, 10, 'a', {'a': 30})
self.assertEqual(r, 0)
def test_warning_status(self):
r = self._analyze(10, 20, 'a', {'a': 15})
self.assertEqual(r, 1)
self.assertEqual(self.output(),
'Warning "a" localhost:2181!|localhost:2181=15;10;20\n')
r = self._analyze(20, 10, 'a', {'a': 15})
self.assertEqual(r, 1)
def test_critical_status(self):
r = self._analyze(10, 20, 'a', {'a': 30})
self.assertEqual(r, 2)
self.assertEqual(self.output(),
'Critical "a" localhost:2181!|localhost:2181=30;10;20\n')
r = self._analyze(20, 10, 'a', {'a': 5})
self.assertEqual(r, 2)
def test_check_a_specific_key_on_all_hosts(self):
class Opts(object):
warning = 10
critical = 20
key = 'latency'
r = NagiosHandler().analyze(Opts(), {
's1:2181': {'latency': 5},
's2:2181': {'latency': 15},
's3:2181': {'latency': 35},
})
self.assertEqual(r, 2)
self.assertEqual(self.output(),
'Critical "latency" s3:2181!|s1:2181=5;10;20 '\
's3:2181=35;10;20 s2:2181=15;10;20\n')
class TestCactiHandler(HandlerTestCase):
class Opts(object):
key = 'a'
leader = False
def __init__(self, leader=False):
self.leader = leader
def test_output_values_for_all_hosts(self):
r = CactiHandler().analyze(TestCactiHandler.Opts(), {
's1:2181':{'a':1},
's2:2181':{'a':2, 'b':3}
})
self.assertEqual(r, None)
self.assertEqual(self.output(), 's1_2181:1 s2_2181:2')
def test_output_single_value_for_leader(self):
r = CactiHandler().analyze(TestCactiHandler.Opts(leader=True), {
's1:2181': {'a':1, 'zk_server_state': 'leader'},
's2:2181': {'a':2}
})
self.assertEqual(r, 0)
self.assertEqual(self.output(), '1\n')
class TestGangliaHandler(unittest.TestCase):
class TestableGangliaHandler(GangliaHandler):
def __init__(self):
GangliaHandler.__init__(self)
self.cli_calls = []
def call(self, cli):
self.cli_calls.append(' '.join(cli))
def test_send_single_metric(self):
class Opts(object):
@property
def gmetric(self): return '/usr/bin/gmetric'
opts = Opts()
h = TestGangliaHandler.TestableGangliaHandler()
h.analyze(opts, {'localhost:2181':{'latency':10}})
cmd = "%s -n latency -v 10 -t uint32" % opts.gmetric
assert cmd in h.cli_calls
if __name__ == '__main__':
unittest.main()
| apache-2.0 |
mccheung/kbengine | kbe/res/scripts/common/Lib/__future__.py | 132 | 4584 | """Record of phased-in incompatible language changes.
Each line is of the form:
FeatureName = "_Feature(" OptionalRelease "," MandatoryRelease ","
CompilerFlag ")"
where, normally, OptionalRelease < MandatoryRelease, and both are 5-tuples
of the same form as sys.version_info:
(PY_MAJOR_VERSION, # the 2 in 2.1.0a3; an int
PY_MINOR_VERSION, # the 1; an int
PY_MICRO_VERSION, # the 0; an int
PY_RELEASE_LEVEL, # "alpha", "beta", "candidate" or "final"; string
PY_RELEASE_SERIAL # the 3; an int
)
OptionalRelease records the first release in which
from __future__ import FeatureName
was accepted.
In the case of MandatoryReleases that have not yet occurred,
MandatoryRelease predicts the release in which the feature will become part
of the language.
Else MandatoryRelease records when the feature became part of the language;
in releases at or after that, modules no longer need
from __future__ import FeatureName
to use the feature in question, but may continue to use such imports.
MandatoryRelease may also be None, meaning that a planned feature got
dropped.
Instances of class _Feature have two corresponding methods,
.getOptionalRelease() and .getMandatoryRelease().
CompilerFlag is the (bitfield) flag that should be passed in the fourth
argument to the builtin function compile() to enable the feature in
dynamically compiled code. This flag is stored in the .compiler_flag
attribute on _Future instances. These values must match the appropriate
#defines of CO_xxx flags in Include/compile.h.
No feature line is ever to be deleted from this file.
"""
all_feature_names = [
"nested_scopes",
"generators",
"division",
"absolute_import",
"with_statement",
"print_function",
"unicode_literals",
"barry_as_FLUFL",
]
__all__ = ["all_feature_names"] + all_feature_names
# The CO_xxx symbols are defined here under the same names used by
# compile.h, so that an editor search will find them here. However,
# they're not exported in __all__, because they don't really belong to
# this module.
CO_NESTED = 0x0010 # nested_scopes
CO_GENERATOR_ALLOWED = 0 # generators (obsolete, was 0x1000)
CO_FUTURE_DIVISION = 0x2000 # division
CO_FUTURE_ABSOLUTE_IMPORT = 0x4000 # perform absolute imports by default
CO_FUTURE_WITH_STATEMENT = 0x8000 # with statement
CO_FUTURE_PRINT_FUNCTION = 0x10000 # print function
CO_FUTURE_UNICODE_LITERALS = 0x20000 # unicode string literals
CO_FUTURE_BARRY_AS_BDFL = 0x40000
class _Feature:
def __init__(self, optionalRelease, mandatoryRelease, compiler_flag):
self.optional = optionalRelease
self.mandatory = mandatoryRelease
self.compiler_flag = compiler_flag
def getOptionalRelease(self):
"""Return first release in which this feature was recognized.
This is a 5-tuple, of the same form as sys.version_info.
"""
return self.optional
def getMandatoryRelease(self):
"""Return release in which this feature will become mandatory.
This is a 5-tuple, of the same form as sys.version_info, or, if
the feature was dropped, is None.
"""
return self.mandatory
def __repr__(self):
return "_Feature" + repr((self.optional,
self.mandatory,
self.compiler_flag))
nested_scopes = _Feature((2, 1, 0, "beta", 1),
(2, 2, 0, "alpha", 0),
CO_NESTED)
generators = _Feature((2, 2, 0, "alpha", 1),
(2, 3, 0, "final", 0),
CO_GENERATOR_ALLOWED)
division = _Feature((2, 2, 0, "alpha", 2),
(3, 0, 0, "alpha", 0),
CO_FUTURE_DIVISION)
absolute_import = _Feature((2, 5, 0, "alpha", 1),
(3, 0, 0, "alpha", 0),
CO_FUTURE_ABSOLUTE_IMPORT)
with_statement = _Feature((2, 5, 0, "alpha", 1),
(2, 6, 0, "alpha", 0),
CO_FUTURE_WITH_STATEMENT)
print_function = _Feature((2, 6, 0, "alpha", 2),
(3, 0, 0, "alpha", 0),
CO_FUTURE_PRINT_FUNCTION)
unicode_literals = _Feature((2, 6, 0, "alpha", 2),
(3, 0, 0, "alpha", 0),
CO_FUTURE_UNICODE_LITERALS)
barry_as_FLUFL = _Feature((3, 1, 0, "alpha", 2),
(3, 9, 0, "alpha", 0),
CO_FUTURE_BARRY_AS_BDFL)
| lgpl-3.0 |
sheagcraig/sal | server/plugins/memory/memory.py | 1 | 1365 | import sal.plugin
GB = 1024 ** 2
MEM_4_GB = 4 * GB
MEM_775_GB = 7.75 * GB
MEM_8_GB = 8 * GB
TITLES = {
'ok': 'Machines with more than 8GB memory',
'warning': 'Machines with between 4GB and 8GB memory',
'alert': 'Machines with less than 4GB memory'}
class Memory(sal.plugin.Widget):
description = 'Installed RAM'
template = 'plugins/traffic_lights.html'
def get_context(self, machines, **kwargs):
context = self.super_get_context(machines, **kwargs)
context['ok_count'] = self._filter(machines, 'ok').count()
context['ok_label'] = '8GB +'
context['warning_count'] = self._filter(machines, 'warning').count()
context['warning_label'] = '4GB +'
context['alert_count'] = self._filter(machines, 'alert').count()
context['alert_label'] = '< 4GB'
return context
def filter(self, machines, data):
if data not in TITLES:
return None, None
return self._filter(machines, data), TITLES[data]
def _filter(self, machines, data):
if data == 'ok':
machines = machines.filter(memory_kb__gte=MEM_8_GB)
elif data == 'warning':
machines = machines.filter(memory_kb__range=[MEM_4_GB, MEM_775_GB])
elif data == 'alert':
machines = machines.filter(memory_kb__lt=MEM_4_GB)
return machines
| apache-2.0 |
vitojph/flws | flws/flwspt.py | 1 | 13249 | #!/usr/bin/env python
# -*- coding: utf-8 -*-
"""
Simple flask-based API to access FreeLing functionalities.
"""
__author__ = "Víctor Peinado"
__email__ = "vitojph@gmail.com"
__date__ = "28/06/2013"
import freeling
from flask import Flask, Response, request
from flask.ext.restful import Api, Resource
import json
# #################################################################
# FreeLing settings (borrowed from freeling-3.0/APIs/python/sample.py)
PUNCTUATION = u""".,;:!? """
## Modify this line to be your FreeLing installation directory
FREELINGDIR = "/usr/local/"
DATA = FREELINGDIR + "share/freeling/"
LANG = "pt"
freeling.util_init_locale("default");
# Create language analyzer
#la=freeling.lang_ident(DATA + "common/lang_ident/ident.dat")
# Create options set for maco analyzer. Default values are Ok, except for data files.
op = freeling.maco_options(LANG)
op.set_active_modules(0,1,1,1,1,1,0,1,1,1,0)
op.set_data_files("",
DATA + LANG + "/locucions.dat",
#DATA + LANG + "/quantities.dat",
"",
DATA + LANG + "/afixos.dat",
DATA + LANG + "/probabilitats.dat",
DATA + LANG + "/dicc.src",
DATA + LANG + "/np.dat",
DATA + "common/punct.dat",
DATA + LANG + "/corrector/corrector.dat")
# Create analyzers
tk = freeling.tokenizer(DATA + LANG + "/tokenizer.dat")
sp = freeling.splitter(DATA + LANG + "/splitter.dat")
mf = freeling.maco(op)
tg = freeling.hmm_tagger(LANG, DATA + LANG + "/tagger.dat", 1, 2)
sen = freeling.senses(DATA+LANG+"/senses.dat")
parser = freeling.chart_parser(DATA + LANG + "/chunker/grammar-chunk.dat")
# #################################################################
# flask API
app = Flask(__name__)
api = Api(app)
# ##############################################################################
def handleParsedTreeAsFL(tree, depth, output):
"""Handles a parsed tree"""
node = tree.get_info()
nch = tree.num_children()
# if node is head and has no children
if nch == 0:
if node.is_head():
w = node.get_word()
output.append("+(%s %s %s)" % (w.get_form(), w.get_lemma(), w.get_tag()))
else:
# if node is head and has children
if node.is_head():
output.append("+%s_[" % (node.get_label()))
else:
# if node has children but isn't head
output.append("%s_[" % (node.get_label()))
# for each children, repeat process
for i in range(nch):
child = tree.nth_child_ref(i)
handleParsedTreeAsFL(child, depth+1, output)
# close node
output.append("]")
return output
# ##############################################################################
def handleParsedTreeAsString(tree, depth, output):
"""Handles a parsed tree"""
node = tree.get_info()
nch = tree.num_children()
parent = tree.get_parent()
# if node is head and has no children
if nch == 0:
if node.is_head():
w = node.get_word()
output.append(u"%s/%s/%s" % (w.get_form(), w.get_lemma(), w.get_tag()))
else:
if depth > 0:
output.append(u"%s(" % node.get_label())
# for each children, repeat process
for i in range(nch):
child = tree.nth_child_ref(i)
handleParsedTreeAsString(child, depth+1, output)
if depth > 0:
output.append(u")")
return output
# ##############################################################################
def handleParsedTreeAsJSON(tree, depth, output):
"""Handles a parsed tree"""
node = tree.get_info()
nch = tree.num_children()
parent = tree.get_parent()
# if node is head and has no children
if nch == 0:
if node.is_head():
w = node.get_word()
output.append(dict(text=w.get_form(), lemma=w.get_lemma(), tag=w.get_tag(), parent=parent.get_info().get_label(), level=depth))
else:
if depth > 0:
output.append(dict(tag=node.get_label(), parent=parent.get_info().get_label(), level=depth))
# for each children, repeat process
for i in range(nch):
child = tree.nth_child_ref(i)
handleParsedTreeAsJSON(child, depth+1, output)
return output
# ##############################################################################
class Splitter(Resource):
"""Splits an input text into sentences."""
def post(self):
text = request.json["texto"]
if text[-1] not in PUNCTUATION:
text = text + "."
tokens = tk.tokenize(text)
sentences = sp.split(tokens, 0)
# output list of sentences
outputSentences = []
for sentence in sentences:
outputTokens = []
for w in sentence.get_words():
outputTokens.append(w.get_form())
outputSentences.append(dict(oracion=" ".join(outputTokens)))
return Response(json.dumps(outputSentences), mimetype="application/json")
class TokenizerSplitter(Resource):
"""Splits an input text into tokenized sentences."""
def post(self):
text = request.json["texto"]
if text[-1] not in PUNCTUATION:
text = text + "."
tokens = tk.tokenize(text)
sentences = sp.split(tokens, 0)
# output list of sentences
outputSentences = []
for sentence in sentences:
outputTokens = []
for w in sentence.get_words():
outputTokens.append(w.get_form())
outputSentences.append(dict(oracion=outputTokens))
return Response(json.dumps(outputSentences), mimetype="application/json")
# ##############################################################################
class NERecognizer(Resource):
"""Recognizes Named Entities from an input text."""
def post(self):
text = request.json["texto"]
if text[-1] not in PUNCTUATION:
text = text + "."
tokens = tk.tokenize(text)
sentences = sp.split(tokens, 0)
sentences = mf.analyze(sentences)
sentences = tg.analyze(sentences)
output = []
for sentence in sentences:
words = sentence.get_words()
for word in words:
# Person (NP00SP0), Geographical location (NP00G00), Organization (NP00O00), and Others (NP00V00)
if word.get_tag() in "NP00SP0 NP00G00 NP00000 NP00V00".split():
entities = []
entities.append(dict(lema=word.get_lemma(), categoria=word.get_tag()))
output.append(dict(palabra=word.get_form(), entidades=entities))
return Response(json.dumps(output), mimetype="application/json")
# ##############################################################################
class DatesQuatitiesRecognizer(Resource):
"""Recognizes dates, currencies, and quatities from an input text."""
def post(self):
text = request.json["texto"]
if text[-1] not in PUNCTUATION:
text = text + "."
tokens = tk.tokenize(text)
sentences = sp.split(tokens, 0)
sentences = mf.analyze(sentences)
sentences = tg.analyze(sentences)
output = []
for sentence in sentences:
words = sentence.get_words()
for word in words:
# dates
tag = word.get_tag()
if tag[0] in "W Z".split():
expression = []
if tag == "W":
expression.append(dict(lema=word.get_lemma(), categoria="temporal"))
else:
if tag == "Z":
category = "numero"
elif tag == "Zd":
category = "partitivo"
elif tag == "Zm":
category = "moneda"
elif tag == "Zp":
category = "porcentaje"
elif tag == "Zu":
category = "magnitud"
else:
category = "numero"
expression.append(dict(lema=word.get_lemma(), categoria=category))
output.append(dict(expresion=word.get_form(), entidades=expression))
return Response(json.dumps(output), mimetype="application/json")
# ##############################################################################
class Tagger(Resource):
"""Performs POS tagging from an input text."""
def post(self):
"""docstring for post"""
text = request.json["texto"]
if text[-1] not in PUNCTUATION:
text = text + "."
tokens = tk.tokenize(text)
sentences = sp.split(tokens, 0)
sentences = mf.analyze(sentences)
sentences = tg.analyze(sentences)
output = []
for sentence in sentences:
words = sentence.get_words()
for word in words:
lemmas = []
lemmas.append(dict(lema=word.get_lemma(), categoria=word.get_tag()))
output.append(dict(palabra=word.get_form(), lemas=lemmas))
return Response(json.dumps(output), mimetype="application/json")
# ##############################################################################
class WSDTagger(Resource):
"""Performs POS tagging and WSD from an input text."""
def post(self):
"""docstring for post"""
text = request.json["texto"]
if text[-1] not in PUNCTUATION:
text = text + "."
tokens = tk.tokenize(text)
sentences = sp.split(tokens, 0)
sentences = mf.analyze(sentences)
sentences = tg.analyze(sentences)
sentences = sen.analyze(sentences)
output = []
for sentence in sentences:
words = sentence.get_words()
for word in words:
lemmas = []
lemmas.append(dict(lema=word.get_lemma(), categoria=word.get_tag()))
# split the senses and get just the synset ID
synsets = []
[synsets.append(synsetID.split(":")[0]) for synsetID in word.get_senses_string().split("/")]
output.append(dict(palabra=word.get_form(), lemas=lemmas, synsets=synsets))
return Response(json.dumps(output), mimetype="application/json")
# ##############################################################################
class Parser(Resource):
"""FreeLing parser with three output formats: freeling-like, stanford-like and jsonified"""
def post(self):
"""docstring for post"""
text = request.json["texto"]
try:
format = request.json["format"]
except KeyError:
format = "json"
if text[-1] not in PUNCTUATION:
text = text + "."
tokens = tk.tokenize(text)
sentences = sp.split(tokens, 0)
sentences = mf.analyze(sentences)
sentences = tg.analyze(sentences)
sentences = sen.analyze(sentences)
sentences = parser.analyze(sentences)
# set up the output format
parsedtree = []
for sentence in sentences:
tree = sentence.get_parse_tree()
if format == "fl":
parsedtree = handleParsedTreeAsFL(tree.begin(), 0, parsedtree)
elif format == "string":
# add the S(entence) tag
parsedtree.append("S(")
parsedtree = handleParsedTreeAsString(tree.begin(), 0, parsedtree)
# close the (S)entence
parsedtree.append(")")
elif format == "json":
# add the S tag with parent ROOT
parsedtree.append(dict(tag="S", parent="ROOT", level=0))
parsedtree = handleParsedTreeAsJSON(tree.begin(), 0, parsedtree)
# format the output accordingly
if format == "fl" or format == "string":
return Response(json.dumps(dict(tree=" ".join(parsedtree))), mimetype="application/json")
elif format == "json":
return Response(json.dumps(parsedtree), mimetype="application/json")
# #############################################################################
# Api resource routing
# split a text into sentences
api.add_resource(Splitter, "/splitter")
# split a text into tokenized sentences
api.add_resource(TokenizerSplitter, "/tokenizersplitter")
# perform PoS tagging from an input text
api.add_resource(Tagger, "/tagger")
# perform PoS tagging and WSD from an input text
api.add_resource(WSDTagger, "/wsdtagger")
# perform NE recognition from an input text
api.add_resource(NERecognizer, "/ner")
# recognizes dates, currencies and quantities
api.add_resource(DatesQuatitiesRecognizer, "/datesquantities")
# returns a parsed tree
api.add_resource(Parser, "/parser")
if __name__ == '__main__':
app.run(debug=True, host="0.0.0.0", port=9999)
| apache-2.0 |
DevinZ1993/regular_2015 | python/cs544/hmm/hmmlearn.py | 4 | 2178 | #! /usr/bin/env python
# -*- coding: utf-8 -*-
import sys, math, codecs
def train(path, pi_smooth, a_smooth):
tags = dict()
with codecs.open(path, 'r', 'utf-8') as fin:
line = fin.readline()
while line:
toks = line.strip().split(' ')
for tok in toks:
tag = tok[tok.rfind('/')+1:]
if tag not in tags:
tags[tag] = 1
else:
tags[tag] += 1
line = fin.readline()
pi = dict((tag, pi_smooth) for tag in tags)
A = dict((tag, dict((ntag, a_smooth) for ntag in tags)) for tag in tags)
B = dict((tag, dict()) for tag in tags)
with codecs.open(path, 'r', 'utf-8') as fin:
line = fin.readline()
while line:
toks = line.strip().split(' ')
for idx, tok in enumerate(toks):
word = tok[:tok.rfind('/')]
tag = tok[tok.rfind('/')+1:]
if word not in B[tag]:
B[tag][word] = 1
else:
B[tag][word] += 1
if 0 == idx:
pi[tag] += 1
else:
A[pre_tag][tag] += 1
pre_tag = tag
line = fin.readline()
sum_of_pi = sum(pi[tag] for tag in pi)
with codecs.open("hmmmodel.txt",'w', 'utf-8') as fout:
for tag, cnt in tags.items():
pi_val = float('-inf') if 0 == pi[tag] else math.log(pi[tag])-math.log(sum_of_pi)
fout.write(tag+" "+str(pi_val)+"\n")
sum_of_a = sum(A[tag][ntag] for ntag in A[tag])
for ntag in A[tag]:
a_val = float('-inf') if 0 == A[tag][ntag] else math.log(A[tag][ntag])-math.log(sum_of_a)
fout.write(ntag+":"+str(a_val)+" ")
fout.write("\n")
sum_of_b = sum(B[tag][word] for word in B[tag])
for word in B[tag]:
b_val = float('-inf') if 0 == B[tag][word] else math.log(B[tag][word])-math.log(sum_of_b)
fout.write(word+":"+str(b_val)+" ")
fout.write("\n")
if __name__ == '__main__':
train(sys.argv[1], 0, 1)
| mpl-2.0 |
android-ia/platform_external_chromium_org | chrome/common/extensions/docs/server2/caching_file_system_test.py | 26 | 11580 | #!/usr/bin/env python
# Copyright (c) 2012 The Chromium Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
import os
import sys
import unittest
from caching_file_system import CachingFileSystem
from extensions_paths import SERVER2
from file_system import FileNotFoundError, StatInfo
from local_file_system import LocalFileSystem
from mock_file_system import MockFileSystem
from object_store_creator import ObjectStoreCreator
from test_file_system import TestFileSystem
from test_object_store import TestObjectStore
def _CreateLocalFs():
return LocalFileSystem.Create(SERVER2, 'test_data', 'file_system/')
class CachingFileSystemTest(unittest.TestCase):
def setUp(self):
# Use this to make sure that every time _CreateCachingFileSystem is called
# the underlying object store data is the same, within each test.
self._object_store_dbs = {}
def _CreateCachingFileSystem(self, fs, start_empty=False):
def store_type_constructor(namespace, start_empty=False):
'''Returns an ObjectStore backed onto test-lifetime-persistent objects
in |_object_store_dbs|.
'''
if namespace not in self._object_store_dbs:
self._object_store_dbs[namespace] = {}
db = self._object_store_dbs[namespace]
if start_empty:
db.clear()
return TestObjectStore(namespace, init=db)
object_store_creator = ObjectStoreCreator(start_empty=start_empty,
store_type=store_type_constructor)
return CachingFileSystem(fs, object_store_creator)
def testReadFiles(self):
file_system = self._CreateCachingFileSystem(
_CreateLocalFs(), start_empty=False)
expected = {
'./test1.txt': 'test1\n',
'./test2.txt': 'test2\n',
'./test3.txt': 'test3\n',
}
self.assertEqual(
expected,
file_system.Read(['./test1.txt', './test2.txt', './test3.txt']).Get())
def testListDir(self):
file_system = self._CreateCachingFileSystem(
_CreateLocalFs(), start_empty=False)
expected = ['dir/'] + ['file%d.html' % i for i in range(7)]
file_system._read_cache.Set(
'list/',
(expected, file_system.Stat('list/').version))
self.assertEqual(expected, sorted(file_system.ReadSingle('list/').Get()))
expected.remove('file0.html')
file_system._read_cache.Set(
'list/',
(expected, file_system.Stat('list/').version))
self.assertEqual(expected, sorted(file_system.ReadSingle('list/').Get()))
def testCaching(self):
test_fs = TestFileSystem({
'bob': {
'bob0': 'bob/bob0 contents',
'bob1': 'bob/bob1 contents',
'bob2': 'bob/bob2 contents',
'bob3': 'bob/bob3 contents',
}
})
mock_fs = MockFileSystem(test_fs)
def create_empty_caching_fs():
return self._CreateCachingFileSystem(mock_fs, start_empty=True)
file_system = create_empty_caching_fs()
# The stat/read should happen before resolving the Future, and resolving
# the future shouldn't do any additional work.
get_future = file_system.ReadSingle('bob/bob0')
self.assertTrue(*mock_fs.CheckAndReset(read_count=1))
self.assertEqual('bob/bob0 contents', get_future.Get())
self.assertTrue(*mock_fs.CheckAndReset(read_resolve_count=1, stat_count=1))
# Resource has been cached, so test resource is not re-fetched.
self.assertEqual('bob/bob0 contents',
file_system.ReadSingle('bob/bob0').Get())
self.assertTrue(*mock_fs.CheckAndReset())
# Test if the Stat version is the same the resource is not re-fetched.
file_system = create_empty_caching_fs()
self.assertEqual('bob/bob0 contents',
file_system.ReadSingle('bob/bob0').Get())
self.assertTrue(*mock_fs.CheckAndReset(stat_count=1))
# Test if there is a newer version, the resource is re-fetched.
file_system = create_empty_caching_fs()
test_fs.IncrementStat();
future = file_system.ReadSingle('bob/bob0')
self.assertTrue(*mock_fs.CheckAndReset(read_count=1, stat_count=1))
self.assertEqual('bob/bob0 contents', future.Get())
self.assertTrue(*mock_fs.CheckAndReset(read_resolve_count=1))
# Test directory and subdirectory stats are cached.
file_system = create_empty_caching_fs()
file_system._stat_cache.Del('bob/bob0')
file_system._read_cache.Del('bob/bob0')
file_system._stat_cache.Del('bob/bob1')
test_fs.IncrementStat();
futures = (file_system.ReadSingle('bob/bob1'),
file_system.ReadSingle('bob/bob0'))
self.assertTrue(*mock_fs.CheckAndReset(read_count=2))
self.assertEqual(('bob/bob1 contents', 'bob/bob0 contents'),
tuple(future.Get() for future in futures))
self.assertTrue(*mock_fs.CheckAndReset(read_resolve_count=2, stat_count=1))
self.assertEqual('bob/bob1 contents',
file_system.ReadSingle('bob/bob1').Get())
self.assertTrue(*mock_fs.CheckAndReset())
# Test a more recent parent directory doesn't force a refetch of children.
file_system = create_empty_caching_fs()
file_system._read_cache.Del('bob/bob0')
file_system._read_cache.Del('bob/bob1')
futures = (file_system.ReadSingle('bob/bob1'),
file_system.ReadSingle('bob/bob2'),
file_system.ReadSingle('bob/bob3'))
self.assertTrue(*mock_fs.CheckAndReset(read_count=3))
self.assertEqual(
('bob/bob1 contents', 'bob/bob2 contents', 'bob/bob3 contents'),
tuple(future.Get() for future in futures))
self.assertTrue(*mock_fs.CheckAndReset(read_resolve_count=3, stat_count=1))
test_fs.IncrementStat(path='bob/bob0')
file_system = create_empty_caching_fs()
self.assertEqual('bob/bob1 contents',
file_system.ReadSingle('bob/bob1').Get())
self.assertEqual('bob/bob2 contents',
file_system.ReadSingle('bob/bob2').Get())
self.assertEqual('bob/bob3 contents',
file_system.ReadSingle('bob/bob3').Get())
self.assertTrue(*mock_fs.CheckAndReset(stat_count=1))
file_system = create_empty_caching_fs()
file_system._stat_cache.Del('bob/bob0')
future = file_system.ReadSingle('bob/bob0')
self.assertTrue(*mock_fs.CheckAndReset(read_count=1))
self.assertEqual('bob/bob0 contents', future.Get())
self.assertTrue(*mock_fs.CheckAndReset(read_resolve_count=1, stat_count=1))
self.assertEqual('bob/bob0 contents',
file_system.ReadSingle('bob/bob0').Get())
self.assertTrue(*mock_fs.CheckAndReset())
# Test skip_not_found caching behavior.
file_system = create_empty_caching_fs()
future = file_system.ReadSingle('bob/no_file', skip_not_found=True)
self.assertTrue(*mock_fs.CheckAndReset(read_count=1))
self.assertEqual(None, future.Get())
self.assertTrue(*mock_fs.CheckAndReset(read_resolve_count=1, stat_count=1))
future = file_system.ReadSingle('bob/no_file', skip_not_found=True)
# There shouldn't be another read/stat from the file system;
# we know the file is not there.
self.assertTrue(*mock_fs.CheckAndReset())
future = file_system.ReadSingle('bob/no_file')
self.assertTrue(*mock_fs.CheckAndReset(read_count=1))
# Even though we cached information about non-existent files,
# trying to read one without specifiying skip_not_found should
# still raise an error.
self.assertRaises(FileNotFoundError, future.Get)
def testCachedStat(self):
test_fs = TestFileSystem({
'bob': {
'bob0': 'bob/bob0 contents',
'bob1': 'bob/bob1 contents'
}
})
mock_fs = MockFileSystem(test_fs)
file_system = self._CreateCachingFileSystem(mock_fs, start_empty=False)
self.assertEqual(StatInfo('0'), file_system.Stat('bob/bob0'))
self.assertTrue(*mock_fs.CheckAndReset(stat_count=1))
self.assertEqual(StatInfo('0'), file_system.Stat('bob/bob0'))
self.assertTrue(*mock_fs.CheckAndReset())
# Caching happens on a directory basis, so reading other files from that
# directory won't result in a stat.
self.assertEqual(StatInfo('0'), file_system.Stat('bob/bob1'))
self.assertEqual(
StatInfo('0', child_versions={'bob0': '0', 'bob1': '0'}),
file_system.Stat('bob/'))
self.assertTrue(*mock_fs.CheckAndReset())
# Even though the stat is bumped, the object store still has it cached so
# this won't update.
test_fs.IncrementStat()
self.assertEqual(StatInfo('0'), file_system.Stat('bob/bob0'))
self.assertEqual(StatInfo('0'), file_system.Stat('bob/bob1'))
self.assertEqual(
StatInfo('0', child_versions={'bob0': '0', 'bob1': '0'}),
file_system.Stat('bob/'))
self.assertTrue(*mock_fs.CheckAndReset())
def testFreshStat(self):
test_fs = TestFileSystem({
'bob': {
'bob0': 'bob/bob0 contents',
'bob1': 'bob/bob1 contents'
}
})
mock_fs = MockFileSystem(test_fs)
def run_expecting_stat(stat):
def run():
file_system = self._CreateCachingFileSystem(mock_fs, start_empty=True)
self.assertEqual(
StatInfo(stat, child_versions={'bob0': stat, 'bob1': stat}),
file_system.Stat('bob/'))
self.assertTrue(*mock_fs.CheckAndReset(stat_count=1))
self.assertEqual(StatInfo(stat), file_system.Stat('bob/bob0'))
self.assertEqual(StatInfo(stat), file_system.Stat('bob/bob0'))
self.assertTrue(*mock_fs.CheckAndReset())
run()
run()
run_expecting_stat('0')
test_fs.IncrementStat()
run_expecting_stat('1')
def testSkipNotFound(self):
caching_fs = self._CreateCachingFileSystem(TestFileSystem({
'bob': {
'bob0': 'bob/bob0 contents',
'bob1': 'bob/bob1 contents'
}
}))
def read_skip_not_found(paths):
return caching_fs.Read(paths, skip_not_found=True).Get()
self.assertEqual({}, read_skip_not_found(('grub',)))
self.assertEqual({}, read_skip_not_found(('bob/bob2',)))
self.assertEqual({
'bob/bob0': 'bob/bob0 contents',
}, read_skip_not_found(('bob/bob0', 'bob/bob2')))
def testWalkCaching(self):
test_fs = TestFileSystem({
'root': {
'file1': 'file1',
'file2': 'file2',
'dir1': {
'dir1_file1': 'dir1_file1',
'dir2': {},
'dir3': {
'dir3_file1': 'dir3_file1',
'dir3_file2': 'dir3_file2'
}
}
}
})
mock_fs = MockFileSystem(test_fs)
file_system = self._CreateCachingFileSystem(mock_fs, start_empty=True)
for walkinfo in file_system.Walk(''):
pass
self.assertTrue(*mock_fs.CheckAndReset(
read_resolve_count=5, read_count=5, stat_count=5))
all_dirs, all_files = [], []
for root, dirs, files in file_system.Walk(''):
all_dirs.extend(dirs)
all_files.extend(files)
self.assertEqual(sorted(['root/', 'dir1/', 'dir2/', 'dir3/']),
sorted(all_dirs))
self.assertEqual(
sorted(['file1', 'file2', 'dir1_file1', 'dir3_file1', 'dir3_file2']),
sorted(all_files))
# All data should be cached.
self.assertTrue(*mock_fs.CheckAndReset())
# Starting from a different root should still pull cached data.
for walkinfo in file_system.Walk('root/dir1/'):
pass
self.assertTrue(*mock_fs.CheckAndReset())
# TODO(ahernandez): Test with a new instance CachingFileSystem so a
# different object store is utilized.
if __name__ == '__main__':
unittest.main()
| bsd-3-clause |
abstract-open-solutions/hr | __unported__/hr_job_hierarchy/hr.py | 27 | 8232 | # -*- coding:utf-8 -*-
#
#
# Copyright (C) 2013 Michael Telahun Makonnen <mmakonnen@gmail.com>.
# All Rights Reserved.
#
# This program is free software: you can redistribute it and/or modify
# it under the terms of the GNU Affero General Public License as published
# by the Free Software Foundation, either version 3 of the License, or
# (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU Affero General Public License for more details.
#
# You should have received a copy of the GNU Affero General Public License
# along with this program. If not, see <http://www.gnu.org/licenses/>.
#
#
from openerp.osv import fields, orm
from openerp.tools.translate import _
import logging
_l = logging.getLogger(__name__)
class hr_job(orm.Model):
_name = 'hr.job'
_inherit = 'hr.job'
def _get_all_child_ids(self, cr, uid, ids, field_name, arg, context=None):
result = dict.fromkeys(ids)
for i in ids:
result[i] = self.search(
cr, uid, [('parent_id', 'child_of', i)], context=context)
return result
_columns = {
'department_manager': fields.boolean(
'Department Manager',
),
'parent_id': fields.many2one(
'hr.job',
'Immediate Superior',
ondelete='cascade',
),
'child_ids': fields.one2many(
'hr.job',
'parent_id',
'Immediate Subordinates',
),
'all_child_ids': fields.function(
_get_all_child_ids,
type='many2many',
relation='hr.job',
),
'parent_left': fields.integer(
'Left Parent',
select=1,
),
'parent_right': fields.integer(
'Right Parent',
select=1,
),
}
_parent_name = "parent_id"
_parent_store = True
_parent_order = 'name'
_order = 'parent_left'
def _check_recursion(self, cr, uid, ids, context=None):
# Copied from product.category
# This is a brute-force approach to the problem, but should be good
# enough.
#
level = 100
while len(ids):
cr.execute(
'select distinct parent_id from hr_job where id IN %s',
(tuple(ids), )
)
ids = filter(None, map(lambda x: x[0], cr.fetchall()))
if not level:
return False
level -= 1
return True
def _rec_message(self, cr, uid, ids, context=None):
return _('Error!\nYou cannot create recursive jobs.')
_constraints = [
(_check_recursion, _rec_message, ['parent_id']),
]
def write(self, cr, uid, ids, vals, context=None):
res = super(hr_job, self).write(cr, uid, ids, vals, context=None)
if isinstance(ids, (int, long)):
ids = [ids]
dept_obj = self.pool.get('hr.department')
if vals.get('department_manager', False):
for di in ids:
job = self.browse(cr, uid, di, context=context)
dept_id = False
if vals.get('department_id', False):
dept_id = vals['department_id']
else:
dept_id = job.department_id.id
employee_id = False
for ee in job.employee_ids:
employee_id = ee.id
if employee_id:
dept_obj.write(
cr, uid, dept_id, {
'manager_id': employee_id,
}, context=context
)
elif vals.get('department_id', False):
for di in ids:
job = self.browse(cr, uid, di, context=context)
if job.department_manager:
employee_id = False
for ee in job.employee_ids:
employee_id = ee.id
dept_obj.write(
cr, uid, vals['department_id'], {
'manager_id': employee_id,
}, context=context
)
elif vals.get('parent_id', False):
ee_obj = self.pool.get('hr.employee')
parent_job = self.browse(
cr, uid, vals['parent_id'], context=context)
parent_id = False
for ee in parent_job.employee_ids:
parent_id = ee.id
for job in self.browse(cr, uid, ids, context=context):
ee_obj.write(
cr, uid, [ee.id for ee in job.employee_ids], {
'parent_id': parent_id,
}, context=context
)
return res
class hr_contract(orm.Model):
_name = 'hr.contract'
_inherit = 'hr.contract'
def create(self, cr, uid, vals, context=None):
res = super(hr_contract, self).create(cr, uid, vals, context=context)
if not vals.get('job_id', False):
return res
ee_obj = self.pool.get('hr.employee')
job = self.pool.get('hr.job').browse(
cr, uid, vals['job_id'], context=context)
# Write the employee's manager
if job and job.parent_id:
parent_id = False
for ee in job.parent_id.employee_ids:
parent_id = ee.id
if parent_id and vals.get('employee_id'):
ee_obj.write(
cr, uid, vals['employee_id'], {'parent_id': parent_id},
context=context)
# Write any employees with jobs that are immediate descendants of this
# job
if job:
job_child_ids = [child.id for child in job.child_ids]
if len(job_child_ids) > 0:
ee_ids = ee_obj.search(
cr, uid, [('job_id', 'in', job_child_ids)])
if len(ee_ids) > 0:
parent_id = False
for ee in job.employee_ids:
parent_id = ee.id
if parent_id:
ee_obj.write(
cr, uid, ee_ids, {
'parent_id': parent_id,
}, context=context
)
return res
def write(self, cr, uid, ids, vals, context=None):
res = super(hr_contract, self).write(
cr, uid, ids, vals, context=context)
if not vals.get('job_id', False):
return res
if isinstance(ids, (int, long)):
ids = [ids]
ee_obj = self.pool.get('hr.employee')
job = self.pool.get('hr.job').browse(
cr, uid, vals['job_id'], context=context)
# Write the employee's manager
if job and job.parent_id:
parent_id = False
for ee in job.parent_id.employee_ids:
parent_id = ee.id
if parent_id:
for contract in self.browse(cr, uid, ids, context=context):
ee_obj.write(cr, uid, contract.employee_id.id, {
'parent_id': parent_id}, context=context)
# Write any employees with jobs that are immediate descendants of this
# job
if job:
job_child_ids = []
[job_child_ids.append(child.id) for child in job.child_ids]
if len(job_child_ids) > 0:
ee_ids = ee_obj.search(
cr, uid, [('job_id', 'in', job_child_ids),
('active', '=', True)])
if len(ee_ids) > 0:
parent_id = False
for ee in job.employee_ids:
parent_id = ee.id
if parent_id:
ee_obj.write(
cr, uid, ee_ids, {
'parent_id': parent_id,
}, context=context
)
return res
| agpl-3.0 |
googleapis/python-workflows | samples/generated_samples/workflows_v1beta_generated_workflows_list_workflows_async.py | 1 | 1909 | # -*- coding: utf-8 -*-
# Copyright 2022 Google LLC
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#
# Generated code. DO NOT EDIT!
#
# Snippet for ListWorkflows
# NOTE: This snippet has been automatically generated for illustrative purposes only.
# It may require modifications to work in your environment.
# To install the latest published package dependency, execute the following:
# python3 -m pip install google-cloud-workflows
# [START workflows_v1beta_generated_Workflows_ListWorkflows_async]
# This snippet has been automatically generated and should be regarded as a
# code template only.
# It will require modifications to work:
# - It may require correct/in-range values for request initialization.
# - It may require specifying regional endpoints when creating the service
# client as shown in:
# https://googleapis.dev/python/google-api-core/latest/client_options.html
from google.cloud import workflows_v1beta
async def sample_list_workflows():
# Create a client
client = workflows_v1beta.WorkflowsAsyncClient()
# Initialize request argument(s)
request = workflows_v1beta.ListWorkflowsRequest(
parent="parent_value",
)
# Make the request
page_result = client.list_workflows(request=request)
# Handle the response
async for response in page_result:
print(response)
# [END workflows_v1beta_generated_Workflows_ListWorkflows_async]
| apache-2.0 |
ryfeus/lambda-packs | LightGBM_sklearn_scipy_numpy/source/scipy/signal/signaltools.py | 4 | 117066 | # Author: Travis Oliphant
# 1999 -- 2002
from __future__ import division, print_function, absolute_import
import operator
import threading
import sys
import timeit
from . import sigtools, dlti
from ._upfirdn import upfirdn, _output_len
from scipy._lib.six import callable
from scipy._lib._version import NumpyVersion
from scipy import fftpack, linalg
from numpy import (allclose, angle, arange, argsort, array, asarray,
atleast_1d, atleast_2d, cast, dot, exp, expand_dims,
iscomplexobj, mean, ndarray, newaxis, ones, pi,
poly, polyadd, polyder, polydiv, polymul, polysub, polyval,
product, r_, ravel, real_if_close, reshape,
roots, sort, take, transpose, unique, where, zeros,
zeros_like)
import numpy as np
import math
from scipy.special import factorial
from .windows import get_window
from ._arraytools import axis_slice, axis_reverse, odd_ext, even_ext, const_ext
from .filter_design import cheby1, _validate_sos
from .fir_filter_design import firwin
if sys.version_info.major >= 3 and sys.version_info.minor >= 5:
from math import gcd
else:
from fractions import gcd
__all__ = ['correlate', 'fftconvolve', 'convolve', 'convolve2d', 'correlate2d',
'order_filter', 'medfilt', 'medfilt2d', 'wiener', 'lfilter',
'lfiltic', 'sosfilt', 'deconvolve', 'hilbert', 'hilbert2',
'cmplx_sort', 'unique_roots', 'invres', 'invresz', 'residue',
'residuez', 'resample', 'resample_poly', 'detrend',
'lfilter_zi', 'sosfilt_zi', 'sosfiltfilt', 'choose_conv_method',
'filtfilt', 'decimate', 'vectorstrength']
_modedict = {'valid': 0, 'same': 1, 'full': 2}
_boundarydict = {'fill': 0, 'pad': 0, 'wrap': 2, 'circular': 2, 'symm': 1,
'symmetric': 1, 'reflect': 4}
_rfft_mt_safe = (NumpyVersion(np.__version__) >= '1.9.0.dev-e24486e')
_rfft_lock = threading.Lock()
def _valfrommode(mode):
try:
val = _modedict[mode]
except KeyError:
if mode not in [0, 1, 2]:
raise ValueError("Acceptable mode flags are 'valid' (0),"
" 'same' (1), or 'full' (2).")
val = mode
return val
def _bvalfromboundary(boundary):
try:
val = _boundarydict[boundary] << 2
except KeyError:
if val not in [0, 1, 2]:
raise ValueError("Acceptable boundary flags are 'fill', 'wrap'"
" (or 'circular'), \n and 'symm'"
" (or 'symmetric').")
val = boundary << 2
return val
def _inputs_swap_needed(mode, shape1, shape2):
"""
If in 'valid' mode, returns whether or not the input arrays need to be
swapped depending on whether `shape1` is at least as large as `shape2` in
every dimension.
This is important for some of the correlation and convolution
implementations in this module, where the larger array input needs to come
before the smaller array input when operating in this mode.
Note that if the mode provided is not 'valid', False is immediately
returned.
"""
if mode == 'valid':
ok1, ok2 = True, True
for d1, d2 in zip(shape1, shape2):
if not d1 >= d2:
ok1 = False
if not d2 >= d1:
ok2 = False
if not (ok1 or ok2):
raise ValueError("For 'valid' mode, one must be at least "
"as large as the other in every dimension")
return not ok1
return False
def correlate(in1, in2, mode='full', method='auto'):
r"""
Cross-correlate two N-dimensional arrays.
Cross-correlate `in1` and `in2`, with the output size determined by the
`mode` argument.
Parameters
----------
in1 : array_like
First input.
in2 : array_like
Second input. Should have the same number of dimensions as `in1`.
mode : str {'full', 'valid', 'same'}, optional
A string indicating the size of the output:
``full``
The output is the full discrete linear cross-correlation
of the inputs. (Default)
``valid``
The output consists only of those elements that do not
rely on the zero-padding. In 'valid' mode, either `in1` or `in2`
must be at least as large as the other in every dimension.
``same``
The output is the same size as `in1`, centered
with respect to the 'full' output.
method : str {'auto', 'direct', 'fft'}, optional
A string indicating which method to use to calculate the correlation.
``direct``
The correlation is determined directly from sums, the definition of
correlation.
``fft``
The Fast Fourier Transform is used to perform the correlation more
quickly (only available for numerical arrays.)
``auto``
Automatically chooses direct or Fourier method based on an estimate
of which is faster (default). See `convolve` Notes for more detail.
.. versionadded:: 0.19.0
Returns
-------
correlate : array
An N-dimensional array containing a subset of the discrete linear
cross-correlation of `in1` with `in2`.
See Also
--------
choose_conv_method : contains more documentation on `method`.
Notes
-----
The correlation z of two d-dimensional arrays x and y is defined as::
z[...,k,...] = sum[..., i_l, ...] x[..., i_l,...] * conj(y[..., i_l - k,...])
This way, if x and y are 1-D arrays and ``z = correlate(x, y, 'full')`` then
.. math::
z[k] = (x * y)(k - N + 1)
= \sum_{l=0}^{||x||-1}x_l y_{l-k+N-1}^{*}
for :math:`k = 0, 1, ..., ||x|| + ||y|| - 2`
where :math:`||x||` is the length of ``x``, :math:`N = \max(||x||,||y||)`,
and :math:`y_m` is 0 when m is outside the range of y.
``method='fft'`` only works for numerical arrays as it relies on
`fftconvolve`. In certain cases (i.e., arrays of objects or when
rounding integers can lose precision), ``method='direct'`` is always used.
Examples
--------
Implement a matched filter using cross-correlation, to recover a signal
that has passed through a noisy channel.
>>> from scipy import signal
>>> sig = np.repeat([0., 1., 1., 0., 1., 0., 0., 1.], 128)
>>> sig_noise = sig + np.random.randn(len(sig))
>>> corr = signal.correlate(sig_noise, np.ones(128), mode='same') / 128
>>> import matplotlib.pyplot as plt
>>> clock = np.arange(64, len(sig), 128)
>>> fig, (ax_orig, ax_noise, ax_corr) = plt.subplots(3, 1, sharex=True)
>>> ax_orig.plot(sig)
>>> ax_orig.plot(clock, sig[clock], 'ro')
>>> ax_orig.set_title('Original signal')
>>> ax_noise.plot(sig_noise)
>>> ax_noise.set_title('Signal with noise')
>>> ax_corr.plot(corr)
>>> ax_corr.plot(clock, corr[clock], 'ro')
>>> ax_corr.axhline(0.5, ls=':')
>>> ax_corr.set_title('Cross-correlated with rectangular pulse')
>>> ax_orig.margins(0, 0.1)
>>> fig.tight_layout()
>>> fig.show()
"""
in1 = asarray(in1)
in2 = asarray(in2)
if in1.ndim == in2.ndim == 0:
return in1 * in2
elif in1.ndim != in2.ndim:
raise ValueError("in1 and in2 should have the same dimensionality")
# Don't use _valfrommode, since correlate should not accept numeric modes
try:
val = _modedict[mode]
except KeyError:
raise ValueError("Acceptable mode flags are 'valid',"
" 'same', or 'full'.")
# this either calls fftconvolve or this function with method=='direct'
if method in ('fft', 'auto'):
return convolve(in1, _reverse_and_conj(in2), mode, method)
# fastpath to faster numpy.correlate for 1d inputs when possible
if _np_conv_ok(in1, in2, mode):
return np.correlate(in1, in2, mode)
# _correlateND is far slower when in2.size > in1.size, so swap them
# and then undo the effect afterward if mode == 'full'. Also, it fails
# with 'valid' mode if in2 is larger than in1, so swap those, too.
# Don't swap inputs for 'same' mode, since shape of in1 matters.
swapped_inputs = ((mode == 'full') and (in2.size > in1.size) or
_inputs_swap_needed(mode, in1.shape, in2.shape))
if swapped_inputs:
in1, in2 = in2, in1
if mode == 'valid':
ps = [i - j + 1 for i, j in zip(in1.shape, in2.shape)]
out = np.empty(ps, in1.dtype)
z = sigtools._correlateND(in1, in2, out, val)
else:
ps = [i + j - 1 for i, j in zip(in1.shape, in2.shape)]
# zero pad input
in1zpadded = np.zeros(ps, in1.dtype)
sc = [slice(0, i) for i in in1.shape]
in1zpadded[sc] = in1.copy()
if mode == 'full':
out = np.empty(ps, in1.dtype)
elif mode == 'same':
out = np.empty(in1.shape, in1.dtype)
z = sigtools._correlateND(in1zpadded, in2, out, val)
if swapped_inputs:
# Reverse and conjugate to undo the effect of swapping inputs
z = _reverse_and_conj(z)
return z
def _centered(arr, newshape):
# Return the center newshape portion of the array.
newshape = asarray(newshape)
currshape = array(arr.shape)
startind = (currshape - newshape) // 2
endind = startind + newshape
myslice = [slice(startind[k], endind[k]) for k in range(len(endind))]
return arr[tuple(myslice)]
def fftconvolve(in1, in2, mode="full"):
"""Convolve two N-dimensional arrays using FFT.
Convolve `in1` and `in2` using the fast Fourier transform method, with
the output size determined by the `mode` argument.
This is generally much faster than `convolve` for large arrays (n > ~500),
but can be slower when only a few output values are needed, and can only
output float arrays (int or object array inputs will be cast to float).
As of v0.19, `convolve` automatically chooses this method or the direct
method based on an estimation of which is faster.
Parameters
----------
in1 : array_like
First input.
in2 : array_like
Second input. Should have the same number of dimensions as `in1`.
If operating in 'valid' mode, either `in1` or `in2` must be
at least as large as the other in every dimension.
mode : str {'full', 'valid', 'same'}, optional
A string indicating the size of the output:
``full``
The output is the full discrete linear convolution
of the inputs. (Default)
``valid``
The output consists only of those elements that do not
rely on the zero-padding.
``same``
The output is the same size as `in1`, centered
with respect to the 'full' output.
Returns
-------
out : array
An N-dimensional array containing a subset of the discrete linear
convolution of `in1` with `in2`.
Examples
--------
Autocorrelation of white noise is an impulse.
>>> from scipy import signal
>>> sig = np.random.randn(1000)
>>> autocorr = signal.fftconvolve(sig, sig[::-1], mode='full')
>>> import matplotlib.pyplot as plt
>>> fig, (ax_orig, ax_mag) = plt.subplots(2, 1)
>>> ax_orig.plot(sig)
>>> ax_orig.set_title('White noise')
>>> ax_mag.plot(np.arange(-len(sig)+1,len(sig)), autocorr)
>>> ax_mag.set_title('Autocorrelation')
>>> fig.tight_layout()
>>> fig.show()
Gaussian blur implemented using FFT convolution. Notice the dark borders
around the image, due to the zero-padding beyond its boundaries.
The `convolve2d` function allows for other types of image boundaries,
but is far slower.
>>> from scipy import misc
>>> face = misc.face(gray=True)
>>> kernel = np.outer(signal.gaussian(70, 8), signal.gaussian(70, 8))
>>> blurred = signal.fftconvolve(face, kernel, mode='same')
>>> fig, (ax_orig, ax_kernel, ax_blurred) = plt.subplots(3, 1,
... figsize=(6, 15))
>>> ax_orig.imshow(face, cmap='gray')
>>> ax_orig.set_title('Original')
>>> ax_orig.set_axis_off()
>>> ax_kernel.imshow(kernel, cmap='gray')
>>> ax_kernel.set_title('Gaussian kernel')
>>> ax_kernel.set_axis_off()
>>> ax_blurred.imshow(blurred, cmap='gray')
>>> ax_blurred.set_title('Blurred')
>>> ax_blurred.set_axis_off()
>>> fig.show()
"""
in1 = asarray(in1)
in2 = asarray(in2)
if in1.ndim == in2.ndim == 0: # scalar inputs
return in1 * in2
elif not in1.ndim == in2.ndim:
raise ValueError("in1 and in2 should have the same dimensionality")
elif in1.size == 0 or in2.size == 0: # empty arrays
return array([])
s1 = array(in1.shape)
s2 = array(in2.shape)
complex_result = (np.issubdtype(in1.dtype, np.complexfloating) or
np.issubdtype(in2.dtype, np.complexfloating))
shape = s1 + s2 - 1
# Check that input sizes are compatible with 'valid' mode
if _inputs_swap_needed(mode, s1, s2):
# Convolution is commutative; order doesn't have any effect on output
in1, s1, in2, s2 = in2, s2, in1, s1
# Speed up FFT by padding to optimal size for FFTPACK
fshape = [fftpack.helper.next_fast_len(int(d)) for d in shape]
fslice = tuple([slice(0, int(sz)) for sz in shape])
# Pre-1.9 NumPy FFT routines are not threadsafe. For older NumPys, make
# sure we only call rfftn/irfftn from one thread at a time.
if not complex_result and (_rfft_mt_safe or _rfft_lock.acquire(False)):
try:
sp1 = np.fft.rfftn(in1, fshape)
sp2 = np.fft.rfftn(in2, fshape)
ret = (np.fft.irfftn(sp1 * sp2, fshape)[fslice].copy())
finally:
if not _rfft_mt_safe:
_rfft_lock.release()
else:
# If we're here, it's either because we need a complex result, or we
# failed to acquire _rfft_lock (meaning rfftn isn't threadsafe and
# is already in use by another thread). In either case, use the
# (threadsafe but slower) SciPy complex-FFT routines instead.
sp1 = fftpack.fftn(in1, fshape)
sp2 = fftpack.fftn(in2, fshape)
ret = fftpack.ifftn(sp1 * sp2)[fslice].copy()
if not complex_result:
ret = ret.real
if mode == "full":
return ret
elif mode == "same":
return _centered(ret, s1)
elif mode == "valid":
return _centered(ret, s1 - s2 + 1)
else:
raise ValueError("Acceptable mode flags are 'valid',"
" 'same', or 'full'.")
def _numeric_arrays(arrays, kinds='buifc'):
"""
See if a list of arrays are all numeric.
Parameters
----------
ndarrays : array or list of arrays
arrays to check if numeric.
numeric_kinds : string-like
The dtypes of the arrays to be checked. If the dtype.kind of
the ndarrays are not in this string the function returns False and
otherwise returns True.
"""
if type(arrays) == ndarray:
return arrays.dtype.kind in kinds
for array_ in arrays:
if array_.dtype.kind not in kinds:
return False
return True
def _prod(iterable):
"""
Product of a list of numbers.
Faster than np.prod for short lists like array shapes.
"""
product = 1
for x in iterable:
product *= x
return product
def _fftconv_faster(x, h, mode):
"""
See if using `fftconvolve` or `_correlateND` is faster. The boolean value
returned depends on the sizes and shapes of the input values.
The big O ratios were found to hold across different machines, which makes
sense as it's the ratio that matters (the effective speed of the computer
is found in both big O constants). Regardless, this had been tuned on an
early 2015 MacBook Pro with 8GB RAM and an Intel i5 processor.
"""
if mode == 'full':
out_shape = [n + k - 1 for n, k in zip(x.shape, h.shape)]
big_O_constant = 10963.92823819 if x.ndim == 1 else 8899.1104874
elif mode == 'same':
out_shape = x.shape
if x.ndim == 1:
if h.size <= x.size:
big_O_constant = 7183.41306773
else:
big_O_constant = 856.78174111
else:
big_O_constant = 34519.21021589
elif mode == 'valid':
out_shape = [n - k + 1 for n, k in zip(x.shape, h.shape)]
big_O_constant = 41954.28006344 if x.ndim == 1 else 66453.24316434
else:
raise ValueError('mode is invalid')
# see whether the Fourier transform convolution method or the direct
# convolution method is faster (discussed in scikit-image PR #1792)
direct_time = (x.size * h.size * _prod(out_shape))
fft_time = sum(n * math.log(n) for n in (x.shape + h.shape +
tuple(out_shape)))
return big_O_constant * fft_time < direct_time
def _reverse_and_conj(x):
"""
Reverse array `x` in all dimensions and perform the complex conjugate
"""
reverse = [slice(None, None, -1)] * x.ndim
return x[reverse].conj()
def _np_conv_ok(volume, kernel, mode):
"""
See if numpy supports convolution of `volume` and `kernel` (i.e. both are
1D ndarrays and of the appropriate shape). Numpy's 'same' mode uses the
size of the larger input, while Scipy's uses the size of the first input.
"""
np_conv_ok = volume.ndim == kernel.ndim == 1
return np_conv_ok and (volume.size >= kernel.size or mode != 'same')
def _timeit_fast(stmt="pass", setup="pass", repeat=3):
"""
Returns the time the statement/function took, in seconds.
Faster, less precise version of IPython's timeit. `stmt` can be a statement
written as a string or a callable.
Will do only 1 loop (like IPython's timeit) with no repetitions
(unlike IPython) for very slow functions. For fast functions, only does
enough loops to take 5 ms, which seems to produce similar results (on
Windows at least), and avoids doing an extraneous cycle that isn't
measured.
"""
timer = timeit.Timer(stmt, setup)
# determine number of calls per rep so total time for 1 rep >= 5 ms
x = 0
for p in range(0, 10):
number = 10**p
x = timer.timeit(number) # seconds
if x >= 5e-3 / 10: # 5 ms for final test, 1/10th that for this one
break
if x > 1: # second
# If it's macroscopic, don't bother with repetitions
best = x
else:
number *= 10
r = timer.repeat(repeat, number)
best = min(r)
sec = best / number
return sec
def choose_conv_method(in1, in2, mode='full', measure=False):
"""
Find the fastest convolution/correlation method.
This primarily exists to be called during the ``method='auto'`` option in
`convolve` and `correlate`, but can also be used when performing many
convolutions of the same input shapes and dtypes, determining
which method to use for all of them, either to avoid the overhead of the
'auto' option or to use accurate real-world measurements.
Parameters
----------
in1 : array_like
The first argument passed into the convolution function.
in2 : array_like
The second argument passed into the convolution function.
mode : str {'full', 'valid', 'same'}, optional
A string indicating the size of the output:
``full``
The output is the full discrete linear convolution
of the inputs. (Default)
``valid``
The output consists only of those elements that do not
rely on the zero-padding.
``same``
The output is the same size as `in1`, centered
with respect to the 'full' output.
measure : bool, optional
If True, run and time the convolution of `in1` and `in2` with both
methods and return the fastest. If False (default), predict the fastest
method using precomputed values.
Returns
-------
method : str
A string indicating which convolution method is fastest, either
'direct' or 'fft'
times : dict, optional
A dictionary containing the times (in seconds) needed for each method.
This value is only returned if ``measure=True``.
See Also
--------
convolve
correlate
Notes
-----
For large n, ``measure=False`` is accurate and can quickly determine the
fastest method to perform the convolution. However, this is not as
accurate for small n (when any dimension in the input or output is small).
In practice, we found that this function estimates the faster method up to
a multiplicative factor of 5 (i.e., the estimated method is *at most* 5
times slower than the fastest method). The estimation values were tuned on
an early 2015 MacBook Pro with 8GB RAM but we found that the prediction
held *fairly* accurately across different machines.
If ``measure=True``, time the convolutions. Because this function uses
`fftconvolve`, an error will be thrown if it does not support the inputs.
There are cases when `fftconvolve` supports the inputs but this function
returns `direct` (e.g., to protect against floating point integer
precision).
.. versionadded:: 0.19
Examples
--------
Estimate the fastest method for a given input:
>>> from scipy import signal
>>> a = np.random.randn(1000)
>>> b = np.random.randn(1000000)
>>> method = signal.choose_conv_method(a, b, mode='same')
>>> method
'fft'
This can then be applied to other arrays of the same dtype and shape:
>>> c = np.random.randn(1000)
>>> d = np.random.randn(1000000)
>>> # `method` works with correlate and convolve
>>> corr1 = signal.correlate(a, b, mode='same', method=method)
>>> corr2 = signal.correlate(c, d, mode='same', method=method)
>>> conv1 = signal.convolve(a, b, mode='same', method=method)
>>> conv2 = signal.convolve(c, d, mode='same', method=method)
"""
volume = asarray(in1)
kernel = asarray(in2)
if measure:
times = {}
for method in ['fft', 'direct']:
times[method] = _timeit_fast(lambda: convolve(volume, kernel,
mode=mode, method=method))
chosen_method = 'fft' if times['fft'] < times['direct'] else 'direct'
return chosen_method, times
# fftconvolve doesn't support complex256
fftconv_unsup = "complex256" if sys.maxsize > 2**32 else "complex192"
if hasattr(np, fftconv_unsup):
if volume.dtype == fftconv_unsup or kernel.dtype == fftconv_unsup:
return 'direct'
# for integer input,
# catch when more precision required than float provides (representing an
# integer as float can lose precision in fftconvolve if larger than 2**52)
if any([_numeric_arrays([x], kinds='ui') for x in [volume, kernel]]):
max_value = int(np.abs(volume).max()) * int(np.abs(kernel).max())
max_value *= int(min(volume.size, kernel.size))
if max_value > 2**np.finfo('float').nmant - 1:
return 'direct'
if _numeric_arrays([volume, kernel], kinds='b'):
return 'direct'
if _numeric_arrays([volume, kernel]):
if _fftconv_faster(volume, kernel, mode):
return 'fft'
return 'direct'
def convolve(in1, in2, mode='full', method='auto'):
"""
Convolve two N-dimensional arrays.
Convolve `in1` and `in2`, with the output size determined by the
`mode` argument.
Parameters
----------
in1 : array_like
First input.
in2 : array_like
Second input. Should have the same number of dimensions as `in1`.
mode : str {'full', 'valid', 'same'}, optional
A string indicating the size of the output:
``full``
The output is the full discrete linear convolution
of the inputs. (Default)
``valid``
The output consists only of those elements that do not
rely on the zero-padding. In 'valid' mode, either `in1` or `in2`
must be at least as large as the other in every dimension.
``same``
The output is the same size as `in1`, centered
with respect to the 'full' output.
method : str {'auto', 'direct', 'fft'}, optional
A string indicating which method to use to calculate the convolution.
``direct``
The convolution is determined directly from sums, the definition of
convolution.
``fft``
The Fourier Transform is used to perform the convolution by calling
`fftconvolve`.
``auto``
Automatically chooses direct or Fourier method based on an estimate
of which is faster (default). See Notes for more detail.
.. versionadded:: 0.19.0
Returns
-------
convolve : array
An N-dimensional array containing a subset of the discrete linear
convolution of `in1` with `in2`.
See Also
--------
numpy.polymul : performs polynomial multiplication (same operation, but
also accepts poly1d objects)
choose_conv_method : chooses the fastest appropriate convolution method
fftconvolve
Notes
-----
By default, `convolve` and `correlate` use ``method='auto'``, which calls
`choose_conv_method` to choose the fastest method using pre-computed
values (`choose_conv_method` can also measure real-world timing with a
keyword argument). Because `fftconvolve` relies on floating point numbers,
there are certain constraints that may force `method=direct` (more detail
in `choose_conv_method` docstring).
Examples
--------
Smooth a square pulse using a Hann window:
>>> from scipy import signal
>>> sig = np.repeat([0., 1., 0.], 100)
>>> win = signal.hann(50)
>>> filtered = signal.convolve(sig, win, mode='same') / sum(win)
>>> import matplotlib.pyplot as plt
>>> fig, (ax_orig, ax_win, ax_filt) = plt.subplots(3, 1, sharex=True)
>>> ax_orig.plot(sig)
>>> ax_orig.set_title('Original pulse')
>>> ax_orig.margins(0, 0.1)
>>> ax_win.plot(win)
>>> ax_win.set_title('Filter impulse response')
>>> ax_win.margins(0, 0.1)
>>> ax_filt.plot(filtered)
>>> ax_filt.set_title('Filtered signal')
>>> ax_filt.margins(0, 0.1)
>>> fig.tight_layout()
>>> fig.show()
"""
volume = asarray(in1)
kernel = asarray(in2)
if volume.ndim == kernel.ndim == 0:
return volume * kernel
if _inputs_swap_needed(mode, volume.shape, kernel.shape):
# Convolution is commutative; order doesn't have any effect on output
volume, kernel = kernel, volume
if method == 'auto':
method = choose_conv_method(volume, kernel, mode=mode)
if method == 'fft':
out = fftconvolve(volume, kernel, mode=mode)
result_type = np.result_type(volume, kernel)
if result_type.kind in {'u', 'i'}:
out = np.around(out)
return out.astype(result_type)
# fastpath to faster numpy.convolve for 1d inputs when possible
if _np_conv_ok(volume, kernel, mode):
return np.convolve(volume, kernel, mode)
return correlate(volume, _reverse_and_conj(kernel), mode, 'direct')
def order_filter(a, domain, rank):
"""
Perform an order filter on an N-dimensional array.
Perform an order filter on the array in. The domain argument acts as a
mask centered over each pixel. The non-zero elements of domain are
used to select elements surrounding each input pixel which are placed
in a list. The list is sorted, and the output for that pixel is the
element corresponding to rank in the sorted list.
Parameters
----------
a : ndarray
The N-dimensional input array.
domain : array_like
A mask array with the same number of dimensions as `a`.
Each dimension should have an odd number of elements.
rank : int
A non-negative integer which selects the element from the
sorted list (0 corresponds to the smallest element, 1 is the
next smallest element, etc.).
Returns
-------
out : ndarray
The results of the order filter in an array with the same
shape as `a`.
Examples
--------
>>> from scipy import signal
>>> x = np.arange(25).reshape(5, 5)
>>> domain = np.identity(3)
>>> x
array([[ 0, 1, 2, 3, 4],
[ 5, 6, 7, 8, 9],
[10, 11, 12, 13, 14],
[15, 16, 17, 18, 19],
[20, 21, 22, 23, 24]])
>>> signal.order_filter(x, domain, 0)
array([[ 0., 0., 0., 0., 0.],
[ 0., 0., 1., 2., 0.],
[ 0., 5., 6., 7., 0.],
[ 0., 10., 11., 12., 0.],
[ 0., 0., 0., 0., 0.]])
>>> signal.order_filter(x, domain, 2)
array([[ 6., 7., 8., 9., 4.],
[ 11., 12., 13., 14., 9.],
[ 16., 17., 18., 19., 14.],
[ 21., 22., 23., 24., 19.],
[ 20., 21., 22., 23., 24.]])
"""
domain = asarray(domain)
size = domain.shape
for k in range(len(size)):
if (size[k] % 2) != 1:
raise ValueError("Each dimension of domain argument "
" should have an odd number of elements.")
return sigtools._order_filterND(a, domain, rank)
def medfilt(volume, kernel_size=None):
"""
Perform a median filter on an N-dimensional array.
Apply a median filter to the input array using a local window-size
given by `kernel_size`.
Parameters
----------
volume : array_like
An N-dimensional input array.
kernel_size : array_like, optional
A scalar or an N-length list giving the size of the median filter
window in each dimension. Elements of `kernel_size` should be odd.
If `kernel_size` is a scalar, then this scalar is used as the size in
each dimension. Default size is 3 for each dimension.
Returns
-------
out : ndarray
An array the same size as input containing the median filtered
result.
"""
volume = atleast_1d(volume)
if kernel_size is None:
kernel_size = [3] * volume.ndim
kernel_size = asarray(kernel_size)
if kernel_size.shape == ():
kernel_size = np.repeat(kernel_size.item(), volume.ndim)
for k in range(volume.ndim):
if (kernel_size[k] % 2) != 1:
raise ValueError("Each element of kernel_size should be odd.")
domain = ones(kernel_size)
numels = product(kernel_size, axis=0)
order = numels // 2
return sigtools._order_filterND(volume, domain, order)
def wiener(im, mysize=None, noise=None):
"""
Perform a Wiener filter on an N-dimensional array.
Apply a Wiener filter to the N-dimensional array `im`.
Parameters
----------
im : ndarray
An N-dimensional array.
mysize : int or array_like, optional
A scalar or an N-length list giving the size of the Wiener filter
window in each dimension. Elements of mysize should be odd.
If mysize is a scalar, then this scalar is used as the size
in each dimension.
noise : float, optional
The noise-power to use. If None, then noise is estimated as the
average of the local variance of the input.
Returns
-------
out : ndarray
Wiener filtered result with the same shape as `im`.
"""
im = asarray(im)
if mysize is None:
mysize = [3] * im.ndim
mysize = asarray(mysize)
if mysize.shape == ():
mysize = np.repeat(mysize.item(), im.ndim)
# Estimate the local mean
lMean = correlate(im, ones(mysize), 'same') / product(mysize, axis=0)
# Estimate the local variance
lVar = (correlate(im ** 2, ones(mysize), 'same') /
product(mysize, axis=0) - lMean ** 2)
# Estimate the noise power if needed.
if noise is None:
noise = mean(ravel(lVar), axis=0)
res = (im - lMean)
res *= (1 - noise / lVar)
res += lMean
out = where(lVar < noise, lMean, res)
return out
def convolve2d(in1, in2, mode='full', boundary='fill', fillvalue=0):
"""
Convolve two 2-dimensional arrays.
Convolve `in1` and `in2` with output size determined by `mode`, and
boundary conditions determined by `boundary` and `fillvalue`.
Parameters
----------
in1 : array_like
First input.
in2 : array_like
Second input. Should have the same number of dimensions as `in1`.
If operating in 'valid' mode, either `in1` or `in2` must be
at least as large as the other in every dimension.
mode : str {'full', 'valid', 'same'}, optional
A string indicating the size of the output:
``full``
The output is the full discrete linear convolution
of the inputs. (Default)
``valid``
The output consists only of those elements that do not
rely on the zero-padding.
``same``
The output is the same size as `in1`, centered
with respect to the 'full' output.
boundary : str {'fill', 'wrap', 'symm'}, optional
A flag indicating how to handle boundaries:
``fill``
pad input arrays with fillvalue. (default)
``wrap``
circular boundary conditions.
``symm``
symmetrical boundary conditions.
fillvalue : scalar, optional
Value to fill pad input arrays with. Default is 0.
Returns
-------
out : ndarray
A 2-dimensional array containing a subset of the discrete linear
convolution of `in1` with `in2`.
Examples
--------
Compute the gradient of an image by 2D convolution with a complex Scharr
operator. (Horizontal operator is real, vertical is imaginary.) Use
symmetric boundary condition to avoid creating edges at the image
boundaries.
>>> from scipy import signal
>>> from scipy import misc
>>> ascent = misc.ascent()
>>> scharr = np.array([[ -3-3j, 0-10j, +3 -3j],
... [-10+0j, 0+ 0j, +10 +0j],
... [ -3+3j, 0+10j, +3 +3j]]) # Gx + j*Gy
>>> grad = signal.convolve2d(ascent, scharr, boundary='symm', mode='same')
>>> import matplotlib.pyplot as plt
>>> fig, (ax_orig, ax_mag, ax_ang) = plt.subplots(3, 1, figsize=(6, 15))
>>> ax_orig.imshow(ascent, cmap='gray')
>>> ax_orig.set_title('Original')
>>> ax_orig.set_axis_off()
>>> ax_mag.imshow(np.absolute(grad), cmap='gray')
>>> ax_mag.set_title('Gradient magnitude')
>>> ax_mag.set_axis_off()
>>> ax_ang.imshow(np.angle(grad), cmap='hsv') # hsv is cyclic, like angles
>>> ax_ang.set_title('Gradient orientation')
>>> ax_ang.set_axis_off()
>>> fig.show()
"""
in1 = asarray(in1)
in2 = asarray(in2)
if not in1.ndim == in2.ndim == 2:
raise ValueError('convolve2d inputs must both be 2D arrays')
if _inputs_swap_needed(mode, in1.shape, in2.shape):
in1, in2 = in2, in1
val = _valfrommode(mode)
bval = _bvalfromboundary(boundary)
out = sigtools._convolve2d(in1, in2, 1, val, bval, fillvalue)
return out
def correlate2d(in1, in2, mode='full', boundary='fill', fillvalue=0):
"""
Cross-correlate two 2-dimensional arrays.
Cross correlate `in1` and `in2` with output size determined by `mode`, and
boundary conditions determined by `boundary` and `fillvalue`.
Parameters
----------
in1 : array_like
First input.
in2 : array_like
Second input. Should have the same number of dimensions as `in1`.
If operating in 'valid' mode, either `in1` or `in2` must be
at least as large as the other in every dimension.
mode : str {'full', 'valid', 'same'}, optional
A string indicating the size of the output:
``full``
The output is the full discrete linear cross-correlation
of the inputs. (Default)
``valid``
The output consists only of those elements that do not
rely on the zero-padding.
``same``
The output is the same size as `in1`, centered
with respect to the 'full' output.
boundary : str {'fill', 'wrap', 'symm'}, optional
A flag indicating how to handle boundaries:
``fill``
pad input arrays with fillvalue. (default)
``wrap``
circular boundary conditions.
``symm``
symmetrical boundary conditions.
fillvalue : scalar, optional
Value to fill pad input arrays with. Default is 0.
Returns
-------
correlate2d : ndarray
A 2-dimensional array containing a subset of the discrete linear
cross-correlation of `in1` with `in2`.
Examples
--------
Use 2D cross-correlation to find the location of a template in a noisy
image:
>>> from scipy import signal
>>> from scipy import misc
>>> face = misc.face(gray=True) - misc.face(gray=True).mean()
>>> template = np.copy(face[300:365, 670:750]) # right eye
>>> template -= template.mean()
>>> face = face + np.random.randn(*face.shape) * 50 # add noise
>>> corr = signal.correlate2d(face, template, boundary='symm', mode='same')
>>> y, x = np.unravel_index(np.argmax(corr), corr.shape) # find the match
>>> import matplotlib.pyplot as plt
>>> fig, (ax_orig, ax_template, ax_corr) = plt.subplots(3, 1,
... figsize=(6, 15))
>>> ax_orig.imshow(face, cmap='gray')
>>> ax_orig.set_title('Original')
>>> ax_orig.set_axis_off()
>>> ax_template.imshow(template, cmap='gray')
>>> ax_template.set_title('Template')
>>> ax_template.set_axis_off()
>>> ax_corr.imshow(corr, cmap='gray')
>>> ax_corr.set_title('Cross-correlation')
>>> ax_corr.set_axis_off()
>>> ax_orig.plot(x, y, 'ro')
>>> fig.show()
"""
in1 = asarray(in1)
in2 = asarray(in2)
if not in1.ndim == in2.ndim == 2:
raise ValueError('correlate2d inputs must both be 2D arrays')
swapped_inputs = _inputs_swap_needed(mode, in1.shape, in2.shape)
if swapped_inputs:
in1, in2 = in2, in1
val = _valfrommode(mode)
bval = _bvalfromboundary(boundary)
out = sigtools._convolve2d(in1, in2, 0, val, bval, fillvalue)
if swapped_inputs:
out = out[::-1, ::-1]
return out
def medfilt2d(input, kernel_size=3):
"""
Median filter a 2-dimensional array.
Apply a median filter to the `input` array using a local window-size
given by `kernel_size` (must be odd).
Parameters
----------
input : array_like
A 2-dimensional input array.
kernel_size : array_like, optional
A scalar or a list of length 2, giving the size of the
median filter window in each dimension. Elements of
`kernel_size` should be odd. If `kernel_size` is a scalar,
then this scalar is used as the size in each dimension.
Default is a kernel of size (3, 3).
Returns
-------
out : ndarray
An array the same size as input containing the median filtered
result.
"""
image = asarray(input)
if kernel_size is None:
kernel_size = [3] * 2
kernel_size = asarray(kernel_size)
if kernel_size.shape == ():
kernel_size = np.repeat(kernel_size.item(), 2)
for size in kernel_size:
if (size % 2) != 1:
raise ValueError("Each element of kernel_size should be odd.")
return sigtools._medfilt2d(image, kernel_size)
def lfilter(b, a, x, axis=-1, zi=None):
"""
Filter data along one-dimension with an IIR or FIR filter.
Filter a data sequence, `x`, using a digital filter. This works for many
fundamental data types (including Object type). The filter is a direct
form II transposed implementation of the standard difference equation
(see Notes).
Parameters
----------
b : array_like
The numerator coefficient vector in a 1-D sequence.
a : array_like
The denominator coefficient vector in a 1-D sequence. If ``a[0]``
is not 1, then both `a` and `b` are normalized by ``a[0]``.
x : array_like
An N-dimensional input array.
axis : int, optional
The axis of the input data array along which to apply the
linear filter. The filter is applied to each subarray along
this axis. Default is -1.
zi : array_like, optional
Initial conditions for the filter delays. It is a vector
(or array of vectors for an N-dimensional input) of length
``max(len(a), len(b)) - 1``. If `zi` is None or is not given then
initial rest is assumed. See `lfiltic` for more information.
Returns
-------
y : array
The output of the digital filter.
zf : array, optional
If `zi` is None, this is not returned, otherwise, `zf` holds the
final filter delay values.
See Also
--------
lfiltic : Construct initial conditions for `lfilter`.
lfilter_zi : Compute initial state (steady state of step response) for
`lfilter`.
filtfilt : A forward-backward filter, to obtain a filter with linear phase.
savgol_filter : A Savitzky-Golay filter.
sosfilt: Filter data using cascaded second-order sections.
sosfiltfilt: A forward-backward filter using second-order sections.
Notes
-----
The filter function is implemented as a direct II transposed structure.
This means that the filter implements::
a[0]*y[n] = b[0]*x[n] + b[1]*x[n-1] + ... + b[M]*x[n-M]
- a[1]*y[n-1] - ... - a[N]*y[n-N]
where `M` is the degree of the numerator, `N` is the degree of the
denominator, and `n` is the sample number. It is implemented using
the following difference equations (assuming M = N)::
a[0]*y[n] = b[0] * x[n] + d[0][n-1]
d[0][n] = b[1] * x[n] - a[1] * y[n] + d[1][n-1]
d[1][n] = b[2] * x[n] - a[2] * y[n] + d[2][n-1]
...
d[N-2][n] = b[N-1]*x[n] - a[N-1]*y[n] + d[N-1][n-1]
d[N-1][n] = b[N] * x[n] - a[N] * y[n]
where `d` are the state variables.
The rational transfer function describing this filter in the
z-transform domain is::
-1 -M
b[0] + b[1]z + ... + b[M] z
Y(z) = -------------------------------- X(z)
-1 -N
a[0] + a[1]z + ... + a[N] z
Examples
--------
Generate a noisy signal to be filtered:
>>> from scipy import signal
>>> import matplotlib.pyplot as plt
>>> t = np.linspace(-1, 1, 201)
>>> x = (np.sin(2*np.pi*0.75*t*(1-t) + 2.1) +
... 0.1*np.sin(2*np.pi*1.25*t + 1) +
... 0.18*np.cos(2*np.pi*3.85*t))
>>> xn = x + np.random.randn(len(t)) * 0.08
Create an order 3 lowpass butterworth filter:
>>> b, a = signal.butter(3, 0.05)
Apply the filter to xn. Use lfilter_zi to choose the initial condition of
the filter:
>>> zi = signal.lfilter_zi(b, a)
>>> z, _ = signal.lfilter(b, a, xn, zi=zi*xn[0])
Apply the filter again, to have a result filtered at an order the same as
filtfilt:
>>> z2, _ = signal.lfilter(b, a, z, zi=zi*z[0])
Use filtfilt to apply the filter:
>>> y = signal.filtfilt(b, a, xn)
Plot the original signal and the various filtered versions:
>>> plt.figure
>>> plt.plot(t, xn, 'b', alpha=0.75)
>>> plt.plot(t, z, 'r--', t, z2, 'r', t, y, 'k')
>>> plt.legend(('noisy signal', 'lfilter, once', 'lfilter, twice',
... 'filtfilt'), loc='best')
>>> plt.grid(True)
>>> plt.show()
"""
a = np.atleast_1d(a)
if len(a) == 1:
# This path only supports types fdgFDGO to mirror _linear_filter below.
# Any of b, a, x, or zi can set the dtype, but there is no default
# casting of other types; instead a NotImplementedError is raised.
b = np.asarray(b)
a = np.asarray(a)
if b.ndim != 1 and a.ndim != 1:
raise ValueError('object of too small depth for desired array')
x = np.asarray(x)
inputs = [b, a, x]
if zi is not None:
# _linear_filter does not broadcast zi, but does do expansion of
# singleton dims.
zi = np.asarray(zi)
if zi.ndim != x.ndim:
raise ValueError('object of too small depth for desired array')
expected_shape = list(x.shape)
expected_shape[axis] = b.shape[0] - 1
expected_shape = tuple(expected_shape)
# check the trivial case where zi is the right shape first
if zi.shape != expected_shape:
strides = zi.ndim * [None]
if axis < 0:
axis += zi.ndim
for k in range(zi.ndim):
if k == axis and zi.shape[k] == expected_shape[k]:
strides[k] = zi.strides[k]
elif k != axis and zi.shape[k] == expected_shape[k]:
strides[k] = zi.strides[k]
elif k != axis and zi.shape[k] == 1:
strides[k] = 0
else:
raise ValueError('Unexpected shape for zi: expected '
'%s, found %s.' %
(expected_shape, zi.shape))
zi = np.lib.stride_tricks.as_strided(zi, expected_shape,
strides)
inputs.append(zi)
dtype = np.result_type(*inputs)
if dtype.char not in 'fdgFDGO':
raise NotImplementedError("input type '%s' not supported" % dtype)
b = np.array(b, dtype=dtype)
a = np.array(a, dtype=dtype, copy=False)
b /= a[0]
x = np.array(x, dtype=dtype, copy=False)
out_full = np.apply_along_axis(lambda y: np.convolve(b, y), axis, x)
ind = out_full.ndim * [slice(None)]
if zi is not None:
ind[axis] = slice(zi.shape[axis])
out_full[ind] += zi
ind[axis] = slice(out_full.shape[axis] - len(b) + 1)
out = out_full[ind]
if zi is None:
return out
else:
ind[axis] = slice(out_full.shape[axis] - len(b) + 1, None)
zf = out_full[ind]
return out, zf
else:
if zi is None:
return sigtools._linear_filter(b, a, x, axis)
else:
return sigtools._linear_filter(b, a, x, axis, zi)
def lfiltic(b, a, y, x=None):
"""
Construct initial conditions for lfilter given input and output vectors.
Given a linear filter (b, a) and initial conditions on the output `y`
and the input `x`, return the initial conditions on the state vector zi
which is used by `lfilter` to generate the output given the input.
Parameters
----------
b : array_like
Linear filter term.
a : array_like
Linear filter term.
y : array_like
Initial conditions.
If ``N = len(a) - 1``, then ``y = {y[-1], y[-2], ..., y[-N]}``.
If `y` is too short, it is padded with zeros.
x : array_like, optional
Initial conditions.
If ``M = len(b) - 1``, then ``x = {x[-1], x[-2], ..., x[-M]}``.
If `x` is not given, its initial conditions are assumed zero.
If `x` is too short, it is padded with zeros.
Returns
-------
zi : ndarray
The state vector ``zi = {z_0[-1], z_1[-1], ..., z_K-1[-1]}``,
where ``K = max(M, N)``.
See Also
--------
lfilter, lfilter_zi
"""
N = np.size(a) - 1
M = np.size(b) - 1
K = max(M, N)
y = asarray(y)
if y.dtype.kind in 'bui':
# ensure calculations are floating point
y = y.astype(np.float64)
zi = zeros(K, y.dtype)
if x is None:
x = zeros(M, y.dtype)
else:
x = asarray(x)
L = np.size(x)
if L < M:
x = r_[x, zeros(M - L)]
L = np.size(y)
if L < N:
y = r_[y, zeros(N - L)]
for m in range(M):
zi[m] = np.sum(b[m + 1:] * x[:M - m], axis=0)
for m in range(N):
zi[m] -= np.sum(a[m + 1:] * y[:N - m], axis=0)
return zi
def deconvolve(signal, divisor):
"""Deconvolves ``divisor`` out of ``signal`` using inverse filtering.
Returns the quotient and remainder such that
``signal = convolve(divisor, quotient) + remainder``
Parameters
----------
signal : array_like
Signal data, typically a recorded signal
divisor : array_like
Divisor data, typically an impulse response or filter that was
applied to the original signal
Returns
-------
quotient : ndarray
Quotient, typically the recovered original signal
remainder : ndarray
Remainder
Examples
--------
Deconvolve a signal that's been filtered:
>>> from scipy import signal
>>> original = [0, 1, 0, 0, 1, 1, 0, 0]
>>> impulse_response = [2, 1]
>>> recorded = signal.convolve(impulse_response, original)
>>> recorded
array([0, 2, 1, 0, 2, 3, 1, 0, 0])
>>> recovered, remainder = signal.deconvolve(recorded, impulse_response)
>>> recovered
array([ 0., 1., 0., 0., 1., 1., 0., 0.])
See Also
--------
numpy.polydiv : performs polynomial division (same operation, but
also accepts poly1d objects)
"""
num = atleast_1d(signal)
den = atleast_1d(divisor)
N = len(num)
D = len(den)
if D > N:
quot = []
rem = num
else:
input = zeros(N - D + 1, float)
input[0] = 1
quot = lfilter(num, den, input)
rem = num - convolve(den, quot, mode='full')
return quot, rem
def hilbert(x, N=None, axis=-1):
"""
Compute the analytic signal, using the Hilbert transform.
The transformation is done along the last axis by default.
Parameters
----------
x : array_like
Signal data. Must be real.
N : int, optional
Number of Fourier components. Default: ``x.shape[axis]``
axis : int, optional
Axis along which to do the transformation. Default: -1.
Returns
-------
xa : ndarray
Analytic signal of `x`, of each 1-D array along `axis`
See Also
--------
scipy.fftpack.hilbert : Return Hilbert transform of a periodic sequence x.
Notes
-----
The analytic signal ``x_a(t)`` of signal ``x(t)`` is:
.. math:: x_a = F^{-1}(F(x) 2U) = x + i y
where `F` is the Fourier transform, `U` the unit step function,
and `y` the Hilbert transform of `x`. [1]_
In other words, the negative half of the frequency spectrum is zeroed
out, turning the real-valued signal into a complex signal. The Hilbert
transformed signal can be obtained from ``np.imag(hilbert(x))``, and the
original signal from ``np.real(hilbert(x))``.
Examples
---------
In this example we use the Hilbert transform to determine the amplitude
envelope and instantaneous frequency of an amplitude-modulated signal.
>>> import numpy as np
>>> import matplotlib.pyplot as plt
>>> from scipy.signal import hilbert, chirp
>>> duration = 1.0
>>> fs = 400.0
>>> samples = int(fs*duration)
>>> t = np.arange(samples) / fs
We create a chirp of which the frequency increases from 20 Hz to 100 Hz and
apply an amplitude modulation.
>>> signal = chirp(t, 20.0, t[-1], 100.0)
>>> signal *= (1.0 + 0.5 * np.sin(2.0*np.pi*3.0*t) )
The amplitude envelope is given by magnitude of the analytic signal. The
instantaneous frequency can be obtained by differentiating the
instantaneous phase in respect to time. The instantaneous phase corresponds
to the phase angle of the analytic signal.
>>> analytic_signal = hilbert(signal)
>>> amplitude_envelope = np.abs(analytic_signal)
>>> instantaneous_phase = np.unwrap(np.angle(analytic_signal))
>>> instantaneous_frequency = (np.diff(instantaneous_phase) /
... (2.0*np.pi) * fs)
>>> fig = plt.figure()
>>> ax0 = fig.add_subplot(211)
>>> ax0.plot(t, signal, label='signal')
>>> ax0.plot(t, amplitude_envelope, label='envelope')
>>> ax0.set_xlabel("time in seconds")
>>> ax0.legend()
>>> ax1 = fig.add_subplot(212)
>>> ax1.plot(t[1:], instantaneous_frequency)
>>> ax1.set_xlabel("time in seconds")
>>> ax1.set_ylim(0.0, 120.0)
References
----------
.. [1] Wikipedia, "Analytic signal".
http://en.wikipedia.org/wiki/Analytic_signal
.. [2] Leon Cohen, "Time-Frequency Analysis", 1995. Chapter 2.
.. [3] Alan V. Oppenheim, Ronald W. Schafer. Discrete-Time Signal
Processing, Third Edition, 2009. Chapter 12.
ISBN 13: 978-1292-02572-8
"""
x = asarray(x)
if iscomplexobj(x):
raise ValueError("x must be real.")
if N is None:
N = x.shape[axis]
if N <= 0:
raise ValueError("N must be positive.")
Xf = fftpack.fft(x, N, axis=axis)
h = zeros(N)
if N % 2 == 0:
h[0] = h[N // 2] = 1
h[1:N // 2] = 2
else:
h[0] = 1
h[1:(N + 1) // 2] = 2
if x.ndim > 1:
ind = [newaxis] * x.ndim
ind[axis] = slice(None)
h = h[ind]
x = fftpack.ifft(Xf * h, axis=axis)
return x
def hilbert2(x, N=None):
"""
Compute the '2-D' analytic signal of `x`
Parameters
----------
x : array_like
2-D signal data.
N : int or tuple of two ints, optional
Number of Fourier components. Default is ``x.shape``
Returns
-------
xa : ndarray
Analytic signal of `x` taken along axes (0,1).
References
----------
.. [1] Wikipedia, "Analytic signal",
http://en.wikipedia.org/wiki/Analytic_signal
"""
x = atleast_2d(x)
if x.ndim > 2:
raise ValueError("x must be 2-D.")
if iscomplexobj(x):
raise ValueError("x must be real.")
if N is None:
N = x.shape
elif isinstance(N, int):
if N <= 0:
raise ValueError("N must be positive.")
N = (N, N)
elif len(N) != 2 or np.any(np.asarray(N) <= 0):
raise ValueError("When given as a tuple, N must hold exactly "
"two positive integers")
Xf = fftpack.fft2(x, N, axes=(0, 1))
h1 = zeros(N[0], 'd')
h2 = zeros(N[1], 'd')
for p in range(2):
h = eval("h%d" % (p + 1))
N1 = N[p]
if N1 % 2 == 0:
h[0] = h[N1 // 2] = 1
h[1:N1 // 2] = 2
else:
h[0] = 1
h[1:(N1 + 1) // 2] = 2
exec("h%d = h" % (p + 1), globals(), locals())
h = h1[:, newaxis] * h2[newaxis, :]
k = x.ndim
while k > 2:
h = h[:, newaxis]
k -= 1
x = fftpack.ifft2(Xf * h, axes=(0, 1))
return x
def cmplx_sort(p):
"""Sort roots based on magnitude.
Parameters
----------
p : array_like
The roots to sort, as a 1-D array.
Returns
-------
p_sorted : ndarray
Sorted roots.
indx : ndarray
Array of indices needed to sort the input `p`.
"""
p = asarray(p)
if iscomplexobj(p):
indx = argsort(abs(p))
else:
indx = argsort(p)
return take(p, indx, 0), indx
def unique_roots(p, tol=1e-3, rtype='min'):
"""
Determine unique roots and their multiplicities from a list of roots.
Parameters
----------
p : array_like
The list of roots.
tol : float, optional
The tolerance for two roots to be considered equal. Default is 1e-3.
rtype : {'max', 'min, 'avg'}, optional
How to determine the returned root if multiple roots are within
`tol` of each other.
- 'max': pick the maximum of those roots.
- 'min': pick the minimum of those roots.
- 'avg': take the average of those roots.
Returns
-------
pout : ndarray
The list of unique roots, sorted from low to high.
mult : ndarray
The multiplicity of each root.
Notes
-----
This utility function is not specific to roots but can be used for any
sequence of values for which uniqueness and multiplicity has to be
determined. For a more general routine, see `numpy.unique`.
Examples
--------
>>> from scipy import signal
>>> vals = [0, 1.3, 1.31, 2.8, 1.25, 2.2, 10.3]
>>> uniq, mult = signal.unique_roots(vals, tol=2e-2, rtype='avg')
Check which roots have multiplicity larger than 1:
>>> uniq[mult > 1]
array([ 1.305])
"""
if rtype in ['max', 'maximum']:
comproot = np.max
elif rtype in ['min', 'minimum']:
comproot = np.min
elif rtype in ['avg', 'mean']:
comproot = np.mean
else:
raise ValueError("`rtype` must be one of "
"{'max', 'maximum', 'min', 'minimum', 'avg', 'mean'}")
p = asarray(p) * 1.0
tol = abs(tol)
p, indx = cmplx_sort(p)
pout = []
mult = []
indx = -1
curp = p[0] + 5 * tol
sameroots = []
for k in range(len(p)):
tr = p[k]
if abs(tr - curp) < tol:
sameroots.append(tr)
curp = comproot(sameroots)
pout[indx] = curp
mult[indx] += 1
else:
pout.append(tr)
curp = tr
sameroots = [tr]
indx += 1
mult.append(1)
return array(pout), array(mult)
def invres(r, p, k, tol=1e-3, rtype='avg'):
"""
Compute b(s) and a(s) from partial fraction expansion.
If `M` is the degree of numerator `b` and `N` the degree of denominator
`a`::
b(s) b[0] s**(M) + b[1] s**(M-1) + ... + b[M]
H(s) = ------ = ------------------------------------------
a(s) a[0] s**(N) + a[1] s**(N-1) + ... + a[N]
then the partial-fraction expansion H(s) is defined as::
r[0] r[1] r[-1]
= -------- + -------- + ... + --------- + k(s)
(s-p[0]) (s-p[1]) (s-p[-1])
If there are any repeated roots (closer together than `tol`), then H(s)
has terms like::
r[i] r[i+1] r[i+n-1]
-------- + ----------- + ... + -----------
(s-p[i]) (s-p[i])**2 (s-p[i])**n
This function is used for polynomials in positive powers of s or z,
such as analog filters or digital filters in controls engineering. For
negative powers of z (typical for digital filters in DSP), use `invresz`.
Parameters
----------
r : array_like
Residues.
p : array_like
Poles.
k : array_like
Coefficients of the direct polynomial term.
tol : float, optional
The tolerance for two roots to be considered equal. Default is 1e-3.
rtype : {'max', 'min, 'avg'}, optional
How to determine the returned root if multiple roots are within
`tol` of each other.
- 'max': pick the maximum of those roots.
- 'min': pick the minimum of those roots.
- 'avg': take the average of those roots.
Returns
-------
b : ndarray
Numerator polynomial coefficients.
a : ndarray
Denominator polynomial coefficients.
See Also
--------
residue, invresz, unique_roots
"""
extra = k
p, indx = cmplx_sort(p)
r = take(r, indx, 0)
pout, mult = unique_roots(p, tol=tol, rtype=rtype)
p = []
for k in range(len(pout)):
p.extend([pout[k]] * mult[k])
a = atleast_1d(poly(p))
if len(extra) > 0:
b = polymul(extra, a)
else:
b = [0]
indx = 0
for k in range(len(pout)):
temp = []
for l in range(len(pout)):
if l != k:
temp.extend([pout[l]] * mult[l])
for m in range(mult[k]):
t2 = temp[:]
t2.extend([pout[k]] * (mult[k] - m - 1))
b = polyadd(b, r[indx] * atleast_1d(poly(t2)))
indx += 1
b = real_if_close(b)
while allclose(b[0], 0, rtol=1e-14) and (b.shape[-1] > 1):
b = b[1:]
return b, a
def residue(b, a, tol=1e-3, rtype='avg'):
"""
Compute partial-fraction expansion of b(s) / a(s).
If `M` is the degree of numerator `b` and `N` the degree of denominator
`a`::
b(s) b[0] s**(M) + b[1] s**(M-1) + ... + b[M]
H(s) = ------ = ------------------------------------------
a(s) a[0] s**(N) + a[1] s**(N-1) + ... + a[N]
then the partial-fraction expansion H(s) is defined as::
r[0] r[1] r[-1]
= -------- + -------- + ... + --------- + k(s)
(s-p[0]) (s-p[1]) (s-p[-1])
If there are any repeated roots (closer together than `tol`), then H(s)
has terms like::
r[i] r[i+1] r[i+n-1]
-------- + ----------- + ... + -----------
(s-p[i]) (s-p[i])**2 (s-p[i])**n
This function is used for polynomials in positive powers of s or z,
such as analog filters or digital filters in controls engineering. For
negative powers of z (typical for digital filters in DSP), use `residuez`.
Parameters
----------
b : array_like
Numerator polynomial coefficients.
a : array_like
Denominator polynomial coefficients.
Returns
-------
r : ndarray
Residues.
p : ndarray
Poles.
k : ndarray
Coefficients of the direct polynomial term.
See Also
--------
invres, residuez, numpy.poly, unique_roots
"""
b, a = map(asarray, (b, a))
rscale = a[0]
k, b = polydiv(b, a)
p = roots(a)
r = p * 0.0
pout, mult = unique_roots(p, tol=tol, rtype=rtype)
p = []
for n in range(len(pout)):
p.extend([pout[n]] * mult[n])
p = asarray(p)
# Compute the residue from the general formula
indx = 0
for n in range(len(pout)):
bn = b.copy()
pn = []
for l in range(len(pout)):
if l != n:
pn.extend([pout[l]] * mult[l])
an = atleast_1d(poly(pn))
# bn(s) / an(s) is (s-po[n])**Nn * b(s) / a(s) where Nn is
# multiplicity of pole at po[n]
sig = mult[n]
for m in range(sig, 0, -1):
if sig > m:
# compute next derivative of bn(s) / an(s)
term1 = polymul(polyder(bn, 1), an)
term2 = polymul(bn, polyder(an, 1))
bn = polysub(term1, term2)
an = polymul(an, an)
r[indx + m - 1] = (polyval(bn, pout[n]) / polyval(an, pout[n]) /
factorial(sig - m))
indx += sig
return r / rscale, p, k
def residuez(b, a, tol=1e-3, rtype='avg'):
"""
Compute partial-fraction expansion of b(z) / a(z).
If `M` is the degree of numerator `b` and `N` the degree of denominator
`a`::
b(z) b[0] + b[1] z**(-1) + ... + b[M] z**(-M)
H(z) = ------ = ------------------------------------------
a(z) a[0] + a[1] z**(-1) + ... + a[N] z**(-N)
then the partial-fraction expansion H(z) is defined as::
r[0] r[-1]
= --------------- + ... + ---------------- + k[0] + k[1]z**(-1) ...
(1-p[0]z**(-1)) (1-p[-1]z**(-1))
If there are any repeated roots (closer than `tol`), then the partial
fraction expansion has terms like::
r[i] r[i+1] r[i+n-1]
-------------- + ------------------ + ... + ------------------
(1-p[i]z**(-1)) (1-p[i]z**(-1))**2 (1-p[i]z**(-1))**n
This function is used for polynomials in negative powers of z,
such as digital filters in DSP. For positive powers, use `residue`.
Parameters
----------
b : array_like
Numerator polynomial coefficients.
a : array_like
Denominator polynomial coefficients.
Returns
-------
r : ndarray
Residues.
p : ndarray
Poles.
k : ndarray
Coefficients of the direct polynomial term.
See Also
--------
invresz, residue, unique_roots
"""
b, a = map(asarray, (b, a))
gain = a[0]
brev, arev = b[::-1], a[::-1]
krev, brev = polydiv(brev, arev)
if krev == []:
k = []
else:
k = krev[::-1]
b = brev[::-1]
p = roots(a)
r = p * 0.0
pout, mult = unique_roots(p, tol=tol, rtype=rtype)
p = []
for n in range(len(pout)):
p.extend([pout[n]] * mult[n])
p = asarray(p)
# Compute the residue from the general formula (for discrete-time)
# the polynomial is in z**(-1) and the multiplication is by terms
# like this (1-p[i] z**(-1))**mult[i]. After differentiation,
# we must divide by (-p[i])**(m-k) as well as (m-k)!
indx = 0
for n in range(len(pout)):
bn = brev.copy()
pn = []
for l in range(len(pout)):
if l != n:
pn.extend([pout[l]] * mult[l])
an = atleast_1d(poly(pn))[::-1]
# bn(z) / an(z) is (1-po[n] z**(-1))**Nn * b(z) / a(z) where Nn is
# multiplicity of pole at po[n] and b(z) and a(z) are polynomials.
sig = mult[n]
for m in range(sig, 0, -1):
if sig > m:
# compute next derivative of bn(s) / an(s)
term1 = polymul(polyder(bn, 1), an)
term2 = polymul(bn, polyder(an, 1))
bn = polysub(term1, term2)
an = polymul(an, an)
r[indx + m - 1] = (polyval(bn, 1.0 / pout[n]) /
polyval(an, 1.0 / pout[n]) /
factorial(sig - m) / (-pout[n]) ** (sig - m))
indx += sig
return r / gain, p, k
def invresz(r, p, k, tol=1e-3, rtype='avg'):
"""
Compute b(z) and a(z) from partial fraction expansion.
If `M` is the degree of numerator `b` and `N` the degree of denominator
`a`::
b(z) b[0] + b[1] z**(-1) + ... + b[M] z**(-M)
H(z) = ------ = ------------------------------------------
a(z) a[0] + a[1] z**(-1) + ... + a[N] z**(-N)
then the partial-fraction expansion H(z) is defined as::
r[0] r[-1]
= --------------- + ... + ---------------- + k[0] + k[1]z**(-1) ...
(1-p[0]z**(-1)) (1-p[-1]z**(-1))
If there are any repeated roots (closer than `tol`), then the partial
fraction expansion has terms like::
r[i] r[i+1] r[i+n-1]
-------------- + ------------------ + ... + ------------------
(1-p[i]z**(-1)) (1-p[i]z**(-1))**2 (1-p[i]z**(-1))**n
This function is used for polynomials in negative powers of z,
such as digital filters in DSP. For positive powers, use `invres`.
Parameters
----------
r : array_like
Residues.
p : array_like
Poles.
k : array_like
Coefficients of the direct polynomial term.
tol : float, optional
The tolerance for two roots to be considered equal. Default is 1e-3.
rtype : {'max', 'min, 'avg'}, optional
How to determine the returned root if multiple roots are within
`tol` of each other.
- 'max': pick the maximum of those roots.
- 'min': pick the minimum of those roots.
- 'avg': take the average of those roots.
Returns
-------
b : ndarray
Numerator polynomial coefficients.
a : ndarray
Denominator polynomial coefficients.
See Also
--------
residuez, unique_roots, invres
"""
extra = asarray(k)
p, indx = cmplx_sort(p)
r = take(r, indx, 0)
pout, mult = unique_roots(p, tol=tol, rtype=rtype)
p = []
for k in range(len(pout)):
p.extend([pout[k]] * mult[k])
a = atleast_1d(poly(p))
if len(extra) > 0:
b = polymul(extra, a)
else:
b = [0]
indx = 0
brev = asarray(b)[::-1]
for k in range(len(pout)):
temp = []
# Construct polynomial which does not include any of this root
for l in range(len(pout)):
if l != k:
temp.extend([pout[l]] * mult[l])
for m in range(mult[k]):
t2 = temp[:]
t2.extend([pout[k]] * (mult[k] - m - 1))
brev = polyadd(brev, (r[indx] * atleast_1d(poly(t2)))[::-1])
indx += 1
b = real_if_close(brev[::-1])
return b, a
def resample(x, num, t=None, axis=0, window=None):
"""
Resample `x` to `num` samples using Fourier method along the given axis.
The resampled signal starts at the same value as `x` but is sampled
with a spacing of ``len(x) / num * (spacing of x)``. Because a
Fourier method is used, the signal is assumed to be periodic.
Parameters
----------
x : array_like
The data to be resampled.
num : int
The number of samples in the resampled signal.
t : array_like, optional
If `t` is given, it is assumed to be the sample positions
associated with the signal data in `x`.
axis : int, optional
The axis of `x` that is resampled. Default is 0.
window : array_like, callable, string, float, or tuple, optional
Specifies the window applied to the signal in the Fourier
domain. See below for details.
Returns
-------
resampled_x or (resampled_x, resampled_t)
Either the resampled array, or, if `t` was given, a tuple
containing the resampled array and the corresponding resampled
positions.
See Also
--------
decimate : Downsample the signal after applying an FIR or IIR filter.
resample_poly : Resample using polyphase filtering and an FIR filter.
Notes
-----
The argument `window` controls a Fourier-domain window that tapers
the Fourier spectrum before zero-padding to alleviate ringing in
the resampled values for sampled signals you didn't intend to be
interpreted as band-limited.
If `window` is a function, then it is called with a vector of inputs
indicating the frequency bins (i.e. fftfreq(x.shape[axis]) ).
If `window` is an array of the same length as `x.shape[axis]` it is
assumed to be the window to be applied directly in the Fourier
domain (with dc and low-frequency first).
For any other type of `window`, the function `scipy.signal.get_window`
is called to generate the window.
The first sample of the returned vector is the same as the first
sample of the input vector. The spacing between samples is changed
from ``dx`` to ``dx * len(x) / num``.
If `t` is not None, then it represents the old sample positions,
and the new sample positions will be returned as well as the new
samples.
As noted, `resample` uses FFT transformations, which can be very
slow if the number of input or output samples is large and prime;
see `scipy.fftpack.fft`.
Examples
--------
Note that the end of the resampled data rises to meet the first
sample of the next cycle:
>>> from scipy import signal
>>> x = np.linspace(0, 10, 20, endpoint=False)
>>> y = np.cos(-x**2/6.0)
>>> f = signal.resample(y, 100)
>>> xnew = np.linspace(0, 10, 100, endpoint=False)
>>> import matplotlib.pyplot as plt
>>> plt.plot(x, y, 'go-', xnew, f, '.-', 10, y[0], 'ro')
>>> plt.legend(['data', 'resampled'], loc='best')
>>> plt.show()
"""
x = asarray(x)
X = fftpack.fft(x, axis=axis)
Nx = x.shape[axis]
if window is not None:
if callable(window):
W = window(fftpack.fftfreq(Nx))
elif isinstance(window, ndarray):
if window.shape != (Nx,):
raise ValueError('window must have the same length as data')
W = window
else:
W = fftpack.ifftshift(get_window(window, Nx))
newshape = [1] * x.ndim
newshape[axis] = len(W)
W.shape = newshape
X = X * W
W.shape = (Nx,)
sl = [slice(None)] * x.ndim
newshape = list(x.shape)
newshape[axis] = num
N = int(np.minimum(num, Nx))
Y = zeros(newshape, 'D')
sl[axis] = slice(0, (N + 1) // 2)
Y[sl] = X[sl]
sl[axis] = slice(-(N - 1) // 2, None)
Y[sl] = X[sl]
if N % 2 == 0: # special treatment if low number of points is even. So far we have set Y[-N/2]=X[-N/2]
if N < Nx: # if downsampling
sl[axis] = slice(N//2,N//2+1,None) # select the component at frequency N/2
Y[sl] += X[sl] # add the component of X at N/2
elif N < num: # if upsampling
sl[axis] = slice(num-N//2,num-N//2+1,None) # select the component at frequency -N/2
Y[sl] /= 2 # halve the component at -N/2
temp = Y[sl]
sl[axis] = slice(N//2,N//2+1,None) # select the component at +N/2
Y[sl] = temp # set that equal to the component at -N/2
y = fftpack.ifft(Y, axis=axis) * (float(num) / float(Nx))
if x.dtype.char not in ['F', 'D']:
y = y.real
if t is None:
return y
else:
new_t = arange(0, num) * (t[1] - t[0]) * Nx / float(num) + t[0]
return y, new_t
def resample_poly(x, up, down, axis=0, window=('kaiser', 5.0)):
"""
Resample `x` along the given axis using polyphase filtering.
The signal `x` is upsampled by the factor `up`, a zero-phase low-pass
FIR filter is applied, and then it is downsampled by the factor `down`.
The resulting sample rate is ``up / down`` times the original sample
rate. Values beyond the boundary of the signal are assumed to be zero
during the filtering step.
Parameters
----------
x : array_like
The data to be resampled.
up : int
The upsampling factor.
down : int
The downsampling factor.
axis : int, optional
The axis of `x` that is resampled. Default is 0.
window : string, tuple, or array_like, optional
Desired window to use to design the low-pass filter, or the FIR filter
coefficients to employ. See below for details.
Returns
-------
resampled_x : array
The resampled array.
See Also
--------
decimate : Downsample the signal after applying an FIR or IIR filter.
resample : Resample up or down using the FFT method.
Notes
-----
This polyphase method will likely be faster than the Fourier method
in `scipy.signal.resample` when the number of samples is large and
prime, or when the number of samples is large and `up` and `down`
share a large greatest common denominator. The length of the FIR
filter used will depend on ``max(up, down) // gcd(up, down)``, and
the number of operations during polyphase filtering will depend on
the filter length and `down` (see `scipy.signal.upfirdn` for details).
The argument `window` specifies the FIR low-pass filter design.
If `window` is an array_like it is assumed to be the FIR filter
coefficients. Note that the FIR filter is applied after the upsampling
step, so it should be designed to operate on a signal at a sampling
frequency higher than the original by a factor of `up//gcd(up, down)`.
This function's output will be centered with respect to this array, so it
is best to pass a symmetric filter with an odd number of samples if, as
is usually the case, a zero-phase filter is desired.
For any other type of `window`, the functions `scipy.signal.get_window`
and `scipy.signal.firwin` are called to generate the appropriate filter
coefficients.
The first sample of the returned vector is the same as the first
sample of the input vector. The spacing between samples is changed
from ``dx`` to ``dx * down / float(up)``.
Examples
--------
Note that the end of the resampled data rises to meet the first
sample of the next cycle for the FFT method, and gets closer to zero
for the polyphase method:
>>> from scipy import signal
>>> x = np.linspace(0, 10, 20, endpoint=False)
>>> y = np.cos(-x**2/6.0)
>>> f_fft = signal.resample(y, 100)
>>> f_poly = signal.resample_poly(y, 100, 20)
>>> xnew = np.linspace(0, 10, 100, endpoint=False)
>>> import matplotlib.pyplot as plt
>>> plt.plot(xnew, f_fft, 'b.-', xnew, f_poly, 'r.-')
>>> plt.plot(x, y, 'ko-')
>>> plt.plot(10, y[0], 'bo', 10, 0., 'ro') # boundaries
>>> plt.legend(['resample', 'resamp_poly', 'data'], loc='best')
>>> plt.show()
"""
x = asarray(x)
if up != int(up):
raise ValueError("up must be an integer")
if down != int(down):
raise ValueError("down must be an integer")
up = int(up)
down = int(down)
if up < 1 or down < 1:
raise ValueError('up and down must be >= 1')
# Determine our up and down factors
# Use a rational approimation to save computation time on really long
# signals
g_ = gcd(up, down)
up //= g_
down //= g_
if up == down == 1:
return x.copy()
n_out = x.shape[axis] * up
n_out = n_out // down + bool(n_out % down)
if isinstance(window, (list, np.ndarray)):
window = array(window) # use array to force a copy (we modify it)
if window.ndim > 1:
raise ValueError('window must be 1-D')
half_len = (window.size - 1) // 2
h = window
else:
# Design a linear-phase low-pass FIR filter
max_rate = max(up, down)
f_c = 1. / max_rate # cutoff of FIR filter (rel. to Nyquist)
half_len = 10 * max_rate # reasonable cutoff for our sinc-like function
h = firwin(2 * half_len + 1, f_c, window=window)
h *= up
# Zero-pad our filter to put the output samples at the center
n_pre_pad = (down - half_len % down)
n_post_pad = 0
n_pre_remove = (half_len + n_pre_pad) // down
# We should rarely need to do this given our filter lengths...
while _output_len(len(h) + n_pre_pad + n_post_pad, x.shape[axis],
up, down) < n_out + n_pre_remove:
n_post_pad += 1
h = np.concatenate((np.zeros(n_pre_pad), h, np.zeros(n_post_pad)))
n_pre_remove_end = n_pre_remove + n_out
# filter then remove excess
y = upfirdn(h, x, up, down, axis=axis)
keep = [slice(None), ]*x.ndim
keep[axis] = slice(n_pre_remove, n_pre_remove_end)
return y[keep]
def vectorstrength(events, period):
'''
Determine the vector strength of the events corresponding to the given
period.
The vector strength is a measure of phase synchrony, how well the
timing of the events is synchronized to a single period of a periodic
signal.
If multiple periods are used, calculate the vector strength of each.
This is called the "resonating vector strength".
Parameters
----------
events : 1D array_like
An array of time points containing the timing of the events.
period : float or array_like
The period of the signal that the events should synchronize to.
The period is in the same units as `events`. It can also be an array
of periods, in which case the outputs are arrays of the same length.
Returns
-------
strength : float or 1D array
The strength of the synchronization. 1.0 is perfect synchronization
and 0.0 is no synchronization. If `period` is an array, this is also
an array with each element containing the vector strength at the
corresponding period.
phase : float or array
The phase that the events are most strongly synchronized to in radians.
If `period` is an array, this is also an array with each element
containing the phase for the corresponding period.
References
----------
van Hemmen, JL, Longtin, A, and Vollmayr, AN. Testing resonating vector
strength: Auditory system, electric fish, and noise.
Chaos 21, 047508 (2011);
:doi:`10.1063/1.3670512`.
van Hemmen, JL. Vector strength after Goldberg, Brown, and von Mises:
biological and mathematical perspectives. Biol Cybern.
2013 Aug;107(4):385-96. :doi:`10.1007/s00422-013-0561-7`.
van Hemmen, JL and Vollmayr, AN. Resonating vector strength: what happens
when we vary the "probing" frequency while keeping the spike times
fixed. Biol Cybern. 2013 Aug;107(4):491-94.
:doi:`10.1007/s00422-013-0560-8`.
'''
events = asarray(events)
period = asarray(period)
if events.ndim > 1:
raise ValueError('events cannot have dimensions more than 1')
if period.ndim > 1:
raise ValueError('period cannot have dimensions more than 1')
# we need to know later if period was originally a scalar
scalarperiod = not period.ndim
events = atleast_2d(events)
period = atleast_2d(period)
if (period <= 0).any():
raise ValueError('periods must be positive')
# this converts the times to vectors
vectors = exp(dot(2j*pi/period.T, events))
# the vector strength is just the magnitude of the mean of the vectors
# the vector phase is the angle of the mean of the vectors
vectormean = mean(vectors, axis=1)
strength = abs(vectormean)
phase = angle(vectormean)
# if the original period was a scalar, return scalars
if scalarperiod:
strength = strength[0]
phase = phase[0]
return strength, phase
def detrend(data, axis=-1, type='linear', bp=0):
"""
Remove linear trend along axis from data.
Parameters
----------
data : array_like
The input data.
axis : int, optional
The axis along which to detrend the data. By default this is the
last axis (-1).
type : {'linear', 'constant'}, optional
The type of detrending. If ``type == 'linear'`` (default),
the result of a linear least-squares fit to `data` is subtracted
from `data`.
If ``type == 'constant'``, only the mean of `data` is subtracted.
bp : array_like of ints, optional
A sequence of break points. If given, an individual linear fit is
performed for each part of `data` between two break points.
Break points are specified as indices into `data`.
Returns
-------
ret : ndarray
The detrended input data.
Examples
--------
>>> from scipy import signal
>>> randgen = np.random.RandomState(9)
>>> npoints = 1000
>>> noise = randgen.randn(npoints)
>>> x = 3 + 2*np.linspace(0, 1, npoints) + noise
>>> (signal.detrend(x) - noise).max() < 0.01
True
"""
if type not in ['linear', 'l', 'constant', 'c']:
raise ValueError("Trend type must be 'linear' or 'constant'.")
data = asarray(data)
dtype = data.dtype.char
if dtype not in 'dfDF':
dtype = 'd'
if type in ['constant', 'c']:
ret = data - expand_dims(mean(data, axis), axis)
return ret
else:
dshape = data.shape
N = dshape[axis]
bp = sort(unique(r_[0, bp, N]))
if np.any(bp > N):
raise ValueError("Breakpoints must be less than length "
"of data along given axis.")
Nreg = len(bp) - 1
# Restructure data so that axis is along first dimension and
# all other dimensions are collapsed into second dimension
rnk = len(dshape)
if axis < 0:
axis = axis + rnk
newdims = r_[axis, 0:axis, axis + 1:rnk]
newdata = reshape(transpose(data, tuple(newdims)),
(N, _prod(dshape) // N))
newdata = newdata.copy() # make sure we have a copy
if newdata.dtype.char not in 'dfDF':
newdata = newdata.astype(dtype)
# Find leastsq fit and remove it for each piece
for m in range(Nreg):
Npts = bp[m + 1] - bp[m]
A = ones((Npts, 2), dtype)
A[:, 0] = cast[dtype](arange(1, Npts + 1) * 1.0 / Npts)
sl = slice(bp[m], bp[m + 1])
coef, resids, rank, s = linalg.lstsq(A, newdata[sl])
newdata[sl] = newdata[sl] - dot(A, coef)
# Put data back in original shape.
tdshape = take(dshape, newdims, 0)
ret = reshape(newdata, tuple(tdshape))
vals = list(range(1, rnk))
olddims = vals[:axis] + [0] + vals[axis:]
ret = transpose(ret, tuple(olddims))
return ret
def lfilter_zi(b, a):
"""
Construct initial conditions for lfilter for step response steady-state.
Compute an initial state `zi` for the `lfilter` function that corresponds
to the steady state of the step response.
A typical use of this function is to set the initial state so that the
output of the filter starts at the same value as the first element of
the signal to be filtered.
Parameters
----------
b, a : array_like (1-D)
The IIR filter coefficients. See `lfilter` for more
information.
Returns
-------
zi : 1-D ndarray
The initial state for the filter.
See Also
--------
lfilter, lfiltic, filtfilt
Notes
-----
A linear filter with order m has a state space representation (A, B, C, D),
for which the output y of the filter can be expressed as::
z(n+1) = A*z(n) + B*x(n)
y(n) = C*z(n) + D*x(n)
where z(n) is a vector of length m, A has shape (m, m), B has shape
(m, 1), C has shape (1, m) and D has shape (1, 1) (assuming x(n) is
a scalar). lfilter_zi solves::
zi = A*zi + B
In other words, it finds the initial condition for which the response
to an input of all ones is a constant.
Given the filter coefficients `a` and `b`, the state space matrices
for the transposed direct form II implementation of the linear filter,
which is the implementation used by scipy.signal.lfilter, are::
A = scipy.linalg.companion(a).T
B = b[1:] - a[1:]*b[0]
assuming `a[0]` is 1.0; if `a[0]` is not 1, `a` and `b` are first
divided by a[0].
Examples
--------
The following code creates a lowpass Butterworth filter. Then it
applies that filter to an array whose values are all 1.0; the
output is also all 1.0, as expected for a lowpass filter. If the
`zi` argument of `lfilter` had not been given, the output would have
shown the transient signal.
>>> from numpy import array, ones
>>> from scipy.signal import lfilter, lfilter_zi, butter
>>> b, a = butter(5, 0.25)
>>> zi = lfilter_zi(b, a)
>>> y, zo = lfilter(b, a, ones(10), zi=zi)
>>> y
array([1., 1., 1., 1., 1., 1., 1., 1., 1., 1.])
Another example:
>>> x = array([0.5, 0.5, 0.5, 0.0, 0.0, 0.0, 0.0])
>>> y, zf = lfilter(b, a, x, zi=zi*x[0])
>>> y
array([ 0.5 , 0.5 , 0.5 , 0.49836039, 0.48610528,
0.44399389, 0.35505241])
Note that the `zi` argument to `lfilter` was computed using
`lfilter_zi` and scaled by `x[0]`. Then the output `y` has no
transient until the input drops from 0.5 to 0.0.
"""
# FIXME: Can this function be replaced with an appropriate
# use of lfiltic? For example, when b,a = butter(N,Wn),
# lfiltic(b, a, y=numpy.ones_like(a), x=numpy.ones_like(b)).
#
# We could use scipy.signal.normalize, but it uses warnings in
# cases where a ValueError is more appropriate, and it allows
# b to be 2D.
b = np.atleast_1d(b)
if b.ndim != 1:
raise ValueError("Numerator b must be 1-D.")
a = np.atleast_1d(a)
if a.ndim != 1:
raise ValueError("Denominator a must be 1-D.")
while len(a) > 1 and a[0] == 0.0:
a = a[1:]
if a.size < 1:
raise ValueError("There must be at least one nonzero `a` coefficient.")
if a[0] != 1.0:
# Normalize the coefficients so a[0] == 1.
b = b / a[0]
a = a / a[0]
n = max(len(a), len(b))
# Pad a or b with zeros so they are the same length.
if len(a) < n:
a = np.r_[a, np.zeros(n - len(a))]
elif len(b) < n:
b = np.r_[b, np.zeros(n - len(b))]
IminusA = np.eye(n - 1) - linalg.companion(a).T
B = b[1:] - a[1:] * b[0]
# Solve zi = A*zi + B
zi = np.linalg.solve(IminusA, B)
# For future reference: we could also use the following
# explicit formulas to solve the linear system:
#
# zi = np.zeros(n - 1)
# zi[0] = B.sum() / IminusA[:,0].sum()
# asum = 1.0
# csum = 0.0
# for k in range(1,n-1):
# asum += a[k]
# csum += b[k] - a[k]*b[0]
# zi[k] = asum*zi[0] - csum
return zi
def sosfilt_zi(sos):
"""
Construct initial conditions for sosfilt for step response steady-state.
Compute an initial state `zi` for the `sosfilt` function that corresponds
to the steady state of the step response.
A typical use of this function is to set the initial state so that the
output of the filter starts at the same value as the first element of
the signal to be filtered.
Parameters
----------
sos : array_like
Array of second-order filter coefficients, must have shape
``(n_sections, 6)``. See `sosfilt` for the SOS filter format
specification.
Returns
-------
zi : ndarray
Initial conditions suitable for use with ``sosfilt``, shape
``(n_sections, 2)``.
See Also
--------
sosfilt, zpk2sos
Notes
-----
.. versionadded:: 0.16.0
Examples
--------
Filter a rectangular pulse that begins at time 0, with and without
the use of the `zi` argument of `scipy.signal.sosfilt`.
>>> from scipy import signal
>>> import matplotlib.pyplot as plt
>>> sos = signal.butter(9, 0.125, output='sos')
>>> zi = signal.sosfilt_zi(sos)
>>> x = (np.arange(250) < 100).astype(int)
>>> f1 = signal.sosfilt(sos, x)
>>> f2, zo = signal.sosfilt(sos, x, zi=zi)
>>> plt.plot(x, 'k--', label='x')
>>> plt.plot(f1, 'b', alpha=0.5, linewidth=2, label='filtered')
>>> plt.plot(f2, 'g', alpha=0.25, linewidth=4, label='filtered with zi')
>>> plt.legend(loc='best')
>>> plt.show()
"""
sos = np.asarray(sos)
if sos.ndim != 2 or sos.shape[1] != 6:
raise ValueError('sos must be shape (n_sections, 6)')
n_sections = sos.shape[0]
zi = np.empty((n_sections, 2))
scale = 1.0
for section in range(n_sections):
b = sos[section, :3]
a = sos[section, 3:]
zi[section] = scale * lfilter_zi(b, a)
# If H(z) = B(z)/A(z) is this section's transfer function, then
# b.sum()/a.sum() is H(1), the gain at omega=0. That's the steady
# state value of this section's step response.
scale *= b.sum() / a.sum()
return zi
def _filtfilt_gust(b, a, x, axis=-1, irlen=None):
"""Forward-backward IIR filter that uses Gustafsson's method.
Apply the IIR filter defined by `(b,a)` to `x` twice, first forward
then backward, using Gustafsson's initial conditions [1]_.
Let ``y_fb`` be the result of filtering first forward and then backward,
and let ``y_bf`` be the result of filtering first backward then forward.
Gustafsson's method is to compute initial conditions for the forward
pass and the backward pass such that ``y_fb == y_bf``.
Parameters
----------
b : scalar or 1-D ndarray
Numerator coefficients of the filter.
a : scalar or 1-D ndarray
Denominator coefficients of the filter.
x : ndarray
Data to be filtered.
axis : int, optional
Axis of `x` to be filtered. Default is -1.
irlen : int or None, optional
The length of the nonnegligible part of the impulse response.
If `irlen` is None, or if the length of the signal is less than
``2 * irlen``, then no part of the impulse response is ignored.
Returns
-------
y : ndarray
The filtered data.
x0 : ndarray
Initial condition for the forward filter.
x1 : ndarray
Initial condition for the backward filter.
Notes
-----
Typically the return values `x0` and `x1` are not needed by the
caller. The intended use of these return values is in unit tests.
References
----------
.. [1] F. Gustaffson. Determining the initial states in forward-backward
filtering. Transactions on Signal Processing, 46(4):988-992, 1996.
"""
# In the comments, "Gustafsson's paper" and [1] refer to the
# paper referenced in the docstring.
b = np.atleast_1d(b)
a = np.atleast_1d(a)
order = max(len(b), len(a)) - 1
if order == 0:
# The filter is just scalar multiplication, with no state.
scale = (b[0] / a[0])**2
y = scale * x
return y, np.array([]), np.array([])
if axis != -1 or axis != x.ndim - 1:
# Move the axis containing the data to the end.
x = np.swapaxes(x, axis, x.ndim - 1)
# n is the number of samples in the data to be filtered.
n = x.shape[-1]
if irlen is None or n <= 2*irlen:
m = n
else:
m = irlen
# Create Obs, the observability matrix (called O in the paper).
# This matrix can be interpreted as the operator that propagates
# an arbitrary initial state to the output, assuming the input is
# zero.
# In Gustafsson's paper, the forward and backward filters are not
# necessarily the same, so he has both O_f and O_b. We use the same
# filter in both directions, so we only need O. The same comment
# applies to S below.
Obs = np.zeros((m, order))
zi = np.zeros(order)
zi[0] = 1
Obs[:, 0] = lfilter(b, a, np.zeros(m), zi=zi)[0]
for k in range(1, order):
Obs[k:, k] = Obs[:-k, 0]
# Obsr is O^R (Gustafsson's notation for row-reversed O)
Obsr = Obs[::-1]
# Create S. S is the matrix that applies the filter to the reversed
# propagated initial conditions. That is,
# out = S.dot(zi)
# is the same as
# tmp, _ = lfilter(b, a, zeros(), zi=zi) # Propagate ICs.
# out = lfilter(b, a, tmp[::-1]) # Reverse and filter.
# Equations (5) & (6) of [1]
S = lfilter(b, a, Obs[::-1], axis=0)
# Sr is S^R (row-reversed S)
Sr = S[::-1]
# M is [(S^R - O), (O^R - S)]
if m == n:
M = np.hstack((Sr - Obs, Obsr - S))
else:
# Matrix described in section IV of [1].
M = np.zeros((2*m, 2*order))
M[:m, :order] = Sr - Obs
M[m:, order:] = Obsr - S
# Naive forward-backward and backward-forward filters.
# These have large transients because the filters use zero initial
# conditions.
y_f = lfilter(b, a, x)
y_fb = lfilter(b, a, y_f[..., ::-1])[..., ::-1]
y_b = lfilter(b, a, x[..., ::-1])[..., ::-1]
y_bf = lfilter(b, a, y_b)
delta_y_bf_fb = y_bf - y_fb
if m == n:
delta = delta_y_bf_fb
else:
start_m = delta_y_bf_fb[..., :m]
end_m = delta_y_bf_fb[..., -m:]
delta = np.concatenate((start_m, end_m), axis=-1)
# ic_opt holds the "optimal" initial conditions.
# The following code computes the result shown in the formula
# of the paper between equations (6) and (7).
if delta.ndim == 1:
ic_opt = linalg.lstsq(M, delta)[0]
else:
# Reshape delta so it can be used as an array of multiple
# right-hand-sides in linalg.lstsq.
delta2d = delta.reshape(-1, delta.shape[-1]).T
ic_opt0 = linalg.lstsq(M, delta2d)[0].T
ic_opt = ic_opt0.reshape(delta.shape[:-1] + (M.shape[-1],))
# Now compute the filtered signal using equation (7) of [1].
# First, form [S^R, O^R] and call it W.
if m == n:
W = np.hstack((Sr, Obsr))
else:
W = np.zeros((2*m, 2*order))
W[:m, :order] = Sr
W[m:, order:] = Obsr
# Equation (7) of [1] says
# Y_fb^opt = Y_fb^0 + W * [x_0^opt; x_{N-1}^opt]
# `wic` is (almost) the product on the right.
# W has shape (m, 2*order), and ic_opt has shape (..., 2*order),
# so we can't use W.dot(ic_opt). Instead, we dot ic_opt with W.T,
# so wic has shape (..., m).
wic = ic_opt.dot(W.T)
# `wic` is "almost" the product of W and the optimal ICs in equation
# (7)--if we're using a truncated impulse response (m < n), `wic`
# contains only the adjustments required for the ends of the signal.
# Here we form y_opt, taking this into account if necessary.
y_opt = y_fb
if m == n:
y_opt += wic
else:
y_opt[..., :m] += wic[..., :m]
y_opt[..., -m:] += wic[..., -m:]
x0 = ic_opt[..., :order]
x1 = ic_opt[..., -order:]
if axis != -1 or axis != x.ndim - 1:
# Restore the data axis to its original position.
x0 = np.swapaxes(x0, axis, x.ndim - 1)
x1 = np.swapaxes(x1, axis, x.ndim - 1)
y_opt = np.swapaxes(y_opt, axis, x.ndim - 1)
return y_opt, x0, x1
def filtfilt(b, a, x, axis=-1, padtype='odd', padlen=None, method='pad',
irlen=None):
"""
Apply a digital filter forward and backward to a signal.
This function applies a linear digital filter twice, once forward and
once backwards. The combined filter has zero phase and a filter order
twice that of the original.
The function provides options for handling the edges of the signal.
Parameters
----------
b : (N,) array_like
The numerator coefficient vector of the filter.
a : (N,) array_like
The denominator coefficient vector of the filter. If ``a[0]``
is not 1, then both `a` and `b` are normalized by ``a[0]``.
x : array_like
The array of data to be filtered.
axis : int, optional
The axis of `x` to which the filter is applied.
Default is -1.
padtype : str or None, optional
Must be 'odd', 'even', 'constant', or None. This determines the
type of extension to use for the padded signal to which the filter
is applied. If `padtype` is None, no padding is used. The default
is 'odd'.
padlen : int or None, optional
The number of elements by which to extend `x` at both ends of
`axis` before applying the filter. This value must be less than
``x.shape[axis] - 1``. ``padlen=0`` implies no padding.
The default value is ``3 * max(len(a), len(b))``.
method : str, optional
Determines the method for handling the edges of the signal, either
"pad" or "gust". When `method` is "pad", the signal is padded; the
type of padding is determined by `padtype` and `padlen`, and `irlen`
is ignored. When `method` is "gust", Gustafsson's method is used,
and `padtype` and `padlen` are ignored.
irlen : int or None, optional
When `method` is "gust", `irlen` specifies the length of the
impulse response of the filter. If `irlen` is None, no part
of the impulse response is ignored. For a long signal, specifying
`irlen` can significantly improve the performance of the filter.
Returns
-------
y : ndarray
The filtered output with the same shape as `x`.
See Also
--------
sosfiltfilt, lfilter_zi, lfilter, lfiltic, savgol_filter, sosfilt
Notes
-----
When `method` is "pad", the function pads the data along the given axis
in one of three ways: odd, even or constant. The odd and even extensions
have the corresponding symmetry about the end point of the data. The
constant extension extends the data with the values at the end points. On
both the forward and backward passes, the initial condition of the
filter is found by using `lfilter_zi` and scaling it by the end point of
the extended data.
When `method` is "gust", Gustafsson's method [1]_ is used. Initial
conditions are chosen for the forward and backward passes so that the
forward-backward filter gives the same result as the backward-forward
filter.
The option to use Gustaffson's method was added in scipy version 0.16.0.
References
----------
.. [1] F. Gustaffson, "Determining the initial states in forward-backward
filtering", Transactions on Signal Processing, Vol. 46, pp. 988-992,
1996.
Examples
--------
The examples will use several functions from `scipy.signal`.
>>> from scipy import signal
>>> import matplotlib.pyplot as plt
First we create a one second signal that is the sum of two pure sine
waves, with frequencies 5 Hz and 250 Hz, sampled at 2000 Hz.
>>> t = np.linspace(0, 1.0, 2001)
>>> xlow = np.sin(2 * np.pi * 5 * t)
>>> xhigh = np.sin(2 * np.pi * 250 * t)
>>> x = xlow + xhigh
Now create a lowpass Butterworth filter with a cutoff of 0.125 times
the Nyquist rate, or 125 Hz, and apply it to ``x`` with `filtfilt`.
The result should be approximately ``xlow``, with no phase shift.
>>> b, a = signal.butter(8, 0.125)
>>> y = signal.filtfilt(b, a, x, padlen=150)
>>> np.abs(y - xlow).max()
9.1086182074789912e-06
We get a fairly clean result for this artificial example because
the odd extension is exact, and with the moderately long padding,
the filter's transients have dissipated by the time the actual data
is reached. In general, transient effects at the edges are
unavoidable.
The following example demonstrates the option ``method="gust"``.
First, create a filter.
>>> b, a = signal.ellip(4, 0.01, 120, 0.125) # Filter to be applied.
>>> np.random.seed(123456)
`sig` is a random input signal to be filtered.
>>> n = 60
>>> sig = np.random.randn(n)**3 + 3*np.random.randn(n).cumsum()
Apply `filtfilt` to `sig`, once using the Gustafsson method, and
once using padding, and plot the results for comparison.
>>> fgust = signal.filtfilt(b, a, sig, method="gust")
>>> fpad = signal.filtfilt(b, a, sig, padlen=50)
>>> plt.plot(sig, 'k-', label='input')
>>> plt.plot(fgust, 'b-', linewidth=4, label='gust')
>>> plt.plot(fpad, 'c-', linewidth=1.5, label='pad')
>>> plt.legend(loc='best')
>>> plt.show()
The `irlen` argument can be used to improve the performance
of Gustafsson's method.
Estimate the impulse response length of the filter.
>>> z, p, k = signal.tf2zpk(b, a)
>>> eps = 1e-9
>>> r = np.max(np.abs(p))
>>> approx_impulse_len = int(np.ceil(np.log(eps) / np.log(r)))
>>> approx_impulse_len
137
Apply the filter to a longer signal, with and without the `irlen`
argument. The difference between `y1` and `y2` is small. For long
signals, using `irlen` gives a significant performance improvement.
>>> x = np.random.randn(5000)
>>> y1 = signal.filtfilt(b, a, x, method='gust')
>>> y2 = signal.filtfilt(b, a, x, method='gust', irlen=approx_impulse_len)
>>> print(np.max(np.abs(y1 - y2)))
1.80056858312e-10
"""
b = np.atleast_1d(b)
a = np.atleast_1d(a)
x = np.asarray(x)
if method not in ["pad", "gust"]:
raise ValueError("method must be 'pad' or 'gust'.")
if method == "gust":
y, z1, z2 = _filtfilt_gust(b, a, x, axis=axis, irlen=irlen)
return y
# method == "pad"
edge, ext = _validate_pad(padtype, padlen, x, axis,
ntaps=max(len(a), len(b)))
# Get the steady state of the filter's step response.
zi = lfilter_zi(b, a)
# Reshape zi and create x0 so that zi*x0 broadcasts
# to the correct value for the 'zi' keyword argument
# to lfilter.
zi_shape = [1] * x.ndim
zi_shape[axis] = zi.size
zi = np.reshape(zi, zi_shape)
x0 = axis_slice(ext, stop=1, axis=axis)
# Forward filter.
(y, zf) = lfilter(b, a, ext, axis=axis, zi=zi * x0)
# Backward filter.
# Create y0 so zi*y0 broadcasts appropriately.
y0 = axis_slice(y, start=-1, axis=axis)
(y, zf) = lfilter(b, a, axis_reverse(y, axis=axis), axis=axis, zi=zi * y0)
# Reverse y.
y = axis_reverse(y, axis=axis)
if edge > 0:
# Slice the actual signal from the extended signal.
y = axis_slice(y, start=edge, stop=-edge, axis=axis)
return y
def _validate_pad(padtype, padlen, x, axis, ntaps):
"""Helper to validate padding for filtfilt"""
if padtype not in ['even', 'odd', 'constant', None]:
raise ValueError(("Unknown value '%s' given to padtype. padtype "
"must be 'even', 'odd', 'constant', or None.") %
padtype)
if padtype is None:
padlen = 0
if padlen is None:
# Original padding; preserved for backwards compatibility.
edge = ntaps * 3
else:
edge = padlen
# x's 'axis' dimension must be bigger than edge.
if x.shape[axis] <= edge:
raise ValueError("The length of the input vector x must be at least "
"padlen, which is %d." % edge)
if padtype is not None and edge > 0:
# Make an extension of length `edge` at each
# end of the input array.
if padtype == 'even':
ext = even_ext(x, edge, axis=axis)
elif padtype == 'odd':
ext = odd_ext(x, edge, axis=axis)
else:
ext = const_ext(x, edge, axis=axis)
else:
ext = x
return edge, ext
def sosfilt(sos, x, axis=-1, zi=None):
"""
Filter data along one dimension using cascaded second-order sections.
Filter a data sequence, `x`, using a digital IIR filter defined by
`sos`. This is implemented by performing `lfilter` for each
second-order section. See `lfilter` for details.
Parameters
----------
sos : array_like
Array of second-order filter coefficients, must have shape
``(n_sections, 6)``. Each row corresponds to a second-order
section, with the first three columns providing the numerator
coefficients and the last three providing the denominator
coefficients.
x : array_like
An N-dimensional input array.
axis : int, optional
The axis of the input data array along which to apply the
linear filter. The filter is applied to each subarray along
this axis. Default is -1.
zi : array_like, optional
Initial conditions for the cascaded filter delays. It is a (at
least 2D) vector of shape ``(n_sections, ..., 2, ...)``, where
``..., 2, ...`` denotes the shape of `x`, but with ``x.shape[axis]``
replaced by 2. If `zi` is None or is not given then initial rest
(i.e. all zeros) is assumed.
Note that these initial conditions are *not* the same as the initial
conditions given by `lfiltic` or `lfilter_zi`.
Returns
-------
y : ndarray
The output of the digital filter.
zf : ndarray, optional
If `zi` is None, this is not returned, otherwise, `zf` holds the
final filter delay values.
See Also
--------
zpk2sos, sos2zpk, sosfilt_zi, sosfiltfilt, sosfreqz
Notes
-----
The filter function is implemented as a series of second-order filters
with direct-form II transposed structure. It is designed to minimize
numerical precision errors for high-order filters.
.. versionadded:: 0.16.0
Examples
--------
Plot a 13th-order filter's impulse response using both `lfilter` and
`sosfilt`, showing the instability that results from trying to do a
13th-order filter in a single stage (the numerical error pushes some poles
outside of the unit circle):
>>> import matplotlib.pyplot as plt
>>> from scipy import signal
>>> b, a = signal.ellip(13, 0.009, 80, 0.05, output='ba')
>>> sos = signal.ellip(13, 0.009, 80, 0.05, output='sos')
>>> x = signal.unit_impulse(700)
>>> y_tf = signal.lfilter(b, a, x)
>>> y_sos = signal.sosfilt(sos, x)
>>> plt.plot(y_tf, 'r', label='TF')
>>> plt.plot(y_sos, 'k', label='SOS')
>>> plt.legend(loc='best')
>>> plt.show()
"""
x = np.asarray(x)
sos, n_sections = _validate_sos(sos)
use_zi = zi is not None
if use_zi:
zi = np.asarray(zi)
x_zi_shape = list(x.shape)
x_zi_shape[axis] = 2
x_zi_shape = tuple([n_sections] + x_zi_shape)
if zi.shape != x_zi_shape:
raise ValueError('Invalid zi shape. With axis=%r, an input with '
'shape %r, and an sos array with %d sections, zi '
'must have shape %r, got %r.' %
(axis, x.shape, n_sections, x_zi_shape, zi.shape))
zf = zeros_like(zi)
for section in range(n_sections):
if use_zi:
x, zf[section] = lfilter(sos[section, :3], sos[section, 3:],
x, axis, zi=zi[section])
else:
x = lfilter(sos[section, :3], sos[section, 3:], x, axis)
out = (x, zf) if use_zi else x
return out
def sosfiltfilt(sos, x, axis=-1, padtype='odd', padlen=None):
"""
A forward-backward digital filter using cascaded second-order sections.
See `filtfilt` for more complete information about this method.
Parameters
----------
sos : array_like
Array of second-order filter coefficients, must have shape
``(n_sections, 6)``. Each row corresponds to a second-order
section, with the first three columns providing the numerator
coefficients and the last three providing the denominator
coefficients.
x : array_like
The array of data to be filtered.
axis : int, optional
The axis of `x` to which the filter is applied.
Default is -1.
padtype : str or None, optional
Must be 'odd', 'even', 'constant', or None. This determines the
type of extension to use for the padded signal to which the filter
is applied. If `padtype` is None, no padding is used. The default
is 'odd'.
padlen : int or None, optional
The number of elements by which to extend `x` at both ends of
`axis` before applying the filter. This value must be less than
``x.shape[axis] - 1``. ``padlen=0`` implies no padding.
The default value is::
3 * (2 * len(sos) + 1 - min((sos[:, 2] == 0).sum(),
(sos[:, 5] == 0).sum()))
The extra subtraction at the end attempts to compensate for poles
and zeros at the origin (e.g. for odd-order filters) to yield
equivalent estimates of `padlen` to those of `filtfilt` for
second-order section filters built with `scipy.signal` functions.
Returns
-------
y : ndarray
The filtered output with the same shape as `x`.
See Also
--------
filtfilt, sosfilt, sosfilt_zi, sosfreqz
Notes
-----
.. versionadded:: 0.18.0
Examples
--------
>>> from scipy.signal import sosfiltfilt, butter
>>> import matplotlib.pyplot as plt
Create an interesting signal to filter.
>>> n = 201
>>> t = np.linspace(0, 1, n)
>>> np.random.seed(123)
>>> x = 1 + (t < 0.5) - 0.25*t**2 + 0.05*np.random.randn(n)
Create a lowpass Butterworth filter, and use it to filter `x`.
>>> sos = butter(4, 0.125, output='sos')
>>> y = sosfiltfilt(sos, x)
For comparison, apply an 8th order filter using `sosfilt`. The filter
is initialized using the mean of the first four values of `x`.
>>> from scipy.signal import sosfilt, sosfilt_zi
>>> sos8 = butter(8, 0.125, output='sos')
>>> zi = x[:4].mean() * sosfilt_zi(sos8)
>>> y2, zo = sosfilt(sos8, x, zi=zi)
Plot the results. Note that the phase of `y` matches the input, while
`y2` has a significant phase delay.
>>> plt.plot(t, x, alpha=0.5, label='x(t)')
>>> plt.plot(t, y, label='y(t)')
>>> plt.plot(t, y2, label='y2(t)')
>>> plt.legend(framealpha=1, shadow=True)
>>> plt.grid(alpha=0.25)
>>> plt.xlabel('t')
>>> plt.show()
"""
sos, n_sections = _validate_sos(sos)
# `method` is "pad"...
ntaps = 2 * n_sections + 1
ntaps -= min((sos[:, 2] == 0).sum(), (sos[:, 5] == 0).sum())
edge, ext = _validate_pad(padtype, padlen, x, axis,
ntaps=ntaps)
# These steps follow the same form as filtfilt with modifications
zi = sosfilt_zi(sos) # shape (n_sections, 2) --> (n_sections, ..., 2, ...)
zi_shape = [1] * x.ndim
zi_shape[axis] = 2
zi.shape = [n_sections] + zi_shape
x_0 = axis_slice(ext, stop=1, axis=axis)
(y, zf) = sosfilt(sos, ext, axis=axis, zi=zi * x_0)
y_0 = axis_slice(y, start=-1, axis=axis)
(y, zf) = sosfilt(sos, axis_reverse(y, axis=axis), axis=axis, zi=zi * y_0)
y = axis_reverse(y, axis=axis)
if edge > 0:
y = axis_slice(y, start=edge, stop=-edge, axis=axis)
return y
def decimate(x, q, n=None, ftype='iir', axis=-1, zero_phase=True):
"""
Downsample the signal after applying an anti-aliasing filter.
By default, an order 8 Chebyshev type I filter is used. A 30 point FIR
filter with Hamming window is used if `ftype` is 'fir'.
Parameters
----------
x : array_like
The signal to be downsampled, as an N-dimensional array.
q : int
The downsampling factor. When using IIR downsampling, it is recommended
to call `decimate` multiple times for downsampling factors higher than
13.
n : int, optional
The order of the filter (1 less than the length for 'fir'). Defaults to
8 for 'iir' and 20 times the downsampling factor for 'fir'.
ftype : str {'iir', 'fir'} or ``dlti`` instance, optional
If 'iir' or 'fir', specifies the type of lowpass filter. If an instance
of an `dlti` object, uses that object to filter before downsampling.
axis : int, optional
The axis along which to decimate.
zero_phase : bool, optional
Prevent phase shift by filtering with `filtfilt` instead of `lfilter`
when using an IIR filter, and shifting the outputs back by the filter's
group delay when using an FIR filter. The default value of ``True`` is
recommended, since a phase shift is generally not desired.
.. versionadded:: 0.18.0
Returns
-------
y : ndarray
The down-sampled signal.
See Also
--------
resample : Resample up or down using the FFT method.
resample_poly : Resample using polyphase filtering and an FIR filter.
Notes
-----
The ``zero_phase`` keyword was added in 0.18.0.
The possibility to use instances of ``dlti`` as ``ftype`` was added in
0.18.0.
"""
x = asarray(x)
q = operator.index(q)
if n is not None:
n = operator.index(n)
if ftype == 'fir':
if n is None:
half_len = 10 * q # reasonable cutoff for our sinc-like function
n = 2 * half_len
b, a = firwin(n+1, 1. / q, window='hamming'), 1.
elif ftype == 'iir':
if n is None:
n = 8
system = dlti(*cheby1(n, 0.05, 0.8 / q))
b, a = system.num, system.den
elif isinstance(ftype, dlti):
system = ftype._as_tf() # Avoids copying if already in TF form
b, a = system.num, system.den
else:
raise ValueError('invalid ftype')
sl = [slice(None)] * x.ndim
a = np.asarray(a)
if a.size == 1: # FIR case
b = b / a
if zero_phase:
y = resample_poly(x, 1, q, axis=axis, window=b)
else:
# upfirdn is generally faster than lfilter by a factor equal to the
# downsampling factor, since it only calculates the needed outputs
n_out = x.shape[axis] // q + bool(x.shape[axis] % q)
y = upfirdn(b, x, up=1, down=q, axis=axis)
sl[axis] = slice(None, n_out, None)
else: # IIR case
if zero_phase:
y = filtfilt(b, a, x, axis=axis)
else:
y = lfilter(b, a, x, axis=axis)
sl[axis] = slice(None, None, q)
return y[sl]
| mit |